hunk
dict | file
stringlengths 0
11.8M
| file_path
stringlengths 2
234
| label
int64 0
1
| commit_url
stringlengths 74
103
| dependency_score
listlengths 5
5
|
---|---|---|---|---|---|
{
"id": 8,
"code_window": [
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-top > .tab-border-top-container {\n",
"\tz-index: 2;\n",
"\ttop: 0;\n",
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-top-color);\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 6;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 219
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { AbstractTreeViewState } from 'vs/base/browser/ui/tree/abstractTree';
import { ObjectTree } from 'vs/base/browser/ui/tree/objectTree';
import { Emitter } from 'vs/base/common/event';
import { FuzzyScore } from 'vs/base/common/filters';
import { Iterable } from 'vs/base/common/iterator';
import { Disposable } from 'vs/base/common/lifecycle';
import { isDefined } from 'vs/base/common/types';
import { ByLocationTestItemElement } from 'vs/workbench/contrib/testing/browser/explorerProjections/hierarchalNodes';
import { IActionableTestTreeElement, ITestTreeProjection, TestExplorerTreeElement, TestItemTreeElement, TestTreeErrorMessage } from 'vs/workbench/contrib/testing/browser/explorerProjections/index';
import { NodeChangeList, NodeRenderDirective, NodeRenderFn, peersHaveChildren } from 'vs/workbench/contrib/testing/browser/explorerProjections/nodeHelper';
import { IComputedStateAndDurationAccessor, refreshComputedState } from 'vs/workbench/contrib/testing/common/getComputedState';
import { InternalTestItem, TestDiffOpType, TestItemExpandState, TestResultState, TestsDiff } from 'vs/workbench/contrib/testing/common/testCollection';
import { ITestResultService } from 'vs/workbench/contrib/testing/common/testResultService';
import { ITestService } from 'vs/workbench/contrib/testing/common/testService';
const computedStateAccessor: IComputedStateAndDurationAccessor<IActionableTestTreeElement> = {
getOwnState: i => i instanceof TestItemTreeElement ? i.ownState : TestResultState.Unset,
getCurrentComputedState: i => i.state,
setComputedState: (i, s) => i.state = s,
getCurrentComputedDuration: i => i.duration,
getOwnDuration: i => i instanceof TestItemTreeElement ? i.ownDuration : undefined,
setComputedDuration: (i, d) => i.duration = d,
getChildren: i => Iterable.filter(
i.children.values(),
(t): t is TestItemTreeElement => t instanceof TestItemTreeElement,
),
*getParents(i) {
for (let parent = i.parent; parent; parent = parent.parent) {
yield parent;
}
},
};
/**
* Projection that lists tests in their traditional tree view.
*/
export class HierarchicalByLocationProjection extends Disposable implements ITestTreeProjection {
private readonly updateEmitter = new Emitter<void>();
protected readonly changes = new NodeChangeList<ByLocationTestItemElement>();
protected readonly items = new Map<string, ByLocationTestItemElement>();
/**
* Gets root elements of the tree.
*/
protected get roots(): Iterable<ByLocationTestItemElement> {
const rootsIt = Iterable.map(this.testService.collection.rootItems, r => this.items.get(r.item.extId));
return Iterable.filter(rootsIt, isDefined);
}
/**
* @inheritdoc
*/
public readonly onUpdate = this.updateEmitter.event;
constructor(
private readonly lastState: AbstractTreeViewState,
@ITestService private readonly testService: ITestService,
@ITestResultService private readonly results: ITestResultService,
) {
super();
this._register(testService.onDidProcessDiff((diff) => this.applyDiff(diff)));
// when test results are cleared, recalculate all state
this._register(results.onResultsChanged((evt) => {
if (!('removed' in evt)) {
return;
}
for (const inTree of [...this.items.values()].sort((a, b) => b.depth - a.depth)) {
const lookup = this.results.getStateById(inTree.test.item.extId)?.[1];
inTree.ownDuration = lookup?.ownDuration;
refreshComputedState(computedStateAccessor, inTree, lookup?.ownComputedState ?? TestResultState.Unset).forEach(this.addUpdated);
}
this.updateEmitter.fire();
}));
// when test states change, reflect in the tree
this._register(results.onTestChanged(({ item: result }) => {
if (result.ownComputedState === TestResultState.Unset) {
const fallback = results.getStateById(result.item.extId);
if (fallback) {
result = fallback[1];
}
}
const item = this.items.get(result.item.extId);
if (!item) {
return;
}
item.retired = result.retired;
item.ownState = result.ownComputedState;
item.ownDuration = result.ownDuration;
// For items without children, always use the computed state. They are
// either leaves (for which it's fine) or nodes where we haven't expanded
// children and should trust whatever the result service gives us.
const explicitComputed = item.children.size ? undefined : result.computedState;
refreshComputedState(computedStateAccessor, item, explicitComputed).forEach(this.addUpdated);
this.addUpdated(item);
this.updateEmitter.fire();
}));
for (const test of testService.collection.all) {
this.storeItem(this.createItem(test));
}
}
/**
* Gets the depth of children to expanded automatically for the node,
*/
protected getRevealDepth(element: ByLocationTestItemElement): number | undefined {
return element.depth === 0 ? 0 : undefined;
}
/**
* @inheritdoc
*/
public getElementByTestId(testId: string): TestItemTreeElement | undefined {
return this.items.get(testId);
}
/**
* @inheritdoc
*/
private applyDiff(diff: TestsDiff) {
for (const op of diff) {
switch (op[0]) {
case TestDiffOpType.Add: {
const item = this.createItem(op[1]);
this.storeItem(item);
break;
}
case TestDiffOpType.Update: {
const patch = op[1];
const existing = this.items.get(patch.extId);
if (!existing) {
break;
}
// parent needs to be re-rendered on an expand update, so that its
// children are rewritten.
const needsParentUpdate = existing.test.expand === TestItemExpandState.NotExpandable && patch.expand;
existing.update(patch);
if (needsParentUpdate) {
this.changes.addedOrRemoved(existing);
} else {
this.changes.updated(existing);
}
break;
}
case TestDiffOpType.Remove: {
const toRemove = this.items.get(op[1]);
if (!toRemove) {
break;
}
this.changes.addedOrRemoved(toRemove);
const queue: Iterable<TestExplorerTreeElement>[] = [[toRemove]];
while (queue.length) {
for (const item of queue.pop()!) {
if (item instanceof ByLocationTestItemElement) {
queue.push(this.unstoreItem(this.items, item));
}
}
}
}
}
}
if (diff.length !== 0) {
this.updateEmitter.fire();
}
}
/**
* @inheritdoc
*/
public applyTo(tree: ObjectTree<TestExplorerTreeElement, FuzzyScore>) {
this.changes.applyTo(tree, this.renderNode, () => this.roots);
}
/**
* @inheritdoc
*/
public expandElement(element: TestItemTreeElement, depth: number): void {
if (!(element instanceof ByLocationTestItemElement)) {
return;
}
if (element.test.expand === TestItemExpandState.NotExpandable) {
return;
}
this.testService.collection.expand(element.test.item.extId, depth);
}
protected createItem(item: InternalTestItem): ByLocationTestItemElement {
const parent = item.parent ? this.items.get(item.parent)! : null;
return new ByLocationTestItemElement(item, parent, n => this.changes.addedOrRemoved(n));
}
protected readonly addUpdated = (item: IActionableTestTreeElement) => {
const cast = item as ByLocationTestItemElement;
this.changes.updated(cast);
};
protected renderNode: NodeRenderFn = (node, recurse) => {
if (node instanceof TestTreeErrorMessage) {
return { element: node };
}
if (node.depth === 0) {
// Omit the test controller root if there are no siblings
if (!peersHaveChildren(node, () => this.roots)) {
return NodeRenderDirective.Concat;
}
// Omit roots that have no child tests
if (node.children.size === 0) {
return NodeRenderDirective.Omit;
}
}
return {
element: node,
collapsible: node.test.expand !== TestItemExpandState.NotExpandable,
collapsed: this.lastState.expanded[node.treeId] !== undefined
? !this.lastState.expanded[node.treeId]
: node.test.expand === TestItemExpandState.Expandable ? true : undefined,
children: recurse(node.children),
};
};
protected unstoreItem(items: Map<string, TestItemTreeElement>, treeElement: ByLocationTestItemElement) {
const parent = treeElement.parent;
parent?.children.delete(treeElement);
items.delete(treeElement.test.item.extId);
if (parent instanceof ByLocationTestItemElement) {
refreshComputedState(computedStateAccessor, parent).forEach(this.addUpdated);
}
return treeElement.children;
}
protected storeItem(treeElement: ByLocationTestItemElement) {
treeElement.parent?.children.add(treeElement);
this.items.set(treeElement.test.item.extId, treeElement);
this.changes.addedOrRemoved(treeElement);
const reveal = this.getRevealDepth(treeElement);
if (reveal !== undefined) {
this.expandElement(treeElement, reveal);
}
const prevState = this.results.getStateById(treeElement.test.item.extId)?.[1];
if (prevState) {
treeElement.retired = prevState.retired;
treeElement.ownState = prevState.computedState;
treeElement.ownDuration = prevState.ownDuration;
refreshComputedState(computedStateAccessor, treeElement).forEach(this.addUpdated);
}
}
}
| src/vs/workbench/contrib/testing/browser/explorerProjections/hierarchalByLocation.ts | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.00017533148638904095,
0.00017083357670344412,
0.00016821046301629394,
0.0001706886978354305,
0.000001609040282346541
]
|
{
"id": 8,
"code_window": [
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-top > .tab-border-top-container {\n",
"\tz-index: 2;\n",
"\ttop: 0;\n",
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-top-color);\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 6;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 219
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { createDecorator } from 'vs/platform/instantiation/common/instantiation';
import { IAuthenticationProvider, SyncStatus, SyncResource, Change, MergeState } from 'vs/platform/userDataSync/common/userDataSync';
import { Event } from 'vs/base/common/event';
import { RawContextKey } from 'vs/platform/contextkey/common/contextkey';
import { localize } from 'vs/nls';
import { URI } from 'vs/base/common/uri';
import { Codicon } from 'vs/base/common/codicons';
import { registerIcon } from 'vs/platform/theme/common/iconRegistry';
export interface IUserDataSyncAccount {
readonly authenticationProviderId: string;
readonly accountName: string;
readonly accountId: string;
}
export interface IUserDataSyncPreview {
readonly onDidChangeResources: Event<ReadonlyArray<IUserDataSyncResource>>;
readonly resources: ReadonlyArray<IUserDataSyncResource>;
accept(syncResource: SyncResource, resource: URI, content?: string | null): Promise<void>;
merge(resource?: URI): Promise<void>;
discard(resource?: URI): Promise<void>;
pull(): Promise<void>;
push(): Promise<void>;
apply(): Promise<void>;
cancel(): Promise<void>;
}
export interface IUserDataSyncResource {
readonly syncResource: SyncResource;
readonly local: URI;
readonly remote: URI;
readonly merged: URI;
readonly accepted: URI;
readonly localChange: Change;
readonly remoteChange: Change;
readonly mergeState: MergeState;
}
export const IUserDataSyncWorkbenchService = createDecorator<IUserDataSyncWorkbenchService>('IUserDataSyncWorkbenchService');
export interface IUserDataSyncWorkbenchService {
_serviceBrand: any;
readonly enabled: boolean;
readonly authenticationProviders: IAuthenticationProvider[];
readonly all: IUserDataSyncAccount[];
readonly current: IUserDataSyncAccount | undefined;
readonly accountStatus: AccountStatus;
readonly onDidChangeAccountStatus: Event<AccountStatus>;
readonly userDataSyncPreview: IUserDataSyncPreview;
turnOn(): Promise<void>;
turnOnUsingCurrentAccount(): Promise<void>;
turnoff(everyWhere: boolean): Promise<void>;
signIn(): Promise<void>;
resetSyncedData(): Promise<void>;
showSyncActivity(): Promise<void>;
syncNow(): Promise<void>;
synchroniseUserDataSyncStoreType(): Promise<void>;
}
export function getSyncAreaLabel(source: SyncResource): string {
switch (source) {
case SyncResource.Settings: return localize('settings', "Settings");
case SyncResource.Keybindings: return localize('keybindings', "Keyboard Shortcuts");
case SyncResource.Snippets: return localize('snippets', "User Snippets");
case SyncResource.Extensions: return localize('extensions', "Extensions");
case SyncResource.GlobalState: return localize('ui state label', "UI State");
}
}
export const enum AccountStatus {
Uninitialized = 'uninitialized',
Unavailable = 'unavailable',
Available = 'available',
}
export const SYNC_TITLE = localize('sync category', "Settings Sync");
export const SYNC_VIEW_ICON = registerIcon('settings-sync-view-icon', Codicon.sync, localize('syncViewIcon', 'View icon of the Settings Sync view.'));
// Contexts
export const CONTEXT_SYNC_STATE = new RawContextKey<string>('syncStatus', SyncStatus.Uninitialized);
export const CONTEXT_SYNC_ENABLEMENT = new RawContextKey<boolean>('syncEnabled', false);
export const CONTEXT_ACCOUNT_STATE = new RawContextKey<string>('userDataSyncAccountStatus', AccountStatus.Uninitialized);
export const CONTEXT_ENABLE_ACTIVITY_VIEWS = new RawContextKey<boolean>(`enableSyncActivityViews`, false);
export const CONTEXT_ENABLE_SYNC_MERGES_VIEW = new RawContextKey<boolean>(`enableSyncMergesView`, false);
// Commands
export const CONFIGURE_SYNC_COMMAND_ID = 'workbench.userDataSync.actions.configure';
export const SHOW_SYNC_LOG_COMMAND_ID = 'workbench.userDataSync.actions.showLog';
// VIEWS
export const SYNC_VIEW_CONTAINER_ID = 'workbench.view.sync';
export const SYNC_MERGES_VIEW_ID = 'workbench.views.sync.merges';
| src/vs/workbench/services/userDataSync/common/userDataSync.ts | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.00048392810276709497,
0.0002120341086992994,
0.000164927463629283,
0.00017270950775127858,
0.00008997817349154502
]
|
{
"id": 9,
"code_window": [
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-top-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-bottom > .tab-border-bottom-container {\n",
"\tz-index: 6;\n",
"\tbottom: 0;\n",
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-bottom-color);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 10;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 226
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
/* Container */
.monaco-workbench .part.editor > .content .editor-group-container {
height: 100%;
}
.monaco-workbench .part.editor > .content .editor-group-container.empty {
opacity: 0.5; /* dimmed to indicate inactive state */
}
.monaco-workbench .part.editor > .content .editor-group-container.empty.active,
.monaco-workbench .part.editor > .content .editor-group-container.empty.dragged-over {
opacity: 1; /* indicate active/dragged-over group through undimmed state */
}
/* Letterpress */
.monaco-workbench .part.editor > .content .editor-group-container > .editor-group-letterpress {
display: none; /* only visible when empty */
}
.monaco-workbench .part.editor > .content .editor-group-container.empty > .editor-group-letterpress {
display: block;
margin: auto;
width: 100%;
height: calc(100% - 70px); /* centered below toolbar */
max-width: 260px;
background-repeat: no-repeat;
background-position: 50% 50%;
background-size: 70% 70%;
}
.monaco-workbench .part.editor > .content.empty .editor-group-container.empty > .editor-group-letterpress {
background-size: 100% 100%; /* larger for empty editor part */
height: 100%; /* no toolbar in this case */
}
/* Title */
.monaco-workbench .part.editor > .content .editor-group-container > .title {
position: relative;
box-sizing: border-box;
overflow: hidden;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title:not(.tabs) {
display: flex; /* when tabs are not shown, use flex layout */
flex-wrap: nowrap;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title.title-border-bottom::after {
content: '';
position: absolute;
bottom: 0;
left: 0;
z-index: 5;
pointer-events: none;
background-color: var(--title-border-bottom-color);
width: 100%;
height: 1px;
}
.monaco-workbench .part.editor > .content .editor-group-container.empty > .title {
display: none;
}
/* Toolbar */
.monaco-workbench .part.editor > .content .editor-group-container > .editor-group-container-toolbar {
display: none;
height: 35px;
}
.monaco-workbench .part.editor > .content:not(.empty) .editor-group-container.empty > .editor-group-container-toolbar {
display: block;
}
.monaco-workbench .part.editor > .content .editor-group-container > .editor-group-container-toolbar .actions-container {
justify-content: flex-end;
}
.monaco-workbench .part.editor > .content .editor-group-container > .editor-group-container-toolbar .action-item {
margin-right: 4px;
}
/* Editor */
.monaco-workbench .part.editor > .content .editor-group-container.empty > .editor-container {
display: none;
}
.monaco-workbench .part.editor > .content .editor-group-container > .editor-container > .editor-instance {
height: 100%;
}
.monaco-workbench .part.editor > .content .grid-view-container {
width: 100%;
height: 100%;
}
| src/vs/workbench/browser/parts/editor/media/editorgroupview.css | 1 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.025686264038085938,
0.003894343739375472,
0.0005486559239216149,
0.0015290938317775726,
0.007004803046584129
]
|
{
"id": 9,
"code_window": [
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-top-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-bottom > .tab-border-bottom-container {\n",
"\tz-index: 6;\n",
"\tbottom: 0;\n",
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-bottom-color);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 10;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 226
} | {
"displayName": "Solarized Light Theme",
"description": "Solarized light theme for Visual Studio Code",
"themeLabel": "Solarized Light"
}
| extensions/theme-solarized-light/package.nls.json | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.00017432478489354253,
0.00017432478489354253,
0.00017432478489354253,
0.00017432478489354253,
0
]
|
{
"id": 9,
"code_window": [
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-top-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-bottom > .tab-border-bottom-container {\n",
"\tz-index: 6;\n",
"\tbottom: 0;\n",
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-bottom-color);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 10;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 226
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { VSBuffer } from 'vs/base/common/buffer';
import { CancellationToken } from 'vs/base/common/cancellation';
import { IStringDictionary } from 'vs/base/common/collections';
import { getErrorMessage } from 'vs/base/common/errors';
import { Event } from 'vs/base/common/event';
import { parse } from 'vs/base/common/json';
import { toFormattedString } from 'vs/base/common/jsonFormatter';
import { isWeb } from 'vs/base/common/platform';
import { URI } from 'vs/base/common/uri';
import { generateUuid } from 'vs/base/common/uuid';
import { IHeaders } from 'vs/base/parts/request/common/request';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { IFileService } from 'vs/platform/files/common/files';
import { ILogService } from 'vs/platform/log/common/log';
import { getServiceMachineId } from 'vs/platform/serviceMachineId/common/serviceMachineId';
import { IStorageService, StorageScope, StorageTarget } from 'vs/platform/storage/common/storage';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { AbstractInitializer, AbstractSynchroniser, IAcceptResult, IMergeResult, IResourcePreview, isSyncData } from 'vs/platform/userDataSync/common/abstractSynchronizer';
import { edit } from 'vs/platform/userDataSync/common/content';
import { merge } from 'vs/platform/userDataSync/common/globalStateMerge';
import { ALL_SYNC_RESOURCES, Change, createSyncHeaders, getEnablementKey, IGlobalState, IRemoteUserData, IStorageValue, ISyncData, ISyncResourceHandle, IUserData, IUserDataSyncBackupStoreService, IUserDataSynchroniser, IUserDataSyncLogService, IUserDataSyncEnablementService, IUserDataSyncStoreService, SyncResource, SYNC_SERVICE_URL_TYPE, UserDataSyncError, UserDataSyncErrorCode, UserDataSyncStoreType, USER_DATA_SYNC_SCHEME } from 'vs/platform/userDataSync/common/userDataSync';
import { UserDataSyncStoreClient } from 'vs/platform/userDataSync/common/userDataSyncStoreService';
const argvStoragePrefx = 'globalState.argv.';
const argvProperties: string[] = ['locale'];
type StorageKeys = { machine: string[], user: string[], unregistered: string[] };
interface IGlobalStateResourceMergeResult extends IAcceptResult {
readonly local: { added: IStringDictionary<IStorageValue>, removed: string[], updated: IStringDictionary<IStorageValue> };
readonly remote: IStringDictionary<IStorageValue> | null;
}
export interface IGlobalStateResourcePreview extends IResourcePreview {
readonly localUserData: IGlobalState;
readonly previewResult: IGlobalStateResourceMergeResult;
readonly storageKeys: StorageKeys;
}
function stringify(globalState: IGlobalState, format: boolean): string {
const storageKeys = globalState.storage ? Object.keys(globalState.storage).sort() : [];
const storage: IStringDictionary<IStorageValue> = {};
storageKeys.forEach(key => storage[key] = globalState.storage[key]);
globalState.storage = storage;
return format ? toFormattedString(globalState, {}) : JSON.stringify(globalState);
}
const GLOBAL_STATE_DATA_VERSION = 1;
/**
* Synchronises global state that includes
* - Global storage with user scope
* - Locale from argv properties
*
* Global storage is synced without checking version just like other resources (settings, keybindings).
* If there is a change in format of the value of a storage key which requires migration then
* Owner of that key should remove that key from user scope and replace that with new user scoped key.
*/
export class GlobalStateSynchroniser extends AbstractSynchroniser implements IUserDataSynchroniser {
private static readonly GLOBAL_STATE_DATA_URI = URI.from({ scheme: USER_DATA_SYNC_SCHEME, authority: 'globalState', path: `/globalState.json` });
protected readonly version: number = GLOBAL_STATE_DATA_VERSION;
private readonly previewResource: URI = this.extUri.joinPath(this.syncPreviewFolder, 'globalState.json');
private readonly localResource: URI = this.previewResource.with({ scheme: USER_DATA_SYNC_SCHEME, authority: 'local' });
private readonly remoteResource: URI = this.previewResource.with({ scheme: USER_DATA_SYNC_SCHEME, authority: 'remote' });
private readonly acceptedResource: URI = this.previewResource.with({ scheme: USER_DATA_SYNC_SCHEME, authority: 'accepted' });
constructor(
@IFileService fileService: IFileService,
@IUserDataSyncStoreService userDataSyncStoreService: IUserDataSyncStoreService,
@IUserDataSyncBackupStoreService userDataSyncBackupStoreService: IUserDataSyncBackupStoreService,
@IUserDataSyncLogService logService: IUserDataSyncLogService,
@IEnvironmentService environmentService: IEnvironmentService,
@IUserDataSyncEnablementService userDataSyncEnablementService: IUserDataSyncEnablementService,
@ITelemetryService telemetryService: ITelemetryService,
@IConfigurationService configurationService: IConfigurationService,
@IStorageService private readonly storageService: IStorageService,
@IUriIdentityService uriIdentityService: IUriIdentityService,
) {
super(SyncResource.GlobalState, fileService, environmentService, storageService, userDataSyncStoreService, userDataSyncBackupStoreService, userDataSyncEnablementService, telemetryService, logService, configurationService, uriIdentityService);
this._register(fileService.watch(this.extUri.dirname(this.environmentService.argvResource)));
this._register(
Event.any(
/* Locale change */
Event.filter(fileService.onDidFilesChange, e => e.contains(this.environmentService.argvResource)),
/* Global storage with user target has changed */
Event.filter(storageService.onDidChangeValue, e => e.scope === StorageScope.GLOBAL && e.target !== undefined ? e.target === StorageTarget.USER : storageService.keys(StorageScope.GLOBAL, StorageTarget.USER).includes(e.key)),
/* Storage key target has changed */
this.storageService.onDidChangeTarget
)((() => this.triggerLocalChange()))
);
}
protected async generateSyncPreview(remoteUserData: IRemoteUserData, lastSyncUserData: IRemoteUserData | null, isRemoteDataFromCurrentMachine: boolean): Promise<IGlobalStateResourcePreview[]> {
const remoteGlobalState: IGlobalState = remoteUserData.syncData ? JSON.parse(remoteUserData.syncData.content) : null;
// Use remote data as last sync data if last sync data does not exist and remote data is from same machine
lastSyncUserData = lastSyncUserData === null && isRemoteDataFromCurrentMachine ? remoteUserData : lastSyncUserData;
const lastSyncGlobalState: IGlobalState | null = lastSyncUserData && lastSyncUserData.syncData ? JSON.parse(lastSyncUserData.syncData.content) : null;
const localGlobalState = await this.getLocalGlobalState();
if (remoteGlobalState) {
this.logService.trace(`${this.syncResourceLogLabel}: Merging remote ui state with local ui state...`);
} else {
this.logService.trace(`${this.syncResourceLogLabel}: Remote ui state does not exist. Synchronizing ui state for the first time.`);
}
const storageKeys = this.getStorageKeys(lastSyncGlobalState);
const { local, remote } = merge(localGlobalState.storage, remoteGlobalState ? remoteGlobalState.storage : null, lastSyncGlobalState ? lastSyncGlobalState.storage : null, storageKeys, this.logService);
const previewResult: IGlobalStateResourceMergeResult = {
content: null,
local,
remote,
localChange: Object.keys(local.added).length > 0 || Object.keys(local.updated).length > 0 || local.removed.length > 0 ? Change.Modified : Change.None,
remoteChange: remote !== null ? Change.Modified : Change.None,
};
return [{
localResource: this.localResource,
localContent: stringify(localGlobalState, false),
localUserData: localGlobalState,
remoteResource: this.remoteResource,
remoteContent: remoteGlobalState ? stringify(remoteGlobalState, false) : null,
previewResource: this.previewResource,
previewResult,
localChange: previewResult.localChange,
remoteChange: previewResult.remoteChange,
acceptedResource: this.acceptedResource,
storageKeys
}];
}
protected async hasRemoteChanged(lastSyncUserData: IRemoteUserData): Promise<boolean> {
const lastSyncGlobalState: IGlobalState | null = lastSyncUserData.syncData ? JSON.parse(lastSyncUserData.syncData.content) : null;
if (lastSyncGlobalState === null) {
return true;
}
const localGlobalState = await this.getLocalGlobalState();
const storageKeys = this.getStorageKeys(lastSyncGlobalState);
const { remote } = merge(localGlobalState.storage, lastSyncGlobalState.storage, lastSyncGlobalState.storage, storageKeys, this.logService);
return remote !== null;
}
protected async getMergeResult(resourcePreview: IGlobalStateResourcePreview, token: CancellationToken): Promise<IMergeResult> {
return { ...resourcePreview.previewResult, hasConflicts: false };
}
protected async getAcceptResult(resourcePreview: IGlobalStateResourcePreview, resource: URI, content: string | null | undefined, token: CancellationToken): Promise<IGlobalStateResourceMergeResult> {
/* Accept local resource */
if (this.extUri.isEqual(resource, this.localResource)) {
return this.acceptLocal(resourcePreview);
}
/* Accept remote resource */
if (this.extUri.isEqual(resource, this.remoteResource)) {
return this.acceptRemote(resourcePreview);
}
/* Accept preview resource */
if (this.extUri.isEqual(resource, this.previewResource)) {
return resourcePreview.previewResult;
}
throw new Error(`Invalid Resource: ${resource.toString()}`);
}
private async acceptLocal(resourcePreview: IGlobalStateResourcePreview): Promise<IGlobalStateResourceMergeResult> {
return {
content: resourcePreview.localContent,
local: { added: {}, removed: [], updated: {} },
remote: resourcePreview.localUserData.storage,
localChange: Change.None,
remoteChange: Change.Modified,
};
}
private async acceptRemote(resourcePreview: IGlobalStateResourcePreview): Promise<IGlobalStateResourceMergeResult> {
if (resourcePreview.remoteContent !== null) {
const remoteGlobalState: IGlobalState = JSON.parse(resourcePreview.remoteContent);
const { local, remote } = merge(resourcePreview.localUserData.storage, remoteGlobalState.storage, null, resourcePreview.storageKeys, this.logService);
return {
content: resourcePreview.remoteContent,
local,
remote,
localChange: Object.keys(local.added).length > 0 || Object.keys(local.updated).length > 0 || local.removed.length > 0 ? Change.Modified : Change.None,
remoteChange: remote !== null ? Change.Modified : Change.None,
};
} else {
return {
content: resourcePreview.remoteContent,
local: { added: {}, removed: [], updated: {} },
remote: null,
localChange: Change.None,
remoteChange: Change.None,
};
}
}
protected async applyResult(remoteUserData: IRemoteUserData, lastSyncUserData: IRemoteUserData | null, resourcePreviews: [IGlobalStateResourcePreview, IGlobalStateResourceMergeResult][], force: boolean): Promise<void> {
let { localUserData } = resourcePreviews[0][0];
let { local, remote, localChange, remoteChange } = resourcePreviews[0][1];
if (localChange === Change.None && remoteChange === Change.None) {
this.logService.info(`${this.syncResourceLogLabel}: No changes found during synchronizing ui state.`);
}
if (localChange !== Change.None) {
// update local
this.logService.trace(`${this.syncResourceLogLabel}: Updating local ui state...`);
await this.backupLocal(JSON.stringify(localUserData));
await this.writeLocalGlobalState(local);
this.logService.info(`${this.syncResourceLogLabel}: Updated local ui state`);
}
if (remoteChange !== Change.None) {
// update remote
this.logService.trace(`${this.syncResourceLogLabel}: Updating remote ui state...`);
const content = JSON.stringify(<IGlobalState>{ storage: remote });
remoteUserData = await this.updateRemoteUserData(content, force ? null : remoteUserData.ref);
this.logService.info(`${this.syncResourceLogLabel}: Updated remote ui state`);
}
if (lastSyncUserData?.ref !== remoteUserData.ref) {
// update last sync
this.logService.trace(`${this.syncResourceLogLabel}: Updating last synchronized ui state...`);
await this.updateLastSyncUserData(remoteUserData);
this.logService.info(`${this.syncResourceLogLabel}: Updated last synchronized ui state`);
}
}
async getAssociatedResources({ uri }: ISyncResourceHandle): Promise<{ resource: URI, comparableResource: URI }[]> {
return [{ resource: this.extUri.joinPath(uri, 'globalState.json'), comparableResource: GlobalStateSynchroniser.GLOBAL_STATE_DATA_URI }];
}
override async resolveContent(uri: URI): Promise<string | null> {
if (this.extUri.isEqual(uri, GlobalStateSynchroniser.GLOBAL_STATE_DATA_URI)) {
const localGlobalState = await this.getLocalGlobalState();
return stringify(localGlobalState, true);
}
if (this.extUri.isEqual(this.remoteResource, uri) || this.extUri.isEqual(this.localResource, uri) || this.extUri.isEqual(this.acceptedResource, uri)) {
const content = await this.resolvePreviewContent(uri);
return content ? stringify(JSON.parse(content), true) : content;
}
let content = await super.resolveContent(uri);
if (content) {
return content;
}
content = await super.resolveContent(this.extUri.dirname(uri));
if (content) {
const syncData = this.parseSyncData(content);
if (syncData) {
switch (this.extUri.basename(uri)) {
case 'globalState.json':
return stringify(JSON.parse(syncData.content), true);
}
}
}
return null;
}
async hasLocalData(): Promise<boolean> {
try {
const { storage } = await this.getLocalGlobalState();
if (Object.keys(storage).length > 1 || storage[`${argvStoragePrefx}.locale`]?.value !== 'en') {
return true;
}
} catch (error) {
/* ignore error */
}
return false;
}
private async getLocalGlobalState(): Promise<IGlobalState> {
const storage: IStringDictionary<IStorageValue> = {};
const argvContent: string = await this.getLocalArgvContent();
const argvValue: IStringDictionary<any> = parse(argvContent);
for (const argvProperty of argvProperties) {
if (argvValue[argvProperty] !== undefined) {
storage[`${argvStoragePrefx}${argvProperty}`] = { version: 1, value: argvValue[argvProperty] };
}
}
for (const key of this.storageService.keys(StorageScope.GLOBAL, StorageTarget.USER)) {
const value = this.storageService.get(key, StorageScope.GLOBAL);
if (value) {
storage[key] = { version: 1, value };
}
}
return { storage };
}
private async getLocalArgvContent(): Promise<string> {
try {
this.logService.debug('GlobalStateSync#getLocalArgvContent', this.environmentService.argvResource);
const content = await this.fileService.readFile(this.environmentService.argvResource);
this.logService.debug('GlobalStateSync#getLocalArgvContent - Resolved', this.environmentService.argvResource);
return content.value.toString();
} catch (error) {
this.logService.debug(getErrorMessage(error));
}
return '{}';
}
private async writeLocalGlobalState({ added, removed, updated }: { added: IStringDictionary<IStorageValue>, updated: IStringDictionary<IStorageValue>, removed: string[] }): Promise<void> {
const argv: IStringDictionary<any> = {};
const updatedStorage: IStringDictionary<any> = {};
const handleUpdatedStorage = (keys: string[], storage?: IStringDictionary<IStorageValue>): void => {
for (const key of keys) {
if (key.startsWith(argvStoragePrefx)) {
argv[key.substring(argvStoragePrefx.length)] = storage ? storage[key].value : undefined;
continue;
}
if (storage) {
const storageValue = storage[key];
if (storageValue.value !== String(this.storageService.get(key, StorageScope.GLOBAL))) {
updatedStorage[key] = storageValue.value;
}
} else {
if (this.storageService.get(key, StorageScope.GLOBAL) !== undefined) {
updatedStorage[key] = undefined;
}
}
}
};
handleUpdatedStorage(Object.keys(added), added);
handleUpdatedStorage(Object.keys(updated), updated);
handleUpdatedStorage(removed);
if (Object.keys(argv).length) {
this.logService.trace(`${this.syncResourceLogLabel}: Updating locale...`);
await this.updateArgv(argv);
this.logService.info(`${this.syncResourceLogLabel}: Updated locale`);
}
const updatedStorageKeys: string[] = Object.keys(updatedStorage);
if (updatedStorageKeys.length) {
this.logService.trace(`${this.syncResourceLogLabel}: Updating global state...`);
for (const key of Object.keys(updatedStorage)) {
this.storageService.store(key, updatedStorage[key], StorageScope.GLOBAL, StorageTarget.USER);
}
this.logService.info(`${this.syncResourceLogLabel}: Updated global state`, Object.keys(updatedStorage));
}
}
private async updateArgv(argv: IStringDictionary<any>): Promise<void> {
const argvContent = await this.getLocalArgvContent();
let content = argvContent;
for (const argvProperty of Object.keys(argv)) {
content = edit(content, [argvProperty], argv[argvProperty], {});
}
if (argvContent !== content) {
this.logService.trace(`${this.syncResourceLogLabel}: Updating locale...`);
await this.fileService.writeFile(this.environmentService.argvResource, VSBuffer.fromString(content));
this.logService.info(`${this.syncResourceLogLabel}: Updated locale.`);
}
}
private getStorageKeys(lastSyncGlobalState: IGlobalState | null): StorageKeys {
const user = this.storageService.keys(StorageScope.GLOBAL, StorageTarget.USER);
const machine = this.storageService.keys(StorageScope.GLOBAL, StorageTarget.MACHINE);
const registered = [...user, ...machine];
const unregistered = lastSyncGlobalState?.storage ? Object.keys(lastSyncGlobalState.storage).filter(key => !key.startsWith(argvStoragePrefx) && !registered.includes(key) && this.storageService.get(key, StorageScope.GLOBAL) !== undefined) : [];
if (!isWeb) {
// Following keys are synced only in web. Do not sync these keys in other platforms
const keysSyncedOnlyInWeb = [...ALL_SYNC_RESOURCES.map(resource => getEnablementKey(resource)), SYNC_SERVICE_URL_TYPE];
unregistered.push(...keysSyncedOnlyInWeb);
machine.push(...keysSyncedOnlyInWeb);
}
return { user, machine, unregistered };
}
}
export class GlobalStateInitializer extends AbstractInitializer {
constructor(
@IStorageService private readonly storageService: IStorageService,
@IFileService fileService: IFileService,
@IEnvironmentService environmentService: IEnvironmentService,
@IUserDataSyncLogService logService: IUserDataSyncLogService,
@IUriIdentityService uriIdentityService: IUriIdentityService,
) {
super(SyncResource.GlobalState, environmentService, logService, fileService, uriIdentityService);
}
async doInitialize(remoteUserData: IRemoteUserData): Promise<void> {
const remoteGlobalState: IGlobalState = remoteUserData.syncData ? JSON.parse(remoteUserData.syncData.content) : null;
if (!remoteGlobalState) {
this.logService.info('Skipping initializing global state because remote global state does not exist.');
return;
}
const argv: IStringDictionary<any> = {};
const storage: IStringDictionary<any> = {};
for (const key of Object.keys(remoteGlobalState.storage)) {
if (key.startsWith(argvStoragePrefx)) {
argv[key.substring(argvStoragePrefx.length)] = remoteGlobalState.storage[key].value;
} else {
if (this.storageService.get(key, StorageScope.GLOBAL) === undefined) {
storage[key] = remoteGlobalState.storage[key].value;
}
}
}
if (Object.keys(argv).length) {
let content = '{}';
try {
const fileContent = await this.fileService.readFile(this.environmentService.argvResource);
content = fileContent.value.toString();
} catch (error) { }
for (const argvProperty of Object.keys(argv)) {
content = edit(content, [argvProperty], argv[argvProperty], {});
}
await this.fileService.writeFile(this.environmentService.argvResource, VSBuffer.fromString(content));
}
if (Object.keys(storage).length) {
for (const key of Object.keys(storage)) {
this.storageService.store(key, storage[key], StorageScope.GLOBAL, StorageTarget.USER);
}
}
}
}
export class UserDataSyncStoreTypeSynchronizer {
constructor(
private readonly userDataSyncStoreClient: UserDataSyncStoreClient,
@IStorageService private readonly storageService: IStorageService,
@IEnvironmentService private readonly environmentService: IEnvironmentService,
@IFileService private readonly fileService: IFileService,
@ILogService private readonly logService: ILogService,
) {
}
getSyncStoreType(userData: IUserData): UserDataSyncStoreType | undefined {
const remoteGlobalState = this.parseGlobalState(userData);
return remoteGlobalState?.storage[SYNC_SERVICE_URL_TYPE]?.value as UserDataSyncStoreType;
}
async sync(userDataSyncStoreType: UserDataSyncStoreType): Promise<void> {
const syncHeaders = createSyncHeaders(generateUuid());
try {
return await this.doSync(userDataSyncStoreType, syncHeaders);
} catch (e) {
if (e instanceof UserDataSyncError) {
switch (e.code) {
case UserDataSyncErrorCode.PreconditionFailed:
this.logService.info(`Failed to synchronize UserDataSyncStoreType as there is a new remote version available. Synchronizing again...`);
return this.doSync(userDataSyncStoreType, syncHeaders);
}
}
throw e;
}
}
private async doSync(userDataSyncStoreType: UserDataSyncStoreType, syncHeaders: IHeaders): Promise<void> {
// Read the global state from remote
const globalStateUserData = await this.userDataSyncStoreClient.read(SyncResource.GlobalState, null, syncHeaders);
const remoteGlobalState = this.parseGlobalState(globalStateUserData) || { storage: {} };
// Update the sync store type
remoteGlobalState.storage[SYNC_SERVICE_URL_TYPE] = { value: userDataSyncStoreType, version: GLOBAL_STATE_DATA_VERSION };
// Write the global state to remote
const machineId = await getServiceMachineId(this.environmentService, this.fileService, this.storageService);
const syncDataToUpdate: ISyncData = { version: GLOBAL_STATE_DATA_VERSION, machineId, content: stringify(remoteGlobalState, false) };
await this.userDataSyncStoreClient.write(SyncResource.GlobalState, JSON.stringify(syncDataToUpdate), globalStateUserData.ref, syncHeaders);
}
private parseGlobalState({ content }: IUserData): IGlobalState | null {
if (!content) {
return null;
}
const syncData = JSON.parse(content);
if (isSyncData(syncData)) {
return syncData ? JSON.parse(syncData.content) : null;
}
throw new Error('Invalid remote data');
}
}
| src/vs/platform/userDataSync/common/globalStateSync.ts | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.0001845459919422865,
0.00017106047016568482,
0.00016420314204879105,
0.00017092018970288336,
0.0000033494750368845416
]
|
{
"id": 9,
"code_window": [
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-top-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-bottom > .tab-border-bottom-container {\n",
"\tz-index: 6;\n",
"\tbottom: 0;\n",
"\theight: 1px;\n",
"\tbackground-color: var(--tab-border-bottom-color);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 10;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 226
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as dom from 'vs/base/browser/dom';
import { IKeyboardEvent } from 'vs/base/browser/keyboardEvent';
import { Checkbox } from 'vs/base/browser/ui/checkbox/checkbox';
import { IContextViewProvider } from 'vs/base/browser/ui/contextview/contextview';
import { HistoryInputBox, IInputBoxStyles } from 'vs/base/browser/ui/inputbox/inputBox';
import { Widget } from 'vs/base/browser/ui/widget';
import { Codicon } from 'vs/base/common/codicons';
import { Emitter, Event as CommonEvent } from 'vs/base/common/event';
import { KeyCode } from 'vs/base/common/keyCodes';
import type { IThemable } from 'vs/base/common/styler';
import * as nls from 'vs/nls';
import { ContextScopedHistoryInputBox } from 'vs/platform/browser/contextScopedHistoryWidget';
import { showHistoryKeybindingHint } from 'vs/platform/browser/historyWidgetKeybindingHint';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { attachCheckboxStyler, attachInputBoxStyler } from 'vs/platform/theme/common/styler';
import { IThemeService } from 'vs/platform/theme/common/themeService';
export interface IOptions {
placeholder?: string;
showPlaceholderOnFocus?: boolean;
tooltip?: string;
width?: number;
ariaLabel?: string;
history?: string[];
}
export class PatternInputWidget extends Widget implements IThemable {
static OPTION_CHANGE: string = 'optionChange';
inputFocusTracker!: dom.IFocusTracker;
private width: number;
private domNode!: HTMLElement;
protected inputBox!: HistoryInputBox;
private _onSubmit = this._register(new Emitter<boolean>());
onSubmit: CommonEvent<boolean /* triggeredOnType */> = this._onSubmit.event;
private _onCancel = this._register(new Emitter<void>());
onCancel: CommonEvent<void> = this._onCancel.event;
constructor(parent: HTMLElement, private contextViewProvider: IContextViewProvider, options: IOptions = Object.create(null),
@IThemeService protected themeService: IThemeService,
@IContextKeyService private readonly contextKeyService: IContextKeyService,
@IConfigurationService protected readonly configurationService: IConfigurationService,
@IKeybindingService private readonly keybindingService: IKeybindingService,
) {
super();
options = {
...{
ariaLabel: nls.localize('defaultLabel', "input")
},
...options,
};
this.width = options.width ?? 100;
this.render(options);
parent.appendChild(this.domNode);
}
override dispose(): void {
super.dispose();
if (this.inputFocusTracker) {
this.inputFocusTracker.dispose();
}
}
setWidth(newWidth: number): void {
this.width = newWidth;
this.domNode.style.width = this.width + 'px';
this.contextViewProvider.layout();
this.setInputWidth();
}
getValue(): string {
return this.inputBox.value;
}
setValue(value: string): void {
if (this.inputBox.value !== value) {
this.inputBox.value = value;
}
}
select(): void {
this.inputBox.select();
}
focus(): void {
this.inputBox.focus();
}
inputHasFocus(): boolean {
return this.inputBox.hasFocus();
}
private setInputWidth(): void {
this.inputBox.width = this.width - this.getSubcontrolsWidth() - 2; // 2 for input box border
}
protected getSubcontrolsWidth(): number {
return 0;
}
getHistory(): string[] {
return this.inputBox.getHistory();
}
clearHistory(): void {
this.inputBox.clearHistory();
}
clear(): void {
this.setValue('');
}
onSearchSubmit(): void {
this.inputBox.addToHistory();
}
showNextTerm() {
this.inputBox.showNextValue();
}
showPreviousTerm() {
this.inputBox.showPreviousValue();
}
style(styles: IInputBoxStyles): void {
this.inputBox.style(styles);
}
private render(options: IOptions): void {
this.domNode = document.createElement('div');
this.domNode.style.width = this.width + 'px';
this.domNode.classList.add('monaco-findInput');
this.inputBox = new ContextScopedHistoryInputBox(this.domNode, this.contextViewProvider, {
placeholder: options.placeholder,
showPlaceholderOnFocus: options.showPlaceholderOnFocus,
tooltip: options.tooltip,
ariaLabel: options.ariaLabel,
validationOptions: {
validation: undefined
},
history: options.history || [],
showHistoryHint: () => showHistoryKeybindingHint(this.keybindingService)
}, this.contextKeyService);
this._register(attachInputBoxStyler(this.inputBox, this.themeService));
this._register(this.inputBox.onDidChange(() => this._onSubmit.fire(true)));
this.inputFocusTracker = dom.trackFocus(this.inputBox.inputElement);
this.onkeyup(this.inputBox.inputElement, (keyboardEvent) => this.onInputKeyUp(keyboardEvent));
const controls = document.createElement('div');
controls.className = 'controls';
this.renderSubcontrols(controls);
this.domNode.appendChild(controls);
this.setInputWidth();
}
protected renderSubcontrols(_controlsDiv: HTMLDivElement): void {
}
private onInputKeyUp(keyboardEvent: IKeyboardEvent) {
switch (keyboardEvent.keyCode) {
case KeyCode.Enter:
this.onSearchSubmit();
this._onSubmit.fire(false);
return;
case KeyCode.Escape:
this._onCancel.fire();
return;
}
}
}
export class IncludePatternInputWidget extends PatternInputWidget {
private _onChangeSearchInEditorsBoxEmitter = this._register(new Emitter<void>());
onChangeSearchInEditorsBox = this._onChangeSearchInEditorsBoxEmitter.event;
constructor(parent: HTMLElement, contextViewProvider: IContextViewProvider, options: IOptions = Object.create(null),
@IThemeService themeService: IThemeService,
@IContextKeyService contextKeyService: IContextKeyService,
@IConfigurationService configurationService: IConfigurationService,
@IKeybindingService keybindingService: IKeybindingService,
) {
super(parent, contextViewProvider, options, themeService, contextKeyService, configurationService, keybindingService);
}
private useSearchInEditorsBox!: Checkbox;
override dispose(): void {
super.dispose();
this.useSearchInEditorsBox.dispose();
}
onlySearchInOpenEditors(): boolean {
return this.useSearchInEditorsBox.checked;
}
setOnlySearchInOpenEditors(value: boolean) {
this.useSearchInEditorsBox.checked = value;
this._onChangeSearchInEditorsBoxEmitter.fire();
}
protected override getSubcontrolsWidth(): number {
return super.getSubcontrolsWidth() + this.useSearchInEditorsBox.width();
}
protected override renderSubcontrols(controlsDiv: HTMLDivElement): void {
this.useSearchInEditorsBox = this._register(new Checkbox({
icon: Codicon.book,
title: nls.localize('onlySearchInOpenEditors', "Search only in Open Editors"),
isChecked: false,
}));
this._register(this.useSearchInEditorsBox.onChange(viaKeyboard => {
this._onChangeSearchInEditorsBoxEmitter.fire();
if (!viaKeyboard) {
this.inputBox.focus();
}
}));
this._register(attachCheckboxStyler(this.useSearchInEditorsBox, this.themeService));
controlsDiv.appendChild(this.useSearchInEditorsBox.domNode);
super.renderSubcontrols(controlsDiv);
}
}
export class ExcludePatternInputWidget extends PatternInputWidget {
private _onChangeIgnoreBoxEmitter = this._register(new Emitter<void>());
onChangeIgnoreBox = this._onChangeIgnoreBoxEmitter.event;
constructor(parent: HTMLElement, contextViewProvider: IContextViewProvider, options: IOptions = Object.create(null),
@IThemeService themeService: IThemeService,
@IContextKeyService contextKeyService: IContextKeyService,
@IConfigurationService configurationService: IConfigurationService,
@IKeybindingService keybindingService: IKeybindingService,
) {
super(parent, contextViewProvider, options, themeService, contextKeyService, configurationService, keybindingService);
}
private useExcludesAndIgnoreFilesBox!: Checkbox;
override dispose(): void {
super.dispose();
this.useExcludesAndIgnoreFilesBox.dispose();
}
useExcludesAndIgnoreFiles(): boolean {
return this.useExcludesAndIgnoreFilesBox.checked;
}
setUseExcludesAndIgnoreFiles(value: boolean) {
this.useExcludesAndIgnoreFilesBox.checked = value;
this._onChangeIgnoreBoxEmitter.fire();
}
protected override getSubcontrolsWidth(): number {
return super.getSubcontrolsWidth() + this.useExcludesAndIgnoreFilesBox.width();
}
protected override renderSubcontrols(controlsDiv: HTMLDivElement): void {
this.useExcludesAndIgnoreFilesBox = this._register(new Checkbox({
icon: Codicon.exclude,
actionClassName: 'useExcludesAndIgnoreFiles',
title: nls.localize('useExcludesAndIgnoreFilesDescription', "Use Exclude Settings and Ignore Files"),
isChecked: true,
}));
this._register(this.useExcludesAndIgnoreFilesBox.onChange(viaKeyboard => {
this._onChangeIgnoreBoxEmitter.fire();
if (!viaKeyboard) {
this.inputBox.focus();
}
}));
this._register(attachCheckboxStyler(this.useExcludesAndIgnoreFilesBox, this.themeService));
controlsDiv.appendChild(this.useExcludesAndIgnoreFilesBox.domNode);
super.renderSubcontrols(controlsDiv);
}
}
| src/vs/workbench/contrib/search/browser/patternInputWidget.ts | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.00031145798857323825,
0.00017509215103928,
0.00016385405615437776,
0.00017087333253584802,
0.00002555347055022139
]
|
{
"id": 10,
"code_window": [
"\tbackground-color: var(--tab-border-bottom-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty-border-top > .tab-border-top-container {\n",
"\tz-index: 2;\n",
"\ttop: 0;\n",
"\theight: 2px;\n",
"\tbackground-color: var(--tab-dirty-border-top-color);\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 6;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 233
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
/*
################################### z-index explainer ###################################
Tabs have various levels of z-index depending on state, typically:
- scrollbar should be above all
- sticky (compact, shrink) tabs need to be above non-sticky tabs for scroll under effect
including non-sticky tabs-top borders, otherwise these borders would not scroll under
(https://github.com/microsoft/vscode/issues/111641)
- bottom-border needs to be above tabs bottom border to win but also support sticky tabs
(https://github.com/microsoft/vscode/issues/99084) <- this currently cannot be done and
is still broken. putting sticky-tabs above tabs bottom border would not render this
border at all for sticky tabs.
On top of that there is 2 borders with a z-index for a general border below tabs
- tabs bottom border
- editor title bottom border (when breadcrumbs are disabled, this border will appear
same as tabs bottom border)
The following tabls shows the current stacking order:
[z-index] [kind]
7 scrollbar
6 active-tab border-bottom
5 tabs, title border bottom
4 sticky-tab
2 active/dirty-tab border top
0 tab
##########################################################################################
*/
/* Title Container */
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container {
display: flex;
position: relative; /* position tabs border bottom or editor actions (when tabs wrap) relative to this container */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container.tabs-border-bottom::after {
content: '';
position: absolute;
bottom: 0;
left: 0;
z-index: 5;
pointer-events: none;
background-color: var(--tabs-border-bottom-color);
width: 100%;
height: 1px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container > .monaco-scrollable-element {
flex: 1;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container > .monaco-scrollable-element .scrollbar {
z-index: 7;
cursor: default;
}
/* Tabs Container */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container {
display: flex;
height: 35px;
scrollbar-width: none; /* Firefox: hide scrollbar */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container.scroll {
overflow: scroll !important;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container.wrapping .tabs-container {
/* Enable wrapping via flex layout and dynamic height */
height: auto;
flex-wrap: wrap;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container::-webkit-scrollbar {
display: none; /* Chrome + Safari: hide scrollbar */
}
/* Tab */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab {
position: relative;
display: flex;
white-space: nowrap;
cursor: pointer;
height: 35px;
box-sizing: border-box;
padding-left: 10px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container.wrapping .tabs-container > .tab:last-child {
margin-right: var(--last-tab-margin-right); /* when tabs wrap, we need a margin away from the absolute positioned editor actions */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container.wrapping .tabs-container > .tab.last-in-row:not(:last-child) {
border-right: 0 !important; /* ensure no border for every last tab in a row except last row (#115046) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.has-icon.tab-actions-right,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.has-icon.tab-actions-off:not(.sticky-compact) {
padding-left: 5px; /* reduce padding when we show icons and are in shrinking mode and tab actions is not left (unless sticky-compact) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit {
width: 120px;
min-width: fit-content;
min-width: -moz-fit-content;
flex-shrink: 0;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container.wrapping .tabs-container > .tab.sizing-fit.last-in-row:not(:last-child) {
flex-grow: 1; /* grow the last tab in a row for a more homogeneous look except for last row (#113801) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink {
min-width: 80px;
flex-basis: 0; /* all tabs are even */
flex-grow: 1; /* all tabs grow even */
max-width: fit-content;
max-width: -moz-fit-content;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit.sticky-compact,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.sticky-compact,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit.sticky-shrink,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.sticky-shrink {
/** Sticky compact/shrink tabs do not scroll in case of overflow and are always above unsticky tabs which scroll under */
position: sticky;
z-index: 4;
/** Sticky compact/shrink tabs are even and never grow */
flex-basis: 0;
flex-grow: 0;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit.sticky-compact,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.sticky-compact {
/** Sticky compact tabs have a fixed width of 38px */
width: 38px;
min-width: 38px;
max-width: 38px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit.sticky-shrink,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.sticky-shrink {
/** Sticky shrink tabs have a fixed width of 80px */
width: 80px;
min-width: 80px;
max-width: 80px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container.disable-sticky-tabs > .tab.sizing-fit.sticky-compact,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container.disable-sticky-tabs > .tab.sizing-shrink.sticky-compact,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container.disable-sticky-tabs > .tab.sizing-fit.sticky-shrink,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container.disable-sticky-tabs > .tab.sizing-shrink.sticky-shrink {
position: static; /** disable sticky positions for sticky compact/shrink tabs if the available space is too little */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-left .action-label {
margin-right: 4px !important;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.tab-actions-left::after,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.tab-actions-off::after {
content: '';
display: flex;
flex: 0;
width: 5px; /* reserve space to hide tab fade when close button is left or off (fixes https://github.com/microsoft/vscode/issues/45728) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.tab-actions-left {
min-width: 80px; /* make more room for close button when it shows to the left */
padding-right: 5px; /* we need less room when sizing is shrink */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dragged {
transform: translate3d(0px, 0px, 0px); /* forces tab to be drawn on a separate layer (fixes https://github.com/microsoft/vscode/issues/18733) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dragged-over div {
pointer-events: none; /* prevents cursor flickering (fixes https://github.com/microsoft/vscode/issues/38753) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-left {
flex-direction: row-reverse;
padding-left: 0;
padding-right: 10px;
}
/* Tab border top/bottom */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab > .tab-border-top-container,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab > .tab-border-bottom-container {
display: none; /* hidden by default until a color is provided (see below) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-top > .tab-border-top-container,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-bottom > .tab-border-bottom-container,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty-border-top > .tab-border-top-container {
display: block;
position: absolute;
left: 0;
pointer-events: none;
width: 100%;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-top > .tab-border-top-container {
z-index: 2;
top: 0;
height: 1px;
background-color: var(--tab-border-top-color);
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active.tab-border-bottom > .tab-border-bottom-container {
z-index: 6;
bottom: 0;
height: 1px;
background-color: var(--tab-border-bottom-color);
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty-border-top > .tab-border-top-container {
z-index: 2;
top: 0;
height: 2px;
background-color: var(--tab-dirty-border-top-color);
}
/* Tab Label */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab .tab-label {
margin-top: auto;
margin-bottom: auto;
line-height: 35px; /* aligns icon and label vertically centered in the tab */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink .tab-label {
position: relative;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink > .tab-label > .monaco-icon-label-container::after {
content: ''; /* enables a linear gradient to overlay the end of the label when tabs overflow */
position: absolute;
right: 0;
width: 5px;
opacity: 1;
padding: 0;
/* the rules below ensure that the gradient does not impact top/bottom borders (https://github.com/microsoft/vscode/issues/115129) */
top: 1px;
bottom: 1px;
height: calc(100% - 2px);
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink:focus > .tab-label > .monaco-icon-label-container::after {
opacity: 0; /* when tab has the focus this shade breaks the tab border (fixes https://github.com/microsoft/vscode/issues/57819) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink > .tab-label.tab-label-has-badge::after {
padding-right: 5px; /* with tab sizing shrink and badges, we want a right-padding because the close button is hidden */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sticky-compact:not(.has-icon) .monaco-icon-label {
text-align: center; /* ensure that sticky-compact tabs without icon have label centered */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit .monaco-icon-label,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-fit .monaco-icon-label > .monaco-icon-label-container {
overflow: visible; /* fixes https://github.com/microsoft/vscode/issues/20182 */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink > .monaco-icon-label > .monaco-icon-label-container {
text-overflow: clip;
flex: none;
}
.monaco-workbench.hc-black .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink > .monaco-icon-label > .monaco-icon-label-container {
text-overflow: ellipsis;
}
/* Tab Actions */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab > .tab-actions {
margin-top: auto;
margin-bottom: auto;
width: 28px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab > .tab-actions > .monaco-action-bar {
width: 28px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-right.sizing-shrink > .tab-actions {
flex: 0;
overflow: hidden; /* let the tab actions be pushed out of view when sizing is set to shrink to make more room */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty.tab-actions-right.sizing-shrink > .tab-actions,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sticky.tab-actions-right.sizing-shrink > .tab-actions,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-right.sizing-shrink:hover > .tab-actions,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-right.sizing-shrink > .tab-actions:focus-within {
overflow: visible; /* ...but still show the tab actions on hover, focus and when dirty or sticky */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off:not(.dirty):not(.sticky) > .tab-actions,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off.sticky-compact > .tab-actions {
display: none; /* hide the tab actions when we are configured to hide it (unless dirty or sticky, but always when sticky-compact) */
}
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab.active > .tab-actions .action-label, /* always show tab actions for active tab */
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab > .tab-actions .action-label:focus, /* always show tab actions on focus */
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab:hover > .tab-actions .action-label, /* always show tab actions on hover */
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab.active:hover > .tab-actions .action-label, /* always show tab actions on hover */
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab.sticky > .tab-actions .action-label, /* always show tab actions for sticky tabs */
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab.dirty > .tab-actions .action-label { /* always show tab actions for dirty tabs */
opacity: 1;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab > .tab-actions .actions-container {
justify-content: center;
}
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab > .tab-actions .action-label.codicon {
color: inherit;
font-size: 16px;
padding: 2px;
width: 16px;
height: 16px;
}
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab.sticky.dirty > .tab-actions .action-label:not(:hover)::before,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sticky.dirty > .tab-actions .action-label:not(:hover)::before {
content: "\ebb2"; /* use `pinned-dirty` icon unicode for sticky-dirty indication */
}
.monaco-workbench .part.editor > .content .editor-group-container.active > .title .tabs-container > .tab.dirty > .tab-actions .action-label:not(:hover)::before,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty > .tab-actions .action-label:not(:hover)::before {
content: "\ea71"; /* use `circle-filled` icon unicode for dirty indication */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active > .tab-actions .action-label,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.active:hover > .tab-actions .action-label,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty > .tab-actions .action-label,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sticky > .tab-actions .action-label,
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab:hover > .tab-actions .action-label {
opacity: 0.5; /* show tab actions dimmed for inactive group */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab > .tab-actions .action-label {
opacity: 0;
}
/* Tab Actions: Off */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off {
padding-right: 10px; /* give a little bit more room if tab actions is off */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.sizing-shrink.tab-actions-off:not(.sticky-compact) {
padding-right: 5px; /* we need less room when sizing is shrink (unless tab is sticky-compact) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off.dirty-border-top > .tab-actions {
display: none; /* hide dirty state when highlightModifiedTabs is enabled and when running without tab actions */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off.dirty:not(.dirty-border-top):not(.sticky-compact),
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off.sticky:not(.sticky-compact) {
padding-right: 0; /* remove extra padding when we are running without tab actions (unless tab is sticky-compact) */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.tab-actions-off > .tab-actions {
pointer-events: none; /* don't allow tab actions to be clicked when running without tab actions */
}
/* Breadcrumbs */
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control {
flex: 1 100%;
height: 22px;
cursor: default;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .monaco-icon-label {
height: 22px;
line-height: 22px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .monaco-icon-label::before {
height: 22px; /* tweak the icon size of the editor labels when icons are enabled */
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .outline-element-icon {
padding-right: 3px;
height: 22px; /* tweak the icon size of the editor labels when icons are enabled */
line-height: 22px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .monaco-breadcrumb-item {
max-width: 80%;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .monaco-breadcrumb-item::before {
width: 16px;
height: 22px;
display: flex;
align-items: center;
justify-content: center;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .monaco-breadcrumb-item:last-child {
padding-right: 8px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-breadcrumbs .breadcrumbs-control .monaco-breadcrumb-item:last-child .codicon:last-child {
display: none; /* hides chevrons when last item */
}
/* Editor Actions Toolbar */
.monaco-workbench .part.editor > .content .editor-group-container > .title .editor-actions {
cursor: default;
flex: initial;
padding: 0 8px 0 4px;
height: 35px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title .editor-actions .action-item {
margin-right: 4px;
}
.monaco-workbench .part.editor > .content .editor-group-container > .title > .tabs-and-actions-container.wrapping .editor-actions {
/* When tabs are wrapped, position the editor actions at the end of the very last row */
position: absolute;
bottom: 0;
right: 0;
}
| src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css | 1 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.7826333045959473,
0.02265309728682041,
0.00016387717914767563,
0.001710335025563836,
0.11515173316001892
]
|
{
"id": 10,
"code_window": [
"\tbackground-color: var(--tab-border-bottom-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty-border-top > .tab-border-top-container {\n",
"\tz-index: 2;\n",
"\ttop: 0;\n",
"\theight: 2px;\n",
"\tbackground-color: var(--tab-dirty-border-top-color);\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 6;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 233
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { isURLDomainTrusted } from 'vs/workbench/contrib/url/browser/trustedDomainsValidator';
import { URI } from 'vs/base/common/uri';
import { extractGitHubRemotesFromGitConfig } from 'vs/workbench/contrib/url/browser/trustedDomains';
function linkAllowedByRules(link: string, rules: string[]) {
assert.ok(isURLDomainTrusted(URI.parse(link), rules), `Link\n${link}\n should be allowed by rules\n${JSON.stringify(rules)}`);
}
function linkNotAllowedByRules(link: string, rules: string[]) {
assert.ok(!isURLDomainTrusted(URI.parse(link), rules), `Link\n${link}\n should NOT be allowed by rules\n${JSON.stringify(rules)}`);
}
suite('GitHub remote extraction', () => {
test('All known formats', () => {
assert.deepStrictEqual(
extractGitHubRemotesFromGitConfig(
`
[remote "1"]
url = [email protected]:sshgit/vscode.git
[remote "2"]
url = [email protected]:ssh/vscode
[remote "3"]
url = https://github.com/httpsgit/vscode.git
[remote "4"]
url = https://github.com/https/vscode`),
[
'https://github.com/sshgit/vscode/',
'https://github.com/ssh/vscode/',
'https://github.com/httpsgit/vscode/',
'https://github.com/https/vscode/'
]);
});
});
suite('Link protection domain matching', () => {
test('simple', () => {
linkNotAllowedByRules('https://x.org', []);
linkAllowedByRules('https://x.org', ['https://x.org']);
linkAllowedByRules('https://x.org/foo', ['https://x.org']);
linkNotAllowedByRules('https://x.org', ['http://x.org']);
linkNotAllowedByRules('http://x.org', ['https://x.org']);
linkNotAllowedByRules('https://www.x.org', ['https://x.org']);
linkAllowedByRules('https://www.x.org', ['https://www.x.org', 'https://y.org']);
});
test('localhost', () => {
linkAllowedByRules('https://127.0.0.1', []);
linkAllowedByRules('https://127.0.0.1:3000', []);
linkAllowedByRules('https://localhost', []);
linkAllowedByRules('https://localhost:3000', []);
});
test('* star', () => {
linkAllowedByRules('https://a.x.org', ['https://*.x.org']);
linkAllowedByRules('https://a.b.x.org', ['https://*.x.org']);
});
test('no scheme', () => {
linkAllowedByRules('https://a.x.org', ['a.x.org']);
linkAllowedByRules('https://a.x.org', ['*.x.org']);
linkAllowedByRules('https://a.b.x.org', ['*.x.org']);
linkAllowedByRules('https://x.org', ['*.x.org']);
});
test('sub paths', () => {
linkAllowedByRules('https://x.org/foo', ['https://x.org/foo']);
linkAllowedByRules('https://x.org/foo/bar', ['https://x.org/foo']);
linkAllowedByRules('https://x.org/foo', ['https://x.org/foo/']);
linkAllowedByRules('https://x.org/foo/bar', ['https://x.org/foo/']);
linkAllowedByRules('https://x.org/foo', ['x.org/foo']);
linkAllowedByRules('https://x.org/foo', ['*.org/foo']);
linkNotAllowedByRules('https://x.org/bar', ['https://x.org/foo']);
linkNotAllowedByRules('https://x.org/bar', ['x.org/foo']);
linkNotAllowedByRules('https://x.org/bar', ['*.org/foo']);
linkAllowedByRules('https://x.org/foo/bar', ['https://x.org/foo']);
linkNotAllowedByRules('https://x.org/foo2', ['https://x.org/foo']);
linkNotAllowedByRules('https://www.x.org/foo', ['https://x.org/foo']);
linkNotAllowedByRules('https://a.x.org/bar', ['https://*.x.org/foo']);
linkNotAllowedByRules('https://a.b.x.org/bar', ['https://*.x.org/foo']);
linkAllowedByRules('https://github.com', ['https://github.com/foo/bar', 'https://github.com']);
});
test('ports', () => {
linkNotAllowedByRules('https://x.org:8080/foo/bar', ['https://x.org:8081/foo']);
linkAllowedByRules('https://x.org:8080/foo/bar', ['https://x.org:*/foo']);
linkAllowedByRules('https://x.org/foo/bar', ['https://x.org:*/foo']);
linkAllowedByRules('https://x.org:8080/foo/bar', ['https://x.org:8080/foo']);
});
test('ip addresses', () => {
linkAllowedByRules('http://192.168.1.7/', ['http://192.168.1.7/']);
linkAllowedByRules('http://192.168.1.7/', ['http://192.168.1.7']);
linkAllowedByRules('http://192.168.1.7/', ['http://192.168.1.*']);
linkNotAllowedByRules('http://192.168.1.7:3000/', ['http://192.168.*.6:*']);
linkAllowedByRules('http://192.168.1.7:3000/', ['http://192.168.1.7:3000/']);
linkAllowedByRules('http://192.168.1.7:3000/', ['http://192.168.1.7:*']);
linkAllowedByRules('http://192.168.1.7:3000/', ['http://192.168.1.*:*']);
linkNotAllowedByRules('http://192.168.1.7:3000/', ['http://192.168.*.6:*']);
});
test('scheme match', () => {
linkAllowedByRules('http://192.168.1.7/', ['http://*']);
linkAllowedByRules('http://twitter.com', ['http://*']);
linkAllowedByRules('http://twitter.com/hello', ['http://*']);
linkNotAllowedByRules('https://192.168.1.7/', ['http://*']);
linkNotAllowedByRules('https://twitter.com/', ['http://*']);
});
test('case normalization', () => {
// https://github.com/microsoft/vscode/issues/99294
linkAllowedByRules('https://github.com/microsoft/vscode/issues/new', ['https://github.com/microsoft']);
linkAllowedByRules('https://github.com/microsoft/vscode/issues/new', ['https://github.com/microsoft']);
});
});
| src/vs/workbench/contrib/url/test/browser/trustedDomains.test.ts | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.00017491102335043252,
0.0001726742193568498,
0.00017092068446800113,
0.00017266569193452597,
0.0000010390955367256538
]
|
{
"id": 10,
"code_window": [
"\tbackground-color: var(--tab-border-bottom-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty-border-top > .tab-border-top-container {\n",
"\tz-index: 2;\n",
"\ttop: 0;\n",
"\theight: 2px;\n",
"\tbackground-color: var(--tab-dirty-border-top-color);\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 6;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 233
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
const gulp = require('gulp');
const path = require('path');
const task = require('./lib/task');
const util = require('./lib/util');
const _ = require('underscore');
const electron = require('gulp-atom-electron');
const { config } = require('./lib/electron');
const filter = require('gulp-filter');
const deps = require('./lib/dependencies');
const root = path.dirname(__dirname);
const BUILD_TARGETS = [
{ platform: 'win32', arch: 'ia32' },
{ platform: 'win32', arch: 'x64' },
{ platform: 'win32', arch: 'arm64' },
{ platform: 'darwin', arch: null, opts: { stats: true } },
{ platform: 'linux', arch: 'ia32' },
{ platform: 'linux', arch: 'x64' },
{ platform: 'linux', arch: 'armhf' },
{ platform: 'linux', arch: 'arm64' },
];
BUILD_TARGETS.forEach(buildTarget => {
const dashed = (str) => (str ? `-${str}` : ``);
const platform = buildTarget.platform;
const arch = buildTarget.arch;
const destinationExe = path.join(path.dirname(root), 'scanbin', `VSCode${dashed(platform)}${dashed(arch)}`, 'bin');
const destinationPdb = path.join(path.dirname(root), 'scanbin', `VSCode${dashed(platform)}${dashed(arch)}`, 'pdb');
const tasks = [];
// removal tasks
tasks.push(util.rimraf(destinationExe), util.rimraf(destinationPdb));
// electron
tasks.push(() => electron.dest(destinationExe, _.extend({}, config, { platform, arch: arch === 'armhf' ? 'arm' : arch })));
// pdbs for windows
if (platform === 'win32') {
tasks.push(
() => electron.dest(destinationPdb, _.extend({}, config, { platform, arch: arch === 'armhf' ? 'arm' : arch, pdbs: true })),
util.rimraf(path.join(destinationExe, 'swiftshader')),
util.rimraf(path.join(destinationExe, 'd3dcompiler_47.dll')));
}
if (platform === 'linux') {
tasks.push(
() => electron.dest(destinationPdb, _.extend({}, config, { platform, arch: arch === 'armhf' ? 'arm' : arch, symbols: true }))
);
}
// node modules
tasks.push(
nodeModules(destinationExe, destinationPdb, platform)
);
const setupSymbolsTask = task.define(`vscode-symbols${dashed(platform)}${dashed(arch)}`,
task.series(...tasks)
);
gulp.task(setupSymbolsTask);
});
function nodeModules(destinationExe, destinationPdb, platform) {
const productionDependencies = deps.getProductionDependencies(root);
const dependenciesSrc = _.flatten(productionDependencies.map(d => path.relative(root, d.path)).map(d => [`${d}/**`, `!${d}/**/{test,tests}/**`]));
const exe = () => {
return gulp.src(dependenciesSrc, { base: '.', dot: true })
.pipe(filter(['**/*.node']))
.pipe(gulp.dest(destinationExe));
};
if (platform === 'win32') {
const pdb = () => {
return gulp.src(dependenciesSrc, { base: '.', dot: true })
.pipe(filter(['**/*.pdb']))
.pipe(gulp.dest(destinationPdb));
};
return gulp.parallel(exe, pdb);
}
if (platform === 'linux') {
const pdb = () => {
return gulp.src(dependenciesSrc, { base: '.', dot: true })
.pipe(filter(['**/*.sym']))
.pipe(gulp.dest(destinationPdb));
};
return gulp.parallel(exe, pdb);
}
return exe;
}
| build/gulpfile.scan.js | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.0001773680851329118,
0.0001726174814393744,
0.0001659816480241716,
0.00017252631369046867,
0.0000027524349661689484
]
|
{
"id": 10,
"code_window": [
"\tbackground-color: var(--tab-border-bottom-color);\n",
"}\n",
"\n",
".monaco-workbench .part.editor > .content .editor-group-container > .title .tabs-container > .tab.dirty-border-top > .tab-border-top-container {\n",
"\tz-index: 2;\n",
"\ttop: 0;\n",
"\theight: 2px;\n",
"\tbackground-color: var(--tab-dirty-border-top-color);\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tz-index: 6;\n"
],
"file_path": "src/vs/workbench/browser/parts/editor/media/tabstitlecontrol.css",
"type": "replace",
"edit_start_line_idx": 233
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as nls from 'vs/nls';
import { MainThreadTunnelServiceShape, IExtHostContext, MainContext, ExtHostContext, ExtHostTunnelServiceShape, CandidatePortSource, PortAttributesProviderSelector } from 'vs/workbench/api/common/extHost.protocol';
import { TunnelDto } from 'vs/workbench/api/common/extHostTunnelService';
import { extHostNamedCustomer } from 'vs/workbench/api/common/extHostCustomers';
import { CandidatePort, IRemoteExplorerService, makeAddress, PORT_AUTO_FORWARD_SETTING, PORT_AUTO_SOURCE_SETTING, PORT_AUTO_SOURCE_SETTING_OUTPUT, PORT_AUTO_SOURCE_SETTING_PROCESS, TunnelSource } from 'vs/workbench/services/remote/common/remoteExplorerService';
import { ITunnelProvider, ITunnelService, TunnelCreationOptions, TunnelProviderFeatures, TunnelOptions, RemoteTunnel, isPortPrivileged, ProvidedPortAttributes, PortAttributesProvider, TunnelProtocol } from 'vs/platform/remote/common/tunnel';
import { Disposable } from 'vs/base/common/lifecycle';
import type { TunnelDescription } from 'vs/platform/remote/common/remoteAuthorityResolver';
import { INotificationService, Severity } from 'vs/platform/notification/common/notification';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { ILogService } from 'vs/platform/log/common/log';
import { IRemoteAgentService } from 'vs/workbench/services/remote/common/remoteAgentService';
import { CancellationToken } from 'vs/base/common/cancellation';
import { Registry } from 'vs/platform/registry/common/platform';
import { IConfigurationRegistry, Extensions as ConfigurationExtensions } from 'vs/platform/configuration/common/configurationRegistry';
@extHostNamedCustomer(MainContext.MainThreadTunnelService)
export class MainThreadTunnelService extends Disposable implements MainThreadTunnelServiceShape, PortAttributesProvider {
private readonly _proxy: ExtHostTunnelServiceShape;
private elevateionRetry: boolean = false;
private portsAttributesProviders: Map<number, PortAttributesProviderSelector> = new Map();
constructor(
extHostContext: IExtHostContext,
@IRemoteExplorerService private readonly remoteExplorerService: IRemoteExplorerService,
@ITunnelService private readonly tunnelService: ITunnelService,
@INotificationService private readonly notificationService: INotificationService,
@IConfigurationService private readonly configurationService: IConfigurationService,
@ILogService private readonly logService: ILogService,
@IRemoteAgentService private readonly remoteAgentService: IRemoteAgentService
) {
super();
this._proxy = extHostContext.getProxy(ExtHostContext.ExtHostTunnelService);
this._register(tunnelService.onTunnelOpened(() => this._proxy.$onDidTunnelsChange()));
this._register(tunnelService.onTunnelClosed(() => this._proxy.$onDidTunnelsChange()));
}
private processFindingEnabled(): boolean {
return (!!this.configurationService.getValue(PORT_AUTO_FORWARD_SETTING) || this.tunnelService.hasTunnelProvider)
&& (this.configurationService.getValue(PORT_AUTO_SOURCE_SETTING) === PORT_AUTO_SOURCE_SETTING_PROCESS);
}
async $setRemoteTunnelService(processId: number): Promise<void> {
this.remoteExplorerService.namedProcesses.set(processId, 'Code Extension Host');
if (this.remoteExplorerService.portsFeaturesEnabled) {
this._proxy.$registerCandidateFinder(this.processFindingEnabled());
} else {
this._register(this.remoteExplorerService.onEnabledPortsFeatures(() => this._proxy.$registerCandidateFinder(this.configurationService.getValue(PORT_AUTO_FORWARD_SETTING))));
}
this._register(this.configurationService.onDidChangeConfiguration(async (e) => {
if (e.affectsConfiguration(PORT_AUTO_FORWARD_SETTING) || e.affectsConfiguration(PORT_AUTO_SOURCE_SETTING)) {
return this._proxy.$registerCandidateFinder(this.processFindingEnabled());
}
}));
this._register(this.tunnelService.onAddedTunnelProvider(() => {
return this._proxy.$registerCandidateFinder(this.processFindingEnabled());
}));
}
private _alreadyRegistered: boolean = false;
async $registerPortsAttributesProvider(selector: PortAttributesProviderSelector, providerHandle: number): Promise<void> {
this.portsAttributesProviders.set(providerHandle, selector);
if (!this._alreadyRegistered) {
this.remoteExplorerService.tunnelModel.addAttributesProvider(this);
this._alreadyRegistered = true;
}
}
async $unregisterPortsAttributesProvider(providerHandle: number): Promise<void> {
this.portsAttributesProviders.delete(providerHandle);
}
async providePortAttributes(ports: number[], pid: number | undefined, commandLine: string | undefined, token: CancellationToken): Promise<ProvidedPortAttributes[]> {
if (this.portsAttributesProviders.size === 0) {
return [];
}
// Check all the selectors to make sure it's worth going to the extension host.
const appropriateHandles = Array.from(this.portsAttributesProviders.entries()).filter(entry => {
const selector = entry[1];
const portRange = selector.portRange;
const portInRange = portRange ? ports.some(port => portRange[0] <= port && port < portRange[1]) : true;
const pidMatches = !selector.pid || (selector.pid === pid);
const commandMatches = !selector.commandMatcher || (commandLine && (commandLine.match(selector.commandMatcher)));
return portInRange && pidMatches && commandMatches;
}).map(entry => entry[0]);
if (appropriateHandles.length === 0) {
return [];
}
return this._proxy.$providePortAttributes(appropriateHandles, ports, pid, commandLine, token);
}
async $openTunnel(tunnelOptions: TunnelOptions, source: string): Promise<TunnelDto | undefined> {
const tunnel = await this.remoteExplorerService.forward({
remote: tunnelOptions.remoteAddress,
local: tunnelOptions.localAddressPort,
name: tunnelOptions.label,
source: {
source: TunnelSource.Extension,
description: source
},
elevateIfNeeded: false
});
if (tunnel) {
if (!this.elevateionRetry
&& (tunnelOptions.localAddressPort !== undefined)
&& (tunnel.tunnelLocalPort !== undefined)
&& isPortPrivileged(tunnelOptions.localAddressPort)
&& (tunnel.tunnelLocalPort !== tunnelOptions.localAddressPort)
&& this.tunnelService.canElevate) {
this.elevationPrompt(tunnelOptions, tunnel, source);
}
return TunnelDto.fromServiceTunnel(tunnel);
}
return undefined;
}
private async elevationPrompt(tunnelOptions: TunnelOptions, tunnel: RemoteTunnel, source: string) {
return this.notificationService.prompt(Severity.Info,
nls.localize('remote.tunnel.openTunnel', "The extension {0} has forwarded port {1}. You'll need to run as superuser to use port {2} locally.", source, tunnelOptions.remoteAddress.port, tunnelOptions.localAddressPort),
[{
label: nls.localize('remote.tunnelsView.elevationButton', "Use Port {0} as Sudo...", tunnel.tunnelRemotePort),
run: async () => {
this.elevateionRetry = true;
await this.remoteExplorerService.close({ host: tunnel.tunnelRemoteHost, port: tunnel.tunnelRemotePort });
await this.remoteExplorerService.forward({
remote: tunnelOptions.remoteAddress,
local: tunnelOptions.localAddressPort,
name: tunnelOptions.label,
source: {
source: TunnelSource.Extension,
description: source
},
elevateIfNeeded: true
});
this.elevateionRetry = false;
}
}]);
}
async $closeTunnel(remote: { host: string, port: number }): Promise<void> {
return this.remoteExplorerService.close(remote);
}
async $getTunnels(): Promise<TunnelDescription[]> {
return (await this.tunnelService.tunnels).map(tunnel => {
return {
remoteAddress: { port: tunnel.tunnelRemotePort, host: tunnel.tunnelRemoteHost },
localAddress: tunnel.localAddress,
privacy: tunnel.privacy,
protocol: tunnel.protocol
};
});
}
async $onFoundNewCandidates(candidates: CandidatePort[]): Promise<void> {
this.remoteExplorerService.onFoundNewCandidates(candidates);
}
async $setTunnelProvider(features?: TunnelProviderFeatures): Promise<void> {
const tunnelProvider: ITunnelProvider = {
forwardPort: (tunnelOptions: TunnelOptions, tunnelCreationOptions: TunnelCreationOptions) => {
const forward = this._proxy.$forwardPort(tunnelOptions, tunnelCreationOptions);
return forward.then(tunnel => {
this.logService.trace(`ForwardedPorts: (MainThreadTunnelService) New tunnel established by tunnel provider: ${tunnel?.remoteAddress.host}:${tunnel?.remoteAddress.port}`);
if (!tunnel) {
return undefined;
}
return {
tunnelRemotePort: tunnel.remoteAddress.port,
tunnelRemoteHost: tunnel.remoteAddress.host,
localAddress: typeof tunnel.localAddress === 'string' ? tunnel.localAddress : makeAddress(tunnel.localAddress.host, tunnel.localAddress.port),
tunnelLocalPort: typeof tunnel.localAddress !== 'string' ? tunnel.localAddress.port : undefined,
public: tunnel.public,
privacy: tunnel.privacy,
protocol: tunnel.protocol ?? TunnelProtocol.Http,
dispose: async (silent?: boolean) => {
this.logService.trace(`ForwardedPorts: (MainThreadTunnelService) Closing tunnel from tunnel provider: ${tunnel?.remoteAddress.host}:${tunnel?.remoteAddress.port}`);
return this._proxy.$closeTunnel({ host: tunnel.remoteAddress.host, port: tunnel.remoteAddress.port }, silent);
}
};
});
}
};
this.tunnelService.setTunnelProvider(tunnelProvider);
if (features) {
this.tunnelService.setTunnelFeatures(features);
}
}
async $setCandidateFilter(): Promise<void> {
this.remoteExplorerService.setCandidateFilter((candidates: CandidatePort[]): Promise<CandidatePort[]> => {
return this._proxy.$applyCandidateFilter(candidates);
});
}
async $setCandidatePortSource(source: CandidatePortSource): Promise<void> {
// Must wait for the remote environment before trying to set settings there.
this.remoteAgentService.getEnvironment().then(() => {
switch (source) {
case CandidatePortSource.None: {
Registry.as<IConfigurationRegistry>(ConfigurationExtensions.Configuration)
.registerDefaultConfigurations([{ overrides: { 'remote.autoForwardPorts': false } }]);
break;
}
case CandidatePortSource.Output: {
Registry.as<IConfigurationRegistry>(ConfigurationExtensions.Configuration)
.registerDefaultConfigurations([{ overrides: { 'remote.autoForwardPortsSource': PORT_AUTO_SOURCE_SETTING_OUTPUT } }]);
break;
}
default: // Do nothing, the defaults for these settings should be used.
}
}).catch(() => {
// The remote failed to get setup. Errors from that area will already be surfaced to the user.
