hunk
dict | file
stringlengths 0
11.8M
| file_path
stringlengths 2
234
| label
int64 0
1
| commit_url
stringlengths 74
103
| dependency_score
sequencelengths 5
5
|
---|---|---|---|---|---|
{
"id": 2,
"code_window": [
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('test', new OneLineRange(1, 1, 5)),\n",
"\t\t\t\tnew TextSearchMatch('this is a test', new OneLineRange(1, 11, 15))),\n",
"\t\t\taRawMatch('/3', new TextSearchMatch('test', lineOneRange))];\n",
"\t\tconst searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tsinon.stub(CellMatch.prototype, 'addContext');\n",
"\n",
"\t\tconst textSearch = sinon.spy(searchService, 'textSearch');\n",
"\t\tconst mdInputCell = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tconst searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 225
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
use std::collections::HashMap;
use crate::{
constants::{PROTOCOL_VERSION, VSCODE_CLI_VERSION},
options::Quality,
update_service::Platform,
};
use serde::{Deserialize, Serialize};
#[derive(Serialize, Debug)]
#[serde(tag = "method", content = "params", rename_all = "camelCase")]
#[allow(non_camel_case_types)]
pub enum ClientRequestMethod<'a> {
servermsg(RefServerMessageParams<'a>),
serverlog(ServerLog<'a>),
makehttpreq(HttpRequestParams<'a>),
version(VersionResponse),
}
#[derive(Deserialize, Debug)]
pub struct HttpBodyParams {
#[serde(with = "serde_bytes")]
pub segment: Vec<u8>,
pub complete: bool,
pub req_id: u32,
}
#[derive(Serialize, Debug)]
pub struct HttpRequestParams<'a> {
pub url: &'a str,
pub method: &'static str,
pub req_id: u32,
}
#[derive(Deserialize, Debug)]
pub struct HttpHeadersParams {
pub status_code: u16,
pub headers: Vec<(String, String)>,
pub req_id: u32,
}
#[derive(Deserialize, Debug)]
pub struct ForwardParams {
pub port: u16,
}
#[derive(Deserialize, Debug)]
pub struct UnforwardParams {
pub port: u16,
}
#[derive(Serialize)]
pub struct ForwardResult {
pub uri: String,
}
#[derive(Deserialize, Debug)]
pub struct ServeParams {
pub socket_id: u16,
pub commit_id: Option<String>,
pub quality: Quality,
pub extensions: Vec<String>,
/// Optional preferred connection token.
#[serde(default)]
pub connection_token: Option<String>,
#[serde(default)]
pub use_local_download: bool,
/// If true, the client and server should gzip servermsg's sent in either direction.
#[serde(default)]
pub compress: bool,
}
#[derive(Deserialize, Serialize, Debug)]
pub struct EmptyObject {}
#[derive(Serialize, Deserialize, Debug)]
pub struct UpdateParams {
pub do_update: bool,
}
#[derive(Deserialize, Debug)]
pub struct ServerMessageParams {
pub i: u16,
#[serde(with = "serde_bytes")]
pub body: Vec<u8>,
}
#[derive(Serialize, Debug)]
pub struct RefServerMessageParams<'a> {
pub i: u16,
#[serde(with = "serde_bytes")]
pub body: &'a [u8],
}
#[derive(Serialize)]
pub struct UpdateResult {
pub up_to_date: bool,
pub did_update: bool,
}
#[derive(Serialize, Debug)]
pub struct ToClientRequest<'a> {
pub id: Option<u32>,
#[serde(flatten)]
pub params: ClientRequestMethod<'a>,
}
#[derive(Debug, Default, Serialize)]
pub struct ServerLog<'a> {
pub line: &'a str,
pub level: u8,
}
#[derive(Serialize)]
pub struct GetHostnameResponse {
pub value: String,
}
#[derive(Serialize)]
pub struct GetEnvResponse {
pub env: HashMap<String, String>,
pub os_platform: &'static str,
pub os_release: String,
}
#[derive(Deserialize)]
pub struct FsStatRequest {
pub path: String,
}
#[derive(Serialize, Default)]
pub struct FsStatResponse {
pub exists: bool,
pub size: Option<u64>,
#[serde(rename = "type")]
pub kind: Option<&'static str>,
}
#[derive(Deserialize, Debug)]
pub struct CallServerHttpParams {
pub path: String,
pub method: String,
pub headers: HashMap<String, String>,
pub body: Option<Vec<u8>>,
}
#[derive(Serialize)]
pub struct CallServerHttpResult {
pub status: u16,
#[serde(with = "serde_bytes")]
pub body: Vec<u8>,
pub headers: HashMap<String, String>,
}
#[derive(Serialize, Debug)]
pub struct VersionResponse {
pub version: &'static str,
pub protocol_version: u32,
}
impl Default for VersionResponse {
fn default() -> Self {
Self {
version: VSCODE_CLI_VERSION.unwrap_or("dev"),
protocol_version: PROTOCOL_VERSION,
}
}
}
#[derive(Deserialize)]
pub struct SpawnParams {
pub command: String,
pub args: Vec<String>,
#[serde(default)]
pub cwd: Option<String>,
#[serde(default)]
pub env: HashMap<String, String>,
}
#[derive(Deserialize)]
pub struct AcquireCliParams {
pub platform: Platform,
pub quality: Quality,
pub commit_id: Option<String>,
#[serde(flatten)]
pub spawn: SpawnParams,
}
#[derive(Serialize)]
pub struct SpawnResult {
pub message: String,
pub exit_code: i32,
}
pub const METHOD_CHALLENGE_ISSUE: &str = "challenge_issue";
pub const METHOD_CHALLENGE_VERIFY: &str = "challenge_verify";
#[derive(Serialize, Deserialize)]
pub struct ChallengeIssueParams {
pub token: Option<String>,
}
#[derive(Serialize, Deserialize)]
pub struct ChallengeIssueResponse {
pub challenge: String,
}
#[derive(Deserialize, Serialize)]
pub struct ChallengeVerifyParams {
pub response: String,
}
pub mod forward_singleton {
use serde::{Deserialize, Serialize};
pub const METHOD_SET_PORTS: &str = "set_ports";
pub type PortList = Vec<u16>;
#[derive(Serialize, Deserialize)]
pub struct SetPortsParams {
pub ports: PortList,
}
#[derive(Serialize, Deserialize)]
pub struct SetPortsResponse {
pub port_format: Option<String>,
}
}
pub mod singleton {
use crate::log;
use serde::{Deserialize, Serialize};
pub const METHOD_RESTART: &str = "restart";
pub const METHOD_SHUTDOWN: &str = "shutdown";
pub const METHOD_STATUS: &str = "status";
pub const METHOD_LOG: &str = "log";
pub const METHOD_LOG_REPLY_DONE: &str = "log_done";
#[derive(Serialize)]
pub struct LogMessage<'a> {
pub level: Option<log::Level>,
pub prefix: &'a str,
pub message: &'a str,
}
#[derive(Deserialize)]
pub struct LogMessageOwned {
pub level: Option<log::Level>,
pub prefix: String,
pub message: String,
}
#[derive(Serialize, Deserialize)]
pub struct Status {
pub tunnel: TunnelState,
}
#[derive(Deserialize, Serialize, Debug)]
pub struct LogReplayFinished {}
#[derive(Deserialize, Serialize, Debug)]
pub enum TunnelState {
Disconnected,
Connected { name: String },
}
}
| cli/src/tunnels/protocol.rs | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.00018977043509949,
0.00017439841758459806,
0.00016354047693312168,
0.00017486169235780835,
0.000004237566827214323
] |
{
"id": 2,
"code_window": [
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('test', new OneLineRange(1, 1, 5)),\n",
"\t\t\t\tnew TextSearchMatch('this is a test', new OneLineRange(1, 11, 15))),\n",
"\t\t\taRawMatch('/3', new TextSearchMatch('test', lineOneRange))];\n",
"\t\tconst searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tsinon.stub(CellMatch.prototype, 'addContext');\n",
"\n",
"\t\tconst textSearch = sinon.spy(searchService, 'textSearch');\n",
"\t\tconst mdInputCell = {\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tconst searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 225
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { CoreNavigationCommands } from 'vs/editor/browser/coreCommands';
import { CursorMove } from 'vs/editor/common/cursor/cursorMoveCommands';
import { Position } from 'vs/editor/common/core/position';
import { Range } from 'vs/editor/common/core/range';
import { Selection } from 'vs/editor/common/core/selection';
import { withTestCodeEditor, ITestCodeEditor } from 'vs/editor/test/browser/testCodeEditor';
import { ViewModel } from 'vs/editor/common/viewModel/viewModelImpl';
suite('Cursor move command test', () => {
const TEXT = [
' \tMy First Line\t ',
'\tMy Second Line',
' Third Line🐶',
'',
'1'
].join('\n');
function executeTest(callback: (editor: ITestCodeEditor, viewModel: ViewModel) => void): void {
withTestCodeEditor(TEXT, {}, (editor, viewModel) => {
callback(editor, viewModel);
});
}
test('move left should move to left character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveLeft(viewModel);
cursorEqual(viewModel, 1, 7);
});
});
test('move left should move to left by n characters', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveLeft(viewModel, 3);
cursorEqual(viewModel, 1, 5);
});
});
test('move left should move to left by half line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveLeft(viewModel, 1, CursorMove.RawUnit.HalfLine);
cursorEqual(viewModel, 1, 1);
});
});
test('move left moves to previous line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 2, 3);
moveLeft(viewModel, 10);
cursorEqual(viewModel, 1, 21);
});
});
test('move right should move to right character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 5);
moveRight(viewModel);
cursorEqual(viewModel, 1, 6);
});
});
test('move right should move to right by n characters', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 2);
moveRight(viewModel, 6);
cursorEqual(viewModel, 1, 8);
});
});
test('move right should move to right by half line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 4);
moveRight(viewModel, 1, CursorMove.RawUnit.HalfLine);
cursorEqual(viewModel, 1, 14);
});
});
test('move right moves to next line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveRight(viewModel, 100);
cursorEqual(viewModel, 2, 1);
});
});
test('move to first character of line from middle', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveToLineStart(viewModel);
cursorEqual(viewModel, 1, 1);
});
});
test('move to first character of line from first non white space character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 6);
moveToLineStart(viewModel);
cursorEqual(viewModel, 1, 1);
});
});
test('move to first character of line from first character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 1);
moveToLineStart(viewModel);
cursorEqual(viewModel, 1, 1);
});
});
test('move to first non white space character of line from middle', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveToLineFirstNonWhitespaceCharacter(viewModel);
cursorEqual(viewModel, 1, 6);
});
});
test('move to first non white space character of line from first non white space character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 6);
moveToLineFirstNonWhitespaceCharacter(viewModel);
cursorEqual(viewModel, 1, 6);
});
});
test('move to first non white space character of line from first character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 1);
moveToLineFirstNonWhitespaceCharacter(viewModel);
cursorEqual(viewModel, 1, 6);
});
});
test('move to end of line from middle', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveToLineEnd(viewModel);
cursorEqual(viewModel, 1, 21);
});
});
test('move to end of line from last non white space character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 19);
moveToLineEnd(viewModel);
cursorEqual(viewModel, 1, 21);
});
});
test('move to end of line from line end', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 21);
moveToLineEnd(viewModel);
cursorEqual(viewModel, 1, 21);
});
});
test('move to last non white space character from middle', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveToLineLastNonWhitespaceCharacter(viewModel);
cursorEqual(viewModel, 1, 19);
});
});
test('move to last non white space character from last non white space character', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 19);
moveToLineLastNonWhitespaceCharacter(viewModel);
cursorEqual(viewModel, 1, 19);
});
});
test('move to last non white space character from line end', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 21);
moveToLineLastNonWhitespaceCharacter(viewModel);
cursorEqual(viewModel, 1, 19);
});
});
test('move to center of line not from center', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 8);
moveToLineCenter(viewModel);
cursorEqual(viewModel, 1, 11);
});
});
test('move to center of line from center', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 11);
moveToLineCenter(viewModel);
cursorEqual(viewModel, 1, 11);
});
});
test('move to center of line from start', () => {
executeTest((editor, viewModel) => {
moveToLineStart(viewModel);
moveToLineCenter(viewModel);
cursorEqual(viewModel, 1, 11);
});
});
test('move to center of line from end', () => {
executeTest((editor, viewModel) => {
moveToLineEnd(viewModel);
moveToLineCenter(viewModel);
cursorEqual(viewModel, 1, 11);
});
});
test('move up by cursor move command', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 3, 5);
cursorEqual(viewModel, 3, 5);
moveUp(viewModel, 2);
cursorEqual(viewModel, 1, 5);
moveUp(viewModel, 1);
cursorEqual(viewModel, 1, 1);
});
});
test('move up by model line cursor move command', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 3, 5);
cursorEqual(viewModel, 3, 5);
moveUpByModelLine(viewModel, 2);
cursorEqual(viewModel, 1, 5);
moveUpByModelLine(viewModel, 1);
cursorEqual(viewModel, 1, 1);
});
});
test('move down by model line cursor move command', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 3, 5);
cursorEqual(viewModel, 3, 5);
moveDownByModelLine(viewModel, 2);
cursorEqual(viewModel, 5, 2);
moveDownByModelLine(viewModel, 1);
cursorEqual(viewModel, 5, 2);
});
});
test('move up with selection by cursor move command', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 3, 5);
cursorEqual(viewModel, 3, 5);
moveUp(viewModel, 1, true);
cursorEqual(viewModel, 2, 2, 3, 5);
moveUp(viewModel, 1, true);
cursorEqual(viewModel, 1, 5, 3, 5);
});
});
test('move up and down with tabs by cursor move command', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 1, 5);
cursorEqual(viewModel, 1, 5);
moveDown(viewModel, 4);
cursorEqual(viewModel, 5, 2);
moveUp(viewModel, 1);
cursorEqual(viewModel, 4, 1);
moveUp(viewModel, 1);
cursorEqual(viewModel, 3, 5);
moveUp(viewModel, 1);
cursorEqual(viewModel, 2, 2);
moveUp(viewModel, 1);
cursorEqual(viewModel, 1, 5);
});
});
test('move up and down with end of lines starting from a long one by cursor move command', () => {
executeTest((editor, viewModel) => {
moveToEndOfLine(viewModel);
cursorEqual(viewModel, 1, 21);
moveToEndOfLine(viewModel);
cursorEqual(viewModel, 1, 21);
moveDown(viewModel, 2);
cursorEqual(viewModel, 3, 17);
moveDown(viewModel, 1);
cursorEqual(viewModel, 4, 1);
moveDown(viewModel, 1);
cursorEqual(viewModel, 5, 2);
moveUp(viewModel, 4);
cursorEqual(viewModel, 1, 21);
});
});
test('move to view top line moves to first visible line if it is first line', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(1, 1, 10, 1);
moveTo(viewModel, 2, 2);
moveToTop(viewModel);
cursorEqual(viewModel, 1, 6);
});
});
test('move to view top line moves to top visible line when first line is not visible', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(2, 1, 10, 1);
moveTo(viewModel, 4, 1);
moveToTop(viewModel);
cursorEqual(viewModel, 2, 2);
});
});
test('move to view top line moves to nth line from top', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(1, 1, 10, 1);
moveTo(viewModel, 4, 1);
moveToTop(viewModel, 3);
cursorEqual(viewModel, 3, 5);
});
});
test('move to view top line moves to last line if n is greater than last visible line number', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(1, 1, 3, 1);
moveTo(viewModel, 2, 2);
moveToTop(viewModel, 4);
cursorEqual(viewModel, 3, 5);
});
});
test('move to view center line moves to the center line', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(3, 1, 3, 1);
moveTo(viewModel, 2, 2);
moveToCenter(viewModel);
cursorEqual(viewModel, 3, 5);
});
});
test('move to view bottom line moves to last visible line if it is last line', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(1, 1, 5, 1);
moveTo(viewModel, 2, 2);
moveToBottom(viewModel);
cursorEqual(viewModel, 5, 1);
});
});
test('move to view bottom line moves to last visible line when last line is not visible', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(2, 1, 3, 1);
moveTo(viewModel, 2, 2);
moveToBottom(viewModel);
cursorEqual(viewModel, 3, 5);
});
});
test('move to view bottom line moves to nth line from bottom', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(1, 1, 5, 1);
moveTo(viewModel, 4, 1);
moveToBottom(viewModel, 3);
cursorEqual(viewModel, 3, 5);
});
});
test('move to view bottom line moves to first line if n is lesser than first visible line number', () => {
executeTest((editor, viewModel) => {
viewModel.getCompletelyVisibleViewRange = () => new Range(2, 1, 5, 1);
moveTo(viewModel, 4, 1);
moveToBottom(viewModel, 5);
cursorEqual(viewModel, 2, 2);
});
});
});
suite('Cursor move by blankline test', () => {
const TEXT = [
' \tMy First Line\t ',
'\tMy Second Line',
' Third Line🐶',
'',
'1',
'2',
'3',
'',
' ',
'a',
'b',
].join('\n');
function executeTest(callback: (editor: ITestCodeEditor, viewModel: ViewModel) => void): void {
withTestCodeEditor(TEXT, {}, (editor, viewModel) => {
callback(editor, viewModel);
});
}
test('move down should move to start of next blank line', () => {
executeTest((editor, viewModel) => {
moveDownByBlankLine(viewModel, false);
cursorEqual(viewModel, 4, 1);
});
});
test('move up should move to start of previous blank line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 7, 1);
moveUpByBlankLine(viewModel, false);
cursorEqual(viewModel, 4, 1);
});
});
test('move down should skip over whitespace if already on blank line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 8, 1);
moveDownByBlankLine(viewModel, false);
cursorEqual(viewModel, 11, 1);
});
});
test('move up should skip over whitespace if already on blank line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 9, 1);
moveUpByBlankLine(viewModel, false);
cursorEqual(viewModel, 4, 1);
});
});
test('move up should go to first column of first line if not empty', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 2, 1);
moveUpByBlankLine(viewModel, false);
cursorEqual(viewModel, 1, 1);
});
});
test('move down should go to first column of last line if not empty', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 10, 1);
moveDownByBlankLine(viewModel, false);
cursorEqual(viewModel, 11, 1);
});
});
test('select down should select to start of next blank line', () => {
executeTest((editor, viewModel) => {
moveDownByBlankLine(viewModel, true);
selectionEqual(viewModel.getSelection(), 4, 1, 1, 1);
});
});
test('select up should select to start of previous blank line', () => {
executeTest((editor, viewModel) => {
moveTo(viewModel, 7, 1);
moveUpByBlankLine(viewModel, true);
selectionEqual(viewModel.getSelection(), 4, 1, 7, 1);
});
});
});
// Move command
function move(viewModel: ViewModel, args: any) {
CoreNavigationCommands.CursorMove.runCoreEditorCommand(viewModel, args);
}
function moveToLineStart(viewModel: ViewModel) {
move(viewModel, { to: CursorMove.RawDirection.WrappedLineStart });
}
function moveToLineFirstNonWhitespaceCharacter(viewModel: ViewModel) {
move(viewModel, { to: CursorMove.RawDirection.WrappedLineFirstNonWhitespaceCharacter });
}
function moveToLineCenter(viewModel: ViewModel) {
move(viewModel, { to: CursorMove.RawDirection.WrappedLineColumnCenter });
}
function moveToLineEnd(viewModel: ViewModel) {
move(viewModel, { to: CursorMove.RawDirection.WrappedLineEnd });
}
function moveToLineLastNonWhitespaceCharacter(viewModel: ViewModel) {
move(viewModel, { to: CursorMove.RawDirection.WrappedLineLastNonWhitespaceCharacter });
}
function moveLeft(viewModel: ViewModel, value?: number, by?: string, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.Left, by: by, value: value, select: select });
}
function moveRight(viewModel: ViewModel, value?: number, by?: string, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.Right, by: by, value: value, select: select });
}
function moveUp(viewModel: ViewModel, noOfLines: number = 1, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.Up, by: CursorMove.RawUnit.WrappedLine, value: noOfLines, select: select });
}
function moveUpByBlankLine(viewModel: ViewModel, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.PrevBlankLine, by: CursorMove.RawUnit.WrappedLine, select: select });
}
function moveUpByModelLine(viewModel: ViewModel, noOfLines: number = 1, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.Up, value: noOfLines, select: select });
}
function moveDown(viewModel: ViewModel, noOfLines: number = 1, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.Down, by: CursorMove.RawUnit.WrappedLine, value: noOfLines, select: select });
}
function moveDownByBlankLine(viewModel: ViewModel, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.NextBlankLine, by: CursorMove.RawUnit.WrappedLine, select: select });
}
function moveDownByModelLine(viewModel: ViewModel, noOfLines: number = 1, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.Down, value: noOfLines, select: select });
}
function moveToTop(viewModel: ViewModel, noOfLines: number = 1, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.ViewPortTop, value: noOfLines, select: select });
}
function moveToCenter(viewModel: ViewModel, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.ViewPortCenter, select: select });
}
function moveToBottom(viewModel: ViewModel, noOfLines: number = 1, select?: boolean) {
move(viewModel, { to: CursorMove.RawDirection.ViewPortBottom, value: noOfLines, select: select });
}
function cursorEqual(viewModel: ViewModel, posLineNumber: number, posColumn: number, selLineNumber: number = posLineNumber, selColumn: number = posColumn) {
positionEqual(viewModel.getPosition(), posLineNumber, posColumn);
selectionEqual(viewModel.getSelection(), posLineNumber, posColumn, selLineNumber, selColumn);
}
function positionEqual(position: Position, lineNumber: number, column: number) {
assert.deepStrictEqual(position, new Position(lineNumber, column), 'position equal');
}
function selectionEqual(selection: Selection, posLineNumber: number, posColumn: number, selLineNumber: number, selColumn: number) {
assert.deepStrictEqual({
selectionStartLineNumber: selection.selectionStartLineNumber,
selectionStartColumn: selection.selectionStartColumn,
positionLineNumber: selection.positionLineNumber,
positionColumn: selection.positionColumn
}, {
selectionStartLineNumber: selLineNumber,
selectionStartColumn: selColumn,
positionLineNumber: posLineNumber,
positionColumn: posColumn
}, 'selection equal');
}
function moveTo(viewModel: ViewModel, lineNumber: number, column: number, inSelectionMode: boolean = false) {
if (inSelectionMode) {
CoreNavigationCommands.MoveToSelect.runCoreEditorCommand(viewModel, {
position: new Position(lineNumber, column)
});
} else {
CoreNavigationCommands.MoveTo.runCoreEditorCommand(viewModel, {
position: new Position(lineNumber, column)
});
}
}
function moveToEndOfLine(viewModel: ViewModel, inSelectionMode: boolean = false) {
if (inSelectionMode) {
CoreNavigationCommands.CursorEndSelect.runCoreEditorCommand(viewModel, {});
} else {
CoreNavigationCommands.CursorEnd.runCoreEditorCommand(viewModel, {});
}
}
| src/vs/editor/test/browser/controller/cursorMoveCommand.test.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.000177535941475071,
0.0001722411107039079,
0.0001646165328565985,
0.00017233230755664408,
0.000002430570930300746
] |
{
"id": 3,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 349
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import * as sinon from 'sinon';
import * as arrays from 'vs/base/common/arrays';
import { DeferredPromise, timeout } from 'vs/base/common/async';
import { CancellationToken, CancellationTokenSource } from 'vs/base/common/cancellation';
import { URI } from 'vs/base/common/uri';
import { Range } from 'vs/editor/common/core/range';
import { IModelService } from 'vs/editor/common/services/model';
import { ModelService } from 'vs/editor/common/services/modelService';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { TestConfigurationService } from 'vs/platform/configuration/test/common/testConfigurationService';
import { TestInstantiationService } from 'vs/platform/instantiation/test/common/instantiationServiceMock';
import { IFileMatch, IFileQuery, IFileSearchStats, IFolderQuery, ISearchComplete, ISearchProgressItem, ISearchQuery, ISearchService, ITextSearchMatch, OneLineRange, QueryType, TextSearchMatch } from 'vs/workbench/services/search/common/search';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { NullTelemetryService } from 'vs/platform/telemetry/common/telemetryUtils';
import { CellMatch, MatchInNotebook, SearchModel } from 'vs/workbench/contrib/search/browser/searchModel';
import { IThemeService } from 'vs/platform/theme/common/themeService';
import { TestThemeService } from 'vs/platform/theme/test/common/testThemeService';
import { FileService } from 'vs/platform/files/common/fileService';
import { ILogService, NullLogService } from 'vs/platform/log/common/log';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { UriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentityService';
import { ILabelService } from 'vs/platform/label/common/label';
import { INotebookEditorService } from 'vs/workbench/contrib/notebook/browser/services/notebookEditorService';
import { IEditorGroupsService } from 'vs/workbench/services/editor/common/editorGroupsService';
import { TestEditorGroupsService } from 'vs/workbench/test/browser/workbenchTestServices';
import { NotebookEditorWidgetService } from 'vs/workbench/contrib/notebook/browser/services/notebookEditorServiceImpl';
import { createFileUriFromPathFromRoot, getRootName } from 'vs/workbench/contrib/search/test/browser/searchTestCommon';
import { ICellMatch, IFileMatchWithCells, contentMatchesToTextSearchMatches, webviewMatchesToTextSearchMatches } from 'vs/workbench/contrib/search/browser/searchNotebookHelpers';
import { CellKind } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { ICellViewModel } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { FindMatch, IReadonlyTextBuffer } from 'vs/editor/common/model';
import { ResourceMap, ResourceSet } from 'vs/base/common/map';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { INotebookSearchService } from 'vs/workbench/contrib/search/browser/notebookSearch';
const nullEvent = new class {
id: number = -1;
topic!: string;
name!: string;
description!: string;
data: any;
startTime!: Date;
stopTime!: Date;
stop(): void {
return;
}
timeTaken(): number {
return -1;
}
};
const lineOneRange = new OneLineRange(1, 0, 1);
suite('SearchModel', () => {
let instantiationService: TestInstantiationService;
const testSearchStats: IFileSearchStats = {
fromCache: false,
resultCount: 1,
type: 'searchProcess',
detailStats: {
fileWalkTime: 0,
cmdTime: 0,
cmdResultCount: 0,
directoriesWalked: 2,
filesWalked: 3
}
};
const folderQueries: IFolderQuery[] = [
{ folder: createFileUriFromPathFromRoot() }
];
setup(() => {
instantiationService = new TestInstantiationService();
instantiationService.stub(ITelemetryService, NullTelemetryService);
instantiationService.stub(ILabelService, { getUriBasenameLabel: (uri: URI) => '' });
instantiationService.stub(INotebookService, { getNotebookTextModels: () => [] });
instantiationService.stub(IModelService, stubModelService(instantiationService));
instantiationService.stub(INotebookEditorService, stubNotebookEditorService(instantiationService));
instantiationService.stub(ISearchService, {});
instantiationService.stub(ISearchService, 'textSearch', Promise.resolve({ results: [] }));
instantiationService.stub(IUriIdentityService, new UriIdentityService(new FileService(new NullLogService())));
instantiationService.stub(ILogService, new NullLogService());
});
teardown(() => sinon.restore());
function searchServiceWithResults(results: IFileMatch[], complete: ISearchComplete | null = null): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void, notebookURIs?: ResourceSet): Promise<ISearchComplete> {
return new Promise(resolve => {
queueMicrotask(() => {
results.forEach(onProgress!);
resolve(complete!);
});
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
return new Promise(resolve => {
queueMicrotask(() => {
resolve({ results: results, messages: [] });
});
});
}
};
}
function searchServiceWithError(error: Error): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void): Promise<ISearchComplete> {
return new Promise((resolve, reject) => {
reject(error);
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
return new Promise((resolve, reject) => {
queueMicrotask(() => {
reject(error);
});
});
}
};
}
function canceleableSearchService(tokenSource: CancellationTokenSource): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void): Promise<ISearchComplete> {
token?.onCancellationRequested(() => tokenSource.cancel());
return new Promise(resolve => {
queueMicrotask(() => {
resolve(<any>{});
});
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
token?.onCancellationRequested(() => tokenSource.cancel());
return new Promise(resolve => {
queueMicrotask(() => {
resolve(<any>{});
});
});
}
};
}
function notebookSearchServiceWithInfo(results: IFileMatchWithCells[], tokenSource: CancellationTokenSource | undefined): INotebookSearchService {
return <INotebookSearchService>{
_serviceBrand: undefined,
notebookSearch(query: ISearchQuery, token: CancellationToken, searchInstanceID: string, onProgress?: (result: ISearchProgressItem) => void, notebookURIs?: ResourceSet): Promise<{ completeData: ISearchComplete; scannedFiles: ResourceSet }> {
token?.onCancellationRequested(() => tokenSource?.cancel());
const localResults = new ResourceMap<IFileMatchWithCells | null>(uri => uri.path);
results.forEach(r => {
localResults.set(r.resource, r);
});
if (onProgress) {
arrays.coalesce([...localResults.values()]).forEach(onProgress);
}
return Promise.resolve(
{
completeData: {
messages: [],
results: arrays.coalesce([...localResults.values()]),
limitHit: false
},
scannedFiles: new ResourceSet([...localResults.keys()]),
});
}
};
}
test('Search Model: Search adds to results', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2', new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
const actual = testObject.searchResult.matches();
assert.strictEqual(2, actual.length);
assert.strictEqual(URI.file(`${getRootName()}/1`).toString(), actual[0].resource.toString());
let actuaMatches = actual[0].matches();
assert.strictEqual(2, actuaMatches.length);
assert.strictEqual('preview 1', actuaMatches[0].text());
assert.ok(new Range(2, 2, 2, 5).equalsRange(actuaMatches[0].range()));
assert.strictEqual('preview 1', actuaMatches[1].text());
assert.ok(new Range(2, 5, 2, 12).equalsRange(actuaMatches[1].range()));
actuaMatches = actual[1].matches();
assert.strictEqual(1, actuaMatches.length);
assert.strictEqual('preview 2', actuaMatches[0].text());
assert.ok(new Range(2, 1, 2, 2).equalsRange(actuaMatches[0].range()));
});
test('Search Model: Search can return notebook results', async () => {
const notebookUri = createFileUriFromPathFromRoot('/1');
const results = [
aRawMatch('/2',
new TextSearchMatch('test', new OneLineRange(1, 1, 5)),
new TextSearchMatch('this is a test', new OneLineRange(1, 11, 15))),
aRawMatch('/3', new TextSearchMatch('test', lineOneRange))];
const searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results));
sinon.stub(CellMatch.prototype, 'addContext');
const textSearch = sinon.spy(searchService, 'textSearch');
const mdInputCell = {
cellKind: CellKind.Markup, textBuffer: <IReadonlyTextBuffer>{
getLineContent(lineNumber: number): string {
if (lineNumber === 1) {
return '# Test';
} else {
return '';
}
}
},
id: 'mdInputCell'
} as ICellViewModel;
const findMatchMds = [new FindMatch(new Range(1, 3, 1, 7), ['Test'])];
const codeCell = {
cellKind: CellKind.Code, textBuffer: <IReadonlyTextBuffer>{
getLineContent(lineNumber: number): string {
if (lineNumber === 1) {
return 'print("test! testing!!")';
} else {
return '';
}
}
},
id: 'codeCell'
} as ICellViewModel;
const findMatchCodeCells =
[new FindMatch(new Range(1, 8, 1, 12), ['test']),
new FindMatch(new Range(1, 14, 1, 18), ['test']),
];
const webviewMatches = [{
index: 0,
searchPreviewInfo: {
line: 'test! testing!!',
range: {
start: 1,
end: 5
}
}
},
{
index: 1,
searchPreviewInfo: {
line: 'test! testing!!',
range: {
start: 7,
end: 11
}
}
}
];
const cellMatchMd: ICellMatch = {
cell: mdInputCell,
index: 0,
contentResults: contentMatchesToTextSearchMatches(findMatchMds, mdInputCell),
webviewResults: []
};
const cellMatchCode: ICellMatch = {
cell: codeCell,
index: 1,
contentResults: contentMatchesToTextSearchMatches(findMatchCodeCells, codeCell),
webviewResults: webviewMatchesToTextSearchMatches(webviewMatches),
};
const notebookSearchService = instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([aRawMatchWithCells('/1', cellMatchMd, cellMatchCode)], undefined));
const notebookSearch = sinon.spy(notebookSearchService, "notebookSearch");
const model: SearchModel = instantiationService.createInstance(SearchModel);
await model.search({ contentPattern: { pattern: 'test' }, type: QueryType.Text, folderQueries });
const actual = model.searchResult.matches();
assert(notebookSearch.calledOnce);
assert(textSearch.getCall(0).args[3]?.size === 1);
assert(textSearch.getCall(0).args[3]?.has(notebookUri)); // ensure that the textsearch knows not to re-source the notebooks
assert.strictEqual(3, actual.length);
assert.strictEqual(URI.file(`${getRootName()}/1`).toString(), actual[0].resource.toString());
const notebookFileMatches = actual[0].matches();
assert.ok(notebookFileMatches[0].range().equalsRange(new Range(1, 3, 1, 7)));
assert.ok(notebookFileMatches[1].range().equalsRange(new Range(1, 8, 1, 12)));
assert.ok(notebookFileMatches[2].range().equalsRange(new Range(1, 14, 1, 18)));
assert.ok(notebookFileMatches[3].range().equalsRange(new Range(1, 2, 1, 6)));
assert.ok(notebookFileMatches[4].range().equalsRange(new Range(1, 8, 1, 12)));
notebookFileMatches.forEach(match => match instanceof MatchInNotebook);
// assert(notebookFileMatches[0] instanceof MatchInNotebook);
assert((notebookFileMatches[0] as MatchInNotebook).cell.id === 'mdInputCell');
assert((notebookFileMatches[1] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[2] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[3] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[4] as MatchInNotebook).cell.id === 'codeCell');
const mdCellMatchProcessed = (notebookFileMatches[0] as MatchInNotebook).cellParent;
const codeCellMatchProcessed = (notebookFileMatches[1] as MatchInNotebook).cellParent;
assert(mdCellMatchProcessed.contentMatches.length === 1);
assert(codeCellMatchProcessed.contentMatches.length === 2);
assert(codeCellMatchProcessed.webviewMatches.length === 2);
assert(mdCellMatchProcessed.contentMatches[0] === notebookFileMatches[0]);
assert(codeCellMatchProcessed.contentMatches[0] === notebookFileMatches[1]);
assert(codeCellMatchProcessed.contentMatches[1] === notebookFileMatches[2]);
assert(codeCellMatchProcessed.webviewMatches[0] === notebookFileMatches[3]);
assert(codeCellMatchProcessed.webviewMatches[1] === notebookFileMatches[4]);
assert.strictEqual(URI.file(`${getRootName()}/2`).toString(), actual[1].resource.toString());
assert.strictEqual(URI.file(`${getRootName()}/3`).toString(), actual[2].resource.toString());
});
test('Search Model: Search reports telemetry on search completed', async () => {
const target = instantiationService.spy(ITelemetryService, 'publicLog');
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2',
new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(target.calledThrice);
assert.ok(target.calledWith('searchResultsFirstRender'));
assert.ok(target.calledWith('searchResultsFinished'));
});
test('Search Model: Search reports timed telemetry on search when progress is not called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithResults([]));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
});
});
});
test('Search Model: Search reports timed telemetry on search when progress is called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithResults(
[aRawMatch('/1', new TextSearchMatch('some preview', lineOneRange))],
{ results: [], stats: testSearchStats, messages: [] }));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => {
return timeout(1).then(() => {
// timeout because promise handlers may run in a different order. We only care that these
// are fired at some point.
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.strictEqual(1, target2.callCount);
});
});
});
test('Search Model: Search reports timed telemetry on search when error is called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithError(new Error('error')));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => { }, () => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.ok(target2.calledOnce);
});
});
});
test('Search Model: Search reports timed telemetry on search when error is cancelled error', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
const deferredPromise = new DeferredPromise<ISearchComplete>();
instantiationService.stub(ISearchService, 'textSearch', deferredPromise.p);
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
deferredPromise.cancel();
return result.then(() => { }, () => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.ok(target2.calledOnce);
});
});
});
test('Search Model: Search results are cleared during search', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2',
new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(!testObject.searchResult.isEmpty());
instantiationService.stub(ISearchService, searchServiceWithResults([]));
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(testObject.searchResult.isEmpty());
});
test('Search Model: Previous search is cancelled when new search is called', async () => {
const tokenSource = new CancellationTokenSource();
instantiationService.stub(ISearchService, canceleableSearchService(tokenSource));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], tokenSource));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
instantiationService.stub(ISearchService, searchServiceWithResults([]));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(tokenSource.token.isCancellationRequested);
});
test('getReplaceString returns proper replace string for regExpressions', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 're' }, type: QueryType.Text, folderQueries });
testObject.replaceString = 'hello';
let match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 're', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 're(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 'r(e)(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 'r(e)(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
testObject.replaceString = 'hello$1';
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('helloe', match.replaceString);
});
function aRawMatch(resource: string, ...results: ITextSearchMatch[]): IFileMatch {
return { resource: createFileUriFromPathFromRoot(resource), results };
}
function aRawMatchWithCells(resource: string, ...cells: ICellMatch[]) {
return { resource: createFileUriFromPathFromRoot(resource), cellResults: cells };
}
function stubModelService(instantiationService: TestInstantiationService): IModelService {
instantiationService.stub(IThemeService, new TestThemeService());
const config = new TestConfigurationService();
config.setUserConfiguration('search', { searchOnType: true });
instantiationService.stub(IConfigurationService, config);
return instantiationService.createInstance(ModelService);
}
function stubNotebookEditorService(instantiationService: TestInstantiationService): INotebookEditorService {
instantiationService.stub(IEditorGroupsService, new TestEditorGroupsService());
return instantiationService.createInstance(NotebookEditorWidgetService);
}
});
| src/vs/workbench/contrib/search/test/browser/searchModel.test.ts | 1 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.9980736970901489,
0.11526063829660416,
0.00016218818200286478,
0.00035497493809089065,
0.29570242762565613
] |
{
"id": 3,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 349
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { ITreeNode } from 'vs/base/browser/ui/tree/tree';
import { WorkbenchObjectTree } from 'vs/platform/list/browser/listService';
import { TestExplorerTreeElement, TestItemTreeElement } from 'vs/workbench/contrib/testing/browser/explorerProjections/index';
import { ISerializedTestTreeCollapseState } from 'vs/workbench/contrib/testing/browser/explorerProjections/testingViewState';
import { TestId } from 'vs/workbench/contrib/testing/common/testId';
export class TestingObjectTree<TFilterData = void> extends WorkbenchObjectTree<TestExplorerTreeElement, TFilterData> {
/**
* Gets a serialized view state for the tree, optimized for storage.
*
* @param updatePreviousState Optional previous state to mutate and update
* instead of creating a new one.
*/
public getOptimizedViewState(updatePreviousState?: ISerializedTestTreeCollapseState): ISerializedTestTreeCollapseState {
const root: ISerializedTestTreeCollapseState = updatePreviousState || {};
/**
* Recursive builder function. Returns whether the subtree has any non-default
* value. Adds itself to the parent children if it does.
*/
const build = (node: ITreeNode<TestExplorerTreeElement | null, unknown>, parent: ISerializedTestTreeCollapseState): boolean => {
if (!(node.element instanceof TestItemTreeElement)) {
return false;
}
const localId = TestId.localId(node.element.test.item.extId);
const inTree = parent.children?.[localId] || {};
// only saved collapsed state if it's not the default (not collapsed, or a root depth)
inTree.collapsed = node.depth === 0 || !node.collapsed ? node.collapsed : undefined;
let hasAnyNonDefaultValue = inTree.collapsed !== undefined;
if (node.children.length) {
for (const child of node.children) {
hasAnyNonDefaultValue = build(child, inTree) || hasAnyNonDefaultValue;
}
}
if (hasAnyNonDefaultValue) {
parent.children ??= {};
parent.children[localId] = inTree;
} else if (parent.children?.hasOwnProperty(localId)) {
delete parent.children[localId];
}
return hasAnyNonDefaultValue;
};
root.children ??= {};
// Controller IDs are hidden if there's only a single test controller, but
// make sure they're added when the tree is built if this is the case, so
// that the later ID lookup works.
for (const node of this.getNode().children) {
if (node.element instanceof TestItemTreeElement) {
if (node.element.test.controllerId === node.element.test.item.extId) {
build(node, root);
} else {
const ctrlNode = root.children[node.element.test.controllerId] ??= { children: {} };
build(node, ctrlNode);
}
}
}
return root;
}
}
| src/vs/workbench/contrib/testing/browser/explorerProjections/testingObjectTree.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.0001739095605444163,
0.0001723151362966746,
0.00016962976951617748,
0.00017227703938260674,
0.0000013498963653546525
] |
{
"id": 3,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 349
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { ITextModel } from 'vs/editor/common/model';
import { URI } from 'vs/base/common/uri';
import { TextResourceEditorInput } from 'vs/workbench/common/editor/textResourceEditorInput';
import { TextResourceEditorModel } from 'vs/workbench/common/editor/textResourceEditorModel';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { workbenchInstantiationService, TestServiceAccessor, ITestTextFileEditorModelManager } from 'vs/workbench/test/browser/workbenchTestServices';
import { toResource } from 'vs/base/test/common/utils';
import { TextFileEditorModel } from 'vs/workbench/services/textfile/common/textFileEditorModel';
import { snapshotToString } from 'vs/workbench/services/textfile/common/textfiles';
import { TextFileEditorModelManager } from 'vs/workbench/services/textfile/common/textFileEditorModelManager';
import { Event } from 'vs/base/common/event';
import { timeout } from 'vs/base/common/async';
import { UntitledTextEditorInput } from 'vs/workbench/services/untitled/common/untitledTextEditorInput';
import { createTextBufferFactory } from 'vs/editor/common/model/textModel';
import { DisposableStore } from 'vs/base/common/lifecycle';
suite('Workbench - TextModelResolverService', () => {
let disposables: DisposableStore;
let instantiationService: IInstantiationService;
let accessor: TestServiceAccessor;
let model: TextFileEditorModel;
setup(() => {
disposables = new DisposableStore();
instantiationService = workbenchInstantiationService(undefined, disposables);
accessor = instantiationService.createInstance(TestServiceAccessor);
});
teardown(() => {
model?.dispose();
(<TextFileEditorModelManager>accessor.textFileService.files).dispose();
disposables.dispose();
});
test('resolve resource', async () => {
const disposable = accessor.textModelResolverService.registerTextModelContentProvider('test', {
provideTextContent: async function (resource: URI): Promise<ITextModel | null> {
if (resource.scheme === 'test') {
const modelContent = 'Hello Test';
const languageSelection = accessor.languageService.createById('json');
return accessor.modelService.createModel(modelContent, languageSelection, resource);
}
return null;
}
});
const resource = URI.from({ scheme: 'test', authority: null!, path: 'thePath' });
const input = instantiationService.createInstance(TextResourceEditorInput, resource, 'The Name', 'The Description', undefined, undefined);
const model = await input.resolve();
assert.ok(model);
assert.strictEqual(snapshotToString(((model as TextResourceEditorModel).createSnapshot()!)), 'Hello Test');
let disposed = false;
const disposedPromise = new Promise<void>(resolve => {
Event.once(model.onWillDispose)(() => {
disposed = true;
resolve();
});
});
input.dispose();
await disposedPromise;
assert.strictEqual(disposed, true);
disposable.dispose();
});
test('resolve file', async function () {
const textModel = instantiationService.createInstance(TextFileEditorModel, toResource.call(this, '/path/file_resolver.txt'), 'utf8', undefined);
(<ITestTextFileEditorModelManager>accessor.textFileService.files).add(textModel.resource, textModel);
await textModel.resolve();
const ref = await accessor.textModelResolverService.createModelReference(textModel.resource);
const model = ref.object;
const editorModel = model.textEditorModel;
assert.ok(editorModel);
assert.strictEqual(editorModel.getValue(), 'Hello Html');
let disposed = false;
Event.once(model.onWillDispose)(() => {
disposed = true;
});
ref.dispose();
await timeout(0); // due to the reference resolving the model first which is async
assert.strictEqual(disposed, true);
});
test('resolved dirty file eventually disposes', async function () {
const textModel = instantiationService.createInstance(TextFileEditorModel, toResource.call(this, '/path/file_resolver.txt'), 'utf8', undefined);
(<ITestTextFileEditorModelManager>accessor.textFileService.files).add(textModel.resource, textModel);
await textModel.resolve();
textModel.updateTextEditorModel(createTextBufferFactory('make dirty'));
const ref = await accessor.textModelResolverService.createModelReference(textModel.resource);
let disposed = false;
Event.once(textModel.onWillDispose)(() => {
disposed = true;
});
ref.dispose();
await timeout(0);
assert.strictEqual(disposed, false); // not disposed because model still dirty
textModel.revert();
await timeout(0);
assert.strictEqual(disposed, true); // now disposed because model got reverted
});
test('resolved dirty file does not dispose when new reference created', async function () {
const textModel = instantiationService.createInstance(TextFileEditorModel, toResource.call(this, '/path/file_resolver.txt'), 'utf8', undefined);
(<ITestTextFileEditorModelManager>accessor.textFileService.files).add(textModel.resource, textModel);
await textModel.resolve();
textModel.updateTextEditorModel(createTextBufferFactory('make dirty'));
const ref1 = await accessor.textModelResolverService.createModelReference(textModel.resource);
let disposed = false;
Event.once(textModel.onWillDispose)(() => {
disposed = true;
});
ref1.dispose();
await timeout(0);
assert.strictEqual(disposed, false); // not disposed because model still dirty
const ref2 = await accessor.textModelResolverService.createModelReference(textModel.resource);
textModel.revert();
await timeout(0);
assert.strictEqual(disposed, false); // not disposed because we got another ref meanwhile
ref2.dispose();
await timeout(0);
assert.strictEqual(disposed, true); // now disposed because last ref got disposed
});
test('resolve untitled', async () => {
const service = accessor.untitledTextEditorService;
const untitledModel = service.create();
const input = instantiationService.createInstance(UntitledTextEditorInput, untitledModel);
await input.resolve();
const ref = await accessor.textModelResolverService.createModelReference(input.resource);
const model = ref.object;
assert.strictEqual(untitledModel, model);
const editorModel = model.textEditorModel;
assert.ok(editorModel);
ref.dispose();
input.dispose();
model.dispose();
});
test('even loading documents should be refcounted', async () => {
let resolveModel!: Function;
const waitForIt = new Promise(resolve => resolveModel = resolve);
const disposable = accessor.textModelResolverService.registerTextModelContentProvider('test', {
provideTextContent: async (resource: URI): Promise<ITextModel> => {
await waitForIt;
const modelContent = 'Hello Test';
const languageSelection = accessor.languageService.createById('json');
return accessor.modelService.createModel(modelContent, languageSelection, resource);
}
});
const uri = URI.from({ scheme: 'test', authority: null!, path: 'thePath' });
const modelRefPromise1 = accessor.textModelResolverService.createModelReference(uri);
const modelRefPromise2 = accessor.textModelResolverService.createModelReference(uri);
resolveModel();
const modelRef1 = await modelRefPromise1;
const model1 = modelRef1.object;
const modelRef2 = await modelRefPromise2;
const model2 = modelRef2.object;
const textModel = model1.textEditorModel;
assert.strictEqual(model1, model2, 'they are the same model');
assert(!textModel.isDisposed(), 'the text model should not be disposed');
modelRef1.dispose();
assert(!textModel.isDisposed(), 'the text model should still not be disposed');
const p1 = new Promise<void>(resolve => textModel.onWillDispose(resolve));
modelRef2.dispose();
await p1;
assert(textModel.isDisposed(), 'the text model should finally be disposed');
disposable.dispose();
});
});
| src/vs/workbench/services/textmodelResolver/test/browser/textModelResolverService.test.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.0032441390212625265,
0.00036043813452124596,
0.0001616789522813633,
0.0001734111865516752,
0.0006692857132293284
] |
{
"id": 3,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 349
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { createDecorator } from 'vs/platform/instantiation/common/instantiation';
import { Event } from 'vs/base/common/event';
import { IDisposable } from 'vs/base/common/lifecycle';
import { IAction } from 'vs/base/common/actions';
import { CancellationToken } from 'vs/base/common/cancellation';
import { URI } from 'vs/base/common/uri';
import { ITerminalCommandSelector, ITerminalOutputMatch, ITerminalOutputMatcher } from 'vs/platform/terminal/common/terminal';
import { ITerminalCommand } from 'vs/platform/terminal/common/capabilities/capabilities';
export const ITerminalQuickFixService = createDecorator<ITerminalQuickFixService>('terminalQuickFixService');
export interface ITerminalQuickFixService {
onDidRegisterProvider: Event<ITerminalQuickFixProviderSelector>;
onDidRegisterCommandSelector: Event<ITerminalCommandSelector>;
onDidUnregisterProvider: Event<string>;
readonly _serviceBrand: undefined;
readonly extensionQuickFixes: Promise<Array<ITerminalCommandSelector>>;
providers: Map<string, ITerminalQuickFixProvider>;
registerQuickFixProvider(id: string, provider: ITerminalQuickFixProvider): IDisposable;
registerCommandSelector(selector: ITerminalCommandSelector): void;
}
export interface ITerminalQuickFixProviderSelector {
selector: ITerminalCommandSelector;
provider: ITerminalQuickFixProvider;
}
export type TerminalQuickFixActionInternal = IAction | ITerminalQuickFixCommandAction | ITerminalQuickFixOpenerAction;
export type TerminalQuickFixCallback = (matchResult: ITerminalCommandMatchResult) => TerminalQuickFixActionInternal[] | TerminalQuickFixActionInternal | undefined;
export type TerminalQuickFixCallbackExtension = (terminalCommand: ITerminalCommand, lines: string[] | undefined, option: ITerminalQuickFixOptions, token: CancellationToken) => Promise<ITerminalQuickFix[] | ITerminalQuickFix | undefined>;
export interface ITerminalQuickFixProvider {
/**
* Provides terminal quick fixes
* @param commandMatchResult The command match result for which to provide quick fixes
* @param token A cancellation token indicating the result is no longer needed
* @return Terminal quick fix(es) if any
*/
provideTerminalQuickFixes(terminalCommand: ITerminalCommand, lines: string[] | undefined, option: ITerminalQuickFixOptions, token: CancellationToken): Promise<ITerminalQuickFix[] | ITerminalQuickFix | undefined>;
}
export enum TerminalQuickFixType {
Command = 0,
Opener = 1,
Port = 2
}
export interface ITerminalQuickFixOptions {
type: 'internal' | 'resolved' | 'unresolved';
id: string;
commandLineMatcher: string | RegExp;
outputMatcher?: ITerminalOutputMatcher;
commandExitResult: 'success' | 'error';
}
export interface ITerminalQuickFix {
type: TerminalQuickFixType;
id: string;
source: string;
}
export interface ITerminalQuickFixCommandAction extends ITerminalQuickFix {
type: TerminalQuickFixType.Command;
terminalCommand: string;
// TODO: Should this depend on whether alt is held?
addNewLine?: boolean;
}
export interface ITerminalQuickFixOpenerAction extends ITerminalQuickFix {
type: TerminalQuickFixType.Opener;
uri: URI;
}
export interface ITerminalCommandMatchResult {
commandLine: string;
commandLineMatch: RegExpMatchArray;
outputMatch?: ITerminalOutputMatch;
}
export interface ITerminalQuickFixInternalOptions extends ITerminalQuickFixOptions {
type: 'internal';
getQuickFixes: TerminalQuickFixCallback;
}
export interface ITerminalQuickFixResolvedExtensionOptions extends ITerminalQuickFixOptions {
type: 'resolved';
getQuickFixes: TerminalQuickFixCallbackExtension;
}
export interface ITerminalQuickFixUnresolvedExtensionOptions extends ITerminalQuickFixOptions {
type: 'unresolved';
}
| src/vs/workbench/contrib/terminalContrib/quickFix/browser/quickFix.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.00017438380746170878,
0.00016966719704214483,
0.00016422646876890212,
0.0001698035339359194,
0.0000043638788156386
] |
{
"id": 5,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n",
"\t\tawait testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results: [] }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 455
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import * as sinon from 'sinon';
import * as arrays from 'vs/base/common/arrays';
import { DeferredPromise, timeout } from 'vs/base/common/async';
import { CancellationToken, CancellationTokenSource } from 'vs/base/common/cancellation';
import { URI } from 'vs/base/common/uri';
import { Range } from 'vs/editor/common/core/range';
import { IModelService } from 'vs/editor/common/services/model';
import { ModelService } from 'vs/editor/common/services/modelService';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { TestConfigurationService } from 'vs/platform/configuration/test/common/testConfigurationService';
import { TestInstantiationService } from 'vs/platform/instantiation/test/common/instantiationServiceMock';
import { IFileMatch, IFileQuery, IFileSearchStats, IFolderQuery, ISearchComplete, ISearchProgressItem, ISearchQuery, ISearchService, ITextSearchMatch, OneLineRange, QueryType, TextSearchMatch } from 'vs/workbench/services/search/common/search';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { NullTelemetryService } from 'vs/platform/telemetry/common/telemetryUtils';
import { CellMatch, MatchInNotebook, SearchModel } from 'vs/workbench/contrib/search/browser/searchModel';
import { IThemeService } from 'vs/platform/theme/common/themeService';
import { TestThemeService } from 'vs/platform/theme/test/common/testThemeService';
import { FileService } from 'vs/platform/files/common/fileService';
import { ILogService, NullLogService } from 'vs/platform/log/common/log';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { UriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentityService';
import { ILabelService } from 'vs/platform/label/common/label';
import { INotebookEditorService } from 'vs/workbench/contrib/notebook/browser/services/notebookEditorService';
import { IEditorGroupsService } from 'vs/workbench/services/editor/common/editorGroupsService';
import { TestEditorGroupsService } from 'vs/workbench/test/browser/workbenchTestServices';
import { NotebookEditorWidgetService } from 'vs/workbench/contrib/notebook/browser/services/notebookEditorServiceImpl';
import { createFileUriFromPathFromRoot, getRootName } from 'vs/workbench/contrib/search/test/browser/searchTestCommon';
import { ICellMatch, IFileMatchWithCells, contentMatchesToTextSearchMatches, webviewMatchesToTextSearchMatches } from 'vs/workbench/contrib/search/browser/searchNotebookHelpers';
import { CellKind } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { ICellViewModel } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { FindMatch, IReadonlyTextBuffer } from 'vs/editor/common/model';
import { ResourceMap, ResourceSet } from 'vs/base/common/map';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { INotebookSearchService } from 'vs/workbench/contrib/search/browser/notebookSearch';
const nullEvent = new class {
id: number = -1;
topic!: string;
name!: string;
description!: string;
data: any;
startTime!: Date;
stopTime!: Date;
stop(): void {
return;
}
timeTaken(): number {
return -1;
}
};
const lineOneRange = new OneLineRange(1, 0, 1);
suite('SearchModel', () => {
let instantiationService: TestInstantiationService;
const testSearchStats: IFileSearchStats = {
fromCache: false,
resultCount: 1,
type: 'searchProcess',
detailStats: {
fileWalkTime: 0,
cmdTime: 0,
cmdResultCount: 0,
directoriesWalked: 2,
filesWalked: 3
}
};
const folderQueries: IFolderQuery[] = [
{ folder: createFileUriFromPathFromRoot() }
];
setup(() => {
instantiationService = new TestInstantiationService();
instantiationService.stub(ITelemetryService, NullTelemetryService);
instantiationService.stub(ILabelService, { getUriBasenameLabel: (uri: URI) => '' });
instantiationService.stub(INotebookService, { getNotebookTextModels: () => [] });
instantiationService.stub(IModelService, stubModelService(instantiationService));
instantiationService.stub(INotebookEditorService, stubNotebookEditorService(instantiationService));
instantiationService.stub(ISearchService, {});
instantiationService.stub(ISearchService, 'textSearch', Promise.resolve({ results: [] }));
instantiationService.stub(IUriIdentityService, new UriIdentityService(new FileService(new NullLogService())));
instantiationService.stub(ILogService, new NullLogService());
});
teardown(() => sinon.restore());
function searchServiceWithResults(results: IFileMatch[], complete: ISearchComplete | null = null): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void, notebookURIs?: ResourceSet): Promise<ISearchComplete> {
return new Promise(resolve => {
queueMicrotask(() => {
results.forEach(onProgress!);
resolve(complete!);
});
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
return new Promise(resolve => {
queueMicrotask(() => {
resolve({ results: results, messages: [] });
});
});
}
};
}
function searchServiceWithError(error: Error): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void): Promise<ISearchComplete> {
return new Promise((resolve, reject) => {
reject(error);
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
return new Promise((resolve, reject) => {
queueMicrotask(() => {
reject(error);
});
});
}
};
}
function canceleableSearchService(tokenSource: CancellationTokenSource): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void): Promise<ISearchComplete> {
token?.onCancellationRequested(() => tokenSource.cancel());
return new Promise(resolve => {
queueMicrotask(() => {
resolve(<any>{});
});
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
token?.onCancellationRequested(() => tokenSource.cancel());
return new Promise(resolve => {
queueMicrotask(() => {
resolve(<any>{});
});
});
}
};
}
function notebookSearchServiceWithInfo(results: IFileMatchWithCells[], tokenSource: CancellationTokenSource | undefined): INotebookSearchService {
return <INotebookSearchService>{
_serviceBrand: undefined,
notebookSearch(query: ISearchQuery, token: CancellationToken, searchInstanceID: string, onProgress?: (result: ISearchProgressItem) => void, notebookURIs?: ResourceSet): Promise<{ completeData: ISearchComplete; scannedFiles: ResourceSet }> {
token?.onCancellationRequested(() => tokenSource?.cancel());
const localResults = new ResourceMap<IFileMatchWithCells | null>(uri => uri.path);
results.forEach(r => {
localResults.set(r.resource, r);
});
if (onProgress) {
arrays.coalesce([...localResults.values()]).forEach(onProgress);
}
return Promise.resolve(
{
completeData: {
messages: [],
results: arrays.coalesce([...localResults.values()]),
limitHit: false
},
scannedFiles: new ResourceSet([...localResults.keys()]),
});
}
};
}
test('Search Model: Search adds to results', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2', new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
const actual = testObject.searchResult.matches();
assert.strictEqual(2, actual.length);
assert.strictEqual(URI.file(`${getRootName()}/1`).toString(), actual[0].resource.toString());
let actuaMatches = actual[0].matches();
assert.strictEqual(2, actuaMatches.length);
assert.strictEqual('preview 1', actuaMatches[0].text());
assert.ok(new Range(2, 2, 2, 5).equalsRange(actuaMatches[0].range()));
assert.strictEqual('preview 1', actuaMatches[1].text());
assert.ok(new Range(2, 5, 2, 12).equalsRange(actuaMatches[1].range()));
actuaMatches = actual[1].matches();
assert.strictEqual(1, actuaMatches.length);
assert.strictEqual('preview 2', actuaMatches[0].text());
assert.ok(new Range(2, 1, 2, 2).equalsRange(actuaMatches[0].range()));
});
test('Search Model: Search can return notebook results', async () => {
const notebookUri = createFileUriFromPathFromRoot('/1');
const results = [
aRawMatch('/2',
new TextSearchMatch('test', new OneLineRange(1, 1, 5)),
new TextSearchMatch('this is a test', new OneLineRange(1, 11, 15))),
aRawMatch('/3', new TextSearchMatch('test', lineOneRange))];
const searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results));
sinon.stub(CellMatch.prototype, 'addContext');
const textSearch = sinon.spy(searchService, 'textSearch');
const mdInputCell = {
cellKind: CellKind.Markup, textBuffer: <IReadonlyTextBuffer>{
getLineContent(lineNumber: number): string {
if (lineNumber === 1) {
return '# Test';
} else {
return '';
}
}
},
id: 'mdInputCell'
} as ICellViewModel;
const findMatchMds = [new FindMatch(new Range(1, 3, 1, 7), ['Test'])];
const codeCell = {
cellKind: CellKind.Code, textBuffer: <IReadonlyTextBuffer>{
getLineContent(lineNumber: number): string {
if (lineNumber === 1) {
return 'print("test! testing!!")';
} else {
return '';
}
}
},
id: 'codeCell'
} as ICellViewModel;
const findMatchCodeCells =
[new FindMatch(new Range(1, 8, 1, 12), ['test']),
new FindMatch(new Range(1, 14, 1, 18), ['test']),
];
const webviewMatches = [{
index: 0,
searchPreviewInfo: {
line: 'test! testing!!',
range: {
start: 1,
end: 5
}
}
},
{
index: 1,
searchPreviewInfo: {
line: 'test! testing!!',
range: {
start: 7,
end: 11
}
}
}
];
const cellMatchMd: ICellMatch = {
cell: mdInputCell,
index: 0,
contentResults: contentMatchesToTextSearchMatches(findMatchMds, mdInputCell),
webviewResults: []
};
const cellMatchCode: ICellMatch = {
cell: codeCell,
index: 1,
contentResults: contentMatchesToTextSearchMatches(findMatchCodeCells, codeCell),
webviewResults: webviewMatchesToTextSearchMatches(webviewMatches),
};
const notebookSearchService = instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([aRawMatchWithCells('/1', cellMatchMd, cellMatchCode)], undefined));
const notebookSearch = sinon.spy(notebookSearchService, "notebookSearch");
const model: SearchModel = instantiationService.createInstance(SearchModel);
await model.search({ contentPattern: { pattern: 'test' }, type: QueryType.Text, folderQueries });
const actual = model.searchResult.matches();
assert(notebookSearch.calledOnce);
assert(textSearch.getCall(0).args[3]?.size === 1);
assert(textSearch.getCall(0).args[3]?.has(notebookUri)); // ensure that the textsearch knows not to re-source the notebooks
assert.strictEqual(3, actual.length);
assert.strictEqual(URI.file(`${getRootName()}/1`).toString(), actual[0].resource.toString());
const notebookFileMatches = actual[0].matches();
assert.ok(notebookFileMatches[0].range().equalsRange(new Range(1, 3, 1, 7)));
assert.ok(notebookFileMatches[1].range().equalsRange(new Range(1, 8, 1, 12)));
assert.ok(notebookFileMatches[2].range().equalsRange(new Range(1, 14, 1, 18)));
assert.ok(notebookFileMatches[3].range().equalsRange(new Range(1, 2, 1, 6)));
assert.ok(notebookFileMatches[4].range().equalsRange(new Range(1, 8, 1, 12)));
notebookFileMatches.forEach(match => match instanceof MatchInNotebook);
// assert(notebookFileMatches[0] instanceof MatchInNotebook);
assert((notebookFileMatches[0] as MatchInNotebook).cell.id === 'mdInputCell');
assert((notebookFileMatches[1] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[2] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[3] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[4] as MatchInNotebook).cell.id === 'codeCell');
const mdCellMatchProcessed = (notebookFileMatches[0] as MatchInNotebook).cellParent;
const codeCellMatchProcessed = (notebookFileMatches[1] as MatchInNotebook).cellParent;
assert(mdCellMatchProcessed.contentMatches.length === 1);
assert(codeCellMatchProcessed.contentMatches.length === 2);
assert(codeCellMatchProcessed.webviewMatches.length === 2);
assert(mdCellMatchProcessed.contentMatches[0] === notebookFileMatches[0]);
assert(codeCellMatchProcessed.contentMatches[0] === notebookFileMatches[1]);
assert(codeCellMatchProcessed.contentMatches[1] === notebookFileMatches[2]);
assert(codeCellMatchProcessed.webviewMatches[0] === notebookFileMatches[3]);
assert(codeCellMatchProcessed.webviewMatches[1] === notebookFileMatches[4]);
assert.strictEqual(URI.file(`${getRootName()}/2`).toString(), actual[1].resource.toString());
assert.strictEqual(URI.file(`${getRootName()}/3`).toString(), actual[2].resource.toString());
});
test('Search Model: Search reports telemetry on search completed', async () => {
const target = instantiationService.spy(ITelemetryService, 'publicLog');
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2',
new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(target.calledThrice);
assert.ok(target.calledWith('searchResultsFirstRender'));
assert.ok(target.calledWith('searchResultsFinished'));
});
test('Search Model: Search reports timed telemetry on search when progress is not called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithResults([]));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
});
});
});
test('Search Model: Search reports timed telemetry on search when progress is called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithResults(
[aRawMatch('/1', new TextSearchMatch('some preview', lineOneRange))],
{ results: [], stats: testSearchStats, messages: [] }));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => {
return timeout(1).then(() => {
// timeout because promise handlers may run in a different order. We only care that these
// are fired at some point.
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.strictEqual(1, target2.callCount);
});
});
});
test('Search Model: Search reports timed telemetry on search when error is called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithError(new Error('error')));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => { }, () => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.ok(target2.calledOnce);
});
});
});
test('Search Model: Search reports timed telemetry on search when error is cancelled error', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
const deferredPromise = new DeferredPromise<ISearchComplete>();
instantiationService.stub(ISearchService, 'textSearch', deferredPromise.p);
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
deferredPromise.cancel();
return result.then(() => { }, () => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.ok(target2.calledOnce);
});
});
});
test('Search Model: Search results are cleared during search', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2',
new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(!testObject.searchResult.isEmpty());
instantiationService.stub(ISearchService, searchServiceWithResults([]));
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(testObject.searchResult.isEmpty());
});
test('Search Model: Previous search is cancelled when new search is called', async () => {
const tokenSource = new CancellationTokenSource();
instantiationService.stub(ISearchService, canceleableSearchService(tokenSource));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], tokenSource));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
instantiationService.stub(ISearchService, searchServiceWithResults([]));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(tokenSource.token.isCancellationRequested);
});
test('getReplaceString returns proper replace string for regExpressions', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 're' }, type: QueryType.Text, folderQueries });
testObject.replaceString = 'hello';
let match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 're', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 're(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 'r(e)(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 'r(e)(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
testObject.replaceString = 'hello$1';
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('helloe', match.replaceString);
});
function aRawMatch(resource: string, ...results: ITextSearchMatch[]): IFileMatch {
return { resource: createFileUriFromPathFromRoot(resource), results };
}
function aRawMatchWithCells(resource: string, ...cells: ICellMatch[]) {
return { resource: createFileUriFromPathFromRoot(resource), cellResults: cells };
}
function stubModelService(instantiationService: TestInstantiationService): IModelService {
instantiationService.stub(IThemeService, new TestThemeService());
const config = new TestConfigurationService();
config.setUserConfiguration('search', { searchOnType: true });
instantiationService.stub(IConfigurationService, config);
return instantiationService.createInstance(ModelService);
}
function stubNotebookEditorService(instantiationService: TestInstantiationService): INotebookEditorService {
instantiationService.stub(IEditorGroupsService, new TestEditorGroupsService());
return instantiationService.createInstance(NotebookEditorWidgetService);
}
});
| src/vs/workbench/contrib/search/test/browser/searchModel.test.ts | 1 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.9983299374580383,
0.19018568098545074,
0.00016037659952417016,
0.0010083976667374372,
0.36279693245887756
] |
{
"id": 5,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n",
"\t\tawait testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results: [] }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 455
} | .PHONY: all
all: echo hello
.PHONY: hello
hello: main.o factorial.o \
hello.o $(first) $($(filter second,second)) \
# This is a long \
comment inside prerequisites.
g++ main.o factorial.o hello.o -o hello
# There are a building steps \
below. And the tab is at the beginning of this line.
main.o: main.cpp
g++ -c main.cpp
factorial.o: factorial.cpp
g++ -c factorial.cpp $(fake_variable)
hello.o: hello.cpp \
$(Colorizing with tabs at the beginning of the second line of prerequisites)
g++ -c hello.cpp -o $@
.PHONY: clean
clean:
rm *o hello
.PHONY: var
var:
# "$$" in a shell means to escape makefile's variable substitution.
some_shell_var=$$(sed -nre 's/some regex with (group)/\1/p')
.PHONY: echo
echo:
echo "#" and '#' in quotes are not comments \
and '\' will be continued
@echo Shell is not printed out, just a message.
@-+-+echo Error will be ignored here; invalidcommand
# And we can see variables are highlited as supposed to be:
@echo '$(CC) $(shell echo "123") -o $@'
@-./point-and-slash-should-not-be-highlighted
define defined
$(info Checking existence of $(1) $(flavor $(1)))
$(if $(filter undefined,$(flavor $(1))),0,1)
endef
ifeq ($(strip $(call defined,TOP_DIR)),0)
$(info TOP_DIR must be set before including paths.mk)
endif
-include $(TOP_DIR)3rdparty.mk
ifeq ($(strip $(call defined,CODIT_DIR)),0)
$(info CODIT_DIR must be set in $(TOP_DIR)3rdparty.mk)
endif
CXXVER_GE480 := $(shell expr `$(CXX) -dumpversion | sed -e 's/\.\([0-9][0-9]\)/\1/g' -e 's/\.\([0-9]\)/0\1/g' -e 's/^[0-9]\{3,4\}$$/&00/'` \>= 40800)
ok := ok
$(info Braces {} in parentheses ({}): ${ok})
${info Parentheses () in braces {()}: $(ok)}
ifeq ("${ok}", "skip")
$(ok))}
else ifeq ("${ok}", "skip")
${ok}})
else
$(info Else: ${ok})
endif
result != echo "'$(ok)' $(shell echo "from inlined shell")"
$(info $(result))
# Below is a test of variable assignment without any spacing.
var=val
var?=val
var:=123
var!=echo val
var:=val \
notvar=butval
var:=$(val:.c=.o)
var:=blah#comment
var?=blah\#not_a_comment
var:=blah\\#comment
var!=blah\\\#not_a_comment
var-$(nested-var)=val
# Spaces in a nested shell will hurt a colorizing of variable,
# but not so much.
var-$(shell printf 2) := val2
$(info Should be 'val2' here: $(var-2))
export a ?= b:c
| extensions/vscode-colorize-tests/test/colorize-fixtures/makefile | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.00017665264022070915,
0.00017360862693749368,
0.00016930529091041535,
0.00017384439706802368,
0.000002177805299652391
] |
{
"id": 5,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n",
"\t\tawait testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results: [] }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 455
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import assert = require('assert');
import { UnchangedRegion } from 'vs/editor/browser/widget/diffEditorWidget2/diffEditorViewModel';
import { LineRange } from 'vs/editor/common/core/lineRange';
import { LineRangeMapping } from 'vs/editor/common/diff/linesDiffComputer';
suite('DiffEditorWidget2', () => {
suite('UnchangedRegion', () => {
function serialize(regions: UnchangedRegion[]): unknown {
return regions.map(r => `${r.originalRange} - ${r.modifiedRange}`);
}
test('Everything changed', () => {
assert.deepStrictEqual(serialize(UnchangedRegion.fromDiffs(
[new LineRangeMapping(new LineRange(1, 10), new LineRange(1, 10), [])],
10,
10,
)), []);
});
test('Nothing changed', () => {
assert.deepStrictEqual(serialize(UnchangedRegion.fromDiffs(
[],
10,
10,
)), [
"[1,11) - [1,11)"
]);
});
test('Change in the middle', () => {
assert.deepStrictEqual(serialize(UnchangedRegion.fromDiffs(
[new LineRangeMapping(new LineRange(50, 60), new LineRange(50, 60), [])],
100,
100,
)), ([
'[1,47) - [1,47)',
'[63,101) - [63,101)'
]));
});
test('Change at the end', () => {
assert.deepStrictEqual(serialize(UnchangedRegion.fromDiffs(
[new LineRangeMapping(new LineRange(99, 100), new LineRange(100, 100), [])],
100,
100,
)), (["[1,96) - [1,96)"]));
});
});
});
| src/vs/editor/test/browser/widget/diffEditorWidget2.test.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.00017493388440925628,
0.00017276545986533165,
0.00016810157103464007,
0.00017350632697343826,
0.0000023403961222356884
] |
{
"id": 5,
"code_window": [
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),\n",
"\t\t\taRawMatch('/2',\n",
"\t\t\t\tnew TextSearchMatch('preview 2', lineOneRange))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n",
"\t\tawait testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results: [] }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 455
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { isNonEmptyArray } from 'vs/base/common/arrays';
import { ExtensionIdentifier, IExtensionDescription } from 'vs/platform/extensions/common/extensions';
import { ILogService } from 'vs/platform/log/common/log';
import { IProductService } from 'vs/platform/product/common/productService';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import { ApiProposalName, allApiProposals } from 'vs/workbench/services/extensions/common/extensionsApiProposals';
export class ExtensionsProposedApi {
private readonly _envEnablesProposedApiForAll: boolean;
private readonly _envEnabledExtensions: Set<string>;
private readonly _productEnabledExtensions: Map<string, string[]>;
constructor(
@ILogService private readonly _logService: ILogService,
@IWorkbenchEnvironmentService private readonly _environmentService: IWorkbenchEnvironmentService,
@IProductService productService: IProductService
) {
this._envEnabledExtensions = new Set((_environmentService.extensionEnabledProposedApi ?? []).map(id => ExtensionIdentifier.toKey(id)));
this._envEnablesProposedApiForAll =
!_environmentService.isBuilt || // always allow proposed API when running out of sources
(_environmentService.isExtensionDevelopment && productService.quality !== 'stable') || // do not allow proposed API against stable builds when developing an extension
(this._envEnabledExtensions.size === 0 && Array.isArray(_environmentService.extensionEnabledProposedApi)); // always allow proposed API if --enable-proposed-api is provided without extension ID
this._productEnabledExtensions = new Map<string, ApiProposalName[]>();
// NEW world - product.json spells out what proposals each extension can use
if (productService.extensionEnabledApiProposals) {
for (const [k, value] of Object.entries(productService.extensionEnabledApiProposals)) {
const key = ExtensionIdentifier.toKey(k);
const proposalNames = value.filter(name => {
if (!allApiProposals[<ApiProposalName>name]) {
_logService.warn(`Via 'product.json#extensionEnabledApiProposals' extension '${key}' wants API proposal '${name}' but that proposal DOES NOT EXIST. Likely, the proposal has been finalized (check 'vscode.d.ts') or was abandoned.`);
return false;
}
return true;
});
this._productEnabledExtensions.set(key, proposalNames);
}
}
}
updateEnabledApiProposals(extensions: IExtensionDescription[]): void {
for (const extension of extensions) {
this.doUpdateEnabledApiProposals(extension);
}
}
private doUpdateEnabledApiProposals(_extension: IExtensionDescription): void {
// this is a trick to make the extension description writeable...
type Writeable<T> = { -readonly [P in keyof T]: Writeable<T[P]> };
const extension = <Writeable<IExtensionDescription>>_extension;
const key = ExtensionIdentifier.toKey(_extension.identifier);
// warn about invalid proposal and remove them from the list
if (isNonEmptyArray(extension.enabledApiProposals)) {
extension.enabledApiProposals = extension.enabledApiProposals.filter(name => {
const result = Boolean(allApiProposals[<ApiProposalName>name]);
if (!result) {
this._logService.error(`Extension '${key}' wants API proposal '${name}' but that proposal DOES NOT EXIST. Likely, the proposal has been finalized (check 'vscode.d.ts') or was abandoned.`);
}
return result;
});
}
if (this._productEnabledExtensions.has(key)) {
// NOTE that proposals that are listed in product.json override whatever is declared in the extension
// itself. This is needed for us to know what proposals are used "in the wild". Merging product.json-proposals
// and extension-proposals would break that.
const productEnabledProposals = this._productEnabledExtensions.get(key)!;
// check for difference between product.json-declaration and package.json-declaration
const productSet = new Set(productEnabledProposals);
const extensionSet = new Set(extension.enabledApiProposals);
const diff = new Set([...extensionSet].filter(a => !productSet.has(a)));
if (diff.size > 0) {
this._logService.error(`Extension '${key}' appears in product.json but enables LESS API proposals than the extension wants.\npackage.json (LOSES): ${[...extensionSet].join(', ')}\nproduct.json (WINS): ${[...productSet].join(', ')}`);
if (this._environmentService.isExtensionDevelopment) {
this._logService.error(`Proceeding with EXTRA proposals (${[...diff].join(', ')}) because extension is in development mode. Still, this EXTENSION WILL BE BROKEN unless product.json is updated.`);
productEnabledProposals.push(...diff);
}
}
extension.enabledApiProposals = productEnabledProposals;
return;
}
if (this._envEnablesProposedApiForAll || this._envEnabledExtensions.has(key)) {
// proposed API usage is not restricted and allowed just like the extension
// has declared it
return;
}
if (!extension.isBuiltin && isNonEmptyArray(extension.enabledApiProposals)) {
// restrictive: extension cannot use proposed API in this context and its declaration is nulled
this._logService.error(`Extension '${extension.identifier.value} CANNOT USE these API proposals '${extension.enabledApiProposals?.join(', ') || '*'}'. You MUST start in extension development mode or use the --enable-proposed-api command line flag`);
extension.enabledApiProposals = [];
}
}
}
| src/vs/workbench/services/extensions/common/extensionsProposedApi.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.0001759207807481289,
0.0001735107507556677,
0.0001691603974904865,
0.00017382226360496134,
0.0000018522613345339778
] |
{
"id": 6,
"code_window": [
"\ttest('getReplaceString returns proper replace string for regExpressions', async () => {\n",
"\t\tconst results = [\n",
"\t\t\taRawMatch('/1',\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 485
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import * as sinon from 'sinon';
import * as arrays from 'vs/base/common/arrays';
import { DeferredPromise, timeout } from 'vs/base/common/async';
import { CancellationToken, CancellationTokenSource } from 'vs/base/common/cancellation';
import { URI } from 'vs/base/common/uri';
import { Range } from 'vs/editor/common/core/range';
import { IModelService } from 'vs/editor/common/services/model';
import { ModelService } from 'vs/editor/common/services/modelService';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { TestConfigurationService } from 'vs/platform/configuration/test/common/testConfigurationService';
import { TestInstantiationService } from 'vs/platform/instantiation/test/common/instantiationServiceMock';
import { IFileMatch, IFileQuery, IFileSearchStats, IFolderQuery, ISearchComplete, ISearchProgressItem, ISearchQuery, ISearchService, ITextSearchMatch, OneLineRange, QueryType, TextSearchMatch } from 'vs/workbench/services/search/common/search';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { NullTelemetryService } from 'vs/platform/telemetry/common/telemetryUtils';
import { CellMatch, MatchInNotebook, SearchModel } from 'vs/workbench/contrib/search/browser/searchModel';
import { IThemeService } from 'vs/platform/theme/common/themeService';
import { TestThemeService } from 'vs/platform/theme/test/common/testThemeService';
import { FileService } from 'vs/platform/files/common/fileService';
import { ILogService, NullLogService } from 'vs/platform/log/common/log';
import { IUriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentity';
import { UriIdentityService } from 'vs/platform/uriIdentity/common/uriIdentityService';
import { ILabelService } from 'vs/platform/label/common/label';
import { INotebookEditorService } from 'vs/workbench/contrib/notebook/browser/services/notebookEditorService';
import { IEditorGroupsService } from 'vs/workbench/services/editor/common/editorGroupsService';
import { TestEditorGroupsService } from 'vs/workbench/test/browser/workbenchTestServices';
import { NotebookEditorWidgetService } from 'vs/workbench/contrib/notebook/browser/services/notebookEditorServiceImpl';
import { createFileUriFromPathFromRoot, getRootName } from 'vs/workbench/contrib/search/test/browser/searchTestCommon';
import { ICellMatch, IFileMatchWithCells, contentMatchesToTextSearchMatches, webviewMatchesToTextSearchMatches } from 'vs/workbench/contrib/search/browser/searchNotebookHelpers';
import { CellKind } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { ICellViewModel } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { FindMatch, IReadonlyTextBuffer } from 'vs/editor/common/model';
import { ResourceMap, ResourceSet } from 'vs/base/common/map';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { INotebookSearchService } from 'vs/workbench/contrib/search/browser/notebookSearch';
const nullEvent = new class {
id: number = -1;
topic!: string;
name!: string;
description!: string;
data: any;
startTime!: Date;
stopTime!: Date;
stop(): void {
return;
}
timeTaken(): number {
return -1;
}
};
const lineOneRange = new OneLineRange(1, 0, 1);
suite('SearchModel', () => {
let instantiationService: TestInstantiationService;
const testSearchStats: IFileSearchStats = {
fromCache: false,
resultCount: 1,
type: 'searchProcess',
detailStats: {
fileWalkTime: 0,
cmdTime: 0,
cmdResultCount: 0,
directoriesWalked: 2,
filesWalked: 3
}
};
const folderQueries: IFolderQuery[] = [
{ folder: createFileUriFromPathFromRoot() }
];
setup(() => {
instantiationService = new TestInstantiationService();
instantiationService.stub(ITelemetryService, NullTelemetryService);
instantiationService.stub(ILabelService, { getUriBasenameLabel: (uri: URI) => '' });
instantiationService.stub(INotebookService, { getNotebookTextModels: () => [] });
instantiationService.stub(IModelService, stubModelService(instantiationService));
instantiationService.stub(INotebookEditorService, stubNotebookEditorService(instantiationService));
instantiationService.stub(ISearchService, {});
instantiationService.stub(ISearchService, 'textSearch', Promise.resolve({ results: [] }));
instantiationService.stub(IUriIdentityService, new UriIdentityService(new FileService(new NullLogService())));
instantiationService.stub(ILogService, new NullLogService());
});
teardown(() => sinon.restore());
function searchServiceWithResults(results: IFileMatch[], complete: ISearchComplete | null = null): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void, notebookURIs?: ResourceSet): Promise<ISearchComplete> {
return new Promise(resolve => {
queueMicrotask(() => {
results.forEach(onProgress!);
resolve(complete!);
});
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
return new Promise(resolve => {
queueMicrotask(() => {
resolve({ results: results, messages: [] });
});
});
}
};
}
function searchServiceWithError(error: Error): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void): Promise<ISearchComplete> {
return new Promise((resolve, reject) => {
reject(error);
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
return new Promise((resolve, reject) => {
queueMicrotask(() => {
reject(error);
});
});
}
};
}
function canceleableSearchService(tokenSource: CancellationTokenSource): ISearchService {
return <ISearchService>{
textSearch(query: ISearchQuery, token?: CancellationToken, onProgress?: (result: ISearchProgressItem) => void): Promise<ISearchComplete> {
token?.onCancellationRequested(() => tokenSource.cancel());
return new Promise(resolve => {
queueMicrotask(() => {
resolve(<any>{});
});
});
},
fileSearch(query: IFileQuery, token?: CancellationToken): Promise<ISearchComplete> {
token?.onCancellationRequested(() => tokenSource.cancel());
return new Promise(resolve => {
queueMicrotask(() => {
resolve(<any>{});
});
});
}
};
}
function notebookSearchServiceWithInfo(results: IFileMatchWithCells[], tokenSource: CancellationTokenSource | undefined): INotebookSearchService {
return <INotebookSearchService>{
_serviceBrand: undefined,
notebookSearch(query: ISearchQuery, token: CancellationToken, searchInstanceID: string, onProgress?: (result: ISearchProgressItem) => void, notebookURIs?: ResourceSet): Promise<{ completeData: ISearchComplete; scannedFiles: ResourceSet }> {
token?.onCancellationRequested(() => tokenSource?.cancel());
const localResults = new ResourceMap<IFileMatchWithCells | null>(uri => uri.path);
results.forEach(r => {
localResults.set(r.resource, r);
});
if (onProgress) {
arrays.coalesce([...localResults.values()]).forEach(onProgress);
}
return Promise.resolve(
{
completeData: {
messages: [],
results: arrays.coalesce([...localResults.values()]),
limitHit: false
},
scannedFiles: new ResourceSet([...localResults.keys()]),
});
}
};
}
test('Search Model: Search adds to results', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2', new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
const actual = testObject.searchResult.matches();
assert.strictEqual(2, actual.length);
assert.strictEqual(URI.file(`${getRootName()}/1`).toString(), actual[0].resource.toString());
let actuaMatches = actual[0].matches();
assert.strictEqual(2, actuaMatches.length);
assert.strictEqual('preview 1', actuaMatches[0].text());
assert.ok(new Range(2, 2, 2, 5).equalsRange(actuaMatches[0].range()));
assert.strictEqual('preview 1', actuaMatches[1].text());
assert.ok(new Range(2, 5, 2, 12).equalsRange(actuaMatches[1].range()));
actuaMatches = actual[1].matches();
assert.strictEqual(1, actuaMatches.length);
assert.strictEqual('preview 2', actuaMatches[0].text());
assert.ok(new Range(2, 1, 2, 2).equalsRange(actuaMatches[0].range()));
});
test('Search Model: Search can return notebook results', async () => {
const notebookUri = createFileUriFromPathFromRoot('/1');
const results = [
aRawMatch('/2',
new TextSearchMatch('test', new OneLineRange(1, 1, 5)),
new TextSearchMatch('this is a test', new OneLineRange(1, 11, 15))),
aRawMatch('/3', new TextSearchMatch('test', lineOneRange))];
const searchService = instantiationService.stub(ISearchService, searchServiceWithResults(results));
sinon.stub(CellMatch.prototype, 'addContext');
const textSearch = sinon.spy(searchService, 'textSearch');
const mdInputCell = {
cellKind: CellKind.Markup, textBuffer: <IReadonlyTextBuffer>{
getLineContent(lineNumber: number): string {
if (lineNumber === 1) {
return '# Test';
} else {
return '';
}
}
},
id: 'mdInputCell'
} as ICellViewModel;
const findMatchMds = [new FindMatch(new Range(1, 3, 1, 7), ['Test'])];
const codeCell = {
cellKind: CellKind.Code, textBuffer: <IReadonlyTextBuffer>{
getLineContent(lineNumber: number): string {
if (lineNumber === 1) {
return 'print("test! testing!!")';
} else {
return '';
}
}
},
id: 'codeCell'
} as ICellViewModel;
const findMatchCodeCells =
[new FindMatch(new Range(1, 8, 1, 12), ['test']),
new FindMatch(new Range(1, 14, 1, 18), ['test']),
];
const webviewMatches = [{
index: 0,
searchPreviewInfo: {
line: 'test! testing!!',
range: {
start: 1,
end: 5
}
}
},
{
index: 1,
searchPreviewInfo: {
line: 'test! testing!!',
range: {
start: 7,
end: 11
}
}
}
];
const cellMatchMd: ICellMatch = {
cell: mdInputCell,
index: 0,
contentResults: contentMatchesToTextSearchMatches(findMatchMds, mdInputCell),
webviewResults: []
};
const cellMatchCode: ICellMatch = {
cell: codeCell,
index: 1,
contentResults: contentMatchesToTextSearchMatches(findMatchCodeCells, codeCell),
webviewResults: webviewMatchesToTextSearchMatches(webviewMatches),
};
const notebookSearchService = instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([aRawMatchWithCells('/1', cellMatchMd, cellMatchCode)], undefined));
const notebookSearch = sinon.spy(notebookSearchService, "notebookSearch");
const model: SearchModel = instantiationService.createInstance(SearchModel);
await model.search({ contentPattern: { pattern: 'test' }, type: QueryType.Text, folderQueries });
const actual = model.searchResult.matches();
assert(notebookSearch.calledOnce);
assert(textSearch.getCall(0).args[3]?.size === 1);
assert(textSearch.getCall(0).args[3]?.has(notebookUri)); // ensure that the textsearch knows not to re-source the notebooks
assert.strictEqual(3, actual.length);
assert.strictEqual(URI.file(`${getRootName()}/1`).toString(), actual[0].resource.toString());
const notebookFileMatches = actual[0].matches();
assert.ok(notebookFileMatches[0].range().equalsRange(new Range(1, 3, 1, 7)));
assert.ok(notebookFileMatches[1].range().equalsRange(new Range(1, 8, 1, 12)));
assert.ok(notebookFileMatches[2].range().equalsRange(new Range(1, 14, 1, 18)));
assert.ok(notebookFileMatches[3].range().equalsRange(new Range(1, 2, 1, 6)));
assert.ok(notebookFileMatches[4].range().equalsRange(new Range(1, 8, 1, 12)));
notebookFileMatches.forEach(match => match instanceof MatchInNotebook);
// assert(notebookFileMatches[0] instanceof MatchInNotebook);
assert((notebookFileMatches[0] as MatchInNotebook).cell.id === 'mdInputCell');
assert((notebookFileMatches[1] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[2] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[3] as MatchInNotebook).cell.id === 'codeCell');
assert((notebookFileMatches[4] as MatchInNotebook).cell.id === 'codeCell');
const mdCellMatchProcessed = (notebookFileMatches[0] as MatchInNotebook).cellParent;
const codeCellMatchProcessed = (notebookFileMatches[1] as MatchInNotebook).cellParent;
assert(mdCellMatchProcessed.contentMatches.length === 1);
assert(codeCellMatchProcessed.contentMatches.length === 2);
assert(codeCellMatchProcessed.webviewMatches.length === 2);
assert(mdCellMatchProcessed.contentMatches[0] === notebookFileMatches[0]);
assert(codeCellMatchProcessed.contentMatches[0] === notebookFileMatches[1]);
assert(codeCellMatchProcessed.contentMatches[1] === notebookFileMatches[2]);
assert(codeCellMatchProcessed.webviewMatches[0] === notebookFileMatches[3]);
assert(codeCellMatchProcessed.webviewMatches[1] === notebookFileMatches[4]);
assert.strictEqual(URI.file(`${getRootName()}/2`).toString(), actual[1].resource.toString());
assert.strictEqual(URI.file(`${getRootName()}/3`).toString(), actual[2].resource.toString());
});
test('Search Model: Search reports telemetry on search completed', async () => {
const target = instantiationService.spy(ITelemetryService, 'publicLog');
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2',
new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(target.calledThrice);
assert.ok(target.calledWith('searchResultsFirstRender'));
assert.ok(target.calledWith('searchResultsFinished'));
});
test('Search Model: Search reports timed telemetry on search when progress is not called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithResults([]));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
});
});
});
test('Search Model: Search reports timed telemetry on search when progress is called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithResults(
[aRawMatch('/1', new TextSearchMatch('some preview', lineOneRange))],
{ results: [], stats: testSearchStats, messages: [] }));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => {
return timeout(1).then(() => {
// timeout because promise handlers may run in a different order. We only care that these
// are fired at some point.
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.strictEqual(1, target2.callCount);
});
});
});
test('Search Model: Search reports timed telemetry on search when error is called', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
instantiationService.stub(ISearchService, searchServiceWithError(new Error('error')));
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
return result.then(() => { }, () => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.ok(target2.calledOnce);
});
});
});
test('Search Model: Search reports timed telemetry on search when error is cancelled error', () => {
const target2 = sinon.spy();
sinon.stub(nullEvent, 'stop').callsFake(target2);
const target1 = sinon.stub().returns(nullEvent);
instantiationService.stub(ITelemetryService, 'publicLog', target1);
const deferredPromise = new DeferredPromise<ISearchComplete>();
instantiationService.stub(ISearchService, 'textSearch', deferredPromise.p);
const testObject = instantiationService.createInstance(SearchModel);
const result = testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
deferredPromise.cancel();
return result.then(() => { }, () => {
return timeout(1).then(() => {
assert.ok(target1.calledWith('searchResultsFirstRender'));
assert.ok(target1.calledWith('searchResultsFinished'));
// assert.ok(target2.calledOnce);
});
});
});
test('Search Model: Search results are cleared during search', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11))),
aRawMatch('/2',
new TextSearchMatch('preview 2', lineOneRange))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(!testObject.searchResult.isEmpty());
instantiationService.stub(ISearchService, searchServiceWithResults([]));
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(testObject.searchResult.isEmpty());
});
test('Search Model: Previous search is cancelled when new search is called', async () => {
const tokenSource = new CancellationTokenSource();
instantiationService.stub(ISearchService, canceleableSearchService(tokenSource));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], tokenSource));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
instantiationService.stub(ISearchService, searchServiceWithResults([]));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
testObject.search({ contentPattern: { pattern: 'somestring' }, type: QueryType.Text, folderQueries });
assert.ok(tokenSource.token.isCancellationRequested);
});
test('getReplaceString returns proper replace string for regExpressions', async () => {
const results = [
aRawMatch('/1',
new TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),
new TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];
instantiationService.stub(ISearchService, searchServiceWithResults(results));
instantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));
const testObject: SearchModel = instantiationService.createInstance(SearchModel);
await testObject.search({ contentPattern: { pattern: 're' }, type: QueryType.Text, folderQueries });
testObject.replaceString = 'hello';
let match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 're', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 're(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 'r(e)(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('hello', match.replaceString);
await testObject.search({ contentPattern: { pattern: 'r(e)(?:vi)', isRegExp: true }, type: QueryType.Text, folderQueries });
testObject.replaceString = 'hello$1';
match = testObject.searchResult.matches()[0].matches()[0];
assert.strictEqual('helloe', match.replaceString);
});
function aRawMatch(resource: string, ...results: ITextSearchMatch[]): IFileMatch {
return { resource: createFileUriFromPathFromRoot(resource), results };
}
function aRawMatchWithCells(resource: string, ...cells: ICellMatch[]) {
return { resource: createFileUriFromPathFromRoot(resource), cellResults: cells };
}
function stubModelService(instantiationService: TestInstantiationService): IModelService {
instantiationService.stub(IThemeService, new TestThemeService());
const config = new TestConfigurationService();
config.setUserConfiguration('search', { searchOnType: true });
instantiationService.stub(IConfigurationService, config);
return instantiationService.createInstance(ModelService);
}
function stubNotebookEditorService(instantiationService: TestInstantiationService): INotebookEditorService {
instantiationService.stub(IEditorGroupsService, new TestEditorGroupsService());
return instantiationService.createInstance(NotebookEditorWidgetService);
}
});
| src/vs/workbench/contrib/search/test/browser/searchModel.test.ts | 1 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.9983620047569275,
0.0953035056591034,
0.0001620678958715871,
0.00027751162997446954,
0.28815212845802307
] |
{
"id": 6,
"code_window": [
"\ttest('getReplaceString returns proper replace string for regExpressions', async () => {\n",
"\t\tconst results = [\n",
"\t\t\taRawMatch('/1',\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 485
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import 'mocha';
import { CancellationTokenSource, CompletionTriggerKind, Selection } from 'vscode';
import { DefaultCompletionItemProvider } from '../defaultCompletionProvider';
import { closeAllEditors, withRandomFileEditor } from './testUtils';
const completionProvider = new DefaultCompletionItemProvider();
suite('Tests for completion in CSS embedded in HTML', () => {
teardown(closeAllEditors);
test('style attribute & attribute value in html', async () => {
await testCompletionProvider('html', '<div style="|"', [{ label: 'padding: ;' }]);
await testCompletionProvider('html', `<div style='|'`, [{ label: 'padding: ;' }]);
await testCompletionProvider('html', `<div style='p|'`, [{ label: 'padding: ;' }]);
await testCompletionProvider('html', `<div style='color: #0|'`, [{ label: '#000000' }]);
});
// https://github.com/microsoft/vscode/issues/79766
test('#79766, correct region determination', async () => {
await testCompletionProvider('html', `<div style="color: #000">di|</div>`, [
{ label: 'div', documentation: `<div>|</div>` }
]);
});
// https://github.com/microsoft/vscode/issues/86941
test('#86941, widows should not be completed', async () => {
await testCompletionProvider('css', `.foo { wi| }`, undefined);
});
// https://github.com/microsoft/vscode/issues/117020
test('#117020, ! at end of abbreviation should have completion', async () => {
await testCompletionProvider('css', `.foo { bdbn!| }`, [
{ label: 'border-bottom: none !important;', documentation: `border-bottom: none !important;` }
]);
});
// https://github.com/microsoft/vscode/issues/138461
test('#138461, JSX array noise', async () => {
await testCompletionProvider('jsx', 'a[i]', undefined);
await testCompletionProvider('jsx', 'Component[a b]', undefined);
await testCompletionProvider('jsx', '[a, b]', undefined);
await testCompletionProvider('jsx', '[a=b]', [
{ label: '<div a="b"></div>', documentation: '<div a="b">|</div>' }
]);
});
});
interface TestCompletionItem {
label: string;
documentation?: string;
}
function testCompletionProvider(fileExtension: string, contents: string, expectedItems: TestCompletionItem[] | undefined): Thenable<boolean> {
const cursorPos = contents.indexOf('|');
const slicedContents = contents.slice(0, cursorPos) + contents.slice(cursorPos + 1);
return withRandomFileEditor(slicedContents, fileExtension, async (editor, _doc) => {
const selection = new Selection(editor.document.positionAt(cursorPos), editor.document.positionAt(cursorPos));
editor.selection = selection;
const cancelSrc = new CancellationTokenSource();
const completionPromise = completionProvider.provideCompletionItems(
editor.document,
editor.selection.active,
cancelSrc.token,
{ triggerKind: CompletionTriggerKind.Invoke, triggerCharacter: undefined }
);
if (!completionPromise) {
return Promise.resolve();
}
const completionList = await completionPromise;
if (!completionList || !completionList.items || !completionList.items.length) {
if (completionList === undefined) {
assert.strictEqual(expectedItems, completionList);
}
return Promise.resolve();
}
assert.strictEqual(expectedItems === undefined, false);
expectedItems!.forEach(eItem => {
const matches = completionList.items.filter(i => i.label === eItem.label);
const match = matches && matches.length > 0 ? matches[0] : undefined;
assert.ok(match, `Didn't find completion item with label ${eItem.label}`);
if (match) {
assert.strictEqual(match.detail, 'Emmet Abbreviation', `Match needs to come from Emmet`);
if (eItem.documentation) {
assert.strictEqual(match.documentation, eItem.documentation, `Emmet completion Documentation doesn't match`);
}
}
});
return Promise.resolve();
});
}
| extensions/emmet/src/test/completion.test.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.00017769956320989877,
0.0001747431088006124,
0.00017200085858348757,
0.00017490201571490616,
0.000001658305791352177
] |
{
"id": 6,
"code_window": [
"\ttest('getReplaceString returns proper replace string for regExpressions', async () => {\n",
"\t\tconst results = [\n",
"\t\t\taRawMatch('/1',\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 485
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Event } from 'vs/base/common/event';
import { ScanCode, ScanCodeUtils } from 'vs/base/common/keyCodes';
import { createDecorator } from 'vs/platform/instantiation/common/instantiation';
import { IKeyboardEvent } from 'vs/platform/keybinding/common/keybinding';
import { IKeyboardMapper } from 'vs/platform/keyboardLayout/common/keyboardMapper';
export const IKeyboardLayoutService = createDecorator<IKeyboardLayoutService>('keyboardLayoutService');
export interface IWindowsKeyMapping {
vkey: string;
value: string;
withShift: string;
withAltGr: string;
withShiftAltGr: string;
}
export interface IWindowsKeyboardMapping {
[code: string]: IWindowsKeyMapping;
}
export interface ILinuxKeyMapping {
value: string;
withShift: string;
withAltGr: string;
withShiftAltGr: string;
}
export interface ILinuxKeyboardMapping {
[code: string]: ILinuxKeyMapping;
}
export interface IMacKeyMapping {
value: string;
valueIsDeadKey: boolean;
withShift: string;
withShiftIsDeadKey: boolean;
withAltGr: string;
withAltGrIsDeadKey: boolean;
withShiftAltGr: string;
withShiftAltGrIsDeadKey: boolean;
}
export interface IMacKeyboardMapping {
[code: string]: IMacKeyMapping;
}
export type IMacLinuxKeyMapping = IMacKeyMapping | ILinuxKeyMapping;
export type IMacLinuxKeyboardMapping = IMacKeyboardMapping | ILinuxKeyboardMapping;
export type IKeyboardMapping = IWindowsKeyboardMapping | ILinuxKeyboardMapping | IMacKeyboardMapping;
export interface IWindowsKeyboardLayoutInfo {
name: string;
id: string;
text: string;
}
export interface ILinuxKeyboardLayoutInfo {
model: string;
group: number;
layout: string;
variant: string;
options: string;
rules: string;
}
export interface IMacKeyboardLayoutInfo {
id: string;
lang: string;
localizedName?: string;
}
export type IKeyboardLayoutInfo = (IWindowsKeyboardLayoutInfo | ILinuxKeyboardLayoutInfo | IMacKeyboardLayoutInfo) & { isUserKeyboardLayout?: boolean; isUSStandard?: true };
export interface IKeyboardLayoutService {
readonly _serviceBrand: undefined;
readonly onDidChangeKeyboardLayout: Event<void>;
getRawKeyboardMapping(): IKeyboardMapping | null;
getCurrentKeyboardLayout(): IKeyboardLayoutInfo | null;
getAllKeyboardLayouts(): IKeyboardLayoutInfo[];
getKeyboardMapper(): IKeyboardMapper;
validateCurrentKeyboardMapping(keyboardEvent: IKeyboardEvent): void;
}
export function areKeyboardLayoutsEqual(a: IKeyboardLayoutInfo | null, b: IKeyboardLayoutInfo | null): boolean {
if (!a || !b) {
return false;
}
if ((<IWindowsKeyboardLayoutInfo>a).name && (<IWindowsKeyboardLayoutInfo>b).name && (<IWindowsKeyboardLayoutInfo>a).name === (<IWindowsKeyboardLayoutInfo>b).name) {
return true;
}
if ((<IMacKeyboardLayoutInfo>a).id && (<IMacKeyboardLayoutInfo>b).id && (<IMacKeyboardLayoutInfo>a).id === (<IMacKeyboardLayoutInfo>b).id) {
return true;
}
if ((<ILinuxKeyboardLayoutInfo>a).model &&
(<ILinuxKeyboardLayoutInfo>b).model &&
(<ILinuxKeyboardLayoutInfo>a).model === (<ILinuxKeyboardLayoutInfo>b).model &&
(<ILinuxKeyboardLayoutInfo>a).layout === (<ILinuxKeyboardLayoutInfo>b).layout
) {
return true;
}
return false;
}
export function parseKeyboardLayoutDescription(layout: IKeyboardLayoutInfo | null): { label: string; description: string } {
if (!layout) {
return { label: '', description: '' };
}
if ((<IWindowsKeyboardLayoutInfo>layout).name) {
// windows
const windowsLayout = <IWindowsKeyboardLayoutInfo>layout;
return {
label: windowsLayout.text,
description: ''
};
}
if ((<IMacKeyboardLayoutInfo>layout).id) {
const macLayout = <IMacKeyboardLayoutInfo>layout;
if (macLayout.localizedName) {
return {
label: macLayout.localizedName,
description: ''
};
}
if (/^com\.apple\.keylayout\./.test(macLayout.id)) {
return {
label: macLayout.id.replace(/^com\.apple\.keylayout\./, '').replace(/-/, ' '),
description: ''
};
}
if (/^.*inputmethod\./.test(macLayout.id)) {
return {
label: macLayout.id.replace(/^.*inputmethod\./, '').replace(/[-\.]/, ' '),
description: `Input Method (${macLayout.lang})`
};
}
return {
label: macLayout.lang,
description: ''
};
}
const linuxLayout = <ILinuxKeyboardLayoutInfo>layout;
return {
label: linuxLayout.layout,
description: ''
};
}
export function getKeyboardLayoutId(layout: IKeyboardLayoutInfo): string {
if ((<IWindowsKeyboardLayoutInfo>layout).name) {
return (<IWindowsKeyboardLayoutInfo>layout).name;
}
if ((<IMacKeyboardLayoutInfo>layout).id) {
return (<IMacKeyboardLayoutInfo>layout).id;
}
return (<ILinuxKeyboardLayoutInfo>layout).layout;
}
function windowsKeyMappingEquals(a: IWindowsKeyMapping, b: IWindowsKeyMapping): boolean {
if (!a && !b) {
return true;
}
if (!a || !b) {
return false;
}
return (
a.vkey === b.vkey
&& a.value === b.value
&& a.withShift === b.withShift
&& a.withAltGr === b.withAltGr
&& a.withShiftAltGr === b.withShiftAltGr
);
}
export function windowsKeyboardMappingEquals(a: IWindowsKeyboardMapping | null, b: IWindowsKeyboardMapping | null): boolean {
if (!a && !b) {
return true;
}
if (!a || !b) {
return false;
}
for (let scanCode = 0; scanCode < ScanCode.MAX_VALUE; scanCode++) {
const strScanCode = ScanCodeUtils.toString(scanCode);
const aEntry = a[strScanCode];
const bEntry = b[strScanCode];
if (!windowsKeyMappingEquals(aEntry, bEntry)) {
return false;
}
}
return true;
}
function macLinuxKeyMappingEquals(a: IMacLinuxKeyMapping, b: IMacLinuxKeyMapping): boolean {
if (!a && !b) {
return true;
}
if (!a || !b) {
return false;
}
return (
a.value === b.value
&& a.withShift === b.withShift
&& a.withAltGr === b.withAltGr
&& a.withShiftAltGr === b.withShiftAltGr
);
}
export function macLinuxKeyboardMappingEquals(a: IMacLinuxKeyboardMapping | null, b: IMacLinuxKeyboardMapping | null): boolean {
if (!a && !b) {
return true;
}
if (!a || !b) {
return false;
}
for (let scanCode = 0; scanCode < ScanCode.MAX_VALUE; scanCode++) {
const strScanCode = ScanCodeUtils.toString(scanCode);
const aEntry = a[strScanCode];
const bEntry = b[strScanCode];
if (!macLinuxKeyMappingEquals(aEntry, bEntry)) {
return false;
}
}
return true;
}
| src/vs/platform/keyboardLayout/common/keyboardLayout.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.00017546261369716376,
0.0001718475978123024,
0.00016521818179171532,
0.00017254980048164725,
0.0000031686258807894774
] |
{
"id": 6,
"code_window": [
"\ttest('getReplaceString returns proper replace string for regExpressions', async () => {\n",
"\t\tconst results = [\n",
"\t\t\taRawMatch('/1',\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 1, 4)),\n",
"\t\t\t\tnew TextSearchMatch('preview 1', new OneLineRange(1, 4, 11)))];\n",
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results));\n",
"\t\tinstantiationService.stub(INotebookSearchService, notebookSearchServiceWithInfo([], undefined));\n",
"\n",
"\t\tconst testObject: SearchModel = instantiationService.createInstance(SearchModel);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tinstantiationService.stub(ISearchService, searchServiceWithResults(results, { limitHit: false, messages: [], results }));\n"
],
"file_path": "src/vs/workbench/contrib/search/test/browser/searchModel.test.ts",
"type": "replace",
"edit_start_line_idx": 485
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { Extensions as ConfigurationExtensions, IConfigurationRegistry } from 'vs/platform/configuration/common/configurationRegistry';
import { Registry } from 'vs/platform/registry/common/platform';
suite('ConfigurationRegistry', () => {
const configurationRegistry = Registry.as<IConfigurationRegistry>(ConfigurationExtensions.Configuration);
test('configuration override', async () => {
configurationRegistry.registerConfiguration({
'id': '_test_default',
'type': 'object',
'properties': {
'config': {
'type': 'object',
}
}
});
configurationRegistry.registerDefaultConfigurations([{ overrides: { 'config': { a: 1, b: 2 } } }]);
configurationRegistry.registerDefaultConfigurations([{ overrides: { '[lang]': { a: 2, c: 3 } } }]);
assert.deepStrictEqual(configurationRegistry.getConfigurationProperties()['config'].default, { a: 1, b: 2 });
assert.deepStrictEqual(configurationRegistry.getConfigurationProperties()['[lang]'].default, { a: 2, c: 3 });
});
test('configuration override defaults - merges defaults', async () => {
configurationRegistry.registerDefaultConfigurations([{ overrides: { '[lang]': { a: 1, b: 2 } } }]);
configurationRegistry.registerDefaultConfigurations([{ overrides: { '[lang]': { a: 2, c: 3 } } }]);
assert.deepStrictEqual(configurationRegistry.getConfigurationProperties()['[lang]'].default, { a: 2, b: 2, c: 3 });
});
test('configuration defaults - overrides defaults', async () => {
configurationRegistry.registerConfiguration({
'id': '_test_default',
'type': 'object',
'properties': {
'config': {
'type': 'object',
}
}
});
configurationRegistry.registerDefaultConfigurations([{ overrides: { 'config': { a: 1, b: 2 } } }]);
configurationRegistry.registerDefaultConfigurations([{ overrides: { 'config': { a: 2, c: 3 } } }]);
assert.deepStrictEqual(configurationRegistry.getConfigurationProperties()['config'].default, { a: 2, c: 3 });
});
test('registering multiple settings with same policy', async () => {
configurationRegistry.registerConfiguration({
'id': '_test_default',
'type': 'object',
'properties': {
'policy1': {
'type': 'object',
policy: {
name: 'policy',
minimumVersion: '1.0.0'
}
},
'policy2': {
'type': 'object',
policy: {
name: 'policy',
minimumVersion: '1.0.0'
}
}
}
});
const actual = configurationRegistry.getConfigurationProperties();
assert.ok(actual['policy1'] !== undefined);
assert.ok(actual['policy2'] === undefined);
});
});
| src/vs/platform/configuration/test/common/configurationRegistry.test.ts | 0 | https://github.com/microsoft/vscode/commit/2bf46260dca5d91b7ac6a1933f37ef25dc0368ca | [
0.0001757186109898612,
0.00017462577670812607,
0.00017195461259689182,
0.00017486896831542253,
0.0000011714744232449448
] |
{
"id": 2,
"code_window": [
"\t\tawait this._webview?.removeMarkdownPreview(cell.id);\n",
"\t}\n",
"\n",
"\tasync updateMarkdownPreviewSelectionState(cell: ICellViewModel, isSelected: boolean): Promise<void> {\n",
"\t\tif (!this.useRenderer) {\n",
"\t\t\t// TODO: handle case where custom renderer is disabled?\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview.isResolved()) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tprivate async updateSelectedMarkdownPreviews(): Promise<void> {\n",
"\t\tif (!this.useRenderer || !this._webview) {\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2069
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as DOM from 'vs/base/browser/dom';
import { raceCancellation } from 'vs/base/common/async';
import { CancellationTokenSource } from 'vs/base/common/cancellation';
import { Disposable, DisposableStore, IDisposable, toDisposable } from 'vs/base/common/lifecycle';
import { CodeEditorWidget } from 'vs/editor/browser/widget/codeEditorWidget';
import { IEditorOptions } from 'vs/editor/common/config/editorOptions';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { EDITOR_BOTTOM_PADDING } from 'vs/workbench/contrib/notebook/browser/constants';
import { CellEditState, CellFocusMode, MarkdownCellRenderTemplate, ICellViewModel, getEditorTopPadding, IActiveNotebookEditor } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { CellFoldingState } from 'vs/workbench/contrib/notebook/browser/contrib/fold/foldingModel';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { ServiceCollection } from 'vs/platform/instantiation/common/serviceCollection';
import { EditorContextKeys } from 'vs/editor/common/editorContextKeys';
import { getExecuteCellPlaceholder, getResizesObserver } from 'vs/workbench/contrib/notebook/browser/view/renderers/cellWidgets';
import { INotebookCellStatusBarService } from 'vs/workbench/contrib/notebook/common/notebookCellStatusBarService';
import { NotebookCellsChangeType } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { collapsedIcon, expandedIcon } from 'vs/workbench/contrib/notebook/browser/notebookIcons';
import { renderIcon } from 'vs/base/browser/ui/iconLabel/iconLabels';
interface IMarkdownRenderStrategy extends IDisposable {
update(): void;
}
class WebviewMarkdownRenderer extends Disposable implements IMarkdownRenderStrategy {
constructor(
readonly notebookEditor: IActiveNotebookEditor,
readonly viewCell: MarkdownCellViewModel
) {
super();
}
update(): void {
this.notebookEditor.createMarkdownPreview(this.viewCell);
}
}
class BuiltinMarkdownRenderer extends Disposable implements IMarkdownRenderStrategy {
private readonly localDisposables = this._register(new DisposableStore());
constructor(
private readonly notebookEditor: IActiveNotebookEditor,
private readonly viewCell: MarkdownCellViewModel,
private readonly container: HTMLElement,
private readonly markdownContainer: HTMLElement,
private readonly editorAccessor: () => CodeEditorWidget | null
) {
super();
this._register(getResizesObserver(this.markdownContainer, undefined, () => {
if (viewCell.editState === CellEditState.Preview) {
this.viewCell.renderedMarkdownHeight = container.clientHeight;
}
})).startObserving();
}
update(): void {
const markdownRenderer = this.viewCell.getMarkdownRenderer();
const renderedHTML = this.viewCell.getHTML();
if (renderedHTML) {
this.markdownContainer.appendChild(renderedHTML);
}
if (this.editorAccessor()) {
// switch from editing mode
this.viewCell.renderedMarkdownHeight = this.container.clientHeight;
this.relayoutCell();
} else {
this.localDisposables.clear();
this.localDisposables.add(markdownRenderer.onDidRenderAsync(() => {
if (this.viewCell.editState === CellEditState.Preview) {
this.viewCell.renderedMarkdownHeight = this.container.clientHeight;
}
this.relayoutCell();
}));
this.localDisposables.add(this.viewCell.textBuffer.onDidChangeContent(() => {
this.markdownContainer.innerText = '';
this.viewCell.clearHTML();
const renderedHTML = this.viewCell.getHTML();
if (renderedHTML) {
this.markdownContainer.appendChild(renderedHTML);
}
}));
this.viewCell.renderedMarkdownHeight = this.container.clientHeight;
this.relayoutCell();
}
}
relayoutCell() {
this.notebookEditor.layoutNotebookCell(this.viewCell, this.viewCell.layoutInfo.totalHeight);
}
}
export class StatefulMarkdownCell extends Disposable {
private editor: CodeEditorWidget | null = null;
private markdownContainer: HTMLElement;
private editorPart: HTMLElement;
private readonly localDisposables = new DisposableStore();
private foldingState: CellFoldingState;
private activeCellRunPlaceholder: IDisposable | null = null;
private useRenderer: boolean = false;
private renderStrategy: IMarkdownRenderStrategy;
constructor(
private readonly notebookEditor: IActiveNotebookEditor,
private readonly viewCell: MarkdownCellViewModel,
private readonly templateData: MarkdownCellRenderTemplate,
private editorOptions: IEditorOptions,
private readonly renderedEditors: Map<ICellViewModel, ICodeEditor | undefined>,
options: { useRenderer: boolean },
@IContextKeyService private readonly contextKeyService: IContextKeyService,
@INotebookCellStatusBarService readonly notebookCellStatusBarService: INotebookCellStatusBarService,
@IInstantiationService private readonly instantiationService: IInstantiationService,
) {
super();
this.markdownContainer = templateData.cellContainer;
this.editorPart = templateData.editorPart;
this.useRenderer = options.useRenderer;
if (this.useRenderer) {
this.templateData.container.classList.toggle('webview-backed-markdown-cell', true);
this.renderStrategy = new WebviewMarkdownRenderer(this.notebookEditor, this.viewCell);
} else {
this.renderStrategy = new BuiltinMarkdownRenderer(this.notebookEditor, this.viewCell, this.templateData.container, this.markdownContainer, () => this.editor);
}
this._register(this.renderStrategy);
this._register(toDisposable(() => renderedEditors.delete(this.viewCell)));
this._register(viewCell.onDidChangeState((e) => {
if (e.editStateChanged) {
this.viewUpdate();
} else if (e.contentChanged) {
this.viewUpdate();
}
}));
this._register(viewCell.model.onDidChangeMetadata(() => {
this.viewUpdate();
}));
const updateForFocusMode = () => {
if (viewCell.focusMode === CellFocusMode.Editor) {
this.focusEditorIfNeeded();
}
templateData.container.classList.toggle('cell-editor-focus', viewCell.focusMode === CellFocusMode.Editor);
};
this._register(viewCell.onDidChangeState((e) => {
if (!e.focusModeChanged) {
return;
}
updateForFocusMode();
}));
updateForFocusMode();
this.foldingState = viewCell.foldingState;
this.setFoldingIndicator();
this._register(viewCell.onDidChangeState((e) => {
if (!e.foldingStateChanged) {
return;
}
const foldingState = viewCell.foldingState;
if (foldingState !== this.foldingState) {
this.foldingState = foldingState;
this.setFoldingIndicator();
}
}));
this._register(viewCell.onDidChangeLayout((e) => {
const layoutInfo = this.editor?.getLayoutInfo();
if (e.outerWidth && this.viewCell.editState === CellEditState.Editing && layoutInfo && layoutInfo.width !== viewCell.layoutInfo.editorWidth) {
this.onCellEditorWidthChange();
} else if (e.totalHeight || e.outerWidth) {
this.relayoutCell();
}
}));
if (this.useRenderer) {
// the markdown preview's height might already be updated after the renderer calls `element.getHeight()`
if (this.viewCell.layoutInfo.totalHeight > 0) {
this.relayoutCell();
}
// Update for selection
this._register(this.notebookEditor.onDidChangeSelection(() => {
const selectedCells = this.notebookEditor.getSelectionViewModels();
// Only show selection if there are more than one cells selected
const isSelected = selectedCells.length > 1 && selectedCells.some(selectedCell => selectedCell === viewCell);
this.notebookEditor.updateMarkdownPreviewSelectionState(viewCell, isSelected);
}));
}
// apply decorations
this._register(viewCell.onCellDecorationsChanged((e) => {
e.added.forEach(options => {
if (options.className) {
if (this.useRenderer) {
this.notebookEditor.deltaCellOutputContainerClassNames(this.viewCell.id, [options.className], []);
} else {
templateData.rootContainer.classList.add(options.className);
}
}
});
e.removed.forEach(options => {
if (options.className) {
if (this.useRenderer) {
this.notebookEditor.deltaCellOutputContainerClassNames(this.viewCell.id, [], [options.className]);
} else {
templateData.rootContainer.classList.remove(options.className);
}
}
});
}));
viewCell.getCellDecorations().forEach(options => {
if (options.className) {
if (this.useRenderer) {
this.notebookEditor.deltaCellOutputContainerClassNames(this.viewCell.id, [options.className], []);
} else {
templateData.rootContainer.classList.add(options.className);
}
}
});
this.viewUpdate();
const updatePlaceholder = () => {
if (
this.notebookEditor.getActiveCell() === this.viewCell
&& !!this.notebookEditor.viewModel.metadata.trusted
) {
// active cell and no run status
if (this.activeCellRunPlaceholder === null) {
// const keybinding = this._keybindingService.lookupKeybinding(EXECUTE_CELL_COMMAND_ID);
const placeholder = getExecuteCellPlaceholder(this.viewCell);
if (!this.notebookCellStatusBarService.getEntries(this.viewCell.uri).find(entry => entry.text === placeholder.text && entry.command === placeholder.command)) {
this.activeCellRunPlaceholder = this.notebookCellStatusBarService.addEntry(placeholder);
this._register(this.activeCellRunPlaceholder);
}
}
return;
}
this.activeCellRunPlaceholder?.dispose();
this.activeCellRunPlaceholder = null;
};
this._register(this.notebookEditor.onDidChangeActiveCell(() => {
updatePlaceholder();
}));
this._register(this.viewCell.model.onDidChangeMetadata(() => {
updatePlaceholder();
}));
this._register(this.notebookEditor.viewModel.notebookDocument.onDidChangeContent(e => {
if (e.rawEvents.find(event => event.kind === NotebookCellsChangeType.ChangeDocumentMetadata)) {
updatePlaceholder();
}
}));
updatePlaceholder();
}
dispose() {
this.localDisposables.dispose();
this.viewCell.detachTextEditor();
super.dispose();
}
private viewUpdate(): void {
if (this.viewCell.metadata?.inputCollapsed) {
this.viewUpdateCollapsed();
} else if (this.viewCell.editState === CellEditState.Editing) {
this.viewUpdateEditing();
} else {
this.viewUpdatePreview();
}
}
private viewUpdateCollapsed(): void {
DOM.show(this.templateData.collapsedPart);
DOM.hide(this.editorPart);
DOM.hide(this.markdownContainer);
this.templateData.container.classList.toggle('collapsed', true);
this.viewCell.renderedMarkdownHeight = 0;
}
private viewUpdateEditing(): void {
// switch to editing mode
let editorHeight: number;
DOM.show(this.editorPart);
DOM.hide(this.markdownContainer);
DOM.hide(this.templateData.collapsedPart);
if (this.useRenderer) {
this.notebookEditor.hideMarkdownPreview(this.viewCell);
}
this.templateData.container.classList.toggle('collapsed', false);
this.templateData.container.classList.toggle('markdown-cell-edit-mode', true);
if (this.editor) {
editorHeight = this.editor.getContentHeight();
// not first time, we don't need to create editor or bind listeners
this.viewCell.attachTextEditor(this.editor);
this.focusEditorIfNeeded();
this.bindEditorListeners(this.editor);
this.editor.layout({
width: this.viewCell.layoutInfo.editorWidth,
height: editorHeight
});
} else {
const width = this.viewCell.layoutInfo.editorWidth;
const lineNum = this.viewCell.lineCount;
const lineHeight = this.viewCell.layoutInfo.fontInfo?.lineHeight || 17;
editorHeight = Math.max(lineNum, 1) * lineHeight + getEditorTopPadding() + EDITOR_BOTTOM_PADDING;
this.templateData.editorContainer.innerText = '';
// create a special context key service that set the inCompositeEditor-contextkey
const editorContextKeyService = this.contextKeyService.createScoped(this.templateData.editorPart);
EditorContextKeys.inCompositeEditor.bindTo(editorContextKeyService).set(true);
const editorInstaService = this.instantiationService.createChild(new ServiceCollection([IContextKeyService, editorContextKeyService]));
this._register(editorContextKeyService);
this.editor = this._register(editorInstaService.createInstance(CodeEditorWidget, this.templateData.editorContainer, {
...this.editorOptions,
dimension: {
width: width,
height: editorHeight
},
// overflowWidgetsDomNode: this.notebookEditor.getOverflowContainerDomNode()
}, {}));
this.templateData.currentEditor = this.editor;
const cts = new CancellationTokenSource();
this._register({ dispose() { cts.dispose(true); } });
raceCancellation(this.viewCell.resolveTextModel(), cts.token).then(model => {
if (!model) {
return;
}
this.editor!.setModel(model);
this.focusEditorIfNeeded();
const realContentHeight = this.editor!.getContentHeight();
if (realContentHeight !== editorHeight) {
this.editor!.layout(
{
width: width,
height: realContentHeight
}
);
editorHeight = realContentHeight;
}
this.viewCell.attachTextEditor(this.editor!);
if (this.viewCell.editState === CellEditState.Editing) {
this.focusEditorIfNeeded();
}
this.bindEditorListeners(this.editor!);
this.viewCell.editorHeight = editorHeight;
});
}
this.viewCell.editorHeight = editorHeight;
this.focusEditorIfNeeded();
this.renderedEditors.set(this.viewCell, this.editor!);
}
private viewUpdatePreview(): void {
this.viewCell.detachTextEditor();
DOM.hide(this.editorPart);
DOM.hide(this.templateData.collapsedPart);
DOM.show(this.markdownContainer);
this.templateData.container.classList.toggle('collapsed', false);
this.templateData.container.classList.toggle('markdown-cell-edit-mode', false);
this.renderedEditors.delete(this.viewCell);
this.markdownContainer.innerText = '';
this.viewCell.clearHTML();
this.renderStrategy.update();
}
private focusEditorIfNeeded() {
if (this.viewCell.focusMode === CellFocusMode.Editor && this.notebookEditor.hasFocus()) {
this.editor?.focus();
}
}
private layoutEditor(dimension: DOM.IDimension): void {
this.editor?.layout(dimension);
this.templateData.statusBar.layout(dimension.width);
}
private onCellEditorWidthChange(): void {
const realContentHeight = this.editor!.getContentHeight();
this.layoutEditor(
{
width: this.viewCell.layoutInfo.editorWidth,
height: realContentHeight
}
);
// LET the content size observer to handle it
// this.viewCell.editorHeight = realContentHeight;
// this.relayoutCell();
}
relayoutCell(): void {
this.notebookEditor.layoutNotebookCell(this.viewCell, this.viewCell.layoutInfo.totalHeight);
}
updateEditorOptions(newValue: IEditorOptions): void {
this.editorOptions = newValue;
if (this.editor) {
this.editor.updateOptions(this.editorOptions);
}
}
setFoldingIndicator() {
switch (this.foldingState) {
case CellFoldingState.None:
this.templateData.foldingIndicator.innerText = '';
break;
case CellFoldingState.Collapsed:
DOM.reset(this.templateData.foldingIndicator, renderIcon(collapsedIcon));
break;
case CellFoldingState.Expanded:
DOM.reset(this.templateData.foldingIndicator, renderIcon(expandedIcon));
break;
default:
break;
}
}
private bindEditorListeners(editor: CodeEditorWidget) {
this.localDisposables.clear();
this.localDisposables.add(editor.onDidContentSizeChange(e => {
const viewLayout = editor.getLayoutInfo();
if (e.contentHeightChanged) {
this.viewCell.editorHeight = e.contentHeight;
editor.layout(
{
width: viewLayout.width,
height: e.contentHeight
}
);
}
}));
this.localDisposables.add(editor.onDidChangeCursorSelection((e) => {
if (e.source === 'restoreState') {
// do not reveal the cell into view if this selection change was caused by restoring editors...
