hunk
dict | file
stringlengths 0
11.8M
| file_path
stringlengths 2
234
| label
int64 0
1
| commit_url
stringlengths 74
103
| dependency_score
sequencelengths 5
5
|
---|---|---|---|---|---|
{
"id": 0,
"code_window": [
" - \"4\"\n",
" - \"5\"\n",
"script:\n",
" - npm run check\n",
"branches:\n",
" only:\n",
" - master"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" - npm run check:lib\n",
" - npm run build\n",
" - npm run check:examples\n"
],
"file_path": ".travis.yml",
"type": "replace",
"edit_start_line_idx": 5
} | import React, { Component, PropTypes } from 'react'
import Todo from './Todo'
export default class TodoList extends Component {
render() {
return (
<ul>
{this.props.todos.map(todo =>
<Todo {...todo}
key={todo.id}
onClick={() => this.props.onTodoClick(todo.id)} />
)}
</ul>
)
}
}
TodoList.propTypes = {
onTodoClick: PropTypes.func.isRequired,
todos: PropTypes.arrayOf(PropTypes.shape({
text: PropTypes.string.isRequired,
completed: PropTypes.bool.isRequired
}).isRequired).isRequired
}
| examples/todos-with-undo/components/TodoList.js | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.00017065230349544436,
0.0001695323153398931,
0.00016743595188017935,
0.0001705086906440556,
0.0000014835118236078415
] |
{
"id": 0,
"code_window": [
" - \"4\"\n",
" - \"5\"\n",
"script:\n",
" - npm run check\n",
"branches:\n",
" only:\n",
" - master"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" - npm run check:lib\n",
" - npm run build\n",
" - npm run check:examples\n"
],
"file_path": ".travis.yml",
"type": "replace",
"edit_start_line_idx": 5
} | # `bindActionCreators(actionCreators, dispatch)`
Turns an object whose values are [action creators](../Glossary.md#action-creator), into an object with the same keys, but with every action creator wrapped into a [`dispatch`](Store.md#dispatch) call so they may be invoked directly.
Normally you should just call [`dispatch`](Store.md#dispatch) directly on your [`Store`](Store.md) instance. If you use Redux with React, [react-redux](https://github.com/gaearon/react-redux) will provide you with the [`dispatch`](Store.md#dispatch) function so you can call it directly, too.
The only use case for `bindActionCreators` is when you want to pass some action creators down to a component that isn’t aware of Redux, and you don’t want to pass [`dispatch`](Store.md#dispatch) or the Redux store to it.
For convenience, you can also pass a single function as the first argument, and get a function in return.
#### Parameters
1. `actionCreators` (*Function* or *Object*): An [action creator](../Glossary.md#action-creator), or an object whose values are action creators.
2. `dispatch` (*Function*): A [`dispatch`](Store.md#dispatch) function available on the [`Store`](Store.md) instance.
#### Returns
(*Function* or *Object*): An object mimicking the original object, but with each function immediately dispatching the action returned by the corresponding action creator. If you passed a function as `actionCreators`, the return value will also be a single function.
#### Example
#### `TodoActionCreators.js`
```js
export function addTodo(text) {
return {
type: 'ADD_TODO',
text
}
}
export function removeTodo(id) {
return {
type: 'REMOVE_TODO',
id
}
}
```
#### `SomeComponent.js`
```js
import { Component } from 'react'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import * as TodoActionCreators from './TodoActionCreators'
console.log(TodoActionCreators)
// {
// addTodo: Function,
// removeTodo: Function
// }
class TodoListContainer extends Component {
componentDidMount() {
// Injected by react-redux:
let { dispatch } = this.props
// Note: this won’t work:
// TodoActionCreators.addTodo('Use Redux')
// You’re just calling a function that creates an action.
// You must dispatch the action, too!
// This will work:
let action = TodoActionCreators.addTodo('Use Redux')
dispatch(action)
}
render() {
// Injected by react-redux:
let { todos, dispatch } = this.props
// Here’s a good use case for bindActionCreators:
// You want a child component to be completely unaware of Redux.
let boundActionCreators = bindActionCreators(TodoActionCreators, dispatch)
console.log(boundActionCreators)
// {
// addTodo: Function,
// removeTodo: Function
// }
return (
<TodoList todos={todos}
{...boundActionCreators} />
)
// An alternative to bindActionCreators is to pass
// just the dispatch function down, but then your child component
// needs to import action creators and know about them.
// return <TodoList todos={todos} dispatch={dispatch} />
}
}
export default connect(
state => ({ todos: state.todos })
)(TodoListContainer)
```
#### Tips
* You might ask: why don’t we bind the action creators to the store instance right away, like in classical Flux? The problem is that this won’t work well with universal apps that need to render on the server. Most likely you want to have a separate store instance per request so you can prepare them with different data, but binding action creators during their definition means you’re stuck with a single store instance for all requests.
* If you use ES5, instead of `import * as` syntax you can just pass `require('./TodoActionCreators')` to `bindActionCreators` as the first argument. The only thing it cares about is that the values of the `actionCreators` arguments are functions. The module system doesn’t matter.
| docs/api/bindActionCreators.md | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.000484649179270491,
0.0001991025055758655,
0.00016553638852201402,
0.00017132566426880658,
0.00009034098911797628
] |
{
"id": 1,
"code_window": [
" \"test:watch\": \"npm test -- --watch\",\n",
" \"test:cov\": \"babel-node $(npm bin)/isparta cover $(npm bin)/_mocha -- --recursive\",\n",
" \"test:examples\": \"babel-node examples/testAll.js\",\n",
" \"check\": \"npm run lint && npm run test && npm run build:examples && npm run test:examples\",\n",
" \"build:lib\": \"babel src --out-dir lib\",\n",
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"check:lib\": \"npm run lint && npm run test\",\n",
" \"check:examples\": \"npm run build:examples && npm run test:examples\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 18
} | {
"name": "redux",
"version": "3.2.1",
"description": "Predictable state container for JavaScript apps",
"main": "lib/index.js",
"jsnext:main": "src/index.js",
"files": [
"dist",
"lib",
"src"
],
"scripts": {
"clean": "rimraf lib dist coverage",
"lint": "eslint src test examples",
"test": "mocha --compilers js:babel-register --recursive",
"test:watch": "npm test -- --watch",
"test:cov": "babel-node $(npm bin)/isparta cover $(npm bin)/_mocha -- --recursive",
"test:examples": "babel-node examples/testAll.js",
"check": "npm run lint && npm run test && npm run build:examples && npm run test:examples",
"build:lib": "babel src --out-dir lib",
"build:umd": "cross-env NODE_ENV=development webpack src/index.js dist/redux.js",
"build:umd:min": "cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js",
"build:examples": "babel-node examples/buildAll.js",
"build": "npm run build:lib && npm run build:umd && npm run build:umd:min",
"prepublish": "npm run clean && npm run build && npm run check && node ./prepublish",
"docs:clean": "rimraf _book",
"docs:prepare": "gitbook install",
"docs:build": "npm run docs:prepare && gitbook build -g rackt/redux",
"docs:watch": "npm run docs:prepare && gitbook serve",
"docs:publish": "npm run docs:clean && npm run docs:build && cp CNAME _book && cd _book && git init && git commit --allow-empty -m 'update book' && git checkout -b gh-pages && touch .nojekyll && git add . && git commit -am 'update book' && git push [email protected]:rackt/redux gh-pages --force"
},
"repository": {
"type": "git",
"url": "https://github.com/rackt/redux.git"
},
"keywords": [
"redux",
"reducer",
"state",
"predictable",
"functional",
"immutable",
"hot",
"live",
"replay",
"flux",
"elm"
],
"authors": [
"Dan Abramov <[email protected]> (https://github.com/gaearon)",
"Andrew Clark <[email protected]> (https://github.com/acdlite)"
],
"license": "MIT",
"bugs": {
"url": "https://github.com/rackt/redux/issues"
},
"homepage": "http://rackt.github.io/redux",
"dependencies": {
"lodash": "^4.2.0",
"loose-envify": "^1.1.0"
},
"devDependencies": {
"babel-cli": "^6.3.15",
"babel-core": "^6.3.15",
"babel-eslint": "^4.1.6",
"babel-loader": "^6.2.0",
"babel-plugin-check-es2015-constants": "^6.3.13",
"babel-plugin-transform-es2015-arrow-functions": "^6.3.13",
"babel-plugin-transform-es2015-block-scoped-functions": "^6.3.13",
"babel-plugin-transform-es2015-block-scoping": "^6.3.13",
"babel-plugin-transform-es2015-classes": "^6.3.13",
"babel-plugin-transform-es2015-computed-properties": "^6.3.13",
"babel-plugin-transform-es2015-destructuring": "^6.3.13",
"babel-plugin-transform-es2015-for-of": "^6.3.13",
"babel-plugin-transform-es2015-function-name": "^6.3.13",
"babel-plugin-transform-es2015-literals": "^6.3.13",
"babel-plugin-transform-es2015-modules-commonjs": "^6.3.13",
"babel-plugin-transform-es2015-object-super": "^6.3.13",
"babel-plugin-transform-es2015-parameters": "^6.3.13",
"babel-plugin-transform-es2015-shorthand-properties": "^6.3.13",
"babel-plugin-transform-es2015-spread": "^6.3.13",
"babel-plugin-transform-es2015-sticky-regex": "^6.3.13",
"babel-plugin-transform-es2015-template-literals": "^6.3.13",
"babel-plugin-transform-es2015-unicode-regex": "^6.3.13",
"babel-plugin-transform-object-rest-spread": "^6.3.13",
"babel-register": "^6.3.13",
"cross-env": "^1.0.7",
"es3ify": "^0.2.0",
"eslint": "^1.10.3",
"eslint-config-rackt": "^1.1.1",
"eslint-plugin-react": "^3.16.1",
"expect": "^1.8.0",
"gitbook-cli": "^0.3.4",
"glob": "^6.0.4",
"isparta": "^4.0.0",
"mocha": "^2.2.5",
"rimraf": "^2.3.4",
"webpack": "^1.9.6"
},
"npmName": "redux",
"npmFileMap": [
{
"basePath": "/dist/",
"files": [
"*.js"
]
}
],
"browserify": {
"transform": [
"loose-envify"
]
}
}
| package.json | 1 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.9926347136497498,
0.08332878351211548,
0.00016233933274634182,
0.00016992157907225192,
0.2741701602935791
] |
{
"id": 1,
"code_window": [
" \"test:watch\": \"npm test -- --watch\",\n",
" \"test:cov\": \"babel-node $(npm bin)/isparta cover $(npm bin)/_mocha -- --recursive\",\n",
" \"test:examples\": \"babel-node examples/testAll.js\",\n",
" \"check\": \"npm run lint && npm run test && npm run build:examples && npm run test:examples\",\n",
" \"build:lib\": \"babel src --out-dir lib\",\n",
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"check:lib\": \"npm run lint && npm run test\",\n",
" \"check:examples\": \"npm run build:examples && npm run test:examples\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 18
} | /**
* Composes single-argument functions from right to left.
*
* @param {...Function} funcs The functions to compose.
* @returns {Function} A function obtained by composing functions from right to
* left. For example, compose(f, g, h) is identical to arg => f(g(h(arg))).
*/
export default function compose(...funcs) {
return (...args) => {
if (funcs.length === 0) {
return args[0]
}
const last = funcs[funcs.length - 1]
const rest = funcs.slice(0, -1)
return rest.reduceRight((composed, f) => f(composed), last(...args))
}
}
| src/compose.js | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.00017559398838784546,
0.00017527502495795488,
0.00017495607607997954,
0.00017527502495795488,
3.1895615393295884e-7
] |
{
"id": 1,
"code_window": [
" \"test:watch\": \"npm test -- --watch\",\n",
" \"test:cov\": \"babel-node $(npm bin)/isparta cover $(npm bin)/_mocha -- --recursive\",\n",
" \"test:examples\": \"babel-node examples/testAll.js\",\n",
" \"check\": \"npm run lint && npm run test && npm run build:examples && npm run test:examples\",\n",
" \"build:lib\": \"babel src --out-dir lib\",\n",
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"check:lib\": \"npm run lint && npm run test\",\n",
" \"check:examples\": \"npm run build:examples && npm run test:examples\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 18
} | import expect from 'expect'
import React from 'react'
import TestUtils from 'react-addons-test-utils'
import Counter from '../../components/Counter'
function setup(value = 0) {
const actions = {
onIncrement: expect.createSpy(),
onDecrement: expect.createSpy()
}
const component = TestUtils.renderIntoDocument(
<Counter value={value} {...actions} />
)
return {
component: component,
actions: actions,
buttons: TestUtils.scryRenderedDOMComponentsWithTag(component, 'button'),
p: TestUtils.findRenderedDOMComponentWithTag(component, 'p')
}
}
describe('Counter component', () => {
it('should display count', () => {
const { p } = setup()
expect(p.textContent).toMatch(/^Clicked: 0 times/)
})
it('first button should call onIncrement', () => {
const { buttons, actions } = setup()
TestUtils.Simulate.click(buttons[0])
expect(actions.onIncrement).toHaveBeenCalled()
})
it('second button should call onDecrement', () => {
const { buttons, actions } = setup()
TestUtils.Simulate.click(buttons[1])
expect(actions.onDecrement).toHaveBeenCalled()
})
it('third button should not call onIncrement if the counter is even', () => {
const { buttons, actions } = setup(42)
TestUtils.Simulate.click(buttons[2])
expect(actions.onIncrement).toNotHaveBeenCalled()
})
it('third button should call onIncrement if the counter is odd', () => {
const { buttons, actions } = setup(43)
TestUtils.Simulate.click(buttons[2])
expect(actions.onIncrement).toHaveBeenCalled()
})
it('fourth button should call onIncrement in a second', (done) => {
const { buttons, actions } = setup()
TestUtils.Simulate.click(buttons[3])
setTimeout(() => {
expect(actions.onIncrement).toHaveBeenCalled()
done()
}, 1000)
})
})
| examples/counter/test/components/Counter.spec.js | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.0001778839941835031,
0.0001750424416968599,
0.00016688572941347957,
0.00017644422769080848,
0.0000034671388675633352
] |
{
"id": 1,
"code_window": [
" \"test:watch\": \"npm test -- --watch\",\n",
" \"test:cov\": \"babel-node $(npm bin)/isparta cover $(npm bin)/_mocha -- --recursive\",\n",
" \"test:examples\": \"babel-node examples/testAll.js\",\n",
" \"check\": \"npm run lint && npm run test && npm run build:examples && npm run test:examples\",\n",
" \"build:lib\": \"babel src --out-dir lib\",\n",
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"check:lib\": \"npm run lint && npm run test\",\n",
" \"check:examples\": \"npm run build:examples && npm run test:examples\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 18
} | # Async Actions
In the [basics guide](../basics/README.md), we built a simple todo application. It was fully synchronous. Every time an action was dispatched, the state was updated immediately.
In this guide, we will build a different, asynchronous application. It will use the Reddit API to show the current headlines for a select subreddit. How does asynchronicity fit into Redux flow?
## Actions
When you call an asynchronous API, there are two crucial moments in time: the moment you start the call, and the moment when you receive an answer (or a timeout).
Each of these two moments can usually require a change in the application state; to do that, you need to dispatch normal actions that will be processed by reducers synchronously. Usually, for any API request you’ll want to dispatch at least three different kinds of actions:
* **An action informing the reducers that the request began.**
The reducers may handle this action by toggling an `isFetching` flag in the state. This way the UI knows it’s time to show a spinner.
* **An action informing the reducers that the request finished successfully.**
The reducers may handle this action by merging the new data into the state they manage and resetting `isFetching`. The UI would hide the spinner, and display the fetched data.
* **An action informing the reducers that the request failed.**
The reducers may handle this action by resetting `isFetching`. Maybe, some reducers will also want to store the error message so the UI can display it.
You may use a dedicated `status` field in your actions:
```js
{ type: 'FETCH_POSTS' }
{ type: 'FETCH_POSTS', status: 'error', error: 'Oops' }
{ type: 'FETCH_POSTS', status: 'success', response: { ... } }
```
Or you can define separate types for them:
```js
{ type: 'FETCH_POSTS_REQUEST' }
{ type: 'FETCH_POSTS_FAILURE', error: 'Oops' }
{ type: 'FETCH_POSTS_SUCCESS', response: { ... } }
```
Choosing whether to use a single action type with flags, or multiple action types, is up to you. It’s a convention you need to decide with your team. Multiple types leave less room for a mistake, but this is not an issue if you generate action creators and reducers with a helper library like [redux-actions](https://github.com/acdlite/redux-actions).
Whatever convention you choose, stick with it throughout the application.
We’ll use separate types in this tutorial.
## Synchronous Action Creators
Let’s start by defining the several synchronous action types and action creators we need in our example app. Here, the user can select a subreddit to display:
#### `actions.js`
```js
export const SELECT_SUBREDDIT = 'SELECT_SUBREDDIT'
export function selectSubreddit(subreddit) {
return {
type: SELECT_SUBREDDIT,
subreddit
}
}
```
They can also press a “refresh” button to update it:
```js
export const INVALIDATE_SUBREDDIT = 'INVALIDATE_SUBREDDIT'
export function invalidateSubreddit(subreddit) {
return {
type: INVALIDATE_SUBREDDIT,
subreddit
}
}
```
These were the actions governed by the user interaction. We will also have another kind of action, governed by the network requests. We will see how to dispatch them later, but for now, we just want to define them.
When it’s time to fetch the posts for some subreddit, we will dispatch a `REQUEST_POSTS` action:
```js
export const REQUEST_POSTS = 'REQUEST_POSTS'
export function requestPosts(subreddit) {
return {
type: REQUEST_POSTS,
subreddit
}
}
```
It is important for it to be separate from `SELECT_SUBREDDIT` or `INVALIDATE_SUBREDDIT`. While they may occur one after another, as the app grows more complex, you might want to fetch some data independently of the user action (for example, to prefetch the most popular subreddits, or to refresh stale data once in a while). You may also want to fetch in response to a route change, so it’s not wise to couple fetching to some particular UI event early on.
Finally, when the network request comes through, we will dispatch `RECEIVE_POSTS`:
```js
export const RECEIVE_POSTS = 'RECEIVE_POSTS'
export function receivePosts(subreddit, json) {
return {
type: RECEIVE_POSTS,
subreddit,
posts: json.data.children.map(child => child.data),
receivedAt: Date.now()
}
}
```
This is all we need to know for now. The particular mechanism to dispatch these actions together with network requests will be discussed later.
>##### Note on Error Handling
>In a real app, you’d also want to dispatch an action on request failure. We won’t implement error handling in this tutorial, but the [real world example](../introduction/Examples.html#real-world) shows one of the possible approaches.
## Designing the State Shape
Just like in the basic tutorial, you’ll need to [design the shape of your application’s state](../basics/Reducers.md#designing-the-state-shape) before rushing into the implementation. With asynchronous code, there is more state to take care of, so we need to think it through.
This part is often confusing to beginners, because it is not immediately clear what information describes the state of an asynchronous application, and how to organize it in a single tree.
We’ll start with the most common use case: lists. Web applications often show lists of things. For example, a list of posts, or a list of friends. You’ll need to figure out what sorts of lists your app can show. You want to store them separately in the state, because this way you can cache them and only fetch again if necessary.
Here’s what the state shape for our “Reddit headlines” app might look like:
```js
{
selectedSubreddit: 'frontend',
postsBySubreddit: {
frontend: {
isFetching: true,
didInvalidate: false,
items: []
},
reactjs: {
isFetching: false,
didInvalidate: false,
lastUpdated: 1439478405547,
items: [
{
id: 42,
title: 'Confusion about Flux and Relay'
},
{
id: 500,
title: 'Creating a Simple Application Using React JS and Flux Architecture'
}
]
}
}
}
```
There are a few important bits here:
* We store each subreddit’s information separately so we can cache every subreddit. When the user switches between them the second time, the update will be instant, and we won’t need to refetch unless we want to. Don’t worry about all these items being in memory: unless you’re dealing with tens of thousands of items, and your user rarely closes the tab, you won’t need any sort of cleanup.
* For every list of items, you’ll want to store `isFetching` to show a spinner, `didInvalidate` so you can later toggle it when the data is stale, `lastUpdated` so you know when it was fetched the last time, and the `items` themselves. In a real app, you’ll also want to store pagination state like `fetchedPageCount` and `nextPageUrl`.
>##### Note on Nested Entities
>In this example, we store the received items together with the pagination information. However, this approach won’t work well if you have nested entities referencing each other, or if you let the user edit items. Imagine the user wants to edit a fetched post, but this post is duplicated in several places in the state tree. This would be really painful to implement.
>If you have nested entities, or if you let users edit received entities, you should keep them separately in the state as if it was a database. In pagination information, you would only refer to them by their IDs. This lets you always keep them up to date. The [real world example](../introduction/Examples.html#real-world) shows this approach, together with [normalizr](https://github.com/gaearon/normalizr) to normalize the nested API responses. With this approach, your state might look like this:
>```js
> {
> selectedSubreddit: 'frontend',
> entities: {
> users: {
> 2: {
> id: 2,
> name: 'Andrew'
> }
> },
> posts: {
> 42: {
> id: 42,
> title: 'Confusion about Flux and Relay',
> author: 2
> },
> 100: {
> id: 100,
> title: 'Creating a Simple Application Using React JS and Flux Architecture',
> author: 2
> }
> }
> },
> postsBySubreddit: {
> frontend: {
> isFetching: true,
> didInvalidate: false,
> items: []
> },
> reactjs: {
> isFetching: false,
> didInvalidate: false,
> lastUpdated: 1439478405547,
> items: [ 42, 100 ]
> }
> }
> }
>```
>In this guide, we won’t normalize entities, but it’s something you should consider for a more dynamic application.
## Handling Actions
Before going into the details of dispatching actions together with network requests, we will write the reducers for the actions we defined above.
>##### Note on Reducer Composition
> Here, we assume that you understand reducer composition with [`combineReducers()`](../api/combineReducers.md), as described in the [Splitting Reducers](../basics/Reducers.md#splitting-reducers) section on the [basics guide](../basics/README.md). If you don’t, please [read it first](../basics/Reducers.md#splitting-reducers).
#### `reducers.js`
```js
import { combineReducers } from 'redux'
import {
SELECT_SUBREDDIT, INVALIDATE_SUBREDDIT,
REQUEST_POSTS, RECEIVE_POSTS
} from '../actions'
function selectedSubreddit(state = 'reactjs', action) {
switch (action.type) {
case SELECT_SUBREDDIT:
return action.subreddit
default:
return state
}
}
function posts(state = {
isFetching: false,
didInvalidate: false,
items: []
}, action) {
switch (action.type) {
case INVALIDATE_SUBREDDIT:
return Object.assign({}, state, {
didInvalidate: true
})
case REQUEST_POSTS:
return Object.assign({}, state, {
isFetching: true,
didInvalidate: false
})
case RECEIVE_POSTS:
return Object.assign({}, state, {
isFetching: false,
didInvalidate: false,
items: action.posts,
lastUpdated: action.receivedAt
})
default:
return state
}
}
function postsBySubreddit(state = {}, action) {
switch (action.type) {
case INVALIDATE_SUBREDDIT:
case RECEIVE_POSTS:
case REQUEST_POSTS:
return Object.assign({}, state, {
[action.subreddit]: posts(state[action.subreddit], action)
})
default:
return state
}
}
const rootReducer = combineReducers({
postsBySubreddit,
selectedSubreddit
})
export default rootReducer
```
In this code, there are two interesting parts:
* We use ES6 computed property syntax so we can update `state[action.subreddit]` with `Object.assign()` in a terse way. This:
```js
return Object.assign({}, state, {
[action.subreddit]: posts(state[action.subreddit], action)
})
```
is equivalent to this:
```js
let nextState = {}
nextState[action.subreddit] = posts(state[action.subreddit], action)
return Object.assign({}, state, nextState)
```
* We extracted `posts(state, action)` that manages the state of a specific post list. This is just [reducer composition](../basics/Reducers.md#splitting-reducers)! It is our choice how to split the reducer into smaller reducers, and in this case, we’re delegating updating items inside an object to a `posts` reducer. The [real world example](../introduction/Examples.html#real-world) goes even further, showing how to create a reducer factory for parameterized pagination reducers.
Remember that reducers are just functions, so you can use functional composition and higher-order functions as much as you feel comfortable.
## Async Action Creators
Finally, how do we use the synchronous action creators we [defined earlier](#synchronous-action-creators) together with network requests? The standard way to do it with Redux is to use the [Redux Thunk middleware](https://github.com/gaearon/redux-thunk). It comes in a separate package called `redux-thunk`. We’ll explain how middleware works in general [later](Middleware.md); for now, there is just one important thing you need to know: by using this specific middleware, an action creator can return a function instead of an action object. This way, the action creator becomes a [thunk](https://en.wikipedia.org/wiki/Thunk).
When an action creator returns a function, that function will get executed by the Redux Thunk middleware. This function doesn’t need to be pure; it is thus allowed to have side effects, including executing asynchronous API calls. The function can also dispatch actions—like those synchronous actions we defined earlier.
We can still define these special thunk action creators inside our `actions.js` file:
#### `actions.js`
```js
import fetch from 'isomorphic-fetch'
export const REQUEST_POSTS = 'REQUEST_POSTS'
function requestPosts(subreddit) {
return {
type: REQUEST_POSTS,
subreddit
}
}
export const RECEIVE_POSTS = 'RECEIVE_POSTS'
function receivePosts(subreddit, json) {
return {
type: RECEIVE_POSTS,
subreddit,
posts: json.data.children.map(child => child.data),
receivedAt: Date.now()
}
}
// Meet our first thunk action creator!
// Though its insides are different, you would use it just like any other action creator:
// store.dispatch(fetchPosts('reactjs'))
export function fetchPosts(subreddit) {
// Thunk middleware knows how to handle functions.
// It passes the dispatch method as an argument to the function,
// thus making it able to dispatch actions itself.
return function (dispatch) {
// First dispatch: the app state is updated to inform
// that the API call is starting.
dispatch(requestPosts(subreddit))
// The function called by the thunk middleware can return a value,
// that is passed on as the return value of the dispatch method.
// In this case, we return a promise to wait for.
// This is not required by thunk middleware, but it is convenient for us.
return fetch(`http://www.reddit.com/r/${subreddit}.json`)
.then(response => response.json())
.then(json =>
// We can dispatch many times!
// Here, we update the app state with the results of the API call.
dispatch(receivePosts(subreddit, json))
)
// In a real world app, you also want to
// catch any error in the network call.
}
}
```
>##### Note on `fetch`
>We use [`fetch` API](https://developer.mozilla.org/en/docs/Web/API/Fetch_API) in the examples. It is a new API for making network requests that replaces `XMLHttpRequest` for most common needs. Because most browsers don’t yet support it natively, we suggest that you use [`isomorphic-fetch`](https://github.com/matthew-andrews/isomorphic-fetch) library:
>```js
// Do this in every file where you use `fetch`
>import fetch from 'isomorphic-fetch'
>```
>Internally, it uses [`whatwg-fetch` polyfill](https://github.com/github/fetch) on the client, and [`node-fetch`](https://github.com/bitinn/node-fetch) on the server, so you won’t need to change API calls if you change your app to be [universal](https://medium.com/@mjackson/universal-javascript-4761051b7ae9).
>Be aware that any `fetch` polyfill assumes a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) polyfill is already present. The easiest way to ensure you have a Promise polyfill is to enable Babel’s ES6 polyfill in your entry point before any other code runs:
>```js
>// Do this once before any other code in your app
>import 'babel-core/polyfill'
>```
How do we include the Redux Thunk middleware in the dispatch mechanism? We use the [`applyMiddleware()`](../api/applyMiddleware.md) store enhancer from Redux, as shown below:
#### `index.js`
```js
import thunkMiddleware from 'redux-thunk'
import createLogger from 'redux-logger'
import { createStore, applyMiddleware } from 'redux'
import { selectSubreddit, fetchPosts } from './actions'
import rootReducer from './reducers'
const loggerMiddleware = createLogger()
const store = createStore(
rootReducer,
applyMiddleware(
thunkMiddleware, // lets us dispatch() functions
loggerMiddleware // neat middleware that logs actions
)
)
store.dispatch(selectSubreddit('reactjs'))
store.dispatch(fetchPosts('reactjs')).then(() =>
console.log(store.getState())
)
```
The nice thing about thunks is that they can dispatch results of each other:
#### `actions.js`
```js
import fetch from 'isomorphic-fetch'
export const REQUEST_POSTS = 'REQUEST_POSTS'
function requestPosts(subreddit) {
return {
type: REQUEST_POSTS,
subreddit
}
}
export const RECEIVE_POSTS = 'RECEIVE_POSTS'
function receivePosts(subreddit, json) {
return {
type: RECEIVE_POSTS,
subreddit,
posts: json.data.children.map(child => child.data),
receivedAt: Date.now()
}
}
function fetchPosts(subreddit) {
return dispatch => {
dispatch(requestPosts(subreddit))
return fetch(`http://www.reddit.com/r/${subreddit}.json`)
.then(response => response.json())
.then(json => dispatch(receivePosts(subreddit, json)))
}
}
function shouldFetchPosts(state, subreddit) {
const posts = state.postsBySubreddit[subreddit]
if (!posts) {
return true
} else if (posts.isFetching) {
return false
} else {
return posts.didInvalidate
}
}
export function fetchPostsIfNeeded(subreddit) {
// Note that the function also receives getState()
// which lets you choose what to dispatch next.
// This is useful for avoiding a network request if
// a cached value is already available.
return (dispatch, getState) => {
if (shouldFetchPosts(getState(), subreddit)) {
// Dispatch a thunk from thunk!
return dispatch(fetchPosts(subreddit))
} else {
// Let the calling code know there's nothing to wait for.
return Promise.resolve()
}
}
}
```
This lets us write more sophisticated async control flow gradually, while the consuming code can stay pretty much the same:
#### `index.js`
```js
store.dispatch(fetchPostsIfNeeded('reactjs')).then(() =>
console.log(store.getState())
)
```
>##### Note about Server Rendering
>Async action creators are especially convenient for server rendering. You can create a store, dispatch a single async action creator that dispatches other async action creators to fetch data for a whole section of your app, and only render after the Promise it returns, completes. Then your store will already be hydrated with the state you need before rendering.
[Thunk middleware](https://github.com/gaearon/redux-thunk) isn’t the only way to orchestrate asynchronous actions in Redux. You can use [redux-promise](https://github.com/acdlite/redux-promise) or [redux-promise-middleware](https://github.com/pburtchaell/redux-promise-middleware) to dispatch Promises instead of functions. You can dispatch Observables with [redux-rx](https://github.com/acdlite/redux-rx). You can even write a custom middleware to describe calls to your API, like the [real world example](../introduction/Examples.html#real-world) does. It is up to you to try a few options, choose a convention you like, and follow it, whether with, or without the middleware.
## Connecting to UI
Dispatching async actions is no different from dispatching synchronous actions, so we won’t discuss this in detail. See [Usage with React](../basics/UsageWithReact.md) for an introduction into using Redux from React components. See [Example: Reddit API](ExampleRedditAPI.md) for the complete source code discussed in this example.
## Next Steps
Read [Async Flow](AsyncFlow.md) to recap how async actions fit into the Redux flow.
| docs/advanced/AsyncActions.md | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.00017976024537347257,
0.00017019231745507568,
0.0001585466816322878,
0.00017057292279787362,
0.000004127431111555779
] |
{
"id": 2,
"code_window": [
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n",
" \"prepublish\": \"npm run clean && npm run build && npm run check && node ./prepublish\",\n",
" \"docs:clean\": \"rimraf _book\",\n",
" \"docs:prepare\": \"gitbook install\",\n",
" \"docs:build\": \"npm run docs:prepare && gitbook build -g rackt/redux\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"prepublish\": \"npm run clean && npm run check:lib && npm run build && node ./prepublish\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 24
} | {
"name": "redux",
"version": "3.2.1",
"description": "Predictable state container for JavaScript apps",
"main": "lib/index.js",
"jsnext:main": "src/index.js",
"files": [
"dist",
"lib",
"src"
],
"scripts": {
"clean": "rimraf lib dist coverage",
"lint": "eslint src test examples",
"test": "mocha --compilers js:babel-register --recursive",
"test:watch": "npm test -- --watch",
"test:cov": "babel-node $(npm bin)/isparta cover $(npm bin)/_mocha -- --recursive",
"test:examples": "babel-node examples/testAll.js",
"check": "npm run lint && npm run test && npm run build:examples && npm run test:examples",
"build:lib": "babel src --out-dir lib",
"build:umd": "cross-env NODE_ENV=development webpack src/index.js dist/redux.js",
"build:umd:min": "cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js",
"build:examples": "babel-node examples/buildAll.js",
"build": "npm run build:lib && npm run build:umd && npm run build:umd:min",
"prepublish": "npm run clean && npm run build && npm run check && node ./prepublish",
"docs:clean": "rimraf _book",
"docs:prepare": "gitbook install",
"docs:build": "npm run docs:prepare && gitbook build -g rackt/redux",
"docs:watch": "npm run docs:prepare && gitbook serve",
"docs:publish": "npm run docs:clean && npm run docs:build && cp CNAME _book && cd _book && git init && git commit --allow-empty -m 'update book' && git checkout -b gh-pages && touch .nojekyll && git add . && git commit -am 'update book' && git push [email protected]:rackt/redux gh-pages --force"
},
"repository": {
"type": "git",
"url": "https://github.com/rackt/redux.git"
},
"keywords": [
"redux",
"reducer",
"state",
"predictable",
"functional",
"immutable",
"hot",
"live",
"replay",
"flux",
"elm"
],
"authors": [
"Dan Abramov <[email protected]> (https://github.com/gaearon)",
"Andrew Clark <[email protected]> (https://github.com/acdlite)"
],
"license": "MIT",
"bugs": {
"url": "https://github.com/rackt/redux/issues"
},
"homepage": "http://rackt.github.io/redux",
"dependencies": {
"lodash": "^4.2.0",
"loose-envify": "^1.1.0"
},
"devDependencies": {
"babel-cli": "^6.3.15",
"babel-core": "^6.3.15",
"babel-eslint": "^4.1.6",
"babel-loader": "^6.2.0",
"babel-plugin-check-es2015-constants": "^6.3.13",
"babel-plugin-transform-es2015-arrow-functions": "^6.3.13",
"babel-plugin-transform-es2015-block-scoped-functions": "^6.3.13",
"babel-plugin-transform-es2015-block-scoping": "^6.3.13",
"babel-plugin-transform-es2015-classes": "^6.3.13",
"babel-plugin-transform-es2015-computed-properties": "^6.3.13",
"babel-plugin-transform-es2015-destructuring": "^6.3.13",
"babel-plugin-transform-es2015-for-of": "^6.3.13",
"babel-plugin-transform-es2015-function-name": "^6.3.13",
"babel-plugin-transform-es2015-literals": "^6.3.13",
"babel-plugin-transform-es2015-modules-commonjs": "^6.3.13",
"babel-plugin-transform-es2015-object-super": "^6.3.13",
"babel-plugin-transform-es2015-parameters": "^6.3.13",
"babel-plugin-transform-es2015-shorthand-properties": "^6.3.13",
"babel-plugin-transform-es2015-spread": "^6.3.13",
"babel-plugin-transform-es2015-sticky-regex": "^6.3.13",
"babel-plugin-transform-es2015-template-literals": "^6.3.13",
"babel-plugin-transform-es2015-unicode-regex": "^6.3.13",
"babel-plugin-transform-object-rest-spread": "^6.3.13",
"babel-register": "^6.3.13",
"cross-env": "^1.0.7",
"es3ify": "^0.2.0",
"eslint": "^1.10.3",
"eslint-config-rackt": "^1.1.1",
"eslint-plugin-react": "^3.16.1",
"expect": "^1.8.0",
"gitbook-cli": "^0.3.4",
"glob": "^6.0.4",
"isparta": "^4.0.0",
"mocha": "^2.2.5",
"rimraf": "^2.3.4",
"webpack": "^1.9.6"
},
"npmName": "redux",
"npmFileMap": [
{
"basePath": "/dist/",
"files": [
"*.js"
]
}
],
"browserify": {
"transform": [
"loose-envify"
]
}
}
| package.json | 1 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.9969034790992737,
0.08324096351861954,
0.00016185169806703925,
0.00016704786685295403,
0.27547961473464966
] |
{
"id": 2,
"code_window": [
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n",
" \"prepublish\": \"npm run clean && npm run build && npm run check && node ./prepublish\",\n",
" \"docs:clean\": \"rimraf _book\",\n",
" \"docs:prepare\": \"gitbook install\",\n",
" \"docs:build\": \"npm run docs:prepare && gitbook build -g rackt/redux\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"prepublish\": \"npm run clean && npm run check:lib && npm run build && node ./prepublish\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 24
} | # EditorConfig helps developers define and maintain
# consistent coding styles between different editors and IDEs.
root = true
[*]
end_of_line = lf
charset = utf-8
trim_trailing_whitespace = true
insert_final_newline = true
indent_style = space
indent_size = 2
| .editorconfig | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.00017355587624479085,
0.00016824915655888617,
0.00016294245142489672,
0.00016824915655888617,
0.0000053067124099470675
] |
{
"id": 2,
"code_window": [
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n",
" \"prepublish\": \"npm run clean && npm run build && npm run check && node ./prepublish\",\n",
" \"docs:clean\": \"rimraf _book\",\n",
" \"docs:prepare\": \"gitbook install\",\n",
" \"docs:build\": \"npm run docs:prepare && gitbook build -g rackt/redux\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"prepublish\": \"npm run clean && npm run check:lib && npm run build && node ./prepublish\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 24
} | {
"name": "redux-shopping-cart-example",
"version": "0.0.0",
"description": "Redux shopping-cart example",
"scripts": {
"start": "node server.js"
},
"repository": {
"type": "git",
"url": "https://github.com/rackt/redux.git"
},
"license": "MIT",
"bugs": {
"url": "https://github.com/rackt/redux/issues"
},
"homepage": "http://rackt.github.io/redux",
"dependencies": {
"babel-polyfill": "^6.3.14",
"react": "^0.14.7",
"react-dom": "^0.14.7",
"react-redux": "^4.1.1",
"redux": "^3.1.2",
"redux-thunk": "^1.0.3"
},
"devDependencies": {
"babel-core": "^6.3.15",
"babel-loader": "^6.2.0",
"babel-preset-es2015": "^6.3.13",
"babel-preset-react": "^6.3.13",
"babel-preset-react-hmre": "^1.0.1",
"express": "^4.13.3",
"json-loader": "^0.5.3",
"redux-logger": "^2.0.1",
"webpack": "^1.9.11",
"webpack-dev-middleware": "^1.2.0",
"webpack-hot-middleware": "^2.2.0"
}
}
| examples/shopping-cart/package.json | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.00017121036944445223,
0.00016642414266243577,
0.00016269311890937388,
0.00016589651932008564,
0.000003632421567090205
] |
{
"id": 2,
"code_window": [
" \"build:umd\": \"cross-env NODE_ENV=development webpack src/index.js dist/redux.js\",\n",
" \"build:umd:min\": \"cross-env NODE_ENV=production webpack src/index.js dist/redux.min.js\",\n",
" \"build:examples\": \"babel-node examples/buildAll.js\",\n",
" \"build\": \"npm run build:lib && npm run build:umd && npm run build:umd:min\",\n",
" \"prepublish\": \"npm run clean && npm run build && npm run check && node ./prepublish\",\n",
" \"docs:clean\": \"rimraf _book\",\n",
" \"docs:prepare\": \"gitbook install\",\n",
" \"docs:build\": \"npm run docs:prepare && gitbook build -g rackt/redux\",\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" \"prepublish\": \"npm run clean && npm run check:lib && npm run build && node ./prepublish\",\n"
],
"file_path": "package.json",
"type": "replace",
"edit_start_line_idx": 24
} | var webpack = require('webpack')
var webpackDevMiddleware = require('webpack-dev-middleware')
var webpackHotMiddleware = require('webpack-hot-middleware')
var config = require('./webpack.config')
var app = new (require('express'))()
var port = 3000
var compiler = webpack(config)
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }))
app.use(webpackHotMiddleware(compiler))
app.get("/", function(req, res) {
res.sendFile(__dirname + '/index.html')
})
app.listen(port, function(error) {
if (error) {
console.error(error)
} else {
console.info("==> 🌎 Listening on port %s. Open up http://localhost:%s/ in your browser.", port, port)
}
})
| examples/async/server.js | 0 | https://github.com/reduxjs/redux/commit/2f11eafb628a908503f499fec2109a24efe96461 | [
0.00020953596686013043,
0.0001841873163357377,
0.00017148436745628715,
0.0001715416001388803,
0.000017924221538123675
] |
{
"id": 0,
"code_window": [
" renderList(iterable(), (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])\n",
" })\n",
"})"
],
"labels": [
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" it('should return empty array when source is undefined', () => {\n",
" expect(\n",
" renderList(undefined, (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual([])\n",
" })\n"
],
"file_path": "packages/runtime-core/__tests__/helpers/renderList.spec.ts",
"type": "add",
"edit_start_line_idx": 43
} | import { renderList } from '../../src/helpers/renderList'
describe('renderList', () => {
it('should render items in an array', () => {
expect(
renderList(['1', '2', '3'], (item, index) => `node ${index}: ${item}`)
).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])
})
it('should render characters of a string', () => {
expect(
renderList('123', (item, index) => `node ${index}: ${item}`)
).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])
})
it('should render integers 1 through N when given a number N', () => {
expect(renderList(3, (item, index) => `node ${index}: ${item}`)).toEqual([
'node 0: 1',
'node 1: 2',
'node 2: 3'
])
})
it('should render properties in an object', () => {
expect(
renderList(
{ a: 1, b: 2, c: 3 },
(item, key, index) => `node ${index}/${key}: ${item}`
)
).toEqual(['node 0/a: 1', 'node 1/b: 2', 'node 2/c: 3'])
})
it('should render an item for entry in an iterable', () => {
const iterable = function*() {
yield 1
yield 2
yield 3
}
expect(
renderList(iterable(), (item, index) => `node ${index}: ${item}`)
).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])
})
})
| packages/runtime-core/__tests__/helpers/renderList.spec.ts | 1 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.9969223141670227,
0.3463389277458191,
0.0013689518673345447,
0.22114704549312592,
0.3495386242866516
] |
{
"id": 0,
"code_window": [
" renderList(iterable(), (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])\n",
" })\n",
"})"
],
"labels": [
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" it('should return empty array when source is undefined', () => {\n",
" expect(\n",
" renderList(undefined, (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual([])\n",
" })\n"
],
"file_path": "packages/runtime-core/__tests__/helpers/renderList.spec.ts",
"type": "add",
"edit_start_line_idx": 43
} | import { ErrorCodes, callWithErrorHandling } from './errorHandling'
import { isArray } from '@vue/shared'
const queue: Function[] = []
const postFlushCbs: Function[] = []
const p = Promise.resolve()
let isFlushing = false
let isFlushPending = false
const RECURSION_LIMIT = 100
type CountMap = Map<Function, number>
export function nextTick(fn?: () => void): Promise<void> {
return fn ? p.then(fn) : p
}
export function queueJob(job: () => void) {
if (!queue.includes(job)) {
queue.push(job)
queueFlush()
}
}
export function queuePostFlushCb(cb: Function | Function[]) {
if (!isArray(cb)) {
postFlushCbs.push(cb)
} else {
postFlushCbs.push(...cb)
}
queueFlush()
}
function queueFlush() {
if (!isFlushing && !isFlushPending) {
isFlushPending = true
nextTick(flushJobs)
}
}
const dedupe = (cbs: Function[]): Function[] => [...new Set(cbs)]
export function flushPostFlushCbs(seen?: CountMap) {
if (postFlushCbs.length) {
const cbs = dedupe(postFlushCbs)
postFlushCbs.length = 0
if (__DEV__) {
seen = seen || new Map()
}
for (let i = 0; i < cbs.length; i++) {
if (__DEV__) {
checkRecursiveUpdates(seen!, cbs[i])
}
cbs[i]()
}
}
}
function flushJobs(seen?: CountMap) {
isFlushPending = false
isFlushing = true
let job
if (__DEV__) {
seen = seen || new Map()
}
while ((job = queue.shift())) {
if (__DEV__) {
checkRecursiveUpdates(seen!, job)
}
callWithErrorHandling(job, null, ErrorCodes.SCHEDULER)
}
flushPostFlushCbs(seen)
isFlushing = false
// some postFlushCb queued jobs!
// keep flushing until it drains.
if (queue.length || postFlushCbs.length) {
flushJobs(seen)
}
}
function checkRecursiveUpdates(seen: CountMap, fn: Function) {
if (!seen.has(fn)) {
seen.set(fn, 1)
} else {
const count = seen.get(fn)!
if (count > RECURSION_LIMIT) {
throw new Error(
'Maximum recursive updates exceeded. ' +
"You may have code that is mutating state in your component's " +
'render function or updated hook or watcher source function.'
)
} else {
seen.set(fn, count + 1)
}
}
}
| packages/runtime-core/src/scheduler.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.00017608625057619065,
0.0001720788422971964,
0.00016597418289165944,
0.00017249463417101651,
0.0000029461409667419503
] |
{
"id": 0,
"code_window": [
" renderList(iterable(), (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])\n",
" })\n",
"})"
],
"labels": [
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" it('should return empty array when source is undefined', () => {\n",
" expect(\n",
" renderList(undefined, (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual([])\n",
" })\n"
],
"file_path": "packages/runtime-core/__tests__/helpers/renderList.spec.ts",
"type": "add",
"edit_start_line_idx": 43
} | The MIT License (MIT)
Copyright (c) 2018-present, Yuxi (Evan) You
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
| packages/compiler-dom/LICENSE | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.00017550945631228387,
0.00017222300812136382,
0.00016879980103112757,
0.00017235975246876478,
0.00000274091144092381
] |
{
"id": 0,
"code_window": [
" renderList(iterable(), (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual(['node 0: 1', 'node 1: 2', 'node 2: 3'])\n",
" })\n",
"})"
],
"labels": [
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" it('should return empty array when source is undefined', () => {\n",
" expect(\n",
" renderList(undefined, (item, index) => `node ${index}: ${item}`)\n",
" ).toEqual([])\n",
" })\n"
],
"file_path": "packages/runtime-core/__tests__/helpers/renderList.spec.ts",
"type": "add",
"edit_start_line_idx": 43
} | import { isArray } from '@vue/shared'
import { TestElement } from './nodeOps'
export function triggerEvent(
el: TestElement,
event: string,
payload: any[] = []
) {
const { eventListeners } = el
if (eventListeners) {
const listener = eventListeners[event]
if (listener) {
if (isArray(listener)) {
for (let i = 0; i < listener.length; i++) {
listener[i](...payload)
}
} else {
listener(...payload)
}
}
}
}
| packages/runtime-test/src/triggerEvent.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.00017474866763222963,
0.0001742952736094594,
0.00017353668226860464,
0.00017460042727179825,
5.397980089583143e-7
] |
{
"id": 1,
"code_window": [
" for (let i = 0, l = keys.length; i < l; i++) {\n",
" const key = keys[i]\n",
" ret[i] = renderItem(source[key], key, i)\n",
" }\n",
" }\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
" } else {\n",
" ret = []\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "add",
"edit_start_line_idx": 33
} | import { VNodeChild } from '../vnode'
import { isArray, isString, isObject } from '@vue/shared'
export function renderList(
source: unknown,
renderItem: (
value: unknown,
key: string | number,
index?: number
) => VNodeChild
): VNodeChild[] {
let ret: VNodeChild[]
if (isArray(source) || isString(source)) {
ret = new Array(source.length)
for (let i = 0, l = source.length; i < l; i++) {
ret[i] = renderItem(source[i], i)
}
} else if (typeof source === 'number') {
ret = new Array(source)
for (let i = 0; i < source; i++) {
ret[i] = renderItem(i + 1, i)
}
} else if (isObject(source)) {
if (source[Symbol.iterator as any]) {
ret = Array.from(source as Iterable<any>, renderItem)
} else {
const keys = Object.keys(source)
ret = new Array(keys.length)
for (let i = 0, l = keys.length; i < l; i++) {
const key = keys[i]
ret[i] = renderItem(source[key], key, i)
}
}
}
return ret!
}
| packages/runtime-core/src/helpers/renderList.ts | 1 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.9977693557739258,
0.505369246006012,
0.0022429097443819046,
0.5107323527336121,
0.49214568734169006
] |
{
"id": 1,
"code_window": [
" for (let i = 0, l = keys.length; i < l; i++) {\n",
" const key = keys[i]\n",
" ret[i] = renderItem(source[key], key, i)\n",
" }\n",
" }\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
" } else {\n",
" ret = []\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "add",
"edit_start_line_idx": 33
} | import { UrlWithStringQuery, parse as uriParse } from 'url'
// TODO use imports instead.
// We need an extra transform context API for injecting arbitrary import
// statements.
export function urlToRequire(url: string): string {
const returnValue = `"${url}"`
const firstChar = url.charAt(0)
if (firstChar === '.' || firstChar === '~' || firstChar === '@') {
if (firstChar === '~') {
const secondChar = url.charAt(1)
url = url.slice(secondChar === '/' ? 2 : 1)
}
const uriParts = parseUriParts(url)
if (!uriParts.hash) {
return `require("${url}")`
} else {
// support uri fragment case by excluding it from
// the require and instead appending it as string;
// assuming that the path part is sufficient according to
// the above caseing(t.i. no protocol-auth-host parts expected)
return `require("${uriParts.path}") + "${uriParts.hash}"`
}
}
return returnValue
}
/**
* vuejs/component-compiler-utils#22 Support uri fragment in transformed require
* @param urlString an url as a string
*/
function parseUriParts(urlString: string): UrlWithStringQuery {
// initialize return value
const returnValue: UrlWithStringQuery = uriParse('')
if (urlString) {
// A TypeError is thrown if urlString is not a string
// @see https://nodejs.org/api/url.html#url_url_parse_urlstring_parsequerystring_slashesdenotehost
if ('string' === typeof urlString) {
// check is an uri
return uriParse(urlString) // take apart the uri
}
}
return returnValue
}
| packages/compiler-sfc/src/templateUtils.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.0001752497482812032,
0.00017311828560195863,
0.00017117446986958385,
0.00017223061877302825,
0.0000016596725345152663
] |
{
"id": 1,
"code_window": [
" for (let i = 0, l = keys.length; i < l; i++) {\n",
" const key = keys[i]\n",
" ret[i] = renderItem(source[key], key, i)\n",
" }\n",
" }\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
" } else {\n",
" ret = []\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "add",
"edit_start_line_idx": 33
} | import { reactive, isReactive, effect, toRaw } from '../../src'
describe('reactivity/collections', () => {
describe('WeakSet', () => {
it('instanceof', () => {
const original = new WeakSet()
const observed = reactive(original)
expect(isReactive(observed)).toBe(true)
expect(original instanceof WeakSet).toBe(true)
expect(observed instanceof WeakSet).toBe(true)
})
it('should observe mutations', () => {
let dummy
const value = {}
const set = reactive(new WeakSet())
effect(() => (dummy = set.has(value)))
expect(dummy).toBe(false)
set.add(value)
expect(dummy).toBe(true)
set.delete(value)
expect(dummy).toBe(false)
})
it('should not observe custom property mutations', () => {
let dummy
const set: any = reactive(new WeakSet())
effect(() => (dummy = set.customProp))
expect(dummy).toBe(undefined)
set.customProp = 'Hello World'
expect(dummy).toBe(undefined)
})
it('should not observe non value changing mutations', () => {
let dummy
const value = {}
const set = reactive(new WeakSet())
const setSpy = jest.fn(() => (dummy = set.has(value)))
effect(setSpy)
expect(dummy).toBe(false)
expect(setSpy).toHaveBeenCalledTimes(1)
set.add(value)
expect(dummy).toBe(true)
expect(setSpy).toHaveBeenCalledTimes(2)
set.add(value)
expect(dummy).toBe(true)
expect(setSpy).toHaveBeenCalledTimes(2)
set.delete(value)
expect(dummy).toBe(false)
expect(setSpy).toHaveBeenCalledTimes(3)
set.delete(value)
expect(dummy).toBe(false)
expect(setSpy).toHaveBeenCalledTimes(3)
})
it('should not observe raw data', () => {
const value = {}
let dummy
const set = reactive(new WeakSet())
effect(() => (dummy = toRaw(set).has(value)))
expect(dummy).toBe(false)
set.add(value)
expect(dummy).toBe(false)
})
it('should not be triggered by raw mutations', () => {
const value = {}
let dummy
const set = reactive(new WeakSet())
effect(() => (dummy = set.has(value)))
expect(dummy).toBe(false)
toRaw(set).add(value)
expect(dummy).toBe(false)
})
it('should not pollute original Set with Proxies', () => {
const set = new WeakSet()
const observed = reactive(set)
const value = reactive({})
observed.add(value)
expect(observed.has(value)).toBe(true)
expect(set.has(value)).toBe(false)
})
})
})
| packages/reactivity/__tests__/collections/WeakSet.spec.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.0001792404509615153,
0.0001772538962541148,
0.00017377728363499045,
0.00017739861505106091,
0.0000015411253571073757
] |
{
"id": 1,
"code_window": [
" for (let i = 0, l = keys.length; i < l; i++) {\n",
" const key = keys[i]\n",
" ret[i] = renderItem(source[key], key, i)\n",
" }\n",
" }\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
" } else {\n",
" ret = []\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "add",
"edit_start_line_idx": 33
} | import { NodeTransform, TransformContext } from '../transform'
import {
NodeTypes,
ElementTypes,
CallExpression,
ObjectExpression,
ElementNode,
DirectiveNode,
ExpressionNode,
ArrayExpression,
createCallExpression,
createArrayExpression,
createObjectProperty,
createSimpleExpression,
createObjectExpression,
Property
} from '../ast'
import { PatchFlags, PatchFlagNames, isSymbol, hyphenate } from '@vue/shared'
import { createCompilerError, ErrorCodes } from '../errors'
import {
CREATE_VNODE,
WITH_DIRECTIVES,
RESOLVE_DIRECTIVE,
RESOLVE_COMPONENT,
RESOLVE_DYNAMIC_COMPONENT,
MERGE_PROPS,
TO_HANDLERS,
PORTAL,
SUSPENSE,
KEEP_ALIVE,
TRANSITION
} from '../runtimeHelpers'
import { getInnerRange, isVSlot, toValidAssetId, findProp } from '../utils'
import { buildSlots } from './vSlot'
import { isStaticNode } from './hoistStatic'
// some directive transforms (e.g. v-model) may return a symbol for runtime
// import, which should be used instead of a resolveDirective call.
const directiveImportMap = new WeakMap<DirectiveNode, symbol>()
const isBuiltInType = (tag: string, expected: string): boolean =>
tag === expected || tag === hyphenate(expected)
// generate a JavaScript AST for this element's codegen
export const transformElement: NodeTransform = (node, context) => {
if (
node.type !== NodeTypes.ELEMENT ||
// handled by transformSlotOutlet
node.tagType === ElementTypes.SLOT ||
// <template v-if/v-for> should have already been replaced
// <templte v-slot> is handled by buildSlots
(node.tagType === ElementTypes.TEMPLATE && node.props.some(isVSlot))
) {
return
}
// perform the work on exit, after all child expressions have been
// processed and merged.
return function postTransformElement() {
const { tag, tagType, props } = node
const isPortal = isBuiltInType(tag, 'Portal')
const isSuspense = isBuiltInType(tag, 'Suspense')
const isKeepAlive = isBuiltInType(tag, 'KeepAlive')
const isTransition = isBuiltInType(tag, 'Transition')
const isComponent = tagType === ElementTypes.COMPONENT
let hasProps = props.length > 0
let patchFlag: number = 0
let runtimeDirectives: DirectiveNode[] | undefined
let dynamicPropNames: string[] | undefined
let dynamicComponent: string | CallExpression | undefined
// handle dynamic component
const isProp = findProp(node, 'is')
if (tag === 'component') {
if (isProp) {
// static <component is="foo" />
if (isProp.type === NodeTypes.ATTRIBUTE) {
const tag = isProp.value && isProp.value.content
if (tag) {
context.helper(RESOLVE_COMPONENT)
context.components.add(tag)
dynamicComponent = toValidAssetId(tag, `component`)
}
}
// dynamic <component :is="asdf" />
else if (isProp.exp) {
dynamicComponent = createCallExpression(
context.helper(RESOLVE_DYNAMIC_COMPONENT),
// _ctx.$ exposes the owner instance of current render function
[isProp.exp, context.prefixIdentifiers ? `_ctx.$` : `$`]
)
}
}
}
let nodeType
if (dynamicComponent) {
nodeType = dynamicComponent
} else if (isPortal) {
nodeType = context.helper(PORTAL)
} else if (isSuspense) {
nodeType = context.helper(SUSPENSE)
} else if (isKeepAlive) {
nodeType = context.helper(KEEP_ALIVE)
} else if (isTransition) {
nodeType = context.helper(TRANSITION)
} else if (isComponent) {
// user component w/ resolve
context.helper(RESOLVE_COMPONENT)
context.components.add(tag)
nodeType = toValidAssetId(tag, `component`)
} else {
// plain element
nodeType = `"${node.tag}"`
}
const args: CallExpression['arguments'] = [nodeType]
// props
if (hasProps) {
const propsBuildResult = buildProps(
node,
context,
// skip reserved "is" prop <component is>
isProp ? node.props.filter(p => p !== isProp) : node.props
)
patchFlag = propsBuildResult.patchFlag
dynamicPropNames = propsBuildResult.dynamicPropNames
runtimeDirectives = propsBuildResult.directives
if (!propsBuildResult.props) {
hasProps = false
} else {
args.push(propsBuildResult.props)
}
}
// children
const hasChildren = node.children.length > 0
if (hasChildren) {
if (!hasProps) {
args.push(`null`)
}
// Portal & KeepAlive should have normal children instead of slots
// Portal is not a real component has dedicated handling in the renderer
// KeepAlive should not track its own deps so that it can be used inside
// Transition
if (isComponent && !isPortal && !isKeepAlive) {
const { slots, hasDynamicSlots } = buildSlots(node, context)
args.push(slots)
if (hasDynamicSlots) {
patchFlag |= PatchFlags.DYNAMIC_SLOTS
}
} else if (node.children.length === 1) {
const child = node.children[0]
const type = child.type
// check for dynamic text children
const hasDynamicTextChild =
type === NodeTypes.INTERPOLATION ||
type === NodeTypes.COMPOUND_EXPRESSION
if (hasDynamicTextChild && !isStaticNode(child)) {
patchFlag |= PatchFlags.TEXT
}
// pass directly if the only child is a text node
// (plain / interpolation / expression)
if (hasDynamicTextChild || type === NodeTypes.TEXT) {
args.push(child)
} else {
args.push(node.children)
}
} else {
args.push(node.children)
}
}
// patchFlag & dynamicPropNames
if (patchFlag !== 0) {
if (!hasChildren) {
if (!hasProps) {
args.push(`null`)
}
args.push(`null`)
}
if (__DEV__) {
const flagNames = Object.keys(PatchFlagNames)
.map(Number)
.filter(n => n > 0 && patchFlag & n)
.map(n => PatchFlagNames[n])
.join(`, `)
args.push(patchFlag + ` /* ${flagNames} */`)
} else {
args.push(patchFlag + '')
}
if (dynamicPropNames && dynamicPropNames.length) {
args.push(stringifyDynamicPropNames(dynamicPropNames))
}
}
const { loc } = node
const vnode = createCallExpression(context.helper(CREATE_VNODE), args, loc)
if (runtimeDirectives && runtimeDirectives.length) {
node.codegenNode = createCallExpression(
context.helper(WITH_DIRECTIVES),
[
vnode,
createArrayExpression(
runtimeDirectives.map(dir => buildDirectiveArgs(dir, context)),
loc
)
],
loc
)
} else {
node.codegenNode = vnode
}
}
}
function stringifyDynamicPropNames(props: string[]): string {
let propsNamesString = `[`
for (let i = 0, l = props.length; i < l; i++) {
propsNamesString += JSON.stringify(props[i])
if (i < l - 1) propsNamesString += ', '
}
return propsNamesString + `]`
}
export type PropsExpression = ObjectExpression | CallExpression | ExpressionNode
export function buildProps(
node: ElementNode,
context: TransformContext,
props: ElementNode['props'] = node.props
): {
props: PropsExpression | undefined
directives: DirectiveNode[]
patchFlag: number
dynamicPropNames: string[]
} {
const elementLoc = node.loc
const isComponent = node.tagType === ElementTypes.COMPONENT
let properties: ObjectExpression['properties'] = []
const mergeArgs: PropsExpression[] = []
const runtimeDirectives: DirectiveNode[] = []
// patchFlag analysis
let patchFlag = 0
let hasRef = false
let hasClassBinding = false
let hasStyleBinding = false
let hasDynamicKeys = false
const dynamicPropNames: string[] = []
const analyzePatchFlag = ({ key, value }: Property) => {
if (key.type === NodeTypes.SIMPLE_EXPRESSION && key.isStatic) {
if (
value.type === NodeTypes.JS_CACHE_EXPRESSION ||
((value.type === NodeTypes.SIMPLE_EXPRESSION ||
value.type === NodeTypes.COMPOUND_EXPRESSION) &&
isStaticNode(value))
) {
return
}
const name = key.content
if (name === 'ref') {
hasRef = true
} else if (name === 'class') {
hasClassBinding = true
} else if (name === 'style') {
hasStyleBinding = true
} else if (name !== 'key') {
dynamicPropNames.push(name)
}
} else {
hasDynamicKeys = true
}
}
for (let i = 0; i < props.length; i++) {
// static attribute
const prop = props[i]
if (prop.type === NodeTypes.ATTRIBUTE) {
const { loc, name, value } = prop
if (name === 'ref') {
hasRef = true
}
properties.push(
createObjectProperty(
createSimpleExpression(
name,
true,
getInnerRange(loc, 0, name.length)
),
createSimpleExpression(
value ? value.content : '',
true,
value ? value.loc : loc
)
)
)
} else {
// directives
const { name, arg, exp, loc } = prop
// skip v-slot - it is handled by its dedicated transform.
if (name === 'slot') {
if (!isComponent) {
context.onError(
createCompilerError(ErrorCodes.X_V_SLOT_MISPLACED, loc)
)
}
continue
}
// skip v-once - it is handled by its dedicated transform.
if (name === 'once') {
continue
}
// special case for v-bind and v-on with no argument
const isBind = name === 'bind'
const isOn = name === 'on'
if (!arg && (isBind || isOn)) {
hasDynamicKeys = true
if (exp) {
if (properties.length) {
mergeArgs.push(
createObjectExpression(dedupeProperties(properties), elementLoc)
)
properties = []
}
if (isBind) {
mergeArgs.push(exp)
} else {
// v-on="obj" -> toHandlers(obj)
mergeArgs.push({
type: NodeTypes.JS_CALL_EXPRESSION,
loc,
callee: context.helper(TO_HANDLERS),
arguments: [exp]
})
}
} else {
context.onError(
createCompilerError(
isBind
? ErrorCodes.X_V_BIND_NO_EXPRESSION
: ErrorCodes.X_V_ON_NO_EXPRESSION,
loc
)
)
}
continue
}
const directiveTransform = context.directiveTransforms[name]
if (directiveTransform) {
// has built-in directive transform.
const { props, needRuntime } = directiveTransform(prop, node, context)
props.forEach(analyzePatchFlag)
properties.push(...props)
if (needRuntime) {
runtimeDirectives.push(prop)
if (isSymbol(needRuntime)) {
directiveImportMap.set(prop, needRuntime)
}
}
} else {
// no built-in transform, this is a user custom directive.
runtimeDirectives.push(prop)
}
}
}
let propsExpression: PropsExpression | undefined = undefined
// has v-bind="object" or v-on="object", wrap with mergeProps
if (mergeArgs.length) {
if (properties.length) {
mergeArgs.push(
createObjectExpression(dedupeProperties(properties), elementLoc)
)
}
if (mergeArgs.length > 1) {
propsExpression = createCallExpression(
context.helper(MERGE_PROPS),
mergeArgs,
elementLoc
)
} else {
// single v-bind with nothing else - no need for a mergeProps call
propsExpression = mergeArgs[0]
}
} else if (properties.length) {
propsExpression = createObjectExpression(
dedupeProperties(properties),
elementLoc
)
}
// patchFlag analysis
if (hasDynamicKeys) {
patchFlag |= PatchFlags.FULL_PROPS
} else {
if (hasClassBinding) {
patchFlag |= PatchFlags.CLASS
}
if (hasStyleBinding) {
patchFlag |= PatchFlags.STYLE
}
if (dynamicPropNames.length) {
patchFlag |= PatchFlags.PROPS
}
}
if (patchFlag === 0 && (hasRef || runtimeDirectives.length > 0)) {
patchFlag |= PatchFlags.NEED_PATCH
}
return {
props: propsExpression,
directives: runtimeDirectives,
patchFlag,
dynamicPropNames
}
}
// Dedupe props in an object literal.