});
}
}
| src/vs/workbench/api/browser/mainThreadTunnelService.ts | 0 | https://github.com/microsoft/vscode/commit/b61f91f54a081567fd9faf501c520172d046e99a | [
0.00017593738448340446,
0.00017107263556681573,
0.00016555030015297234,
0.0001713480451144278,
0.000002661485723365331
]
|
{
"id": 0,
"code_window": [
"\n",
" const currentItem = views.value.find((v) => v.id === evt.item.id)\n",
"\n",
" if (!currentItem || !currentItem.id) return\n",
"\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : {}) as ViewType\n",
" const nextItem = (nextEl ? views.value.find((v) => v.id === nextEl.id) : {}) as ViewType\n",
"\n",
" let nextOrder: number\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set default order value as 0 if item not found\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : { order: 0 }) as ViewType\n"
],
"file_path": "packages/nc-gui/components/dashboard/TreeView/ViewsList.vue",
"type": "replace",
"edit_start_line_idx": 160
} | import { type ColumnType, type SelectOptionsType, UITypes, type ViewType } from 'nocodb-sdk'
import type { Ref } from 'vue'
import { GROUP_BY_VARS, ref, storeToRefs, useApi, useBase, useViewColumnsOrThrow } from '#imports'
import type { Group, GroupNestedIn, Row } from '#imports'
export const useViewGroupBy = (view: Ref<ViewType | undefined>, where?: ComputedRef<string | undefined>) => {
const { api } = useApi()
const { appInfo } = useGlobal()
const { base } = storeToRefs(useBase())
const { sharedView, fetchSharedViewData } = useSharedView()
const meta = inject(MetaInj)
const { gridViewCols } = useViewColumnsOrThrow()
const groupBy = computed<{ column: ColumnType; sort: string; order?: number }[]>(() => {
const tempGroupBy: { column: ColumnType; sort: string; order?: number }[] = []
Object.values(gridViewCols.value).forEach((col) => {
if (col.group_by) {
const column = meta?.value.columns?.find((f) => f.id === col.fk_column_id)
if (column) {
tempGroupBy.push({
column,
sort: col.group_by_sort || 'asc',
order: col.group_by_order || 1,
})
}
}
})
tempGroupBy.sort((a, b) => (a.order ?? Infinity) - (b.order ?? Infinity))
return tempGroupBy
})
const isGroupBy = computed(() => !!groupBy.value.length)
const { isUIAllowed } = useRoles()
const { sorts, nestedFilters } = useSmartsheetStoreOrThrow()
const isPublic = inject(IsPublicInj, ref(false))
const reloadViewDataHook = inject(ReloadViewDataHookInj, createEventHook())
const groupByGroupLimit = computed(() => {
return appInfo.value.defaultGroupByLimit?.limitGroup || 10
})
const groupByRecordLimit = computed(() => {
return appInfo.value.defaultGroupByLimit?.limitRecord || 10
})
const rootGroup = ref<Group>({
key: 'root',
color: 'root',
count: 0,
column: {} as any,
nestedIn: [],
paginationData: { page: 1, pageSize: groupByGroupLimit.value },
nested: true,
children: [],
root: true,
})
async function groupWrapperChangePage(page: number, groupWrapper?: Group) {
groupWrapper = groupWrapper || rootGroup.value
if (!groupWrapper) return
groupWrapper.paginationData.page = page
await loadGroups(
{
offset: (page - 1) * (groupWrapper.paginationData.pageSize || groupByGroupLimit.value),
} as any,
groupWrapper,
)
}
const formatData = (list: Record<string, any>[]) =>
list.map((row) => ({
row: { ...row },
oldRow: { ...row },
rowMeta: {},
}))
const valueToTitle = (value: string, col: ColumnType) => {
if (col.uidt === UITypes.Checkbox) {
return value ? GROUP_BY_VARS.TRUE : GROUP_BY_VARS.FALSE
}
if ([UITypes.User, UITypes.CreatedBy, UITypes.LastModifiedBy].includes(col.uidt as UITypes)) {
if (!value) {
return GROUP_BY_VARS.NULL
}
}
// convert to JSON string if non-string value
if (value && typeof value === 'object') {
value = JSON.stringify(value)
}
return value ?? GROUP_BY_VARS.NULL
}
const colors = ref(enumColor.light)
const nextGroupColor = ref(colors.value[0])
const getNextColor = () => {
const tempColor = nextGroupColor.value
const index = colors.value.indexOf(nextGroupColor.value)
if (index === colors.value.length - 1) {
nextGroupColor.value = colors.value[0]
} else {
nextGroupColor.value = colors.value[index + 1]
}
return tempColor
}
const findKeyColor = (key?: string, col?: ColumnType): string => {
if (col) {
switch (col.uidt) {
case UITypes.MultiSelect: {
const keys = key?.split(',') || []
const colors = []
for (const k of keys) {
const option = (col.colOptions as SelectOptionsType).options?.find((o) => o.title === k)
if (option) {
colors.push(option.color)
}
}
return colors.join(',')
}
case UITypes.SingleSelect: {
const option = (col.colOptions as SelectOptionsType).options?.find((o) => o.title === key)
if (option) {
return option.color || getNextColor()
}
return 'gray'
}
case UITypes.Checkbox: {
if (key) {
return themeColors.success
}
return themeColors.error
}
default:
return key ? getNextColor() : 'gray'
}
}
return key ? getNextColor() : 'gray'
}
const calculateNestedWhere = (nestedIn: GroupNestedIn[], existing = '') => {
return nestedIn.reduce((acc, curr) => {
if (curr.key === GROUP_BY_VARS.NULL) {
acc += `${acc.length ? '~and' : ''}(${curr.title},gb_null)`
} else if (curr.column_uidt === UITypes.Checkbox) {
acc += `${acc.length ? '~and' : ''}(${curr.title},${curr.key === GROUP_BY_VARS.TRUE ? 'checked' : 'notchecked'})`
} else if ([UITypes.Date, UITypes.DateTime].includes(curr.column_uidt as UITypes)) {
acc += `${acc.length ? '~and' : ''}(${curr.title},eq,exactDate,${curr.key})`
} else if ([UITypes.User, UITypes.CreatedBy, UITypes.LastModifiedBy].includes(curr.column_uidt as UITypes)) {
try {
const value = JSON.parse(curr.key)
acc += `${acc.length ? '~and' : ''}(${curr.title},gb_eq,${(Array.isArray(value) ? value : [value])
.map((v: any) => v.id)
.join(',')})`
} catch (e) {
console.error(e)
}
} else {
acc += `${acc.length ? '~and' : ''}(${curr.title},gb_eq,${curr.key})`
}
return acc
}, existing)
}
async function loadGroups(params: any = {}, group?: Group) {
group = group || rootGroup.value
if (!base?.value?.id || !view.value?.id || !view.value?.fk_model_id || !group) return
if (groupBy.value.length === 0) {
group.children = []
return
}
if (group.nestedIn.length > groupBy.value.length) return
if (group.nestedIn.length === 0) nextGroupColor.value = colors.value[0]
const groupby = groupBy.value[group.nestedIn.length]
const nestedWhere = calculateNestedWhere(group.nestedIn, where?.value)
if (!groupby || !groupby.column.title) return
if (isPublic.value && !sharedView.value?.uuid) {
return
}
const response = !isPublic.value
? await api.dbViewRow.groupBy('noco', base.value.id, view.value.fk_model_id, view.value.id, {
offset: ((group.paginationData.page ?? 0) - 1) * (group.paginationData.pageSize ?? groupByGroupLimit.value),
limit: group.paginationData.pageSize ?? groupByGroupLimit.value,
...params,
...(isUIAllowed('sortSync') ? {} : { sortArrJson: JSON.stringify(sorts.value) }),
...(isUIAllowed('filterSync') ? {} : { filterArrJson: JSON.stringify(nestedFilters.value) }),
where: `${nestedWhere}`,
sort: `${groupby.sort === 'desc' ? '-' : ''}${groupby.column.title}`,
column_name: groupby.column.title,
} as any)
: await api.public.dataGroupBy(sharedView.value!.uuid!, {
offset: ((group.paginationData.page ?? 0) - 1) * (group.paginationData.pageSize ?? groupByGroupLimit.value),
limit: group.paginationData.pageSize ?? groupByGroupLimit.value,
...params,
where: nestedWhere,
sort: `${groupby.sort === 'desc' ? '-' : ''}${groupby.column.title}`,
column_name: groupby.column.title,
sortsArr: sorts.value,
filtersArr: nestedFilters.value,
})
const tempList: Group[] = response.list.reduce((acc: Group[], curr: Record<string, any>) => {
const keyExists = acc.find(
(a) => a.key === valueToTitle(curr[groupby.column.column_name!] ?? curr[groupby.column.title!], groupby.column),
)
if (keyExists) {
keyExists.count += +curr.count
keyExists.paginationData = { page: 1, pageSize: groupByGroupLimit.value, totalRows: keyExists.count }
return acc
}
if (groupby.column.title && groupby.column.uidt) {
acc.push({
key: valueToTitle(curr[groupby.column.title!], groupby.column),
column: groupby.column,
count: +curr.count,
color: findKeyColor(curr[groupby.column.title!], groupby.column),
nestedIn: [
...group!.nestedIn,
{
title: groupby.column.title,
column_name: groupby.column.title!,
key: valueToTitle(curr[groupby.column.title!], groupby.column),
column_uidt: groupby.column.uidt,
},
],
paginationData: {
page: 1,
pageSize: group!.nestedIn.length < groupBy.value.length - 1 ? groupByGroupLimit.value : groupByRecordLimit.value,
totalRows: +curr.count,
},
nested: group!.nestedIn.length < groupBy.value.length - 1,
})
}
return acc
}, [])
if (!group.children) group.children = []
for (const temp of tempList) {
const keyExists = group.children?.find((a) => a.key === temp.key)
if (keyExists) {
temp.paginationData = {
page: keyExists.paginationData.page || temp.paginationData.page,
pageSize: keyExists.paginationData.pageSize || temp.paginationData.pageSize,
totalRows: temp.count,
}
temp.color = keyExists.color
// update group
Object.assign(keyExists, temp)
continue
}
group.children.push(temp)
}
// clear rest of the children
group.children = group.children.filter((c) => tempList.find((t) => t.key === c.key))
if (group.count <= (group.paginationData.pageSize ?? groupByGroupLimit.value)) {
group.children.sort((a, b) => {
const orderA = tempList.findIndex((t) => t.key === a.key)
const orderB = tempList.findIndex((t) => t.key === b.key)
return orderA - orderB
})
}
group.paginationData = response.pageInfo
// to cater the case like when querying with a non-zero offset
// the result page may point to the target page where the actual returned data don't display on
const expectedPage = Math.max(1, Math.ceil(group.paginationData.totalRows! / group.paginationData.pageSize!))
if (expectedPage < group.paginationData.page!) {
await groupWrapperChangePage(expectedPage, group)
}
}
async function loadGroupData(group: Group, force = false) {
if (!base?.value?.id || !view.value?.id || !view.value?.fk_model_id) return
if (group.children && !force) return
if (!group.paginationData) {
group.paginationData = { page: 1, pageSize: groupByRecordLimit.value }
}
const nestedWhere = calculateNestedWhere(group.nestedIn, where?.value)
const query = {
offset: ((group.paginationData.page ?? 0) - 1) * (group.paginationData.pageSize ?? groupByRecordLimit.value),
limit: group.paginationData.pageSize ?? groupByRecordLimit.value,
where: `${nestedWhere}`,
}
const response = !isPublic.value
? await api.dbViewRow.list('noco', base.value.id, view.value.fk_model_id, view.value.id, {
...query,
...(isUIAllowed('sortSync') ? {} : { sortArrJson: JSON.stringify(sorts.value) }),
...(isUIAllowed('filterSync') ? {} : { filterArrJson: JSON.stringify(nestedFilters.value) }),
} as any)
: await fetchSharedViewData({ sortsArr: sorts.value, filtersArr: nestedFilters.value, ...query })
group.count = response.pageInfo.totalRows ?? 0
group.rows = formatData(response.list)
group.paginationData = response.pageInfo
}
const loadGroupPage = async (group: Group, p: number) => {
if (!group.paginationData) {
group.paginationData = { page: 1, pageSize: groupByRecordLimit.value }
}
group.paginationData.page = p
await loadGroupData(group, true)
}
const refreshNested = (group?: Group, nestLevel = 0) => {
group = group || rootGroup.value
if (!group) return
if (nestLevel < groupBy.value.length) {
group.nested = true
} else {
group.nested = false
}
if (group.nested) {
if (group?.rows) {
group.rows = []
}
} else {
if (group?.children) {
group.children = []
}
}
if (nestLevel > groupBy.value.length) return
for (const child of group.children || []) {
refreshNested(child, nestLevel + 1)
}
}
watch(
() => groupBy.value.length,
async () => {
if (groupBy.value.length > 0) {
rootGroup.value.paginationData = { page: 1, pageSize: groupByGroupLimit.value }
rootGroup.value.column = {} as any
await loadGroups()
refreshNested()
nextTick(() => reloadViewDataHook?.trigger())
}
},
{ immediate: true },
)
const findGroupByNestedIn = (nestedIn: GroupNestedIn[], group?: Group, nestLevel = 0): Group => {
group = group || rootGroup.value
if (nestLevel >= nestedIn.length) return group
const child = group.children?.find((g) => g.key === nestedIn[nestLevel].key)
if (child) {
if (child.nested) {
return findGroupByNestedIn(nestedIn, child, nestLevel + 1)
}
return child
}
return group
}
const parentGroup = (group: Group) => {
const parent = findGroupByNestedIn(group.nestedIn.slice(0, -1))
return parent
}
const modifyCount = (group: Group, countEffect: number) => {
if (!group) return
group.count += countEffect
// remove group if count is 0
if (group.count === 0) {
const parent = parentGroup(group)
if (parent) {
parent.children = parent.children?.filter((c) => c.key !== group.key)
}
}
if (group.root) return
modifyCount(parentGroup(group), countEffect)
}
const findGroupForRow = (row: Row, group?: Group, nestLevel = 0): { found: boolean; group: Group } => {
group = group || rootGroup.value
if (group.nested) {
const child = group.children?.find((g) => {
if (!groupBy.value[nestLevel].column.title) return undefined
return g.key === valueToTitle(row.row[groupBy.value[nestLevel].column.title!], groupBy.value[nestLevel].column)
})
if (child) {
return findGroupForRow(row, child, nestLevel + 1)
}
return { found: false, group }
}
return { found: true, group }
}
const redistributeRows = (group?: Group) => {
group = group || rootGroup.value
if (!group) return
if (!group.nested && group.rows) {
group.rows.forEach((row) => {
const properGroup = findGroupForRow(row)
if (properGroup.found) {
if (properGroup.group !== group) {
if (properGroup.group) {
properGroup.group.rows?.push(row)
modifyCount(properGroup.group, 1)
}
if (group) {
group.rows?.splice(group!.rows.indexOf(row), 1)
modifyCount(group, -1)
}
}
} else {
if (group) {
group.rows?.splice(group!.rows.indexOf(row), 1)
modifyCount(group, -1)
}
if (properGroup.group?.children) loadGroups({}, properGroup.group)
}
})
} else {
group.children?.forEach((g) => redistributeRows(g))
}
}
return { rootGroup, groupBy, isGroupBy, loadGroups, loadGroupData, loadGroupPage, groupWrapperChangePage, redistributeRows }
}
| packages/nc-gui/composables/useViewGroupBy.ts | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00020517781376838684,
0.0001726421614876017,
0.00016490435518790036,
0.000172332365764305,
0.000006310249318630667
]
|
{
"id": 0,
"code_window": [
"\n",
" const currentItem = views.value.find((v) => v.id === evt.item.id)\n",
"\n",
" if (!currentItem || !currentItem.id) return\n",
"\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : {}) as ViewType\n",
" const nextItem = (nextEl ? views.value.find((v) => v.id === nextEl.id) : {}) as ViewType\n",
"\n",
" let nextOrder: number\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set default order value as 0 if item not found\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : { order: 0 }) as ViewType\n"
],
"file_path": "packages/nc-gui/components/dashboard/TreeView/ViewsList.vue",
"type": "replace",
"edit_start_line_idx": 160
} | import { PluginCategory, XcActionType, XcType } from 'nocodb-sdk';
import S3Plugin from './S3Plugin';
import type { XcPluginConfig } from 'nc-plugin';
const config: XcPluginConfig = {
builder: S3Plugin,
title: 'S3',
version: '0.0.1',
logo: 'plugins/s3.png',
description:
'Amazon Simple Storage Service (Amazon S3) is an object storage service that offers industry-leading scalability, data availability, security, and performance.',
inputs: {
title: 'Configure Amazon S3',
items: [
{
key: 'bucket',
label: 'Bucket Name',
placeholder: 'Bucket Name',
type: XcType.SingleLineText,
required: true,
},
{
key: 'region',
label: 'Region',
placeholder: 'Region',
type: XcType.SingleLineText,
required: true,
},
{
key: 'access_key',
label: 'Access Key',
placeholder: 'Access Key',
type: XcType.SingleLineText,
required: true,
},
{
key: 'access_secret',
label: 'Access Secret',
placeholder: 'Access Secret',
type: XcType.Password,
required: true,
},
],
actions: [
{
label: 'Test',
placeholder: 'Test',
key: 'test',
actionType: XcActionType.TEST,
type: XcType.Button,
},
{
label: 'Save',
placeholder: 'Save',
key: 'save',
actionType: XcActionType.SUBMIT,
type: XcType.Button,
},
],
msgOnInstall:
'Successfully installed and attachment will be stored in AWS S3',
msgOnUninstall: '',
},
category: PluginCategory.STORAGE,
tags: 'Storage',
};
export default config;
| packages/nocodb/src/plugins/s3/index.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017708689847495407,
0.0001744791807141155,
0.00016912426508497447,
0.00017520882829558104,
0.000002486258608769276
]
|
{
"id": 0,
"code_window": [
"\n",
" const currentItem = views.value.find((v) => v.id === evt.item.id)\n",
"\n",
" if (!currentItem || !currentItem.id) return\n",
"\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : {}) as ViewType\n",
" const nextItem = (nextEl ? views.value.find((v) => v.id === nextEl.id) : {}) as ViewType\n",
"\n",
" let nextOrder: number\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set default order value as 0 if item not found\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : { order: 0 }) as ViewType\n"
],
"file_path": "packages/nc-gui/components/dashboard/TreeView/ViewsList.vue",
"type": "replace",
"edit_start_line_idx": 160
} | <script lang="ts" setup>
import type { ColumnType, LinkToAnotherRecordType } from 'nocodb-sdk'
import { RelationTypes, UITypes, isCreatedOrLastModifiedByCol, isLinksOrLTAR, isSystemColumn } from 'nocodb-sdk'
const props = defineProps<{
// As we need to focus search box when the parent is opened
isParentOpen: boolean
}>()
const emits = defineEmits(['created'])
const { isParentOpen } = toRefs(props)
const inputRef = ref()
const search = ref('')
const activeFieldIndex = ref(-1)
const activeView = inject(ActiveViewInj, ref())
const meta = inject(MetaInj, ref())
const { showSystemFields, metaColumnById } = useViewColumnsOrThrow(activeView, meta)
const { sorts } = useViewSorts(activeView)
const options = computed<ColumnType[]>(
() =>
meta.value?.columns
?.filter((c: ColumnType) => {
if (c.uidt === UITypes.Links) {
return true
}
if (isSystemColumn(metaColumnById?.value?.[c.id!])) {
if (isCreatedOrLastModifiedByCol(c)) {
/** ignore created by and last modified by system field */
return false
}
return (
/** hide system columns if not enabled */
showSystemFields.value
)
} else if (c.uidt === UITypes.QrCode || c.uidt === UITypes.Barcode || c.uidt === UITypes.ID) {
return false
} else {
/** ignore hasmany and manytomany relations if it's using within sort menu */
return !(isLinksOrLTAR(c) && (c.colOptions as LinkToAnotherRecordType).type !== RelationTypes.BELONGS_TO)
/** ignore virtual fields which are system fields ( mm relation ) and qr code fields */
}
})
.filter((c: ColumnType) => !sorts.value.find((s) => s.fk_column_id === c.id))
.filter((c: ColumnType) => c.title?.toLowerCase().includes(search.value.toLowerCase())) ?? [],
)
const onClick = (column: ColumnType) => {
emits('created', column)
}
watch(
isParentOpen,
() => {
if (!isParentOpen.value) return
setTimeout(() => {
inputRef.value?.focus()
}, 100)
},
{
immediate: true,
},
)
onMounted(() => {
search.value = ''
activeFieldIndex.value = -1
})
const onArrowDown = () => {
activeFieldIndex.value = Math.min(activeFieldIndex.value + 1, options.value.length - 1)
}
const onArrowUp = () => {
activeFieldIndex.value = Math.max(activeFieldIndex.value - 1, 0)
}
</script>
<template>
<div
class="flex flex-col w-full pt-4 pb-2 min-w-64 nc-sort-create-modal"
@keydown.arrow-down.prevent="onArrowDown"
@keydown.arrow-up.prevent="onArrowUp"
@keydown.enter.prevent="onClick(options[activeFieldIndex])"
>
<div class="flex pb-3 px-4 border-b-1 border-gray-100">
<input ref="inputRef" v-model="search" class="w-full focus:outline-none" :placeholder="$t('msg.selectFieldToSort')" />
</div>
<div class="flex-col w-full max-h-100 max-w-76 nc-scrollbar-md !overflow-y-auto">
<div v-if="!options.length" class="flex text-gray-500 px-4 py-2.25">{{ $t('general.empty') }}</div>
<div
v-for="(option, index) in options"
:key="index"
v-e="['c:sort:add:column:select']"
class="flex flex-row h-10 items-center gap-x-1.5 px-2.5 rounded-md m-1.5 hover:bg-gray-100 cursor-pointer nc-sort-column-search-item"
:class="{
'bg-gray-100': activeFieldIndex === index,
}"
@click="onClick(option)"
>
<SmartsheetHeaderIcon :column="option" />
<NcTooltip class="truncate" show-on-truncate-only>
<template #title> {{ option.title }}</template>
<template #default>
{{ option.title }}
</template>
</NcTooltip>
</div>
</div>
</div>
</template>
| packages/nc-gui/components/smartsheet/toolbar/CreateSort.vue | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017642640159465373,
0.00017131914501078427,
0.00016616960056126118,
0.00017170001228805631,
0.000002524938508940977
]
|
{
"id": 0,
"code_window": [
"\n",
" const currentItem = views.value.find((v) => v.id === evt.item.id)\n",
"\n",
" if (!currentItem || !currentItem.id) return\n",
"\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : {}) as ViewType\n",
" const nextItem = (nextEl ? views.value.find((v) => v.id === nextEl.id) : {}) as ViewType\n",
"\n",
" let nextOrder: number\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set default order value as 0 if item not found\n",
" const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : { order: 0 }) as ViewType\n"
],
"file_path": "packages/nc-gui/components/dashboard/TreeView/ViewsList.vue",
"type": "replace",
"edit_start_line_idx": 160
} | import { Injectable } from '@nestjs/common';
import { AuthGuard } from '@nestjs/passport';
import { lastValueFrom, Observable } from 'rxjs';
import { extractRolesObj } from 'nocodb-sdk';
import type { Request } from 'express';
import type { ExecutionContext } from '@nestjs/common';
import { JwtStrategy } from '~/strategies/jwt.strategy';
@Injectable()
export class GlobalGuard extends AuthGuard(['jwt']) {
constructor(private jwtStrategy: JwtStrategy) {
super();
}
async canActivate(context: ExecutionContext) {
let result;
const req = context.switchToHttp().getRequest();
if (req.headers?.['xc-auth']) {
try {
result = await this.extractBoolVal(super.canActivate(context));
} catch (e) {
console.log(e);
}
}
if (result && !req.headers['xc-shared-base-id']) {
if (
req.path.indexOf('/user/me') === -1 &&
req.header('xc-preview') &&
['owner', 'creator'].some((role) => req.user.roles?.[role])
) {
return (req.user = {
...req.user,
isAuthorized: true,
roles: extractRolesObj(req.header('xc-preview')),
});
}
}
if (result) return true;
if (req.headers['xc-token']) {
let canActivate = false;
try {
const guard = new (AuthGuard('authtoken'))(context);
canActivate = await this.extractBoolVal(guard.canActivate(context));
} catch {}
if (canActivate) {
return this.authenticate(req, {
...req.user,
isAuthorized: true,
roles: req.user.roles,
});
}
} else if (req.headers['xc-shared-base-id']) {
let canActivate = false;
try {
const guard = new (AuthGuard('base-view'))(context);
canActivate = await this.extractBoolVal(guard.canActivate(context));
} catch {}
if (canActivate) {
return this.authenticate(req, {
...req.user,
isAuthorized: true,
isPublicBase: true,
});
}
}
// If JWT authentication fails, use the fallback strategy to set a default user
return await this.authenticate(req);
}
private async authenticate(
req: Request,
user: any = {
roles: {
guest: true,
},
},
): Promise<any> {
const u = await this.jwtStrategy.validate(req, user);
req.user = u;
return true;
}
async extractBoolVal(
canActivate: boolean | Promise<boolean> | Observable<boolean>,
) {
if (canActivate instanceof Observable) {
return lastValueFrom(canActivate);
} else if (
typeof canActivate === 'boolean' ||
canActivate instanceof Promise
) {
return canActivate;
}
}
}
| packages/nocodb/src/guards/global/global.guard.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0001758585567586124,
0.00017216824926435947,
0.00016973346646409482,
0.00017270637908950448,
0.0000017039827753251302
]
|
{
"id": 1,
"code_window": [
" // horizontally scroll to the end of the kanban container\n",
" // when a new option is added within kanban view\n",
" nextTick(() => {\n",
" if (shouldScrollToRight.value) {\n",
" kanbanContainerRef.value.scrollTo({\n",
" left: kanbanContainerRef.value.scrollWidth,\n",
" behavior: 'smooth',\n",
" })\n",
" // reset shouldScrollToRight\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (shouldScrollToRight.value && kanbanContainerRef.value) {\n"
],
"file_path": "packages/nc-gui/components/smartsheet/Kanban.vue",
"type": "replace",
"edit_start_line_idx": 369
} | import type { ColumnType } from 'nocodb-sdk'
export const useColumnDrag = ({
fields,
tableBodyEl,
gridWrapper,
}: {
fields: Ref<ColumnType[]>
tableBodyEl: Ref<HTMLElement | undefined>
gridWrapper: Ref<HTMLElement | undefined>
}) => {
const { eventBus } = useSmartsheetStoreOrThrow()
const { addUndo, defineViewScope } = useUndoRedo()
const { activeView } = storeToRefs(useViewsStore())
const { gridViewCols, updateGridViewColumn } = useViewColumnsOrThrow()
const { leftSidebarWidth } = storeToRefs(useSidebarStore())
const { width } = useWindowSize()
const draggedCol = ref<ColumnType | null>(null)
const dragColPlaceholderDomRef = ref<HTMLElement | null>(null)
const toBeDroppedColId = ref<string | null>(null)
const reorderColumn = async (colId: string, toColId: string) => {
const toBeReorderedViewCol = gridViewCols.value[colId]
const toViewCol = gridViewCols.value[toColId]!
const toColIndex = fields.value.findIndex((f) => f.id === toColId)
const nextToColField = toColIndex < fields.value.length - 1 ? fields.value[toColIndex + 1] : null
const nextToViewCol = nextToColField ? gridViewCols.value[nextToColField.id!] : null
const lastCol = fields.value[fields.value.length - 1]
const lastViewCol = gridViewCols.value[lastCol.id!]
// if nextToViewCol/toViewCol is null, return
if (nextToViewCol === null || lastViewCol === null) return
const newOrder = nextToViewCol ? toViewCol.order! + (nextToViewCol.order! - toViewCol.order!) / 2 : lastViewCol.order! + 1
const oldOrder = toBeReorderedViewCol.order
toBeReorderedViewCol.order = newOrder
addUndo({
undo: {
fn: async () => {
if (!fields.value) return
toBeReorderedViewCol.order = oldOrder
await updateGridViewColumn(colId, { order: oldOrder } as any)
eventBus.emit(SmartsheetStoreEvents.FIELD_RELOAD)
},
args: [],
},
redo: {
fn: async () => {
if (!fields.value) return
toBeReorderedViewCol.order = newOrder
await updateGridViewColumn(colId, { order: newOrder } as any)
eventBus.emit(SmartsheetStoreEvents.FIELD_RELOAD)
},
args: [],
},
scope: defineViewScope({ view: activeView.value }),
})
await updateGridViewColumn(colId, { order: newOrder } as any, true)
eventBus.emit(SmartsheetStoreEvents.FIELD_RELOAD)
}
const onDragStart = (colId: string, e: DragEvent) => {
if (!e.dataTransfer) return
const dom = document.querySelector('[data-testid="drag-icon-placeholder"]')
e.dataTransfer.dropEffect = 'none'
e.dataTransfer.effectAllowed = 'none'
e.dataTransfer.setDragImage(dom!, 10, 10)
e.dataTransfer.clearData()
e.dataTransfer.setData('text/plain', colId)
draggedCol.value = fields.value.find((f) => f.id === colId) ?? null
const remInPx = parseFloat(getComputedStyle(document.documentElement).fontSize)
const placeholderHeight = tableBodyEl.value?.getBoundingClientRect().height ?? 6.1 * remInPx
dragColPlaceholderDomRef.value!.style.height = `${placeholderHeight}px`
const x = e.clientX - leftSidebarWidth.value
if (x >= 0 && dragColPlaceholderDomRef.value) {
dragColPlaceholderDomRef.value.style.left = `${x.toString()}px`
}
}
const onDrag = (e: DragEvent) => {
e.preventDefault()
if (!e.dataTransfer) return
if (!draggedCol.value) return
if (!dragColPlaceholderDomRef.value) return
if (e.clientX === 0) {
dragColPlaceholderDomRef.value!.style.left = `0px`
dragColPlaceholderDomRef.value!.style.height = '0px'
reorderColumn(draggedCol.value!.id!, toBeDroppedColId.value!)
draggedCol.value = null
toBeDroppedColId.value = null
return
}
const y = dragColPlaceholderDomRef.value!.getBoundingClientRect().top
const domsUnderMouse = document.elementsFromPoint(e.clientX, y)
const columnDom = domsUnderMouse.find((dom) => dom.classList.contains('nc-grid-column-header'))
if (columnDom) {
toBeDroppedColId.value = columnDom?.getAttribute('data-col') ?? null
}
const x = e.clientX - leftSidebarWidth.value
if (x >= 0) {
dragColPlaceholderDomRef.value.style.left = `${x.toString()}px`
}
const remInPx = parseFloat(getComputedStyle(document.documentElement).fontSize)
if (x < leftSidebarWidth.value + 1 * remInPx) {
setTimeout(() => {
gridWrapper.value!.scrollLeft -= 2.5
}, 250)
} else if (width.value - x - leftSidebarWidth.value < 15 * remInPx) {
setTimeout(() => {
gridWrapper.value!.scrollLeft += 2.5
}, 250)
}
}
return {
onDrag,
onDragStart,
draggedCol,
dragColPlaceholderDomRef,
toBeDroppedColId,
}
}
| packages/nc-gui/components/smartsheet/grid/useColumnDrag.ts | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017650060181040317,
0.00016921127098612487,
0.0001631216873647645,
0.00016924402734730393,
0.0000035923051200370537
]
|
{
"id": 1,
"code_window": [
" // horizontally scroll to the end of the kanban container\n",
" // when a new option is added within kanban view\n",
" nextTick(() => {\n",
" if (shouldScrollToRight.value) {\n",
" kanbanContainerRef.value.scrollTo({\n",
" left: kanbanContainerRef.value.scrollWidth,\n",
" behavior: 'smooth',\n",
" })\n",
" // reset shouldScrollToRight\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (shouldScrollToRight.value && kanbanContainerRef.value) {\n"
],
"file_path": "packages/nc-gui/components/smartsheet/Kanban.vue",
"type": "replace",
"edit_start_line_idx": 369
} | <script setup lang="ts">
import type { FilterReqType, HookReqType, HookType } from 'nocodb-sdk'
import {
MetaInj,
extractSdkResponseErrorMsg,
iconMap,
inject,
message,
onMounted,
parseProp,
ref,
useI18n,
useNuxtApp,
} from '#imports'
const emit = defineEmits(['edit', 'add'])
const { t } = useI18n()
const { $api, $e } = useNuxtApp()
const hooks = ref<HookType[]>([])
const meta = inject(MetaInj, ref())
async function loadHooksList() {
try {
const hookList = (await $api.dbTableWebhook.list(meta.value?.id as string)).list
hooks.value = hookList.map((hook) => {
hook.notification = parseProp(hook.notification)
return hook
})
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
}
async function deleteHook(item: HookType, index: number) {
Modal.confirm({
title: `Do you want to delete '${item.title}'?`,
wrapClassName: 'nc-modal-hook-delete',
okText: 'Yes',
okType: 'danger',
cancelText: 'No',
async onOk() {
try {
if (item.id) {
await $api.dbTableWebhook.delete(item.id)
hooks.value.splice(index, 1)
} else {
hooks.value.splice(index, 1)
}
// Hook deleted successfully
message.success(t('msg.success.webhookDeleted'))
if (!hooks.value.length) {
hooks.value = []
}
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
$e('a:webhook:delete')
},
})
}
async function copyHook(hook: HookType) {
try {
const newHook = await $api.dbTableWebhook.create(hook.fk_model_id!, {
...hook,
title: `${hook.title} - Copy`,
active: false,
} as HookReqType)
if (newHook) {
$e('a:webhook:copy')
// create the corresponding filters
const hookFilters = (await $api.dbTableWebhookFilter.read(hook.id!, {})).list
for (const hookFilter of hookFilters) {
await $api.dbTableWebhookFilter.create(newHook.id!, {
comparison_op: hookFilter.comparison_op,
comparison_sub_op: hookFilter.comparison_sub_op,
fk_column_id: hookFilter.fk_column_id,
fk_parent_id: hookFilter.fk_parent_id,
is_group: hookFilter.is_group,
logical_op: hookFilter.logical_op,
value: hookFilter.value,
} as FilterReqType)
}
newHook.notification = parseProp(newHook.notification)
hooks.value = [newHook, ...hooks.value]
}
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
}
onMounted(() => {
loadHooksList()
})
</script>
<template>
<div class="">
<div class="mb-2">
<div class="float-left font-bold text-xl mt-2 mb-4">{{ meta?.title }} : Webhooks</div>
<a-button
v-e="['c:webhook:add']"
class="float-right !rounded-md nc-btn-create-webhook"
type="primary"
size="middle"
@click="emit('add')"
>
{{ $t('activity.addWebhook') }}
</a-button>
</div>
<a-divider />
<div v-if="hooks.length" class="">
<a-list item-layout="horizontal" :data-source="hooks" class="cursor-pointer scrollbar-thin-primary">
<template #renderItem="{ item, index }">
<a-list-item class="p-2 nc-hook" @click="emit('edit', item)">
<a-list-item-meta>
<template #description>
<span class="uppercase"> {{ item.event }} {{ item.operation.replace(/[A-Z]/g, ' $&') }}</span>
</template>
<template #title>
<div class="text-xl normal-case">
<span class="text-gray-400 text-sm"> ({{ item.version }}) </span>
{{ item.title }}
</div>
</template>
<template #avatar>
<div class="px-2">
<component :is="iconMap.hook" class="text-xl" />
</div>
<div class="px-2 text-white rounded" :class="{ 'bg-green-500': item.active, 'bg-gray-500': !item.active }">
{{ item.active ? 'ON' : 'OFF' }}
</div>
</template>
</a-list-item-meta>
<template #extra>
<div>
<!-- Notify Via -->
<div class="mr-2">{{ $t('labels.notifyVia') }} : {{ item?.notification?.type }}</div>
<div class="float-right pt-2 pr-1">
<a-tooltip v-if="item.version === 'v2'" placement="left">
<template #title>
{{ $t('activity.copyWebhook') }}
</template>
<component :is="iconMap.copy" class="text-xl nc-hook-copy-icon" @click.stop="copyHook(item)" />
</a-tooltip>
<a-tooltip placement="left">
<template #title>
{{ $t('activity.deleteWebhook') }}
</template>
<component :is="iconMap.delete" class="text-xl nc-hook-delete-icon" @click.stop="deleteHook(item, index)" />
</a-tooltip>
</div>
</div>
</template>
</a-list-item>
</template>
</a-list>
</div>
<div v-else class="min-h-[75vh]">
<div class="p-4 bg-gray-100 text-gray-600">
Webhooks list is empty, create new webhook by clicking 'Create webhook' button.
</div>
</div>
</div>
</template>
| packages/nc-gui/components/webhook/List.vue | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017660498269833624,
0.00017287211085204035,
0.00016754789976403117,
0.00017342982755508274,
0.0000018862350543713546
]
|
{
"id": 1,
"code_window": [
" // horizontally scroll to the end of the kanban container\n",
" // when a new option is added within kanban view\n",
" nextTick(() => {\n",
" if (shouldScrollToRight.value) {\n",
" kanbanContainerRef.value.scrollTo({\n",
" left: kanbanContainerRef.value.scrollWidth,\n",
" behavior: 'smooth',\n",
" })\n",
" // reset shouldScrollToRight\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (shouldScrollToRight.value && kanbanContainerRef.value) {\n"
],
"file_path": "packages/nc-gui/components/smartsheet/Kanban.vue",
"type": "replace",
"edit_start_line_idx": 369
} | // import ses from '../../v1-legacy/plugins/ses';
import type { Knex } from 'knex';
const up = async (knex: Knex) => {
await knex('nc_plugins').del().where({ title: 'SES' });
};
const down = async (_: Knex) => {
// await knex('nc_plugins').insert([ses]);
};
export { up, down };
| packages/nocodb/src/meta/migrations/v1/nc_011_remove_old_ses_plugin.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0001779133453965187,
0.00016994503675960004,
0.0001619767426745966,
0.00016994503675960004,
0.00000796830136096105
]
|
{
"id": 1,
"code_window": [
" // horizontally scroll to the end of the kanban container\n",
" // when a new option is added within kanban view\n",
" nextTick(() => {\n",
" if (shouldScrollToRight.value) {\n",
" kanbanContainerRef.value.scrollTo({\n",
" left: kanbanContainerRef.value.scrollWidth,\n",
" behavior: 'smooth',\n",
" })\n",
" // reset shouldScrollToRight\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (shouldScrollToRight.value && kanbanContainerRef.value) {\n"
],
"file_path": "packages/nc-gui/components/smartsheet/Kanban.vue",
"type": "replace",
"edit_start_line_idx": 369
} | <script setup lang="ts">
import {
ActiveViewInj,
AllFiltersInj,
IsLockedInj,
computed,
iconMap,
inject,
ref,
useGlobal,
useMenuCloseOnEsc,
useSmartsheetStoreOrThrow,
useViewFilters,
watch,
} from '#imports'
const isLocked = inject(IsLockedInj, ref(false))
const activeView = inject(ActiveViewInj, ref())
const { isMobileMode } = useGlobal()
const filterComp = ref<typeof ColumnFilter>()
const { nestedFilters } = useSmartsheetStoreOrThrow()
// todo: avoid duplicate api call by keeping a filter store
const { nonDeletedFilters, loadFilters } = useViewFilters(
activeView!,
undefined,
computed(() => true),
() => false,
nestedFilters.value,
true,
)
const filtersLength = ref(0)
watch(
() => activeView?.value?.id,
async (viewId) => {
if (viewId) {
await loadFilters()
filtersLength.value = nonDeletedFilters.value.length || 0
}
},
{ immediate: true },
)
const open = ref(false)
const allFilters = ref({})
provide(AllFiltersInj, allFilters)
useMenuCloseOnEsc(open)
</script>
<template>
<NcDropdown
v-model:visible="open"
:trigger="['click']"
overlay-class-name="nc-dropdown-filter-menu nc-toolbar-dropdown"
class="!xs:hidden"
>
<div :class="{ 'nc-active-btn': filtersLength }">
<a-button v-e="['c:filter']" class="nc-filter-menu-btn nc-toolbar-btn txt-sm" :disabled="isLocked">
<div class="flex items-center gap-2">
<component :is="iconMap.filter" class="h-4 w-4" />
<!-- Filter -->
<span v-if="!isMobileMode" class="text-capitalize !text-sm font-medium">{{ $t('activity.filter') }}</span>
<span v-if="filtersLength" class="bg-brand-50 text-brand-500 py-1 px-2 text-md rounded-md">{{ filtersLength }}</span>
</div>
</a-button>
</div>
<template #overlay>
<SmartsheetToolbarColumnFilter
ref="filterComp"
class="nc-table-toolbar-menu"
:auto-save="true"
data-testid="nc-filter-menu"
@update:filters-length="filtersLength = $event"
>
</SmartsheetToolbarColumnFilter>
</template>
</NcDropdown>
</template>
| packages/nc-gui/components/smartsheet/toolbar/ColumnFilterMenu.vue | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017357393517158926,
0.00017080568068195134,
0.00016798269643913954,
0.000170685671037063,
0.0000015297288200599723
]
|
{
"id": 2,
"code_window": [
"// const optionsMagic = async () => {\n",
"// await _optionsMagic(base, formState, getNextColor, options.value, renderedOptions.value)\n",
"// }\n",
"\n",
"const syncOptions = () => {\n",
" vModel.value.colOptions.options = options.value\n",
" .filter((op) => op.status !== 'remove')\n",
" .sort((a, b) => {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set initial colOptions if not set\n",
" vModel.value.colOptions = vModel.value.colOptions || {}\n"
],
"file_path": "packages/nc-gui/components/smartsheet/column/SelectOptions.vue",
"type": "add",
"edit_start_line_idx": 178
} | import { type ColumnType } from 'nocodb-sdk'
import type { PropType } from '@vue/runtime-core'
import {
ColumnInj,
computed,
defineComponent,
h,
iconMap,
inject,
isAttachment,
isBoolean,
isCurrency,
isDate,
isDateTime,
isDecimal,
isDuration,
isEmail,
isFloat,
isGeoData,
isGeometry,
isInt,
isJSON,
isPercent,
isPhoneNumber,
isPrimaryKey,
isRating,
isSet,
isSingleSelect,
isSpecificDBType,
isString,
isTextArea,
isTime,
isURL,
isUser,
isYear,
storeToRefs,
toRef,
useBase,
} from '#imports'
const renderIcon = (column: ColumnType, abstractType: any) => {
if (isPrimaryKey(column)) {
return iconMap.key
} else if (isSpecificDBType(column)) {
return iconMap.specificDbType
} else if (isJSON(column)) {
return iconMap.json
} else if (isDate(column, abstractType)) {
return iconMap.calendar
} else if (isDateTime(column, abstractType)) {
return iconMap.datetime
} else if (isGeoData(column)) {
return iconMap.geoData
} else if (isSet(column)) {
return iconMap.multiSelect
} else if (isSingleSelect(column)) {
return iconMap.singleSelect
} else if (isBoolean(column, abstractType)) {
return iconMap.boolean
} else if (isTextArea(column)) {
return iconMap.longText
} else if (isEmail(column)) {
return iconMap.email
} else if (isYear(column, abstractType)) {
return iconMap.calendar
} else if (isTime(column, abstractType)) {
return iconMap.clock
} else if (isRating(column)) {
return iconMap.rating
} else if (isAttachment(column)) {
return iconMap.image
} else if (isDecimal(column)) {
return iconMap.decimal
} else if (isPhoneNumber(column)) {
return iconMap.phone
} else if (isURL(column)) {
return iconMap.web
} else if (isCurrency(column)) {
return iconMap.currency
} else if (isDuration(column)) {
return iconMap.duration
} else if (isPercent(column)) {
return iconMap.percent
} else if (isGeometry(column)) {
return iconMap.calculator
} else if (isUser(column)) {
if ((column.meta as { is_multi?: boolean; notify?: boolean })?.is_multi) {
return iconMap.phUsers
}
return iconMap.phUser
} else if (isInt(column, abstractType) || isFloat(column, abstractType)) {
return iconMap.number
} else if (isString(column, abstractType)) {
return iconMap.text
} else {
return iconMap.generic
}
}
export default defineComponent({
name: 'CellIcon',
props: {
columnMeta: {
type: Object as PropType<ColumnType>,
required: false,
},
},
setup(props) {
const columnMeta = toRef(props, 'columnMeta')
const injectedColumn = inject(ColumnInj, columnMeta)
const column = computed(() => columnMeta.value ?? injectedColumn.value)
const { sqlUis } = storeToRefs(useBase())
const sqlUi = ref(column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0])
const abstractType = computed(() => column.value && sqlUi.value.getAbstractType(column.value))
return () => {
if (!column.value && !columnMeta.value) return null
return h(renderIcon((columnMeta.value ?? column.value)!, abstractType.value), {
class: 'text-inherit mx-1',
})
}
},
})
| packages/nc-gui/components/smartsheet/header/CellIcon.ts | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017668279178906232,
0.00017330753325950354,
0.00016752106603235006,
0.0001732110686134547,
0.0000026521245217736578
]
|
{
"id": 2,
"code_window": [
"// const optionsMagic = async () => {\n",
"// await _optionsMagic(base, formState, getNextColor, options.value, renderedOptions.value)\n",
"// }\n",
"\n",
"const syncOptions = () => {\n",
" vModel.value.colOptions.options = options.value\n",
" .filter((op) => op.status !== 'remove')\n",
" .sort((a, b) => {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set initial colOptions if not set\n",
" vModel.value.colOptions = vModel.value.colOptions || {}\n"
],
"file_path": "packages/nc-gui/components/smartsheet/column/SelectOptions.vue",
"type": "add",
"edit_start_line_idx": 178
} | GNU AFFERO GENERAL PUBLIC LICENSE
Version 3, 19 November 2007
Copyright (C) 2007 Free Software Foundation, Inc. <http://fsf.org/>
Everyone is permitted to copy and distribute verbatim copies
of this license document, but changing it is not allowed.
Preamble
The GNU Affero General Public License is a free, copyleft license for
software and other kinds of works, specifically designed to ensure
cooperation with the community in the case of network server software.
The licenses for most software and other practical works are designed
to take away your freedom to share and change the works. By contrast,
our General Public Licenses are intended to guarantee your freedom to
share and change all versions of a program--to make sure it remains free
software for all its users.
When we speak of free software, we are referring to freedom, not
price. Our General Public Licenses are designed to make sure that you
have the freedom to distribute copies of free software (and charge for
them if you wish), that you receive source code or can get it if you
want it, that you can change the software or use pieces of it in new
free programs, and that you know you can do these things.
Developers that use our General Public Licenses protect your rights
with two steps: (1) assert copyright on the software, and (2) offer
you this License which gives you legal permission to copy, distribute
and/or modify the software.
A secondary benefit of defending all users' freedom is that
improvements made in alternate versions of the program, if they
receive widespread use, become available for other developers to
incorporate. Many developers of free software are heartened and
encouraged by the resulting cooperation. However, in the case of
software used on network servers, this result may fail to come about.
The GNU General Public License permits making a modified version and
letting the public access it on a server without ever releasing its
source code to the public.
The GNU Affero General Public License is designed specifically to
ensure that, in such cases, the modified source code becomes available
to the community. It requires the operator of a network server to
provide the source code of the modified version running there to the
users of that server. Therefore, public use of a modified version, on
a publicly accessible server, gives the public access to the source
code of the modified version.
An older license, called the Affero General Public License and
published by Affero, was designed to accomplish similar goals. This is
a different license, not a version of the Affero GPL, but Affero has
released a new version of the Affero GPL which permits relicensing under
this license.
The precise terms and conditions for copying, distribution and
modification follow.
TERMS AND CONDITIONS
0. Definitions.
"This License" refers to version 3 of the GNU Affero General Public License.
"Copyright" also means copyright-like laws that apply to other kinds of
works, such as semiconductor masks.
"The Program" refers to any copyrightable work licensed under this
License. Each licensee is addressed as "you". "Licensees" and
"recipients" may be individuals or organizations.
To "modify" a work means to copy from or adapt all or part of the work
in a fashion requiring copyright permission, other than the making of an
exact copy. The resulting work is called a "modified version" of the
earlier work or a work "based on" the earlier work.
A "covered work" means either the unmodified Program or a work based
on the Program.
To "propagate" a work means to do anything with it that, without
permission, would make you directly or secondarily liable for
infringement under applicable copyright law, except executing it on a
computer or modifying a private copy. Propagation includes copying,
distribution (with or without modification), making available to the
public, and in some countries other activities as well.
To "convey" a work means any kind of propagation that enables other
parties to make or receive copies. Mere interaction with a user through
a computer network, with no transfer of a copy, is not conveying.
An interactive user interface displays "Appropriate Legal Notices"
to the extent that it includes a convenient and prominently visible
feature that (1) displays an appropriate copyright notice, and (2)
tells the user that there is no warranty for the work (except to the
extent that warranties are provided), that licensees may convey the
work under this License, and how to view a copy of this License. If
the interface presents a list of user commands or options, such as a
menu, a prominent item in the list meets this criterion.
1. Source Code.
The "source code" for a work means the preferred form of the work
for making modifications to it. "Object code" means any non-source
form of a work.
A "Standard Interface" means an interface that either is an official
standard defined by a recognized standards body, or, in the case of
interfaces specified for a particular programming language, one that
is widely used among developers working in that language.
The "System Libraries" of an executable work include anything, other
than the work as a whole, that (a) is included in the normal form of
packaging a Major Component, but which is not part of that Major
Component, and (b) serves only to enable use of the work with that
Major Component, or to implement a Standard Interface for which an
implementation is available to the public in source code form. A
"Major Component", in this context, means a major essential component
(kernel, window system, and so on) of the specific operating system
(if any) on which the executable work runs, or a compiler used to
produce the work, or an object code interpreter used to run it.
The "Corresponding Source" for a work in object code form means all
the source code needed to generate, install, and (for an executable
work) run the object code and to modify the work, including scripts to
control those activities. However, it does not include the work's
System Libraries, or general-purpose tools or generally available free
programs which are used unmodified in performing those activities but
which are not part of the work. For example, Corresponding Source
includes interface definition files associated with source files for
the work, and the source code for shared libraries and dynamically
linked subprograms that the work is specifically designed to require,
such as by intimate data communication or control flow between those
subprograms and other parts of the work.
The Corresponding Source need not include anything that users
can regenerate automatically from other parts of the Corresponding
Source.
The Corresponding Source for a work in source code form is that
same work.
2. Basic Permissions.
All rights granted under this License are granted for the term of
copyright on the Program, and are irrevocable provided the stated
conditions are met. This License explicitly affirms your unlimited
permission to run the unmodified Program. The output from running a
covered work is covered by this License only if the output, given its
content, constitutes a covered work. This License acknowledges your
rights of fair use or other equivalent, as provided by copyright law.
You may make, run and propagate covered works that you do not
convey, without conditions so long as your license otherwise remains
in force. You may convey covered works to others for the sole purpose
of having them make modifications exclusively for you, or provide you
with facilities for running those works, provided that you comply with
the terms of this License in conveying all material for which you do
not control copyright. Those thus making or running the covered works
for you must do so exclusively on your behalf, under your direction
and control, on terms that prohibit them from making any copies of
your copyrighted material outside their relationship with you.
Conveying under any other circumstances is permitted solely under
the conditions stated below. Sublicensing is not allowed; section 10
makes it unnecessary.
3. Protecting Users' Legal Rights From Anti-Circumvention Law.
No covered work shall be deemed part of an effective technological
measure under any applicable law fulfilling obligations under article
11 of the WIPO copyright treaty adopted on 20 December 1996, or
similar laws prohibiting or restricting circumvention of such
measures.
When you convey a covered work, you waive any legal power to forbid
circumvention of technological measures to the extent such circumvention
is effected by exercising rights under this License with respect to
the covered work, and you disclaim any intention to limit operation or
modification of the work as a means of enforcing, against the work's
users, your or third parties' legal rights to forbid circumvention of
technological measures.
4. Conveying Verbatim Copies.
You may convey verbatim copies of the Program's source code as you
receive it, in any medium, provided that you conspicuously and
appropriately publish on each copy an appropriate copyright notice;
keep intact all notices stating that this License and any
non-permissive terms added in accord with section 7 apply to the code;
keep intact all notices of the absence of any warranty; and give all
recipients a copy of this License along with the Program.
You may charge any price or no price for each copy that you convey,
and you may offer support or warranty protection for a fee.
5. Conveying Modified Source Versions.
You may convey a work based on the Program, or the modifications to
produce it from the Program, in the form of source code under the
terms of section 4, provided that you also meet all of these conditions:
a) The work must carry prominent notices stating that you modified
it, and giving a relevant date.
b) The work must carry prominent notices stating that it is
released under this License and any conditions added under section
7. This requirement modifies the requirement in section 4 to
"keep intact all notices".
c) You must license the entire work, as a whole, under this
License to anyone who comes into possession of a copy. This
License will therefore apply, along with any applicable section 7
additional terms, to the whole of the work, and all its parts,
regardless of how they are packaged. This License gives no
permission to license the work in any other way, but it does not
invalidate such permission if you have separately received it.
d) If the work has interactive user interfaces, each must display
Appropriate Legal Notices; however, if the Program has interactive
interfaces that do not display Appropriate Legal Notices, your
work need not make them do so.
A compilation of a covered work with other separate and independent
works, which are not by their nature extensions of the covered work,
and which are not combined with it such as to form a larger program,
in or on a volume of a storage or distribution medium, is called an
"aggregate" if the compilation and its resulting copyright are not
used to limit the access or legal rights of the compilation's users
beyond what the individual works permit. Inclusion of a covered work
in an aggregate does not cause this License to apply to the other
parts of the aggregate.
6. Conveying Non-Source Forms.
You may convey a covered work in object code form under the terms
of sections 4 and 5, provided that you also convey the
machine-readable Corresponding Source under the terms of this License,
in one of these ways:
a) Convey the object code in, or embodied in, a physical product
(including a physical distribution medium), accompanied by the
Corresponding Source fixed on a durable physical medium
customarily used for software interchange.
b) Convey the object code in, or embodied in, a physical product
(including a physical distribution medium), accompanied by a
written offer, valid for at least three years and valid for as
long as you offer spare parts or customer support for that product
model, to give anyone who possesses the object code either (1) a
copy of the Corresponding Source for all the software in the
product that is covered by this License, on a durable physical
medium customarily used for software interchange, for a price no
more than your reasonable cost of physically performing this
conveying of source, or (2) access to copy the
Corresponding Source from a network server at no charge.
c) Convey individual copies of the object code with a copy of the
written offer to provide the Corresponding Source. This
alternative is allowed only occasionally and noncommercially, and
only if you received the object code with such an offer, in accord
with subsection 6b.
d) Convey the object code by offering access from a designated
place (gratis or for a charge), and offer equivalent access to the
Corresponding Source in the same way through the same place at no
further charge. You need not require recipients to copy the
Corresponding Source along with the object code. If the place to
copy the object code is a network server, the Corresponding Source
may be on a different server (operated by you or a third party)
that supports equivalent copying facilities, provided you maintain
clear directions next to the object code saying where to find the
Corresponding Source. Regardless of what server hosts the
Corresponding Source, you remain obligated to ensure that it is
available for as long as needed to satisfy these requirements.
e) Convey the object code using peer-to-peer transmission, provided
you inform other peers where the object code and Corresponding
Source of the work are being offered to the general public at no
charge under subsection 6d.
A separable portion of the object code, whose source code is excluded
from the Corresponding Source as a System Library, need not be
included in conveying the object code work.
A "User Product" is either (1) a "consumer product", which means any
tangible personal property which is normally used for personal, family,
or household purposes, or (2) anything designed or sold for incorporation
into a dwelling. In determining whether a product is a consumer product,
doubtful cases shall be resolved in favor of coverage. For a particular
product received by a particular user, "normally used" refers to a
typical or common use of that class of product, regardless of the status
of the particular user or of the way in which the particular user
actually uses, or expects or is expected to use, the product. A product
is a consumer product regardless of whether the product has substantial
commercial, industrial or non-consumer uses, unless such uses represent
the only significant mode of use of the product.
"Installation Information" for a User Product means any methods,
procedures, authorization keys, or other information required to install
and execute modified versions of a covered work in that User Product from
a modified version of its Corresponding Source. The information must
suffice to ensure that the continued functioning of the modified object
code is in no case prevented or interfered with solely because
modification has been made.
If you convey an object code work under this section in, or with, or
specifically for use in, a User Product, and the conveying occurs as
part of a transaction in which the right of possession and use of the
User Product is transferred to the recipient in perpetuity or for a
fixed term (regardless of how the transaction is characterized), the
Corresponding Source conveyed under this section must be accompanied
by the Installation Information. But this requirement does not apply
if neither you nor any third party retains the ability to install
modified object code on the User Product (for example, the work has
been installed in ROM).
The requirement to provide Installation Information does not include a
requirement to continue to provide support service, warranty, or updates
for a work that has been modified or installed by the recipient, or for
the User Product in which it has been modified or installed. Access to a
network may be denied when the modification itself materially and
adversely affects the operation of the network or violates the rules and
protocols for communication across the network.
Corresponding Source conveyed, and Installation Information provided,
in accord with this section must be in a format that is publicly
documented (and with an implementation available to the public in
source code form), and must require no special password or key for
unpacking, reading or copying.
7. Additional Terms.
"Additional permissions" are terms that supplement the terms of this
License by making exceptions from one or more of its conditions.
Additional permissions that are applicable to the entire Program shall
be treated as though they were included in this License, to the extent
that they are valid under applicable law. If additional permissions
apply only to part of the Program, that part may be used separately
under those permissions, but the entire Program remains governed by
this License without regard to the additional permissions.
When you convey a copy of a covered work, you may at your option
remove any additional permissions from that copy, or from any part of
it. (Additional permissions may be written to require their own
removal in certain cases when you modify the work.) You may place
additional permissions on material, added by you to a covered work,
for which you have or can give appropriate copyright permission.
Notwithstanding any other provision of this License, for material you
add to a covered work, you may (if authorized by the copyright holders of
that material) supplement the terms of this License with terms:
a) Disclaiming warranty or limiting liability differently from the
terms of sections 15 and 16 of this License; or
b) Requiring preservation of specified reasonable legal notices or
author attributions in that material or in the Appropriate Legal
Notices displayed by works containing it; or
c) Prohibiting misrepresentation of the origin of that material, or
requiring that modified versions of such material be marked in
reasonable ways as different from the original version; or
d) Limiting the use for publicity purposes of names of licensors or
authors of the material; or
e) Declining to grant rights under trademark law for use of some
trade names, trademarks, or service marks; or
f) Requiring indemnification of licensors and authors of that
material by anyone who conveys the material (or modified versions of
it) with contractual assumptions of liability to the recipient, for
any liability that these contractual assumptions directly impose on
those licensors and authors.
All other non-permissive additional terms are considered "further
restrictions" within the meaning of section 10. If the Program as you
received it, or any part of it, contains a notice stating that it is
governed by this License along with a term that is a further
restriction, you may remove that term. If a license document contains
a further restriction but permits relicensing or conveying under this
License, you may add to a covered work material governed by the terms
of that license document, provided that the further restriction does
not survive such relicensing or conveying.
If you add terms to a covered work in accord with this section, you
must place, in the relevant source files, a statement of the
additional terms that apply to those files, or a notice indicating
where to find the applicable terms.
Additional terms, permissive or non-permissive, may be stated in the
form of a separately written license, or stated as exceptions;
the above requirements apply either way.
8. Termination.
You may not propagate or modify a covered work except as expressly
provided under this License. Any attempt otherwise to propagate or
modify it is void, and will automatically terminate your rights under
this License (including any patent licenses granted under the third
paragraph of section 11).
However, if you cease all violation of this License, then your
license from a particular copyright holder is reinstated (a)
provisionally, unless and until the copyright holder explicitly and
finally terminates your license, and (b) permanently, if the copyright
holder fails to notify you of the violation by some reasonable means
prior to 60 days after the cessation.
Moreover, your license from a particular copyright holder is
reinstated permanently if the copyright holder notifies you of the
violation by some reasonable means, this is the first time you have
received notice of violation of this License (for any work) from that
copyright holder, and you cure the violation prior to 30 days after
your receipt of the notice.
Termination of your rights under this section does not terminate the
licenses of parties who have received copies or rights from you under
this License. If your rights have been terminated and not permanently
reinstated, you do not qualify to receive new licenses for the same
material under section 10.
9. Acceptance Not Required for Having Copies.
You are not required to accept this License in order to receive or
run a copy of the Program. Ancillary propagation of a covered work
occurring solely as a consequence of using peer-to-peer transmission
to receive a copy likewise does not require acceptance. However,
nothing other than this License grants you permission to propagate or
modify any covered work. These actions infringe copyright if you do
not accept this License. Therefore, by modifying or propagating a
covered work, you indicate your acceptance of this License to do so.
10. Automatic Licensing of Downstream Recipients.
Each time you convey a covered work, the recipient automatically
receives a license from the original licensors, to run, modify and
propagate that work, subject to this License. You are not responsible
for enforcing compliance by third parties with this License.
An "entity transaction" is a transaction transferring control of an
organization, or substantially all assets of one, or subdividing an
organization, or merging organizations. If propagation of a covered
work results from an entity transaction, each party to that
transaction who receives a copy of the work also receives whatever
licenses to the work the party's predecessor in interest had or could
give under the previous paragraph, plus a right to possession of the
Corresponding Source of the work from the predecessor in interest, if
the predecessor has it or can get it with reasonable efforts.
You may not impose any further restrictions on the exercise of the
rights granted or affirmed under this License. For example, you may
not impose a license fee, royalty, or other charge for exercise of
rights granted under this License, and you may not initiate litigation
(including a cross-claim or counterclaim in a lawsuit) alleging that
any patent claim is infringed by making, using, selling, offering for
sale, or importing the Program or any portion of it.
11. Patents.
A "contributor" is a copyright holder who authorizes use under this
License of the Program or a work on which the Program is based. The
work thus licensed is called the contributor's "contributor version".
A contributor's "essential patent claims" are all patent claims
owned or controlled by the contributor, whether already acquired or
hereafter acquired, that would be infringed by some manner, permitted
by this License, of making, using, or selling its contributor version,
but do not include claims that would be infringed only as a
consequence of further modification of the contributor version. For
purposes of this definition, "control" includes the right to grant
patent sublicenses in a manner consistent with the requirements of
this License.
Each contributor grants you a non-exclusive, worldwide, royalty-free
patent license under the contributor's essential patent claims, to
make, use, sell, offer for sale, import and otherwise run, modify and
propagate the contents of its contributor version.
In the following three paragraphs, a "patent license" is any express
agreement or commitment, however denominated, not to enforce a patent
(such as an express permission to practice a patent or covenant not to
sue for patent infringement). To "grant" such a patent license to a
party means to make such an agreement or commitment not to enforce a
patent against the party.
If you convey a covered work, knowingly relying on a patent license,
and the Corresponding Source of the work is not available for anyone
to copy, free of charge and under the terms of this License, through a
publicly available network server or other readily accessible means,
then you must either (1) cause the Corresponding Source to be so
available, or (2) arrange to deprive yourself of the benefit of the
patent license for this particular work, or (3) arrange, in a manner
consistent with the requirements of this License, to extend the patent
license to downstream recipients. "Knowingly relying" means you have
actual knowledge that, but for the patent license, your conveying the
covered work in a country, or your recipient's use of the covered work
in a country, would infringe one or more identifiable patents in that
country that you have reason to believe are valid.
If, pursuant to or in connection with a single transaction or
arrangement, you convey, or propagate by procuring conveyance of, a
covered work, and grant a patent license to some of the parties
receiving the covered work authorizing them to use, propagate, modify
or convey a specific copy of the covered work, then the patent license
you grant is automatically extended to all recipients of the covered
work and works based on it.
A patent license is "discriminatory" if it does not include within
the scope of its coverage, prohibits the exercise of, or is
conditioned on the non-exercise of one or more of the rights that are
specifically granted under this License. You may not convey a covered
work if you are a party to an arrangement with a third party that is
in the business of distributing software, under which you make payment
to the third party based on the extent of your activity of conveying
the work, and under which the third party grants, to any of the
parties who would receive the covered work from you, a discriminatory
patent license (a) in connection with copies of the covered work
conveyed by you (or copies made from those copies), or (b) primarily
for and in connection with specific products or compilations that
contain the covered work, unless you entered into that arrangement,
or that patent license was granted, prior to 28 March 2007.
Nothing in this License shall be construed as excluding or limiting
any implied license or other defenses to infringement that may
otherwise be available to you under applicable patent law.
12. No Surrender of Others' Freedom.
If conditions are imposed on you (whether by court order, agreement or
otherwise) that contradict the conditions of this License, they do not
excuse you from the conditions of this License. If you cannot convey a
covered work so as to satisfy simultaneously your obligations under this
License and any other pertinent obligations, then as a consequence you may
not convey it at all. For example, if you agree to terms that obligate you
to collect a royalty for further conveying from those to whom you convey
the Program, the only way you could satisfy both those terms and this
License would be to refrain entirely from conveying the Program.
13. Remote Network Interaction; Use with the GNU General Public License.
Notwithstanding any other provision of this License, if you modify the
Program, your modified version must prominently offer all users
interacting with it remotely through a computer network (if your version
supports such interaction) an opportunity to receive the Corresponding
Source of your version by providing access to the Corresponding Source
from a network server at no charge, through some standard or customary
means of facilitating copying of software. This Corresponding Source
shall include the Corresponding Source for any work covered by version 3
of the GNU General Public License that is incorporated pursuant to the
following paragraph.
Notwithstanding any other provision of this License, you have
permission to link or combine any covered work with a work licensed
under version 3 of the GNU General Public License into a single
combined work, and to convey the resulting work. The terms of this
License will continue to apply to the part which is the covered work,
but the work with which it is combined will remain governed by version
3 of the GNU General Public License.
14. Revised Versions of this License.
The Free Software Foundation may publish revised and/or new versions of
the GNU Affero General Public License from time to time. Such new versions
will be similar in spirit to the present version, but may differ in detail to
address new problems or concerns.
Each version is given a distinguishing version number. If the
Program specifies that a certain numbered version of the GNU Affero General
Public License "or any later version" applies to it, you have the
option of following the terms and conditions either of that numbered
version or of any later version published by the Free Software
Foundation. If the Program does not specify a version number of the
GNU Affero General Public License, you may choose any version ever published
by the Free Software Foundation.
If the Program specifies that a proxy can decide which future
versions of the GNU Affero General Public License can be used, that proxy's
public statement of acceptance of a version permanently authorizes you
to choose that version for the Program.
Later license versions may give you additional or different
permissions. However, no additional obligations are imposed on any
author or copyright holder as a result of your choosing to follow a
later version.
15. Disclaimer of Warranty.
THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY
APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT
HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY
OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO,
THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM
IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF
ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
16. Limitation of Liability.
IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS
THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY
GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE
USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF
DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD
PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS),
EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF
SUCH DAMAGES.
17. Interpretation of Sections 15 and 16.
If the disclaimer of warranty and limitation of liability provided
above cannot be given local legal effect according to their terms,
reviewing courts shall apply local law that most closely approximates
an absolute waiver of all civil liability in connection with the
Program, unless a warranty or assumption of liability accompanies a
copy of the Program in return for a fee.
END OF TERMS AND CONDITIONS
How to Apply These Terms to Your New Programs
If you develop a new program, and you want it to be of the greatest
possible use to the public, the best way to achieve this is to make it
free software which everyone can redistribute and change under these terms.
To do so, attach the following notices to the program. It is safest
to attach them to the start of each source file to most effectively
state the exclusion of warranty; and each file should have at least
the "copyright" line and a pointer to where the full notice is found.
<one line to give the program's name and a brief idea of what it does.>
Copyright (C) <year> <name of author>
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU Affero General Public License as published
by the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Affero General Public License for more details.
You should have received a copy of the GNU Affero General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
Also add information on how to contact you by electronic and paper mail.
If your software can interact with users remotely through a computer
network, you should also make sure that it provides a way for users to
get its source. For example, if your program is a web application, its
interface could display a "Source" link that leads users to an archive
of the code. There are many ways you could offer source, and different
solutions will be better for different programs; see section 13 for the
specific requirements.
You should also get your employer (if you work as a programmer) or school,
if any, to sign a "copyright disclaimer" for the program, if necessary.
For more information on this, and how to apply and follow the GNU AGPL, see
<http://www.gnu.org/licenses/>. | packages/nc-cli/LICENSE | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00018002181604970247,
0.00017429600120522082,
0.00016334193060174584,
0.00017517374362796545,
0.0000037725847050751327
]
|
{
"id": 2,
"code_window": [
"// const optionsMagic = async () => {\n",
"// await _optionsMagic(base, formState, getNextColor, options.value, renderedOptions.value)\n",
"// }\n",
"\n",
"const syncOptions = () => {\n",
" vModel.value.colOptions.options = options.value\n",
" .filter((op) => op.status !== 'remove')\n",
" .sort((a, b) => {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set initial colOptions if not set\n",
" vModel.value.colOptions = vModel.value.colOptions || {}\n"
],
"file_path": "packages/nc-gui/components/smartsheet/column/SelectOptions.vue",
"type": "add",
"edit_start_line_idx": 178
} | import { Test } from '@nestjs/testing';
import { PublicDatasExportService } from './public-datas-export.service';
import type { TestingModule } from '@nestjs/testing';
describe('PublicDatasExportService', () => {
let service: PublicDatasExportService;
beforeEach(async () => {
const module: TestingModule = await Test.createTestingModule({
providers: [PublicDatasExportService],
}).compile();
service = module.get<PublicDatasExportService>(PublicDatasExportService);
});
it('should be defined', () => {
expect(service).toBeDefined();
});
});
| packages/nocodb/src/services/public-datas-export.service.spec.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017199201101902872,
0.00017062161350622773,
0.00016925121599342674,
0.00017062161350622773,
0.0000013703975128009915
]
|
{
"id": 2,
"code_window": [
"// const optionsMagic = async () => {\n",
"// await _optionsMagic(base, formState, getNextColor, options.value, renderedOptions.value)\n",
"// }\n",
"\n",
"const syncOptions = () => {\n",
" vModel.value.colOptions.options = options.value\n",
" .filter((op) => op.status !== 'remove')\n",
" .sort((a, b) => {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" // set initial colOptions if not set\n",
" vModel.value.colOptions = vModel.value.colOptions || {}\n"
],
"file_path": "packages/nc-gui/components/smartsheet/column/SelectOptions.vue",
"type": "add",
"edit_start_line_idx": 178
} | {
"name": "noco-docs",
"version": "1.1.0",
"private": true,
"author": {
"name": "NocoDB Inc",
"url": "https://nocodb.com/"
},
"homepage": "https://github.com/nocodb/nocodb",
"license": "AGPL-3.0-or-later",
"repository": {
"type": "git",
"url": "https://github.com/nocodb/nocodb.git"
},
"bugs": {
"url": "https://github.com/nocodb/nocodb/issues"
},
"scripts": {
"docusaurus": "docusaurus",
"start": "docusaurus start",
"build": "docusaurus build --out-dir dist",
"generate": "docusaurus build --out-dir dist",
"swizzle": "docusaurus swizzle",
"clear": "docusaurus clear",
"serve": "docusaurus serve --dir dist",
"write-translations": "docusaurus write-translations",
"write-heading-ids": "docusaurus write-heading-ids",
"typecheck": "tsc"
},
"dependencies": {
"@docusaurus/core": "3.0.1",
"@docusaurus/plugin-client-redirects": "3.0.1",
"@docusaurus/plugin-ideal-image": "3.0.1",
"@docusaurus/plugin-sitemap": "3.0.1",
"@docusaurus/preset-classic": "3.0.1",
"@mdx-js/react": "^3.0.0",
"clsx": "^1.2.1",
"docusaurus-plugin-sass": "^0.2.5",
"docusaurus-theme-search-typesense": "^0.14.1",
"nc-analytics": "^0.0.7",
"plugin-image-zoom": "github:flexanalytics/plugin-image-zoom",
"prism-react-renderer": "^1.3.5",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"sass": "^1.69.7"
},
"devDependencies": {
"@docusaurus/module-type-aliases": "3.0.1",
"@tsconfig/docusaurus": "^1.0.7",
"typescript": "^4.9.5"
},
"browserslist": {
"production": [
">0.5%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
},
"engines": {
"node": ">=16.14.2"
}
}
| packages/noco-docs/package.json | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017566497263032943,
0.00017230756930075586,
0.00017012201715260744,
0.00017147061589639634,
0.00000195684697246179
]
|
{
"id": 3,
"code_window": [
" return _fields?.value ?? []\n",
"})\n",
"\n",
"const hiddenFields = computed(() => {\n",
" return (meta.value.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n",
"})\n",
"\n",
"const showHiddenFields = ref(false)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // todo: figure out when meta.value is undefined\n",
" return (meta.value?.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/expanded-form/index.vue",
"type": "replace",
"edit_start_line_idx": 118
} | <script lang="ts" setup>
import type { ViewType } from 'nocodb-sdk'
import { ViewTypes } from 'nocodb-sdk'
import type { SortableEvent } from 'sortablejs'
import Sortable from 'sortablejs'
import type { Menu as AntMenu } from 'ant-design-vue'
import {
extractSdkResponseErrorMsg,
isDefaultBase,
message,
onMounted,
parseProp,
ref,
resolveComponent,
useApi,
useCommandPalette,
useDialog,
useNuxtApp,
useUndoRedo,
viewTypeAlias,
watch,
} from '#imports'
interface Emits {
(event: 'openModal', data: { type: ViewTypes; title?: string; copyViewId?: string; groupingFieldColumnId?: string }): void
(event: 'deleted'): void
}
const emits = defineEmits<Emits>()
const base = inject(ProjectInj)!
const table = inject(SidebarTableInj)!
const { isLeftSidebarOpen } = storeToRefs(useSidebarStore())
const { activeTableId } = storeToRefs(useTablesStore())
const { isUIAllowed } = useRoles()
const { isMobileMode } = useGlobal()
const { $e } = useNuxtApp()
const { t } = useI18n()
const { viewsByTable, activeView, allRecentViews } = storeToRefs(useViewsStore())
const { navigateToTable } = useTablesStore()
const views = computed(() => viewsByTable.value.get(table.value.id!)?.filter((v) => !v.is_default) ?? [])
const { api } = useApi()
const { refreshCommandPalette } = useCommandPalette()
const { addUndo, defineModelScope } = useUndoRedo()
const { navigateToView, loadViews, removeFromRecentViews } = useViewsStore()
/** Selected view(s) for menu */
const selected = ref<string[]>([])
/** dragging renamable view items */
const dragging = ref(false)
const menuRef = ref<typeof AntMenu>()
const isMarked = ref<string | false>(false)
/** Watch currently active view, so we can mark it in the menu */
watch(activeView, (nextActiveView) => {
if (nextActiveView && nextActiveView.id) {
selected.value = [nextActiveView.id]
}
})
/** shortly mark an item after sorting */
function markItem(id: string) {
isMarked.value = id
setTimeout(() => {
isMarked.value = false
}, 300)
}
const isDefaultSource = computed(() => {
const source = base.value?.sources?.find((b) => b.id === table.value.source_id)
if (!source) return false
return isDefaultBase(source)
})
/** validate view title */
function validate(view: ViewType) {
if (!view.title || view.title.trim().length < 0) {
return t('msg.error.viewNameRequired')
}
if (views.value.some((v) => v.title === view.title && v.id !== view.id)) {
return t('msg.error.viewNameDuplicate')
}
return true
}
let sortable: Sortable
function onSortStart(evt: SortableEvent) {
evt.stopImmediatePropagation()
evt.preventDefault()
dragging.value = true
}
async function onSortEnd(evt: SortableEvent, undo = false) {
if (!undo) {
evt.stopImmediatePropagation()
evt.preventDefault()
dragging.value = false
}
if (views.value.length < 2) return
const { newIndex = 0, oldIndex = 0 } = evt
if (newIndex === oldIndex) return
if (!undo) {
addUndo({
redo: {
fn: async () => {
const ord = sortable.toArray()
const temp = ord.splice(oldIndex, 1)
ord.splice(newIndex, 0, temp[0])
sortable.sort(ord)
await onSortEnd(evt, true)
},
args: [],
},
undo: {
fn: async () => {
const ord = sortable.toArray()
const temp = ord.splice(newIndex, 1)
ord.splice(oldIndex, 0, temp[0])
sortable.sort(ord)
await onSortEnd({ ...evt, oldIndex: newIndex, newIndex: oldIndex }, true)
},
args: [],
},
scope: defineModelScope({ view: activeView.value }),
})
}
const children = evt.to.children as unknown as HTMLLIElement[]
const previousEl = children[newIndex - 1]
const nextEl = children[newIndex + 1]
const currentItem = views.value.find((v) => v.id === evt.item.id)
if (!currentItem || !currentItem.id) return
const previousItem = (previousEl ? views.value.find((v) => v.id === previousEl.id) ?? { order: 0 } : {}) as ViewType
const nextItem = (nextEl ? views.value.find((v) => v.id === nextEl.id) : {}) as ViewType
let nextOrder: number
// set new order value based on the new order of the items
if (views.value.length - 1 === newIndex) {
nextOrder = parseFloat(String(previousItem.order)) + 1
} else if (newIndex === 0) {
nextOrder = parseFloat(String(nextItem.order)) / 2
} else {
nextOrder = (parseFloat(String(previousItem.order)) + parseFloat(String(nextItem.order))) / 2
}
const _nextOrder = !isNaN(Number(nextOrder)) ? nextOrder : oldIndex
currentItem.order = _nextOrder
await api.dbView.update(currentItem.id, { order: _nextOrder })
markItem(currentItem.id)
$e('a:view:reorder')
}
const initSortable = (el: HTMLElement) => {
if (sortable) sortable.destroy()
if (isMobileMode.value) return
sortable = new Sortable(el, {
// handle: '.nc-drag-icon',
ghostClass: 'ghost',
onStart: onSortStart,
onEnd: onSortEnd,
})
}
onMounted(() => menuRef.value && initSortable(menuRef.value.$el))
/** Navigate to view by changing url param */
async function changeView(view: ViewType) {
await navigateToView({
view,
tableId: table.value.id!,
baseId: base.value.id!,
hardReload: view.type === ViewTypes.FORM && selected.value[0] === view.id,
doNotSwitchTab: true,
})
if (isMobileMode.value) {
isLeftSidebarOpen.value = false
}
}
/** Rename a view */
async function onRename(view: ViewType, originalTitle?: string, undo = false) {
try {
await api.dbView.update(view.id!, {
title: view.title,
order: view.order,
})
navigateToView({
view,
tableId: table.value.id!,
baseId: base.value.id!,
hardReload: view.type === ViewTypes.FORM && selected.value[0] === view.id,
})
refreshCommandPalette()
if (!undo) {
addUndo({
redo: {
fn: (v: ViewType, title: string) => {
const tempTitle = v.title
v.title = title
onRename(v, tempTitle, true)
},
args: [view, view.title],
},
undo: {
fn: (v: ViewType, title: string) => {
const tempTitle = v.title
v.title = title
onRename(v, tempTitle, true)
},
args: [view, originalTitle],
},
scope: defineModelScope({ view: activeView.value }),
})
}
// update view name in recent views
allRecentViews.value = allRecentViews.value.map((rv) => {
if (rv.viewId === view.id && rv.tableID === view.fk_model_id) {
rv.viewName = view.title
}
return rv
})
// View renamed successfully
// message.success(t('msg.success.viewRenamed'))
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
}
/** Open delete modal */
function openDeleteDialog(view: ViewType) {
const isOpen = ref(true)
const { close } = useDialog(resolveComponent('DlgViewDelete'), {
'modelValue': isOpen,
'view': view,
'onUpdate:modelValue': closeDialog,
'onDeleted': async () => {
closeDialog()
emits('deleted')
removeFromRecentViews({ viewId: view.id, tableId: view.fk_model_id, baseId: base.value.id })
refreshCommandPalette()
if (activeView.value?.id === view.id) {
navigateToTable({
tableId: table.value.id!,
baseId: base.value.id!,
})
}
await loadViews({
tableId: table.value.id!,
force: true,
})
const activeNonDefaultViews = viewsByTable.value.get(table.value.id!)?.filter((v) => !v.is_default) ?? []
table.value.meta = {
...(table.value.meta as object),
hasNonDefaultViews: activeNonDefaultViews.length > 1,
}
},
})
function closeDialog() {
isOpen.value = false
close(1000)
}
}
const setIcon = async (icon: string, view: ViewType) => {
try {
// modify the icon property in meta
view.meta = {
...parseProp(view.meta),
icon,
}
api.dbView.update(view.id as string, {
meta: view.meta,
})
$e('a:view:icon:sidebar', { icon })
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
}
function onOpenModal({
title = '',
type,
copyViewId,
groupingFieldColumnId,
}: {
title?: string
type: ViewTypes
copyViewId?: string
groupingFieldColumnId?: string
}) {
const isOpen = ref(true)
const { close } = useDialog(resolveComponent('DlgViewCreate'), {
'modelValue': isOpen,
title,
type,
'tableId': table.value.id,
'selectedViewId': copyViewId,
groupingFieldColumnId,
'views': views,
'onUpdate:modelValue': closeDialog,
'onCreated': async (view: ViewType) => {
closeDialog()
refreshCommandPalette()
await loadViews({
force: true,
tableId: table.value.id!,
})
navigateToView({
view,
tableId: table.value.id!,
baseId: base.value.id!,
hardReload: view.type === ViewTypes.FORM && selected.value[0] === view.id,
})
$e('a:view:create', { view: view.type })
},
})
function closeDialog() {
isOpen.value = false
close(1000)
}
}
</script>
<template>
<a-menu
ref="menuRef"
:class="{ dragging }"
class="nc-views-menu flex flex-col w-full !border-r-0 !bg-inherit"
:selected-keys="selected"
>
<DashboardTreeViewCreateViewBtn
v-if="isUIAllowed('viewCreateOrEdit')"
:class="{
'!pl-18 !xs:(pl-19.75)': isDefaultSource,
'!pl-23.5 !xs:(pl-27)': !isDefaultSource,
}"
:align-left-level="isDefaultSource ? 1 : 2"
>
<div
role="button"
class="nc-create-view-btn flex flex-row items-center cursor-pointer rounded-md w-full"
:class="{
'text-brand-500 hover:text-brand-600': activeTableId === table.id,
'text-gray-500 hover:text-brand-500': activeTableId !== table.id,
}"
>
<div class="flex flex-row items-center pl-1.25 !py-1.5 text-inherit">
<GeneralIcon icon="plus" />
<div class="pl-1.75">
{{
$t('general.createEntity', {
entity: $t('objects.view'),
})
}}
</div>
</div>
</div>
</DashboardTreeViewCreateViewBtn>
<template v-if="views.length">
<DashboardTreeViewViewsNode
v-for="view of views"
:id="view.id"
:key="view.id"
:view="view"
:on-validate="validate"
:table="table"
class="nc-view-item !rounded-md !px-0.75 !py-0.5 w-full transition-all ease-in duration-100"
:class="{
'bg-gray-200': isMarked === view.id,
'active': activeView?.id === view.id,
[`nc-${view.type ? viewTypeAlias[view.type] : undefined || view.type}-view-item`]: true,
}"
:data-view-id="view.id"
@change-view="changeView"
@open-modal="onOpenModal"
@delete="openDeleteDialog"
@rename="onRename"
@select-icon="setIcon($event, view)"
/>
</template>
</a-menu>
</template>
<style lang="scss">
.nc-views-menu {
.ghost,
.ghost > * {
@apply !pointer-events-none;
}
&.dragging {
.nc-icon {
@apply !hidden;
}
.nc-view-icon {
@apply !block;
}
}
.ant-menu-item:not(.sortable-chosen) {
@apply color-transition;
}
.ant-menu-title-content {
@apply !w-full;
}
.sortable-chosen {
@apply !bg-gray-200;
}
.active {
@apply !bg-primary-selected font-medium;
}
}
</style>
| packages/nc-gui/components/dashboard/TreeView/ViewsList.vue | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0030233587604016066,
0.0002637572179082781,
0.00016482040518894792,
0.00017139496048912406,
0.0004338152357377112
]
|
{
"id": 3,
"code_window": [
" return _fields?.value ?? []\n",
"})\n",
"\n",
"const hiddenFields = computed(() => {\n",
" return (meta.value.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n",
"})\n",
"\n",
"const showHiddenFields = ref(false)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // todo: figure out when meta.value is undefined\n",
" return (meta.value?.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/expanded-form/index.vue",
"type": "replace",
"edit_start_line_idx": 118
} | ---
title: 'Upgrading'
description: 'Upgrading NocoDB : Docker, Node and Homebrew!'
---
By default, if `NC_DB` is not specified upon [installation](/0.109.7/getting-started/installation), then SQLite will be used to store metadata. We suggest users to separate the metadata and user data in different databases as pictured in our [architecture](/engineering/architecture).
## Docker
### Find, Stop & Delete NocoDB Docker Container
```bash
# find NocoDB container ID
docker ps
# stop NocoDB container
docker stop <YOUR_CONTAINER_ID>
# delete NocoDB container
docker rm <YOUR_CONTAINER_ID>
```
Note: Deleting your docker container without setting `NC_DB` or mounting to a persistent volume for a default SQLite database will result in losing your data. See examples below.
### Find & Remove NocoDB Docker Image
```bash
# find NocoDB image
docker images
# delete NocoDB image
docker rmi <YOUR_IMAGE_ID>
```
### Pull the latest NocoDB image with same environment variables
```bash
docker run -d -p 8080:8080 \
-e NC_DB="<YOUR_NC_DB_URL>" \
-e NC_AUTH_JWT_SECRET="<YOUR_NC_AUTH_JWT_SECRET_IF_GIVEN>" \
nocodb/nocodb:latest
```
Updating nocodb docker container is similar to updating [any other docker containers](https://www.whitesourcesoftware.com/free-developer-tools/blog/update-docker-images/).
### Example: Docker Upgrade
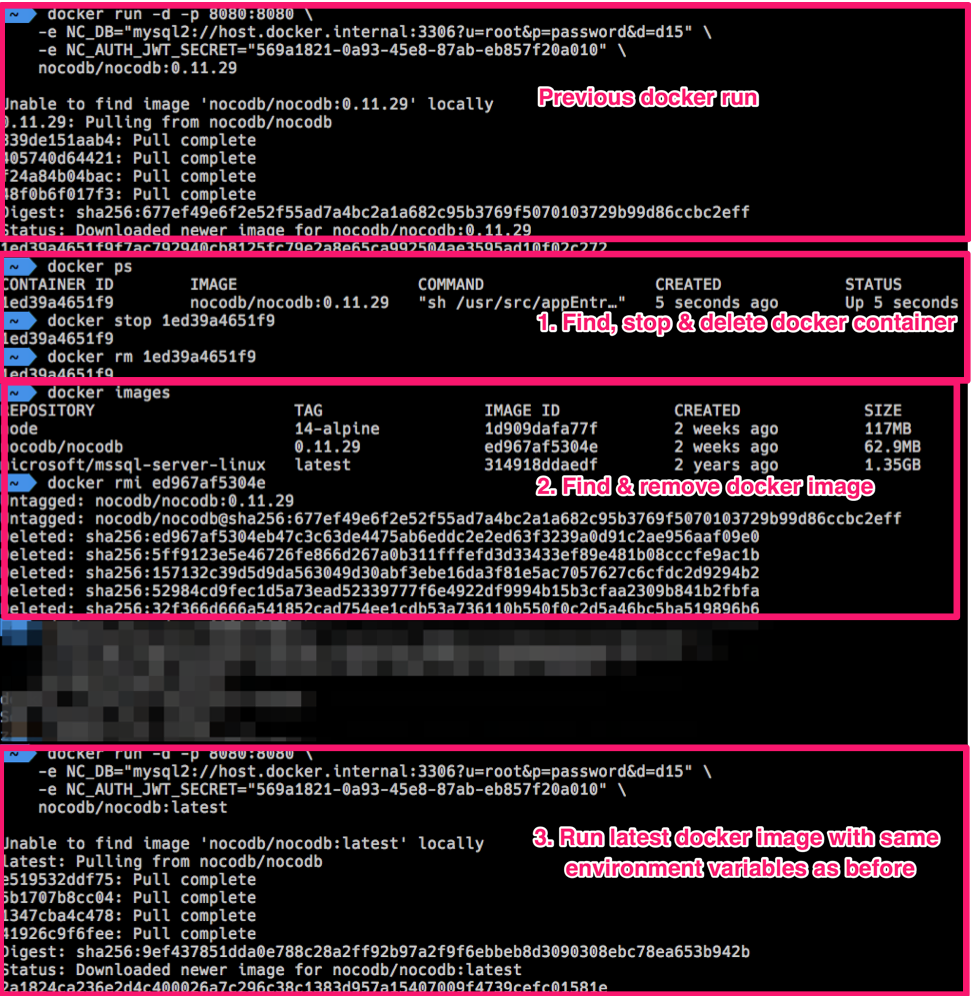
## Node
Updating docker container is similar to updating a npm package.
From your root folder
#### Uninstall NocoDB package
```bash
npm uninstall nocodb
```
#### Install NocoDB package
```bash
npm install --save nocodb
```
## Homebrew
Run following commands to upgrade Homebrew Nocodb version.
