hunk
dict | file
stringlengths 0
11.8M
| file_path
stringlengths 2
234
| label
int64 0
1
| commit_url
stringlengths 74
103
| dependency_score
sequencelengths 5
5
|
---|---|---|---|---|---|
{
"id": 1,
"code_window": [
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n",
" * // Create a new app (will auto-add extract plugin to renderer)\n",
" * const app = new Application();\n",
" *\n",
" * // Draw a red circle\n",
" * const graphics = new Graphics()\n",
" * .beginFill(0xFF0000)\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a new application (extract will be auto-added to renderer)\n"
],
"file_path": "packages/extract/src/Extract.ts",
"type": "replace",
"edit_start_line_idx": 17
} | # @pixi/filter-color-matrix
## Installation
```bash
npm install @pixi/filter-color-matrix
```
## Usage
```js
import '@pixi/filter-color-matrix';
``` | packages/filter-color-matrix/README.md | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017005244444590062,
0.0001687374897301197,
0.0001674225350143388,
0.0001687374897301197,
0.0000013149547157809138
] |
{
"id": 1,
"code_window": [
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n",
" * // Create a new app (will auto-add extract plugin to renderer)\n",
" * const app = new Application();\n",
" *\n",
" * // Draw a red circle\n",
" * const graphics = new Graphics()\n",
" * .beginFill(0xFF0000)\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a new application (extract will be auto-added to renderer)\n"
],
"file_path": "packages/extract/src/Extract.ts",
"type": "replace",
"edit_start_line_idx": 17
} | # package.lock
packages/**/package-lock.json
# sublime text files
*.sublime*
*.*~*.TMP
# temp files
.DS_Store
Thumbs.db
Desktop.ini
npm-debug.log
lerna-debug.log
.eslintcache
# project files
.project
# vim swap files
*.sw*
# emacs temp files
*~
\#*#
# project ignores
!.gitkeep
*__temp
node_modules
docs
coverage
coverage.lcov
.nyc_output
dist
lib
bundles/*/*.d.ts
out
packages/**/index.d.ts
# jetBrains IDE ignores
.idea
# VSCode ignores
.vscode
pixi.code-workspace
# IntelliJ
*.iml
# webdoc api
dist/docs/pixi.api.json
| .gitignore | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017332237621303648,
0.00017088184540625662,
0.00016633748600725085,
0.00017182539158966392,
0.000002517320126571576
] |
{
"id": 2,
"code_window": [
" * const graphics = new Graphics()\n",
" * .beginFill(0xFF0000)\n",
" * .drawCircle(0, 0, 50);\n",
" *\n",
" * // Render the graphics as an HTMLImageElement\n",
" * const image = app.renderer.plugins.image(graphics);\n",
" * document.body.appendChild(image);\n",
" * @memberof PIXI\n",
" */\n",
"\n",
"export class Extract implements ISystem\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" * const image = await app.renderer.extract.image(graphics);\n"
],
"file_path": "packages/extract/src/Extract.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { BaseTexture, extensions, ExtensionType } from '@pixi/core';
import { Graphics } from '@pixi/graphics';
import { BasePrepare } from './BasePrepare';
import type { ExtensionMetadata, IRenderer, ISystem, Renderer } from '@pixi/core';
import type { IDisplayObjectExtended } from './BasePrepare';
/**
* Built-in hook to upload PIXI.Texture objects to the GPU.
* @private
* @param renderer - instance of the webgl renderer
* @param item - Item to check
* @returns If item was uploaded.
*/
function uploadBaseTextures(renderer: IRenderer | BasePrepare, item: IDisplayObjectExtended | BaseTexture): boolean
{
if (item instanceof BaseTexture)
{
// if the texture already has a GL texture, then the texture has been prepared or rendered
// before now. If the texture changed, then the changer should be calling texture.update() which
// reuploads the texture without need for preparing it again
if (!item._glTextures[(renderer as Renderer).CONTEXT_UID])
{
(renderer as Renderer).texture.bind(item);
}
return true;
}
return false;
}
/**
* Built-in hook to upload PIXI.Graphics to the GPU.
* @private
* @param renderer - instance of the webgl renderer
* @param item - Item to check
* @returns If item was uploaded.
*/
function uploadGraphics(renderer: IRenderer | BasePrepare, item: IDisplayObjectExtended): boolean
{
if (!(item instanceof Graphics))
{
return false;
}
const { geometry } = item;
// update dirty graphics to get batches
item.finishPoly();
geometry.updateBatches();
const { batches } = geometry;
// upload all textures found in styles
for (let i = 0; i < batches.length; i++)
{
const { texture } = batches[i].style;
if (texture)
{
uploadBaseTextures(renderer, texture.baseTexture);
}
}
// if its not batchable - update vao for particular shader
if (!geometry.batchable)
{
(renderer as Renderer).geometry.bind(geometry, (item as any)._resolveDirectShader((renderer as Renderer)));
}
return true;
}
/**
* Built-in hook to find graphics.
* @private
* @param item - Display object to check
* @param queue - Collection of items to upload
* @returns if a PIXI.Graphics object was found.
*/
function findGraphics(item: IDisplayObjectExtended, queue: Array<any>): boolean
{
if (item instanceof Graphics)
{
queue.push(item);
return true;
}
return false;
}
/**
* The prepare plugin provides renderer-specific plugins for pre-rendering DisplayObjects. These plugins are useful for
* asynchronously preparing and uploading to the GPU assets, textures, graphics waiting to be displayed.
*
* Do not instantiate this plugin directly. It is available from the `renderer.plugins` property.
* See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.
* @example
* import { Application, Graphics } from 'pixi.js';
*
* // Create a new application
* const app = new Application();
* document.body.appendChild(app.view);
*
* // Don't start rendering right away
* app.stop();
*
* // create a display object
* const rect = new Graphics()
* .beginFill(0x00ff00)
* .drawRect(40, 40, 200, 200);
*
* // Add to the stage
* app.stage.addChild(rect);
*
* // Don't start rendering until the graphic is uploaded to the GPU
* app.renderer.prepare.upload(app.stage, () => {
* app.start();
* });
* @memberof PIXI
*/
export class Prepare extends BasePrepare implements ISystem
{
/** @ignore */
static extension: ExtensionMetadata = {
name: 'prepare',
type: ExtensionType.RendererSystem,
};
/**
* @param {PIXI.Renderer} renderer - A reference to the current renderer
*/
constructor(renderer: Renderer)
{
super(renderer);
this.uploadHookHelper = this.renderer;
// Add textures and graphics to upload
this.registerFindHook(findGraphics);
this.registerUploadHook(uploadBaseTextures);
this.registerUploadHook(uploadGraphics);
}
}
extensions.add(Prepare);
| packages/prepare/src/Prepare.ts | 1 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.0027808467857539654,
0.000423526915255934,
0.00017040743841789663,
0.000195082655409351,
0.0006389942136593163
] |
{
"id": 2,
"code_window": [
" * const graphics = new Graphics()\n",
" * .beginFill(0xFF0000)\n",
" * .drawCircle(0, 0, 50);\n",
" *\n",
" * // Render the graphics as an HTMLImageElement\n",
" * const image = app.renderer.plugins.image(graphics);\n",
" * document.body.appendChild(image);\n",
" * @memberof PIXI\n",
" */\n",
"\n",
"export class Extract implements ISystem\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" * const image = await app.renderer.extract.image(graphics);\n"
],
"file_path": "packages/extract/src/Extract.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { extensions, ExtensionType, Texture } from '@pixi/core';
import type { CacheParser } from '../CacheParser';
export const cacheTextureArray: CacheParser<Texture[]> = {
extension: ExtensionType.CacheParser,
test: (asset: any[]) => Array.isArray(asset) && asset.every((t) => t instanceof Texture),
getCacheableAssets: (keys: string[], asset: Texture[]) =>
{
const out: Record<string, Texture> = {};
keys.forEach((key: string) =>
{
asset.forEach((item: Texture, i: number) =>
{
out[key + (i === 0 ? '' : i + 1)] = item;
});
});
return out;
}
};
extensions.add(cacheTextureArray);
| packages/assets/src/cache/parsers/cacheTextureArray.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017280389147344977,
0.00016891038103494793,
0.00016520086501259357,
0.00016872641572263092,
0.0000031066474548424594
] |
{
"id": 2,
"code_window": [
" * const graphics = new Graphics()\n",
" * .beginFill(0xFF0000)\n",
" * .drawCircle(0, 0, 50);\n",
" *\n",
" * // Render the graphics as an HTMLImageElement\n",
" * const image = app.renderer.plugins.image(graphics);\n",
" * document.body.appendChild(image);\n",
" * @memberof PIXI\n",
" */\n",
"\n",
"export class Extract implements ISystem\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" * const image = await app.renderer.extract.image(graphics);\n"
],
"file_path": "packages/extract/src/Extract.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { defaultFilterVertex, Filter } from '@pixi/core';
import fragment from './noise.frag';
/**
* A Noise effect filter.
*
* original filter: https://github.com/evanw/glfx.js/blob/master/src/filters/adjust/noise.js
* @memberof PIXI
* @author Vico @vicocotea
*/
export class NoiseFilter extends Filter
{
/**
* @param {number} [noise=0.5] - The noise intensity, should be a normalized value in the range [0, 1].
* @param {number} [seed] - A random seed for the noise generation. Default is `Math.random()`.
*/
constructor(noise = 0.5, seed = Math.random())
{
super(defaultFilterVertex, fragment, {
uNoise: 0,
uSeed: 0,
});
this.noise = noise;
this.seed = seed;
}
/**
* The amount of noise to apply, this value should be in the range (0, 1].
* @default 0.5
*/
get noise(): number
{
return this.uniforms.uNoise;
}
set noise(value: number)
{
this.uniforms.uNoise = value;
}
/** A seed value to apply to the random noise generation. `Math.random()` is a good value to use. */
get seed(): number
{
return this.uniforms.uSeed;
}
set seed(value: number)
{
this.uniforms.uSeed = value;
}
}
| packages/filter-noise/src/NoiseFilter.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017676351126283407,
0.00017073441995307803,
0.00016587451682426035,
0.00016964366659522057,
0.000003461992491793353
] |
{
"id": 2,
"code_window": [
" * const graphics = new Graphics()\n",
" * .beginFill(0xFF0000)\n",
" * .drawCircle(0, 0, 50);\n",
" *\n",
" * // Render the graphics as an HTMLImageElement\n",
" * const image = app.renderer.plugins.image(graphics);\n",
" * document.body.appendChild(image);\n",
" * @memberof PIXI\n",
" */\n",
"\n",
"export class Extract implements ISystem\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" * const image = await app.renderer.extract.image(graphics);\n"
],
"file_path": "packages/extract/src/Extract.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { Rectangle } from '@pixi/core';
import type { IPointData, Matrix, Transform } from '@pixi/core';
/**
* 'Builder' pattern for bounds rectangles.
*
* This could be called an Axis-Aligned Bounding Box.
* It is not an actual shape. It is a mutable thing; no 'EMPTY' or those kind of problems.
* @memberof PIXI
*/
export class Bounds
{
/** @default Infinity */
public minX: number;
/** @default Infinity */
public minY: number;
/** @default -Infinity */
public maxX: number;
/** @default -Infinity */
public maxY: number;
public rect: Rectangle;
/**
* It is updated to _boundsID of corresponding object to keep bounds in sync with content.
* Updated from outside, thus public modifier.
*/
public updateID: number;
constructor()
{
this.minX = Infinity;
this.minY = Infinity;
this.maxX = -Infinity;
this.maxY = -Infinity;
this.rect = null;
this.updateID = -1;
}
/**
* Checks if bounds are empty.
* @returns - True if empty.
*/
isEmpty(): boolean
{
return this.minX > this.maxX || this.minY > this.maxY;
}
/** Clears the bounds and resets. */
clear(): void
{
this.minX = Infinity;
this.minY = Infinity;
this.maxX = -Infinity;
this.maxY = -Infinity;
}
/**
* Can return Rectangle.EMPTY constant, either construct new rectangle, either use your rectangle
* It is not guaranteed that it will return tempRect
* @param rect - Temporary object will be used if AABB is not empty
* @returns - A rectangle of the bounds
*/
getRectangle(rect?: Rectangle): Rectangle
{
if (this.minX > this.maxX || this.minY > this.maxY)
{
return Rectangle.EMPTY;
}
rect = rect || new Rectangle(0, 0, 1, 1);
rect.x = this.minX;
rect.y = this.minY;
rect.width = this.maxX - this.minX;
rect.height = this.maxY - this.minY;
return rect;
}
/**
* This function should be inlined when its possible.
* @param point - The point to add.
*/
addPoint(point: IPointData): void
{
this.minX = Math.min(this.minX, point.x);
this.maxX = Math.max(this.maxX, point.x);
this.minY = Math.min(this.minY, point.y);
this.maxY = Math.max(this.maxY, point.y);
}
/**
* Adds a point, after transformed. This should be inlined when its possible.
* @param matrix
* @param point
*/
addPointMatrix(matrix: Matrix, point: IPointData): void
{
const { a, b, c, d, tx, ty } = matrix;
const x = (a * point.x) + (c * point.y) + tx;
const y = (b * point.x) + (d * point.y) + ty;
this.minX = Math.min(this.minX, x);
this.maxX = Math.max(this.maxX, x);
this.minY = Math.min(this.minY, y);
this.maxY = Math.max(this.maxY, y);
}
/**
* Adds a quad, not transformed
* @param vertices - The verts to add.
*/
addQuad(vertices: Float32Array): void
{
let minX = this.minX;
let minY = this.minY;
let maxX = this.maxX;
let maxY = this.maxY;
let x = vertices[0];
let y = vertices[1];
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
x = vertices[2];
y = vertices[3];
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
x = vertices[4];
y = vertices[5];
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
x = vertices[6];
y = vertices[7];
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
this.minX = minX;
this.minY = minY;
this.maxX = maxX;
this.maxY = maxY;
}
/**
* Adds sprite frame, transformed.
* @param transform - transform to apply
* @param x0 - left X of frame
* @param y0 - top Y of frame
* @param x1 - right X of frame
* @param y1 - bottom Y of frame
*/
addFrame(transform: Transform, x0: number, y0: number, x1: number, y1: number): void
{
this.addFrameMatrix(transform.worldTransform, x0, y0, x1, y1);
}
/**
* Adds sprite frame, multiplied by matrix
* @param matrix - matrix to apply
* @param x0 - left X of frame
* @param y0 - top Y of frame
* @param x1 - right X of frame
* @param y1 - bottom Y of frame
*/
addFrameMatrix(matrix: Matrix, x0: number, y0: number, x1: number, y1: number): void
{
const a = matrix.a;
const b = matrix.b;
const c = matrix.c;
const d = matrix.d;
const tx = matrix.tx;
const ty = matrix.ty;
let minX = this.minX;
let minY = this.minY;
let maxX = this.maxX;
let maxY = this.maxY;
let x = (a * x0) + (c * y0) + tx;
let y = (b * x0) + (d * y0) + ty;
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
x = (a * x1) + (c * y0) + tx;
y = (b * x1) + (d * y0) + ty;
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
x = (a * x0) + (c * y1) + tx;
y = (b * x0) + (d * y1) + ty;
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
x = (a * x1) + (c * y1) + tx;
y = (b * x1) + (d * y1) + ty;
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
this.minX = minX;
this.minY = minY;
this.maxX = maxX;
this.maxY = maxY;
}
/**
* Adds screen vertices from array
* @param vertexData - calculated vertices
* @param beginOffset - begin offset
* @param endOffset - end offset, excluded
*/
addVertexData(vertexData: Float32Array, beginOffset: number, endOffset: number): void
{
let minX = this.minX;
let minY = this.minY;
let maxX = this.maxX;
let maxY = this.maxY;
for (let i = beginOffset; i < endOffset; i += 2)
{
const x = vertexData[i];
const y = vertexData[i + 1];
minX = x < minX ? x : minX;
minY = y < minY ? y : minY;
maxX = x > maxX ? x : maxX;
maxY = y > maxY ? y : maxY;
}
this.minX = minX;
this.minY = minY;
this.maxX = maxX;
this.maxY = maxY;
}
/**
* Add an array of mesh vertices
* @param transform - mesh transform
* @param vertices - mesh coordinates in array
* @param beginOffset - begin offset
* @param endOffset - end offset, excluded
*/
addVertices(transform: Transform, vertices: Float32Array, beginOffset: number, endOffset: number): void
{
this.addVerticesMatrix(transform.worldTransform, vertices, beginOffset, endOffset);
}
/**
* Add an array of mesh vertices.
* @param matrix - mesh matrix
* @param vertices - mesh coordinates in array
* @param beginOffset - begin offset
* @param endOffset - end offset, excluded
* @param padX - x padding
* @param padY - y padding
*/
addVerticesMatrix(matrix: Matrix, vertices: Float32Array, beginOffset: number,
endOffset: number, padX = 0, padY = padX): void
{
const a = matrix.a;
const b = matrix.b;
const c = matrix.c;
const d = matrix.d;
const tx = matrix.tx;
const ty = matrix.ty;
let minX = this.minX;
let minY = this.minY;
let maxX = this.maxX;
let maxY = this.maxY;
for (let i = beginOffset; i < endOffset; i += 2)
{
const rawX = vertices[i];
const rawY = vertices[i + 1];
const x = (a * rawX) + (c * rawY) + tx;
const y = (d * rawY) + (b * rawX) + ty;
minX = Math.min(minX, x - padX);
maxX = Math.max(maxX, x + padX);
minY = Math.min(minY, y - padY);
maxY = Math.max(maxY, y + padY);
}
this.minX = minX;
this.minY = minY;
this.maxX = maxX;
this.maxY = maxY;
}
/**
* Adds other {@link Bounds}.
* @param bounds - The Bounds to be added
*/
addBounds(bounds: Bounds): void
{
const minX = this.minX;
const minY = this.minY;
const maxX = this.maxX;
const maxY = this.maxY;
this.minX = bounds.minX < minX ? bounds.minX : minX;
this.minY = bounds.minY < minY ? bounds.minY : minY;
this.maxX = bounds.maxX > maxX ? bounds.maxX : maxX;
this.maxY = bounds.maxY > maxY ? bounds.maxY : maxY;
}
/**
* Adds other Bounds, masked with Bounds.
* @param bounds - The Bounds to be added.
* @param mask - TODO
*/
addBoundsMask(bounds: Bounds, mask: Bounds): void
{
const _minX = bounds.minX > mask.minX ? bounds.minX : mask.minX;
const _minY = bounds.minY > mask.minY ? bounds.minY : mask.minY;
const _maxX = bounds.maxX < mask.maxX ? bounds.maxX : mask.maxX;
const _maxY = bounds.maxY < mask.maxY ? bounds.maxY : mask.maxY;
if (_minX <= _maxX && _minY <= _maxY)
{
const minX = this.minX;
const minY = this.minY;
const maxX = this.maxX;
const maxY = this.maxY;
this.minX = _minX < minX ? _minX : minX;
this.minY = _minY < minY ? _minY : minY;
this.maxX = _maxX > maxX ? _maxX : maxX;
this.maxY = _maxY > maxY ? _maxY : maxY;
}
}
/**
* Adds other Bounds, multiplied by matrix. Bounds shouldn't be empty.
* @param bounds - other bounds
* @param matrix - multiplicator
*/
addBoundsMatrix(bounds: Bounds, matrix: Matrix): void
{
this.addFrameMatrix(matrix, bounds.minX, bounds.minY, bounds.maxX, bounds.maxY);
}
/**
* Adds other Bounds, masked with Rectangle.
* @param bounds - TODO
* @param area - TODO
*/
addBoundsArea(bounds: Bounds, area: Rectangle): void
{
const _minX = bounds.minX > area.x ? bounds.minX : area.x;
const _minY = bounds.minY > area.y ? bounds.minY : area.y;
const _maxX = bounds.maxX < area.x + area.width ? bounds.maxX : (area.x + area.width);
const _maxY = bounds.maxY < area.y + area.height ? bounds.maxY : (area.y + area.height);
if (_minX <= _maxX && _minY <= _maxY)
{
const minX = this.minX;
const minY = this.minY;
const maxX = this.maxX;
const maxY = this.maxY;
this.minX = _minX < minX ? _minX : minX;
this.minY = _minY < minY ? _minY : minY;
this.maxX = _maxX > maxX ? _maxX : maxX;
this.maxY = _maxY > maxY ? _maxY : maxY;
}
}
/**
* Pads bounds object, making it grow in all directions.
* If paddingY is omitted, both paddingX and paddingY will be set to paddingX.
* @param paddingX - The horizontal padding amount.
* @param paddingY - The vertical padding amount.
*/
pad(paddingX = 0, paddingY = paddingX): void
{
if (!this.isEmpty())
{
this.minX -= paddingX;
this.maxX += paddingX;
this.minY -= paddingY;
this.maxY += paddingY;
}
}
/**
* Adds padded frame. (x0, y0) should be strictly less than (x1, y1)
* @param x0 - left X of frame
* @param y0 - top Y of frame
* @param x1 - right X of frame
* @param y1 - bottom Y of frame
* @param padX - padding X
* @param padY - padding Y
*/
addFramePad(x0: number, y0: number, x1: number, y1: number, padX: number, padY: number): void
{
x0 -= padX;
y0 -= padY;
x1 += padX;
y1 += padY;
this.minX = this.minX < x0 ? this.minX : x0;
this.maxX = this.maxX > x1 ? this.maxX : x1;
this.minY = this.minY < y0 ? this.minY : y0;
this.maxY = this.maxY > y1 ? this.maxY : y1;
}
}
| packages/display/src/Bounds.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017605250468477607,
0.0001702803565422073,
0.00016400316962972283,
0.00017038825899362564,
0.0000026475802314962493
] |
{
"id": 3,
"code_window": [
"/**\n",
" * The prepare plugin provides renderer-specific plugins for pre-rendering DisplayObjects. These plugins are useful for\n",
" * asynchronously preparing and uploading to the GPU assets, textures, graphics waiting to be displayed.\n",
" *\n",
" * Do not instantiate this plugin directly. It is available from the `renderer.plugins` property.\n",
" * See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.\n",
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * Do not instantiate this plugin directly. It is available from the `renderer.prepare` property.\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 97
} | import { extensions, ExtensionType, Rectangle, RenderTexture, utils } from '@pixi/core';
import type { ExtensionMetadata, ICanvas, ISystem, Renderer } from '@pixi/core';
import type { DisplayObject } from '@pixi/display';
const TEMP_RECT = new Rectangle();
const BYTES_PER_PIXEL = 4;
/**
* This class provides renderer-specific plugins for exporting content from a renderer.
* For instance, these plugins can be used for saving an Image, Canvas element or for exporting the raw image data (pixels).
*
* Do not instantiate these plugins directly. It is available from the `renderer.plugins` property.
* See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.
* @example
* import { Application, Graphics } from 'pixi.js';
*
* // Create a new app (will auto-add extract plugin to renderer)
* const app = new Application();
*
* // Draw a red circle
* const graphics = new Graphics()
* .beginFill(0xFF0000)
* .drawCircle(0, 0, 50);
*
* // Render the graphics as an HTMLImageElement
* const image = app.renderer.plugins.image(graphics);
* document.body.appendChild(image);
* @memberof PIXI
*/
export class Extract implements ISystem
{
/** @ignore */
static extension: ExtensionMetadata = {
name: 'extract',
type: ExtensionType.RendererSystem,
};
private renderer: Renderer;
/**
* @param renderer - A reference to the current renderer
*/
constructor(renderer: Renderer)
{
this.renderer = renderer;
}
/**
* Will return a HTML Image of the target
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param format - Image format, e.g. "image/jpeg" or "image/webp".
* @param quality - JPEG or Webp compression from 0 to 1. Default is 0.92.
* @returns - HTML Image of the target
*/
public async image(target: DisplayObject | RenderTexture, format?: string, quality?: number): Promise<HTMLImageElement>
{
const image = new Image();
image.src = await this.base64(target, format, quality);
return image;
}
/**
* Will return a base64 encoded string of this target. It works by calling
* `Extract.getCanvas` and then running toDataURL on that.
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param format - Image format, e.g. "image/jpeg" or "image/webp".
* @param quality - JPEG or Webp compression from 0 to 1. Default is 0.92.
* @returns - A base64 encoded string of the texture.
*/
public async base64(target: DisplayObject | RenderTexture, format?: string, quality?: number): Promise<string>
{
const canvas = this.canvas(target);
if (canvas.toDataURL !== undefined)
{
return canvas.toDataURL(format, quality);
}
if (canvas.convertToBlob !== undefined)
{
const blob = await canvas.convertToBlob({ type: format, quality });
return await new Promise<string>((resolve) =>
{
const reader = new FileReader();
reader.onload = () => resolve(reader.result as string);
reader.readAsDataURL(blob);
});
}
throw new Error('Extract.base64() requires ICanvas.toDataURL or ICanvas.convertToBlob to be implemented');
}
/**
* Creates a Canvas element, renders this target to it and then returns it.
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param frame - The frame the extraction is restricted to.
* @returns - A Canvas element with the texture rendered on.
*/
public canvas(target: DisplayObject | RenderTexture, frame?: Rectangle): ICanvas
{
const renderer = this.renderer;
let resolution;
let flipY = false;
let renderTexture;
let generated = false;
if (target)
{
if (target instanceof RenderTexture)
{
renderTexture = target;
}
else
{
renderTexture = this.renderer.generateTexture(target);
generated = true;
}
}
if (renderTexture)
{
resolution = renderTexture.baseTexture.resolution;
frame = frame ?? renderTexture.frame;
flipY = false;
renderer.renderTexture.bind(renderTexture);
}
else
{
resolution = renderer.resolution;
if (!frame)
{
frame = TEMP_RECT;
frame.width = renderer.width;
frame.height = renderer.height;
}
flipY = true;
renderer.renderTexture.bind(null);
}
const width = Math.round(frame.width * resolution);
const height = Math.round(frame.height * resolution);
let canvasBuffer = new utils.CanvasRenderTarget(width, height, 1);
const webglPixels = new Uint8Array(BYTES_PER_PIXEL * width * height);
// read pixels to the array
const gl = renderer.gl;
gl.readPixels(
Math.round(frame.x * resolution),
Math.round(frame.y * resolution),
width,
height,
gl.RGBA,
gl.UNSIGNED_BYTE,
webglPixels
);
// add the pixels to the canvas
const canvasData = canvasBuffer.context.getImageData(0, 0, width, height);
Extract.arrayPostDivide(webglPixels, canvasData.data);
canvasBuffer.context.putImageData(canvasData, 0, 0);
// pulling pixels
if (flipY)
{
const target = new utils.CanvasRenderTarget(canvasBuffer.width, canvasBuffer.height, 1);
target.context.scale(1, -1);
// we can't render to itself because we should be empty before render.
target.context.drawImage(canvasBuffer.canvas, 0, -height);
canvasBuffer.destroy();
canvasBuffer = target;
}
if (generated)
{
renderTexture.destroy(true);
}
// send the canvas back..
return canvasBuffer.canvas;
}
/**
* Will return a one-dimensional array containing the pixel data of the entire texture in RGBA
* order, with integer values between 0 and 255 (included).
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param frame - The frame the extraction is restricted to.
* @returns - One-dimensional array containing the pixel data of the entire texture
*/
public pixels(target?: DisplayObject | RenderTexture, frame?: Rectangle): Uint8Array
{
const renderer = this.renderer;
let resolution;
let renderTexture;
let generated = false;
if (target)
{
if (target instanceof RenderTexture)
{
renderTexture = target;
}
else
{
renderTexture = this.renderer.generateTexture(target);
generated = true;
}
}
if (renderTexture)
{
resolution = renderTexture.baseTexture.resolution;
frame = frame ?? renderTexture.frame;
renderer.renderTexture.bind(renderTexture);
}
else
{
resolution = renderer.resolution;
if (!frame)
{
frame = TEMP_RECT;
frame.width = renderer.width;
frame.height = renderer.height;
}
renderer.renderTexture.bind(null);
}
const width = Math.round(frame.width * resolution);
const height = Math.round(frame.height * resolution);
const webglPixels = new Uint8Array(BYTES_PER_PIXEL * width * height);
// read pixels to the array
const gl = renderer.gl;
gl.readPixels(
Math.round(frame.x * resolution),
Math.round(frame.y * resolution),
width,
height,
gl.RGBA,
gl.UNSIGNED_BYTE,
webglPixels
);
if (generated)
{
renderTexture.destroy(true);
}
Extract.arrayPostDivide(webglPixels, webglPixels);
return webglPixels;
}
/** Destroys the extract. */
public destroy(): void
{
this.renderer = null;
}
/**
* Takes premultiplied pixel data and produces regular pixel data
* @private
* @param pixels - array of pixel data
* @param out - output array
*/
static arrayPostDivide(
pixels: number[] | Uint8Array | Uint8ClampedArray, out: number[] | Uint8Array | Uint8ClampedArray
): void
{
for (let i = 0; i < pixels.length; i += 4)
{
const alpha = out[i + 3] = pixels[i + 3];
if (alpha !== 0)
{
out[i] = Math.round(Math.min(pixels[i] * 255.0 / alpha, 255.0));
out[i + 1] = Math.round(Math.min(pixels[i + 1] * 255.0 / alpha, 255.0));
out[i + 2] = Math.round(Math.min(pixels[i + 2] * 255.0 / alpha, 255.0));
}
else
{
out[i] = pixels[i];
out[i + 1] = pixels[i + 1];
out[i + 2] = pixels[i + 2];
}
}
}
}
extensions.add(Extract);
| packages/extract/src/Extract.ts | 1 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.0004860885674133897,
0.00019898930622730404,
0.00016536901239305735,
0.00018304993864148855,
0.000056120221415767446
] |
{
"id": 3,
"code_window": [
"/**\n",
" * The prepare plugin provides renderer-specific plugins for pre-rendering DisplayObjects. These plugins are useful for\n",
" * asynchronously preparing and uploading to the GPU assets, textures, graphics waiting to be displayed.\n",
" *\n",
" * Do not instantiate this plugin directly. It is available from the `renderer.plugins` property.\n",
" * See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.\n",
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * Do not instantiate this plugin directly. It is available from the `renderer.prepare` property.\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 97
} | {
"name": "@pixi/canvas-particle-container",
"version": "7.1.0",
"main": "lib/index.js",
"module": "lib/index.mjs",
"types": "lib/index.d.ts",
"exports": {
".": {
"import": {
"types": "./lib/index.d.ts",
"default": "./lib/index.mjs"
},
"require": {
"types": "./lib/index.d.ts",
"default": "./lib/index.js"
}
}
},
"description": "Canvas mixin for the particles package",
"author": "Mat Groves",
"contributors": [
"Matt Karl <[email protected]>"
],
"homepage": "http://pixijs.com/",
"bugs": "https://github.com/pixijs/pixijs/issues",
"license": "MIT",
"repository": {
"type": "git",
"url": "https://github.com/pixijs/pixijs.git"
},
"publishConfig": {
"access": "public"
},
"files": [
"lib",
"*.d.ts"
],
"pixiRequirements": [
"@pixi/particle-container"
]
}
| packages/canvas-particle-container/package.json | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017860971274785697,
0.00017135315283667296,
0.00016395063721574843,
0.00017324884538538754,
0.000005902408702240791
] |
{
"id": 3,
"code_window": [
"/**\n",
" * The prepare plugin provides renderer-specific plugins for pre-rendering DisplayObjects. These plugins are useful for\n",
" * asynchronously preparing and uploading to the GPU assets, textures, graphics waiting to be displayed.\n",
" *\n",
" * Do not instantiate this plugin directly. It is available from the `renderer.plugins` property.\n",
" * See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.\n",
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * Do not instantiate this plugin directly. It is available from the `renderer.prepare` property.\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 97
} | import { Cache, loadTextures, loadTxt } from '@pixi/assets';
import { utils } from '@pixi/core';
import { BitmapFont, loadBitmapFont } from '@pixi/text-bitmap';
import { Loader } from '../../assets/src/loader/Loader';
import type { ImageResource, Texture } from '@pixi/core';
describe('BitmapFontLoader', () =>
{
let loader: Loader;
const serverPath = process.env.GITHUB_ACTIONS
? `https://raw.githubusercontent.com/pixijs/pixijs/${process.env.GITHUB_SHA}/packages/text-bitmap/test/resources/`
: 'http://localhost:8080/text-bitmap/test/resources/';
beforeEach(() =>
{
Cache.reset();
loader.reset();
});
afterEach(() =>
{
for (const font in BitmapFont.available)
{
delete BitmapFont.available[font];
}
for (const baseTexture in utils.BaseTextureCache)
{
delete utils.BaseTextureCache[baseTexture];
}
for (const texture in utils.TextureCache)
{
delete utils.TextureCache[texture];
}
});
beforeAll(() =>
{
loader = new Loader();
loader['_parsers'].push(loadTxt, loadTextures, loadBitmapFont);
});
it('should do nothing if the resource is not XML/TXT font data', async () =>
{
expect(loadBitmapFont.test('notAFont.png')).toBe(false);
expect(await loadBitmapFont.testParse('notAFont.png')).toBe(false);
});
it('should do nothing if the resource is not properly formatted', async () =>
{
expect(await loadBitmapFont.testParse(
'<?xml version="1.0"?><!DOCTYPE WISHES<!ELEMENT WISHES (to, from)><Wishes >Have a good day!!</WISHES >'
)).toBe(false);
expect(await loadBitmapFont.testParse(
'abcdefg'
)).toBe(false);
});
it('should properly register bitmap font', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}font.fnt`);
expect(font).toBeObject();
expect(BitmapFont.available.font).toEqual(font);
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charATexture.frame.x).toEqual(2);
expect(charATexture.frame.y).toEqual(2);
expect(charATexture.frame.width).toEqual(19);
expect(charATexture.frame.height).toEqual(20);
const charB = font.chars['B'.charCodeAt(0)];
const charBTexture = charB.texture as Texture<ImageResource>;
expect(charB).toBeDefined();
expect(charBTexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charBTexture.frame.x).toEqual(2);
expect(charBTexture.frame.y).toEqual(24);
expect(charBTexture.frame.width).toEqual(15);
expect(charBTexture.frame.height).toEqual(20);
const charC = font.chars['C'.charCodeAt(0)];
const charCTexture = charC.texture as Texture<ImageResource>;
expect(charC).toBeDefined();
expect(charCTexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charCTexture.frame.x).toEqual(23);
expect(charCTexture.frame.y).toEqual(2);
expect(charCTexture.frame.width).toEqual(18);
expect(charCTexture.frame.height).toEqual(20);
const charD = font.chars['D'.charCodeAt(0)];
const charDTexture = charD.texture as Texture<ImageResource>;
expect(charD).toBeDefined();
expect(charDTexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charDTexture.frame.x).toEqual(19);
expect(charDTexture.frame.y).toEqual(24);
expect(charDTexture.frame.width).toEqual(17);
expect(charDTexture.frame.height).toEqual(20);
const charE = font.chars['E'.charCodeAt(0)];
expect(charE).toBeUndefined();
});
it('should properly register bitmap font based on txt data', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}bmtxt-test.txt`);
expect(font).toBeObject();
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}bmGlyph-test.png`);
expect(charATexture.frame.x).toEqual(1);
expect(charATexture.frame.y).toEqual(179);
expect(charATexture.frame.width).toEqual(38);
expect(charATexture.frame.height).toEqual(28);
const charB = font.chars['B'.charCodeAt(0)];
const charBTexture = charB.texture as Texture<ImageResource>;
expect(charB).toBeDefined();
expect(charBTexture.baseTexture.resource.src).toEqual(`${serverPath}bmGlyph-test.png`);
expect(charBTexture.frame.x).toEqual(52);
expect(charBTexture.frame.y).toEqual(146);
expect(charBTexture.frame.width).toEqual(34);
expect(charBTexture.frame.height).toEqual(28);
const charC = font.chars['C'.charCodeAt(0)];
const charCTexture = charC.texture as Texture<ImageResource>;
expect(charC).toBeDefined();
expect(charCTexture.baseTexture.resource.src).toEqual(`${serverPath}bmGlyph-test.png`);
expect(charCTexture.frame.x).toEqual(52);
expect(charCTexture.frame.y).toEqual(117);
expect(charCTexture.frame.width).toEqual(34);
expect(charCTexture.frame.height).toEqual(28);
const charD = font.chars['D'.charCodeAt(0)];
const charDTexture = charD.texture as Texture<ImageResource>;
expect(charD).toBeDefined();
expect(charDTexture.baseTexture.resource.src).toEqual(`${serverPath}bmGlyph-test.png`);
expect(charDTexture.frame.x).toEqual(52);
expect(charDTexture.frame.y).toEqual(88);
expect(charDTexture.frame.width).toEqual(34);
expect(charDTexture.frame.height).toEqual(28);
const charUndefined = font.chars['£'.charCodeAt(0)];
expect(charUndefined).toBeUndefined();
});
it('should properly register bitmap font based on text format', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}font-text.fnt`);
expect(font).toBeObject();
expect(BitmapFont.available.fontText).toEqual(font);
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charATexture.frame.x).toEqual(2);
expect(charATexture.frame.y).toEqual(2);
expect(charATexture.frame.width).toEqual(19);
expect(charATexture.frame.height).toEqual(20);
const charB = font.chars['B'.charCodeAt(0)];
const charBTexture = charB.texture as Texture<ImageResource>;
expect(charB).toBeDefined();
expect(charBTexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charBTexture.frame.x).toEqual(2);
expect(charBTexture.frame.y).toEqual(24);
expect(charBTexture.frame.width).toEqual(15);
expect(charBTexture.frame.height).toEqual(20);
const charC = font.chars['C'.charCodeAt(0)];
const charCTexture = charC.texture as Texture<ImageResource>;
expect(charC).toBeDefined();
expect(charCTexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charCTexture.frame.x).toEqual(23);
expect(charCTexture.frame.y).toEqual(2);
expect(charCTexture.frame.width).toEqual(18);
expect(charCTexture.frame.height).toEqual(20);
const charD = font.chars['D'.charCodeAt(0)];
const charDTexture = charD.texture as Texture<ImageResource>;
expect(charD).toBeDefined();
expect(charDTexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charDTexture.frame.x).toEqual(19);
expect(charDTexture.frame.y).toEqual(24);
expect(charDTexture.frame.width).toEqual(17);
expect(charDTexture.frame.height).toEqual(20);
const charE = font.chars['E'.charCodeAt(0)];
expect(charE).toBeUndefined();
});
it('should properly register bitmap font with url params', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}font-text.fnt?version=1.0.0`);
expect(font).toBeObject();
expect(BitmapFont.available.fontText).toEqual(font);
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}font.png?version=1.0.0`);
expect(charATexture.frame.x).toEqual(2);
expect(charATexture.frame.y).toEqual(2);
expect(charATexture.frame.width).toEqual(19);
expect(charATexture.frame.height).toEqual(20);
});
it('should properly register SCALED bitmap font', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}[email protected]`);
expect(font).toBeObject();
expect(BitmapFont.available.font).toEqual(font);
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}[email protected]`);
expect(charATexture.frame.x).toEqual(4); // 2 / 0.5
expect(charATexture.frame.y).toEqual(4); // 2 / 0.5
expect(charATexture.frame.width).toEqual(38); // 19 / 0.5
expect(charATexture.frame.height).toEqual(40); // 20 / 0.5
const charB = font.chars['B'.charCodeAt(0)];
const charBTexture = charB.texture as Texture<ImageResource>;
expect(charB).toBeDefined();
expect(charBTexture.baseTexture.resource.src).toEqual(`${serverPath}[email protected]`);
expect(charBTexture.frame.x).toEqual(4); // 2 / 0.5
expect(charBTexture.frame.y).toEqual(48); // 24 / 0.5
expect(charBTexture.frame.width).toEqual(30); // 15 / 0.5
expect(charBTexture.frame.height).toEqual(40); // 20 / 0.5
const charC = font.chars['C'.charCodeAt(0)];
const charCTexture = charC.texture as Texture<ImageResource>;
expect(charC).toBeDefined();
expect(charCTexture.baseTexture.resource.src).toEqual(`${serverPath}[email protected]`);
expect(charCTexture.frame.x).toEqual(46); // 23 / 0.5
expect(charCTexture.frame.y).toEqual(4); // 2 / 0.5
expect(charCTexture.frame.width).toEqual(36); // 18 / 0.5
expect(charCTexture.frame.height).toEqual(40); // 20 / 0.5
const charD = font.chars['D'.charCodeAt(0)];
const charDTexture = charD.texture as Texture<ImageResource>;
expect(charD).toBeDefined();
expect(charDTexture.baseTexture.resource.src).toEqual(`${serverPath}[email protected]`);
expect(charDTexture.frame.x).toEqual(38); // 19 / 0.5
expect(charDTexture.frame.y).toEqual(48); // 24 / 0.5
expect(charDTexture.frame.width).toEqual(34); // 17 / 0.5
expect(charDTexture.frame.height).toEqual(40); // 20 / 0.5
const charE = font.chars['E'.charCodeAt(0)];
expect(charE).toBeUndefined();
});
it('should properly register bitmap font having more than one texture', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}split_font.fnt`);
expect(font).toBeObject();
expect(BitmapFont.available.split_font).toEqual(font);
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
let src = charATexture.baseTexture.resource.src;
src = src.substring(src.length - 17);
expect(src).toEqual('split_font_ab.png');
expect(charATexture.frame.x).toEqual(2);
expect(charATexture.frame.y).toEqual(2);
expect(charATexture.frame.width).toEqual(19);
expect(charATexture.frame.height).toEqual(20);
const charB = font.chars['B'.charCodeAt(0)];
const charBTexture = charB.texture as Texture<ImageResource>;
expect(charB).toBeDefined();
src = charBTexture.baseTexture.resource.src;
src = src.substring(src.length - 17);
expect(src).toEqual('split_font_ab.png');
expect(charBTexture.frame.x).toEqual(2);
expect(charBTexture.frame.y).toEqual(24);
expect(charBTexture.frame.width).toEqual(15);
expect(charBTexture.frame.height).toEqual(20);
const charC = font.chars['C'.charCodeAt(0)];
const charCTexture = charC.texture as Texture<ImageResource>;
expect(charC).toBeDefined();
src = charCTexture.baseTexture.resource.src;
src = src.substring(src.length - 17);
expect(src).toEqual('split_font_cd.png');
expect(charCTexture.frame.x).toEqual(2);
expect(charCTexture.frame.y).toEqual(2);
expect(charCTexture.frame.width).toEqual(18);
expect(charCTexture.frame.height).toEqual(20);
const charD = font.chars['D'.charCodeAt(0)];
const charDTexture = charD.texture as Texture<ImageResource>;
expect(charD).toBeDefined();
src = charDTexture.baseTexture.resource.src;
src = src.substring(src.length - 17);
expect(src).toEqual('split_font_cd.png');
expect(charDTexture.frame.x).toEqual(2);
expect(charDTexture.frame.y).toEqual(24);
expect(charDTexture.frame.width).toEqual(17);
expect(charDTexture.frame.height).toEqual(20);
const charE = font.chars['E'.charCodeAt(0)];
expect(charE).toBeUndefined();
});
it('should split fonts if page IDs are in chronological order', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}split_font2.fnt`);
const charA = font.chars['A'.charCodeAt(0)];
const charC = font.chars['C'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
const charCTexture = charC.texture as Texture<ImageResource>;
expect(charA.page).toEqual(0);
expect(charC.page).toEqual(1);
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}split_font_ab.png`);
expect(charCTexture.baseTexture.resource.src).toEqual(`${serverPath}split_font_cd.png`);
});
it('should set the texture to NPM on SDF fonts', async () =>
{
const sdfFont = await loader.load<BitmapFont>(`${serverPath}sdf.fnt`);
const msdfFont = await loader.load<BitmapFont>(`${serverPath}msdf.fnt`);
const regularFont = await loader.load<BitmapFont>(`${serverPath}font-text.fnt`);
expect(sdfFont.chars['A'.charCodeAt(0)].texture.baseTexture.alphaMode).toEqual(0);
expect(msdfFont.chars['A'.charCodeAt(0)].texture.baseTexture.alphaMode).toEqual(0);
expect(regularFont.chars['A'.charCodeAt(0)].texture.baseTexture.alphaMode).not.toEqual(0);
});
it('should set the distanceFieldType correctly', async () =>
{
const sdfFont = await loader.load<BitmapFont>(`${serverPath}sdf.fnt`);
const msdfFont = await loader.load<BitmapFont>(`${serverPath}msdf.fnt`);
const regularFont = await loader.load<BitmapFont>(`${serverPath}font-text.fnt`);
expect(sdfFont.distanceFieldType).toEqual('sdf');
expect(msdfFont.distanceFieldType).toEqual('msdf');
expect(regularFont.distanceFieldType).toEqual('none');
});
it('should properly register bitmap font with random placed arguments into info tag', async () =>
{
const font = await loader.load<BitmapFont>(`${serverPath}font-random-args.fnt`);
expect(font).toBeObject();
expect(BitmapFont.available.font).toEqual(font);
expect(font).toHaveProperty('chars');
const charA = font.chars['A'.charCodeAt(0)];
const charATexture = charA.texture as Texture<ImageResource>;
expect(charA).toBeDefined();
expect(charATexture.baseTexture.resource.src).toEqual(`${serverPath}font.png`);
expect(charATexture.frame.x).toEqual(2);
expect(charATexture.frame.y).toEqual(2);
expect(charATexture.frame.width).toEqual(19);
expect(charATexture.frame.height).toEqual(20);
});
it('should unload a bitmap font', async () =>
{
const loader = new Loader();
loader['_parsers'].push(loadTextures, loadBitmapFont);
const bitmapFont = await loader.load<BitmapFont>(`${serverPath}desyrel.xml`);
expect(bitmapFont).toBeInstanceOf(BitmapFont);
await loader.unload(`${serverPath}desyrel.xml`);
expect(bitmapFont.pageTextures).toBe(null);
});
});
| packages/text-bitmap/test/BitmapFontLoader.tests.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.000172294516232796,
0.00016530818538740277,
0.00016351552039850503,
0.00016489562403876334,
0.0000016053171520979959
] |
{
"id": 3,
"code_window": [
"/**\n",
" * The prepare plugin provides renderer-specific plugins for pre-rendering DisplayObjects. These plugins are useful for\n",
" * asynchronously preparing and uploading to the GPU assets, textures, graphics waiting to be displayed.\n",
" *\n",
" * Do not instantiate this plugin directly. It is available from the `renderer.plugins` property.\n",
" * See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.\n",
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * Do not instantiate this plugin directly. It is available from the `renderer.prepare` property.\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 97
} | import { BUFFER_TYPE } from '@pixi/constants';
import { Runner } from '@pixi/runner';
import type { GLBuffer } from './GLBuffer';
let UID = 0;
/* eslint-disable max-len */
/**
* Marks places in PixiJS where you can pass Float32Array, UInt32Array, any typed arrays, and ArrayBuffer
*
* Same as ArrayBuffer in typescript lib, defined here just for documentation
*/
// eslint-disable-next-line @typescript-eslint/no-empty-interface
export interface IArrayBuffer extends ArrayBuffer
{
}
/**
* PixiJS classes use this type instead of ArrayBuffer and typed arrays
* to support expressions like `geometry.buffers[0].data[0] = position.x`.
*
* Gives access to indexing and `length` field
* - @popelyshev: If data is actually ArrayBuffer and throws Exception on indexing - its user problem :)
*/
export interface ITypedArray extends IArrayBuffer
{
readonly length: number;
[index: number]: number;
readonly BYTES_PER_ELEMENT: number;
}
/**
* A wrapper for data so that it can be used and uploaded by WebGL
* @memberof PIXI
*/
export class Buffer
{
/**
* The data in the buffer, as a typed array
* @type {PIXI.IArrayBuffer}
*/
public data: ITypedArray;
/**
* The type of buffer this is, one of:
* + ELEMENT_ARRAY_BUFFER - used as an index buffer
* + ARRAY_BUFFER - used as an attribute buffer
* + UNIFORM_BUFFER - used as a uniform buffer (if available)
*/
public type: BUFFER_TYPE;
public static: boolean;
public id: number;
disposeRunner: Runner;
/**
* A map of renderer IDs to webgl buffer
* @private
* @type {Object<number, GLBuffer>}
*/
_glBuffers: {[key: number]: GLBuffer};
_updateID: number;
/**
* @param {PIXI.IArrayBuffer} data - the data to store in the buffer.
* @param _static - `true` for static buffer
* @param index - `true` for index buffer
*/
constructor(data?: IArrayBuffer, _static = true, index = false)
{
this.data = (data || new Float32Array(1)) as ITypedArray;
this._glBuffers = {};
this._updateID = 0;
this.index = index;
this.static = _static;
this.id = UID++;
this.disposeRunner = new Runner('disposeBuffer');
}
// TODO could explore flagging only a partial upload?
/**
* Flags this buffer as requiring an upload to the GPU.
* @param {PIXI.IArrayBuffer|number[]} [data] - the data to update in the buffer.
*/
update(data?: IArrayBuffer | Array<number>): void
{
if (data instanceof Array)
{
data = new Float32Array(data);
}
this.data = (data as ITypedArray) || this.data;
this._updateID++;
}
/** Disposes WebGL resources that are connected to this geometry. */
dispose(): void
{
this.disposeRunner.emit(this, false);
}
/** Destroys the buffer. */
destroy(): void
{
this.dispose();
this.data = null;
}
/**
* Flags whether this is an index buffer.
*
* Index buffers are of type `ELEMENT_ARRAY_BUFFER`. Note that setting this property to false will make
* the buffer of type `ARRAY_BUFFER`.
*
* For backwards compatibility.
*/
set index(value: boolean)
{
this.type = value ? BUFFER_TYPE.ELEMENT_ARRAY_BUFFER : BUFFER_TYPE.ARRAY_BUFFER;
}
get index(): boolean
{
return this.type === BUFFER_TYPE.ELEMENT_ARRAY_BUFFER;
}
/**
* Helper function that creates a buffer based on an array or TypedArray
* @param {ArrayBufferView | number[]} data - the TypedArray that the buffer will store. If this is a regular Array it will be converted to a Float32Array.
* @returns - A new Buffer based on the data provided.
*/
static from(data: IArrayBuffer | number[]): Buffer
{
if (data instanceof Array)
{
data = new Float32Array(data);
}
return new Buffer(data);
}
}
| packages/core/src/geometry/Buffer.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.0002570025098975748,
0.00018237593758385628,
0.00016864680219441652,
0.00017494171333964914,
0.00002230267637060024
] |
{
"id": 4,
"code_window": [
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n",
" * // Create a new application\n",
" * const app = new Application();\n",
" * document.body.appendChild(app.view);\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a new application (prepare will be auto-added to renderer)\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 102
} | import { extensions, ExtensionType, Rectangle, RenderTexture, utils } from '@pixi/core';
import type { ExtensionMetadata, ICanvas, ISystem, Renderer } from '@pixi/core';
import type { DisplayObject } from '@pixi/display';
const TEMP_RECT = new Rectangle();
const BYTES_PER_PIXEL = 4;
/**
* This class provides renderer-specific plugins for exporting content from a renderer.
* For instance, these plugins can be used for saving an Image, Canvas element or for exporting the raw image data (pixels).
*
* Do not instantiate these plugins directly. It is available from the `renderer.plugins` property.
* See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.
* @example
* import { Application, Graphics } from 'pixi.js';
*
* // Create a new app (will auto-add extract plugin to renderer)
* const app = new Application();
*
* // Draw a red circle
* const graphics = new Graphics()
* .beginFill(0xFF0000)
* .drawCircle(0, 0, 50);
*
* // Render the graphics as an HTMLImageElement
* const image = app.renderer.plugins.image(graphics);
* document.body.appendChild(image);
* @memberof PIXI
*/
export class Extract implements ISystem
{
/** @ignore */
static extension: ExtensionMetadata = {
name: 'extract',
type: ExtensionType.RendererSystem,
};
private renderer: Renderer;
/**
* @param renderer - A reference to the current renderer
*/
constructor(renderer: Renderer)
{
this.renderer = renderer;
}
/**
* Will return a HTML Image of the target
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param format - Image format, e.g. "image/jpeg" or "image/webp".
* @param quality - JPEG or Webp compression from 0 to 1. Default is 0.92.
* @returns - HTML Image of the target
*/
public async image(target: DisplayObject | RenderTexture, format?: string, quality?: number): Promise<HTMLImageElement>
{
const image = new Image();
image.src = await this.base64(target, format, quality);
return image;
}
/**
* Will return a base64 encoded string of this target. It works by calling
* `Extract.getCanvas` and then running toDataURL on that.
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param format - Image format, e.g. "image/jpeg" or "image/webp".
* @param quality - JPEG or Webp compression from 0 to 1. Default is 0.92.
* @returns - A base64 encoded string of the texture.
*/
public async base64(target: DisplayObject | RenderTexture, format?: string, quality?: number): Promise<string>
{
const canvas = this.canvas(target);
if (canvas.toDataURL !== undefined)
{
return canvas.toDataURL(format, quality);
}
if (canvas.convertToBlob !== undefined)
{
const blob = await canvas.convertToBlob({ type: format, quality });
return await new Promise<string>((resolve) =>
{
const reader = new FileReader();
reader.onload = () => resolve(reader.result as string);
reader.readAsDataURL(blob);
});
}
throw new Error('Extract.base64() requires ICanvas.toDataURL or ICanvas.convertToBlob to be implemented');
}
/**
* Creates a Canvas element, renders this target to it and then returns it.
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param frame - The frame the extraction is restricted to.
* @returns - A Canvas element with the texture rendered on.
*/
public canvas(target: DisplayObject | RenderTexture, frame?: Rectangle): ICanvas
{
const renderer = this.renderer;
let resolution;
let flipY = false;
let renderTexture;
let generated = false;
if (target)
{
if (target instanceof RenderTexture)
{
renderTexture = target;
}
else
{
renderTexture = this.renderer.generateTexture(target);
generated = true;
}
}
if (renderTexture)
{
resolution = renderTexture.baseTexture.resolution;
frame = frame ?? renderTexture.frame;
flipY = false;
renderer.renderTexture.bind(renderTexture);
}
else
{
resolution = renderer.resolution;
if (!frame)
{
frame = TEMP_RECT;
frame.width = renderer.width;
frame.height = renderer.height;
}
flipY = true;
renderer.renderTexture.bind(null);
}
const width = Math.round(frame.width * resolution);
const height = Math.round(frame.height * resolution);
let canvasBuffer = new utils.CanvasRenderTarget(width, height, 1);
const webglPixels = new Uint8Array(BYTES_PER_PIXEL * width * height);
// read pixels to the array
const gl = renderer.gl;
gl.readPixels(
Math.round(frame.x * resolution),
Math.round(frame.y * resolution),
width,
height,
gl.RGBA,
gl.UNSIGNED_BYTE,
webglPixels
);
// add the pixels to the canvas
const canvasData = canvasBuffer.context.getImageData(0, 0, width, height);
Extract.arrayPostDivide(webglPixels, canvasData.data);
canvasBuffer.context.putImageData(canvasData, 0, 0);
// pulling pixels
if (flipY)
{
const target = new utils.CanvasRenderTarget(canvasBuffer.width, canvasBuffer.height, 1);
target.context.scale(1, -1);
// we can't render to itself because we should be empty before render.
target.context.drawImage(canvasBuffer.canvas, 0, -height);
canvasBuffer.destroy();
canvasBuffer = target;
}
if (generated)
{
renderTexture.destroy(true);
}
// send the canvas back..
return canvasBuffer.canvas;
}
/**
* Will return a one-dimensional array containing the pixel data of the entire texture in RGBA
* order, with integer values between 0 and 255 (included).
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param frame - The frame the extraction is restricted to.
* @returns - One-dimensional array containing the pixel data of the entire texture
*/
public pixels(target?: DisplayObject | RenderTexture, frame?: Rectangle): Uint8Array
{
const renderer = this.renderer;
let resolution;
let renderTexture;
let generated = false;
if (target)
{
if (target instanceof RenderTexture)
{
renderTexture = target;
}
else
{
renderTexture = this.renderer.generateTexture(target);
generated = true;
}
}
if (renderTexture)
{
resolution = renderTexture.baseTexture.resolution;
frame = frame ?? renderTexture.frame;
renderer.renderTexture.bind(renderTexture);
}
else
{
resolution = renderer.resolution;
if (!frame)
{
frame = TEMP_RECT;
frame.width = renderer.width;
frame.height = renderer.height;
}
renderer.renderTexture.bind(null);
}
const width = Math.round(frame.width * resolution);
const height = Math.round(frame.height * resolution);
const webglPixels = new Uint8Array(BYTES_PER_PIXEL * width * height);
// read pixels to the array
const gl = renderer.gl;
gl.readPixels(
Math.round(frame.x * resolution),
Math.round(frame.y * resolution),
width,
height,
gl.RGBA,
gl.UNSIGNED_BYTE,
webglPixels
);
if (generated)
{
renderTexture.destroy(true);
}
Extract.arrayPostDivide(webglPixels, webglPixels);
return webglPixels;
}
/** Destroys the extract. */
public destroy(): void
{
this.renderer = null;
}
/**
* Takes premultiplied pixel data and produces regular pixel data
* @private
* @param pixels - array of pixel data
* @param out - output array
*/
static arrayPostDivide(
pixels: number[] | Uint8Array | Uint8ClampedArray, out: number[] | Uint8Array | Uint8ClampedArray
): void
{
for (let i = 0; i < pixels.length; i += 4)
{
const alpha = out[i + 3] = pixels[i + 3];
if (alpha !== 0)
{
out[i] = Math.round(Math.min(pixels[i] * 255.0 / alpha, 255.0));
out[i + 1] = Math.round(Math.min(pixels[i + 1] * 255.0 / alpha, 255.0));
out[i + 2] = Math.round(Math.min(pixels[i + 2] * 255.0 / alpha, 255.0));
}
else
{
out[i] = pixels[i];
out[i + 1] = pixels[i + 1];
out[i + 2] = pixels[i + 2];
}
}
}
}
extensions.add(Extract);
| packages/extract/src/Extract.ts | 1 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.9963815212249756,
0.031840190291404724,
0.00016428348317276686,
0.00017075735377147794,
0.17326264083385468
] |
{
"id": 4,
"code_window": [
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n",
" * // Create a new application\n",
" * const app = new Application();\n",
" * document.body.appendChild(app.view);\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a new application (prepare will be auto-added to renderer)\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 102
} | The MIT License
Copyright (c) 2013-2023 Mathew Groves, Chad Engler
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
| packages/canvas-renderer/LICENSE | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017469302110839635,
0.00017097506497520953,
0.00016464086365886033,
0.00017359131015837193,
0.000004501482635532739
] |
{
"id": 4,
"code_window": [
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n",
" * // Create a new application\n",
" * const app = new Application();\n",
" * document.body.appendChild(app.view);\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a new application (prepare will be auto-added to renderer)\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 102
} | The MIT License
Copyright (c) 2013-2023 Mathew Groves, Chad Engler
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
| bundles/pixi.js-webworker/LICENSE | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017469302110839635,
0.00017097506497520953,
0.00016464086365886033,
0.00017359131015837193,
0.000004501482635532739
] |
{
"id": 4,
"code_window": [
" * @example\n",
" * import { Application, Graphics } from 'pixi.js';\n",
" *\n",
" * // Create a new application\n",
" * const app = new Application();\n",
" * document.body.appendChild(app.view);\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a new application (prepare will be auto-added to renderer)\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 102
} | @pixi/webworker
=============
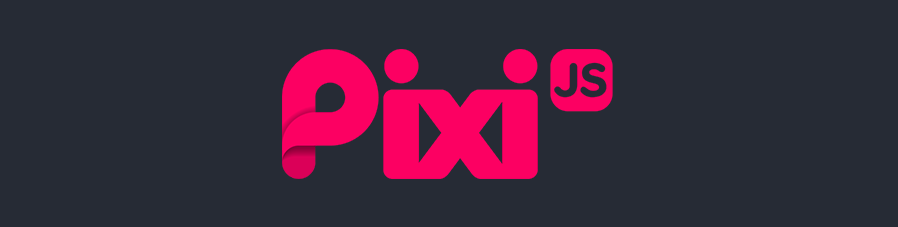
The aim of this project is to provide a fast lightweight 2D library that works
across all devices. The PixiJS renderer allows everyone to enjoy the power of
hardware acceleration without prior knowledge of WebGL. Also, it's fast. Really fast.
**We are now a part of the [Open Collective](https://opencollective.com/pixijs) and with your support you can help us make PixiJS even better. To make a donation, simply click the button below and we'll love you forever!**
<div align="center">
<a href="https://opencollective.com/pixijs/donate" target="_blank">
<img src="https://opencollective.com/pixijs/donate/[email protected]?color=blue" width=250 />
</a>
</div>
### Setup
PixiJS can be installed with [npm](https://docs.npmjs.com/getting-started/what-is-npm) to integrate with [Webpack](https://webpack.js.org/), [Browserify](http://browserify.org/), [Rollup](https://rollupjs.org/), [Electron](https://electron.atom.io/), [NW.js](https://nwjs.io/) or other module backed environments.
#### Install
```
npm install @pixi/webworker
```
There is no default export. The correct way to import PixiJS is:
```js
import * as PIXI from '@pixi/webworker';
```
#### CDN Install
Via jsDelivr:
```js
importScripts('https://cdn.jsdelivr.net/npm/[email protected]/dist/pixi-webworker.min.js');
```
Or via unpkg:
```js
importScripts('https://unpkg.com/[email protected]/dist/pixi-webworker.min.js');
```
### Basic Usage Example
In `index.js`:
```js
// Create a canvas element and insert it into DOM
const width = 800, height = 600;
const resolution = window.devicePixelRatio;
const canvas = document.createElement('canvas');
canvas.style.width = `${ width }px`;
canvas.style.height = `${ height }px`;
document.body.appendChild(canvas);
// Create the worker
const worker = new Worker('worker.js', { type: 'module' });
// Transfer canvas to the worker
const view = canvas.transferControlToOffscreen();
worker.postMessage({ width, height, resolution, view }, [view]);
```
In `worker.js`:
```js
import { Application, Assets, Sprite } from '@pixi/webworker';
self.onmessage = async e => {
// Recieve OffscreenCanvas from index.js
const { width, height, resolution, view } = e.data;
// The application will create a renderer using WebGL, if possible,
// with a fallback to a canvas render. It will also setup the ticker
// and the root stage PIXI.Container
const app = new Application({ width, height, resolution, view });
// load the texture we need
const texture = await Assets.load('assets/bunny.png');
// This creates a texture from a 'bunny.png' image
const bunny = new Sprite(texture);
// Setup the position of the bunny
bunny.x = app.renderer.width / 2;
bunny.y = app.renderer.height / 2;
// Rotate around the center
bunny.anchor.x = 0.5;
bunny.anchor.y = 0.5;
// Add the bunny to the scene we are building
app.stage.addChild(bunny);
// Listen for frame updates
app.ticker.add(() => {
// each frame we spin the bunny around a bit
bunny.rotation += 0.01;
});
}
```
### License
This content is released under the (http://opensource.org/licenses/MIT) MIT License.
| bundles/pixi.js-webworker/README.md | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.9285326600074768,
0.0847783163189888,
0.00016270227206405252,
0.00018335136701352894,
0.266819030046463
] |
{
"id": 5,
"code_window": [
" *\n",
" * // Don't start rendering right away\n",
" * app.stop();\n",
" *\n",
" * // create a display object\n",
" * const rect = new Graphics()\n",
" * .beginFill(0x00ff00)\n",
" * .drawRect(40, 40, 200, 200);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a display object\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 109
} | import { extensions, ExtensionType, Rectangle, RenderTexture, utils } from '@pixi/core';
import type { ExtensionMetadata, ICanvas, ISystem, Renderer } from '@pixi/core';
import type { DisplayObject } from '@pixi/display';
const TEMP_RECT = new Rectangle();
const BYTES_PER_PIXEL = 4;
/**
* This class provides renderer-specific plugins for exporting content from a renderer.
* For instance, these plugins can be used for saving an Image, Canvas element or for exporting the raw image data (pixels).
*
* Do not instantiate these plugins directly. It is available from the `renderer.plugins` property.
* See {@link PIXI.CanvasRenderer#plugins} or {@link PIXI.Renderer#plugins}.
* @example
* import { Application, Graphics } from 'pixi.js';
*
* // Create a new app (will auto-add extract plugin to renderer)
* const app = new Application();
*
* // Draw a red circle
* const graphics = new Graphics()
* .beginFill(0xFF0000)
* .drawCircle(0, 0, 50);
*
* // Render the graphics as an HTMLImageElement
* const image = app.renderer.plugins.image(graphics);
* document.body.appendChild(image);
* @memberof PIXI
*/
export class Extract implements ISystem
{
/** @ignore */
static extension: ExtensionMetadata = {
name: 'extract',
type: ExtensionType.RendererSystem,
};
private renderer: Renderer;
/**
* @param renderer - A reference to the current renderer
*/
constructor(renderer: Renderer)
{
this.renderer = renderer;
}
/**
* Will return a HTML Image of the target
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param format - Image format, e.g. "image/jpeg" or "image/webp".
* @param quality - JPEG or Webp compression from 0 to 1. Default is 0.92.
* @returns - HTML Image of the target
*/
public async image(target: DisplayObject | RenderTexture, format?: string, quality?: number): Promise<HTMLImageElement>
{
const image = new Image();
image.src = await this.base64(target, format, quality);
return image;
}
/**
* Will return a base64 encoded string of this target. It works by calling
* `Extract.getCanvas` and then running toDataURL on that.
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param format - Image format, e.g. "image/jpeg" or "image/webp".
* @param quality - JPEG or Webp compression from 0 to 1. Default is 0.92.
* @returns - A base64 encoded string of the texture.
*/
public async base64(target: DisplayObject | RenderTexture, format?: string, quality?: number): Promise<string>
{
const canvas = this.canvas(target);
if (canvas.toDataURL !== undefined)
{
return canvas.toDataURL(format, quality);
}
if (canvas.convertToBlob !== undefined)
{
const blob = await canvas.convertToBlob({ type: format, quality });
return await new Promise<string>((resolve) =>
{
const reader = new FileReader();
reader.onload = () => resolve(reader.result as string);
reader.readAsDataURL(blob);
});
}
throw new Error('Extract.base64() requires ICanvas.toDataURL or ICanvas.convertToBlob to be implemented');
}
/**
* Creates a Canvas element, renders this target to it and then returns it.
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param frame - The frame the extraction is restricted to.
* @returns - A Canvas element with the texture rendered on.
*/
public canvas(target: DisplayObject | RenderTexture, frame?: Rectangle): ICanvas
{
const renderer = this.renderer;
let resolution;
let flipY = false;
let renderTexture;
let generated = false;
if (target)
{
if (target instanceof RenderTexture)
{
renderTexture = target;
}
else
{
renderTexture = this.renderer.generateTexture(target);
generated = true;
}
}
if (renderTexture)
{
resolution = renderTexture.baseTexture.resolution;
frame = frame ?? renderTexture.frame;
flipY = false;
renderer.renderTexture.bind(renderTexture);
}
else
{
resolution = renderer.resolution;
if (!frame)
{
frame = TEMP_RECT;
frame.width = renderer.width;
frame.height = renderer.height;
}
flipY = true;
renderer.renderTexture.bind(null);
}
const width = Math.round(frame.width * resolution);
const height = Math.round(frame.height * resolution);
let canvasBuffer = new utils.CanvasRenderTarget(width, height, 1);
const webglPixels = new Uint8Array(BYTES_PER_PIXEL * width * height);
// read pixels to the array
const gl = renderer.gl;
gl.readPixels(
Math.round(frame.x * resolution),
Math.round(frame.y * resolution),
width,
height,
gl.RGBA,
gl.UNSIGNED_BYTE,
webglPixels
);
// add the pixels to the canvas
const canvasData = canvasBuffer.context.getImageData(0, 0, width, height);
Extract.arrayPostDivide(webglPixels, canvasData.data);
canvasBuffer.context.putImageData(canvasData, 0, 0);
// pulling pixels
if (flipY)
{
const target = new utils.CanvasRenderTarget(canvasBuffer.width, canvasBuffer.height, 1);
target.context.scale(1, -1);
// we can't render to itself because we should be empty before render.
target.context.drawImage(canvasBuffer.canvas, 0, -height);
canvasBuffer.destroy();
canvasBuffer = target;
}
if (generated)
{
renderTexture.destroy(true);
}
// send the canvas back..
return canvasBuffer.canvas;
}
/**
* Will return a one-dimensional array containing the pixel data of the entire texture in RGBA
* order, with integer values between 0 and 255 (included).
* @param target - A displayObject or renderTexture
* to convert. If left empty will use the main renderer
* @param frame - The frame the extraction is restricted to.
* @returns - One-dimensional array containing the pixel data of the entire texture
*/
public pixels(target?: DisplayObject | RenderTexture, frame?: Rectangle): Uint8Array
{
const renderer = this.renderer;
let resolution;
let renderTexture;
let generated = false;
if (target)
{
if (target instanceof RenderTexture)
{
renderTexture = target;
}
else
{
renderTexture = this.renderer.generateTexture(target);
generated = true;
}
}
if (renderTexture)
{
resolution = renderTexture.baseTexture.resolution;
frame = frame ?? renderTexture.frame;
renderer.renderTexture.bind(renderTexture);
}
else
{
resolution = renderer.resolution;
if (!frame)
{
frame = TEMP_RECT;
frame.width = renderer.width;
frame.height = renderer.height;
}
renderer.renderTexture.bind(null);
}
const width = Math.round(frame.width * resolution);
const height = Math.round(frame.height * resolution);
const webglPixels = new Uint8Array(BYTES_PER_PIXEL * width * height);
// read pixels to the array
const gl = renderer.gl;
gl.readPixels(
Math.round(frame.x * resolution),
Math.round(frame.y * resolution),
width,
height,
gl.RGBA,
gl.UNSIGNED_BYTE,
webglPixels
);
if (generated)
{
renderTexture.destroy(true);
}
Extract.arrayPostDivide(webglPixels, webglPixels);
return webglPixels;
}
/** Destroys the extract. */
public destroy(): void
{
this.renderer = null;
}
/**
* Takes premultiplied pixel data and produces regular pixel data
* @private
* @param pixels - array of pixel data
* @param out - output array
*/
static arrayPostDivide(
pixels: number[] | Uint8Array | Uint8ClampedArray, out: number[] | Uint8Array | Uint8ClampedArray
): void
{
for (let i = 0; i < pixels.length; i += 4)
{
const alpha = out[i + 3] = pixels[i + 3];
if (alpha !== 0)
{
out[i] = Math.round(Math.min(pixels[i] * 255.0 / alpha, 255.0));
out[i + 1] = Math.round(Math.min(pixels[i + 1] * 255.0 / alpha, 255.0));
out[i + 2] = Math.round(Math.min(pixels[i + 2] * 255.0 / alpha, 255.0));
}
else
{
out[i] = pixels[i];
out[i + 1] = pixels[i + 1];
out[i + 2] = pixels[i + 2];
}
}
}
}
extensions.add(Extract);
| packages/extract/src/Extract.ts | 1 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.005860177334398031,
0.0004232220526318997,
0.0001677869149716571,
0.00016971422883216292,
0.00105158647056669
] |
{
"id": 5,
"code_window": [
" *\n",
" * // Don't start rendering right away\n",
" * app.stop();\n",
" *\n",
" * // create a display object\n",
" * const rect = new Graphics()\n",
" * .beginFill(0x00ff00)\n",
" * .drawRect(40, 40, 200, 200);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a display object\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 109
} | import { Rectangle } from '@pixi/core';
/**
* Determines whether the `other` Rectangle is contained within `this` Rectangle object.