// Literal duplicated attributes would have been warned during the parse phase,
// however, it's possible to encounter duplicated `onXXX` handlers with different
// modifiers. We also need to merge static and dynamic class / style attributes.
// - onXXX handlers / style: merge into array
// - class: merge into single expression with concatenation
function dedupeProperties(properties: Property[]): Property[] {
const knownProps: Map<string, Property> = new Map()
const deduped: Property[] = []
for (let i = 0; i < properties.length; i++) {
const prop = properties[i]
// dynamic keys are always allowed
if (prop.key.type === NodeTypes.COMPOUND_EXPRESSION || !prop.key.isStatic) {
deduped.push(prop)
continue
}
const name = prop.key.content
const existing = knownProps.get(name)
if (existing) {
if (
name === 'style' ||
name === 'class' ||
name.startsWith('on') ||
name.startsWith('vnode')
) {
mergeAsArray(existing, prop)
}
// unexpected duplicate, should have emitted error during parse
} else {
knownProps.set(name, prop)
deduped.push(prop)
}
}
return deduped
}
function mergeAsArray(existing: Property, incoming: Property) {
if (existing.value.type === NodeTypes.JS_ARRAY_EXPRESSION) {
existing.value.elements.push(incoming.value)
} else {
existing.value = createArrayExpression(
[existing.value, incoming.value],
existing.loc
)
}
}
function buildDirectiveArgs(
dir: DirectiveNode,
context: TransformContext
): ArrayExpression {
const dirArgs: ArrayExpression['elements'] = []
const runtime = directiveImportMap.get(dir)
if (runtime) {
context.helper(runtime)
dirArgs.push(context.helperString(runtime))
} else {
// inject statement for resolving directive
context.helper(RESOLVE_DIRECTIVE)
context.directives.add(dir.name)
dirArgs.push(toValidAssetId(dir.name, `directive`))
}
const { loc } = dir
if (dir.exp) dirArgs.push(dir.exp)
if (dir.arg) {
if (!dir.exp) {
dirArgs.push(`void 0`)
}
dirArgs.push(dir.arg)
}
if (Object.keys(dir.modifiers).length) {
if (!dir.arg) {
if (!dir.exp) {
dirArgs.push(`void 0`)
}
dirArgs.push(`void 0`)
}
const trueExpression = createSimpleExpression(`true`, false, loc)
dirArgs.push(
createObjectExpression(
dir.modifiers.map(modifier =>
createObjectProperty(modifier, trueExpression)
),
loc
)
)
}
return createArrayExpression(dirArgs, dir.loc)
}
| packages/compiler-core/src/transforms/transformElement.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.002915708115324378,
0.0003075720160268247,
0.00016528955893591046,
0.0001741178857628256,
0.0005497217061929405
] |
{
"id": 2,
"code_window": [
" }\n",
" return ret!\n",
"}"
],
"labels": [
"keep",
"replace",
"keep"
],
"after_edit": [
" return ret\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "replace",
"edit_start_line_idx": 34
} | import { VNodeChild } from '../vnode'
import { isArray, isString, isObject } from '@vue/shared'
export function renderList(
source: unknown,
renderItem: (
value: unknown,
key: string | number,
index?: number
) => VNodeChild
): VNodeChild[] {
let ret: VNodeChild[]
if (isArray(source) || isString(source)) {
ret = new Array(source.length)
for (let i = 0, l = source.length; i < l; i++) {
ret[i] = renderItem(source[i], i)
}
} else if (typeof source === 'number') {
ret = new Array(source)
for (let i = 0; i < source; i++) {
ret[i] = renderItem(i + 1, i)
}
} else if (isObject(source)) {
if (source[Symbol.iterator as any]) {
ret = Array.from(source as Iterable<any>, renderItem)
} else {
const keys = Object.keys(source)
ret = new Array(keys.length)
for (let i = 0, l = keys.length; i < l; i++) {
const key = keys[i]
ret[i] = renderItem(source[key], key, i)
}
}
}
return ret!
}
| packages/runtime-core/src/helpers/renderList.ts | 1 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.8535976409912109,
0.21735668182373047,
0.0001677651598583907,
0.007830670103430748,
0.3673502504825592
] |
{
"id": 2,
"code_window": [
" }\n",
" return ret!\n",
"}"
],
"labels": [
"keep",
"replace",
"keep"
],
"after_edit": [
" return ret\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "replace",
"edit_start_line_idx": 34
} | {
"extends": "../../api-extractor.json",
"mainEntryPointFilePath": "./dist/packages/<unscopedPackageName>/src/index.d.ts",
"dtsRollup": {
"untrimmedFilePath": "./dist/<unscopedPackageName>.d.ts"
}
}
| packages/runtime-core/api-extractor.json | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.00016855790454428643,
0.00016855790454428643,
0.00016855790454428643,
0.00016855790454428643,
0
] |
{
"id": 2,
"code_window": [
" }\n",
" return ret!\n",
"}"
],
"labels": [
"keep",
"replace",
"keep"
],
"after_edit": [
" return ret\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "replace",
"edit_start_line_idx": 34
} | import { ComponentInternalInstance, currentInstance } from './component'
import { VNode, NormalizedChildren, normalizeVNode, VNodeChild } from './vnode'
import { isArray, isFunction, EMPTY_OBJ } from '@vue/shared'
import { ShapeFlags } from './shapeFlags'
import { warn } from './warning'
import { isKeepAlive } from './components/KeepAlive'
export type Slot = (...args: any[]) => VNode[]
export type InternalSlots = {
[name: string]: Slot
}
export type Slots = Readonly<InternalSlots>
export type RawSlots = {
[name: string]: unknown
// manual render fn hint to skip forced children updates
$stable?: boolean
// internal, indicates compiler generated slots = can skip normalization
_compiled?: boolean
}
const normalizeSlotValue = (value: unknown): VNode[] =>
isArray(value)
? value.map(normalizeVNode)
: [normalizeVNode(value as VNodeChild)]
const normalizeSlot = (key: string, rawSlot: Function): Slot => (
props: any
) => {
if (__DEV__ && currentInstance != null) {
warn(
`Slot "${key}" invoked outside of the render function: ` +
`this will not track dependencies used in the slot. ` +
`Invoke the slot function inside the render function instead.`
)
}
return normalizeSlotValue(rawSlot(props))
}
export function resolveSlots(
instance: ComponentInternalInstance,
children: NormalizedChildren
) {
let slots: InternalSlots | void
if (instance.vnode.shapeFlag & ShapeFlags.SLOTS_CHILDREN) {
const rawSlots = children as RawSlots
if (rawSlots._compiled) {
// pre-normalized slots object generated by compiler
slots = children as Slots
} else {
slots = {}
for (const key in rawSlots) {
if (key === '$stable') continue
const value = rawSlots[key]
if (isFunction(value)) {
slots[key] = normalizeSlot(key, value)
} else if (value != null) {
if (__DEV__) {
warn(
`Non-function value encountered for slot "${key}". ` +
`Prefer function slots for better performance.`
)
}
const normalized = normalizeSlotValue(value)
slots[key] = () => normalized
}
}
}
} else if (children !== null) {
// non slot object children (direct value) passed to a component
if (__DEV__ && !isKeepAlive(instance.vnode)) {
warn(
`Non-function value encountered for default slot. ` +
`Prefer function slots for better performance.`
)
}
const normalized = normalizeSlotValue(children)
slots = { default: () => normalized }
}
instance.slots = slots || EMPTY_OBJ
}
| packages/runtime-core/src/componentSlots.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.00021570370881818235,
0.00017346517415717244,
0.00016583973774686456,
0.00016831762332003564,
0.000015008774425950833
] |
{
"id": 2,
"code_window": [
" }\n",
" return ret!\n",
"}"
],
"labels": [
"keep",
"replace",
"keep"
],
"after_edit": [
" return ret\n"
],
"file_path": "packages/runtime-core/src/helpers/renderList.ts",
"type": "replace",
"edit_start_line_idx": 34
} | import { isString, hyphenate, capitalize } from '@vue/shared'
import { camelize } from '@vue/runtime-core'
type Style = string | Partial<CSSStyleDeclaration> | null
export function patchStyle(el: Element, prev: Style, next: Style) {
const style = (el as HTMLElement).style
if (!next) {
el.removeAttribute('style')
} else if (isString(next)) {
style.cssText = next
} else {
for (const key in next) {
setStyle(style, key, next[key] as string)
}
if (prev && !isString(prev)) {
for (const key in prev) {
if (!next[key]) {
setStyle(style, key, '')
}
}
}
}
}
const importantRE = /\s*!important$/
function setStyle(style: CSSStyleDeclaration, name: string, val: string) {
if (name.startsWith('--')) {
// custom property definition
style.setProperty(name, val)
} else {
const prefixed = autoPrefix(style, name)
if (importantRE.test(val)) {
// !important
style.setProperty(
hyphenate(prefixed),
val.replace(importantRE, ''),
'important'
)
} else {
style[prefixed as any] = val
}
}
}
const prefixes = ['Webkit', 'Moz', 'ms']
const prefixCache: Record<string, string> = {}
function autoPrefix(style: CSSStyleDeclaration, rawName: string): string {
const cached = prefixCache[rawName]
if (cached) {
return cached
}
let name = camelize(rawName)
if (name !== 'filter' && name in style) {
return (prefixCache[rawName] = name)
}
name = capitalize(name)
for (let i = 0; i < prefixes.length; i++) {
const prefixed = prefixes[i] + name
if (prefixed in style) {
return (prefixCache[rawName] = prefixed)
}
}
return rawName
}
| packages/runtime-dom/src/modules/style.ts | 0 | https://github.com/vuejs/core/commit/d4f4c7c4d48f64f093a0204e83097eaf01936d25 | [
0.00019360303122084588,
0.00017540434782858938,
0.00016839902673382312,
0.00016943964874371886,
0.00000978468688117573
] |
{
"id": 0,
"code_window": [
"import riotGenerator from './generators/RIOT';\n",
"import preactGenerator from './generators/PREACT';\n",
"import svelteGenerator from './generators/SVELTE';\n",
"import raxGenerator from './generators/RAX';\n",
"import { warn } from './warn';\n",
"\n",
"const logger = console;\n",
"\n",
"type CommandOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { JsPackageManagerFactory } from './js-package-manager/JsPackageManagerFactory';\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "add",
"edit_start_line_idx": 38
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.9978173971176147,
0.17243745923042297,
0.00016615487402305007,
0.0009596741292625666,
0.3466745615005493
] |
{
"id": 0,
"code_window": [
"import riotGenerator from './generators/RIOT';\n",
"import preactGenerator from './generators/PREACT';\n",
"import svelteGenerator from './generators/SVELTE';\n",
"import raxGenerator from './generators/RAX';\n",
"import { warn } from './warn';\n",
"\n",
"const logger = console;\n",
"\n",
"type CommandOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { JsPackageManagerFactory } from './js-package-manager/JsPackageManagerFactory';\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "add",
"edit_start_line_idx": 38
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`storiesof-to-csf transforms correctly using "export-destructuring.input.js" data 1`] = `
"/* eslint-disable import/no-extraneous-dependencies */
import React from 'react';
import ComponentRow from './ComponentRow';
import * as SpecRowStories from './SpecRow.stories';
export const { actions } = SpecRowStories;
export default {
title: 'ComponentRow',
excludeStories: ['actions'],
};
export const Pending = () => (
<ComponentRow snapshots={snapshots.pending} buildNumber={2} {...actions} />
);
Pending.story = {
name: 'pending',
};"
`;
| lib/codemod/src/transforms/__testfixtures__/storiesof-to-csf/export-destructuring.output.snapshot | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017315437435172498,
0.00017221270536538213,
0.0001712954544927925,
0.00017218825814779848,
7.590975883431383e-7
] |
{
"id": 0,
"code_window": [
"import riotGenerator from './generators/RIOT';\n",
"import preactGenerator from './generators/PREACT';\n",
"import svelteGenerator from './generators/SVELTE';\n",
"import raxGenerator from './generators/RAX';\n",
"import { warn } from './warn';\n",
"\n",
"const logger = console;\n",
"\n",
"type CommandOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { JsPackageManagerFactory } from './js-package-manager/JsPackageManagerFactory';\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "add",
"edit_start_line_idx": 38
} | <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" id="Layer_1" x="0" y="0" enable-background="new 0 0 320.1 99.9" version="1.1" viewBox="0 0 320.1 99.9" xml:space="preserve"><path fill="#FF5A5F" d="M168.7,25.1c0,3.6-2.9,6.5-6.5,6.5s-6.5-2.9-6.5-6.5s2.8-6.5,6.5-6.5C165.9,18.7,168.7,21.6,168.7,25.1z M141.9,38.2c0,0.6,0,1.6,0,1.6s-3.1-4-9.7-4c-10.9,0-19.4,8.3-19.4,19.8c0,11.4,8.4,19.8,19.4,19.8c6.7,0,9.7-4.1,9.7-4.1v1.7 c0,0.8,0.6,1.4,1.4,1.4h8.1V36.8c0,0-7.4,0-8.1,0C142.5,36.8,141.9,37.5,141.9,38.2z M141.9,62.3c-1.5,2.2-4.5,4.1-8.1,4.1 c-6.4,0-11.3-4-11.3-10.8s4.9-10.8,11.3-10.8c3.5,0,6.7,2,8.1,4.1V62.3z M157.4,36.8h9.6v37.6h-9.6V36.8z M300.8,35.8 c-6.6,0-9.7,4-9.7,4V18.7h-9.6v55.7c0,0,7.4,0,8.1,0c0.8,0,1.4-0.7,1.4-1.4v-1.7l0,0c0,0,3.1,4.1,9.7,4.1c10.9,0,19.4-8.4,19.4-19.8 C320.1,44.2,311.6,35.8,300.8,35.8z M299.2,66.3c-3.7,0-6.6-1.9-8.1-4.1V48.8c1.5-2,4.7-4.1,8.1-4.1c6.4,0,11.3,4,11.3,10.8 S305.6,66.3,299.2,66.3z M276.5,52.1v22.4h-9.6V53.2c0-6.2-2-8.7-7.4-8.7c-2.9,0-5.9,1.5-7.8,3.7v26.2h-9.6V36.8h7.6 c0.8,0,1.4,0.7,1.4,1.4v1.6c2.8-2.9,6.5-4,10.2-4c4.2,0,7.7,1.2,10.5,3.6C275.2,42.2,276.5,45.8,276.5,52.1z M218.8,35.8 c-6.6,0-9.7,4-9.7,4V18.7h-9.6v55.7c0,0,7.4,0,8.1,0c0.8,0,1.4-0.7,1.4-1.4v-1.7l0,0c0,0,3.1,4.1,9.7,4.1c10.9,0,19.4-8.4,19.4-19.8 C238.2,44.2,229.7,35.8,218.8,35.8z M217.2,66.3c-3.7,0-6.6-1.9-8.1-4.1V48.8c1.5-2,4.7-4.1,8.1-4.1c6.4,0,11.3,4,11.3,10.8 S223.6,66.3,217.2,66.3z M191.2,35.8c2.9,0,4.4,0.5,4.4,0.5v8.9c0,0-8-2.7-13,3v26.3h-9.6V36.8c0,0,7.4,0,8.1,0 c0.8,0,1.4,0.7,1.4,1.4v1.6C184.3,37.7,188.2,35.8,191.2,35.8z M91.5,71c-0.5-1.2-1-2.5-1.5-3.6c-0.8-1.8-1.6-3.5-2.3-5.1l-0.1-0.1 c-6.9-15-14.3-30.2-22.1-45.2l-0.3-0.6c-0.8-1.5-1.6-3.1-2.4-4.7c-1-1.8-2-3.7-3.6-5.5C56,2.2,51.4,0,46.5,0c-5,0-9.5,2.2-12.8,6 c-1.5,1.8-2.6,3.7-3.6,5.5c-0.8,1.6-1.6,3.2-2.4,4.7l-0.3,0.6C19.7,31.8,12.2,47,5.3,62l-0.1,0.2c-0.7,1.6-1.5,3.3-2.3,5.1 c-0.5,1.1-1,2.3-1.5,3.6c-1.3,3.7-1.7,7.2-1.2,10.8c1.1,7.5,6.1,13.8,13,16.6c2.6,1.1,5.3,1.6,8.1,1.6c0.8,0,1.8-0.1,2.6-0.2 c3.3-0.4,6.7-1.5,10-3.4c4.1-2.3,8-5.6,12.4-10.4c4.4,4.8,8.4,8.1,12.4,10.4c3.3,1.9,6.7,3,10,3.4c0.8,0.1,1.8,0.2,2.6,0.2 c2.8,0,5.6-0.5,8.1-1.6c7-2.8,11.9-9.2,13-16.6C93.2,78.2,92.8,74.7,91.5,71z M46.4,76.2c-5.4-6.8-8.9-13.2-10.1-18.6 c-0.5-2.3-0.6-4.3-0.3-6.1c0.2-1.6,0.8-3,1.6-4.2c1.9-2.7,5.1-4.4,8.8-4.4c3.7,0,7,1.6,8.8,4.4c0.8,1.2,1.4,2.6,1.6,4.2 c0.3,1.8,0.2,3.9-0.3,6.1C55.3,62.9,51.8,69.3,46.4,76.2z M86.3,80.9c-0.7,5.2-4.2,9.7-9.1,11.7c-2.4,1-5,1.3-7.6,1 c-2.5-0.3-5-1.1-7.6-2.6c-3.6-2-7.2-5.1-11.4-9.7c6.6-8.1,10.6-15.5,12.1-22.1c0.7-3.1,0.8-5.9,0.5-8.5c-0.4-2.5-1.3-4.8-2.7-6.8 c-3.1-4.5-8.3-7.1-14.1-7.1s-11,2.7-14.1,7.1c-1.4,2-2.3,4.3-2.7,6.8c-0.4,2.6-0.3,5.5,0.5,8.5c1.5,6.6,5.6,14.1,12.1,22.2 c-4.1,4.6-7.8,7.7-11.4,9.7c-2.6,1.5-5.1,2.3-7.6,2.6c-2.7,0.3-5.3-0.1-7.6-1c-4.9-2-8.4-6.5-9.1-11.7c-0.3-2.5-0.1-5,0.9-7.8 c0.3-1,0.8-2,1.3-3.2c0.7-1.6,1.5-3.3,2.3-5l0.1-0.2c6.9-14.9,14.3-30.1,22-44.9l0.3-0.6c0.8-1.5,1.6-3.1,2.4-4.6 c0.8-1.6,1.7-3.1,2.8-4.4c2.1-2.4,4.9-3.7,8-3.7c3.1,0,5.9,1.3,8,3.7c1.1,1.3,2,2.8,2.8,4.4c0.8,1.5,1.6,3.1,2.4,4.6l0.3,0.6 C67.7,34.8,75.1,50,82,64.9L82,65c0.8,1.6,1.5,3.4,2.3,5c0.5,1.2,1,2.2,1.3,3.2C86.4,75.8,86.7,78.3,86.3,80.9z"/></svg> | docs/src/pages/logos/airbnb.svg | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001701434375718236,
0.0001701434375718236,
0.0001701434375718236,
0.0001701434375718236,
0
] |
{
"id": 0,
"code_window": [
"import riotGenerator from './generators/RIOT';\n",
"import preactGenerator from './generators/PREACT';\n",
"import svelteGenerator from './generators/SVELTE';\n",
"import raxGenerator from './generators/RAX';\n",
"import { warn } from './warn';\n",
"\n",
"const logger = console;\n",
"\n",
"type CommandOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { JsPackageManagerFactory } from './js-package-manager/JsPackageManagerFactory';\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "add",
"edit_start_line_idx": 38
} | <!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Aurelia</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<base href="/">
</head>
<body>
<app></app>
</body>
</html>
| examples/aurelia-kitchen-sink/index.ejs | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017188086349051446,
0.00017182138981297612,
0.00017176190158352256,
0.00017182138981297612,
5.948095349594951e-8
] |
{
"id": 1,
"code_window": [
"};\n",
"\n",
"const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {\n",
" const useYarn = Boolean(options.useNpm !== true) && hasYarn();\n",
"\n",
" const npmOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" const packageManager = JsPackageManagerFactory.getPackageManager(options.useNpm);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 53
} | export abstract class JsPackageManager {
public abstract initPackageJson(): void;
}
| lib/cli/src/js-package-manager/JsPackageManager.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00016829629021231085,
0.00016829629021231085,
0.00016829629021231085,
0.00016829629021231085,
0
] |
{
"id": 1,
"code_window": [
"};\n",
"\n",
"const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {\n",
" const useYarn = Boolean(options.useNpm !== true) && hasYarn();\n",
"\n",
" const npmOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" const packageManager = JsPackageManagerFactory.getPackageManager(options.useNpm);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 53
} | # Storybook Core
This package contains common data structures and bootstrapping capabilities
used among the different frameworks
(React, RN, Vue, Ember, Angular, etc).
## Preview
The files in `src/client/preview` alongside the `@storybook/client-api` package form the "API" of the preview (iframe) side of Storybook. Here is a brief overview of the architecture:
Each framework (e.g. `@storybook/react` / `@storybook/angular` / et al.) initializes the preview by calling into `src/client/preview/start.ts`, passing a `render` function that will be used to render stories.
The `start` module initiaizes all the submodules:
- `StoryStore` (from `@storybook/client-api`) - stores the stories and their state as well as the current selection or error.
- `ClientApi` (from `@storybook/client-api`) - provides the entry point for `storiesOf()` API calls; re-exported by each framework.
- `ConfigApi` (from `@storybook/client-api`) - provides the configure API (wrapped by `loadCsf` below).
- `StoryRenderer` - controls the HTML that is rendered in the preview (calling the `render` function with the current story at appropriate times).
- `url.js` - controls the URL in the preview and sets the selection based on it.
- `loadCsf` - loads CSF files from `require.context()` calls and uses `ClientApi` to load them into the store.
Each module uses the channel to communicate with each other and the manager. Each module also has direct access to the story store.
### Events on startup
The store can only be changed during "configuration". The `ConfigApi` will call `store.startConfiguration()`, then the user code (or `loadCsf`'s loader) which will use client API to load up stories. At the end of the user's code the `ConfigApi` will call `store.finishConfiguration()`. At this point the `SET_STORIES` event is emitted and the stories are transmitted to the manager.
The `SET_CURRENT_STORY` "command" event can be used to set the selection on the store. However only once this has been recieved _and_ configuration is over will the store use the `RENDER_CURRENT_STORY` to tell the `StoryRenderer` to render it.
| lib/core/README.md | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.000279100495390594,
0.0002051772316917777,
0.00016552061424590647,
0.0001709105708869174,
0.00005231794057181105
] |
{
"id": 1,
"code_window": [
"};\n",
"\n",
"const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {\n",
" const useYarn = Boolean(options.useNpm !== true) && hasYarn();\n",
"\n",
" const npmOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" const packageManager = JsPackageManagerFactory.getPackageManager(options.useNpm);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 53
} | module.exports = require('../dist/frameworks/common/index');
| addons/docs/react/index.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017327064415439963,
0.00017327064415439963,
0.00017327064415439963,
0.00017327064415439963,
0
] |
{
"id": 1,
"code_window": [
"};\n",
"\n",
"const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {\n",
" const useYarn = Boolean(options.useNpm !== true) && hasYarn();\n",
"\n",
" const npmOptions = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" const packageManager = JsPackageManagerFactory.getPackageManager(options.useNpm);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 53
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`csf-hoist-story-annotations transforms correctly using "basic.input.js" data 1`] = `
"import React from 'react';
import Button from './Button';
import { action } from '@storybook/addon-actions';
export default {
title: 'Button',
};
export const story1 = () => <Button label=\\"Story 1\\" />;
export const story2 = () => <Button label=\\"Story 2\\" onClick={action('click')} />;
story2.storyName = 'second story';
export const story3 = () => (
<div>
<Button label=\\"The Button\\" onClick={action('onClick')} />
<br />
</div>
);
const baz = 17;
story3.storyName = 'complex story';
story3.parameters = { foo: { bar: baz } };
story3.decorators = [(storyFn) => <bar>{storyFn}</bar>];"
`;
| lib/codemod/src/transforms/__testfixtures__/csf-hoist-story-annotations/basic.output.snapshot | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017246726201847196,
0.0001720048749120906,
0.0001712502125883475,
0.00017229716468136758,
5.381295977713307e-7
] |
{
"id": 2,
"code_window": [
"\n",
" const npmOptions = {\n",
" useYarn,\n",
" installAsDevDependencies: true,\n",
" skipInstall: options.skipInstall,\n",
" };\n",
"\n",
" const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" useYarn: Boolean(options.useNpm !== true) && hasYarn(),\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 56
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.9989908337593079,
0.4346367120742798,
0.0001715753023745492,
0.00046463136095553637,
0.49217942357063293
] |
{
"id": 2,
"code_window": [
"\n",
" const npmOptions = {\n",
" useYarn,\n",
" installAsDevDependencies: true,\n",
" skipInstall: options.skipInstall,\n",
" };\n",
"\n",
" const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" useYarn: Boolean(options.useNpm !== true) && hasYarn(),\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 56
} | import React from 'react';
import { Root as App } from './index';
import { PrettyFakeProvider, FakeProvider } from './FakeProvider';
import Provider from './provider';
export default {
title: 'UI/App',
component: App,
parameters: {
layout: 'fullscreen',
},
};
export const Default = () => <App provider={(new FakeProvider() as unknown) as Provider} />;
export const LoadingState = () => (
<App provider={(new PrettyFakeProvider() as unknown) as Provider} />
);
| lib/ui/src/app.stories.tsx | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017703759658616036,
0.0001745863410178572,
0.0001721350708976388,
0.0001745863410178572,
0.000002451262844260782
] |
{
"id": 2,
"code_window": [
"\n",
" const npmOptions = {\n",
" useYarn,\n",
" installAsDevDependencies: true,\n",
" skipInstall: options.skipInstall,\n",
" };\n",
"\n",
" const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" useYarn: Boolean(options.useNpm !== true) && hasYarn(),\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 56
} | import React, { FunctionComponent } from 'react';
import { styled } from '@storybook/theming';
import { transparentize } from 'polished';
import { getBlockBackgroundStyle } from './BlockBackgroundStyles';
import { ResetWrapper } from '../typography/DocumentFormatting';
const ItemTitle = styled.div(({ theme }) => ({
fontWeight: theme.typography.weight.bold,
color: theme.color.defaultText,
}));
const ItemSubtitle = styled.div(({ theme }) => ({
color:
theme.base === 'light'
? transparentize(0.2, theme.color.defaultText)
: transparentize(0.6, theme.color.defaultText),
}));
const ItemDescription = styled.div({
flex: '0 0 30%',
lineHeight: '20px',
marginTop: 5,
});
const SwatchLabel = styled.div(({ theme }) => ({
flex: 1,
textAlign: 'center',
fontFamily: theme.typography.fonts.mono,
fontSize: theme.typography.size.s1,
lineHeight: 1,
overflow: 'hidden',
color:
theme.base === 'light'
? transparentize(0.4, theme.color.defaultText)
: transparentize(0.6, theme.color.defaultText),
'> div': {
display: 'inline-block',
overflow: 'hidden',
maxWidth: '100%',
textOverflow: 'ellipsis',
},
span: {
display: 'block',
marginTop: 2,
},
}));
const SwatchLabels = styled.div({
display: 'flex',
flexDirection: 'row',
});
const Swatch = styled.div({
flex: 1,
});
const SwatchColors = styled.div(({ theme }) => ({
...getBlockBackgroundStyle(theme),
display: 'flex',
flexDirection: 'row',
height: 50,
marginBottom: 5,
overflow: 'hidden',
}));
const SwatchSpecimen = styled.div({
display: 'flex',
flexDirection: 'column',
flex: 1,
position: 'relative',
marginBottom: 30,
});
const Swatches = styled.div({
flex: 1,
display: 'flex',
flexDirection: 'row',
});
const Item = styled.div({
display: 'flex',
alignItems: 'flex-start',
});
const ListName = styled.div({
flex: '0 0 30%',
});
const ListSwatches = styled.div({
flex: 1,
});
const ListHeading = styled.div(({ theme }) => ({
display: 'flex',
flexDirection: 'row',
alignItems: 'center',
paddingBottom: 20,
fontWeight: theme.typography.weight.bold,
color:
theme.base === 'light'
? transparentize(0.4, theme.color.defaultText)
: transparentize(0.6, theme.color.defaultText),
}));
const List = styled.div(({ theme }) => ({
fontSize: theme.typography.size.s2,
lineHeight: `20px`,
display: 'flex',
flexDirection: 'column',
}));
type Colors = string[] | { [key: string]: string };
interface ColorProps {
title: string;
subtitle: string;
colors: Colors;
}
function renderSwatch(color: string) {
return (
<Swatch
key={color}
title={color}
style={{
background: color,
}}
/>
);
}
function renderSwatchLabel(color: string, colorDescription?: string) {
return (
<SwatchLabel key={color} title={color}>
<div>
{color}
{colorDescription && <span>{colorDescription}</span>}
</div>
</SwatchLabel>
);
}
function renderSwatchSpecimen(colors: Colors) {
if (Array.isArray(colors)) {
return (
<SwatchSpecimen>
<SwatchColors>{colors.map((color) => renderSwatch(color))}</SwatchColors>
<SwatchLabels>{colors.map((color) => renderSwatchLabel(color))}</SwatchLabels>
</SwatchSpecimen>
);
}
return (
<SwatchSpecimen>
<SwatchColors>{Object.values(colors).map((color) => renderSwatch(color))}</SwatchColors>
<SwatchLabels>
{Object.keys(colors).map((color) => renderSwatchLabel(color, colors[color]))}
</SwatchLabels>
</SwatchSpecimen>
);
}
/**
* A single color row your styleguide showing title, subtitle and one or more colors, used
* as a child of `ColorPalette`.
*/
export const ColorItem: FunctionComponent<ColorProps> = ({ title, subtitle, colors }) => {
return (
<Item>
<ItemDescription>
<ItemTitle>{title}</ItemTitle>
<ItemSubtitle>{subtitle}</ItemSubtitle>
</ItemDescription>
<Swatches>{renderSwatchSpecimen(colors)}</Swatches>
</Item>
);
};
/**
* Styleguide documentation for colors, including names, captions, and color swatches,
* all specified as `ColorItem` children of this wrapper component.
*/
export const ColorPalette: FunctionComponent = ({ children, ...props }) => (
<ResetWrapper>
<List {...props} className="docblock-colorpalette">
<ListHeading>
<ListName>Name</ListName>
<ListSwatches>Swatches</ListSwatches>
</ListHeading>
{children}
</List>
</ResetWrapper>
);
| lib/components/src/blocks/ColorPalette.tsx | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.000179243681486696,
0.00017582238069735467,
0.00016871387197170407,
0.0001760833547450602,
0.000002470228082529502
] |
{
"id": 2,
"code_window": [
"\n",
" const npmOptions = {\n",
" useYarn,\n",
" installAsDevDependencies: true,\n",
" skipInstall: options.skipInstall,\n",
" };\n",
"\n",
" const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" useYarn: Boolean(options.useNpm !== true) && hasYarn(),\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 56
} | import { patchNode } from './parse-helpers';
import getParser from './parsers';
import {
splitSTORYOF,
findAddsMap,
splitExports,
popParametersObjectFromDefaultExport,
findExportsMap as generateExportsMap,
} from './traverse-helpers';
function isUglyComment(comment, uglyCommentsRegex) {
return uglyCommentsRegex.some((regex) => regex.test(comment));
}
function generateSourceWithoutUglyComments(source, { comments, uglyCommentsRegex }) {
let lastIndex = 0;
const parts = [source];
comments
.filter((comment) => isUglyComment(comment.value.trim(), uglyCommentsRegex))
.map(patchNode)
.forEach((comment) => {
parts.pop();
const start = source.slice(lastIndex, comment.start);
const end = source.slice(comment.end);
parts.push(start, end);
lastIndex = comment.end;
});
return parts.join('');
}
function prettifyCode(source, { prettierConfig, parser, filepath }) {
let config = prettierConfig;
let foundParser = null;
if (parser === 'flow') foundParser = 'flow';
if (parser === 'javascript' || /jsx?/.test(parser)) foundParser = 'javascript';
if (parser === 'typescript' || /tsx?/.test(parser)) foundParser = 'typescript';
if (!config.parser) {
config = {
...prettierConfig,
};
} else if (filepath) {
config = {
...prettierConfig,
filepath,
};
} else {
config = {
...prettierConfig,
};
}
try {
return getParser(foundParser || 'javascript').format(source, config);
} catch (e) {
// Can fail when the source is a JSON
return source;
}
}
const ADD_PARAMETERS_STATEMENT =
'.addParameters({ storySource: { source: __STORY__, locationsMap: __LOCATIONS_MAP__ } })';
const applyExportDecoratorStatement = (part) =>
part.declaration.isVariableDeclaration
? ` ${part.source};`
: ` const ${part.declaration.ident} = ${part.source};`;
export function generateSourceWithDecorators(source, ast) {
const { comments = [] } = ast;
const partsUsingStoryOfToken = splitSTORYOF(ast, source);
if (partsUsingStoryOfToken.length > 1) {
const newSource = partsUsingStoryOfToken.join(ADD_PARAMETERS_STATEMENT);
return {
storyOfTokenFound: true,
changed: partsUsingStoryOfToken.length > 1,
source: newSource,
comments,
};
}
const partsUsingExports = splitExports(ast, source);
const newSource = partsUsingExports
.map((part, i) => (i % 2 === 0 ? part.source : applyExportDecoratorStatement(part)))
.join('');
return {
exportTokenFound: true,
changed: partsUsingExports.length > 1,
source: newSource,
comments,
};
}
export function generateSourceWithoutDecorators(source, ast) {
const { comments = [] } = ast;
return {
changed: true,
source,
comments,
};
}
export function generateAddsMap(ast, storiesOfIdentifiers) {
return findAddsMap(ast, storiesOfIdentifiers);
}
export function generateStoriesLocationsMap(ast, storiesOfIdentifiers) {
const usingAddsMap = generateAddsMap(ast, storiesOfIdentifiers);
const addsMap = usingAddsMap;
if (Object.keys(addsMap).length > 0) {
return usingAddsMap;
}
const usingExportsMap = generateExportsMap(ast);
return usingExportsMap || usingAddsMap;
}
export function generateStorySource({ source, ...options }) {
let storySource = source;
storySource = generateSourceWithoutUglyComments(storySource, options);
storySource = prettifyCode(storySource, options);
return storySource;
}
export function generateSourcesInExportedParameters(source, ast, additionalParameters) {
const {
splicedSource,
parametersSliceOfCode,
indexWhereToAppend,
foundParametersProperty,
} = popParametersObjectFromDefaultExport(source, ast);
if (indexWhereToAppend !== -1) {
const additionalParametersAsJson = JSON.stringify({ storySource: additionalParameters }).slice(
0,
-1
);
const propertyDeclaration = foundParametersProperty ? '' : 'parameters: ';
const comma = foundParametersProperty ? '' : ',';
const newParameters = `${propertyDeclaration}${additionalParametersAsJson},${parametersSliceOfCode.substring(
1
)}${comma}`;
const additionalComma = comma === ',' ? '' : ',';
const result = `${splicedSource.substring(
0,
indexWhereToAppend
)}${newParameters}${additionalComma}${splicedSource.substring(indexWhereToAppend)}`;
return result;
}
return source;
}
| lib/source-loader/src/abstract-syntax-tree/generate-helpers.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0002106684260070324,
0.0001786825159797445,
0.00016324913303833455,
0.0001749690854921937,
0.000012428795344021637
] |
{
"id": 3,
"code_window": [
" const generatorOptions = {\n",
" storyFormat: options.storyFormat || defaultStoryFormat,\n",
" };\n",
"\n",
" const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';\n",
"\n",
" const end = () => {\n",
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 71
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.9990248680114746,
0.43561461567878723,
0.00017161424329970032,
0.0031816582195460796,
0.492763876914978
] |
{
"id": 3,
"code_window": [
" const generatorOptions = {\n",
" storyFormat: options.storyFormat || defaultStoryFormat,\n",
" };\n",
"\n",
" const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';\n",
"\n",
" const end = () => {\n",
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 71
} | lib/cli/test/fixtures/angular-cli-v7/src/assets/.gitkeep | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001778434670995921,
0.0001778434670995921,
0.0001778434670995921,
0.0001778434670995921,
0
] |
|
{
"id": 3,
"code_window": [
" const generatorOptions = {\n",
" storyFormat: options.storyFormat || defaultStoryFormat,\n",
" };\n",
"\n",
" const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';\n",
"\n",
" const end = () => {\n",
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 71
} | import { addParameters } from '@storybook/client-api';
import { extractArgTypes, extractComponentDescription } from './jsondoc';
addParameters({
docs: {
iframeHeight: 80,
extractArgTypes,
extractComponentDescription,
},
});
| addons/docs/src/frameworks/ember/config.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001778434670995921,
0.00017687960644252598,
0.0001759157603373751,
0.00017687960644252598,
9.638533811084926e-7
] |
{
"id": 3,
"code_window": [
" const generatorOptions = {\n",
" storyFormat: options.storyFormat || defaultStoryFormat,\n",
" };\n",
"\n",
" const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';\n",
"\n",
" const end = () => {\n",
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 71
} | import React from 'react';
import { render, waitFor, fireEvent, act } from '@testing-library/react';
import { ThemeProvider, themes, convert } from '@storybook/theming';
import * as api from '@storybook/api';
import { A11YPanel } from './A11YPanel';
import { EVENTS } from '../constants';
jest.mock('@storybook/api');
const mockedApi = api as jest.Mocked<typeof api>;
const axeResult = {
incomplete: [
{
id: 'color-contrast',
impact: 'serious',
tags: ['cat.color', 'wcag2aa', 'wcag143'],
description:
'Ensures the contrast between foreground and background colors meets WCAG 2 AA contrast ratio thresholds',
help: 'Elements must have sufficient color contrast',
helpUrl: 'https://dequeuniversity.com/rules/axe/3.2/color-contrast?application=axeAPI',
nodes: [],
},
],
passes: [
{
id: 'aria-allowed-attr',
impact: null,
tags: ['cat.aria', 'wcag2a', 'wcag412'],
description: "Ensures ARIA attributes are allowed for an element's role",
help: 'Elements must only use allowed ARIA attributes',
helpUrl: 'https://dequeuniversity.com/rules/axe/3.2/aria-allowed-attr?application=axeAPI',
nodes: [],
},
],
violations: [
{
id: 'color-contrast',
impact: 'serious',
tags: ['cat.color', 'wcag2aa', 'wcag143'],
description:
'Ensures the contrast between foreground and background colors meets WCAG 2 AA contrast ratio thresholds',
help: 'Elements must have sufficient color contrast',
helpUrl: 'https://dequeuniversity.com/rules/axe/3.2/color-contrast?application=axeAPI',
nodes: [],
},
],
};
function ThemedA11YPanel() {
return (
<ThemeProvider theme={convert(themes.light)}>
<A11YPanel />
</ThemeProvider>
);
}
describe('A11YPanel', () => {
beforeEach(() => {
mockedApi.useChannel.mockReset();
mockedApi.useParameter.mockReset();
mockedApi.useStorybookState.mockReset();
mockedApi.useAddonState.mockReset();
mockedApi.useChannel.mockReturnValue(jest.fn());
mockedApi.useParameter.mockReturnValue({ manual: false });
const state: Partial<api.State> = { storyId: 'jest' };
// Lazy to mock entire state
mockedApi.useStorybookState.mockReturnValue(state as any);
mockedApi.useAddonState.mockImplementation(React.useState);
});
it('should render', () => {
const { container } = render(<A11YPanel />);
expect(container.firstChild).toBeTruthy();
});
it('should register event listener on mount', () => {
render(<A11YPanel />);
expect(mockedApi.useChannel).toHaveBeenCalledWith(
expect.objectContaining({
[EVENTS.RESULT]: expect.any(Function),
[EVENTS.ERROR]: expect.any(Function),
})
);
});
it('should handle "initial" status', () => {
const { getByText } = render(<A11YPanel />);
expect(getByText(/Initializing/)).toBeTruthy();
});
it('should handle "manual" status', async () => {
mockedApi.useParameter.mockReturnValue({ manual: true });
const { getByText } = render(<ThemedA11YPanel />);
await waitFor(() => {
expect(getByText(/Manually run the accessibility scan/)).toBeTruthy();
});
});
describe('running', () => {
it('should handle "running" status', async () => {
const emit = jest.fn();
mockedApi.useChannel.mockReturnValue(emit);
mockedApi.useParameter.mockReturnValue({ manual: true });
const { getByRole, getByText } = render(<ThemedA11YPanel />);
await waitFor(() => {
const button = getByRole('button', { name: 'Run test' });
fireEvent.click(button);
});
await waitFor(() => {
expect(getByText(/Please wait while the accessibility scan is running/)).toBeTruthy();
expect(emit).toHaveBeenCalledWith(EVENTS.MANUAL, 'jest');
});
});
it('should set running status on event', async () => {
const { getByText } = render(<ThemedA11YPanel />);
const useChannelArgs = mockedApi.useChannel.mock.calls[0][0];
act(() => useChannelArgs[EVENTS.RUNNING]());
await waitFor(() => {
expect(getByText(/Please wait while the accessibility scan is running/)).toBeTruthy();
});
});
});
it('should handle "ran" status', async () => {
const { getByText } = render(<ThemedA11YPanel />);
const useChannelArgs = mockedApi.useChannel.mock.calls[0][0];
act(() => useChannelArgs[EVENTS.RESULT](axeResult));
await waitFor(() => {
expect(getByText(/Tests completed/)).toBeTruthy();
expect(getByText(/Violations/)).toBeTruthy();
expect(getByText(/Passes/)).toBeTruthy();
expect(getByText(/Incomplete/)).toBeTruthy();
});
});
});
| addons/a11y/src/components/A11YPanel.test.tsx | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.000179377049789764,
0.00017536827363073826,
0.00016932499420363456,
0.00017575504898559302,
0.0000024400615075137466
] |
{
"id": 4,
"code_window": [
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n",
"\n",
" logger.log('\\nTo run your storybook, type:\\n');\n",
" codeLog([runStorybookCommand]);\n",
" logger.log('\\nFor more information visit:', chalk.cyan('https://storybook.js.org'));\n",
"\n",
" // Add a new line for the clear visibility.\n",
" logger.log();\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" codeLog([packageManager.getRunStorybookCommand()]);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 79
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.9651334285736084,
0.032936546951532364,
0.00016510668501723558,
0.0003065281198360026,
0.1676541268825531
] |
{
"id": 4,
"code_window": [
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n",
"\n",
" logger.log('\\nTo run your storybook, type:\\n');\n",
" codeLog([runStorybookCommand]);\n",
" logger.log('\\nFor more information visit:', chalk.cyan('https://storybook.js.org'));\n",
"\n",
" // Add a new line for the clear visibility.\n",
" logger.log();\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" codeLog([packageManager.getRunStorybookCommand()]);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 79
} | import { action } from '@storybook/addon-actions';
import Button from '../components/action-button/index.marko';
export default {
title: 'Addons/Actions/Button',
parameters: {
component: Button,
options: {
panelPosition: 'right',
},
},
};
export const Simple = () => ({
component: Button,
input: { click: action('action logged!') },
});
| examples/marko-cli/src/stories/addon-actions.stories.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017379882046952844,
0.00017278814630117267,
0.0001717774721328169,
0.00017278814630117267,
0.000001010674168355763
] |
{
"id": 4,
"code_window": [
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n",
"\n",
" logger.log('\\nTo run your storybook, type:\\n');\n",
" codeLog([runStorybookCommand]);\n",
" logger.log('\\nFor more information visit:', chalk.cyan('https://storybook.js.org'));\n",
"\n",
" // Add a new line for the clear visibility.\n",
" logger.log();\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" codeLog([packageManager.getRunStorybookCommand()]);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 79
} | {
"name": "storybook",
"version": "6.0.0-beta.23",
"description": "Storybook CLI",
"keywords": [
"storybook"
],
"homepage": "https://github.com/storybookjs/storybook/tree/master/lib/cli",
"bugs": {
"url": "https://github.com/storybookjs/storybook/issues"
},
"repository": {
"type": "git",
"url": "https://github.com/storybookjs/storybook.git",
"directory": "lib/cli"
},
"license": "MIT",
"bin": {
"sb": "./index.js",
"storybook": "./index.js"
},
"scripts": {
"prepare": "node ../../scripts/prepare.js"
},
"dependencies": {
"@storybook/cli": "6.0.0-beta.23"
},
"publishConfig": {
"access": "public"
}
}
| lib/cli-storybook/package.json | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017110641056206077,
0.00016828658408485353,
0.00016519312339369208,
0.0001684233866399154,
0.0000021978075892548077
] |
{
"id": 4,
"code_window": [
" if (!options.skipInstall) {\n",
" installDepsFromPackageJson(npmOptions);\n",
" }\n",
"\n",
" logger.log('\\nTo run your storybook, type:\\n');\n",
" codeLog([runStorybookCommand]);\n",
" logger.log('\\nFor more information visit:', chalk.cyan('https://storybook.js.org'));\n",
"\n",
" // Add a new line for the clear visibility.\n",
" logger.log();\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" codeLog([packageManager.getRunStorybookCommand()]);\n"
],
"file_path": "lib/cli/src/initiate.ts",
"type": "replace",
"edit_start_line_idx": 79
} | import { Story, Preview, Meta } from '@storybook/addon-docs/blocks';
import { action } from '@storybook/addon-actions';
# Storybook Docs for HTML
<Meta title="Addons/Docs" />
## Story definition
Hallelujah! HTML is working out of the box without modification.
How you like them apples?!
<Story name="heading" height="100px">
{'<h1>Hello World</h1>'}
</Story>
## Function stories
<Story name="function" height="100px">
{() => {
const btn = document.createElement('button');
btn.innerHTML = 'Hello Button';
btn.addEventListener('click', action('Click'));
return btn;
}}
</Story>
## Custom source
<Preview>
<Story name="custom source" height="100px" parameters={{ docs: { source: { code: 'hello' } } }}>
{'<h1>Custom source</h1>'}
</Story>
</Preview>
## Transformed source
<Preview>
<Story
name="transformed source"
height="100px"
parameters={{ docs: { transformSource: (src) => `transformed: ${src}` } }}
>
{'<h1>Some source</h1>'}
</Story>
</Preview>
## Story reference
You can also reference an existing story from within your MDX file.
<Story id="welcome--welcome" height="800px" />
| examples/html-kitchen-sink/stories/addon-docs.stories.mdx | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.000196660213987343,
0.0001745222689351067,
0.00016684802540112287,
0.00017082408885471523,
0.000010117469173565041
] |
{
"id": 5,
"code_window": [
"export abstract class JsPackageManager {\n",
" public abstract initPackageJson(): void;\n",
"}"
],
"labels": [
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" public abstract getRunStorybookCommand(): string;\n"
],
"file_path": "lib/cli/src/js-package-manager/JsPackageManager.ts",
"type": "add",
"edit_start_line_idx": 2
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.5701200366020203,
0.019593950361013412,
0.00016682400018908083,
0.0001702423469396308,
0.09918060153722763
] |
{
"id": 5,
"code_window": [
"export abstract class JsPackageManager {\n",
" public abstract initPackageJson(): void;\n",
"}"
],
"labels": [
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" public abstract getRunStorybookCommand(): string;\n"
],
"file_path": "lib/cli/src/js-package-manager/JsPackageManager.ts",
"type": "add",
"edit_start_line_idx": 2
} | module.exports = {
stories: ['../src/stories/welcome.stories', '../src/stories/**/button.stories.js'],
addons: [
'@storybook/preset-create-react-app',
'@storybook/addon-actions',
'@storybook/addon-links',
],
};
| examples/cra-react15/.storybook/main.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001686704345047474,
0.0001686704345047474,
0.0001686704345047474,
0.0001686704345047474,
0
] |
{
"id": 5,
"code_window": [
"export abstract class JsPackageManager {\n",
" public abstract initPackageJson(): void;\n",
"}"
],
"labels": [
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" public abstract getRunStorybookCommand(): string;\n"
],
"file_path": "lib/cli/src/js-package-manager/JsPackageManager.ts",
"type": "add",
"edit_start_line_idx": 2
} | import { Component, Input } from '@angular/core';
@Component({
selector: `storybook-base-button`,
template: ` <button>{{ label }}</button> `,
})
export class BaseButtonComponent {
@Input()
label: string;
}
| examples/angular-cli/src/stories/inheritance/base-button.component.ts | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00018290855223312974,
0.00017746486992109567,
0.0001720211876090616,
0.00017746486992109567,
0.0000054436823120340705
] |
{
"id": 5,
"code_window": [
"export abstract class JsPackageManager {\n",
" public abstract initPackageJson(): void;\n",
"}"
],
"labels": [
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" public abstract getRunStorybookCommand(): string;\n"
],
"file_path": "lib/cli/src/js-package-manager/JsPackageManager.ts",
"type": "add",
"edit_start_line_idx": 2
} | import React, { Fragment, memo } from 'react';
import { useAddonState, useChannel } from '@storybook/api';
import { ActionBar } from '@storybook/components';
import { ADDON_ID, EVENTS } from './constants';
type Results = string[];
interface ContentProps {
results: Results;
}
const Content = memo(({ results }: ContentProps) => (
<Fragment>
{results.length ? (
<ol>
{results.map((i, index) => (
// eslint-disable-next-line react/no-array-index-key
<li key={index}>{i}</li>
))}
</ol>
) : null}
</Fragment>
));
export const Panel = () => {
const [results, setState] = useAddonState<Results>(ADDON_ID, []);
const emit = useChannel({
[EVENTS.RESULT]: (newResults: Results) => setState(newResults),
});
return (
<Fragment>
<Content results={results} />
<ActionBar
key="actionbar"
actionItems={[
{ title: 'emit', onClick: () => emit(EVENTS.REQUEST) },
{ title: 'setState', onClick: () => setState(['foo']) },
{
title: 'setState with options',
onClick: () => setState(['bar'], { persistence: 'session' }),
},
{ title: 'setState with function', onClick: () => setState((s) => [...s, 'baz']) },
]}
/>
</Fragment>
);
};
| dev-kits/addon-roundtrip/src/panel.tsx | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017039442900568247,
0.00016934837913140655,
0.00016850985412020236,
0.00016927463002502918,
7.266398256433604e-7
] |
{
"id": 6,
"code_window": [
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'npm run storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/NPMProxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import spawn from 'cross-spawn';
import { JsPackageManager } from './JsPackageManager';
export class Yarn1Proxy extends JsPackageManager {
initPackageJson() {
const results = spawn.sync('yarn', ['init', '-y'], {
cwd: process.cwd(),
env: process.env,
stdio: 'pipe',
encoding: 'utf-8',
});
return results.stdout;
}
}
| lib/cli/src/js-package-manager/Yarn1Proxy.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.01098659448325634,
0.00701109180226922,
0.003035588888451457,
0.00701109180226922,
0.003975503146648407
] |
{
"id": 6,
"code_window": [
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'npm run storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/NPMProxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import path from 'path';
import {
isDefaultProjectSet,
editStorybookTsConfig,
getAngularAppTsConfigJson,
getAngularAppTsConfigPath,
} from './angular-helpers';
import {
retrievePackageJson,
getVersionedPackages,
writePackageJson,
getBabelDependencies,
installDependencies,
writeFileAsJson,
copyTemplate,
} from '../../helpers';
import { StoryFormat } from '../../project_types';
import { NpmOptions } from '../../NpmOptions';
import { Generator, GeneratorOptions } from '../Generator';
async function addDependencies(npmOptions: NpmOptions, { storyFormat }: GeneratorOptions) {
const packages = [
'@storybook/angular',
'@storybook/addon-actions',
'@storybook/addon-links',
'@storybook/addons',
];
if (storyFormat === StoryFormat.MDX) {
packages.push('@storybook/addon-docs');
}
const versionedPackages = await getVersionedPackages(npmOptions, ...packages);
const packageJson = await retrievePackageJson();
packageJson.dependencies = packageJson.dependencies || {};
packageJson.devDependencies = packageJson.devDependencies || {};
packageJson.scripts = packageJson.scripts || {};
packageJson.scripts.storybook = 'start-storybook -p 6006';
packageJson.scripts['build-storybook'] = 'build-storybook';
writePackageJson(packageJson);
const babelDependencies = await getBabelDependencies(npmOptions, packageJson);
installDependencies({ ...npmOptions, packageJson }, [...versionedPackages, ...babelDependencies]);
}
function editAngularAppTsConfig() {
const tsConfigJson = getAngularAppTsConfigJson();
const glob = '**/*.stories.ts';
if (!tsConfigJson) {
return;
}
const { exclude = [] } = tsConfigJson;
if (exclude.includes(glob)) {
return;
}
tsConfigJson.exclude = [...exclude, glob];
writeFileAsJson(getAngularAppTsConfigPath(), tsConfigJson);
}
const generator: Generator = async (npmOptions, { storyFormat }) => {
if (!isDefaultProjectSet()) {
throw new Error(
'Could not find a default project in your Angular workspace.\nSet a defaultProject in your angular.json and re-run the installation.'
);
}
copyTemplate(__dirname, storyFormat);
await addDependencies(npmOptions, { storyFormat });
editAngularAppTsConfig();
editStorybookTsConfig(path.resolve('./.storybook/tsconfig.json'));
};
export default generator;
| lib/cli/src/generators/ANGULAR/index.ts | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0002464040298946202,
0.0001804292551241815,
0.00016922589566092938,
0.0001716528640827164,
0.000023408214474329725
] |
{
"id": 6,
"code_window": [
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'npm run storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/NPMProxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import React from 'react';
import { actions as makeActions } from '@storybook/addon-actions';
import { Search } from './Search';
export default {
component: Search,
title: 'UI/Sidebar/Search',
decorators: [(storyFn: any) => <div style={{ width: '240px' }}>{storyFn()}</div>],
};
const actions = makeActions('onChange');
const pureActions = { ...actions, ...makeActions('onSetFocussed') };
export const simple = () => <Search {...actions} />;
export const focussed = () => <Search defaultFocussed {...pureActions} />;
export const filledIn = () => <Search defaultValue="Searchstring" {...pureActions} />;
| lib/ui/src/components/sidebar/Search.stories.tsx | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001730436342768371,
0.0001723773020785302,
0.0001717109844321385,
0.0001723773020785302,
6.66324922349304e-7
] |
{
"id": 6,
"code_window": [
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'npm run storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/NPMProxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`react component properties 9626-js-default-values 1`] = `
Object {
"rows": Array [
Object {
"defaultValue": Object {
"detail": undefined,
"summary": "'Beta'",
},
"description": undefined,
"name": "title",
"required": false,
"type": Object {
"detail": undefined,
"summary": "unknown",
},
},
],
}
`;
| addons/docs/src/frameworks/react/__testfixtures__/9626-js-default-values/properties.snapshot | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001775566051946953,
0.00017560040578246117,
0.0001742943568388,
0.00017495024076197296,
0.0000014089232536207419
] |
{
"id": 7,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn1Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001793705450836569,
0.00017441317322663963,
0.00017009038128890097,
0.00017479454982094467,
0.0000020997654246457387
] |
{
"id": 7,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn1Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`render a riot element can accept a constructor 1`] = `
<simpletest hacked="true"
test="with a parameter"
>
<div>
HACKED : true ; simple test (with a parameter). Oh, by the way (value is mapped to riotValue)
</div>
</simpletest>
`;
exports[`render a riot element can nest several tags 1`] = `
<matriochka>
<div>
<tag1>
<div>
Inside tag1:
<ul>
<li>
<tag2>
<div>
Inside tag2:
<ul>
<li>
<tag3>
<div>
Inside tag3:
<ul>
<li>
<tag4>
<div>
Inside tag4:
<ul>
<li>
<tag5>
<div>
Inside tag5:
<ul>
<li>
Content
</li>
</ul>
</div>
</tag5>
</li>
</ul>
</div>
</tag4>
</li>
</ul>
</div>
</tag3>
</li>
</ul>
</div>
</tag2>
</li>
</ul>
</div>
</tag1>
</div>
</matriochka>
`;
exports[`render a riot element can template some vars 1`] = `
<simpletest test="with a parameter">
<div>
simple test (with a parameter). Oh, by the way (value is mapped to riotValue)
</div>
</simpletest>
`;
| app/riot/src/client/preview/__snapshots__/render-riot.test.js.snap | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.000178274538484402,
0.00017258309526368976,
0.00016949202108662575,
0.00017170017235912383,
0.000002904203483922174
] |
{
"id": 7,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn1Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | <!--The content below is only a placeholder and can be replaced.-->
<div style="text-align:center">
<h1>
Welcome to {{ title }}!