```bash
# Update the local homebrew formulas
brew update
# Upgrade nocodb package
brew upgrade nocodb
```
| packages/noco-docs/versioned_docs/version-0.109.7/020.getting-started/030.upgrading.md | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017345414380542934,
0.00016909572877921164,
0.00016459048492833972,
0.00016938027692958713,
0.000002487169012965751
]
|
{
"id": 3,
"code_window": [
" return _fields?.value ?? []\n",
"})\n",
"\n",
"const hiddenFields = computed(() => {\n",
" return (meta.value.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n",
"})\n",
"\n",
"const showHiddenFields = ref(false)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // todo: figure out when meta.value is undefined\n",
" return (meta.value?.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/expanded-form/index.vue",
"type": "replace",
"edit_start_line_idx": 118
} | <script lang="ts" setup>
import { Pane, Splitpanes } from 'splitpanes'
import 'splitpanes/dist/splitpanes.css'
const router = useRouter()
const route = router.currentRoute
const { isMobileMode } = storeToRefs(useConfigStore())
const {
isLeftSidebarOpen,
leftSidebarWidthPercent,
leftSideBarSize: sideBarSize,
leftSidebarState: sidebarState,
mobileNormalizedSidebarSize,
} = storeToRefs(useSidebarStore())
const wrapperRef = ref<HTMLDivElement>()
const animationDuration = 250
const viewportWidth = ref(window.innerWidth)
const currentSidebarSize = computed({
get: () => sideBarSize.value.current,
set: (val) => {
sideBarSize.value.current = val
sideBarSize.value.old = val
},
})
const { handleSidebarOpenOnMobileForNonViews } = useConfigStore()
const contentSize = computed(() => 100 - sideBarSize.value.current)
const mobileNormalizedContentSize = computed(() => {
if (isMobileMode.value) {
return isLeftSidebarOpen.value ? 0 : 100
}
return contentSize.value
})
const sidebarWidth = computed(() =>
isMobileMode.value ? viewportWidth.value : (sideBarSize.value.old * viewportWidth.value) / 100,
)
watch(currentSidebarSize, () => {
leftSidebarWidthPercent.value = currentSidebarSize.value
})
watch(isLeftSidebarOpen, () => {
sideBarSize.value.current = sideBarSize.value.old
if (isLeftSidebarOpen.value) {
setTimeout(() => (sidebarState.value = 'openStart'), 0)
setTimeout(() => (sidebarState.value = 'openEnd'), animationDuration)
} else {
sideBarSize.value.old = sideBarSize.value.current
sideBarSize.value.current = 0
sidebarState.value = 'hiddenStart'
setTimeout(() => {
sidebarState.value = 'hiddenEnd'
}, animationDuration)
}
})
function handleMouseMove(e: MouseEvent) {
if (isMobileMode.value) return
if (!wrapperRef.value) return
if (sidebarState.value === 'openEnd') return
if (e.clientX < 4 && ['hiddenEnd', 'peekCloseEnd'].includes(sidebarState.value)) {
sidebarState.value = 'peekOpenStart'
setTimeout(() => {
sidebarState.value = 'peekOpenEnd'
}, animationDuration)
} else if (e.clientX > sidebarWidth.value + 10 && sidebarState.value === 'peekOpenEnd') {
sidebarState.value = 'peekCloseOpen'
setTimeout(() => {
sidebarState.value = 'peekCloseEnd'
}, animationDuration)
}
}
function onWindowResize() {
viewportWidth.value = window.innerWidth
onResize(currentSidebarSize.value)
}
onMounted(() => {
document.addEventListener('mousemove', handleMouseMove)
window.addEventListener('resize', onWindowResize)
})
onBeforeUnmount(() => {
document.removeEventListener('mousemove', handleMouseMove)
window.removeEventListener('resize', onWindowResize)
})
watch(route, () => {
if (route.value.name === 'index-index') {
isLeftSidebarOpen.value = true
}
})
watch(isMobileMode, () => {
isLeftSidebarOpen.value = !isMobileMode.value
})
watch(sidebarState, () => {
if (sidebarState.value === 'peekCloseEnd') {
setTimeout(() => {
sidebarState.value = 'hiddenEnd'
}, animationDuration)
}
})
onMounted(() => {
handleSidebarOpenOnMobileForNonViews()
})
const remToPx = (rem: number) => {
const fontSize = parseFloat(getComputedStyle(document.documentElement).fontSize)
return rem * fontSize
}
function onResize(widthPercent: any) {
if (isMobileMode.value) return
const width = (widthPercent * viewportWidth.value) / 100
const fontSize = parseFloat(getComputedStyle(document.documentElement).fontSize)
const widthRem = width / fontSize
if (widthRem < 16) {
sideBarSize.value.old = ((16 * fontSize) / viewportWidth.value) * 100
if (isLeftSidebarOpen.value) sideBarSize.value.current = sideBarSize.value.old
return
} else if (widthRem > 23.5) {
sideBarSize.value.old = ((23.5 * fontSize) / viewportWidth.value) * 100
if (isLeftSidebarOpen.value) sideBarSize.value.current = sideBarSize.value.old
return
}
sideBarSize.value.old = widthPercent
sideBarSize.value.current = sideBarSize.value.old
}
const normalizedWidth = computed(() => {
const maxSize = remToPx(23.5)
const minSize = remToPx(16)
if (sidebarWidth.value > maxSize) {
return maxSize
} else if (sidebarWidth.value < minSize) {
return minSize
} else {
return sidebarWidth.value
}
})
</script>
<template>
<Splitpanes
class="nc-sidebar-content-resizable-wrapper !w-screen h-full"
:class="{
'hide-resize-bar': !isLeftSidebarOpen || sidebarState === 'openStart',
}"
@resize="(event: any) => onResize(event[0].size)"
>
<Pane
min-size="15%"
:size="mobileNormalizedSidebarSize"
max-size="40%"
class="nc-sidebar-splitpane !sm:max-w-94 relative !overflow-visible flex"
:style="{
width: `${mobileNormalizedSidebarSize}%`,
}"
>
<div
ref="wrapperRef"
class="nc-sidebar-wrapper relative flex flex-col h-full justify-center !sm:(max-w-94) absolute overflow-visible"
:class="{
'mobile': isMobileMode,
'minimized-height': !isLeftSidebarOpen,
'hide-sidebar': ['hiddenStart', 'hiddenEnd', 'peekCloseEnd'].includes(sidebarState),
}"
:style="{
width: sidebarState === 'hiddenEnd' ? '0px' : `${sidebarWidth}px`,
minWidth: sidebarState === 'hiddenEnd' ? '0px' : `${normalizedWidth}px`,
}"
>
<slot name="sidebar" />
</div>
</Pane>
<Pane
:size="mobileNormalizedContentSize"
class="flex-grow"
:style="{
'min-width': `${100 - mobileNormalizedSidebarSize}%`,
}"
>
<slot name="content" />
</Pane>
</Splitpanes>
</template>
<style lang="scss">
.nc-sidebar-wrapper.minimized-height > * {
@apply h-4/5 pb-2 !(rounded-r-lg border-1 border-gray-200 shadow-lg);
width: calc(100% + 4px);
}
.mobile.nc-sidebar-wrapper.minimized-height > * {
@apply !h-full;
}
.nc-sidebar-wrapper > * {
transition: all 0.2s ease-in-out;
@apply z-10 absolute;
}
.nc-sidebar-wrapper.hide-sidebar {
@apply !min-w-0;
> * {
@apply opacity-0;
z-index: -1 !important;
transform: translateX(-100%);
}
}
/** Split pane CSS */
.nc-sidebar-content-resizable-wrapper > {
.splitpanes__splitter {
@apply !w-0 relative overflow-visible;
}
.splitpanes__splitter:before {
@apply bg-gray-200 w-0.25 absolute left-0 top-0 h-full z-40;
content: '';
}
.splitpanes__splitter:hover:before {
@apply bg-scrollbar;
width: 3px !important;
left: 0px;
}
.splitpanes--dragging .splitpanes__splitter:before {
@apply bg-scrollbar;
width: 3px !important;
left: 0px;
}
.splitpanes--dragging .splitpanes__splitter {
@apply w-1 mr-0;
}
}
.nc-sidebar-content-resizable-wrapper.hide-resize-bar > {
.splitpanes__splitter {
cursor: default !important;
opacity: 0 !important;
background-color: transparent !important;
}
}
.splitpanes__pane {
transition: width 0.15s ease-in-out !important;
}
.splitpanes--dragging {
cursor: col-resize;
> .splitpanes__pane {
transition: none !important;
}
}
</style>
| packages/nc-gui/components/dashboard/View.vue | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0004106055712327361,
0.0001955548214027658,
0.0001653811486903578,
0.00017204695905093104,
0.00005714630242437124
]
|
{
"id": 3,
"code_window": [
" return _fields?.value ?? []\n",
"})\n",
"\n",
"const hiddenFields = computed(() => {\n",
" return (meta.value.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n",
"})\n",
"\n",
"const showHiddenFields = ref(false)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" // todo: figure out when meta.value is undefined\n",
" return (meta.value?.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/expanded-form/index.vue",
"type": "replace",
"edit_start_line_idx": 118
} | /node_modules/
#build
src/**.js
.idea/*
coverage
.nyc_output
*.log
yarn.lock
/lib/dist/
| packages/nc-lib-gui/.gitignore | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0001761661987984553,
0.00017503206618130207,
0.00017389794811606407,
0.00017503206618130207,
0.0000011341253411956131
]
|
{
"id": 4,
"code_window": [
" const reorderColumn = async (colId: string, toColId: string) => {\n",
" const toBeReorderedViewCol = gridViewCols.value[colId]\n",
"\n",
" const toViewCol = gridViewCols.value[toColId]!\n",
" const toColIndex = fields.value.findIndex((f) => f.id === toColId)\n",
"\n",
" const nextToColField = toColIndex < fields.value.length - 1 ? fields.value[toColIndex + 1] : null\n",
" const nextToViewCol = nextToColField ? gridViewCols.value[nextToColField.id!] : null\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const toViewCol = gridViewCols.value[toColId]\n",
"\n",
" // if toBeReorderedViewCol/toViewCol is null, return\n",
" if (!toBeReorderedViewCol || !toViewCol) return\n",
"\n"
],
"file_path": "packages/nc-gui/components/smartsheet/grid/useColumnDrag.ts",
"type": "replace",
"edit_start_line_idx": 27
} | <script setup lang="ts">
import type { ColumnType, TableType, ViewType } from 'nocodb-sdk'
import {
ViewTypes,
isCreatedOrLastModifiedByCol,
isCreatedOrLastModifiedTimeCol,
isLinksOrLTAR,
isSystemColumn,
isVirtualCol,
} from 'nocodb-sdk'
import type { Ref } from 'vue'
import MdiChevronDown from '~icons/mdi/chevron-down'
import {
CellClickHookInj,
FieldsInj,
IsExpandedFormOpenInj,
IsKanbanInj,
IsPublicInj,
MetaInj,
ReloadRowDataHookInj,
computedInject,
createEventHook,
iconMap,
inject,
message,
provide,
ref,
toRef,
useActiveKeyupListener,
useProvideExpandedFormStore,
useProvideSmartsheetStore,
useRoles,
useRouter,
useVModel,
watch,
} from '#imports'
interface Props {
modelValue?: boolean
state?: Record<string, any> | null
meta: TableType
loadRow?: boolean
useMetaFields?: boolean
row?: Row
rowId?: string
view?: ViewType
showNextPrevIcons?: boolean
firstRow?: boolean
lastRow?: boolean
closeAfterSave?: boolean
newRecordHeader?: string
}
const props = defineProps<Props>()
const emits = defineEmits(['update:modelValue', 'cancel', 'next', 'prev', 'createdRecord'])
const { activeView } = storeToRefs(useViewsStore())
const key = ref(0)
const wrapper = ref()
const { dashboardUrl } = useDashboard()
const { copy } = useClipboard()
const { isMobileMode } = useGlobal()
const { t } = useI18n()
const rowId = toRef(props, 'rowId')
const row = toRef(props, 'row')
const state = toRef(props, 'state')
const meta = toRef(props, 'meta')
const islastRow = toRef(props, 'lastRow')
const isFirstRow = toRef(props, 'firstRow')
const route = useRoute()
const router = useRouter()
const isPublic = inject(IsPublicInj, ref(false))
// to check if a expanded form which is not yet saved exist or not
const isUnsavedFormExist = ref(false)
const isRecordLinkCopied = ref(false)
const { isUIAllowed } = useRoles()
const readOnly = computed(() => !isUIAllowed('dataEdit') || isPublic.value)
const expandedFormScrollWrapper = ref()
const reloadTrigger = inject(ReloadRowDataHookInj, createEventHook())
const { addOrEditStackRow } = useKanbanViewStoreOrThrow()
const { isExpandedFormCommentMode } = storeToRefs(useConfigStore())
// override cell click hook to avoid unexpected behavior at form fields
provide(CellClickHookInj, undefined)
const fields = computedInject(FieldsInj, (_fields) => {
if (props.useMetaFields) {
return (meta.value.columns ?? []).filter((col) => !isSystemColumn(col))
}
return _fields?.value ?? []
})
const hiddenFields = computed(() => {
return (meta.value.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))
})
const showHiddenFields = ref(false)
const toggleHiddenFields = () => {
showHiddenFields.value = !showHiddenFields.value
}
const isKanban = inject(IsKanbanInj, ref(false))
provide(MetaInj, meta)
const isLoading = ref(true)
const {
commentsDrawer,
changedColumns,
deleteRowById,
displayValue,
state: rowState,
isNew,
loadRow: _loadRow,
primaryKey,
saveRowAndStay,
row: _row,
save: _save,
loadCommentsAndLogs,
clearColumns,
} = useProvideExpandedFormStore(meta, row)
const duplicatingRowInProgress = ref(false)
useProvideSmartsheetStore(ref({}) as Ref<ViewType>, meta)
watch(
state,
() => {
if (state.value) {
rowState.value = state.value
} else {
rowState.value = {}
}
},
{ immediate: true },
)
const isExpanded = useVModel(props, 'modelValue', emits, {
defaultValue: false,
})
const onClose = () => {
if (!isUIAllowed('dataEdit')) {
isExpanded.value = false
} else if (changedColumns.value.size > 0) {
isCloseModalOpen.value = true
} else {
if (_row.value?.rowMeta?.new) emits('cancel')
isExpanded.value = false
}
}
const onDuplicateRow = () => {
duplicatingRowInProgress.value = true
isUnsavedFormExist.value = true
const oldRow = { ..._row.value.row }
delete oldRow.ncRecordId
const newRow = Object.assign(
{},
{
row: oldRow,
oldRow: {},
rowMeta: { new: true },
},
)
setTimeout(async () => {
_row.value = newRow
duplicatingRowInProgress.value = false
message.success(t('msg.success.rowDuplicatedWithoutSavedYet'))
}, 500)
}
const save = async () => {
let kanbanClbk
if (activeView.value?.type === ViewTypes.KANBAN) {
kanbanClbk = (row: any, isNewRow: boolean) => {
addOrEditStackRow(row, isNewRow)
}
}
if (isNew.value) {
await _save(rowState.value, undefined, {
kanbanClbk,
})
reloadTrigger?.trigger()
} else {
await _save(undefined, undefined, {
kanbanClbk,
})
_loadRow()
reloadTrigger?.trigger()
}
isUnsavedFormExist.value = false
if (props.closeAfterSave) {
isExpanded.value = false
}
emits('createdRecord', _row.value.row)
}
const isPreventChangeModalOpen = ref(false)
const isCloseModalOpen = ref(false)
const discardPreventModal = () => {
// when user click on next or previous button
if (isPreventChangeModalOpen.value) {
emits('next')
if (_row.value?.rowMeta?.new) emits('cancel')
isPreventChangeModalOpen.value = false
}
// when user click on close button
if (isCloseModalOpen.value) {
isCloseModalOpen.value = false
if (_row.value?.rowMeta?.new) emits('cancel')
isExpanded.value = false
}
// clearing all new modifed change on close
clearColumns()
}
const onNext = async () => {
if (changedColumns.value.size > 0) {
isPreventChangeModalOpen.value = true
return
}
emits('next')
}
const copyRecordUrl = async () => {
await copy(
encodeURI(
`${dashboardUrl?.value}#/${route.params.typeOrId}/${route.params.baseId}/${meta.value?.id}${
props.view ? `/${props.view.title}` : ''
}?rowId=${primaryKey.value}`,
),
)
isRecordLinkCopied.value = true
}
const saveChanges = async () => {
if (isPreventChangeModalOpen.value) {
isUnsavedFormExist.value = false
await save()
emits('next')
isPreventChangeModalOpen.value = false
}
if (isCloseModalOpen.value) {
isCloseModalOpen.value = false
await save()
isExpanded.value = false
}
}
const reloadParentRowHook = inject(ReloadRowDataHookInj, createEventHook())
// override reload trigger and use it to reload grid and the form itself
const reloadHook = createEventHook()
reloadHook.on(() => {
reloadParentRowHook?.trigger(false)
if (isNew.value) return
_loadRow(null, true)
})
provide(ReloadRowDataHookInj, reloadHook)
if (isKanban.value) {
// adding column titles to changedColumns if they are preset
for (const [k, v] of Object.entries(_row.value.row)) {
if (v) {
changedColumns.value.add(k)
}
}
}
provide(IsExpandedFormOpenInj, isExpanded)
const cellWrapperEl = ref()
onMounted(async () => {
isRecordLinkCopied.value = false
isLoading.value = true
const focusFirstCell = !isExpandedFormCommentMode.value
if (props.loadRow) {
await _loadRow()
await loadCommentsAndLogs()
}
if (props.rowId) {
try {
await _loadRow(props.rowId)
await loadCommentsAndLogs()
} catch (e: any) {
if (e.response?.status === 404) {
message.error(t('msg.noRecordFound'))
router.replace({ query: {} })
} else throw e
}
}
isLoading.value = false
if (focusFirstCell) {
setTimeout(() => {
cellWrapperEl.value?.$el?.querySelector('input,select,textarea')?.focus()
}, 300)
}
})
const addNewRow = () => {
setTimeout(async () => {
_row.value = {
row: {},
oldRow: {},
rowMeta: { new: true },
}
rowState.value = {}
key.value++
isExpanded.value = true
}, 500)
}
// attach keyboard listeners to switch between rows
// using alt + left/right arrow keys
useActiveKeyupListener(
isExpanded,
async (e: KeyboardEvent) => {
if (!e.altKey) return
if (e.key === 'ArrowLeft') {
e.stopPropagation()
emits('prev')
} else if (e.key === 'ArrowRight') {
e.stopPropagation()
onNext()
}
// on alt + s save record
else if (e.code === 'KeyS') {
// remove focus from the active input if any
;(document.activeElement as HTMLElement)?.blur()
e.stopPropagation()
if (isNew.value) {
await _save(rowState.value)
reloadHook?.trigger(null)
} else {
await save()
reloadHook?.trigger(null)
}
if (!saveRowAndStay.value) {
onClose()
}
// on alt + n create new record
} else if (e.code === 'KeyN') {
// remove focus from the active input if any to avoid unwanted input
;(document.activeElement as HTMLInputElement)?.blur?.()
if (changedColumns.value.size > 0) {
await Modal.confirm({
title: t('msg.saveChanges'),
okText: t('general.save'),
cancelText: t('labels.discard'),
onOk: async () => {
await save()
reloadHook?.trigger(null)
addNewRow()
},
onCancel: () => {
addNewRow()
},
})
} else if (isNew.value) {
await Modal.confirm({
title: 'Do you want to save the record?',
okText: t('general.save'),
cancelText: t('labels.discard'),
onOk: async () => {
await _save(rowState.value)
reloadHook?.trigger(null)
addNewRow()
},
onCancel: () => {
addNewRow()
},
})
} else {
addNewRow()
}
}
},
{ immediate: true },
)
const showDeleteRowModal = ref(false)
const onDeleteRowClick = () => {
showDeleteRowModal.value = true
}
const onConfirmDeleteRowClick = async () => {
showDeleteRowModal.value = false
await deleteRowById(primaryKey.value)
message.success(t('msg.rowDeleted'))
reloadTrigger.trigger()
onClose()
showDeleteRowModal.value = false
}
watch(rowId, async (nRow) => {
await _loadRow(nRow)
await loadCommentsAndLogs()
})
const showRightSections = computed(() => {
return !isNew.value && commentsDrawer.value && isUIAllowed('commentList')
})
const preventModalStatus = computed({
get: () => isCloseModalOpen.value || isPreventChangeModalOpen.value,
set: (v) => {
isCloseModalOpen.value = v
},
})
const onIsExpandedUpdate = (v: boolean) => {
let isDropdownOpen = false
document.querySelectorAll('.ant-select-dropdown').forEach((el) => {
isDropdownOpen = isDropdownOpen || el.checkVisibility()
})
if (isDropdownOpen) return
if (changedColumns.value.size === 0 && !isUnsavedFormExist.value) {
isExpanded.value = v
} else if (!v && isUIAllowed('dataEdit')) {
preventModalStatus.value = true
} else {
isExpanded.value = v
}
}
const isReadOnlyVirtualCell = (column: ColumnType) => {
return (
isRollup(column) ||
isFormula(column) ||
isBarcode(column) ||
isLookup(column) ||
isQrCode(column) ||
isSystemColumn(column) ||
isCreatedOrLastModifiedTimeCol(column) ||
isCreatedOrLastModifiedByCol(column)
)
}
// Small hack. We need to scroll to the bottom of the form after its mounted and back to top.
// So that tab to next row works properly, as otherwise browser will focus to save button
// when we reach to the bottom of the visual scrollable area, not the actual bottom of the form
watch([expandedFormScrollWrapper, isLoading], () => {
if (isMobileMode.value) return
const expandedFormScrollWrapperEl = expandedFormScrollWrapper.value
if (expandedFormScrollWrapperEl && !isLoading.value) {
const height = expandedFormScrollWrapperEl.scrollHeight
expandedFormScrollWrapperEl.scrollTop = height
setTimeout(() => {
expandedFormScrollWrapperEl.scrollTop = 0
}, 125)
}
})
</script>
<script lang="ts">
export default {
name: 'ExpandedForm',
}
</script>
<template>
<NcModal
:visible="isExpanded"
:footer="null"
:width="commentsDrawer && isUIAllowed('commentList') ? 'min(80vw,1280px)' : 'min(80vw,1280px)'"
:body-style="{ padding: 0 }"
:closable="false"
size="small"
class="nc-drawer-expanded-form"
:class="{ active: isExpanded }"
@update:visible="onIsExpandedUpdate"
>
<div class="h-[85vh] xs:(max-h-full) max-h-215 flex flex-col p-6">
<div class="flex h-9.5 flex-shrink-0 w-full items-center nc-expanded-form-header relative mb-4 justify-between">
<template v-if="!isMobileMode">
<div class="flex gap-3 w-100">
<div class="flex gap-2">
<NcButton
v-if="props.showNextPrevIcons"
:disabled="isFirstRow"
type="secondary"
class="nc-prev-arrow !w-10"
@click="$emit('prev')"
>
<MdiChevronUp class="text-md" />
</NcButton>
<NcButton
v-if="props.showNextPrevIcons"
:disabled="islastRow"
type="secondary"
class="nc-next-arrow !w-10"
@click="onNext"
>
<MdiChevronDown class="text-md" />
</NcButton>
</div>
<div v-if="isLoading">
<a-skeleton-input class="!h-8 !sm:mr-14 !w-52 mt-1 !rounded-md !overflow-hidden" active size="small" />
</div>
<div
v-if="row.rowMeta?.new || props.newRecordHeader"
class="flex items-center truncate font-bold text-gray-800 text-xl"
>
{{ props.newRecordHeader ?? $t('activity.newRecord') }}
</div>
<div v-else-if="displayValue && !row.rowMeta?.new" class="flex items-center font-bold text-gray-800 text-xl w-64">
<span class="truncate">
{{ displayValue }}
</span>
</div>
</div>
<div class="flex gap-2">
<NcButton
v-if="!isNew && rowId"
type="secondary"
class="!xs:hidden text-gray-700"
:disabled="isLoading"
@click="copyRecordUrl()"
>
<div v-e="['c:row-expand:copy-url']" data-testid="nc-expanded-form-copy-url" class="flex gap-2 items-center">
<component :is="iconMap.check" v-if="isRecordLinkCopied" class="cursor-pointer nc-duplicate-row" />
<component :is="iconMap.link" v-else class="cursor-pointer nc-duplicate-row" />
{{ isRecordLinkCopied ? $t('labels.copiedRecordURL') : $t('labels.copyRecordURL') }}
</div>
</NcButton>
<NcDropdown v-if="!isNew" placement="bottomRight">
<NcButton type="secondary" class="nc-expand-form-more-actions w-10" :disabled="isLoading">
<GeneralIcon icon="threeDotVertical" class="text-md" :class="isLoading ? 'text-gray-300' : 'text-gray-700'" />
</NcButton>
<template #overlay>
<NcMenu>
<NcMenuItem class="text-gray-700" @click="_loadRow()">
<div v-e="['c:row-expand:reload']" class="flex gap-2 items-center" data-testid="nc-expanded-form-reload">
<component :is="iconMap.reload" class="cursor-pointer" />
{{ $t('general.reload') }}
</div>
</NcMenuItem>
<NcMenuItem v-if="isUIAllowed('dataEdit')" class="text-gray-700" @click="!isNew ? onDuplicateRow() : () => {}">
<div
v-e="['c:row-expand:duplicate']"
data-testid="nc-expanded-form-duplicate"
class="flex gap-2 items-center"
>
<component :is="iconMap.copy" class="cursor-pointer nc-duplicate-row" />
<span class="-ml-0.25">
{{ $t('labels.duplicateRecord') }}
</span>
</div>
</NcMenuItem>
<NcDivider v-if="isUIAllowed('dataEdit')" />
<NcMenuItem
v-if="isUIAllowed('dataEdit')"
class="!text-red-500 !hover:bg-red-50"
@click="!isNew && onDeleteRowClick()"
>
<div v-e="['c:row-expand:delete']" data-testid="nc-expanded-form-delete" class="flex gap-2 items-center">
<component :is="iconMap.delete" class="cursor-pointer nc-delete-row" />
<span class="-ml-0.25">
{{ $t('activity.deleteRecord') }}
</span>
</div>
</NcMenuItem>
</NcMenu>
</template>
</NcDropdown>
<NcButton
type="secondary"
class="nc-expand-form-close-btn w-10"
data-testid="nc-expanded-form-close"
@click="onClose"
>
<GeneralIcon icon="close" class="text-md text-gray-700" />
</NcButton>
</div>
</template>
<template v-else>
<div class="flex flex-row w-full">
<NcButton
v-if="props.showNextPrevIcons && !isFirstRow"
v-e="['c:row-expand:prev']"
type="secondary"
class="nc-prev-arrow !w-10"
@click="$emit('prev')"
>
<GeneralIcon icon="arrowLeft" class="text-lg text-gray-700" />
</NcButton>
<div v-else class="min-w-10.5"></div>
<div class="flex flex-grow justify-center items-center font-semibold text-lg">
<div>{{ meta.title }}</div>
</div>
<NcButton
v-if="props.showNextPrevIcons && !islastRow"
v-e="['c:row-expand:next']"
type="secondary"
class="nc-next-arrow !w-10"
@click="onNext"
>
<GeneralIcon icon="arrowRight" class="text-lg text-gray-700" />
</NcButton>
<div v-else class="min-w-10.5"></div>
</div>
</template>
</div>
<div ref="wrapper" class="flex flex-grow flex-row h-[calc(100%-4rem)] w-full gap-4">
<div
class="flex xs:w-full flex-col border-1 rounded-xl overflow-hidden border-gray-200 xs:(border-0 rounded-none)"
:class="{
'w-full': !showRightSections,
'w-2/3': showRightSections,
}"
>
<div
ref="expandedFormScrollWrapper"
class="flex flex-col flex-grow mt-2 h-full max-h-full nc-scrollbar-md pb-6 items-center w-full bg-white p-4 xs:p-0"
>
<div
v-for="(col, i) of fields"
v-show="isFormula(col) || !isVirtualCol(col) || !isNew || isLinksOrLTAR(col)"
:key="col.title"
class="nc-expanded-form-row mt-2 py-2 xs:w-full"
:class="`nc-expand-col-${col.title}`"
:col-id="col.id"
:data-testid="`nc-expand-col-${col.title}`"
>
<div class="flex items-start flex-row sm:(gap-x-6) xs:(flex-col w-full) nc-expanded-cell min-h-10">
<div class="w-48 xs:(w-full) mt-0.25 !h-[35px]">
<LazySmartsheetHeaderVirtualCell
v-if="isVirtualCol(col)"
class="nc-expanded-cell-header h-full"
:column="col"
/>
<LazySmartsheetHeaderCell v-else class="nc-expanded-cell-header" :column="col" />
</div>
<template v-if="isLoading">
<div
v-if="isMobileMode"
class="!h-8.5 !xs:h-12 !xs:bg-white sm:mr-21 w-60 mt-0.75 !rounded-lg !overflow-hidden"
></div>
<a-skeleton-input
v-else
class="!h-8.5 !xs:h-9.5 !xs:bg-white sm:mr-21 !w-60 mt-0.75 !rounded-lg !overflow-hidden"
active
size="small"
/>
</template>
<template v-else>
<SmartsheetDivDataCell
v-if="col.title"
:ref="i ? null : (el: any) => (cellWrapperEl = el)"
class="bg-white w-80 xs:w-full px-1 sm:min-h-[35px] xs:min-h-13 flex items-center relative"
:class="{
'!bg-gray-50 !px-0 !select-text nc-system-field': isReadOnlyVirtualCell(col),
}"
>
<LazySmartsheetVirtualCell
v-if="isVirtualCol(col)"
v-model="_row.row[col.title]"
:row="_row"
:column="col"
:class="{
'px-1': isReadOnlyVirtualCell(col),
}"
:read-only="readOnly"
/>
<LazySmartsheetCell
v-else
v-model="_row.row[col.title]"
:column="col"
:edit-enabled="true"
:active="true"
:read-only="readOnly"
@update:model-value="changedColumns.add(col.title)"
/>
</SmartsheetDivDataCell>
</template>
</div>
</div>
<div v-if="hiddenFields.length > 0" class="flex w-full sm:px-12 xs:(px-1 mt-2) items-center py-3">
<div class="flex-grow h-px mr-1 bg-gray-100"></div>
<NcButton
type="secondary"
:size="isMobileMode ? 'medium' : 'small'"
class="flex-shrink-1 !text-sm"
@click="toggleHiddenFields"
>
{{ showHiddenFields ? `Hide ${hiddenFields.length} hidden` : `Show ${hiddenFields.length} hidden` }}
{{ hiddenFields.length > 1 ? `fields` : `field` }}
<MdiChevronDown class="ml-1" :class="showHiddenFields ? 'transform rotate-180' : ''" />
</NcButton>
<div class="flex-grow h-px ml-1 bg-gray-100"></div>
</div>
<div v-if="hiddenFields.length > 0 && showHiddenFields" class="flex flex-col w-full mb-3 items-center">
<div
v-for="(col, i) of hiddenFields"
v-show="isFormula(col) || !isVirtualCol(col) || !isNew || isLinksOrLTAR(col)"
:key="col.title"
class="sm:(mt-2) py-2 xs:w-full"
:class="`nc-expand-col-${col.title}`"
:data-testid="`nc-expand-col-${col.title}`"
>
<div class="sm:gap-x-6 flex sm:flex-row xs:(flex-col) items-start min-h-10">
<div class="sm:w-48 xs:w-full scale-110 !h-[35px]">
<LazySmartsheetHeaderVirtualCell v-if="isVirtualCol(col)" :column="col" class="nc-expanded-cell-header" />
<LazySmartsheetHeaderCell v-else class="nc-expanded-cell-header" :column="col" />
</div>
<template v-if="isLoading">
<div
v-if="isMobileMode"
class="!h-8.5 !xs:h-9.5 !xs:bg-white sm:mr-21 w-60 mt-0.75 !rounded-lg !overflow-hidden"
></div>
<a-skeleton-input
v-else
class="!h-8.5 !xs:h-12 !xs:bg-white sm:mr-21 w-60 mt-0.75 !rounded-lg !overflow-hidden"
active
size="small"
/>
</template>
<template v-else>
<LazySmartsheetDivDataCell
v-if="col.title"
:ref="i ? null : (el: any) => (cellWrapperEl = el)"
class="bg-white rounded-lg w-80 border-1 overflow-hidden border-gray-200 px-1 sm:min-h-[35px] xs:min-h-13 flex items-center relative"
>
<LazySmartsheetVirtualCell
v-if="isVirtualCol(col)"
v-model="_row.row[col.title]"
:row="_row"
:column="col"
:read-only="readOnly"
/>
<LazySmartsheetCell
v-else
v-model="_row.row[col.title]"
:column="col"
:edit-enabled="true"
:active="true"
:read-only="readOnly"
@update:model-value="changedColumns.add(col.title)"
/>
</LazySmartsheetDivDataCell>
</template>
</div>
</div>
</div>
</div>
<div
v-if="isUIAllowed('dataEdit')"
class="w-full h-16 border-t-1 border-gray-200 bg-white flex items-center justify-end p-3 xs:(p-0 mt-4 border-t-0 gap-x-4 justify-between)"
>
<NcDropdown v-if="!isNew && isMobileMode" placement="bottomRight">
<NcButton type="secondary" class="nc-expand-form-more-actions w-10" :disabled="isLoading">
<GeneralIcon icon="threeDotVertical" class="text-md" :class="isLoading ? 'text-gray-300' : 'text-gray-700'" />
</NcButton>
<template #overlay>
<NcMenu>
<NcMenuItem class="text-gray-700" @click="_loadRow()">
<div v-e="['c:row-expand:reload']" class="flex gap-2 items-center" data-testid="nc-expanded-form-reload">
<component :is="iconMap.reload" class="cursor-pointer" />
{{ $t('general.reload') }}
</div>
</NcMenuItem>
<NcMenuItem v-if="rowId" class="text-gray-700" @click="!isNew ? copyRecordUrl() : () => {}">
<div v-e="['c:row-expand:copy-url']" data-testid="nc-expanded-form-copy-url" class="flex gap-2 items-center">
<component :is="iconMap.link" class="cursor-pointer nc-duplicate-row" />
{{ $t('labels.copyRecordURL') }}
</div>
</NcMenuItem>
<NcDivider />
<NcMenuItem
v-if="isUIAllowed('dataEdit') && !isNew"
v-e="['c:row-expand:delete']"
class="!text-red-500 !hover:bg-red-50"
@click="!isNew && onDeleteRowClick()"
>
<div data-testid="nc-expanded-form-delete">
<component :is="iconMap.delete" class="cursor-pointer nc-delete-row" />
Delete record
</div>
</NcMenuItem>
</NcMenu>
</template>
</NcDropdown>
<div class="flex flex-row gap-x-3">
<NcButton
v-if="isMobileMode"
type="secondary"
size="medium"
data-testid="nc-expanded-form-save"
class="nc-expand-form-save-btn !xs:(text-base)"
@click="onClose"
>
<div class="px-1">Close</div>
</NcButton>
<NcButton
v-e="['c:row-expand:save']"
data-testid="nc-expanded-form-save"
type="primary"
size="medium"
class="nc-expand-form-save-btn !xs:(text-base)"
:disabled="changedColumns.size === 0 && !isUnsavedFormExist"
@click="save"
>
<div class="xs:px-1">Save</div>
</NcButton>
</div>
</div>
</div>
<div
v-if="showRightSections"
class="nc-comments-drawer border-1 relative border-gray-200 w-1/3 max-w-125 bg-gray-50 rounded-xl min-w-0 overflow-hidden h-full xs:hidden"
:class="{ active: commentsDrawer && isUIAllowed('commentList') }"
>
<SmartsheetExpandedFormComments :loading="isLoading" />
</div>
</div>
</div>
</NcModal>
<GeneralDeleteModal v-model:visible="showDeleteRowModal" entity-name="Record" :on-delete="() => onConfirmDeleteRowClick()">
<template #entity-preview>
<span>
<div class="flex flex-row items-center py-2.25 px-2.5 bg-gray-50 rounded-lg text-gray-700 mb-4">
<div class="capitalize text-ellipsis overflow-hidden select-none w-full pl-1.75 break-keep whitespace-nowrap">
{{ displayValue }}
</div>
</div>
</span>
</template>
</GeneralDeleteModal>
<!-- Prevent unsaved change modal -->
<NcModal v-model:visible="preventModalStatus" size="small">
<div class="">
<div class="flex flex-row items-center gap-x-2 text-base font-bold">
{{ $t('tooltip.saveChanges') }}
</div>
<div class="flex font-medium mt-2">
{{ $t('activity.doYouWantToSaveTheChanges') }}
</div>
<div class="flex flex-row justify-end gap-x-2 mt-5">
<NcButton type="secondary" @click="discardPreventModal">{{ $t('labels.discard') }}</NcButton>
<NcButton key="submit" type="primary" label="Rename Table" loading-label="Renaming Table" @click="saveChanges">
{{ $t('tooltip.saveChanges') }}
</NcButton>
</div>
</div>
</NcModal>
</template>
<style lang="scss">
.nc-drawer-expanded-form {
@apply xs:my-0;
}
.nc-expanded-cell {
input {
@apply xs:(h-12 text-base);
}
}
.nc-expanded-cell-header {
@apply w-full text-gray-500 xs:(text-gray-600 mb-2);
}
.nc-expanded-cell-header > :nth-child(2) {
@apply !text-sm !xs:text-base;
}
.nc-expanded-cell-header > :first-child {
@apply !text-xl;
}
.nc-drawer-expanded-form .nc-modal {
@apply !p-0;
}
</style>
<style lang="scss" scoped>
:deep(.ant-select-selector) {
@apply !xs:(h-full);
}
:deep(.ant-select-selection-item) {
@apply !xs:(mt-1.75 ml-1);
}
.nc-data-cell:focus-within {
@apply !border-1 !border-brand-500 !rounded-lg !shadow-none !ring-0;
}
:deep(.nc-system-field input) {
@apply bg-transparent;
}
</style>
| packages/nc-gui/components/smartsheet/expanded-form/index.vue | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0015549532836303115,
0.00018492656818125397,
0.0001639423571759835,
0.00017047958681359887,
0.0001413379650330171
]
|
{
"id": 4,
"code_window": [
" const reorderColumn = async (colId: string, toColId: string) => {\n",
" const toBeReorderedViewCol = gridViewCols.value[colId]\n",
"\n",
" const toViewCol = gridViewCols.value[toColId]!\n",
" const toColIndex = fields.value.findIndex((f) => f.id === toColId)\n",
"\n",
" const nextToColField = toColIndex < fields.value.length - 1 ? fields.value[toColIndex + 1] : null\n",
" const nextToViewCol = nextToColField ? gridViewCols.value[nextToColField.id!] : null\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const toViewCol = gridViewCols.value[toColId]\n",
"\n",
" // if toBeReorderedViewCol/toViewCol is null, return\n",
" if (!toBeReorderedViewCol || !toViewCol) return\n",
"\n"
],
"file_path": "packages/nc-gui/components/smartsheet/grid/useColumnDrag.ts",
"type": "replace",
"edit_start_line_idx": 27
} | import debug from 'debug';
import { JOBS_QUEUE } from '~/interface/Jobs';
const debugLog = debug('nc:jobs:timings');
export const initTime = function () {
return {
hrTime: process.hrtime(),
};
};
export const elapsedTime = function (
time: { hrTime: [number, number] },
label?: string,
context?: string,
) {
const elapsedS = process.hrtime(time.hrTime)[0].toFixed(3);
const elapsedMs = process.hrtime(time.hrTime)[1] / 1000000;
if (label)
debugLog(
`${label}: ${elapsedS}s ${elapsedMs}ms`,
`${JOBS_QUEUE}${context ? `:${context}` : ''}`,
);
time.hrTime = process.hrtime();
};
| packages/nocodb/src/modules/jobs/helpers.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017491652397438884,
0.00017268460942432284,
0.00016973591118585318,
0.00017340137856081128,
0.000002174858082071296
]
|
{
"id": 4,
"code_window": [
" const reorderColumn = async (colId: string, toColId: string) => {\n",
" const toBeReorderedViewCol = gridViewCols.value[colId]\n",
"\n",
" const toViewCol = gridViewCols.value[toColId]!\n",
" const toColIndex = fields.value.findIndex((f) => f.id === toColId)\n",
"\n",
" const nextToColField = toColIndex < fields.value.length - 1 ? fields.value[toColIndex + 1] : null\n",
" const nextToViewCol = nextToColField ? gridViewCols.value[nextToColField.id!] : null\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const toViewCol = gridViewCols.value[toColId]\n",
"\n",
" // if toBeReorderedViewCol/toViewCol is null, return\n",
" if (!toBeReorderedViewCol || !toViewCol) return\n",
"\n"
],
"file_path": "packages/nc-gui/components/smartsheet/grid/useColumnDrag.ts",
"type": "replace",
"edit_start_line_idx": 27
} | {
"label": "Fields",
"collapsible": true,
"collapsed": true,
"link": {
"type": "generated-index"
}
} | packages/noco-docs/docs/070.fields/_category_.json | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0001710949873086065,
0.0001710949873086065,
0.0001710949873086065,
0.0001710949873086065,
0
]
|
{
"id": 4,
"code_window": [
" const reorderColumn = async (colId: string, toColId: string) => {\n",
" const toBeReorderedViewCol = gridViewCols.value[colId]\n",
"\n",
" const toViewCol = gridViewCols.value[toColId]!\n",
" const toColIndex = fields.value.findIndex((f) => f.id === toColId)\n",
"\n",
" const nextToColField = toColIndex < fields.value.length - 1 ? fields.value[toColIndex + 1] : null\n",
" const nextToViewCol = nextToColField ? gridViewCols.value[nextToColField.id!] : null\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const toViewCol = gridViewCols.value[toColId]\n",
"\n",
" // if toBeReorderedViewCol/toViewCol is null, return\n",
" if (!toBeReorderedViewCol || !toViewCol) return\n",
"\n"
],
"file_path": "packages/nc-gui/components/smartsheet/grid/useColumnDrag.ts",
"type": "replace",
"edit_start_line_idx": 27
} | ---
title: "Formulas"
description: "NocoDB Formulas Syntaxes and Functions"
---
## Adding formula column
<img width="990" alt="image" src="https://user-images.githubusercontent.com/35857179/189108950-fba76e31-8ae4-4108-916b-e413c841f451.png" />
### 1. Click on '+' (Add column)
### 2. Populate column Name
### 3. Select column Type as 'Formula'
### 4. Insert required formula
- You can use explicit numerical values/ strings as needed, e.g. `123` (numeric) or `"123"` (string).
- You can reference column names in equation with `{}`, e.g. `{column_name}`, if the column name conflicts with literals
- Table below lists supported formula & associated syntax
- Nested formula (formula equation referring to another formula column) is supported
### 5. Click on 'Save'
## Editing formula column
Unlike other column types, formula cells cannot be modified by double-clicking since the value is generated based on the formula. Instead, the vaule can be changed by updating the formula in the column setting.
<img width="253" alt="image" src="https://user-images.githubusercontent.com/35857179/189109486-4d41f2b7-0a19-46ef-8bb4-a8d1aabd3592.png" />
## Available Formula Features
### Numeric Functions
| Name | Syntax | Sample | Output |
|-------------|----------------------------|----------------------------------|------------------------------------------------------------------|
| **ABS** | `ABS(value)` | `ABS({Column})` | Absolute value of the input parameter |
| **ADD** | `ADD(value1,[value2,...])` | `ADD({Column1}, {Column2})` | Sum of input parameters |
| **AVG** | `AVG(value1,[value2,...])` | `AVG({Column1}, {Column2})` | Average of input parameters |
| **CEILING** | `CEILING(value)` | `CEILING({Column})` | Rounded next largest integer value of input parameter |
| **EXP** | `EXP(value)` | `EXP({Column})` | Exponential value of input parameter (`e^x`) |
| **FLOOR** | `FLOOR(value)` | `FLOOR({Column})` | Rounded largest integer less than or equal to input parameter |
| **INT** | `INT(value)` | `INT({Column})` | Integer value of input parameter |
| **LOG** | `LOG([base], value)` | `LOG(10, {Column})` | Logarithm of input parameter to the base (default = e) specified |
| **MAX** | `MAX(value1,[value2,...])` | `MAX({Column1}, {Column2}, {Column3})` | Maximum value amongst input parameters |
| **MIN** | `MIN(value1,[value2,...])` | `MIN({Column1}, {Column2}, {Column3})` | Minimum value amongst input parameters |
| **MOD** | `MOD(value1, value2)` | `MOD({Column}, 2)` | Remainder after integer division of input parameters |
| **POWER** | `POWER(base, exponent)` | `POWER({Column}, 3)` | `base` to the `exponent` power, as in `base ^ exponent` |
| **ROUND** | `ROUND(value, precision)` | `ROUND({Column}, 3)` | Round input `value` to decimal place specified by `precision` (Nearest integer if `precision` not provided) |
| **SQRT** | `SQRT(value)` | `SQRT({Column})` | Square root of the input parameter |
### Numeric Operators
| Operator | Sample | Description |
| -------- | ----------------------- | -------------------------------- |
| `+` | `{Column1} + {Column2} + 2` | Addition of numeric values |
| `-` | `{Column1} - {Column2}` | Subtraction of numeric values |
| `*` | `{Column1} * {Column2}` | Multiplication of numeric values |
| `/` | `{Column1} / {Column2}` | Division of numeric values |
:::tip
To change the order of arithmetic operation, you can use round bracket parantheses (). <br/>
Example: `({Column1} + ({Column2} * {Column3}) / (3 - $Column4$ ))`
:::
### String Functions
| Name | Syntax | Sample | Output |
|-------------|----------------------------------|---------------------------------|---------------------------------------------------------------------------|
| **CONCAT** | `CONCAT(str1, [str2,...])` | `CONCAT({Column1}, ' ', {Column2})` | Concatenated string of input parameters |
| **LEFT** | `LEFT(str1, n)` | `LEFT({Column}, 3)` | `n` characters from the beginning of input parameter |
| **LEN** | `LEN(str)` | `LEN({Column})` | Input parameter character length |
| **LOWER** | `LOWER(str)` | `LOWER({Column})` | Lower case converted string of input parameter |
| **MID** | `MID(str, position, [count])` | `MID({Column}, 3, 2)` | Alias for `SUBSTR` |
| **REPEAT** | `REPEAT(str, count)` | `REPEAT({Column}, 2)` | Specified copies of the input parameter string concatenated together |
| **REPLACE** | `REPLACE(str, srchStr, rplcStr)` | `REPLACE({Column}, 'int', 'num')` | String, after replacing all occurrences of `srchStr` with `rplcStr` |
| **RIGHT** | `RIGHT(str, n)` | `RIGHT({Column}, 3)` | `n` characters from the end of input parameter |
| **SEARCH** | `SEARCH(str, srchStr)` | `SEARCH({Column}, 'str')` | Index of `srchStr` specified if found, 0 otherwise |
| **SUBSTR** | `SUBTR(str, position, [count])` | `SUBSTR({Column}, 3, 2)` | Substring of length 'count' of input string, from the postition specified |
| **TRIM** | `TRIM(str)` | `TRIM({Column})` | Remove trailing and leading whitespaces from input parameter |
| **UPPER** | `UPPER(str)` | `UPPER({Column})` | Upper case converted string of input parameter |
| **URL** | `URL(str)` | `URL({Column})` | Convert to a hyperlink if it is a valid URL |
### Date Functions
| Name | Syntax | Sample | Output | Remark |
|---|---|---|---|---|
| **NOW** | `NOW()` | `NOW()` | 2022-05-19 17:20:43 | Returns the current time and day |
| | `IF(NOW() < {DATE_COL}, "true", "false")` | `IF(NOW() < date, "true", "false")` | If current date is less than `{DATE_COL}`, it returns true. Otherwise, it returns false. | DateTime columns and negative values are supported. |
| **DATEADD** | `DATEADD(date \| datetime, value, ["day" \| "week" \| "month" \| "year"])` | `DATEADD(date, 1, 'day')` | Supposing `{DATE_COL}` is 2022-03-14. The result is 2022-03-15. | DateTime columns and negative values are supported. Example: `DATEADD(DATE_TIME_COL, -1, 'day')` |
| | | `DATEADD(date, 1, 'week')` | Supposing `{DATE_COL}` is 2022-03-14 03:14. The result is 2022-03-21 03:14. | DateTime columns and negative values are supported. Example: `DATEADD(DATE_TIME_COL, -1, 'week')` |
| | | `DATEADD(date, 1, 'month')` | Supposing `{DATE_COL}` is 2022-03-14 03:14. The result is 2022-04-14 03:14. | DateTime columns and negative values are supported. Example: `DATEADD(DATE_TIME_COL, -1, 'month')` |
| | | `DATEADD(date, 1, 'year')` | Supposing `{DATE_COL}` is 2022-03-14 03:14. The result is 2023-03-14 03:14. | DateTime columns and negative values are supported. Example: `DATEADD(DATE_TIME_COL, -1, 'year')` |
| | | `IF(NOW() < DATEADD(date,10,'day'), "true", "false")` | If the current date is less than `{DATE_COL}` plus 10 days, it returns true. Otherwise, it returns false. | DateTime columns and negative values are supported. |
| | | `IF(NOW() < DATEADD(date,10,'day'), "true", "false")` | If the current date is less than `{DATE_COL}` plus 10 days, it returns true. Otherwise, it returns false. | DateTime columns and negative values are supported. |
| **DATETIME_DIFF** | `DATETIME_DIFF(date, date, ["milliseconds" \| "ms" \| "seconds" \| "s" \| "minutes" \| "m" \| "hours" \| "h" \| "days" \| "d" \| "weeks" \| "w" \| "months" \| "M" \| "quarters" \| "Q" \| "years" \| "y"])` | `DATETIME_DIFF("2022/10/14", "2022/10/15", "second")` | Supposing `{DATE_COL_1}` is 2017-08-25 and `{DATE_COL_2}` is 2011-08-25. The result is 86400. | Compares two dates and returns the difference in the unit specified. Positive integers indicate the second date being in the past compared to the first and vice versa for negative ones. |
| | | `WEEKDAY(NOW(), "sunday")` | If today is Monday, it returns 1 | Get the week day of NOW() with the first day set as sunday |
| **WEEKDAY** | `WEEKDAY(date, [startDayOfWeek])` | `WEEKDAY(NOW())` | If today is Monday, it returns 0 | Returns the day of the week as an integer between 0 and 6 inclusive starting from Monday by default. You can optionally change the start day of the week by specifying in the second argument |
| | | `WEEKDAY(NOW(), "sunday")` | If today is Monday, it returns 1 | Get the week day of NOW() with the first day set as sunday |
### Logical Operators
| Operator | Sample | Description |
| -------- | -------------------- | ------------------------ |
| `<` | `{Column1} < {Column2}` | Less than |
| `>` | `{Column1} > {Column2}` | Greater than |
| `<=` | `{Column1} <= {Column2}` | Less than or equal to |
| `>=` | `{Column1} >= {Column2}` | Greater than or equal to |
| `==` | `{Column1} == {Column2}` | Equal to |
| `!=` | `{Column1} != {Column2}` | Not equal to |
### Conditional Expressions
| Name | Syntax | Sample | Output |
|------------|------------------------------------------------|---------------------------------------------|-------------------------------------------------------------|
| **IF** | `IF(expr, successCase, elseCase)` | `IF({Column} > 1, Value1, Value2)` | successCase if `expr` evaluates to TRUE, elseCase otherwise |
| **SWITCH** | `SWITCH(expr, [pattern, value, ..., default])` | `SWITCH({Column}, 1, 'One', 2, 'Two', '--')` | Switch case value based on `expr` output |
| **AND** | `AND(expr1, [expr2,...])` | `AND({Column} > 2, {Column} < 10)` | TRUE if all `expr` evaluate to TRUE |
| **OR** | `OR(expr1, [expr2,...])` | `OR({Column} > 2, {Column} < 10)` | TRUE if at least one `expr` evaluates to TRUE |
Logical operators, along with Numerical operators can be used to build conditional `expressions`.
Examples:
```
IF({marksSecured} > 80, "GradeA", "GradeB")
```
```
SWITCH({quarterNumber},
1, 'Jan-Mar',
2, 'Apr-Jun',
3, 'Jul-Sep',
4, 'Oct-Dec',
'INVALID'
)
``` | packages/noco-docs/versioned_docs/version-0.109.7/030.setup-and-usages/090.formulas.md | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0001776265271473676,
0.00016948247503023595,
0.00016418294399045408,
0.00017003071843646467,
0.0000032458945042890264
]
|
{
"id": 5,
"code_window": [
"\n",
" const column = computed(() => columnMeta.value ?? injectedColumn.value)\n",
"\n",
" const { sqlUis } = storeToRefs(useBase())\n",
"\n",
" const sqlUi = ref(column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0])\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" const sqlUi = computed(() =>\n",
" column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0],\n",
" )\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 117
} | import { type ColumnType } from 'nocodb-sdk'
import type { PropType } from '@vue/runtime-core'
import {
ColumnInj,
computed,
defineComponent,
h,
iconMap,
inject,
isAttachment,
isBoolean,
isCurrency,
isDate,
isDateTime,
isDecimal,
isDuration,
isEmail,
isFloat,
isGeoData,
isGeometry,
isInt,
isJSON,
isPercent,
isPhoneNumber,
isPrimaryKey,
isRating,
isSet,
isSingleSelect,
isSpecificDBType,
isString,
isTextArea,
isTime,
isURL,
isUser,
isYear,
storeToRefs,
toRef,
useBase,
} from '#imports'
const renderIcon = (column: ColumnType, abstractType: any) => {
if (isPrimaryKey(column)) {
return iconMap.key
} else if (isSpecificDBType(column)) {
return iconMap.specificDbType
} else if (isJSON(column)) {
return iconMap.json
} else if (isDate(column, abstractType)) {
return iconMap.calendar
} else if (isDateTime(column, abstractType)) {
return iconMap.datetime
} else if (isGeoData(column)) {
return iconMap.geoData
} else if (isSet(column)) {
return iconMap.multiSelect
} else if (isSingleSelect(column)) {
return iconMap.singleSelect
} else if (isBoolean(column, abstractType)) {
return iconMap.boolean
} else if (isTextArea(column)) {
return iconMap.longText
} else if (isEmail(column)) {
return iconMap.email
} else if (isYear(column, abstractType)) {
return iconMap.calendar
} else if (isTime(column, abstractType)) {
return iconMap.clock
} else if (isRating(column)) {
return iconMap.rating
} else if (isAttachment(column)) {
return iconMap.image
} else if (isDecimal(column)) {
return iconMap.decimal
} else if (isPhoneNumber(column)) {
return iconMap.phone
} else if (isURL(column)) {
return iconMap.web
} else if (isCurrency(column)) {
return iconMap.currency
} else if (isDuration(column)) {
return iconMap.duration
} else if (isPercent(column)) {
return iconMap.percent
} else if (isGeometry(column)) {
return iconMap.calculator
} else if (isUser(column)) {
if ((column.meta as { is_multi?: boolean; notify?: boolean })?.is_multi) {
return iconMap.phUsers
}
return iconMap.phUser
} else if (isInt(column, abstractType) || isFloat(column, abstractType)) {
return iconMap.number
} else if (isString(column, abstractType)) {
return iconMap.text
} else {
return iconMap.generic
}
}
export default defineComponent({
name: 'CellIcon',
props: {
columnMeta: {
type: Object as PropType<ColumnType>,
required: false,
},
},
setup(props) {
const columnMeta = toRef(props, 'columnMeta')
const injectedColumn = inject(ColumnInj, columnMeta)
const column = computed(() => columnMeta.value ?? injectedColumn.value)
const { sqlUis } = storeToRefs(useBase())
const sqlUi = ref(column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0])
const abstractType = computed(() => column.value && sqlUi.value.getAbstractType(column.value))
return () => {
if (!column.value && !columnMeta.value) return null
return h(renderIcon((columnMeta.value ?? column.value)!, abstractType.value), {
class: 'text-inherit mx-1',
})
}
},
})
| packages/nc-gui/components/smartsheet/header/CellIcon.ts | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.9981997013092041,
0.14151301980018616,
0.0001706542680040002,
0.001293169567361474,
0.34313756227493286
]
|
{
"id": 5,
"code_window": [
"\n",
" const column = computed(() => columnMeta.value ?? injectedColumn.value)\n",
"\n",
" const { sqlUis } = storeToRefs(useBase())\n",
"\n",
" const sqlUi = ref(column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0])\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" const sqlUi = computed(() =>\n",
" column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0],\n",
" )\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 117
} | import { Injectable } from '@nestjs/common';
import { AppEvents } from 'nocodb-sdk';
import type { ViewColumnReqType, ViewColumnUpdateReqType } from 'nocodb-sdk';
import type { NcRequest } from '~/interface/config';
import { AppHooksService } from '~/services/app-hooks/app-hooks.service';
import { validatePayload } from '~/helpers';
import { View } from '~/models';
@Injectable()
export class ViewColumnsService {
constructor(private appHooksService: AppHooksService) {}
async columnList(param: { viewId: string }) {
return await View.getColumns(param.viewId, undefined);
}
async columnAdd(param: {
viewId: string;
column: ViewColumnReqType;
req: NcRequest;
}) {
validatePayload(
'swagger.json#/components/schemas/ViewColumnReq',
param.column,
);
const viewColumn = await View.insertOrUpdateColumn(
param.viewId,
param.column.fk_column_id,
{
order: param.column.order,
show: param.column.show,
},
);
this.appHooksService.emit(AppEvents.VIEW_COLUMN_CREATE, {
viewColumn,
req: param.req,
});
return viewColumn;
}
async columnUpdate(param: {
viewId: string;
columnId: string;
column: ViewColumnUpdateReqType;
req: NcRequest;
}) {
validatePayload(
'swagger.json#/components/schemas/ViewColumnUpdateReq',
param.column,
);
const result = await View.updateColumn(
param.viewId,
param.columnId,
param.column,
);
this.appHooksService.emit(AppEvents.VIEW_COLUMN_UPDATE, {
viewColumn: param.column,
req: param.req,
});
return result;
}
}
| packages/nocodb/src/services/view-columns.service.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0010196455987170339,
0.0003302468976471573,
0.00017451272287871689,
0.00021415996889118105,
0.0002848152944352478
]
|
{
"id": 5,
"code_window": [
"\n",
" const column = computed(() => columnMeta.value ?? injectedColumn.value)\n",
"\n",
" const { sqlUis } = storeToRefs(useBase())\n",
"\n",
" const sqlUi = ref(column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0])\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" const sqlUi = computed(() =>\n",
" column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0],\n",
" )\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 117
} | import { MetaTable, MetaTableOldV2, orderedMetaTables } from '~/utils/globals';
const up = async (knex) => {
await knex.schema.createTable(MetaTableOldV2.PROJECT, (table) => {
table.string('id', 128).primary();
table.string('title');
table.string('prefix');
table.string('status');
table.text('description');
table.text('meta');
table.string('color');
table.string('uuid');
table.string('password');
table.string('roles');
table.boolean('deleted').defaultTo(false);
table.boolean('is_meta');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTableOldV2.BASES, (table) => {
table.string('id', 20).primary().notNullable();
// todo: foreign key
// table.string('project_id', 128);
table.string('project_id', 128);
table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('alias');
table.text('config');
table.text('meta');
table.boolean('is_meta');
table.string('type');
table.string('inflection_column');
table.string('inflection_table');
// todo: type
// table.text('ssl');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.MODELS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('table_name');
table.string('title');
table.string('type').defaultTo('table');
table.text('meta', 'mediumtext');
table.text('schema', 'text');
table.boolean('enabled').defaultTo(true);
table.boolean('mm').defaultTo(false);
table.string('tags');
table.boolean('pinned');
table.boolean('deleted');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.COLUMNS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_model_id', 20);
table.foreign('fk_model_id').references(`${MetaTable.MODELS}.id`);
table.string('title');
table.string('column_name');
// todo: decide type
table.string('uidt');
table.string('dt');
table.string('np');
table.string('ns');
table.string('clen');
table.string('cop');
table.boolean('pk');
table.boolean('pv');
table.boolean('rqd');
table.boolean('un');
table.string('ct');
table.boolean('ai');
table.boolean('unique');
table.string('cdf');
table.string('cc');
table.string('csn');
table.string('dtx');
table.string('dtxp');
table.string('dtxs');
table.boolean('au');
// todo: normalise
table.text('validate');
//todo: virtual, real, etc
table.boolean('virtual');
table.boolean('deleted');
table.boolean('system').defaultTo(false);
table.float('order');
table.timestamps(true, true);
});
// await knex.schema.createTable(MetaTable.COLUMN_VALIDATIONS, table => {
// table
// .string('id', 20)
// .primary()
// .notNullable();
// })
/*
await knex.schema.createTable('nc_col_props_v2', table => {
table
.string('id', 20)
.primary()
.notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_column_id',20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('cn');
// todo: decide type
table.string('uidt');
table.string('dt');
table.string('np');
table.string('ns');
table.string('clen');
table.string('cop');
table.boolean('pk');
table.boolean('rqd');
table.boolean('un');
table.string('ct');
table.boolean('ai');
table.boolean('unique');
table.string('ctf');
table.string('cc');
table.string('csn');
table.string('dtx');
table.string('dtxp');
table.string('dtxs');
table.boolean('au');
table.timestamps(true, true);
// table.index(['db_alias', 'tn']);
});
*/
await knex.schema.createTable(MetaTable.COL_RELATIONS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('ref_db_alias');
table.string('type');
table.boolean('virtual');
table.string('db_type');
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('fk_related_model_id', 20);
table.foreign('fk_related_model_id').references(`${MetaTable.MODELS}.id`);
// fk_rel_column_id
// fk_rel_ref_column_id
table.string('fk_child_column_id', 20);
table.foreign('fk_child_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('fk_parent_column_id', 20);
table.foreign('fk_parent_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('fk_mm_model_id', 20);
table.foreign('fk_mm_model_id').references(`${MetaTable.MODELS}.id`);
table.string('fk_mm_child_column_id', 20);
table
.foreign('fk_mm_child_column_id')
.references(`${MetaTable.COLUMNS}.id`);
table.string('fk_mm_parent_column_id', 20);
table
.foreign('fk_mm_parent_column_id')
.references(`${MetaTable.COLUMNS}.id`);
table.string('ur');
table.string('dr');
table.string('fk_index_name');
table.boolean('deleted');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.COL_SELECT_OPTIONS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('title');
table.string('color');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.COL_LOOKUP, (table) => {
table.string('id', 20).primary().notNullable();
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
// todo: refer relation column
// table.string('fk_child_column_id',20);
// table.foreign('fk_child_column_id').references(`${MetaTable.COLUMNS}.id`);
// table.string('fk_parent_column_id',20);
// table.foreign('fk_parent_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('fk_relation_column_id', 20);
table
.foreign('fk_relation_column_id')
.references(`${MetaTable.COLUMNS}.id`);
table.string('fk_lookup_column_id', 20);
table.foreign('fk_lookup_column_id').references(`${MetaTable.COLUMNS}.id`);
table.boolean('deleted');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.COL_ROLLUP, (table) => {
table.string('id', 20).primary().notNullable();
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('fk_relation_column_id', 20);
table
.foreign('fk_relation_column_id')
.references(`${MetaTable.COLUMNS}.id`);
table.string('fk_rollup_column_id', 20);
table.foreign('fk_rollup_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('rollup_function');
table.boolean('deleted');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.COL_FORMULA, (table) => {
table.string('id', 20).primary().notNullable();
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.text('formula').notNullable();
table.text('formula_raw');
table.text('error');
table.boolean('deleted');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.VIEWS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_model_id', 20);
table.foreign('fk_model_id').references(`${MetaTable.MODELS}.id`);
table.string('title');
table.integer('type');
table.boolean('is_default');
table.boolean('show_system_fields');
table.string('lock_type').defaultTo('collaborative');
table.string('uuid');
table.string('password');
// todo: type
table.boolean('show');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.HOOKS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_model_id', 20);
table.foreign('fk_model_id').references(`${MetaTable.MODELS}.id`);
table.string('title');
table.string('description', 255);
table.string('env').defaultTo('all');
table.string('type');
table.string('event');
table.string('operation');
table.boolean('async').defaultTo(false);
table.boolean('payload').defaultTo(true);
table.text('url', 'text');
table.text('headers', 'text');
// todo: normalise
table.boolean('condition').defaultTo(false);
table.text('notification', 'text');
table.integer('retries').defaultTo(0);
table.integer('retry_interval').defaultTo(60000);
table.integer('timeout').defaultTo(60000);
table.boolean('active').defaultTo(true);
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.HOOK_LOGS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_hook_id', 20);
// table.foreign('fk_hook_id').references(`${MetaTable.HOOKS}.id`);
table.string('type');
table.string('event');
table.string('operation');
table.boolean('test_call').defaultTo(true);
table.boolean('payload').defaultTo(true);
table.text('conditions');
table.text('notification', 'text');
table.string('error_code');
table.string('error_message');
table.text('error', 'text');
table.integer('execution_time');
table.string('response');
table.string('triggered_by');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.FILTER_EXP, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20);
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.string('fk_hook_id', 20);
table.foreign('fk_hook_id').references(`${MetaTable.HOOKS}.id`);
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('fk_parent_id', 20);
table.foreign('fk_parent_id').references(`${MetaTable.FILTER_EXP}.id`);
table.string('logical_op');
table.string('comparison_op');
table.string('value');
table.boolean('is_group');
table.float('order');
table.timestamps(true, true);
});
// await knex.schema.createTable(MetaTable.HOOK_FILTER_EXP, table => {
// table
// .string('id', 20)
// .primary()
// .notNullable();
//
// table.string('base_id', 20);
// // table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
// table.string('project_id', 128);
// // table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
//
// table.string('fk_hook_id', 20);
// table.foreign('fk_hook_id').references(`${MetaTable.HOOKS}.id`);
// table.string('fk_column_id', 20);
// table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
//
// table.string('fk_parent_id', 20);
// table.foreign('fk_parent_id').references(`${MetaTable.HOOK_FILTER_EXP}.id`);
//
// table.string('logical_op');
// table.string('comparison_op');
// table.string('value');
// table.boolean('is_group');
//
// table.float('order');
// table.timestamps(true, true);
// });
await knex.schema.createTable(MetaTable.SORT, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20);
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('direction').defaultTo(false);
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.SHARED_VIEWS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('fk_view_id', 20);
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.text('meta', 'mediumtext');
// todo:
table.text('query_params', 'mediumtext');
table.string('view_id');
table.boolean('show_all_fields');
table.boolean('allow_copy');
table.string('password');
table.boolean('deleted');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.FORM_VIEW, (table) => {
// table
// .string('id', 20)
// .primary()
// .notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20).primary();
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.string('heading');
table.string('subheading');
table.string('success_msg');
table.string('redirect_url');
table.string('redirect_after_secs');
table.string('email');
table.boolean('submit_another_form');
table.boolean('show_blank_form');
table.string('uuid');
table.string('banner_image_url');
table.string('logo_url');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.FORM_VIEW_COLUMNS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20);
table.foreign('fk_view_id').references(`${MetaTable.FORM_VIEW}.fk_view_id`);
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
// todo: type
table.string('uuid');
table.string('label');
table.string('help');
table.string('description');
table.boolean('required');
table.boolean('show');
table.float('order');
// todo : condition
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.GALLERY_VIEW, (table) => {
// table
// .string('id', 20)
// .primary()
// .notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20).primary();
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
// todo: type
table.boolean('next_enabled');
table.boolean('prev_enabled');
table.integer('cover_image_idx');
table.string('fk_cover_image_col_id', 20);
table
.foreign('fk_cover_image_col_id')
.references(`${MetaTable.COLUMNS}.id`);
table.string('cover_image');
table.string('restrict_types');
table.string('restrict_size');
table.string('restrict_number');
table.boolean('public');
table.string('dimensions');
table.string('responsive_columns');
// todo : condition
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.GALLERY_VIEW_COLUMNS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20);
table
.foreign('fk_view_id')
.references(`${MetaTable.GALLERY_VIEW}.fk_view_id`);
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('uuid');
// todo: type
table.string('label');
table.string('help');
table.boolean('show');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.GRID_VIEW, (table) => {
table.string('fk_view_id', 20).primary();
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('uuid');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.GRID_VIEW_COLUMNS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('fk_view_id', 20);
table.foreign('fk_view_id').references(`${MetaTable.GRID_VIEW}.fk_view_id`);
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('uuid');
// todo: type
table.string('label');
table.string('help');
table.string('width').defaultTo('200px');
table.boolean('show');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.KANBAN_VIEW, (table) => {
table.string('fk_view_id', 20).primary();
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.boolean('show');
table.float('order');
table.string('uuid');
table.string('title');
// todo: type
table.boolean('public');
table.string('password');
table.boolean('show_all_fields');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.KANBAN_VIEW_COLUMNS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_view_id', 20);
table
.foreign('fk_view_id')
.references(`${MetaTable.KANBAN_VIEW}.fk_view_id`);
table.string('fk_column_id', 20);
table.foreign('fk_column_id').references(`${MetaTable.COLUMNS}.id`);
table.string('uuid');
// todo: type
table.string('label');
table.string('help');
table.boolean('show');
table.float('order');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.USERS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('email');
table.string('password', 255);
table.string('salt', 255);
table.string('firstname');
table.string('lastname');
table.string('username');
table.string('refresh_token', 255);
table.string('invite_token', 255);
table.string('invite_token_expires', 255);
table.timestamp('reset_password_expires');
table.string('reset_password_token', 255);
table.string('email_verification_token', 255);
table.boolean('email_verified');
table.string('roles', 255).defaultTo('editor');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTableOldV2.PROJECT_USERS, (table) => {
table.string('project_id', 128);
table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_user_id', 20);
table.foreign('fk_user_id').references(`${MetaTable.USERS}.id`);
table.text('roles');
table.boolean('starred');
table.boolean('pinned');
table.string('group');
table.string('color');
table.float('order');
table.float('hidden');
table.timestamp('opened_date');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.ORGS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('title');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.TEAMS, (table) => {
table.string('id', 20).primary().notNullable();
table.string('title');
table.string('org_id', 20);
table.foreign('org_id').references(`${MetaTable.ORGS}.id`);
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.TEAM_USERS, (table) => {
table.string('org_id', 20);
table.foreign('org_id').references(`${MetaTable.ORGS}.id`);
table.string('user_id', 20);
table.foreign('user_id').references(`${MetaTable.USERS}.id`);
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.AUDIT, (table) => {
table.string('id', 20).primary().notNullable();
table.string('user');
table.string('ip');
table.string('base_id', 20);
table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
table.string('fk_model_id', 20);
table.foreign('fk_model_id').references(`${MetaTable.MODELS}.id`);
table.string('row_id').index();
/* op_type - AUTH, DATA, SQL, META */
table.string('op_type');
table.string('op_sub_type');
table.string('status');
table.text('description');
table.text('details');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.PLUGIN, (table) => {
table.string('id', 20).primary().notNullable();
table.string('title', 45);
table.text('description');
table.boolean('active').defaultTo(false);
table.float('rating');
table.string('version');
table.string('docs');
table.string('status').defaultTo('install');
table.string('status_details');
table.string('logo');
table.string('icon');
table.string('tags');
table.string('category');
table.text('input_schema');
table.text('input');
table.string('creator');
table.string('creator_website');
table.string('price');
table.timestamps(true, true);
});
await knex.schema.createTable(MetaTable.MODEL_ROLE_VISIBILITY, (table) => {
table.string('id', 20).primary().notNullable();
table.string('base_id', 20);
// table.foreign('base_id').references(`${MetaTableOldV2.BASES}.id`);
table.string('project_id', 128);
// table.foreign('project_id').references(`${MetaTableOldV2.PROJECT}.id`);
// table.string('fk_model_id', 20);
// table.foreign('fk_model_id').references(`${MetaTable.MODELS}.id`);
table.string('fk_view_id', 20);
table.foreign('fk_view_id').references(`${MetaTable.VIEWS}.id`);
table.string('role', 45);
table.boolean('disabled').defaultTo(true);
table.timestamps(true, true);
});
// await knex('nc_plugins').insert([
// googleAuth,
// ses,
// cache
// // ee,
// // brand,
// ]);
};
const down = async (knex) => {
for (const tableName of orderedMetaTables) {
await knex.schema.dropTable(tableName);
}
};
export { up, down };
| packages/nocodb/src/meta/migrations/v2/nc_011.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017947096785064787,
0.00017370688146911561,
0.0001636850938666612,
0.000173900683876127,
0.000003610939529608004
]
|
{
"id": 5,
"code_window": [
"\n",
" const column = computed(() => columnMeta.value ?? injectedColumn.value)\n",
"\n",
" const { sqlUis } = storeToRefs(useBase())\n",
"\n",
" const sqlUi = ref(column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0])\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" const sqlUi = computed(() =>\n",
" column.value?.source_id ? sqlUis.value[column.value?.source_id] : Object.values(sqlUis.value)[0],\n",
" )\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 117
} | <svg width="16" height="16" viewBox="0 0 16 16" fill="none" xmlns="http://www.w3.org/2000/svg">
<path d="M7.33333 12.6667C10.2789 12.6667 12.6667 10.2789 12.6667 7.33333C12.6667 4.38781 10.2789 2 7.33333 2C4.38781 2 2 4.38781 2 7.33333C2 10.2789 4.38781 12.6667 7.33333 12.6667Z" stroke="currentColor" stroke-width="1.33333" stroke-linecap="round" stroke-linejoin="round"/>
<path d="M14.0001 13.9996L11.1001 11.0996" stroke="currentColor" stroke-width="1.33333" stroke-linecap="round" stroke-linejoin="round"/>
</svg>
| packages/nc-gui/assets/nc-icons/search.svg | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017499993555247784,
0.00017499993555247784,
0.00017499993555247784,
0.00017499993555247784,
0
]
|
{
"id": 6,
"code_window": [
"\n",
" const abstractType = computed(() => column.value && sqlUi.value.getAbstractType(column.value))\n",
"\n",
" return () => {\n",
" if (!column.value && !columnMeta.value) return null\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" const abstractType = computed(() => column.value && sqlUi.value?.getAbstractType(column.value))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 119
} | <script setup lang="ts">
import type { ColumnType, TableType, ViewType } from 'nocodb-sdk'
import {
ViewTypes,
isCreatedOrLastModifiedByCol,
isCreatedOrLastModifiedTimeCol,
isLinksOrLTAR,
isSystemColumn,
isVirtualCol,
} from 'nocodb-sdk'
import type { Ref } from 'vue'
import MdiChevronDown from '~icons/mdi/chevron-down'
import {
CellClickHookInj,
FieldsInj,
IsExpandedFormOpenInj,
IsKanbanInj,
IsPublicInj,
MetaInj,
ReloadRowDataHookInj,
computedInject,
createEventHook,
iconMap,
inject,
message,
provide,
ref,
toRef,
useActiveKeyupListener,
useProvideExpandedFormStore,
useProvideSmartsheetStore,
useRoles,
useRouter,
useVModel,
watch,
} from '#imports'
interface Props {
modelValue?: boolean
state?: Record<string, any> | null
meta: TableType
loadRow?: boolean
useMetaFields?: boolean
row?: Row
rowId?: string
view?: ViewType
showNextPrevIcons?: boolean
firstRow?: boolean
lastRow?: boolean
closeAfterSave?: boolean
newRecordHeader?: string
}
const props = defineProps<Props>()
const emits = defineEmits(['update:modelValue', 'cancel', 'next', 'prev', 'createdRecord'])
const { activeView } = storeToRefs(useViewsStore())
const key = ref(0)
const wrapper = ref()
const { dashboardUrl } = useDashboard()
const { copy } = useClipboard()
const { isMobileMode } = useGlobal()
const { t } = useI18n()
const rowId = toRef(props, 'rowId')
const row = toRef(props, 'row')
const state = toRef(props, 'state')
const meta = toRef(props, 'meta')
const islastRow = toRef(props, 'lastRow')
const isFirstRow = toRef(props, 'firstRow')
const route = useRoute()
const router = useRouter()
const isPublic = inject(IsPublicInj, ref(false))
// to check if a expanded form which is not yet saved exist or not
const isUnsavedFormExist = ref(false)
const isRecordLinkCopied = ref(false)
const { isUIAllowed } = useRoles()
const readOnly = computed(() => !isUIAllowed('dataEdit') || isPublic.value)
const expandedFormScrollWrapper = ref()
const reloadTrigger = inject(ReloadRowDataHookInj, createEventHook())
const { addOrEditStackRow } = useKanbanViewStoreOrThrow()
const { isExpandedFormCommentMode } = storeToRefs(useConfigStore())
// override cell click hook to avoid unexpected behavior at form fields
provide(CellClickHookInj, undefined)
const fields = computedInject(FieldsInj, (_fields) => {
if (props.useMetaFields) {
return (meta.value.columns ?? []).filter((col) => !isSystemColumn(col))
}
return _fields?.value ?? []
})
const hiddenFields = computed(() => {
return (meta.value.columns ?? []).filter((col) => !fields.value?.includes(col)).filter((col) => !isSystemColumn(col))
})
const showHiddenFields = ref(false)
const toggleHiddenFields = () => {
showHiddenFields.value = !showHiddenFields.value
}
const isKanban = inject(IsKanbanInj, ref(false))
provide(MetaInj, meta)
const isLoading = ref(true)
const {
commentsDrawer,
changedColumns,
deleteRowById,
displayValue,
state: rowState,
isNew,
loadRow: _loadRow,
primaryKey,
saveRowAndStay,
row: _row,
save: _save,
loadCommentsAndLogs,
clearColumns,
} = useProvideExpandedFormStore(meta, row)
const duplicatingRowInProgress = ref(false)
useProvideSmartsheetStore(ref({}) as Ref<ViewType>, meta)
watch(
state,
() => {
if (state.value) {
rowState.value = state.value
} else {
rowState.value = {}
}
},
{ immediate: true },
)
const isExpanded = useVModel(props, 'modelValue', emits, {
defaultValue: false,
})
const onClose = () => {
if (!isUIAllowed('dataEdit')) {
isExpanded.value = false
} else if (changedColumns.value.size > 0) {
isCloseModalOpen.value = true
} else {
if (_row.value?.rowMeta?.new) emits('cancel')
isExpanded.value = false
}
}
const onDuplicateRow = () => {
duplicatingRowInProgress.value = true
isUnsavedFormExist.value = true
const oldRow = { ..._row.value.row }
delete oldRow.ncRecordId
const newRow = Object.assign(
{},
{
row: oldRow,
oldRow: {},
rowMeta: { new: true },
},
)
setTimeout(async () => {
_row.value = newRow
duplicatingRowInProgress.value = false
message.success(t('msg.success.rowDuplicatedWithoutSavedYet'))
}, 500)
}
const save = async () => {
let kanbanClbk
if (activeView.value?.type === ViewTypes.KANBAN) {
kanbanClbk = (row: any, isNewRow: boolean) => {
addOrEditStackRow(row, isNewRow)
}
}
if (isNew.value) {
await _save(rowState.value, undefined, {
kanbanClbk,
})
reloadTrigger?.trigger()
} else {
await _save(undefined, undefined, {
kanbanClbk,
})
_loadRow()
reloadTrigger?.trigger()
}
isUnsavedFormExist.value = false
if (props.closeAfterSave) {
isExpanded.value = false
}
emits('createdRecord', _row.value.row)
}
const isPreventChangeModalOpen = ref(false)
const isCloseModalOpen = ref(false)
const discardPreventModal = () => {
// when user click on next or previous button
if (isPreventChangeModalOpen.value) {
emits('next')
if (_row.value?.rowMeta?.new) emits('cancel')
isPreventChangeModalOpen.value = false
}
// when user click on close button
if (isCloseModalOpen.value) {
isCloseModalOpen.value = false
if (_row.value?.rowMeta?.new) emits('cancel')
isExpanded.value = false
}
// clearing all new modifed change on close
clearColumns()
}
const onNext = async () => {
if (changedColumns.value.size > 0) {
isPreventChangeModalOpen.value = true
return
}
emits('next')
}
const copyRecordUrl = async () => {
await copy(
encodeURI(
`${dashboardUrl?.value}#/${route.params.typeOrId}/${route.params.baseId}/${meta.value?.id}${
props.view ? `/${props.view.title}` : ''
}?rowId=${primaryKey.value}`,
),
)
isRecordLinkCopied.value = true
}
const saveChanges = async () => {
if (isPreventChangeModalOpen.value) {
isUnsavedFormExist.value = false
await save()
emits('next')
isPreventChangeModalOpen.value = false
}
if (isCloseModalOpen.value) {
isCloseModalOpen.value = false
await save()
isExpanded.value = false
}
}
const reloadParentRowHook = inject(ReloadRowDataHookInj, createEventHook())
// override reload trigger and use it to reload grid and the form itself
const reloadHook = createEventHook()
reloadHook.on(() => {
reloadParentRowHook?.trigger(false)
if (isNew.value) return
_loadRow(null, true)
})
provide(ReloadRowDataHookInj, reloadHook)
if (isKanban.value) {
// adding column titles to changedColumns if they are preset
for (const [k, v] of Object.entries(_row.value.row)) {
if (v) {
changedColumns.value.add(k)
}
}
}
provide(IsExpandedFormOpenInj, isExpanded)
const cellWrapperEl = ref()
onMounted(async () => {
isRecordLinkCopied.value = false
isLoading.value = true
const focusFirstCell = !isExpandedFormCommentMode.value
if (props.loadRow) {
await _loadRow()
await loadCommentsAndLogs()
}
if (props.rowId) {
try {
await _loadRow(props.rowId)
await loadCommentsAndLogs()
} catch (e: any) {
if (e.response?.status === 404) {
message.error(t('msg.noRecordFound'))
router.replace({ query: {} })
} else throw e
}
}
isLoading.value = false
if (focusFirstCell) {
setTimeout(() => {
cellWrapperEl.value?.$el?.querySelector('input,select,textarea')?.focus()
}, 300)
}
})
const addNewRow = () => {
setTimeout(async () => {
_row.value = {
row: {},
oldRow: {},
rowMeta: { new: true },
}
rowState.value = {}
key.value++
isExpanded.value = true
}, 500)
}
// attach keyboard listeners to switch between rows
// using alt + left/right arrow keys
useActiveKeyupListener(
isExpanded,
async (e: KeyboardEvent) => {
if (!e.altKey) return
if (e.key === 'ArrowLeft') {
e.stopPropagation()
emits('prev')
} else if (e.key === 'ArrowRight') {
e.stopPropagation()
onNext()
}
// on alt + s save record
else if (e.code === 'KeyS') {
// remove focus from the active input if any
;(document.activeElement as HTMLElement)?.blur()
e.stopPropagation()
if (isNew.value) {
await _save(rowState.value)
reloadHook?.trigger(null)
} else {
await save()
reloadHook?.trigger(null)
}
if (!saveRowAndStay.value) {
onClose()
}
// on alt + n create new record
} else if (e.code === 'KeyN') {
// remove focus from the active input if any to avoid unwanted input
;(document.activeElement as HTMLInputElement)?.blur?.()
if (changedColumns.value.size > 0) {
await Modal.confirm({
title: t('msg.saveChanges'),
okText: t('general.save'),
cancelText: t('labels.discard'),
onOk: async () => {
await save()
reloadHook?.trigger(null)
addNewRow()
},
onCancel: () => {
addNewRow()
},
})
} else if (isNew.value) {
await Modal.confirm({
title: 'Do you want to save the record?',
okText: t('general.save'),
cancelText: t('labels.discard'),
onOk: async () => {
await _save(rowState.value)
reloadHook?.trigger(null)
addNewRow()
},
onCancel: () => {
addNewRow()
},
})
} else {
addNewRow()
}
}
},
{ immediate: true },
)
const showDeleteRowModal = ref(false)
const onDeleteRowClick = () => {
showDeleteRowModal.value = true
}
const onConfirmDeleteRowClick = async () => {
showDeleteRowModal.value = false
await deleteRowById(primaryKey.value)
message.success(t('msg.rowDeleted'))
reloadTrigger.trigger()
onClose()
showDeleteRowModal.value = false
}
watch(rowId, async (nRow) => {
await _loadRow(nRow)
await loadCommentsAndLogs()
})
const showRightSections = computed(() => {
return !isNew.value && commentsDrawer.value && isUIAllowed('commentList')
})
const preventModalStatus = computed({
get: () => isCloseModalOpen.value || isPreventChangeModalOpen.value,
set: (v) => {
isCloseModalOpen.value = v
},
})
const onIsExpandedUpdate = (v: boolean) => {
let isDropdownOpen = false
document.querySelectorAll('.ant-select-dropdown').forEach((el) => {
isDropdownOpen = isDropdownOpen || el.checkVisibility()
})
if (isDropdownOpen) return
if (changedColumns.value.size === 0 && !isUnsavedFormExist.value) {
isExpanded.value = v
} else if (!v && isUIAllowed('dataEdit')) {
preventModalStatus.value = true
} else {
isExpanded.value = v
}
}
const isReadOnlyVirtualCell = (column: ColumnType) => {
return (
isRollup(column) ||
isFormula(column) ||
isBarcode(column) ||
isLookup(column) ||
isQrCode(column) ||
isSystemColumn(column) ||
isCreatedOrLastModifiedTimeCol(column) ||
isCreatedOrLastModifiedByCol(column)
)
}
// Small hack. We need to scroll to the bottom of the form after its mounted and back to top.