return;
}
const primarySelection = editor.getSelection();
if (primarySelection) {
this.notebookEditor.revealLineInViewAsync(this.viewCell, primarySelection.positionLineNumber);
}
}));
const updateFocusMode = () => this.viewCell.focusMode = editor.hasWidgetFocus() ? CellFocusMode.Editor : CellFocusMode.Container;
this.localDisposables.add(editor.onDidFocusEditorWidget(() => {
updateFocusMode();
}));
this.localDisposables.add(editor.onDidBlurEditorWidget(() => {
// this is for a special case:
// users click the status bar empty space, which we will then focus the editor
// so we don't want to update the focus state too eagerly
if (document.activeElement?.contains(this.templateData.container)) {
setTimeout(() => {
updateFocusMode();
}, 300);
} else {
updateFocusMode();
}
}));
updateFocusMode();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/markdownCell.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.9991599321365356,
0.019888250157237053,
0.00016324508760590106,
0.00017004368419293314,
0.13584968447685242
] |
{
"id": 2,
"code_window": [
"\t\tawait this._webview?.removeMarkdownPreview(cell.id);\n",
"\t}\n",
"\n",
"\tasync updateMarkdownPreviewSelectionState(cell: ICellViewModel, isSelected: boolean): Promise<void> {\n",
"\t\tif (!this.useRenderer) {\n",
"\t\t\t// TODO: handle case where custom renderer is disabled?\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview.isResolved()) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tprivate async updateSelectedMarkdownPreviews(): Promise<void> {\n",
"\t\tif (!this.useRenderer || !this._webview) {\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2069
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/css!./media/keybindings';
import * as nls from 'vs/nls';
import { OS } from 'vs/base/common/platform';
import { Disposable, toDisposable, DisposableStore } from 'vs/base/common/lifecycle';
import { Event, Emitter } from 'vs/base/common/event';
import { KeybindingLabel } from 'vs/base/browser/ui/keybindingLabel/keybindingLabel';
import { Widget } from 'vs/base/browser/ui/widget';
import { ResolvedKeybinding, KeyCode } from 'vs/base/common/keyCodes';
import * as dom from 'vs/base/browser/dom';
import { IKeyboardEvent, StandardKeyboardEvent } from 'vs/base/browser/keyboardEvent';
import { FastDomNode, createFastDomNode } from 'vs/base/browser/fastDomNode';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { IContextViewService } from 'vs/platform/contextview/browser/contextView';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { ICodeEditor, IOverlayWidget, IOverlayWidgetPosition } from 'vs/editor/browser/editorBrowser';
import { attachInputBoxStyler, attachStylerCallback } from 'vs/platform/theme/common/styler';
import { IThemeService } from 'vs/platform/theme/common/themeService';
import { editorWidgetBackground, editorWidgetForeground, widgetShadow } from 'vs/platform/theme/common/colorRegistry';
import { ScrollType } from 'vs/editor/common/editorCommon';
import { SearchWidget, SearchOptions } from 'vs/workbench/contrib/preferences/browser/preferencesWidgets';
import { withNullAsUndefined } from 'vs/base/common/types';
import { timeout } from 'vs/base/common/async';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
export interface KeybindingsSearchOptions extends SearchOptions {
recordEnter?: boolean;
quoteRecordedKeys?: boolean;
}
export class KeybindingsSearchWidget extends SearchWidget {
private _firstPart: ResolvedKeybinding | null;
private _chordPart: ResolvedKeybinding | null;
private _inputValue: string;
private readonly recordDisposables = this._register(new DisposableStore());
private _onKeybinding = this._register(new Emitter<[ResolvedKeybinding | null, ResolvedKeybinding | null]>());
readonly onKeybinding: Event<[ResolvedKeybinding | null, ResolvedKeybinding | null]> = this._onKeybinding.event;
private _onEnter = this._register(new Emitter<void>());
readonly onEnter: Event<void> = this._onEnter.event;
private _onEscape = this._register(new Emitter<void>());
readonly onEscape: Event<void> = this._onEscape.event;
private _onBlur = this._register(new Emitter<void>());
readonly onBlur: Event<void> = this._onBlur.event;
constructor(parent: HTMLElement, options: KeybindingsSearchOptions,
@IContextViewService contextViewService: IContextViewService,
@IKeybindingService private readonly keybindingService: IKeybindingService,
@IInstantiationService instantiationService: IInstantiationService,
@IThemeService themeService: IThemeService,
@IContextKeyService contextKeyService: IContextKeyService
) {
super(parent, options, contextViewService, instantiationService, themeService, contextKeyService);
this._register(attachInputBoxStyler(this.inputBox, themeService));
this._register(toDisposable(() => this.stopRecordingKeys()));
this._firstPart = null;
this._chordPart = null;
this._inputValue = '';
this._reset();
}
clear(): void {
this._reset();
super.clear();
}
startRecordingKeys(): void {
this.recordDisposables.add(dom.addDisposableListener(this.inputBox.inputElement, dom.EventType.KEY_DOWN, (e: KeyboardEvent) => this._onKeyDown(new StandardKeyboardEvent(e))));
this.recordDisposables.add(dom.addDisposableListener(this.inputBox.inputElement, dom.EventType.BLUR, () => this._onBlur.fire()));
this.recordDisposables.add(dom.addDisposableListener(this.inputBox.inputElement, dom.EventType.INPUT, () => {
// Prevent other characters from showing up
this.setInputValue(this._inputValue);
}));
}
stopRecordingKeys(): void {
this._reset();
this.recordDisposables.clear();
}
setInputValue(value: string): void {
this._inputValue = value;
this.inputBox.value = this._inputValue;
}
private _reset() {
this._firstPart = null;
this._chordPart = null;
}
private _onKeyDown(keyboardEvent: IKeyboardEvent): void {
keyboardEvent.preventDefault();
keyboardEvent.stopPropagation();
const options = this.options as KeybindingsSearchOptions;
if (!options.recordEnter && keyboardEvent.equals(KeyCode.Enter)) {
this._onEnter.fire();
return;
}
if (keyboardEvent.equals(KeyCode.Escape)) {
this._onEscape.fire();
return;
}
this.printKeybinding(keyboardEvent);
}
private printKeybinding(keyboardEvent: IKeyboardEvent): void {
const keybinding = this.keybindingService.resolveKeyboardEvent(keyboardEvent);
const info = `code: ${keyboardEvent.browserEvent.code}, keyCode: ${keyboardEvent.browserEvent.keyCode}, key: ${keyboardEvent.browserEvent.key} => UI: ${keybinding.getAriaLabel()}, user settings: ${keybinding.getUserSettingsLabel()}, dispatch: ${keybinding.getDispatchParts()[0]}`;
const options = this.options as KeybindingsSearchOptions;
const hasFirstPart = (this._firstPart && this._firstPart.getDispatchParts()[0] !== null);
const hasChordPart = (this._chordPart && this._chordPart.getDispatchParts()[0] !== null);
if (hasFirstPart && hasChordPart) {
// Reset
this._firstPart = keybinding;
this._chordPart = null;
} else if (!hasFirstPart) {
this._firstPart = keybinding;
} else {
this._chordPart = keybinding;
}
let value = '';
if (this._firstPart) {
value = (this._firstPart.getUserSettingsLabel() || '');
}
if (this._chordPart) {
value = value + ' ' + this._chordPart.getUserSettingsLabel();
}
this.setInputValue(options.quoteRecordedKeys ? `"${value}"` : value);
this.inputBox.inputElement.title = info;
this._onKeybinding.fire([this._firstPart, this._chordPart]);
}
}
export class DefineKeybindingWidget extends Widget {
private static readonly WIDTH = 400;
private static readonly HEIGHT = 110;
private _domNode: FastDomNode<HTMLElement>;
private _keybindingInputWidget: KeybindingsSearchWidget;
private _outputNode: HTMLElement;
private _showExistingKeybindingsNode: HTMLElement;
private _firstPart: ResolvedKeybinding | null = null;
private _chordPart: ResolvedKeybinding | null = null;
private _isVisible: boolean = false;
private _onHide = this._register(new Emitter<void>());
private _onDidChange = this._register(new Emitter<string>());
onDidChange: Event<string> = this._onDidChange.event;
private _onShowExistingKeybindings = this._register(new Emitter<string | null>());
readonly onShowExistingKeybidings: Event<string | null> = this._onShowExistingKeybindings.event;
constructor(
parent: HTMLElement | null,
@IInstantiationService private readonly instantiationService: IInstantiationService,
@IThemeService private readonly themeService: IThemeService
) {
super();
this._domNode = createFastDomNode(document.createElement('div'));
this._domNode.setDisplay('none');
this._domNode.setClassName('defineKeybindingWidget');
this._domNode.setWidth(DefineKeybindingWidget.WIDTH);
this._domNode.setHeight(DefineKeybindingWidget.HEIGHT);
const message = nls.localize('defineKeybinding.initial', "Press desired key combination and then press ENTER.");
dom.append(this._domNode.domNode, dom.$('.message', undefined, message));
this._register(attachStylerCallback(this.themeService, { editorWidgetBackground, editorWidgetForeground, widgetShadow }, colors => {
if (colors.editorWidgetBackground) {
this._domNode.domNode.style.backgroundColor = colors.editorWidgetBackground.toString();
} else {
this._domNode.domNode.style.backgroundColor = '';
}
if (colors.editorWidgetForeground) {
this._domNode.domNode.style.color = colors.editorWidgetForeground.toString();
} else {
this._domNode.domNode.style.color = '';
}
if (colors.widgetShadow) {
this._domNode.domNode.style.boxShadow = `0 2px 8px ${colors.widgetShadow}`;
} else {
this._domNode.domNode.style.boxShadow = '';
}
}));
this._keybindingInputWidget = this._register(this.instantiationService.createInstance(KeybindingsSearchWidget, this._domNode.domNode, { ariaLabel: message, history: [] }));
this._keybindingInputWidget.startRecordingKeys();
this._register(this._keybindingInputWidget.onKeybinding(keybinding => this.onKeybinding(keybinding)));
this._register(this._keybindingInputWidget.onEnter(() => this.hide()));
this._register(this._keybindingInputWidget.onEscape(() => this.onCancel()));
this._register(this._keybindingInputWidget.onBlur(() => this.onCancel()));
this._outputNode = dom.append(this._domNode.domNode, dom.$('.output'));
this._showExistingKeybindingsNode = dom.append(this._domNode.domNode, dom.$('.existing'));
if (parent) {
dom.append(parent, this._domNode.domNode);
}
}
get domNode(): HTMLElement {
return this._domNode.domNode;
}
define(): Promise<string | null> {
this._keybindingInputWidget.clear();
return new Promise<string | null>(async (c) => {
if (!this._isVisible) {
this._isVisible = true;
this._domNode.setDisplay('block');
this._firstPart = null;
this._chordPart = null;
this._keybindingInputWidget.setInputValue('');
dom.clearNode(this._outputNode);
dom.clearNode(this._showExistingKeybindingsNode);
// Input is not getting focus without timeout in safari
// https://github.com/microsoft/vscode/issues/108817
await timeout(0);
this._keybindingInputWidget.focus();
}
const disposable = this._onHide.event(() => {
c(this.getUserSettingsLabel());
disposable.dispose();
});
});
}
layout(layout: dom.Dimension): void {
const top = Math.round((layout.height - DefineKeybindingWidget.HEIGHT) / 2);
this._domNode.setTop(top);
const left = Math.round((layout.width - DefineKeybindingWidget.WIDTH) / 2);
this._domNode.setLeft(left);
}
printExisting(numberOfExisting: number): void {
if (numberOfExisting > 0) {
const existingElement = dom.$('span.existingText');
const text = numberOfExisting === 1 ? nls.localize('defineKeybinding.oneExists', "1 existing command has this keybinding", numberOfExisting) : nls.localize('defineKeybinding.existing', "{0} existing commands have this keybinding", numberOfExisting);
dom.append(existingElement, document.createTextNode(text));
this._showExistingKeybindingsNode.appendChild(existingElement);
existingElement.onmousedown = (e) => { e.preventDefault(); };
existingElement.onmouseup = (e) => { e.preventDefault(); };
existingElement.onclick = () => { this._onShowExistingKeybindings.fire(this.getUserSettingsLabel()); };
}
}
private onKeybinding(keybinding: [ResolvedKeybinding | null, ResolvedKeybinding | null]): void {
const [firstPart, chordPart] = keybinding;
this._firstPart = firstPart;
this._chordPart = chordPart;
dom.clearNode(this._outputNode);
dom.clearNode(this._showExistingKeybindingsNode);
new KeybindingLabel(this._outputNode, OS).set(withNullAsUndefined(this._firstPart));
if (this._chordPart) {
this._outputNode.appendChild(document.createTextNode(nls.localize('defineKeybinding.chordsTo', "chord to")));
new KeybindingLabel(this._outputNode, OS).set(this._chordPart);
}
const label = this.getUserSettingsLabel();
if (label) {
this._onDidChange.fire(label);
}
}
private getUserSettingsLabel(): string | null {
let label: string | null = null;
if (this._firstPart) {
label = this._firstPart.getUserSettingsLabel();
if (this._chordPart) {
label = label + ' ' + this._chordPart.getUserSettingsLabel();
}
}
return label;
}
private onCancel(): void {
this._firstPart = null;
this._chordPart = null;
this.hide();
}
private hide(): void {
this._domNode.setDisplay('none');
this._isVisible = false;
this._onHide.fire();
}
}
export class DefineKeybindingOverlayWidget extends Disposable implements IOverlayWidget {
private static readonly ID = 'editor.contrib.defineKeybindingWidget';
private readonly _widget: DefineKeybindingWidget;
constructor(private _editor: ICodeEditor,
@IInstantiationService instantiationService: IInstantiationService
) {
super();
this._widget = instantiationService.createInstance(DefineKeybindingWidget, null);
this._editor.addOverlayWidget(this);
}
getId(): string {
return DefineKeybindingOverlayWidget.ID;
}
getDomNode(): HTMLElement {
return this._widget.domNode;
}
getPosition(): IOverlayWidgetPosition {
return {
preference: null
};
}
dispose(): void {
this._editor.removeOverlayWidget(this);
super.dispose();
}
start(): Promise<string | null> {
if (this._editor.hasModel()) {
this._editor.revealPositionInCenterIfOutsideViewport(this._editor.getPosition(), ScrollType.Smooth);
}
const layoutInfo = this._editor.getLayoutInfo();
this._widget.layout(new dom.Dimension(layoutInfo.width, layoutInfo.height));
return this._widget.define();
}
}
| src/vs/workbench/contrib/preferences/browser/keybindingWidgets.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017613898671697825,
0.00017032033065333962,
0.00016080259229056537,
0.00017104831931646913,
0.000003675589823615155
] |
{
"id": 2,
"code_window": [
"\t\tawait this._webview?.removeMarkdownPreview(cell.id);\n",
"\t}\n",
"\n",
"\tasync updateMarkdownPreviewSelectionState(cell: ICellViewModel, isSelected: boolean): Promise<void> {\n",
"\t\tif (!this.useRenderer) {\n",
"\t\t\t// TODO: handle case where custom renderer is disabled?\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview.isResolved()) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tprivate async updateSelectedMarkdownPreviews(): Promise<void> {\n",
"\t\tif (!this.useRenderer || !this._webview) {\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2069
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Registry } from 'vs/platform/registry/common/platform';
import { IQuickAccessRegistry, Extensions } from 'vs/platform/quickinput/common/quickAccess';
import { QuickCommandNLS } from 'vs/editor/common/standaloneStrings';
import { ICommandQuickPick } from 'vs/platform/quickinput/browser/commandsQuickAccess';
import { ICodeEditorService } from 'vs/editor/browser/services/codeEditorService';
import { AbstractEditorCommandsQuickAccessProvider } from 'vs/editor/contrib/quickAccess/commandsQuickAccess';
import { IEditor } from 'vs/editor/common/editorCommon';
import { withNullAsUndefined } from 'vs/base/common/types';
import { IInstantiationService, ServicesAccessor } from 'vs/platform/instantiation/common/instantiation';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { ICommandService } from 'vs/platform/commands/common/commands';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { INotificationService } from 'vs/platform/notification/common/notification';
import { EditorAction, registerEditorAction } from 'vs/editor/browser/editorExtensions';
import { EditorContextKeys } from 'vs/editor/common/editorContextKeys';
import { KeyCode } from 'vs/base/common/keyCodes';
import { KeybindingWeight } from 'vs/platform/keybinding/common/keybindingsRegistry';
import { IQuickInputService } from 'vs/platform/quickinput/common/quickInput';
export class StandaloneCommandsQuickAccessProvider extends AbstractEditorCommandsQuickAccessProvider {
protected get activeTextEditorControl(): IEditor | undefined { return withNullAsUndefined(this.codeEditorService.getFocusedCodeEditor()); }
constructor(
@IInstantiationService instantiationService: IInstantiationService,
@ICodeEditorService private readonly codeEditorService: ICodeEditorService,
@IKeybindingService keybindingService: IKeybindingService,
@ICommandService commandService: ICommandService,
@ITelemetryService telemetryService: ITelemetryService,
@INotificationService notificationService: INotificationService
) {
super({ showAlias: false }, instantiationService, keybindingService, commandService, telemetryService, notificationService);
}
protected async getCommandPicks(): Promise<Array<ICommandQuickPick>> {
return this.getCodeEditorCommandPicks();
}
}
Registry.as<IQuickAccessRegistry>(Extensions.Quickaccess).registerQuickAccessProvider({
ctor: StandaloneCommandsQuickAccessProvider,
prefix: StandaloneCommandsQuickAccessProvider.PREFIX,
helpEntries: [{ description: QuickCommandNLS.quickCommandHelp, needsEditor: true }]
});
export class GotoLineAction extends EditorAction {
constructor() {
super({
id: 'editor.action.quickCommand',
label: QuickCommandNLS.quickCommandActionLabel,
alias: 'Command Palette',
precondition: undefined,
kbOpts: {
kbExpr: EditorContextKeys.focus,
primary: KeyCode.F1,
weight: KeybindingWeight.EditorContrib
},
contextMenuOpts: {
group: 'z_commands',
order: 1
}
});
}
run(accessor: ServicesAccessor): void {
accessor.get(IQuickInputService).quickAccess.show(StandaloneCommandsQuickAccessProvider.PREFIX);
}
}
registerEditorAction(GotoLineAction);
| src/vs/editor/standalone/browser/quickAccess/standaloneCommandsQuickAccess.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017622901941649616,
0.0001724342000670731,
0.00016914992011152208,
0.0001726891496218741,
0.000002288216592205572
] |
{
"id": 2,
"code_window": [
"\t\tawait this._webview?.removeMarkdownPreview(cell.id);\n",
"\t}\n",
"\n",
"\tasync updateMarkdownPreviewSelectionState(cell: ICellViewModel, isSelected: boolean): Promise<void> {\n",
"\t\tif (!this.useRenderer) {\n",
"\t\t\t// TODO: handle case where custom renderer is disabled?\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this._webview.isResolved()) {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\tprivate async updateSelectedMarkdownPreviews(): Promise<void> {\n",
"\t\tif (!this.useRenderer || !this._webview) {\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2069
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Event as BaseEvent, Emitter } from 'vs/base/common/event';
export type EventHandler = HTMLElement | HTMLDocument | Window;
export interface IDomEvent {
<K extends keyof HTMLElementEventMap>(element: EventHandler, type: K, useCapture?: boolean): BaseEvent<HTMLElementEventMap[K]>;
(element: EventHandler, type: string, useCapture?: boolean): BaseEvent<unknown>;
}
export const domEvent: IDomEvent = (element: EventHandler, type: string, useCapture?: boolean) => {
const fn = (e: Event) => emitter.fire(e);
const emitter = new Emitter<Event>({
onFirstListenerAdd: () => {
element.addEventListener(type, fn, useCapture);
},
onLastListenerRemove: () => {
element.removeEventListener(type, fn, useCapture);
}
});
return emitter.event;
};
export interface CancellableEvent {
preventDefault(): void;
stopPropagation(): void;
}
export function stopEvent<T extends CancellableEvent>(event: T): T {
event.preventDefault();
event.stopPropagation();
return event;
}
export function stop<T extends CancellableEvent>(event: BaseEvent<T>): BaseEvent<T> {
return BaseEvent.map(event, stopEvent);
}
| src/vs/base/browser/event.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0001760828890837729,
0.0001724417379591614,
0.00016877839516382664,
0.00017175059474539012,
0.0000024820174076012336
] |
{
"id": 3,
"code_window": [
"\t\t\tawait this._resolveWebview();\n",
"\t\t}\n",
"\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelectionState(cell.id, isSelected);\n",
"\t}\n",
"\n",
"\tasync createOutput(cell: CodeCellViewModel, output: IInsetRenderOutput, offset: number): Promise<void> {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tconst selectedCells = this.getSelectionViewModels().map(cell => cell.id);\n",
"\n",
"\t\t// Only show selection when there is more than 1 cell selected\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelections(selectedCells.length > 1 ? selectedCells : []);\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2083
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { VSBuffer } from 'vs/base/common/buffer';
import { Emitter, Event } from 'vs/base/common/event';
import { Disposable } from 'vs/base/common/lifecycle';
import { getExtensionForMimeType } from 'vs/base/common/mime';
import { FileAccess, Schemas } from 'vs/base/common/network';
import { isWeb } from 'vs/base/common/platform';
import { dirname, joinPath } from 'vs/base/common/resources';
import { URI } from 'vs/base/common/uri';
import * as UUID from 'vs/base/common/uuid';
import { IFileDialogService } from 'vs/platform/dialogs/common/dialogs';
import { IFileService } from 'vs/platform/files/common/files';
import { IOpenerService, matchesScheme } from 'vs/platform/opener/common/opener';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
import { CellEditState, ICellOutputViewModel, ICommonCellInfo, ICommonNotebookEditor, IDisplayOutputLayoutUpdateRequest, IDisplayOutputViewModel, IGenericCellViewModel, IInsetRenderOutput, RenderOutputType } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { preloadsScriptStr } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads';
import { transformWebviewThemeVars } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewThemeMapping';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { INotebookRendererInfo } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { IWebviewService, WebviewContentPurpose, WebviewElement } from 'vs/workbench/contrib/webview/browser/webview';
import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import * as nls from 'vs/nls';
interface BaseToWebviewMessage {
readonly __vscode_notebook_message: true;
}
export interface WebviewIntialized extends BaseToWebviewMessage {
type: 'initialized';
}
export interface DimensionUpdate {
id: string;
init?: boolean;
data: { height: number };
isOutput?: boolean;
}
export interface IDimensionMessage extends BaseToWebviewMessage {
type: 'dimension';
updates: readonly DimensionUpdate[];
}
export interface IMouseEnterMessage extends BaseToWebviewMessage {
type: 'mouseenter';
id: string;
}
export interface IMouseLeaveMessage extends BaseToWebviewMessage {
type: 'mouseleave';
id: string;
}
export interface IOutputFocusMessage extends BaseToWebviewMessage {
type: 'outputFocus';
id: string;
}
export interface IOutputBlurMessage extends BaseToWebviewMessage {
type: 'outputBlur';
id: string;
}
export interface IWheelMessage extends BaseToWebviewMessage {
type: 'did-scroll-wheel';
payload: any;
}
export interface IScrollAckMessage extends BaseToWebviewMessage {
type: 'scroll-ack';
data: { top: number };
version: number;
}
export interface IBlurOutputMessage extends BaseToWebviewMessage {
type: 'focus-editor';
id: string;
focusNext?: boolean;
}
export interface IClickedDataUrlMessage extends BaseToWebviewMessage {
type: 'clicked-data-url';
data: string | ArrayBuffer | null;
downloadName?: string;
}
export interface IClickMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'clickMarkdownPreview';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly metaKey: boolean;
readonly shiftKey: boolean;
}
export interface IMouseEnterMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseEnterMarkdownPreview';
cellId: string;
}
export interface IMouseLeaveMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseLeaveMarkdownPreview';
cellId: string;
}
export interface IToggleMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'toggleMarkdownPreview';
cellId: string;
}
export interface ICellDragStartMessage extends BaseToWebviewMessage {
type: 'cell-drag-start';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragMessage extends BaseToWebviewMessage {
type: 'cell-drag';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDropMessage extends BaseToWebviewMessage {
readonly type: 'cell-drop';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragEndMessage extends BaseToWebviewMessage {
readonly type: 'cell-drag-end';
readonly cellId: string;
}
export interface IInitializedMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'initializedMarkdownPreview';
}
export interface IClearMessage {
type: 'clear';
}
export interface IOutputRequestMetadata {
/**
* Additional attributes of a cell metadata.
*/
custom?: { [key: string]: unknown };
}
export interface IOutputRequestDto {
/**
* { mime_type: value }
*/
data: { [key: string]: unknown; }
metadata?: IOutputRequestMetadata;
outputId: string;
}
export interface ICreationRequestMessage {
type: 'html';
content:
| { type: RenderOutputType.Html; htmlContent: string }
| { type: RenderOutputType.Extension; outputId: string; value: unknown; metadata: unknown; mimeType: string };
cellId: string;
outputId: string;
top: number;
left: number;
requiredPreloads: ReadonlyArray<IPreloadResource>;
initiallyHidden?: boolean;
apiNamespace?: string | undefined;
}
export interface IContentWidgetTopRequest {
id: string;
top: number;
}
export interface IViewScrollTopRequestMessage {
type: 'view-scroll';
forceDisplay: boolean;
widgets: IContentWidgetTopRequest[];
}
export interface IViewScrollMarkdownRequestMessage {
type: 'view-scroll-markdown';
cells: { id: string; top: number }[];
}
export interface IScrollRequestMessage {
type: 'scroll';
id: string;
top: number;
widgetTop?: number;
version: number;
}
export interface IClearOutputRequestMessage {
type: 'clearOutput';
cellId: string;
outputId: string;
cellUri: string;
apiNamespace: string | undefined;
}
export interface IHideOutputMessage {
type: 'hideOutput';
outputId: string;
cellId: string;
}
export interface IShowOutputMessage {
type: 'showOutput';
cellId: string;
outputId: string;
top: number;
}
export interface IFocusOutputMessage {
type: 'focus-output';
cellId: string;
}
export interface IPreloadResource {
originalUri: string;
uri: string;
}
export interface IUpdatePreloadResourceMessage {
type: 'preload';
resources: IPreloadResource[];
source: 'renderer' | 'kernel';
}
export interface IUpdateDecorationsMessage {
type: 'decorations';
cellId: string;
addedClassNames: string[];
removedClassNames: string[];
}
export interface ICustomRendererMessage extends BaseToWebviewMessage {
type: 'customRendererMessage';
rendererId: string;
message: unknown;
}
export interface ICreateMarkdownMessage {
type: 'createMarkdownPreview',
id: string;
handle: number;
content: string;
top: number;
}
export interface IRemoveMarkdownMessage {
type: 'removeMarkdownPreview',
id: string;
}
export interface IHideMarkdownMessage {
type: 'hideMarkdownPreview';
id: string;
}
export interface IUnhideMarkdownMessage {
type: 'unhideMarkdownPreview';
id: string;
}
export interface IShowMarkdownMessage {
type: 'showMarkdownPreview',
id: string;
handle: number;
content: string | undefined;
top: number;
}
export interface IUpdateMarkdownPreviewSelectionState {
readonly type: 'updateMarkdownPreviewSelectionState',
readonly id: string;
readonly isSelected: boolean;
}
export interface IInitializeMarkdownMessage {
type: 'initializeMarkdownPreview';
cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>;
}
export type FromWebviewMessage =
| WebviewIntialized
| IDimensionMessage
| IMouseEnterMessage
| IMouseLeaveMessage
| IOutputFocusMessage
| IOutputBlurMessage
| IWheelMessage
| IScrollAckMessage
| IBlurOutputMessage
| ICustomRendererMessage
| IClickedDataUrlMessage
| IClickMarkdownPreviewMessage
| IMouseEnterMarkdownPreviewMessage
| IMouseLeaveMarkdownPreviewMessage
| IToggleMarkdownPreviewMessage
| ICellDragStartMessage
| ICellDragMessage
| ICellDropMessage
| ICellDragEndMessage
| IInitializedMarkdownPreviewMessage
;
export type ToWebviewMessage =
| IClearMessage
| IFocusOutputMessage
| ICreationRequestMessage
| IViewScrollTopRequestMessage
| IScrollRequestMessage
| IClearOutputRequestMessage
| IHideOutputMessage
| IShowOutputMessage
| IUpdatePreloadResourceMessage
| IUpdateDecorationsMessage
| ICustomRendererMessage
| ICreateMarkdownMessage
| IRemoveMarkdownMessage
| IShowMarkdownMessage
| IHideMarkdownMessage
| IUnhideMarkdownMessage
| IUpdateMarkdownPreviewSelectionState
| IInitializeMarkdownMessage
| IViewScrollMarkdownRequestMessage;
export type AnyMessage = FromWebviewMessage | ToWebviewMessage;
export interface ICachedInset<K extends ICommonCellInfo> {
outputId: string;
cellInfo: K;
renderer?: INotebookRendererInfo;
cachedCreation: ICreationRequestMessage;
}
function html(strings: TemplateStringsArray, ...values: any[]): string {
let str = '';
strings.forEach((string, i) => {
str += string + (values[i] || '');
});
return str;
}
export interface INotebookWebviewMessage {
message: unknown;
forRenderer?: string;
}
export interface IResolvedBackLayerWebview {
webview: WebviewElement;
}
export class BackLayerWebView<T extends ICommonCellInfo> extends Disposable {
element: HTMLElement;
webview: WebviewElement | undefined = undefined;
insetMapping: Map<IDisplayOutputViewModel, ICachedInset<T>> = new Map();
readonly markdownPreviewMapping = new Map<string, { version: number, visible: boolean }>();
hiddenInsetMapping: Set<IDisplayOutputViewModel> = new Set();
reversedInsetMapping: Map<string, IDisplayOutputViewModel> = new Map();
localResourceRootsCache: URI[] | undefined = undefined;
rendererRootsCache: URI[] = [];
kernelRootsCache: URI[] = [];
private readonly _onMessage = this._register(new Emitter<INotebookWebviewMessage>());
private readonly _preloadsCache = new Set<string>();
public readonly onMessage: Event<INotebookWebviewMessage> = this._onMessage.event;
private _loaded!: Promise<void>;
private _initalized?: Promise<void>;
private _disposed = false;
constructor(
public notebookEditor: ICommonNotebookEditor,
public id: string,
public documentUri: URI,
public options: {
outputNodePadding: number,
outputNodeLeftPadding: number,
previewNodePadding: number,
leftMargin: number,
cellMargin: number,
runGutter: number,
},
@IWebviewService readonly webviewService: IWebviewService,
@IOpenerService readonly openerService: IOpenerService,
@INotebookService private readonly notebookService: INotebookService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IWorkbenchEnvironmentService private readonly environmentService: IWorkbenchEnvironmentService,
@IFileDialogService private readonly fileDialogService: IFileDialogService,
@IFileService private readonly fileService: IFileService,
) {
super();
this.element = document.createElement('div');
this.element.style.height = '1400px';
this.element.style.position = 'absolute';
}
private generateContent(coreDependencies: string, baseUrl: string) {
const markdownRenderersSrc = this.getMarkdownRendererScripts();
return html`
<html lang="en">
<head>
<meta charset="UTF-8">
<base href="${baseUrl}/"/>
<!--
Markdown previews are rendered using a shadow dom and are not effected by normal css.
Insert this style node into all preview shadow doms for styling.
-->
<template id="preview-styles">
<style>
img {
max-width: 100%;
max-height: 100%;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
a:focus,
input:focus,
select:focus,
textarea:focus {
outline: 1px solid -webkit-focus-ring-color;
outline-offset: -1px;
}
hr {
border: 0;
height: 2px;
border-bottom: 2px solid;
}
h1 {
font-size: 26px;
padding-bottom: 8px;
line-height: 31px;
border-bottom-width: 1px;
border-bottom-style: solid;
border-color: var(--vscode-foreground);
margin: 0;
margin-bottom: 13px;
}
h2 {
font-size: 19px;
margin: 0;
margin-bottom: 10px;
}
h1,
h2,
h3 {
font-weight: normal;
}
div {
width: 100%;
}
/* Adjust margin of first item in markdown cell */
*:first-child {
margin-top: 0px;
}
/* h1 tags don't need top margin */
h1:first-child {
margin-top: 0;
}
/* Removes bottom margin when only one item exists in markdown cell */
*:only-child,
*:last-child {
margin-bottom: 0;
padding-bottom: 0;
}
/* makes all markdown cells consistent */
div {
min-height: ${this.options.previewNodePadding * 2}px;
}
table {
border-collapse: collapse;
border-spacing: 0;
}
table th,
table td {
border: 1px solid;
}
table > thead > tr > th {
text-align: left;
border-bottom: 1px solid;
}
table > thead > tr > th,
table > thead > tr > td,
table > tbody > tr > th,
table > tbody > tr > td {
padding: 5px 10px;
}
table > tbody > tr + tr > td {
border-top: 1px solid;
}
blockquote {
margin: 0 7px 0 5px;
padding: 0 16px 0 10px;
border-left-width: 5px;
border-left-style: solid;
}
code,
.code {
font-family: var(--monaco-monospace-font);
font-size: 1em;
line-height: 1.357em;
}
.code {
white-space: pre-wrap;
}
.latex-block {
display: block;
}
.latex {
vertical-align: middle;
display: inline-block;
}
.latex img,
.latex-block img {
filter: brightness(0) invert(0)
}
dragging {
background-color: var(--vscode-editor-background);
}
</style>
</template>
<style>
#container > div > div.output {
width: calc(100% - ${this.options.leftMargin + (this.options.cellMargin * 2) + this.options.runGutter}px);
margin-left: ${this.options.leftMargin + this.options.runGutter}px;
padding: ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodeLeftPadding}px;
box-sizing: border-box;
background-color: var(--vscode-notebook-outputContainerBackgroundColor);
}
#container > div > div.preview {
width: 100%;
box-sizing: border-box;
white-space: nowrap;
overflow: hidden;
user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
white-space: initial;
cursor: grab;
}
#container > div > div.preview.emptyMarkdownCell::before {
content: "${nls.localize('notebook.emptyMarkdownPlaceholder', "Empty markdown cell, double click or press enter to edit.")}";
font-style: italic;
opacity: 0.6;
}
/* markdown */
#container > div > div.preview {
color: var(--vscode-foreground);
width: 100%;
padding-left: ${this.options.leftMargin}px;
padding-top: ${this.options.previewNodePadding}px;
padding-bottom: ${this.options.previewNodePadding}px;
}
#container > div > div.preview.selected {
background: var(--vscode-notebook-selectedCellBackground);
}
#container > div > div.preview.dragging {
background-color: var(--vscode-editor-background);
}
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex img,
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex-block img {
filter: brightness(0) invert(1)
}
#container > div.nb-symbolHighlight > div {
background-color: var(--vscode-notebook-symbolHighlightBackground);
}
#container > div.nb-cellDeleted > div {
background-color: var(--vscode-diffEditor-removedTextBackground);
}
#container > div.nb-cellAdded > div {
background-color: var(--vscode-diffEditor-insertedTextBackground);
}
#container > div > div:not(.preview) > div {
overflow-x: scroll;
}
body {
padding: 0px;
height: 100%;
width: 100%;
}
table, thead, tr, th, td, tbody {
border: none !important;
border-color: transparent;
border-spacing: 0;
border-collapse: collapse;
}
table {
width: 100%;
}
table, th, tr {
text-align: left !important;
}
thead {
font-weight: bold;
background-color: rgba(130, 130, 130, 0.16);
}
th, td {
padding: 4px 8px;
}
tr:nth-child(even) {
background-color: rgba(130, 130, 130, 0.08);
}
tbody th {
font-weight: normal;
}
</style>
</head>
<body style="overflow: hidden;">
<script>
self.require = {};
</script>
${coreDependencies}
<div id='container' class="widgetarea" style="position: absolute;width:100%;top: 0px"></div>
<script>${preloadsScriptStr({
outputNodePadding: this.options.outputNodePadding,
outputNodeLeftPadding: this.options.outputNodeLeftPadding,
previewNodePadding: this.options.previewNodePadding,
leftMargin: this.options.leftMargin
})}</script>
${markdownRenderersSrc}
</body>
</html>`;
}
private getMarkdownRendererScripts() {
const markdownRenderers = this.notebookService.getMarkdownRendererInfo();
return markdownRenderers
.sort((a, b) => {
// prefer built-in extension
if (a.extensionIsBuiltin) {
return b.extensionIsBuiltin ? 0 : -1;
}
return b.extensionIsBuiltin ? 1 : -1;
})
.map(renderer => {
return asWebviewUri(this.environmentService, this.id, renderer.entrypoint);
})
.map(src => `<script src="${src}"></script>`)
.join('\n');
}
postRendererMessage(rendererId: string, message: any) {
this._sendMessageToWebview({
__vscode_notebook_message: true,
type: 'customRendererMessage',
message,
rendererId
});
}
private resolveOutputId(id: string): { cellInfo: T, output: ICellOutputViewModel } | undefined {
const output = this.reversedInsetMapping.get(id);
if (!output) {
return;
}
const cellInfo = this.insetMapping.get(output)!.cellInfo;
return { cellInfo, output };
}
isResolved(): this is IResolvedBackLayerWebview {
return !!this.webview;
}
async createWebview(): Promise<void> {
let coreDependencies = '';
let resolveFunc: () => void;
this._initalized = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const baseUrl = asWebviewUri(this.environmentService, this.id, dirname(this.documentUri));
if (!isWeb) {
const loaderUri = FileAccess.asFileUri('vs/loader.js', require);
const loader = asWebviewUri(this.environmentService, this.id, loaderUri);
coreDependencies = `<script src="${loader}"></script><script>
var requirejs = (function() {
return require;
}());
</script>`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
} else {
const loaderUri = FileAccess.asBrowserUri('vs/loader.js', require);
fetch(loaderUri.toString(true)).then(async response => {
if (response.status !== 200) {
throw new Error(response.statusText);
}
const loaderJs = await response.text();
coreDependencies = `
<script>
${loaderJs}
</script>
<script>
var requirejs = (function() {
return require;
}());
</script>
`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
});
}
await this._initalized;
}
private async _initialize(content: string) {
if (!document.body.contains(this.element)) {
throw new Error('Element is already detached from the DOM tree');
}
this.webview = this._createInset(this.webviewService, content);
this.webview.mountTo(this.element);
this._register(this.webview);
this._register(this.webview.onDidClickLink(link => {
if (this._disposed) {
return;
}
if (!link) {
return;
}
if (matchesScheme(link, Schemas.http) || matchesScheme(link, Schemas.https) || matchesScheme(link, Schemas.mailto)
|| matchesScheme(link, Schemas.command)) {
this.openerService.open(link, { fromUserGesture: true, allowContributedOpeners: true });
}
}));
this._register(this.webview.onDidReload(() => {
if (this._disposed) {
return;
}
let renderers = new Set<INotebookRendererInfo>();
for (const inset of this.insetMapping.values()) {
if (inset.renderer) {
renderers.add(inset.renderer);
}
}
this._preloadsCache.clear();
this.updateRendererPreloads(renderers);
for (const [output, inset] of this.insetMapping.entries()) {
this._sendMessageToWebview({ ...inset.cachedCreation, initiallyHidden: this.hiddenInsetMapping.has(output) });
}
}));
this._register(this.webview.onMessage((message) => {
const data: FromWebviewMessage | { readonly __vscode_notebook_message: undefined } = message.message;
if (this._disposed) {
return;
}
if (!data.__vscode_notebook_message) {
this._onMessage.fire({ message: data });
return;
}
switch (data.type) {
case 'dimension':
{
for (const update of data.updates) {
if (update.isOutput) {
const height = update.data.height;
const outputHeight = height;
const resolvedResult = this.resolveOutputId(update.id);
if (resolvedResult) {
const { cellInfo, output } = resolvedResult;
this.notebookEditor.updateOutputHeight(cellInfo, output, outputHeight, !!update.init, 'webview#dimension');
}
} else {
const cellId = update.id.substr(0, update.id.length - '_preview'.length);
this.notebookEditor.updateMarkdownCellHeight(cellId, update.data.height, !!update.init);
}
}
break;
}
case 'mouseenter':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = true;
}
}
break;
}
case 'mouseleave':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = false;
}
}
break;
}
case 'outputFocus':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = true;
}
}
break;
}
case 'outputBlur':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = false;
}
}
break;
}
case 'scroll-ack':
{
// const date = new Date();
// const top = data.data.top;
// console.log('ack top ', top, ' version: ', data.version, ' - ', date.getMinutes() + ':' + date.getSeconds() + ':' + date.getMilliseconds());
break;
}
case 'did-scroll-wheel':
{
this.notebookEditor.triggerScroll({
...data.payload,
preventDefault: () => { },
stopPropagation: () => { }
});
break;
}
case 'focus-editor':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (!latestCell) {
return;
}
if (data.focusNext) {
this.notebookEditor.focusNextNotebookCell(latestCell, 'editor');
} else {
this.notebookEditor.focusNotebookCell(latestCell, 'editor');
}
}
break;
}
case 'clicked-data-url':
{
this._onDidClickDataLink(data);
break;
}
case 'customRendererMessage':
{
this._onMessage.fire({ message: data.message, forRenderer: data.rendererId });
break;
}
case 'clickMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
if (data.shiftKey || data.metaKey) {
// Add to selection
this.notebookEditor.toggleNotebookCellSelection(cell);
} else {
// Normal click
this.notebookEditor.focusNotebookCell(cell, 'container', { skipReveal: true });
}
}
break;
}
case 'toggleMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
this.notebookEditor.setMarkdownCellEditState(data.cellId, CellEditState.Editing);
this.notebookEditor.focusNotebookCell(cell, 'editor', { skipReveal: true });
}
break;
}
case 'mouseEnterMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = true;
}
break;
}
case 'mouseLeaveMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = false;
}
break;
}
case 'cell-drag-start':
{
this.notebookEditor.markdownCellDragStart(data.cellId, data.position);
break;
}
case 'cell-drag':
{
this.notebookEditor.markdownCellDrag(data.cellId, data.position);
break;
}
case 'cell-drop':
{
this.notebookEditor.markdownCellDrop(data.cellId, {
clientY: data.position.clientY,
ctrlKey: data.ctrlKey,
altKey: data.altKey,
});
break;
}
case 'cell-drag-end':
{
this.notebookEditor.markdownCellDragEnd(data.cellId);
break;
}
}
}));
}
private async _onDidClickDataLink(event: IClickedDataUrlMessage): Promise<void> {
if (typeof event.data !== 'string') {
return;
}
const [splitStart, splitData] = event.data.split(';base64,');
if (!splitData || !splitStart) {
return;
}
const defaultDir = dirname(this.documentUri);
let defaultName: string;
if (event.downloadName) {
defaultName = event.downloadName;
} else {
const mimeType = splitStart.replace(/^data:/, '');
const candidateExtension = mimeType && getExtensionForMimeType(mimeType);
defaultName = candidateExtension ? `download${candidateExtension}` : 'download';
}
const defaultUri = joinPath(defaultDir, defaultName);
const newFileUri = await this.fileDialogService.showSaveDialog({
defaultUri
});
if (!newFileUri) {
return;
}
const decoded = atob(splitData);
const typedArray = new Uint8Array(decoded.length);
for (let i = 0; i < decoded.length; i++) {
typedArray[i] = decoded.charCodeAt(i);
}
const buff = VSBuffer.wrap(typedArray);
await this.fileService.writeFile(newFileUri, buff);
await this.openerService.open(newFileUri);
}
private _createInset(webviewService: IWebviewService, content: string) {
const rootPath = isWeb ? FileAccess.asBrowserUri('', require) : FileAccess.asFileUri('', require);
const workspaceFolders = this.contextService.getWorkspace().folders.map(x => x.uri);
this.localResourceRootsCache = [
...this.notebookService.getNotebookProviderResourceRoots(),
...this.notebookService.getMarkdownRendererInfo().map(x => dirname(x.entrypoint)),
...workspaceFolders,
rootPath,
];
const webview = webviewService.createWebviewElement(this.id, {
purpose: WebviewContentPurpose.NotebookRenderer,
enableFindWidget: false,
transformCssVariables: transformWebviewThemeVars,
}, {
allowMultipleAPIAcquire: true,
allowScripts: true,
localResourceRoots: this.localResourceRootsCache
}, undefined);
let resolveFunc: () => void;
this._loaded = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const dispose = webview.onMessage((message) => {
const data: FromWebviewMessage = message.message;
if (data.__vscode_notebook_message && data.type === 'initialized') {
resolveFunc();
dispose.dispose();
}
});
webview.html = content;
return webview;
}
shouldUpdateInset(cell: IGenericCellViewModel, output: ICellOutputViewModel, cellTop: number) {
if (this._disposed) {
return;
}
if (cell.metadata?.outputCollapsed) {
return false;
}
const outputCache = this.insetMapping.get(output)!;
const outputIndex = cell.outputsViewModels.indexOf(output);
const outputOffset = cellTop + cell.getOutputOffset(outputIndex);
if (this.hiddenInsetMapping.has(output)) {
return true;
}
if (outputOffset === outputCache.cachedCreation.top) {
return false;
}
return true;
}
updateMarkdownScrollTop(items: { id: string, top: number }[]) {
if (this._disposed || !items.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll-markdown',
cells: items
});
}
updateViewScrollTop(forceDisplay: boolean, items: IDisplayOutputLayoutUpdateRequest[]) {
if (!items.length) {
return;
}
const widgets = coalesce(items.map((item): IContentWidgetTopRequest | undefined => {
const outputCache = this.insetMapping.get(item.output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
const outputOffset = item.outputOffset;
outputCache.cachedCreation.top = outputOffset;
this.hiddenInsetMapping.delete(item.output);
return {
id: id,
top: outputOffset,
};
}));
if (!widgets.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll',
forceDisplay,
widgets: widgets
});
}
private async createMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (this.markdownPreviewMapping.has(cellId)) {
console.error('Trying to create markdown preview that already exists');
return;
}
const initialTop = cellTop;
this.markdownPreviewMapping.set(cellId, { version: contentVersion, visible: true });
this._sendMessageToWebview({
type: 'createMarkdownPreview',
id: cellId,
handle: cellHandle,
content: content,
top: initialTop,
});
}
async showMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
return this.createMarkdownPreview(cellId, cellHandle, content, cellTop, contentVersion);
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error('Try to show a preview that does not exist');
return;
}
if (entry.version !== contentVersion || !entry.visible) {
this._sendMessageToWebview({
type: 'showMarkdownPreview',
id: cellId,
handle: cellHandle,
// If the content has not changed, we still want to make sure the
// preview is visible but don't need to send anything over
content: entry.version === contentVersion ? undefined : content,
top: cellTop
});
}
entry.version = contentVersion;
entry.visible = true;
}
async hideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
// TODO: this currently seems expected on first load
// console.error(`Try to hide a preview that does not exist: ${cellId}`);
return;
}
if (entry.visible) {
this._sendMessageToWebview({
type: 'hideMarkdownPreview',
id: cellId
});
entry.visible = false;
}
}
async unhideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error(`Try to unhide a preview that does not exist: ${cellId}`);
return;
}
if (!entry.visible) {
this._sendMessageToWebview({
type: 'unhideMarkdownPreview',
id: cellId
});
entry.visible = true;
}
}
async removeMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
console.error(`Try to delete a preview that does not exist: ${cellId}`);
return;
}
this.markdownPreviewMapping.delete(cellId);
this._sendMessageToWebview({
type: 'removeMarkdownPreview',
id: cellId
});
}
async updateMarkdownPreviewSelectionState(cellId: any, isSelected: boolean) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
// TODO: this currently seems expected on first load
// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);
return;
}
this._sendMessageToWebview({
type: 'updateMarkdownPreviewSelectionState',
id: cellId,
isSelected
});
}
async initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {
await this._loaded;
if (this._disposed) {
return;
}
// TODO: use proper handler
const p = new Promise<void>(resolve => {
this.webview?.onMessage(e => {
if (e.message.type === 'initializedMarkdownPreview') {
resolve();
}
});
});
for (const cell of cells) {
this.markdownPreviewMapping.set(cell.cellId, { version: 0, visible: false });
}
this._sendMessageToWebview({
type: 'initializeMarkdownPreview',
cells: cells,
});
await p;
}
async createOutput(cellInfo: T, content: IInsetRenderOutput, cellTop: number, offset: number) {
if (this._disposed) {
return;
}
const initialTop = cellTop + offset;
if (this.insetMapping.has(content.source)) {
const outputCache = this.insetMapping.get(content.source);
if (outputCache) {
this.hiddenInsetMapping.delete(content.source);
this._sendMessageToWebview({
type: 'showOutput',
cellId: outputCache.cellInfo.cellId,
outputId: outputCache.outputId,
top: initialTop
});
return;
}
}
const messageBase = {
type: 'html',
cellId: cellInfo.cellId,
top: initialTop,
left: 0,
requiredPreloads: [],
} as const;
let message: ICreationRequestMessage;
let renderer: INotebookRendererInfo | undefined;
if (content.type === RenderOutputType.Extension) {
const output = content.source.model;
renderer = content.renderer;
const outputDto = output.outputs.find(op => op.mime === content.mimeType);
message = {
...messageBase,
outputId: output.outputId,
apiNamespace: content.renderer.id,
requiredPreloads: await this.updateRendererPreloads([content.renderer]),
content: {
type: RenderOutputType.Extension,
outputId: output.outputId,
mimeType: content.mimeType,
value: outputDto?.value,
metadata: outputDto?.metadata,
},
};
} else {
message = {
...messageBase,
outputId: UUID.generateUuid(),
content: {
type: content.type,
htmlContent: content.htmlContent,
}
};
}
this._sendMessageToWebview(message);
this.insetMapping.set(content.source, { outputId: message.outputId, cellInfo: cellInfo, renderer, cachedCreation: message });
this.hiddenInsetMapping.delete(content.source);
this.reversedInsetMapping.set(message.outputId, content.source);
}
removeInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
this._sendMessageToWebview({
type: 'clearOutput',
apiNamespace: outputCache.cachedCreation.apiNamespace,
cellUri: outputCache.cellInfo.cellUri.toString(),
outputId: id,
cellId: outputCache.cellInfo.cellId
});
this.insetMapping.delete(output);
this.reversedInsetMapping.delete(id);
}
hideInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
this.hiddenInsetMapping.add(output);
this._sendMessageToWebview({
type: 'hideOutput',
outputId: outputCache.outputId,
cellId: outputCache.cellInfo.cellId,
});
}
clearInsets() {
if (this._disposed) {
return;
}
this._sendMessageToWebview({
type: 'clear'
});
this.insetMapping = new Map();
this.reversedInsetMapping = new Map();
}
focusWebview() {
if (this._disposed) {
return;
}
this.webview?.focus();
}
focusOutput(cellId: string) {
if (this._disposed) {
return;
}
this.webview?.focus();
setTimeout(() => { // Need this, or focus decoration is not shown. No clue.
this._sendMessageToWebview({
type: 'focus-output',
cellId,
});
}, 50);
}
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]) {
this._sendMessageToWebview({
type: 'decorations',
cellId,
addedClassNames: added,
removedClassNames: removed
});
}
async updateKernelPreloads(extensionLocations: URI[], preloads: URI[]) {
if (this._disposed) {
return;
}
await this._loaded;
const resources: IPreloadResource[] = [];
for (const preload of preloads) {
const uri = this.environmentService.isExtensionDevelopment && (preload.scheme === 'http' || preload.scheme === 'https')
? preload : asWebviewUri(this.environmentService, this.id, preload);
if (!this._preloadsCache.has(uri.toString())) {
resources.push({ uri: uri.toString(), originalUri: preload.toString() });
this._preloadsCache.add(uri.toString());
}
}
if (!resources.length) {
return;
}
this.kernelRootsCache = [...extensionLocations, ...this.kernelRootsCache];
this._updatePreloads(resources, 'kernel');
}
async updateRendererPreloads(renderers: Iterable<INotebookRendererInfo>) {
if (this._disposed) {
return [];
}
await this._loaded;
const requiredPreloads: IPreloadResource[] = [];
const resources: IPreloadResource[] = [];
const extensionLocations: URI[] = [];
for (const rendererInfo of renderers) {
extensionLocations.push(rendererInfo.extensionLocation);
for (const preload of [rendererInfo.entrypoint, ...rendererInfo.preloads]) {
const uri = asWebviewUri(this.environmentService, this.id, preload);
const resource: IPreloadResource = { uri: uri.toString(), originalUri: preload.toString() };
requiredPreloads.push(resource);
if (!this._preloadsCache.has(uri.toString())) {
resources.push(resource);
this._preloadsCache.add(uri.toString());
}
}
}
if (!resources.length) {
return requiredPreloads;
}
this.rendererRootsCache = extensionLocations;
this._updatePreloads(resources, 'renderer');
return requiredPreloads;
}
private _updatePreloads(resources: IPreloadResource[], source: 'renderer' | 'kernel') {
if (!this.webview) {
return;
}
const mixedResourceRoots = [...(this.localResourceRootsCache || []), ...this.rendererRootsCache, ...this.kernelRootsCache];
this.webview.localResourcesRoot = mixedResourceRoots;
this._sendMessageToWebview({
type: 'preload',
resources: resources,
source: source
});
}
private _sendMessageToWebview(message: ToWebviewMessage) {
if (this._disposed) {
return;
}
this.webview?.postMessage(message);
}
clearPreloadsCache() {
this._preloadsCache.clear();
}
dispose() {
this._disposed = true;
this.webview?.dispose();
super.dispose();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.1892659217119217,
0.0020241038873791695,
0.00016454167780466378,
0.00017508439486846328,
0.01517180074006319
] |
{
"id": 3,
"code_window": [
"\t\t\tawait this._resolveWebview();\n",
"\t\t}\n",
"\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelectionState(cell.id, isSelected);\n",
"\t}\n",
"\n",
"\tasync createOutput(cell: CodeCellViewModel, output: IInsetRenderOutput, offset: number): Promise<void> {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tconst selectedCells = this.getSelectionViewModels().map(cell => cell.id);\n",
"\n",
"\t\t// Only show selection when there is more than 1 cell selected\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelections(selectedCells.length > 1 ? selectedCells : []);\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2083
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { globals, INodeProcess, IProcessEnvironment } from 'vs/base/common/platform';
import { ISandboxConfiguration } from 'vs/base/parts/sandbox/common/sandboxTypes';
import { ProcessMemoryInfo, CrashReporter, IpcRenderer, WebFrame } from 'vs/base/parts/sandbox/electron-sandbox/electronTypes';
/**
* In sandboxed renderers we cannot expose all of the `process` global of node.js
*/
export interface ISandboxNodeProcess extends INodeProcess {
/**
* The process.platform property returns a string identifying the operating system platform
* on which the Node.js process is running.
*/
readonly platform: string;
/**
* The process.arch property returns a string identifying the CPU architecture
* on which the Node.js process is running.
*/
readonly arch: string;
/**
* The type will always be `renderer`.
*/
readonly type: string;
/**
* Whether the process is sandboxed or not.
*/
readonly sandboxed: boolean;
/**
* A list of versions for the current node.js/electron configuration.
*/
readonly versions: { [key: string]: string | undefined };
/**
* The process.env property returns an object containing the user environment.
*/
readonly env: IProcessEnvironment;
/**
* The `execPath` will be the location of the executable of this application.
*/
readonly execPath: string;
/**
* A listener on the process. Only a small subset of listener types are allowed.
*/
on: (type: string, callback: Function) => void;
/**
* The current working directory of the process.
*/
cwd: () => string;
/**
* Resolves with a ProcessMemoryInfo
*
* Returns an object giving memory usage statistics about the current process. Note
* that all statistics are reported in Kilobytes. This api should be called after
* app ready.
*
* Chromium does not provide `residentSet` value for macOS. This is because macOS
* performs in-memory compression of pages that haven't been recently used. As a
* result the resident set size value is not what one would expect. `private`
* memory is more representative of the actual pre-compression memory usage of the
* process on macOS.
*/
getProcessMemoryInfo: () => Promise<ProcessMemoryInfo>;
/**
* A custom method we add to `process`: Resolve the true process environment to use and
* apply it to `process.env`.
*
* There are different layers of environment that will apply:
* - `process.env`: this is the actual environment of the process before this method
* - `shellEnv` : if the program was not started from a terminal, we resolve all shell
* variables to get the same experience as if the program was started from
* a terminal (Linux, macOS)
* - `userEnv` : this is instance specific environment, e.g. if the user started the program
* from a terminal and changed certain variables
*
* The order of overwrites is `process.env` < `shellEnv` < `userEnv`.
*
* It is critical that every process awaits this method early on startup to get the right
* set of environment in `process.env`.
*/
resolveEnv(): Promise<void>;
/**
* Returns a process environment that includes any shell environment even if the application
* was not started from a shell / terminal / console.
*/
getShellEnv(): Promise<IProcessEnvironment>;
}
export interface IpcMessagePort {
/**
* Establish a connection via `MessagePort` to a target. The main process
* will need to transfer the port over to the `channelResponse` after listening
* to `channelRequest` with a payload of `requestNonce` so that the
* source can correlate the response.
*
* The source should install a `window.on('message')` listener, ensuring `e.data`
* matches `requestNonce`, `e.source` matches `window` and then receiving the
* `MessagePort` via `e.ports[0]`.
*/
connect(channelRequest: string, channelResponse: string, requestNonce: string): void;
}
export interface ISandboxContext {
/**
* A configuration object made accessible from the main side
* to configure the sandbox browser window.
*/
configuration: Promise<ISandboxConfiguration>;
}
export const ipcRenderer: IpcRenderer = globals.vscode.ipcRenderer;
export const ipcMessagePort: IpcMessagePort = globals.vscode.ipcMessagePort;
export const webFrame: WebFrame = globals.vscode.webFrame;
export const crashReporter: CrashReporter = globals.vscode.crashReporter;
export const process: ISandboxNodeProcess = globals.vscode.process;
export const context: ISandboxContext = globals.vscode.context;
| src/vs/base/parts/sandbox/electron-sandbox/globals.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0019897001329809427,
0.00029975036159157753,
0.00016213250637520105,
0.00017121891141869128,
0.0004687308392021805
] |
{
"id": 3,
"code_window": [
"\t\t\tawait this._resolveWebview();\n",
"\t\t}\n",
"\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelectionState(cell.id, isSelected);\n",
"\t}\n",
"\n",
"\tasync createOutput(cell: CodeCellViewModel, output: IInsetRenderOutput, offset: number): Promise<void> {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tconst selectedCells = this.getSelectionViewModels().map(cell => cell.id);\n",
"\n",
"\t\t// Only show selection when there is more than 1 cell selected\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelections(selectedCells.length > 1 ? selectedCells : []);\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2083
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
.monaco-workbench .debug-toolbar {
position: absolute;
z-index: 1000;
height: 32px;
display: flex;
padding-left: 7px;
}
.monaco-workbench .debug-toolbar .monaco-action-bar .action-item {
height: 32px;
}
.monaco-workbench .debug-toolbar .monaco-action-bar .action-item.select-container {
margin-right: 7px;
}
.monaco-workbench .debug-toolbar .monaco-action-bar .action-item.select-container .monaco-select-box,
.monaco-workbench .start-debug-action-item .select-container .monaco-select-box {
padding: 0 22px 0 6px;
}
.monaco-workbench .debug-toolbar .drag-area {
cursor: grab;
height: 32px;
width: 16px;
opacity: 0.5;
display: flex;
align-items: center;
justify-content: center;
}
.monaco-workbench .debug-toolbar .drag-area.dragged {
cursor: grabbing;
}
.monaco-workbench .debug-toolbar .monaco-action-bar .action-item .action-label {
width: 32px;
height: 32px;
margin-right: 0;
background-size: 16px;
background-position: center center;
background-repeat: no-repeat;
display: flex;
align-items: center;
justify-content: center;
}
| src/vs/workbench/contrib/debug/browser/media/debugToolBar.css | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0001807776279747486,
0.00017565461166668683,
0.0001726813497953117,
0.00017500203102827072,
0.0000028295621632423718
] |
{
"id": 3,
"code_window": [
"\t\t\tawait this._resolveWebview();\n",
"\t\t}\n",
"\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelectionState(cell.id, isSelected);\n",
"\t}\n",
"\n",
"\tasync createOutput(cell: CodeCellViewModel, output: IInsetRenderOutput, offset: number): Promise<void> {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\tconst selectedCells = this.getSelectionViewModels().map(cell => cell.id);\n",
"\n",
"\t\t// Only show selection when there is more than 1 cell selected\n",
"\t\tawait this._webview?.updateMarkdownPreviewSelections(selectedCells.length > 1 ? selectedCells : []);\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts",
"type": "replace",
"edit_start_line_idx": 2083
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { renderLabelWithIcons } from 'vs/base/browser/ui/iconLabel/iconLabels';
suite('renderLabelWithIcons', () => {
test('no icons', () => {
const result = renderLabelWithIcons(' hello World .');
assert.strictEqual(elementsToString(result), ' hello World .');
});
test('icons only', () => {
const result = renderLabelWithIcons('$(alert)');
assert.strictEqual(elementsToString(result), '<span class="codicon codicon-alert"></span>');
});
test('icon and non-icon strings', () => {
const result = renderLabelWithIcons(` $(alert) Unresponsive`);
assert.strictEqual(elementsToString(result), ' <span class="codicon codicon-alert"></span> Unresponsive');
});
test('multiple icons', () => {
const result = renderLabelWithIcons('$(check)$(error)');
assert.strictEqual(elementsToString(result), '<span class="codicon codicon-check"></span><span class="codicon codicon-error"></span>');
});
test('escaped icons', () => {
const result = renderLabelWithIcons('\\$(escaped)');
assert.strictEqual(elementsToString(result), '$(escaped)');
});
test('icon with animation', () => {
const result = renderLabelWithIcons('$(zip~anim)');
assert.strictEqual(elementsToString(result), '<span class="codicon codicon-zip codicon-modifier-anim"></span>');
});
const elementsToString = (elements: Array<HTMLElement | string>): string => {
return elements
.map(elem => elem instanceof HTMLElement ? elem.outerHTML : elem)
.reduce((a, b) => a + b, '');
};
});
| src/vs/base/test/browser/iconLabels.test.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0001802160550141707,
0.00017602166917640716,
0.00017293675045948476,
0.00017578918777871877,
0.000002346818519072258
] |
{
"id": 4,
"code_window": [
"import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';\n",
"import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';\n",
"import * as nls from 'vs/nls';\n",
"\n",
"interface BaseToWebviewMessage {\n",
"\treadonly __vscode_notebook_message: true;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { coalesce } from 'vs/base/common/arrays';\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "add",
"edit_start_line_idx": 28
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { VSBuffer } from 'vs/base/common/buffer';
import { Emitter, Event } from 'vs/base/common/event';
import { Disposable } from 'vs/base/common/lifecycle';
import { getExtensionForMimeType } from 'vs/base/common/mime';
import { FileAccess, Schemas } from 'vs/base/common/network';
import { isWeb } from 'vs/base/common/platform';
import { dirname, joinPath } from 'vs/base/common/resources';
import { URI } from 'vs/base/common/uri';
import * as UUID from 'vs/base/common/uuid';
import { IFileDialogService } from 'vs/platform/dialogs/common/dialogs';
import { IFileService } from 'vs/platform/files/common/files';
import { IOpenerService, matchesScheme } from 'vs/platform/opener/common/opener';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
import { CellEditState, ICellOutputViewModel, ICommonCellInfo, ICommonNotebookEditor, IDisplayOutputLayoutUpdateRequest, IDisplayOutputViewModel, IGenericCellViewModel, IInsetRenderOutput, RenderOutputType } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { preloadsScriptStr } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads';
import { transformWebviewThemeVars } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewThemeMapping';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { INotebookRendererInfo } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { IWebviewService, WebviewContentPurpose, WebviewElement } from 'vs/workbench/contrib/webview/browser/webview';
import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import * as nls from 'vs/nls';
interface BaseToWebviewMessage {
readonly __vscode_notebook_message: true;
}
export interface WebviewIntialized extends BaseToWebviewMessage {
type: 'initialized';
}
export interface DimensionUpdate {
id: string;
init?: boolean;
data: { height: number };
isOutput?: boolean;
}
export interface IDimensionMessage extends BaseToWebviewMessage {
type: 'dimension';
updates: readonly DimensionUpdate[];
}
export interface IMouseEnterMessage extends BaseToWebviewMessage {
type: 'mouseenter';
id: string;
}
export interface IMouseLeaveMessage extends BaseToWebviewMessage {
type: 'mouseleave';
id: string;
}
export interface IOutputFocusMessage extends BaseToWebviewMessage {
type: 'outputFocus';
id: string;
}
export interface IOutputBlurMessage extends BaseToWebviewMessage {
type: 'outputBlur';
id: string;
}
export interface IWheelMessage extends BaseToWebviewMessage {
type: 'did-scroll-wheel';
payload: any;
}
export interface IScrollAckMessage extends BaseToWebviewMessage {
type: 'scroll-ack';
data: { top: number };
version: number;
}
export interface IBlurOutputMessage extends BaseToWebviewMessage {
type: 'focus-editor';
id: string;
focusNext?: boolean;
}
export interface IClickedDataUrlMessage extends BaseToWebviewMessage {
type: 'clicked-data-url';
data: string | ArrayBuffer | null;
downloadName?: string;
}
export interface IClickMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'clickMarkdownPreview';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly metaKey: boolean;
readonly shiftKey: boolean;
}
export interface IMouseEnterMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseEnterMarkdownPreview';
cellId: string;
}
export interface IMouseLeaveMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseLeaveMarkdownPreview';
cellId: string;
}
export interface IToggleMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'toggleMarkdownPreview';
cellId: string;
}
export interface ICellDragStartMessage extends BaseToWebviewMessage {
type: 'cell-drag-start';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragMessage extends BaseToWebviewMessage {
type: 'cell-drag';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDropMessage extends BaseToWebviewMessage {
readonly type: 'cell-drop';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragEndMessage extends BaseToWebviewMessage {
readonly type: 'cell-drag-end';
readonly cellId: string;
}
export interface IInitializedMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'initializedMarkdownPreview';
}
export interface IClearMessage {
type: 'clear';
}
export interface IOutputRequestMetadata {
/**
* Additional attributes of a cell metadata.
*/
custom?: { [key: string]: unknown };
}
export interface IOutputRequestDto {
/**
* { mime_type: value }
*/
data: { [key: string]: unknown; }
metadata?: IOutputRequestMetadata;
outputId: string;
}
export interface ICreationRequestMessage {
type: 'html';
content:
| { type: RenderOutputType.Html; htmlContent: string }
| { type: RenderOutputType.Extension; outputId: string; value: unknown; metadata: unknown; mimeType: string };
cellId: string;
outputId: string;
top: number;
left: number;
requiredPreloads: ReadonlyArray<IPreloadResource>;
initiallyHidden?: boolean;
apiNamespace?: string | undefined;
}
export interface IContentWidgetTopRequest {
id: string;
top: number;
}
export interface IViewScrollTopRequestMessage {
type: 'view-scroll';
forceDisplay: boolean;
widgets: IContentWidgetTopRequest[];
}
export interface IViewScrollMarkdownRequestMessage {
type: 'view-scroll-markdown';
cells: { id: string; top: number }[];
}
export interface IScrollRequestMessage {
type: 'scroll';
id: string;
top: number;
widgetTop?: number;
version: number;
}
export interface IClearOutputRequestMessage {
type: 'clearOutput';
cellId: string;
outputId: string;
cellUri: string;
apiNamespace: string | undefined;
}
export interface IHideOutputMessage {
type: 'hideOutput';
outputId: string;
cellId: string;
}
export interface IShowOutputMessage {
type: 'showOutput';
cellId: string;
outputId: string;
top: number;
}
export interface IFocusOutputMessage {
type: 'focus-output';
cellId: string;
}
export interface IPreloadResource {
originalUri: string;
uri: string;
}
export interface IUpdatePreloadResourceMessage {
type: 'preload';
resources: IPreloadResource[];
source: 'renderer' | 'kernel';
}
export interface IUpdateDecorationsMessage {
type: 'decorations';
cellId: string;
addedClassNames: string[];
removedClassNames: string[];
}
export interface ICustomRendererMessage extends BaseToWebviewMessage {
type: 'customRendererMessage';
rendererId: string;
message: unknown;
}
export interface ICreateMarkdownMessage {
type: 'createMarkdownPreview',
id: string;
handle: number;
content: string;
top: number;
}
export interface IRemoveMarkdownMessage {
type: 'removeMarkdownPreview',
id: string;
}
export interface IHideMarkdownMessage {
type: 'hideMarkdownPreview';
id: string;
}
export interface IUnhideMarkdownMessage {
type: 'unhideMarkdownPreview';
id: string;
}
export interface IShowMarkdownMessage {
type: 'showMarkdownPreview',
id: string;
handle: number;
content: string | undefined;
top: number;
}
export interface IUpdateMarkdownPreviewSelectionState {
readonly type: 'updateMarkdownPreviewSelectionState',
readonly id: string;
readonly isSelected: boolean;
}
export interface IInitializeMarkdownMessage {
type: 'initializeMarkdownPreview';
cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>;
}
export type FromWebviewMessage =
| WebviewIntialized
| IDimensionMessage
| IMouseEnterMessage
| IMouseLeaveMessage
| IOutputFocusMessage
| IOutputBlurMessage
| IWheelMessage
| IScrollAckMessage
| IBlurOutputMessage
| ICustomRendererMessage
| IClickedDataUrlMessage
| IClickMarkdownPreviewMessage
| IMouseEnterMarkdownPreviewMessage
| IMouseLeaveMarkdownPreviewMessage
| IToggleMarkdownPreviewMessage
| ICellDragStartMessage
| ICellDragMessage
| ICellDropMessage
| ICellDragEndMessage
| IInitializedMarkdownPreviewMessage
;
export type ToWebviewMessage =
| IClearMessage
| IFocusOutputMessage
| ICreationRequestMessage
| IViewScrollTopRequestMessage
| IScrollRequestMessage
| IClearOutputRequestMessage
| IHideOutputMessage
| IShowOutputMessage
| IUpdatePreloadResourceMessage
| IUpdateDecorationsMessage
| ICustomRendererMessage
| ICreateMarkdownMessage
| IRemoveMarkdownMessage
| IShowMarkdownMessage
| IHideMarkdownMessage
| IUnhideMarkdownMessage
| IUpdateMarkdownPreviewSelectionState
| IInitializeMarkdownMessage
| IViewScrollMarkdownRequestMessage;
export type AnyMessage = FromWebviewMessage | ToWebviewMessage;
export interface ICachedInset<K extends ICommonCellInfo> {
outputId: string;
cellInfo: K;
renderer?: INotebookRendererInfo;
cachedCreation: ICreationRequestMessage;
}
function html(strings: TemplateStringsArray, ...values: any[]): string {
let str = '';
strings.forEach((string, i) => {
str += string + (values[i] || '');
});
return str;
}
export interface INotebookWebviewMessage {
message: unknown;
forRenderer?: string;
}
export interface IResolvedBackLayerWebview {
webview: WebviewElement;
}
export class BackLayerWebView<T extends ICommonCellInfo> extends Disposable {
element: HTMLElement;
webview: WebviewElement | undefined = undefined;
insetMapping: Map<IDisplayOutputViewModel, ICachedInset<T>> = new Map();
readonly markdownPreviewMapping = new Map<string, { version: number, visible: boolean }>();
hiddenInsetMapping: Set<IDisplayOutputViewModel> = new Set();
reversedInsetMapping: Map<string, IDisplayOutputViewModel> = new Map();
localResourceRootsCache: URI[] | undefined = undefined;
rendererRootsCache: URI[] = [];
kernelRootsCache: URI[] = [];
private readonly _onMessage = this._register(new Emitter<INotebookWebviewMessage>());
private readonly _preloadsCache = new Set<string>();
public readonly onMessage: Event<INotebookWebviewMessage> = this._onMessage.event;
private _loaded!: Promise<void>;
private _initalized?: Promise<void>;
private _disposed = false;
constructor(
public notebookEditor: ICommonNotebookEditor,
public id: string,
public documentUri: URI,
public options: {
outputNodePadding: number,
outputNodeLeftPadding: number,
previewNodePadding: number,
leftMargin: number,
cellMargin: number,
runGutter: number,
},
@IWebviewService readonly webviewService: IWebviewService,
@IOpenerService readonly openerService: IOpenerService,
@INotebookService private readonly notebookService: INotebookService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IWorkbenchEnvironmentService private readonly environmentService: IWorkbenchEnvironmentService,
@IFileDialogService private readonly fileDialogService: IFileDialogService,
@IFileService private readonly fileService: IFileService,
) {
super();
this.element = document.createElement('div');
this.element.style.height = '1400px';
this.element.style.position = 'absolute';
}
private generateContent(coreDependencies: string, baseUrl: string) {
const markdownRenderersSrc = this.getMarkdownRendererScripts();
return html`
<html lang="en">
<head>
<meta charset="UTF-8">
<base href="${baseUrl}/"/>
<!--
Markdown previews are rendered using a shadow dom and are not effected by normal css.
Insert this style node into all preview shadow doms for styling.
-->
<template id="preview-styles">
<style>
img {
max-width: 100%;
max-height: 100%;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
a:focus,
input:focus,
select:focus,
textarea:focus {
outline: 1px solid -webkit-focus-ring-color;
outline-offset: -1px;
}
hr {
border: 0;
height: 2px;
border-bottom: 2px solid;
}
h1 {
font-size: 26px;
padding-bottom: 8px;
line-height: 31px;
border-bottom-width: 1px;
border-bottom-style: solid;
border-color: var(--vscode-foreground);
margin: 0;
margin-bottom: 13px;
}
h2 {
font-size: 19px;
margin: 0;
margin-bottom: 10px;
}
h1,
h2,
h3 {
font-weight: normal;
}
div {
width: 100%;
}
/* Adjust margin of first item in markdown cell */
*:first-child {
margin-top: 0px;
}
/* h1 tags don't need top margin */
h1:first-child {
margin-top: 0;
}
/* Removes bottom margin when only one item exists in markdown cell */
*:only-child,
*:last-child {
margin-bottom: 0;
padding-bottom: 0;
}
/* makes all markdown cells consistent */
div {
min-height: ${this.options.previewNodePadding * 2}px;
}
table {
border-collapse: collapse;
border-spacing: 0;
}
table th,
table td {
border: 1px solid;
}
table > thead > tr > th {
text-align: left;
border-bottom: 1px solid;
}
table > thead > tr > th,
table > thead > tr > td,
table > tbody > tr > th,
table > tbody > tr > td {
padding: 5px 10px;
}
table > tbody > tr + tr > td {
border-top: 1px solid;
}
blockquote {
margin: 0 7px 0 5px;
padding: 0 16px 0 10px;
border-left-width: 5px;
border-left-style: solid;
}
code,
.code {
font-family: var(--monaco-monospace-font);
font-size: 1em;
line-height: 1.357em;
}
.code {
white-space: pre-wrap;
}
.latex-block {
display: block;
}
.latex {
vertical-align: middle;
display: inline-block;
}
.latex img,
.latex-block img {
filter: brightness(0) invert(0)
}
dragging {
background-color: var(--vscode-editor-background);
}
</style>
</template>
<style>
#container > div > div.output {
width: calc(100% - ${this.options.leftMargin + (this.options.cellMargin * 2) + this.options.runGutter}px);
margin-left: ${this.options.leftMargin + this.options.runGutter}px;
padding: ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodeLeftPadding}px;
box-sizing: border-box;
background-color: var(--vscode-notebook-outputContainerBackgroundColor);
}
#container > div > div.preview {
width: 100%;
box-sizing: border-box;
white-space: nowrap;
overflow: hidden;
user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
white-space: initial;
cursor: grab;
}
#container > div > div.preview.emptyMarkdownCell::before {
content: "${nls.localize('notebook.emptyMarkdownPlaceholder', "Empty markdown cell, double click or press enter to edit.")}";
font-style: italic;
opacity: 0.6;
}
/* markdown */
#container > div > div.preview {
color: var(--vscode-foreground);
width: 100%;
padding-left: ${this.options.leftMargin}px;
padding-top: ${this.options.previewNodePadding}px;
padding-bottom: ${this.options.previewNodePadding}px;
}
#container > div > div.preview.selected {
background: var(--vscode-notebook-selectedCellBackground);
}
#container > div > div.preview.dragging {
background-color: var(--vscode-editor-background);
}
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex img,
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex-block img {
filter: brightness(0) invert(1)
}
#container > div.nb-symbolHighlight > div {
background-color: var(--vscode-notebook-symbolHighlightBackground);
}
#container > div.nb-cellDeleted > div {
background-color: var(--vscode-diffEditor-removedTextBackground);
}
#container > div.nb-cellAdded > div {
background-color: var(--vscode-diffEditor-insertedTextBackground);
}
#container > div > div:not(.preview) > div {
overflow-x: scroll;
}
body {
padding: 0px;
height: 100%;
width: 100%;
}
table, thead, tr, th, td, tbody {
border: none !important;
border-color: transparent;
border-spacing: 0;
border-collapse: collapse;
}
table {
width: 100%;
}
table, th, tr {
text-align: left !important;
}
thead {
font-weight: bold;
background-color: rgba(130, 130, 130, 0.16);
}
th, td {
padding: 4px 8px;
}
tr:nth-child(even) {
background-color: rgba(130, 130, 130, 0.08);
}
tbody th {
font-weight: normal;
}
</style>
</head>
<body style="overflow: hidden;">
<script>
self.require = {};
</script>
${coreDependencies}
<div id='container' class="widgetarea" style="position: absolute;width:100%;top: 0px"></div>
<script>${preloadsScriptStr({
outputNodePadding: this.options.outputNodePadding,
outputNodeLeftPadding: this.options.outputNodeLeftPadding,
previewNodePadding: this.options.previewNodePadding,
leftMargin: this.options.leftMargin
})}</script>
${markdownRenderersSrc}
</body>
</html>`;
}
private getMarkdownRendererScripts() {
const markdownRenderers = this.notebookService.getMarkdownRendererInfo();
return markdownRenderers
.sort((a, b) => {
// prefer built-in extension
if (a.extensionIsBuiltin) {
return b.extensionIsBuiltin ? 0 : -1;
}
return b.extensionIsBuiltin ? 1 : -1;
})
.map(renderer => {
return asWebviewUri(this.environmentService, this.id, renderer.entrypoint);
})
.map(src => `<script src="${src}"></script>`)
.join('\n');
}
postRendererMessage(rendererId: string, message: any) {
this._sendMessageToWebview({
__vscode_notebook_message: true,
type: 'customRendererMessage',
message,
rendererId
});
}
private resolveOutputId(id: string): { cellInfo: T, output: ICellOutputViewModel } | undefined {
const output = this.reversedInsetMapping.get(id);
if (!output) {
return;
}
const cellInfo = this.insetMapping.get(output)!.cellInfo;
return { cellInfo, output };
}
isResolved(): this is IResolvedBackLayerWebview {
return !!this.webview;
}
async createWebview(): Promise<void> {
let coreDependencies = '';
let resolveFunc: () => void;
this._initalized = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const baseUrl = asWebviewUri(this.environmentService, this.id, dirname(this.documentUri));
if (!isWeb) {
const loaderUri = FileAccess.asFileUri('vs/loader.js', require);
const loader = asWebviewUri(this.environmentService, this.id, loaderUri);
coreDependencies = `<script src="${loader}"></script><script>
var requirejs = (function() {
return require;
}());
</script>`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
} else {
const loaderUri = FileAccess.asBrowserUri('vs/loader.js', require);
fetch(loaderUri.toString(true)).then(async response => {
if (response.status !== 200) {
throw new Error(response.statusText);
}
const loaderJs = await response.text();
coreDependencies = `
<script>
${loaderJs}
</script>
<script>
var requirejs = (function() {
return require;
}());
</script>
`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
});
}
await this._initalized;
}
private async _initialize(content: string) {
if (!document.body.contains(this.element)) {
throw new Error('Element is already detached from the DOM tree');
}
this.webview = this._createInset(this.webviewService, content);
this.webview.mountTo(this.element);
this._register(this.webview);
this._register(this.webview.onDidClickLink(link => {
if (this._disposed) {
return;
}
if (!link) {
return;
}
if (matchesScheme(link, Schemas.http) || matchesScheme(link, Schemas.https) || matchesScheme(link, Schemas.mailto)
|| matchesScheme(link, Schemas.command)) {
this.openerService.open(link, { fromUserGesture: true, allowContributedOpeners: true });
}
}));
this._register(this.webview.onDidReload(() => {
if (this._disposed) {
return;
}
let renderers = new Set<INotebookRendererInfo>();
for (const inset of this.insetMapping.values()) {
if (inset.renderer) {
renderers.add(inset.renderer);
}
}
this._preloadsCache.clear();
this.updateRendererPreloads(renderers);
for (const [output, inset] of this.insetMapping.entries()) {
this._sendMessageToWebview({ ...inset.cachedCreation, initiallyHidden: this.hiddenInsetMapping.has(output) });
}
}));
this._register(this.webview.onMessage((message) => {
const data: FromWebviewMessage | { readonly __vscode_notebook_message: undefined } = message.message;
if (this._disposed) {
return;
}
if (!data.__vscode_notebook_message) {
this._onMessage.fire({ message: data });
return;
}
switch (data.type) {
case 'dimension':
{
for (const update of data.updates) {
if (update.isOutput) {
const height = update.data.height;
const outputHeight = height;
const resolvedResult = this.resolveOutputId(update.id);
if (resolvedResult) {
const { cellInfo, output } = resolvedResult;
this.notebookEditor.updateOutputHeight(cellInfo, output, outputHeight, !!update.init, 'webview#dimension');
}
} else {
const cellId = update.id.substr(0, update.id.length - '_preview'.length);
this.notebookEditor.updateMarkdownCellHeight(cellId, update.data.height, !!update.init);
}
}
break;
}
case 'mouseenter':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = true;
}
}
break;
}
case 'mouseleave':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = false;
}
}
break;
}
case 'outputFocus':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = true;
}
}
break;
}
case 'outputBlur':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = false;
}
}
break;
}
case 'scroll-ack':
{
// const date = new Date();
// const top = data.data.top;
// console.log('ack top ', top, ' version: ', data.version, ' - ', date.getMinutes() + ':' + date.getSeconds() + ':' + date.getMilliseconds());
break;
}
case 'did-scroll-wheel':
{
this.notebookEditor.triggerScroll({
...data.payload,
preventDefault: () => { },
stopPropagation: () => { }
});
break;
}
case 'focus-editor':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (!latestCell) {
return;
}
if (data.focusNext) {
this.notebookEditor.focusNextNotebookCell(latestCell, 'editor');
} else {
this.notebookEditor.focusNotebookCell(latestCell, 'editor');
}
}
break;
}
case 'clicked-data-url':
{
this._onDidClickDataLink(data);
break;
}
case 'customRendererMessage':
{
this._onMessage.fire({ message: data.message, forRenderer: data.rendererId });
break;
}
case 'clickMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
if (data.shiftKey || data.metaKey) {
// Add to selection
this.notebookEditor.toggleNotebookCellSelection(cell);
} else {
// Normal click
this.notebookEditor.focusNotebookCell(cell, 'container', { skipReveal: true });
}
}
break;
}
case 'toggleMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
this.notebookEditor.setMarkdownCellEditState(data.cellId, CellEditState.Editing);
this.notebookEditor.focusNotebookCell(cell, 'editor', { skipReveal: true });
}
break;
}
case 'mouseEnterMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = true;
}
break;
}
case 'mouseLeaveMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = false;
}
break;
}
case 'cell-drag-start':
{
this.notebookEditor.markdownCellDragStart(data.cellId, data.position);
break;
}
case 'cell-drag':
{
this.notebookEditor.markdownCellDrag(data.cellId, data.position);
break;
}
case 'cell-drop':
{
this.notebookEditor.markdownCellDrop(data.cellId, {
clientY: data.position.clientY,
ctrlKey: data.ctrlKey,
altKey: data.altKey,
});
break;
}
case 'cell-drag-end':
{
this.notebookEditor.markdownCellDragEnd(data.cellId);
break;
}
}
}));
}
private async _onDidClickDataLink(event: IClickedDataUrlMessage): Promise<void> {
if (typeof event.data !== 'string') {
return;
}
const [splitStart, splitData] = event.data.split(';base64,');
if (!splitData || !splitStart) {
return;
}
const defaultDir = dirname(this.documentUri);
let defaultName: string;
if (event.downloadName) {
defaultName = event.downloadName;
} else {
const mimeType = splitStart.replace(/^data:/, '');
const candidateExtension = mimeType && getExtensionForMimeType(mimeType);
defaultName = candidateExtension ? `download${candidateExtension}` : 'download';
}
const defaultUri = joinPath(defaultDir, defaultName);
const newFileUri = await this.fileDialogService.showSaveDialog({
defaultUri
});
if (!newFileUri) {
return;
}
const decoded = atob(splitData);
const typedArray = new Uint8Array(decoded.length);
for (let i = 0; i < decoded.length; i++) {
typedArray[i] = decoded.charCodeAt(i);
}
const buff = VSBuffer.wrap(typedArray);
await this.fileService.writeFile(newFileUri, buff);
await this.openerService.open(newFileUri);
}
private _createInset(webviewService: IWebviewService, content: string) {
const rootPath = isWeb ? FileAccess.asBrowserUri('', require) : FileAccess.asFileUri('', require);
const workspaceFolders = this.contextService.getWorkspace().folders.map(x => x.uri);
this.localResourceRootsCache = [
...this.notebookService.getNotebookProviderResourceRoots(),
...this.notebookService.getMarkdownRendererInfo().map(x => dirname(x.entrypoint)),
...workspaceFolders,
rootPath,
];
const webview = webviewService.createWebviewElement(this.id, {
purpose: WebviewContentPurpose.NotebookRenderer,
enableFindWidget: false,
transformCssVariables: transformWebviewThemeVars,
}, {
allowMultipleAPIAcquire: true,
allowScripts: true,
localResourceRoots: this.localResourceRootsCache
}, undefined);
let resolveFunc: () => void;
this._loaded = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const dispose = webview.onMessage((message) => {
const data: FromWebviewMessage = message.message;
if (data.__vscode_notebook_message && data.type === 'initialized') {
resolveFunc();
dispose.dispose();
}
});
webview.html = content;
return webview;
}
shouldUpdateInset(cell: IGenericCellViewModel, output: ICellOutputViewModel, cellTop: number) {
if (this._disposed) {
return;
}
if (cell.metadata?.outputCollapsed) {
return false;
}
const outputCache = this.insetMapping.get(output)!;
const outputIndex = cell.outputsViewModels.indexOf(output);
const outputOffset = cellTop + cell.getOutputOffset(outputIndex);
if (this.hiddenInsetMapping.has(output)) {
return true;
}
if (outputOffset === outputCache.cachedCreation.top) {
return false;
}
return true;
}
updateMarkdownScrollTop(items: { id: string, top: number }[]) {
if (this._disposed || !items.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll-markdown',
cells: items
});
}
updateViewScrollTop(forceDisplay: boolean, items: IDisplayOutputLayoutUpdateRequest[]) {
if (!items.length) {
return;
}
const widgets = coalesce(items.map((item): IContentWidgetTopRequest | undefined => {
const outputCache = this.insetMapping.get(item.output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
const outputOffset = item.outputOffset;
outputCache.cachedCreation.top = outputOffset;
this.hiddenInsetMapping.delete(item.output);
return {
id: id,
top: outputOffset,
};
}));
if (!widgets.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll',
forceDisplay,
widgets: widgets
});
}
private async createMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (this.markdownPreviewMapping.has(cellId)) {
console.error('Trying to create markdown preview that already exists');
return;
}
const initialTop = cellTop;
this.markdownPreviewMapping.set(cellId, { version: contentVersion, visible: true });
this._sendMessageToWebview({
type: 'createMarkdownPreview',
id: cellId,
handle: cellHandle,
content: content,
top: initialTop,
});
}
async showMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
return this.createMarkdownPreview(cellId, cellHandle, content, cellTop, contentVersion);
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error('Try to show a preview that does not exist');
return;
}
if (entry.version !== contentVersion || !entry.visible) {
this._sendMessageToWebview({
type: 'showMarkdownPreview',
id: cellId,
handle: cellHandle,
// If the content has not changed, we still want to make sure the
// preview is visible but don't need to send anything over
content: entry.version === contentVersion ? undefined : content,
top: cellTop
});
}
entry.version = contentVersion;
entry.visible = true;
}
async hideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
// TODO: this currently seems expected on first load
// console.error(`Try to hide a preview that does not exist: ${cellId}`);
return;
}
if (entry.visible) {
this._sendMessageToWebview({
type: 'hideMarkdownPreview',
id: cellId
});
entry.visible = false;
}
}
async unhideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error(`Try to unhide a preview that does not exist: ${cellId}`);
return;
}
if (!entry.visible) {
this._sendMessageToWebview({
type: 'unhideMarkdownPreview',
id: cellId
});
entry.visible = true;
}
}
async removeMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
console.error(`Try to delete a preview that does not exist: ${cellId}`);
return;
}
this.markdownPreviewMapping.delete(cellId);
this._sendMessageToWebview({
type: 'removeMarkdownPreview',
id: cellId
});
}
async updateMarkdownPreviewSelectionState(cellId: any, isSelected: boolean) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
// TODO: this currently seems expected on first load
// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);
return;
}
this._sendMessageToWebview({
type: 'updateMarkdownPreviewSelectionState',
id: cellId,
isSelected
});
}
async initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {
await this._loaded;
if (this._disposed) {
return;
}
// TODO: use proper handler
const p = new Promise<void>(resolve => {
this.webview?.onMessage(e => {
if (e.message.type === 'initializedMarkdownPreview') {
resolve();
}
});
});
for (const cell of cells) {
this.markdownPreviewMapping.set(cell.cellId, { version: 0, visible: false });
}
this._sendMessageToWebview({
type: 'initializeMarkdownPreview',
cells: cells,
});
await p;
}
async createOutput(cellInfo: T, content: IInsetRenderOutput, cellTop: number, offset: number) {
if (this._disposed) {
return;
}
const initialTop = cellTop + offset;
if (this.insetMapping.has(content.source)) {
const outputCache = this.insetMapping.get(content.source);
if (outputCache) {
this.hiddenInsetMapping.delete(content.source);
this._sendMessageToWebview({
type: 'showOutput',
cellId: outputCache.cellInfo.cellId,
outputId: outputCache.outputId,
top: initialTop
});
return;
}
}
const messageBase = {
type: 'html',
cellId: cellInfo.cellId,
top: initialTop,
left: 0,
requiredPreloads: [],
} as const;
let message: ICreationRequestMessage;
let renderer: INotebookRendererInfo | undefined;
if (content.type === RenderOutputType.Extension) {
const output = content.source.model;
renderer = content.renderer;
const outputDto = output.outputs.find(op => op.mime === content.mimeType);
message = {
...messageBase,
outputId: output.outputId,
apiNamespace: content.renderer.id,
requiredPreloads: await this.updateRendererPreloads([content.renderer]),
content: {
type: RenderOutputType.Extension,
outputId: output.outputId,
mimeType: content.mimeType,
value: outputDto?.value,
metadata: outputDto?.metadata,
},
};
} else {
message = {
...messageBase,
outputId: UUID.generateUuid(),
content: {
type: content.type,
htmlContent: content.htmlContent,
}
};
}
this._sendMessageToWebview(message);
this.insetMapping.set(content.source, { outputId: message.outputId, cellInfo: cellInfo, renderer, cachedCreation: message });
this.hiddenInsetMapping.delete(content.source);
this.reversedInsetMapping.set(message.outputId, content.source);
}
removeInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
this._sendMessageToWebview({
type: 'clearOutput',
apiNamespace: outputCache.cachedCreation.apiNamespace,
cellUri: outputCache.cellInfo.cellUri.toString(),
outputId: id,
cellId: outputCache.cellInfo.cellId
});
this.insetMapping.delete(output);
this.reversedInsetMapping.delete(id);
}
hideInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
this.hiddenInsetMapping.add(output);
this._sendMessageToWebview({
type: 'hideOutput',
outputId: outputCache.outputId,
cellId: outputCache.cellInfo.cellId,
});
}
clearInsets() {
if (this._disposed) {
return;
}
this._sendMessageToWebview({
type: 'clear'
});
this.insetMapping = new Map();
this.reversedInsetMapping = new Map();
}
focusWebview() {
if (this._disposed) {
return;
}
this.webview?.focus();
}
focusOutput(cellId: string) {
if (this._disposed) {
return;
}
this.webview?.focus();
setTimeout(() => { // Need this, or focus decoration is not shown. No clue.
this._sendMessageToWebview({
type: 'focus-output',
cellId,
});
}, 50);
}
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]) {
this._sendMessageToWebview({
type: 'decorations',
cellId,
addedClassNames: added,
removedClassNames: removed
});
}
async updateKernelPreloads(extensionLocations: URI[], preloads: URI[]) {
if (this._disposed) {
return;
}
await this._loaded;
const resources: IPreloadResource[] = [];
for (const preload of preloads) {
const uri = this.environmentService.isExtensionDevelopment && (preload.scheme === 'http' || preload.scheme === 'https')
? preload : asWebviewUri(this.environmentService, this.id, preload);
if (!this._preloadsCache.has(uri.toString())) {
resources.push({ uri: uri.toString(), originalUri: preload.toString() });
this._preloadsCache.add(uri.toString());
}
}
if (!resources.length) {
return;
}
this.kernelRootsCache = [...extensionLocations, ...this.kernelRootsCache];
this._updatePreloads(resources, 'kernel');
}
async updateRendererPreloads(renderers: Iterable<INotebookRendererInfo>) {
if (this._disposed) {
return [];
}
await this._loaded;
const requiredPreloads: IPreloadResource[] = [];
const resources: IPreloadResource[] = [];
const extensionLocations: URI[] = [];
for (const rendererInfo of renderers) {
extensionLocations.push(rendererInfo.extensionLocation);
for (const preload of [rendererInfo.entrypoint, ...rendererInfo.preloads]) {
const uri = asWebviewUri(this.environmentService, this.id, preload);
const resource: IPreloadResource = { uri: uri.toString(), originalUri: preload.toString() };
requiredPreloads.push(resource);
if (!this._preloadsCache.has(uri.toString())) {
resources.push(resource);
this._preloadsCache.add(uri.toString());
}
}
}
if (!resources.length) {
return requiredPreloads;
}
this.rendererRootsCache = extensionLocations;
this._updatePreloads(resources, 'renderer');
return requiredPreloads;
}
private _updatePreloads(resources: IPreloadResource[], source: 'renderer' | 'kernel') {
if (!this.webview) {
return;
}
const mixedResourceRoots = [...(this.localResourceRootsCache || []), ...this.rendererRootsCache, ...this.kernelRootsCache];
this.webview.localResourcesRoot = mixedResourceRoots;
this._sendMessageToWebview({
type: 'preload',
resources: resources,
source: source
});
}
private _sendMessageToWebview(message: ToWebviewMessage) {
if (this._disposed) {
return;
}
this.webview?.postMessage(message);
}
clearPreloadsCache() {
this._preloadsCache.clear();
}
dispose() {
this._disposed = true;
this.webview?.dispose();
super.dispose();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.9994081258773804,
0.1317502111196518,
0.00016585932462476194,
0.00018106399511452764,
0.3277618885040283
] |
{
"id": 4,
"code_window": [
"import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';\n",
"import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';\n",
"import * as nls from 'vs/nls';\n",
"\n",
"interface BaseToWebviewMessage {\n",
"\treadonly __vscode_notebook_message: true;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { coalesce } from 'vs/base/common/arrays';\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "add",
"edit_start_line_idx": 28
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { KeyboardLayoutContribution } from 'vs/workbench/services/keybinding/browser/keyboardLayouts/_.contribution';
KeyboardLayoutContribution.INSTANCE.registerKeyboardLayout({
layout: { id: 'com.apple.keylayout.Dvorak', localizedName: 'Dvorak', lang: 'en' },
secondaryLayouts: [],
mapping: {
KeyA: ['a', 'A', 'å', 'Å', 0],
KeyB: ['x', 'X', '≈', '˛', 0],
KeyC: ['j', 'J', '∆', 'Ô', 0],
KeyD: ['e', 'E', '´', '´', 4],
KeyE: ['.', '>', '≥', '˘', 0],
KeyF: ['u', 'U', '¨', '¨', 4],
KeyG: ['i', 'I', 'ˆ', 'ˆ', 4],
KeyH: ['d', 'D', '∂', 'Î', 0],
KeyI: ['c', 'C', 'ç', 'Ç', 0],
KeyJ: ['h', 'H', '˙', 'Ó', 0],
KeyK: ['t', 'T', '†', 'ˇ', 0],
KeyL: ['n', 'N', '˜', '˜', 4],
KeyM: ['m', 'M', 'µ', 'Â', 0],
KeyN: ['b', 'B', '∫', 'ı', 0],
KeyO: ['r', 'R', '®', '‰', 0],
KeyP: ['l', 'L', '¬', 'Ò', 0],
KeyQ: ['\'', '"', 'æ', 'Æ', 0],
KeyR: ['p', 'P', 'π', '∏', 0],
KeyS: ['o', 'O', 'ø', 'Ø', 0],
KeyT: ['y', 'Y', '¥', 'Á', 0],
KeyU: ['g', 'G', '©', '˝', 0],
KeyV: ['k', 'K', '˚', '', 0],
KeyW: [',', '<', '≤', '¯', 0],
KeyX: ['q', 'Q', 'œ', 'Œ', 0],
KeyY: ['f', 'F', 'ƒ', 'Ï', 0],
KeyZ: [';', ':', '…', 'Ú', 0],
Digit1: ['1', '!', '¡', '⁄', 0],
Digit2: ['2', '@', '™', '€', 0],
Digit3: ['3', '#', '£', '‹', 0],
Digit4: ['4', '$', '¢', '›', 0],
Digit5: ['5', '%', '∞', 'fi', 0],
Digit6: ['6', '^', '§', 'fl', 0],
Digit7: ['7', '&', '¶', '‡', 0],
Digit8: ['8', '*', '•', '°', 0],
Digit9: ['9', '(', 'ª', '·', 0],
Digit0: ['0', ')', 'º', '‚', 0],
Enter: [],
Escape: [],
Backspace: [],
Tab: [],
Space: [' ', ' ', ' ', ' ', 0],
Minus: ['[', '{', '“', '”', 0],
Equal: [']', '}', '‘', '’', 0],
BracketLeft: ['/', '?', '÷', '¿', 0],
BracketRight: ['=', '+', '≠', '±', 0],
Backslash: ['\\', '|', '«', '»', 0],
Semicolon: ['s', 'S', 'ß', 'Í', 0],
Quote: ['-', '_', '–', '—', 0],
Backquote: ['`', '~', '`', '`', 4],
Comma: ['w', 'W', '∑', '„', 0],
Period: ['v', 'V', '√', '◊', 0],
Slash: ['z', 'Z', 'Ω', '¸', 0],
CapsLock: [],
F1: [],
F2: [],
F3: [],
F4: [],
F5: [],
F6: [],
F7: [],
F8: [],
F9: [],
F10: [],
F11: [],
F12: [],
Insert: [],
Home: [],
PageUp: [],
Delete: [],
End: [],
PageDown: [],
ArrowRight: [],
ArrowLeft: [],
ArrowDown: [],
ArrowUp: [],
NumLock: [],
NumpadDivide: ['/', '/', '/', '/', 0],
NumpadMultiply: ['*', '*', '*', '*', 0],
NumpadSubtract: ['-', '-', '-', '-', 0],
NumpadAdd: ['+', '+', '+', '+', 0],
NumpadEnter: [],
Numpad1: ['1', '1', '1', '1', 0],
Numpad2: ['2', '2', '2', '2', 0],
Numpad3: ['3', '3', '3', '3', 0],
Numpad4: ['4', '4', '4', '4', 0],
Numpad5: ['5', '5', '5', '5', 0],
Numpad6: ['6', '6', '6', '6', 0],
Numpad7: ['7', '7', '7', '7', 0],
Numpad8: ['8', '8', '8', '8', 0],
Numpad9: ['9', '9', '9', '9', 0],
Numpad0: ['0', '0', '0', '0', 0],
NumpadDecimal: ['.', '.', '.', '.', 0],
IntlBackslash: ['§', '±', '§', '±', 0],
ContextMenu: [],
NumpadEqual: ['=', '=', '=', '=', 0],
F13: [],
F14: [],
F15: [],
F16: [],
F17: [],
F18: [],
F19: [],
F20: [],
AudioVolumeMute: [],
AudioVolumeUp: ['', '=', '', '=', 0],
AudioVolumeDown: [],
NumpadComma: [],
IntlRo: [],
KanaMode: [],
IntlYen: [],
ControlLeft: [],
ShiftLeft: [],
AltLeft: [],
MetaLeft: [],
ControlRight: [],
ShiftRight: [],
AltRight: [],
MetaRight: []
}
});
| src/vs/workbench/services/keybinding/browser/keyboardLayouts/dvorak.darwin.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017408774874638766,
0.00016899892943911254,
0.0001677122199907899,
0.00016849277017172426,
0.0000015672219433326973
] |
{
"id": 4,
"code_window": [
"import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';\n",
"import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';\n",
"import * as nls from 'vs/nls';\n",
"\n",
"interface BaseToWebviewMessage {\n",
"\treadonly __vscode_notebook_message: true;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { coalesce } from 'vs/base/common/arrays';\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "add",
"edit_start_line_idx": 28
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as strings from 'vs/base/common/strings';
import * as platform from 'vs/base/common/platform';
import * as buffer from 'vs/base/common/buffer';
declare const TextDecoder: {
prototype: TextDecoder;
new(label?: string): TextDecoder;
};
interface TextDecoder {
decode(view: Uint16Array): string;
}
export interface IStringBuilder {
build(): string;
reset(): void;
write1(charCode: number): void;
appendASCII(charCode: number): void;
appendASCIIString(str: string): void;
}
let _utf16LE_TextDecoder: TextDecoder | null;
function getUTF16LE_TextDecoder(): TextDecoder {
if (!_utf16LE_TextDecoder) {
_utf16LE_TextDecoder = new TextDecoder('UTF-16LE');
}
return _utf16LE_TextDecoder;
}
let _utf16BE_TextDecoder: TextDecoder | null;
function getUTF16BE_TextDecoder(): TextDecoder {
if (!_utf16BE_TextDecoder) {
_utf16BE_TextDecoder = new TextDecoder('UTF-16BE');
}
return _utf16BE_TextDecoder;
}
let _platformTextDecoder: TextDecoder | null;
export function getPlatformTextDecoder(): TextDecoder {
if (!_platformTextDecoder) {
_platformTextDecoder = platform.isLittleEndian() ? getUTF16LE_TextDecoder() : getUTF16BE_TextDecoder();
}
return _platformTextDecoder;
}
export const hasTextDecoder = (typeof TextDecoder !== 'undefined');
export let createStringBuilder: (capacity: number) => IStringBuilder;
export let decodeUTF16LE: (source: Uint8Array, offset: number, len: number) => string;
if (hasTextDecoder) {
createStringBuilder = (capacity) => new StringBuilder(capacity);
decodeUTF16LE = standardDecodeUTF16LE;
} else {
createStringBuilder = (capacity) => new CompatStringBuilder();
decodeUTF16LE = compatDecodeUTF16LE;
}
function standardDecodeUTF16LE(source: Uint8Array, offset: number, len: number): string {
const view = new Uint16Array(source.buffer, offset, len);
if (len > 0 && (view[0] === 0xFEFF || view[0] === 0xFFFE)) {
// UTF16 sometimes starts with a BOM https://de.wikipedia.org/wiki/Byte_Order_Mark
// It looks like TextDecoder.decode will eat up a leading BOM (0xFEFF or 0xFFFE)
// We don't want that behavior because we know the string is UTF16LE and the BOM should be maintained
// So we use the manual decoder
return compatDecodeUTF16LE(source, offset, len);
}
return getUTF16LE_TextDecoder().decode(view);
}
function compatDecodeUTF16LE(source: Uint8Array, offset: number, len: number): string {
let result: string[] = [];
let resultLen = 0;
for (let i = 0; i < len; i++) {
const charCode = buffer.readUInt16LE(source, offset); offset += 2;
result[resultLen++] = String.fromCharCode(charCode);
}
return result.join('');
}
class StringBuilder implements IStringBuilder {
private readonly _capacity: number;
private readonly _buffer: Uint16Array;
private _completedStrings: string[] | null;
private _bufferLength: number;
constructor(capacity: number) {
this._capacity = capacity | 0;
this._buffer = new Uint16Array(this._capacity);
this._completedStrings = null;
this._bufferLength = 0;
}
public reset(): void {
this._completedStrings = null;
this._bufferLength = 0;
}
public build(): string {
if (this._completedStrings !== null) {
this._flushBuffer();
return this._completedStrings.join('');
}
return this._buildBuffer();
}
private _buildBuffer(): string {
if (this._bufferLength === 0) {
return '';
}
const view = new Uint16Array(this._buffer.buffer, 0, this._bufferLength);
return getPlatformTextDecoder().decode(view);
}
private _flushBuffer(): void {
const bufferString = this._buildBuffer();
this._bufferLength = 0;
if (this._completedStrings === null) {
this._completedStrings = [bufferString];
} else {
this._completedStrings[this._completedStrings.length] = bufferString;
}
}
public write1(charCode: number): void {
const remainingSpace = this._capacity - this._bufferLength;
if (remainingSpace <= 1) {
if (remainingSpace === 0 || strings.isHighSurrogate(charCode)) {
this._flushBuffer();
}
}
this._buffer[this._bufferLength++] = charCode;
}
public appendASCII(charCode: number): void {
if (this._bufferLength === this._capacity) {
// buffer is full
this._flushBuffer();
}
this._buffer[this._bufferLength++] = charCode;
}
public appendASCIIString(str: string): void {
const strLen = str.length;
if (this._bufferLength + strLen >= this._capacity) {
// This string does not fit in the remaining buffer space
this._flushBuffer();
this._completedStrings![this._completedStrings!.length] = str;
return;
}
for (let i = 0; i < strLen; i++) {
this._buffer[this._bufferLength++] = str.charCodeAt(i);
}
}
}
class CompatStringBuilder implements IStringBuilder {
private _pieces: string[];
private _piecesLen: number;
constructor() {
this._pieces = [];
this._piecesLen = 0;
}
public reset(): void {
this._pieces = [];
this._piecesLen = 0;
}
public build(): string {
return this._pieces.join('');
}
public write1(charCode: number): void {
this._pieces[this._piecesLen++] = String.fromCharCode(charCode);
}
public appendASCII(charCode: number): void {
this._pieces[this._piecesLen++] = String.fromCharCode(charCode);
}
public appendASCIIString(str: string): void {
this._pieces[this._piecesLen++] = str;
}
}
| src/vs/editor/common/core/stringBuilder.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0002582008019089699,
0.00017399044008925557,
0.00016548337589483708,
0.0001680823479546234,
0.00001949517900357023
] |
{
"id": 4,
"code_window": [
"import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';\n",
"import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';\n",
"import * as nls from 'vs/nls';\n",
"\n",
"interface BaseToWebviewMessage {\n",
"\treadonly __vscode_notebook_message: true;\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
"import { coalesce } from 'vs/base/common/arrays';\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "add",
"edit_start_line_idx": 28
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { Token, TokenizationResult, TokenizationResult2 } from 'vs/editor/common/core/token';
import { ColorId, FontStyle, IState, LanguageId, LanguageIdentifier, MetadataConsts, StandardTokenType } from 'vs/editor/common/modes';
class NullStateImpl implements IState {
public clone(): IState {
return this;
}
public equals(other: IState): boolean {
return (this === other);
}
}
export const NULL_STATE: IState = new NullStateImpl();
export const NULL_MODE_ID = 'vs.editor.nullMode';
export const NULL_LANGUAGE_IDENTIFIER = new LanguageIdentifier(NULL_MODE_ID, LanguageId.Null);
export function nullTokenize(modeId: string, buffer: string, state: IState, deltaOffset: number): TokenizationResult {
return new TokenizationResult([new Token(deltaOffset, '', modeId)], state);
}
export function nullTokenize2(languageId: LanguageId, buffer: string, state: IState | null, deltaOffset: number): TokenizationResult2 {
let tokens = new Uint32Array(2);
tokens[0] = deltaOffset;
tokens[1] = (
(languageId << MetadataConsts.LANGUAGEID_OFFSET)
| (StandardTokenType.Other << MetadataConsts.TOKEN_TYPE_OFFSET)
| (FontStyle.None << MetadataConsts.FONT_STYLE_OFFSET)
| (ColorId.DefaultForeground << MetadataConsts.FOREGROUND_OFFSET)
| (ColorId.DefaultBackground << MetadataConsts.BACKGROUND_OFFSET)
) >>> 0;
return new TokenizationResult2(tokens, state === null ? NULL_STATE : state);
}
| src/vs/editor/common/modes/nullMode.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017224391922354698,
0.0001702061708783731,
0.0001677344407653436,
0.00017070730973500758,
0.0000015307771263906034
] |
{
"id": 5,
"code_window": [
"\tcontent: string | undefined;\n",
"\ttop: number;\n",
"}\n",
"\n",
"export interface IUpdateMarkdownPreviewSelectionState {\n",
"\treadonly type: 'updateMarkdownPreviewSelectionState',\n",
"\treadonly id: string;\n",
"\treadonly isSelected: boolean;\n",
"}\n",
"\n",
"export interface IInitializeMarkdownMessage {\n",
"\ttype: 'initializeMarkdownPreview';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IUpdateSelectedMarkdownPreviews {\n",
"\treadonly type: 'updateSelectedMarkdownPreviews',\n",
"\treadonly selectedCellIds: readonly string[]\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 290
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { VSBuffer } from 'vs/base/common/buffer';
import { Emitter, Event } from 'vs/base/common/event';
import { Disposable } from 'vs/base/common/lifecycle';
import { getExtensionForMimeType } from 'vs/base/common/mime';
import { FileAccess, Schemas } from 'vs/base/common/network';
import { isWeb } from 'vs/base/common/platform';
import { dirname, joinPath } from 'vs/base/common/resources';
import { URI } from 'vs/base/common/uri';
import * as UUID from 'vs/base/common/uuid';
import { IFileDialogService } from 'vs/platform/dialogs/common/dialogs';
import { IFileService } from 'vs/platform/files/common/files';
import { IOpenerService, matchesScheme } from 'vs/platform/opener/common/opener';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
import { CellEditState, ICellOutputViewModel, ICommonCellInfo, ICommonNotebookEditor, IDisplayOutputLayoutUpdateRequest, IDisplayOutputViewModel, IGenericCellViewModel, IInsetRenderOutput, RenderOutputType } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { preloadsScriptStr } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads';
import { transformWebviewThemeVars } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewThemeMapping';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { INotebookRendererInfo } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { IWebviewService, WebviewContentPurpose, WebviewElement } from 'vs/workbench/contrib/webview/browser/webview';
import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import * as nls from 'vs/nls';
interface BaseToWebviewMessage {
readonly __vscode_notebook_message: true;
}
export interface WebviewIntialized extends BaseToWebviewMessage {
type: 'initialized';
}
export interface DimensionUpdate {
id: string;
init?: boolean;
data: { height: number };
isOutput?: boolean;
}
export interface IDimensionMessage extends BaseToWebviewMessage {
type: 'dimension';
updates: readonly DimensionUpdate[];
}
export interface IMouseEnterMessage extends BaseToWebviewMessage {
type: 'mouseenter';
id: string;
}
export interface IMouseLeaveMessage extends BaseToWebviewMessage {
type: 'mouseleave';
id: string;
}
export interface IOutputFocusMessage extends BaseToWebviewMessage {
type: 'outputFocus';
id: string;
}
export interface IOutputBlurMessage extends BaseToWebviewMessage {
type: 'outputBlur';
id: string;
}
export interface IWheelMessage extends BaseToWebviewMessage {
type: 'did-scroll-wheel';
payload: any;
}
export interface IScrollAckMessage extends BaseToWebviewMessage {
type: 'scroll-ack';
data: { top: number };
version: number;
}
export interface IBlurOutputMessage extends BaseToWebviewMessage {
type: 'focus-editor';
id: string;
focusNext?: boolean;
}
export interface IClickedDataUrlMessage extends BaseToWebviewMessage {
type: 'clicked-data-url';
data: string | ArrayBuffer | null;
downloadName?: string;
}
export interface IClickMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'clickMarkdownPreview';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly metaKey: boolean;
readonly shiftKey: boolean;
}
export interface IMouseEnterMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseEnterMarkdownPreview';
cellId: string;
}
export interface IMouseLeaveMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseLeaveMarkdownPreview';
cellId: string;
}
export interface IToggleMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'toggleMarkdownPreview';
cellId: string;
}
export interface ICellDragStartMessage extends BaseToWebviewMessage {
type: 'cell-drag-start';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragMessage extends BaseToWebviewMessage {
type: 'cell-drag';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDropMessage extends BaseToWebviewMessage {
readonly type: 'cell-drop';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragEndMessage extends BaseToWebviewMessage {
readonly type: 'cell-drag-end';
readonly cellId: string;
}
export interface IInitializedMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'initializedMarkdownPreview';
}
export interface IClearMessage {
type: 'clear';
}
export interface IOutputRequestMetadata {
/**
* Additional attributes of a cell metadata.