</h1>
<img width="300" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
</div>
<h2>Here are some links to help you start: </h2>
<ul>
<li>
<h2><a target="_blank" rel="noopener" href="https://angular.io/tutorial">Tour of Heroes</a></h2>
</li>
<li>
<h2><a target="_blank" rel="noopener" href="https://github.com/angular/angular-cli/wiki">CLI Documentation</a></h2>
</li>
<li>
<h2><a target="_blank" rel="noopener" href="https://blog.angular.io/">Angular blog</a></h2>
</li>
</ul>
| lib/cli/test/fixtures/angular-cli-v6/src/app/app.component.html | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00021564160124398768,
0.00019042303028982133,
0.00017625709006097168,
0.00017937037046067417,
0.000017877466234494932
] |
{
"id": 7,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn1Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`Storyshots Custom/Providers Simple 1`] = `
<storybook-dynamic-app-root
cfr={[Function CodegenComponentFactoryResolver]}
data={[Function Object]}
target={[Function ViewContainerRef_]}
>
<storybook-simple-service-component>
<p>
Static name:
</p>
<ul>
</ul>
</storybook-simple-service-component>
</storybook-dynamic-app-root>
`;
exports[`Storyshots Custom/Providers With knobs 1`] = `
<storybook-dynamic-app-root
cfr={[Function CodegenComponentFactoryResolver]}
data={[Function Object]}
target={[Function ViewContainerRef_]}
>
<storybook-simple-service-component>
<p>
Dynamic knob:
</p>
<ul>
</ul>
</storybook-simple-service-component>
</storybook-dynamic-app-root>
`;
| examples/angular-cli/src/stories/__snapshots__/custom-providers.stories.storyshot | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017740986368153244,
0.00017171198851428926,
0.00016550483996979892,
0.00017196658882312477,
0.000004268276825314388
] |
{
"id": 8,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn2Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import { UpdateNotifier, IPackage } from 'update-notifier';
import chalk from 'chalk';
import inquirer from 'inquirer';
import { detect, isStorybookInstalled, detectLanguage } from './detect';
import { hasYarn } from './has_yarn';
import {
installableProjectTypes,
ProjectType,
StoryFormat,
SupportedLanguage,
} from './project_types';
import {
commandLog,
codeLog,
paddedLog,
installDepsFromPackageJson,
getPackageJson,
} from './helpers';
import angularGenerator from './generators/ANGULAR';
import emberGenerator from './generators/EMBER';
import meteorGenerator from './generators/METEOR';
import reactGenerator from './generators/REACT';
import reactNativeGenerator from './generators/REACT_NATIVE';
import reactScriptsGenerator from './generators/REACT_SCRIPTS';
import sfcVueGenerator from './generators/SFC_VUE';
import updateOrganisationsGenerator from './generators/UPDATE_PACKAGE_ORGANIZATIONS';
import vueGenerator from './generators/VUE';
import webpackReactGenerator from './generators/WEBPACK_REACT';
import mithrilGenerator from './generators/MITHRIL';
import marionetteGenerator from './generators/MARIONETTE';
import markoGenerator from './generators/MARKO';
import htmlGenerator from './generators/HTML';
import webComponentsGenerator from './generators/WEB-COMPONENTS';
import riotGenerator from './generators/RIOT';
import preactGenerator from './generators/PREACT';
import svelteGenerator from './generators/SVELTE';
import raxGenerator from './generators/RAX';
import { warn } from './warn';
const logger = console;
type CommandOptions = {
useNpm?: boolean;
type?: any;
force?: any;
html?: boolean;
skipInstall?: boolean;
storyFormat?: StoryFormat;
parser?: string;
yes?: boolean;
};
const installStorybook = (projectType: ProjectType, options: CommandOptions): Promise<void> => {
const useYarn = Boolean(options.useNpm !== true) && hasYarn();
const npmOptions = {
useYarn,
installAsDevDependencies: true,
skipInstall: options.skipInstall,
};
const hasTSDependency = detectLanguage() === SupportedLanguage.TYPESCRIPT;
warn({ hasTSDependency });
const defaultStoryFormat = hasTSDependency ? StoryFormat.CSF_TYPESCRIPT : StoryFormat.CSF;
const generatorOptions = {
storyFormat: options.storyFormat || defaultStoryFormat,
};
const runStorybookCommand = useYarn ? 'yarn storybook' : 'npm run storybook';
const end = () => {
if (!options.skipInstall) {
installDepsFromPackageJson(npmOptions);
}
logger.log('\nTo run your storybook, type:\n');
codeLog([runStorybookCommand]);
logger.log('\nFor more information visit:', chalk.cyan('https://storybook.js.org'));
// Add a new line for the clear visibility.
logger.log();
};
const REACT_NATIVE_DISCUSSION =
'https://github.com/storybookjs/storybook/blob/master/app/react-native/docs/manual-setup.md';
const runGenerator: () => Promise<void> = () => {
switch (projectType) {
case ProjectType.ALREADY_HAS_STORYBOOK:
logger.log();
paddedLog('There seems to be a storybook already available in this project.');
paddedLog('Apply following command to force:\n');
codeLog(['sb init [options] -f']);
// Add a new line for the clear visibility.
logger.log();
return Promise.resolve();
case ProjectType.UPDATE_PACKAGE_ORGANIZATIONS:
return updateOrganisationsGenerator(options.parser, npmOptions)
.then(() => null) // commmandLog doesn't like to see output
.then(commandLog('Upgrading your project to the new storybook packages.'))
.then(end);
case ProjectType.REACT_SCRIPTS:
return reactScriptsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Create React App" based project'))
.then(end);
case ProjectType.REACT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" app'))
.then(end);
case ProjectType.REACT_NATIVE: {
return (options.yes
? Promise.resolve({ server: true })
: (inquirer.prompt([
{
type: 'confirm',
name: 'server',
message:
'Do you want to install dependencies necessary to run storybook server? You can manually do it later by install @storybook/react-native-server',
default: false,
},
]) as Promise<{ server: boolean }>)
)
.then(({ server }) => reactNativeGenerator(npmOptions, server, generatorOptions))
.then(commandLog('Adding storybook support to your "React Native" app'))
.then(end)
.then(() => {
logger.log(chalk.red('NOTE: installation is not 100% automated.'));
logger.log(`To quickly run storybook, replace contents of your app entry with:\n`);
codeLog(["export default from './storybook';"]);
logger.log('\n For more in depth setup instructions, see:\n');
logger.log(chalk.cyan(REACT_NATIVE_DISCUSSION));
logger.log();
});
}
case ProjectType.METEOR:
return meteorGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Meteor" app'))
.then(end);
case ProjectType.WEBPACK_REACT:
return webpackReactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Webpack React" app'))
.then(end);
case ProjectType.REACT_PROJECT:
return reactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "React" library'))
.then(end);
case ProjectType.SFC_VUE:
return sfcVueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Single File Components Vue" app'))
.then(end);
case ProjectType.VUE:
return vueGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Vue" app'))
.then(end);
case ProjectType.ANGULAR:
return angularGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Angular" app'))
.then(end);
case ProjectType.EMBER:
return emberGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Ember" app'))
.then(end);
case ProjectType.MITHRIL:
return mithrilGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Mithril" app'))
.then(end);
case ProjectType.MARIONETTE:
return marionetteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marionette.js" app'))
.then(end);
case ProjectType.MARKO:
return markoGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Marko" app'))
.then(end);
case ProjectType.HTML:
return htmlGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "HTML" app'))
.then(end);
case ProjectType.WEB_COMPONENTS:
return webComponentsGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "web components" app'))
.then(end);
case ProjectType.RIOT:
return riotGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "riot.js" app'))
.then(end);
case ProjectType.PREACT:
return preactGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Preact" app'))
.then(end);
case ProjectType.SVELTE:
return svelteGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Svelte" app'))
.then(end);
case ProjectType.RAX:
return raxGenerator(npmOptions, generatorOptions)
.then(commandLog('Adding storybook support to your "Rax" app'))
.then(end);
default:
paddedLog(`We couldn't detect your project type. (code: ${projectType})`);
paddedLog(
'You can specify a project type explicitly via `sb init --type <type>` or follow some of the slow start guides: https://storybook.js.org/basics/slow-start-guide/'
);
// Add a new line for the clear visibility.
logger.log();
return projectTypeInquirer(options);
}
};
return runGenerator().catch((ex) => {
logger.error(`\n ${chalk.red(ex.stack)}`);
process.exit(1);
});
};
const projectTypeInquirer = async (options: { yes?: boolean }) => {
const manualAnswer = options.yes
? true
: await inquirer.prompt([
{
type: 'confirm',
name: 'manual',
message: 'Do you want to manually choose a Storybook project type to install?',
default: false,
},
]);
if (manualAnswer !== true && manualAnswer.manual) {
const frameworkAnswer = await inquirer.prompt([
{
type: 'list',
name: 'manualFramework',
message: 'Please choose a project type from the following list:',
choices: installableProjectTypes.map((type) => type.toUpperCase()),
},
]);
return installStorybook(frameworkAnswer.manualFramework, options);
}
return Promise.resolve();
};
export default function (options: CommandOptions, pkg: IPackage): Promise<void> {
const welcomeMessage = 'sb init - the simplest way to add a storybook to your project.';
logger.log(chalk.inverse(`\n ${welcomeMessage} \n`));
// Update notify code.
new UpdateNotifier({
pkg,
updateCheckInterval: 1000 * 60 * 60, // every hour (we could increase this later on.)
}).notify();
let projectType;
const projectTypeProvided = options.type;
const infoText = projectTypeProvided
? 'Installing Storybook for user specified project type'
: 'Detecting project type';
const done = commandLog(infoText);
try {
if (projectTypeProvided) {
if (installableProjectTypes.includes(options.type)) {
const storybookInstalled = isStorybookInstalled(getPackageJson(), options.force);
projectType = storybookInstalled
? ProjectType.ALREADY_HAS_STORYBOOK
: options.type.toUpperCase();
} else {
done(`The provided project type was not recognized by Storybook.`);
logger.log(`\nThe project types currently supported by Storybook are:\n`);
installableProjectTypes.sort().forEach((framework) => paddedLog(`- ${framework}`));
logger.log();
process.exit(1);
}
} else {
projectType = detect(options);
}
} catch (ex) {
done(ex.message);
process.exit(1);
}
done();
return installStorybook(projectType, options);
}
| lib/cli/src/initiate.ts | 1 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.0001793705450836569,
0.00017441317322663963,
0.00017009038128890097,
0.00017479454982094467,
0.0000020997654246457387
] |
{
"id": 8,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn2Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import { Component, Input } from '@angular/core';
@Component({
selector: 'storybook-simple-knobs-component',
template: `
<div>I am {{ name }} and I'm {{ age }} years old.</div>
<div>Phone Number: {{ phoneNumber }}</div>
`,
})
export class SimpleKnobsComponent {
@Input()
name;
@Input()
age;
@Input()
phoneNumber;
}
| examples/angular-cli/src/stories/knobs.component.ts | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017767210374586284,
0.00017585064051672816,
0.00017402917728759348,
0.00017585064051672816,
0.0000018214632291346788
] |
{
"id": 8,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn2Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | module.exports = {
stories: ['../stories/**/*.stories.js'],
}
| lib/cli/test/fixtures/react_babel_pkg_json/.storybook/main.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017640049918554723,
0.00017640049918554723,
0.00017640049918554723,
0.00017640049918554723,
0
] |
{
"id": 8,
"code_window": [
" stdio: 'pipe',\n",
" encoding: 'utf-8',\n",
" });\n",
" return results.stdout;\n",
" }\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
" getRunStorybookCommand(): string {\n",
" return 'yarn storybook';\n",
" }\n"
],
"file_path": "lib/cli/src/js-package-manager/Yarn2Proxy.ts",
"type": "add",
"edit_start_line_idx": 13
} | import WelcomeView from './views/WelcomeView.svelte';
export default {
title: 'Welcome',
};
export const Welcome = () => ({
Component: WelcomeView,
});
| examples/svelte-kitchen-sink/src/stories/welcome.stories.js | 0 | https://github.com/storybookjs/storybook/commit/100ffac29c46a67d28b014e09aad5fffb0bc2b9d | [
0.00017577808466739953,
0.00017577808466739953,
0.00017577808466739953,
0.00017577808466739953,
0
] |
{
"id": 0,
"code_window": [
"<template>\n",
" <div class=\"flex gap-2\">\n",
" <SmartsheetToolbarLockMenu />\n",
"\n",
" <div class=\"dot\" />\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" <slot name=\"start\" />\n",
"\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 2
} | <template>
<div class="flex gap-2">
<SmartsheetToolbarLockMenu />
<div class="dot" />
<SmartsheetToolbarReload />
<div class="dot" />
<SmartsheetToolbarAddRow />
<div class="dot" />
<SmartsheetToolbarDeleteTable />
<div class="dot" />
<SmartsheetToolbarToggleDrawer />
</div>
</template>
<style scoped>
:deep(.nc-toolbar-btn) {
@apply border-0 !text-xs font-semibold px-2;
}
.dot {
@apply w-[3px] h-[3px] bg-gray-300 rounded-full;
}
</style>
| packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue | 1 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.9768801927566528,
0.25217345356941223,
0.00017418837524019182,
0.01581977680325508,
0.4185819923877716
] |
{
"id": 0,
"code_window": [
"<template>\n",
" <div class=\"flex gap-2\">\n",
" <SmartsheetToolbarLockMenu />\n",
"\n",
" <div class=\"dot\" />\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" <slot name=\"start\" />\n",
"\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 2
} | <template>
<div class="">
<div v-if="loading" class="pr-4">
<v-skeleton-loader type="text" class="mx-2 mt-4 mr-14" />
<v-skeleton-loader type="text@10" class="mx-2 mt-4" />
<v-skeleton-loader type="text@2" class="mx-2 mt-4 mr-14" />
</div>
<template v-else>
<v-list dense>
<v-list-item dense>
<v-list-item-subtitle>
<span class="caption" @dblclick="counterLoc++">Categories</span>
</v-list-item-subtitle>
</v-list-item>
<v-list-item-group v-model="category">
<v-list-item v-for="c in categories" :key="c.category" :value="c.category" dense>
<v-list-item-title>
<span :class="{ 'font-weight-black': category === c.category }" class="body-1">
{{ c.category }}
</span>
<span class="grey--text">({{ c.count }})</span>
</v-list-item-title>
</v-list-item>
</v-list-item-group>
</v-list>
<!-- v-if="counter > 4"-->
<v-btn class="ml-4" color="grey" small outlined @click="showTemplateEditor">
<v-icon class="mr-1" small> mdi-plus </v-icon> New template
</v-btn>
<v-tooltip bottom>
<template #activator="{ on }">
<v-btn
class="ml-4 mt-4"
color="grey"
small
outlined
v-on="on"
@click="$toast.info('Happy hacking!').goAway(3000)"
>
<v-icon small class="mr-1"> mdi-file-excel-outline </v-icon>
Import
</v-btn>
</template>
<span class="caption">Create templates from multiple Excel files</span>
</v-tooltip>
<v-text-field
v-if="$store.state.templateE > 3"
v-model="t"
autocomplete="new-password"
name="nc"
outlined
dense
:full-width="false"
style="width: 135px"
type="password"
class="caption mt-4 ml-1 mr-3 ml-4"
hide-details
/>
</template>
</div>
</template>
<script>
// import categories from './templates.categories'
export default {
name: 'Categories',
props: { value: String, counter: Number },
data: () => ({
categories: [],
loading: false,
}),
computed: {
counterLoc: {
get() {
return this.$store.state.templateE;
},
set(c) {
this.$store.commit('mutTemplateE', c);
},
},
category: {
get() {
return this.value;
},
set(v) {
this.$emit('input', v);
},
},
t: {
get() {
return this.$store.state.template;
},
set(t) {
this.$store.commit('mutTemplate', t);
},
},
},
created() {
this.loadCategories();
},
methods: {
async loadCategories() {
this.loading = true;
try {
const res = await this.$axios.get(`${process.env.NC_API_URL}/api/v1/nc/templates/categories`);
this.categories = res.data;
} catch (e) {
console.log(e);
}
this.loading = false;
},
showTemplateEditor() {
this.$emit('showTemplateEditor');
},
},
};
</script>
<style scoped></style>
| packages/nc-gui/components/templates/Categories.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00027684515225701034,
0.00017746951198205352,
0.00016517737822141498,
0.00016867509111762047,
0.000028815838959417306
] |
{
"id": 0,
"code_window": [
"<template>\n",
" <div class=\"flex gap-2\">\n",
" <SmartsheetToolbarLockMenu />\n",
"\n",
" <div class=\"dot\" />\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" <slot name=\"start\" />\n",
"\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 2
} | const {expect} = require('chai');
const models = require('./models')
const country = models.country;
const city = models.city;
//desribe group of tests done
describe('xc-data-mapper tests', function () {
before(function (done) {
//start appln
done();
});
after(function (done) {
//stop appln
done();
});
beforeEach(function (done) {
//init common variables for each test
done();
});
afterEach(function (done) {
//term common variables for each test
done();
});
it('create, read, update, delete', async function () {
let data = await country.insert({country: 'xc'});
expect(data['country']).to.equal('xc');
data = await country.readByPk(data.country_id);
expect(data['country']).to.equal('xc');
data.country = 'xgc'
const updated = await country.updateByPk(data.country_id, data);
expect(updated).to.equal(1);
data.country = 'xgc'
const deleted = await country.delByPk(data.country_id);
expect(deleted).to.equal(1);
});
it('create : transaction + commit', async function () {
let trx = null;
try {
trx = await country.transaction();
let data = await country.insert({country: 'xc'}, trx);
expect(data['country']).to.equal('xc');
let find = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(find['country']).to.equal(undefined);
await country.commit(trx);
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal(data.country);
const deleted = await country.delByPk(data.country_id);
expect(deleted).to.equal(1);
} catch (e) {
if (trx)
await country.rollback(trx)
console.log(e);
throw e;
}
});
it('create: transaction + rollback ', async function () {
let trx = null;
let data = null;
try {
trx = await country.transaction();
data = await country.insert({country: 'xc'}, trx);
expect(data['country']).to.equal('xc');
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal(undefined);
await country.rollback(trx);
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal(undefined);
} catch (e) {
await country.rollback(trx)
console.log(e);
throw e;
} finally {
}
});
it('update : transaction + commit', async function () {
let trx = null;
let data = null;
try {
let data = await country.insert({country: 'xc'});
trx = await country.transaction();
data.country = 'xgc'
const updated = await country.updateByPk(data.country_id, data, trx);
await country.commit(trx);
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal('xgc');
} catch (e) {
await country.rollback(trx)
console.log(e);
throw e;
} finally {
if (data)
await country.delByPk(data.country_id);
}
});
it('update: transaction + rollback ', async function () {
let trx = null;
let data = null;
try {
let data = await country.insert({country: 'xc'});
trx = await country.transaction();
data.country = 'xgc'
const updated = await country.updateByPk(data.country_id, data, trx);
await country.rollback(trx);
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal('xc');
} catch (e) {
await country.rollback(trx)
console.log(e);
throw e;
} finally {
if (data)
await country.delByPk(data.country_id);
}
});
it('delete : transaction + commit', async function () {
let trx = null;
let data = null;
try {
let data = await country.insert({country: 'xc'});
trx = await country.transaction();
const deleted = await country.delByPk(data.country_id, trx);
await country.commit(trx);
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal(undefined);
data = null;
} catch (e) {
await country.rollback(trx)
console.log(e);
throw e;
} finally {
if (data)
await country.delByPk(data.country_id);
}
});
it('delete: transaction + rollback ', async function () {
let trx = null;
let data = null;
try {
let data = await country.insert({country: 'xc'});
trx = await country.transaction();
const deleted = await country.delByPk(data.country_id, trx);
await country.rollback(trx);
data = await country.findOne({where: `(country_id,eq,${data.country_id})`});
expect(data['country']).to.equal('xc');
} catch (e) {
await country.rollback(trx)
console.log(e);
throw e;
} finally {
if (data)
await country.delByPk(data.country_id);
}
});
it('read invalid', async function () {
data = await country.readByPk('xys');
expect(Object.keys(data).length).to.equal(0);
});
it('list', async function () {
let data = await country.list({})
expect(data.length).to.not.equal(0)
});
it('list + fields', async function () {
let data = await country.list({fields: 'country'})
expect(Object.keys(data[0]).length).to.equal(1)
expect(Object.keys(data[0])[0]).to.equal('country')
data = await country.list({f: 'country'})
expect(Object.keys(data[0]).length).to.equal(1)
expect(Object.keys(data[0])[0]).to.equal('country')
data = await country.list({f: 'country_id,country'})
expect(Object.keys(data[0]).length).to.equal(2)
expect(Object.keys(data[0])[0]).to.equal('country_id')
expect(Object.keys(data[0])[1]).to.equal('country')
});
it('list + limit', async function () {
let data = await country.list({limit: 2})
expect(data.length).to.equal(2)
data = await country.list({l: 2})
expect(data.length).to.equal(2)
});
it('list + offset', async function () {
let data = await country.list({offset: 1})
expect(data[0]['country']).to.not.equal('Afghanistan')
});
it('list + where', async function () {
let data = await country.list({where: '(country,eq,India)'})
expect(data.length).to.equal(1)
data = await country.list({w: '(country,eq,India)'})
expect(data.length).to.equal(1)
});
it('list + sort', async function () {
let data = await country.list({sort: 'country'})
expect(data[0]['country']).to.equal('Afghanistan')
data = await country.list({sort: '-country'})
expect(data[0]['country']).to.equal('Zambia')
});
it('list + sort', async function () {
let data = await country.list({sort: 'country'})
expect(data[0]['country']).to.equal('Afghanistan')
data = await country.list({sort: '-country'})
expect(data[0]['country']).to.equal('Zambia')
});
it('findOne', async function () {
let data = await country.findOne({where: '(country,eq,India)'})
expect(data['country']).to.equal('India')
data = await country.findOne({sort: '-country'})
expect(data['country']).to.equal('Zambia')
data = await country.findOne({offset: '1'})
expect(data['country']).not.to.equal('Afghanistan')
data = await country.findOne()
expect(data['country']).to.equal('Afghanistan')
});
it('count', async function () {
let data = await country.countByPk({});
expect(data['count']).to.be.above(100)
});
it('groupBy', async function () {
let data = await city.groupBy({cn: 'country_id', limit: 2});
expect(data[0]['count']).to.not.equal(0);
expect(data.length).to.be.equal(2);
data = await city.groupBy({cn: 'country_id', having: '(count,gt,50)'});
expect(data.length).to.be.equal(2);
});
it('aggregate', async function () {
let data = await city.aggregate({cn: 'country_id', fields: 'country_id', func: 'count,sum,avg'});
expect(data[0]['count']).to.not.equal(0);
expect(data[0]['sum']).to.not.equal(0);
expect(data[0]['avg']).to.not.equal(0);
data = await city.aggregate({
column_name:'country_id',
fields: 'country_id',
func: 'count,sum,avg',
having: '(count,gt,50)'
});
expect(data.length).to.be.equal(2);
});
it('distinct', async function () {
let data = await city.distinct({cn: 'country_id'});
expect(Object.keys(data[0]).length).to.be.equal(1);
expect(data[0]['country_id']).to.not.equal(0);
data = await city.distinct({cn: 'country_id', fields: 'city'});
expect(Object.keys(data[0]).length).to.be.equal(2);
});
it('distribution', async function () {
let data = await city.distribution({cn: 'country_id', steps: '0,20,40,100'});
expect(data.length).to.be.equal(3);
expect(data[0]['range']).to.be.equal('0-20');
expect(data[0]['count']).to.be.above(70);
expect(data[1]['range']).to.be.equal('21-40');
expect(data[1]['count']).to.be.equal(100);
expect(data[2]['range']).to.be.equal('41-100');
data = await city.distribution({cn: 'country_id', step: 20, min: 0, max: 100});
expect(data.length).to.be.equal(5);
expect(data[0]['range']).to.be.equal('0-20');
expect(data[0]['count']).to.be.above(70);
expect(data[3]['count']).to.be.equal(106);
});
it('hasManyList', async function () {
let data = await country.hasManyList({childs: 'city'})
for (let i = 0; i < data.length; ++i) {
expect(data[i].city.length).to.not.equal(0)
expect(Object.keys(data[i]).length).to.be.equal(4)
expect(Object.keys(data[i].city[0]).length).to.be.equal(4)
}
data = await country.hasManyList({childs: 'city', fields: 'country', fields1: 'city'})
for (let i = 0; i < data.length; ++i) {
expect(data[i].city.length).to.not.equal(0)
expect(Object.keys(data[i]).length).to.be.equal(3)
expect(Object.keys(data[i].city[0]).length).to.be.equal(2)
}
data = await models.film.hasManyList({childs: 'inventory.film_category'})
for (let i = 0; i < data.length; ++i) {
expect(data[i].inventory.length).to.not.equal(0)
expect(data[i].film_category.length).to.not.equal(0)
}
});
it('belongsTo', async function () {
let data = await city.belongsTo({parents: 'country'})
for (let i = 0; i < data.length; ++i) {
expect(data[i].country).to.not.equal(null)
}
});
it('hasManyListGQL', async function () {
let data = await country.hasManyListGQL({ids: [1, 2], child: 'city'})
expect(data['1'].length).to.not.equal(0)
expect(data['2'].length).to.not.equal(0)
});
it('bulk - create, update, delete', async function () {
// let data = await country.insertb([{country:'IN'}, {country:'US'}])
// console.log(data);
});
it('update and delete with where', async function () {
let data = await country.insertb([{country: 'abc'}, {country: 'abc'}])
let updateCount = await country.update({
data: {country: 'abcd'},
where: '(country,eq,abc)'
});
expect(updateCount).to.be.equal(2);
let deleteCount = await country.del({
where: '(country,eq,abcd)'
});
expect(deleteCount).to.be.equal(2);
});
it('xc condition test', async function () {
let data = await country.list({condition: {country_id: {eq: '34'}}})
expect(data.length).to.be.equal(1);
expect(data[0].country_id).to.be.equal(34);
data = await country.list({condition: {_or: [{country: {like: 'Ind%'}}, {country: {like: '%dia'}}]}})
const pattern = /^ind|dia$/i;
for (const {country} of data) {
expect(pattern.test(country)).to.be.equal(true);
}
});
});
| packages/nocodb/src/lib/db/sql-data-mapper/__tests__/sql.test.js | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00017587802722118795,
0.00017157978436443955,
0.00016364747716579586,
0.00017202695016749203,
0.000002528136519686086
] |
{
"id": 0,
"code_window": [
"<template>\n",
" <div class=\"flex gap-2\">\n",
" <SmartsheetToolbarLockMenu />\n",
"\n",
" <div class=\"dot\" />\n"
],
"labels": [
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" <slot name=\"start\" />\n",
"\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 2
} | import UITypes from '../UITypes';
import { IDType } from './index';
const dbTypes = [
'bigint',
'binary',
'bit',
'char',
'date',
'datetime',
'datetime2',
'datetimeoffset',
'decimal',
'float',
'geography',
'geometry',
'heirarchyid',
'image',
'int',
'money',
'nchar',
'ntext',
'numeric',
'nvarchar',
'real',
'json',
'smalldatetime',
'smallint',
'smallmoney',
'sql_variant',
'sysname',
'text',
'time',
'timestamp',
'tinyint',
'uniqueidentifier',
'varbinary',
'xml',
'varchar',
];
export class MssqlUi {
static getNewTableColumns() {
return [
{
column_name: 'id',
title: 'Id',
dt: 'int',
dtx: 'integer',
ct: 'int(11)',
nrqd: false,
rqd: true,
ck: false,
pk: true,
un: false,
ai: true,
cdf: null,
clen: null,
np: null,
ns: 0,
dtxp: '',
dtxs: '',
altered: 1,
uidt: 'ID',
uip: '',
uicn: '',
},
{
column_name: 'title',
title: 'Title',
dt: 'varchar',
dtx: 'specificType',
ct: 'varchar(45)',
nrqd: true,
rqd: false,
ck: false,
pk: false,
un: false,
ai: false,
cdf: null,
clen: 45,
np: null,
ns: null,
dtxp: '45',
dtxs: '',
altered: 1,
uidt: 'SingleLineText',
uip: '',
uicn: '',
},
{
column_name: 'created_at',
title: 'CreatedAt',
dt: 'datetime',
dtx: 'specificType',
ct: 'varchar(45)',
nrqd: true,
rqd: false,
ck: false,
pk: false,
un: false,
ai: false,
cdf: 'GETDATE()',
clen: 45,
np: null,
ns: null,
dtxp: '',
dtxs: '',
altered: 1,
uidt: UITypes.DateTime,
uip: '',
uicn: '',
},
{
column_name: 'updated_at',
title: 'UpdatedAt',
dt: 'datetime',
dtx: 'specificType',
ct: 'varchar(45)',
nrqd: true,
rqd: false,
ck: false,
pk: false,
un: false,
ai: false,
au: true,
cdf: 'GETDATE()',
clen: 45,
np: null,
ns: null,
dtxp: '',
dtxs: '',
altered: 1,
uidt: UITypes.DateTime,
uip: '',
uicn: '',
},
];
}
static getNewColumn(suffix) {
return {
column_name: 'title' + suffix,
dt: 'varchar',
dtx: 'specificType',
ct: 'varchar(45)',
nrqd: true,
rqd: false,
ck: false,
pk: false,
un: false,
ai: false,
cdf: null,
clen: 45,
np: null,
ns: null,
dtxp: '45',
dtxs: '',
altered: 1,
uidt: 'SingleLineText',
uip: '',
uicn: '',
};
}
// static getDefaultLengthForDatatype(type) {
// switch (type) {
// case "int":
// return 11;
// break;
// case "tinyint":
// return 1;
// break;
// case "smallint":
// return 5;
// break;
//
// case "mediumint":
// return 9;
// break;
// case "bigint":
// return 20;
// break;
// case "bit":
// return 64;
// break;
// case "boolean":
// return '';
// break;
// case "float":
// return 12;
// break;
// case "decimal":
// return 10;
// break;
// case "double":
// return 22;
// break;
// case "serial":
// return 20;
// break;
// case "date":
// return '';
// break;
// case "datetime":
// case "timestamp":
// return 6;
// break;
// case "time":
// return '';
// break;
// case "year":
// return '';
// break;
// case "char":
// return 255;
// break;
// case "varchar":
// return 45;
// break;
// case "nchar":
// return 255;
// break;
// case "text":
// return '';
// break;
// case "tinytext":
// return '';
// break;
// case "mediumtext":
// return '';
// break;
// case "longtext":
// return ''
// break;
// case "binary":
// return 255;
// break;
// case "varbinary":
// return 65500;
// break;
// case "blob":
// return '';
// break;
// case "tinyblob":
// return '';
// break;
// case "mediumblob":
// return '';
// break;
// case "longblob":
// return '';
// break;
// case "enum":
// return '\'a\',\'b\'';
// break;
// case "set":
// return '\'a\',\'b\'';
// break;
// case "geometry":
// return '';
// case "point":
// return '';
// case "linestring":
// return '';
// case "polygon":
// return '';
// case "multipoint":
// return '';
// case "multilinestring":
// return '';
// case "multipolygon":
// return '';
// case "json":
// return ''
// break;
//
// }
//
// }
static getDefaultLengthForDatatype(type) {
switch (type) {
case 'bigint':
return '';
case 'binary':
return '';
case 'bit':
return '';
case 'char':
return '';
case 'date':
return '';
case 'datetime':
return '';
case 'datetime2':
return '';
case 'datetimeoffset':
return '';
case 'decimal':
return '';
case 'float':
return '';
case 'geography':
return '';
case 'geometry':
return '';
case 'heirarchyid':
return '';
case 'image':
return '';
case 'int':
return '';
case 'money':
return '';
case 'nchar':
return '';
case 'ntext':
return '';
case 'numeric':
return '';
case 'nvarchar':
return 255;
case 'real':
return '';
case 'json':
return '';
case 'smalldatetime':
return '';
case 'smallint':
return '';
case 'smallmoney':
return '';
case 'sql_variant':
return '';
case 'sysname':
return '';
case 'text':
return '';
case 'time':
return '';
case 'timestamp':
return '';
case 'tinyint':
return '';
case 'uniqueidentifier':
return '';
case 'varbinary':
return '';
case 'xml':
return '';
case 'varchar':
return '';
default:
return '';
}
}
static getDefaultLengthIsDisabled(type) {
switch (type) {
case 'nvarchar':
return false;
case 'bigint':
case 'binary':
case 'bit':
case 'char':
case 'date':
case 'datetime':
case 'datetime2':
case 'datetimeoffset':
case 'decimal':
case 'float':
case 'geography':
case 'geometry':
case 'heirarchyid':
case 'image':
case 'int':
case 'money':
case 'nchar':
case 'ntext':
case 'numeric':
case 'nvarchar':
case 'real':
case 'json':
case 'smalldatetime':
case 'smallint':
case 'smallmoney':
case 'sql_variant':
case 'sysname':
case 'text':
case 'time':
case 'timestamp':
case 'tinyint':
case 'uniqueidentifier':
case 'varbinary':
case 'xml':
return true;
case 'varchar':
return false;
default:
return true;
}
}
static getDefaultValueForDatatype(type) {
switch (type) {
case 'bigint':
return 'eg: ';
case 'binary':
return 'eg: ';
case 'bit':
return 'eg: ';
case 'char':
return 'eg: ';
case 'date':
return 'eg: ';
case 'datetime':
return 'eg: ';
case 'datetime2':
return 'eg: ';
case 'datetimeoffset':
return 'eg: ';
case 'decimal':
return 'eg: ';
case 'float':
return 'eg: ';
case 'geography':
return 'eg: ';
case 'geometry':
return 'eg: ';
case 'heirarchyid':
return 'eg: ';
case 'image':
return 'eg: ';
case 'int':
return 'eg: ';
case 'money':
return 'eg: ';
case 'nchar':
return 'eg: ';
case 'ntext':
return 'eg: ';
case 'numeric':
return 'eg: ';
case 'nvarchar':
return 'eg: ';
case 'real':
return 'eg: ';
case 'json':
return 'eg: ';
case 'smalldatetime':
return 'eg: ';
case 'smallint':
return 'eg: ';
case 'smallmoney':
return 'eg: ';
case 'sql_variant':
return 'eg: ';
case 'sysname':
return 'eg: ';
case 'text':
return 'eg: ';
case 'time':
return 'eg: ';
case 'timestamp':
return 'eg: ';
case 'tinyint':
return 'eg: ';
case 'uniqueidentifier':
return 'eg: ';
case 'varbinary':
return 'eg: ';
case 'xml':
return 'eg: ';
case 'varchar':
return 'eg: ';
default:
return '';
}
}
static getDefaultScaleForDatatype(type) {
switch (type) {
case 'bigint':
return '';
case 'binary':
return '';
case 'bit':
return '';
case 'char':
return '';
case 'date':
return '';
case 'datetime':
return '';
case 'datetime2':
return '';
case 'datetimeoffset':
return '';
case 'decimal':
return '';
case 'float':
return '';
case 'geography':
return '';
case 'geometry':
return '';
case 'heirarchyid':
return '';
case 'image':
return '';
case 'int':
return '';
case 'money':
return '';
case 'nchar':
return '';
case 'ntext':
return '';
case 'numeric':
return '';
case 'nvarchar':
return '';
case 'real':
return '';
case 'json':
return '';
case 'smalldatetime':
return '';
case 'smallint':
return '';
case 'smallmoney':
return '';
case 'sql_variant':
return '';
case 'sysname':
return '';
case 'text':
return '';
case 'time':
return '';
case 'timestamp':
return '';
case 'tinyint':
return '';
case 'uniqueidentifier':
return '';
case 'varbinary':
return '';
case 'xml':
return '';
case 'varchar':
return '';
default:
return '';
}
}
static colPropAIDisabled(col, columns) {
// console.log(col);
if (
col.dt === 'int4' ||
col.dt === 'integer' ||
col.dt === 'bigint' ||
col.dt === 'smallint'
) {
for (let i = 0; i < columns.length; ++i) {
if (columns[i].cn !== col.cn && columns[i].ai) {
return true;
}
}
return false;
} else {
return true;
}
}
static colPropUNDisabled(_col) {
// console.log(col);
return true;
// if (col.dt === 'int' ||
// col.dt === 'tinyint' ||
// col.dt === 'smallint' ||
// col.dt === 'mediumint' ||
// col.dt === 'bigint') {
// return false;
// } else {
// return true;
// }
}
static onCheckboxChangeAI(col) {
console.log(col);
if (
col.dt === 'int' ||
col.dt === 'bigint' ||
col.dt === 'smallint' ||
col.dt === 'tinyint'
) {
col.altered = col.altered || 2;
}
// if (!col.ai) {
// col.dtx = 'specificType'
// } else {
// col.dtx = ''
// }
}
static showScale(_columnObj) {
return false;
}
static removeUnsigned(columns) {
for (let i = 0; i < columns.length; ++i) {
if (
columns[i].altered === 1 &&
!(
columns[i].dt === 'int' ||
columns[i].dt === 'bigint' ||
columns[i].dt === 'tinyint' ||
columns[i].dt === 'smallint' ||
columns[i].dt === 'mediumint'
)
) {
columns[i].un = false;
console.log('>> resetting unsigned value', columns[i].cn);
}
console.log(columns[i].cn);
}
}
static columnEditable(colObj) {
return colObj.tn !== '_evolutions' || colObj.tn !== 'nc_evolutions';
}
static extractFunctionName(query) {
const reg =
/^\s*CREATE\s+(?:OR\s+REPLACE\s*)?\s*FUNCTION\s+(?:[\w\d_]+\.)?([\w_\d]+)/i;
const match = query.match(reg);
return match && match[1];
}
static extractProcedureName(query) {
const reg =
/^\s*CREATE\s+(?:OR\s+REPLACE\s*)?\s*PROCEDURE\s+(?:[\w\d_]+\.)?([\w_\d]+)/i;
const match = query.match(reg);
return match && match[1];
}
static handleRawOutput(result, headers) {
console.log(result);
if (Array.isArray(result) && result[0]) {
const keys = Object.keys(result[0]);
// set headers before settings result
for (let i = 0; i < keys.length; i++) {
const text = keys[i];
headers.push({ text, value: text, sortable: false });
}
} else if (result === undefined) {
headers.push({ text: 'Message', value: 'message', sortable: false });
result = [{ message: 'Success' }];
}
return result;
}
static splitQueries(query) {
/***
* we are splitting based on semicolon
* there are mechanism to escape semicolon within single/double quotes(string)
*/
return query.match(/\b("[^"]*;[^"]*"|'[^']*;[^']*'|[^;])*;/g);
}
/**
* if sql statement is SELECT - it limits to a number
* @param args
* @returns {string|*}
*/
sanitiseQuery(args) {
let q = args.query.trim().split(';');
if (q[0].startsWith('Select')) {
q = q[0] + ` LIMIT 0,${args.limit ? args.limit : 100};`;
} else if (q[0].startsWith('select')) {
q = q[0] + ` LIMIT 0,${args.limit ? args.limit : 100};`;
} else if (q[0].startsWith('SELECT')) {
q = q[0] + ` LIMIT 0,${args.limit ? args.limit : 100};`;
} else {
return args.query;
}
return q;
}
static getColumnsFromJson(json, tn) {
const columns = [];
try {
if (typeof json === 'object' && !Array.isArray(json)) {
const keys = Object.keys(json);
for (let i = 0; i < keys.length; ++i) {
const column = {
dp: null,
tn,
column_name: keys[i],
cno: keys[i],
np: 10,
ns: 0,
clen: null,
cop: 1,
pk: false,
nrqd: false,
rqd: false,
un: false,
ct: 'int(11) unsigned',
ai: false,
unique: false,
cdf: null,
cc: '',
csn: null,
dtx: 'specificType',
dtxp: null,
dtxs: 0,
altered: 1,
};
switch (typeof json[keys[i]]) {
case 'number':
if (Number.isInteger(json[keys[i]])) {
if (MssqlUi.isValidTimestamp(keys[i], json[keys[i]])) {
Object.assign(column, {
dt: 'timestamp',
});
} else {
Object.assign(column, {
dt: 'int',
np: 10,
ns: 0,
});
}
} else {
Object.assign(column, {
dt: 'float',
np: 10,
ns: 2,
dtxp: '11',
dtxs: 2,
});
}
break;
case 'string':
if (MssqlUi.isValidDate(json[keys[i]])) {
Object.assign(column, {
dt: 'datetime',
});
} else if (json[keys[i]].length <= 255) {
Object.assign(column, {
dt: 'varchar',
np: 255,
ns: 0,
dtxp: '255',
});
} else {
Object.assign(column, {
dt: 'text',
});
}
break;
case 'boolean':
Object.assign(column, {
dt: 'bit',
np: null,
ns: 0,
});
break;
case 'object':
Object.assign(column, {
dt: 'varchar',
np: 255,
ns: 0,
dtxp: '255',
});
break;
default:
break;
}
columns.push(column);
}
}
} catch (e) {
console.log('Error in getColumnsFromJson', e);
}
return columns;
}
static isValidTimestamp(key, value) {
if (typeof value !== 'number') {
return false;
}
return new Date(value).getTime() > 0 && /(?:_|(?=A))[aA]t$/.test(key);
}
static isValidDate(value) {
return new Date(value).getTime() > 0;
}
static onCheckboxChangeAU(col) {
console.log(col);
// if (1) {
col.altered = col.altered || 2;
// }
if (col.au) {
col.cdf = 'GETDATE()';
}
// if (!col.ai) {
// col.dtx = 'specificType'
// } else {
// col.dtx = ''
// }
}
static colPropAuDisabled(col) {
if (col.altered !== 1) {
return true;
}
switch (col.dt) {
case 'date':
case 'datetime':
case 'datetime2':
case 'datetimeoffset':
case 'time':
case 'timestamp':
return false;
default:
return true;
}
}
static getAbstractType(col): any {
switch ((col.dt || col.dt).toLowerCase()) {
case 'bigint':
case 'smallint':
case 'bit':
case 'tinyint':
case 'int':
return 'integer';
case 'binary':
return 'string';
case 'char':
return 'string';
case 'date':
return 'date';
case 'datetime':
case 'datetime2':
case 'smalldatetime':
case 'datetimeoffset':
return 'datetime';
case 'decimal':
case 'float':
return 'float';
case 'geography':
case 'geometry':
case 'heirarchyid':
case 'image':
return 'string';
case 'money':
case 'nchar':
return 'string';
case 'ntext':
return 'text';
case 'numeric':
return 'float';
case 'nvarchar':
return 'string';
case 'real':
return 'float';
case 'json':
return 'json';
case 'smallmoney':
case 'sql_variant':
case 'sysname':
return 'string';
case 'text':
return 'text';
case 'time':
return 'time';
case 'timestamp':
return 'timestamp';
case 'uniqueidentifier':
case 'varbinary':
case 'xml':
return 'string';
case 'varchar':
return 'string';
}
return 'string';
}
static getUIType(col): any {
switch (this.getAbstractType(col)) {
case 'integer':
return 'Number';
case 'boolean':
return 'Checkbox';
case 'float':
return 'Decimal';
case 'date':
return 'Date';
case 'datetime':
return 'CreateTime';
case 'time':
return 'Time';
case 'year':
return 'Year';
case 'string':
return 'SingleLineText';
case 'text':
return 'LongText';
case 'blob':
return 'Attachment';
case 'enum':
return 'SingleSelect';
case 'set':
return 'MultiSelect';
case 'json':
return 'LongText';
}
}
static getDataTypeForUiType(
col: { uidt: UITypes },
idType?: IDType
): {
readonly dt: string;
readonly [key: string]: any;
} {
const colProp: any = {};
switch (col.uidt) {
case 'ID':
{
const isAutoIncId = idType === 'AI';
const isAutoGenId = idType === 'AG';
colProp.dt = isAutoGenId ? 'varchar' : 'int';
colProp.pk = true;
colProp.un = isAutoIncId;
colProp.ai = isAutoIncId;
colProp.rqd = true;
colProp.meta = isAutoGenId ? { ag: 'nc' } : undefined;
}
break;
case 'ForeignKey':
colProp.dt = 'varchar';
break;
case 'SingleLineText':
colProp.dt = 'varchar';
break;
case 'LongText':
colProp.dt = 'text';
break;
case 'Attachment':
colProp.dt = 'text';
break;
case 'Checkbox':
colProp.dt = 'tinyint';
colProp.dtxp = 1;
break;
case 'MultiSelect':
colProp.dt = 'text';
break;
case 'SingleSelect':
colProp.dt = 'text';
break;
case 'Collaborator':
colProp.dt = 'varchar';
break;
case 'Date':
colProp.dt = 'varchar';
break;
case 'Year':
colProp.dt = 'int';
break;
case 'Time':
colProp.dt = 'time';
break;
case 'PhoneNumber':
colProp.dt = 'varchar';
colProp.validate = {
func: ['isMobilePhone'],
args: [''],
msg: ['Validation failed : isMobilePhone'],
};
break;
case 'Email':
colProp.dt = 'varchar';
colProp.validate = {
func: ['isEmail'],
args: [''],
msg: ['Validation failed : isEmail'],
};
break;
case 'URL':
colProp.dt = 'varchar';
colProp.validate = {
func: ['isURL'],
args: [''],
msg: ['Validation failed : isURL'],
};
break;
case 'Number':
colProp.dt = 'int';
break;
case 'Decimal':
colProp.dt = 'decimal';
break;
case 'Currency':
colProp.dt = 'decimal';
colProp.validate = {
func: ['isCurrency'],
args: [''],
msg: ['Validation failed : isCurrency'],
};
break;
case 'Percent':
colProp.dt = 'double';
break;
case 'Duration':
colProp.dt = 'decimal';
break;
case 'Rating':
colProp.dt = 'int';
break;
case 'Formula':
colProp.dt = 'varchar';
break;
case 'Rollup':
colProp.dt = 'varchar';
break;
case 'Count':
colProp.dt = 'int';
break;
case 'Lookup':
colProp.dt = 'varchar';
break;
case 'DateTime':
colProp.dt = 'datetimeoffset';
break;
case 'CreateTime':
colProp.dt = 'datetime';
break;
case 'LastModifiedTime':
colProp.dt = 'datetime';
break;
case 'AutoNumber':
colProp.dt = 'int';
break;
case 'Barcode':
colProp.dt = 'varchar';
break;
case 'Button':
colProp.dt = 'varchar';
break;
default:
colProp.dt = 'varchar';
break;
}
return colProp;
}
static getDataTypeListForUiType(col, idType: IDType) {
switch (col.uidt) {
case 'ID':
if (idType === 'AG') {
return ['char', 'ntext', 'text', 'varchar', 'nvarchar'];
} else if (idType === 'AI') {
return ['int', 'bigint', 'bit', 'smallint', 'tinyint'];
} else {
return dbTypes;
}
case 'ForeignKey':
return dbTypes;
case 'SingleLineText':
case 'LongText':
case 'Attachment':
case 'Collaborator':
return ['char', 'ntext', 'text', 'varchar', 'nvarchar'];
case 'JSON':
return ['text', 'ntext'];
case 'Checkbox':
return ['bigint', 'bit', 'int', 'tinyint'];
case 'MultiSelect':
return ['text', 'ntext'];
case 'SingleSelect':
return ['text', 'ntext'];
case 'Year':
return ['int'];
case 'Time':
return ['time'];
case 'PhoneNumber':
case 'Email':
return ['varchar'];
case 'URL':
return ['varchar', 'text'];
case 'Number':
return [
'int',
'bigint',
'bit',
'decimal',
'float',
'numeric',
'real',
'smallint',
'tinyint',
];
case 'Decimal':
return ['decimal', 'float'];
case 'Currency':
return [
'int',
'bigint',
'bit',
'decimal',
'float',
'numeric',
'real',
'smallint',
'tinyint',
];
case 'Percent':
return [
'int',
'bigint',
'bit',
'decimal',
'float',
'numeric',
'real',
'smallint',
'tinyint',
];
case 'Duration':
return [
'int',
'bigint',
'bit',
'decimal',
'float',
'numeric',
'real',
'smallint',
'tinyint',
];
case 'Rating':
return [
'int',
'bigint',
'bit',
'decimal',
'float',
'numeric',
'real',
'smallint',
'tinyint',
];
case 'Formula':
return ['text', 'ntext', 'varchar', 'nvarchar'];
case 'Rollup':
return ['varchar'];
case 'Count':
return ['int', 'bigint', 'smallint', 'tinyint'];
case 'Lookup':
return ['varchar'];
case 'Date':
return ['date'];
case 'DateTime':
case 'CreateTime':
case 'LastModifiedTime':
return [
'datetimeoffset',
'datetime2',
// 'datetime'
];
case 'AutoNumber':
return ['int', 'bigint', 'smallint', 'tinyint'];
case 'Barcode':
return ['varchar'];
case 'Geometry':
return ['geometry'];
case 'Button':
default:
return dbTypes;
}
}
static getUnsupportedFnList() {
return [];
}
}
// module.exports = PgUiHelp;
/**
* @copyright Copyright (c) 2021, Xgene Cloud Ltd
*
* @author Naveen MR <[email protected]>
* @author Pranav C Balan <[email protected]>
*
* @license GNU AGPL version 3 or any later version
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*
*/
| packages/nocodb-sdk/src/lib/sqlUi/MssqlUi.ts | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00017811906582210213,
0.00017262196342926472,
0.00016639442765153944,
0.0001734460238367319,
0.0000027356047667126404
] |
{
"id": 1,
"code_window": [
"\n",
" <div class=\"dot\" />\n",
"\n",
" <SmartsheetToolbarToggleDrawer />\n",
" </div>\n",
"</template>\n",
"\n",
"<style scoped>\n",
":deep(.nc-toolbar-btn) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
" <slot name=\"end\" />\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 19
} | <script setup lang="ts">
import type { FormType, GalleryType, GridType, KanbanType, ViewTypes } from 'nocodb-sdk'
import MenuTop from './MenuTop.vue'
import MenuBottom from './MenuBottom.vue'
import Toolbar from './Toolbar.vue'
import { inject, provide, ref, useApi, useViews, watch } from '#imports'
import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'
const meta = inject(MetaInj, ref())
const activeView = inject(ActiveViewInj, ref())
const { views, loadViews } = useViews(meta)
const { api } = useApi()
provide(ViewListInj, views)
/** Sidebar visible */
const drawerOpen = inject('navDrawerOpen', ref(true))
/** View type to create from modal */
let viewCreateType = $ref<ViewTypes>()
/** View title to create from modal (when duplicating) */
let viewCreateTitle = $ref('')
/** is view creation modal open */
let modalOpen = $ref(false)
/** Watch current views and on change set the next active view */
watch(
views,
(nextViews) => {
if (nextViews.length) {
activeView.value = nextViews[0]
}
},
{ immediate: true },
)
/** Open view creation modal */
function openModal({ type, title = '' }: { type: ViewTypes; title: string }) {
modalOpen = true
viewCreateType = type
viewCreateTitle = title
}
/** Handle view creation */
function onCreate(view: GridType | FormType | KanbanType | GalleryType) {
views.value.push(view)
activeView.value = view
modalOpen = false
}
</script>
<template>
<a-layout-sider class="shadow !mt-[-9px]" style="height: calc(100% + 9px)" theme="light" :width="drawerOpen ? 250 : 50">
<Toolbar v-if="drawerOpen" class="flex items-center py-3 px-3 justify-between border-b-1" />
<Toolbar v-else class="py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]" />
<div v-if="drawerOpen" class="flex-1 flex flex-col">
<MenuTop @open-modal="openModal" @deleted="loadViews" @sorted="loadViews" />
<a-divider class="my-2" />
<MenuBottom @open-modal="openModal" />
</div>
<dlg-view-create v-if="views" v-model="modalOpen" :title="viewCreateTitle" :type="viewCreateType" @created="onCreate" />
</a-layout-sider>
</template>
<style scoped>
:deep(.ant-menu-title-content) {
@apply w-full;
}
:deep(.ant-layout-sider-children) {
@apply flex flex-col;
}
</style>
| packages/nc-gui-v2/components/smartsheet/sidebar/index.vue | 1 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.16498051583766937,
0.018488090485334396,
0.00016792872338555753,
0.00017250757082365453,
0.051792893558740616
] |
{
"id": 1,
"code_window": [
"\n",
" <div class=\"dot\" />\n",
"\n",
" <SmartsheetToolbarToggleDrawer />\n",
" </div>\n",
"</template>\n",
"\n",
"<style scoped>\n",
":deep(.nc-toolbar-btn) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
" <slot name=\"end\" />\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 19
} | ---
title: 'Metadata'
description: 'Metadata'
position: 600
category: 'Product'
menuTitle: 'Metadata'
---
<announcement></announcement>
To go to the Meta Management Portal, click ``Project Metadata`` under ``Settings`` on the leftmost menu.
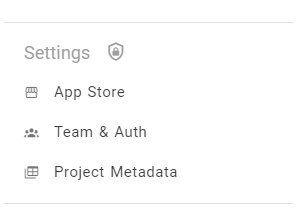
## Project Metadata
The metadata is stored in meta directory in project level, database level, and API level.
Under ``Project Metadata``, you can perform the following operations.
- Export all metadata from the meta tables to meta directory
- Import all metadata from the meta directory to meta tables
- Export project meta to zip file and download
- Import project meta zip file and restart
- Clear all metadata from meta tables
<alert>
Import won't work with zip files exported from the older version of apps (< 0.11.6). <br>
Import / Export will only transfer metadata and files related to the project and not any table data in the project.
</alert>
## Migration example
### Export metadata
Source project : Under ``Meta Management`` tab, select ``Export zip``, click ``Submit``. This step extracts project metadata and stores it in compressed (zip) format

### Import metadata
Destination project : Under ``Meta Management`` tab, select ``Import zip``, select ``meta.zip`` file stored in previous step. This step imports project metadata from compressed file (zip) selected and restarts project.
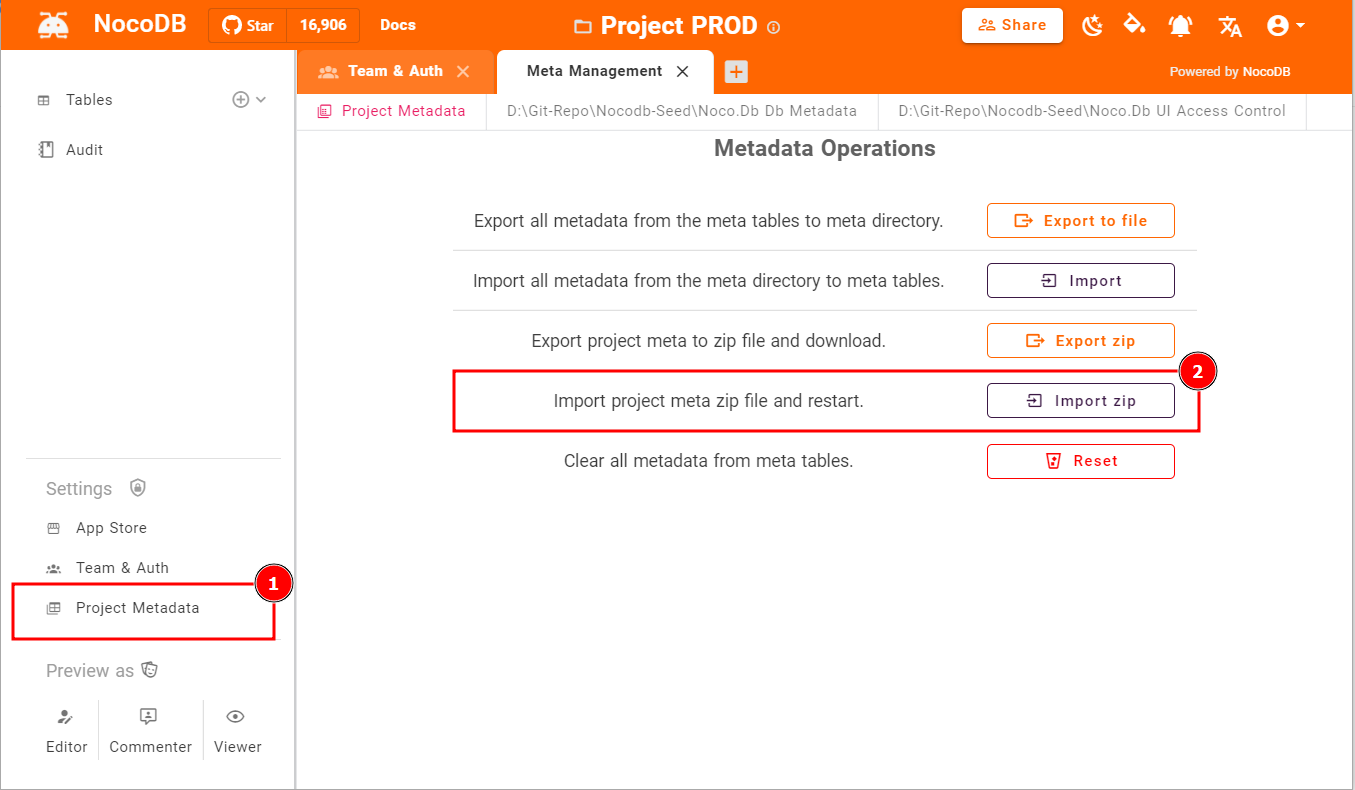
---
## Database Metadata
Under ``DB Metadata``, You can manage your models. For example, if you do not want to expose some APIs, you can untick those under APIs here.
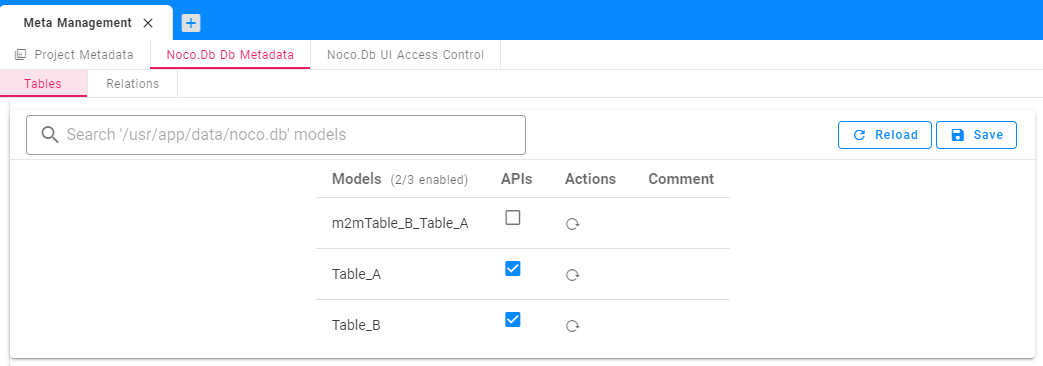
The relations of all tables are listed under ``Relations``.
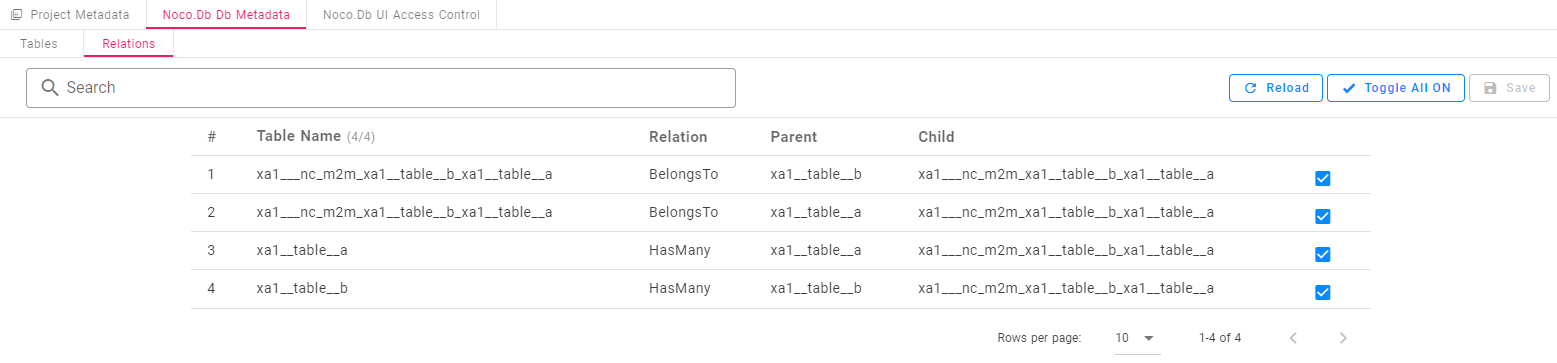
## UI Access Control
You can control the access to each table and relation by roles.