* Rectangles that occupy the same space are considered to be containing each other.
* Rectangles without area (width or height equal to zero) can't contain anything,
* not even other arealess rectangles.
*
* _Note: Only available with **@pixi/math-extras**._
* @method containsRect
* @memberof PIXI.Rectangle#
* @param {Rectangle} other - The Rectangle to fit inside `this`.
* @returns {boolean} A value of `true` if `this` Rectangle contains `other`; otherwise `false`.
*/
Rectangle.prototype.containsRect = function containsRect(other: Rectangle): boolean
{
if (other.width <= 0 || other.height <= 0)
{
return other.x > this.x && other.y > this.y && other.right < this.right && other.bottom < this.bottom;
}
return other.x >= this.x && other.y >= this.y && other.right <= this.right && other.bottom <= this.bottom;
};
/**
* Accepts `other` Rectangle and returns true if the given Rectangle is equal to `this` Rectangle.
*
* _Note: Only available with **@pixi/math-extras**._
* @method equals
* @memberof PIXI.Rectangle#
* @param {Rectangle} other - The Rectangle to compare with `this`
* @returns {boolean} Returns true if all `x`, `y`, `width`, and `height` are equal.
*/
Rectangle.prototype.equals = function equals(other: Rectangle): boolean
{
if (other === this)
{
return true;
}
return other && this.x === other.x && this.y === other.y && this.width === other.width && this.height === other.height;
};
/**
* If the area of the intersection between the Rectangles `other` and `this` is not zero,
* returns the area of intersection as a Rectangle object. Otherwise, return an empty Rectangle
* with its properties set to zero.
* Rectangles without area (width or height equal to zero) can't intersect or be intersected
* and will always return an empty rectangle with its properties set to zero.
*
* _Note: Only available with **@pixi/math-extras**._
* @method intersection
* @memberof PIXI.Rectangle#
* @param {Rectangle} other - The Rectangle to intersect with `this`.
* @param {Rectangle} [outRect] - A Rectangle object in which to store the value,
* optional (otherwise will create a new Rectangle).
* @returns {Rectangle} The intersection of `this` and `other`.
*/
Rectangle.prototype.intersection = function intersection<T extends Rectangle>(other: Rectangle, outRect?: T): T
{
if (!outRect)
{
(outRect as any) = new Rectangle();
}
const x0 = this.x < other.x ? other.x : this.x;
const x1 = this.right > other.right ? other.right : this.right;
if (x1 <= x0)
{
outRect.x = outRect.y = outRect.width = outRect.height = 0;
return outRect;
}
const y0 = this.y < other.y ? other.y : this.y;
const y1 = this.bottom > other.bottom ? other.bottom : this.bottom;
if (y1 <= y0)
{
outRect.x = outRect.y = outRect.width = outRect.height = 0;
return outRect;
}
outRect.x = x0;
outRect.y = y0;
outRect.width = x1 - x0;
outRect.height = y1 - y0;
return outRect;
};
/**
* Adds `this` and `other` Rectangles together to create a new Rectangle object filling
* the horizontal and vertical space between the two rectangles.
*
* _Note: Only available with **@pixi/math-extras**._
* @method union
* @memberof PIXI.Rectangle#
* @param {Rectangle} other - The Rectangle to unite with `this`.
* @param {Rectangle} [outRect] - A Rectangle object in which to store the value,
* optional (otherwise will create a new Rectangle).
* @returns {Rectangle} The union of `this` and `other`.
*/
Rectangle.prototype.union = function union<T extends Rectangle>(other: Rectangle, outRect?: T): T
{
if (!outRect)
{
(outRect as any) = new Rectangle();
}
const x1 = Math.min(this.x, other.x);
const x2 = Math.max(this.x + this.width, other.x + other.width);
const y1 = Math.min(this.y, other.y);
const y2 = Math.max(this.y + this.height, other.y + other.height);
outRect.x = x1;
outRect.y = y1;
outRect.width = x2 - x1;
outRect.height = y2 - y1;
return outRect;
};
| packages/math-extras/src/rectangleExtras.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00026575528318062425,
0.00017576491518411785,
0.0001652304781600833,
0.0001672549988143146,
0.000026152434656978585
] |
{
"id": 5,
"code_window": [
" *\n",
" * // Don't start rendering right away\n",
" * app.stop();\n",
" *\n",
" * // create a display object\n",
" * const rect = new Graphics()\n",
" * .beginFill(0x00ff00)\n",
" * .drawRect(40, 40, 200, 200);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a display object\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 109
} | import { TranscoderWorkerWrapper } from './TranscoderWorkerWrapper';
import type { BASIS_FORMATS } from './Basis';
import type { ITranscodeResponse } from './TranscoderWorkerWrapper';
/**
* Worker class for transcoding *.basis files in background threads.
*
* To enable asynchronous transcoding, you need to provide the URL to the basis_universal transcoding
* library.
* @memberof PIXI.BasisParser
*/
export class TranscoderWorker
{
// IMPLEMENTATION NOTE: TranscoderWorker tracks transcoding requests with a requestID; the worker can be issued
// multiple requests (once it is initialized) and the response contains the requestID of the triggering request. Based on
// the response, the transcodeAsync promise is fulfilled or rejected.
// TODO: Publish our own @pixi/basis package & set default URL to jsdelivr/cdnjs
/** URL for the script containing the basis_universal library. */
static bindingURL: string;
static jsSource: string;
static wasmSource: ArrayBuffer;
private static _onTranscoderInitializedResolve: () => void;
/** a promise that when reslved means the transcoder is ready to be used */
public static onTranscoderInitialized = new Promise<void>((resolve) =>
{
TranscoderWorker._onTranscoderInitializedResolve = resolve;
});
isInit: boolean;
load: number;
requests: { [id: number]: {
resolve: (data: ITranscodeResponse) => void,
reject: () => void
} } = {};
private static _workerURL: string;
private static _tempID = 0;
/** Generated URL for the transcoder worker script. */
static get workerURL(): string
{
if (!TranscoderWorker._workerURL)
{
let workerSource = TranscoderWorkerWrapper.toString();
const beginIndex = workerSource.indexOf('{');
const endIndex = workerSource.lastIndexOf('}');
workerSource = workerSource.slice(beginIndex + 1, endIndex);
if (TranscoderWorker.jsSource)
{
workerSource = `${TranscoderWorker.jsSource}\n${workerSource}`;
}
TranscoderWorker._workerURL = URL.createObjectURL(new Blob([workerSource]));
}
return TranscoderWorker._workerURL;
}
protected worker: Worker;
protected initPromise: Promise<void>;
protected onInit: () => void;
constructor()
{
this.isInit = false;
this.load = 0;
this.initPromise = new Promise((resolve) => { this.onInit = resolve; });
if (!TranscoderWorker.wasmSource)
{
console.warn('resources.BasisResource.TranscoderWorker has not been given the transcoder WASM binary!');
}
this.worker = new Worker(TranscoderWorker.workerURL);
this.worker.onmessage = this.onMessage;
this.worker.postMessage({
type: 'init',
jsSource: TranscoderWorker.jsSource,
wasmSource: TranscoderWorker.wasmSource
});
}
/** @returns a promise that is resolved when the web-worker is initialized */
initAsync(): Promise<void>
{
return this.initPromise;
}
/**
* Creates a promise that will resolve when the transcoding of a *.basis file is complete.
* @param basisData - *.basis file contents
* @param rgbaFormat - transcoding format for RGBA files
* @param rgbFormat - transcoding format for RGB files
* @returns a promise that is resolved with the transcoding response of the web-worker
*/
async transcodeAsync(
basisData: Uint8Array,
rgbaFormat: BASIS_FORMATS,
rgbFormat: BASIS_FORMATS
): Promise<ITranscodeResponse>
{
++this.load;
const requestID = TranscoderWorker._tempID++;
const requestPromise = new Promise((resolve: (data: ITranscodeResponse) => void, reject: () => void) =>
{
this.requests[requestID] = {
resolve,
reject
};
});
this.worker.postMessage({
requestID,
basisData,
rgbaFormat,
rgbFormat,
type: 'transcode'
});
return requestPromise;
}
/**
* Handles responses from the web-worker
* @param e - a message event containing the transcoded response
*/
protected onMessage = (e: MessageEvent): void =>
{
const data = e.data as ITranscodeResponse;
if (data.type === 'init')
{
if (!data.success)
{
throw new Error('BasisResource.TranscoderWorker failed to initialize.');
}
this.isInit = true;
this.onInit();
}
else if (data.type === 'transcode')
{
--this.load;
const requestID = data.requestID;
if (data.success)
{
this.requests[requestID].resolve(data);
}
else
{
this.requests[requestID].reject();
}
delete this.requests[requestID];
}
};
/**
* Loads the transcoder source code
* @param jsURL - URL to the javascript basis transcoder
* @param wasmURL - URL to the wasm basis transcoder
* @returns A promise that resolves when both the js and wasm transcoders have been loaded.
*/
static loadTranscoder(jsURL: string, wasmURL: string): Promise<[void, void]>
{
const jsPromise = fetch(jsURL)
.then((res: Response) => res.text())
.then((text: string) => { TranscoderWorker.jsSource = text; });
const wasmPromise = fetch(wasmURL)
.then((res: Response) => res.arrayBuffer())
.then((arrayBuffer: ArrayBuffer) => { TranscoderWorker.wasmSource = arrayBuffer; });
return Promise.all([jsPromise, wasmPromise]).then((data) =>
{
this._onTranscoderInitializedResolve();
return data;
});
}
/**
* Set the transcoder source code directly
* @param jsSource - source for the javascript basis transcoder
* @param wasmSource - source for the wasm basis transcoder
*/
static setTranscoder(jsSource: string, wasmSource: ArrayBuffer): void
{
TranscoderWorker.jsSource = jsSource;
TranscoderWorker.wasmSource = wasmSource;
}
}
| packages/basis/src/TranscoderWorker.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00018119646119885147,
0.00016996238264255226,
0.00016467351815663278,
0.00017010775627568364,
0.000003343162461533211
] |
{
"id": 5,
"code_window": [
" *\n",
" * // Don't start rendering right away\n",
" * app.stop();\n",
" *\n",
" * // create a display object\n",
" * const rect = new Graphics()\n",
" * .beginFill(0x00ff00)\n",
" * .drawRect(40, 40, 200, 200);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" * // Create a display object\n"
],
"file_path": "packages/prepare/src/Prepare.ts",
"type": "replace",
"edit_start_line_idx": 109
} | /// <reference path="../global.d.ts" />
import {
ArcUtils,
BATCH_POOL,
BatchPart,
BezierUtils,
buildCircle,
buildLine,
buildPoly,
buildRectangle,
buildRoundedRectangle,
DRAW_CALL_POOL,
FILL_COMMANDS,
QuadraticUtils,
} from './utils';
import type { SHAPES } from '@pixi/core';
import type { BatchDrawCall } from '@pixi/core/';
import type { IShapeBuildCommand } from './utils/IShapeBuildCommand';
export * from './const';
export * from './Graphics';
export * from './GraphicsData';
export * from './GraphicsGeometry';
export * from './styles/FillStyle';
export * from './styles/LineStyle';
export const graphicsUtils = {
buildPoly: buildPoly as IShapeBuildCommand,
buildCircle: buildCircle as IShapeBuildCommand,
buildRectangle: buildRectangle as IShapeBuildCommand,
buildRoundedRectangle: buildRoundedRectangle as IShapeBuildCommand,
buildLine,
ArcUtils,
BezierUtils,
QuadraticUtils,
BatchPart,
FILL_COMMANDS: FILL_COMMANDS as Record<SHAPES, IShapeBuildCommand>,
BATCH_POOL: BATCH_POOL as Array<BatchPart>,
DRAW_CALL_POOL: DRAW_CALL_POOL as Array<BatchDrawCall>
};
| packages/graphics/src/index.ts | 0 | https://github.com/pixijs/pixijs/commit/bacf0de3195b04aedc050c7afcaeb9d42bf6289b | [
0.00017378739721607417,
0.00016897558816708624,
0.00016703187429811805,
0.00016812674584798515,
0.000002452380613249261
] |
{
"id": 0,
"code_window": [
"const {execSync} = require('child_process');\n",
"\n",
"console.log(`Rebuilding installer...`);\n",
"try {\n",
" execSync('npm run tsc-installer');\n",
"} catch (e) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" execSync('npm run tsc-installer', {\n",
" stdio: ['inherit', 'inherit', 'inherit'],\n",
" });\n"
],
"file_path": "install-from-github.js",
"type": "replace",
"edit_start_line_idx": 23
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// This file is only run when someone installs via the github repo
const {execSync} = require('child_process');
console.log(`Rebuilding installer...`);
try {
execSync('npm run tsc-installer');
} catch (e) {
}
console.log(`Downloading browsers...`);
const { installBrowsersWithProgressBar } = require('./lib/install/installer');
installBrowsersWithProgressBar().catch(e => {
console.error(`Failed to install browsers, caused by\n${e.stack}`);
process.exit(1);
});
console.log(`Done. Use "npm run watch" to compile.`);
| install-from-github.js | 1 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.9951637983322144,
0.4955655336380005,
0.00017431663582101464,
0.493461936712265,
0.49539029598236084
] |
{
"id": 0,
"code_window": [
"const {execSync} = require('child_process');\n",
"\n",
"console.log(`Rebuilding installer...`);\n",
"try {\n",
" execSync('npm run tsc-installer');\n",
"} catch (e) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" execSync('npm run tsc-installer', {\n",
" stdio: ['inherit', 'inherit', 'inherit'],\n",
" });\n"
],
"file_path": "install-from-github.js",
"type": "replace",
"edit_start_line_idx": 23
} | # Tool for printing .exe and .dll dependencies on Windows
This is similar to `ldd` on linux in that loads specified files and tries to
resolve all DLLs referenced by it, printing in the formar `<lib name> => <full path> | "no found"`
To minimize dependencies we link all C runtime libraries statically, there is
still one dynamic dependency on `dbghelp.dll` which is supposed to be preinstalled
on all Windows machines.
## Build instructions
Open `PrintDeps.sln` solution in Visual Studio 2019 and build `x64/Release` configuration. We
currently commit output binary into `bin/PrintDeps.exe` and bundle it in every npm. | browser_patches/winldd/README.md | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.00016761837468948215,
0.00016600082744844258,
0.00016438329475931823,
0.00016600082744844258,
0.00000161753996508196
] |
{
"id": 0,
"code_window": [
"const {execSync} = require('child_process');\n",
"\n",
"console.log(`Rebuilding installer...`);\n",
"try {\n",
" execSync('npm run tsc-installer');\n",
"} catch (e) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" execSync('npm run tsc-installer', {\n",
" stdio: ['inherit', 'inherit', 'inherit'],\n",
" });\n"
],
"file_path": "install-from-github.js",
"type": "replace",
"edit_start_line_idx": 23
} | <style>
body {
display: flex;
flex-direction: column;
height: 400px;
margin: 8px;
}
body iframe {
flex-grow: 1;
flex-shrink: 1;
border: 0;
}
</style>
<iframe src='./frame.html' name='uno'></iframe>
<iframe src='./frame.html' name='dos'></iframe>
| test/assets/frames/two-frames.html | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.0001751520176185295,
0.00017462644609622657,
0.00017410086002200842,
0.00017462644609622657,
5.255787982605398e-7
] |
{
"id": 0,
"code_window": [
"const {execSync} = require('child_process');\n",
"\n",
"console.log(`Rebuilding installer...`);\n",
"try {\n",
" execSync('npm run tsc-installer');\n",
"} catch (e) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" execSync('npm run tsc-installer', {\n",
" stdio: ['inherit', 'inherit', 'inherit'],\n",
" });\n"
],
"file_path": "install-from-github.js",
"type": "replace",
"edit_start_line_idx": 23
} | ---
id: dialogs
title: "Dialogs"
---
Playwright can interact with the web page dialogs such as [`alert`](https://developer.mozilla.org/en-US/docs/Web/API/Window/alert), [`confirm`](https://developer.mozilla.org/en-US/docs/Web/API/Window/confirm), [`prompt`](https://developer.mozilla.org/en-US/docs/Web/API/Window/prompt) as well as [`beforeunload`](https://developer.mozilla.org/en-US/docs/Web/API/Window/beforeunload_event) confirmation.
<!-- TOC -->
## alert(), confirm(), prompt() dialogs
By default, dialogs are auto-dismissed by Playwright, so you don't have to handle them. However, you can register a dialog handler before the action that triggers the dialog to accept or decline it.
```js
page.on('dialog', dialog => dialog.accept());
await page.click('button');
```
```java
page.onDialog(dialog -> dialog.accept());
page.click("button");
```
```python async
page.on("dialog", lambda dialog: dialog.accept())
await page.click("button")
```
```python sync
page.on("dialog", lambda dialog: dialog.accept())
page.click("button")
```
:::note
[`event: Page.dialog`] listener **must handle** the dialog. Otherwise your action will stall, be it [`method: Page.click`], [`method: Page.evaluate`] or any other. That's because dialogs in Web are modal and block further page execution until they are handled.
:::
As a result, following snippet will never resolve:
:::warning
WRONG!
:::
```js
page.on('dialog', dialog => console.log(dialog.message()));
await page.click('button'); // Will hang here
```
```java
page.onDialog(dialog -> System.out.println(dialog.message()));
page.click("button"); // Will hang here
```
```python async
page.on("dialog", lambda dialog: print(dialog.message))
await page.click("button") # Will hang here
```
```python sync
page.on("dialog", lambda dialog: print(dialog.message))
page.click("button") # Will hang here
```
:::note
If there is no listener for [`event: Page.dialog`], all dialogs are automatically dismissed.
:::
### API reference
- [Dialog]
- [`method: Dialog.accept`]
- [`method: Dialog.dismiss`]
## beforeunload dialog
When [`method: Page.close`] is invoked with the truthy [`option: runBeforeUnload`] value, it page runs its unload handlers. This is the only case when [`method: Page.close`] does not wait for the page to actually close, because it might be that the page stays open in the end of the operation.
You can register a dialog handler to handle the beforeunload dialog yourself:
```js
page.on('dialog', async dialog => {
assert(dialog.type() === 'beforeunload');
await dialog.dismiss();
});
await page.close({runBeforeUnload: true});
```
```java
page.onDialog(dialog -> {
assertEquals("beforeunload", dialog.type());
dialog.dismiss();
});
page.close(new Page.CloseOptions().setRunBeforeUnload(true));
```
```python async
async def handle_dialog(dialog):
assert dialog.type == 'beforeunload'
await dialog.dismiss()
page.on('dialog', lambda: handle_dialog)
await page.close(run_before_unload=True)
```
```python sync
def handle_dialog(dialog):
assert dialog.type == 'beforeunload'
dialog.dismiss()
page.on('dialog', lambda: handle_dialog)
page.close(run_before_unload=True)
```
| docs/src/dialogs.md | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.00017265336646232754,
0.00016975948528852314,
0.00016441104526165873,
0.00017041919636540115,
0.000002765269300653017
] |
{
"id": 1,
"code_window": [
"} catch (e) {\n",
"}\n",
"\n",
"console.log(`Downloading browsers...`);\n",
"const { installBrowsersWithProgressBar } = require('./lib/install/installer');\n",
"installBrowsersWithProgressBar().catch(e => {\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" process.exit(1);\n"
],
"file_path": "install-from-github.js",
"type": "add",
"edit_start_line_idx": 25
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// This file is only run when someone installs via the github repo
const {execSync} = require('child_process');
console.log(`Rebuilding installer...`);
try {
execSync('npm run tsc-installer');
} catch (e) {
}
console.log(`Downloading browsers...`);
const { installBrowsersWithProgressBar } = require('./lib/install/installer');
installBrowsersWithProgressBar().catch(e => {
console.error(`Failed to install browsers, caused by\n${e.stack}`);
process.exit(1);
});
console.log(`Done. Use "npm run watch" to compile.`);
| install-from-github.js | 1 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.998307466506958,
0.2502070665359497,
0.00017519712855573744,
0.0011728209210559726,
0.431916743516922
] |
{
"id": 1,
"code_window": [
"} catch (e) {\n",
"}\n",
"\n",
"console.log(`Downloading browsers...`);\n",
"const { installBrowsersWithProgressBar } = require('./lib/install/installer');\n",
"installBrowsersWithProgressBar().catch(e => {\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" process.exit(1);\n"
],
"file_path": "install-from-github.js",
"type": "add",
"edit_start_line_idx": 25
} | <style>
body {
padding: 10px;
}
/*# sourceURL=nicename.css */
</style>
| test/assets/csscoverage/sourceurl.html | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.0001718998682918027,
0.0001718998682918027,
0.0001718998682918027,
0.0001718998682918027,
0
] |
{
"id": 1,
"code_window": [
"} catch (e) {\n",
"}\n",
"\n",
"console.log(`Downloading browsers...`);\n",
"const { installBrowsersWithProgressBar } = require('./lib/install/installer');\n",
"installBrowsersWithProgressBar().catch(e => {\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" process.exit(1);\n"
],
"file_path": "install-from-github.js",
"type": "add",
"edit_start_line_idx": 25
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { it, expect } from './fixtures';
import { attachFrame } from './utils';
import type { ElementHandle } from '..';
it('exposeBinding should work', async ({page}) => {
let bindingSource;
await page.exposeBinding('add', (source, a, b) => {
bindingSource = source;
return a + b;
});
const result = await page.evaluate(async function() {
return window['add'](5, 6);
});
expect(bindingSource.context).toBe(page.context());
expect(bindingSource.page).toBe(page);
expect(bindingSource.frame).toBe(page.mainFrame());
expect(result).toEqual(11);
});
it('should work', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return a * b;
});
const result = await page.evaluate(async function() {
return await window['compute'](9, 4);
});
expect(result).toBe(36);
});
it('should work with handles and complex objects', async ({page, server}) => {
const fooHandle = await page.evaluateHandle(() => {
window['fooValue'] = { bar: 2 };
return window['fooValue'];
});
await page.exposeFunction('handle', () => {
return [{ foo: fooHandle }];
});
const equals = await page.evaluate(async function() {
const value = await window['handle']();
const [{ foo }] = value;
return foo === window['fooValue'];
});
expect(equals).toBe(true);
});
it('should throw exception in page context', async ({page, server}) => {
await page.exposeFunction('woof', function() {
throw new Error('WOOF WOOF');
});
const {message, stack} = await page.evaluate(async () => {
try {
await window['woof']();
} catch (e) {
return {message: e.message, stack: e.stack};
}
});
expect(message).toBe('WOOF WOOF');
expect(stack).toContain(__filename);
});
it('should support throwing "null"', async ({page, server}) => {
await page.exposeFunction('woof', function() {
throw null;
});
const thrown = await page.evaluate(async () => {
try {
await window['woof']();
} catch (e) {
return e;
}
});
expect(thrown).toBe(null);
});
it('should be callable from-inside addInitScript', async ({page, server}) => {
let called = false;
await page.exposeFunction('woof', function() {
called = true;
});
await page.addInitScript(() => window['woof']());
await page.reload();
expect(called).toBe(true);
});
it('should survive navigation', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return a * b;
});
await page.goto(server.EMPTY_PAGE);
const result = await page.evaluate(async function() {
return await window['compute'](9, 4);
});
expect(result).toBe(36);
});
it('should await returned promise', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
const result = await page.evaluate(async function() {
return await window['compute'](3, 5);
});
expect(result).toBe(15);
});
it('should work on frames', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
await page.goto(server.PREFIX + '/frames/nested-frames.html');
const frame = page.frames()[1];
const result = await frame.evaluate(async function() {
return await window['compute'](3, 5);
});
expect(result).toBe(15);
});
it('should work on frames before navigation', async ({page, server}) => {
await page.goto(server.PREFIX + '/frames/nested-frames.html');
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
const frame = page.frames()[1];
const result = await frame.evaluate(async function() {
return await window['compute'](3, 5);
});
expect(result).toBe(15);
});
it('should work after cross origin navigation', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
await page.exposeFunction('compute', function(a, b) {
return a * b;
});
await page.goto(server.CROSS_PROCESS_PREFIX + '/empty.html');
const result = await page.evaluate(async function() {
return await window['compute'](9, 4);
});
expect(result).toBe(36);
});
it('should work with complex objects', async ({page, server}) => {
await page.exposeFunction('complexObject', function(a, b) {
return {x: a.x + b.x};
});
const result = await page.evaluate(async () => window['complexObject']({x: 5}, {x: 2}));
expect(result.x).toBe(7);
});
it('exposeBindingHandle should work', async ({page}) => {
let target;
await page.exposeBinding('logme', (source, t) => {
target = t;
return 17;
}, { handle: true });
const result = await page.evaluate(async function() {
return window['logme']({ foo: 42 });
});
expect(await target.evaluate(x => x.foo)).toBe(42);
expect(result).toEqual(17);
});
it('exposeBindingHandle should not throw during navigation', async ({page, server}) => {
await page.exposeBinding('logme', (source, t) => {
return 17;
}, { handle: true });
await page.goto(server.EMPTY_PAGE);
await Promise.all([
page.evaluate(async url => {
window['logme']({ foo: 42 });
window.location.href = url;
}, server.PREFIX + '/one-style.html'),
page.waitForNavigation({ waitUntil: 'load' }),
]);
});
it('should throw for duplicate registrations', async ({page}) => {
await page.exposeFunction('foo', () => {});
const error = await page.exposeFunction('foo', () => {}).catch(e => e);
expect(error.message).toContain('page.exposeFunction: Function "foo" has been already registered');
});
it('exposeBindingHandle should throw for multiple arguments', async ({page}) => {
await page.exposeBinding('logme', (source, t) => {
return 17;
}, { handle: true });
expect(await page.evaluate(async function() {
return window['logme']({ foo: 42 });
})).toBe(17);
expect(await page.evaluate(async function() {
return window['logme']({ foo: 42 }, undefined, undefined);
})).toBe(17);
expect(await page.evaluate(async function() {
return window['logme'](undefined, undefined, undefined);
})).toBe(17);
const error = await page.evaluate(async function() {
return window['logme'](1, 2);
}).catch(e => e);
expect(error.message).toContain('exposeBindingHandle supports a single argument, 2 received');
});
it('should not result in unhandled rejection', async ({page}) => {
const closedPromise = page.waitForEvent('close');
await page.exposeFunction('foo', async () => {
await page.close();
});
await page.evaluate(() => {
setTimeout(() => (window as any).foo(), 0);
return undefined;
});
await closedPromise;
// Make a round-trip to be sure we did not throw immediately after closing.
expect(await page.evaluate('1 + 1').catch(e => e)).toBeInstanceOf(Error);
});
it('should work with internal bindings', (test, { mode, browserName }) => {
test.skip(mode !== 'default');
test.skip(browserName !== 'chromium');
}, async ({page, toImpl, server}) => {
const implPage: import('../src/server/page').Page = toImpl(page);
let foo;
await implPage.exposeBinding('foo', false, ({}, arg) => {
foo = arg;
}, 'utility');
expect(await page.evaluate('!!window.foo')).toBe(false);
expect(await implPage.mainFrame().evaluateExpression('!!window.foo', false, {}, 'utility')).toBe(true);
expect(foo).toBe(undefined);
await implPage.mainFrame().evaluateExpression('window.foo(123)', false, {}, 'utility');
expect(foo).toBe(123);
// should work after reload
await page.goto(server.EMPTY_PAGE);
expect(await page.evaluate('!!window.foo')).toBe(false);
await implPage.mainFrame().evaluateExpression('window.foo(456)', false, {}, 'utility');
expect(foo).toBe(456);
// should work inside frames
const frame = await attachFrame(page, 'myframe', server.CROSS_PROCESS_PREFIX + '/empty.html');
expect(await frame.evaluate('!!window.foo')).toBe(false);
const implFrame: import('../src/server/frames').Frame = toImpl(frame);
await implFrame.evaluateExpression('window.foo(789)', false, {}, 'utility');
expect(foo).toBe(789);
});
it('exposeBinding(handle) should work with element handles', async ({ page}) => {
let cb;
const promise = new Promise(f => cb = f);
await page.exposeBinding('clicked', async (source, element: ElementHandle) => {
cb(await element.innerText().catch(e => e));
}, { handle: true });
await page.goto('about:blank');
await page.setContent(`
<script>
document.addEventListener('click', event => window.clicked(event.target));
</script>
<div id="a1">Click me</div>
`);
await page.click('#a1');
expect(await promise).toBe('Click me');
});
it('should work with setContent', async ({page, server}) => {
await page.exposeFunction('compute', function(a, b) {
return Promise.resolve(a * b);
});
await page.setContent('<script>window.result = compute(3, 2)</script>');
expect(await page.evaluate('window.result')).toBe(6);
});
| test/page-expose-function.spec.ts | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.00017777649918571115,
0.00017277308506891131,
0.00016606759163551033,
0.00017306227528024465,
0.0000034261192922713235
] |
{
"id": 1,
"code_window": [
"} catch (e) {\n",
"}\n",
"\n",
"console.log(`Downloading browsers...`);\n",
"const { installBrowsersWithProgressBar } = require('./lib/install/installer');\n",
"installBrowsersWithProgressBar().catch(e => {\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" process.exit(1);\n"
],
"file_path": "install-from-github.js",
"type": "add",
"edit_start_line_idx": 25
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { isUnderTest } from '../utils/utils';
export class ValidationError extends Error {}
export type Validator = (arg: any, path: string) => any;
export const tNumber: Validator = (arg: any, path: string) => {
if (arg instanceof Number)
return arg.valueOf();
if (typeof arg === 'number')
return arg;
throw new ValidationError(`${path}: expected number, got ${typeof arg}`);
};
export const tBoolean: Validator = (arg: any, path: string) => {
if (arg instanceof Boolean)
return arg.valueOf();
if (typeof arg === 'boolean')
return arg;
throw new ValidationError(`${path}: expected boolean, got ${typeof arg}`);
};
export const tString: Validator = (arg: any, path: string) => {
if (arg instanceof String)
return arg.valueOf();
if (typeof arg === 'string')
return arg;
throw new ValidationError(`${path}: expected string, got ${typeof arg}`);
};
export const tBinary: Validator = (arg: any, path: string) => {
if (arg instanceof String)
return arg.valueOf();
if (typeof arg === 'string')
return arg;
throw new ValidationError(`${path}: expected base64-encoded buffer, got ${typeof arg}`);
};
export const tUndefined: Validator = (arg: any, path: string) => {
if (Object.is(arg, undefined))
return arg;
throw new ValidationError(`${path}: expected undefined, got ${typeof arg}`);
};
export const tAny: Validator = (arg: any, path: string) => {
return arg;
};
export const tOptional = (v: Validator): Validator => {
return (arg: any, path: string) => {
if (Object.is(arg, undefined))
return arg;
return v(arg, path);
};
};
export const tArray = (v: Validator): Validator => {
return (arg: any, path: string) => {
if (!Array.isArray(arg))
throw new ValidationError(`${path}: expected array, got ${typeof arg}`);
return arg.map((x, index) => v(x, path + '[' + index + ']'));
};
};
export const tObject = (s: { [key: string]: Validator }): Validator => {
return (arg: any, path: string) => {
if (Object.is(arg, null))
throw new ValidationError(`${path}: expected object, got null`);
if (typeof arg !== 'object')
throw new ValidationError(`${path}: expected object, got ${typeof arg}`);
const result: any = {};
for (const [key, v] of Object.entries(s)) {
const value = v(arg[key], path ? path + '.' + key : key);
if (!Object.is(value, undefined))
result[key] = value;
}
if (isUnderTest()) {
for (const [key, value] of Object.entries(arg)) {
if (key.startsWith('__testHook'))
result[key] = value;
}
}
return result;
};
};
export const tEnum = (e: string[]): Validator => {
return (arg: any, path: string) => {
if (!e.includes(arg))
throw new ValidationError(`${path}: expected one of (${e.join('|')})`);
return arg;
};
};
| src/protocol/validatorPrimitives.ts | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.0001765893102856353,
0.00017203528841491789,
0.0001672564394539222,
0.00017245873459614813,
0.00000250092443820904
] |
{
"id": 2,
"code_window": [
"export function makeWaitForNextTask() {\n",
" // As of Mar 2021, Electorn v12 doesn't create new task with `setImmediate` despite\n",
" // using Node 14 internally, so we fallback to `setTimeout(0)` instead.\n",
" // @see https://github.com/electron/electron/issues/28261\n",
" if (process.versions.electron)\n",
" return (callback: () => void) => setTimeout(callback, 0);\n",
" if (parseInt(process.versions.node, 10) >= 11)\n",
" return setImmediate;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if ((process.versions as any).electron)\n"
],
"file_path": "src/utils/utils.ts",
"type": "replace",
"edit_start_line_idx": 29
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// This file is only run when someone installs via the github repo
const {execSync} = require('child_process');
console.log(`Rebuilding installer...`);
try {
execSync('npm run tsc-installer');
} catch (e) {
}
console.log(`Downloading browsers...`);
const { installBrowsersWithProgressBar } = require('./lib/install/installer');
installBrowsersWithProgressBar().catch(e => {
console.error(`Failed to install browsers, caused by\n${e.stack}`);
process.exit(1);
});
console.log(`Done. Use "npm run watch" to compile.`);
| install-from-github.js | 1 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.00017473750631324947,
0.00017155897512566298,
0.00016867590602487326,
0.00017141125863417983,
0.000002173097527702339
] |
{
"id": 2,
"code_window": [
"export function makeWaitForNextTask() {\n",
" // As of Mar 2021, Electorn v12 doesn't create new task with `setImmediate` despite\n",
" // using Node 14 internally, so we fallback to `setTimeout(0)` instead.\n",
" // @see https://github.com/electron/electron/issues/28261\n",
" if (process.versions.electron)\n",
" return (callback: () => void) => setTimeout(callback, 0);\n",
" if (parseInt(process.versions.node, 10) >= 11)\n",
" return setImmediate;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if ((process.versions as any).electron)\n"
],
"file_path": "src/utils/utils.ts",
"type": "replace",
"edit_start_line_idx": 29
} | /*
Copyright (c) Microsoft Corporation.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
.source-tab {
flex: auto;
position: relative;
overflow: hidden;
display: flex;
flex-direction: row;
}
| src/web/traceViewer/ui/sourceTab.css | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.0001794047566363588,
0.00017771458078641444,
0.00017443002434447408,
0.00017930900503415614,
0.0000023228712962009013
] |
{
"id": 2,
"code_window": [
"export function makeWaitForNextTask() {\n",
" // As of Mar 2021, Electorn v12 doesn't create new task with `setImmediate` despite\n",
" // using Node 14 internally, so we fallback to `setTimeout(0)` instead.\n",
" // @see https://github.com/electron/electron/issues/28261\n",
" if (process.versions.electron)\n",
" return (callback: () => void) => setTimeout(callback, 0);\n",
" if (parseInt(process.versions.node, 10) >= 11)\n",
" return setImmediate;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if ((process.versions as any).electron)\n"
],
"file_path": "src/utils/utils.ts",
"type": "replace",
"edit_start_line_idx": 29
} | GNU LESSER GENERAL PUBLIC LICENSE
Version 2.1, February 1999
Copyright (C) 1991, 1999 Free Software Foundation, Inc.
51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
Everyone is permitted to copy and distribute verbatim copies
of this license document, but changing it is not allowed.
[This is the first released version of the Lesser GPL. It also counts
as the successor of the GNU Library Public License, version 2, hence
the version number 2.1.]
Preamble
The licenses for most software are designed to take away your
freedom to share and change it. By contrast, the GNU General Public
Licenses are intended to guarantee your freedom to share and change
free software--to make sure the software is free for all its users.
This license, the Lesser General Public License, applies to some
specially designated software packages--typically libraries--of the
Free Software Foundation and other authors who decide to use it. You
can use it too, but we suggest you first think carefully about whether
this license or the ordinary General Public License is the better
strategy to use in any particular case, based on the explanations below.
When we speak of free software, we are referring to freedom of use,
not price. Our General Public Licenses are designed to make sure that
you have the freedom to distribute copies of free software (and charge
for this service if you wish); that you receive source code or can get
it if you want it; that you can change the software and use pieces of
it in new free programs; and that you are informed that you can do
these things.
To protect your rights, we need to make restrictions that forbid
distributors to deny you these rights or to ask you to surrender these
rights. These restrictions translate to certain responsibilities for
you if you distribute copies of the library or if you modify it.
For example, if you distribute copies of the library, whether gratis
or for a fee, you must give the recipients all the rights that we gave
you. You must make sure that they, too, receive or can get the source
code. If you link other code with the library, you must provide
complete object files to the recipients, so that they can relink them
with the library after making changes to the library and recompiling
it. And you must show them these terms so they know their rights.
We protect your rights with a two-step method: (1) we copyright the
library, and (2) we offer you this license, which gives you legal
permission to copy, distribute and/or modify the library.
To protect each distributor, we want to make it very clear that
there is no warranty for the free library. Also, if the library is
modified by someone else and passed on, the recipients should know
that what they have is not the original version, so that the original
author's reputation will not be affected by problems that might be
introduced by others.
Finally, software patents pose a constant threat to the existence of
any free program. We wish to make sure that a company cannot
effectively restrict the users of a free program by obtaining a
restrictive license from a patent holder. Therefore, we insist that
any patent license obtained for a version of the library must be
consistent with the full freedom of use specified in this license.
Most GNU software, including some libraries, is covered by the
ordinary GNU General Public License. This license, the GNU Lesser
General Public License, applies to certain designated libraries, and
is quite different from the ordinary General Public License. We use
this license for certain libraries in order to permit linking those
libraries into non-free programs.
When a program is linked with a library, whether statically or using
a shared library, the combination of the two is legally speaking a
combined work, a derivative of the original library. The ordinary
General Public License therefore permits such linking only if the
entire combination fits its criteria of freedom. The Lesser General
Public License permits more lax criteria for linking other code with
the library.
We call this license the "Lesser" General Public License because it
does Less to protect the user's freedom than the ordinary General
Public License. It also provides other free software developers Less
of an advantage over competing non-free programs. These disadvantages
are the reason we use the ordinary General Public License for many
libraries. However, the Lesser license provides advantages in certain
special circumstances.
For example, on rare occasions, there may be a special need to
encourage the widest possible use of a certain library, so that it becomes
a de-facto standard. To achieve this, non-free programs must be
allowed to use the library. A more frequent case is that a free
library does the same job as widely used non-free libraries. In this
case, there is little to gain by limiting the free library to free
software only, so we use the Lesser General Public License.
In other cases, permission to use a particular library in non-free
programs enables a greater number of people to use a large body of
free software. For example, permission to use the GNU C Library in
non-free programs enables many more people to use the whole GNU
operating system, as well as its variant, the GNU/Linux operating
system.
Although the Lesser General Public License is Less protective of the
users' freedom, it does ensure that the user of a program that is
linked with the Library has the freedom and the wherewithal to run
that program using a modified version of the Library.
The precise terms and conditions for copying, distribution and
modification follow. Pay close attention to the difference between a
"work based on the library" and a "work that uses the library". The
former contains code derived from the library, whereas the latter must
be combined with the library in order to run.
GNU LESSER GENERAL PUBLIC LICENSE
TERMS AND CONDITIONS FOR COPYING, DISTRIBUTION AND MODIFICATION
0. This License Agreement applies to any software library or other
program which contains a notice placed by the copyright holder or
other authorized party saying it may be distributed under the terms of
this Lesser General Public License (also called "this License").
Each licensee is addressed as "you".
A "library" means a collection of software functions and/or data
prepared so as to be conveniently linked with application programs
(which use some of those functions and data) to form executables.
The "Library", below, refers to any such software library or work
which has been distributed under these terms. A "work based on the
Library" means either the Library or any derivative work under
copyright law: that is to say, a work containing the Library or a
portion of it, either verbatim or with modifications and/or translated
straightforwardly into another language. (Hereinafter, translation is
included without limitation in the term "modification".)
"Source code" for a work means the preferred form of the work for
making modifications to it. For a library, complete source code means
all the source code for all modules it contains, plus any associated
interface definition files, plus the scripts used to control compilation
and installation of the library.
Activities other than copying, distribution and modification are not
covered by this License; they are outside its scope. The act of
running a program using the Library is not restricted, and output from
such a program is covered only if its contents constitute a work based
on the Library (independent of the use of the Library in a tool for
writing it). Whether that is true depends on what the Library does
and what the program that uses the Library does.
1. You may copy and distribute verbatim copies of the Library's
complete source code as you receive it, in any medium, provided that
you conspicuously and appropriately publish on each copy an
appropriate copyright notice and disclaimer of warranty; keep intact
all the notices that refer to this License and to the absence of any
warranty; and distribute a copy of this License along with the
Library.
You may charge a fee for the physical act of transferring a copy,
and you may at your option offer warranty protection in exchange for a
fee.
2. You may modify your copy or copies of the Library or any portion
of it, thus forming a work based on the Library, and copy and
distribute such modifications or work under the terms of Section 1
above, provided that you also meet all of these conditions:
a) The modified work must itself be a software library.
b) You must cause the files modified to carry prominent notices
stating that you changed the files and the date of any change.
c) You must cause the whole of the work to be licensed at no
charge to all third parties under the terms of this License.
d) If a facility in the modified Library refers to a function or a
table of data to be supplied by an application program that uses
the facility, other than as an argument passed when the facility
is invoked, then you must make a good faith effort to ensure that,
in the event an application does not supply such function or
table, the facility still operates, and performs whatever part of
its purpose remains meaningful.
(For example, a function in a library to compute square roots has
a purpose that is entirely well-defined independent of the
application. Therefore, Subsection 2d requires that any
application-supplied function or table used by this function must
be optional: if the application does not supply it, the square
root function must still compute square roots.)
These requirements apply to the modified work as a whole. If
identifiable sections of that work are not derived from the Library,
and can be reasonably considered independent and separate works in
themselves, then this License, and its terms, do not apply to those
sections when you distribute them as separate works. But when you
distribute the same sections as part of a whole which is a work based
on the Library, the distribution of the whole must be on the terms of
this License, whose permissions for other licensees extend to the
entire whole, and thus to each and every part regardless of who wrote
it.
Thus, it is not the intent of this section to claim rights or contest
your rights to work written entirely by you; rather, the intent is to
exercise the right to control the distribution of derivative or
collective works based on the Library.
In addition, mere aggregation of another work not based on the Library
with the Library (or with a work based on the Library) on a volume of
a storage or distribution medium does not bring the other work under
the scope of this License.
3. You may opt to apply the terms of the ordinary GNU General Public
License instead of this License to a given copy of the Library. To do
this, you must alter all the notices that refer to this License, so
that they refer to the ordinary GNU General Public License, version 2,
instead of to this License. (If a newer version than version 2 of the
ordinary GNU General Public License has appeared, then you can specify
that version instead if you wish.) Do not make any other change in
these notices.
Once this change is made in a given copy, it is irreversible for
that copy, so the ordinary GNU General Public License applies to all
subsequent copies and derivative works made from that copy.
This option is useful when you wish to copy part of the code of
the Library into a program that is not a library.
4. You may copy and distribute the Library (or a portion or
derivative of it, under Section 2) in object code or executable form
under the terms of Sections 1 and 2 above provided that you accompany
it with the complete corresponding machine-readable source code, which
must be distributed under the terms of Sections 1 and 2 above on a
medium customarily used for software interchange.
If distribution of object code is made by offering access to copy
from a designated place, then offering equivalent access to copy the
source code from the same place satisfies the requirement to
distribute the source code, even though third parties are not
compelled to copy the source along with the object code.
5. A program that contains no derivative of any portion of the
Library, but is designed to work with the Library by being compiled or
linked with it, is called a "work that uses the Library". Such a
work, in isolation, is not a derivative work of the Library, and
therefore falls outside the scope of this License.
However, linking a "work that uses the Library" with the Library
creates an executable that is a derivative of the Library (because it
contains portions of the Library), rather than a "work that uses the
library". The executable is therefore covered by this License.
Section 6 states terms for distribution of such executables.
When a "work that uses the Library" uses material from a header file
that is part of the Library, the object code for the work may be a
derivative work of the Library even though the source code is not.
Whether this is true is especially significant if the work can be
linked without the Library, or if the work is itself a library. The
threshold for this to be true is not precisely defined by law.
If such an object file uses only numerical parameters, data
structure layouts and accessors, and small macros and small inline
functions (ten lines or less in length), then the use of the object
file is unrestricted, regardless of whether it is legally a derivative
work. (Executables containing this object code plus portions of the
Library will still fall under Section 6.)
Otherwise, if the work is a derivative of the Library, you may
distribute the object code for the work under the terms of Section 6.
Any executables containing that work also fall under Section 6,
whether or not they are linked directly with the Library itself.
6. As an exception to the Sections above, you may also combine or
link a "work that uses the Library" with the Library to produce a
work containing portions of the Library, and distribute that work
under terms of your choice, provided that the terms permit
modification of the work for the customer's own use and reverse
engineering for debugging such modifications.
You must give prominent notice with each copy of the work that the
Library is used in it and that the Library and its use are covered by
this License. You must supply a copy of this License. If the work
during execution displays copyright notices, you must include the
copyright notice for the Library among them, as well as a reference
directing the user to the copy of this License. Also, you must do one
of these things:
a) Accompany the work with the complete corresponding
machine-readable source code for the Library including whatever
changes were used in the work (which must be distributed under
Sections 1 and 2 above); and, if the work is an executable linked
with the Library, with the complete machine-readable "work that
uses the Library", as object code and/or source code, so that the
user can modify the Library and then relink to produce a modified
executable containing the modified Library. (It is understood
that the user who changes the contents of definitions files in the
Library will not necessarily be able to recompile the application
to use the modified definitions.)
b) Use a suitable shared library mechanism for linking with the
Library. A suitable mechanism is one that (1) uses at run time a
copy of the library already present on the user's computer system,
rather than copying library functions into the executable, and (2)
will operate properly with a modified version of the library, if
the user installs one, as long as the modified version is
interface-compatible with the version that the work was made with.
c) Accompany the work with a written offer, valid for at
least three years, to give the same user the materials
specified in Subsection 6a, above, for a charge no more
than the cost of performing this distribution.
d) If distribution of the work is made by offering access to copy
from a designated place, offer equivalent access to copy the above
specified materials from the same place.
e) Verify that the user has already received a copy of these
materials or that you have already sent this user a copy.
For an executable, the required form of the "work that uses the
Library" must include any data and utility programs needed for
reproducing the executable from it. However, as a special exception,
the materials to be distributed need not include anything that is
normally distributed (in either source or binary form) with the major
components (compiler, kernel, and so on) of the operating system on
which the executable runs, unless that component itself accompanies
the executable.
It may happen that this requirement contradicts the license
restrictions of other proprietary libraries that do not normally
accompany the operating system. Such a contradiction means you cannot
use both them and the Library together in an executable that you
distribute.
7. You may place library facilities that are a work based on the
Library side-by-side in a single library together with other library
facilities not covered by this License, and distribute such a combined
library, provided that the separate distribution of the work based on
the Library and of the other library facilities is otherwise
permitted, and provided that you do these two things:
a) Accompany the combined library with a copy of the same work
based on the Library, uncombined with any other library
facilities. This must be distributed under the terms of the
Sections above.
b) Give prominent notice with the combined library of the fact
that part of it is a work based on the Library, and explaining
where to find the accompanying uncombined form of the same work.
8. You may not copy, modify, sublicense, link with, or distribute
the Library except as expressly provided under this License. Any
attempt otherwise to copy, modify, sublicense, link with, or
distribute the Library is void, and will automatically terminate your
rights under this License. However, parties who have received copies,
or rights, from you under this License will not have their licenses
terminated so long as such parties remain in full compliance.
9. You are not required to accept this License, since you have not
signed it. However, nothing else grants you permission to modify or
distribute the Library or its derivative works. These actions are
prohibited by law if you do not accept this License. Therefore, by
modifying or distributing the Library (or any work based on the
Library), you indicate your acceptance of this License to do so, and
all its terms and conditions for copying, distributing or modifying
the Library or works based on it.
10. Each time you redistribute the Library (or any work based on the
Library), the recipient automatically receives a license from the
original licensor to copy, distribute, link with or modify the Library
subject to these terms and conditions. You may not impose any further
restrictions on the recipients' exercise of the rights granted herein.
You are not responsible for enforcing compliance by third parties with
this License.
11. If, as a consequence of a court judgment or allegation of patent
infringement or for any other reason (not limited to patent issues),
conditions are imposed on you (whether by court order, agreement or
otherwise) that contradict the conditions of this License, they do not
excuse you from the conditions of this License. If you cannot
distribute so as to satisfy simultaneously your obligations under this
License and any other pertinent obligations, then as a consequence you
may not distribute the Library at all. For example, if a patent
license would not permit royalty-free redistribution of the Library by
all those who receive copies directly or indirectly through you, then
the only way you could satisfy both it and this License would be to
refrain entirely from distribution of the Library.
If any portion of this section is held invalid or unenforceable under any
particular circumstance, the balance of the section is intended to apply,
and the section as a whole is intended to apply in other circumstances.
It is not the purpose of this section to induce you to infringe any
patents or other property right claims or to contest validity of any
such claims; this section has the sole purpose of protecting the
integrity of the free software distribution system which is
implemented by public license practices. Many people have made
generous contributions to the wide range of software distributed
through that system in reliance on consistent application of that
system; it is up to the author/donor to decide if he or she is willing
to distribute software through any other system and a licensee cannot
impose that choice.
This section is intended to make thoroughly clear what is believed to
be a consequence of the rest of this License.
12. If the distribution and/or use of the Library is restricted in
certain countries either by patents or by copyrighted interfaces, the
original copyright holder who places the Library under this License may add
an explicit geographical distribution limitation excluding those countries,
so that distribution is permitted only in or among countries not thus
excluded. In such case, this License incorporates the limitation as if
written in the body of this License.
13. The Free Software Foundation may publish revised and/or new
versions of the Lesser General Public License from time to time.
Such new versions will be similar in spirit to the present version,
but may differ in detail to address new problems or concerns.
Each version is given a distinguishing version number. If the Library
specifies a version number of this License which applies to it and
"any later version", you have the option of following the terms and
conditions either of that version or of any later version published by
the Free Software Foundation. If the Library does not specify a
license version number, you may choose any version ever published by
the Free Software Foundation.
14. If you wish to incorporate parts of the Library into other free
programs whose distribution conditions are incompatible with these,
write to the author to ask for permission. For software which is
copyrighted by the Free Software Foundation, write to the Free
Software Foundation; we sometimes make exceptions for this. Our
decision will be guided by the two goals of preserving the free status
of all derivatives of our free software and of promoting the sharing
and reuse of software generally.
NO WARRANTY
15. BECAUSE THE LIBRARY IS LICENSED FREE OF CHARGE, THERE IS NO
WARRANTY FOR THE LIBRARY, TO THE EXTENT PERMITTED BY APPLICABLE LAW.
EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT HOLDERS AND/OR
OTHER PARTIES PROVIDE THE LIBRARY "AS IS" WITHOUT WARRANTY OF ANY
KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE
LIBRARY IS WITH YOU. SHOULD THE LIBRARY PROVE DEFECTIVE, YOU ASSUME
THE COST OF ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
16. IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN
WRITING WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MAY MODIFY
AND/OR REDISTRIBUTE THE LIBRARY AS PERMITTED ABOVE, BE LIABLE TO YOU
FOR DAMAGES, INCLUDING ANY GENERAL, SPECIAL, INCIDENTAL OR
CONSEQUENTIAL DAMAGES ARISING OUT OF THE USE OR INABILITY TO USE THE
LIBRARY (INCLUDING BUT NOT LIMITED TO LOSS OF DATA OR DATA BEING
RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD PARTIES OR A
FAILURE OF THE LIBRARY TO OPERATE WITH ANY OTHER SOFTWARE), EVEN IF
SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH
DAMAGES.
END OF TERMS AND CONDITIONS
How to Apply These Terms to Your New Libraries
If you develop a new library, and you want it to be of the greatest
possible use to the public, we recommend making it free software that
everyone can redistribute and change. You can do so by permitting
redistribution under these terms (or, alternatively, under the terms of the
ordinary General Public License).
To apply these terms, attach the following notices to the library. It is
safest to attach them to the start of each source file to most effectively
convey the exclusion of warranty; and each file should have at least the
"copyright" line and a pointer to where the full notice is found.
<one line to give the library's name and a brief idea of what it does.>
Copyright (C) <year> <name of author>
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
Also add information on how to contact you by electronic and paper mail.
You should also get your employer (if you work as a programmer) or your
school, if any, to sign a "copyright disclaimer" for the library, if
necessary. Here is a sample; alter the names:
Yoyodyne, Inc., hereby disclaims all copyright interest in the
library `Frob' (a library for tweaking knobs) written by James Random Hacker.
<signature of Ty Coon>, 1 April 1990
Ty Coon, President of Vice
That's all there is to it!
| browser_patches/ffmpeg/ffmpeg-license/COPYING.LGPLv2.1 | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.0001816460571717471,
0.0001741656888043508,
0.00015773935592733324,
0.0001749735965859145,
0.000004942795385431964
] |
{
"id": 2,
"code_window": [
"export function makeWaitForNextTask() {\n",
" // As of Mar 2021, Electorn v12 doesn't create new task with `setImmediate` despite\n",
" // using Node 14 internally, so we fallback to `setTimeout(0)` instead.\n",
" // @see https://github.com/electron/electron/issues/28261\n",
" if (process.versions.electron)\n",
" return (callback: () => void) => setTimeout(callback, 0);\n",
" if (parseInt(process.versions.node, 10) >= 11)\n",
" return setImmediate;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if ((process.versions as any).electron)\n"
],
"file_path": "src/utils/utils.ts",
"type": "replace",
"edit_start_line_idx": 29
} | /**
* Copyright 2018 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { it, expect } from './fixtures';
import type { Frame } from '..';
import { TestServer } from '../utils/testserver';
it('should navigate to empty page with networkidle', async ({page, server}) => {
const response = await page.goto(server.EMPTY_PAGE, { waitUntil: 'networkidle' });
expect(response.status()).toBe(200);
});
async function networkIdleTest(frame: Frame, server: TestServer, action: () => Promise<any>, isSetContent?: boolean) {
const waitForRequest = (suffix: string) => {
return Promise.all([
server.waitForRequest(suffix),
frame.page().waitForRequest(server.PREFIX + suffix),
]);
};
let responseA, responseB;
// Hold on to a bunch of requests without answering.
server.setRoute('/fetch-request-a.js', (req, res) => responseA = res);
const firstFetchResourceRequested = waitForRequest('/fetch-request-a.js');
server.setRoute('/fetch-request-b.js', (req, res) => responseB = res);
const secondFetchResourceRequested = waitForRequest('/fetch-request-b.js');
const waitForLoadPromise = isSetContent ? Promise.resolve() : frame.waitForNavigation({ waitUntil: 'load' });
// Navigate to a page which loads immediately and then does a bunch of
// requests via javascript's fetch method.
const actionPromise = action();
// Track when the action gets completed.
let actionFinished = false;
actionPromise.then(() => actionFinished = true);
// Wait for the frame's 'load' event.
await waitForLoadPromise;
expect(actionFinished).toBe(false);
// Wait for the initial resource to be requested.
await firstFetchResourceRequested;
expect(actionFinished).toBe(false);
// Trigger the second request.
await frame.page().evaluate(() => window['fetchSecond']());
// Finish the first request.
responseA.statusCode = 404;
responseA.end(`File not found`);
// Wait for the second round to be requested.
await secondFetchResourceRequested;
expect(actionFinished).toBe(false);
// Finishing the second response should trigger networkidle.
let timerTriggered = false;
const timer = setTimeout(() => timerTriggered = true, 500);
responseB.statusCode = 404;
responseB.end(`File not found`);
const response = await actionPromise;
clearTimeout(timer);
expect(timerTriggered).toBe(true);
if (!isSetContent)
expect(response.ok()).toBe(true);
}
it('should wait for networkidle to succeed navigation', async ({page, server}) => {
await networkIdleTest(page.mainFrame(), server, () => {
return page.goto(server.PREFIX + '/networkidle.html', { waitUntil: 'networkidle' });
});
});
it('should wait for networkidle to succeed navigation with request from previous navigation', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
server.setRoute('/foo.js', () => {});
await page.setContent(`<script>fetch('foo.js');</script>`);
await networkIdleTest(page.mainFrame(), server, () => {
return page.goto(server.PREFIX + '/networkidle.html', { waitUntil: 'networkidle' });
});
});
it('should wait for networkidle in waitForNavigation', async ({page, server}) => {
await networkIdleTest(page.mainFrame(), server, () => {
const promise = page.waitForNavigation({ waitUntil: 'networkidle' });
page.goto(server.PREFIX + '/networkidle.html');
return promise;
});
});
it('should wait for networkidle in setContent', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
await networkIdleTest(page.mainFrame(), server, () => {
return page.setContent(`<script src='networkidle.js'></script>`, { waitUntil: 'networkidle' });
}, true);
});
it('should wait for networkidle in setContent with request from previous navigation', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
server.setRoute('/foo.js', () => {});
await page.setContent(`<script>fetch('foo.js');</script>`);
await networkIdleTest(page.mainFrame(), server, () => {
return page.setContent(`<script src='networkidle.js'></script>`, { waitUntil: 'networkidle' });
}, true);
});
it('should wait for networkidle when navigating iframe', async ({page, server}) => {
await page.goto(server.PREFIX + '/frames/one-frame.html');
const frame = page.mainFrame().childFrames()[0];
await networkIdleTest(frame, server, () => frame.goto(server.PREFIX + '/networkidle.html', { waitUntil: 'networkidle' }));
});
it('should wait for networkidle in setContent from the child frame', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
await networkIdleTest(page.mainFrame(), server, () => {
return page.setContent(`<iframe src='networkidle.html'></iframe>`, { waitUntil: 'networkidle' });
}, true);
});
it('should wait for networkidle from the child frame', async ({page, server}) => {
await networkIdleTest(page.mainFrame(), server, () => {
return page.goto(server.PREFIX + '/networkidle-frame.html', { waitUntil: 'networkidle' });
});
});
it('should wait for networkidle from the popup', async ({page, server}) => {
await page.goto(server.EMPTY_PAGE);
await page.setContent(`
<button id=box1 onclick="window.open('./popup/popup.html')">Button1</button>
<button id=box2 onclick="window.open('./popup/popup.html')">Button2</button>
<button id=box3 onclick="window.open('./popup/popup.html')">Button3</button>
<button id=box4 onclick="window.open('./popup/popup.html')">Button4</button>
<button id=box5 onclick="window.open('./popup/popup.html')">Button5</button>
`);
for (let i = 1; i < 6; ++i) {
const [popup] = await Promise.all([
page.waitForEvent('popup'),
page.click('#box' + i)
]);
await popup.waitForLoadState('networkidle');
}
});
| test/page-network-idle.spec.ts | 0 | https://github.com/microsoft/playwright/commit/ec6453d1b2e93439595aceb6454448a96668a00d | [
0.00017745859804563224,
0.00017217572894878685,
0.00016567455895710737,
0.00017293053679168224,
0.000002796322633003001
] |
{
"id": 0,
"code_window": [
" {\n",
" allowIndexSignaturePropertyAccess: true,\n",
" },\n",
" ],\n",
" },\n",
" overrides: [\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" '@typescript-eslint/no-restricted-imports': [\n",
" 'error',\n",
" {\n",
" paths: [\n",
" {\n",
" name: 'vite',\n",
" message: 'Please dynamically import from vite instead, to force the use of ESM',\n",
" allowTypeImports: true,\n",
" },\n",
" ],\n",
" },\n",
" ],\n"
],
"file_path": "code/.eslintrc.js",
"type": "add",
"edit_start_line_idx": 36
} | import type { Options, Presets } from '@storybook/types';
import { loadConfigFromFile } from 'vite';
import { commonConfig } from './vite-config';
jest.mock('vite', () => ({
...jest.requireActual('vite'),
loadConfigFromFile: jest.fn(async () => ({})),
}));
const loadConfigFromFileMock = jest.mocked(loadConfigFromFile);
const dummyOptions: Options = {
configType: 'DEVELOPMENT',
configDir: '',
packageJson: {},
presets: {
apply: async (key: string) =>
({
framework: {
name: '',
},
addons: [],
core: {
builder: {},
},
options: {},
}[key]),
} as Presets,
presetsList: [],
};
describe('commonConfig', () => {
it('should preserve default envPrefix', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: {},
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['VITE_', 'STORYBOOK_']);
});
it('should preserve custom envPrefix string', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: { envPrefix: 'SECRET_' },
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['SECRET_', 'STORYBOOK_']);
});
it('should preserve custom envPrefix array', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: { envPrefix: ['SECRET_', 'VUE_'] },
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['SECRET_', 'VUE_', 'STORYBOOK_']);
});
});
| code/builders/builder-vite/src/vite-config.test.ts | 1 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.0002583781024441123,
0.00018029000784736127,
0.00016611938190180808,
0.00016712810611352324,
0.000031898467568680644
] |
{
"id": 0,
"code_window": [
" {\n",
" allowIndexSignaturePropertyAccess: true,\n",
" },\n",
" ],\n",
" },\n",
" overrides: [\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" '@typescript-eslint/no-restricted-imports': [\n",
" 'error',\n",
" {\n",
" paths: [\n",
" {\n",
" name: 'vite',\n",
" message: 'Please dynamically import from vite instead, to force the use of ESM',\n",
" allowTypeImports: true,\n",
" },\n",
" ],\n",
" },\n",
" ],\n"
],
"file_path": "code/.eslintrc.js",
"type": "add",
"edit_start_line_idx": 36
} | ```shell
pnpm add --save-dev @storybook/addon-coverage
```
| docs/snippets/common/storybook-coverage-addon-install.pnpm.js.mdx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00016731690266169608,
0.00016731690266169608,
0.00016731690266169608,
0.00016731690266169608,
0
] |
{
"id": 0,
"code_window": [
" {\n",
" allowIndexSignaturePropertyAccess: true,\n",
" },\n",
" ],\n",
" },\n",
" overrides: [\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" '@typescript-eslint/no-restricted-imports': [\n",
" 'error',\n",
" {\n",
" paths: [\n",
" {\n",
" name: 'vite',\n",
" message: 'Please dynamically import from vite instead, to force the use of ESM',\n",
" allowTypeImports: true,\n",
" },\n",
" ],\n",
" },\n",
" ],\n"
],
"file_path": "code/.eslintrc.js",
"type": "add",
"edit_start_line_idx": 36
} | ```ts
// MyComponent.stories.ts
import type { Meta } from '@storybook/web-components';
const meta: Meta = {
title: 'Path/To/MyComponent',
component: 'my-component',
decorators: [ ... ],
parameters: { ... },
};
export default meta;
```
| docs/snippets/web-components/my-component-story-mandatory-export.ts.mdx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00016974221216514707,
0.00016810823581181467,
0.00016647425945848227,
0.00016810823581181467,
0.0000016339763533324003
] |
{
"id": 0,
"code_window": [
" {\n",
" allowIndexSignaturePropertyAccess: true,\n",
" },\n",
" ],\n",
" },\n",
" overrides: [\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" '@typescript-eslint/no-restricted-imports': [\n",
" 'error',\n",
" {\n",
" paths: [\n",
" {\n",
" name: 'vite',\n",
" message: 'Please dynamically import from vite instead, to force the use of ESM',\n",
" allowTypeImports: true,\n",
" },\n",
" ],\n",
" },\n",
" ],\n"
],
"file_path": "code/.eslintrc.js",
"type": "add",
"edit_start_line_idx": 36
} | ```ts
// Button.stories.ts|tsx
import type { Meta, StoryObj } from '@storybook/react';
import { Button } from './Button';
const meta: Meta<typeof Button> = {
component: Button,
};
export default meta;
type Story = StoryObj<typeof Button>;
export const Primary: Story = {
args: {
backgroundColor: '#ff0',
label: 'Button',
},
};
export const Secondary: Story = {
args: {
...Primary.args,
label: '😄👍😍💯',
},
};
export const Tertiary: Story = {
args: {
...Primary.args,
label: '📚📕📈🤓',
},
};
```
| docs/snippets/react/button-story-using-args.ts.mdx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00016936181054916233,
0.00016789406072348356,
0.00016648347082082182,
0.00016786549531389028,
0.0000010701724022510462
] |
{
"id": 1,
"code_window": [
"import type { Options, Presets } from '@storybook/types';\n",
"import { loadConfigFromFile } from 'vite';\n",
"import { commonConfig } from './vite-config';\n",
"\n",
"jest.mock('vite', () => ({\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/builders/builder-vite/src/vite-config.test.ts",
"type": "add",
"edit_start_line_idx": 1
} | import { type Plugin } from 'vite';
export function configOverrides() {
return {
// SvelteKit sets SSR, we need it to be false when building
name: 'storybook:sveltekit-overrides',
apply: 'build',
config: () => {
return { build: { ssr: false } };
},
} satisfies Plugin;
}
| code/frameworks/sveltekit/src/plugins/config-overrides.ts | 1 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00024678235058672726,
0.0002106017927872017,
0.00017442123498767614,
0.0002106017927872017,
0.00003618055779952556
] |
{
"id": 1,
"code_window": [
"import type { Options, Presets } from '@storybook/types';\n",
"import { loadConfigFromFile } from 'vite';\n",
"import { commonConfig } from './vite-config';\n",
"\n",
"jest.mock('vite', () => ({\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/builders/builder-vite/src/vite-config.test.ts",
"type": "add",
"edit_start_line_idx": 1
} | import React, { useMemo } from 'react';
import { HeadManagerContext } from 'next/dist/shared/lib/head-manager-context.shared-runtime';
import initHeadManager from 'next/dist/client/head-manager';
type HeadManagerValue = {
updateHead?: ((state: JSX.Element[]) => void) | undefined;
mountedInstances?: Set<unknown>;
updateScripts?: ((state: any) => void) | undefined;
scripts?: any;
getIsSsr?: () => boolean;
appDir?: boolean | undefined;
nonce?: string | undefined;
};
const HeadManagerProvider: React.FC = ({ children }) => {
const headManager: HeadManagerValue = useMemo(initHeadManager, []);
headManager.getIsSsr = () => false;
return <HeadManagerContext.Provider value={headManager}>{children}</HeadManagerContext.Provider>;
};
export default HeadManagerProvider;
| code/frameworks/nextjs/src/head-manager/head-manager-provider.tsx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00017568777548149228,
0.00017431878950446844,
0.00017308240057900548,
0.00017418622155673802,
0.0000010677631507860497
] |
{
"id": 1,
"code_window": [
"import type { Options, Presets } from '@storybook/types';\n",
"import { loadConfigFromFile } from 'vite';\n",
"import { commonConfig } from './vite-config';\n",
"\n",
"jest.mock('vite', () => ({\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/builders/builder-vite/src/vite-config.test.ts",
"type": "add",
"edit_start_line_idx": 1
} | import { html } from 'lit';
import './demo-wc-card';
export default {
component: 'demo-wc-card',
render: ({ backSide, header, rows, prefix }) =>
html`
<demo-wc-card .backSide="${backSide}" .header="${header}" .rows="${rows}"
><span slot="prefix">${prefix}</span>A simple card</demo-wc-card
>
`,
};
export const Front = {
args: { backSide: false, header: undefined, rows: [] },
};
export const Back = {
args: { backSide: true, header: undefined, rows: [] },
};
export const Prefix = {
args: { backSide: false, prefix: 'prefix:', header: 'my header', rows: [] },
};
| code/renderers/web-components/template/stories/demo-wc-card.stories.js | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00017412792658433318,
0.00017204094910994172,
0.0001687447656877339,
0.00017325016960967332,
0.000002358142864977708
] |
{
"id": 1,
"code_window": [
"import type { Options, Presets } from '@storybook/types';\n",
"import { loadConfigFromFile } from 'vite';\n",
"import { commonConfig } from './vite-config';\n",
"\n",
"jest.mock('vite', () => ({\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/builders/builder-vite/src/vite-config.test.ts",
"type": "add",
"edit_start_line_idx": 1
} | ```js
// MyComponent.stories.js|jsx
import { MyComponent } from './MyComponent';
export default {
component: MyComponent,
tags: ['skip-test'], // 👈 Provides the `skip-test` tag to all stories in this file
};
export const SkipStory = {
//👇 Adds the `skip-test` tag to this story to allow it to be skipped in the tests when enabled in the test-runner configuration
tags: ['skip-test'],
};
```
| docs/snippets/common/my-component-skip-tags.story.js.mdx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00016685343871358782,
0.00016680342378094792,
0.0001667533942963928,
0.00016680342378094792,
5.002220859751105e-8
] |
{
"id": 2,
"code_window": [
"import { type Plugin } from 'vite';\n",
"\n",
"export function configOverrides() {\n",
" return {\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"import type { Plugin } from 'vite';\n"
],
"file_path": "code/frameworks/sveltekit/src/plugins/config-overrides.ts",
"type": "replace",
"edit_start_line_idx": 0
} | import type { Options, Presets } from '@storybook/types';
import { loadConfigFromFile } from 'vite';
import { commonConfig } from './vite-config';
jest.mock('vite', () => ({
...jest.requireActual('vite'),
loadConfigFromFile: jest.fn(async () => ({})),
}));
const loadConfigFromFileMock = jest.mocked(loadConfigFromFile);
const dummyOptions: Options = {
configType: 'DEVELOPMENT',
configDir: '',
packageJson: {},
presets: {
apply: async (key: string) =>
({
framework: {
name: '',
},
addons: [],
core: {
builder: {},
},
options: {},
}[key]),
} as Presets,
presetsList: [],
};
describe('commonConfig', () => {
it('should preserve default envPrefix', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: {},
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['VITE_', 'STORYBOOK_']);
});
it('should preserve custom envPrefix string', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: { envPrefix: 'SECRET_' },
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['SECRET_', 'STORYBOOK_']);
});
it('should preserve custom envPrefix array', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: { envPrefix: ['SECRET_', 'VUE_'] },
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['SECRET_', 'VUE_', 'STORYBOOK_']);
});
});
| code/builders/builder-vite/src/vite-config.test.ts | 1 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.0002951768401544541,
0.00019453754066489637,
0.00016578692884650081,
0.00016971825971268117,
0.00004585590795613825
] |
{
"id": 2,
"code_window": [
"import { type Plugin } from 'vite';\n",
"\n",
"export function configOverrides() {\n",
" return {\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"import type { Plugin } from 'vite';\n"
],
"file_path": "code/frameworks/sveltekit/src/plugins/config-overrides.ts",
"type": "replace",
"edit_start_line_idx": 0
} | # Storybook Telemetry
Storybook collects completely anonymous telemetry data about general usage. Participation in this program is optional and it’s easy to opt-out.