*/
custom?: { [key: string]: unknown };
}
export interface IOutputRequestDto {
/**
* { mime_type: value }
*/
data: { [key: string]: unknown; }
metadata?: IOutputRequestMetadata;
outputId: string;
}
export interface ICreationRequestMessage {
type: 'html';
content:
| { type: RenderOutputType.Html; htmlContent: string }
| { type: RenderOutputType.Extension; outputId: string; value: unknown; metadata: unknown; mimeType: string };
cellId: string;
outputId: string;
top: number;
left: number;
requiredPreloads: ReadonlyArray<IPreloadResource>;
initiallyHidden?: boolean;
apiNamespace?: string | undefined;
}
export interface IContentWidgetTopRequest {
id: string;
top: number;
}
export interface IViewScrollTopRequestMessage {
type: 'view-scroll';
forceDisplay: boolean;
widgets: IContentWidgetTopRequest[];
}
export interface IViewScrollMarkdownRequestMessage {
type: 'view-scroll-markdown';
cells: { id: string; top: number }[];
}
export interface IScrollRequestMessage {
type: 'scroll';
id: string;
top: number;
widgetTop?: number;
version: number;
}
export interface IClearOutputRequestMessage {
type: 'clearOutput';
cellId: string;
outputId: string;
cellUri: string;
apiNamespace: string | undefined;
}
export interface IHideOutputMessage {
type: 'hideOutput';
outputId: string;
cellId: string;
}
export interface IShowOutputMessage {
type: 'showOutput';
cellId: string;
outputId: string;
top: number;
}
export interface IFocusOutputMessage {
type: 'focus-output';
cellId: string;
}
export interface IPreloadResource {
originalUri: string;
uri: string;
}
export interface IUpdatePreloadResourceMessage {
type: 'preload';
resources: IPreloadResource[];
source: 'renderer' | 'kernel';
}
export interface IUpdateDecorationsMessage {
type: 'decorations';
cellId: string;
addedClassNames: string[];
removedClassNames: string[];
}
export interface ICustomRendererMessage extends BaseToWebviewMessage {
type: 'customRendererMessage';
rendererId: string;
message: unknown;
}
export interface ICreateMarkdownMessage {
type: 'createMarkdownPreview',
id: string;
handle: number;
content: string;
top: number;
}
export interface IRemoveMarkdownMessage {
type: 'removeMarkdownPreview',
id: string;
}
export interface IHideMarkdownMessage {
type: 'hideMarkdownPreview';
id: string;
}
export interface IUnhideMarkdownMessage {
type: 'unhideMarkdownPreview';
id: string;
}
export interface IShowMarkdownMessage {
type: 'showMarkdownPreview',
id: string;
handle: number;
content: string | undefined;
top: number;
}
export interface IUpdateMarkdownPreviewSelectionState {
readonly type: 'updateMarkdownPreviewSelectionState',
readonly id: string;
readonly isSelected: boolean;
}
export interface IInitializeMarkdownMessage {
type: 'initializeMarkdownPreview';
cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>;
}
export type FromWebviewMessage =
| WebviewIntialized
| IDimensionMessage
| IMouseEnterMessage
| IMouseLeaveMessage
| IOutputFocusMessage
| IOutputBlurMessage
| IWheelMessage
| IScrollAckMessage
| IBlurOutputMessage
| ICustomRendererMessage
| IClickedDataUrlMessage
| IClickMarkdownPreviewMessage
| IMouseEnterMarkdownPreviewMessage
| IMouseLeaveMarkdownPreviewMessage
| IToggleMarkdownPreviewMessage
| ICellDragStartMessage
| ICellDragMessage
| ICellDropMessage
| ICellDragEndMessage
| IInitializedMarkdownPreviewMessage
;
export type ToWebviewMessage =
| IClearMessage
| IFocusOutputMessage
| ICreationRequestMessage
| IViewScrollTopRequestMessage
| IScrollRequestMessage
| IClearOutputRequestMessage
| IHideOutputMessage
| IShowOutputMessage
| IUpdatePreloadResourceMessage
| IUpdateDecorationsMessage
| ICustomRendererMessage
| ICreateMarkdownMessage
| IRemoveMarkdownMessage
| IShowMarkdownMessage
| IHideMarkdownMessage
| IUnhideMarkdownMessage
| IUpdateMarkdownPreviewSelectionState
| IInitializeMarkdownMessage
| IViewScrollMarkdownRequestMessage;
export type AnyMessage = FromWebviewMessage | ToWebviewMessage;
export interface ICachedInset<K extends ICommonCellInfo> {
outputId: string;
cellInfo: K;
renderer?: INotebookRendererInfo;
cachedCreation: ICreationRequestMessage;
}
function html(strings: TemplateStringsArray, ...values: any[]): string {
let str = '';
strings.forEach((string, i) => {
str += string + (values[i] || '');
});
return str;
}
export interface INotebookWebviewMessage {
message: unknown;
forRenderer?: string;
}
export interface IResolvedBackLayerWebview {
webview: WebviewElement;
}
export class BackLayerWebView<T extends ICommonCellInfo> extends Disposable {
element: HTMLElement;
webview: WebviewElement | undefined = undefined;
insetMapping: Map<IDisplayOutputViewModel, ICachedInset<T>> = new Map();
readonly markdownPreviewMapping = new Map<string, { version: number, visible: boolean }>();
hiddenInsetMapping: Set<IDisplayOutputViewModel> = new Set();
reversedInsetMapping: Map<string, IDisplayOutputViewModel> = new Map();
localResourceRootsCache: URI[] | undefined = undefined;
rendererRootsCache: URI[] = [];
kernelRootsCache: URI[] = [];
private readonly _onMessage = this._register(new Emitter<INotebookWebviewMessage>());
private readonly _preloadsCache = new Set<string>();
public readonly onMessage: Event<INotebookWebviewMessage> = this._onMessage.event;
private _loaded!: Promise<void>;
private _initalized?: Promise<void>;
private _disposed = false;
constructor(
public notebookEditor: ICommonNotebookEditor,
public id: string,
public documentUri: URI,
public options: {
outputNodePadding: number,
outputNodeLeftPadding: number,
previewNodePadding: number,
leftMargin: number,
cellMargin: number,
runGutter: number,
},
@IWebviewService readonly webviewService: IWebviewService,
@IOpenerService readonly openerService: IOpenerService,
@INotebookService private readonly notebookService: INotebookService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IWorkbenchEnvironmentService private readonly environmentService: IWorkbenchEnvironmentService,
@IFileDialogService private readonly fileDialogService: IFileDialogService,
@IFileService private readonly fileService: IFileService,
) {
super();
this.element = document.createElement('div');
this.element.style.height = '1400px';
this.element.style.position = 'absolute';
}
private generateContent(coreDependencies: string, baseUrl: string) {
const markdownRenderersSrc = this.getMarkdownRendererScripts();
return html`
<html lang="en">
<head>
<meta charset="UTF-8">
<base href="${baseUrl}/"/>
<!--
Markdown previews are rendered using a shadow dom and are not effected by normal css.
Insert this style node into all preview shadow doms for styling.
-->
<template id="preview-styles">
<style>
img {
max-width: 100%;
max-height: 100%;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
a:focus,
input:focus,
select:focus,
textarea:focus {
outline: 1px solid -webkit-focus-ring-color;
outline-offset: -1px;
}
hr {
border: 0;
height: 2px;
border-bottom: 2px solid;
}
h1 {
font-size: 26px;
padding-bottom: 8px;
line-height: 31px;
border-bottom-width: 1px;
border-bottom-style: solid;
border-color: var(--vscode-foreground);
margin: 0;
margin-bottom: 13px;
}
h2 {
font-size: 19px;
margin: 0;
margin-bottom: 10px;
}
h1,
h2,
h3 {
font-weight: normal;
}
div {
width: 100%;
}
/* Adjust margin of first item in markdown cell */
*:first-child {
margin-top: 0px;
}
/* h1 tags don't need top margin */
h1:first-child {
margin-top: 0;
}
/* Removes bottom margin when only one item exists in markdown cell */
*:only-child,
*:last-child {
margin-bottom: 0;
padding-bottom: 0;
}
/* makes all markdown cells consistent */
div {
min-height: ${this.options.previewNodePadding * 2}px;
}
table {
border-collapse: collapse;
border-spacing: 0;
}
table th,
table td {
border: 1px solid;
}
table > thead > tr > th {
text-align: left;
border-bottom: 1px solid;
}
table > thead > tr > th,
table > thead > tr > td,
table > tbody > tr > th,
table > tbody > tr > td {
padding: 5px 10px;
}
table > tbody > tr + tr > td {
border-top: 1px solid;
}
blockquote {
margin: 0 7px 0 5px;
padding: 0 16px 0 10px;
border-left-width: 5px;
border-left-style: solid;
}
code,
.code {
font-family: var(--monaco-monospace-font);
font-size: 1em;
line-height: 1.357em;
}
.code {
white-space: pre-wrap;
}
.latex-block {
display: block;
}
.latex {
vertical-align: middle;
display: inline-block;
}
.latex img,
.latex-block img {
filter: brightness(0) invert(0)
}
dragging {
background-color: var(--vscode-editor-background);
}
</style>
</template>
<style>
#container > div > div.output {
width: calc(100% - ${this.options.leftMargin + (this.options.cellMargin * 2) + this.options.runGutter}px);
margin-left: ${this.options.leftMargin + this.options.runGutter}px;
padding: ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodeLeftPadding}px;
box-sizing: border-box;
background-color: var(--vscode-notebook-outputContainerBackgroundColor);
}
#container > div > div.preview {
width: 100%;
box-sizing: border-box;
white-space: nowrap;
overflow: hidden;
user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
white-space: initial;
cursor: grab;
}
#container > div > div.preview.emptyMarkdownCell::before {
content: "${nls.localize('notebook.emptyMarkdownPlaceholder', "Empty markdown cell, double click or press enter to edit.")}";
font-style: italic;
opacity: 0.6;
}
/* markdown */
#container > div > div.preview {
color: var(--vscode-foreground);
width: 100%;
padding-left: ${this.options.leftMargin}px;
padding-top: ${this.options.previewNodePadding}px;
padding-bottom: ${this.options.previewNodePadding}px;
}
#container > div > div.preview.selected {
background: var(--vscode-notebook-selectedCellBackground);
}
#container > div > div.preview.dragging {
background-color: var(--vscode-editor-background);
}
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex img,
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex-block img {
filter: brightness(0) invert(1)
}
#container > div.nb-symbolHighlight > div {
background-color: var(--vscode-notebook-symbolHighlightBackground);
}
#container > div.nb-cellDeleted > div {
background-color: var(--vscode-diffEditor-removedTextBackground);
}
#container > div.nb-cellAdded > div {
background-color: var(--vscode-diffEditor-insertedTextBackground);
}
#container > div > div:not(.preview) > div {
overflow-x: scroll;
}
body {
padding: 0px;
height: 100%;
width: 100%;
}
table, thead, tr, th, td, tbody {
border: none !important;
border-color: transparent;
border-spacing: 0;
border-collapse: collapse;
}
table {
width: 100%;
}
table, th, tr {
text-align: left !important;
}
thead {
font-weight: bold;
background-color: rgba(130, 130, 130, 0.16);
}
th, td {
padding: 4px 8px;
}
tr:nth-child(even) {
background-color: rgba(130, 130, 130, 0.08);
}
tbody th {
font-weight: normal;
}
</style>
</head>
<body style="overflow: hidden;">
<script>
self.require = {};
</script>
${coreDependencies}
<div id='container' class="widgetarea" style="position: absolute;width:100%;top: 0px"></div>
<script>${preloadsScriptStr({
outputNodePadding: this.options.outputNodePadding,
outputNodeLeftPadding: this.options.outputNodeLeftPadding,
previewNodePadding: this.options.previewNodePadding,
leftMargin: this.options.leftMargin
})}</script>
${markdownRenderersSrc}
</body>
</html>`;
}
private getMarkdownRendererScripts() {
const markdownRenderers = this.notebookService.getMarkdownRendererInfo();
return markdownRenderers
.sort((a, b) => {
// prefer built-in extension
if (a.extensionIsBuiltin) {
return b.extensionIsBuiltin ? 0 : -1;
}
return b.extensionIsBuiltin ? 1 : -1;
})
.map(renderer => {
return asWebviewUri(this.environmentService, this.id, renderer.entrypoint);
})
.map(src => `<script src="${src}"></script>`)
.join('\n');
}
postRendererMessage(rendererId: string, message: any) {
this._sendMessageToWebview({
__vscode_notebook_message: true,
type: 'customRendererMessage',
message,
rendererId
});
}
private resolveOutputId(id: string): { cellInfo: T, output: ICellOutputViewModel } | undefined {
const output = this.reversedInsetMapping.get(id);
if (!output) {
return;
}
const cellInfo = this.insetMapping.get(output)!.cellInfo;
return { cellInfo, output };
}
isResolved(): this is IResolvedBackLayerWebview {
return !!this.webview;
}
async createWebview(): Promise<void> {
let coreDependencies = '';
let resolveFunc: () => void;
this._initalized = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const baseUrl = asWebviewUri(this.environmentService, this.id, dirname(this.documentUri));
if (!isWeb) {
const loaderUri = FileAccess.asFileUri('vs/loader.js', require);
const loader = asWebviewUri(this.environmentService, this.id, loaderUri);
coreDependencies = `<script src="${loader}"></script><script>
var requirejs = (function() {
return require;
}());
</script>`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
} else {
const loaderUri = FileAccess.asBrowserUri('vs/loader.js', require);
fetch(loaderUri.toString(true)).then(async response => {
if (response.status !== 200) {
throw new Error(response.statusText);
}
const loaderJs = await response.text();
coreDependencies = `
<script>
${loaderJs}
</script>
<script>
var requirejs = (function() {
return require;
}());
</script>
`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
});
}
await this._initalized;
}
private async _initialize(content: string) {
if (!document.body.contains(this.element)) {
throw new Error('Element is already detached from the DOM tree');
}
this.webview = this._createInset(this.webviewService, content);
this.webview.mountTo(this.element);
this._register(this.webview);
this._register(this.webview.onDidClickLink(link => {
if (this._disposed) {
return;
}
if (!link) {
return;
}
if (matchesScheme(link, Schemas.http) || matchesScheme(link, Schemas.https) || matchesScheme(link, Schemas.mailto)
|| matchesScheme(link, Schemas.command)) {
this.openerService.open(link, { fromUserGesture: true, allowContributedOpeners: true });
}
}));
this._register(this.webview.onDidReload(() => {
if (this._disposed) {
return;
}
let renderers = new Set<INotebookRendererInfo>();
for (const inset of this.insetMapping.values()) {
if (inset.renderer) {
renderers.add(inset.renderer);
}
}
this._preloadsCache.clear();
this.updateRendererPreloads(renderers);
for (const [output, inset] of this.insetMapping.entries()) {
this._sendMessageToWebview({ ...inset.cachedCreation, initiallyHidden: this.hiddenInsetMapping.has(output) });
}
}));
this._register(this.webview.onMessage((message) => {
const data: FromWebviewMessage | { readonly __vscode_notebook_message: undefined } = message.message;
if (this._disposed) {
return;
}
if (!data.__vscode_notebook_message) {
this._onMessage.fire({ message: data });
return;
}
switch (data.type) {
case 'dimension':
{
for (const update of data.updates) {
if (update.isOutput) {
const height = update.data.height;
const outputHeight = height;
const resolvedResult = this.resolveOutputId(update.id);
if (resolvedResult) {
const { cellInfo, output } = resolvedResult;
this.notebookEditor.updateOutputHeight(cellInfo, output, outputHeight, !!update.init, 'webview#dimension');
}
} else {
const cellId = update.id.substr(0, update.id.length - '_preview'.length);
this.notebookEditor.updateMarkdownCellHeight(cellId, update.data.height, !!update.init);
}
}
break;
}
case 'mouseenter':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = true;
}
}
break;
}
case 'mouseleave':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = false;
}
}
break;
}
case 'outputFocus':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = true;
}
}
break;
}
case 'outputBlur':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = false;
}
}
break;
}
case 'scroll-ack':
{
// const date = new Date();
// const top = data.data.top;
// console.log('ack top ', top, ' version: ', data.version, ' - ', date.getMinutes() + ':' + date.getSeconds() + ':' + date.getMilliseconds());
break;
}
case 'did-scroll-wheel':
{
this.notebookEditor.triggerScroll({
...data.payload,
preventDefault: () => { },
stopPropagation: () => { }
});
break;
}
case 'focus-editor':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (!latestCell) {
return;
}
if (data.focusNext) {
this.notebookEditor.focusNextNotebookCell(latestCell, 'editor');
} else {
this.notebookEditor.focusNotebookCell(latestCell, 'editor');
}
}
break;
}
case 'clicked-data-url':
{
this._onDidClickDataLink(data);
break;
}
case 'customRendererMessage':
{
this._onMessage.fire({ message: data.message, forRenderer: data.rendererId });
break;
}
case 'clickMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
if (data.shiftKey || data.metaKey) {
// Add to selection
this.notebookEditor.toggleNotebookCellSelection(cell);
} else {
// Normal click
this.notebookEditor.focusNotebookCell(cell, 'container', { skipReveal: true });
}
}
break;
}
case 'toggleMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
this.notebookEditor.setMarkdownCellEditState(data.cellId, CellEditState.Editing);
this.notebookEditor.focusNotebookCell(cell, 'editor', { skipReveal: true });
}
break;
}
case 'mouseEnterMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = true;
}
break;
}
case 'mouseLeaveMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = false;
}
break;
}
case 'cell-drag-start':
{
this.notebookEditor.markdownCellDragStart(data.cellId, data.position);
break;
}
case 'cell-drag':
{
this.notebookEditor.markdownCellDrag(data.cellId, data.position);
break;
}
case 'cell-drop':
{
this.notebookEditor.markdownCellDrop(data.cellId, {
clientY: data.position.clientY,
ctrlKey: data.ctrlKey,
altKey: data.altKey,
});
break;
}
case 'cell-drag-end':
{
this.notebookEditor.markdownCellDragEnd(data.cellId);
break;
}
}
}));
}
private async _onDidClickDataLink(event: IClickedDataUrlMessage): Promise<void> {
if (typeof event.data !== 'string') {
return;
}
const [splitStart, splitData] = event.data.split(';base64,');
if (!splitData || !splitStart) {
return;
}
const defaultDir = dirname(this.documentUri);
let defaultName: string;
if (event.downloadName) {
defaultName = event.downloadName;
} else {
const mimeType = splitStart.replace(/^data:/, '');
const candidateExtension = mimeType && getExtensionForMimeType(mimeType);
defaultName = candidateExtension ? `download${candidateExtension}` : 'download';
}
const defaultUri = joinPath(defaultDir, defaultName);
const newFileUri = await this.fileDialogService.showSaveDialog({
defaultUri
});
if (!newFileUri) {
return;
}
const decoded = atob(splitData);
const typedArray = new Uint8Array(decoded.length);
for (let i = 0; i < decoded.length; i++) {
typedArray[i] = decoded.charCodeAt(i);
}
const buff = VSBuffer.wrap(typedArray);
await this.fileService.writeFile(newFileUri, buff);
await this.openerService.open(newFileUri);
}
private _createInset(webviewService: IWebviewService, content: string) {
const rootPath = isWeb ? FileAccess.asBrowserUri('', require) : FileAccess.asFileUri('', require);
const workspaceFolders = this.contextService.getWorkspace().folders.map(x => x.uri);
this.localResourceRootsCache = [
...this.notebookService.getNotebookProviderResourceRoots(),
...this.notebookService.getMarkdownRendererInfo().map(x => dirname(x.entrypoint)),
...workspaceFolders,
rootPath,
];
const webview = webviewService.createWebviewElement(this.id, {
purpose: WebviewContentPurpose.NotebookRenderer,
enableFindWidget: false,
transformCssVariables: transformWebviewThemeVars,
}, {
allowMultipleAPIAcquire: true,
allowScripts: true,
localResourceRoots: this.localResourceRootsCache
}, undefined);
let resolveFunc: () => void;
this._loaded = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const dispose = webview.onMessage((message) => {
const data: FromWebviewMessage = message.message;
if (data.__vscode_notebook_message && data.type === 'initialized') {
resolveFunc();
dispose.dispose();
}
});
webview.html = content;
return webview;
}
shouldUpdateInset(cell: IGenericCellViewModel, output: ICellOutputViewModel, cellTop: number) {
if (this._disposed) {
return;
}
if (cell.metadata?.outputCollapsed) {
return false;
}
const outputCache = this.insetMapping.get(output)!;
const outputIndex = cell.outputsViewModels.indexOf(output);
const outputOffset = cellTop + cell.getOutputOffset(outputIndex);
if (this.hiddenInsetMapping.has(output)) {
return true;
}
if (outputOffset === outputCache.cachedCreation.top) {
return false;
}
return true;
}
updateMarkdownScrollTop(items: { id: string, top: number }[]) {
if (this._disposed || !items.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll-markdown',
cells: items
});
}
updateViewScrollTop(forceDisplay: boolean, items: IDisplayOutputLayoutUpdateRequest[]) {
if (!items.length) {
return;
}
const widgets = coalesce(items.map((item): IContentWidgetTopRequest | undefined => {
const outputCache = this.insetMapping.get(item.output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
const outputOffset = item.outputOffset;
outputCache.cachedCreation.top = outputOffset;
this.hiddenInsetMapping.delete(item.output);
return {
id: id,
top: outputOffset,
};
}));
if (!widgets.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll',
forceDisplay,
widgets: widgets
});
}
private async createMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (this.markdownPreviewMapping.has(cellId)) {
console.error('Trying to create markdown preview that already exists');
return;
}
const initialTop = cellTop;
this.markdownPreviewMapping.set(cellId, { version: contentVersion, visible: true });
this._sendMessageToWebview({
type: 'createMarkdownPreview',
id: cellId,
handle: cellHandle,
content: content,
top: initialTop,
});
}
async showMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
return this.createMarkdownPreview(cellId, cellHandle, content, cellTop, contentVersion);
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error('Try to show a preview that does not exist');
return;
}
if (entry.version !== contentVersion || !entry.visible) {
this._sendMessageToWebview({
type: 'showMarkdownPreview',
id: cellId,
handle: cellHandle,
// If the content has not changed, we still want to make sure the
// preview is visible but don't need to send anything over
content: entry.version === contentVersion ? undefined : content,
top: cellTop
});
}
entry.version = contentVersion;
entry.visible = true;
}
async hideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
// TODO: this currently seems expected on first load
// console.error(`Try to hide a preview that does not exist: ${cellId}`);
return;
}
if (entry.visible) {
this._sendMessageToWebview({
type: 'hideMarkdownPreview',
id: cellId
});
entry.visible = false;
}
}
async unhideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error(`Try to unhide a preview that does not exist: ${cellId}`);
return;
}
if (!entry.visible) {
this._sendMessageToWebview({
type: 'unhideMarkdownPreview',
id: cellId
});
entry.visible = true;
}
}
async removeMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
console.error(`Try to delete a preview that does not exist: ${cellId}`);
return;
}
this.markdownPreviewMapping.delete(cellId);
this._sendMessageToWebview({
type: 'removeMarkdownPreview',
id: cellId
});
}
async updateMarkdownPreviewSelectionState(cellId: any, isSelected: boolean) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
// TODO: this currently seems expected on first load
// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);
return;
}
this._sendMessageToWebview({
type: 'updateMarkdownPreviewSelectionState',
id: cellId,
isSelected
});
}
async initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {
await this._loaded;
if (this._disposed) {
return;
}
// TODO: use proper handler
const p = new Promise<void>(resolve => {
this.webview?.onMessage(e => {
if (e.message.type === 'initializedMarkdownPreview') {
resolve();
}
});
});
for (const cell of cells) {
this.markdownPreviewMapping.set(cell.cellId, { version: 0, visible: false });
}
this._sendMessageToWebview({
type: 'initializeMarkdownPreview',
cells: cells,
});
await p;
}
async createOutput(cellInfo: T, content: IInsetRenderOutput, cellTop: number, offset: number) {
if (this._disposed) {
return;
}
const initialTop = cellTop + offset;
if (this.insetMapping.has(content.source)) {
const outputCache = this.insetMapping.get(content.source);
if (outputCache) {
this.hiddenInsetMapping.delete(content.source);
this._sendMessageToWebview({
type: 'showOutput',
cellId: outputCache.cellInfo.cellId,
outputId: outputCache.outputId,
top: initialTop
});
return;
}
}
const messageBase = {
type: 'html',
cellId: cellInfo.cellId,
top: initialTop,
left: 0,
requiredPreloads: [],
} as const;
let message: ICreationRequestMessage;
let renderer: INotebookRendererInfo | undefined;
if (content.type === RenderOutputType.Extension) {
const output = content.source.model;
renderer = content.renderer;
const outputDto = output.outputs.find(op => op.mime === content.mimeType);
message = {
...messageBase,
outputId: output.outputId,
apiNamespace: content.renderer.id,
requiredPreloads: await this.updateRendererPreloads([content.renderer]),
content: {
type: RenderOutputType.Extension,
outputId: output.outputId,
mimeType: content.mimeType,
value: outputDto?.value,
metadata: outputDto?.metadata,
},
};
} else {
message = {
...messageBase,
outputId: UUID.generateUuid(),
content: {
type: content.type,
htmlContent: content.htmlContent,
}
};
}
this._sendMessageToWebview(message);
this.insetMapping.set(content.source, { outputId: message.outputId, cellInfo: cellInfo, renderer, cachedCreation: message });
this.hiddenInsetMapping.delete(content.source);
this.reversedInsetMapping.set(message.outputId, content.source);
}
removeInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
this._sendMessageToWebview({
type: 'clearOutput',
apiNamespace: outputCache.cachedCreation.apiNamespace,
cellUri: outputCache.cellInfo.cellUri.toString(),
outputId: id,
cellId: outputCache.cellInfo.cellId
});
this.insetMapping.delete(output);
this.reversedInsetMapping.delete(id);
}
hideInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
this.hiddenInsetMapping.add(output);
this._sendMessageToWebview({
type: 'hideOutput',
outputId: outputCache.outputId,
cellId: outputCache.cellInfo.cellId,
});
}
clearInsets() {
if (this._disposed) {
return;
}
this._sendMessageToWebview({
type: 'clear'
});
this.insetMapping = new Map();
this.reversedInsetMapping = new Map();
}
focusWebview() {
if (this._disposed) {
return;
}
this.webview?.focus();
}
focusOutput(cellId: string) {
if (this._disposed) {
return;
}
this.webview?.focus();
setTimeout(() => { // Need this, or focus decoration is not shown. No clue.
this._sendMessageToWebview({
type: 'focus-output',
cellId,
});
}, 50);
}
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]) {
this._sendMessageToWebview({
type: 'decorations',
cellId,
addedClassNames: added,
removedClassNames: removed
});
}
async updateKernelPreloads(extensionLocations: URI[], preloads: URI[]) {
if (this._disposed) {
return;
}
await this._loaded;
const resources: IPreloadResource[] = [];
for (const preload of preloads) {
const uri = this.environmentService.isExtensionDevelopment && (preload.scheme === 'http' || preload.scheme === 'https')
? preload : asWebviewUri(this.environmentService, this.id, preload);
if (!this._preloadsCache.has(uri.toString())) {
resources.push({ uri: uri.toString(), originalUri: preload.toString() });
this._preloadsCache.add(uri.toString());
}
}
if (!resources.length) {
return;
}
this.kernelRootsCache = [...extensionLocations, ...this.kernelRootsCache];
this._updatePreloads(resources, 'kernel');
}
async updateRendererPreloads(renderers: Iterable<INotebookRendererInfo>) {
if (this._disposed) {
return [];
}
await this._loaded;
const requiredPreloads: IPreloadResource[] = [];
const resources: IPreloadResource[] = [];
const extensionLocations: URI[] = [];
for (const rendererInfo of renderers) {
extensionLocations.push(rendererInfo.extensionLocation);
for (const preload of [rendererInfo.entrypoint, ...rendererInfo.preloads]) {
const uri = asWebviewUri(this.environmentService, this.id, preload);
const resource: IPreloadResource = { uri: uri.toString(), originalUri: preload.toString() };
requiredPreloads.push(resource);
if (!this._preloadsCache.has(uri.toString())) {
resources.push(resource);
this._preloadsCache.add(uri.toString());
}
}
}
if (!resources.length) {
return requiredPreloads;
}
this.rendererRootsCache = extensionLocations;
this._updatePreloads(resources, 'renderer');
return requiredPreloads;
}
private _updatePreloads(resources: IPreloadResource[], source: 'renderer' | 'kernel') {
if (!this.webview) {
return;
}
const mixedResourceRoots = [...(this.localResourceRootsCache || []), ...this.rendererRootsCache, ...this.kernelRootsCache];
this.webview.localResourcesRoot = mixedResourceRoots;
this._sendMessageToWebview({
type: 'preload',
resources: resources,
source: source
});
}
private _sendMessageToWebview(message: ToWebviewMessage) {
if (this._disposed) {
return;
}
this.webview?.postMessage(message);
}
clearPreloadsCache() {
this._preloadsCache.clear();
}
dispose() {
this._disposed = true;
this.webview?.dispose();
super.dispose();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.9983093738555908,
0.019650695845484734,
0.00016410343232564628,
0.0001725323818391189,
0.12493468075990677
] |
{
"id": 5,
"code_window": [
"\tcontent: string | undefined;\n",
"\ttop: number;\n",
"}\n",
"\n",
"export interface IUpdateMarkdownPreviewSelectionState {\n",
"\treadonly type: 'updateMarkdownPreviewSelectionState',\n",
"\treadonly id: string;\n",
"\treadonly isSelected: boolean;\n",
"}\n",
"\n",
"export interface IInitializeMarkdownMessage {\n",
"\ttype: 'initializeMarkdownPreview';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IUpdateSelectedMarkdownPreviews {\n",
"\treadonly type: 'updateSelectedMarkdownPreviews',\n",
"\treadonly selectedCellIds: readonly string[]\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 290
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { globals, INodeProcess, IProcessEnvironment } from 'vs/base/common/platform';
import { ISandboxConfiguration } from 'vs/base/parts/sandbox/common/sandboxTypes';
import { ProcessMemoryInfo, CrashReporter, IpcRenderer, WebFrame } from 'vs/base/parts/sandbox/electron-sandbox/electronTypes';
/**
* In sandboxed renderers we cannot expose all of the `process` global of node.js
*/
export interface ISandboxNodeProcess extends INodeProcess {
/**
* The process.platform property returns a string identifying the operating system platform
* on which the Node.js process is running.
*/
readonly platform: string;
/**
* The process.arch property returns a string identifying the CPU architecture
* on which the Node.js process is running.
*/
readonly arch: string;
/**
* The type will always be `renderer`.
*/
readonly type: string;
/**
* Whether the process is sandboxed or not.
*/
readonly sandboxed: boolean;
/**
* A list of versions for the current node.js/electron configuration.
*/
readonly versions: { [key: string]: string | undefined };
/**
* The process.env property returns an object containing the user environment.
*/
readonly env: IProcessEnvironment;
/**
* The `execPath` will be the location of the executable of this application.
*/
readonly execPath: string;
/**
* A listener on the process. Only a small subset of listener types are allowed.
*/
on: (type: string, callback: Function) => void;
/**
* The current working directory of the process.
*/
cwd: () => string;
/**
* Resolves with a ProcessMemoryInfo
*
* Returns an object giving memory usage statistics about the current process. Note
* that all statistics are reported in Kilobytes. This api should be called after
* app ready.
*
* Chromium does not provide `residentSet` value for macOS. This is because macOS
* performs in-memory compression of pages that haven't been recently used. As a
* result the resident set size value is not what one would expect. `private`
* memory is more representative of the actual pre-compression memory usage of the
* process on macOS.
*/
getProcessMemoryInfo: () => Promise<ProcessMemoryInfo>;
/**
* A custom method we add to `process`: Resolve the true process environment to use and
* apply it to `process.env`.
*
* There are different layers of environment that will apply:
* - `process.env`: this is the actual environment of the process before this method
* - `shellEnv` : if the program was not started from a terminal, we resolve all shell
* variables to get the same experience as if the program was started from
* a terminal (Linux, macOS)
* - `userEnv` : this is instance specific environment, e.g. if the user started the program
* from a terminal and changed certain variables
*
* The order of overwrites is `process.env` < `shellEnv` < `userEnv`.
*
* It is critical that every process awaits this method early on startup to get the right
* set of environment in `process.env`.
*/
resolveEnv(): Promise<void>;
/**
* Returns a process environment that includes any shell environment even if the application
* was not started from a shell / terminal / console.
*/
getShellEnv(): Promise<IProcessEnvironment>;
}
export interface IpcMessagePort {
/**
* Establish a connection via `MessagePort` to a target. The main process
* will need to transfer the port over to the `channelResponse` after listening
* to `channelRequest` with a payload of `requestNonce` so that the
* source can correlate the response.
*
* The source should install a `window.on('message')` listener, ensuring `e.data`
* matches `requestNonce`, `e.source` matches `window` and then receiving the
* `MessagePort` via `e.ports[0]`.
*/
connect(channelRequest: string, channelResponse: string, requestNonce: string): void;
}
export interface ISandboxContext {
/**
* A configuration object made accessible from the main side
* to configure the sandbox browser window.
*/
configuration: Promise<ISandboxConfiguration>;
}
export const ipcRenderer: IpcRenderer = globals.vscode.ipcRenderer;
export const ipcMessagePort: IpcMessagePort = globals.vscode.ipcMessagePort;
export const webFrame: WebFrame = globals.vscode.webFrame;
export const crashReporter: CrashReporter = globals.vscode.crashReporter;
export const process: ISandboxNodeProcess = globals.vscode.process;
export const context: ISandboxContext = globals.vscode.context;
| src/vs/base/parts/sandbox/electron-sandbox/globals.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00021016484242863953,
0.00017497710359748453,
0.00016189321468118578,
0.00017102525453083217,
0.000012157202945672907
] |
{
"id": 5,
"code_window": [
"\tcontent: string | undefined;\n",
"\ttop: number;\n",
"}\n",
"\n",
"export interface IUpdateMarkdownPreviewSelectionState {\n",
"\treadonly type: 'updateMarkdownPreviewSelectionState',\n",
"\treadonly id: string;\n",
"\treadonly isSelected: boolean;\n",
"}\n",
"\n",
"export interface IInitializeMarkdownMessage {\n",
"\ttype: 'initializeMarkdownPreview';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IUpdateSelectedMarkdownPreviews {\n",
"\treadonly type: 'updateSelectedMarkdownPreviews',\n",
"\treadonly selectedCellIds: readonly string[]\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 290
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { URI, UriComponents } from 'vs/base/common/uri';
import { IListService } from 'vs/platform/list/browser/listService';
import { OpenEditor, SortOrder } from 'vs/workbench/contrib/files/common/files';
import { EditorResourceAccessor, SideBySideEditor, IEditorIdentifier, EditorInput, IEditorInputSerializer } from 'vs/workbench/common/editor';
import { List } from 'vs/base/browser/ui/list/listWidget';
import { IEditorService } from 'vs/workbench/services/editor/common/editorService';
import { ExplorerItem } from 'vs/workbench/contrib/files/common/explorerModel';
import { coalesce } from 'vs/base/common/arrays';
import { AsyncDataTree } from 'vs/base/browser/ui/tree/asyncDataTree';
import { IEditorGroupsService } from 'vs/workbench/services/editor/common/editorGroupsService';
import { IEditableData } from 'vs/workbench/common/views';
import { createDecorator, IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { ResourceFileEdit } from 'vs/editor/browser/services/bulkEditService';
import { ProgressLocation } from 'vs/platform/progress/common/progress';
import { FileEditorInput } from 'vs/workbench/contrib/files/common/editors/fileEditorInput';
import { isEqual } from 'vs/base/common/resources';
interface ISerializedFileEditorInput {
resourceJSON: UriComponents;
preferredResourceJSON?: UriComponents;
name?: string;
description?: string;
encoding?: string;
modeId?: string;
}
export class FileEditorInputSerializer implements IEditorInputSerializer {
canSerialize(editorInput: EditorInput): boolean {
return true;
}
serialize(editorInput: EditorInput): string {
const fileEditorInput = <FileEditorInput>editorInput;
const resource = fileEditorInput.resource;
const preferredResource = fileEditorInput.preferredResource;
const serializedFileEditorInput: ISerializedFileEditorInput = {
resourceJSON: resource.toJSON(),
preferredResourceJSON: isEqual(resource, preferredResource) ? undefined : preferredResource, // only storing preferredResource if it differs from the resource
name: fileEditorInput.getPreferredName(),
description: fileEditorInput.getPreferredDescription(),
encoding: fileEditorInput.getEncoding(),
modeId: fileEditorInput.getPreferredMode() // only using the preferred user associated mode here if available to not store redundant data
};
return JSON.stringify(serializedFileEditorInput);
}
deserialize(instantiationService: IInstantiationService, serializedEditorInput: string): FileEditorInput {
return instantiationService.invokeFunction<FileEditorInput>(accessor => {
const serializedFileEditorInput: ISerializedFileEditorInput = JSON.parse(serializedEditorInput);
const resource = URI.revive(serializedFileEditorInput.resourceJSON);
const preferredResource = URI.revive(serializedFileEditorInput.preferredResourceJSON);
const name = serializedFileEditorInput.name;
const description = serializedFileEditorInput.description;
const encoding = serializedFileEditorInput.encoding;
const mode = serializedFileEditorInput.modeId;
const fileEditorInput = accessor.get(IEditorService).createEditorInput({ resource, label: name, description, encoding, mode, forceFile: true }) as FileEditorInput;
if (preferredResource) {
fileEditorInput.setPreferredResource(preferredResource);
}
return fileEditorInput;
});
}
}
export interface IExplorerService {
readonly _serviceBrand: undefined;
readonly roots: ExplorerItem[];
readonly sortOrder: SortOrder;
getContext(respectMultiSelection: boolean): ExplorerItem[];
hasViewFocus(): boolean;
setEditable(stat: ExplorerItem, data: IEditableData | null): Promise<void>;
getEditable(): { stat: ExplorerItem, data: IEditableData } | undefined;
getEditableData(stat: ExplorerItem): IEditableData | undefined;
// If undefined is passed checks if any element is currently being edited.
isEditable(stat: ExplorerItem | undefined): boolean;
findClosest(resource: URI): ExplorerItem | null;
findClosestRoot(resource: URI): ExplorerItem | null;
refresh(): Promise<void>;
setToCopy(stats: ExplorerItem[], cut: boolean): Promise<void>;
isCut(stat: ExplorerItem): boolean;
applyBulkEdit(edit: ResourceFileEdit[], options: { undoLabel: string, progressLabel: string, confirmBeforeUndo?: boolean, progressLocation?: ProgressLocation.Explorer | ProgressLocation.Window }): Promise<void>;
/**
* Selects and reveal the file element provided by the given resource if its found in the explorer.
* Will try to resolve the path in case the explorer is not yet expanded to the file yet.
*/
select(resource: URI, reveal?: boolean | string): Promise<void>;
registerView(contextAndRefreshProvider: IExplorerView): void;
}
export const IExplorerService = createDecorator<IExplorerService>('explorerService');
export interface IExplorerView {
getContext(respectMultiSelection: boolean): ExplorerItem[];
refresh(recursive: boolean, item?: ExplorerItem): Promise<void>;
selectResource(resource: URI | undefined, reveal?: boolean | string): Promise<void>;
setTreeInput(): Promise<void>;
itemsCopied(tats: ExplorerItem[], cut: boolean, previousCut: ExplorerItem[] | undefined): void;
setEditable(stat: ExplorerItem, isEditing: boolean): Promise<void>;
focusNeighbourIfItemFocused(item: ExplorerItem): void;
isItemVisible(item: ExplorerItem): boolean;
hasFocus(): boolean;
}
function getFocus(listService: IListService): unknown | undefined {
let list = listService.lastFocusedList;
if (list?.getHTMLElement() === document.activeElement) {
let focus: unknown;
if (list instanceof List) {
const focused = list.getFocusedElements();
if (focused.length) {
focus = focused[0];
}
} else if (list instanceof AsyncDataTree) {
const focused = list.getFocus();
if (focused.length) {
focus = focused[0];
}
}
return focus;
}
return undefined;
}
// Commands can get executed from a command palette, from a context menu or from some list using a keybinding
// To cover all these cases we need to properly compute the resource on which the command is being executed
export function getResourceForCommand(resource: URI | object | undefined, listService: IListService, editorService: IEditorService): URI | undefined {
if (URI.isUri(resource)) {
return resource;
}
const focus = getFocus(listService);
if (focus instanceof ExplorerItem) {
return focus.resource;
} else if (focus instanceof OpenEditor) {
return focus.getResource();
}
return EditorResourceAccessor.getOriginalUri(editorService.activeEditor, { supportSideBySide: SideBySideEditor.PRIMARY });
}
export function getMultiSelectedResources(resource: URI | object | undefined, listService: IListService, editorService: IEditorService, explorerService: IExplorerService): Array<URI> {
const list = listService.lastFocusedList;
if (list?.getHTMLElement() === document.activeElement) {
// Explorer
if (list instanceof AsyncDataTree && list.getFocus().every(item => item instanceof ExplorerItem)) {
// Explorer
const context = explorerService.getContext(true);
if (context.length) {
return context.map(c => c.resource);
}
}
// Open editors view
if (list instanceof List) {
const selection = coalesce(list.getSelectedElements().filter(s => s instanceof OpenEditor).map((oe: OpenEditor) => oe.getResource()));
const focusedElements = list.getFocusedElements();
const focus = focusedElements.length ? focusedElements[0] : undefined;
let mainUriStr: string | undefined = undefined;
if (URI.isUri(resource)) {
mainUriStr = resource.toString();
} else if (focus instanceof OpenEditor) {
const focusedResource = focus.getResource();
mainUriStr = focusedResource ? focusedResource.toString() : undefined;
}
// We only respect the selection if it contains the main element.
if (selection.some(s => s.toString() === mainUriStr)) {
return selection;
}
}
}
const result = getResourceForCommand(resource, listService, editorService);
return !!result ? [result] : [];
}
export function getOpenEditorsViewMultiSelection(listService: IListService, editorGroupService: IEditorGroupsService): Array<IEditorIdentifier> | undefined {
const list = listService.lastFocusedList;
if (list?.getHTMLElement() === document.activeElement) {
// Open editors view
if (list instanceof List) {
const selection = coalesce(list.getSelectedElements().filter(s => s instanceof OpenEditor));
const focusedElements = list.getFocusedElements();
const focus = focusedElements.length ? focusedElements[0] : undefined;
let mainEditor: IEditorIdentifier | undefined = undefined;
if (focus instanceof OpenEditor) {
mainEditor = focus;
}
// We only respect the selection if it contains the main element.
if (selection.some(s => s === mainEditor)) {
return selection;
}
}
}
return undefined;
}
| src/vs/workbench/contrib/files/browser/files.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0016484821680933237,
0.0002441051765345037,
0.00016599951777607203,
0.0001720848522381857,
0.00030708781559951603
] |
{
"id": 5,
"code_window": [
"\tcontent: string | undefined;\n",
"\ttop: number;\n",
"}\n",
"\n",
"export interface IUpdateMarkdownPreviewSelectionState {\n",
"\treadonly type: 'updateMarkdownPreviewSelectionState',\n",
"\treadonly id: string;\n",
"\treadonly isSelected: boolean;\n",
"}\n",
"\n",
"export interface IInitializeMarkdownMessage {\n",
"\ttype: 'initializeMarkdownPreview';\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"export interface IUpdateSelectedMarkdownPreviews {\n",
"\treadonly type: 'updateSelectedMarkdownPreviews',\n",
"\treadonly selectedCellIds: readonly string[]\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 290
} | #define RootLicenseFileName FileExists(RepoDir + '\LICENSE.rtf') ? 'LICENSE.rtf' : 'LICENSE.txt'
#define LocalizedLanguageFile(Language = "") \
DirExists(RepoDir + "\licenses") && Language != "" \
? ('; LicenseFile: "' + RepoDir + '\licenses\LICENSE-' + Language + '.rtf"') \
: '; LicenseFile: "' + RepoDir + '\' + RootLicenseFileName + '"'
[Setup]
AppId={#AppId}
AppName={#NameLong}
AppVerName={#NameVersion}
AppPublisher=Microsoft Corporation
AppPublisherURL=https://code.visualstudio.com/
AppSupportURL=https://code.visualstudio.com/
AppUpdatesURL=https://code.visualstudio.com/
DefaultGroupName={#NameLong}
AllowNoIcons=yes
OutputDir={#OutputDir}
OutputBaseFilename=VSCodeSetup
Compression=lzma
SolidCompression=yes
AppMutex={code:GetAppMutex}
SetupMutex={#AppMutex}setup
WizardImageFile="{#RepoDir}\resources\win32\inno-big-100.bmp,{#RepoDir}\resources\win32\inno-big-125.bmp,{#RepoDir}\resources\win32\inno-big-150.bmp,{#RepoDir}\resources\win32\inno-big-175.bmp,{#RepoDir}\resources\win32\inno-big-200.bmp,{#RepoDir}\resources\win32\inno-big-225.bmp,{#RepoDir}\resources\win32\inno-big-250.bmp"
WizardSmallImageFile="{#RepoDir}\resources\win32\inno-small-100.bmp,{#RepoDir}\resources\win32\inno-small-125.bmp,{#RepoDir}\resources\win32\inno-small-150.bmp,{#RepoDir}\resources\win32\inno-small-175.bmp,{#RepoDir}\resources\win32\inno-small-200.bmp,{#RepoDir}\resources\win32\inno-small-225.bmp,{#RepoDir}\resources\win32\inno-small-250.bmp"
SetupIconFile={#RepoDir}\resources\win32\code.ico
UninstallDisplayIcon={app}\{#ExeBasename}.exe
ChangesEnvironment=true
ChangesAssociations=true
MinVersion=6.1.7600
SourceDir={#SourceDir}
AppVersion={#Version}
VersionInfoVersion={#RawVersion}
ShowLanguageDialog=auto
ArchitecturesAllowed={#ArchitecturesAllowed}
ArchitecturesInstallIn64BitMode={#ArchitecturesInstallIn64BitMode}
WizardStyle=modern
#ifdef Sign
SignTool=esrp
#endif
#if "user" == InstallTarget
DefaultDirName={userpf}\{#DirName}
PrivilegesRequired=lowest
#else
DefaultDirName={pf}\{#DirName}
#endif
[Languages]
Name: "english"; MessagesFile: "compiler:Default.isl,{#RepoDir}\build\win32\i18n\messages.en.isl" {#LocalizedLanguageFile}
Name: "german"; MessagesFile: "compiler:Languages\German.isl,{#RepoDir}\build\win32\i18n\messages.de.isl" {#LocalizedLanguageFile("deu")}
Name: "spanish"; MessagesFile: "compiler:Languages\Spanish.isl,{#RepoDir}\build\win32\i18n\messages.es.isl" {#LocalizedLanguageFile("esp")}
Name: "french"; MessagesFile: "compiler:Languages\French.isl,{#RepoDir}\build\win32\i18n\messages.fr.isl" {#LocalizedLanguageFile("fra")}
Name: "italian"; MessagesFile: "compiler:Languages\Italian.isl,{#RepoDir}\build\win32\i18n\messages.it.isl" {#LocalizedLanguageFile("ita")}
Name: "japanese"; MessagesFile: "compiler:Languages\Japanese.isl,{#RepoDir}\build\win32\i18n\messages.ja.isl" {#LocalizedLanguageFile("jpn")}
Name: "russian"; MessagesFile: "compiler:Languages\Russian.isl,{#RepoDir}\build\win32\i18n\messages.ru.isl" {#LocalizedLanguageFile("rus")}
Name: "korean"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.ko.isl,{#RepoDir}\build\win32\i18n\messages.ko.isl" {#LocalizedLanguageFile("kor")}
Name: "simplifiedChinese"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.zh-cn.isl,{#RepoDir}\build\win32\i18n\messages.zh-cn.isl" {#LocalizedLanguageFile("chs")}
Name: "traditionalChinese"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.zh-tw.isl,{#RepoDir}\build\win32\i18n\messages.zh-tw.isl" {#LocalizedLanguageFile("cht")}
Name: "brazilianPortuguese"; MessagesFile: "compiler:Languages\BrazilianPortuguese.isl,{#RepoDir}\build\win32\i18n\messages.pt-br.isl" {#LocalizedLanguageFile("ptb")}
Name: "hungarian"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.hu.isl,{#RepoDir}\build\win32\i18n\messages.hu.isl" {#LocalizedLanguageFile("hun")}
Name: "turkish"; MessagesFile: "compiler:Languages\Turkish.isl,{#RepoDir}\build\win32\i18n\messages.tr.isl" {#LocalizedLanguageFile("trk")}
[InstallDelete]
Type: filesandordirs; Name: "{app}\resources\app\out"; Check: IsNotUpdate
Type: filesandordirs; Name: "{app}\resources\app\plugins"; Check: IsNotUpdate
Type: filesandordirs; Name: "{app}\resources\app\extensions"; Check: IsNotUpdate
Type: filesandordirs; Name: "{app}\resources\app\node_modules"; Check: IsNotUpdate
Type: filesandordirs; Name: "{app}\resources\app\node_modules.asar.unpacked"; Check: IsNotUpdate
Type: files; Name: "{app}\resources\app\node_modules.asar"; Check: IsNotUpdate
Type: files; Name: "{app}\resources\app\Credits_45.0.2454.85.html"; Check: IsNotUpdate
[UninstallDelete]
Type: filesandordirs; Name: "{app}\_"
[Tasks]
Name: "desktopicon"; Description: "{cm:CreateDesktopIcon}"; GroupDescription: "{cm:AdditionalIcons}"; Flags: unchecked
Name: "quicklaunchicon"; Description: "{cm:CreateQuickLaunchIcon}"; GroupDescription: "{cm:AdditionalIcons}"; Flags: unchecked; OnlyBelowVersion: 0,6.1
Name: "addcontextmenufiles"; Description: "{cm:AddContextMenuFiles,{#NameShort}}"; GroupDescription: "{cm:Other}"; Flags: unchecked
Name: "addcontextmenufolders"; Description: "{cm:AddContextMenuFolders,{#NameShort}}"; GroupDescription: "{cm:Other}"; Flags: unchecked
Name: "associatewithfiles"; Description: "{cm:AssociateWithFiles,{#NameShort}}"; GroupDescription: "{cm:Other}"; Flags: unchecked
Name: "addtopath"; Description: "{cm:AddToPath}"; GroupDescription: "{cm:Other}"
Name: "runcode"; Description: "{cm:RunAfter,{#NameShort}}"; GroupDescription: "{cm:Other}"; Check: WizardSilent
[Files]
Source: "*"; Excludes: "\CodeSignSummary*.md,\tools,\tools\*,\resources\app\product.json"; DestDir: "{code:GetDestDir}"; Flags: ignoreversion recursesubdirs createallsubdirs
Source: "tools\*"; DestDir: "{app}\tools"; Flags: ignoreversion
Source: "{#ProductJsonPath}"; DestDir: "{code:GetDestDir}\resources\app"; Flags: ignoreversion
[Icons]
Name: "{group}\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; AppUserModelID: "{#AppUserId}"
Name: "{autodesktop}\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; Tasks: desktopicon; AppUserModelID: "{#AppUserId}"
Name: "{userappdata}\Microsoft\Internet Explorer\Quick Launch\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; Tasks: quicklaunchicon; AppUserModelID: "{#AppUserId}"
[Run]
Filename: "{app}\{#ExeBasename}.exe"; Description: "{cm:LaunchProgram,{#NameLong}}"; Tasks: runcode; Flags: nowait postinstall; Check: ShouldRunAfterUpdate
Filename: "{app}\{#ExeBasename}.exe"; Description: "{cm:LaunchProgram,{#NameLong}}"; Flags: nowait postinstall; Check: WizardNotSilent
[Registry]
#if "user" == InstallTarget
#define SoftwareClassesRootKey "HKCU"
#else
#define SoftwareClassesRootKey "HKLM"
#endif
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ascx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ascx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.ascx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ascx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,ASCX}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ascx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ascx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\xml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ascx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ascx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.asp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.asp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.asp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.asp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,ASP}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.asp"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.asp\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.asp\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.asp\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.aspx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.aspx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.aspx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.aspx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,ASPX}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.aspx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.aspx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.aspx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.aspx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bash"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Bash}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash_login\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash_login\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bash_login"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_login"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Bash Login}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_login"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_login\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_login\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_login\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash_logout\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash_logout\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bash_logout"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_logout"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Bash Logout}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_logout"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_logout\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_logout\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_logout\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash_profile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bash_profile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bash_profile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_profile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Bash Profile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_profile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_profile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_profile\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bash_profile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bashrc\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bashrc\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bashrc"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bashrc"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Bash RC}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bashrc"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bashrc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bashrc\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bashrc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bib\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bib\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bib"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bib"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,BibTeX}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bib"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bib\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bib\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bib\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bowerrc\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.bowerrc\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.bowerrc"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Bower RC}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\bower.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c++\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c++\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.c++"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.c"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\c.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cc\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cc\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cc"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cjs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cjs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cjs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JavaScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\javascript.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.clj\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.clj\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.clj"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clj"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Clojure}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clj"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clj\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clj\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clj\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cljs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cljs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cljs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,ClojureScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cljx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cljx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cljx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,CLJX}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cljx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.clojure\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.clojure\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.clojure"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clojure"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Clojure}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clojure"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clojure\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clojure\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clojure\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.code-workspace\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.code-workspace\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.code-workspace"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.code-workspace"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Code Workspace}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.code"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.code-workspace\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.code-workspace\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.code-workspace\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.coffee\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.coffee\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.coffee"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.coffee"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,CoffeeScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.coffee"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.coffee\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.coffee\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.coffee\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.config\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.config\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.config"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Configuration}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.containerfile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.containerfile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.containerfile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Containerfile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cpp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cpp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cpp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cpp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cpp"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cpp\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cpp\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cpp\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C#}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\csharp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cshtml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cshtml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cshtml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cshtml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,CSHTML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cshtml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cshtml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cshtml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cshtml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.csproj\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.csproj\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.csproj"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csproj"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C# Project}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csproj"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csproj\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\xml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csproj\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csproj\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.css\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.css\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.css"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.css"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,CSS}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.css"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.css\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\css.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.css\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.css\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.csx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.csx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.csx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C# Script}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\csharp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.csx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ctp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ctp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.ctp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ctp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,CakePHP Template}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ctp"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ctp\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ctp\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ctp\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cxx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cxx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cxx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cxx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cxx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cxx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cxx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cxx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.dockerfile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.dockerfile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.dockerfile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dockerfile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Dockerfile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dockerfile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dockerfile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dockerfile\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dockerfile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.dot\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.dot\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.dot"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dot"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Dot}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dot"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dot\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dot\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dot\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.dtd\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.dtd\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.dtd"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dtd"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Document Type Definition}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dtd"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dtd\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\xml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dtd\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.dtd\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.editorconfig\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.editorconfig\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.editorconfig"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.editorconfig"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Editor Config}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.editorconfig"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.editorconfig\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.editorconfig\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.editorconfig\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.edn\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.edn\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.edn"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.edn"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Extensible Data Notation}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.edn"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.edn\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.edn\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.edn\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.eyaml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.eyaml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.eyaml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyaml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Hiera Eyaml}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyaml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyaml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\yaml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyaml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyaml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.eyml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.eyml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.eyml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Hiera Eyaml}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\yaml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.eyml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.fs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,F#}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fsi\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fsi\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.fsi"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsi"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,F# Signature}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsi"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsi\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsi\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsi\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fsscript\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fsscript\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.fsscript"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsscript"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,F# Script}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsscript"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsscript\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsscript\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsscript\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fsx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.fsx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.fsx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,F# Script}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.fsx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gemspec\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gemspec\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.gemspec"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gemspec"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Gemspec}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gemspec"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gemspec\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\ruby.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gemspec\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gemspec\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gitattributes\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gitattributes\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.gitattributes"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitattributes"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Git Attributes}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitattributes"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitattributes"; ValueType: string; ValueName: "AlwaysShowExt"; ValueData: ""; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitattributes\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitattributes\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitattributes\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gitconfig\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gitconfig\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.gitconfig"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitconfig"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Git Config}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitconfig"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitconfig"; ValueType: string; ValueName: "AlwaysShowExt"; ValueData: ""; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitconfig\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitconfig\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitconfig\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gitignore\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.gitignore\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.gitignore"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitignore"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Git Ignore}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitignore"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitignore"; ValueType: string; ValueName: "AlwaysShowExt"; ValueData: ""; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitignore\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitignore\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.gitignore\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.go\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.go\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.go"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.go"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Go}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.go"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.go\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\go.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.go\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.go\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.h\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.h\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.h"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\c.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.handlebars\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.handlebars\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.handlebars"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.handlebars"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Handlebars}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.handlebars"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.handlebars\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.handlebars\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.handlebars\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hbs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hbs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.hbs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Handlebars}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.h++\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.h++\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.h++"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++ Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hh\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hh\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.hh"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hh"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++ Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hh"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hh\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hh\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hh\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hpp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hpp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.hpp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hpp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++ Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hpp"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hpp\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hpp\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hpp\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.htm\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.htm\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.htm"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.htm"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,HTML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.htm"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.htm\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.htm\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.htm\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.html\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.html\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.html"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.html"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,HTML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.html"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.html\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.html\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.html\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hxx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hxx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.hxx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hxx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++ Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hxx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hxx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hxx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hxx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ini\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ini\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.ini"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ini"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,INI}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ini"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ini\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ini\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ini\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jade\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jade\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jade"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jade"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Jade}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jade"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jade\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\jade.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jade\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jade\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jav\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jav\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jav"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jav"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Java}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jav"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jav\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\java.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jav\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jav\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.java\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.java\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.java"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.java"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Java}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.java"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.java\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\java.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.java\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.java\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.js\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.js\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.js"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.js"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JavaScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.js"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.js\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\javascript.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.js\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.js\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jsx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jsx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jsx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JavaScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\react.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jscsrc\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jscsrc\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jscsrc"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jscsrc"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JSCS RC}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jscsrc"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jscsrc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\javascript.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jscsrc\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jscsrc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jshintrc\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jshintrc\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jshintrc"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshintrc"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JSHint RC}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshintrc"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshintrc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\javascript.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshintrc\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshintrc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jshtm\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jshtm\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jshtm"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshtm"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JavaScript HTML Template}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshtm"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshtm\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshtm\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jshtm\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.json\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.json\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.json"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.json"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JSON}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.json"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.json\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\json.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.json\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.json\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jsp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.jsp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.jsp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Java Server Pages}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsp"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsp\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsp\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.jsp\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.less\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.less\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.less"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.less"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,LESS}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.less"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.less\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\less.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.less\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.less\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.lua\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.lua\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.lua"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.lua"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Lua}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.lua"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.lua\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.lua\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.lua\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.m\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.m\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.m"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.m"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Objective C}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.m"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.m\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.m\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.m\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.makefile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.makefile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.makefile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.makefile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Makefile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.makefile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.makefile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.makefile\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.makefile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.markdown\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.markdown\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.markdown"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.markdown"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.markdown"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.markdown\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.markdown\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.markdown\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.md\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.md\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.md"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.md"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.md"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.md\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.md\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.md\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdoc\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdoc\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mdoc"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdoc"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,MDoc}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdoc"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdoc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdoc\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdoc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdown\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdown\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mdown"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdown"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdown"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdown\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdown\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdown\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdtext\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdtext\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mdtext"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtext"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtext"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtext\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtext\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtext\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdtxt\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdtxt\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mdtxt"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtxt"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtxt"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtxt\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtxt\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdtxt\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdwn\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mdwn\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mdwn"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdwn"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdwn"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdwn\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdwn\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mdwn\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mkd\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mkd\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mkd"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkd"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkd"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkd\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkd\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkd\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mkdn\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mkdn\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mkdn"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkdn"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Markdown}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkdn"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkdn\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\markdown.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkdn\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mkdn\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.ml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,OCaml}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mli\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mli\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mli"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mli"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,OCaml}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mli"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mli\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mli\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mli\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mjs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.mjs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.mjs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mjs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JavaScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mjs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mjs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\javascript.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mjs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.mjs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.npmignore\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.npmignore\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.npmignore"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.npmignore"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,NPM Ignore}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.npmignore"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.npmignore"; ValueType: string; ValueName: "AlwaysShowExt"; ValueData: ""; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.npmignore\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.npmignore\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.npmignore\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.php\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.php\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.php"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.php"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,PHP}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.php"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.php\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\php.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.php\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.php\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.phtml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.phtml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.phtml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.phtml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,PHP HTML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.phtml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.phtml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.phtml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.phtml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pl\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pl\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.pl"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pl6\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pl6\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.pl6"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl6"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl 6}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl6"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl6\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl6\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pl6\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pm\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pm\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.pm"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl Module}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pm6\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pm6\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.pm6"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm6"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl 6 Module}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm6"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm6\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm6\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pm6\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pod\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pod\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.pod"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pod"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl POD}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pod"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pod\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pod\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pod\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.pp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.pp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pp"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pp\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pp\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.pp\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.profile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.profile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.profile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.profile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Profile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.profile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.profile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.profile\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.profile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.properties\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.properties\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.properties"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.properties"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Properties}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.properties"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.properties\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.properties\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.properties\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ps1\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ps1\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.ps1"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ps1"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,PowerShell}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ps1"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ps1\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\powershell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ps1\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ps1\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.psd1\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.psd1\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.psd1"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psd1"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,PowerShell Module Manifest}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psd1"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psd1\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\powershell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psd1\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psd1\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.psgi\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.psgi\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.psgi"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psgi"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl CGI}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psgi"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psgi\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psgi\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psgi\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.psm1\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.psm1\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.psm1"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psm1"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,PowerShell Module}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psm1"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psm1\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\powershell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psm1\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.psm1\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.py\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.py\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.py"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.py"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Python}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.py"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.py\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\python.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.py\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.py\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.r\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.r\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.r"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.r"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,R}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.r"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.r\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.r\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.r\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rb\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rb\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.rb"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rb"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Ruby}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rb"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rb\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\ruby.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rb\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rb\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rhistory\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rhistory\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.rhistory"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rhistory"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,R History}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rhistory"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rhistory\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rhistory\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rhistory\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rprofile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rprofile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.rprofile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rprofile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,R Profile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rprofile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rprofile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rprofile\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rprofile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.rs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Rust}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rt\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.rt\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.rt"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rt"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Rich Text}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rt"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rt\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rt\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.rt\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.scss\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.scss\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.scss"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.scss"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Sass}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.scss"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.scss\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\sass.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.scss\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.scss\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.sh\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.sh\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.sh"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sh"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,SH}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sh"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sh\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sh\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sh\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.shtml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.shtml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.shtml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.shtml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,SHTML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.shtml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.shtml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\html.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.shtml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.shtml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.sql\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.sql\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.sql"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sql"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,SQL}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sql"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sql\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\sql.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sql\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.sql\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.svg\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.svg\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.svg"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svg"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,SVG}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svg"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svg\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svg\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svg\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.svgz\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.svgz\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.svgz"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svgz"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,SVGZ}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svgz"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svgz\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svgz\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.svgz\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.t\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.t\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.t"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.t"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Perl}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.t"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.t\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.t\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.t\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.tex\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.tex\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.tex"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tex"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,LaTeX}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tex"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tex\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tex\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tex\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ts\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.ts\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.ts"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ts"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,TypeScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ts"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ts\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\typescript.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ts\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.ts\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.tsx\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.tsx\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.tsx"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tsx"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,TypeScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tsx"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tsx\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\react.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tsx\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.tsx\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.txt\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.txt\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.txt"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.txt"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Text}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.txt"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.txt\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.txt\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.txt\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.vb\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.vb\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.vb"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vb"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Visual Basic}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vb"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vb\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vb\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vb\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.vue\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.vue\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.vue"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vue"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,VUE}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vue"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vue\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\vue.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vue\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.vue\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.wxi\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.wxi\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.wxi"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxi"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,WiX Include}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxi"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxi\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxi\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxi\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.wxl\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.wxl\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.wxl"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxl"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,WiX Localization}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxl"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxl\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxl\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxl\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.wxs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.wxs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.wxs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,WiX}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxs\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.wxs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.xaml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.xaml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.xaml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xaml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,XAML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xaml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xaml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\xml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xaml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xaml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.xml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.xml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.xml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,XML}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\xml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.xml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.yaml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.yaml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.yaml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yaml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Yaml}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yaml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yaml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\yaml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yaml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yaml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.yml\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.yml\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.yml"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yml"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Yaml}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yml"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yml\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\yaml.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yml\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.yml\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.zsh\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.zsh\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.zsh"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.zsh"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,ZSH}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.zsh"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.zsh\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\shell.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.zsh\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.zsh\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}SourceFile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,{#NameLong}}"; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}SourceFile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}SourceFile\shell\open"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"""
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}SourceFile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBasename}.exe"; ValueType: none; ValueName: ""; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBasename}.exe\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBasename}.exe\shell\open"; ValueType: string; ValueName: "Icon"; ValueData: """{app}\{#ExeBasename}.exe"""
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBasename}.exe\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufiles; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: addcontextmenufiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%V"""; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%V"""; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%V"""; Tasks: addcontextmenufolders
; Environment
#if "user" == InstallTarget
#define EnvironmentRootKey "HKCU"
#define EnvironmentKey "Environment"
#define Uninstall64RootKey "HKCU64"
#define Uninstall32RootKey "HKCU32"
#else
#define EnvironmentRootKey "HKLM"
#define EnvironmentKey "System\CurrentControlSet\Control\Session Manager\Environment"
#define Uninstall64RootKey "HKLM64"
#define Uninstall32RootKey "HKLM32"
#endif
Root: {#EnvironmentRootKey}; Subkey: "{#EnvironmentKey}"; ValueType: expandsz; ValueName: "Path"; ValueData: "{olddata};{app}\bin"; Tasks: addtopath; Check: NeedsAddPath(ExpandConstant('{app}\bin'))
[Code]
// Don't allow installing conflicting architectures
function InitializeSetup(): Boolean;
var
RegKey: String;
ThisArch: String;
AltArch: String;
begin
Result := True;
#if "user" == InstallTarget
if not WizardSilent() and IsAdmin() then begin
if MsgBox('This User Installer is not meant to be run as an Administrator. If you would like to install VS Code for all users in this system, download the System Installer instead from https://code.visualstudio.com. Are you sure you want to continue?', mbError, MB_OKCANCEL) = IDCANCEL then begin
Result := False;
end;
end;
#endif
#if "user" == InstallTarget
#if "ia32" == Arch || "arm64" == Arch
#define IncompatibleArchRootKey "HKLM32"
#else
#define IncompatibleArchRootKey "HKLM64"
#endif
if Result and not WizardSilent() then begin
RegKey := 'SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall\' + copy('{#IncompatibleTargetAppId}', 2, 38) + '_is1';
if RegKeyExists({#IncompatibleArchRootKey}, RegKey) then begin
if MsgBox('{#NameShort} is already installed on this system for all users. We recommend first uninstalling that version before installing this one. Are you sure you want to continue the installation?', mbConfirmation, MB_YESNO) = IDNO then begin
Result := False;
end;
end;
end;
#endif
if Result and IsWin64 then begin
RegKey := 'SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall\' + copy('{#IncompatibleArchAppId}', 2, 38) + '_is1';
if '{#Arch}' = 'ia32' then begin
Result := not RegKeyExists({#Uninstall64RootKey}, RegKey);
ThisArch := '32';
AltArch := '64';
end else begin
Result := not RegKeyExists({#Uninstall32RootKey}, RegKey);
ThisArch := '64';
AltArch := '32';
end;
if not Result and not WizardSilent() then begin
MsgBox('Please uninstall the ' + AltArch + '-bit version of {#NameShort} before installing this ' + ThisArch + '-bit version.', mbInformation, MB_OK);
end;
end;
end;
function WizardNotSilent(): Boolean;
begin
Result := not WizardSilent();
end;
// Updates
function IsBackgroundUpdate(): Boolean;
begin
Result := ExpandConstant('{param:update|false}') <> 'false';
end;
function IsNotUpdate(): Boolean;
begin
Result := not IsBackgroundUpdate();
end;
// VS Code will create a flag file before the update starts (/update=C:\foo\bar)
// - if the file exists at this point, the user quit Code before the update finished, so don't start Code after update
// - otherwise, the user has accepted to apply the update and Code should start
function LockFileExists(): Boolean;
begin
Result := FileExists(ExpandConstant('{param:update}'))
end;
function ShouldRunAfterUpdate(): Boolean;
begin
if IsBackgroundUpdate() then
Result := not LockFileExists()
else
Result := True;
end;
function GetAppMutex(Value: string): string;
begin
if IsBackgroundUpdate() then
Result := ''
else
Result := '{#AppMutex}';
end;
function GetDestDir(Value: string): string;
begin
if IsBackgroundUpdate() then
Result := ExpandConstant('{app}\_')
else
Result := ExpandConstant('{app}');
end;
function BoolToStr(Value: Boolean): String;
begin
if Value then
Result := 'true'
else
Result := 'false';
end;
procedure CurStepChanged(CurStep: TSetupStep);
var
UpdateResultCode: Integer;
begin
if IsBackgroundUpdate() and (CurStep = ssPostInstall) then
begin
CreateMutex('{#AppMutex}-ready');
while (CheckForMutexes('{#AppMutex}')) do
begin
Log('Application is still running, waiting');
Sleep(1000);
end;
Exec(ExpandConstant('{app}\tools\inno_updater.exe'), ExpandConstant('"{app}\{#ExeBasename}.exe" ' + BoolToStr(LockFileExists())), '', SW_SHOW, ewWaitUntilTerminated, UpdateResultCode);
end;
end;
// https://stackoverflow.com/a/23838239/261019
procedure Explode(var Dest: TArrayOfString; Text: String; Separator: String);
var
i, p: Integer;
begin
i := 0;
repeat
SetArrayLength(Dest, i+1);
p := Pos(Separator,Text);
if p > 0 then begin
Dest[i] := Copy(Text, 1, p-1);
Text := Copy(Text, p + Length(Separator), Length(Text));
i := i + 1;
end else begin
Dest[i] := Text;
Text := '';
end;
until Length(Text)=0;
end;
function NeedsAddPath(Param: string): boolean;
var
OrigPath: string;
begin
if not RegQueryStringValue({#EnvironmentRootKey}, '{#EnvironmentKey}', 'Path', OrigPath)
then begin
Result := True;
exit;
end;
Result := Pos(';' + Param + ';', ';' + OrigPath + ';') = 0;
end;
procedure CurUninstallStepChanged(CurUninstallStep: TUninstallStep);
var
Path: string;
VSCodePath: string;
Parts: TArrayOfString;
NewPath: string;
i: Integer;
begin
if not CurUninstallStep = usUninstall then begin
exit;
end;
if not RegQueryStringValue({#EnvironmentRootKey}, '{#EnvironmentKey}', 'Path', Path)
then begin
exit;
end;
NewPath := '';
VSCodePath := ExpandConstant('{app}\bin')
Explode(Parts, Path, ';');
for i:=0 to GetArrayLength(Parts)-1 do begin
if CompareText(Parts[i], VSCodePath) <> 0 then begin
NewPath := NewPath + Parts[i];
if i < GetArrayLength(Parts) - 1 then begin
NewPath := NewPath + ';';
end;
end;
end;
RegWriteExpandStringValue({#EnvironmentRootKey}, '{#EnvironmentKey}', 'Path', NewPath);
end;
#ifdef Debug
#expr SaveToFile(AddBackslash(SourcePath) + "code-processed.iss")
#endif
| build/win32/code.iss | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00022912757412996143,
0.0001677810651017353,
0.00016222064732573926,
0.00016675004735589027,
0.000006333241344691487
] |
{
"id": 6,
"code_window": [
"\t| ICreateMarkdownMessage\n",
"\t| IRemoveMarkdownMessage\n",
"\t| IShowMarkdownMessage\n",
"\t| IHideMarkdownMessage\n",
"\t| IUnhideMarkdownMessage\n",
"\t| IUpdateMarkdownPreviewSelectionState\n",
"\t| IInitializeMarkdownMessage\n",
"\t| IViewScrollMarkdownRequestMessage;\n",
"\n",
"export type AnyMessage = FromWebviewMessage | ToWebviewMessage;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t| IUpdateSelectedMarkdownPreviews\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 340
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { VSBuffer } from 'vs/base/common/buffer';
import { Emitter, Event } from 'vs/base/common/event';
import { Disposable } from 'vs/base/common/lifecycle';
import { getExtensionForMimeType } from 'vs/base/common/mime';
import { FileAccess, Schemas } from 'vs/base/common/network';
import { isWeb } from 'vs/base/common/platform';
import { dirname, joinPath } from 'vs/base/common/resources';
import { URI } from 'vs/base/common/uri';
import * as UUID from 'vs/base/common/uuid';
import { IFileDialogService } from 'vs/platform/dialogs/common/dialogs';
import { IFileService } from 'vs/platform/files/common/files';
import { IOpenerService, matchesScheme } from 'vs/platform/opener/common/opener';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
import { CellEditState, ICellOutputViewModel, ICommonCellInfo, ICommonNotebookEditor, IDisplayOutputLayoutUpdateRequest, IDisplayOutputViewModel, IGenericCellViewModel, IInsetRenderOutput, RenderOutputType } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { preloadsScriptStr } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads';
import { transformWebviewThemeVars } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewThemeMapping';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { INotebookRendererInfo } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { IWebviewService, WebviewContentPurpose, WebviewElement } from 'vs/workbench/contrib/webview/browser/webview';
import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import * as nls from 'vs/nls';
interface BaseToWebviewMessage {
readonly __vscode_notebook_message: true;
}
export interface WebviewIntialized extends BaseToWebviewMessage {
type: 'initialized';
}
export interface DimensionUpdate {
id: string;
init?: boolean;
data: { height: number };
isOutput?: boolean;
}
export interface IDimensionMessage extends BaseToWebviewMessage {
type: 'dimension';
updates: readonly DimensionUpdate[];
}
export interface IMouseEnterMessage extends BaseToWebviewMessage {
type: 'mouseenter';
id: string;
}
export interface IMouseLeaveMessage extends BaseToWebviewMessage {
type: 'mouseleave';
id: string;
}
export interface IOutputFocusMessage extends BaseToWebviewMessage {
type: 'outputFocus';
id: string;
}
export interface IOutputBlurMessage extends BaseToWebviewMessage {
type: 'outputBlur';
id: string;
}
export interface IWheelMessage extends BaseToWebviewMessage {
type: 'did-scroll-wheel';
payload: any;
}
export interface IScrollAckMessage extends BaseToWebviewMessage {
type: 'scroll-ack';
data: { top: number };
version: number;
}
export interface IBlurOutputMessage extends BaseToWebviewMessage {
type: 'focus-editor';
id: string;
focusNext?: boolean;
}
export interface IClickedDataUrlMessage extends BaseToWebviewMessage {
type: 'clicked-data-url';
data: string | ArrayBuffer | null;
downloadName?: string;
}
export interface IClickMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'clickMarkdownPreview';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly metaKey: boolean;
readonly shiftKey: boolean;
}
export interface IMouseEnterMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseEnterMarkdownPreview';
cellId: string;
}
export interface IMouseLeaveMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseLeaveMarkdownPreview';
cellId: string;
}
export interface IToggleMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'toggleMarkdownPreview';
cellId: string;
}
export interface ICellDragStartMessage extends BaseToWebviewMessage {
type: 'cell-drag-start';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragMessage extends BaseToWebviewMessage {
type: 'cell-drag';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDropMessage extends BaseToWebviewMessage {
readonly type: 'cell-drop';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragEndMessage extends BaseToWebviewMessage {
readonly type: 'cell-drag-end';
readonly cellId: string;
}
export interface IInitializedMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'initializedMarkdownPreview';
}
export interface IClearMessage {
type: 'clear';
}
export interface IOutputRequestMetadata {
/**
* Additional attributes of a cell metadata.
*/
custom?: { [key: string]: unknown };
}
export interface IOutputRequestDto {
/**
* { mime_type: value }
*/
data: { [key: string]: unknown; }
metadata?: IOutputRequestMetadata;
outputId: string;
}
export interface ICreationRequestMessage {
type: 'html';
content:
| { type: RenderOutputType.Html; htmlContent: string }
| { type: RenderOutputType.Extension; outputId: string; value: unknown; metadata: unknown; mimeType: string };
cellId: string;
outputId: string;
top: number;
left: number;
requiredPreloads: ReadonlyArray<IPreloadResource>;
initiallyHidden?: boolean;
apiNamespace?: string | undefined;
}
export interface IContentWidgetTopRequest {
id: string;
top: number;
}
export interface IViewScrollTopRequestMessage {
type: 'view-scroll';
forceDisplay: boolean;
widgets: IContentWidgetTopRequest[];
}
export interface IViewScrollMarkdownRequestMessage {
type: 'view-scroll-markdown';
cells: { id: string; top: number }[];
}
export interface IScrollRequestMessage {
type: 'scroll';
id: string;
top: number;
widgetTop?: number;
version: number;
}
export interface IClearOutputRequestMessage {
type: 'clearOutput';
cellId: string;
outputId: string;
cellUri: string;
apiNamespace: string | undefined;
}
export interface IHideOutputMessage {
type: 'hideOutput';
outputId: string;
cellId: string;
}
export interface IShowOutputMessage {
type: 'showOutput';
cellId: string;
outputId: string;
top: number;
}
export interface IFocusOutputMessage {
type: 'focus-output';
cellId: string;
}
export interface IPreloadResource {
originalUri: string;
uri: string;
}
export interface IUpdatePreloadResourceMessage {
type: 'preload';
resources: IPreloadResource[];
source: 'renderer' | 'kernel';
}
export interface IUpdateDecorationsMessage {
type: 'decorations';
cellId: string;
addedClassNames: string[];
removedClassNames: string[];
}
export interface ICustomRendererMessage extends BaseToWebviewMessage {
type: 'customRendererMessage';
rendererId: string;
message: unknown;
}
export interface ICreateMarkdownMessage {
type: 'createMarkdownPreview',
id: string;
handle: number;
content: string;
top: number;
}
export interface IRemoveMarkdownMessage {
type: 'removeMarkdownPreview',
id: string;
}
export interface IHideMarkdownMessage {
type: 'hideMarkdownPreview';
id: string;
}
export interface IUnhideMarkdownMessage {
type: 'unhideMarkdownPreview';
id: string;
}
export interface IShowMarkdownMessage {
type: 'showMarkdownPreview',
id: string;
handle: number;
content: string | undefined;
top: number;
}
export interface IUpdateMarkdownPreviewSelectionState {
readonly type: 'updateMarkdownPreviewSelectionState',
readonly id: string;
readonly isSelected: boolean;
}
export interface IInitializeMarkdownMessage {
type: 'initializeMarkdownPreview';
cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>;
}
export type FromWebviewMessage =
| WebviewIntialized
| IDimensionMessage
| IMouseEnterMessage
| IMouseLeaveMessage
| IOutputFocusMessage
| IOutputBlurMessage
| IWheelMessage
| IScrollAckMessage
| IBlurOutputMessage
| ICustomRendererMessage
| IClickedDataUrlMessage
| IClickMarkdownPreviewMessage
| IMouseEnterMarkdownPreviewMessage
| IMouseLeaveMarkdownPreviewMessage
| IToggleMarkdownPreviewMessage
| ICellDragStartMessage
| ICellDragMessage
| ICellDropMessage
| ICellDragEndMessage
| IInitializedMarkdownPreviewMessage
;
export type ToWebviewMessage =
| IClearMessage
| IFocusOutputMessage
| ICreationRequestMessage
| IViewScrollTopRequestMessage
| IScrollRequestMessage
| IClearOutputRequestMessage
| IHideOutputMessage
| IShowOutputMessage
| IUpdatePreloadResourceMessage
| IUpdateDecorationsMessage
| ICustomRendererMessage
| ICreateMarkdownMessage
| IRemoveMarkdownMessage
| IShowMarkdownMessage
| IHideMarkdownMessage
| IUnhideMarkdownMessage
| IUpdateMarkdownPreviewSelectionState
| IInitializeMarkdownMessage
| IViewScrollMarkdownRequestMessage;
export type AnyMessage = FromWebviewMessage | ToWebviewMessage;
export interface ICachedInset<K extends ICommonCellInfo> {
outputId: string;
cellInfo: K;
renderer?: INotebookRendererInfo;
cachedCreation: ICreationRequestMessage;
}
function html(strings: TemplateStringsArray, ...values: any[]): string {
let str = '';
strings.forEach((string, i) => {
str += string + (values[i] || '');
});
return str;
}
export interface INotebookWebviewMessage {
message: unknown;
forRenderer?: string;
}
export interface IResolvedBackLayerWebview {
webview: WebviewElement;
}
export class BackLayerWebView<T extends ICommonCellInfo> extends Disposable {
element: HTMLElement;
webview: WebviewElement | undefined = undefined;
insetMapping: Map<IDisplayOutputViewModel, ICachedInset<T>> = new Map();
readonly markdownPreviewMapping = new Map<string, { version: number, visible: boolean }>();
hiddenInsetMapping: Set<IDisplayOutputViewModel> = new Set();
reversedInsetMapping: Map<string, IDisplayOutputViewModel> = new Map();
localResourceRootsCache: URI[] | undefined = undefined;
rendererRootsCache: URI[] = [];
kernelRootsCache: URI[] = [];
private readonly _onMessage = this._register(new Emitter<INotebookWebviewMessage>());
private readonly _preloadsCache = new Set<string>();
public readonly onMessage: Event<INotebookWebviewMessage> = this._onMessage.event;
private _loaded!: Promise<void>;
private _initalized?: Promise<void>;
private _disposed = false;
constructor(
public notebookEditor: ICommonNotebookEditor,
public id: string,
public documentUri: URI,
public options: {
outputNodePadding: number,
outputNodeLeftPadding: number,
previewNodePadding: number,
leftMargin: number,
cellMargin: number,
runGutter: number,
},
@IWebviewService readonly webviewService: IWebviewService,
@IOpenerService readonly openerService: IOpenerService,
@INotebookService private readonly notebookService: INotebookService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IWorkbenchEnvironmentService private readonly environmentService: IWorkbenchEnvironmentService,
@IFileDialogService private readonly fileDialogService: IFileDialogService,
@IFileService private readonly fileService: IFileService,
) {
super();
this.element = document.createElement('div');
this.element.style.height = '1400px';
this.element.style.position = 'absolute';
}
private generateContent(coreDependencies: string, baseUrl: string) {
const markdownRenderersSrc = this.getMarkdownRendererScripts();
return html`
<html lang="en">
<head>
<meta charset="UTF-8">
<base href="${baseUrl}/"/>
<!--
Markdown previews are rendered using a shadow dom and are not effected by normal css.
Insert this style node into all preview shadow doms for styling.