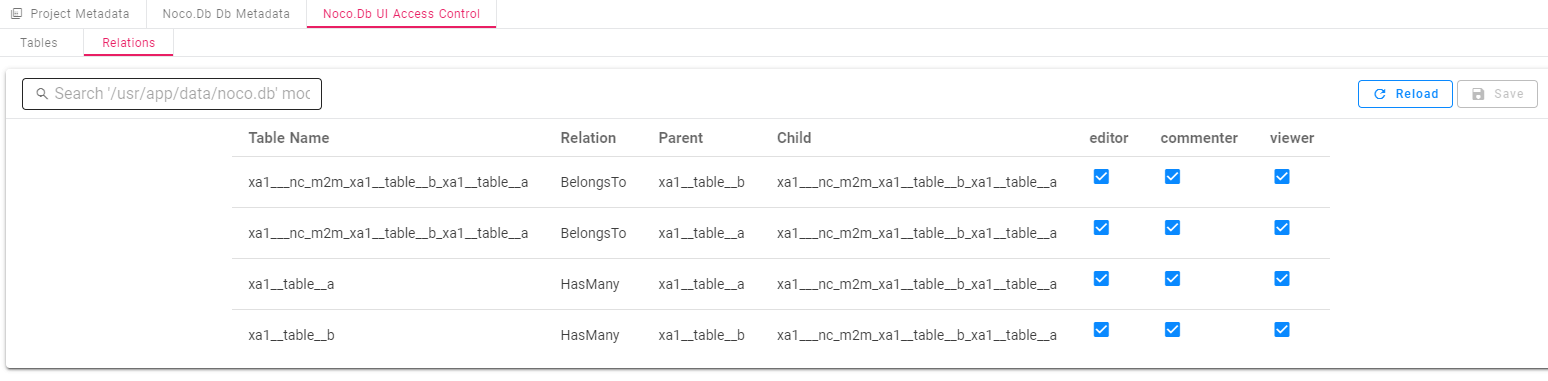
| packages/noco-docs-prev/content/en/setup-and-usages/meta-management.md | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.000174066168256104,
0.00016943344962783158,
0.00016479604528285563,
0.0001696480467217043,
0.0000029917896426923107
] |
{
"id": 1,
"code_window": [
"\n",
" <div class=\"dot\" />\n",
"\n",
" <SmartsheetToolbarToggleDrawer />\n",
" </div>\n",
"</template>\n",
"\n",
"<style scoped>\n",
":deep(.nc-toolbar-btn) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
" <slot name=\"end\" />\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 19
} | import {
MssqlUi,
MysqlUi,
OracleUi,
PgUi,
SqliteUi,
SqlUiFactory,
UITypes,
} from 'nocodb-sdk';
export default class NcTemplateParser {
sqlUi:
| typeof MysqlUi
| typeof MssqlUi
| typeof PgUi
| typeof OracleUi
| typeof SqliteUi;
private _tables: any[];
private client: string;
private _relations: any[];
private _m2mRelations: any[];
private _virtualColumns: { [tn: string]: any[] };
private prefix: string;
private template: any;
constructor({ client, template, prefix = '' }) {
this.client = client;
this.sqlUi = SqlUiFactory.create({ client });
this.template = template;
this.prefix = prefix;
}
public parse(template?: any): any {
const tables = [];
this.template = template || this.template;
const tableTemplates = this.template.tables.map((tableTemplate) => {
const t = {
...tableTemplate,
tn: this.getTable(tableTemplate.tn),
_tn: tableTemplate._tn || tableTemplate.tn,
};
const table = this.extractTable(t);
tables.push(table);
return t;
});
this._tables = tables;
for (const tableTemplate of tableTemplates) {
this.extractRelations(tableTemplate);
this.extractVirtualColumns(tableTemplate);
}
}
private extractTable(tableTemplate) {
if (!tableTemplate?.tn) {
throw Error('Missing table name in template');
}
const defaultColumns = this.sqlUi
.getNewTableColumns()
.filter(
(column) =>
column.cn !== 'title' &&
(column.uidt !== 'ID' ||
tableTemplate.columns.every((c) => c.uidt !== 'ID'))
);
return {
tn: tableTemplate.tn,
_tn: tableTemplate._tn,
columns: [
defaultColumns[0],
...this.extractTableColumns(tableTemplate.columns),
...defaultColumns.slice(1),
],
};
}
private extractTableColumns(tableColumns: any[]) {
const columns = [];
for (const tableColumn of tableColumns) {
if (!tableColumn?.cn) {
throw Error('Missing column name in template');
}
switch (tableColumn.uidt) {
// case UITypes.ForeignKey:
// // todo :
// this.extractRelations(tableColumn, 'bt');
// break;
// case UITypes.LinkToAnotherRecord:
// // todo :
// this.extractRelations(tableColumn, 'hm');
// // this.extractRelations(tableColumn, 'mm');
// break;
default:
{
const colProp = this.sqlUi.getDataTypeForUiType(tableColumn);
columns.push({
...this.sqlUi.getNewColumn(''),
rqd: false,
pk: false,
ai: false,
cdf: null,
un: false,
dtx: 'specificType',
dtxp: this.sqlUi.getDefaultLengthForDatatype(colProp.dt),
dtxs: this.sqlUi.getDefaultScaleForDatatype(colProp.dt),
...colProp,
_cn: tableColumn.cn,
...tableColumn,
});
}
break;
}
}
return columns;
}
protected extractRelations(tableTemplate) {
if (!this._relations) this._relations = [];
if (!this._m2mRelations) this._m2mRelations = [];
for (const hasMany of tableTemplate.hasMany || []) {
const childTable = this.tables.find(
(table) => table.tn === this.getTable(hasMany.tn)
);
const parentTable = this.tables.find(
(table) => table.tn === tableTemplate.tn
);
const parentPrimaryColumn = parentTable.columns.find(
(column) => column.uidt === UITypes.ID
);
//
// // if duplicate relation ignore
// if (
// this._relations.some(rl => {
// return (
// (rl.childTable === childTable.tn &&
// rl.parentTable === parentTable.tn) ||
// (rl.parentTable === childTable.tn &&
// rl.childTable === parentTable.tn)
// );
// })
// ) {
// continue;f
// }
// add a column in child table
const childColumnName = `${tableTemplate.tn}_id`;
childTable.columns.push({
column_name: childColumnName,
_cn: childColumnName,
rqd: false,
pk: false,
ai: false,
cdf: null,
dt: parentPrimaryColumn.dt,
dtxp: parentPrimaryColumn.dtxp,
dtxs: parentPrimaryColumn.dtxs,
un: parentPrimaryColumn.un,
altered: 1,
});
// add relation create entry
this._relations.push({
childColumn: childColumnName,
childTable: childTable.tn,
onDelete: 'NO ACTION',
onUpdate: 'NO ACTION',
parentColumn: parentPrimaryColumn.cn,
parentTable: tableTemplate.tn,
type: this.client === 'sqlite3' ? 'virtual' : 'real',
updateRelation: false,
});
}
for (const manyToMany of tableTemplate.manyToMany || []) {
// @ts-ignore
const childTable = this.tables.find(
(table) => table.tn === this.getTable(manyToMany.rtn)
);
const parentTable = this.tables.find(
(table) => table.tn === tableTemplate.tn
);
const parentPrimaryColumn = parentTable.columns.find(
(column) => column.uidt === UITypes.ID
);
const childPrimaryColumn = childTable.columns.find(
(column) => column.uidt === UITypes.ID
);
// if duplicate relation ignore
if (
this._m2mRelations.some((mm) => {
return (
(mm.childTable === childTable.tn &&
mm.parentTable === parentTable.tn) ||
(mm.parentTable === childTable.tn &&
mm.childTable === parentTable.tn)
);
})
) {
continue;
}
// add many to many relation create entry
this._m2mRelations.push({
alias: 'title8',
childColumn: childPrimaryColumn.cn,
childTable: childTable.tn,
onDelete: 'NO ACTION',
onUpdate: 'NO ACTION',
parentColumn: parentPrimaryColumn.cn,
parentTable: parentTable.tn,
type: this.client === 'sqlite3' ? 'virtual' : 'real',
updateRelation: false,
});
}
}
private extractVirtualColumns(tableMeta) {
if (!this._virtualColumns) this._virtualColumns = {};
const virtualColumns = [];
for (const v of tableMeta.v || []) {
const v1 = { ...v };
if (v.rl) {
v1.rl.rlttn = v1.rl.rltn;
v1.rl.rltn = this.getTable(v1.rl.rltn);
} else if (v.lk) {
v1.lk._ltn = v1.lk.ltn;
v1.lk.ltn = this.getTable(v1.lk.ltn);
}
virtualColumns.push(v1);
}
this.virtualColumns[tableMeta.tn] = virtualColumns;
}
get tables(): any[] {
return this._tables;
}
get relations(): any[] {
return this._relations;
}
get m2mRelations(): any[] {
return this._m2mRelations;
}
get virtualColumns(): { [tn: string]: any[] } {
return this._virtualColumns;
}
private getTable(tn) {
return `${this.prefix}${tn}`;
}
}
| packages/nocodb/src/lib/v1-legacy/templates/NcTemplateParser.ts | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.0006832952494733036,
0.00022266503947321326,
0.00016724081069696695,
0.0001732116797938943,
0.00011658964649541304
] |
{
"id": 1,
"code_window": [
"\n",
" <div class=\"dot\" />\n",
"\n",
" <SmartsheetToolbarToggleDrawer />\n",
" </div>\n",
"</template>\n",
"\n",
"<style scoped>\n",
":deep(.nc-toolbar-btn) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
" <slot name=\"end\" />\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue",
"type": "add",
"edit_start_line_idx": 19
} | <template>
<div class="h-100" style="min-height: 500px">
<v-toolbar v-if="!viewMode" class="elevation-0">
<slot name="toolbar" :valid="valid">
<v-tooltip bottom>
<template #activator="{ on }">
<v-btn small outlined v-on="on" @click="$toast.info('Happy hacking!').goAway(3000)">
<v-icon small class="mr-1"> mdi-file-excel-outline </v-icon>
Import
</v-btn>
</template>
<span class="caption">Create template from Excel</span>
</v-tooltip>
<v-spacer />
<v-icon class="mr-3" @click="helpModal = true"> mdi-information-outline </v-icon>
<v-btn small outlined class="mr-1" @click="project = { tables: [] }">
<v-icon small> mdi-close </v-icon>
Reset
</v-btn>
<v-btn small outlined class="mr-1" @click="createTableClick">
<v-icon small> mdi-plus </v-icon>
New table
</v-btn>
<v-btn color="primary" outlined small class="mr-1" :loading="loading" :disabled="loading" @click="saveTemplate">
{{ id || localId ? 'Update in' : 'Submit to' }} NocoDB
</v-btn>
</slot>
</v-toolbar>
<v-divider class="mt-6" />
<v-container class="text-center" style="height: calc(100% - 64px); overflow-y: auto">
<v-form ref="form" v-model="valid">
<v-row fluid class="justify-center">
<v-col cols="12">
<v-card class="elevation-0">
<v-card-text>
<p v-if="project.tables && quickImportType === 'excel'" class="caption grey--text mt-4">
{{ project.tables.length }} sheet{{ project.tables.length > 1 ? 's' : '' }}
available for import
</p>
<v-expansion-panels
v-if="project.tables && project.tables.length"
v-model="expansionPanel"
:multiple="viewMode"
accordion
>
<v-expansion-panel v-for="(table, i) in project.tables" :key="i">
<v-expansion-panel-header :id="`tn_${table.table_name}`">
<v-text-field
v-if="editableTn[i]"
:value="table.table_name"
class="font-weight-bold"
style="max-width: 300px"
outlinedk
autofocus
dense
hide-details
@input="e => onTableNameUpdate(table, e)"
@click="e => viewMode || e.stopPropagation()"
@blur="$set(editableTn, i, false)"
@keydown.enter="$set(editableTn, i, false)"
/>
<span
v-else
class="font-weight-bold"
@click="e => viewMode || (e.stopPropagation(), $set(editableTn, i, true))"
>
<v-icon color="primary lighten-1">mdi-table</v-icon>
{{ table.table_name }}
</span>
<v-spacer />
<v-tooltip bottom>
<template #activator="{ on }">
<v-icon
v-if="!viewMode && project.tables.length > 1"
class="flex-grow-0 mr-2"
small
color="grey"
@click.stop="deleteTable(i)"
v-on="on"
>
mdi-delete-outline
</v-icon>
</template>
<!-- TODO: i18n -->
<span>Delete Table</span>
</v-tooltip>
</v-expansion-panel-header>
<v-expansion-panel-content>
<!-- <v-toolbar>
<v-spacer></v-spacer>
<v-btn outlined small @click='showColCreateDialog(table,i)'>New Column
</v-btn>
</v-toolbar>-->
<template>
<v-simple-table v-if="table.columns.length" dense class="my-4">
<thead>
<tr>
<th class="caption text-left pa-1">
<!--Column Name-->
{{ $t('labels.columnName') }}
</th>
<th class="caption text-left pa-1" colspan="4">
<!--Column Type-->
{{ $t('labels.columnType') }}
</th>
<th />
<!-- <th class='text-center'>Related Table</th>-->
<!-- <th class='text-center'>Related Column</th>-->
</tr>
</thead>
<tbody>
<tr v-for="(col, j) in table.columns" :key="j" :data-exp="i">
<td class="pa-1 text-left" :style="{ width: viewMode ? '33%' : '15%' }">
<span v-if="viewMode" class="body-1">
{{ col.column_name }}
</span>
<v-text-field
v-else
:ref="`cn_${table.table_name}_${j}`"
:value="col.column_name"
outlined
dense
class="caption"
:placeholder="$t('labels.columnName')"
hide-details="auto"
:rules="[
v => !!v || 'Column name required',
v =>
!table.columns.some(c => c !== col && c.column_name === v) ||
'Duplicate column not allowed',
]"
@input="e => onColumnNameUpdate(col, e, table.table_name)"
/>
</td>
<template v-if="viewMode">
<td
:style="{
width: (viewMode && isRelation(col)) || isLookupOrRollup(col) ? '33%' : '',
}"
:colspan="isRelation(col) || isLookupOrRollup(col) ? 3 : 5"
class="text-left"
>
<v-icon small>
{{ getIcon(col.uidt) }}
</v-icon>
<span class="caption">{{ col.uidt }}</span>
</td>
<td v-if="isRelation(col) || isLookupOrRollup(col)" class="text-left">
<span
v-if="isRelation(col)"
class="caption pointer primary--text"
@click="navigateToTable(col.ref_table_name)"
>
{{ col.ref_table_name }}
</span>
<template v-else-if="isLookup(col)">
<span
class="caption pointer primary--text"
@click="navigateToTable(col.ref_table_name && col.ref_table_name.table_name)"
>
{{ col.ref_table_name && col.ref_table_name.table_name }}
</span>
<span class="caption">({{ col.ref_column_name }})</span>
</template>
<template v-else-if="isRollup(col)">
<span
class="caption pointer primary--text"
@click="navigateToTable(col.ref_table_name && col.ref_table_name.table_name)"
>
{{ col.ref_table_name && col.ref_table_name.table_name }}
</span>
<span class="caption">({{ col.fn }})</span>
</template>
</td>
</template>
<template v-else>
<td class="pa-1 text-left" style="width: 200px; max-width: 200px">
<v-autocomplete
:ref="`uidt_${table.table_name}_${j}`"
style="max-width: 200px"
:value="col.uidt"
placeholder="Column Data Type"
outlined
dense
class="caption"
hide-details="auto"
:rules="[v => !!v || 'Column data type required']"
:items="
col.uidt === 'ForeignKey'
? [
...uiTypes,
{
name: 'ForeignKey',
icon: 'mdi-link-variant',
virtual: 1,
},
]
: uiTypes
"
item-text="name"
item-value="name"
@input="v => onUidtChange(col.uidt, v, col, table)"
>
<template #item="{ item: { name } }">
<v-chip v-if="colors[name]" :color="colors[name]" small>
{{ name }}
</v-chip>
<span v-else class="caption">{{ name }}</span>
</template>
<template #selection="{ item: { name } }">
<v-chip v-if="colors[name]" :color="colors[name]" small style="max-width: 100px">
{{ name }}
</v-chip>
<span v-else class="caption">{{ name }}</span>
</template>
</v-autocomplete>
</td>
<template v-if="isRelation(col) || isLookupOrRollup(col)">
<td class="pa-1 text-left">
<v-autocomplete
:value="col.ref_table_name"
placeholder="Related table"
outlined
class="caption"
dense
hide-details="auto"
:rules="[v => !!v || 'Related table name required', ...getRules(col, table)]"
:items="
isLookupOrRollup(col)
? getRelatedTables(table.table_name, isRollup(col))
: project.tables
"
:item-text="
t => (isLookupOrRollup(col) ? `${t.table_name} (${t.type})` : t.table_name)
"
:item-value="t => (isLookupOrRollup(col) ? t : t.table_name)"
:value-comparator="compareRel"
@input="v => onRtnChange(col.ref_table_name, v, col, table)"
/>
</td>
<td v-if="isRelation(col)" class="pa-1">
<template v-if="col.uidt !== 'ForeignKey'">
<span v-if="viewMode" class="caption">
<!-- {{ col.type }}-->
</span>
<v-autocomplete
v-else
:value="col.type"
placeholder="Relation Type"
outlined
class="caption"
dense
hide-details="auto"
:rules="[v => !!v || 'Relation type required']"
:items="[
{ text: 'Many To Many', value: 'mm' },
{ text: 'Has Many', value: 'hm' },
]"
@input="v => onRTypeChange(col.type, v, col, table)"
/>
</template>
</td>
<td v-if="isLookupOrRollup(col)" class="pa-1">
<span v-if="viewMode" class="caption">
{{ col.ref_column_name }}
</span>
<v-autocomplete
v-else
v-model="col.ref_column_name"
placeholder="Related table column"
outlined
dense
class="caption"
hide-details="auto"
:rules="[v => !!v || 'Related column name required']"
:items="
(
project.tables.find(
t =>
t.table_name ===
((col.ref_table_name && col.ref_table_name.table_name) ||
col.ref_table_name)
) || { columns: [] }
).columns.filter(v => !isVirtual(v))
"
item-text="column_name"
item-value="column_name"
/>
</td>
<td v-if="isRollup(col)" class="pa-1">
<span v-if="viewMode" class="caption">
{{ col.fn }}
</span>
<v-autocomplete
v-else
v-model="col.fn"
placeholder="Rollup function"
outlined
dense
class="caption"
hide-details="auto"
:rules="[v => !!v || 'Rollup aggregate function name required']"
:items="rollupFnList"
/>
</td>
</template>
<template v-if="isSelect(col)">
<td class="pa-1 text-left" colspan="2">
<span v-if="viewMode" class="caption">
{{ col.dtxp }}
</span>
<v-text-field
v-model="col.dtxp"
placeholder="Select options"
outlined
class="caption"
dense
hide-details
/>
</td>
</template>
<td
v-if="!isRollup(col)"
:colspan="
isLookupOrRollup(col) || isRelation(col) || isSelect(col)
? isRollup(col)
? 0
: 1
: 3
"
/>
<td style="max-width: 50px; width: 50px">
<v-tooltip v-if="!viewMode && j == 0" bottom>
<template #activator="{ on }">
<x-icon small class="mr-1" color="primary" v-on="on"> mdi-key-star </x-icon>
</template>
<!-- TODO: i18n -->
<span>Primary Value</span>
</v-tooltip>
<v-tooltip v-else bottom>
<template #activator="{ on }">
<v-icon
class="flex-grow-0"
small
color="grey"
@click.stop="deleteTableColumn(i, j, col, table)"
v-on="on"
>
mdi-delete-outline
</v-icon>
</template>
<!-- TODO: i18n -->
<span>Delete Column</span>
</v-tooltip>
</td>
</template>
</tr>
</tbody>
</v-simple-table>
<div v-if="!viewMode" class="text-center">
<v-tooltip bottom>
<template #activator="{ on }">
<v-icon class="mx-2" small @click="addNewColumnRow(table, 'Number')" v-on="on">
{{ getIcon('Number') }}
</v-icon>
</template>
<!-- TODO: i18n -->
<span>Add Number Column</span>
</v-tooltip>
<v-tooltip bottom>
<template #activator="{ on }">
<v-icon class="mx-2" small @click="addNewColumnRow(table, 'SingleLineText')" v-on="on">
{{ getIcon('SingleLineText') }}
</v-icon>
</template>
<!-- TODO: i18n -->
<span>Add SingleLineText Column</span>
</v-tooltip>
<v-tooltip bottom>
<template #activator="{ on }">
<v-icon class="mx-2" small @click="addNewColumnRow(table, 'LongText')" v-on="on">
{{ getIcon('LongText') }}
</v-icon>
</template>
<!-- TODO: i18n -->
<span>Add LongText Column</span>
</v-tooltip>
<!-- <v-tooltip bottom>
<template #activator="{ on }">
<v-icon
class="mx-2"
small
@click="addNewColumnRow(table, 'LinkToAnotherRecord')"
v-on="on"
>
{{ getIcon("LinkToAnotherRecord") }}
</v-icon>
</template>
<span>Add LinkToAnotherRecord Column</span>
</v-tooltip>
<v-tooltip bottom>
<template #activator="{ on }">
<v-icon
class="mx-2"
small
@click="addNewColumnRow(table, 'Lookup')"
v-on="on"
>
{{ getIcon("Lookup") }}
</v-icon>
</template>
<span>Add Lookup Column</span>
</v-tooltip>
<v-tooltip bottom>
<template #activator="{ on }">
<v-icon
class="mx-2"
small
@click="addNewColumnRow(table, 'Rollup')"
v-on="on"
>
{{ getIcon("Rollup") }}
</v-icon>
</template>
<span>Add Rollup Column</span>
</v-tooltip> -->
<v-tooltip bottom>
<template #activator="{ on }">
<v-btn class="mx-2" small @click="addNewColumnRow(table)" v-on="on"> + column </v-btn>
</template>
<!-- TODO: i18n -->
<span>Add Other Column</span>
</v-tooltip>
</div>
</template>
</v-expansion-panel-content>
</v-expansion-panel>
</v-expansion-panels>
<!-- Disable Gradient Generator at time being -->
<!-- <div v-if="!viewMode" class="mx-auto" style="max-width: 600px">
<template v-if="!quickImport">
<gradient-generator
v-model="project.image_url"
class="d-100 mt-4"
/>
<v-row>
<v-col>
<v-text-field
v-model="project.category"
:rules="[(v) => !!v || 'Category name required']"
class="caption"
outlined
dense
label="Project Category"
/>
</v-col>
<v-col>
<v-text-field
v-model="project.tags"
class="caption"
outlined
dense
label="Project Tags"
/>
</v-col>
</v-row>
<div>
<v-textarea
v-model="project.description"
class="caption"
outlined
dense
label="Project Description"
@click="counter++"
/>
</div>
</template>
</div> -->
</v-card-text>
</v-card>
</v-col>
</v-row>
</v-form>
</v-container>
<v-dialog v-model="createTablesDialog" max-width="500">
<v-card>
<v-card-title>
<!--Enter table name-->
{{ $t('msg.info.enterTableName') }}
</v-card-title>
<v-card-text>
<v-text-field
v-model="tableNamesInput"
autofocus
hide-details
dense
outlined
label="Enter comma separated table names"
@keydown.enter="addTables"
/>
</v-card-text>
<v-card-actions>
<v-spacer />
<v-btn outlined small @click="createTablesDialog = false">
<!-- Cancel -->
{{ $t('general.cancel') }}
</v-btn>
<v-btn outlined color="primary" small @click="addTables">
<!-- Save -->
{{ $t('general.save') }}
</v-btn>
</v-card-actions>
</v-card>
</v-dialog>
<v-dialog v-model="createTableColumnsDialog" max-width="500">
<v-card>
<v-card-title>Enter column name</v-card-title>
<v-card-text>
<v-text-field
v-model="columnNamesInput"
autofocus
dense
outlined
hide-details
label="Enter comma separated column names"
@keydown.enter="addColumns"
/>
</v-card-text>
<v-card-actions>
<v-spacer />
<v-btn outlined small @click="createTableColumnsDialog = false">
<!-- Cancel -->
{{ $t('general.cancel') }}
</v-btn>
<v-btn outlined color="primary" small @click="addColumns">
<!-- Save -->
{{ $t('general.save') }}
</v-btn>
</v-card-actions>
</v-card>
</v-dialog>
<help v-model="helpModal" />
<v-tooltip v-if="!viewMode" left>
<template #activator="{ on }">
<v-btn fixed fab large color="primary" right style="top: 45%" @click="createTableClick" v-on="on">
<v-icon>mdi-plus</v-icon>
</v-btn>
</template>
<span class="caption">
<!--Add new table-->
{{ $t('tooltip.addTable') }}
</span>
</v-tooltip>
</div>
</template>
<script>
import { isVirtualCol } from 'nocodb-sdk';
import { uiTypes, getUIDTIcon, UITypes } from '~/components/project/spreadsheet/helpers/uiTypes';
import GradientGenerator from '~/components/templates/GradientGenerator';
import Help from '~/components/templates/Help';
const LinkToAnotherRecord = 'LinkToAnotherRecord';
const Lookup = 'Lookup';
const Rollup = 'Rollup';
const defaultColProp = {};
export default {
name: 'TemplateEditor',
components: { Help, GradientGenerator },
props: {
id: [Number, String],
viewMode: Boolean,
projectTemplate: Object,
quickImport: Boolean,
quickImportType: String,
},
data: () => ({
loading: false,
localId: null,
valid: false,
url: '',
githubConfigForm: false,
helpModal: false,
editableTn: {},
expansionPanel: 0,
project: {
name: 'Project name',
tables: [],
},
tableNamesInput: '',
columnNamesInput: '',
createTablesDialog: false,
createTableColumnsDialog: false,
selectedTable: null,
uiTypes: uiTypes.filter(t => !isVirtualCol(t.name)),
rollupFnList: [
{ text: 'count', value: 'count' },
{ text: 'min', value: 'min' },
{ text: 'max', value: 'max' },
{ text: 'avg', value: 'avg' },
{ text: 'min', value: 'min' },
{ text: 'sum', value: 'sum' },
{ text: 'countDistinct', value: 'countDistinct' },
{ text: 'sumDistinct', value: 'sumDistinct' },
{ text: 'avgDistinct', value: 'avgDistinct' },
],
colors: {
LinkToAnotherRecord: 'blue lighten-5',
Rollup: 'pink lighten-5',
Lookup: 'green lighten-5',
},
}),
computed: {
counter: {
get() {
return this.$store.state.templateC;
},
set(c) {
this.$store.commit('mutTemplateC', c);
},
},
updateFilename() {
return this.url && this.url.split('/').pop();
},
},
watch: {
project: {
deep: true,
handler() {
const template = {
...this.project,
tables: (this.project.tables || []).map(t => {
const table = {
...t,
columns: [],
hasMany: [],
manyToMany: [],
belongsTo: [],
v: [],
};
for (const column of t.columns || []) {
if (this.isRelation(column)) {
if (column.type === 'hm') {
table.hasMany.push({
table_name: column.ref_table_name,
title: column.column_name,
});
} else if (column.type === 'mm') {
table.manyToMany.push({
ref_table_name: column.ref_table_name,
title: column.column_name,
});
} else if (column.uidt === UITypes.ForeignKey) {
table.belongsTo.push({
table_name: column.ref_table_name,
title: column.column_name,
});
}
} else if (this.isLookup(column)) {
if (column.ref_table_name) {
table.v.push({
title: column.column_name,
lk: {
ltn: column.ref_table_name.table_name,
type: column.ref_table_name.type,
lcn: column.ref_column_name,
},
});
}
} else if (this.isRollup(column)) {
if (column.ref_table_name) {
table.v.push({
title: column.column_name,
rl: {
rltn: column.ref_table_name.table_name,
rlcn: column.ref_column_name,
type: column.ref_table_name.type,
fn: column.fn,
},
});
}
} else {
table.columns.push(column);
}
}
return table;
}),
};
this.$emit('update:projectTemplate', template);
},
},
},
created() {
document.addEventListener('keydown', this.handleKeyDown);
},
destroyed() {
document.removeEventListener('keydown', this.handleKeyDown);
},
mounted() {
this.parseAndLoadTemplate();
const input = this.$refs.projec && this.$refs.project.$el.querySelector('input');
if (input) {
input.focus();
input.select();
}
},
methods: {
createTableClick() {
this.createTablesDialog = true;
this.$e('c:table:create:navdraw');
},
parseAndLoadTemplate() {
if (this.projectTemplate) {
this.parseTemplate(this.projectTemplate);
this.expansionPanel = Array.from({ length: this.project.tables.length }, (_, i) => i);
}
},
getIcon(type) {
return getUIDTIcon(type);
},
getRelatedTables(tableName, rollup = false) {
const tables = [];
for (const t of this.projectTemplate.tables) {
if (tableName === t.table_name) {
for (const hm of t.hasMany) {
const rTable = this.project.tables.find(t1 => t1.table_name === hm.table_name);
tables.push({
...rTable,
type: 'hm',
});
}
for (const mm of t.manyToMany) {
const rTable = this.project.tables.find(t1 => t1.table_name === mm.ref_table_name);
tables.push({
...rTable,
type: 'mm',
});
}
} else {
for (const hm of t.hasMany) {
if (hm.table_name === tableName && !rollup) {
tables.push({
...t,
type: 'bt',
});
}
}
for (const mm of t.manyToMany) {
if (mm.ref_table_name === tableName) {
tables.push({
...t,
type: 'mm',
});
}
}
}
}
return tables;
},
validateAndFocus() {
if (!this.$refs.form.validate()) {
const input = this.$el.querySelector('.v-input.error--text');
this.expansionPanel =
input &&
input.parentElement &&
input.parentElement.parentElement &&
+input.parentElement.parentElement.dataset.exp;
setTimeout(() => {
input.querySelector('input,select').focus();
}, 500);
return false;
}
return true;
},
deleteTable(i) {
const deleteTable = this.project.tables[i];
for (const table of this.project.tables) {
if (table === deleteTable) {
continue;
}
table.columns = table.columns.filter(c => c.ref_table_name !== deleteTable.table_name);
}
this.project.tables.splice(i, 1);
},
deleteTableColumn(i, j, col, table) {
const deleteTable = this.project.tables[i];
const deleteColumn = deleteTable.columns[j];
let rTable, index;
// if relation column, delete the corresponding relation from other table
if (col.uidt === UITypes.LinkToAnotherRecord) {
if (col.type === 'hm') {
rTable = this.project.tables.find(t => t.table_name === col.ref_table_name);
index =
rTable &&
rTable.columns.findIndex(c => c.uidt === UITypes.ForeignKey && c.ref_table_name === table.table_name);
} else if (col.type === 'mm') {
rTable = this.project.tables.find(t => t.table_name === col.ref_table_name);
index =
rTable &&
rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'mm'
);
}
} else if (col.uidt === UITypes.ForeignKey) {
rTable = this.project.tables.find(t => t.table_name === col.ref_table_name);
index =
rTable &&
rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'hm'
);
}
if (rTable && index > -1) {
rTable.columns.splice(index, 1);
}
for (const table of this.project.tables) {
if (table === deleteTable) {
continue;
}
table.columns = table.columns.filter(
c => c.ref_table_name !== deleteTable.table_name || c.ref_column_name !== deleteColumn.column_name
);
}
deleteTable.columns.splice(j, 1);
},
addTables() {
if (!this.tableNamesInput) {
return;
}
// todo: fix
const re = /(?:^|,\s*)(\w+)(?:\(((\w+)(?:\s*,\s*\w+)?)?\)){0,1}(?=\s*,|\s*$)/g;
let m;
// eslint-disable-next-line no-cond-assign
while ((m = re.exec(this.tableNamesInput))) {
if (this.project.tables.some(t => t.table_name === m[1])) {
this.$toast.info(`Table '${m[1]}' is already exist`).goAway(1000);
continue;
}
this.project.tables.push({
table_name: m[1],
columns: (m[2] ? m[2].split(/\s*,\s*/) : [])
.map(col => ({
column_name: col,
...defaultColProp,
}))
.filter((v, i, arr) => i === arr.findIndex(c => c.column_name === v.column_name)),
});
}
this.createTablesDialog = false;
this.tableNamesInput = '';
},
compareRel(a, b) {
return ((a && a.table_name) || a) === ((b && b.table_name) || b) && (a && a.type) === (b && b.type);
},
addColumns() {
if (!this.columnNamesInput) {
return;
}
const table = this.project.tables[this.expansionPanel];
for (const col of this.columnNamesInput.split(/\s*,\s*/)) {
if (table.columns.some(c => c.column_name === col)) {
this.$toast.info(`Column '${col}' is already exist`).goAway(1000);
continue;
}
table.columns.push({
column_name: col,
...defaultColProp,
});
}
this.columnNamesInput = '';
this.createTableColumnsDialog = false;
this.$nextTick(() => {
const input = this.$refs[`uidt_${table.table_name}_${table.columns.length - 1}`][0].$el.querySelector('input');
input.focus();
this.$nextTick(() => {
input.select();
});
});
},
showColCreateDialog(table) {
this.createTableColumnsDialog = true;
this.selectedTable = table;
},
isRelation(col) {
return col.uidt === 'LinkToAnotherRecord' || col.uidt === 'ForeignKey';
},
isLookup(col) {
return col.uidt === 'Lookup';
},
isRollup(col) {
return col.uidt === 'Rollup';
},
isVirtual(col) {
return col && uiTypes.some(ut => ut.name === col.uidt && ut.virtual);
},
isLookupOrRollup(col) {
return this.isLookup(col) || this.isRollup(col);
},
isSelect(col) {
return col.uidt === 'MultiSelect' || col.uidt === 'SingleSelect';
},
addNewColumnRow(table, uidt) {
table.columns.push({
column_name: `title${table.columns.length + 1}`,
...defaultColProp,
uidt,
...(uidt === LinkToAnotherRecord
? {
type: 'mm',
}
: {}),
});
this.$nextTick(() => {
const input = this.$refs[`cn_${table.table_name}_${table.columns.length - 1}`][0].$el.querySelector('input');
input.focus();
input.select();
});
},
async handleKeyDown({ metaKey, key, altKey, shiftKey, ctrlKey }) {
if (!(metaKey && ctrlKey) && !(altKey && shiftKey)) {
return;
}
switch (key && key.toLowerCase()) {
case 't':
this.createTablesDialog = true;
break;
case 'c':
this.createTableColumnsDialog = true;
break;
case 'a':
this.addNewColumnRow(this.project.tables[this.expansionPanel]);
break;
case 'j':
this.copyJSON();
break;
case 's':
await this.saveTemplate();
break;
case 'arrowup':
this.expansionPanel = this.expansionPanel ? --this.expansionPanel : this.project.tables.length - 1;
break;
case 'arrowdown':
this.expansionPanel = ++this.expansionPanel % this.project.tables.length;
break;
case '1':
this.addNewColumnRow(this.project.tables[this.expansionPanel], 'Number');
break;
case '2':
this.addNewColumnRow(this.project.tables[this.expansionPanel], 'SingleLineText');
break;
case '3':
this.addNewColumnRow(this.project.tables[this.expansionPanel], 'LongText');
break;
case '4':
this.addNewColumnRow(this.project.tables[this.expansionPanel], 'LinkToAnotherRecord');
break;
case '5':
this.addNewColumnRow(this.project.tables[this.expansionPanel], 'Lookup');
break;
case '6':
this.addNewColumnRow(this.project.tables[this.expansionPanel], 'Rollup');
break;
}
},
copyJSON() {
if (!this.validateAndFocus()) {
this.$toast.info('Please fill all the required column!').goAway(5000);
return;
}
const el = document.createElement('textarea');
el.addEventListener('focusin', e => e.stopPropagation());
el.value = JSON.stringify(this.projectTemplate, null, 2);
el.style = { position: 'absolute', left: '-9999px' };
document.body.appendChild(el);
el.select();
document.execCommand('copy');
document.body.removeChild(el);
this.$toast.success('Successfully copied JSON data to clipboard!').goAway(3000);
return true;
},
openUrl() {
window.open(this.url, '_blank');
},
async loadUrl() {
try {
let template = (await this.$axios.get(this.url)).data;
if (typeof template === 'string') {
template = JSON.parse(template);
}
this.parseTemplate(template);
} catch (e) {
this.$toast.error(e.message).goAway(5000);
}
},
parseTemplate({ tables = [], ...rest }) {
const parsedTemplate = {
...rest,
tables: tables.map(({ manyToMany = [], hasMany = [], belongsTo = [], v = [], columns = [], ...rest }) => ({
...rest,
columns: [
...columns,
...manyToMany.map(mm => ({
column_name: mm.title || `${rest.table_name} <=> ${mm.ref_table_name}`,
uidt: LinkToAnotherRecord,
type: 'mm',
...mm,
})),
...hasMany.map(hm => ({
column_name: hm.title || `${rest.table_name} => ${hm.table_name}`,
uidt: LinkToAnotherRecord,
type: 'hm',
ref_table_name: hm.table_name,
...hm,
})),
...belongsTo.map(bt => ({
column_name: bt.title || `${rest.table_name} => ${bt.ref_table_name}`,
uidt: UITypes.ForeignKey,
ref_table_name: bt.table_name,
...bt,
})),
...v.map(v => {
const res = {
column_name: v.title,
ref_table_name: {
...v,
},
};
if (v.lk) {
res.uidt = Lookup;
res.ref_table_name.table_name = v.lk.ltn;
res.ref_column_name = v.lk.lcn;
res.ref_table_name.type = v.lk.type;
} else if (v.rl) {
res.uidt = Rollup;
res.ref_table_name.table_name = v.rl.rltn;
res.ref_column_name = v.rl.rlcn;
res.ref_table_name.type = v.rl.type;
res.fn = v.rl.fn;
}
return res;
}),
],
})),
};
this.project = parsedTemplate;
},
async projectTemplateCreate() {
if (!this.validateAndFocus()) {
this.$toast.info('Please fill all the required column!').goAway(5000);
return;
}
try {
const githubConfig = this.$store.state.github;
// const token = await models.store.where({ key: 'GITHUB_TOKEN' }).first()
// const branch = await models.store.where({ key: 'GITHUB_BRANCH' }).first()
// const filePath = await models.store.where({ key: 'GITHUB_FILE_PATH' }).first()
// const templateRepo = await models.store.where({ key: 'PROJECT_TEMPLATES_REPO' }).first()
if (!githubConfig.token || !githubConfig.repo) {
throw new Error('Missing token or template path');
}
const data = JSON.stringify(this.projectTemplate, 0, 2);
const filename = this.updateFilename || `${this.projectTemplate.name}_${Date.now()}.json`;
const filePath = `${githubConfig.filePath ? githubConfig.filePath + '/' : ''}${filename}`;
const apiPath = `https://api.github.com/repos/${githubConfig.repo}/contents/${filePath}`;
let sha;
if (this.updateFilename) {
const {
data: { sha: _sha },
} = await this.$axios({
url: `https://api.github.com/repos/${githubConfig.repo}/contents/${filePath}`,
method: 'get',
headers: {
Authorization: 'token ' + githubConfig.token,
},
});
sha = _sha;
}
await this.$axios({
url: apiPath,
method: 'put',
headers: {
'Content-Type': 'application/json',
Authorization: 'token ' + githubConfig.token,
},
data: {
message: `templates : init template ${filename}`,
content: Base64.encode(data),
sha,
branch: githubConfig.branch,
},
});
this.url = `https://raw.githubusercontent.com/${githubConfig.repo}/${githubConfig.branch}/${filePath}`;
this.$toast.success('Template generated and saved successfully!').goAway(4000);
} catch (e) {
this.$toast.error(e.message).goAway(5000);
}
},
navigateToTable(tn) {
const index = this.projectTemplate.tables.findIndex(t => t.table_name === tn);
if (Array.isArray(this.expansionPanel)) {
this.expansionPanel.push(index);
} else {
this.expansionPanel = index;
}
this.$nextTick(() => {
const accord = this.$el.querySelector(`#tn_${tn}`);
accord.focus();
accord.scrollIntoView();
});
},
async saveTemplate() {
this.loading = true;
try {
if (this.id || this.localId) {
await this.$axios.put(
`${process.env.NC_API_URL}/api/v1/nc/templates/${this.id || this.localId}`,
this.projectTemplate,
{
params: {
token: this.$store.state.template,
},
}
);
this.$toast.success('Template updated successfully').goAway(3000);
} else if (!this.$store.state.template) {
if (!this.copyJSON()) {
return;
}
this.$toast.info('Initiating Github for template').goAway(3000);
const res = await this.$axios.post(
`${process.env.NC_API_URL}/api/v1/projectTemplateCreate`,
this.projectTemplate
);
this.$toast.success('Initiated Github successfully').goAway(3000);
window.open(res.data.path, '_blank');
} else {
const res = await this.$axios.post(`${process.env.NC_API_URL}/api/v1/nc/templates`, this.projectTemplate, {
params: {
token: this.$store.state.template,
},
});
this.localId = res.data.id;
this.$toast.success('Template updated successfully').goAway(3000);
}
this.$emit('saved');
} catch (e) {
this.$toast.error(e.message).goAway(3000);
} finally {
this.loading = false;
}
},
getRules(col, table) {
return v =>
col.uidt !== UITypes.LinkToAnotherRecord ||
!table.columns.some(
c =>
c !== col &&
c.uidt === UITypes.LinkToAnotherRecord &&
c.type === col.type &&
c.ref_table_name === col.ref_table_name
) ||
'Duplicate relation is not allowed';
},
onTableNameUpdate(oldTable, newVal) {
const oldVal = oldTable.table_name;
this.$set(oldTable, 'table_name', newVal);
for (const table of this.project.tables) {
for (const col of table.columns) {
if (col.uidt === UITypes.LinkToAnotherRecord) {
if (col.ref_table_name === oldVal) {
this.$set(col, 'ref_table_name', newVal);
}
} else if (col.uidt === UITypes.Rollup || col.uidt === UITypes.Lookup) {
if (col.ref_table_name && col.ref_table_name.table_name === oldVal) {
this.$set(col.ref_table_name, 'table_name', newVal);
}
}
}
}
},
onColumnNameUpdate(oldCol, newVal, tn) {
const oldVal = oldCol.column_name;
this.$set(oldCol, 'column_name', newVal);
for (const table of this.project.tables) {
for (const col of table.columns) {
if (col.uidt === UITypes.Rollup || col.uidt === UITypes.Lookup) {
if (col.ref_table_name && col.ref_column_name === oldVal && col.ref_table_name.table_name === tn) {
this.$set(col, 'ref_column_name', newVal);
}
}
}
}
},
async onRtnChange(oldVal, newVal, col, table) {
this.$set(col, 'ref_table_name', newVal);
await this.$nextTick();
if (col.uidt !== UITypes.LinkToAnotherRecord && col.uidt !== UITypes.ForeignKey) {
return;
}
if (oldVal) {
const rTable = this.project.tables.find(t => t.table_name === oldVal);
// delete relation from other table if exist
let index = -1;
if (col.uidt === UITypes.LinkToAnotherRecord && col.type === 'mm') {
index = rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'mm'
);
} else if (col.uidt === UITypes.LinkToAnotherRecord && col.type === 'hm') {
index = rTable.columns.findIndex(c => c.uidt === UITypes.ForeignKey && c.ref_table_name === table.table_name);
} else if (col.uidt === UITypes.ForeignKey) {
index = rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'hm'
);
}
if (index > -1) {
rTable.columns.splice(index, 1);
}
}
if (newVal) {
const rTable = this.project.tables.find(t => t.table_name === newVal);
// check relation relation exist in other table
// if not create a relation
if (col.uidt === UITypes.LinkToAnotherRecord && col.type === 'mm') {
if (
!rTable.columns.find(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'mm'
)
) {
rTable.columns.push({
column_name: `title${rTable.columns.length + 1}`,
uidt: UITypes.LinkToAnotherRecord,
type: 'mm',
ref_table_name: table.table_name,
});
}
} else if (col.uidt === UITypes.LinkToAnotherRecord && col.type === 'hm') {
if (!rTable.columns.find(c => c.uidt === UITypes.ForeignKey && c.ref_table_name === table.table_name)) {
rTable.columns.push({
column_name: `title${rTable.columns.length + 1}`,
uidt: UITypes.ForeignKey,
ref_table_name: table.table_name,
});
}
} else if (col.uidt === UITypes.ForeignKey) {
if (
!rTable.columns.find(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'hm'
)
) {
rTable.columns.push({
column_name: `title${rTable.columns.length + 1}`,
uidt: UITypes.LinkToAnotherRecord,
type: 'hm',
ref_table_name: table.table_name,
});
}
}
}
},
onRTypeChange(oldType, newType, col, table) {
this.$set(col, 'type', newType);
const rTable = this.project.tables.find(t => t.table_name === col.ref_table_name);
let index = -1;
// find column and update relation
// or create a new column
if (oldType === 'hm') {
index = rTable.columns.findIndex(c => c.uidt === UITypes.ForeignKey && c.ref_table_name === table.table_name);
} else if (oldType === 'mm') {
index = rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'mm'
);
}
const rCol = index === -1 ? { column_name: `title${rTable.columns.length + 1}` } : { ...rTable.columns[index] };
index = index === -1 ? rTable.columns.length : index;
if (newType === 'mm') {
rCol.type = 'mm';
rCol.uidt = UITypes.LinkToAnotherRecord;
} else if (newType === 'hm') {
rCol.type = 'bt';
rCol.uidt = UITypes.ForeignKey;
}
rCol.ref_table_name = table.table_name;
this.$set(rTable.columns, index, rCol);
},
onUidtChange(oldVal, newVal, col, table) {
this.$set(col, 'uidt', newVal);
this.$set(col, 'dtxp', undefined);
// delete relation column from other table
// if previous type is relation
let index = -1;
let rTable;
if (oldVal === UITypes.LinkToAnotherRecord) {
rTable = this.project.tables.find(t => t.table_name === col.ref_table_name);
if (rTable) {
if (col.type === 'hm') {
index = rTable.columns.findIndex(
c => c.uidt === UITypes.ForeignKey && c.ref_table_name === table.table_name
);
} else if (col.type === 'mm') {
index = rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'mm'
);
}
}
} else if (oldVal === UITypes.ForeignKey) {
rTable = this.project.tables.find(t => t.table_name === col.ref_table_name);
if (rTable) {
index = rTable.columns.findIndex(
c => c.uidt === UITypes.LinkToAnotherRecord && c.ref_table_name === table.table_name && c.type === 'hm'
);
}
}
if (rTable && index > -1) {
rTable.columns.splice(index, 1);
}
col.ref_table_name = undefined;
col.type = undefined;
col.ref_column_name = undefined;
if (col.uidt === LinkToAnotherRecord) {
col.type = col.type || 'mm';
}
},
},
};
</script>
<style scoped>
/deep/ .v-select__selections {
flex-wrap: nowrap;
}
</style>
| packages/nc-gui/components/templates/Editor.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.0013462776551023126,
0.00023327255621552467,
0.00016345473704859614,
0.00017224524344783276,
0.00017523921269457787
] |
{
"id": 2,
"code_window": [
"import MenuBottom from './MenuBottom.vue'\n",
"import Toolbar from './Toolbar.vue'\n",
"import { inject, provide, ref, useApi, useViews, watch } from '#imports'\n",
"import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'\n",
"\n",
"const meta = inject(MetaInj, ref())\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import MdiXml from '~icons/mdi/xml'\n",
"import MdiHook from '~icons/mdi/hook'\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 7
} | <script setup lang="ts">
import type { FormType, GalleryType, GridType, KanbanType, ViewTypes } from 'nocodb-sdk'
import MenuTop from './MenuTop.vue'
import MenuBottom from './MenuBottom.vue'
import Toolbar from './Toolbar.vue'
import { inject, provide, ref, useApi, useViews, watch } from '#imports'
import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'
const meta = inject(MetaInj, ref())
const activeView = inject(ActiveViewInj, ref())
const { views, loadViews } = useViews(meta)
const { api } = useApi()
provide(ViewListInj, views)
/** Sidebar visible */
const drawerOpen = inject('navDrawerOpen', ref(true))
/** View type to create from modal */
let viewCreateType = $ref<ViewTypes>()
/** View title to create from modal (when duplicating) */
let viewCreateTitle = $ref('')
/** is view creation modal open */
let modalOpen = $ref(false)
/** Watch current views and on change set the next active view */
watch(
views,
(nextViews) => {
if (nextViews.length) {
activeView.value = nextViews[0]
}
},
{ immediate: true },
)
/** Open view creation modal */
function openModal({ type, title = '' }: { type: ViewTypes; title: string }) {
modalOpen = true
viewCreateType = type
viewCreateTitle = title
}
/** Handle view creation */
function onCreate(view: GridType | FormType | KanbanType | GalleryType) {
views.value.push(view)
activeView.value = view
modalOpen = false
}
</script>
<template>
<a-layout-sider class="shadow !mt-[-9px]" style="height: calc(100% + 9px)" theme="light" :width="drawerOpen ? 250 : 50">
<Toolbar v-if="drawerOpen" class="flex items-center py-3 px-3 justify-between border-b-1" />
<Toolbar v-else class="py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]" />
<div v-if="drawerOpen" class="flex-1 flex flex-col">
<MenuTop @open-modal="openModal" @deleted="loadViews" @sorted="loadViews" />
<a-divider class="my-2" />
<MenuBottom @open-modal="openModal" />
</div>
<dlg-view-create v-if="views" v-model="modalOpen" :title="viewCreateTitle" :type="viewCreateType" @created="onCreate" />
</a-layout-sider>
</template>
<style scoped>
:deep(.ant-menu-title-content) {
@apply w-full;
}
:deep(.ant-layout-sider-children) {
@apply flex flex-col;
}
</style>
| packages/nc-gui-v2/components/smartsheet/sidebar/index.vue | 1 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.9982000589370728,
0.2219301015138626,
0.00016517910989932716,
0.00017782939539756626,
0.41482987999916077
] |
{
"id": 2,
"code_window": [
"import MenuBottom from './MenuBottom.vue'\n",
"import Toolbar from './Toolbar.vue'\n",
"import { inject, provide, ref, useApi, useViews, watch } from '#imports'\n",
"import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'\n",
"\n",
"const meta = inject(MetaInj, ref())\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import MdiXml from '~icons/mdi/xml'\n",
"import MdiHook from '~icons/mdi/hook'\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 7
} | // import path from "path";
// import mkdirp from "mkdirp";
// import {promisify} from "util";
//
// import ejs from "ejs";
// import fs from "fs";
// import js_beautify from "js-beautify";
// import fsExtra from "fs-extra";
// import md5 from "md5";
// import dayjs from "dayjs";import Emit from "../../sql-migrator/util/emit";
// const beautify = js_beautify.js;
import Debug from '../../util/Debug';
import Emit from '../../util/emit';
class BaseRender {
protected dir: any;
protected filename: any;
protected ctx: any;
protected evt: any;
protected log: any;
protected ejsContent: any;
/**
* Class responsible for rendering code
*
* @param {string} - dir - dir where file will be rendered
* @param {string} - filename - filename of file to be rendered
* @param {Object} - ctx - context to render this file
*/
constructor({ dir = '', filename = '', ctx }) {
this.dir = dir;
this.filename = filename;
this.ctx = ctx;
this.evt = new Emit();
this.log = new Debug('BaseRender');
}
emit(data) {
this.log.api(data);
this.evt.evt.emit('UI', {
status: 0,
data: `File : ${data}`,
});
}
emitW(data) {
this.log.warn(data);
this.evt.evt.emit('UI', {
status: 1,
data: `File : ${data}`,
});
}
emitE(data) {
this.log.error(data);
this.evt.evt.emit('UI', {
status: -1,
data: `File : ${data}`,
});
}
/**
* Function that prepares data to be used in template
* This should be strictly overridden by deriving class
*
* @returns {Promise<void>}
*/
async prepare() {
console.log('BaseRender::prepare -> Should be overriden');
}
/**
* Renders the ejs code template using the data sent
* @param {String} - ejsPath - path to ejs file template
* @param {Object} - ejsData - data to be rendered
* @param {Boolean} - force - on true overwrites the file
* @returns {Promise<void>}
*/
/*
async render(obj) {
const {ejsPath, ejsData, force = false, writeFile = true} = obj;
const {ejsContent} = this;
try {
const fileExists = await promisify(fs.exists)(path.join(this.dir, this.filename));
/!* file exists and *!/
if (writeFile && fileExists && !force)
return;
/!* ejs render the file *!/
let generatedCode = null;
generatedCode = ejs.render(ejsContent, {data: ejsData});
if (!process.env.TS_ENABLED) {
/!* prettify the received file *!/
generatedCode = beautify(generatedCode, {indent_size: 2, space_in_empty_paren: true});
} else {
generatedCode = beautify(generatedCode, {indent_size: 2, space_in_empty_paren: true});
}
if (writeFile) {
/!* create dir if not exists *!/
await promisify(mkdirp)(this.dir);
/!* Take a backup of the file *!/
if (force && fileExists) {
/!* newFileName = oldFileName + Date + extension *!/
let newFileName = this.filename.split('.')
const extension = newFileName.pop();
newFileName.push(dayjs().format('YYMMDD_HHmmss'));
newFileName.push(extension);
newFileName = newFileName.join('.');
if (md5(generatedCode) === md5(fs.readFileSync(path.join(this.dir, this.filename)))) return;
await fsExtra.copy(path.join(this.dir, this.filename), path.join(this.dir, newFileName));
}
/!* create file *!/
fs.writeFileSync(path.join(this.dir, this.filename), generatedCode, 'utf8');
} else {
return generatedCode;
}
} catch (e) {
console.log(`Error rendering template ${ejsPath} in ${this.dir}/${this.filename}\n\n`, e);
throw e;
}
}
*/
}
export default BaseRender;
| packages/nocodb/src/lib/db/sql-mgr/code/BaseRender.ts | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00017807507538236678,
0.00017389818094670773,
0.000167272228281945,
0.00017409960855729878,
0.0000029607706437673187
] |
{
"id": 2,
"code_window": [
"import MenuBottom from './MenuBottom.vue'\n",
"import Toolbar from './Toolbar.vue'\n",
"import { inject, provide, ref, useApi, useViews, watch } from '#imports'\n",
"import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'\n",
"\n",
"const meta = inject(MetaInj, ref())\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import MdiXml from '~icons/mdi/xml'\n",
"import MdiHook from '~icons/mdi/hook'\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 7
} | <script setup lang="ts">
interface Props {
modelValue: number
}
const { modelValue: value } = defineProps<Props>()
const emit = defineEmits(['update:modelValue'])
const editEnabled = inject<boolean>('editEnabled')
const root = ref<HTMLInputElement>()
const localState = computed({
get: () => value,
set: (val) => emit('update:modelValue', val),
})
onMounted(() => {
root.value?.focus()
})
</script>
<template>
<input v-if="editEnabled" ref="root" v-model="localState" type="number" />
<span v-else>{{ localState }}</span>
</template>
<style scoped>
input {
outline: none;
width: 100%;
height: 100%;
color: var(--v-textColor-base);
}
</style>
| packages/nc-gui-v2/components/cell/Integer.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00017603521700948477,
0.0001730669755488634,
0.00017069933528546244,
0.00017276668222621083,
0.000001988090161830769
] |
{
"id": 2,
"code_window": [
"import MenuBottom from './MenuBottom.vue'\n",
"import Toolbar from './Toolbar.vue'\n",
"import { inject, provide, ref, useApi, useViews, watch } from '#imports'\n",
"import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'\n",
"\n",
"const meta = inject(MetaInj, ref())\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import MdiXml from '~icons/mdi/xml'\n",
"import MdiHook from '~icons/mdi/hook'\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 7
} | <script setup lang="ts">
import type { ColumnType } from 'nocodb-sdk'
import ItemChip from './components/ItemChip.vue'
import { ColumnInj } from '~/context'
import useBelongsTo from '~/composables/useBelongsTo'
const column = inject(ColumnInj)
const value = inject('value')
const active = false
const localState = null
const { parentMeta, loadParentMeta, primaryValueProp } = useBelongsTo(column as ColumnType)
await loadParentMeta()
// import ApiFactory from '@/components/project/spreadsheet/apis/apiFactory'
/* import { RelationTypes, UITypes, isSystemColumn } from 'nocodb-sdk'
import ListItems from '~/components/project/spreadsheet/components/virtualCell/components/ListItems'
import ListChildItems from '~/components/project/spreadsheet/components/virtualCell/components/ListChildItems'
import ItemChip from '~/components/project/spreadsheet/components/virtualCell/components/ItemChip'
import { parseIfInteger } from '@/helpers'
export default {
name: 'BelongsToCell',
components: { ListChildItems, ItemChip, ListItems },
props: {
isLocked: Boolean,
breadcrumbs: {
type: Array,
default() {
return []
},
},
isForm: Boolean,
value: [Array, Object],
meta: [Object],
nodes: [Object],
row: [Object],
api: [Object, Function],
sqlUi: [Object, Function],
active: Boolean,
isNew: Boolean,
disabledColumns: Object,
isPublic: Boolean,
metas: Object,
password: String,
column: Object,
},
data: () => ({
newRecordModal: false,
parentListModal: false,
// parentMeta: null,
list: null,
childList: null,
dialogShow: false,
confirmAction: null,
confirmMessage: '',
selectedParent: null,
isNewParent: false,
expandFormModal: false,
localState: null,
pid: null,
}),
computed: {
parentMeta() {
return this.metas
? this.metas[this.column.colOptions.fk_related_model_id]
: this.$store.state.meta.metas[this.column.colOptions.fk_related_model_id]
},
// todo : optimize
parentApi() {},
parentId() {
return (
this.pid ??