For more information visit: [storybook.js.org/telemetry](https://storybook.js.org/telemetry)
| code/lib/telemetry/README.md | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00017186315380968153,
0.00017186315380968153,
0.00017186315380968153,
0.00017186315380968153,
0
] |
{
"id": 2,
"code_window": [
"import { type Plugin } from 'vite';\n",
"\n",
"export function configOverrides() {\n",
" return {\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"import type { Plugin } from 'vite';\n"
],
"file_path": "code/frameworks/sveltekit/src/plugins/config-overrides.ts",
"type": "replace",
"edit_start_line_idx": 0
} | import { logger } from '@storybook/node-logger';
import type { Options, StorybookConfig } from '@storybook/types';
import { getDirectoryFromWorkingDir } from '@storybook/core-common';
import { ConflictingStaticDirConfigError } from '@storybook/core-events/server-errors';
import chalk from 'chalk';
import type { Router } from 'express';
import express from 'express';
import { pathExists } from 'fs-extra';
import path, { basename, isAbsolute } from 'path';
import isEqual from 'lodash/isEqual.js';
import { dedent } from 'ts-dedent';
import { defaultStaticDirs } from './constants';
export async function useStatics(router: Router, options: Options) {
const staticDirs =
(await options.presets.apply<StorybookConfig['staticDirs']>('staticDirs')) ?? [];
const faviconPath = await options.presets.apply<string>('favicon');
if (options.staticDir && !isEqual(staticDirs, defaultStaticDirs)) {
throw new ConflictingStaticDirConfigError();
}
const statics = [
...staticDirs.map((dir) => (typeof dir === 'string' ? dir : `${dir.from}:${dir.to}`)),
...(options.staticDir || []),
];
if (statics && statics.length > 0) {
await Promise.all(
statics.map(async (dir) => {
try {
const normalizedDir =
staticDirs && !isAbsolute(dir)
? getDirectoryFromWorkingDir({
configDir: options.configDir,
workingDir: process.cwd(),
directory: dir,
})
: dir;
const { staticDir, staticPath, targetEndpoint } = await parseStaticDir(normalizedDir);
// Don't log for the internal static dir
if (!targetEndpoint.startsWith('/sb-')) {
logger.info(
chalk`=> Serving static files from {cyan ${staticDir}} at {cyan ${targetEndpoint}}`
);
}
router.use(targetEndpoint, express.static(staticPath, { index: false }));
} catch (e) {
if (e instanceof Error) logger.warn(e.message);
}
})
);
}
router.get(`/${basename(faviconPath)}`, (req, res) => res.sendFile(faviconPath));
}
export const parseStaticDir = async (arg: string) => {
// Split on last index of ':', for Windows compatibility (e.g. 'C:\some\dir:\foo')
const lastColonIndex = arg.lastIndexOf(':');
const isWindowsAbsolute = path.win32.isAbsolute(arg);
const isWindowsRawDirOnly = isWindowsAbsolute && lastColonIndex === 1; // e.g. 'C:\some\dir'
const splitIndex = lastColonIndex !== -1 && !isWindowsRawDirOnly ? lastColonIndex : arg.length;
const targetRaw = arg.substring(splitIndex + 1) || '/';
const target = targetRaw.split(path.sep).join(path.posix.sep); // Ensure target has forward-slash path
const rawDir = arg.substring(0, splitIndex);
const staticDir = path.isAbsolute(rawDir) ? rawDir : `./${rawDir}`;
const staticPath = path.resolve(staticDir);
const targetDir = target.replace(/^\/?/, './');
const targetEndpoint = targetDir.substring(1);
if (!(await pathExists(staticPath))) {
throw new Error(
dedent(chalk`
Failed to load static files, no such directory: {cyan ${staticPath}}
Make sure this directory exists, or omit the {bold -s (--static-dir)} option.
`)
);
}
return { staticDir, staticPath, targetDir, targetEndpoint };
};
| code/lib/core-server/src/utils/server-statics.ts | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00035968469455838203,
0.00019003944180440158,
0.00016527240222785622,
0.00016934647283051163,
0.00006001937072142027
] |
{
"id": 2,
"code_window": [
"import { type Plugin } from 'vite';\n",
"\n",
"export function configOverrides() {\n",
" return {\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"import type { Plugin } from 'vite';\n"
],
"file_path": "code/frameworks/sveltekit/src/plugins/config-overrides.ts",
"type": "replace",
"edit_start_line_idx": 0
} | ```html
<!-- YourPage.vue -->
<template>
<PageLayout :user="user">
<DocumentHeader :document="document" />
<DocumentList :documents="subdocuments" />
</PageLayout>
</template>
<script>
import PageLayout from './PageLayout';
import DocumentHeader from './DocumentHeader';
import DocumentList from './DocumentList';
export default {
name: 'DocumentScreen',
components: { PageLayout, DocumentHeader, DocumentList },
props: {
user: {
type: String,
default: 'N/A',
},
document: {
type: Object,
default: () => ({
id: 1,
title: 'A document',
content: 'Lorem Ipsum',
}),
},
subdocuments: {
type: Array,
default: () => [],
},
},
};
</script>
```
| docs/snippets/vue/simple-page-implementation.2.js.mdx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00017168249178212136,
0.00016998255159705877,
0.00016766760381869972,
0.00017029006266966462,
0.000001456140694244823
] |
{
"id": 3,
"code_window": [
"import path from 'path';\n",
"import pluginTurbosnap from 'vite-plugin-turbosnap';\n",
"import { mergeConfig } from 'vite';\n",
"import type { StorybookConfig } from '../../frameworks/react-vite';\n",
"\n",
"const isBlocksOnly = process.env.STORYBOOK_BLOCKS_ONLY === 'true';\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/ui/.storybook/main.ts",
"type": "add",
"edit_start_line_idx": 2
} | import type { Options, Presets } from '@storybook/types';
import { loadConfigFromFile } from 'vite';
import { commonConfig } from './vite-config';
jest.mock('vite', () => ({
...jest.requireActual('vite'),
loadConfigFromFile: jest.fn(async () => ({})),
}));
const loadConfigFromFileMock = jest.mocked(loadConfigFromFile);
const dummyOptions: Options = {
configType: 'DEVELOPMENT',
configDir: '',
packageJson: {},
presets: {
apply: async (key: string) =>
({
framework: {
name: '',
},
addons: [],
core: {
builder: {},
},
options: {},
}[key]),
} as Presets,
presetsList: [],
};
describe('commonConfig', () => {
it('should preserve default envPrefix', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: {},
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['VITE_', 'STORYBOOK_']);
});
it('should preserve custom envPrefix string', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: { envPrefix: 'SECRET_' },
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['SECRET_', 'STORYBOOK_']);
});
it('should preserve custom envPrefix array', async () => {
loadConfigFromFileMock.mockReturnValueOnce(
Promise.resolve({
config: { envPrefix: ['SECRET_', 'VUE_'] },
path: '',
dependencies: [],
})
);
const config = await commonConfig(dummyOptions, 'development');
expect(config.envPrefix).toStrictEqual(['SECRET_', 'VUE_', 'STORYBOOK_']);
});
});
| code/builders/builder-vite/src/vite-config.test.ts | 1 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00023670455266255885,
0.00018395588267594576,
0.00016373083053622395,
0.0001689543860265985,
0.00002712505556701217
] |
{
"id": 3,
"code_window": [
"import path from 'path';\n",
"import pluginTurbosnap from 'vite-plugin-turbosnap';\n",
"import { mergeConfig } from 'vite';\n",
"import type { StorybookConfig } from '../../frameworks/react-vite';\n",
"\n",
"const isBlocksOnly = process.env.STORYBOOK_BLOCKS_ONLY === 'true';\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/ui/.storybook/main.ts",
"type": "add",
"edit_start_line_idx": 2
} | /* eslint-disable jest/no-disabled-tests */
import { test, expect } from '@playwright/test';
import process from 'process';
import { SbPage } from './util';
const storybookUrl = process.env.STORYBOOK_URL || 'http://localhost:8001';
const templateName = process.env.STORYBOOK_TEMPLATE_NAME || '';
test.describe('addon-interactions', () => {
test.beforeEach(async ({ page }) => {
await page.goto(storybookUrl);
await new SbPage(page).waitUntilLoaded();
});
test('should have interactions', async ({ page }) => {
// templateName is e.g. 'vue-cli/default-js'
test.skip(
// eslint-disable-next-line jest/valid-title
/^(lit)/i.test(`${templateName}`),
`Skipping ${templateName}, which does not support addon-interactions`
);
const sbPage = new SbPage(page);
await sbPage.navigateToStory('example/page', 'logged-in');
await sbPage.viewAddonPanel('Interactions');
const welcome = await sbPage.previewRoot().locator('.welcome');
await expect(welcome).toContainText('Welcome, Jane Doe!');
const interactionsTab = await page.locator('#tabbutton-storybook-interactions-panel');
await expect(interactionsTab).toContainText(/(\d)/);
await expect(interactionsTab).toBeVisible();
const panel = sbPage.panelContent();
await expect(panel).toContainText(/Pass/);
await expect(panel).toContainText(/userEvent.click/);
await expect(panel).toBeVisible();
const done = await panel.locator('[data-testid=icon-done]').nth(0);
await expect(done).toBeVisible();
});
test('should step through interactions', async ({ page }) => {
// templateName is e.g. 'vue-cli/default-js'
test.skip(
// eslint-disable-next-line jest/valid-title
/^(lit)/i.test(`${templateName}`),
`Skipping ${templateName}, which does not support addon-interactions`
);
const sbPage = new SbPage(page);
await sbPage.deepLinkToStory(storybookUrl, 'addons/interactions/basics', 'type-and-clear');
await sbPage.viewAddonPanel('Interactions');
// Test initial state - Interactions have run, count is correct and values are as expected
const formInput = await sbPage.previewRoot().locator('#interaction-test-form input');
await expect(formInput).toHaveValue('final value');
const interactionsTab = await page.locator('#tabbutton-storybook-interactions-panel');
await expect(interactionsTab.getByText('3')).toBeVisible();
await expect(interactionsTab).toBeVisible();
await expect(interactionsTab).toBeVisible();
const panel = sbPage.panelContent();
const runStatusBadge = await panel.locator('[aria-label="Status of the test run"]');
await expect(runStatusBadge).toContainText(/Pass/);
await expect(panel).toContainText(/"initial value"/);
await expect(panel).toContainText(/clear/);
await expect(panel).toContainText(/"final value"/);
await expect(panel).toBeVisible();
// Test interactions debugger - Stepping through works, count is correct and values are as expected
const interactionsRow = await panel.locator('[aria-label="Interaction step"]');
await interactionsRow.first().isVisible();
await expect(await interactionsRow.count()).toEqual(3);
const firstInteraction = interactionsRow.first();
await firstInteraction.click();
await expect(runStatusBadge).toContainText(/Runs/);
await expect(formInput).toHaveValue('initial value');
const goForwardBtn = await panel.locator('[aria-label="Go forward"]');
await goForwardBtn.click();
await expect(formInput).toHaveValue('');
await goForwardBtn.click();
await expect(formInput).toHaveValue('final value');
await expect(runStatusBadge).toContainText(/Pass/);
// Test rerun state (from addon panel) - Interactions have rerun, count is correct and values are as expected
const rerunInteractionButton = await panel.locator('[aria-label="Rerun"]');
await rerunInteractionButton.click();
await expect(formInput).toHaveValue('final value');
await interactionsRow.first().isVisible();
await interactionsRow.nth(1).isVisible();
await interactionsRow.nth(2).isVisible();
await expect(interactionsTab.getByText('3')).toBeVisible();
await expect(interactionsTab).toBeVisible();
await expect(interactionsTab.getByText('3')).toBeVisible();
// Test remount state (from toolbar) - Interactions have rerun, count is correct and values are as expected
const remountComponentButton = await page.locator('[title="Remount component"]');
await remountComponentButton.click();
await interactionsRow.first().isVisible();
await interactionsRow.nth(1).isVisible();
await interactionsRow.nth(2).isVisible();
await expect(interactionsTab.getByText('3')).toBeVisible();
await expect(interactionsTab).toBeVisible();
await expect(interactionsTab).toBeVisible();
await expect(formInput).toHaveValue('final value');
});
});
| code/e2e-tests/addon-interactions.spec.ts | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.0002453278284519911,
0.00018077126878779382,
0.00016610608145128936,
0.00017662857135292143,
0.000019848259398713708
] |
{
"id": 3,
"code_window": [
"import path from 'path';\n",
"import pluginTurbosnap from 'vite-plugin-turbosnap';\n",
"import { mergeConfig } from 'vite';\n",
"import type { StorybookConfig } from '../../frameworks/react-vite';\n",
"\n",
"const isBlocksOnly = process.env.STORYBOOK_BLOCKS_ONLY === 'true';\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/ui/.storybook/main.ts",
"type": "add",
"edit_start_line_idx": 2
} | import type { FC } from 'react';
import React from 'react';
import { transparentize } from 'polished';
import { withReset } from '@storybook/components';
import type { CSSObject } from '@storybook/theming';
import { styled } from '@storybook/theming';
/**
* This selector styles all raw elements inside the DocsPage like this example with a `<div/>`:
* :where(div:not(.sb-unstyled, .sb-anchor, .sb-unstyled div, .sb-unstyled div))
*
* 1. ':where': ensures this has a specificity of 0, making it easier to override.
* 3. 'div:not(...)': selects all div elements that are not...
* 4. '.sb-anchor': Ensures anchors are not styled, which would have led to inheritable styles bleeding all the way down to stories
* 5. '.sb-unstyled, .sb-unstyled div': any element with sb-unstyled class, or descendants thereof
* 6. .sb-unstyled is an escape hatch that allows the user to opt-out of the default styles
* by wrapping their content in an element with the 'sb-unstyled' class or the <Unstyled /> block.
*
* Most Storybook doc blocks has the sb-unstyled class to opt-out of the default styles.
*/
const toGlobalSelector = (element: string): string =>
`& :where(${element}:not(.sb-anchor, .sb-unstyled, .sb-unstyled ${element}))`;
const breakpoint = 600;
export const Title = styled.h1(withReset, ({ theme }) => ({
color: theme.color.defaultText,
fontSize: theme.typography.size.m3,
fontWeight: theme.typography.weight.bold,
lineHeight: '32px',
[`@media (min-width: ${breakpoint}px)`]: {
fontSize: theme.typography.size.l1,
lineHeight: '36px',
marginBottom: '16px',
},
}));
export const Subtitle = styled.h2(withReset, ({ theme }) => ({
fontWeight: theme.typography.weight.regular,
fontSize: theme.typography.size.s3,
lineHeight: '20px',
borderBottom: 'none',
marginBottom: 15,
[`@media (min-width: ${breakpoint}px)`]: {
fontSize: theme.typography.size.m1,
lineHeight: '28px',
marginBottom: 24,
},
color: transparentize(0.25, theme.color.defaultText),
}));
// @ts-expect-error don't know why it doesn't accept our returned styles. if we add `...{}` anywhere to the returned object it stops erroring
export const DocsContent = styled.div(({ theme }) => {
const reset = {
fontFamily: theme.typography.fonts.base,
fontSize: theme.typography.size.s3,
margin: 0,
WebkitFontSmoothing: 'antialiased',
MozOsxFontSmoothing: 'grayscale',
WebkitTapHighlightColor: 'rgba(0, 0, 0, 0)',
WebkitOverflowScrolling: 'touch' as CSSObject['WebkitOverflowScrolling'],
};
const headers = {
margin: '20px 0 8px',
padding: 0,
cursor: 'text',
position: 'relative',
color: theme.color.defaultText,
'&:first-of-type': {
marginTop: 0,
paddingTop: 0,
},
'&:hover a.anchor': {
textDecoration: 'none',
},
'& code': {
fontSize: 'inherit',
},
};
const code = {
lineHeight: 1,
margin: '0 2px',
padding: '3px 5px',
whiteSpace: 'nowrap',
borderRadius: 3,
fontSize: theme.typography.size.s2 - 1,
border:
theme.base === 'light'
? `1px solid ${theme.color.mediumlight}`
: `1px solid ${theme.color.darker}`,
color:
theme.base === 'light'
? transparentize(0.1, theme.color.defaultText)
: transparentize(0.3, theme.color.defaultText),
backgroundColor: theme.base === 'light' ? theme.color.lighter : theme.color.border,
};
return {
maxWidth: 1000,
width: '100%',
[toGlobalSelector('a')]: {
...reset,
fontSize: 'inherit',
lineHeight: '24px',
color: theme.color.secondary,
textDecoration: 'none',
'&.absent': {
color: '#cc0000',
},
'&.anchor': {
display: 'block',
paddingLeft: 30,
marginLeft: -30,
cursor: 'pointer',
position: 'absolute',
top: 0,
left: 0,
bottom: 0,
},
},
[toGlobalSelector('blockquote')]: {
...reset,
margin: '16px 0',
borderLeft: `4px solid ${theme.color.medium}`,
padding: '0 15px',
color: theme.color.dark,
'& > :first-of-type': {
marginTop: 0,
},
'& > :last-child': {
marginBottom: 0,
},
},
[toGlobalSelector('div')]: reset,
[toGlobalSelector('dl')]: {
...reset,
margin: '16px 0',
padding: 0,
'& dt': {
fontSize: '14px',
fontWeight: 'bold',
fontStyle: 'italic',
padding: 0,
margin: '16px 0 4px',
},
'& dt:first-of-type': {
padding: 0,
},
'& dt > :first-of-type': {
marginTop: 0,
},
'& dt > :last-child': {
marginBottom: 0,
},
'& dd': {
margin: '0 0 16px',
padding: '0 15px',
},
'& dd > :first-of-type': {
marginTop: 0,
},
'& dd > :last-child': {
marginBottom: 0,
},
},
[toGlobalSelector('h1')]: {
...reset,
...headers,
fontSize: `${theme.typography.size.l1}px`,
fontWeight: theme.typography.weight.bold,
},
[toGlobalSelector('h2')]: {
...reset,
...headers,
fontSize: `${theme.typography.size.m2}px`,
paddingBottom: 4,
borderBottom: `1px solid ${theme.appBorderColor}`,
},
[toGlobalSelector('h3')]: {
...reset,
...headers,
fontSize: `${theme.typography.size.m1}px`,
fontWeight: theme.typography.weight.bold,
},
[toGlobalSelector('h4')]: {
...reset,
...headers,
fontSize: `${theme.typography.size.s3}px`,
},
[toGlobalSelector('h5')]: {
...reset,
...headers,
fontSize: `${theme.typography.size.s2}px`,
},
[toGlobalSelector('h6')]: {
...reset,
...headers,
fontSize: `${theme.typography.size.s2}px`,
color: theme.color.dark,
},
[toGlobalSelector('hr')]: {
border: '0 none',
borderTop: `1px solid ${theme.appBorderColor}`,
height: 4,
padding: 0,
},
[toGlobalSelector('img')]: {
maxWidth: '100%',
},
[toGlobalSelector('li')]: {
...reset,
fontSize: theme.typography.size.s2,
color: theme.color.defaultText,
lineHeight: '24px',
'& + li': {
marginTop: '.25em',
},
'& ul, & ol': {
marginTop: '.25em',
marginBottom: 0,
},
'& code': code,
},
[toGlobalSelector('ol')]: {
...reset,
margin: '16px 0',
paddingLeft: 30,
'& :first-of-type': {
marginTop: 0,
},
'& :last-child': {
marginBottom: 0,
},
},
[toGlobalSelector('p')]: {
...reset,
margin: '16px 0',
fontSize: theme.typography.size.s2,
lineHeight: '24px',
color: theme.color.defaultText,
'& code': code,
},
[toGlobalSelector('pre')]: {
...reset,
// reset
fontFamily: theme.typography.fonts.mono,
WebkitFontSmoothing: 'antialiased',
MozOsxFontSmoothing: 'grayscale',
lineHeight: '18px',
padding: '11px 1rem',
whiteSpace: 'pre-wrap',
color: 'inherit',
borderRadius: 3,
margin: '1rem 0',
'&:not(.prismjs)': {
background: 'transparent',
border: 'none',
borderRadius: 0,
padding: 0,
margin: 0,
},
'& pre, &.prismjs': {
padding: 15,
margin: 0,
whiteSpace: 'pre-wrap',
color: 'inherit',
fontSize: '13px',
lineHeight: '19px',
code: {
color: 'inherit',
fontSize: 'inherit',
},
},
'& code': {
whiteSpace: 'pre',
},
'& code, & tt': {
border: 'none',
},
},
[toGlobalSelector('span')]: {
...reset,
'&.frame': {
display: 'block',
overflow: 'hidden',
'& > span': {
border: `1px solid ${theme.color.medium}`,
display: 'block',
float: 'left',
overflow: 'hidden',
margin: '13px 0 0',
padding: 7,
width: 'auto',
},
'& span img': {
display: 'block',
float: 'left',
},
'& span span': {
clear: 'both',
color: theme.color.darkest,
display: 'block',
padding: '5px 0 0',
},
},
'&.align-center': {
display: 'block',
overflow: 'hidden',
clear: 'both',
'& > span': {
display: 'block',
overflow: 'hidden',
margin: '13px auto 0',
textAlign: 'center',
},
'& span img': {
margin: '0 auto',
textAlign: 'center',
},
},
'&.align-right': {
display: 'block',
overflow: 'hidden',
clear: 'both',
'& > span': {
display: 'block',
overflow: 'hidden',
margin: '13px 0 0',
textAlign: 'right',
},
'& span img': {
margin: 0,
textAlign: 'right',
},
},
'&.float-left': {
display: 'block',
marginRight: 13,
overflow: 'hidden',
float: 'left',
'& span': {
margin: '13px 0 0',
},
},
'&.float-right': {
display: 'block',
marginLeft: 13,
overflow: 'hidden',
float: 'right',
'& > span': {
display: 'block',
overflow: 'hidden',
margin: '13px auto 0',
textAlign: 'right',
},
},
},
[toGlobalSelector('table')]: {
...reset,
margin: '16px 0',
fontSize: theme.typography.size.s2,
lineHeight: '24px',
padding: 0,
borderCollapse: 'collapse',
'& tr': {
borderTop: `1px solid ${theme.appBorderColor}`,
backgroundColor: theme.appContentBg,
margin: 0,
padding: 0,
},
'& tr:nth-of-type(2n)': {
backgroundColor: theme.base === 'dark' ? theme.color.darker : theme.color.lighter,
},
'& tr th': {
fontWeight: 'bold',
color: theme.color.defaultText,
border: `1px solid ${theme.appBorderColor}`,
margin: 0,
padding: '6px 13px',
},
'& tr td': {
border: `1px solid ${theme.appBorderColor}`,
color: theme.color.defaultText,
margin: 0,
padding: '6px 13px',
},
'& tr th :first-of-type, & tr td :first-of-type': {
marginTop: 0,
},
'& tr th :last-child, & tr td :last-child': {
marginBottom: 0,
},
},
[toGlobalSelector('ul')]: {
...reset,
margin: '16px 0',
paddingLeft: 30,
'& :first-of-type': {
marginTop: 0,
},
'& :last-child': {
marginBottom: 0,
},
listStyle: 'disc',
},
};
});
export const DocsWrapper = styled.div(({ theme }) => ({
background: theme.background.content,
display: 'flex',
justifyContent: 'center',
padding: '4rem 20px',
minHeight: '100vh',
boxSizing: 'border-box',
gap: '3rem',
[`@media (min-width: ${breakpoint}px)`]: {},
}));
interface DocsPageWrapperProps {
children?: React.ReactNode;
toc?: React.ReactNode;
}
export const DocsPageWrapper: FC<DocsPageWrapperProps> = ({ children, toc }) => (
<DocsWrapper className="sbdocs sbdocs-wrapper">
<DocsContent className="sbdocs sbdocs-content">{children}</DocsContent>
{toc}
</DocsWrapper>
);
| code/ui/blocks/src/components/DocsPage.tsx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00018032950174529105,
0.00017440285591874272,
0.00016531618894077837,
0.0001750199735397473,
0.000003197790192643879
] |
{
"id": 3,
"code_window": [
"import path from 'path';\n",
"import pluginTurbosnap from 'vite-plugin-turbosnap';\n",
"import { mergeConfig } from 'vite';\n",
"import type { StorybookConfig } from '../../frameworks/react-vite';\n",
"\n",
"const isBlocksOnly = process.env.STORYBOOK_BLOCKS_ONLY === 'true';\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// eslint-disable-next-line @typescript-eslint/no-restricted-imports\n"
],
"file_path": "code/ui/.storybook/main.ts",
"type": "add",
"edit_start_line_idx": 2
} | ```ts
// .storybook/test-runner.ts
import type { TestRunnerConfig } from '@storybook/test-runner';
import { waitForPageReady } from '@storybook/test-runner';
import { toMatchImageSnapshot } from 'jest-image-snapshot';
const customSnapshotsDir = `${process.cwd()}/__snapshots__`;
const config: TestRunnerConfig = {
setup() {
expect.extend({ toMatchImageSnapshot });
},
async postVisit(page, context) {
// Awaits for the page to be loaded and available including assets (e.g., fonts)
await waitForPageReady(page);
// Generates a snapshot file based on the story identifier
const image = await page.screenshot();
expect(image).toMatchImageSnapshot({
customSnapshotsDir,
customSnapshotIdentifier: context.id,
});
},
};
export default config;
```
| docs/snippets/common/test-runner-waitpageready.ts.mdx | 0 | https://github.com/storybookjs/storybook/commit/3fc9be5f3f03a54f535845427c597aa92676b53c | [
0.00020146813767496496,
0.00018531309615354985,
0.00017087098967749625,
0.00018360013200435787,
0.000012549820894491859
] |
{
"id": 0,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"#includeAll(vs/editor/standalone/browser/standaloneEditor;modes.=>languages.;editorCommon.=>):\n",
"#include(vs/editor/standalone/common/standaloneThemeService): BuiltinTheme, IStandaloneThemeData, IColors\n",
"#include(vs/editor/common/modes/supports/tokenization): ITokenThemeRule\n",
"#include(vs/editor/common/services/webWorker): MonacoWebWorker, IWebWorkerOptions\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"#include(vs/editor/browser/widget/diffNavigator): IDiffNavigator\n"
],
"file_path": "build/monaco/monaco.d.ts.recipe",
"type": "replace",
"edit_start_line_idx": 45
} |
// This file is adding references to various symbols which should not be removed via tree shaking
import { ServiceIdentifier } from './vs/platform/instantiation/common/instantiation';
import { IHighlight } from './vs/base/parts/quickopen/browser/quickOpenModel';
import { SimpleWorkerClient, create as create1 } from './vs/base/common/worker/simpleWorker';
import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';
import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';
import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';
import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';
import * as editorAPI from './vs/editor/editor.api';
(function () {
var a: any;
var b: any;
a = (<DiffNavigator>b).previous; // IDiffNavigator
a = (<ServiceIdentifier<any>>b).type;
a = (<IHighlight>b).start;
a = (<IHighlight>b).end;
a = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient
a = create1;
a = create2;
a = (<DocumentRangeFormattingEditProvider>b).extensionId;
// injection madness
a = (<SyncDescriptor0<any>>b).ctor;
a = (<SyncDescriptor0<any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor2<any, any, any>>b).ctor;
a = (<SyncDescriptor2<any, any, any>>b).bind;
a = (<SyncDescriptor3<any, any, any, any>>b).ctor;
a = (<SyncDescriptor3<any, any, any, any>>b).bind;
a = (<SyncDescriptor4<any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor4<any, any, any, any, any>>b).bind;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).bind;
// exported API
a = editorAPI.CancellationTokenSource;
a = editorAPI.Emitter;
a = editorAPI.KeyCode;
a = editorAPI.KeyMod;
a = editorAPI.Position;
a = editorAPI.Range;
a = editorAPI.Selection;
a = editorAPI.SelectionDirection;
a = editorAPI.MarkerSeverity;
a = editorAPI.MarkerTag;
a = editorAPI.Uri;
a = editorAPI.Token;
a = editorAPI.editor;
a = editorAPI.languages;
})();
| build/monaco/monaco.usage.recipe | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0007038225303404033,
0.0002562347799539566,
0.00016326314653269947,
0.00017719398601911962,
0.00018357856606598943
] |
{
"id": 0,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"#includeAll(vs/editor/standalone/browser/standaloneEditor;modes.=>languages.;editorCommon.=>):\n",
"#include(vs/editor/standalone/common/standaloneThemeService): BuiltinTheme, IStandaloneThemeData, IColors\n",
"#include(vs/editor/common/modes/supports/tokenization): ITokenThemeRule\n",
"#include(vs/editor/common/services/webWorker): MonacoWebWorker, IWebWorkerOptions\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"#include(vs/editor/browser/widget/diffNavigator): IDiffNavigator\n"
],
"file_path": "build/monaco/monaco.d.ts.recipe",
"type": "replace",
"edit_start_line_idx": 45
} | #!/bin/sh
echo '' | extensions/git/src/askpass-empty.sh | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.000170675411936827,
0.000170675411936827,
0.000170675411936827,
0.000170675411936827,
0
] |
{
"id": 0,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"#includeAll(vs/editor/standalone/browser/standaloneEditor;modes.=>languages.;editorCommon.=>):\n",
"#include(vs/editor/standalone/common/standaloneThemeService): BuiltinTheme, IStandaloneThemeData, IColors\n",
"#include(vs/editor/common/modes/supports/tokenization): ITokenThemeRule\n",
"#include(vs/editor/common/services/webWorker): MonacoWebWorker, IWebWorkerOptions\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"#include(vs/editor/browser/widget/diffNavigator): IDiffNavigator\n"
],
"file_path": "build/monaco/monaco.d.ts.recipe",
"type": "replace",
"edit_start_line_idx": 45
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as vscode from 'vscode';
import { Command } from '../commandManager';
import { PreviewSecuritySelector } from '../security';
import { isMarkdownFile } from '../util/file';
import { MarkdownPreviewManager } from '../features/previewManager';
export class ShowPreviewSecuritySelectorCommand implements Command {
public readonly id = 'markdown.showPreviewSecuritySelector';
public constructor(
private readonly previewSecuritySelector: PreviewSecuritySelector,
private readonly previewManager: MarkdownPreviewManager
) { }
public execute(resource: string | undefined) {
if (this.previewManager.activePreviewResource) {
this.previewSecuritySelector.showSecuritySelectorForResource(this.previewManager.activePreviewResource);
} else if (resource) {
const source = vscode.Uri.parse(resource);
this.previewSecuritySelector.showSecuritySelectorForResource(source.query ? vscode.Uri.parse(source.query) : source);
} else if (vscode.window.activeTextEditor && isMarkdownFile(vscode.window.activeTextEditor.document)) {
this.previewSecuritySelector.showSecuritySelectorForResource(vscode.window.activeTextEditor.document.uri);
}
}
} | extensions/markdown-language-features/src/commands/showPreviewSecuritySelector.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017730738909449428,
0.00017351849237456918,
0.00016695719386916608,
0.00017490467871539295,
0.000004186561909591546
] |
{
"id": 0,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"#includeAll(vs/editor/standalone/browser/standaloneEditor;modes.=>languages.;editorCommon.=>):\n",
"#include(vs/editor/standalone/common/standaloneThemeService): BuiltinTheme, IStandaloneThemeData, IColors\n",
"#include(vs/editor/common/modes/supports/tokenization): ITokenThemeRule\n",
"#include(vs/editor/common/services/webWorker): MonacoWebWorker, IWebWorkerOptions\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"#include(vs/editor/browser/widget/diffNavigator): IDiffNavigator\n"
],
"file_path": "build/monaco/monaco.d.ts.recipe",
"type": "replace",
"edit_start_line_idx": 45
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'mocha';
import * as assert from 'assert';
import { Selection } from 'vscode';
import { withRandomFileEditor, closeAllEditors } from './testUtils';
import { fetchEditPoint } from '../editPoint';
import { fetchSelectItem } from '../selectItem';
import { balanceOut, balanceIn } from '../balance';
suite('Tests for Next/Previous Select/Edit point and Balance actions', () => {
teardown(closeAllEditors);
const cssContents = `
.boo {
margin: 20px 10px;
background-image: url('tryme.png');
}
.boo .hoo {
margin: 10px;
}
`;
const scssContents = `
.boo {
margin: 20px 10px;
background-image: url('tryme.png');
.boo .hoo {
margin: 10px;
}
}
`;
const htmlContents = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
</head>
<body>
<div>
\t\t
</div>
<div class="header">
<ul class="nav main">
<li class="item1">Item 1</li>
<li class="item2">Item 2</li>
</ul>
</div>
</body>
</html>
`;
test('Emmet Next/Prev Edit point in html file', function (): any {
return withRandomFileEditor(htmlContents, '.html', (editor, _) => {
editor.selections = [new Selection(1, 5, 1, 5)];
let expectedNextEditPoints: [number, number][] = [[4, 16], [6, 8], [10, 2], [20, 0]];
expectedNextEditPoints.forEach(([line, col]) => {
fetchEditPoint('next');
testSelection(editor.selection, col, line);
});
let expectedPrevEditPoints = [[10, 2], [6, 8], [4, 16], [0, 0]];
expectedPrevEditPoints.forEach(([line, col]) => {
fetchEditPoint('prev');
testSelection(editor.selection, col, line);
});
return Promise.resolve();
});
});
test('Emmet Select Next/Prev Item in html file', function (): any {
return withRandomFileEditor(htmlContents, '.html', (editor, _) => {
editor.selections = [new Selection(2, 2, 2, 2)];
let expectedNextItemPoints: [number, number, number][] = [
[2, 1, 5], // html
[2, 6, 15], // lang="en"
[2, 12, 14], // en
[3, 1, 5], // head
[4, 2, 6], // meta
[4, 7, 17], // charset=""
[5, 2, 6], // meta
[5, 7, 22], // name="viewport"
[5, 13, 21], // viewport
[5, 23, 70], // content="width=device-width, initial-scale=1.0"
[5, 32, 69], // width=device-width, initial-scale=1.0
[5, 32, 51], // width=device-width,
[5, 52, 69], // initial-scale=1.0
[6, 2, 7] // title
];
expectedNextItemPoints.forEach(([line, colstart, colend]) => {
fetchSelectItem('next');
testSelection(editor.selection, colstart, line, colend);
});
editor.selections = [new Selection(6, 15, 6, 15)];
expectedNextItemPoints.reverse().forEach(([line, colstart, colend]) => {
fetchSelectItem('prev');
testSelection(editor.selection, colstart, line, colend);
});
return Promise.resolve();
});
});
test('Emmet Select Next/Prev item at boundary', function(): any {
return withRandomFileEditor(htmlContents, '.html', (editor, _) => {
editor.selections = [new Selection(4, 1, 4, 1)];
fetchSelectItem('next');
testSelection(editor.selection, 2, 4, 6);
editor.selections = [new Selection(4, 1, 4, 1)];
fetchSelectItem('prev');
testSelection(editor.selection, 1, 3, 5);
return Promise.resolve();
});
});
test('Emmet Next/Prev Item in html template', function (): any {
const templateContents = `
<script type="text/template">
<div class="header">
<ul class="nav main">
</ul>
</div>
</script>
`;
return withRandomFileEditor(templateContents, '.html', (editor, _) => {
editor.selections = [new Selection(2, 2, 2, 2)];
let expectedNextItemPoints: [number, number, number][] = [
[2, 2, 5], // div
[2, 6, 20], // class="header"
[2, 13, 19], // header
[3, 3, 5], // ul
[3, 6, 22], // class="nav main"
[3, 13, 21], // nav main
[3, 13, 16], // nav
[3, 17, 21], // main
];
expectedNextItemPoints.forEach(([line, colstart, colend]) => {
fetchSelectItem('next');
testSelection(editor.selection, colstart, line, colend);
});
editor.selections = [new Selection(4, 1, 4, 1)];
expectedNextItemPoints.reverse().forEach(([line, colstart, colend]) => {
fetchSelectItem('prev');
testSelection(editor.selection, colstart, line, colend);
});
return Promise.resolve();
});
});
test('Emmet Select Next/Prev Item in css file', function (): any {
return withRandomFileEditor(cssContents, '.css', (editor, _) => {
editor.selections = [new Selection(0, 0, 0, 0)];
let expectedNextItemPoints: [number, number, number][] = [
[1, 0, 4], // .boo
[2, 1, 19], // margin: 20px 10px;
[2, 9, 18], // 20px 10px
[2, 9, 13], // 20px
[2, 14, 18], // 10px
[3, 1, 36], // background-image: url('tryme.png');
[3, 19, 35], // url('tryme.png')
[6, 0, 9], // .boo .hoo
[7, 1, 14], // margin: 10px;
[7, 9, 13], // 10px
];
expectedNextItemPoints.forEach(([line, colstart, colend]) => {
fetchSelectItem('next');
testSelection(editor.selection, colstart, line, colend);
});
editor.selections = [new Selection(9, 0, 9, 0)];
expectedNextItemPoints.reverse().forEach(([line, colstart, colend]) => {
fetchSelectItem('prev');
testSelection(editor.selection, colstart, line, colend);
});
return Promise.resolve();
});
});
test('Emmet Select Next/Prev Item in scss file with nested rules', function (): any {
return withRandomFileEditor(scssContents, '.scss', (editor, _) => {
editor.selections = [new Selection(0, 0, 0, 0)];
let expectedNextItemPoints: [number, number, number][] = [
[1, 0, 4], // .boo
[2, 1, 19], // margin: 20px 10px;
[2, 9, 18], // 20px 10px
[2, 9, 13], // 20px
[2, 14, 18], // 10px
[3, 1, 36], // background-image: url('tryme.png');
[3, 19, 35], // url('tryme.png')
[5, 1, 10], // .boo .hoo
[6, 2, 15], // margin: 10px;
[6, 10, 14], // 10px
];
expectedNextItemPoints.forEach(([line, colstart, colend]) => {
fetchSelectItem('next');
testSelection(editor.selection, colstart, line, colend);
});
editor.selections = [new Selection(8, 0, 8, 0)];
expectedNextItemPoints.reverse().forEach(([line, colstart, colend]) => {
fetchSelectItem('prev');
testSelection(editor.selection, colstart, line, colend);
});
return Promise.resolve();
});
});
test('Emmet Balance Out in html file', function (): any {
return withRandomFileEditor(htmlContents, 'html', (editor, _) => {
editor.selections = [new Selection(14, 6, 14, 10)];
let expectedBalanceOutRanges: [number, number, number, number][] = [
[14, 3, 14, 32], // <li class="item1">Item 1</li>
[13, 23, 16, 2], // inner contents of <ul class="nav main">
[13, 2, 16, 7], // outer contents of <ul class="nav main">
[12, 21, 17, 1], // inner contents of <div class="header">
[12, 1, 17, 7], // outer contents of <div class="header">
[8, 6, 18, 0], // inner contents of <body>
[8, 0, 18, 7], // outer contents of <body>
[2, 16, 19, 0], // inner contents of <html>
[2, 0, 19, 7], // outer contents of <html>
];
expectedBalanceOutRanges.forEach(([linestart, colstart, lineend, colend]) => {
balanceOut();
testSelection(editor.selection, colstart, linestart, colend, lineend);
});
editor.selections = [new Selection(12, 7, 12, 7)];
let expectedBalanceInRanges: [number, number, number, number][] = [
[12, 21, 17, 1], // inner contents of <div class="header">
[13, 2, 16, 7], // outer contents of <ul class="nav main">
[13, 23, 16, 2], // inner contents of <ul class="nav main">
[14, 3, 14, 32], // <li class="item1">Item 1</li>
[14, 21, 14, 27] // Item 1
];
expectedBalanceInRanges.forEach(([linestart, colstart, lineend, colend]) => {
balanceIn();
testSelection(editor.selection, colstart, linestart, colend, lineend);
});
return Promise.resolve();
});
});
test('Emmet Balance In using the same stack as Balance out in html file', function (): any {
return withRandomFileEditor(htmlContents, 'html', (editor, _) => {
editor.selections = [new Selection(15, 6, 15, 10)];
let expectedBalanceOutRanges: [number, number, number, number][] = [
[15, 3, 15, 32], // <li class="item1">Item 2</li>
[13, 23, 16, 2], // inner contents of <ul class="nav main">
[13, 2, 16, 7], // outer contents of <ul class="nav main">
[12, 21, 17, 1], // inner contents of <div class="header">
[12, 1, 17, 7], // outer contents of <div class="header">
[8, 6, 18, 0], // inner contents of <body>
[8, 0, 18, 7], // outer contents of <body>
[2, 16, 19, 0], // inner contents of <html>
[2, 0, 19, 7], // outer contents of <html>
];
expectedBalanceOutRanges.forEach(([linestart, colstart, lineend, colend]) => {
balanceOut();
testSelection(editor.selection, colstart, linestart, colend, lineend);
});
expectedBalanceOutRanges.reverse().forEach(([linestart, colstart, lineend, colend]) => {
testSelection(editor.selection, colstart, linestart, colend, lineend);
balanceIn();
});
return Promise.resolve();
});
});
test('Emmet Balance In when selection doesnt span entire node or its inner contents', function (): any {
return withRandomFileEditor(htmlContents, 'html', (editor, _) => {
editor.selection = new Selection(13, 7, 13, 10); // Inside the open tag of <ul class="nav main">
balanceIn();
testSelection(editor.selection, 23, 13, 2, 16); // inner contents of <ul class="nav main">
editor.selection = new Selection(16, 4, 16, 5); // Inside the open close of <ul class="nav main">
balanceIn();
testSelection(editor.selection, 23, 13, 2, 16); // inner contents of <ul class="nav main">
editor.selection = new Selection(13, 7, 14, 2); // Inside the open tag of <ul class="nav main"> and the next line
balanceIn();
testSelection(editor.selection, 23, 13, 2, 16); // inner contents of <ul class="nav main">
return Promise.resolve();
});
});
test('Emmet Balance In/Out in html template', function (): any {
const htmlTemplate = `
<script type="text/html">
<div class="header">
<ul class="nav main">
<li class="item1">Item 1</li>
<li class="item2">Item 2</li>
</ul>
</div>
</script>`;
return withRandomFileEditor(htmlTemplate, 'html', (editor, _) => {
editor.selections = [new Selection(5, 24, 5, 24)];
let expectedBalanceOutRanges: [number, number, number, number][] = [
[5, 20, 5, 26], // <li class="item1">``Item 2''</li>
[5, 2, 5, 31], // ``<li class="item1">Item 2</li>''
[3, 22, 6, 1], // inner contents of ul
[3, 1, 6, 6], // outer contents of ul
[2, 20, 7, 0], // inner contents of div
[2, 0, 7, 6], // outer contents of div
];
expectedBalanceOutRanges.forEach(([linestart, colstart, lineend, colend]) => {
balanceOut();
testSelection(editor.selection, colstart, linestart, colend, lineend);
});
expectedBalanceOutRanges.pop();
expectedBalanceOutRanges.reverse().forEach(([linestart, colstart, lineend, colend]) => {
balanceIn();
testSelection(editor.selection, colstart, linestart, colend, lineend);
});
return Promise.resolve();
});
});
});
function testSelection(selection: Selection, startChar: number, startline: number, endChar?: number, endLine?: number) {
assert.equal(selection.anchor.line, startline);
assert.equal(selection.anchor.character, startChar);
if (!endLine && endLine !== 0) {
assert.equal(selection.isSingleLine, true);
} else {
assert.equal(selection.active.line, endLine);
}
if (!endChar && endChar !== 0) {
assert.equal(selection.isEmpty, true);
} else {
assert.equal(selection.active.character, endChar);
}
} | extensions/emmet/src/test/editPointSelectItemBalance.test.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0002608787326607853,
0.000181754759978503,
0.00016673558275215328,
0.00017577373364474624,
0.000018514960174798034
] |
{
"id": 2,
"code_window": [
"import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';\n",
"import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';\n",
"import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';\n",
"import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';\n",
"import * as editorAPI from './vs/editor/editor.api';\n",
"\n",
"(function () {\n",
"\tvar a: any;\n"
],
"labels": [
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 8
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/css!./standalone-tokens';
import { IDisposable } from 'vs/base/common/lifecycle';
import { URI } from 'vs/base/common/uri';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { ICodeEditorService } from 'vs/editor/browser/services/codeEditorService';
import { OpenerService } from 'vs/editor/browser/services/openerService';
import { DiffNavigator } from 'vs/editor/browser/widget/diffNavigator';
import { ConfigurationChangedEvent } from 'vs/editor/common/config/editorOptions';
import { BareFontInfo, FontInfo } from 'vs/editor/common/config/fontInfo';
import { Token } from 'vs/editor/common/core/token';
import * as editorCommon from 'vs/editor/common/editorCommon';
import { FindMatch, ITextModel, TextModelResolvedOptions } from 'vs/editor/common/model';
import * as modes from 'vs/editor/common/modes';
import { NULL_STATE, nullTokenize } from 'vs/editor/common/modes/nullMode';
import { IEditorWorkerService } from 'vs/editor/common/services/editorWorkerService';
import { ILanguageSelection } from 'vs/editor/common/services/modeService';
import { ITextModelService } from 'vs/editor/common/services/resolverService';
import { IWebWorkerOptions, MonacoWebWorker, createWebWorker as actualCreateWebWorker } from 'vs/editor/common/services/webWorker';
import * as standaloneEnums from 'vs/editor/common/standalone/standaloneEnums';
import { Colorizer, IColorizerElementOptions, IColorizerOptions } from 'vs/editor/standalone/browser/colorizer';
import { SimpleEditorModelResolverService } from 'vs/editor/standalone/browser/simpleServices';
import { IDiffEditorConstructionOptions, IStandaloneEditorConstructionOptions, IStandaloneCodeEditor, IStandaloneDiffEditor, StandaloneDiffEditor, StandaloneEditor } from 'vs/editor/standalone/browser/standaloneCodeEditor';
import { DynamicStandaloneServices, IEditorOverrideServices, StaticServices } from 'vs/editor/standalone/browser/standaloneServices';
import { IStandaloneThemeData, IStandaloneThemeService } from 'vs/editor/standalone/common/standaloneThemeService';
import { ICommandService } from 'vs/platform/commands/common/commands';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { IContextViewService, IContextMenuService } from 'vs/platform/contextview/browser/contextView';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { IMarker, IMarkerData } from 'vs/platform/markers/common/markers';
import { INotificationService } from 'vs/platform/notification/common/notification';
import { IOpenerService } from 'vs/platform/opener/common/opener';
import { IAccessibilityService } from 'vs/platform/accessibility/common/accessibility';
import { clearAllFontInfos } from 'vs/editor/browser/config/configuration';
import { IEditorProgressService } from 'vs/platform/progress/common/progress';
type Omit<T, K extends keyof T> = Pick<T, Exclude<keyof T, K>>;
function withAllStandaloneServices<T extends editorCommon.IEditor>(domElement: HTMLElement, override: IEditorOverrideServices, callback: (services: DynamicStandaloneServices) => T): T {
let services = new DynamicStandaloneServices(domElement, override);
let simpleEditorModelResolverService: SimpleEditorModelResolverService | null = null;
if (!services.has(ITextModelService)) {
simpleEditorModelResolverService = new SimpleEditorModelResolverService();
services.set(ITextModelService, simpleEditorModelResolverService);
}
if (!services.has(IOpenerService)) {
services.set(IOpenerService, new OpenerService(services.get(ICodeEditorService), services.get(ICommandService)));
}
let result = callback(services);
if (simpleEditorModelResolverService) {
simpleEditorModelResolverService.setEditor(result);
}
return result;
}
/**
* Create a new editor under `domElement`.
* `domElement` should be empty (not contain other dom nodes).
* The editor will read the size of `domElement`.
*/
export function create(domElement: HTMLElement, options?: IStandaloneEditorConstructionOptions, override?: IEditorOverrideServices): IStandaloneCodeEditor {
return withAllStandaloneServices(domElement, override || {}, (services) => {
return new StandaloneEditor(
domElement,
options,
services,
services.get(IInstantiationService),
services.get(ICodeEditorService),
services.get(ICommandService),
services.get(IContextKeyService),
services.get(IKeybindingService),
services.get(IContextViewService),
services.get(IStandaloneThemeService),
services.get(INotificationService),
services.get(IConfigurationService),
services.get(IAccessibilityService)
);
});
}
/**
* Emitted when an editor is created.
* Creating a diff editor might cause this listener to be invoked with the two editors.
* @event
*/
export function onDidCreateEditor(listener: (codeEditor: ICodeEditor) => void): IDisposable {
return StaticServices.codeEditorService.get().onCodeEditorAdd((editor) => {
listener(<ICodeEditor>editor);
});
}
/**
* Create a new diff editor under `domElement`.
* `domElement` should be empty (not contain other dom nodes).
* The editor will read the size of `domElement`.
*/
export function createDiffEditor(domElement: HTMLElement, options?: IDiffEditorConstructionOptions, override?: IEditorOverrideServices): IStandaloneDiffEditor {
return withAllStandaloneServices(domElement, override || {}, (services) => {
return new StandaloneDiffEditor(
domElement,
options,
services,
services.get(IInstantiationService),
services.get(IContextKeyService),
services.get(IKeybindingService),
services.get(IContextViewService),
services.get(IEditorWorkerService),
services.get(ICodeEditorService),
services.get(IStandaloneThemeService),
services.get(INotificationService),
services.get(IConfigurationService),
services.get(IContextMenuService),
services.get(IEditorProgressService),
null
);
});
}
export interface IDiffNavigator {
canNavigate(): boolean;
next(): void;
previous(): void;
dispose(): void;
}
export interface IDiffNavigatorOptions {
readonly followsCaret?: boolean;
readonly ignoreCharChanges?: boolean;
readonly alwaysRevealFirst?: boolean;
}
export function createDiffNavigator(diffEditor: IStandaloneDiffEditor, opts?: IDiffNavigatorOptions): IDiffNavigator {
return new DiffNavigator(diffEditor, opts);
}
function doCreateModel(value: string, languageSelection: ILanguageSelection, uri?: URI): ITextModel {
return StaticServices.modelService.get().createModel(value, languageSelection, uri);
}
/**
* Create a new editor model.
* You can specify the language that should be set for this model or let the language be inferred from the `uri`.
*/
export function createModel(value: string, language?: string, uri?: URI): ITextModel {
value = value || '';
if (!language) {
let firstLF = value.indexOf('\n');
let firstLine = value;
if (firstLF !== -1) {
firstLine = value.substring(0, firstLF);
}
return doCreateModel(value, StaticServices.modeService.get().createByFilepathOrFirstLine(uri || null, firstLine), uri);
}
return doCreateModel(value, StaticServices.modeService.get().create(language), uri);
}
/**
* Change the language for a model.
*/
export function setModelLanguage(model: ITextModel, languageId: string): void {
StaticServices.modelService.get().setMode(model, StaticServices.modeService.get().create(languageId));
}
/**
* Set the markers for a model.
*/
export function setModelMarkers(model: ITextModel, owner: string, markers: IMarkerData[]): void {
if (model) {
StaticServices.markerService.get().changeOne(owner, model.uri, markers);
}
}
/**
* Get markers for owner and/or resource
*
* @returns list of markers
*/
export function getModelMarkers(filter: { owner?: string, resource?: URI, take?: number }): IMarker[] {
return StaticServices.markerService.get().read(filter);
}
/**
* Get the model that has `uri` if it exists.
*/
export function getModel(uri: URI): ITextModel | null {
return StaticServices.modelService.get().getModel(uri);
}
/**
* Get all the created models.
*/
export function getModels(): ITextModel[] {
return StaticServices.modelService.get().getModels();
}
/**
* Emitted when a model is created.
* @event
*/
export function onDidCreateModel(listener: (model: ITextModel) => void): IDisposable {
return StaticServices.modelService.get().onModelAdded(listener);
}
/**
* Emitted right before a model is disposed.
* @event
*/
export function onWillDisposeModel(listener: (model: ITextModel) => void): IDisposable {
return StaticServices.modelService.get().onModelRemoved(listener);
}
/**
* Emitted when a different language is set to a model.
* @event
*/
export function onDidChangeModelLanguage(listener: (e: { readonly model: ITextModel; readonly oldLanguage: string; }) => void): IDisposable {
return StaticServices.modelService.get().onModelModeChanged((e) => {
listener({
model: e.model,
oldLanguage: e.oldModeId
});
});
}
/**
* Create a new web worker that has model syncing capabilities built in.
* Specify an AMD module to load that will `create` an object that will be proxied.
*/
export function createWebWorker<T>(opts: IWebWorkerOptions): MonacoWebWorker<T> {
return actualCreateWebWorker<T>(StaticServices.modelService.get(), opts);
}
/**
* Colorize the contents of `domNode` using attribute `data-lang`.
*/
export function colorizeElement(domNode: HTMLElement, options: IColorizerElementOptions): Promise<void> {
return Colorizer.colorizeElement(StaticServices.standaloneThemeService.get(), StaticServices.modeService.get(), domNode, options);
}
/**
* Colorize `text` using language `languageId`.
*/
export function colorize(text: string, languageId: string, options: IColorizerOptions): Promise<string> {
return Colorizer.colorize(StaticServices.modeService.get(), text, languageId, options);
}
/**
* Colorize a line in a model.
*/
export function colorizeModelLine(model: ITextModel, lineNumber: number, tabSize: number = 4): string {
return Colorizer.colorizeModelLine(model, lineNumber, tabSize);
}
/**
* @internal
*/
function getSafeTokenizationSupport(language: string): Omit<modes.ITokenizationSupport, 'tokenize2'> {
let tokenizationSupport = modes.TokenizationRegistry.get(language);
if (tokenizationSupport) {
return tokenizationSupport;
}
return {
getInitialState: () => NULL_STATE,
tokenize: (line: string, state: modes.IState, deltaOffset: number) => nullTokenize(language, line, state, deltaOffset)
};
}
/**
* Tokenize `text` using language `languageId`
*/
export function tokenize(text: string, languageId: string): Token[][] {
let modeService = StaticServices.modeService.get();
// Needed in order to get the mode registered for subsequent look-ups
modeService.triggerMode(languageId);
let tokenizationSupport = getSafeTokenizationSupport(languageId);
let lines = text.split(/\r\n|\r|\n/);
let result: Token[][] = [];
let state = tokenizationSupport.getInitialState();
for (let i = 0, len = lines.length; i < len; i++) {
let line = lines[i];
let tokenizationResult = tokenizationSupport.tokenize(line, state, 0);
result[i] = tokenizationResult.tokens;
state = tokenizationResult.endState;
}
return result;
}
/**
* Define a new theme or update an existing theme.
*/
export function defineTheme(themeName: string, themeData: IStandaloneThemeData): void {
StaticServices.standaloneThemeService.get().defineTheme(themeName, themeData);
}
/**
* Switches to a theme.
*/
export function setTheme(themeName: string): void {
StaticServices.standaloneThemeService.get().setTheme(themeName);
}
/**
* Clears all cached font measurements and triggers re-measurement.
*/
export function remeasureFonts(): void {
clearAllFontInfos();
}
/**
* @internal
*/
export function createMonacoEditorAPI(): typeof monaco.editor {
return {
// methods
create: <any>create,
onDidCreateEditor: <any>onDidCreateEditor,
createDiffEditor: <any>createDiffEditor,
createDiffNavigator: <any>createDiffNavigator,
createModel: <any>createModel,
setModelLanguage: <any>setModelLanguage,
setModelMarkers: <any>setModelMarkers,
getModelMarkers: <any>getModelMarkers,
getModels: <any>getModels,
getModel: <any>getModel,
onDidCreateModel: <any>onDidCreateModel,
onWillDisposeModel: <any>onWillDisposeModel,
onDidChangeModelLanguage: <any>onDidChangeModelLanguage,
createWebWorker: <any>createWebWorker,
colorizeElement: <any>colorizeElement,
colorize: <any>colorize,
colorizeModelLine: <any>colorizeModelLine,
tokenize: <any>tokenize,
defineTheme: <any>defineTheme,
setTheme: <any>setTheme,
remeasureFonts: remeasureFonts,
// enums
AccessibilitySupport: standaloneEnums.AccessibilitySupport,
ScrollbarVisibility: standaloneEnums.ScrollbarVisibility,
WrappingIndent: standaloneEnums.WrappingIndent,
OverviewRulerLane: standaloneEnums.OverviewRulerLane,
MinimapPosition: standaloneEnums.MinimapPosition,
EndOfLinePreference: standaloneEnums.EndOfLinePreference,
DefaultEndOfLine: standaloneEnums.DefaultEndOfLine,
EndOfLineSequence: standaloneEnums.EndOfLineSequence,
TrackedRangeStickiness: standaloneEnums.TrackedRangeStickiness,
CursorChangeReason: standaloneEnums.CursorChangeReason,
MouseTargetType: standaloneEnums.MouseTargetType,
TextEditorCursorStyle: standaloneEnums.TextEditorCursorStyle,
TextEditorCursorBlinkingStyle: standaloneEnums.TextEditorCursorBlinkingStyle,
ContentWidgetPositionPreference: standaloneEnums.ContentWidgetPositionPreference,
OverlayWidgetPositionPreference: standaloneEnums.OverlayWidgetPositionPreference,
RenderMinimap: standaloneEnums.RenderMinimap,
ScrollType: standaloneEnums.ScrollType,
RenderLineNumbersType: standaloneEnums.RenderLineNumbersType,
// classes
ConfigurationChangedEvent: <any>ConfigurationChangedEvent,
BareFontInfo: <any>BareFontInfo,
FontInfo: <any>FontInfo,
TextModelResolvedOptions: <any>TextModelResolvedOptions,
FindMatch: <any>FindMatch,
// vars
EditorType: editorCommon.EditorType,
};
}
| src/vs/editor/standalone/browser/standaloneEditor.ts | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.001388426753692329,
0.000220599613385275,
0.00015990216343197972,
0.0001733134558890015,
0.00019865093054249883
] |
{
"id": 2,
"code_window": [
"import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';\n",
"import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';\n",
"import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';\n",
"import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';\n",
"import * as editorAPI from './vs/editor/editor.api';\n",
"\n",
"(function () {\n",
"\tvar a: any;\n"
],
"labels": [
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 8
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { window, tasks, Disposable, TaskDefinition, Task, EventEmitter, CustomExecution, Pseudoterminal, TaskScope, commands, Task2 } from 'vscode';
suite('workspace-namespace', () => {
suite('Tasks', () => {
let disposables: Disposable[] = [];
teardown(() => {
disposables.forEach(d => d.dispose());
disposables.length = 0;
});
test('CustomExecution task should start and shutdown successfully', (done) => {
interface CustomTestingTaskDefinition extends TaskDefinition {
/**
* One of the task properties. This can be used to customize the task in the tasks.json
*/
customProp1: string;
}
const taskType: string = 'customTesting';
const taskName = 'First custom task';
let isPseudoterminalClosed = false;
disposables.push(window.onDidOpenTerminal(term => {
disposables.push(window.onDidWriteTerminalData(e => {
try {
assert.equal(e.data, 'testing\r\n');
} catch (e) {
done(e);
}
disposables.push(window.onDidCloseTerminal(() => {
try {
// Pseudoterminal.close should have fired by now, additionally we want
// to make sure all events are flushed before continuing with more tests
assert.ok(isPseudoterminalClosed);
} catch (e) {
done(e);
return;
}
done();
}));
term.dispose();
}));
}));
disposables.push(tasks.registerTaskProvider(taskType, {
provideTasks: () => {
const result: Task[] = [];
const kind: CustomTestingTaskDefinition = {
type: taskType,
customProp1: 'testing task one'
};
const writeEmitter = new EventEmitter<string>();
const execution = new CustomExecution((): Thenable<Pseudoterminal> => {
const pty: Pseudoterminal = {
onDidWrite: writeEmitter.event,
open: () => writeEmitter.fire('testing\r\n'),
close: () => isPseudoterminalClosed = true
};
return Promise.resolve(pty);
});
const task = new Task2(kind, TaskScope.Workspace, taskName, taskType, execution);
result.push(task);
return result;
},
resolveTask(_task: Task): Task | undefined {
try {
assert.fail('resolveTask should not trigger during the test');
} catch (e) {
done(e);
}
return undefined;
}
}));
commands.executeCommand('workbench.action.tasks.runTask', `${taskType}: ${taskName}`);
});
});
});
| extensions/vscode-api-tests/src/singlefolder-tests/workspace.tasks.test.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017593972734175622,
0.0001739081781124696,
0.0001720641739666462,
0.00017433741595596075,
0.0000012099447985747247
] |
{
"id": 2,
"code_window": [
"import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';\n",
"import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';\n",
"import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';\n",
"import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';\n",
"import * as editorAPI from './vs/editor/editor.api';\n",
"\n",
"(function () {\n",
"\tvar a: any;\n"
],
"labels": [
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 8
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { KeyChord, KeyCode, KeyMod, SimpleKeybinding, createKeybinding } from 'vs/base/common/keyCodes';
import { OperatingSystem } from 'vs/base/common/platform';
import { ScanCode, ScanCodeBinding } from 'vs/base/common/scanCode';
import { IWindowsKeyboardMapping, WindowsKeyboardMapper } from 'vs/workbench/services/keybinding/common/windowsKeyboardMapper';
import { IResolvedKeybinding, assertMapping, assertResolveKeybinding, assertResolveKeyboardEvent, assertResolveUserBinding, readRawMapping } from 'vs/workbench/services/keybinding/test/keyboardMapperTestUtils';
const WRITE_FILE_IF_DIFFERENT = false;
async function createKeyboardMapper(isUSStandard: boolean, file: string): Promise<WindowsKeyboardMapper> {
const rawMappings = await readRawMapping<IWindowsKeyboardMapping>(file);
return new WindowsKeyboardMapper(isUSStandard, rawMappings);
}
function _assertResolveKeybinding(mapper: WindowsKeyboardMapper, k: number, expected: IResolvedKeybinding[]): void {
const keyBinding = createKeybinding(k, OperatingSystem.Windows);
assertResolveKeybinding(mapper, keyBinding!, expected);
}
suite('keyboardMapper - WINDOWS de_ch', () => {
let mapper: WindowsKeyboardMapper;
suiteSetup(async () => {
mapper = await createKeyboardMapper(false, 'win_de_ch');
});
test('mapping', () => {
return assertMapping(WRITE_FILE_IF_DIFFERENT, mapper, 'win_de_ch.txt');
});
test('resolveKeybinding Ctrl+A', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.KEY_A,
[{
label: 'Ctrl+A',
ariaLabel: 'Control+A',
electronAccelerator: 'Ctrl+A',
userSettingsLabel: 'ctrl+a',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+A'],
}]
);
});
test('resolveKeybinding Ctrl+Z', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.KEY_Z,
[{
label: 'Ctrl+Z',
ariaLabel: 'Control+Z',
electronAccelerator: 'Ctrl+Z',
userSettingsLabel: 'ctrl+z',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+Z'],
}]
);
});
test('resolveKeyboardEvent Ctrl+Z', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.KEY_Z,
code: null!
},
{
label: 'Ctrl+Z',
ariaLabel: 'Control+Z',
electronAccelerator: 'Ctrl+Z',
userSettingsLabel: 'ctrl+z',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+Z'],
}
);
});
test('resolveKeybinding Ctrl+]', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.US_CLOSE_SQUARE_BRACKET,
[{
label: 'Ctrl+^',
ariaLabel: 'Control+^',
electronAccelerator: 'Ctrl+]',
userSettingsLabel: 'ctrl+oem_6',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['ctrl+]'],
}]
);
});
test('resolveKeyboardEvent Ctrl+]', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.US_CLOSE_SQUARE_BRACKET,
code: null!
},
{
label: 'Ctrl+^',
ariaLabel: 'Control+^',
electronAccelerator: 'Ctrl+]',
userSettingsLabel: 'ctrl+oem_6',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['ctrl+]'],
}
);
});
test('resolveKeybinding Shift+]', () => {
_assertResolveKeybinding(
mapper,
KeyMod.Shift | KeyCode.US_CLOSE_SQUARE_BRACKET,
[{
label: 'Shift+^',
ariaLabel: 'Shift+^',
electronAccelerator: 'Shift+]',
userSettingsLabel: 'shift+oem_6',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['shift+]'],
}]
);
});
test('resolveKeybinding Ctrl+/', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.US_SLASH,
[{
label: 'Ctrl+§',
ariaLabel: 'Control+§',
electronAccelerator: 'Ctrl+/',
userSettingsLabel: 'ctrl+oem_2',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['ctrl+/'],
}]
);
});
test('resolveKeybinding Ctrl+Shift+/', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.US_SLASH,
[{
label: 'Ctrl+Shift+§',
ariaLabel: 'Control+Shift+§',
electronAccelerator: 'Ctrl+Shift+/',
userSettingsLabel: 'ctrl+shift+oem_2',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['ctrl+shift+/'],
}]
);
});
test('resolveKeybinding Ctrl+K Ctrl+\\', () => {
_assertResolveKeybinding(
mapper,
KeyChord(KeyMod.CtrlCmd | KeyCode.KEY_K, KeyMod.CtrlCmd | KeyCode.US_BACKSLASH),
[{
label: 'Ctrl+K Ctrl+ä',
ariaLabel: 'Control+K Control+ä',
electronAccelerator: null,
userSettingsLabel: 'ctrl+k ctrl+oem_5',
isWYSIWYG: false,
isChord: true,
dispatchParts: ['ctrl+K', 'ctrl+\\'],
}]
);
});
test('resolveKeybinding Ctrl+K Ctrl+=', () => {
_assertResolveKeybinding(
mapper,
KeyChord(KeyMod.CtrlCmd | KeyCode.KEY_K, KeyMod.CtrlCmd | KeyCode.US_EQUAL),
[]
);
});
test('resolveKeybinding Ctrl+DownArrow', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.DownArrow,
[{
label: 'Ctrl+DownArrow',
ariaLabel: 'Control+DownArrow',
electronAccelerator: 'Ctrl+Down',
userSettingsLabel: 'ctrl+down',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+DownArrow'],
}]
);
});
test('resolveKeybinding Ctrl+NUMPAD_0', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.NUMPAD_0,
[{
label: 'Ctrl+NumPad0',
ariaLabel: 'Control+NumPad0',
electronAccelerator: null,
userSettingsLabel: 'ctrl+numpad0',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+NumPad0'],
}]
);
});
test('resolveKeybinding Ctrl+Home', () => {
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.Home,
[{
label: 'Ctrl+Home',
ariaLabel: 'Control+Home',
electronAccelerator: 'Ctrl+Home',
userSettingsLabel: 'ctrl+home',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+Home'],
}]
);
});
test('resolveKeyboardEvent Ctrl+Home', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.Home,
code: null!
},
{
label: 'Ctrl+Home',
ariaLabel: 'Control+Home',
electronAccelerator: 'Ctrl+Home',
userSettingsLabel: 'ctrl+home',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+Home'],
}
);
});
test('resolveUserBinding empty', () => {
assertResolveUserBinding(mapper, [], []);
});
test('resolveUserBinding Ctrl+[Comma] Ctrl+/', () => {
assertResolveUserBinding(
mapper, [
new ScanCodeBinding(true, false, false, false, ScanCode.Comma),
new SimpleKeybinding(true, false, false, false, KeyCode.US_SLASH),
],
[{
label: 'Ctrl+, Ctrl+§',
ariaLabel: 'Control+, Control+§',
electronAccelerator: null,
userSettingsLabel: 'ctrl+oem_comma ctrl+oem_2',
isWYSIWYG: false,
isChord: true,
dispatchParts: ['ctrl+,', 'ctrl+/'],
}]
);
});
test('resolveKeyboardEvent Modifier only Ctrl+', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.Ctrl,
code: null!
},
{
label: 'Ctrl+',
ariaLabel: 'Control+',
electronAccelerator: null,
userSettingsLabel: 'ctrl+',
isWYSIWYG: true,
isChord: false,
dispatchParts: [null],
}
);
});
});
suite('keyboardMapper - WINDOWS en_us', () => {
let mapper: WindowsKeyboardMapper;
suiteSetup(async () => {
mapper = await createKeyboardMapper(true, 'win_en_us');
});
test('mapping', () => {
return assertMapping(WRITE_FILE_IF_DIFFERENT, mapper, 'win_en_us.txt');
});
test('resolveKeybinding Ctrl+K Ctrl+\\', () => {
_assertResolveKeybinding(
mapper,
KeyChord(KeyMod.CtrlCmd | KeyCode.KEY_K, KeyMod.CtrlCmd | KeyCode.US_BACKSLASH),
[{
label: 'Ctrl+K Ctrl+\\',
ariaLabel: 'Control+K Control+\\',
electronAccelerator: null,
userSettingsLabel: 'ctrl+k ctrl+\\',
isWYSIWYG: true,
isChord: true,
dispatchParts: ['ctrl+K', 'ctrl+\\'],
}]
);
});
test('resolveUserBinding Ctrl+[Comma] Ctrl+/', () => {
assertResolveUserBinding(
mapper, [
new ScanCodeBinding(true, false, false, false, ScanCode.Comma),
new SimpleKeybinding(true, false, false, false, KeyCode.US_SLASH),
],
[{
label: 'Ctrl+, Ctrl+/',
ariaLabel: 'Control+, Control+/',
electronAccelerator: null,
userSettingsLabel: 'ctrl+, ctrl+/',
isWYSIWYG: true,
isChord: true,
dispatchParts: ['ctrl+,', 'ctrl+/'],
}]
);
});
test('resolveUserBinding Ctrl+[Comma]', () => {
assertResolveUserBinding(
mapper, [
new ScanCodeBinding(true, false, false, false, ScanCode.Comma),
],
[{
label: 'Ctrl+,',
ariaLabel: 'Control+,',
electronAccelerator: 'Ctrl+,',
userSettingsLabel: 'ctrl+,',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+,'],
}]
);
});
test('resolveKeyboardEvent Modifier only Ctrl+', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.Ctrl,
code: null!
},
{
label: 'Ctrl+',
ariaLabel: 'Control+',
electronAccelerator: null,
userSettingsLabel: 'ctrl+',
isWYSIWYG: true,
isChord: false,
dispatchParts: [null],
}
);
});
});
suite('keyboardMapper - WINDOWS por_ptb', () => {
let mapper: WindowsKeyboardMapper;
suiteSetup(async () => {
mapper = await createKeyboardMapper(false, 'win_por_ptb');
});
test('mapping', () => {
return assertMapping(WRITE_FILE_IF_DIFFERENT, mapper, 'win_por_ptb.txt');
});
test('resolveKeyboardEvent Ctrl+[IntlRo]', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.ABNT_C1,
code: null!
},
{
label: 'Ctrl+/',
ariaLabel: 'Control+/',
electronAccelerator: 'Ctrl+ABNT_C1',
userSettingsLabel: 'ctrl+abnt_c1',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['ctrl+ABNT_C1'],
}
);
});
test('resolveKeyboardEvent Ctrl+[NumpadComma]', () => {
assertResolveKeyboardEvent(
mapper,
{
_standardKeyboardEventBrand: true,
ctrlKey: true,
shiftKey: false,
altKey: false,
metaKey: false,
keyCode: KeyCode.ABNT_C2,
code: null!