-->
<template id="preview-styles">
<style>
img {
max-width: 100%;
max-height: 100%;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
a:focus,
input:focus,
select:focus,
textarea:focus {
outline: 1px solid -webkit-focus-ring-color;
outline-offset: -1px;
}
hr {
border: 0;
height: 2px;
border-bottom: 2px solid;
}
h1 {
font-size: 26px;
padding-bottom: 8px;
line-height: 31px;
border-bottom-width: 1px;
border-bottom-style: solid;
border-color: var(--vscode-foreground);
margin: 0;
margin-bottom: 13px;
}
h2 {
font-size: 19px;
margin: 0;
margin-bottom: 10px;
}
h1,
h2,
h3 {
font-weight: normal;
}
div {
width: 100%;
}
/* Adjust margin of first item in markdown cell */
*:first-child {
margin-top: 0px;
}
/* h1 tags don't need top margin */
h1:first-child {
margin-top: 0;
}
/* Removes bottom margin when only one item exists in markdown cell */
*:only-child,
*:last-child {
margin-bottom: 0;
padding-bottom: 0;
}
/* makes all markdown cells consistent */
div {
min-height: ${this.options.previewNodePadding * 2}px;
}
table {
border-collapse: collapse;
border-spacing: 0;
}
table th,
table td {
border: 1px solid;
}
table > thead > tr > th {
text-align: left;
border-bottom: 1px solid;
}
table > thead > tr > th,
table > thead > tr > td,
table > tbody > tr > th,
table > tbody > tr > td {
padding: 5px 10px;
}
table > tbody > tr + tr > td {
border-top: 1px solid;
}
blockquote {
margin: 0 7px 0 5px;
padding: 0 16px 0 10px;
border-left-width: 5px;
border-left-style: solid;
}
code,
.code {
font-family: var(--monaco-monospace-font);
font-size: 1em;
line-height: 1.357em;
}
.code {
white-space: pre-wrap;
}
.latex-block {
display: block;
}
.latex {
vertical-align: middle;
display: inline-block;
}
.latex img,
.latex-block img {
filter: brightness(0) invert(0)
}
dragging {
background-color: var(--vscode-editor-background);
}
</style>
</template>
<style>
#container > div > div.output {
width: calc(100% - ${this.options.leftMargin + (this.options.cellMargin * 2) + this.options.runGutter}px);
margin-left: ${this.options.leftMargin + this.options.runGutter}px;
padding: ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodeLeftPadding}px;
box-sizing: border-box;
background-color: var(--vscode-notebook-outputContainerBackgroundColor);
}
#container > div > div.preview {
width: 100%;
box-sizing: border-box;
white-space: nowrap;
overflow: hidden;
user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
white-space: initial;
cursor: grab;
}
#container > div > div.preview.emptyMarkdownCell::before {
content: "${nls.localize('notebook.emptyMarkdownPlaceholder', "Empty markdown cell, double click or press enter to edit.")}";
font-style: italic;
opacity: 0.6;
}
/* markdown */
#container > div > div.preview {
color: var(--vscode-foreground);
width: 100%;
padding-left: ${this.options.leftMargin}px;
padding-top: ${this.options.previewNodePadding}px;
padding-bottom: ${this.options.previewNodePadding}px;
}
#container > div > div.preview.selected {
background: var(--vscode-notebook-selectedCellBackground);
}
#container > div > div.preview.dragging {
background-color: var(--vscode-editor-background);
}
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex img,
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex-block img {
filter: brightness(0) invert(1)
}
#container > div.nb-symbolHighlight > div {
background-color: var(--vscode-notebook-symbolHighlightBackground);
}
#container > div.nb-cellDeleted > div {
background-color: var(--vscode-diffEditor-removedTextBackground);
}
#container > div.nb-cellAdded > div {
background-color: var(--vscode-diffEditor-insertedTextBackground);
}
#container > div > div:not(.preview) > div {
overflow-x: scroll;
}
body {
padding: 0px;
height: 100%;
width: 100%;
}
table, thead, tr, th, td, tbody {
border: none !important;
border-color: transparent;
border-spacing: 0;
border-collapse: collapse;
}
table {
width: 100%;
}
table, th, tr {
text-align: left !important;
}
thead {
font-weight: bold;
background-color: rgba(130, 130, 130, 0.16);
}
th, td {
padding: 4px 8px;
}
tr:nth-child(even) {
background-color: rgba(130, 130, 130, 0.08);
}
tbody th {
font-weight: normal;
}
</style>
</head>
<body style="overflow: hidden;">
<script>
self.require = {};
</script>
${coreDependencies}
<div id='container' class="widgetarea" style="position: absolute;width:100%;top: 0px"></div>
<script>${preloadsScriptStr({
outputNodePadding: this.options.outputNodePadding,
outputNodeLeftPadding: this.options.outputNodeLeftPadding,
previewNodePadding: this.options.previewNodePadding,
leftMargin: this.options.leftMargin
})}</script>
${markdownRenderersSrc}
</body>
</html>`;
}
private getMarkdownRendererScripts() {
const markdownRenderers = this.notebookService.getMarkdownRendererInfo();
return markdownRenderers
.sort((a, b) => {
// prefer built-in extension
if (a.extensionIsBuiltin) {
return b.extensionIsBuiltin ? 0 : -1;
}
return b.extensionIsBuiltin ? 1 : -1;
})
.map(renderer => {
return asWebviewUri(this.environmentService, this.id, renderer.entrypoint);
})
.map(src => `<script src="${src}"></script>`)
.join('\n');
}
postRendererMessage(rendererId: string, message: any) {
this._sendMessageToWebview({
__vscode_notebook_message: true,
type: 'customRendererMessage',
message,
rendererId
});
}
private resolveOutputId(id: string): { cellInfo: T, output: ICellOutputViewModel } | undefined {
const output = this.reversedInsetMapping.get(id);
if (!output) {
return;
}
const cellInfo = this.insetMapping.get(output)!.cellInfo;
return { cellInfo, output };
}
isResolved(): this is IResolvedBackLayerWebview {
return !!this.webview;
}
async createWebview(): Promise<void> {
let coreDependencies = '';
let resolveFunc: () => void;
this._initalized = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const baseUrl = asWebviewUri(this.environmentService, this.id, dirname(this.documentUri));
if (!isWeb) {
const loaderUri = FileAccess.asFileUri('vs/loader.js', require);
const loader = asWebviewUri(this.environmentService, this.id, loaderUri);
coreDependencies = `<script src="${loader}"></script><script>
var requirejs = (function() {
return require;
}());
</script>`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
} else {
const loaderUri = FileAccess.asBrowserUri('vs/loader.js', require);
fetch(loaderUri.toString(true)).then(async response => {
if (response.status !== 200) {
throw new Error(response.statusText);
}
const loaderJs = await response.text();
coreDependencies = `
<script>
${loaderJs}
</script>
<script>
var requirejs = (function() {
return require;
}());
</script>
`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
});
}
await this._initalized;
}
private async _initialize(content: string) {
if (!document.body.contains(this.element)) {
throw new Error('Element is already detached from the DOM tree');
}
this.webview = this._createInset(this.webviewService, content);
this.webview.mountTo(this.element);
this._register(this.webview);
this._register(this.webview.onDidClickLink(link => {
if (this._disposed) {
return;
}
if (!link) {
return;
}
if (matchesScheme(link, Schemas.http) || matchesScheme(link, Schemas.https) || matchesScheme(link, Schemas.mailto)
|| matchesScheme(link, Schemas.command)) {
this.openerService.open(link, { fromUserGesture: true, allowContributedOpeners: true });
}
}));
this._register(this.webview.onDidReload(() => {
if (this._disposed) {
return;
}
let renderers = new Set<INotebookRendererInfo>();
for (const inset of this.insetMapping.values()) {
if (inset.renderer) {
renderers.add(inset.renderer);
}
}
this._preloadsCache.clear();
this.updateRendererPreloads(renderers);
for (const [output, inset] of this.insetMapping.entries()) {
this._sendMessageToWebview({ ...inset.cachedCreation, initiallyHidden: this.hiddenInsetMapping.has(output) });
}
}));
this._register(this.webview.onMessage((message) => {
const data: FromWebviewMessage | { readonly __vscode_notebook_message: undefined } = message.message;
if (this._disposed) {
return;
}
if (!data.__vscode_notebook_message) {
this._onMessage.fire({ message: data });
return;
}
switch (data.type) {
case 'dimension':
{
for (const update of data.updates) {
if (update.isOutput) {
const height = update.data.height;
const outputHeight = height;
const resolvedResult = this.resolveOutputId(update.id);
if (resolvedResult) {
const { cellInfo, output } = resolvedResult;
this.notebookEditor.updateOutputHeight(cellInfo, output, outputHeight, !!update.init, 'webview#dimension');
}
} else {
const cellId = update.id.substr(0, update.id.length - '_preview'.length);
this.notebookEditor.updateMarkdownCellHeight(cellId, update.data.height, !!update.init);
}
}
break;
}
case 'mouseenter':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = true;
}
}
break;
}
case 'mouseleave':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = false;
}
}
break;
}
case 'outputFocus':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = true;
}
}
break;
}
case 'outputBlur':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = false;
}
}
break;
}
case 'scroll-ack':
{
// const date = new Date();
// const top = data.data.top;
// console.log('ack top ', top, ' version: ', data.version, ' - ', date.getMinutes() + ':' + date.getSeconds() + ':' + date.getMilliseconds());
break;
}
case 'did-scroll-wheel':
{
this.notebookEditor.triggerScroll({
...data.payload,
preventDefault: () => { },
stopPropagation: () => { }
});
break;
}
case 'focus-editor':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (!latestCell) {
return;
}
if (data.focusNext) {
this.notebookEditor.focusNextNotebookCell(latestCell, 'editor');
} else {
this.notebookEditor.focusNotebookCell(latestCell, 'editor');
}
}
break;
}
case 'clicked-data-url':
{
this._onDidClickDataLink(data);
break;
}
case 'customRendererMessage':
{
this._onMessage.fire({ message: data.message, forRenderer: data.rendererId });
break;
}
case 'clickMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
if (data.shiftKey || data.metaKey) {
// Add to selection
this.notebookEditor.toggleNotebookCellSelection(cell);
} else {
// Normal click
this.notebookEditor.focusNotebookCell(cell, 'container', { skipReveal: true });
}
}
break;
}
case 'toggleMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
this.notebookEditor.setMarkdownCellEditState(data.cellId, CellEditState.Editing);
this.notebookEditor.focusNotebookCell(cell, 'editor', { skipReveal: true });
}
break;
}
case 'mouseEnterMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = true;
}
break;
}
case 'mouseLeaveMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = false;
}
break;
}
case 'cell-drag-start':
{
this.notebookEditor.markdownCellDragStart(data.cellId, data.position);
break;
}
case 'cell-drag':
{
this.notebookEditor.markdownCellDrag(data.cellId, data.position);
break;
}
case 'cell-drop':
{
this.notebookEditor.markdownCellDrop(data.cellId, {
clientY: data.position.clientY,
ctrlKey: data.ctrlKey,
altKey: data.altKey,
});
break;
}
case 'cell-drag-end':
{
this.notebookEditor.markdownCellDragEnd(data.cellId);
break;
}
}
}));
}
private async _onDidClickDataLink(event: IClickedDataUrlMessage): Promise<void> {
if (typeof event.data !== 'string') {
return;
}
const [splitStart, splitData] = event.data.split(';base64,');
if (!splitData || !splitStart) {
return;
}
const defaultDir = dirname(this.documentUri);
let defaultName: string;
if (event.downloadName) {
defaultName = event.downloadName;
} else {
const mimeType = splitStart.replace(/^data:/, '');
const candidateExtension = mimeType && getExtensionForMimeType(mimeType);
defaultName = candidateExtension ? `download${candidateExtension}` : 'download';
}
const defaultUri = joinPath(defaultDir, defaultName);
const newFileUri = await this.fileDialogService.showSaveDialog({
defaultUri
});
if (!newFileUri) {
return;
}
const decoded = atob(splitData);
const typedArray = new Uint8Array(decoded.length);
for (let i = 0; i < decoded.length; i++) {
typedArray[i] = decoded.charCodeAt(i);
}
const buff = VSBuffer.wrap(typedArray);
await this.fileService.writeFile(newFileUri, buff);
await this.openerService.open(newFileUri);
}
private _createInset(webviewService: IWebviewService, content: string) {
const rootPath = isWeb ? FileAccess.asBrowserUri('', require) : FileAccess.asFileUri('', require);
const workspaceFolders = this.contextService.getWorkspace().folders.map(x => x.uri);
this.localResourceRootsCache = [
...this.notebookService.getNotebookProviderResourceRoots(),
...this.notebookService.getMarkdownRendererInfo().map(x => dirname(x.entrypoint)),
...workspaceFolders,
rootPath,
];
const webview = webviewService.createWebviewElement(this.id, {
purpose: WebviewContentPurpose.NotebookRenderer,
enableFindWidget: false,
transformCssVariables: transformWebviewThemeVars,
}, {
allowMultipleAPIAcquire: true,
allowScripts: true,
localResourceRoots: this.localResourceRootsCache
}, undefined);
let resolveFunc: () => void;
this._loaded = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const dispose = webview.onMessage((message) => {
const data: FromWebviewMessage = message.message;
if (data.__vscode_notebook_message && data.type === 'initialized') {
resolveFunc();
dispose.dispose();
}
});
webview.html = content;
return webview;
}
shouldUpdateInset(cell: IGenericCellViewModel, output: ICellOutputViewModel, cellTop: number) {
if (this._disposed) {
return;
}
if (cell.metadata?.outputCollapsed) {
return false;
}
const outputCache = this.insetMapping.get(output)!;
const outputIndex = cell.outputsViewModels.indexOf(output);
const outputOffset = cellTop + cell.getOutputOffset(outputIndex);
if (this.hiddenInsetMapping.has(output)) {
return true;
}
if (outputOffset === outputCache.cachedCreation.top) {
return false;
}
return true;
}
updateMarkdownScrollTop(items: { id: string, top: number }[]) {
if (this._disposed || !items.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll-markdown',
cells: items
});
}
updateViewScrollTop(forceDisplay: boolean, items: IDisplayOutputLayoutUpdateRequest[]) {
if (!items.length) {
return;
}
const widgets = coalesce(items.map((item): IContentWidgetTopRequest | undefined => {
const outputCache = this.insetMapping.get(item.output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
const outputOffset = item.outputOffset;
outputCache.cachedCreation.top = outputOffset;
this.hiddenInsetMapping.delete(item.output);
return {
id: id,
top: outputOffset,
};
}));
if (!widgets.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll',
forceDisplay,
widgets: widgets
});
}
private async createMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (this.markdownPreviewMapping.has(cellId)) {
console.error('Trying to create markdown preview that already exists');
return;
}
const initialTop = cellTop;
this.markdownPreviewMapping.set(cellId, { version: contentVersion, visible: true });
this._sendMessageToWebview({
type: 'createMarkdownPreview',
id: cellId,
handle: cellHandle,
content: content,
top: initialTop,
});
}
async showMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
return this.createMarkdownPreview(cellId, cellHandle, content, cellTop, contentVersion);
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error('Try to show a preview that does not exist');
return;
}
if (entry.version !== contentVersion || !entry.visible) {
this._sendMessageToWebview({
type: 'showMarkdownPreview',
id: cellId,
handle: cellHandle,
// If the content has not changed, we still want to make sure the
// preview is visible but don't need to send anything over
content: entry.version === contentVersion ? undefined : content,
top: cellTop
});
}
entry.version = contentVersion;
entry.visible = true;
}
async hideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
// TODO: this currently seems expected on first load
// console.error(`Try to hide a preview that does not exist: ${cellId}`);
return;
}
if (entry.visible) {
this._sendMessageToWebview({
type: 'hideMarkdownPreview',
id: cellId
});
entry.visible = false;
}
}
async unhideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error(`Try to unhide a preview that does not exist: ${cellId}`);
return;
}
if (!entry.visible) {
this._sendMessageToWebview({
type: 'unhideMarkdownPreview',
id: cellId
});
entry.visible = true;
}
}
async removeMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
console.error(`Try to delete a preview that does not exist: ${cellId}`);
return;
}
this.markdownPreviewMapping.delete(cellId);
this._sendMessageToWebview({
type: 'removeMarkdownPreview',
id: cellId
});
}
async updateMarkdownPreviewSelectionState(cellId: any, isSelected: boolean) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
// TODO: this currently seems expected on first load
// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);
return;
}
this._sendMessageToWebview({
type: 'updateMarkdownPreviewSelectionState',
id: cellId,
isSelected
});
}
async initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {
await this._loaded;
if (this._disposed) {
return;
}
// TODO: use proper handler
const p = new Promise<void>(resolve => {
this.webview?.onMessage(e => {
if (e.message.type === 'initializedMarkdownPreview') {
resolve();
}
});
});
for (const cell of cells) {
this.markdownPreviewMapping.set(cell.cellId, { version: 0, visible: false });
}
this._sendMessageToWebview({
type: 'initializeMarkdownPreview',
cells: cells,
});
await p;
}
async createOutput(cellInfo: T, content: IInsetRenderOutput, cellTop: number, offset: number) {
if (this._disposed) {
return;
}
const initialTop = cellTop + offset;
if (this.insetMapping.has(content.source)) {
const outputCache = this.insetMapping.get(content.source);
if (outputCache) {
this.hiddenInsetMapping.delete(content.source);
this._sendMessageToWebview({
type: 'showOutput',
cellId: outputCache.cellInfo.cellId,
outputId: outputCache.outputId,
top: initialTop
});
return;
}
}
const messageBase = {
type: 'html',
cellId: cellInfo.cellId,
top: initialTop,
left: 0,
requiredPreloads: [],
} as const;
let message: ICreationRequestMessage;
let renderer: INotebookRendererInfo | undefined;
if (content.type === RenderOutputType.Extension) {
const output = content.source.model;
renderer = content.renderer;
const outputDto = output.outputs.find(op => op.mime === content.mimeType);
message = {
...messageBase,
outputId: output.outputId,
apiNamespace: content.renderer.id,
requiredPreloads: await this.updateRendererPreloads([content.renderer]),
content: {
type: RenderOutputType.Extension,
outputId: output.outputId,
mimeType: content.mimeType,
value: outputDto?.value,
metadata: outputDto?.metadata,
},
};
} else {
message = {
...messageBase,
outputId: UUID.generateUuid(),
content: {
type: content.type,
htmlContent: content.htmlContent,
}
};
}
this._sendMessageToWebview(message);
this.insetMapping.set(content.source, { outputId: message.outputId, cellInfo: cellInfo, renderer, cachedCreation: message });
this.hiddenInsetMapping.delete(content.source);
this.reversedInsetMapping.set(message.outputId, content.source);
}
removeInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
this._sendMessageToWebview({
type: 'clearOutput',
apiNamespace: outputCache.cachedCreation.apiNamespace,
cellUri: outputCache.cellInfo.cellUri.toString(),
outputId: id,
cellId: outputCache.cellInfo.cellId
});
this.insetMapping.delete(output);
this.reversedInsetMapping.delete(id);
}
hideInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
this.hiddenInsetMapping.add(output);
this._sendMessageToWebview({
type: 'hideOutput',
outputId: outputCache.outputId,
cellId: outputCache.cellInfo.cellId,
});
}
clearInsets() {
if (this._disposed) {
return;
}
this._sendMessageToWebview({
type: 'clear'
});
this.insetMapping = new Map();
this.reversedInsetMapping = new Map();
}
focusWebview() {
if (this._disposed) {
return;
}
this.webview?.focus();
}
focusOutput(cellId: string) {
if (this._disposed) {
return;
}
this.webview?.focus();
setTimeout(() => { // Need this, or focus decoration is not shown. No clue.
this._sendMessageToWebview({
type: 'focus-output',
cellId,
});
}, 50);
}
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]) {
this._sendMessageToWebview({
type: 'decorations',
cellId,
addedClassNames: added,
removedClassNames: removed
});
}
async updateKernelPreloads(extensionLocations: URI[], preloads: URI[]) {
if (this._disposed) {
return;
}
await this._loaded;
const resources: IPreloadResource[] = [];
for (const preload of preloads) {
const uri = this.environmentService.isExtensionDevelopment && (preload.scheme === 'http' || preload.scheme === 'https')
? preload : asWebviewUri(this.environmentService, this.id, preload);
if (!this._preloadsCache.has(uri.toString())) {
resources.push({ uri: uri.toString(), originalUri: preload.toString() });
this._preloadsCache.add(uri.toString());
}
}
if (!resources.length) {
return;
}
this.kernelRootsCache = [...extensionLocations, ...this.kernelRootsCache];
this._updatePreloads(resources, 'kernel');
}
async updateRendererPreloads(renderers: Iterable<INotebookRendererInfo>) {
if (this._disposed) {
return [];
}
await this._loaded;
const requiredPreloads: IPreloadResource[] = [];
const resources: IPreloadResource[] = [];
const extensionLocations: URI[] = [];
for (const rendererInfo of renderers) {
extensionLocations.push(rendererInfo.extensionLocation);
for (const preload of [rendererInfo.entrypoint, ...rendererInfo.preloads]) {
const uri = asWebviewUri(this.environmentService, this.id, preload);
const resource: IPreloadResource = { uri: uri.toString(), originalUri: preload.toString() };
requiredPreloads.push(resource);
if (!this._preloadsCache.has(uri.toString())) {
resources.push(resource);
this._preloadsCache.add(uri.toString());
}
}
}
if (!resources.length) {
return requiredPreloads;
}
this.rendererRootsCache = extensionLocations;
this._updatePreloads(resources, 'renderer');
return requiredPreloads;
}
private _updatePreloads(resources: IPreloadResource[], source: 'renderer' | 'kernel') {
if (!this.webview) {
return;
}
const mixedResourceRoots = [...(this.localResourceRootsCache || []), ...this.rendererRootsCache, ...this.kernelRootsCache];
this.webview.localResourcesRoot = mixedResourceRoots;
this._sendMessageToWebview({
type: 'preload',
resources: resources,
source: source
});
}
private _sendMessageToWebview(message: ToWebviewMessage) {
if (this._disposed) {
return;
}
this.webview?.postMessage(message);
}
clearPreloadsCache() {
this._preloadsCache.clear();
}
dispose() {
this._disposed = true;
this.webview?.dispose();
super.dispose();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.9973135590553284,
0.007607243023812771,
0.0001626230077818036,
0.00017292371194344014,
0.0794157162308693
] |
{
"id": 6,
"code_window": [
"\t| ICreateMarkdownMessage\n",
"\t| IRemoveMarkdownMessage\n",
"\t| IShowMarkdownMessage\n",
"\t| IHideMarkdownMessage\n",
"\t| IUnhideMarkdownMessage\n",
"\t| IUpdateMarkdownPreviewSelectionState\n",
"\t| IInitializeMarkdownMessage\n",
"\t| IViewScrollMarkdownRequestMessage;\n",
"\n",
"export type AnyMessage = FromWebviewMessage | ToWebviewMessage;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t| IUpdateSelectedMarkdownPreviews\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 340
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { ExtHostTreeViewsShape, IExtHostContext } from 'vs/workbench/api/common/extHost.protocol';
import { mock } from 'vs/base/test/common/mock';
import { ITreeItem, IViewsRegistry, Extensions, ViewContainerLocation, IViewContainersRegistry, ITreeViewDescriptor, ITreeView, ViewContainer, IViewDescriptorService, TreeItemCollapsibleState } from 'vs/workbench/common/views';
import { NullLogService } from 'vs/platform/log/common/log';
import { MainThreadTreeViews } from 'vs/workbench/api/browser/mainThreadTreeViews';
import { TestViewsService, workbenchInstantiationService } from 'vs/workbench/test/browser/workbenchTestServices';
import { TestExtensionService } from 'vs/workbench/test/common/workbenchTestServices';
import { TestNotificationService } from 'vs/platform/notification/test/common/testNotificationService';
import { Registry } from 'vs/platform/registry/common/platform';
import { SyncDescriptor } from 'vs/platform/instantiation/common/descriptors';
import { TestInstantiationService } from 'vs/platform/instantiation/test/common/instantiationServiceMock';
import { ViewDescriptorService } from 'vs/workbench/services/views/browser/viewDescriptorService';
import { CustomTreeView } from 'vs/workbench/browser/parts/views/treeView';
import { ExtensionHostKind } from 'vs/workbench/services/extensions/common/extensions';
suite('MainThreadHostTreeView', function () {
const testTreeViewId = 'testTreeView';
const customValue = 'customValue';
const ViewsRegistry = Registry.as<IViewsRegistry>(Extensions.ViewsRegistry);
interface CustomTreeItem extends ITreeItem {
customProp: string;
}
class MockExtHostTreeViewsShape extends mock<ExtHostTreeViewsShape>() {
async $getChildren(treeViewId: string, treeItemHandle?: string): Promise<ITreeItem[]> {
return [<CustomTreeItem>{ handle: 'testItem1', collapsibleState: TreeItemCollapsibleState.Expanded, customProp: customValue }];
}
async $hasResolve(): Promise<boolean> {
return false;
}
$setVisible(): void { }
}
let container: ViewContainer;
let mainThreadTreeViews: MainThreadTreeViews;
let extHostTreeViewsShape: MockExtHostTreeViewsShape;
setup(async () => {
const instantiationService: TestInstantiationService = <TestInstantiationService>workbenchInstantiationService();
const viewDescriptorService = instantiationService.createInstance(ViewDescriptorService);
instantiationService.stub(IViewDescriptorService, viewDescriptorService);
container = Registry.as<IViewContainersRegistry>(Extensions.ViewContainersRegistry).registerViewContainer({ id: 'testContainer', title: 'test', ctorDescriptor: new SyncDescriptor(<any>{}) }, ViewContainerLocation.Sidebar);
const viewDescriptor: ITreeViewDescriptor = {
id: testTreeViewId,
ctorDescriptor: null!,
name: 'Test View 1',
treeView: instantiationService.createInstance(CustomTreeView, 'testTree', 'Test Title'),
};
ViewsRegistry.registerViews([viewDescriptor], container);
const testExtensionService = new TestExtensionService();
extHostTreeViewsShape = new MockExtHostTreeViewsShape();
mainThreadTreeViews = new MainThreadTreeViews(
new class implements IExtHostContext {
remoteAuthority = '';
extensionHostKind = ExtensionHostKind.LocalProcess;
assertRegistered() { }
set(v: any): any { return null; }
getProxy(): any {
return extHostTreeViewsShape;
}
drain(): any { return null; }
}, new TestViewsService(), new TestNotificationService(), testExtensionService, new NullLogService());
mainThreadTreeViews.$registerTreeViewDataProvider(testTreeViewId, { showCollapseAll: false, canSelectMany: false });
await testExtensionService.whenInstalledExtensionsRegistered();
});
teardown(() => {
ViewsRegistry.deregisterViews(ViewsRegistry.getViews(container), container);
});
test('getChildren keeps custom properties', async () => {
const treeView: ITreeView = (<ITreeViewDescriptor>ViewsRegistry.getView(testTreeViewId)).treeView;
const children = await treeView.dataProvider?.getChildren({ handle: 'root', collapsibleState: TreeItemCollapsibleState.Expanded });
assert(children!.length === 1, 'Exactly one child should be returned');
assert((<CustomTreeItem>children![0]).customProp === customValue, 'Tree Items should keep custom properties');
});
});
| src/vs/workbench/test/browser/api/mainThreadTreeViews.test.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017366067913826555,
0.00017138157272711396,
0.00016600522212684155,
0.00017260249296668917,
0.0000025135723262792453
] |
{
"id": 6,
"code_window": [
"\t| ICreateMarkdownMessage\n",
"\t| IRemoveMarkdownMessage\n",
"\t| IShowMarkdownMessage\n",
"\t| IHideMarkdownMessage\n",
"\t| IUnhideMarkdownMessage\n",
"\t| IUpdateMarkdownPreviewSelectionState\n",
"\t| IInitializeMarkdownMessage\n",
"\t| IViewScrollMarkdownRequestMessage;\n",
"\n",
"export type AnyMessage = FromWebviewMessage | ToWebviewMessage;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t| IUpdateSelectedMarkdownPreviews\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 340
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IViewletViewOptions } from 'vs/workbench/browser/parts/views/viewsViewlet';
import { IThemeService } from 'vs/platform/theme/common/themeService';
import { IKeybindingService } from 'vs/platform/keybinding/common/keybinding';
import { IContextMenuService } from 'vs/platform/contextview/browser/contextView';
import { IConfigurationService } from 'vs/platform/configuration/common/configuration';
import { IContextKeyService, RawContextKey, IContextKey, ContextKeyExpr } from 'vs/platform/contextkey/common/contextkey';
import { localize } from 'vs/nls';
import { IDebugService, CONTEXT_DEBUGGERS_AVAILABLE } from 'vs/workbench/contrib/debug/common/debug';
import { IEditorService } from 'vs/workbench/services/editor/common/editorService';
import { ViewPane } from 'vs/workbench/browser/parts/views/viewPane';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { IViewDescriptorService, IViewsRegistry, Extensions, ViewContentGroups } from 'vs/workbench/common/views';
import { Registry } from 'vs/platform/registry/common/platform';
import { IOpenerService } from 'vs/platform/opener/common/opener';
import { WorkbenchStateContext } from 'vs/workbench/browser/contextkeys';
import { OpenFolderAction, OpenFileAction, OpenFileFolderAction } from 'vs/workbench/browser/actions/workspaceActions';
import { isMacintosh } from 'vs/base/common/platform';
import { isCodeEditor } from 'vs/editor/browser/editorBrowser';
import { IStorageService, StorageScope, StorageTarget } from 'vs/platform/storage/common/storage';
import { ITelemetryService } from 'vs/platform/telemetry/common/telemetry';
import { DisposableStore } from 'vs/base/common/lifecycle';
import { SELECT_AND_START_ID, DEBUG_CONFIGURE_COMMAND_ID, DEBUG_START_COMMAND_ID } from 'vs/workbench/contrib/debug/browser/debugCommands';
const debugStartLanguageKey = 'debugStartLanguage';
const CONTEXT_DEBUG_START_LANGUAGE = new RawContextKey<string>(debugStartLanguageKey, undefined);
const CONTEXT_DEBUGGER_INTERESTED_IN_ACTIVE_EDITOR = new RawContextKey<boolean>('debuggerInterestedInActiveEditor', false);
export class WelcomeView extends ViewPane {
static ID = 'workbench.debug.welcome';
static LABEL = localize('run', "Run");
private debugStartLanguageContext: IContextKey<string | undefined>;
private debuggerInterestedContext: IContextKey<boolean>;
constructor(
options: IViewletViewOptions,
@IThemeService themeService: IThemeService,
@IKeybindingService keybindingService: IKeybindingService,
@IContextMenuService contextMenuService: IContextMenuService,
@IConfigurationService configurationService: IConfigurationService,
@IContextKeyService contextKeyService: IContextKeyService,
@IDebugService private readonly debugService: IDebugService,
@IEditorService private readonly editorService: IEditorService,
@IInstantiationService instantiationService: IInstantiationService,
@IViewDescriptorService viewDescriptorService: IViewDescriptorService,
@IOpenerService openerService: IOpenerService,
@IStorageService storageSevice: IStorageService,
@ITelemetryService telemetryService: ITelemetryService,
) {
super(options, keybindingService, contextMenuService, configurationService, contextKeyService, viewDescriptorService, instantiationService, openerService, themeService, telemetryService);
this.debugStartLanguageContext = CONTEXT_DEBUG_START_LANGUAGE.bindTo(contextKeyService);
this.debuggerInterestedContext = CONTEXT_DEBUGGER_INTERESTED_IN_ACTIVE_EDITOR.bindTo(contextKeyService);
const lastSetLanguage = storageSevice.get(debugStartLanguageKey, StorageScope.WORKSPACE);
this.debugStartLanguageContext.set(lastSetLanguage);
const setContextKey = () => {
const editorControl = this.editorService.activeTextEditorControl;
if (isCodeEditor(editorControl)) {
const model = editorControl.getModel();
const language = model ? model.getLanguageIdentifier().language : undefined;
if (language && this.debugService.getAdapterManager().isDebuggerInterestedInLanguage(language)) {
this.debugStartLanguageContext.set(language);
this.debuggerInterestedContext.set(true);
storageSevice.store(debugStartLanguageKey, language, StorageScope.WORKSPACE, StorageTarget.MACHINE);
return;
}
}
this.debuggerInterestedContext.set(false);
};
const disposables = new DisposableStore();
this._register(disposables);
this._register(editorService.onDidActiveEditorChange(() => {
disposables.clear();
const editorControl = this.editorService.activeTextEditorControl;
if (isCodeEditor(editorControl)) {
disposables.add(editorControl.onDidChangeModelLanguage(setContextKey));
}
setContextKey();
}));
this._register(this.debugService.getAdapterManager().onDidRegisterDebugger(setContextKey));
this._register(this.onDidChangeBodyVisibility(visible => {
if (visible) {
setContextKey();
}
}));
setContextKey();
const debugKeybinding = this.keybindingService.lookupKeybinding(DEBUG_START_COMMAND_ID);
debugKeybindingLabel = debugKeybinding ? ` (${debugKeybinding.getLabel()})` : '';
}
shouldShowWelcome(): boolean {
return true;
}
}
const viewsRegistry = Registry.as<IViewsRegistry>(Extensions.ViewsRegistry);
viewsRegistry.registerViewWelcomeContent(WelcomeView.ID, {
content: localize({ key: 'openAFileWhichCanBeDebugged', comment: ['Please do not translate the word "commmand", it is part of our internal syntax which must not change'] },
"[Open a file](command:{0}) which can be debugged or run.", isMacintosh ? OpenFileFolderAction.ID : OpenFileAction.ID),
when: ContextKeyExpr.and(CONTEXT_DEBUGGERS_AVAILABLE, CONTEXT_DEBUGGER_INTERESTED_IN_ACTIVE_EDITOR.toNegated()),
group: ViewContentGroups.Open
});
let debugKeybindingLabel = '';
viewsRegistry.registerViewWelcomeContent(WelcomeView.ID, {
content: localize({ key: 'runAndDebugAction', comment: ['Please do not translate the word "commmand", it is part of our internal syntax which must not change'] },
"[Run and Debug{0}](command:{1})", debugKeybindingLabel, DEBUG_START_COMMAND_ID),
when: CONTEXT_DEBUGGERS_AVAILABLE,
group: ViewContentGroups.Debug
});
viewsRegistry.registerViewWelcomeContent(WelcomeView.ID, {
content: localize({ key: 'detectThenRunAndDebug', comment: ['Please do not translate the word "commmand", it is part of our internal syntax which must not change'] },
"[Show](command:{0}) all automatic debug configurations.", SELECT_AND_START_ID),
when: CONTEXT_DEBUGGERS_AVAILABLE,
group: ViewContentGroups.Debug,
order: 10
});
viewsRegistry.registerViewWelcomeContent(WelcomeView.ID, {
content: localize({ key: 'customizeRunAndDebug', comment: ['Please do not translate the word "commmand", it is part of our internal syntax which must not change'] },
"To customize Run and Debug [create a launch.json file](command:{0}).", DEBUG_CONFIGURE_COMMAND_ID),
when: ContextKeyExpr.and(CONTEXT_DEBUGGERS_AVAILABLE, WorkbenchStateContext.notEqualsTo('empty')),
group: ViewContentGroups.Debug
});
viewsRegistry.registerViewWelcomeContent(WelcomeView.ID, {
content: localize({ key: 'customizeRunAndDebugOpenFolder', comment: ['Please do not translate the word "commmand", it is part of our internal syntax which must not change'] },
"To customize Run and Debug, [open a folder](command:{0}) and create a launch.json file.", isMacintosh ? OpenFileFolderAction.ID : OpenFolderAction.ID),
when: ContextKeyExpr.and(CONTEXT_DEBUGGERS_AVAILABLE, WorkbenchStateContext.isEqualTo('empty')),
group: ViewContentGroups.Debug
});
| src/vs/workbench/contrib/debug/browser/welcomeView.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017339643090963364,
0.0001706054899841547,
0.00016613886691629887,
0.00017113822104874998,
0.000002063508418359561
] |
{
"id": 6,
"code_window": [
"\t| ICreateMarkdownMessage\n",
"\t| IRemoveMarkdownMessage\n",
"\t| IShowMarkdownMessage\n",
"\t| IHideMarkdownMessage\n",
"\t| IUnhideMarkdownMessage\n",
"\t| IUpdateMarkdownPreviewSelectionState\n",
"\t| IInitializeMarkdownMessage\n",
"\t| IViewScrollMarkdownRequestMessage;\n",
"\n",
"export type AnyMessage = FromWebviewMessage | ToWebviewMessage;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t| IUpdateSelectedMarkdownPreviews\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 340
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as vscode from 'vscode';
import { Disposable } from '../util/dispose';
import { isMarkdownFile } from '../util/file';
import { Lazy, lazy } from '../util/lazy';
import MDDocumentSymbolProvider from './documentSymbolProvider';
import { SkinnyTextDocument, SkinnyTextLine } from '../tableOfContentsProvider';
export interface WorkspaceMarkdownDocumentProvider {
getAllMarkdownDocuments(): Thenable<Iterable<SkinnyTextDocument>>;
readonly onDidChangeMarkdownDocument: vscode.Event<SkinnyTextDocument>;
readonly onDidCreateMarkdownDocument: vscode.Event<SkinnyTextDocument>;
readonly onDidDeleteMarkdownDocument: vscode.Event<vscode.Uri>;
}
class VSCodeWorkspaceMarkdownDocumentProvider extends Disposable implements WorkspaceMarkdownDocumentProvider {
private readonly _onDidChangeMarkdownDocumentEmitter = this._register(new vscode.EventEmitter<SkinnyTextDocument>());
private readonly _onDidCreateMarkdownDocumentEmitter = this._register(new vscode.EventEmitter<SkinnyTextDocument>());
private readonly _onDidDeleteMarkdownDocumentEmitter = this._register(new vscode.EventEmitter<vscode.Uri>());
private _watcher: vscode.FileSystemWatcher | undefined;
async getAllMarkdownDocuments() {
const resources = await vscode.workspace.findFiles('**/*.md', '**/node_modules/**');
const docs = await Promise.all(resources.map(doc => this.getMarkdownDocument(doc)));
return docs.filter(doc => !!doc) as SkinnyTextDocument[];
}
public get onDidChangeMarkdownDocument() {
this.ensureWatcher();
return this._onDidChangeMarkdownDocumentEmitter.event;
}
public get onDidCreateMarkdownDocument() {
this.ensureWatcher();
return this._onDidCreateMarkdownDocumentEmitter.event;
}
public get onDidDeleteMarkdownDocument() {
this.ensureWatcher();
return this._onDidDeleteMarkdownDocumentEmitter.event;
}
private ensureWatcher(): void {
if (this._watcher) {
return;
}
this._watcher = this._register(vscode.workspace.createFileSystemWatcher('**/*.md'));
this._watcher.onDidChange(async resource => {
const document = await this.getMarkdownDocument(resource);
if (document) {
this._onDidChangeMarkdownDocumentEmitter.fire(document);
}
}, null, this._disposables);
this._watcher.onDidCreate(async resource => {
const document = await this.getMarkdownDocument(resource);
if (document) {
this._onDidCreateMarkdownDocumentEmitter.fire(document);
}
}, null, this._disposables);
this._watcher.onDidDelete(async resource => {
this._onDidDeleteMarkdownDocumentEmitter.fire(resource);
}, null, this._disposables);
vscode.workspace.onDidChangeTextDocument(e => {
if (isMarkdownFile(e.document)) {
this._onDidChangeMarkdownDocumentEmitter.fire(e.document);
}
}, null, this._disposables);
}
private async getMarkdownDocument(resource: vscode.Uri): Promise<SkinnyTextDocument | undefined> {
const matchingDocuments = vscode.workspace.textDocuments.filter((doc) => doc.uri.toString() === resource.toString());
if (matchingDocuments.length !== 0) {
return matchingDocuments[0];
}
const bytes = await vscode.workspace.fs.readFile(resource);
// We assume that markdown is in UTF-8
const text = Buffer.from(bytes).toString('utf-8');
const lines: SkinnyTextLine[] = [];
const parts = text.split(/(\r?\n)/);
const lineCount = Math.floor(parts.length / 2) + 1;
for (let line = 0; line < lineCount; line++) {
lines.push({
text: parts[line * 2]
});
}
return {
uri: resource,
version: 0,
lineCount: lineCount,
lineAt: (index) => {
return lines[index];
},
getText: () => {
return text;
}
};
}
}
export default class MarkdownWorkspaceSymbolProvider extends Disposable implements vscode.WorkspaceSymbolProvider {
private _symbolCache = new Map<string, Lazy<Thenable<vscode.SymbolInformation[]>>>();
private _symbolCachePopulated: boolean = false;
public constructor(
private _symbolProvider: MDDocumentSymbolProvider,
private _workspaceMarkdownDocumentProvider: WorkspaceMarkdownDocumentProvider = new VSCodeWorkspaceMarkdownDocumentProvider()
) {
super();
}
public async provideWorkspaceSymbols(query: string): Promise<vscode.SymbolInformation[]> {
if (!this._symbolCachePopulated) {
await this.populateSymbolCache();
this._symbolCachePopulated = true;
this._workspaceMarkdownDocumentProvider.onDidChangeMarkdownDocument(this.onDidChangeDocument, this, this._disposables);
this._workspaceMarkdownDocumentProvider.onDidCreateMarkdownDocument(this.onDidChangeDocument, this, this._disposables);
this._workspaceMarkdownDocumentProvider.onDidDeleteMarkdownDocument(this.onDidDeleteDocument, this, this._disposables);
}
const allSymbolsSets = await Promise.all(Array.from(this._symbolCache.values()).map(x => x.value));
const allSymbols = allSymbolsSets.flat();
return allSymbols.filter(symbolInformation => symbolInformation.name.toLowerCase().indexOf(query.toLowerCase()) !== -1);
}
public async populateSymbolCache(): Promise<void> {
const markdownDocumentUris = await this._workspaceMarkdownDocumentProvider.getAllMarkdownDocuments();
for (const document of markdownDocumentUris) {
this._symbolCache.set(document.uri.fsPath, this.getSymbols(document));
}
}
private getSymbols(document: SkinnyTextDocument): Lazy<Thenable<vscode.SymbolInformation[]>> {
return lazy(async () => {
return this._symbolProvider.provideDocumentSymbolInformation(document);
});
}
private onDidChangeDocument(document: SkinnyTextDocument) {
this._symbolCache.set(document.uri.fsPath, this.getSymbols(document));
}
private onDidDeleteDocument(resource: vscode.Uri) {
this._symbolCache.delete(resource.fsPath);
}
}
| extensions/markdown-language-features/src/features/workspaceSymbolProvider.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0007252476061694324,
0.00024507148191332817,
0.00016359380970243365,
0.00017290787945967168,
0.00014540247502736747
] |
{
"id": 8,
"code_window": [
"\t\tif (this._disposed) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this.markdownPreviewMapping.has(cellId)) {\n",
"\t\t\t// TODO: this currently seems expected on first load\n",
"\t\t\t// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tthis._sendMessageToWebview({\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1273
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { VSBuffer } from 'vs/base/common/buffer';
import { Emitter, Event } from 'vs/base/common/event';
import { Disposable } from 'vs/base/common/lifecycle';
import { getExtensionForMimeType } from 'vs/base/common/mime';
import { FileAccess, Schemas } from 'vs/base/common/network';
import { isWeb } from 'vs/base/common/platform';
import { dirname, joinPath } from 'vs/base/common/resources';
import { URI } from 'vs/base/common/uri';
import * as UUID from 'vs/base/common/uuid';
import { IFileDialogService } from 'vs/platform/dialogs/common/dialogs';
import { IFileService } from 'vs/platform/files/common/files';
import { IOpenerService, matchesScheme } from 'vs/platform/opener/common/opener';
import { IWorkspaceContextService } from 'vs/platform/workspace/common/workspace';
import { CellEditState, ICellOutputViewModel, ICommonCellInfo, ICommonNotebookEditor, IDisplayOutputLayoutUpdateRequest, IDisplayOutputViewModel, IGenericCellViewModel, IInsetRenderOutput, RenderOutputType } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { preloadsScriptStr } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads';
import { transformWebviewThemeVars } from 'vs/workbench/contrib/notebook/browser/view/renderers/webviewThemeMapping';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { INotebookRendererInfo } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { INotebookService } from 'vs/workbench/contrib/notebook/common/notebookService';
import { IWebviewService, WebviewContentPurpose, WebviewElement } from 'vs/workbench/contrib/webview/browser/webview';
import { asWebviewUri } from 'vs/workbench/contrib/webview/common/webviewUri';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import * as nls from 'vs/nls';
interface BaseToWebviewMessage {
readonly __vscode_notebook_message: true;
}
export interface WebviewIntialized extends BaseToWebviewMessage {
type: 'initialized';
}
export interface DimensionUpdate {
id: string;
init?: boolean;
data: { height: number };
isOutput?: boolean;
}
export interface IDimensionMessage extends BaseToWebviewMessage {
type: 'dimension';
updates: readonly DimensionUpdate[];
}
export interface IMouseEnterMessage extends BaseToWebviewMessage {
type: 'mouseenter';
id: string;
}
export interface IMouseLeaveMessage extends BaseToWebviewMessage {
type: 'mouseleave';
id: string;
}
export interface IOutputFocusMessage extends BaseToWebviewMessage {
type: 'outputFocus';
id: string;
}
export interface IOutputBlurMessage extends BaseToWebviewMessage {
type: 'outputBlur';
id: string;
}
export interface IWheelMessage extends BaseToWebviewMessage {
type: 'did-scroll-wheel';
payload: any;
}
export interface IScrollAckMessage extends BaseToWebviewMessage {
type: 'scroll-ack';
data: { top: number };
version: number;
}
export interface IBlurOutputMessage extends BaseToWebviewMessage {
type: 'focus-editor';
id: string;
focusNext?: boolean;
}
export interface IClickedDataUrlMessage extends BaseToWebviewMessage {
type: 'clicked-data-url';
data: string | ArrayBuffer | null;
downloadName?: string;
}
export interface IClickMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'clickMarkdownPreview';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly metaKey: boolean;
readonly shiftKey: boolean;
}
export interface IMouseEnterMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseEnterMarkdownPreview';
cellId: string;
}
export interface IMouseLeaveMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'mouseLeaveMarkdownPreview';
cellId: string;
}
export interface IToggleMarkdownPreviewMessage extends BaseToWebviewMessage {
type: 'toggleMarkdownPreview';
cellId: string;
}
export interface ICellDragStartMessage extends BaseToWebviewMessage {
type: 'cell-drag-start';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragMessage extends BaseToWebviewMessage {
type: 'cell-drag';
readonly cellId: string;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDropMessage extends BaseToWebviewMessage {
readonly type: 'cell-drop';
readonly cellId: string;
readonly ctrlKey: boolean
readonly altKey: boolean;
readonly position: {
readonly clientY: number;
};
}
export interface ICellDragEndMessage extends BaseToWebviewMessage {
readonly type: 'cell-drag-end';
readonly cellId: string;
}
export interface IInitializedMarkdownPreviewMessage extends BaseToWebviewMessage {
readonly type: 'initializedMarkdownPreview';
}
export interface IClearMessage {
type: 'clear';
}
export interface IOutputRequestMetadata {
/**
* Additional attributes of a cell metadata.
*/
custom?: { [key: string]: unknown };
}
export interface IOutputRequestDto {
/**
* { mime_type: value }
*/
data: { [key: string]: unknown; }
metadata?: IOutputRequestMetadata;
outputId: string;
}
export interface ICreationRequestMessage {
type: 'html';
content:
| { type: RenderOutputType.Html; htmlContent: string }
| { type: RenderOutputType.Extension; outputId: string; value: unknown; metadata: unknown; mimeType: string };
cellId: string;
outputId: string;
top: number;
left: number;
requiredPreloads: ReadonlyArray<IPreloadResource>;
initiallyHidden?: boolean;
apiNamespace?: string | undefined;
}
export interface IContentWidgetTopRequest {
id: string;
top: number;
}
export interface IViewScrollTopRequestMessage {
type: 'view-scroll';
forceDisplay: boolean;
widgets: IContentWidgetTopRequest[];
}
export interface IViewScrollMarkdownRequestMessage {
type: 'view-scroll-markdown';
cells: { id: string; top: number }[];
}
export interface IScrollRequestMessage {
type: 'scroll';
id: string;
top: number;
widgetTop?: number;
version: number;
}
export interface IClearOutputRequestMessage {
type: 'clearOutput';
cellId: string;
outputId: string;
cellUri: string;
apiNamespace: string | undefined;
}
export interface IHideOutputMessage {
type: 'hideOutput';
outputId: string;
cellId: string;
}
export interface IShowOutputMessage {
type: 'showOutput';
cellId: string;
outputId: string;
top: number;
}
export interface IFocusOutputMessage {
type: 'focus-output';
cellId: string;
}
export interface IPreloadResource {
originalUri: string;
uri: string;
}
export interface IUpdatePreloadResourceMessage {
type: 'preload';
resources: IPreloadResource[];
source: 'renderer' | 'kernel';
}
export interface IUpdateDecorationsMessage {
type: 'decorations';
cellId: string;
addedClassNames: string[];
removedClassNames: string[];
}
export interface ICustomRendererMessage extends BaseToWebviewMessage {
type: 'customRendererMessage';
rendererId: string;
message: unknown;
}
export interface ICreateMarkdownMessage {
type: 'createMarkdownPreview',
id: string;
handle: number;
content: string;
top: number;
}
export interface IRemoveMarkdownMessage {
type: 'removeMarkdownPreview',
id: string;
}
export interface IHideMarkdownMessage {
type: 'hideMarkdownPreview';
id: string;
}
export interface IUnhideMarkdownMessage {
type: 'unhideMarkdownPreview';
id: string;
}
export interface IShowMarkdownMessage {
type: 'showMarkdownPreview',
id: string;
handle: number;
content: string | undefined;
top: number;
}
export interface IUpdateMarkdownPreviewSelectionState {
readonly type: 'updateMarkdownPreviewSelectionState',
readonly id: string;
readonly isSelected: boolean;
}
export interface IInitializeMarkdownMessage {
type: 'initializeMarkdownPreview';
cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>;
}
export type FromWebviewMessage =
| WebviewIntialized
| IDimensionMessage
| IMouseEnterMessage
| IMouseLeaveMessage
| IOutputFocusMessage
| IOutputBlurMessage
| IWheelMessage
| IScrollAckMessage
| IBlurOutputMessage
| ICustomRendererMessage
| IClickedDataUrlMessage
| IClickMarkdownPreviewMessage
| IMouseEnterMarkdownPreviewMessage
| IMouseLeaveMarkdownPreviewMessage
| IToggleMarkdownPreviewMessage
| ICellDragStartMessage
| ICellDragMessage
| ICellDropMessage
| ICellDragEndMessage
| IInitializedMarkdownPreviewMessage
;
export type ToWebviewMessage =
| IClearMessage
| IFocusOutputMessage
| ICreationRequestMessage
| IViewScrollTopRequestMessage
| IScrollRequestMessage
| IClearOutputRequestMessage
| IHideOutputMessage
| IShowOutputMessage
| IUpdatePreloadResourceMessage
| IUpdateDecorationsMessage
| ICustomRendererMessage
| ICreateMarkdownMessage
| IRemoveMarkdownMessage
| IShowMarkdownMessage
| IHideMarkdownMessage
| IUnhideMarkdownMessage
| IUpdateMarkdownPreviewSelectionState
| IInitializeMarkdownMessage
| IViewScrollMarkdownRequestMessage;
export type AnyMessage = FromWebviewMessage | ToWebviewMessage;
export interface ICachedInset<K extends ICommonCellInfo> {
outputId: string;
cellInfo: K;
renderer?: INotebookRendererInfo;
cachedCreation: ICreationRequestMessage;
}
function html(strings: TemplateStringsArray, ...values: any[]): string {
let str = '';
strings.forEach((string, i) => {
str += string + (values[i] || '');
});
return str;
}
export interface INotebookWebviewMessage {
message: unknown;
forRenderer?: string;
}
export interface IResolvedBackLayerWebview {
webview: WebviewElement;
}
export class BackLayerWebView<T extends ICommonCellInfo> extends Disposable {
element: HTMLElement;
webview: WebviewElement | undefined = undefined;
insetMapping: Map<IDisplayOutputViewModel, ICachedInset<T>> = new Map();
readonly markdownPreviewMapping = new Map<string, { version: number, visible: boolean }>();
hiddenInsetMapping: Set<IDisplayOutputViewModel> = new Set();
reversedInsetMapping: Map<string, IDisplayOutputViewModel> = new Map();
localResourceRootsCache: URI[] | undefined = undefined;
rendererRootsCache: URI[] = [];
kernelRootsCache: URI[] = [];
private readonly _onMessage = this._register(new Emitter<INotebookWebviewMessage>());
private readonly _preloadsCache = new Set<string>();
public readonly onMessage: Event<INotebookWebviewMessage> = this._onMessage.event;
private _loaded!: Promise<void>;
private _initalized?: Promise<void>;
private _disposed = false;
constructor(
public notebookEditor: ICommonNotebookEditor,
public id: string,
public documentUri: URI,
public options: {
outputNodePadding: number,
outputNodeLeftPadding: number,
previewNodePadding: number,
leftMargin: number,
cellMargin: number,
runGutter: number,
},
@IWebviewService readonly webviewService: IWebviewService,
@IOpenerService readonly openerService: IOpenerService,
@INotebookService private readonly notebookService: INotebookService,
@IWorkspaceContextService private readonly contextService: IWorkspaceContextService,
@IWorkbenchEnvironmentService private readonly environmentService: IWorkbenchEnvironmentService,
@IFileDialogService private readonly fileDialogService: IFileDialogService,
@IFileService private readonly fileService: IFileService,
) {
super();
this.element = document.createElement('div');
this.element.style.height = '1400px';
this.element.style.position = 'absolute';
}
private generateContent(coreDependencies: string, baseUrl: string) {
const markdownRenderersSrc = this.getMarkdownRendererScripts();
return html`
<html lang="en">
<head>
<meta charset="UTF-8">
<base href="${baseUrl}/"/>
<!--
Markdown previews are rendered using a shadow dom and are not effected by normal css.
Insert this style node into all preview shadow doms for styling.
-->
<template id="preview-styles">
<style>
img {
max-width: 100%;
max-height: 100%;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
a:focus,
input:focus,
select:focus,
textarea:focus {
outline: 1px solid -webkit-focus-ring-color;
outline-offset: -1px;
}
hr {
border: 0;
height: 2px;
border-bottom: 2px solid;
}
h1 {
font-size: 26px;
padding-bottom: 8px;
line-height: 31px;
border-bottom-width: 1px;
border-bottom-style: solid;
border-color: var(--vscode-foreground);
margin: 0;
margin-bottom: 13px;
}
h2 {
font-size: 19px;
margin: 0;
margin-bottom: 10px;
}
h1,
h2,
h3 {
font-weight: normal;
}
div {
width: 100%;
}
/* Adjust margin of first item in markdown cell */
*:first-child {
margin-top: 0px;
}
/* h1 tags don't need top margin */
h1:first-child {
margin-top: 0;
}
/* Removes bottom margin when only one item exists in markdown cell */
*:only-child,
*:last-child {
margin-bottom: 0;
padding-bottom: 0;
}
/* makes all markdown cells consistent */
div {
min-height: ${this.options.previewNodePadding * 2}px;
}
table {
border-collapse: collapse;
border-spacing: 0;
}
table th,
table td {
border: 1px solid;
}
table > thead > tr > th {
text-align: left;
border-bottom: 1px solid;
}
table > thead > tr > th,
table > thead > tr > td,
table > tbody > tr > th,
table > tbody > tr > td {
padding: 5px 10px;
}
table > tbody > tr + tr > td {
border-top: 1px solid;
}
blockquote {
margin: 0 7px 0 5px;
padding: 0 16px 0 10px;
border-left-width: 5px;
border-left-style: solid;
}
code,
.code {
font-family: var(--monaco-monospace-font);
font-size: 1em;
line-height: 1.357em;
}
.code {
white-space: pre-wrap;
}
.latex-block {
display: block;
}
.latex {
vertical-align: middle;
display: inline-block;
}
.latex img,
.latex-block img {
filter: brightness(0) invert(0)
}
dragging {
background-color: var(--vscode-editor-background);
}
</style>
</template>
<style>
#container > div > div.output {
width: calc(100% - ${this.options.leftMargin + (this.options.cellMargin * 2) + this.options.runGutter}px);
margin-left: ${this.options.leftMargin + this.options.runGutter}px;
padding: ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodePadding}px ${this.options.outputNodeLeftPadding}px;
box-sizing: border-box;
background-color: var(--vscode-notebook-outputContainerBackgroundColor);
}
#container > div > div.preview {
width: 100%;
box-sizing: border-box;
white-space: nowrap;
overflow: hidden;
user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
white-space: initial;
cursor: grab;
}
#container > div > div.preview.emptyMarkdownCell::before {
content: "${nls.localize('notebook.emptyMarkdownPlaceholder', "Empty markdown cell, double click or press enter to edit.")}";
font-style: italic;
opacity: 0.6;
}
/* markdown */
#container > div > div.preview {
color: var(--vscode-foreground);
width: 100%;
padding-left: ${this.options.leftMargin}px;
padding-top: ${this.options.previewNodePadding}px;
padding-bottom: ${this.options.previewNodePadding}px;
}
#container > div > div.preview.selected {
background: var(--vscode-notebook-selectedCellBackground);
}
#container > div > div.preview.dragging {
background-color: var(--vscode-editor-background);
}
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex img,
.monaco-workbench.vs-dark .notebookOverlay .cell.markdown .latex-block img {
filter: brightness(0) invert(1)
}
#container > div.nb-symbolHighlight > div {
background-color: var(--vscode-notebook-symbolHighlightBackground);
}
#container > div.nb-cellDeleted > div {
background-color: var(--vscode-diffEditor-removedTextBackground);
}
#container > div.nb-cellAdded > div {
background-color: var(--vscode-diffEditor-insertedTextBackground);
}
#container > div > div:not(.preview) > div {
overflow-x: scroll;
}
body {
padding: 0px;
height: 100%;
width: 100%;
}
table, thead, tr, th, td, tbody {
border: none !important;
border-color: transparent;
border-spacing: 0;
border-collapse: collapse;
}
table {
width: 100%;
}
table, th, tr {
text-align: left !important;
}
thead {
font-weight: bold;
background-color: rgba(130, 130, 130, 0.16);
}
th, td {
padding: 4px 8px;
}
tr:nth-child(even) {
background-color: rgba(130, 130, 130, 0.08);
}
tbody th {
font-weight: normal;
}
</style>
</head>
<body style="overflow: hidden;">
<script>
self.require = {};
</script>
${coreDependencies}
<div id='container' class="widgetarea" style="position: absolute;width:100%;top: 0px"></div>
<script>${preloadsScriptStr({
outputNodePadding: this.options.outputNodePadding,
outputNodeLeftPadding: this.options.outputNodeLeftPadding,
previewNodePadding: this.options.previewNodePadding,
leftMargin: this.options.leftMargin
})}</script>
${markdownRenderersSrc}
</body>
</html>`;
}
private getMarkdownRendererScripts() {
const markdownRenderers = this.notebookService.getMarkdownRendererInfo();
return markdownRenderers
.sort((a, b) => {
// prefer built-in extension
if (a.extensionIsBuiltin) {
return b.extensionIsBuiltin ? 0 : -1;
}
return b.extensionIsBuiltin ? 1 : -1;
})
.map(renderer => {
return asWebviewUri(this.environmentService, this.id, renderer.entrypoint);
})
.map(src => `<script src="${src}"></script>`)
.join('\n');
}
postRendererMessage(rendererId: string, message: any) {
this._sendMessageToWebview({
__vscode_notebook_message: true,
type: 'customRendererMessage',
message,
rendererId
});
}
private resolveOutputId(id: string): { cellInfo: T, output: ICellOutputViewModel } | undefined {
const output = this.reversedInsetMapping.get(id);
if (!output) {
return;
}
const cellInfo = this.insetMapping.get(output)!.cellInfo;
return { cellInfo, output };
}
isResolved(): this is IResolvedBackLayerWebview {
return !!this.webview;
}
async createWebview(): Promise<void> {
let coreDependencies = '';
let resolveFunc: () => void;
this._initalized = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const baseUrl = asWebviewUri(this.environmentService, this.id, dirname(this.documentUri));
if (!isWeb) {
const loaderUri = FileAccess.asFileUri('vs/loader.js', require);
const loader = asWebviewUri(this.environmentService, this.id, loaderUri);
coreDependencies = `<script src="${loader}"></script><script>
var requirejs = (function() {
return require;
}());
</script>`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
} else {
const loaderUri = FileAccess.asBrowserUri('vs/loader.js', require);
fetch(loaderUri.toString(true)).then(async response => {
if (response.status !== 200) {
throw new Error(response.statusText);
}
const loaderJs = await response.text();
coreDependencies = `
<script>
${loaderJs}
</script>
<script>
var requirejs = (function() {
return require;
}());
</script>
`;
const htmlContent = this.generateContent(coreDependencies, baseUrl.toString());
this._initialize(htmlContent);
resolveFunc!();
});
}
await this._initalized;
}
private async _initialize(content: string) {
if (!document.body.contains(this.element)) {
throw new Error('Element is already detached from the DOM tree');
}
this.webview = this._createInset(this.webviewService, content);
this.webview.mountTo(this.element);
this._register(this.webview);
this._register(this.webview.onDidClickLink(link => {
if (this._disposed) {
return;
}
if (!link) {
return;
}
if (matchesScheme(link, Schemas.http) || matchesScheme(link, Schemas.https) || matchesScheme(link, Schemas.mailto)
|| matchesScheme(link, Schemas.command)) {
this.openerService.open(link, { fromUserGesture: true, allowContributedOpeners: true });
}
}));
this._register(this.webview.onDidReload(() => {
if (this._disposed) {
return;
}
let renderers = new Set<INotebookRendererInfo>();
for (const inset of this.insetMapping.values()) {
if (inset.renderer) {
renderers.add(inset.renderer);
}
}
this._preloadsCache.clear();
this.updateRendererPreloads(renderers);
for (const [output, inset] of this.insetMapping.entries()) {
this._sendMessageToWebview({ ...inset.cachedCreation, initiallyHidden: this.hiddenInsetMapping.has(output) });
}
}));
this._register(this.webview.onMessage((message) => {
const data: FromWebviewMessage | { readonly __vscode_notebook_message: undefined } = message.message;
if (this._disposed) {
return;
}
if (!data.__vscode_notebook_message) {
this._onMessage.fire({ message: data });
return;
}
switch (data.type) {
case 'dimension':
{
for (const update of data.updates) {
if (update.isOutput) {
const height = update.data.height;
const outputHeight = height;
const resolvedResult = this.resolveOutputId(update.id);
if (resolvedResult) {
const { cellInfo, output } = resolvedResult;
this.notebookEditor.updateOutputHeight(cellInfo, output, outputHeight, !!update.init, 'webview#dimension');
}
} else {
const cellId = update.id.substr(0, update.id.length - '_preview'.length);
this.notebookEditor.updateMarkdownCellHeight(cellId, update.data.height, !!update.init);
}
}
break;
}
case 'mouseenter':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = true;
}
}
break;
}
case 'mouseleave':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsHovered = false;
}
}
break;
}
case 'outputFocus':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = true;
}
}
break;
}
case 'outputBlur':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (latestCell) {
latestCell.outputIsFocused = false;
}
}
break;
}
case 'scroll-ack':
{
// const date = new Date();
// const top = data.data.top;
// console.log('ack top ', top, ' version: ', data.version, ' - ', date.getMinutes() + ':' + date.getSeconds() + ':' + date.getMilliseconds());
break;
}
case 'did-scroll-wheel':
{
this.notebookEditor.triggerScroll({
...data.payload,
preventDefault: () => { },
stopPropagation: () => { }
});
break;
}
case 'focus-editor':
{
const resolvedResult = this.resolveOutputId(data.id);
if (resolvedResult) {
const latestCell = this.notebookEditor.getCellByInfo(resolvedResult.cellInfo);
if (!latestCell) {
return;
}
if (data.focusNext) {
this.notebookEditor.focusNextNotebookCell(latestCell, 'editor');
} else {
this.notebookEditor.focusNotebookCell(latestCell, 'editor');
}
}
break;
}
case 'clicked-data-url':
{
this._onDidClickDataLink(data);
break;
}
case 'customRendererMessage':
{
this._onMessage.fire({ message: data.message, forRenderer: data.rendererId });
break;
}
case 'clickMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
if (data.shiftKey || data.metaKey) {
// Add to selection
this.notebookEditor.toggleNotebookCellSelection(cell);
} else {
// Normal click
this.notebookEditor.focusNotebookCell(cell, 'container', { skipReveal: true });
}
}
break;
}
case 'toggleMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell) {
this.notebookEditor.setMarkdownCellEditState(data.cellId, CellEditState.Editing);
this.notebookEditor.focusNotebookCell(cell, 'editor', { skipReveal: true });
}
break;
}
case 'mouseEnterMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = true;
}
break;
}
case 'mouseLeaveMarkdownPreview':
{
const cell = this.notebookEditor.getCellById(data.cellId);
if (cell instanceof MarkdownCellViewModel) {
cell.cellIsHovered = false;
}
break;
}
case 'cell-drag-start':
{
this.notebookEditor.markdownCellDragStart(data.cellId, data.position);
break;
}
case 'cell-drag':
{
this.notebookEditor.markdownCellDrag(data.cellId, data.position);
break;
}
case 'cell-drop':
{
this.notebookEditor.markdownCellDrop(data.cellId, {
clientY: data.position.clientY,
ctrlKey: data.ctrlKey,
altKey: data.altKey,
});
break;
}
case 'cell-drag-end':
{
this.notebookEditor.markdownCellDragEnd(data.cellId);
break;
}
}
}));
}
private async _onDidClickDataLink(event: IClickedDataUrlMessage): Promise<void> {
if (typeof event.data !== 'string') {
return;
}
const [splitStart, splitData] = event.data.split(';base64,');
if (!splitData || !splitStart) {
return;
}
const defaultDir = dirname(this.documentUri);
let defaultName: string;
if (event.downloadName) {
defaultName = event.downloadName;
} else {
const mimeType = splitStart.replace(/^data:/, '');
const candidateExtension = mimeType && getExtensionForMimeType(mimeType);
defaultName = candidateExtension ? `download${candidateExtension}` : 'download';
}
const defaultUri = joinPath(defaultDir, defaultName);
const newFileUri = await this.fileDialogService.showSaveDialog({
defaultUri
});
if (!newFileUri) {
return;
}
const decoded = atob(splitData);
const typedArray = new Uint8Array(decoded.length);
for (let i = 0; i < decoded.length; i++) {
typedArray[i] = decoded.charCodeAt(i);
}
const buff = VSBuffer.wrap(typedArray);
await this.fileService.writeFile(newFileUri, buff);
await this.openerService.open(newFileUri);
}
private _createInset(webviewService: IWebviewService, content: string) {
const rootPath = isWeb ? FileAccess.asBrowserUri('', require) : FileAccess.asFileUri('', require);
const workspaceFolders = this.contextService.getWorkspace().folders.map(x => x.uri);
this.localResourceRootsCache = [
...this.notebookService.getNotebookProviderResourceRoots(),
...this.notebookService.getMarkdownRendererInfo().map(x => dirname(x.entrypoint)),
...workspaceFolders,
rootPath,
];
const webview = webviewService.createWebviewElement(this.id, {
purpose: WebviewContentPurpose.NotebookRenderer,
enableFindWidget: false,
transformCssVariables: transformWebviewThemeVars,
}, {
allowMultipleAPIAcquire: true,
allowScripts: true,
localResourceRoots: this.localResourceRootsCache
}, undefined);
let resolveFunc: () => void;
this._loaded = new Promise<void>((resolve, reject) => {
resolveFunc = resolve;
});
const dispose = webview.onMessage((message) => {
const data: FromWebviewMessage = message.message;
if (data.__vscode_notebook_message && data.type === 'initialized') {
resolveFunc();
dispose.dispose();
}
});
webview.html = content;
return webview;
}
shouldUpdateInset(cell: IGenericCellViewModel, output: ICellOutputViewModel, cellTop: number) {
if (this._disposed) {
return;
}
if (cell.metadata?.outputCollapsed) {
return false;
}
const outputCache = this.insetMapping.get(output)!;
const outputIndex = cell.outputsViewModels.indexOf(output);
const outputOffset = cellTop + cell.getOutputOffset(outputIndex);
if (this.hiddenInsetMapping.has(output)) {
return true;
}
if (outputOffset === outputCache.cachedCreation.top) {
return false;
}
return true;
}
updateMarkdownScrollTop(items: { id: string, top: number }[]) {
if (this._disposed || !items.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll-markdown',
cells: items
});
}
updateViewScrollTop(forceDisplay: boolean, items: IDisplayOutputLayoutUpdateRequest[]) {
if (!items.length) {
return;
}
const widgets = coalesce(items.map((item): IContentWidgetTopRequest | undefined => {
const outputCache = this.insetMapping.get(item.output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
const outputOffset = item.outputOffset;
outputCache.cachedCreation.top = outputOffset;
this.hiddenInsetMapping.delete(item.output);
return {
id: id,
top: outputOffset,
};
}));
if (!widgets.length) {
return;
}
this._sendMessageToWebview({
type: 'view-scroll',
forceDisplay,
widgets: widgets
});
}
private async createMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (this.markdownPreviewMapping.has(cellId)) {
console.error('Trying to create markdown preview that already exists');
return;
}
const initialTop = cellTop;
this.markdownPreviewMapping.set(cellId, { version: contentVersion, visible: true });
this._sendMessageToWebview({
type: 'createMarkdownPreview',
id: cellId,
handle: cellHandle,
content: content,
top: initialTop,
});
}
async showMarkdownPreview(cellId: string, cellHandle: number, content: string, cellTop: number, contentVersion: number) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
return this.createMarkdownPreview(cellId, cellHandle, content, cellTop, contentVersion);
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error('Try to show a preview that does not exist');
return;
}
if (entry.version !== contentVersion || !entry.visible) {
this._sendMessageToWebview({
type: 'showMarkdownPreview',
id: cellId,
handle: cellHandle,
// If the content has not changed, we still want to make sure the
// preview is visible but don't need to send anything over
content: entry.version === contentVersion ? undefined : content,
top: cellTop
});
}
entry.version = contentVersion;
entry.visible = true;
}
async hideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
// TODO: this currently seems expected on first load
// console.error(`Try to hide a preview that does not exist: ${cellId}`);
return;
}
if (entry.visible) {
this._sendMessageToWebview({
type: 'hideMarkdownPreview',
id: cellId
});
entry.visible = false;
}
}
async unhideMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
const entry = this.markdownPreviewMapping.get(cellId);
if (!entry) {
console.error(`Try to unhide a preview that does not exist: ${cellId}`);
return;
}
if (!entry.visible) {
this._sendMessageToWebview({
type: 'unhideMarkdownPreview',
id: cellId
});
entry.visible = true;
}
}
async removeMarkdownPreview(cellId: string,) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
console.error(`Try to delete a preview that does not exist: ${cellId}`);
return;
}
this.markdownPreviewMapping.delete(cellId);
this._sendMessageToWebview({
type: 'removeMarkdownPreview',
id: cellId
});
}
async updateMarkdownPreviewSelectionState(cellId: any, isSelected: boolean) {
if (this._disposed) {
return;
}
if (!this.markdownPreviewMapping.has(cellId)) {
// TODO: this currently seems expected on first load
// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);
return;
}
this._sendMessageToWebview({
type: 'updateMarkdownPreviewSelectionState',
id: cellId,
isSelected
});
}
async initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {
await this._loaded;
if (this._disposed) {
return;
}
// TODO: use proper handler
const p = new Promise<void>(resolve => {
this.webview?.onMessage(e => {
if (e.message.type === 'initializedMarkdownPreview') {
resolve();
}
});
});
for (const cell of cells) {
this.markdownPreviewMapping.set(cell.cellId, { version: 0, visible: false });
}
this._sendMessageToWebview({
type: 'initializeMarkdownPreview',
cells: cells,
});
await p;
}
async createOutput(cellInfo: T, content: IInsetRenderOutput, cellTop: number, offset: number) {
if (this._disposed) {
return;
}
const initialTop = cellTop + offset;
if (this.insetMapping.has(content.source)) {
const outputCache = this.insetMapping.get(content.source);
if (outputCache) {
this.hiddenInsetMapping.delete(content.source);
this._sendMessageToWebview({
type: 'showOutput',
cellId: outputCache.cellInfo.cellId,
outputId: outputCache.outputId,
top: initialTop
});
return;
}
}
const messageBase = {
type: 'html',
cellId: cellInfo.cellId,
top: initialTop,
left: 0,
requiredPreloads: [],
} as const;
let message: ICreationRequestMessage;
let renderer: INotebookRendererInfo | undefined;
if (content.type === RenderOutputType.Extension) {
const output = content.source.model;
renderer = content.renderer;
const outputDto = output.outputs.find(op => op.mime === content.mimeType);
message = {
...messageBase,
outputId: output.outputId,
apiNamespace: content.renderer.id,
requiredPreloads: await this.updateRendererPreloads([content.renderer]),
content: {
type: RenderOutputType.Extension,
outputId: output.outputId,
mimeType: content.mimeType,
value: outputDto?.value,
metadata: outputDto?.metadata,
},
};
} else {
message = {
...messageBase,
outputId: UUID.generateUuid(),
content: {
type: content.type,
htmlContent: content.htmlContent,
}
};
}
this._sendMessageToWebview(message);
this.insetMapping.set(content.source, { outputId: message.outputId, cellInfo: cellInfo, renderer, cachedCreation: message });
this.hiddenInsetMapping.delete(content.source);
this.reversedInsetMapping.set(message.outputId, content.source);
}
removeInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
const id = outputCache.outputId;
this._sendMessageToWebview({
type: 'clearOutput',
apiNamespace: outputCache.cachedCreation.apiNamespace,
cellUri: outputCache.cellInfo.cellUri.toString(),
outputId: id,
cellId: outputCache.cellInfo.cellId
});
this.insetMapping.delete(output);
this.reversedInsetMapping.delete(id);
}
hideInset(output: ICellOutputViewModel) {
if (this._disposed) {
return;
}
const outputCache = this.insetMapping.get(output);
if (!outputCache) {
return;
}
this.hiddenInsetMapping.add(output);
this._sendMessageToWebview({
type: 'hideOutput',
outputId: outputCache.outputId,
cellId: outputCache.cellInfo.cellId,
});
}
clearInsets() {
if (this._disposed) {
return;
}
this._sendMessageToWebview({
type: 'clear'
});
this.insetMapping = new Map();
this.reversedInsetMapping = new Map();
}
focusWebview() {
if (this._disposed) {
return;
}
this.webview?.focus();
}
focusOutput(cellId: string) {
if (this._disposed) {
return;
}
this.webview?.focus();
setTimeout(() => { // Need this, or focus decoration is not shown. No clue.
this._sendMessageToWebview({
type: 'focus-output',
cellId,
});
}, 50);
}
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]) {
this._sendMessageToWebview({
type: 'decorations',
cellId,
addedClassNames: added,
removedClassNames: removed
});
}
async updateKernelPreloads(extensionLocations: URI[], preloads: URI[]) {
if (this._disposed) {
return;
}
await this._loaded;
const resources: IPreloadResource[] = [];
for (const preload of preloads) {
const uri = this.environmentService.isExtensionDevelopment && (preload.scheme === 'http' || preload.scheme === 'https')
? preload : asWebviewUri(this.environmentService, this.id, preload);
if (!this._preloadsCache.has(uri.toString())) {
resources.push({ uri: uri.toString(), originalUri: preload.toString() });
this._preloadsCache.add(uri.toString());
}
}
if (!resources.length) {
return;
}
this.kernelRootsCache = [...extensionLocations, ...this.kernelRootsCache];
this._updatePreloads(resources, 'kernel');
}
async updateRendererPreloads(renderers: Iterable<INotebookRendererInfo>) {
if (this._disposed) {
return [];
}
await this._loaded;
const requiredPreloads: IPreloadResource[] = [];
const resources: IPreloadResource[] = [];
const extensionLocations: URI[] = [];
for (const rendererInfo of renderers) {
extensionLocations.push(rendererInfo.extensionLocation);
for (const preload of [rendererInfo.entrypoint, ...rendererInfo.preloads]) {
const uri = asWebviewUri(this.environmentService, this.id, preload);
const resource: IPreloadResource = { uri: uri.toString(), originalUri: preload.toString() };
requiredPreloads.push(resource);
if (!this._preloadsCache.has(uri.toString())) {
resources.push(resource);
this._preloadsCache.add(uri.toString());
}
}
}
if (!resources.length) {
return requiredPreloads;
}
this.rendererRootsCache = extensionLocations;
this._updatePreloads(resources, 'renderer');
return requiredPreloads;
}
private _updatePreloads(resources: IPreloadResource[], source: 'renderer' | 'kernel') {
if (!this.webview) {
return;
}
const mixedResourceRoots = [...(this.localResourceRootsCache || []), ...this.rendererRootsCache, ...this.kernelRootsCache];
this.webview.localResourcesRoot = mixedResourceRoots;
this._sendMessageToWebview({
type: 'preload',
resources: resources,
source: source
});
}
private _sendMessageToWebview(message: ToWebviewMessage) {
if (this._disposed) {
return;
}
this.webview?.postMessage(message);
}
clearPreloadsCache() {
this._preloadsCache.clear();
}
dispose() {
this._disposed = true;
this.webview?.dispose();
super.dispose();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.9963526725769043,
0.011325844563543797,
0.000163417324074544,
0.00021029653726145625,
0.08429892361164093
] |
{
"id": 8,
"code_window": [
"\t\tif (this._disposed) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this.markdownPreviewMapping.has(cellId)) {\n",
"\t\t\t// TODO: this currently seems expected on first load\n",
"\t\t\t// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tthis._sendMessageToWebview({\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1273
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
'use strict';
//@ts-check
(function () {
/**
* @returns {{mark(name:string):void, getMarks():{name:string, startTime:number}[]}}
*/
function _definePolyfillMarks(timeOrigin) {
const _data = [];
if (typeof timeOrigin === 'number') {
_data.push('code/timeOrigin', timeOrigin);
}
function mark(name) {
_data.push(name, Date.now());
}
function getMarks() {
const result = [];
for (let i = 0; i < _data.length; i += 2) {
result.push({
name: _data[i],
startTime: _data[i + 1],
});
}
return result;
}
return { mark, getMarks };
}
/**
* @returns {{mark(name:string):void, getMarks():{name:string, startTime:number}[]}}
*/
function _define() {
if (typeof performance === 'object' && typeof performance.mark === 'function') {
// in a browser context, reuse performance-util
if (typeof performance.timeOrigin !== 'number' && !performance.timing) {
// safari & webworker: because there is no timeOrigin and no workaround
// we use the `Date.now`-based polyfill.
return _definePolyfillMarks();
} else {
// use "native" performance for mark and getMarks
return {
mark(name) {
performance.mark(name);
},
getMarks() {
let timeOrigin = performance.timeOrigin;
if (typeof timeOrigin !== 'number') {
// safari: there is no timerOrigin but in renderers there is the timing-property
// see https://bugs.webkit.org/show_bug.cgi?id=174862
timeOrigin = performance.timing.navigationStart || performance.timing.redirectStart || performance.timing.fetchStart;
}
const result = [{ name: 'code/timeOrigin', startTime: Math.round(timeOrigin) }];
for (const entry of performance.getEntriesByType('mark')) {
result.push({
name: entry.name,
startTime: Math.round(timeOrigin + entry.startTime)
});
}
return result;
}
};
}
} else if (typeof process === 'object') {
// node.js: use the normal polyfill but add the timeOrigin
// from the node perf_hooks API as very first mark
const timeOrigin = Math.round((require.nodeRequire || require)('perf_hooks').performance.timeOrigin);
return _definePolyfillMarks(timeOrigin);
} else {
// unknown environment
console.trace('perf-util loaded in UNKNOWN environment');
return _definePolyfillMarks();
}
}
function _factory(sharedObj) {
if (!sharedObj.MonacoPerformanceMarks) {
sharedObj.MonacoPerformanceMarks = _define();
}
return sharedObj.MonacoPerformanceMarks;
}
// This module can be loaded in an amd and commonjs-context.
// Because we want both instances to use the same perf-data
// we store them globally
// eslint-disable-next-line no-var
var sharedObj;
if (typeof global === 'object') {
// nodejs
sharedObj = global;
} else if (typeof self === 'object') {
// browser
sharedObj = self;
} else {
sharedObj = {};
}
if (typeof define === 'function') {
// amd
define([], function () { return _factory(sharedObj); });
} else if (typeof module === 'object' && typeof module.exports === 'object') {
// commonjs
module.exports = _factory(sharedObj);
} else {
console.trace('perf-util defined in UNKNOWN context (neither requirejs or commonjs)');
sharedObj.perf = _factory(sharedObj);
}
})();
| src/vs/base/common/performance.js | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017296758596785367,
0.00016868238162714988,
0.00016158986545633525,
0.00016836609574966133,
0.0000032060065677796956
] |
{
"id": 8,
"code_window": [
"\t\tif (this._disposed) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this.markdownPreviewMapping.has(cellId)) {\n",
"\t\t\t// TODO: this currently seems expected on first load\n",
"\t\t\t// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tthis._sendMessageToWebview({\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1273
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as nls from 'vs/nls';
import { Codicon } from 'vs/base/common/codicons';
import { registerIcon } from 'vs/platform/theme/common/iconRegistry';
export const getStartedIcon = registerIcon('remote-explorer-get-started', Codicon.star, nls.localize('getStartedIcon', 'Getting started icon in the remote explorer view.'));
export const documentationIcon = registerIcon('remote-explorer-documentation', Codicon.book, nls.localize('documentationIcon', 'Documentation icon in the remote explorer view.'));
export const feedbackIcon = registerIcon('remote-explorer-feedback', Codicon.twitter, nls.localize('feedbackIcon', 'Feedback icon in the remote explorer view.'));
export const reviewIssuesIcon = registerIcon('remote-explorer-review-issues', Codicon.issues, nls.localize('reviewIssuesIcon', 'Review issue icon in the remote explorer view.'));
export const reportIssuesIcon = registerIcon('remote-explorer-report-issues', Codicon.comment, nls.localize('reportIssuesIcon', 'Report issue icon in the remote explorer view.'));
export const remoteExplorerViewIcon = registerIcon('remote-explorer-view-icon', Codicon.remoteExplorer, nls.localize('remoteExplorerViewIcon', 'View icon of the remote explorer view.'));
export const portsViewIcon = registerIcon('ports-view-icon', Codicon.plug, nls.localize('portsViewIcon', 'View icon of the remote ports view.'));
export const portIcon = registerIcon('ports-view-icon', Codicon.plug, nls.localize('portIcon', 'Icon representing a remote port.'));
export const privatePortIcon = registerIcon('private-ports-view-icon', Codicon.lock, nls.localize('privatePortIcon', 'Icon representing a private remote port.'));
export const publicPortIcon = registerIcon('public-ports-view-icon', Codicon.eye, nls.localize('publicPortIcon', 'Icon representing a public remote port.'));
export const forwardPortIcon = registerIcon('ports-forward-icon', Codicon.plus, nls.localize('forwardPortIcon', 'Icon for the forward action.'));
export const stopForwardIcon = registerIcon('ports-stop-forward-icon', Codicon.x, nls.localize('stopForwardIcon', 'Icon for the stop forwarding action.'));
export const openBrowserIcon = registerIcon('ports-open-browser-icon', Codicon.globe, nls.localize('openBrowserIcon', 'Icon for the open browser action.'));
export const openPreviewIcon = registerIcon('ports-open-preview-icon', Codicon.openPreview, nls.localize('openPreviewIcon', 'Icon for the open preview action.'));
export const copyAddressIcon = registerIcon('ports-copy-address-icon', Codicon.clippy, nls.localize('copyAddressIcon', 'Icon for the copy local address action.'));
export const labelPortIcon = registerIcon('ports-label-icon', Codicon.tag, nls.localize('labelPortIcon', 'Icon for the label port action.'));
export const forwardedPortWithoutProcessIcon = registerIcon('ports-forwarded-without-process-icon', Codicon.circleOutline, nls.localize('forwardedPortWithoutProcessIcon', 'Icon for forwarded ports that don\'t have a running process.'));
export const forwardedPortWithProcessIcon = registerIcon('ports-forwarded-with-process-icon', Codicon.circleFilled, nls.localize('forwardedPortWithProcessIcon', 'Icon for forwarded ports that do have a running process.'));
| src/vs/workbench/contrib/remote/browser/remoteIcons.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017593636584933847,
0.00017049568123184144,
0.0001644881849642843,
0.0001707790797809139,
0.0000040925583562057
] |
{
"id": 8,
"code_window": [
"\t\tif (this._disposed) {\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tif (!this.markdownPreviewMapping.has(cellId)) {\n",
"\t\t\t// TODO: this currently seems expected on first load\n",
"\t\t\t// console.error(`Try to update selection state for preview that does not exist: ${cellId}`);\n",
"\t\t\treturn;\n",
"\t\t}\n",
"\n",
"\t\tthis._sendMessageToWebview({\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1273
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { isWindows } from 'vs/base/common/platform';
import { startsWithIgnoreCase, equalsIgnoreCase, rtrim } from 'vs/base/common/strings';
import { CharCode } from 'vs/base/common/charCode';
import { sep, posix, isAbsolute, join, normalize } from 'vs/base/common/path';
import { isNumber } from 'vs/base/common/types';
export function isPathSeparator(code: number) {
return code === CharCode.Slash || code === CharCode.Backslash;
}
/**
* Takes a Windows OS path and changes backward slashes to forward slashes.
* This should only be done for OS paths from Windows (or user provided paths potentially from Windows).
* Using it on a Linux or MaxOS path might change it.
*/
export function toSlashes(osPath: string) {
return osPath.replace(/[\\/]/g, posix.sep);
}
/**
* Computes the _root_ this path, like `getRoot('c:\files') === c:\`,
* `getRoot('files:///files/path') === files:///`,
* or `getRoot('\\server\shares\path') === \\server\shares\`
*/
export function getRoot(path: string, sep: string = posix.sep): string {
if (!path) {
return '';
}
const len = path.length;
const firstLetter = path.charCodeAt(0);
if (isPathSeparator(firstLetter)) {
if (isPathSeparator(path.charCodeAt(1))) {
// UNC candidate \\localhost\shares\ddd
// ^^^^^^^^^^^^^^^^^^^
if (!isPathSeparator(path.charCodeAt(2))) {
let pos = 3;
const start = pos;
for (; pos < len; pos++) {
if (isPathSeparator(path.charCodeAt(pos))) {
break;
}
}
if (start !== pos && !isPathSeparator(path.charCodeAt(pos + 1))) {
pos += 1;
for (; pos < len; pos++) {
if (isPathSeparator(path.charCodeAt(pos))) {
return path.slice(0, pos + 1) // consume this separator
.replace(/[\\/]/g, sep);
}
}
}
}
}
// /user/far
// ^
return sep;
} else if (isWindowsDriveLetter(firstLetter)) {
// check for windows drive letter c:\ or c:
if (path.charCodeAt(1) === CharCode.Colon) {
if (isPathSeparator(path.charCodeAt(2))) {
// C:\fff
// ^^^
return path.slice(0, 2) + sep;
} else {
// C:
// ^^
return path.slice(0, 2);
}
}
}
// check for URI
// scheme://authority/path
// ^^^^^^^^^^^^^^^^^^^
let pos = path.indexOf('://');
if (pos !== -1) {
pos += 3; // 3 -> "://".length
for (; pos < len; pos++) {
if (isPathSeparator(path.charCodeAt(pos))) {
return path.slice(0, pos + 1); // consume this separator
}
}
}
return '';
}
/**
* Check if the path follows this pattern: `\\hostname\sharename`.
*
* @see https://msdn.microsoft.com/en-us/library/gg465305.aspx
* @return A boolean indication if the path is a UNC path, on none-windows
* always false.
*/
export function isUNC(path: string): boolean {
if (!isWindows) {
// UNC is a windows concept
return false;
}
if (!path || path.length < 5) {
// at least \\a\b
return false;
}
let code = path.charCodeAt(0);
if (code !== CharCode.Backslash) {
return false;
}
code = path.charCodeAt(1);
if (code !== CharCode.Backslash) {
return false;
}
let pos = 2;
const start = pos;
for (; pos < path.length; pos++) {
code = path.charCodeAt(pos);
if (code === CharCode.Backslash) {
break;
}
}
if (start === pos) {
return false;
}
code = path.charCodeAt(pos + 1);
if (isNaN(code) || code === CharCode.Backslash) {
return false;
}
return true;
}
// Reference: https://en.wikipedia.org/wiki/Filename
const WINDOWS_INVALID_FILE_CHARS = /[\\/:\*\?"<>\|]/g;
const UNIX_INVALID_FILE_CHARS = /[\\/]/g;
const WINDOWS_FORBIDDEN_NAMES = /^(con|prn|aux|clock\$|nul|lpt[0-9]|com[0-9])(\.(.*?))?$/i;
export function isValidBasename(name: string | null | undefined, isWindowsOS: boolean = isWindows): boolean {
const invalidFileChars = isWindowsOS ? WINDOWS_INVALID_FILE_CHARS : UNIX_INVALID_FILE_CHARS;
if (!name || name.length === 0 || /^\s+$/.test(name)) {
return false; // require a name that is not just whitespace
}
invalidFileChars.lastIndex = 0; // the holy grail of software development
if (invalidFileChars.test(name)) {
return false; // check for certain invalid file characters
}
if (isWindowsOS && WINDOWS_FORBIDDEN_NAMES.test(name)) {
return false; // check for certain invalid file names
}
if (name === '.' || name === '..') {
return false; // check for reserved values
}
if (isWindowsOS && name[name.length - 1] === '.') {
return false; // Windows: file cannot end with a "."
}
if (isWindowsOS && name.length !== name.trim().length) {
return false; // Windows: file cannot end with a whitespace
}
if (name.length > 255) {
return false; // most file systems do not allow files > 255 length
}
return true;
}
export function isEqual(pathA: string, pathB: string, ignoreCase?: boolean): boolean {
const identityEquals = (pathA === pathB);
if (!ignoreCase || identityEquals) {
return identityEquals;
}
if (!pathA || !pathB) {
return false;
}
return equalsIgnoreCase(pathA, pathB);
}
export function isEqualOrParent(base: string, parentCandidate: string, ignoreCase?: boolean, separator = sep): boolean {
if (base === parentCandidate) {
return true;
}
if (!base || !parentCandidate) {
return false;
}
if (parentCandidate.length > base.length) {
return false;
}
if (ignoreCase) {
const beginsWith = startsWithIgnoreCase(base, parentCandidate);
if (!beginsWith) {
return false;
}
if (parentCandidate.length === base.length) {
return true; // same path, different casing
}
let sepOffset = parentCandidate.length;
if (parentCandidate.charAt(parentCandidate.length - 1) === separator) {
sepOffset--; // adjust the expected sep offset in case our candidate already ends in separator character
}
return base.charAt(sepOffset) === separator;
}
if (parentCandidate.charAt(parentCandidate.length - 1) !== separator) {
parentCandidate += separator;
}
return base.indexOf(parentCandidate) === 0;
}
export function isWindowsDriveLetter(char0: number): boolean {
return char0 >= CharCode.A && char0 <= CharCode.Z || char0 >= CharCode.a && char0 <= CharCode.z;
}
export function sanitizeFilePath(candidate: string, cwd: string): string {
// Special case: allow to open a drive letter without trailing backslash
if (isWindows && candidate.endsWith(':')) {
candidate += sep;
}
// Ensure absolute
if (!isAbsolute(candidate)) {
candidate = join(cwd, candidate);
}
// Ensure normalized
candidate = normalize(candidate);
// Ensure no trailing slash/backslash
if (isWindows) {
candidate = rtrim(candidate, sep);
// Special case: allow to open drive root ('C:\')
if (candidate.endsWith(':')) {
candidate += sep;
}
} else {
candidate = rtrim(candidate, sep);
// Special case: allow to open root ('/')
if (!candidate) {
candidate = sep;
}
}
return candidate;
}
export function isRootOrDriveLetter(path: string): boolean {
const pathNormalized = normalize(path);
if (isWindows) {
if (path.length > 3) {
return false;
}
return hasDriveLetter(pathNormalized) &&
(path.length === 2 || pathNormalized.charCodeAt(2) === CharCode.Backslash);
}
return pathNormalized === posix.sep;
}
export function hasDriveLetter(path: string): boolean {
if (isWindows) {
return isWindowsDriveLetter(path.charCodeAt(0)) && path.charCodeAt(1) === CharCode.Colon;
}
return false;
}
export function getDriveLetter(path: string): string | undefined {
return hasDriveLetter(path) ? path[0] : undefined;
}
export function indexOfPath(path: string, candidate: string, ignoreCase?: boolean): number {
if (candidate.length > path.length) {
return -1;
}
if (path === candidate) {
return 0;
}
if (ignoreCase) {
path = path.toLowerCase();
candidate = candidate.toLowerCase();
}
return path.indexOf(candidate);
}
export interface IPathWithLineAndColumn {
path: string;
line?: number;
column?: number;
}
export function parseLineAndColumnAware(rawPath: string): IPathWithLineAndColumn {
const segments = rawPath.split(':'); // C:\file.txt:<line>:<column>
let path: string | undefined = undefined;
let line: number | undefined = undefined;
let column: number | undefined = undefined;
segments.forEach(segment => {
const segmentAsNumber = Number(segment);
if (!isNumber(segmentAsNumber)) {
path = !!path ? [path, segment].join(':') : segment; // a colon can well be part of a path (e.g. C:\...)
} else if (line === undefined) {
line = segmentAsNumber;
} else if (column === undefined) {
column = segmentAsNumber;
}
});
if (!path) {
throw new Error('Format for `--goto` should be: `FILE:LINE(:COLUMN)`');
}
return {
path,
line: line !== undefined ? line : undefined,
column: column !== undefined ? column : line !== undefined ? 1 : undefined // if we have a line, make sure column is also set
};
}
| src/vs/base/common/extpath.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00020091449550818652,
0.00017344136722385883,
0.00016526514082215726,
0.00017377892800141126,
0.000005389021225710167
] |
{
"id": 9,
"code_window": [
"\t\tthis._sendMessageToWebview({\n",
"\t\t\ttype: 'updateMarkdownPreviewSelectionState',\n",
"\t\t\tid: cellId,\n",
"\t\t\tisSelected\n",
"\t\t});\n",
"\t}\n",
"\n",
"\tasync initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {\n",
"\t\tawait this._loaded;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\ttype: 'updateSelectedMarkdownPreviews',\n",
"\t\t\tselectedCellIds: selectedCellsIds.filter(id => this.markdownPreviewMapping.has(id)),\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1280
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IMouseWheelEvent } from 'vs/base/browser/mouseEvent';
import { IListContextMenuEvent, IListEvent, IListMouseEvent } from 'vs/base/browser/ui/list/list';
import { IListOptions, IListStyles } from 'vs/base/browser/ui/list/listWidget';
import { ProgressBar } from 'vs/base/browser/ui/progressbar/progressbar';
import { ToolBar } from 'vs/base/browser/ui/toolbar/toolbar';
import { Emitter, Event } from 'vs/base/common/event';
import { DisposableStore, IDisposable } from 'vs/base/common/lifecycle';
import { ScrollEvent } from 'vs/base/common/scrollable';
import { URI } from 'vs/base/common/uri';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { BareFontInfo } from 'vs/editor/common/config/fontInfo';
import { IPosition } from 'vs/editor/common/core/position';
import { Range } from 'vs/editor/common/core/range';
import { FindMatch, IReadonlyTextBuffer, ITextModel } from 'vs/editor/common/model';
import { ContextKeyExpr, RawContextKey, IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { OutputRenderer } from 'vs/workbench/contrib/notebook/browser/view/output/outputRenderer';
import { RunStateRenderer, TimerRenderer } from 'vs/workbench/contrib/notebook/browser/view/renderers/cellRenderer';
import { CellViewModel, IModelDecorationsChangeAccessor, NotebookViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/notebookViewModel';
import { NotebookCellTextModel } from 'vs/workbench/contrib/notebook/common/model/notebookCellTextModel';
import { CellKind, NotebookCellMetadata, NotebookDocumentMetadata, INotebookKernel, ICellRange, IOrderedMimeType, INotebookRendererInfo, ICellOutput, IOutputItemDto, cellRangesToIndexes, reduceRanges } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { Webview } from 'vs/workbench/contrib/webview/browser/webview';
import { NotebookTextModel } from 'vs/workbench/contrib/notebook/common/model/notebookTextModel';
import { IMenu } from 'vs/platform/actions/common/actions';
import { EditorOptions, IEditorPane } from 'vs/workbench/common/editor';
import { IResourceEditorInput } from 'vs/platform/editor/common/editor';
import { IConstructorSignature1 } from 'vs/platform/instantiation/common/instantiation';
import { CellEditorStatusBar } from 'vs/workbench/contrib/notebook/browser/view/renderers/cellWidgets';
//#region Context Keys
export const KEYBINDING_CONTEXT_NOTEBOOK_FIND_WIDGET_FOCUSED = new RawContextKey<boolean>('notebookFindWidgetFocused', false);
// Is Notebook
export const NOTEBOOK_IS_ACTIVE_EDITOR = ContextKeyExpr.equals('activeEditor', 'workbench.editor.notebook');
// Editor keys
export const NOTEBOOK_EDITOR_FOCUSED = new RawContextKey<boolean>('notebookEditorFocused', false);
export const NOTEBOOK_EDITOR_OPEN = new RawContextKey<boolean>('notebookEditorOpen', false);
export const NOTEBOOK_CELL_LIST_FOCUSED = new RawContextKey<boolean>('notebookCellListFocused', false);
export const NOTEBOOK_OUTPUT_FOCUSED = new RawContextKey<boolean>('notebookOutputFocused', false);
export const NOTEBOOK_EDITOR_EDITABLE = new RawContextKey<boolean>('notebookEditable', true);
export const NOTEBOOK_HAS_RUNNING_CELL = new RawContextKey<boolean>('notebookHasRunningCell', false);
// Diff Editor Keys
export const IN_NOTEBOOK_TEXT_DIFF_EDITOR = new RawContextKey<boolean>('isInNotebookTextDiffEditor', false);
// Cell keys
export const NOTEBOOK_VIEW_TYPE = new RawContextKey<string>('notebookViewType', undefined);
export const NOTEBOOK_CELL_TYPE = new RawContextKey<string>('notebookCellType', undefined); // code, markdown
export const NOTEBOOK_CELL_EDITABLE = new RawContextKey<boolean>('notebookCellEditable', false); // bool
export const NOTEBOOK_CELL_FOCUSED = new RawContextKey<boolean>('notebookCellFocused', false); // bool
export const NOTEBOOK_CELL_EDITOR_FOCUSED = new RawContextKey<boolean>('notebookCellEditorFocused', false); // bool
export const NOTEBOOK_CELL_MARKDOWN_EDIT_MODE = new RawContextKey<boolean>('notebookCellMarkdownEditMode', false); // bool
export type NotebookCellExecutionStateContext = 'idle' | 'pending' | 'executing' | 'succeeded' | 'failed';
export const NOTEBOOK_CELL_EXECUTION_STATE = new RawContextKey<NotebookCellExecutionStateContext>('notebookCellExecutionState', undefined);
export const NOTEBOOK_CELL_HAS_OUTPUTS = new RawContextKey<boolean>('notebookCellHasOutputs', false); // bool
export const NOTEBOOK_CELL_INPUT_COLLAPSED = new RawContextKey<boolean>('notebookCellInputIsCollapsed', false); // bool
export const NOTEBOOK_CELL_OUTPUT_COLLAPSED = new RawContextKey<boolean>('notebookCellOutputIsCollapsed', false); // bool
// Kernels
export const NOTEBOOK_HAS_MULTIPLE_KERNELS = new RawContextKey<boolean>('notebookHasMultipleKernels', false);
export const NOTEBOOK_KERNEL_COUNT = new RawContextKey<number>('notebookKernelCount', 0);
export const NOTEBOOK_INTERRUPTIBLE_KERNEL = new RawContextKey<boolean>('notebookInterruptibleKernel', false);
//#endregion
//#region Shared commands
export const EXPAND_CELL_INPUT_COMMAND_ID = 'notebook.cell.expandCellInput';
export const EXECUTE_CELL_COMMAND_ID = 'notebook.cell.execute';
//#endregion
//#region Output related types
export const enum RenderOutputType {
Mainframe,
Html,
Extension
}
export interface IRenderMainframeOutput {
type: RenderOutputType.Mainframe;
supportAppend?: boolean;
}
export interface IRenderPlainHtmlOutput {
type: RenderOutputType.Html;
source: IDisplayOutputViewModel;
htmlContent: string;
}
export interface IRenderOutputViaExtension {
type: RenderOutputType.Extension;
source: IDisplayOutputViewModel;
mimeType: string;
renderer: INotebookRendererInfo;
}
export type IInsetRenderOutput = IRenderPlainHtmlOutput | IRenderOutputViaExtension;
export type IRenderOutput = IRenderMainframeOutput | IInsetRenderOutput;
export interface ICellOutputViewModel {
cellViewModel: IGenericCellViewModel;
/**
* When rendering an output, `model` should always be used as we convert legacy `text/error` output to `display_data` output under the hood.
*/
model: ICellOutput;
resolveMimeTypes(textModel: NotebookTextModel): [readonly IOrderedMimeType[], number];
pickedMimeType: number;
supportAppend(): boolean;
toRawJSON(): any;
}
export interface IDisplayOutputViewModel extends ICellOutputViewModel {
resolveMimeTypes(textModel: NotebookTextModel): [readonly IOrderedMimeType[], number];
pickedMimeType: number;
}
//#endregion
//#region Shared types between the Notebook Editor and Notebook Diff Editor, they are mostly used for output rendering
export interface IGenericCellViewModel {
id: string;
handle: number;
uri: URI;
metadata: NotebookCellMetadata | undefined;
outputIsHovered: boolean;
outputIsFocused: boolean;
outputsViewModels: ICellOutputViewModel[];
getOutputOffset(index: number): number;
updateOutputHeight(index: number, height: number, source?: string): void;
}
export interface IDisplayOutputLayoutUpdateRequest {
output: IDisplayOutputViewModel;
cellTop: number;
outputOffset: number;
}
export interface ICommonCellInfo {
cellId: string;
cellHandle: number;
cellUri: URI;
}
export interface INotebookCellOutputLayoutInfo {
width: number;
height: number;
fontInfo: BareFontInfo;
}
export interface IFocusNotebookCellOptions {
readonly skipReveal?: boolean;
}
export interface ICommonNotebookEditor {
getCellOutputLayoutInfo(cell: IGenericCellViewModel): INotebookCellOutputLayoutInfo;
triggerScroll(event: IMouseWheelEvent): void;
getCellByInfo(cellInfo: ICommonCellInfo): IGenericCellViewModel;
getCellById(cellId: string): IGenericCellViewModel | undefined;
toggleNotebookCellSelection(cell: IGenericCellViewModel): void;
focusNotebookCell(cell: IGenericCellViewModel, focus: 'editor' | 'container' | 'output', options?: IFocusNotebookCellOptions): void;
focusNextNotebookCell(cell: IGenericCellViewModel, focus: 'editor' | 'container' | 'output'): void;
updateOutputHeight(cellInfo: ICommonCellInfo, output: IDisplayOutputViewModel, height: number, isInit: boolean, source?: string): void;
updateMarkdownCellHeight(cellId: string, height: number, isInit: boolean): void;
setMarkdownCellEditState(cellId: string, editState: CellEditState): void;
markdownCellDragStart(cellId: string, position: { clientY: number }): void;
markdownCellDrag(cellId: string, position: { clientY: number }): void;
markdownCellDrop(cellId: string, position: { clientY: number, ctrlKey: boolean, altKey: boolean }): void;
markdownCellDragEnd(cellId: string): void;
}
//#endregion
export interface NotebookLayoutInfo {
width: number;
height: number;
fontInfo: BareFontInfo;
}
export interface NotebookLayoutChangeEvent {
width?: boolean;
height?: boolean;
fontInfo?: boolean;
}
export enum CodeCellLayoutState {
Uninitialized,
Estimated,
FromCache,
Measured
}
export interface CodeCellLayoutInfo {
readonly fontInfo: BareFontInfo | null;
readonly editorHeight: number;
readonly editorWidth: number;
readonly totalHeight: number;
readonly outputContainerOffset: number;
readonly outputTotalHeight: number;
readonly outputShowMoreContainerHeight: number;
readonly outputShowMoreContainerOffset: number;
readonly indicatorHeight: number;
readonly bottomToolbarOffset: number;
readonly layoutState: CodeCellLayoutState;
}
export interface CodeCellLayoutChangeEvent {
source?: string;
editorHeight?: boolean;
outputHeight?: boolean;
outputShowMoreContainerHeight?: number;
totalHeight?: boolean;
outerWidth?: number;
font?: BareFontInfo;
}
export interface MarkdownCellLayoutInfo {
readonly fontInfo: BareFontInfo | null;
readonly editorWidth: number;
readonly editorHeight: number;
readonly bottomToolbarOffset: number;
readonly totalHeight: number;
}
export interface MarkdownCellLayoutChangeEvent {
font?: BareFontInfo;
outerWidth?: number;
totalHeight?: number;
}
export interface ICellViewModel extends IGenericCellViewModel {
readonly model: NotebookCellTextModel;
readonly id: string;
readonly textBuffer: IReadonlyTextBuffer;
readonly layoutInfo: { totalHeight: number; };
readonly onDidChangeLayout: Event<any>;
dragging: boolean;
handle: number;
uri: URI;
language: string;
cellKind: CellKind;
editState: CellEditState;
focusMode: CellFocusMode;
outputIsHovered: boolean;
getText(): string;
getTextLength(): number;
getHeight(lineHeight: number): number;
metadata: NotebookCellMetadata | undefined;
textModel: ITextModel | undefined;
hasModel(): this is IEditableCellViewModel;
resolveTextModel(): Promise<ITextModel>;
getEvaluatedMetadata(documentMetadata: NotebookDocumentMetadata | undefined): NotebookCellMetadata;
getSelectionsStartPosition(): IPosition[] | undefined;
getCellDecorations(): INotebookCellDecorationOptions[];
}
export interface IEditableCellViewModel extends ICellViewModel {
textModel: ITextModel;
}
export interface INotebookEditorMouseEvent {
readonly event: MouseEvent;
readonly target: CellViewModel;
}
export interface INotebookEditorContribution {
/**
* Dispose this contribution.
*/
dispose(): void;
/**
* Store view state.
*/
saveViewState?(): unknown;
/**
* Restore view state.
*/
restoreViewState?(state: unknown): void;
}
export interface INotebookCellDecorationOptions {
className?: string;
gutterClassName?: string;
outputClassName?: string;
topClassName?: string;
}
export interface INotebookDeltaDecoration {
handle: number;
options: INotebookCellDecorationOptions;
}
export class NotebookEditorOptions extends EditorOptions {
readonly cellOptions?: IResourceEditorInput;
readonly cellSelections?: ICellRange[];
constructor(options: Partial<NotebookEditorOptions>) {
super();
this.overwrite(options);
this.cellOptions = options.cellOptions;
this.cellSelections = options.cellSelections;
}
with(options: Partial<NotebookEditorOptions>): NotebookEditorOptions {
return new NotebookEditorOptions({ ...this, ...options });
}
}
export type INotebookEditorContributionCtor = IConstructorSignature1<INotebookEditor, INotebookEditorContribution>;
export interface INotebookEditorContributionDescription {
id: string;
ctor: INotebookEditorContributionCtor;
}
export interface INotebookEditorCreationOptions {
readonly isEmbedded?: boolean;
readonly contributions?: INotebookEditorContributionDescription[];
}
export interface IActiveNotebookEditor extends INotebookEditor {
viewModel: NotebookViewModel;
getFocus(): ICellRange;
}
export const NOTEBOOK_EDITOR_ID = 'workbench.editor.notebook';
export const NOTEBOOK_DIFF_EDITOR_ID = 'workbench.editor.notebookTextDiffEditor';
export interface INotebookEditor extends ICommonNotebookEditor {
// from the old IEditor
readonly onDidChangeVisibleRanges: Event<void>;
readonly onDidChangeSelection: Event<void>;
getSelections(): ICellRange[];
visibleRanges: ICellRange[];
textModel?: NotebookTextModel;
getId(): string;
hasFocus(): boolean;
isEmbedded: boolean;
cursorNavigationMode: boolean;
/**
* Notebook view model attached to the current editor
*/
viewModel: NotebookViewModel | undefined;
hasModel(): this is IActiveNotebookEditor;
/**
* An event emitted when the model of this editor has changed.
* @event
*/
readonly onDidChangeModel: Event<NotebookTextModel | undefined>;
readonly onDidFocusEditorWidget: Event<void>;
activeKernel: INotebookKernel | undefined;
multipleKernelsAvailable: boolean;
readonly onDidScroll: Event<void>;
readonly onDidChangeAvailableKernels: Event<void>;
readonly onDidChangeKernel: Event<void>;
readonly onDidChangeActiveCell: Event<void>;
isDisposed: boolean;
dispose(): void;
getId(): string;
getDomNode(): HTMLElement;
getOverflowContainerDomNode(): HTMLElement;
getInnerWebview(): Webview | undefined;
getSelectionViewModels(): ICellViewModel[];
/**
* Focus the notebook editor cell list
*/
focus(): void;
hasFocus(): boolean;
hasWebviewFocus(): boolean;
hasOutputTextSelection(): boolean;
setOptions(options: NotebookEditorOptions | undefined): Promise<void>;
/**
* Select & focus cell
*/
focusElement(cell: ICellViewModel): void;
/**
* Layout info for the notebook editor
*/
getLayoutInfo(): NotebookLayoutInfo;
getVisibleRangesPlusViewportAboveBelow(): ICellRange[];
/**
* Fetch the output renderers for notebook outputs.
*/
getOutputRenderer(): OutputRenderer;
/**
* Fetch the contributed kernels for this notebook
*/
beginComputeContributedKernels(): Promise<INotebookKernel[]>;
/**
* Insert a new cell around `cell`
*/
insertNotebookCell(cell: ICellViewModel | undefined, type: CellKind, direction?: 'above' | 'below', initialText?: string, ui?: boolean): CellViewModel | null;
/**
* Split a given cell into multiple cells of the same type using the selection start positions.
*/
splitNotebookCell(cell: ICellViewModel): Promise<CellViewModel[] | null>;
/**
* Delete a cell from the notebook
*/
deleteNotebookCell(cell: ICellViewModel): Promise<boolean>;
/**
* Move a cell up one spot
*/
moveCellUp(cell: ICellViewModel): Promise<ICellViewModel | null>;
/**
* Move a cell down one spot
*/
moveCellDown(cell: ICellViewModel): Promise<ICellViewModel | null>;
/**
* Move a cell to a specific position
*/
moveCellsToIdx(index: number, length: number, toIdx: number): Promise<ICellViewModel | null>;
/**
* Focus the container of a cell (the monaco editor inside is not focused).
*/
focusNotebookCell(cell: ICellViewModel, focus: 'editor' | 'container' | 'output'): void;
focusNextNotebookCell(cell: ICellViewModel, focus: 'editor' | 'container' | 'output'): void;
/**
* Execute the given notebook cell
*/
executeNotebookCell(cell: ICellViewModel): Promise<void>;
/**
* Cancel the cell execution
*/
cancelNotebookCellExecution(cell: ICellViewModel): void;
/**
* Executes all notebook cells in order
*/
executeNotebook(): Promise<void>;
/**
* Cancel the notebook execution
*/
cancelNotebookExecution(): void;
/**
* Get current active cell
*/
getActiveCell(): ICellViewModel | undefined;
/**
* Layout the cell with a new height
*/
layoutNotebookCell(cell: ICellViewModel, height: number): Promise<void>;
createMarkdownPreview(cell: ICellViewModel): Promise<void>;
unhideMarkdownPreview(cell: ICellViewModel): Promise<void>;
hideMarkdownPreview(cell: ICellViewModel): Promise<void>;
removeMarkdownPreview(cell: ICellViewModel): Promise<void>;
updateMarkdownPreviewSelectionState(cell: ICellViewModel, isSelected: boolean): Promise<void>;
/**
* Render the output in webview layer
*/
createOutput(cell: ICellViewModel, output: IInsetRenderOutput, offset: number): Promise<void>;
/**
* Remove the output from the webview layer
*/
removeInset(output: IDisplayOutputViewModel): void;
/**
* Hide the inset in the webview layer without removing it
*/
hideInset(output: IDisplayOutputViewModel): void;
/**
* Send message to the webview for outputs.
*/
postMessage(forRendererId: string | undefined, message: any): void;
/**
* Remove class name on the notebook editor root DOM node.
*/
addClassName(className: string): void;
/**
* Remove class name on the notebook editor root DOM node.
*/
removeClassName(className: string): void;
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]): void;
/**
* Trigger the editor to scroll from scroll event programmatically
*/
triggerScroll(event: IMouseWheelEvent): void;
/**
* The range will be revealed with as little scrolling as possible.
*/
revealCellRangeInView(range: ICellRange): void;
/**
* Reveal cell into viewport.
*/
revealInView(cell: ICellViewModel): void;
/**
* Reveal cell into the top of viewport.
*/
revealInViewAtTop(cell: ICellViewModel): void;
/**
* Reveal cell into viewport center.
*/
revealInCenter(cell: ICellViewModel): void;
/**
* Reveal cell into viewport center if cell is currently out of the viewport.
*/
revealInCenterIfOutsideViewport(cell: ICellViewModel): void;
/**
* Reveal a line in notebook cell into viewport with minimal scrolling.
*/
revealLineInViewAsync(cell: ICellViewModel, line: number): Promise<void>;
/**
* Reveal a line in notebook cell into viewport center.
*/
revealLineInCenterAsync(cell: ICellViewModel, line: number): Promise<void>;
/**
* Reveal a line in notebook cell into viewport center.
*/
revealLineInCenterIfOutsideViewportAsync(cell: ICellViewModel, line: number): Promise<void>;
/**
* Reveal a range in notebook cell into viewport with minimal scrolling.
*/
revealRangeInViewAsync(cell: ICellViewModel, range: Range): Promise<void>;
/**
* Reveal a range in notebook cell into viewport center.
*/
revealRangeInCenterAsync(cell: ICellViewModel, range: Range): Promise<void>;
/**
* Reveal a range in notebook cell into viewport center.
*/
revealRangeInCenterIfOutsideViewportAsync(cell: ICellViewModel, range: Range): Promise<void>;
/**
* Get the view index of a cell
*/
getViewIndex(cell: ICellViewModel): number;
/**
* Get the view height of a cell (from the list view)
*/
getViewHeight(cell: ICellViewModel): number;
/**
* @param startIndex Inclusive
* @param endIndex Exclusive
*/
getCellRangeFromViewRange(startIndex: number, endIndex: number): ICellRange | undefined;
/**
* @param startIndex Inclusive
* @param endIndex Exclusive
*/
getCellsFromViewRange(startIndex: number, endIndex: number): ICellViewModel[];
/**
* Set hidden areas on cell text models.
*/
setHiddenAreas(_ranges: ICellRange[]): boolean;
setCellEditorSelection(cell: ICellViewModel, selection: Range): void;
deltaCellDecorations(oldDecorations: string[], newDecorations: INotebookDeltaDecoration[]): string[];
/**
* Change the decorations on cells.
* The notebook is virtualized and this method should be called to create/delete editor decorations safely.
*/
changeModelDecorations<T>(callback: (changeAccessor: IModelDecorationsChangeAccessor) => T): T | null;
setEditorDecorations(key: string, range: ICellRange): void;
removeEditorDecorations(key: string): void;
/**
* An event emitted on a "mouseup".
* @event
*/
onMouseUp(listener: (e: INotebookEditorMouseEvent) => void): IDisposable;
/**
* An event emitted on a "mousedown".
* @event
*/
onMouseDown(listener: (e: INotebookEditorMouseEvent) => void): IDisposable;
/**
* Get a contribution of this editor.
* @id Unique identifier of the contribution.
* @return The contribution or null if contribution not found.
*/
getContribution<T extends INotebookEditorContribution>(id: string): T;
getCellByInfo(cellInfo: ICommonCellInfo): ICellViewModel;
getCellById(cellId: string): ICellViewModel | undefined;
updateOutputHeight(cellInfo: ICommonCellInfo, output: IDisplayOutputViewModel, height: number, isInit: boolean, source?: string): void;
}
export interface INotebookCellList {
isDisposed: boolean;
viewModel: NotebookViewModel | null;
readonly contextKeyService: IContextKeyService;
element(index: number): ICellViewModel | undefined;
elementAt(position: number): ICellViewModel | undefined;
elementHeight(element: ICellViewModel): number;
onWillScroll: Event<ScrollEvent>;
onDidScroll: Event<ScrollEvent>;
onDidChangeFocus: Event<IListEvent<ICellViewModel>>;
onDidChangeContentHeight: Event<number>;
onDidChangeVisibleRanges: Event<void>;
visibleRanges: ICellRange[];
scrollTop: number;
scrollHeight: number;
scrollLeft: number;
length: number;
rowsContainer: HTMLElement;
readonly onDidRemoveOutput: Event<ICellOutputViewModel>;
readonly onDidHideOutput: Event<ICellOutputViewModel>;
readonly onDidRemoveCellFromView: Event<ICellViewModel>;
readonly onMouseUp: Event<IListMouseEvent<CellViewModel>>;
readonly onMouseDown: Event<IListMouseEvent<CellViewModel>>;
readonly onContextMenu: Event<IListContextMenuEvent<CellViewModel>>;
detachViewModel(): void;
attachViewModel(viewModel: NotebookViewModel): void;
clear(): void;
getViewIndex(cell: ICellViewModel): number | undefined;
getViewIndex2(modelIndex: number): number | undefined;
getModelIndex(cell: CellViewModel): number | undefined;
getModelIndex2(viewIndex: number): number | undefined;
getVisibleRangesPlusViewportAboveBelow(): ICellRange[];
focusElement(element: ICellViewModel): void;
selectElements(elements: ICellViewModel[]): void;
getFocusedElements(): ICellViewModel[];
getSelectedElements(): ICellViewModel[];
revealElementsInView(range: ICellRange): void;
revealElementInView(element: ICellViewModel): void;
revealElementInViewAtTop(element: ICellViewModel): void;
revealElementInCenterIfOutsideViewport(element: ICellViewModel): void;
revealElementInCenter(element: ICellViewModel): void;
revealElementInCenterIfOutsideViewportAsync(element: ICellViewModel): Promise<void>;
revealElementLineInViewAsync(element: ICellViewModel, line: number): Promise<void>;
revealElementLineInCenterAsync(element: ICellViewModel, line: number): Promise<void>;
revealElementLineInCenterIfOutsideViewportAsync(element: ICellViewModel, line: number): Promise<void>;
revealElementRangeInViewAsync(element: ICellViewModel, range: Range): Promise<void>;
revealElementRangeInCenterAsync(element: ICellViewModel, range: Range): Promise<void>;
revealElementRangeInCenterIfOutsideViewportAsync(element: ICellViewModel, range: Range): Promise<void>;
setHiddenAreas(_ranges: ICellRange[], triggerViewUpdate: boolean): boolean;
domElementOfElement(element: ICellViewModel): HTMLElement | null;
focusView(): void;
getAbsoluteTopOfElement(element: ICellViewModel): number;
triggerScrollFromMouseWheelEvent(browserEvent: IMouseWheelEvent): void;
updateElementHeight2(element: ICellViewModel, size: number): void;
domFocus(): void;
setCellSelection(element: ICellViewModel, range: Range): void;
style(styles: IListStyles): void;
getRenderHeight(): number;
updateOptions(options: IListOptions<ICellViewModel>): void;
layout(height?: number, width?: number): void;
dispose(): void;
}
export interface BaseCellRenderTemplate {
rootContainer: HTMLElement;
editorPart: HTMLElement;
collapsedPart: HTMLElement;
expandButton: HTMLElement;
contextKeyService: IContextKeyService;
container: HTMLElement;
cellContainer: HTMLElement;
decorationContainer: HTMLElement;
toolbar: ToolBar;
deleteToolbar: ToolBar;
betweenCellToolbar: ToolBar;
focusIndicatorLeft: HTMLElement;
focusIndicatorRight: HTMLElement;
disposables: DisposableStore;
elementDisposables: DisposableStore;
bottomCellContainer: HTMLElement;
currentRenderedCell?: ICellViewModel;
statusBar: CellEditorStatusBar;
titleMenu: IMenu;
toJSON: () => object;
}
export interface MarkdownCellRenderTemplate extends BaseCellRenderTemplate {
editorContainer: HTMLElement;
foldingIndicator: HTMLElement;
focusIndicatorBottom: HTMLElement;
currentEditor?: ICodeEditor;
readonly useRenderer: boolean;
}
export interface CodeCellRenderTemplate extends BaseCellRenderTemplate {
cellRunState: RunStateRenderer;
runToolbar: ToolBar;
runButtonContainer: HTMLElement;
executionOrderLabel: HTMLElement;
outputContainer: HTMLElement;
outputShowMoreContainer: HTMLElement;
focusSinkElement: HTMLElement;
editor: ICodeEditor;
progressBar: ProgressBar;
timer: TimerRenderer;
focusIndicatorRight: HTMLElement;
focusIndicatorBottom: HTMLElement;
dragHandle: HTMLElement;
}
export function isCodeCellRenderTemplate(templateData: BaseCellRenderTemplate): templateData is CodeCellRenderTemplate {
return !!(templateData as CodeCellRenderTemplate).runToolbar;
}
export interface IOutputTransformContribution {
getMimetypes(): string[];
/**
* Dispose this contribution.
*/
dispose(): void;
/**
* Returns contents to place in the webview inset, or the {@link IRenderNoOutput}.
* This call is allowed to have side effects, such as placing output
* directly into the container element.
*/
render(output: ICellOutputViewModel, items: IOutputItemDto[], container: HTMLElement, notebookUri: URI | undefined): IRenderOutput;
}
export interface CellFindMatch {
cell: CellViewModel;
matches: FindMatch[];
}
export enum CellRevealType {
Line,
Range
}
export enum CellRevealPosition {
Top,
Center,
Bottom
}
export enum CellEditState {
/**
* Default state.
* For markdown cell, it's Markdown preview.
* For code cell, the browser focus should be on the container instead of the editor
*/
Preview,
/**
* Eding mode. Source for markdown or code is rendered in editors and the state will be persistent.