(this.value &&
this.parentMeta &&
this.parentMeta.columns
.filter((c) => c.pk)
.map((c) => this.value[c.title])
.join('___'))
)
},
rowId() {
return (
this.row &&
this.meta &&
this.meta.columns
.filter((c) => c.pk)
.map((c) => this.row[c.title])
.join('___')
)
},
parentPrimaryCol() {
return this.parentMeta && (this.parentMeta.columns.find((c) => c.pv) || {}).title
},
parentPrimaryKey() {
return this.parentMeta && (this.parentMeta.columns.find((c) => c.pk) || {}).title
},
parentReferenceKey() {
return (
this.parentMeta && (this.parentMeta.columns.find((c) => c.id === this.column.colOptions.fk_parent_column_id) || {}).title
)
},
btWhereClause() {
// if parent reference key is pk, then filter out the selected value
// else, filter out the selected value + empty values (as we can't set an empty value)
const prk = this.parentReferenceKey
const selectedValue =
this.meta && this.meta.columns
? this.meta.columns
.filter((c) => c.id === this.column.colOptions.fk_child_column_id)
.map((c) => this.row[c.title] || '')
.join('___')
: ''
return `(${prk},not,${selectedValue})~or(${prk},is,null)`
},
parentQueryParams() {
if (!this.parentMeta) {
return {}
}
// todo: use reduce
return {}
},
parentAvailableColumns() {
if (!this.parentMeta) {
return []
}
const columns = []
if (this.parentMeta.columns) {
columns.push(...this.parentMeta.columns.filter((c) => !isSystemColumn(c)))
}
return columns
},
// todo:
form() {
return this.selectedParent && !this.isPublic
? () => import('~/components/project/spreadsheet/components/ExpandedForm')
: 'span'
},
cellValue() {
if (this.value || this.localState) {
if (this.parentMeta && this.parentPrimaryCol) {
return (this.value || this.localState)[this.parentPrimaryCol]
}
return Object.values(this.value || this.localState)[1]
}
return null
},
},
watch: {
isNew(n, o) {
if (!n && o) {
this.localState = null
this.$emit('update:localState', this.localState)
}
},
},
async mounted() {
if (this.isNew && this.value) {
this.localState = this.value
}
if (this.isForm) {
await this.loadParentMeta()
}
},
created() {
this.loadParentMeta()
},
methods: {
async onParentSave(parent) {
if (this.isNewParent) {
await this.addChildToParent(parent)
} else {
this.$emit('loadTableData')
}
},
async insertAndMapNewParentRecord() {
await this.loadParentMeta()
this.newRecordModal = false
this.isNewParent = true
this.selectedParent = {
[(
this.parentMeta.columns.find(
(c) =>
c.uidt === UITypes.LinkToAnotherRecord &&
c.colOptions &&
this.column.colOptions &&
c.colOptions.fk_child_column_id === this.column.colOptions.fk_child_column_id &&
c.colOptions.fk_parent_column_id === this.column.colOptions.fk_parent_column_id &&
c.colOptions.type === RelationTypes.HAS_MANY,
) || {}
).title]: [this.row],
}
this.expandFormModal = true
},
async unlink(parent) {
const column = this.meta.columns.find((c) => c.id === this.column.colOptions.fk_child_column_id)
const _cn = column.title
if (this.isNew) {
this.$emit('updateCol', this.row, _cn, null)
this.localState = null
this.$emit('update:localState', this.localState)
return
}
if (column.rqd) {
this.$toast.info('Unlink is not possible, instead map to another parent.').goAway(3000)
return
}
const id = this.meta.columns
.filter((c) => c.pk)
.map((c) => this.row[c.title])
.join('___')
// todo: audit
await this.$api.dbTableRow.nestedRemove(
NOCO,
this.projectName,
this.meta.title,
id,
'bt',
this.column.title,
parent[this.parentPrimaryKey],
)
this.$emit('loadTableData')
if (this.isForm && this.$refs.childList) {
this.$refs.childList.loadData()
}
},
async showParentListModal() {
this.parentListModal = true
await this.loadParentMeta()
const pid = this.meta.columns
.filter((c) => c.pk)
.map((c) => this.row[c.title])
.join('___')
const _cn = this.parentMeta.columns.find((c) => c.column_name === this.hm.column_name).title
this.childList = await this.parentApi.paginatedList({
where: `(${_cn},eq,${pid})`,
})
},
async removeChild(child) {
this.dialogShow = true
this.confirmMessage = 'Do you want to delete the record?'
this.confirmAction = async (act) => {
if (act === 'hideDialog') {
this.dialogShow = false
} else {
const id = this.parentMeta.columns
.filter((c) => c.pk)
.map((c) => child[c.title])
.join('___')
await this.parentApi.delete(id)
this.pid = null
this.dialogShow = false
this.$emit('loadTableData')
if (this.isForm && this.$refs.childList) {
this.$refs.childList.loadData()
}
}
}
},
async loadParentMeta() {
// todo: optimize
if (!this.parentMeta) {
await this.$store.dispatch('meta/ActLoadMeta', {
env: this.nodes.env,
dbAlias: this.nodes.dbAlias,
id: this.column.colOptions.fk_related_model_id,
})
}
},
async showNewRecordModal() {
await this.loadParentMeta()
this.newRecordModal = true
},
async addChildToParent(parent) {
const pid = this._extractRowId(parent, this.parentMeta)
const id = this._extractRowId(this.row, this.meta)
const _cn = this.meta.columns.find((c) => c.id === this.column.colOptions.fk_child_column_id).title
if (this.isNew) {
const _rcn = this.parentMeta.columns.find((c) => c.id === this.column.colOptions.fk_parent_column_id).title
this.localState = parent
this.$emit('update:localState', this.localState)
this.$emit('updateCol', this.row, _cn, parent[_rcn])
this.newRecordModal = false
return
}
await this.$api.dbTableRow.nestedAdd(NOCO, this.projectName, this.meta.title, id, 'bt', this.column.title, pid)
this.pid = pid
this.newRecordModal = false
this.$emit('loadTableData')
if (this.isForm && this.$refs.childList) {
this.$refs.childList.loadData()
}
},
async editParent(parent) {
await this.loadParentMeta()
this.isNewParent = false
this.selectedParent = parent
this.expandFormModal = true
setTimeout(() => {
this.$refs.expandedForm && this.$refs.expandedForm.reload()
}, 500)
},
},
} */
</script>
<template>
<div class="d-flex d-100 chips-wrapper" :class="{ active }">
<!-- <template v-if="!isForm"> -->
<div class="chips d-flex align-center img-container flex-grow-1 hm-items">
<template v-if="value || localState">
<ItemChip :active="active" :item="value" :value="value[primaryValueProp]" />
<!-- :readonly="isLocked || (isPublic && !isForm)"
@edit="editParent"
@unlink="unlink" -->
</template>
</div>
<!-- <div
v-if="!isLocked && _isUIAllowed('xcDatatableEditable') && (isForm || !isPublic)"
class="action align-center justify-center px-1 flex-shrink-1"
:class="{ 'd-none': !active, 'd-flex': active }"
>
<x-icon small :color="['primary', 'grey']" @click="showNewRecordModal">
{{ value ? 'mdi-arrow-expand' : 'mdi-plus' }}
</x-icon>
</div> -->
<!-- </template> -->
<!-- <ListItems
v-if="newRecordModal"
:key="parentId"
v-model="newRecordModal"
:size="10"
:meta="parentMeta"
:column="column"
:primary-col="parentPrimaryCol"
:primary-key="parentPrimaryKey"
:parent-meta="meta"
:api="parentApi"
:query-params="{
...parentQueryParams,
where: isNew ? null : `${btWhereClause}`,
}"
:is-public="isPublic"
:tn="bt && bt.rtn"
:password="password"
:row-id="rowId"
@add-new-record="insertAndMapNewParentRecord"
@add="addChildToParent"
/>
<ListChildItems
v-if="parentMeta && isForm"
ref="childList"
:is-form="isForm"
:local-state="localState ? [localState] : []"
:is-new="isNew"
:size="10"
:parent-meta="parentMeta"
:meta="parentMeta"
:primary-col="parentPrimaryCol"
:primary-key="parentPrimaryKey"
:api="parentApi"
:query-params="{
...parentQueryParams,
where: `(${parentPrimaryKey},eq,${parentId})`,
}"
:bt="value"
:is-public="isPublic"
:row-id="parentId"
@new-record="showNewRecordModal"
@edit="editParent"
@unlink="unlink"
/>
<v-dialog
v-if="!isPublic && selectedParent"
v-model="expandFormModal"
:overlay-opacity="0.8"
width="1000px"
max-width="100%"
class="mx-auto"
>
<component
:is="form"
v-if="selectedParent"
ref="expandedForm"
v-model="selectedParent"
v-model:is-new="isNewParent"
:db-alias="nodes.dbAlias"
:has-many="parentMeta.hasMany"
:belongs-to="parentMeta.belongsTo"
:table="parentMeta.table_name"
:old-row="{ ...selectedParent }"
:meta="parentMeta"
:sql-ui="sqlUi"
:primary-value-column="parentPrimaryCol"
:api="parentApi"
:available-columns="parentAvailableColumns"
:nodes="nodes"
:query-params="parentQueryParams"
icon-color="warning"
:breadcrumbs="breadcrumbs"
@cancel="
selectedParent = null
expandFormModal = false
"
@input="onParentSave"
/>
</v-dialog> -->
</div>
</template>
<style scoped lang="scss">
.items-container {
overflow-x: visible;
max-height: min(500px, 60vh);
overflow-y: auto;
}
.primary-value {
.primary-key {
display: none;
margin-left: 0.5em;
}
&:hover .primary-key {
display: inline;
}
}
.child-card {
cursor: pointer;
&:hover {
box-shadow: 0 0 0.2em var(--v-textColor-lighten5);
}
}
.hm-items {
flex-wrap: wrap;
row-gap: 3px;
gap: 3px;
margin: 3px auto;
}
.chips-wrapper {
.chips {
max-width: 100%;
}
&.active {
.chips {
max-width: calc(100% - 22px);
}
}
}
</style>
<!--
/**
* @copyright Copyright (c) 2021, Xgene Cloud Ltd
*
* @author Naveen MR <[email protected]>
* @author Pranav C Balan <[email protected]>
* @author Md Ishtiaque Zafar <[email protected]>
* @author Wing-Kam Wong <[email protected]>
*
* @license GNU AGPL version 3 or any later version
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*
*/
-->
| packages/nc-gui-v2/components/virtual-cell/BelongsTo.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00040514214197173715,
0.00018511951202526689,
0.00016351848898921162,
0.00017439354269299656,
0.00004621150947059505
] |
{
"id": 3,
"code_window": [
"\n",
"<template>\n",
" <a-layout-sider class=\"shadow !mt-[-9px]\" style=\"height: calc(100% + 9px)\" theme=\"light\" :width=\"drawerOpen ? 250 : 50\">\n",
" <Toolbar v-if=\"drawerOpen\" class=\"flex items-center py-3 px-3 justify-between border-b-1\" />\n",
"\n",
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\" />\n",
"\n",
" <div v-if=\"drawerOpen\" class=\"flex-1 flex flex-col\">\n",
" <MenuTop @open-modal=\"openModal\" @deleted=\"loadViews\" @sorted=\"loadViews\" />\n",
"\n",
" <a-divider class=\"my-2\" />\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\">\n",
" <template #start>\n",
" <MdiHook class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
"\n",
" <MdiXml class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
" </template>\n",
" </Toolbar>\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "replace",
"edit_start_line_idx": 60
} | <template>
<div class="flex gap-2">
<SmartsheetToolbarLockMenu />
<div class="dot" />
<SmartsheetToolbarReload />
<div class="dot" />
<SmartsheetToolbarAddRow />
<div class="dot" />
<SmartsheetToolbarDeleteTable />
<div class="dot" />
<SmartsheetToolbarToggleDrawer />
</div>
</template>
<style scoped>
:deep(.nc-toolbar-btn) {
@apply border-0 !text-xs font-semibold px-2;
}
.dot {
@apply w-[3px] h-[3px] bg-gray-300 rounded-full;
}
</style>
| packages/nc-gui-v2/components/smartsheet/sidebar/Toolbar.vue | 1 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.0006308371666818857,
0.0003044494951609522,
0.00016556245100218803,
0.00021069917420390993,
0.00019044541113544255
] |
{
"id": 3,
"code_window": [
"\n",
"<template>\n",
" <a-layout-sider class=\"shadow !mt-[-9px]\" style=\"height: calc(100% + 9px)\" theme=\"light\" :width=\"drawerOpen ? 250 : 50\">\n",
" <Toolbar v-if=\"drawerOpen\" class=\"flex items-center py-3 px-3 justify-between border-b-1\" />\n",
"\n",
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\" />\n",
"\n",
" <div v-if=\"drawerOpen\" class=\"flex-1 flex flex-col\">\n",
" <MenuTop @open-modal=\"openModal\" @deleted=\"loadViews\" @sorted=\"loadViews\" />\n",
"\n",
" <a-divider class=\"my-2\" />\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\">\n",
" <template #start>\n",
" <MdiHook class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
"\n",
" <MdiXml class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
" </template>\n",
" </Toolbar>\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "replace",
"edit_start_line_idx": 60
} | <template>
<v-container fluid class="wrapper">
<v-row>
<v-col cols="6">
<v-autocomplete
v-model="relation.parentTable"
:rules="[v => !!v || 'Reference Table required']"
outlined
class="caption"
hide-details
:loading="isRefTablesLoading"
label="Reference Table"
:full-width="false"
:items="refTables"
item-text="title"
item-value="table_name"
required
dense
@change="loadColumnList"
/>
</v-col>
<v-col cols="6">
<v-autocomplete
ref="parentColumnRef"
v-model="relation.parentColumn"
:rules="[v => !!v || 'Reference Column required']"
outlined
class="caption"
hide-details
:loading="isRefColumnsLoading"
label="Reference Column"
:full-width="false"
:items="refColumns"
item-text="title"
item-value="column_name"
required
dense
@change="onColumnSelect"
/>
</v-col>
</v-row>
<v-row>
<v-col cols="6">
<v-autocomplete
v-model="relation.onUpdate"
outlined
class="caption"
hide-details
label="On Update"
:full-width="false"
:items="onUpdateDeleteOptions"
required
dense
:disabled="relation.type !== 'real'"
/>
</v-col>
<v-col cols="6">
<v-autocomplete
v-model="relation.onDelete"
outlined
class="caption"
hide-details
label="On Delete"
:full-width="false"
:items="onUpdateDeleteOptions"
required
dense
:disabled="relation.type !== 'real'"
/>
</v-col>
</v-row>
<v-row>
<v-col>
<v-checkbox
v-model="relation.type"
:disabled="isSQLite"
false-value="real"
true-value="virtual"
label="Virtual Relation"
:full-width="false"
required
class="mt-0"
dense
/>
</v-col>
</v-row>
</v-container>
</template>
<script>
export default {
name: 'RelationOptions',
props: ['nodes', 'column', 'isSQLite', 'isMSSQL', 'alias'],
data: () => ({
refTables: [],
refColumns: [],
relation: {},
isRefTablesLoading: false,
isRefColumnsLoading: false,
}),
computed: {
onUpdateDeleteOptions() {
if (this.isMSSQL) {
return ['NO ACTION'];
}
return ['NO ACTION', 'CASCADE', 'RESTRICT', 'SET NULL', 'SET DEFAULT'];
},
},
watch: {
'column.column_name'(c) {
this.$set(this.relation, 'childColumn', c);
},
isSQLite(v) {
this.$set(this.relation, 'type', v ? 'virtual' : 'real');
},
},
async created() {
await this.loadTablesList();
this.relation = {
childColumn: this.column.column_name,
childTable: this.nodes.table_name,
parentTable: this.column.rtn || '',
parentColumn: this.column.rcn || '',
onDelete: 'NO ACTION',
onUpdate: 'NO ACTION',
updateRelation: !!this.column.rtn,
type: this.isSQLite ? 'virtual' : 'real',
};
},
mounted() {
this.$set(this.relation, 'type', this.isSqlite ? 'virtual' : 'real');
},
methods: {
async loadColumnList() {
if (!this.relation.parentTable) {
return;
}
this.isRefColumnsLoading = true;
const result = await this.$store.dispatch('sqlMgr/ActSqlOp', [
{
env: this.nodes.env,
dbAlias: this.nodes.dbAlias,
},
'columnList',
{ table_name: this.relation.parentTable },
]);
const columns = result.data.list;
this.refColumns = JSON.parse(JSON.stringify(columns));
if (this.relation.updateRelation && !this.relationColumnChanged) {
// only first time when editing add defaault value to this field
this.relation.parentColumn = this.column.rcn;
this.relationColumnChanged = true;
} else {
// find pk column and assign to parentColumn
const pkKeyColumns = this.refColumns.filter(el => el.pk);
this.relation.parentColumn = (pkKeyColumns[0] || {}).column_name || '';
}
this.onColumnSelect();
this.isRefColumnsLoading = false;
},
async loadTablesList() {
this.isRefTablesLoading = true;
const result = await this.$store.dispatch('sqlMgr/ActSqlOp', [
{
env: this.nodes.env,
dbAlias: this.nodes.dbAlias,
},
'tableList',
]);
this.refTables = result.data.list.map(({ table_name, title }) => ({ table_name, title }));
this.isRefTablesLoading = false;
},
async saveRelation() {
// try {
await this.$store.dispatch('sqlMgr/ActSqlOpPlus', [
{
env: this.nodes.env,
dbAlias: this.nodes.dbAlias,
},
this.relation.type === 'real' && !this.isSQLite ? 'relationCreate' : 'xcVirtualRelationCreate',
{ alias: this.alias, ...this.relation },
]);
},
onColumnSelect() {
const col = this.refColumns.find(c => this.relation.parentColumn === c.column_name);
this.$emit('onColumnSelect', col);
},
},
};
</script>
<style scoped>
.wrapper {
border: solid 2px #7f828b33;
border-radius: 4px;
}
/deep/ .v-input__append-inner {
margin-top: 4px !important;
}
</style>
| packages/nc-gui/components/project/spreadsheet/components/editColumn/RelationOptions.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00022245559375733137,
0.00017281854525208473,
0.00016400785534642637,
0.00017087995365727693,
0.00001137861909228377
] |
{
"id": 3,
"code_window": [
"\n",
"<template>\n",
" <a-layout-sider class=\"shadow !mt-[-9px]\" style=\"height: calc(100% + 9px)\" theme=\"light\" :width=\"drawerOpen ? 250 : 50\">\n",
" <Toolbar v-if=\"drawerOpen\" class=\"flex items-center py-3 px-3 justify-between border-b-1\" />\n",
"\n",
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\" />\n",
"\n",
" <div v-if=\"drawerOpen\" class=\"flex-1 flex flex-col\">\n",
" <MenuTop @open-modal=\"openModal\" @deleted=\"loadViews\" @sorted=\"loadViews\" />\n",
"\n",
" <a-divider class=\"my-2\" />\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\">\n",
" <template #start>\n",
" <MdiHook class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
"\n",
" <MdiXml class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
" </template>\n",
" </Toolbar>\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "replace",
"edit_start_line_idx": 60
} | <script lang="ts" setup>
import { onMounted, onUpdated } from '@vue/runtime-core'
import type { Form } from 'ant-design-vue'
import { useToast } from 'vue-toastification'
import { nextTick, ref } from '#imports'
import { navigateTo, useNuxtApp } from '#app'
import { extractSdkResponseErrorMsg } from '~/utils/errorUtils'
import { projectTitleValidator } from '~/utils/validation'
import MaterialSymbolsRocketLaunchOutline from '~icons/material-symbols/rocket-launch-outline'
const name = ref('')
const loading = ref(false)
const valid = ref(false)
const { $api, $state, $e } = useNuxtApp()
const toast = useToast()
const nameValidationRules = [
{
required: true,
message: 'Project name is required',
},
projectTitleValidator,
]
const formState = reactive({
title: '',
})
const createProject = async () => {
$e('a:project:create:xcdb')
loading.value = true
try {
const result = await $api.project.create({
title: formState.title,
})
await navigateTo(`/nc/${result.id}`)
} catch (e: any) {
toast.error(await extractSdkResponseErrorMsg(e))
}
loading.value = false
}
const form = ref<typeof Form>()
// hide sidebar
$state.sidebarOpen.value = false
// select and focus title field on load
onMounted(async () => {
nextTick(() => {
// todo: replace setTimeout and follow better approach
setTimeout(() => {
const input = form.value?.$el?.querySelector('input[type=text]')
input.setSelectionRange(0, formState.title.length)
input.focus()
}, 500)
})
})
</script>
<template>
<a-card class="w-[500px] mx-auto !mt-100px shadow-md">
<h3 class="text-3xl text-center font-semibold mb-2">{{ $t('activity.createProject') }}</h3>
<a-form ref="form" :model="formState" name="basic" layout="vertical" autocomplete="off" @finish="createProject">
<a-form-item :label="$t('labels.projName')" name="title" :rules="nameValidationRules" class="my-10 mx-10">
<a-input v-model:value="formState.title" name="title" class="nc-metadb-project-name" />
</a-form-item>
<a-form-item style="text-align: center" class="mt-2">
<a-button type="primary" html-type="submit">
<div class="flex items-center">
<MaterialSymbolsRocketLaunchOutline class="mr-1" />
{{ $t('general.create') }}
</div>
</a-button>
</a-form-item>
</a-form>
</a-card>
</template>
| packages/nc-gui-v2/pages/project/index/create.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.000552492099814117,
0.0002206339850090444,
0.00016410827811341733,
0.0001711643417365849,
0.00011938461830141023
] |
{
"id": 3,
"code_window": [
"\n",
"<template>\n",
" <a-layout-sider class=\"shadow !mt-[-9px]\" style=\"height: calc(100% + 9px)\" theme=\"light\" :width=\"drawerOpen ? 250 : 50\">\n",
" <Toolbar v-if=\"drawerOpen\" class=\"flex items-center py-3 px-3 justify-between border-b-1\" />\n",
"\n",
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\" />\n",
"\n",
" <div v-if=\"drawerOpen\" class=\"flex-1 flex flex-col\">\n",
" <MenuTop @open-modal=\"openModal\" @deleted=\"loadViews\" @sorted=\"loadViews\" />\n",
"\n",
" <a-divider class=\"my-2\" />\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <Toolbar v-else class=\"py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]\">\n",
" <template #start>\n",
" <MdiHook class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
"\n",
" <MdiXml class=\"rounded text-xl p-1 text-gray-500 hover:(text-white bg-pink-500 shadow)\" />\n",
"\n",
" <div class=\"dot\" />\n",
" </template>\n",
" </Toolbar>\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "replace",
"edit_start_line_idx": 60
} | <script lang="ts" setup>
import { useI18n } from 'vue-i18n'
import { message } from 'ant-design-vue'
import { extractSdkResponseErrorMsg } from '~/utils/errorUtils'
import { useNuxtApp } from '#app'
import { reactive, ref } from '#imports'
import MaterialSymbolsWarning from '~icons/material-symbols/warning'
import MdiKeyChange from '~icons/mdi/key-change'
const { $api, $state } = useNuxtApp()
const { t } = useI18n()
const formValidator = ref()
let error = $ref<string | null>(null)
const form = reactive({
currentPassword: '',
password: '',
passwordRepeat: '',
})
const formRules = {
currentPassword: [
// Current password is required
{ required: true, message: t('msg.error.signUpRules.passwdRequired') },
],
password: [
// Password is required
{ required: true, message: t('msg.error.signUpRules.passwdRequired') },
{ min: 8, message: t('msg.error.signUpRules.passwdLength') },
],
passwordRepeat: [
// PasswordRepeat is required
{ required: true, message: t('msg.error.signUpRules.passwdRequired') },
// Passwords match
{
validator: (_: unknown, v: string) => {
return new Promise((resolve, reject) => {
if (form.password === form.passwordRepeat) return resolve(true)
reject(new Error(t('msg.error.signUpRules.passwdMismatch')))
})
},
message: t('msg.error.signUpRules.passwdMismatch'),
},
],
}
const passwordChange = async () => {
const valid = formValidator.value.validate()
if (!valid) return
error = null
try {
const { msg } = await $api.auth.passwordChange({
currentPassword: form.currentPassword,
newPassword: form.password,
})
message.success(msg)
} catch (e: any) {
error = await extractSdkResponseErrorMsg(e)
}
}
const resetError = () => {
if (error) {
error = null
}
}
</script>
<template>
<a-form ref="formValidator" layout="vertical" :model="form" class="change-password h-full w-full" @finish="passwordChange">
<div
class="md:relative flex flex-col gap-2 w-full h-full p-8 lg:(max-w-1/2)"
:class="{ 'mx-auto': !$state.sidebarOpen.value }"
>
<h1 class="prose-2xl font-bold mb-4">{{ $t('activity.changePwd') }}</h1>
<Transition name="layout">
<div v-if="error" class="self-center mb-4 bg-red-500 text-white rounded-lg w-3/4 p-1">
<div class="flex items-center gap-2 justify-center"><MaterialSymbolsWarning /> {{ error }}</div>
</div>
</Transition>
<a-form-item :label="$t('placeholder.password.current')" name="currentPassword" :rules="formRules.currentPassword">
<a-input-password
v-model:value="form.currentPassword"
size="large"
class="password"
:placeholder="$t('placeholder.password.current')"
@focus="resetError"
/>
</a-form-item>
<a-form-item :label="$t('placeholder.password.new')" name="password" :rules="formRules.password">
<a-input-password
v-model:value="form.password"
size="large"
class="password"
:placeholder="$t('placeholder.password.new')"
@focus="resetError"
/>
</a-form-item>
<a-form-item :label="$t('placeholder.password.confirm')" name="passwordRepeat" :rules="formRules.passwordRepeat">
<a-input-password
v-model:value="form.passwordRepeat"
size="large"
class="password"
:placeholder="$t('placeholder.password.confirm')"
@focus="resetError"
/>
</a-form-item>
<div class="flex flex-wrap gap-4 items-center mt-4 md:justify-between w-full">
<button class="submit" type="submit">
<span class="flex items-center gap-2"><MdiKeyChange /> {{ $t('activity.changePwd') }}</span>
</button>
</div>
</div>
</a-form>
</template>
<style lang="scss">
.change-password {
.ant-input-affix-wrapper,
.ant-input {
@apply dark:(!bg-gray-700 !text-white) !appearance-none my-1 border-1 border-solid border-primary/50 rounded;
}
.password {
input {
@apply !border-none;
}
.ant-input-password-icon {
@apply dark:!text-white;
}
}
.submit {
@apply ml-1 border border-gray-300 rounded-lg p-4 bg-gray-100/50 text-white bg-primary hover:bg-primary/75 dark:(!bg-secondary/75 hover:!bg-secondary/50);
}
}
</style>
| packages/nc-gui-v2/pages/index/user/index/index.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00034303433494642377,
0.0002018548984779045,
0.00016638095257803798,
0.00017268117517232895,
0.00006233242311282083
] |
{
"id": 4,
"code_window": [
"}\n",
"\n",
":deep(.ant-layout-sider-children) {\n",
" @apply flex flex-col;\n",
"}\n",
"</style>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
".dot {\n",
" @apply w-[3px] h-[3px] bg-gray-300 rounded-full;\n",
"}\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 82
} | <script setup lang="ts">
import type { FormType, GalleryType, GridType, KanbanType, ViewTypes } from 'nocodb-sdk'
import MenuTop from './MenuTop.vue'
import MenuBottom from './MenuBottom.vue'
import Toolbar from './Toolbar.vue'
import { inject, provide, ref, useApi, useViews, watch } from '#imports'
import { ActiveViewInj, MetaInj, ViewListInj } from '~/context'
const meta = inject(MetaInj, ref())
const activeView = inject(ActiveViewInj, ref())
const { views, loadViews } = useViews(meta)
const { api } = useApi()
provide(ViewListInj, views)
/** Sidebar visible */
const drawerOpen = inject('navDrawerOpen', ref(true))
/** View type to create from modal */
let viewCreateType = $ref<ViewTypes>()
/** View title to create from modal (when duplicating) */
let viewCreateTitle = $ref('')
/** is view creation modal open */
let modalOpen = $ref(false)
/** Watch current views and on change set the next active view */
watch(
views,
(nextViews) => {
if (nextViews.length) {
activeView.value = nextViews[0]
}
},
{ immediate: true },
)
/** Open view creation modal */
function openModal({ type, title = '' }: { type: ViewTypes; title: string }) {
modalOpen = true
viewCreateType = type
viewCreateTitle = title
}
/** Handle view creation */
function onCreate(view: GridType | FormType | KanbanType | GalleryType) {
views.value.push(view)
activeView.value = view
modalOpen = false
}
</script>
<template>
<a-layout-sider class="shadow !mt-[-9px]" style="height: calc(100% + 9px)" theme="light" :width="drawerOpen ? 250 : 50">
<Toolbar v-if="drawerOpen" class="flex items-center py-3 px-3 justify-between border-b-1" />
<Toolbar v-else class="py-3 px-2 max-w-[50px] flex !flex-col-reverse gap-4 items-center mt-[-1px]" />
<div v-if="drawerOpen" class="flex-1 flex flex-col">
<MenuTop @open-modal="openModal" @deleted="loadViews" @sorted="loadViews" />
<a-divider class="my-2" />
<MenuBottom @open-modal="openModal" />
</div>
<dlg-view-create v-if="views" v-model="modalOpen" :title="viewCreateTitle" :type="viewCreateType" @created="onCreate" />
</a-layout-sider>
</template>
<style scoped>
:deep(.ant-menu-title-content) {
@apply w-full;
}
:deep(.ant-layout-sider-children) {
@apply flex flex-col;
}
</style>
| packages/nc-gui-v2/components/smartsheet/sidebar/index.vue | 1 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.01957849971950054,
0.003411907237023115,
0.0001674541417742148,
0.0001712982339086011,
0.0061903963796794415
] |
{
"id": 4,
"code_window": [
"}\n",
"\n",
":deep(.ant-layout-sider-children) {\n",
" @apply flex flex-col;\n",
"}\n",
"</style>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
".dot {\n",
" @apply w-[3px] h-[3px] bg-gray-300 rounded-full;\n",
"}\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 82
} | <template>
<div>
<v-icon v-if="column.pk" color="warning" x-small class="mr-1"> mdi-key-variant </v-icon>
<v-icon v-else-if="uiDatatypeIcon" small class="mr-1">
{{ uiDatatypeIcon }}
</v-icon>
<v-icon v-else-if="isForeignKey" color="purple" small class="mr-1"> mdi-link-variant </v-icon>
<span v-else-if="isInt" class="font-weight-bold mr-1" style="font-size: 15px">#</span>
<v-icon v-else-if="isFloat" color="grey" class="mr-1 mt-n1"> mdi-decimal </v-icon>
<v-icon v-else-if="isDate" color="grey" small class="mr-1"> mdi-calendar </v-icon>
<v-icon v-else-if="isDateTime" color="grey" small class="mr-1"> mdi-calendar-clock </v-icon>
<v-icon v-else-if="isSet" color="grey" small class="mr-1"> mdi-checkbox-multiple-marked </v-icon>
<v-icon v-else-if="isEnum" color="grey" small class="mr-1"> mdi-radiobox-marked </v-icon>
<v-icon v-else-if="isBoolean" color="grey" small class="mr-1"> mdi-check-box-outline </v-icon>
<v-icon v-else-if="isString" color="grey" class=""> mdi-alpha-a </v-icon>
<v-icon v-else-if="isTextArea" color="grey" small class="mr-1"> mdi-card-text-outline </v-icon>
</div>
</template>
<script>
import cell from '@/components/project/spreadsheet/mixins/cell';
export default {
name: 'FieldsMenuItem',
mixins: [cell],
props: {
sqlUi: [Object, Function],
column: Object,
},
};
</script>
<style scoped></style>
| packages/nc-gui/components/project/spreadsheet/components/FieldsMenuItem.vue | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.0001859505573520437,
0.00017291121184825897,
0.00016595884517300874,
0.00016986772243399173,
0.000007764410838717595
] |
{
"id": 4,
"code_window": [
"}\n",
"\n",
":deep(.ant-layout-sider-children) {\n",
" @apply flex flex-col;\n",
"}\n",
"</style>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
".dot {\n",
" @apply w-[3px] h-[3px] bg-gray-300 rounded-full;\n",
"}\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 82
} | import { HookType } from 'nocodb-sdk';
import {
CacheDelDirection,
CacheGetType,
CacheScope,
MetaTable,
} from '../utils/globals';
import Noco from '../Noco';
import Model from './Model';
import NocoCache from '../cache/NocoCache';
import Filter from './Filter';
import HookFilter from './HookFilter';
export default class Hook implements HookType {
id?: string;
fk_model_id?: string;
title?: string;
description?: string;
env?: string;
type?: string;
event?: 'After' | 'Before';
operation?: 'insert' | 'delete' | 'update';
async?: boolean;
payload?: string;
url?: string;
headers?: string;
condition?: boolean;
notification?: string;
retries?: number;
retry_interval?: number;
timeout?: number;
active?: boolean;
project_id?: string;
base_id?: string;
constructor(hook: Partial<Hook>) {
Object.assign(this, hook);
}
public static async get(hookId: string, ncMeta = Noco.ncMeta) {
let hook =
hookId &&
(await NocoCache.get(
`${CacheScope.HOOK}:${hookId}`,
CacheGetType.TYPE_OBJECT
));
if (!hook) {
hook = await ncMeta.metaGet2(null, null, MetaTable.HOOKS, hookId);
await NocoCache.set(`${CacheScope.HOOK}:${hookId}`, hook);
}
return hook && new Hook(hook);
}
public async getFilters(ncMeta = Noco.ncMeta) {
return await Filter.rootFilterListByHook({ hookId: this.id }, ncMeta);
}
// public static async insert(hook: Partial<Hook>) {
// const { id } = await ncMeta.metaInsert2(null, null, MetaTable.HOOKS, {
// // user: hook.user,
// // ip: hook.ip,
// // base_id: hook.base_id,
// // project_id: hook.project_id,
// // row_id: hook.row_id,
// // fk_model_id: hook.fk_model_id,
// // op_type: hook.op_type,
// // op_sub_type: hook.op_sub_type,
// // status: hook.status,
// // description: hook.description,
// // details: hook.details
// });
//
// return this.get(id);
// }
static async list(
param: {
fk_model_id: string;
event?: 'after' | 'before';
operation?: 'insert' | 'delete' | 'update';
},
ncMeta = Noco.ncMeta
) {
let hooks = await NocoCache.getList(CacheScope.HOOK, [param.fk_model_id]);
if (!hooks.length) {
hooks = await ncMeta.metaList(null, null, MetaTable.HOOKS, {
condition: {
fk_model_id: param.fk_model_id,
// ...(param.event ? { event: param.event?.toLowerCase?.() } : {}),
// ...(param.operation
// ? { operation: param.operation?.toLowerCase?.() }
// : {})
},
orderBy: {
created_at: 'asc',
},
});
await NocoCache.setList(CacheScope.HOOK, [param.fk_model_id], hooks);
}
// filter event & operation
if (param.event) {
hooks = hooks.filter(
(h) => h.event?.toLowerCase() === param.event?.toLowerCase()
);
}
if (param.operation) {
hooks = hooks.filter(
(h) => h.operation?.toLowerCase() === param.operation?.toLowerCase()
);
}
return hooks?.map((h) => new Hook(h));
}
public static async insert(
hook: Partial<
Hook & {
created_at?;
updated_at?;
}
>,
ncMeta = Noco.ncMeta
) {
const insertObj = {
fk_model_id: hook.fk_model_id,
title: hook.title,
description: hook.description,
env: hook.env,
type: hook.type,
event: hook.event?.toLowerCase?.(),
operation: hook.operation?.toLowerCase?.(),
async: hook.async,
payload: !!hook.payload,
url: hook.url,
headers: hook.headers,
condition: hook.condition,
notification:
hook.notification && typeof hook.notification === 'object'
? JSON.stringify(hook.notification)
: hook.notification,
retries: hook.retries,
retry_interval: hook.retry_interval,
timeout: hook.timeout,
active: hook.active,
project_id: hook.project_id,
base_id: hook.base_id,
created_at: hook.created_at,
updated_at: hook.updated_at,
};
if (!(hook.project_id && hook.base_id)) {
const model = await Model.getByIdOrName({ id: hook.fk_model_id }, ncMeta);
insertObj.project_id = model.project_id;
insertObj.base_id = model.base_id;
}
const { id } = await ncMeta.metaInsert2(
null,
null,
MetaTable.HOOKS,
insertObj
);
await NocoCache.appendToList(
CacheScope.HOOK,
[hook.fk_model_id],
`${CacheScope.HOOK}:${id}`
);
return this.get(id, ncMeta);
}
public static async update(
hookId: string,
hook: Partial<Hook>,
ncMeta = Noco.ncMeta
) {
const updateObj = {
title: hook.title,
description: hook.description,
env: hook.env,
type: hook.type,
event: hook.event?.toLowerCase?.(),
operation: hook.operation?.toLowerCase?.(),
async: hook.async,
payload: !!hook.payload,
url: hook.url,
headers: hook.headers,
condition: !!hook.condition,
notification:
hook.notification && typeof hook.notification === 'object'
? JSON.stringify(hook.notification)
: hook.notification,
retries: hook.retries,
retry_interval: hook.retry_interval,
timeout: hook.timeout,
active: hook.active,
};
// get existing cache
const key = `${CacheScope.HOOK}:${hookId}`;
let o = await NocoCache.get(key, CacheGetType.TYPE_OBJECT);
if (o) {
// update data
o = { ...o, ...updateObj };
o.notification = updateObj.notification;
// set cache
await NocoCache.set(key, o);
}
// set meta
await ncMeta.metaUpdate(null, null, MetaTable.HOOKS, updateObj, hookId);
return this.get(hookId, ncMeta);
}
static async delete(hookId: any, ncMeta = Noco.ncMeta) {
// Delete Hook Filters
const filterList = await ncMeta.metaList2(
null,
null,
MetaTable.FILTER_EXP,
{
condition: { fk_hook_id: hookId },
}
);
for (const filter of filterList) {
await NocoCache.deepDel(
CacheScope.FILTER_EXP,
`${CacheScope.FILTER_EXP}:${filter.id}`,
CacheDelDirection.CHILD_TO_PARENT
);
await HookFilter.delete(filter.id);
}
// Delete Hook
await NocoCache.deepDel(
CacheScope.HOOK,
`${CacheScope.HOOK}:${hookId}`,
CacheDelDirection.CHILD_TO_PARENT
);
return await ncMeta.metaDelete(null, null, MetaTable.HOOKS, hookId);
}
}
| packages/nocodb/src/lib/models/Hook.ts | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.00025687782908789814,
0.0001722585438983515,
0.00016450513794552535,
0.00016852060798555613,
0.00001749685179674998
] |
{
"id": 4,
"code_window": [
"}\n",
"\n",
":deep(.ant-layout-sider-children) {\n",
" @apply flex flex-col;\n",
"}\n",
"</style>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep"
],
"after_edit": [
"\n",
".dot {\n",
" @apply w-[3px] h-[3px] bg-gray-300 rounded-full;\n",
"}\n"
],
"file_path": "packages/nc-gui-v2/components/smartsheet/sidebar/index.vue",
"type": "add",
"edit_start_line_idx": 82
} | import { Request, Response, Router } from 'express';
import Model from '../../models/Model';
import { PagedResponseImpl } from '../helpers/PagedResponse';
import { Tele } from 'nc-help';
import {
AuditOperationSubTypes,
AuditOperationTypes,
isVirtualCol,
ModelTypes,
TableListType,
TableReqType,
TableType,
UITypes,
} from 'nocodb-sdk';
import ProjectMgrv2 from '../../db/sql-mgr/v2/ProjectMgrv2';
import Project from '../../models/Project';
import Audit from '../../models/Audit';
import ncMetaAclMw from '../helpers/ncMetaAclMw';
import { xcVisibilityMetaGet } from './modelVisibilityApis';
import View from '../../models/View';
import getColumnPropsFromUIDT from '../helpers/getColumnPropsFromUIDT';
import mapDefaultPrimaryValue from '../helpers/mapDefaultPrimaryValue';
import { NcError } from '../helpers/catchError';
import getTableNameAlias, { getColumnNameAlias } from '../helpers/getTableName';
import Column from '../../models/Column';
import NcConnectionMgrv2 from '../../utils/common/NcConnectionMgrv2';
import getColumnUiType from '../helpers/getColumnUiType';
import LinkToAnotherRecordColumn from '../../models/LinkToAnotherRecordColumn';
import { metaApiMetrics } from '../helpers/apiMetrics';
export async function tableGet(req: Request, res: Response<TableType>) {
const table = await Model.getWithInfo({
id: req.params.tableId,
});
// todo: optimise
const viewList = <View[]>await xcVisibilityMetaGet(table.project_id, [table]);
//await View.list(req.params.tableId)
table.views = viewList.filter((table: any) => {
return Object.keys((req as any).session?.passport?.user?.roles).some(
(role) =>
(req as any)?.session?.passport?.user?.roles[role] &&
!table.disabled[role]
);
});
res.json(table);
}
export async function tableReorder(req: Request, res: Response) {
res.json(Model.updateOrder(req.params.tableId, req.body.order));
}
export async function tableList(req: Request, res: Response<TableListType>) {
const viewList = await xcVisibilityMetaGet(req.params.projectId);
// todo: optimise
const tableViewMapping = viewList.reduce((o, view: any) => {
o[view.fk_model_id] = o[view.fk_model_id] || 0;
if (
Object.keys((req as any).session?.passport?.user?.roles).some(
(role) =>
(req as any)?.session?.passport?.user?.roles[role] &&
!view.disabled[role]
)
) {
o[view.fk_model_id]++;
}
return o;
}, {});
const tableList = (
await Model.list({
project_id: req.params.projectId,
base_id: req.params.baseId,
})
).filter((t) => tableViewMapping[t.id]);
res // todo: pagination
.json(
new PagedResponseImpl(
req.query?.includeM2M
? tableList
: (tableList.filter((t) => !t.mm) as Model[])
)
);
}
export async function tableCreate(req: Request<any, any, TableReqType>, res) {
const project = await Project.getWithInfo(req.params.projectId);
const base = project.bases[0];
if (!req.body.table_name) {
NcError.badRequest(
'Missing table name `table_name` property in request body'
);
}
if (project.prefix) {
if (!req.body.table_name.startsWith(project.prefix)) {
req.body.table_name = `${project.prefix}_${req.body.table_name}`;
}
}
// validate table name
if (/^\s+|\s+$/.test(req.body.table_name)) {
NcError.badRequest(
'Leading or trailing whitespace not allowed in table names'
);
}
if (
!(await Model.checkTitleAvailable({
table_name: req.body.table_name,
project_id: project.id,
base_id: base.id,
}))
) {
NcError.badRequest('Duplicate table name');
}
if (!req.body.title) {
req.body.title = getTableNameAlias(
req.body.table_name,
project.prefix,
base
);
}
if (
!(await Model.checkAliasAvailable({
title: req.body.title,
project_id: project.id,
base_id: base.id,
}))
) {
NcError.badRequest('Duplicate table alias');
}
const sqlMgr = await ProjectMgrv2.getSqlMgr(project);
const sqlClient = NcConnectionMgrv2.getSqlClient(base);
let tableNameLengthLimit = 255;
const sqlClientType = sqlClient.clientType;
if (sqlClientType === 'mysql2' || sqlClientType === 'mysql') {
tableNameLengthLimit = 64;
} else if (sqlClientType === 'pg') {
tableNameLengthLimit = 63;
} else if (sqlClientType === 'mssql') {
tableNameLengthLimit = 128;
}
if (req.body.table_name.length > tableNameLengthLimit) {
NcError.badRequest(`Table name exceeds ${tableNameLengthLimit} characters`);
}
req.body.columns = req.body.columns?.map((c) => ({
...getColumnPropsFromUIDT(c as any, base),
cn: c.column_name,
}));
await sqlMgr.sqlOpPlus(base, 'tableCreate', {
...req.body,
tn: req.body.table_name,
});
const columns: Array<
Omit<Column, 'column_name' | 'title'> & {
cn: string;
system?: boolean;
}
> = (await sqlClient.columnList({ tn: req.body.table_name }))?.data?.list;
const tables = await Model.list({
project_id: project.id,
base_id: base.id,
});
Audit.insert({
project_id: project.id,
op_type: AuditOperationTypes.TABLE,
op_sub_type: AuditOperationSubTypes.CREATED,
user: (req as any)?.user?.email,
description: `created table ${req.body.table_name} with alias ${req.body.title} `,
ip: (req as any).clientIp,
}).then(() => {});
mapDefaultPrimaryValue(req.body.columns);
Tele.emit('evt', { evt_type: 'table:created' });
res.json(
await Model.insert(project.id, base.id, {
...req.body,
columns: columns.map((c, i) => {
const colMetaFromReq = req.body?.columns?.find(
(c1) => c.cn === c1.column_name
);
return {
...colMetaFromReq,
uidt: colMetaFromReq?.uidt || c.uidt || getColumnUiType(base, c),
...c,
dtxp: [UITypes.MultiSelect, UITypes.SingleSelect].includes(
colMetaFromReq.uidt as any
)
? colMetaFromReq.dtxp
: c.dtxp,
title: colMetaFromReq?.title || getColumnNameAlias(c.cn, base),
column_name: c.cn,
order: i + 1,
};
}),
order: +(tables?.pop()?.order ?? 0) + 1,
})
);
}
export async function tableUpdate(req: Request<any, any>, res) {
const model = await Model.get(req.params.tableId);
if (
!(await Model.checkAliasAvailable({
title: req.body.title,
project_id: model.project_id,
base_id: model.base_id,
exclude_id: req.params.tableId,
}))
) {
NcError.badRequest('Duplicate table name');
}
await Model.updateAlias(req.params.tableId, req.body.title);
Tele.emit('evt', { evt_type: 'table:updated' });
res.json({ msg: 'success' });
}
export async function tableDelete(req: Request, res: Response) {
const table = await Model.getByIdOrName({ id: req.params.tableId });
await table.getColumns();
const relationColumns = table.columns.filter(
(c) => c.uidt === UITypes.LinkToAnotherRecord
);
if (relationColumns?.length) {
const referredTables = await Promise.all(
relationColumns.map(async (c) =>
c
.getColOptions<LinkToAnotherRecordColumn>()
.then((opt) => opt.getRelatedTable())
.then()
)
);
NcError.badRequest(
`Table can't be deleted since Table is being referred in following tables : ${referredTables.join(
', '
)}. Delete LinkToAnotherRecord columns and try again.`
);
}
const project = await Project.getWithInfo(table.project_id);
const base = project.bases.find((b) => b.id === table.base_id);
const sqlMgr = await ProjectMgrv2.getSqlMgr(project);
(table as any).tn = table.table_name;
table.columns = table.columns.filter((c) => !isVirtualCol(c));
table.columns.forEach((c) => {
(c as any).cn = c.column_name;
});
if (table.type === ModelTypes.TABLE) {
await sqlMgr.sqlOpPlus(base, 'tableDelete', table);
} else if (table.type === ModelTypes.VIEW) {
await sqlMgr.sqlOpPlus(base, 'viewDelete', {
...table,
view_name: table.table_name,
});
}
Audit.insert({
project_id: project.id,
op_type: AuditOperationTypes.TABLE,
op_sub_type: AuditOperationSubTypes.DELETED,
user: (req as any)?.user?.email,
description: `Deleted ${table.type} ${table.table_name} with alias ${table.title} `,
ip: (req as any).clientIp,
}).then(() => {});
Tele.emit('evt', { evt_type: 'table:deleted' });
res.json(await table.delete());
}
const router = Router({ mergeParams: true });
router.get(
'/api/v1/db/meta/projects/:projectId/tables',
metaApiMetrics,
ncMetaAclMw(tableList, 'tableList')
);
router.post(
'/api/v1/db/meta/projects/:projectId/tables',
metaApiMetrics,
ncMetaAclMw(tableCreate, 'tableCreate')
);
router.get(
'/api/v1/db/meta/tables/:tableId',
metaApiMetrics,
ncMetaAclMw(tableGet, 'tableGet')
);
router.patch(
'/api/v1/db/meta/tables/:tableId',
metaApiMetrics,
ncMetaAclMw(tableUpdate, 'tableUpdate')
);
router.delete(
'/api/v1/db/meta/tables/:tableId',
metaApiMetrics,
ncMetaAclMw(tableDelete, 'tableDelete')
);
router.post(
'/api/v1/db/meta/tables/:tableId/reorder',
metaApiMetrics,
ncMetaAclMw(tableReorder, 'tableReorder')
);
export default router;
| packages/nocodb/src/lib/meta/api/tableApis.ts | 0 | https://github.com/nocodb/nocodb/commit/4c2a5454172fbe13186d5c115e8099eac0c5c903 | [
0.0008219711016863585,
0.00018855577218346298,
0.0001628060854272917,
0.00016857654554769397,
0.00011201082088518888
] |
{
"id": 0,
"code_window": [
"\ttop: initial !important;\n",
"\tleft: initial !important;\n",
"\tbottom: 0 !important;\n",
"\tright: 0 !important;\n",
"}*/\n",
".monaco-editor .inputarea.ime-input {\n",
"\tz-index: 10;\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tcolor: black !important;\n",
"\tbackground: white !important;\n",
"\tline-height: 15px !important;\n",
"\tfont-size: 14px !important;\n"
],
"file_path": "src/vs/editor/browser/controller/textAreaHandler.css",
"type": "add",
"edit_start_line_idx": 26
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import * as nls from 'vs/nls';
import * as strings from 'vs/base/common/strings';
import { onUnexpectedError } from 'vs/base/common/errors';
import { CursorCollection } from 'vs/editor/common/controller/cursorCollection';
import { Position } from 'vs/editor/common/core/position';
import { Range } from 'vs/editor/common/core/range';
import { Selection, SelectionDirection, ISelection } from 'vs/editor/common/core/selection';
import * as editorCommon from 'vs/editor/common/editorCommon';
import { CursorColumns, CursorConfiguration, EditOperationResult, CursorContext, CursorState, RevealTarget, IColumnSelectData, ICursors } from 'vs/editor/common/controller/cursorCommon';
import { LanguageConfigurationRegistry } from 'vs/editor/common/modes/languageConfigurationRegistry';
import { DeleteOperations } from 'vs/editor/common/controller/cursorDeleteOperations';
import { TypeOperations } from 'vs/editor/common/controller/cursorTypeOperations';
import { TextModelEventType, ModelRawContentChangedEvent, RawContentChangedType } from 'vs/editor/common/model/textModelEvents';
import { CursorChangeReason } from 'vs/editor/common/controller/cursorEvents';
import { IViewModel } from "vs/editor/common/viewModel/viewModel";
import * as viewEvents from 'vs/editor/common/view/viewEvents';
import Event, { Emitter } from 'vs/base/common/event';
function containsLineMappingChanged(events: viewEvents.ViewEvent[]): boolean {
for (let i = 0, len = events.length; i < len; i++) {
if (events[i].type === viewEvents.ViewEventType.ViewLineMappingChanged) {
return true;
}
}
return false;
}
export class CursorStateChangedEvent {
/**
* The new selections.
* The primary selection is always at index 0.
*/
readonly selections: Selection[];
/**
* Source of the call that caused the event.
*/
readonly source: string;
/**
* Reason.
*/
readonly reason: CursorChangeReason;
constructor(selections: Selection[], source: string, reason: CursorChangeReason) {
this.selections = selections;
this.source = source;
this.reason = reason;
}
}
export class Cursor extends viewEvents.ViewEventEmitter implements ICursors {
private readonly _onDidChange: Emitter<CursorStateChangedEvent> = this._register(new Emitter<CursorStateChangedEvent>());
public readonly onDidChange: Event<CursorStateChangedEvent> = this._onDidChange.event;
private readonly _configuration: editorCommon.IConfiguration;
private readonly _model: editorCommon.IModel;
private readonly _viewModel: IViewModel;
public context: CursorContext;
private _cursors: CursorCollection;
private _isHandling: boolean;
private _isDoingComposition: boolean;
private _columnSelectData: IColumnSelectData;
constructor(configuration: editorCommon.IConfiguration, model: editorCommon.IModel, viewModel: IViewModel) {
super();
this._configuration = configuration;
this._model = model;
this._viewModel = viewModel;
this.context = new CursorContext(this._configuration, this._model, this._viewModel);
this._cursors = new CursorCollection(this.context);
this._isHandling = false;
this._isDoingComposition = false;
this._columnSelectData = null;
this._register(this._model.addBulkListener((events) => {
if (this._isHandling) {
return;
}
let hadContentChange = false;
let hadFlushEvent = false;
for (let i = 0, len = events.length; i < len; i++) {
const event = events[i];
const eventType = event.type;
if (eventType === TextModelEventType.ModelRawContentChanged2) {
hadContentChange = true;
const rawChangeEvent = <ModelRawContentChangedEvent>event.data;
hadFlushEvent = hadFlushEvent || rawChangeEvent.containsEvent(RawContentChangedType.Flush);
}
}
if (!hadContentChange) {
return;
}
this._onModelContentChanged(hadFlushEvent);
}));
this._register(viewModel.addEventListener((events: viewEvents.ViewEvent[]) => {
if (!containsLineMappingChanged(events)) {
return;
}
// Ensure valid state
this.setStates('viewModel', CursorChangeReason.NotSet, this.getAll());
}));
const updateCursorContext = () => {
this.context = new CursorContext(this._configuration, this._model, this._viewModel);
this._cursors.updateContext(this.context);
};
this._register(this._model.onDidChangeLanguage((e) => {
updateCursorContext();
}));
this._register(LanguageConfigurationRegistry.onDidChange(() => {
// TODO@Alex: react only if certain supports changed? (and if my model's mode changed)
updateCursorContext();
}));
this._register(model.onDidChangeOptions(() => {
updateCursorContext();
}));
this._register(this._configuration.onDidChange((e) => {
if (CursorConfiguration.shouldRecreate(e)) {
updateCursorContext();
}
}));
}
public dispose(): void {
this._cursors.dispose();
super.dispose();
}
// ------ some getters/setters
public getPrimaryCursor(): CursorState {
return this._cursors.getPrimaryCursor();
}
public getLastAddedCursorIndex(): number {
return this._cursors.getLastAddedCursorIndex();
}
public getAll(): CursorState[] {
return this._cursors.getAll();
}
private static _somethingChanged(oldSelections: Selection[], oldViewSelections: Selection[], newSelections: Selection[], newViewSelections: Selection[]): boolean {
if (oldSelections.length !== newSelections.length) {
return true;
}
for (let i = 0, len = oldSelections.length; i < len; i++) {
if (!oldSelections[i].equalsSelection(newSelections[i])) {
return true;
}
}
for (let i = 0, len = oldViewSelections.length; i < len; i++) {
if (!oldViewSelections[i].equalsSelection(newViewSelections[i])) {
return true;
}
}
return false;
}
public setStates(source: string, reason: CursorChangeReason, states: CursorState[]): void {
const oldSelections = this._cursors.getSelections();
const oldViewSelections = this._cursors.getViewSelections();
this._cursors.setStates(states);
this._cursors.normalize();
this._columnSelectData = null;
const newSelections = this._cursors.getSelections();
const newViewSelections = this._cursors.getViewSelections();
if (Cursor._somethingChanged(oldSelections, oldViewSelections, newSelections, newViewSelections)) {
this._emitStateChanged(source, reason);
}
}
public setColumnSelectData(columnSelectData: IColumnSelectData): void {
this._columnSelectData = columnSelectData;
}
public reveal(horizontal: boolean, target: RevealTarget): void {
this._revealRange(target, viewEvents.VerticalRevealType.Simple, horizontal);
}
public revealRange(revealHorizontal: boolean, modelRange: Range, viewRange: Range, verticalType: viewEvents.VerticalRevealType) {
this.emitCursorRevealRange(modelRange, viewRange, verticalType, revealHorizontal);
}
public scrollTo(desiredScrollTop: number): void {
this._viewModel.viewLayout.setScrollPosition({
scrollTop: desiredScrollTop
});
}
public saveState(): editorCommon.ICursorState[] {
let result: editorCommon.ICursorState[] = [];
const selections = this._cursors.getSelections();
for (let i = 0, len = selections.length; i < len; i++) {
const selection = selections[i];
result.push({
inSelectionMode: !selection.isEmpty(),
selectionStart: {
lineNumber: selection.selectionStartLineNumber,
column: selection.selectionStartColumn,
},
position: {
lineNumber: selection.positionLineNumber,
column: selection.positionColumn,
}
});
}
return result;
}
public restoreState(states: editorCommon.ICursorState[]): void {
let desiredSelections: ISelection[] = [];
for (let i = 0, len = states.length; i < len; i++) {
const state = states[i];
let positionLineNumber = 1;
let positionColumn = 1;
// Avoid missing properties on the literal
if (state.position && state.position.lineNumber) {
positionLineNumber = state.position.lineNumber;
}
if (state.position && state.position.column) {
positionColumn = state.position.column;
}
let selectionStartLineNumber = positionLineNumber;
let selectionStartColumn = positionColumn;
// Avoid missing properties on the literal
if (state.selectionStart && state.selectionStart.lineNumber) {
selectionStartLineNumber = state.selectionStart.lineNumber;
}
if (state.selectionStart && state.selectionStart.column) {
selectionStartColumn = state.selectionStart.column;
}
desiredSelections.push({
selectionStartLineNumber: selectionStartLineNumber,
selectionStartColumn: selectionStartColumn,
positionLineNumber: positionLineNumber,
positionColumn: positionColumn
});
}
this.setStates('restoreState', CursorChangeReason.NotSet, CursorState.fromModelSelections(desiredSelections));
this.reveal(true, RevealTarget.Primary);
}
private _onModelContentChanged(hadFlushEvent: boolean): void {
if (hadFlushEvent) {
// a model.setValue() was called
this._cursors.dispose();
this._cursors = new CursorCollection(this.context);
this._emitStateChanged('model', CursorChangeReason.ContentFlush);
} else {
const selectionsFromMarkers = this._cursors.readSelectionFromMarkers();
this.setStates('modelChange', CursorChangeReason.RecoverFromMarkers, CursorState.fromModelSelections(selectionsFromMarkers));
}
}
public getSelection(): Selection {
return this._cursors.getPrimaryCursor().modelState.selection;
}
public getColumnSelectData(): IColumnSelectData {
if (this._columnSelectData) {
return this._columnSelectData;
}
const primaryCursor = this._cursors.getPrimaryCursor();
const primaryPos = primaryCursor.viewState.position;
return {
toViewLineNumber: primaryPos.lineNumber,
toViewVisualColumn: CursorColumns.visibleColumnFromColumn2(this.context.config, this.context.viewModel, primaryPos)
};
}
public getSelections(): Selection[] {
return this._cursors.getSelections();
}
public getPosition(): Position {
return this._cursors.getPrimaryCursor().modelState.position;
}
public setSelections(source: string, selections: ISelection[]): void {
this.setStates(source, CursorChangeReason.NotSet, CursorState.fromModelSelections(selections));
}
// ------ auxiliary handling logic
private _executeEditOperation(opResult: EditOperationResult): void {
if (!opResult) {
// Nothing to execute
return;
}
if (this._configuration.editor.readOnly) {
// Cannot execute when read only
return;
}
if (opResult.shouldPushStackElementBefore) {
this._model.pushStackElement();
}
const result = CommandExecutor.executeCommands(this._model, this._cursors.getSelections(), opResult.commands);
if (result) {
// The commands were applied correctly
this._interpretCommandResult(result);
}
if (opResult.shouldPushStackElementAfter) {
this._model.pushStackElement();
}
}
private _interpretCommandResult(cursorState: Selection[]): void {
if (!cursorState || cursorState.length === 0) {
return;
}
this._columnSelectData = null;
this._cursors.setSelections(cursorState);
this._cursors.normalize();
}
// -----------------------------------------------------------------------------------------------------------
// ----- emitting events
private _emitStateChanged(source: string, reason: CursorChangeReason): void {
source = source || 'keyboard';
const positions = this._cursors.getPositions();
let isInEditableRange: boolean = true;
if (this._model.hasEditableRange()) {
const editableRange = this._model.getEditableRange();
if (!editableRange.containsPosition(positions[0])) {
isInEditableRange = false;
}
}
const selections = this._cursors.getSelections();
const viewSelections = this._cursors.getViewSelections();
this._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));
this._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);
}
private _revealRange(revealTarget: RevealTarget, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean): void {
const positions = this._cursors.getPositions();
const viewPositions = this._cursors.getViewPositions();
let position = positions[0];
let viewPosition = viewPositions[0];
if (revealTarget === RevealTarget.TopMost) {
for (let i = 1; i < positions.length; i++) {
if (positions[i].isBefore(position)) {
position = positions[i];
viewPosition = viewPositions[i];
}
}
} else if (revealTarget === RevealTarget.BottomMost) {
for (let i = 1; i < positions.length; i++) {
if (position.isBeforeOrEqual(positions[i])) {
position = positions[i];
viewPosition = viewPositions[i];
}
}
} else {
if (positions.length > 1) {
// no revealing!
return;
}
}
const range = new Range(position.lineNumber, position.column, position.lineNumber, position.column);
const viewRange = new Range(viewPosition.lineNumber, viewPosition.column, viewPosition.lineNumber, viewPosition.column);
this.emitCursorRevealRange(range, viewRange, verticalType, revealHorizontal);
}
public emitCursorRevealRange(range: Range, viewRange: Range, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean) {
// Ensure event has viewRange
if (!viewRange) {
viewRange = this.context.convertModelRangeToViewRange(range);
}
this._emit([new viewEvents.ViewRevealRangeRequestEvent(viewRange, verticalType, revealHorizontal)]);
}
// -----------------------------------------------------------------------------------------------------------
// ----- handlers beyond this point
public trigger(source: string, handlerId: string, payload: any): void {
const H = editorCommon.Handler;
if (handlerId === H.CompositionStart) {
this._isDoingComposition = true;
return;
}
if (handlerId === H.CompositionEnd) {
this._isDoingComposition = false;
return;
}
const oldSelections = this._cursors.getSelections();
const oldViewSelections = this._cursors.getViewSelections();
let cursorChangeReason = CursorChangeReason.NotSet;
// ensure valid state on all cursors
this._cursors.ensureValidState();
this._isHandling = true;
try {
switch (handlerId) {
case H.Type:
this._type(source, <string>payload.text);
break;
case H.ReplacePreviousChar:
this._replacePreviousChar(<string>payload.text, <number>payload.replaceCharCnt);
break;
case H.Paste:
cursorChangeReason = CursorChangeReason.Paste;
this._paste(<string>payload.text, <boolean>payload.pasteOnNewLine);
break;
case H.Cut:
this._cut();
break;
case H.Undo:
cursorChangeReason = CursorChangeReason.Undo;
this._interpretCommandResult(this._model.undo());
break;
case H.Redo:
cursorChangeReason = CursorChangeReason.Redo;
this._interpretCommandResult(this._model.redo());
break;
case H.ExecuteCommand:
this._externalExecuteCommand(<editorCommon.ICommand>payload);
break;
case H.ExecuteCommands:
this._externalExecuteCommands(<editorCommon.ICommand[]>payload);
break;
}
} catch (err) {
onUnexpectedError(err);
}
this._isHandling = false;
const newSelections = this._cursors.getSelections();
const newViewSelections = this._cursors.getViewSelections();
if (Cursor._somethingChanged(oldSelections, oldViewSelections, newSelections, newViewSelections)) {
this._emitStateChanged(source, cursorChangeReason);
this._revealRange(RevealTarget.Primary, viewEvents.VerticalRevealType.Simple, true);
}
}
private _type(source: string, text: string): void {
if (!this._isDoingComposition && source === 'keyboard') {
// If this event is coming straight from the keyboard, look for electric characters and enter
for (let i = 0, len = text.length; i < len; i++) {
let charCode = text.charCodeAt(i);
let chr: string;
if (strings.isHighSurrogate(charCode) && i + 1 < len) {
chr = text.charAt(i) + text.charAt(i + 1);
i++;
} else {
chr = text.charAt(i);
}
// Here we must interpret each typed character individually, that's why we create a new context
this._executeEditOperation(TypeOperations.typeWithInterceptors(this.context.config, this.context.model, this.getSelections(), chr));
}
} else {
this._executeEditOperation(TypeOperations.typeWithoutInterceptors(this.context.config, this.context.model, this.getSelections(), text));
}
}
private _replacePreviousChar(text: string, replaceCharCnt: number): void {
this._executeEditOperation(TypeOperations.replacePreviousChar(this.context.config, this.context.model, this.getSelections(), text, replaceCharCnt));
}
private _paste(text: string, pasteOnNewLine: boolean): void {
this._executeEditOperation(TypeOperations.paste(this.context.config, this.context.model, this.getSelections(), pasteOnNewLine, text));
}
private _cut(): void {
this._executeEditOperation(DeleteOperations.cut(this.context.config, this.context.model, this.getSelections()));
}
private _externalExecuteCommand(command: editorCommon.ICommand): void {
this._cursors.killSecondaryCursors();
this._executeEditOperation(new EditOperationResult([command], {
shouldPushStackElementBefore: false,
shouldPushStackElementAfter: false
}));
}
private _externalExecuteCommands(commands: editorCommon.ICommand[]): void {
this._executeEditOperation(new EditOperationResult(commands, {
shouldPushStackElementBefore: false,
shouldPushStackElementAfter: false
}));
}
}
interface IExecContext {
readonly model: editorCommon.IModel;
readonly selectionsBefore: Selection[];
readonly selectionStartMarkers: string[];
readonly positionMarkers: string[];
}
interface ICommandData {
operations: editorCommon.IIdentifiedSingleEditOperation[];
hadTrackedEditOperation: boolean;
}
interface ICommandsData {
operations: editorCommon.IIdentifiedSingleEditOperation[];
hadTrackedEditOperation: boolean;
}
class CommandExecutor {
public static executeCommands(model: editorCommon.IModel, selectionsBefore: Selection[], commands: editorCommon.ICommand[]): Selection[] {
const ctx: IExecContext = {
model: model,
selectionsBefore: selectionsBefore,
selectionStartMarkers: [],
positionMarkers: []
};
const result = this._innerExecuteCommands(ctx, commands);
for (let i = 0; i < ctx.selectionStartMarkers.length; i++) {
ctx.model._removeMarker(ctx.selectionStartMarkers[i]);
ctx.model._removeMarker(ctx.positionMarkers[i]);
}
return result;
}
private static _innerExecuteCommands(ctx: IExecContext, commands: editorCommon.ICommand[]): Selection[] {
if (this._arrayIsEmpty(commands)) {
return null;
}
const commandsData = this._getEditOperations(ctx, commands);
if (commandsData.operations.length === 0) {
return null;
}
const rawOperations = commandsData.operations;
const editableRange = ctx.model.getEditableRange();
const editableRangeStart = editableRange.getStartPosition();
const editableRangeEnd = editableRange.getEndPosition();
for (let i = 0, len = rawOperations.length; i < len; i++) {
const operationRange = rawOperations[i].range;
if (!editableRangeStart.isBeforeOrEqual(operationRange.getStartPosition()) || !operationRange.getEndPosition().isBeforeOrEqual(editableRangeEnd)) {
// These commands are outside of the editable range
return null;
}
}
const loserCursorsMap = this._getLoserCursorMap(rawOperations);
if (loserCursorsMap.hasOwnProperty('0')) {
// These commands are very messed up
console.warn('Ignoring commands');
return null;
}
// Remove operations belonging to losing cursors
let filteredOperations: editorCommon.IIdentifiedSingleEditOperation[] = [];
for (let i = 0, len = rawOperations.length; i < len; i++) {
if (!loserCursorsMap.hasOwnProperty(rawOperations[i].identifier.major.toString())) {
filteredOperations.push(rawOperations[i]);
}
}
// TODO@Alex: find a better way to do this.