},
{
label: 'Ctrl+.',
ariaLabel: 'Control+.',
electronAccelerator: 'Ctrl+ABNT_C2',
userSettingsLabel: 'ctrl+abnt_c2',
isWYSIWYG: false,
isChord: false,
dispatchParts: ['ctrl+ABNT_C2'],
}
);
});
});
suite('keyboardMapper - WINDOWS ru', () => {
let mapper: WindowsKeyboardMapper;
suiteSetup(async () => {
mapper = await createKeyboardMapper(false, 'win_ru');
});
test('mapping', () => {
return assertMapping(WRITE_FILE_IF_DIFFERENT, mapper, 'win_ru.txt');
});
test('issue ##24361: resolveKeybinding Ctrl+K Ctrl+K', () => {
_assertResolveKeybinding(
mapper,
KeyChord(KeyMod.CtrlCmd | KeyCode.KEY_K, KeyMod.CtrlCmd | KeyCode.KEY_K),
[{
label: 'Ctrl+K Ctrl+K',
ariaLabel: 'Control+K Control+K',
electronAccelerator: null,
userSettingsLabel: 'ctrl+k ctrl+k',
isWYSIWYG: true,
isChord: true,
dispatchParts: ['ctrl+K', 'ctrl+K'],
}]
);
});
});
suite('keyboardMapper - misc', () => {
test('issue #23513: Toggle Sidebar Visibility and Go to Line display same key mapping in Arabic keyboard', () => {
const mapper = new WindowsKeyboardMapper(false, {
'KeyB': {
'vkey': 'VK_B',
'value': 'لا',
'withShift': 'لآ',
'withAltGr': '',
'withShiftAltGr': ''
},
'KeyG': {
'vkey': 'VK_G',
'value': 'ل',
'withShift': 'لأ',
'withAltGr': '',
'withShiftAltGr': ''
}
});
_assertResolveKeybinding(
mapper,
KeyMod.CtrlCmd | KeyCode.KEY_B,
[{
label: 'Ctrl+B',
ariaLabel: 'Control+B',
electronAccelerator: 'Ctrl+B',
userSettingsLabel: 'ctrl+b',
isWYSIWYG: true,
isChord: false,
dispatchParts: ['ctrl+B'],
}]
);
});
});
| src/vs/workbench/services/keybinding/test/windowsKeyboardMapper.test.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017626953194849193,
0.00017287494847550988,
0.00016740673163440078,
0.00017309273243881762,
0.0000016071818436103058
] |
{
"id": 2,
"code_window": [
"import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';\n",
"import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';\n",
"import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';\n",
"import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';\n",
"import * as editorAPI from './vs/editor/editor.api';\n",
"\n",
"(function () {\n",
"\tvar a: any;\n"
],
"labels": [
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 8
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import Severity from 'vs/base/common/severity';
import * as vscode from 'vscode';
import { MainContext, MainThreadMessageServiceShape, MainThreadMessageOptions, IMainContext } from './extHost.protocol';
import { IExtensionDescription } from 'vs/platform/extensions/common/extensions';
import { ILogService } from 'vs/platform/log/common/log';
function isMessageItem(item: any): item is vscode.MessageItem {
return item && item.title;
}
export class ExtHostMessageService {
private _proxy: MainThreadMessageServiceShape;
constructor(
mainContext: IMainContext,
@ILogService private readonly _logService: ILogService
) {
this._proxy = mainContext.getProxy(MainContext.MainThreadMessageService);
}
showMessage(extension: IExtensionDescription, severity: Severity, message: string, optionsOrFirstItem: vscode.MessageOptions | string, rest: string[]): Promise<string | undefined>;
showMessage(extension: IExtensionDescription, severity: Severity, message: string, optionsOrFirstItem: vscode.MessageOptions | vscode.MessageItem, rest: vscode.MessageItem[]): Promise<vscode.MessageItem | undefined>;
showMessage(extension: IExtensionDescription, severity: Severity, message: string, optionsOrFirstItem: vscode.MessageOptions | vscode.MessageItem | string, rest: Array<vscode.MessageItem | string>): Promise<string | vscode.MessageItem | undefined>;
showMessage(extension: IExtensionDescription, severity: Severity, message: string, optionsOrFirstItem: vscode.MessageOptions | string | vscode.MessageItem, rest: Array<string | vscode.MessageItem>): Promise<string | vscode.MessageItem | undefined> {
const options: MainThreadMessageOptions = { extension };
let items: (string | vscode.MessageItem)[];
if (typeof optionsOrFirstItem === 'string' || isMessageItem(optionsOrFirstItem)) {
items = [optionsOrFirstItem, ...rest];
} else {
options.modal = optionsOrFirstItem && optionsOrFirstItem.modal;
items = rest;
}
const commands: { title: string; isCloseAffordance: boolean; handle: number; }[] = [];
for (let handle = 0; handle < items.length; handle++) {
const command = items[handle];
if (typeof command === 'string') {
commands.push({ title: command, handle, isCloseAffordance: false });
} else if (typeof command === 'object') {
let { title, isCloseAffordance } = command;
commands.push({ title, isCloseAffordance: !!isCloseAffordance, handle });
} else {
this._logService.warn('Invalid message item:', command);
}
}
return this._proxy.$showMessage(severity, message, options, commands).then(handle => {
if (typeof handle === 'number') {
return items[handle];
}
return undefined;
});
}
}
| src/vs/workbench/api/common/extHostMessageService.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0001759630540618673,
0.00017389595450367779,
0.00016994513862300664,
0.00017463874246459454,
0.000002152195747839869
] |
{
"id": 3,
"code_window": [
"\n",
"(function () {\n",
"\tvar a: any;\n",
"\tvar b: any;\n",
"\ta = (<DiffNavigator>b).previous; // IDiffNavigator\n",
"\ta = (<ServiceIdentifier<any>>b).type;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 15
} |
// This file is adding references to various symbols which should not be removed via tree shaking
import { ServiceIdentifier } from './vs/platform/instantiation/common/instantiation';
import { IHighlight } from './vs/base/parts/quickopen/browser/quickOpenModel';
import { SimpleWorkerClient, create as create1 } from './vs/base/common/worker/simpleWorker';
import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';
import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';
import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';
import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';
import * as editorAPI from './vs/editor/editor.api';
(function () {
var a: any;
var b: any;
a = (<DiffNavigator>b).previous; // IDiffNavigator
a = (<ServiceIdentifier<any>>b).type;
a = (<IHighlight>b).start;
a = (<IHighlight>b).end;
a = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient
a = create1;
a = create2;
a = (<DocumentRangeFormattingEditProvider>b).extensionId;
// injection madness
a = (<SyncDescriptor0<any>>b).ctor;
a = (<SyncDescriptor0<any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor2<any, any, any>>b).ctor;
a = (<SyncDescriptor2<any, any, any>>b).bind;
a = (<SyncDescriptor3<any, any, any, any>>b).ctor;
a = (<SyncDescriptor3<any, any, any, any>>b).bind;
a = (<SyncDescriptor4<any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor4<any, any, any, any, any>>b).bind;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).bind;
// exported API
a = editorAPI.CancellationTokenSource;
a = editorAPI.Emitter;
a = editorAPI.KeyCode;
a = editorAPI.KeyMod;
a = editorAPI.Position;
a = editorAPI.Range;
a = editorAPI.Selection;
a = editorAPI.SelectionDirection;
a = editorAPI.MarkerSeverity;
a = editorAPI.MarkerTag;
a = editorAPI.Uri;
a = editorAPI.Token;
a = editorAPI.editor;
a = editorAPI.languages;
})();
| build/monaco/monaco.usage.recipe | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.9977574944496155,
0.5682757496833801,
0.00031874774140305817,
0.9880863428115845,
0.4898836016654968
] |
{
"id": 3,
"code_window": [
"\n",
"(function () {\n",
"\tvar a: any;\n",
"\tvar b: any;\n",
"\ta = (<DiffNavigator>b).previous; // IDiffNavigator\n",
"\ta = (<ServiceIdentifier<any>>b).type;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 15
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { GestureEvent } from 'vs/base/browser/touch';
import { IKeyboardEvent } from 'vs/base/browser/keyboardEvent';
import { IDragAndDropData } from 'vs/base/browser/dnd';
export interface IListVirtualDelegate<T> {
getHeight(element: T): number;
getTemplateId(element: T): string;
hasDynamicHeight?(element: T): boolean;
setDynamicHeight?(element: T, height: number): void;
}
export interface IListRenderer<T, TTemplateData> {
templateId: string;
renderTemplate(container: HTMLElement): TTemplateData;
renderElement(element: T, index: number, templateData: TTemplateData, height: number | undefined): void;
disposeElement?(element: T, index: number, templateData: TTemplateData, height: number | undefined): void;
disposeTemplate(templateData: TTemplateData): void;
}
export interface IListEvent<T> {
elements: T[];
indexes: number[];
browserEvent?: UIEvent;
}
export interface IListMouseEvent<T> {
browserEvent: MouseEvent;
element: T | undefined;
index: number | undefined;
}
export interface IListTouchEvent<T> {
browserEvent: TouchEvent;
element: T | undefined;
index: number | undefined;
}
export interface IListGestureEvent<T> {
browserEvent: GestureEvent;
element: T | undefined;
index: number | undefined;
}
export interface IListDragEvent<T> {
browserEvent: DragEvent;
element: T | undefined;
index: number | undefined;
}
export interface IListContextMenuEvent<T> {
browserEvent: UIEvent;
element: T | undefined;
index: number | undefined;
anchor: HTMLElement | { x: number; y: number; };
}
export interface IIdentityProvider<T> {
getId(element: T): { toString(): string; };
}
export enum ListAriaRootRole {
/** default tree structure role */
TREE = 'tree',
/** role='tree' can interfere with screenreaders reading nested elements inside the tree row. Use FORM in that case. */
FORM = 'form'
}
export interface IKeyboardNavigationLabelProvider<T> {
/**
* Return a keyboard navigation label which will be used by the
* list for filtering/navigating. Return `undefined` to make an
* element always match.
*/
getKeyboardNavigationLabel(element: T): { toString(): string | undefined; } | undefined;
}
export interface IKeyboardNavigationDelegate {
mightProducePrintableCharacter(event: IKeyboardEvent): boolean;
}
export const enum ListDragOverEffect {
Copy,
Move
}
export interface IListDragOverReaction {
accept: boolean;
effect?: ListDragOverEffect;
feedback?: number[]; // use -1 for entire list
}
export const ListDragOverReactions = {
reject(): IListDragOverReaction { return { accept: false }; },
accept(): IListDragOverReaction { return { accept: true }; },
};
export interface IListDragAndDrop<T> {
getDragURI(element: T): string | null;
getDragLabel?(elements: T[]): string | undefined;
onDragStart?(data: IDragAndDropData, originalEvent: DragEvent): void;
onDragOver(data: IDragAndDropData, targetElement: T | undefined, targetIndex: number | undefined, originalEvent: DragEvent): boolean | IListDragOverReaction;
drop(data: IDragAndDropData, targetElement: T | undefined, targetIndex: number | undefined, originalEvent: DragEvent): void;
}
export class ListError extends Error {
constructor(user: string, message: string) {
super(`ListError [${user}] ${message}`);
}
}
export abstract class CachedListVirtualDelegate<T extends object> implements IListVirtualDelegate<T> {
private cache = new WeakMap<T, number>();
getHeight(element: T): number {
return this.cache.get(element) ?? this.estimateHeight(element);
}
protected abstract estimateHeight(element: T): number;
abstract getTemplateId(element: T): string;
setDynamicHeight(element: T, height: number): void {
this.cache.set(element, height);
}
}
| src/vs/base/browser/ui/list/list.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00038241673610173166,
0.00018990456010214984,
0.00016841154138091952,
0.00017444556578993797,
0.000053753519750898704
] |
{
"id": 3,
"code_window": [
"\n",
"(function () {\n",
"\tvar a: any;\n",
"\tvar b: any;\n",
"\ta = (<DiffNavigator>b).previous; // IDiffNavigator\n",
"\ta = (<ServiceIdentifier<any>>b).type;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 15
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { ProxyIdentifier, IRPCProtocol } from 'vs/workbench/services/extensions/common/proxyIdentifier';
import { createDecorator } from 'vs/platform/instantiation/common/instantiation';
export const IExtHostRpcService = createDecorator<IExtHostRpcService>('IExtHostRpcService');
export interface IExtHostRpcService extends IRPCProtocol {
_serviceBrand: undefined;
}
export class ExtHostRpcService implements IExtHostRpcService {
readonly _serviceBrand: undefined;
readonly getProxy: <T>(identifier: ProxyIdentifier<T>) => T;
readonly set: <T, R extends T> (identifier: ProxyIdentifier<T>, instance: R) => R;
readonly assertRegistered: (identifiers: ProxyIdentifier<any>[]) => void;
constructor(rpcProtocol: IRPCProtocol) {
this.getProxy = rpcProtocol.getProxy.bind(rpcProtocol);
this.set = rpcProtocol.set.bind(rpcProtocol);
this.assertRegistered = rpcProtocol.assertRegistered.bind(rpcProtocol);
}
}
| src/vs/workbench/api/common/extHostRpcService.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0006572363199666142,
0.00033219988108612597,
0.00016407403745688498,
0.00017528937314637005,
0.00022988105774857104
] |
{
"id": 3,
"code_window": [
"\n",
"(function () {\n",
"\tvar a: any;\n",
"\tvar b: any;\n",
"\ta = (<DiffNavigator>b).previous; // IDiffNavigator\n",
"\ta = (<ServiceIdentifier<any>>b).type;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 15
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { KeyboardLayoutContribution } from 'vs/workbench/services/keybinding/browser/keyboardLayouts/_.contribution';
KeyboardLayoutContribution.INSTANCE.registerKeyboardLayout({
layout: { model: 'pc105', layout: 'us', variant: '', options: '', rules: 'evdev', isUSStandard: true },
secondaryLayouts: [
{ model: 'pc105', layout: 'cn', variant: '', options: '', rules: 'evdev' },
],
mapping: {
Sleep: [],
WakeUp: [],
KeyA: ['a', 'A', 'a', 'A', 0],
KeyB: ['b', 'B', 'b', 'B', 0],
KeyC: ['c', 'C', 'c', 'C', 0],
KeyD: ['d', 'D', 'd', 'D', 0],
KeyE: ['e', 'E', 'e', 'E', 0],
KeyF: ['f', 'F', 'f', 'F', 0],
KeyG: ['g', 'G', 'g', 'G', 0],
KeyH: ['h', 'H', 'h', 'H', 0],
KeyI: ['i', 'I', 'i', 'I', 0],
KeyJ: ['j', 'J', 'j', 'J', 0],
KeyK: ['k', 'K', 'k', 'K', 0],
KeyL: ['l', 'L', 'l', 'L', 0],
KeyM: ['m', 'M', 'm', 'M', 0],
KeyN: ['n', 'N', 'n', 'N', 0],
KeyO: ['o', 'O', 'o', 'O', 0],
KeyP: ['p', 'P', 'p', 'P', 0],
KeyQ: ['q', 'Q', 'q', 'Q', 0],
KeyR: ['r', 'R', 'r', 'R', 0],
KeyS: ['s', 'S', 's', 'S', 0],
KeyT: ['t', 'T', 't', 'T', 0],
KeyU: ['u', 'U', 'u', 'U', 0],
KeyV: ['v', 'V', 'v', 'V', 0],
KeyW: ['w', 'W', 'w', 'W', 0],
KeyX: ['x', 'X', 'x', 'X', 0],
KeyY: ['y', 'Y', 'y', 'Y', 0],
KeyZ: ['z', 'Z', 'z', 'Z', 0],
Digit1: ['1', '!', '1', '!', 0],
Digit2: ['2', '@', '2', '@', 0],
Digit3: ['3', '#', '3', '#', 0],
Digit4: ['4', '$', '4', '$', 0],
Digit5: ['5', '%', '5', '%', 0],
Digit6: ['6', '^', '6', '^', 0],
Digit7: ['7', '&', '7', '&', 0],
Digit8: ['8', '*', '8', '*', 0],
Digit9: ['9', '(', '9', '(', 0],
Digit0: ['0', ')', '0', ')', 0],
Enter: ['\r', '\r', '\r', '\r', 0],
Escape: ['\u001b', '\u001b', '\u001b', '\u001b', 0],
Backspace: ['\b', '\b', '\b', '\b', 0],
Tab: ['\t', '', '\t', '', 0],
Space: [' ', ' ', ' ', ' ', 0],
Minus: ['-', '_', '-', '_', 0],
Equal: ['=', '+', '=', '+', 0],
BracketLeft: ['[', '{', '[', '{', 0],
BracketRight: [']', '}', ']', '}', 0],
Backslash: ['\\', '|', '\\', '|', 0],
Semicolon: [';', ':', ';', ':', 0],
Quote: ['\'', '"', '\'', '"', 0],
Backquote: ['`', '~', '`', '~', 0],
Comma: [',', '<', ',', '<', 0],
Period: ['.', '>', '.', '>', 0],
Slash: ['/', '?', '/', '?', 0],
CapsLock: [],
F1: [],
F2: [],
F3: [],
F4: [],
F5: [],
F6: [],
F7: [],
F8: [],
F9: [],
F10: [],
F11: [],
F12: [],
PrintScreen: [],
ScrollLock: [],
Pause: [],
Insert: [],
Home: [],
PageUp: [],
Delete: ['', '', '', '', 0],
End: [],
PageDown: [],
ArrowRight: [],
ArrowLeft: [],
ArrowDown: [],
ArrowUp: [],
NumLock: [],
NumpadDivide: ['/', '/', '/', '/', 0],
NumpadMultiply: ['*', '*', '*', '*', 0],
NumpadSubtract: ['-', '-', '-', '-', 0],
NumpadAdd: ['+', '+', '+', '+', 0],
NumpadEnter: ['\r', '\r', '\r', '\r', 0],
Numpad1: ['', '1', '', '1', 0],
Numpad2: ['', '2', '', '2', 0],
Numpad3: ['', '3', '', '3', 0],
Numpad4: ['', '4', '', '4', 0],
Numpad5: ['', '5', '', '5', 0],
Numpad6: ['', '6', '', '6', 0],
Numpad7: ['', '7', '', '7', 0],
Numpad8: ['', '8', '', '8', 0],
Numpad9: ['', '9', '', '9', 0],
Numpad0: ['', '0', '', '0', 0],
NumpadDecimal: ['', '.', '', '.', 0],
IntlBackslash: ['<', '>', '|', '¦', 0],
ContextMenu: [],
Power: [],
NumpadEqual: ['=', '=', '=', '=', 0],
F13: [],
F14: [],
F15: [],
F16: [],
F17: [],
F18: [],
F19: [],
F20: [],
F21: [],
F22: [],
F23: [],
F24: [],
Open: [],
Help: [],
Select: [],
Again: [],
Undo: [],
Cut: [],
Copy: [],
Paste: [],
Find: [],
AudioVolumeMute: [],
AudioVolumeUp: [],
AudioVolumeDown: [],
NumpadComma: ['.', '.', '.', '.', 0],
IntlRo: [],
KanaMode: [],
IntlYen: [],
Convert: [],
NonConvert: [],
Lang1: [],
Lang2: [],
Lang3: [],
Lang4: [],
Lang5: [],
NumpadParenLeft: ['(', '(', '(', '(', 0],
NumpadParenRight: [')', ')', ')', ')', 0],
ControlLeft: [],
ShiftLeft: [],
AltLeft: [],
MetaLeft: [],
ControlRight: [],
ShiftRight: [],
AltRight: [],
MetaRight: [],
BrightnessUp: [],
BrightnessDown: [],
MediaPlay: [],
MediaRecord: [],
MediaFastForward: [],
MediaRewind: [],
MediaTrackNext: [],
MediaTrackPrevious: [],
MediaStop: [],
Eject: [],
MediaPlayPause: [],
MediaSelect: [],
LaunchMail: [],
LaunchApp2: [],
LaunchApp1: [],
SelectTask: [],
LaunchScreenSaver: [],
BrowserSearch: [],
BrowserHome: [],
BrowserBack: [],
BrowserForward: [],
BrowserStop: [],
BrowserRefresh: [],
BrowserFavorites: [],
MailReply: [],
MailForward: [],
MailSend: []
}
});
| src/vs/workbench/services/keybinding/browser/keyboardLayouts/en.linux.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00018003760487772524,
0.0001725379697745666,
0.0001664461597101763,
0.00017267413204535842,
0.0000032318821467924863
] |
{
"id": 4,
"code_window": [
"\ta = (<ServiceIdentifier<any>>b).type;\n",
"\ta = (<IHighlight>b).start;\n",
"\ta = (<IHighlight>b).end;\n",
"\ta = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient\n",
"\ta = create1;\n",
"\ta = create2;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 17
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as nls from 'vs/nls';
import * as types from 'vs/base/common/types';
import { URI } from 'vs/base/common/uri';
import { ITree, IActionProvider } from 'vs/base/parts/tree/browser/tree';
import { IconLabel, IIconLabelValueOptions } from 'vs/base/browser/ui/iconLabel/iconLabel';
import { IQuickNavigateConfiguration, IModel, IDataSource, IFilter, IAccessiblityProvider, IRenderer, IRunner, Mode, IEntryRunContext } from 'vs/base/parts/quickopen/common/quickOpen';
import { IAction, IActionRunner } from 'vs/base/common/actions';
import { ActionBar } from 'vs/base/browser/ui/actionbar/actionbar';
import { HighlightedLabel } from 'vs/base/browser/ui/highlightedlabel/highlightedLabel';
import * as DOM from 'vs/base/browser/dom';
import { IQuickOpenStyles } from 'vs/base/parts/quickopen/browser/quickOpenWidget';
import { KeybindingLabel } from 'vs/base/browser/ui/keybindingLabel/keybindingLabel';
import { OS } from 'vs/base/common/platform';
import { ResolvedKeybinding } from 'vs/base/common/keyCodes';
import { IItemAccessor } from 'vs/base/parts/quickopen/common/quickOpenScorer';
import { coalesce } from 'vs/base/common/arrays';
export interface IContext {
event: any;
quickNavigateConfiguration: IQuickNavigateConfiguration;
}
export interface IHighlight {
start: number;
end: number;
}
let IDS = 0;
export class QuickOpenItemAccessorClass implements IItemAccessor<QuickOpenEntry> {
getItemLabel(entry: QuickOpenEntry): string | null {
return types.withUndefinedAsNull(entry.getLabel());
}
getItemDescription(entry: QuickOpenEntry): string | null {
return types.withUndefinedAsNull(entry.getDescription());
}
getItemPath(entry: QuickOpenEntry): string | undefined {
const resource = entry.getResource();
return resource ? resource.fsPath : undefined;
}
}
export const QuickOpenItemAccessor = new QuickOpenItemAccessorClass();
export class QuickOpenEntry {
private id: string;
private labelHighlights?: IHighlight[];
private descriptionHighlights?: IHighlight[];
private detailHighlights?: IHighlight[];
private hidden: boolean | undefined;
constructor(highlights: IHighlight[] = []) {
this.id = (IDS++).toString();
this.labelHighlights = highlights;
this.descriptionHighlights = [];
}
/**
* A unique identifier for the entry
*/
getId(): string {
return this.id;
}
/**
* The label of the entry to identify it from others in the list
*/
getLabel(): string | undefined {
return undefined;
}
/**
* The options for the label to use for this entry
*/
getLabelOptions(): IIconLabelValueOptions | undefined {
return undefined;
}
/**
* The label of the entry to use when a screen reader wants to read about the entry
*/
getAriaLabel(): string {
return coalesce([this.getLabel(), this.getDescription(), this.getDetail()])
.join(', ');
}
/**
* Detail information about the entry that is optional and can be shown below the label
*/
getDetail(): string | undefined {
return undefined;
}
/**
* The icon of the entry to identify it from others in the list
*/
getIcon(): string | undefined {
return undefined;
}
/**
* A secondary description that is optional and can be shown right to the label
*/
getDescription(): string | undefined {
return undefined;
}
/**
* A tooltip to show when hovering over the entry.
*/
getTooltip(): string | undefined {
return undefined;
}
/**
* A tooltip to show when hovering over the description portion of the entry.
*/
getDescriptionTooltip(): string | undefined {
return undefined;
}
/**
* An optional keybinding to show for an entry.
*/
getKeybinding(): ResolvedKeybinding | undefined {
return undefined;
}
/**
* A resource for this entry. Resource URIs can be used to compare different kinds of entries and group
* them together.
*/
getResource(): URI | undefined {
return undefined;
}
/**
* Allows to reuse the same model while filtering. Hidden entries will not show up in the viewer.
*/
isHidden(): boolean {
return !!this.hidden;
}
/**
* Allows to reuse the same model while filtering. Hidden entries will not show up in the viewer.
*/
setHidden(hidden: boolean): void {
this.hidden = hidden;
}
/**
* Allows to set highlight ranges that should show up for the entry label and optionally description if set.
*/
setHighlights(labelHighlights?: IHighlight[], descriptionHighlights?: IHighlight[], detailHighlights?: IHighlight[]): void {
this.labelHighlights = labelHighlights;
this.descriptionHighlights = descriptionHighlights;
this.detailHighlights = detailHighlights;
}
/**
* Allows to return highlight ranges that should show up for the entry label and description.
*/
getHighlights(): [IHighlight[] | undefined /* Label */, IHighlight[] | undefined /* Description */, IHighlight[] | undefined /* Detail */] {
return [this.labelHighlights, this.descriptionHighlights, this.detailHighlights];
}
/**
* Called when the entry is selected for opening. Returns a boolean value indicating if an action was performed or not.
* The mode parameter gives an indication if the element is previewed (using arrow keys) or opened.
*
* The context parameter provides additional context information how the run was triggered.
*/
run(mode: Mode, context: IEntryRunContext): boolean {
return false;
}
/**
* Determines if this quick open entry should merge with the editor history in quick open. If set to true
* and the resource of this entry is the same as the resource for an editor history, it will not show up
* because it is considered to be a duplicate of an editor history.
*/
mergeWithEditorHistory(): boolean {
return false;
}
}
export class QuickOpenEntryGroup extends QuickOpenEntry {
private entry?: QuickOpenEntry;
private groupLabel?: string;
private withBorder?: boolean;
constructor(entry?: QuickOpenEntry, groupLabel?: string, withBorder?: boolean) {
super();
this.entry = entry;
this.groupLabel = groupLabel;
this.withBorder = withBorder;
}
/**
* The label of the group or null if none.
*/
getGroupLabel(): string | undefined {
return this.groupLabel;
}
setGroupLabel(groupLabel: string | undefined): void {
this.groupLabel = groupLabel;
}
/**
* Whether to show a border on top of the group entry or not.
*/
showBorder(): boolean {
return !!this.withBorder;
}
setShowBorder(showBorder: boolean): void {
this.withBorder = showBorder;
}
getLabel(): string | undefined {
return this.entry ? this.entry.getLabel() : super.getLabel();
}
getLabelOptions(): IIconLabelValueOptions | undefined {
return this.entry ? this.entry.getLabelOptions() : super.getLabelOptions();
}
getAriaLabel(): string {
return this.entry ? this.entry.getAriaLabel() : super.getAriaLabel();
}
getDetail(): string | undefined {
return this.entry ? this.entry.getDetail() : super.getDetail();
}
getResource(): URI | undefined {
return this.entry ? this.entry.getResource() : super.getResource();
}
getIcon(): string | undefined {
return this.entry ? this.entry.getIcon() : super.getIcon();
}
getDescription(): string | undefined {
return this.entry ? this.entry.getDescription() : super.getDescription();
}
getEntry(): QuickOpenEntry | undefined {
return this.entry;
}
getHighlights(): [IHighlight[] | undefined, IHighlight[] | undefined, IHighlight[] | undefined] {
return this.entry ? this.entry.getHighlights() : super.getHighlights();
}
isHidden(): boolean {
return this.entry ? this.entry.isHidden() : super.isHidden();
}
setHighlights(labelHighlights?: IHighlight[], descriptionHighlights?: IHighlight[], detailHighlights?: IHighlight[]): void {
this.entry ? this.entry.setHighlights(labelHighlights, descriptionHighlights, detailHighlights) : super.setHighlights(labelHighlights, descriptionHighlights, detailHighlights);
}
setHidden(hidden: boolean): void {
this.entry ? this.entry.setHidden(hidden) : super.setHidden(hidden);
}
run(mode: Mode, context: IEntryRunContext): boolean {
return this.entry ? this.entry.run(mode, context) : super.run(mode, context);
}
}
class NoActionProvider implements IActionProvider {
hasActions(tree: ITree, element: any): boolean {
return false;
}
getActions(tree: ITree, element: any): IAction[] | null {
return null;
}
}
export interface IQuickOpenEntryTemplateData {
container: HTMLElement;
entry: HTMLElement;
icon: HTMLSpanElement;
label: IconLabel;
detail: HighlightedLabel;
keybinding: KeybindingLabel;
actionBar: ActionBar;
}
export interface IQuickOpenEntryGroupTemplateData extends IQuickOpenEntryTemplateData {
group?: HTMLDivElement;
}
const templateEntry = 'quickOpenEntry';
const templateEntryGroup = 'quickOpenEntryGroup';
class Renderer implements IRenderer<QuickOpenEntry> {
private actionProvider: IActionProvider;
private actionRunner?: IActionRunner;
constructor(actionProvider: IActionProvider = new NoActionProvider(), actionRunner?: IActionRunner) {
this.actionProvider = actionProvider;
this.actionRunner = actionRunner;
}
getHeight(entry: QuickOpenEntry): number {
if (entry.getDetail()) {
return 44;
}
return 22;
}
getTemplateId(entry: QuickOpenEntry): string {
if (entry instanceof QuickOpenEntryGroup) {
return templateEntryGroup;
}
return templateEntry;
}
renderTemplate(templateId: string, container: HTMLElement, styles: IQuickOpenStyles): IQuickOpenEntryGroupTemplateData {
const entryContainer = document.createElement('div');
DOM.addClass(entryContainer, 'sub-content');
container.appendChild(entryContainer);
// Entry
const row1 = DOM.$('.quick-open-row');
const row2 = DOM.$('.quick-open-row');
const entry = DOM.$('.quick-open-entry', undefined, row1, row2);
entryContainer.appendChild(entry);
// Icon
const icon = document.createElement('span');
row1.appendChild(icon);
// Label
const label = new IconLabel(row1, { supportHighlights: true, supportDescriptionHighlights: true, supportCodicons: true });
// Keybinding
const keybindingContainer = document.createElement('span');
row1.appendChild(keybindingContainer);
DOM.addClass(keybindingContainer, 'quick-open-entry-keybinding');
const keybinding = new KeybindingLabel(keybindingContainer, OS);
// Detail
const detailContainer = document.createElement('div');
row2.appendChild(detailContainer);
DOM.addClass(detailContainer, 'quick-open-entry-meta');
const detail = new HighlightedLabel(detailContainer, true);
// Entry Group
let group: HTMLDivElement | undefined;
if (templateId === templateEntryGroup) {
group = document.createElement('div');
DOM.addClass(group, 'results-group');
container.appendChild(group);
}
// Actions
DOM.addClass(container, 'actions');
const actionBarContainer = document.createElement('div');
DOM.addClass(actionBarContainer, 'primary-action-bar');
container.appendChild(actionBarContainer);
const actionBar = new ActionBar(actionBarContainer, {
actionRunner: this.actionRunner
});
return {
container,
entry,
icon,
label,
detail,
keybinding,
group,
actionBar
};
}
renderElement(entry: QuickOpenEntry, templateId: string, data: IQuickOpenEntryGroupTemplateData, styles: IQuickOpenStyles): void {
// Action Bar
if (this.actionProvider.hasActions(null, entry)) {
DOM.addClass(data.container, 'has-actions');
} else {
DOM.removeClass(data.container, 'has-actions');
}
data.actionBar.context = entry; // make sure the context is the current element
const actions = this.actionProvider.getActions(null, entry);
if (data.actionBar.isEmpty() && actions && actions.length > 0) {
data.actionBar.push(actions, { icon: true, label: false });
} else if (!data.actionBar.isEmpty() && (!actions || actions.length === 0)) {
data.actionBar.clear();
}
// Entry group class
if (entry instanceof QuickOpenEntryGroup && entry.getGroupLabel()) {
DOM.addClass(data.container, 'has-group-label');
} else {
DOM.removeClass(data.container, 'has-group-label');
}
// Entry group
if (entry instanceof QuickOpenEntryGroup) {
const group = <QuickOpenEntryGroup>entry;
const groupData = data;
// Border
if (group.showBorder()) {
DOM.addClass(groupData.container, 'results-group-separator');
if (styles.pickerGroupBorder) {
groupData.container.style.borderTopColor = styles.pickerGroupBorder.toString();
}
} else {
DOM.removeClass(groupData.container, 'results-group-separator');
groupData.container.style.borderTopColor = '';
}
// Group Label
const groupLabel = group.getGroupLabel() || '';
if (groupData.group) {
groupData.group.textContent = groupLabel;
if (styles.pickerGroupForeground) {
groupData.group.style.color = styles.pickerGroupForeground.toString();
}
}
}
// Normal Entry
if (entry instanceof QuickOpenEntry) {
const [labelHighlights, descriptionHighlights, detailHighlights] = entry.getHighlights();
// Icon
const iconClass = entry.getIcon() ? ('quick-open-entry-icon ' + entry.getIcon()) : '';
data.icon.className = iconClass;
// Label
const options: IIconLabelValueOptions = entry.getLabelOptions() || Object.create(null);
options.matches = labelHighlights || [];
options.title = entry.getTooltip();
options.descriptionTitle = entry.getDescriptionTooltip() || entry.getDescription(); // tooltip over description because it could overflow
options.descriptionMatches = descriptionHighlights || [];
data.label.setLabel(types.withNullAsUndefined(entry.getLabel()), entry.getDescription(), options);
// Meta
data.detail.set(entry.getDetail(), detailHighlights);
// Keybinding
data.keybinding.set(entry.getKeybinding()!);
}
}
disposeTemplate(templateId: string, templateData: IQuickOpenEntryGroupTemplateData): void {
templateData.actionBar.dispose();
templateData.actionBar = null!;
templateData.container = null!;
templateData.entry = null!;
templateData.keybinding = null!;
templateData.detail = null!;
templateData.group = null!;
templateData.icon = null!;
templateData.label.dispose();
templateData.label = null!;
}
}
export class QuickOpenModel implements
IModel<QuickOpenEntry>,
IDataSource<QuickOpenEntry>,
IFilter<QuickOpenEntry>,
IRunner<QuickOpenEntry>,
IAccessiblityProvider<QuickOpenEntry>
{
private _entries: QuickOpenEntry[];
private _dataSource: IDataSource<QuickOpenEntry>;
private _renderer: IRenderer<QuickOpenEntry>;
private _filter: IFilter<QuickOpenEntry>;
private _runner: IRunner<QuickOpenEntry>;
private _accessibilityProvider: IAccessiblityProvider<QuickOpenEntry>;
constructor(entries: QuickOpenEntry[] = [], actionProvider: IActionProvider = new NoActionProvider()) {
this._entries = entries;
this._dataSource = this;
this._renderer = new Renderer(actionProvider);
this._filter = this;
this._runner = this;
this._accessibilityProvider = this;
}
get entries() { return this._entries; }
get dataSource() { return this._dataSource; }
get renderer() { return this._renderer; }
get filter() { return this._filter; }
get runner() { return this._runner; }
get accessibilityProvider() { return this._accessibilityProvider; }
set entries(entries: QuickOpenEntry[]) {
this._entries = entries;
}
/**
* Adds entries that should show up in the quick open viewer.
*/
addEntries(entries: QuickOpenEntry[]): void {
if (types.isArray(entries)) {
this._entries = this._entries.concat(entries);
}
}
/**
* Set the entries that should show up in the quick open viewer.
*/
setEntries(entries: QuickOpenEntry[]): void {
if (types.isArray(entries)) {
this._entries = entries;
}
}
/**
* Get the entries that should show up in the quick open viewer.
*
* @visibleOnly optional parameter to only return visible entries
*/
getEntries(visibleOnly?: boolean): QuickOpenEntry[] {
if (visibleOnly) {
return this._entries.filter((e) => !e.isHidden());
}
return this._entries;
}
getId(entry: QuickOpenEntry): string {
return entry.getId();
}
getLabel(entry: QuickOpenEntry): string | null {
return types.withUndefinedAsNull(entry.getLabel());
}
getAriaLabel(entry: QuickOpenEntry): string {
const ariaLabel = entry.getAriaLabel();
if (ariaLabel) {
return nls.localize('quickOpenAriaLabelEntry', "{0}, picker", entry.getAriaLabel());
}
return nls.localize('quickOpenAriaLabel', "picker");
}
isVisible(entry: QuickOpenEntry): boolean {
return !entry.isHidden();
}
run(entry: QuickOpenEntry, mode: Mode, context: IEntryRunContext): boolean {
return entry.run(mode, context);
}
}
| src/vs/base/parts/quickopen/browser/quickOpenModel.ts | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0022814851254224777,
0.0002167072525480762,
0.00016322857118211687,
0.000175979730556719,
0.0002757734037004411
] |
{
"id": 4,
"code_window": [
"\ta = (<ServiceIdentifier<any>>b).type;\n",
"\ta = (<IHighlight>b).start;\n",
"\ta = (<IHighlight>b).end;\n",
"\ta = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient\n",
"\ta = create1;\n",
"\ta = create2;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 17
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import product from 'vs/platform/product/common/product';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { ILifecycleMainService } from 'vs/platform/lifecycle/electron-main/lifecycleMainService';
import { State, IUpdate, AvailableForDownload, UpdateType } from 'vs/platform/update/common/update';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { ILogService } from 'vs/platform/log/common/log';
import { createUpdateURL, AbstractUpdateService, UpdateNotAvailableClassification } from 'vs/platform/update/electron-main/abstractUpdateService';
import { IRequestService, asJson } from 'vs/platform/request/common/request';
import { shell } from 'electron';
import { CancellationToken } from 'vs/base/common/cancellation';
export class LinuxUpdateService extends AbstractUpdateService {
_serviceBrand: undefined;
constructor(
@ILifecycleMainService lifecycleMainService: ILifecycleMainService,
@IConfigurationService configurationService: IConfigurationService,
@ITelemetryService private readonly telemetryService: ITelemetryService,
@IEnvironmentService environmentService: IEnvironmentService,
@IRequestService requestService: IRequestService,
@ILogService logService: ILogService
) {
super(lifecycleMainService, configurationService, environmentService, requestService, logService);
}
protected buildUpdateFeedUrl(quality: string): string {
return createUpdateURL(`linux-${process.arch}`, quality);
}
protected doCheckForUpdates(context: any): void {
if (!this.url) {
return;
}
this.setState(State.CheckingForUpdates(context));
this.requestService.request({ url: this.url }, CancellationToken.None)
.then<IUpdate | null>(asJson)
.then(update => {
if (!update || !update.url || !update.version || !update.productVersion) {
this.telemetryService.publicLog2<{ explicit: boolean }, UpdateNotAvailableClassification>('update:notAvailable', { explicit: !!context });
this.setState(State.Idle(UpdateType.Archive));
} else {
this.setState(State.AvailableForDownload(update));
}
})
.then(undefined, err => {
this.logService.error(err);
this.telemetryService.publicLog2<{ explicit: boolean }, UpdateNotAvailableClassification>('update:notAvailable', { explicit: !!context });
// only show message when explicitly checking for updates
const message: string | undefined = !!context ? (err.message || err) : undefined;
this.setState(State.Idle(UpdateType.Archive, message));
});
}
protected async doDownloadUpdate(state: AvailableForDownload): Promise<void> {
// Use the download URL if available as we don't currently detect the package type that was
// installed and the website download page is more useful than the tarball generally.
if (product.downloadUrl && product.downloadUrl.length > 0) {
shell.openExternal(product.downloadUrl);
} else if (state.update.url) {
shell.openExternal(state.update.url);
}
this.setState(State.Idle(UpdateType.Archive));
}
}
| src/vs/platform/update/electron-main/updateService.linux.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017799714987631887,
0.00017643626779317856,
0.0001735773985274136,
0.00017657779972068965,
0.0000013344774743018206
] |
{
"id": 4,
"code_window": [
"\ta = (<ServiceIdentifier<any>>b).type;\n",
"\ta = (<IHighlight>b).start;\n",
"\ta = (<IHighlight>b).end;\n",
"\ta = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient\n",
"\ta = create1;\n",
"\ta = create2;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 17
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as cp from 'child_process';
import * as env from 'vs/base/common/platform';
import { getSystemShell } from 'vs/workbench/contrib/terminal/node/terminal';
import { WindowsExternalTerminalService, MacExternalTerminalService, LinuxExternalTerminalService } from 'vs/workbench/contrib/externalTerminal/node/externalTerminalService';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IExternalTerminalService } from 'vs/workbench/contrib/externalTerminal/common/externalTerminal';
import { ExtHostConfigProvider } from 'vs/workbench/api/common/extHostConfiguration';
let externalTerminalService: IExternalTerminalService | undefined = undefined;
export function runInExternalTerminal(args: DebugProtocol.RunInTerminalRequestArguments, configProvider: ExtHostConfigProvider): void {
if (!externalTerminalService) {
if (env.isWindows) {
externalTerminalService = new WindowsExternalTerminalService(<IConfigurationService><unknown>undefined);
} else if (env.isMacintosh) {
externalTerminalService = new MacExternalTerminalService(<IConfigurationService><unknown>undefined);
} else if (env.isLinux) {
externalTerminalService = new LinuxExternalTerminalService(<IConfigurationService><unknown>undefined);
}
}
if (externalTerminalService) {
const config = configProvider.getConfiguration('terminal');
externalTerminalService.runInTerminal(args.title!, args.cwd, args.args, args.env || {}, config.external || {});
}
}
function spawnAsPromised(command: string, args: string[]): Promise<string> {
return new Promise((resolve, reject) => {
let stdout = '';
const child = cp.spawn(command, args);
if (child.pid) {
child.stdout.on('data', (data: Buffer) => {
stdout += data.toString();
});
}
child.on('error', err => {
reject(err);
});
child.on('close', code => {
resolve(stdout);
});
});
}
export function hasChildProcesses(processId: number): Promise<boolean> {
if (processId) {
// if shell has at least one child process, assume that shell is busy
if (env.isWindows) {
return spawnAsPromised('wmic', ['process', 'get', 'ParentProcessId']).then(stdout => {
const pids = stdout.split('\r\n');
return pids.some(p => parseInt(p) === processId);
}, error => {
return true;
});
} else {
return spawnAsPromised('/usr/bin/pgrep', ['-lP', String(processId)]).then(stdout => {
const r = stdout.trim();
if (r.length === 0 || r.indexOf(' tmux') >= 0) { // ignore 'tmux'; see #43683
return false;
} else {
return true;
}
}, error => {
return true;
});
}
}
// fall back to safe side
return Promise.resolve(true);
}
const enum ShellType { cmd, powershell, bash }
export function prepareCommand(args: DebugProtocol.RunInTerminalRequestArguments, shell: string, configProvider: ExtHostConfigProvider): string {
let shellType = env.isWindows ? ShellType.cmd : ShellType.bash; // pick a good default
if (shell) {
const config = configProvider.getConfiguration('terminal');
// get the shell configuration for the current platform
const shell_config = config.integrated.shell;
if (env.isWindows) {
shell = shell_config.windows || getSystemShell(env.Platform.Windows);
} else if (env.isLinux) {
shell = shell_config.linux || getSystemShell(env.Platform.Linux);
} else if (env.isMacintosh) {
shell = shell_config.osx || getSystemShell(env.Platform.Mac);
} else {
throw new Error('Unknown platform');
}
}
// try to determine the shell type
shell = shell.trim().toLowerCase();
if (shell.indexOf('powershell') >= 0 || shell.indexOf('pwsh') >= 0) {
shellType = ShellType.powershell;
} else if (shell.indexOf('cmd.exe') >= 0) {
shellType = ShellType.cmd;
} else if (shell.indexOf('bash') >= 0) {
shellType = ShellType.bash;
}
let quote: (s: string) => string;
let command = '';
switch (shellType) {
case ShellType.powershell:
quote = (s: string) => {
s = s.replace(/\'/g, '\'\'');
if (s.length > 0 && s.charAt(s.length - 1) === '\\') {
return `'${s}\\'`;
}
return `'${s}'`;
};
if (args.cwd) {
command += `cd '${args.cwd}'; `;
}
if (args.env) {
for (let key in args.env) {
const value = args.env[key];
if (value === null) {
command += `Remove-Item env:${key}; `;
} else {
command += `\${env:${key}}='${value}'; `;
}
}
}
if (args.args && args.args.length > 0) {
const cmd = quote(args.args.shift()!);
command += (cmd[0] === '\'') ? `& ${cmd} ` : `${cmd} `;
for (let a of args.args) {
command += `${quote(a)} `;
}
}
break;
case ShellType.cmd:
quote = (s: string) => {
s = s.replace(/\"/g, '""');
return (s.indexOf(' ') >= 0 || s.indexOf('"') >= 0 || s.length === 0) ? `"${s}"` : s;
};
if (args.cwd) {
command += `cd ${quote(args.cwd)} && `;
}
if (args.env) {
command += 'cmd /C "';
for (let key in args.env) {
let value = args.env[key];
if (value === null) {
command += `set "${key}=" && `;
} else {
value = value.replace(/[\^\&\|\<\>]/g, s => `^${s}`);
command += `set "${key}=${value}" && `;
}
}
}
for (let a of args.args) {
command += `${quote(a)} `;
}
if (args.env) {
command += '"';
}
break;
case ShellType.bash:
quote = (s: string) => {
s = s.replace(/([\"\\])/g, '\\$1');
return (s.indexOf(' ') >= 0 || s.length === 0) ? `"${s}"` : s;
};
const hardQuote = (s: string) => {
return /[^\w@%\/+=,.:^-]/.test(s) ? `'${s.replace(/'/g, '\'\\\'\'')}'` : s;
};
if (args.cwd) {
command += `cd ${quote(args.cwd)} ; `;
}
if (args.env) {
command += 'env';
for (let key in args.env) {
const value = args.env[key];
if (value === null) {
command += ` -u ${hardQuote(key)}`;
} else {
command += ` ${hardQuote(`${key}=${value}`)}`;
}
}
command += ' ';
}
for (let a of args.args) {
command += `${quote(a)} `;
}
break;
}
return command;
}
| src/vs/workbench/contrib/debug/node/terminals.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.002480667782947421,
0.00028083790675736964,
0.00016685629088897258,
0.00017617642879486084,
0.0004800540627911687
] |
{
"id": 4,
"code_window": [
"\ta = (<ServiceIdentifier<any>>b).type;\n",
"\ta = (<IHighlight>b).start;\n",
"\ta = (<IHighlight>b).end;\n",
"\ta = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient\n",
"\ta = create1;\n",
"\ta = create2;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 17
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IDisposable, dispose, DisposableStore } from 'vs/base/common/lifecycle';
export enum WidgetVerticalAlignment {
Bottom,
Top
}
const WIDGET_HEIGHT = 29;
export class TerminalWidgetManager implements IDisposable {
private _container: HTMLElement | undefined;
private _xtermViewport: HTMLElement | undefined;
private _messageWidget: MessageWidget | undefined;
private readonly _messageListeners = new DisposableStore();
constructor(
terminalWrapper: HTMLElement
) {
this._container = document.createElement('div');
this._container.classList.add('terminal-widget-overlay');
terminalWrapper.appendChild(this._container);
this._initTerminalHeightWatcher(terminalWrapper);
}
public dispose(): void {
if (this._container && this._container.parentElement) {
this._container.parentElement.removeChild(this._container);
this._container = undefined;
}
this._xtermViewport = undefined;
this._messageListeners.dispose();
}
private _initTerminalHeightWatcher(terminalWrapper: HTMLElement) {
// Watch the xterm.js viewport for style changes and do a layout if it changes
this._xtermViewport = <HTMLElement>terminalWrapper.querySelector('.xterm-viewport');
if (!this._xtermViewport) {
return;
}
const mutationObserver = new MutationObserver(() => this._refreshHeight());
mutationObserver.observe(this._xtermViewport, { attributes: true, attributeFilter: ['style'] });
}
public showMessage(left: number, y: number, text: string, verticalAlignment: WidgetVerticalAlignment = WidgetVerticalAlignment.Bottom): void {
if (!this._container) {
return;
}
dispose(this._messageWidget);
this._messageListeners.clear();
this._messageWidget = new MessageWidget(this._container, left, y, text, verticalAlignment);
}
public closeMessage(): void {
this._messageListeners.clear();
if (this._messageWidget) {
this._messageListeners.add(MessageWidget.fadeOut(this._messageWidget));
}
}
private _refreshHeight(): void {
if (!this._container || !this._xtermViewport) {
return;
}
this._container.style.height = this._xtermViewport.style.height;
}
}
class MessageWidget {
private _domNode: HTMLDivElement;
public get left(): number { return this._left; }
public get y(): number { return this._y; }
public get text(): string { return this._text; }
public get domNode(): HTMLElement { return this._domNode; }
public get verticalAlignment(): WidgetVerticalAlignment { return this._verticalAlignment; }
public static fadeOut(messageWidget: MessageWidget): IDisposable {
let handle: any;
const dispose = () => {
messageWidget.dispose();
clearTimeout(handle);
messageWidget.domNode.removeEventListener('animationend', dispose);
};
handle = setTimeout(dispose, 110);
messageWidget.domNode.addEventListener('animationend', dispose);
messageWidget.domNode.classList.add('fadeOut');
return { dispose };
}
constructor(
private _container: HTMLElement,
private _left: number,
private _y: number,
private _text: string,
private _verticalAlignment: WidgetVerticalAlignment
) {
this._domNode = document.createElement('div');
this._domNode.style.position = 'absolute';
this._domNode.style.left = `${_left}px`;
if (this.verticalAlignment === WidgetVerticalAlignment.Top) {
// Y position is to the top of the widget
this._domNode.style.bottom = `${Math.max(_y, WIDGET_HEIGHT) - WIDGET_HEIGHT}px`;
} else {
// Y position is to the bottom of the widget
this._domNode.style.bottom = `${Math.min(_y, _container.offsetHeight - WIDGET_HEIGHT)}px`;
}
this._domNode.classList.add('terminal-message-widget', 'fadeIn');
this._domNode.textContent = _text;
this._container.appendChild(this._domNode);
}
public dispose(): void {
if (this.domNode.parentElement === this._container) {
this._container.removeChild(this.domNode);
}
}
}
| src/vs/workbench/contrib/terminal/browser/terminalWidgetManager.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017929822206497192,
0.00017494136409368366,
0.0001675816165516153,
0.00017484916315879673,
0.0000032757418466644594
] |
{
"id": 5,
"code_window": [
"\ta = create1;\n",
"\ta = create2;\n",
"\ta = (<DocumentRangeFormattingEditProvider>b).extensionId;\n",
"\n",
"\t// injection madness\n",
"\ta = (<SyncDescriptor0<any>>b).ctor;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 22
} |
// This file is adding references to various symbols which should not be removed via tree shaking
import { ServiceIdentifier } from './vs/platform/instantiation/common/instantiation';
import { IHighlight } from './vs/base/parts/quickopen/browser/quickOpenModel';
import { SimpleWorkerClient, create as create1 } from './vs/base/common/worker/simpleWorker';
import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';
import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';
import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';
import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';
import * as editorAPI from './vs/editor/editor.api';
(function () {
var a: any;
var b: any;
a = (<DiffNavigator>b).previous; // IDiffNavigator
a = (<ServiceIdentifier<any>>b).type;
a = (<IHighlight>b).start;
a = (<IHighlight>b).end;
a = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient
a = create1;
a = create2;
a = (<DocumentRangeFormattingEditProvider>b).extensionId;
// injection madness
a = (<SyncDescriptor0<any>>b).ctor;
a = (<SyncDescriptor0<any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor2<any, any, any>>b).ctor;
a = (<SyncDescriptor2<any, any, any>>b).bind;
a = (<SyncDescriptor3<any, any, any, any>>b).ctor;
a = (<SyncDescriptor3<any, any, any, any>>b).bind;
a = (<SyncDescriptor4<any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor4<any, any, any, any, any>>b).bind;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).bind;
// exported API
a = editorAPI.CancellationTokenSource;
a = editorAPI.Emitter;
a = editorAPI.KeyCode;
a = editorAPI.KeyMod;
a = editorAPI.Position;
a = editorAPI.Range;
a = editorAPI.Selection;
a = editorAPI.SelectionDirection;
a = editorAPI.MarkerSeverity;
a = editorAPI.MarkerTag;
a = editorAPI.Uri;
a = editorAPI.Token;
a = editorAPI.editor;
a = editorAPI.languages;
})();
| build/monaco/monaco.usage.recipe | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.9980402588844299,
0.1456054449081421,
0.0021444049198180437,
0.0039322273805737495,
0.34800633788108826
] |
{
"id": 5,
"code_window": [
"\ta = create1;\n",
"\ta = create2;\n",
"\ta = (<DocumentRangeFormattingEditProvider>b).extensionId;\n",
"\n",
"\t// injection madness\n",
"\ta = (<SyncDescriptor0<any>>b).ctor;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 22
} | # THIS IS AN AUTOGENERATED FILE. DO NOT EDIT THIS FILE DIRECTLY.
# yarn lockfile v1
nan@^2.14.0:
version "2.14.0"
resolved "https://registry.yarnpkg.com/nan/-/nan-2.14.0.tgz#7818f722027b2459a86f0295d434d1fc2336c52c"
integrity sha512-INOFj37C7k3AfaNTtX8RhsTw7qRy7eLET14cROi9+5HAVbbHuIWUHEauBv5qT4Av2tWasiTY1Jw6puUNqRJXQg==
onigasm-umd@^2.2.2:
version "2.2.2"
resolved "https://registry.yarnpkg.com/onigasm-umd/-/onigasm-umd-2.2.2.tgz#b989d762df61f899a3052ac794a50bd93fe20257"
integrity sha512-v2eMOJu7iE444L2iJN+U6s6s5S0y7oj/N0DAkrd6wokRtTVoq/v/yaDI1lIqFrTeJbNtqNzYvguDF5yNzW3Rvw==
oniguruma@^7.2.0:
version "7.2.0"
resolved "https://registry.yarnpkg.com/oniguruma/-/oniguruma-7.2.0.tgz#c9a59c1ea7b9fe67e237a02e02139b638856f3af"
integrity sha512-bh+ZLdykY1sdIx8jBp2zpLbVFDBc3XmKH4Ceo2lijNaN1WhEqtnpqFlmtCbRuDB17nJ58RAUStVwfW8e8uEbnA==
dependencies:
nan "^2.14.0"
semver-umd@^5.5.3:
version "5.5.3"
resolved "https://registry.yarnpkg.com/semver-umd/-/semver-umd-5.5.3.tgz#b64d7a2d4f5a717b369d56e31940a38e47e34d1e"
integrity sha512-HOnQrn2iKnVe/xlqCTzMXQdvSz3rPbD0DmQXYuQ+oK1dpptGFfPghonQrx5JHl2O7EJwDqtQnjhE7ME23q6ngw==
vscode-textmate@^4.3.0:
version "4.3.0"
resolved "https://registry.yarnpkg.com/vscode-textmate/-/vscode-textmate-4.3.0.tgz#6e1f0f273d84148cfa1e9c7ed85bd16c974f9f61"
integrity sha512-MhEZ3hvxOVuYGsrRzW/PZLDR2VdtG2+V6TIKPvmE9JT+RAq/OtPlrFd1+ZQwBefoHEhjRNuRJ0OktcFezuxPmg==
dependencies:
oniguruma "^7.2.0"
[email protected]:
version "0.4.0-beta4"
resolved "https://registry.yarnpkg.com/xterm-addon-search/-/xterm-addon-search-0.4.0-beta4.tgz#7762ea342c6b4f5e824d83466bd93793c9d7d779"
integrity sha512-TIbEBVhydGIxcyu/CfKJbD+BKHisMGbkAfaWlCPaWis2Xmw8yE7CKrCPn+lhZYl1MdjDVEmb8lQI6WetbC2OZA==
[email protected]:
version "0.2.1"
resolved "https://registry.yarnpkg.com/xterm-addon-web-links/-/xterm-addon-web-links-0.2.1.tgz#6d1f2ce613e09870badf17615e7a1170a31542b2"
integrity sha512-2KnHtiq0IG7hfwv3jw2/jQeH1RBk2d5CH4zvgwQe00rLofSJqSfgnJ7gwowxxpGHrpbPr6Lv4AmH/joaNw2+HQ==
[email protected]:
version "4.3.0-beta17"
resolved "https://registry.yarnpkg.com/xterm/-/xterm-4.3.0-beta17.tgz#c038cc00cb5be33d2a5f083255c329d9ed186565"
integrity sha512-Lgz7vL12MBKJSgK/UXJF22Yw+uEXEE7YZDWfUD+/jbHAKN4geLQJ/Y/b3gxjLL020dnYZuayfKOr2KWdhKsmCA==
| remote/web/yarn.lock | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0001665931922616437,
0.00016494952433276922,
0.00016359458095394075,
0.00016464493819512427,
0.0000011646840221146704
] |
{
"id": 5,
"code_window": [
"\ta = create1;\n",
"\ta = create2;\n",
"\ta = (<DocumentRangeFormattingEditProvider>b).extensionId;\n",
"\n",
"\t// injection madness\n",
"\ta = (<SyncDescriptor0<any>>b).ctor;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 22
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IChannel, IServerChannel } from 'vs/base/parts/ipc/common/ipc';
import { Event } from 'vs/base/common/event';
import { IRequestService } from 'vs/platform/request/common/request';
import { IRequestOptions, IRequestContext, IHeaders } from 'vs/base/parts/request/common/request';
import { CancellationToken } from 'vs/base/common/cancellation';
import { VSBuffer, bufferToStream, streamToBuffer } from 'vs/base/common/buffer';
type RequestResponse = [
{
headers: IHeaders;
statusCode?: number;
},
VSBuffer
];
export class RequestChannel implements IServerChannel {
constructor(private readonly service: IRequestService) { }
listen(context: any, event: string): Event<any> {
throw new Error('Invalid listen');
}
call(context: any, command: string, args?: any): Promise<any> {
switch (command) {
case 'request': return this.service.request(args[0], CancellationToken.None)
.then(async ({ res, stream }) => {
const buffer = await streamToBuffer(stream);
return <RequestResponse>[{ statusCode: res.statusCode, headers: res.headers }, buffer];
});
}
throw new Error('Invalid call');
}
}
export class RequestChannelClient {
_serviceBrand: undefined;
constructor(private readonly channel: IChannel) { }
async request(options: IRequestOptions, token: CancellationToken): Promise<IRequestContext> {
const [res, buffer] = await this.channel.call<RequestResponse>('request', [options]);
return { res, stream: bufferToStream(buffer) };
}
}
| src/vs/platform/request/common/requestIpc.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00018112632096745074,
0.00017126962484326214,
0.00016543934179935604,
0.00016951259749475867,
0.0000056755784498818684
] |
{
"id": 5,
"code_window": [
"\ta = create1;\n",
"\ta = create2;\n",
"\ta = (<DocumentRangeFormattingEditProvider>b).extensionId;\n",
"\n",
"\t// injection madness\n",
"\ta = (<SyncDescriptor0<any>>b).ctor;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "build/monaco/monaco.usage.recipe",
"type": "replace",
"edit_start_line_idx": 22
} | // See https://go.microsoft.com/fwlink/?LinkId=733558
// for the documentation about the tasks.json format
{
"version": "2.0.0",
"tasks": [
{
"type": "npm",
"script": "compile",
"problemMatcher": "$tsc-watch",
"isBackground": true,
"presentation": {
"reveal": "never"
},
"group": {
"kind": "build",
"isDefault": true
}
}
]
} | extensions/json-language-features/server/.vscode/tasks.json | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017972425848711282,
0.00017683912301436067,
0.0001752749813022092,
0.0001755181438056752,
0.0000020425090951903258
] |
{
"id": 6,
"code_window": [
"import { ResolvedKeybinding } from 'vs/base/common/keyCodes';\n",
"import { IItemAccessor } from 'vs/base/parts/quickopen/common/quickOpenScorer';\n",
"import { coalesce } from 'vs/base/common/arrays';\n",
"\n",
"export interface IContext {\n",
"\tevent: any;\n",
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { IMatch } from 'vs/base/common/filters';\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "add",
"edit_start_line_idx": 21
} |
// This file is adding references to various symbols which should not be removed via tree shaking
import { ServiceIdentifier } from './vs/platform/instantiation/common/instantiation';
import { IHighlight } from './vs/base/parts/quickopen/browser/quickOpenModel';
import { SimpleWorkerClient, create as create1 } from './vs/base/common/worker/simpleWorker';
import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';
import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';
import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';
import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';
import * as editorAPI from './vs/editor/editor.api';
(function () {
var a: any;
var b: any;
a = (<DiffNavigator>b).previous; // IDiffNavigator
a = (<ServiceIdentifier<any>>b).type;
a = (<IHighlight>b).start;
a = (<IHighlight>b).end;
a = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient
a = create1;
a = create2;
a = (<DocumentRangeFormattingEditProvider>b).extensionId;
// injection madness
a = (<SyncDescriptor0<any>>b).ctor;
a = (<SyncDescriptor0<any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor2<any, any, any>>b).ctor;
a = (<SyncDescriptor2<any, any, any>>b).bind;
a = (<SyncDescriptor3<any, any, any, any>>b).ctor;
a = (<SyncDescriptor3<any, any, any, any>>b).bind;
a = (<SyncDescriptor4<any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor4<any, any, any, any, any>>b).bind;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).bind;
// exported API
a = editorAPI.CancellationTokenSource;
a = editorAPI.Emitter;
a = editorAPI.KeyCode;
a = editorAPI.KeyMod;
a = editorAPI.Position;
a = editorAPI.Range;
a = editorAPI.Selection;
a = editorAPI.SelectionDirection;
a = editorAPI.MarkerSeverity;
a = editorAPI.MarkerTag;
a = editorAPI.Uri;
a = editorAPI.Token;
a = editorAPI.editor;
a = editorAPI.languages;
})();
| build/monaco/monaco.usage.recipe | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0018025175668299198,
0.00040407711640000343,
0.00015983158664312214,
0.0001745167246554047,
0.000570939970202744
] |
{
"id": 6,
"code_window": [
"import { ResolvedKeybinding } from 'vs/base/common/keyCodes';\n",
"import { IItemAccessor } from 'vs/base/parts/quickopen/common/quickOpenScorer';\n",
"import { coalesce } from 'vs/base/common/arrays';\n",
"\n",
"export interface IContext {\n",
"\tevent: any;\n",
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { IMatch } from 'vs/base/common/filters';\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "add",
"edit_start_line_idx": 21
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Emitter } from 'vs/base/common/event';
import { parse } from 'vs/base/common/json';
import { Disposable } from 'vs/base/common/lifecycle';
import * as network from 'vs/base/common/network';
import { assign } from 'vs/base/common/objects';
import * as strings from 'vs/base/common/strings';
import { URI } from 'vs/base/common/uri';
import { getCodeEditor, ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { EditOperation } from 'vs/editor/common/core/editOperation';
import { IPosition, Position } from 'vs/editor/common/core/position';
import { ITextModel } from 'vs/editor/common/model';
import { IModelService } from 'vs/editor/common/services/modelService';
import { IModeService } from 'vs/editor/common/services/modeService';
import { ITextModelService } from 'vs/editor/common/services/resolverService';
import * as nls from 'vs/nls';
import { ConfigurationTarget, IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IEditorOptions } from 'vs/platform/editor/common/editor';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { FileOperationError, FileOperationResult } from 'vs/platform/files/common/files';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { ILabelService } from 'vs/platform/label/common/label';
import { INotificationService } from 'vs/platform/notification/common/notification';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { IWorkspaceContextService, WorkbenchState } from 'vs/platform/workspace/common/workspace';
import { EditorInput, IEditor } from 'vs/workbench/common/editor';
import { IJSONEditingService } from 'vs/workbench/services/configuration/common/jsonEditing';
import { IEditorService } from 'vs/workbench/services/editor/common/editorService';
import { GroupDirection, IEditorGroup, IEditorGroupsService } from 'vs/workbench/services/editor/common/editorGroupsService';
import { DEFAULT_SETTINGS_EDITOR_SETTING, FOLDER_SETTINGS_PATH, getSettingsTargetName, IPreferencesEditorModel, IPreferencesService, ISetting, ISettingsEditorOptions, SettingsEditorOptions, USE_SPLIT_JSON_SETTING } from 'vs/workbench/services/preferences/common/preferences';
import { DefaultPreferencesEditorInput, KeybindingsEditorInput, PreferencesEditorInput, SettingsEditor2Input } from 'vs/workbench/services/preferences/common/preferencesEditorInput';
import { defaultKeybindingsContents, DefaultKeybindingsEditorModel, DefaultSettings, DefaultSettingsEditorModel, Settings2EditorModel, SettingsEditorModel, WorkspaceConfigurationEditorModel, DefaultRawSettingsEditorModel } from 'vs/workbench/services/preferences/common/preferencesModels';
import { registerSingleton } from 'vs/platform/instantiation/common/extensions';
import { IRemoteAgentService } from 'vs/workbench/services/remote/common/remoteAgentService';
import { ITextFileService } from 'vs/workbench/services/textfile/common/textfiles';
import { withNullAsUndefined } from 'vs/base/common/types';
const emptyEditableSettingsContent = '{\n}';
export class PreferencesService extends Disposable implements IPreferencesService {
_serviceBrand: undefined;
private lastOpenedSettingsInput: PreferencesEditorInput | null = null;
private readonly _onDispose = this._register(new Emitter<void>());
private _defaultUserSettingsUriCounter = 0;
private _defaultUserSettingsContentModel: DefaultSettings | undefined;
private _defaultWorkspaceSettingsUriCounter = 0;
private _defaultWorkspaceSettingsContentModel: DefaultSettings | undefined;
private _defaultFolderSettingsUriCounter = 0;
private _defaultFolderSettingsContentModel: DefaultSettings | undefined;
constructor(
@IEditorService private readonly editorService: IEditorService,
@IEditorGroupsService private readonly editorGroupService: IEditorGroupsService,
@ITextFileService private readonly textFileService: ITextFileService,
@IConfigurationService private readonly configurationService: IConfigurationService,
@INotificationService private readonly notificationService: INotificationService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IInstantiationService private readonly instantiationService: IInstantiationService,
@IEnvironmentService private readonly environmentService: IEnvironmentService,
@ITelemetryService private readonly telemetryService: ITelemetryService,
@ITextModelService private readonly textModelResolverService: ITextModelService,
@IKeybindingService keybindingService: IKeybindingService,
@IModelService private readonly modelService: IModelService,
@IJSONEditingService private readonly jsonEditingService: IJSONEditingService,
@IModeService private readonly modeService: IModeService,
@ILabelService private readonly labelService: ILabelService,
@IRemoteAgentService private readonly remoteAgentService: IRemoteAgentService
) {
super();
// The default keybindings.json updates based on keyboard layouts, so here we make sure
// if a model has been given out we update it accordingly.
this._register(keybindingService.onDidUpdateKeybindings(() => {
const model = modelService.getModel(this.defaultKeybindingsResource);
if (!model) {
// model has not been given out => nothing to do
return;
}
modelService.updateModel(model, defaultKeybindingsContents(keybindingService));
}));
}
readonly defaultKeybindingsResource = URI.from({ scheme: network.Schemas.vscode, authority: 'defaultsettings', path: '/keybindings.json' });
private readonly defaultSettingsRawResource = URI.from({ scheme: network.Schemas.vscode, authority: 'defaultsettings', path: '/defaultSettings.json' });
get userSettingsResource(): URI {
return this.environmentService.settingsResource;
}
get workspaceSettingsResource(): URI | null {
if (this.contextService.getWorkbenchState() === WorkbenchState.EMPTY) {
return null;
}
const workspace = this.contextService.getWorkspace();
return workspace.configuration || workspace.folders[0].toResource(FOLDER_SETTINGS_PATH);
}
get settingsEditor2Input(): SettingsEditor2Input {
return this.instantiationService.createInstance(SettingsEditor2Input);
}
getFolderSettingsResource(resource: URI): URI | null {
const folder = this.contextService.getWorkspaceFolder(resource);
return folder ? folder.toResource(FOLDER_SETTINGS_PATH) : null;
}
resolveModel(uri: URI): Promise<ITextModel | null> {
if (this.isDefaultSettingsResource(uri)) {
const target = this.getConfigurationTargetFromDefaultSettingsResource(uri);
const languageSelection = this.modeService.create('jsonc');
const model = this._register(this.modelService.createModel('', languageSelection, uri));
let defaultSettings: DefaultSettings | undefined;
this.configurationService.onDidChangeConfiguration(e => {
if (e.source === ConfigurationTarget.DEFAULT) {
const model = this.modelService.getModel(uri);
if (!model) {
// model has not been given out => nothing to do
return;
}
defaultSettings = this.getDefaultSettings(target);
this.modelService.updateModel(model, defaultSettings.getContent(true));
defaultSettings._onDidChange.fire();
}
});
// Check if Default settings is already created and updated in above promise
if (!defaultSettings) {
defaultSettings = this.getDefaultSettings(target);
this.modelService.updateModel(model, defaultSettings.getContent(true));
}
return Promise.resolve(model);
}
if (this.defaultSettingsRawResource.toString() === uri.toString()) {
const defaultRawSettingsEditorModel = this.instantiationService.createInstance(DefaultRawSettingsEditorModel, this.getDefaultSettings(ConfigurationTarget.USER_LOCAL));
const languageSelection = this.modeService.create('jsonc');
const model = this._register(this.modelService.createModel(defaultRawSettingsEditorModel.content, languageSelection, uri));
return Promise.resolve(model);
}
if (this.defaultKeybindingsResource.toString() === uri.toString()) {
const defaultKeybindingsEditorModel = this.instantiationService.createInstance(DefaultKeybindingsEditorModel, uri);
const languageSelection = this.modeService.create('jsonc');
const model = this._register(this.modelService.createModel(defaultKeybindingsEditorModel.content, languageSelection, uri));
return Promise.resolve(model);
}
return Promise.resolve(null);
}
async createPreferencesEditorModel(uri: URI): Promise<IPreferencesEditorModel<any> | null> {
if (this.isDefaultSettingsResource(uri)) {
return this.createDefaultSettingsEditorModel(uri);
}
if (this.userSettingsResource.toString() === uri.toString()) {
return this.createEditableSettingsEditorModel(ConfigurationTarget.USER_LOCAL, uri);
}
const workspaceSettingsUri = await this.getEditableSettingsURI(ConfigurationTarget.WORKSPACE);
if (workspaceSettingsUri && workspaceSettingsUri.toString() === uri.toString()) {
return this.createEditableSettingsEditorModel(ConfigurationTarget.WORKSPACE, workspaceSettingsUri);
}
if (this.contextService.getWorkbenchState() === WorkbenchState.WORKSPACE) {
const settingsUri = await this.getEditableSettingsURI(ConfigurationTarget.WORKSPACE_FOLDER, uri);
if (settingsUri && settingsUri.toString() === uri.toString()) {
return this.createEditableSettingsEditorModel(ConfigurationTarget.WORKSPACE_FOLDER, uri);
}
}
const remoteEnvironment = await this.remoteAgentService.getEnvironment();
const remoteSettingsUri = remoteEnvironment ? remoteEnvironment.settingsPath : null;
if (remoteSettingsUri && remoteSettingsUri.toString() === uri.toString()) {
return this.createEditableSettingsEditorModel(ConfigurationTarget.USER_REMOTE, uri);
}
return null;
}
openRawDefaultSettings(): Promise<IEditor | undefined> {
return this.editorService.openEditor({ resource: this.defaultSettingsRawResource });
}
openRawUserSettings(): Promise<IEditor | undefined> {
return this.editorService.openEditor({ resource: this.userSettingsResource });
}
openSettings(jsonEditor: boolean | undefined, query: string | undefined): Promise<IEditor | undefined> {
jsonEditor = typeof jsonEditor === 'undefined' ?