*/
Editing
}
export enum CellFocusMode {
Container,
Editor
}
export enum CursorAtBoundary {
None,
Top,
Bottom,
Both
}
export interface CellViewModelStateChangeEvent {
readonly metadataChanged?: boolean;
readonly runStateChanged?: boolean;
readonly selectionChanged?: boolean;
readonly focusModeChanged?: boolean;
readonly editStateChanged?: boolean;
readonly languageChanged?: boolean;
readonly foldingStateChanged?: boolean;
readonly contentChanged?: boolean;
readonly outputIsHoveredChanged?: boolean;
readonly outputIsFocusedChanged?: boolean;
readonly cellIsHoveredChanged?: boolean;
}
export function cellRangesEqual(a: ICellRange[], b: ICellRange[]) {
a = reduceCellRanges(a);
b = reduceCellRanges(b);
if (a.length !== b.length) {
return false;
}
for (let i = 0; i < a.length; i++) {
if (a[i].start !== b[i].start || a[i].end !== b[i].end) {
return false;
}
}
return true;
}
/**
* @param _ranges
*/
export function reduceCellRanges(_ranges: ICellRange[]): ICellRange[] {
if (!_ranges.length) {
return [];
}
const ranges = _ranges.sort((a, b) => a.start - b.start);
const result: ICellRange[] = [];
let currentRangeStart = ranges[0].start;
let currentRangeEnd = ranges[0].end + 1;
for (let i = 0, len = ranges.length; i < len; i++) {
const range = ranges[i];
if (range.start > currentRangeEnd) {
result.push({ start: currentRangeStart, end: currentRangeEnd - 1 });
currentRangeStart = range.start;
currentRangeEnd = range.end + 1;
} else if (range.end + 1 > currentRangeEnd) {
currentRangeEnd = range.end + 1;
}
}
result.push({ start: currentRangeStart, end: currentRangeEnd - 1 });
return result;
}
export function getVisibleCells(cells: CellViewModel[], hiddenRanges: ICellRange[]) {
if (!hiddenRanges.length) {
return cells;
}
let start = 0;
let hiddenRangeIndex = 0;
const result: CellViewModel[] = [];
while (start < cells.length && hiddenRangeIndex < hiddenRanges.length) {
if (start < hiddenRanges[hiddenRangeIndex].start) {
result.push(...cells.slice(start, hiddenRanges[hiddenRangeIndex].start));
}
start = hiddenRanges[hiddenRangeIndex].end + 1;
hiddenRangeIndex++;
}
if (start < cells.length) {
result.push(...cells.slice(start));
}
return result;
}
export function getNotebookEditorFromEditorPane(editorPane?: IEditorPane): INotebookEditor | undefined {
return editorPane?.getId() === NOTEBOOK_EDITOR_ID ? editorPane.getControl() as INotebookEditor | undefined : undefined;
}
let EDITOR_TOP_PADDING = 12;
const editorTopPaddingChangeEmitter = new Emitter<void>();
export const EditorTopPaddingChangeEvent = editorTopPaddingChangeEmitter.event;
export function updateEditorTopPadding(top: number) {
EDITOR_TOP_PADDING = top;
editorTopPaddingChangeEmitter.fire();
}
export function getEditorTopPadding() {
return EDITOR_TOP_PADDING;
}
/**
* ranges: model selections
* this will convert model selections to view indexes first, and then include the hidden ranges in the list view
*/
export function expandCellRangesWithHiddenCells(editor: INotebookEditor, viewModel: NotebookViewModel, ranges: ICellRange[]) {
// assuming ranges are sorted and no overlap
const indexes = cellRangesToIndexes(ranges);
let modelRanges: ICellRange[] = [];
indexes.forEach(index => {
const viewCell = viewModel.viewCells[index];
if (!viewCell) {
return;
}
const viewIndex = editor.getViewIndex(viewCell);
if (viewIndex < 0) {
return;
}
const nextViewIndex = viewIndex + 1;
const range = editor.getCellRangeFromViewRange(viewIndex, nextViewIndex);
if (range) {
modelRanges.push(range);
}
});
return reduceRanges(modelRanges);
}
/**
* Return a set of ranges for the cells matching the given predicate
*/
export function getRanges(cells: ICellViewModel[], included: (cell: ICellViewModel) => boolean): ICellRange[] {
const ranges: ICellRange[] = [];
let currentRange: ICellRange | undefined;
cells.forEach((cell, idx) => {
if (included(cell)) {
if (!currentRange) {
currentRange = { start: idx, end: idx + 1 };
ranges.push(currentRange);
} else {
currentRange.end = idx + 1;
}
} else {
currentRange = undefined;
}
});
return ranges;
}
| src/vs/workbench/contrib/notebook/browser/notebookBrowser.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.006491679698228836,
0.0002878431405406445,
0.00016130752919707447,
0.00017047843721229583,
0.0006640192004851997
] |
{
"id": 9,
"code_window": [
"\t\tthis._sendMessageToWebview({\n",
"\t\t\ttype: 'updateMarkdownPreviewSelectionState',\n",
"\t\t\tid: cellId,\n",
"\t\t\tisSelected\n",
"\t\t});\n",
"\t}\n",
"\n",
"\tasync initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {\n",
"\t\tawait this._loaded;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\ttype: 'updateSelectedMarkdownPreviews',\n",
"\t\t\tselectedCellIds: selectedCellsIds.filter(id => this.markdownPreviewMapping.has(id)),\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1280
} | # Gulp - Automate and enhance your workflow
**Notice:** This extension is bundled with Visual Studio Code. It can be disabled but not uninstalled.
## Features
This extension supports running [Gulp](https://gulpjs.com/) tasks defined in a `gulpfile.{js,ts}` file as [VS Code tasks](https://code.visualstudio.com/docs/editor/tasks). Gulp tasks with the name 'build', 'compile', or 'watch' are treated as build tasks.
To run Gulp tasks, use the **Tasks** menu.
## Settings
- `gulp.autoDetect` - Enable detecting tasks from `gulpfile.{js,ts}` files, the default is `on`.
| extensions/gulp/README.md | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017449891311116517,
0.00017044413834810257,
0.00016638934903312474,
0.00017044413834810257,
0.0000040547820390202105
] |
{
"id": 9,
"code_window": [
"\t\tthis._sendMessageToWebview({\n",
"\t\t\ttype: 'updateMarkdownPreviewSelectionState',\n",
"\t\t\tid: cellId,\n",
"\t\t\tisSelected\n",
"\t\t});\n",
"\t}\n",
"\n",
"\tasync initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {\n",
"\t\tawait this._loaded;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\ttype: 'updateSelectedMarkdownPreviews',\n",
"\t\t\tselectedCellIds: selectedCellsIds.filter(id => this.markdownPreviewMapping.has(id)),\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1280
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as strings from 'vs/base/common/strings';
import { Position } from 'vs/editor/common/core/position';
import { Range } from 'vs/editor/common/core/range';
import { EndOfLinePreference } from 'vs/editor/common/model';
export const _debugComposition = false;
export interface ITextAreaWrapper {
getValue(): string;
setValue(reason: string, value: string): void;
getSelectionStart(): number;
getSelectionEnd(): number;
setSelectionRange(reason: string, selectionStart: number, selectionEnd: number): void;
}
export interface ISimpleModel {
getLineCount(): number;
getLineMaxColumn(lineNumber: number): number;
getValueInRange(range: Range, eol: EndOfLinePreference): string;
}
export interface ITypeData {
text: string;
replacePrevCharCnt: number;
replaceNextCharCnt: number;
positionDelta: number;
}
export class TextAreaState {
public static readonly EMPTY = new TextAreaState('', 0, 0, null, null);
public readonly value: string;
public readonly selectionStart: number;
public readonly selectionEnd: number;
public readonly selectionStartPosition: Position | null;
public readonly selectionEndPosition: Position | null;
constructor(value: string, selectionStart: number, selectionEnd: number, selectionStartPosition: Position | null, selectionEndPosition: Position | null) {
this.value = value;
this.selectionStart = selectionStart;
this.selectionEnd = selectionEnd;
this.selectionStartPosition = selectionStartPosition;
this.selectionEndPosition = selectionEndPosition;
}
public toString(): string {
return '[ <' + this.value + '>, selectionStart: ' + this.selectionStart + ', selectionEnd: ' + this.selectionEnd + ']';
}
public static readFromTextArea(textArea: ITextAreaWrapper): TextAreaState {
return new TextAreaState(textArea.getValue(), textArea.getSelectionStart(), textArea.getSelectionEnd(), null, null);
}
public collapseSelection(): TextAreaState {
return new TextAreaState(this.value, this.value.length, this.value.length, null, null);
}
public writeToTextArea(reason: string, textArea: ITextAreaWrapper, select: boolean): void {
if (_debugComposition) {
console.log('writeToTextArea ' + reason + ': ' + this.toString());
}
textArea.setValue(reason, this.value);
if (select) {
textArea.setSelectionRange(reason, this.selectionStart, this.selectionEnd);
}
}
public deduceEditorPosition(offset: number): [Position | null, number, number] {
if (offset <= this.selectionStart) {
const str = this.value.substring(offset, this.selectionStart);
return this._finishDeduceEditorPosition(this.selectionStartPosition, str, -1);
}
if (offset >= this.selectionEnd) {
const str = this.value.substring(this.selectionEnd, offset);
return this._finishDeduceEditorPosition(this.selectionEndPosition, str, 1);
}
const str1 = this.value.substring(this.selectionStart, offset);
if (str1.indexOf(String.fromCharCode(8230)) === -1) {
return this._finishDeduceEditorPosition(this.selectionStartPosition, str1, 1);
}
const str2 = this.value.substring(offset, this.selectionEnd);
return this._finishDeduceEditorPosition(this.selectionEndPosition, str2, -1);
}
private _finishDeduceEditorPosition(anchor: Position | null, deltaText: string, signum: number): [Position | null, number, number] {
let lineFeedCnt = 0;
let lastLineFeedIndex = -1;
while ((lastLineFeedIndex = deltaText.indexOf('\n', lastLineFeedIndex + 1)) !== -1) {
lineFeedCnt++;
}
return [anchor, signum * deltaText.length, lineFeedCnt];
}
public static selectedText(text: string): TextAreaState {
return new TextAreaState(text, 0, text.length, null, null);
}
public static deduceInput(previousState: TextAreaState, currentState: TextAreaState, couldBeEmojiInput: boolean): ITypeData {
if (!previousState) {
// This is the EMPTY state
return {
text: '',
replacePrevCharCnt: 0,
replaceNextCharCnt: 0,
positionDelta: 0
};
}
if (_debugComposition) {
console.log('------------------------deduceInput');
console.log('PREVIOUS STATE: ' + previousState.toString());
console.log('CURRENT STATE: ' + currentState.toString());
}
let previousValue = previousState.value;
let previousSelectionStart = previousState.selectionStart;
let previousSelectionEnd = previousState.selectionEnd;
let currentValue = currentState.value;
let currentSelectionStart = currentState.selectionStart;
let currentSelectionEnd = currentState.selectionEnd;
// Strip the previous suffix from the value (without interfering with the current selection)
const previousSuffix = previousValue.substring(previousSelectionEnd);
const currentSuffix = currentValue.substring(currentSelectionEnd);
const suffixLength = strings.commonSuffixLength(previousSuffix, currentSuffix);
currentValue = currentValue.substring(0, currentValue.length - suffixLength);
previousValue = previousValue.substring(0, previousValue.length - suffixLength);
const previousPrefix = previousValue.substring(0, previousSelectionStart);
const currentPrefix = currentValue.substring(0, currentSelectionStart);
const prefixLength = strings.commonPrefixLength(previousPrefix, currentPrefix);
currentValue = currentValue.substring(prefixLength);
previousValue = previousValue.substring(prefixLength);
currentSelectionStart -= prefixLength;
previousSelectionStart -= prefixLength;
currentSelectionEnd -= prefixLength;
previousSelectionEnd -= prefixLength;
if (_debugComposition) {
console.log('AFTER DIFFING PREVIOUS STATE: <' + previousValue + '>, selectionStart: ' + previousSelectionStart + ', selectionEnd: ' + previousSelectionEnd);
console.log('AFTER DIFFING CURRENT STATE: <' + currentValue + '>, selectionStart: ' + currentSelectionStart + ', selectionEnd: ' + currentSelectionEnd);
}
if (couldBeEmojiInput && currentSelectionStart === currentSelectionEnd && previousValue.length > 0) {
// on OSX, emojis from the emoji picker are inserted at random locations
// the only hints we can use is that the selection is immediately after the inserted emoji
// and that none of the old text has been deleted
let potentialEmojiInput: string | null = null;
if (currentSelectionStart === currentValue.length) {
// emoji potentially inserted "somewhere" after the previous selection => it should appear at the end of `currentValue`
if (currentValue.startsWith(previousValue)) {
// only if all of the old text is accounted for
potentialEmojiInput = currentValue.substring(previousValue.length);
}
} else {
// emoji potentially inserted "somewhere" before the previous selection => it should appear at the start of `currentValue`
if (currentValue.endsWith(previousValue)) {
// only if all of the old text is accounted for
potentialEmojiInput = currentValue.substring(0, currentValue.length - previousValue.length);
}
}
if (potentialEmojiInput !== null && potentialEmojiInput.length > 0) {
// now we check that this is indeed an emoji
// emojis can grow quite long, so a length check is of no help
// e.g. 1F3F4 E0067 E0062 E0065 E006E E0067 E007F ; fully-qualified # 🏴 England
// Oftentimes, emojis use Variation Selector-16 (U+FE0F), so that is a good hint
// http://emojipedia.org/variation-selector-16/
// > An invisible codepoint which specifies that the preceding character
// > should be displayed with emoji presentation. Only required if the
// > preceding character defaults to text presentation.
if (/\uFE0F/.test(potentialEmojiInput) || strings.containsEmoji(potentialEmojiInput)) {
return {
text: potentialEmojiInput,
replacePrevCharCnt: 0,
replaceNextCharCnt: 0,
positionDelta: 0
};
}
}
}
if (currentSelectionStart === currentSelectionEnd) {
// composition accept case (noticed in FF + Japanese)
// [blahblah] => blahblah|
if (
previousValue === currentValue
&& previousSelectionStart === 0
&& previousSelectionEnd === previousValue.length
&& currentSelectionStart === currentValue.length
&& currentValue.indexOf('\n') === -1
) {
if (strings.containsFullWidthCharacter(currentValue)) {
return {
text: '',
replacePrevCharCnt: 0,
replaceNextCharCnt: 0,
positionDelta: 0
};
}
}
// no current selection
const replacePreviousCharacters = (previousPrefix.length - prefixLength);
if (_debugComposition) {
console.log('REMOVE PREVIOUS: ' + (previousPrefix.length - prefixLength) + ' chars');
}
return {
text: currentValue,
replacePrevCharCnt: replacePreviousCharacters,
replaceNextCharCnt: 0,
positionDelta: 0
};
}
// there is a current selection => composition case
const replacePreviousCharacters = previousSelectionEnd - previousSelectionStart;
return {
text: currentValue,
replacePrevCharCnt: replacePreviousCharacters,
replaceNextCharCnt: 0,
positionDelta: 0
};
}
public static deduceAndroidCompositionInput(previousState: TextAreaState, currentState: TextAreaState): ITypeData {
if (!previousState) {
// This is the EMPTY state
return {
text: '',
replacePrevCharCnt: 0,
replaceNextCharCnt: 0,
positionDelta: 0
};
}
if (_debugComposition) {
console.log('------------------------deduceAndroidCompositionInput');
console.log('PREVIOUS STATE: ' + previousState.toString());
console.log('CURRENT STATE: ' + currentState.toString());
}
if (previousState.value === currentState.value) {
return {
text: '',
replacePrevCharCnt: 0,
replaceNextCharCnt: 0,
positionDelta: currentState.selectionEnd - previousState.selectionEnd
};
}
const prefixLength = Math.min(strings.commonPrefixLength(previousState.value, currentState.value), previousState.selectionEnd);
const suffixLength = Math.min(strings.commonSuffixLength(previousState.value, currentState.value), previousState.value.length - previousState.selectionEnd);
const previousValue = previousState.value.substring(prefixLength, previousState.value.length - suffixLength);
const currentValue = currentState.value.substring(prefixLength, currentState.value.length - suffixLength);
const previousSelectionStart = previousState.selectionStart - prefixLength;
const previousSelectionEnd = previousState.selectionEnd - prefixLength;
const currentSelectionStart = currentState.selectionStart - prefixLength;
const currentSelectionEnd = currentState.selectionEnd - prefixLength;
if (_debugComposition) {
console.log('AFTER DIFFING PREVIOUS STATE: <' + previousValue + '>, selectionStart: ' + previousSelectionStart + ', selectionEnd: ' + previousSelectionEnd);
console.log('AFTER DIFFING CURRENT STATE: <' + currentValue + '>, selectionStart: ' + currentSelectionStart + ', selectionEnd: ' + currentSelectionEnd);
}
return {
text: currentValue,
replacePrevCharCnt: previousSelectionEnd,
replaceNextCharCnt: previousValue.length - previousSelectionEnd,
positionDelta: currentSelectionEnd - currentValue.length
};
}
}
export class PagedScreenReaderStrategy {
private static _getPageOfLine(lineNumber: number, linesPerPage: number): number {
return Math.floor((lineNumber - 1) / linesPerPage);
}
private static _getRangeForPage(page: number, linesPerPage: number): Range {
const offset = page * linesPerPage;
const startLineNumber = offset + 1;
const endLineNumber = offset + linesPerPage;
return new Range(startLineNumber, 1, endLineNumber + 1, 1);
}
public static fromEditorSelection(previousState: TextAreaState, model: ISimpleModel, selection: Range, linesPerPage: number, trimLongText: boolean): TextAreaState {
const selectionStartPage = PagedScreenReaderStrategy._getPageOfLine(selection.startLineNumber, linesPerPage);
const selectionStartPageRange = PagedScreenReaderStrategy._getRangeForPage(selectionStartPage, linesPerPage);
const selectionEndPage = PagedScreenReaderStrategy._getPageOfLine(selection.endLineNumber, linesPerPage);
const selectionEndPageRange = PagedScreenReaderStrategy._getRangeForPage(selectionEndPage, linesPerPage);
const pretextRange = selectionStartPageRange.intersectRanges(new Range(1, 1, selection.startLineNumber, selection.startColumn))!;
let pretext = model.getValueInRange(pretextRange, EndOfLinePreference.LF);
const lastLine = model.getLineCount();
const lastLineMaxColumn = model.getLineMaxColumn(lastLine);
const posttextRange = selectionEndPageRange.intersectRanges(new Range(selection.endLineNumber, selection.endColumn, lastLine, lastLineMaxColumn))!;
let posttext = model.getValueInRange(posttextRange, EndOfLinePreference.LF);
let text: string;
if (selectionStartPage === selectionEndPage || selectionStartPage + 1 === selectionEndPage) {
// take full selection
text = model.getValueInRange(selection, EndOfLinePreference.LF);
} else {
const selectionRange1 = selectionStartPageRange.intersectRanges(selection)!;
const selectionRange2 = selectionEndPageRange.intersectRanges(selection)!;
text = (
model.getValueInRange(selectionRange1, EndOfLinePreference.LF)
+ String.fromCharCode(8230)
+ model.getValueInRange(selectionRange2, EndOfLinePreference.LF)
);
}
// Chromium handles very poorly text even of a few thousand chars
// Cut text to avoid stalling the entire UI
if (trimLongText) {
const LIMIT_CHARS = 500;
if (pretext.length > LIMIT_CHARS) {
pretext = pretext.substring(pretext.length - LIMIT_CHARS, pretext.length);
}
if (posttext.length > LIMIT_CHARS) {
posttext = posttext.substring(0, LIMIT_CHARS);
}
if (text.length > 2 * LIMIT_CHARS) {
text = text.substring(0, LIMIT_CHARS) + String.fromCharCode(8230) + text.substring(text.length - LIMIT_CHARS, text.length);
}
}
return new TextAreaState(pretext + text + posttext, pretext.length, pretext.length + text.length, new Position(selection.startLineNumber, selection.startColumn), new Position(selection.endLineNumber, selection.endColumn));
}
}
| src/vs/editor/browser/controller/textAreaState.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00026487826835364103,
0.00017559163097757846,
0.00016110030992422253,
0.00017237306747119874,
0.000018250473658554256
] |
{
"id": 9,
"code_window": [
"\t\tthis._sendMessageToWebview({\n",
"\t\t\ttype: 'updateMarkdownPreviewSelectionState',\n",
"\t\t\tid: cellId,\n",
"\t\t\tisSelected\n",
"\t\t});\n",
"\t}\n",
"\n",
"\tasync initializeMarkdown(cells: Array<{ cellId: string, cellHandle: number, content: string, offset: number }>) {\n",
"\t\tawait this._loaded;\n"
],
"labels": [
"keep",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"\t\t\ttype: 'updateSelectedMarkdownPreviews',\n",
"\t\t\tselectedCellIds: selectedCellsIds.filter(id => this.markdownPreviewMapping.has(id)),\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/backLayerWebView.ts",
"type": "replace",
"edit_start_line_idx": 1280
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { ILogService } from 'vs/platform/log/common/log';
import { IProductService } from 'vs/platform/product/common/productService';
import { ISignService } from 'vs/platform/sign/common/sign';
import { IRemoteAgentService } from 'vs/workbench/services/remote/common/remoteAgentService';
import { BaseTunnelService } from 'vs/platform/remote/node/tunnelService';
import { nodeSocketFactory } from 'vs/platform/remote/node/nodeSocketFactory';
import { IWorkbenchEnvironmentService } from 'vs/workbench/services/environment/common/environmentService';
import { URI } from 'vs/base/common/uri';
import { registerSingleton } from 'vs/platform/instantiation/common/extensions';
import { ITunnelService } from 'vs/platform/remote/common/tunnel';
export class TunnelService extends BaseTunnelService {
public constructor(
@ILogService logService: ILogService,
@ISignService signService: ISignService,
@IProductService productService: IProductService,
@IRemoteAgentService _remoteAgentService: IRemoteAgentService,
@IWorkbenchEnvironmentService private environmentService: IWorkbenchEnvironmentService
) {
super(nodeSocketFactory, logService, signService, productService);
}
canTunnel(uri: URI): boolean {
return super.canTunnel(uri) && !!this.environmentService.remoteAuthority;
}
}
registerSingleton(ITunnelService, TunnelService);
| src/vs/workbench/services/remote/electron-browser/tunnelServiceImpl.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017732683045323938,
0.00017447677964810282,
0.0001716648112051189,
0.00017445774574298412,
0.0000020845736798946746
] |
{
"id": 10,
"code_window": [
"\t\tif (this.useRenderer) {\n",
"\t\t\t// the markdown preview's height might already be updated after the renderer calls `element.getHeight()`\n",
"\t\t\tif (this.viewCell.layoutInfo.totalHeight > 0) {\n",
"\t\t\t\tthis.relayoutCell();\n",
"\t\t\t}\n",
"\n",
"\t\t\t// Update for selection\n",
"\t\t\tthis._register(this.notebookEditor.onDidChangeSelection(() => {\n",
"\t\t\t\tconst selectedCells = this.notebookEditor.getSelectionViewModels();\n",
"\n",
"\t\t\t\t// Only show selection if there are more than one cells selected\n",
"\t\t\t\tconst isSelected = selectedCells.length > 1 && selectedCells.some(selectedCell => selectedCell === viewCell);\n",
"\t\t\t\tthis.notebookEditor.updateMarkdownPreviewSelectionState(viewCell, isSelected);\n",
"\t\t\t}));\n",
"\t\t}\n",
"\n",
"\t\t// apply decorations\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/markdownCell.ts",
"type": "replace",
"edit_start_line_idx": 200
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as DOM from 'vs/base/browser/dom';
import { raceCancellation } from 'vs/base/common/async';
import { CancellationTokenSource } from 'vs/base/common/cancellation';
import { Disposable, DisposableStore, IDisposable, toDisposable } from 'vs/base/common/lifecycle';
import { CodeEditorWidget } from 'vs/editor/browser/widget/codeEditorWidget';
import { IEditorOptions } from 'vs/editor/common/config/editorOptions';
import { IInstantiationService } from 'vs/platform/instantiation/common/instantiation';
import { EDITOR_BOTTOM_PADDING } from 'vs/workbench/contrib/notebook/browser/constants';
import { CellEditState, CellFocusMode, MarkdownCellRenderTemplate, ICellViewModel, getEditorTopPadding, IActiveNotebookEditor } from 'vs/workbench/contrib/notebook/browser/notebookBrowser';
import { CellFoldingState } from 'vs/workbench/contrib/notebook/browser/contrib/fold/foldingModel';
import { MarkdownCellViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/markdownCellViewModel';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { ServiceCollection } from 'vs/platform/instantiation/common/serviceCollection';
import { EditorContextKeys } from 'vs/editor/common/editorContextKeys';
import { getExecuteCellPlaceholder, getResizesObserver } from 'vs/workbench/contrib/notebook/browser/view/renderers/cellWidgets';
import { INotebookCellStatusBarService } from 'vs/workbench/contrib/notebook/common/notebookCellStatusBarService';
import { NotebookCellsChangeType } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { collapsedIcon, expandedIcon } from 'vs/workbench/contrib/notebook/browser/notebookIcons';
import { renderIcon } from 'vs/base/browser/ui/iconLabel/iconLabels';
interface IMarkdownRenderStrategy extends IDisposable {
update(): void;
}
class WebviewMarkdownRenderer extends Disposable implements IMarkdownRenderStrategy {
constructor(
readonly notebookEditor: IActiveNotebookEditor,
readonly viewCell: MarkdownCellViewModel
) {
super();
}
update(): void {
this.notebookEditor.createMarkdownPreview(this.viewCell);
}
}
class BuiltinMarkdownRenderer extends Disposable implements IMarkdownRenderStrategy {
private readonly localDisposables = this._register(new DisposableStore());
constructor(
private readonly notebookEditor: IActiveNotebookEditor,
private readonly viewCell: MarkdownCellViewModel,
private readonly container: HTMLElement,
private readonly markdownContainer: HTMLElement,
private readonly editorAccessor: () => CodeEditorWidget | null
) {
super();
this._register(getResizesObserver(this.markdownContainer, undefined, () => {
if (viewCell.editState === CellEditState.Preview) {
this.viewCell.renderedMarkdownHeight = container.clientHeight;
}
})).startObserving();
}
update(): void {
const markdownRenderer = this.viewCell.getMarkdownRenderer();
const renderedHTML = this.viewCell.getHTML();
if (renderedHTML) {
this.markdownContainer.appendChild(renderedHTML);
}
if (this.editorAccessor()) {
// switch from editing mode
this.viewCell.renderedMarkdownHeight = this.container.clientHeight;
this.relayoutCell();
} else {
this.localDisposables.clear();
this.localDisposables.add(markdownRenderer.onDidRenderAsync(() => {
if (this.viewCell.editState === CellEditState.Preview) {
this.viewCell.renderedMarkdownHeight = this.container.clientHeight;
}
this.relayoutCell();
}));
this.localDisposables.add(this.viewCell.textBuffer.onDidChangeContent(() => {
this.markdownContainer.innerText = '';
this.viewCell.clearHTML();
const renderedHTML = this.viewCell.getHTML();
if (renderedHTML) {
this.markdownContainer.appendChild(renderedHTML);
}
}));
this.viewCell.renderedMarkdownHeight = this.container.clientHeight;
this.relayoutCell();
}
}
relayoutCell() {
this.notebookEditor.layoutNotebookCell(this.viewCell, this.viewCell.layoutInfo.totalHeight);
}
}
export class StatefulMarkdownCell extends Disposable {
private editor: CodeEditorWidget | null = null;
private markdownContainer: HTMLElement;
private editorPart: HTMLElement;
private readonly localDisposables = new DisposableStore();
private foldingState: CellFoldingState;
private activeCellRunPlaceholder: IDisposable | null = null;
private useRenderer: boolean = false;
private renderStrategy: IMarkdownRenderStrategy;
constructor(
private readonly notebookEditor: IActiveNotebookEditor,
private readonly viewCell: MarkdownCellViewModel,
private readonly templateData: MarkdownCellRenderTemplate,
private editorOptions: IEditorOptions,
private readonly renderedEditors: Map<ICellViewModel, ICodeEditor | undefined>,
options: { useRenderer: boolean },
@IContextKeyService private readonly contextKeyService: IContextKeyService,
@INotebookCellStatusBarService readonly notebookCellStatusBarService: INotebookCellStatusBarService,
@IInstantiationService private readonly instantiationService: IInstantiationService,
) {
super();
this.markdownContainer = templateData.cellContainer;
this.editorPart = templateData.editorPart;
this.useRenderer = options.useRenderer;
if (this.useRenderer) {
this.templateData.container.classList.toggle('webview-backed-markdown-cell', true);
this.renderStrategy = new WebviewMarkdownRenderer(this.notebookEditor, this.viewCell);
} else {
this.renderStrategy = new BuiltinMarkdownRenderer(this.notebookEditor, this.viewCell, this.templateData.container, this.markdownContainer, () => this.editor);
}
this._register(this.renderStrategy);
this._register(toDisposable(() => renderedEditors.delete(this.viewCell)));
this._register(viewCell.onDidChangeState((e) => {
if (e.editStateChanged) {
this.viewUpdate();
} else if (e.contentChanged) {
this.viewUpdate();
}
}));
this._register(viewCell.model.onDidChangeMetadata(() => {
this.viewUpdate();
}));
const updateForFocusMode = () => {
if (viewCell.focusMode === CellFocusMode.Editor) {
this.focusEditorIfNeeded();
}
templateData.container.classList.toggle('cell-editor-focus', viewCell.focusMode === CellFocusMode.Editor);
};
this._register(viewCell.onDidChangeState((e) => {
if (!e.focusModeChanged) {
return;
}
updateForFocusMode();
}));
updateForFocusMode();
this.foldingState = viewCell.foldingState;
this.setFoldingIndicator();
this._register(viewCell.onDidChangeState((e) => {
if (!e.foldingStateChanged) {
return;
}
const foldingState = viewCell.foldingState;
if (foldingState !== this.foldingState) {
this.foldingState = foldingState;
this.setFoldingIndicator();
}
}));
this._register(viewCell.onDidChangeLayout((e) => {
const layoutInfo = this.editor?.getLayoutInfo();
if (e.outerWidth && this.viewCell.editState === CellEditState.Editing && layoutInfo && layoutInfo.width !== viewCell.layoutInfo.editorWidth) {
this.onCellEditorWidthChange();
} else if (e.totalHeight || e.outerWidth) {
this.relayoutCell();
}
}));
if (this.useRenderer) {
// the markdown preview's height might already be updated after the renderer calls `element.getHeight()`
if (this.viewCell.layoutInfo.totalHeight > 0) {
this.relayoutCell();
}
// Update for selection
this._register(this.notebookEditor.onDidChangeSelection(() => {
const selectedCells = this.notebookEditor.getSelectionViewModels();
// Only show selection if there are more than one cells selected
const isSelected = selectedCells.length > 1 && selectedCells.some(selectedCell => selectedCell === viewCell);
this.notebookEditor.updateMarkdownPreviewSelectionState(viewCell, isSelected);
}));
}
// apply decorations
this._register(viewCell.onCellDecorationsChanged((e) => {
e.added.forEach(options => {
if (options.className) {
if (this.useRenderer) {
this.notebookEditor.deltaCellOutputContainerClassNames(this.viewCell.id, [options.className], []);
} else {
templateData.rootContainer.classList.add(options.className);
}
}
});
e.removed.forEach(options => {
if (options.className) {
if (this.useRenderer) {
this.notebookEditor.deltaCellOutputContainerClassNames(this.viewCell.id, [], [options.className]);
} else {
templateData.rootContainer.classList.remove(options.className);
}
}
});
}));
viewCell.getCellDecorations().forEach(options => {
if (options.className) {
if (this.useRenderer) {
this.notebookEditor.deltaCellOutputContainerClassNames(this.viewCell.id, [options.className], []);
} else {
templateData.rootContainer.classList.add(options.className);
}
}
});
this.viewUpdate();
const updatePlaceholder = () => {
if (
this.notebookEditor.getActiveCell() === this.viewCell
&& !!this.notebookEditor.viewModel.metadata.trusted
) {
// active cell and no run status
if (this.activeCellRunPlaceholder === null) {
// const keybinding = this._keybindingService.lookupKeybinding(EXECUTE_CELL_COMMAND_ID);
const placeholder = getExecuteCellPlaceholder(this.viewCell);
if (!this.notebookCellStatusBarService.getEntries(this.viewCell.uri).find(entry => entry.text === placeholder.text && entry.command === placeholder.command)) {
this.activeCellRunPlaceholder = this.notebookCellStatusBarService.addEntry(placeholder);
this._register(this.activeCellRunPlaceholder);
}
}
return;
}
this.activeCellRunPlaceholder?.dispose();
this.activeCellRunPlaceholder = null;
};
this._register(this.notebookEditor.onDidChangeActiveCell(() => {
updatePlaceholder();
}));
this._register(this.viewCell.model.onDidChangeMetadata(() => {
updatePlaceholder();
}));
this._register(this.notebookEditor.viewModel.notebookDocument.onDidChangeContent(e => {
if (e.rawEvents.find(event => event.kind === NotebookCellsChangeType.ChangeDocumentMetadata)) {
updatePlaceholder();
}
}));
updatePlaceholder();
}
dispose() {
this.localDisposables.dispose();
this.viewCell.detachTextEditor();
super.dispose();
}
private viewUpdate(): void {
if (this.viewCell.metadata?.inputCollapsed) {
this.viewUpdateCollapsed();
} else if (this.viewCell.editState === CellEditState.Editing) {
this.viewUpdateEditing();
} else {
this.viewUpdatePreview();
}
}
private viewUpdateCollapsed(): void {
DOM.show(this.templateData.collapsedPart);
DOM.hide(this.editorPart);
DOM.hide(this.markdownContainer);
this.templateData.container.classList.toggle('collapsed', true);
this.viewCell.renderedMarkdownHeight = 0;
}
private viewUpdateEditing(): void {
// switch to editing mode
let editorHeight: number;
DOM.show(this.editorPart);
DOM.hide(this.markdownContainer);
DOM.hide(this.templateData.collapsedPart);
if (this.useRenderer) {
this.notebookEditor.hideMarkdownPreview(this.viewCell);
}
this.templateData.container.classList.toggle('collapsed', false);
this.templateData.container.classList.toggle('markdown-cell-edit-mode', true);
if (this.editor) {
editorHeight = this.editor.getContentHeight();
// not first time, we don't need to create editor or bind listeners
this.viewCell.attachTextEditor(this.editor);
this.focusEditorIfNeeded();
this.bindEditorListeners(this.editor);
this.editor.layout({
width: this.viewCell.layoutInfo.editorWidth,
height: editorHeight
});
} else {
const width = this.viewCell.layoutInfo.editorWidth;
const lineNum = this.viewCell.lineCount;
const lineHeight = this.viewCell.layoutInfo.fontInfo?.lineHeight || 17;
editorHeight = Math.max(lineNum, 1) * lineHeight + getEditorTopPadding() + EDITOR_BOTTOM_PADDING;
this.templateData.editorContainer.innerText = '';
// create a special context key service that set the inCompositeEditor-contextkey
const editorContextKeyService = this.contextKeyService.createScoped(this.templateData.editorPart);
EditorContextKeys.inCompositeEditor.bindTo(editorContextKeyService).set(true);
const editorInstaService = this.instantiationService.createChild(new ServiceCollection([IContextKeyService, editorContextKeyService]));
this._register(editorContextKeyService);
this.editor = this._register(editorInstaService.createInstance(CodeEditorWidget, this.templateData.editorContainer, {
...this.editorOptions,
dimension: {
width: width,
height: editorHeight
},
// overflowWidgetsDomNode: this.notebookEditor.getOverflowContainerDomNode()
}, {}));
this.templateData.currentEditor = this.editor;
const cts = new CancellationTokenSource();
this._register({ dispose() { cts.dispose(true); } });
raceCancellation(this.viewCell.resolveTextModel(), cts.token).then(model => {
if (!model) {
return;
}
this.editor!.setModel(model);
this.focusEditorIfNeeded();
const realContentHeight = this.editor!.getContentHeight();
if (realContentHeight !== editorHeight) {
this.editor!.layout(
{
width: width,
height: realContentHeight
}
);
editorHeight = realContentHeight;
}
this.viewCell.attachTextEditor(this.editor!);
if (this.viewCell.editState === CellEditState.Editing) {
this.focusEditorIfNeeded();
}
this.bindEditorListeners(this.editor!);
this.viewCell.editorHeight = editorHeight;
});
}
this.viewCell.editorHeight = editorHeight;
this.focusEditorIfNeeded();
this.renderedEditors.set(this.viewCell, this.editor!);
}
private viewUpdatePreview(): void {
this.viewCell.detachTextEditor();
DOM.hide(this.editorPart);
DOM.hide(this.templateData.collapsedPart);
DOM.show(this.markdownContainer);
this.templateData.container.classList.toggle('collapsed', false);
this.templateData.container.classList.toggle('markdown-cell-edit-mode', false);
this.renderedEditors.delete(this.viewCell);
this.markdownContainer.innerText = '';
this.viewCell.clearHTML();
this.renderStrategy.update();
}
private focusEditorIfNeeded() {
if (this.viewCell.focusMode === CellFocusMode.Editor && this.notebookEditor.hasFocus()) {
this.editor?.focus();
}
}
private layoutEditor(dimension: DOM.IDimension): void {
this.editor?.layout(dimension);
this.templateData.statusBar.layout(dimension.width);
}
private onCellEditorWidthChange(): void {
const realContentHeight = this.editor!.getContentHeight();
this.layoutEditor(
{
width: this.viewCell.layoutInfo.editorWidth,
height: realContentHeight
}
);
// LET the content size observer to handle it
// this.viewCell.editorHeight = realContentHeight;
// this.relayoutCell();
}
relayoutCell(): void {
this.notebookEditor.layoutNotebookCell(this.viewCell, this.viewCell.layoutInfo.totalHeight);
}
updateEditorOptions(newValue: IEditorOptions): void {
this.editorOptions = newValue;
if (this.editor) {
this.editor.updateOptions(this.editorOptions);
}
}
setFoldingIndicator() {
switch (this.foldingState) {
case CellFoldingState.None:
this.templateData.foldingIndicator.innerText = '';
break;
case CellFoldingState.Collapsed:
DOM.reset(this.templateData.foldingIndicator, renderIcon(collapsedIcon));
break;
case CellFoldingState.Expanded:
DOM.reset(this.templateData.foldingIndicator, renderIcon(expandedIcon));
break;
default:
break;
}
}
private bindEditorListeners(editor: CodeEditorWidget) {
this.localDisposables.clear();
this.localDisposables.add(editor.onDidContentSizeChange(e => {
const viewLayout = editor.getLayoutInfo();
if (e.contentHeightChanged) {
this.viewCell.editorHeight = e.contentHeight;
editor.layout(
{
width: viewLayout.width,
height: e.contentHeight
}
);
}
}));
this.localDisposables.add(editor.onDidChangeCursorSelection((e) => {
if (e.source === 'restoreState') {
// do not reveal the cell into view if this selection change was caused by restoring editors...
return;
}
const primarySelection = editor.getSelection();
if (primarySelection) {
this.notebookEditor.revealLineInViewAsync(this.viewCell, primarySelection.positionLineNumber);
}
}));
const updateFocusMode = () => this.viewCell.focusMode = editor.hasWidgetFocus() ? CellFocusMode.Editor : CellFocusMode.Container;
this.localDisposables.add(editor.onDidFocusEditorWidget(() => {
updateFocusMode();
}));
this.localDisposables.add(editor.onDidBlurEditorWidget(() => {
// this is for a special case:
// users click the status bar empty space, which we will then focus the editor
// so we don't want to update the focus state too eagerly
if (document.activeElement?.contains(this.templateData.container)) {
setTimeout(() => {
updateFocusMode();
}, 300);
} else {
updateFocusMode();
}
}));
updateFocusMode();
}
}
| src/vs/workbench/contrib/notebook/browser/view/renderers/markdownCell.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.998439610004425,
0.039580099284648895,
0.0001663382863625884,
0.001455687335692346,
0.1865186095237732
] |
{
"id": 10,
"code_window": [
"\t\tif (this.useRenderer) {\n",
"\t\t\t// the markdown preview's height might already be updated after the renderer calls `element.getHeight()`\n",
"\t\t\tif (this.viewCell.layoutInfo.totalHeight > 0) {\n",
"\t\t\t\tthis.relayoutCell();\n",
"\t\t\t}\n",
"\n",
"\t\t\t// Update for selection\n",
"\t\t\tthis._register(this.notebookEditor.onDidChangeSelection(() => {\n",
"\t\t\t\tconst selectedCells = this.notebookEditor.getSelectionViewModels();\n",
"\n",
"\t\t\t\t// Only show selection if there are more than one cells selected\n",
"\t\t\t\tconst isSelected = selectedCells.length > 1 && selectedCells.some(selectedCell => selectedCell === viewCell);\n",
"\t\t\t\tthis.notebookEditor.updateMarkdownPreviewSelectionState(viewCell, isSelected);\n",
"\t\t\t}));\n",
"\t\t}\n",
"\n",
"\t\t// apply decorations\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/markdownCell.ts",
"type": "replace",
"edit_start_line_idx": 200
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
export interface ITask<T> {
(): T;
}
export class Delayer<T> {
public defaultDelay: number;
private timeout: any; // Timer
private completionPromise: Promise<T | null> | null;
private onSuccess: ((value: T | PromiseLike<T> | undefined) => void) | null;
private task: ITask<T> | null;
constructor(defaultDelay: number) {
this.defaultDelay = defaultDelay;
this.timeout = null;
this.completionPromise = null;
this.onSuccess = null;
this.task = null;
}
public trigger(task: ITask<T>, delay: number = this.defaultDelay): Promise<T | null> {
this.task = task;
if (delay >= 0) {
this.cancelTimeout();
}
if (!this.completionPromise) {
this.completionPromise = new Promise<T | undefined>((resolve) => {
this.onSuccess = resolve;
}).then(() => {
this.completionPromise = null;
this.onSuccess = null;
const result = this.task && this.task();
this.task = null;
return result;
});
}
if (delay >= 0 || this.timeout === null) {
this.timeout = setTimeout(() => {
this.timeout = null;
if (this.onSuccess) {
this.onSuccess(undefined);
}
}, delay >= 0 ? delay : this.defaultDelay);
}
return this.completionPromise;
}
private cancelTimeout(): void {
if (this.timeout !== null) {
clearTimeout(this.timeout);
this.timeout = null;
}
}
}
| extensions/typescript-language-features/src/utils/async.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017402683442924172,
0.00017067312728613615,
0.00016760989092290401,
0.0001705919421510771,
0.000002078916622849647
] |
{
"id": 10,
"code_window": [
"\t\tif (this.useRenderer) {\n",
"\t\t\t// the markdown preview's height might already be updated after the renderer calls `element.getHeight()`\n",
"\t\t\tif (this.viewCell.layoutInfo.totalHeight > 0) {\n",
"\t\t\t\tthis.relayoutCell();\n",
"\t\t\t}\n",
"\n",
"\t\t\t// Update for selection\n",
"\t\t\tthis._register(this.notebookEditor.onDidChangeSelection(() => {\n",
"\t\t\t\tconst selectedCells = this.notebookEditor.getSelectionViewModels();\n",
"\n",
"\t\t\t\t// Only show selection if there are more than one cells selected\n",
"\t\t\t\tconst isSelected = selectedCells.length > 1 && selectedCells.some(selectedCell => selectedCell === viewCell);\n",
"\t\t\t\tthis.notebookEditor.updateMarkdownPreviewSelectionState(viewCell, isSelected);\n",
"\t\t\t}));\n",
"\t\t}\n",
"\n",
"\t\t// apply decorations\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/markdownCell.ts",
"type": "replace",
"edit_start_line_idx": 200
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { MainContext, MainThreadStorageShape, ExtHostStorageShape } from './extHost.protocol';
import { Emitter } from 'vs/base/common/event';
import { IExtHostRpcService } from 'vs/workbench/api/common/extHostRpcService';
import { createDecorator } from 'vs/platform/instantiation/common/instantiation';
import { IExtensionIdWithVersion } from 'vs/platform/userDataSync/common/extensionsStorageSync';
export interface IStorageChangeEvent {
shared: boolean;
key: string;
value: object;
}
export class ExtHostStorage implements ExtHostStorageShape {
readonly _serviceBrand: undefined;
private _proxy: MainThreadStorageShape;
private readonly _onDidChangeStorage = new Emitter<IStorageChangeEvent>();
readonly onDidChangeStorage = this._onDidChangeStorage.event;
constructor(mainContext: IExtHostRpcService) {
this._proxy = mainContext.getProxy(MainContext.MainThreadStorage);
}
registerExtensionStorageKeysToSync(extension: IExtensionIdWithVersion, keys: string[]): void {
this._proxy.$registerExtensionStorageKeysToSync(extension, keys);
}
getValue<T>(shared: boolean, key: string, defaultValue?: T): Promise<T | undefined> {
return this._proxy.$getValue<T>(shared, key).then(value => value || defaultValue);
}
setValue(shared: boolean, key: string, value: object): Promise<void> {
return this._proxy.$setValue(shared, key, value);
}
$acceptValue(shared: boolean, key: string, value: object): void {
this._onDidChangeStorage.fire({ shared, key, value });
}
}
export interface IExtHostStorage extends ExtHostStorage { }
export const IExtHostStorage = createDecorator<IExtHostStorage>('IExtHostStorage');
| src/vs/workbench/api/common/extHostStorage.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0003390589263290167,
0.00020364162628538907,
0.0001647599128773436,
0.00017269107047468424,
0.00006778605893487111
] |
{
"id": 10,
"code_window": [
"\t\tif (this.useRenderer) {\n",
"\t\t\t// the markdown preview's height might already be updated after the renderer calls `element.getHeight()`\n",
"\t\t\tif (this.viewCell.layoutInfo.totalHeight > 0) {\n",
"\t\t\t\tthis.relayoutCell();\n",
"\t\t\t}\n",
"\n",
"\t\t\t// Update for selection\n",
"\t\t\tthis._register(this.notebookEditor.onDidChangeSelection(() => {\n",
"\t\t\t\tconst selectedCells = this.notebookEditor.getSelectionViewModels();\n",
"\n",
"\t\t\t\t// Only show selection if there are more than one cells selected\n",
"\t\t\t\tconst isSelected = selectedCells.length > 1 && selectedCells.some(selectedCell => selectedCell === viewCell);\n",
"\t\t\t\tthis.notebookEditor.updateMarkdownPreviewSelectionState(viewCell, isSelected);\n",
"\t\t\t}));\n",
"\t\t}\n",
"\n",
"\t\t// apply decorations\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/markdownCell.ts",
"type": "replace",
"edit_start_line_idx": 200
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { StandardTokenType } from 'vs/editor/common/modes';
import { CharacterPairSupport } from 'vs/editor/common/modes/supports/characterPair';
import { TokenText, createFakeScopedLineTokens } from 'vs/editor/test/common/modesTestUtils';
import { StandardAutoClosingPairConditional } from 'vs/editor/common/modes/languageConfiguration';
suite('CharacterPairSupport', () => {
test('only autoClosingPairs', () => {
let characaterPairSupport = new CharacterPairSupport({ autoClosingPairs: [{ open: 'a', close: 'b' }] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), [new StandardAutoClosingPairConditional({ open: 'a', close: 'b' })]);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), [new StandardAutoClosingPairConditional({ open: 'a', close: 'b' })]);
});
test('only empty autoClosingPairs', () => {
let characaterPairSupport = new CharacterPairSupport({ autoClosingPairs: [] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), []);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), []);
});
test('only brackets', () => {
let characaterPairSupport = new CharacterPairSupport({ brackets: [['a', 'b']] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), [new StandardAutoClosingPairConditional({ open: 'a', close: 'b' })]);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), [new StandardAutoClosingPairConditional({ open: 'a', close: 'b' })]);
});
test('only empty brackets', () => {
let characaterPairSupport = new CharacterPairSupport({ brackets: [] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), []);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), []);
});
test('only surroundingPairs', () => {
let characaterPairSupport = new CharacterPairSupport({ surroundingPairs: [{ open: 'a', close: 'b' }] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), []);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), [{ open: 'a', close: 'b' }]);
});
test('only empty surroundingPairs', () => {
let characaterPairSupport = new CharacterPairSupport({ surroundingPairs: [] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), []);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), []);
});
test('brackets is ignored when having autoClosingPairs', () => {
let characaterPairSupport = new CharacterPairSupport({ autoClosingPairs: [], brackets: [['a', 'b']] });
assert.deepStrictEqual(characaterPairSupport.getAutoClosingPairs(), []);
assert.deepStrictEqual(characaterPairSupport.getSurroundingPairs(), []);
});
function findAutoClosingPair(characterPairSupport: CharacterPairSupport, character: string): StandardAutoClosingPairConditional | undefined {
return characterPairSupport.getAutoClosingPairs().find(autoClosingPair => autoClosingPair.open === character);
}
function testShouldAutoClose(characterPairSupport: CharacterPairSupport, line: TokenText[], character: string, column: number): boolean {
const autoClosingPair = findAutoClosingPair(characterPairSupport, character);
if (!autoClosingPair) {
return false;
}
return CharacterPairSupport.shouldAutoClosePair(autoClosingPair, createFakeScopedLineTokens(line), column);
}
test('shouldAutoClosePair in empty line', () => {
let sup = new CharacterPairSupport({ autoClosingPairs: [{ open: '{', close: '}', notIn: ['string', 'comment'] }] });
assert.strictEqual(testShouldAutoClose(sup, [], 'a', 1), false);
assert.strictEqual(testShouldAutoClose(sup, [], '{', 1), true);
});
test('shouldAutoClosePair in not interesting line 1', () => {
let sup = new CharacterPairSupport({ autoClosingPairs: [{ open: '{', close: '}', notIn: ['string', 'comment'] }] });
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'do', type: StandardTokenType.Other }], '{', 3), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'do', type: StandardTokenType.Other }], 'a', 3), false);
});
test('shouldAutoClosePair in not interesting line 2', () => {
let sup = new CharacterPairSupport({ autoClosingPairs: [{ open: '{', close: '}' }] });
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'do', type: StandardTokenType.String }], '{', 3), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'do', type: StandardTokenType.String }], 'a', 3), false);
});
test('shouldAutoClosePair in interesting line 1', () => {
let sup = new CharacterPairSupport({ autoClosingPairs: [{ open: '{', close: '}', notIn: ['string', 'comment'] }] });
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], '{', 1), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], 'a', 1), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], '{', 2), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], 'a', 2), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], '{', 3), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], 'a', 3), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], '{', 4), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: '"a"', type: StandardTokenType.String }], 'a', 4), false);
});
test('shouldAutoClosePair in interesting line 2', () => {
let sup = new CharacterPairSupport({ autoClosingPairs: [{ open: '{', close: '}', notIn: ['string', 'comment'] }] });
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 1), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 1), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 2), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 2), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 3), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 3), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 4), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 4), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 5), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 5), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 6), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 6), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], '{', 7), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: 'x=', type: StandardTokenType.Other }, { text: '"a"', type: StandardTokenType.String }, { text: ';', type: StandardTokenType.Other }], 'a', 7), false);
});
test('shouldAutoClosePair in interesting line 3', () => {
let sup = new CharacterPairSupport({ autoClosingPairs: [{ open: '{', close: '}', notIn: ['string', 'comment'] }] });
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], '{', 1), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], 'a', 1), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], '{', 2), true);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], 'a', 2), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], '{', 3), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], 'a', 3), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], '{', 4), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], 'a', 4), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], '{', 5), false);
assert.strictEqual(testShouldAutoClose(sup, [{ text: ' ', type: StandardTokenType.Other }, { text: '//a', type: StandardTokenType.Comment }], 'a', 5), false);
});
});
| src/vs/editor/test/common/modes/supports/characterPair.test.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017638418648857623,
0.0001733398821670562,
0.00016621127724647522,
0.00017352262511849403,
0.0000022407502910937183
] |
{
"id": 11,
"code_window": [
"\t\t\t\t\t\tcellContainer.parentElement?.removeChild(cellContainer);\n",
"\t\t\t\t\t}\n",
"\t\t\t\t}\n",
"\t\t\t\tbreak;\n",
"\t\t\tcase 'updateMarkdownPreviewSelectionState':\n",
"\t\t\t\t{\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t\tcase 'updateSelectedMarkdownPreviews':\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads.ts",
"type": "replace",
"edit_start_line_idx": 552
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { IMouseWheelEvent } from 'vs/base/browser/mouseEvent';
import { IListContextMenuEvent, IListEvent, IListMouseEvent } from 'vs/base/browser/ui/list/list';
import { IListOptions, IListStyles } from 'vs/base/browser/ui/list/listWidget';
import { ProgressBar } from 'vs/base/browser/ui/progressbar/progressbar';
import { ToolBar } from 'vs/base/browser/ui/toolbar/toolbar';
import { Emitter, Event } from 'vs/base/common/event';
import { DisposableStore, IDisposable } from 'vs/base/common/lifecycle';
import { ScrollEvent } from 'vs/base/common/scrollable';
import { URI } from 'vs/base/common/uri';
import { ICodeEditor } from 'vs/editor/browser/editorBrowser';
import { BareFontInfo } from 'vs/editor/common/config/fontInfo';
import { IPosition } from 'vs/editor/common/core/position';
import { Range } from 'vs/editor/common/core/range';
import { FindMatch, IReadonlyTextBuffer, ITextModel } from 'vs/editor/common/model';
import { ContextKeyExpr, RawContextKey, IContextKeyService } from 'vs/platform/contextkey/common/contextkey';
import { OutputRenderer } from 'vs/workbench/contrib/notebook/browser/view/output/outputRenderer';
import { RunStateRenderer, TimerRenderer } from 'vs/workbench/contrib/notebook/browser/view/renderers/cellRenderer';
import { CellViewModel, IModelDecorationsChangeAccessor, NotebookViewModel } from 'vs/workbench/contrib/notebook/browser/viewModel/notebookViewModel';
import { NotebookCellTextModel } from 'vs/workbench/contrib/notebook/common/model/notebookCellTextModel';
import { CellKind, NotebookCellMetadata, NotebookDocumentMetadata, INotebookKernel, ICellRange, IOrderedMimeType, INotebookRendererInfo, ICellOutput, IOutputItemDto, cellRangesToIndexes, reduceRanges } from 'vs/workbench/contrib/notebook/common/notebookCommon';
import { Webview } from 'vs/workbench/contrib/webview/browser/webview';
import { NotebookTextModel } from 'vs/workbench/contrib/notebook/common/model/notebookTextModel';
import { IMenu } from 'vs/platform/actions/common/actions';
import { EditorOptions, IEditorPane } from 'vs/workbench/common/editor';
import { IResourceEditorInput } from 'vs/platform/editor/common/editor';
import { IConstructorSignature1 } from 'vs/platform/instantiation/common/instantiation';
import { CellEditorStatusBar } from 'vs/workbench/contrib/notebook/browser/view/renderers/cellWidgets';
//#region Context Keys
export const KEYBINDING_CONTEXT_NOTEBOOK_FIND_WIDGET_FOCUSED = new RawContextKey<boolean>('notebookFindWidgetFocused', false);
// Is Notebook
export const NOTEBOOK_IS_ACTIVE_EDITOR = ContextKeyExpr.equals('activeEditor', 'workbench.editor.notebook');
// Editor keys
export const NOTEBOOK_EDITOR_FOCUSED = new RawContextKey<boolean>('notebookEditorFocused', false);
export const NOTEBOOK_EDITOR_OPEN = new RawContextKey<boolean>('notebookEditorOpen', false);
export const NOTEBOOK_CELL_LIST_FOCUSED = new RawContextKey<boolean>('notebookCellListFocused', false);
export const NOTEBOOK_OUTPUT_FOCUSED = new RawContextKey<boolean>('notebookOutputFocused', false);
export const NOTEBOOK_EDITOR_EDITABLE = new RawContextKey<boolean>('notebookEditable', true);
export const NOTEBOOK_HAS_RUNNING_CELL = new RawContextKey<boolean>('notebookHasRunningCell', false);
// Diff Editor Keys
export const IN_NOTEBOOK_TEXT_DIFF_EDITOR = new RawContextKey<boolean>('isInNotebookTextDiffEditor', false);
// Cell keys
export const NOTEBOOK_VIEW_TYPE = new RawContextKey<string>('notebookViewType', undefined);
export const NOTEBOOK_CELL_TYPE = new RawContextKey<string>('notebookCellType', undefined); // code, markdown
export const NOTEBOOK_CELL_EDITABLE = new RawContextKey<boolean>('notebookCellEditable', false); // bool
export const NOTEBOOK_CELL_FOCUSED = new RawContextKey<boolean>('notebookCellFocused', false); // bool
export const NOTEBOOK_CELL_EDITOR_FOCUSED = new RawContextKey<boolean>('notebookCellEditorFocused', false); // bool
export const NOTEBOOK_CELL_MARKDOWN_EDIT_MODE = new RawContextKey<boolean>('notebookCellMarkdownEditMode', false); // bool
export type NotebookCellExecutionStateContext = 'idle' | 'pending' | 'executing' | 'succeeded' | 'failed';
export const NOTEBOOK_CELL_EXECUTION_STATE = new RawContextKey<NotebookCellExecutionStateContext>('notebookCellExecutionState', undefined);
export const NOTEBOOK_CELL_HAS_OUTPUTS = new RawContextKey<boolean>('notebookCellHasOutputs', false); // bool
export const NOTEBOOK_CELL_INPUT_COLLAPSED = new RawContextKey<boolean>('notebookCellInputIsCollapsed', false); // bool
export const NOTEBOOK_CELL_OUTPUT_COLLAPSED = new RawContextKey<boolean>('notebookCellOutputIsCollapsed', false); // bool
// Kernels
export const NOTEBOOK_HAS_MULTIPLE_KERNELS = new RawContextKey<boolean>('notebookHasMultipleKernels', false);
export const NOTEBOOK_KERNEL_COUNT = new RawContextKey<number>('notebookKernelCount', 0);
export const NOTEBOOK_INTERRUPTIBLE_KERNEL = new RawContextKey<boolean>('notebookInterruptibleKernel', false);
//#endregion
//#region Shared commands
export const EXPAND_CELL_INPUT_COMMAND_ID = 'notebook.cell.expandCellInput';
export const EXECUTE_CELL_COMMAND_ID = 'notebook.cell.execute';
//#endregion
//#region Output related types
export const enum RenderOutputType {
Mainframe,
Html,
Extension
}
export interface IRenderMainframeOutput {
type: RenderOutputType.Mainframe;
supportAppend?: boolean;
}
export interface IRenderPlainHtmlOutput {
type: RenderOutputType.Html;
source: IDisplayOutputViewModel;
htmlContent: string;
}
export interface IRenderOutputViaExtension {
type: RenderOutputType.Extension;
source: IDisplayOutputViewModel;
mimeType: string;
renderer: INotebookRendererInfo;
}
export type IInsetRenderOutput = IRenderPlainHtmlOutput | IRenderOutputViaExtension;
export type IRenderOutput = IRenderMainframeOutput | IInsetRenderOutput;
export interface ICellOutputViewModel {
cellViewModel: IGenericCellViewModel;
/**
* When rendering an output, `model` should always be used as we convert legacy `text/error` output to `display_data` output under the hood.
*/
model: ICellOutput;
resolveMimeTypes(textModel: NotebookTextModel): [readonly IOrderedMimeType[], number];
pickedMimeType: number;
supportAppend(): boolean;
toRawJSON(): any;
}
export interface IDisplayOutputViewModel extends ICellOutputViewModel {
resolveMimeTypes(textModel: NotebookTextModel): [readonly IOrderedMimeType[], number];
pickedMimeType: number;
}
//#endregion
//#region Shared types between the Notebook Editor and Notebook Diff Editor, they are mostly used for output rendering
export interface IGenericCellViewModel {
id: string;
handle: number;
uri: URI;
metadata: NotebookCellMetadata | undefined;
outputIsHovered: boolean;
outputIsFocused: boolean;
outputsViewModels: ICellOutputViewModel[];
getOutputOffset(index: number): number;
updateOutputHeight(index: number, height: number, source?: string): void;
}
export interface IDisplayOutputLayoutUpdateRequest {
output: IDisplayOutputViewModel;
cellTop: number;
outputOffset: number;
}
export interface ICommonCellInfo {
cellId: string;
cellHandle: number;
cellUri: URI;
}
export interface INotebookCellOutputLayoutInfo {
width: number;
height: number;
fontInfo: BareFontInfo;
}
export interface IFocusNotebookCellOptions {
readonly skipReveal?: boolean;
}
export interface ICommonNotebookEditor {
getCellOutputLayoutInfo(cell: IGenericCellViewModel): INotebookCellOutputLayoutInfo;
triggerScroll(event: IMouseWheelEvent): void;
getCellByInfo(cellInfo: ICommonCellInfo): IGenericCellViewModel;
getCellById(cellId: string): IGenericCellViewModel | undefined;
toggleNotebookCellSelection(cell: IGenericCellViewModel): void;
focusNotebookCell(cell: IGenericCellViewModel, focus: 'editor' | 'container' | 'output', options?: IFocusNotebookCellOptions): void;
focusNextNotebookCell(cell: IGenericCellViewModel, focus: 'editor' | 'container' | 'output'): void;
updateOutputHeight(cellInfo: ICommonCellInfo, output: IDisplayOutputViewModel, height: number, isInit: boolean, source?: string): void;
updateMarkdownCellHeight(cellId: string, height: number, isInit: boolean): void;
setMarkdownCellEditState(cellId: string, editState: CellEditState): void;
markdownCellDragStart(cellId: string, position: { clientY: number }): void;
markdownCellDrag(cellId: string, position: { clientY: number }): void;
markdownCellDrop(cellId: string, position: { clientY: number, ctrlKey: boolean, altKey: boolean }): void;
markdownCellDragEnd(cellId: string): void;
}
//#endregion
export interface NotebookLayoutInfo {
width: number;
height: number;
fontInfo: BareFontInfo;
}
export interface NotebookLayoutChangeEvent {
width?: boolean;
height?: boolean;
fontInfo?: boolean;
}
export enum CodeCellLayoutState {
Uninitialized,
Estimated,
FromCache,
Measured
}
export interface CodeCellLayoutInfo {
readonly fontInfo: BareFontInfo | null;
readonly editorHeight: number;
readonly editorWidth: number;
readonly totalHeight: number;
readonly outputContainerOffset: number;
readonly outputTotalHeight: number;
readonly outputShowMoreContainerHeight: number;
readonly outputShowMoreContainerOffset: number;
readonly indicatorHeight: number;
readonly bottomToolbarOffset: number;
readonly layoutState: CodeCellLayoutState;
}
export interface CodeCellLayoutChangeEvent {
source?: string;
editorHeight?: boolean;
outputHeight?: boolean;
outputShowMoreContainerHeight?: number;
totalHeight?: boolean;
outerWidth?: number;
font?: BareFontInfo;
}
export interface MarkdownCellLayoutInfo {
readonly fontInfo: BareFontInfo | null;
readonly editorWidth: number;
readonly editorHeight: number;
readonly bottomToolbarOffset: number;
readonly totalHeight: number;
}
export interface MarkdownCellLayoutChangeEvent {
font?: BareFontInfo;
outerWidth?: number;
totalHeight?: number;
}
export interface ICellViewModel extends IGenericCellViewModel {
readonly model: NotebookCellTextModel;
readonly id: string;
readonly textBuffer: IReadonlyTextBuffer;
readonly layoutInfo: { totalHeight: number; };
readonly onDidChangeLayout: Event<any>;
dragging: boolean;
handle: number;
uri: URI;
language: string;
cellKind: CellKind;
editState: CellEditState;
focusMode: CellFocusMode;
outputIsHovered: boolean;
getText(): string;
getTextLength(): number;
getHeight(lineHeight: number): number;
metadata: NotebookCellMetadata | undefined;
textModel: ITextModel | undefined;
hasModel(): this is IEditableCellViewModel;
resolveTextModel(): Promise<ITextModel>;
getEvaluatedMetadata(documentMetadata: NotebookDocumentMetadata | undefined): NotebookCellMetadata;
getSelectionsStartPosition(): IPosition[] | undefined;
getCellDecorations(): INotebookCellDecorationOptions[];
}
export interface IEditableCellViewModel extends ICellViewModel {
textModel: ITextModel;
}
export interface INotebookEditorMouseEvent {
readonly event: MouseEvent;
readonly target: CellViewModel;
}
export interface INotebookEditorContribution {
/**
* Dispose this contribution.
*/
dispose(): void;
/**
* Store view state.
*/
saveViewState?(): unknown;
/**
* Restore view state.
*/
restoreViewState?(state: unknown): void;
}
export interface INotebookCellDecorationOptions {
className?: string;
gutterClassName?: string;
outputClassName?: string;
topClassName?: string;
}
export interface INotebookDeltaDecoration {
handle: number;
options: INotebookCellDecorationOptions;
}
export class NotebookEditorOptions extends EditorOptions {
readonly cellOptions?: IResourceEditorInput;
readonly cellSelections?: ICellRange[];
constructor(options: Partial<NotebookEditorOptions>) {
super();
this.overwrite(options);
this.cellOptions = options.cellOptions;
this.cellSelections = options.cellSelections;
}
with(options: Partial<NotebookEditorOptions>): NotebookEditorOptions {
return new NotebookEditorOptions({ ...this, ...options });
}
}
export type INotebookEditorContributionCtor = IConstructorSignature1<INotebookEditor, INotebookEditorContribution>;
export interface INotebookEditorContributionDescription {
id: string;
ctor: INotebookEditorContributionCtor;
}
export interface INotebookEditorCreationOptions {
readonly isEmbedded?: boolean;
readonly contributions?: INotebookEditorContributionDescription[];
}
export interface IActiveNotebookEditor extends INotebookEditor {
viewModel: NotebookViewModel;
getFocus(): ICellRange;
}
export const NOTEBOOK_EDITOR_ID = 'workbench.editor.notebook';
export const NOTEBOOK_DIFF_EDITOR_ID = 'workbench.editor.notebookTextDiffEditor';
export interface INotebookEditor extends ICommonNotebookEditor {
// from the old IEditor
readonly onDidChangeVisibleRanges: Event<void>;
readonly onDidChangeSelection: Event<void>;
getSelections(): ICellRange[];
visibleRanges: ICellRange[];
textModel?: NotebookTextModel;
getId(): string;
hasFocus(): boolean;
isEmbedded: boolean;
cursorNavigationMode: boolean;
/**
* Notebook view model attached to the current editor
*/
viewModel: NotebookViewModel | undefined;
hasModel(): this is IActiveNotebookEditor;
/**
* An event emitted when the model of this editor has changed.
* @event
*/
readonly onDidChangeModel: Event<NotebookTextModel | undefined>;
readonly onDidFocusEditorWidget: Event<void>;
activeKernel: INotebookKernel | undefined;
multipleKernelsAvailable: boolean;
readonly onDidScroll: Event<void>;
readonly onDidChangeAvailableKernels: Event<void>;
readonly onDidChangeKernel: Event<void>;
readonly onDidChangeActiveCell: Event<void>;
isDisposed: boolean;
dispose(): void;
getId(): string;
getDomNode(): HTMLElement;
getOverflowContainerDomNode(): HTMLElement;
getInnerWebview(): Webview | undefined;
getSelectionViewModels(): ICellViewModel[];
/**
* Focus the notebook editor cell list
*/
focus(): void;
hasFocus(): boolean;
hasWebviewFocus(): boolean;
hasOutputTextSelection(): boolean;
setOptions(options: NotebookEditorOptions | undefined): Promise<void>;
/**
* Select & focus cell
*/
focusElement(cell: ICellViewModel): void;
/**
* Layout info for the notebook editor
*/
getLayoutInfo(): NotebookLayoutInfo;
getVisibleRangesPlusViewportAboveBelow(): ICellRange[];
/**
* Fetch the output renderers for notebook outputs.
*/
getOutputRenderer(): OutputRenderer;
/**
* Fetch the contributed kernels for this notebook
*/
beginComputeContributedKernels(): Promise<INotebookKernel[]>;
/**
* Insert a new cell around `cell`
*/
insertNotebookCell(cell: ICellViewModel | undefined, type: CellKind, direction?: 'above' | 'below', initialText?: string, ui?: boolean): CellViewModel | null;
/**
* Split a given cell into multiple cells of the same type using the selection start positions.
*/
splitNotebookCell(cell: ICellViewModel): Promise<CellViewModel[] | null>;
/**
* Delete a cell from the notebook
*/
deleteNotebookCell(cell: ICellViewModel): Promise<boolean>;
/**
* Move a cell up one spot
*/
moveCellUp(cell: ICellViewModel): Promise<ICellViewModel | null>;
/**
* Move a cell down one spot
*/
moveCellDown(cell: ICellViewModel): Promise<ICellViewModel | null>;
/**
* Move a cell to a specific position
*/
moveCellsToIdx(index: number, length: number, toIdx: number): Promise<ICellViewModel | null>;
/**
* Focus the container of a cell (the monaco editor inside is not focused).
*/
focusNotebookCell(cell: ICellViewModel, focus: 'editor' | 'container' | 'output'): void;
focusNextNotebookCell(cell: ICellViewModel, focus: 'editor' | 'container' | 'output'): void;
/**
* Execute the given notebook cell
*/
executeNotebookCell(cell: ICellViewModel): Promise<void>;
/**
* Cancel the cell execution
*/
cancelNotebookCellExecution(cell: ICellViewModel): void;
/**
* Executes all notebook cells in order
*/
executeNotebook(): Promise<void>;
/**
* Cancel the notebook execution
*/
cancelNotebookExecution(): void;
/**
* Get current active cell
*/
getActiveCell(): ICellViewModel | undefined;
/**
* Layout the cell with a new height
*/
layoutNotebookCell(cell: ICellViewModel, height: number): Promise<void>;
createMarkdownPreview(cell: ICellViewModel): Promise<void>;
unhideMarkdownPreview(cell: ICellViewModel): Promise<void>;
hideMarkdownPreview(cell: ICellViewModel): Promise<void>;
removeMarkdownPreview(cell: ICellViewModel): Promise<void>;
updateMarkdownPreviewSelectionState(cell: ICellViewModel, isSelected: boolean): Promise<void>;
/**
* Render the output in webview layer
*/
createOutput(cell: ICellViewModel, output: IInsetRenderOutput, offset: number): Promise<void>;
/**
* Remove the output from the webview layer
*/
removeInset(output: IDisplayOutputViewModel): void;
/**
* Hide the inset in the webview layer without removing it
*/
hideInset(output: IDisplayOutputViewModel): void;
/**
* Send message to the webview for outputs.
*/
postMessage(forRendererId: string | undefined, message: any): void;
/**
* Remove class name on the notebook editor root DOM node.
*/
addClassName(className: string): void;
/**
* Remove class name on the notebook editor root DOM node.
*/
removeClassName(className: string): void;
deltaCellOutputContainerClassNames(cellId: string, added: string[], removed: string[]): void;
/**
* Trigger the editor to scroll from scroll event programmatically
*/
triggerScroll(event: IMouseWheelEvent): void;
/**
* The range will be revealed with as little scrolling as possible.
*/
revealCellRangeInView(range: ICellRange): void;
/**
* Reveal cell into viewport.
*/
revealInView(cell: ICellViewModel): void;
/**
* Reveal cell into the top of viewport.
*/
revealInViewAtTop(cell: ICellViewModel): void;
/**
* Reveal cell into viewport center.
*/
revealInCenter(cell: ICellViewModel): void;
/**
* Reveal cell into viewport center if cell is currently out of the viewport.
*/
revealInCenterIfOutsideViewport(cell: ICellViewModel): void;
/**
* Reveal a line in notebook cell into viewport with minimal scrolling.
*/
revealLineInViewAsync(cell: ICellViewModel, line: number): Promise<void>;
/**
* Reveal a line in notebook cell into viewport center.
*/
revealLineInCenterAsync(cell: ICellViewModel, line: number): Promise<void>;
/**
* Reveal a line in notebook cell into viewport center.
*/
revealLineInCenterIfOutsideViewportAsync(cell: ICellViewModel, line: number): Promise<void>;
/**
* Reveal a range in notebook cell into viewport with minimal scrolling.
*/
revealRangeInViewAsync(cell: ICellViewModel, range: Range): Promise<void>;
/**
* Reveal a range in notebook cell into viewport center.
*/
revealRangeInCenterAsync(cell: ICellViewModel, range: Range): Promise<void>;
/**
* Reveal a range in notebook cell into viewport center.
*/
revealRangeInCenterIfOutsideViewportAsync(cell: ICellViewModel, range: Range): Promise<void>;
/**
* Get the view index of a cell
*/
getViewIndex(cell: ICellViewModel): number;
/**
* Get the view height of a cell (from the list view)
*/
getViewHeight(cell: ICellViewModel): number;
/**
* @param startIndex Inclusive
* @param endIndex Exclusive
*/
getCellRangeFromViewRange(startIndex: number, endIndex: number): ICellRange | undefined;
/**
* @param startIndex Inclusive
* @param endIndex Exclusive
*/
getCellsFromViewRange(startIndex: number, endIndex: number): ICellViewModel[];
/**
* Set hidden areas on cell text models.
*/
setHiddenAreas(_ranges: ICellRange[]): boolean;
setCellEditorSelection(cell: ICellViewModel, selection: Range): void;
deltaCellDecorations(oldDecorations: string[], newDecorations: INotebookDeltaDecoration[]): string[];
/**
* Change the decorations on cells.
* The notebook is virtualized and this method should be called to create/delete editor decorations safely.
*/
changeModelDecorations<T>(callback: (changeAccessor: IModelDecorationsChangeAccessor) => T): T | null;
setEditorDecorations(key: string, range: ICellRange): void;
removeEditorDecorations(key: string): void;
/**
* An event emitted on a "mouseup".
* @event
*/
onMouseUp(listener: (e: INotebookEditorMouseEvent) => void): IDisposable;
/**
* An event emitted on a "mousedown".
* @event
*/
onMouseDown(listener: (e: INotebookEditorMouseEvent) => void): IDisposable;
/**
* Get a contribution of this editor.
* @id Unique identifier of the contribution.
* @return The contribution or null if contribution not found.
*/
getContribution<T extends INotebookEditorContribution>(id: string): T;
getCellByInfo(cellInfo: ICommonCellInfo): ICellViewModel;
getCellById(cellId: string): ICellViewModel | undefined;
updateOutputHeight(cellInfo: ICommonCellInfo, output: IDisplayOutputViewModel, height: number, isInit: boolean, source?: string): void;
}
export interface INotebookCellList {
isDisposed: boolean;
viewModel: NotebookViewModel | null;
readonly contextKeyService: IContextKeyService;
element(index: number): ICellViewModel | undefined;
elementAt(position: number): ICellViewModel | undefined;
elementHeight(element: ICellViewModel): number;
onWillScroll: Event<ScrollEvent>;
onDidScroll: Event<ScrollEvent>;
onDidChangeFocus: Event<IListEvent<ICellViewModel>>;
onDidChangeContentHeight: Event<number>;
onDidChangeVisibleRanges: Event<void>;
visibleRanges: ICellRange[];
scrollTop: number;
scrollHeight: number;
scrollLeft: number;
length: number;
rowsContainer: HTMLElement;
readonly onDidRemoveOutput: Event<ICellOutputViewModel>;
readonly onDidHideOutput: Event<ICellOutputViewModel>;
readonly onDidRemoveCellFromView: Event<ICellViewModel>;
readonly onMouseUp: Event<IListMouseEvent<CellViewModel>>;
readonly onMouseDown: Event<IListMouseEvent<CellViewModel>>;
readonly onContextMenu: Event<IListContextMenuEvent<CellViewModel>>;
detachViewModel(): void;
attachViewModel(viewModel: NotebookViewModel): void;
clear(): void;
getViewIndex(cell: ICellViewModel): number | undefined;
getViewIndex2(modelIndex: number): number | undefined;
getModelIndex(cell: CellViewModel): number | undefined;
getModelIndex2(viewIndex: number): number | undefined;
getVisibleRangesPlusViewportAboveBelow(): ICellRange[];
focusElement(element: ICellViewModel): void;
selectElements(elements: ICellViewModel[]): void;
getFocusedElements(): ICellViewModel[];
getSelectedElements(): ICellViewModel[];
revealElementsInView(range: ICellRange): void;
revealElementInView(element: ICellViewModel): void;
revealElementInViewAtTop(element: ICellViewModel): void;
revealElementInCenterIfOutsideViewport(element: ICellViewModel): void;
revealElementInCenter(element: ICellViewModel): void;
revealElementInCenterIfOutsideViewportAsync(element: ICellViewModel): Promise<void>;
revealElementLineInViewAsync(element: ICellViewModel, line: number): Promise<void>;
revealElementLineInCenterAsync(element: ICellViewModel, line: number): Promise<void>;
revealElementLineInCenterIfOutsideViewportAsync(element: ICellViewModel, line: number): Promise<void>;
revealElementRangeInViewAsync(element: ICellViewModel, range: Range): Promise<void>;
revealElementRangeInCenterAsync(element: ICellViewModel, range: Range): Promise<void>;
revealElementRangeInCenterIfOutsideViewportAsync(element: ICellViewModel, range: Range): Promise<void>;
setHiddenAreas(_ranges: ICellRange[], triggerViewUpdate: boolean): boolean;
domElementOfElement(element: ICellViewModel): HTMLElement | null;
focusView(): void;
getAbsoluteTopOfElement(element: ICellViewModel): number;
triggerScrollFromMouseWheelEvent(browserEvent: IMouseWheelEvent): void;
updateElementHeight2(element: ICellViewModel, size: number): void;
domFocus(): void;
setCellSelection(element: ICellViewModel, range: Range): void;
style(styles: IListStyles): void;
getRenderHeight(): number;
updateOptions(options: IListOptions<ICellViewModel>): void;
layout(height?: number, width?: number): void;
dispose(): void;
}
export interface BaseCellRenderTemplate {
rootContainer: HTMLElement;
editorPart: HTMLElement;
collapsedPart: HTMLElement;
expandButton: HTMLElement;
contextKeyService: IContextKeyService;
container: HTMLElement;
cellContainer: HTMLElement;
decorationContainer: HTMLElement;
toolbar: ToolBar;
deleteToolbar: ToolBar;
betweenCellToolbar: ToolBar;
focusIndicatorLeft: HTMLElement;
focusIndicatorRight: HTMLElement;
disposables: DisposableStore;
elementDisposables: DisposableStore;
bottomCellContainer: HTMLElement;
currentRenderedCell?: ICellViewModel;
statusBar: CellEditorStatusBar;
titleMenu: IMenu;
toJSON: () => object;
}
export interface MarkdownCellRenderTemplate extends BaseCellRenderTemplate {
editorContainer: HTMLElement;
foldingIndicator: HTMLElement;
focusIndicatorBottom: HTMLElement;
currentEditor?: ICodeEditor;
readonly useRenderer: boolean;
}
export interface CodeCellRenderTemplate extends BaseCellRenderTemplate {
cellRunState: RunStateRenderer;
runToolbar: ToolBar;
runButtonContainer: HTMLElement;
executionOrderLabel: HTMLElement;
outputContainer: HTMLElement;
outputShowMoreContainer: HTMLElement;
focusSinkElement: HTMLElement;
editor: ICodeEditor;
progressBar: ProgressBar;
timer: TimerRenderer;
focusIndicatorRight: HTMLElement;
focusIndicatorBottom: HTMLElement;
dragHandle: HTMLElement;
}
export function isCodeCellRenderTemplate(templateData: BaseCellRenderTemplate): templateData is CodeCellRenderTemplate {
return !!(templateData as CodeCellRenderTemplate).runToolbar;
}
export interface IOutputTransformContribution {
getMimetypes(): string[];
/**
* Dispose this contribution.
*/
dispose(): void;
/**
* Returns contents to place in the webview inset, or the {@link IRenderNoOutput}.
* This call is allowed to have side effects, such as placing output
* directly into the container element.
*/
render(output: ICellOutputViewModel, items: IOutputItemDto[], container: HTMLElement, notebookUri: URI | undefined): IRenderOutput;
}
export interface CellFindMatch {
cell: CellViewModel;
matches: FindMatch[];
}
export enum CellRevealType {
Line,
Range
}
export enum CellRevealPosition {
Top,
Center,
Bottom
}
export enum CellEditState {
/**
* Default state.
* For markdown cell, it's Markdown preview.
* For code cell, the browser focus should be on the container instead of the editor
*/
Preview,
/**
* Eding mode. Source for markdown or code is rendered in editors and the state will be persistent.
*/
Editing
}
export enum CellFocusMode {
Container,
Editor
}
export enum CursorAtBoundary {
None,
Top,
Bottom,
Both
}
export interface CellViewModelStateChangeEvent {
readonly metadataChanged?: boolean;
readonly runStateChanged?: boolean;
readonly selectionChanged?: boolean;
readonly focusModeChanged?: boolean;
readonly editStateChanged?: boolean;
readonly languageChanged?: boolean;
readonly foldingStateChanged?: boolean;
readonly contentChanged?: boolean;
readonly outputIsHoveredChanged?: boolean;
readonly outputIsFocusedChanged?: boolean;
readonly cellIsHoveredChanged?: boolean;
}
export function cellRangesEqual(a: ICellRange[], b: ICellRange[]) {
a = reduceCellRanges(a);
b = reduceCellRanges(b);
if (a.length !== b.length) {
return false;
}
for (let i = 0; i < a.length; i++) {
if (a[i].start !== b[i].start || a[i].end !== b[i].end) {
return false;
}
}
return true;
}
/**
* @param _ranges
*/
export function reduceCellRanges(_ranges: ICellRange[]): ICellRange[] {
if (!_ranges.length) {
return [];
}
const ranges = _ranges.sort((a, b) => a.start - b.start);
const result: ICellRange[] = [];
let currentRangeStart = ranges[0].start;
let currentRangeEnd = ranges[0].end + 1;
for (let i = 0, len = ranges.length; i < len; i++) {
const range = ranges[i];
if (range.start > currentRangeEnd) {
result.push({ start: currentRangeStart, end: currentRangeEnd - 1 });
currentRangeStart = range.start;
currentRangeEnd = range.end + 1;
} else if (range.end + 1 > currentRangeEnd) {
currentRangeEnd = range.end + 1;
}
}
result.push({ start: currentRangeStart, end: currentRangeEnd - 1 });
return result;
}
export function getVisibleCells(cells: CellViewModel[], hiddenRanges: ICellRange[]) {
if (!hiddenRanges.length) {
return cells;
}
let start = 0;
let hiddenRangeIndex = 0;
const result: CellViewModel[] = [];
while (start < cells.length && hiddenRangeIndex < hiddenRanges.length) {
if (start < hiddenRanges[hiddenRangeIndex].start) {
result.push(...cells.slice(start, hiddenRanges[hiddenRangeIndex].start));
}
start = hiddenRanges[hiddenRangeIndex].end + 1;
hiddenRangeIndex++;
}
if (start < cells.length) {
result.push(...cells.slice(start));
}
return result;
}
export function getNotebookEditorFromEditorPane(editorPane?: IEditorPane): INotebookEditor | undefined {
return editorPane?.getId() === NOTEBOOK_EDITOR_ID ? editorPane.getControl() as INotebookEditor | undefined : undefined;
}
let EDITOR_TOP_PADDING = 12;
const editorTopPaddingChangeEmitter = new Emitter<void>();
export const EditorTopPaddingChangeEvent = editorTopPaddingChangeEmitter.event;
export function updateEditorTopPadding(top: number) {
EDITOR_TOP_PADDING = top;
editorTopPaddingChangeEmitter.fire();
}
export function getEditorTopPadding() {
return EDITOR_TOP_PADDING;
}
/**
* ranges: model selections
* this will convert model selections to view indexes first, and then include the hidden ranges in the list view
*/
export function expandCellRangesWithHiddenCells(editor: INotebookEditor, viewModel: NotebookViewModel, ranges: ICellRange[]) {
// assuming ranges are sorted and no overlap
const indexes = cellRangesToIndexes(ranges);
let modelRanges: ICellRange[] = [];
indexes.forEach(index => {
const viewCell = viewModel.viewCells[index];
if (!viewCell) {
return;
}
const viewIndex = editor.getViewIndex(viewCell);
if (viewIndex < 0) {
return;
}
const nextViewIndex = viewIndex + 1;
const range = editor.getCellRangeFromViewRange(viewIndex, nextViewIndex);
if (range) {
modelRanges.push(range);
}
});
return reduceRanges(modelRanges);
}
/**
* Return a set of ranges for the cells matching the given predicate
*/
export function getRanges(cells: ICellViewModel[], included: (cell: ICellViewModel) => boolean): ICellRange[] {
const ranges: ICellRange[] = [];
let currentRange: ICellRange | undefined;
cells.forEach((cell, idx) => {
if (included(cell)) {
if (!currentRange) {
currentRange = { start: idx, end: idx + 1 };
ranges.push(currentRange);
} else {
currentRange.end = idx + 1;
}
} else {
currentRange = undefined;
}
});
return ranges;
}
| src/vs/workbench/contrib/notebook/browser/notebookBrowser.ts | 1 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.008005735464394093,
0.0003240216465201229,
0.00016451171541120857,
0.00017036146891769022,
0.0008481223485432565
] |
{
"id": 11,
"code_window": [
"\t\t\t\t\t\tcellContainer.parentElement?.removeChild(cellContainer);\n",
"\t\t\t\t\t}\n",
"\t\t\t\t}\n",
"\t\t\t\tbreak;\n",
"\t\t\tcase 'updateMarkdownPreviewSelectionState':\n",
"\t\t\t\t{\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t\tcase 'updateSelectedMarkdownPreviews':\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads.ts",
"type": "replace",
"edit_start_line_idx": 552
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import * as assert from 'assert';
import { URI } from 'vs/base/common/uri';
import { score } from 'vs/editor/common/modes/languageSelector';
suite('LanguageSelector', function () {
let model = {
language: 'farboo',
uri: URI.parse('file:///testbed/file.fb')
};
test('score, invalid selector', function () {
assert.strictEqual(score({}, model.uri, model.language, true), 0);
assert.strictEqual(score(undefined!, model.uri, model.language, true), 0);
assert.strictEqual(score(null!, model.uri, model.language, true), 0);
assert.strictEqual(score('', model.uri, model.language, true), 0);
});
test('score, any language', function () {
assert.strictEqual(score({ language: '*' }, model.uri, model.language, true), 5);
assert.strictEqual(score('*', model.uri, model.language, true), 5);
assert.strictEqual(score('*', URI.parse('foo:bar'), model.language, true), 5);
assert.strictEqual(score('farboo', URI.parse('foo:bar'), model.language, true), 10);
});
test('score, default schemes', function () {
const uri = URI.parse('git:foo/file.txt');
const language = 'farboo';
assert.strictEqual(score('*', uri, language, true), 5);
assert.strictEqual(score('farboo', uri, language, true), 10);
assert.strictEqual(score({ language: 'farboo', scheme: '' }, uri, language, true), 10);
assert.strictEqual(score({ language: 'farboo', scheme: 'git' }, uri, language, true), 10);
assert.strictEqual(score({ language: 'farboo', scheme: '*' }, uri, language, true), 10);
assert.strictEqual(score({ language: 'farboo' }, uri, language, true), 10);
assert.strictEqual(score({ language: '*' }, uri, language, true), 5);
assert.strictEqual(score({ scheme: '*' }, uri, language, true), 5);
assert.strictEqual(score({ scheme: 'git' }, uri, language, true), 10);
});
test('score, filter', function () {
assert.strictEqual(score('farboo', model.uri, model.language, true), 10);
assert.strictEqual(score({ language: 'farboo' }, model.uri, model.language, true), 10);
assert.strictEqual(score({ language: 'farboo', scheme: 'file' }, model.uri, model.language, true), 10);
assert.strictEqual(score({ language: 'farboo', scheme: 'http' }, model.uri, model.language, true), 0);
assert.strictEqual(score({ pattern: '**/*.fb' }, model.uri, model.language, true), 10);
assert.strictEqual(score({ pattern: '**/*.fb', scheme: 'file' }, model.uri, model.language, true), 10);
assert.strictEqual(score({ pattern: '**/*.fb' }, URI.parse('foo:bar'), model.language, true), 0);
assert.strictEqual(score({ pattern: '**/*.fb', scheme: 'foo' }, URI.parse('foo:bar'), model.language, true), 0);
let doc = {
uri: URI.parse('git:/my/file.js'),
langId: 'javascript'
};
assert.strictEqual(score('javascript', doc.uri, doc.langId, true), 10); // 0;
assert.strictEqual(score({ language: 'javascript', scheme: 'git' }, doc.uri, doc.langId, true), 10); // 10;
assert.strictEqual(score('*', doc.uri, doc.langId, true), 5); // 5
assert.strictEqual(score('fooLang', doc.uri, doc.langId, true), 0); // 0
assert.strictEqual(score(['fooLang', '*'], doc.uri, doc.langId, true), 5); // 5
});
test('score, max(filters)', function () {
let match = { language: 'farboo', scheme: 'file' };
let fail = { language: 'farboo', scheme: 'http' };
assert.strictEqual(score(match, model.uri, model.language, true), 10);
assert.strictEqual(score(fail, model.uri, model.language, true), 0);
assert.strictEqual(score([match, fail], model.uri, model.language, true), 10);
assert.strictEqual(score([fail, fail], model.uri, model.language, true), 0);
assert.strictEqual(score(['farboo', '*'], model.uri, model.language, true), 10);
assert.strictEqual(score(['*', 'farboo'], model.uri, model.language, true), 10);
});
test('score hasAccessToAllModels', function () {
let doc = {
uri: URI.parse('file:/my/file.js'),
langId: 'javascript'
};
assert.strictEqual(score('javascript', doc.uri, doc.langId, false), 0);
assert.strictEqual(score({ language: 'javascript', scheme: 'file' }, doc.uri, doc.langId, false), 0);
assert.strictEqual(score('*', doc.uri, doc.langId, false), 0);
assert.strictEqual(score('fooLang', doc.uri, doc.langId, false), 0);
assert.strictEqual(score(['fooLang', '*'], doc.uri, doc.langId, false), 0);
assert.strictEqual(score({ language: 'javascript', scheme: 'file', hasAccessToAllModels: true }, doc.uri, doc.langId, false), 10);
assert.strictEqual(score(['fooLang', '*', { language: '*', hasAccessToAllModels: true }], doc.uri, doc.langId, false), 5);
});
test('Document selector match - unexpected result value #60232', function () {
let selector = {
language: 'json',
scheme: 'file',
pattern: '**/*.interface.json'
};
let value = score(selector, URI.parse('file:///C:/Users/zlhe/Desktop/test.interface.json'), 'json', true);
assert.strictEqual(value, 10);
});
test('Document selector match - platform paths #99938', function () {
let selector = {
pattern: {
base: '/home/user/Desktop',
pattern: '*.json'
}
};
let value = score(selector, URI.file('/home/user/Desktop/test.json'), 'json', true);
assert.strictEqual(value, 10);
});
});
| src/vs/editor/test/common/modes/languageSelector.test.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00017951839254237711,
0.0001741180894896388,
0.00016939573106355965,
0.00017378285701852292,
0.0000025764875317690894
] |
{
"id": 11,
"code_window": [
"\t\t\t\t\t\tcellContainer.parentElement?.removeChild(cellContainer);\n",
"\t\t\t\t\t}\n",
"\t\t\t\t}\n",
"\t\t\t\tbreak;\n",
"\t\t\tcase 'updateMarkdownPreviewSelectionState':\n",
"\t\t\t\t{\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t\tcase 'updateSelectedMarkdownPreviews':\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads.ts",
"type": "replace",
"edit_start_line_idx": 552
} | /*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import 'vs/workbench/contrib/markers/browser/markersFileDecorations';
import { ContextKeyEqualsExpr, ContextKeyExpr } from 'vs/platform/contextkey/common/contextkey';
import { Extensions, IConfigurationRegistry } from 'vs/platform/configuration/common/configurationRegistry';
import { CATEGORIES } from 'vs/workbench/common/actions';
import { KeybindingsRegistry, KeybindingWeight } from 'vs/platform/keybinding/common/keybindingsRegistry';
import { KeyCode, KeyMod } from 'vs/base/common/keyCodes';
import { localize } from 'vs/nls';
import { Marker, RelatedInformation } from 'vs/workbench/contrib/markers/browser/markersModel';
import { MarkersView } from 'vs/workbench/contrib/markers/browser/markersView';
import { MenuId, registerAction2, Action2 } from 'vs/platform/actions/common/actions';
import { Registry } from 'vs/platform/registry/common/platform';
import Constants from 'vs/workbench/contrib/markers/browser/constants';
import Messages from 'vs/workbench/contrib/markers/browser/messages';
import { IWorkbenchContributionsRegistry, Extensions as WorkbenchExtensions, IWorkbenchContribution } from 'vs/workbench/common/contributions';
import { ActivityUpdater, IMarkersView } from 'vs/workbench/contrib/markers/browser/markers';
import { LifecyclePhase } from 'vs/workbench/services/lifecycle/common/lifecycle';
import { IClipboardService } from 'vs/platform/clipboard/common/clipboardService';
import { Disposable } from 'vs/base/common/lifecycle';
import { IStatusbarEntryAccessor, IStatusbarService, StatusbarAlignment, IStatusbarEntry } from 'vs/workbench/services/statusbar/common/statusbar';
import { IMarkerService, MarkerStatistics } from 'vs/platform/markers/common/markers';
import { ViewContainer, IViewContainersRegistry, Extensions as ViewContainerExtensions, ViewContainerLocation, IViewsRegistry, IViewsService, getVisbileViewContextKey, FocusedViewContext } from 'vs/workbench/common/views';
import { ViewPaneContainer } from 'vs/workbench/browser/parts/views/viewPaneContainer';
import { SyncDescriptor } from 'vs/platform/instantiation/common/descriptors';
import { ServicesAccessor } from 'vs/platform/instantiation/common/instantiation';
import { Codicon } from 'vs/base/common/codicons';
import { registerIcon } from 'vs/platform/theme/common/iconRegistry';
import { ViewAction } from 'vs/workbench/browser/parts/views/viewPane';
KeybindingsRegistry.registerCommandAndKeybindingRule({
id: Constants.MARKER_OPEN_ACTION_ID,
weight: KeybindingWeight.WorkbenchContrib,
when: ContextKeyExpr.and(Constants.MarkerFocusContextKey),
primary: KeyCode.Enter,
mac: {
primary: KeyCode.Enter,
secondary: [KeyMod.CtrlCmd | KeyCode.DownArrow]
},
handler: (accessor, args: any) => {
const markersView = accessor.get(IViewsService).getActiveViewWithId<MarkersView>(Constants.MARKERS_VIEW_ID)!;
markersView.openFileAtElement(markersView.getFocusElement(), false, false, true);
}
});
KeybindingsRegistry.registerCommandAndKeybindingRule({
id: Constants.MARKER_OPEN_SIDE_ACTION_ID,
weight: KeybindingWeight.WorkbenchContrib,
when: ContextKeyExpr.and(Constants.MarkerFocusContextKey),
primary: KeyMod.CtrlCmd | KeyCode.Enter,
mac: {
primary: KeyMod.WinCtrl | KeyCode.Enter
},
handler: (accessor, args: any) => {
const markersView = accessor.get(IViewsService).getActiveViewWithId<MarkersView>(Constants.MARKERS_VIEW_ID)!;
markersView.openFileAtElement(markersView.getFocusElement(), false, true, true);
}
});
KeybindingsRegistry.registerCommandAndKeybindingRule({
id: Constants.MARKER_SHOW_PANEL_ID,
weight: KeybindingWeight.WorkbenchContrib,
when: undefined,
primary: undefined,
handler: async (accessor, args: any) => {
await accessor.get(IViewsService).openView(Constants.MARKERS_VIEW_ID);
}
});
KeybindingsRegistry.registerCommandAndKeybindingRule({
id: Constants.MARKER_SHOW_QUICK_FIX,
weight: KeybindingWeight.WorkbenchContrib,
when: Constants.MarkerFocusContextKey,
primary: KeyMod.CtrlCmd | KeyCode.US_DOT,
handler: (accessor, args: any) => {
const markersView = accessor.get(IViewsService).getActiveViewWithId<MarkersView>(Constants.MARKERS_VIEW_ID)!;
const focusedElement = markersView.getFocusElement();
if (focusedElement instanceof Marker) {
markersView.showQuickFixes(focusedElement);
}
}
});
// configuration
Registry.as<IConfigurationRegistry>(Extensions.Configuration).registerConfiguration({
'id': 'problems',
'order': 101,
'title': Messages.PROBLEMS_PANEL_CONFIGURATION_TITLE,
'type': 'object',
'properties': {
'problems.autoReveal': {
'description': Messages.PROBLEMS_PANEL_CONFIGURATION_AUTO_REVEAL,
'type': 'boolean',
'default': true
},
'problems.showCurrentInStatus': {
'description': Messages.PROBLEMS_PANEL_CONFIGURATION_SHOW_CURRENT_STATUS,
'type': 'boolean',
'default': false
}
}
});
const markersViewIcon = registerIcon('markers-view-icon', Codicon.warning, localize('markersViewIcon', 'View icon of the markers view.'));
// markers view container
const VIEW_CONTAINER: ViewContainer = Registry.as<IViewContainersRegistry>(ViewContainerExtensions.ViewContainersRegistry).registerViewContainer({
id: Constants.MARKERS_CONTAINER_ID,
title: Messages.MARKERS_PANEL_TITLE_PROBLEMS,
icon: markersViewIcon,
hideIfEmpty: true,
order: 0,
ctorDescriptor: new SyncDescriptor(ViewPaneContainer, [Constants.MARKERS_CONTAINER_ID, { mergeViewWithContainerWhenSingleView: true, donotShowContainerTitleWhenMergedWithContainer: true }]),
storageId: Constants.MARKERS_VIEW_STORAGE_ID,
}, ViewContainerLocation.Panel, { donotRegisterOpenCommand: true });
Registry.as<IViewsRegistry>(ViewContainerExtensions.ViewsRegistry).registerViews([{
id: Constants.MARKERS_VIEW_ID,
containerIcon: markersViewIcon,
name: Messages.MARKERS_PANEL_TITLE_PROBLEMS,
canToggleVisibility: false,
canMoveView: true,
ctorDescriptor: new SyncDescriptor(MarkersView),
openCommandActionDescriptor: {
id: 'workbench.actions.view.problems',
mnemonicTitle: localize({ key: 'miMarker', comment: ['&& denotes a mnemonic'] }, "&&Problems"),
keybindings: { primary: KeyMod.CtrlCmd | KeyMod.Shift | KeyCode.KEY_M },
order: 0,
}
}], VIEW_CONTAINER);
// workbench
const workbenchRegistry = Registry.as<IWorkbenchContributionsRegistry>(WorkbenchExtensions.Workbench);
workbenchRegistry.registerWorkbenchContribution(ActivityUpdater, LifecyclePhase.Restored);
// actions
registerAction2(class extends Action2 {
constructor() {
super({
id: 'workbench.action.problems.focus',
title: { value: Messages.MARKERS_PANEL_SHOW_LABEL, original: 'Focus Problems (Errors, Warnings, Infos)' },
category: CATEGORIES.View.value,
f1: true,
});
}
async run(accessor: ServicesAccessor): Promise<void> {
accessor.get(IViewsService).openView(Constants.MARKERS_VIEW_ID, true);
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.MARKER_COPY_ACTION_ID,
title: { value: localize('copyMarker', "Copy"), original: 'Copy' },
menu: {
id: MenuId.ProblemsPanelContext,
when: Constants.MarkerFocusContextKey,
group: 'navigation'
},
keybinding: {
weight: KeybindingWeight.WorkbenchContrib,
primary: KeyMod.CtrlCmd | KeyCode.KEY_C,
when: Constants.MarkerFocusContextKey
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
const clipboardService = serviceAccessor.get(IClipboardService);
const element = markersView.getFocusElement();
if (element instanceof Marker) {
await clipboardService.writeText(`${element}`);
}
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.MARKER_COPY_MESSAGE_ACTION_ID,
title: { value: localize('copyMessage', "Copy Message"), original: 'Copy Message' },
menu: {
id: MenuId.ProblemsPanelContext,
when: Constants.MarkerFocusContextKey,
group: 'navigation'
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
const clipboardService = serviceAccessor.get(IClipboardService);
const element = markersView.getFocusElement();
if (element instanceof Marker) {
await clipboardService.writeText(element.marker.message);
}
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.RELATED_INFORMATION_COPY_MESSAGE_ACTION_ID,
title: { value: localize('copyMessage', "Copy Message"), original: 'Copy Message' },
menu: {
id: MenuId.ProblemsPanelContext,
when: Constants.RelatedInformationFocusContextKey,
group: 'navigation'
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
const clipboardService = serviceAccessor.get(IClipboardService);
const element = markersView.getFocusElement();
if (element instanceof RelatedInformation) {
await clipboardService.writeText(element.raw.message);
}
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.FOCUS_PROBLEMS_FROM_FILTER,
title: localize('focusProblemsList', "Focus problems view"),
keybinding: {
when: Constants.MarkerViewFilterFocusContextKey,
weight: KeybindingWeight.WorkbenchContrib,
primary: KeyMod.CtrlCmd | KeyCode.DownArrow
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
markersView.focus();
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.MARKERS_VIEW_FOCUS_FILTER,
title: localize('focusProblemsFilter', "Focus problems filter"),
keybinding: {
when: FocusedViewContext.isEqualTo(Constants.MARKERS_VIEW_ID),
weight: KeybindingWeight.WorkbenchContrib,
primary: KeyMod.CtrlCmd | KeyCode.KEY_F
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
markersView.focusFilter();
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.MARKERS_VIEW_SHOW_MULTILINE_MESSAGE,
title: { value: localize('show multiline', "Show message in multiple lines"), original: 'Problems: Show message in multiple lines' },
category: localize('problems', "Problems"),
menu: {
id: MenuId.CommandPalette,
when: ContextKeyExpr.has(getVisbileViewContextKey(Constants.MARKERS_VIEW_ID))
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
markersView.setMultiline(true);
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.MARKERS_VIEW_SHOW_SINGLELINE_MESSAGE,
title: { value: localize('show singleline', "Show message in single line"), original: 'Problems: Show message in single line' },
category: localize('problems', "Problems"),
menu: {
id: MenuId.CommandPalette,
when: ContextKeyExpr.has(getVisbileViewContextKey(Constants.MARKERS_VIEW_ID))
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
markersView.setMultiline(false);
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: Constants.MARKERS_VIEW_CLEAR_FILTER_TEXT,
title: localize('clearFiltersText', "Clear filters text"),
category: localize('problems', "Problems"),
keybinding: {
when: Constants.MarkerViewFilterFocusContextKey,
weight: KeybindingWeight.WorkbenchContrib,
},
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, markersView: IMarkersView): Promise<void> {
markersView.clearFilterText();
}
});
registerAction2(class extends ViewAction<IMarkersView> {
constructor() {
super({
id: `workbench.actions.treeView.${Constants.MARKERS_VIEW_ID}.collapseAll`,
title: localize('collapseAll', "Collapse All"),
menu: {
id: MenuId.ViewTitle,
when: ContextKeyEqualsExpr.create('view', Constants.MARKERS_VIEW_ID),
group: 'navigation',
order: 2,
},
icon: Codicon.collapseAll,
viewId: Constants.MARKERS_VIEW_ID
});
}
async runInView(serviceAccessor: ServicesAccessor, view: IMarkersView): Promise<void> {
return view.collapseAll();
}
});
registerAction2(class extends Action2 {
constructor() {
super({
id: `workbench.actions.treeView.${Constants.MARKERS_VIEW_ID}.filter`,
title: localize('filter', "Filter"),
menu: {
id: MenuId.ViewTitle,
when: ContextKeyExpr.and(ContextKeyEqualsExpr.create('view', Constants.MARKERS_VIEW_ID), Constants.MarkersViewSmallLayoutContextKey.negate()),
group: 'navigation',
order: 1,
},
});
}
async run(): Promise<void> { }
});
registerAction2(class extends Action2 {
constructor() {
super({
id: Constants.TOGGLE_MARKERS_VIEW_ACTION_ID,
title: Messages.MARKERS_PANEL_TOGGLE_LABEL,
});
}
async run(accessor: ServicesAccessor): Promise<void> {
const viewsService = accessor.get(IViewsService);
if (viewsService.isViewVisible(Constants.MARKERS_VIEW_ID)) {
viewsService.closeView(Constants.MARKERS_VIEW_ID);
} else {
viewsService.openView(Constants.MARKERS_VIEW_ID, true);
}
}
});
class MarkersStatusBarContributions extends Disposable implements IWorkbenchContribution {
private markersStatusItem: IStatusbarEntryAccessor;
constructor(
@IMarkerService private readonly markerService: IMarkerService,
@IStatusbarService private readonly statusbarService: IStatusbarService
) {
super();
this.markersStatusItem = this._register(this.statusbarService.addEntry(this.getMarkersItem(), 'status.problems', localize('status.problems', "Problems"), StatusbarAlignment.LEFT, 50 /* Medium Priority */));
this.markerService.onMarkerChanged(() => this.markersStatusItem.update(this.getMarkersItem()));
}
private getMarkersItem(): IStatusbarEntry {
const markersStatistics = this.markerService.getStatistics();
const tooltip = this.getMarkersTooltip(markersStatistics);
return {
text: this.getMarkersText(markersStatistics),
ariaLabel: tooltip,
tooltip,
command: 'workbench.actions.view.toggleProblems'
};
}
private getMarkersTooltip(stats: MarkerStatistics): string {
const errorTitle = (n: number) => localize('totalErrors', "{0} Errors", n);
const warningTitle = (n: number) => localize('totalWarnings', "{0} Warnings", n);
const infoTitle = (n: number) => localize('totalInfos', "{0} Infos", n);
const titles: string[] = [];
if (stats.errors > 0) {
titles.push(errorTitle(stats.errors));
}
if (stats.warnings > 0) {
titles.push(warningTitle(stats.warnings));
}
if (stats.infos > 0) {
titles.push(infoTitle(stats.infos));
}
if (titles.length === 0) {
return localize('noProblems', "No Problems");
}
return titles.join(', ');
}
private getMarkersText(stats: MarkerStatistics): string {
const problemsText: string[] = [];
// Errors
problemsText.push('$(error) ' + this.packNumber(stats.errors));
// Warnings
problemsText.push('$(warning) ' + this.packNumber(stats.warnings));
// Info (only if any)
if (stats.infos > 0) {
problemsText.push('$(info) ' + this.packNumber(stats.infos));
}
return problemsText.join(' ');
}
private packNumber(n: number): string {
const manyProblems = localize('manyProblems', "10K+");
return n > 9999 ? manyProblems : n > 999 ? n.toString().charAt(0) + 'K' : n.toString();
}
}
workbenchRegistry.registerWorkbenchContribution(MarkersStatusBarContributions, LifecyclePhase.Restored);
| src/vs/workbench/contrib/markers/browser/markers.contribution.ts | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.00044318888103589416,
0.00017698630108498037,
0.00016468719695694745,
0.0001709995704004541,
0.00004022675784653984
] |
{
"id": 11,
"code_window": [
"\t\t\t\t\t\tcellContainer.parentElement?.removeChild(cellContainer);\n",
"\t\t\t\t\t}\n",
"\t\t\t\t}\n",
"\t\t\t\tbreak;\n",
"\t\t\tcase 'updateMarkdownPreviewSelectionState':\n",
"\t\t\t\t{\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"\t\t\tcase 'updateSelectedMarkdownPreviews':\n"
],
"file_path": "src/vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads.ts",
"type": "replace",
"edit_start_line_idx": 552
} | {
"comments": {
"lineComment": "#"
},
"brackets": [
["{", "}"],
["[", "]"],
["(", ")"]
],
"autoClosingPairs": [
["{", "}"],
["[", "]"],
["(", ")"],
{ "open": "\"", "close": "\"", "notIn": ["string"] },
{ "open": "'", "close": "'", "notIn": ["string"] },
{ "open": "`", "close": "`", "notIn": ["string"] }
],
"surroundingPairs": [
["{", "}"],
["[", "]"],
["(", ")"],
["\"", "\""],
["'", "'"],
["`", "`"]
],
"folding": {
"markers": {
"start": "^(?:(?:=pod\\s*$)|(?:\\s*#region\\b))",
"end": "^(?:(?:=cut\\s*$)|(?:\\s*#endregion\\b))"
}
}
}
| extensions/perl/perl.language-configuration.json | 0 | https://github.com/microsoft/vscode/commit/0e5ecf116f0e27d111448c15ffbfa11320a39d08 | [
0.0001795783027773723,
0.00017288979142904282,
0.0001679231208981946,
0.00017202884191647172,
0.000004268476914148778
] |
{
"id": 0,
"code_window": [
" eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),\n",
" eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),\n",
" eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId, event.delay, event.targetIsCurrentFrame)),\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 381
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import path from 'path';
import { PNG, jpegjs } from '../../utilsBundle';
import { splitErrorMessage } from '../../utils/stackTrace';
import { assert, createGuid, debugAssert, headersArrayToObject } from '../../utils';
import { hostPlatform } from '../../utils/hostPlatform';
import type * as accessibility from '../accessibility';
import * as dialog from '../dialog';
import * as dom from '../dom';
import type * as frames from '../frames';
import type { RegisteredListener } from '../../utils/eventsHelper';
import { eventsHelper } from '../../utils/eventsHelper';
import { helper } from '../helper';
import type { JSHandle } from '../javascript';
import * as network from '../network';
import type { PageBinding, PageDelegate } from '../page';
import { Page } from '../page';
import type { Progress } from '../progress';
import type * as types from '../types';
import type { Protocol } from './protocol';
import { getAccessibilityTree } from './wkAccessibility';
import type { WKBrowserContext } from './wkBrowser';
import { WKSession } from './wkConnection';
import { WKExecutionContext } from './wkExecutionContext';
import { RawKeyboardImpl, RawMouseImpl, RawTouchscreenImpl } from './wkInput';
import { WKInterceptableRequest, WKRouteImpl } from './wkInterceptableRequest';
import { WKProvisionalPage } from './wkProvisionalPage';
import { WKWorkers } from './wkWorkers';
import { debugLogger } from '../../common/debugLogger';
import { ManualPromise } from '../../utils/manualPromise';
const UTILITY_WORLD_NAME = '__playwright_utility_world__';
export class WKPage implements PageDelegate {
readonly rawMouse: RawMouseImpl;
readonly rawKeyboard: RawKeyboardImpl;
readonly rawTouchscreen: RawTouchscreenImpl;
_session: WKSession;
private _provisionalPage: WKProvisionalPage | null = null;
readonly _page: Page;
private readonly _pagePromise = new ManualPromise<Page | Error>();
private readonly _pageProxySession: WKSession;
readonly _opener: WKPage | null;
private readonly _requestIdToRequest = new Map<string, WKInterceptableRequest>();
private readonly _workers: WKWorkers;
private readonly _contextIdToContext: Map<number, dom.FrameExecutionContext>;
private _mainFrameContextId?: number;
private _sessionListeners: RegisteredListener[] = [];
private _eventListeners: RegisteredListener[];
readonly _browserContext: WKBrowserContext;
_initializedPage: Page | null = null;
private _firstNonInitialNavigationCommittedPromise: Promise<void>;
private _firstNonInitialNavigationCommittedFulfill = () => {};
_firstNonInitialNavigationCommittedReject = (e: Error) => {};
private _lastConsoleMessage: { derivedType: string, text: string, handles: JSHandle[]; count: number, location: types.ConsoleMessageLocation; } | null = null;
private readonly _requestIdToResponseReceivedPayloadEvent = new Map<string, Protocol.Network.responseReceivedPayload>();
// Holds window features for the next popup being opened via window.open,
// until the popup page proxy arrives.
private _nextWindowOpenPopupFeatures?: string[];
private _recordingVideoFile: string | null = null;
private _screencastGeneration: number = 0;
constructor(browserContext: WKBrowserContext, pageProxySession: WKSession, opener: WKPage | null) {
this._pageProxySession = pageProxySession;
this._opener = opener;
this.rawKeyboard = new RawKeyboardImpl(pageProxySession);
this.rawMouse = new RawMouseImpl(pageProxySession);
this.rawTouchscreen = new RawTouchscreenImpl(pageProxySession);
this._contextIdToContext = new Map();
this._page = new Page(this, browserContext);
this.rawMouse.setPage(this._page);
this._workers = new WKWorkers(this._page);
this._session = undefined as any as WKSession;
this._browserContext = browserContext;
this._page.on(Page.Events.FrameDetached, (frame: frames.Frame) => this._removeContextsForFrame(frame, false));
this._eventListeners = [
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetCreated', this._onTargetCreated.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetDestroyed', this._onTargetDestroyed.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.dispatchMessageFromTarget', this._onDispatchMessageFromTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.didCommitProvisionalTarget', this._onDidCommitProvisionalTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Screencast.screencastFrame', this._onScreencastFrame.bind(this)),
];
this._firstNonInitialNavigationCommittedPromise = new Promise((f, r) => {
this._firstNonInitialNavigationCommittedFulfill = f;
this._firstNonInitialNavigationCommittedReject = r;
});
if (opener && !browserContext._options.noDefaultViewport && opener._nextWindowOpenPopupFeatures) {
const viewportSize = helper.getViewportSizeFromWindowFeatures(opener._nextWindowOpenPopupFeatures);
opener._nextWindowOpenPopupFeatures = undefined;
if (viewportSize)
this._page._emulatedSize = { viewport: viewportSize, screen: viewportSize };
}
}
potentiallyUninitializedPage(): Page {
return this._page;
}
private async _initializePageProxySession() {
if (this._page._browserContext.isSettingStorageState())
return;
const promises: Promise<any>[] = [
this._pageProxySession.send('Dialog.enable'),
this._pageProxySession.send('Emulation.setActiveAndFocused', { active: true }),
];
const contextOptions = this._browserContext._options;
if (contextOptions.javaScriptEnabled === false)
promises.push(this._pageProxySession.send('Emulation.setJavaScriptEnabled', { enabled: false }));
promises.push(this._updateViewport());
promises.push(this.updateHttpCredentials());
if (this._browserContext._permissions.size) {
for (const [key, value] of this._browserContext._permissions)
promises.push(this._grantPermissions(key, value));
}
if (this._browserContext._options.recordVideo) {
const outputFile = path.join(this._browserContext._options.recordVideo.dir, createGuid() + '.webm');
promises.push(this._browserContext._ensureVideosPath().then(() => {
return this._startVideo({
// validateBrowserContextOptions ensures correct video size.