// give the hint that edit operations are tracked to the model
if (commandsData.hadTrackedEditOperation && filteredOperations.length > 0) {
filteredOperations[0]._isTracked = true;
}
const selectionsAfter = ctx.model.pushEditOperations(ctx.selectionsBefore, filteredOperations, (inverseEditOperations: editorCommon.IIdentifiedSingleEditOperation[]): Selection[] => {
let groupedInverseEditOperations: editorCommon.IIdentifiedSingleEditOperation[][] = [];
for (let i = 0; i < ctx.selectionsBefore.length; i++) {
groupedInverseEditOperations[i] = [];
}
for (let i = 0; i < inverseEditOperations.length; i++) {
const op = inverseEditOperations[i];
if (!op.identifier) {
// perhaps auto whitespace trim edits
continue;
}
groupedInverseEditOperations[op.identifier.major].push(op);
}
const minorBasedSorter = (a: editorCommon.IIdentifiedSingleEditOperation, b: editorCommon.IIdentifiedSingleEditOperation) => {
return a.identifier.minor - b.identifier.minor;
};
let cursorSelections: Selection[] = [];
for (let i = 0; i < ctx.selectionsBefore.length; i++) {
if (groupedInverseEditOperations[i].length > 0) {
groupedInverseEditOperations[i].sort(minorBasedSorter);
cursorSelections[i] = commands[i].computeCursorState(ctx.model, {
getInverseEditOperations: () => {
return groupedInverseEditOperations[i];
},
getTrackedSelection: (id: string) => {
const idx = parseInt(id, 10);
const selectionStartMarker = ctx.model._getMarker(ctx.selectionStartMarkers[idx]);
const positionMarker = ctx.model._getMarker(ctx.positionMarkers[idx]);
return new Selection(selectionStartMarker.lineNumber, selectionStartMarker.column, positionMarker.lineNumber, positionMarker.column);
}
});
} else {
cursorSelections[i] = ctx.selectionsBefore[i];
}
}
return cursorSelections;
});
// Extract losing cursors
let losingCursors: number[] = [];
for (let losingCursorIndex in loserCursorsMap) {
if (loserCursorsMap.hasOwnProperty(losingCursorIndex)) {
losingCursors.push(parseInt(losingCursorIndex, 10));
}
}
// Sort losing cursors descending
losingCursors.sort((a: number, b: number): number => {
return b - a;
});
// Remove losing cursors
for (let i = 0; i < losingCursors.length; i++) {
selectionsAfter.splice(losingCursors[i], 1);
}
return selectionsAfter;
}
private static _arrayIsEmpty(commands: editorCommon.ICommand[]): boolean {
for (let i = 0, len = commands.length; i < len; i++) {
if (commands[i]) {
return false;
}
}
return true;
}
private static _getEditOperations(ctx: IExecContext, commands: editorCommon.ICommand[]): ICommandsData {
let operations: editorCommon.IIdentifiedSingleEditOperation[] = [];
let hadTrackedEditOperation: boolean = false;
for (let i = 0, len = commands.length; i < len; i++) {
if (commands[i]) {
const r = this._getEditOperationsFromCommand(ctx, i, commands[i]);
operations = operations.concat(r.operations);
hadTrackedEditOperation = hadTrackedEditOperation || r.hadTrackedEditOperation;
}
}
return {
operations: operations,
hadTrackedEditOperation: hadTrackedEditOperation
};
}
private static _getEditOperationsFromCommand(ctx: IExecContext, majorIdentifier: number, command: editorCommon.ICommand): ICommandData {
// This method acts as a transaction, if the command fails
// everything it has done is ignored
let operations: editorCommon.IIdentifiedSingleEditOperation[] = [];
let operationMinor = 0;
const addEditOperation = (selection: Range, text: string) => {
if (selection.isEmpty() && text === '') {
// This command wants to add a no-op => no thank you
return;
}
operations.push({
identifier: {
major: majorIdentifier,
minor: operationMinor++
},
range: selection,
text: text,
forceMoveMarkers: false,
isAutoWhitespaceEdit: command.insertsAutoWhitespace
});
};
let hadTrackedEditOperation = false;
const addTrackedEditOperation = (selection: Range, text: string) => {
hadTrackedEditOperation = true;
addEditOperation(selection, text);
};
const trackSelection = (selection: Selection, trackPreviousOnEmpty?: boolean) => {
let selectionMarkerStickToPreviousCharacter: boolean;
let positionMarkerStickToPreviousCharacter: boolean;
if (selection.isEmpty()) {
// Try to lock it with surrounding text
if (typeof trackPreviousOnEmpty === 'boolean') {
selectionMarkerStickToPreviousCharacter = trackPreviousOnEmpty;
positionMarkerStickToPreviousCharacter = trackPreviousOnEmpty;
} else {
const maxLineColumn = ctx.model.getLineMaxColumn(selection.startLineNumber);
if (selection.startColumn === maxLineColumn) {
selectionMarkerStickToPreviousCharacter = true;
positionMarkerStickToPreviousCharacter = true;
} else {
selectionMarkerStickToPreviousCharacter = false;
positionMarkerStickToPreviousCharacter = false;
}
}
} else {
if (selection.getDirection() === SelectionDirection.LTR) {
selectionMarkerStickToPreviousCharacter = false;
positionMarkerStickToPreviousCharacter = true;
} else {
selectionMarkerStickToPreviousCharacter = true;
positionMarkerStickToPreviousCharacter = false;
}
}
const l = ctx.selectionStartMarkers.length;
ctx.selectionStartMarkers[l] = ctx.model._addMarker(0, selection.selectionStartLineNumber, selection.selectionStartColumn, selectionMarkerStickToPreviousCharacter);
ctx.positionMarkers[l] = ctx.model._addMarker(0, selection.positionLineNumber, selection.positionColumn, positionMarkerStickToPreviousCharacter);
return l.toString();
};
const editOperationBuilder: editorCommon.IEditOperationBuilder = {
addEditOperation: addEditOperation,
addTrackedEditOperation: addTrackedEditOperation,
trackSelection: trackSelection
};
try {
command.getEditOperations(ctx.model, editOperationBuilder);
} catch (e) {
e.friendlyMessage = nls.localize('corrupt.commands', "Unexpected exception while executing command.");
onUnexpectedError(e);
return {
operations: [],
hadTrackedEditOperation: false
};
}
return {
operations: operations,
hadTrackedEditOperation: hadTrackedEditOperation
};
}
private static _getLoserCursorMap(operations: editorCommon.IIdentifiedSingleEditOperation[]): { [index: string]: boolean; } {
// This is destructive on the array
operations = operations.slice(0);
// Sort operations with last one first
operations.sort((a: editorCommon.IIdentifiedSingleEditOperation, b: editorCommon.IIdentifiedSingleEditOperation): number => {
// Note the minus!
return -(Range.compareRangesUsingEnds(a.range, b.range));
});
// Operations can not overlap!
let loserCursorsMap: { [index: string]: boolean; } = {};
for (let i = 1; i < operations.length; i++) {
const previousOp = operations[i - 1];
const currentOp = operations[i];
if (previousOp.range.getStartPosition().isBefore(currentOp.range.getEndPosition())) {
let loserMajor: number;
if (previousOp.identifier.major > currentOp.identifier.major) {
// previousOp loses the battle
loserMajor = previousOp.identifier.major;
} else {
loserMajor = currentOp.identifier.major;
}
loserCursorsMap[loserMajor.toString()] = true;
for (let j = 0; j < operations.length; j++) {
if (operations[j].identifier.major === loserMajor) {
operations.splice(j, 1);
if (j < i) {
i--;
}
j--;
}
}
if (i > 0) {
i--;
}
}
}
return loserCursorsMap;
}
}
| src/vs/editor/common/controller/cursor.ts | 1 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00017689770902507007,
0.0001713475357973948,
0.0001626816374482587,
0.00017186679178848863,
0.0000031415399917023024
] |
{
"id": 0,
"code_window": [
"\ttop: initial !important;\n",
"\tleft: initial !important;\n",
"\tbottom: 0 !important;\n",
"\tright: 0 !important;\n",
"}*/\n",
".monaco-editor .inputarea.ime-input {\n",
"\tz-index: 10;\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tcolor: black !important;\n",
"\tbackground: white !important;\n",
"\tline-height: 15px !important;\n",
"\tfont-size: 14px !important;\n"
],
"file_path": "src/vs/editor/browser/controller/textAreaHandler.css",
"type": "add",
"edit_start_line_idx": 26
} | {
"tabSize": 4,
"indentSize": 4,
"newLineCharacter": "\n",
"convertTabsToSpaces": false,
"insertSpaceAfterCommaDelimiter": true,
"insertSpaceAfterSemicolonInForStatements": true,
"insertSpaceBeforeAndAfterBinaryOperators": true,
"insertSpaceAfterKeywordsInControlFlowStatements": true,
"insertSpaceAfterFunctionKeywordForAnonymousFunctions": true,
"insertSpaceAfterOpeningAndBeforeClosingNonemptyParenthesis": false,
"insertSpaceAfterOpeningAndBeforeClosingNonemptyBrackets": false,
"insertSpaceAfterOpeningAndBeforeClosingTemplateStringBraces": false,
"insertSpaceBeforeFunctionParenthesis": false,
"placeOpenBraceOnNewLineForFunctions": false,
"placeOpenBraceOnNewLineForControlBlocks": false
}
| tsfmt.json | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00017213392129633576,
0.0001706027251202613,
0.0001690715434961021,
0.0001706027251202613,
0.000001531188900116831
] |
{
"id": 0,
"code_window": [
"\ttop: initial !important;\n",
"\tleft: initial !important;\n",
"\tbottom: 0 !important;\n",
"\tright: 0 !important;\n",
"}*/\n",
".monaco-editor .inputarea.ime-input {\n",
"\tz-index: 10;\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tcolor: black !important;\n",
"\tbackground: white !important;\n",
"\tline-height: 15px !important;\n",
"\tfont-size: 14px !important;\n"
],
"file_path": "src/vs/editor/browser/controller/textAreaHandler.css",
"type": "add",
"edit_start_line_idx": 26
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import { createConnection, IConnection, TextDocuments, InitializeParams, InitializeResult, RequestType, DocumentRangeFormattingRequest, Disposable, DocumentSelector } from 'vscode-languageserver';
import { DocumentContext } from 'vscode-html-languageservice';
import { TextDocument, Diagnostic, DocumentLink, Range, SymbolInformation } from 'vscode-languageserver-types';
import { getLanguageModes, LanguageModes } from './modes/languageModes';
import { format } from './modes/formatting';
import { pushAll } from './utils/arrays';
import * as url from 'url';
import * as path from 'path';
import uri from 'vscode-uri';
import * as nls from 'vscode-nls';
nls.config(process.env['VSCODE_NLS_CONFIG']);
namespace ColorSymbolRequest {
export const type: RequestType<string, Range[], any, any> = new RequestType('css/colorSymbols');
}
// Create a connection for the server
let connection: IConnection = createConnection();
console.log = connection.console.log.bind(connection.console);
console.error = connection.console.error.bind(connection.console);
// Create a simple text document manager. The text document manager
// supports full document sync only
let documents: TextDocuments = new TextDocuments();
// Make the text document manager listen on the connection
// for open, change and close text document events
documents.listen(connection);
let workspacePath: string;
var languageModes: LanguageModes;
var settings: any = {};
let clientSnippetSupport = false;
let clientDynamicRegisterSupport = false;
// After the server has started the client sends an initilize request. The server receives
// in the passed params the rootPath of the workspace plus the client capabilites
connection.onInitialize((params: InitializeParams): InitializeResult => {
let initializationOptions = params.initializationOptions;
workspacePath = params.rootPath;
languageModes = getLanguageModes(initializationOptions ? initializationOptions.embeddedLanguages : { css: true, javascript: true });
documents.onDidClose(e => {
languageModes.onDocumentRemoved(e.document);
});
connection.onShutdown(() => {
languageModes.dispose();
});
function hasClientCapability(...keys: string[]) {
let c = params.capabilities;
for (let i = 0; c && i < keys.length; i++) {
c = c[keys[i]];
}
return !!c;
}
clientSnippetSupport = hasClientCapability('textDocument', 'completion', 'completionItem', 'snippetSupport');
clientDynamicRegisterSupport = hasClientCapability('workspace', 'symbol', 'dynamicRegistration');
return {
capabilities: {
// Tell the client that the server works in FULL text document sync mode
textDocumentSync: documents.syncKind,
completionProvider: clientSnippetSupport ? { resolveProvider: true, triggerCharacters: ['.', ':', '<', '"', '=', '/'] } : null,
hoverProvider: true,
documentHighlightProvider: true,
documentRangeFormattingProvider: false,
documentLinkProvider: { resolveProvider: false },
documentSymbolProvider: true,
definitionProvider: true,
signatureHelpProvider: { triggerCharacters: ['('] },
referencesProvider: true,
}
};
});
let validation = {
html: true,
css: true,
javascript: true
};
let formatterRegistration: Thenable<Disposable> = null;
// The settings have changed. Is send on server activation as well.
connection.onDidChangeConfiguration((change) => {
settings = change.settings;
let validationSettings = settings && settings.html && settings.html.validate || {};
validation.css = validationSettings.styles !== false;
validation.javascript = validationSettings.scripts !== false;
languageModes.getAllModes().forEach(m => {
if (m.configure) {
m.configure(change.settings);
}
});
documents.all().forEach(triggerValidation);
// dynamically enable & disable the formatter
if (clientDynamicRegisterSupport) {
let enableFormatter = settings && settings.html && settings.html.format && settings.html.format.enable;
if (enableFormatter) {
if (!formatterRegistration) {
let documentSelector: DocumentSelector = [{ language: 'html' }, { language: 'handlebars' }]; // don't register razor, the formatter does more harm than good
formatterRegistration = connection.client.register(DocumentRangeFormattingRequest.type, { documentSelector });
}
} else if (formatterRegistration) {
formatterRegistration.then(r => r.dispose());
formatterRegistration = null;
}
}
});
let pendingValidationRequests: { [uri: string]: NodeJS.Timer } = {};
const validationDelayMs = 200;
// The content of a text document has changed. This event is emitted
// when the text document first opened or when its content has changed.
documents.onDidChangeContent(change => {
triggerValidation(change.document);
});
// a document has closed: clear all diagnostics
documents.onDidClose(event => {
cleanPendingValidation(event.document);
connection.sendDiagnostics({ uri: event.document.uri, diagnostics: [] });
});
function cleanPendingValidation(textDocument: TextDocument): void {
let request = pendingValidationRequests[textDocument.uri];
if (request) {
clearTimeout(request);
delete pendingValidationRequests[textDocument.uri];
}
}
function triggerValidation(textDocument: TextDocument): void {
cleanPendingValidation(textDocument);
pendingValidationRequests[textDocument.uri] = setTimeout(() => {
delete pendingValidationRequests[textDocument.uri];
validateTextDocument(textDocument);
}, validationDelayMs);
}
function validateTextDocument(textDocument: TextDocument): void {
let diagnostics: Diagnostic[] = [];
if (textDocument.languageId === 'html') {
languageModes.getAllModesInDocument(textDocument).forEach(mode => {
if (mode.doValidation && validation[mode.getId()]) {
pushAll(diagnostics, mode.doValidation(textDocument));
}
});
}
connection.sendDiagnostics({ uri: textDocument.uri, diagnostics });
}
connection.onCompletion(textDocumentPosition => {
let document = documents.get(textDocumentPosition.textDocument.uri);
let mode = languageModes.getModeAtPosition(document, textDocumentPosition.position);
if (mode && mode.doComplete) {
if (mode.getId() !== 'html') {
connection.telemetry.logEvent({ key: 'html.embbedded.complete', value: { languageId: mode.getId() } });
}
return mode.doComplete(document, textDocumentPosition.position);
}
return { isIncomplete: true, items: [] };
});
connection.onCompletionResolve(item => {
let data = item.data;
if (data && data.languageId && data.uri) {
let mode = languageModes.getMode(data.languageId);
let document = documents.get(data.uri);
if (mode && mode.doResolve && document) {
return mode.doResolve(document, item);
}
}
return item;
});
connection.onHover(textDocumentPosition => {
let document = documents.get(textDocumentPosition.textDocument.uri);
let mode = languageModes.getModeAtPosition(document, textDocumentPosition.position);
if (mode && mode.doHover) {
return mode.doHover(document, textDocumentPosition.position);
}
return null;
});
connection.onDocumentHighlight(documentHighlightParams => {
let document = documents.get(documentHighlightParams.textDocument.uri);
let mode = languageModes.getModeAtPosition(document, documentHighlightParams.position);
if (mode && mode.findDocumentHighlight) {
return mode.findDocumentHighlight(document, documentHighlightParams.position);
}
return [];
});
connection.onDefinition(definitionParams => {
let document = documents.get(definitionParams.textDocument.uri);
let mode = languageModes.getModeAtPosition(document, definitionParams.position);
if (mode && mode.findDefinition) {
return mode.findDefinition(document, definitionParams.position);
}
return [];
});
connection.onReferences(referenceParams => {
let document = documents.get(referenceParams.textDocument.uri);
let mode = languageModes.getModeAtPosition(document, referenceParams.position);
if (mode && mode.findReferences) {
return mode.findReferences(document, referenceParams.position);
}
return [];
});
connection.onSignatureHelp(signatureHelpParms => {
let document = documents.get(signatureHelpParms.textDocument.uri);
let mode = languageModes.getModeAtPosition(document, signatureHelpParms.position);
if (mode && mode.doSignatureHelp) {
return mode.doSignatureHelp(document, signatureHelpParms.position);
}
return null;
});
connection.onDocumentRangeFormatting(formatParams => {
let document = documents.get(formatParams.textDocument.uri);
let unformattedTags: string = settings && settings.html && settings.html.format && settings.html.format.unformatted || '';
let enabledModes = { css: !unformattedTags.match(/\bstyle\b/), javascript: !unformattedTags.match(/\bscript\b/) };
return format(languageModes, document, formatParams.range, formatParams.options, enabledModes);
});
connection.onDocumentLinks(documentLinkParam => {
let document = documents.get(documentLinkParam.textDocument.uri);
let documentContext: DocumentContext = {
resolveReference: (ref, base) => {
if (base) {
ref = url.resolve(base, ref);
}
if (workspacePath && ref[0] === '/') {
return uri.file(path.join(workspacePath, ref)).toString();
}
return url.resolve(document.uri, ref);
},
};
let links: DocumentLink[] = [];
languageModes.getAllModesInDocument(document).forEach(m => {
if (m.findDocumentLinks) {
pushAll(links, m.findDocumentLinks(document, documentContext));
}
});
return links;
});
connection.onDocumentSymbol(documentSymbolParms => {
let document = documents.get(documentSymbolParms.textDocument.uri);
let symbols: SymbolInformation[] = [];
languageModes.getAllModesInDocument(document).forEach(m => {
if (m.findDocumentSymbols) {
pushAll(symbols, m.findDocumentSymbols(document));
}
});
return symbols;
});
connection.onRequest(ColorSymbolRequest.type, uri => {
let ranges: Range[] = [];
let document = documents.get(uri);
if (document) {
languageModes.getAllModesInDocument(document).forEach(m => {
if (m.findColorSymbols) {
pushAll(ranges, m.findColorSymbols(document));
}
});
}
return ranges;
});
// Listen on the connection
connection.listen(); | extensions/html/server/src/htmlServerMain.ts | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00017894791380967945,
0.0001727033668430522,
0.0001646912714932114,
0.00017335204756818712,
0.0000030543296816176735
] |
{
"id": 0,
"code_window": [
"\ttop: initial !important;\n",
"\tleft: initial !important;\n",
"\tbottom: 0 !important;\n",
"\tright: 0 !important;\n",
"}*/\n",
".monaco-editor .inputarea.ime-input {\n",
"\tz-index: 10;\n",
"}"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tcolor: black !important;\n",
"\tbackground: white !important;\n",
"\tline-height: 15px !important;\n",
"\tfont-size: 14px !important;\n"
],
"file_path": "src/vs/editor/browser/controller/textAreaHandler.css",
"type": "add",
"edit_start_line_idx": 26
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
// Do not edit this file. It is machine generated.
{
"ok": "OK",
"pathNotExistTitle": "Il percorso non esiste",
"pathNotExistDetail": "Il percorso '{0}' sembra non esistere più sul disco.",
"accessibilityOptionsWindowTitle": "Opzioni accessibilità",
"reopen": "Riapri",
"wait": "Continua ad attendere",
"close": "Chiudi",
"appStalled": "La finestra non risponde",
"appStalledDetail": "È possibile riaprire la finestra, chiuderla oppure attendere.",
"appCrashed": "Si è verificato un arresto anomalo della finestra",
"appCrashedDetail": "Ci scusiamo per l'inconveniente. Per riprendere dal punto in cui si è verificata l'interruzione, riaprire la finestra.",
"newWindow": "Nuova finestra",
"newWindowDesc": "Apre una nuova finestra",
"recentFolders": "Cartelle recenti",
"folderDesc": "{0} {1}"
} | i18n/ita/src/vs/code/electron-main/windows.i18n.json | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00017370140994898975,
0.0001728013885440305,
0.000171216408489272,
0.0001734863908495754,
0.000001124192749557551
] |
{
"id": 1,
"code_window": [
"\n",
"\t\tconst selections = this._cursors.getSelections();\n",
"\t\tconst viewSelections = this._cursors.getViewSelections();\n",
"\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n",
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t// Let the view get the event first.\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "replace",
"edit_start_line_idx": 374
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
.monaco-editor .inputarea {
min-width: 0;
min-height: 0;
margin: 0;
padding: 0;
position: absolute;
outline: none !important;
resize: none;
border: none;
overflow: hidden;
color: transparent;
background-color: transparent;
}
/*.monaco-editor .inputarea {
position: fixed !important;
width: 800px !important;
height: 500px !important;
top: initial !important;
left: initial !important;
bottom: 0 !important;
right: 0 !important;
}*/
.monaco-editor .inputarea.ime-input {
z-index: 10;
}
| src/vs/editor/browser/controller/textAreaHandler.css | 1 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00017771750572137535,
0.00017679716984275728,
0.0001750740484567359,
0.00017719855532050133,
0.000001017506406242319
] |
{
"id": 1,
"code_window": [
"\n",
"\t\tconst selections = this._cursors.getSelections();\n",
"\t\tconst viewSelections = this._cursors.getViewSelections();\n",
"\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n",
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t// Let the view get the event first.\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "replace",
"edit_start_line_idx": 374
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
// Do not edit this file. It is machine generated.
{
"vscode.extension.contributes.grammars": "Добавляет разметчики TextMate.",
"vscode.extension.contributes.grammars.language": "Идентификатор языка, для которого добавляется этот синтаксис.",
"vscode.extension.contributes.grammars.scopeName": "Имя области TextMate, используемое в файле tmLanguage.",
"vscode.extension.contributes.grammars.path": "Путь к файлу tmLanguage. Путь указывается относительно папки расширения и обычно начинается с \"./syntaxes/\".",
"vscode.extension.contributes.grammars.embeddedLanguages": "Сопоставление имени области и идентификатора языка, если грамматика содержит внедренные языки.",
"vscode.extension.contributes.grammars.injectTo": "Список имен языковых областей, в которые вставляется эта грамматика."
} | i18n/rus/src/vs/editor/node/textMate/TMGrammars.i18n.json | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.0001698390260571614,
0.00016951381985563785,
0.0001691886136541143,
0.00016951381985563785,
3.252062015235424e-7
] |
{
"id": 1,
"code_window": [
"\n",
"\t\tconst selections = this._cursors.getSelections();\n",
"\t\tconst viewSelections = this._cursors.getViewSelections();\n",
"\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n",
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t// Let the view get the event first.\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "replace",
"edit_start_line_idx": 374
} | <svg xmlns="http://www.w3.org/2000/svg" width="300" height="40"><path d="M288.483 33c-.772 0-1.497-.123-2.153-.365-.678-.253-1.27-.617-1.76-1.084-.5-.475-.892-1.049-1.163-1.704-.27-.644-.407-1.371-.407-2.158 0-.517.061-1.018.178-1.49.116-.47.29-.925.516-1.348.225-.422.508-.815.844-1.167.334-.352.717-.656 1.139-.905.416-.246.881-.44 1.38-.576.493-.134 1.026-.202 1.587-.202.705 0 1.382.109 2.013.324.642.217 1.218.538 1.708.955.501.425.903.948 1.193 1.556.294.623.442 1.316.442 2.064 0 .619-.09 1.185-.268 1.679-.178.492-.42.92-.721 1.275-.331.377-.699.658-1.104.847l-.048.022v1.53l-.587.266c-.128.059-.288.117-.474.179-.193.062-.404.114-.645.159-.229.04-.477.076-.753.103-.27.027-.578.04-.917.04z" fill="#2D2D2D"/><path d="M291.716 24.041c-.396-.336-.856-.593-1.384-.771-.527-.18-1.09-.271-1.689-.271-.473 0-.912.055-1.324.167-.414.112-.791.27-1.135.473-.342.202-.65.446-.922.733-.273.286-.502.602-.686.949-.186.347-.33.722-.428 1.119-.1.399-.148.814-.148 1.247 0 .652.109 1.247.332 1.776.219.531.53.984.928 1.361.396.378.871.667 1.416.87.548.202 1.152.304 1.808.304.302 0 .577-.011.823-.035.246-.023.468-.056.664-.091.195-.036.366-.078.514-.125l.375-.14v-.854l-.463.184c-.16.056-.336.104-.521.143-.188.037-.387.069-.604.089-.213.024-.448.034-.7.034-.562 0-1.064-.088-1.509-.264-.442-.176-.816-.421-1.125-.731-.309-.314-.545-.687-.708-1.124-.161-.435-.243-.913-.243-1.432 0-.545.09-1.053.273-1.522.182-.471.435-.879.758-1.225.324-.345.708-.617 1.155-.815.446-.196.934-.294 1.457-.294.419 0 .798.044 1.122.136.329.091.62.215.871.369.254.158.465.339.643.547.179.209.324.432.438.667.113.237.193.48.246.731.051.254.076.5.076.741 0 .344-.033.653-.102.926-.068.274-.158.503-.269.694-.11.189-.239.335-.386.434s-.295.148-.453.148l-.215-.045c-.066-.029-.119-.08-.166-.156-.046-.075-.082-.177-.107-.306-.025-.126-.039-.292-.039-.492l.018-.325.041-.53.055-.644.058-.647.048-.546.027-.344h-.919l-.054.6h-.021c-.025-.103-.07-.195-.136-.281-.063-.083-.141-.155-.233-.216-.091-.061-.193-.106-.307-.141-.115-.033-.238-.048-.369-.048-.337 0-.646.07-.924.216-.281.144-.518.344-.721.599-.201.254-.355.556-.465.905-.115.35-.17.726-.17 1.134 0 .344.045.645.135.901.088.26.211.473.359.646.153.171.329.3.534.382.2.086.415.129.641.129.176 0 .342-.027.499-.081.154-.052.302-.13.432-.232.134-.104.248-.23.348-.38.102-.149.182-.323.236-.52h.027c0 .376.101.674.307.893.207.22.502.33.889.33.292 0 .58-.064.863-.198.283-.132.536-.328.762-.586.223-.262.404-.583.543-.966.138-.384.208-.83.208-1.34 0-.605-.117-1.15-.345-1.634-.231-.482-.546-.891-.939-1.225m-2.368 3.774c-.056.277-.136.517-.246.719-.109.203-.246.363-.407.481-.163.115-.354.176-.572.176-.12 0-.236-.025-.344-.078-.108-.052-.206-.13-.289-.232-.081-.103-.148-.234-.198-.39-.046-.156-.07-.337-.07-.547 0-.237.027-.481.08-.729.056-.247.137-.473.25-.677.109-.2.25-.363.416-.492.165-.127.361-.191.582-.191.123 0 .234.021.34.063.107.042.198.107.279.196.08.087.145.197.189.33.043.134.07.294.07.48 0 .317-.031.615-.08.891" fill="#C5C5C5"/><path d="M288.483 13c-.772 0-1.497-.123-2.153-.365-.678-.253-1.27-.617-1.76-1.084-.5-.475-.892-1.049-1.163-1.704-.269-.644-.407-1.371-.407-2.159 0-.517.061-1.018.178-1.49.116-.47.29-.925.516-1.348.225-.422.508-.815.844-1.167.334-.352.717-.656 1.139-.905.416-.246.881-.44 1.38-.576.492-.134 1.025-.202 1.586-.202.705 0 1.382.109 2.013.324.642.217 1.218.538 1.708.955.501.425.903.948 1.193 1.556.295.624.443 1.317.443 2.065 0 .619-.09 1.185-.268 1.679-.178.492-.42.92-.721 1.275-.331.377-.699.658-1.104.847l-.048.022v1.53l-.587.266c-.128.059-.288.117-.474.179-.193.062-.404.114-.645.159-.229.04-.477.076-.753.103-.27.027-.578.04-.917.04z" fill="#F3F3F3"/><path d="M291.716 4.041c-.396-.336-.856-.593-1.384-.771-.527-.179-1.09-.27-1.689-.27-.473 0-.912.055-1.324.167-.414.112-.791.27-1.135.473-.342.202-.65.446-.922.733-.273.286-.502.602-.686.949-.186.347-.33.722-.428 1.119-.099.4-.148.815-.148 1.247 0 .652.109 1.247.332 1.776.219.531.53.984.928 1.361.396.378.871.667 1.416.87.548.202 1.152.304 1.808.304.302 0 .577-.011.823-.035.246-.023.468-.056.664-.091.195-.036.366-.078.514-.125l.375-.14v-.854l-.463.184c-.16.056-.336.104-.521.143-.188.037-.387.069-.604.089-.213.024-.448.034-.7.034-.562 0-1.064-.088-1.509-.264-.442-.176-.816-.421-1.125-.731-.309-.314-.545-.687-.708-1.124-.161-.435-.243-.913-.243-1.432 0-.545.09-1.053.273-1.522.182-.471.435-.879.758-1.225.324-.345.708-.617 1.155-.815.446-.196.934-.294 1.457-.294.419 0 .798.044 1.122.136.329.091.62.215.871.369.254.158.465.339.643.547.179.209.324.432.438.667.113.237.193.48.246.731.051.254.076.5.076.741 0 .344-.033.653-.102.926-.068.274-.158.503-.269.694-.11.189-.239.335-.386.434s-.295.148-.453.148l-.215-.045c-.066-.029-.119-.08-.166-.156-.046-.075-.082-.177-.107-.306-.025-.126-.039-.292-.039-.492l.018-.325.041-.53.055-.644.058-.647.048-.546.027-.344h-.919l-.054.6h-.021c-.025-.103-.07-.195-.136-.281-.063-.083-.141-.155-.233-.216-.091-.061-.193-.106-.307-.141-.115-.033-.238-.048-.369-.048-.337 0-.646.07-.924.216-.281.144-.518.344-.721.599-.201.254-.355.556-.465.905-.115.35-.17.726-.17 1.134 0 .344.045.645.135.901.088.26.211.473.359.646.153.171.329.3.534.382.2.086.415.129.641.129.176 0 .342-.027.499-.081.154-.052.302-.13.432-.232.134-.104.248-.23.348-.38.102-.149.182-.323.236-.52h.027c0 .376.101.674.307.893.207.22.502.33.889.33.292 0 .58-.064.863-.198.283-.132.536-.328.762-.586.223-.262.404-.583.543-.966.138-.385.208-.831.208-1.341 0-.605-.117-1.15-.345-1.634-.231-.482-.546-.891-.939-1.225m-2.368 3.774c-.056.277-.136.517-.246.719-.109.203-.246.363-.407.481-.163.115-.354.176-.572.176-.12 0-.236-.025-.344-.078-.108-.052-.206-.13-.289-.232-.081-.103-.148-.234-.198-.39-.046-.156-.07-.337-.07-.547 0-.237.027-.481.08-.729.056-.247.137-.473.25-.677.109-.2.25-.363.416-.492.165-.127.361-.191.582-.191.123 0 .234.021.34.063.107.042.198.107.279.196.08.087.145.197.189.33.043.134.07.294.07.48 0 .317-.031.615-.08.891" fill="#424242"/><path d="M264 37v-14h8.625l3.375 3.556v10.444h-12z" fill="#2D2D2D"/><path d="M272 24h-7v12h10v-9l-3-3zm2 11h-8v-10h5v3h3v7z" fill="#C5C5C5"/><polygon points="266,25 271,25 271,28 274,28 274,35 266,35" fill="#2D2D2D"/><path d="M264 17v-14h8.625l3.375 3.556v10.444h-12z" fill="#F3F3F3"/><path d="M272 4h-7v12h10v-9l-3-3zm2 11h-8v-10h5v3h3v7z" fill="#424242"/><polygon points="266,5 271,5 271,8 274,8 274,15 266,15" fill="#F0EFF1"/><polygon points="247,34 247,30 245,30 245,26 255,26 255,34" fill="#2D2D2D"/><path d="M254 29h-8v-2h8v2zm0 1h-6v1h6v-1zm0 2h-6v1h6v-1z" fill="#C5C5C5"/><polygon points="247,14 247,10 245,10 245,6 255,6 255,14" fill="#F3F3F3"/><path d="M254 9h-8v-2h8v2zm0 1h-6v1h6v-1zm0 2h-6v1h6v-1z" fill="#424242"/><path d="M230.5 22c-4.143 0-7.5 3.357-7.5 7.5s3.357 7.5 7.5 7.5 7.5-3.357 7.5-7.5-3.357-7.5-7.5-7.5zm0 11c-1.933 0-3.5-1.566-3.5-3.5s1.567-3.5 3.5-3.5 3.5 1.566 3.5 3.5-1.567 3.5-3.5 3.5z" fill="#2D2D2D"/><path d="M224.025 29c.108-1.418.669-2.708 1.542-3.726l1.431 1.431c-.516.646-.851 1.43-.947 2.295h-2.026zm2.973 3.295c-.516-.646-.851-1.43-.947-2.295h-2.025c.108 1.418.669 2.707 1.542 3.726l1.43-1.431zm4.002-9.27v2.025c.865.097 1.649.432 2.295.947l1.431-1.431c-1.018-.872-2.308-1.432-3.726-1.541zm-3.295 2.973c.646-.516 1.43-.851 2.295-.947v-2.025c-1.418.108-2.708.669-3.726 1.542l1.431 1.43zm6.297.707c.516.646.851 1.43.947 2.295h2.025c-.108-1.418-.669-2.708-1.542-3.726l-1.43 1.431zm-4.002 7.244c-.865-.097-1.649-.432-2.295-.947l-1.431 1.431c1.018.873 2.307 1.434 3.726 1.542v-2.026zm4.949-3.949c-.097.865-.432 1.648-.947 2.295l1.431 1.431c.873-1.019 1.434-2.308 1.542-3.726h-2.026zm-1.654 3.002c-.646.516-1.43.851-2.295.947v2.025c1.419-.108 2.708-.669 3.726-1.542l-1.431-1.43z" fill="#C5C5C5"/><path d="M230.5 2c-4.143 0-7.5 3.358-7.5 7.5 0 4.143 3.357 7.5 7.5 7.5s7.5-3.357 7.5-7.5c0-4.142-3.357-7.5-7.5-7.5zm0 11c-1.933 0-3.5-1.566-3.5-3.5 0-1.933 1.567-3.5 3.5-3.5s3.5 1.567 3.5 3.5c0 1.934-1.567 3.5-3.5 3.5z" fill="#F3F3F3"/><path d="M224.025 9c.108-1.418.669-2.708 1.542-3.726l1.431 1.431c-.516.646-.851 1.43-.947 2.294h-2.026zm2.973 3.295c-.516-.646-.851-1.43-.947-2.295h-2.025c.108 1.418.669 2.707 1.542 3.726l1.43-1.431zm4.002-9.27v2.025c.865.097 1.649.432 2.295.948l1.431-1.431c-1.018-.873-2.308-1.433-3.726-1.542zm-3.295 2.974c.646-.516 1.43-.851 2.295-.948v-2.026c-1.418.108-2.708.669-3.726 1.542l1.431 1.432zm6.297.707c.516.646.851 1.43.947 2.294h2.025c-.108-1.418-.669-2.708-1.542-3.726l-1.43 1.432zm-4.002 7.243c-.865-.097-1.649-.432-2.295-.947l-1.431 1.431c1.018.873 2.307 1.434 3.726 1.542v-2.026zm4.949-3.949c-.097.865-.432 1.648-.947 2.295l1.431 1.431c.873-1.019 1.434-2.308 1.542-3.726h-2.026zm-1.654 3.002c-.646.516-1.43.851-2.295.947v2.025c1.419-.108 2.708-.669 3.726-1.542l-1.431-1.43z" fill="#424242"/><rect x="202" y="23" width="16" height="14" fill="#2D2D2D"/><path d="M203 24v12h14v-12h-14zm13 11h-12v-10h12v10zm-6-7v-1h-1v5h3v-4h-2zm1 3h-1v-2h1v2zm3-2v2h1v1h-2v-4h2v1h-1zm-6-1v4h-3v-2h1v1h1v-1h-1v-1h-1v-1h3z" fill="#C5C5C5"/><path d="M210 29h1v2h-1v-2zm-3 2v-1h-1v1h1zm9-6v10h-12v-10h12zm-8 3h-3v1h1v1h-1v2h3v-4zm4 0h-2v-1h-1v5h3v-4zm3 0h-2v4h2v-1h-1v-2h1v-1z" fill="#2D2D2D"/><rect x="202" y="3" width="16" height="14" fill="#F3F3F3"/><path d="M203 4v12h14v-12h-14zm13 11h-12v-10h12v10zm-6-7v-1h-1v5h3v-4h-2zm1 3h-1v-2h1v2zm3-2v2h1v1h-2v-4h2v1h-1zm-6-1v4h-3v-2h1v1h1v-1h-1v-1h-1v-1h3z" fill="#424242"/><path d="M210 9h1v2h-1v-2zm-3 2v-1h-1v1h1zm9-6v10h-12v-10h12zm-8 3h-3v1h1v1h-1v2h3v-4zm4 0h-2v-1h-1v5h3v-4zm3 0h-2v4h2v-1h-1v-2h1v-1z" fill="#F0EFF1"/><path d="M196.652 32.5c.811-.537 1.348-1.457 1.348-2.5 0-1.654-1.346-3-3-3-.771 0-1.468.301-2 .779v-5.779h-11v12h3.764l-1.452.727 1.481 1.48c.322.322.803.5 1.354.5.436 0 .897-.111 1.301-.313l3.144-1.572c.134.053.271.098.414.127l-.005.051c0 1.654 1.346 3 3 3s3-1.346 3-3c-.001-1.043-.538-1.963-1.349-2.5z" fill="#2D2D2D"/><path d="M195 33c-.293 0-.569.066-.82.18l-.25-.25c.042-.137.07-.279.07-.43s-.028-.293-.07-.43l.25-.25c.251.113.527.18.82.18 1.104 0 2-.896 2-2 0-1.105-.896-2-2-2s-2 .895-2 2c0 .293.066.568.18.82l-.25.25c-.137-.043-.279-.07-.43-.07-.337 0-.645.115-.895.303l-2.607-1.305-.999-.5c-.552-.275-1.223-.275-1.499.002l-.5.5 5 2.5-5 2.5.5.5c.276.275.947.275 1.5 0l1-.5 2.605-1.303c.25.188.558.303.895.303.15 0 .293-.029.43-.07l.25.25c-.114.25-.18.527-.18.82 0 1.104.896 2 2 2s2-.896 2-2c0-1.105-.896-2-2-2zm0-4c.553 0 1 .447 1 1 0 .551-.447 1-1 1s-1-.449-1-1c0-.553.447-1 1-1zm-2.5 4c-.276 0-.5-.225-.5-.5 0-.277.224-.5.5-.5s.5.223.5.5c0 .275-.224.5-.5.5zm2.5 3c-.553 0-1-.449-1-1 0-.553.447-1 1-1s1 .447 1 1c0 .551-.447 1-1 1zm-3-13v7.051c-.142.029-.279.07-.413.123l-.587-.174v-6h-7v7h-1v-8h9zm-8 10h-1v-1h1v1zm2-1h-1v1h1v-1zm2 0h-1v1h1v-1z" fill="#C5C5C5"/><path d="M185.793 28.793l-1.793 1.207v-6h7v5.381l-2.554-.777c-.816-.409-1.99-.475-2.653.189zm-.793 2.207h.764l-.764-.383v.383zm11 4c0 .551-.447 1-1 1s-1-.449-1-1c0-.553.447-1 1-1s1 .447 1 1zm-3.5-3c-.276 0-.5.223-.5.5 0 .275.224.5.5.5s.5-.225.5-.5c0-.277-.224-.5-.5-.5zm2.5-3c-.553 0-1 .447-1 1 0 .551.447 1 1 1s1-.449 1-1c0-.553-.447-1-1-1z" fill="#2D2D2D"/><path d="M196.652 12.5c.811-.538 1.348-1.458 1.348-2.5 0-1.654-1.346-3-3-3-.771 0-1.468.301-2 .779v-5.779h-11v12h3.764l-1.452.727 1.481 1.48c.322.322.803.5 1.354.5.436 0 .897-.111 1.301-.313l3.144-1.572c.134.053.271.098.414.127l-.005.051c0 1.654 1.346 3 3 3s3-1.346 3-3c-.001-1.043-.538-1.963-1.349-2.5z" fill="#F3F3F3"/><path d="M195 13c-.293 0-.569.066-.82.18l-.25-.25c.042-.137.07-.279.07-.43s-.028-.293-.07-.43l.25-.25c.251.113.527.18.82.18 1.104 0 2-.896 2-2 0-1.105-.896-2-2-2s-2 .895-2 2c0 .293.066.568.18.82l-.25.25c-.137-.043-.279-.07-.43-.07-.337 0-.645.115-.895.303l-2.607-1.304-.999-.5c-.552-.275-1.223-.275-1.499.002l-.5.499 5 2.5-5 2.5.5.5c.276.275.947.275 1.5 0l1-.5 2.605-1.303c.25.188.558.303.895.303.15 0 .293-.029.43-.07l.25.25c-.113.25-.18.527-.18.82 0 1.104.896 2 2 2s2-.896 2-2c0-1.106-.896-2-2-2zm0-4c.553 0 1 .447 1 1 0 .551-.447 1-1 1s-1-.449-1-1c0-.553.447-1 1-1zm-2.5 4c-.276 0-.5-.225-.5-.5 0-.277.224-.5.5-.5s.5.223.5.5c0 .275-.224.5-.5.5zm2.5 3c-.553 0-1-.449-1-1 0-.553.447-1 1-1s1 .447 1 1c0 .55-.447 1-1 1zm-3-13v7.051c-.142.029-.279.07-.413.123l-.587-.174v-6h-7v7h-1v-8h9zm-8 10h-1v-1h1v1zm2-1h-1v1h1v-1zm2 0h-1v1h1v-1z" fill="#424242"/><path d="M185.793 8.793l-1.793 1.207v-6h7v5.381l-2.554-.777c-.816-.409-1.99-.475-2.653.189zm-.793 2.207h.764l-.764-.383v.383zm11 4c0 .551-.447 1-1 1s-1-.449-1-1c0-.553.447-1 1-1s1 .447 1 1zm-3.5-3c-.276 0-.5.223-.5.5 0 .275.224.5.5.5s.5-.225.5-.5c0-.278-.224-.5-.5-.5zm2.5-3c-.553 0-1 .447-1 1 0 .551.447 1 1 1s1-.449 1-1c0-.553-.447-1-1-1z" fill="#F0EFF1"/><path d="M178 27v-3h-7v-1h-9v14h13v-3h3v-3h-1v-3h-6v-1h7zm-8 7v-3h1v3h-1z" fill="#2D2D2D"/><path d="M177 26h-5v-1h5v1zm-1 3h-2v1h2v-1zm-4 0h-9v1h9v-1zm2 6h-11v1h11v-1zm-5-3h-6v1h6v-1zm8 0h-5v1h5v-1zm-7-8v3h-7v-3h7zm-1 1h-5v1h5v-1z" fill="#C5C5C5"/><rect x="164" y="25" width="5" height="1" fill="#2D2D2D"/><path d="M178 7v-3h-7v-1h-9v14h13v-3h3v-3h-1v-3h-6v-1h7zm-8 7v-3h1v3h-1z" fill="#F3F3F3"/><path d="M177 6h-5v-1h5v1zm-1 3h-2v1h2v-1zm-4 0h-9v1h9v-1zm2 6h-11v1h11v-1zm-5-3h-6v1h6v-1zm8 0h-5v1h5v-1zm-7-8v3h-7v-3h7zm-1 1h-5v1h5v-1z" fill="#424242"/><rect x="164" y="5" width="5" height="1" fill="#F0EFF1"/><polygon points="154.414,24 149.586,24 148,25.586 148,28 144,28 144,35 152,35 152,31 154.414,31 156,29.414 156,25.586" fill="#2D2D2D"/><g fill="#75BEFF"><path d="M154 25h-4l-1 1v2h5v1h-2v1h2l1-1v-3l-1-1zm0 2h-4v-1h4v1zM145 34h6v-5h-6v5zm1-3h4v1h-4v-1z"/></g><g fill="#2D2D2D"><rect x="146" y="31" width="4" height="1"/><rect x="150" y="26" width="4" height="1"/><rect x="152" y="28" width="2" height="1"/></g><polygon points="154.414,4 149.586,4 148,5.586 148,8 144,8 144,15 152,15 152,11 154.414,11 156,9.414 156,5.586" fill="#F3F3F3"/><g fill="#00539C"><path d="M154 5h-4l-1 1v2h5v1h-2v1h2l1-1v-3l-1-1zm0 2h-4v-1h4v1zM145 14h6v-5h-6v5zm1-3h4v1h-4v-1z"/></g><g fill="#F0EFF1"><rect x="146" y="11" width="4" height="1"/><rect x="150" y="6" width="4" height="1"/><rect x="152" y="8" width="2" height="1"/></g><path d="M138 24h-15v4h-1v8h8v-6h8v-6zm-11 9h-2v-2h2v2z" fill="#2D2D2D"/><path d="M137 29h-7v-1h-6v-3h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v4zm-12 1v-1h-2v6h2v-1h-1v-4h1zm2 4v1h2v-6h-2v1h1v4h-1z" fill="#C5C5C5"/><path d="M125 27v-2h1v2h-1zm3 0v-2h-1v2h1zm2 0v-2h-1v2h1zm2 0v-2h-1v2h1zm2 0v-2h-1v2h1zm2 0v-2h-1v2h1z" fill="#2D2D2D"/><path d="M138 4h-15v4h-1v8h8v-6h8v-6zm-11 9h-2v-2h2v2z" fill="#F3F3F3"/><path d="M137 9h-7v-1h-6v-3h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v2h1v-2h1v4zm-12 1v-1h-2v6h2v-1h-1v-4h1zm2 4v1h2v-6h-2v1h1v4h-1z" fill="#424242"/><path d="M125 7v-2h1v2h-1zm3 0v-2h-1v2h1zm2 0v-2h-1v2h1zm2 0v-2h-1v2h1zm2 0v-2h-1v2h1zm2 0v-2h-1v2h1z" fill="#F0EFF1"/><path d="M110.449 23c-1.637 0-3.075.797-3.987 2.012l.001.002c-.628.836-1.014 1.863-1.014 2.986 0 .469.067.933.2 1.385l-2.907 2.908c-.687.686-1.253 2.161 0 3.414.609.609 1.244.736 1.67.736.958 0 1.621-.613 1.744-.736l2.907-2.908c.453.133.917.201 1.386.201 1.123 0 2.149-.387 2.985-1.014l.002.001c1.216-.912 2.013-2.352 2.013-3.987 0-2.762-2.238-5-5-5z" fill="#2D2D2D"/><path d="M114.09 26.359l-2.641 2.641-2-2 2.641-2.641c-.502-.227-1.055-.359-1.641-.359-2.209 0-4 1.791-4 4 0 .586.133 1.139.359 1.64l-3.359 3.36s-1 1 0 2h2l3.359-3.36c.502.227 1.055.36 1.641.36 2.209 0 4-1.791 4-4 0-.586-.133-1.139-.359-1.641z" fill="#C5C5C5"/><path d="M110.449 3c-1.637 0-3.075.797-3.987 2.012l.001.002c-.628.836-1.014 1.863-1.014 2.986 0 .469.067.933.2 1.385l-2.907 2.908c-.687.686-1.253 2.161 0 3.414.609.609 1.244.736 1.67.736.958 0 1.621-.613 1.744-.736l2.907-2.908c.453.133.917.201 1.386.201 1.123 0 2.149-.387 2.985-1.014l.002.001c1.216-.912 2.013-2.352 2.013-3.987 0-2.762-2.238-5-5-5z" fill="#F3F3F3"/><path d="M114.09 6.359l-2.641 2.641-2-2 2.641-2.641c-.502-.226-1.055-.359-1.641-.359-2.209 0-4 1.791-4 4 0 .586.133 1.139.359 1.64l-3.359 3.36s-1 1 0 2h2l3.359-3.36c.502.227 1.055.36 1.641.36 2.209 0 4-1.791 4-4 0-.586-.133-1.139-.359-1.641z" fill="#424242"/><path d="M89 33h1v-1c0-.537.741-1.613 1-2-.259-.389-1-1.467-1-2v-1h-1v-3h1c1.969.021 3 1.277 3 3v1l1 1v2l-1 1v1c0 1.709-1.031 2.979-3 3h-1v-3zm-2 0h-1v-1c0-.537-.741-1.613-1-2 .259-.389 1-1.467 1-2v-1h1v-3h-1c-1.969.021-3 1.277-3 3v1l-1 1v2l1 1v1c0 1.709 1.317 2.979 3.286 3h.714v-3z" fill="#2D2D2D"/><path d="M91 33v-1c0-.834.496-1.738 1-2-.504-.27-1-1.168-1-2v-1c0-.84-.584-1-1-1v-1c2.083 0 2 1.166 2 2v1c0 .969.703.98 1 1v2c-.322.02-1 .053-1 1v1c0 .834.083 2-2 2v-1c.833 0 1-1 1-1zm-6 0v-1c0-.834-.496-1.738-1-2 .504-.27 1-1.168 1-2v-1c0-.84.584-1 1-1v-1c-2.083 0-2 1.166-2 2v1c0 .969-.703.98-1 1v2c.322.02 1 .053 1 1v1c0 .834-.083 2 2 2v-1c-.833 0-1-1-1-1z" fill="#C5C5C5"/><path d="M89 13h1v-1c0-.537.741-1.613 1-2-.259-.389-1-1.467-1-2v-1h-1v-3h1c1.969.021 3 1.277 3 3v1l1 1v2l-1 1v1c0 1.709-1.031 2.979-3 3h-1v-3zm-2 0h-1v-1c0-.537-.741-1.613-1-2 .259-.389 1-1.467 1-2v-1h1v-3h-1c-1.969.021-3 1.277-3 3v1l-1 1v2l1 1v1c0 1.709 1.317 2.979 3.286 3h.714v-3z" fill="#F3F3F3"/><path d="M91 13v-1c0-.834.496-1.738 1-2-.504-.27-1-1.168-1-2v-1c0-.84-.584-1-1-1v-1c2.083 0 2 1.166 2 2v1c0 .969.703.98 1 1v2c-.322.02-1 .053-1 1v1c0 .834.083 2-2 2v-1c.833 0 1-1 1-1zm-6 0v-1c0-.834-.496-1.738-1-2 .504-.27 1-1.168 1-2v-1c0-.84.584-1 1-1v-1c-2.083 0-2 1.166-2 2v1c0 .969-.703.98-1 1v2c.322.02 1 .053 1 1v1c0 .834-.083 2 2 2v-1c-.833 0-1-1-1-1z" fill="#424242"/><path d="M73.5 34c-1.914 0-3.601-1.242-4.227-3h-1.683c-.524.91-1.503 1.5-2.591 1.5-1.654 0-3-1.346-3-3s1.346-3 3-3c1.088 0 2.066.588 2.591 1.5h1.683c.626-1.76 2.313-3 4.227-3 2.481 0 4.5 2.018 4.5 4.5 0 2.48-2.019 4.5-4.5 4.5z" fill="#2D2D2D"/><path d="M73.5 26c-1.759 0-3.204 1.308-3.449 3h-3.122c-.223-.861-.998-1.5-1.929-1.5-1.104 0-2 .895-2 2 0 1.104.896 2 2 2 .931 0 1.706-.639 1.929-1.5h3.122c.245 1.691 1.69 3 3.449 3 1.93 0 3.5-1.57 3.5-3.5 0-1.931-1.57-3.5-3.5-3.5zm0 5c-.827 0-1.5-.674-1.5-1.5 0-.828.673-1.5 1.5-1.5s1.5.672 1.5 1.5c0 .826-.673 1.5-1.5 1.5z" fill="#75BEFF"/><circle cx="73.5" cy="29.5" r="1.5" fill="#2D2D2D"/><path d="M73.5 14c-1.914 0-3.601-1.242-4.227-3h-1.683c-.524.91-1.503 1.5-2.591 1.5-1.654 0-3-1.346-3-3s1.346-3 3-3c1.088 0 2.066.588 2.591 1.5h1.683c.626-1.76 2.313-3 4.227-3 2.481 0 4.5 2.018 4.5 4.5 0 2.48-2.019 4.5-4.5 4.5z" fill="#F3F3F3"/><path d="M73.5 6c-1.759 0-3.204 1.308-3.449 3h-3.122c-.223-.861-.998-1.5-1.929-1.5-1.104 0-2 .895-2 2 0 1.104.896 2 2 2 .931 0 1.706-.639 1.929-1.5h3.122c.245 1.691 1.69 3 3.449 3 1.93 0 3.5-1.57 3.5-3.5 0-1.931-1.57-3.5-3.5-3.5zm0 5c-.827 0-1.5-.674-1.5-1.5 0-.828.673-1.5 1.5-1.5s1.5.672 1.5 1.5c0 .826-.673 1.5-1.5 1.5z" fill="#00539C"/><circle cx="73.5" cy="9.5" r="1.5" fill="#F0EFF1"/><path d="M58 28.586l-3-3-1.414 1.414h-2.172l1-1-4-4h-.828l-5.586 5.586v.828l4 4 2.414-2.414h.586v5h1.586l3 3h.828l3.586-3.586v-.828l-2.086-2.086 2.086-2.086v-.828z" fill="#2D2D2D"/><polygon points="53.998,33.002 51,33 51,29 53,29 52,30 54,32 57,29 55,27 54,28 49,28 51,26 48,23 43,28 46,31 48,29 50,29 50,34 53,34 52,35 54,37 57,34 55,32" fill="#C27D1A"/><path d="M58 8.586l-3-3-1.414 1.414h-2.172l1-1-4-4h-.828l-5.586 5.586v.828l4 4 2.414-2.414h.586v5h1.586l3 3h.828l3.586-3.586v-.828l-2.086-2.086 2.086-2.086v-.828z" fill="#F3F3F3"/><polygon points="53.998,13.002 51,13 51,9 53,9 52,10 54,12 57,9 55,7 54,8 49,8 51,6 48,3 43,8 46,11 48,9 50,9 50,14 53,14 52,15 54,17 57,14 55,12" fill="#C27D1A"/><path d="M29.263 24l4.737 2.369v5.236l-6.791 3.395h-.42l-4.789-2.395v-5.236l6.739-3.369h.524z" fill="#2D2D2D"/><path d="M23 28v4l4 2 6-3v-4l-4-2-6 3zm4 1l-2-1 4-2 2 1-4 2z" fill="#75BEFF"/><path d="M29 26l2 1-4 2-2-1 4-2z" fill="#2D2D2D"/><path d="M29.263 4l4.737 2.369v5.236l-6.791 3.395h-.42l-4.789-2.395v-5.236l6.739-3.369h.524z" fill="#F3F3F3"/><path d="M23 8v4l4 2 6-3v-4l-4-2-6 3zm4 1l-2-1 4-2 2 1-4 2z" fill="#00539C"/><path d="M29 6l2 1-4 2-2-1 4-2z" fill="#F0EFF1"/><polygon points="2,27.308 2,32.692 7.209,36 7.791,36 13,32.692 13,27.308 7.791,24 7.209,24" fill="#2D2D2D"/><path d="M7.5 25l-4.5 2.857v4.285l4.5 2.858 4.5-2.857v-4.285l-4.5-2.858zm-.5 8.498l-3-1.905v-2.815l3 1.905v2.815zm-2.358-5.498l2.858-1.815 2.858 1.815-2.858 1.815-2.858-1.815zm6.358 3.593l-3 1.905v-2.815l3-1.905v2.815z" fill="#B180D7"/><polygon points="10.358,28 7.5,29.815 4.642,28 7.5,26.185" fill="#2D2D2D"/><polygon points="4,28.777 7,30.683 7,33.498 4,31.593" fill="#2D2D2D"/><polygon points="8,33.498 8,30.683 11,28.777 11,31.593" fill="#2D2D2D"/><polygon points="2,7.308 2,12.692 7.209,16 7.791,16 13,12.692 13,7.308 7.791,4 7.209,4" fill="#F3F3F3"/><path d="M7.5 5l-4.5 2.857v4.285l4.5 2.858 4.5-2.857v-4.286l-4.5-2.857zm-.5 8.498l-3-1.905v-2.816l3 1.905v2.816zm-2.358-5.498l2.858-1.815 2.858 1.815-2.858 1.815-2.858-1.815zm6.358 3.593l-3 1.905v-2.815l3-1.905v2.815z" fill="#652D90"/><polygon points="10.358,8 7.5,9.815 4.642,8 7.5,6.185" fill="#F0EFF1"/><polygon points="4,8.777 7,10.683 7,13.498 4,11.593" fill="#F0EFF1"/><polygon points="8,13.498 8,10.683 11,8.777 11,11.593" fill="#F0EFF1"/></svg> | src/vs/editor/contrib/quickOpen/browser/symbol-sprite.svg | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.000262220564763993,
0.000262220564763993,
0.000262220564763993,
0.000262220564763993,
0
] |
{
"id": 1,
"code_window": [
"\n",
"\t\tconst selections = this._cursors.getSelections();\n",
"\t\tconst viewSelections = this._cursors.getViewSelections();\n",
"\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n",
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t// Let the view get the event first.\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "replace",
"edit_start_line_idx": 374
} | <svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 16 16"><style>.icon-canvas-transparent{opacity:0;fill:#252526}.icon-vs-out{fill:#252526}.icon-vs-bg{fill:#c5c5c5}.icon-vs-fg{fill:#2b282e}.icon-vs-action-blue{fill:#75beff}</style><path class="icon-canvas-transparent" d="M16 16H0V0h16v16z" id="canvas"/><path class="icon-vs-out" d="M2.879 14L1 12.121V3.879L2.879 2h10.242L15 3.879v8.242L13.121 14H2.879z" id="outline"/><path class="icon-vs-fg" d="M12.293 4H3.707L3 4.707v6.586l.707.707h8.586l.707-.707V4.707L12.293 4zM11 10H5V9h6v1zm0-3H5V6h6v1z" id="iconFg"/><g id="iconBg"><path class="icon-vs-bg" d="M12.707 13H3.293L2 11.707V4.293L3.293 3h9.414L14 4.293v7.414L12.707 13zm-9-1h8.586l.707-.707V4.707L12.293 4H3.707L3 4.707v6.586l.707.707z"/><path class="icon-vs-action-blue" d="M11 7H5V6h6v1zm0 2H5v1h6V9z"/></g></svg> | src/vs/editor/contrib/suggest/browser/media/Constant_16x_inverse.svg | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00031101054628379643,
0.00031101054628379643,
0.00031101054628379643,
0.00031101054628379643,
0
] |
{
"id": 2,
"code_window": [
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n",
"\t}\n",
"\n",
"\tprivate _revealRange(revealTarget: RevealTarget, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean): void {\n",
"\t\tconst positions = this._cursors.getPositions();\n",
"\t\tconst viewPositions = this._cursors.getViewPositions();\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
"\t\t// Only after the view has been notified, let the rest of the world know...\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "add",
"edit_start_line_idx": 376
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
import * as nls from 'vs/nls';
import * as strings from 'vs/base/common/strings';
import { onUnexpectedError } from 'vs/base/common/errors';
import { CursorCollection } from 'vs/editor/common/controller/cursorCollection';
import { Position } from 'vs/editor/common/core/position';
import { Range } from 'vs/editor/common/core/range';
import { Selection, SelectionDirection, ISelection } from 'vs/editor/common/core/selection';
import * as editorCommon from 'vs/editor/common/editorCommon';
import { CursorColumns, CursorConfiguration, EditOperationResult, CursorContext, CursorState, RevealTarget, IColumnSelectData, ICursors } from 'vs/editor/common/controller/cursorCommon';
import { LanguageConfigurationRegistry } from 'vs/editor/common/modes/languageConfigurationRegistry';
import { DeleteOperations } from 'vs/editor/common/controller/cursorDeleteOperations';
import { TypeOperations } from 'vs/editor/common/controller/cursorTypeOperations';
import { TextModelEventType, ModelRawContentChangedEvent, RawContentChangedType } from 'vs/editor/common/model/textModelEvents';
import { CursorChangeReason } from 'vs/editor/common/controller/cursorEvents';
import { IViewModel } from "vs/editor/common/viewModel/viewModel";
import * as viewEvents from 'vs/editor/common/view/viewEvents';
import Event, { Emitter } from 'vs/base/common/event';
function containsLineMappingChanged(events: viewEvents.ViewEvent[]): boolean {
for (let i = 0, len = events.length; i < len; i++) {
if (events[i].type === viewEvents.ViewEventType.ViewLineMappingChanged) {
return true;
}
}
return false;
}
export class CursorStateChangedEvent {
/**
* The new selections.
* The primary selection is always at index 0.
*/
readonly selections: Selection[];
/**
* Source of the call that caused the event.
*/
readonly source: string;
/**
* Reason.