this.configurationService.getValue('workbench.settings.editor') === 'json' :
jsonEditor;
if (!jsonEditor) {
return this.openSettings2({ query: query });
}
const editorInput = this.getActiveSettingsEditorInput() || this.lastOpenedSettingsInput;
const resource = editorInput ? editorInput.master.getResource()! : this.userSettingsResource;
const target = this.getConfigurationTargetFromSettingsResource(resource);
return this.openOrSwitchSettings(target, resource, { query: query });
}
private openSettings2(options?: ISettingsEditorOptions): Promise<IEditor> {
const input = this.settingsEditor2Input;
return this.editorService.openEditor(input, options ? SettingsEditorOptions.create(options) : undefined)
.then(() => this.editorGroupService.activeGroup.activeControl!);
}
openGlobalSettings(jsonEditor?: boolean, options?: ISettingsEditorOptions, group?: IEditorGroup): Promise<IEditor | undefined> {
jsonEditor = typeof jsonEditor === 'undefined' ?
this.configurationService.getValue('workbench.settings.editor') === 'json' :
jsonEditor;
return jsonEditor ?
this.openOrSwitchSettings(ConfigurationTarget.USER_LOCAL, this.userSettingsResource, options, group) :
this.openOrSwitchSettings2(ConfigurationTarget.USER_LOCAL, undefined, options, group);
}
async openRemoteSettings(): Promise<IEditor | undefined> {
const environment = await this.remoteAgentService.getEnvironment();
if (environment) {
await this.createIfNotExists(environment.settingsPath, emptyEditableSettingsContent);
return this.editorService.openEditor({ resource: environment.settingsPath, options: { pinned: true, revealIfOpened: true } });
}
return undefined;
}
openWorkspaceSettings(jsonEditor?: boolean, options?: ISettingsEditorOptions, group?: IEditorGroup): Promise<IEditor | undefined> {
jsonEditor = typeof jsonEditor === 'undefined' ?
this.configurationService.getValue('workbench.settings.editor') === 'json' :
jsonEditor;
if (!this.workspaceSettingsResource) {
this.notificationService.info(nls.localize('openFolderFirst', "Open a folder first to create workspace settings"));
return Promise.reject(null);
}
return jsonEditor ?
this.openOrSwitchSettings(ConfigurationTarget.WORKSPACE, this.workspaceSettingsResource, options, group) :
this.openOrSwitchSettings2(ConfigurationTarget.WORKSPACE, undefined, options, group);
}
async openFolderSettings(folder: URI, jsonEditor?: boolean, options?: ISettingsEditorOptions, group?: IEditorGroup): Promise<IEditor | undefined> {
jsonEditor = typeof jsonEditor === 'undefined' ?
this.configurationService.getValue('workbench.settings.editor') === 'json' :
jsonEditor;
const folderSettingsUri = await this.getEditableSettingsURI(ConfigurationTarget.WORKSPACE_FOLDER, folder);
if (jsonEditor) {
if (folderSettingsUri) {
return this.openOrSwitchSettings(ConfigurationTarget.WORKSPACE_FOLDER, folderSettingsUri, options, group);
}
return Promise.reject(`Invalid folder URI - ${folder.toString()}`);
}
return this.openOrSwitchSettings2(ConfigurationTarget.WORKSPACE_FOLDER, folder, options, group);
}
switchSettings(target: ConfigurationTarget, resource: URI, jsonEditor?: boolean): Promise<void> {
if (!jsonEditor) {
return this.doOpenSettings2(target, resource).then(() => undefined);
}
const activeControl = this.editorService.activeControl;
if (activeControl && activeControl.input instanceof PreferencesEditorInput) {
return this.doSwitchSettings(target, resource, activeControl.input, activeControl.group).then(() => undefined);
} else {
return this.doOpenSettings(target, resource).then(() => undefined);
}
}
openGlobalKeybindingSettings(textual: boolean): Promise<void> {
type OpenKeybindingsClassification = {
textual: { classification: 'SystemMetaData', purpose: 'FeatureInsight', isMeasurement: true };
};
this.telemetryService.publicLog2<{ textual: boolean }, OpenKeybindingsClassification>('openKeybindings', { textual });
if (textual) {
const emptyContents = '// ' + nls.localize('emptyKeybindingsHeader', "Place your key bindings in this file to override the defaults") + '\n[\n]';
const editableKeybindings = this.environmentService.keybindingsResource;
const openDefaultKeybindings = !!this.configurationService.getValue('workbench.settings.openDefaultKeybindings');
// Create as needed and open in editor
return this.createIfNotExists(editableKeybindings, emptyContents).then(() => {
if (openDefaultKeybindings) {
const activeEditorGroup = this.editorGroupService.activeGroup;
const sideEditorGroup = this.editorGroupService.addGroup(activeEditorGroup.id, GroupDirection.RIGHT);
return Promise.all([
this.editorService.openEditor({ resource: this.defaultKeybindingsResource, options: { pinned: true, preserveFocus: true, revealIfOpened: true }, label: nls.localize('defaultKeybindings', "Default Keybindings"), description: '' }),
this.editorService.openEditor({ resource: editableKeybindings, options: { pinned: true, revealIfOpened: true } }, sideEditorGroup.id)
]).then(editors => undefined);
} else {
return this.editorService.openEditor({ resource: editableKeybindings, options: { pinned: true, revealIfOpened: true } }).then(() => undefined);
}
});
}
return this.editorService.openEditor(this.instantiationService.createInstance(KeybindingsEditorInput), { pinned: true, revealIfOpened: true }).then(() => undefined);
}
openDefaultKeybindingsFile(): Promise<IEditor | undefined> {
return this.editorService.openEditor({ resource: this.defaultKeybindingsResource, label: nls.localize('defaultKeybindings', "Default Keybindings") });
}
configureSettingsForLanguage(language: string): void {
this.openGlobalSettings(true)
.then(editor => this.createPreferencesEditorModel(this.userSettingsResource)
.then((settingsModel: IPreferencesEditorModel<ISetting>) => {
const codeEditor = editor ? getCodeEditor(editor.getControl()) : null;
if (codeEditor) {
this.addLanguageOverrideEntry(language, settingsModel, codeEditor)
.then(position => {
if (codeEditor && position) {
codeEditor.setPosition(position);
codeEditor.revealLine(position.lineNumber);
codeEditor.focus();
}
});
}
}));
}
private openOrSwitchSettings(configurationTarget: ConfigurationTarget, resource: URI, options?: ISettingsEditorOptions, group: IEditorGroup = this.editorGroupService.activeGroup): Promise<IEditor | undefined> {
const editorInput = this.getActiveSettingsEditorInput(group);
if (editorInput) {
const editorInputResource = editorInput.master.getResource();
if (editorInputResource && editorInputResource.fsPath !== resource.fsPath) {
return this.doSwitchSettings(configurationTarget, resource, editorInput, group, options);
}
}
return this.doOpenSettings(configurationTarget, resource, options, group);
}
private openOrSwitchSettings2(configurationTarget: ConfigurationTarget, folderUri?: URI, options?: ISettingsEditorOptions, group: IEditorGroup = this.editorGroupService.activeGroup): Promise<IEditor | undefined> {
return this.doOpenSettings2(configurationTarget, folderUri, options, group);
}
private doOpenSettings(configurationTarget: ConfigurationTarget, resource: URI, options?: ISettingsEditorOptions, group?: IEditorGroup): Promise<IEditor | undefined> {
const openSplitJSON = !!this.configurationService.getValue(USE_SPLIT_JSON_SETTING);
if (openSplitJSON) {
return this.doOpenSplitJSON(configurationTarget, resource, options, group);
}
const openDefaultSettings = !!this.configurationService.getValue(DEFAULT_SETTINGS_EDITOR_SETTING);
return this.getOrCreateEditableSettingsEditorInput(configurationTarget, resource)
.then(editableSettingsEditorInput => {
if (!options) {
options = { pinned: true };
} else {
options = assign(options, { pinned: true });
}
if (openDefaultSettings) {
const activeEditorGroup = this.editorGroupService.activeGroup;
const sideEditorGroup = this.editorGroupService.addGroup(activeEditorGroup.id, GroupDirection.RIGHT);
return Promise.all([
this.editorService.openEditor({ resource: this.defaultSettingsRawResource, options: { pinned: true, preserveFocus: true, revealIfOpened: true }, label: nls.localize('defaultSettings', "Default Settings"), description: '' }),
this.editorService.openEditor(editableSettingsEditorInput, { pinned: true, revealIfOpened: true }, sideEditorGroup.id)
]).then(([defaultEditor, editor]) => withNullAsUndefined(editor));
} else {
return this.editorService.openEditor(editableSettingsEditorInput, SettingsEditorOptions.create(options), group);
}
});
}
private doOpenSplitJSON(configurationTarget: ConfigurationTarget, resource: URI, options?: ISettingsEditorOptions, group?: IEditorGroup): Promise<IEditor | undefined> {
return this.getOrCreateEditableSettingsEditorInput(configurationTarget, resource)
.then(editableSettingsEditorInput => {
if (!options) {
options = { pinned: true };
} else {
options = assign(options, { pinned: true });
}
const defaultPreferencesEditorInput = this.instantiationService.createInstance(DefaultPreferencesEditorInput, this.getDefaultSettingsResource(configurationTarget));
const preferencesEditorInput = new PreferencesEditorInput(this.getPreferencesEditorInputName(configurationTarget, resource), editableSettingsEditorInput.getDescription(), defaultPreferencesEditorInput, <EditorInput>editableSettingsEditorInput);
this.lastOpenedSettingsInput = preferencesEditorInput;
return this.editorService.openEditor(preferencesEditorInput, SettingsEditorOptions.create(options), group);
});
}
public createSettings2EditorModel(): Settings2EditorModel {
return this.instantiationService.createInstance(Settings2EditorModel, this.getDefaultSettings(ConfigurationTarget.USER_LOCAL));
}
private doOpenSettings2(target: ConfigurationTarget, folderUri: URI | undefined, options?: IEditorOptions, group?: IEditorGroup): Promise<IEditor | undefined> {
const input = this.settingsEditor2Input;
const settingsOptions: ISettingsEditorOptions = {
...options,
target,
folderUri
};
return this.editorService.openEditor(input, SettingsEditorOptions.create(settingsOptions), group);
}
private async doSwitchSettings(target: ConfigurationTarget, resource: URI, input: PreferencesEditorInput, group: IEditorGroup, options?: ISettingsEditorOptions): Promise<IEditor> {
const settingsURI = await this.getEditableSettingsURI(target, resource);
if (!settingsURI) {
return Promise.reject(`Invalid settings URI - ${resource.toString()}`);
}
return this.getOrCreateEditableSettingsEditorInput(target, settingsURI)
.then(toInput => {
return group.openEditor(input).then(() => {
const replaceWith = new PreferencesEditorInput(this.getPreferencesEditorInputName(target, resource), toInput.getDescription(), this.instantiationService.createInstance(DefaultPreferencesEditorInput, this.getDefaultSettingsResource(target)), toInput);
return group.replaceEditors([{
editor: input,
replacement: replaceWith,
options: options ? SettingsEditorOptions.create(options) : undefined
}]).then(() => {
this.lastOpenedSettingsInput = replaceWith;
return group.activeControl!;
});
});
});
}
private getActiveSettingsEditorInput(group: IEditorGroup = this.editorGroupService.activeGroup): PreferencesEditorInput {
return <PreferencesEditorInput>group.editors.filter(e => e instanceof PreferencesEditorInput)[0];
}
private getConfigurationTargetFromSettingsResource(resource: URI): ConfigurationTarget {
if (this.userSettingsResource.toString() === resource.toString()) {
return ConfigurationTarget.USER_LOCAL;
}
const workspaceSettingsResource = this.workspaceSettingsResource;
if (workspaceSettingsResource && workspaceSettingsResource.toString() === resource.toString()) {
return ConfigurationTarget.WORKSPACE;
}
const folder = this.contextService.getWorkspaceFolder(resource);
if (folder) {
return ConfigurationTarget.WORKSPACE_FOLDER;
}
return ConfigurationTarget.USER_LOCAL;
}
private getConfigurationTargetFromDefaultSettingsResource(uri: URI) {
return this.isDefaultWorkspaceSettingsResource(uri) ?
ConfigurationTarget.WORKSPACE :
this.isDefaultFolderSettingsResource(uri) ?
ConfigurationTarget.WORKSPACE_FOLDER :
ConfigurationTarget.USER_LOCAL;
}
private isDefaultSettingsResource(uri: URI): boolean {
return this.isDefaultUserSettingsResource(uri) || this.isDefaultWorkspaceSettingsResource(uri) || this.isDefaultFolderSettingsResource(uri);
}
private isDefaultUserSettingsResource(uri: URI): boolean {
return uri.authority === 'defaultsettings' && uri.scheme === network.Schemas.vscode && !!uri.path.match(/\/(\d+\/)?settings\.json$/);
}
private isDefaultWorkspaceSettingsResource(uri: URI): boolean {
return uri.authority === 'defaultsettings' && uri.scheme === network.Schemas.vscode && !!uri.path.match(/\/(\d+\/)?workspaceSettings\.json$/);
}
private isDefaultFolderSettingsResource(uri: URI): boolean {
return uri.authority === 'defaultsettings' && uri.scheme === network.Schemas.vscode && !!uri.path.match(/\/(\d+\/)?resourceSettings\.json$/);
}
private getDefaultSettingsResource(configurationTarget: ConfigurationTarget): URI {
switch (configurationTarget) {
case ConfigurationTarget.WORKSPACE:
return URI.from({ scheme: network.Schemas.vscode, authority: 'defaultsettings', path: `/${this._defaultWorkspaceSettingsUriCounter++}/workspaceSettings.json` });
case ConfigurationTarget.WORKSPACE_FOLDER:
return URI.from({ scheme: network.Schemas.vscode, authority: 'defaultsettings', path: `/${this._defaultFolderSettingsUriCounter++}/resourceSettings.json` });
}
return URI.from({ scheme: network.Schemas.vscode, authority: 'defaultsettings', path: `/${this._defaultUserSettingsUriCounter++}/settings.json` });
}
private getPreferencesEditorInputName(target: ConfigurationTarget, resource: URI): string {
const name = getSettingsTargetName(target, resource, this.contextService);
return target === ConfigurationTarget.WORKSPACE_FOLDER ? nls.localize('folderSettingsName', "{0} (Folder Settings)", name) : name;
}
private getOrCreateEditableSettingsEditorInput(target: ConfigurationTarget, resource: URI): Promise<EditorInput> {
return this.createSettingsIfNotExists(target, resource)
.then(() => <EditorInput>this.editorService.createInput({ resource }));
}
private createEditableSettingsEditorModel(configurationTarget: ConfigurationTarget, settingsUri: URI): Promise<SettingsEditorModel> {
const workspace = this.contextService.getWorkspace();
if (workspace.configuration && workspace.configuration.toString() === settingsUri.toString()) {
return this.textModelResolverService.createModelReference(settingsUri)
.then(reference => this.instantiationService.createInstance(WorkspaceConfigurationEditorModel, reference, configurationTarget));
}
return this.textModelResolverService.createModelReference(settingsUri)
.then(reference => this.instantiationService.createInstance(SettingsEditorModel, reference, configurationTarget));
}
private createDefaultSettingsEditorModel(defaultSettingsUri: URI): Promise<DefaultSettingsEditorModel> {
return this.textModelResolverService.createModelReference(defaultSettingsUri)
.then(reference => {
const target = this.getConfigurationTargetFromDefaultSettingsResource(defaultSettingsUri);
return this.instantiationService.createInstance(DefaultSettingsEditorModel, defaultSettingsUri, reference, this.getDefaultSettings(target));
});
}
private getDefaultSettings(target: ConfigurationTarget): DefaultSettings {
if (target === ConfigurationTarget.WORKSPACE) {
if (!this._defaultWorkspaceSettingsContentModel) {
this._defaultWorkspaceSettingsContentModel = new DefaultSettings(this.getMostCommonlyUsedSettings(), target);
}
return this._defaultWorkspaceSettingsContentModel;
}
if (target === ConfigurationTarget.WORKSPACE_FOLDER) {
if (!this._defaultFolderSettingsContentModel) {
this._defaultFolderSettingsContentModel = new DefaultSettings(this.getMostCommonlyUsedSettings(), target);
}
return this._defaultFolderSettingsContentModel;
}
if (!this._defaultUserSettingsContentModel) {
this._defaultUserSettingsContentModel = new DefaultSettings(this.getMostCommonlyUsedSettings(), target);
}
return this._defaultUserSettingsContentModel;
}
private async getEditableSettingsURI(configurationTarget: ConfigurationTarget, resource?: URI): Promise<URI | null> {
switch (configurationTarget) {
case ConfigurationTarget.USER:
case ConfigurationTarget.USER_LOCAL:
return this.userSettingsResource;
case ConfigurationTarget.USER_REMOTE:
const remoteEnvironment = await this.remoteAgentService.getEnvironment();
return remoteEnvironment ? remoteEnvironment.settingsPath : null;
case ConfigurationTarget.WORKSPACE:
return this.workspaceSettingsResource;
case ConfigurationTarget.WORKSPACE_FOLDER:
if (resource) {
return this.getFolderSettingsResource(resource);
}
}
return null;
}
private createSettingsIfNotExists(target: ConfigurationTarget, resource: URI): Promise<void> {
if (this.contextService.getWorkbenchState() === WorkbenchState.WORKSPACE && target === ConfigurationTarget.WORKSPACE) {
const workspaceConfig = this.contextService.getWorkspace().configuration;
if (!workspaceConfig) {
return Promise.resolve(undefined);
}
return this.textFileService.read(workspaceConfig)
.then(content => {
if (Object.keys(parse(content.value)).indexOf('settings') === -1) {
return this.jsonEditingService.write(resource, [{ key: 'settings', value: {} }], true).then(undefined, () => { });
}
return undefined;
});
}
return this.createIfNotExists(resource, emptyEditableSettingsContent).then(() => { });
}
private createIfNotExists(resource: URI, contents: string): Promise<any> {
return this.textFileService.read(resource, { acceptTextOnly: true }).then(undefined, error => {
if ((<FileOperationError>error).fileOperationResult === FileOperationResult.FILE_NOT_FOUND) {
return this.textFileService.write(resource, contents).then(undefined, error => {
return Promise.reject(new Error(nls.localize('fail.createSettings', "Unable to create '{0}' ({1}).", this.labelService.getUriLabel(resource, { relative: true }), error)));
});
}
return Promise.reject(error);
});
}
private getMostCommonlyUsedSettings(): string[] {
return [
'files.autoSave',
'editor.fontSize',
'editor.fontFamily',
'editor.tabSize',
'editor.renderWhitespace',
'editor.cursorStyle',
'editor.multiCursorModifier',
'editor.insertSpaces',
'editor.wordWrap',
'files.exclude',
'files.associations'
];
}
private addLanguageOverrideEntry(language: string, settingsModel: IPreferencesEditorModel<ISetting>, codeEditor: ICodeEditor): Promise<IPosition | null> {
const languageKey = `[${language}]`;
let setting = settingsModel.getPreference(languageKey);
const model = codeEditor.getModel();
if (model) {
const configuration = this.configurationService.getValue<{ editor: { tabSize: number; insertSpaces: boolean } }>();
const eol = model.getEOL();
if (setting) {
if (setting.overrides && setting.overrides.length) {
const lastSetting = setting.overrides[setting.overrides.length - 1];
return Promise.resolve({ lineNumber: lastSetting.valueRange.endLineNumber, column: model.getLineMaxColumn(lastSetting.valueRange.endLineNumber) });
}
return Promise.resolve({ lineNumber: setting.valueRange.startLineNumber, column: setting.valueRange.startColumn + 1 });
}
return this.configurationService.updateValue(languageKey, {}, ConfigurationTarget.USER)
.then(() => {
setting = settingsModel.getPreference(languageKey);
if (setting) {
let content = eol + this.spaces(2, configuration.editor) + eol + this.spaces(1, configuration.editor);
let editOperation = EditOperation.insert(new Position(setting.valueRange.endLineNumber, setting.valueRange.endColumn - 1), content);
model.pushEditOperations([], [editOperation], () => []);
let lineNumber = setting.valueRange.endLineNumber + 1;
settingsModel.dispose();
return { lineNumber, column: model.getLineMaxColumn(lineNumber) };
}
return null;
});
}
return Promise.resolve(null);
}
private spaces(count: number, { tabSize, insertSpaces }: { tabSize: number; insertSpaces: boolean }): string {
return insertSpaces ? strings.repeat(' ', tabSize * count) : strings.repeat('\t', count);
}
public dispose(): void {
this._onDispose.fire();
super.dispose();
}
}
registerSingleton(IPreferencesService, PreferencesService);
| src/vs/workbench/services/preferences/browser/preferencesService.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0004945398541167378,
0.00017843070963863283,
0.0001633671490708366,
0.00017456882051192224,
0.000039973892853595316
] |
{
"id": 6,
"code_window": [
"import { ResolvedKeybinding } from 'vs/base/common/keyCodes';\n",
"import { IItemAccessor } from 'vs/base/parts/quickopen/common/quickOpenScorer';\n",
"import { coalesce } from 'vs/base/common/arrays';\n",
"\n",
"export interface IContext {\n",
"\tevent: any;\n",
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { IMatch } from 'vs/base/common/filters';\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "add",
"edit_start_line_idx": 21
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IDisposable } from 'vs/base/common/lifecycle';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { IMenuService, MenuId, IMenu } from 'vs/platform/actions/common/actions';
import { IAction } from 'vs/base/common/actions';
import { IContextMenuService } from 'vs/platform/contextview/browser/contextView';
import { Comment, CommentThread } from 'vs/editor/common/modes';
import { createAndFillInContextMenuActions } from 'vs/platform/actions/browser/menuEntryActionViewItem';
export class CommentMenus implements IDisposable {
constructor(
@IMenuService private readonly menuService: IMenuService,
@IContextMenuService private readonly contextMenuService: IContextMenuService
) { }
getCommentThreadTitleActions(commentThread: CommentThread, contextKeyService: IContextKeyService): IMenu {
return this.getMenu(MenuId.CommentThreadTitle, contextKeyService);
}
getCommentThreadActions(commentThread: CommentThread, contextKeyService: IContextKeyService): IMenu {
return this.getMenu(MenuId.CommentThreadActions, contextKeyService);
}
getCommentTitleActions(comment: Comment, contextKeyService: IContextKeyService): IMenu {
return this.getMenu(MenuId.CommentTitle, contextKeyService);
}
getCommentActions(comment: Comment, contextKeyService: IContextKeyService): IMenu {
return this.getMenu(MenuId.CommentActions, contextKeyService);
}
private getMenu(menuId: MenuId, contextKeyService: IContextKeyService): IMenu {
const menu = this.menuService.createMenu(menuId, contextKeyService);
const primary: IAction[] = [];
const secondary: IAction[] = [];
const result = { primary, secondary };
createAndFillInContextMenuActions(menu, { shouldForwardArgs: true }, result, this.contextMenuService, g => /^inline/.test(g));
return menu;
}
dispose(): void {
}
}
| src/vs/workbench/contrib/comments/browser/commentMenus.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.8998459577560425,
0.2993136942386627,
0.00017462756659369916,
0.0005860925884917378,
0.42275768518447876
] |
{
"id": 6,
"code_window": [
"import { ResolvedKeybinding } from 'vs/base/common/keyCodes';\n",
"import { IItemAccessor } from 'vs/base/parts/quickopen/common/quickOpenScorer';\n",
"import { coalesce } from 'vs/base/common/arrays';\n",
"\n",
"export interface IContext {\n",
"\tevent: any;\n",
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { IMatch } from 'vs/base/common/filters';\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "add",
"edit_start_line_idx": 21
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
.monaco-quick-open-widget .monaco-tree .monaco-tree-row .monaco-highlighted-label .highlight,
.monaco-quick-open-widget .monaco-list .monaco-list-row .monaco-highlighted-label .highlight {
color: #0066BF;
}
.vs-dark .monaco-quick-open-widget .monaco-tree .monaco-tree-row .monaco-highlighted-label .highlight,
.vs-dark .monaco-quick-open-widget .monaco-list .monaco-list-row .monaco-highlighted-label .highlight {
color: #0097fb;
}
.hc-black .monaco-quick-open-widget .monaco-tree .monaco-tree-row .monaco-highlighted-label .highlight,
.hc-black .monaco-quick-open-widget .monaco-list .monaco-list-row .monaco-highlighted-label .highlight {
color: #F38518;
} | src/vs/editor/standalone/browser/quickOpen/editorQuickOpen.css | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017654284602031112,
0.00017528186435811222,
0.00017402088269591331,
0.00017528186435811222,
0.0000012609816621989012
] |
{
"id": 7,
"code_window": [
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n",
"\n",
"export interface IHighlight {\n",
"\tstart: number;\n",
"\tend: number;\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IHighlight extends IMatch {\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "replace",
"edit_start_line_idx": 27
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
declare namespace monaco {
// THIS IS A GENERATED FILE. DO NOT EDIT DIRECTLY.
export type Thenable<T> = PromiseLike<T>;
export interface IDisposable {
dispose(): void;
}
export interface IEvent<T> {
(listener: (e: T) => any, thisArg?: any): IDisposable;
}
/**
* A helper that allows to emit and listen to typed events
*/
export class Emitter<T> {
constructor();
readonly event: Event<T>;
fire(event: T): void;
dispose(): void;
}
#include(vs/platform/markers/common/markers): MarkerTag, MarkerSeverity
#include(vs/base/common/cancellation): CancellationTokenSource, CancellationToken
#include(vs/base/common/uri): URI, UriComponents
#include(vs/base/common/keyCodes): KeyCode
#include(vs/editor/common/standalone/standaloneBase): KeyMod
#include(vs/base/common/htmlContent): IMarkdownString
#include(vs/base/browser/keyboardEvent): IKeyboardEvent
#include(vs/base/browser/mouseEvent): IMouseEvent
#include(vs/editor/common/editorCommon): IScrollEvent
#include(vs/editor/common/core/position): IPosition, Position
#include(vs/editor/common/core/range): IRange, Range
#include(vs/editor/common/core/selection): ISelection, Selection, SelectionDirection
#include(vs/editor/common/core/token): Token
}
declare namespace monaco.editor {
#includeAll(vs/editor/standalone/browser/standaloneEditor;modes.=>languages.;editorCommon.=>):
#include(vs/editor/standalone/common/standaloneThemeService): BuiltinTheme, IStandaloneThemeData, IColors
#include(vs/editor/common/modes/supports/tokenization): ITokenThemeRule
#include(vs/editor/common/services/webWorker): MonacoWebWorker, IWebWorkerOptions
#include(vs/editor/standalone/browser/standaloneCodeEditor): IActionDescriptor, IStandaloneEditorConstructionOptions, IDiffEditorConstructionOptions, IStandaloneCodeEditor, IStandaloneDiffEditor
export interface ICommandHandler {
(...args: any[]): void;
}
#include(vs/platform/contextkey/common/contextkey): IContextKey
#include(vs/editor/standalone/browser/standaloneServices): IEditorOverrideServices
#include(vs/platform/markers/common/markers): IMarker, IMarkerData, IRelatedInformation
#include(vs/editor/standalone/browser/colorizer): IColorizerOptions, IColorizerElementOptions
#include(vs/base/common/scrollable): ScrollbarVisibility
#include(vs/platform/theme/common/themeService): ThemeColor
#includeAll(vs/editor/common/model;LanguageIdentifier=>languages.LanguageIdentifier): IScrollEvent
#includeAll(vs/editor/common/editorCommon;editorOptions.=>): IScrollEvent
#includeAll(vs/editor/common/model/textModelEvents):
#includeAll(vs/editor/common/controller/cursorEvents):
#include(vs/platform/accessibility/common/accessibility): AccessibilitySupport
#includeAll(vs/editor/common/config/editorOptions):
#includeAll(vs/editor/browser/editorBrowser;editorCommon.=>;editorOptions.=>):
#include(vs/editor/common/config/fontInfo): FontInfo, BareFontInfo
//compatibility:
export type IReadOnlyModel = ITextModel;
export type IModel = ITextModel;
}
declare namespace monaco.languages {
#includeAll(vs/editor/standalone/browser/standaloneLanguages;modes.=>;editorCommon.=>editor.;model.=>editor.;IMarkerData=>editor.IMarkerData):
#includeAll(vs/editor/common/modes/languageConfiguration):
#includeAll(vs/editor/common/modes;editorCommon.IRange=>IRange;editorCommon.IPosition=>IPosition;editorCommon.=>editor.;IMarkerData=>editor.IMarkerData;model.=>editor.):
#include(vs/editor/common/services/modeService): ILanguageExtensionPoint
#includeAll(vs/editor/standalone/common/monarch/monarchTypes):
}
declare namespace monaco.worker {
#includeAll(vs/editor/common/services/editorSimpleWorker;):
}
//dtsv=2
| build/monaco/monaco.d.ts.recipe | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017835698963608593,
0.00017271740944124758,
0.0001667374890530482,
0.00017211381054949015,
0.000003604882749641547
] |
{
"id": 7,
"code_window": [
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n",
"\n",
"export interface IHighlight {\n",
"\tstart: number;\n",
"\tend: number;\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IHighlight extends IMatch {\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "replace",
"edit_start_line_idx": 27
} | struct Foo {
Foo();
int a;
int b;
int c;
};
Foo::Foo()
: a(1),
// b(2),
c(3) {}
| extensions/cpp/test/colorize-fixtures/test-80644.cpp | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00018012536747846752,
0.00017631251830607653,
0.00017249966913368553,
0.00017631251830607653,
0.0000038128491723909974
] |
{
"id": 7,
"code_window": [
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n",
"\n",
"export interface IHighlight {\n",
"\tstart: number;\n",
"\tend: number;\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IHighlight extends IMatch {\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "replace",
"edit_start_line_idx": 27
} | 'use strict';
var M;
(function (M) {
var C = (function () {
function C() {
}
return C;
})();
(function (x, property, number) {
if (property === undefined) { property = w; }
var local = 1;
// unresolved symbol because x is local
//self.x++;
self.w--; // ok because w is a property
property;
f = function (y) {
return y + x + local + w + self.w;
};
function sum(z) {
return z + f(z) + w + self.w;
}
});
})(M || (M = {}));
var c = new M.C(12, 5);
| src/vs/workbench/services/search/test/node/fixtures/examples/small.js | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.000705058453604579,
0.0003500524035189301,
0.00017253321129828691,
0.00017256563296541572,
0.00025102717336267233
] |
{
"id": 7,
"code_window": [
"\tquickNavigateConfiguration: IQuickNavigateConfiguration;\n",
"}\n",
"\n",
"export interface IHighlight {\n",
"\tstart: number;\n",
"\tend: number;\n",
"}\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IHighlight extends IMatch {\n"
],
"file_path": "src/vs/base/parts/quickopen/browser/quickOpenModel.ts",
"type": "replace",
"edit_start_line_idx": 27
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Registry } from 'vs/platform/registry/common/platform';
import { IPanel } from 'vs/workbench/common/panel';
import { Composite, CompositeDescriptor, CompositeRegistry } from 'vs/workbench/browser/composite';
import { Action } from 'vs/base/common/actions';
import { IPanelService } from 'vs/workbench/services/panel/common/panelService';
import { IWorkbenchLayoutService, Parts } from 'vs/workbench/services/layout/browser/layoutService';
import { IConstructorSignature0 } from 'vs/platform/instantiation/common/instantiation';
import { isAncestor } from 'vs/base/browser/dom';
import { assertIsDefined } from 'vs/base/common/types';
export abstract class Panel extends Composite implements IPanel { }
/**
* A panel descriptor is a leightweight descriptor of a panel in the workbench.
*/
export class PanelDescriptor extends CompositeDescriptor<Panel> {
constructor(ctor: IConstructorSignature0<Panel>, id: string, name: string, cssClass?: string, order?: number, _commandId?: string) {
super(ctor, id, name, cssClass, order, _commandId);
}
}
export class PanelRegistry extends CompositeRegistry<Panel> {
private defaultPanelId: string | undefined;
/**
* Registers a panel to the platform.
*/
registerPanel(descriptor: PanelDescriptor): void {
super.registerComposite(descriptor);
}
/**
* Deregisters a panel to the platform.
*/
deregisterPanel(id: string): void {
super.deregisterComposite(id);
}
/**
* Returns a panel by id.
*/
getPanel(id: string): PanelDescriptor | undefined {
return this.getComposite(id);
}
/**
* Returns an array of registered panels known to the platform.
*/
getPanels(): PanelDescriptor[] {
return this.getComposites();
}
/**
* Sets the id of the panel that should open on startup by default.
*/
setDefaultPanelId(id: string): void {
this.defaultPanelId = id;
}
/**
* Gets the id of the panel that should open on startup by default.
*/
getDefaultPanelId(): string {
return assertIsDefined(this.defaultPanelId);
}
/**
* Find out if a panel exists with the provided ID.
*/
hasPanel(id: string): boolean {
return this.getPanels().some(panel => panel.id === id);
}
}
/**
* A reusable action to toggle a panel with a specific id depending on focus.
*/
export abstract class TogglePanelAction extends Action {
constructor(
id: string,
label: string,
private readonly panelId: string,
protected panelService: IPanelService,
private layoutService: IWorkbenchLayoutService,
cssClass?: string
) {
super(id, label, cssClass);
}
run(): Promise<any> {
if (this.isPanelFocused()) {
this.layoutService.setPanelHidden(true);
} else {
this.panelService.openPanel(this.panelId, true);
}
return Promise.resolve();
}
private isPanelActive(): boolean {
const activePanel = this.panelService.getActivePanel();
return activePanel?.getId() === this.panelId;
}
private isPanelFocused(): boolean {
const activeElement = document.activeElement;
const panelPart = this.layoutService.getContainer(Parts.PANEL_PART);
return !!(this.isPanelActive() && activeElement && panelPart && isAncestor(activeElement, panelPart));
}
}
export const Extensions = {
Panels: 'workbench.contributions.panels'
};
Registry.add(Extensions.Panels, new PanelRegistry());
| src/vs/workbench/browser/panel.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0024208042304962873,
0.00036476299283094704,
0.00016290329222101718,
0.00017102077254094183,
0.0005977026303298771
] |
{
"id": 9,
"code_window": [
"/**\n",
" * Create a new diff navigator for the provided diff editor.\n",
" */\n",
"export class DiffNavigator extends Disposable {\n",
"\n",
"\tprivate readonly _editor: IDiffEditor;\n",
"\tprivate readonly _options: Options;\n",
"\tprivate readonly _onDidUpdate = this._register(new Emitter<this>());\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export class DiffNavigator extends Disposable implements IDiffNavigator {\n"
],
"file_path": "src/vs/editor/browser/widget/diffNavigator.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/css!./standalone-tokens';
import { IDisposable } from 'vs/base/common/lifecycle';
import { URI } from 'vs/base/common/uri';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { ICodeEditorService } from 'vs/editor/browser/services/codeEditorService';
import { OpenerService } from 'vs/editor/browser/services/openerService';
import { DiffNavigator } from 'vs/editor/browser/widget/diffNavigator';
import { ConfigurationChangedEvent } from 'vs/editor/common/config/editorOptions';
import { BareFontInfo, FontInfo } from 'vs/editor/common/config/fontInfo';
import { Token } from 'vs/editor/common/core/token';
import * as editorCommon from 'vs/editor/common/editorCommon';
import { FindMatch, ITextModel, TextModelResolvedOptions } from 'vs/editor/common/model';
import * as modes from 'vs/editor/common/modes';
import { NULL_STATE, nullTokenize } from 'vs/editor/common/modes/nullMode';
import { IEditorWorkerService } from 'vs/editor/common/services/editorWorkerService';
import { ILanguageSelection } from 'vs/editor/common/services/modeService';
import { ITextModelService } from 'vs/editor/common/services/resolverService';
import { IWebWorkerOptions, MonacoWebWorker, createWebWorker as actualCreateWebWorker } from 'vs/editor/common/services/webWorker';
import * as standaloneEnums from 'vs/editor/common/standalone/standaloneEnums';
import { Colorizer, IColorizerElementOptions, IColorizerOptions } from 'vs/editor/standalone/browser/colorizer';
import { SimpleEditorModelResolverService } from 'vs/editor/standalone/browser/simpleServices';
import { IDiffEditorConstructionOptions, IStandaloneEditorConstructionOptions, IStandaloneCodeEditor, IStandaloneDiffEditor, StandaloneDiffEditor, StandaloneEditor } from 'vs/editor/standalone/browser/standaloneCodeEditor';
import { DynamicStandaloneServices, IEditorOverrideServices, StaticServices } from 'vs/editor/standalone/browser/standaloneServices';
import { IStandaloneThemeData, IStandaloneThemeService } from 'vs/editor/standalone/common/standaloneThemeService';
import { ICommandService } from 'vs/platform/commands/common/commands';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { IContextViewService, IContextMenuService } from 'vs/platform/contextview/browser/contextView';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { IMarker, IMarkerData } from 'vs/platform/markers/common/markers';
import { INotificationService } from 'vs/platform/notification/common/notification';
import { IOpenerService } from 'vs/platform/opener/common/opener';
import { IAccessibilityService } from 'vs/platform/accessibility/common/accessibility';
import { clearAllFontInfos } from 'vs/editor/browser/config/configuration';
import { IEditorProgressService } from 'vs/platform/progress/common/progress';
type Omit<T, K extends keyof T> = Pick<T, Exclude<keyof T, K>>;
function withAllStandaloneServices<T extends editorCommon.IEditor>(domElement: HTMLElement, override: IEditorOverrideServices, callback: (services: DynamicStandaloneServices) => T): T {
let services = new DynamicStandaloneServices(domElement, override);
let simpleEditorModelResolverService: SimpleEditorModelResolverService | null = null;
if (!services.has(ITextModelService)) {
simpleEditorModelResolverService = new SimpleEditorModelResolverService();
services.set(ITextModelService, simpleEditorModelResolverService);
}
if (!services.has(IOpenerService)) {
services.set(IOpenerService, new OpenerService(services.get(ICodeEditorService), services.get(ICommandService)));
}
let result = callback(services);
if (simpleEditorModelResolverService) {
simpleEditorModelResolverService.setEditor(result);
}
return result;
}
/**
* Create a new editor under `domElement`.
* `domElement` should be empty (not contain other dom nodes).
* The editor will read the size of `domElement`.
*/
export function create(domElement: HTMLElement, options?: IStandaloneEditorConstructionOptions, override?: IEditorOverrideServices): IStandaloneCodeEditor {
return withAllStandaloneServices(domElement, override || {}, (services) => {
return new StandaloneEditor(
domElement,
options,
services,
services.get(IInstantiationService),
services.get(ICodeEditorService),
services.get(ICommandService),
services.get(IContextKeyService),
services.get(IKeybindingService),
services.get(IContextViewService),
services.get(IStandaloneThemeService),
services.get(INotificationService),
services.get(IConfigurationService),
services.get(IAccessibilityService)
);
});
}
/**
* Emitted when an editor is created.
* Creating a diff editor might cause this listener to be invoked with the two editors.
* @event
*/
export function onDidCreateEditor(listener: (codeEditor: ICodeEditor) => void): IDisposable {
return StaticServices.codeEditorService.get().onCodeEditorAdd((editor) => {
listener(<ICodeEditor>editor);
});
}
/**
* Create a new diff editor under `domElement`.
* `domElement` should be empty (not contain other dom nodes).
* The editor will read the size of `domElement`.
*/
export function createDiffEditor(domElement: HTMLElement, options?: IDiffEditorConstructionOptions, override?: IEditorOverrideServices): IStandaloneDiffEditor {
return withAllStandaloneServices(domElement, override || {}, (services) => {
return new StandaloneDiffEditor(
domElement,
options,
services,
services.get(IInstantiationService),
services.get(IContextKeyService),
services.get(IKeybindingService),
services.get(IContextViewService),
services.get(IEditorWorkerService),
services.get(ICodeEditorService),
services.get(IStandaloneThemeService),
services.get(INotificationService),
services.get(IConfigurationService),
services.get(IContextMenuService),
services.get(IEditorProgressService),
null
);
});
}
export interface IDiffNavigator {
canNavigate(): boolean;
next(): void;
previous(): void;
dispose(): void;
}
export interface IDiffNavigatorOptions {
readonly followsCaret?: boolean;
readonly ignoreCharChanges?: boolean;
readonly alwaysRevealFirst?: boolean;
}
export function createDiffNavigator(diffEditor: IStandaloneDiffEditor, opts?: IDiffNavigatorOptions): IDiffNavigator {
return new DiffNavigator(diffEditor, opts);
}
function doCreateModel(value: string, languageSelection: ILanguageSelection, uri?: URI): ITextModel {
return StaticServices.modelService.get().createModel(value, languageSelection, uri);
}
/**
* Create a new editor model.
* You can specify the language that should be set for this model or let the language be inferred from the `uri`.
*/
export function createModel(value: string, language?: string, uri?: URI): ITextModel {
value = value || '';
if (!language) {
let firstLF = value.indexOf('\n');
let firstLine = value;
if (firstLF !== -1) {
firstLine = value.substring(0, firstLF);
}
return doCreateModel(value, StaticServices.modeService.get().createByFilepathOrFirstLine(uri || null, firstLine), uri);
}
return doCreateModel(value, StaticServices.modeService.get().create(language), uri);
}
/**
* Change the language for a model.
*/
export function setModelLanguage(model: ITextModel, languageId: string): void {
StaticServices.modelService.get().setMode(model, StaticServices.modeService.get().create(languageId));
}
/**
* Set the markers for a model.
*/
export function setModelMarkers(model: ITextModel, owner: string, markers: IMarkerData[]): void {
if (model) {
StaticServices.markerService.get().changeOne(owner, model.uri, markers);
}
}
/**
* Get markers for owner and/or resource
*
* @returns list of markers
*/
export function getModelMarkers(filter: { owner?: string, resource?: URI, take?: number }): IMarker[] {
return StaticServices.markerService.get().read(filter);
}
/**
* Get the model that has `uri` if it exists.
*/
export function getModel(uri: URI): ITextModel | null {
return StaticServices.modelService.get().getModel(uri);
}
/**
* Get all the created models.
*/
export function getModels(): ITextModel[] {
return StaticServices.modelService.get().getModels();
}
/**
* Emitted when a model is created.
* @event
*/
export function onDidCreateModel(listener: (model: ITextModel) => void): IDisposable {
return StaticServices.modelService.get().onModelAdded(listener);
}
/**
* Emitted right before a model is disposed.
* @event
*/
export function onWillDisposeModel(listener: (model: ITextModel) => void): IDisposable {
return StaticServices.modelService.get().onModelRemoved(listener);
}
/**
* Emitted when a different language is set to a model.
* @event
*/
export function onDidChangeModelLanguage(listener: (e: { readonly model: ITextModel; readonly oldLanguage: string; }) => void): IDisposable {
return StaticServices.modelService.get().onModelModeChanged((e) => {
listener({
model: e.model,
oldLanguage: e.oldModeId
});
});
}
/**
* Create a new web worker that has model syncing capabilities built in.
* Specify an AMD module to load that will `create` an object that will be proxied.
*/
export function createWebWorker<T>(opts: IWebWorkerOptions): MonacoWebWorker<T> {
return actualCreateWebWorker<T>(StaticServices.modelService.get(), opts);
}
/**
* Colorize the contents of `domNode` using attribute `data-lang`.
*/
export function colorizeElement(domNode: HTMLElement, options: IColorizerElementOptions): Promise<void> {
return Colorizer.colorizeElement(StaticServices.standaloneThemeService.get(), StaticServices.modeService.get(), domNode, options);
}
/**
* Colorize `text` using language `languageId`.
*/
export function colorize(text: string, languageId: string, options: IColorizerOptions): Promise<string> {
return Colorizer.colorize(StaticServices.modeService.get(), text, languageId, options);
}
/**
* Colorize a line in a model.
*/
export function colorizeModelLine(model: ITextModel, lineNumber: number, tabSize: number = 4): string {
return Colorizer.colorizeModelLine(model, lineNumber, tabSize);
}
/**
* @internal
*/
function getSafeTokenizationSupport(language: string): Omit<modes.ITokenizationSupport, 'tokenize2'> {
let tokenizationSupport = modes.TokenizationRegistry.get(language);
if (tokenizationSupport) {
return tokenizationSupport;
}
return {
getInitialState: () => NULL_STATE,
tokenize: (line: string, state: modes.IState, deltaOffset: number) => nullTokenize(language, line, state, deltaOffset)
};
}
/**
* Tokenize `text` using language `languageId`
*/
export function tokenize(text: string, languageId: string): Token[][] {
let modeService = StaticServices.modeService.get();
// Needed in order to get the mode registered for subsequent look-ups
modeService.triggerMode(languageId);
let tokenizationSupport = getSafeTokenizationSupport(languageId);
let lines = text.split(/\r\n|\r|\n/);
let result: Token[][] = [];
let state = tokenizationSupport.getInitialState();
for (let i = 0, len = lines.length; i < len; i++) {
let line = lines[i];
let tokenizationResult = tokenizationSupport.tokenize(line, state, 0);
result[i] = tokenizationResult.tokens;
state = tokenizationResult.endState;
}
return result;
}
/**
* Define a new theme or update an existing theme.
*/
export function defineTheme(themeName: string, themeData: IStandaloneThemeData): void {
StaticServices.standaloneThemeService.get().defineTheme(themeName, themeData);
}
/**
* Switches to a theme.
*/
export function setTheme(themeName: string): void {
StaticServices.standaloneThemeService.get().setTheme(themeName);
}
/**
* Clears all cached font measurements and triggers re-measurement.
*/
export function remeasureFonts(): void {
clearAllFontInfos();
}
/**
* @internal
*/
export function createMonacoEditorAPI(): typeof monaco.editor {
return {
// methods
create: <any>create,
onDidCreateEditor: <any>onDidCreateEditor,
createDiffEditor: <any>createDiffEditor,
createDiffNavigator: <any>createDiffNavigator,
createModel: <any>createModel,
setModelLanguage: <any>setModelLanguage,
setModelMarkers: <any>setModelMarkers,
getModelMarkers: <any>getModelMarkers,
getModels: <any>getModels,
getModel: <any>getModel,
onDidCreateModel: <any>onDidCreateModel,
onWillDisposeModel: <any>onWillDisposeModel,
onDidChangeModelLanguage: <any>onDidChangeModelLanguage,
createWebWorker: <any>createWebWorker,
colorizeElement: <any>colorizeElement,
colorize: <any>colorize,
colorizeModelLine: <any>colorizeModelLine,
tokenize: <any>tokenize,
defineTheme: <any>defineTheme,
setTheme: <any>setTheme,
remeasureFonts: remeasureFonts,
// enums
AccessibilitySupport: standaloneEnums.AccessibilitySupport,
ScrollbarVisibility: standaloneEnums.ScrollbarVisibility,
WrappingIndent: standaloneEnums.WrappingIndent,
OverviewRulerLane: standaloneEnums.OverviewRulerLane,
MinimapPosition: standaloneEnums.MinimapPosition,
EndOfLinePreference: standaloneEnums.EndOfLinePreference,
DefaultEndOfLine: standaloneEnums.DefaultEndOfLine,
EndOfLineSequence: standaloneEnums.EndOfLineSequence,
TrackedRangeStickiness: standaloneEnums.TrackedRangeStickiness,
CursorChangeReason: standaloneEnums.CursorChangeReason,
MouseTargetType: standaloneEnums.MouseTargetType,
TextEditorCursorStyle: standaloneEnums.TextEditorCursorStyle,
TextEditorCursorBlinkingStyle: standaloneEnums.TextEditorCursorBlinkingStyle,
ContentWidgetPositionPreference: standaloneEnums.ContentWidgetPositionPreference,
OverlayWidgetPositionPreference: standaloneEnums.OverlayWidgetPositionPreference,
RenderMinimap: standaloneEnums.RenderMinimap,
ScrollType: standaloneEnums.ScrollType,
RenderLineNumbersType: standaloneEnums.RenderLineNumbersType,
// classes
ConfigurationChangedEvent: <any>ConfigurationChangedEvent,
BareFontInfo: <any>BareFontInfo,
FontInfo: <any>FontInfo,
TextModelResolvedOptions: <any>TextModelResolvedOptions,
FindMatch: <any>FindMatch,
// vars
EditorType: editorCommon.EditorType,
};
}
| src/vs/editor/standalone/browser/standaloneEditor.ts | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.9987438321113586,
0.053120601922273636,
0.0001610676117707044,
0.00017408543499186635,
0.2197754681110382
] |
{
"id": 9,
"code_window": [
"/**\n",
" * Create a new diff navigator for the provided diff editor.\n",
" */\n",
"export class DiffNavigator extends Disposable {\n",
"\n",
"\tprivate readonly _editor: IDiffEditor;\n",
"\tprivate readonly _options: Options;\n",
"\tprivate readonly _onDidUpdate = this._register(new Emitter<this>());\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export class DiffNavigator extends Disposable implements IDiffNavigator {\n"
],
"file_path": "src/vs/editor/browser/widget/diffNavigator.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { ResponseError, ErrorCodes, CancellationToken } from 'vscode-languageserver';
export function formatError(message: string, err: any): string {
if (err instanceof Error) {
let error = <Error>err;
return `${message}: ${error.message}\n${error.stack}`;
} else if (typeof err === 'string') {
return `${message}: ${err}`;
} else if (err) {
return `${message}: ${err.toString()}`;
}
return message;
}
export function runSafeAsync<T>(func: () => Thenable<T>, errorVal: T, errorMessage: string, token: CancellationToken): Thenable<T | ResponseError<any>> {
return new Promise<T | ResponseError<any>>((resolve) => {
setImmediate(() => {
if (token.isCancellationRequested) {
resolve(cancelValue());
}
return func().then(result => {
if (token.isCancellationRequested) {
resolve(cancelValue());
return;
} else {
resolve(result);
}
}, e => {
console.error(formatError(errorMessage, e));
resolve(errorVal);
});
});
});
}
export function runSafe<T, E>(func: () => T, errorVal: T, errorMessage: string, token: CancellationToken): Thenable<T | ResponseError<E>> {
return new Promise<T | ResponseError<E>>((resolve) => {
setImmediate(() => {
if (token.isCancellationRequested) {
resolve(cancelValue());
} else {
try {
let result = func();
if (token.isCancellationRequested) {
resolve(cancelValue());
return;
} else {
resolve(result);
}
} catch (e) {
console.error(formatError(errorMessage, e));
resolve(errorVal);
}
}
});
});
}
function cancelValue<E>() {
return new ResponseError<E>(ErrorCodes.RequestCancelled, 'Request cancelled');
}
| extensions/css-language-features/server/src/utils/runner.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017568677139934152,
0.00017351974383927882,
0.00016943870286922902,
0.00017308439419139177,
0.000002033810005741543
] |
{
"id": 9,
"code_window": [
"/**\n",
" * Create a new diff navigator for the provided diff editor.\n",
" */\n",
"export class DiffNavigator extends Disposable {\n",
"\n",
"\tprivate readonly _editor: IDiffEditor;\n",
"\tprivate readonly _options: Options;\n",
"\tprivate readonly _onDidUpdate = this._register(new Emitter<this>());\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export class DiffNavigator extends Disposable implements IDiffNavigator {\n"
],
"file_path": "src/vs/editor/browser/widget/diffNavigator.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Event } from 'vs/base/common/event';
import { LanguageId } from 'vs/editor/common/modes';
import { createDecorator } from 'vs/platform/instantiation/common/instantiation';
export const ITextMateService = createDecorator<ITextMateService>('textMateService');
export interface ITextMateService {
_serviceBrand: undefined;
onDidEncounterLanguage: Event<LanguageId>;
createGrammar(modeId: string): Promise<IGrammar>;
}
// -------------- Types "liberated" from vscode-textmate due to usage in /common/
export const enum StandardTokenType {
Other = 0,
Comment = 1,
String = 2,
RegEx = 4,
}
/**
* A grammar
*/
export interface IGrammar {
/**
* Tokenize `lineText` using previous line state `prevState`.
*/
tokenizeLine(lineText: string, prevState: StackElement | null): ITokenizeLineResult;
/**
* Tokenize `lineText` using previous line state `prevState`.
* The result contains the tokens in binary format, resolved with the following information:
* - language
* - token type (regex, string, comment, other)
* - font style
* - foreground color
* - background color
* e.g. for getting the languageId: `(metadata & MetadataConsts.LANGUAGEID_MASK) >>> MetadataConsts.LANGUAGEID_OFFSET`
*/
tokenizeLine2(lineText: string, prevState: StackElement | null): ITokenizeLineResult2;
}
export interface ITokenizeLineResult {
readonly tokens: IToken[];
/**
* The `prevState` to be passed on to the next line tokenization.
*/
readonly ruleStack: StackElement;
}
/**
* Helpers to manage the "collapsed" metadata of an entire StackElement stack.
* The following assumptions have been made:
* - languageId < 256 => needs 8 bits
* - unique color count < 512 => needs 9 bits
*
* The binary format is:
* - -------------------------------------------
* 3322 2222 2222 1111 1111 1100 0000 0000
* 1098 7654 3210 9876 5432 1098 7654 3210
* - -------------------------------------------
* xxxx xxxx xxxx xxxx xxxx xxxx xxxx xxxx
* bbbb bbbb bfff ffff ffFF FTTT LLLL LLLL
* - -------------------------------------------
* - L = LanguageId (8 bits)
* - T = StandardTokenType (3 bits)
* - F = FontStyle (3 bits)
* - f = foreground color (9 bits)
* - b = background color (9 bits)
*/
export const enum MetadataConsts {
LANGUAGEID_MASK = 255,
TOKEN_TYPE_MASK = 1792,
FONT_STYLE_MASK = 14336,
FOREGROUND_MASK = 8372224,
BACKGROUND_MASK = 4286578688,
LANGUAGEID_OFFSET = 0,
TOKEN_TYPE_OFFSET = 8,
FONT_STYLE_OFFSET = 11,
FOREGROUND_OFFSET = 14,
BACKGROUND_OFFSET = 23,
}
export interface ITokenizeLineResult2 {
/**
* The tokens in binary format. Each token occupies two array indices. For token i:
* - at offset 2*i => startIndex
* - at offset 2*i + 1 => metadata
*
*/
readonly tokens: Uint32Array;
/**
* The `prevState` to be passed on to the next line tokenization.
*/
readonly ruleStack: StackElement;
}
export interface IToken {
startIndex: number;
readonly endIndex: number;
readonly scopes: string[];
}
/**
* **IMPORTANT** - Immutable!
*/
export interface StackElement {
_stackElementBrand: void;
readonly depth: number;
clone(): StackElement;
equals(other: StackElement): boolean;
}
// -------------- End Types "liberated" from vscode-textmate due to usage in /common/
| src/vs/workbench/services/textMate/common/textMateService.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00019276620878372341,
0.00017178883717861027,
0.0001653047074796632,
0.00017099826072808355,
0.000006859798304503784
] |
{
"id": 9,
"code_window": [
"/**\n",
" * Create a new diff navigator for the provided diff editor.\n",
" */\n",
"export class DiffNavigator extends Disposable {\n",
"\n",
"\tprivate readonly _editor: IDiffEditor;\n",
"\tprivate readonly _options: Options;\n",
"\tprivate readonly _onDidUpdate = this._register(new Emitter<this>());\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export class DiffNavigator extends Disposable implements IDiffNavigator {\n"
],
"file_path": "src/vs/editor/browser/widget/diffNavigator.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as path from 'path';
import * as vscode from 'vscode';
import * as nls from 'vscode-nls';
import { OpenDocumentLinkCommand } from '../commands/openDocumentLink';
import { getUriForLinkWithKnownExternalScheme, isOfScheme, Schemes } from '../util/links';
const localize = nls.loadMessageBundle();
function parseLink(
document: vscode.TextDocument,
link: string,
base: string
): { uri: vscode.Uri, tooltip?: string } {
const externalSchemeUri = getUriForLinkWithKnownExternalScheme(link);
if (externalSchemeUri) {
// Normalize VS Code links to target currently running version
if (isOfScheme(Schemes.vscode, link) || isOfScheme(Schemes['vscode-insiders'], link)) {
return { uri: vscode.Uri.parse(link).with({ scheme: vscode.env.uriScheme }) };
}
return { uri: externalSchemeUri };
}
// Assume it must be an relative or absolute file path
// Use a fake scheme to avoid parse warnings
const tempUri = vscode.Uri.parse(`vscode-resource:${link}`);
let resourcePath = tempUri.path;
if (!tempUri.path && document.uri.scheme === 'file') {
resourcePath = document.uri.path;
} else if (tempUri.path[0] === '/') {
const root = vscode.workspace.getWorkspaceFolder(document.uri);
if (root) {
resourcePath = path.join(root.uri.fsPath, tempUri.path);
}
} else {
resourcePath = base ? path.join(base, tempUri.path) : tempUri.path;
}
return {
uri: OpenDocumentLinkCommand.createCommandUri(document.uri, resourcePath, tempUri.fragment),
tooltip: localize('documentLink.tooltip', 'Follow link')
};
}
function matchAll(
pattern: RegExp,
text: string
): Array<RegExpMatchArray> {
const out: RegExpMatchArray[] = [];
pattern.lastIndex = 0;
let match: RegExpMatchArray | null;
while ((match = pattern.exec(text))) {
out.push(match);
}
return out;
}
function extractDocumentLink(
document: vscode.TextDocument,
base: string,
pre: number,
link: string,
matchIndex: number | undefined
): vscode.DocumentLink | undefined {
const offset = (matchIndex || 0) + pre;
const linkStart = document.positionAt(offset);
const linkEnd = document.positionAt(offset + link.length);
try {
const { uri, tooltip } = parseLink(document, link, base);
const documentLink = new vscode.DocumentLink(
new vscode.Range(linkStart, linkEnd),
uri);
documentLink.tooltip = tooltip;
return documentLink;
} catch (e) {
return undefined;
}
}
export default class LinkProvider implements vscode.DocumentLinkProvider {
private readonly linkPattern = /(\[((!\[[^\]]*?\]\(\s*)([^\s\(\)]+?)\s*\)\]|(?:\\\]|[^\]])*\])\(\s*)(([^\s\(\)]|\(\S*?\))+)\s*(".*?")?\)/g;
private readonly referenceLinkPattern = /(\[((?:\\\]|[^\]])+)\]\[\s*?)([^\s\]]*?)\]/g;
private readonly definitionPattern = /^([\t ]*\[((?:\\\]|[^\]])+)\]:\s*)(\S+)/gm;
public provideDocumentLinks(
document: vscode.TextDocument,
_token: vscode.CancellationToken
): vscode.DocumentLink[] {
const base = document.uri.scheme === 'file' ? path.dirname(document.uri.fsPath) : '';
const text = document.getText();
return [
...this.providerInlineLinks(text, document, base),
...this.provideReferenceLinks(text, document, base)
];
}
private providerInlineLinks(
text: string,
document: vscode.TextDocument,
base: string
): vscode.DocumentLink[] {
const results: vscode.DocumentLink[] = [];
for (const match of matchAll(this.linkPattern, text)) {
const matchImage = match[4] && extractDocumentLink(document, base, match[3].length + 1, match[4], match.index);
if (matchImage) {
results.push(matchImage);
}
const matchLink = extractDocumentLink(document, base, match[1].length, match[5], match.index);
if (matchLink) {
results.push(matchLink);
}
}
return results;
}
private provideReferenceLinks(
text: string,
document: vscode.TextDocument,
base: string
): vscode.DocumentLink[] {
const results: vscode.DocumentLink[] = [];
const definitions = this.getDefinitions(text, document);
for (const match of matchAll(this.referenceLinkPattern, text)) {
let linkStart: vscode.Position;
let linkEnd: vscode.Position;
let reference = match[3];
if (reference) { // [text][ref]
const pre = match[1];
const offset = (match.index || 0) + pre.length;
linkStart = document.positionAt(offset);
linkEnd = document.positionAt(offset + reference.length);
} else if (match[2]) { // [ref][]
reference = match[2];
const offset = (match.index || 0) + 1;
linkStart = document.positionAt(offset);
linkEnd = document.positionAt(offset + match[2].length);
} else {
continue;
}
try {
const link = definitions.get(reference);
if (link) {
results.push(new vscode.DocumentLink(
new vscode.Range(linkStart, linkEnd),
vscode.Uri.parse(`command:_markdown.moveCursorToPosition?${encodeURIComponent(JSON.stringify([link.linkRange.start.line, link.linkRange.start.character]))}`)));
}
} catch (e) {
// noop
}
}
for (const definition of definitions.values()) {
try {
const { uri } = parseLink(document, definition.link, base);
results.push(new vscode.DocumentLink(definition.linkRange, uri));
} catch (e) {
// noop
}
}
return results;
}
private getDefinitions(text: string, document: vscode.TextDocument) {
const out = new Map<string, { link: string, linkRange: vscode.Range }>();
for (const match of matchAll(this.definitionPattern, text)) {
const pre = match[1];
const reference = match[2];
const link = match[3].trim();
const offset = (match.index || 0) + pre.length;
const linkStart = document.positionAt(offset);
const linkEnd = document.positionAt(offset + link.length);
out.set(reference, {
link: link,
linkRange: new vscode.Range(linkStart, linkEnd)
});
}
return out;
}
}
| extensions/markdown-language-features/src/features/documentLinkProvider.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017786533862818033,
0.00017468865553382784,
0.00016579404473304749,
0.00017523387214168906,
0.0000030511062050209148
] |
{
"id": 11,
"code_window": [
"\t\t\tnull\n",
"\t\t);\n",
"\t});\n",
"}\n",
"\n",
"export interface IDiffNavigator {\n",
"\tcanNavigate(): boolean;\n",
"\tnext(): void;\n",
"\tprevious(): void;\n",
"\tdispose(): void;\n",
"}\n",
"\n",
"export interface IDiffNavigatorOptions {\n",
"\treadonly followsCaret?: boolean;\n",
"\treadonly ignoreCharChanges?: boolean;\n",
"\treadonly alwaysRevealFirst?: boolean;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/editor/standalone/browser/standaloneEditor.ts",
"type": "replace",
"edit_start_line_idx": 129
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/css!./standalone-tokens';
import { IDisposable } from 'vs/base/common/lifecycle';
import { URI } from 'vs/base/common/uri';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { ICodeEditorService } from 'vs/editor/browser/services/codeEditorService';
import { OpenerService } from 'vs/editor/browser/services/openerService';
import { DiffNavigator } from 'vs/editor/browser/widget/diffNavigator';
import { ConfigurationChangedEvent } from 'vs/editor/common/config/editorOptions';
import { BareFontInfo, FontInfo } from 'vs/editor/common/config/fontInfo';
import { Token } from 'vs/editor/common/core/token';
import * as editorCommon from 'vs/editor/common/editorCommon';
import { FindMatch, ITextModel, TextModelResolvedOptions } from 'vs/editor/common/model';
import * as modes from 'vs/editor/common/modes';
import { NULL_STATE, nullTokenize } from 'vs/editor/common/modes/nullMode';
import { IEditorWorkerService } from 'vs/editor/common/services/editorWorkerService';
import { ILanguageSelection } from 'vs/editor/common/services/modeService';
import { ITextModelService } from 'vs/editor/common/services/resolverService';
import { IWebWorkerOptions, MonacoWebWorker, createWebWorker as actualCreateWebWorker } from 'vs/editor/common/services/webWorker';
import * as standaloneEnums from 'vs/editor/common/standalone/standaloneEnums';
import { Colorizer, IColorizerElementOptions, IColorizerOptions } from 'vs/editor/standalone/browser/colorizer';
import { SimpleEditorModelResolverService } from 'vs/editor/standalone/browser/simpleServices';
import { IDiffEditorConstructionOptions, IStandaloneEditorConstructionOptions, IStandaloneCodeEditor, IStandaloneDiffEditor, StandaloneDiffEditor, StandaloneEditor } from 'vs/editor/standalone/browser/standaloneCodeEditor';
import { DynamicStandaloneServices, IEditorOverrideServices, StaticServices } from 'vs/editor/standalone/browser/standaloneServices';
import { IStandaloneThemeData, IStandaloneThemeService } from 'vs/editor/standalone/common/standaloneThemeService';
import { ICommandService } from 'vs/platform/commands/common/commands';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { IContextViewService, IContextMenuService } from 'vs/platform/contextview/browser/contextView';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { IMarker, IMarkerData } from 'vs/platform/markers/common/markers';
import { INotificationService } from 'vs/platform/notification/common/notification';
import { IOpenerService } from 'vs/platform/opener/common/opener';
import { IAccessibilityService } from 'vs/platform/accessibility/common/accessibility';
import { clearAllFontInfos } from 'vs/editor/browser/config/configuration';
import { IEditorProgressService } from 'vs/platform/progress/common/progress';
type Omit<T, K extends keyof T> = Pick<T, Exclude<keyof T, K>>;
function withAllStandaloneServices<T extends editorCommon.IEditor>(domElement: HTMLElement, override: IEditorOverrideServices, callback: (services: DynamicStandaloneServices) => T): T {
let services = new DynamicStandaloneServices(domElement, override);
let simpleEditorModelResolverService: SimpleEditorModelResolverService | null = null;
if (!services.has(ITextModelService)) {
simpleEditorModelResolverService = new SimpleEditorModelResolverService();
services.set(ITextModelService, simpleEditorModelResolverService);
}
if (!services.has(IOpenerService)) {
services.set(IOpenerService, new OpenerService(services.get(ICodeEditorService), services.get(ICommandService)));
}
let result = callback(services);
if (simpleEditorModelResolverService) {
simpleEditorModelResolverService.setEditor(result);
}
return result;
}
/**
* Create a new editor under `domElement`.
* `domElement` should be empty (not contain other dom nodes).
* The editor will read the size of `domElement`.
*/
export function create(domElement: HTMLElement, options?: IStandaloneEditorConstructionOptions, override?: IEditorOverrideServices): IStandaloneCodeEditor {
return withAllStandaloneServices(domElement, override || {}, (services) => {
return new StandaloneEditor(
domElement,
options,
services,
services.get(IInstantiationService),
services.get(ICodeEditorService),
services.get(ICommandService),
services.get(IContextKeyService),
services.get(IKeybindingService),
services.get(IContextViewService),
services.get(IStandaloneThemeService),
services.get(INotificationService),
services.get(IConfigurationService),
services.get(IAccessibilityService)
);
});
}
/**
* Emitted when an editor is created.
* Creating a diff editor might cause this listener to be invoked with the two editors.
* @event
*/
export function onDidCreateEditor(listener: (codeEditor: ICodeEditor) => void): IDisposable {
return StaticServices.codeEditorService.get().onCodeEditorAdd((editor) => {
listener(<ICodeEditor>editor);
});
}
/**
* Create a new diff editor under `domElement`.
* `domElement` should be empty (not contain other dom nodes).
* The editor will read the size of `domElement`.
*/
export function createDiffEditor(domElement: HTMLElement, options?: IDiffEditorConstructionOptions, override?: IEditorOverrideServices): IStandaloneDiffEditor {
return withAllStandaloneServices(domElement, override || {}, (services) => {
return new StandaloneDiffEditor(
domElement,
options,
services,
services.get(IInstantiationService),
services.get(IContextKeyService),
services.get(IKeybindingService),
services.get(IContextViewService),
services.get(IEditorWorkerService),
services.get(ICodeEditorService),
services.get(IStandaloneThemeService),
services.get(INotificationService),
services.get(IConfigurationService),
services.get(IContextMenuService),
services.get(IEditorProgressService),
null
);
});
}
export interface IDiffNavigator {
canNavigate(): boolean;
next(): void;
previous(): void;
dispose(): void;
}
export interface IDiffNavigatorOptions {
readonly followsCaret?: boolean;
readonly ignoreCharChanges?: boolean;
readonly alwaysRevealFirst?: boolean;
}
export function createDiffNavigator(diffEditor: IStandaloneDiffEditor, opts?: IDiffNavigatorOptions): IDiffNavigator {
return new DiffNavigator(diffEditor, opts);
}
function doCreateModel(value: string, languageSelection: ILanguageSelection, uri?: URI): ITextModel {
return StaticServices.modelService.get().createModel(value, languageSelection, uri);
}
/**
* Create a new editor model.
* You can specify the language that should be set for this model or let the language be inferred from the `uri`.
*/
export function createModel(value: string, language?: string, uri?: URI): ITextModel {
value = value || '';
if (!language) {
let firstLF = value.indexOf('\n');
let firstLine = value;
if (firstLF !== -1) {
firstLine = value.substring(0, firstLF);
}
return doCreateModel(value, StaticServices.modeService.get().createByFilepathOrFirstLine(uri || null, firstLine), uri);
}
return doCreateModel(value, StaticServices.modeService.get().create(language), uri);
}
/**
* Change the language for a model.
*/
export function setModelLanguage(model: ITextModel, languageId: string): void {
StaticServices.modelService.get().setMode(model, StaticServices.modeService.get().create(languageId));
}
/**
* Set the markers for a model.
*/
export function setModelMarkers(model: ITextModel, owner: string, markers: IMarkerData[]): void {
if (model) {
StaticServices.markerService.get().changeOne(owner, model.uri, markers);
}
}
/**
* Get markers for owner and/or resource
*
* @returns list of markers
*/
export function getModelMarkers(filter: { owner?: string, resource?: URI, take?: number }): IMarker[] {
return StaticServices.markerService.get().read(filter);
}
/**
* Get the model that has `uri` if it exists.
*/
export function getModel(uri: URI): ITextModel | null {
return StaticServices.modelService.get().getModel(uri);
}
/**
* Get all the created models.
*/
export function getModels(): ITextModel[] {
return StaticServices.modelService.get().getModels();
}
/**
* Emitted when a model is created.
* @event
*/
export function onDidCreateModel(listener: (model: ITextModel) => void): IDisposable {
return StaticServices.modelService.get().onModelAdded(listener);
}
/**
* Emitted right before a model is disposed.
* @event
*/
export function onWillDisposeModel(listener: (model: ITextModel) => void): IDisposable {
return StaticServices.modelService.get().onModelRemoved(listener);
}
/**
* Emitted when a different language is set to a model.
* @event
*/
export function onDidChangeModelLanguage(listener: (e: { readonly model: ITextModel; readonly oldLanguage: string; }) => void): IDisposable {
return StaticServices.modelService.get().onModelModeChanged((e) => {
listener({
model: e.model,
oldLanguage: e.oldModeId
});
});
}
/**
* Create a new web worker that has model syncing capabilities built in.
* Specify an AMD module to load that will `create` an object that will be proxied.