...this._browserContext._options.recordVideo!.size!,
outputFile,
});
}));
}
await Promise.all(promises);
}
private _setSession(session: WKSession) {
eventsHelper.removeEventListeners(this._sessionListeners);
this._session = session;
this.rawKeyboard.setSession(session);
this.rawMouse.setSession(session);
this._addSessionListeners();
this._workers.setSession(session);
}
// This method is called for provisional targets as well. The session passed as the parameter
// may be different from the current session and may be destroyed without becoming current.
async _initializeSession(session: WKSession, provisional: boolean, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
await this._initializeSessionMayThrow(session, resourceTreeHandler).catch(e => {
// Provisional session can be disposed at any time, for example due to new navigation initiating
// a new provisional page.
if (provisional && session.isDisposed())
return;
// Swallow initialization errors due to newer target swap in,
// since we will reinitialize again.
if (this._session === session)
throw e;
});
}
private async _initializeSessionMayThrow(session: WKSession, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
const [, frameTree] = await Promise.all([
// Page agent must be enabled before Runtime.
session.send('Page.enable'),
session.send('Page.getResourceTree'),
] as const);
resourceTreeHandler(frameTree);
const promises: Promise<any>[] = [
// Resource tree should be received before first execution context.
session.send('Runtime.enable'),
session.send('Page.createUserWorld', { name: UTILITY_WORLD_NAME }).catch(_ => {}), // Worlds are per-process
session.send('Console.enable'),
session.send('Network.enable'),
this._workers.initializeSession(session)
];
if (this._page.needsRequestInterception()) {
promises.push(session.send('Network.setInterceptionEnabled', { enabled: true }));
promises.push(session.send('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }));
}
if (this._page._browserContext.isSettingStorageState()) {
await Promise.all(promises);
return;
}
const contextOptions = this._browserContext._options;
if (contextOptions.userAgent)
promises.push(this.updateUserAgent());
const emulatedMedia = this._page.emulatedMedia();
if (emulatedMedia.media || emulatedMedia.colorScheme || emulatedMedia.reducedMotion || emulatedMedia.forcedColors)
promises.push(WKPage._setEmulateMedia(session, emulatedMedia.media, emulatedMedia.colorScheme, emulatedMedia.reducedMotion, emulatedMedia.forcedColors));
for (const binding of this._page.allBindings())
promises.push(session.send('Runtime.addBinding', { name: binding.name }));
const bootstrapScript = this._calculateBootstrapScript();
if (bootstrapScript.length)
promises.push(session.send('Page.setBootstrapScript', { source: bootstrapScript }));
this._page.frames().map(frame => frame.evaluateExpression(bootstrapScript, false, undefined).catch(e => {}));
if (contextOptions.bypassCSP)
promises.push(session.send('Page.setBypassCSP', { enabled: true }));
const emulatedSize = this._page.emulatedSize();
if (emulatedSize) {
promises.push(session.send('Page.setScreenSizeOverride', {
width: emulatedSize.screen.width,
height: emulatedSize.screen.height,
}));
}
promises.push(this.updateEmulateMedia());
promises.push(session.send('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) }));
if (contextOptions.offline)
promises.push(session.send('Network.setEmulateOfflineState', { offline: true }));
promises.push(session.send('Page.setTouchEmulationEnabled', { enabled: !!contextOptions.hasTouch }));
if (contextOptions.timezoneId) {
promises.push(session.send('Page.setTimeZone', { timeZone: contextOptions.timezoneId }).
catch(e => { throw new Error(`Invalid timezone ID: ${contextOptions.timezoneId}`); }));
}
if (this._page.fileChooserIntercepted())
promises.push(session.send('Page.setInterceptFileChooserDialog', { enabled: true }));
promises.push(session.send('Page.overrideSetting', { setting: 'DeviceOrientationEventEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'FullScreenEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'NotificationsEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'PointerLockEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeMonthEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeWeekEnabled' as any, value: contextOptions.isMobile }));
await Promise.all(promises);
}
private _onDidCommitProvisionalTarget(event: Protocol.Target.didCommitProvisionalTargetPayload) {
const { oldTargetId, newTargetId } = event;
assert(this._provisionalPage);
assert(this._provisionalPage._session.sessionId === newTargetId, 'Unknown new target: ' + newTargetId);
assert(this._session.sessionId === oldTargetId, 'Unknown old target: ' + oldTargetId);
const newSession = this._provisionalPage._session;
this._provisionalPage.commit();
this._provisionalPage.dispose();
this._provisionalPage = null;
this._setSession(newSession);
}
private _onTargetDestroyed(event: Protocol.Target.targetDestroyedPayload) {
const { targetId, crashed } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId) {
this._provisionalPage._session.dispose(false);
this._provisionalPage.dispose();
this._provisionalPage = null;
} else if (this._session.sessionId === targetId) {
this._session.dispose(false);
eventsHelper.removeEventListeners(this._sessionListeners);
if (crashed) {
this._session.markAsCrashed();
this._page._didCrash();
}
}
}
didClose() {
this._page._didClose();
}
dispose(disconnected: boolean) {
this._pageProxySession.dispose(disconnected);
eventsHelper.removeEventListeners(this._sessionListeners);
eventsHelper.removeEventListeners(this._eventListeners);
if (this._session)
this._session.dispose(disconnected);
if (this._provisionalPage) {
this._provisionalPage._session.dispose(disconnected);
this._provisionalPage.dispose();
this._provisionalPage = null;
}
this._page._didDisconnect();
this._firstNonInitialNavigationCommittedReject(new Error('Page closed'));
}
dispatchMessageToSession(message: any) {
this._pageProxySession.dispatchMessage(message);
}
handleProvisionalLoadFailed(event: Protocol.Playwright.provisionalLoadFailedPayload) {
if (!this._initializedPage) {
this._firstNonInitialNavigationCommittedReject(new Error('Initial load failed'));
return;
}
if (!this._provisionalPage)
return;
let errorText = event.error;
if (errorText.includes('cancelled'))
errorText += '; maybe frame was detached?';
this._page._frameManager.frameAbortedNavigation(this._page.mainFrame()._id, errorText, event.loaderId);
}
handleWindowOpen(event: Protocol.Playwright.windowOpenPayload) {
debugAssert(!this._nextWindowOpenPopupFeatures);
this._nextWindowOpenPopupFeatures = event.windowFeatures;
}
async pageOrError(): Promise<Page | Error> {
return this._pagePromise;
}
private async _onTargetCreated(event: Protocol.Target.targetCreatedPayload) {
const { targetInfo } = event;
const session = new WKSession(this._pageProxySession.connection, targetInfo.targetId, `Target closed`, (message: any) => {
this._pageProxySession.send('Target.sendMessageToTarget', {
message: JSON.stringify(message), targetId: targetInfo.targetId
}).catch(e => {
session.dispatchMessage({ id: message.id, error: { message: e.message } });
});
});
assert(targetInfo.type === 'page', 'Only page targets are expected in WebKit, received: ' + targetInfo.type);
if (!targetInfo.isProvisional) {
assert(!this._initializedPage);
let pageOrError: Page | Error;
try {
this._setSession(session);
await Promise.all([
this._initializePageProxySession(),
this._initializeSession(session, false, ({ frameTree }) => this._handleFrameTree(frameTree)),
]);
pageOrError = this._page;
} catch (e) {
pageOrError = e;
}
if (targetInfo.isPaused)
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
if ((pageOrError instanceof Page) && this._page.mainFrame().url() === '') {
try {
// Initial empty page has an empty url. We should wait until the first real url has been loaded,
// even if that url is about:blank. This is especially important for popups, where we need the
// actual url before interacting with it.
await this._firstNonInitialNavigationCommittedPromise;
} catch (e) {
pageOrError = e;
}
} else {
// Avoid rejection on disconnect.
this._firstNonInitialNavigationCommittedPromise.catch(() => {});
}
await this._page.initOpener(this._opener);
// Note: it is important to call |reportAsNew| before resolving pageOrError promise,
// so that anyone who awaits pageOrError got a ready and reported page.
this._initializedPage = pageOrError instanceof Page ? pageOrError : null;
this._page.reportAsNew(pageOrError instanceof Page ? undefined : pageOrError);
this._pagePromise.resolve(pageOrError);
} else {
assert(targetInfo.isProvisional);
assert(!this._provisionalPage);
this._provisionalPage = new WKProvisionalPage(session, this);
if (targetInfo.isPaused) {
this._provisionalPage.initializationPromise.then(() => {
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
});
}
}
}
private _onDispatchMessageFromTarget(event: Protocol.Target.dispatchMessageFromTargetPayload) {
const { targetId, message } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId)
this._provisionalPage._session.dispatchMessage(JSON.parse(message));
else if (this._session.sessionId === targetId)
this._session.dispatchMessage(JSON.parse(message));
else
throw new Error('Unknown target: ' + targetId);
}
private _addSessionListeners() {
this._sessionListeners = [
eventsHelper.addEventListener(this._session, 'Page.frameNavigated', event => this._onFrameNavigated(event.frame, false)),
eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),
eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),
eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),
eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),
eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),
eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),
eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),
eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),
eventsHelper.addEventListener(this._session, 'Console.messageRepeatCountUpdated', event => this._onConsoleRepeatCountUpdated(event)),
eventsHelper.addEventListener(this._pageProxySession, 'Dialog.javascriptDialogOpening', event => this._onDialog(event)),
eventsHelper.addEventListener(this._session, 'Page.fileChooserOpened', event => this._onFileChooserOpened(event)),
eventsHelper.addEventListener(this._session, 'Network.requestWillBeSent', e => this._onRequestWillBeSent(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.requestIntercepted', e => this._onRequestIntercepted(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.responseReceived', e => this._onResponseReceived(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFinished', e => this._onLoadingFinished(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFailed', e => this._onLoadingFailed(e)),
eventsHelper.addEventListener(this._session, 'Network.webSocketCreated', e => this._page._frameManager.onWebSocketCreated(e.requestId, e.url)),
eventsHelper.addEventListener(this._session, 'Network.webSocketWillSendHandshakeRequest', e => this._page._frameManager.onWebSocketRequest(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketHandshakeResponseReceived', e => this._page._frameManager.onWebSocketResponse(e.requestId, e.response.status, e.response.statusText)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameSent', e => e.response.payloadData && this._page._frameManager.onWebSocketFrameSent(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameReceived', e => e.response.payloadData && this._page._frameManager.webSocketFrameReceived(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketClosed', e => this._page._frameManager.webSocketClosed(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameError', e => this._page._frameManager.webSocketError(e.requestId, e.errorMessage)),
];
}
private async _updateState<T extends keyof Protocol.CommandParameters>(
method: T,
params?: Protocol.CommandParameters[T]
): Promise<void> {
await this._forAllSessions(session => session.send(method, params).then());
}
private async _forAllSessions(callback: ((session: WKSession) => Promise<void>)): Promise<void> {
const sessions = [
this._session
];
// If the state changes during provisional load, push it to the provisional page
// as well to always be in sync with the backend.
if (this._provisionalPage)
sessions.push(this._provisionalPage._session);
await Promise.all(sessions.map(session => callback(session).catch(e => {})));
}
private _onWillCheckNavigationPolicy(frameId: string) {
// It may happen that new policy check occurs while there is an ongoing
// provisional load, in this case it should be safe to ignore it as it will
// either:
// - end up canceled, e.g. ctrl+click opening link in new tab, having no effect
// on this page
// - start new provisional load which we will miss in our signal trackers but
// we certainly won't hang waiting for it to finish and there is high chance
// that the current provisional page will commit navigation canceling the new
// one.
if (this._provisionalPage)
return;
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _onDidCheckNavigationPolicy(frameId: string, cancel?: boolean) {
if (!cancel)
return;
// This is a cross-process navigation that is canceled in the original page and continues in
// the provisional page. Bail out as we are tracking it.
if (this._provisionalPage)
return;
this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');
}
private _onFrameScheduledNavigation(frameId: string) {
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {
this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);
this._onFrameNavigated(frameTree.frame, true);
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'domcontentloaded');
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'load');
if (!frameTree.childFrames)
return;
for (const child of frameTree.childFrames)
this._handleFrameTree(child);
}
_onFrameAttached(frameId: string, parentFrameId: string | null): frames.Frame {
return this._page._frameManager.frameAttached(frameId, parentFrameId);
}
private _onFrameNavigated(framePayload: Protocol.Page.Frame, initial: boolean) {
const frame = this._page._frameManager.frame(framePayload.id);
assert(frame);
this._removeContextsForFrame(frame, true);
if (!framePayload.parentId)
this._workers.clear();
this._page._frameManager.frameCommittedNewDocumentNavigation(framePayload.id, framePayload.url, framePayload.name || '', framePayload.loaderId, initial);
if (!initial)
this._firstNonInitialNavigationCommittedFulfill();
}
private _onFrameNavigatedWithinDocument(frameId: string, url: string) {
this._page._frameManager.frameCommittedSameDocumentNavigation(frameId, url);
}
private _onFrameDetached(frameId: string) {
this._page._frameManager.frameDetached(frameId);
}
private _removeContextsForFrame(frame: frames.Frame, notifyFrame: boolean) {
for (const [contextId, context] of this._contextIdToContext) {
if (context.frame === frame) {
this._contextIdToContext.delete(contextId);
if (notifyFrame)
frame._contextDestroyed(context);
}
}
}
private _onExecutionContextCreated(contextPayload: Protocol.Runtime.ExecutionContextDescription) {
if (this._contextIdToContext.has(contextPayload.id))
return;
const frame = this._page._frameManager.frame(contextPayload.frameId);
if (!frame)
return;
const delegate = new WKExecutionContext(this._session, contextPayload.id);
let worldName: types.World|null = null;
if (contextPayload.type === 'normal')
worldName = 'main';
else if (contextPayload.type === 'user' && contextPayload.name === UTILITY_WORLD_NAME)
worldName = 'utility';
const context = new dom.FrameExecutionContext(delegate, frame, worldName);
(context as any)[contextDelegateSymbol] = delegate;
if (worldName)
frame._contextCreated(worldName, context);
if (contextPayload.type === 'normal' && frame === this._page.mainFrame())
this._mainFrameContextId = contextPayload.id;
this._contextIdToContext.set(contextPayload.id, context);
}
private async _onBindingCalled(contextId: Protocol.Runtime.ExecutionContextId, argument: string) {
const pageOrError = await this.pageOrError();
if (!(pageOrError instanceof Error)) {
const context = this._contextIdToContext.get(contextId);
if (context)
await this._page._onBindingCalled(argument, context);
}
}
async navigateFrame(frame: frames.Frame, url: string, referrer: string | undefined): Promise<frames.GotoResult> {
if (this._pageProxySession.isDisposed())
throw new Error('Target closed');
const pageProxyId = this._pageProxySession.sessionId;
const result = await this._pageProxySession.connection.browserSession.send('Playwright.navigate', { url, pageProxyId, frameId: frame._id, referrer });
return { newDocumentId: result.loaderId };
}
private _onConsoleMessage(event: Protocol.Console.messageAddedPayload) {
// Note: do no introduce await in this function, otherwise we lose the ordering.
// For example, frame.setContent relies on this.
const { type, level, text, parameters, url, line: lineNumber, column: columnNumber, source } = event.message;
if (level === 'error' && source === 'javascript') {
const { name, message } = splitErrorMessage(text);
let stack: string;
if (event.message.stackTrace) {
stack = text + '\n' + event.message.stackTrace.callFrames.map(callFrame => {
return ` at ${callFrame.functionName || 'unknown'} (${callFrame.url}:${callFrame.lineNumber}:${callFrame.columnNumber})`;
}).join('\n');
} else {
stack = '';
}
const error = new Error(message);
error.stack = stack;
error.name = name;
this._page.firePageError(error);
return;
}
let derivedType: string = type || '';
if (type === 'log')
derivedType = level;
else if (type === 'timing')
derivedType = 'timeEnd';
const handles: JSHandle[] = [];
for (const p of parameters || []) {
let context: dom.FrameExecutionContext | undefined;
if (p.objectId) {
const objectId = JSON.parse(p.objectId);
context = this._contextIdToContext.get(objectId.injectedScriptId);
} else {
context = this._contextIdToContext.get(this._mainFrameContextId!);
}
if (!context)
return;
handles.push(context.createHandle(p));
}
this._lastConsoleMessage = {
derivedType,
text,
handles,
count: 0,
location: {
url: url || '',
lineNumber: (lineNumber || 1) - 1,
columnNumber: (columnNumber || 1) - 1,
}
};
this._onConsoleRepeatCountUpdated({ count: 1 });
}
_onConsoleRepeatCountUpdated(event: Protocol.Console.messageRepeatCountUpdatedPayload) {
if (this._lastConsoleMessage) {
const {
derivedType,
text,
handles,
count,
location
} = this._lastConsoleMessage;
for (let i = count; i < event.count; ++i)
this._page._addConsoleMessage(derivedType, handles, location, handles.length ? undefined : text);
this._lastConsoleMessage.count = event.count;
}
}
_onDialog(event: Protocol.Dialog.javascriptDialogOpeningPayload) {
this._page.emit(Page.Events.Dialog, new dialog.Dialog(
this._page,
event.type as dialog.DialogType,
event.message,
async (accept: boolean, promptText?: string) => {
await this._pageProxySession.send('Dialog.handleJavaScriptDialog', { accept, promptText });
},
event.defaultPrompt));
}
private async _onFileChooserOpened(event: {frameId: Protocol.Network.FrameId, element: Protocol.Runtime.RemoteObject}) {
let handle;
try {
const context = await this._page._frameManager.frame(event.frameId)!._mainContext();
handle = context.createHandle(event.element).asElement()!;
} catch (e) {
// During async processing, frame/context may go away. We should not throw.
return;
}
await this._page._onFileChooserOpened(handle);
}
private static async _setEmulateMedia(session: WKSession, mediaType: types.MediaType, colorScheme: types.ColorScheme, reducedMotion: types.ReducedMotion, forcedColors: types.ForcedColors): Promise<void> {
const promises = [];
promises.push(session.send('Page.setEmulatedMedia', { media: mediaType === 'no-override' ? '' : mediaType }));
let appearance: any = undefined;
switch (colorScheme) {
case 'light': appearance = 'Light'; break;
case 'dark': appearance = 'Dark'; break;
case 'no-override': appearance = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersColorScheme', value: appearance }));
let reducedMotionWk: any = undefined;
switch (reducedMotion) {
case 'reduce': reducedMotionWk = 'Reduce'; break;
case 'no-preference': reducedMotionWk = 'NoPreference'; break;
case 'no-override': reducedMotionWk = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersReducedMotion', value: reducedMotionWk }));
let forcedColorsWk: any = undefined;
switch (forcedColors) {
case 'active': forcedColorsWk = 'Active'; break;
case 'none': forcedColorsWk = 'None'; break;
case 'no-override': forcedColorsWk = undefined; break;
}
promises.push(session.send('Page.setForcedColors', { forcedColors: forcedColorsWk }));
await Promise.all(promises);
}
async updateExtraHTTPHeaders(): Promise<void> {
await this._updateState('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) });
}
_calculateExtraHTTPHeaders(): types.HeadersArray {
const locale = this._browserContext._options.locale;
const headers = network.mergeHeaders([
this._browserContext._options.extraHTTPHeaders,
this._page.extraHTTPHeaders(),
locale ? network.singleHeader('Accept-Language', locale) : undefined,
]);
return headers;
}
async updateEmulateMedia(): Promise<void> {
const emulatedMedia = this._page.emulatedMedia();
const colorScheme = emulatedMedia.colorScheme;
const reducedMotion = emulatedMedia.reducedMotion;
const forcedColors = emulatedMedia.forcedColors;
await this._forAllSessions(session => WKPage._setEmulateMedia(session, emulatedMedia.media, colorScheme, reducedMotion, forcedColors));
}
async updateEmulatedViewportSize(): Promise<void> {
await this._updateViewport();
}
async updateUserAgent(): Promise<void> {
const contextOptions = this._browserContext._options;
this._updateState('Page.overrideUserAgent', { value: contextOptions.userAgent });
}
async bringToFront(): Promise<void> {
this._pageProxySession.send('Target.activate', {
targetId: this._session.sessionId
});
}
async _updateViewport(): Promise<void> {
const options = this._browserContext._options;
const deviceSize = this._page.emulatedSize();
if (deviceSize === null)
return;
const viewportSize = deviceSize.viewport;
const screenSize = deviceSize.screen;
const promises: Promise<any>[] = [
this._pageProxySession.send('Emulation.setDeviceMetricsOverride', {
width: viewportSize.width,
height: viewportSize.height,
fixedLayout: !!options.isMobile,
deviceScaleFactor: options.deviceScaleFactor || 1
}),
this._session.send('Page.setScreenSizeOverride', {
width: screenSize.width,
height: screenSize.height,
}),
];
if (options.isMobile) {
const angle = viewportSize.width > viewportSize.height ? 90 : 0;
promises.push(this._session.send('Page.setOrientationOverride', { angle }));
}
await Promise.all(promises);
}
async updateRequestInterception(): Promise<void> {
const enabled = this._page.needsRequestInterception();
await Promise.all([
this._updateState('Network.setInterceptionEnabled', { enabled }),
this._updateState('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }),
]);
}
async updateOffline() {
await this._updateState('Network.setEmulateOfflineState', { offline: !!this._browserContext._options.offline });
}
async updateHttpCredentials() {
const credentials = this._browserContext._options.httpCredentials || { username: '', password: '' };
await this._pageProxySession.send('Emulation.setAuthCredentials', { username: credentials.username, password: credentials.password });
}
async updateFileChooserInterception() {
const enabled = this._page.fileChooserIntercepted();
await this._session.send('Page.setInterceptFileChooserDialog', { enabled }).catch(() => {}); // target can be closed.
}
async reload(): Promise<void> {
await this._session.send('Page.reload');
}
goBack(): Promise<boolean> {
return this._session.send('Page.goBack').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goBack): Failed to go`))
return false;
throw error;
});
}
goForward(): Promise<boolean> {
return this._session.send('Page.goForward').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goForward): Failed to go`))
return false;
throw error;
});
}
async exposeBinding(binding: PageBinding): Promise<void> {
this._session.send('Runtime.addBinding', { name: binding.name });
await this._updateBootstrapScript();
await Promise.all(this._page.frames().map(frame => frame.evaluateExpression(binding.source, false, {}).catch(e => {})));
}
async removeExposedBindings(): Promise<void> {
await this._updateBootstrapScript();
}
async addInitScript(script: string): Promise<void> {
await this._updateBootstrapScript();
}
async removeInitScripts() {
await this._updateBootstrapScript();
}
private _calculateBootstrapScript(): string {
const scripts: string[] = [];
if (!this._page.context()._options.isMobile) {
scripts.push('delete window.orientation');
scripts.push('delete window.ondevicemotion');
scripts.push('delete window.ondeviceorientation');
}
for (const binding of this._page.allBindings())
scripts.push(binding.source);
scripts.push(...this._browserContext.initScripts);
scripts.push(...this._page.initScripts);
return scripts.join(';\n');
}
async _updateBootstrapScript(): Promise<void> {
await this._updateState('Page.setBootstrapScript', { source: this._calculateBootstrapScript() });
}
async closePage(runBeforeUnload: boolean): Promise<void> {
await this._stopVideo();
await this._pageProxySession.sendMayFail('Target.close', {
targetId: this._session.sessionId,
runBeforeUnload
});
}
async setBackgroundColor(color?: { r: number; g: number; b: number; a: number; }): Promise<void> {
await this._session.send('Page.setDefaultBackgroundColorOverride', { color });
}
private _toolbarHeight(): number {
if (this._page._browserContext._browser?.options.headful)
return hostPlatform === 'mac10.15' ? 55 : 59;
return 0;
}
private async _startVideo(options: types.PageScreencastOptions): Promise<void> {
assert(!this._recordingVideoFile);
const { screencastId } = await this._pageProxySession.send('Screencast.startVideo', {
file: options.outputFile,
width: options.width,
height: options.height,
toolbarHeight: this._toolbarHeight()
});
this._recordingVideoFile = options.outputFile;
this._browserContext._browser._videoStarted(this._browserContext, screencastId, options.outputFile, this.pageOrError());
}
async _stopVideo(): Promise<void> {
if (!this._recordingVideoFile)
return;
await this._pageProxySession.sendMayFail('Screencast.stopVideo');
this._recordingVideoFile = null;
}
private validateScreenshotDimension(side: number, omitDeviceScaleFactor: boolean) {
// Cairo based implementations (Linux and Windows) have hard limit of 32767
// (see https://github.com/microsoft/playwright/issues/16727).
if (process.platform === 'darwin')
return;
if (!omitDeviceScaleFactor && this._page._browserContext._options.deviceScaleFactor)
side = Math.ceil(side * this._page._browserContext._options.deviceScaleFactor);
if (side > 32767)
throw new Error('Cannot take screenshot larger than 32767 pixels on any dimension');
}
async takeScreenshot(progress: Progress, format: string, documentRect: types.Rect | undefined, viewportRect: types.Rect | undefined, quality: number | undefined, fitsViewport: boolean, scale: 'css' | 'device'): Promise<Buffer> {
const rect = (documentRect || viewportRect)!;
const omitDeviceScaleFactor = scale === 'css';
this.validateScreenshotDimension(rect.width, omitDeviceScaleFactor);
this.validateScreenshotDimension(rect.height, omitDeviceScaleFactor);
const result = await this._session.send('Page.snapshotRect', { ...rect, coordinateSystem: documentRect ? 'Page' : 'Viewport', omitDeviceScaleFactor });
const prefix = 'data:image/png;base64,';
let buffer = Buffer.from(result.dataURL.substr(prefix.length), 'base64');
if (format === 'jpeg')
buffer = jpegjs.encode(PNG.sync.read(buffer), quality).data;
return buffer;
}
async getContentFrame(handle: dom.ElementHandle): Promise<frames.Frame | null> {
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
if (!nodeInfo.contentFrameId)
return null;
return this._page._frameManager.frame(nodeInfo.contentFrameId);
}
async getOwnerFrame(handle: dom.ElementHandle): Promise<string | null> {
if (!handle._objectId)
return null;
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
return nodeInfo.ownerFrameId || null;
}
isElementHandle(remoteObject: any): boolean {
return (remoteObject as Protocol.Runtime.RemoteObject).subtype === 'node';
}
async getBoundingBox(handle: dom.ElementHandle): Promise<types.Rect | null> {
const quads = await this.getContentQuads(handle);
if (!quads || !quads.length)
return null;
let minX = Infinity;
let maxX = -Infinity;
let minY = Infinity;
let maxY = -Infinity;
for (const quad of quads) {
for (const point of quad) {
minX = Math.min(minX, point.x);
maxX = Math.max(maxX, point.x);
minY = Math.min(minY, point.y);
maxY = Math.max(maxY, point.y);
}
}
return { x: minX, y: minY, width: maxX - minX, height: maxY - minY };
}
async scrollRectIntoViewIfNeeded(handle: dom.ElementHandle, rect?: types.Rect): Promise<'error:notvisible' | 'error:notconnected' | 'done'> {
return await this._session.send('DOM.scrollIntoViewIfNeeded', {
objectId: handle._objectId,
rect,
}).then(() => 'done' as const).catch(e => {
if (e instanceof Error && e.message.includes('Node does not have a layout object'))
return 'error:notvisible';
if (e instanceof Error && e.message.includes('Node is detached from document'))
return 'error:notconnected';
throw e;
});
}
async setScreencastOptions(options: { width: number, height: number, quality: number } | null): Promise<void> {
if (options) {
const so = { ...options, toolbarHeight: this._toolbarHeight() };
const { generation } = await this._pageProxySession.send('Screencast.startScreencast', so);
this._screencastGeneration = generation;
} else {
await this._pageProxySession.send('Screencast.stopScreencast');
}
}
private _onScreencastFrame(event: Protocol.Screencast.screencastFramePayload) {
const generation = this._screencastGeneration;
this._page.throttleScreencastFrameAck(() => {
this._pageProxySession.send('Screencast.screencastFrameAck', { generation }).catch(e => debugLogger.log('error', e));
});
const buffer = Buffer.from(event.data, 'base64');
this._page.emit(Page.Events.ScreencastFrame, {
buffer,
width: event.deviceWidth,
height: event.deviceHeight,
});
}
rafCountForStablePosition(): number {
return process.platform === 'win32' ? 5 : 1;
}
async getContentQuads(handle: dom.ElementHandle): Promise<types.Quad[] | null> {
const result = await this._session.sendMayFail('DOM.getContentQuads', {
objectId: handle._objectId
});
if (!result)
return null;
return result.quads.map(quad => [
{ x: quad[0], y: quad[1] },
{ x: quad[2], y: quad[3] },
{ x: quad[4], y: quad[5] },
{ x: quad[6], y: quad[7] }
]);
}
async setInputFiles(handle: dom.ElementHandle<HTMLInputElement>, files: types.FilePayload[]): Promise<void> {
const objectId = handle._objectId;
const protocolFiles = files.map(file => ({
name: file.name,
type: file.mimeType,
data: file.buffer,
}));
await this._session.send('DOM.setInputFiles', { objectId, files: protocolFiles });
}
async setInputFilePaths(handle: dom.ElementHandle<HTMLInputElement>, paths: string[]): Promise<void> {
const pageProxyId = this._pageProxySession.sessionId;
const objectId = handle._objectId;
await Promise.all([
this._pageProxySession.connection.browserSession.send('Playwright.grantFileReadAccess', { pageProxyId, paths }),
this._session.send('DOM.setInputFiles', { objectId, paths })
]);
}
async adoptElementHandle<T extends Node>(handle: dom.ElementHandle<T>, to: dom.FrameExecutionContext): Promise<dom.ElementHandle<T>> {
const result = await this._session.sendMayFail('DOM.resolveNode', {
objectId: handle._objectId,
executionContextId: ((to as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error(dom.kUnableToAdoptErrorMessage);
return to.createHandle(result.object) as dom.ElementHandle<T>;
}
async getAccessibilityTree(needle?: dom.ElementHandle): Promise<{tree: accessibility.AXNode, needle: accessibility.AXNode | null}> {
return getAccessibilityTree(this._session, needle);
}
async inputActionEpilogue(): Promise<void> {
}
async getFrameElement(frame: frames.Frame): Promise<dom.ElementHandle> {
const parent = frame.parentFrame();
if (!parent)
throw new Error('Frame has been detached.');
const context = await parent._mainContext();
const result = await this._session.send('DOM.resolveNode', {
frameId: frame._id,
executionContextId: ((context as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error('Frame has been detached.');
return context.createHandle(result.object) as dom.ElementHandle;
}
_onRequestWillBeSent(session: WKSession, event: Protocol.Network.requestWillBeSentPayload) {
if (event.request.url.startsWith('data:'))
return;
let redirectedFrom: WKInterceptableRequest | null = null;
if (event.redirectResponse) {
const request = this._requestIdToRequest.get(event.requestId);
// If we connect late to the target, we could have missed the requestWillBeSent event.
if (request) {
this._handleRequestRedirect(request, event.redirectResponse, event.timestamp);
redirectedFrom = request;
}
}
const frame = redirectedFrom ? redirectedFrom.request.frame() : this._page._frameManager.frame(event.frameId);
// sometimes we get stray network events for detached frames
// TODO(einbinder) why?
if (!frame)
return;
// TODO(einbinder) this will fail if we are an XHR document request
const isNavigationRequest = event.type === 'Document';
const documentId = isNavigationRequest ? event.loaderId : undefined;
let route = null;
// We do not support intercepting redirects.
if (this._page.needsRequestInterception() && !redirectedFrom)
route = new WKRouteImpl(session, event.requestId);
const request = new WKInterceptableRequest(session, route, frame, event, redirectedFrom, documentId);
this._requestIdToRequest.set(event.requestId, request);
this._page._frameManager.requestStarted(request.request, route || undefined);
}
private _handleRequestRedirect(request: WKInterceptableRequest, responsePayload: Protocol.Network.Response, timestamp: number) {
const response = request.createResponse(responsePayload);
response._securityDetailsFinished();
response._serverAddrFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(responsePayload.timing ? helper.secondsToRoundishMillis(timestamp - request._timestamp) : -1);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.requestReceivedResponse(response);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onRequestIntercepted(session: WKSession, event: Protocol.Network.requestInterceptedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
if (!request) {
session.sendMayFail('Network.interceptRequestWithError', { errorType: 'Cancellation', requestId: event.requestId });
return;
}
// There is no point in waiting for the raw headers in Network.responseReceived when intercepting.
// Use provisional headers as raw headers, so that client can call allHeaders() from the route handler.
request.request.setRawRequestHeaders(null);
if (!request._route) {
// Intercepted, although we do not intend to allow interception.
// Just continue.
session.sendMayFail('Network.interceptWithRequest', { requestId: request._requestId });
} else {
request._route._requestInterceptedPromise.resolve();
}
}
_onResponseReceived(event: Protocol.Network.responseReceivedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// FileUpload sends a response without a matching request.
if (!request)
return;
this._requestIdToResponseReceivedPayloadEvent.set(request._requestId, event);
const response = request.createResponse(event.response);
this._page._frameManager.requestReceivedResponse(response);
if (response.status() === 204) {
this._onLoadingFailed({
requestId: event.requestId,
errorText: 'Aborted: 204 No Content',
timestamp: event.timestamp
});
}
}
_onLoadingFinished(event: Protocol.Network.loadingFinishedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
// Under certain conditions we never get the Network.responseReceived
// event from protocol. @see https://crbug.com/883475
const response = request.request._existingResponse();
if (response) {
const responseReceivedPayload = this._requestIdToResponseReceivedPayloadEvent.get(request._requestId);
response._serverAddrFinished(parseRemoteAddress(event?.metrics?.remoteAddress));
response._securityDetailsFinished({
protocol: isLoadedSecurely(response.url(), response.timing()) ? event.metrics?.securityConnection?.protocol : undefined,
subjectName: responseReceivedPayload?.response.security?.certificate?.subject,
validFrom: responseReceivedPayload?.response.security?.certificate?.validFrom,
validTo: responseReceivedPayload?.response.security?.certificate?.validUntil,
});
if (event.metrics?.protocol)
response._setHttpVersion(event.metrics.protocol);
response.setEncodedBodySize(event.metrics?.responseBodyBytesReceived ?? null);
response.setResponseHeadersSize(event.metrics?.responseHeaderBytesReceived ?? null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToResponseReceivedPayloadEvent.delete(request._requestId);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onLoadingFailed(event: Protocol.Network.loadingFailedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
const response = request.request._existingResponse();
if (response) {
response._serverAddrFinished();
response._securityDetailsFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToRequest.delete(request._requestId);
request.request._setFailureText(event.errorText);
this._page._frameManager.requestFailed(request.request, event.errorText.includes('cancelled'));
}
async _grantPermissions(origin: string, permissions: string[]) {
const webPermissionToProtocol = new Map<string, string>([
['geolocation', 'geolocation'],
]);
const filtered = permissions.map(permission => {
const protocolPermission = webPermissionToProtocol.get(permission);
if (!protocolPermission)
throw new Error('Unknown permission: ' + permission);
return protocolPermission;
});
await this._pageProxySession.send('Emulation.grantPermissions', { origin, permissions: filtered });
}
async _clearPermissions() {
await this._pageProxySession.send('Emulation.resetPermissions', {});
}
}
/**
* WebKit Remote Addresses look like:
*
* macOS:
* ::1.8911
* 2606:2800:220:1:248:1893:25c8:1946.443
* 127.0.0.1:8000
*
* ubuntu:
* ::1:8907
* 127.0.0.1:8000
*
* NB: They look IPv4 and IPv6's with ports but use an alternative notation.
*/
function parseRemoteAddress(value?: string) {
if (!value)
return;
try {
const colon = value.lastIndexOf(':');
const dot = value.lastIndexOf('.');
if (dot < 0) { // IPv6ish:port
return {
ipAddress: `[${value.slice(0, colon)}]`,
port: +value.slice(colon + 1)
};
}
if (colon > dot) { // IPv4:port
const [address, port] = value.split(':');
return {
ipAddress: address,
port: +port,
};
} else { // IPv6ish.port
const [address, port] = value.split('.');
return {
ipAddress: `[${address}]`,
port: +port,
};
}
} catch (_) {}
}
/**
* Adapted from Source/WebInspectorUI/UserInterface/Models/Resource.js in
* WebKit codebase.
*/
function isLoadedSecurely(url: string, timing: network.ResourceTiming) {
try {
const u = new URL(url);
if (u.protocol !== 'https:' && u.protocol !== 'wss:' && u.protocol !== 'sftp:')
return false;
if (timing.secureConnectionStart === -1 && timing.connectStart !== -1)
return false;
return true;
} catch (_) {}
}
const contextDelegateSymbol = Symbol('delegate');
| packages/playwright-core/src/server/webkit/wkPage.ts | 1 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.07136273384094238,
0.004249129444360733,
0.00015973088738974184,
0.002190173137933016,
0.007437525317072868
] |
{
"id": 0,
"code_window": [
" eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),\n",
" eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),\n",
" eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId, event.delay, event.targetIsCurrentFrame)),\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 381
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import fs from 'fs';
import path from 'path';
import type { FullConfig, TestCase, Suite, TestResult, TestError, TestStep, FullResult, Location, Reporter, JSONReport, JSONReportSuite, JSONReportSpec, JSONReportTest, JSONReportTestResult, JSONReportTestStep, JSONReportError } from '../../types/testReporter';
import { formatError, prepareErrorStack } from './base';
import { MultiMap } from 'playwright-core/lib/utils';
import { assert } from 'playwright-core/lib/utils';
export function toPosixPath(aPath: string): string {
return aPath.split(path.sep).join(path.posix.sep);
}
class JSONReporter implements Reporter {
config!: FullConfig;
suite!: Suite;
private _errors: TestError[] = [];
private _outputFile: string | undefined;
constructor(options: { outputFile?: string } = {}) {
this._outputFile = options.outputFile || reportOutputNameFromEnv();
}
printsToStdio() {
return !this._outputFile;
}
onBegin(config: FullConfig, suite: Suite) {
this.config = config;
this.suite = suite;
}
onError(error: TestError): void {
this._errors.push(error);
}
async onEnd(result: FullResult) {
outputReport(this._serializeReport(), this.config, this._outputFile);
}
private _serializeReport(): JSONReport {
return {
config: {
...removePrivateFields(this.config),
rootDir: toPosixPath(this.config.rootDir),
projects: this.config.projects.map(project => {
return {
outputDir: toPosixPath(project.outputDir),
repeatEach: project.repeatEach,
retries: project.retries,
metadata: project.metadata,
id: (project as any)._id,
name: project.name,
testDir: toPosixPath(project.testDir),
testIgnore: serializePatterns(project.testIgnore),
testMatch: serializePatterns(project.testMatch),
timeout: project.timeout,
};
})
},
suites: this._mergeSuites(this.suite.suites),
errors: this._errors
};
}
private _mergeSuites(suites: Suite[]): JSONReportSuite[] {
const fileSuites = new MultiMap<string, JSONReportSuite>();
for (const projectSuite of suites) {
const projectId = (projectSuite.project() as any)._id;
const projectName = projectSuite.project()!.name;
for (const fileSuite of projectSuite.suites) {
const file = fileSuite.location!.file;
const serialized = this._serializeSuite(projectId, projectName, fileSuite);
if (serialized)
fileSuites.set(file, serialized);
}
}
const results: JSONReportSuite[] = [];
for (const [, suites] of fileSuites) {
const result: JSONReportSuite = {
title: suites[0].title,
file: suites[0].file,
column: 0,
line: 0,
specs: [],
};
for (const suite of suites)
this._mergeTestsFromSuite(result, suite);
results.push(result);
}
return results;
}
private _relativeLocation(location: Location | undefined): Location {
if (!location)
return { file: '', line: 0, column: 0 };
return {
file: toPosixPath(path.relative(this.config.rootDir, location.file)),
line: location.line,
column: location.column,
};
}
private _locationMatches(s1: JSONReportSuite | JSONReportSpec, s2: JSONReportSuite | JSONReportSpec) {
return s1.file === s2.file && s1.line === s2.line && s1.column === s2.column;
}
private _mergeTestsFromSuite(to: JSONReportSuite, from: JSONReportSuite) {
for (const fromSuite of from.suites || []) {
const toSuite = (to.suites || []).find(s => s.title === fromSuite.title && this._locationMatches(s, fromSuite));
if (toSuite) {
this._mergeTestsFromSuite(toSuite, fromSuite);
} else {
if (!to.suites)
to.suites = [];
to.suites.push(fromSuite);
}
}
for (const spec of from.specs || []) {
const toSpec = to.specs.find(s => s.title === spec.title && s.file === toPosixPath(path.relative(this.config.rootDir, spec.file)) && s.line === spec.line && s.column === spec.column);
if (toSpec)
toSpec.tests.push(...spec.tests);
else
to.specs.push(spec);
}
}
private _serializeSuite(projectId: string, projectName: string, suite: Suite): null | JSONReportSuite {
if (!suite.allTests().length)
return null;
const suites = suite.suites.map(suite => this._serializeSuite(projectId, projectName, suite)).filter(s => s) as JSONReportSuite[];
return {
title: suite.title,
...this._relativeLocation(suite.location),
specs: suite.tests.map(test => this._serializeTestSpec(projectId, projectName, test)),
suites: suites.length ? suites : undefined,
};
}
private _serializeTestSpec(projectId: string, projectName: string, test: TestCase): JSONReportSpec {
return {
title: test.title,
ok: test.ok(),
tags: (test.title.match(/@[\S]+/g) || []).map(t => t.substring(1)),
tests: [this._serializeTest(projectId, projectName, test)],
id: test.id,
...this._relativeLocation(test.location),
};
}
private _serializeTest(projectId: string, projectName: string, test: TestCase): JSONReportTest {
return {
timeout: test.timeout,
annotations: test.annotations,
expectedStatus: test.expectedStatus,
projectId,
projectName,
results: test.results.map(r => this._serializeTestResult(r, test)),
status: test.outcome(),
};
}
private _serializeTestResult(result: TestResult, test: TestCase): JSONReportTestResult {
const steps = result.steps.filter(s => s.category === 'test.step');
const jsonResult: JSONReportTestResult = {
workerIndex: result.workerIndex,
status: result.status,
duration: result.duration,
error: result.error,
errors: result.errors.map(e => this._serializeError(e)),
stdout: result.stdout.map(s => stdioEntry(s)),
stderr: result.stderr.map(s => stdioEntry(s)),
retry: result.retry,
steps: steps.length ? steps.map(s => this._serializeTestStep(s)) : undefined,
startTime: result.startTime,
attachments: result.attachments.map(a => ({
name: a.name,
contentType: a.contentType,
path: a.path,
body: a.body?.toString('base64')
})),
};
if (result.error?.stack)
jsonResult.errorLocation = prepareErrorStack(result.error.stack).location;
return jsonResult;
}
private _serializeError(error: TestError): JSONReportError {
return formatError(this.config, error, true);
}
private _serializeTestStep(step: TestStep): JSONReportTestStep {
const steps = step.steps.filter(s => s.category === 'test.step');
return {
title: step.title,
duration: step.duration,
error: step.error,
steps: steps.length ? steps.map(s => this._serializeTestStep(s)) : undefined,
};
}
}
function outputReport(report: JSONReport, config: FullConfig, outputFile: string | undefined) {
const reportString = JSON.stringify(report, undefined, 2);
if (outputFile) {
assert(config.configFile || path.isAbsolute(outputFile), 'Expected fully resolved path if not using config file.');
outputFile = config.configFile ? path.resolve(path.dirname(config.configFile), outputFile) : outputFile;
fs.mkdirSync(path.dirname(outputFile), { recursive: true });
fs.writeFileSync(outputFile, reportString);
} else {
console.log(reportString);
}
}
function stdioEntry(s: string | Buffer): any {
if (typeof s === 'string')
return { text: s };
return { buffer: s.toString('base64') };
}
function removePrivateFields(config: FullConfig): FullConfig {
return Object.fromEntries(Object.entries(config).filter(([name, value]) => !name.startsWith('_'))) as FullConfig;
}
function reportOutputNameFromEnv(): string | undefined {
if (process.env[`PLAYWRIGHT_JSON_OUTPUT_NAME`])
return path.resolve(process.cwd(), process.env[`PLAYWRIGHT_JSON_OUTPUT_NAME`]);
return undefined;
}
export function serializePatterns(patterns: string | RegExp | (string | RegExp)[]): string[] {
if (!Array.isArray(patterns))
patterns = [patterns];
return patterns.map(s => s.toString());
}
export default JSONReporter;
| packages/playwright-test/src/reporters/json.ts | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.0003288431034889072,
0.0001747845089994371,
0.00016036847955547273,
0.00016553813475184143,
0.0000321871048072353
] |
{
"id": 0,
"code_window": [
" eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),\n",
" eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),\n",
" eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId, event.delay, event.targetIsCurrentFrame)),\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 381
} | export default function LoginPage() {
return <main>Login</main>
}
| tests/components/ct-react-vite/src/pages/LoginPage.tsx | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.007175013422966003,
0.007175013422966003,
0.007175013422966003,
0.007175013422966003,
0
] |
{
"id": 0,
"code_window": [
" eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),\n",
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),\n",
" eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),\n",
" eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),\n",
" eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),\n",
" eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId, event.delay, event.targetIsCurrentFrame)),\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 381
} | Web platform tests are copied from https://github.com/web-platform-tests/wpt.
Includes:
- `LICENSE.md`
- `accname/name*` test files
- `accname/foo.jpg`
- `wai-aria/scripts/manual.css`
Modified:
- `wai-aria/scripts/ATTAcomm.js` contains our own harness to avoid modifying test files
| tests/assets/wpt/README.md | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.0003810135822277516,
0.00027078751008957624,
0.00016056142339948565,
0.00027078751008957624,
0.00011022607941413298
] |
{
"id": 1,
"code_window": [
" if (this._provisionalPage)\n",
" return;\n",
" this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');\n",
" }\n",
"\n",
" private _onFrameScheduledNavigation(frameId: string) {\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n",
" }\n",
"\n",
" private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {\n",
" this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);\n",
" this._onFrameNavigated(frameTree.frame, true);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" private _onFrameScheduledNavigation(frameId: string, delay: number, targetIsCurrentFrame: boolean) {\n",
" if (targetIsCurrentFrame)\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 447
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import path from 'path';
import { PNG, jpegjs } from '../../utilsBundle';
import { splitErrorMessage } from '../../utils/stackTrace';
import { assert, createGuid, debugAssert, headersArrayToObject } from '../../utils';
import { hostPlatform } from '../../utils/hostPlatform';
import type * as accessibility from '../accessibility';
import * as dialog from '../dialog';
import * as dom from '../dom';
import type * as frames from '../frames';
import type { RegisteredListener } from '../../utils/eventsHelper';
import { eventsHelper } from '../../utils/eventsHelper';
import { helper } from '../helper';
import type { JSHandle } from '../javascript';
import * as network from '../network';
import type { PageBinding, PageDelegate } from '../page';
import { Page } from '../page';
import type { Progress } from '../progress';
import type * as types from '../types';
import type { Protocol } from './protocol';
import { getAccessibilityTree } from './wkAccessibility';
import type { WKBrowserContext } from './wkBrowser';
import { WKSession } from './wkConnection';
import { WKExecutionContext } from './wkExecutionContext';
import { RawKeyboardImpl, RawMouseImpl, RawTouchscreenImpl } from './wkInput';
import { WKInterceptableRequest, WKRouteImpl } from './wkInterceptableRequest';
import { WKProvisionalPage } from './wkProvisionalPage';
import { WKWorkers } from './wkWorkers';
import { debugLogger } from '../../common/debugLogger';
import { ManualPromise } from '../../utils/manualPromise';
const UTILITY_WORLD_NAME = '__playwright_utility_world__';
export class WKPage implements PageDelegate {
readonly rawMouse: RawMouseImpl;
readonly rawKeyboard: RawKeyboardImpl;
readonly rawTouchscreen: RawTouchscreenImpl;
_session: WKSession;
private _provisionalPage: WKProvisionalPage | null = null;
readonly _page: Page;
private readonly _pagePromise = new ManualPromise<Page | Error>();
private readonly _pageProxySession: WKSession;
readonly _opener: WKPage | null;
private readonly _requestIdToRequest = new Map<string, WKInterceptableRequest>();
private readonly _workers: WKWorkers;
private readonly _contextIdToContext: Map<number, dom.FrameExecutionContext>;
private _mainFrameContextId?: number;
private _sessionListeners: RegisteredListener[] = [];
private _eventListeners: RegisteredListener[];
readonly _browserContext: WKBrowserContext;
_initializedPage: Page | null = null;
private _firstNonInitialNavigationCommittedPromise: Promise<void>;
private _firstNonInitialNavigationCommittedFulfill = () => {};
_firstNonInitialNavigationCommittedReject = (e: Error) => {};
private _lastConsoleMessage: { derivedType: string, text: string, handles: JSHandle[]; count: number, location: types.ConsoleMessageLocation; } | null = null;
private readonly _requestIdToResponseReceivedPayloadEvent = new Map<string, Protocol.Network.responseReceivedPayload>();
// Holds window features for the next popup being opened via window.open,
// until the popup page proxy arrives.
private _nextWindowOpenPopupFeatures?: string[];
private _recordingVideoFile: string | null = null;
private _screencastGeneration: number = 0;
constructor(browserContext: WKBrowserContext, pageProxySession: WKSession, opener: WKPage | null) {
this._pageProxySession = pageProxySession;
this._opener = opener;
this.rawKeyboard = new RawKeyboardImpl(pageProxySession);
this.rawMouse = new RawMouseImpl(pageProxySession);
this.rawTouchscreen = new RawTouchscreenImpl(pageProxySession);
this._contextIdToContext = new Map();
this._page = new Page(this, browserContext);
this.rawMouse.setPage(this._page);
this._workers = new WKWorkers(this._page);
this._session = undefined as any as WKSession;
this._browserContext = browserContext;
this._page.on(Page.Events.FrameDetached, (frame: frames.Frame) => this._removeContextsForFrame(frame, false));
this._eventListeners = [
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetCreated', this._onTargetCreated.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetDestroyed', this._onTargetDestroyed.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.dispatchMessageFromTarget', this._onDispatchMessageFromTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.didCommitProvisionalTarget', this._onDidCommitProvisionalTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Screencast.screencastFrame', this._onScreencastFrame.bind(this)),
];
this._firstNonInitialNavigationCommittedPromise = new Promise((f, r) => {
this._firstNonInitialNavigationCommittedFulfill = f;
this._firstNonInitialNavigationCommittedReject = r;
});
if (opener && !browserContext._options.noDefaultViewport && opener._nextWindowOpenPopupFeatures) {
const viewportSize = helper.getViewportSizeFromWindowFeatures(opener._nextWindowOpenPopupFeatures);
opener._nextWindowOpenPopupFeatures = undefined;
if (viewportSize)
this._page._emulatedSize = { viewport: viewportSize, screen: viewportSize };
}
}
potentiallyUninitializedPage(): Page {
return this._page;
}
private async _initializePageProxySession() {
if (this._page._browserContext.isSettingStorageState())
return;
const promises: Promise<any>[] = [
this._pageProxySession.send('Dialog.enable'),
this._pageProxySession.send('Emulation.setActiveAndFocused', { active: true }),
];
const contextOptions = this._browserContext._options;
if (contextOptions.javaScriptEnabled === false)
promises.push(this._pageProxySession.send('Emulation.setJavaScriptEnabled', { enabled: false }));
promises.push(this._updateViewport());
promises.push(this.updateHttpCredentials());
if (this._browserContext._permissions.size) {
for (const [key, value] of this._browserContext._permissions)
promises.push(this._grantPermissions(key, value));
}
if (this._browserContext._options.recordVideo) {
const outputFile = path.join(this._browserContext._options.recordVideo.dir, createGuid() + '.webm');
promises.push(this._browserContext._ensureVideosPath().then(() => {
return this._startVideo({
// validateBrowserContextOptions ensures correct video size.
...this._browserContext._options.recordVideo!.size!,
outputFile,
});
}));
}
await Promise.all(promises);
}
private _setSession(session: WKSession) {
eventsHelper.removeEventListeners(this._sessionListeners);
this._session = session;
this.rawKeyboard.setSession(session);
this.rawMouse.setSession(session);
this._addSessionListeners();
this._workers.setSession(session);
}
// This method is called for provisional targets as well. The session passed as the parameter
// may be different from the current session and may be destroyed without becoming current.
async _initializeSession(session: WKSession, provisional: boolean, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
await this._initializeSessionMayThrow(session, resourceTreeHandler).catch(e => {
// Provisional session can be disposed at any time, for example due to new navigation initiating
// a new provisional page.
if (provisional && session.isDisposed())
return;
// Swallow initialization errors due to newer target swap in,
// since we will reinitialize again.
if (this._session === session)
throw e;
});
}
private async _initializeSessionMayThrow(session: WKSession, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
const [, frameTree] = await Promise.all([
// Page agent must be enabled before Runtime.
session.send('Page.enable'),
session.send('Page.getResourceTree'),
] as const);
resourceTreeHandler(frameTree);
const promises: Promise<any>[] = [
// Resource tree should be received before first execution context.
session.send('Runtime.enable'),
session.send('Page.createUserWorld', { name: UTILITY_WORLD_NAME }).catch(_ => {}), // Worlds are per-process
session.send('Console.enable'),
session.send('Network.enable'),
this._workers.initializeSession(session)
];
if (this._page.needsRequestInterception()) {
promises.push(session.send('Network.setInterceptionEnabled', { enabled: true }));
promises.push(session.send('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }));
}
if (this._page._browserContext.isSettingStorageState()) {
await Promise.all(promises);
return;
}
const contextOptions = this._browserContext._options;
if (contextOptions.userAgent)
promises.push(this.updateUserAgent());
const emulatedMedia = this._page.emulatedMedia();
if (emulatedMedia.media || emulatedMedia.colorScheme || emulatedMedia.reducedMotion || emulatedMedia.forcedColors)
promises.push(WKPage._setEmulateMedia(session, emulatedMedia.media, emulatedMedia.colorScheme, emulatedMedia.reducedMotion, emulatedMedia.forcedColors));
for (const binding of this._page.allBindings())
promises.push(session.send('Runtime.addBinding', { name: binding.name }));
const bootstrapScript = this._calculateBootstrapScript();
if (bootstrapScript.length)
promises.push(session.send('Page.setBootstrapScript', { source: bootstrapScript }));
this._page.frames().map(frame => frame.evaluateExpression(bootstrapScript, false, undefined).catch(e => {}));
if (contextOptions.bypassCSP)
promises.push(session.send('Page.setBypassCSP', { enabled: true }));
const emulatedSize = this._page.emulatedSize();
if (emulatedSize) {
promises.push(session.send('Page.setScreenSizeOverride', {
width: emulatedSize.screen.width,
height: emulatedSize.screen.height,
}));
}
promises.push(this.updateEmulateMedia());
promises.push(session.send('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) }));
if (contextOptions.offline)
promises.push(session.send('Network.setEmulateOfflineState', { offline: true }));
promises.push(session.send('Page.setTouchEmulationEnabled', { enabled: !!contextOptions.hasTouch }));
if (contextOptions.timezoneId) {
promises.push(session.send('Page.setTimeZone', { timeZone: contextOptions.timezoneId }).
catch(e => { throw new Error(`Invalid timezone ID: ${contextOptions.timezoneId}`); }));
}
if (this._page.fileChooserIntercepted())
promises.push(session.send('Page.setInterceptFileChooserDialog', { enabled: true }));
promises.push(session.send('Page.overrideSetting', { setting: 'DeviceOrientationEventEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'FullScreenEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'NotificationsEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'PointerLockEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeMonthEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeWeekEnabled' as any, value: contextOptions.isMobile }));
await Promise.all(promises);
}
private _onDidCommitProvisionalTarget(event: Protocol.Target.didCommitProvisionalTargetPayload) {
const { oldTargetId, newTargetId } = event;
assert(this._provisionalPage);
assert(this._provisionalPage._session.sessionId === newTargetId, 'Unknown new target: ' + newTargetId);
assert(this._session.sessionId === oldTargetId, 'Unknown old target: ' + oldTargetId);
const newSession = this._provisionalPage._session;
this._provisionalPage.commit();
this._provisionalPage.dispose();
this._provisionalPage = null;
this._setSession(newSession);
}
private _onTargetDestroyed(event: Protocol.Target.targetDestroyedPayload) {
const { targetId, crashed } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId) {
this._provisionalPage._session.dispose(false);
this._provisionalPage.dispose();
this._provisionalPage = null;
} else if (this._session.sessionId === targetId) {
this._session.dispose(false);
eventsHelper.removeEventListeners(this._sessionListeners);
if (crashed) {
this._session.markAsCrashed();
this._page._didCrash();
}
}
}
didClose() {
this._page._didClose();
}
dispose(disconnected: boolean) {
this._pageProxySession.dispose(disconnected);
eventsHelper.removeEventListeners(this._sessionListeners);
eventsHelper.removeEventListeners(this._eventListeners);
if (this._session)
this._session.dispose(disconnected);
if (this._provisionalPage) {
this._provisionalPage._session.dispose(disconnected);
this._provisionalPage.dispose();
this._provisionalPage = null;
}
this._page._didDisconnect();
this._firstNonInitialNavigationCommittedReject(new Error('Page closed'));
}
dispatchMessageToSession(message: any) {
this._pageProxySession.dispatchMessage(message);
}
handleProvisionalLoadFailed(event: Protocol.Playwright.provisionalLoadFailedPayload) {
if (!this._initializedPage) {
this._firstNonInitialNavigationCommittedReject(new Error('Initial load failed'));
return;
}
if (!this._provisionalPage)
return;
let errorText = event.error;
if (errorText.includes('cancelled'))
errorText += '; maybe frame was detached?';
this._page._frameManager.frameAbortedNavigation(this._page.mainFrame()._id, errorText, event.loaderId);
}
handleWindowOpen(event: Protocol.Playwright.windowOpenPayload) {
debugAssert(!this._nextWindowOpenPopupFeatures);
this._nextWindowOpenPopupFeatures = event.windowFeatures;
}
async pageOrError(): Promise<Page | Error> {
return this._pagePromise;
}
private async _onTargetCreated(event: Protocol.Target.targetCreatedPayload) {
const { targetInfo } = event;
const session = new WKSession(this._pageProxySession.connection, targetInfo.targetId, `Target closed`, (message: any) => {
this._pageProxySession.send('Target.sendMessageToTarget', {
message: JSON.stringify(message), targetId: targetInfo.targetId
}).catch(e => {
session.dispatchMessage({ id: message.id, error: { message: e.message } });
});
});
assert(targetInfo.type === 'page', 'Only page targets are expected in WebKit, received: ' + targetInfo.type);
if (!targetInfo.isProvisional) {
assert(!this._initializedPage);
let pageOrError: Page | Error;
try {
this._setSession(session);
await Promise.all([
this._initializePageProxySession(),
this._initializeSession(session, false, ({ frameTree }) => this._handleFrameTree(frameTree)),
]);
pageOrError = this._page;
} catch (e) {
pageOrError = e;
}
if (targetInfo.isPaused)
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
if ((pageOrError instanceof Page) && this._page.mainFrame().url() === '') {
try {
// Initial empty page has an empty url. We should wait until the first real url has been loaded,
// even if that url is about:blank. This is especially important for popups, where we need the
// actual url before interacting with it.
await this._firstNonInitialNavigationCommittedPromise;
} catch (e) {
pageOrError = e;
}
} else {
// Avoid rejection on disconnect.
this._firstNonInitialNavigationCommittedPromise.catch(() => {});
}
await this._page.initOpener(this._opener);
// Note: it is important to call |reportAsNew| before resolving pageOrError promise,
// so that anyone who awaits pageOrError got a ready and reported page.
this._initializedPage = pageOrError instanceof Page ? pageOrError : null;
this._page.reportAsNew(pageOrError instanceof Page ? undefined : pageOrError);
this._pagePromise.resolve(pageOrError);
} else {
assert(targetInfo.isProvisional);
assert(!this._provisionalPage);
this._provisionalPage = new WKProvisionalPage(session, this);
if (targetInfo.isPaused) {
this._provisionalPage.initializationPromise.then(() => {
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
});
}
}
}
private _onDispatchMessageFromTarget(event: Protocol.Target.dispatchMessageFromTargetPayload) {
const { targetId, message } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId)
this._provisionalPage._session.dispatchMessage(JSON.parse(message));
else if (this._session.sessionId === targetId)
this._session.dispatchMessage(JSON.parse(message));
else
throw new Error('Unknown target: ' + targetId);
}
private _addSessionListeners() {
this._sessionListeners = [
eventsHelper.addEventListener(this._session, 'Page.frameNavigated', event => this._onFrameNavigated(event.frame, false)),
eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),
eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),
eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),
eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),
eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),
eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),
eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),
eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),
eventsHelper.addEventListener(this._session, 'Console.messageRepeatCountUpdated', event => this._onConsoleRepeatCountUpdated(event)),
eventsHelper.addEventListener(this._pageProxySession, 'Dialog.javascriptDialogOpening', event => this._onDialog(event)),
eventsHelper.addEventListener(this._session, 'Page.fileChooserOpened', event => this._onFileChooserOpened(event)),
eventsHelper.addEventListener(this._session, 'Network.requestWillBeSent', e => this._onRequestWillBeSent(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.requestIntercepted', e => this._onRequestIntercepted(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.responseReceived', e => this._onResponseReceived(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFinished', e => this._onLoadingFinished(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFailed', e => this._onLoadingFailed(e)),
eventsHelper.addEventListener(this._session, 'Network.webSocketCreated', e => this._page._frameManager.onWebSocketCreated(e.requestId, e.url)),
eventsHelper.addEventListener(this._session, 'Network.webSocketWillSendHandshakeRequest', e => this._page._frameManager.onWebSocketRequest(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketHandshakeResponseReceived', e => this._page._frameManager.onWebSocketResponse(e.requestId, e.response.status, e.response.statusText)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameSent', e => e.response.payloadData && this._page._frameManager.onWebSocketFrameSent(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameReceived', e => e.response.payloadData && this._page._frameManager.webSocketFrameReceived(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketClosed', e => this._page._frameManager.webSocketClosed(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameError', e => this._page._frameManager.webSocketError(e.requestId, e.errorMessage)),
];
}
private async _updateState<T extends keyof Protocol.CommandParameters>(
method: T,
params?: Protocol.CommandParameters[T]
): Promise<void> {
await this._forAllSessions(session => session.send(method, params).then());
}
private async _forAllSessions(callback: ((session: WKSession) => Promise<void>)): Promise<void> {
const sessions = [
this._session
];
// If the state changes during provisional load, push it to the provisional page
// as well to always be in sync with the backend.
if (this._provisionalPage)
sessions.push(this._provisionalPage._session);
await Promise.all(sessions.map(session => callback(session).catch(e => {})));
}
private _onWillCheckNavigationPolicy(frameId: string) {
// It may happen that new policy check occurs while there is an ongoing
// provisional load, in this case it should be safe to ignore it as it will
// either:
// - end up canceled, e.g. ctrl+click opening link in new tab, having no effect
// on this page
// - start new provisional load which we will miss in our signal trackers but
// we certainly won't hang waiting for it to finish and there is high chance
// that the current provisional page will commit navigation canceling the new
// one.
if (this._provisionalPage)
return;
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _onDidCheckNavigationPolicy(frameId: string, cancel?: boolean) {
if (!cancel)
return;
// This is a cross-process navigation that is canceled in the original page and continues in
// the provisional page. Bail out as we are tracking it.
if (this._provisionalPage)
return;
this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');
}
private _onFrameScheduledNavigation(frameId: string) {
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {
this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);
this._onFrameNavigated(frameTree.frame, true);
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'domcontentloaded');
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'load');
if (!frameTree.childFrames)
return;
for (const child of frameTree.childFrames)
this._handleFrameTree(child);
}
_onFrameAttached(frameId: string, parentFrameId: string | null): frames.Frame {
return this._page._frameManager.frameAttached(frameId, parentFrameId);
}
private _onFrameNavigated(framePayload: Protocol.Page.Frame, initial: boolean) {
const frame = this._page._frameManager.frame(framePayload.id);
assert(frame);
this._removeContextsForFrame(frame, true);
if (!framePayload.parentId)
this._workers.clear();
this._page._frameManager.frameCommittedNewDocumentNavigation(framePayload.id, framePayload.url, framePayload.name || '', framePayload.loaderId, initial);
if (!initial)
this._firstNonInitialNavigationCommittedFulfill();
}
private _onFrameNavigatedWithinDocument(frameId: string, url: string) {
this._page._frameManager.frameCommittedSameDocumentNavigation(frameId, url);
}
private _onFrameDetached(frameId: string) {
this._page._frameManager.frameDetached(frameId);
}
private _removeContextsForFrame(frame: frames.Frame, notifyFrame: boolean) {
for (const [contextId, context] of this._contextIdToContext) {
if (context.frame === frame) {
this._contextIdToContext.delete(contextId);
if (notifyFrame)
frame._contextDestroyed(context);
}
}
}
private _onExecutionContextCreated(contextPayload: Protocol.Runtime.ExecutionContextDescription) {
if (this._contextIdToContext.has(contextPayload.id))
return;
const frame = this._page._frameManager.frame(contextPayload.frameId);
if (!frame)
return;
const delegate = new WKExecutionContext(this._session, contextPayload.id);
let worldName: types.World|null = null;
if (contextPayload.type === 'normal')
worldName = 'main';
else if (contextPayload.type === 'user' && contextPayload.name === UTILITY_WORLD_NAME)
worldName = 'utility';
const context = new dom.FrameExecutionContext(delegate, frame, worldName);
(context as any)[contextDelegateSymbol] = delegate;
if (worldName)
frame._contextCreated(worldName, context);
if (contextPayload.type === 'normal' && frame === this._page.mainFrame())
this._mainFrameContextId = contextPayload.id;
this._contextIdToContext.set(contextPayload.id, context);
}
private async _onBindingCalled(contextId: Protocol.Runtime.ExecutionContextId, argument: string) {
const pageOrError = await this.pageOrError();
if (!(pageOrError instanceof Error)) {
const context = this._contextIdToContext.get(contextId);
if (context)
await this._page._onBindingCalled(argument, context);
}
}
async navigateFrame(frame: frames.Frame, url: string, referrer: string | undefined): Promise<frames.GotoResult> {
if (this._pageProxySession.isDisposed())
throw new Error('Target closed');
const pageProxyId = this._pageProxySession.sessionId;
const result = await this._pageProxySession.connection.browserSession.send('Playwright.navigate', { url, pageProxyId, frameId: frame._id, referrer });
return { newDocumentId: result.loaderId };
}
private _onConsoleMessage(event: Protocol.Console.messageAddedPayload) {
// Note: do no introduce await in this function, otherwise we lose the ordering.
// For example, frame.setContent relies on this.
const { type, level, text, parameters, url, line: lineNumber, column: columnNumber, source } = event.message;
if (level === 'error' && source === 'javascript') {
const { name, message } = splitErrorMessage(text);
let stack: string;
if (event.message.stackTrace) {
stack = text + '\n' + event.message.stackTrace.callFrames.map(callFrame => {
return ` at ${callFrame.functionName || 'unknown'} (${callFrame.url}:${callFrame.lineNumber}:${callFrame.columnNumber})`;
}).join('\n');
} else {
stack = '';
}
const error = new Error(message);
error.stack = stack;
error.name = name;
this._page.firePageError(error);
return;
}
let derivedType: string = type || '';
if (type === 'log')
derivedType = level;
else if (type === 'timing')
derivedType = 'timeEnd';
const handles: JSHandle[] = [];
for (const p of parameters || []) {
let context: dom.FrameExecutionContext | undefined;
if (p.objectId) {
const objectId = JSON.parse(p.objectId);
context = this._contextIdToContext.get(objectId.injectedScriptId);
} else {
context = this._contextIdToContext.get(this._mainFrameContextId!);
}
if (!context)
return;
handles.push(context.createHandle(p));
}
this._lastConsoleMessage = {
derivedType,
text,
handles,
count: 0,
location: {
url: url || '',
lineNumber: (lineNumber || 1) - 1,
columnNumber: (columnNumber || 1) - 1,
}
};
this._onConsoleRepeatCountUpdated({ count: 1 });
}
_onConsoleRepeatCountUpdated(event: Protocol.Console.messageRepeatCountUpdatedPayload) {
if (this._lastConsoleMessage) {
const {
derivedType,
text,
handles,
count,
location
} = this._lastConsoleMessage;
for (let i = count; i < event.count; ++i)
this._page._addConsoleMessage(derivedType, handles, location, handles.length ? undefined : text);
this._lastConsoleMessage.count = event.count;
}
}
_onDialog(event: Protocol.Dialog.javascriptDialogOpeningPayload) {
this._page.emit(Page.Events.Dialog, new dialog.Dialog(
this._page,
event.type as dialog.DialogType,
event.message,
async (accept: boolean, promptText?: string) => {
await this._pageProxySession.send('Dialog.handleJavaScriptDialog', { accept, promptText });
},
event.defaultPrompt));
}
private async _onFileChooserOpened(event: {frameId: Protocol.Network.FrameId, element: Protocol.Runtime.RemoteObject}) {
let handle;
try {
const context = await this._page._frameManager.frame(event.frameId)!._mainContext();
handle = context.createHandle(event.element).asElement()!;
} catch (e) {
// During async processing, frame/context may go away. We should not throw.
return;
}
await this._page._onFileChooserOpened(handle);
}
private static async _setEmulateMedia(session: WKSession, mediaType: types.MediaType, colorScheme: types.ColorScheme, reducedMotion: types.ReducedMotion, forcedColors: types.ForcedColors): Promise<void> {
const promises = [];
promises.push(session.send('Page.setEmulatedMedia', { media: mediaType === 'no-override' ? '' : mediaType }));
let appearance: any = undefined;
switch (colorScheme) {
case 'light': appearance = 'Light'; break;
case 'dark': appearance = 'Dark'; break;
case 'no-override': appearance = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersColorScheme', value: appearance }));
let reducedMotionWk: any = undefined;
switch (reducedMotion) {
case 'reduce': reducedMotionWk = 'Reduce'; break;
case 'no-preference': reducedMotionWk = 'NoPreference'; break;
case 'no-override': reducedMotionWk = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersReducedMotion', value: reducedMotionWk }));
let forcedColorsWk: any = undefined;
switch (forcedColors) {
case 'active': forcedColorsWk = 'Active'; break;
case 'none': forcedColorsWk = 'None'; break;
case 'no-override': forcedColorsWk = undefined; break;
}
promises.push(session.send('Page.setForcedColors', { forcedColors: forcedColorsWk }));
await Promise.all(promises);
}
async updateExtraHTTPHeaders(): Promise<void> {
await this._updateState('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) });
}
_calculateExtraHTTPHeaders(): types.HeadersArray {
const locale = this._browserContext._options.locale;
const headers = network.mergeHeaders([
this._browserContext._options.extraHTTPHeaders,
this._page.extraHTTPHeaders(),
locale ? network.singleHeader('Accept-Language', locale) : undefined,
]);
return headers;
}
async updateEmulateMedia(): Promise<void> {
const emulatedMedia = this._page.emulatedMedia();
const colorScheme = emulatedMedia.colorScheme;
const reducedMotion = emulatedMedia.reducedMotion;
const forcedColors = emulatedMedia.forcedColors;
await this._forAllSessions(session => WKPage._setEmulateMedia(session, emulatedMedia.media, colorScheme, reducedMotion, forcedColors));
}
async updateEmulatedViewportSize(): Promise<void> {
await this._updateViewport();
}
async updateUserAgent(): Promise<void> {
const contextOptions = this._browserContext._options;
this._updateState('Page.overrideUserAgent', { value: contextOptions.userAgent });
}
async bringToFront(): Promise<void> {
this._pageProxySession.send('Target.activate', {
targetId: this._session.sessionId
});
}
async _updateViewport(): Promise<void> {
const options = this._browserContext._options;
const deviceSize = this._page.emulatedSize();
if (deviceSize === null)
return;
const viewportSize = deviceSize.viewport;
const screenSize = deviceSize.screen;
const promises: Promise<any>[] = [
this._pageProxySession.send('Emulation.setDeviceMetricsOverride', {
width: viewportSize.width,
height: viewportSize.height,
fixedLayout: !!options.isMobile,
deviceScaleFactor: options.deviceScaleFactor || 1
}),
this._session.send('Page.setScreenSizeOverride', {
width: screenSize.width,
height: screenSize.height,
}),
];
if (options.isMobile) {
const angle = viewportSize.width > viewportSize.height ? 90 : 0;
promises.push(this._session.send('Page.setOrientationOverride', { angle }));
}
await Promise.all(promises);
}
async updateRequestInterception(): Promise<void> {
const enabled = this._page.needsRequestInterception();
await Promise.all([
this._updateState('Network.setInterceptionEnabled', { enabled }),
this._updateState('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }),
]);
}
async updateOffline() {
await this._updateState('Network.setEmulateOfflineState', { offline: !!this._browserContext._options.offline });
}
async updateHttpCredentials() {
const credentials = this._browserContext._options.httpCredentials || { username: '', password: '' };
await this._pageProxySession.send('Emulation.setAuthCredentials', { username: credentials.username, password: credentials.password });
}
async updateFileChooserInterception() {
const enabled = this._page.fileChooserIntercepted();
await this._session.send('Page.setInterceptFileChooserDialog', { enabled }).catch(() => {}); // target can be closed.
}
async reload(): Promise<void> {
await this._session.send('Page.reload');
}
goBack(): Promise<boolean> {
return this._session.send('Page.goBack').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goBack): Failed to go`))
return false;
throw error;
});
}
goForward(): Promise<boolean> {
return this._session.send('Page.goForward').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goForward): Failed to go`))
return false;
throw error;
});
}
async exposeBinding(binding: PageBinding): Promise<void> {
this._session.send('Runtime.addBinding', { name: binding.name });
await this._updateBootstrapScript();
await Promise.all(this._page.frames().map(frame => frame.evaluateExpression(binding.source, false, {}).catch(e => {})));
}
async removeExposedBindings(): Promise<void> {
await this._updateBootstrapScript();
}
async addInitScript(script: string): Promise<void> {
await this._updateBootstrapScript();
}
async removeInitScripts() {
await this._updateBootstrapScript();
}
private _calculateBootstrapScript(): string {
const scripts: string[] = [];
if (!this._page.context()._options.isMobile) {
scripts.push('delete window.orientation');
scripts.push('delete window.ondevicemotion');
scripts.push('delete window.ondeviceorientation');
}
for (const binding of this._page.allBindings())
scripts.push(binding.source);
scripts.push(...this._browserContext.initScripts);
scripts.push(...this._page.initScripts);
return scripts.join(';\n');
}
async _updateBootstrapScript(): Promise<void> {
await this._updateState('Page.setBootstrapScript', { source: this._calculateBootstrapScript() });
}
async closePage(runBeforeUnload: boolean): Promise<void> {
await this._stopVideo();
await this._pageProxySession.sendMayFail('Target.close', {
targetId: this._session.sessionId,
runBeforeUnload
});
}
async setBackgroundColor(color?: { r: number; g: number; b: number; a: number; }): Promise<void> {
await this._session.send('Page.setDefaultBackgroundColorOverride', { color });
}
private _toolbarHeight(): number {
if (this._page._browserContext._browser?.options.headful)
return hostPlatform === 'mac10.15' ? 55 : 59;
return 0;
}
private async _startVideo(options: types.PageScreencastOptions): Promise<void> {
assert(!this._recordingVideoFile);
const { screencastId } = await this._pageProxySession.send('Screencast.startVideo', {
file: options.outputFile,
width: options.width,
height: options.height,
toolbarHeight: this._toolbarHeight()
});
this._recordingVideoFile = options.outputFile;
this._browserContext._browser._videoStarted(this._browserContext, screencastId, options.outputFile, this.pageOrError());
}
async _stopVideo(): Promise<void> {
if (!this._recordingVideoFile)
return;
await this._pageProxySession.sendMayFail('Screencast.stopVideo');
this._recordingVideoFile = null;
}
private validateScreenshotDimension(side: number, omitDeviceScaleFactor: boolean) {
// Cairo based implementations (Linux and Windows) have hard limit of 32767
// (see https://github.com/microsoft/playwright/issues/16727).
if (process.platform === 'darwin')
return;
if (!omitDeviceScaleFactor && this._page._browserContext._options.deviceScaleFactor)
side = Math.ceil(side * this._page._browserContext._options.deviceScaleFactor);
if (side > 32767)
throw new Error('Cannot take screenshot larger than 32767 pixels on any dimension');
}
async takeScreenshot(progress: Progress, format: string, documentRect: types.Rect | undefined, viewportRect: types.Rect | undefined, quality: number | undefined, fitsViewport: boolean, scale: 'css' | 'device'): Promise<Buffer> {
const rect = (documentRect || viewportRect)!;
const omitDeviceScaleFactor = scale === 'css';
this.validateScreenshotDimension(rect.width, omitDeviceScaleFactor);
this.validateScreenshotDimension(rect.height, omitDeviceScaleFactor);
const result = await this._session.send('Page.snapshotRect', { ...rect, coordinateSystem: documentRect ? 'Page' : 'Viewport', omitDeviceScaleFactor });
const prefix = 'data:image/png;base64,';
let buffer = Buffer.from(result.dataURL.substr(prefix.length), 'base64');
if (format === 'jpeg')
buffer = jpegjs.encode(PNG.sync.read(buffer), quality).data;
return buffer;
}
async getContentFrame(handle: dom.ElementHandle): Promise<frames.Frame | null> {
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
if (!nodeInfo.contentFrameId)
return null;
return this._page._frameManager.frame(nodeInfo.contentFrameId);
}
async getOwnerFrame(handle: dom.ElementHandle): Promise<string | null> {
if (!handle._objectId)
return null;
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
return nodeInfo.ownerFrameId || null;
}
isElementHandle(remoteObject: any): boolean {
return (remoteObject as Protocol.Runtime.RemoteObject).subtype === 'node';
}
async getBoundingBox(handle: dom.ElementHandle): Promise<types.Rect | null> {
const quads = await this.getContentQuads(handle);
if (!quads || !quads.length)
return null;
let minX = Infinity;
let maxX = -Infinity;
let minY = Infinity;
let maxY = -Infinity;
for (const quad of quads) {
for (const point of quad) {
minX = Math.min(minX, point.x);
maxX = Math.max(maxX, point.x);
minY = Math.min(minY, point.y);
maxY = Math.max(maxY, point.y);
}
}
return { x: minX, y: minY, width: maxX - minX, height: maxY - minY };
}
async scrollRectIntoViewIfNeeded(handle: dom.ElementHandle, rect?: types.Rect): Promise<'error:notvisible' | 'error:notconnected' | 'done'> {
return await this._session.send('DOM.scrollIntoViewIfNeeded', {
objectId: handle._objectId,
rect,
}).then(() => 'done' as const).catch(e => {
if (e instanceof Error && e.message.includes('Node does not have a layout object'))
return 'error:notvisible';
if (e instanceof Error && e.message.includes('Node is detached from document'))
return 'error:notconnected';
throw e;
});
}
async setScreencastOptions(options: { width: number, height: number, quality: number } | null): Promise<void> {
if (options) {
const so = { ...options, toolbarHeight: this._toolbarHeight() };
const { generation } = await this._pageProxySession.send('Screencast.startScreencast', so);
this._screencastGeneration = generation;
} else {
await this._pageProxySession.send('Screencast.stopScreencast');
}
}
private _onScreencastFrame(event: Protocol.Screencast.screencastFramePayload) {
const generation = this._screencastGeneration;
this._page.throttleScreencastFrameAck(() => {
this._pageProxySession.send('Screencast.screencastFrameAck', { generation }).catch(e => debugLogger.log('error', e));
});
const buffer = Buffer.from(event.data, 'base64');
this._page.emit(Page.Events.ScreencastFrame, {
buffer,
width: event.deviceWidth,
height: event.deviceHeight,
});
}
rafCountForStablePosition(): number {
return process.platform === 'win32' ? 5 : 1;
}
async getContentQuads(handle: dom.ElementHandle): Promise<types.Quad[] | null> {
const result = await this._session.sendMayFail('DOM.getContentQuads', {
objectId: handle._objectId
});
if (!result)
return null;
return result.quads.map(quad => [
{ x: quad[0], y: quad[1] },
{ x: quad[2], y: quad[3] },
{ x: quad[4], y: quad[5] },
{ x: quad[6], y: quad[7] }
]);
}
async setInputFiles(handle: dom.ElementHandle<HTMLInputElement>, files: types.FilePayload[]): Promise<void> {
const objectId = handle._objectId;
const protocolFiles = files.map(file => ({
name: file.name,
type: file.mimeType,
data: file.buffer,
}));
await this._session.send('DOM.setInputFiles', { objectId, files: protocolFiles });
}
async setInputFilePaths(handle: dom.ElementHandle<HTMLInputElement>, paths: string[]): Promise<void> {
const pageProxyId = this._pageProxySession.sessionId;
const objectId = handle._objectId;
await Promise.all([
this._pageProxySession.connection.browserSession.send('Playwright.grantFileReadAccess', { pageProxyId, paths }),
this._session.send('DOM.setInputFiles', { objectId, paths })
]);
}
async adoptElementHandle<T extends Node>(handle: dom.ElementHandle<T>, to: dom.FrameExecutionContext): Promise<dom.ElementHandle<T>> {
const result = await this._session.sendMayFail('DOM.resolveNode', {
objectId: handle._objectId,
executionContextId: ((to as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error(dom.kUnableToAdoptErrorMessage);
return to.createHandle(result.object) as dom.ElementHandle<T>;
}
async getAccessibilityTree(needle?: dom.ElementHandle): Promise<{tree: accessibility.AXNode, needle: accessibility.AXNode | null}> {
return getAccessibilityTree(this._session, needle);
}
async inputActionEpilogue(): Promise<void> {
}
async getFrameElement(frame: frames.Frame): Promise<dom.ElementHandle> {
const parent = frame.parentFrame();
if (!parent)
throw new Error('Frame has been detached.');
const context = await parent._mainContext();
const result = await this._session.send('DOM.resolveNode', {
frameId: frame._id,
executionContextId: ((context as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error('Frame has been detached.');
return context.createHandle(result.object) as dom.ElementHandle;
}
_onRequestWillBeSent(session: WKSession, event: Protocol.Network.requestWillBeSentPayload) {
if (event.request.url.startsWith('data:'))
return;
let redirectedFrom: WKInterceptableRequest | null = null;
if (event.redirectResponse) {
const request = this._requestIdToRequest.get(event.requestId);
// If we connect late to the target, we could have missed the requestWillBeSent event.
if (request) {
this._handleRequestRedirect(request, event.redirectResponse, event.timestamp);
redirectedFrom = request;
}
}
const frame = redirectedFrom ? redirectedFrom.request.frame() : this._page._frameManager.frame(event.frameId);
// sometimes we get stray network events for detached frames
// TODO(einbinder) why?
if (!frame)
return;
// TODO(einbinder) this will fail if we are an XHR document request
const isNavigationRequest = event.type === 'Document';
const documentId = isNavigationRequest ? event.loaderId : undefined;
let route = null;
// We do not support intercepting redirects.
if (this._page.needsRequestInterception() && !redirectedFrom)
route = new WKRouteImpl(session, event.requestId);
const request = new WKInterceptableRequest(session, route, frame, event, redirectedFrom, documentId);
this._requestIdToRequest.set(event.requestId, request);
this._page._frameManager.requestStarted(request.request, route || undefined);
}
private _handleRequestRedirect(request: WKInterceptableRequest, responsePayload: Protocol.Network.Response, timestamp: number) {
const response = request.createResponse(responsePayload);
response._securityDetailsFinished();
response._serverAddrFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(responsePayload.timing ? helper.secondsToRoundishMillis(timestamp - request._timestamp) : -1);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.requestReceivedResponse(response);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onRequestIntercepted(session: WKSession, event: Protocol.Network.requestInterceptedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
if (!request) {
session.sendMayFail('Network.interceptRequestWithError', { errorType: 'Cancellation', requestId: event.requestId });
return;
}
// There is no point in waiting for the raw headers in Network.responseReceived when intercepting.
// Use provisional headers as raw headers, so that client can call allHeaders() from the route handler.
request.request.setRawRequestHeaders(null);
if (!request._route) {
// Intercepted, although we do not intend to allow interception.
// Just continue.
session.sendMayFail('Network.interceptWithRequest', { requestId: request._requestId });
} else {
request._route._requestInterceptedPromise.resolve();
}
}
_onResponseReceived(event: Protocol.Network.responseReceivedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// FileUpload sends a response without a matching request.
if (!request)
return;
this._requestIdToResponseReceivedPayloadEvent.set(request._requestId, event);
const response = request.createResponse(event.response);
this._page._frameManager.requestReceivedResponse(response);
if (response.status() === 204) {
this._onLoadingFailed({
requestId: event.requestId,
errorText: 'Aborted: 204 No Content',
timestamp: event.timestamp
});
}
}
_onLoadingFinished(event: Protocol.Network.loadingFinishedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
// Under certain conditions we never get the Network.responseReceived
// event from protocol. @see https://crbug.com/883475
const response = request.request._existingResponse();
if (response) {
const responseReceivedPayload = this._requestIdToResponseReceivedPayloadEvent.get(request._requestId);
response._serverAddrFinished(parseRemoteAddress(event?.metrics?.remoteAddress));
response._securityDetailsFinished({
protocol: isLoadedSecurely(response.url(), response.timing()) ? event.metrics?.securityConnection?.protocol : undefined,
subjectName: responseReceivedPayload?.response.security?.certificate?.subject,
validFrom: responseReceivedPayload?.response.security?.certificate?.validFrom,
validTo: responseReceivedPayload?.response.security?.certificate?.validUntil,
});
if (event.metrics?.protocol)
response._setHttpVersion(event.metrics.protocol);
response.setEncodedBodySize(event.metrics?.responseBodyBytesReceived ?? null);
response.setResponseHeadersSize(event.metrics?.responseHeaderBytesReceived ?? null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToResponseReceivedPayloadEvent.delete(request._requestId);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onLoadingFailed(event: Protocol.Network.loadingFailedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
const response = request.request._existingResponse();
if (response) {
response._serverAddrFinished();
response._securityDetailsFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToRequest.delete(request._requestId);
request.request._setFailureText(event.errorText);
this._page._frameManager.requestFailed(request.request, event.errorText.includes('cancelled'));
}
async _grantPermissions(origin: string, permissions: string[]) {
const webPermissionToProtocol = new Map<string, string>([
['geolocation', 'geolocation'],
]);
const filtered = permissions.map(permission => {
const protocolPermission = webPermissionToProtocol.get(permission);
if (!protocolPermission)
throw new Error('Unknown permission: ' + permission);
return protocolPermission;
});
await this._pageProxySession.send('Emulation.grantPermissions', { origin, permissions: filtered });
}
async _clearPermissions() {
await this._pageProxySession.send('Emulation.resetPermissions', {});
}
}
/**
* WebKit Remote Addresses look like:
*
* macOS:
* ::1.8911
* 2606:2800:220:1:248:1893:25c8:1946.443
* 127.0.0.1:8000
*
* ubuntu:
* ::1:8907
* 127.0.0.1:8000
*
* NB: They look IPv4 and IPv6's with ports but use an alternative notation.
*/
function parseRemoteAddress(value?: string) {
if (!value)
return;
try {
const colon = value.lastIndexOf(':');
const dot = value.lastIndexOf('.');
if (dot < 0) { // IPv6ish:port
return {
ipAddress: `[${value.slice(0, colon)}]`,
port: +value.slice(colon + 1)
};
}
if (colon > dot) { // IPv4:port
const [address, port] = value.split(':');
return {
ipAddress: address,
port: +port,
};
} else { // IPv6ish.port
const [address, port] = value.split('.');
return {
ipAddress: `[${address}]`,
port: +port,
};
}
} catch (_) {}
}
/**
* Adapted from Source/WebInspectorUI/UserInterface/Models/Resource.js in
* WebKit codebase.
*/
function isLoadedSecurely(url: string, timing: network.ResourceTiming) {
try {
const u = new URL(url);
if (u.protocol !== 'https:' && u.protocol !== 'wss:' && u.protocol !== 'sftp:')
return false;
if (timing.secureConnectionStart === -1 && timing.connectStart !== -1)
return false;
return true;
} catch (_) {}
}
const contextDelegateSymbol = Symbol('delegate');
| packages/playwright-core/src/server/webkit/wkPage.ts | 1 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.9991695880889893,
0.04911564663052559,
0.00016290298663079739,
0.00021894092787988484,
0.2007564902305603
] |
{
"id": 1,
"code_window": [
" if (this._provisionalPage)\n",
" return;\n",
" this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');\n",
" }\n",
"\n",
" private _onFrameScheduledNavigation(frameId: string) {\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n",
" }\n",
"\n",
" private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {\n",
" this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);\n",
" this._onFrameNavigated(frameTree.frame, true);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" private _onFrameScheduledNavigation(frameId: string, delay: number, targetIsCurrentFrame: boolean) {\n",
" if (targetIsCurrentFrame)\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 447
} | ---
id: ci
title: "Continuous Integration"
---
Playwright tests can be executed in CI environments. We have created sample
configurations for common CI providers.
<!-- TOC -->
## Introduction
3 steps to get your tests running on CI:
1. **Ensure CI agent can run browsers**: Use [our Docker image](./docker.md)
in Linux agents or install your dependencies using the [CLI](./cli.md#install-system-dependencies).
1. **Install Playwright**:
```bash js
# Install NPM packages
npm ci
# or
npm install
# Install Playwright browsers and dependencies
npx playwright install --with-deps
```
```bash python
pip install playwright
playwright install --with-deps
```
```bash java
mvn exec:java -e -D exec.mainClass=com.microsoft.playwright.CLI -D exec.args="install --with-deps"
```
```bash csharp
pwsh bin/Debug/netX/playwright.ps1 install --with-deps
```
1. **Run your tests**:
```bash js
npx playwright test
```
```bash python
pytest
```
```bash java
mvn test
```
```bash csharp
dotnet test
```
## CI configurations
The [Command line tools](./cli.md#install-system-dependencies) can be used to install all operating system dependencies on GitHub Actions.
### GitHub Actions
```yml js
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Install Playwright
run: npx playwright install --with-deps
- name: Run your tests
run: npx playwright test
- name: Upload test results
if: always()
uses: actions/upload-artifact@v3
with:
name: playwright-report
path: playwright-report
```
```yml python
steps:
- uses: actions/checkout@v3
- name: Set up Python
uses: actions/setup-python@v4
with:
python-version: '3.11'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r local-requirements.txt
pip install -e .
- name: Ensure browsers are installed
run: python -m playwright install --with-deps
- name: Run your tests
run: pytest
```
```yml java
steps:
- uses: actions/checkout@v3
- uses: actions/setup-java@v3
with:
distribution: 'temurin'
java-version: '17'
- name: Build & Install
run: mvn -B install -D skipTests --no-transfer-progress
- name: Install Playwright
run: mvn exec:java -e -D exec.mainClass=com.microsoft.playwright.CLI -D exec.args="install --with-deps"
- name: Run tests
run: mvn test
```
```yml csharp
steps:
- uses: actions/checkout@v3
- name: Setup dotnet
uses: actions/setup-dotnet@v3
with:
dotnet-version: 6.0.x
- run: dotnet build
- name: Ensure browsers are installed
run: pwsh bin\Debug\net6.0\playwright.ps1 install --with-deps
- name: Run your tests
run: dotnet test
```
We run [our tests](https://github.com/microsoft/playwright/blob/main/.github/workflows/tests_secondary.yml) on GitHub Actions, across a matrix of 3 platforms (Windows, Linux, macOS) and 3 browsers (Chromium, Firefox, WebKit).
### GitHub Actions on deployment
This will start the tests after a [GitHub Deployment](https://developer.github.com/v3/repos/deployments/) went into the `success` state.
Services like Vercel use this pattern so you can run your end-to-end tests on their deployed environment.
```yml
name: Playwright Tests
on:
deployment_status:
jobs:
test:
timeout-minutes: 60
runs-on: ubuntu-latest
if: github.event.deployment_status.state == 'success'
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: '18.x'
- name: Install dependencies
run: npm ci
- name: Install Playwright
run: npx playwright install --with-deps
- name: Run Playwright tests
run: npx playwright test
env:
# This might depend on your test-runner/language binding
PLAYWRIGHT_TEST_BASE_URL: ${{ github.event.deployment_status.target_url }}
```
### Docker
We have a [pre-built Docker image](./docker.md) which can either be used directly, or as a reference to update your existing Docker definitions.
Suggested configuration
1. Using `--ipc=host` is also recommended when using Chromium—without it Chromium can run out of memory
and crash. Learn more about this option in [Docker docs](https://docs.docker.com/engine/reference/run/#ipc-settings---ipc).
1. Seeing other weird errors when launching Chromium? Try running your container
with `docker run --cap-add=SYS_ADMIN` when developing locally.
1. Using `--init` Docker flag or [dumb-init](https://github.com/Yelp/dumb-init) is recommended to avoid special
treatment for processes with PID=1. This is a common reason for zombie processes.
### GitHub Actions (via containers)
GitHub Actions support [running jobs in a container](https://docs.github.com/en/actions/using-jobs/running-jobs-in-a-container) by using the [`jobs.<job_id>.container`](https://docs.github.com/en/actions/using-workflows/workflow-syntax-for-github-actions#jobsjob_idcontainer) option.
```yml js
jobs:
playwright:
name: 'Playwright Tests'
runs-on: ubuntu-latest
container:
image: mcr.microsoft.com/playwright:v1.31.0-focal
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Run your tests
run: npx playwright test
```
```yml python
jobs:
playwright:
name: 'Playwright Tests'
runs-on: ubuntu-latest
container:
image: mcr.microsoft.com/playwright:v1.31.0-focal
steps:
- uses: actions/checkout@v3
- name: Set up Python
uses: actions/setup-python@v4
with:
python-version: '3.11'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r local-requirements.txt
pip install -e .
- name: Ensure browsers are installed
run: python -m playwright install --with-deps
- name: Run your tests
run: pytest
```
```yml java
jobs:
playwright:
name: 'Playwright Tests'
runs-on: ubuntu-latest
container:
image: mcr.microsoft.com/playwright:v1.31.0-focal
steps:
- uses: actions/checkout@v3
- uses: actions/setup-java@v3
with:
distribution: 'temurin'
java-version: '17'
- name: Build & Install
run: mvn -B install -D skipTests --no-transfer-progress
- name: Install Playwright
run: mvn exec:java -e -D exec.mainClass=com.microsoft.playwright.CLI -D exec.args="install --with-deps"
- name: Run tests
run: mvn test
```
```yml csharp
jobs:
playwright:
name: 'Playwright Tests'
runs-on: ubuntu-latest
container:
image: mcr.microsoft.com/playwright:v1.31.0-focal
steps:
- uses: actions/checkout@v3
- name: Setup dotnet
uses: actions/setup-dotnet@v3
with:
dotnet-version: 6.0.x
- run: dotnet build
- name: Ensure browsers are installed
run: pwsh bin\Debug\net6.0\playwright.ps1 install --with-deps
- name: Run your tests
run: dotnet test
```
#### Sharding
* langs: js
GitHub Actions supports [sharding tests between multiple jobs](https://docs.github.com/en/actions/using-jobs/using-a-matrix-for-your-jobs) using the [`jobs.<job_id>.strategy.matrix`](https://docs.github.com/en/actions/using-workflows/workflow-syntax-for-github-actions#jobsjob_idstrategymatrix) option. The `matrix` option will run a separate job for every possible combination of the provided options. In the example below, we have 2 `project` values, 10 `shardIndex` values and 1 `shardTotal` value, resulting in a total of 20 jobs to be run.
```yml js
jobs:
playwright:
name: 'Playwright Tests - ${{ matrix.project }} - Shard ${{ matrix.shardIndex }} of ${{ matrix.shardTotal }}'
runs-on: ubuntu-latest
container:
image: mcr.microsoft.com/playwright:v1.31.0-focal
strategy:
fail-fast: false
matrix:
project: [chromium, webkit]
shardIndex: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
shardTotal: [10]
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Run your tests
run: npx playwright test --project=${{ matrix.project }} --shard=${{ matrix.shardIndex }}/${{ matrix.shardTotal }}
```
> Note: The `${{ <expression> }}` is the [expression](https://docs.github.com/en/actions/learn-github-actions/expressions) syntax that allows accessing the current [context](https://docs.github.com/en/actions/learn-github-actions/contexts). In this example, we are using the [`matrix`](https://docs.github.com/en/actions/learn-github-actions/contexts#matrix-context) context to set the job variants.
### Azure Pipelines
For Windows or macOS agents, no additional configuration required, just install Playwright and run your tests.
For Linux agents, you can use [our Docker container](./docker.md) with Azure
Pipelines support [running containerized
jobs](https://docs.microsoft.com/en-us/azure/devops/pipelines/process/container-phases?view=azure-devops).
Alternatively, you can use [Command line tools](./cli.md#install-system-dependencies) to install all necessary dependencies.
For running the Playwright tests use this pipeline task:
```yml
jobs:
- deployment: Run_E2E_Tests
pool:
vmImage: ubuntu-20.04
container: mcr.microsoft.com/playwright:v1.31.0-focal
environment: testing
strategy:
runOnce:
deploy:
steps:
- checkout: self
- task: Bash@3
displayName: 'Run Playwright tests'
inputs:
workingDirectory: 'my-e2e-tests'
targetType: 'inline'
failOnStderr: true
env:
CI: true
script: |
npm ci
npx playwright test
```
This will make the pipeline run fail if any of the playwright tests fails.
If you also want to integrate the test results with Azure DevOps, use the task `PublishTestResults` task like so:
```yml
jobs:
- deployment: Run_E2E_Tests
pool:
vmImage: ubuntu-20.04
container: mcr.microsoft.com/playwright:v1.31.0-focal
environment: testing
strategy:
runOnce:
deploy:
steps:
- checkout: self
- task: Bash@3
displayName: 'Run Playwright tests'
inputs:
workingDirectory: 'my-e2e-tests'
targetType: 'inline'
failOnStderr: true
env:
CI: true
script: |
npm ci
npx playwright test
- task: PublishTestResults@2
displayName: 'Publish test results'
inputs:
searchFolder: 'my-e2e-tests/test-results'
testResultsFormat: 'JUnit'
testResultsFiles: 'e2e-junit-results.xml'
mergeTestResults: true
failTaskOnFailedTests: true
testRunTitle: 'My End-To-End Tests'
condition: succeededOrFailed()
```
Note: The JUnit reporter needs to be configured accordingly via
```ts
["junit", { outputFile: "test-results/e2e-junit-results.xml" }]
```
in `playwright.config.ts`.
### CircleCI
Running Playwright on CircleCI is very similar to running on GitHub Actions. In order to specify the pre-built Playwright [Docker image](./docker.md) , simply modify the agent definition with `docker:` in your config like so:
```yml
executors:
pw-focal-development:
docker:
- image: mcr.microsoft.com/playwright:v1.31.0-focal
```
Note: When using the docker agent definition, you are specifying the resource class of where playwright runs to the 'medium' tier [here](https://circleci.com/docs/configuration-reference?#docker-execution-environment). The default behavior of Playwright is to set the number of workers to the detected core count (2 in the case of the medium tier). Overriding the number of workers to greater than this number will cause unnecessary timeouts and failures.
Similarly, If you’re using Playwright through Jest, then you may encounter an error spawning child processes:
```
[00:00.0] jest args: --e2e --spec --max-workers=36
Error: spawn ENOMEM
at ChildProcess.spawn (internal/child_process.js:394:11)
```
This is likely caused by Jest autodetecting the number of processes on the entire machine (`36`) rather than the number allowed to your container (`2`). To fix this, set `jest --maxWorkers=2` in your test command.
#### Sharding in CircleCI
Sharding in CircleCI is indexed with 0 which means that you will need to override the default parallelism ENV VARS. The following example demonstrates how to run Playwright with a CircleCI Parallelism of 4 by adding 1 to the `CIRCLE_NODE_INDEX` to pass into the `--shard` cli arg.
```yml
playwright-job-name:
executor: pw-focal-development
parallelism: 4
steps:
- run: SHARD="$((${CIRCLE_NODE_INDEX}+1))"; npx playwright test -- --shard=${SHARD}/${CIRCLE_NODE_TOTAL}
```
### Jenkins
Jenkins supports Docker agents for pipelines. Use the [Playwright Docker image](./docker.md)
to run tests on Jenkins.
```groovy
pipeline {
agent { docker { image 'mcr.microsoft.com/playwright:v1.31.0-focal' } }
stages {
stage('e2e-tests') {
steps {
// Depends on your language / test framework
sh 'npm install'
sh 'npx playwright test'
}
}
}
}
```
### Bitbucket Pipelines
Bitbucket Pipelines can use public [Docker images as build environments](https://confluence.atlassian.com/bitbucket/use-docker-images-as-build-environments-792298897.html). To run Playwright tests on Bitbucket, use our public Docker image ([see Dockerfile](./docker.md)).
```yml
image: mcr.microsoft.com/playwright:v1.31.0-focal
```
### GitLab CI
To run Playwright tests on GitLab, use our public Docker image ([see Dockerfile](./docker.md)).
```yml
stages:
- test
tests:
stage: test
image: mcr.microsoft.com/playwright:v1.31.0-focal
script:
...
```
#### Sharding
* langs: js
GitLab CI supports [sharding tests between multiple jobs](https://docs.gitlab.com/ee/ci/jobs/job_control.html#parallelize-large-jobs) using the [parallel](https://docs.gitlab.com/ee/ci/yaml/index.html#parallel) keyword. The test job will be split into multiple smaller jobs that run in parallel. Parallel jobs are named sequentially from `job_name 1/N` to `job_name N/N`.
```yml
stages:
- test
tests:
stage: test
image: mcr.microsoft.com/playwright:v1.31.0-focal
parallel: 7
script:
- npm ci
- npx playwright test --shard=$CI_NODE_INDEX/$CI_NODE_TOTAL
```
GitLab CI also supports sharding tests between multiple jobs using the [parallel:matrix](https://docs.gitlab.com/ee/ci/yaml/index.html#parallelmatrix) option. The test job will run multiple times in parallel in a single pipeline, but with different variable values for each instance of the job. In the example below, we have 2 `PROJECT` values, 10 `SHARD_INDEX` values and 1 `SHARD_TOTAL` value, resulting in a total of 20 jobs to be run.
```yml
stages:
- test
tests:
stage: test
image: mcr.microsoft.com/playwright:v1.31.0-focal
parallel:
matrix:
- PROJECT: ['chromium', 'webkit']
SHARD_INDEX: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
SHARD_TOTAL: 10
script:
- npm ci
- npx playwright test --project=$PROJECT --shard=$SHARD_INDEX/$SHARD_TOTAL
```
## Caching browsers
By default, Playwright downloads browser binaries when the Playwright NPM package
is installed. The NPM packages have a `postinstall` hook that downloads the browser
binaries. This behavior can be [customized with environment variables](./browsers.md#managing-browser-binaries).
Caching browsers on CI is **strictly optional**: The `postinstall` hooks should
execute and download the browser binaries on every run.
#### Exception: `node_modules` are cached (Node-specific)
Most CI providers cache the [npm-cache](https://docs.npmjs.com/cli-commands/cache.html)
directory (located at `$HOME/.npm`). If your CI pipelines caches the `node_modules`
directory and you run `npm install` (instead of `npm ci`), the default configuration
**will not work**. This is because the `npm install` step will find the Playwright NPM
package on disk and not execute the `postinstall` step.
> Travis CI automatically caches `node_modules` if your repo does not have a
`package-lock.json` file.
This behavior can be fixed with one of the following approaches:
1. Move to caching `$HOME/.npm` or the npm-cache directory. (This is the default
behavior in most CI providers.)
1. Set `PLAYWRIGHT_BROWSERS_PATH=0` as the environment variable before running
`npm install`. This will download the browser binaries in the `node_modules`
directory and cache them with the package code. See [managing browser binaries](./browsers.md#managing-browser-binaries).
1. Use `npm ci` (instead of `npm install`) which forces a clean install: by
removing the existing `node_modules` directory. See [npm docs](https://docs.npmjs.com/cli/ci.html).
1. Cache the browser binaries, with the steps below.
#### Directories to cache
With the default behavior, Playwright downloads the browser binaries in the following
directories:
- `%USERPROFILE%\AppData\Local\ms-playwright` on Windows
- `~/Library/Caches/ms-playwright` on MacOS
- `~/.cache/ms-playwright` on Linux
To cache the browser downloads between CI runs, cache this location in your CI
configuration, against a hash of the Playwright version.
## Debugging browser launches
Playwright supports the `DEBUG` environment variable to output debug logs during execution. Setting it to `pw:browser*` is helpful while debugging `Error: Failed to launch browser` errors.
```bash js
DEBUG=pw:browser* npx playwright test
```
```bash python
DEBUG=pw:browser* pytest
```
## Running headed
By default, Playwright launches browsers in headless mode. This can be changed by passing a flag when the browser is launched.
```js
// Works across chromium, firefox and webkit
const { chromium } = require('playwright');
const browser = await chromium.launch({ headless: false });
```
```java
// Works across chromium, firefox and webkit
import com.microsoft.playwright.*;
public class Example {
public static void main(String[] args) {
try (Playwright playwright = Playwright.create()) {
BrowserType chromium = playwright.chromium();
Browser browser = chromium.launch(new BrowserType.LaunchOptions().setHeadless(false));
}
}
}
```
```python async
import asyncio
from playwright.async_api import async_playwright
async def main():
async with async_playwright() as p:
# Works across chromium, firefox and webkit
browser = await p.chromium.launch(headless=False)
asyncio.run(main())
```
```python sync
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
# Works across chromium, firefox and webkit
browser = p.chromium.launch(headless=False)
```
```csharp
using Microsoft.Playwright;
using var playwright = await Playwright.CreateAsync();
await playwright.Chromium.LaunchAsync(new()
{
Headless = false
});
```
On Linux agents, headed execution requires [Xvfb](https://en.wikipedia.org/wiki/Xvfb) to be installed. Our [Docker image](./docker.md) and GitHub Action have Xvfb pre-installed. To run browsers in headed mode with Xvfb, add `xvfb-run` before the Node.js command.