// So that tab to next row works properly, as otherwise browser will focus to save button
// when we reach to the bottom of the visual scrollable area, not the actual bottom of the form
watch([expandedFormScrollWrapper, isLoading], () => {
if (isMobileMode.value) return
const expandedFormScrollWrapperEl = expandedFormScrollWrapper.value
if (expandedFormScrollWrapperEl && !isLoading.value) {
const height = expandedFormScrollWrapperEl.scrollHeight
expandedFormScrollWrapperEl.scrollTop = height
setTimeout(() => {
expandedFormScrollWrapperEl.scrollTop = 0
}, 125)
}
})
</script>
<script lang="ts">
export default {
name: 'ExpandedForm',
}
</script>
<template>
<NcModal
:visible="isExpanded"
:footer="null"
:width="commentsDrawer && isUIAllowed('commentList') ? 'min(80vw,1280px)' : 'min(80vw,1280px)'"
:body-style="{ padding: 0 }"
:closable="false"
size="small"
class="nc-drawer-expanded-form"
:class="{ active: isExpanded }"
@update:visible="onIsExpandedUpdate"
>
<div class="h-[85vh] xs:(max-h-full) max-h-215 flex flex-col p-6">
<div class="flex h-9.5 flex-shrink-0 w-full items-center nc-expanded-form-header relative mb-4 justify-between">
<template v-if="!isMobileMode">
<div class="flex gap-3 w-100">
<div class="flex gap-2">
<NcButton
v-if="props.showNextPrevIcons"
:disabled="isFirstRow"
type="secondary"
class="nc-prev-arrow !w-10"
@click="$emit('prev')"
>
<MdiChevronUp class="text-md" />
</NcButton>
<NcButton
v-if="props.showNextPrevIcons"
:disabled="islastRow"
type="secondary"
class="nc-next-arrow !w-10"
@click="onNext"
>
<MdiChevronDown class="text-md" />
</NcButton>
</div>
<div v-if="isLoading">
<a-skeleton-input class="!h-8 !sm:mr-14 !w-52 mt-1 !rounded-md !overflow-hidden" active size="small" />
</div>
<div
v-if="row.rowMeta?.new || props.newRecordHeader"
class="flex items-center truncate font-bold text-gray-800 text-xl"
>
{{ props.newRecordHeader ?? $t('activity.newRecord') }}
</div>
<div v-else-if="displayValue && !row.rowMeta?.new" class="flex items-center font-bold text-gray-800 text-xl w-64">
<span class="truncate">
{{ displayValue }}
</span>
</div>
</div>
<div class="flex gap-2">
<NcButton
v-if="!isNew && rowId"
type="secondary"
class="!xs:hidden text-gray-700"
:disabled="isLoading"
@click="copyRecordUrl()"
>
<div v-e="['c:row-expand:copy-url']" data-testid="nc-expanded-form-copy-url" class="flex gap-2 items-center">
<component :is="iconMap.check" v-if="isRecordLinkCopied" class="cursor-pointer nc-duplicate-row" />
<component :is="iconMap.link" v-else class="cursor-pointer nc-duplicate-row" />
{{ isRecordLinkCopied ? $t('labels.copiedRecordURL') : $t('labels.copyRecordURL') }}
</div>
</NcButton>
<NcDropdown v-if="!isNew" placement="bottomRight">
<NcButton type="secondary" class="nc-expand-form-more-actions w-10" :disabled="isLoading">
<GeneralIcon icon="threeDotVertical" class="text-md" :class="isLoading ? 'text-gray-300' : 'text-gray-700'" />
</NcButton>
<template #overlay>
<NcMenu>
<NcMenuItem class="text-gray-700" @click="_loadRow()">
<div v-e="['c:row-expand:reload']" class="flex gap-2 items-center" data-testid="nc-expanded-form-reload">
<component :is="iconMap.reload" class="cursor-pointer" />
{{ $t('general.reload') }}
</div>
</NcMenuItem>
<NcMenuItem v-if="isUIAllowed('dataEdit')" class="text-gray-700" @click="!isNew ? onDuplicateRow() : () => {}">
<div
v-e="['c:row-expand:duplicate']"
data-testid="nc-expanded-form-duplicate"
class="flex gap-2 items-center"
>
<component :is="iconMap.copy" class="cursor-pointer nc-duplicate-row" />
<span class="-ml-0.25">
{{ $t('labels.duplicateRecord') }}
</span>
</div>
</NcMenuItem>
<NcDivider v-if="isUIAllowed('dataEdit')" />
<NcMenuItem
v-if="isUIAllowed('dataEdit')"
class="!text-red-500 !hover:bg-red-50"
@click="!isNew && onDeleteRowClick()"
>
<div v-e="['c:row-expand:delete']" data-testid="nc-expanded-form-delete" class="flex gap-2 items-center">
<component :is="iconMap.delete" class="cursor-pointer nc-delete-row" />
<span class="-ml-0.25">
{{ $t('activity.deleteRecord') }}
</span>
</div>
</NcMenuItem>
</NcMenu>
</template>
</NcDropdown>
<NcButton
type="secondary"
class="nc-expand-form-close-btn w-10"
data-testid="nc-expanded-form-close"
@click="onClose"
>
<GeneralIcon icon="close" class="text-md text-gray-700" />
</NcButton>
</div>
</template>
<template v-else>
<div class="flex flex-row w-full">
<NcButton
v-if="props.showNextPrevIcons && !isFirstRow"
v-e="['c:row-expand:prev']"
type="secondary"
class="nc-prev-arrow !w-10"
@click="$emit('prev')"
>
<GeneralIcon icon="arrowLeft" class="text-lg text-gray-700" />
</NcButton>
<div v-else class="min-w-10.5"></div>
<div class="flex flex-grow justify-center items-center font-semibold text-lg">
<div>{{ meta.title }}</div>
</div>
<NcButton
v-if="props.showNextPrevIcons && !islastRow"
v-e="['c:row-expand:next']"
type="secondary"
class="nc-next-arrow !w-10"
@click="onNext"
>
<GeneralIcon icon="arrowRight" class="text-lg text-gray-700" />
</NcButton>
<div v-else class="min-w-10.5"></div>
</div>
</template>
</div>
<div ref="wrapper" class="flex flex-grow flex-row h-[calc(100%-4rem)] w-full gap-4">
<div
class="flex xs:w-full flex-col border-1 rounded-xl overflow-hidden border-gray-200 xs:(border-0 rounded-none)"
:class="{
'w-full': !showRightSections,
'w-2/3': showRightSections,
}"
>
<div
ref="expandedFormScrollWrapper"
class="flex flex-col flex-grow mt-2 h-full max-h-full nc-scrollbar-md pb-6 items-center w-full bg-white p-4 xs:p-0"
>
<div
v-for="(col, i) of fields"
v-show="isFormula(col) || !isVirtualCol(col) || !isNew || isLinksOrLTAR(col)"
:key="col.title"
class="nc-expanded-form-row mt-2 py-2 xs:w-full"
:class="`nc-expand-col-${col.title}`"
:col-id="col.id"
:data-testid="`nc-expand-col-${col.title}`"
>
<div class="flex items-start flex-row sm:(gap-x-6) xs:(flex-col w-full) nc-expanded-cell min-h-10">
<div class="w-48 xs:(w-full) mt-0.25 !h-[35px]">
<LazySmartsheetHeaderVirtualCell
v-if="isVirtualCol(col)"
class="nc-expanded-cell-header h-full"
:column="col"
/>
<LazySmartsheetHeaderCell v-else class="nc-expanded-cell-header" :column="col" />
</div>
<template v-if="isLoading">
<div
v-if="isMobileMode"
class="!h-8.5 !xs:h-12 !xs:bg-white sm:mr-21 w-60 mt-0.75 !rounded-lg !overflow-hidden"
></div>
<a-skeleton-input
v-else
class="!h-8.5 !xs:h-9.5 !xs:bg-white sm:mr-21 !w-60 mt-0.75 !rounded-lg !overflow-hidden"
active
size="small"
/>
</template>
<template v-else>
<SmartsheetDivDataCell
v-if="col.title"
:ref="i ? null : (el: any) => (cellWrapperEl = el)"
class="bg-white w-80 xs:w-full px-1 sm:min-h-[35px] xs:min-h-13 flex items-center relative"
:class="{
'!bg-gray-50 !px-0 !select-text nc-system-field': isReadOnlyVirtualCell(col),
}"
>
<LazySmartsheetVirtualCell
v-if="isVirtualCol(col)"
v-model="_row.row[col.title]"
:row="_row"
:column="col"
:class="{
'px-1': isReadOnlyVirtualCell(col),
}"
:read-only="readOnly"
/>
<LazySmartsheetCell
v-else
v-model="_row.row[col.title]"
:column="col"
:edit-enabled="true"
:active="true"
:read-only="readOnly"
@update:model-value="changedColumns.add(col.title)"
/>
</SmartsheetDivDataCell>
</template>
</div>
</div>
<div v-if="hiddenFields.length > 0" class="flex w-full sm:px-12 xs:(px-1 mt-2) items-center py-3">
<div class="flex-grow h-px mr-1 bg-gray-100"></div>
<NcButton
type="secondary"
:size="isMobileMode ? 'medium' : 'small'"
class="flex-shrink-1 !text-sm"
@click="toggleHiddenFields"
>
{{ showHiddenFields ? `Hide ${hiddenFields.length} hidden` : `Show ${hiddenFields.length} hidden` }}
{{ hiddenFields.length > 1 ? `fields` : `field` }}
<MdiChevronDown class="ml-1" :class="showHiddenFields ? 'transform rotate-180' : ''" />
</NcButton>
<div class="flex-grow h-px ml-1 bg-gray-100"></div>
</div>
<div v-if="hiddenFields.length > 0 && showHiddenFields" class="flex flex-col w-full mb-3 items-center">
<div
v-for="(col, i) of hiddenFields"
v-show="isFormula(col) || !isVirtualCol(col) || !isNew || isLinksOrLTAR(col)"
:key="col.title"
class="sm:(mt-2) py-2 xs:w-full"
:class="`nc-expand-col-${col.title}`"
:data-testid="`nc-expand-col-${col.title}`"
>
<div class="sm:gap-x-6 flex sm:flex-row xs:(flex-col) items-start min-h-10">
<div class="sm:w-48 xs:w-full scale-110 !h-[35px]">
<LazySmartsheetHeaderVirtualCell v-if="isVirtualCol(col)" :column="col" class="nc-expanded-cell-header" />
<LazySmartsheetHeaderCell v-else class="nc-expanded-cell-header" :column="col" />
</div>
<template v-if="isLoading">
<div
v-if="isMobileMode"
class="!h-8.5 !xs:h-9.5 !xs:bg-white sm:mr-21 w-60 mt-0.75 !rounded-lg !overflow-hidden"
></div>
<a-skeleton-input
v-else
class="!h-8.5 !xs:h-12 !xs:bg-white sm:mr-21 w-60 mt-0.75 !rounded-lg !overflow-hidden"
active
size="small"
/>
</template>
<template v-else>
<LazySmartsheetDivDataCell
v-if="col.title"
:ref="i ? null : (el: any) => (cellWrapperEl = el)"
class="bg-white rounded-lg w-80 border-1 overflow-hidden border-gray-200 px-1 sm:min-h-[35px] xs:min-h-13 flex items-center relative"
>
<LazySmartsheetVirtualCell
v-if="isVirtualCol(col)"
v-model="_row.row[col.title]"
:row="_row"
:column="col"
:read-only="readOnly"
/>
<LazySmartsheetCell
v-else
v-model="_row.row[col.title]"
:column="col"
:edit-enabled="true"
:active="true"
:read-only="readOnly"
@update:model-value="changedColumns.add(col.title)"
/>
</LazySmartsheetDivDataCell>
</template>
</div>
</div>
</div>
</div>
<div
v-if="isUIAllowed('dataEdit')"
class="w-full h-16 border-t-1 border-gray-200 bg-white flex items-center justify-end p-3 xs:(p-0 mt-4 border-t-0 gap-x-4 justify-between)"
>
<NcDropdown v-if="!isNew && isMobileMode" placement="bottomRight">
<NcButton type="secondary" class="nc-expand-form-more-actions w-10" :disabled="isLoading">
<GeneralIcon icon="threeDotVertical" class="text-md" :class="isLoading ? 'text-gray-300' : 'text-gray-700'" />
</NcButton>
<template #overlay>
<NcMenu>
<NcMenuItem class="text-gray-700" @click="_loadRow()">
<div v-e="['c:row-expand:reload']" class="flex gap-2 items-center" data-testid="nc-expanded-form-reload">
<component :is="iconMap.reload" class="cursor-pointer" />
{{ $t('general.reload') }}
</div>
</NcMenuItem>
<NcMenuItem v-if="rowId" class="text-gray-700" @click="!isNew ? copyRecordUrl() : () => {}">
<div v-e="['c:row-expand:copy-url']" data-testid="nc-expanded-form-copy-url" class="flex gap-2 items-center">
<component :is="iconMap.link" class="cursor-pointer nc-duplicate-row" />
{{ $t('labels.copyRecordURL') }}
</div>
</NcMenuItem>
<NcDivider />
<NcMenuItem
v-if="isUIAllowed('dataEdit') && !isNew"
v-e="['c:row-expand:delete']"
class="!text-red-500 !hover:bg-red-50"
@click="!isNew && onDeleteRowClick()"
>
<div data-testid="nc-expanded-form-delete">
<component :is="iconMap.delete" class="cursor-pointer nc-delete-row" />
Delete record
</div>
</NcMenuItem>
</NcMenu>
</template>
</NcDropdown>
<div class="flex flex-row gap-x-3">
<NcButton
v-if="isMobileMode"
type="secondary"
size="medium"
data-testid="nc-expanded-form-save"
class="nc-expand-form-save-btn !xs:(text-base)"
@click="onClose"
>
<div class="px-1">Close</div>
</NcButton>
<NcButton
v-e="['c:row-expand:save']"
data-testid="nc-expanded-form-save"
type="primary"
size="medium"
class="nc-expand-form-save-btn !xs:(text-base)"
:disabled="changedColumns.size === 0 && !isUnsavedFormExist"
@click="save"
>
<div class="xs:px-1">Save</div>
</NcButton>
</div>
</div>
</div>
<div
v-if="showRightSections"
class="nc-comments-drawer border-1 relative border-gray-200 w-1/3 max-w-125 bg-gray-50 rounded-xl min-w-0 overflow-hidden h-full xs:hidden"
:class="{ active: commentsDrawer && isUIAllowed('commentList') }"
>
<SmartsheetExpandedFormComments :loading="isLoading" />
</div>
</div>
</div>
</NcModal>
<GeneralDeleteModal v-model:visible="showDeleteRowModal" entity-name="Record" :on-delete="() => onConfirmDeleteRowClick()">
<template #entity-preview>
<span>
<div class="flex flex-row items-center py-2.25 px-2.5 bg-gray-50 rounded-lg text-gray-700 mb-4">
<div class="capitalize text-ellipsis overflow-hidden select-none w-full pl-1.75 break-keep whitespace-nowrap">
{{ displayValue }}
</div>
</div>
</span>
</template>
</GeneralDeleteModal>
<!-- Prevent unsaved change modal -->
<NcModal v-model:visible="preventModalStatus" size="small">
<div class="">
<div class="flex flex-row items-center gap-x-2 text-base font-bold">
{{ $t('tooltip.saveChanges') }}
</div>
<div class="flex font-medium mt-2">
{{ $t('activity.doYouWantToSaveTheChanges') }}
</div>
<div class="flex flex-row justify-end gap-x-2 mt-5">
<NcButton type="secondary" @click="discardPreventModal">{{ $t('labels.discard') }}</NcButton>
<NcButton key="submit" type="primary" label="Rename Table" loading-label="Renaming Table" @click="saveChanges">
{{ $t('tooltip.saveChanges') }}
</NcButton>
</div>
</div>
</NcModal>
</template>
<style lang="scss">
.nc-drawer-expanded-form {
@apply xs:my-0;
}
.nc-expanded-cell {
input {
@apply xs:(h-12 text-base);
}
}
.nc-expanded-cell-header {
@apply w-full text-gray-500 xs:(text-gray-600 mb-2);
}
.nc-expanded-cell-header > :nth-child(2) {
@apply !text-sm !xs:text-base;
}
.nc-expanded-cell-header > :first-child {
@apply !text-xl;
}
.nc-drawer-expanded-form .nc-modal {
@apply !p-0;
}
</style>
<style lang="scss" scoped>
:deep(.ant-select-selector) {
@apply !xs:(h-full);
}
:deep(.ant-select-selection-item) {
@apply !xs:(mt-1.75 ml-1);
}
.nc-data-cell:focus-within {
@apply !border-1 !border-brand-500 !rounded-lg !shadow-none !ring-0;
}
:deep(.nc-system-field input) {
@apply bg-transparent;
}
</style>
| packages/nc-gui/components/smartsheet/expanded-form/index.vue | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0022254304494708776,
0.00020900994422845542,
0.00016129952564369887,
0.00017116287199314684,
0.00023945257999002934
]
|
{
"id": 6,
"code_window": [
"\n",
" const abstractType = computed(() => column.value && sqlUi.value.getAbstractType(column.value))\n",
"\n",
" return () => {\n",
" if (!column.value && !columnMeta.value) return null\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" const abstractType = computed(() => column.value && sqlUi.value?.getAbstractType(column.value))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 119
} | version: '3'
x-uffizzi:
ingress:
service: nocodb
port: 8080
services:
postgres:
image: postgres
environment:
POSTGRES_PASSWORD: password
POSTGRES_USER: postgres
POSTGRES_DB: root_db
deploy:
resources:
limits:
memory: 500M
mssql:
image: "mcr.microsoft.com/mssql/server:2017-latest"
environment:
ACCEPT_EULA: "Y"
SA_PASSWORD: Password123.
deploy:
resources:
limits:
memory: 1000M
mysql:
environment:
MYSQL_DATABASE: root_db
MYSQL_PASSWORD: password
MYSQL_ROOT_PASSWORD: password
MYSQL_USER: noco
image: "mysql:8.0.35"
deploy:
resources:
limits:
memory: 500M
nocodb:
image: "${NOCODB_IMAGE}"
ports:
- "8080:8080"
entrypoint: /bin/sh
command: ["-c", "apk add wait4ports && wait4ports tcp://localhost:5432 && /usr/src/appEntry/start.sh"]
environment:
NC_DB: "pg://localhost:5432?u=postgres&p=password&d=root_db"
NC_ADMIN_EMAIL: [email protected]
NC_ADMIN_PASSWORD: password
deploy:
resources:
limits:
memory: 500M | .github/uffizzi/docker-compose.uffizzi.yml | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017855367332231253,
0.0001719984138617292,
0.00016608073201496154,
0.00017062723054550588,
0.0000043671366256603505
]
|
{
"id": 6,
"code_window": [
"\n",
" const abstractType = computed(() => column.value && sqlUi.value.getAbstractType(column.value))\n",
"\n",
" return () => {\n",
" if (!column.value && !columnMeta.value) return null\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" const abstractType = computed(() => column.value && sqlUi.value?.getAbstractType(column.value))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 119
} | import BasePage from '../../Base';
import { GridPage } from './index';
export class RowPageObject extends BasePage {
readonly grid: GridPage;
constructor(grid: GridPage) {
super(grid.rootPage);
this.grid = grid;
}
get() {
return this.rootPage.locator('tr.nc-grid-row');
}
getRecord(index: number) {
return this.get().nth(index);
}
// style="height: 3rem;"
async getRecordHeight(index: number) {
const record = this.getRecord(index);
const style = await record.getAttribute('style');
return style.split(':')[1].split(';')[0].trim();
}
}
| tests/playwright/pages/Dashboard/Grid/Row.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017448008293285966,
0.00017288750677835196,
0.0001697590487310663,
0.00017442338867112994,
0.000002212275148849585
]
|
{
"id": 6,
"code_window": [
"\n",
" const abstractType = computed(() => column.value && sqlUi.value.getAbstractType(column.value))\n",
"\n",
" return () => {\n",
" if (!column.value && !columnMeta.value) return null\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" const abstractType = computed(() => column.value && sqlUi.value?.getAbstractType(column.value))\n"
],
"file_path": "packages/nc-gui/components/smartsheet/header/CellIcon.ts",
"type": "replace",
"edit_start_line_idx": 119
} | <script lang="ts" setup>
import type { ColumnType, KanbanType, ViewType } from 'nocodb-sdk'
import { ViewTypes } from 'nocodb-sdk'
import tinycolor from 'tinycolor2'
import { useMetas } from '#imports'
const { view: _view, $api } = useSmartsheetStoreOrThrow()
const { $e } = useNuxtApp()
const { getBaseUrl, appInfo } = useGlobal()
const { dashboardUrl } = useDashboard()
const viewStore = useViewsStore()
const { metas } = useMetas()
const workspaceStore = useWorkspace()
const isLocked = inject(IsLockedInj, ref(false))
const isUpdating = ref({
public: false,
password: false,
download: false,
})
const activeView = computed<(ViewType & { meta: object & Record<string, any> }) | undefined>({
get: () => {
if (typeof _view.value?.meta === 'string') {
_view.value.meta = JSON.parse(_view.value.meta)
}
return _view.value as ViewType & { meta: object }
},
set: (value: ViewType | undefined) => {
if (typeof _view.value?.meta === 'string') {
_view.value!.meta = JSON.parse((_view.value.meta as string)!)
}
if (typeof value?.meta === 'string') {
value!.meta = JSON.parse(value.meta as string)
}
_view.value = value
},
})
const url = computed(() => {
return sharedViewUrl() ?? ''
})
const passwordProtectedLocal = ref(false)
const passwordProtected = computed(() => {
return !!activeView.value?.password || passwordProtectedLocal.value
})
const password = computed({
get: () => (passwordProtected.value ? activeView.value?.password ?? '' : ''),
set: async (value) => {
if (!activeView.value) return
activeView.value = { ...(activeView.value as any), password: passwordProtected.value ? value : null }
updateSharedView()
},
})
const viewTheme = computed({
get: () => !!activeView.value?.meta.withTheme,
set: (withTheme) => {
if (!activeView.value?.meta) return
activeView.value.meta = {
...activeView.value.meta,
withTheme,
}
saveTheme()
},
})
const togglePasswordProtected = async () => {
passwordProtectedLocal.value = !passwordProtected.value
if (!activeView.value) return
if (isUpdating.value.password) return
isUpdating.value.password = true
try {
if (passwordProtected.value) {
activeView.value = { ...(activeView.value as any), password: null }
} else {
activeView.value = { ...(activeView.value as any), password: '' }
}
await updateSharedView()
} finally {
isUpdating.value.password = false
}
}
const withRTL = computed({
get: () => {
if (!activeView.value?.meta) return false
if (typeof activeView.value?.meta === 'string') {
activeView.value.meta = JSON.parse(activeView.value.meta)
}
return !!(activeView.value?.meta as any)?.rtl
},
set: (rtl) => {
if (!activeView.value?.meta) return
if (typeof activeView.value?.meta === 'string') {
activeView.value.meta = JSON.parse(activeView.value.meta)
}
activeView.value.meta = { ...(activeView.value.meta as any), rtl }
updateSharedView()
},
})
const allowCSVDownload = computed({
get: () => !!(activeView.value?.meta as any)?.allowCSVDownload,
set: async (allow) => {
if (!activeView.value?.meta) return
isUpdating.value.download = true
try {
activeView.value.meta = { ...activeView.value.meta, allowCSVDownload: allow }
await saveAllowCSVDownload()
} finally {
isUpdating.value.download = false
}
},
})
const surveyMode = computed({
get: () => !!activeView.value?.meta.surveyMode,
set: (survey) => {
if (!activeView.value?.meta) return
activeView.value.meta = { ...activeView.value.meta, surveyMode: survey }
saveSurveyMode()
},
})
function sharedViewUrl() {
if (!activeView.value) return
let viewType
switch (activeView.value.type) {
case ViewTypes.FORM:
viewType = 'form'
break
case ViewTypes.KANBAN:
viewType = 'kanban'
break
case ViewTypes.GALLERY:
viewType = 'gallery'
break
case ViewTypes.MAP:
viewType = 'map'
break
default:
viewType = 'view'
}
// get base url for workspace
const baseUrl = getBaseUrl(workspaceStore.activeWorkspaceId)
let dashboardUrl1 = dashboardUrl.value
if (baseUrl) {
dashboardUrl1 = `${baseUrl}${appInfo.value?.dashboardPath}`
}
return encodeURI(`${dashboardUrl1}#/nc/${viewType}/${activeView.value.uuid}`)
}
const toggleViewShare = async () => {
if (!activeView.value?.id) return
if (activeView.value?.uuid) {
await $api.dbViewShare.delete(activeView.value.id)
activeView.value = { ...activeView.value, uuid: undefined, password: undefined }
} else {
const response = await $api.dbViewShare.create(activeView.value.id)
activeView.value = { ...activeView.value, ...(response as any) }
if (activeView.value!.type === ViewTypes.KANBAN) {
// extract grouping column meta
const groupingFieldColumn = metas.value[viewStore.activeView!.fk_model_id].columns!.find(
(col: ColumnType) => col.id === ((viewStore.activeView!.view! as KanbanType).fk_grp_col_id! as string),
)
activeView.value!.meta = { ...activeView.value!.meta, groupingFieldColumn }
await updateSharedView()
}
}
}
const toggleShare = async () => {
if (isUpdating.value.public) return
isUpdating.value.public = true
try {
return await toggleViewShare()
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
} finally {
isUpdating.value.public = false
}
}
async function saveAllowCSVDownload() {
isUpdating.value.download = true
try {
await updateSharedView()
$e(`a:view:share:${allowCSVDownload.value ? 'enable' : 'disable'}-csv-download`)
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
isUpdating.value.download = false
}
async function saveSurveyMode() {
await updateSharedView()
$e(`a:view:share:${surveyMode.value ? 'enable' : 'disable'}-survey-mode`)
}
async function saveTheme() {
await updateSharedView()
$e(`a:view:share:${viewTheme.value ? 'enable' : 'disable'}-theme`)
}
async function updateSharedView() {
try {
if (!activeView.value?.meta) return
const meta = activeView.value.meta
await $api.dbViewShare.update(activeView.value.id!, {
meta,
password: activeView.value.password,
})
} catch (e: any) {
message.error(await extractSdkResponseErrorMsg(e))
}
return true
}
function onChangeTheme(color: string) {
if (!activeView.value?.meta) return
const tcolor = tinycolor(color)
if (tcolor.isValid()) {
const complement = tcolor.complement()
activeView.value.meta.theme = {
primaryColor: color,
accentColor: complement.toHex8String(),
}
saveTheme()
}
}
const isPublicShared = computed(() => {
return !!activeView.value?.uuid
})
</script>
<template>
<div class="flex flex-col py-2 px-3 mb-1">
<div class="flex flex-col w-full mt-2.5 px-3 py-2.5 border-gray-200 border-1 rounded-md gap-y-2">
<div class="flex flex-row w-full justify-between py-0.5">
<div class="text-gray-900 font-medium">{{ $t('activity.enabledPublicViewing') }}</div>
<a-switch
v-e="['c:share:view:enable:toggle']"
data-testid="share-view-toggle"
:checked="isPublicShared"
:loading="isUpdating.public"
class="share-view-toggle !mt-0.25"
:disabled="isLocked"
@click="toggleShare"
/>
</div>
<template v-if="isPublicShared">
<div class="mt-0.5 border-t-1 border-gray-100 pt-3">
<GeneralCopyUrl v-model:url="url" />
</div>
<div class="flex flex-col justify-between mt-1 py-2 px-3 bg-gray-50 rounded-md">
<div class="flex flex-row justify-between">
<div class="flex text-black">{{ $t('activity.restrictAccessWithPassword') }}</div>
<a-switch
v-e="['c:share:view:password:toggle']"
data-testid="share-password-toggle"
:checked="passwordProtected"
:loading="isUpdating.password"
class="share-password-toggle !mt-0.25"
@click="togglePasswordProtected"
/>
</div>
<Transition name="layout" mode="out-in">
<div v-if="passwordProtected" class="flex gap-2 mt-2 w-2/3">
<a-input-password
v-model:value="password"
data-testid="nc-modal-share-view__password"
class="!rounded-lg !py-1 !bg-white"
size="small"
type="password"
:placeholder="$t('placeholder.password.enter')"
/>
</div>
</Transition>
</div>
<div class="flex flex-col justify-between gap-y-3 mt-1 py-2 px-3 bg-gray-50 rounded-md">
<div
v-if="
activeView &&
(activeView.type === ViewTypes.GRID ||
activeView.type === ViewTypes.KANBAN ||
activeView.type === ViewTypes.GALLERY ||
activeView.type === ViewTypes.MAP)
"
class="flex flex-row justify-between"
>
<div class="flex text-black">{{ $t('activity.allowDownload') }}</div>
<a-switch
v-model:checked="allowCSVDownload"
v-e="['c:share:view:allow-csv-download:toggle']"
data-testid="share-download-toggle"
:loading="isUpdating.download"
class="public-password-toggle !mt-0.25"
/>
</div>
<div v-if="activeView?.type === ViewTypes.FORM" class="flex flex-row justify-between">
<div class="text-black">{{ $t('activity.surveyMode') }}</div>
<a-switch
v-model:checked="surveyMode"
v-e="['c:share:view:surver-mode:toggle']"
data-testid="nc-modal-share-view__surveyMode"
>
</a-switch>
</div>
<div v-if="activeView?.type === ViewTypes.FORM && isEeUI" class="flex flex-row justify-between">
<div class="text-black">{{ $t('activity.rtlOrientation') }}</div>
<a-switch
v-model:checked="withRTL"
v-e="['c:share:view:rtl-orientation:toggle']"
data-testid="nc-modal-share-view__RTL"
>
</a-switch>
</div>
<div v-if="activeView?.type === ViewTypes.FORM" class="flex flex-col justify-between gap-y-1 bg-gray-50 rounded-md">
<div class="flex flex-row justify-between">
<div class="text-black">{{ $t('activity.useTheme') }}</div>
<a-switch
v-e="['c:share:view:theme:toggle']"
data-testid="share-theme-toggle"
:checked="viewTheme"
:loading="isUpdating.password"
class="share-theme-toggle !mt-0.25"
@click="viewTheme = !viewTheme"
/>
</div>
<Transition name="layout" mode="out-in">
<div v-if="viewTheme" class="flex -ml-1">
<LazyGeneralColorPicker
data-testid="nc-modal-share-view__theme-picker"
class="!p-0 !bg-inherit"
:model-value="activeView?.meta?.theme?.primaryColor"
:colors="baseThemeColors"
:row-size="9"
:advanced="false"
@input="onChangeTheme"
/>
</div>
</Transition>
</div>
</div>
</template>
</div>
</div>
</template>
<style lang="scss">
.docs-share-public-toggle {
height: 1.25rem !important;
min-width: 2.4rem !important;
width: 2.4rem !important;
line-height: 1rem;
.ant-switch-handle {
height: 1rem !important;
min-width: 1rem !important;
line-height: 0.8rem !important;
}
.ant-switch-inner {
height: 1rem !important;
min-width: 1rem !important;
line-height: 1rem !important;
}
}
</style>
| packages/nc-gui/components/dlg/share-and-collaborate/SharePage.vue | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00022112544684205204,
0.00017388901324011385,
0.00016578486247453839,
0.00017258997831959277,
0.000009730903911986388
]
|
{
"id": 7,
"code_window": [
"\n",
" const groupBy = computed<{ column: ColumnType; sort: string; order?: number }[]>(() => {\n",
" const tempGroupBy: { column: ColumnType; sort: string; order?: number }[] = []\n",
" Object.values(gridViewCols.value).forEach((col) => {\n",
" if (col.group_by) {\n",
" const column = meta?.value.columns?.find((f) => f.id === col.fk_column_id)\n",
" if (column) {\n",
" tempGroupBy.push({\n",
" column,\n",
" sort: col.group_by_sort || 'asc',\n",
" order: col.group_by_order || 1,\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const column = meta?.value?.columns?.find((f) => f.id === col.fk_column_id)\n"
],
"file_path": "packages/nc-gui/composables/useViewGroupBy.ts",
"type": "replace",
"edit_start_line_idx": 22
} | import type { ColumnType } from 'nocodb-sdk'
export const useColumnDrag = ({
fields,
tableBodyEl,
gridWrapper,
}: {
fields: Ref<ColumnType[]>
tableBodyEl: Ref<HTMLElement | undefined>
gridWrapper: Ref<HTMLElement | undefined>
}) => {
const { eventBus } = useSmartsheetStoreOrThrow()
const { addUndo, defineViewScope } = useUndoRedo()
const { activeView } = storeToRefs(useViewsStore())
const { gridViewCols, updateGridViewColumn } = useViewColumnsOrThrow()
const { leftSidebarWidth } = storeToRefs(useSidebarStore())
const { width } = useWindowSize()
const draggedCol = ref<ColumnType | null>(null)
const dragColPlaceholderDomRef = ref<HTMLElement | null>(null)
const toBeDroppedColId = ref<string | null>(null)
const reorderColumn = async (colId: string, toColId: string) => {
const toBeReorderedViewCol = gridViewCols.value[colId]
const toViewCol = gridViewCols.value[toColId]!
const toColIndex = fields.value.findIndex((f) => f.id === toColId)
const nextToColField = toColIndex < fields.value.length - 1 ? fields.value[toColIndex + 1] : null
const nextToViewCol = nextToColField ? gridViewCols.value[nextToColField.id!] : null
const lastCol = fields.value[fields.value.length - 1]
const lastViewCol = gridViewCols.value[lastCol.id!]
// if nextToViewCol/toViewCol is null, return
if (nextToViewCol === null || lastViewCol === null) return
const newOrder = nextToViewCol ? toViewCol.order! + (nextToViewCol.order! - toViewCol.order!) / 2 : lastViewCol.order! + 1
const oldOrder = toBeReorderedViewCol.order
toBeReorderedViewCol.order = newOrder
addUndo({
undo: {
fn: async () => {
if (!fields.value) return
toBeReorderedViewCol.order = oldOrder
await updateGridViewColumn(colId, { order: oldOrder } as any)
eventBus.emit(SmartsheetStoreEvents.FIELD_RELOAD)
},
args: [],
},
redo: {
fn: async () => {
if (!fields.value) return
toBeReorderedViewCol.order = newOrder
await updateGridViewColumn(colId, { order: newOrder } as any)
eventBus.emit(SmartsheetStoreEvents.FIELD_RELOAD)
},
args: [],
},
scope: defineViewScope({ view: activeView.value }),
})
await updateGridViewColumn(colId, { order: newOrder } as any, true)
eventBus.emit(SmartsheetStoreEvents.FIELD_RELOAD)
}
const onDragStart = (colId: string, e: DragEvent) => {
if (!e.dataTransfer) return
const dom = document.querySelector('[data-testid="drag-icon-placeholder"]')
e.dataTransfer.dropEffect = 'none'
e.dataTransfer.effectAllowed = 'none'
e.dataTransfer.setDragImage(dom!, 10, 10)
e.dataTransfer.clearData()
e.dataTransfer.setData('text/plain', colId)
draggedCol.value = fields.value.find((f) => f.id === colId) ?? null
const remInPx = parseFloat(getComputedStyle(document.documentElement).fontSize)
const placeholderHeight = tableBodyEl.value?.getBoundingClientRect().height ?? 6.1 * remInPx
dragColPlaceholderDomRef.value!.style.height = `${placeholderHeight}px`
const x = e.clientX - leftSidebarWidth.value
if (x >= 0 && dragColPlaceholderDomRef.value) {
dragColPlaceholderDomRef.value.style.left = `${x.toString()}px`
}
}
const onDrag = (e: DragEvent) => {
e.preventDefault()
if (!e.dataTransfer) return
if (!draggedCol.value) return
if (!dragColPlaceholderDomRef.value) return
if (e.clientX === 0) {
dragColPlaceholderDomRef.value!.style.left = `0px`
dragColPlaceholderDomRef.value!.style.height = '0px'
reorderColumn(draggedCol.value!.id!, toBeDroppedColId.value!)
draggedCol.value = null
toBeDroppedColId.value = null
return
}
const y = dragColPlaceholderDomRef.value!.getBoundingClientRect().top
const domsUnderMouse = document.elementsFromPoint(e.clientX, y)
const columnDom = domsUnderMouse.find((dom) => dom.classList.contains('nc-grid-column-header'))
if (columnDom) {
toBeDroppedColId.value = columnDom?.getAttribute('data-col') ?? null
}
const x = e.clientX - leftSidebarWidth.value
if (x >= 0) {
dragColPlaceholderDomRef.value.style.left = `${x.toString()}px`
}
const remInPx = parseFloat(getComputedStyle(document.documentElement).fontSize)
if (x < leftSidebarWidth.value + 1 * remInPx) {
setTimeout(() => {
gridWrapper.value!.scrollLeft -= 2.5
}, 250)
} else if (width.value - x - leftSidebarWidth.value < 15 * remInPx) {
setTimeout(() => {
gridWrapper.value!.scrollLeft += 2.5
}, 250)
}
}
return {
onDrag,
onDragStart,
draggedCol,
dragColPlaceholderDomRef,
toBeDroppedColId,
}
}
| packages/nc-gui/components/smartsheet/grid/useColumnDrag.ts | 1 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.011692248284816742,
0.0013512281002476811,
0.00016427424270659685,
0.00017578763072378933,
0.0029844294767826796
]
|
{
"id": 7,
"code_window": [
"\n",
" const groupBy = computed<{ column: ColumnType; sort: string; order?: number }[]>(() => {\n",
" const tempGroupBy: { column: ColumnType; sort: string; order?: number }[] = []\n",
" Object.values(gridViewCols.value).forEach((col) => {\n",
" if (col.group_by) {\n",
" const column = meta?.value.columns?.find((f) => f.id === col.fk_column_id)\n",
" if (column) {\n",
" tempGroupBy.push({\n",
" column,\n",
" sort: col.group_by_sort || 'asc',\n",
" order: col.group_by_order || 1,\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const column = meta?.value?.columns?.find((f) => f.id === col.fk_column_id)\n"
],
"file_path": "packages/nc-gui/composables/useViewGroupBy.ts",
"type": "replace",
"edit_start_line_idx": 22
} | <svg width="17" height="16" viewBox="0 0 17 16" fill="none" xmlns="http://www.w3.org/2000/svg">
<g clip-path="url(#clip0_1409_68546)">
<path d="M12.3571 5.96842L4.64282 10.4219" stroke="#1F293A" stroke-width="1.33333" stroke-linecap="round" stroke-linejoin="round"/>
<rect x="1.15184" width="9.06208" height="9.06208" rx="1.335" transform="matrix(0.866044 -0.499967 0.866044 0.499967 -0.345705 8.77119)" stroke="#1F293A" stroke-width="1.33"/>
<path d="M4 6.33984L11.7143 10.7933" stroke="#1F293A" stroke-width="1.33333" stroke-linecap="round" stroke-linejoin="round"/>
</g>
<defs>
<clipPath id="clip0_1409_68546">
<rect width="16" height="16" fill="white" transform="translate(0.5)"/>
</clipPath>
</defs>
</svg>
| packages/nc-gui/assets/nc-icons/table.svg | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.00017193576786667109,
0.00016853901615832,
0.00016514226444996893,
0.00016853901615832,
0.0000033967517083510756
]
|
{
"id": 7,
"code_window": [
"\n",
" const groupBy = computed<{ column: ColumnType; sort: string; order?: number }[]>(() => {\n",
" const tempGroupBy: { column: ColumnType; sort: string; order?: number }[] = []\n",
" Object.values(gridViewCols.value).forEach((col) => {\n",
" if (col.group_by) {\n",
" const column = meta?.value.columns?.find((f) => f.id === col.fk_column_id)\n",
" if (column) {\n",
" tempGroupBy.push({\n",
" column,\n",
" sort: col.group_by_sort || 'asc',\n",
" order: col.group_by_order || 1,\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const column = meta?.value?.columns?.find((f) => f.id === col.fk_column_id)\n"
],
"file_path": "packages/nc-gui/composables/useViewGroupBy.ts",
"type": "replace",
"edit_start_line_idx": 22
} | import { expect, Locator } from '@playwright/test';
import { DashboardPage } from '../../index';
import BasePage from '../../../Base';
import { getTextExcludeIconText } from '../../../../tests/utils/general';
import { isEE } from '../../../../setup/db';
import { NcContext } from '../../../../setup';
export class LeftSidebarPage extends BasePage {
readonly base: any;
readonly dashboard: DashboardPage;
readonly btn_workspace: Locator;
readonly btn_newProject: Locator;
readonly btn_cmdK: Locator;
readonly btn_teamAndSettings: Locator;
readonly modal_workspace: Locator;
constructor(dashboard: DashboardPage) {
super(dashboard.rootPage);
this.dashboard = dashboard;
this.btn_workspace = this.get().locator('.nc-workspace-menu');
this.btn_newProject = this.get().locator('[data-testid="nc-sidebar-create-base-btn"]');
this.btn_cmdK = this.get().locator('[data-testid="nc-sidebar-search-btn"]');
this.btn_teamAndSettings = this.get().locator('[data-testid="nc-sidebar-team-settings-btn"]');
this.modal_workspace = this.rootPage.locator('.nc-dropdown-workspace-menu');
}
get() {
return this.dashboard.get().locator('.nc-sidebar');
}
async createProject({ title, context }: { title: string; context: NcContext }) {
title = isEE() ? title : `nc-${context.workerId}-${title}`;
await this.btn_newProject.click();
await this.rootPage.locator('.ant-modal-content:has-text(" Create Base")').waitFor();
await this.rootPage.locator('.ant-modal-content:has-text(" Create Base")').locator('input').fill(title);
await this.rootPage
.locator('.ant-modal-content:has-text(" Create Base")')
.locator('button.ant-btn-primary')
.click();
}
async clickTeamAndSettings() {
await this.btn_teamAndSettings.click();
}
async clickWorkspace() {
await this.btn_workspace.click();
}
async clickHome() {}
async getWorkspaceName() {
return await this.btn_workspace.getAttribute('data-workspace-title');
}
async verifyWorkspaceName({ title }: { title: string }) {
await expect(this.btn_workspace.locator('.nc-workspace-title')).toHaveText(title);
}
async createWorkspace({ title }: { title: string }) {
await this.clickWorkspace();
await this.modal_workspace.locator('.ant-dropdown-menu-item:has-text("Create New Workspace")').waitFor();
await this.modal_workspace.locator('.ant-dropdown-menu-item:has-text("Create New Workspace")').click();
const inputModal = this.rootPage.locator('div.ant-modal.active');
await inputModal.waitFor();
await inputModal.locator('input').clear();
await inputModal.locator('input').fill(title);
await inputModal.locator('button.ant-btn-primary').click();
}
async verifyWorkspaceCount({ count }: { count: number }) {
await this.clickWorkspace();
// TODO: THere is one extra html attribute
await expect(this.rootPage.getByTestId('nc-workspace-list')).toHaveCount(count + 1);
}
async getWorkspaceList() {
const ws = [];
await this.clickWorkspace();
const nodes = this.modal_workspace.locator('[data-testid="nc-workspace-list"]');
for (let i = 0; i < (await nodes.count()); i++) {
ws.push(await getTextExcludeIconText(nodes.nth(i)));
}
ws.push(await this.getWorkspaceName());
await this.rootPage.keyboard.press('Escape');
return ws;
}
async openWorkspace(param: { title: any }) {
await this.clickWorkspace();
const nodes = this.modal_workspace.locator('[data-testid="nc-workspace-list"]');
await this.rootPage.waitForTimeout(2000);
for (let i = 0; i < (await nodes.count()); i++) {
const text = await getTextExcludeIconText(nodes.nth(i));
if (text.toLowerCase() === param.title.toLowerCase()) {
await nodes.nth(i).click({ force: true });
break;
}
}
await this.rootPage.keyboard.press('Escape');
await this.rootPage.waitForTimeout(3500);
}
}
| tests/playwright/pages/Dashboard/common/LeftSidebar/index.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0001757246209308505,
0.00017278945597354323,
0.00016924412921071053,
0.00017231909441761672,
0.0000019081223854300333
]
|
{
"id": 7,
"code_window": [
"\n",
" const groupBy = computed<{ column: ColumnType; sort: string; order?: number }[]>(() => {\n",
" const tempGroupBy: { column: ColumnType; sort: string; order?: number }[] = []\n",
" Object.values(gridViewCols.value).forEach((col) => {\n",
" if (col.group_by) {\n",
" const column = meta?.value.columns?.find((f) => f.id === col.fk_column_id)\n",
" if (column) {\n",
" tempGroupBy.push({\n",
" column,\n",
" sort: col.group_by_sort || 'asc',\n",
" order: col.group_by_order || 1,\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const column = meta?.value?.columns?.find((f) => f.id === col.fk_column_id)\n"
],
"file_path": "packages/nc-gui/composables/useViewGroupBy.ts",
"type": "replace",
"edit_start_line_idx": 22
} | import { UITypes } from 'nocodb-sdk';
import type { BoolType, FilterType } from 'nocodb-sdk';
import type { COMPARISON_OPS, COMPARISON_SUB_OPS } from '~/utils/globals';
import Model from '~/models/Model';
import Column from '~/models/Column';
import Hook from '~/models/Hook';
import View from '~/models/View';
import Noco from '~/Noco';
import {
CacheDelDirection,
CacheGetType,
CacheScope,
MetaTable,
} from '~/utils/globals';
import NocoCache from '~/cache/NocoCache';
import { NcError } from '~/helpers/catchError';
import { extractProps } from '~/helpers/extractProps';
export default class Filter implements FilterType {
id: string;
fk_model_id?: string;
fk_view_id?: string;
fk_hook_id?: string;
fk_column_id?: string;
fk_parent_id?: string;
comparison_op?: (typeof COMPARISON_OPS)[number];
comparison_sub_op?: (typeof COMPARISON_SUB_OPS)[number];
value?: string;
logical_op?: 'and' | 'or' | 'not';
is_group?: BoolType;
children?: Filter[];
base_id?: string;
source_id?: string;
column?: Column;
constructor(data: Filter | FilterType) {
Object.assign(this, data);
}
public static castType(filter: Filter): Filter {
return filter && new Filter(filter);
}
public castType(filter: Filter): Filter {
return filter && new Filter(filter);
}
public async getModel(ncMeta = Noco.ncMeta): Promise<Model> {
return this.fk_view_id
? (await View.get(this.fk_view_id, ncMeta)).getModel(ncMeta)
: Model.getByIdOrName(
{
id: this.fk_model_id,
},
ncMeta,
);
}
public static async insert(
filter: Partial<FilterType> & { order?: number },
ncMeta = Noco.ncMeta,
) {
const insertObj = extractProps(filter, [
'id',
'fk_view_id',
'fk_hook_id',
'fk_column_id',
'comparison_op',
'comparison_sub_op',
'value',
'fk_parent_id',
'is_group',
'logical_op',
'base_id',
'source_id',
'order',
]);
const referencedModelColName = filter.fk_hook_id
? 'fk_hook_id'
: 'fk_view_id';
insertObj.order = await ncMeta.metaGetNextOrder(MetaTable.FILTER_EXP, {
[referencedModelColName]: filter[referencedModelColName],
});
if (!(filter.base_id && filter.source_id)) {
let model: { base_id?: string; source_id?: string };
if (filter.fk_view_id) {
model = await View.get(filter.fk_view_id, ncMeta);
} else if (filter.fk_hook_id) {
model = await Hook.get(filter.fk_hook_id, ncMeta);
} else if (filter.fk_column_id) {
model = await Column.get({ colId: filter.fk_column_id }, ncMeta);
} else {
NcError.badRequest('Invalid filter');
}
if (model != null) {
insertObj.base_id = model.base_id;
insertObj.source_id = model.source_id;
}
}
const row = await ncMeta.metaInsert2(
null,
null,
MetaTable.FILTER_EXP,
insertObj,
);
if (filter?.children?.length) {
await Promise.all(
filter.children.map((f) =>
this.insert(
{
...f,
fk_parent_id: row.id,
[filter.fk_hook_id ? 'fk_hook_id' : 'fk_view_id']:
filter.fk_hook_id ? filter.fk_hook_id : filter.fk_view_id,
},
ncMeta,
),
),
);
}
return await this.redisPostInsert(row.id, filter, ncMeta);
}
static async redisPostInsert(
id,
filter: Partial<FilterType>,
ncMeta = Noco.ncMeta,
) {
if (!(id && (filter.fk_view_id || filter.fk_hook_id))) {
throw new Error(
`Mandatory fields missing in FITLER_EXP cache population : id(${id}), fk_view_id(${filter.fk_view_id}), fk_hook_id(${filter.fk_hook_id})`,
);
}
const key = `${CacheScope.FILTER_EXP}:${id}`;
let value = await NocoCache.get(key, CacheGetType.TYPE_OBJECT);
if (!value) {
/* get from db */
value = await ncMeta.metaGet2(null, null, MetaTable.FILTER_EXP, id);
// pushing calls for Promise.all
const p = [];
/* store in redis */
p.push(NocoCache.set(key, value));
/* append key to relevant lists */
if (filter.fk_view_id) {
p.push(
NocoCache.appendToList(
CacheScope.FILTER_EXP,
[filter.fk_view_id],
key,
),
);
}
if (filter.fk_hook_id) {
p.push(
NocoCache.appendToList(
CacheScope.FILTER_EXP,
[filter.fk_hook_id],
key,
),
);
}
if (filter.fk_parent_id) {
if (filter.fk_view_id) {
p.push(
NocoCache.appendToList(
CacheScope.FILTER_EXP,
[filter.fk_view_id, filter.fk_parent_id],
key,
),
);
}
if (filter.fk_hook_id) {
p.push(
NocoCache.appendToList(
CacheScope.FILTER_EXP,
[filter.fk_hook_id, filter.fk_parent_id],
key,
),
);
}
p.push(
NocoCache.appendToList(
CacheScope.FILTER_EXP,
[filter.fk_parent_id],
key,
),
);
}
if (filter.fk_column_id) {
p.push(
NocoCache.appendToList(
CacheScope.FILTER_EXP,
[filter.fk_column_id],
key,
),
);
}
await Promise.all(p);
}
// on new filter creation delete any optimised single query cache
{
// if not a view filter then no need to delete
if (filter.fk_view_id) {
const view = await View.get(filter.fk_view_id, ncMeta);
await NocoCache.delAll(
CacheScope.SINGLE_QUERY,
`${view.fk_model_id}:${view.id}:*`,
);
}
}
return this.castType(value);
}
static async update(id, filter: Partial<Filter>, ncMeta = Noco.ncMeta) {
const updateObj = extractProps(filter, [
'fk_column_id',
'comparison_op',
'comparison_sub_op',
'value',
'fk_parent_id',
'is_group',
'logical_op',
]);
if (typeof updateObj.value === 'string')
updateObj.value = updateObj.value.slice(0, 255);
// get existing cache
const key = `${CacheScope.FILTER_EXP}:${id}`;
let o = await NocoCache.get(key, CacheGetType.TYPE_OBJECT);
// update alias
if (o) {
o = { ...o, ...updateObj };
// set cache
await NocoCache.set(key, o);
}
// set meta
const res = await ncMeta.metaUpdate(
null,
null,
MetaTable.FILTER_EXP,
updateObj,
id,
);
// on update delete any optimised single query cache
{
const filter = await this.get(id, ncMeta);
// if not a view filter then no need to delete
if (filter.fk_view_id) {
const view = await View.get(filter.fk_view_id, ncMeta);
await NocoCache.delAll(
CacheScope.SINGLE_QUERY,
`${view.fk_model_id}:${view.id}:*`,
);
}
}
return res;
}
static async delete(id: string, ncMeta = Noco.ncMeta) {
const filter = await this.get(id, ncMeta);
const deleteRecursively = async (filter: Filter) => {
if (!filter) return;
for (const f of (await filter?.getChildren()) || [])
await deleteRecursively(f);
await ncMeta.metaDelete(null, null, MetaTable.FILTER_EXP, filter.id);
await NocoCache.deepDel(
CacheScope.FILTER_EXP,
`${CacheScope.FILTER_EXP}:${filter.id}`,
CacheDelDirection.CHILD_TO_PARENT,
);
};
await deleteRecursively(filter);
// delete any optimised single query cache
{
// if not a view filter then no need to delete
if (filter.fk_view_id) {
const view = await View.get(filter.fk_view_id, ncMeta);
await NocoCache.delAll(
CacheScope.SINGLE_QUERY,
`${view.fk_model_id}:${view.id}:*`,
);
}
}
}
public getColumn(): Promise<Column> {
if (!this.fk_column_id) return null;
return Column.get({
colId: this.fk_column_id,
});
}
public async getGroup(ncMeta = Noco.ncMeta): Promise<Filter> {
if (!this.fk_parent_id) return null;
let filterObj = await NocoCache.get(
`${CacheScope.FILTER_EXP}:${this.fk_parent_id}`,
2,
);
if (!filterObj) {
filterObj = await ncMeta.metaGet2(null, null, MetaTable.FILTER_EXP, {
id: this.fk_parent_id,
});
await NocoCache.set(
`${CacheScope.FILTER_EXP}:${this.fk_parent_id}`,
filterObj,
);
}
return this.castType(filterObj);
}
public async getChildren(ncMeta = Noco.ncMeta): Promise<Filter[]> {
if (this.children) return this.children;
if (!this.is_group) return null;
const cachedList = await NocoCache.getList(CacheScope.FILTER_EXP, [
this.id,
]);
let { list: childFilters } = cachedList;
const { isNoneList } = cachedList;
if (!isNoneList && !childFilters.length) {
childFilters = await ncMeta.metaList2(null, null, MetaTable.FILTER_EXP, {
condition: {
fk_parent_id: this.id,
},
orderBy: {
order: 'asc',
},
});
await NocoCache.setList(CacheScope.FILTER_EXP, [this.id], childFilters);
}
return childFilters && childFilters.map((f) => this.castType(f));
}
// public static async getFilter({
// viewId
// }: {
// viewId: string;
// }): Promise<Filter> {
// if (!viewId) return null;
//
// const filterObj = await ncMeta.metaGet2(
// null,
// null,
// MetaTable.FILTER_EXP,
// { fk_view_id: viewId, fk_parent_id: null }
// );
// return filterObj && new Filter(filterObj);
// }
public static async getFilterObject(
{
viewId,
hookId,
}: {
viewId?: string;
hookId?: string;
},
ncMeta = Noco.ncMeta,
): Promise<FilterType> {
const cachedList = await NocoCache.getList(CacheScope.FILTER_EXP, [
viewId || hookId,
]);
let { list: filters } = cachedList;
const { isNoneList } = cachedList;
if (!isNoneList && !filters.length) {
filters = await ncMeta.metaList2(null, null, MetaTable.FILTER_EXP, {
condition: viewId ? { fk_view_id: viewId } : { fk_hook_id: hookId },
orderBy: {
order: 'asc',
},
});
await NocoCache.setList(
CacheScope.FILTER_EXP,
[viewId || hookId],
filters,
);
}
const result: FilterType = {
is_group: true,
children: [],
logical_op: 'and',
};
const grouped = {};
const idFilterMapping = {};
for (const filter of filters) {
if (!filter._fk_parent_id) {
result.children.push(filter);
idFilterMapping[result.id] = result;
} else {
grouped[filter._fk_parent_id] = grouped[filter._fk_parent_id] || [];
grouped[filter._fk_parent_id].push(filter);
idFilterMapping[filter.id] = filter;
filter.column = await new Filter(filter).getColumn();
if (filter.column?.uidt === UITypes.LinkToAnotherRecord) {
}
}
}
for (const [id, children] of Object.entries(grouped)) {
if (idFilterMapping?.[id]) idFilterMapping[id].children = children;
}
// if (!result) {
// return (await Filter.insert({
// fk_view_id: viewId,
// is_group: true,
// logical_op: 'AND'
// })) as any;
// }
return result;
}
static async deleteAll(viewId: string, ncMeta = Noco.ncMeta) {
const filter = await this.getFilterObject({ viewId }, ncMeta);
const deleteRecursively = async (filter) => {
if (!filter) return;
for (const f of filter?.children || []) await deleteRecursively(f);
if (filter.id) {
await ncMeta.metaDelete(null, null, MetaTable.FILTER_EXP, filter.id);
await NocoCache.deepDel(
CacheScope.FILTER_EXP,
`${CacheScope.FILTER_EXP}:${filter.id}`,
CacheDelDirection.CHILD_TO_PARENT,
);
}
};
await deleteRecursively(filter);
// on update delete any optimised single query cache
{
const view = await View.get(viewId, ncMeta);
await NocoCache.delAll(
CacheScope.SINGLE_QUERY,
`${view.fk_model_id}:${view.id}:*`,
);
}
}
static async deleteAllByHook(hookId: string, ncMeta = Noco.ncMeta) {
const filter = await this.getFilterObject({ hookId }, ncMeta);
const deleteRecursively = async (filter) => {
if (!filter) return;
for (const f of filter?.children || []) await deleteRecursively(f);
if (filter.id) {
await ncMeta.metaDelete(null, null, MetaTable.FILTER_EXP, filter.id);
await NocoCache.deepDel(
CacheScope.FILTER_EXP,
`${CacheScope.FILTER_EXP}:${filter.id}`,
CacheDelDirection.CHILD_TO_PARENT,
);
}
};
await deleteRecursively(filter);
}
public static async get(id: string, ncMeta = Noco.ncMeta) {
let filterObj =
id &&
(await NocoCache.get(
`${CacheScope.FILTER_EXP}:${id}`,
CacheGetType.TYPE_OBJECT,
));
if (!filterObj) {
filterObj = await ncMeta.metaGet2(null, null, MetaTable.FILTER_EXP, {
id,
});
await NocoCache.set(`${CacheScope.FILTER_EXP}:${id}`, filterObj);
}
return this.castType(filterObj);
}
static async rootFilterList(
{ viewId }: { viewId: any },
ncMeta = Noco.ncMeta,
) {
const cachedList = await NocoCache.getList(CacheScope.FILTER_EXP, [viewId]);
let { list: filterObjs } = cachedList;
const { isNoneList } = cachedList;
if (!isNoneList && !filterObjs.length) {
filterObjs = await ncMeta.metaList2(null, null, MetaTable.FILTER_EXP, {
condition: { fk_view_id: viewId },
orderBy: {
order: 'asc',
},
});
await NocoCache.setList(CacheScope.FILTER_EXP, [viewId], filterObjs);
}
return filterObjs
?.filter((f) => !f.fk_parent_id)
?.map((f) => this.castType(f));
}
static async rootFilterListByHook(
{ hookId }: { hookId: any },
ncMeta = Noco.ncMeta,
) {
const cachedList = await NocoCache.getList(CacheScope.FILTER_EXP, [hookId]);
let { list: filterObjs } = cachedList;
const { isNoneList } = cachedList;
if (!isNoneList && !filterObjs.length) {
filterObjs = await ncMeta.metaList2(null, null, MetaTable.FILTER_EXP, {
condition: { fk_hook_id: hookId },
orderBy: {
order: 'asc',
},
});
await NocoCache.setList(CacheScope.FILTER_EXP, [hookId], filterObjs);
}
return filterObjs?.map((f) => this.castType(f));
}
static async parentFilterList(
{
parentId,
}: {
parentId: any;
},
ncMeta = Noco.ncMeta,
) {
const cachedList = await NocoCache.getList(CacheScope.FILTER_EXP, [
parentId,
]);
let { list: filterObjs } = cachedList;
const { isNoneList } = cachedList;
if (!isNoneList && !filterObjs.length) {
filterObjs = await ncMeta.metaList2(null, null, MetaTable.FILTER_EXP, {
condition: {
fk_parent_id: parentId,
// fk_view_id: viewId,
},
orderBy: {
order: 'asc',
},
});
await NocoCache.setList(CacheScope.FILTER_EXP, [parentId], filterObjs);
}
return filterObjs?.map((f) => this.castType(f));
}
static async parentFilterListByHook(
{
hookId,
parentId,
}: {
hookId: any;
parentId: any;
},
ncMeta = Noco.ncMeta,
) {
const cachedList = await NocoCache.getList(CacheScope.FILTER_EXP, [
hookId,
parentId,
]);
let { list: filterObjs } = cachedList;
const { isNoneList } = cachedList;
if (!isNoneList && !filterObjs.length) {
filterObjs = await ncMeta.metaList2(null, null, MetaTable.FILTER_EXP, {
condition: {
fk_parent_id: parentId,
fk_hook_id: hookId,
},
orderBy: {
order: 'asc',
},
});
await NocoCache.setList(
CacheScope.FILTER_EXP,
[hookId, parentId],
filterObjs,
);
}
return filterObjs?.map((f) => this.castType(f));
}
static async hasEmptyOrNullFilters(baseId: string, ncMeta = Noco.ncMeta) {
const emptyOrNullFilterObjs = await ncMeta.metaList2(
null,
null,
MetaTable.FILTER_EXP,
{
condition: {
base_id: baseId,
},
xcCondition: {
_or: [
{
comparison_op: {
eq: 'null',
},
},
{
comparison_op: {
eq: 'notnull',
},
},
{
comparison_op: {
eq: 'empty',
},
},
{
comparison_op: {
eq: 'notempty',
},
},
],
},
},
);
return emptyOrNullFilterObjs.length > 0;
}
}
| packages/nocodb/src/models/Filter.ts | 0 | https://github.com/nocodb/nocodb/commit/fd0803ccc887b0e7c03e496015f5a5a414800075 | [
0.0046800607815384865,
0.0002748904807958752,
0.00016110186697915196,
0.00017042287799995393,
0.0005677356384694576
]
|
{
"id": 0,
"code_window": [
" }\n",
" scene.scale = scale;\n",
" };\n",
"\n",
" return (\n",
" <TransformWrapper\n",
" doubleClick={{ mode: 'reset' }}\n",
" ref={scene.transformComponentRef}\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!scene.shouldPanZoom || scene.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!scene.contextMenuVisible && !scene.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "add",
"edit_start_line_idx": 21
} | import React from 'react';
import { TransformWrapper, TransformComponent, ReactZoomPanPinchRef } from 'react-zoom-pan-pinch';
import { config } from '@grafana/runtime';
import { Scene } from './scene';
type SceneTransformWrapperProps = {
scene: Scene;
children: React.ReactNode;
};
export const SceneTransformWrapper = ({ scene, children: sceneDiv }: SceneTransformWrapperProps) => {
const onZoom = (zoomPanPinchRef: ReactZoomPanPinchRef) => {
const scale = zoomPanPinchRef.state.scale;
if (scene.moveable && scale > 0) {
scene.moveable.zoom = 1 / scale;
}
scene.scale = scale;
};
return (
<TransformWrapper
doubleClick={{ mode: 'reset' }}
ref={scene.transformComponentRef}
onZoom={onZoom}
onTransformed={(_, state) => {
scene.scale = state.scale;
}}
limitToBounds={true}
disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}
panning={{ allowLeftClickPan: false }}
>
<TransformComponent>{sceneDiv}</TransformComponent>
</TransformWrapper>
);
};
| public/app/features/canvas/runtime/SceneTransformWrapper.tsx | 1 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.9806675910949707,
0.24722890555858612,
0.001354818930849433,
0.0034465992357581854,
0.4234539568424225
]
|
{
"id": 0,
"code_window": [
" }\n",
" scene.scale = scale;\n",
" };\n",
"\n",
" return (\n",
" <TransformWrapper\n",
" doubleClick={{ mode: 'reset' }}\n",
" ref={scene.transformComponentRef}\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!scene.shouldPanZoom || scene.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!scene.contextMenuVisible && !scene.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "add",
"edit_start_line_idx": 21
} | import { render, screen, fireEvent, waitFor } from '@testing-library/react';
import userEvent from '@testing-library/user-event';
import React from 'react';
import { TestProvider } from 'test/helpers/TestProvider';
import { ServiceAccountCreatePage, Props } from './ServiceAccountCreatePage';
const postMock = jest.fn().mockResolvedValue({});
const patchMock = jest.fn().mockResolvedValue({});
const putMock = jest.fn().mockResolvedValue({});
jest.mock('@grafana/runtime', () => ({
...jest.requireActual('@grafana/runtime'),
getBackendSrv: () => ({
post: postMock,
patch: patchMock,
put: putMock,
}),
config: {
loginError: false,
buildInfo: {
version: 'v1.0',
commit: '1',
env: 'production',
edition: 'Open Source',
},
licenseInfo: {
stateInfo: '',
licenseUrl: '',
},
appSubUrl: '',
featureToggles: {},
},
}));
jest.mock('app/core/core', () => ({
contextSrv: {
licensedAccessControlEnabled: () => false,
hasPermission: () => true,
hasPermissionInMetadata: () => true,
user: { orgId: 1 },
fetchUserPermissions: () => Promise.resolve(),
},
}));
const setup = (propOverrides: Partial<Props>) => {
const props: Props = {
navModel: {
main: {
text: 'Configuration',
},
node: {
text: 'Service accounts',
},
},
};
Object.assign(props, propOverrides);
render(
<TestProvider>
<ServiceAccountCreatePage {...props} />
</TestProvider>
);
};
describe('ServiceAccountCreatePage tests', () => {
it('Should display service account create page', () => {
setup({});
expect(screen.getByRole('button', { name: 'Create' })).toBeInTheDocument();
});
it('Should fire form validation error if name is not set', async () => {
setup({});
fireEvent.click(screen.getByRole('button', { name: 'Create' }));
expect(await screen.findByText('Display name is required')).toBeInTheDocument();
});
it('Should call API with proper params when creating new service account', async () => {
setup({});
await userEvent.type(screen.getByLabelText('Display name *'), 'Data source scavenger');
fireEvent.click(screen.getByRole('button', { name: 'Create' }));
await waitFor(() =>
expect(postMock).toHaveBeenCalledWith('/api/serviceaccounts/', {
name: 'Data source scavenger',
role: 'Viewer',
})
);
});
});
| public/app/features/serviceaccounts/ServiceAccountCreatePage.test.tsx | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017932659829966724,
0.00017433674656786025,
0.00017143451259471476,
0.00017392980225849897,
0.00000236226833294495
]
|
{
"id": 0,
"code_window": [
" }\n",
" scene.scale = scale;\n",
" };\n",
"\n",
" return (\n",
" <TransformWrapper\n",
" doubleClick={{ mode: 'reset' }}\n",
" ref={scene.transformComponentRef}\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!scene.shouldPanZoom || scene.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!scene.contextMenuVisible && !scene.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "add",
"edit_start_line_idx": 21
} | ---
_build:
list: false
labels:
products:
- cloud
- enterprise
- oss
title: Release notes for Grafana 8.0.3
---
<!-- Auto generated by update changelog github action -->
# Release notes for Grafana 8.0.3
### Features and enhancements
- **Alerting:** Increase alertmanager_conf column if MySQL. [#35657](https://github.com/grafana/grafana/pull/35657), [@kylebrandt](https://github.com/kylebrandt)
- **Time series/Bar chart panel:** Handle infinite numbers as nulls when converting to plot array. [#35638](https://github.com/grafana/grafana/pull/35638), [@dprokop](https://github.com/dprokop)
- **TimeSeries:** Ensure series overrides that contain color are migrated, and migrate the previous `fieldConfig` when changing the panel type. [#35676](https://github.com/grafana/grafana/pull/35676), [@ashharrison90](https://github.com/ashharrison90)
- **ValueMappings:** Improve singlestat value mappings migration. [#35578](https://github.com/grafana/grafana/pull/35578), [@dprokop](https://github.com/dprokop)
### Bug fixes
- **Annotations:** Fix annotation line and marker colors. [#35608](https://github.com/grafana/grafana/pull/35608), [@torkelo](https://github.com/torkelo)
- **AzureMonitor:** Fix KQL template variable queries without default workspace. [#35836](https://github.com/grafana/grafana/pull/35836), [@joshhunt](https://github.com/joshhunt)
- **CloudWatch/Logs:** Fix missing response data for log queries. [#35724](https://github.com/grafana/grafana/pull/35724), [@aocenas](https://github.com/aocenas)
- **Elasticsearch:** Restore previous field naming strategy when using variables. [#35624](https://github.com/grafana/grafana/pull/35624), [@Elfo404](https://github.com/Elfo404)
- **LibraryPanels:** Fix crash in library panels list when panel plugin is not found. [#35907](https://github.com/grafana/grafana/pull/35907), [@torkelo](https://github.com/torkelo)
- **LogsPanel:** Fix performance drop when moving logs panel in dashboard. [#35379](https://github.com/grafana/grafana/pull/35379), [@aocenas](https://github.com/aocenas)
- **Loki:** Parse log levels when ANSI coloring is enabled. [#35607](https://github.com/grafana/grafana/pull/35607), [@olbo98](https://github.com/olbo98)
- **MSSQL:** Fix issue with hidden queries still being executed. [#35787](https://github.com/grafana/grafana/pull/35787), [@torkelo](https://github.com/torkelo)
- **PanelEdit:** Display the VisualizationPicker that was not displayed if a panel has an unknown panel plugin. [#35831](https://github.com/grafana/grafana/pull/35831), [@jackw](https://github.com/jackw)
- **Plugins:** Fix loading symbolically linked plugins. [#35635](https://github.com/grafana/grafana/pull/35635), [@domasx2](https://github.com/domasx2)
- **Prometheus:** Fix issue where legend name was replaced with name Value in stat and gauge panels. [#35863](https://github.com/grafana/grafana/pull/35863), [@torkelo](https://github.com/torkelo)
- **State Timeline:** Fix crash when hovering over panel. [#35692](https://github.com/grafana/grafana/pull/35692), [@hugohaggmark](https://github.com/hugohaggmark)
| docs/sources/release-notes/release-notes-8-0-3.md | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017268727242480963,
0.00017055954958777875,
0.0001657793327467516,
0.00017188579658977687,
0.0000027803866942122113
]
|
{
"id": 0,
"code_window": [
" }\n",
" scene.scale = scale;\n",
" };\n",
"\n",
" return (\n",
" <TransformWrapper\n",
" doubleClick={{ mode: 'reset' }}\n",
" ref={scene.transformComponentRef}\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!scene.shouldPanZoom || scene.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!scene.contextMenuVisible && !scene.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "add",
"edit_start_line_idx": 21
} | [
{
"alias": "",
"bucketAggs": [
{
"field": "@timestamp",
"id": "2",
"settings": {
"interval": "1m"
},
"type": "date_histogram"
}
],
"datasource": {
"type": "elasticsearch",
"uid": "uid"
},
"datasourceId": 42,
"metrics": [
{
"id": "1",
"settings": {
"metrics": [
"float"
],
"order": "desc",
"orderBy": "float"
},
"type": "top_metrics"
}
],
"query": "",
"refId": "A"
}
]
| pkg/tsdb/elasticsearch/testdata_response/metric_top_metrics.queries.json | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017624600150156766,
0.00017449106962885708,
0.00017323448264505714,
0.00017424189718440175,
0.000001116177827498177
]
|
{
"id": 1,
"code_window": [
" limitToBounds={true}\n",
" disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}\n",
" panning={{ allowLeftClickPan: false }}\n",
" >\n",
" <TransformComponent>{sceneDiv}</TransformComponent>\n",
" </TransformWrapper>\n",
" );\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <TransformComponent>\n",
" {/* The <div> element has child elements that allow for mouse events, so we need to disable the linter rule */}\n",
" {/* eslint-disable-next-line jsx-a11y/no-static-element-interactions */}\n",
" <div onMouseDown={onSceneContainerMouseDown}>{sceneDiv}</div>\n",
" </TransformComponent>\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "replace",
"edit_start_line_idx": 33
} | import React from 'react';
import { TransformWrapper, TransformComponent, ReactZoomPanPinchRef } from 'react-zoom-pan-pinch';
import { config } from '@grafana/runtime';
import { Scene } from './scene';
type SceneTransformWrapperProps = {
scene: Scene;
children: React.ReactNode;
};
export const SceneTransformWrapper = ({ scene, children: sceneDiv }: SceneTransformWrapperProps) => {
const onZoom = (zoomPanPinchRef: ReactZoomPanPinchRef) => {
const scale = zoomPanPinchRef.state.scale;
if (scene.moveable && scale > 0) {
scene.moveable.zoom = 1 / scale;
}
scene.scale = scale;
};
return (
<TransformWrapper
doubleClick={{ mode: 'reset' }}
ref={scene.transformComponentRef}
onZoom={onZoom}
onTransformed={(_, state) => {
scene.scale = state.scale;
}}
limitToBounds={true}
disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}
panning={{ allowLeftClickPan: false }}
>
<TransformComponent>{sceneDiv}</TransformComponent>
</TransformWrapper>
);
};
| public/app/features/canvas/runtime/SceneTransformWrapper.tsx | 1 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.8228617906570435,
0.2097661793231964,
0.0006501897587440908,
0.007776367478072643,
0.3539896011352539
]
|
{
"id": 1,
"code_window": [
" limitToBounds={true}\n",
" disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}\n",
" panning={{ allowLeftClickPan: false }}\n",
" >\n",
" <TransformComponent>{sceneDiv}</TransformComponent>\n",
" </TransformWrapper>\n",
" );\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <TransformComponent>\n",
" {/* The <div> element has child elements that allow for mouse events, so we need to disable the linter rule */}\n",
" {/* eslint-disable-next-line jsx-a11y/no-static-element-interactions */}\n",
" <div onMouseDown={onSceneContainerMouseDown}>{sceneDiv}</div>\n",
" </TransformComponent>\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "replace",
"edit_start_line_idx": 33
} | import { useEffect } from 'react';
import { Unsubscribable } from 'rxjs';
import { getAppEvents } from '@grafana/runtime';
import { useGrafana } from 'app/core/context/GrafanaContext';
import { useDispatch } from 'app/types';
import { AbsoluteTimeEvent, CopyTimeEvent, PasteTimeEvent, ShiftTimeEvent, ZoomOutEvent } from 'app/types/events';
import {
copyTimeRangeToClipboard,
makeAbsoluteTime,
pasteTimeRangeFromClipboard,
shiftTime,
zoomOut,
} from '../state/time';
export function useKeyboardShortcuts() {
const { keybindings } = useGrafana();
const dispatch = useDispatch();
useEffect(() => {
keybindings.setupTimeRangeBindings(false);
const tearDown: Unsubscribable[] = [];
tearDown.push(
getAppEvents().subscribe(AbsoluteTimeEvent, () => {
dispatch(makeAbsoluteTime());
})
);
tearDown.push(
getAppEvents().subscribe(ShiftTimeEvent, (event) => {
dispatch(shiftTime(event.payload.direction));
})
);
tearDown.push(
getAppEvents().subscribe(ZoomOutEvent, (event) => {
dispatch(zoomOut(event.payload.scale));
})
);
tearDown.push(
getAppEvents().subscribe(CopyTimeEvent, () => {
dispatch(copyTimeRangeToClipboard());
})
);
tearDown.push(
getAppEvents().subscribe(PasteTimeEvent, () => {
dispatch(pasteTimeRangeFromClipboard());
})
);
return () => {
tearDown.forEach((u) => u.unsubscribe());
};
}, [dispatch, keybindings]);
}
| public/app/features/explore/hooks/useKeyboardShortcuts.ts | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017730081162881106,
0.0001741079322528094,
0.00016739172860980034,
0.00017479565576650202,
0.0000031402041713590734
]
|
{
"id": 1,
"code_window": [
" limitToBounds={true}\n",
" disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}\n",
" panning={{ allowLeftClickPan: false }}\n",
" >\n",
" <TransformComponent>{sceneDiv}</TransformComponent>\n",
" </TransformWrapper>\n",
" );\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <TransformComponent>\n",
" {/* The <div> element has child elements that allow for mouse events, so we need to disable the linter rule */}\n",
" {/* eslint-disable-next-line jsx-a11y/no-static-element-interactions */}\n",
" <div onMouseDown={onSceneContainerMouseDown}>{sceneDiv}</div>\n",
" </TransformComponent>\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "replace",
"edit_start_line_idx": 33
} | package state_test
import (
"bytes"
"context"
"errors"
"fmt"
"math"
"math/rand"
"sort"
"strings"
"testing"
"time"
"github.com/benbjohnson/clock"
"github.com/google/go-cmp/cmp"
"github.com/google/go-cmp/cmp/cmpopts"
"github.com/grafana/grafana-plugin-sdk-go/data"
"github.com/prometheus/client_golang/prometheus"
"github.com/prometheus/client_golang/prometheus/testutil"
"github.com/stretchr/testify/assert"
"github.com/stretchr/testify/require"
"golang.org/x/exp/slices"
"github.com/grafana/grafana/pkg/expr"
"github.com/grafana/grafana/pkg/infra/log"
"github.com/grafana/grafana/pkg/infra/tracing"
"github.com/grafana/grafana/pkg/services/annotations"
"github.com/grafana/grafana/pkg/services/annotations/annotationstest"
"github.com/grafana/grafana/pkg/services/dashboards"
"github.com/grafana/grafana/pkg/services/ngalert/eval"
"github.com/grafana/grafana/pkg/services/ngalert/metrics"
"github.com/grafana/grafana/pkg/services/ngalert/models"
"github.com/grafana/grafana/pkg/services/ngalert/state"
"github.com/grafana/grafana/pkg/services/ngalert/state/historian"
"github.com/grafana/grafana/pkg/services/ngalert/tests"
"github.com/grafana/grafana/pkg/util"
)
func TestWarmStateCache(t *testing.T) {
evaluationTime, err := time.Parse("2006-01-02", "2021-03-25")
require.NoError(t, err)
ctx := context.Background()
_, dbstore := tests.SetupTestEnv(t, 1)
const mainOrgID int64 = 1
rule := tests.CreateTestAlertRule(t, ctx, dbstore, 600, mainOrgID)
expectedEntries := []*state.State{
{
AlertRuleUID: rule.UID,
OrgID: rule.OrgID,
Labels: data.Labels{"test1": "testValue1"},
State: eval.Normal,
Results: []state.Evaluation{
{EvaluationTime: evaluationTime, EvaluationState: eval.Normal},
},
StartsAt: evaluationTime.Add(-1 * time.Minute),
EndsAt: evaluationTime.Add(1 * time.Minute),
LastEvaluationTime: evaluationTime,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
ResultFingerprint: data.Fingerprint(math.MaxUint64),
}, {
AlertRuleUID: rule.UID,
OrgID: rule.OrgID,
Labels: data.Labels{"test2": "testValue2"},
State: eval.Alerting,
Results: []state.Evaluation{
{EvaluationTime: evaluationTime, EvaluationState: eval.Alerting},
},
StartsAt: evaluationTime.Add(-1 * time.Minute),
EndsAt: evaluationTime.Add(1 * time.Minute),
LastEvaluationTime: evaluationTime,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
ResultFingerprint: data.Fingerprint(math.MaxUint64 - 1),
},
{
AlertRuleUID: rule.UID,
OrgID: rule.OrgID,
Labels: data.Labels{"test3": "testValue3"},
State: eval.NoData,
Results: []state.Evaluation{
{EvaluationTime: evaluationTime, EvaluationState: eval.NoData},
},
StartsAt: evaluationTime.Add(-1 * time.Minute),
EndsAt: evaluationTime.Add(1 * time.Minute),
LastEvaluationTime: evaluationTime,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
ResultFingerprint: data.Fingerprint(0),
},
{
AlertRuleUID: rule.UID,
OrgID: rule.OrgID,
Labels: data.Labels{"test4": "testValue4"},
State: eval.Error,
Results: []state.Evaluation{
{EvaluationTime: evaluationTime, EvaluationState: eval.Error},
},
StartsAt: evaluationTime.Add(-1 * time.Minute),
EndsAt: evaluationTime.Add(1 * time.Minute),
LastEvaluationTime: evaluationTime,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
ResultFingerprint: data.Fingerprint(1),
},
{
AlertRuleUID: rule.UID,
OrgID: rule.OrgID,
Labels: data.Labels{"test5": "testValue5"},
State: eval.Pending,
Results: []state.Evaluation{
{EvaluationTime: evaluationTime, EvaluationState: eval.Pending},
},
StartsAt: evaluationTime.Add(-1 * time.Minute),
EndsAt: evaluationTime.Add(1 * time.Minute),
LastEvaluationTime: evaluationTime,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
ResultFingerprint: data.Fingerprint(2),
},
}
instances := make([]models.AlertInstance, 0)
labels := models.InstanceLabels{"test1": "testValue1"}
_, hash, _ := labels.StringAndHash()
instances = append(instances, models.AlertInstance{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash,
},
CurrentState: models.InstanceStateNormal,
LastEvalTime: evaluationTime,
CurrentStateSince: evaluationTime.Add(-1 * time.Minute),
CurrentStateEnd: evaluationTime.Add(1 * time.Minute),
Labels: labels,
ResultFingerprint: data.Fingerprint(math.MaxUint64).String(),
})
labels = models.InstanceLabels{"test2": "testValue2"}
_, hash, _ = labels.StringAndHash()
instances = append(instances, models.AlertInstance{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash,
},
CurrentState: models.InstanceStateFiring,
LastEvalTime: evaluationTime,
CurrentStateSince: evaluationTime.Add(-1 * time.Minute),
CurrentStateEnd: evaluationTime.Add(1 * time.Minute),
Labels: labels,
ResultFingerprint: data.Fingerprint(math.MaxUint64 - 1).String(),
})
labels = models.InstanceLabels{"test3": "testValue3"}
_, hash, _ = labels.StringAndHash()
instances = append(instances, models.AlertInstance{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash,
},
CurrentState: models.InstanceStateNoData,
LastEvalTime: evaluationTime,
CurrentStateSince: evaluationTime.Add(-1 * time.Minute),
CurrentStateEnd: evaluationTime.Add(1 * time.Minute),
Labels: labels,
ResultFingerprint: data.Fingerprint(0).String(),
})
labels = models.InstanceLabels{"test4": "testValue4"}
_, hash, _ = labels.StringAndHash()
instances = append(instances, models.AlertInstance{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash,
},
CurrentState: models.InstanceStateError,
LastEvalTime: evaluationTime,
CurrentStateSince: evaluationTime.Add(-1 * time.Minute),
CurrentStateEnd: evaluationTime.Add(1 * time.Minute),
Labels: labels,
ResultFingerprint: data.Fingerprint(1).String(),
})
labels = models.InstanceLabels{"test5": "testValue5"}
_, hash, _ = labels.StringAndHash()
instances = append(instances, models.AlertInstance{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash,
},
CurrentState: models.InstanceStatePending,
LastEvalTime: evaluationTime,
CurrentStateSince: evaluationTime.Add(-1 * time.Minute),
CurrentStateEnd: evaluationTime.Add(1 * time.Minute),
Labels: labels,
ResultFingerprint: data.Fingerprint(2).String(),
})
for _, instance := range instances {
_ = dbstore.SaveAlertInstance(ctx, instance)
}
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: dbstore,
Images: &state.NoopImageService{},
Clock: clock.NewMock(),
Historian: &state.FakeHistorian{},
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
st.Warm(ctx, dbstore)
t.Run("instance cache has expected entries", func(t *testing.T) {
for _, entry := range expectedEntries {
setCacheID(entry)
cacheEntry := st.Get(entry.OrgID, entry.AlertRuleUID, entry.CacheID)
if diff := cmp.Diff(entry, cacheEntry, cmpopts.IgnoreFields(state.State{}, "Results")); diff != "" {
t.Errorf("Result mismatch (-want +got):\n%s", diff)
t.FailNow()
}
}
})
}
func TestDashboardAnnotations(t *testing.T) {
evaluationTime, err := time.Parse("2006-01-02", "2022-01-01")
require.NoError(t, err)
ctx := context.Background()
_, dbstore := tests.SetupTestEnv(t, 1)
fakeAnnoRepo := annotationstest.NewFakeAnnotationsRepo()
historianMetrics := metrics.NewHistorianMetrics(prometheus.NewRegistry(), metrics.Subsystem)
store := historian.NewAnnotationStore(fakeAnnoRepo, &dashboards.FakeDashboardService{}, historianMetrics)
hist := historian.NewAnnotationBackend(store, nil, historianMetrics)
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: dbstore,
Images: &state.NoopImageService{},
Clock: clock.New(),
Historian: hist,
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
const mainOrgID int64 = 1
rule := tests.CreateTestAlertRuleWithLabels(t, ctx, dbstore, 600, mainOrgID, map[string]string{
"test1": "testValue1",
"test2": "{{ $labels.instance_label }}",
})
st.Warm(ctx, dbstore)
bValue := float64(42)
cValue := float64(1)
_ = st.ProcessEvalResults(ctx, evaluationTime, rule, eval.Results{{
Instance: data.Labels{"instance_label": "testValue2"},
State: eval.Alerting,
EvaluatedAt: evaluationTime,
Values: map[string]eval.NumberValueCapture{
"B": {Var: "B", Value: &bValue, Labels: data.Labels{"job": "prometheus"}},
"C": {Var: "C", Value: &cValue, Labels: data.Labels{"job": "prometheus"}},
},
}}, data.Labels{
"alertname": rule.Title,
})
expected := []string{rule.Title + " {alertname=" + rule.Title + ", instance_label=testValue2, test1=testValue1, test2=testValue2} - B=42.000000, C=1.000000"}
sort.Strings(expected)
require.Eventuallyf(t, func() bool {
var actual []string
for _, next := range fakeAnnoRepo.Items() {
actual = append(actual, next.Text)
}
sort.Strings(actual)
if len(expected) != len(actual) {
return false
}
for i := 0; i < len(expected); i++ {
if expected[i] != actual[i] {
return false
}
}
return true
}, time.Second, 100*time.Millisecond, "unexpected annotations")
}
func TestProcessEvalResults(t *testing.T) {
evaluationDuration := 10 * time.Millisecond
evaluationInterval := 10 * time.Second
t1 := time.Time{}.Add(evaluationInterval)
tn := func(n int) time.Time {
return t1.Add(time.Duration(n-1) * evaluationInterval)
}
t2 := tn(2)
t3 := tn(3)
baseRule := &models.AlertRule{
OrgID: 1,
Title: "test_title",
UID: "test_alert_rule_uid",
Data: []models.AlertQuery{{
RefID: "A",
DatasourceUID: "datasource_uid_1",
}, {
RefID: "B",
DatasourceUID: expr.DatasourceType,
}},
NamespaceUID: "test_namespace_uid",
Annotations: map[string]string{"annotation": "test"},
Labels: map[string]string{"label": "test"},
IntervalSeconds: int64(evaluationInterval.Seconds()),
NoDataState: models.NoData,
ExecErrState: models.ErrorErrState,
}
newEvaluation := func(evalTime time.Time, evalState eval.State) state.Evaluation {
return state.Evaluation{
EvaluationTime: evalTime,
EvaluationState: evalState,
Values: make(map[string]*float64),
}
}
baseRuleWith := func(mutators ...models.AlertRuleMutator) *models.AlertRule {
r := models.CopyRule(baseRule)
for _, mutator := range mutators {
mutator(r)
}
return r
}
newResult := func(mutators ...eval.ResultMutator) eval.Result {
r := eval.Result{
State: eval.Normal,
EvaluationDuration: evaluationDuration,
}
for _, mutator := range mutators {
mutator(&r)
}
return r
}
labels1 := data.Labels{
"instance_label": "test-1",
}
labels2 := data.Labels{
"instance_label": "test-2",
}
systemLabels := data.Labels{
"system": "owned",
}
noDataLabels := data.Labels{
"datasource_uid": "1",
"ref_id": "A",
}
labels := map[string]data.Labels{
"system + rule": mergeLabels(baseRule.Labels, systemLabels),
"system + rule + labels1": mergeLabels(mergeLabels(labels1, baseRule.Labels), systemLabels),
"system + rule + labels2": mergeLabels(mergeLabels(labels2, baseRule.Labels), systemLabels),
"system + rule + no-data": mergeLabels(mergeLabels(noDataLabels, baseRule.Labels), systemLabels),
}
// keep it separate to make code folding work correctly.
type testCase struct {
desc string
alertRule *models.AlertRule
evalResults map[time.Time]eval.Results
expectedStates []*state.State
expectedAnnotations int
}
testCases := []testCase{
{
desc: "a cache entry is correctly created",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
},
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t1,
},
},
},
{
desc: "two results create two correct cache entries",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels2)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t1,
},
{
Labels: labels["system + rule + labels2"],
ResultFingerprint: labels2.Fingerprint(),
State: eval.Alerting,
Results: []state.Evaluation{
newEvaluation(t1, eval.Alerting),
},
StartsAt: t1,
EndsAt: t1.Add(state.ResendDelay * 4),
LastEvaluationTime: t1,
},
},
},
{
desc: "state is maintained",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
tn(6): {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
},
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(tn(6), eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: tn(6),
},
},
},
{
desc: "normal -> alerting transition when For is unset",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Alerting,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.Alerting),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> alerting when For is set",
alertRule: baseRuleWith(models.WithForNTimes(2)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t3: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 2,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Alerting,
Results: []state.Evaluation{
newEvaluation(t3, eval.Alerting),
newEvaluation(tn(4), eval.Alerting),
},
StartsAt: tn(4),
EndsAt: tn(4).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(4),
},
},
},
{
desc: "normal -> alerting -> noData -> alerting when For is set",
alertRule: baseRuleWith(models.WithForNTimes(2)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t3: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)), // TODO fix it because NoData does not have labels
},
tn(4): {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
tn(5): {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 3, // Normal -> Pending, Pending -> NoData, NoData -> Pending
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Pending,
Results: []state.Evaluation{
newEvaluation(tn(4), eval.Alerting),
newEvaluation(tn(5), eval.Alerting),
},
StartsAt: tn(4),
EndsAt: tn(4).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(5),
},
},
},
{
desc: "pending -> alerting -> noData when For is set and NoDataState is NoData",
alertRule: baseRuleWith(models.WithForNTimes(2)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t3: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 3,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.NoData,
Results: []state.Evaluation{
newEvaluation(t3, eval.Alerting),
newEvaluation(tn(4), eval.NoData),
},
StartsAt: tn(4),
EndsAt: tn(4).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(4),
},
},
},
{
desc: "normal -> pending when For is set but not exceeded and first result is normal",
alertRule: baseRuleWith(models.WithForNTimes(2)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Pending,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.Alerting),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> pending when For is set but not exceeded and first result is alerting",
alertRule: baseRuleWith(models.WithForNTimes(6)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Pending,
Results: []state.Evaluation{
newEvaluation(t1, eval.Alerting),
newEvaluation(t2, eval.Alerting),
},
StartsAt: t1,
EndsAt: t1.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> pending when For is set but not exceeded, result is NoData and NoDataState is alerting",
alertRule: baseRuleWith(models.WithForNTimes(6), models.WithNoDataExecAs(models.Alerting)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Pending,
StateReason: eval.NoData.String(),
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.NoData),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> alerting when For is exceeded, result is NoData and NoDataState is alerting",
alertRule: baseRuleWith(models.WithForNTimes(3), models.WithNoDataExecAs(models.Alerting)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)), // TODO fix it because nodata has no labels of regular result
},
t3: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)),
},
tn(5): {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 2,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Alerting,
StateReason: eval.NoData.String(),
Results: []state.Evaluation{
newEvaluation(t3, eval.NoData),
newEvaluation(tn(4), eval.NoData),
newEvaluation(tn(5), eval.NoData),
},
StartsAt: tn(5),
EndsAt: tn(5).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(5),
},
},
},
{
desc: "normal -> nodata when result is NoData and NoDataState is nodata",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.NoData,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.NoData),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> nodata no labels when result is NoData and NoDataState is nodata", // TODO should be broken in https://github.com/grafana/grafana/pull/68142
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(nil)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t1,
},
{
Labels: labels["system + rule"],
ResultFingerprint: data.Labels{}.Fingerprint(),
State: eval.NoData,
Results: []state.Evaluation{
newEvaluation(t2, eval.NoData),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal (multi-dimensional) -> nodata no labels when result is NoData and NoDataState is nodata",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels2)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(data.Labels{})),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t1,
},
{
Labels: labels["system + rule + labels2"],
ResultFingerprint: labels2.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t1,
},
{
Labels: labels["system + rule"],
ResultFingerprint: data.Labels{}.Fingerprint(),
State: eval.NoData,
Results: []state.Evaluation{
newEvaluation(t2, eval.NoData),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> nodata no labels -> normal when result is NoData and NoDataState is nodata",
alertRule: baseRule,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(noDataLabels)),
},
t3: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t3, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t3,
},
{
Labels: labels["system + rule + no-data"],
ResultFingerprint: noDataLabels.Fingerprint(),
State: eval.NoData,
Results: []state.Evaluation{
newEvaluation(t2, eval.NoData),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> normal when result is NoData and NoDataState is ok",
alertRule: baseRuleWith(models.WithNoDataExecAs(models.OK)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)), // TODO fix it because NoData does not have same labels
},
},
expectedAnnotations: 0,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
StateReason: eval.NoData.String(),
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.NoData),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> pending when For is set but not exceeded, result is Error and ExecErrState is Alerting",
alertRule: baseRuleWith(models.WithForNTimes(6), models.WithErrorExecAs(models.AlertingErrState)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Pending,
StateReason: eval.Error.String(),
Error: errors.New("with_state_error"),
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.Error),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> alerting when For is exceeded, result is Error and ExecErrState is Alerting",
alertRule: baseRuleWith(models.WithForNTimes(3), models.WithErrorExecAs(models.AlertingErrState)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)),
},
t3: {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)),
},
tn(5): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 2,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Alerting,
StateReason: eval.Error.String(),
Error: errors.New("with_state_error"),
Results: []state.Evaluation{
newEvaluation(t3, eval.Error),
newEvaluation(tn(4), eval.Error),
newEvaluation(tn(5), eval.Error),
},
StartsAt: tn(5),
EndsAt: tn(5).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(5),
},
},
},
{
desc: "normal -> error when result is Error and ExecErrState is Error",
alertRule: baseRuleWith(models.WithForNTimes(6), models.WithErrorExecAs(models.ErrorErrState)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithError(expr.MakeQueryError("A", "datasource_uid_1", errors.New("this is an error"))), eval.WithLabels(labels1)), // TODO fix it because error labels are different
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
CacheID: func() string {
lbls := models.InstanceLabels(labels["system + rule + labels1"])
r, err := lbls.StringKey()
if err != nil {
panic(err)
}
return r
}(),
Labels: mergeLabels(labels["system + rule + labels1"], data.Labels{
"datasource_uid": "datasource_uid_1",
"ref_id": "A",
}),
ResultFingerprint: labels1.Fingerprint(),
State: eval.Error,
Error: expr.MakeQueryError("A", "datasource_uid_1", errors.New("this is an error")),
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.Error),
},
StartsAt: t2,
EndsAt: t2.Add(state.ResendDelay * 4),
LastEvaluationTime: t2,
EvaluationDuration: evaluationDuration,
Annotations: map[string]string{"annotation": "test", "Error": "[sse.dataQueryError] failed to execute query [A]: this is an error"},
},
},
},
{
desc: "normal -> normal when result is Error and ExecErrState is OK",
alertRule: baseRuleWith(models.WithForNTimes(6), models.WithErrorExecAs(models.OkErrState)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithError(expr.MakeQueryError("A", "datasource_uid_1", errors.New("this is an error"))), eval.WithLabels(labels1)), // TODO fix it because error labels are different
},
},
expectedAnnotations: 1,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
StateReason: eval.Error.String(),
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
newEvaluation(t2, eval.Error),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t2,
},
},
},
{
desc: "alerting -> normal when result is Error and ExecErrState is OK",
alertRule: baseRuleWith(models.WithForNTimes(6), models.WithErrorExecAs(models.OkErrState)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithError(expr.MakeQueryError("A", "datasource_uid_1", errors.New("this is an error"))), eval.WithLabels(labels1)), // TODO fix it because error labels are different
},
},
expectedAnnotations: 2,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Normal,
StateReason: eval.Error.String(),
Results: []state.Evaluation{
newEvaluation(t1, eval.Alerting),
newEvaluation(t2, eval.Error),
},
StartsAt: t2,
EndsAt: t2,
LastEvaluationTime: t2,
},
},
},
{
desc: "normal -> alerting -> error when result is Error and ExecErrorState is Error",
alertRule: baseRuleWith(models.WithForNTimes(2)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t2: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
t3: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)), // TODO this is not how error result is created
},
tn(5): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)), // TODO this is not how error result is created
},
tn(6): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)), // TODO this is not how error result is created
},
},
expectedAnnotations: 3,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Error,
Error: fmt.Errorf("with_state_error"),
Results: []state.Evaluation{
newEvaluation(tn(5), eval.Error),
newEvaluation(tn(6), eval.Error),
},
StartsAt: tn(4),
EndsAt: tn(6).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(6),
},
},
},
{
desc: "normal -> alerting -> error -> alerting - it should clear the error",
alertRule: baseRuleWith(models.WithForNTimes(3)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
tn(5): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)), // TODO fix it
},
tn(8): {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
},
expectedAnnotations: 3,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.Pending,
Results: []state.Evaluation{
newEvaluation(tn(4), eval.Alerting),
newEvaluation(tn(5), eval.Error),
newEvaluation(tn(8), eval.Alerting),
},
StartsAt: tn(8),
EndsAt: tn(8).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(8),
},
},
},
{
desc: "normal -> alerting -> error -> no data - it should clear the error",
alertRule: baseRuleWith(models.WithForNTimes(3)),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(labels1)),
},
tn(4): {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(labels1)),
},
tn(5): {
newResult(eval.WithState(eval.Error), eval.WithLabels(labels1)), // TODO FIX it
},
tn(6): {
newResult(eval.WithState(eval.NoData), eval.WithLabels(labels1)), // TODO fix it because it's not possible
},
},
expectedAnnotations: 3,
expectedStates: []*state.State{
{
Labels: labels["system + rule + labels1"],
ResultFingerprint: labels1.Fingerprint(),
State: eval.NoData,
Results: []state.Evaluation{
newEvaluation(tn(4), eval.Alerting),
newEvaluation(tn(5), eval.Error),
newEvaluation(tn(6), eval.NoData),
},
StartsAt: tn(6),
EndsAt: tn(6).Add(state.ResendDelay * 4),
LastEvaluationTime: tn(6),
},
},
},
{
desc: "template is correctly expanded",
alertRule: baseRuleWith(
models.WithAnnotations(map[string]string{"summary": "{{$labels.pod}} is down in {{$labels.cluster}} cluster -> {{$labels.namespace}} namespace"}),
models.WithLabels(map[string]string{"label": "test", "job": "{{$labels.namespace}}/{{$labels.pod}}"}),
),
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Normal), eval.WithLabels(data.Labels{
"cluster": "us-central-1",
"namespace": "prod",
"pod": "grafana",
})),
},
},
expectedStates: []*state.State{
{
Labels: mergeLabels(systemLabels, data.Labels{
"cluster": "us-central-1",
"namespace": "prod",
"pod": "grafana",
"label": "test",
"job": "prod/grafana",
}),
State: eval.Normal,
Results: []state.Evaluation{
newEvaluation(t1, eval.Normal),
},
StartsAt: t1,
EndsAt: t1,
LastEvaluationTime: t1,
EvaluationDuration: evaluationDuration,
Annotations: map[string]string{"summary": "grafana is down in us-central-1 cluster -> prod namespace"},
ResultFingerprint: data.Labels{
"cluster": "us-central-1",
"namespace": "prod",
"pod": "grafana",
}.Fingerprint(),
},
},
},
{
desc: "classic condition, execution Error as Error (alerting -> query error -> alerting)",
alertRule: baseRuleWith(models.WithErrorExecAs(models.ErrorErrState)),
expectedAnnotations: 3,
evalResults: map[time.Time]eval.Results{
t1: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(data.Labels{})),
},
t2: {
newResult(eval.WithError(expr.MakeQueryError("A", "test-datasource-uid", errors.New("this is an error"))), eval.WithLabels(data.Labels{})),
},
t3: {
newResult(eval.WithState(eval.Alerting), eval.WithLabels(data.Labels{})),
},
},
expectedStates: []*state.State{
{
Labels: labels["system + rule"],
ResultFingerprint: data.Labels{}.Fingerprint(),
State: eval.Alerting,
Results: []state.Evaluation{
newEvaluation(t1, eval.Alerting),
newEvaluation(t2, eval.Error),
newEvaluation(t3, eval.Alerting),
},
StartsAt: t3,
EndsAt: t3.Add(state.ResendDelay * 4),
LastEvaluationTime: t3,
},
},
},
}
for _, tc := range testCases {
t.Run(tc.desc, func(t *testing.T) {
fakeAnnoRepo := annotationstest.NewFakeAnnotationsRepo()
reg := prometheus.NewPedanticRegistry()
stateMetrics := metrics.NewStateMetrics(reg)
m := metrics.NewHistorianMetrics(prometheus.NewRegistry(), metrics.Subsystem)
store := historian.NewAnnotationStore(fakeAnnoRepo, &dashboards.FakeDashboardService{}, m)
hist := historian.NewAnnotationBackend(store, nil, m)
clk := clock.NewMock()
cfg := state.ManagerCfg{
Metrics: stateMetrics,
ExternalURL: nil,
InstanceStore: &state.FakeInstanceStore{},
Images: &state.NotAvailableImageService{},
Clock: clk,
Historian: hist,
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
evals := make([]time.Time, 0, len(tc.evalResults))
for evalTime := range tc.evalResults {
evals = append(evals, evalTime)
}
slices.SortFunc(evals, func(a, b time.Time) bool {
return a.Before(b)
})
results := 0
for _, evalTime := range evals {
res := tc.evalResults[evalTime]
for i := 0; i < len(res); i++ {
res[i].EvaluatedAt = evalTime
}
clk.Set(evalTime)
_ = st.ProcessEvalResults(context.Background(), evalTime, tc.alertRule, res, systemLabels)
results += len(res)
}
states := st.GetStatesForRuleUID(tc.alertRule.OrgID, tc.alertRule.UID)
assert.Len(t, states, len(tc.expectedStates))
expectedStates := make(map[string]*state.State, len(tc.expectedStates))
for _, s := range tc.expectedStates {
// patch all optional fields of the expected state
setCacheID(s)
if s.AlertRuleUID == "" {
s.AlertRuleUID = tc.alertRule.UID
}
if s.OrgID == 0 {
s.OrgID = tc.alertRule.OrgID
}
if s.Annotations == nil {
s.Annotations = tc.alertRule.Annotations
}
if s.EvaluationDuration == 0 {
s.EvaluationDuration = evaluationDuration
}
if s.Values == nil {
s.Values = make(map[string]float64)
}
expectedStates[s.CacheID] = s
}
for _, actual := range states {
expected, ok := expectedStates[actual.CacheID]
if !ok {
assert.Failf(t, "state is not expected", "State: %#v", actual)
continue
}
delete(expectedStates, actual.CacheID)
if !assert.ObjectsAreEqual(expected, actual) {
assert.Failf(t, "expected and actual states are not equal", "Diff: %s", cmp.Diff(expected, actual, cmpopts.EquateErrors()))
}
}
if len(expectedStates) > 0 {
vals := make([]state.State, 0, len(expectedStates))
for _, s := range expectedStates {
vals = append(vals, *s)
}
assert.Failf(t, "some expected states do not exist", "States: %#v", vals)
}
require.Eventuallyf(t, func() bool {
return tc.expectedAnnotations == fakeAnnoRepo.Len()
}, time.Second, 100*time.Millisecond, "%d annotations are present, expected %d. We have %+v", fakeAnnoRepo.Len(), tc.expectedAnnotations, printAllAnnotations(fakeAnnoRepo.Items()))
expectedMetric := fmt.Sprintf(
`# HELP grafana_alerting_state_calculation_duration_seconds The duration of calculation of a single state.