*/
export function createWebWorker<T>(opts: IWebWorkerOptions): MonacoWebWorker<T> {
return actualCreateWebWorker<T>(StaticServices.modelService.get(), opts);
}
/**
* Colorize the contents of `domNode` using attribute `data-lang`.
*/
export function colorizeElement(domNode: HTMLElement, options: IColorizerElementOptions): Promise<void> {
return Colorizer.colorizeElement(StaticServices.standaloneThemeService.get(), StaticServices.modeService.get(), domNode, options);
}
/**
* Colorize `text` using language `languageId`.
*/
export function colorize(text: string, languageId: string, options: IColorizerOptions): Promise<string> {
return Colorizer.colorize(StaticServices.modeService.get(), text, languageId, options);
}
/**
* Colorize a line in a model.
*/
export function colorizeModelLine(model: ITextModel, lineNumber: number, tabSize: number = 4): string {
return Colorizer.colorizeModelLine(model, lineNumber, tabSize);
}
/**
* @internal
*/
function getSafeTokenizationSupport(language: string): Omit<modes.ITokenizationSupport, 'tokenize2'> {
let tokenizationSupport = modes.TokenizationRegistry.get(language);
if (tokenizationSupport) {
return tokenizationSupport;
}
return {
getInitialState: () => NULL_STATE,
tokenize: (line: string, state: modes.IState, deltaOffset: number) => nullTokenize(language, line, state, deltaOffset)
};
}
/**
* Tokenize `text` using language `languageId`
*/
export function tokenize(text: string, languageId: string): Token[][] {
let modeService = StaticServices.modeService.get();
// Needed in order to get the mode registered for subsequent look-ups
modeService.triggerMode(languageId);
let tokenizationSupport = getSafeTokenizationSupport(languageId);
let lines = text.split(/\r\n|\r|\n/);
let result: Token[][] = [];
let state = tokenizationSupport.getInitialState();
for (let i = 0, len = lines.length; i < len; i++) {
let line = lines[i];
let tokenizationResult = tokenizationSupport.tokenize(line, state, 0);
result[i] = tokenizationResult.tokens;
state = tokenizationResult.endState;
}
return result;
}
/**
* Define a new theme or update an existing theme.
*/
export function defineTheme(themeName: string, themeData: IStandaloneThemeData): void {
StaticServices.standaloneThemeService.get().defineTheme(themeName, themeData);
}
/**
* Switches to a theme.
*/
export function setTheme(themeName: string): void {
StaticServices.standaloneThemeService.get().setTheme(themeName);
}
/**
* Clears all cached font measurements and triggers re-measurement.
*/
export function remeasureFonts(): void {
clearAllFontInfos();
}
/**
* @internal
*/
export function createMonacoEditorAPI(): typeof monaco.editor {
return {
// methods
create: <any>create,
onDidCreateEditor: <any>onDidCreateEditor,
createDiffEditor: <any>createDiffEditor,
createDiffNavigator: <any>createDiffNavigator,
createModel: <any>createModel,
setModelLanguage: <any>setModelLanguage,
setModelMarkers: <any>setModelMarkers,
getModelMarkers: <any>getModelMarkers,
getModels: <any>getModels,
getModel: <any>getModel,
onDidCreateModel: <any>onDidCreateModel,
onWillDisposeModel: <any>onWillDisposeModel,
onDidChangeModelLanguage: <any>onDidChangeModelLanguage,
createWebWorker: <any>createWebWorker,
colorizeElement: <any>colorizeElement,
colorize: <any>colorize,
colorizeModelLine: <any>colorizeModelLine,
tokenize: <any>tokenize,
defineTheme: <any>defineTheme,
setTheme: <any>setTheme,
remeasureFonts: remeasureFonts,
// enums
AccessibilitySupport: standaloneEnums.AccessibilitySupport,
ScrollbarVisibility: standaloneEnums.ScrollbarVisibility,
WrappingIndent: standaloneEnums.WrappingIndent,
OverviewRulerLane: standaloneEnums.OverviewRulerLane,
MinimapPosition: standaloneEnums.MinimapPosition,
EndOfLinePreference: standaloneEnums.EndOfLinePreference,
DefaultEndOfLine: standaloneEnums.DefaultEndOfLine,
EndOfLineSequence: standaloneEnums.EndOfLineSequence,
TrackedRangeStickiness: standaloneEnums.TrackedRangeStickiness,
CursorChangeReason: standaloneEnums.CursorChangeReason,
MouseTargetType: standaloneEnums.MouseTargetType,
TextEditorCursorStyle: standaloneEnums.TextEditorCursorStyle,
TextEditorCursorBlinkingStyle: standaloneEnums.TextEditorCursorBlinkingStyle,
ContentWidgetPositionPreference: standaloneEnums.ContentWidgetPositionPreference,
OverlayWidgetPositionPreference: standaloneEnums.OverlayWidgetPositionPreference,
RenderMinimap: standaloneEnums.RenderMinimap,
ScrollType: standaloneEnums.ScrollType,
RenderLineNumbersType: standaloneEnums.RenderLineNumbersType,
// classes
ConfigurationChangedEvent: <any>ConfigurationChangedEvent,
BareFontInfo: <any>BareFontInfo,
FontInfo: <any>FontInfo,
TextModelResolvedOptions: <any>TextModelResolvedOptions,
FindMatch: <any>FindMatch,
// vars
EditorType: editorCommon.EditorType,
};
}
| src/vs/editor/standalone/browser/standaloneEditor.ts | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.9990547299385071,
0.05207931622862816,
0.00016144540859386325,
0.00017325181397609413,
0.21999217569828033
] |
{
"id": 11,
"code_window": [
"\t\t\tnull\n",
"\t\t);\n",
"\t});\n",
"}\n",
"\n",
"export interface IDiffNavigator {\n",
"\tcanNavigate(): boolean;\n",
"\tnext(): void;\n",
"\tprevious(): void;\n",
"\tdispose(): void;\n",
"}\n",
"\n",
"export interface IDiffNavigatorOptions {\n",
"\treadonly followsCaret?: boolean;\n",
"\treadonly ignoreCharChanges?: boolean;\n",
"\treadonly alwaysRevealFirst?: boolean;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/editor/standalone/browser/standaloneEditor.ts",
"type": "replace",
"edit_start_line_idx": 129
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
/**
* Extracted from json.ts to keep json nls free.
*/
import { localize } from 'vs/nls';
import { ParseErrorCode } from './json';
export function getParseErrorMessage(errorCode: ParseErrorCode): string {
switch (errorCode) {
case ParseErrorCode.InvalidSymbol: return localize('error.invalidSymbol', 'Invalid symbol');
case ParseErrorCode.InvalidNumberFormat: return localize('error.invalidNumberFormat', 'Invalid number format');
case ParseErrorCode.PropertyNameExpected: return localize('error.propertyNameExpected', 'Property name expected');
case ParseErrorCode.ValueExpected: return localize('error.valueExpected', 'Value expected');
case ParseErrorCode.ColonExpected: return localize('error.colonExpected', 'Colon expected');
case ParseErrorCode.CommaExpected: return localize('error.commaExpected', 'Comma expected');
case ParseErrorCode.CloseBraceExpected: return localize('error.closeBraceExpected', 'Closing brace expected');
case ParseErrorCode.CloseBracketExpected: return localize('error.closeBracketExpected', 'Closing bracket expected');
case ParseErrorCode.EndOfFileExpected: return localize('error.endOfFileExpected', 'End of file expected');
default:
return '';
}
} | src/vs/base/common/jsonErrorMessages.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.000177245688973926,
0.00017489783931523561,
0.00017190609651152045,
0.00017554174701217562,
0.0000022269216515269363
] |
{
"id": 11,
"code_window": [
"\t\t\tnull\n",
"\t\t);\n",
"\t});\n",
"}\n",
"\n",
"export interface IDiffNavigator {\n",
"\tcanNavigate(): boolean;\n",
"\tnext(): void;\n",
"\tprevious(): void;\n",
"\tdispose(): void;\n",
"}\n",
"\n",
"export interface IDiffNavigatorOptions {\n",
"\treadonly followsCaret?: boolean;\n",
"\treadonly ignoreCharChanges?: boolean;\n",
"\treadonly alwaysRevealFirst?: boolean;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/editor/standalone/browser/standaloneEditor.ts",
"type": "replace",
"edit_start_line_idx": 129
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as crypto from 'crypto';
import { IFileService, IResolveFileResult, IFileStat } from 'vs/platform/files/common/files';
import { IWorkspaceContextService, WorkbenchState, IWorkspace } from 'vs/platform/workspace/common/workspace';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import { IWindowConfiguration } from 'vs/platform/windows/common/windows';
import { IHostService } from 'vs/workbench/services/host/browser/host';
import { INotificationService, NeverShowAgainScope, INeverShowAgainOptions } from 'vs/platform/notification/common/notification';
import { IQuickInputService, IQuickPickItem } from 'vs/platform/quickinput/common/quickInput';
import { ITextFileService, ITextFileContent } from 'vs/workbench/services/textfile/common/textfiles';
import { URI } from 'vs/base/common/uri';
import { Schemas } from 'vs/base/common/network';
import { hasWorkspaceFileExtension } from 'vs/platform/workspaces/common/workspaces';
import { localize } from 'vs/nls';
import Severity from 'vs/base/common/severity';
import { joinPath } from 'vs/base/common/resources';
import { registerSingleton } from 'vs/platform/instantiation/common/extensions';
import { IWorkspaceTagsService, Tags } from 'vs/workbench/contrib/tags/common/workspaceTags';
import { getHashedRemotesFromConfig } from 'vs/workbench/contrib/tags/electron-browser/workspaceTags';
import { IProductService } from 'vs/platform/product/common/productService';
const ModulesToLookFor = [
// Packages that suggest a node server
'express',
'sails',
'koa',
'hapi',
'socket.io',
'restify',
// JS frameworks
'react',
'react-native',
'rnpm-plugin-windows',
'@angular/core',
'@ionic',
'vue',
'tns-core-modules',
// Other interesting packages
'aws-sdk',
'aws-amplify',
'azure',
'azure-storage',
'firebase',
'@google-cloud/common',
'heroku-cli',
//Office and Sharepoint packages
'@microsoft/office-js',
'@microsoft/office-js-helpers',
'@types/office-js',
'@types/office-runtime',
'office-ui-fabric-react',
'@uifabric/icons',
'@uifabric/merge-styles',
'@uifabric/styling',
'@uifabric/experiments',
'@uifabric/utilities',
'@microsoft/rush',
'lerna',
'just-task',
'beachball'
];
const PyModulesToLookFor = [
'azure',
'azure-storage-common',
'azure-storage-blob',
'azure-storage-file',
'azure-storage-queue',
'azure-shell',
'azure-cosmos',
'azure-devtools',
'azure-elasticluster',
'azure-eventgrid',
'azure-functions',
'azure-graphrbac',
'azure-keyvault',
'azure-loganalytics',
'azure-monitor',
'azure-servicebus',
'azure-servicefabric',
'azure-storage',
'azure-translator',
'azure-iothub-device-client',
'adal',
'pydocumentdb',
'botbuilder-core',
'botbuilder-schema',
'botframework-connector'
];
export class WorkspaceTagsService implements IWorkspaceTagsService {
_serviceBrand: undefined;
private _tags: Tags | undefined;
constructor(
@IFileService private readonly fileService: IFileService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IWorkbenchEnvironmentService private readonly environmentService: IWorkbenchEnvironmentService,
@IProductService private readonly productService: IProductService,
@IHostService private readonly hostService: IHostService,
@INotificationService private readonly notificationService: INotificationService,
@IQuickInputService private readonly quickInputService: IQuickInputService,
@ITextFileService private readonly textFileService: ITextFileService
) { }
async getTags(): Promise<Tags> {
if (!this._tags) {
this._tags = await this.resolveWorkspaceTags(this.environmentService.configuration, rootFiles => this.handleWorkspaceFiles(rootFiles));
}
return this._tags;
}
getTelemetryWorkspaceId(workspace: IWorkspace, state: WorkbenchState): string | undefined {
function createHash(uri: URI): string {
return crypto.createHash('sha1').update(uri.scheme === Schemas.file ? uri.fsPath : uri.toString()).digest('hex');
}
let workspaceId: string | undefined;
switch (state) {
case WorkbenchState.EMPTY:
workspaceId = undefined;
break;
case WorkbenchState.FOLDER:
workspaceId = createHash(workspace.folders[0].uri);
break;
case WorkbenchState.WORKSPACE:
if (workspace.configuration) {
workspaceId = createHash(workspace.configuration);
}
}
return workspaceId;
}
getHashedRemotesFromUri(workspaceUri: URI, stripEndingDotGit: boolean = false): Promise<string[]> {
const path = workspaceUri.path;
const uri = workspaceUri.with({ path: `${path !== '/' ? path : ''}/.git/config` });
return this.fileService.exists(uri).then(exists => {
if (!exists) {
return [];
}
return this.textFileService.read(uri, { acceptTextOnly: true }).then(
content => getHashedRemotesFromConfig(content.value, stripEndingDotGit),
err => [] // ignore missing or binary file
);
});
}
/* __GDPR__FRAGMENT__
"WorkspaceTags" : {
"workbench.filesToOpenOrCreate" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workbench.filesToDiff" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.id" : { "classification": "SystemMetaData", "purpose": "FeatureInsight" },
"workspace.roots" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.empty" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.grunt" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.gulp" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.jake" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.tsconfig" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.jsconfig" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.config.xml" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.vsc.extension" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.asp<NUMBER>" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.sln" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.unity" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.express" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.sails" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.koa" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.hapi" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.socket.io" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.restify" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.rnpm-plugin-windows" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.react" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@angular/core" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.vue" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.aws-sdk" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.aws-amplify-sdk" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.azure" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.azure-storage" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@google-cloud/common" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.firebase" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.heroku-cli" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@microsoft/office-js" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@microsoft/office-js-helpers" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@types/office-js" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@types/office-runtime" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.office-ui-fabric-react" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@uifabric/icons" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@uifabric/merge-styles" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@uifabric/styling" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@uifabric/experiments" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@uifabric/utilities" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.@microsoft/rush" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.lerna" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.just-task" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.npm.beachball" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.bower" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.yeoman.code.ext" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.cordova.high" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.cordova.low" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.xamarin.android" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.xamarin.ios" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.android.cpp" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.reactNative" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.ionic" : { "classification" : "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": "true" },
"workspace.nativeScript" : { "classification" : "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": "true" },
"workspace.java.pom" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.requirements" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.requirements.star" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.Pipfile" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.conda" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.any-azure" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-storage-common" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-storage-blob" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-storage-file" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-storage-queue" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-mgmt" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-shell" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.pulumi-azure" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-cosmos" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-devtools" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-elasticluster" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-eventgrid" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-functions" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-graphrbac" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-keyvault" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-loganalytics" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-monitor" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-servicebus" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-servicefabric" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-storage" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-translator" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-iothub-device-client" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-ml" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.azure-cognitiveservices" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.adal" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.pydocumentdb" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.botbuilder-core" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.botbuilder-schema" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true },
"workspace.py.botframework-connector" : { "classification": "SystemMetaData", "purpose": "FeatureInsight", "isMeasurement": true }
}
*/
private resolveWorkspaceTags(configuration: IWindowConfiguration, participant?: (rootFiles: string[]) => void): Promise<Tags> {
const tags: Tags = Object.create(null);
const state = this.contextService.getWorkbenchState();
const workspace = this.contextService.getWorkspace();
tags['workspace.id'] = this.getTelemetryWorkspaceId(workspace, state);
const { filesToOpenOrCreate, filesToDiff } = configuration;
tags['workbench.filesToOpenOrCreate'] = filesToOpenOrCreate && filesToOpenOrCreate.length || 0;
tags['workbench.filesToDiff'] = filesToDiff && filesToDiff.length || 0;
const isEmpty = state === WorkbenchState.EMPTY;
tags['workspace.roots'] = isEmpty ? 0 : workspace.folders.length;
tags['workspace.empty'] = isEmpty;
const folders = !isEmpty ? workspace.folders.map(folder => folder.uri) : this.productService.quality !== 'stable' && this.findFolders(configuration);
if (!folders || !folders.length || !this.fileService) {
return Promise.resolve(tags);
}
return this.fileService.resolveAll(folders.map(resource => ({ resource }))).then((files: IResolveFileResult[]) => {
const names = (<IFileStat[]>[]).concat(...files.map(result => result.success ? (result.stat!.children || []) : [])).map(c => c.name);
const nameSet = names.reduce((s, n) => s.add(n.toLowerCase()), new Set());
if (participant) {
participant(names);
}
tags['workspace.grunt'] = nameSet.has('gruntfile.js');
tags['workspace.gulp'] = nameSet.has('gulpfile.js');
tags['workspace.jake'] = nameSet.has('jakefile.js');
tags['workspace.tsconfig'] = nameSet.has('tsconfig.json');
tags['workspace.jsconfig'] = nameSet.has('jsconfig.json');
tags['workspace.config.xml'] = nameSet.has('config.xml');
tags['workspace.vsc.extension'] = nameSet.has('vsc-extension-quickstart.md');
tags['workspace.ASP5'] = nameSet.has('project.json') && this.searchArray(names, /^.+\.cs$/i);
tags['workspace.sln'] = this.searchArray(names, /^.+\.sln$|^.+\.csproj$/i);
tags['workspace.unity'] = nameSet.has('assets') && nameSet.has('library') && nameSet.has('projectsettings');
tags['workspace.npm'] = nameSet.has('package.json') || nameSet.has('node_modules');
tags['workspace.bower'] = nameSet.has('bower.json') || nameSet.has('bower_components');
tags['workspace.java.pom'] = nameSet.has('pom.xml');
tags['workspace.yeoman.code.ext'] = nameSet.has('vsc-extension-quickstart.md');
tags['workspace.py.requirements'] = nameSet.has('requirements.txt');
tags['workspace.py.requirements.star'] = this.searchArray(names, /^(.*)requirements(.*)\.txt$/i);
tags['workspace.py.Pipfile'] = nameSet.has('pipfile');
tags['workspace.py.conda'] = this.searchArray(names, /^environment(\.yml$|\.yaml$)/i);
const mainActivity = nameSet.has('mainactivity.cs') || nameSet.has('mainactivity.fs');
const appDelegate = nameSet.has('appdelegate.cs') || nameSet.has('appdelegate.fs');
const androidManifest = nameSet.has('androidmanifest.xml');
const platforms = nameSet.has('platforms');
const plugins = nameSet.has('plugins');
const www = nameSet.has('www');
const properties = nameSet.has('properties');
const resources = nameSet.has('resources');
const jni = nameSet.has('jni');
if (tags['workspace.config.xml'] &&
!tags['workspace.language.cs'] && !tags['workspace.language.vb'] && !tags['workspace.language.aspx']) {
if (platforms && plugins && www) {
tags['workspace.cordova.high'] = true;
} else {
tags['workspace.cordova.low'] = true;
}
}
if (tags['workspace.config.xml'] &&
!tags['workspace.language.cs'] && !tags['workspace.language.vb'] && !tags['workspace.language.aspx']) {
if (nameSet.has('ionic.config.json')) {
tags['workspace.ionic'] = true;
}
}
if (mainActivity && properties && resources) {
tags['workspace.xamarin.android'] = true;
}
if (appDelegate && resources) {
tags['workspace.xamarin.ios'] = true;
}
if (androidManifest && jni) {
tags['workspace.android.cpp'] = true;
}
function getFilePromises(filename: string, fileService: IFileService, textFileService: ITextFileService, contentHandler: (content: ITextFileContent) => void): Promise<void>[] {
return !nameSet.has(filename) ? [] : (folders as URI[]).map(workspaceUri => {
const uri = workspaceUri.with({ path: `${workspaceUri.path !== '/' ? workspaceUri.path : ''}/${filename}` });
return fileService.exists(uri).then(exists => {
if (!exists) {
return undefined;
}
return textFileService.read(uri, { acceptTextOnly: true }).then(contentHandler);
}, err => {
// Ignore missing file
});
});
}
function addPythonTags(packageName: string): void {
if (PyModulesToLookFor.indexOf(packageName) > -1) {
tags['workspace.py.' + packageName] = true;
}
// cognitive services has a lot of tiny packages. e.g. 'azure-cognitiveservices-search-autosuggest'
if (packageName.indexOf('azure-cognitiveservices') > -1) {
tags['workspace.py.azure-cognitiveservices'] = true;
}
if (packageName.indexOf('azure-mgmt') > -1) {
tags['workspace.py.azure-mgmt'] = true;
}
if (packageName.indexOf('azure-ml') > -1) {
tags['workspace.py.azure-ml'] = true;
}
if (!tags['workspace.py.any-azure']) {
tags['workspace.py.any-azure'] = /azure/i.test(packageName);
}
}
const requirementsTxtPromises = getFilePromises('requirements.txt', this.fileService, this.textFileService, content => {
const dependencies: string[] = content.value.split(/\r\n|\r|\n/);
for (let dependency of dependencies) {
// Dependencies in requirements.txt can have 3 formats: `foo==3.1, foo>=3.1, foo`
const format1 = dependency.split('==');
const format2 = dependency.split('>=');
const packageName = (format1.length === 2 ? format1[0] : format2[0]).trim();
addPythonTags(packageName);
}
});
const pipfilePromises = getFilePromises('pipfile', this.fileService, this.textFileService, content => {
let dependencies: string[] = content.value.split(/\r\n|\r|\n/);
// We're only interested in the '[packages]' section of the Pipfile
dependencies = dependencies.slice(dependencies.indexOf('[packages]') + 1);
for (let dependency of dependencies) {
if (dependency.trim().indexOf('[') > -1) {
break;
}
// All dependencies in Pipfiles follow the format: `<package> = <version, or git repo, or something else>`
if (dependency.indexOf('=') === -1) {
continue;
}
const packageName = dependency.split('=')[0].trim();
addPythonTags(packageName);
}
});
const packageJsonPromises = getFilePromises('package.json', this.fileService, this.textFileService, content => {
try {
const packageJsonContents = JSON.parse(content.value);
let dependencies = packageJsonContents['dependencies'];
let devDependencies = packageJsonContents['devDependencies'];
for (let module of ModulesToLookFor) {
if ('react-native' === module) {
if ((dependencies && dependencies[module]) || (devDependencies && devDependencies[module])) {
tags['workspace.reactNative'] = true;
}
} else if ('tns-core-modules' === module) {
if ((dependencies && dependencies[module]) || (devDependencies && devDependencies[module])) {
tags['workspace.nativescript'] = true;
}
} else {
if ((dependencies && dependencies[module]) || (devDependencies && devDependencies[module])) {
tags['workspace.npm.' + module] = true;
}
}
}
}
catch (e) {
// Ignore errors when resolving file or parsing file contents
}
});
return Promise.all([...packageJsonPromises, ...requirementsTxtPromises, ...pipfilePromises]).then(() => tags);
});
}
private handleWorkspaceFiles(rootFiles: string[]): void {
const state = this.contextService.getWorkbenchState();
const workspace = this.contextService.getWorkspace();
// Handle top-level workspace files for local single folder workspace
if (state === WorkbenchState.FOLDER) {
const workspaceFiles = rootFiles.filter(hasWorkspaceFileExtension);
if (workspaceFiles.length > 0) {
this.doHandleWorkspaceFiles(workspace.folders[0].uri, workspaceFiles);
}
}
}
private doHandleWorkspaceFiles(folder: URI, workspaces: string[]): void {
const neverShowAgain: INeverShowAgainOptions = { id: 'workspaces.dontPromptToOpen', scope: NeverShowAgainScope.WORKSPACE, isSecondary: true };
// Prompt to open one workspace
if (workspaces.length === 1) {
const workspaceFile = workspaces[0];
this.notificationService.prompt(Severity.Info, localize('workspaceFound', "This folder contains a workspace file '{0}'. Do you want to open it? [Learn more]({1}) about workspace files.", workspaceFile, 'https://go.microsoft.com/fwlink/?linkid=2025315'), [{
label: localize('openWorkspace', "Open Workspace"),
run: () => this.hostService.openWindow([{ workspaceUri: joinPath(folder, workspaceFile) }])
}], { neverShowAgain });
}
// Prompt to select a workspace from many
else if (workspaces.length > 1) {
this.notificationService.prompt(Severity.Info, localize('workspacesFound', "This folder contains multiple workspace files. Do you want to open one? [Learn more]({0}) about workspace files.", 'https://go.microsoft.com/fwlink/?linkid=2025315'), [{
label: localize('selectWorkspace', "Select Workspace"),
run: () => {
this.quickInputService.pick(
workspaces.map(workspace => ({ label: workspace } as IQuickPickItem)),
{ placeHolder: localize('selectToOpen', "Select a workspace to open") }).then(pick => {
if (pick) {
this.hostService.openWindow([{ workspaceUri: joinPath(folder, pick.label) }]);
}
});
}
}], { neverShowAgain });
}
}
private findFolders(configuration: IWindowConfiguration): URI[] | undefined {
const folder = this.findFolder(configuration);
return folder && [folder];
}
private findFolder({ filesToOpenOrCreate, filesToDiff }: IWindowConfiguration): URI | undefined {
if (filesToOpenOrCreate && filesToOpenOrCreate.length) {
return this.parentURI(filesToOpenOrCreate[0].fileUri);
} else if (filesToDiff && filesToDiff.length) {
return this.parentURI(filesToDiff[0].fileUri);
}
return undefined;
}
private parentURI(uri: URI | undefined): URI | undefined {
if (!uri) {
return undefined;
}
const path = uri.path;
const i = path.lastIndexOf('/');
return i !== -1 ? uri.with({ path: path.substr(0, i) }) : undefined;
}
private searchArray(arr: string[], regEx: RegExp): boolean | undefined {
return arr.some(v => v.search(regEx) > -1) || undefined;
}
}
registerSingleton(IWorkspaceTagsService, WorkspaceTagsService, true);
| src/vs/workbench/contrib/tags/electron-browser/workspaceTagsService.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017722338088788092,
0.00017270652460865676,
0.00016646584845148027,
0.0001733412209432572,
0.000002822226633725222
] |
{
"id": 11,
"code_window": [
"\t\t\tnull\n",
"\t\t);\n",
"\t});\n",
"}\n",
"\n",
"export interface IDiffNavigator {\n",
"\tcanNavigate(): boolean;\n",
"\tnext(): void;\n",
"\tprevious(): void;\n",
"\tdispose(): void;\n",
"}\n",
"\n",
"export interface IDiffNavigatorOptions {\n",
"\treadonly followsCaret?: boolean;\n",
"\treadonly ignoreCharChanges?: boolean;\n",
"\treadonly alwaysRevealFirst?: boolean;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/editor/standalone/browser/standaloneEditor.ts",
"type": "replace",
"edit_start_line_idx": 129
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as nls from 'vs/nls';
import { IDiffEditor } from 'vs/editor/browser/editorBrowser';
import { registerDiffEditorContribution } from 'vs/editor/browser/editorExtensions';
import { IDiffEditorContribution } from 'vs/editor/common/editorCommon';
import { Disposable, IDisposable } from 'vs/base/common/lifecycle';
import { FloatingClickWidget } from 'vs/workbench/browser/parts/editor/editorWidgets';
import { IDiffComputationResult } from 'vs/editor/common/services/editorWorkerService';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IConfigurationService, ConfigurationTarget } from 'vs/platform/configuration/common/configuration';
import { INotificationService, Severity } from 'vs/platform/notification/common/notification';
const enum WidgetState {
Hidden,
HintWhitespace
}
class DiffEditorHelperContribution extends Disposable implements IDiffEditorContribution {
public static ID = 'editor.contrib.diffEditorHelper';
private _helperWidget: FloatingClickWidget | null;
private _helperWidgetListener: IDisposable | null;
private _state: WidgetState;
constructor(
private readonly _diffEditor: IDiffEditor,
@IInstantiationService private readonly _instantiationService: IInstantiationService,
@IConfigurationService private readonly _configurationService: IConfigurationService,
@INotificationService private readonly _notificationService: INotificationService,
) {
super();
this._helperWidget = null;
this._helperWidgetListener = null;
this._state = WidgetState.Hidden;
this._register(this._diffEditor.onDidUpdateDiff(() => {
const diffComputationResult = this._diffEditor.getDiffComputationResult();
this._setState(this._deduceState(diffComputationResult));
if (diffComputationResult && diffComputationResult.quitEarly) {
this._notificationService.prompt(
Severity.Warning,
nls.localize('hintTimeout', "The diff algorithm was stopped early (after {0} ms.)", this._diffEditor.maxComputationTime),
[{
label: nls.localize('removeTimeout', "Remove limit"),
run: () => {
this._configurationService.updateValue('diffEditor.maxComputationTime', 0, ConfigurationTarget.USER);
}
}],
{}
);
}
}));
}
private _deduceState(diffComputationResult: IDiffComputationResult | null): WidgetState {
if (!diffComputationResult) {
return WidgetState.Hidden;
}
if (this._diffEditor.ignoreTrimWhitespace && diffComputationResult.changes.length === 0 && !diffComputationResult.identical) {
return WidgetState.HintWhitespace;
}
return WidgetState.Hidden;
}
private _setState(newState: WidgetState) {
if (this._state === newState) {
return;
}
this._state = newState;
if (this._helperWidgetListener) {
this._helperWidgetListener.dispose();
this._helperWidgetListener = null;
}
if (this._helperWidget) {
this._helperWidget.dispose();
this._helperWidget = null;
}
if (this._state === WidgetState.HintWhitespace) {
this._helperWidget = this._instantiationService.createInstance(FloatingClickWidget, this._diffEditor.getModifiedEditor(), nls.localize('hintWhitespace', "Show Whitespace Differences"), null);
this._helperWidgetListener = this._helperWidget.onClick(() => this._onDidClickHelperWidget());
this._helperWidget.render();
}
}
private _onDidClickHelperWidget(): void {
if (this._state === WidgetState.HintWhitespace) {
this._configurationService.updateValue('diffEditor.ignoreTrimWhitespace', false, ConfigurationTarget.USER);
}
}
dispose(): void {
super.dispose();
}
}
registerDiffEditorContribution(DiffEditorHelperContribution.ID, DiffEditorHelperContribution);
| src/vs/workbench/contrib/codeEditor/browser/diffEditorHelper.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0006575101288035512,
0.0002261882327729836,
0.0001619134418433532,
0.00017104280414059758,
0.0001390475663356483
] |
{
"id": 12,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"\n",
"\t/**\n",
"\t * Create a new editor under `domElement`.\n",
"\t * `domElement` should be empty (not contain other dom nodes).\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\texport interface IDiffNavigator {\n",
"\t\tcanNavigate(): boolean;\n",
"\t\tnext(): void;\n",
"\t\tprevious(): void;\n",
"\t\tdispose(): void;\n",
"\t}\n"
],
"file_path": "src/vs/monaco.d.ts",
"type": "add",
"edit_start_line_idx": 804
} |
// This file is adding references to various symbols which should not be removed via tree shaking
import { ServiceIdentifier } from './vs/platform/instantiation/common/instantiation';
import { IHighlight } from './vs/base/parts/quickopen/browser/quickOpenModel';
import { SimpleWorkerClient, create as create1 } from './vs/base/common/worker/simpleWorker';
import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';
import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';
import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';
import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';
import * as editorAPI from './vs/editor/editor.api';
(function () {
var a: any;
var b: any;
a = (<DiffNavigator>b).previous; // IDiffNavigator
a = (<ServiceIdentifier<any>>b).type;
a = (<IHighlight>b).start;
a = (<IHighlight>b).end;
a = (<SimpleWorkerClient<any, any>>b).getProxyObject; // IWorkerClient
a = create1;
a = create2;
a = (<DocumentRangeFormattingEditProvider>b).extensionId;
// injection madness
a = (<SyncDescriptor0<any>>b).ctor;
a = (<SyncDescriptor0<any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor1<any, any>>b).ctor;
a = (<SyncDescriptor1<any, any>>b).bind;
a = (<SyncDescriptor2<any, any, any>>b).ctor;
a = (<SyncDescriptor2<any, any, any>>b).bind;
a = (<SyncDescriptor3<any, any, any, any>>b).ctor;
a = (<SyncDescriptor3<any, any, any, any>>b).bind;
a = (<SyncDescriptor4<any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor4<any, any, any, any, any>>b).bind;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor5<any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor6<any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor7<any, any, any, any, any, any, any, any>>b).bind;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).ctor;
a = (<SyncDescriptor8<any, any, any, any, any, any, any, any, any>>b).bind;
// exported API
a = editorAPI.CancellationTokenSource;
a = editorAPI.Emitter;
a = editorAPI.KeyCode;
a = editorAPI.KeyMod;
a = editorAPI.Position;
a = editorAPI.Range;
a = editorAPI.Selection;
a = editorAPI.SelectionDirection;
a = editorAPI.MarkerSeverity;
a = editorAPI.MarkerTag;
a = editorAPI.Uri;
a = editorAPI.Token;
a = editorAPI.editor;
a = editorAPI.languages;
})();
| build/monaco/monaco.usage.recipe | 1 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.0003564534126780927,
0.00019653353956528008,
0.0001662635913817212,
0.0001700491993688047,
0.00006531828694278374
] |
{
"id": 12,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"\n",
"\t/**\n",
"\t * Create a new editor under `domElement`.\n",
"\t * `domElement` should be empty (not contain other dom nodes).\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\texport interface IDiffNavigator {\n",
"\t\tcanNavigate(): boolean;\n",
"\t\tnext(): void;\n",
"\t\tprevious(): void;\n",
"\t\tdispose(): void;\n",
"\t}\n"
],
"file_path": "src/vs/monaco.d.ts",
"type": "add",
"edit_start_line_idx": 804
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { URI, UriComponents } from 'vs/base/common/uri';
import { Event, Emitter } from 'vs/base/common/event';
import { debounce } from 'vs/base/common/decorators';
import { DisposableStore, MutableDisposable } from 'vs/base/common/lifecycle';
import { asPromise } from 'vs/base/common/async';
import { ExtHostCommands } from 'vs/workbench/api/common/extHostCommands';
import { MainContext, MainThreadSCMShape, SCMRawResource, SCMRawResourceSplice, SCMRawResourceSplices, IMainContext, ExtHostSCMShape, ICommandDto } from './extHost.protocol';
import { sortedDiff, equals } from 'vs/base/common/arrays';
import { comparePaths } from 'vs/base/common/comparers';
import * as vscode from 'vscode';
import { ISplice } from 'vs/base/common/sequence';
import { ILogService } from 'vs/platform/log/common/log';
import { CancellationToken } from 'vs/base/common/cancellation';
import { ExtensionIdentifier, IExtensionDescription } from 'vs/platform/extensions/common/extensions';
type ProviderHandle = number;
type GroupHandle = number;
type ResourceStateHandle = number;
function getIconPath(decorations?: vscode.SourceControlResourceThemableDecorations): string | undefined {
if (!decorations) {
return undefined;
} else if (typeof decorations.iconPath === 'string') {
return URI.file(decorations.iconPath).toString();
} else if (decorations.iconPath) {
return `${decorations.iconPath}`;
}
return undefined;
}
function compareResourceThemableDecorations(a: vscode.SourceControlResourceThemableDecorations, b: vscode.SourceControlResourceThemableDecorations): number {
if (!a.iconPath && !b.iconPath) {
return 0;
} else if (!a.iconPath) {
return -1;
} else if (!b.iconPath) {
return 1;
}
const aPath = typeof a.iconPath === 'string' ? a.iconPath : a.iconPath.fsPath;
const bPath = typeof b.iconPath === 'string' ? b.iconPath : b.iconPath.fsPath;
return comparePaths(aPath, bPath);
}
function compareResourceStatesDecorations(a: vscode.SourceControlResourceDecorations, b: vscode.SourceControlResourceDecorations): number {
let result = 0;
if (a.strikeThrough !== b.strikeThrough) {
return a.strikeThrough ? 1 : -1;
}
if (a.faded !== b.faded) {
return a.faded ? 1 : -1;
}
if (a.tooltip !== b.tooltip) {
return (a.tooltip || '').localeCompare(b.tooltip || '');
}
result = compareResourceThemableDecorations(a, b);
if (result !== 0) {
return result;
}
if (a.light && b.light) {
result = compareResourceThemableDecorations(a.light, b.light);
} else if (a.light) {
return 1;
} else if (b.light) {
return -1;
}
if (result !== 0) {
return result;
}
if (a.dark && b.dark) {
result = compareResourceThemableDecorations(a.dark, b.dark);
} else if (a.dark) {
return 1;
} else if (b.dark) {
return -1;
}
return result;
}
function compareResourceStates(a: vscode.SourceControlResourceState, b: vscode.SourceControlResourceState): number {
let result = comparePaths(a.resourceUri.fsPath, b.resourceUri.fsPath, true);
if (result !== 0) {
return result;
}
if (a.decorations && b.decorations) {
result = compareResourceStatesDecorations(a.decorations, b.decorations);
} else if (a.decorations) {
return 1;
} else if (b.decorations) {
return -1;
}
return result;
}
function compareArgs(a: any[], b: any[]): boolean {
for (let i = 0; i < a.length; i++) {
if (a[i] !== b[i]) {
return false;
}
}
return true;
}
function commandEquals(a: vscode.Command, b: vscode.Command): boolean {
return a.command === b.command
&& a.title === b.title
&& a.tooltip === b.tooltip
&& (a.arguments && b.arguments ? compareArgs(a.arguments, b.arguments) : a.arguments === b.arguments);
}
function commandListEquals(a: readonly vscode.Command[], b: readonly vscode.Command[]): boolean {
return equals(a, b, commandEquals);
}
export interface IValidateInput {
(value: string, cursorPosition: number): vscode.ProviderResult<vscode.SourceControlInputBoxValidation | undefined | null>;
}
export class ExtHostSCMInputBox implements vscode.SourceControlInputBox {
private _value: string = '';
get value(): string {
return this._value;
}
set value(value: string) {
this._proxy.$setInputBoxValue(this._sourceControlHandle, value);
this.updateValue(value);
}
private readonly _onDidChange = new Emitter<string>();
get onDidChange(): Event<string> {
return this._onDidChange.event;
}
private _placeholder: string = '';
get placeholder(): string {
return this._placeholder;
}
set placeholder(placeholder: string) {
this._proxy.$setInputBoxPlaceholder(this._sourceControlHandle, placeholder);
this._placeholder = placeholder;
}
private _validateInput: IValidateInput | undefined;
get validateInput(): IValidateInput | undefined {
if (!this._extension.enableProposedApi) {
throw new Error(`[${this._extension.identifier.value}]: Proposed API is only available when running out of dev or with the following command line switch: --enable-proposed-api ${this._extension.identifier.value}`);
}
return this._validateInput;
}
set validateInput(fn: IValidateInput | undefined) {
if (!this._extension.enableProposedApi) {
throw new Error(`[${this._extension.identifier.value}]: Proposed API is only available when running out of dev or with the following command line switch: --enable-proposed-api ${this._extension.identifier.value}`);
}
if (fn && typeof fn !== 'function') {
throw new Error(`[${this._extension.identifier.value}]: Invalid SCM input box validation function`);
}
this._validateInput = fn;
this._proxy.$setValidationProviderIsEnabled(this._sourceControlHandle, !!fn);
}
private _visible: boolean = true;
get visible(): boolean {
return this._visible;
}
set visible(visible: boolean) {
visible = !!visible;
this._visible = visible;
this._proxy.$setInputBoxVisibility(this._sourceControlHandle, visible);
}
constructor(private _extension: IExtensionDescription, private _proxy: MainThreadSCMShape, private _sourceControlHandle: number) {
// noop
}
$onInputBoxValueChange(value: string): void {
this.updateValue(value);
}
private updateValue(value: string): void {
this._value = value;
this._onDidChange.fire(value);
}
}
class ExtHostSourceControlResourceGroup implements vscode.SourceControlResourceGroup {
private static _handlePool: number = 0;
private _resourceHandlePool: number = 0;
private _resourceStates: vscode.SourceControlResourceState[] = [];
private _resourceStatesMap: Map<ResourceStateHandle, vscode.SourceControlResourceState> = new Map<ResourceStateHandle, vscode.SourceControlResourceState>();
private _resourceStatesCommandsMap: Map<ResourceStateHandle, vscode.Command> = new Map<ResourceStateHandle, vscode.Command>();
private readonly _onDidUpdateResourceStates = new Emitter<void>();
readonly onDidUpdateResourceStates = this._onDidUpdateResourceStates.event;
private readonly _onDidDispose = new Emitter<void>();
readonly onDidDispose = this._onDidDispose.event;
private _handlesSnapshot: number[] = [];
private _resourceSnapshot: vscode.SourceControlResourceState[] = [];
get id(): string { return this._id; }
get label(): string { return this._label; }
set label(label: string) {
this._label = label;
this._proxy.$updateGroupLabel(this._sourceControlHandle, this.handle, label);
}
private _hideWhenEmpty: boolean | undefined = undefined;
get hideWhenEmpty(): boolean | undefined { return this._hideWhenEmpty; }
set hideWhenEmpty(hideWhenEmpty: boolean | undefined) {
this._hideWhenEmpty = hideWhenEmpty;
this._proxy.$updateGroup(this._sourceControlHandle, this.handle, { hideWhenEmpty });
}
get resourceStates(): vscode.SourceControlResourceState[] { return [...this._resourceStates]; }
set resourceStates(resources: vscode.SourceControlResourceState[]) {
this._resourceStates = [...resources];
this._onDidUpdateResourceStates.fire();
}
readonly handle = ExtHostSourceControlResourceGroup._handlePool++;
constructor(
private _proxy: MainThreadSCMShape,
private _commands: ExtHostCommands,
private _sourceControlHandle: number,
private _id: string,
private _label: string,
) {
this._proxy.$registerGroup(_sourceControlHandle, this.handle, _id, _label);
}
getResourceState(handle: number): vscode.SourceControlResourceState | undefined {
return this._resourceStatesMap.get(handle);
}
$executeResourceCommand(handle: number): Promise<void> {
const command = this._resourceStatesCommandsMap.get(handle);
if (!command) {
return Promise.resolve(undefined);
}
return asPromise(() => this._commands.executeCommand(command.command, ...(command.arguments || [])));
}
_takeResourceStateSnapshot(): SCMRawResourceSplice[] {
const snapshot = [...this._resourceStates].sort(compareResourceStates);
const diffs = sortedDiff(this._resourceSnapshot, snapshot, compareResourceStates);
const splices = diffs.map<ISplice<{ rawResource: SCMRawResource, handle: number }>>(diff => {
const toInsert = diff.toInsert.map(r => {
const handle = this._resourceHandlePool++;
this._resourceStatesMap.set(handle, r);
const sourceUri = r.resourceUri;
const iconPath = getIconPath(r.decorations);
const lightIconPath = r.decorations && getIconPath(r.decorations.light) || iconPath;
const darkIconPath = r.decorations && getIconPath(r.decorations.dark) || iconPath;
const icons: string[] = [];
if (r.command) {
this._resourceStatesCommandsMap.set(handle, r.command);
}
if (lightIconPath) {
icons.push(lightIconPath);
}
if (darkIconPath && (darkIconPath !== lightIconPath)) {
icons.push(darkIconPath);
}
const tooltip = (r.decorations && r.decorations.tooltip) || '';
const strikeThrough = r.decorations && !!r.decorations.strikeThrough;
const faded = r.decorations && !!r.decorations.faded;
const rawResource = [handle, <UriComponents>sourceUri, icons, tooltip, strikeThrough, faded] as SCMRawResource;
return { rawResource, handle };
});
return { start: diff.start, deleteCount: diff.deleteCount, toInsert };
});
const rawResourceSplices = splices
.map(({ start, deleteCount, toInsert }) => [start, deleteCount, toInsert.map(i => i.rawResource)] as SCMRawResourceSplice);
const reverseSplices = splices.reverse();
for (const { start, deleteCount, toInsert } of reverseSplices) {
const handles = toInsert.map(i => i.handle);
const handlesToDelete = this._handlesSnapshot.splice(start, deleteCount, ...handles);
for (const handle of handlesToDelete) {
this._resourceStatesMap.delete(handle);
this._resourceStatesCommandsMap.delete(handle);
}
}
this._resourceSnapshot = snapshot;
return rawResourceSplices;
}
dispose(): void {
this._proxy.$unregisterGroup(this._sourceControlHandle, this.handle);
this._onDidDispose.fire();
}
}
class ExtHostSourceControl implements vscode.SourceControl {
private static _handlePool: number = 0;
private _groups: Map<GroupHandle, ExtHostSourceControlResourceGroup> = new Map<GroupHandle, ExtHostSourceControlResourceGroup>();
get id(): string {
return this._id;
}
get label(): string {
return this._label;
}
get rootUri(): vscode.Uri | undefined {
return this._rootUri;
}
private _inputBox: ExtHostSCMInputBox;
get inputBox(): ExtHostSCMInputBox { return this._inputBox; }
private _count: number | undefined = undefined;
get count(): number | undefined {
return this._count;
}
set count(count: number | undefined) {
if (this._count === count) {
return;
}
this._count = count;
this._proxy.$updateSourceControl(this.handle, { count });
}
private _quickDiffProvider: vscode.QuickDiffProvider | undefined = undefined;
get quickDiffProvider(): vscode.QuickDiffProvider | undefined {
return this._quickDiffProvider;
}
set quickDiffProvider(quickDiffProvider: vscode.QuickDiffProvider | undefined) {
this._quickDiffProvider = quickDiffProvider;
this._proxy.$updateSourceControl(this.handle, { hasQuickDiffProvider: !!quickDiffProvider });
}
private _commitTemplate: string | undefined = undefined;
get commitTemplate(): string | undefined {
return this._commitTemplate;
}
set commitTemplate(commitTemplate: string | undefined) {
if (commitTemplate === this._commitTemplate) {
return;
}
this._commitTemplate = commitTemplate;
this._proxy.$updateSourceControl(this.handle, { commitTemplate });
}
private _acceptInputDisposables = new MutableDisposable<DisposableStore>();
private _acceptInputCommand: vscode.Command | undefined = undefined;
get acceptInputCommand(): vscode.Command | undefined {
return this._acceptInputCommand;
}
set acceptInputCommand(acceptInputCommand: vscode.Command | undefined) {
this._acceptInputDisposables.value = new DisposableStore();
this._acceptInputCommand = acceptInputCommand;
const internal = this._commands.converter.toInternal(acceptInputCommand, this._acceptInputDisposables.value);
this._proxy.$updateSourceControl(this.handle, { acceptInputCommand: internal });
}
private _statusBarDisposables = new MutableDisposable<DisposableStore>();
private _statusBarCommands: vscode.Command[] | undefined = undefined;
get statusBarCommands(): vscode.Command[] | undefined {
return this._statusBarCommands;
}
set statusBarCommands(statusBarCommands: vscode.Command[] | undefined) {
if (this._statusBarCommands && statusBarCommands && commandListEquals(this._statusBarCommands, statusBarCommands)) {
return;
}
this._statusBarDisposables.value = new DisposableStore();
this._statusBarCommands = statusBarCommands;
const internal = (statusBarCommands || []).map(c => this._commands.converter.toInternal(c, this._statusBarDisposables.value!)) as ICommandDto[];
this._proxy.$updateSourceControl(this.handle, { statusBarCommands: internal });
}
private _selected: boolean = false;
get selected(): boolean {
return this._selected;
}
private readonly _onDidChangeSelection = new Emitter<boolean>();
readonly onDidChangeSelection = this._onDidChangeSelection.event;
private handle: number = ExtHostSourceControl._handlePool++;
constructor(
_extension: IExtensionDescription,
private _proxy: MainThreadSCMShape,
private _commands: ExtHostCommands,
private _id: string,
private _label: string,
private _rootUri?: vscode.Uri
) {
this._inputBox = new ExtHostSCMInputBox(_extension, this._proxy, this.handle);
this._proxy.$registerSourceControl(this.handle, _id, _label, _rootUri);
}
private updatedResourceGroups = new Set<ExtHostSourceControlResourceGroup>();
createResourceGroup(id: string, label: string): ExtHostSourceControlResourceGroup {
const group = new ExtHostSourceControlResourceGroup(this._proxy, this._commands, this.handle, id, label);
const updateListener = group.onDidUpdateResourceStates(() => {
this.updatedResourceGroups.add(group);
this.eventuallyUpdateResourceStates();
});
Event.once(group.onDidDispose)(() => {
this.updatedResourceGroups.delete(group);
updateListener.dispose();
this._groups.delete(group.handle);
});
this._groups.set(group.handle, group);
return group;
}
@debounce(100)
eventuallyUpdateResourceStates(): void {
const splices: SCMRawResourceSplices[] = [];
this.updatedResourceGroups.forEach(group => {
const snapshot = group._takeResourceStateSnapshot();
if (snapshot.length === 0) {
return;
}
splices.push([group.handle, snapshot]);
});
if (splices.length > 0) {
this._proxy.$spliceResourceStates(this.handle, splices);
}
this.updatedResourceGroups.clear();
}
getResourceGroup(handle: GroupHandle): ExtHostSourceControlResourceGroup | undefined {
return this._groups.get(handle);
}
setSelectionState(selected: boolean): void {
this._selected = selected;
this._onDidChangeSelection.fire(selected);
}
dispose(): void {
this._acceptInputDisposables.dispose();
this._statusBarDisposables.dispose();
this._groups.forEach(group => group.dispose());
this._proxy.$unregisterSourceControl(this.handle);
}
}
export class ExtHostSCM implements ExtHostSCMShape {
private static _handlePool: number = 0;
private _proxy: MainThreadSCMShape;
private _sourceControls: Map<ProviderHandle, ExtHostSourceControl> = new Map<ProviderHandle, ExtHostSourceControl>();
private _sourceControlsByExtension: Map<string, ExtHostSourceControl[]> = new Map<string, ExtHostSourceControl[]>();
private readonly _onDidChangeActiveProvider = new Emitter<vscode.SourceControl>();
get onDidChangeActiveProvider(): Event<vscode.SourceControl> { return this._onDidChangeActiveProvider.event; }
private _selectedSourceControlHandles = new Set<number>();
constructor(
mainContext: IMainContext,
private _commands: ExtHostCommands,
@ILogService private readonly logService: ILogService
) {
this._proxy = mainContext.getProxy(MainContext.MainThreadSCM);
_commands.registerArgumentProcessor({
processArgument: arg => {
if (arg && arg.$mid === 3) {
const sourceControl = this._sourceControls.get(arg.sourceControlHandle);
if (!sourceControl) {
return arg;
}
const group = sourceControl.getResourceGroup(arg.groupHandle);
if (!group) {
return arg;
}
return group.getResourceState(arg.handle);
} else if (arg && arg.$mid === 4) {
const sourceControl = this._sourceControls.get(arg.sourceControlHandle);
if (!sourceControl) {
return arg;
}
return sourceControl.getResourceGroup(arg.groupHandle);
} else if (arg && arg.$mid === 5) {
const sourceControl = this._sourceControls.get(arg.handle);
if (!sourceControl) {
return arg;
}
return sourceControl;
}
return arg;
}
});
}
createSourceControl(extension: IExtensionDescription, id: string, label: string, rootUri: vscode.Uri | undefined): vscode.SourceControl {
this.logService.trace('ExtHostSCM#createSourceControl', extension.identifier.value, id, label, rootUri);
const handle = ExtHostSCM._handlePool++;
const sourceControl = new ExtHostSourceControl(extension, this._proxy, this._commands, id, label, rootUri);
this._sourceControls.set(handle, sourceControl);
const sourceControls = this._sourceControlsByExtension.get(ExtensionIdentifier.toKey(extension.identifier)) || [];
sourceControls.push(sourceControl);
this._sourceControlsByExtension.set(ExtensionIdentifier.toKey(extension.identifier), sourceControls);
return sourceControl;
}
// Deprecated
getLastInputBox(extension: IExtensionDescription): ExtHostSCMInputBox | undefined {
this.logService.trace('ExtHostSCM#getLastInputBox', extension.identifier.value);
const sourceControls = this._sourceControlsByExtension.get(ExtensionIdentifier.toKey(extension.identifier));
const sourceControl = sourceControls && sourceControls[sourceControls.length - 1];
return sourceControl && sourceControl.inputBox;
}
$provideOriginalResource(sourceControlHandle: number, uriComponents: UriComponents, token: CancellationToken): Promise<UriComponents | null> {
const uri = URI.revive(uriComponents);
this.logService.trace('ExtHostSCM#$provideOriginalResource', sourceControlHandle, uri.toString());
const sourceControl = this._sourceControls.get(sourceControlHandle);
if (!sourceControl || !sourceControl.quickDiffProvider || !sourceControl.quickDiffProvider.provideOriginalResource) {
return Promise.resolve(null);
}
return asPromise(() => sourceControl.quickDiffProvider!.provideOriginalResource!(uri, token))
.then<UriComponents | null>(r => r || null);
}
$onInputBoxValueChange(sourceControlHandle: number, value: string): Promise<void> {
this.logService.trace('ExtHostSCM#$onInputBoxValueChange', sourceControlHandle);
const sourceControl = this._sourceControls.get(sourceControlHandle);
if (!sourceControl) {
return Promise.resolve(undefined);
}
sourceControl.inputBox.$onInputBoxValueChange(value);
return Promise.resolve(undefined);
}
$executeResourceCommand(sourceControlHandle: number, groupHandle: number, handle: number): Promise<void> {
this.logService.trace('ExtHostSCM#$executeResourceCommand', sourceControlHandle, groupHandle, handle);
const sourceControl = this._sourceControls.get(sourceControlHandle);
if (!sourceControl) {
return Promise.resolve(undefined);
}
const group = sourceControl.getResourceGroup(groupHandle);
if (!group) {
return Promise.resolve(undefined);
}
return group.$executeResourceCommand(handle);
}
$validateInput(sourceControlHandle: number, value: string, cursorPosition: number): Promise<[string, number] | undefined> {
this.logService.trace('ExtHostSCM#$validateInput', sourceControlHandle);
const sourceControl = this._sourceControls.get(sourceControlHandle);
if (!sourceControl) {
return Promise.resolve(undefined);
}
if (!sourceControl.inputBox.validateInput) {
return Promise.resolve(undefined);
}
return asPromise(() => sourceControl.inputBox.validateInput!(value, cursorPosition)).then(result => {
if (!result) {
return Promise.resolve(undefined);
}
return Promise.resolve<[string, number]>([result.message, result.type]);
});
}
$setSelectedSourceControls(selectedSourceControlHandles: number[]): Promise<void> {
this.logService.trace('ExtHostSCM#$setSelectedSourceControls', selectedSourceControlHandles);
const set = new Set<number>();
for (const handle of selectedSourceControlHandles) {
set.add(handle);
}
set.forEach(handle => {
if (!this._selectedSourceControlHandles.has(handle)) {
const sourceControl = this._sourceControls.get(handle);
if (!sourceControl) {
return;
}
sourceControl.setSelectionState(true);
}
});
this._selectedSourceControlHandles.forEach(handle => {
if (!set.has(handle)) {
const sourceControl = this._sourceControls.get(handle);
if (!sourceControl) {
return;
}
sourceControl.setSelectionState(false);
}
});
this._selectedSourceControlHandles = set;
return Promise.resolve(undefined);
}
}
| src/vs/workbench/api/common/extHostSCM.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00017119811673182994,
0.00016816169954836369,
0.0001651523489272222,
0.00016812706599012017,
0.0000013768541293757153
] |
{
"id": 12,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"\n",
"\t/**\n",
"\t * Create a new editor under `domElement`.\n",
"\t * `domElement` should be empty (not contain other dom nodes).\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\texport interface IDiffNavigator {\n",
"\t\tcanNavigate(): boolean;\n",
"\t\tnext(): void;\n",
"\t\tprevious(): void;\n",
"\t\tdispose(): void;\n",
"\t}\n"
],
"file_path": "src/vs/monaco.d.ts",
"type": "add",
"edit_start_line_idx": 804
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { getLocation, parse, visit } from 'jsonc-parser';
import * as path from 'path';
import * as vscode from 'vscode';
import * as nls from 'vscode-nls';
import { SettingsDocument } from './settingsDocumentHelper';
const localize = nls.loadMessageBundle();
const fadedDecoration = vscode.window.createTextEditorDecorationType({
light: {
color: '#757575'
},
dark: {
color: '#878787'
}
});
let pendingLaunchJsonDecoration: NodeJS.Timer;
export function activate(context: vscode.ExtensionContext): void {
//settings.json suggestions
context.subscriptions.push(registerSettingsCompletions());
//extensions suggestions
context.subscriptions.push(...registerExtensionsCompletions());
// launch.json variable suggestions
context.subscriptions.push(registerVariableCompletions('**/launch.json'));
// task.json variable suggestions
context.subscriptions.push(registerVariableCompletions('**/tasks.json'));
// launch.json decorations
context.subscriptions.push(vscode.window.onDidChangeActiveTextEditor(editor => updateLaunchJsonDecorations(editor), null, context.subscriptions));
context.subscriptions.push(vscode.workspace.onDidChangeTextDocument(event => {
if (vscode.window.activeTextEditor && event.document === vscode.window.activeTextEditor.document) {
if (pendingLaunchJsonDecoration) {
clearTimeout(pendingLaunchJsonDecoration);
}
pendingLaunchJsonDecoration = setTimeout(() => updateLaunchJsonDecorations(vscode.window.activeTextEditor), 1000);
}
}, null, context.subscriptions));
updateLaunchJsonDecorations(vscode.window.activeTextEditor);
}
function registerSettingsCompletions(): vscode.Disposable {
return vscode.languages.registerCompletionItemProvider({ language: 'jsonc', pattern: '**/settings.json' }, {
provideCompletionItems(document, position, token) {
return new SettingsDocument(document).provideCompletionItems(position, token);
}
});
}
function registerVariableCompletions(pattern: string): vscode.Disposable {
return vscode.languages.registerCompletionItemProvider({ language: 'jsonc', pattern }, {
provideCompletionItems(document, position, _token) {
const location = getLocation(document.getText(), document.offsetAt(position));
if (!location.isAtPropertyKey && location.previousNode && location.previousNode.type === 'string') {
const indexOf$ = document.lineAt(position.line).text.indexOf('$');
const startPosition = indexOf$ >= 0 ? new vscode.Position(position.line, indexOf$) : position;
return [
{ label: 'workspaceFolder', detail: localize('workspaceFolder', "The path of the folder opened in VS Code") },
{ label: 'workspaceFolderBasename', detail: localize('workspaceFolderBasename', "The name of the folder opened in VS Code without any slashes (/)") },
{ label: 'relativeFile', detail: localize('relativeFile', "The current opened file relative to ${workspaceFolder}") },
{ label: 'relativeFileDirname', detail: localize('relativeFileDirname', "The current opened file's dirname relative to ${workspaceFolder}") },
{ label: 'file', detail: localize('file', "The current opened file") },
{ label: 'cwd', detail: localize('cwd', "The task runner's current working directory on startup") },
{ label: 'lineNumber', detail: localize('lineNumber', "The current selected line number in the active file") },
{ label: 'selectedText', detail: localize('selectedText', "The current selected text in the active file") },
{ label: 'fileDirname', detail: localize('fileDirname', "The current opened file's dirname") },
{ label: 'fileExtname', detail: localize('fileExtname', "The current opened file's extension") },
{ label: 'fileBasename', detail: localize('fileBasename', "The current opened file's basename") },
{ label: 'fileBasenameNoExtension', detail: localize('fileBasenameNoExtension', "The current opened file's basename with no file extension") },
{ label: 'defaultBuildTask', detail: localize('defaultBuildTask', "The name of the default build task. If there is not a single default build task then a quick pick is shown to choose the build task.") },
].map(variable => ({
label: '${' + variable.label + '}',
range: new vscode.Range(startPosition, position),
detail: variable.detail
}));
}
return [];
}
});
}
interface IExtensionsContent {
recommendations: string[];
}
function registerExtensionsCompletions(): vscode.Disposable[] {
return [registerExtensionsCompletionsInExtensionsDocument(), registerExtensionsCompletionsInWorkspaceConfigurationDocument()];
}
function registerExtensionsCompletionsInExtensionsDocument(): vscode.Disposable {
return vscode.languages.registerCompletionItemProvider({ pattern: '**/extensions.json' }, {
provideCompletionItems(document, position, _token) {
const location = getLocation(document.getText(), document.offsetAt(position));
const range = document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
if (location.path[0] === 'recommendations') {
const extensionsContent = <IExtensionsContent>parse(document.getText());
return provideInstalledExtensionProposals(extensionsContent, range);
}
return [];
}
});
}
function registerExtensionsCompletionsInWorkspaceConfigurationDocument(): vscode.Disposable {
return vscode.languages.registerCompletionItemProvider({ pattern: '**/*.code-workspace' }, {
provideCompletionItems(document, position, _token) {
const location = getLocation(document.getText(), document.offsetAt(position));
const range = document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
if (location.path[0] === 'extensions' && location.path[1] === 'recommendations') {
const extensionsContent = <IExtensionsContent>parse(document.getText())['extensions'];
return provideInstalledExtensionProposals(extensionsContent, range);
}
return [];
}
});
}
function provideInstalledExtensionProposals(extensionsContent: IExtensionsContent, range: vscode.Range): vscode.ProviderResult<vscode.CompletionItem[] | vscode.CompletionList> {
const alreadyEnteredExtensions = extensionsContent && extensionsContent.recommendations || [];
if (Array.isArray(alreadyEnteredExtensions)) {
const knownExtensionProposals = vscode.extensions.all.filter(e =>
!(e.id.startsWith('vscode.')
|| e.id === 'Microsoft.vscode-markdown'
|| alreadyEnteredExtensions.indexOf(e.id) > -1));
if (knownExtensionProposals.length) {
return knownExtensionProposals.map(e => {
const item = new vscode.CompletionItem(e.id);
const insertText = `"${e.id}"`;
item.kind = vscode.CompletionItemKind.Value;
item.insertText = insertText;
item.range = range;
item.filterText = insertText;
return item;
});
} else {
const example = new vscode.CompletionItem(localize('exampleExtension', "Example"));
example.insertText = '"vscode.csharp"';
example.kind = vscode.CompletionItemKind.Value;
example.range = range;
return [example];
}
}
return undefined;
}
function updateLaunchJsonDecorations(editor: vscode.TextEditor | undefined): void {
if (!editor || path.basename(editor.document.fileName) !== 'launch.json') {
return;
}
const ranges: vscode.Range[] = [];
let addPropertyAndValue = false;
let depthInArray = 0;
visit(editor.document.getText(), {
onObjectProperty: (property, offset, length) => {
// Decorate attributes which are unlikely to be edited by the user.