```bash js
xvfb-run node index.js
```
```bash python
xvfb-run python test.py
```
| docs/src/ci.md | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00017693027621135116,
0.00017214642139151692,
0.0001635997323319316,
0.00017263012705370784,
0.000003331533434902667
] |
{
"id": 1,
"code_window": [
" if (this._provisionalPage)\n",
" return;\n",
" this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');\n",
" }\n",
"\n",
" private _onFrameScheduledNavigation(frameId: string) {\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n",
" }\n",
"\n",
" private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {\n",
" this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);\n",
" this._onFrameNavigated(frameTree.frame, true);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" private _onFrameScheduledNavigation(frameId: string, delay: number, targetIsCurrentFrame: boolean) {\n",
" if (targetIsCurrentFrame)\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 447
} | name: "infra"
on:
push:
branches:
- main
- release-*
pull_request:
branches:
- main
- release-*
jobs:
doc-and-lint:
name: "docs & lint"
runs-on: ubuntu-20.04
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: 14
- run: npm i -g npm@8
- run: npm ci
- run: npm run build
- run: npx playwright install-deps
- run: npx playwright install
- run: npm run lint
- name: Verify clean tree
run: |
if [[ -n $(git status -s) ]]; then
echo "ERROR: tree is dirty after npm run build:"
git diff
exit 1
fi
- name: Audit prod NPM dependencies
run: npm audit --omit dev
| .github/workflows/infra.yml | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00017599946295376867,
0.0001748199574649334,
0.00017355935415253043,
0.00017486049910075963,
0.000001165328967545065
] |
{
"id": 1,
"code_window": [
" if (this._provisionalPage)\n",
" return;\n",
" this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');\n",
" }\n",
"\n",
" private _onFrameScheduledNavigation(frameId: string) {\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n",
" }\n",
"\n",
" private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {\n",
" this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);\n",
" this._onFrameNavigated(frameTree.frame, true);\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" private _onFrameScheduledNavigation(frameId: string, delay: number, targetIsCurrentFrame: boolean) {\n",
" if (targetIsCurrentFrame)\n",
" this._page._frameManager.frameRequestedNavigation(frameId);\n"
],
"file_path": "packages/playwright-core/src/server/webkit/wkPage.ts",
"type": "replace",
"edit_start_line_idx": 447
} | name: "electron"
on:
push:
branches:
- main
- release-*
pull_request:
paths-ignore:
- 'browser_patches/**'
- 'docs/**'
types: [ labeled ]
branches:
- main
- release-*
env:
# Force terminal colors. @see https://www.npmjs.com/package/colors
FORCE_COLOR: 1
FLAKINESS_CONNECTION_STRING: ${{ secrets.FLAKINESS_CONNECTION_STRING }}
jobs:
test_electron:
name: ${{ matrix.os }}
strategy:
fail-fast: false
matrix:
os: [ubuntu-latest, macos-latest, windows-latest]
runs-on: ${{ matrix.os }}
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: 14
- run: npm i -g npm@8
- run: npm ci
env:
PLAYWRIGHT_SKIP_BROWSER_DOWNLOAD: 1
- run: npm run build
- run: npx playwright install --with-deps chromium
- run: xvfb-run --auto-servernum --server-args="-screen 0 1280x960x24" -- npm run etest
if: matrix.os == 'ubuntu-latest'
- run: npm run etest
if: matrix.os != 'ubuntu-latest'
- run: node tests/config/checkCoverage.js electron
if: always() && matrix.os == 'ubuntu-latest'
- run: ./utils/upload_flakiness_dashboard.sh ./test-results/report.json
if: always()
shell: bash
- uses: actions/upload-artifact@v3
if: always() && matrix.os == 'ubuntu-latest'
with:
name: electron-linux-test-results
path: test-results
| .github/workflows/tests_electron.yml | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.0001768731017364189,
0.00017487374134361744,
0.00017316181038040668,
0.0001747473725117743,
0.000001131771455220587
] |
{
"id": 2,
"code_window": [
" await page.goto(server.EMPTY_PAGE);\n",
" await page.click('\"Click me\"');\n",
"});\n",
"\n",
"it('should work with _blank target in form', async ({ page, server, browserName }) => {\n",
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"it('should work with _blank target in form', async ({ page, server }) => {\n"
],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import path from 'path';
import { PNG, jpegjs } from '../../utilsBundle';
import { splitErrorMessage } from '../../utils/stackTrace';
import { assert, createGuid, debugAssert, headersArrayToObject } from '../../utils';
import { hostPlatform } from '../../utils/hostPlatform';
import type * as accessibility from '../accessibility';
import * as dialog from '../dialog';
import * as dom from '../dom';
import type * as frames from '../frames';
import type { RegisteredListener } from '../../utils/eventsHelper';
import { eventsHelper } from '../../utils/eventsHelper';
import { helper } from '../helper';
import type { JSHandle } from '../javascript';
import * as network from '../network';
import type { PageBinding, PageDelegate } from '../page';
import { Page } from '../page';
import type { Progress } from '../progress';
import type * as types from '../types';
import type { Protocol } from './protocol';
import { getAccessibilityTree } from './wkAccessibility';
import type { WKBrowserContext } from './wkBrowser';
import { WKSession } from './wkConnection';
import { WKExecutionContext } from './wkExecutionContext';
import { RawKeyboardImpl, RawMouseImpl, RawTouchscreenImpl } from './wkInput';
import { WKInterceptableRequest, WKRouteImpl } from './wkInterceptableRequest';
import { WKProvisionalPage } from './wkProvisionalPage';
import { WKWorkers } from './wkWorkers';
import { debugLogger } from '../../common/debugLogger';
import { ManualPromise } from '../../utils/manualPromise';
const UTILITY_WORLD_NAME = '__playwright_utility_world__';
export class WKPage implements PageDelegate {
readonly rawMouse: RawMouseImpl;
readonly rawKeyboard: RawKeyboardImpl;
readonly rawTouchscreen: RawTouchscreenImpl;
_session: WKSession;
private _provisionalPage: WKProvisionalPage | null = null;
readonly _page: Page;
private readonly _pagePromise = new ManualPromise<Page | Error>();
private readonly _pageProxySession: WKSession;
readonly _opener: WKPage | null;
private readonly _requestIdToRequest = new Map<string, WKInterceptableRequest>();
private readonly _workers: WKWorkers;
private readonly _contextIdToContext: Map<number, dom.FrameExecutionContext>;
private _mainFrameContextId?: number;
private _sessionListeners: RegisteredListener[] = [];
private _eventListeners: RegisteredListener[];
readonly _browserContext: WKBrowserContext;
_initializedPage: Page | null = null;
private _firstNonInitialNavigationCommittedPromise: Promise<void>;
private _firstNonInitialNavigationCommittedFulfill = () => {};
_firstNonInitialNavigationCommittedReject = (e: Error) => {};
private _lastConsoleMessage: { derivedType: string, text: string, handles: JSHandle[]; count: number, location: types.ConsoleMessageLocation; } | null = null;
private readonly _requestIdToResponseReceivedPayloadEvent = new Map<string, Protocol.Network.responseReceivedPayload>();
// Holds window features for the next popup being opened via window.open,
// until the popup page proxy arrives.
private _nextWindowOpenPopupFeatures?: string[];
private _recordingVideoFile: string | null = null;
private _screencastGeneration: number = 0;
constructor(browserContext: WKBrowserContext, pageProxySession: WKSession, opener: WKPage | null) {
this._pageProxySession = pageProxySession;
this._opener = opener;
this.rawKeyboard = new RawKeyboardImpl(pageProxySession);
this.rawMouse = new RawMouseImpl(pageProxySession);
this.rawTouchscreen = new RawTouchscreenImpl(pageProxySession);
this._contextIdToContext = new Map();
this._page = new Page(this, browserContext);
this.rawMouse.setPage(this._page);
this._workers = new WKWorkers(this._page);
this._session = undefined as any as WKSession;
this._browserContext = browserContext;
this._page.on(Page.Events.FrameDetached, (frame: frames.Frame) => this._removeContextsForFrame(frame, false));
this._eventListeners = [
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetCreated', this._onTargetCreated.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetDestroyed', this._onTargetDestroyed.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.dispatchMessageFromTarget', this._onDispatchMessageFromTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.didCommitProvisionalTarget', this._onDidCommitProvisionalTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Screencast.screencastFrame', this._onScreencastFrame.bind(this)),
];
this._firstNonInitialNavigationCommittedPromise = new Promise((f, r) => {
this._firstNonInitialNavigationCommittedFulfill = f;
this._firstNonInitialNavigationCommittedReject = r;
});
if (opener && !browserContext._options.noDefaultViewport && opener._nextWindowOpenPopupFeatures) {
const viewportSize = helper.getViewportSizeFromWindowFeatures(opener._nextWindowOpenPopupFeatures);
opener._nextWindowOpenPopupFeatures = undefined;
if (viewportSize)
this._page._emulatedSize = { viewport: viewportSize, screen: viewportSize };
}
}
potentiallyUninitializedPage(): Page {
return this._page;
}
private async _initializePageProxySession() {
if (this._page._browserContext.isSettingStorageState())
return;
const promises: Promise<any>[] = [
this._pageProxySession.send('Dialog.enable'),
this._pageProxySession.send('Emulation.setActiveAndFocused', { active: true }),
];
const contextOptions = this._browserContext._options;
if (contextOptions.javaScriptEnabled === false)
promises.push(this._pageProxySession.send('Emulation.setJavaScriptEnabled', { enabled: false }));
promises.push(this._updateViewport());
promises.push(this.updateHttpCredentials());
if (this._browserContext._permissions.size) {
for (const [key, value] of this._browserContext._permissions)
promises.push(this._grantPermissions(key, value));
}
if (this._browserContext._options.recordVideo) {
const outputFile = path.join(this._browserContext._options.recordVideo.dir, createGuid() + '.webm');
promises.push(this._browserContext._ensureVideosPath().then(() => {
return this._startVideo({
// validateBrowserContextOptions ensures correct video size.
...this._browserContext._options.recordVideo!.size!,
outputFile,
});
}));
}
await Promise.all(promises);
}
private _setSession(session: WKSession) {
eventsHelper.removeEventListeners(this._sessionListeners);
this._session = session;
this.rawKeyboard.setSession(session);
this.rawMouse.setSession(session);
this._addSessionListeners();
this._workers.setSession(session);
}
// This method is called for provisional targets as well. The session passed as the parameter
// may be different from the current session and may be destroyed without becoming current.
async _initializeSession(session: WKSession, provisional: boolean, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
await this._initializeSessionMayThrow(session, resourceTreeHandler).catch(e => {
// Provisional session can be disposed at any time, for example due to new navigation initiating
// a new provisional page.
if (provisional && session.isDisposed())
return;
// Swallow initialization errors due to newer target swap in,
// since we will reinitialize again.
if (this._session === session)
throw e;
});
}
private async _initializeSessionMayThrow(session: WKSession, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
const [, frameTree] = await Promise.all([
// Page agent must be enabled before Runtime.
session.send('Page.enable'),
session.send('Page.getResourceTree'),
] as const);
resourceTreeHandler(frameTree);
const promises: Promise<any>[] = [
// Resource tree should be received before first execution context.
session.send('Runtime.enable'),
session.send('Page.createUserWorld', { name: UTILITY_WORLD_NAME }).catch(_ => {}), // Worlds are per-process
session.send('Console.enable'),
session.send('Network.enable'),
this._workers.initializeSession(session)
];
if (this._page.needsRequestInterception()) {
promises.push(session.send('Network.setInterceptionEnabled', { enabled: true }));
promises.push(session.send('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }));
}
if (this._page._browserContext.isSettingStorageState()) {
await Promise.all(promises);
return;
}
const contextOptions = this._browserContext._options;
if (contextOptions.userAgent)
promises.push(this.updateUserAgent());
const emulatedMedia = this._page.emulatedMedia();
if (emulatedMedia.media || emulatedMedia.colorScheme || emulatedMedia.reducedMotion || emulatedMedia.forcedColors)
promises.push(WKPage._setEmulateMedia(session, emulatedMedia.media, emulatedMedia.colorScheme, emulatedMedia.reducedMotion, emulatedMedia.forcedColors));
for (const binding of this._page.allBindings())
promises.push(session.send('Runtime.addBinding', { name: binding.name }));
const bootstrapScript = this._calculateBootstrapScript();
if (bootstrapScript.length)
promises.push(session.send('Page.setBootstrapScript', { source: bootstrapScript }));
this._page.frames().map(frame => frame.evaluateExpression(bootstrapScript, false, undefined).catch(e => {}));
if (contextOptions.bypassCSP)
promises.push(session.send('Page.setBypassCSP', { enabled: true }));
const emulatedSize = this._page.emulatedSize();
if (emulatedSize) {
promises.push(session.send('Page.setScreenSizeOverride', {
width: emulatedSize.screen.width,
height: emulatedSize.screen.height,
}));
}
promises.push(this.updateEmulateMedia());
promises.push(session.send('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) }));
if (contextOptions.offline)
promises.push(session.send('Network.setEmulateOfflineState', { offline: true }));
promises.push(session.send('Page.setTouchEmulationEnabled', { enabled: !!contextOptions.hasTouch }));
if (contextOptions.timezoneId) {
promises.push(session.send('Page.setTimeZone', { timeZone: contextOptions.timezoneId }).
catch(e => { throw new Error(`Invalid timezone ID: ${contextOptions.timezoneId}`); }));
}
if (this._page.fileChooserIntercepted())
promises.push(session.send('Page.setInterceptFileChooserDialog', { enabled: true }));
promises.push(session.send('Page.overrideSetting', { setting: 'DeviceOrientationEventEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'FullScreenEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'NotificationsEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'PointerLockEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeMonthEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeWeekEnabled' as any, value: contextOptions.isMobile }));
await Promise.all(promises);
}
private _onDidCommitProvisionalTarget(event: Protocol.Target.didCommitProvisionalTargetPayload) {
const { oldTargetId, newTargetId } = event;
assert(this._provisionalPage);
assert(this._provisionalPage._session.sessionId === newTargetId, 'Unknown new target: ' + newTargetId);
assert(this._session.sessionId === oldTargetId, 'Unknown old target: ' + oldTargetId);
const newSession = this._provisionalPage._session;
this._provisionalPage.commit();
this._provisionalPage.dispose();
this._provisionalPage = null;
this._setSession(newSession);
}
private _onTargetDestroyed(event: Protocol.Target.targetDestroyedPayload) {
const { targetId, crashed } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId) {
this._provisionalPage._session.dispose(false);
this._provisionalPage.dispose();
this._provisionalPage = null;
} else if (this._session.sessionId === targetId) {
this._session.dispose(false);
eventsHelper.removeEventListeners(this._sessionListeners);
if (crashed) {
this._session.markAsCrashed();
this._page._didCrash();
}
}
}
didClose() {
this._page._didClose();
}
dispose(disconnected: boolean) {
this._pageProxySession.dispose(disconnected);
eventsHelper.removeEventListeners(this._sessionListeners);
eventsHelper.removeEventListeners(this._eventListeners);
if (this._session)
this._session.dispose(disconnected);
if (this._provisionalPage) {
this._provisionalPage._session.dispose(disconnected);
this._provisionalPage.dispose();
this._provisionalPage = null;
}
this._page._didDisconnect();
this._firstNonInitialNavigationCommittedReject(new Error('Page closed'));
}
dispatchMessageToSession(message: any) {
this._pageProxySession.dispatchMessage(message);
}
handleProvisionalLoadFailed(event: Protocol.Playwright.provisionalLoadFailedPayload) {
if (!this._initializedPage) {
this._firstNonInitialNavigationCommittedReject(new Error('Initial load failed'));
return;
}
if (!this._provisionalPage)
return;
let errorText = event.error;
if (errorText.includes('cancelled'))
errorText += '; maybe frame was detached?';
this._page._frameManager.frameAbortedNavigation(this._page.mainFrame()._id, errorText, event.loaderId);
}
handleWindowOpen(event: Protocol.Playwright.windowOpenPayload) {
debugAssert(!this._nextWindowOpenPopupFeatures);
this._nextWindowOpenPopupFeatures = event.windowFeatures;
}
async pageOrError(): Promise<Page | Error> {
return this._pagePromise;
}
private async _onTargetCreated(event: Protocol.Target.targetCreatedPayload) {
const { targetInfo } = event;
const session = new WKSession(this._pageProxySession.connection, targetInfo.targetId, `Target closed`, (message: any) => {
this._pageProxySession.send('Target.sendMessageToTarget', {
message: JSON.stringify(message), targetId: targetInfo.targetId
}).catch(e => {
session.dispatchMessage({ id: message.id, error: { message: e.message } });
});
});
assert(targetInfo.type === 'page', 'Only page targets are expected in WebKit, received: ' + targetInfo.type);
if (!targetInfo.isProvisional) {
assert(!this._initializedPage);
let pageOrError: Page | Error;
try {
this._setSession(session);
await Promise.all([
this._initializePageProxySession(),
this._initializeSession(session, false, ({ frameTree }) => this._handleFrameTree(frameTree)),
]);
pageOrError = this._page;
} catch (e) {
pageOrError = e;
}
if (targetInfo.isPaused)
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
if ((pageOrError instanceof Page) && this._page.mainFrame().url() === '') {
try {
// Initial empty page has an empty url. We should wait until the first real url has been loaded,
// even if that url is about:blank. This is especially important for popups, where we need the
// actual url before interacting with it.
await this._firstNonInitialNavigationCommittedPromise;
} catch (e) {
pageOrError = e;
}
} else {
// Avoid rejection on disconnect.
this._firstNonInitialNavigationCommittedPromise.catch(() => {});
}
await this._page.initOpener(this._opener);
// Note: it is important to call |reportAsNew| before resolving pageOrError promise,
// so that anyone who awaits pageOrError got a ready and reported page.
this._initializedPage = pageOrError instanceof Page ? pageOrError : null;
this._page.reportAsNew(pageOrError instanceof Page ? undefined : pageOrError);
this._pagePromise.resolve(pageOrError);
} else {
assert(targetInfo.isProvisional);
assert(!this._provisionalPage);
this._provisionalPage = new WKProvisionalPage(session, this);
if (targetInfo.isPaused) {
this._provisionalPage.initializationPromise.then(() => {
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
});
}
}
}
private _onDispatchMessageFromTarget(event: Protocol.Target.dispatchMessageFromTargetPayload) {
const { targetId, message } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId)
this._provisionalPage._session.dispatchMessage(JSON.parse(message));
else if (this._session.sessionId === targetId)
this._session.dispatchMessage(JSON.parse(message));
else
throw new Error('Unknown target: ' + targetId);
}
private _addSessionListeners() {
this._sessionListeners = [
eventsHelper.addEventListener(this._session, 'Page.frameNavigated', event => this._onFrameNavigated(event.frame, false)),
eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),
eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),
eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),
eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),
eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),
eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),
eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),
eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),
eventsHelper.addEventListener(this._session, 'Console.messageRepeatCountUpdated', event => this._onConsoleRepeatCountUpdated(event)),
eventsHelper.addEventListener(this._pageProxySession, 'Dialog.javascriptDialogOpening', event => this._onDialog(event)),
eventsHelper.addEventListener(this._session, 'Page.fileChooserOpened', event => this._onFileChooserOpened(event)),
eventsHelper.addEventListener(this._session, 'Network.requestWillBeSent', e => this._onRequestWillBeSent(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.requestIntercepted', e => this._onRequestIntercepted(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.responseReceived', e => this._onResponseReceived(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFinished', e => this._onLoadingFinished(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFailed', e => this._onLoadingFailed(e)),
eventsHelper.addEventListener(this._session, 'Network.webSocketCreated', e => this._page._frameManager.onWebSocketCreated(e.requestId, e.url)),
eventsHelper.addEventListener(this._session, 'Network.webSocketWillSendHandshakeRequest', e => this._page._frameManager.onWebSocketRequest(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketHandshakeResponseReceived', e => this._page._frameManager.onWebSocketResponse(e.requestId, e.response.status, e.response.statusText)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameSent', e => e.response.payloadData && this._page._frameManager.onWebSocketFrameSent(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameReceived', e => e.response.payloadData && this._page._frameManager.webSocketFrameReceived(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketClosed', e => this._page._frameManager.webSocketClosed(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameError', e => this._page._frameManager.webSocketError(e.requestId, e.errorMessage)),
];
}
private async _updateState<T extends keyof Protocol.CommandParameters>(
method: T,
params?: Protocol.CommandParameters[T]
): Promise<void> {
await this._forAllSessions(session => session.send(method, params).then());
}
private async _forAllSessions(callback: ((session: WKSession) => Promise<void>)): Promise<void> {
const sessions = [
this._session
];
// If the state changes during provisional load, push it to the provisional page
// as well to always be in sync with the backend.
if (this._provisionalPage)
sessions.push(this._provisionalPage._session);
await Promise.all(sessions.map(session => callback(session).catch(e => {})));
}
private _onWillCheckNavigationPolicy(frameId: string) {
// It may happen that new policy check occurs while there is an ongoing
// provisional load, in this case it should be safe to ignore it as it will
// either:
// - end up canceled, e.g. ctrl+click opening link in new tab, having no effect
// on this page
// - start new provisional load which we will miss in our signal trackers but
// we certainly won't hang waiting for it to finish and there is high chance
// that the current provisional page will commit navigation canceling the new
// one.
if (this._provisionalPage)
return;
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _onDidCheckNavigationPolicy(frameId: string, cancel?: boolean) {
if (!cancel)
return;
// This is a cross-process navigation that is canceled in the original page and continues in
// the provisional page. Bail out as we are tracking it.
if (this._provisionalPage)
return;
this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');
}
private _onFrameScheduledNavigation(frameId: string) {
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {
this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);
this._onFrameNavigated(frameTree.frame, true);
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'domcontentloaded');
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'load');
if (!frameTree.childFrames)
return;
for (const child of frameTree.childFrames)
this._handleFrameTree(child);
}
_onFrameAttached(frameId: string, parentFrameId: string | null): frames.Frame {
return this._page._frameManager.frameAttached(frameId, parentFrameId);
}
private _onFrameNavigated(framePayload: Protocol.Page.Frame, initial: boolean) {
const frame = this._page._frameManager.frame(framePayload.id);
assert(frame);
this._removeContextsForFrame(frame, true);
if (!framePayload.parentId)
this._workers.clear();
this._page._frameManager.frameCommittedNewDocumentNavigation(framePayload.id, framePayload.url, framePayload.name || '', framePayload.loaderId, initial);
if (!initial)
this._firstNonInitialNavigationCommittedFulfill();
}
private _onFrameNavigatedWithinDocument(frameId: string, url: string) {
this._page._frameManager.frameCommittedSameDocumentNavigation(frameId, url);
}
private _onFrameDetached(frameId: string) {
this._page._frameManager.frameDetached(frameId);
}
private _removeContextsForFrame(frame: frames.Frame, notifyFrame: boolean) {
for (const [contextId, context] of this._contextIdToContext) {
if (context.frame === frame) {
this._contextIdToContext.delete(contextId);
if (notifyFrame)
frame._contextDestroyed(context);
}
}
}
private _onExecutionContextCreated(contextPayload: Protocol.Runtime.ExecutionContextDescription) {
if (this._contextIdToContext.has(contextPayload.id))
return;
const frame = this._page._frameManager.frame(contextPayload.frameId);
if (!frame)
return;
const delegate = new WKExecutionContext(this._session, contextPayload.id);
let worldName: types.World|null = null;
if (contextPayload.type === 'normal')
worldName = 'main';
else if (contextPayload.type === 'user' && contextPayload.name === UTILITY_WORLD_NAME)
worldName = 'utility';
const context = new dom.FrameExecutionContext(delegate, frame, worldName);
(context as any)[contextDelegateSymbol] = delegate;
if (worldName)
frame._contextCreated(worldName, context);
if (contextPayload.type === 'normal' && frame === this._page.mainFrame())
this._mainFrameContextId = contextPayload.id;
this._contextIdToContext.set(contextPayload.id, context);
}
private async _onBindingCalled(contextId: Protocol.Runtime.ExecutionContextId, argument: string) {
const pageOrError = await this.pageOrError();
if (!(pageOrError instanceof Error)) {
const context = this._contextIdToContext.get(contextId);
if (context)
await this._page._onBindingCalled(argument, context);
}
}
async navigateFrame(frame: frames.Frame, url: string, referrer: string | undefined): Promise<frames.GotoResult> {
if (this._pageProxySession.isDisposed())
throw new Error('Target closed');
const pageProxyId = this._pageProxySession.sessionId;
const result = await this._pageProxySession.connection.browserSession.send('Playwright.navigate', { url, pageProxyId, frameId: frame._id, referrer });
return { newDocumentId: result.loaderId };
}
private _onConsoleMessage(event: Protocol.Console.messageAddedPayload) {
// Note: do no introduce await in this function, otherwise we lose the ordering.
// For example, frame.setContent relies on this.
const { type, level, text, parameters, url, line: lineNumber, column: columnNumber, source } = event.message;
if (level === 'error' && source === 'javascript') {
const { name, message } = splitErrorMessage(text);
let stack: string;
if (event.message.stackTrace) {
stack = text + '\n' + event.message.stackTrace.callFrames.map(callFrame => {
return ` at ${callFrame.functionName || 'unknown'} (${callFrame.url}:${callFrame.lineNumber}:${callFrame.columnNumber})`;
}).join('\n');
} else {
stack = '';
}
const error = new Error(message);
error.stack = stack;
error.name = name;
this._page.firePageError(error);
return;
}
let derivedType: string = type || '';
if (type === 'log')
derivedType = level;
else if (type === 'timing')
derivedType = 'timeEnd';
const handles: JSHandle[] = [];
for (const p of parameters || []) {
let context: dom.FrameExecutionContext | undefined;
if (p.objectId) {
const objectId = JSON.parse(p.objectId);
context = this._contextIdToContext.get(objectId.injectedScriptId);
} else {
context = this._contextIdToContext.get(this._mainFrameContextId!);
}
if (!context)
return;
handles.push(context.createHandle(p));
}
this._lastConsoleMessage = {
derivedType,
text,
handles,
count: 0,
location: {
url: url || '',
lineNumber: (lineNumber || 1) - 1,
columnNumber: (columnNumber || 1) - 1,
}
};
this._onConsoleRepeatCountUpdated({ count: 1 });
}
_onConsoleRepeatCountUpdated(event: Protocol.Console.messageRepeatCountUpdatedPayload) {
if (this._lastConsoleMessage) {
const {
derivedType,
text,
handles,
count,
location
} = this._lastConsoleMessage;
for (let i = count; i < event.count; ++i)
this._page._addConsoleMessage(derivedType, handles, location, handles.length ? undefined : text);
this._lastConsoleMessage.count = event.count;
}
}
_onDialog(event: Protocol.Dialog.javascriptDialogOpeningPayload) {
this._page.emit(Page.Events.Dialog, new dialog.Dialog(
this._page,
event.type as dialog.DialogType,
event.message,
async (accept: boolean, promptText?: string) => {
await this._pageProxySession.send('Dialog.handleJavaScriptDialog', { accept, promptText });
},
event.defaultPrompt));
}
private async _onFileChooserOpened(event: {frameId: Protocol.Network.FrameId, element: Protocol.Runtime.RemoteObject}) {
let handle;
try {
const context = await this._page._frameManager.frame(event.frameId)!._mainContext();
handle = context.createHandle(event.element).asElement()!;
} catch (e) {
// During async processing, frame/context may go away. We should not throw.
return;
}
await this._page._onFileChooserOpened(handle);
}
private static async _setEmulateMedia(session: WKSession, mediaType: types.MediaType, colorScheme: types.ColorScheme, reducedMotion: types.ReducedMotion, forcedColors: types.ForcedColors): Promise<void> {
const promises = [];
promises.push(session.send('Page.setEmulatedMedia', { media: mediaType === 'no-override' ? '' : mediaType }));
let appearance: any = undefined;
switch (colorScheme) {
case 'light': appearance = 'Light'; break;
case 'dark': appearance = 'Dark'; break;
case 'no-override': appearance = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersColorScheme', value: appearance }));
let reducedMotionWk: any = undefined;
switch (reducedMotion) {
case 'reduce': reducedMotionWk = 'Reduce'; break;
case 'no-preference': reducedMotionWk = 'NoPreference'; break;
case 'no-override': reducedMotionWk = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersReducedMotion', value: reducedMotionWk }));
let forcedColorsWk: any = undefined;
switch (forcedColors) {
case 'active': forcedColorsWk = 'Active'; break;
case 'none': forcedColorsWk = 'None'; break;
case 'no-override': forcedColorsWk = undefined; break;
}
promises.push(session.send('Page.setForcedColors', { forcedColors: forcedColorsWk }));
await Promise.all(promises);
}
async updateExtraHTTPHeaders(): Promise<void> {
await this._updateState('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) });
}
_calculateExtraHTTPHeaders(): types.HeadersArray {
const locale = this._browserContext._options.locale;
const headers = network.mergeHeaders([
this._browserContext._options.extraHTTPHeaders,
this._page.extraHTTPHeaders(),
locale ? network.singleHeader('Accept-Language', locale) : undefined,
]);
return headers;
}
async updateEmulateMedia(): Promise<void> {
const emulatedMedia = this._page.emulatedMedia();
const colorScheme = emulatedMedia.colorScheme;
const reducedMotion = emulatedMedia.reducedMotion;
const forcedColors = emulatedMedia.forcedColors;
await this._forAllSessions(session => WKPage._setEmulateMedia(session, emulatedMedia.media, colorScheme, reducedMotion, forcedColors));
}
async updateEmulatedViewportSize(): Promise<void> {
await this._updateViewport();
}
async updateUserAgent(): Promise<void> {
const contextOptions = this._browserContext._options;
this._updateState('Page.overrideUserAgent', { value: contextOptions.userAgent });
}
async bringToFront(): Promise<void> {
this._pageProxySession.send('Target.activate', {
targetId: this._session.sessionId
});
}
async _updateViewport(): Promise<void> {
const options = this._browserContext._options;
const deviceSize = this._page.emulatedSize();
if (deviceSize === null)
return;
const viewportSize = deviceSize.viewport;
const screenSize = deviceSize.screen;
const promises: Promise<any>[] = [
this._pageProxySession.send('Emulation.setDeviceMetricsOverride', {
width: viewportSize.width,
height: viewportSize.height,
fixedLayout: !!options.isMobile,
deviceScaleFactor: options.deviceScaleFactor || 1
}),
this._session.send('Page.setScreenSizeOverride', {
width: screenSize.width,
height: screenSize.height,
}),
];
if (options.isMobile) {
const angle = viewportSize.width > viewportSize.height ? 90 : 0;
promises.push(this._session.send('Page.setOrientationOverride', { angle }));
}
await Promise.all(promises);
}
async updateRequestInterception(): Promise<void> {
const enabled = this._page.needsRequestInterception();
await Promise.all([
this._updateState('Network.setInterceptionEnabled', { enabled }),
this._updateState('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }),
]);
}
async updateOffline() {
await this._updateState('Network.setEmulateOfflineState', { offline: !!this._browserContext._options.offline });
}
async updateHttpCredentials() {
const credentials = this._browserContext._options.httpCredentials || { username: '', password: '' };
await this._pageProxySession.send('Emulation.setAuthCredentials', { username: credentials.username, password: credentials.password });
}
async updateFileChooserInterception() {
const enabled = this._page.fileChooserIntercepted();
await this._session.send('Page.setInterceptFileChooserDialog', { enabled }).catch(() => {}); // target can be closed.
}
async reload(): Promise<void> {
await this._session.send('Page.reload');
}
goBack(): Promise<boolean> {
return this._session.send('Page.goBack').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goBack): Failed to go`))
return false;
throw error;
});
}
goForward(): Promise<boolean> {
return this._session.send('Page.goForward').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goForward): Failed to go`))
return false;
throw error;
});
}
async exposeBinding(binding: PageBinding): Promise<void> {
this._session.send('Runtime.addBinding', { name: binding.name });
await this._updateBootstrapScript();
await Promise.all(this._page.frames().map(frame => frame.evaluateExpression(binding.source, false, {}).catch(e => {})));
}
async removeExposedBindings(): Promise<void> {
await this._updateBootstrapScript();
}
async addInitScript(script: string): Promise<void> {
await this._updateBootstrapScript();
}
async removeInitScripts() {
await this._updateBootstrapScript();
}
private _calculateBootstrapScript(): string {
const scripts: string[] = [];
if (!this._page.context()._options.isMobile) {
scripts.push('delete window.orientation');
scripts.push('delete window.ondevicemotion');
scripts.push('delete window.ondeviceorientation');
}
for (const binding of this._page.allBindings())
scripts.push(binding.source);
scripts.push(...this._browserContext.initScripts);
scripts.push(...this._page.initScripts);
return scripts.join(';\n');
}
async _updateBootstrapScript(): Promise<void> {
await this._updateState('Page.setBootstrapScript', { source: this._calculateBootstrapScript() });
}
async closePage(runBeforeUnload: boolean): Promise<void> {
await this._stopVideo();
await this._pageProxySession.sendMayFail('Target.close', {
targetId: this._session.sessionId,
runBeforeUnload
});
}
async setBackgroundColor(color?: { r: number; g: number; b: number; a: number; }): Promise<void> {
await this._session.send('Page.setDefaultBackgroundColorOverride', { color });
}
private _toolbarHeight(): number {
if (this._page._browserContext._browser?.options.headful)
return hostPlatform === 'mac10.15' ? 55 : 59;
return 0;
}
private async _startVideo(options: types.PageScreencastOptions): Promise<void> {
assert(!this._recordingVideoFile);
const { screencastId } = await this._pageProxySession.send('Screencast.startVideo', {
file: options.outputFile,
width: options.width,
height: options.height,
toolbarHeight: this._toolbarHeight()
});
this._recordingVideoFile = options.outputFile;
this._browserContext._browser._videoStarted(this._browserContext, screencastId, options.outputFile, this.pageOrError());
}
async _stopVideo(): Promise<void> {
if (!this._recordingVideoFile)
return;
await this._pageProxySession.sendMayFail('Screencast.stopVideo');
this._recordingVideoFile = null;
}
private validateScreenshotDimension(side: number, omitDeviceScaleFactor: boolean) {
// Cairo based implementations (Linux and Windows) have hard limit of 32767
// (see https://github.com/microsoft/playwright/issues/16727).
if (process.platform === 'darwin')
return;
if (!omitDeviceScaleFactor && this._page._browserContext._options.deviceScaleFactor)
side = Math.ceil(side * this._page._browserContext._options.deviceScaleFactor);
if (side > 32767)
throw new Error('Cannot take screenshot larger than 32767 pixels on any dimension');
}
async takeScreenshot(progress: Progress, format: string, documentRect: types.Rect | undefined, viewportRect: types.Rect | undefined, quality: number | undefined, fitsViewport: boolean, scale: 'css' | 'device'): Promise<Buffer> {
const rect = (documentRect || viewportRect)!;
const omitDeviceScaleFactor = scale === 'css';
this.validateScreenshotDimension(rect.width, omitDeviceScaleFactor);
this.validateScreenshotDimension(rect.height, omitDeviceScaleFactor);
const result = await this._session.send('Page.snapshotRect', { ...rect, coordinateSystem: documentRect ? 'Page' : 'Viewport', omitDeviceScaleFactor });
const prefix = 'data:image/png;base64,';
let buffer = Buffer.from(result.dataURL.substr(prefix.length), 'base64');
if (format === 'jpeg')
buffer = jpegjs.encode(PNG.sync.read(buffer), quality).data;
return buffer;
}
async getContentFrame(handle: dom.ElementHandle): Promise<frames.Frame | null> {
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
if (!nodeInfo.contentFrameId)
return null;
return this._page._frameManager.frame(nodeInfo.contentFrameId);
}
async getOwnerFrame(handle: dom.ElementHandle): Promise<string | null> {
if (!handle._objectId)
return null;
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
return nodeInfo.ownerFrameId || null;
}
isElementHandle(remoteObject: any): boolean {
return (remoteObject as Protocol.Runtime.RemoteObject).subtype === 'node';
}
async getBoundingBox(handle: dom.ElementHandle): Promise<types.Rect | null> {
const quads = await this.getContentQuads(handle);
if (!quads || !quads.length)
return null;
let minX = Infinity;
let maxX = -Infinity;
let minY = Infinity;
let maxY = -Infinity;
for (const quad of quads) {
for (const point of quad) {
minX = Math.min(minX, point.x);
maxX = Math.max(maxX, point.x);
minY = Math.min(minY, point.y);
maxY = Math.max(maxY, point.y);
}
}
return { x: minX, y: minY, width: maxX - minX, height: maxY - minY };
}
async scrollRectIntoViewIfNeeded(handle: dom.ElementHandle, rect?: types.Rect): Promise<'error:notvisible' | 'error:notconnected' | 'done'> {
return await this._session.send('DOM.scrollIntoViewIfNeeded', {
objectId: handle._objectId,
rect,
}).then(() => 'done' as const).catch(e => {
if (e instanceof Error && e.message.includes('Node does not have a layout object'))
return 'error:notvisible';
if (e instanceof Error && e.message.includes('Node is detached from document'))
return 'error:notconnected';
throw e;
});
}
async setScreencastOptions(options: { width: number, height: number, quality: number } | null): Promise<void> {
if (options) {
const so = { ...options, toolbarHeight: this._toolbarHeight() };
const { generation } = await this._pageProxySession.send('Screencast.startScreencast', so);
this._screencastGeneration = generation;
} else {
await this._pageProxySession.send('Screencast.stopScreencast');
}
}
private _onScreencastFrame(event: Protocol.Screencast.screencastFramePayload) {
const generation = this._screencastGeneration;
this._page.throttleScreencastFrameAck(() => {
this._pageProxySession.send('Screencast.screencastFrameAck', { generation }).catch(e => debugLogger.log('error', e));
});
const buffer = Buffer.from(event.data, 'base64');
this._page.emit(Page.Events.ScreencastFrame, {
buffer,
width: event.deviceWidth,
height: event.deviceHeight,
});
}
rafCountForStablePosition(): number {
return process.platform === 'win32' ? 5 : 1;
}
async getContentQuads(handle: dom.ElementHandle): Promise<types.Quad[] | null> {
const result = await this._session.sendMayFail('DOM.getContentQuads', {
objectId: handle._objectId
});
if (!result)
return null;
return result.quads.map(quad => [
{ x: quad[0], y: quad[1] },
{ x: quad[2], y: quad[3] },
{ x: quad[4], y: quad[5] },
{ x: quad[6], y: quad[7] }
]);
}
async setInputFiles(handle: dom.ElementHandle<HTMLInputElement>, files: types.FilePayload[]): Promise<void> {
const objectId = handle._objectId;
const protocolFiles = files.map(file => ({
name: file.name,
type: file.mimeType,
data: file.buffer,
}));
await this._session.send('DOM.setInputFiles', { objectId, files: protocolFiles });
}
async setInputFilePaths(handle: dom.ElementHandle<HTMLInputElement>, paths: string[]): Promise<void> {
const pageProxyId = this._pageProxySession.sessionId;
const objectId = handle._objectId;
await Promise.all([
this._pageProxySession.connection.browserSession.send('Playwright.grantFileReadAccess', { pageProxyId, paths }),
this._session.send('DOM.setInputFiles', { objectId, paths })
]);
}
async adoptElementHandle<T extends Node>(handle: dom.ElementHandle<T>, to: dom.FrameExecutionContext): Promise<dom.ElementHandle<T>> {
const result = await this._session.sendMayFail('DOM.resolveNode', {
objectId: handle._objectId,
executionContextId: ((to as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error(dom.kUnableToAdoptErrorMessage);
return to.createHandle(result.object) as dom.ElementHandle<T>;
}
async getAccessibilityTree(needle?: dom.ElementHandle): Promise<{tree: accessibility.AXNode, needle: accessibility.AXNode | null}> {
return getAccessibilityTree(this._session, needle);
}
async inputActionEpilogue(): Promise<void> {
}
async getFrameElement(frame: frames.Frame): Promise<dom.ElementHandle> {
const parent = frame.parentFrame();
if (!parent)
throw new Error('Frame has been detached.');
const context = await parent._mainContext();
const result = await this._session.send('DOM.resolveNode', {
frameId: frame._id,
executionContextId: ((context as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error('Frame has been detached.');
return context.createHandle(result.object) as dom.ElementHandle;
}
_onRequestWillBeSent(session: WKSession, event: Protocol.Network.requestWillBeSentPayload) {
if (event.request.url.startsWith('data:'))
return;
let redirectedFrom: WKInterceptableRequest | null = null;
if (event.redirectResponse) {
const request = this._requestIdToRequest.get(event.requestId);
// If we connect late to the target, we could have missed the requestWillBeSent event.
if (request) {
this._handleRequestRedirect(request, event.redirectResponse, event.timestamp);
redirectedFrom = request;
}
}
const frame = redirectedFrom ? redirectedFrom.request.frame() : this._page._frameManager.frame(event.frameId);
// sometimes we get stray network events for detached frames
// TODO(einbinder) why?
if (!frame)
return;
// TODO(einbinder) this will fail if we are an XHR document request
const isNavigationRequest = event.type === 'Document';
const documentId = isNavigationRequest ? event.loaderId : undefined;
let route = null;
// We do not support intercepting redirects.
if (this._page.needsRequestInterception() && !redirectedFrom)
route = new WKRouteImpl(session, event.requestId);
const request = new WKInterceptableRequest(session, route, frame, event, redirectedFrom, documentId);
this._requestIdToRequest.set(event.requestId, request);
this._page._frameManager.requestStarted(request.request, route || undefined);
}
private _handleRequestRedirect(request: WKInterceptableRequest, responsePayload: Protocol.Network.Response, timestamp: number) {
const response = request.createResponse(responsePayload);
response._securityDetailsFinished();
response._serverAddrFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(responsePayload.timing ? helper.secondsToRoundishMillis(timestamp - request._timestamp) : -1);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.requestReceivedResponse(response);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onRequestIntercepted(session: WKSession, event: Protocol.Network.requestInterceptedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
if (!request) {
session.sendMayFail('Network.interceptRequestWithError', { errorType: 'Cancellation', requestId: event.requestId });
return;
}
// There is no point in waiting for the raw headers in Network.responseReceived when intercepting.
// Use provisional headers as raw headers, so that client can call allHeaders() from the route handler.
request.request.setRawRequestHeaders(null);
if (!request._route) {
// Intercepted, although we do not intend to allow interception.
// Just continue.
session.sendMayFail('Network.interceptWithRequest', { requestId: request._requestId });
} else {
request._route._requestInterceptedPromise.resolve();
}
}
_onResponseReceived(event: Protocol.Network.responseReceivedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// FileUpload sends a response without a matching request.
if (!request)
return;
this._requestIdToResponseReceivedPayloadEvent.set(request._requestId, event);
const response = request.createResponse(event.response);
this._page._frameManager.requestReceivedResponse(response);
if (response.status() === 204) {
this._onLoadingFailed({
requestId: event.requestId,
errorText: 'Aborted: 204 No Content',
timestamp: event.timestamp
});
}
}
_onLoadingFinished(event: Protocol.Network.loadingFinishedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
// Under certain conditions we never get the Network.responseReceived
// event from protocol. @see https://crbug.com/883475
const response = request.request._existingResponse();
if (response) {
const responseReceivedPayload = this._requestIdToResponseReceivedPayloadEvent.get(request._requestId);
response._serverAddrFinished(parseRemoteAddress(event?.metrics?.remoteAddress));
response._securityDetailsFinished({
protocol: isLoadedSecurely(response.url(), response.timing()) ? event.metrics?.securityConnection?.protocol : undefined,
subjectName: responseReceivedPayload?.response.security?.certificate?.subject,
validFrom: responseReceivedPayload?.response.security?.certificate?.validFrom,
validTo: responseReceivedPayload?.response.security?.certificate?.validUntil,
});
if (event.metrics?.protocol)
response._setHttpVersion(event.metrics.protocol);
response.setEncodedBodySize(event.metrics?.responseBodyBytesReceived ?? null);
response.setResponseHeadersSize(event.metrics?.responseHeaderBytesReceived ?? null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToResponseReceivedPayloadEvent.delete(request._requestId);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onLoadingFailed(event: Protocol.Network.loadingFailedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
const response = request.request._existingResponse();
if (response) {
response._serverAddrFinished();
response._securityDetailsFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToRequest.delete(request._requestId);
request.request._setFailureText(event.errorText);
this._page._frameManager.requestFailed(request.request, event.errorText.includes('cancelled'));
}
async _grantPermissions(origin: string, permissions: string[]) {
const webPermissionToProtocol = new Map<string, string>([
['geolocation', 'geolocation'],
]);
const filtered = permissions.map(permission => {
const protocolPermission = webPermissionToProtocol.get(permission);
if (!protocolPermission)
throw new Error('Unknown permission: ' + permission);
return protocolPermission;
});
await this._pageProxySession.send('Emulation.grantPermissions', { origin, permissions: filtered });
}
async _clearPermissions() {
await this._pageProxySession.send('Emulation.resetPermissions', {});
}
}
/**
* WebKit Remote Addresses look like:
*
* macOS:
* ::1.8911
* 2606:2800:220:1:248:1893:25c8:1946.443
* 127.0.0.1:8000
*
* ubuntu:
* ::1:8907
* 127.0.0.1:8000
*
* NB: They look IPv4 and IPv6's with ports but use an alternative notation.
*/
function parseRemoteAddress(value?: string) {
if (!value)
return;
try {
const colon = value.lastIndexOf(':');
const dot = value.lastIndexOf('.');
if (dot < 0) { // IPv6ish:port
return {
ipAddress: `[${value.slice(0, colon)}]`,
port: +value.slice(colon + 1)
};
}
if (colon > dot) { // IPv4:port
const [address, port] = value.split(':');
return {
ipAddress: address,
port: +port,
};
} else { // IPv6ish.port
const [address, port] = value.split('.');
return {
ipAddress: `[${address}]`,
port: +port,
};
}
} catch (_) {}
}
/**
* Adapted from Source/WebInspectorUI/UserInterface/Models/Resource.js in
* WebKit codebase.
*/
function isLoadedSecurely(url: string, timing: network.ResourceTiming) {
try {
const u = new URL(url);
if (u.protocol !== 'https:' && u.protocol !== 'wss:' && u.protocol !== 'sftp:')
return false;
if (timing.secureConnectionStart === -1 && timing.connectStart !== -1)
return false;
return true;
} catch (_) {}
}
const contextDelegateSymbol = Symbol('delegate');
| packages/playwright-core/src/server/webkit/wkPage.ts | 1 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.0018159463070333004,
0.00019839878950733691,
0.0001639132824493572,
0.00017112016212195158,
0.00015508529031649232
] |
{
"id": 2,
"code_window": [
" await page.goto(server.EMPTY_PAGE);\n",
" await page.click('\"Click me\"');\n",
"});\n",
"\n",
"it('should work with _blank target in form', async ({ page, server, browserName }) => {\n",
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"it('should work with _blank target in form', async ({ page, server }) => {\n"
],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
const t = {};
t.String = function(x, details = {}, path = ['<root>']) {
if (typeof x === 'string' || typeof x === 'String')
return true;
details.error = `Expected "${path.join('.')}" to be |string|; found |${typeof x}| \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Number = function(x, details = {}, path = ['<root>']) {
if (typeof x === 'number')
return true;
details.error = `Expected "${path.join('.')}" to be |number|; found |${typeof x}| \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Boolean = function(x, details = {}, path = ['<root>']) {
if (typeof x === 'boolean')
return true;
details.error = `Expected "${path.join('.')}" to be |boolean|; found |${typeof x}| \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Null = function(x, details = {}, path = ['<root>']) {
if (Object.is(x, null))
return true;
details.error = `Expected "${path.join('.')}" to be \`null\`; found \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Undefined = function(x, details = {}, path = ['<root>']) {
if (Object.is(x, undefined))
return true;
details.error = `Expected "${path.join('.')}" to be \`undefined\`; found \`${JSON.stringify(x)}\` instead.`;
return false;
}
t.Any = x => true,
t.Enum = function(values) {
return function(x, details = {}, path = ['<root>']) {
if (values.indexOf(x) !== -1)
return true;
details.error = `Expected "${path.join('.')}" to be one of [${values.join(', ')}]; found \`${JSON.stringify(x)}\` (${typeof x}) instead.`;
return false;
}
}
t.Nullable = function(scheme) {
return function(x, details = {}, path = ['<root>']) {
if (Object.is(x, null))
return true;
return checkScheme(scheme, x, details, path);
}
}
t.Optional = function(scheme) {
return function(x, details = {}, path = ['<root>']) {
if (Object.is(x, undefined))
return true;
return checkScheme(scheme, x, details, path);
}
}
t.Array = function(scheme) {
return function(x, details = {}, path = ['<root>']) {
if (!Array.isArray(x)) {
details.error = `Expected "${path.join('.')}" to be an array; found \`${JSON.stringify(x)}\` (${typeof x}) instead.`;
return false;
}
const lastPathElement = path[path.length - 1];
for (let i = 0; i < x.length; ++i) {
path[path.length - 1] = lastPathElement + `[${i}]`;
if (!checkScheme(scheme, x[i], details, path))
return false;
}
path[path.length - 1] = lastPathElement;
return true;
}
}
t.Recursive = function(types, schemeName) {
return function(x, details = {}, path = ['<root>']) {
const scheme = types[schemeName];
return checkScheme(scheme, x, details, path);
}
}
function beauty(path, obj) {
if (path.length === 1)
return `object ${JSON.stringify(obj, null, 2)}`;
return `property "${path.join('.')}" - ${JSON.stringify(obj, null, 2)}`;
}
function checkScheme(scheme, x, details = {}, path = ['<root>']) {
if (!scheme)
throw new Error(`ILLDEFINED SCHEME: ${path.join('.')}`);
if (typeof scheme === 'object') {
if (!x) {
details.error = `Object "${path.join('.')}" is undefined, but has some scheme`;
return false;
}
for (const [propertyName, aScheme] of Object.entries(scheme)) {
path.push(propertyName);
const result = checkScheme(aScheme, x[propertyName], details, path);
path.pop();
if (!result)
return false;
}
for (const propertyName of Object.keys(x)) {
if (!scheme[propertyName]) {
path.push(propertyName);
details.error = `Found ${beauty(path, x[propertyName])} which is not described in this scheme`;
return false;
}
}
return true;
}
return scheme(x, details, path);
}
/*
function test(scheme, obj) {
const details = {};
if (!checkScheme(scheme, obj, details)) {
dump(`FAILED: ${JSON.stringify(obj)}
details.error: ${details.error}
`);
} else {
dump(`SUCCESS: ${JSON.stringify(obj)}
`);
}
}
test(t.Array(t.String), ['a', 'b', 2, 'c']);
test(t.Either(t.String, t.Number), {});
*/
this.t = t;
this.checkScheme = checkScheme;
this.EXPORTED_SYMBOLS = ['t', 'checkScheme'];
| browser_patches/firefox/juggler/protocol/PrimitiveTypes.js | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00017452353495173156,
0.00017203128663823009,
0.00016931805294007063,
0.0001719803549349308,
0.0000015522394960498787
] |
{
"id": 2,
"code_window": [
" await page.goto(server.EMPTY_PAGE);\n",
" await page.click('\"Click me\"');\n",
"});\n",
"\n",
"it('should work with _blank target in form', async ({ page, server, browserName }) => {\n",
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"it('should work with _blank target in form', async ({ page, server }) => {\n"
],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 35
} | # class: AndroidInput
* since: v1.9
* langs: js
## async method: AndroidInput.drag
* since: v1.9
Performs a drag between [`param: from`] and [`param: to`] points.
### param: AndroidInput.drag.from
* since: v1.9
- `from` <[Object]>
- `x` <[float]>
- `y` <[float]>
The start point of the drag.
### param: AndroidInput.drag.to
* since: v1.9
- `to` <[Object]>
- `x` <[float]>
- `y` <[float]>
The end point of the drag.
### param: AndroidInput.drag.steps
* since: v1.9
- `steps` <[int]>
The number of steps in the drag. Each step takes 5 milliseconds to complete.
## async method: AndroidInput.press
* since: v1.9
Presses the [`param: key`].
### param: AndroidInput.press.key
* since: v1.9
- `key` <[AndroidKey]>
Key to press.
## async method: AndroidInput.swipe
* since: v1.9
Swipes following the path defined by [`param: segments`].
### param: AndroidInput.swipe.from
* since: v1.9
- `from` <[Object]>
- `x` <[float]>
- `y` <[float]>
The point to start swiping from.
### param: AndroidInput.swipe.segments
* since: v1.9
- `segments` <[Array]<[Object]>>
- `x` <[float]>
- `y` <[float]>
Points following the [`param: from`] point in the swipe gesture.
### param: AndroidInput.swipe.steps
* since: v1.9
- `steps` <[int]>
The number of steps for each segment. Each step takes 5 milliseconds to complete, so 100 steps means half a second per each segment.
## async method: AndroidInput.tap
* since: v1.9
Taps at the specified [`param: point`].
### param: AndroidInput.tap.point
* since: v1.9
- `point` <[Object]>
- `x` <[float]>
- `y` <[float]>
The point to tap at.
## async method: AndroidInput.type
* since: v1.9
Types [`param: text`] into currently focused widget.
### param: AndroidInput.type.text
* since: v1.9
- `text` <[string]>
Text to type.
| docs/src/api/class-androidinput.md | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.0001734045217745006,
0.0001713672827463597,
0.00016540907381568104,
0.00017230339290108532,
0.0000022879403331899084
] |
{
"id": 2,
"code_window": [
" await page.goto(server.EMPTY_PAGE);\n",
" await page.click('\"Click me\"');\n",
"});\n",
"\n",
"it('should work with _blank target in form', async ({ page, server, browserName }) => {\n",
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
"it('should work with _blank target in form', async ({ page, server }) => {\n"
],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 35
} | /**
* Copyright Microsoft Corporation. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import fs from 'fs';
import path from 'path';
import { monotonicTime } from 'playwright-core/lib/utils';
import type { TestInfoError, TestInfo, TestStatus } from '../../types/test';
import type { StepBeginPayload, StepEndPayload, WorkerInitParams } from './ipc';
import type { TestCase } from './test';
import { TimeoutManager } from './timeoutManager';
import type { Annotation, FullConfigInternal, FullProjectInternal, TestStepInternal } from './types';
import { getContainedPath, normalizeAndSaveAttachment, sanitizeForFilePath, serializeError, trimLongString } from '../util';
export class TestInfoImpl implements TestInfo {
private _onStepBegin: (payload: StepBeginPayload) => void;
private _onStepEnd: (payload: StepEndPayload) => void;
readonly _test: TestCase;
readonly _timeoutManager: TimeoutManager;
readonly _startTime: number;
readonly _startWallTime: number;
private _hasHardError: boolean = false;
readonly _onTestFailureImmediateCallbacks = new Map<() => Promise<void>, string>(); // fn -> title
_didTimeout = false;
_lastStepId = 0;
// ------------ TestInfo fields ------------
readonly repeatEachIndex: number;
readonly retry: number;
readonly workerIndex: number;
readonly parallelIndex: number;
readonly project: FullProjectInternal;
config: FullConfigInternal;
readonly title: string;
readonly titlePath: string[];
readonly file: string;
readonly line: number;
readonly column: number;
readonly fn: Function;
expectedStatus: TestStatus;
duration: number = 0;
readonly annotations: Annotation[] = [];
readonly attachments: TestInfo['attachments'] = [];
status: TestStatus = 'passed';
readonly stdout: TestInfo['stdout'] = [];
readonly stderr: TestInfo['stderr'] = [];
snapshotSuffix: string = '';
readonly outputDir: string;
readonly snapshotDir: string;
errors: TestInfoError[] = [];
currentStep: TestStepInternal | undefined;
get error(): TestInfoError | undefined {
return this.errors[0];
}
set error(e: TestInfoError | undefined) {
if (e === undefined)
throw new Error('Cannot assign testInfo.error undefined value!');
this.errors[0] = e;
}
get timeout(): number {
return this._timeoutManager.defaultSlotTimings().timeout;
}
set timeout(timeout: number) {
// Ignored.
}
constructor(
config: FullConfigInternal,
project: FullProjectInternal,
workerParams: WorkerInitParams,
test: TestCase,
retry: number,
onStepBegin: (payload: StepBeginPayload) => void,
onStepEnd: (payload: StepEndPayload) => void,
) {
this._test = test;
this._onStepBegin = onStepBegin;
this._onStepEnd = onStepEnd;
this._startTime = monotonicTime();
this._startWallTime = Date.now();
this.repeatEachIndex = workerParams.repeatEachIndex;
this.retry = retry;
this.workerIndex = workerParams.workerIndex;
this.parallelIndex = workerParams.parallelIndex;
this.project = project;
this.config = config;
this.title = test.title;
this.titlePath = test.titlePath();
this.file = test.location.file;
this.line = test.location.line;
this.column = test.location.column;
this.fn = test.fn;
this.expectedStatus = test.expectedStatus;
this._timeoutManager = new TimeoutManager(this.project.timeout);
this.outputDir = (() => {
const relativeTestFilePath = path.relative(this.project.testDir, test._requireFile.replace(/\.(spec|test)\.(js|ts|mjs)$/, ''));
const sanitizedRelativePath = relativeTestFilePath.replace(process.platform === 'win32' ? new RegExp('\\\\', 'g') : new RegExp('/', 'g'), '-');
const fullTitleWithoutSpec = test.titlePath().slice(1).join(' ');
let testOutputDir = trimLongString(sanitizedRelativePath + '-' + sanitizeForFilePath(fullTitleWithoutSpec));
if (project._id)
testOutputDir += '-' + sanitizeForFilePath(project._id);
if (this.retry)
testOutputDir += '-retry' + this.retry;
if (this.repeatEachIndex)
testOutputDir += '-repeat' + this.repeatEachIndex;
return path.join(this.project.outputDir, testOutputDir);
})();
this.snapshotDir = (() => {
const relativeTestFilePath = path.relative(this.project.testDir, test._requireFile);
return path.join(this.project.snapshotDir, relativeTestFilePath + '-snapshots');
})();
}
private _modifier(type: 'skip' | 'fail' | 'fixme' | 'slow', modifierArgs: [arg?: any, description?: string]) {
if (typeof modifierArgs[1] === 'function') {
throw new Error([
'It looks like you are calling test.skip() inside the test and pass a callback.',
'Pass a condition instead and optional description instead:',
`test('my test', async ({ page, isMobile }) => {`,
` test.skip(isMobile, 'This test is not applicable on mobile');`,
`});`,
].join('\n'));
}
if (modifierArgs.length >= 1 && !modifierArgs[0])
return;
const description = modifierArgs[1];
this.annotations.push({ type, description });
if (type === 'slow') {
this._timeoutManager.slow();
} else if (type === 'skip' || type === 'fixme') {
this.expectedStatus = 'skipped';
throw new SkipError('Test is skipped: ' + (description || ''));
} else if (type === 'fail') {
if (this.expectedStatus !== 'skipped')
this.expectedStatus = 'failed';
}
}
async _runWithTimeout(cb: () => Promise<any>): Promise<void> {
const timeoutError = await this._timeoutManager.runWithTimeout(cb);
// Do not overwrite existing failure upon hook/teardown timeout.
if (timeoutError && !this._didTimeout) {
this._didTimeout = true;
this.errors.push(timeoutError);
if (this.status === 'passed' || this.status === 'skipped')
this.status = 'timedOut';
}
this.duration = this._timeoutManager.defaultSlotTimings().elapsed | 0;
}
async _runFn(fn: Function, skips?: 'allowSkips'): Promise<TestInfoError | undefined> {
try {
await fn();
} catch (error) {
if (skips === 'allowSkips' && error instanceof SkipError) {
if (this.status === 'passed')
this.status = 'skipped';
} else {
const serialized = serializeError(error);
this._failWithError(serialized, true /* isHardError */);
return serialized;
}
}
}
_addStep(data: Omit<TestStepInternal, 'complete'>): TestStepInternal {
const stepId = `${data.category}@${data.title}@${++this._lastStepId}`;
let callbackHandled = false;
const firstErrorIndex = this.errors.length;
const step: TestStepInternal = {
...data,
complete: result => {
if (callbackHandled)
return;
callbackHandled = true;
let error: TestInfoError | undefined;
if (result.error instanceof Error) {
// Step function threw an error.
error = serializeError(result.error);
} else if (result.error) {
// Internal API step reported an error.
error = result.error;
} else {
// There was some other error (porbably soft expect) during step execution.
// Report step as failed to make it easier to spot.
error = this.errors[firstErrorIndex];
}
const payload: StepEndPayload = {
testId: this._test.id,
refinedTitle: step.refinedTitle,
stepId,
wallTime: Date.now(),
error,
};
this._onStepEnd(payload);
}
};
const hasLocation = data.location && !data.location.file.includes('@playwright');
// Sanitize location that comes from user land, it might have extra properties.
const location = data.location && hasLocation ? { file: data.location.file, line: data.location.line, column: data.location.column } : undefined;
const payload: StepBeginPayload = {
testId: this._test.id,
stepId,
...data,
location,
wallTime: Date.now(),
};
this._onStepBegin(payload);
return step;
}
_failWithError(error: TestInfoError, isHardError: boolean) {
// Do not overwrite any previous hard errors.
// Some (but not all) scenarios include:
// - expect() that fails after uncaught exception.
// - fail after the timeout, e.g. due to fixture teardown.
if (isHardError && this._hasHardError)
return;
if (isHardError)
this._hasHardError = true;
if (this.status === 'passed' || this.status === 'skipped')
this.status = 'failed';
this.errors.push(error);
}
async _runAsStep<T>(cb: () => Promise<T>, stepInfo: Omit<TestStepInternal, 'complete'>): Promise<T> {
const step = this._addStep(stepInfo);
try {
const result = await cb();
step.complete({});
return result;
} catch (e) {
step.complete({ error: e instanceof SkipError ? undefined : serializeError(e) });
throw e;
}
}
_isFailure() {
return this.status !== 'skipped' && this.status !== this.expectedStatus;
}
// ------------ TestInfo methods ------------
async attach(name: string, options: { path?: string, body?: string | Buffer, contentType?: string } = {}) {
this.attachments.push(await normalizeAndSaveAttachment(this.outputPath(), name, options));
}
outputPath(...pathSegments: string[]){
fs.mkdirSync(this.outputDir, { recursive: true });
const joinedPath = path.join(...pathSegments);
const outputPath = getContainedPath(this.outputDir, joinedPath);
if (outputPath)
return outputPath;
throw new Error(`The outputPath is not allowed outside of the parent directory. Please fix the defined path.\n\n\toutputPath: ${joinedPath}`);
}
_fsSanitizedTestName() {
const fullTitleWithoutSpec = this.titlePath.slice(1).join(' ');
return sanitizeForFilePath(trimLongString(fullTitleWithoutSpec));
}
snapshotPath(...pathSegments: string[]) {
const subPath = path.join(...pathSegments);
const parsedSubPath = path.parse(subPath);
const relativeTestFilePath = path.relative(this.project.testDir, this._test._requireFile);
const parsedRelativeTestFilePath = path.parse(relativeTestFilePath);
const projectNamePathSegment = sanitizeForFilePath(this.project.name);
const snapshotPath = this.project.snapshotPathTemplate
.replace(/\{(.)?testDir\}/g, '$1' + this.project.testDir)
.replace(/\{(.)?snapshotDir\}/g, '$1' + this.project.snapshotDir)
.replace(/\{(.)?snapshotSuffix\}/g, this.snapshotSuffix ? '$1' + this.snapshotSuffix : '')
.replace(/\{(.)?testFileDir\}/g, '$1' + parsedRelativeTestFilePath.dir)
.replace(/\{(.)?platform\}/g, '$1' + process.platform)
.replace(/\{(.)?projectName\}/g, projectNamePathSegment ? '$1' + projectNamePathSegment : '')
.replace(/\{(.)?testName\}/g, '$1' + this._fsSanitizedTestName())
.replace(/\{(.)?testFileName\}/g, '$1' + parsedRelativeTestFilePath.base)
.replace(/\{(.)?testFilePath\}/g, '$1' + relativeTestFilePath)
.replace(/\{(.)?arg\}/g, '$1' + path.join(parsedSubPath.dir, parsedSubPath.name))
.replace(/\{(.)?ext\}/g, parsedSubPath.ext ? '$1' + parsedSubPath.ext : '');
return path.normalize(path.resolve(this.config._configDir, snapshotPath));
}
skip(...args: [arg?: any, description?: string]) {
this._modifier('skip', args);
}
fixme(...args: [arg?: any, description?: string]) {
this._modifier('fixme', args);
}
fail(...args: [arg?: any, description?: string]) {
this._modifier('fail', args);
}
slow(...args: [arg?: any, description?: string]) {
this._modifier('slow', args);
}
setTimeout(timeout: number) {
this._timeoutManager.setTimeout(timeout);
}
}
class SkipError extends Error {
}
| packages/playwright-test/src/common/testInfo.ts | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00019210016762372106,
0.0001721280423225835,
0.00016523804515600204,
0.00017141956777777523,
0.000004804987838724628
] |
{
"id": 3,
"code_window": [
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n",
" it.fixme(browserName === 'webkit');\n",
" server.setRoute('/done.html?', (req, res) => {\n",
" res.end(`Done`);\n",
" });\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 37
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import path from 'path';
import { PNG, jpegjs } from '../../utilsBundle';
import { splitErrorMessage } from '../../utils/stackTrace';
import { assert, createGuid, debugAssert, headersArrayToObject } from '../../utils';
import { hostPlatform } from '../../utils/hostPlatform';
import type * as accessibility from '../accessibility';
import * as dialog from '../dialog';
import * as dom from '../dom';
import type * as frames from '../frames';
import type { RegisteredListener } from '../../utils/eventsHelper';
import { eventsHelper } from '../../utils/eventsHelper';
import { helper } from '../helper';
import type { JSHandle } from '../javascript';
import * as network from '../network';
import type { PageBinding, PageDelegate } from '../page';
import { Page } from '../page';
import type { Progress } from '../progress';
import type * as types from '../types';
import type { Protocol } from './protocol';
import { getAccessibilityTree } from './wkAccessibility';
import type { WKBrowserContext } from './wkBrowser';
import { WKSession } from './wkConnection';
import { WKExecutionContext } from './wkExecutionContext';
import { RawKeyboardImpl, RawMouseImpl, RawTouchscreenImpl } from './wkInput';
import { WKInterceptableRequest, WKRouteImpl } from './wkInterceptableRequest';
import { WKProvisionalPage } from './wkProvisionalPage';
import { WKWorkers } from './wkWorkers';
import { debugLogger } from '../../common/debugLogger';
import { ManualPromise } from '../../utils/manualPromise';
const UTILITY_WORLD_NAME = '__playwright_utility_world__';
export class WKPage implements PageDelegate {
readonly rawMouse: RawMouseImpl;
readonly rawKeyboard: RawKeyboardImpl;
readonly rawTouchscreen: RawTouchscreenImpl;
_session: WKSession;
private _provisionalPage: WKProvisionalPage | null = null;
readonly _page: Page;
private readonly _pagePromise = new ManualPromise<Page | Error>();
private readonly _pageProxySession: WKSession;
readonly _opener: WKPage | null;
private readonly _requestIdToRequest = new Map<string, WKInterceptableRequest>();
private readonly _workers: WKWorkers;
private readonly _contextIdToContext: Map<number, dom.FrameExecutionContext>;
private _mainFrameContextId?: number;
private _sessionListeners: RegisteredListener[] = [];
private _eventListeners: RegisteredListener[];
readonly _browserContext: WKBrowserContext;
_initializedPage: Page | null = null;
private _firstNonInitialNavigationCommittedPromise: Promise<void>;
private _firstNonInitialNavigationCommittedFulfill = () => {};
_firstNonInitialNavigationCommittedReject = (e: Error) => {};
private _lastConsoleMessage: { derivedType: string, text: string, handles: JSHandle[]; count: number, location: types.ConsoleMessageLocation; } | null = null;
private readonly _requestIdToResponseReceivedPayloadEvent = new Map<string, Protocol.Network.responseReceivedPayload>();
// Holds window features for the next popup being opened via window.open,
// until the popup page proxy arrives.
private _nextWindowOpenPopupFeatures?: string[];
private _recordingVideoFile: string | null = null;
private _screencastGeneration: number = 0;
constructor(browserContext: WKBrowserContext, pageProxySession: WKSession, opener: WKPage | null) {
this._pageProxySession = pageProxySession;
this._opener = opener;
this.rawKeyboard = new RawKeyboardImpl(pageProxySession);
this.rawMouse = new RawMouseImpl(pageProxySession);
this.rawTouchscreen = new RawTouchscreenImpl(pageProxySession);
this._contextIdToContext = new Map();
this._page = new Page(this, browserContext);
this.rawMouse.setPage(this._page);
this._workers = new WKWorkers(this._page);
this._session = undefined as any as WKSession;
this._browserContext = browserContext;
this._page.on(Page.Events.FrameDetached, (frame: frames.Frame) => this._removeContextsForFrame(frame, false));
this._eventListeners = [
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetCreated', this._onTargetCreated.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.targetDestroyed', this._onTargetDestroyed.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.dispatchMessageFromTarget', this._onDispatchMessageFromTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Target.didCommitProvisionalTarget', this._onDidCommitProvisionalTarget.bind(this)),
eventsHelper.addEventListener(this._pageProxySession, 'Screencast.screencastFrame', this._onScreencastFrame.bind(this)),
];
this._firstNonInitialNavigationCommittedPromise = new Promise((f, r) => {
this._firstNonInitialNavigationCommittedFulfill = f;
this._firstNonInitialNavigationCommittedReject = r;
});
if (opener && !browserContext._options.noDefaultViewport && opener._nextWindowOpenPopupFeatures) {
const viewportSize = helper.getViewportSizeFromWindowFeatures(opener._nextWindowOpenPopupFeatures);
opener._nextWindowOpenPopupFeatures = undefined;
if (viewportSize)
this._page._emulatedSize = { viewport: viewportSize, screen: viewportSize };
}
}
potentiallyUninitializedPage(): Page {
return this._page;
}
private async _initializePageProxySession() {
if (this._page._browserContext.isSettingStorageState())
return;
const promises: Promise<any>[] = [
this._pageProxySession.send('Dialog.enable'),
this._pageProxySession.send('Emulation.setActiveAndFocused', { active: true }),
];
const contextOptions = this._browserContext._options;
if (contextOptions.javaScriptEnabled === false)
promises.push(this._pageProxySession.send('Emulation.setJavaScriptEnabled', { enabled: false }));
promises.push(this._updateViewport());
promises.push(this.updateHttpCredentials());
if (this._browserContext._permissions.size) {
for (const [key, value] of this._browserContext._permissions)
promises.push(this._grantPermissions(key, value));
}
if (this._browserContext._options.recordVideo) {
const outputFile = path.join(this._browserContext._options.recordVideo.dir, createGuid() + '.webm');
promises.push(this._browserContext._ensureVideosPath().then(() => {
return this._startVideo({
// validateBrowserContextOptions ensures correct video size.
...this._browserContext._options.recordVideo!.size!,
outputFile,
});
}));
}
await Promise.all(promises);
}
private _setSession(session: WKSession) {
eventsHelper.removeEventListeners(this._sessionListeners);
this._session = session;
this.rawKeyboard.setSession(session);
this.rawMouse.setSession(session);
this._addSessionListeners();
this._workers.setSession(session);
}
// This method is called for provisional targets as well. The session passed as the parameter
// may be different from the current session and may be destroyed without becoming current.
async _initializeSession(session: WKSession, provisional: boolean, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
await this._initializeSessionMayThrow(session, resourceTreeHandler).catch(e => {
// Provisional session can be disposed at any time, for example due to new navigation initiating
// a new provisional page.
if (provisional && session.isDisposed())
return;
// Swallow initialization errors due to newer target swap in,
// since we will reinitialize again.
if (this._session === session)
throw e;
});
}
private async _initializeSessionMayThrow(session: WKSession, resourceTreeHandler: (r: Protocol.Page.getResourceTreeReturnValue) => void) {
const [, frameTree] = await Promise.all([
// Page agent must be enabled before Runtime.
session.send('Page.enable'),
session.send('Page.getResourceTree'),
] as const);
resourceTreeHandler(frameTree);
const promises: Promise<any>[] = [
// Resource tree should be received before first execution context.
session.send('Runtime.enable'),
session.send('Page.createUserWorld', { name: UTILITY_WORLD_NAME }).catch(_ => {}), // Worlds are per-process
session.send('Console.enable'),
session.send('Network.enable'),
this._workers.initializeSession(session)
];
if (this._page.needsRequestInterception()) {
promises.push(session.send('Network.setInterceptionEnabled', { enabled: true }));
promises.push(session.send('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }));
}
if (this._page._browserContext.isSettingStorageState()) {
await Promise.all(promises);
return;
}
const contextOptions = this._browserContext._options;
if (contextOptions.userAgent)
promises.push(this.updateUserAgent());
const emulatedMedia = this._page.emulatedMedia();
if (emulatedMedia.media || emulatedMedia.colorScheme || emulatedMedia.reducedMotion || emulatedMedia.forcedColors)
promises.push(WKPage._setEmulateMedia(session, emulatedMedia.media, emulatedMedia.colorScheme, emulatedMedia.reducedMotion, emulatedMedia.forcedColors));
for (const binding of this._page.allBindings())
promises.push(session.send('Runtime.addBinding', { name: binding.name }));
const bootstrapScript = this._calculateBootstrapScript();
if (bootstrapScript.length)
promises.push(session.send('Page.setBootstrapScript', { source: bootstrapScript }));
this._page.frames().map(frame => frame.evaluateExpression(bootstrapScript, false, undefined).catch(e => {}));
if (contextOptions.bypassCSP)
promises.push(session.send('Page.setBypassCSP', { enabled: true }));
const emulatedSize = this._page.emulatedSize();
if (emulatedSize) {
promises.push(session.send('Page.setScreenSizeOverride', {
width: emulatedSize.screen.width,
height: emulatedSize.screen.height,
}));
}
promises.push(this.updateEmulateMedia());
promises.push(session.send('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) }));
if (contextOptions.offline)
promises.push(session.send('Network.setEmulateOfflineState', { offline: true }));
promises.push(session.send('Page.setTouchEmulationEnabled', { enabled: !!contextOptions.hasTouch }));
if (contextOptions.timezoneId) {
promises.push(session.send('Page.setTimeZone', { timeZone: contextOptions.timezoneId }).
catch(e => { throw new Error(`Invalid timezone ID: ${contextOptions.timezoneId}`); }));
}
if (this._page.fileChooserIntercepted())
promises.push(session.send('Page.setInterceptFileChooserDialog', { enabled: true }));
promises.push(session.send('Page.overrideSetting', { setting: 'DeviceOrientationEventEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'FullScreenEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'NotificationsEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'PointerLockEnabled' as any, value: !contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeMonthEnabled' as any, value: contextOptions.isMobile }));
promises.push(session.send('Page.overrideSetting', { setting: 'InputTypeWeekEnabled' as any, value: contextOptions.isMobile }));
await Promise.all(promises);
}
private _onDidCommitProvisionalTarget(event: Protocol.Target.didCommitProvisionalTargetPayload) {
const { oldTargetId, newTargetId } = event;
assert(this._provisionalPage);
assert(this._provisionalPage._session.sessionId === newTargetId, 'Unknown new target: ' + newTargetId);
assert(this._session.sessionId === oldTargetId, 'Unknown old target: ' + oldTargetId);
const newSession = this._provisionalPage._session;
this._provisionalPage.commit();
this._provisionalPage.dispose();
this._provisionalPage = null;
this._setSession(newSession);
}
private _onTargetDestroyed(event: Protocol.Target.targetDestroyedPayload) {
const { targetId, crashed } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId) {
this._provisionalPage._session.dispose(false);
this._provisionalPage.dispose();
this._provisionalPage = null;
} else if (this._session.sessionId === targetId) {
this._session.dispose(false);
eventsHelper.removeEventListeners(this._sessionListeners);
if (crashed) {
this._session.markAsCrashed();
this._page._didCrash();
}
}
}
didClose() {
this._page._didClose();
}
dispose(disconnected: boolean) {
this._pageProxySession.dispose(disconnected);
eventsHelper.removeEventListeners(this._sessionListeners);
eventsHelper.removeEventListeners(this._eventListeners);
if (this._session)
this._session.dispose(disconnected);
if (this._provisionalPage) {
this._provisionalPage._session.dispose(disconnected);
this._provisionalPage.dispose();
this._provisionalPage = null;
}
this._page._didDisconnect();
this._firstNonInitialNavigationCommittedReject(new Error('Page closed'));
}
dispatchMessageToSession(message: any) {
this._pageProxySession.dispatchMessage(message);
}
handleProvisionalLoadFailed(event: Protocol.Playwright.provisionalLoadFailedPayload) {
if (!this._initializedPage) {
this._firstNonInitialNavigationCommittedReject(new Error('Initial load failed'));
return;
}
if (!this._provisionalPage)
return;
let errorText = event.error;
if (errorText.includes('cancelled'))
errorText += '; maybe frame was detached?';
this._page._frameManager.frameAbortedNavigation(this._page.mainFrame()._id, errorText, event.loaderId);
}
handleWindowOpen(event: Protocol.Playwright.windowOpenPayload) {
debugAssert(!this._nextWindowOpenPopupFeatures);
this._nextWindowOpenPopupFeatures = event.windowFeatures;
}
async pageOrError(): Promise<Page | Error> {
return this._pagePromise;
}
private async _onTargetCreated(event: Protocol.Target.targetCreatedPayload) {
const { targetInfo } = event;
const session = new WKSession(this._pageProxySession.connection, targetInfo.targetId, `Target closed`, (message: any) => {
this._pageProxySession.send('Target.sendMessageToTarget', {
message: JSON.stringify(message), targetId: targetInfo.targetId
}).catch(e => {
session.dispatchMessage({ id: message.id, error: { message: e.message } });
});
});
assert(targetInfo.type === 'page', 'Only page targets are expected in WebKit, received: ' + targetInfo.type);
if (!targetInfo.isProvisional) {
assert(!this._initializedPage);
let pageOrError: Page | Error;
try {
this._setSession(session);
await Promise.all([
this._initializePageProxySession(),
this._initializeSession(session, false, ({ frameTree }) => this._handleFrameTree(frameTree)),
]);
pageOrError = this._page;
} catch (e) {
pageOrError = e;
}
if (targetInfo.isPaused)
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
if ((pageOrError instanceof Page) && this._page.mainFrame().url() === '') {
try {
// Initial empty page has an empty url. We should wait until the first real url has been loaded,
// even if that url is about:blank. This is especially important for popups, where we need the
// actual url before interacting with it.
await this._firstNonInitialNavigationCommittedPromise;
} catch (e) {
pageOrError = e;
}
} else {
// Avoid rejection on disconnect.
this._firstNonInitialNavigationCommittedPromise.catch(() => {});
}
await this._page.initOpener(this._opener);
// Note: it is important to call |reportAsNew| before resolving pageOrError promise,
// so that anyone who awaits pageOrError got a ready and reported page.
this._initializedPage = pageOrError instanceof Page ? pageOrError : null;
this._page.reportAsNew(pageOrError instanceof Page ? undefined : pageOrError);
this._pagePromise.resolve(pageOrError);
} else {
assert(targetInfo.isProvisional);
assert(!this._provisionalPage);
this._provisionalPage = new WKProvisionalPage(session, this);
if (targetInfo.isPaused) {
this._provisionalPage.initializationPromise.then(() => {
this._pageProxySession.sendMayFail('Target.resume', { targetId: targetInfo.targetId });
});
}
}
}
private _onDispatchMessageFromTarget(event: Protocol.Target.dispatchMessageFromTargetPayload) {
const { targetId, message } = event;
if (this._provisionalPage && this._provisionalPage._session.sessionId === targetId)
this._provisionalPage._session.dispatchMessage(JSON.parse(message));
else if (this._session.sessionId === targetId)
this._session.dispatchMessage(JSON.parse(message));
else
throw new Error('Unknown target: ' + targetId);
}
private _addSessionListeners() {
this._sessionListeners = [
eventsHelper.addEventListener(this._session, 'Page.frameNavigated', event => this._onFrameNavigated(event.frame, false)),
eventsHelper.addEventListener(this._session, 'Page.navigatedWithinDocument', event => this._onFrameNavigatedWithinDocument(event.frameId, event.url)),
eventsHelper.addEventListener(this._session, 'Page.frameAttached', event => this._onFrameAttached(event.frameId, event.parentFrameId)),
eventsHelper.addEventListener(this._session, 'Page.frameDetached', event => this._onFrameDetached(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.willCheckNavigationPolicy', event => this._onWillCheckNavigationPolicy(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.didCheckNavigationPolicy', event => this._onDidCheckNavigationPolicy(event.frameId, event.cancel)),
eventsHelper.addEventListener(this._session, 'Page.frameScheduledNavigation', event => this._onFrameScheduledNavigation(event.frameId)),
eventsHelper.addEventListener(this._session, 'Page.loadEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'load')),
eventsHelper.addEventListener(this._session, 'Page.domContentEventFired', event => this._page._frameManager.frameLifecycleEvent(event.frameId, 'domcontentloaded')),
eventsHelper.addEventListener(this._session, 'Runtime.executionContextCreated', event => this._onExecutionContextCreated(event.context)),
eventsHelper.addEventListener(this._session, 'Runtime.bindingCalled', event => this._onBindingCalled(event.contextId, event.argument)),
eventsHelper.addEventListener(this._session, 'Console.messageAdded', event => this._onConsoleMessage(event)),
eventsHelper.addEventListener(this._session, 'Console.messageRepeatCountUpdated', event => this._onConsoleRepeatCountUpdated(event)),
eventsHelper.addEventListener(this._pageProxySession, 'Dialog.javascriptDialogOpening', event => this._onDialog(event)),
eventsHelper.addEventListener(this._session, 'Page.fileChooserOpened', event => this._onFileChooserOpened(event)),
eventsHelper.addEventListener(this._session, 'Network.requestWillBeSent', e => this._onRequestWillBeSent(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.requestIntercepted', e => this._onRequestIntercepted(this._session, e)),
eventsHelper.addEventListener(this._session, 'Network.responseReceived', e => this._onResponseReceived(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFinished', e => this._onLoadingFinished(e)),
eventsHelper.addEventListener(this._session, 'Network.loadingFailed', e => this._onLoadingFailed(e)),
eventsHelper.addEventListener(this._session, 'Network.webSocketCreated', e => this._page._frameManager.onWebSocketCreated(e.requestId, e.url)),
eventsHelper.addEventListener(this._session, 'Network.webSocketWillSendHandshakeRequest', e => this._page._frameManager.onWebSocketRequest(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketHandshakeResponseReceived', e => this._page._frameManager.onWebSocketResponse(e.requestId, e.response.status, e.response.statusText)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameSent', e => e.response.payloadData && this._page._frameManager.onWebSocketFrameSent(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameReceived', e => e.response.payloadData && this._page._frameManager.webSocketFrameReceived(e.requestId, e.response.opcode, e.response.payloadData)),
eventsHelper.addEventListener(this._session, 'Network.webSocketClosed', e => this._page._frameManager.webSocketClosed(e.requestId)),
eventsHelper.addEventListener(this._session, 'Network.webSocketFrameError', e => this._page._frameManager.webSocketError(e.requestId, e.errorMessage)),
];
}
private async _updateState<T extends keyof Protocol.CommandParameters>(
method: T,
params?: Protocol.CommandParameters[T]
): Promise<void> {
await this._forAllSessions(session => session.send(method, params).then());
}
private async _forAllSessions(callback: ((session: WKSession) => Promise<void>)): Promise<void> {
const sessions = [
this._session
];
// If the state changes during provisional load, push it to the provisional page
// as well to always be in sync with the backend.
if (this._provisionalPage)
sessions.push(this._provisionalPage._session);
await Promise.all(sessions.map(session => callback(session).catch(e => {})));
}
private _onWillCheckNavigationPolicy(frameId: string) {
// It may happen that new policy check occurs while there is an ongoing
// provisional load, in this case it should be safe to ignore it as it will
// either:
// - end up canceled, e.g. ctrl+click opening link in new tab, having no effect
// on this page
// - start new provisional load which we will miss in our signal trackers but
// we certainly won't hang waiting for it to finish and there is high chance
// that the current provisional page will commit navigation canceling the new
// one.
if (this._provisionalPage)
return;
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _onDidCheckNavigationPolicy(frameId: string, cancel?: boolean) {
if (!cancel)
return;
// This is a cross-process navigation that is canceled in the original page and continues in
// the provisional page. Bail out as we are tracking it.
if (this._provisionalPage)
return;
this._page._frameManager.frameAbortedNavigation(frameId, 'Navigation canceled by policy check');
}
private _onFrameScheduledNavigation(frameId: string) {
this._page._frameManager.frameRequestedNavigation(frameId);
}
private _handleFrameTree(frameTree: Protocol.Page.FrameResourceTree) {
this._onFrameAttached(frameTree.frame.id, frameTree.frame.parentId || null);
this._onFrameNavigated(frameTree.frame, true);
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'domcontentloaded');
this._page._frameManager.frameLifecycleEvent(frameTree.frame.id, 'load');
if (!frameTree.childFrames)
return;
for (const child of frameTree.childFrames)
this._handleFrameTree(child);
}
_onFrameAttached(frameId: string, parentFrameId: string | null): frames.Frame {
return this._page._frameManager.frameAttached(frameId, parentFrameId);
}
private _onFrameNavigated(framePayload: Protocol.Page.Frame, initial: boolean) {
const frame = this._page._frameManager.frame(framePayload.id);
assert(frame);
this._removeContextsForFrame(frame, true);
if (!framePayload.parentId)
this._workers.clear();
this._page._frameManager.frameCommittedNewDocumentNavigation(framePayload.id, framePayload.url, framePayload.name || '', framePayload.loaderId, initial);
if (!initial)
this._firstNonInitialNavigationCommittedFulfill();
}
private _onFrameNavigatedWithinDocument(frameId: string, url: string) {
this._page._frameManager.frameCommittedSameDocumentNavigation(frameId, url);
}
private _onFrameDetached(frameId: string) {
this._page._frameManager.frameDetached(frameId);
}
private _removeContextsForFrame(frame: frames.Frame, notifyFrame: boolean) {
for (const [contextId, context] of this._contextIdToContext) {
if (context.frame === frame) {
this._contextIdToContext.delete(contextId);
if (notifyFrame)
frame._contextDestroyed(context);
}
}
}
private _onExecutionContextCreated(contextPayload: Protocol.Runtime.ExecutionContextDescription) {
if (this._contextIdToContext.has(contextPayload.id))
return;
const frame = this._page._frameManager.frame(contextPayload.frameId);
if (!frame)
return;
const delegate = new WKExecutionContext(this._session, contextPayload.id);
let worldName: types.World|null = null;
if (contextPayload.type === 'normal')
worldName = 'main';
else if (contextPayload.type === 'user' && contextPayload.name === UTILITY_WORLD_NAME)
worldName = 'utility';
const context = new dom.FrameExecutionContext(delegate, frame, worldName);
(context as any)[contextDelegateSymbol] = delegate;
if (worldName)
frame._contextCreated(worldName, context);
if (contextPayload.type === 'normal' && frame === this._page.mainFrame())
this._mainFrameContextId = contextPayload.id;
this._contextIdToContext.set(contextPayload.id, context);
}
private async _onBindingCalled(contextId: Protocol.Runtime.ExecutionContextId, argument: string) {
const pageOrError = await this.pageOrError();
if (!(pageOrError instanceof Error)) {
const context = this._contextIdToContext.get(contextId);
if (context)
await this._page._onBindingCalled(argument, context);
}
}
async navigateFrame(frame: frames.Frame, url: string, referrer: string | undefined): Promise<frames.GotoResult> {
if (this._pageProxySession.isDisposed())
throw new Error('Target closed');
const pageProxyId = this._pageProxySession.sessionId;
const result = await this._pageProxySession.connection.browserSession.send('Playwright.navigate', { url, pageProxyId, frameId: frame._id, referrer });
return { newDocumentId: result.loaderId };
}
private _onConsoleMessage(event: Protocol.Console.messageAddedPayload) {
// Note: do no introduce await in this function, otherwise we lose the ordering.
// For example, frame.setContent relies on this.
const { type, level, text, parameters, url, line: lineNumber, column: columnNumber, source } = event.message;
if (level === 'error' && source === 'javascript') {
const { name, message } = splitErrorMessage(text);
let stack: string;
if (event.message.stackTrace) {
stack = text + '\n' + event.message.stackTrace.callFrames.map(callFrame => {
return ` at ${callFrame.functionName || 'unknown'} (${callFrame.url}:${callFrame.lineNumber}:${callFrame.columnNumber})`;
}).join('\n');
} else {
stack = '';
}
const error = new Error(message);
error.stack = stack;
error.name = name;
this._page.firePageError(error);
return;
}
let derivedType: string = type || '';
if (type === 'log')
derivedType = level;
else if (type === 'timing')
derivedType = 'timeEnd';
const handles: JSHandle[] = [];
for (const p of parameters || []) {
let context: dom.FrameExecutionContext | undefined;
if (p.objectId) {
const objectId = JSON.parse(p.objectId);
context = this._contextIdToContext.get(objectId.injectedScriptId);
} else {
context = this._contextIdToContext.get(this._mainFrameContextId!);
}
if (!context)
return;
handles.push(context.createHandle(p));
}
this._lastConsoleMessage = {
derivedType,
text,
handles,
count: 0,
location: {
url: url || '',
lineNumber: (lineNumber || 1) - 1,
columnNumber: (columnNumber || 1) - 1,
}
};
this._onConsoleRepeatCountUpdated({ count: 1 });
}
_onConsoleRepeatCountUpdated(event: Protocol.Console.messageRepeatCountUpdatedPayload) {
if (this._lastConsoleMessage) {
const {
derivedType,
text,
handles,
count,
location
} = this._lastConsoleMessage;
for (let i = count; i < event.count; ++i)
this._page._addConsoleMessage(derivedType, handles, location, handles.length ? undefined : text);
this._lastConsoleMessage.count = event.count;
}
}
_onDialog(event: Protocol.Dialog.javascriptDialogOpeningPayload) {
this._page.emit(Page.Events.Dialog, new dialog.Dialog(
this._page,
event.type as dialog.DialogType,
event.message,
async (accept: boolean, promptText?: string) => {
await this._pageProxySession.send('Dialog.handleJavaScriptDialog', { accept, promptText });
},
event.defaultPrompt));
}
private async _onFileChooserOpened(event: {frameId: Protocol.Network.FrameId, element: Protocol.Runtime.RemoteObject}) {
let handle;
try {
const context = await this._page._frameManager.frame(event.frameId)!._mainContext();
handle = context.createHandle(event.element).asElement()!;
} catch (e) {
// During async processing, frame/context may go away. We should not throw.
return;
}
await this._page._onFileChooserOpened(handle);
}
private static async _setEmulateMedia(session: WKSession, mediaType: types.MediaType, colorScheme: types.ColorScheme, reducedMotion: types.ReducedMotion, forcedColors: types.ForcedColors): Promise<void> {
const promises = [];
promises.push(session.send('Page.setEmulatedMedia', { media: mediaType === 'no-override' ? '' : mediaType }));
let appearance: any = undefined;
switch (colorScheme) {
case 'light': appearance = 'Light'; break;
case 'dark': appearance = 'Dark'; break;
case 'no-override': appearance = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersColorScheme', value: appearance }));
let reducedMotionWk: any = undefined;
switch (reducedMotion) {
case 'reduce': reducedMotionWk = 'Reduce'; break;
case 'no-preference': reducedMotionWk = 'NoPreference'; break;
case 'no-override': reducedMotionWk = undefined; break;
}
promises.push(session.send('Page.overrideUserPreference', { name: 'PrefersReducedMotion', value: reducedMotionWk }));
let forcedColorsWk: any = undefined;
switch (forcedColors) {
case 'active': forcedColorsWk = 'Active'; break;
case 'none': forcedColorsWk = 'None'; break;
case 'no-override': forcedColorsWk = undefined; break;
}
promises.push(session.send('Page.setForcedColors', { forcedColors: forcedColorsWk }));
await Promise.all(promises);
}
async updateExtraHTTPHeaders(): Promise<void> {
await this._updateState('Network.setExtraHTTPHeaders', { headers: headersArrayToObject(this._calculateExtraHTTPHeaders(), false /* lowerCase */) });
}
_calculateExtraHTTPHeaders(): types.HeadersArray {
const locale = this._browserContext._options.locale;
const headers = network.mergeHeaders([
this._browserContext._options.extraHTTPHeaders,
this._page.extraHTTPHeaders(),
locale ? network.singleHeader('Accept-Language', locale) : undefined,
]);
return headers;
}
async updateEmulateMedia(): Promise<void> {
const emulatedMedia = this._page.emulatedMedia();
const colorScheme = emulatedMedia.colorScheme;
const reducedMotion = emulatedMedia.reducedMotion;
const forcedColors = emulatedMedia.forcedColors;
await this._forAllSessions(session => WKPage._setEmulateMedia(session, emulatedMedia.media, colorScheme, reducedMotion, forcedColors));
}
async updateEmulatedViewportSize(): Promise<void> {
await this._updateViewport();
}
async updateUserAgent(): Promise<void> {
const contextOptions = this._browserContext._options;
this._updateState('Page.overrideUserAgent', { value: contextOptions.userAgent });
}
async bringToFront(): Promise<void> {
this._pageProxySession.send('Target.activate', {
targetId: this._session.sessionId
});
}
async _updateViewport(): Promise<void> {
const options = this._browserContext._options;
const deviceSize = this._page.emulatedSize();
if (deviceSize === null)
return;
const viewportSize = deviceSize.viewport;
const screenSize = deviceSize.screen;
const promises: Promise<any>[] = [
this._pageProxySession.send('Emulation.setDeviceMetricsOverride', {
width: viewportSize.width,
height: viewportSize.height,
fixedLayout: !!options.isMobile,
deviceScaleFactor: options.deviceScaleFactor || 1
}),
this._session.send('Page.setScreenSizeOverride', {
width: screenSize.width,
height: screenSize.height,
}),
];
if (options.isMobile) {
const angle = viewportSize.width > viewportSize.height ? 90 : 0;
promises.push(this._session.send('Page.setOrientationOverride', { angle }));
}
await Promise.all(promises);
}
async updateRequestInterception(): Promise<void> {
const enabled = this._page.needsRequestInterception();
await Promise.all([
this._updateState('Network.setInterceptionEnabled', { enabled }),
this._updateState('Network.addInterception', { url: '.*', stage: 'request', isRegex: true }),
]);
}
async updateOffline() {
await this._updateState('Network.setEmulateOfflineState', { offline: !!this._browserContext._options.offline });
}
async updateHttpCredentials() {
const credentials = this._browserContext._options.httpCredentials || { username: '', password: '' };
await this._pageProxySession.send('Emulation.setAuthCredentials', { username: credentials.username, password: credentials.password });
}
async updateFileChooserInterception() {
const enabled = this._page.fileChooserIntercepted();
await this._session.send('Page.setInterceptFileChooserDialog', { enabled }).catch(() => {}); // target can be closed.
}
async reload(): Promise<void> {
await this._session.send('Page.reload');
}
goBack(): Promise<boolean> {
return this._session.send('Page.goBack').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goBack): Failed to go`))
return false;
throw error;
});
}
goForward(): Promise<boolean> {
return this._session.send('Page.goForward').then(() => true).catch(error => {
if (error instanceof Error && error.message.includes(`Protocol error (Page.goForward): Failed to go`))
return false;
throw error;
});
}
async exposeBinding(binding: PageBinding): Promise<void> {
this._session.send('Runtime.addBinding', { name: binding.name });
await this._updateBootstrapScript();
await Promise.all(this._page.frames().map(frame => frame.evaluateExpression(binding.source, false, {}).catch(e => {})));
}
async removeExposedBindings(): Promise<void> {
await this._updateBootstrapScript();
}
async addInitScript(script: string): Promise<void> {
await this._updateBootstrapScript();
}
async removeInitScripts() {
await this._updateBootstrapScript();
}
private _calculateBootstrapScript(): string {
const scripts: string[] = [];
if (!this._page.context()._options.isMobile) {
scripts.push('delete window.orientation');
scripts.push('delete window.ondevicemotion');
scripts.push('delete window.ondeviceorientation');
}
for (const binding of this._page.allBindings())
scripts.push(binding.source);
scripts.push(...this._browserContext.initScripts);
scripts.push(...this._page.initScripts);
return scripts.join(';\n');
}
async _updateBootstrapScript(): Promise<void> {
await this._updateState('Page.setBootstrapScript', { source: this._calculateBootstrapScript() });
}
async closePage(runBeforeUnload: boolean): Promise<void> {
await this._stopVideo();
await this._pageProxySession.sendMayFail('Target.close', {
targetId: this._session.sessionId,
runBeforeUnload
});
}
async setBackgroundColor(color?: { r: number; g: number; b: number; a: number; }): Promise<void> {
await this._session.send('Page.setDefaultBackgroundColorOverride', { color });
}
private _toolbarHeight(): number {
if (this._page._browserContext._browser?.options.headful)
return hostPlatform === 'mac10.15' ? 55 : 59;
return 0;
}
private async _startVideo(options: types.PageScreencastOptions): Promise<void> {
assert(!this._recordingVideoFile);
const { screencastId } = await this._pageProxySession.send('Screencast.startVideo', {
file: options.outputFile,
width: options.width,
height: options.height,
toolbarHeight: this._toolbarHeight()
});
this._recordingVideoFile = options.outputFile;
this._browserContext._browser._videoStarted(this._browserContext, screencastId, options.outputFile, this.pageOrError());
}
async _stopVideo(): Promise<void> {
if (!this._recordingVideoFile)
return;
await this._pageProxySession.sendMayFail('Screencast.stopVideo');
this._recordingVideoFile = null;
}
private validateScreenshotDimension(side: number, omitDeviceScaleFactor: boolean) {
// Cairo based implementations (Linux and Windows) have hard limit of 32767
// (see https://github.com/microsoft/playwright/issues/16727).
if (process.platform === 'darwin')
return;
if (!omitDeviceScaleFactor && this._page._browserContext._options.deviceScaleFactor)
side = Math.ceil(side * this._page._browserContext._options.deviceScaleFactor);
if (side > 32767)
throw new Error('Cannot take screenshot larger than 32767 pixels on any dimension');
}
async takeScreenshot(progress: Progress, format: string, documentRect: types.Rect | undefined, viewportRect: types.Rect | undefined, quality: number | undefined, fitsViewport: boolean, scale: 'css' | 'device'): Promise<Buffer> {
const rect = (documentRect || viewportRect)!;
const omitDeviceScaleFactor = scale === 'css';
this.validateScreenshotDimension(rect.width, omitDeviceScaleFactor);
this.validateScreenshotDimension(rect.height, omitDeviceScaleFactor);
const result = await this._session.send('Page.snapshotRect', { ...rect, coordinateSystem: documentRect ? 'Page' : 'Viewport', omitDeviceScaleFactor });
const prefix = 'data:image/png;base64,';
let buffer = Buffer.from(result.dataURL.substr(prefix.length), 'base64');
if (format === 'jpeg')
buffer = jpegjs.encode(PNG.sync.read(buffer), quality).data;
return buffer;
}
async getContentFrame(handle: dom.ElementHandle): Promise<frames.Frame | null> {
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
if (!nodeInfo.contentFrameId)
return null;
return this._page._frameManager.frame(nodeInfo.contentFrameId);
}
async getOwnerFrame(handle: dom.ElementHandle): Promise<string | null> {
if (!handle._objectId)
return null;
const nodeInfo = await this._session.send('DOM.describeNode', {
objectId: handle._objectId
});
return nodeInfo.ownerFrameId || null;
}
isElementHandle(remoteObject: any): boolean {
return (remoteObject as Protocol.Runtime.RemoteObject).subtype === 'node';
}
async getBoundingBox(handle: dom.ElementHandle): Promise<types.Rect | null> {
const quads = await this.getContentQuads(handle);
if (!quads || !quads.length)
return null;
let minX = Infinity;
let maxX = -Infinity;
let minY = Infinity;
let maxY = -Infinity;
for (const quad of quads) {
for (const point of quad) {
minX = Math.min(minX, point.x);
maxX = Math.max(maxX, point.x);
minY = Math.min(minY, point.y);
maxY = Math.max(maxY, point.y);
}
}
return { x: minX, y: minY, width: maxX - minX, height: maxY - minY };
}
async scrollRectIntoViewIfNeeded(handle: dom.ElementHandle, rect?: types.Rect): Promise<'error:notvisible' | 'error:notconnected' | 'done'> {
return await this._session.send('DOM.scrollIntoViewIfNeeded', {
objectId: handle._objectId,
rect,
}).then(() => 'done' as const).catch(e => {
if (e instanceof Error && e.message.includes('Node does not have a layout object'))
return 'error:notvisible';
if (e instanceof Error && e.message.includes('Node is detached from document'))
return 'error:notconnected';
throw e;
});
}
async setScreencastOptions(options: { width: number, height: number, quality: number } | null): Promise<void> {
if (options) {
const so = { ...options, toolbarHeight: this._toolbarHeight() };
const { generation } = await this._pageProxySession.send('Screencast.startScreencast', so);
this._screencastGeneration = generation;
} else {
await this._pageProxySession.send('Screencast.stopScreencast');
}
}
private _onScreencastFrame(event: Protocol.Screencast.screencastFramePayload) {
const generation = this._screencastGeneration;
this._page.throttleScreencastFrameAck(() => {
this._pageProxySession.send('Screencast.screencastFrameAck', { generation }).catch(e => debugLogger.log('error', e));
});
const buffer = Buffer.from(event.data, 'base64');
this._page.emit(Page.Events.ScreencastFrame, {
buffer,
width: event.deviceWidth,
height: event.deviceHeight,
});
}
rafCountForStablePosition(): number {
return process.platform === 'win32' ? 5 : 1;
}
async getContentQuads(handle: dom.ElementHandle): Promise<types.Quad[] | null> {
const result = await this._session.sendMayFail('DOM.getContentQuads', {
objectId: handle._objectId
});
if (!result)
return null;
return result.quads.map(quad => [
{ x: quad[0], y: quad[1] },
{ x: quad[2], y: quad[3] },
{ x: quad[4], y: quad[5] },
{ x: quad[6], y: quad[7] }
]);
}
async setInputFiles(handle: dom.ElementHandle<HTMLInputElement>, files: types.FilePayload[]): Promise<void> {
const objectId = handle._objectId;
const protocolFiles = files.map(file => ({
name: file.name,
type: file.mimeType,
data: file.buffer,
}));
await this._session.send('DOM.setInputFiles', { objectId, files: protocolFiles });
}
async setInputFilePaths(handle: dom.ElementHandle<HTMLInputElement>, paths: string[]): Promise<void> {
const pageProxyId = this._pageProxySession.sessionId;
const objectId = handle._objectId;
await Promise.all([
this._pageProxySession.connection.browserSession.send('Playwright.grantFileReadAccess', { pageProxyId, paths }),
this._session.send('DOM.setInputFiles', { objectId, paths })
]);
}
async adoptElementHandle<T extends Node>(handle: dom.ElementHandle<T>, to: dom.FrameExecutionContext): Promise<dom.ElementHandle<T>> {
const result = await this._session.sendMayFail('DOM.resolveNode', {
objectId: handle._objectId,
executionContextId: ((to as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error(dom.kUnableToAdoptErrorMessage);
return to.createHandle(result.object) as dom.ElementHandle<T>;
}
async getAccessibilityTree(needle?: dom.ElementHandle): Promise<{tree: accessibility.AXNode, needle: accessibility.AXNode | null}> {
return getAccessibilityTree(this._session, needle);
}
async inputActionEpilogue(): Promise<void> {
}
async getFrameElement(frame: frames.Frame): Promise<dom.ElementHandle> {
const parent = frame.parentFrame();
if (!parent)
throw new Error('Frame has been detached.');
const context = await parent._mainContext();
const result = await this._session.send('DOM.resolveNode', {
frameId: frame._id,
executionContextId: ((context as any)[contextDelegateSymbol] as WKExecutionContext)._contextId
});
if (!result || result.object.subtype === 'null')
throw new Error('Frame has been detached.');
return context.createHandle(result.object) as dom.ElementHandle;
}
_onRequestWillBeSent(session: WKSession, event: Protocol.Network.requestWillBeSentPayload) {
if (event.request.url.startsWith('data:'))
return;
let redirectedFrom: WKInterceptableRequest | null = null;
if (event.redirectResponse) {
const request = this._requestIdToRequest.get(event.requestId);
// If we connect late to the target, we could have missed the requestWillBeSent event.
if (request) {
this._handleRequestRedirect(request, event.redirectResponse, event.timestamp);
redirectedFrom = request;
}
}
const frame = redirectedFrom ? redirectedFrom.request.frame() : this._page._frameManager.frame(event.frameId);
// sometimes we get stray network events for detached frames
// TODO(einbinder) why?
if (!frame)
return;
// TODO(einbinder) this will fail if we are an XHR document request
const isNavigationRequest = event.type === 'Document';
const documentId = isNavigationRequest ? event.loaderId : undefined;
let route = null;
// We do not support intercepting redirects.
if (this._page.needsRequestInterception() && !redirectedFrom)
route = new WKRouteImpl(session, event.requestId);
const request = new WKInterceptableRequest(session, route, frame, event, redirectedFrom, documentId);
this._requestIdToRequest.set(event.requestId, request);
this._page._frameManager.requestStarted(request.request, route || undefined);
}
private _handleRequestRedirect(request: WKInterceptableRequest, responsePayload: Protocol.Network.Response, timestamp: number) {
const response = request.createResponse(responsePayload);
response._securityDetailsFinished();
response._serverAddrFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(responsePayload.timing ? helper.secondsToRoundishMillis(timestamp - request._timestamp) : -1);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.requestReceivedResponse(response);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onRequestIntercepted(session: WKSession, event: Protocol.Network.requestInterceptedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
if (!request) {
session.sendMayFail('Network.interceptRequestWithError', { errorType: 'Cancellation', requestId: event.requestId });
return;
}
// There is no point in waiting for the raw headers in Network.responseReceived when intercepting.
// Use provisional headers as raw headers, so that client can call allHeaders() from the route handler.
request.request.setRawRequestHeaders(null);
if (!request._route) {
// Intercepted, although we do not intend to allow interception.
// Just continue.
session.sendMayFail('Network.interceptWithRequest', { requestId: request._requestId });
} else {
request._route._requestInterceptedPromise.resolve();
}
}
_onResponseReceived(event: Protocol.Network.responseReceivedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// FileUpload sends a response without a matching request.
if (!request)
return;
this._requestIdToResponseReceivedPayloadEvent.set(request._requestId, event);
const response = request.createResponse(event.response);
this._page._frameManager.requestReceivedResponse(response);
if (response.status() === 204) {
this._onLoadingFailed({
requestId: event.requestId,
errorText: 'Aborted: 204 No Content',
timestamp: event.timestamp
});
}
}
_onLoadingFinished(event: Protocol.Network.loadingFinishedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
// Under certain conditions we never get the Network.responseReceived
// event from protocol. @see https://crbug.com/883475
const response = request.request._existingResponse();
if (response) {
const responseReceivedPayload = this._requestIdToResponseReceivedPayloadEvent.get(request._requestId);
response._serverAddrFinished(parseRemoteAddress(event?.metrics?.remoteAddress));
response._securityDetailsFinished({
protocol: isLoadedSecurely(response.url(), response.timing()) ? event.metrics?.securityConnection?.protocol : undefined,
subjectName: responseReceivedPayload?.response.security?.certificate?.subject,
validFrom: responseReceivedPayload?.response.security?.certificate?.validFrom,
validTo: responseReceivedPayload?.response.security?.certificate?.validUntil,
});
if (event.metrics?.protocol)
response._setHttpVersion(event.metrics.protocol);
response.setEncodedBodySize(event.metrics?.responseBodyBytesReceived ?? null);
response.setResponseHeadersSize(event.metrics?.responseHeaderBytesReceived ?? null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToResponseReceivedPayloadEvent.delete(request._requestId);
this._requestIdToRequest.delete(request._requestId);
this._page._frameManager.reportRequestFinished(request.request, response);
}
_onLoadingFailed(event: Protocol.Network.loadingFailedPayload) {
const request = this._requestIdToRequest.get(event.requestId);
// For certain requestIds we never receive requestWillBeSent event.
// @see https://crbug.com/750469
if (!request)
return;
const response = request.request._existingResponse();
if (response) {
response._serverAddrFinished();
response._securityDetailsFinished();
response.setResponseHeadersSize(null);
response.setEncodedBodySize(null);
response._requestFinished(helper.secondsToRoundishMillis(event.timestamp - request._timestamp));
} else {
// Use provisional headers if we didn't have the response with raw headers.
request.request.setRawRequestHeaders(null);
}
this._requestIdToRequest.delete(request._requestId);
request.request._setFailureText(event.errorText);
this._page._frameManager.requestFailed(request.request, event.errorText.includes('cancelled'));
}
async _grantPermissions(origin: string, permissions: string[]) {
const webPermissionToProtocol = new Map<string, string>([
['geolocation', 'geolocation'],
]);
const filtered = permissions.map(permission => {
const protocolPermission = webPermissionToProtocol.get(permission);
if (!protocolPermission)
throw new Error('Unknown permission: ' + permission);
return protocolPermission;
});
await this._pageProxySession.send('Emulation.grantPermissions', { origin, permissions: filtered });
}
async _clearPermissions() {
await this._pageProxySession.send('Emulation.resetPermissions', {});
}
}
/**
* WebKit Remote Addresses look like:
*
* macOS:
* ::1.8911
* 2606:2800:220:1:248:1893:25c8:1946.443
* 127.0.0.1:8000
*
* ubuntu:
* ::1:8907
* 127.0.0.1:8000
*
* NB: They look IPv4 and IPv6's with ports but use an alternative notation.
*/
function parseRemoteAddress(value?: string) {
if (!value)
return;
try {
const colon = value.lastIndexOf(':');
const dot = value.lastIndexOf('.');
if (dot < 0) { // IPv6ish:port
return {
ipAddress: `[${value.slice(0, colon)}]`,
port: +value.slice(colon + 1)
};
}
if (colon > dot) { // IPv4:port
const [address, port] = value.split(':');
return {
ipAddress: address,
port: +port,
};
} else { // IPv6ish.port
const [address, port] = value.split('.');
return {
ipAddress: `[${address}]`,
port: +port,
};
}
} catch (_) {}
}
/**
* Adapted from Source/WebInspectorUI/UserInterface/Models/Resource.js in
* WebKit codebase.
*/
function isLoadedSecurely(url: string, timing: network.ResourceTiming) {
try {
const u = new URL(url);
if (u.protocol !== 'https:' && u.protocol !== 'wss:' && u.protocol !== 'sftp:')
return false;
if (timing.secureConnectionStart === -1 && timing.connectStart !== -1)
return false;
return true;
} catch (_) {}
}
const contextDelegateSymbol = Symbol('delegate');
| packages/playwright-core/src/server/webkit/wkPage.ts | 1 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00021281265071593225,
0.0001729276409605518,
0.00016504025552421808,
0.0001729793002596125,
0.000004952776635036571
] |
{
"id": 3,
"code_window": [
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n",
" it.fixme(browserName === 'webkit');\n",
" server.setRoute('/done.html?', (req, res) => {\n",
" res.end(`Done`);\n",
" });\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 37
} | #!/bin/bash
set -e
set -x
rm -rf "/Applications/Google Chrome.app"
cd /tmp
curl -o ./googlechrome.dmg -k https://dl.google.com/chrome/mac/universal/stable/GGRO/googlechrome.dmg
hdiutil attach -nobrowse -quiet -noautofsck -noautoopen -mountpoint /Volumes/googlechrome.dmg ./googlechrome.dmg
cp -rf "/Volumes/googlechrome.dmg/Google Chrome.app" /Applications
hdiutil detach /Volumes/googlechrome.dmg
rm -rf /tmp/googlechrome.dmg
/Applications/Google\ Chrome.app/Contents/MacOS/Google\ Chrome --version
| packages/playwright-core/bin/reinstall_chrome_stable_mac.sh | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00017385632963851094,
0.0001712920202407986,
0.00016872771084308624,
0.0001712920202407986,
0.00000256430939771235
] |
{
"id": 3,
"code_window": [
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n",
" it.fixme(browserName === 'webkit');\n",
" server.setRoute('/done.html?', (req, res) => {\n",
" res.end(`Done`);\n",
" });\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 37
} | /**
* Copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import type { BabelFileResult } from '../../bundles/babel/node_modules/@types/babel__core';
export const codeFrameColumns: typeof import('../../bundles/babel/node_modules/@types/babel__code-frame').codeFrameColumns = require('./babelBundleImpl').codeFrameColumns;
export const declare: typeof import('../../bundles/babel/node_modules/@types/babel__helper-plugin-utils').declare = require('./babelBundleImpl').declare;
export const types: typeof import('../../bundles/babel/node_modules/@types/babel__core').types = require('./babelBundleImpl').types;
export const parse: typeof import('../../bundles/babel/node_modules/@babel/parser/typings/babel-parser').parse = require('./babelBundleImpl').parse;
export const traverse: typeof import('../../bundles/babel/node_modules/@types/babel__traverse').default = require('./babelBundleImpl').traverse;
export type BabelTransformFunction = (filename: string, isTypeScript: boolean, isModule: boolean, scriptPreprocessor: string | undefined, additionalPlugin: [string]) => BabelFileResult;
export const babelTransform: BabelTransformFunction = require('./babelBundleImpl').babelTransform;
export type { NodePath, types as T } from '../../bundles/babel/node_modules/@types/babel__core';
export type { BabelAPI } from '../../bundles/babel/node_modules/@types/babel__helper-plugin-utils';
| packages/playwright-test/src/common/babelBundle.ts | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.00017726648366078734,
0.00017655629198998213,
0.00017619659774936736,
0.00017620582366362214,
5.021885840506002e-7
] |
{
"id": 3,
"code_window": [
" it.info().annotations.push({ type: 'issue', description: 'https://github.com/microsoft/playwright/issues/18392' });\n",
" it.fixme(browserName === 'webkit');\n",
" server.setRoute('/done.html?', (req, res) => {\n",
" res.end(`Done`);\n",
" });\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [],
"file_path": "tests/page/page-navigation.spec.ts",
"type": "replace",
"edit_start_line_idx": 37
} | /**
* Copyright 2017 Google Inc. All rights reserved.