# TYPE grafana_alerting_state_calculation_duration_seconds histogram
grafana_alerting_state_calculation_duration_seconds_bucket{le="0.01"} %[1]d
grafana_alerting_state_calculation_duration_seconds_bucket{le="0.1"} %[1]d
grafana_alerting_state_calculation_duration_seconds_bucket{le="1"} %[1]d
grafana_alerting_state_calculation_duration_seconds_bucket{le="2"} %[1]d
grafana_alerting_state_calculation_duration_seconds_bucket{le="5"} %[1]d
grafana_alerting_state_calculation_duration_seconds_bucket{le="10"} %[1]d
grafana_alerting_state_calculation_duration_seconds_bucket{le="+Inf"} %[1]d
grafana_alerting_state_calculation_duration_seconds_sum 0
grafana_alerting_state_calculation_duration_seconds_count %[1]d
`, results)
err := testutil.GatherAndCompare(reg, bytes.NewBufferString(expectedMetric), "grafana_alerting_state_calculation_duration_seconds", "grafana_alerting_state_calculation_total")
require.NoError(t, err)
})
}
t.Run("should save state to database", func(t *testing.T) {
instanceStore := &state.FakeInstanceStore{}
clk := clock.New()
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: instanceStore,
Images: &state.NotAvailableImageService{},
Clock: clk,
Historian: &state.FakeHistorian{},
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
MaxStateSaveConcurrency: 1,
}
statePersister := state.NewSyncStatePersisiter(log.New("ngalert.state.manager.persist"), cfg)
st := state.NewManager(cfg, statePersister)
rule := models.AlertRuleGen()()
var results = eval.GenerateResults(rand.Intn(4)+1, eval.ResultGen(eval.WithEvaluatedAt(clk.Now())))
states := st.ProcessEvalResults(context.Background(), clk.Now(), rule, results, make(data.Labels))
require.NotEmpty(t, states)
savedStates := make(map[string]models.AlertInstance)
for _, op := range instanceStore.RecordedOps {
switch q := op.(type) {
case models.AlertInstance:
cacheId, err := q.Labels.StringKey()
require.NoError(t, err)
savedStates[cacheId] = q
}
}
require.Len(t, savedStates, len(states))
for _, s := range states {
require.Contains(t, savedStates, s.CacheID)
}
})
}
func printAllAnnotations(annos map[int64]annotations.Item) string {
b := strings.Builder{}
b.WriteRune('[')
idx := make([]int64, 0, len(annos))
for id := range annos {
idx = append(idx, id)
}
slices.Sort(idx)
for idx, id := range idx {
if idx > 0 {
b.WriteRune(',')
}
b.WriteString(fmt.Sprintf("%s: %s -> %s", time.UnixMilli(annos[id].Epoch).Format(time.TimeOnly), annos[id].PrevState, annos[id].NewState))
}
b.WriteRune(']')
return b.String()
}
func TestStaleResultsHandler(t *testing.T) {
evaluationTime := time.Now()
interval := time.Minute
ctx := context.Background()
_, dbstore := tests.SetupTestEnv(t, 1)
const mainOrgID int64 = 1
rule := tests.CreateTestAlertRule(t, ctx, dbstore, int64(interval.Seconds()), mainOrgID)
lastEval := evaluationTime.Add(-2 * interval)
labels1 := models.InstanceLabels{"test1": "testValue1"}
_, hash1, _ := labels1.StringAndHash()
labels2 := models.InstanceLabels{"test2": "testValue2"}
_, hash2, _ := labels2.StringAndHash()
instances := []models.AlertInstance{
{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash1,
},
CurrentState: models.InstanceStateNormal,
Labels: labels1,
LastEvalTime: lastEval,
CurrentStateSince: lastEval,
CurrentStateEnd: lastEval.Add(3 * interval),
},
{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash2,
},
CurrentState: models.InstanceStateFiring,
Labels: labels2,
LastEvalTime: lastEval,
CurrentStateSince: lastEval,
CurrentStateEnd: lastEval.Add(3 * interval),
},
}
for _, instance := range instances {
_ = dbstore.SaveAlertInstance(ctx, instance)
}
testCases := []struct {
desc string
evalResults []eval.Results
expectedStates []*state.State
startingStateCount int
finalStateCount int
}{
{
desc: "stale cache entries are removed",
evalResults: []eval.Results{
{
eval.Result{
Instance: data.Labels{"test1": "testValue1"},
State: eval.Normal,
EvaluatedAt: evaluationTime,
},
},
},
expectedStates: []*state.State{
{
AlertRuleUID: rule.UID,
OrgID: 1,
Labels: data.Labels{
"__alert_rule_namespace_uid__": "namespace",
"__alert_rule_uid__": rule.UID,
"alertname": rule.Title,
"test1": "testValue1",
},
Values: make(map[string]float64),
State: eval.Normal,
Results: []state.Evaluation{
{
EvaluationTime: evaluationTime,
EvaluationState: eval.Normal,
Values: make(map[string]*float64),
Condition: "A",
},
},
StartsAt: evaluationTime,
EndsAt: evaluationTime,
LastEvaluationTime: evaluationTime,
EvaluationDuration: 0,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
ResultFingerprint: data.Labels{"test1": "testValue1"}.Fingerprint(),
},
},
startingStateCount: 2,
finalStateCount: 1,
},
}
for _, tc := range testCases {
ctx := context.Background()
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: dbstore,
Images: &state.NoopImageService{},
Clock: clock.New(),
Historian: &state.FakeHistorian{},
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
st.Warm(ctx, dbstore)
existingStatesForRule := st.GetStatesForRuleUID(rule.OrgID, rule.UID)
// We have loaded the expected number of entries from the db
assert.Equal(t, tc.startingStateCount, len(existingStatesForRule))
for _, res := range tc.evalResults {
evalTime := evaluationTime
for _, re := range res {
if re.EvaluatedAt.After(evalTime) {
evalTime = re.EvaluatedAt
}
}
st.ProcessEvalResults(context.Background(), evalTime, rule, res, data.Labels{
"alertname": rule.Title,
"__alert_rule_namespace_uid__": rule.NamespaceUID,
"__alert_rule_uid__": rule.UID,
})
for _, s := range tc.expectedStates {
setCacheID(s)
cachedState := st.Get(s.OrgID, s.AlertRuleUID, s.CacheID)
assert.Equal(t, s, cachedState)
}
}
existingStatesForRule = st.GetStatesForRuleUID(rule.OrgID, rule.UID)
// The expected number of state entries remains after results are processed
assert.Equal(t, tc.finalStateCount, len(existingStatesForRule))
}
}
func TestStaleResults(t *testing.T) {
getCacheID := func(t *testing.T, rule *models.AlertRule, result eval.Result) string {
t.Helper()
labels := data.Labels{}
for key, value := range rule.Labels {
labels[key] = value
}
for key, value := range result.Instance {
labels[key] = value
}
lbls := models.InstanceLabels(labels)
key, err := lbls.StringKey()
require.NoError(t, err)
return key
}
checkExpectedStates := func(t *testing.T, actual []*state.State, expected map[string]struct{}) map[string]*state.State {
t.Helper()
result := make(map[string]*state.State)
require.Len(t, actual, len(expected))
for _, currentState := range actual {
_, ok := expected[currentState.CacheID]
result[currentState.CacheID] = currentState
require.Truef(t, ok, "State %s is not expected. States: %v", currentState.CacheID, expected)
}
return result
}
checkExpectedStateTransitions := func(t *testing.T, actual []state.StateTransition, expected map[string]struct{}) {
t.Helper()
require.Len(t, actual, len(expected))
for _, currentState := range actual {
_, ok := expected[currentState.CacheID]
require.Truef(t, ok, "State %s is not expected. States: %v", currentState.CacheID, expected)
}
}
ctx := context.Background()
clk := clock.NewMock()
store := &state.FakeInstanceStore{}
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: store,
Images: &state.NoopImageService{},
Clock: clk,
Historian: &state.FakeHistorian{},
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
rule := models.AlertRuleGen(models.WithFor(0))()
initResults := eval.Results{
eval.ResultGen(eval.WithEvaluatedAt(clk.Now()))(),
eval.ResultGen(eval.WithState(eval.Alerting), eval.WithEvaluatedAt(clk.Now()))(),
eval.ResultGen(eval.WithState(eval.Normal), eval.WithEvaluatedAt(clk.Now()))(),
}
state1 := getCacheID(t, rule, initResults[0])
state2 := getCacheID(t, rule, initResults[1])
state3 := getCacheID(t, rule, initResults[2])
initStates := map[string]struct{}{
state1: {},
state2: {},
state3: {},
}
// Init
processed := st.ProcessEvalResults(ctx, clk.Now(), rule, initResults, nil)
checkExpectedStateTransitions(t, processed, initStates)
currentStates := st.GetStatesForRuleUID(rule.OrgID, rule.UID)
statesMap := checkExpectedStates(t, currentStates, initStates)
require.Equal(t, eval.Alerting, statesMap[state2].State) // make sure the state is alerting because we need it to be resolved later
staleDuration := 2 * time.Duration(rule.IntervalSeconds) * time.Second
clk.Add(staleDuration)
result := initResults[0]
result.EvaluatedAt = clk.Now()
results := eval.Results{
result,
}
var expectedStaleKeys []models.AlertInstanceKey
t.Run("should mark missing states as stale", func(t *testing.T) {
processed = st.ProcessEvalResults(ctx, clk.Now(), rule, results, nil)
checkExpectedStateTransitions(t, processed, initStates)
for _, s := range processed {
if s.CacheID == state1 {
continue
}
assert.Equal(t, eval.Normal, s.State.State)
assert.Equal(t, models.StateReasonMissingSeries, s.StateReason)
assert.Equal(t, clk.Now(), s.EndsAt)
if s.CacheID == state2 {
assert.Truef(t, s.Resolved, "Returned stale state should have Resolved set to true")
}
key, err := s.GetAlertInstanceKey()
require.NoError(t, err)
expectedStaleKeys = append(expectedStaleKeys, key)
}
})
t.Run("should remove stale states from cache", func(t *testing.T) {
currentStates = st.GetStatesForRuleUID(rule.OrgID, rule.UID)
checkExpectedStates(t, currentStates, map[string]struct{}{
getCacheID(t, rule, results[0]): {},
})
})
t.Run("should delete stale states from the database", func(t *testing.T) {
for _, op := range store.RecordedOps {
switch q := op.(type) {
case state.FakeInstanceStoreOp:
keys, ok := q.Args[1].([]models.AlertInstanceKey)
require.Truef(t, ok, "Failed to parse fake store operations")
require.Len(t, keys, 2)
require.EqualValues(t, expectedStaleKeys, keys)
}
}
})
}
func TestDeleteStateByRuleUID(t *testing.T) {
interval := time.Minute
ctx := context.Background()
_, dbstore := tests.SetupTestEnv(t, 1)
const mainOrgID int64 = 1
rule := tests.CreateTestAlertRule(t, ctx, dbstore, int64(interval.Seconds()), mainOrgID)
labels1 := models.InstanceLabels{"test1": "testValue1"}
_, hash1, _ := labels1.StringAndHash()
labels2 := models.InstanceLabels{"test2": "testValue2"}
_, hash2, _ := labels2.StringAndHash()
instances := []models.AlertInstance{
{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash1,
},
CurrentState: models.InstanceStateNormal,
Labels: labels1,
},
{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash2,
},
CurrentState: models.InstanceStateFiring,
Labels: labels2,
},
}
for _, instance := range instances {
_ = dbstore.SaveAlertInstance(ctx, instance)
}
testCases := []struct {
desc string
instanceStore state.InstanceStore
expectedStates []*state.State
startingStateCacheCount int
finalStateCacheCount int
startingInstanceDBCount int
finalInstanceDBCount int
}{
{
desc: "all states/instances are removed from cache and DB",
instanceStore: dbstore,
expectedStates: []*state.State{
{
AlertRuleUID: rule.UID,
OrgID: 1,
Labels: data.Labels{"test1": "testValue1"},
State: eval.Normal,
EvaluationDuration: 0,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
},
{
AlertRuleUID: rule.UID,
OrgID: 1,
Labels: data.Labels{"test2": "testValue2"},
State: eval.Alerting,
EvaluationDuration: 0,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
},
},
startingStateCacheCount: 2,
finalStateCacheCount: 0,
startingInstanceDBCount: 2,
finalInstanceDBCount: 0,
},
}
for _, tc := range testCases {
expectedStatesMap := make(map[string]*state.State, len(tc.expectedStates))
for _, expectedState := range tc.expectedStates {
s := setCacheID(expectedState)
expectedStatesMap[s.CacheID] = s
}
t.Run(tc.desc, func(t *testing.T) {
ctx := context.Background()
clk := clock.NewMock()
clk.Set(time.Now())
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: dbstore,
Images: &state.NoopImageService{},
Clock: clk,
Historian: &state.FakeHistorian{},
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
st.Warm(ctx, dbstore)
q := &models.ListAlertInstancesQuery{RuleOrgID: rule.OrgID, RuleUID: rule.UID}
alerts, _ := dbstore.ListAlertInstances(ctx, q)
existingStatesForRule := st.GetStatesForRuleUID(rule.OrgID, rule.UID)
// We have loaded the expected number of entries from the db
assert.Equal(t, tc.startingStateCacheCount, len(existingStatesForRule))
assert.Equal(t, tc.startingInstanceDBCount, len(alerts))
expectedReason := util.GenerateShortUID()
transitions := st.DeleteStateByRuleUID(ctx, rule.GetKey(), expectedReason)
// Check that the deleted states are the same as the ones that were in cache
assert.Equal(t, tc.startingStateCacheCount, len(transitions))
for _, s := range transitions {
assert.Contains(t, expectedStatesMap, s.CacheID)
oldState := expectedStatesMap[s.CacheID]
assert.Equal(t, oldState.State, s.PreviousState)
assert.Equal(t, oldState.StateReason, s.PreviousStateReason)
assert.Equal(t, eval.Normal, s.State.State)
assert.Equal(t, expectedReason, s.StateReason)
if oldState.State == eval.Normal {
assert.Equal(t, oldState.StartsAt, s.StartsAt)
assert.False(t, s.Resolved)
} else {
assert.Equal(t, clk.Now(), s.StartsAt)
if oldState.State == eval.Alerting {
assert.True(t, s.Resolved)
}
}
assert.Equal(t, clk.Now(), s.EndsAt)
}
q = &models.ListAlertInstancesQuery{RuleOrgID: rule.OrgID, RuleUID: rule.UID}
alertInstances, _ := dbstore.ListAlertInstances(ctx, q)
existingStatesForRule = st.GetStatesForRuleUID(rule.OrgID, rule.UID)
// The expected number of state entries remains after states are deleted
assert.Equal(t, tc.finalStateCacheCount, len(existingStatesForRule))
assert.Equal(t, tc.finalInstanceDBCount, len(alertInstances))
})
}
}
func TestResetStateByRuleUID(t *testing.T) {
interval := time.Minute
ctx := context.Background()
_, dbstore := tests.SetupTestEnv(t, 1)
const mainOrgID int64 = 1
rule := tests.CreateTestAlertRule(t, ctx, dbstore, int64(interval.Seconds()), mainOrgID)
labels1 := models.InstanceLabels{"test1": "testValue1"}
_, hash1, _ := labels1.StringAndHash()
labels2 := models.InstanceLabels{"test2": "testValue2"}
_, hash2, _ := labels2.StringAndHash()
instances := []models.AlertInstance{
{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash1,
},
CurrentState: models.InstanceStateNormal,
Labels: labels1,
},
{
AlertInstanceKey: models.AlertInstanceKey{
RuleOrgID: rule.OrgID,
RuleUID: rule.UID,
LabelsHash: hash2,
},
CurrentState: models.InstanceStateFiring,
Labels: labels2,
},
}
for _, instance := range instances {
_ = dbstore.SaveAlertInstance(ctx, instance)
}
testCases := []struct {
desc string
instanceStore state.InstanceStore
expectedStates []*state.State
startingStateCacheCount int
finalStateCacheCount int
startingInstanceDBCount int
finalInstanceDBCount int
newHistorianEntriesCount int
}{
{
desc: "all states/instances are removed from cache and DB and saved in historian",
instanceStore: dbstore,
expectedStates: []*state.State{
{
AlertRuleUID: rule.UID,
OrgID: 1,
Labels: data.Labels{"test1": "testValue1"},
State: eval.Normal,
EvaluationDuration: 0,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
},
{
AlertRuleUID: rule.UID,
OrgID: 1,
Labels: data.Labels{"test2": "testValue2"},
State: eval.Alerting,
EvaluationDuration: 0,
Annotations: map[string]string{"testAnnoKey": "testAnnoValue"},
},
},
startingStateCacheCount: 2,
finalStateCacheCount: 0,
startingInstanceDBCount: 2,
finalInstanceDBCount: 0,
newHistorianEntriesCount: 2,
},
}
for _, tc := range testCases {
expectedStatesMap := stateSliceToMap(tc.expectedStates)
t.Run(tc.desc, func(t *testing.T) {
ctx := context.Background()
fakeHistorian := &state.FakeHistorian{StateTransitions: make([]state.StateTransition, 0)}
clk := clock.NewMock()
clk.Set(time.Now())
cfg := state.ManagerCfg{
Metrics: metrics.NewNGAlert(prometheus.NewPedanticRegistry()).GetStateMetrics(),
ExternalURL: nil,
InstanceStore: dbstore,
Images: &state.NoopImageService{},
Clock: clk,
Historian: fakeHistorian,
Tracer: tracing.InitializeTracerForTest(),
Log: log.New("ngalert.state.manager"),
}
st := state.NewManager(cfg, state.NewNoopPersister())
st.Warm(ctx, dbstore)
q := &models.ListAlertInstancesQuery{RuleOrgID: rule.OrgID, RuleUID: rule.UID}
alerts, _ := dbstore.ListAlertInstances(ctx, q)
existingStatesForRule := st.GetStatesForRuleUID(rule.OrgID, rule.UID)
// We have loaded the expected number of entries from the db
assert.Equal(t, tc.startingStateCacheCount, len(existingStatesForRule))
assert.Equal(t, tc.startingInstanceDBCount, len(alerts))
transitions := st.ResetStateByRuleUID(ctx, rule, models.StateReasonPaused)
// Check that the deleted states are the same as the ones that were in cache
assert.Equal(t, tc.startingStateCacheCount, len(transitions))
for _, s := range transitions {
assert.Contains(t, expectedStatesMap, s.CacheID)
oldState := expectedStatesMap[s.CacheID]
assert.Equal(t, oldState.State, s.PreviousState)
assert.Equal(t, oldState.StateReason, s.PreviousStateReason)
assert.Equal(t, eval.Normal, s.State.State)
assert.Equal(t, models.StateReasonPaused, s.StateReason)
if oldState.State == eval.Normal {
assert.Equal(t, oldState.StartsAt, s.StartsAt)
assert.False(t, s.Resolved)
} else {
assert.Equal(t, clk.Now(), s.StartsAt)
if oldState.State == eval.Alerting {
assert.True(t, s.Resolved)
}
}
assert.Equal(t, clk.Now(), s.EndsAt)
}
// Check if both entries have been added to the historian
assert.Equal(t, tc.newHistorianEntriesCount, len(fakeHistorian.StateTransitions))
assert.Equal(t, transitions, fakeHistorian.StateTransitions)
q = &models.ListAlertInstancesQuery{RuleOrgID: rule.OrgID, RuleUID: rule.UID}
alertInstances, _ := dbstore.ListAlertInstances(ctx, q)
existingStatesForRule = st.GetStatesForRuleUID(rule.OrgID, rule.UID)
// The expected number of state entries remains after states are deleted
assert.Equal(t, tc.finalStateCacheCount, len(existingStatesForRule))
assert.Equal(t, tc.finalInstanceDBCount, len(alertInstances))
})
}
}
func setCacheID(s *state.State) *state.State {
if s.CacheID != "" {
return s
}
il := models.InstanceLabels(s.Labels)
id, err := il.StringKey()
if err != nil {
panic(err)
}
s.CacheID = id
return s
}
func stateSliceToMap(states []*state.State) map[string]*state.State {
result := make(map[string]*state.State, len(states))
for _, s := range states {
setCacheID(s)
result[s.CacheID] = s
}
return result
}
func mergeLabels(a, b data.Labels) data.Labels {
result := make(data.Labels, len(a)+len(b))
for k, v := range a {
result[k] = v
}
for k, v := range b {
result[k] = v
}
return result
}
| pkg/services/ngalert/state/manager_test.go | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017895337077789009,
0.00017463062249589711,
0.00016343571769539267,
0.00017517332162242383,
0.0000025465392354817595
]
|
{
"id": 1,
"code_window": [
" limitToBounds={true}\n",
" disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}\n",
" panning={{ allowLeftClickPan: false }}\n",
" >\n",
" <TransformComponent>{sceneDiv}</TransformComponent>\n",
" </TransformWrapper>\n",
" );\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <TransformComponent>\n",
" {/* The <div> element has child elements that allow for mouse events, so we need to disable the linter rule */}\n",
" {/* eslint-disable-next-line jsx-a11y/no-static-element-interactions */}\n",
" <div onMouseDown={onSceneContainerMouseDown}>{sceneDiv}</div>\n",
" </TransformComponent>\n"
],
"file_path": "public/app/features/canvas/runtime/SceneTransformWrapper.tsx",
"type": "replace",
"edit_start_line_idx": 33
} | import { ComparisonOperation } from '@grafana/schema';
import { compareValues } from './compareValues';
describe('compare values', () => {
it('simple comparisons', () => {
expect(compareValues(null, ComparisonOperation.EQ, null)).toEqual(true);
expect(compareValues(null, ComparisonOperation.NEQ, null)).toEqual(false);
expect(compareValues(1, ComparisonOperation.GT, 2)).toEqual(false);
expect(compareValues(2, ComparisonOperation.GT, 1)).toEqual(true);
expect(compareValues(1, ComparisonOperation.GTE, 2)).toEqual(false);
expect(compareValues(2, ComparisonOperation.GTE, 1)).toEqual(true);
expect(compareValues(1, ComparisonOperation.LT, 2)).toEqual(true);
expect(compareValues(2, ComparisonOperation.LT, 1)).toEqual(false);
expect(compareValues(1, ComparisonOperation.LTE, 2)).toEqual(true);
expect(compareValues(2, ComparisonOperation.LTE, 1)).toEqual(false);
expect(compareValues(1, ComparisonOperation.EQ, 1)).toEqual(true);
expect(compareValues(1, ComparisonOperation.LTE, 1)).toEqual(true);
expect(compareValues(1, ComparisonOperation.GTE, 1)).toEqual(true);
});
});
| packages/grafana-data/src/transformations/matchers/compareValues.test.ts | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017699741874821484,
0.00017638255667407066,
0.0001754898694343865,
0.00017666038183961064,
6.460476811298577e-7
]
|
{
"id": 2,
"code_window": [
"\n",
" render() {\n",
" const isTooltipValid = (this.tooltip?.element?.data?.links?.length ?? 0) > 0;\n",
" const canShowElementTooltip = !this.isEditingEnabled && isTooltipValid;\n",
"\n",
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!this.shouldPanZoom || this.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!this.contextMenuVisible && !this.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n",
" const sceneDiv = (\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 670
} | import { css } from '@emotion/css';
import Moveable from 'moveable';
import React, { createRef, CSSProperties, RefObject } from 'react';
import { ReactZoomPanPinchContentRef } from 'react-zoom-pan-pinch';
import { BehaviorSubject, ReplaySubject, Subject, Subscription } from 'rxjs';
import { first } from 'rxjs/operators';
import Selecto from 'selecto';
import { AppEvents, GrafanaTheme2, PanelData } from '@grafana/data';
import { locationService } from '@grafana/runtime/src';
import {
ColorDimensionConfig,
ResourceDimensionConfig,
ScalarDimensionConfig,
ScaleDimensionConfig,
TextDimensionConfig,
} from '@grafana/schema';
import { Portal, stylesFactory } from '@grafana/ui';
import { config } from 'app/core/config';
import { CanvasFrameOptions, DEFAULT_CANVAS_ELEMENT_CONFIG } from 'app/features/canvas';
import { DimensionContext } from 'app/features/dimensions';
import {
getColorDimensionFromData,
getResourceDimensionFromData,
getScalarDimensionFromData,
getScaleDimensionFromData,
getTextDimensionFromData,
} from 'app/features/dimensions/utils';
import { CanvasContextMenu } from 'app/plugins/panel/canvas/components/CanvasContextMenu';
import { CanvasTooltip } from 'app/plugins/panel/canvas/components/CanvasTooltip';
import { CONNECTION_ANCHOR_DIV_ID } from 'app/plugins/panel/canvas/components/connections/ConnectionAnchors';
import { Connections } from 'app/plugins/panel/canvas/components/connections/Connections';
import { AnchorPoint, CanvasTooltipPayload, LayerActionID } from 'app/plugins/panel/canvas/types';
import { getParent, getTransformInstance } from 'app/plugins/panel/canvas/utils';
import appEvents from '../../../core/app_events';
import { CanvasPanel } from '../../../plugins/panel/canvas/CanvasPanel';
import { HorizontalConstraint, Placement, VerticalConstraint } from '../types';
import { SceneTransformWrapper } from './SceneTransformWrapper';
import { constraintViewable, dimensionViewable, settingsViewable } from './ables';
import { ElementState } from './element';
import { FrameState } from './frame';
import { RootElement } from './root';
export interface SelectionParams {
targets: Array<HTMLElement | SVGElement>;
frame?: FrameState;
}
export class Scene {
styles = getStyles(config.theme2);
readonly selection = new ReplaySubject<ElementState[]>(1);
readonly moved = new Subject<number>(); // called after resize/drag for editor updates
readonly byName = new Map<string, ElementState>();
root: RootElement;
revId = 0;
width = 0;
height = 0;
scale = 1;
style: CSSProperties = {};
data?: PanelData;
selecto?: Selecto;
moveable?: Moveable;
div?: HTMLDivElement;
connections: Connections;
currentLayer?: FrameState;
isEditingEnabled?: boolean;
shouldShowAdvancedTypes?: boolean;
shouldPanZoom?: boolean;
skipNextSelectionBroadcast = false;
ignoreDataUpdate = false;
panel: CanvasPanel;
contextMenuVisible?: boolean;
contextMenuOnVisibilityChange = (visible: boolean) => {
this.contextMenuVisible = visible;
const transformInstance = getTransformInstance(this);
if (transformInstance) {
if (visible) {
transformInstance.setup.disabled = true;
} else {
transformInstance.setup.disabled = false;
}
}
};
isPanelEditing = locationService.getSearchObject().editPanel !== undefined;
inlineEditingCallback?: () => void;
setBackgroundCallback?: (anchorPoint: AnchorPoint) => void;
tooltipCallback?: (tooltip: CanvasTooltipPayload | undefined) => void;
tooltip?: CanvasTooltipPayload;
moveableActionCallback?: (moved: boolean) => void;
readonly editModeEnabled = new BehaviorSubject<boolean>(false);
subscription: Subscription;
targetsToSelect = new Set<HTMLDivElement>();
transformComponentRef: RefObject<ReactZoomPanPinchContentRef> | undefined;
constructor(
cfg: CanvasFrameOptions,
enableEditing: boolean,
showAdvancedTypes: boolean,
panZoom: boolean,
public onSave: (cfg: CanvasFrameOptions) => void,
panel: CanvasPanel
) {
this.root = this.load(cfg, enableEditing, showAdvancedTypes, panZoom);
this.subscription = this.editModeEnabled.subscribe((open) => {
if (!this.moveable || !this.isEditingEnabled) {
return;
}
this.moveable.draggable = !open;
});
this.panel = panel;
this.connections = new Connections(this);
this.transformComponentRef = createRef();
}
getNextElementName = (isFrame = false) => {
const label = isFrame ? 'Frame' : 'Element';
let idx = this.byName.size + 1;
const max = idx + 100;
while (true && idx < max) {
const name = `${label} ${idx++}`;
if (!this.byName.has(name)) {
return name;
}
}
return `${label} ${Date.now()}`;
};
canRename = (v: string) => {
return !this.byName.has(v);
};
load(cfg: CanvasFrameOptions, enableEditing: boolean, showAdvancedTypes: boolean, panZoom: boolean) {
this.root = new RootElement(
cfg ?? {
type: 'frame',
elements: [DEFAULT_CANVAS_ELEMENT_CONFIG],
},
this,
this.save // callback when changes are made
);
this.isEditingEnabled = enableEditing;
this.shouldShowAdvancedTypes = showAdvancedTypes;
this.shouldPanZoom = panZoom;
setTimeout(() => {
if (this.div) {
// If editing is enabled, clear selecto instance
const destroySelecto = enableEditing;
this.initMoveable(destroySelecto, enableEditing);
this.currentLayer = this.root;
this.selection.next([]);
this.connections.select(undefined);
this.connections.updateState();
}
});
return this.root;
}
context: DimensionContext = {
getColor: (color: ColorDimensionConfig) => getColorDimensionFromData(this.data, color),
getScale: (scale: ScaleDimensionConfig) => getScaleDimensionFromData(this.data, scale),
getScalar: (scalar: ScalarDimensionConfig) => getScalarDimensionFromData(this.data, scalar),
getText: (text: TextDimensionConfig) => getTextDimensionFromData(this.data, text),
getResource: (res: ResourceDimensionConfig) => getResourceDimensionFromData(this.data, res),
getPanelData: () => this.data,
};
updateData(data: PanelData) {
this.data = data;
this.root.updateData(this.context);
}
updateSize(width: number, height: number) {
this.width = width;
this.height = height;
this.style = { width, height };
if (this.selecto?.getSelectedTargets().length) {
this.clearCurrentSelection();
}
}
frameSelection() {
this.selection.pipe(first()).subscribe((currentSelectedElements) => {
const currentLayer = currentSelectedElements[0].parent!;
const newLayer = new FrameState(
{
type: 'frame',
name: this.getNextElementName(true),
elements: [],
},
this,
currentSelectedElements[0].parent
);
const framePlacement = this.generateFrameContainer(currentSelectedElements);
newLayer.options.placement = framePlacement;
currentSelectedElements.forEach((element: ElementState) => {
const elementContainer = element.div?.getBoundingClientRect();
element.setPlacementFromConstraint(elementContainer, framePlacement as DOMRect);
currentLayer.doAction(LayerActionID.Delete, element);
newLayer.doAction(LayerActionID.Duplicate, element, false, false);
});
newLayer.setPlacementFromConstraint(framePlacement as DOMRect, currentLayer.div?.getBoundingClientRect());
currentLayer.elements.push(newLayer);
this.byName.set(newLayer.getName(), newLayer);
this.save();
});
}
private generateFrameContainer = (elements: ElementState[]): Placement => {
let minTop = Infinity;
let minLeft = Infinity;
let maxRight = 0;
let maxBottom = 0;
elements.forEach((element: ElementState) => {
const elementContainer = element.div?.getBoundingClientRect();
if (!elementContainer) {
return;
}
if (minTop > elementContainer.top) {
minTop = elementContainer.top;
}
if (minLeft > elementContainer.left) {
minLeft = elementContainer.left;
}
if (maxRight < elementContainer.right) {
maxRight = elementContainer.right;
}
if (maxBottom < elementContainer.bottom) {
maxBottom = elementContainer.bottom;
}
});
return {
top: minTop,
left: minLeft,
width: maxRight - minLeft,
height: maxBottom - minTop,
};
};
clearCurrentSelection(skipNextSelectionBroadcast = false) {
this.skipNextSelectionBroadcast = skipNextSelectionBroadcast;
let event: MouseEvent = new MouseEvent('click');
this.selecto?.clickTarget(event, this.div);
}
updateCurrentLayer(newLayer: FrameState) {
this.currentLayer = newLayer;
this.clearCurrentSelection();
this.save();
}
save = (updateMoveable = false) => {
this.onSave(this.root.getSaveModel());
if (updateMoveable) {
setTimeout(() => {
if (this.div) {
this.initMoveable(true, this.isEditingEnabled);
}
});
}
};
findElementByTarget = (target: Element): ElementState | undefined => {
// We will probably want to add memoization to this as we are calling on drag / resize
const stack = [...this.root.elements];
while (stack.length > 0) {
const currentElement = stack.shift();
if (currentElement && currentElement.div && currentElement.div === target) {
return currentElement;
}
const nestedElements = currentElement instanceof FrameState ? currentElement.elements : [];
for (const nestedElement of nestedElements) {
stack.unshift(nestedElement);
}
}
return undefined;
};
setNonTargetPointerEvents = (target: Element, disablePointerEvents: boolean) => {
const stack = [...this.root.elements];
while (stack.length > 0) {
const currentElement = stack.shift();
if (currentElement && currentElement.div && currentElement.div !== target) {
currentElement.applyLayoutStylesToDiv(disablePointerEvents);
}
const nestedElements = currentElement instanceof FrameState ? currentElement.elements : [];
for (const nestedElement of nestedElements) {
stack.unshift(nestedElement);
}
}
};
setRef = (sceneContainer: HTMLDivElement) => {
this.div = sceneContainer;
};
select = (selection: SelectionParams) => {
if (this.selecto) {
this.selecto.setSelectedTargets(selection.targets);
this.updateSelection(selection);
this.editModeEnabled.next(false);
// Hide connection anchors on programmatic select
if (this.connections.connectionAnchorDiv) {
this.connections.connectionAnchorDiv.style.display = 'none';
}
}
};
private updateSelection = (selection: SelectionParams) => {
this.moveable!.target = selection.targets;
if (this.skipNextSelectionBroadcast) {
this.skipNextSelectionBroadcast = false;
return;
}
if (selection.frame) {
this.selection.next([selection.frame]);
} else {
const s = selection.targets.map((t) => this.findElementByTarget(t)!);
this.selection.next(s);
}
};
private generateTargetElements = (rootElements: ElementState[]): HTMLDivElement[] => {
let targetElements: HTMLDivElement[] = [];
const stack = [...rootElements];
while (stack.length > 0) {
const currentElement = stack.shift();
if (currentElement && currentElement.div) {
targetElements.push(currentElement.div);
}
const nestedElements = currentElement instanceof FrameState ? currentElement.elements : [];
for (const nestedElement of nestedElements) {
stack.unshift(nestedElement);
}
}
return targetElements;
};
initMoveable = (destroySelecto = false, allowChanges = true) => {
const targetElements = this.generateTargetElements(this.root.elements);
if (destroySelecto && this.selecto) {
this.selecto.destroy();
}
this.selecto = new Selecto({
container: this.div,
rootContainer: getParent(this),
selectableTargets: targetElements,
toggleContinueSelect: 'shift',
selectFromInside: false,
hitRate: 0,
});
this.moveable = new Moveable(this.div!, {
draggable: allowChanges && !this.editModeEnabled.getValue(),
resizable: allowChanges,
ables: [dimensionViewable, constraintViewable(this), settingsViewable(this)],
props: {
dimensionViewable: allowChanges,
constraintViewable: allowChanges,
settingsViewable: allowChanges,
},
origin: false,
className: this.styles.selected,
})
.on('click', (event) => {
const targetedElement = this.findElementByTarget(event.target);
let elementSupportsEditing = false;
if (targetedElement) {
elementSupportsEditing = targetedElement.item.hasEditMode ?? false;
}
if (event.isDouble && allowChanges && !this.editModeEnabled.getValue() && elementSupportsEditing) {
this.editModeEnabled.next(true);
}
})
.on('clickGroup', (event) => {
this.selecto!.clickTarget(event.inputEvent, event.inputTarget);
})
.on('dragStart', (event) => {
this.ignoreDataUpdate = true;
this.setNonTargetPointerEvents(event.target, true);
})
.on('dragGroupStart', (event) => {
this.ignoreDataUpdate = true;
})
.on('drag', (event) => {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
targetedElement.applyDrag(event);
if (this.connections.connectionsNeedUpdate(targetedElement) && this.moveableActionCallback) {
this.moveableActionCallback(true);
}
}
})
.on('dragGroup', (e) => {
let needsUpdate = false;
for (let event of e.events) {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
targetedElement.applyDrag(event);
if (!needsUpdate) {
needsUpdate = this.connections.connectionsNeedUpdate(targetedElement);
}
}
}
if (needsUpdate && this.moveableActionCallback) {
this.moveableActionCallback(true);
}
})
.on('dragGroupEnd', (e) => {
e.events.forEach((event) => {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
if (targetedElement) {
targetedElement.setPlacementFromConstraint(undefined, undefined, this.scale);
}
}
});
this.moved.next(Date.now());
this.ignoreDataUpdate = false;
})
.on('dragEnd', (event) => {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
targetedElement.setPlacementFromConstraint(undefined, undefined, this.scale);
}
this.moved.next(Date.now());
this.ignoreDataUpdate = false;
this.setNonTargetPointerEvents(event.target, false);
})
.on('resizeStart', (event) => {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
targetedElement.tempConstraint = { ...targetedElement.options.constraint };
targetedElement.options.constraint = {
vertical: VerticalConstraint.Top,
horizontal: HorizontalConstraint.Left,
};
targetedElement.setPlacementFromConstraint(undefined, undefined, this.scale);
}
})
.on('resize', (event) => {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
targetedElement.applyResize(event, this.scale);
if (this.connections.connectionsNeedUpdate(targetedElement) && this.moveableActionCallback) {
this.moveableActionCallback(true);
}
}
this.moved.next(Date.now()); // TODO only on end
})
.on('resizeGroup', (e) => {
let needsUpdate = false;
for (let event of e.events) {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
targetedElement.applyResize(event);
if (!needsUpdate) {
needsUpdate = this.connections.connectionsNeedUpdate(targetedElement);
}
}
}
if (needsUpdate && this.moveableActionCallback) {
this.moveableActionCallback(true);
}
this.moved.next(Date.now()); // TODO only on end
})
.on('resizeEnd', (event) => {
const targetedElement = this.findElementByTarget(event.target);
if (targetedElement) {
if (targetedElement.tempConstraint) {
targetedElement.options.constraint = targetedElement.tempConstraint;
targetedElement.tempConstraint = undefined;
}
targetedElement.setPlacementFromConstraint(undefined, undefined, this.scale);
}
});
let targets: Array<HTMLElement | SVGElement> = [];
this.selecto!.on('dragStart', (event) => {
const selectedTarget = event.inputEvent.target;
// If selected target is a connection control, eject to handle connection event
if (selectedTarget.id === CONNECTION_ANCHOR_DIV_ID) {
this.connections.handleConnectionDragStart(selectedTarget, event.inputEvent.clientX, event.inputEvent.clientY);
event.stop();
return;
}
const isTargetMoveableElement =
this.moveable!.isMoveableElement(selectedTarget) ||
targets.some((target) => target === selectedTarget || target.contains(selectedTarget));
const isTargetAlreadySelected = this.selecto
?.getSelectedTargets()
.includes(selectedTarget.parentElement.parentElement);
// Apply grabbing cursor while dragging, applyLayoutStylesToDiv() resets it to grab when done
if (
this.isEditingEnabled &&
!this.editModeEnabled.getValue() &&
isTargetMoveableElement &&
this.selecto?.getSelectedTargets().length
) {
this.selecto.getSelectedTargets()[0].style.cursor = 'grabbing';
}
if (isTargetMoveableElement || isTargetAlreadySelected || !this.isEditingEnabled) {
// Prevent drawing selection box when selected target is a moveable element or already selected
event.stop();
}
})
.on('select', () => {
this.editModeEnabled.next(false);
// Hide connection anchors on select
if (this.connections.connectionAnchorDiv) {
this.connections.connectionAnchorDiv.style.display = 'none';
}
})
.on('selectEnd', (event) => {
targets = event.selected;
this.updateSelection({ targets });
if (event.isDragStart) {
if (this.isEditingEnabled && !this.editModeEnabled.getValue() && this.selecto?.getSelectedTargets().length) {
this.selecto.getSelectedTargets()[0].style.cursor = 'grabbing';
}
event.inputEvent.preventDefault();
event.data.timer = setTimeout(() => {
this.moveable!.dragStart(event.inputEvent);
});
}
})
.on('dragEnd', (event) => {
clearTimeout(event.data.timer);
});
};
reorderElements = (src: ElementState, dest: ElementState, dragToGap: boolean, destPosition: number) => {
switch (dragToGap) {
case true:
switch (destPosition) {
case -1:
// top of the tree
if (src.parent instanceof FrameState) {
// move outside the frame
if (dest.parent) {
this.updateElements(src, dest.parent, dest.parent.elements.length);
src.updateData(dest.parent.scene.context);
}
} else {
dest.parent?.reorderTree(src, dest, true);
}
break;
default:
if (dest.parent) {
this.updateElements(src, dest.parent, dest.parent.elements.indexOf(dest));
src.updateData(dest.parent.scene.context);
}
break;
}
break;
case false:
if (dest instanceof FrameState) {
if (src.parent === dest) {
// same frame parent
src.parent?.reorderTree(src, dest, true);
} else {
this.updateElements(src, dest);
src.updateData(dest.scene.context);
}
} else if (src.parent === dest.parent) {
src.parent?.reorderTree(src, dest);
} else {
if (dest.parent) {
this.updateElements(src, dest.parent);
src.updateData(dest.parent.scene.context);
}
}
break;
}
};
private updateElements = (src: ElementState, dest: FrameState | RootElement, idx: number | null = null) => {
src.parent?.doAction(LayerActionID.Delete, src);
src.parent = dest;
const elementContainer = src.div?.getBoundingClientRect();
src.setPlacementFromConstraint(elementContainer, dest.div?.getBoundingClientRect());
const destIndex = idx ?? dest.elements.length - 1;
dest.elements.splice(destIndex, 0, src);
dest.scene.save();
dest.reinitializeMoveable();
};
addToSelection = () => {
try {
let selection: SelectionParams = { targets: [] };
selection.targets = [...this.targetsToSelect];
this.select(selection);
} catch (error) {
appEvents.emit(AppEvents.alertError, ['Unable to add to selection']);
}
};
render() {
const isTooltipValid = (this.tooltip?.element?.data?.links?.length ?? 0) > 0;
const canShowElementTooltip = !this.isEditingEnabled && isTooltipValid;
const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {
// If pan and zoom is disabled or context menu is visible, don't pan
if ((!this.shouldPanZoom || this.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {
e.preventDefault();
e.stopPropagation();
}
// If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan
if (!this.contextMenuVisible && !this.isPanelEditing && e.button === 2 && !e.ctrlKey) {
e.preventDefault();
e.stopPropagation();
}
};
const sceneDiv = (
// The <div> element has child elements that allow for mouse events, so we need to disable the linter rule
// eslint-disable-next-line jsx-a11y/no-static-element-interactions
<div
key={this.revId}
className={this.styles.wrap}
style={this.style}
ref={this.setRef}
onMouseDown={onSceneContainerMouseDown}
>
{this.connections.render()}
{this.root.render()}
{this.isEditingEnabled && (
<Portal>
<CanvasContextMenu
scene={this}
panel={this.panel}
onVisibilityChange={this.contextMenuOnVisibilityChange}
/>
</Portal>
)}
{canShowElementTooltip && (
<Portal>
<CanvasTooltip scene={this} />
</Portal>
)}
</div>
);
return config.featureToggles.canvasPanelPanZoom ? (
<SceneTransformWrapper scene={this}>{sceneDiv}</SceneTransformWrapper>
) : (
sceneDiv
);
}
}
const getStyles = stylesFactory((theme: GrafanaTheme2) => ({
wrap: css`
overflow: hidden;
position: relative;
`,
selected: css`
z-index: 999 !important;
`,
}));
| public/app/features/canvas/runtime/scene.tsx | 1 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.9987516403198242,
0.07312210649251938,
0.00016059052722994238,
0.00017005865811370313,
0.2525152862071991
]
|
{
"id": 2,
"code_window": [
"\n",
" render() {\n",
" const isTooltipValid = (this.tooltip?.element?.data?.links?.length ?? 0) > 0;\n",
" const canShowElementTooltip = !this.isEditingEnabled && isTooltipValid;\n",
"\n",
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!this.shouldPanZoom || this.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!this.contextMenuVisible && !this.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n",
" const sceneDiv = (\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 670
} | export * from './logAnalyticsMetadata';
export * from './query';
export * from './types';
| public/app/plugins/datasource/azuremonitor/types/index.ts | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00016956475155893713,
0.00016956475155893713,
0.00016956475155893713,
0.00016956475155893713,
0
]
|
{
"id": 2,
"code_window": [
"\n",
" render() {\n",
" const isTooltipValid = (this.tooltip?.element?.data?.links?.length ?? 0) > 0;\n",
" const canShowElementTooltip = !this.isEditingEnabled && isTooltipValid;\n",
"\n",
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!this.shouldPanZoom || this.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!this.contextMenuVisible && !this.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n",
" const sceneDiv = (\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 670
} | <svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24"><path d="M11,11.72V15a1,1,0,0,0,2,0V11.72A2,2,0,0,0,14,10a2,2,0,0,0-4,0A2,2,0,0,0,11,11.72ZM21,2H3A1,1,0,0,0,2,3V21a1,1,0,0,0,1,1H21a1,1,0,0,0,1-1V3A1,1,0,0,0,21,2ZM20,20H4V4H20Z"/></svg> | public/img/icons/unicons/keyhole-square-full.svg | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.000171498759300448,
0.000171498759300448,
0.000171498759300448,
0.000171498759300448,
0
]
|
{
"id": 2,
"code_window": [
"\n",
" render() {\n",
" const isTooltipValid = (this.tooltip?.element?.data?.links?.length ?? 0) > 0;\n",
" const canShowElementTooltip = !this.isEditingEnabled && isTooltipValid;\n",
"\n",
" const onSceneContainerMouseDown = (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => {\n",
" // If pan and zoom is disabled or context menu is visible, don't pan\n",
" if ((!this.shouldPanZoom || this.contextMenuVisible) && (e.button === 1 || (e.button === 2 && e.ctrlKey))) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
"\n",
" // If context menu is hidden, ignore left mouse or non-ctrl right mouse for pan\n",
" if (!this.contextMenuVisible && !this.isPanelEditing && e.button === 2 && !e.ctrlKey) {\n",
" e.preventDefault();\n",
" e.stopPropagation();\n",
" }\n",
" };\n",
"\n",
" const sceneDiv = (\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 670
} | <?xml version="1.0" encoding="utf-8"?>
<!-- Generator: Adobe Illustrator 20.1.0, SVG Export Plug-In . SVG Version: 6.00 Build 0) -->
<svg version="1.1" id="Layer_1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" x="0px" y="0px"
width="64px" height="64px" viewBox="0 0 64 64" style="enable-background:new 0 0 64 64;" xml:space="preserve">
<style type="text/css">
.st0{fill:#E3E3E3;}
</style>
<g>
<path class="st0" d="M41.5,35.5c-0.1-0.1-0.2-0.1-0.4-0.2c-1.2-0.7-1-2.6-0.7-3.8c0.9-1,1.8-2.5,2.2-5.2c0.1-0.7,0.4-1.3,0.8-1.9
c0.8-1.1,1.2-2.5,1.2-4c0-1.1-0.3-2.1-0.8-2.9c-0.1-0.2-0.3-0.4-0.4-0.5c0.6-1.6,1.3-4,0.7-6.6C43.1,6,37.1,4,32,4
c-4.6,0-8.1,1.6-9.5,4.3c-1.2,0.6-1.9,1.5-2.3,2.2c-1.1,2.2-0.4,4.8,0.3,6.6c-0.2,0.2-0.3,0.4-0.4,0.6c-0.5,0.8-0.8,1.8-0.8,3
c0,1.4,0.4,2.9,1.2,3.9c0.4,0.5,0.7,1.2,0.8,1.9c0.3,2.6,1.3,4.2,2.3,5.2c0.3,1.1,0.5,3-0.7,3.7c-0.1,0.1-0.2,0.1-0.3,0.2
C8.6,41.5,8.5,44,8.5,45v7.6c0,4.1,3.3,7.4,7.4,7.4h15.8h11.4h4.9c4.1,0,7.4-3.3,7.4-7.4V45C55.6,44,55.5,41.5,41.5,35.5z"/>
</g>
</svg>
| public/img/icons_dark_theme/icon_user.svg | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017258389561902732,
0.00017086585285142064,
0.0001691478246357292,
0.00017086585285142064,
0.0000017180354916490614
]
|
{
"id": 3,
"code_window": [
" const sceneDiv = (\n",
" // The <div> element has child elements that allow for mouse events, so we need to disable the linter rule\n",
" // eslint-disable-next-line jsx-a11y/no-static-element-interactions\n",
" <div\n",
" key={this.revId}\n",
" className={this.styles.wrap}\n",
" style={this.style}\n",
" ref={this.setRef}\n",
" onMouseDown={onSceneContainerMouseDown}\n",
" >\n",
" {this.connections.render()}\n",
" {this.root.render()}\n",
" {this.isEditingEnabled && (\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <div key={this.revId} className={this.styles.wrap} style={this.style} ref={this.setRef}>\n"
],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 685
} | import React from 'react';
import { TransformWrapper, TransformComponent, ReactZoomPanPinchRef } from 'react-zoom-pan-pinch';
import { config } from '@grafana/runtime';
import { Scene } from './scene';
type SceneTransformWrapperProps = {
scene: Scene;
children: React.ReactNode;
};
export const SceneTransformWrapper = ({ scene, children: sceneDiv }: SceneTransformWrapperProps) => {
const onZoom = (zoomPanPinchRef: ReactZoomPanPinchRef) => {
const scale = zoomPanPinchRef.state.scale;
if (scene.moveable && scale > 0) {
scene.moveable.zoom = 1 / scale;
}
scene.scale = scale;
};
return (
<TransformWrapper
doubleClick={{ mode: 'reset' }}
ref={scene.transformComponentRef}
onZoom={onZoom}
onTransformed={(_, state) => {
scene.scale = state.scale;
}}
limitToBounds={true}
disabled={!config.featureToggles.canvasPanelPanZoom || !scene.shouldPanZoom}
panning={{ allowLeftClickPan: false }}
>
<TransformComponent>{sceneDiv}</TransformComponent>
</TransformWrapper>
);
};
| public/app/features/canvas/runtime/SceneTransformWrapper.tsx | 1 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.009591050446033478,
0.003212129231542349,
0.00016330408107023686,
0.001547081395983696,
0.003850729903206229
]
|
{
"id": 3,
"code_window": [
" const sceneDiv = (\n",
" // The <div> element has child elements that allow for mouse events, so we need to disable the linter rule\n",
" // eslint-disable-next-line jsx-a11y/no-static-element-interactions\n",
" <div\n",
" key={this.revId}\n",
" className={this.styles.wrap}\n",
" style={this.style}\n",
" ref={this.setRef}\n",
" onMouseDown={onSceneContainerMouseDown}\n",
" >\n",
" {this.connections.render()}\n",
" {this.root.render()}\n",
" {this.isEditingEnabled && (\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <div key={this.revId} className={this.styles.wrap} style={this.style} ref={this.setRef}>\n"
],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 685
} | import { invert } from 'lodash';
import { Token } from 'prismjs';
import {
AbstractLabelMatcher,
AbstractLabelOperator,
AbstractQuery,
DataQuery,
dateMath,
DateTime,
incrRoundDn,
TimeRange,
} from '@grafana/data';
import { addLabelToQuery } from './add_label_to_query';
import { SUGGESTIONS_LIMIT } from './language_provider';
import { PROMETHEUS_QUERY_BUILDER_MAX_RESULTS } from './querybuilder/components/MetricSelect';
import { PrometheusCacheLevel, PromMetricsMetadata, PromMetricsMetadataItem } from './types';
export const processHistogramMetrics = (metrics: string[]) => {
const resultSet: Set<string> = new Set();
const regexp = new RegExp('_bucket($|:)');
for (let index = 0; index < metrics.length; index++) {
const metric = metrics[index];
const isHistogramValue = regexp.test(metric);
if (isHistogramValue) {
resultSet.add(metric);
}
}
return [...resultSet];
};
export function processLabels(labels: Array<{ [key: string]: string }>, withName = false) {
// For processing we are going to use sets as they have significantly better performance than arrays
// After we process labels, we will convert sets to arrays and return object with label values in arrays
const valueSet: { [key: string]: Set<string> } = {};
labels.forEach((label) => {
const { __name__, ...rest } = label;
if (withName) {
valueSet['__name__'] = valueSet['__name__'] || new Set();
if (!valueSet['__name__'].has(__name__)) {
valueSet['__name__'].add(__name__);
}
}
Object.keys(rest).forEach((key) => {
if (!valueSet[key]) {
valueSet[key] = new Set();
}
if (!valueSet[key].has(rest[key])) {
valueSet[key].add(rest[key]);
}
});
});
// valueArray that we are going to return in the object
const valueArray: { [key: string]: string[] } = {};
limitSuggestions(Object.keys(valueSet)).forEach((key) => {
valueArray[key] = limitSuggestions(Array.from(valueSet[key]));
});
return { values: valueArray, keys: Object.keys(valueArray) };
}
// const cleanSelectorRegexp = /\{(\w+="[^"\n]*?")(,\w+="[^"\n]*?")*\}/;
export const selectorRegexp = /\{[^}]*?(\}|$)/;
export const labelRegexp = /\b(\w+)(!?=~?)("[^"\n]*?")/g;
export function parseSelector(query: string, cursorOffset = 1): { labelKeys: any[]; selector: string } {
if (!query.match(selectorRegexp)) {
// Special matcher for metrics
if (query.match(/^[A-Za-z:][\w:]*$/)) {
return {
selector: `{__name__="${query}"}`,
labelKeys: ['__name__'],
};
}
throw new Error('Query must contain a selector: ' + query);
}
// Check if inside a selector
const prefix = query.slice(0, cursorOffset);
const prefixOpen = prefix.lastIndexOf('{');
const prefixClose = prefix.lastIndexOf('}');
if (prefixOpen === -1) {
throw new Error('Not inside selector, missing open brace: ' + prefix);
}
if (prefixClose > -1 && prefixClose > prefixOpen) {
throw new Error('Not inside selector, previous selector already closed: ' + prefix);
}
const suffix = query.slice(cursorOffset);
const suffixCloseIndex = suffix.indexOf('}');
const suffixClose = suffixCloseIndex + cursorOffset;
const suffixOpenIndex = suffix.indexOf('{');
const suffixOpen = suffixOpenIndex + cursorOffset;
if (suffixClose === -1) {
throw new Error('Not inside selector, missing closing brace in suffix: ' + suffix);
}
if (suffixOpenIndex > -1 && suffixOpen < suffixClose) {
throw new Error('Not inside selector, next selector opens before this one closed: ' + suffix);
}
// Extract clean labels to form clean selector, incomplete labels are dropped
const selector = query.slice(prefixOpen, suffixClose);
const labels: { [key: string]: { value: string; operator: string } } = {};
selector.replace(labelRegexp, (label, key, operator, value) => {
const labelOffset = query.indexOf(label);
const valueStart = labelOffset + key.length + operator.length + 1;
const valueEnd = labelOffset + key.length + operator.length + value.length - 1;
// Skip label if cursor is in value
if (cursorOffset < valueStart || cursorOffset > valueEnd) {
labels[key] = { value, operator };
}
return '';
});
// Add metric if there is one before the selector
const metricPrefix = query.slice(0, prefixOpen);
const metricMatch = metricPrefix.match(/[A-Za-z:][\w:]*$/);
if (metricMatch) {
labels['__name__'] = { value: `"${metricMatch[0]}"`, operator: '=' };
}
// Build sorted selector
const labelKeys = Object.keys(labels).sort();
const cleanSelector = labelKeys.map((key) => `${key}${labels[key].operator}${labels[key].value}`).join(',');
const selectorString = ['{', cleanSelector, '}'].join('');
return { labelKeys, selector: selectorString };
}
export function expandRecordingRules(query: string, mapping: { [name: string]: string }): string {
const ruleNames = Object.keys(mapping);
const rulesRegex = new RegExp(`(\\s|^)(${ruleNames.join('|')})(\\s|$|\\(|\\[|\\{)`, 'ig');
const expandedQuery = query.replace(rulesRegex, (match, pre, name, post) => `${pre}${mapping[name]}${post}`);
// Split query into array, so if query uses operators, we can correctly add labels to each individual part.
const queryArray = expandedQuery.split(/(\+|\-|\*|\/|\%|\^)/);
// Regex that matches occurrences of ){ or }{ or ]{ which is a sign of incorrecly added labels.
const invalidLabelsRegex = /(\)\{|\}\{|\]\{)/;
const correctlyExpandedQueryArray = queryArray.map((query) => {
return addLabelsToExpression(query, invalidLabelsRegex);
});
return correctlyExpandedQueryArray.join('');
}
function addLabelsToExpression(expr: string, invalidLabelsRegexp: RegExp) {
const match = expr.match(invalidLabelsRegexp);
if (!match) {
return expr;
}
// Split query into 2 parts - before the invalidLabelsRegex match and after.
const indexOfRegexMatch = match.index ?? 0;
const exprBeforeRegexMatch = expr.slice(0, indexOfRegexMatch + 1);
const exprAfterRegexMatch = expr.slice(indexOfRegexMatch + 1);
// Create arrayOfLabelObjects with label objects that have key, operator and value.
const arrayOfLabelObjects: Array<{ key: string; operator: string; value: string }> = [];
exprAfterRegexMatch.replace(labelRegexp, (label, key, operator, value) => {
arrayOfLabelObjects.push({ key, operator, value });
return '';
});
// Loop through all label objects and add them to query.
// As a starting point we have valid query without the labels.
let result = exprBeforeRegexMatch;
arrayOfLabelObjects.filter(Boolean).forEach((obj) => {
// Remove extra set of quotes from obj.value
const value = obj.value.slice(1, -1);
result = addLabelToQuery(result, obj.key, value, obj.operator);
});
return result;
}
/**
* Adds metadata for synthetic metrics for which the API does not provide metadata.
* See https://github.com/grafana/grafana/issues/22337 for details.
*
* @param metadata HELP and TYPE metadata from /api/v1/metadata
*/
export function fixSummariesMetadata(metadata: { [metric: string]: PromMetricsMetadataItem[] }): PromMetricsMetadata {
if (!metadata) {
return metadata;
}
const baseMetadata: PromMetricsMetadata = {};
const summaryMetadata: PromMetricsMetadata = {};
for (const metric in metadata) {
// NOTE: based on prometheus-documentation, we can receive
// multiple metadata-entries for the given metric, it seems
// it happens when the same metric is on multiple targets
// and their help-text differs
// (https://prometheus.io/docs/prometheus/latest/querying/api/#querying-metric-metadata)
// for now we just use the first entry.
const item = metadata[metric][0];
baseMetadata[metric] = item;
if (item.type === 'histogram') {
summaryMetadata[`${metric}_bucket`] = {
type: 'counter',
help: `Cumulative counters for the observation buckets (${item.help})`,
};
summaryMetadata[`${metric}_count`] = {
type: 'counter',
help: `Count of events that have been observed for the histogram metric (${item.help})`,
};
summaryMetadata[`${metric}_sum`] = {
type: 'counter',
help: `Total sum of all observed values for the histogram metric (${item.help})`,
};
}
if (item.type === 'summary') {
summaryMetadata[`${metric}_count`] = {
type: 'counter',
help: `Count of events that have been observed for the base metric (${item.help})`,
};
summaryMetadata[`${metric}_sum`] = {
type: 'counter',
help: `Total sum of all observed values for the base metric (${item.help})`,
};
}
}
// Synthetic series
const syntheticMetadata: PromMetricsMetadata = {};
syntheticMetadata['ALERTS'] = {
type: 'counter',
help: 'Time series showing pending and firing alerts. The sample value is set to 1 as long as the alert is in the indicated active (pending or firing) state.',
};
return { ...baseMetadata, ...summaryMetadata, ...syntheticMetadata };
}
export function roundMsToMin(milliseconds: number): number {
return roundSecToMin(milliseconds / 1000);
}
export function roundSecToMin(seconds: number): number {
return Math.floor(seconds / 60);
}
// Returns number of minutes rounded up to the nearest nth minute
export function roundSecToNextMin(seconds: number, secondsToRound = 1): number {
return Math.ceil(seconds / 60) - (Math.ceil(seconds / 60) % secondsToRound);
}
export function limitSuggestions(items: string[]) {
return items.slice(0, SUGGESTIONS_LIMIT);
}
export function addLimitInfo(items: any[] | undefined): string {
return items && items.length >= SUGGESTIONS_LIMIT ? `, limited to the first ${SUGGESTIONS_LIMIT} received items` : '';
}
// NOTE: the following 2 exported functions are very similar to the prometheus*Escape
// functions in datasource.ts, but they are not exactly the same algorithm, and we found
// no way to reuse one in the another or vice versa.
// Prometheus regular-expressions use the RE2 syntax (https://github.com/google/re2/wiki/Syntax),
// so every character that matches something in that list has to be escaped.
// the list of metacharacters is: *+?()|\.[]{}^$
// we make a javascript regular expression that matches those characters:
const RE2_METACHARACTERS = /[*+?()|\\.\[\]{}^$]/g;
function escapePrometheusRegexp(value: string): string {
return value.replace(RE2_METACHARACTERS, '\\$&');
}
// based on the openmetrics-documentation, the 3 symbols we have to handle are:
// - \n ... the newline character
// - \ ... the backslash character
// - " ... the double-quote character
export function escapeLabelValueInExactSelector(labelValue: string): string {
return labelValue.replace(/\\/g, '\\\\').replace(/\n/g, '\\n').replace(/"/g, '\\"');
}
export function escapeLabelValueInRegexSelector(labelValue: string): string {
return escapeLabelValueInExactSelector(escapePrometheusRegexp(labelValue));
}
const FromPromLikeMap: Record<string, AbstractLabelOperator> = {
'=': AbstractLabelOperator.Equal,
'!=': AbstractLabelOperator.NotEqual,
'=~': AbstractLabelOperator.EqualRegEx,
'!~': AbstractLabelOperator.NotEqualRegEx,
};
const ToPromLikeMap: Record<AbstractLabelOperator, string> = invert(FromPromLikeMap) as Record<
AbstractLabelOperator,
string
>;
export function toPromLikeExpr(labelBasedQuery: AbstractQuery): string {
const expr = labelBasedQuery.labelMatchers
.map((selector: AbstractLabelMatcher) => {
const operator = ToPromLikeMap[selector.operator];
if (operator) {
return `${selector.name}${operator}"${selector.value}"`;
} else {
return '';
}
})
.filter((e: string) => e !== '')
.join(', ');
return expr ? `{${expr}}` : '';
}
export function toPromLikeQuery(labelBasedQuery: AbstractQuery): PromLikeQuery {
return {
refId: labelBasedQuery.refId,
expr: toPromLikeExpr(labelBasedQuery),
range: true,
};
}
export interface PromLikeQuery extends DataQuery {
expr: string;
range: boolean;
}
function getMaybeTokenStringContent(token: Token): string {
if (typeof token.content === 'string') {
return token.content;
}
return '';
}
export function extractLabelMatchers(tokens: Array<string | Token>): AbstractLabelMatcher[] {
const labelMatchers: AbstractLabelMatcher[] = [];
for (const token of tokens) {
if (!(token instanceof Token)) {
continue;
}
if (token.type === 'context-labels') {
let labelKey = '';
let labelValue = '';
let labelOperator = '';
const contentTokens = Array.isArray(token.content) ? token.content : [token.content];
for (let currentToken of contentTokens) {
if (typeof currentToken === 'string') {
let currentStr: string;
currentStr = currentToken;
if (currentStr === '=' || currentStr === '!=' || currentStr === '=~' || currentStr === '!~') {
labelOperator = currentStr;
}
} else if (currentToken instanceof Token) {
switch (currentToken.type) {
case 'label-key':
labelKey = getMaybeTokenStringContent(currentToken);
break;
case 'label-value':
labelValue = getMaybeTokenStringContent(currentToken);
labelValue = labelValue.substring(1, labelValue.length - 1);
const labelComparator = FromPromLikeMap[labelOperator];
if (labelComparator) {
labelMatchers.push({ name: labelKey, operator: labelComparator, value: labelValue });
}
break;
}
}
}
}
}
return labelMatchers;
}
/**
* Calculates new interval "snapped" to the closest Nth minute, depending on cache level datasource setting
* @param cacheLevel
* @param range
*/
export function getRangeSnapInterval(
cacheLevel: PrometheusCacheLevel,
range: TimeRange
): { start: string; end: string } {
// Don't round the range if we're not caching
if (cacheLevel === PrometheusCacheLevel.None) {
return {
start: getPrometheusTime(range.from, false).toString(),
end: getPrometheusTime(range.to, true).toString(),
};
}
// Otherwise round down to the nearest nth minute for the start time
const startTime = getPrometheusTime(range.from, false);
// const startTimeQuantizedSeconds = roundSecToLastMin(startTime, getClientCacheDurationInMinutes(cacheLevel)) * 60;
const startTimeQuantizedSeconds = incrRoundDn(startTime, getClientCacheDurationInMinutes(cacheLevel) * 60);
// And round up to the nearest nth minute for the end time
const endTime = getPrometheusTime(range.to, true);
const endTimeQuantizedSeconds = roundSecToNextMin(endTime, getClientCacheDurationInMinutes(cacheLevel)) * 60;
// If the interval was too short, we could have rounded both start and end to the same time, if so let's add one step to the end
if (startTimeQuantizedSeconds === endTimeQuantizedSeconds) {
const endTimePlusOneStep = endTimeQuantizedSeconds + getClientCacheDurationInMinutes(cacheLevel) * 60;
return { start: startTimeQuantizedSeconds.toString(), end: endTimePlusOneStep.toString() };
}
const start = startTimeQuantizedSeconds.toString();
const end = endTimeQuantizedSeconds.toString();
return { start, end };
}
export function getClientCacheDurationInMinutes(cacheLevel: PrometheusCacheLevel) {
switch (cacheLevel) {
case PrometheusCacheLevel.Medium:
return 10;
case PrometheusCacheLevel.High:
return 60;
default:
return 1;
}
}
export function getPrometheusTime(date: string | DateTime, roundUp: boolean) {
if (typeof date === 'string') {
date = dateMath.parse(date, roundUp)!;
}
return Math.ceil(date.valueOf() / 1000);
}
export function truncateResult<T>(array: T[], limit?: number): T[] {
if (limit === undefined) {
limit = PROMETHEUS_QUERY_BUILDER_MAX_RESULTS;
}
array.length = Math.min(array.length, limit);
return array;
}
| public/app/plugins/datasource/prometheus/language_utils.ts | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017806420510169119,
0.00017361917707603425,
0.00016788358334451914,
0.0001736517297104001,
0.000002365985437791096
]
|
{
"id": 3,
"code_window": [
" const sceneDiv = (\n",
" // The <div> element has child elements that allow for mouse events, so we need to disable the linter rule\n",
" // eslint-disable-next-line jsx-a11y/no-static-element-interactions\n",
" <div\n",
" key={this.revId}\n",
" className={this.styles.wrap}\n",
" style={this.style}\n",
" ref={this.setRef}\n",
" onMouseDown={onSceneContainerMouseDown}\n",
" >\n",
" {this.connections.render()}\n",
" {this.root.render()}\n",
" {this.isEditingEnabled && (\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <div key={this.revId} className={this.styles.wrap} style={this.style} ref={this.setRef}>\n"
],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 685
} | import { omit } from 'lodash';
import {
DataQuery,
DataSourceInstanceSettings,
DataSourceRef,
getDefaultRelativeTimeRange,
IntervalValues,
rangeUtil,
RelativeTimeRange,
ScopedVars,
TimeRange,
} from '@grafana/data';
import { getDataSourceSrv } from '@grafana/runtime';
import { ExpressionDatasourceRef } from '@grafana/runtime/src/utils/DataSourceWithBackend';
import { DataSourceJsonData } from '@grafana/schema';
import { getNextRefIdChar } from 'app/core/utils/query';
import { DashboardModel, PanelModel } from 'app/features/dashboard/state';
import { ExpressionDatasourceUID, ExpressionQuery, ExpressionQueryType } from 'app/features/expressions/types';
import { LokiQuery } from 'app/plugins/datasource/loki/types';
import { PromQuery } from 'app/plugins/datasource/prometheus/types';
import { RuleWithLocation } from 'app/types/unified-alerting';
import {
AlertDataQuery,
AlertQuery,
Annotations,
GrafanaAlertStateDecision,
GrafanaNotificationSettings,
GrafanaRuleDefinition,
Labels,
PostableRuleGrafanaRuleDTO,
RulerAlertingRuleDTO,
RulerRecordingRuleDTO,
RulerRuleDTO,
} from 'app/types/unified-alerting-dto';
import { EvalFunction } from '../../state/alertDef';
import { AlertManagerManualRouting, ContactPoint, RuleFormType, RuleFormValues } from '../types/rule-form';
import { getRulesAccess } from './access-control';
import { Annotation, defaultAnnotations } from './constants';
import { getDefaultOrFirstCompatibleDataSource, GRAFANA_RULES_SOURCE_NAME, isGrafanaRulesSource } from './datasource';
import { arrayToRecord, recordToArray } from './misc';
import { isAlertingRulerRule, isGrafanaRulerRule, isRecordingRulerRule } from './rules';
import { parseInterval } from './time';
export type PromOrLokiQuery = PromQuery | LokiQuery;
export const MINUTE = '1m';
export const getDefaultFormValues = (): RuleFormValues => {
const { canCreateGrafanaRules, canCreateCloudRules } = getRulesAccess();
return Object.freeze({
name: '',
uid: '',
labels: [{ key: '', value: '' }],
annotations: defaultAnnotations,
dataSourceName: null,
type: canCreateGrafanaRules ? RuleFormType.grafana : canCreateCloudRules ? RuleFormType.cloudAlerting : undefined, // viewers can't create prom alerts
group: '',
// grafana
folder: null,
queries: [],
recordingRulesQueries: [],
condition: '',
noDataState: GrafanaAlertStateDecision.NoData,
execErrState: GrafanaAlertStateDecision.Error,
evaluateFor: '5m',
evaluateEvery: MINUTE,
manualRouting: false, // let's decide this later
contactPoints: {},
overrideGrouping: false,
overrideTimings: false,
muteTimeIntervals: [],
// cortex / loki
namespace: '',
expression: '',
forTime: 1,
forTimeUnit: 'm',
});
};
export function formValuesToRulerRuleDTO(values: RuleFormValues): RulerRuleDTO {
const { name, expression, forTime, forTimeUnit, keepFiringForTime, keepFiringForTimeUnit, type } = values;
if (type === RuleFormType.cloudAlerting) {
let keepFiringFor: string | undefined;
if (keepFiringForTime && keepFiringForTimeUnit) {
keepFiringFor = `${keepFiringForTime}${keepFiringForTimeUnit}`;
}
return {
alert: name,
for: `${forTime}${forTimeUnit}`,
keep_firing_for: keepFiringFor,
annotations: arrayToRecord(values.annotations || []),
labels: arrayToRecord(values.labels || []),
expr: expression,
};
} else if (type === RuleFormType.cloudRecording) {
return {
record: name,
labels: arrayToRecord(values.labels || []),
expr: expression,
};
}
throw new Error(`unexpected rule type: ${type}`);
}
export function listifyLabelsOrAnnotations(
item: Labels | Annotations | undefined,
addEmpty: boolean
): Array<{ key: string; value: string }> {
const list = [...recordToArray(item || {})];
if (addEmpty) {
list.push({ key: '', value: '' });
}
return list;
}
//make sure default annotations are always shown in order even if empty
export function normalizeDefaultAnnotations(annotations: Array<{ key: string; value: string }>) {
const orderedAnnotations = [...annotations];
const defaultAnnotationKeys = defaultAnnotations.map((annotation) => annotation.key);
defaultAnnotationKeys.forEach((defaultAnnotationKey, index) => {
const fieldIndex = orderedAnnotations.findIndex((field) => field.key === defaultAnnotationKey);
if (fieldIndex === -1) {
//add the default annotation if abstent
const emptyValue = { key: defaultAnnotationKey, value: '' };
orderedAnnotations.splice(index, 0, emptyValue);
} else if (fieldIndex !== index) {
//move it to the correct position if present
orderedAnnotations.splice(index, 0, orderedAnnotations.splice(fieldIndex, 1)[0]);
}
});
return orderedAnnotations;
}
export function getNotificationSettingsForDTO(
manualRouting: boolean,
contactPoints?: AlertManagerManualRouting
): GrafanaNotificationSettings | undefined {
if (contactPoints?.grafana?.selectedContactPoint && manualRouting) {
return {
receiver: contactPoints?.grafana?.selectedContactPoint,
mute_timings: contactPoints?.grafana?.muteTimeIntervals,
group_by: contactPoints?.grafana?.overrideGrouping ? contactPoints?.grafana?.groupBy : undefined,
group_wait: contactPoints?.grafana?.overrideTimings ? contactPoints?.grafana?.groupWaitValue : undefined,
group_interval: contactPoints?.grafana?.overrideTimings ? contactPoints?.grafana?.groupIntervalValue : undefined,
repeat_interval: contactPoints?.grafana?.overrideTimings
? contactPoints?.grafana?.repeatIntervalValue
: undefined,
};
}
return undefined;
}
export function formValuesToRulerGrafanaRuleDTO(values: RuleFormValues): PostableRuleGrafanaRuleDTO {
const { name, condition, noDataState, execErrState, evaluateFor, queries, isPaused, contactPoints, manualRouting } =
values;
if (condition) {
const notificationSettings: GrafanaNotificationSettings | undefined = getNotificationSettingsForDTO(
manualRouting,
contactPoints
);
return {
grafana_alert: {
title: name,
condition,
no_data_state: noDataState,
exec_err_state: execErrState,
data: queries.map(fixBothInstantAndRangeQuery),
is_paused: Boolean(isPaused),
notification_settings: notificationSettings,
},
for: evaluateFor,
annotations: arrayToRecord(values.annotations || []),
labels: arrayToRecord(values.labels || []),
};
}
throw new Error('Cannot create rule without specifying alert condition');
}
export function getContactPointsFromDTO(ga: GrafanaRuleDefinition): AlertManagerManualRouting | undefined {
const contactPoint: ContactPoint | undefined = ga.notification_settings
? {
selectedContactPoint: ga.notification_settings.receiver,
muteTimeIntervals: ga.notification_settings.mute_timings ?? [],
overrideGrouping: Boolean(ga.notification_settings?.group_by),
overrideTimings: Boolean(ga.notification_settings.group_wait),
groupBy: ga.notification_settings.group_by || [],
groupWaitValue: ga.notification_settings.group_wait || '',
groupIntervalValue: ga.notification_settings.group_interval || '',
repeatIntervalValue: ga.notification_settings.repeat_interval || '',
}
: undefined;
const routingSettings: AlertManagerManualRouting | undefined = contactPoint
? {
[GRAFANA_RULES_SOURCE_NAME]: contactPoint,
}
: undefined;
return routingSettings;
}
export function rulerRuleToFormValues(ruleWithLocation: RuleWithLocation): RuleFormValues {
const { ruleSourceName, namespace, group, rule } = ruleWithLocation;
const defaultFormValues = getDefaultFormValues();
if (isGrafanaRulesSource(ruleSourceName)) {
if (isGrafanaRulerRule(rule)) {
const ga = rule.grafana_alert;
const routingSettings: AlertManagerManualRouting | undefined = getContactPointsFromDTO(ga);
return {
...defaultFormValues,
name: ga.title,
type: RuleFormType.grafana,
group: group.name,
evaluateEvery: group.interval || defaultFormValues.evaluateEvery,
evaluateFor: rule.for || '0',
noDataState: ga.no_data_state,
execErrState: ga.exec_err_state,
queries: ga.data,
condition: ga.condition,
annotations: normalizeDefaultAnnotations(listifyLabelsOrAnnotations(rule.annotations, false)),
labels: listifyLabelsOrAnnotations(rule.labels, true),
folder: { title: namespace, uid: ga.namespace_uid },
isPaused: ga.is_paused,
contactPoints: routingSettings,
manualRouting: Boolean(routingSettings),
// next line is for testing
// manualRouting: true,
// contactPoints: {
// grafana: {
// selectedContactPoint: "contact_point_5",
// muteTimeIntervals: [
// "mute timing 1"
// ],
// overrideGrouping: true,
// overrideTimings: true,
// "groupBy": [
// "..."