// Only decorate "configurations" if it is not inside an array (compounds have a configurations property which should not be decorated).
addPropertyAndValue = property === 'version' || property === 'type' || property === 'request' || property === 'compounds' || (property === 'configurations' && depthInArray === 0);
if (addPropertyAndValue) {
ranges.push(new vscode.Range(editor.document.positionAt(offset), editor.document.positionAt(offset + length)));
}
},
onLiteralValue: (_value, offset, length) => {
if (addPropertyAndValue) {
ranges.push(new vscode.Range(editor.document.positionAt(offset), editor.document.positionAt(offset + length)));
}
},
onArrayBegin: (_offset: number, _length: number) => {
depthInArray++;
},
onArrayEnd: (_offset: number, _length: number) => {
depthInArray--;
}
});
editor.setDecorations(fadedDecoration, ranges);
}
vscode.languages.registerDocumentSymbolProvider({ pattern: '**/launch.json', language: 'jsonc' }, {
provideDocumentSymbols(document: vscode.TextDocument, _token: vscode.CancellationToken): vscode.ProviderResult<vscode.SymbolInformation[]> {
const result: vscode.SymbolInformation[] = [];
let name: string = '';
let lastProperty = '';
let startOffset = 0;
let depthInObjects = 0;
visit(document.getText(), {
onObjectProperty: (property, _offset, _length) => {
lastProperty = property;
},
onLiteralValue: (value: any, _offset: number, _length: number) => {
if (lastProperty === 'name') {
name = value;
}
},
onObjectBegin: (offset: number, _length: number) => {
depthInObjects++;
if (depthInObjects === 2) {
startOffset = offset;
}
},
onObjectEnd: (offset: number, _length: number) => {
if (name && depthInObjects === 2) {
result.push(new vscode.SymbolInformation(name, vscode.SymbolKind.Object, new vscode.Range(document.positionAt(startOffset), document.positionAt(offset))));
}
depthInObjects--;
},
});
return result;
}
}, { label: 'Launch Targets' });
| extensions/configuration-editing/src/extension.ts | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00027345301350578666,
0.00017762641073204577,
0.00016501729260198772,
0.00016952920123003423,
0.000024425355150015093
] |
{
"id": 12,
"code_window": [
"}\n",
"\n",
"declare namespace monaco.editor {\n",
"\n",
"\n",
"\t/**\n",
"\t * Create a new editor under `domElement`.\n",
"\t * `domElement` should be empty (not contain other dom nodes).\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\texport interface IDiffNavigator {\n",
"\t\tcanNavigate(): boolean;\n",
"\t\tnext(): void;\n",
"\t\tprevious(): void;\n",
"\t\tdispose(): void;\n",
"\t}\n"
],
"file_path": "src/vs/monaco.d.ts",
"type": "add",
"edit_start_line_idx": 804
} | <!-- Copyright (C) Microsoft Corporation. All rights reserved. -->
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="Content-Security-Policy" content="default-src 'none'; img-src 'self' https: data:; media-src 'none'; child-src 'self'; object-src 'self'; script-src 'self'; style-src 'self' 'unsafe-inline'; connect-src 'self' https:; font-src 'self' https:;">
</head>
<body aria-label="">
<table id="process-list"></table>
</body>
<!-- Startup via processExplorer.js -->
<script src="processExplorer.js"></script>
</html> | src/vs/code/electron-browser/processExplorer/processExplorer.html | 0 | https://github.com/microsoft/vscode/commit/27770ed1b52617d16c671f1909f9d759cdebcdf1 | [
0.00016865193902049214,
0.00016840858734212816,
0.00016816523566376418,
0.00016840858734212816,
2.4335167836397886e-7
] |
{
"id": 0,
"code_window": [
"/* A helper that can be used to show a placeholder when creating a new stat */\n",
"export class NewStatPlaceholder extends ExplorerItem {\n",
"\n",
"\tprivate static ID = 0;\n",
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tpublic static NAME = '';\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "add",
"edit_start_line_idx": 380
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import 'vs/css!./media/fileactions';
import { TPromise } from 'vs/base/common/winjs.base';
import * as nls from 'vs/nls';
import { isWindows, isLinux } from 'vs/base/common/platform';
import { sequence, ITask, always } from 'vs/base/common/async';
import * as paths from 'vs/base/common/paths';
import * as resources from 'vs/base/common/resources';
import URI from 'vs/base/common/uri';
import { posix } from 'path';
import * as errors from 'vs/base/common/errors';
import { toErrorMessage } from 'vs/base/common/errorMessage';
import * as strings from 'vs/base/common/strings';
import * as diagnostics from 'vs/base/common/diagnostics';
import { Action, IAction } from 'vs/base/common/actions';
import { MessageType, IInputValidator } from 'vs/base/browser/ui/inputbox/inputBox';
import { ITree, IHighlightEvent } from 'vs/base/parts/tree/browser/tree';
import { dispose, IDisposable } from 'vs/base/common/lifecycle';
import { VIEWLET_ID } from 'vs/workbench/parts/files/common/files';
import { ITextFileService } from 'vs/workbench/services/textfile/common/textfiles';
import { IFileService, IFileStat } from 'vs/platform/files/common/files';
import { toResource } from 'vs/workbench/common/editor';
import { ExplorerItem, Model, NewStatPlaceholder } from 'vs/workbench/parts/files/common/explorerModel';
import { ExplorerView } from 'vs/workbench/parts/files/electron-browser/views/explorerView';
import { ExplorerViewlet } from 'vs/workbench/parts/files/electron-browser/explorerViewlet';
import { IUntitledEditorService } from 'vs/workbench/services/untitled/common/untitledEditorService';
import { IWorkbenchEditorService } from 'vs/workbench/services/editor/common/editorService';
import { CollapseAction } from 'vs/workbench/browser/viewlet';
import { IEditorGroupService } from 'vs/workbench/services/group/common/groupService';
import { IQuickOpenService } from 'vs/platform/quickOpen/common/quickOpen';
import { IViewletService } from 'vs/workbench/services/viewlet/browser/viewlet';
import { IUntitledResourceInput } from 'vs/platform/editor/common/editor';
import { IInstantiationService, ServicesAccessor, IConstructorSignature2 } from 'vs/platform/instantiation/common/instantiation';
import { ITextModel } from 'vs/editor/common/model';
import { IBackupFileService } from 'vs/workbench/services/backup/common/backup';
import { IWindowsService } from 'vs/platform/windows/common/windows';
import { COPY_PATH_COMMAND_ID, REVEAL_IN_EXPLORER_COMMAND_ID, SAVE_ALL_COMMAND_ID, SAVE_ALL_LABEL, SAVE_ALL_IN_GROUP_COMMAND_ID } from 'vs/workbench/parts/files/electron-browser/fileCommands';
import { ITextModelService, ITextModelContentProvider } from 'vs/editor/common/services/resolverService';
import { IConfigurationService, ConfigurationTarget } from 'vs/platform/configuration/common/configuration';
import { once } from 'vs/base/common/event';
import { IClipboardService } from 'vs/platform/clipboard/common/clipboardService';
import { IModeService } from 'vs/editor/common/services/modeService';
import { IModelService } from 'vs/editor/common/services/modelService';
import { ICommandService, CommandsRegistry } from 'vs/platform/commands/common/commands';
import { IListService, ListWidget } from 'vs/platform/list/browser/listService';
import { RawContextKey, IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { Schemas } from 'vs/base/common/network';
import { IDialogService, IConfirmationResult, IConfirmation, getConfirmMessage } from 'vs/platform/dialogs/common/dialogs';
import { INotificationService, Severity } from 'vs/platform/notification/common/notification';
export interface IEditableData {
action: IAction;
validator: IInputValidator;
}
export interface IFileViewletState {
getEditableData(stat: ExplorerItem): IEditableData;
setEditable(stat: ExplorerItem, editableData: IEditableData): void;
clearEditable(stat: ExplorerItem): void;
}
export const NEW_FILE_COMMAND_ID = 'explorer.newFile';
export const NEW_FILE_LABEL = nls.localize('newFile', "New File");
export const NEW_FOLDER_COMMAND_ID = 'explorer.newFolder';
export const NEW_FOLDER_LABEL = nls.localize('newFolder', "New Folder");
export const TRIGGER_RENAME_LABEL = nls.localize('rename', "Rename");
export const MOVE_FILE_TO_TRASH_LABEL = nls.localize('delete', "Delete");
export const COPY_FILE_LABEL = nls.localize('copyFile', "Copy");
export const PASTE_FILE_LABEL = nls.localize('pasteFile', "Paste");
export const FileCopiedContext = new RawContextKey<boolean>('fileCopied', false);
export class BaseErrorReportingAction extends Action {
constructor(
id: string,
label: string,
private _notificationService: INotificationService
) {
super(id, label);
}
public get notificationService() {
return this._notificationService;
}
protected onError(error: any): void {
if (error.message === 'string') {
error = error.message;
}
this._notificationService.error(toErrorMessage(error, false));
}
protected onErrorWithRetry(error: any, retry: () => TPromise<any>): void {
this._notificationService.prompt(Severity.Error, toErrorMessage(error, false),
[{
label: nls.localize('retry', "Retry"),
run: () => retry()
}]
);
}
}
export class BaseFileAction extends BaseErrorReportingAction {
public element: ExplorerItem;
constructor(
id: string,
label: string,
@IFileService protected fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService protected textFileService: ITextFileService
) {
super(id, label, notificationService);
this.enabled = false;
}
_isEnabled(): boolean {
return true;
}
_updateEnablement(): void {
this.enabled = !!(this.fileService && this._isEnabled());
}
}
class TriggerRenameFileAction extends BaseFileAction {
public static readonly ID = 'renameFile';
private tree: ITree;
private renameAction: BaseRenameAction;
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IInstantiationService instantiationService: IInstantiationService
) {
super(TriggerRenameFileAction.ID, TRIGGER_RENAME_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this.element = element;
this.renameAction = instantiationService.createInstance(RenameFileAction, element);
this._updateEnablement();
}
public validateFileName(name: string): string {
const names: string[] = name.split(/[\\/]/).filter(part => !!part);
if (names.length > 1) { // error only occurs on multi-path
const comparer = isLinux ? strings.compare : strings.compareIgnoreCase;
if (comparer(names[0], this.element.name) === 0) {
return nls.localize('renameWhenSourcePathIsParentOfTargetError', "Please use the 'New Folder' or 'New File' command to add children to an existing folder");
}
}
return this.renameAction.validateFileName(this.element.parent, name);
}
public run(context?: any): TPromise<any> {
if (!context) {
return TPromise.wrapError(new Error('No context provided to BaseEnableFileRenameAction.'));
}
const viewletState = <IFileViewletState>context.viewletState;
if (!viewletState) {
return TPromise.wrapError(new Error('Invalid viewlet state provided to BaseEnableFileRenameAction.'));
}
const stat = <ExplorerItem>context.stat;
if (!stat) {
return TPromise.wrapError(new Error('Invalid stat provided to BaseEnableFileRenameAction.'));
}
viewletState.setEditable(stat, {
action: this.renameAction,
validator: (value) => {
const message = this.validateFileName(value);
if (!message) {
return null;
}
return {
content: message,
formatContent: true,
type: MessageType.ERROR
};
}
});
this.tree.refresh(stat, false).then(() => {
this.tree.setHighlight(stat);
const unbind = this.tree.onDidChangeHighlight((e: IHighlightEvent) => {
if (!e.highlight) {
viewletState.clearEditable(stat);
this.tree.refresh(stat).done(null, errors.onUnexpectedError);
unbind.dispose();
}
});
}).done(null, errors.onUnexpectedError);
return void 0;
}
}
export abstract class BaseRenameAction extends BaseFileAction {
constructor(
id: string,
label: string,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(id, label, fileService, notificationService, textFileService);
this.element = element;
}
public run(context?: any): TPromise<any> {
if (!context) {
return TPromise.wrapError(new Error('No context provided to BaseRenameFileAction.'));
}
let name = <string>context.value;
if (!name) {
return TPromise.wrapError(new Error('No new name provided to BaseRenameFileAction.'));
}
// Automatically trim whitespaces and trailing dots to produce nice file names
name = getWellFormedFileName(name);
const existingName = getWellFormedFileName(this.element.name);
// Return early if name is invalid or didn't change
if (name === existingName || this.validateFileName(this.element.parent, name)) {
return TPromise.as(null);
}
// Call function and Emit Event through viewer
const promise = this.runAction(name).then(null, (error: any) => {
this.onError(error);
});
return promise;
}
public validateFileName(parent: ExplorerItem, name: string): string {
let source = this.element.name;
let target = name;
if (!isLinux) { // allow rename of same file also when case differs (e.g. Game.js => game.js)
source = source.toLowerCase();
target = target.toLowerCase();
}
if (getWellFormedFileName(source) === getWellFormedFileName(target)) {
return null;
}
return validateFileName(parent, name, false);
}
public abstract runAction(newName: string): TPromise<any>;
}
class RenameFileAction extends BaseRenameAction {
public static readonly ID = 'workbench.files.action.renameFile';
constructor(
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IBackupFileService private backupFileService: IBackupFileService
) {
super(RenameFileAction.ID, nls.localize('rename', "Rename"), element, fileService, notificationService, textFileService);
this._updateEnablement();
}
public runAction(newName: string): TPromise<any> {
const dirty = this.textFileService.getDirty().filter(d => resources.isEqualOrParent(d, this.element.resource, !isLinux /* ignorecase */));
const dirtyRenamed: URI[] = [];
return TPromise.join(dirty.map(d => {
let renamed: URI;
// If the dirty file itself got moved, just reparent it to the target folder
const targetPath = paths.join(this.element.parent.resource.path, newName);
if (this.element.resource.toString() === d.toString()) {
renamed = this.element.parent.resource.with({ path: targetPath });
}
// Otherwise, a parent of the dirty resource got moved, so we have to reparent more complicated. Example:
else {
renamed = this.element.parent.resource.with({ path: paths.join(targetPath, d.path.substr(this.element.resource.path.length + 1)) });
}
dirtyRenamed.push(renamed);
const model = this.textFileService.models.get(d);
return this.backupFileService.backupResource(renamed, model.createSnapshot(), model.getVersionId());
}))
// 2. soft revert all dirty since we have backed up their contents
.then(() => this.textFileService.revertAll(dirty, { soft: true /* do not attempt to load content from disk */ }))
// 3.) run the rename operation
.then(() => this.fileService.rename(this.element.resource, newName).then(null, (error: Error) => {
return TPromise.join(dirtyRenamed.map(d => this.backupFileService.discardResourceBackup(d))).then(() => {
this.onErrorWithRetry(error, () => this.runAction(newName));
});
}))
// 4.) resolve those that were dirty to load their previous dirty contents from disk
.then(() => {
return TPromise.join(dirtyRenamed.map(t => this.textFileService.models.loadOrCreate(t)));
});
}
}
/* Base New File/Folder Action */
export class BaseNewAction extends BaseFileAction {
private presetFolder: ExplorerItem;
private tree: ITree;
private isFile: boolean;
private renameAction: BaseRenameAction;
constructor(
id: string,
label: string,
tree: ITree,
isFile: boolean,
editableAction: BaseRenameAction,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(id, label, fileService, notificationService, textFileService);
if (element) {
this.presetFolder = element.isDirectory ? element : element.parent;
}
this.tree = tree;
this.isFile = isFile;
this.renameAction = editableAction;
}
public run(context?: any): TPromise<any> {
if (!context) {
return TPromise.wrapError(new Error('No context provided to BaseNewAction.'));
}
const viewletState = <IFileViewletState>context.viewletState;
if (!viewletState) {
return TPromise.wrapError(new Error('Invalid viewlet state provided to BaseNewAction.'));
}
let folder = this.presetFolder;
if (!folder) {
const focus = <ExplorerItem>this.tree.getFocus();
if (focus) {
folder = focus.isDirectory ? focus : focus.parent;
} else {
const input: ExplorerItem | Model = this.tree.getInput();
folder = input instanceof Model ? input.roots[0] : input;
}
}
if (!folder) {
return TPromise.wrapError(new Error('Invalid parent folder to create.'));
}
return this.tree.reveal(folder, 0.5).then(() => {
return this.tree.expand(folder).then(() => {
const stat = NewStatPlaceholder.addNewStatPlaceholder(folder, !this.isFile);
this.renameAction.element = stat;
viewletState.setEditable(stat, {
action: this.renameAction,
validator: (value) => {
const message = this.renameAction.validateFileName(folder, value);
if (!message) {
return null;
}
return {
content: message,
formatContent: true,
type: MessageType.ERROR
};
}
});
return this.tree.refresh(folder).then(() => {
return this.tree.expand(folder).then(() => {
return this.tree.reveal(stat, 0.5).then(() => {
this.tree.setHighlight(stat);
const unbind = this.tree.onDidChangeHighlight((e: IHighlightEvent) => {
if (!e.highlight) {
stat.destroy();
this.tree.refresh(folder).done(null, errors.onUnexpectedError);
unbind.dispose();
}
});
});
});
});
});
});
}
}
/* New File */
export class NewFileAction extends BaseNewAction {
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IInstantiationService instantiationService: IInstantiationService
) {
super('explorer.newFile', NEW_FILE_LABEL, tree, true, instantiationService.createInstance(CreateFileAction, element), null, fileService, notificationService, textFileService);
this.class = 'explorer-action new-file';
this._updateEnablement();
}
}
/* New Folder */
export class NewFolderAction extends BaseNewAction {
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IInstantiationService instantiationService: IInstantiationService
) {
super('explorer.newFolder', NEW_FOLDER_LABEL, tree, false, instantiationService.createInstance(CreateFolderAction, element), null, fileService, notificationService, textFileService);
this.class = 'explorer-action new-folder';
this._updateEnablement();
}
}
/* Create new file from anywhere: Open untitled */
export class GlobalNewUntitledFileAction extends Action {
public static readonly ID = 'workbench.action.files.newUntitledFile';
public static readonly LABEL = nls.localize('newUntitledFile', "New Untitled File");
constructor(
id: string,
label: string,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.editorService.openEditor({ options: { pinned: true } } as IUntitledResourceInput); // untitled are always pinned
}
}
/* Create New File/Folder (only used internally by explorerViewer) */
export abstract class BaseCreateAction extends BaseRenameAction {
public validateFileName(parent: ExplorerItem, name: string): string {
if (this.element instanceof NewStatPlaceholder) {
return validateFileName(parent, name, false);
}
return super.validateFileName(parent, name);
}
}
/* Create New File (only used internally by explorerViewer) */
class CreateFileAction extends BaseCreateAction {
public static readonly ID = 'workbench.files.action.createFileFromExplorer';
public static readonly LABEL = nls.localize('createNewFile', "New File");
constructor(
element: ExplorerItem,
@IFileService fileService: IFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(CreateFileAction.ID, CreateFileAction.LABEL, element, fileService, notificationService, textFileService);
this._updateEnablement();
}
public runAction(fileName: string): TPromise<any> {
const resource = this.element.parent.resource;
return this.fileService.createFile(resource.with({ path: paths.join(resource.path, fileName) })).then(stat => {
return this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } });
}, (error) => {
this.onErrorWithRetry(error, () => this.runAction(fileName));
});
}
}
/* Create New Folder (only used internally by explorerViewer) */
class CreateFolderAction extends BaseCreateAction {
public static readonly ID = 'workbench.files.action.createFolderFromExplorer';
public static readonly LABEL = nls.localize('createNewFolder', "New Folder");
constructor(
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(CreateFolderAction.ID, CreateFolderAction.LABEL, null, fileService, notificationService, textFileService);
this._updateEnablement();
}
public runAction(fileName: string): TPromise<any> {
const resource = this.element.parent.resource;
return this.fileService.createFolder(resource.with({ path: paths.join(resource.path, fileName) })).then(null, (error) => {
this.onErrorWithRetry(error, () => this.runAction(fileName));
});
}
}
class BaseDeleteFileAction extends BaseFileAction {
private static readonly CONFIRM_DELETE_SETTING_KEY = 'explorer.confirmDelete';
private skipConfirm: boolean;
constructor(
private tree: ITree,
private elements: ExplorerItem[],
private useTrash: boolean,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@IDialogService private dialogService: IDialogService,
@ITextFileService textFileService: ITextFileService,
@IConfigurationService private configurationService: IConfigurationService
) {
super('moveFileToTrash', MOVE_FILE_TO_TRASH_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this.useTrash = useTrash && elements.every(e => !paths.isUNC(e.resource.fsPath)); // on UNC shares there is no trash
this._updateEnablement();
}
public run(): TPromise<any> {
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
let primaryButton: string;
if (this.useTrash) {
primaryButton = isWindows ? nls.localize('deleteButtonLabelRecycleBin', "&&Move to Recycle Bin") : nls.localize({ key: 'deleteButtonLabelTrash', comment: ['&& denotes a mnemonic'] }, "&&Move to Trash");
} else {
primaryButton = nls.localize({ key: 'deleteButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Delete");
}
const distinctElements = resources.distinctParents(this.elements, e => e.resource);
// Handle dirty
let confirmDirtyPromise: TPromise<boolean> = TPromise.as(true);
const dirty = this.textFileService.getDirty().filter(d => distinctElements.some(e => resources.isEqualOrParent(d, e.resource, !isLinux /* ignorecase */)));
if (dirty.length) {
let message: string;
if (distinctElements.length > 1) {
message = nls.localize('dirtyMessageFilesDelete', "You are deleting files with unsaved changes. Do you want to continue?");
} else if (distinctElements[0].isDirectory) {
if (dirty.length === 1) {
message = nls.localize('dirtyMessageFolderOneDelete', "You are deleting a folder with unsaved changes in 1 file. Do you want to continue?");
} else {
message = nls.localize('dirtyMessageFolderDelete', "You are deleting a folder with unsaved changes in {0} files. Do you want to continue?", dirty.length);
}
} else {
message = nls.localize('dirtyMessageFileDelete', "You are deleting a file with unsaved changes. Do you want to continue?");
}
confirmDirtyPromise = this.dialogService.confirm({
message,
type: 'warning',
detail: nls.localize('dirtyWarning', "Your changes will be lost if you don't save them."),
primaryButton
}).then(res => {
if (!res.confirmed) {
return false;
}
this.skipConfirm = true; // since we already asked for confirmation
return this.textFileService.revertAll(dirty).then(() => true);
});
}
// Check if file is dirty in editor and save it to avoid data loss
return confirmDirtyPromise.then(confirmed => {
if (!confirmed) {
return null;
}
let confirmDeletePromise: TPromise<IConfirmationResult>;
// Check if we need to ask for confirmation at all
if (this.skipConfirm || (this.useTrash && this.configurationService.getValue<boolean>(BaseDeleteFileAction.CONFIRM_DELETE_SETTING_KEY) === false)) {
confirmDeletePromise = TPromise.as({ confirmed: true } as IConfirmationResult);
}
// Confirm for moving to trash
else if (this.useTrash) {
const message = distinctElements.length > 1 ? getConfirmMessage(nls.localize('confirmMoveTrashMessageMultiple', "Are you sure you want to delete the following {0} files?", distinctElements.length), distinctElements.map(e => e.resource))
: distinctElements[0].isDirectory ? nls.localize('confirmMoveTrashMessageFolder', "Are you sure you want to delete '{0}' and its contents?", distinctElements[0].name)
: nls.localize('confirmMoveTrashMessageFile', "Are you sure you want to delete '{0}'?", distinctElements[0].name);
confirmDeletePromise = this.dialogService.confirm({
message,
detail: isWindows ? nls.localize('undoBin', "You can restore from the Recycle Bin.") : nls.localize('undoTrash', "You can restore from the Trash."),
primaryButton,
checkbox: {
label: nls.localize('doNotAskAgain', "Do not ask me again")
},
type: 'question'
});
}
// Confirm for deleting permanently
else {
const message = distinctElements.length > 1 ? getConfirmMessage(nls.localize('confirmDeleteMessageMultiple', "Are you sure you want to permanently delete the following {0} files?", distinctElements.length), distinctElements.map(e => e.resource))
: distinctElements[0].isDirectory ? nls.localize('confirmDeleteMessageFolder', "Are you sure you want to permanently delete '{0}' and its contents?", distinctElements[0].name)
: nls.localize('confirmDeleteMessageFile', "Are you sure you want to permanently delete '{0}'?", distinctElements[0].name);
confirmDeletePromise = this.dialogService.confirm({
message,
detail: nls.localize('irreversible', "This action is irreversible!"),
primaryButton,
type: 'warning'
});
}
return confirmDeletePromise.then(confirmation => {
// Check for confirmation checkbox
let updateConfirmSettingsPromise: TPromise<void> = TPromise.as(void 0);
if (confirmation.confirmed && confirmation.checkboxChecked === true) {
updateConfirmSettingsPromise = this.configurationService.updateValue(BaseDeleteFileAction.CONFIRM_DELETE_SETTING_KEY, false, ConfigurationTarget.USER);
}
return updateConfirmSettingsPromise.then(() => {
// Check for confirmation
if (!confirmation.confirmed) {
return TPromise.as(null);
}
// Call function
const servicePromise = TPromise.join(distinctElements.map(e => this.fileService.del(e.resource, this.useTrash))).then(() => {
if (distinctElements[0].parent) {
this.tree.setFocus(distinctElements[0].parent); // move focus to parent
}
}, (error: any) => {
// Handle error to delete file(s) from a modal confirmation dialog
let errorMessage: string;
let detailMessage: string;
let primaryButton: string;
if (this.useTrash) {
errorMessage = isWindows ? nls.localize('binFailed', "Failed to delete using the Recycle Bin. Do you want to permanently delete instead?") : nls.localize('trashFailed', "Failed to delete using the Trash. Do you want to permanently delete instead?");
detailMessage = nls.localize('irreversible', "This action is irreversible!");
primaryButton = nls.localize({ key: 'deletePermanentlyButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Delete Permanently");
} else {
errorMessage = toErrorMessage(error, false);
primaryButton = nls.localize({ key: 'retryButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Retry");
}
return this.dialogService.confirm({
message: errorMessage,
detail: detailMessage,
type: 'warning',
primaryButton
}).then(res => {
// Focus back to tree
this.tree.domFocus();
if (res.confirmed) {
if (this.useTrash) {
this.useTrash = false; // Delete Permanently
}
this.skipConfirm = true;
return this.run();
}
return TPromise.as(void 0);
});
});
return servicePromise;
});
});
});
}
}
/* Add File */
export class AddFilesAction extends BaseFileAction {
private tree: ITree;
constructor(
tree: ITree,
element: ExplorerItem,
clazz: string,
@IFileService fileService: IFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@IDialogService private dialogService: IDialogService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super('workbench.files.action.addFile', nls.localize('addFiles', "Add Files"), fileService, notificationService, textFileService);
this.tree = tree;
this.element = element;
if (clazz) {
this.class = clazz;
}
this._updateEnablement();
}
public run(resources: URI[]): TPromise<any> {
const addPromise = TPromise.as(null).then(() => {
if (resources && resources.length > 0) {
// Find parent to add to
let targetElement: ExplorerItem;
if (this.element) {
targetElement = this.element;
} else {
const input: ExplorerItem | Model = this.tree.getInput();
targetElement = this.tree.getFocus() || (input instanceof Model ? input.roots[0] : input);
}
if (!targetElement.isDirectory) {
targetElement = targetElement.parent;
}
// Resolve target to check for name collisions and ask user
return this.fileService.resolveFile(targetElement.resource).then((targetStat: IFileStat) => {
// Check for name collisions
const targetNames = new Set<string>();
targetStat.children.forEach((child) => {
targetNames.add(isLinux ? child.name : child.name.toLowerCase());
});
let overwritePromise: TPromise<IConfirmationResult> = TPromise.as({ confirmed: true });
if (resources.some(resource => {
return targetNames.has(isLinux ? paths.basename(resource.fsPath) : paths.basename(resource.fsPath).toLowerCase());
})) {
const confirm: IConfirmation = {
message: nls.localize('confirmOverwrite', "A file or folder with the same name already exists in the destination folder. Do you want to replace it?"),
detail: nls.localize('irreversible', "This action is irreversible!"),
primaryButton: nls.localize({ key: 'replaceButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Replace"),
type: 'warning'
};
overwritePromise = this.dialogService.confirm(confirm);
}
return overwritePromise.then(res => {
if (!res.confirmed) {
return void 0;
}
// Run add in sequence
const addPromisesFactory: ITask<TPromise<void>>[] = [];
resources.forEach(resource => {
addPromisesFactory.push(() => {
const sourceFile = resource;
const targetFile = targetElement.resource.with({ path: paths.join(targetElement.resource.path, paths.basename(sourceFile.path)) });
// if the target exists and is dirty, make sure to revert it. otherwise the dirty contents
// of the target file would replace the contents of the added file. since we already
// confirmed the overwrite before, this is OK.
let revertPromise = TPromise.wrap(null);
if (this.textFileService.isDirty(targetFile)) {
revertPromise = this.textFileService.revertAll([targetFile], { soft: true });
}
return revertPromise.then(() => {
const target = targetElement.resource.with({ path: posix.join(targetElement.resource.path, posix.basename(sourceFile.path)) });
return this.fileService.copyFile(sourceFile, target, true).then(stat => {
// if we only add one file, just open it directly
if (resources.length === 1) {
this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } }).done(null, errors.onUnexpectedError);
}
}, error => this.onError(error));
});
});
});
return sequence(addPromisesFactory);
});
});
}
return void 0;
});
return addPromise.then(() => {
this.tree.clearHighlight();
}, (error: any) => {
this.onError(error);
this.tree.clearHighlight();
});
}
}
// Copy File/Folder
class CopyFileAction extends BaseFileAction {
private tree: ITree;
constructor(
tree: ITree,
private elements: ExplorerItem[],
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IContextKeyService contextKeyService: IContextKeyService,
@IClipboardService private clipboardService: IClipboardService
) {
super('filesExplorer.copy', COPY_FILE_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this._updateEnablement();
}
public run(): TPromise<any> {
// Write to clipboard as file/folder to copy
this.clipboardService.writeFiles(this.elements.map(e => e.resource));
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
this.tree.domFocus();
return TPromise.as(null);
}
}
// Paste File/Folder
class PasteFileAction extends BaseFileAction {
public static readonly ID = 'filesExplorer.paste';
private tree: ITree;
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService
) {
super(PasteFileAction.ID, PASTE_FILE_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this.element = element;
if (!this.element) {
const input: ExplorerItem | Model = this.tree.getInput();
this.element = input instanceof Model ? input.roots[0] : input;
}
this._updateEnablement();
}
public run(fileToPaste: URI): TPromise<any> {
// Check if target is ancestor of pasted folder
if (this.element.resource.toString() !== fileToPaste.toString() && resources.isEqualOrParent(this.element.resource, fileToPaste, !isLinux /* ignorecase */)) {
throw new Error(nls.localize('fileIsAncestor', "File to paste is an ancestor of the destination folder"));
}
return this.fileService.resolveFile(fileToPaste).then(fileToPasteStat => {
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
// Find target
let target: ExplorerItem;
if (this.element.resource.toString() === fileToPaste.toString()) {
target = this.element.parent;
} else {
target = this.element.isDirectory ? this.element : this.element.parent;
}
const targetFile = findValidPasteFileTarget(target, { resource: fileToPaste, isDirectory: fileToPasteStat.isDirectory });
// Copy File
return this.fileService.copyFile(fileToPaste, targetFile).then(stat => {
if (!stat.isDirectory) {
return this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } });
}
return void 0;
}, error => this.onError(error)).then(() => {
this.tree.domFocus();
});
}, error => {
this.onError(new Error(nls.localize('fileDeleted', "File to paste was deleted or moved meanwhile")));
});
}
}
// Duplicate File/Folder
export class DuplicateFileAction extends BaseFileAction {
private tree: ITree;
private target: ExplorerItem;
constructor(
tree: ITree,
fileToDuplicate: ExplorerItem,
target: ExplorerItem,
@IFileService fileService: IFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super('workbench.files.action.duplicateFile', nls.localize('duplicateFile', "Duplicate"), fileService, notificationService, textFileService);
this.tree = tree;
this.element = fileToDuplicate;
this.target = (target && target.isDirectory) ? target : fileToDuplicate.parent;
this._updateEnablement();
}
public run(): TPromise<any> {
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
// Copy File
const result = this.fileService.copyFile(this.element.resource, findValidPasteFileTarget(this.target, { resource: this.element.resource, isDirectory: this.element.isDirectory })).then(stat => {
if (!stat.isDirectory) {
return this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } });
}
return void 0;
}, error => this.onError(error));
return result;
}
}
function findValidPasteFileTarget(targetFolder: ExplorerItem, fileToPaste: { resource: URI, isDirectory?: boolean }): URI {
let name = resources.basenameOrAuthority(fileToPaste.resource);
let candidate = targetFolder.resource.with({ path: paths.join(targetFolder.resource.path, name) });
while (true) {
if (!targetFolder.root.find(candidate)) {
break;
}
name = incrementFileName(name, fileToPaste.isDirectory);
candidate = targetFolder.resource.with({ path: paths.join(targetFolder.resource.path, name) });
}
return candidate;
}
function incrementFileName(name: string, isFolder: boolean): string {
// file.1.txt=>file.2.txt
if (!isFolder && name.match(/(.*\.)(\d+)(\..*)$/)) {
return name.replace(/(.*\.)(\d+)(\..*)$/, (match, g1?, g2?, g3?) => { return g1 + (parseInt(g2) + 1) + g3; });
}
// file.txt=>file.1.txt
const lastIndexOfDot = name.lastIndexOf('.');
if (!isFolder && lastIndexOfDot >= 0) {
return strings.format('{0}.1{1}', name.substr(0, lastIndexOfDot), name.substr(lastIndexOfDot));
}
// folder.1=>folder.2
if (isFolder && name.match(/(\d+)$/)) {
return name.replace(/(\d+)$/, (match: string, ...groups: any[]) => { return String(parseInt(groups[0]) + 1); });
}
// file/folder=>file.1/folder.1
return strings.format('{0}.1', name);
}
// Global Compare with
export class GlobalCompareResourcesAction extends Action {
public static readonly ID = 'workbench.files.action.compareFileWith';
public static readonly LABEL = nls.localize('globalCompareFile', "Compare Active File With...");
constructor(
id: string,
label: string,
@IQuickOpenService private quickOpenService: IQuickOpenService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService private notificationService: INotificationService,
@IEditorGroupService private editorGroupService: IEditorGroupService
) {
super(id, label);
}
public run(): TPromise<any> {
const activeInput = this.editorService.getActiveEditorInput();
const activeResource = activeInput ? activeInput.getResource() : void 0;
if (activeResource) {
// Compare with next editor that opens
const unbind = once(this.editorGroupService.onEditorOpening)(e => {
const resource = e.input.getResource();
if (resource) {
e.prevent(() => {
return this.editorService.openEditor({
leftResource: activeResource,
rightResource: resource
});
});
}
});
// Bring up quick open
this.quickOpenService.show('', { autoFocus: { autoFocusSecondEntry: true } }).then(() => {
unbind.dispose(); // make sure to unbind if quick open is closing
});
} else {
this.notificationService.info(nls.localize('openFileToCompare', "Open a file first to compare it with another file."));
}
return TPromise.as(true);
}
}
// Refresh Explorer Viewer
export class RefreshViewExplorerAction extends Action {
constructor(explorerView: ExplorerView, clazz: string) {
super('workbench.files.action.refreshFilesExplorer', nls.localize('refresh', "Refresh"), clazz, true, (context: any) => explorerView.refresh());
}
}
export abstract class BaseSaveAllAction extends BaseErrorReportingAction {
private toDispose: IDisposable[];
private lastIsDirty: boolean;
constructor(
id: string,
label: string,
@ITextFileService private textFileService: ITextFileService,
@IUntitledEditorService private untitledEditorService: IUntitledEditorService,
@ICommandService protected commandService: ICommandService,
@INotificationService notificationService: INotificationService,
) {
super(id, label, notificationService);
this.toDispose = [];
this.lastIsDirty = this.textFileService.isDirty();
this.enabled = this.lastIsDirty;
this.registerListeners();
}
protected abstract includeUntitled(): boolean;
protected abstract doRun(context: any): TPromise<any>;
private registerListeners(): void {
// listen to files being changed locally
this.toDispose.push(this.textFileService.models.onModelsDirty(e => this.updateEnablement(true)));
this.toDispose.push(this.textFileService.models.onModelsSaved(e => this.updateEnablement(false)));
this.toDispose.push(this.textFileService.models.onModelsReverted(e => this.updateEnablement(false)));
this.toDispose.push(this.textFileService.models.onModelsSaveError(e => this.updateEnablement(true)));
if (this.includeUntitled()) {
this.toDispose.push(this.untitledEditorService.onDidChangeDirty(resource => this.updateEnablement(this.untitledEditorService.isDirty(resource))));
}
}
private updateEnablement(isDirty: boolean): void {
if (this.lastIsDirty !== isDirty) {
this.enabled = this.textFileService.isDirty();
this.lastIsDirty = this.enabled;
}
}
public run(context?: any): TPromise<boolean> {
return this.doRun(context).then(() => true, error => {
this.onError(error);
return null;
});
}
public dispose(): void {
this.toDispose = dispose(this.toDispose);
super.dispose();
}
}
export class SaveAllAction extends BaseSaveAllAction {
public static readonly ID = 'workbench.action.files.saveAll';
public static readonly LABEL = SAVE_ALL_LABEL;
public get class(): string {
return 'explorer-action save-all';
}
protected doRun(context: any): TPromise<any> {
return this.commandService.executeCommand(SAVE_ALL_COMMAND_ID);
}
protected includeUntitled(): boolean {
return true;
}
}
export class SaveAllInGroupAction extends BaseSaveAllAction {
public static readonly ID = 'workbench.files.action.saveAllInGroup';
public static readonly LABEL = nls.localize('saveAllInGroup', "Save All in Group");
public get class(): string {
return 'explorer-action save-all';
}
protected doRun(context: any): TPromise<any> {
return this.commandService.executeCommand(SAVE_ALL_IN_GROUP_COMMAND_ID);
}
protected includeUntitled(): boolean {
return true;
}
}
export class FocusOpenEditorsView extends Action {
public static readonly ID = 'workbench.files.action.focusOpenEditorsView';
public static readonly LABEL = nls.localize({ key: 'focusOpenEditors', comment: ['Open is an adjective'] }, "Focus on Open Editors View");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const openEditorsView = viewlet.getOpenEditorsView();
if (openEditorsView) {
openEditorsView.setExpanded(true);
openEditorsView.getList().domFocus();
}
});
}
}
export class FocusFilesExplorer extends Action {
public static readonly ID = 'workbench.files.action.focusFilesExplorer';
public static readonly LABEL = nls.localize('focusFilesExplorer', "Focus on Files Explorer");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const view = viewlet.getExplorerView();
if (view) {
view.setExpanded(true);
view.getViewer().domFocus();
}
});
}
}
export class ShowActiveFileInExplorer extends Action {
public static readonly ID = 'workbench.files.action.showActiveFileInExplorer';
public static readonly LABEL = nls.localize('showInExplorer', "Reveal Active File in Side Bar");
constructor(
id: string,
label: string,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService private notificationService: INotificationService,
@ICommandService private commandService: ICommandService
) {
super(id, label);
}
public run(): TPromise<any> {
const resource = toResource(this.editorService.getActiveEditorInput(), { supportSideBySide: true });
if (resource) {
this.commandService.executeCommand(REVEAL_IN_EXPLORER_COMMAND_ID, resource);
} else {
this.notificationService.info(nls.localize('openFileToShow', "Open a file first to show it in the explorer"));
}
return TPromise.as(true);
}
}
export class CollapseExplorerView extends Action {
public static readonly ID = 'workbench.files.action.collapseExplorerFolders';
public static readonly LABEL = nls.localize('collapseExplorerFolders', "Collapse Folders in Explorer");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const explorerView = viewlet.getExplorerView();
if (explorerView) {
const viewer = explorerView.getViewer();
if (viewer) {
const action = new CollapseAction(viewer, true, null);
action.run().done();
action.dispose();
}
}
});
}
}
export class RefreshExplorerView extends Action {
public static readonly ID = 'workbench.files.action.refreshFilesExplorer';
public static readonly LABEL = nls.localize('refreshExplorer', "Refresh Explorer");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const explorerView = viewlet.getExplorerView();
if (explorerView) {
explorerView.refresh();
}
});
}
}
export class ShowOpenedFileInNewWindow extends Action {
public static readonly ID = 'workbench.action.files.showOpenedFileInNewWindow';
public static readonly LABEL = nls.localize('openFileInNewWindow', "Open Active File in New Window");
constructor(
id: string,
label: string,
@IWindowsService private windowsService: IWindowsService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService private notificationService: INotificationService,
) {
super(id, label);
}
public run(): TPromise<any> {
const fileResource = toResource(this.editorService.getActiveEditorInput(), { supportSideBySide: true, filter: Schemas.file /* todo@remote */ });
if (fileResource) {
this.windowsService.openWindow([fileResource.fsPath], { forceNewWindow: true, forceOpenWorkspaceAsFile: true });
} else {
this.notificationService.info(nls.localize('openFileToShowInNewWindow', "Open a file first to open in new window"));
}
return TPromise.as(true);
}
}
export class CopyPathAction extends Action {
public static readonly LABEL = nls.localize('copyPath', "Copy Path");
constructor(
private resource: URI,
@ICommandService private commandService: ICommandService
) {
super('copyFilePath', CopyPathAction.LABEL);
this.order = 140;
}
public run(): TPromise<any> {
return this.commandService.executeCommand(COPY_PATH_COMMAND_ID, this.resource);
}
}
export function validateFileName(parent: ExplorerItem, name: string, allowOverwriting: boolean = false): string {
// Produce a well formed file name
name = getWellFormedFileName(name);
// Name not provided
if (!name || name.length === 0 || /^\s+$/.test(name)) {
return nls.localize('emptyFileNameError', "A file or folder name must be provided.");
}
// Relative paths only
if (name[0] === '/' || name[0] === '\\') {
return nls.localize('fileNameStartsWithSlashError', "A file or folder name cannot start with a slash.");
}
const names: string[] = name.split(/[\\/]/).filter(part => !!part);
const analyzedPath = analyzePath(parent, names);
// Do not allow to overwrite existing file
if (!allowOverwriting && analyzedPath.fullPathAlreadyExists) {
return nls.localize('fileNameExistsError', "A file or folder **{0}** already exists at this location. Please choose a different name.", name);
}
// A file must always be a leaf
if (analyzedPath.lastExistingPathSegment.isFile) {
return nls.localize('fileUsedAsFolderError', "**{0}** is a file and cannot have any descendants.", analyzedPath.lastExistingPathSegment.name);
}
// Invalid File name
if (names.some((folderName) => !paths.isValidBasename(folderName))) {
return nls.localize('invalidFileNameError', "The name **{0}** is not valid as a file or folder name. Please choose a different name.", trimLongName(name));
}
// Max length restriction (on Windows)
if (isWindows) {
const fullPathLength = name.length + parent.resource.fsPath.length + 1 /* path segment */;
if (fullPathLength > 255) {
return nls.localize('filePathTooLongError', "The name **{0}** results in a path that is too long. Please choose a shorter name.", trimLongName(name));
}
}
return null;
}
function analyzePath(parent: ExplorerItem, pathNames: string[]): { fullPathAlreadyExists: boolean; lastExistingPathSegment: { isFile: boolean; name: string; } } {
let lastExistingPathSegment = { isFile: false, name: '' };
for (const name of pathNames) {
const { exists, child } = alreadyExists(parent, name);
if (exists) {
lastExistingPathSegment = { isFile: !child.isDirectory, name };
parent = child;
} else {
return { fullPathAlreadyExists: false, lastExistingPathSegment };
}
}
return { fullPathAlreadyExists: true, lastExistingPathSegment };
}
function alreadyExists(parent: ExplorerItem, name: string): { exists: boolean, child: ExplorerItem | undefined } {
let duplicateChild: ExplorerItem;
if (parent && parent.isDirectory) {
duplicateChild = parent.getChild(name);
return { exists: !!duplicateChild, child: duplicateChild };
}
return { exists: false, child: undefined };
}
function trimLongName(name: string): string {
if (name && name.length > 255) {
return `${name.substr(0, 255)}...`;
}
return name;
}
export function getWellFormedFileName(filename: string): string {
if (!filename) {
return filename;
}
// Trim tabs
filename = strings.trim(filename, '\t');
// Remove trailing dots, slashes, and spaces
filename = strings.rtrim(filename, '.');
filename = strings.rtrim(filename, ' ');
filename = strings.rtrim(filename, '/');
filename = strings.rtrim(filename, '\\');
return filename;
}
export class CompareWithClipboardAction extends Action {
public static readonly ID = 'workbench.files.action.compareWithClipboard';
public static readonly LABEL = nls.localize('compareWithClipboard', "Compare Active File with Clipboard");
private static readonly SCHEME = 'clipboardCompare';
private registrationDisposal: IDisposable;
constructor(
id: string,
label: string,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@IInstantiationService private instantiationService: IInstantiationService,
@ITextModelService private textModelService: ITextModelService,
@IFileService private fileService: IFileService
) {
super(id, label);
this.enabled = true;
}
public run(): TPromise<any> {
const resource: URI = toResource(this.editorService.getActiveEditorInput(), { supportSideBySide: true });
if (resource && (this.fileService.canHandleResource(resource) || resource.scheme === Schemas.untitled)) {
if (!this.registrationDisposal) {
const provider = this.instantiationService.createInstance(ClipboardContentProvider);
this.registrationDisposal = this.textModelService.registerTextModelContentProvider(CompareWithClipboardAction.SCHEME, provider);
}
const name = paths.basename(resource.fsPath);
const editorLabel = nls.localize('clipboardComparisonLabel', "Clipboard ↔ {0}", name);
const cleanUp = () => {
this.registrationDisposal = dispose(this.registrationDisposal);
};
return always(this.editorService.openEditor({ leftResource: URI.from({ scheme: CompareWithClipboardAction.SCHEME, path: resource.fsPath }), rightResource: resource, label: editorLabel }), cleanUp);
}
return TPromise.as(true);
}
public dispose(): void {
super.dispose();
this.registrationDisposal = dispose(this.registrationDisposal);
}
}
class ClipboardContentProvider implements ITextModelContentProvider {
constructor(
@IClipboardService private clipboardService: IClipboardService,
@IModeService private modeService: IModeService,
@IModelService private modelService: IModelService
) { }
provideTextContent(resource: URI): TPromise<ITextModel> {
const model = this.modelService.createModel(this.clipboardService.readText(), this.modeService.getOrCreateMode('text/plain'), resource);
return TPromise.as(model);
}
}
// Diagnostics support
let diag: (...args: any[]) => void;
if (!diag) {
diag = diagnostics.register('FileActionsDiagnostics', function (...args: any[]) {
console.log(args[1] + ' - ' + args[0] + ' (time: ' + args[2].getTime() + ' [' + args[2].toUTCString() + '])');
});
}
interface IExplorerContext {
viewletState: IFileViewletState;
stat: ExplorerItem;
selection: ExplorerItem[];
}
function getContext(listWidget: ListWidget, viewletService: IViewletService): IExplorerContext {
// These commands can only be triggered when explorer viewlet is visible so get it using the active viewlet
const tree = <ITree>listWidget;
const stat = tree.getFocus();
const selection = tree.getSelection();
// Only respect the selection if user clicked inside it (focus belongs to it)
return { stat, selection: selection && selection.indexOf(stat) >= 0 ? selection : [], viewletState: (<ExplorerViewlet>viewletService.getActiveViewlet()).getViewletState() };
}
// TODO@isidor these commands are calling into actions due to the complex inheritance action structure.
// It should be the other way around, that actions call into commands.
function openExplorerAndRunAction(accessor: ServicesAccessor, constructor: IConstructorSignature2<ITree, ExplorerItem, Action>): TPromise<any> {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const viewletService = accessor.get(IViewletService);
const activeViewlet = viewletService.getActiveViewlet();
let explorerPromise = TPromise.as(activeViewlet);
if (!activeViewlet || activeViewlet.getId() !== VIEWLET_ID) {
explorerPromise = viewletService.openViewlet(VIEWLET_ID, true);
}
return explorerPromise.then((explorer: ExplorerViewlet) => {
const explorerView = explorer.getExplorerView();
if (explorerView && explorerView.isVisible() && explorerView.isExpanded()) {
explorerView.focus();
const explorerContext = getContext(listService.lastFocusedList, viewletService);
const action = instantationService.createInstance(constructor, listService.lastFocusedList, explorerContext.stat);
return action.run(explorerContext);
}
return undefined;
});
}
CommandsRegistry.registerCommand({
id: NEW_FILE_COMMAND_ID,
handler: (accessor) => {
return openExplorerAndRunAction(accessor, NewFileAction);
}
});
CommandsRegistry.registerCommand({
id: NEW_FOLDER_COMMAND_ID,
handler: (accessor) => {
return openExplorerAndRunAction(accessor, NewFolderAction);
}
});
export const renameHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const renameAction = instantationService.createInstance(TriggerRenameFileAction, listService.lastFocusedList, explorerContext.stat);
return renameAction.run(explorerContext);
};
export const moveFileToTrashHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const stats = explorerContext.selection.length > 1 ? explorerContext.selection : [explorerContext.stat];
const moveFileToTrashAction = instantationService.createInstance(BaseDeleteFileAction, listService.lastFocusedList, stats, true);
return moveFileToTrashAction.run();
};
export const deleteFileHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const stats = explorerContext.selection.length > 1 ? explorerContext.selection : [explorerContext.stat];
const deleteFileAction = instantationService.createInstance(BaseDeleteFileAction, listService.lastFocusedList, stats, false);
return deleteFileAction.run();
};
export const copyFileHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const stats = explorerContext.selection.length > 1 ? explorerContext.selection : [explorerContext.stat];
const copyFileAction = instantationService.createInstance(CopyFileAction, listService.lastFocusedList, stats);
return copyFileAction.run();
};
export const pasteFileHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const clipboardService = accessor.get(IClipboardService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
return TPromise.join(resources.distinctParents(clipboardService.readFiles(), r => r).map(toCopy => {
const pasteFileAction = instantationService.createInstance(PasteFileAction, listService.lastFocusedList, explorerContext.stat);
return pasteFileAction.run(toCopy);
}));
};
| src/vs/workbench/parts/files/electron-browser/fileActions.ts | 1 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.9992921352386475,
0.03126710280776024,
0.00015871686628088355,
0.00017236097482964396,
0.16892574727535248
] |
{
"id": 0,
"code_window": [
"/* A helper that can be used to show a placeholder when creating a new stat */\n",
"export class NewStatPlaceholder extends ExplorerItem {\n",
"\n",
"\tprivate static ID = 0;\n",
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tpublic static NAME = '';\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "add",
"edit_start_line_idx": 380
} | // ATTENTION - THIS DIRECTORY CONTAINS THIRD PARTY OPEN SOURCE MATERIALS:
[{
"name": "expand-abbreviation",
"version": "0.5.8",
"license": "MIT",
"repositoryURL": "https://github.com/emmetio/expand-abbreviation"
}
]
| extensions/emmet/OSSREADME.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00016709096962586045,
0.00016709096962586045,
0.00016709096962586045,
0.00016709096962586045,
0
] |
{
"id": 0,
"code_window": [
"/* A helper that can be used to show a placeholder when creating a new stat */\n",
"export class NewStatPlaceholder extends ExplorerItem {\n",
"\n",
"\tprivate static ID = 0;\n",
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tpublic static NAME = '';\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "add",
"edit_start_line_idx": 380
} | {
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the MIT License. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"editorWalkThrough.arrowUp": "Desplazar hacia arriba (línea)",
"editorWalkThrough.arrowDown": "Desplazar hacia abajo (línea)",
"editorWalkThrough.pageUp": "Desplazar hacia arriba (página)",
"editorWalkThrough.pageDown": "Desplazar hacia abajo (página)"
} | i18n/esn/src/vs/workbench/parts/welcome/walkThrough/electron-browser/walkThroughActions.i18n.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00017427591956220567,
0.00017286068759858608,
0.0001714454556349665,
0.00017286068759858608,
0.0000014152319636195898
] |
{
"id": 0,
"code_window": [
"/* A helper that can be used to show a placeholder when creating a new stat */\n",
"export class NewStatPlaceholder extends ExplorerItem {\n",
"\n",
"\tprivate static ID = 0;\n",
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tpublic static NAME = '';\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "add",
"edit_start_line_idx": 380
} | {
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the MIT License. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"releaseNotesInputName": "Sürüm Notları: {0}",
"unassigned": "atanmamış"
} | i18n/trk/src/vs/workbench/parts/update/electron-browser/releaseNotesEditor.i18n.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00017745116201695055,
0.00017599941929802299,
0.0001745476620271802,
0.00017599941929802299,
0.0000014517499948851764
] |
{
"id": 1,
"code_window": [
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n",
"\n",
"\tconstructor(isDirectory: boolean, root: ExplorerItem) {\n",
"\t\tsuper(URI.file(''), root, false, false, '');\n",
"\n",
"\t\tthis.id = NewStatPlaceholder.ID++;\n",
"\t\tthis.isDirectoryResolved = isDirectory;\n",
"\t\tthis.directoryPlaceholder = isDirectory;\n",
"\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tsuper(URI.file(''), root, false, false, NewStatPlaceholder.NAME);\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "replace",
"edit_start_line_idx": 386
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import 'vs/css!./media/fileactions';
import { TPromise } from 'vs/base/common/winjs.base';
import * as nls from 'vs/nls';
import { isWindows, isLinux } from 'vs/base/common/platform';
import { sequence, ITask, always } from 'vs/base/common/async';
import * as paths from 'vs/base/common/paths';
import * as resources from 'vs/base/common/resources';
import URI from 'vs/base/common/uri';
import { posix } from 'path';
import * as errors from 'vs/base/common/errors';
import { toErrorMessage } from 'vs/base/common/errorMessage';
import * as strings from 'vs/base/common/strings';
import * as diagnostics from 'vs/base/common/diagnostics';
import { Action, IAction } from 'vs/base/common/actions';
import { MessageType, IInputValidator } from 'vs/base/browser/ui/inputbox/inputBox';
import { ITree, IHighlightEvent } from 'vs/base/parts/tree/browser/tree';
import { dispose, IDisposable } from 'vs/base/common/lifecycle';
import { VIEWLET_ID } from 'vs/workbench/parts/files/common/files';
import { ITextFileService } from 'vs/workbench/services/textfile/common/textfiles';
import { IFileService, IFileStat } from 'vs/platform/files/common/files';
import { toResource } from 'vs/workbench/common/editor';
import { ExplorerItem, Model, NewStatPlaceholder } from 'vs/workbench/parts/files/common/explorerModel';
import { ExplorerView } from 'vs/workbench/parts/files/electron-browser/views/explorerView';
import { ExplorerViewlet } from 'vs/workbench/parts/files/electron-browser/explorerViewlet';
import { IUntitledEditorService } from 'vs/workbench/services/untitled/common/untitledEditorService';
import { IWorkbenchEditorService } from 'vs/workbench/services/editor/common/editorService';
import { CollapseAction } from 'vs/workbench/browser/viewlet';
import { IEditorGroupService } from 'vs/workbench/services/group/common/groupService';
import { IQuickOpenService } from 'vs/platform/quickOpen/common/quickOpen';
import { IViewletService } from 'vs/workbench/services/viewlet/browser/viewlet';
import { IUntitledResourceInput } from 'vs/platform/editor/common/editor';
import { IInstantiationService, ServicesAccessor, IConstructorSignature2 } from 'vs/platform/instantiation/common/instantiation';
import { ITextModel } from 'vs/editor/common/model';
import { IBackupFileService } from 'vs/workbench/services/backup/common/backup';
import { IWindowsService } from 'vs/platform/windows/common/windows';
import { COPY_PATH_COMMAND_ID, REVEAL_IN_EXPLORER_COMMAND_ID, SAVE_ALL_COMMAND_ID, SAVE_ALL_LABEL, SAVE_ALL_IN_GROUP_COMMAND_ID } from 'vs/workbench/parts/files/electron-browser/fileCommands';
import { ITextModelService, ITextModelContentProvider } from 'vs/editor/common/services/resolverService';
import { IConfigurationService, ConfigurationTarget } from 'vs/platform/configuration/common/configuration';
import { once } from 'vs/base/common/event';
import { IClipboardService } from 'vs/platform/clipboard/common/clipboardService';
import { IModeService } from 'vs/editor/common/services/modeService';
import { IModelService } from 'vs/editor/common/services/modelService';
import { ICommandService, CommandsRegistry } from 'vs/platform/commands/common/commands';
import { IListService, ListWidget } from 'vs/platform/list/browser/listService';
import { RawContextKey, IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { Schemas } from 'vs/base/common/network';
import { IDialogService, IConfirmationResult, IConfirmation, getConfirmMessage } from 'vs/platform/dialogs/common/dialogs';
import { INotificationService, Severity } from 'vs/platform/notification/common/notification';
export interface IEditableData {
action: IAction;
validator: IInputValidator;
}
export interface IFileViewletState {
getEditableData(stat: ExplorerItem): IEditableData;
setEditable(stat: ExplorerItem, editableData: IEditableData): void;
clearEditable(stat: ExplorerItem): void;
}
export const NEW_FILE_COMMAND_ID = 'explorer.newFile';
export const NEW_FILE_LABEL = nls.localize('newFile', "New File");
export const NEW_FOLDER_COMMAND_ID = 'explorer.newFolder';
export const NEW_FOLDER_LABEL = nls.localize('newFolder', "New Folder");
export const TRIGGER_RENAME_LABEL = nls.localize('rename', "Rename");
export const MOVE_FILE_TO_TRASH_LABEL = nls.localize('delete', "Delete");
export const COPY_FILE_LABEL = nls.localize('copyFile', "Copy");
export const PASTE_FILE_LABEL = nls.localize('pasteFile', "Paste");
export const FileCopiedContext = new RawContextKey<boolean>('fileCopied', false);
export class BaseErrorReportingAction extends Action {
constructor(
id: string,
label: string,
private _notificationService: INotificationService
) {
super(id, label);
}
public get notificationService() {
return this._notificationService;
}
protected onError(error: any): void {
if (error.message === 'string') {
error = error.message;
}
this._notificationService.error(toErrorMessage(error, false));
}
protected onErrorWithRetry(error: any, retry: () => TPromise<any>): void {
this._notificationService.prompt(Severity.Error, toErrorMessage(error, false),
[{
label: nls.localize('retry', "Retry"),
run: () => retry()
}]
);
}
}
export class BaseFileAction extends BaseErrorReportingAction {
public element: ExplorerItem;
constructor(
id: string,
label: string,
@IFileService protected fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService protected textFileService: ITextFileService
) {
super(id, label, notificationService);
this.enabled = false;
}
_isEnabled(): boolean {
return true;
}
_updateEnablement(): void {
this.enabled = !!(this.fileService && this._isEnabled());
}
}
class TriggerRenameFileAction extends BaseFileAction {
public static readonly ID = 'renameFile';
private tree: ITree;
private renameAction: BaseRenameAction;
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IInstantiationService instantiationService: IInstantiationService
) {
super(TriggerRenameFileAction.ID, TRIGGER_RENAME_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this.element = element;
this.renameAction = instantiationService.createInstance(RenameFileAction, element);
this._updateEnablement();
}
public validateFileName(name: string): string {
const names: string[] = name.split(/[\\/]/).filter(part => !!part);
if (names.length > 1) { // error only occurs on multi-path
const comparer = isLinux ? strings.compare : strings.compareIgnoreCase;
if (comparer(names[0], this.element.name) === 0) {
return nls.localize('renameWhenSourcePathIsParentOfTargetError', "Please use the 'New Folder' or 'New File' command to add children to an existing folder");
}
}
return this.renameAction.validateFileName(this.element.parent, name);
}
public run(context?: any): TPromise<any> {
if (!context) {
return TPromise.wrapError(new Error('No context provided to BaseEnableFileRenameAction.'));
}
const viewletState = <IFileViewletState>context.viewletState;
if (!viewletState) {
return TPromise.wrapError(new Error('Invalid viewlet state provided to BaseEnableFileRenameAction.'));
}
const stat = <ExplorerItem>context.stat;
if (!stat) {
return TPromise.wrapError(new Error('Invalid stat provided to BaseEnableFileRenameAction.'));
}
viewletState.setEditable(stat, {
action: this.renameAction,
validator: (value) => {
const message = this.validateFileName(value);
if (!message) {
return null;
}
return {
content: message,
formatContent: true,
type: MessageType.ERROR
};
}
});
this.tree.refresh(stat, false).then(() => {
this.tree.setHighlight(stat);
const unbind = this.tree.onDidChangeHighlight((e: IHighlightEvent) => {
if (!e.highlight) {
viewletState.clearEditable(stat);
this.tree.refresh(stat).done(null, errors.onUnexpectedError);
unbind.dispose();
}
});
}).done(null, errors.onUnexpectedError);
return void 0;
}
}
export abstract class BaseRenameAction extends BaseFileAction {
constructor(
id: string,
label: string,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(id, label, fileService, notificationService, textFileService);
this.element = element;
}
public run(context?: any): TPromise<any> {
if (!context) {
return TPromise.wrapError(new Error('No context provided to BaseRenameFileAction.'));
}
let name = <string>context.value;
if (!name) {
return TPromise.wrapError(new Error('No new name provided to BaseRenameFileAction.'));
}
// Automatically trim whitespaces and trailing dots to produce nice file names
name = getWellFormedFileName(name);
const existingName = getWellFormedFileName(this.element.name);
// Return early if name is invalid or didn't change
if (name === existingName || this.validateFileName(this.element.parent, name)) {
return TPromise.as(null);
}
// Call function and Emit Event through viewer
const promise = this.runAction(name).then(null, (error: any) => {
this.onError(error);
});
return promise;
}
public validateFileName(parent: ExplorerItem, name: string): string {
let source = this.element.name;
let target = name;
if (!isLinux) { // allow rename of same file also when case differs (e.g. Game.js => game.js)
source = source.toLowerCase();
target = target.toLowerCase();
}
if (getWellFormedFileName(source) === getWellFormedFileName(target)) {
return null;
}
return validateFileName(parent, name, false);
}
public abstract runAction(newName: string): TPromise<any>;
}
class RenameFileAction extends BaseRenameAction {
public static readonly ID = 'workbench.files.action.renameFile';
constructor(
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IBackupFileService private backupFileService: IBackupFileService
) {
super(RenameFileAction.ID, nls.localize('rename', "Rename"), element, fileService, notificationService, textFileService);
this._updateEnablement();
}
public runAction(newName: string): TPromise<any> {
const dirty = this.textFileService.getDirty().filter(d => resources.isEqualOrParent(d, this.element.resource, !isLinux /* ignorecase */));
const dirtyRenamed: URI[] = [];
return TPromise.join(dirty.map(d => {
let renamed: URI;
// If the dirty file itself got moved, just reparent it to the target folder
const targetPath = paths.join(this.element.parent.resource.path, newName);
if (this.element.resource.toString() === d.toString()) {
renamed = this.element.parent.resource.with({ path: targetPath });
}
// Otherwise, a parent of the dirty resource got moved, so we have to reparent more complicated. Example:
else {
renamed = this.element.parent.resource.with({ path: paths.join(targetPath, d.path.substr(this.element.resource.path.length + 1)) });
}
dirtyRenamed.push(renamed);
const model = this.textFileService.models.get(d);
return this.backupFileService.backupResource(renamed, model.createSnapshot(), model.getVersionId());
}))
// 2. soft revert all dirty since we have backed up their contents
.then(() => this.textFileService.revertAll(dirty, { soft: true /* do not attempt to load content from disk */ }))
// 3.) run the rename operation
.then(() => this.fileService.rename(this.element.resource, newName).then(null, (error: Error) => {
return TPromise.join(dirtyRenamed.map(d => this.backupFileService.discardResourceBackup(d))).then(() => {
this.onErrorWithRetry(error, () => this.runAction(newName));
});
}))
// 4.) resolve those that were dirty to load their previous dirty contents from disk
.then(() => {
return TPromise.join(dirtyRenamed.map(t => this.textFileService.models.loadOrCreate(t)));
});
}
}
/* Base New File/Folder Action */
export class BaseNewAction extends BaseFileAction {
private presetFolder: ExplorerItem;
private tree: ITree;
private isFile: boolean;
private renameAction: BaseRenameAction;
constructor(
id: string,
label: string,
tree: ITree,
isFile: boolean,
editableAction: BaseRenameAction,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(id, label, fileService, notificationService, textFileService);
if (element) {
this.presetFolder = element.isDirectory ? element : element.parent;
}
this.tree = tree;
this.isFile = isFile;
this.renameAction = editableAction;
}
public run(context?: any): TPromise<any> {
if (!context) {
return TPromise.wrapError(new Error('No context provided to BaseNewAction.'));
}
const viewletState = <IFileViewletState>context.viewletState;
if (!viewletState) {
return TPromise.wrapError(new Error('Invalid viewlet state provided to BaseNewAction.'));
}
let folder = this.presetFolder;
if (!folder) {
const focus = <ExplorerItem>this.tree.getFocus();
if (focus) {
folder = focus.isDirectory ? focus : focus.parent;
} else {
const input: ExplorerItem | Model = this.tree.getInput();
folder = input instanceof Model ? input.roots[0] : input;
}
}
if (!folder) {
return TPromise.wrapError(new Error('Invalid parent folder to create.'));
}
return this.tree.reveal(folder, 0.5).then(() => {
return this.tree.expand(folder).then(() => {
const stat = NewStatPlaceholder.addNewStatPlaceholder(folder, !this.isFile);
this.renameAction.element = stat;
viewletState.setEditable(stat, {
action: this.renameAction,
validator: (value) => {
const message = this.renameAction.validateFileName(folder, value);
if (!message) {
return null;
}
return {
content: message,
formatContent: true,
type: MessageType.ERROR
};
}
});
return this.tree.refresh(folder).then(() => {
return this.tree.expand(folder).then(() => {
return this.tree.reveal(stat, 0.5).then(() => {
this.tree.setHighlight(stat);
const unbind = this.tree.onDidChangeHighlight((e: IHighlightEvent) => {
if (!e.highlight) {
stat.destroy();
this.tree.refresh(folder).done(null, errors.onUnexpectedError);
unbind.dispose();
}
});
});
});
});
});
});
}
}
/* New File */
export class NewFileAction extends BaseNewAction {
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IInstantiationService instantiationService: IInstantiationService
) {
super('explorer.newFile', NEW_FILE_LABEL, tree, true, instantiationService.createInstance(CreateFileAction, element), null, fileService, notificationService, textFileService);
this.class = 'explorer-action new-file';
this._updateEnablement();
}
}
/* New Folder */
export class NewFolderAction extends BaseNewAction {
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IInstantiationService instantiationService: IInstantiationService
) {
super('explorer.newFolder', NEW_FOLDER_LABEL, tree, false, instantiationService.createInstance(CreateFolderAction, element), null, fileService, notificationService, textFileService);
this.class = 'explorer-action new-folder';
this._updateEnablement();
}
}
/* Create new file from anywhere: Open untitled */
export class GlobalNewUntitledFileAction extends Action {
public static readonly ID = 'workbench.action.files.newUntitledFile';
public static readonly LABEL = nls.localize('newUntitledFile', "New Untitled File");
constructor(
id: string,
label: string,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.editorService.openEditor({ options: { pinned: true } } as IUntitledResourceInput); // untitled are always pinned
}
}
/* Create New File/Folder (only used internally by explorerViewer) */
export abstract class BaseCreateAction extends BaseRenameAction {
public validateFileName(parent: ExplorerItem, name: string): string {
if (this.element instanceof NewStatPlaceholder) {
return validateFileName(parent, name, false);
}
return super.validateFileName(parent, name);
}
}
/* Create New File (only used internally by explorerViewer) */
class CreateFileAction extends BaseCreateAction {
public static readonly ID = 'workbench.files.action.createFileFromExplorer';
public static readonly LABEL = nls.localize('createNewFile', "New File");
constructor(
element: ExplorerItem,
@IFileService fileService: IFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(CreateFileAction.ID, CreateFileAction.LABEL, element, fileService, notificationService, textFileService);
this._updateEnablement();
}
public runAction(fileName: string): TPromise<any> {
const resource = this.element.parent.resource;
return this.fileService.createFile(resource.with({ path: paths.join(resource.path, fileName) })).then(stat => {
return this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } });
}, (error) => {
this.onErrorWithRetry(error, () => this.runAction(fileName));
});
}
}
/* Create New Folder (only used internally by explorerViewer) */
class CreateFolderAction extends BaseCreateAction {
public static readonly ID = 'workbench.files.action.createFolderFromExplorer';
public static readonly LABEL = nls.localize('createNewFolder', "New Folder");
constructor(
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super(CreateFolderAction.ID, CreateFolderAction.LABEL, null, fileService, notificationService, textFileService);
this._updateEnablement();
}
public runAction(fileName: string): TPromise<any> {
const resource = this.element.parent.resource;
return this.fileService.createFolder(resource.with({ path: paths.join(resource.path, fileName) })).then(null, (error) => {
this.onErrorWithRetry(error, () => this.runAction(fileName));
});
}
}
class BaseDeleteFileAction extends BaseFileAction {
private static readonly CONFIRM_DELETE_SETTING_KEY = 'explorer.confirmDelete';
private skipConfirm: boolean;
constructor(
private tree: ITree,
private elements: ExplorerItem[],
private useTrash: boolean,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@IDialogService private dialogService: IDialogService,
@ITextFileService textFileService: ITextFileService,
@IConfigurationService private configurationService: IConfigurationService
) {
super('moveFileToTrash', MOVE_FILE_TO_TRASH_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this.useTrash = useTrash && elements.every(e => !paths.isUNC(e.resource.fsPath)); // on UNC shares there is no trash
this._updateEnablement();
}
public run(): TPromise<any> {
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
let primaryButton: string;
if (this.useTrash) {
primaryButton = isWindows ? nls.localize('deleteButtonLabelRecycleBin', "&&Move to Recycle Bin") : nls.localize({ key: 'deleteButtonLabelTrash', comment: ['&& denotes a mnemonic'] }, "&&Move to Trash");
} else {
primaryButton = nls.localize({ key: 'deleteButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Delete");
}
const distinctElements = resources.distinctParents(this.elements, e => e.resource);
// Handle dirty
let confirmDirtyPromise: TPromise<boolean> = TPromise.as(true);
const dirty = this.textFileService.getDirty().filter(d => distinctElements.some(e => resources.isEqualOrParent(d, e.resource, !isLinux /* ignorecase */)));
if (dirty.length) {
let message: string;
if (distinctElements.length > 1) {
message = nls.localize('dirtyMessageFilesDelete', "You are deleting files with unsaved changes. Do you want to continue?");
} else if (distinctElements[0].isDirectory) {
if (dirty.length === 1) {
message = nls.localize('dirtyMessageFolderOneDelete', "You are deleting a folder with unsaved changes in 1 file. Do you want to continue?");
} else {
message = nls.localize('dirtyMessageFolderDelete', "You are deleting a folder with unsaved changes in {0} files. Do you want to continue?", dirty.length);
}
} else {
message = nls.localize('dirtyMessageFileDelete', "You are deleting a file with unsaved changes. Do you want to continue?");
}
confirmDirtyPromise = this.dialogService.confirm({
message,
type: 'warning',
detail: nls.localize('dirtyWarning', "Your changes will be lost if you don't save them."),
primaryButton
}).then(res => {
if (!res.confirmed) {
return false;
}
this.skipConfirm = true; // since we already asked for confirmation
return this.textFileService.revertAll(dirty).then(() => true);
});
}
// Check if file is dirty in editor and save it to avoid data loss
return confirmDirtyPromise.then(confirmed => {
if (!confirmed) {
return null;
}
let confirmDeletePromise: TPromise<IConfirmationResult>;
// Check if we need to ask for confirmation at all
if (this.skipConfirm || (this.useTrash && this.configurationService.getValue<boolean>(BaseDeleteFileAction.CONFIRM_DELETE_SETTING_KEY) === false)) {
confirmDeletePromise = TPromise.as({ confirmed: true } as IConfirmationResult);
}
// Confirm for moving to trash
else if (this.useTrash) {
const message = distinctElements.length > 1 ? getConfirmMessage(nls.localize('confirmMoveTrashMessageMultiple', "Are you sure you want to delete the following {0} files?", distinctElements.length), distinctElements.map(e => e.resource))
: distinctElements[0].isDirectory ? nls.localize('confirmMoveTrashMessageFolder', "Are you sure you want to delete '{0}' and its contents?", distinctElements[0].name)
: nls.localize('confirmMoveTrashMessageFile', "Are you sure you want to delete '{0}'?", distinctElements[0].name);
confirmDeletePromise = this.dialogService.confirm({
message,
detail: isWindows ? nls.localize('undoBin', "You can restore from the Recycle Bin.") : nls.localize('undoTrash', "You can restore from the Trash."),
primaryButton,
checkbox: {
label: nls.localize('doNotAskAgain', "Do not ask me again")
},
type: 'question'
});
}
// Confirm for deleting permanently
else {
const message = distinctElements.length > 1 ? getConfirmMessage(nls.localize('confirmDeleteMessageMultiple', "Are you sure you want to permanently delete the following {0} files?", distinctElements.length), distinctElements.map(e => e.resource))
: distinctElements[0].isDirectory ? nls.localize('confirmDeleteMessageFolder', "Are you sure you want to permanently delete '{0}' and its contents?", distinctElements[0].name)
: nls.localize('confirmDeleteMessageFile', "Are you sure you want to permanently delete '{0}'?", distinctElements[0].name);
confirmDeletePromise = this.dialogService.confirm({
message,
detail: nls.localize('irreversible', "This action is irreversible!"),
primaryButton,
type: 'warning'
});
}
return confirmDeletePromise.then(confirmation => {
// Check for confirmation checkbox
let updateConfirmSettingsPromise: TPromise<void> = TPromise.as(void 0);
if (confirmation.confirmed && confirmation.checkboxChecked === true) {
updateConfirmSettingsPromise = this.configurationService.updateValue(BaseDeleteFileAction.CONFIRM_DELETE_SETTING_KEY, false, ConfigurationTarget.USER);
}
return updateConfirmSettingsPromise.then(() => {
// Check for confirmation
if (!confirmation.confirmed) {
return TPromise.as(null);
}
// Call function
const servicePromise = TPromise.join(distinctElements.map(e => this.fileService.del(e.resource, this.useTrash))).then(() => {
if (distinctElements[0].parent) {
this.tree.setFocus(distinctElements[0].parent); // move focus to parent
}
}, (error: any) => {
// Handle error to delete file(s) from a modal confirmation dialog
let errorMessage: string;
let detailMessage: string;
let primaryButton: string;
if (this.useTrash) {
errorMessage = isWindows ? nls.localize('binFailed', "Failed to delete using the Recycle Bin. Do you want to permanently delete instead?") : nls.localize('trashFailed', "Failed to delete using the Trash. Do you want to permanently delete instead?");
detailMessage = nls.localize('irreversible', "This action is irreversible!");
primaryButton = nls.localize({ key: 'deletePermanentlyButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Delete Permanently");
} else {
errorMessage = toErrorMessage(error, false);
primaryButton = nls.localize({ key: 'retryButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Retry");
}
return this.dialogService.confirm({
message: errorMessage,
detail: detailMessage,
type: 'warning',
primaryButton
}).then(res => {
// Focus back to tree
this.tree.domFocus();
if (res.confirmed) {
if (this.useTrash) {
this.useTrash = false; // Delete Permanently
}
this.skipConfirm = true;
return this.run();
}
return TPromise.as(void 0);
});
});
return servicePromise;
});
});
});
}
}
/* Add File */
export class AddFilesAction extends BaseFileAction {
private tree: ITree;
constructor(
tree: ITree,
element: ExplorerItem,
clazz: string,
@IFileService fileService: IFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@IDialogService private dialogService: IDialogService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super('workbench.files.action.addFile', nls.localize('addFiles', "Add Files"), fileService, notificationService, textFileService);
this.tree = tree;
this.element = element;
if (clazz) {
this.class = clazz;
}
this._updateEnablement();
}
public run(resources: URI[]): TPromise<any> {
const addPromise = TPromise.as(null).then(() => {
if (resources && resources.length > 0) {
// Find parent to add to
let targetElement: ExplorerItem;
if (this.element) {
targetElement = this.element;
} else {
const input: ExplorerItem | Model = this.tree.getInput();
targetElement = this.tree.getFocus() || (input instanceof Model ? input.roots[0] : input);
}
if (!targetElement.isDirectory) {
targetElement = targetElement.parent;
}
// Resolve target to check for name collisions and ask user
return this.fileService.resolveFile(targetElement.resource).then((targetStat: IFileStat) => {
// Check for name collisions
const targetNames = new Set<string>();
targetStat.children.forEach((child) => {
targetNames.add(isLinux ? child.name : child.name.toLowerCase());
});
let overwritePromise: TPromise<IConfirmationResult> = TPromise.as({ confirmed: true });
if (resources.some(resource => {
return targetNames.has(isLinux ? paths.basename(resource.fsPath) : paths.basename(resource.fsPath).toLowerCase());
})) {
const confirm: IConfirmation = {
message: nls.localize('confirmOverwrite', "A file or folder with the same name already exists in the destination folder. Do you want to replace it?"),
detail: nls.localize('irreversible', "This action is irreversible!"),
primaryButton: nls.localize({ key: 'replaceButtonLabel', comment: ['&& denotes a mnemonic'] }, "&&Replace"),
type: 'warning'
};
overwritePromise = this.dialogService.confirm(confirm);
}
return overwritePromise.then(res => {
if (!res.confirmed) {
return void 0;
}
// Run add in sequence
const addPromisesFactory: ITask<TPromise<void>>[] = [];
resources.forEach(resource => {
addPromisesFactory.push(() => {
const sourceFile = resource;
const targetFile = targetElement.resource.with({ path: paths.join(targetElement.resource.path, paths.basename(sourceFile.path)) });
// if the target exists and is dirty, make sure to revert it. otherwise the dirty contents
// of the target file would replace the contents of the added file. since we already
// confirmed the overwrite before, this is OK.