*/
readonly reason: CursorChangeReason;
constructor(selections: Selection[], source: string, reason: CursorChangeReason) {
this.selections = selections;
this.source = source;
this.reason = reason;
}
}
export class Cursor extends viewEvents.ViewEventEmitter implements ICursors {
private readonly _onDidChange: Emitter<CursorStateChangedEvent> = this._register(new Emitter<CursorStateChangedEvent>());
public readonly onDidChange: Event<CursorStateChangedEvent> = this._onDidChange.event;
private readonly _configuration: editorCommon.IConfiguration;
private readonly _model: editorCommon.IModel;
private readonly _viewModel: IViewModel;
public context: CursorContext;
private _cursors: CursorCollection;
private _isHandling: boolean;
private _isDoingComposition: boolean;
private _columnSelectData: IColumnSelectData;
constructor(configuration: editorCommon.IConfiguration, model: editorCommon.IModel, viewModel: IViewModel) {
super();
this._configuration = configuration;
this._model = model;
this._viewModel = viewModel;
this.context = new CursorContext(this._configuration, this._model, this._viewModel);
this._cursors = new CursorCollection(this.context);
this._isHandling = false;
this._isDoingComposition = false;
this._columnSelectData = null;
this._register(this._model.addBulkListener((events) => {
if (this._isHandling) {
return;
}
let hadContentChange = false;
let hadFlushEvent = false;
for (let i = 0, len = events.length; i < len; i++) {
const event = events[i];
const eventType = event.type;
if (eventType === TextModelEventType.ModelRawContentChanged2) {
hadContentChange = true;
const rawChangeEvent = <ModelRawContentChangedEvent>event.data;
hadFlushEvent = hadFlushEvent || rawChangeEvent.containsEvent(RawContentChangedType.Flush);
}
}
if (!hadContentChange) {
return;
}
this._onModelContentChanged(hadFlushEvent);
}));
this._register(viewModel.addEventListener((events: viewEvents.ViewEvent[]) => {
if (!containsLineMappingChanged(events)) {
return;
}
// Ensure valid state
this.setStates('viewModel', CursorChangeReason.NotSet, this.getAll());
}));
const updateCursorContext = () => {
this.context = new CursorContext(this._configuration, this._model, this._viewModel);
this._cursors.updateContext(this.context);
};
this._register(this._model.onDidChangeLanguage((e) => {
updateCursorContext();
}));
this._register(LanguageConfigurationRegistry.onDidChange(() => {
// TODO@Alex: react only if certain supports changed? (and if my model's mode changed)
updateCursorContext();
}));
this._register(model.onDidChangeOptions(() => {
updateCursorContext();
}));
this._register(this._configuration.onDidChange((e) => {
if (CursorConfiguration.shouldRecreate(e)) {
updateCursorContext();
}
}));
}
public dispose(): void {
this._cursors.dispose();
super.dispose();
}
// ------ some getters/setters
public getPrimaryCursor(): CursorState {
return this._cursors.getPrimaryCursor();
}
public getLastAddedCursorIndex(): number {
return this._cursors.getLastAddedCursorIndex();
}
public getAll(): CursorState[] {
return this._cursors.getAll();
}
private static _somethingChanged(oldSelections: Selection[], oldViewSelections: Selection[], newSelections: Selection[], newViewSelections: Selection[]): boolean {
if (oldSelections.length !== newSelections.length) {
return true;
}
for (let i = 0, len = oldSelections.length; i < len; i++) {
if (!oldSelections[i].equalsSelection(newSelections[i])) {
return true;
}
}
for (let i = 0, len = oldViewSelections.length; i < len; i++) {
if (!oldViewSelections[i].equalsSelection(newViewSelections[i])) {
return true;
}
}
return false;
}
public setStates(source: string, reason: CursorChangeReason, states: CursorState[]): void {
const oldSelections = this._cursors.getSelections();
const oldViewSelections = this._cursors.getViewSelections();
this._cursors.setStates(states);
this._cursors.normalize();
this._columnSelectData = null;
const newSelections = this._cursors.getSelections();
const newViewSelections = this._cursors.getViewSelections();
if (Cursor._somethingChanged(oldSelections, oldViewSelections, newSelections, newViewSelections)) {
this._emitStateChanged(source, reason);
}
}
public setColumnSelectData(columnSelectData: IColumnSelectData): void {
this._columnSelectData = columnSelectData;
}
public reveal(horizontal: boolean, target: RevealTarget): void {
this._revealRange(target, viewEvents.VerticalRevealType.Simple, horizontal);
}
public revealRange(revealHorizontal: boolean, modelRange: Range, viewRange: Range, verticalType: viewEvents.VerticalRevealType) {
this.emitCursorRevealRange(modelRange, viewRange, verticalType, revealHorizontal);
}
public scrollTo(desiredScrollTop: number): void {
this._viewModel.viewLayout.setScrollPosition({
scrollTop: desiredScrollTop
});
}
public saveState(): editorCommon.ICursorState[] {
let result: editorCommon.ICursorState[] = [];
const selections = this._cursors.getSelections();
for (let i = 0, len = selections.length; i < len; i++) {
const selection = selections[i];
result.push({
inSelectionMode: !selection.isEmpty(),
selectionStart: {
lineNumber: selection.selectionStartLineNumber,
column: selection.selectionStartColumn,
},
position: {
lineNumber: selection.positionLineNumber,
column: selection.positionColumn,
}
});
}
return result;
}
public restoreState(states: editorCommon.ICursorState[]): void {
let desiredSelections: ISelection[] = [];
for (let i = 0, len = states.length; i < len; i++) {
const state = states[i];
let positionLineNumber = 1;
let positionColumn = 1;
// Avoid missing properties on the literal
if (state.position && state.position.lineNumber) {
positionLineNumber = state.position.lineNumber;
}
if (state.position && state.position.column) {
positionColumn = state.position.column;
}
let selectionStartLineNumber = positionLineNumber;
let selectionStartColumn = positionColumn;
// Avoid missing properties on the literal
if (state.selectionStart && state.selectionStart.lineNumber) {
selectionStartLineNumber = state.selectionStart.lineNumber;
}
if (state.selectionStart && state.selectionStart.column) {
selectionStartColumn = state.selectionStart.column;
}
desiredSelections.push({
selectionStartLineNumber: selectionStartLineNumber,
selectionStartColumn: selectionStartColumn,
positionLineNumber: positionLineNumber,
positionColumn: positionColumn
});
}
this.setStates('restoreState', CursorChangeReason.NotSet, CursorState.fromModelSelections(desiredSelections));
this.reveal(true, RevealTarget.Primary);
}
private _onModelContentChanged(hadFlushEvent: boolean): void {
if (hadFlushEvent) {
// a model.setValue() was called
this._cursors.dispose();
this._cursors = new CursorCollection(this.context);
this._emitStateChanged('model', CursorChangeReason.ContentFlush);
} else {
const selectionsFromMarkers = this._cursors.readSelectionFromMarkers();
this.setStates('modelChange', CursorChangeReason.RecoverFromMarkers, CursorState.fromModelSelections(selectionsFromMarkers));
}
}
public getSelection(): Selection {
return this._cursors.getPrimaryCursor().modelState.selection;
}
public getColumnSelectData(): IColumnSelectData {
if (this._columnSelectData) {
return this._columnSelectData;
}
const primaryCursor = this._cursors.getPrimaryCursor();
const primaryPos = primaryCursor.viewState.position;
return {
toViewLineNumber: primaryPos.lineNumber,
toViewVisualColumn: CursorColumns.visibleColumnFromColumn2(this.context.config, this.context.viewModel, primaryPos)
};
}
public getSelections(): Selection[] {
return this._cursors.getSelections();
}
public getPosition(): Position {
return this._cursors.getPrimaryCursor().modelState.position;
}
public setSelections(source: string, selections: ISelection[]): void {
this.setStates(source, CursorChangeReason.NotSet, CursorState.fromModelSelections(selections));
}
// ------ auxiliary handling logic
private _executeEditOperation(opResult: EditOperationResult): void {
if (!opResult) {
// Nothing to execute
return;
}
if (this._configuration.editor.readOnly) {
// Cannot execute when read only
return;
}
if (opResult.shouldPushStackElementBefore) {
this._model.pushStackElement();
}
const result = CommandExecutor.executeCommands(this._model, this._cursors.getSelections(), opResult.commands);
if (result) {
// The commands were applied correctly
this._interpretCommandResult(result);
}
if (opResult.shouldPushStackElementAfter) {
this._model.pushStackElement();
}
}
private _interpretCommandResult(cursorState: Selection[]): void {
if (!cursorState || cursorState.length === 0) {
return;
}
this._columnSelectData = null;
this._cursors.setSelections(cursorState);
this._cursors.normalize();
}
// -----------------------------------------------------------------------------------------------------------
// ----- emitting events
private _emitStateChanged(source: string, reason: CursorChangeReason): void {
source = source || 'keyboard';
const positions = this._cursors.getPositions();
let isInEditableRange: boolean = true;
if (this._model.hasEditableRange()) {
const editableRange = this._model.getEditableRange();
if (!editableRange.containsPosition(positions[0])) {
isInEditableRange = false;
}
}
const selections = this._cursors.getSelections();
const viewSelections = this._cursors.getViewSelections();
this._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));
this._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);
}
private _revealRange(revealTarget: RevealTarget, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean): void {
const positions = this._cursors.getPositions();
const viewPositions = this._cursors.getViewPositions();
let position = positions[0];
let viewPosition = viewPositions[0];
if (revealTarget === RevealTarget.TopMost) {
for (let i = 1; i < positions.length; i++) {
if (positions[i].isBefore(position)) {
position = positions[i];
viewPosition = viewPositions[i];
}
}
} else if (revealTarget === RevealTarget.BottomMost) {
for (let i = 1; i < positions.length; i++) {
if (position.isBeforeOrEqual(positions[i])) {
position = positions[i];
viewPosition = viewPositions[i];
}
}
} else {
if (positions.length > 1) {
// no revealing!
return;
}
}
const range = new Range(position.lineNumber, position.column, position.lineNumber, position.column);
const viewRange = new Range(viewPosition.lineNumber, viewPosition.column, viewPosition.lineNumber, viewPosition.column);
this.emitCursorRevealRange(range, viewRange, verticalType, revealHorizontal);
}
public emitCursorRevealRange(range: Range, viewRange: Range, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean) {
// Ensure event has viewRange
if (!viewRange) {
viewRange = this.context.convertModelRangeToViewRange(range);
}
this._emit([new viewEvents.ViewRevealRangeRequestEvent(viewRange, verticalType, revealHorizontal)]);
}
// -----------------------------------------------------------------------------------------------------------
// ----- handlers beyond this point
public trigger(source: string, handlerId: string, payload: any): void {
const H = editorCommon.Handler;
if (handlerId === H.CompositionStart) {
this._isDoingComposition = true;
return;
}
if (handlerId === H.CompositionEnd) {
this._isDoingComposition = false;
return;
}
const oldSelections = this._cursors.getSelections();
const oldViewSelections = this._cursors.getViewSelections();
let cursorChangeReason = CursorChangeReason.NotSet;
// ensure valid state on all cursors
this._cursors.ensureValidState();
this._isHandling = true;
try {
switch (handlerId) {
case H.Type:
this._type(source, <string>payload.text);
break;
case H.ReplacePreviousChar:
this._replacePreviousChar(<string>payload.text, <number>payload.replaceCharCnt);
break;
case H.Paste:
cursorChangeReason = CursorChangeReason.Paste;
this._paste(<string>payload.text, <boolean>payload.pasteOnNewLine);
break;
case H.Cut:
this._cut();
break;
case H.Undo:
cursorChangeReason = CursorChangeReason.Undo;
this._interpretCommandResult(this._model.undo());
break;
case H.Redo:
cursorChangeReason = CursorChangeReason.Redo;
this._interpretCommandResult(this._model.redo());
break;
case H.ExecuteCommand:
this._externalExecuteCommand(<editorCommon.ICommand>payload);
break;
case H.ExecuteCommands:
this._externalExecuteCommands(<editorCommon.ICommand[]>payload);
break;
}
} catch (err) {
onUnexpectedError(err);
}
this._isHandling = false;
const newSelections = this._cursors.getSelections();
const newViewSelections = this._cursors.getViewSelections();
if (Cursor._somethingChanged(oldSelections, oldViewSelections, newSelections, newViewSelections)) {
this._emitStateChanged(source, cursorChangeReason);
this._revealRange(RevealTarget.Primary, viewEvents.VerticalRevealType.Simple, true);
}
}
private _type(source: string, text: string): void {
if (!this._isDoingComposition && source === 'keyboard') {
// If this event is coming straight from the keyboard, look for electric characters and enter
for (let i = 0, len = text.length; i < len; i++) {
let charCode = text.charCodeAt(i);
let chr: string;
if (strings.isHighSurrogate(charCode) && i + 1 < len) {
chr = text.charAt(i) + text.charAt(i + 1);
i++;
} else {
chr = text.charAt(i);
}
// Here we must interpret each typed character individually, that's why we create a new context
this._executeEditOperation(TypeOperations.typeWithInterceptors(this.context.config, this.context.model, this.getSelections(), chr));
}
} else {
this._executeEditOperation(TypeOperations.typeWithoutInterceptors(this.context.config, this.context.model, this.getSelections(), text));
}
}
private _replacePreviousChar(text: string, replaceCharCnt: number): void {
this._executeEditOperation(TypeOperations.replacePreviousChar(this.context.config, this.context.model, this.getSelections(), text, replaceCharCnt));
}
private _paste(text: string, pasteOnNewLine: boolean): void {
this._executeEditOperation(TypeOperations.paste(this.context.config, this.context.model, this.getSelections(), pasteOnNewLine, text));
}
private _cut(): void {
this._executeEditOperation(DeleteOperations.cut(this.context.config, this.context.model, this.getSelections()));
}
private _externalExecuteCommand(command: editorCommon.ICommand): void {
this._cursors.killSecondaryCursors();
this._executeEditOperation(new EditOperationResult([command], {
shouldPushStackElementBefore: false,
shouldPushStackElementAfter: false
}));
}
private _externalExecuteCommands(commands: editorCommon.ICommand[]): void {
this._executeEditOperation(new EditOperationResult(commands, {
shouldPushStackElementBefore: false,
shouldPushStackElementAfter: false
}));
}
}
interface IExecContext {
readonly model: editorCommon.IModel;
readonly selectionsBefore: Selection[];
readonly selectionStartMarkers: string[];
readonly positionMarkers: string[];
}
interface ICommandData {
operations: editorCommon.IIdentifiedSingleEditOperation[];
hadTrackedEditOperation: boolean;
}
interface ICommandsData {
operations: editorCommon.IIdentifiedSingleEditOperation[];
hadTrackedEditOperation: boolean;
}
class CommandExecutor {
public static executeCommands(model: editorCommon.IModel, selectionsBefore: Selection[], commands: editorCommon.ICommand[]): Selection[] {
const ctx: IExecContext = {
model: model,
selectionsBefore: selectionsBefore,
selectionStartMarkers: [],
positionMarkers: []
};
const result = this._innerExecuteCommands(ctx, commands);
for (let i = 0; i < ctx.selectionStartMarkers.length; i++) {
ctx.model._removeMarker(ctx.selectionStartMarkers[i]);
ctx.model._removeMarker(ctx.positionMarkers[i]);
}
return result;
}
private static _innerExecuteCommands(ctx: IExecContext, commands: editorCommon.ICommand[]): Selection[] {
if (this._arrayIsEmpty(commands)) {
return null;
}
const commandsData = this._getEditOperations(ctx, commands);
if (commandsData.operations.length === 0) {
return null;
}
const rawOperations = commandsData.operations;
const editableRange = ctx.model.getEditableRange();
const editableRangeStart = editableRange.getStartPosition();
const editableRangeEnd = editableRange.getEndPosition();
for (let i = 0, len = rawOperations.length; i < len; i++) {
const operationRange = rawOperations[i].range;
if (!editableRangeStart.isBeforeOrEqual(operationRange.getStartPosition()) || !operationRange.getEndPosition().isBeforeOrEqual(editableRangeEnd)) {
// These commands are outside of the editable range
return null;
}
}
const loserCursorsMap = this._getLoserCursorMap(rawOperations);
if (loserCursorsMap.hasOwnProperty('0')) {
// These commands are very messed up
console.warn('Ignoring commands');
return null;
}
// Remove operations belonging to losing cursors
let filteredOperations: editorCommon.IIdentifiedSingleEditOperation[] = [];
for (let i = 0, len = rawOperations.length; i < len; i++) {
if (!loserCursorsMap.hasOwnProperty(rawOperations[i].identifier.major.toString())) {
filteredOperations.push(rawOperations[i]);
}
}
// TODO@Alex: find a better way to do this.
// give the hint that edit operations are tracked to the model
if (commandsData.hadTrackedEditOperation && filteredOperations.length > 0) {
filteredOperations[0]._isTracked = true;
}
const selectionsAfter = ctx.model.pushEditOperations(ctx.selectionsBefore, filteredOperations, (inverseEditOperations: editorCommon.IIdentifiedSingleEditOperation[]): Selection[] => {
let groupedInverseEditOperations: editorCommon.IIdentifiedSingleEditOperation[][] = [];
for (let i = 0; i < ctx.selectionsBefore.length; i++) {
groupedInverseEditOperations[i] = [];
}
for (let i = 0; i < inverseEditOperations.length; i++) {
const op = inverseEditOperations[i];
if (!op.identifier) {
// perhaps auto whitespace trim edits
continue;
}
groupedInverseEditOperations[op.identifier.major].push(op);
}
const minorBasedSorter = (a: editorCommon.IIdentifiedSingleEditOperation, b: editorCommon.IIdentifiedSingleEditOperation) => {
return a.identifier.minor - b.identifier.minor;
};
let cursorSelections: Selection[] = [];
for (let i = 0; i < ctx.selectionsBefore.length; i++) {
if (groupedInverseEditOperations[i].length > 0) {
groupedInverseEditOperations[i].sort(minorBasedSorter);
cursorSelections[i] = commands[i].computeCursorState(ctx.model, {
getInverseEditOperations: () => {
return groupedInverseEditOperations[i];
},
getTrackedSelection: (id: string) => {
const idx = parseInt(id, 10);
const selectionStartMarker = ctx.model._getMarker(ctx.selectionStartMarkers[idx]);
const positionMarker = ctx.model._getMarker(ctx.positionMarkers[idx]);
return new Selection(selectionStartMarker.lineNumber, selectionStartMarker.column, positionMarker.lineNumber, positionMarker.column);
}
});
} else {
cursorSelections[i] = ctx.selectionsBefore[i];
}
}
return cursorSelections;
});
// Extract losing cursors
let losingCursors: number[] = [];
for (let losingCursorIndex in loserCursorsMap) {
if (loserCursorsMap.hasOwnProperty(losingCursorIndex)) {
losingCursors.push(parseInt(losingCursorIndex, 10));
}
}
// Sort losing cursors descending
losingCursors.sort((a: number, b: number): number => {
return b - a;
});
// Remove losing cursors
for (let i = 0; i < losingCursors.length; i++) {
selectionsAfter.splice(losingCursors[i], 1);
}
return selectionsAfter;
}
private static _arrayIsEmpty(commands: editorCommon.ICommand[]): boolean {
for (let i = 0, len = commands.length; i < len; i++) {
if (commands[i]) {
return false;
}
}
return true;
}
private static _getEditOperations(ctx: IExecContext, commands: editorCommon.ICommand[]): ICommandsData {
let operations: editorCommon.IIdentifiedSingleEditOperation[] = [];
let hadTrackedEditOperation: boolean = false;
for (let i = 0, len = commands.length; i < len; i++) {
if (commands[i]) {
const r = this._getEditOperationsFromCommand(ctx, i, commands[i]);
operations = operations.concat(r.operations);
hadTrackedEditOperation = hadTrackedEditOperation || r.hadTrackedEditOperation;
}
}
return {
operations: operations,
hadTrackedEditOperation: hadTrackedEditOperation
};
}
private static _getEditOperationsFromCommand(ctx: IExecContext, majorIdentifier: number, command: editorCommon.ICommand): ICommandData {
// This method acts as a transaction, if the command fails
// everything it has done is ignored
let operations: editorCommon.IIdentifiedSingleEditOperation[] = [];
let operationMinor = 0;
const addEditOperation = (selection: Range, text: string) => {
if (selection.isEmpty() && text === '') {
// This command wants to add a no-op => no thank you
return;
}
operations.push({
identifier: {
major: majorIdentifier,
minor: operationMinor++
},
range: selection,
text: text,
forceMoveMarkers: false,
isAutoWhitespaceEdit: command.insertsAutoWhitespace
});
};
let hadTrackedEditOperation = false;
const addTrackedEditOperation = (selection: Range, text: string) => {
hadTrackedEditOperation = true;
addEditOperation(selection, text);
};
const trackSelection = (selection: Selection, trackPreviousOnEmpty?: boolean) => {
let selectionMarkerStickToPreviousCharacter: boolean;
let positionMarkerStickToPreviousCharacter: boolean;
if (selection.isEmpty()) {
// Try to lock it with surrounding text
if (typeof trackPreviousOnEmpty === 'boolean') {
selectionMarkerStickToPreviousCharacter = trackPreviousOnEmpty;
positionMarkerStickToPreviousCharacter = trackPreviousOnEmpty;
} else {
const maxLineColumn = ctx.model.getLineMaxColumn(selection.startLineNumber);
if (selection.startColumn === maxLineColumn) {
selectionMarkerStickToPreviousCharacter = true;
positionMarkerStickToPreviousCharacter = true;
} else {
selectionMarkerStickToPreviousCharacter = false;
positionMarkerStickToPreviousCharacter = false;
}
}
} else {
if (selection.getDirection() === SelectionDirection.LTR) {
selectionMarkerStickToPreviousCharacter = false;
positionMarkerStickToPreviousCharacter = true;
} else {
selectionMarkerStickToPreviousCharacter = true;
positionMarkerStickToPreviousCharacter = false;
}
}
const l = ctx.selectionStartMarkers.length;
ctx.selectionStartMarkers[l] = ctx.model._addMarker(0, selection.selectionStartLineNumber, selection.selectionStartColumn, selectionMarkerStickToPreviousCharacter);
ctx.positionMarkers[l] = ctx.model._addMarker(0, selection.positionLineNumber, selection.positionColumn, positionMarkerStickToPreviousCharacter);
return l.toString();
};
const editOperationBuilder: editorCommon.IEditOperationBuilder = {
addEditOperation: addEditOperation,
addTrackedEditOperation: addTrackedEditOperation,
trackSelection: trackSelection
};
try {
command.getEditOperations(ctx.model, editOperationBuilder);
} catch (e) {
e.friendlyMessage = nls.localize('corrupt.commands', "Unexpected exception while executing command.");
onUnexpectedError(e);
return {
operations: [],
hadTrackedEditOperation: false
};
}
return {
operations: operations,
hadTrackedEditOperation: hadTrackedEditOperation
};
}
private static _getLoserCursorMap(operations: editorCommon.IIdentifiedSingleEditOperation[]): { [index: string]: boolean; } {
// This is destructive on the array
operations = operations.slice(0);
// Sort operations with last one first
operations.sort((a: editorCommon.IIdentifiedSingleEditOperation, b: editorCommon.IIdentifiedSingleEditOperation): number => {
// Note the minus!
return -(Range.compareRangesUsingEnds(a.range, b.range));
});
// Operations can not overlap!
let loserCursorsMap: { [index: string]: boolean; } = {};
for (let i = 1; i < operations.length; i++) {
const previousOp = operations[i - 1];
const currentOp = operations[i];
if (previousOp.range.getStartPosition().isBefore(currentOp.range.getEndPosition())) {
let loserMajor: number;
if (previousOp.identifier.major > currentOp.identifier.major) {
// previousOp loses the battle
loserMajor = previousOp.identifier.major;
} else {
loserMajor = currentOp.identifier.major;
}
loserCursorsMap[loserMajor.toString()] = true;
for (let j = 0; j < operations.length; j++) {
if (operations[j].identifier.major === loserMajor) {
operations.splice(j, 1);
if (j < i) {
i--;
}
j--;
}
}
if (i > 0) {
i--;
}
}
}
return loserCursorsMap;
}
}
| src/vs/editor/common/controller/cursor.ts | 1 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.9990548491477966,
0.09599438309669495,
0.00016634668281767517,
0.0002101898135151714,
0.2877565622329712
] |
{
"id": 2,
"code_window": [
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n",
"\t}\n",
"\n",
"\tprivate _revealRange(revealTarget: RevealTarget, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean): void {\n",
"\t\tconst positions = this._cursors.getPositions();\n",
"\t\tconst viewPositions = this._cursors.getViewPositions();\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
"\t\t// Only after the view has been notified, let the rest of the world know...\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "add",
"edit_start_line_idx": 376
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
// Do not edit this file. It is machine generated.
{
"entryAriaLabel": "{0},符號選擇器",
"symbols": "符號結果",
"noSymbolsMatching": "沒有相符的符號",
"noSymbolsWithoutInput": "輸入以搜尋符號"
} | i18n/cht/src/vs/workbench/parts/search/browser/openSymbolHandler.i18n.json | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.0001746798661770299,
0.0001738769351504743,
0.00017307398957200348,
0.0001738769351504743,
8.029382456697931e-7
] |
{
"id": 2,
"code_window": [
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n",
"\t}\n",
"\n",
"\tprivate _revealRange(revealTarget: RevealTarget, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean): void {\n",
"\t\tconst positions = this._cursors.getPositions();\n",
"\t\tconst viewPositions = this._cursors.getViewPositions();\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
"\t\t// Only after the view has been notified, let the rest of the world know...\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "add",
"edit_start_line_idx": 376
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
// Do not edit this file. It is machine generated.
{
"commandsHandlerDescriptionDefault": "Befehle anzeigen und ausführen",
"gotoLineDescriptionMac": "Gehe zu Zeile",
"gotoLineDescriptionWin": "Gehe zu Zeile",
"gotoSymbolDescription": "Gehe zu Symbol in Datei",
"gotoSymbolDescriptionScoped": "Gehe zu Symbol in Datei nach Kategorie",
"helpDescription": "Hilfe anzeigen",
"viewPickerDescription": "Ansicht öffnen"
} | i18n/deu/src/vs/workbench/parts/quickopen/browser/quickopen.contribution.i18n.json | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00017632365052122623,
0.00017534091603010893,
0.00017435819609090686,
0.00017534091603010893,
9.827272151596844e-7
] |
{
"id": 2,
"code_window": [
"\t\tthis._emit([new viewEvents.ViewCursorStateChangedEvent(viewSelections, isInEditableRange)]);\n",
"\t}\n",
"\n",
"\tprivate _revealRange(revealTarget: RevealTarget, verticalType: viewEvents.VerticalRevealType, revealHorizontal: boolean): void {\n",
"\t\tconst positions = this._cursors.getPositions();\n",
"\t\tconst viewPositions = this._cursors.getViewPositions();\n"
],
"labels": [
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\n",
"\t\t// Only after the view has been notified, let the rest of the world know...\n",
"\t\tthis._onDidChange.fire(new CursorStateChangedEvent(selections, source, reason));\n"
],
"file_path": "src/vs/editor/common/controller/cursor.ts",
"type": "add",
"edit_start_line_idx": 376
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/css!./media/scrollbar';
import 'vs/css!./media/terminal';
import 'vs/css!./media/xterm';
import 'vs/css!./media/widgets';
import * as panel from 'vs/workbench/browser/panel';
import * as platform from 'vs/base/common/platform';
import nls = require('vs/nls');
import { Extensions, IConfigurationRegistry } from 'vs/platform/configuration/common/configurationRegistry';
import { GlobalQuickOpenAction } from 'vs/workbench/browser/parts/quickopen/quickopen.contribution';
import { ITerminalService, KEYBINDING_CONTEXT_TERMINAL_FOCUS, KEYBINDING_CONTEXT_TERMINAL_TEXT_SELECTED, TERMINAL_PANEL_ID, TERMINAL_DEFAULT_RIGHT_CLICK_COPY_PASTE, TerminalCursorStyle } from 'vs/workbench/parts/terminal/common/terminal';
import { TERMINAL_DEFAULT_SHELL_LINUX, TERMINAL_DEFAULT_SHELL_OSX, TERMINAL_DEFAULT_SHELL_WINDOWS } from 'vs/workbench/parts/terminal/electron-browser/terminal';
import { IWorkbenchActionRegistry, Extensions as ActionExtensions } from 'vs/workbench/common/actionRegistry';
import { KeyCode, KeyMod } from 'vs/base/common/keyCodes';
import { ContextKeyExpr } from 'vs/platform/contextkey/common/contextkey';
import { KillTerminalAction, CopyTerminalSelectionAction, CreateNewTerminalAction, FocusActiveTerminalAction, FocusNextTerminalAction, FocusPreviousTerminalAction, FocusTerminalAtIndexAction, SelectDefaultShellWindowsTerminalAction, RunSelectedTextInTerminalAction, RunActiveFileInTerminalAction, ScrollDownTerminalAction, ScrollDownPageTerminalAction, ScrollToBottomTerminalAction, ScrollUpTerminalAction, ScrollUpPageTerminalAction, ScrollToTopTerminalAction, TerminalPasteAction, ToggleTerminalAction, ClearTerminalAction, AllowWorkspaceShellTerminalCommand, DisallowWorkspaceShellTerminalCommand } from 'vs/workbench/parts/terminal/electron-browser/terminalActions';
import { Registry } from 'vs/platform/platform';
import { ShowAllCommandsAction } from 'vs/workbench/parts/quickopen/browser/commandsHandler';
import { SyncActionDescriptor } from 'vs/platform/actions/common/actions';
import { TerminalService } from 'vs/workbench/parts/terminal/electron-browser/terminalService';
import { ToggleTabFocusModeAction } from 'vs/editor/contrib/toggleTabFocusMode/common/toggleTabFocusMode';
import { registerSingleton } from 'vs/platform/instantiation/common/extensions';
import debugActions = require('vs/workbench/parts/debug/browser/debugActions');
import { KeybindingsRegistry } from 'vs/platform/keybinding/common/keybindingsRegistry';
import { OpenNextRecentlyUsedEditorInGroupAction, OpenPreviousRecentlyUsedEditorInGroupAction, FocusActiveGroupAction, FocusFirstGroupAction, FocusSecondGroupAction, FocusThirdGroupAction } from 'vs/workbench/browser/parts/editor/editorActions';
import { EDITOR_FONT_DEFAULTS } from "vs/editor/common/config/editorOptions";
import { registerColors } from './terminalColorRegistry';
let configurationRegistry = <IConfigurationRegistry>Registry.as(Extensions.Configuration);
configurationRegistry.registerConfiguration({
'id': 'terminal',
'order': 100,
'title': nls.localize('terminalIntegratedConfigurationTitle', "Integrated Terminal"),
'type': 'object',
'properties': {
'terminal.integrated.shell.linux': {
'description': nls.localize('terminal.integrated.shell.linux', "The path of the shell that the terminal uses on Linux."),
'type': 'string',
'default': TERMINAL_DEFAULT_SHELL_LINUX
},
'terminal.integrated.shellArgs.linux': {
'description': nls.localize('terminal.integrated.shellArgs.linux', "The command line arguments to use when on the Linux terminal."),
'type': 'array',
'items': {
'type': 'string'
},
'default': []
},
'terminal.integrated.shell.osx': {
'description': nls.localize('terminal.integrated.shell.osx', "The path of the shell that the terminal uses on OS X."),
'type': 'string',
'default': TERMINAL_DEFAULT_SHELL_OSX
},
'terminal.integrated.shellArgs.osx': {
'description': nls.localize('terminal.integrated.shellArgs.osx', "The command line arguments to use when on the OS X terminal."),
'type': 'array',
'items': {
'type': 'string'
},
// Unlike on Linux, ~/.profile is not sourced when logging into a macOS session. This
// is the reason terminals on macOS typically run login shells by default which set up
// the environment. See http://unix.stackexchange.com/a/119675/115410
'default': ['-l']
},
'terminal.integrated.shell.windows': {
'description': nls.localize('terminal.integrated.shell.windows', "The path of the shell that the terminal uses on Windows. When using shells shipped with Windows (cmd, PowerShell or Bash on Ubuntu), prefer C:\\Windows\\sysnative over C:\\Windows\\System32 to use the 64-bit versions."),
'type': 'string',
'default': TERMINAL_DEFAULT_SHELL_WINDOWS
},
'terminal.integrated.shellArgs.windows': {
'description': nls.localize('terminal.integrated.shellArgs.windows', "The command line arguments to use when on the Windows terminal."),
'type': 'array',
'items': {
'type': 'string'
},
'default': []
},
'terminal.integrated.rightClickCopyPaste': {
'description': nls.localize('terminal.integrated.rightClickCopyPaste', "When set, this will prevent the context menu from appearing when right clicking within the terminal, instead it will copy when there is a selection and paste when there is no selection."),
'type': 'boolean',
'default': TERMINAL_DEFAULT_RIGHT_CLICK_COPY_PASTE
},
'terminal.integrated.fontFamily': {
'description': nls.localize('terminal.integrated.fontFamily', "Controls the font family of the terminal, this defaults to editor.fontFamily's value."),
'type': 'string'
},
'terminal.integrated.fontLigatures': {
'description': nls.localize('terminal.integrated.fontLigatures', "Controls whether font ligatures are enabled in the terminal."),
'type': 'boolean',
'default': false
},
'terminal.integrated.fontSize': {
'description': nls.localize('terminal.integrated.fontSize', "Controls the font size in pixels of the terminal."),
'type': 'number',
'default': EDITOR_FONT_DEFAULTS.fontSize
},
'terminal.integrated.lineHeight': {
'description': nls.localize('terminal.integrated.lineHeight', "Controls the line height of the terminal, this number is multipled by the terminal font size to get the actual line-height in pixels."),
'type': 'number',
'default': 1.2
},
'terminal.integrated.enableBold': {
'type': 'boolean',
'description': nls.localize('terminal.integrated.enableBold', "Whether to enable bold text within the terminal, this requires support from the terminal shell."),
'default': true
},
'terminal.integrated.cursorBlinking': {
'description': nls.localize('terminal.integrated.cursorBlinking', "Controls whether the terminal cursor blinks."),
'type': 'boolean',
'default': false
},
'terminal.integrated.cursorStyle': {
'description': nls.localize('terminal.integrated.cursorStyle', "Controls the style of terminal cursor."),
'enum': [TerminalCursorStyle.BLOCK, TerminalCursorStyle.LINE, TerminalCursorStyle.UNDERLINE],
'default': TerminalCursorStyle.BLOCK
},
'terminal.integrated.scrollback': {
'description': nls.localize('terminal.integrated.scrollback', "Controls the maximum amount of lines the terminal keeps in its buffer."),
'type': 'number',
'default': 1000
},
'terminal.integrated.setLocaleVariables': {
'description': nls.localize('terminal.integrated.setLocaleVariables', "Controls whether locale variables are set at startup of the terminal, this defaults to true on OS X, false on other platforms."),
'type': 'boolean',
'default': platform.isMacintosh
},
'terminal.integrated.cwd': {
'description': nls.localize('terminal.integrated.cwd', "An explicit start path where the terminal will be launched, this is used as the current working directory (cwd) for the shell process. This may be particularly useful in workspace settings if the root directory is not a convenient cwd."),
'type': 'string',
'default': undefined
},
'terminal.integrated.confirmOnExit': {
'description': nls.localize('terminal.integrated.confirmOnExit', "Whether to confirm on exit if there are active terminal sessions."),
'type': 'boolean',
'default': false
},
'terminal.integrated.commandsToSkipShell': {
'description': nls.localize('terminal.integrated.commandsToSkipShell', "A set of command IDs whose keybindings will not be sent to the shell and instead always be handled by Code. This allows the use of keybindings that would normally be consumed by the shell to act the same as when the terminal is not focused, for example ctrl+p to launch Quick Open."),
'type': 'array',
'items': {
'type': 'string'
},
'default': [
ToggleTabFocusModeAction.ID,
FocusActiveGroupAction.ID,
GlobalQuickOpenAction.ID,
ShowAllCommandsAction.ID,
CreateNewTerminalAction.ID,
CopyTerminalSelectionAction.ID,
KillTerminalAction.ID,
FocusActiveTerminalAction.ID,
FocusPreviousTerminalAction.ID,
FocusNextTerminalAction.ID,
FocusTerminalAtIndexAction.getId(1),
FocusTerminalAtIndexAction.getId(2),
FocusTerminalAtIndexAction.getId(3),
FocusTerminalAtIndexAction.getId(4),
FocusTerminalAtIndexAction.getId(5),
FocusTerminalAtIndexAction.getId(6),
FocusTerminalAtIndexAction.getId(7),
FocusTerminalAtIndexAction.getId(8),
FocusTerminalAtIndexAction.getId(9),
TerminalPasteAction.ID,
RunSelectedTextInTerminalAction.ID,
RunActiveFileInTerminalAction.ID,
ToggleTerminalAction.ID,
ScrollDownTerminalAction.ID,
ScrollDownPageTerminalAction.ID,
ScrollToBottomTerminalAction.ID,
ScrollUpTerminalAction.ID,
ScrollUpPageTerminalAction.ID,
ScrollToTopTerminalAction.ID,
ClearTerminalAction.ID,
debugActions.StartAction.ID,
debugActions.StopAction.ID,
debugActions.RunAction.ID,
debugActions.RestartAction.ID,
debugActions.ContinueAction.ID,
debugActions.PauseAction.ID,
OpenNextRecentlyUsedEditorInGroupAction.ID,
OpenPreviousRecentlyUsedEditorInGroupAction.ID,
FocusFirstGroupAction.ID,
FocusSecondGroupAction.ID,
FocusThirdGroupAction.ID
].sort()
}
}
});
registerSingleton(ITerminalService, TerminalService);
(<panel.PanelRegistry>Registry.as(panel.Extensions.Panels)).registerPanel(new panel.PanelDescriptor(
'vs/workbench/parts/terminal/electron-browser/terminalPanel',
'TerminalPanel',
TERMINAL_PANEL_ID,
nls.localize('terminal', "Terminal"),
'terminal',
40,
ToggleTerminalAction.ID
));
// On mac cmd+` is reserved to cycle between windows, that's why the keybindings use WinCtrl
const category = nls.localize('terminalCategory', "Terminal");
let actionRegistry = <IWorkbenchActionRegistry>Registry.as(ActionExtensions.WorkbenchActions);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(KillTerminalAction, KillTerminalAction.ID, KillTerminalAction.LABEL), 'Terminal: Kill the Active Terminal Instance', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(CopyTerminalSelectionAction, CopyTerminalSelectionAction.ID, CopyTerminalSelectionAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.KEY_C,
linux: { primary: KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.KEY_C },
// Don't apply to Mac since cmd+c works
mac: { primary: null }
}, ContextKeyExpr.and(KEYBINDING_CONTEXT_TERMINAL_TEXT_SELECTED, KEYBINDING_CONTEXT_TERMINAL_FOCUS)), 'Terminal: Copy Selection', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(CreateNewTerminalAction, CreateNewTerminalAction.ID, CreateNewTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.US_BACKTICK,
mac: { primary: KeyMod.WinCtrl | KeyMod.Shift | KeyCode.US_BACKTICK }
}), 'Terminal: Create New Integrated Terminal', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(FocusActiveTerminalAction, FocusActiveTerminalAction.ID, FocusActiveTerminalAction.LABEL), 'Terminal: Focus Active Terminal', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(FocusNextTerminalAction, FocusNextTerminalAction.ID, FocusNextTerminalAction.LABEL), 'Terminal: Focus Next Terminal', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(FocusPreviousTerminalAction, FocusPreviousTerminalAction.ID, FocusPreviousTerminalAction.LABEL), 'Terminal: Focus Previous Terminal', category);
for (let i = 1; i < 10; i++) {
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(FocusTerminalAtIndexAction, FocusTerminalAtIndexAction.getId(i), FocusTerminalAtIndexAction.getLabel(i)), 'Terminal: Focus Terminal ' + i, category);
}
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(TerminalPasteAction, TerminalPasteAction.ID, TerminalPasteAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.KEY_V,
linux: { primary: KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.KEY_V },
// Don't apply to Mac since cmd+v works
mac: { primary: null }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Paste into Active Terminal', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(RunSelectedTextInTerminalAction, RunSelectedTextInTerminalAction.ID, RunSelectedTextInTerminalAction.LABEL), 'Terminal: Run Selected Text In Active Terminal', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(RunActiveFileInTerminalAction, RunActiveFileInTerminalAction.ID, RunActiveFileInTerminalAction.LABEL), 'Terminal: Run Active File In Active Terminal', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ToggleTerminalAction, ToggleTerminalAction.ID, ToggleTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.US_BACKTICK,
mac: { primary: KeyMod.WinCtrl | KeyCode.US_BACKTICK }
}), 'View: Toggle Integrated Terminal', nls.localize('viewCategory', "View"));
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ScrollDownTerminalAction, ScrollDownTerminalAction.ID, ScrollDownTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.DownArrow,
linux: { primary: KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.DownArrow }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Scroll Down (Line)', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ScrollDownPageTerminalAction, ScrollDownPageTerminalAction.ID, ScrollDownPageTerminalAction.LABEL, {
primary: KeyMod.Shift | KeyCode.PageDown,
mac: { primary: KeyCode.PageDown }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Scroll Down (Page)', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ScrollToBottomTerminalAction, ScrollToBottomTerminalAction.ID, ScrollToBottomTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.End,
linux: { primary: KeyMod.Shift | KeyCode.End }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Scroll to Bottom', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ScrollUpTerminalAction, ScrollUpTerminalAction.ID, ScrollUpTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.UpArrow,
linux: { primary: KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.UpArrow },
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Scroll Up (Line)', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ScrollUpPageTerminalAction, ScrollUpPageTerminalAction.ID, ScrollUpPageTerminalAction.LABEL, {
primary: KeyMod.Shift | KeyCode.PageUp,
mac: { primary: KeyCode.PageUp }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Scroll Up (Page)', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ScrollToTopTerminalAction, ScrollToTopTerminalAction.ID, ScrollToTopTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.Home,
linux: { primary: KeyMod.Shift | KeyCode.Home }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS), 'Terminal: Scroll to Top', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(ClearTerminalAction, ClearTerminalAction.ID, ClearTerminalAction.LABEL, {
primary: KeyMod.CtrlCmd | KeyCode.KEY_K,
linux: { primary: null }
}, KEYBINDING_CONTEXT_TERMINAL_FOCUS, KeybindingsRegistry.WEIGHT.workbenchContrib(1)), 'Terminal: Clear', category);
if (platform.isWindows) {
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(SelectDefaultShellWindowsTerminalAction, SelectDefaultShellWindowsTerminalAction.ID, SelectDefaultShellWindowsTerminalAction.LABEL), 'Terminal: Select Default Shell', category);
}
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(AllowWorkspaceShellTerminalCommand, AllowWorkspaceShellTerminalCommand.ID, AllowWorkspaceShellTerminalCommand.LABEL), 'Terminal: Allow Workspace Shell Configuration', category);
actionRegistry.registerWorkbenchAction(new SyncActionDescriptor(DisallowWorkspaceShellTerminalCommand, DisallowWorkspaceShellTerminalCommand.ID, DisallowWorkspaceShellTerminalCommand.LABEL), 'Terminal: Disallow Workspace Shell Configuration', category);
registerColors();
| src/vs/workbench/parts/terminal/electron-browser/terminal.contribution.ts | 0 | https://github.com/microsoft/vscode/commit/8d30b30eea0a17055a1e60cf7d65870290604c76 | [
0.00018477275443729013,
0.00017260720778722316,
0.0001632877392694354,
0.0001723745372146368,
0.000004046147296321578
] |
{
"id": 0,
"code_window": [
" }\n",
"\n",
" render() {\n",
" const { text } = this.state;\n",
" const textAfterFormatted = text ? text.trim().replace(/\\n/g, '<br />') : '';\n",
"\n",
" return (\n",
" <Panel\n",
" className=\"addon-notes-container\"\n",
" dangerouslySetInnerHTML={{ __html: textAfterFormatted }}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const textAfterFormatted = text\n",
" ? text\n",
" .trim()\n",
" .replace(/(<\\S+.*>)\\n/g, '$1')\n",
" .replace(/\\n/g, '<br />')\n",
" : '';\n"
],
"file_path": "addons/notes/src/register.js",
"type": "replace",
"edit_start_line_idx": 47
} | import React from 'react';
import PropTypes from 'prop-types';
import addons from '@storybook/addons';
import styled from 'react-emotion';
const Panel = styled('div')({
padding: 10,
boxSizing: 'border-box',
width: '100%',
});
export class Notes extends React.Component {
constructor(...args) {
super(...args);
this.state = { text: '' };
this.onAddNotes = this.onAddNotes.bind(this);
}
componentDidMount() {
const { channel, api } = this.props;
// Listen to the notes and render it.
channel.on('storybook/notes/add_notes', this.onAddNotes);
// Clear the current notes on every story change.
this.stopListeningOnStory = api.onStory(() => {
this.onAddNotes('');
});
}
// This is some cleanup tasks when the Notes panel is unmounting.
componentWillUnmount() {
if (this.stopListeningOnStory) {
this.stopListeningOnStory();
}
this.unmounted = true;
const { channel } = this.props;
channel.removeListener('storybook/notes/add_notes', this.onAddNotes);
}
onAddNotes(text) {
this.setState({ text });
}
render() {
const { text } = this.state;
const textAfterFormatted = text ? text.trim().replace(/\n/g, '<br />') : '';
return (
<Panel
className="addon-notes-container"
dangerouslySetInnerHTML={{ __html: textAfterFormatted }}
/>
);
}
}
Notes.propTypes = {
// eslint-disable-next-line react/forbid-prop-types
channel: PropTypes.object,
// eslint-disable-next-line react/forbid-prop-types
api: PropTypes.object,
};
Notes.defaultProps = {
channel: {},
api: {},
};
// Register the addon with a unique name.
addons.register('storybook/notes', api => {
// Also need to set a unique name to the panel.
addons.addPanel('storybook/notes/panel', {
title: 'Notes',
render: () => <Notes channel={addons.getChannel()} api={api} />,
});
});
| addons/notes/src/register.js | 1 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.9985296726226807,
0.2561027705669403,
0.0001725018082652241,
0.004987808875739574,
0.4287722408771515
] |
{
"id": 0,
"code_window": [
" }\n",
"\n",
" render() {\n",
" const { text } = this.state;\n",
" const textAfterFormatted = text ? text.trim().replace(/\\n/g, '<br />') : '';\n",
"\n",
" return (\n",
" <Panel\n",
" className=\"addon-notes-container\"\n",
" dangerouslySetInnerHTML={{ __html: textAfterFormatted }}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const textAfterFormatted = text\n",
" ? text\n",
" .trim()\n",
" .replace(/(<\\S+.*>)\\n/g, '$1')\n",
" .replace(/\\n/g, '<br />')\n",
" : '';\n"
],
"file_path": "addons/notes/src/register.js",
"type": "replace",
"edit_start_line_idx": 47
} | import '@storybook/addon-storysource/register';
import '@storybook/addon-actions/register';
import '@storybook/addon-links/register';
import '@storybook/addon-notes/register';
import '@storybook/addon-knobs/register';
import '@storybook/addon-options/register';
import '@storybook/addon-jest/register';
import '@storybook/addon-backgrounds/register';
| examples/angular-cli/.storybook/addons.js | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.00016619036614429206,
0.00016619036614429206,
0.00016619036614429206,
0.00016619036614429206,
0
] |
{
"id": 0,
"code_window": [
" }\n",
"\n",
" render() {\n",
" const { text } = this.state;\n",
" const textAfterFormatted = text ? text.trim().replace(/\\n/g, '<br />') : '';\n",
"\n",
" return (\n",
" <Panel\n",
" className=\"addon-notes-container\"\n",
" dangerouslySetInnerHTML={{ __html: textAfterFormatted }}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const textAfterFormatted = text\n",
" ? text\n",
" .trim()\n",
" .replace(/(<\\S+.*>)\\n/g, '$1')\n",
" .replace(/\\n/g, '<br />')\n",
" : '';\n"
],
"file_path": "addons/notes/src/register.js",
"type": "replace",
"edit_start_line_idx": 47
} | <svg xmlns="http://www.w3.org/2000/svg" width="596" height="180"><rect id="backgroundrect" width="100%" height="100%" x="0" y="0" fill="none" stroke="none" class=""/><g class="currentLayer"><title>Layer 1</title><g class="" id="svg_5"><g fill="#2E3133" id="svg_1" class=""><path d="M360.4999953150749,154.73703964948652 v-13.1 h6.5 v13.1 h-6.5 zM426.9999953150749,109.63703964948655 c-0.2,-2.8 -1,-5.4 -2.2,-7.6 c-1.2,-2.2 -2.8,-4 -4.8,-5.5 s-4.2,-2.6 -6.9,-3.3 c-2.6,-0.7000000000000006 -5.4,-1.1 -8.3,-1.1 c-4,0 -7.7,0.9 -10.8,2.4 c-3.2,1.6 -5.8,3.8 -7.8,6.6 c-2.1,2.7 -3.7,5.9 -4.8,9.4 s-1.6,7.1 -1.6,10.9 c0,4.4 0.5,8.4 1.5,12.1 c1,3.7 2.6,7 4.7,9.7 c2.1,2.7 4.8,4.9 7.8,6.4 c3.2,1.5 6.9,2.3 11,2.3 c3.1,0 5.9,-0.5 8.4,-1.5 c2.6,-1 4.9,-2.4 6.9,-4.2 c2,-1.8 3.7,-3.9 4.9,-6.4 s2.1,-5.3 2.6,-8.2 h4.8 c-0.5,3.9 -1.6,7.3 -3.2,10.4 c-1.6,3.1 -3.7,5.6 -6.1,7.7 c-2.4,2.1 -5.1,3.7 -8.2,4.8 s-6.4,1.6 -9.9,1.6 c-5.4,0 -9.9,-1 -13.7,-2.8 c-3.8,-2 -6.9,-4.4 -9.3,-7.7 c-2.4,-3.2 -4.2,-6.9 -5.4,-11.1 c-1.1,-4.2 -1.7000000000000002,-8.6 -1.7000000000000002,-13.1 s0.6000000000000006,-8.9 2,-13 c1.3,-4 3.2,-7.6 5.8,-10.6 s5.6,-5.5 9.4,-7.2 c3.7,-1.8 8,-2.7 12.9,-2.7 c3.5,0 6.9,0.5 9.9,1.3 c3.1,0.9 5.9,2.2 8.2,4 c2.4,1.8 4.4,4 5.9,6.7 s2.4,5.9 2.8,9.7 h-4.7 zM439.4999953150749,122.1370396494865 c0,-4.7 0.6000000000000006,-9.1 2,-13.1 c1.3,-4 3.2,-7.7 5.8,-10.9 c2.6,-3.2 5.6,-5.6 9.4,-7.5 c3.7,-1.8 8,-2.7 12.9,-2.7 c4.8,0 9.1,0.9 12.9,2.7 c3.7,1.8 6.9,4.3 9.4,7.5 c2.6,3.2 4.4,6.7 5.8,10.9 c1.3,4.2 2,8.4 2,13.1 c0,4.7 -0.6000000000000006,9.1 -2,13.2 c-1.3,4.2 -3.2,7.7 -5.8,10.9 c-2.6,3.1 -5.6,5.5 -9.4,7.3 c-3.7,1.8 -8,2.7 -12.9,2.7 c-4.8,0 -9.1,-0.9 -12.9,-2.7 c-3.7,-1.8 -6.9,-4.3 -9.4,-7.3 s-4.5,-6.7 -5.8,-10.9 c-1.4,-4.1 -2,-8.6 -2,-13.2 zm4.7,0 c0,3.9 0.5,7.6 1.6,11.3 c1.1,3.5 2.7,6.7 4.8,9.5 c2.1,2.8 4.8,5 7.8,6.6 c3.2,1.6 6.7,2.4 10.8,2.4 s7.7,-0.9 10.8,-2.4 c3.2,-1.6 5.8,-3.9 7.8,-6.6 c2.1,-2.8 3.7,-6 4.8,-9.5 s1.6,-7.3 1.6,-11.3 s-0.6000000000000006,-7.6 -1.6,-11.3 c-1.1,-3.5 -2.7,-6.7 -4.8,-9.5 c-2.1,-2.8 -4.8,-5 -7.8,-6.6 c-3.2,-1.6 -6.7,-2.4 -10.8,-2.4 s-7.7,0.9 -10.8,2.4 c-3.2,1.6 -5.8,3.9 -7.8,6.6 c-2.1,2.8 -3.7,6 -4.8,9.5 c-1,3.7 -1.6,7.4 -1.6,11.3 zM509.5999953150749,89.53703964948653 h4.8 v13.3 h0.2 c0.5,-2.1 1.3,-3.9 2.7,-5.8 c1.2,-1.8 2.8,-3.4 4.7,-4.8 c1.8,-1.3 3.8,-2.4 6.1,-3.2 c2.2,-0.7000000000000006 4.7,-1.1 7.1,-1.1 c3.2,0 5.9,0.4 8.1,1.2 c2.3,0.9 4.3,1.8 5.9,3.2 s2.9,2.8 3.8,4.4 c1,1.6 1.6,3.3 2,5 h0.2 c2,-4.5 4.5,-8 7.8,-10.3 c3.3,-2.3 7.6,-3.5 12.9,-3.5 c2.9,0 5.8,0.5 8.2,1.3 c2.4,0.9 4.7,2.2 6.5,4 s3.2,4.2 4.3,6.9 c1,2.8 1.5,6.1 1.5,9.9 v44.4 h-4.9 v-44.7 c0,-4.2 -0.6000000000000006,-7.3 -2,-9.8 c-1.2,-2.3 -2.8,-4.2 -4.5,-5.4 c-1.7000000000000002,-1.2 -3.4,-2 -5.3,-2.3 c-1.7000000000000002,-0.2 -3.1,-0.5 -4,-0.5 c-2.9,0 -5.8,0.5 -8.2,1.5 c-2.4,1 -4.7,2.6 -6.5,4.5 c-1.8,2.1 -3.2,4.5 -4.3,7.6 c-1,3.1 -1.5,6.5 -1.5,10.3 v38.8 h-4.8 v-44.7 c0,-4 -0.6000000000000006,-7.3 -1.8,-9.7 c-1.2,-2.4 -2.7,-4.2 -4.3,-5.4 s-3.3,-2 -5.1,-2.3 c-1.7000000000000002,-0.4 -3.1,-0.5 -4.2,-0.5 c-2.3,0 -4.7,0.5 -7.1,1.3 c-2.4,1 -4.7,2.3 -6.7,4.3 s-3.7,4.4 -4.9,7.5 s-2,6.6 -2,10.8 v38.7 h-4.8 V89.33703964948654 h0.1 z" id="svg_2"/></g><g class="" id="svg_6"><path fill="#FFCB29" d="M199.6999953150749,22.33703964948654 c-7,3.7 -9.5,9.8 -9.5,26.7 c0,0 3.5,-3.4 8.7,-5.3 c3.8,-1.3 7,-3.4 8.9,-4.8 c5.9,-4.3 6.7,-9.8 6.7,-19.1 c0,0 -9.6,-0.3000000000000003 -14.8,2.5 z" id="svg_3" class=""/><path fill="#2E3133" d="M158.6999953150749,26.33703964948654 c-5.6,5 -7.3,12.9 -7.3,12.9 L132.59999531507492,111.43703964948656 l-15.5,-59.2 c-1.5,-6.2 -4.3,-14.1 -8.6,-19.3 c-5.4,-6.6 -16.5,-7 -17.7,-7 s-12.4,0.4 -17.9,7.1 c-4.3,5.3 -7.1,13.1 -8.6,19.3 l-15.5,59.2 l-18.6,-72.3 s-1.6,-8 -7.3,-12.9 c-9.2,-8.1 -22.9,-6.4 -22.9,-6.4 L35.699995315074915,155.33703964948654 s11.8,0.9 17.7,-2.2 c7.7,-3.9 11.5,-7 16.2,-25.5 c4.2,-16.4 15.9,-64.6 17,-68.1 c0.5,-1.7000000000000002 1.2,-5.8 4.2,-5.8 c3.1,0 3.7,4 4.2,5.8 c1.1,3.4 12.7,51.7 17,68.1 c4.7,18.4 8.4,21.4 16.2,25.5 c5.9,3.1 17.7,2.2 17.7,2.2 L181.59999531507492,20.03703964948653 s-13.7,-1.7000000000000002 -22.9,6.3 zM214.4999953150749,42.03703964948653 s-2.2,3.4 -7.3,6.4 c-3.3,1.8 -6.4,3.1 -9.8,4.7 c-5.6,2.7 -7.2,5.8 -7.2,10.4 V155.33703964948654 s9.1,1.1 14.9,-1.8 c7.6,-3.9 9.3,-7.6 9.4,-24.5 v-87 zM303.69999531507494,87.93703964948656 l45.3,-67.6 s-19.1,-3.3 -28.6,5.4 c-6.1,5.5 -12.9,15.4 -12.9,15.4 L290.89999531507493,65.33703964948654 c-0.9,1.2 -1.8,2.6 -3.5,2.6 s-2.8,-1.3 -3.5,-2.6 l-16.8,-24.1 s-6.7,-9.9 -12.9,-15.4 c-9.4,-8.7 -28.6,-5.4 -28.6,-5.4 l45.4,67.4 l-45.4,67.3 s20,2.6 29.4,-6.1 c6.1,-5.5 12,-14.6 12,-14.6 L283.5999953150749,110.33703964948654 c0.9,-1.2 1.8,-2.6 3.5,-2.6 s2.8,1.3 3.5,2.6 l16.6,24.1 s6.2,8.9 12.2,14.6 c9.4,8.7 29.1,6.1 29.1,6.1 l-44.8,-67.2 z" id="svg_4" class=""/></g></g></g></svg> | docs/src/pages/logos/wix.svg | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.00021188089158385992,
0.00021188089158385992,
0.00021188089158385992,
0.00021188089158385992,
0
] |
{
"id": 0,
"code_window": [
" }\n",
"\n",
" render() {\n",
" const { text } = this.state;\n",
" const textAfterFormatted = text ? text.trim().replace(/\\n/g, '<br />') : '';\n",
"\n",
" return (\n",
" <Panel\n",
" className=\"addon-notes-container\"\n",
" dangerouslySetInnerHTML={{ __html: textAfterFormatted }}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const textAfterFormatted = text\n",
" ? text\n",
" .trim()\n",
" .replace(/(<\\S+.*>)\\n/g, '$1')\n",
" .replace(/\\n/g, '<br />')\n",
" : '';\n"
],
"file_path": "addons/notes/src/register.js",
"type": "replace",
"edit_start_line_idx": 47
} | {
"short_name": "React App",
"name": "Create React App Sample",
"icons": [
{
"src": "favicon.ico",
"sizes": "192x192",
"type": "image/png"
}
],
"start_url": "./index.html",
"display": "standalone",
"theme_color": "#000000",
"background_color": "#ffffff"
}
| lib/cli/test/snapshots/update_package_organisations/public/manifest.json | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.0001761529128998518,
0.00017426552949473262,
0.00017237814608961344,
0.00017426552949473262,
0.0000018873834051191807
] |
{
"id": 1,
"code_window": [
" )\n",
"~~~\n",
" `;\n",
"\n",
"storiesOf('Addons|Notes', module)\n",
" .addDecorator(withNotes)\n",
" .add('withNotes', baseStory, {\n",
" notes:\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"const markdownTable = `\n",
"| Column1 | Column2 | Column3 |\n",
"|---------|---------|---------|\n",
"| Row1.1 | Row1.2 | Row1.3 |\n",
"| Row2.1 | Row2.2 | Row2.3 |\n",
"| Row3.1 | Row3.2 | Row3.3 |\n",
"| Row4.1 | Row4.2 | Row4.3 |\n",
"`;\n",
"\n"
],
"file_path": "examples/official-storybook/stories/addon-notes.stories.js",
"type": "add",
"edit_start_line_idx": 28
} | import React from 'react';
import PropTypes from 'prop-types';
import addons from '@storybook/addons';
import styled from 'react-emotion';
const Panel = styled('div')({
padding: 10,
boxSizing: 'border-box',
width: '100%',
});
export class Notes extends React.Component {
constructor(...args) {
super(...args);
this.state = { text: '' };
this.onAddNotes = this.onAddNotes.bind(this);
}
componentDidMount() {
const { channel, api } = this.props;
// Listen to the notes and render it.
channel.on('storybook/notes/add_notes', this.onAddNotes);
// Clear the current notes on every story change.
this.stopListeningOnStory = api.onStory(() => {
this.onAddNotes('');
});
}
// This is some cleanup tasks when the Notes panel is unmounting.
componentWillUnmount() {
if (this.stopListeningOnStory) {
this.stopListeningOnStory();
}
this.unmounted = true;
const { channel } = this.props;
channel.removeListener('storybook/notes/add_notes', this.onAddNotes);
}
onAddNotes(text) {
this.setState({ text });
}
render() {
const { text } = this.state;
const textAfterFormatted = text ? text.trim().replace(/\n/g, '<br />') : '';
return (
<Panel
className="addon-notes-container"
dangerouslySetInnerHTML={{ __html: textAfterFormatted }}
/>
);
}
}
Notes.propTypes = {
// eslint-disable-next-line react/forbid-prop-types
channel: PropTypes.object,
// eslint-disable-next-line react/forbid-prop-types
api: PropTypes.object,
};
Notes.defaultProps = {
channel: {},
api: {},
};
// Register the addon with a unique name.
addons.register('storybook/notes', api => {
// Also need to set a unique name to the panel.
addons.addPanel('storybook/notes/panel', {
title: 'Notes',
render: () => <Notes channel={addons.getChannel()} api={api} />,
});
});
| addons/notes/src/register.js | 1 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.0020049079321324825,
0.0004816027940250933,
0.00016702120774425566,
0.0002594950492493808,
0.0005791352596133947
] |
{
"id": 1,
"code_window": [
" )\n",
"~~~\n",
" `;\n",
"\n",
"storiesOf('Addons|Notes', module)\n",
" .addDecorator(withNotes)\n",
" .add('withNotes', baseStory, {\n",
" notes:\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"const markdownTable = `\n",
"| Column1 | Column2 | Column3 |\n",
"|---------|---------|---------|\n",
"| Row1.1 | Row1.2 | Row1.3 |\n",
"| Row2.1 | Row2.2 | Row2.3 |\n",
"| Row3.1 | Row3.2 | Row3.3 |\n",
"| Row4.1 | Row4.2 | Row4.3 |\n",
"`;\n",
"\n"
],
"file_path": "examples/official-storybook/stories/addon-notes.stories.js",
"type": "add",
"edit_start_line_idx": 28
} | // !$*UTF8*$!