// ],
// groupWaitValue: "35s",
// groupIntervalValue: "6m",
// repeatIntervalValue: "5h"
// }
// }
};
} else {
throw new Error('Unexpected type of rule for grafana rules source');
}
} else {
if (isAlertingRulerRule(rule)) {
const datasourceUid = getDataSourceSrv().getInstanceSettings(ruleSourceName)?.uid ?? '';
const defaultQuery = {
refId: 'A',
datasourceUid,
queryType: '',
relativeTimeRange: getDefaultRelativeTimeRange(),
expr: rule.expr,
model: {
refId: 'A',
hide: false,
expr: rule.expr,
},
};
const alertingRuleValues = alertingRulerRuleToRuleForm(rule);
return {
...defaultFormValues,
...alertingRuleValues,
queries: [defaultQuery],
annotations: normalizeDefaultAnnotations(listifyLabelsOrAnnotations(rule.annotations, false)),
type: RuleFormType.cloudAlerting,
dataSourceName: ruleSourceName,
namespace,
group: group.name,
};
} else if (isRecordingRulerRule(rule)) {
const recordingRuleValues = recordingRulerRuleToRuleForm(rule);
return {
...defaultFormValues,
...recordingRuleValues,
type: RuleFormType.cloudRecording,
dataSourceName: ruleSourceName,
namespace,
group: group.name,
};
} else {
throw new Error('Unexpected type of rule for cloud rules source');
}
}
}
export function alertingRulerRuleToRuleForm(
rule: RulerAlertingRuleDTO
): Pick<
RuleFormValues,
| 'name'
| 'forTime'
| 'forTimeUnit'
| 'keepFiringForTime'
| 'keepFiringForTimeUnit'
| 'expression'
| 'annotations'
| 'labels'
> {
const defaultFormValues = getDefaultFormValues();
const [forTime, forTimeUnit] = rule.for
? parseInterval(rule.for)
: [defaultFormValues.forTime, defaultFormValues.forTimeUnit];
const [keepFiringForTime, keepFiringForTimeUnit] = rule.keep_firing_for
? parseInterval(rule.keep_firing_for)
: [defaultFormValues.keepFiringForTime, defaultFormValues.keepFiringForTimeUnit];
return {
name: rule.alert,
expression: rule.expr,
forTime,
forTimeUnit,
keepFiringForTime,
keepFiringForTimeUnit,
annotations: listifyLabelsOrAnnotations(rule.annotations, false),
labels: listifyLabelsOrAnnotations(rule.labels, true),
};
}
export function recordingRulerRuleToRuleForm(
rule: RulerRecordingRuleDTO
): Pick<RuleFormValues, 'name' | 'expression' | 'labels'> {
return {
name: rule.record,
expression: rule.expr,
labels: listifyLabelsOrAnnotations(rule.labels, true),
};
}
export const getDefaultQueries = (): AlertQuery[] => {
const dataSource = getDefaultOrFirstCompatibleDataSource();
if (!dataSource) {
return [...getDefaultExpressions('A', 'B')];
}
const relativeTimeRange = getDefaultRelativeTimeRange();
return [
{
refId: 'A',
datasourceUid: dataSource.uid,
queryType: '',
relativeTimeRange,
model: {
refId: 'A',
},
},
...getDefaultExpressions('B', 'C'),
];
};
export const getDefaultRecordingRulesQueries = (
rulesSourcesWithRuler: Array<DataSourceInstanceSettings<DataSourceJsonData>>
): AlertQuery[] => {
const relativeTimeRange = getDefaultRelativeTimeRange();
return [
{
refId: 'A',
datasourceUid: rulesSourcesWithRuler[0]?.uid || '',
queryType: '',
relativeTimeRange,
model: {
refId: 'A',
},
},
];
};
const getDefaultExpressions = (...refIds: [string, string]): AlertQuery[] => {
const refOne = refIds[0];
const refTwo = refIds[1];
const reduceExpression: ExpressionQuery = {
refId: refIds[0],
type: ExpressionQueryType.reduce,
datasource: {
uid: ExpressionDatasourceUID,
type: ExpressionDatasourceRef.type,
},
conditions: [
{
type: 'query',
evaluator: {
params: [],
type: EvalFunction.IsAbove,
},
operator: {
type: 'and',
},
query: {
params: [refOne],
},
reducer: {
params: [],
type: 'last',
},
},
],
reducer: 'last',
expression: 'A',
};
const thresholdExpression: ExpressionQuery = {
refId: refTwo,
type: ExpressionQueryType.threshold,
datasource: {
uid: ExpressionDatasourceUID,
type: ExpressionDatasourceRef.type,
},
conditions: [
{
type: 'query',
evaluator: {
params: [0],
type: EvalFunction.IsAbove,
},
operator: {
type: 'and',
},
query: {
params: [refTwo],
},
reducer: {
params: [],
type: 'last',
},
},
],
expression: refOne,
};
return [
{
refId: refOne,
datasourceUid: ExpressionDatasourceUID,
queryType: '',
model: reduceExpression,
},
{
refId: refTwo,
datasourceUid: ExpressionDatasourceUID,
queryType: '',
model: thresholdExpression,
},
];
};
const dataQueriesToGrafanaQueries = async (
queries: DataQuery[],
relativeTimeRange: RelativeTimeRange,
scopedVars: ScopedVars | {},
panelDataSourceRef?: DataSourceRef,
maxDataPoints?: number,
minInterval?: string
): Promise<AlertQuery[]> => {
const result: AlertQuery[] = [];
for (const target of queries) {
const datasource = await getDataSourceSrv().get(target.datasource?.uid ? target.datasource : panelDataSourceRef);
const dsRef = { uid: datasource.uid, type: datasource.type };
const range = rangeUtil.relativeToTimeRange(relativeTimeRange);
const { interval, intervalMs } = getIntervals(range, minInterval ?? datasource.interval, maxDataPoints);
const queryVariables = {
__interval: { text: interval, value: interval },
__interval_ms: { text: intervalMs, value: intervalMs },
...scopedVars,
};
const interpolatedTarget = datasource.interpolateVariablesInQueries
? await datasource.interpolateVariablesInQueries([target], queryVariables)[0]
: target;
// expressions
if (dsRef.uid === ExpressionDatasourceUID) {
const newQuery: AlertQuery = {
refId: interpolatedTarget.refId,
queryType: '',
relativeTimeRange,
datasourceUid: ExpressionDatasourceUID,
model: interpolatedTarget,
};
result.push(newQuery);
// queries
} else {
const datasourceSettings = getDataSourceSrv().getInstanceSettings(dsRef);
if (datasourceSettings && datasourceSettings.meta.alerting) {
const newQuery: AlertQuery = {
refId: interpolatedTarget.refId,
queryType: interpolatedTarget.queryType ?? '',
relativeTimeRange,
datasourceUid: datasourceSettings.uid,
model: {
...interpolatedTarget,
maxDataPoints,
intervalMs,
},
};
result.push(newQuery);
}
}
}
return result;
};
export const panelToRuleFormValues = async (
panel: PanelModel,
dashboard: DashboardModel
): Promise<Partial<RuleFormValues> | undefined> => {
const { targets } = panel;
if (!panel.id || !dashboard.uid) {
return undefined;
}
const relativeTimeRange = rangeUtil.timeRangeToRelative(rangeUtil.convertRawToRange(dashboard.time));
const queries = await dataQueriesToGrafanaQueries(
targets,
relativeTimeRange,
panel.scopedVars || {},
panel.datasource ?? undefined,
panel.maxDataPoints ?? undefined,
panel.interval ?? undefined
);
// if no alerting capable queries are found, can't create a rule
if (!queries.length || !queries.find((query) => query.datasourceUid !== ExpressionDatasourceUID)) {
return undefined;
}
if (!queries.find((query) => query.datasourceUid === ExpressionDatasourceUID)) {
const [reduceExpression, _thresholdExpression] = getDefaultExpressions(getNextRefIdChar(queries), '-');
queries.push(reduceExpression);
const [_reduceExpression, thresholdExpression] = getDefaultExpressions(
reduceExpression.refId,
getNextRefIdChar(queries)
);
queries.push(thresholdExpression);
}
const { folderTitle, folderUid } = dashboard.meta;
const formValues = {
type: RuleFormType.grafana,
folder:
folderUid && folderTitle
? {
uid: folderUid,
title: folderTitle,
}
: undefined,
queries,
name: panel.title,
condition: queries[queries.length - 1].refId,
annotations: [
{
key: Annotation.dashboardUID,
value: dashboard.uid,
},
{
key: Annotation.panelID,
value: String(panel.id),
},
],
};
return formValues;
};
export function getIntervals(range: TimeRange, lowLimit?: string, resolution?: number): IntervalValues {
if (!resolution) {
if (lowLimit && rangeUtil.intervalToMs(lowLimit) > 1000) {
return {
interval: lowLimit,
intervalMs: rangeUtil.intervalToMs(lowLimit),
};
}
return { interval: '1s', intervalMs: 1000 };
}
return rangeUtil.calculateInterval(range, resolution, lowLimit);
}
export function fixBothInstantAndRangeQuery(query: AlertQuery) {
const model = query.model;
if (!isPromQuery(model)) {
return query;
}
const isBothInstantAndRange = model.instant && model.range;
if (isBothInstantAndRange) {
return { ...query, model: { ...model, range: true, instant: false } };
}
return query;
}
function isPromQuery(model: AlertDataQuery): model is PromQuery {
return 'expr' in model && 'instant' in model && 'range' in model;
}
export function isPromOrLokiQuery(model: AlertDataQuery): model is PromOrLokiQuery {
return 'expr' in model;
}
// the backend will always execute "hidden" queries, so we have no choice but to remove the property in the front-end
// to avoid confusion. The query editor shows them as "disabled" and that's a different semantic meaning.
// furthermore the "AlertingQueryRunner" calls `filterQuery` on each data source and those will skip running queries that are "hidden"."
// It seems like we have no choice but to act like "hidden" queries don't exist in alerting.
export const ignoreHiddenQueries = (ruleDefinition: RuleFormValues): RuleFormValues => {
return {
...ruleDefinition,
queries: ruleDefinition.queries?.map((query) => omit(query, 'model.hide')),
};
};
export function formValuesFromExistingRule(rule: RuleWithLocation<RulerRuleDTO>) {
return ignoreHiddenQueries(rulerRuleToFormValues(rule));
}
| public/app/features/alerting/unified/utils/rule-form.ts | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00018114325939677656,
0.00017271142860408872,
0.00016223920101765543,
0.00017341726925224066,
0.0000035504992865753593
]
|
{
"id": 3,
"code_window": [
" const sceneDiv = (\n",
" // The <div> element has child elements that allow for mouse events, so we need to disable the linter rule\n",
" // eslint-disable-next-line jsx-a11y/no-static-element-interactions\n",
" <div\n",
" key={this.revId}\n",
" className={this.styles.wrap}\n",
" style={this.style}\n",
" ref={this.setRef}\n",
" onMouseDown={onSceneContainerMouseDown}\n",
" >\n",
" {this.connections.render()}\n",
" {this.root.render()}\n",
" {this.isEditingEnabled && (\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" <div key={this.revId} className={this.styles.wrap} style={this.style} ref={this.setRef}>\n"
],
"file_path": "public/app/features/canvas/runtime/scene.tsx",
"type": "replace",
"edit_start_line_idx": 685
} | package v0alpha1
import (
metav1 "k8s.io/apimachinery/pkg/apis/meta/v1"
"k8s.io/apimachinery/pkg/runtime"
"k8s.io/apimachinery/pkg/runtime/schema"
common "github.com/grafana/grafana/pkg/apis/common/v0alpha1"
)
const (
GROUP = "service.grafana.app"
VERSION = "v0alpha1"
APIVERSION = GROUP + "/" + VERSION
)
var ExternalNameResourceInfo = common.NewResourceInfo(GROUP, VERSION,
"externalnames", "externalname", "ExternalName",
func() runtime.Object { return &ExternalName{} },
func() runtime.Object { return &ExternalNameList{} },
)
var (
// SchemeGroupVersion is group version used to register these objects
SchemeGroupVersion = schema.GroupVersion{Group: GROUP, Version: VERSION}
// SchemaBuilder is used by standard codegen
SchemeBuilder runtime.SchemeBuilder
localSchemeBuilder = &SchemeBuilder
AddToScheme = localSchemeBuilder.AddToScheme
)
func init() {
localSchemeBuilder.Register(addKnownTypes)
}
// Adds the list of known types to the given scheme.
func addKnownTypes(scheme *runtime.Scheme) error {
scheme.AddKnownTypes(SchemeGroupVersion,
&ExternalName{},
&ExternalNameList{},
)
metav1.AddToGroupVersion(scheme, SchemeGroupVersion)
return nil
}
// Resource takes an unqualified resource and returns a Group qualified GroupResource
func Resource(resource string) schema.GroupResource {
return SchemeGroupVersion.WithResource(resource).GroupResource()
}
| pkg/apis/service/v0alpha1/register.go | 0 | https://github.com/grafana/grafana/commit/8784052ccfb6d72a40307556bf1f40fc621f68b6 | [
0.00017391685105394572,
0.00016917713219299912,
0.00016121742373798043,
0.0001699195709079504,
0.000004273040303814923
]
|
{
"id": 0,
"code_window": [
" }\n",
"}\n",
"\n",
"function validateLaunchOptions<Options extends types.LaunchOptions>(options: Options): Options {\n",
" const { devtools = false } = options;\n",
" let { headless = !devtools } = options;\n",
" if (debugMode())\n",
" headless = false;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" let { headless = !devtools, downloadsPath } = options;\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 273
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { playwrightTest as it, expect } from './config/browserTest';
import fs from 'fs';
it.describe('downloads path', () => {
it.beforeEach(async ({server}) => {
server.setRoute('/download', (req, res) => {
res.setHeader('Content-Type', 'application/octet-stream');
res.setHeader('Content-Disposition', 'attachment; filename=file.txt');
res.end(`Hello world`);
});
});
it('should keep downloadsPath folder', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage();
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
expect(download.url()).toBe(`${server.PREFIX}/download`);
expect(download.suggestedFilename()).toBe(`file.txt`);
await download.path().catch(e => void 0);
await page.close();
await downloadsBrowser.close();
expect(fs.existsSync(testInfo.outputPath(''))).toBeTruthy();
});
it('should delete downloads when context closes', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage({ acceptDownloads: true });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
const path = await download.path();
expect(fs.existsSync(path)).toBeTruthy();
await page.close();
expect(fs.existsSync(path)).toBeFalsy();
await downloadsBrowser.close();
});
it('should report downloads in downloadsPath folder', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage({ acceptDownloads: true });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
const path = await download.path();
expect(path.startsWith(testInfo.outputPath(''))).toBeTruthy();
await page.close();
await downloadsBrowser.close();
});
it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {
const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a'),
]);
expect(download.url()).toBe(`${server.PREFIX}/download`);
expect(download.suggestedFilename()).toBe(`file.txt`);
const path = await download.path();
expect(path.startsWith(testInfo.outputPath(''))).toBeTruthy();
await context.close();
});
it('should delete downloads when persistent context closes', async ({launchPersistent, server}, testInfo) => {
const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a'),
]);
const path = await download.path();
expect(fs.existsSync(path)).toBeTruthy();
await context.close();
expect(fs.existsSync(path)).toBeFalsy();
});
});
| tests/downloads-path.spec.ts | 1 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.00017943316197488457,
0.0001734054967528209,
0.00016506685642525554,
0.0001757167628966272,
0.000004913560587738175
]
|
{
"id": 0,
"code_window": [
" }\n",
"}\n",
"\n",
"function validateLaunchOptions<Options extends types.LaunchOptions>(options: Options): Options {\n",
" const { devtools = false } = options;\n",
" let { headless = !devtools } = options;\n",
" if (debugMode())\n",
" headless = false;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" let { headless = !devtools, downloadsPath } = options;\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 273
} | # This Source Code Form is subject to the terms of the Mozilla Public
# License, v. 2.0. If a copy of the MPL was not distributed with this
# file, You can obtain one at http://mozilla.org/MPL/2.0/.
juggler.jar:
% content juggler %content/
content/Helper.js (Helper.js)
content/NetworkObserver.js (NetworkObserver.js)
content/TargetRegistry.js (TargetRegistry.js)
content/SimpleChannel.js (SimpleChannel.js)
content/protocol/PrimitiveTypes.js (protocol/PrimitiveTypes.js)
content/protocol/Protocol.js (protocol/Protocol.js)
content/protocol/Dispatcher.js (protocol/Dispatcher.js)
content/protocol/PageHandler.js (protocol/PageHandler.js)
content/protocol/BrowserHandler.js (protocol/BrowserHandler.js)
content/content/main.js (content/main.js)
content/content/FrameTree.js (content/FrameTree.js)
content/content/PageAgent.js (content/PageAgent.js)
content/content/Runtime.js (content/Runtime.js)
content/content/WorkerMain.js (content/WorkerMain.js)
content/content/hidden-scrollbars.css (content/hidden-scrollbars.css)
| browser_patches/firefox-stable/juggler/jar.mn | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.000176378627656959,
0.00017180595023091882,
0.00016746915935073048,
0.000171570063685067,
0.0000036410976917977678
]
|
{
"id": 0,
"code_window": [
" }\n",
"}\n",
"\n",
"function validateLaunchOptions<Options extends types.LaunchOptions>(options: Options): Options {\n",
" const { devtools = false } = options;\n",
" let { headless = !devtools } = options;\n",
" if (debugMode())\n",
" headless = false;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" let { headless = !devtools, downloadsPath } = options;\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 273
} | /**
* Copyright 2018 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { test as it, expect } from './config/pageTest';
it('should work', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
const button = await page.$('button');
await button.click();
expect(await page.evaluate(() => window['result'])).toBe('Clicked');
});
it('should work with Node removed', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
await page.evaluate(() => delete window['Node']);
const button = await page.$('button');
await button.click();
expect(await page.evaluate(() => window['result'])).toBe('Clicked');
});
it('should work for Shadow DOM v1', async ({ page, server }) => {
await page.goto(server.PREFIX + '/shadow.html');
const buttonHandle = await page.evaluateHandle(() => window['button'] as HTMLButtonElement);
await buttonHandle.click();
expect(await page.evaluate('clicked')).toBe(true);
});
it('should work for TextNodes', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
const buttonTextNode = await page.evaluateHandle(() => document.querySelector('button').firstChild);
await buttonTextNode.click();
expect(await page.evaluate(() => window['result'])).toBe('Clicked');
});
it('should throw for detached nodes', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
const button = await page.$('button');
await page.evaluate(button => button.remove(), button);
let error = null;
await button.click().catch(err => error = err);
expect(error.message).toContain('Element is not attached to the DOM');
});
it('should throw for hidden nodes with force', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
const button = await page.$('button');
await page.evaluate(button => button.style.display = 'none', button);
const error = await button.click({ force: true }).catch(err => err);
expect(error.message).toContain('Element is not visible');
});
it('should throw for recursively hidden nodes with force', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
const button = await page.$('button');
await page.evaluate(button => button.parentElement.style.display = 'none', button);
const error = await button.click({ force: true }).catch(err => err);
expect(error.message).toContain('Element is not visible');
});
it('should throw for <br> elements with force', async ({ page, server }) => {
await page.setContent('hello<br>goodbye');
const br = await page.$('br');
const error = await br.click({ force: true }).catch(err => err);
expect(error.message).toContain('Element is outside of the viewport');
});
it('should double click the button', async ({ page, server }) => {
await page.goto(server.PREFIX + '/input/button.html');
await page.evaluate(() => {
window['double'] = false;
const button = document.querySelector('button');
button.addEventListener('dblclick', event => {
window['double'] = true;
});
});
const button = await page.$('button');
await button.dblclick();
expect(await page.evaluate('double')).toBe(true);
expect(await page.evaluate('result')).toBe('Clicked');
});
| tests/elementhandle-click.spec.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.0001789114176062867,
0.00017514568753540516,
0.00017066924192477018,
0.00017480607493780553,
0.000002347346480746637
]
|
{
"id": 0,
"code_window": [
" }\n",
"}\n",
"\n",
"function validateLaunchOptions<Options extends types.LaunchOptions>(options: Options): Options {\n",
" const { devtools = false } = options;\n",
" let { headless = !devtools } = options;\n",
" if (debugMode())\n",
" headless = false;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" let { headless = !devtools, downloadsPath } = options;\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 273
} | /*
Copyright (c) Microsoft Corporation.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
.workbench {
contain: size;
user-select: none;
}
.workbench .header {
display: flex;
background-color: #000;
flex: none;
flex-basis: 48px;
line-height: 48px;
font-size: 16px;
color: white;
}
.workbench tab-content {
padding: 25px;
contain: size;
}
.workbench tab-strip {
margin-left: calc(-1*var(--sidebar-width));
padding-left: var(--sidebar-width);
box-shadow: var(--box-shadow);
}
.workbench .logo {
font-size: 20px;
margin-left: 16px;
}
.workbench .product {
font-weight: 600;
margin-left: 16px;
}
.workbench .spacer {
flex: auto;
}
tab-strip {
background-color: var(--light-background);
}
| src/web/traceViewer/ui/workbench.css | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.00017900014063343406,
0.00017551692144479603,
0.00016985507681965828,
0.00017554192163515836,
0.0000031331753689300967
]
|
{
"id": 1,
"code_window": [
" if (debugMode())\n",
" headless = false;\n",
" return { ...options, devtools, headless };\n",
"}"
],
"labels": [
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (downloadsPath && !path.isAbsolute(downloadsPath))\n",
" downloadsPath = path.join(process.cwd(), downloadsPath);\n",
" return { ...options, devtools, headless, downloadsPath };\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 276
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { playwrightTest as it, expect } from './config/browserTest';
import fs from 'fs';
it.describe('downloads path', () => {
it.beforeEach(async ({server}) => {
server.setRoute('/download', (req, res) => {
res.setHeader('Content-Type', 'application/octet-stream');
res.setHeader('Content-Disposition', 'attachment; filename=file.txt');
res.end(`Hello world`);
});
});
it('should keep downloadsPath folder', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage();
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
expect(download.url()).toBe(`${server.PREFIX}/download`);
expect(download.suggestedFilename()).toBe(`file.txt`);
await download.path().catch(e => void 0);
await page.close();
await downloadsBrowser.close();
expect(fs.existsSync(testInfo.outputPath(''))).toBeTruthy();
});
it('should delete downloads when context closes', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage({ acceptDownloads: true });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
const path = await download.path();
expect(fs.existsSync(path)).toBeTruthy();
await page.close();
expect(fs.existsSync(path)).toBeFalsy();
await downloadsBrowser.close();
});
it('should report downloads in downloadsPath folder', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage({ acceptDownloads: true });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
const path = await download.path();
expect(path.startsWith(testInfo.outputPath(''))).toBeTruthy();
await page.close();
await downloadsBrowser.close();
});
it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {
const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a'),
]);
expect(download.url()).toBe(`${server.PREFIX}/download`);
expect(download.suggestedFilename()).toBe(`file.txt`);
const path = await download.path();
expect(path.startsWith(testInfo.outputPath(''))).toBeTruthy();
await context.close();
});
it('should delete downloads when persistent context closes', async ({launchPersistent, server}, testInfo) => {
const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a'),
]);
const path = await download.path();
expect(fs.existsSync(path)).toBeTruthy();
await context.close();
expect(fs.existsSync(path)).toBeFalsy();
});
});
| tests/downloads-path.spec.ts | 1 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.00018448334594722837,
0.00017420916992705315,
0.00016694015357643366,
0.00017247431969735771,
0.000004439851181814447
]
|
{
"id": 1,
"code_window": [
" if (debugMode())\n",
" headless = false;\n",
" return { ...options, devtools, headless };\n",
"}"
],
"labels": [
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (downloadsPath && !path.isAbsolute(downloadsPath))\n",
" downloadsPath = path.join(process.cwd(), downloadsPath);\n",
" return { ...options, devtools, headless, downloadsPath };\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 276
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { CRSession } from './crConnection';
import { Protocol } from './protocol';
import fs from 'fs';
import * as util from 'util';
import * as types from '../types';
import { mkdirIfNeeded } from '../../utils/utils';
import { splitErrorMessage } from '../../utils/stackTrace';
export function getExceptionMessage(exceptionDetails: Protocol.Runtime.ExceptionDetails): string {
if (exceptionDetails.exception)
return exceptionDetails.exception.description || String(exceptionDetails.exception.value);
let message = exceptionDetails.text;
if (exceptionDetails.stackTrace) {
for (const callframe of exceptionDetails.stackTrace.callFrames) {
const location = callframe.url + ':' + callframe.lineNumber + ':' + callframe.columnNumber;
const functionName = callframe.functionName || '<anonymous>';
message += `\n at ${functionName} (${location})`;
}
}
return message;
}
export async function releaseObject(client: CRSession, objectId: string) {
await client.send('Runtime.releaseObject', { objectId }).catch(error => {});
}
export async function readProtocolStream(client: CRSession, handle: string, path: string | null): Promise<Buffer> {
let eof = false;
let fd: number | undefined;
if (path) {
await mkdirIfNeeded(path);
fd = await util.promisify(fs.open)(path, 'w');
}
const bufs = [];
while (!eof) {
const response = await client.send('IO.read', {handle});
eof = response.eof;
const buf = Buffer.from(response.data, response.base64Encoded ? 'base64' : undefined);
bufs.push(buf);
if (path)
await util.promisify(fs.write)(fd!, buf);
}
if (path)
await util.promisify(fs.close)(fd!);
await client.send('IO.close', {handle});
return Buffer.concat(bufs);
}
export function toConsoleMessageLocation(stackTrace: Protocol.Runtime.StackTrace | undefined): types.ConsoleMessageLocation {
return stackTrace && stackTrace.callFrames.length ? {
url: stackTrace.callFrames[0].url,
lineNumber: stackTrace.callFrames[0].lineNumber,
columnNumber: stackTrace.callFrames[0].columnNumber,
} : { url: '', lineNumber: 0, columnNumber: 0 };
}
export function exceptionToError(exceptionDetails: Protocol.Runtime.ExceptionDetails): Error {
const messageWithStack = getExceptionMessage(exceptionDetails);
const lines = messageWithStack.split('\n');
const firstStackTraceLine = lines.findIndex(line => line.startsWith(' at'));
let messageWithName = '';
let stack = '';
if (firstStackTraceLine === -1) {
messageWithName = messageWithStack;
} else {
messageWithName = lines.slice(0, firstStackTraceLine).join('\n');
stack = messageWithStack;
}
const {name, message} = splitErrorMessage(messageWithName);
const err = new Error(message);
err.stack = stack;
err.name = name;
return err;
}
| src/server/chromium/crProtocolHelper.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.0001777385186869651,
0.00017280651081819087,
0.0001681883295532316,
0.0001722802990116179,
0.0000032015152555686655
]
|
{
"id": 1,
"code_window": [
" if (debugMode())\n",
" headless = false;\n",
" return { ...options, devtools, headless };\n",
"}"
],
"labels": [
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (downloadsPath && !path.isAbsolute(downloadsPath))\n",
" downloadsPath = path.join(process.cwd(), downloadsPath);\n",
" return { ...options, devtools, headless, downloadsPath };\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 276
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { test as it, expect } from './config/pageTest';
import { attachFrame } from './config/utils';
import type { ElementHandle } from '../index';
it('exposeBinding should work', async ({page}) => {
let bindingSource;
await page.exposeBinding('add', (source, a, b) => {
bindingSource = source;
return a + b;
});
const result = await page.evaluate(async function() {
return window['add'](5, 6);
});
expect(bindingSource.context).toBe(page.context());
expect(bindingSource.page).toBe(page);
expect(bindingSource.frame).toBe(page.mainFrame());
expect(result).toEqual(11);
});
it('should work', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return a * b;
});
const result = await page.evaluate(async function() {
return await window['compute'](9, 4);
});
expect(result).toBe(36);
});
it('should work with handles and complex objects', async ({page, server}) => {
const fooHandle = await page.evaluateHandle(() => {
window['fooValue'] = { bar: 2 };
return window['fooValue'];
});
await page.exposeFunction('handle', () => {
return [{ foo: fooHandle }];
});
const equals = await page.evaluate(async function() {
const value = await window['handle']();
const [{ foo }] = value;
return foo === window['fooValue'];
});
expect(equals).toBe(true);
});
it('should throw exception in page context', async ({page, server}) => {
await page.exposeFunction('woof', function() {
throw new Error('WOOF WOOF');
});
const {message, stack} = await page.evaluate(async () => {
try {
await window['woof']();
} catch (e) {
return {message: e.message, stack: e.stack};
}
});
expect(message).toBe('WOOF WOOF');
expect(stack).toContain(__filename);
});
it('should support throwing "null"', async ({page, server}) => {
await page.exposeFunction('woof', function() {
throw null;
});
const thrown = await page.evaluate(async () => {
try {
await window['woof']();
} catch (e) {
return e;
}
});
expect(thrown).toBe(null);
});
it('should be callable from-inside addInitScript', async ({page, server}) => {
let called = false;
await page.exposeFunction('woof', function() {
called = true;
});
await page.addInitScript(() => window['woof']());
await page.reload();
expect(called).toBe(true);
});
it('should survive navigation', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return a * b;
});
await page.goto(server.EMPTY_PAGE);
const result = await page.evaluate(async function() {
return await window['compute'](9, 4);
});
expect(result).toBe(36);
});
it('should await returned promise', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
const result = await page.evaluate(async function() {
return await window['compute'](3, 5);
});
expect(result).toBe(15);
});
it('should work on frames', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
await page.goto(server.PREFIX + '/frames/nested-frames.html');
const frame = page.frames()[1];
const result = await frame.evaluate(async function() {
return await window['compute'](3, 5);
});
expect(result).toBe(15);
});
it('should work on frames before navigation', async ({page, server}) => {
await page.goto(server.PREFIX + '/frames/nested-frames.html');
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
const frame = page.frames()[1];
const result = await frame.evaluate(async function() {
return await window['compute'](3, 5);
});
expect(result).toBe(15);
});
it('should work after cross origin navigation', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
await page.exposeFunction('compute', function(a, b) {
return a * b;
});
await page.goto(server.CROSS_PROCESS_PREFIX + '/empty.html');
const result = await page.evaluate(async function() {
return await window['compute'](9, 4);
});
expect(result).toBe(36);
});
it('should work with complex objects', async ({page, server}) => {
await page.exposeFunction('complexObject', function(a, b) {
return {x: a.x + b.x};
});
const result = await page.evaluate(async () => window['complexObject']({x: 5}, {x: 2}));
expect(result.x).toBe(7);
});
it('exposeBindingHandle should work', async ({page}) => {
let target;
await page.exposeBinding('logme', (source, t) => {
target = t;
return 17;
}, { handle: true });
const result = await page.evaluate(async function() {
return window['logme']({ foo: 42 });
});
expect(await target.evaluate(x => x.foo)).toBe(42);
expect(result).toEqual(17);
});
it('exposeBindingHandle should not throw during navigation', async ({page, server}) => {
await page.exposeBinding('logme', (source, t) => {
return 17;
}, { handle: true });
await page.goto(server.EMPTY_PAGE);
await Promise.all([
page.evaluate(async url => {
window['logme']({ foo: 42 });
window.location.href = url;
}, server.PREFIX + '/one-style.html'),
page.waitForNavigation({ waitUntil: 'load' }),
]);
});
it('should throw for duplicate registrations', async ({page}) => {
await page.exposeFunction('foo', () => {});
const error = await page.exposeFunction('foo', () => {}).catch(e => e);
expect(error.message).toContain('page.exposeFunction: Function "foo" has been already registered');
});
it('exposeBindingHandle should throw for multiple arguments', async ({page}) => {
await page.exposeBinding('logme', (source, t) => {
return 17;
}, { handle: true });
expect(await page.evaluate(async function() {
return window['logme']({ foo: 42 });
})).toBe(17);
expect(await page.evaluate(async function() {
return window['logme']({ foo: 42 }, undefined, undefined);
})).toBe(17);
expect(await page.evaluate(async function() {
return window['logme'](undefined, undefined, undefined);
})).toBe(17);
const error = await page.evaluate(async function() {
return window['logme'](1, 2);
}).catch(e => e);
expect(error.message).toContain('exposeBindingHandle supports a single argument, 2 received');
});
it('should not result in unhandled rejection', async ({page, isAndroid}) => {
it.fixme(isAndroid);
const closedPromise = page.waitForEvent('close');
await page.exposeFunction('foo', async () => {
await page.close();
});
await page.evaluate(() => {
setTimeout(() => (window as any).foo(), 0);
return undefined;
});
await closedPromise;
// Make a round-trip to be sure we did not throw immediately after closing.
expect(await page.evaluate('1 + 1').catch(e => e)).toBeInstanceOf(Error);
});
it('should work with internal bindings', async ({page, toImpl, server, mode, browserName, isElectron, isAndroid}) => {
it.skip(mode !== 'default');
it.skip(browserName !== 'chromium');
it.skip(isAndroid);
it.skip(isElectron);
const implPage: import('../src/server/page').Page = toImpl(page);
let foo;
await implPage.exposeBinding('foo', false, ({}, arg) => {
foo = arg;
}, 'utility');
expect(await page.evaluate('!!window.foo')).toBe(false);
expect(await implPage.mainFrame().evaluateExpression('!!window.foo', false, {}, 'utility')).toBe(true);
expect(foo).toBe(undefined);
await implPage.mainFrame().evaluateExpression('window.foo(123)', false, {}, 'utility');
expect(foo).toBe(123);
// should work after reload
await page.goto(server.EMPTY_PAGE);
expect(await page.evaluate('!!window.foo')).toBe(false);
await implPage.mainFrame().evaluateExpression('window.foo(456)', false, {}, 'utility');
expect(foo).toBe(456);
// should work inside frames
const frame = await attachFrame(page, 'myframe', server.CROSS_PROCESS_PREFIX + '/empty.html');
expect(await frame.evaluate('!!window.foo')).toBe(false);
const implFrame: import('../src/server/frames').Frame = toImpl(frame);
await implFrame.evaluateExpression('window.foo(789)', false, {}, 'utility');
expect(foo).toBe(789);
});
it('exposeBinding(handle) should work with element handles', async ({ page}) => {
let cb;
const promise = new Promise(f => cb = f);
await page.exposeBinding('clicked', async (source, element: ElementHandle) => {
cb(await element.innerText().catch(e => e));
}, { handle: true });
await page.goto('about:blank');
await page.setContent(`
<script>
document.addEventListener('click', event => window.clicked(event.target));
</script>
<div id="a1">Click me</div>
`);
await page.click('#a1');
expect(await promise).toBe('Click me');
});
it('should work with setContent', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
await page.setContent('<script>window.result = compute(3, 2)</script>');
expect(await page.evaluate('window.result')).toBe(6);
});
| tests/page-expose-function.spec.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.00017789094999898225,
0.00017404163372702897,
0.00016979483189061284,
0.00017461858806200325,
0.000002180727278755512
]
|
{
"id": 1,
"code_window": [
" if (debugMode())\n",
" headless = false;\n",
" return { ...options, devtools, headless };\n",
"}"
],
"labels": [
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (downloadsPath && !path.isAbsolute(downloadsPath))\n",
" downloadsPath = path.join(process.cwd(), downloadsPath);\n",
" return { ...options, devtools, headless, downloadsPath };\n"
],
"file_path": "src/server/browserType.ts",
"type": "replace",
"edit_start_line_idx": 276
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the 'License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { ConsoleMessage } from '../server/console';
import * as channels from '../protocol/channels';
import { Dispatcher, DispatcherScope } from './dispatcher';
import { ElementHandleDispatcher } from './elementHandlerDispatcher';
export class ConsoleMessageDispatcher extends Dispatcher<ConsoleMessage, channels.ConsoleMessageInitializer> implements channels.ConsoleMessageChannel {
constructor(scope: DispatcherScope, message: ConsoleMessage) {
super(scope, message, 'ConsoleMessage', {
type: message.type(),
text: message.text(),
args: message.args().map(a => ElementHandleDispatcher.fromJSHandle(scope, a)),
location: message.location(),
});
}
}
| src/dispatchers/consoleMessageDispatcher.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.0001874210429377854,
0.00018160667968913913,
0.00017690277309156954,
0.0001810514513636008,
0.0000044939374674868304
]
|
{
"id": 2,
"code_window": [
"\n",
"import { playwrightTest as it, expect } from './config/browserTest';\n",
"import fs from 'fs';\n",
"\n",
"it.describe('downloads path', () => {\n",
" it.beforeEach(async ({server}) => {\n",
" server.setRoute('/download', (req, res) => {\n",
" res.setHeader('Content-Type', 'application/octet-stream');\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import path from 'path';\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 18
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { playwrightTest as it, expect } from './config/browserTest';
import fs from 'fs';
it.describe('downloads path', () => {
it.beforeEach(async ({server}) => {
server.setRoute('/download', (req, res) => {
res.setHeader('Content-Type', 'application/octet-stream');
res.setHeader('Content-Disposition', 'attachment; filename=file.txt');
res.end(`Hello world`);
});
});
it('should keep downloadsPath folder', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage();
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
expect(download.url()).toBe(`${server.PREFIX}/download`);
expect(download.suggestedFilename()).toBe(`file.txt`);
await download.path().catch(e => void 0);
await page.close();
await downloadsBrowser.close();
expect(fs.existsSync(testInfo.outputPath(''))).toBeTruthy();
});
it('should delete downloads when context closes', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage({ acceptDownloads: true });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
const path = await download.path();
expect(fs.existsSync(path)).toBeTruthy();
await page.close();
expect(fs.existsSync(path)).toBeFalsy();
await downloadsBrowser.close();
});
it('should report downloads in downloadsPath folder', async ({browserType, browserOptions, server}, testInfo) => {
const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: testInfo.outputPath('') });
const page = await downloadsBrowser.newPage({ acceptDownloads: true });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a')
]);
const path = await download.path();
expect(path.startsWith(testInfo.outputPath(''))).toBeTruthy();
await page.close();
await downloadsBrowser.close();
});
it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {
const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a'),
]);
expect(download.url()).toBe(`${server.PREFIX}/download`);
expect(download.suggestedFilename()).toBe(`file.txt`);
const path = await download.path();
expect(path.startsWith(testInfo.outputPath(''))).toBeTruthy();
await context.close();
});
it('should delete downloads when persistent context closes', async ({launchPersistent, server}, testInfo) => {
const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });
await page.setContent(`<a href="${server.PREFIX}/download">download</a>`);
const [ download ] = await Promise.all([
page.waitForEvent('download'),
page.click('a'),
]);
const path = await download.path();
expect(fs.existsSync(path)).toBeTruthy();
await context.close();
expect(fs.existsSync(path)).toBeFalsy();
});
});
| tests/downloads-path.spec.ts | 1 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.9940667152404785,
0.0979657918214798,
0.00017296972509939224,
0.0024512314703315496,
0.28390127420425415
]
|
{
"id": 2,
"code_window": [
"\n",
"import { playwrightTest as it, expect } from './config/browserTest';\n",
"import fs from 'fs';\n",
"\n",
"it.describe('downloads path', () => {\n",
" it.beforeEach(async ({server}) => {\n",
" server.setRoute('/download', (req, res) => {\n",
" res.setHeader('Content-Type', 'application/octet-stream');\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import path from 'path';\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 18
} | /**
* Copyright 2018 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { contextTest as it } from './config/browserTest';
it('should load svg favicon with prefer-color-scheme', async ({page, server, browserName, browserChannel, headful, asset}) => {
it.skip(!headful && browserName !== 'firefox', 'headless browsers, except firefox, do not request favicons');
it.skip(headful && browserName === 'webkit' && !browserChannel, 'playwright headful webkit does not have a favicon feature');
// Browsers aggresively cache favicons, so force bust with the
// `d` parameter to make iterating on this test more predictable and isolated.
const favicon = `/favicon.svg?d=${Date.now()}`;
server.setRoute(favicon, (req, res) => {
server.serveFile(req, res, asset('media-query-prefers-color-scheme.svg'));
});
server.setRoute('/page.html', (_, res) => {
res.end(`
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<link rel="icon" type="image/svg+xml" href="${favicon}">
<title>SVG Favicon Test</title>
</head>
<body>
favicons
</body>
</html>
`);
});
await Promise.all([
server.waitForRequest(favicon),
page.goto(server.PREFIX + '/page.html'),
]);
// Add artificial delay since, just because we saw the request for the favicon,
// it does not mean the browser has processed it. There's not a great way to
// hook into something like "favicon is fully displayed" event, so hopefully
// 500ms is enough, but not too much!
await page.waitForTimeout(500);
// Text still being around ensures we haven't actually lost our browser to a crash.
await page.waitForSelector('text=favicons');
});
| tests/favicon.spec.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.0004214139480609447,
0.0002568280615378171,
0.00017115456284955144,
0.0001879309129435569,
0.00010834575368789956
]
|
{
"id": 2,
"code_window": [
"\n",
"import { playwrightTest as it, expect } from './config/browserTest';\n",
"import fs from 'fs';\n",
"\n",
"it.describe('downloads path', () => {\n",
" it.beforeEach(async ({server}) => {\n",
" server.setRoute('/download', (req, res) => {\n",
" res.setHeader('Content-Type', 'application/octet-stream');\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import path from 'path';\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 18
} | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
const t = {};
t.String = function(x, details = {}, path = ['<root>']) {
if (typeof x === 'string' || typeof x === 'String')
return true;
details.error = `Expected "${path.join('.')}" to be |string|; found |${typeof x}| \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Number = function(x, details = {}, path = ['<root>']) {
if (typeof x === 'number')
return true;
details.error = `Expected "${path.join('.')}" to be |number|; found |${typeof x}| \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Boolean = function(x, details = {}, path = ['<root>']) {
if (typeof x === 'boolean')
return true;
details.error = `Expected "${path.join('.')}" to be |boolean|; found |${typeof x}| \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Null = function(x, details = {}, path = ['<root>']) {
if (Object.is(x, null))
return true;
details.error = `Expected "${path.join('.')}" to be \`null\`; found \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Undefined = function(x, details = {}, path = ['<root>']) {
if (Object.is(x, undefined))
return true;
details.error = `Expected "${path.join('.')}" to be \`undefined\`; found \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Any = x => true,
t.Enum = function(values) {
return function(x, details = {}, path = ['<root>']) {
if (values.indexOf(x) !== -1)
return true;
details.error = `Expected "${path.join('.')}" to be one of [${values.join(', ')}]; found \`${JSON.stringify(x)}\` (${typeof x}) instead.`;
return false;
}
}
t.Nullable = function(scheme) {
return function(x, details = {}, path = ['<root>']) {
if (Object.is(x, null))
return true;
return checkScheme(scheme, x, details, path);
}
}
t.Optional = function(scheme) {
return function(x, details = {}, path = ['<root>']) {
if (Object.is(x, undefined))
return true;
return checkScheme(scheme, x, details, path);
}
}
t.Array = function(scheme) {
return function(x, details = {}, path = ['<root>']) {
if (!Array.isArray(x)) {
details.error = `Expected "${path.join('.')}" to be an array; found \`${JSON.stringify(x)}\` (${typeof x}) instead.`;
return false;
}
const lastPathElement = path[path.length - 1];
for (let i = 0; i < x.length; ++i) {
path[path.length - 1] = lastPathElement + `[${i}]`;
if (!checkScheme(scheme, x[i], details, path))
return false;
}
path[path.length - 1] = lastPathElement;
return true;
}
}
t.Recursive = function(types, schemeName) {
return function(x, details = {}, path = ['<root>']) {
const scheme = types[schemeName];
return checkScheme(scheme, x, details, path);
}
}
function beauty(path, obj) {
if (path.length === 1)
return `object ${JSON.stringify(obj, null, 2)}`;
return `property "${path.join('.')}" - ${JSON.stringify(obj, null, 2)}`;
}
function checkScheme(scheme, x, details = {}, path = ['<root>']) {
if (!scheme)
throw new Error(`ILLDEFINED SCHEME: ${path.join('.')}`);
if (typeof scheme === 'object') {
if (!x) {
details.error = `Object "${path.join('.')}" is undefined, but has some scheme`;
return false;
}
for (const [propertyName, aScheme] of Object.entries(scheme)) {
path.push(propertyName);
const result = checkScheme(aScheme, x[propertyName], details, path);
path.pop();
if (!result)
return false;
}
for (const propertyName of Object.keys(x)) {
if (!scheme[propertyName]) {
path.push(propertyName);
details.error = `Found ${beauty(path, x[propertyName])} which is not described in this scheme`;
return false;
}
}
return true;
}
return scheme(x, details, path);
}
/*
function test(scheme, obj) {
const details = {};
if (!checkScheme(scheme, obj, details)) {
dump(`FAILED: ${JSON.stringify(obj)}
details.error: ${details.error}
`);
} else {
dump(`SUCCESS: ${JSON.stringify(obj)}
`);
}
}
test(t.Array(t.String), ['a', 'b', 2, 'c']);
test(t.Either(t.String, t.Number), {});
*/
this.t = t;
this.checkScheme = checkScheme;
this.EXPORTED_SYMBOLS = ['t', 'checkScheme'];
| browser_patches/firefox-stable/juggler/protocol/PrimitiveTypes.js | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.00017688877414911985,
0.00017302986816503108,
0.00016562228847760707,
0.0001733383978717029,
0.0000029207865281932754
]
|
{
"id": 2,
"code_window": [
"\n",
"import { playwrightTest as it, expect } from './config/browserTest';\n",
"import fs from 'fs';\n",
"\n",
"it.describe('downloads path', () => {\n",
" it.beforeEach(async ({server}) => {\n",
" server.setRoute('/download', (req, res) => {\n",
" res.setHeader('Content-Type', 'application/octet-stream');\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import path from 'path';\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 18
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the 'License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { Dispatcher, DispatcherScope } from './dispatcher';
import * as channels from '../protocol/channels';
import { Selectors } from '../server/selectors';
export class SelectorsDispatcher extends Dispatcher<Selectors, channels.SelectorsInitializer> implements channels.SelectorsChannel {
constructor(scope: DispatcherScope, selectors: Selectors) {
super(scope, selectors, 'Selectors', {});
}
async register(params: channels.SelectorsRegisterParams): Promise<void> {
await this._object.register(params.name, params.source, params.contentScript);
}
}
| src/dispatchers/selectorsDispatcher.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.0001771990500856191,
0.00017413035675417632,
0.0001698772539384663,
0.00017531476623844355,
0.000003104222287220182
]
|
{
"id": 3,
"code_window": [
" await downloadsBrowser.close();\n",
" });\n",
"\n",
" it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {\n",
" const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" it('should report downloads in downloadsPath folder with a relative path', async ({browserType, browserOptions, server}, testInfo) => {\n",
" const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: path.relative(process.cwd(), testInfo.outputPath('')) });\n",
" const page = await downloadsBrowser.newPage({ acceptDownloads: true });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n",
" const [ download ] = await Promise.all([\n",
" page.waitForEvent('download'),\n",
" page.click('a')\n",
" ]);\n",
" const downloadPath = await download.path();\n",
" expect(downloadPath.startsWith(testInfo.outputPath(''))).toBeTruthy();\n",
" await page.close();\n",
" await downloadsBrowser.close();\n",
" });\n",
"\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 73
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import fs from 'fs';
import * as os from 'os';
import path from 'path';
import * as util from 'util';
import { BrowserContext, normalizeProxySettings, validateBrowserContextOptions } from './browserContext';
import * as registry from '../utils/registry';
import { ConnectionTransport, WebSocketTransport } from './transport';
import { BrowserOptions, Browser, BrowserProcess, PlaywrightOptions } from './browser';
import { launchProcess, Env, envArrayToObject } from './processLauncher';
import { PipeTransport } from './pipeTransport';
import { Progress, ProgressController } from './progress';
import * as types from './types';
import { DEFAULT_TIMEOUT, TimeoutSettings } from '../utils/timeoutSettings';
import { validateHostRequirements } from './validateDependencies';
import { debugMode } from '../utils/utils';
import { helper } from './helper';
import { RecentLogsCollector } from '../utils/debugLogger';
import { CallMetadata, SdkObject } from './instrumentation';
const mkdirAsync = util.promisify(fs.mkdir);
const mkdtempAsync = util.promisify(fs.mkdtemp);
const existsAsync = (path: string): Promise<boolean> => new Promise(resolve => fs.stat(path, err => resolve(!err)));
const DOWNLOADS_FOLDER = path.join(os.tmpdir(), 'playwright_downloads-');
export abstract class BrowserType extends SdkObject {
private _name: registry.BrowserName;
readonly _registry: registry.Registry;
readonly _playwrightOptions: PlaywrightOptions;
constructor(browserName: registry.BrowserName, playwrightOptions: PlaywrightOptions) {
super(playwrightOptions.rootSdkObject, 'browser-type');
this.attribution.browserType = this;
this._playwrightOptions = playwrightOptions;
this._name = browserName;
this._registry = playwrightOptions.registry;
}
executablePath(channel?: types.BrowserChannel): string {
return this._registry.executablePath(this._name) || '';
}
name(): string {
return this._name;
}
async launch(metadata: CallMetadata, options: types.LaunchOptions, protocolLogger?: types.ProtocolLogger): Promise<Browser> {
options = validateLaunchOptions(options);
const controller = new ProgressController(metadata, this);
controller.setLogName('browser');
const browser = await controller.run(progress => {
return this._innerLaunchWithRetries(progress, options, undefined, helper.debugProtocolLogger(protocolLogger)).catch(e => { throw this._rewriteStartupError(e); });
}, TimeoutSettings.timeout(options));
return browser;
}
async launchPersistentContext(metadata: CallMetadata, userDataDir: string, options: types.LaunchPersistentOptions): Promise<BrowserContext> {
options = validateLaunchOptions(options);
const controller = new ProgressController(metadata, this);
const persistent: types.BrowserContextOptions = options;
controller.setLogName('browser');
const browser = await controller.run(progress => {
return this._innerLaunchWithRetries(progress, options, persistent, helper.debugProtocolLogger(), userDataDir).catch(e => { throw this._rewriteStartupError(e); });
}, TimeoutSettings.timeout(options));
return browser._defaultContext!;
}
async _innerLaunchWithRetries(progress: Progress, options: types.LaunchOptions, persistent: types.BrowserContextOptions | undefined, protocolLogger: types.ProtocolLogger, userDataDir?: string): Promise<Browser> {
try {
return this._innerLaunch(progress, options, persistent, protocolLogger, userDataDir);
} catch (error) {
// @see https://github.com/microsoft/playwright/issues/5214
const errorMessage = typeof error === 'object' && typeof error.message === 'string' ? error.message : '';
if (errorMessage.includes('Inconsistency detected by ld.so')) {
progress.log(`<restarting browser due to hitting race condition in glibc>`);
return this._innerLaunch(progress, options, persistent, protocolLogger, userDataDir);
}
throw error;
}
}
async _innerLaunch(progress: Progress, options: types.LaunchOptions, persistent: types.BrowserContextOptions | undefined, protocolLogger: types.ProtocolLogger, userDataDir?: string): Promise<Browser> {
options.proxy = options.proxy ? normalizeProxySettings(options.proxy) : undefined;
const browserLogsCollector = new RecentLogsCollector();
const { browserProcess, downloadsPath, transport } = await this._launchProcess(progress, options, !!persistent, browserLogsCollector, userDataDir);
if ((options as any).__testHookBeforeCreateBrowser)
await (options as any).__testHookBeforeCreateBrowser();
const browserOptions: BrowserOptions = {
...this._playwrightOptions,
name: this._name,
isChromium: this._name === 'chromium',
channel: options.channel,
slowMo: options.slowMo,
persistent,
headful: !options.headless,
downloadsPath,
browserProcess,
customExecutablePath: options.executablePath,
proxy: options.proxy,
protocolLogger,
browserLogsCollector,
wsEndpoint: options.useWebSocket ? (transport as WebSocketTransport).wsEndpoint : undefined,
traceDir: options._traceDir,
};
if (persistent)
validateBrowserContextOptions(persistent, browserOptions);
copyTestHooks(options, browserOptions);
const browser = await this._connectToTransport(transport, browserOptions);
// We assume no control when using custom arguments, and do not prepare the default context in that case.
if (persistent && !options.ignoreAllDefaultArgs)
await browser._defaultContext!._loadDefaultContext(progress);
return browser;
}
private async _launchProcess(progress: Progress, options: types.LaunchOptions, isPersistent: boolean, browserLogsCollector: RecentLogsCollector, userDataDir?: string): Promise<{ browserProcess: BrowserProcess, downloadsPath: string, transport: ConnectionTransport }> {
const {
ignoreDefaultArgs,
ignoreAllDefaultArgs,
args = [],
executablePath = null,
handleSIGINT = true,
handleSIGTERM = true,
handleSIGHUP = true,
} = options;
const env = options.env ? envArrayToObject(options.env) : process.env;
const tempDirectories = [];
const ensurePath = async (tmpPrefix: string, pathFromOptions?: string) => {
let dir;
if (pathFromOptions) {
dir = pathFromOptions;
await mkdirAsync(pathFromOptions, { recursive: true });
} else {
dir = await mkdtempAsync(tmpPrefix);
tempDirectories.push(dir);
}
return dir;
};
// TODO: add downloadsPath to newContext().
const downloadsPath = await ensurePath(DOWNLOADS_FOLDER, options.downloadsPath);
if (!userDataDir) {
userDataDir = await mkdtempAsync(path.join(os.tmpdir(), `playwright_${this._name}dev_profile-`));
tempDirectories.push(userDataDir);
}
const browserArguments = [];
if (ignoreAllDefaultArgs)
browserArguments.push(...args);
else if (ignoreDefaultArgs)
browserArguments.push(...this._defaultArgs(options, isPersistent, userDataDir).filter(arg => ignoreDefaultArgs.indexOf(arg) === -1));
else
browserArguments.push(...this._defaultArgs(options, isPersistent, userDataDir));
const executable = executablePath || this.executablePath(options.channel);
if (!executable)
throw new Error(`No executable path is specified. Pass "executablePath" option directly.`);
if (!(await existsAsync(executable))) {
const errorMessageLines = [`Failed to launch ${this._name} because executable doesn't exist at ${executable}`];
// If we tried using stock downloaded browser, suggest re-installing playwright.
if (!executablePath)
errorMessageLines.push(`Try re-installing playwright with "npm install playwright"`);
throw new Error(errorMessageLines.join('\n'));
}
// Only validate dependencies for downloadable browsers.
if (!executablePath && !options.channel)
await validateHostRequirements(this._registry, this._name);
else if (!executablePath && options.channel && this._registry.isSupportedBrowser(options.channel))
await validateHostRequirements(this._registry, options.channel as registry.BrowserName);
let wsEndpointCallback: ((wsEndpoint: string) => void) | undefined;
const wsEndpoint = options.useWebSocket ? new Promise<string>(f => wsEndpointCallback = f) : undefined;
// Note: it is important to define these variables before launchProcess, so that we don't get
// "Cannot access 'browserServer' before initialization" if something went wrong.
let transport: ConnectionTransport | undefined = undefined;
let browserProcess: BrowserProcess | undefined = undefined;
const { launchedProcess, gracefullyClose, kill } = await launchProcess({
executablePath: executable,
args: browserArguments,
env: this._amendEnvironment(env, userDataDir, executable, browserArguments),
handleSIGINT,
handleSIGTERM,
handleSIGHUP,
log: (message: string) => {
if (wsEndpointCallback) {
const match = message.match(/DevTools listening on (.*)/);
if (match)
wsEndpointCallback(match[1]);
}
progress.log(message);
browserLogsCollector.log(message);
},
stdio: 'pipe',
tempDirectories,
attemptToGracefullyClose: async () => {
if ((options as any).__testHookGracefullyClose)
await (options as any).__testHookGracefullyClose();
// We try to gracefully close to prevent crash reporting and core dumps.
// Note that it's fine to reuse the pipe transport, since
// our connection ignores kBrowserCloseMessageId.
this._attemptToGracefullyCloseBrowser(transport!);
},
onExit: (exitCode, signal) => {
if (browserProcess && browserProcess.onclose)
browserProcess.onclose(exitCode, signal);
},
});
async function closeOrKill(timeout: number): Promise<void> {
let timer: NodeJS.Timer;
try {
await Promise.race([
gracefullyClose(),
new Promise((resolve, reject) => timer = setTimeout(reject, timeout)),
]);
} catch (ignored) {
await kill().catch(ignored => {}); // Make sure to await actual process exit.
} finally {
clearTimeout(timer!);
}
}
browserProcess = {
onclose: undefined,
process: launchedProcess,
close: () => closeOrKill((options as any).__testHookBrowserCloseTimeout || DEFAULT_TIMEOUT),
kill
};
progress.cleanupWhenAborted(() => closeOrKill(progress.timeUntilDeadline()));
if (options.useWebSocket) {
transport = await WebSocketTransport.connect(progress, await wsEndpoint!);
} else {
const stdio = launchedProcess.stdio as unknown as [NodeJS.ReadableStream, NodeJS.WritableStream, NodeJS.WritableStream, NodeJS.WritableStream, NodeJS.ReadableStream];
transport = new PipeTransport(stdio[3], stdio[4]);
}
return { browserProcess, downloadsPath, transport };
}
async connectOverCDP(metadata: CallMetadata, endpointURL: string, options: { slowMo?: number, sdkLanguage: string }, timeout?: number): Promise<Browser> {
throw new Error('CDP connections are only supported by Chromium');
}
abstract _defaultArgs(options: types.LaunchOptions, isPersistent: boolean, userDataDir: string): string[];
abstract _connectToTransport(transport: ConnectionTransport, options: BrowserOptions): Promise<Browser>;
abstract _amendEnvironment(env: Env, userDataDir: string, executable: string, browserArguments: string[]): Env;
abstract _rewriteStartupError(error: Error): Error;
abstract _attemptToGracefullyCloseBrowser(transport: ConnectionTransport): void;
}
function copyTestHooks(from: object, to: object) {
for (const [key, value] of Object.entries(from)) {
if (key.startsWith('__testHook'))
(to as any)[key] = value;
}
}
function validateLaunchOptions<Options extends types.LaunchOptions>(options: Options): Options {
const { devtools = false } = options;
let { headless = !devtools } = options;
if (debugMode())
headless = false;
return { ...options, devtools, headless };
}
| src/server/browserType.ts | 1 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.003312367480248213,
0.00048591798986308277,
0.00016350398072972894,
0.00017029447189997882,
0.000848588824737817
]
|
{
"id": 3,
"code_window": [
" await downloadsBrowser.close();\n",
" });\n",
"\n",
" it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {\n",
" const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" it('should report downloads in downloadsPath folder with a relative path', async ({browserType, browserOptions, server}, testInfo) => {\n",
" const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: path.relative(process.cwd(), testInfo.outputPath('')) });\n",
" const page = await downloadsBrowser.newPage({ acceptDownloads: true });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n",
" const [ download ] = await Promise.all([\n",
" page.waitForEvent('download'),\n",
" page.click('a')\n",
" ]);\n",
" const downloadPath = await download.path();\n",
" expect(downloadPath.startsWith(testInfo.outputPath(''))).toBeTruthy();\n",
" await page.close();\n",
" await downloadsBrowser.close();\n",
" });\n",
"\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 73
} | /*
* Copyright (c) 2010, The WebM Project authors. All rights reserved.
* Copyright (c) 2013 The Chromium Authors. All rights reserved.
* Copyright (C) 2020 Microsoft Corporation.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
#include "ScreencastEncoder.h"
#include <algorithm>
#include <libyuv.h>
#include <vpx/vp8.h>
#include <vpx/vp8cx.h>
#include <vpx/vpx_encoder.h>
#include "nsIThread.h"
#include "nsThreadUtils.h"
#include "WebMFileWriter.h"
#include "webrtc/api/video/video_frame.h"
namespace mozilla {
namespace {
// Number of timebase unints per one frame.
constexpr int timeScale = 1000;
// Defines the dimension of a macro block. This is used to compute the active
// map for the encoder.
const int kMacroBlockSize = 16;
void createImage(unsigned int width, unsigned int height,
std::unique_ptr<vpx_image_t>& out_image,
std::unique_ptr<uint8_t[]>& out_image_buffer,
int& out_buffer_size) {
std::unique_ptr<vpx_image_t> image(new vpx_image_t());
memset(image.get(), 0, sizeof(vpx_image_t));
// libvpx seems to require both to be assigned.
image->d_w = width;
image->w = width;
image->d_h = height;
image->h = height;
// I420
image->fmt = VPX_IMG_FMT_YV12;
image->x_chroma_shift = 1;
image->y_chroma_shift = 1;
// libyuv's fast-path requires 16-byte aligned pointers and strides, so pad
// the Y, U and V planes' strides to multiples of 16 bytes.
const int y_stride = ((image->w - 1) & ~15) + 16;
const int uv_unaligned_stride = y_stride >> image->x_chroma_shift;
const int uv_stride = ((uv_unaligned_stride - 1) & ~15) + 16;
// libvpx accesses the source image in macro blocks, and will over-read
// if the image is not padded out to the next macroblock: crbug.com/119633.
// Pad the Y, U and V planes' height out to compensate.
// Assuming macroblocks are 16x16, aligning the planes' strides above also
// macroblock aligned them.
static_assert(kMacroBlockSize == 16, "macroblock_size_not_16");
const int y_rows = ((image->h - 1) & ~(kMacroBlockSize-1)) + kMacroBlockSize;
const int uv_rows = y_rows >> image->y_chroma_shift;
// Allocate a YUV buffer large enough for the aligned data & padding.
out_buffer_size = y_stride * y_rows + 2*uv_stride * uv_rows;
std::unique_ptr<uint8_t[]> image_buffer(new uint8_t[out_buffer_size]);
// Reset image value to 128 so we just need to fill in the y plane.
memset(image_buffer.get(), 128, out_buffer_size);
// Fill in the information for |image_|.
unsigned char* uchar_buffer =
reinterpret_cast<unsigned char*>(image_buffer.get());
image->planes[0] = uchar_buffer;
image->planes[1] = image->planes[0] + y_stride * y_rows;
image->planes[2] = image->planes[1] + uv_stride * uv_rows;
image->stride[0] = y_stride;
image->stride[1] = uv_stride;
image->stride[2] = uv_stride;
out_image = std::move(image);
out_image_buffer = std::move(image_buffer);
}
} // namespace
class ScreencastEncoder::VPXFrame {
public:
VPXFrame(rtc::scoped_refptr<webrtc::VideoFrameBuffer>&& buffer, Maybe<double> scale, const gfx::IntMargin& margin)
: m_frameBuffer(std::move(buffer))
, m_scale(scale)
, m_margin(margin)
{ }
void setDuration(TimeDuration duration) { m_duration = duration; }
TimeDuration duration() const { return m_duration; }
void convertToVpxImage(vpx_image_t* image)
{
if (m_frameBuffer->type() != webrtc::VideoFrameBuffer::Type::kI420) {
fprintf(stderr, "convertToVpxImage unexpected frame buffer type: %d\n", m_frameBuffer->type());
return;
}
auto src = m_frameBuffer->GetI420();
const int y_stride = image->stride[VPX_PLANE_Y];
MOZ_ASSERT(image->stride[VPX_PLANE_U] == image->stride[VPX_PLANE_V]);
const int uv_stride = image->stride[1];
uint8_t* y_data = image->planes[VPX_PLANE_Y];
uint8_t* u_data = image->planes[VPX_PLANE_U];
uint8_t* v_data = image->planes[VPX_PLANE_V];
double src_width = src->width() - m_margin.LeftRight();
double src_height = src->height() - m_margin.top;
if (m_scale || (src_width > image->w || src_height > image->h)) {
double scale = m_scale ? m_scale.value() : std::min(image->w / src_width, image->h / src_height);
double dst_width = src_width * scale;
if (dst_width > image->w) {
src_width *= image->w / dst_width;
dst_width = image->w;
}
double dst_height = src_height * scale;
if (dst_height > image->h) {
src_height *= image->h / dst_height;
dst_height = image->h;
}
libyuv::I420Scale(src->DataY() + m_margin.top * src->StrideY() + m_margin.left, src->StrideY(),
src->DataU() + (m_margin.top * src->StrideU() + m_margin.left) / 2, src->StrideU(),
src->DataV() + (m_margin.top * src->StrideV() + m_margin.left) / 2, src->StrideV(),
src_width, src_height,
y_data, y_stride,
u_data, uv_stride,
v_data, uv_stride,
dst_width, dst_height,
libyuv::kFilterBilinear);
} else {
int width = std::min<int>(image->w, src_width);
int height = std::min<int>(image->h, src_height);
libyuv::I420Copy(src->DataY() + m_margin.top * src->StrideY() + m_margin.left, src->StrideY(),
src->DataU() + (m_margin.top * src->StrideU() + m_margin.left) / 2, src->StrideU(),
src->DataV() + (m_margin.top * src->StrideV() + m_margin.left) / 2, src->StrideV(),
y_data, y_stride,
u_data, uv_stride,
v_data, uv_stride,
width, height);
}
}
private:
rtc::scoped_refptr<webrtc::VideoFrameBuffer> m_frameBuffer;
Maybe<double> m_scale;
gfx::IntMargin m_margin;
TimeDuration m_duration;
};
class ScreencastEncoder::VPXCodec {
public:
VPXCodec(vpx_codec_ctx_t codec, vpx_codec_enc_cfg_t cfg, FILE* file)
: m_codec(codec)
, m_cfg(cfg)
, m_file(file)
, m_writer(new WebMFileWriter(file, &m_cfg))
{
nsresult rv = NS_NewNamedThread("Screencast enc", getter_AddRefs(m_encoderQueue));
if (rv != NS_OK) {
fprintf(stderr, "ScreencastEncoder::VPXCodec failed to spawn thread %d\n", rv);
return;
}
createImage(cfg.g_w, cfg.g_h, m_image, m_imageBuffer, m_imageBufferSize);
}
~VPXCodec() {
m_encoderQueue->Shutdown();
m_encoderQueue = nullptr;
}
void encodeFrameAsync(std::unique_ptr<VPXFrame>&& frame)
{
m_encoderQueue->Dispatch(NS_NewRunnableFunction("VPXCodec::encodeFrameAsync", [this, frame = std::move(frame)] {
memset(m_imageBuffer.get(), 128, m_imageBufferSize);
frame->convertToVpxImage(m_image.get());
double frameCount = frame->duration().ToSeconds() * fps;
// For long duration repeat frame at 1 fps to ensure last frame duration is short enough.
// TODO: figure out why simply passing duration doesn't work well.
for (;frameCount > 1.5; frameCount -= 1) {
encodeFrame(m_image.get(), timeScale);
}
encodeFrame(m_image.get(), std::max<int>(1, frameCount * timeScale));
}));
}
void finishAsync(std::function<void()>&& callback)
{
m_encoderQueue->Dispatch(NS_NewRunnableFunction("VPXCodec::finishAsync", [this, callback = std::move(callback)] {
finish();
callback();
}));
}
private:
bool encodeFrame(vpx_image_t *img, int duration)
{
vpx_codec_iter_t iter = nullptr;
const vpx_codec_cx_pkt_t *pkt = nullptr;
int flags = 0;
const vpx_codec_err_t res = vpx_codec_encode(&m_codec, img, m_pts, duration, flags, VPX_DL_REALTIME);
if (res != VPX_CODEC_OK) {
fprintf(stderr, "Failed to encode frame: %s\n", vpx_codec_error(&m_codec));
return false;
}
bool gotPkts = false;
while ((pkt = vpx_codec_get_cx_data(&m_codec, &iter)) != nullptr) {
gotPkts = true;
if (pkt->kind == VPX_CODEC_CX_FRAME_PKT) {
m_writer->writeFrame(pkt);
++m_frameCount;
// fprintf(stderr, " #%03d %spts=%" PRId64 " sz=%zd\n", m_frameCount, (pkt->data.frame.flags & VPX_FRAME_IS_KEY) != 0 ? "[K] " : "", pkt->data.frame.pts, pkt->data.frame.sz);
m_pts += pkt->data.frame.duration;
}
}
return gotPkts;
}
void finish()
{
// Flush encoder.
while (encodeFrame(nullptr, 1))
++m_frameCount;
m_writer->finish();
fclose(m_file);
// fprintf(stderr, "ScreencastEncoder::finish %d frames\n", m_frameCount);
}
RefPtr<nsIThread> m_encoderQueue;
vpx_codec_ctx_t m_codec;
vpx_codec_enc_cfg_t m_cfg;
FILE* m_file { nullptr };
std::unique_ptr<WebMFileWriter> m_writer;
int m_frameCount { 0 };
int64_t m_pts { 0 };
std::unique_ptr<uint8_t[]> m_imageBuffer;
int m_imageBufferSize { 0 };
std::unique_ptr<vpx_image_t> m_image;
};
ScreencastEncoder::ScreencastEncoder(std::unique_ptr<VPXCodec>&& vpxCodec, Maybe<double> scale, const gfx::IntMargin& margin)
: m_vpxCodec(std::move(vpxCodec))
, m_scale(scale)
, m_margin(margin)
{
}
ScreencastEncoder::~ScreencastEncoder()
{
}
RefPtr<ScreencastEncoder> ScreencastEncoder::create(nsCString& errorString, const nsCString& filePath, int width, int height, Maybe<double> scale, const gfx::IntMargin& margin)
{
vpx_codec_iface_t* codec_interface = vpx_codec_vp8_cx();
if (!codec_interface) {
errorString = "Codec not found.";
return nullptr;
}
if (width <= 0 || height <= 0 || (width % 2) != 0 || (height % 2) != 0) {
errorString.AppendPrintf("Invalid frame size: %dx%d", width, height);
return nullptr;
}
vpx_codec_enc_cfg_t cfg;
memset(&cfg, 0, sizeof(cfg));
vpx_codec_err_t error = vpx_codec_enc_config_default(codec_interface, &cfg, 0);
if (error) {
errorString.AppendPrintf("Failed to get default codec config: %s", vpx_codec_err_to_string(error));
return nullptr;
}
cfg.g_w = width;
cfg.g_h = height;
cfg.g_timebase.num = 1;
cfg.g_timebase.den = fps * timeScale;
cfg.g_error_resilient = VPX_ERROR_RESILIENT_DEFAULT;
vpx_codec_ctx_t codec;
if (vpx_codec_enc_init(&codec, codec_interface, &cfg, 0)) {
errorString.AppendPrintf("Failed to initialize encoder: %s", vpx_codec_error(&codec));
return nullptr;
}
FILE* file = fopen(filePath.get(), "wb");
if (!file) {
errorString.AppendPrintf("Failed to open file '%s' for writing: %s", filePath.get(), strerror(errno));
return nullptr;
}
std::unique_ptr<VPXCodec> vpxCodec(new VPXCodec(codec, cfg, file));
// fprintf(stderr, "ScreencastEncoder initialized with: %s\n", vpx_codec_iface_name(codec_interface));
return new ScreencastEncoder(std::move(vpxCodec), scale, margin);
}
void ScreencastEncoder::flushLastFrame()
{
TimeStamp now = TimeStamp::Now();
if (m_lastFrameTimestamp) {
// If previous frame encoding failed for some rason leave the timestampt intact.
if (!m_lastFrame)
return;
m_lastFrame->setDuration(now - m_lastFrameTimestamp);
m_vpxCodec->encodeFrameAsync(std::move(m_lastFrame));
}
m_lastFrameTimestamp = now;
}
void ScreencastEncoder::encodeFrame(const webrtc::VideoFrame& videoFrame)
{
// fprintf(stderr, "ScreencastEncoder::encodeFrame\n");
flushLastFrame();
m_lastFrame = std::make_unique<VPXFrame>(videoFrame.video_frame_buffer(), m_scale, m_margin);
}
void ScreencastEncoder::finish(std::function<void()>&& callback)
{
if (!m_vpxCodec) {
callback();
return;
}
flushLastFrame();
m_vpxCodec->finishAsync([callback = std::move(callback)] () mutable {
NS_DispatchToMainThread(NS_NewRunnableFunction("ScreencastEncoder::finish callback", std::move(callback)));
});
}
} // namespace mozilla
| browser_patches/firefox/juggler/screencast/ScreencastEncoder.cpp | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.0003273345355410129,
0.00017621876031626016,
0.00016554271860513836,
0.00017249197117052972,
0.000024995086278067902
]
|
{
"id": 3,
"code_window": [
" await downloadsBrowser.close();\n",
" });\n",
"\n",
" it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {\n",
" const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" it('should report downloads in downloadsPath folder with a relative path', async ({browserType, browserOptions, server}, testInfo) => {\n",
" const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: path.relative(process.cwd(), testInfo.outputPath('')) });\n",
" const page = await downloadsBrowser.newPage({ acceptDownloads: true });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n",
" const [ download ] = await Promise.all([\n",
" page.waitForEvent('download'),\n",
" page.click('a')\n",
" ]);\n",
" const downloadPath = await download.path();\n",
" expect(downloadPath.startsWith(testInfo.outputPath(''))).toBeTruthy();\n",
" await page.close();\n",
" await downloadsBrowser.close();\n",
" });\n",
"\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 73
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { test as it, expect } from './config/pageTest';
import util from 'util';
it('should work', async ({page}) => {
let message = null;
page.once('console', m => message = m);
await Promise.all([
page.evaluate(() => console.log('hello', 5, {foo: 'bar'})),
page.waitForEvent('console')
]);
expect(message.text()).toEqual('hello 5 JSHandle@object');
expect(message.type()).toEqual('log');
expect(await message.args()[0].jsonValue()).toEqual('hello');
expect(await message.args()[1].jsonValue()).toEqual(5);
expect(await message.args()[2].jsonValue()).toEqual({foo: 'bar'});
});
it('should emit same log twice', async ({page}) => {
const messages = [];
page.on('console', m => messages.push(m.text()));
await page.evaluate(() => { for (let i = 0; i < 2; ++i) console.log('hello'); });
expect(messages).toEqual(['hello', 'hello']);
});
it('should use text() for inspection', async ({page}) => {
let text;
const inspect = value => {
text = util.inspect(value);
};
page.on('console', inspect);
await page.evaluate(() => console.log('Hello world'));
expect(text).toEqual('Hello world');
});
it('should work for different console API calls', async ({page}) => {
const messages = [];
page.on('console', msg => messages.push(msg));
// All console events will be reported before `page.evaluate` is finished.
await page.evaluate(() => {
// A pair of time/timeEnd generates only one Console API call.
console.time('calling console.time');
console.timeEnd('calling console.time');
console.trace('calling console.trace');
console.dir('calling console.dir');
console.warn('calling console.warn');
console.error('calling console.error');
console.log(Promise.resolve('should not wait until resolved!'));
});
expect(messages.map(msg => msg.type())).toEqual([
'timeEnd', 'trace', 'dir', 'warning', 'error', 'log'
]);
expect(messages[0].text()).toContain('calling console.time');
expect(messages.slice(1).map(msg => msg.text())).toEqual([
'calling console.trace',
'calling console.dir',
'calling console.warn',
'calling console.error',
'JSHandle@promise',
]);
});
it('should not fail for window object', async ({page}) => {
let message = null;
page.once('console', msg => message = msg);
await Promise.all([
page.evaluate(() => console.error(window)),
page.waitForEvent('console')
]);
expect(message.text()).toBe('JSHandle@object');
});
it('should trigger correct Log', async ({page, server}) => {
await page.goto('about:blank');
const [message] = await Promise.all([
page.waitForEvent('console'),
page.evaluate(async url => fetch(url).catch(e => {}), server.EMPTY_PAGE)
]);
expect(message.text()).toContain('Access-Control-Allow-Origin');
expect(message.type()).toEqual('error');
});
it('should have location for console API calls', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
const [message] = await Promise.all([
page.waitForEvent('console', m => m.text() === 'yellow'),
page.goto(server.PREFIX + '/consolelog.html'),
]);
expect(message.type()).toBe('log');
const location = message.location();
// Engines have different column notion.
delete location.columnNumber;
expect(location).toEqual({
url: server.PREFIX + '/consolelog.html',
lineNumber: 7,
});
});
it('should not throw when there are console messages in detached iframes', async ({page, server}) => {
// @see https://github.com/GoogleChrome/puppeteer/issues/3865
await page.goto(server.EMPTY_PAGE);
const [popup] = await Promise.all([
page.waitForEvent('popup'),
page.evaluate(async () => {
// 1. Create a popup that Playwright is not connected to.
const win = window.open('');
window['_popup'] = win;
if (window.document.readyState !== 'complete')
await new Promise(f => window.addEventListener('load', f));
// 2. In this popup, create an iframe that console.logs a message.
win.document.body.innerHTML = `<iframe src='/consolelog.html'></iframe>`;
const frame = win.document.querySelector('iframe');
if (!frame.contentDocument || frame.contentDocument.readyState !== 'complete')
await new Promise(f => frame.addEventListener('load', f));
// 3. After that, remove the iframe.
frame.remove();
}),
]);
// 4. Connect to the popup and make sure it doesn't throw.
expect(await popup.evaluate('1 + 1')).toBe(2);
});
| tests/page-event-console.spec.ts | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.9761469960212708,
0.34601789712905884,
0.00016260315896943212,
0.0031972802244126797,
0.43915194272994995
]
|
{
"id": 3,
"code_window": [
" await downloadsBrowser.close();\n",
" });\n",
"\n",
" it('should accept downloads in persistent context', async ({launchPersistent, server}, testInfo) => {\n",
" const { context, page } = await launchPersistent({ acceptDownloads: true, downloadsPath: testInfo.outputPath('') });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" it('should report downloads in downloadsPath folder with a relative path', async ({browserType, browserOptions, server}, testInfo) => {\n",
" const downloadsBrowser = await browserType.launch({ ...browserOptions, downloadsPath: path.relative(process.cwd(), testInfo.outputPath('')) });\n",
" const page = await downloadsBrowser.newPage({ acceptDownloads: true });\n",
" await page.setContent(`<a href=\"${server.PREFIX}/download\">download</a>`);\n",
" const [ download ] = await Promise.all([\n",
" page.waitForEvent('download'),\n",
" page.click('a')\n",
" ]);\n",
" const downloadPath = await download.path();\n",
" expect(downloadPath.startsWith(testInfo.outputPath(''))).toBeTruthy();\n",
" await page.close();\n",
" await downloadsBrowser.close();\n",
" });\n",
"\n"
],
"file_path": "tests/downloads-path.spec.ts",
"type": "add",
"edit_start_line_idx": 73
} | output
| packages/.gitignore | 0 | https://github.com/microsoft/playwright/commit/ab850afb451a0223b4d2649348293d09e1f1ff83 | [
0.00029109904426150024,
0.00029109904426150024,
0.00029109904426150024,
0.00029109904426150024,
0
]
|
{
"id": 0,
"code_window": [
"\t\t\tif (opts.recursive && opts.excludes.length === 0) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\topts.excludes.push(key);\n",
"\t\t\t\t\t\t}\n",
"\t\t\t\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 279
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { DisposableMap, DisposableStore } from 'vs/base/common/lifecycle';
import { FileOperation, IFileService, IFilesConfiguration, IWatchOptions } from 'vs/platform/files/common/files';
import { extHostNamedCustomer, IExtHostContext } from 'vs/workbench/services/extensions/common/extHostCustomers';
import { ExtHostContext, ExtHostFileSystemEventServiceShape, MainContext, MainThreadFileSystemEventServiceShape } from '../common/extHost.protocol';
import { localize } from 'vs/nls';
import { IWorkingCopyFileOperationParticipant, IWorkingCopyFileService, SourceTargetPair, IFileOperationUndoRedoInfo } from 'vs/workbench/services/workingCopy/common/workingCopyFileService';
import { IBulkEditService } from 'vs/editor/browser/services/bulkEditService';
import { IProgressService, ProgressLocation } from 'vs/platform/progress/common/progress';
import { raceCancellation } from 'vs/base/common/async';
import { CancellationToken, CancellationTokenSource } from 'vs/base/common/cancellation';
import { IDialogService } from 'vs/platform/dialogs/common/dialogs';
import Severity from 'vs/base/common/severity';
import { IStorageService, StorageScope, StorageTarget } from 'vs/platform/storage/common/storage';
import { Action2, registerAction2 } from 'vs/platform/actions/common/actions';
import { ServicesAccessor } from 'vs/platform/instantiation/common/instantiation';
import { ILogService } from 'vs/platform/log/common/log';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { reviveWorkspaceEditDto } from 'vs/workbench/api/browser/mainThreadBulkEdits';
import { GLOBSTAR } from 'vs/base/common/glob';
import { rtrim } from 'vs/base/common/strings';
import { UriComponents, URI } from 'vs/base/common/uri';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { normalizeWatcherPattern } from 'vs/platform/files/common/watcher';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
@extHostNamedCustomer(MainContext.MainThreadFileSystemEventService)
export class MainThreadFileSystemEventService implements MainThreadFileSystemEventServiceShape {
static readonly MementoKeyAdditionalEdits = `file.particpants.additionalEdits`;
private readonly _proxy: ExtHostFileSystemEventServiceShape;
private readonly _listener = new DisposableStore();
private readonly _watches = new DisposableMap<number>();
constructor(
extHostContext: IExtHostContext,
@IFileService private readonly _fileService: IFileService,
@IWorkingCopyFileService workingCopyFileService: IWorkingCopyFileService,
@IBulkEditService bulkEditService: IBulkEditService,
@IProgressService progressService: IProgressService,
@IDialogService dialogService: IDialogService,
@IStorageService storageService: IStorageService,
@ILogService logService: ILogService,
@IEnvironmentService envService: IEnvironmentService,
@IUriIdentityService uriIdentService: IUriIdentityService,
@IWorkspaceContextService private readonly _contextService: IWorkspaceContextService,
@ILogService private readonly _logService: ILogService,
@IConfigurationService private readonly _configurationService: IConfigurationService
) {
this._proxy = extHostContext.getProxy(ExtHostContext.ExtHostFileSystemEventService);
this._listener.add(_fileService.onDidFilesChange(event => {
this._proxy.$onFileEvent({
created: event.rawAdded,
changed: event.rawUpdated,
deleted: event.rawDeleted
});
}));
const that = this;
const fileOperationParticipant = new class implements IWorkingCopyFileOperationParticipant {
async participate(files: SourceTargetPair[], operation: FileOperation, undoInfo: IFileOperationUndoRedoInfo | undefined, timeout: number, token: CancellationToken) {
if (undoInfo?.isUndoing) {
return;
}
const cts = new CancellationTokenSource(token);
const timer = setTimeout(() => cts.cancel(), timeout);
const data = await progressService.withProgress({
location: ProgressLocation.Notification,
title: this._progressLabel(operation),
cancellable: true,
delay: Math.min(timeout / 2, 3000)
}, () => {
// race extension host event delivery against timeout AND user-cancel
const onWillEvent = that._proxy.$onWillRunFileOperation(operation, files, timeout, cts.token);
return raceCancellation(onWillEvent, cts.token);
}, () => {
// user-cancel
cts.cancel();
}).finally(() => {
cts.dispose();
clearTimeout(timer);
});
if (!data || data.edit.edits.length === 0) {
// cancelled, no reply, or no edits
return;
}
const needsConfirmation = data.edit.edits.some(edit => edit.metadata?.needsConfirmation);
let showPreview = storageService.getBoolean(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, StorageScope.PROFILE);
if (envService.extensionTestsLocationURI) {
// don't show dialog in tests
showPreview = false;
}
if (showPreview === undefined) {
// show a user facing message
let message: string;
if (data.extensionNames.length === 1) {
if (operation === FileOperation.CREATE) {
message = localize('ask.1.create', "Extension '{0}' wants to make refactoring changes with this file creation", data.extensionNames[0]);
} else if (operation === FileOperation.COPY) {
message = localize('ask.1.copy', "Extension '{0}' wants to make refactoring changes with this file copy", data.extensionNames[0]);
} else if (operation === FileOperation.MOVE) {
message = localize('ask.1.move', "Extension '{0}' wants to make refactoring changes with this file move", data.extensionNames[0]);
} else /* if (operation === FileOperation.DELETE) */ {
message = localize('ask.1.delete', "Extension '{0}' wants to make refactoring changes with this file deletion", data.extensionNames[0]);
}
} else {
if (operation === FileOperation.CREATE) {
message = localize({ key: 'ask.N.create', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file creation", data.extensionNames.length);
} else if (operation === FileOperation.COPY) {
message = localize({ key: 'ask.N.copy', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file copy", data.extensionNames.length);
} else if (operation === FileOperation.MOVE) {
message = localize({ key: 'ask.N.move', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file move", data.extensionNames.length);
} else /* if (operation === FileOperation.DELETE) */ {
message = localize({ key: 'ask.N.delete', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file deletion", data.extensionNames.length);
}
}
if (needsConfirmation) {
// edit which needs confirmation -> always show dialog
const { confirmed } = await dialogService.confirm({
type: Severity.Info,
message,
primaryButton: localize('preview', "Show &&Preview"),
cancelButton: localize('cancel', "Skip Changes")
});
showPreview = true;
if (!confirmed) {
// no changes wanted
return;
}
} else {
// choice
enum Choice {
OK = 0,
Preview = 1,
Cancel = 2
}
const { result, checkboxChecked } = await dialogService.prompt<Choice>({
type: Severity.Info,
message,
buttons: [
{
label: localize({ key: 'ok', comment: ['&& denotes a mnemonic'] }, "&&OK"),
run: () => Choice.OK
},
{
label: localize({ key: 'preview', comment: ['&& denotes a mnemonic'] }, "Show &&Preview"),
run: () => Choice.Preview
}
],
cancelButton: {
label: localize('cancel', "Skip Changes"),
run: () => Choice.Cancel
},
checkbox: { label: localize('again', "Do not ask me again") }
});
if (result === Choice.Cancel) {
// no changes wanted, don't persist cancel option
return;
}
showPreview = result === Choice.Preview;
if (checkboxChecked) {
storageService.store(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, showPreview, StorageScope.PROFILE, StorageTarget.USER);
}
}
}
logService.info('[onWill-handler] applying additional workspace edit from extensions', data.extensionNames);
await bulkEditService.apply(
reviveWorkspaceEditDto(data.edit, uriIdentService),
{ undoRedoGroupId: undoInfo?.undoRedoGroupId, showPreview }
);
}
private _progressLabel(operation: FileOperation): string {
switch (operation) {
case FileOperation.CREATE:
return localize('msg-create', "Running 'File Create' participants...");
case FileOperation.MOVE:
return localize('msg-rename', "Running 'File Rename' participants...");
case FileOperation.COPY:
return localize('msg-copy', "Running 'File Copy' participants...");
case FileOperation.DELETE:
return localize('msg-delete', "Running 'File Delete' participants...");
case FileOperation.WRITE:
return localize('msg-write', "Running 'File Write' participants...");
}
}
};
// BEFORE file operation
this._listener.add(workingCopyFileService.addFileOperationParticipant(fileOperationParticipant));
// AFTER file operation
this._listener.add(workingCopyFileService.onDidRunWorkingCopyFileOperation(e => this._proxy.$onDidRunFileOperation(e.operation, e.files)));
}
async $watch(extensionId: string, session: number, resource: UriComponents, unvalidatedOpts: IWatchOptions, correlate: boolean): Promise<void> {
const uri = URI.revive(resource);
const opts: IWatchOptions = {
...unvalidatedOpts
};
// Convert a recursive watcher to a flat watcher if the path
// turns out to not be a folder. Recursive watching is only
// possible on folders, so we help all file watchers by checking
// early.
if (opts.recursive) {
try {
const stat = await this._fileService.stat(uri);
if (!stat.isDirectory) {
opts.recursive = false;
}
} catch (error) {
this._logService.error(`MainThreadFileSystemEventService#$watch(): failed to stat a resource for file watching (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session}): ${error}`);
}
}
// Correlated file watching is taken as is
if (correlate) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): request to start watching correlated (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
const watcherDisposables = new DisposableStore();
const subscription = watcherDisposables.add(this._fileService.createWatcher(uri, opts));
watcherDisposables.add(subscription.onDidChange(event => {
this._proxy.$onFileEvent({
session,
created: event.rawAdded,
changed: event.rawUpdated,
deleted: event.rawDeleted
});
}));
this._watches.set(session, watcherDisposables);
}
// Uncorrelated file watching gets special treatment
else {
// Refuse to watch anything that is already watched via
// our workspace watchers in case the request is a
// recursive file watcher and does not opt-in to event
// correlation via specific exclude rules.
// Still allow for non-recursive watch requests as a way
// to bypass configured exclude rules though
// (see https://github.com/microsoft/vscode/issues/146066)
const workspaceFolder = this._contextService.getWorkspaceFolder(uri);
if (workspaceFolder && opts.recursive) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): ignoring request to start watching because path is inside workspace (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
return;
}
this._logService.trace(`MainThreadFileSystemEventService#$watch(): request to start watching uncorrelated (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
// Automatically add `files.watcherExclude` patterns when watching
// recursively to give users a chance to configure exclude rules
// for reducing the overhead of watching recursively
if (opts.recursive && opts.excludes.length === 0) {
const config = this._configurationService.getValue<IFilesConfiguration>();
if (config.files?.watcherExclude) {
for (const key in config.files.watcherExclude) {
if (config.files.watcherExclude[key] === true) {
opts.excludes.push(key);
}
}
}
}
// Non-recursive watching inside the workspace will overlap with
// our standard workspace watchers. To prevent duplicate events,
// we only want to include events for files that are otherwise
// excluded via `files.watcherExclude`. As such, we configure
// to include each configured exclude pattern so that only those
// events are reported that are otherwise excluded.
// However, we cannot just use the pattern as is, because a pattern
// such as `bar` for a exclude, will work to exclude any of
// `<workspace path>/bar` but will not work as include for files within
// `bar` unless a suffix of `/**` if added.