let revertPromise = TPromise.wrap(null);
if (this.textFileService.isDirty(targetFile)) {
revertPromise = this.textFileService.revertAll([targetFile], { soft: true });
}
return revertPromise.then(() => {
const target = targetElement.resource.with({ path: posix.join(targetElement.resource.path, posix.basename(sourceFile.path)) });
return this.fileService.copyFile(sourceFile, target, true).then(stat => {
// if we only add one file, just open it directly
if (resources.length === 1) {
this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } }).done(null, errors.onUnexpectedError);
}
}, error => this.onError(error));
});
});
});
return sequence(addPromisesFactory);
});
});
}
return void 0;
});
return addPromise.then(() => {
this.tree.clearHighlight();
}, (error: any) => {
this.onError(error);
this.tree.clearHighlight();
});
}
}
// Copy File/Folder
class CopyFileAction extends BaseFileAction {
private tree: ITree;
constructor(
tree: ITree,
private elements: ExplorerItem[],
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IContextKeyService contextKeyService: IContextKeyService,
@IClipboardService private clipboardService: IClipboardService
) {
super('filesExplorer.copy', COPY_FILE_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this._updateEnablement();
}
public run(): TPromise<any> {
// Write to clipboard as file/folder to copy
this.clipboardService.writeFiles(this.elements.map(e => e.resource));
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
this.tree.domFocus();
return TPromise.as(null);
}
}
// Paste File/Folder
class PasteFileAction extends BaseFileAction {
public static readonly ID = 'filesExplorer.paste';
private tree: ITree;
constructor(
tree: ITree,
element: ExplorerItem,
@IFileService fileService: IFileService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService
) {
super(PasteFileAction.ID, PASTE_FILE_LABEL, fileService, notificationService, textFileService);
this.tree = tree;
this.element = element;
if (!this.element) {
const input: ExplorerItem | Model = this.tree.getInput();
this.element = input instanceof Model ? input.roots[0] : input;
}
this._updateEnablement();
}
public run(fileToPaste: URI): TPromise<any> {
// Check if target is ancestor of pasted folder
if (this.element.resource.toString() !== fileToPaste.toString() && resources.isEqualOrParent(this.element.resource, fileToPaste, !isLinux /* ignorecase */)) {
throw new Error(nls.localize('fileIsAncestor', "File to paste is an ancestor of the destination folder"));
}
return this.fileService.resolveFile(fileToPaste).then(fileToPasteStat => {
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
// Find target
let target: ExplorerItem;
if (this.element.resource.toString() === fileToPaste.toString()) {
target = this.element.parent;
} else {
target = this.element.isDirectory ? this.element : this.element.parent;
}
const targetFile = findValidPasteFileTarget(target, { resource: fileToPaste, isDirectory: fileToPasteStat.isDirectory });
// Copy File
return this.fileService.copyFile(fileToPaste, targetFile).then(stat => {
if (!stat.isDirectory) {
return this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } });
}
return void 0;
}, error => this.onError(error)).then(() => {
this.tree.domFocus();
});
}, error => {
this.onError(new Error(nls.localize('fileDeleted', "File to paste was deleted or moved meanwhile")));
});
}
}
// Duplicate File/Folder
export class DuplicateFileAction extends BaseFileAction {
private tree: ITree;
private target: ExplorerItem;
constructor(
tree: ITree,
fileToDuplicate: ExplorerItem,
target: ExplorerItem,
@IFileService fileService: IFileService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService notificationService: INotificationService,
@ITextFileService textFileService: ITextFileService
) {
super('workbench.files.action.duplicateFile', nls.localize('duplicateFile', "Duplicate"), fileService, notificationService, textFileService);
this.tree = tree;
this.element = fileToDuplicate;
this.target = (target && target.isDirectory) ? target : fileToDuplicate.parent;
this._updateEnablement();
}
public run(): TPromise<any> {
// Remove highlight
if (this.tree) {
this.tree.clearHighlight();
}
// Copy File
const result = this.fileService.copyFile(this.element.resource, findValidPasteFileTarget(this.target, { resource: this.element.resource, isDirectory: this.element.isDirectory })).then(stat => {
if (!stat.isDirectory) {
return this.editorService.openEditor({ resource: stat.resource, options: { pinned: true } });
}
return void 0;
}, error => this.onError(error));
return result;
}
}
function findValidPasteFileTarget(targetFolder: ExplorerItem, fileToPaste: { resource: URI, isDirectory?: boolean }): URI {
let name = resources.basenameOrAuthority(fileToPaste.resource);
let candidate = targetFolder.resource.with({ path: paths.join(targetFolder.resource.path, name) });
while (true) {
if (!targetFolder.root.find(candidate)) {
break;
}
name = incrementFileName(name, fileToPaste.isDirectory);
candidate = targetFolder.resource.with({ path: paths.join(targetFolder.resource.path, name) });
}
return candidate;
}
function incrementFileName(name: string, isFolder: boolean): string {
// file.1.txt=>file.2.txt
if (!isFolder && name.match(/(.*\.)(\d+)(\..*)$/)) {
return name.replace(/(.*\.)(\d+)(\..*)$/, (match, g1?, g2?, g3?) => { return g1 + (parseInt(g2) + 1) + g3; });
}
// file.txt=>file.1.txt
const lastIndexOfDot = name.lastIndexOf('.');
if (!isFolder && lastIndexOfDot >= 0) {
return strings.format('{0}.1{1}', name.substr(0, lastIndexOfDot), name.substr(lastIndexOfDot));
}
// folder.1=>folder.2
if (isFolder && name.match(/(\d+)$/)) {
return name.replace(/(\d+)$/, (match: string, ...groups: any[]) => { return String(parseInt(groups[0]) + 1); });
}
// file/folder=>file.1/folder.1
return strings.format('{0}.1', name);
}
// Global Compare with
export class GlobalCompareResourcesAction extends Action {
public static readonly ID = 'workbench.files.action.compareFileWith';
public static readonly LABEL = nls.localize('globalCompareFile', "Compare Active File With...");
constructor(
id: string,
label: string,
@IQuickOpenService private quickOpenService: IQuickOpenService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService private notificationService: INotificationService,
@IEditorGroupService private editorGroupService: IEditorGroupService
) {
super(id, label);
}
public run(): TPromise<any> {
const activeInput = this.editorService.getActiveEditorInput();
const activeResource = activeInput ? activeInput.getResource() : void 0;
if (activeResource) {
// Compare with next editor that opens
const unbind = once(this.editorGroupService.onEditorOpening)(e => {
const resource = e.input.getResource();
if (resource) {
e.prevent(() => {
return this.editorService.openEditor({
leftResource: activeResource,
rightResource: resource
});
});
}
});
// Bring up quick open
this.quickOpenService.show('', { autoFocus: { autoFocusSecondEntry: true } }).then(() => {
unbind.dispose(); // make sure to unbind if quick open is closing
});
} else {
this.notificationService.info(nls.localize('openFileToCompare', "Open a file first to compare it with another file."));
}
return TPromise.as(true);
}
}
// Refresh Explorer Viewer
export class RefreshViewExplorerAction extends Action {
constructor(explorerView: ExplorerView, clazz: string) {
super('workbench.files.action.refreshFilesExplorer', nls.localize('refresh', "Refresh"), clazz, true, (context: any) => explorerView.refresh());
}
}
export abstract class BaseSaveAllAction extends BaseErrorReportingAction {
private toDispose: IDisposable[];
private lastIsDirty: boolean;
constructor(
id: string,
label: string,
@ITextFileService private textFileService: ITextFileService,
@IUntitledEditorService private untitledEditorService: IUntitledEditorService,
@ICommandService protected commandService: ICommandService,
@INotificationService notificationService: INotificationService,
) {
super(id, label, notificationService);
this.toDispose = [];
this.lastIsDirty = this.textFileService.isDirty();
this.enabled = this.lastIsDirty;
this.registerListeners();
}
protected abstract includeUntitled(): boolean;
protected abstract doRun(context: any): TPromise<any>;
private registerListeners(): void {
// listen to files being changed locally
this.toDispose.push(this.textFileService.models.onModelsDirty(e => this.updateEnablement(true)));
this.toDispose.push(this.textFileService.models.onModelsSaved(e => this.updateEnablement(false)));
this.toDispose.push(this.textFileService.models.onModelsReverted(e => this.updateEnablement(false)));
this.toDispose.push(this.textFileService.models.onModelsSaveError(e => this.updateEnablement(true)));
if (this.includeUntitled()) {
this.toDispose.push(this.untitledEditorService.onDidChangeDirty(resource => this.updateEnablement(this.untitledEditorService.isDirty(resource))));
}
}
private updateEnablement(isDirty: boolean): void {
if (this.lastIsDirty !== isDirty) {
this.enabled = this.textFileService.isDirty();
this.lastIsDirty = this.enabled;
}
}
public run(context?: any): TPromise<boolean> {
return this.doRun(context).then(() => true, error => {
this.onError(error);
return null;
});
}
public dispose(): void {
this.toDispose = dispose(this.toDispose);
super.dispose();
}
}
export class SaveAllAction extends BaseSaveAllAction {
public static readonly ID = 'workbench.action.files.saveAll';
public static readonly LABEL = SAVE_ALL_LABEL;
public get class(): string {
return 'explorer-action save-all';
}
protected doRun(context: any): TPromise<any> {
return this.commandService.executeCommand(SAVE_ALL_COMMAND_ID);
}
protected includeUntitled(): boolean {
return true;
}
}
export class SaveAllInGroupAction extends BaseSaveAllAction {
public static readonly ID = 'workbench.files.action.saveAllInGroup';
public static readonly LABEL = nls.localize('saveAllInGroup', "Save All in Group");
public get class(): string {
return 'explorer-action save-all';
}
protected doRun(context: any): TPromise<any> {
return this.commandService.executeCommand(SAVE_ALL_IN_GROUP_COMMAND_ID);
}
protected includeUntitled(): boolean {
return true;
}
}
export class FocusOpenEditorsView extends Action {
public static readonly ID = 'workbench.files.action.focusOpenEditorsView';
public static readonly LABEL = nls.localize({ key: 'focusOpenEditors', comment: ['Open is an adjective'] }, "Focus on Open Editors View");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const openEditorsView = viewlet.getOpenEditorsView();
if (openEditorsView) {
openEditorsView.setExpanded(true);
openEditorsView.getList().domFocus();
}
});
}
}
export class FocusFilesExplorer extends Action {
public static readonly ID = 'workbench.files.action.focusFilesExplorer';
public static readonly LABEL = nls.localize('focusFilesExplorer', "Focus on Files Explorer");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const view = viewlet.getExplorerView();
if (view) {
view.setExpanded(true);
view.getViewer().domFocus();
}
});
}
}
export class ShowActiveFileInExplorer extends Action {
public static readonly ID = 'workbench.files.action.showActiveFileInExplorer';
public static readonly LABEL = nls.localize('showInExplorer', "Reveal Active File in Side Bar");
constructor(
id: string,
label: string,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService private notificationService: INotificationService,
@ICommandService private commandService: ICommandService
) {
super(id, label);
}
public run(): TPromise<any> {
const resource = toResource(this.editorService.getActiveEditorInput(), { supportSideBySide: true });
if (resource) {
this.commandService.executeCommand(REVEAL_IN_EXPLORER_COMMAND_ID, resource);
} else {
this.notificationService.info(nls.localize('openFileToShow', "Open a file first to show it in the explorer"));
}
return TPromise.as(true);
}
}
export class CollapseExplorerView extends Action {
public static readonly ID = 'workbench.files.action.collapseExplorerFolders';
public static readonly LABEL = nls.localize('collapseExplorerFolders', "Collapse Folders in Explorer");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const explorerView = viewlet.getExplorerView();
if (explorerView) {
const viewer = explorerView.getViewer();
if (viewer) {
const action = new CollapseAction(viewer, true, null);
action.run().done();
action.dispose();
}
}
});
}
}
export class RefreshExplorerView extends Action {
public static readonly ID = 'workbench.files.action.refreshFilesExplorer';
public static readonly LABEL = nls.localize('refreshExplorer', "Refresh Explorer");
constructor(
id: string,
label: string,
@IViewletService private viewletService: IViewletService
) {
super(id, label);
}
public run(): TPromise<any> {
return this.viewletService.openViewlet(VIEWLET_ID, true).then((viewlet: ExplorerViewlet) => {
const explorerView = viewlet.getExplorerView();
if (explorerView) {
explorerView.refresh();
}
});
}
}
export class ShowOpenedFileInNewWindow extends Action {
public static readonly ID = 'workbench.action.files.showOpenedFileInNewWindow';
public static readonly LABEL = nls.localize('openFileInNewWindow', "Open Active File in New Window");
constructor(
id: string,
label: string,
@IWindowsService private windowsService: IWindowsService,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@INotificationService private notificationService: INotificationService,
) {
super(id, label);
}
public run(): TPromise<any> {
const fileResource = toResource(this.editorService.getActiveEditorInput(), { supportSideBySide: true, filter: Schemas.file /* todo@remote */ });
if (fileResource) {
this.windowsService.openWindow([fileResource.fsPath], { forceNewWindow: true, forceOpenWorkspaceAsFile: true });
} else {
this.notificationService.info(nls.localize('openFileToShowInNewWindow', "Open a file first to open in new window"));
}
return TPromise.as(true);
}
}
export class CopyPathAction extends Action {
public static readonly LABEL = nls.localize('copyPath', "Copy Path");
constructor(
private resource: URI,
@ICommandService private commandService: ICommandService
) {
super('copyFilePath', CopyPathAction.LABEL);
this.order = 140;
}
public run(): TPromise<any> {
return this.commandService.executeCommand(COPY_PATH_COMMAND_ID, this.resource);
}
}
export function validateFileName(parent: ExplorerItem, name: string, allowOverwriting: boolean = false): string {
// Produce a well formed file name
name = getWellFormedFileName(name);
// Name not provided
if (!name || name.length === 0 || /^\s+$/.test(name)) {
return nls.localize('emptyFileNameError', "A file or folder name must be provided.");
}
// Relative paths only
if (name[0] === '/' || name[0] === '\\') {
return nls.localize('fileNameStartsWithSlashError', "A file or folder name cannot start with a slash.");
}
const names: string[] = name.split(/[\\/]/).filter(part => !!part);
const analyzedPath = analyzePath(parent, names);
// Do not allow to overwrite existing file
if (!allowOverwriting && analyzedPath.fullPathAlreadyExists) {
return nls.localize('fileNameExistsError', "A file or folder **{0}** already exists at this location. Please choose a different name.", name);
}
// A file must always be a leaf
if (analyzedPath.lastExistingPathSegment.isFile) {
return nls.localize('fileUsedAsFolderError', "**{0}** is a file and cannot have any descendants.", analyzedPath.lastExistingPathSegment.name);
}
// Invalid File name
if (names.some((folderName) => !paths.isValidBasename(folderName))) {
return nls.localize('invalidFileNameError', "The name **{0}** is not valid as a file or folder name. Please choose a different name.", trimLongName(name));
}
// Max length restriction (on Windows)
if (isWindows) {
const fullPathLength = name.length + parent.resource.fsPath.length + 1 /* path segment */;
if (fullPathLength > 255) {
return nls.localize('filePathTooLongError', "The name **{0}** results in a path that is too long. Please choose a shorter name.", trimLongName(name));
}
}
return null;
}
function analyzePath(parent: ExplorerItem, pathNames: string[]): { fullPathAlreadyExists: boolean; lastExistingPathSegment: { isFile: boolean; name: string; } } {
let lastExistingPathSegment = { isFile: false, name: '' };
for (const name of pathNames) {
const { exists, child } = alreadyExists(parent, name);
if (exists) {
lastExistingPathSegment = { isFile: !child.isDirectory, name };
parent = child;
} else {
return { fullPathAlreadyExists: false, lastExistingPathSegment };
}
}
return { fullPathAlreadyExists: true, lastExistingPathSegment };
}
function alreadyExists(parent: ExplorerItem, name: string): { exists: boolean, child: ExplorerItem | undefined } {
let duplicateChild: ExplorerItem;
if (parent && parent.isDirectory) {
duplicateChild = parent.getChild(name);
return { exists: !!duplicateChild, child: duplicateChild };
}
return { exists: false, child: undefined };
}
function trimLongName(name: string): string {
if (name && name.length > 255) {
return `${name.substr(0, 255)}...`;
}
return name;
}
export function getWellFormedFileName(filename: string): string {
if (!filename) {
return filename;
}
// Trim tabs
filename = strings.trim(filename, '\t');
// Remove trailing dots, slashes, and spaces
filename = strings.rtrim(filename, '.');
filename = strings.rtrim(filename, ' ');
filename = strings.rtrim(filename, '/');
filename = strings.rtrim(filename, '\\');
return filename;
}
export class CompareWithClipboardAction extends Action {
public static readonly ID = 'workbench.files.action.compareWithClipboard';
public static readonly LABEL = nls.localize('compareWithClipboard', "Compare Active File with Clipboard");
private static readonly SCHEME = 'clipboardCompare';
private registrationDisposal: IDisposable;
constructor(
id: string,
label: string,
@IWorkbenchEditorService private editorService: IWorkbenchEditorService,
@IInstantiationService private instantiationService: IInstantiationService,
@ITextModelService private textModelService: ITextModelService,
@IFileService private fileService: IFileService
) {
super(id, label);
this.enabled = true;
}
public run(): TPromise<any> {
const resource: URI = toResource(this.editorService.getActiveEditorInput(), { supportSideBySide: true });
if (resource && (this.fileService.canHandleResource(resource) || resource.scheme === Schemas.untitled)) {
if (!this.registrationDisposal) {
const provider = this.instantiationService.createInstance(ClipboardContentProvider);
this.registrationDisposal = this.textModelService.registerTextModelContentProvider(CompareWithClipboardAction.SCHEME, provider);
}
const name = paths.basename(resource.fsPath);
const editorLabel = nls.localize('clipboardComparisonLabel', "Clipboard ↔ {0}", name);
const cleanUp = () => {
this.registrationDisposal = dispose(this.registrationDisposal);
};
return always(this.editorService.openEditor({ leftResource: URI.from({ scheme: CompareWithClipboardAction.SCHEME, path: resource.fsPath }), rightResource: resource, label: editorLabel }), cleanUp);
}
return TPromise.as(true);
}
public dispose(): void {
super.dispose();
this.registrationDisposal = dispose(this.registrationDisposal);
}
}
class ClipboardContentProvider implements ITextModelContentProvider {
constructor(
@IClipboardService private clipboardService: IClipboardService,
@IModeService private modeService: IModeService,
@IModelService private modelService: IModelService
) { }
provideTextContent(resource: URI): TPromise<ITextModel> {
const model = this.modelService.createModel(this.clipboardService.readText(), this.modeService.getOrCreateMode('text/plain'), resource);
return TPromise.as(model);
}
}
// Diagnostics support
let diag: (...args: any[]) => void;
if (!diag) {
diag = diagnostics.register('FileActionsDiagnostics', function (...args: any[]) {
console.log(args[1] + ' - ' + args[0] + ' (time: ' + args[2].getTime() + ' [' + args[2].toUTCString() + '])');
});
}
interface IExplorerContext {
viewletState: IFileViewletState;
stat: ExplorerItem;
selection: ExplorerItem[];
}
function getContext(listWidget: ListWidget, viewletService: IViewletService): IExplorerContext {
// These commands can only be triggered when explorer viewlet is visible so get it using the active viewlet
const tree = <ITree>listWidget;
const stat = tree.getFocus();
const selection = tree.getSelection();
// Only respect the selection if user clicked inside it (focus belongs to it)
return { stat, selection: selection && selection.indexOf(stat) >= 0 ? selection : [], viewletState: (<ExplorerViewlet>viewletService.getActiveViewlet()).getViewletState() };
}
// TODO@isidor these commands are calling into actions due to the complex inheritance action structure.
// It should be the other way around, that actions call into commands.
function openExplorerAndRunAction(accessor: ServicesAccessor, constructor: IConstructorSignature2<ITree, ExplorerItem, Action>): TPromise<any> {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const viewletService = accessor.get(IViewletService);
const activeViewlet = viewletService.getActiveViewlet();
let explorerPromise = TPromise.as(activeViewlet);
if (!activeViewlet || activeViewlet.getId() !== VIEWLET_ID) {
explorerPromise = viewletService.openViewlet(VIEWLET_ID, true);
}
return explorerPromise.then((explorer: ExplorerViewlet) => {
const explorerView = explorer.getExplorerView();
if (explorerView && explorerView.isVisible() && explorerView.isExpanded()) {
explorerView.focus();
const explorerContext = getContext(listService.lastFocusedList, viewletService);
const action = instantationService.createInstance(constructor, listService.lastFocusedList, explorerContext.stat);
return action.run(explorerContext);
}
return undefined;
});
}
CommandsRegistry.registerCommand({
id: NEW_FILE_COMMAND_ID,
handler: (accessor) => {
return openExplorerAndRunAction(accessor, NewFileAction);
}
});
CommandsRegistry.registerCommand({
id: NEW_FOLDER_COMMAND_ID,
handler: (accessor) => {
return openExplorerAndRunAction(accessor, NewFolderAction);
}
});
export const renameHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const renameAction = instantationService.createInstance(TriggerRenameFileAction, listService.lastFocusedList, explorerContext.stat);
return renameAction.run(explorerContext);
};
export const moveFileToTrashHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const stats = explorerContext.selection.length > 1 ? explorerContext.selection : [explorerContext.stat];
const moveFileToTrashAction = instantationService.createInstance(BaseDeleteFileAction, listService.lastFocusedList, stats, true);
return moveFileToTrashAction.run();
};
export const deleteFileHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const stats = explorerContext.selection.length > 1 ? explorerContext.selection : [explorerContext.stat];
const deleteFileAction = instantationService.createInstance(BaseDeleteFileAction, listService.lastFocusedList, stats, false);
return deleteFileAction.run();
};
export const copyFileHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
const stats = explorerContext.selection.length > 1 ? explorerContext.selection : [explorerContext.stat];
const copyFileAction = instantationService.createInstance(CopyFileAction, listService.lastFocusedList, stats);
return copyFileAction.run();
};
export const pasteFileHandler = (accessor: ServicesAccessor) => {
const instantationService = accessor.get(IInstantiationService);
const listService = accessor.get(IListService);
const clipboardService = accessor.get(IClipboardService);
const explorerContext = getContext(listService.lastFocusedList, accessor.get(IViewletService));
return TPromise.join(resources.distinctParents(clipboardService.readFiles(), r => r).map(toCopy => {
const pasteFileAction = instantationService.createInstance(PasteFileAction, listService.lastFocusedList, explorerContext.stat);
return pasteFileAction.run(toCopy);
}));
};
| src/vs/workbench/parts/files/electron-browser/fileActions.ts | 1 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.016746312379837036,
0.00045190509990788996,
0.00016397674335166812,
0.00017219228902831674,
0.0015151515835896134
] |
{
"id": 1,
"code_window": [
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n",
"\n",
"\tconstructor(isDirectory: boolean, root: ExplorerItem) {\n",
"\t\tsuper(URI.file(''), root, false, false, '');\n",
"\n",
"\t\tthis.id = NewStatPlaceholder.ID++;\n",
"\t\tthis.isDirectoryResolved = isDirectory;\n",
"\t\tthis.directoryPlaceholder = isDirectory;\n",
"\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tsuper(URI.file(''), root, false, false, NewStatPlaceholder.NAME);\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "replace",
"edit_start_line_idx": 386
} | {
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the MIT License. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"indentationToSpaces": "インデントをスペースに変換",
"indentationToTabs": "インデントをタブに変換",
"configuredTabSize": "構成されたタブのサイズ",
"selectTabWidth": "現在のファイルのタブのサイズを選択",
"indentUsingTabs": "タブによるインデント",
"indentUsingSpaces": "スペースによるインデント",
"detectIndentation": "内容からインデントを検出",
"editor.reindentlines": "行の再インデント"
} | i18n/jpn/src/vs/editor/contrib/indentation/indentation.i18n.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.0001747903152136132,
0.0001712919765850529,
0.0001677936379564926,
0.0001712919765850529,
0.0000034983386285603046
] |
{
"id": 1,
"code_window": [
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n",
"\n",
"\tconstructor(isDirectory: boolean, root: ExplorerItem) {\n",
"\t\tsuper(URI.file(''), root, false, false, '');\n",
"\n",
"\t\tthis.id = NewStatPlaceholder.ID++;\n",
"\t\tthis.isDirectoryResolved = isDirectory;\n",
"\t\tthis.directoryPlaceholder = isDirectory;\n",
"\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tsuper(URI.file(''), root, false, false, NewStatPlaceholder.NAME);\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "replace",
"edit_start_line_idx": 386
} | {
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the MIT License. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"vscode.extension.contributes.grammars": "TextMate-tokenizálókat szolgáltat.",
"vscode.extension.contributes.grammars.language": "Annak a nyelvnek az azonosítója, amely számára szolgáltatva van ez a szintaxis.",
"vscode.extension.contributes.grammars.scopeName": "A tmLanguage-fájl által használt TextMate-hatókör neve.",
"vscode.extension.contributes.grammars.path": "A tmLanguage-fájl elérési útja. Az elérési út relatív a kiegészítő mappájához képest, és általában './syntaxes/'-zal kezdődik.",
"vscode.extension.contributes.grammars.embeddedLanguages": "Hatókörnevek leképezése nyelvazonosítókra, ha a nyelvtan tartalmaz beágyazott nyelveket.",
"vscode.extension.contributes.grammars.tokenTypes": "Hatókörnevek leképezése tokentípusokra.",
"vscode.extension.contributes.grammars.injectTo": "Azon nyelvi hatókörök nevei, ahová be lesz ágyazva ez a nyelvtan."
} | i18n/hun/src/vs/workbench/services/textMate/electron-browser/TMGrammars.i18n.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00017582353029865772,
0.00017473407206125557,
0.0001736445992719382,
0.00017473407206125557,
0.0000010894655133597553
] |
{
"id": 1,
"code_window": [
"\n",
"\tprivate id: number;\n",
"\tprivate directoryPlaceholder: boolean;\n",
"\n",
"\tconstructor(isDirectory: boolean, root: ExplorerItem) {\n",
"\t\tsuper(URI.file(''), root, false, false, '');\n",
"\n",
"\t\tthis.id = NewStatPlaceholder.ID++;\n",
"\t\tthis.isDirectoryResolved = isDirectory;\n",
"\t\tthis.directoryPlaceholder = isDirectory;\n",
"\t}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tsuper(URI.file(''), root, false, false, NewStatPlaceholder.NAME);\n"
],
"file_path": "src/vs/workbench/parts/files/common/explorerModel.ts",
"type": "replace",
"edit_start_line_idx": 386
} | FROM ubuntu
MAINTAINER Kimbro Staken
RUN apt-get install -y software-properties-common python
RUN add-apt-repository ppa:chris-lea/node.js
RUN echo "deb http://us.archive.ubuntu.com/ubuntu/ precise universe" >> /etc/apt/sources.list
RUN apt-get update
RUN apt-get install -y nodejs
#RUN apt-get install -y nodejs=0.6.12~dfsg1-1ubuntu1
RUN mkdir /var/www
ADD app.js /var/www/app.js
CMD ["/usr/bin/node", "/var/www/app.js"] | extensions/docker/test/colorize-fixtures/Dockerfile | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00017291351105086505,
0.00017132081848103553,
0.000169728125911206,
0.00017132081848103553,
0.0000015926925698295236
] |
{
"id": 2,
"code_window": [
"\n",
"\t\tif (!folder) {\n",
"\t\t\treturn TPromise.wrapError(new Error('Invalid parent folder to create.'));\n",
"\t\t}\n",
"\n",
"\t\treturn this.tree.reveal(folder, 0.5).then(() => {\n",
"\t\t\treturn this.tree.expand(folder).then(() => {\n",
"\t\t\t\tconst stat = NewStatPlaceholder.addNewStatPlaceholder(folder, !this.isFile);\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tif (!!folder.getChild(NewStatPlaceholder.NAME)) {\n",
"\t\t\t// Do not allow to creatae a new file/folder while in the process of creating a new file/folder #47606\n",
"\t\t\treturn TPromise.as(new Error('Parent folder is already in the process of creating a file'));\n",
"\t\t}\n"
],
"file_path": "src/vs/workbench/parts/files/electron-browser/fileActions.ts",
"type": "add",
"edit_start_line_idx": 393
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import URI from 'vs/base/common/uri';
import * as paths from 'vs/base/common/paths';
import * as resources from 'vs/base/common/resources';
import { ResourceMap } from 'vs/base/common/map';
import { isLinux } from 'vs/base/common/platform';
import { IFileStat } from 'vs/platform/files/common/files';
import { IEditorInput } from 'vs/platform/editor/common/editor';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
import { IEditorGroup, toResource, IEditorIdentifier } from 'vs/workbench/common/editor';
import { IDisposable, dispose } from 'vs/base/common/lifecycle';
import { getPathLabel } from 'vs/base/common/labels';
import { Schemas } from 'vs/base/common/network';
import { startsWith, startsWithIgnoreCase, equalsIgnoreCase } from 'vs/base/common/strings';
export class Model {
private _roots: ExplorerItem[];
private _listener: IDisposable;
constructor(@IWorkspaceContextService private contextService: IWorkspaceContextService) {
const setRoots = () => this._roots = this.contextService.getWorkspace().folders.map(folder => {
const root = new ExplorerItem(folder.uri, undefined);
root.name = folder.name;
return root;
});
this._listener = this.contextService.onDidChangeWorkspaceFolders(() => setRoots());
setRoots();
}
public get roots(): ExplorerItem[] {
return this._roots;
}
/**
* Returns an array of child stat from this stat that matches with the provided path.
* Starts matching from the first root.
* Will return empty array in case the FileStat does not exist.
*/
public findAll(resource: URI): ExplorerItem[] {
return this.roots.map(root => root.find(resource)).filter(stat => !!stat);
}
/**
* Returns a FileStat that matches the passed resource.
* In case multiple FileStat are matching the resource (same folder opened multiple times) returns the FileStat that has the closest root.
* Will return null in case the FileStat does not exist.
*/
public findClosest(resource: URI): ExplorerItem {
const folder = this.contextService.getWorkspaceFolder(resource);
if (folder) {
const root = this.roots.filter(r => r.resource.toString() === folder.uri.toString()).pop();
if (root) {
return root.find(resource);
}
}
return null;
}
public dispose(): void {
this._listener = dispose(this._listener);
}
}
export class ExplorerItem {
public resource: URI;
public name: string;
public mtime: number;
public etag: string;
private _isDirectory: boolean;
private _isSymbolicLink: boolean;
private children: Map<string, ExplorerItem>;
public parent: ExplorerItem;
public isDirectoryResolved: boolean;
constructor(resource: URI, public root: ExplorerItem, isSymbolicLink?: boolean, isDirectory?: boolean, name: string = getPathLabel(resource), mtime?: number, etag?: string) {
this.resource = resource;
this.name = name;
this.isDirectory = !!isDirectory;
this._isSymbolicLink = !!isSymbolicLink;
this.etag = etag;
this.mtime = mtime;
if (!this.root) {
this.root = this;
}
this.isDirectoryResolved = false;
}
public get isSymbolicLink(): boolean {
return this._isSymbolicLink;
}
public get isDirectory(): boolean {
return this._isDirectory;
}
public set isDirectory(value: boolean) {
if (value !== this._isDirectory) {
this._isDirectory = value;
if (this._isDirectory) {
this.children = new Map<string, ExplorerItem>();
} else {
this.children = undefined;
}
}
}
public get nonexistentRoot(): boolean {
return this.isRoot && !this.isDirectoryResolved && this.isDirectory;
}
public getId(): string {
return this.resource.toString();
}
public get isRoot(): boolean {
return this.resource.toString() === this.root.resource.toString();
}
public static create(raw: IFileStat, root: ExplorerItem, resolveTo?: URI[]): ExplorerItem {
const stat = new ExplorerItem(raw.resource, root, raw.isSymbolicLink, raw.isDirectory, raw.name, raw.mtime, raw.etag);
// Recursively add children if present
if (stat.isDirectory) {
// isDirectoryResolved is a very important indicator in the stat model that tells if the folder was fully resolved
// the folder is fully resolved if either it has a list of children or the client requested this by using the resolveTo
// array of resource path to resolve.
stat.isDirectoryResolved = !!raw.children || (!!resolveTo && resolveTo.some((r) => {
return resources.isEqualOrParent(r, stat.resource, !isLinux /* ignorecase */);
}));
// Recurse into children
if (raw.children) {
for (let i = 0, len = raw.children.length; i < len; i++) {
const child = ExplorerItem.create(raw.children[i], root, resolveTo);
child.parent = stat;
stat.addChild(child);
}
}
}
return stat;
}
/**
* Merges the stat which was resolved from the disk with the local stat by copying over properties
* and children. The merge will only consider resolved stat elements to avoid overwriting data which
* exists locally.
*/
public static mergeLocalWithDisk(disk: ExplorerItem, local: ExplorerItem): void {
if (disk.resource.toString() !== local.resource.toString()) {
return; // Merging only supported for stats with the same resource
}
// Stop merging when a folder is not resolved to avoid loosing local data
const mergingDirectories = disk.isDirectory || local.isDirectory;
if (mergingDirectories && local.isDirectoryResolved && !disk.isDirectoryResolved) {
return;
}
// Properties
local.resource = disk.resource;
local.name = disk.name;
local.isDirectory = disk.isDirectory;
local.mtime = disk.mtime;
local.isDirectoryResolved = disk.isDirectoryResolved;
local._isSymbolicLink = disk.isSymbolicLink;
// Merge Children if resolved
if (mergingDirectories && disk.isDirectoryResolved) {
// Map resource => stat
const oldLocalChildren = new ResourceMap<ExplorerItem>();
if (local.children) {
local.children.forEach(child => {
oldLocalChildren.set(child.resource, child);
});
}
// Clear current children
local.children = new Map<string, ExplorerItem>();
// Merge received children
disk.children.forEach(diskChild => {
const formerLocalChild = oldLocalChildren.get(diskChild.resource);
// Existing child: merge
if (formerLocalChild) {
ExplorerItem.mergeLocalWithDisk(diskChild, formerLocalChild);
formerLocalChild.parent = local;
local.addChild(formerLocalChild);
}
// New child: add
else {
diskChild.parent = local;
local.addChild(diskChild);
}
});
}
}
/**
* Adds a child element to this folder.
*/
public addChild(child: ExplorerItem): void {
// Inherit some parent properties to child
child.parent = this;
child.updateResource(false);
this.children.set(this.getPlatformAwareName(child.name), child);
}
public getChild(name: string): ExplorerItem {
if (!this.children) {
return undefined;
}
return this.children.get(this.getPlatformAwareName(name));
}
/**
* Only use this method if you need all the children since it converts a map to an array
*/
public getChildrenArray(): ExplorerItem[] {
if (!this.children) {
return undefined;
}
const items: ExplorerItem[] = [];
this.children.forEach(child => {
items.push(child);
});
return items;
}
public getChildrenCount(): number {
if (!this.children) {
return 0;
}
return this.children.size;
}
public getChildrenNames(): string[] {
if (!this.children) {
return [];
}
const names: string[] = [];
this.children.forEach(child => {
names.push(child.name);
});
return names;
}
/**
* Removes a child element from this folder.
*/
public removeChild(child: ExplorerItem): void {
this.children.delete(this.getPlatformAwareName(child.name));
}
private getPlatformAwareName(name: string): string {
return (isLinux || !name) ? name : name.toLowerCase();
}
/**
* Moves this element under a new parent element.
*/
public move(newParent: ExplorerItem, fnBetweenStates?: (callback: () => void) => void, fnDone?: () => void): void {
if (!fnBetweenStates) {
fnBetweenStates = (cb: () => void) => { cb(); };
}
this.parent.removeChild(this);
fnBetweenStates(() => {
newParent.removeChild(this); // make sure to remove any previous version of the file if any
newParent.addChild(this);
this.updateResource(true);
if (fnDone) {
fnDone();
}
});
}
private updateResource(recursive: boolean): void {
this.resource = this.parent.resource.with({ path: paths.join(this.parent.resource.path, this.name) });
if (recursive) {
if (this.isDirectory && this.children) {
this.children.forEach(child => {
child.updateResource(true);
});
}
}
}
/**
* Tells this stat that it was renamed. This requires changes to all children of this stat (if any)
* so that the path property can be updated properly.
*/
public rename(renamedStat: { name: string, mtime: number }): void {
// Merge a subset of Properties that can change on rename
this.name = renamedStat.name;
this.mtime = renamedStat.mtime;
// Update Paths including children
this.updateResource(true);
}
/**
* Returns a child stat from this stat that matches with the provided path.
* Will return "null" in case the child does not exist.
*/
public find(resource: URI): ExplorerItem {
// Return if path found
if (resource && this.resource.scheme === resource.scheme && this.resource.authority === resource.authority &&
(isLinux ? startsWith(resource.path, this.resource.path) : startsWithIgnoreCase(resource.path, this.resource.path))
) {
return this.findByPath(resource.path, this.resource.path.length);
}
return null; //Unable to find
}
private findByPath(path: string, index: number): ExplorerItem {
if (this.resource.path === path) {
return this;
}
if (!isLinux && equalsIgnoreCase(this.resource.path, path)) {
return this;
}
if (this.children) {
// Ignore separtor to more easily deduct the next name to search
while (index < path.length && path[index] === paths.sep) {
index++;
}
let indexOfNextSep = path.indexOf(paths.sep, index);
if (indexOfNextSep === -1) {
// If there is no separator take the remainder of the path
indexOfNextSep = path.length;
}
// The name to search is between two separators
const name = path.substring(index, indexOfNextSep);
const child = this.children.get(this.getPlatformAwareName(name));
if (child) {
// We found a child with the given name, search inside it
return child.findByPath(path, indexOfNextSep);
}
}
return null;
}
}
/* A helper that can be used to show a placeholder when creating a new stat */
export class NewStatPlaceholder extends ExplorerItem {
private static ID = 0;
private id: number;
private directoryPlaceholder: boolean;
constructor(isDirectory: boolean, root: ExplorerItem) {
super(URI.file(''), root, false, false, '');
this.id = NewStatPlaceholder.ID++;
this.isDirectoryResolved = isDirectory;
this.directoryPlaceholder = isDirectory;
}
public destroy(): void {
this.parent.removeChild(this);
this.isDirectoryResolved = void 0;
this.name = void 0;
this.isDirectory = void 0;
this.mtime = void 0;
}
public getId(): string {
return `new-stat-placeholder:${this.id}:${this.parent.resource.toString()}`;
}
public isDirectoryPlaceholder(): boolean {
return this.directoryPlaceholder;
}
public addChild(child: NewStatPlaceholder): void {
throw new Error('Can\'t perform operations in NewStatPlaceholder.');
}
public removeChild(child: NewStatPlaceholder): void {
throw new Error('Can\'t perform operations in NewStatPlaceholder.');
}
public move(newParent: NewStatPlaceholder): void {
throw new Error('Can\'t perform operations in NewStatPlaceholder.');
}
public rename(renamedStat: NewStatPlaceholder): void {
throw new Error('Can\'t perform operations in NewStatPlaceholder.');
}
public find(resource: URI): NewStatPlaceholder {
return null;
}
public static addNewStatPlaceholder(parent: ExplorerItem, isDirectory: boolean): NewStatPlaceholder {
const child = new NewStatPlaceholder(isDirectory, parent.root);
// Inherit some parent properties to child
child.parent = parent;
parent.addChild(child);
return child;
}
}
export class OpenEditor implements IEditorIdentifier {
constructor(private _editor: IEditorInput, private _group: IEditorGroup) {
// noop
}
public get editor() {
return this._editor;
}
public get editorIndex() {
return this._group.indexOf(this.editor);
}
public get group() {
return this._group;
}
public getId(): string {
return `openeditor:${this.group.id}:${this.group.indexOf(this.editor)}:${this.editor.getName()}:${this.editor.getDescription()}`;
}
public isPreview(): boolean {
return this.group.isPreview(this.editor);
}
public isUntitled(): boolean {
return !!toResource(this.editor, { supportSideBySide: true, filter: Schemas.untitled });
}
public isDirty(): boolean {
return this.editor.isDirty();
}
public getResource(): URI {
return toResource(this.editor, { supportSideBySide: true });
}
}
| src/vs/workbench/parts/files/common/explorerModel.ts | 1 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.9882481694221497,
0.03512986749410629,
0.00016204039275180548,
0.00017182074952870607,
0.16896548867225647
] |
{
"id": 2,
"code_window": [
"\n",
"\t\tif (!folder) {\n",
"\t\t\treturn TPromise.wrapError(new Error('Invalid parent folder to create.'));\n",
"\t\t}\n",
"\n",
"\t\treturn this.tree.reveal(folder, 0.5).then(() => {\n",
"\t\t\treturn this.tree.expand(folder).then(() => {\n",
"\t\t\t\tconst stat = NewStatPlaceholder.addNewStatPlaceholder(folder, !this.isFile);\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tif (!!folder.getChild(NewStatPlaceholder.NAME)) {\n",
"\t\t\t// Do not allow to creatae a new file/folder while in the process of creating a new file/folder #47606\n",
"\t\t\treturn TPromise.as(new Error('Parent folder is already in the process of creating a file'));\n",
"\t\t}\n"
],
"file_path": "src/vs/workbench/parts/files/electron-browser/fileActions.ts",
"type": "add",
"edit_start_line_idx": 393
} | {
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the MIT License. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"unknownSource": "Bilinmeyen Kaynak"
} | i18n/trk/src/vs/workbench/parts/debug/common/debugSource.i18n.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00017699808813631535,
0.00017454646877013147,
0.0001720948494039476,
0.00017454646877013147,
0.000002451619366183877
] |
{
"id": 2,
"code_window": [
"\n",
"\t\tif (!folder) {\n",
"\t\t\treturn TPromise.wrapError(new Error('Invalid parent folder to create.'));\n",
"\t\t}\n",
"\n",
"\t\treturn this.tree.reveal(folder, 0.5).then(() => {\n",
"\t\t\treturn this.tree.expand(folder).then(() => {\n",
"\t\t\t\tconst stat = NewStatPlaceholder.addNewStatPlaceholder(folder, !this.isFile);\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tif (!!folder.getChild(NewStatPlaceholder.NAME)) {\n",
"\t\t\t// Do not allow to creatae a new file/folder while in the process of creating a new file/folder #47606\n",
"\t\t\treturn TPromise.as(new Error('Parent folder is already in the process of creating a file'));\n",
"\t\t}\n"
],
"file_path": "src/vs/workbench/parts/files/electron-browser/fileActions.ts",
"type": "add",
"edit_start_line_idx": 393
} | {
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the MIT License. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"toggleRenderControlCharacters": "Visualizza: Attiva/Disattiva caratteri di controllo"
} | i18n/ita/src/vs/workbench/parts/codeEditor/electron-browser/toggleRenderControlCharacter.i18n.json | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.00017593419761396945,
0.00017401452350895852,
0.0001720948494039476,
0.00017401452350895852,
0.0000019196741050109267
] |
{
"id": 2,
"code_window": [
"\n",
"\t\tif (!folder) {\n",
"\t\t\treturn TPromise.wrapError(new Error('Invalid parent folder to create.'));\n",
"\t\t}\n",
"\n",
"\t\treturn this.tree.reveal(folder, 0.5).then(() => {\n",
"\t\t\treturn this.tree.expand(folder).then(() => {\n",
"\t\t\t\tconst stat = NewStatPlaceholder.addNewStatPlaceholder(folder, !this.isFile);\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tif (!!folder.getChild(NewStatPlaceholder.NAME)) {\n",
"\t\t\t// Do not allow to creatae a new file/folder while in the process of creating a new file/folder #47606\n",
"\t\t\treturn TPromise.as(new Error('Parent folder is already in the process of creating a file'));\n",
"\t\t}\n"
],
"file_path": "src/vs/workbench/parts/files/electron-browser/fileActions.ts",
"type": "add",
"edit_start_line_idx": 393
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import 'vs/css!./inspectTMScopes';
import * as nls from 'vs/nls';
import * as dom from 'vs/base/browser/dom';
import { Disposable } from 'vs/base/common/lifecycle';
import { escape } from 'vs/base/common/strings';
import { KeyCode } from 'vs/base/common/keyCodes';
import { Position } from 'vs/editor/common/core/position';
import { IEditorContribution } from 'vs/editor/common/editorCommon';
import { ITextModel } from 'vs/editor/common/model';
import { registerEditorAction, registerEditorContribution, EditorAction, ServicesAccessor } from 'vs/editor/browser/editorExtensions';
import { ICodeEditor, ContentWidgetPositionPreference, IContentWidget, IContentWidgetPosition } from 'vs/editor/browser/editorBrowser';
import { TPromise } from 'vs/base/common/winjs.base';
import { IGrammar, StackElement, IToken } from 'vscode-textmate';
import { ITextMateService } from 'vs/workbench/services/textMate/electron-browser/textMateService';
import { IModeService } from 'vs/editor/common/services/modeService';
import { TokenizationRegistry, LanguageIdentifier, FontStyle, StandardTokenType, TokenMetadata } from 'vs/editor/common/modes';
import { CharCode } from 'vs/base/common/charCode';
import { findMatchingThemeRule } from 'vs/workbench/services/textMate/electron-browser/TMHelper';
import { IWorkbenchThemeService } from 'vs/workbench/services/themes/common/workbenchThemeService';
import { Color } from 'vs/base/common/color';
import { registerThemingParticipant, HIGH_CONTRAST } from 'vs/platform/theme/common/themeService';
import { editorHoverBackground, editorHoverBorder } from 'vs/platform/theme/common/colorRegistry';
import { INotificationService } from 'vs/platform/notification/common/notification';
class InspectTMScopesController extends Disposable implements IEditorContribution {
private static readonly ID = 'editor.contrib.inspectTMScopes';
public static get(editor: ICodeEditor): InspectTMScopesController {
return editor.getContribution<InspectTMScopesController>(InspectTMScopesController.ID);
}
private _editor: ICodeEditor;
private _textMateService: ITextMateService;
private _themeService: IWorkbenchThemeService;
private _modeService: IModeService;
private _notificationService: INotificationService;
private _widget: InspectTMScopesWidget;
constructor(
editor: ICodeEditor,
@ITextMateService textMateService: ITextMateService,
@IModeService modeService: IModeService,
@IWorkbenchThemeService themeService: IWorkbenchThemeService,
@INotificationService notificationService: INotificationService
) {
super();
this._editor = editor;
this._textMateService = textMateService;
this._themeService = themeService;
this._modeService = modeService;
this._notificationService = notificationService;
this._widget = null;
this._register(this._editor.onDidChangeModel((e) => this.stop()));
this._register(this._editor.onDidChangeModelLanguage((e) => this.stop()));
this._register(this._editor.onKeyUp((e) => e.keyCode === KeyCode.Escape && this.stop()));
}
public getId(): string {
return InspectTMScopesController.ID;
}
public dispose(): void {
this.stop();
super.dispose();
}
public launch(): void {
if (this._widget) {
return;
}
if (!this._editor.getModel()) {
return;
}
this._widget = new InspectTMScopesWidget(this._editor, this._textMateService, this._modeService, this._themeService, this._notificationService);
}
public stop(): void {
if (this._widget) {
this._widget.dispose();
this._widget = null;
}
}
public toggle(): void {
if (!this._widget) {
this.launch();
} else {
this.stop();
}
}
}
class InspectTMScopes extends EditorAction {
constructor() {
super({
id: 'editor.action.inspectTMScopes',
label: nls.localize('inspectTMScopes', "Developer: Inspect TM Scopes"),
alias: 'Developer: Inspect TM Scopes',
precondition: null
});
}
public run(accessor: ServicesAccessor, editor: ICodeEditor): void {
let controller = InspectTMScopesController.get(editor);
if (controller) {
controller.toggle();
}
}
}
interface ICompleteLineTokenization {
startState: StackElement;
tokens1: IToken[];
tokens2: Uint32Array;
endState: StackElement;
}
interface IDecodedMetadata {
languageIdentifier: LanguageIdentifier;
tokenType: StandardTokenType;
fontStyle: FontStyle;
foreground: Color;
background: Color;
}
function renderTokenText(tokenText: string): string {
if (tokenText.length > 40) {
tokenText = tokenText.substr(0, 20) + '…' + tokenText.substr(tokenText.length - 20);
}
let result: string = '';
for (let charIndex = 0, len = tokenText.length; charIndex < len; charIndex++) {
let charCode = tokenText.charCodeAt(charIndex);
switch (charCode) {
case CharCode.Tab:
result += '→';
break;
case CharCode.Space:
result += '·';
break;
case CharCode.LessThan:
result += '<';
break;
case CharCode.GreaterThan:
result += '>';
break;
case CharCode.Ampersand:
result += '&';
break;
default:
result += String.fromCharCode(charCode);
}
}
return result;
}
class InspectTMScopesWidget extends Disposable implements IContentWidget {
private static readonly _ID = 'editor.contrib.inspectTMScopesWidget';
// Editor.IContentWidget.allowEditorOverflow
public readonly allowEditorOverflow = true;
private _isDisposed: boolean;
private readonly _editor: ICodeEditor;
private readonly _modeService: IModeService;
private readonly _themeService: IWorkbenchThemeService;
private readonly _notificationService: INotificationService;
private readonly _model: ITextModel;
private readonly _domNode: HTMLElement;
private readonly _grammar: TPromise<IGrammar>;
constructor(
editor: ICodeEditor,
textMateService: ITextMateService,
modeService: IModeService,
themeService: IWorkbenchThemeService,
notificationService: INotificationService
) {
super();
this._isDisposed = false;
this._editor = editor;
this._modeService = modeService;
this._themeService = themeService;
this._notificationService = notificationService;
this._model = this._editor.getModel();
this._domNode = document.createElement('div');
this._domNode.className = 'tm-inspect-widget';
this._grammar = textMateService.createGrammar(this._model.getLanguageIdentifier().language);
this._beginCompute(this._editor.getPosition());
this._register(this._editor.onDidChangeCursorPosition((e) => this._beginCompute(this._editor.getPosition())));
this._editor.addContentWidget(this);
}
public dispose(): void {
this._isDisposed = true;
this._editor.removeContentWidget(this);
super.dispose();
}
public getId(): string {
return InspectTMScopesWidget._ID;
}
private _beginCompute(position: Position): void {
dom.clearNode(this._domNode);
this._domNode.appendChild(document.createTextNode(nls.localize('inspectTMScopesWidget.loading', "Loading...")));
this._grammar.then(
(grammar) => this._compute(grammar, position),
(err) => {
this._notificationService.warn(err);
setTimeout(() => {
InspectTMScopesController.get(this._editor).stop();
});
}
);
}
private _compute(grammar: IGrammar, position: Position): void {
if (this._isDisposed) {
return;
}
let data = this._getTokensAtLine(grammar, position.lineNumber);
let token1Index = 0;
for (let i = data.tokens1.length - 1; i >= 0; i--) {
let t = data.tokens1[i];
if (position.column - 1 >= t.startIndex) {
token1Index = i;
break;
}
}
let token2Index = 0;
for (let i = (data.tokens2.length >>> 1); i >= 0; i--) {
if (position.column - 1 >= data.tokens2[(i << 1)]) {
token2Index = i;
break;
}
}
let result = '';
let tokenStartIndex = data.tokens1[token1Index].startIndex;
let tokenEndIndex = data.tokens1[token1Index].endIndex;
let tokenText = this._model.getLineContent(position.lineNumber).substring(tokenStartIndex, tokenEndIndex);
result += `<h2 class="tm-token">${renderTokenText(tokenText)}<span class="tm-token-length">(${tokenText.length} ${tokenText.length === 1 ? 'char' : 'chars'})</span></h2>`;
result += `<hr class="tm-metadata-separator" style="clear:both"/>`;
let metadata = this._decodeMetadata(data.tokens2[(token2Index << 1) + 1]);
result += `<table class="tm-metadata-table"><tbody>`;
result += `<tr><td class="tm-metadata-key">language</td><td class="tm-metadata-value">${escape(metadata.languageIdentifier.language)}</td>`;
result += `<tr><td class="tm-metadata-key">token type</td><td class="tm-metadata-value">${this._tokenTypeToString(metadata.tokenType)}</td>`;
result += `<tr><td class="tm-metadata-key">font style</td><td class="tm-metadata-value">${this._fontStyleToString(metadata.fontStyle)}</td>`;
result += `<tr><td class="tm-metadata-key">foreground</td><td class="tm-metadata-value">${Color.Format.CSS.formatHexA(metadata.foreground)}</td>`;
result += `<tr><td class="tm-metadata-key">background</td><td class="tm-metadata-value">${Color.Format.CSS.formatHexA(metadata.background)}</td>`;
result += `</tbody></table>`;
let theme = this._themeService.getColorTheme();
result += `<hr class="tm-metadata-separator"/>`;
let matchingRule = findMatchingThemeRule(theme, data.tokens1[token1Index].scopes, false);
if (matchingRule) {
result += `<code class="tm-theme-selector">${matchingRule.rawSelector}\n${JSON.stringify(matchingRule.settings, null, '\t')}</code>`;
} else {
result += `<span class="tm-theme-selector">No theme selector.</span>`;
}
result += `<hr class="tm-metadata-separator"/>`;
result += `<ul>`;
for (let i = data.tokens1[token1Index].scopes.length - 1; i >= 0; i--) {
result += `<li>${escape(data.tokens1[token1Index].scopes[i])}</li>`;
}
result += `</ul>`;
this._domNode.innerHTML = result;
this._editor.layoutContentWidget(this);
}
private _decodeMetadata(metadata: number): IDecodedMetadata {
let colorMap = TokenizationRegistry.getColorMap();
let languageId = TokenMetadata.getLanguageId(metadata);
let tokenType = TokenMetadata.getTokenType(metadata);
let fontStyle = TokenMetadata.getFontStyle(metadata);
let foreground = TokenMetadata.getForeground(metadata);
let background = TokenMetadata.getBackground(metadata);
return {
languageIdentifier: this._modeService.getLanguageIdentifier(languageId),
tokenType: tokenType,
fontStyle: fontStyle,
foreground: colorMap[foreground],
background: colorMap[background]
};
}
private _tokenTypeToString(tokenType: StandardTokenType): string {
switch (tokenType) {
case StandardTokenType.Other: return 'Other';
case StandardTokenType.Comment: return 'Comment';
case StandardTokenType.String: return 'String';
case StandardTokenType.RegEx: return 'RegEx';
}
return '??';
}
private _fontStyleToString(fontStyle: FontStyle): string {
let r = '';
if (fontStyle & FontStyle.Italic) {
r += 'italic ';
}
if (fontStyle & FontStyle.Bold) {
r += 'bold ';
}
if (fontStyle & FontStyle.Underline) {
r += 'underline ';
}
if (r.length === 0) {
r = '---';
}
return r;
}
private _getTokensAtLine(grammar: IGrammar, lineNumber: number): ICompleteLineTokenization {
let stateBeforeLine = this._getStateBeforeLine(grammar, lineNumber);
let tokenizationResult1 = grammar.tokenizeLine(this._model.getLineContent(lineNumber), stateBeforeLine);
let tokenizationResult2 = grammar.tokenizeLine2(this._model.getLineContent(lineNumber), stateBeforeLine);
return {
startState: stateBeforeLine,
tokens1: tokenizationResult1.tokens,
tokens2: tokenizationResult2.tokens,
endState: tokenizationResult1.ruleStack
};
}
private _getStateBeforeLine(grammar: IGrammar, lineNumber: number): StackElement {
let state: StackElement = null;
for (let i = 1; i < lineNumber; i++) {
let tokenizationResult = grammar.tokenizeLine(this._model.getLineContent(i), state);
state = tokenizationResult.ruleStack;
}
return state;
}
public getDomNode(): HTMLElement {
return this._domNode;
}
public getPosition(): IContentWidgetPosition {
return {
position: this._editor.getPosition(),
preference: [ContentWidgetPositionPreference.BELOW, ContentWidgetPositionPreference.ABOVE]
};
}
}
registerEditorContribution(InspectTMScopesController);
registerEditorAction(InspectTMScopes);
registerThemingParticipant((theme, collector) => {
let border = theme.getColor(editorHoverBorder);
if (border) {
let borderWidth = theme.type === HIGH_CONTRAST ? 2 : 1;
collector.addRule(`.monaco-editor .tm-inspect-widget { border: ${borderWidth}px solid ${border}; }`);
collector.addRule(`.monaco-editor .tm-inspect-widget .tm-metadata-separator { background-color: ${border}; }`);
}
let background = theme.getColor(editorHoverBackground);
if (background) {
collector.addRule(`.monaco-editor .tm-inspect-widget { background-color: ${background}; }`);
}
});
| src/vs/workbench/parts/codeEditor/electron-browser/textMate/inspectTMScopes.ts | 0 | https://github.com/microsoft/vscode/commit/db9cddaa6eef3309ea28990fe1931de10ddd48dd | [
0.0001786262437235564,
0.00017348215624224395,
0.00016576905909460038,
0.00017325131921097636,
0.000002505801376173622
] |
{
"id": 0,
"code_window": [
"import LabeledList from '../common/LabeledList';\n",
"import TraceName from '../common/TraceName';\n",
"import { getTraceName } from '../model/trace-viewer';\n",
"import { TNil } from '../types';\n",
"import { Trace } from '../types/trace';\n",
"import { formatDatetime, formatDuration } from '../utils/date';\n",
"import { getTraceLinks } from '../model/link-patterns';\n",
"\n",
"import ExternalLinks from '../common/ExternalLinks';\n",
"import { createStyle } from '../Theme';\n",
"import { uTxMuted } from '../uberUtilityStyles';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { formatDuration } from '../utils/date';\n"
],
"file_path": "packages/jaeger-ui-components/src/TracePageHeader/TracePageHeader.tsx",
"type": "replace",
"edit_start_line_idx": 28
} | // Copyright (c) 2017 Uber Technologies, Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import * as React from 'react';
import { get as _get, maxBy as _maxBy, values as _values } from 'lodash';
import MdKeyboardArrowRight from 'react-icons/lib/md/keyboard-arrow-right';
import { css } from '@emotion/css';
import cx from 'classnames';
import SpanGraph from './SpanGraph';
import TracePageSearchBar from './TracePageSearchBar';
import { autoColor, Theme, TUpdateViewRangeTimeFunction, useTheme, ViewRange, ViewRangeTimeUpdate } from '..';
import LabeledList from '../common/LabeledList';
import TraceName from '../common/TraceName';
import { getTraceName } from '../model/trace-viewer';
import { TNil } from '../types';
import { Trace } from '../types/trace';
import { formatDatetime, formatDuration } from '../utils/date';
import { getTraceLinks } from '../model/link-patterns';
import ExternalLinks from '../common/ExternalLinks';
import { createStyle } from '../Theme';
import { uTxMuted } from '../uberUtilityStyles';
const getStyles = createStyle((theme: Theme) => {
return {
TracePageHeader: css`
label: TracePageHeader;
& > :first-child {
border-bottom: 1px solid ${autoColor(theme, '#e8e8e8')};
}
& > :nth-child(2) {
background-color: ${autoColor(theme, '#eee')};
border-bottom: 1px solid ${autoColor(theme, '#e4e4e4')};
}
& > :last-child {
border-bottom: 1px solid ${autoColor(theme, '#ccc')};
}
`,
TracePageHeaderTitleRow: css`
label: TracePageHeaderTitleRow;
align-items: center;
display: flex;
`,
TracePageHeaderBack: css`
label: TracePageHeaderBack;
align-items: center;
align-self: stretch;
background-color: #fafafa;
border-bottom: 1px solid #ddd;
border-right: 1px solid #ddd;
color: inherit;
display: flex;
font-size: 1.4rem;
padding: 0 1rem;
margin-bottom: -1px;
&:hover {
background-color: #f0f0f0;
border-color: #ccc;
}
`,
TracePageHeaderTitleLink: css`
label: TracePageHeaderTitleLink;
align-items: center;
display: flex;
flex: 1;
&:hover * {
text-decoration: underline;
}
&:hover > *,
&:hover small {
text-decoration: none;
}
`,
TracePageHeaderDetailToggle: css`
label: TracePageHeaderDetailToggle;
font-size: 2.5rem;
transition: transform 0.07s ease-out;
`,
TracePageHeaderDetailToggleExpanded: css`
label: TracePageHeaderDetailToggleExpanded;
transform: rotate(90deg);
`,
TracePageHeaderTitle: css`
label: TracePageHeaderTitle;
color: inherit;
flex: 1;
font-size: 1.7em;
line-height: 1em;
margin: 0 0 0 0.5em;
padding-bottom: 0.5em;
`,
TracePageHeaderTitleCollapsible: css`
label: TracePageHeaderTitleCollapsible;
margin-left: 0;
`,
TracePageHeaderOverviewItems: css`
label: TracePageHeaderOverviewItems;
border-bottom: 1px solid #e4e4e4;
padding: 0.25rem 0.5rem !important;
`,
TracePageHeaderOverviewItemValueDetail: cx(
css`
label: TracePageHeaderOverviewItemValueDetail;
color: #aaa;
`,
'trace-item-value-detail'
),
TracePageHeaderOverviewItemValue: css`
label: TracePageHeaderOverviewItemValue;
&:hover > .trace-item-value-detail {
color: unset;
}
`,
TracePageHeaderArchiveIcon: css`
label: TracePageHeaderArchiveIcon;
font-size: 1.78em;
margin-right: 0.15em;
`,
TracePageHeaderTraceId: css`
label: TracePageHeaderTraceId;
white-space: nowrap;
`,
};
});
type TracePageHeaderEmbedProps = {
canCollapse: boolean;
clearSearch: () => void;
focusUiFindMatches: () => void;
hideMap: boolean;
hideSummary: boolean;
nextResult: () => void;
onSlimViewClicked: () => void;
onTraceGraphViewClicked: () => void;
prevResult: () => void;
resultCount: number;
slimView: boolean;
textFilter: string | TNil;
trace: Trace;
traceGraphView: boolean;
updateNextViewRangeTime: (update: ViewRangeTimeUpdate) => void;
updateViewRangeTime: TUpdateViewRangeTimeFunction;
viewRange: ViewRange;
searchValue: string;
onSearchValueChange: (value: string) => void;
hideSearchButtons?: boolean;
};
export const HEADER_ITEMS = [
{
key: 'timestamp',
label: 'Trace Start',
renderer(trace: Trace, styles: ReturnType<typeof getStyles>) {
const dateStr = formatDatetime(trace.startTime);
const match = dateStr.match(/^(.+)(:\d\d\.\d+)$/);
return match ? (
<span className={styles.TracePageHeaderOverviewItemValue}>
{match[1]}
<span className={styles.TracePageHeaderOverviewItemValueDetail}>{match[2]}</span>
</span>
) : (
dateStr
);
},
},
{
key: 'duration',
label: 'Duration',
renderer: (trace: Trace) => formatDuration(trace.duration),
},
{
key: 'service-count',
label: 'Services',
renderer: (trace: Trace) => new Set(_values(trace.processes).map((p) => p.serviceName)).size,
},
{
key: 'depth',
label: 'Depth',
renderer: (trace: Trace) => _get(_maxBy(trace.spans, 'depth'), 'depth', 0) + 1,
},
{
key: 'span-count',
label: 'Total Spans',
renderer: (trace: Trace) => trace.spans.length,
},
];
export default function TracePageHeader(props: TracePageHeaderEmbedProps) {
const {
canCollapse,
clearSearch,
focusUiFindMatches,
hideMap,
hideSummary,
nextResult,
onSlimViewClicked,
prevResult,
resultCount,
slimView,
textFilter,
trace,
traceGraphView,
updateNextViewRangeTime,
updateViewRangeTime,
viewRange,
searchValue,
onSearchValueChange,
hideSearchButtons,
} = props;
const styles = getStyles(useTheme());
const links = React.useMemo(() => {
if (!trace) {
return [];
}
return getTraceLinks(trace);
}, [trace]);
if (!trace) {
return null;
}
const summaryItems =
!hideSummary &&
!slimView &&
HEADER_ITEMS.map((item) => {
const { renderer, ...rest } = item;
return { ...rest, value: renderer(trace, styles) };
});
const title = (
<h1 className={cx(styles.TracePageHeaderTitle, canCollapse && styles.TracePageHeaderTitleCollapsible)}>
<TraceName traceName={getTraceName(trace.spans)} />{' '}
<small className={cx(styles.TracePageHeaderTraceId, uTxMuted)}>{trace.traceID}</small>
</h1>
);
return (
<header className={styles.TracePageHeader}>
<div className={styles.TracePageHeaderTitleRow}>
{links && links.length > 0 && <ExternalLinks links={links} className={styles.TracePageHeaderBack} />}
{canCollapse ? (
<a
className={styles.TracePageHeaderTitleLink}
onClick={onSlimViewClicked}
role="switch"
aria-checked={!slimView}
>
<MdKeyboardArrowRight
className={cx(
styles.TracePageHeaderDetailToggle,
!slimView && styles.TracePageHeaderDetailToggleExpanded
)}
/>
{title}
</a>
) : (
title
)}
<TracePageSearchBar
clearSearch={clearSearch}
focusUiFindMatches={focusUiFindMatches}
nextResult={nextResult}
prevResult={prevResult}
resultCount={resultCount}
textFilter={textFilter}
navigable={!traceGraphView}
searchValue={searchValue}
onSearchValueChange={onSearchValueChange}
hideSearchButtons={hideSearchButtons}
/>
</div>
{summaryItems && <LabeledList className={styles.TracePageHeaderOverviewItems} items={summaryItems} />}
{!hideMap && !slimView && (
<SpanGraph
trace={trace}
viewRange={viewRange}
updateNextViewRangeTime={updateNextViewRangeTime}
updateViewRangeTime={updateViewRangeTime}
/>
)}
</header>
);
}
| packages/jaeger-ui-components/src/TracePageHeader/TracePageHeader.tsx | 1 | https://github.com/grafana/grafana/commit/b89f8fdfcbf70dc3a2c3a7208d12fea87f7979a0 | [
0.8419848084449768,
0.02929016202688217,
0.00016437780868727714,
0.0001729304640321061,
0.1509653776884079
] |
{
"id": 0,
"code_window": [
"import LabeledList from '../common/LabeledList';\n",
"import TraceName from '../common/TraceName';\n",
"import { getTraceName } from '../model/trace-viewer';\n",
"import { TNil } from '../types';\n",
"import { Trace } from '../types/trace';\n",
"import { formatDatetime, formatDuration } from '../utils/date';\n",
"import { getTraceLinks } from '../model/link-patterns';\n",
"\n",
"import ExternalLinks from '../common/ExternalLinks';\n",
"import { createStyle } from '../Theme';\n",
"import { uTxMuted } from '../uberUtilityStyles';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { formatDuration } from '../utils/date';\n"
],
"file_path": "packages/jaeger-ui-components/src/TracePageHeader/TracePageHeader.tsx",
"type": "replace",
"edit_start_line_idx": 28
} | package migrator
import (
"errors"
"fmt"
"strings"
"github.com/grafana/grafana/pkg/util/errutil"
"github.com/mattn/go-sqlite3"
"xorm.io/xorm"
)
type SQLite3 struct {
BaseDialect
}
func NewSQLite3Dialect(engine *xorm.Engine) Dialect {
d := SQLite3{}
d.BaseDialect.dialect = &d
d.BaseDialect.engine = engine
d.BaseDialect.driverName = SQLite
return &d
}
func (db *SQLite3) SupportEngine() bool {
return false
}
func (db *SQLite3) Quote(name string) string {
return "`" + name + "`"
}
func (db *SQLite3) AutoIncrStr() string {
return "AUTOINCREMENT"
}
func (db *SQLite3) BooleanStr(value bool) string {
if value {
return "1"
}
return "0"
}
func (db *SQLite3) DateTimeFunc(value string) string {
return "datetime(" + value + ")"
}
func (db *SQLite3) SQLType(c *Column) string {
switch c.Type {
case DB_Date, DB_DateTime, DB_TimeStamp, DB_Time:
return DB_DateTime
case DB_TimeStampz:
return DB_Text
case DB_Char, DB_Varchar, DB_NVarchar, DB_TinyText, DB_Text, DB_MediumText, DB_LongText:
return DB_Text
case DB_Bit, DB_TinyInt, DB_SmallInt, DB_MediumInt, DB_Int, DB_Integer, DB_BigInt, DB_Bool:
return DB_Integer
case DB_Float, DB_Double, DB_Real:
return DB_Real
case DB_Decimal, DB_Numeric:
return DB_Numeric
case DB_TinyBlob, DB_Blob, DB_MediumBlob, DB_LongBlob, DB_Bytea, DB_Binary, DB_VarBinary:
return DB_Blob
case DB_Serial, DB_BigSerial:
c.IsPrimaryKey = true
c.IsAutoIncrement = true
c.Nullable = false
return DB_Integer
default:
return c.Type
}
}
func (db *SQLite3) IndexCheckSQL(tableName, indexName string) (string, []interface{}) {
args := []interface{}{tableName, indexName}
sql := "SELECT 1 FROM " + db.Quote("sqlite_master") + " WHERE " + db.Quote("type") + "='index' AND " + db.Quote("tbl_name") + "=? AND " + db.Quote("name") + "=?"
return sql, args
}
func (db *SQLite3) DropIndexSQL(tableName string, index *Index) string {
quote := db.Quote
// var unique string
idxName := index.XName(tableName)
return fmt.Sprintf("DROP INDEX %v", quote(idxName))
}
func (db *SQLite3) CleanDB() error {
return nil
}
// TruncateDBTables deletes all data from all the tables and resets the sequences.
// A special case is the dashboard_acl table where we keep the default permissions.
func (db *SQLite3) TruncateDBTables() error {
tables, err := db.engine.DBMetas()
if err != nil {
return err
}
sess := db.engine.NewSession()
defer sess.Close()
for _, table := range tables {
switch table.Name {
case "migration_log":
continue
case "dashboard_acl":
// keep default dashboard permissions
if _, err := sess.Exec(fmt.Sprintf("DELETE FROM %q WHERE dashboard_id != -1 AND org_id != -1;", table.Name)); err != nil {
return errutil.Wrapf(err, "failed to truncate table %q", table.Name)
}
if _, err := sess.Exec("UPDATE sqlite_sequence SET seq = 2 WHERE name = '%s';", table.Name); err != nil {
return errutil.Wrapf(err, "failed to cleanup sqlite_sequence")
}
default:
if _, err := sess.Exec(fmt.Sprintf("DELETE FROM %s;", table.Name)); err != nil {
return errutil.Wrapf(err, "failed to truncate table %q", table.Name)
}
}
}
if _, err := sess.Exec("UPDATE sqlite_sequence SET seq = 0 WHERE name != 'dashboard_acl';"); err != nil {
return errutil.Wrapf(err, "failed to cleanup sqlite_sequence")
}
return nil
}
func (db *SQLite3) isThisError(err error, errcode int) bool {
var driverErr sqlite3.Error
if errors.As(err, &driverErr) {
if int(driverErr.ExtendedCode) == errcode {
return true
}
}
return false
}
func (db *SQLite3) ErrorMessage(err error) string {
var driverErr sqlite3.Error
if errors.As(err, &driverErr) {
return driverErr.Error()
}
return ""
}
func (db *SQLite3) IsUniqueConstraintViolation(err error) bool {
return db.isThisError(err, int(sqlite3.ErrConstraintUnique))
}
func (db *SQLite3) IsDeadlock(err error) bool {
return false // No deadlock
}
// UpsertSQL returns the upsert sql statement for SQLite dialect
func (db *SQLite3) UpsertSQL(tableName string, keyCols, updateCols []string) string {
columnsStr := strings.Builder{}
onConflictStr := strings.Builder{}
colPlaceHoldersStr := strings.Builder{}
setStr := strings.Builder{}
const separator = ", "
separatorVar := separator
for i, c := range updateCols {
if i == len(updateCols)-1 {
separatorVar = ""
}
columnsStr.WriteString(fmt.Sprintf("%s%s", db.Quote(c), separatorVar))
colPlaceHoldersStr.WriteString(fmt.Sprintf("?%s", separatorVar))
setStr.WriteString(fmt.Sprintf("%s=excluded.%s%s", db.Quote(c), db.Quote(c), separatorVar))
}
separatorVar = separator
for i, c := range keyCols {
if i == len(keyCols)-1 {
separatorVar = ""
}
onConflictStr.WriteString(fmt.Sprintf("%s%s", db.Quote(c), separatorVar))
}
s := fmt.Sprintf(`INSERT INTO %s (%s) VALUES (%s) ON CONFLICT(%s) DO UPDATE SET %s`,
tableName,
columnsStr.String(),
colPlaceHoldersStr.String(),
onConflictStr.String(),
setStr.String(),
)
return s
}
| pkg/services/sqlstore/migrator/sqlite_dialect.go | 0 | https://github.com/grafana/grafana/commit/b89f8fdfcbf70dc3a2c3a7208d12fea87f7979a0 | [
0.0004858166794292629,
0.00018996377184521407,
0.00016324804164469242,
0.00017209304496645927,
0.00007044235098874196
] |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.