* Modifications copyright (c) Microsoft Corporation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import { WKBrowser } from '../webkit/wkBrowser';
import type { Env } from '../../utils/processLauncher';
import path from 'path';
import { kBrowserCloseMessageId } from './wkConnection';
import { BrowserType, kNoXServerRunningError } from '../browserType';
import type { ConnectionTransport } from '../transport';
import type { BrowserOptions, PlaywrightOptions } from '../browser';
import type * as types from '../types';
import { rewriteErrorMessage } from '../../utils/stackTrace';
import { wrapInASCIIBox } from '../../utils';
export class WebKit extends BrowserType {
constructor(playwrightOptions: PlaywrightOptions) {
super('webkit', playwrightOptions);
}
_connectToTransport(transport: ConnectionTransport, options: BrowserOptions): Promise<WKBrowser> {
return WKBrowser.connect(transport, options);
}
_amendEnvironment(env: Env, userDataDir: string, executable: string, browserArguments: string[]): Env {
return { ...env, CURL_COOKIE_JAR_PATH: path.join(userDataDir, 'cookiejar.db') };
}
_rewriteStartupError(error: Error): Error {
if (error.message.includes('cannot open display'))
return rewriteErrorMessage(error, '\n' + wrapInASCIIBox(kNoXServerRunningError, 1));
return error;
}
_attemptToGracefullyCloseBrowser(transport: ConnectionTransport): void {
transport.send({ method: 'Playwright.close', params: {}, id: kBrowserCloseMessageId });
}
_defaultArgs(options: types.LaunchOptions, isPersistent: boolean, userDataDir: string): string[] {
const { args = [], proxy, headless } = options;
const userDataDirArg = args.find(arg => arg.startsWith('--user-data-dir'));
if (userDataDirArg)
throw new Error('Pass userDataDir parameter to `browserType.launchPersistentContext(userDataDir, ...)` instead of specifying --user-data-dir argument');
if (args.find(arg => !arg.startsWith('-')))
throw new Error('Arguments can not specify page to be opened');
const webkitArguments = ['--inspector-pipe'];
if (process.platform === 'win32')
webkitArguments.push('--disable-accelerated-compositing');
if (headless)
webkitArguments.push('--headless');
if (isPersistent)
webkitArguments.push(`--user-data-dir=${userDataDir}`);
else
webkitArguments.push(`--no-startup-window`);
if (proxy) {
if (process.platform === 'darwin') {
webkitArguments.push(`--proxy=${proxy.server}`);
if (proxy.bypass)
webkitArguments.push(`--proxy-bypass-list=${proxy.bypass}`);
} else if (process.platform === 'linux') {
webkitArguments.push(`--proxy=${proxy.server}`);
if (proxy.bypass)
webkitArguments.push(...proxy.bypass.split(',').map(t => `--ignore-host=${t}`));
} else if (process.platform === 'win32') {
// Enable socks5 hostname resolution on Windows. Workaround can be removed once fixed upstream.
// See https://github.com/microsoft/playwright/issues/20451
webkitArguments.push(`--curl-proxy=${proxy.server.replace(/^socks5:\/\//, 'socks5h://')}`);
if (proxy.bypass)
webkitArguments.push(`--curl-noproxy=${proxy.bypass}`);
}
}
webkitArguments.push(...args);
if (isPersistent)
webkitArguments.push('about:blank');
return webkitArguments;
}
}
| packages/playwright-core/src/server/webkit/webkit.ts | 0 | https://github.com/microsoft/playwright/commit/cffe7b65e3ffb713a1f7138d5e881cbba20a569c | [
0.0002322211948921904,
0.00017683437908999622,
0.00016536176553927362,
0.00017053185729309916,
0.000018969818484038115
] |
{
"id": 0,
"code_window": [
".append(readStream, {name: 'archiver.d.ts'});\n",
"\n",
"archiver.directory('./path', './someOtherPath');\n",
"archiver.directory('./path', { name: \"testName\" });\n",
"archiver.directory('./', \"\", {});\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 37
} | import * as Archiver from 'archiver';
import * as fs from 'fs';
const options: Archiver.ArchiverOptions = {
statConcurrency: 1,
allowHalfOpen: true,
readableObjectMode: true,
writeableObjectMode: true,
decodeStrings: true,
encoding: 'test',
highWaterMark: 1,
objectmode: true,
comment: 'test',
forceLocalTime: true,
forceZip64: true,
store: true,
zlib: {},
gzip: true,
gzipOptions: {},
};
Archiver('zip', options);
const archiver = Archiver.create('zip');
const writeStream = fs.createWriteStream('./archiver.d.ts');
const readStream = fs.createReadStream('./archiver.d.ts');
archiver.abort();
archiver.pipe(writeStream);
archiver.append(readStream, { name: 'archiver.d.ts' });
archiver.append(readStream, {name: 'archiver.d.ts'})
.append(readStream, {name: 'archiver.d.ts'});
archiver.directory('./path', './someOtherPath');
archiver.directory('./path', { name: "testName" });
archiver.directory('./', "", {});
archiver.directory('./', { name: 'test' }, {});
archiver.append(readStream, {
name: "sub/folder.xml"
});
archiver.glob("**", {
cwd: 'path/to/files',
});
archiver.glob('./path', {}, {});
archiver.file('./path', { name: 'test' });
archiver.setFormat('zip');
archiver.setModule(() => {});
archiver.pointer();
archiver.use(() => {});
archiver.finalize().then();
archiver.symlink('./path', './target');
| types/archiver/archiver-tests.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.9833547472953796,
0.14522209763526917,
0.00017851947632152587,
0.0027849781326949596,
0.3422130346298218
] |
{
"id": 0,
"code_window": [
".append(readStream, {name: 'archiver.d.ts'});\n",
"\n",
"archiver.directory('./path', './someOtherPath');\n",
"archiver.directory('./path', { name: \"testName\" });\n",
"archiver.directory('./', \"\", {});\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 37
} | sap.ui.getCore().attachInit(function () {
new sap.m.Text({
text: "Hello World"
}).placeAt("content");
});
sap.ui.getCore().attachInit(function () {
new sap.ui.core.mvc.XMLView({
viewName: "sap.ui.demo.wt.App"
}).placeAt("content");
});
class Ctrl extends sap.ui.core.mvc.Controller {
constructor(private JSONModel: typeof sap.ui.model.json.JSONModel) {
super(undefined);
}
public onShowHello(): void {
// show a native JavaScript alert
alert("Hello World");
}
public onInit() {
// set data model on view
var oData = {
recipient: {
name: "World"
}
};
var oModel = new this.JSONModel(oData);
this.getView().setModel(oModel);
}
}
sap.ui.define([
"sap/ui/core/mvc/Controller"
], function (Controller: typeof sap.ui.core.mvc.Controller, JSONModel: typeof sap.ui.model.json.JSONModel) {
"use strict";
return new Ctrl(JSONModel);
});
namespace ectr_web.controller {
export class BaseController extends sap.ui.core.mvc.Controller {
constructor() {
super(undefined);
}
public getRouter() {
return (<sap.ui.core.UIComponent>this.getOwnerComponent()).getRouter();
}
public getJSONModel(name: string) {
return <sap.ui.model.json.JSONModel>this.getView().getModel(name);
}
public getModel(name: string) {
return this.getView().getModel(name);
}
}
}
var oTable = new sap.m.Table({
editable: false,
toolbar: new sap.m.Toolbar({
items: [
new sap.m.Button({
text: "Create user",
press: function () {
},
}),
]
}),
});
const lbl = new sap.m.Label(undefined);
lbl.setText('text');
const col = new sap.m.Column();
var oUploadDialog = new sap.m.Dialog(undefined);
oUploadDialog.setTitle("Upload photo");
(<sap.ui.model.odata.ODataModel>sap.ui.getCore().getModel(undefined)).refreshSecurityToken();
// prepare the FileUploader control
var oFileUploader = new sap.ui.unified.FileUploader({
headerParameters: [
new sap.ui.unified.FileUploaderParameter({
name: "x-csrf-token",
value: (<sap.ui.model.odata.ODataModel>sap.ui.getCore().getModel(undefined)).getHeaders()['x-csrf-token']
}),
],
uploadComplete: function (oEvent: sap.ui.base.Event) {
var sResponse = oEvent.getParameter("response");
if (sResponse) {
oUploadDialog.close();
sap.m.MessageBox.show("Return Code: " + sResponse);
}
}
});
// create a button to trigger the upload
var oTriggerButton = new sap.m.Button({
text: 'Upload',
press: function () {
// call the upload method
oFileUploader.insertHeaderParameter(new sap.ui.unified.FileUploaderParameter({ name: "slug", value: oFileUploader.getValue() }), 0);
oFileUploader.upload();
}
});
oUploadDialog.addContent(oFileUploader);
oUploadDialog.addContent(oTriggerButton);
oUploadDialog.open(); | types/openui5/openui5-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.0001787436194717884,
0.00017629438661970198,
0.00017431065498385578,
0.00017592613585293293,
0.0000014378857713381876
] |
{
"id": 0,
"code_window": [
".append(readStream, {name: 'archiver.d.ts'});\n",
"\n",
"archiver.directory('./path', './someOtherPath');\n",
"archiver.directory('./path', { name: \"testName\" });\n",
"archiver.directory('./', \"\", {});\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 37
} | // Type definitions for is-root-path 1.0
// Project: https://github.com/sindresorhus/is-root-path#readme
// Definitions by: Mohamed Hegazy <https://github.com/mhegazy>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
export = is_root_path;
declare function is_root_path(pth: string): boolean;
| types/is-root-path/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00016745734319556504,
0.00016745734319556504,
0.00016745734319556504,
0.00016745734319556504,
0
] |
{
"id": 0,
"code_window": [
".append(readStream, {name: 'archiver.d.ts'});\n",
"\n",
"archiver.directory('./path', './someOtherPath');\n",
"archiver.directory('./path', { name: \"testName\" });\n",
"archiver.directory('./', \"\", {});\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 37
} | // Type definitions for promised-temp 0.1
// Project: https://www.npmjs.com/package/promised-temp, https://github.com/mikaturunen/promised-temp
// Definitions by: Saqib Rokadia <https://github.com/rokadias>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
/// <reference types="node" />
import * as fs from 'fs';
import { AffixOptions, OpenFile, Stats } from "temp";
export { AffixOptions, OpenFile, Stats } from "temp";
interface TempStatic {
dir: string;
track(value?: boolean): TempStatic;
path(affixes?: string | AffixOptions, defaultPrefix?: string): string;
mkdir(affixes?: string | AffixOptions): Promise<string>;
open(affixes?: string | AffixOptions): Promise<OpenFile>;
cleanup(): Promise<boolean | Stats>;
createWriteStream(affixes?: string | AffixOptions): Promise<fs.WriteStream>;
}
declare var PromisedTemp: TempStatic;
export default PromisedTemp;
| types/promised-temp/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.0001998209481826052,
0.00018381676636636257,
0.0001741973392199725,
0.00017743202624842525,
0.000011393450222385582
] |
{
"id": 1,
"code_window": [
"archiver.directory('./', \"\", {});\n",
"archiver.directory('./', { name: 'test' }, {});\n",
"\n",
"archiver.append(readStream, {\n",
" name: \"sub/folder.xml\"\n",
"});\n",
"\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"archiver.directory('./', false, { name: 'test' });\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => {\n",
" entry.name = \"foobar\";\n",
" return entry;\n",
"});\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => false);\n"
],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 39
} | import * as Archiver from 'archiver';
import * as fs from 'fs';
const options: Archiver.ArchiverOptions = {
statConcurrency: 1,
allowHalfOpen: true,
readableObjectMode: true,
writeableObjectMode: true,
decodeStrings: true,
encoding: 'test',
highWaterMark: 1,
objectmode: true,
comment: 'test',
forceLocalTime: true,
forceZip64: true,
store: true,
zlib: {},
gzip: true,
gzipOptions: {},
};
Archiver('zip', options);
const archiver = Archiver.create('zip');
const writeStream = fs.createWriteStream('./archiver.d.ts');
const readStream = fs.createReadStream('./archiver.d.ts');
archiver.abort();
archiver.pipe(writeStream);
archiver.append(readStream, { name: 'archiver.d.ts' });
archiver.append(readStream, {name: 'archiver.d.ts'})
.append(readStream, {name: 'archiver.d.ts'});
archiver.directory('./path', './someOtherPath');
archiver.directory('./path', { name: "testName" });
archiver.directory('./', "", {});
archiver.directory('./', { name: 'test' }, {});
archiver.append(readStream, {
name: "sub/folder.xml"
});
archiver.glob("**", {
cwd: 'path/to/files',
});
archiver.glob('./path', {}, {});
archiver.file('./path', { name: 'test' });
archiver.setFormat('zip');
archiver.setModule(() => {});
archiver.pointer();
archiver.use(() => {});
archiver.finalize().then();
archiver.symlink('./path', './target');
| types/archiver/archiver-tests.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.6866233348846436,
0.1036766767501831,
0.0001743716566124931,
0.0025718475226312876,
0.23811157047748566
] |
{
"id": 1,
"code_window": [
"archiver.directory('./', \"\", {});\n",
"archiver.directory('./', { name: 'test' }, {});\n",
"\n",
"archiver.append(readStream, {\n",
" name: \"sub/folder.xml\"\n",
"});\n",
"\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"archiver.directory('./', false, { name: 'test' });\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => {\n",
" entry.name = \"foobar\";\n",
" return entry;\n",
"});\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => false);\n"
],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 39
} | // Type definitions for vitalsigns 0.4.3
// Project: https://github.com/TomFrost/node-vitalsigns
// Definitions by: Cyril Schumacher <https://github.com/cyrilschumacher>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
///<reference types="express"/>
declare module "vitalsigns" {
import { RequestHandler } from "express";
namespace vitalsigns {
/**
* Contraint.
* @interface
*/
export interface Constraint {
/**
* The comparator to use when comparing the field's value with the constraint value.
* Valid comparators are: 'equal', 'greater', and 'less'.
* @type {string}
*/
comparator: string;
/**
* The name of the field to be constrained.
* @type {string}
*/
field: string;
/**
* The name of the monitor containing the field to be constrained.
* @type {string}
*/
monitor: string;
/**
* true to negate the outcome of the comparison; false or omitted to use the comparison result.
* @type {boolean}
*/
negate?: boolean;
/**
* The value against which the field should be compared.
* @type {any}
*/
value: any;
}
/**
* Constraint wrapper.
* @interface
*/
export interface ConstraintWrapper {
equals?: (num: number) => ConstraintWrapper;
greaterThan?: (num: number) => ConstraintWrapper;
lessThan?: (num: number) => ConstraintWrapper;
not?: ConstraintWrapper;
}
/**
* Options.
* @interface
*/
export interface Options {
/**
* Number of milliseconds to wait between automatic health checks.
* @type {number|boolean}
*/
autoCheck?: number | boolean;
/**
* HTTP response code to send back in the VitalSigns.
* @type {number}
*/
httpHealthy?: number;
/**
* HTTP response code to send back in the VitalSigns.
* @type {number}
*/
httpUnhealthy?: number;
}
export interface Monitor {
/**
* Connections.
* @type {any}
*/
connections: any;
}
/**
* Monitor field.
* @interface
*/
export interface MonitorField {
/**
* Name.
* @type {string}
*/
name?: string;
/**
* Units.
* @type {string}
*/
units?: string;
}
/**
* Report options.
* @interface
*/
interface ReportOptions {
/**
* true to flatten the report object down to a single level by concatenating nested key names; false to keep the default hierarchical format.
* @type {boolean}
*/
flatten?: boolean;
/**
* If flatten is true, this string will be used to separate key names when they are concatenated together.
* @type {boolean}
*/
separator?: string;
}
}
/**
* VitalSigns instance.
*/
class VitalSigns {
/**
* Constructor.
* @constructors
* @param {Options} [options] Options.
*/
constructor (options?: vitalsigns.Options);
/**
* Pushes a health constraint onto this instance's constraint array.
* Health constraints define scenarios in which VitalSigns will consider the application to be in an unhealthy state.
* @param {} constraint A constraint.
*/
addConstraint(): void;
/**
* Destroys this VitalSigns instance.
*/
destroy(): void;
/**
* Gets a request handler.
* @type {RequestHandler}
*/
express: RequestHandler;
/**
* Retrieves an array of human-readable messages that define the specific health constraints that failed when running the last health check.
* @returns {Array<string>} An array of failure messages.
*/
getFailed(): Array<string>;
/**
* Gets a report of all monitors, their fields, and the values of those fields, compiled into Javascript object form. Additionally, a 'healthy' field is
* attached. This field will be boolean true if all health constraints passed; false otherwise.
* @param {ReportOptions} [options] A mapping of options to customize this report.
* @return {Object} The full health report.
*/
getReport(options?: vitalsigns.ReportOptions): {};
/**
* Generates a health report and checks each health constraint against it. Any constraints that fail will be added to the 'failed' array in the form of
* a human-readable failure message, which can be retrieved with {@link #getFailed}.
* @param {Object} [report] A report object on which to run the health constraints. If omitted, this function will generate a health report automatically.
* @return {boolean} true if all health constraints passed; false otherwise.
*/
isHealthy(report?: {}): boolean;
/**
* Registers monitor.
* @param {string} monitorName A monitor name.
* @param {MonitorField} [field] Options.
*/
monitor(monitor: string | vitalsigns.Monitor | {}, field?: vitalsigns.MonitorField): void;
/**
* Defines a new health constraint in a chainable, more easily readable format.
* When called with the monitor name and field name of concern, a wrapper is
* returned that allows the constraint to be built out with function calls.
* @param {string} monitorName A monitor name.
* @param {string} fieldName A field name.
* @return {ConstraintWrapper} The constraint wrapper.
*/
unhealthyWhen: (monitorName: string, fieldName: string) => vitalsigns.ConstraintWrapper;
}
export = VitalSigns;
}
| types/vitalsigns/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017666239000391215,
0.00017275952268391848,
0.00016798748401924968,
0.00017325363296549767,
0.0000024985304207802983
] |
{
"id": 1,
"code_window": [
"archiver.directory('./', \"\", {});\n",
"archiver.directory('./', { name: 'test' }, {});\n",
"\n",
"archiver.append(readStream, {\n",
" name: \"sub/folder.xml\"\n",
"});\n",
"\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"archiver.directory('./', false, { name: 'test' });\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => {\n",
" entry.name = \"foobar\";\n",
" return entry;\n",
"});\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => false);\n"
],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 39
} | import * as React from 'react';
import { IconBaseProps } from 'react-icon-base';
export default class TiCameraOutline extends React.Component<IconBaseProps> { }
| types/react-icons/lib/ti/camera-outline.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017621928418520838,
0.00017621928418520838,
0.00017621928418520838,
0.00017621928418520838,
0
] |
{
"id": 1,
"code_window": [
"archiver.directory('./', \"\", {});\n",
"archiver.directory('./', { name: 'test' }, {});\n",
"\n",
"archiver.append(readStream, {\n",
" name: \"sub/folder.xml\"\n",
"});\n",
"\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"archiver.directory('./', false, { name: 'test' });\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => {\n",
" entry.name = \"foobar\";\n",
" return entry;\n",
"});\n",
"archiver.directory('./', false, (entry: Archiver.EntryData) => false);\n"
],
"file_path": "types/archiver/archiver-tests.ts",
"type": "replace",
"edit_start_line_idx": 39
} | {
"compilerOptions": {
"module": "commonjs",
"lib": [
"es6"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": false,
"strictFunctionTypes": true,
"baseUrl": "../",
"typeRoots": [
"../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
},
"files": [
"index.d.ts",
"rss-tests.ts"
]
} | types/rss/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017677007417660207,
0.00017434816982131451,
0.0001728524948703125,
0.00017342189676128328,
0.000001728259917399555
] |
{
"id": 2,
"code_window": [
" prefix?: string;\n",
" stats?: string;\n",
" }\n",
"\n",
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** A function that lets you either opt out of including an entry (by returning false), or modify the contents of an entry as it is added (by returning an EntryData) */\n",
" type EntryDataFunction = (entry: EntryData) => false | EntryData;\n",
"\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "add",
"edit_start_line_idx": 23
} | // Type definitions for archiver 2.0
// Project: https://github.com/archiverjs/node-archiver
// Definitions by: Esri <https://github.com/archiverjs/node-archiver>, Dolan Miu <https://github.com/dolanmiu>, Crevil <https://github.com/crevil>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
import * as stream from 'stream';
import * as glob from 'glob';
import { ZlibOptions } from 'zlib';
declare function archiver(format: archiver.Format, options?: archiver.ArchiverOptions): archiver.Archiver;
declare namespace archiver {
type Format = 'zip' | 'tar';
function create(format: string, options?: ArchiverOptions): Archiver;
function registerFormat(format: string, module: Function): void;
interface EntryData {
name?: string;
prefix?: string;
stats?: string;
}
interface Archiver extends stream.Transform {
abort(): this;
append(source: stream.Readable | Buffer | string, name?: EntryData): this;
directory(dirpath: string, options: EntryData | string, data?: EntryData): this;
file(filename: string, data: EntryData): this;
glob(pattern: string, options?: glob.IOptions, data?: EntryData): this;
finalize(): Promise<void>;
setFormat(format: string): this;
setModule(module: Function): this;
pointer(): number;
use(plugin: Function): this;
symlink(filepath: string, target: string): this;
}
type ArchiverOptions = CoreOptions & TransformOptions & ZipOptions & TarOptions;
interface CoreOptions {
statConcurrency?: number;
}
interface TransformOptions {
allowHalfOpen?: boolean;
readableObjectMode?: boolean;
writeableObjectMode?: boolean;
decodeStrings?: boolean;
encoding?: string;
highWaterMark?: number;
objectmode?: boolean;
}
interface ZipOptions {
comment?: string;
forceLocalTime?: boolean;
forceZip64?: boolean;
store?: boolean;
zlib?: ZlibOptions;
}
interface TarOptions {
gzip?: boolean;
gzipOptions?: ZlibOptions;
}
}
export = archiver;
| types/archiver/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.9992192983627319,
0.5458256006240845,
0.0001692668884061277,
0.6862988471984863,
0.4572032690048218
] |
{
"id": 2,
"code_window": [
" prefix?: string;\n",
" stats?: string;\n",
" }\n",
"\n",
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** A function that lets you either opt out of including an entry (by returning false), or modify the contents of an entry as it is added (by returning an EntryData) */\n",
" type EntryDataFunction = (entry: EntryData) => false | EntryData;\n",
"\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "add",
"edit_start_line_idx": 23
} | {
"compilerOptions": {
"module": "commonjs",
"lib": [
"es6",
"dom"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": false,
"strictFunctionTypes": true,
"baseUrl": "../",
"typeRoots": [
"../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
},
"files": [
"index.d.ts",
"meteor-jboulhous-dev-tests.ts"
]
} | types/meteor-jboulhous-dev/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017626446788199246,
0.00017525919247418642,
0.00017461940296925604,
0.00017489369201939553,
7.196063620540372e-7
] |
{
"id": 2,
"code_window": [
" prefix?: string;\n",
" stats?: string;\n",
" }\n",
"\n",
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** A function that lets you either opt out of including an entry (by returning false), or modify the contents of an entry as it is added (by returning an EntryData) */\n",
" type EntryDataFunction = (entry: EntryData) => false | EntryData;\n",
"\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "add",
"edit_start_line_idx": 23
} | {
"compilerOptions": {
"module": "commonjs",
"lib": [
"es6",
"dom"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": false,
"strictFunctionTypes": true,
"baseUrl": "../",
"typeRoots": [
"../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
},
"files": [
"index.d.ts",
"cordova-plugin-qrscanner-tests.ts"
]
} | types/cordova-plugin-qrscanner/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017567822942510247,
0.00017506377480458468,
0.00017461940296925604,
0.00017489369201939553,
4.4868286863675166e-7
] |
{
"id": 2,
"code_window": [
" prefix?: string;\n",
" stats?: string;\n",
" }\n",
"\n",
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** A function that lets you either opt out of including an entry (by returning false), or modify the contents of an entry as it is added (by returning an EntryData) */\n",
" type EntryDataFunction = (entry: EntryData) => false | EntryData;\n",
"\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "add",
"edit_start_line_idx": 23
} | import * as React from 'react';
import { IconBaseProps } from 'react-icon-base';
export default class IoPaperclip extends React.Component<IconBaseProps> { }
| types/react-icons/lib/io/paperclip.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017979624681174755,
0.00017979624681174755,
0.00017979624681174755,
0.00017979624681174755,
0
] |
{
"id": 3,
"code_window": [
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n",
" directory(dirpath: string, options: EntryData | string, data?: EntryData): this;\n",
"\n",
" file(filename: string, data: EntryData): this;\n",
" glob(pattern: string, options?: glob.IOptions, data?: EntryData): this;\n",
" finalize(): Promise<void>;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** if false is passed for destpath, the path of a chunk of data in the archive is set to the root */\n",
" directory(dirpath: string, destpath: false | string, data?: EntryData | EntryDataFunction): this;\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "replace",
"edit_start_line_idx": 27
} | // Type definitions for archiver 2.0
// Project: https://github.com/archiverjs/node-archiver
// Definitions by: Esri <https://github.com/archiverjs/node-archiver>, Dolan Miu <https://github.com/dolanmiu>, Crevil <https://github.com/crevil>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
import * as stream from 'stream';
import * as glob from 'glob';
import { ZlibOptions } from 'zlib';
declare function archiver(format: archiver.Format, options?: archiver.ArchiverOptions): archiver.Archiver;
declare namespace archiver {
type Format = 'zip' | 'tar';
function create(format: string, options?: ArchiverOptions): Archiver;
function registerFormat(format: string, module: Function): void;
interface EntryData {
name?: string;
prefix?: string;
stats?: string;
}
interface Archiver extends stream.Transform {
abort(): this;
append(source: stream.Readable | Buffer | string, name?: EntryData): this;
directory(dirpath: string, options: EntryData | string, data?: EntryData): this;
file(filename: string, data: EntryData): this;
glob(pattern: string, options?: glob.IOptions, data?: EntryData): this;
finalize(): Promise<void>;
setFormat(format: string): this;
setModule(module: Function): this;
pointer(): number;
use(plugin: Function): this;
symlink(filepath: string, target: string): this;
}
type ArchiverOptions = CoreOptions & TransformOptions & ZipOptions & TarOptions;
interface CoreOptions {
statConcurrency?: number;
}
interface TransformOptions {
allowHalfOpen?: boolean;
readableObjectMode?: boolean;
writeableObjectMode?: boolean;
decodeStrings?: boolean;
encoding?: string;
highWaterMark?: number;
objectmode?: boolean;
}
interface ZipOptions {
comment?: string;
forceLocalTime?: boolean;
forceZip64?: boolean;
store?: boolean;
zlib?: ZlibOptions;
}
interface TarOptions {
gzip?: boolean;
gzipOptions?: ZlibOptions;
}
}
export = archiver;
| types/archiver/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.9985077977180481,
0.4501767158508301,
0.0001676621614024043,
0.32271459698677063,
0.4382161796092987
] |
{
"id": 3,
"code_window": [
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n",
" directory(dirpath: string, options: EntryData | string, data?: EntryData): this;\n",
"\n",
" file(filename: string, data: EntryData): this;\n",
" glob(pattern: string, options?: glob.IOptions, data?: EntryData): this;\n",
" finalize(): Promise<void>;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** if false is passed for destpath, the path of a chunk of data in the archive is set to the root */\n",
" directory(dirpath: string, destpath: false | string, data?: EntryData | EntryDataFunction): this;\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "replace",
"edit_start_line_idx": 27
} | import { take } from "lodash";
export default take;
| types/lodash-es/take.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017547815514262766,
0.00017547815514262766,
0.00017547815514262766,
0.00017547815514262766,
0
] |
{
"id": 3,
"code_window": [
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n",
" directory(dirpath: string, options: EntryData | string, data?: EntryData): this;\n",
"\n",
" file(filename: string, data: EntryData): this;\n",
" glob(pattern: string, options?: glob.IOptions, data?: EntryData): this;\n",
" finalize(): Promise<void>;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** if false is passed for destpath, the path of a chunk of data in the archive is set to the root */\n",
" directory(dirpath: string, destpath: false | string, data?: EntryData | EntryDataFunction): this;\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "replace",
"edit_start_line_idx": 27
} | /**
* Created by stefansteinhart on 30.01.15.
*/
import mdns = require('mdns')
var ad:mdns.Advertisement = mdns.createAdvertisement(mdns.tcp('http'), 4321);
ad.start();
var browser = mdns.createBrowser(mdns.tcp('http'));
browser.on('serviceUp', function(service:mdns.Service) {
console.log("service up: ", service);
});
browser.on('serviceDown', function(service:mdns.Service) {
console.log("service down: ", service);
});
browser.start();
var r0 = mdns.tcp('http') // string form: _http._tcp
, r1 = mdns.udp('osc', 'api-v1') // string form: _osc._udp,_api-v1
, r2 = new mdns.ServiceType('http', 'tcp') // string form: _http._tcp
, r3 = mdns.makeServiceType('https', 'tcp') // string form: _https._tcp
;
var txt_record = {
name: 'bacon'
, chunky: true
, strips: 5
};
var ad:mdns.Advertisement = mdns.createAdvertisement(mdns.tcp('http'), 4321, {txtRecord: txt_record});
var sequence = [
mdns.rst.DNSServiceResolve()
, mdns.rst.DNSServiceGetAddrInfo({families: [4] })
];
var browser = mdns.createBrowser(mdns.tcp('http'), {resolverSequence: sequence});
interface HammerTimeService extends mdns.Service {
hammerTime:Date;
}
function MCHammer(options:any) {
options = options || {};
return function MCHammer(service:HammerTimeService, next:()=>void) {
console.log('STOP!');
setTimeout(function() {
console.log('hammertime...');
service.hammerTime = new Date();
next();
}, options.delay || 1000);
}
}
var browser = mdns.createBrowser( mdns.tcp('http')
, { networkInterface: mdns.loopbackInterface()});
var ad:mdns.Advertisement;
function createAdvertisement() {
try {
ad = mdns.createAdvertisement(mdns.tcp('http'), 1337);
ad.on('error', handleError);
ad.start();
} catch (ex) {
handleError(ex);
}
}
function handleError(error:mdns.DnsSdError) {
switch (error.errorCode) {
case mdns.kDNSServiceErr_Unknown:
console.warn(error);
setTimeout(createAdvertisement, 5000);
break;
default:
throw error;
}
} | types/mdns/mdns-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00017745184595696628,
0.0001741787273203954,
0.00017089591710828245,
0.0001743086613714695,
0.000002029357119681663
] |
{
"id": 3,
"code_window": [
" interface Archiver extends stream.Transform {\n",
" abort(): this;\n",
" append(source: stream.Readable | Buffer | string, name?: EntryData): this;\n",
"\n",
" directory(dirpath: string, options: EntryData | string, data?: EntryData): this;\n",
"\n",
" file(filename: string, data: EntryData): this;\n",
" glob(pattern: string, options?: glob.IOptions, data?: EntryData): this;\n",
" finalize(): Promise<void>;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /** if false is passed for destpath, the path of a chunk of data in the archive is set to the root */\n",
" directory(dirpath: string, destpath: false | string, data?: EntryData | EntryDataFunction): this;\n"
],
"file_path": "types/archiver/index.d.ts",
"type": "replace",
"edit_start_line_idx": 27
} | import * as React from 'react';
import { IconBaseProps } from 'react-icon-base';
export default class GoFileDirectory extends React.Component<IconBaseProps> { }
| types/react-icons/lib/go/file-directory.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/71bfd778611b63df5a9315b924bacde41ca19e16 | [
0.00016922927170526236,
0.00016922927170526236,
0.00016922927170526236,
0.00016922927170526236,
0
] |
{
"id": 0,
"code_window": [
"\n",
" if (filterStack.length === 1)\n",
" {\n",
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.renderTexture;\n",
" }\n",
"\n",
" filterStack.push(state);\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.current;\n"
],
"file_path": "packages/core/src/filters/FilterSystem.js",
"type": "replace",
"edit_start_line_idx": 205
} | import System from '../System';
import { Rectangle } from '@pixi/math';
const tempRect = new Rectangle();
/**
* System plugin to the renderer to manage render textures.
*
* @class
* @extends PIXI.System
* @memberof PIXI.systems
*/
export default class RenderTextureSystem extends System
{
/**
* @param {PIXI.Renderer} renderer - The renderer this System works for.
*/
constructor(renderer)
{
super(renderer);
/**
* The clear background color as rgba
* @member {number[]}
*/
this.clearColor = renderer._backgroundColorRgba;
// TODO move this property somewhere else!
/**
* List of masks for the StencilSystem
* @member {PIXI.Graphics[]}
* @readonly
*/
this.defaultMaskStack = [];
// empty render texture?
/**
* Render texture
* @member {PIXI.RenderTexture}
* @readonly
*/
this.renderTexture = null;
/**
* Source frame
* @member {PIXI.Rectangle}
* @readonly
*/
this.sourceFrame = new Rectangle();
/**
* Destination frame
* @member {PIXI.Rectangle}
* @readonly
*/
this.destinationFrame = new Rectangle();
}
/**
* Bind the current render texture
* @param {PIXI.RenderTexture} renderTexture
* @param {PIXI.Rectangle} sourceFrame
* @param {PIXI.Rectangle} destinationFrame
*/
bind(renderTexture, sourceFrame, destinationFrame)
{
// TODO - do we want this??
if (this.renderTexture === renderTexture) return;
this.renderTexture = renderTexture;
const renderer = this.renderer;
let resolution;
if (renderTexture)
{
const baseTexture = renderTexture.baseTexture;
resolution = baseTexture.resolution;
if (!destinationFrame)
{
tempRect.width = baseTexture.realWidth;
tempRect.height = baseTexture.realHeight;
destinationFrame = tempRect;
}
if (!sourceFrame)
{
sourceFrame = destinationFrame;
}
this.renderer.framebuffer.bind(baseTexture.framebuffer, destinationFrame);
this.renderer.projection.update(destinationFrame, sourceFrame, resolution, false);
this.renderer.stencil.setMaskStack(baseTexture.stencilMaskStack);
}
else
{
resolution = this.renderer.resolution;
// TODO these validation checks happen deeper down..
// thing they can be avoided..
if (!destinationFrame)
{
tempRect.width = renderer.width;
tempRect.height = renderer.height;
destinationFrame = tempRect;
}
if (!sourceFrame)
{
sourceFrame = destinationFrame;
}
renderer.framebuffer.bind(null, destinationFrame);
// TODO store this..
this.renderer.projection.update(destinationFrame, sourceFrame, resolution, true);
this.renderer.stencil.setMaskStack(this.defaultMaskStack);
}
this.sourceFrame.copyFrom(sourceFrame);
this.destinationFrame.x = destinationFrame.x / resolution;
this.destinationFrame.y = destinationFrame.y / resolution;
this.destinationFrame.width = destinationFrame.width / resolution;
this.destinationFrame.height = destinationFrame.height / resolution;
}
/**
* Erases the render texture and fills the drawing area with a colour
*
* @param {number[]} [clearColor] - The color as rgba, default to use the renderer backgroundColor
* @return {PIXI.Renderer} Returns itself.
*/
clear(clearColor)
{
if (this.renderTexture)
{
clearColor = clearColor || this.renderTexture.baseTexture.clearColor;
}
else
{
clearColor = clearColor || this.clearColor;
}
this.renderer.framebuffer.clear(clearColor[0], clearColor[1], clearColor[2], clearColor[3]);
}
resize()// screenWidth, screenHeight)
{
// resize the root only!
this.bind(null);
}
}
| packages/core/src/renderTexture/RenderTextureSystem.js | 1 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.005040344316512346,
0.0006755004869773984,
0.0001651995989959687,
0.00019651796901598573,
0.0012206960236653686
] |
{
"id": 0,
"code_window": [
"\n",
" if (filterStack.length === 1)\n",
" {\n",
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.renderTexture;\n",
" }\n",
"\n",
" filterStack.push(state);\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.current;\n"
],
"file_path": "packages/core/src/filters/FilterSystem.js",
"type": "replace",
"edit_start_line_idx": 205
} | PixiJS — The HTML5 Creation Engine
=============
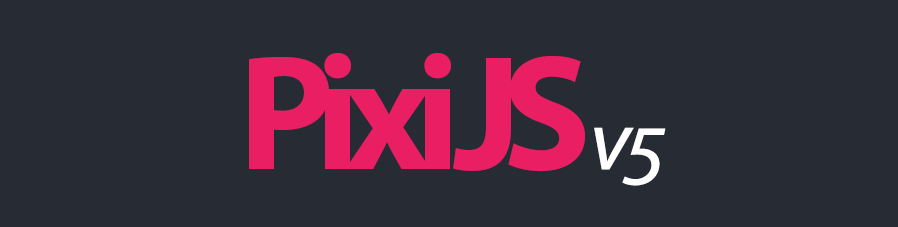
The aim of this project is to provide a fast lightweight 2D library that works
across all devices. The PixiJS renderer allows everyone to enjoy the power of
hardware acceleration without prior knowledge of WebGL. Also, it's fast. Really fast.
**Your support helps us make PixiJS even better. Make your pledge on [Patreon](https://www.patreon.com/user?u=2384552&ty=h&u=2384552) and we'll love you forever!**
This package is the same as **pixi.js**, but provides fallback support for browsers that do not support WebGL or more modern JavaScript features.
### Setup
PixiJS can be installed with [npm](https://docs.npmjs.com/getting-started/what-is-npm) to integration with [Webpack](https://webpack.js.org/), [Browserify](http://browserify.org/), [Rollup](https://rollupjs.org/), [Electron](https://electron.atom.io/), [NW.js](https://nwjs.io/) or other module backed environments.
#### Install
```
npm install pixi.js-legacy
```
There is no default export. The correct way to import PixiJS is:
```js
import * as PIXI from 'pixi.js-legacy'
```
### Basic Usage Example
```js
// The application will create a renderer using WebGL, if possible,
// with a fallback to a canvas render. It will also setup the ticker
// and the root stage PIXI.Container.
const app = new PIXI.Application();
// The application will create a canvas element for you that you
// can then insert into the DOM.
document.body.appendChild(app.view);
// load the texture we need
PIXI.loader.add('bunny', 'bunny.png').load((loader, resources) => {
// This creates a texture from a 'bunny.png' image.
const bunny = new PIXI.Sprite(resources.bunny.texture);
// Setup the position of the bunny
bunny.x = app.renderer.width / 2;
bunny.y = app.renderer.height / 2;
// Rotate around the center
bunny.anchor.x = 0.5;
bunny.anchor.y = 0.5;
// Add the bunny to the scene we are building.
app.stage.addChild(bunny);
// Listen for frame updates
app.ticker.add(() => {
// each frame we spin the bunny around a bit
bunny.rotation += 0.01;
});
});
```
### License
This content is released under the (http://opensource.org/licenses/MIT) MIT License.
[](https://github.com/igrigorik/ga-beacon)
| bundles/pixi.js-legacy/README.md | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017466738063376397,
0.00016764846805017442,
0.00016131367010530084,
0.0001660153502598405,
0.000005608310402749339
] |
{
"id": 0,
"code_window": [
"\n",
" if (filterStack.length === 1)\n",
" {\n",
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.renderTexture;\n",
" }\n",
"\n",
" filterStack.push(state);\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.current;\n"
],
"file_path": "packages/core/src/filters/FilterSystem.js",
"type": "replace",
"edit_start_line_idx": 205
} | {
"name": "@pixi/sprite-animated",
"version": "5.0.0-rc",
"main": "lib/sprite-animated.js",
"module": "lib/sprite-animated.es.js",
"description": "Sprite Animations as depicted by playing a series of Textures",
"author": "Mat Groves",
"contributors": [
"Matt Karl <[email protected]>"
],
"homepage": "http://pixijs.com/",
"bugs": "https://github.com/pixijs/pixi.js/issues",
"license": "MIT",
"repository": {
"type": "git",
"url": "https://github.com/pixijs/pixi.js.git"
},
"publishConfig": {
"access": "public"
},
"scripts": {
"test": "floss --path test"
},
"files": [
"lib"
],
"dependencies": {
"@pixi/core": "^5.0.0-rc",
"@pixi/sprite": "^5.0.0-rc",
"@pixi/ticker": "^5.0.0-rc"
},
"devDependencies": {
"floss": "^2.1.5"
}
}
| packages/sprite-animated/package.json | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017648930952418596,
0.00017474431660957634,
0.00017343445506412536,
0.00017452676547691226,
0.0000013121215260980534
] |
{
"id": 0,
"code_window": [
"\n",
" if (filterStack.length === 1)\n",
" {\n",
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.renderTexture;\n",
" }\n",
"\n",
" filterStack.push(state);\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.defaultFilterStack[0].renderTexture = renderer.renderTexture.current;\n"
],
"file_path": "packages/core/src/filters/FilterSystem.js",
"type": "replace",
"edit_start_line_idx": 205
} | import { Resource } from 'resource-loader';
/**
* Reference to **{@link https://github.com/englercj/resource-loader
* resource-loader}**'s Resource class.
* @see http://englercj.github.io/resource-loader/Resource.html
* @class LoaderResource
* @memberof PIXI
*/
export const LoaderResource = Resource;
export { default as Loader } from './Loader';
export { default as TextureLoader } from './TextureLoader';
export { default as AppLoaderPlugin } from './AppLoaderPlugin';
| packages/loaders/src/index.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017043750267475843,
0.00016832415712997317,
0.0001662108115851879,
0.00016832415712997317,
0.000002113345544785261
] |
{
"id": 1,
"code_window": [
" * Render texture\n",
" * @member {PIXI.RenderTexture}\n",
" * @readonly\n",
" */\n",
" this.renderTexture = null;\n",
"\n",
" /**\n",
" * Source frame\n",
" * @member {PIXI.Rectangle}\n",
" * @readonly\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.current = null;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 42
} | import System from '../System';
import { Rectangle } from '@pixi/math';
const tempRect = new Rectangle();
/**
* System plugin to the renderer to manage render textures.
*
* @class
* @extends PIXI.System
* @memberof PIXI.systems
*/
export default class RenderTextureSystem extends System
{
/**
* @param {PIXI.Renderer} renderer - The renderer this System works for.
*/
constructor(renderer)
{
super(renderer);
/**
* The clear background color as rgba
* @member {number[]}
*/
this.clearColor = renderer._backgroundColorRgba;
// TODO move this property somewhere else!
/**
* List of masks for the StencilSystem
* @member {PIXI.Graphics[]}
* @readonly
*/
this.defaultMaskStack = [];
// empty render texture?
/**
* Render texture
* @member {PIXI.RenderTexture}
* @readonly
*/
this.renderTexture = null;
/**
* Source frame
* @member {PIXI.Rectangle}
* @readonly
*/
this.sourceFrame = new Rectangle();
/**
* Destination frame
* @member {PIXI.Rectangle}
* @readonly
*/
this.destinationFrame = new Rectangle();
}
/**
* Bind the current render texture
* @param {PIXI.RenderTexture} renderTexture
* @param {PIXI.Rectangle} sourceFrame
* @param {PIXI.Rectangle} destinationFrame
*/
bind(renderTexture, sourceFrame, destinationFrame)
{
// TODO - do we want this??
if (this.renderTexture === renderTexture) return;
this.renderTexture = renderTexture;
const renderer = this.renderer;
let resolution;
if (renderTexture)
{
const baseTexture = renderTexture.baseTexture;
resolution = baseTexture.resolution;
if (!destinationFrame)
{
tempRect.width = baseTexture.realWidth;
tempRect.height = baseTexture.realHeight;
destinationFrame = tempRect;
}
if (!sourceFrame)
{
sourceFrame = destinationFrame;
}
this.renderer.framebuffer.bind(baseTexture.framebuffer, destinationFrame);
this.renderer.projection.update(destinationFrame, sourceFrame, resolution, false);
this.renderer.stencil.setMaskStack(baseTexture.stencilMaskStack);
}
else
{
resolution = this.renderer.resolution;
// TODO these validation checks happen deeper down..
// thing they can be avoided..
if (!destinationFrame)
{
tempRect.width = renderer.width;
tempRect.height = renderer.height;
destinationFrame = tempRect;
}
if (!sourceFrame)
{
sourceFrame = destinationFrame;
}
renderer.framebuffer.bind(null, destinationFrame);
// TODO store this..
this.renderer.projection.update(destinationFrame, sourceFrame, resolution, true);
this.renderer.stencil.setMaskStack(this.defaultMaskStack);
}
this.sourceFrame.copyFrom(sourceFrame);
this.destinationFrame.x = destinationFrame.x / resolution;
this.destinationFrame.y = destinationFrame.y / resolution;
this.destinationFrame.width = destinationFrame.width / resolution;
this.destinationFrame.height = destinationFrame.height / resolution;
}
/**
* Erases the render texture and fills the drawing area with a colour
*
* @param {number[]} [clearColor] - The color as rgba, default to use the renderer backgroundColor
* @return {PIXI.Renderer} Returns itself.
*/
clear(clearColor)
{
if (this.renderTexture)
{
clearColor = clearColor || this.renderTexture.baseTexture.clearColor;
}
else
{
clearColor = clearColor || this.clearColor;
}
this.renderer.framebuffer.clear(clearColor[0], clearColor[1], clearColor[2], clearColor[3]);
}
resize()// screenWidth, screenHeight)
{
// resize the root only!
this.bind(null);
}
}
| packages/core/src/renderTexture/RenderTextureSystem.js | 1 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.9854076504707336,
0.0583064928650856,
0.00016583246178925037,
0.00019862687622662634,
0.23177550733089447
] |
{
"id": 1,
"code_window": [
" * Render texture\n",
" * @member {PIXI.RenderTexture}\n",
" * @readonly\n",
" */\n",
" this.renderTexture = null;\n",
"\n",
" /**\n",
" * Source frame\n",
" * @member {PIXI.Rectangle}\n",
" * @readonly\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.current = null;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 42
} | {
"name": "@pixi/filter-displacement",
"version": "5.0.0-rc",
"main": "lib/filter-displacement.js",
"module": "lib/filter-displacement.es.js",
"description": "Filter that allows offsetting of pixel values to create warping effects",
"author": "Mat Groves",
"contributors": [
"Matt Karl <[email protected]>"
],
"homepage": "http://pixijs.com/",
"bugs": "https://github.com/pixijs/pixi.js/issues",
"license": "MIT",
"repository": {
"type": "git",
"url": "https://github.com/pixijs/pixi.js.git"
},
"publishConfig": {
"access": "public"
},
"files": [
"lib"
],
"dependencies": {
"@pixi/core": "^5.0.0-rc",
"@pixi/math": "^5.0.0-rc"
},
"devDependencies": {
"floss": "^2.1.5"
}
}
| packages/filters/filter-displacement/package.json | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017629069043323398,
0.0001742718304740265,
0.0001717833656584844,
0.00017450661107432097,
0.0000016664151871736976
] |
{
"id": 1,
"code_window": [
" * Render texture\n",
" * @member {PIXI.RenderTexture}\n",
" * @readonly\n",
" */\n",
" this.renderTexture = null;\n",
"\n",
" /**\n",
" * Source frame\n",
" * @member {PIXI.Rectangle}\n",
" * @readonly\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.current = null;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 42
} | export { default as AlphaFilter } from './AlphaFilter';
| packages/filters/filter-alpha/src/index.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00016653427155688405,
0.00016653427155688405,
0.00016653427155688405,
0.00016653427155688405,
0
] |
{
"id": 1,
"code_window": [
" * Render texture\n",
" * @member {PIXI.RenderTexture}\n",
" * @readonly\n",
" */\n",
" this.renderTexture = null;\n",
"\n",
" /**\n",
" * Source frame\n",
" * @member {PIXI.Rectangle}\n",
" * @readonly\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" this.current = null;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 42
} | import { LoaderResource } from '@pixi/loaders';
import BitmapText from './BitmapText';
/**
* {@link PIXI.Loader Loader} middleware for loading
* bitmap-based fonts suitable for using with {@link PIXI.BitmapText}.
* @class
* @memberof PIXI
* @implements PIXI.ILoaderPlugin
*/
export default class BitmapFontLoader
{
/**
* Register a BitmapText font from loader resource.
*
* @param {PIXI.LoaderResource} resource - Loader resource.
* @param {PIXI.Texture} texture - Reference to texture.
*/
static parse(resource, texture)
{
resource.bitmapFont = BitmapText.registerFont(resource.data, texture);
}
/**
* Called when the plugin is installed.
*
* @see PIXI.Loader.registerPlugin
*/
static add()
{
LoaderResource.setExtensionXhrType('fnt', LoaderResource.XHR_RESPONSE_TYPE.DOCUMENT);
}
/**
* Replacement for NodeJS's path.dirname
* @private
* @param {string} url Path to get directory for
*/
static dirname(url)
{
const dir = url
.replace(/\/$/, '') // replace trailing slash
.replace(/\/[^\/]*$/, ''); // remove everything after the last
// File request is relative, use current directory
if (dir === url)
{
return '.';
}
// Started with a slash
else if (dir === '')
{
return '/';
}
return dir;
}
/**
* Called after a resource is loaded.
* @see PIXI.Loader.loaderMiddleware
* @param {PIXI.LoaderResource} resource
* @param {function} next
*/
static use(resource, next)
{
// skip if no data or not xml data
if (!resource.data || resource.type !== LoaderResource.TYPE.XML)
{
next();
return;
}
// skip if not bitmap font data, using some silly duck-typing
if (resource.data.getElementsByTagName('page').length === 0
|| resource.data.getElementsByTagName('info').length === 0
|| resource.data.getElementsByTagName('info')[0].getAttribute('face') === null
)
{
next();
return;
}
let xmlUrl = !resource.isDataUrl ? BitmapFontLoader.dirname(resource.url) : '';
if (resource.isDataUrl)
{
if (xmlUrl === '.')
{
xmlUrl = '';
}
if (this.baseUrl && xmlUrl)
{
// if baseurl has a trailing slash then add one to xmlUrl so the replace works below
if (this.baseUrl.charAt(this.baseUrl.length - 1) === '/')
{
xmlUrl += '/';
}
}
}
// remove baseUrl from xmlUrl
xmlUrl = xmlUrl.replace(this.baseUrl, '');
// if there is an xmlUrl now, it needs a trailing slash. Ensure that it does if the string isn't empty.
if (xmlUrl && xmlUrl.charAt(xmlUrl.length - 1) !== '/')
{
xmlUrl += '/';
}
const pages = resource.data.getElementsByTagName('page');
const textures = {};
// Handle completed, when the number of textures
// load is the same number as references in the fnt file
const completed = (page) =>
{
textures[page.metadata.pageFile] = page.texture;
if (Object.keys(textures).length === pages.length)
{
BitmapFontLoader.parse(resource, textures);
next();
}
};
for (let i = 0; i < pages.length; ++i)
{
const pageFile = pages[i].getAttribute('file');
const url = xmlUrl + pageFile;
let exists = false;
// incase the image is loaded outside
// using the same loader, resource will be available
for (const name in this.resources)
{
const bitmapResource = this.resources[name];
if (bitmapResource.url === url)
{
bitmapResource.metadata.pageFile = pageFile;
if (bitmapResource.texture)
{
completed(bitmapResource);
}
else
{
bitmapResource.onAfterMiddleware.add(completed);
}
exists = true;
break;
}
}
// texture is not loaded, we'll attempt to add
// it to the load and add the texture to the list
if (!exists)
{
// Standard loading options for images
const options = {
crossOrigin: resource.crossOrigin,
loadType: LoaderResource.LOAD_TYPE.IMAGE,
metadata: Object.assign(
{ pageFile },
resource.metadata.imageMetadata
),
parentResource: resource,
};
this.add(url, options, completed);
}
}
}
}
| packages/text-bitmap/src/BitmapFontLoader.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.0001732063974486664,
0.00016911508282646537,
0.0001658749970374629,
0.00016945404058787972,
0.0000019264675756858196
] |
{
"id": 2,
"code_window": [
" * @param {PIXI.Rectangle} destinationFrame\n",
" */\n",
" bind(renderTexture, sourceFrame, destinationFrame)\n",
" {\n",
" // TODO - do we want this??\n",
" if (this.renderTexture === renderTexture) return;\n",
" this.renderTexture = renderTexture;\n",
"\n",
" const renderer = this.renderer;\n",
"\n",
" let resolution;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (this.current === renderTexture) return;\n",
" this.current = renderTexture;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 68
} | import System from '../System';
import { Rectangle } from '@pixi/math';
const tempRect = new Rectangle();
/**
* System plugin to the renderer to manage render textures.
*
* @class
* @extends PIXI.System
* @memberof PIXI.systems
*/
export default class RenderTextureSystem extends System
{
/**
* @param {PIXI.Renderer} renderer - The renderer this System works for.
*/
constructor(renderer)
{
super(renderer);
/**
* The clear background color as rgba
* @member {number[]}
*/
this.clearColor = renderer._backgroundColorRgba;
// TODO move this property somewhere else!
/**
* List of masks for the StencilSystem
* @member {PIXI.Graphics[]}
* @readonly
*/
this.defaultMaskStack = [];
// empty render texture?
/**
* Render texture
* @member {PIXI.RenderTexture}
* @readonly
*/
this.renderTexture = null;
/**
* Source frame
* @member {PIXI.Rectangle}
* @readonly
*/
this.sourceFrame = new Rectangle();
/**
* Destination frame
* @member {PIXI.Rectangle}
* @readonly
*/
this.destinationFrame = new Rectangle();
}
/**
* Bind the current render texture
* @param {PIXI.RenderTexture} renderTexture
* @param {PIXI.Rectangle} sourceFrame
* @param {PIXI.Rectangle} destinationFrame
*/
bind(renderTexture, sourceFrame, destinationFrame)
{
// TODO - do we want this??
if (this.renderTexture === renderTexture) return;
this.renderTexture = renderTexture;
const renderer = this.renderer;
let resolution;
if (renderTexture)
{
const baseTexture = renderTexture.baseTexture;
resolution = baseTexture.resolution;
if (!destinationFrame)
{
tempRect.width = baseTexture.realWidth;
tempRect.height = baseTexture.realHeight;
destinationFrame = tempRect;
}
if (!sourceFrame)
{
sourceFrame = destinationFrame;
}
this.renderer.framebuffer.bind(baseTexture.framebuffer, destinationFrame);
this.renderer.projection.update(destinationFrame, sourceFrame, resolution, false);
this.renderer.stencil.setMaskStack(baseTexture.stencilMaskStack);
}
else
{
resolution = this.renderer.resolution;
// TODO these validation checks happen deeper down..
// thing they can be avoided..
if (!destinationFrame)
{
tempRect.width = renderer.width;
tempRect.height = renderer.height;
destinationFrame = tempRect;
}
if (!sourceFrame)
{
sourceFrame = destinationFrame;
}
renderer.framebuffer.bind(null, destinationFrame);
// TODO store this..
this.renderer.projection.update(destinationFrame, sourceFrame, resolution, true);
this.renderer.stencil.setMaskStack(this.defaultMaskStack);
}
this.sourceFrame.copyFrom(sourceFrame);
this.destinationFrame.x = destinationFrame.x / resolution;
this.destinationFrame.y = destinationFrame.y / resolution;
this.destinationFrame.width = destinationFrame.width / resolution;
this.destinationFrame.height = destinationFrame.height / resolution;
}
/**
* Erases the render texture and fills the drawing area with a colour
*
* @param {number[]} [clearColor] - The color as rgba, default to use the renderer backgroundColor
* @return {PIXI.Renderer} Returns itself.
*/
clear(clearColor)
{
if (this.renderTexture)
{
clearColor = clearColor || this.renderTexture.baseTexture.clearColor;
}
else
{
clearColor = clearColor || this.clearColor;
}
this.renderer.framebuffer.clear(clearColor[0], clearColor[1], clearColor[2], clearColor[3]);
}
resize()// screenWidth, screenHeight)
{
// resize the root only!
this.bind(null);
}
}
| packages/core/src/renderTexture/RenderTextureSystem.js | 1 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.9986250400543213,
0.5144314765930176,
0.00016437906015198678,
0.7368090748786926,
0.48231247067451477
] |
{
"id": 2,
"code_window": [
" * @param {PIXI.Rectangle} destinationFrame\n",
" */\n",
" bind(renderTexture, sourceFrame, destinationFrame)\n",
" {\n",
" // TODO - do we want this??\n",
" if (this.renderTexture === renderTexture) return;\n",
" this.renderTexture = renderTexture;\n",
"\n",
" const renderer = this.renderer;\n",
"\n",
" let resolution;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (this.current === renderTexture) return;\n",
" this.current = renderTexture;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 68
} | const { BaseTextureCache, TextureCache } = require('@pixi/utils');
const { Rectangle, Point } = require('@pixi/math');
const { BaseTexture, Texture } = require('../');
const URL = 'foo.png';
const NAME = 'foo';
const NAME2 = 'bar';
function cleanCache()
{
delete BaseTextureCache[URL];
delete BaseTextureCache[NAME];
delete BaseTextureCache[NAME2];
delete TextureCache[URL];
delete TextureCache[NAME];
delete TextureCache[NAME2];
}
describe('PIXI.Texture', function ()
{
it('should register Texture from Loader', function ()
{
cleanCache();
const image = new Image();
const texture = Texture.fromLoader(image, URL, NAME);
expect(texture.baseTexture.resource.url).to.equal('foo.png');
expect(TextureCache[NAME]).to.equal(texture);
expect(BaseTextureCache[NAME]).to.equal(texture.baseTexture);
expect(TextureCache[URL]).to.equal(texture);
expect(BaseTextureCache[URL]).to.equal(texture.baseTexture);
});
it('should remove Texture from cache on destroy', function ()
{
cleanCache();
const texture = new Texture(new BaseTexture());
Texture.addToCache(texture, NAME);
Texture.addToCache(texture, NAME2);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(texture.textureCacheIds.indexOf(NAME2)).to.equal(1);
expect(TextureCache[NAME]).to.equal(texture);
expect(TextureCache[NAME2]).to.equal(texture);
texture.destroy();
expect(texture.textureCacheIds).to.equal(null);
expect(TextureCache[NAME]).to.equal(undefined);
expect(TextureCache[NAME2]).to.equal(undefined);
});
it('should be added to the texture cache correctly, '
+ 'and should remove only itself, not effecting the base texture and its cache', function ()
{
cleanCache();
const texture = new Texture(new BaseTexture());
BaseTexture.addToCache(texture.baseTexture, NAME);
Texture.addToCache(texture, NAME);
expect(texture.baseTexture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(BaseTextureCache[NAME]).to.equal(texture.baseTexture);
expect(TextureCache[NAME]).to.equal(texture);
Texture.removeFromCache(NAME);
expect(texture.baseTexture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(-1);
expect(BaseTextureCache[NAME]).to.equal(texture.baseTexture);
expect(TextureCache[NAME]).to.equal(undefined);
});
it('should remove Texture from entire cache using removeFromCache (by Texture instance)', function ()
{
cleanCache();
const texture = new Texture(new BaseTexture());
Texture.addToCache(texture, NAME);
Texture.addToCache(texture, NAME2);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(texture.textureCacheIds.indexOf(NAME2)).to.equal(1);
expect(TextureCache[NAME]).to.equal(texture);
expect(TextureCache[NAME2]).to.equal(texture);
Texture.removeFromCache(texture);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(-1);
expect(texture.textureCacheIds.indexOf(NAME2)).to.equal(-1);
expect(TextureCache[NAME]).to.equal(undefined);
expect(TextureCache[NAME2]).to.equal(undefined);
});
it('should remove Texture from single cache entry using removeFromCache (by id)', function ()
{
cleanCache();
const texture = new Texture(new BaseTexture());
Texture.addToCache(texture, NAME);
Texture.addToCache(texture, NAME2);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(texture.textureCacheIds.indexOf(NAME2)).to.equal(1);
expect(TextureCache[NAME]).to.equal(texture);
expect(TextureCache[NAME2]).to.equal(texture);
Texture.removeFromCache(NAME);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(-1);
expect(texture.textureCacheIds.indexOf(NAME2)).to.equal(0);
expect(TextureCache[NAME]).to.equal(undefined);
expect(TextureCache[NAME2]).to.equal(texture);
});
it('should not remove Texture from cache if Texture instance has been replaced', function ()
{
cleanCache();
const texture = new Texture(new BaseTexture());
const texture2 = new Texture(new BaseTexture());
Texture.addToCache(texture, NAME);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(TextureCache[NAME]).to.equal(texture);
Texture.addToCache(texture2, NAME);
expect(texture2.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(TextureCache[NAME]).to.equal(texture2);
Texture.removeFromCache(texture);
expect(texture.textureCacheIds.indexOf(NAME)).to.equal(-1);
expect(texture2.textureCacheIds.indexOf(NAME)).to.equal(0);
expect(TextureCache[NAME]).to.equal(texture2);
});
it('destroying a destroyed texture should not throw an error', function ()
{
const texture = new Texture(new BaseTexture());
texture.destroy(true);
texture.destroy(true);
});
it('should clone a texture', function ()
{
const baseTexture = new BaseTexture();
const frame = new Rectangle();
const orig = new Rectangle();
const trim = new Rectangle();
const rotate = 2;
const anchor = new Point(1, 0.5);
const texture = new Texture(baseTexture, frame, orig, trim, rotate, anchor);
const clone = texture.clone();
expect(clone.baseTexture).to.equal(baseTexture);
expect(clone.defaultAnchor.x).to.equal(texture.defaultAnchor.x);
expect(clone.defaultAnchor.y).to.equal(texture.defaultAnchor.y);
expect(clone.frame).to.equal(texture.frame);
expect(clone.trim).to.equal(texture.trim);
expect(clone.orig).to.equal(texture.orig);
expect(clone.rotate).to.equal(texture.rotate);
clone.destroy();
texture.destroy(true);
});
});
| packages/core/test/Texture.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.0021743515972048044,
0.00029479325166903436,
0.0001633057981962338,
0.0001716004917398095,
0.0004705663595814258
] |
{
"id": 2,
"code_window": [
" * @param {PIXI.Rectangle} destinationFrame\n",
" */\n",
" bind(renderTexture, sourceFrame, destinationFrame)\n",
" {\n",
" // TODO - do we want this??\n",
" if (this.renderTexture === renderTexture) return;\n",
" this.renderTexture = renderTexture;\n",
"\n",
" const renderer = this.renderer;\n",
"\n",
" let resolution;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (this.current === renderTexture) return;\n",
" this.current = renderTexture;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 68
} | {
"source": {
"include": [
"bundles",
"packages"
],
"excludePattern": "(node_modules|lib|dist|test|deprecated|canvas)"
},
"plugins": [
"@pixi/jsdoc-template/plugins/es6-fix"
],
"opts": {
"recurse": true,
"destination": "./dist/types",
"outFile": "pixi.js.d.ts",
"template": "./node_modules/tsd-jsdoc/dist"
}
}
| types/jsdoc.conf.json | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017303191998507828,
0.00017180673603434116,
0.00017058155208360404,
0.00017180673603434116,
0.0000012251839507371187
] |
{
"id": 2,
"code_window": [
" * @param {PIXI.Rectangle} destinationFrame\n",
" */\n",
" bind(renderTexture, sourceFrame, destinationFrame)\n",
" {\n",
" // TODO - do we want this??\n",
" if (this.renderTexture === renderTexture) return;\n",
" this.renderTexture = renderTexture;\n",
"\n",
" const renderer = this.renderer;\n",
"\n",
" let resolution;\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (this.current === renderTexture) return;\n",
" this.current = renderTexture;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 68
} | import AbstractRenderer from './AbstractRenderer';
import { sayHello } from '@pixi/utils';
import MaskSystem from './mask/MaskSystem';
import StencilSystem from './mask/StencilSystem';
import FilterSystem from './filters/FilterSystem';
import FramebufferSystem from './framebuffer/FramebufferSystem';
import RenderTextureSystem from './renderTexture/RenderTextureSystem';
import TextureSystem from './textures/TextureSystem';
import ProjectionSystem from './projection/ProjectionSystem';
import StateSystem from './state/StateSystem';
import GeometrySystem from './geometry/GeometrySystem';
import ShaderSystem from './shader/ShaderSystem';
import ContextSystem from './context/ContextSystem';
import BatchSystem from './batch/BatchSystem';
import TextureGCSystem from './textures/TextureGCSystem';
import { RENDERER_TYPE } from '@pixi/constants';
import UniformGroup from './shader/UniformGroup';
import { Matrix } from '@pixi/math';
import Runner from 'mini-runner';
/**
* The Renderer draws the scene and all its content onto a WebGL enabled canvas.
*
* This renderer should be used for browsers that support WebGL.
*
* This renderer works by automatically managing WebGLBatchesm, so no need for Sprite Batches or Sprite Clouds.
* Don't forget to add the view to your DOM or you will not see anything!
*
* @class
* @memberof PIXI
* @extends PIXI.AbstractRenderer
*/
export default class Renderer extends AbstractRenderer
{
/**
* @param {object} [options] - The optional renderer parameters.
* @param {number} [options.width=800] - The width of the screen.
* @param {number} [options.height=600] - The height of the screen.
* @param {HTMLCanvasElement} [options.view] - The canvas to use as a view, optional.
* @param {boolean} [options.transparent=false] - If the render view is transparent.
* @param {boolean} [options.autoDensity=false] - Resizes renderer view in CSS pixels to allow for
* resolutions other than 1.
* @param {boolean} [options.antialias=false] - Sets antialias. If not available natively then FXAA
* antialiasing is used.
* @param {boolean} [options.forceFXAA=false] - Forces FXAA antialiasing to be used over native.
* FXAA is faster, but may not always look as great.
* @param {number} [options.resolution=1] - The resolution / device pixel ratio of the renderer.
* The resolution of the renderer retina would be 2.
* @param {boolean} [options.clearBeforeRender=true] - This sets if the renderer will clear
* the canvas or not before the new render pass. If you wish to set this to false, you *must* set
* preserveDrawingBuffer to `true`.
* @param {boolean} [options.preserveDrawingBuffer=false] - Enables drawing buffer preservation,
* enable this if you need to call toDataUrl on the WebGL context.
* @param {number} [options.backgroundColor=0x000000] - The background color of the rendered area
* (shown if not transparent).
* @param {string} [options.powerPreference] - Parameter passed to WebGL context, set to "high-performance"
* for devices with dual graphics card.
* @param {object} [options.context] If WebGL context already exists, all parameters must be taken from it.
*/
constructor(options = {}, arg2, arg3)
{
super('WebGL', options, arg2, arg3);
// the options will have been modified here in the super constructor with pixi's default settings..
options = this.options;
/**
* The type of this renderer as a standardized const
*
* @member {number}
* @see PIXI.RENDERER_TYPE
*/
this.type = RENDERER_TYPE.WEBGL;
// this will be set by the contextSystem (this.context)
this.gl = null;
this.CONTEXT_UID = 0;
// TODO legacy!
/**
* Internal signal instances of **mini-runner**, these
* are assigned to each system created.
* @see https://github.com/GoodBoyDigital/mini-runner
* @name PIXI.Renderer#runners
* @private
* @type {object}
* @readonly
* @property {Runner} destroy - Destroy runner
* @property {Runner} contextChange - Context change runner
* @property {Runner} reset - Reset runner
* @property {Runner} update - Update runner
* @property {Runner} postrender - Post-render runner
* @property {Runner} prerender - Pre-render runner
* @property {Runner} resize - Resize runner
*/
this.runners = {
destroy: new Runner('destroy'),
contextChange: new Runner('contextChange', 1),
reset: new Runner('reset'),
update: new Runner('update'),
postrender: new Runner('postrender'),
prerender: new Runner('prerender'),
resize: new Runner('resize', 2),
};
/**
* Global uniforms
* @member {PIXI.UniformGroup}
*/
this.globalUniforms = new UniformGroup({
projectionMatrix: new Matrix(),
}, true);
/**
* Mask system instance
* @member {PIXI.systems.MaskSystem} mask
* @memberof PIXI.Renderer#
* @readonly
*/
this.addSystem(MaskSystem, 'mask')
/**
* Context system instance
* @member {PIXI.systems.ContextSystem} context
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(ContextSystem, 'context')
/**
* State system instance
* @member {PIXI.systems.StateSystem} state
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(StateSystem, 'state')
/**
* Shader system instance
* @member {PIXI.systems.ShaderSystem} shader
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(ShaderSystem, 'shader')
/**
* Texture system instance
* @member {PIXI.systems.TextureSystem} texture
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(TextureSystem, 'texture')
/**
* Geometry system instance
* @member {PIXI.systems.GeometrySystem} geometry
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(GeometrySystem, 'geometry')
/**
* Framebuffer system instance
* @member {PIXI.systems.FramebufferSystem} framebuffer
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(FramebufferSystem, 'framebuffer')
/**
* Stencil system instance
* @member {PIXI.systems.StencilSystem} stencil
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(StencilSystem, 'stencil')
/**
* Projection system instance
* @member {PIXI.systems.ProjectionSystem} projection
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(ProjectionSystem, 'projection')
/**
* Texture garbage collector system instance
* @member {PIXI.systems.TextureGCSystem} textureGC
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(TextureGCSystem, 'textureGC')
/**
* Filter system instance
* @member {PIXI.systems.FilterSystem} filter
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(FilterSystem, 'filter')
/**
* RenderTexture system instance
* @member {PIXI.systems.RenderTextureSystem} renderTexture
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(RenderTextureSystem, 'renderTexture')
/**
* Batch system instance
* @member {PIXI.systems.BatchSystem} batch
* @memberof PIXI.Renderer#
* @readonly
*/
.addSystem(BatchSystem, 'batch');
this.initPlugins(Renderer.__plugins);
/**
* The options passed in to create a new WebGL context.
*/
if (options.context)
{
this.context.initFromContext(options.context);
}
else
{
this.context.initFromOptions({
alpha: this.transparent,
antialias: options.antialias,
premultipliedAlpha: this.transparent && this.transparent !== 'notMultiplied',
stencil: true,
preserveDrawingBuffer: options.preserveDrawingBuffer,
powerPreference: this.options.powerPreference,
});
}
/**
* Flag if we are rendering to the screen vs renderTexture
* @member {boolean}
* @readonly
* @default true
*/
this.renderingToScreen = true;
sayHello(this.context.webGLVersion === 2 ? 'WebGL 2' : 'WebGL 1');
this.resize(this.options.width, this.options.height);
}
/**
* Add a new system to the renderer.
* @param {Function} ClassRef - Class reference
* @param {string} [name] - Property name for system, if not specified
* will use a static `name` property on the class itself. This
* name will be assigned as s property on the Renderer so make
* sure it doesn't collide with properties on Renderer.
* @return {PIXI.Renderer} Return instance of renderer
*/
addSystem(ClassRef, name)
{
if (!name)
{
name = ClassRef.name;
}
const system = new ClassRef(this);
if (this[name])
{
throw new Error(`Whoops! The name "${name}" is already in use`);
}
this[name] = system;
for (const i in this.runners)
{
this.runners[i].add(system);
}
/**
* Fired after rendering finishes.
*
* @event PIXI.Renderer#postrender
*/
/**
* Fired before rendering starts.
*
* @event PIXI.Renderer#prerender
*/
/**
* Fired when the WebGL context is set.
*
* @event PIXI.Renderer#context
* @param {WebGLRenderingContext} gl - WebGL context.
*/
return this;
}
/**
* Renders the object to its WebGL view
*
* @param {PIXI.DisplayObject} displayObject - The object to be rendered.
* @param {PIXI.RenderTexture} [renderTexture] - The render texture to render to.
* @param {boolean} [clear=true] - Should the canvas be cleared before the new render.
* @param {PIXI.Matrix} [transform] - A transform to apply to the render texture before rendering.
* @param {boolean} [skipUpdateTransform=false] - Should we skip the update transform pass?
*/
render(displayObject, renderTexture, clear, transform, skipUpdateTransform)
{
// can be handy to know!
this.renderingToScreen = !renderTexture;
this.runners.prerender.run();
this.emit('prerender');
// no point rendering if our context has been blown up!
if (this.context.isLost)
{
return;
}
if (!renderTexture)
{
this._lastObjectRendered = displayObject;
}
if (!skipUpdateTransform)
{
// update the scene graph
const cacheParent = displayObject.parent;
displayObject.parent = this._tempDisplayObjectParent;
displayObject.updateTransform();
displayObject.parent = cacheParent;
// displayObject.hitArea = //TODO add a temp hit area
}
this.renderTexture.bind(renderTexture);
this.batch.currentRenderer.start();
if (clear !== undefined ? clear : this.clearBeforeRender)
{
this.renderTexture.clear();
}
displayObject.render(this);
// apply transform..
this.batch.currentRenderer.flush();
if (renderTexture)
{
renderTexture.baseTexture.update();
}
this.runners.postrender.run();
this.emit('postrender');
}
/**
* Resizes the WebGL view to the specified width and height.
*
* @param {number} screenWidth - The new width of the screen.
* @param {number} screenHeight - The new height of the screen.
*/
resize(screenWidth, screenHeight)
{
AbstractRenderer.prototype.resize.call(this, screenWidth, screenHeight);
this.runners.resize.run(screenWidth, screenHeight);
}
/**
* Resets the WebGL state so you can render things however you fancy!
*
* @return {PIXI.Renderer} Returns itself.
*/
reset()
{
this.runners.reset.run();
return this;
}
/**
* Clear the frame buffer
*/
clear()
{
this.framebuffer.bind();
this.framebuffer.clear();
}
/**
* Removes everything from the renderer (event listeners, spritebatch, etc...)
*
* @param {boolean} [removeView=false] - Removes the Canvas element from the DOM.
* See: https://github.com/pixijs/pixi.js/issues/2233
*/
destroy(removeView)
{
this.runners.destroy.run();
// call base destroy
super.destroy(removeView);
// TODO nullify all the managers..
this.gl = null;
}
/**
* Collection of installed plugins. These are included by default in PIXI, but can be excluded
* by creating a custom build. Consult the README for more information about creating custom
* builds and excluding plugins.
* @name PIXI.Renderer#plugins
* @type {object}
* @readonly
* @property {PIXI.accessibility.AccessibilityManager} accessibility Support tabbing interactive elements.
* @property {PIXI.extract.Extract} extract Extract image data from renderer.
* @property {PIXI.interaction.InteractionManager} interaction Handles mouse, touch and pointer events.
* @property {PIXI.prepare.Prepare} prepare Pre-render display objects.
*/
/**
* Adds a plugin to the renderer.
*
* @method
* @param {string} pluginName - The name of the plugin.
* @param {Function} ctor - The constructor function or class for the plugin.
*/
static registerPlugin(pluginName, ctor)
{
Renderer.__plugins = Renderer.__plugins || {};
Renderer.__plugins[pluginName] = ctor;
}
}
| packages/core/src/Renderer.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.8682035803794861,
0.01993854157626629,
0.00016023183707147837,
0.00017010420560836792,
0.12935927510261536
] |
{
"id": 3,
"code_window": [
" */\n",
" clear(clearColor)\n",
" {\n",
" if (this.renderTexture)\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (this.current)\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 142
} | import System from '../System';
import RenderTexture from '../renderTexture/RenderTexture';
import Quad from '../utils/Quad';
import QuadUv from '../utils/QuadUv';
import { Rectangle } from '@pixi/math';
import * as filterTransforms from './filterTransforms';
import { nextPow2 } from '@pixi/utils';
import UniformGroup from '../shader/UniformGroup';
import { DRAW_MODES } from '@pixi/constants';
/**
* System plugin to the renderer to manage filter states.
*
* @class
* @private
*/
class FilterState
{
constructor()
{
this.renderTexture = null;
/**
* Target of the filters
* We store for case when custom filter wants to know the element it was applied on
* @member {PIXI.DisplayObject}
* @private
*/
this.target = null;
/**
* Compatibility with PixiJS v4 filters
* @member {boolean}
* @default false
* @private
*/
this.legacy = false;
/**
* Resolution of filters
* @member {number}
* @default 1
* @private
*/
this.resolution = 1;
// next three fields are created only for root
// re-assigned for everything else
/**
* Source frame
* @member {PIXI.Rectangle}
* @private
*/
this.sourceFrame = new Rectangle();
/**
* Destination frame
* @member {PIXI.Rectangle}
* @private
*/
this.destinationFrame = new Rectangle();
/**
* Collection of filters
* @member {PIXI.Filter[]}
* @private
*/
this.filters = [];
}
/**
* clears the state
* @private
*/
clear()
{
this.target = null;
this.filters = null;
this.renderTexture = null;
}
}
const screenKey = 'screen';
/**
* System plugin to the renderer to manage the filters.
*
* @class
* @memberof PIXI.systems
* @extends PIXI.System
*/
export default class FilterSystem extends System
{
/**
* @param {PIXI.Renderer} renderer - The renderer this System works for.
*/
constructor(renderer)
{
super(renderer);
/**
* List of filters for the FilterSystem
* @member {Object[]}
* @readonly
*/
this.defaultFilterStack = [{}];
/**
* stores a bunch of PO2 textures used for filtering
* @member {Object}
*/
this.texturePool = {};
/**
* a pool for storing filter states, save us creating new ones each tick
* @member {Object[]}
*/
this.statePool = [];
/**
* A very simple geometry used when drawing a filter effect to the screen
* @member {PIXI.Quad}
*/
this.quad = new Quad();
/**
* Quad UVs
* @member {PIXI.QuadUv}
*/
this.quadUv = new QuadUv();
/**
* Temporary rect for maths
* @type {PIXI.Rectangle}
*/
this.tempRect = new Rectangle();
/**
* Active state
* @member {object}
*/
this.activeState = {};
/**
* This uniform group is attached to filter uniforms when used
* @member {PIXI.UniformGroup}
* @property {PIXI.Rectangle} outputFrame
* @property {Float32Array} inputSize
* @property {Float32Array} inputPixel
* @property {Float32Array} inputClamp
* @property {Number} resolution
* @property {Float32Array} filterArea
* @property {Fload32Array} filterClamp
*/
this.globalUniforms = new UniformGroup({
outputFrame: this.tempRect,
inputSize: new Float32Array(4),
inputPixel: new Float32Array(4),
inputClamp: new Float32Array(4),
resolution: 1,
// legacy variables
filterArea: new Float32Array(4),
filterClamp: new Float32Array(4),
}, true);
this._pixelsWidth = renderer.view.width;
this._pixelsHeight = renderer.view.height;
}
/**
* Adds a new filter to the System.
*
* @param {PIXI.DisplayObject} target - The target of the filter to render.
* @param {PIXI.Filter[]} filters - The filters to apply.
*/
push(target, filters)
{
const renderer = this.renderer;
const filterStack = this.defaultFilterStack;
const state = this.statePool.pop() || new FilterState();
let resolution = filters[0].resolution;
let padding = filters[0].padding;
let autoFit = filters[0].autoFit;
let legacy = filters[0].legacy;
for (let i = 1; i < filters.length; i++)
{
const filter = filters[i];
// lets use the lowest resolution..
resolution = Math.min(resolution, filter.resolution);
// and the largest amount of padding!
padding = Math.max(padding, filter.padding);
// only auto fit if all filters are autofit
autoFit = autoFit || filter.autoFit;
legacy = legacy || filter.legacy;
}
if (filterStack.length === 1)
{
this.defaultFilterStack[0].renderTexture = renderer.renderTexture.renderTexture;
}
filterStack.push(state);
state.resolution = resolution;
state.legacy = legacy;
state.target = target;
state.sourceFrame.copyFrom(target.filterArea || target.getBounds(true));
state.sourceFrame.pad(padding);
if (autoFit)
{
state.sourceFrame.fit(this.renderer.renderTexture.sourceFrame);
}
// round to whole number based on resolution
state.sourceFrame.ceil(resolution);
state.renderTexture = this.getOptimalFilterTexture(state.sourceFrame.width, state.sourceFrame.height, resolution);
state.filters = filters;
state.destinationFrame.width = state.renderTexture.width;
state.destinationFrame.height = state.renderTexture.height;
state.renderTexture.filterFrame = state.sourceFrame;
renderer.renderTexture.bind(state.renderTexture, state.sourceFrame);// /, state.destinationFrame);
renderer.renderTexture.clear();
}
/**
* Pops off the filter and applies it.
*
*/
pop()
{
const filterStack = this.defaultFilterStack;
const state = filterStack.pop();
const filters = state.filters;
this.activeState = state;
const globalUniforms = this.globalUniforms.uniforms;
globalUniforms.outputFrame = state.sourceFrame;
globalUniforms.resolution = state.resolution;
const inputSize = globalUniforms.inputSize;
const inputPixel = globalUniforms.inputPixel;
const inputClamp = globalUniforms.inputClamp;
inputSize[0] = state.destinationFrame.width;
inputSize[1] = state.destinationFrame.height;
inputSize[2] = 1.0 / inputSize[0];
inputSize[3] = 1.0 / inputSize[1];
inputPixel[0] = inputSize[0] * state.resolution;
inputPixel[1] = inputSize[1] * state.resolution;
inputPixel[2] = 1.0 / inputPixel[0];
inputPixel[3] = 1.0 / inputPixel[1];
inputClamp[0] = 0.5 * inputPixel[2];
inputClamp[1] = 0.5 * inputPixel[3];
inputClamp[2] = (state.sourceFrame.width * inputSize[2]) - (0.5 * inputPixel[2]);
inputClamp[3] = (state.sourceFrame.height * inputSize[3]) - (0.5 * inputPixel[3]);
// only update the rect if its legacy..
if (state.legacy)
{
const filterArea = globalUniforms.filterArea;
filterArea[0] = state.destinationFrame.width;
filterArea[1] = state.destinationFrame.height;
filterArea[2] = state.sourceFrame.x;
filterArea[3] = state.sourceFrame.y;
globalUniforms.filterClamp = globalUniforms.inputClamp;
}
this.globalUniforms.update();
const lastState = filterStack[filterStack.length - 1];
if (filters.length === 1)
{
filters[0].apply(this, state.renderTexture, lastState.renderTexture, false, state);
this.returnFilterTexture(state.renderTexture);
}
else
{
let flip = state.renderTexture;
let flop = this.getOptimalFilterTexture(
flip.width,
flip.height,
state.resolution
);
flop.filterFrame = flip.filterFrame;
let i = 0;
for (i = 0; i < filters.length - 1; ++i)
{
filters[i].apply(this, flip, flop, true, state);
const t = flip;
flip = flop;
flop = t;
}
filters[i].apply(this, flip, lastState.renderTexture, false, state);
this.returnFilterTexture(flip);
this.returnFilterTexture(flop);
}
state.clear();
this.statePool.push(state);
}
/**
* Draws a filter.
*
* @param {PIXI.Filter} filter - The filter to draw.
* @param {PIXI.RenderTexture} input - The input render target.
* @param {PIXI.RenderTexture} output - The target to output to.
* @param {boolean} clear - Should the output be cleared before rendering to it
*/
applyFilter(filter, input, output, clear)
{
const renderer = this.renderer;
renderer.renderTexture.bind(output, output ? output.filterFrame : null);
if (clear)
{
// gl.disable(gl.SCISSOR_TEST);
renderer.renderTexture.clear();
// gl.enable(gl.SCISSOR_TEST);
}
// set the uniforms..
filter.uniforms.uSampler = input;
filter.uniforms.filterGlobals = this.globalUniforms;
// TODO make it so that the order of this does not matter..
// because it does at the moment cos of global uniforms.
// they need to get resynced
renderer.state.setState(filter.state);
renderer.shader.bind(filter);
if (filter.legacy)
{
this.quadUv.map(input._frame, input.filterFrame);
renderer.geometry.bind(this.quadUv);
renderer.geometry.draw(DRAW_MODES.TRIANGLES);
}
else
{
renderer.geometry.bind(this.quad);
renderer.geometry.draw(DRAW_MODES.TRIANGLE_STRIP);
}
}
/**
* Calculates the mapped matrix.
*
* TODO playing around here.. this is temporary - (will end up in the shader)
* this returns a matrix that will normalize map filter cords in the filter to screen space
*
* @param {PIXI.Matrix} outputMatrix - the matrix to output to.
* @return {PIXI.Matrix} The mapped matrix.
*/
calculateScreenSpaceMatrix(outputMatrix)
{
const currentState = this.activeState;
return filterTransforms.calculateScreenSpaceMatrix(
outputMatrix,
currentState.sourceFrame,
currentState.destinationFrame
);
}
/**
* This will map the filter coord so that a texture can be used based on the transform of a sprite
*
* @param {PIXI.Matrix} outputMatrix - The matrix to output to.
* @param {PIXI.Sprite} sprite - The sprite to map to.
* @return {PIXI.Matrix} The mapped matrix.
*/
calculateSpriteMatrix(outputMatrix, sprite)
{
const currentState = this.activeState;
return filterTransforms.calculateSpriteMatrix(
outputMatrix,
currentState.sourceFrame,
currentState.destinationFrame,
sprite
);
}
/**
* Destroys this Filter System.
*
* @param {boolean} [contextLost=false] context was lost, do not free shaders
*
*/
destroy(contextLost = false)
{
if (!contextLost)
{
this.emptyPool();
}
else
{
this.texturePool = {};
}
}
/**
* Gets a Power-of-Two render texture or fullScreen texture
*
* TODO move to a separate class could be on renderer?
*
* @protected
* @param {number} minWidth - The minimum width of the render texture in real pixels.
* @param {number} minHeight - The minimum height of the render texture in real pixels.
* @param {number} [resolution=1] - The resolution of the render texture.
* @return {PIXI.RenderTexture} The new render texture.
*/
getOptimalFilterTexture(minWidth, minHeight, resolution = 1)
{
let key = screenKey;
minWidth *= resolution;
minHeight *= resolution;
if (minWidth !== this._pixelsWidth || minHeight !== this._pixelsHeight)
{
minWidth = nextPow2(minWidth);
minHeight = nextPow2(minHeight);
key = ((minWidth & 0xFFFF) << 16) | (minHeight & 0xFFFF);
}
if (!this.texturePool[key])
{
this.texturePool[key] = [];
}
let renderTexture = this.texturePool[key].pop();
if (!renderTexture)
{
// temporary bypass cache..
// internally - this will cause a texture to be bound..
renderTexture = RenderTexture.create({
width: minWidth / resolution,
height: minHeight / resolution,
resolution,
});
}
renderTexture.filterPoolKey = key;
return renderTexture;
}
/**
* Gets extra render texture to use inside current filter
*
* @param {number} resolution resolution of the renderTexture
* @returns {PIXI.RenderTexture}
*/
getFilterTexture(resolution)
{
const rt = this.activeState.renderTexture;
const filterTexture = this.getOptimalFilterTexture(rt.width, rt.height, resolution || rt.baseTexture.resolution);
filterTexture.filterFrame = rt.filterFrame;
return filterTexture;
}
/**
* Frees a render texture back into the pool.
*
* @param {PIXI.RenderTexture} renderTexture - The renderTarget to free
*/
returnFilterTexture(renderTexture)
{
const key = renderTexture.filterPoolKey;
renderTexture.filterFrame = null;
this.texturePool[key].push(renderTexture);
}
/**
* Empties the texture pool.
*
*/
emptyPool()
{
for (const i in this.texturePool)
{
const textures = this.texturePool[i];
if (textures)
{
for (let j = 0; j < textures.length; j++)
{
textures[j].destroy(true);
}
}
}
this.texturePool = {};
}
resize()
{
const textures = this.texturePool[screenKey];
if (textures)
{
for (let j = 0; j < textures.length; j++)
{
textures[j].destroy(true);
}
}
this.texturePool[screenKey] = [];
this._pixelsWidth = this.renderer.view.width;
this._pixelsHeight = this.renderer.view.height;
}
}
| packages/core/src/filters/FilterSystem.js | 1 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.826849102973938,
0.017492149025201797,
0.00016447564121335745,
0.00017367668624501675,
0.10969620943069458
] |
{
"id": 3,
"code_window": [
" */\n",
" clear(clearColor)\n",
" {\n",
" if (this.renderTexture)\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (this.current)\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 142
} | const { Spritesheet } = require('../');
const { BaseTexture, Texture } = require('@pixi/core');
const path = require('path');
describe('PIXI.Spritesheet', function ()
{
before(function ()
{
this.resources = path.join(__dirname, 'resources');
this.validate = function (spritesheet, done)
{
spritesheet.parse(function (textures)
{
const id = 'goldmine_10_5.png';
const width = Math.floor(spritesheet.data.frames[id].frame.w);
const height = Math.floor(spritesheet.data.frames[id].frame.h);
expect(Object.keys(textures).length).to.equal(5);
expect(Object.keys(spritesheet.textures).length).to.equal(5);
expect(textures[id]).to.be.an.instanceof(Texture);
expect(textures[id].width).to.equal(width / spritesheet.resolution);
expect(textures[id].height).to.equal(height / spritesheet.resolution);
expect(textures[id].defaultAnchor.x).to.equal(0);
expect(textures[id].defaultAnchor.y).to.equal(0);
expect(textures[id].textureCacheIds.indexOf(id)).to.equal(0);
expect(this.animations).to.have.property('star').that.is.an('array');
expect(this.animations.star.length).to.equal(4);
expect(this.animations.star[0].defaultAnchor.x).to.equal(0.5);
expect(this.animations.star[0].defaultAnchor.y).to.equal(0.5);
spritesheet.destroy(true);
expect(spritesheet.textures).to.be.null;
expect(spritesheet.baseTexture).to.be.null;
done();
});
};
this.parseFrame = function (frameData, callback)
{
const data = {
frames: { frame: frameData },
meta: { scale: 1 },
};
const baseTexture = BaseTexture.from(
document.createElement('canvas')
);
baseTexture.imageUrl = 'test.png';
const sheet = new Spritesheet(baseTexture, data);
sheet.parse(() =>
{
const { frame } = sheet.textures;
expect(frame).to.be.instanceof(Texture);
callback(frame);
sheet.destroy(true);
});
};
});
it('should exist on PIXI', function ()
{
expect(Spritesheet).to.be.a.function;
expect(Spritesheet.BATCH_SIZE).to.be.a.number;
});
it('should create an instance', function ()
{
const baseTexture = new BaseTexture();
const data = {
frames: {},
meta: {},
};
const spritesheet = new Spritesheet(baseTexture, data);
expect(spritesheet.data).to.equal(data);
expect(spritesheet.baseTexture).to.equal(baseTexture);
expect(spritesheet.resolution).to.equal(1);
spritesheet.destroy(true);
});
it('should create instance with scale resolution', function (done)
{
const data = require(path.resolve(this.resources, 'building1.json')); // eslint-disable-line global-require
const image = new Image();
image.src = path.join(this.resources, data.meta.image);
image.onload = () =>
{
const baseTexture = new BaseTexture(image, null, 1);
const spritesheet = new Spritesheet(baseTexture, data);
expect(data).to.be.an.object;
expect(data.meta.image).to.equal('building1.png');
expect(spritesheet.resolution).to.equal(0.5);
this.validate(spritesheet, done);
};
});
it('should create instance with BaseTexture source scale', function (done)
{
const data = require(path.resolve(this.resources, 'building1.json')); // eslint-disable-line global-require
const baseTexture = BaseTexture.from(data.meta.image);// , undefined, undefined, 1.5);
const spritesheet = new Spritesheet(baseTexture, data);
expect(data).to.be.an.object;
expect(data.meta.image).to.equal('building1.png');
expect(spritesheet.resolution).to.equal(0.5);
this.validate(spritesheet, done);
});
it('should create instance with filename resolution', function (done)
{
const uri = path.resolve(this.resources, '[email protected]');
const data = require(uri); // eslint-disable-line global-require
const image = new Image();
image.src = path.join(this.resources, data.meta.image);
image.onload = () =>
{
const baseTexture = new BaseTexture(image, { resolution: 1 });
const spritesheet = new Spritesheet(baseTexture, data, uri);
expect(data).to.be.an.object;
expect(data.meta.image).to.equal('[email protected]');
expect(spritesheet.resolution).to.equal(2);
this.validate(spritesheet, done);
};
});
it('should parse full data untrimmed', function (done)
{
const data = {
frame: { x: 0, y: 0, w: 14, h: 16 },
rotated: false,
trimmed: false,
spriteSourceSize: { x: 0, y: 0, w: 14, h: 16 },
sourceSize: { w: 14, h: 16 },
};
this.parseFrame(data, (texture) =>
{
expect(texture.width).to.equal(14);
expect(texture.height).to.equal(16);
done();
});
});
it('should parse texture from trimmed', function (done)
{
const data = {
frame: { x: 0, y: 28, w: 14, h: 14 },
rotated: false,
trimmed: true,
spriteSourceSize: { x: 0, y: 0, w: 40, h: 20 },
sourceSize: { w: 40, h: 20 },
};
this.parseFrame(data, (texture) =>
{
expect(texture.width).to.equal(40);
expect(texture.height).to.equal(20);
done();
});
});
it('should parse texture from minimal data', function (done)
{
const data = { frame: { x: 0, y: 0, w: 14, h: 14 } };
this.parseFrame(data, (texture) =>
{
expect(texture.width).to.equal(14);
expect(texture.height).to.equal(14);
done();
});
});
it('should parse texture without trimmed or sourceSize', function (done)
{
const data = {
frame: { x: 0, y: 14, w: 14, h: 14 },
rotated: false,
trimmed: false,
spriteSourceSize: { x: 0, y: 0, w: 20, h: 30 },
};
this.parseFrame(data, (texture) =>
{
expect(texture.width).to.equal(14);
expect(texture.height).to.equal(14);
done();
});
});
it('should parse as trimmed if spriteSourceSize is set', function (done)
{
// shoebox format
const data = {
frame: { x: 0, y: 0, w: 14, h: 16 },
spriteSourceSize: { x: 0, y: 0, w: 120, h: 100 },
sourceSize: { w: 120, h: 100 },
};
this.parseFrame(data, (texture) =>
{
expect(texture.width).to.equal(120);
expect(texture.height).to.equal(100);
done();
});
});
});
| packages/spritesheet/test/Spritesheet.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.0004329355142544955,
0.00020467923604883254,
0.00016409653471782804,
0.00017259508604183793,
0.00006867728370707482
] |
{
"id": 3,
"code_window": [
" */\n",
" clear(clearColor)\n",
" {\n",
" if (this.renderTexture)\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (this.current)\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 142
} | import { settings } from '@pixi/settings';
/**
* The prefix that denotes a URL is for a retina asset.
*
* @static
* @name RETINA_PREFIX
* @memberof PIXI.settings
* @type {RegExp}
* @default /@([0-9\.]+)x/
* @example `@2x`
*/
settings.RETINA_PREFIX = /@([0-9\.]+)x/;
export { settings };
| packages/utils/src/settings.js | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017280818428844213,
0.00017055857460945845,
0.00016830896493047476,
0.00017055857460945845,
0.0000022496096789836884
] |
{
"id": 3,
"code_window": [
" */\n",
" clear(clearColor)\n",
" {\n",
" if (this.renderTexture)\n",
" {\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" if (this.current)\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 142
} | The MIT License
Copyright (c) 2013-2018 Mathew Groves, Chad Engler
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE. | packages/canvas/canvas-prepare/LICENSE | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.00017204547475557774,
0.00017127691535279155,
0.00017015007324516773,
0.00017163518350571394,
8.142097840391216e-7
] |
{
"id": 4,
"code_window": [
" {\n",
" clearColor = clearColor || this.renderTexture.baseTexture.clearColor;\n",
" }\n",
" else\n",
" {\n",
" clearColor = clearColor || this.clearColor;\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" clearColor = clearColor || this.current.baseTexture.clearColor;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 144
} | import System from '../System';
import RenderTexture from '../renderTexture/RenderTexture';
import Quad from '../utils/Quad';
import QuadUv from '../utils/QuadUv';
import { Rectangle } from '@pixi/math';
import * as filterTransforms from './filterTransforms';
import { nextPow2 } from '@pixi/utils';
import UniformGroup from '../shader/UniformGroup';
import { DRAW_MODES } from '@pixi/constants';
/**
* System plugin to the renderer to manage filter states.
*
* @class
* @private
*/
class FilterState
{
constructor()
{
this.renderTexture = null;
/**
* Target of the filters
* We store for case when custom filter wants to know the element it was applied on
* @member {PIXI.DisplayObject}
* @private
*/
this.target = null;
/**
* Compatibility with PixiJS v4 filters
* @member {boolean}
* @default false
* @private
*/
this.legacy = false;
/**
* Resolution of filters
* @member {number}
* @default 1
* @private
*/
this.resolution = 1;
// next three fields are created only for root
// re-assigned for everything else
/**
* Source frame
* @member {PIXI.Rectangle}
* @private
*/
this.sourceFrame = new Rectangle();
/**
* Destination frame
* @member {PIXI.Rectangle}
* @private
*/
this.destinationFrame = new Rectangle();
/**
* Collection of filters
* @member {PIXI.Filter[]}
* @private
*/
this.filters = [];
}
/**
* clears the state
* @private
*/
clear()
{
this.target = null;
this.filters = null;
this.renderTexture = null;
}
}
const screenKey = 'screen';
/**
* System plugin to the renderer to manage the filters.
*
* @class
* @memberof PIXI.systems
* @extends PIXI.System
*/
export default class FilterSystem extends System
{
/**
* @param {PIXI.Renderer} renderer - The renderer this System works for.
*/
constructor(renderer)
{
super(renderer);
/**
* List of filters for the FilterSystem
* @member {Object[]}
* @readonly
*/
this.defaultFilterStack = [{}];
/**
* stores a bunch of PO2 textures used for filtering
* @member {Object}
*/
this.texturePool = {};
/**
* a pool for storing filter states, save us creating new ones each tick
* @member {Object[]}
*/
this.statePool = [];
/**
* A very simple geometry used when drawing a filter effect to the screen
* @member {PIXI.Quad}
*/
this.quad = new Quad();
/**
* Quad UVs
* @member {PIXI.QuadUv}
*/
this.quadUv = new QuadUv();
/**
* Temporary rect for maths
* @type {PIXI.Rectangle}
*/
this.tempRect = new Rectangle();
/**
* Active state
* @member {object}
*/
this.activeState = {};
/**
* This uniform group is attached to filter uniforms when used
* @member {PIXI.UniformGroup}
* @property {PIXI.Rectangle} outputFrame
* @property {Float32Array} inputSize
* @property {Float32Array} inputPixel
* @property {Float32Array} inputClamp
* @property {Number} resolution
* @property {Float32Array} filterArea
* @property {Fload32Array} filterClamp
*/
this.globalUniforms = new UniformGroup({
outputFrame: this.tempRect,
inputSize: new Float32Array(4),
inputPixel: new Float32Array(4),
inputClamp: new Float32Array(4),
resolution: 1,
// legacy variables
filterArea: new Float32Array(4),
filterClamp: new Float32Array(4),
}, true);
this._pixelsWidth = renderer.view.width;
this._pixelsHeight = renderer.view.height;
}
/**
* Adds a new filter to the System.
*
* @param {PIXI.DisplayObject} target - The target of the filter to render.
* @param {PIXI.Filter[]} filters - The filters to apply.
*/
push(target, filters)
{
const renderer = this.renderer;
const filterStack = this.defaultFilterStack;
const state = this.statePool.pop() || new FilterState();
let resolution = filters[0].resolution;
let padding = filters[0].padding;
let autoFit = filters[0].autoFit;
let legacy = filters[0].legacy;
for (let i = 1; i < filters.length; i++)
{
const filter = filters[i];
// lets use the lowest resolution..
resolution = Math.min(resolution, filter.resolution);
// and the largest amount of padding!
padding = Math.max(padding, filter.padding);
// only auto fit if all filters are autofit
autoFit = autoFit || filter.autoFit;
legacy = legacy || filter.legacy;
}
if (filterStack.length === 1)
{
this.defaultFilterStack[0].renderTexture = renderer.renderTexture.renderTexture;
}
filterStack.push(state);
state.resolution = resolution;
state.legacy = legacy;
state.target = target;
state.sourceFrame.copyFrom(target.filterArea || target.getBounds(true));
state.sourceFrame.pad(padding);
if (autoFit)
{
state.sourceFrame.fit(this.renderer.renderTexture.sourceFrame);
}
// round to whole number based on resolution
state.sourceFrame.ceil(resolution);
state.renderTexture = this.getOptimalFilterTexture(state.sourceFrame.width, state.sourceFrame.height, resolution);
state.filters = filters;
state.destinationFrame.width = state.renderTexture.width;
state.destinationFrame.height = state.renderTexture.height;
state.renderTexture.filterFrame = state.sourceFrame;
renderer.renderTexture.bind(state.renderTexture, state.sourceFrame);// /, state.destinationFrame);
renderer.renderTexture.clear();
}
/**
* Pops off the filter and applies it.
*
*/
pop()
{
const filterStack = this.defaultFilterStack;
const state = filterStack.pop();
const filters = state.filters;
this.activeState = state;
const globalUniforms = this.globalUniforms.uniforms;
globalUniforms.outputFrame = state.sourceFrame;
globalUniforms.resolution = state.resolution;
const inputSize = globalUniforms.inputSize;
const inputPixel = globalUniforms.inputPixel;
const inputClamp = globalUniforms.inputClamp;
inputSize[0] = state.destinationFrame.width;
inputSize[1] = state.destinationFrame.height;
inputSize[2] = 1.0 / inputSize[0];
inputSize[3] = 1.0 / inputSize[1];
inputPixel[0] = inputSize[0] * state.resolution;
inputPixel[1] = inputSize[1] * state.resolution;
inputPixel[2] = 1.0 / inputPixel[0];
inputPixel[3] = 1.0 / inputPixel[1];
inputClamp[0] = 0.5 * inputPixel[2];
inputClamp[1] = 0.5 * inputPixel[3];
inputClamp[2] = (state.sourceFrame.width * inputSize[2]) - (0.5 * inputPixel[2]);
inputClamp[3] = (state.sourceFrame.height * inputSize[3]) - (0.5 * inputPixel[3]);
// only update the rect if its legacy..
if (state.legacy)
{
const filterArea = globalUniforms.filterArea;
filterArea[0] = state.destinationFrame.width;
filterArea[1] = state.destinationFrame.height;
filterArea[2] = state.sourceFrame.x;
filterArea[3] = state.sourceFrame.y;
globalUniforms.filterClamp = globalUniforms.inputClamp;
}
this.globalUniforms.update();
const lastState = filterStack[filterStack.length - 1];
if (filters.length === 1)
{
filters[0].apply(this, state.renderTexture, lastState.renderTexture, false, state);
this.returnFilterTexture(state.renderTexture);
}
else
{
let flip = state.renderTexture;
let flop = this.getOptimalFilterTexture(
flip.width,
flip.height,
state.resolution
);
flop.filterFrame = flip.filterFrame;
let i = 0;
for (i = 0; i < filters.length - 1; ++i)
{
filters[i].apply(this, flip, flop, true, state);
const t = flip;
flip = flop;
flop = t;
}
filters[i].apply(this, flip, lastState.renderTexture, false, state);
this.returnFilterTexture(flip);
this.returnFilterTexture(flop);
}
state.clear();
this.statePool.push(state);
}
/**
* Draws a filter.
*
* @param {PIXI.Filter} filter - The filter to draw.
* @param {PIXI.RenderTexture} input - The input render target.
* @param {PIXI.RenderTexture} output - The target to output to.
* @param {boolean} clear - Should the output be cleared before rendering to it
*/
applyFilter(filter, input, output, clear)
{
const renderer = this.renderer;
renderer.renderTexture.bind(output, output ? output.filterFrame : null);
if (clear)
{
// gl.disable(gl.SCISSOR_TEST);
renderer.renderTexture.clear();
// gl.enable(gl.SCISSOR_TEST);
}
// set the uniforms..
filter.uniforms.uSampler = input;
filter.uniforms.filterGlobals = this.globalUniforms;
// TODO make it so that the order of this does not matter..
// because it does at the moment cos of global uniforms.
// they need to get resynced
renderer.state.setState(filter.state);
renderer.shader.bind(filter);
if (filter.legacy)
{
this.quadUv.map(input._frame, input.filterFrame);
renderer.geometry.bind(this.quadUv);
renderer.geometry.draw(DRAW_MODES.TRIANGLES);
}
else
{
renderer.geometry.bind(this.quad);
renderer.geometry.draw(DRAW_MODES.TRIANGLE_STRIP);
}
}
/**
* Calculates the mapped matrix.
*
* TODO playing around here.. this is temporary - (will end up in the shader)
* this returns a matrix that will normalize map filter cords in the filter to screen space
*
* @param {PIXI.Matrix} outputMatrix - the matrix to output to.
* @return {PIXI.Matrix} The mapped matrix.
*/
calculateScreenSpaceMatrix(outputMatrix)
{
const currentState = this.activeState;
return filterTransforms.calculateScreenSpaceMatrix(
outputMatrix,
currentState.sourceFrame,
currentState.destinationFrame
);
}
/**
* This will map the filter coord so that a texture can be used based on the transform of a sprite
*
* @param {PIXI.Matrix} outputMatrix - The matrix to output to.
* @param {PIXI.Sprite} sprite - The sprite to map to.
* @return {PIXI.Matrix} The mapped matrix.
*/
calculateSpriteMatrix(outputMatrix, sprite)
{
const currentState = this.activeState;
return filterTransforms.calculateSpriteMatrix(
outputMatrix,
currentState.sourceFrame,
currentState.destinationFrame,
sprite
);
}
/**
* Destroys this Filter System.
*
* @param {boolean} [contextLost=false] context was lost, do not free shaders
*
*/
destroy(contextLost = false)
{
if (!contextLost)
{
this.emptyPool();
}
else
{
this.texturePool = {};
}
}
/**
* Gets a Power-of-Two render texture or fullScreen texture
*
* TODO move to a separate class could be on renderer?
*
* @protected
* @param {number} minWidth - The minimum width of the render texture in real pixels.
* @param {number} minHeight - The minimum height of the render texture in real pixels.
* @param {number} [resolution=1] - The resolution of the render texture.
* @return {PIXI.RenderTexture} The new render texture.
*/
getOptimalFilterTexture(minWidth, minHeight, resolution = 1)
{
let key = screenKey;
minWidth *= resolution;
minHeight *= resolution;
if (minWidth !== this._pixelsWidth || minHeight !== this._pixelsHeight)
{
minWidth = nextPow2(minWidth);
minHeight = nextPow2(minHeight);
key = ((minWidth & 0xFFFF) << 16) | (minHeight & 0xFFFF);
}
if (!this.texturePool[key])
{
this.texturePool[key] = [];
}
let renderTexture = this.texturePool[key].pop();
if (!renderTexture)
{
// temporary bypass cache..
// internally - this will cause a texture to be bound..
renderTexture = RenderTexture.create({
width: minWidth / resolution,
height: minHeight / resolution,
resolution,
});
}
renderTexture.filterPoolKey = key;
return renderTexture;
}
/**
* Gets extra render texture to use inside current filter
*
* @param {number} resolution resolution of the renderTexture
* @returns {PIXI.RenderTexture}
*/
getFilterTexture(resolution)
{
const rt = this.activeState.renderTexture;
const filterTexture = this.getOptimalFilterTexture(rt.width, rt.height, resolution || rt.baseTexture.resolution);
filterTexture.filterFrame = rt.filterFrame;
return filterTexture;
}
/**
* Frees a render texture back into the pool.
*
* @param {PIXI.RenderTexture} renderTexture - The renderTarget to free
*/
returnFilterTexture(renderTexture)
{
const key = renderTexture.filterPoolKey;
renderTexture.filterFrame = null;
this.texturePool[key].push(renderTexture);
}
/**
* Empties the texture pool.
*
*/
emptyPool()
{
for (const i in this.texturePool)
{
const textures = this.texturePool[i];
if (textures)
{
for (let j = 0; j < textures.length; j++)
{
textures[j].destroy(true);
}
}
}
this.texturePool = {};
}
resize()
{
const textures = this.texturePool[screenKey];
if (textures)
{
for (let j = 0; j < textures.length; j++)
{
textures[j].destroy(true);
}
}
this.texturePool[screenKey] = [];
this._pixelsWidth = this.renderer.view.width;
this._pixelsHeight = this.renderer.view.height;
}
}
| packages/core/src/filters/FilterSystem.js | 1 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.0021305507980287075,
0.00025953599833883345,
0.00016350428631994873,
0.00017267928342334926,
0.0002964196028187871
] |
{
"id": 4,
"code_window": [
" {\n",
" clearColor = clearColor || this.renderTexture.baseTexture.clearColor;\n",
" }\n",
" else\n",
" {\n",
" clearColor = clearColor || this.clearColor;\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" clearColor = clearColor || this.current.baseTexture.clearColor;\n"
],
"file_path": "packages/core/src/renderTexture/RenderTextureSystem.js",
"type": "replace",
"edit_start_line_idx": 144
} | <font>
<info face="split_font2" size="24" bold="0" italic="0" charset="" unicode="" stretchH="100" smooth="1" aa="1" padding="2,2,2,2" spacing="0,0" outline="0"/>
<common lineHeight="27" base="18" scaleW="22" scaleH="46" pages="2" packed="0"/>
<pages>
<page id="1" file="split_font_cd.png"/>
<page id="0" file="split_font_ab.png"/>
</pages>
<chars count="5">
<char id="65" x="2" y="2" width="19" height="20" xoffset="0" yoffset="0" xadvance="16" page="0" chnl="15"/>
<char id="66" x="2" y="24" width="15" height="20" xoffset="2" yoffset="0" xadvance="16" page="0" chnl="15"/>
<char id="67" x="2" y="2" width="18" height="20" xoffset="1" yoffset="0" xadvance="17" page="1" chnl="15"/>
<char id="68" x="2" y="24" width="17" height="20" xoffset="2" yoffset="0" xadvance="17" page="1" chnl="15"/>
</chars>
<kernings count="0"/>
</font> | packages/text-bitmap/test/resources/split_font2.fnt | 0 | https://github.com/pixijs/pixijs/commit/f2e54200dddb2f78ad1e4f7573aae46dad7d5494 | [
0.0001755993434926495,
0.0001704006572254002,
0.00016520197095815092,
0.0001704006572254002,
0.000005198686267249286
] |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.