// (https://github.com/microsoft/vscode/issues/148245)
else if (workspaceFolder) {
const config = this._configurationService.getValue<IFilesConfiguration>();
if (config.files?.watcherExclude) {
for (const key in config.files.watcherExclude) {
if (config.files.watcherExclude[key] === true) {
if (!opts.includes) {
opts.includes = [];
}
const includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;
opts.includes.push(normalizeWatcherPattern(workspaceFolder.uri.fsPath, includePattern));
}
}
}
// Still ignore watch request if there are actually no configured
// exclude rules, because in that case our default recursive watcher
// should be able to take care of all events.
if (!opts.includes || opts.includes.length === 0) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): ignoring request to start watching because path is inside workspace and no excludes are configured (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
return;
}
}
const subscription = this._fileService.watch(uri, opts);
this._watches.set(session, subscription);
}
}
$unwatch(session: number): void {
if (this._watches.has(session)) {
this._logService.trace(`MainThreadFileSystemEventService#$unwatch(): request to stop watching (session: ${session})`);
this._watches.deleteAndDispose(session);
}
}
dispose(): void {
this._listener.dispose();
this._watches.dispose();
}
}
registerAction2(class ResetMemento extends Action2 {
constructor() {
super({
id: 'files.participants.resetChoice',
title: {
value: localize('label', "Reset choice for 'File operation needs preview'"),
original: `Reset choice for 'File operation needs preview'`
},
f1: true
});
}
run(accessor: ServicesAccessor) {
accessor.get(IStorageService).remove(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, StorageScope.PROFILE);
}
});
| src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts | 1 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.9978066086769104,
0.1112275943160057,
0.0001616157533135265,
0.0001728349889162928,
0.31298601627349854
]
|
{
"id": 0,
"code_window": [
"\t\t\tif (opts.recursive && opts.excludes.length === 0) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\topts.excludes.push(key);\n",
"\t\t\t\t\t\t}\n",
"\t\t\t\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 279
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { $, createStyleSheet, h, isInShadowDOM, reset } from 'vs/base/browser/dom';
import { renderLabelWithIcons } from 'vs/base/browser/ui/iconLabel/iconLabels';
import { hash } from 'vs/base/common/hash';
import { Disposable, IDisposable } from 'vs/base/common/lifecycle';
import { autorun, derived, IObservable, transaction } from 'vs/base/common/observable';
import { ICodeEditor, IViewZoneChangeAccessor } from 'vs/editor/browser/editorBrowser';
import { EditorOption, EDITOR_FONT_DEFAULTS } from 'vs/editor/common/config/editorOptions';
import { localize } from 'vs/nls';
import { ModifiedBaseRange, ModifiedBaseRangeState, ModifiedBaseRangeStateKind } from 'vs/workbench/contrib/mergeEditor/browser/model/modifiedBaseRange';
import { FixedZoneWidget } from 'vs/workbench/contrib/mergeEditor/browser/view/fixedZoneWidget';
import { MergeEditorViewModel } from 'vs/workbench/contrib/mergeEditor/browser/view/viewModel';
export class ConflictActionsFactory extends Disposable {
private readonly _styleClassName: string;
private readonly _styleElement: HTMLStyleElement;
constructor(private readonly _editor: ICodeEditor) {
super();
this._register(this._editor.onDidChangeConfiguration((e) => {
if (e.hasChanged(EditorOption.fontInfo) || e.hasChanged(EditorOption.codeLensFontSize) || e.hasChanged(EditorOption.codeLensFontFamily)) {
this._updateLensStyle();
}
}));
this._styleClassName = '_conflictActionsFactory_' + hash(this._editor.getId()).toString(16);
this._styleElement = createStyleSheet(
isInShadowDOM(this._editor.getContainerDomNode())
? this._editor.getContainerDomNode()
: undefined, undefined, this._store
);
this._updateLensStyle();
}
private _updateLensStyle(): void {
const { codeLensHeight, fontSize } = this._getLayoutInfo();
const fontFamily = this._editor.getOption(EditorOption.codeLensFontFamily);
const editorFontInfo = this._editor.getOption(EditorOption.fontInfo);
const fontFamilyVar = `--codelens-font-family${this._styleClassName}`;
const fontFeaturesVar = `--codelens-font-features${this._styleClassName}`;
let newStyle = `
.${this._styleClassName} { line-height: ${codeLensHeight}px; font-size: ${fontSize}px; padding-right: ${Math.round(fontSize * 0.5)}px; font-feature-settings: var(${fontFeaturesVar}) }
.monaco-workbench .${this._styleClassName} span.codicon { line-height: ${codeLensHeight}px; font-size: ${fontSize}px; }
`;
if (fontFamily) {
newStyle += `${this._styleClassName} { font-family: var(${fontFamilyVar}), ${EDITOR_FONT_DEFAULTS.fontFamily}}`;
}
this._styleElement.textContent = newStyle;
this._editor.getContainerDomNode().style.setProperty(fontFamilyVar, fontFamily ?? 'inherit');
this._editor.getContainerDomNode().style.setProperty(fontFeaturesVar, editorFontInfo.fontFeatureSettings);
}
private _getLayoutInfo() {
const lineHeightFactor = Math.max(1.3, this._editor.getOption(EditorOption.lineHeight) / this._editor.getOption(EditorOption.fontSize));
let fontSize = this._editor.getOption(EditorOption.codeLensFontSize);
if (!fontSize || fontSize < 5) {
fontSize = (this._editor.getOption(EditorOption.fontSize) * .9) | 0;
}
return {
fontSize,
codeLensHeight: (fontSize * lineHeightFactor) | 0,
};
}
public createWidget(viewZoneChangeAccessor: IViewZoneChangeAccessor, lineNumber: number, items: IObservable<IContentWidgetAction[]>, viewZoneIdsToCleanUp: string[]): IDisposable {
const layoutInfo = this._getLayoutInfo();
return new ActionsContentWidget(
this._editor,
viewZoneChangeAccessor,
lineNumber,
layoutInfo.codeLensHeight + 2,
this._styleClassName,
items,
viewZoneIdsToCleanUp,
);
}
}
export class ActionsSource {
constructor(
private readonly viewModel: MergeEditorViewModel,
private readonly modifiedBaseRange: ModifiedBaseRange,
) {
}
private getItemsInput(inputNumber: 1 | 2): IObservable<IContentWidgetAction[]> {
return derived(reader => {
/** @description items */
const viewModel = this.viewModel;
const modifiedBaseRange = this.modifiedBaseRange;
if (!viewModel.model.hasBaseRange(modifiedBaseRange)) {
return [];
}
const state = viewModel.model.getState(modifiedBaseRange).read(reader);
const handled = viewModel.model.isHandled(modifiedBaseRange).read(reader);
const model = viewModel.model;
const result: IContentWidgetAction[] = [];
const inputData = inputNumber === 1 ? viewModel.model.input1 : viewModel.model.input2;
const showNonConflictingChanges = viewModel.showNonConflictingChanges.read(reader);
if (!modifiedBaseRange.isConflicting && handled && !showNonConflictingChanges) {
return [];
}
const otherInputNumber = inputNumber === 1 ? 2 : 1;
if (state.kind !== ModifiedBaseRangeStateKind.unrecognized && !state.isInputIncluded(inputNumber)) {
if (!state.isInputIncluded(otherInputNumber) || !this.viewModel.shouldUseAppendInsteadOfAccept.read(reader)) {
result.push(
command(localize('accept', "Accept {0}", inputData.title), async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
state.withInputValue(inputNumber, true, false),
inputNumber,
tx
);
model.telemetry.reportAcceptInvoked(inputNumber, state.includesInput(otherInputNumber));
});
}, localize('acceptTooltip', "Accept {0} in the result document.", inputData.title))
);
if (modifiedBaseRange.canBeCombined) {
const commandName = modifiedBaseRange.isOrderRelevant
? localize('acceptBoth0First', "Accept Combination ({0} First)", inputData.title)
: localize('acceptBoth', "Accept Combination");
result.push(
command(commandName, async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
ModifiedBaseRangeState.base
.withInputValue(inputNumber, true)
.withInputValue(otherInputNumber, true, true),
true,
tx
);
model.telemetry.reportSmartCombinationInvoked(state.includesInput(otherInputNumber));
});
}, localize('acceptBothTooltip', "Accept an automatic combination of both sides in the result document.")),
);
}
} else {
result.push(
command(localize('append', "Append {0}", inputData.title), async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
state.withInputValue(inputNumber, true, false),
inputNumber,
tx
);
model.telemetry.reportAcceptInvoked(inputNumber, state.includesInput(otherInputNumber));
});
}, localize('appendTooltip', "Append {0} to the result document.", inputData.title))
);
if (modifiedBaseRange.canBeCombined) {
result.push(
command(localize('combine', "Accept Combination", inputData.title), async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
state.withInputValue(inputNumber, true, true),
inputNumber,
tx
);
model.telemetry.reportSmartCombinationInvoked(state.includesInput(otherInputNumber));
});
}, localize('acceptBothTooltip', "Accept an automatic combination of both sides in the result document.")),
);
}
}
if (!model.isInputHandled(modifiedBaseRange, inputNumber).read(reader)) {
result.push(
command(
localize('ignore', 'Ignore'),
async () => {
transaction((tx) => {
model.setInputHandled(modifiedBaseRange, inputNumber, true, tx);
});
},
localize('markAsHandledTooltip', "Don't take this side of the conflict.")
)
);
}
}
return result;
});
}
public readonly itemsInput1 = this.getItemsInput(1);
public readonly itemsInput2 = this.getItemsInput(2);
public readonly resultItems = derived(this, reader => {
const viewModel = this.viewModel;
const modifiedBaseRange = this.modifiedBaseRange;
const state = viewModel.model.getState(modifiedBaseRange).read(reader);
const model = viewModel.model;
const result: IContentWidgetAction[] = [];
if (state.kind === ModifiedBaseRangeStateKind.unrecognized) {
result.push({
text: localize('manualResolution', "Manual Resolution"),
tooltip: localize('manualResolutionTooltip', "This conflict has been resolved manually."),
});
} else if (state.kind === ModifiedBaseRangeStateKind.base) {
result.push({
text: localize('noChangesAccepted', 'No Changes Accepted'),
tooltip: localize(
'noChangesAcceptedTooltip',
'The current resolution of this conflict equals the common ancestor of both the right and left changes.'
),
});
} else {
const labels = [];
if (state.includesInput1) {
labels.push(model.input1.title);
}
if (state.includesInput2) {
labels.push(model.input2.title);
}
if (state.kind === ModifiedBaseRangeStateKind.both && state.firstInput === 2) {
labels.reverse();
}
result.push({
text: `${labels.join(' + ')}`
});
}
const stateToggles: IContentWidgetAction[] = [];
if (state.includesInput1) {
stateToggles.push(
command(
localize('remove', 'Remove {0}', model.input1.title),
async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
state.withInputValue(1, false),
true,
tx
);
model.telemetry.reportRemoveInvoked(1, state.includesInput(2));
});
},
localize('removeTooltip', 'Remove {0} from the result document.', model.input1.title)
)
);
}
if (state.includesInput2) {
stateToggles.push(
command(
localize('remove', 'Remove {0}', model.input2.title),
async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
state.withInputValue(2, false),
true,
tx
);
model.telemetry.reportRemoveInvoked(2, state.includesInput(1));
});
},
localize('removeTooltip', 'Remove {0} from the result document.', model.input2.title)
)
);
}
if (
state.kind === ModifiedBaseRangeStateKind.both &&
state.firstInput === 2
) {
stateToggles.reverse();
}
result.push(...stateToggles);
if (state.kind === ModifiedBaseRangeStateKind.unrecognized) {
result.push(
command(
localize('resetToBase', 'Reset to base'),
async () => {
transaction((tx) => {
model.setState(
modifiedBaseRange,
ModifiedBaseRangeState.base,
true,
tx
);
model.telemetry.reportResetToBaseInvoked();
});
},
localize('resetToBaseTooltip', 'Reset this conflict to the common ancestor of both the right and left changes.')
)
);
}
return result;
});
public readonly isEmpty = derived(this, reader => {
return this.itemsInput1.read(reader).length + this.itemsInput2.read(reader).length + this.resultItems.read(reader).length === 0;
});
public readonly inputIsEmpty = derived(this, reader => {
return this.itemsInput1.read(reader).length + this.itemsInput2.read(reader).length === 0;
});
}
function command(title: string, action: () => Promise<void>, tooltip?: string): IContentWidgetAction {
return {
text: title,
action,
tooltip,
};
}
export interface IContentWidgetAction {
text: string;
tooltip?: string;
action?: () => Promise<void>;
}
class ActionsContentWidget extends FixedZoneWidget {
private readonly _domNode = h('div.merge-editor-conflict-actions').root;
constructor(
editor: ICodeEditor,
viewZoneAccessor: IViewZoneChangeAccessor,
afterLineNumber: number,
height: number,
className: string,
items: IObservable<IContentWidgetAction[]>,
viewZoneIdsToCleanUp: string[],
) {
super(editor, viewZoneAccessor, afterLineNumber, height, viewZoneIdsToCleanUp);
this.widgetDomNode.appendChild(this._domNode);
this._domNode.classList.add(className);
this._register(autorun(reader => {
/** @description update commands */
const i = items.read(reader);
this.setState(i);
}));
}
private setState(items: IContentWidgetAction[]) {
const children: HTMLElement[] = [];
let isFirst = true;
for (const item of items) {
if (isFirst) {
isFirst = false;
} else {
children.push($('span', undefined, '\u00a0|\u00a0'));
}
const title = renderLabelWithIcons(item.text);
if (item.action) {
children.push($('a', { title: item.tooltip, role: 'button', onclick: () => item.action!() }, ...title));
} else {
children.push($('span', { title: item.tooltip }, ...title));
}
}
reset(this._domNode, ...children);
}
}
| src/vs/workbench/contrib/mergeEditor/browser/view/conflictActions.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017881265375763178,
0.0001727566123008728,
0.00016727030742913485,
0.00017325181397609413,
0.000002309705678271712
]
|
{
"id": 0,
"code_window": [
"\t\t\tif (opts.recursive && opts.excludes.length === 0) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\topts.excludes.push(key);\n",
"\t\t\t\t\t\t}\n",
"\t\t\t\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 279
} | {
"original": {
"content": "import { KeyCode, KeyMod } from 'vs/base/common/keyCodes';\nimport { Disposable } from 'vs/base/common/lifecycle';\nimport { ICodeEditor } from 'vs/editor/browser/editorBrowser';\nimport { EditorAction, EditorCommand, registerEditorAction, registerEditorCommand, registerEditorContribution, ServicesAccessor } from 'vs/editor/browser/editorExtensions';\nimport { IEditorContribution } from 'vs/editor/common/editorCommon';\nimport { EditorContextKeys } from 'vs/editor/common/editorContextKeys';\nimport * as languages from 'vs/editor/common/languages';\nimport { TriggerContext } from 'vs/editor/contrib/parameterHints/browser/parameterHintsModel';\nimport { Context } from 'vs/editor/contrib/parameterHints/browser/provideSignatureHelp';\nimport * as nls from 'vs/nls';\nimport { ContextKeyExpr } from 'vs/platform/contextkey/common/contextkey';\nimport { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';\nimport { KeybindingWeight } from 'vs/platform/keybinding/common/keybindingsRegistry';\nimport { ParameterHintsWidget } from './parameterHintsWidget';\n",
"fileName": "./1.tst"
},
"modified": {
"content": "import { KeyCode, KeyMod } from 'vs/base/common/keyCodes';\nimport { Lazy } from 'vs/base/common/lazy';\nimport { Disposable } from 'vs/base/common/lifecycle';\nimport { ICodeEditor } from 'vs/editor/browser/editorBrowser';\nimport { EditorAction, EditorCommand, registerEditorAction, registerEditorCommand, registerEditorContribution, ServicesAccessor } from 'vs/editor/browser/editorExtensions';\nimport { IEditorContribution } from 'vs/editor/common/editorCommon';\nimport { EditorContextKeys } from 'vs/editor/common/editorContextKeys';\nimport * as languages from 'vs/editor/common/languages';\nimport { ILanguageFeaturesService } from 'vs/editor/common/services/languageFeatures';\nimport { ParameterHintsModel, TriggerContext } from 'vs/editor/contrib/parameterHints/browser/parameterHintsModel';\nimport { Context } from 'vs/editor/contrib/parameterHints/browser/provideSignatureHelp';\nimport * as nls from 'vs/nls';\nimport { ContextKeyExpr } from 'vs/platform/contextkey/common/contextkey';\nimport { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';\nimport { KeybindingWeight } from 'vs/platform/keybinding/common/keybindingsRegistry';\nimport { ParameterHintsWidget } from './parameterHintsWidget';\n",
"fileName": "./2.tst"
},
"diffs": [
{
"originalRange": "[2,2)",
"modifiedRange": "[2,3)",
"innerChanges": [
{
"originalRange": "[2,1 -> 2,1]",
"modifiedRange": "[2,1 -> 3,1]"
}
]
},
{
"originalRange": "[8,9)",
"modifiedRange": "[9,11)",
"innerChanges": [
{
"originalRange": "[8,9 -> 8,9]",
"modifiedRange": "[9,9 -> 10,30]"
}
]
}
]
} | src/vs/editor/test/node/diffing/fixtures/ts-unfragmented-diffing/advanced.expected.diff.json | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017443702381569892,
0.00017314219439867884,
0.0001718085986794904,
0.00017316160665359348,
9.48273054746096e-7
]
|
{
"id": 0,
"code_window": [
"\t\t\tif (opts.recursive && opts.excludes.length === 0) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\topts.excludes.push(key);\n",
"\t\t\t\t\t\t}\n",
"\t\t\t\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 279
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
declare module 'vscode' {
// https://github.com/microsoft/vscode/issues/185269
export interface SourceControl {
historyProvider?: SourceControlHistoryProvider;
}
export interface SourceControlHistoryProvider {
currentHistoryItemGroup?: SourceControlHistoryItemGroup;
/**
* Fires when the current history item group changes after
* a user action (ex: commit, checkout, fetch, pull, push)
*/
onDidChangeCurrentHistoryItemGroup: Event<void>;
/**
* Fires when the history item groups change (ex: commit, push, fetch)
*/
// onDidChangeHistoryItemGroups: Event<SourceControlHistoryChangeEvent>;
provideHistoryItems(historyItemGroupId: string, options: SourceControlHistoryOptions, token: CancellationToken): ProviderResult<SourceControlHistoryItem[]>;
provideHistoryItemSummary?(historyItemId: string, historyItemParentId: string | undefined, token: CancellationToken): ProviderResult<SourceControlHistoryItem>;
provideHistoryItemChanges(historyItemId: string, historyItemParentId: string | undefined, token: CancellationToken): ProviderResult<SourceControlHistoryItemChange[]>;
resolveHistoryItemGroupCommonAncestor(historyItemGroupId1: string, historyItemGroupId2: string | undefined, token: CancellationToken): ProviderResult<{ id: string; ahead: number; behind: number }>;
}
export interface SourceControlHistoryOptions {
readonly cursor?: string;
readonly limit?: number | { id?: string };
}
export interface SourceControlHistoryItemGroup {
readonly id: string;
readonly label: string;
readonly base?: Omit<SourceControlRemoteHistoryItemGroup, 'base'>;
}
export interface SourceControlRemoteHistoryItemGroup {
readonly id: string;
readonly label: string;
}
export interface SourceControlHistoryItemStatistics {
readonly files: number;
readonly insertions: number;
readonly deletions: number;
}
export interface SourceControlHistoryItem {
readonly id: string;
readonly parentIds: string[];
readonly label: string;
readonly description?: string;
readonly icon?: Uri | { light: Uri; dark: Uri } | ThemeIcon;
readonly timestamp?: number;
readonly statistics?: SourceControlHistoryItemStatistics;
}
export interface SourceControlHistoryItemChange {
readonly uri: Uri;
readonly originalUri: Uri | undefined;
readonly modifiedUri: Uri | undefined;
readonly renameUri: Uri | undefined;
}
// export interface SourceControlHistoryChangeEvent {
// readonly added: Iterable<SourceControlHistoryItemGroup>;
// readonly removed: Iterable<SourceControlHistoryItemGroup>;
// readonly modified: Iterable<SourceControlHistoryItemGroup>;
// }
}
| src/vscode-dts/vscode.proposed.scmHistoryProvider.d.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017643194587435573,
0.00017207702330779284,
0.000165937643032521,
0.00017283936904277653,
0.0000035686730370798614
]
|
{
"id": 1,
"code_window": [
"\t\t\telse if (workspaceFolder) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\tif (!opts.includes) {\n",
"\t\t\t\t\t\t\t\topts.includes = [];\n",
"\t\t\t\t\t\t\t}\n",
"\n",
"\t\t\t\t\t\t\tconst includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 301
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { DisposableMap, DisposableStore } from 'vs/base/common/lifecycle';
import { FileOperation, IFileService, IFilesConfiguration, IWatchOptions } from 'vs/platform/files/common/files';
import { extHostNamedCustomer, IExtHostContext } from 'vs/workbench/services/extensions/common/extHostCustomers';
import { ExtHostContext, ExtHostFileSystemEventServiceShape, MainContext, MainThreadFileSystemEventServiceShape } from '../common/extHost.protocol';
import { localize } from 'vs/nls';
import { IWorkingCopyFileOperationParticipant, IWorkingCopyFileService, SourceTargetPair, IFileOperationUndoRedoInfo } from 'vs/workbench/services/workingCopy/common/workingCopyFileService';
import { IBulkEditService } from 'vs/editor/browser/services/bulkEditService';
import { IProgressService, ProgressLocation } from 'vs/platform/progress/common/progress';
import { raceCancellation } from 'vs/base/common/async';
import { CancellationToken, CancellationTokenSource } from 'vs/base/common/cancellation';
import { IDialogService } from 'vs/platform/dialogs/common/dialogs';
import Severity from 'vs/base/common/severity';
import { IStorageService, StorageScope, StorageTarget } from 'vs/platform/storage/common/storage';
import { Action2, registerAction2 } from 'vs/platform/actions/common/actions';
import { ServicesAccessor } from 'vs/platform/instantiation/common/instantiation';
import { ILogService } from 'vs/platform/log/common/log';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { reviveWorkspaceEditDto } from 'vs/workbench/api/browser/mainThreadBulkEdits';
import { GLOBSTAR } from 'vs/base/common/glob';
import { rtrim } from 'vs/base/common/strings';
import { UriComponents, URI } from 'vs/base/common/uri';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { normalizeWatcherPattern } from 'vs/platform/files/common/watcher';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
@extHostNamedCustomer(MainContext.MainThreadFileSystemEventService)
export class MainThreadFileSystemEventService implements MainThreadFileSystemEventServiceShape {
static readonly MementoKeyAdditionalEdits = `file.particpants.additionalEdits`;
private readonly _proxy: ExtHostFileSystemEventServiceShape;
private readonly _listener = new DisposableStore();
private readonly _watches = new DisposableMap<number>();
constructor(
extHostContext: IExtHostContext,
@IFileService private readonly _fileService: IFileService,
@IWorkingCopyFileService workingCopyFileService: IWorkingCopyFileService,
@IBulkEditService bulkEditService: IBulkEditService,
@IProgressService progressService: IProgressService,
@IDialogService dialogService: IDialogService,
@IStorageService storageService: IStorageService,
@ILogService logService: ILogService,
@IEnvironmentService envService: IEnvironmentService,
@IUriIdentityService uriIdentService: IUriIdentityService,
@IWorkspaceContextService private readonly _contextService: IWorkspaceContextService,
@ILogService private readonly _logService: ILogService,
@IConfigurationService private readonly _configurationService: IConfigurationService
) {
this._proxy = extHostContext.getProxy(ExtHostContext.ExtHostFileSystemEventService);
this._listener.add(_fileService.onDidFilesChange(event => {
this._proxy.$onFileEvent({
created: event.rawAdded,
changed: event.rawUpdated,
deleted: event.rawDeleted
});
}));
const that = this;
const fileOperationParticipant = new class implements IWorkingCopyFileOperationParticipant {
async participate(files: SourceTargetPair[], operation: FileOperation, undoInfo: IFileOperationUndoRedoInfo | undefined, timeout: number, token: CancellationToken) {
if (undoInfo?.isUndoing) {
return;
}
const cts = new CancellationTokenSource(token);
const timer = setTimeout(() => cts.cancel(), timeout);
const data = await progressService.withProgress({
location: ProgressLocation.Notification,
title: this._progressLabel(operation),
cancellable: true,
delay: Math.min(timeout / 2, 3000)
}, () => {
// race extension host event delivery against timeout AND user-cancel
const onWillEvent = that._proxy.$onWillRunFileOperation(operation, files, timeout, cts.token);
return raceCancellation(onWillEvent, cts.token);
}, () => {
// user-cancel
cts.cancel();
}).finally(() => {
cts.dispose();
clearTimeout(timer);
});
if (!data || data.edit.edits.length === 0) {
// cancelled, no reply, or no edits
return;
}
const needsConfirmation = data.edit.edits.some(edit => edit.metadata?.needsConfirmation);
let showPreview = storageService.getBoolean(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, StorageScope.PROFILE);
if (envService.extensionTestsLocationURI) {
// don't show dialog in tests
showPreview = false;
}
if (showPreview === undefined) {
// show a user facing message
let message: string;
if (data.extensionNames.length === 1) {
if (operation === FileOperation.CREATE) {
message = localize('ask.1.create', "Extension '{0}' wants to make refactoring changes with this file creation", data.extensionNames[0]);
} else if (operation === FileOperation.COPY) {
message = localize('ask.1.copy', "Extension '{0}' wants to make refactoring changes with this file copy", data.extensionNames[0]);
} else if (operation === FileOperation.MOVE) {
message = localize('ask.1.move', "Extension '{0}' wants to make refactoring changes with this file move", data.extensionNames[0]);
} else /* if (operation === FileOperation.DELETE) */ {
message = localize('ask.1.delete', "Extension '{0}' wants to make refactoring changes with this file deletion", data.extensionNames[0]);
}
} else {
if (operation === FileOperation.CREATE) {
message = localize({ key: 'ask.N.create', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file creation", data.extensionNames.length);
} else if (operation === FileOperation.COPY) {
message = localize({ key: 'ask.N.copy', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file copy", data.extensionNames.length);
} else if (operation === FileOperation.MOVE) {
message = localize({ key: 'ask.N.move', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file move", data.extensionNames.length);
} else /* if (operation === FileOperation.DELETE) */ {
message = localize({ key: 'ask.N.delete', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file deletion", data.extensionNames.length);
}
}
if (needsConfirmation) {
// edit which needs confirmation -> always show dialog
const { confirmed } = await dialogService.confirm({
type: Severity.Info,
message,
primaryButton: localize('preview', "Show &&Preview"),
cancelButton: localize('cancel', "Skip Changes")
});
showPreview = true;
if (!confirmed) {
// no changes wanted
return;
}
} else {
// choice
enum Choice {
OK = 0,
Preview = 1,
Cancel = 2
}
const { result, checkboxChecked } = await dialogService.prompt<Choice>({
type: Severity.Info,
message,
buttons: [
{
label: localize({ key: 'ok', comment: ['&& denotes a mnemonic'] }, "&&OK"),
run: () => Choice.OK
},
{
label: localize({ key: 'preview', comment: ['&& denotes a mnemonic'] }, "Show &&Preview"),
run: () => Choice.Preview
}
],
cancelButton: {
label: localize('cancel', "Skip Changes"),
run: () => Choice.Cancel
},
checkbox: { label: localize('again', "Do not ask me again") }
});
if (result === Choice.Cancel) {
// no changes wanted, don't persist cancel option
return;
}
showPreview = result === Choice.Preview;
if (checkboxChecked) {
storageService.store(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, showPreview, StorageScope.PROFILE, StorageTarget.USER);
}
}
}
logService.info('[onWill-handler] applying additional workspace edit from extensions', data.extensionNames);
await bulkEditService.apply(
reviveWorkspaceEditDto(data.edit, uriIdentService),
{ undoRedoGroupId: undoInfo?.undoRedoGroupId, showPreview }
);
}
private _progressLabel(operation: FileOperation): string {
switch (operation) {
case FileOperation.CREATE:
return localize('msg-create', "Running 'File Create' participants...");
case FileOperation.MOVE:
return localize('msg-rename', "Running 'File Rename' participants...");
case FileOperation.COPY:
return localize('msg-copy', "Running 'File Copy' participants...");
case FileOperation.DELETE:
return localize('msg-delete', "Running 'File Delete' participants...");
case FileOperation.WRITE:
return localize('msg-write', "Running 'File Write' participants...");
}
}
};
// BEFORE file operation
this._listener.add(workingCopyFileService.addFileOperationParticipant(fileOperationParticipant));
// AFTER file operation
this._listener.add(workingCopyFileService.onDidRunWorkingCopyFileOperation(e => this._proxy.$onDidRunFileOperation(e.operation, e.files)));
}
async $watch(extensionId: string, session: number, resource: UriComponents, unvalidatedOpts: IWatchOptions, correlate: boolean): Promise<void> {
const uri = URI.revive(resource);
const opts: IWatchOptions = {
...unvalidatedOpts
};
// Convert a recursive watcher to a flat watcher if the path
// turns out to not be a folder. Recursive watching is only
// possible on folders, so we help all file watchers by checking
// early.
if (opts.recursive) {
try {
const stat = await this._fileService.stat(uri);
if (!stat.isDirectory) {
opts.recursive = false;
}
} catch (error) {
this._logService.error(`MainThreadFileSystemEventService#$watch(): failed to stat a resource for file watching (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session}): ${error}`);
}
}
// Correlated file watching is taken as is
if (correlate) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): request to start watching correlated (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
const watcherDisposables = new DisposableStore();
const subscription = watcherDisposables.add(this._fileService.createWatcher(uri, opts));
watcherDisposables.add(subscription.onDidChange(event => {
this._proxy.$onFileEvent({
session,
created: event.rawAdded,
changed: event.rawUpdated,
deleted: event.rawDeleted
});
}));
this._watches.set(session, watcherDisposables);
}
// Uncorrelated file watching gets special treatment
else {
// Refuse to watch anything that is already watched via
// our workspace watchers in case the request is a
// recursive file watcher and does not opt-in to event
// correlation via specific exclude rules.
// Still allow for non-recursive watch requests as a way
// to bypass configured exclude rules though
// (see https://github.com/microsoft/vscode/issues/146066)
const workspaceFolder = this._contextService.getWorkspaceFolder(uri);
if (workspaceFolder && opts.recursive) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): ignoring request to start watching because path is inside workspace (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
return;
}
this._logService.trace(`MainThreadFileSystemEventService#$watch(): request to start watching uncorrelated (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
// Automatically add `files.watcherExclude` patterns when watching
// recursively to give users a chance to configure exclude rules
// for reducing the overhead of watching recursively
if (opts.recursive && opts.excludes.length === 0) {
const config = this._configurationService.getValue<IFilesConfiguration>();
if (config.files?.watcherExclude) {
for (const key in config.files.watcherExclude) {
if (config.files.watcherExclude[key] === true) {
opts.excludes.push(key);
}
}
}
}
// Non-recursive watching inside the workspace will overlap with
// our standard workspace watchers. To prevent duplicate events,
// we only want to include events for files that are otherwise
// excluded via `files.watcherExclude`. As such, we configure
// to include each configured exclude pattern so that only those
// events are reported that are otherwise excluded.
// However, we cannot just use the pattern as is, because a pattern
// such as `bar` for a exclude, will work to exclude any of
// `<workspace path>/bar` but will not work as include for files within
// `bar` unless a suffix of `/**` if added.
// (https://github.com/microsoft/vscode/issues/148245)
else if (workspaceFolder) {
const config = this._configurationService.getValue<IFilesConfiguration>();
if (config.files?.watcherExclude) {
for (const key in config.files.watcherExclude) {
if (config.files.watcherExclude[key] === true) {
if (!opts.includes) {
opts.includes = [];
}
const includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;
opts.includes.push(normalizeWatcherPattern(workspaceFolder.uri.fsPath, includePattern));
}
}
}
// Still ignore watch request if there are actually no configured
// exclude rules, because in that case our default recursive watcher
// should be able to take care of all events.
if (!opts.includes || opts.includes.length === 0) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): ignoring request to start watching because path is inside workspace and no excludes are configured (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
return;
}
}
const subscription = this._fileService.watch(uri, opts);
this._watches.set(session, subscription);
}
}
$unwatch(session: number): void {
if (this._watches.has(session)) {
this._logService.trace(`MainThreadFileSystemEventService#$unwatch(): request to stop watching (session: ${session})`);
this._watches.deleteAndDispose(session);
}
}
dispose(): void {
this._listener.dispose();
this._watches.dispose();
}
}
registerAction2(class ResetMemento extends Action2 {
constructor() {
super({
id: 'files.participants.resetChoice',
title: {
value: localize('label', "Reset choice for 'File operation needs preview'"),
original: `Reset choice for 'File operation needs preview'`
},
f1: true
});
}
run(accessor: ServicesAccessor) {
accessor.get(IStorageService).remove(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, StorageScope.PROFILE);
}
});
| src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts | 1 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.9981649518013,
0.11102935671806335,
0.0001634419459151104,
0.000173906737472862,
0.313188761472702
]
|
{
"id": 1,
"code_window": [
"\t\t\telse if (workspaceFolder) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\tif (!opts.includes) {\n",
"\t\t\t\t\t\t\t\topts.includes = [];\n",
"\t\t\t\t\t\t\t}\n",
"\n",
"\t\t\t\t\t\t\tconst includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 301
} | # theme-seti
This is an icon theme that uses the icons from [`seti-ui`](https://github.com/jesseweed/seti-ui).
## Updating icons
There is script that can be used to update icons, [./build/update-icon-theme.js](build/update-icon-theme.js).
To run this script, run `npm run update` from the `theme-seti` directory.
This can be run in one of two ways: looking at a local copy of `seti-ui` for icons, or getting them straight from GitHub.
If you want to run it from a local copy of `seti-ui`, first clone [`seti-ui`](https://github.com/jesseweed/seti-ui) to the folder next to your `vscode` repo (from the `theme-seti` directory, `../../`).
Then, inside the `set-ui` directory, run `npm install` followed by `npm run prepublishOnly`. This will generate updated icons.
If you want to download the icons straight from GitHub, change the `FROM_DISK` variable to `false` inside of `update-icon-theme.js`.
### Languages not shipped with `vscode`
Languages that are not shipped with `vscode` must be added to the `nonBuiltInLanguages` object inside of `update-icon-theme.js`.
These should match [the file mapping in `seti-ui`](https://github.com/jesseweed/seti-ui/blob/master/styles/components/icons/mapping.less).
Please try and keep this list in alphabetical order! Thank you.
## Previewing icons
There is a [`./icons/preview.html`](./icons/preview.html) file that can be opened to see all of the icons included in the theme.
Note that to view this, it needs to be hosted by a web server.
When updating icons, it is always a good idea to make sure that they work properly by looking at this page.
When submitting a PR that updates these icons, a screenshot of the preview page should accompany it.
| extensions/theme-seti/README.md | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017410219879820943,
0.00016993976896628737,
0.00016814839909784496,
0.00016875423898454756,
0.0000024349064915440977
]
|
{
"id": 1,
"code_window": [
"\t\t\telse if (workspaceFolder) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\tif (!opts.includes) {\n",
"\t\t\t\t\t\t\t\topts.includes = [];\n",
"\t\t\t\t\t\t\t}\n",
"\n",
"\t\t\t\t\t\t\tconst includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 301
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IPointerHandlerHelper } from 'vs/editor/browser/controller/mouseHandler';
import { IMouseTargetContentEmptyData, IMouseTargetMarginData, IMouseTarget, IMouseTargetContentEmpty, IMouseTargetContentText, IMouseTargetContentWidget, IMouseTargetMargin, IMouseTargetOutsideEditor, IMouseTargetOverlayWidget, IMouseTargetScrollbar, IMouseTargetTextarea, IMouseTargetUnknown, IMouseTargetViewZone, IMouseTargetContentTextData, IMouseTargetViewZoneData, MouseTargetType } from 'vs/editor/browser/editorBrowser';
import { ClientCoordinates, EditorMouseEvent, EditorPagePosition, PageCoordinates, CoordinatesRelativeToEditor } from 'vs/editor/browser/editorDom';
import { PartFingerprint, PartFingerprints } from 'vs/editor/browser/view/viewPart';
import { ViewLine } from 'vs/editor/browser/viewParts/lines/viewLine';
import { IViewCursorRenderData } from 'vs/editor/browser/viewParts/viewCursors/viewCursor';
import { EditorLayoutInfo, EditorOption } from 'vs/editor/common/config/editorOptions';
import { Position } from 'vs/editor/common/core/position';
import { Range as EditorRange } from 'vs/editor/common/core/range';
import { HorizontalPosition } from 'vs/editor/browser/view/renderingContext';
import { ViewContext } from 'vs/editor/common/viewModel/viewContext';
import { IViewModel } from 'vs/editor/common/viewModel';
import { CursorColumns } from 'vs/editor/common/core/cursorColumns';
import * as dom from 'vs/base/browser/dom';
import { AtomicTabMoveOperations, Direction } from 'vs/editor/common/cursor/cursorAtomicMoveOperations';
import { PositionAffinity } from 'vs/editor/common/model';
import { InjectedText } from 'vs/editor/common/modelLineProjectionData';
import { Mutable } from 'vs/base/common/types';
const enum HitTestResultType {
Unknown,
Content,
}
class UnknownHitTestResult {
readonly type = HitTestResultType.Unknown;
constructor(
readonly hitTarget: HTMLElement | null = null
) { }
}
class ContentHitTestResult {
readonly type = HitTestResultType.Content;
constructor(
readonly position: Position,
readonly spanNode: HTMLElement,
readonly injectedText: InjectedText | null,
) { }
}
type HitTestResult = UnknownHitTestResult | ContentHitTestResult;
namespace HitTestResult {
export function createFromDOMInfo(ctx: HitTestContext, spanNode: HTMLElement, offset: number): HitTestResult {
const position = ctx.getPositionFromDOMInfo(spanNode, offset);
if (position) {
return new ContentHitTestResult(position, spanNode, null);
}
return new UnknownHitTestResult(spanNode);
}
}
export class PointerHandlerLastRenderData {
constructor(
public readonly lastViewCursorsRenderData: IViewCursorRenderData[],
public readonly lastTextareaPosition: Position | null
) { }
}
export class MouseTarget {
private static _deduceRage(position: Position): EditorRange;
private static _deduceRage(position: Position, range: EditorRange | null): EditorRange;
private static _deduceRage(position: Position | null): EditorRange | null;
private static _deduceRage(position: Position | null, range: EditorRange | null = null): EditorRange | null {
if (!range && position) {
return new EditorRange(position.lineNumber, position.column, position.lineNumber, position.column);
}
return range ?? null;
}
public static createUnknown(element: HTMLElement | null, mouseColumn: number, position: Position | null): IMouseTargetUnknown {
return { type: MouseTargetType.UNKNOWN, element, mouseColumn, position, range: this._deduceRage(position) };
}
public static createTextarea(element: HTMLElement | null, mouseColumn: number): IMouseTargetTextarea {
return { type: MouseTargetType.TEXTAREA, element, mouseColumn, position: null, range: null };
}
public static createMargin(type: MouseTargetType.GUTTER_GLYPH_MARGIN | MouseTargetType.GUTTER_LINE_NUMBERS | MouseTargetType.GUTTER_LINE_DECORATIONS, element: HTMLElement | null, mouseColumn: number, position: Position, range: EditorRange, detail: IMouseTargetMarginData): IMouseTargetMargin {
return { type, element, mouseColumn, position, range, detail };
}
public static createViewZone(type: MouseTargetType.GUTTER_VIEW_ZONE | MouseTargetType.CONTENT_VIEW_ZONE, element: HTMLElement | null, mouseColumn: number, position: Position, detail: IMouseTargetViewZoneData): IMouseTargetViewZone {
return { type, element, mouseColumn, position, range: this._deduceRage(position), detail };
}
public static createContentText(element: HTMLElement | null, mouseColumn: number, position: Position, range: EditorRange | null, detail: IMouseTargetContentTextData): IMouseTargetContentText {
return { type: MouseTargetType.CONTENT_TEXT, element, mouseColumn, position, range: this._deduceRage(position, range), detail };
}
public static createContentEmpty(element: HTMLElement | null, mouseColumn: number, position: Position, detail: IMouseTargetContentEmptyData): IMouseTargetContentEmpty {
return { type: MouseTargetType.CONTENT_EMPTY, element, mouseColumn, position, range: this._deduceRage(position), detail };
}
public static createContentWidget(element: HTMLElement | null, mouseColumn: number, detail: string): IMouseTargetContentWidget {
return { type: MouseTargetType.CONTENT_WIDGET, element, mouseColumn, position: null, range: null, detail };
}
public static createScrollbar(element: HTMLElement | null, mouseColumn: number, position: Position): IMouseTargetScrollbar {
return { type: MouseTargetType.SCROLLBAR, element, mouseColumn, position, range: this._deduceRage(position) };
}
public static createOverlayWidget(element: HTMLElement | null, mouseColumn: number, detail: string): IMouseTargetOverlayWidget {
return { type: MouseTargetType.OVERLAY_WIDGET, element, mouseColumn, position: null, range: null, detail };
}
public static createOutsideEditor(mouseColumn: number, position: Position, outsidePosition: 'above' | 'below' | 'left' | 'right', outsideDistance: number): IMouseTargetOutsideEditor {
return { type: MouseTargetType.OUTSIDE_EDITOR, element: null, mouseColumn, position, range: this._deduceRage(position), outsidePosition, outsideDistance };
}
private static _typeToString(type: MouseTargetType): string {
if (type === MouseTargetType.TEXTAREA) {
return 'TEXTAREA';
}
if (type === MouseTargetType.GUTTER_GLYPH_MARGIN) {
return 'GUTTER_GLYPH_MARGIN';
}
if (type === MouseTargetType.GUTTER_LINE_NUMBERS) {
return 'GUTTER_LINE_NUMBERS';
}
if (type === MouseTargetType.GUTTER_LINE_DECORATIONS) {
return 'GUTTER_LINE_DECORATIONS';
}
if (type === MouseTargetType.GUTTER_VIEW_ZONE) {
return 'GUTTER_VIEW_ZONE';
}
if (type === MouseTargetType.CONTENT_TEXT) {
return 'CONTENT_TEXT';
}
if (type === MouseTargetType.CONTENT_EMPTY) {
return 'CONTENT_EMPTY';
}
if (type === MouseTargetType.CONTENT_VIEW_ZONE) {
return 'CONTENT_VIEW_ZONE';
}
if (type === MouseTargetType.CONTENT_WIDGET) {
return 'CONTENT_WIDGET';
}
if (type === MouseTargetType.OVERVIEW_RULER) {
return 'OVERVIEW_RULER';
}
if (type === MouseTargetType.SCROLLBAR) {
return 'SCROLLBAR';
}
if (type === MouseTargetType.OVERLAY_WIDGET) {
return 'OVERLAY_WIDGET';
}
return 'UNKNOWN';
}
public static toString(target: IMouseTarget): string {
return this._typeToString(target.type) + ': ' + target.position + ' - ' + target.range + ' - ' + JSON.stringify((<any>target).detail);
}
}
class ElementPath {
public static isTextArea(path: Uint8Array): boolean {
return (
path.length === 2
&& path[0] === PartFingerprint.OverflowGuard
&& path[1] === PartFingerprint.TextArea
);
}
public static isChildOfViewLines(path: Uint8Array): boolean {
return (
path.length >= 4
&& path[0] === PartFingerprint.OverflowGuard
&& path[3] === PartFingerprint.ViewLines
);
}
public static isStrictChildOfViewLines(path: Uint8Array): boolean {
return (
path.length > 4
&& path[0] === PartFingerprint.OverflowGuard
&& path[3] === PartFingerprint.ViewLines
);
}
public static isChildOfScrollableElement(path: Uint8Array): boolean {
return (
path.length >= 2
&& path[0] === PartFingerprint.OverflowGuard
&& path[1] === PartFingerprint.ScrollableElement
);
}
public static isChildOfMinimap(path: Uint8Array): boolean {
return (
path.length >= 2
&& path[0] === PartFingerprint.OverflowGuard
&& path[1] === PartFingerprint.Minimap
);
}
public static isChildOfContentWidgets(path: Uint8Array): boolean {
return (
path.length >= 4
&& path[0] === PartFingerprint.OverflowGuard
&& path[3] === PartFingerprint.ContentWidgets
);
}
public static isChildOfOverflowGuard(path: Uint8Array): boolean {
return (
path.length >= 1
&& path[0] === PartFingerprint.OverflowGuard
);
}
public static isChildOfOverflowingContentWidgets(path: Uint8Array): boolean {
return (
path.length >= 1
&& path[0] === PartFingerprint.OverflowingContentWidgets
);
}
public static isChildOfOverlayWidgets(path: Uint8Array): boolean {
return (
path.length >= 2
&& path[0] === PartFingerprint.OverflowGuard
&& path[1] === PartFingerprint.OverlayWidgets
);
}
public static isChildOfOverflowingOverlayWidgets(path: Uint8Array): boolean {
return (
path.length >= 1
&& path[0] === PartFingerprint.OverflowingOverlayWidgets
);
}
}
export class HitTestContext {
public readonly viewModel: IViewModel;
public readonly layoutInfo: EditorLayoutInfo;
public readonly viewDomNode: HTMLElement;
public readonly lineHeight: number;
public readonly stickyTabStops: boolean;
public readonly typicalHalfwidthCharacterWidth: number;
public readonly lastRenderData: PointerHandlerLastRenderData;
private readonly _context: ViewContext;
private readonly _viewHelper: IPointerHandlerHelper;
constructor(context: ViewContext, viewHelper: IPointerHandlerHelper, lastRenderData: PointerHandlerLastRenderData) {
this.viewModel = context.viewModel;
const options = context.configuration.options;
this.layoutInfo = options.get(EditorOption.layoutInfo);
this.viewDomNode = viewHelper.viewDomNode;
this.lineHeight = options.get(EditorOption.lineHeight);
this.stickyTabStops = options.get(EditorOption.stickyTabStops);
this.typicalHalfwidthCharacterWidth = options.get(EditorOption.fontInfo).typicalHalfwidthCharacterWidth;
this.lastRenderData = lastRenderData;
this._context = context;
this._viewHelper = viewHelper;
}
public getZoneAtCoord(mouseVerticalOffset: number): IMouseTargetViewZoneData | null {
return HitTestContext.getZoneAtCoord(this._context, mouseVerticalOffset);
}
public static getZoneAtCoord(context: ViewContext, mouseVerticalOffset: number): IMouseTargetViewZoneData | null {
// The target is either a view zone or the empty space after the last view-line
const viewZoneWhitespace = context.viewLayout.getWhitespaceAtVerticalOffset(mouseVerticalOffset);
if (viewZoneWhitespace) {
const viewZoneMiddle = viewZoneWhitespace.verticalOffset + viewZoneWhitespace.height / 2;
const lineCount = context.viewModel.getLineCount();
let positionBefore: Position | null = null;
let position: Position | null;
let positionAfter: Position | null = null;
if (viewZoneWhitespace.afterLineNumber !== lineCount) {
// There are more lines after this view zone
positionAfter = new Position(viewZoneWhitespace.afterLineNumber + 1, 1);
}
if (viewZoneWhitespace.afterLineNumber > 0) {
// There are more lines above this view zone
positionBefore = new Position(viewZoneWhitespace.afterLineNumber, context.viewModel.getLineMaxColumn(viewZoneWhitespace.afterLineNumber));
}
if (positionAfter === null) {
position = positionBefore;
} else if (positionBefore === null) {
position = positionAfter;
} else if (mouseVerticalOffset < viewZoneMiddle) {
position = positionBefore;
} else {
position = positionAfter;
}
return {
viewZoneId: viewZoneWhitespace.id,
afterLineNumber: viewZoneWhitespace.afterLineNumber,
positionBefore: positionBefore,
positionAfter: positionAfter,
position: position!
};
}
return null;
}
public getFullLineRangeAtCoord(mouseVerticalOffset: number): { range: EditorRange; isAfterLines: boolean } {
if (this._context.viewLayout.isAfterLines(mouseVerticalOffset)) {
// Below the last line
const lineNumber = this._context.viewModel.getLineCount();
const maxLineColumn = this._context.viewModel.getLineMaxColumn(lineNumber);
return {
range: new EditorRange(lineNumber, maxLineColumn, lineNumber, maxLineColumn),
isAfterLines: true
};
}
const lineNumber = this._context.viewLayout.getLineNumberAtVerticalOffset(mouseVerticalOffset);
const maxLineColumn = this._context.viewModel.getLineMaxColumn(lineNumber);
return {
range: new EditorRange(lineNumber, 1, lineNumber, maxLineColumn),
isAfterLines: false
};
}
public getLineNumberAtVerticalOffset(mouseVerticalOffset: number): number {
return this._context.viewLayout.getLineNumberAtVerticalOffset(mouseVerticalOffset);
}
public isAfterLines(mouseVerticalOffset: number): boolean {
return this._context.viewLayout.isAfterLines(mouseVerticalOffset);
}
public isInTopPadding(mouseVerticalOffset: number): boolean {
return this._context.viewLayout.isInTopPadding(mouseVerticalOffset);
}
public isInBottomPadding(mouseVerticalOffset: number): boolean {
return this._context.viewLayout.isInBottomPadding(mouseVerticalOffset);
}
public getVerticalOffsetForLineNumber(lineNumber: number): number {
return this._context.viewLayout.getVerticalOffsetForLineNumber(lineNumber);
}
public findAttribute(element: Element, attr: string): string | null {
return HitTestContext._findAttribute(element, attr, this._viewHelper.viewDomNode);
}
private static _findAttribute(element: Element, attr: string, stopAt: Element): string | null {
while (element && element !== element.ownerDocument.body) {
if (element.hasAttribute && element.hasAttribute(attr)) {
return element.getAttribute(attr);
}
if (element === stopAt) {
return null;
}
element = <Element>element.parentNode;
}
return null;
}
public getLineWidth(lineNumber: number): number {
return this._viewHelper.getLineWidth(lineNumber);
}
public visibleRangeForPosition(lineNumber: number, column: number): HorizontalPosition | null {
return this._viewHelper.visibleRangeForPosition(lineNumber, column);
}
public getPositionFromDOMInfo(spanNode: HTMLElement, offset: number): Position | null {
return this._viewHelper.getPositionFromDOMInfo(spanNode, offset);
}
public getCurrentScrollTop(): number {
return this._context.viewLayout.getCurrentScrollTop();
}
public getCurrentScrollLeft(): number {
return this._context.viewLayout.getCurrentScrollLeft();
}
}
abstract class BareHitTestRequest {
public readonly editorPos: EditorPagePosition;
public readonly pos: PageCoordinates;
public readonly relativePos: CoordinatesRelativeToEditor;
public readonly mouseVerticalOffset: number;
public readonly isInMarginArea: boolean;
public readonly isInContentArea: boolean;
public readonly mouseContentHorizontalOffset: number;
protected readonly mouseColumn: number;
constructor(ctx: HitTestContext, editorPos: EditorPagePosition, pos: PageCoordinates, relativePos: CoordinatesRelativeToEditor) {
this.editorPos = editorPos;
this.pos = pos;
this.relativePos = relativePos;
this.mouseVerticalOffset = Math.max(0, ctx.getCurrentScrollTop() + this.relativePos.y);
this.mouseContentHorizontalOffset = ctx.getCurrentScrollLeft() + this.relativePos.x - ctx.layoutInfo.contentLeft;
this.isInMarginArea = (this.relativePos.x < ctx.layoutInfo.contentLeft && this.relativePos.x >= ctx.layoutInfo.glyphMarginLeft);
this.isInContentArea = !this.isInMarginArea;
this.mouseColumn = Math.max(0, MouseTargetFactory._getMouseColumn(this.mouseContentHorizontalOffset, ctx.typicalHalfwidthCharacterWidth));
}
}
class HitTestRequest extends BareHitTestRequest {
private readonly _ctx: HitTestContext;
public readonly target: HTMLElement | null;
public readonly targetPath: Uint8Array;
constructor(ctx: HitTestContext, editorPos: EditorPagePosition, pos: PageCoordinates, relativePos: CoordinatesRelativeToEditor, target: HTMLElement | null) {
super(ctx, editorPos, pos, relativePos);
this._ctx = ctx;
if (target) {
this.target = target;
this.targetPath = PartFingerprints.collect(target, ctx.viewDomNode);
} else {
this.target = null;
this.targetPath = new Uint8Array(0);
}
}
public override toString(): string {
return `pos(${this.pos.x},${this.pos.y}), editorPos(${this.editorPos.x},${this.editorPos.y}), relativePos(${this.relativePos.x},${this.relativePos.y}), mouseVerticalOffset: ${this.mouseVerticalOffset}, mouseContentHorizontalOffset: ${this.mouseContentHorizontalOffset}\n\ttarget: ${this.target ? (<HTMLElement>this.target).outerHTML : null}`;
}
private _getMouseColumn(position: Position | null = null): number {
if (position && position.column < this._ctx.viewModel.getLineMaxColumn(position.lineNumber)) {
// Most likely, the line contains foreign decorations...
return CursorColumns.visibleColumnFromColumn(this._ctx.viewModel.getLineContent(position.lineNumber), position.column, this._ctx.viewModel.model.getOptions().tabSize) + 1;
}
return this.mouseColumn;
}
public fulfillUnknown(position: Position | null = null): IMouseTargetUnknown {
return MouseTarget.createUnknown(this.target, this._getMouseColumn(position), position);
}
public fulfillTextarea(): IMouseTargetTextarea {
return MouseTarget.createTextarea(this.target, this._getMouseColumn());
}
public fulfillMargin(type: MouseTargetType.GUTTER_GLYPH_MARGIN | MouseTargetType.GUTTER_LINE_NUMBERS | MouseTargetType.GUTTER_LINE_DECORATIONS, position: Position, range: EditorRange, detail: IMouseTargetMarginData): IMouseTargetMargin {
return MouseTarget.createMargin(type, this.target, this._getMouseColumn(position), position, range, detail);
}
public fulfillViewZone(type: MouseTargetType.GUTTER_VIEW_ZONE | MouseTargetType.CONTENT_VIEW_ZONE, position: Position, detail: IMouseTargetViewZoneData): IMouseTargetViewZone {
return MouseTarget.createViewZone(type, this.target, this._getMouseColumn(position), position, detail);
}
public fulfillContentText(position: Position, range: EditorRange | null, detail: IMouseTargetContentTextData): IMouseTargetContentText {
return MouseTarget.createContentText(this.target, this._getMouseColumn(position), position, range, detail);
}
public fulfillContentEmpty(position: Position, detail: IMouseTargetContentEmptyData): IMouseTargetContentEmpty {
return MouseTarget.createContentEmpty(this.target, this._getMouseColumn(position), position, detail);
}
public fulfillContentWidget(detail: string): IMouseTargetContentWidget {
return MouseTarget.createContentWidget(this.target, this._getMouseColumn(), detail);
}
public fulfillScrollbar(position: Position): IMouseTargetScrollbar {
return MouseTarget.createScrollbar(this.target, this._getMouseColumn(position), position);
}
public fulfillOverlayWidget(detail: string): IMouseTargetOverlayWidget {
return MouseTarget.createOverlayWidget(this.target, this._getMouseColumn(), detail);
}
public withTarget(target: HTMLElement | null): HitTestRequest {
return new HitTestRequest(this._ctx, this.editorPos, this.pos, this.relativePos, target);
}
}
interface ResolvedHitTestRequest extends HitTestRequest {
readonly target: HTMLElement;
}
const EMPTY_CONTENT_AFTER_LINES: IMouseTargetContentEmptyData = { isAfterLines: true };
function createEmptyContentDataInLines(horizontalDistanceToText: number): IMouseTargetContentEmptyData {
return {
isAfterLines: false,
horizontalDistanceToText: horizontalDistanceToText
};
}
export class MouseTargetFactory {
private readonly _context: ViewContext;
private readonly _viewHelper: IPointerHandlerHelper;
constructor(context: ViewContext, viewHelper: IPointerHandlerHelper) {
this._context = context;
this._viewHelper = viewHelper;
}
public mouseTargetIsWidget(e: EditorMouseEvent): boolean {
const t = <Element>e.target;
const path = PartFingerprints.collect(t, this._viewHelper.viewDomNode);
// Is it a content widget?
if (ElementPath.isChildOfContentWidgets(path) || ElementPath.isChildOfOverflowingContentWidgets(path)) {
return true;
}
// Is it an overlay widget?
if (ElementPath.isChildOfOverlayWidgets(path) || ElementPath.isChildOfOverflowingOverlayWidgets(path)) {
return true;
}
return false;
}
public createMouseTarget(lastRenderData: PointerHandlerLastRenderData, editorPos: EditorPagePosition, pos: PageCoordinates, relativePos: CoordinatesRelativeToEditor, target: HTMLElement | null): IMouseTarget {
const ctx = new HitTestContext(this._context, this._viewHelper, lastRenderData);
const request = new HitTestRequest(ctx, editorPos, pos, relativePos, target);
try {
const r = MouseTargetFactory._createMouseTarget(ctx, request, false);
if (r.type === MouseTargetType.CONTENT_TEXT) {
// Snap to the nearest soft tab boundary if atomic soft tabs are enabled.
if (ctx.stickyTabStops && r.position !== null) {
const position = MouseTargetFactory._snapToSoftTabBoundary(r.position, ctx.viewModel);
const range = EditorRange.fromPositions(position, position).plusRange(r.range);
return request.fulfillContentText(position, range, r.detail);
}
}
// console.log(MouseTarget.toString(r));
return r;
} catch (err) {
// console.log(err);
return request.fulfillUnknown();
}
}
private static _createMouseTarget(ctx: HitTestContext, request: HitTestRequest, domHitTestExecuted: boolean): IMouseTarget {
// console.log(`${domHitTestExecuted ? '=>' : ''}CAME IN REQUEST: ${request}`);
// First ensure the request has a target
if (request.target === null) {
if (domHitTestExecuted) {
// Still no target... and we have already executed hit test...
return request.fulfillUnknown();
}
const hitTestResult = MouseTargetFactory._doHitTest(ctx, request);
if (hitTestResult.type === HitTestResultType.Content) {
return MouseTargetFactory.createMouseTargetFromHitTestPosition(ctx, request, hitTestResult.spanNode, hitTestResult.position, hitTestResult.injectedText);
}
return this._createMouseTarget(ctx, request.withTarget(hitTestResult.hitTarget), true);
}
// we know for a fact that request.target is not null
const resolvedRequest = <ResolvedHitTestRequest>request;
let result: IMouseTarget | null = null;
if (!ElementPath.isChildOfOverflowGuard(request.targetPath) && !ElementPath.isChildOfOverflowingContentWidgets(request.targetPath) && !ElementPath.isChildOfOverflowingOverlayWidgets(request.targetPath)) {
// We only render dom nodes inside the overflow guard or in the overflowing content widgets
result = result || request.fulfillUnknown();
}
result = result || MouseTargetFactory._hitTestContentWidget(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestOverlayWidget(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestMinimap(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestScrollbarSlider(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestViewZone(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestMargin(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestViewCursor(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestTextArea(ctx, resolvedRequest);
result = result || MouseTargetFactory._hitTestViewLines(ctx, resolvedRequest, domHitTestExecuted);
result = result || MouseTargetFactory._hitTestScrollbar(ctx, resolvedRequest);
return (result || request.fulfillUnknown());
}
private static _hitTestContentWidget(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
// Is it a content widget?
if (ElementPath.isChildOfContentWidgets(request.targetPath) || ElementPath.isChildOfOverflowingContentWidgets(request.targetPath)) {
const widgetId = ctx.findAttribute(request.target, 'widgetId');
if (widgetId) {
return request.fulfillContentWidget(widgetId);
} else {
return request.fulfillUnknown();
}
}
return null;
}
private static _hitTestOverlayWidget(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
// Is it an overlay widget?
if (ElementPath.isChildOfOverlayWidgets(request.targetPath) || ElementPath.isChildOfOverflowingOverlayWidgets(request.targetPath)) {
const widgetId = ctx.findAttribute(request.target, 'widgetId');
if (widgetId) {
return request.fulfillOverlayWidget(widgetId);
} else {
return request.fulfillUnknown();
}
}
return null;
}
private static _hitTestViewCursor(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
if (request.target) {
// Check if we've hit a painted cursor
const lastViewCursorsRenderData = ctx.lastRenderData.lastViewCursorsRenderData;
for (const d of lastViewCursorsRenderData) {
if (request.target === d.domNode) {
return request.fulfillContentText(d.position, null, { mightBeForeignElement: false, injectedText: null });
}
}
}
if (request.isInContentArea) {
// Edge has a bug when hit-testing the exact position of a cursor,
// instead of returning the correct dom node, it returns the
// first or last rendered view line dom node, therefore help it out
// and first check if we are on top of a cursor
const lastViewCursorsRenderData = ctx.lastRenderData.lastViewCursorsRenderData;
const mouseContentHorizontalOffset = request.mouseContentHorizontalOffset;
const mouseVerticalOffset = request.mouseVerticalOffset;
for (const d of lastViewCursorsRenderData) {
if (mouseContentHorizontalOffset < d.contentLeft) {
// mouse position is to the left of the cursor
continue;
}
if (mouseContentHorizontalOffset > d.contentLeft + d.width) {
// mouse position is to the right of the cursor
continue;
}
const cursorVerticalOffset = ctx.getVerticalOffsetForLineNumber(d.position.lineNumber);
if (
cursorVerticalOffset <= mouseVerticalOffset
&& mouseVerticalOffset <= cursorVerticalOffset + d.height
) {
return request.fulfillContentText(d.position, null, { mightBeForeignElement: false, injectedText: null });
}
}
}
return null;
}
private static _hitTestViewZone(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
const viewZoneData = ctx.getZoneAtCoord(request.mouseVerticalOffset);
if (viewZoneData) {
const mouseTargetType = (request.isInContentArea ? MouseTargetType.CONTENT_VIEW_ZONE : MouseTargetType.GUTTER_VIEW_ZONE);
return request.fulfillViewZone(mouseTargetType, viewZoneData.position, viewZoneData);
}
return null;
}
private static _hitTestTextArea(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
// Is it the textarea?
if (ElementPath.isTextArea(request.targetPath)) {
if (ctx.lastRenderData.lastTextareaPosition) {
return request.fulfillContentText(ctx.lastRenderData.lastTextareaPosition, null, { mightBeForeignElement: false, injectedText: null });
}
return request.fulfillTextarea();
}
return null;
}
private static _hitTestMargin(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
if (request.isInMarginArea) {
const res = ctx.getFullLineRangeAtCoord(request.mouseVerticalOffset);
const pos = res.range.getStartPosition();
let offset = Math.abs(request.relativePos.x);
const detail: Mutable<IMouseTargetMarginData> = {
isAfterLines: res.isAfterLines,
glyphMarginLeft: ctx.layoutInfo.glyphMarginLeft,
glyphMarginWidth: ctx.layoutInfo.glyphMarginWidth,
lineNumbersWidth: ctx.layoutInfo.lineNumbersWidth,
offsetX: offset
};
offset -= ctx.layoutInfo.glyphMarginLeft;
if (offset <= ctx.layoutInfo.glyphMarginWidth) {
// On the glyph margin
const modelCoordinate = ctx.viewModel.coordinatesConverter.convertViewPositionToModelPosition(res.range.getStartPosition());
const lanes = ctx.viewModel.glyphLanes.getLanesAtLine(modelCoordinate.lineNumber);
detail.glyphMarginLane = lanes[Math.floor(offset / ctx.lineHeight)];
return request.fulfillMargin(MouseTargetType.GUTTER_GLYPH_MARGIN, pos, res.range, detail);
}
offset -= ctx.layoutInfo.glyphMarginWidth;
if (offset <= ctx.layoutInfo.lineNumbersWidth) {
// On the line numbers
return request.fulfillMargin(MouseTargetType.GUTTER_LINE_NUMBERS, pos, res.range, detail);
}
offset -= ctx.layoutInfo.lineNumbersWidth;
// On the line decorations
return request.fulfillMargin(MouseTargetType.GUTTER_LINE_DECORATIONS, pos, res.range, detail);
}
return null;
}
private static _hitTestViewLines(ctx: HitTestContext, request: ResolvedHitTestRequest, domHitTestExecuted: boolean): IMouseTarget | null {
if (!ElementPath.isChildOfViewLines(request.targetPath)) {
return null;
}
if (ctx.isInTopPadding(request.mouseVerticalOffset)) {
return request.fulfillContentEmpty(new Position(1, 1), EMPTY_CONTENT_AFTER_LINES);
}
// Check if it is below any lines and any view zones
if (ctx.isAfterLines(request.mouseVerticalOffset) || ctx.isInBottomPadding(request.mouseVerticalOffset)) {
// This most likely indicates it happened after the last view-line
const lineCount = ctx.viewModel.getLineCount();
const maxLineColumn = ctx.viewModel.getLineMaxColumn(lineCount);
return request.fulfillContentEmpty(new Position(lineCount, maxLineColumn), EMPTY_CONTENT_AFTER_LINES);
}
if (domHitTestExecuted) {
// Check if we are hitting a view-line (can happen in the case of inline decorations on empty lines)
// See https://github.com/microsoft/vscode/issues/46942
if (ElementPath.isStrictChildOfViewLines(request.targetPath)) {
const lineNumber = ctx.getLineNumberAtVerticalOffset(request.mouseVerticalOffset);
if (ctx.viewModel.getLineLength(lineNumber) === 0) {
const lineWidth = ctx.getLineWidth(lineNumber);
const detail = createEmptyContentDataInLines(request.mouseContentHorizontalOffset - lineWidth);
return request.fulfillContentEmpty(new Position(lineNumber, 1), detail);
}
const lineWidth = ctx.getLineWidth(lineNumber);
if (request.mouseContentHorizontalOffset >= lineWidth) {
const detail = createEmptyContentDataInLines(request.mouseContentHorizontalOffset - lineWidth);
const pos = new Position(lineNumber, ctx.viewModel.getLineMaxColumn(lineNumber));
return request.fulfillContentEmpty(pos, detail);
}
}
// We have already executed hit test...
return request.fulfillUnknown();
}
const hitTestResult = MouseTargetFactory._doHitTest(ctx, request);
if (hitTestResult.type === HitTestResultType.Content) {
return MouseTargetFactory.createMouseTargetFromHitTestPosition(ctx, request, hitTestResult.spanNode, hitTestResult.position, hitTestResult.injectedText);
}
return this._createMouseTarget(ctx, request.withTarget(hitTestResult.hitTarget), true);
}
private static _hitTestMinimap(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
if (ElementPath.isChildOfMinimap(request.targetPath)) {
const possibleLineNumber = ctx.getLineNumberAtVerticalOffset(request.mouseVerticalOffset);
const maxColumn = ctx.viewModel.getLineMaxColumn(possibleLineNumber);
return request.fulfillScrollbar(new Position(possibleLineNumber, maxColumn));
}
return null;
}
private static _hitTestScrollbarSlider(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
if (ElementPath.isChildOfScrollableElement(request.targetPath)) {
if (request.target && request.target.nodeType === 1) {
const className = request.target.className;
if (className && /\b(slider|scrollbar)\b/.test(className)) {
const possibleLineNumber = ctx.getLineNumberAtVerticalOffset(request.mouseVerticalOffset);
const maxColumn = ctx.viewModel.getLineMaxColumn(possibleLineNumber);
return request.fulfillScrollbar(new Position(possibleLineNumber, maxColumn));
}
}
}
return null;
}
private static _hitTestScrollbar(ctx: HitTestContext, request: ResolvedHitTestRequest): IMouseTarget | null {
// Is it the overview ruler?
// Is it a child of the scrollable element?
if (ElementPath.isChildOfScrollableElement(request.targetPath)) {
const possibleLineNumber = ctx.getLineNumberAtVerticalOffset(request.mouseVerticalOffset);
const maxColumn = ctx.viewModel.getLineMaxColumn(possibleLineNumber);
return request.fulfillScrollbar(new Position(possibleLineNumber, maxColumn));
}
return null;
}
public getMouseColumn(relativePos: CoordinatesRelativeToEditor): number {
const options = this._context.configuration.options;
const layoutInfo = options.get(EditorOption.layoutInfo);
const mouseContentHorizontalOffset = this._context.viewLayout.getCurrentScrollLeft() + relativePos.x - layoutInfo.contentLeft;
return MouseTargetFactory._getMouseColumn(mouseContentHorizontalOffset, options.get(EditorOption.fontInfo).typicalHalfwidthCharacterWidth);
}
public static _getMouseColumn(mouseContentHorizontalOffset: number, typicalHalfwidthCharacterWidth: number): number {
if (mouseContentHorizontalOffset < 0) {
return 1;
}
const chars = Math.round(mouseContentHorizontalOffset / typicalHalfwidthCharacterWidth);
return (chars + 1);
}
private static createMouseTargetFromHitTestPosition(ctx: HitTestContext, request: HitTestRequest, spanNode: HTMLElement, pos: Position, injectedText: InjectedText | null): IMouseTarget {
const lineNumber = pos.lineNumber;
const column = pos.column;
const lineWidth = ctx.getLineWidth(lineNumber);
if (request.mouseContentHorizontalOffset > lineWidth) {
const detail = createEmptyContentDataInLines(request.mouseContentHorizontalOffset - lineWidth);
return request.fulfillContentEmpty(pos, detail);
}
const visibleRange = ctx.visibleRangeForPosition(lineNumber, column);
if (!visibleRange) {
return request.fulfillUnknown(pos);
}
const columnHorizontalOffset = visibleRange.left;
if (Math.abs(request.mouseContentHorizontalOffset - columnHorizontalOffset) < 1) {
return request.fulfillContentText(pos, null, { mightBeForeignElement: !!injectedText, injectedText });
}
// Let's define a, b, c and check if the offset is in between them...
interface OffsetColumn { offset: number; column: number }
const points: OffsetColumn[] = [];
points.push({ offset: visibleRange.left, column: column });
if (column > 1) {
const visibleRange = ctx.visibleRangeForPosition(lineNumber, column - 1);
if (visibleRange) {
points.push({ offset: visibleRange.left, column: column - 1 });
}
}
const lineMaxColumn = ctx.viewModel.getLineMaxColumn(lineNumber);
if (column < lineMaxColumn) {
const visibleRange = ctx.visibleRangeForPosition(lineNumber, column + 1);
if (visibleRange) {
points.push({ offset: visibleRange.left, column: column + 1 });
}
}
points.sort((a, b) => a.offset - b.offset);
const mouseCoordinates = request.pos.toClientCoordinates(dom.getWindow(ctx.viewDomNode));
const spanNodeClientRect = spanNode.getBoundingClientRect();
const mouseIsOverSpanNode = (spanNodeClientRect.left <= mouseCoordinates.clientX && mouseCoordinates.clientX <= spanNodeClientRect.right);
let rng: EditorRange | null = null;
for (let i = 1; i < points.length; i++) {
const prev = points[i - 1];
const curr = points[i];
if (prev.offset <= request.mouseContentHorizontalOffset && request.mouseContentHorizontalOffset <= curr.offset) {
rng = new EditorRange(lineNumber, prev.column, lineNumber, curr.column);
// See https://github.com/microsoft/vscode/issues/152819
// Due to the use of zwj, the browser's hit test result is skewed towards the left
// Here we try to correct that if the mouse horizontal offset is closer to the right than the left
const prevDelta = Math.abs(prev.offset - request.mouseContentHorizontalOffset);
const nextDelta = Math.abs(curr.offset - request.mouseContentHorizontalOffset);
pos = (
prevDelta < nextDelta
? new Position(lineNumber, prev.column)
: new Position(lineNumber, curr.column)
);
break;
}
}
return request.fulfillContentText(pos, rng, { mightBeForeignElement: !mouseIsOverSpanNode || !!injectedText, injectedText });
}
/**
* Most probably WebKit browsers and Edge
*/
private static _doHitTestWithCaretRangeFromPoint(ctx: HitTestContext, request: BareHitTestRequest): HitTestResult {
// In Chrome, especially on Linux it is possible to click between lines,
// so try to adjust the `hity` below so that it lands in the center of a line
const lineNumber = ctx.getLineNumberAtVerticalOffset(request.mouseVerticalOffset);
const lineStartVerticalOffset = ctx.getVerticalOffsetForLineNumber(lineNumber);
const lineEndVerticalOffset = lineStartVerticalOffset + ctx.lineHeight;
const isBelowLastLine = (
lineNumber === ctx.viewModel.getLineCount()
&& request.mouseVerticalOffset > lineEndVerticalOffset
);
if (!isBelowLastLine) {
const lineCenteredVerticalOffset = Math.floor((lineStartVerticalOffset + lineEndVerticalOffset) / 2);
let adjustedPageY = request.pos.y + (lineCenteredVerticalOffset - request.mouseVerticalOffset);
if (adjustedPageY <= request.editorPos.y) {
adjustedPageY = request.editorPos.y + 1;
}
if (adjustedPageY >= request.editorPos.y + request.editorPos.height) {
adjustedPageY = request.editorPos.y + request.editorPos.height - 1;
}
const adjustedPage = new PageCoordinates(request.pos.x, adjustedPageY);
const r = this._actualDoHitTestWithCaretRangeFromPoint(ctx, adjustedPage.toClientCoordinates(dom.getWindow(ctx.viewDomNode)));
if (r.type === HitTestResultType.Content) {
return r;
}
}
// Also try to hit test without the adjustment (for the edge cases that we are near the top or bottom)
return this._actualDoHitTestWithCaretRangeFromPoint(ctx, request.pos.toClientCoordinates(dom.getWindow(ctx.viewDomNode)));
}
private static _actualDoHitTestWithCaretRangeFromPoint(ctx: HitTestContext, coords: ClientCoordinates): HitTestResult {
const shadowRoot = dom.getShadowRoot(ctx.viewDomNode);
let range: Range;
if (shadowRoot) {
if (typeof (<any>shadowRoot).caretRangeFromPoint === 'undefined') {
range = shadowCaretRangeFromPoint(shadowRoot, coords.clientX, coords.clientY);
} else {
range = (<any>shadowRoot).caretRangeFromPoint(coords.clientX, coords.clientY);
}
} else {
range = (<any>ctx.viewDomNode.ownerDocument).caretRangeFromPoint(coords.clientX, coords.clientY);
}
if (!range || !range.startContainer) {
return new UnknownHitTestResult();
}
// Chrome always hits a TEXT_NODE, while Edge sometimes hits a token span
const startContainer = range.startContainer;
if (startContainer.nodeType === startContainer.TEXT_NODE) {
// startContainer is expected to be the token text
const parent1 = startContainer.parentNode; // expected to be the token span
const parent2 = parent1 ? parent1.parentNode : null; // expected to be the view line container span
const parent3 = parent2 ? parent2.parentNode : null; // expected to be the view line div
const parent3ClassName = parent3 && parent3.nodeType === parent3.ELEMENT_NODE ? (<HTMLElement>parent3).className : null;
if (parent3ClassName === ViewLine.CLASS_NAME) {
return HitTestResult.createFromDOMInfo(ctx, <HTMLElement>parent1, range.startOffset);
} else {
return new UnknownHitTestResult(<HTMLElement>startContainer.parentNode);
}
} else if (startContainer.nodeType === startContainer.ELEMENT_NODE) {
// startContainer is expected to be the token span
const parent1 = startContainer.parentNode; // expected to be the view line container span
const parent2 = parent1 ? parent1.parentNode : null; // expected to be the view line div
const parent2ClassName = parent2 && parent2.nodeType === parent2.ELEMENT_NODE ? (<HTMLElement>parent2).className : null;
if (parent2ClassName === ViewLine.CLASS_NAME) {
return HitTestResult.createFromDOMInfo(ctx, <HTMLElement>startContainer, (<HTMLElement>startContainer).textContent!.length);
} else {
return new UnknownHitTestResult(<HTMLElement>startContainer);
}
}
return new UnknownHitTestResult();
}
/**
* Most probably Gecko
*/
private static _doHitTestWithCaretPositionFromPoint(ctx: HitTestContext, coords: ClientCoordinates): HitTestResult {
const hitResult: { offsetNode: Node; offset: number } = (<any>ctx.viewDomNode.ownerDocument).caretPositionFromPoint(coords.clientX, coords.clientY);
if (hitResult.offsetNode.nodeType === hitResult.offsetNode.TEXT_NODE) {
// offsetNode is expected to be the token text
const parent1 = hitResult.offsetNode.parentNode; // expected to be the token span
const parent2 = parent1 ? parent1.parentNode : null; // expected to be the view line container span
const parent3 = parent2 ? parent2.parentNode : null; // expected to be the view line div
const parent3ClassName = parent3 && parent3.nodeType === parent3.ELEMENT_NODE ? (<HTMLElement>parent3).className : null;
if (parent3ClassName === ViewLine.CLASS_NAME) {
return HitTestResult.createFromDOMInfo(ctx, <HTMLElement>hitResult.offsetNode.parentNode, hitResult.offset);
} else {
return new UnknownHitTestResult(<HTMLElement>hitResult.offsetNode.parentNode);
}
}
// For inline decorations, Gecko sometimes returns the `<span>` of the line and the offset is the `<span>` with the inline decoration
// Some other times, it returns the `<span>` with the inline decoration
if (hitResult.offsetNode.nodeType === hitResult.offsetNode.ELEMENT_NODE) {
const parent1 = hitResult.offsetNode.parentNode;
const parent1ClassName = parent1 && parent1.nodeType === parent1.ELEMENT_NODE ? (<HTMLElement>parent1).className : null;
const parent2 = parent1 ? parent1.parentNode : null;
const parent2ClassName = parent2 && parent2.nodeType === parent2.ELEMENT_NODE ? (<HTMLElement>parent2).className : null;
if (parent1ClassName === ViewLine.CLASS_NAME) {
// it returned the `<span>` of the line and the offset is the `<span>` with the inline decoration
const tokenSpan = hitResult.offsetNode.childNodes[Math.min(hitResult.offset, hitResult.offsetNode.childNodes.length - 1)];
if (tokenSpan) {
return HitTestResult.createFromDOMInfo(ctx, <HTMLElement>tokenSpan, 0);
}
} else if (parent2ClassName === ViewLine.CLASS_NAME) {
// it returned the `<span>` with the inline decoration
return HitTestResult.createFromDOMInfo(ctx, <HTMLElement>hitResult.offsetNode, 0);
}
}
return new UnknownHitTestResult(<HTMLElement>hitResult.offsetNode);
}
private static _snapToSoftTabBoundary(position: Position, viewModel: IViewModel): Position {
const lineContent = viewModel.getLineContent(position.lineNumber);
const { tabSize } = viewModel.model.getOptions();
const newPosition = AtomicTabMoveOperations.atomicPosition(lineContent, position.column - 1, tabSize, Direction.Nearest);
if (newPosition !== -1) {
return new Position(position.lineNumber, newPosition + 1);
}
return position;
}
private static _doHitTest(ctx: HitTestContext, request: BareHitTestRequest): HitTestResult {
let result: HitTestResult = new UnknownHitTestResult();
if (typeof (<any>ctx.viewDomNode.ownerDocument).caretRangeFromPoint === 'function') {
result = this._doHitTestWithCaretRangeFromPoint(ctx, request);
} else if ((<any>ctx.viewDomNode.ownerDocument).caretPositionFromPoint) {
result = this._doHitTestWithCaretPositionFromPoint(ctx, request.pos.toClientCoordinates(dom.getWindow(ctx.viewDomNode)));
}
if (result.type === HitTestResultType.Content) {
const injectedText = ctx.viewModel.getInjectedTextAt(result.position);
const normalizedPosition = ctx.viewModel.normalizePosition(result.position, PositionAffinity.None);
if (injectedText || !normalizedPosition.equals(result.position)) {
result = new ContentHitTestResult(normalizedPosition, result.spanNode, injectedText);
}
}
return result;
}
}
function shadowCaretRangeFromPoint(shadowRoot: ShadowRoot, x: number, y: number): Range {
const range = document.createRange();
// Get the element under the point
let el: Element | null = (<any>shadowRoot).elementFromPoint(x, y);
if (el !== null) {
// Get the last child of the element until its firstChild is a text node
// This assumes that the pointer is on the right of the line, out of the tokens
// and that we want to get the offset of the last token of the line
while (el && el.firstChild && el.firstChild.nodeType !== el.firstChild.TEXT_NODE && el.lastChild && el.lastChild.firstChild) {
el = <Element>el.lastChild;
}
// Grab its rect
const rect = el.getBoundingClientRect();
// And its font (the computed shorthand font property might be empty, see #3217)
const elWindow = dom.getWindow(el);
const fontStyle = elWindow.getComputedStyle(el, null).getPropertyValue('font-style');
const fontVariant = elWindow.getComputedStyle(el, null).getPropertyValue('font-variant');
const fontWeight = elWindow.getComputedStyle(el, null).getPropertyValue('font-weight');
const fontSize = elWindow.getComputedStyle(el, null).getPropertyValue('font-size');
const lineHeight = elWindow.getComputedStyle(el, null).getPropertyValue('line-height');
const fontFamily = elWindow.getComputedStyle(el, null).getPropertyValue('font-family');
const font = `${fontStyle} ${fontVariant} ${fontWeight} ${fontSize}/${lineHeight} ${fontFamily}`;
// And also its txt content
const text = (el as any).innerText;
// Position the pixel cursor at the left of the element
let pixelCursor = rect.left;
let offset = 0;
let step: number;
// If the point is on the right of the box put the cursor after the last character
if (x > rect.left + rect.width) {
offset = text.length;
} else {
const charWidthReader = CharWidthReader.getInstance();
// Goes through all the characters of the innerText, and checks if the x of the point
// belongs to the character.
for (let i = 0; i < text.length + 1; i++) {
// The step is half the width of the character
step = charWidthReader.getCharWidth(text.charAt(i), font) / 2;
// Move to the center of the character
pixelCursor += step;
// If the x of the point is smaller that the position of the cursor, the point is over that character
if (x < pixelCursor) {
offset = i;
break;
}
// Move between the current character and the next
pixelCursor += step;
}
}
// Creates a range with the text node of the element and set the offset found
range.setStart(el.firstChild!, offset);
range.setEnd(el.firstChild!, offset);
}
return range;
}
class CharWidthReader {
private static _INSTANCE: CharWidthReader | null = null;
public static getInstance(): CharWidthReader {
if (!CharWidthReader._INSTANCE) {
CharWidthReader._INSTANCE = new CharWidthReader();
}
return CharWidthReader._INSTANCE;
}
private readonly _cache: { [cacheKey: string]: number };
private readonly _canvas: HTMLCanvasElement;
private constructor() {
this._cache = {};
this._canvas = document.createElement('canvas');
}
public getCharWidth(char: string, font: string): number {
const cacheKey = char + font;
if (this._cache[cacheKey]) {
return this._cache[cacheKey];
}
const context = this._canvas.getContext('2d')!;
context.font = font;
const metrics = context.measureText(char);
const width = metrics.width;
this._cache[cacheKey] = width;
return width;
}
}
| src/vs/editor/browser/controller/mouseTarget.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.0002859230153262615,
0.00017482713155914098,
0.00016130074800457805,
0.0001744236215017736,
0.000010796024071169086
]
|
{
"id": 1,
"code_window": [
"\t\t\telse if (workspaceFolder) {\n",
"\t\t\t\tconst config = this._configurationService.getValue<IFilesConfiguration>();\n",
"\t\t\t\tif (config.files?.watcherExclude) {\n",
"\t\t\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\t\t\tif (!opts.includes) {\n",
"\t\t\t\t\t\t\t\topts.includes = [];\n",
"\t\t\t\t\t\t\t}\n",
"\n",
"\t\t\t\t\t\t\tconst includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts",
"type": "replace",
"edit_start_line_idx": 301
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { URI } from 'vs/base/common/uri';
import { IJSONEditingService, IJSONValue } from 'vs/workbench/services/configuration/common/jsonEditing';
export class TestJSONEditingService implements IJSONEditingService {
_serviceBrand: any;
async write(resource: URI, values: IJSONValue[], save: boolean): Promise<void> { }
}
| src/vs/workbench/services/configuration/test/common/testServices.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017587868205737323,
0.0001734232355374843,
0.00017096778901759535,
0.0001734232355374843,
0.0000024554465198889375
]
|
{
"id": 2,
"code_window": [
"\t\t// Compute the watcher exclude rules from configuration\n",
"\t\tconst excludes: string[] = [];\n",
"\t\tconst config = this.configurationService.getValue<IFilesConfiguration>({ resource: workspace.uri });\n",
"\t\tif (config.files?.watcherExclude) {\n",
"\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\texcludes.push(key);\n",
"\t\t\t\t}\n",
"\t\t\t}\n",
"\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/contrib/files/browser/workspaceWatcher.ts",
"type": "replace",
"edit_start_line_idx": 111
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { DisposableMap, DisposableStore } from 'vs/base/common/lifecycle';
import { FileOperation, IFileService, IFilesConfiguration, IWatchOptions } from 'vs/platform/files/common/files';
import { extHostNamedCustomer, IExtHostContext } from 'vs/workbench/services/extensions/common/extHostCustomers';
import { ExtHostContext, ExtHostFileSystemEventServiceShape, MainContext, MainThreadFileSystemEventServiceShape } from '../common/extHost.protocol';
import { localize } from 'vs/nls';
import { IWorkingCopyFileOperationParticipant, IWorkingCopyFileService, SourceTargetPair, IFileOperationUndoRedoInfo } from 'vs/workbench/services/workingCopy/common/workingCopyFileService';
import { IBulkEditService } from 'vs/editor/browser/services/bulkEditService';
import { IProgressService, ProgressLocation } from 'vs/platform/progress/common/progress';
import { raceCancellation } from 'vs/base/common/async';
import { CancellationToken, CancellationTokenSource } from 'vs/base/common/cancellation';
import { IDialogService } from 'vs/platform/dialogs/common/dialogs';
import Severity from 'vs/base/common/severity';
import { IStorageService, StorageScope, StorageTarget } from 'vs/platform/storage/common/storage';
import { Action2, registerAction2 } from 'vs/platform/actions/common/actions';
import { ServicesAccessor } from 'vs/platform/instantiation/common/instantiation';
import { ILogService } from 'vs/platform/log/common/log';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { reviveWorkspaceEditDto } from 'vs/workbench/api/browser/mainThreadBulkEdits';
import { GLOBSTAR } from 'vs/base/common/glob';
import { rtrim } from 'vs/base/common/strings';
import { UriComponents, URI } from 'vs/base/common/uri';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { normalizeWatcherPattern } from 'vs/platform/files/common/watcher';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
@extHostNamedCustomer(MainContext.MainThreadFileSystemEventService)
export class MainThreadFileSystemEventService implements MainThreadFileSystemEventServiceShape {
static readonly MementoKeyAdditionalEdits = `file.particpants.additionalEdits`;
private readonly _proxy: ExtHostFileSystemEventServiceShape;
private readonly _listener = new DisposableStore();
private readonly _watches = new DisposableMap<number>();
constructor(
extHostContext: IExtHostContext,
@IFileService private readonly _fileService: IFileService,
@IWorkingCopyFileService workingCopyFileService: IWorkingCopyFileService,
@IBulkEditService bulkEditService: IBulkEditService,
@IProgressService progressService: IProgressService,
@IDialogService dialogService: IDialogService,
@IStorageService storageService: IStorageService,
@ILogService logService: ILogService,
@IEnvironmentService envService: IEnvironmentService,
@IUriIdentityService uriIdentService: IUriIdentityService,
@IWorkspaceContextService private readonly _contextService: IWorkspaceContextService,
@ILogService private readonly _logService: ILogService,
@IConfigurationService private readonly _configurationService: IConfigurationService
) {
this._proxy = extHostContext.getProxy(ExtHostContext.ExtHostFileSystemEventService);
this._listener.add(_fileService.onDidFilesChange(event => {
this._proxy.$onFileEvent({
created: event.rawAdded,
changed: event.rawUpdated,
deleted: event.rawDeleted
});
}));
const that = this;
const fileOperationParticipant = new class implements IWorkingCopyFileOperationParticipant {
async participate(files: SourceTargetPair[], operation: FileOperation, undoInfo: IFileOperationUndoRedoInfo | undefined, timeout: number, token: CancellationToken) {
if (undoInfo?.isUndoing) {
return;
}
const cts = new CancellationTokenSource(token);
const timer = setTimeout(() => cts.cancel(), timeout);
const data = await progressService.withProgress({
location: ProgressLocation.Notification,
title: this._progressLabel(operation),
cancellable: true,
delay: Math.min(timeout / 2, 3000)
}, () => {
// race extension host event delivery against timeout AND user-cancel
const onWillEvent = that._proxy.$onWillRunFileOperation(operation, files, timeout, cts.token);
return raceCancellation(onWillEvent, cts.token);
}, () => {
// user-cancel
cts.cancel();
}).finally(() => {
cts.dispose();
clearTimeout(timer);
});
if (!data || data.edit.edits.length === 0) {
// cancelled, no reply, or no edits
return;
}
const needsConfirmation = data.edit.edits.some(edit => edit.metadata?.needsConfirmation);
let showPreview = storageService.getBoolean(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, StorageScope.PROFILE);
if (envService.extensionTestsLocationURI) {
// don't show dialog in tests
showPreview = false;
}
if (showPreview === undefined) {
// show a user facing message
let message: string;
if (data.extensionNames.length === 1) {
if (operation === FileOperation.CREATE) {
message = localize('ask.1.create', "Extension '{0}' wants to make refactoring changes with this file creation", data.extensionNames[0]);
} else if (operation === FileOperation.COPY) {
message = localize('ask.1.copy', "Extension '{0}' wants to make refactoring changes with this file copy", data.extensionNames[0]);
} else if (operation === FileOperation.MOVE) {
message = localize('ask.1.move', "Extension '{0}' wants to make refactoring changes with this file move", data.extensionNames[0]);
} else /* if (operation === FileOperation.DELETE) */ {
message = localize('ask.1.delete', "Extension '{0}' wants to make refactoring changes with this file deletion", data.extensionNames[0]);
}
} else {
if (operation === FileOperation.CREATE) {
message = localize({ key: 'ask.N.create', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file creation", data.extensionNames.length);
} else if (operation === FileOperation.COPY) {
message = localize({ key: 'ask.N.copy', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file copy", data.extensionNames.length);
} else if (operation === FileOperation.MOVE) {
message = localize({ key: 'ask.N.move', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file move", data.extensionNames.length);
} else /* if (operation === FileOperation.DELETE) */ {
message = localize({ key: 'ask.N.delete', comment: ['{0} is a number, e.g "3 extensions want..."'] }, "{0} extensions want to make refactoring changes with this file deletion", data.extensionNames.length);
}
}
if (needsConfirmation) {
// edit which needs confirmation -> always show dialog
const { confirmed } = await dialogService.confirm({
type: Severity.Info,
message,
primaryButton: localize('preview', "Show &&Preview"),
cancelButton: localize('cancel', "Skip Changes")
});
showPreview = true;
if (!confirmed) {
// no changes wanted
return;
}
} else {
// choice
enum Choice {
OK = 0,
Preview = 1,
Cancel = 2
}
const { result, checkboxChecked } = await dialogService.prompt<Choice>({
type: Severity.Info,
message,
buttons: [
{
label: localize({ key: 'ok', comment: ['&& denotes a mnemonic'] }, "&&OK"),
run: () => Choice.OK
},
{
label: localize({ key: 'preview', comment: ['&& denotes a mnemonic'] }, "Show &&Preview"),
run: () => Choice.Preview
}
],
cancelButton: {
label: localize('cancel', "Skip Changes"),
run: () => Choice.Cancel
},
checkbox: { label: localize('again', "Do not ask me again") }
});
if (result === Choice.Cancel) {
// no changes wanted, don't persist cancel option
return;
}
showPreview = result === Choice.Preview;
if (checkboxChecked) {
storageService.store(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, showPreview, StorageScope.PROFILE, StorageTarget.USER);
}
}
}
logService.info('[onWill-handler] applying additional workspace edit from extensions', data.extensionNames);
await bulkEditService.apply(
reviveWorkspaceEditDto(data.edit, uriIdentService),
{ undoRedoGroupId: undoInfo?.undoRedoGroupId, showPreview }
);
}
private _progressLabel(operation: FileOperation): string {
switch (operation) {
case FileOperation.CREATE:
return localize('msg-create', "Running 'File Create' participants...");
case FileOperation.MOVE:
return localize('msg-rename', "Running 'File Rename' participants...");
case FileOperation.COPY:
return localize('msg-copy', "Running 'File Copy' participants...");
case FileOperation.DELETE:
return localize('msg-delete', "Running 'File Delete' participants...");
case FileOperation.WRITE:
return localize('msg-write', "Running 'File Write' participants...");
}
}
};
// BEFORE file operation
this._listener.add(workingCopyFileService.addFileOperationParticipant(fileOperationParticipant));
// AFTER file operation
this._listener.add(workingCopyFileService.onDidRunWorkingCopyFileOperation(e => this._proxy.$onDidRunFileOperation(e.operation, e.files)));
}
async $watch(extensionId: string, session: number, resource: UriComponents, unvalidatedOpts: IWatchOptions, correlate: boolean): Promise<void> {
const uri = URI.revive(resource);
const opts: IWatchOptions = {
...unvalidatedOpts
};
// Convert a recursive watcher to a flat watcher if the path
// turns out to not be a folder. Recursive watching is only
// possible on folders, so we help all file watchers by checking
// early.
if (opts.recursive) {
try {
const stat = await this._fileService.stat(uri);
if (!stat.isDirectory) {
opts.recursive = false;
}
} catch (error) {
this._logService.error(`MainThreadFileSystemEventService#$watch(): failed to stat a resource for file watching (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session}): ${error}`);
}
}
// Correlated file watching is taken as is
if (correlate) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): request to start watching correlated (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
const watcherDisposables = new DisposableStore();
const subscription = watcherDisposables.add(this._fileService.createWatcher(uri, opts));
watcherDisposables.add(subscription.onDidChange(event => {
this._proxy.$onFileEvent({
session,
created: event.rawAdded,
changed: event.rawUpdated,
deleted: event.rawDeleted
});
}));
this._watches.set(session, watcherDisposables);
}
// Uncorrelated file watching gets special treatment
else {
// Refuse to watch anything that is already watched via
// our workspace watchers in case the request is a
// recursive file watcher and does not opt-in to event
// correlation via specific exclude rules.