{
archiveVersion = 1;
classes = {
};
objectVersion = 46;
objects = {
/* Begin PBXBuildFile section */
00C302E51ABCBA2D00DB3ED1 /* libRCTActionSheet.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 00C302AC1ABCB8CE00DB3ED1 /* libRCTActionSheet.a */; };
00C302E71ABCBA2D00DB3ED1 /* libRCTGeolocation.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 00C302BA1ABCB90400DB3ED1 /* libRCTGeolocation.a */; };
00C302E81ABCBA2D00DB3ED1 /* libRCTImage.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 00C302C01ABCB91800DB3ED1 /* libRCTImage.a */; };
00C302E91ABCBA2D00DB3ED1 /* libRCTNetwork.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 00C302DC1ABCB9D200DB3ED1 /* libRCTNetwork.a */; };
00C302EA1ABCBA2D00DB3ED1 /* libRCTVibration.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 00C302E41ABCB9EE00DB3ED1 /* libRCTVibration.a */; };
00E356F31AD99517003FC87E /* ReactNativeTypescriptTests.m in Sources */ = {isa = PBXBuildFile; fileRef = 00E356F21AD99517003FC87E /* ReactNativeTypescriptTests.m */; };
133E29F31AD74F7200F7D852 /* libRCTLinking.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 78C398B91ACF4ADC00677621 /* libRCTLinking.a */; };
139105C61AF99C1200B5F7CC /* libRCTSettings.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 139105C11AF99BAD00B5F7CC /* libRCTSettings.a */; };
139FDEF61B0652A700C62182 /* libRCTWebSocket.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 139FDEF41B06529B00C62182 /* libRCTWebSocket.a */; };
13B07FBC1A68108700A75B9A /* AppDelegate.m in Sources */ = {isa = PBXBuildFile; fileRef = 13B07FB01A68108700A75B9A /* AppDelegate.m */; };
13B07FBD1A68108700A75B9A /* LaunchScreen.xib in Resources */ = {isa = PBXBuildFile; fileRef = 13B07FB11A68108700A75B9A /* LaunchScreen.xib */; };
13B07FBF1A68108700A75B9A /* Images.xcassets in Resources */ = {isa = PBXBuildFile; fileRef = 13B07FB51A68108700A75B9A /* Images.xcassets */; };
13B07FC11A68108700A75B9A /* main.m in Sources */ = {isa = PBXBuildFile; fileRef = 13B07FB71A68108700A75B9A /* main.m */; };
140ED2AC1D01E1AD002B40FF /* libReact.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 146834041AC3E56700842450 /* libReact.a */; };
146834051AC3E58100842450 /* libReact.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 146834041AC3E56700842450 /* libReact.a */; };
2D02E4BC1E0B4A80006451C7 /* AppDelegate.m in Sources */ = {isa = PBXBuildFile; fileRef = 13B07FB01A68108700A75B9A /* AppDelegate.m */; };
2D02E4BD1E0B4A84006451C7 /* Images.xcassets in Resources */ = {isa = PBXBuildFile; fileRef = 13B07FB51A68108700A75B9A /* Images.xcassets */; };
2D02E4BF1E0B4AB3006451C7 /* main.m in Sources */ = {isa = PBXBuildFile; fileRef = 13B07FB71A68108700A75B9A /* main.m */; };
2D02E4C21E0B4AEC006451C7 /* libRCTAnimation.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 5E9157351DD0AC6500FF2AA8 /* libRCTAnimation.a */; };
2D02E4C31E0B4AEC006451C7 /* libRCTImage-tvOS.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 3DAD3E841DF850E9000B6D8A /* libRCTImage-tvOS.a */; };
2D02E4C41E0B4AEC006451C7 /* libRCTLinking-tvOS.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 3DAD3E881DF850E9000B6D8A /* libRCTLinking-tvOS.a */; };
2D02E4C51E0B4AEC006451C7 /* libRCTNetwork-tvOS.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 3DAD3E8C1DF850E9000B6D8A /* libRCTNetwork-tvOS.a */; };
2D02E4C61E0B4AEC006451C7 /* libRCTSettings-tvOS.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 3DAD3E901DF850E9000B6D8A /* libRCTSettings-tvOS.a */; };
2D02E4C71E0B4AEC006451C7 /* libRCTText-tvOS.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 3DAD3E941DF850E9000B6D8A /* libRCTText-tvOS.a */; };
2D02E4C81E0B4AEC006451C7 /* libRCTWebSocket-tvOS.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 3DAD3E991DF850E9000B6D8A /* libRCTWebSocket-tvOS.a */; };
2D16E6881FA4F8E400B85C8A /* libReact.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 2D16E6891FA4F8E400B85C8A /* libReact.a */; };
2DCD954D1E0B4F2C00145EB5 /* ReactNativeTypescriptTests.m in Sources */ = {isa = PBXBuildFile; fileRef = 00E356F21AD99517003FC87E /* ReactNativeTypescriptTests.m */; };
5E9157361DD0AC6A00FF2AA8 /* libRCTAnimation.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 5E9157331DD0AC6500FF2AA8 /* libRCTAnimation.a */; };
832341BD1AAA6AB300B99B32 /* libRCTText.a in Frameworks */ = {isa = PBXBuildFile; fileRef = 832341B51AAA6A8300B99B32 /* libRCTText.a */; };
ADBDB9381DFEBF1600ED6528 /* libRCTBlob.a in Frameworks */ = {isa = PBXBuildFile; fileRef = ADBDB9271DFEBF0700ED6528 /* libRCTBlob.a */; };
/* End PBXBuildFile section */
/* Begin PBXContainerItemProxy section */
00C302AB1ABCB8CE00DB3ED1 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302A71ABCB8CE00DB3ED1 /* RCTActionSheet.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 134814201AA4EA6300B7C361;
remoteInfo = RCTActionSheet;
};
00C302B91ABCB90400DB3ED1 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302B51ABCB90400DB3ED1 /* RCTGeolocation.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 134814201AA4EA6300B7C361;
remoteInfo = RCTGeolocation;
};
00C302BF1ABCB91800DB3ED1 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302BB1ABCB91800DB3ED1 /* RCTImage.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 58B5115D1A9E6B3D00147676;
remoteInfo = RCTImage;
};
00C302DB1ABCB9D200DB3ED1 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302D31ABCB9D200DB3ED1 /* RCTNetwork.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 58B511DB1A9E6C8500147676;
remoteInfo = RCTNetwork;
};
00C302E31ABCB9EE00DB3ED1 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302DF1ABCB9EE00DB3ED1 /* RCTVibration.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 832C81801AAF6DEF007FA2F7;
remoteInfo = RCTVibration;
};
00E356F41AD99517003FC87E /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 83CBB9F71A601CBA00E9B192 /* Project object */;
proxyType = 1;
remoteGlobalIDString = 13B07F861A680F5B00A75B9A;
remoteInfo = ReactNativeTypescript;
};
139105C01AF99BAD00B5F7CC /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 139105B61AF99BAD00B5F7CC /* RCTSettings.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 134814201AA4EA6300B7C361;
remoteInfo = RCTSettings;
};
139FDEF31B06529B00C62182 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3C86DF461ADF2C930047B81A;
remoteInfo = RCTWebSocket;
};
146834031AC3E56700842450 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 83CBBA2E1A601D0E00E9B192;
remoteInfo = React;
};
2D02E4911E0B4A5D006451C7 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 83CBB9F71A601CBA00E9B192 /* Project object */;
proxyType = 1;
remoteGlobalIDString = 2D02E47A1E0B4A5D006451C7;
remoteInfo = "ReactNativeTypescript-tvOS";
};
2D16E6711FA4F8DC00B85C8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = ADBDB91F1DFEBF0600ED6528 /* RCTBlob.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = ADD01A681E09402E00F6D226;
remoteInfo = "RCTBlob-tvOS";
};
2D16E6831FA4F8DC00B85C8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3DBE0D001F3B181A0099AA32;
remoteInfo = fishhook;
};
2D16E6851FA4F8DC00B85C8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3DBE0D0D1F3B181C0099AA32;
remoteInfo = "fishhook-tvOS";
};
3DAD3E831DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302BB1ABCB91800DB3ED1 /* RCTImage.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A283A1D9B042B00D4039D;
remoteInfo = "RCTImage-tvOS";
};
3DAD3E871DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 78C398B01ACF4ADC00677621 /* RCTLinking.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A28471D9B043800D4039D;
remoteInfo = "RCTLinking-tvOS";
};
3DAD3E8B1DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 00C302D31ABCB9D200DB3ED1 /* RCTNetwork.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A28541D9B044C00D4039D;
remoteInfo = "RCTNetwork-tvOS";
};
3DAD3E8F1DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 139105B61AF99BAD00B5F7CC /* RCTSettings.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A28611D9B046600D4039D;
remoteInfo = "RCTSettings-tvOS";
};
3DAD3E931DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 832341B01AAA6A8300B99B32 /* RCTText.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A287B1D9B048500D4039D;
remoteInfo = "RCTText-tvOS";
};
3DAD3E981DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A28881D9B049200D4039D;
remoteInfo = "RCTWebSocket-tvOS";
};
3DAD3EA21DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A28131D9B038B00D4039D;
remoteInfo = "React-tvOS";
};
3DAD3EA41DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3D3C059A1DE3340900C268FA;
remoteInfo = yoga;
};
3DAD3EA61DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3D3C06751DE3340C00C268FA;
remoteInfo = "yoga-tvOS";
};
3DAD3EA81DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3D3CD9251DE5FBEC00167DC4;
remoteInfo = cxxreact;
};
3DAD3EAA1DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3D3CD9321DE5FBEE00167DC4;
remoteInfo = "cxxreact-tvOS";
};
3DAD3EAC1DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3D3CD90B1DE5FBD600167DC4;
remoteInfo = jschelpers;
};
3DAD3EAE1DF850E9000B6D8A /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 146833FF1AC3E56700842450 /* React.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 3D3CD9181DE5FBD800167DC4;
remoteInfo = "jschelpers-tvOS";
};
5E9157321DD0AC6500FF2AA8 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 5E91572D1DD0AC6500FF2AA8 /* RCTAnimation.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 134814201AA4EA6300B7C361;
remoteInfo = RCTAnimation;
};
5E9157341DD0AC6500FF2AA8 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 5E91572D1DD0AC6500FF2AA8 /* RCTAnimation.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 2D2A28201D9B03D100D4039D;
remoteInfo = "RCTAnimation-tvOS";
};
78C398B81ACF4ADC00677621 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 78C398B01ACF4ADC00677621 /* RCTLinking.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 134814201AA4EA6300B7C361;
remoteInfo = RCTLinking;
};
832341B41AAA6A8300B99B32 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = 832341B01AAA6A8300B99B32 /* RCTText.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 58B5119B1A9E6C1200147676;
remoteInfo = RCTText;
};
ADBDB9261DFEBF0700ED6528 /* PBXContainerItemProxy */ = {
isa = PBXContainerItemProxy;
containerPortal = ADBDB91F1DFEBF0600ED6528 /* RCTBlob.xcodeproj */;
proxyType = 2;
remoteGlobalIDString = 358F4ED71D1E81A9004DF814;
remoteInfo = RCTBlob;
};
/* End PBXContainerItemProxy section */
/* Begin PBXFileReference section */
008F07F21AC5B25A0029DE68 /* main.jsbundle */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = text; path = main.jsbundle; sourceTree = "<group>"; };
00C302A71ABCB8CE00DB3ED1 /* RCTActionSheet.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTActionSheet.xcodeproj; path = "../node_modules/react-native/Libraries/ActionSheetIOS/RCTActionSheet.xcodeproj"; sourceTree = "<group>"; };
00C302B51ABCB90400DB3ED1 /* RCTGeolocation.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTGeolocation.xcodeproj; path = "../node_modules/react-native/Libraries/Geolocation/RCTGeolocation.xcodeproj"; sourceTree = "<group>"; };
00C302BB1ABCB91800DB3ED1 /* RCTImage.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTImage.xcodeproj; path = "../node_modules/react-native/Libraries/Image/RCTImage.xcodeproj"; sourceTree = "<group>"; };
00C302D31ABCB9D200DB3ED1 /* RCTNetwork.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTNetwork.xcodeproj; path = "../node_modules/react-native/Libraries/Network/RCTNetwork.xcodeproj"; sourceTree = "<group>"; };
00C302DF1ABCB9EE00DB3ED1 /* RCTVibration.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTVibration.xcodeproj; path = "../node_modules/react-native/Libraries/Vibration/RCTVibration.xcodeproj"; sourceTree = "<group>"; };
00E356EE1AD99517003FC87E /* ReactNativeTypescriptTests.xctest */ = {isa = PBXFileReference; explicitFileType = wrapper.cfbundle; includeInIndex = 0; path = ReactNativeTypescriptTests.xctest; sourceTree = BUILT_PRODUCTS_DIR; };
00E356F11AD99517003FC87E /* Info.plist */ = {isa = PBXFileReference; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = "<group>"; };
00E356F21AD99517003FC87E /* ReactNativeTypescriptTests.m */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.c.objc; path = ReactNativeTypescriptTests.m; sourceTree = "<group>"; };
139105B61AF99BAD00B5F7CC /* RCTSettings.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTSettings.xcodeproj; path = "../node_modules/react-native/Libraries/Settings/RCTSettings.xcodeproj"; sourceTree = "<group>"; };
139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTWebSocket.xcodeproj; path = "../node_modules/react-native/Libraries/WebSocket/RCTWebSocket.xcodeproj"; sourceTree = "<group>"; };
13B07F961A680F5B00A75B9A /* ReactNativeTypescript.app */ = {isa = PBXFileReference; explicitFileType = wrapper.application; includeInIndex = 0; path = ReactNativeTypescript.app; sourceTree = BUILT_PRODUCTS_DIR; };
13B07FAF1A68108700A75B9A /* AppDelegate.h */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.h; name = AppDelegate.h; path = ReactNativeTypescript/AppDelegate.h; sourceTree = "<group>"; };
13B07FB01A68108700A75B9A /* AppDelegate.m */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.objc; name = AppDelegate.m; path = ReactNativeTypescript/AppDelegate.m; sourceTree = "<group>"; };
13B07FB21A68108700A75B9A /* Base */ = {isa = PBXFileReference; lastKnownFileType = file.xib; name = Base; path = Base.lproj/LaunchScreen.xib; sourceTree = "<group>"; };
13B07FB51A68108700A75B9A /* Images.xcassets */ = {isa = PBXFileReference; lastKnownFileType = folder.assetcatalog; name = Images.xcassets; path = ReactNativeTypescript/Images.xcassets; sourceTree = "<group>"; };
13B07FB61A68108700A75B9A /* Info.plist */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = text.plist.xml; name = Info.plist; path = ReactNativeTypescript/Info.plist; sourceTree = "<group>"; };
13B07FB71A68108700A75B9A /* main.m */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.objc; name = main.m; path = ReactNativeTypescript/main.m; sourceTree = "<group>"; };
146833FF1AC3E56700842450 /* React.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = React.xcodeproj; path = "../node_modules/react-native/React/React.xcodeproj"; sourceTree = "<group>"; };
2D02E47B1E0B4A5D006451C7 /* ReactNativeTypescript-tvOS.app */ = {isa = PBXFileReference; explicitFileType = wrapper.application; includeInIndex = 0; path = "ReactNativeTypescript-tvOS.app"; sourceTree = BUILT_PRODUCTS_DIR; };
2D02E4901E0B4A5D006451C7 /* ReactNativeTypescript-tvOSTests.xctest */ = {isa = PBXFileReference; explicitFileType = wrapper.cfbundle; includeInIndex = 0; path = "ReactNativeTypescript-tvOSTests.xctest"; sourceTree = BUILT_PRODUCTS_DIR; };
2D16E6891FA4F8E400B85C8A /* libReact.a */ = {isa = PBXFileReference; explicitFileType = archive.ar; path = libReact.a; sourceTree = BUILT_PRODUCTS_DIR; };
5E91572D1DD0AC6500FF2AA8 /* RCTAnimation.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTAnimation.xcodeproj; path = "../node_modules/react-native/Libraries/NativeAnimation/RCTAnimation.xcodeproj"; sourceTree = "<group>"; };
78C398B01ACF4ADC00677621 /* RCTLinking.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTLinking.xcodeproj; path = "../node_modules/react-native/Libraries/LinkingIOS/RCTLinking.xcodeproj"; sourceTree = "<group>"; };
832341B01AAA6A8300B99B32 /* RCTText.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTText.xcodeproj; path = "../node_modules/react-native/Libraries/Text/RCTText.xcodeproj"; sourceTree = "<group>"; };
ADBDB91F1DFEBF0600ED6528 /* RCTBlob.xcodeproj */ = {isa = PBXFileReference; lastKnownFileType = "wrapper.pb-project"; name = RCTBlob.xcodeproj; path = "../node_modules/react-native/Libraries/Blob/RCTBlob.xcodeproj"; sourceTree = "<group>"; };
/* End PBXFileReference section */
/* Begin PBXFrameworksBuildPhase section */
00E356EB1AD99517003FC87E /* Frameworks */ = {
isa = PBXFrameworksBuildPhase;
buildActionMask = 2147483647;
files = (
140ED2AC1D01E1AD002B40FF /* libReact.a in Frameworks */,
);
runOnlyForDeploymentPostprocessing = 0;
};
13B07F8C1A680F5B00A75B9A /* Frameworks */ = {
isa = PBXFrameworksBuildPhase;
buildActionMask = 2147483647;
files = (
ADBDB9381DFEBF1600ED6528 /* libRCTBlob.a in Frameworks */,
5E9157361DD0AC6A00FF2AA8 /* libRCTAnimation.a in Frameworks */,
146834051AC3E58100842450 /* libReact.a in Frameworks */,
5E9157361DD0AC6A00FF2AA8 /* libRCTAnimation.a in Frameworks */,
00C302E51ABCBA2D00DB3ED1 /* libRCTActionSheet.a in Frameworks */,
00C302E71ABCBA2D00DB3ED1 /* libRCTGeolocation.a in Frameworks */,
00C302E81ABCBA2D00DB3ED1 /* libRCTImage.a in Frameworks */,
133E29F31AD74F7200F7D852 /* libRCTLinking.a in Frameworks */,
00C302E91ABCBA2D00DB3ED1 /* libRCTNetwork.a in Frameworks */,
139105C61AF99C1200B5F7CC /* libRCTSettings.a in Frameworks */,
832341BD1AAA6AB300B99B32 /* libRCTText.a in Frameworks */,
00C302EA1ABCBA2D00DB3ED1 /* libRCTVibration.a in Frameworks */,
139FDEF61B0652A700C62182 /* libRCTWebSocket.a in Frameworks */,
);
runOnlyForDeploymentPostprocessing = 0;
};
2D02E4781E0B4A5D006451C7 /* Frameworks */ = {
isa = PBXFrameworksBuildPhase;
buildActionMask = 2147483647;
files = (
2D16E6881FA4F8E400B85C8A /* libReact.a in Frameworks */,
2D02E4C21E0B4AEC006451C7 /* libRCTAnimation.a in Frameworks */,
2D02E4C31E0B4AEC006451C7 /* libRCTImage-tvOS.a in Frameworks */,
2D02E4C41E0B4AEC006451C7 /* libRCTLinking-tvOS.a in Frameworks */,
2D02E4C51E0B4AEC006451C7 /* libRCTNetwork-tvOS.a in Frameworks */,
2D02E4C61E0B4AEC006451C7 /* libRCTSettings-tvOS.a in Frameworks */,
2D02E4C71E0B4AEC006451C7 /* libRCTText-tvOS.a in Frameworks */,
2D02E4C81E0B4AEC006451C7 /* libRCTWebSocket-tvOS.a in Frameworks */,
);
runOnlyForDeploymentPostprocessing = 0;
};
2D02E48D1E0B4A5D006451C7 /* Frameworks */ = {
isa = PBXFrameworksBuildPhase;
buildActionMask = 2147483647;
files = (
);
runOnlyForDeploymentPostprocessing = 0;
};
/* End PBXFrameworksBuildPhase section */
/* Begin PBXGroup section */
00C302A81ABCB8CE00DB3ED1 /* Products */ = {
isa = PBXGroup;
children = (
00C302AC1ABCB8CE00DB3ED1 /* libRCTActionSheet.a */,
);
name = Products;
sourceTree = "<group>";
};
00C302B61ABCB90400DB3ED1 /* Products */ = {
isa = PBXGroup;
children = (
00C302BA1ABCB90400DB3ED1 /* libRCTGeolocation.a */,
);
name = Products;
sourceTree = "<group>";
};
00C302BC1ABCB91800DB3ED1 /* Products */ = {
isa = PBXGroup;
children = (
00C302C01ABCB91800DB3ED1 /* libRCTImage.a */,
3DAD3E841DF850E9000B6D8A /* libRCTImage-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
00C302D41ABCB9D200DB3ED1 /* Products */ = {
isa = PBXGroup;
children = (
00C302DC1ABCB9D200DB3ED1 /* libRCTNetwork.a */,
3DAD3E8C1DF850E9000B6D8A /* libRCTNetwork-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
00C302E01ABCB9EE00DB3ED1 /* Products */ = {
isa = PBXGroup;
children = (
00C302E41ABCB9EE00DB3ED1 /* libRCTVibration.a */,
);
name = Products;
sourceTree = "<group>";
};
00E356EF1AD99517003FC87E /* ReactNativeTypescriptTests */ = {
isa = PBXGroup;
children = (
00E356F21AD99517003FC87E /* ReactNativeTypescriptTests.m */,
00E356F01AD99517003FC87E /* Supporting Files */,
);
path = ReactNativeTypescriptTests;
sourceTree = "<group>";
};
00E356F01AD99517003FC87E /* Supporting Files */ = {
isa = PBXGroup;
children = (
00E356F11AD99517003FC87E /* Info.plist */,
);
name = "Supporting Files";
sourceTree = "<group>";
};
139105B71AF99BAD00B5F7CC /* Products */ = {
isa = PBXGroup;
children = (
139105C11AF99BAD00B5F7CC /* libRCTSettings.a */,
3DAD3E901DF850E9000B6D8A /* libRCTSettings-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
139FDEE71B06529A00C62182 /* Products */ = {
isa = PBXGroup;
children = (
139FDEF41B06529B00C62182 /* libRCTWebSocket.a */,
3DAD3E991DF850E9000B6D8A /* libRCTWebSocket-tvOS.a */,
2D16E6841FA4F8DC00B85C8A /* libfishhook.a */,
2D16E6861FA4F8DC00B85C8A /* libfishhook-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
13B07FAE1A68108700A75B9A /* ReactNativeTypescript */ = {
isa = PBXGroup;
children = (
008F07F21AC5B25A0029DE68 /* main.jsbundle */,
13B07FAF1A68108700A75B9A /* AppDelegate.h */,
13B07FB01A68108700A75B9A /* AppDelegate.m */,
13B07FB51A68108700A75B9A /* Images.xcassets */,
13B07FB61A68108700A75B9A /* Info.plist */,
13B07FB11A68108700A75B9A /* LaunchScreen.xib */,
13B07FB71A68108700A75B9A /* main.m */,
);
name = ReactNativeTypescript;
sourceTree = "<group>";
};
146834001AC3E56700842450 /* Products */ = {
isa = PBXGroup;
children = (
146834041AC3E56700842450 /* libReact.a */,
3DAD3EA51DF850E9000B6D8A /* libyoga.a */,
3DAD3EA71DF850E9000B6D8A /* libyoga.a */,
3DAD3EA91DF850E9000B6D8A /* libcxxreact.a */,
3DAD3EAB1DF850E9000B6D8A /* libcxxreact.a */,
3DAD3EAD1DF850E9000B6D8A /* libjschelpers.a */,
3DAD3EAF1DF850E9000B6D8A /* libjschelpers.a */,
3DAD3EA31DF850E9000B6D8A /* libReact-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
2D16E6871FA4F8E400B85C8A /* Frameworks */ = {
isa = PBXGroup;
children = (
2D16E6891FA4F8E400B85C8A /* libReact.a */,
);
name = Frameworks;
sourceTree = "<group>";
};
5E91572E1DD0AC6500FF2AA8 /* Products */ = {
isa = PBXGroup;
children = (
5E9157331DD0AC6500FF2AA8 /* libRCTAnimation.a */,
5E9157351DD0AC6500FF2AA8 /* libRCTAnimation.a */,
);
name = Products;
sourceTree = "<group>";
};
78C398B11ACF4ADC00677621 /* Products */ = {
isa = PBXGroup;
children = (
78C398B91ACF4ADC00677621 /* libRCTLinking.a */,
3DAD3E881DF850E9000B6D8A /* libRCTLinking-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
832341AE1AAA6A7D00B99B32 /* Libraries */ = {
isa = PBXGroup;
children = (
5E91572D1DD0AC6500FF2AA8 /* RCTAnimation.xcodeproj */,
146833FF1AC3E56700842450 /* React.xcodeproj */,
00C302A71ABCB8CE00DB3ED1 /* RCTActionSheet.xcodeproj */,
ADBDB91F1DFEBF0600ED6528 /* RCTBlob.xcodeproj */,
00C302B51ABCB90400DB3ED1 /* RCTGeolocation.xcodeproj */,
00C302BB1ABCB91800DB3ED1 /* RCTImage.xcodeproj */,
78C398B01ACF4ADC00677621 /* RCTLinking.xcodeproj */,
00C302D31ABCB9D200DB3ED1 /* RCTNetwork.xcodeproj */,
139105B61AF99BAD00B5F7CC /* RCTSettings.xcodeproj */,
832341B01AAA6A8300B99B32 /* RCTText.xcodeproj */,
00C302DF1ABCB9EE00DB3ED1 /* RCTVibration.xcodeproj */,
139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */,
);
name = Libraries;
sourceTree = "<group>";
};
832341B11AAA6A8300B99B32 /* Products */ = {
isa = PBXGroup;
children = (
832341B51AAA6A8300B99B32 /* libRCTText.a */,
3DAD3E941DF850E9000B6D8A /* libRCTText-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
83CBB9F61A601CBA00E9B192 = {
isa = PBXGroup;
children = (
13B07FAE1A68108700A75B9A /* ReactNativeTypescript */,
832341AE1AAA6A7D00B99B32 /* Libraries */,
00E356EF1AD99517003FC87E /* ReactNativeTypescriptTests */,
83CBBA001A601CBA00E9B192 /* Products */,
2D16E6871FA4F8E400B85C8A /* Frameworks */,
);
indentWidth = 2;
sourceTree = "<group>";
tabWidth = 2;
usesTabs = 0;
};
83CBBA001A601CBA00E9B192 /* Products */ = {
isa = PBXGroup;
children = (
13B07F961A680F5B00A75B9A /* ReactNativeTypescript.app */,
00E356EE1AD99517003FC87E /* ReactNativeTypescriptTests.xctest */,
2D02E47B1E0B4A5D006451C7 /* ReactNativeTypescript-tvOS.app */,
2D02E4901E0B4A5D006451C7 /* ReactNativeTypescript-tvOSTests.xctest */,
);
name = Products;
sourceTree = "<group>";
};
ADBDB9201DFEBF0600ED6528 /* Products */ = {
isa = PBXGroup;
children = (
ADBDB9271DFEBF0700ED6528 /* libRCTBlob.a */,
2D16E6721FA4F8DC00B85C8A /* libRCTBlob-tvOS.a */,
);
name = Products;
sourceTree = "<group>";
};
/* End PBXGroup section */
/* Begin PBXNativeTarget section */
00E356ED1AD99517003FC87E /* ReactNativeTypescriptTests */ = {
isa = PBXNativeTarget;
buildConfigurationList = 00E357021AD99517003FC87E /* Build configuration list for PBXNativeTarget "ReactNativeTypescriptTests" */;
buildPhases = (
00E356EA1AD99517003FC87E /* Sources */,
00E356EB1AD99517003FC87E /* Frameworks */,
00E356EC1AD99517003FC87E /* Resources */,
);
buildRules = (
);
dependencies = (
00E356F51AD99517003FC87E /* PBXTargetDependency */,
);
name = ReactNativeTypescriptTests;
productName = ReactNativeTypescriptTests;
productReference = 00E356EE1AD99517003FC87E /* ReactNativeTypescriptTests.xctest */;
productType = "com.apple.product-type.bundle.unit-test";
};
13B07F861A680F5B00A75B9A /* ReactNativeTypescript */ = {
isa = PBXNativeTarget;
buildConfigurationList = 13B07F931A680F5B00A75B9A /* Build configuration list for PBXNativeTarget "ReactNativeTypescript" */;
buildPhases = (
13B07F871A680F5B00A75B9A /* Sources */,
13B07F8C1A680F5B00A75B9A /* Frameworks */,
13B07F8E1A680F5B00A75B9A /* Resources */,
00DD1BFF1BD5951E006B06BC /* Bundle React Native code and images */,
);
buildRules = (
);
dependencies = (
);
name = ReactNativeTypescript;
productName = "Hello World";
productReference = 13B07F961A680F5B00A75B9A /* ReactNativeTypescript.app */;
productType = "com.apple.product-type.application";
};
2D02E47A1E0B4A5D006451C7 /* ReactNativeTypescript-tvOS */ = {
isa = PBXNativeTarget;
buildConfigurationList = 2D02E4BA1E0B4A5E006451C7 /* Build configuration list for PBXNativeTarget "ReactNativeTypescript-tvOS" */;
buildPhases = (
2D02E4771E0B4A5D006451C7 /* Sources */,
2D02E4781E0B4A5D006451C7 /* Frameworks */,
2D02E4791E0B4A5D006451C7 /* Resources */,
2D02E4CB1E0B4B27006451C7 /* Bundle React Native Code And Images */,
);
buildRules = (
);
dependencies = (
);
name = "ReactNativeTypescript-tvOS";
productName = "ReactNativeTypescript-tvOS";
productReference = 2D02E47B1E0B4A5D006451C7 /* ReactNativeTypescript-tvOS.app */;
productType = "com.apple.product-type.application";
};
2D02E48F1E0B4A5D006451C7 /* ReactNativeTypescript-tvOSTests */ = {
isa = PBXNativeTarget;
buildConfigurationList = 2D02E4BB1E0B4A5E006451C7 /* Build configuration list for PBXNativeTarget "ReactNativeTypescript-tvOSTests" */;
buildPhases = (
2D02E48C1E0B4A5D006451C7 /* Sources */,
2D02E48D1E0B4A5D006451C7 /* Frameworks */,
2D02E48E1E0B4A5D006451C7 /* Resources */,
);
buildRules = (
);
dependencies = (
2D02E4921E0B4A5D006451C7 /* PBXTargetDependency */,
);
name = "ReactNativeTypescript-tvOSTests";
productName = "ReactNativeTypescript-tvOSTests";
productReference = 2D02E4901E0B4A5D006451C7 /* ReactNativeTypescript-tvOSTests.xctest */;
productType = "com.apple.product-type.bundle.unit-test";
};
/* End PBXNativeTarget section */
/* Begin PBXProject section */
83CBB9F71A601CBA00E9B192 /* Project object */ = {
isa = PBXProject;
attributes = {
LastUpgradeCheck = 0610;
ORGANIZATIONNAME = Facebook;
TargetAttributes = {
00E356ED1AD99517003FC87E = {
CreatedOnToolsVersion = 6.2;
TestTargetID = 13B07F861A680F5B00A75B9A;
};
2D02E47A1E0B4A5D006451C7 = {
CreatedOnToolsVersion = 8.2.1;
ProvisioningStyle = Automatic;
};
2D02E48F1E0B4A5D006451C7 = {
CreatedOnToolsVersion = 8.2.1;
ProvisioningStyle = Automatic;
TestTargetID = 2D02E47A1E0B4A5D006451C7;
};
};
};
buildConfigurationList = 83CBB9FA1A601CBA00E9B192 /* Build configuration list for PBXProject "ReactNativeTypescript" */;
compatibilityVersion = "Xcode 3.2";
developmentRegion = English;
hasScannedForEncodings = 0;
knownRegions = (
en,
Base,
);
mainGroup = 83CBB9F61A601CBA00E9B192;
productRefGroup = 83CBBA001A601CBA00E9B192 /* Products */;
projectDirPath = "";
projectReferences = (
{
ProductGroup = 00C302A81ABCB8CE00DB3ED1 /* Products */;
ProjectRef = 00C302A71ABCB8CE00DB3ED1 /* RCTActionSheet.xcodeproj */;
},
{
ProductGroup = 5E91572E1DD0AC6500FF2AA8 /* Products */;
ProjectRef = 5E91572D1DD0AC6500FF2AA8 /* RCTAnimation.xcodeproj */;
},
{
ProductGroup = ADBDB9201DFEBF0600ED6528 /* Products */;
ProjectRef = ADBDB91F1DFEBF0600ED6528 /* RCTBlob.xcodeproj */;
},
{
ProductGroup = 00C302B61ABCB90400DB3ED1 /* Products */;
ProjectRef = 00C302B51ABCB90400DB3ED1 /* RCTGeolocation.xcodeproj */;
},
{
ProductGroup = 00C302BC1ABCB91800DB3ED1 /* Products */;
ProjectRef = 00C302BB1ABCB91800DB3ED1 /* RCTImage.xcodeproj */;
},
{
ProductGroup = 78C398B11ACF4ADC00677621 /* Products */;
ProjectRef = 78C398B01ACF4ADC00677621 /* RCTLinking.xcodeproj */;
},
{
ProductGroup = 00C302D41ABCB9D200DB3ED1 /* Products */;
ProjectRef = 00C302D31ABCB9D200DB3ED1 /* RCTNetwork.xcodeproj */;
},
{
ProductGroup = 139105B71AF99BAD00B5F7CC /* Products */;
ProjectRef = 139105B61AF99BAD00B5F7CC /* RCTSettings.xcodeproj */;
},
{
ProductGroup = 832341B11AAA6A8300B99B32 /* Products */;
ProjectRef = 832341B01AAA6A8300B99B32 /* RCTText.xcodeproj */;
},
{
ProductGroup = 00C302E01ABCB9EE00DB3ED1 /* Products */;
ProjectRef = 00C302DF1ABCB9EE00DB3ED1 /* RCTVibration.xcodeproj */;
},
{
ProductGroup = 139FDEE71B06529A00C62182 /* Products */;
ProjectRef = 139FDEE61B06529A00C62182 /* RCTWebSocket.xcodeproj */;
},
{
ProductGroup = 146834001AC3E56700842450 /* Products */;
ProjectRef = 146833FF1AC3E56700842450 /* React.xcodeproj */;
},
);
projectRoot = "";
targets = (
13B07F861A680F5B00A75B9A /* ReactNativeTypescript */,
00E356ED1AD99517003FC87E /* ReactNativeTypescriptTests */,
2D02E47A1E0B4A5D006451C7 /* ReactNativeTypescript-tvOS */,
2D02E48F1E0B4A5D006451C7 /* ReactNativeTypescript-tvOSTests */,
);
};
/* End PBXProject section */
/* Begin PBXReferenceProxy section */
00C302AC1ABCB8CE00DB3ED1 /* libRCTActionSheet.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTActionSheet.a;
remoteRef = 00C302AB1ABCB8CE00DB3ED1 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
00C302BA1ABCB90400DB3ED1 /* libRCTGeolocation.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTGeolocation.a;
remoteRef = 00C302B91ABCB90400DB3ED1 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
00C302C01ABCB91800DB3ED1 /* libRCTImage.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTImage.a;
remoteRef = 00C302BF1ABCB91800DB3ED1 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
00C302DC1ABCB9D200DB3ED1 /* libRCTNetwork.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTNetwork.a;
remoteRef = 00C302DB1ABCB9D200DB3ED1 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
00C302E41ABCB9EE00DB3ED1 /* libRCTVibration.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTVibration.a;
remoteRef = 00C302E31ABCB9EE00DB3ED1 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
139105C11AF99BAD00B5F7CC /* libRCTSettings.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTSettings.a;
remoteRef = 139105C01AF99BAD00B5F7CC /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
139FDEF41B06529B00C62182 /* libRCTWebSocket.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTWebSocket.a;
remoteRef = 139FDEF31B06529B00C62182 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
146834041AC3E56700842450 /* libReact.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libReact.a;
remoteRef = 146834031AC3E56700842450 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
2D16E6721FA4F8DC00B85C8A /* libRCTBlob-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTBlob-tvOS.a";
remoteRef = 2D16E6711FA4F8DC00B85C8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
2D16E6841FA4F8DC00B85C8A /* libfishhook.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libfishhook.a;
remoteRef = 2D16E6831FA4F8DC00B85C8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
2D16E6861FA4F8DC00B85C8A /* libfishhook-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libfishhook-tvOS.a";
remoteRef = 2D16E6851FA4F8DC00B85C8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3E841DF850E9000B6D8A /* libRCTImage-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTImage-tvOS.a";
remoteRef = 3DAD3E831DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3E881DF850E9000B6D8A /* libRCTLinking-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTLinking-tvOS.a";
remoteRef = 3DAD3E871DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3E8C1DF850E9000B6D8A /* libRCTNetwork-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTNetwork-tvOS.a";
remoteRef = 3DAD3E8B1DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3E901DF850E9000B6D8A /* libRCTSettings-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTSettings-tvOS.a";
remoteRef = 3DAD3E8F1DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3E941DF850E9000B6D8A /* libRCTText-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTText-tvOS.a";
remoteRef = 3DAD3E931DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3E991DF850E9000B6D8A /* libRCTWebSocket-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libRCTWebSocket-tvOS.a";
remoteRef = 3DAD3E981DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EA31DF850E9000B6D8A /* libReact-tvOS.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = "libReact-tvOS.a";
remoteRef = 3DAD3EA21DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EA51DF850E9000B6D8A /* libyoga.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libyoga.a;
remoteRef = 3DAD3EA41DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EA71DF850E9000B6D8A /* libyoga.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libyoga.a;
remoteRef = 3DAD3EA61DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EA91DF850E9000B6D8A /* libcxxreact.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libcxxreact.a;
remoteRef = 3DAD3EA81DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EAB1DF850E9000B6D8A /* libcxxreact.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libcxxreact.a;
remoteRef = 3DAD3EAA1DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EAD1DF850E9000B6D8A /* libjschelpers.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libjschelpers.a;
remoteRef = 3DAD3EAC1DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
3DAD3EAF1DF850E9000B6D8A /* libjschelpers.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libjschelpers.a;
remoteRef = 3DAD3EAE1DF850E9000B6D8A /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
5E9157331DD0AC6500FF2AA8 /* libRCTAnimation.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTAnimation.a;
remoteRef = 5E9157321DD0AC6500FF2AA8 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
5E9157351DD0AC6500FF2AA8 /* libRCTAnimation.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTAnimation.a;
remoteRef = 5E9157341DD0AC6500FF2AA8 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
78C398B91ACF4ADC00677621 /* libRCTLinking.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTLinking.a;
remoteRef = 78C398B81ACF4ADC00677621 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
832341B51AAA6A8300B99B32 /* libRCTText.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTText.a;
remoteRef = 832341B41AAA6A8300B99B32 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
ADBDB9271DFEBF0700ED6528 /* libRCTBlob.a */ = {
isa = PBXReferenceProxy;
fileType = archive.ar;
path = libRCTBlob.a;
remoteRef = ADBDB9261DFEBF0700ED6528 /* PBXContainerItemProxy */;
sourceTree = BUILT_PRODUCTS_DIR;
};
/* End PBXReferenceProxy section */
/* Begin PBXResourcesBuildPhase section */
00E356EC1AD99517003FC87E /* Resources */ = {
isa = PBXResourcesBuildPhase;
buildActionMask = 2147483647;
files = (
);
runOnlyForDeploymentPostprocessing = 0;
};
13B07F8E1A680F5B00A75B9A /* Resources */ = {
isa = PBXResourcesBuildPhase;
buildActionMask = 2147483647;
files = (
13B07FBF1A68108700A75B9A /* Images.xcassets in Resources */,
13B07FBD1A68108700A75B9A /* LaunchScreen.xib in Resources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
2D02E4791E0B4A5D006451C7 /* Resources */ = {
isa = PBXResourcesBuildPhase;
buildActionMask = 2147483647;
files = (
2D02E4BD1E0B4A84006451C7 /* Images.xcassets in Resources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
2D02E48E1E0B4A5D006451C7 /* Resources */ = {
isa = PBXResourcesBuildPhase;
buildActionMask = 2147483647;
files = (
);
runOnlyForDeploymentPostprocessing = 0;
};
/* End PBXResourcesBuildPhase section */
/* Begin PBXShellScriptBuildPhase section */
00DD1BFF1BD5951E006B06BC /* Bundle React Native code and images */ = {
isa = PBXShellScriptBuildPhase;
buildActionMask = 2147483647;
files = (
);
inputPaths = (
);
name = "Bundle React Native code and images";
outputPaths = (
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
shellScript = "export NODE_BINARY=node\n../node_modules/react-native/scripts/react-native-xcode.sh";
};
2D02E4CB1E0B4B27006451C7 /* Bundle React Native Code And Images */ = {
isa = PBXShellScriptBuildPhase;
buildActionMask = 2147483647;
files = (
);
inputPaths = (
);
name = "Bundle React Native Code And Images";
outputPaths = (
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
shellScript = "export NODE_BINARY=node\n../node_modules/react-native/scripts/react-native-xcode.sh";
};
/* End PBXShellScriptBuildPhase section */
/* Begin PBXSourcesBuildPhase section */
00E356EA1AD99517003FC87E /* Sources */ = {
isa = PBXSourcesBuildPhase;
buildActionMask = 2147483647;
files = (
00E356F31AD99517003FC87E /* ReactNativeTypescriptTests.m in Sources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
13B07F871A680F5B00A75B9A /* Sources */ = {
isa = PBXSourcesBuildPhase;
buildActionMask = 2147483647;
files = (
13B07FBC1A68108700A75B9A /* AppDelegate.m in Sources */,
13B07FC11A68108700A75B9A /* main.m in Sources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
2D02E4771E0B4A5D006451C7 /* Sources */ = {
isa = PBXSourcesBuildPhase;
buildActionMask = 2147483647;
files = (
2D02E4BF1E0B4AB3006451C7 /* main.m in Sources */,
2D02E4BC1E0B4A80006451C7 /* AppDelegate.m in Sources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
2D02E48C1E0B4A5D006451C7 /* Sources */ = {
isa = PBXSourcesBuildPhase;
buildActionMask = 2147483647;
files = (
2DCD954D1E0B4F2C00145EB5 /* ReactNativeTypescriptTests.m in Sources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
/* End PBXSourcesBuildPhase section */
/* Begin PBXTargetDependency section */
00E356F51AD99517003FC87E /* PBXTargetDependency */ = {
isa = PBXTargetDependency;
target = 13B07F861A680F5B00A75B9A /* ReactNativeTypescript */;
targetProxy = 00E356F41AD99517003FC87E /* PBXContainerItemProxy */;
};
2D02E4921E0B4A5D006451C7 /* PBXTargetDependency */ = {
isa = PBXTargetDependency;
target = 2D02E47A1E0B4A5D006451C7 /* ReactNativeTypescript-tvOS */;
targetProxy = 2D02E4911E0B4A5D006451C7 /* PBXContainerItemProxy */;
};
/* End PBXTargetDependency section */
/* Begin PBXVariantGroup section */
13B07FB11A68108700A75B9A /* LaunchScreen.xib */ = {
isa = PBXVariantGroup;
children = (
13B07FB21A68108700A75B9A /* Base */,
);
name = LaunchScreen.xib;
path = ReactNativeTypescript;
sourceTree = "<group>";
};
/* End PBXVariantGroup section */
/* Begin XCBuildConfiguration section */
00E356F61AD99517003FC87E /* Debug */ = {
isa = XCBuildConfiguration;
buildSettings = {
BUNDLE_LOADER = "$(TEST_HOST)";
GCC_PREPROCESSOR_DEFINITIONS = (
"DEBUG=1",
"$(inherited)",
);
INFOPLIST_FILE = ReactNativeTypescriptTests/Info.plist;
IPHONEOS_DEPLOYMENT_TARGET = 8.0;
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
OTHER_LDFLAGS = (
"-ObjC",
"-lc++",
);
PRODUCT_NAME = "$(TARGET_NAME)";
TEST_HOST = "$(BUILT_PRODUCTS_DIR)/ReactNativeTypescript.app/ReactNativeTypescript";
};
name = Debug;
};
00E356F71AD99517003FC87E /* Release */ = {
isa = XCBuildConfiguration;
buildSettings = {
BUNDLE_LOADER = "$(TEST_HOST)";
COPY_PHASE_STRIP = NO;
INFOPLIST_FILE = ReactNativeTypescriptTests/Info.plist;
IPHONEOS_DEPLOYMENT_TARGET = 8.0;
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
OTHER_LDFLAGS = (
"-ObjC",
"-lc++",
);
PRODUCT_NAME = "$(TARGET_NAME)";
TEST_HOST = "$(BUILT_PRODUCTS_DIR)/ReactNativeTypescript.app/ReactNativeTypescript";
};
name = Release;
};
13B07F941A680F5B00A75B9A /* Debug */ = {
isa = XCBuildConfiguration;
buildSettings = {
ASSETCATALOG_COMPILER_APPICON_NAME = AppIcon;
CURRENT_PROJECT_VERSION = 1;
DEAD_CODE_STRIPPING = NO;
INFOPLIST_FILE = ReactNativeTypescript/Info.plist;
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks";
OTHER_LDFLAGS = (
"$(inherited)",
"-ObjC",
"-lc++",
);
PRODUCT_NAME = ReactNativeTypescript;
VERSIONING_SYSTEM = "apple-generic";
};
name = Debug;
};
13B07F951A680F5B00A75B9A /* Release */ = {
isa = XCBuildConfiguration;
buildSettings = {
ASSETCATALOG_COMPILER_APPICON_NAME = AppIcon;
CURRENT_PROJECT_VERSION = 1;
INFOPLIST_FILE = ReactNativeTypescript/Info.plist;
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks";
OTHER_LDFLAGS = (
"$(inherited)",
"-ObjC",
"-lc++",
);
PRODUCT_NAME = ReactNativeTypescript;
VERSIONING_SYSTEM = "apple-generic";
};
name = Release;
};
2D02E4971E0B4A5E006451C7 /* Debug */ = {
isa = XCBuildConfiguration;
buildSettings = {
ASSETCATALOG_COMPILER_APPICON_NAME = "App Icon & Top Shelf Image";
ASSETCATALOG_COMPILER_LAUNCHIMAGE_NAME = LaunchImage;
CLANG_ANALYZER_NONNULL = YES;
CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
CLANG_WARN_INFINITE_RECURSION = YES;
CLANG_WARN_SUSPICIOUS_MOVE = YES;
DEBUG_INFORMATION_FORMAT = dwarf;
ENABLE_TESTABILITY = YES;
GCC_NO_COMMON_BLOCKS = YES;
INFOPLIST_FILE = "ReactNativeTypescript-tvOS/Info.plist";
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks";
OTHER_LDFLAGS = (
"-ObjC",
"-lc++",
);
PRODUCT_BUNDLE_IDENTIFIER = "com.facebook.REACT.ReactNativeTypescript-tvOS";
PRODUCT_NAME = "$(TARGET_NAME)";
SDKROOT = appletvos;
TARGETED_DEVICE_FAMILY = 3;
TVOS_DEPLOYMENT_TARGET = 9.2;
};
name = Debug;
};
2D02E4981E0B4A5E006451C7 /* Release */ = {
isa = XCBuildConfiguration;
buildSettings = {
ASSETCATALOG_COMPILER_APPICON_NAME = "App Icon & Top Shelf Image";
ASSETCATALOG_COMPILER_LAUNCHIMAGE_NAME = LaunchImage;
CLANG_ANALYZER_NONNULL = YES;
CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
CLANG_WARN_INFINITE_RECURSION = YES;
CLANG_WARN_SUSPICIOUS_MOVE = YES;
COPY_PHASE_STRIP = NO;
DEBUG_INFORMATION_FORMAT = "dwarf-with-dsym";
GCC_NO_COMMON_BLOCKS = YES;
INFOPLIST_FILE = "ReactNativeTypescript-tvOS/Info.plist";
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks";
OTHER_LDFLAGS = (
"-ObjC",
"-lc++",
);
PRODUCT_BUNDLE_IDENTIFIER = "com.facebook.REACT.ReactNativeTypescript-tvOS";
PRODUCT_NAME = "$(TARGET_NAME)";
SDKROOT = appletvos;
TARGETED_DEVICE_FAMILY = 3;
TVOS_DEPLOYMENT_TARGET = 9.2;
};
name = Release;
};
2D02E4991E0B4A5E006451C7 /* Debug */ = {
isa = XCBuildConfiguration;
buildSettings = {
BUNDLE_LOADER = "$(TEST_HOST)";
CLANG_ANALYZER_NONNULL = YES;
CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
CLANG_WARN_INFINITE_RECURSION = YES;
CLANG_WARN_SUSPICIOUS_MOVE = YES;
DEBUG_INFORMATION_FORMAT = dwarf;
ENABLE_TESTABILITY = YES;
GCC_NO_COMMON_BLOCKS = YES;
INFOPLIST_FILE = "ReactNativeTypescript-tvOSTests/Info.plist";
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
PRODUCT_BUNDLE_IDENTIFIER = "com.facebook.REACT.ReactNativeTypescript-tvOSTests";
PRODUCT_NAME = "$(TARGET_NAME)";
SDKROOT = appletvos;
TEST_HOST = "$(BUILT_PRODUCTS_DIR)/ReactNativeTypescript-tvOS.app/ReactNativeTypescript-tvOS";
TVOS_DEPLOYMENT_TARGET = 10.1;
};
name = Debug;
};
2D02E49A1E0B4A5E006451C7 /* Release */ = {
isa = XCBuildConfiguration;
buildSettings = {
BUNDLE_LOADER = "$(TEST_HOST)";
CLANG_ANALYZER_NONNULL = YES;
CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
CLANG_WARN_INFINITE_RECURSION = YES;
CLANG_WARN_SUSPICIOUS_MOVE = YES;
COPY_PHASE_STRIP = NO;
DEBUG_INFORMATION_FORMAT = "dwarf-with-dsym";
GCC_NO_COMMON_BLOCKS = YES;
INFOPLIST_FILE = "ReactNativeTypescript-tvOSTests/Info.plist";
LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
PRODUCT_BUNDLE_IDENTIFIER = "com.facebook.REACT.ReactNativeTypescript-tvOSTests";
PRODUCT_NAME = "$(TARGET_NAME)";
SDKROOT = appletvos;
TEST_HOST = "$(BUILT_PRODUCTS_DIR)/ReactNativeTypescript-tvOS.app/ReactNativeTypescript-tvOS";
TVOS_DEPLOYMENT_TARGET = 10.1;
};
name = Release;
};
83CBBA201A601CBA00E9B192 /* Debug */ = {
isa = XCBuildConfiguration;
buildSettings = {
ALWAYS_SEARCH_USER_PATHS = NO;
CLANG_CXX_LANGUAGE_STANDARD = "gnu++0x";
CLANG_CXX_LIBRARY = "libc++";
CLANG_ENABLE_MODULES = YES;
CLANG_ENABLE_OBJC_ARC = YES;
CLANG_WARN_BOOL_CONVERSION = YES;
CLANG_WARN_CONSTANT_CONVERSION = YES;
CLANG_WARN_DIRECT_OBJC_ISA_USAGE = YES_ERROR;
CLANG_WARN_EMPTY_BODY = YES;
CLANG_WARN_ENUM_CONVERSION = YES;
CLANG_WARN_INT_CONVERSION = YES;
CLANG_WARN_OBJC_ROOT_CLASS = YES_ERROR;
CLANG_WARN_UNREACHABLE_CODE = YES;
CLANG_WARN__DUPLICATE_METHOD_MATCH = YES;
"CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "iPhone Developer";
COPY_PHASE_STRIP = NO;
ENABLE_STRICT_OBJC_MSGSEND = YES;
GCC_C_LANGUAGE_STANDARD = gnu99;
GCC_DYNAMIC_NO_PIC = NO;
GCC_OPTIMIZATION_LEVEL = 0;
GCC_PREPROCESSOR_DEFINITIONS = (
"DEBUG=1",
"$(inherited)",
);
GCC_SYMBOLS_PRIVATE_EXTERN = NO;
GCC_WARN_64_TO_32_BIT_CONVERSION = YES;
GCC_WARN_ABOUT_RETURN_TYPE = YES_ERROR;
GCC_WARN_UNDECLARED_SELECTOR = YES;
GCC_WARN_UNINITIALIZED_AUTOS = YES_AGGRESSIVE;
GCC_WARN_UNUSED_FUNCTION = YES;
GCC_WARN_UNUSED_VARIABLE = YES;
IPHONEOS_DEPLOYMENT_TARGET = 8.0;
MTL_ENABLE_DEBUG_INFO = YES;
ONLY_ACTIVE_ARCH = YES;
SDKROOT = iphoneos;
};
name = Debug;
};
83CBBA211A601CBA00E9B192 /* Release */ = {
isa = XCBuildConfiguration;
buildSettings = {
ALWAYS_SEARCH_USER_PATHS = NO;
CLANG_CXX_LANGUAGE_STANDARD = "gnu++0x";
CLANG_CXX_LIBRARY = "libc++";
CLANG_ENABLE_MODULES = YES;
CLANG_ENABLE_OBJC_ARC = YES;
CLANG_WARN_BOOL_CONVERSION = YES;
CLANG_WARN_CONSTANT_CONVERSION = YES;
CLANG_WARN_DIRECT_OBJC_ISA_USAGE = YES_ERROR;
CLANG_WARN_EMPTY_BODY = YES;
CLANG_WARN_ENUM_CONVERSION = YES;
CLANG_WARN_INT_CONVERSION = YES;
CLANG_WARN_OBJC_ROOT_CLASS = YES_ERROR;
CLANG_WARN_UNREACHABLE_CODE = YES;
CLANG_WARN__DUPLICATE_METHOD_MATCH = YES;
"CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "iPhone Developer";
COPY_PHASE_STRIP = YES;
ENABLE_NS_ASSERTIONS = NO;
ENABLE_STRICT_OBJC_MSGSEND = YES;
GCC_C_LANGUAGE_STANDARD = gnu99;
GCC_WARN_64_TO_32_BIT_CONVERSION = YES;
GCC_WARN_ABOUT_RETURN_TYPE = YES_ERROR;
GCC_WARN_UNDECLARED_SELECTOR = YES;
GCC_WARN_UNINITIALIZED_AUTOS = YES_AGGRESSIVE;
GCC_WARN_UNUSED_FUNCTION = YES;
GCC_WARN_UNUSED_VARIABLE = YES;
IPHONEOS_DEPLOYMENT_TARGET = 8.0;
MTL_ENABLE_DEBUG_INFO = NO;
SDKROOT = iphoneos;
VALIDATE_PRODUCT = YES;
};
name = Release;
};
/* End XCBuildConfiguration section */
/* Begin XCConfigurationList section */
00E357021AD99517003FC87E /* Build configuration list for PBXNativeTarget "ReactNativeTypescriptTests" */ = {
isa = XCConfigurationList;
buildConfigurations = (
00E356F61AD99517003FC87E /* Debug */,
00E356F71AD99517003FC87E /* Release */,
);
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
13B07F931A680F5B00A75B9A /* Build configuration list for PBXNativeTarget "ReactNativeTypescript" */ = {
isa = XCConfigurationList;
buildConfigurations = (
13B07F941A680F5B00A75B9A /* Debug */,
13B07F951A680F5B00A75B9A /* Release */,
);
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
2D02E4BA1E0B4A5E006451C7 /* Build configuration list for PBXNativeTarget "ReactNativeTypescript-tvOS" */ = {
isa = XCConfigurationList;
buildConfigurations = (
2D02E4971E0B4A5E006451C7 /* Debug */,
2D02E4981E0B4A5E006451C7 /* Release */,
);
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
2D02E4BB1E0B4A5E006451C7 /* Build configuration list for PBXNativeTarget "ReactNativeTypescript-tvOSTests" */ = {
isa = XCConfigurationList;
buildConfigurations = (
2D02E4991E0B4A5E006451C7 /* Debug */,
2D02E49A1E0B4A5E006451C7 /* Release */,
);
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
83CBB9FA1A601CBA00E9B192 /* Build configuration list for PBXProject "ReactNativeTypescript" */ = {
isa = XCConfigurationList;
buildConfigurations = (
83CBBA201A601CBA00E9B192 /* Debug */,
83CBBA211A601CBA00E9B192 /* Release */,
);
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
/* End XCConfigurationList section */
};
rootObject = 83CBB9F71A601CBA00E9B192 /* Project object */;
}
| examples/react-native-typescript/ios/ReactNativeTypescript.xcodeproj/project.pbxproj | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.003089227946475148,
0.00019783196330536157,
0.00016454623255413026,
0.00017069430032279342,
0.00025402387836948037
] |
{
"id": 1,
"code_window": [
" )\n",
"~~~\n",
" `;\n",
"\n",
"storiesOf('Addons|Notes', module)\n",
" .addDecorator(withNotes)\n",
" .add('withNotes', baseStory, {\n",
" notes:\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"const markdownTable = `\n",
"| Column1 | Column2 | Column3 |\n",
"|---------|---------|---------|\n",
"| Row1.1 | Row1.2 | Row1.3 |\n",
"| Row2.1 | Row2.2 | Row2.3 |\n",
"| Row3.1 | Row3.2 | Row3.3 |\n",
"| Row4.1 | Row4.2 | Row4.3 |\n",
"`;\n",
"\n"
],
"file_path": "examples/official-storybook/stories/addon-notes.stories.js",
"type": "add",
"edit_start_line_idx": 28
} | import { uselessMixin } from './useless-mixin';
export const separatedButton = superClass =>
class SeparatedButton extends uselessMixin(superClass) {
static get is() {
return 'separated-button';
}
static get properties() {
return {
title: {
type: String,
value: 'Click me:',
},
counter: {
type: Number,
value: 0,
},
};
}
async handleTap() {
this.counter += await Promise.resolve(1);
this.someMethod();
}
};
| examples/polymer-cli/src/separated-button/separated-button.js | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.0001770313538145274,
0.0001744124456308782,
0.00017142633441835642,
0.00017477966321166605,
0.0000023029249405226437
] |
{
"id": 1,
"code_window": [
" )\n",
"~~~\n",
" `;\n",
"\n",
"storiesOf('Addons|Notes', module)\n",
" .addDecorator(withNotes)\n",
" .add('withNotes', baseStory, {\n",
" notes:\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"const markdownTable = `\n",
"| Column1 | Column2 | Column3 |\n",
"|---------|---------|---------|\n",
"| Row1.1 | Row1.2 | Row1.3 |\n",
"| Row2.1 | Row2.2 | Row2.3 |\n",
"| Row3.1 | Row3.2 | Row3.3 |\n",
"| Row4.1 | Row4.2 | Row4.3 |\n",
"`;\n",
"\n"
],
"file_path": "examples/official-storybook/stories/addon-notes.stories.js",
"type": "add",
"edit_start_line_idx": 28
} | import { defineTest } from 'jscodeshift/dist/testUtils';
defineTest(
__dirname,
'update-organisation-name',
null,
'update-organisation-name/update-organisation-name'
);
| lib/codemod/src/transforms/__tests__/update-organisation-name.test.js | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.00017652567476034164,
0.00017652567476034164,
0.00017652567476034164,
0.00017652567476034164,
0
] |
{
"id": 2,
"code_window": [
" })\n",
" .add('withNotes rendering inline, github-flavored markdown', baseStory, {\n",
" notes: { markdown: markdownString },\n",
" })\n",
" .add('using decorator arguments, withNotes', withNotes('Notes into withNotes')(baseStory))\n",
" .add(\n",
" 'using decorator arguments, withMarkdownNotes',\n",
" withMarkdownNotes(markdownString)(baseStory)\n",
" );\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace"
],
"after_edit": [
" .add('using decorator arguments, withMarkdownNotes', withMarkdownNotes(markdownString)(baseStory))\n",
" .add('with a markdown table', baseStory, {\n",
" notes: { markdown: markdownTable },\n",
" });"
],
"file_path": "examples/official-storybook/stories/addon-notes.stories.js",
"type": "replace",
"edit_start_line_idx": 41
} | import React from 'react';
import PropTypes from 'prop-types';
import addons from '@storybook/addons';
import styled from 'react-emotion';
const Panel = styled('div')({
padding: 10,
boxSizing: 'border-box',
width: '100%',
});
export class Notes extends React.Component {
constructor(...args) {
super(...args);
this.state = { text: '' };
this.onAddNotes = this.onAddNotes.bind(this);
}
componentDidMount() {
const { channel, api } = this.props;
// Listen to the notes and render it.
channel.on('storybook/notes/add_notes', this.onAddNotes);
// Clear the current notes on every story change.
this.stopListeningOnStory = api.onStory(() => {
this.onAddNotes('');
});
}
// This is some cleanup tasks when the Notes panel is unmounting.
componentWillUnmount() {
if (this.stopListeningOnStory) {
this.stopListeningOnStory();
}
this.unmounted = true;
const { channel } = this.props;
channel.removeListener('storybook/notes/add_notes', this.onAddNotes);
}
onAddNotes(text) {
this.setState({ text });
}
render() {
const { text } = this.state;
const textAfterFormatted = text ? text.trim().replace(/\n/g, '<br />') : '';
return (
<Panel
className="addon-notes-container"
dangerouslySetInnerHTML={{ __html: textAfterFormatted }}
/>
);
}
}
Notes.propTypes = {
// eslint-disable-next-line react/forbid-prop-types
channel: PropTypes.object,
// eslint-disable-next-line react/forbid-prop-types
api: PropTypes.object,
};
Notes.defaultProps = {
channel: {},
api: {},
};
// Register the addon with a unique name.
addons.register('storybook/notes', api => {
// Also need to set a unique name to the panel.
addons.addPanel('storybook/notes/panel', {
title: 'Notes',
render: () => <Notes channel={addons.getChannel()} api={api} />,
});
});
| addons/notes/src/register.js | 1 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.0016298377886414528,
0.00044891724246554077,
0.00017516205844003707,
0.0002209982048952952,
0.00046410958748310804
] |
{
"id": 2,
"code_window": [
" })\n",
" .add('withNotes rendering inline, github-flavored markdown', baseStory, {\n",
" notes: { markdown: markdownString },\n",
" })\n",
" .add('using decorator arguments, withNotes', withNotes('Notes into withNotes')(baseStory))\n",
" .add(\n",
" 'using decorator arguments, withMarkdownNotes',\n",
" withMarkdownNotes(markdownString)(baseStory)\n",
" );\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace"
],
"after_edit": [
" .add('using decorator arguments, withMarkdownNotes', withMarkdownNotes(markdownString)(baseStory))\n",
" .add('with a markdown table', baseStory, {\n",
" notes: { markdown: markdownTable },\n",
" });"
],
"file_path": "examples/official-storybook/stories/addon-notes.stories.js",
"type": "replace",
"edit_start_line_idx": 41
} | import React from 'react';
import { storiesOf } from '@storybook/react';
import { linkTo } from '@storybook/addon-links';
import { Welcome } from '@storybook/react/demo';
storiesOf('Welcome', module).add('to Storybook', () => <Welcome showApp={linkTo('Button')} />);
| addons/storyshots/stories/required_with_context/Welcome.stories.js | 0 | https://github.com/storybookjs/storybook/commit/ddc4e71fc285b8aa6bc9fef93683eb732d4f511d | [
0.00017437980568502098,
0.00017437980568502098,
0.00017437980568502098,
0.00017437980568502098,
0
] |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.