// Still allow for non-recursive watch requests as a way
// to bypass configured exclude rules though
// (see https://github.com/microsoft/vscode/issues/146066)
const workspaceFolder = this._contextService.getWorkspaceFolder(uri);
if (workspaceFolder && opts.recursive) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): ignoring request to start watching because path is inside workspace (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
return;
}
this._logService.trace(`MainThreadFileSystemEventService#$watch(): request to start watching uncorrelated (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
// Automatically add `files.watcherExclude` patterns when watching
// recursively to give users a chance to configure exclude rules
// for reducing the overhead of watching recursively
if (opts.recursive && opts.excludes.length === 0) {
const config = this._configurationService.getValue<IFilesConfiguration>();
if (config.files?.watcherExclude) {
for (const key in config.files.watcherExclude) {
if (config.files.watcherExclude[key] === true) {
opts.excludes.push(key);
}
}
}
}
// Non-recursive watching inside the workspace will overlap with
// our standard workspace watchers. To prevent duplicate events,
// we only want to include events for files that are otherwise
// excluded via `files.watcherExclude`. As such, we configure
// to include each configured exclude pattern so that only those
// events are reported that are otherwise excluded.
// However, we cannot just use the pattern as is, because a pattern
// such as `bar` for a exclude, will work to exclude any of
// `<workspace path>/bar` but will not work as include for files within
// `bar` unless a suffix of `/**` if added.
// (https://github.com/microsoft/vscode/issues/148245)
else if (workspaceFolder) {
const config = this._configurationService.getValue<IFilesConfiguration>();
if (config.files?.watcherExclude) {
for (const key in config.files.watcherExclude) {
if (config.files.watcherExclude[key] === true) {
if (!opts.includes) {
opts.includes = [];
}
const includePattern = `${rtrim(key, '/')}/${GLOBSTAR}`;
opts.includes.push(normalizeWatcherPattern(workspaceFolder.uri.fsPath, includePattern));
}
}
}
// Still ignore watch request if there are actually no configured
// exclude rules, because in that case our default recursive watcher
// should be able to take care of all events.
if (!opts.includes || opts.includes.length === 0) {
this._logService.trace(`MainThreadFileSystemEventService#$watch(): ignoring request to start watching because path is inside workspace and no excludes are configured (extension: ${extensionId}, path: ${uri.toString(true)}, recursive: ${opts.recursive}, session: ${session})`);
return;
}
}
const subscription = this._fileService.watch(uri, opts);
this._watches.set(session, subscription);
}
}
$unwatch(session: number): void {
if (this._watches.has(session)) {
this._logService.trace(`MainThreadFileSystemEventService#$unwatch(): request to stop watching (session: ${session})`);
this._watches.deleteAndDispose(session);
}
}
dispose(): void {
this._listener.dispose();
this._watches.dispose();
}
}
registerAction2(class ResetMemento extends Action2 {
constructor() {
super({
id: 'files.participants.resetChoice',
title: {
value: localize('label', "Reset choice for 'File operation needs preview'"),
original: `Reset choice for 'File operation needs preview'`
},
f1: true
});
}
run(accessor: ServicesAccessor) {
accessor.get(IStorageService).remove(MainThreadFileSystemEventService.MementoKeyAdditionalEdits, StorageScope.PROFILE);
}
});
| src/vs/workbench/api/browser/mainThreadFileSystemEventService.ts | 1 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.9961361289024353,
0.10897153615951538,
0.00016239986871369183,
0.00017309191753156483,
0.30775266885757446
]
|
{
"id": 2,
"code_window": [
"\t\t// Compute the watcher exclude rules from configuration\n",
"\t\tconst excludes: string[] = [];\n",
"\t\tconst config = this.configurationService.getValue<IFilesConfiguration>({ resource: workspace.uri });\n",
"\t\tif (config.files?.watcherExclude) {\n",
"\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\texcludes.push(key);\n",
"\t\t\t\t}\n",
"\t\t\t}\n",
"\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/contrib/files/browser/workspaceWatcher.ts",
"type": "replace",
"edit_start_line_idx": 111
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/css!./media/paneCompositePart';
import { Event } from 'vs/base/common/event';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IProgressIndicator } from 'vs/platform/progress/common/progress';
import { Extensions, PaneComposite, PaneCompositeDescriptor, PaneCompositeRegistry } from 'vs/workbench/browser/panecomposite';
import { IPaneComposite } from 'vs/workbench/common/panecomposite';
import { IViewDescriptorService, ViewContainerLocation } from 'vs/workbench/common/views';
import { DisposableStore, MutableDisposable } from 'vs/base/common/lifecycle';
import { IView } from 'vs/base/browser/ui/grid/grid';
import { IWorkbenchLayoutService, Parts } from 'vs/workbench/services/layout/browser/layoutService';
import { CompositePart, ICompositeTitleLabel } from 'vs/workbench/browser/parts/compositePart';
import { IPaneCompositeBarOptions, PaneCompositeBar } from 'vs/workbench/browser/parts/paneCompositeBar';
import { Dimension, EventHelper, trackFocus, $, addDisposableListener, EventType, prepend, getWindow } from 'vs/base/browser/dom';
import { Registry } from 'vs/platform/registry/common/platform';
import { INotificationService } from 'vs/platform/notification/common/notification';
import { IStorageService } from 'vs/platform/storage/common/storage';
import { IContextMenuService } from 'vs/platform/contextview/browser/contextView';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { IThemeService } from 'vs/platform/theme/common/themeService';
import { IContextKey, IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { IExtensionService } from 'vs/workbench/services/extensions/common/extensions';
import { IComposite } from 'vs/workbench/common/composite';
import { localize } from 'vs/nls';
import { CompositeDragAndDropObserver, toggleDropEffect } from 'vs/workbench/browser/dnd';
import { EDITOR_DRAG_AND_DROP_BACKGROUND } from 'vs/workbench/common/theme';
import { IPartOptions } from 'vs/workbench/browser/part';
import { ToolBar } from 'vs/base/browser/ui/toolbar/toolbar';
import { CompositeMenuActions } from 'vs/workbench/browser/actions';
import { IMenuService, MenuId } from 'vs/platform/actions/common/actions';
import { ActionsOrientation, prepareActions } from 'vs/base/browser/ui/actionbar/actionbar';
import { Gesture, EventType as GestureEventType } from 'vs/base/browser/touch';
import { StandardMouseEvent } from 'vs/base/browser/mouseEvent';
import { IAction, SubmenuAction } from 'vs/base/common/actions';
import { Composite } from 'vs/workbench/browser/composite';
import { ViewsSubMenu } from 'vs/workbench/browser/parts/views/viewPaneContainer';
import { createAndFillInActionBarActions } from 'vs/platform/actions/browser/menuEntryActionViewItem';
export interface IPaneCompositePart extends IView {
readonly partId: Parts.PANEL_PART | Parts.AUXILIARYBAR_PART | Parts.SIDEBAR_PART;
readonly onDidPaneCompositeOpen: Event<IPaneComposite>;
readonly onDidPaneCompositeClose: Event<IPaneComposite>;
/**
* Opens a viewlet with the given identifier and pass keyboard focus to it if specified.
*/
openPaneComposite(id: string | undefined, focus?: boolean): Promise<IPaneComposite | undefined>;
/**
* Returns the current active viewlet if any.
*/
getActivePaneComposite(): IPaneComposite | undefined;
/**
* Returns the viewlet by id.
*/
getPaneComposite(id: string): PaneCompositeDescriptor | undefined;
/**
* Returns all enabled viewlets
*/
getPaneComposites(): PaneCompositeDescriptor[];
/**
* Returns the progress indicator for the side bar.
*/
getProgressIndicator(id: string): IProgressIndicator | undefined;
/**
* Hide the active viewlet.
*/
hideActivePaneComposite(): void;
/**
* Return the last active viewlet id.
*/
getLastActivePaneCompositeId(): string;
/**
* Returns id of pinned view containers following the visual order.
*/
getPinnedPaneCompositeIds(): string[];
/**
* Returns id of visible view containers following the visual order.
*/
getVisiblePaneCompositeIds(): string[];
}
export abstract class AbstractPaneCompositePart extends CompositePart<PaneComposite> implements IPaneCompositePart {
private static readonly MIN_COMPOSITE_BAR_WIDTH = 50;
get snap(): boolean {
// Always allow snapping closed
// Only allow dragging open if the panel contains view containers
return this.layoutService.isVisible(this.partId) || !!this.paneCompositeBar.value?.getVisiblePaneCompositeIds().length;
}
get onDidPaneCompositeOpen(): Event<IPaneComposite> { return Event.map(this.onDidCompositeOpen.event, compositeEvent => <IPaneComposite>compositeEvent.composite); }
readonly onDidPaneCompositeClose = this.onDidCompositeClose.event as Event<IPaneComposite>;
private readonly location: ViewContainerLocation;
private titleContainer: HTMLElement | undefined;
private paneCompositeBarContainer: HTMLElement | undefined;
private paneCompositeBar = this._register(new MutableDisposable<PaneCompositeBar>());
private emptyPaneMessageElement: HTMLElement | undefined;
private globalToolBar: ToolBar | undefined;
private readonly globalActions: CompositeMenuActions;
private blockOpening = false;
protected contentDimension: Dimension | undefined;
constructor(
readonly partId: Parts.PANEL_PART | Parts.AUXILIARYBAR_PART | Parts.SIDEBAR_PART,
partOptions: IPartOptions,
activePaneCompositeSettingsKey: string,
private readonly activePaneContextKey: IContextKey<string>,
private paneFocusContextKey: IContextKey<boolean>,
nameForTelemetry: string,
compositeCSSClass: string,
titleForegroundColor: string | undefined,
@INotificationService notificationService: INotificationService,
@IStorageService storageService: IStorageService,
@IContextMenuService contextMenuService: IContextMenuService,
@IWorkbenchLayoutService layoutService: IWorkbenchLayoutService,
@IKeybindingService keybindingService: IKeybindingService,
@IInstantiationService instantiationService: IInstantiationService,
@IThemeService themeService: IThemeService,
@IViewDescriptorService private readonly viewDescriptorService: IViewDescriptorService,
@IContextKeyService protected readonly contextKeyService: IContextKeyService,
@IExtensionService private readonly extensionService: IExtensionService,
@IMenuService protected readonly menuService: IMenuService,
) {
let location = ViewContainerLocation.Sidebar;
let registryId = Extensions.Viewlets;
let globalActionsMenuId = MenuId.SidebarTitle;
if (partId === Parts.PANEL_PART) {
location = ViewContainerLocation.Panel;
registryId = Extensions.Panels;
globalActionsMenuId = MenuId.PanelTitle;
} else if (partId === Parts.AUXILIARYBAR_PART) {
location = ViewContainerLocation.AuxiliaryBar;
registryId = Extensions.Auxiliary;
globalActionsMenuId = MenuId.AuxiliaryBarTitle;
}
super(
notificationService,
storageService,
contextMenuService,
layoutService,
keybindingService,
instantiationService,
themeService,
Registry.as<PaneCompositeRegistry>(registryId),
activePaneCompositeSettingsKey,
viewDescriptorService.getDefaultViewContainer(location)?.id || '',
nameForTelemetry,
compositeCSSClass,
titleForegroundColor,
partId,
partOptions
);
this.location = location;
this.globalActions = this._register(this.instantiationService.createInstance(CompositeMenuActions, globalActionsMenuId, undefined, undefined));
this.registerListeners();
}
private registerListeners(): void {
this._register(this.onDidPaneCompositeOpen(composite => this.onDidOpen(composite)));
this._register(this.onDidPaneCompositeClose(this.onDidClose, this));
this._register(this.globalActions.onDidChange(() => this.updateGlobalToolbarActions()));
this._register(this.registry.onDidDeregister(async (viewletDescriptor: PaneCompositeDescriptor) => {
const activeContainers = this.viewDescriptorService.getViewContainersByLocation(this.location)
.filter(container => this.viewDescriptorService.getViewContainerModel(container).activeViewDescriptors.length > 0);
if (activeContainers.length) {
if (this.getActiveComposite()?.getId() === viewletDescriptor.id) {
const defaultViewletId = this.viewDescriptorService.getDefaultViewContainer(this.location)?.id;
const containerToOpen = activeContainers.filter(c => c.id === defaultViewletId)[0] || activeContainers[0];
await this.openPaneComposite(containerToOpen.id);
}
} else {
this.layoutService.setPartHidden(true, this.partId);
}
this.removeComposite(viewletDescriptor.id);
}));
this._register(this.extensionService.onDidRegisterExtensions(() => {
this.layoutCompositeBar();
}));
}
private onDidOpen(composite: IComposite): void {
this.activePaneContextKey.set(composite.getId());
}
private onDidClose(composite: IComposite): void {
const id = composite.getId();
if (this.activePaneContextKey.get() === id) {
this.activePaneContextKey.reset();
}
}
protected override showComposite(composite: Composite): void {
super.showComposite(composite);
this.layoutCompositeBar();
this.layoutEmptyMessage();
}
protected override hideActiveComposite(): Composite | undefined {
const composite = super.hideActiveComposite();
this.layoutCompositeBar();
this.layoutEmptyMessage();
return composite;
}
override create(parent: HTMLElement): void {
this.element = parent;
this.element.classList.add('pane-composite-part');
super.create(parent);
const contentArea = this.getContentArea();
if (contentArea) {
this.createEmptyPaneMessage(contentArea);
}
const focusTracker = this._register(trackFocus(parent));
this._register(focusTracker.onDidFocus(() => this.paneFocusContextKey.set(true)));
this._register(focusTracker.onDidBlur(() => this.paneFocusContextKey.set(false)));
}
private createEmptyPaneMessage(parent: HTMLElement): void {
this.emptyPaneMessageElement = document.createElement('div');
this.emptyPaneMessageElement.classList.add('empty-pane-message-area');
const messageElement = document.createElement('div');
messageElement.classList.add('empty-pane-message');
messageElement.innerText = localize('pane.emptyMessage', "Drag a view here to display.");
this.emptyPaneMessageElement.appendChild(messageElement);
parent.appendChild(this.emptyPaneMessageElement);
this._register(CompositeDragAndDropObserver.INSTANCE.registerTarget(this.emptyPaneMessageElement, {
onDragOver: (e) => {
EventHelper.stop(e.eventData, true);
if (this.paneCompositeBar.value) {
const validDropTarget = this.paneCompositeBar.value.dndHandler.onDragEnter(e.dragAndDropData, undefined, e.eventData);
toggleDropEffect(e.eventData.dataTransfer, 'move', validDropTarget);
}
},
onDragEnter: (e) => {
EventHelper.stop(e.eventData, true);
if (this.paneCompositeBar.value) {
const validDropTarget = this.paneCompositeBar.value.dndHandler.onDragEnter(e.dragAndDropData, undefined, e.eventData);
this.emptyPaneMessageElement!.style.backgroundColor = validDropTarget ? this.theme.getColor(EDITOR_DRAG_AND_DROP_BACKGROUND)?.toString() || '' : '';
}
},
onDragLeave: (e) => {
EventHelper.stop(e.eventData, true);
this.emptyPaneMessageElement!.style.backgroundColor = '';
},
onDragEnd: (e) => {
EventHelper.stop(e.eventData, true);
this.emptyPaneMessageElement!.style.backgroundColor = '';
},
onDrop: (e) => {
EventHelper.stop(e.eventData, true);
this.emptyPaneMessageElement!.style.backgroundColor = '';
if (this.paneCompositeBar.value) {
this.paneCompositeBar.value.dndHandler.drop(e.dragAndDropData, undefined, e.eventData);
}
},
}));
}
protected override createTitleArea(parent: HTMLElement): HTMLElement {
const titleArea = super.createTitleArea(parent);
this._register(addDisposableListener(titleArea, EventType.CONTEXT_MENU, e => {
this.onTitleAreaContextMenu(new StandardMouseEvent(getWindow(titleArea), e));
}));
this._register(Gesture.addTarget(titleArea));
this._register(addDisposableListener(titleArea, GestureEventType.Contextmenu, e => {
this.onTitleAreaContextMenu(new StandardMouseEvent(getWindow(titleArea), e));
}));
const globalTitleActionsContainer = titleArea.appendChild($('.global-actions'));
// Global Actions Toolbar
this.globalToolBar = this._register(new ToolBar(globalTitleActionsContainer, this.contextMenuService, {
actionViewItemProvider: action => this.actionViewItemProvider(action),
orientation: ActionsOrientation.HORIZONTAL,
getKeyBinding: action => this.keybindingService.lookupKeybinding(action.id),
anchorAlignmentProvider: () => this.getTitleAreaDropDownAnchorAlignment(),
toggleMenuTitle: localize('moreActions', "More Actions...")
}));
this.updateGlobalToolbarActions();
return titleArea;
}
protected override createTitleLabel(parent: HTMLElement): ICompositeTitleLabel {
this.titleContainer = parent;
const titleLabel = super.createTitleLabel(parent);
this.titleLabelElement!.draggable = true;
const draggedItemProvider = (): { type: 'view' | 'composite'; id: string } => {
const activeViewlet = this.getActivePaneComposite()!;
return { type: 'composite', id: activeViewlet.getId() };
};
this._register(CompositeDragAndDropObserver.INSTANCE.registerDraggable(this.titleLabelElement!, draggedItemProvider, {}));
this.updateTitleArea();
return titleLabel;
}
protected updateTitleArea(): void {
if (!this.titleContainer) {
return;
}
if (this.shouldShowCompositeBar()) {
if (!this.paneCompositeBar.value) {
this.titleContainer.classList.add('has-composite-bar');
this.paneCompositeBarContainer = prepend(this.titleContainer, $('.composite-bar-container'));
this.paneCompositeBar.value = this.createCompisteBar();
this.paneCompositeBar.value.create(this.paneCompositeBarContainer);
}
} else {
this.titleContainer.classList.remove('has-composite-bar');
this.paneCompositeBarContainer?.remove();
this.paneCompositeBarContainer = undefined;
this.paneCompositeBar.clear();
}
}
protected createCompisteBar(): PaneCompositeBar {
return this.instantiationService.createInstance(PaneCompositeBar, this.getCompositeBarOptions(), this.partId, this);
}
protected override onTitleAreaUpdate(compositeId: string): void {
super.onTitleAreaUpdate(compositeId);
// If title actions change, relayout the composite bar
this.layoutCompositeBar();
}
async openPaneComposite(id?: string, focus?: boolean): Promise<PaneComposite | undefined> {
if (typeof id === 'string' && this.getPaneComposite(id)) {
return this.doOpenPaneComposite(id, focus);
}
await this.extensionService.whenInstalledExtensionsRegistered();
if (typeof id === 'string' && this.getPaneComposite(id)) {
return this.doOpenPaneComposite(id, focus);
}
return undefined;
}
private doOpenPaneComposite(id: string, focus?: boolean): PaneComposite | undefined {
if (this.blockOpening) {
return undefined; // Workaround against a potential race condition
}
if (!this.layoutService.isVisible(this.partId)) {
try {
this.blockOpening = true;
this.layoutService.setPartHidden(false, this.partId);
} finally {
this.blockOpening = false;
}
}
return this.openComposite(id, focus) as PaneComposite;
}
getPaneComposite(id: string): PaneCompositeDescriptor | undefined {
return (this.registry as PaneCompositeRegistry).getPaneComposite(id);
}
getPaneComposites(): PaneCompositeDescriptor[] {
return (this.registry as PaneCompositeRegistry).getPaneComposites()
.sort((v1, v2) => {
if (typeof v1.order !== 'number') {
return 1;
}
if (typeof v2.order !== 'number') {
return -1;
}
return v1.order - v2.order;
});
}
getPinnedPaneCompositeIds(): string[] {
return this.paneCompositeBar.value?.getPinnedPaneCompositeIds() ?? [];
}
getVisiblePaneCompositeIds(): string[] {
return this.paneCompositeBar.value?.getVisiblePaneCompositeIds() ?? [];
}
getActivePaneComposite(): IPaneComposite | undefined {
return <IPaneComposite>this.getActiveComposite();
}
getLastActivePaneCompositeId(): string {
return this.getLastActiveCompositeId();
}
hideActivePaneComposite(): void {
if (this.layoutService.isVisible(this.partId)) {
this.layoutService.setPartHidden(true, this.partId);
}
this.hideActiveComposite();
}
protected focusComositeBar(): void {
this.paneCompositeBar.value?.focus();
}
override layout(width: number, height: number, top: number, left: number): void {
if (!this.layoutService.isVisible(this.partId)) {
return;
}
this.contentDimension = new Dimension(width, height);
// Layout contents
super.layout(this.contentDimension.width, this.contentDimension.height, top, left);
// Layout composite bar
this.layoutCompositeBar();
// Add empty pane message
this.layoutEmptyMessage();
}
private layoutCompositeBar(): void {
if (this.contentDimension && this.dimension && this.paneCompositeBar.value) {
let availableWidth = this.contentDimension.width - 16; // take padding into account
if (this.toolBar) {
availableWidth = Math.max(AbstractPaneCompositePart.MIN_COMPOSITE_BAR_WIDTH, availableWidth - this.getToolbarWidth());
}
this.paneCompositeBar.value.layout(availableWidth, this.dimension.height);
}
}
private layoutEmptyMessage(): void {
this.emptyPaneMessageElement?.classList.toggle('visible', !this.getActiveComposite());
}
private updateGlobalToolbarActions(): void {
const primaryActions = this.globalActions.getPrimaryActions();
const secondaryActions = this.globalActions.getSecondaryActions();
this.globalToolBar?.setActions(prepareActions(primaryActions), prepareActions(secondaryActions));
}
protected getToolbarWidth(): number {
const activePane = this.getActivePaneComposite();
if (!activePane || !this.toolBar) {
return 0;
}
return this.toolBar.getItemsWidth() + 5 + (this.globalToolBar?.getItemsWidth() ?? 0); // 5px toolBar padding-left
}
private onTitleAreaContextMenu(event: StandardMouseEvent): void {
if (this.shouldShowCompositeBar() && this.paneCompositeBar.value) {
const actions: IAction[] = [...this.paneCompositeBar.value.getContextMenuActions()];
if (actions.length) {
this.contextMenuService.showContextMenu({
getAnchor: () => event,
getActions: () => actions,
skipTelemetry: true
});
}
} else {
const activePaneComposite = this.getActivePaneComposite() as PaneComposite;
const activePaneCompositeActions = activePaneComposite ? activePaneComposite.getContextMenuActions() : [];
if (activePaneCompositeActions.length) {
this.contextMenuService.showContextMenu({
getAnchor: () => event,
getActions: () => activePaneCompositeActions,
getActionViewItem: action => this.actionViewItemProvider(action),
actionRunner: activePaneComposite.getActionRunner(),
skipTelemetry: true
});
}
}
}
protected getViewsSubmenuAction(): SubmenuAction | undefined {
const viewPaneContainer = (this.getActivePaneComposite() as PaneComposite)?.getViewPaneContainer();
if (viewPaneContainer) {
const disposables = new DisposableStore();
const viewsActions: IAction[] = [];
const scopedContextKeyService = disposables.add(this.contextKeyService.createScoped(this.element));
scopedContextKeyService.createKey('viewContainer', viewPaneContainer.viewContainer.id);
const menu = disposables.add(this.menuService.createMenu(ViewsSubMenu, scopedContextKeyService));
createAndFillInActionBarActions(menu, { shouldForwardArgs: true, renderShortTitle: true }, { primary: viewsActions, secondary: [] }, () => true);
disposables.dispose();
return viewsActions.length > 1 && viewsActions.some(a => a.enabled) ? new SubmenuAction('views', localize('views', "Views"), viewsActions) : undefined;
}
return undefined;
}
protected abstract shouldShowCompositeBar(): boolean;
protected abstract getCompositeBarOptions(): IPaneCompositeBarOptions;
}
| src/vs/workbench/browser/parts/paneCompositePart.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017841123917605728,
0.00017405809194315225,
0.00016499935009051114,
0.00017463557014707476,
0.000002612723847050802
]
|
{
"id": 2,
"code_window": [
"\t\t// Compute the watcher exclude rules from configuration\n",
"\t\tconst excludes: string[] = [];\n",
"\t\tconst config = this.configurationService.getValue<IFilesConfiguration>({ resource: workspace.uri });\n",
"\t\tif (config.files?.watcherExclude) {\n",
"\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\texcludes.push(key);\n",
"\t\t\t\t}\n",
"\t\t\t}\n",
"\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/contrib/files/browser/workspaceWatcher.ts",
"type": "replace",
"edit_start_line_idx": 111
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { TextDocument } from 'vscode-languageserver';
export interface LanguageModelCache<T> {
get(document: TextDocument): T;
onDocumentRemoved(document: TextDocument): void;
dispose(): void;
}
export function getLanguageModelCache<T>(maxEntries: number, cleanupIntervalTimeInSec: number, parse: (document: TextDocument) => T): LanguageModelCache<T> {
let languageModels: { [uri: string]: { version: number; languageId: string; cTime: number; languageModel: T } } = {};
let nModels = 0;
let cleanupInterval: NodeJS.Timer | undefined = undefined;
if (cleanupIntervalTimeInSec > 0) {
cleanupInterval = setInterval(() => {
const cutoffTime = Date.now() - cleanupIntervalTimeInSec * 1000;
const uris = Object.keys(languageModels);
for (const uri of uris) {
const languageModelInfo = languageModels[uri];
if (languageModelInfo.cTime < cutoffTime) {
delete languageModels[uri];
nModels--;
}
}
}, cleanupIntervalTimeInSec * 1000);
}
return {
get(document: TextDocument): T {
const version = document.version;
const languageId = document.languageId;
const languageModelInfo = languageModels[document.uri];
if (languageModelInfo && languageModelInfo.version === version && languageModelInfo.languageId === languageId) {
languageModelInfo.cTime = Date.now();
return languageModelInfo.languageModel;
}
const languageModel = parse(document);
languageModels[document.uri] = { languageModel, version, languageId, cTime: Date.now() };
if (!languageModelInfo) {
nModels++;
}
if (nModels === maxEntries) {
let oldestTime = Number.MAX_VALUE;
let oldestUri = null;
for (const uri in languageModels) {
const languageModelInfo = languageModels[uri];
if (languageModelInfo.cTime < oldestTime) {
oldestUri = uri;
oldestTime = languageModelInfo.cTime;
}
}
if (oldestUri) {
delete languageModels[oldestUri];
nModels--;
}
}
return languageModel;
},
onDocumentRemoved(document: TextDocument) {
const uri = document.uri;
if (languageModels[uri]) {
delete languageModels[uri];
nModels--;
}
},
dispose() {
if (typeof cleanupInterval !== 'undefined') {
clearInterval(cleanupInterval);
cleanupInterval = undefined;
languageModels = {};
nModels = 0;
}
}
};
} | extensions/json-language-features/server/src/languageModelCache.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017851419397629797,
0.00017515980289317667,
0.00017320159531664103,
0.00017496207146905363,
0.000001627961069061712
]
|
{
"id": 2,
"code_window": [
"\t\t// Compute the watcher exclude rules from configuration\n",
"\t\tconst excludes: string[] = [];\n",
"\t\tconst config = this.configurationService.getValue<IFilesConfiguration>({ resource: workspace.uri });\n",
"\t\tif (config.files?.watcherExclude) {\n",
"\t\t\tfor (const key in config.files.watcherExclude) {\n",
"\t\t\t\tif (config.files.watcherExclude[key] === true) {\n",
"\t\t\t\t\texcludes.push(key);\n",
"\t\t\t\t}\n",
"\t\t\t}\n",
"\t\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\t\tif (key && config.files.watcherExclude[key] === true) {\n"
],
"file_path": "src/vs/workbench/contrib/files/browser/workspaceWatcher.ts",
"type": "replace",
"edit_start_line_idx": 111
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Position } from 'vs/editor/common/core/position';
import { Selection } from 'vs/editor/common/core/selection';
/**
* Describes the reason the cursor has changed its position.
*/
export const enum CursorChangeReason {
/**
* Unknown or not set.
*/
NotSet = 0,
/**
* A `model.setValue()` was called.
*/
ContentFlush = 1,
/**
* The `model` has been changed outside of this cursor and the cursor recovers its position from associated markers.
*/
RecoverFromMarkers = 2,
/**
* There was an explicit user gesture.
*/
Explicit = 3,
/**
* There was a Paste.
*/
Paste = 4,
/**
* There was an Undo.
*/
Undo = 5,
/**
* There was a Redo.
*/
Redo = 6,
}
/**
* An event describing that the cursor position has changed.
*/
export interface ICursorPositionChangedEvent {
/**
* Primary cursor's position.
*/
readonly position: Position;
/**
* Secondary cursors' position.
*/
readonly secondaryPositions: Position[];
/**
* Reason.
*/
readonly reason: CursorChangeReason;
/**
* Source of the call that caused the event.
*/
readonly source: string;
}
/**
* An event describing that the cursor selection has changed.
*/
export interface ICursorSelectionChangedEvent {
/**
* The primary selection.
*/
readonly selection: Selection;
/**
* The secondary selections.
*/
readonly secondarySelections: Selection[];
/**
* The model version id.
*/
readonly modelVersionId: number;
/**
* The old selections.
*/
readonly oldSelections: Selection[] | null;
/**
* The model version id the that `oldSelections` refer to.
*/
readonly oldModelVersionId: number;
/**
* Source of the call that caused the event.
*/
readonly source: string;
/**
* Reason.
*/
readonly reason: CursorChangeReason;
}
| src/vs/editor/common/cursorEvents.ts | 0 | https://github.com/microsoft/vscode/commit/ba2cf46e20df3edf77bdd905acde3e175d985f70 | [
0.00017772514547687024,
0.0001739950675982982,
0.00015969615196809173,
0.00017556914826855063,
0.000005020615844841814
]
|
{
"id": 0,
"code_window": [
" </Row>\n",
" </Modal.Body>\n",
" <Modal.Footer>\n",
" <Button\n",
" type=\"submit\"\n",
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "add",
"edit_start_line_idx": 264
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/* eslint camelcase: 0 */
import React from 'react';
import PropTypes from 'prop-types';
import { connect } from 'react-redux';
import {
Alert,
Button,
FormControl,
FormGroup,
ControlLabel,
Modal,
Radio,
} from 'react-bootstrap';
import { CreatableSelect } from 'src/components/Select/SupersetStyledSelect';
import { t } from '@superset-ui/translation';
import ReactMarkdown from 'react-markdown';
import { EXPLORE_ONLY_VIZ_TYPE } from '../constants';
const propTypes = {
can_overwrite: PropTypes.bool,
onHide: PropTypes.func.isRequired,
actions: PropTypes.object.isRequired,
form_data: PropTypes.object,
userId: PropTypes.string.isRequired,
dashboards: PropTypes.array.isRequired,
alert: PropTypes.string,
slice: PropTypes.object,
datasource: PropTypes.object,
};
// Session storage key for recent dashboard
const SK_DASHBOARD_ID = 'save_chart_recent_dashboard';
const SELECT_PLACEHOLDER = t('**Select** a dashboard OR **create** a new one');
class SaveModal extends React.Component {
constructor(props) {
super(props);
this.state = {
saveToDashboardId: null,
newSliceName: props.sliceName,
dashboards: [],
alert: null,
action: props.can_overwrite ? 'overwrite' : 'saveas',
vizType: props.form_data.viz_type,
};
this.onDashboardSelectChange = this.onDashboardSelectChange.bind(this);
this.onSliceNameChange = this.onSliceNameChange.bind(this);
}
componentDidMount() {
this.props.actions.fetchDashboards(this.props.userId).then(() => {
const dashboardIds = this.props.dashboards.map(
dashboard => dashboard.value,
);
let recentDashboard = sessionStorage.getItem(SK_DASHBOARD_ID);
recentDashboard = recentDashboard && parseInt(recentDashboard, 10);
if (
recentDashboard !== null &&
dashboardIds.indexOf(recentDashboard) !== -1
) {
this.setState({
saveToDashboardId: recentDashboard,
});
}
});
}
onSliceNameChange(event) {
this.setState({ newSliceName: event.target.value });
}
onDashboardSelectChange(event) {
const newDashboardName = event ? event.label : null;
const saveToDashboardId =
event && typeof event.value === 'number' ? event.value : null;
this.setState({ saveToDashboardId, newDashboardName });
}
changeAction(action) {
this.setState({ action });
}
saveOrOverwrite(gotodash) {
this.setState({ alert: null });
this.props.actions.removeSaveModalAlert();
const sliceParams = {};
if (this.props.slice && this.props.slice.slice_id) {
sliceParams.slice_id = this.props.slice.slice_id;
}
if (sliceParams.action === 'saveas') {
if (this.state.newSliceName === '') {
this.setState({ alert: t('Please enter a chart name') });
return;
}
}
sliceParams.action = this.state.action;
sliceParams.slice_name = this.state.newSliceName;
sliceParams.save_to_dashboard_id = this.state.saveToDashboardId;
sliceParams.new_dashboard_name = this.state.newDashboardName;
this.props.actions
.saveSlice(this.props.form_data, sliceParams)
.then(({ data }) => {
if (data.dashboard_id === null) {
sessionStorage.removeItem(SK_DASHBOARD_ID);
} else {
sessionStorage.setItem(SK_DASHBOARD_ID, data.dashboard_id);
}
// Go to new slice url or dashboard url
const url = gotodash ? data.dashboard_url : data.slice.slice_url;
window.location.assign(url);
});
this.props.onHide();
}
removeAlert() {
if (this.props.alert) {
this.props.actions.removeSaveModalAlert();
}
this.setState({ alert: null });
}
render() {
const canNotSaveToDash =
EXPLORE_ONLY_VIZ_TYPE.indexOf(this.state.vizType) > -1;
return (
<Modal show onHide={this.props.onHide}>
<Modal.Header closeButton>
<Modal.Title>{t('Save Chart')}</Modal.Title>
</Modal.Header>
<Modal.Body>
{(this.state.alert || this.props.alert) && (
<Alert>
{this.state.alert ? this.state.alert : this.props.alert}
<i
role="button"
tabIndex={0}
className="fa fa-close pull-right"
onClick={this.removeAlert.bind(this)}
style={{ cursor: 'pointer' }}
/>
</Alert>
)}
<FormGroup>
<Radio
id="overwrite-radio"
inline
disabled={!(this.props.can_overwrite && this.props.slice)}
checked={this.state.action === 'overwrite'}
onChange={this.changeAction.bind(this, 'overwrite')}
>
{t('Save (Overwrite)')}
</Radio>
<Radio
id="saveas-radio"
inline
checked={this.state.action === 'saveas'}
onChange={this.changeAction.bind(this, 'saveas')}
>
{' '}
{t('Save as ...')}
</Radio>
</FormGroup>
<hr />
<FormGroup>
<ControlLabel>{t('Chart name')}</ControlLabel>
<FormControl
name="new_slice_name"
type="text"
bsSize="sm"
placeholder="Name"
value={this.state.newSliceName}
onChange={this.onSliceNameChange}
/>
</FormGroup>
<FormGroup>
<ControlLabel>{t('Add to dashboard')}</ControlLabel>
<CreatableSelect
id="dashboard-creatable-select"
className="save-modal-selector"
options={this.props.dashboards}
clearable
creatable
onChange={this.onDashboardSelectChange}
autoSize={false}
value={
this.state.saveToDashboardId || this.state.newDashboardName
}
placeholder={
// Using markdown to allow for good i18n
<ReactMarkdown
source={SELECT_PLACEHOLDER}
renderers={{ paragraph: 'span' }}
/>
}
/>
</FormGroup>
</Modal.Body>
<Modal.Footer>
<div className="float-right">
<Button
type="button"
id="btn_cancel"
bsSize="sm"
onClick={this.props.onHide}
>
{t('Cancel')}
</Button>
<Button
type="button"
id="btn_modal_save_goto_dash"
bsSize="sm"
disabled={canNotSaveToDash || !this.state.newDashboardName}
onClick={this.saveOrOverwrite.bind(this, true)}
>
{t('Save & go to dashboard')}
</Button>
<Button
type="button"
id="btn_modal_save"
bsSize="sm"
bsStyle="primary"
onClick={this.saveOrOverwrite.bind(this, false)}
>
{t('Save')}
</Button>
</div>
</Modal.Footer>
</Modal>
);
}
}
SaveModal.propTypes = propTypes;
function mapStateToProps({ explore, saveModal }) {
return {
datasource: explore.datasource,
slice: explore.slice,
can_overwrite: explore.can_overwrite,
userId: explore.user_id,
dashboards: saveModal.dashboards,
alert: saveModal.saveModalAlert,
};
}
export { SaveModal };
export default connect(mapStateToProps, () => ({}))(SaveModal);
| superset-frontend/src/explore/components/SaveModal.jsx | 1 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00975429080426693,
0.0010270212078467011,
0.00016617562505416572,
0.00017341444618068635,
0.0022374573163688183
]
|
{
"id": 0,
"code_window": [
" </Row>\n",
" </Modal.Body>\n",
" <Modal.Footer>\n",
" <Button\n",
" type=\"submit\"\n",
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "add",
"edit_start_line_idx": 264
} | # Licensed to the Apache Software Foundation (ASF) under one
# or more contributor license agreements. See the NOTICE file
# distributed with this work for additional information
# regarding copyright ownership. The ASF licenses this file
# to you under the Apache License, Version 2.0 (the
# "License"); you may not use this file except in compliance
# with the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing,
# software distributed under the License is distributed on an
# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
# KIND, either express or implied. See the License for the
# specific language governing permissions and limitations
# under the License.
from datetime import datetime
from typing import Optional
from urllib import parse
from sqlalchemy.engine.url import URL
from superset.db_engine_specs.base import BaseEngineSpec
from superset.utils import core as utils
class DrillEngineSpec(BaseEngineSpec):
"""Engine spec for Apache Drill"""
engine = "drill"
_time_grain_expressions = {
None: "{col}",
"PT1S": "NEARESTDATE({col}, 'SECOND')",
"PT1M": "NEARESTDATE({col}, 'MINUTE')",
"PT15M": "NEARESTDATE({col}, 'QUARTER_HOUR')",
"PT0.5H": "NEARESTDATE({col}, 'HALF_HOUR')",
"PT1H": "NEARESTDATE({col}, 'HOUR')",
"P1D": "NEARESTDATE({col}, 'DAY')",
"P1W": "NEARESTDATE({col}, 'WEEK_SUNDAY')",
"P1M": "NEARESTDATE({col}, 'MONTH')",
"P0.25Y": "NEARESTDATE({col}, 'QUARTER')",
"P1Y": "NEARESTDATE({col}, 'YEAR')",
}
# Returns a function to convert a Unix timestamp in milliseconds to a date
@classmethod
def epoch_to_dttm(cls) -> str:
return cls.epoch_ms_to_dttm().replace("{col}", "({col}*1000)")
@classmethod
def epoch_ms_to_dttm(cls) -> str:
return "TO_DATE({col})"
@classmethod
def convert_dttm(cls, target_type: str, dttm: datetime) -> Optional[str]:
tt = target_type.upper()
if tt == utils.TemporalType.DATE:
return f"TO_DATE('{dttm.date().isoformat()}', 'yyyy-MM-dd')"
if tt == utils.TemporalType.TIMESTAMP:
return f"""TO_TIMESTAMP('{dttm.isoformat(sep=" ", timespec="seconds")}', 'yyyy-MM-dd HH:mm:ss')""" # pylint: disable=line-too-long
return None
@classmethod
def adjust_database_uri(cls, uri: URL, selected_schema: Optional[str]) -> None:
if selected_schema:
uri.database = parse.quote(selected_schema, safe="")
| superset/db_engine_specs/drill.py | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00020035379566252232,
0.0001780155289452523,
0.0001716381375445053,
0.0001749143557390198,
0.000009323649464931805
]
|
{
"id": 0,
"code_window": [
" </Row>\n",
" </Modal.Body>\n",
" <Modal.Footer>\n",
" <Button\n",
" type=\"submit\"\n",
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "add",
"edit_start_line_idx": 264
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import React from 'react';
import PropTypes from 'prop-types';
import { Popover, OverlayTrigger } from 'react-bootstrap';
import { t } from '@superset-ui/translation';
import { isFeatureEnabled, FeatureFlag } from 'src/featureFlags';
import Button from '../../components/Button';
import CopyToClipboard from '../../components/CopyToClipboard';
import { storeQuery } from '../../utils/common';
import getClientErrorObject from '../../utils/getClientErrorObject';
import withToasts from '../../messageToasts/enhancers/withToasts';
const propTypes = {
queryEditor: PropTypes.shape({
dbId: PropTypes.number,
title: PropTypes.string,
schema: PropTypes.string,
autorun: PropTypes.bool,
sql: PropTypes.string,
remoteId: PropTypes.number,
}).isRequired,
addDangerToast: PropTypes.func.isRequired,
};
class ShareSqlLabQuery extends React.Component {
constructor(props) {
super(props);
this.state = {
shortUrl: t('Loading ...'),
};
this.getCopyUrl = this.getCopyUrl.bind(this);
}
getCopyUrl() {
if (isFeatureEnabled(FeatureFlag.SHARE_QUERIES_VIA_KV_STORE)) {
return this.getCopyUrlForKvStore();
}
return this.getCopyUrlForSavedQuery();
}
getCopyUrlForKvStore() {
const { dbId, title, schema, autorun, sql } = this.props.queryEditor;
const sharedQuery = { dbId, title, schema, autorun, sql };
return storeQuery(sharedQuery)
.then(shortUrl => {
this.setState({ shortUrl });
})
.catch(response => {
getClientErrorObject(response).then(({ error }) => {
this.props.addDangerToast(error);
this.setState({ shortUrl: t('Error') });
});
});
}
getCopyUrlForSavedQuery() {
let savedQueryToastContent;
if (this.props.queryEditor.remoteId) {
savedQueryToastContent = `${
window.location.origin + window.location.pathname
}?savedQueryId=${this.props.queryEditor.remoteId}`;
this.setState({ shortUrl: savedQueryToastContent });
} else {
savedQueryToastContent = t('Please save the query to enable sharing');
this.setState({ shortUrl: savedQueryToastContent });
}
}
renderPopover() {
return (
<Popover id="sqllab-shareurl-popover">
<CopyToClipboard
text={this.state.shortUrl || t('Loading ...')}
copyNode={
<i className="fa fa-clipboard" title={t('Copy to clipboard')} />
}
/>
</Popover>
);
}
render() {
return (
<OverlayTrigger
trigger="click"
placement="top"
onEnter={this.getCopyUrl}
rootClose
shouldUpdatePosition
overlay={this.renderPopover()}
>
<Button bsSize="small" className="toggleSave">
<i className="fa fa-share" /> {t('Share')}
</Button>
</OverlayTrigger>
);
}
}
ShareSqlLabQuery.propTypes = propTypes;
export default withToasts(ShareSqlLabQuery);
| superset-frontend/src/SqlLab/components/ShareSqlLabQuery.jsx | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00023420414072461426,
0.00017769864643923938,
0.00016831987886689603,
0.00017407845007255673,
0.00001647520730330143
]
|
{
"id": 0,
"code_window": [
" </Row>\n",
" </Modal.Body>\n",
" <Modal.Footer>\n",
" <Button\n",
" type=\"submit\"\n",
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "add",
"edit_start_line_idx": 264
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(<App />, document.getElementById('app'));
| superset-frontend/src/profile/index.tsx | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00017661474703345448,
0.0001716722472338006,
0.00016405258793383837,
0.0001743493921821937,
0.000005466705715662101
]
|
{
"id": 1,
"code_window": [
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n",
" className=\"m-r-5\"\n",
" disabled={!owners || submitting}\n",
" >\n",
" {t('Save')}\n",
" </Button>\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" disabled={!owners || submitting || !name}\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 269
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import React, { useState, useEffect, useRef } from 'react';
import {
Button,
Modal,
Row,
Col,
FormControl,
FormGroup,
// @ts-ignore
} from 'react-bootstrap';
// @ts-ignore
import Dialog from 'react-bootstrap-dialog';
import { OptionsType } from 'react-select/src/types';
import { AsyncSelect } from 'src/components/Select';
import rison from 'rison';
import { t } from '@superset-ui/translation';
import { SupersetClient } from '@superset-ui/connection';
import Chart from 'src/types/Chart';
import FormLabel from 'src/components/FormLabel';
import getClientErrorObject from '../../utils/getClientErrorObject';
export type Slice = {
slice_id: number;
slice_name: string;
description: string | null;
cache_timeout: number | null;
};
type InternalProps = {
slice: Slice;
onHide: () => void;
onSave: (chart: Chart) => void;
};
type OwnerOption = {
label: string;
value: number;
};
export type WrapperProps = InternalProps & {
show: boolean;
animation?: boolean; // for the modal
};
export default function PropertiesModalWrapper({
show,
onHide,
animation,
slice,
onSave,
}: WrapperProps) {
// The wrapper is a separate component so that hooks only run when the modal opens
return (
<Modal show={show} onHide={onHide} animation={animation} bsSize="large">
<PropertiesModal slice={slice} onHide={onHide} onSave={onSave} />
</Modal>
);
}
function PropertiesModal({ slice, onHide, onSave }: InternalProps) {
const [submitting, setSubmitting] = useState(false);
const errorDialog = useRef<any>(null);
// values of form inputs
const [name, setName] = useState(slice.slice_name || '');
const [description, setDescription] = useState(slice.description || '');
const [cacheTimeout, setCacheTimeout] = useState(
slice.cache_timeout != null ? slice.cache_timeout : '',
);
const [owners, setOwners] = useState<OptionsType<OwnerOption> | null>(null);
function showError({ error, statusText }: any) {
errorDialog.current.show({
title: 'Error',
bsSize: 'medium',
bsStyle: 'danger',
actions: [Dialog.DefaultAction('Ok', () => {}, 'btn-danger')],
body: error || statusText || t('An error has occurred'),
});
}
async function fetchChartData() {
try {
const response = await SupersetClient.get({
endpoint: `/api/v1/chart/${slice.slice_id}`,
});
const chart = response.json.result;
setOwners(
chart.owners.map((owner: any) => ({
value: owner.id,
label: `${owner.first_name} ${owner.last_name}`,
})),
);
} catch (response) {
const clientError = await getClientErrorObject(response);
showError(clientError);
}
}
// get the owners of this slice
useEffect(() => {
fetchChartData();
}, []);
const loadOptions = (input = '') => {
const query = rison.encode({
filter: input,
});
return SupersetClient.get({
endpoint: `/api/v1/chart/related/owners?q=${query}`,
}).then(
response => {
const { result } = response.json;
return result.map((item: any) => ({
value: item.value,
label: item.text,
}));
},
badResponse => {
getClientErrorObject(badResponse).then(showError);
return [];
},
);
};
const onSubmit = async (event: React.FormEvent) => {
event.stopPropagation();
event.preventDefault();
setSubmitting(true);
const payload: { [key: string]: any } = {
slice_name: name || null,
description: description || null,
cache_timeout: cacheTimeout || null,
};
if (owners) {
payload.owners = owners.map(o => o.value);
}
try {
const res = await SupersetClient.put({
endpoint: `/api/v1/chart/${slice.slice_id}`,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(payload),
});
// update the redux state
const updatedChart = {
...res.json.result,
id: slice.slice_id,
};
onSave(updatedChart);
onHide();
} catch (res) {
const clientError = await getClientErrorObject(res);
showError(clientError);
}
setSubmitting(false);
};
return (
<form onSubmit={onSubmit}>
<Modal.Header closeButton>
<Modal.Title>Edit Chart Properties</Modal.Title>
</Modal.Header>
<Modal.Body>
<Row>
<Col md={6}>
<h3>{t('Basic Information')}</h3>
<FormGroup>
<FormLabel htmlFor="name" required>
{t('Name')}
</FormLabel>
<FormControl
name="name"
type="text"
bsSize="sm"
value={name}
// @ts-ignore
onChange={(event: React.ChangeEvent<HTMLInputElement>) =>
setName(event.target.value)
}
/>
</FormGroup>
<FormGroup>
<FormLabel htmlFor="description">{t('Description')}</FormLabel>
<FormControl
name="description"
type="text"
componentClass="textarea"
bsSize="sm"
value={description}
// @ts-ignore
onChange={(event: React.ChangeEvent<HTMLInputElement>) =>
setDescription(event.target.value)
}
style={{ maxWidth: '100%' }}
/>
<p className="help-block">
{t(
'The description can be displayed as widget headers in the dashboard view. Supports markdown.',
)}
</p>
</FormGroup>
</Col>
<Col md={6}>
<h3>{t('Configuration')}</h3>
<FormGroup>
<FormLabel htmlFor="cacheTimeout">{t('Cache Timeout')}</FormLabel>
<FormControl
name="cacheTimeout"
type="text"
bsSize="sm"
value={cacheTimeout}
// @ts-ignore
onChange={(event: React.ChangeEvent<HTMLInputElement>) =>
setCacheTimeout(event.target.value.replace(/[^0-9]/, ''))
}
/>
<p className="help-block">
{t(
'Duration (in seconds) of the caching timeout for this chart. Note this defaults to the datasource/table timeout if undefined.',
)}
</p>
</FormGroup>
<h3 style={{ marginTop: '1em' }}>{t('Access')}</h3>
<FormGroup>
<FormLabel htmlFor="owners">{t('Owners')}</FormLabel>
<AsyncSelect
isMulti
name="owners"
value={owners || []}
loadOptions={loadOptions}
defaultOptions // load options on render
cacheOptions
onChange={setOwners}
disabled={!owners}
filterOption={null} // options are filtered at the api
/>
<p className="help-block">
{t(
'A list of users who can alter the chart. Searchable by name or username.',
)}
</p>
</FormGroup>
</Col>
</Row>
</Modal.Body>
<Modal.Footer>
<Button
type="submit"
bsSize="sm"
bsStyle="primary"
className="m-r-5"
disabled={!owners || submitting}
>
{t('Save')}
</Button>
<Button type="button" bsSize="sm" onClick={onHide}>
{t('Cancel')}
</Button>
<Dialog ref={errorDialog} />
</Modal.Footer>
</form>
);
}
| superset-frontend/src/explore/components/PropertiesModal.tsx | 1 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.9676498770713806,
0.03465815633535385,
0.00016451407282147557,
0.0004104551044292748,
0.17633113265037537
]
|
{
"id": 1,
"code_window": [
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n",
" className=\"m-r-5\"\n",
" disabled={!owners || submitting}\n",
" >\n",
" {t('Save')}\n",
" </Button>\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" disabled={!owners || submitting || !name}\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 269
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import React, { SVGProps } from 'react';
import { ReactComponent as CancelXIcon } from 'images/icons/cancel-x.svg';
import { ReactComponent as CheckIcon } from 'images/icons/check.svg';
import { ReactComponent as CircleCheckIcon } from 'images/icons/circle-check.svg';
import { ReactComponent as CircleCheckSolidIcon } from 'images/icons/circle-check-solid.svg';
import { ReactComponent as CheckboxHalfIcon } from 'images/icons/checkbox-half.svg';
import { ReactComponent as CheckboxOffIcon } from 'images/icons/checkbox-off.svg';
import { ReactComponent as CheckboxOnIcon } from 'images/icons/checkbox-on.svg';
import { ReactComponent as CloseIcon } from 'images/icons/close.svg';
import { ReactComponent as CompassIcon } from 'images/icons/compass.svg';
import { ReactComponent as DatasetPhysicalIcon } from 'images/icons/dataset_physical.svg';
import { ReactComponent as DatasetVirtualIcon } from 'images/icons/dataset_virtual.svg';
import { ReactComponent as ErrorIcon } from 'images/icons/error.svg';
import { ReactComponent as PencilIcon } from 'images/icons/pencil.svg';
import { ReactComponent as SearchIcon } from 'images/icons/search.svg';
import { ReactComponent as SortAscIcon } from 'images/icons/sort-asc.svg';
import { ReactComponent as SortDescIcon } from 'images/icons/sort-desc.svg';
import { ReactComponent as SortIcon } from 'images/icons/sort.svg';
import { ReactComponent as TrashIcon } from 'images/icons/trash.svg';
import { ReactComponent as WarningIcon } from 'images/icons/warning.svg';
import { ReactComponent as ShareIcon } from 'images/icons/share.svg';
type IconName =
| 'cancel-x'
| 'check'
| 'checkbox-half'
| 'checkbox-off'
| 'checkbox-on'
| 'close'
| 'circle-check'
| 'circle-check-solid'
| 'compass'
| 'dataset-physical'
| 'dataset-virtual'
| 'error'
| 'pencil'
| 'search'
| 'sort'
| 'sort-asc'
| 'sort-desc'
| 'trash'
| 'share'
| 'warning';
const iconsRegistry: Record<
IconName,
React.ComponentType<SVGProps<SVGSVGElement>>
> = {
'cancel-x': CancelXIcon,
'checkbox-half': CheckboxHalfIcon,
'checkbox-off': CheckboxOffIcon,
'checkbox-on': CheckboxOnIcon,
'circle-check': CircleCheckIcon,
'circle-check-solid': CircleCheckSolidIcon,
'dataset-physical': DatasetPhysicalIcon,
'dataset-virtual': DatasetVirtualIcon,
'sort-asc': SortAscIcon,
'sort-desc': SortDescIcon,
check: CheckIcon,
close: CloseIcon,
compass: CompassIcon,
error: ErrorIcon,
pencil: PencilIcon,
search: SearchIcon,
sort: SortIcon,
trash: TrashIcon,
warning: WarningIcon,
share: ShareIcon,
};
interface IconProps extends SVGProps<SVGSVGElement> {
name: IconName;
}
const Icon = ({ name, color = '#666666', ...rest }: IconProps) => {
const Component = iconsRegistry[name];
return <Component color={color} data-test={name} {...rest} />;
};
export default Icon;
| superset-frontend/src/components/Icon.tsx | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00022098107729107141,
0.00017938113887794316,
0.00016976729966700077,
0.00017529880278743804,
0.000014055371138965711
]
|
{
"id": 1,
"code_window": [
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n",
" className=\"m-r-5\"\n",
" disabled={!owners || submitting}\n",
" >\n",
" {t('Save')}\n",
" </Button>\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" disabled={!owners || submitting || !name}\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 269
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import React from 'react';
import PropTypes from 'prop-types';
import { t } from '@superset-ui/translation';
import ErrorMessageWithStackTrace from './ErrorMessage/ErrorMessageWithStackTrace';
const propTypes = {
children: PropTypes.node.isRequired,
onError: PropTypes.func,
showMessage: PropTypes.bool,
};
const defaultProps = {
onError: () => {},
showMessage: true,
};
export default class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { error: null, info: null };
}
componentDidCatch(error, info) {
this.props.onError(error, info);
this.setState({ error, info });
}
render() {
const { error, info } = this.state;
if (error) {
const firstLine = error.toString();
const message = (
<span>
<strong>{t('Unexpected error')}</strong>
{firstLine ? `: ${firstLine}` : ''}
</span>
);
if (this.props.showMessage) {
return (
<ErrorMessageWithStackTrace
message={message}
stackTrace={info ? info.componentStack : null}
/>
);
}
return null;
}
return this.props.children;
}
}
ErrorBoundary.propTypes = propTypes;
ErrorBoundary.defaultProps = defaultProps;
| superset-frontend/src/components/ErrorBoundary.jsx | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.0001751433446770534,
0.00016773364041000605,
0.00016153394244611263,
0.00016676135419402272,
0.000004544665898720268
]
|
{
"id": 1,
"code_window": [
" bsSize=\"sm\"\n",
" bsStyle=\"primary\"\n",
" className=\"m-r-5\"\n",
" disabled={!owners || submitting}\n",
" >\n",
" {t('Save')}\n",
" </Button>\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" disabled={!owners || submitting || !name}\n"
],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 269
} | # Licensed to the Apache Software Foundation (ASF) under one
# or more contributor license agreements. See the NOTICE file
# distributed with this work for additional information
# regarding copyright ownership. The ASF licenses this file
# to you under the Apache License, Version 2.0 (the
# "License"); you may not use this file except in compliance
# with the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing,
# software distributed under the License is distributed on an
# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
# KIND, either express or implied. See the License for the
# specific language governing permissions and limitations
# under the License.
# pylint: disable=too-few-public-methods
import json
import logging
from typing import Any, Dict, List, Optional, Union
from urllib import request
from urllib.error import URLError
from celery.utils.log import get_task_logger
from sqlalchemy import and_, func
from superset import app, db
from superset.extensions import celery_app
from superset.models.core import Log
from superset.models.dashboard import Dashboard
from superset.models.slice import Slice
from superset.models.tags import Tag, TaggedObject
from superset.utils.core import parse_human_datetime
from superset.views.utils import build_extra_filters
logger = get_task_logger(__name__)
logger.setLevel(logging.INFO)
def get_form_data(
chart_id: int, dashboard: Optional[Dashboard] = None
) -> Dict[str, Any]:
"""
Build `form_data` for chart GET request from dashboard's `default_filters`.
When a dashboard has `default_filters` they need to be added as extra
filters in the GET request for charts.
"""
form_data: Dict[str, Any] = {"slice_id": chart_id}
if dashboard is None or not dashboard.json_metadata:
return form_data
json_metadata = json.loads(dashboard.json_metadata)
default_filters = json.loads(json_metadata.get("default_filters", "null"))
if not default_filters:
return form_data
filter_scopes = json_metadata.get("filter_scopes", {})
layout = json.loads(dashboard.position_json or "{}")
if (
isinstance(layout, dict)
and isinstance(filter_scopes, dict)
and isinstance(default_filters, dict)
):
extra_filters = build_extra_filters(
layout, filter_scopes, default_filters, chart_id
)
if extra_filters:
form_data["extra_filters"] = extra_filters
return form_data
def get_url(chart: Slice, extra_filters: Optional[Dict[str, Any]] = None) -> str:
"""Return external URL for warming up a given chart/table cache."""
with app.test_request_context():
baseurl = (
"{SUPERSET_WEBSERVER_PROTOCOL}://"
"{SUPERSET_WEBSERVER_ADDRESS}:"
"{SUPERSET_WEBSERVER_PORT}".format(**app.config)
)
return f"{baseurl}{chart.get_explore_url(overrides=extra_filters)}"
class Strategy:
"""
A cache warm up strategy.
Each strategy defines a `get_urls` method that returns a list of URLs to
be fetched from the `/superset/warm_up_cache/` endpoint.
Strategies can be configured in `superset/config.py`:
CELERYBEAT_SCHEDULE = {
'cache-warmup-hourly': {
'task': 'cache-warmup',
'schedule': crontab(minute=1, hour='*'), # @hourly
'kwargs': {
'strategy_name': 'top_n_dashboards',
'top_n': 10,
'since': '7 days ago',
},
},
}
"""
def __init__(self) -> None:
pass
def get_urls(self) -> List[str]:
raise NotImplementedError("Subclasses must implement get_urls!")
class DummyStrategy(Strategy):
"""
Warm up all charts.
This is a dummy strategy that will fetch all charts. Can be configured by:
CELERYBEAT_SCHEDULE = {
'cache-warmup-hourly': {
'task': 'cache-warmup',
'schedule': crontab(minute=1, hour='*'), # @hourly
'kwargs': {'strategy_name': 'dummy'},
},
}
"""
name = "dummy"
def get_urls(self) -> List[str]:
charts = db.session.query(Slice).all()
return [get_url(chart) for chart in charts]
class TopNDashboardsStrategy(Strategy):
"""
Warm up charts in the top-n dashboards.
CELERYBEAT_SCHEDULE = {
'cache-warmup-hourly': {
'task': 'cache-warmup',
'schedule': crontab(minute=1, hour='*'), # @hourly
'kwargs': {
'strategy_name': 'top_n_dashboards',
'top_n': 5,
'since': '7 days ago',
},
},
}
"""
name = "top_n_dashboards"
def __init__(self, top_n: int = 5, since: str = "7 days ago") -> None:
super(TopNDashboardsStrategy, self).__init__()
self.top_n = top_n
self.since = parse_human_datetime(since) if since else None
def get_urls(self) -> List[str]:
urls = []
records = (
db.session.query(Log.dashboard_id, func.count(Log.dashboard_id))
.filter(and_(Log.dashboard_id.isnot(None), Log.dttm >= self.since))
.group_by(Log.dashboard_id)
.order_by(func.count(Log.dashboard_id).desc())
.limit(self.top_n)
.all()
)
dash_ids = [record.dashboard_id for record in records]
dashboards = (
db.session.query(Dashboard).filter(Dashboard.id.in_(dash_ids)).all()
)
for dashboard in dashboards:
for chart in dashboard.slices:
form_data_with_filters = get_form_data(chart.id, dashboard)
urls.append(get_url(chart, form_data_with_filters))
return urls
class DashboardTagsStrategy(Strategy):
"""
Warm up charts in dashboards with custom tags.
CELERYBEAT_SCHEDULE = {
'cache-warmup-hourly': {
'task': 'cache-warmup',
'schedule': crontab(minute=1, hour='*'), # @hourly
'kwargs': {
'strategy_name': 'dashboard_tags',
'tags': ['core', 'warmup'],
},
},
}
"""
name = "dashboard_tags"
def __init__(self, tags: Optional[List[str]] = None) -> None:
super(DashboardTagsStrategy, self).__init__()
self.tags = tags or []
def get_urls(self) -> List[str]:
urls = []
tags = db.session.query(Tag).filter(Tag.name.in_(self.tags)).all()
tag_ids = [tag.id for tag in tags]
# add dashboards that are tagged
tagged_objects = (
db.session.query(TaggedObject)
.filter(
and_(
TaggedObject.object_type == "dashboard",
TaggedObject.tag_id.in_(tag_ids),
)
)
.all()
)
dash_ids = [tagged_object.object_id for tagged_object in tagged_objects]
tagged_dashboards = db.session.query(Dashboard).filter(
Dashboard.id.in_(dash_ids)
)
for dashboard in tagged_dashboards:
for chart in dashboard.slices:
urls.append(get_url(chart))
# add charts that are tagged
tagged_objects = (
db.session.query(TaggedObject)
.filter(
and_(
TaggedObject.object_type == "chart",
TaggedObject.tag_id.in_(tag_ids),
)
)
.all()
)
chart_ids = [tagged_object.object_id for tagged_object in tagged_objects]
tagged_charts = db.session.query(Slice).filter(Slice.id.in_(chart_ids))
for chart in tagged_charts:
urls.append(get_url(chart))
return urls
strategies = [DummyStrategy, TopNDashboardsStrategy, DashboardTagsStrategy]
@celery_app.task(name="cache-warmup")
def cache_warmup(
strategy_name: str, *args: Any, **kwargs: Any
) -> Union[Dict[str, List[str]], str]:
"""
Warm up cache.
This task periodically hits charts to warm up the cache.
"""
logger.info("Loading strategy")
class_ = None
for class_ in strategies:
if class_.name == strategy_name: # type: ignore
break
else:
message = f"No strategy {strategy_name} found!"
logger.error(message)
return message
logger.info("Loading %s", class_.__name__)
try:
strategy = class_(*args, **kwargs)
logger.info("Success!")
except TypeError:
message = "Error loading strategy!"
logger.exception(message)
return message
results: Dict[str, List[str]] = {"success": [], "errors": []}
for url in strategy.get_urls():
try:
logger.info("Fetching %s", url)
request.urlopen(url)
results["success"].append(url)
except URLError:
logger.exception("Error warming up cache!")
results["errors"].append(url)
return results
| superset/tasks/cache.py | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.005488530267030001,
0.00036442180862650275,
0.00016532644804101437,
0.00017084492719732225,
0.0009529055678285658
]
|
{
"id": 2,
"code_window": [
" >\n",
" {t('Save')}\n",
" </Button>\n",
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n",
" <Dialog ref={errorDialog} />\n",
" </Modal.Footer>\n",
" </form>\n",
" );\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 273
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/* eslint camelcase: 0 */
import React from 'react';
import PropTypes from 'prop-types';
import { connect } from 'react-redux';
import {
Alert,
Button,
FormControl,
FormGroup,
ControlLabel,
Modal,
Radio,
} from 'react-bootstrap';
import { CreatableSelect } from 'src/components/Select/SupersetStyledSelect';
import { t } from '@superset-ui/translation';
import ReactMarkdown from 'react-markdown';
import { EXPLORE_ONLY_VIZ_TYPE } from '../constants';
const propTypes = {
can_overwrite: PropTypes.bool,
onHide: PropTypes.func.isRequired,
actions: PropTypes.object.isRequired,
form_data: PropTypes.object,
userId: PropTypes.string.isRequired,
dashboards: PropTypes.array.isRequired,
alert: PropTypes.string,
slice: PropTypes.object,
datasource: PropTypes.object,
};
// Session storage key for recent dashboard
const SK_DASHBOARD_ID = 'save_chart_recent_dashboard';
const SELECT_PLACEHOLDER = t('**Select** a dashboard OR **create** a new one');
class SaveModal extends React.Component {
constructor(props) {
super(props);
this.state = {
saveToDashboardId: null,
newSliceName: props.sliceName,
dashboards: [],
alert: null,
action: props.can_overwrite ? 'overwrite' : 'saveas',
vizType: props.form_data.viz_type,
};
this.onDashboardSelectChange = this.onDashboardSelectChange.bind(this);
this.onSliceNameChange = this.onSliceNameChange.bind(this);
}
componentDidMount() {
this.props.actions.fetchDashboards(this.props.userId).then(() => {
const dashboardIds = this.props.dashboards.map(
dashboard => dashboard.value,
);
let recentDashboard = sessionStorage.getItem(SK_DASHBOARD_ID);
recentDashboard = recentDashboard && parseInt(recentDashboard, 10);
if (
recentDashboard !== null &&
dashboardIds.indexOf(recentDashboard) !== -1
) {
this.setState({
saveToDashboardId: recentDashboard,
});
}
});
}
onSliceNameChange(event) {
this.setState({ newSliceName: event.target.value });
}
onDashboardSelectChange(event) {
const newDashboardName = event ? event.label : null;
const saveToDashboardId =
event && typeof event.value === 'number' ? event.value : null;
this.setState({ saveToDashboardId, newDashboardName });
}
changeAction(action) {
this.setState({ action });
}
saveOrOverwrite(gotodash) {
this.setState({ alert: null });
this.props.actions.removeSaveModalAlert();
const sliceParams = {};
if (this.props.slice && this.props.slice.slice_id) {
sliceParams.slice_id = this.props.slice.slice_id;
}
if (sliceParams.action === 'saveas') {
if (this.state.newSliceName === '') {
this.setState({ alert: t('Please enter a chart name') });
return;
}
}
sliceParams.action = this.state.action;
sliceParams.slice_name = this.state.newSliceName;
sliceParams.save_to_dashboard_id = this.state.saveToDashboardId;
sliceParams.new_dashboard_name = this.state.newDashboardName;
this.props.actions
.saveSlice(this.props.form_data, sliceParams)
.then(({ data }) => {
if (data.dashboard_id === null) {
sessionStorage.removeItem(SK_DASHBOARD_ID);
} else {
sessionStorage.setItem(SK_DASHBOARD_ID, data.dashboard_id);
}
// Go to new slice url or dashboard url
const url = gotodash ? data.dashboard_url : data.slice.slice_url;
window.location.assign(url);
});
this.props.onHide();
}
removeAlert() {
if (this.props.alert) {
this.props.actions.removeSaveModalAlert();
}
this.setState({ alert: null });
}
render() {
const canNotSaveToDash =
EXPLORE_ONLY_VIZ_TYPE.indexOf(this.state.vizType) > -1;
return (
<Modal show onHide={this.props.onHide}>
<Modal.Header closeButton>
<Modal.Title>{t('Save Chart')}</Modal.Title>
</Modal.Header>
<Modal.Body>
{(this.state.alert || this.props.alert) && (
<Alert>
{this.state.alert ? this.state.alert : this.props.alert}
<i
role="button"
tabIndex={0}
className="fa fa-close pull-right"
onClick={this.removeAlert.bind(this)}
style={{ cursor: 'pointer' }}
/>
</Alert>
)}
<FormGroup>
<Radio
id="overwrite-radio"
inline
disabled={!(this.props.can_overwrite && this.props.slice)}
checked={this.state.action === 'overwrite'}
onChange={this.changeAction.bind(this, 'overwrite')}
>
{t('Save (Overwrite)')}
</Radio>
<Radio
id="saveas-radio"
inline
checked={this.state.action === 'saveas'}
onChange={this.changeAction.bind(this, 'saveas')}
>
{' '}
{t('Save as ...')}
</Radio>
</FormGroup>
<hr />
<FormGroup>
<ControlLabel>{t('Chart name')}</ControlLabel>
<FormControl
name="new_slice_name"
type="text"
bsSize="sm"
placeholder="Name"
value={this.state.newSliceName}
onChange={this.onSliceNameChange}
/>
</FormGroup>
<FormGroup>
<ControlLabel>{t('Add to dashboard')}</ControlLabel>
<CreatableSelect
id="dashboard-creatable-select"
className="save-modal-selector"
options={this.props.dashboards}
clearable
creatable
onChange={this.onDashboardSelectChange}
autoSize={false}
value={
this.state.saveToDashboardId || this.state.newDashboardName
}
placeholder={
// Using markdown to allow for good i18n
<ReactMarkdown
source={SELECT_PLACEHOLDER}
renderers={{ paragraph: 'span' }}
/>
}
/>
</FormGroup>
</Modal.Body>
<Modal.Footer>
<div className="float-right">
<Button
type="button"
id="btn_cancel"
bsSize="sm"
onClick={this.props.onHide}
>
{t('Cancel')}
</Button>
<Button
type="button"
id="btn_modal_save_goto_dash"
bsSize="sm"
disabled={canNotSaveToDash || !this.state.newDashboardName}
onClick={this.saveOrOverwrite.bind(this, true)}
>
{t('Save & go to dashboard')}
</Button>
<Button
type="button"
id="btn_modal_save"
bsSize="sm"
bsStyle="primary"
onClick={this.saveOrOverwrite.bind(this, false)}
>
{t('Save')}
</Button>
</div>
</Modal.Footer>
</Modal>
);
}
}
SaveModal.propTypes = propTypes;
function mapStateToProps({ explore, saveModal }) {
return {
datasource: explore.datasource,
slice: explore.slice,
can_overwrite: explore.can_overwrite,
userId: explore.user_id,
dashboards: saveModal.dashboards,
alert: saveModal.saveModalAlert,
};
}
export { SaveModal };
export default connect(mapStateToProps, () => ({}))(SaveModal);
| superset-frontend/src/explore/components/SaveModal.jsx | 1 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.14296722412109375,
0.0067495666444301605,
0.00016138522187247872,
0.00017436583584640175,
0.027167100459337234
]
|
{
"id": 2,
"code_window": [
" >\n",
" {t('Save')}\n",
" </Button>\n",
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n",
" <Dialog ref={errorDialog} />\n",
" </Modal.Footer>\n",
" </form>\n",
" );\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 273
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import { hot } from 'react-hot-loader/root';
import React from 'react';
import { Provider } from 'react-redux';
import { supersetTheme, ThemeProvider } from '@superset-ui/style';
import setupApp from '../setup/setupApp';
import setupPlugins from '../setup/setupPlugins';
import DashboardContainer from './containers/Dashboard';
setupApp();
setupPlugins();
const App = ({ store }) => (
<Provider store={store}>
<ThemeProvider theme={supersetTheme}>
<DashboardContainer />
</ThemeProvider>
</Provider>
);
export default hot(App);
| superset-frontend/src/dashboard/App.jsx | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00017461857351008803,
0.00017064818530343473,
0.00015978740702848881,
0.00017409338033758104,
0.000006282130016188603
]
|
{
"id": 2,
"code_window": [
" >\n",
" {t('Save')}\n",
" </Button>\n",
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n",
" <Dialog ref={errorDialog} />\n",
" </Modal.Footer>\n",
" </form>\n",
" );\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 273
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import React from 'react';
import PropTypes from 'prop-types';
import { FormControl, OverlayTrigger, Tooltip } from 'react-bootstrap';
import AdhocMetric from '../AdhocMetric';
const propTypes = {
adhocMetric: PropTypes.instanceOf(AdhocMetric),
onChange: PropTypes.func.isRequired,
};
export default class AdhocMetricEditPopoverTitle extends React.Component {
constructor(props) {
super(props);
this.onMouseOver = this.onMouseOver.bind(this);
this.onMouseOut = this.onMouseOut.bind(this);
this.onClick = this.onClick.bind(this);
this.onBlur = this.onBlur.bind(this);
this.state = {
isHovered: false,
isEditable: false,
};
}
onMouseOver() {
this.setState({ isHovered: true });
}
onMouseOut() {
this.setState({ isHovered: false });
}
onClick() {
this.setState({ isEditable: true });
}
onBlur() {
this.setState({ isEditable: false });
}
render() {
const { adhocMetric, onChange } = this.props;
const editPrompt = (
<Tooltip id="edit-metric-label-tooltip">Click to edit label</Tooltip>
);
return this.state.isEditable ? (
<FormControl
className="metric-edit-popover-label-input"
type="text"
placeholder={adhocMetric.label}
value={adhocMetric.hasCustomLabel ? adhocMetric.label : ''}
autoFocus
onChange={onChange}
data-test="AdhocMetricEditTitle#input"
/>
) : (
<OverlayTrigger
placement="top"
overlay={editPrompt}
onMouseOver={this.onMouseOver}
onMouseOut={this.onMouseOut}
onClick={this.onClick}
onBlur={this.onBlur}
className="AdhocMetricEditPopoverTitle"
>
<span
className="inline-editable"
data-test="AdhocMetricEditTitle#trigger"
>
{adhocMetric.hasCustomLabel ? adhocMetric.label : 'My Metric'}
<i
className="fa fa-pencil"
style={{ color: this.state.isHovered ? 'black' : 'grey' }}
/>
</span>
</OverlayTrigger>
);
}
}
AdhocMetricEditPopoverTitle.propTypes = propTypes;
| superset-frontend/src/explore/components/AdhocMetricEditPopoverTitle.jsx | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.0019780369475483894,
0.00033597511355765164,
0.00016628991579636931,
0.00017348291294183582,
0.0005192719399929047
]
|
{
"id": 2,
"code_window": [
" >\n",
" {t('Save')}\n",
" </Button>\n",
" <Button type=\"button\" bsSize=\"sm\" onClick={onHide}>\n",
" {t('Cancel')}\n",
" </Button>\n",
" <Dialog ref={errorDialog} />\n",
" </Modal.Footer>\n",
" </form>\n",
" );\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "superset-frontend/src/explore/components/PropertiesModal.tsx",
"type": "replace",
"edit_start_line_idx": 273
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import shortid from 'shortid';
import {
CHART_TYPE,
COLUMN_TYPE,
DIVIDER_TYPE,
HEADER_TYPE,
MARKDOWN_TYPE,
ROW_TYPE,
TABS_TYPE,
TAB_TYPE,
} from './componentTypes';
import {
MEDIUM_HEADER,
BACKGROUND_TRANSPARENT,
GRID_DEFAULT_CHART_WIDTH,
} from './constants';
const typeToDefaultMetaData = {
[CHART_TYPE]: { width: GRID_DEFAULT_CHART_WIDTH, height: 50 },
[COLUMN_TYPE]: {
width: GRID_DEFAULT_CHART_WIDTH,
background: BACKGROUND_TRANSPARENT,
},
[DIVIDER_TYPE]: null,
[HEADER_TYPE]: {
text: 'New header',
headerSize: MEDIUM_HEADER,
background: BACKGROUND_TRANSPARENT,
},
[MARKDOWN_TYPE]: { width: GRID_DEFAULT_CHART_WIDTH, height: 50 },
[ROW_TYPE]: { background: BACKGROUND_TRANSPARENT },
[TABS_TYPE]: null,
[TAB_TYPE]: { text: 'New Tab' },
};
function uuid(type) {
return `${type}-${shortid.generate()}`;
}
export default function entityFactory(type, meta, parents = []) {
return {
type,
id: uuid(type),
children: [],
parents,
meta: {
...typeToDefaultMetaData[type],
...meta,
},
};
}
| superset-frontend/src/dashboard/util/newComponentFactory.js | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00020428982679732144,
0.00017660457524470985,
0.00016939168563112617,
0.00017433009634260088,
0.00001068261462933151
]
|
{
"id": 3,
"code_window": [
"import {\n",
" Alert,\n",
" Button,\n",
" FormControl,\n",
" FormGroup,\n",
" ControlLabel,\n",
" Modal,\n",
" Radio,\n",
"} from 'react-bootstrap';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "superset-frontend/src/explore/components/SaveModal.jsx",
"type": "replace",
"edit_start_line_idx": 27
} | /**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/* eslint camelcase: 0 */
import React from 'react';
import PropTypes from 'prop-types';
import { connect } from 'react-redux';
import {
Alert,
Button,
FormControl,
FormGroup,
ControlLabel,
Modal,
Radio,
} from 'react-bootstrap';
import { CreatableSelect } from 'src/components/Select/SupersetStyledSelect';
import { t } from '@superset-ui/translation';
import ReactMarkdown from 'react-markdown';
import { EXPLORE_ONLY_VIZ_TYPE } from '../constants';
const propTypes = {
can_overwrite: PropTypes.bool,
onHide: PropTypes.func.isRequired,
actions: PropTypes.object.isRequired,
form_data: PropTypes.object,
userId: PropTypes.string.isRequired,
dashboards: PropTypes.array.isRequired,
alert: PropTypes.string,
slice: PropTypes.object,
datasource: PropTypes.object,
};
// Session storage key for recent dashboard
const SK_DASHBOARD_ID = 'save_chart_recent_dashboard';
const SELECT_PLACEHOLDER = t('**Select** a dashboard OR **create** a new one');
class SaveModal extends React.Component {
constructor(props) {
super(props);
this.state = {
saveToDashboardId: null,
newSliceName: props.sliceName,
dashboards: [],
alert: null,
action: props.can_overwrite ? 'overwrite' : 'saveas',
vizType: props.form_data.viz_type,
};
this.onDashboardSelectChange = this.onDashboardSelectChange.bind(this);
this.onSliceNameChange = this.onSliceNameChange.bind(this);
}
componentDidMount() {
this.props.actions.fetchDashboards(this.props.userId).then(() => {
const dashboardIds = this.props.dashboards.map(
dashboard => dashboard.value,
);
let recentDashboard = sessionStorage.getItem(SK_DASHBOARD_ID);
recentDashboard = recentDashboard && parseInt(recentDashboard, 10);
if (
recentDashboard !== null &&
dashboardIds.indexOf(recentDashboard) !== -1
) {
this.setState({
saveToDashboardId: recentDashboard,
});
}
});
}
onSliceNameChange(event) {
this.setState({ newSliceName: event.target.value });
}
onDashboardSelectChange(event) {
const newDashboardName = event ? event.label : null;
const saveToDashboardId =
event && typeof event.value === 'number' ? event.value : null;
this.setState({ saveToDashboardId, newDashboardName });
}
changeAction(action) {
this.setState({ action });
}
saveOrOverwrite(gotodash) {
this.setState({ alert: null });
this.props.actions.removeSaveModalAlert();
const sliceParams = {};
if (this.props.slice && this.props.slice.slice_id) {
sliceParams.slice_id = this.props.slice.slice_id;
}
if (sliceParams.action === 'saveas') {
if (this.state.newSliceName === '') {
this.setState({ alert: t('Please enter a chart name') });
return;
}
}
sliceParams.action = this.state.action;
sliceParams.slice_name = this.state.newSliceName;
sliceParams.save_to_dashboard_id = this.state.saveToDashboardId;
sliceParams.new_dashboard_name = this.state.newDashboardName;
this.props.actions
.saveSlice(this.props.form_data, sliceParams)
.then(({ data }) => {
if (data.dashboard_id === null) {
sessionStorage.removeItem(SK_DASHBOARD_ID);
} else {
sessionStorage.setItem(SK_DASHBOARD_ID, data.dashboard_id);
}
// Go to new slice url or dashboard url
const url = gotodash ? data.dashboard_url : data.slice.slice_url;
window.location.assign(url);
});
this.props.onHide();
}
removeAlert() {
if (this.props.alert) {
this.props.actions.removeSaveModalAlert();
}
this.setState({ alert: null });
}
render() {
const canNotSaveToDash =
EXPLORE_ONLY_VIZ_TYPE.indexOf(this.state.vizType) > -1;
return (
<Modal show onHide={this.props.onHide}>
<Modal.Header closeButton>
<Modal.Title>{t('Save Chart')}</Modal.Title>
</Modal.Header>
<Modal.Body>
{(this.state.alert || this.props.alert) && (
<Alert>
{this.state.alert ? this.state.alert : this.props.alert}
<i
role="button"
tabIndex={0}
className="fa fa-close pull-right"
onClick={this.removeAlert.bind(this)}
style={{ cursor: 'pointer' }}
/>
</Alert>
)}
<FormGroup>
<Radio
id="overwrite-radio"
inline
disabled={!(this.props.can_overwrite && this.props.slice)}
checked={this.state.action === 'overwrite'}
onChange={this.changeAction.bind(this, 'overwrite')}
>
{t('Save (Overwrite)')}
</Radio>
<Radio
id="saveas-radio"
inline
checked={this.state.action === 'saveas'}
onChange={this.changeAction.bind(this, 'saveas')}
>
{' '}
{t('Save as ...')}
</Radio>
</FormGroup>
<hr />
<FormGroup>
<ControlLabel>{t('Chart name')}</ControlLabel>
<FormControl
name="new_slice_name"
type="text"
bsSize="sm"
placeholder="Name"
value={this.state.newSliceName}
onChange={this.onSliceNameChange}
/>
</FormGroup>
<FormGroup>
<ControlLabel>{t('Add to dashboard')}</ControlLabel>
<CreatableSelect
id="dashboard-creatable-select"
className="save-modal-selector"
options={this.props.dashboards}
clearable
creatable
onChange={this.onDashboardSelectChange}
autoSize={false}
value={
this.state.saveToDashboardId || this.state.newDashboardName
}
placeholder={
// Using markdown to allow for good i18n
<ReactMarkdown
source={SELECT_PLACEHOLDER}
renderers={{ paragraph: 'span' }}
/>
}
/>
</FormGroup>
</Modal.Body>
<Modal.Footer>
<div className="float-right">
<Button
type="button"
id="btn_cancel"
bsSize="sm"
onClick={this.props.onHide}
>
{t('Cancel')}
</Button>
<Button
type="button"
id="btn_modal_save_goto_dash"
bsSize="sm"
disabled={canNotSaveToDash || !this.state.newDashboardName}
onClick={this.saveOrOverwrite.bind(this, true)}
>
{t('Save & go to dashboard')}
</Button>
<Button
type="button"
id="btn_modal_save"
bsSize="sm"
bsStyle="primary"
onClick={this.saveOrOverwrite.bind(this, false)}
>
{t('Save')}
</Button>
</div>
</Modal.Footer>
</Modal>
);
}
}
SaveModal.propTypes = propTypes;
function mapStateToProps({ explore, saveModal }) {
return {
datasource: explore.datasource,
slice: explore.slice,
can_overwrite: explore.can_overwrite,
userId: explore.user_id,
dashboards: saveModal.dashboards,
alert: saveModal.saveModalAlert,
};
}
export { SaveModal };
export default connect(mapStateToProps, () => ({}))(SaveModal);
| superset-frontend/src/explore/components/SaveModal.jsx | 1 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.9232280850410461,
0.03518400713801384,
0.0001657028478803113,
0.0002303500659763813,
0.17416663467884064
]
|
{
"id": 3,
"code_window": [
"import {\n",
" Alert,\n",
" Button,\n",
" FormControl,\n",
" FormGroup,\n",
" ControlLabel,\n",
" Modal,\n",
" Radio,\n",
"} from 'react-bootstrap';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "superset-frontend/src/explore/components/SaveModal.jsx",
"type": "replace",
"edit_start_line_idx": 27
} | # Licensed to the Apache Software Foundation (ASF) under one
# or more contributor license agreements. See the NOTICE file
# distributed with this work for additional information
# regarding copyright ownership. The ASF licenses this file
# to you under the Apache License, Version 2.0 (the
# "License"); you may not use this file except in compliance
# with the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing,
# software distributed under the License is distributed on an
# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
# KIND, either express or implied. See the License for the
# specific language governing permissions and limitations
# under the License.
"""Fix wrong constraint on table columns
Revision ID: 1226819ee0e3
Revises: 956a063c52b3
Create Date: 2016-05-27 15:03:32.980343
"""
import logging
from alembic import op
from superset import db
from superset.utils.core import generic_find_constraint_name
# revision identifiers, used by Alembic.
revision = "1226819ee0e3"
down_revision = "956a063c52b3"
naming_convention = {
"fk": "fk_%(table_name)s_%(column_0_name)s_%(referred_table_name)s"
}
def find_constraint_name(upgrade=True):
cols = {"column_name"} if upgrade else {"datasource_name"}
return generic_find_constraint_name(
table="columns", columns=cols, referenced="datasources", database=db
)
def upgrade():
try:
constraint = find_constraint_name()
with op.batch_alter_table(
"columns", naming_convention=naming_convention
) as batch_op:
if constraint:
batch_op.drop_constraint(constraint, type_="foreignkey")
batch_op.create_foreign_key(
"fk_columns_datasource_name_datasources",
"datasources",
["datasource_name"],
["datasource_name"],
)
except:
logging.warning("Could not find or drop constraint on `columns`")
def downgrade():
constraint = find_constraint_name(False) or "fk_columns_datasource_name_datasources"
with op.batch_alter_table(
"columns", naming_convention=naming_convention
) as batch_op:
batch_op.drop_constraint(constraint, type_="foreignkey")
batch_op.create_foreign_key(
"fk_columns_column_name_datasources",
"datasources",
["column_name"],
["datasource_name"],
)
| superset/migrations/versions/1226819ee0e3_fix_wrong_constraint_on_table_columns.py | 0 | https://github.com/apache/superset/commit/9eab29aeaacc9e7346649ca46730d49f2fe0b4ef | [
0.00017786143871489912,
0.00017106566519942135,
0.0001671469071879983,
0.00016924834926612675,
0.000003813556304521626
]
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.