hunk
dict | file
stringlengths 0
11.8M
| file_path
stringlengths 2
234
| label
int64 0
1
| commit_url
stringlengths 74
103
| dependency_score
sequencelengths 5
5
|
---|---|---|---|---|---|
{
"id": 8,
"code_window": [
" $config: any\n",
" }\n",
" }\n",
"}\n",
"\n",
"// type export required to turn this into a module for TS augmentation purposes\n",
"export type A = {}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace"
],
"after_edit": [
"// export required to turn this into a module for TS augmentation purposes\n",
"export { }"
],
"file_path": "packages/nitro/src/runtime/types.d.ts",
"type": "replace",
"edit_start_line_idx": 9
} | import { Worker } from 'worker_threads'
import chokidar, { FSWatcher } from 'chokidar'
import debounce from 'debounce'
import { stat } from 'fs-extra'
import { createApp, Middleware } from 'h3'
import { createProxy } from 'http-proxy'
import { listen, Listener, ListenOptions } from 'listhen'
import servePlaceholder from 'serve-placeholder'
import serveStatic from 'serve-static'
import { resolve } from 'upath'
import type { Server } from 'connect'
import type { NitroContext } from '../context'
export function createDevServer (nitroContext: NitroContext) {
// Worker
const workerEntry = resolve(nitroContext.output.dir, nitroContext.output.serverDir, 'index.js')
let pendingWorker: Worker | null
let activeWorker: Worker
let workerAddress: string | null
async function reload () {
if (pendingWorker) {
await pendingWorker.terminate()
workerAddress = null
pendingWorker = null
}
if (!(await stat(workerEntry)).isFile) {
throw new Error('Entry not found: ' + workerEntry)
}
return new Promise((resolve, reject) => {
const worker = pendingWorker = new Worker(workerEntry)
worker.once('exit', (code) => {
if (code) {
reject(new Error('[worker] exited with code: ' + code))
}
})
worker.on('error', (err) => {
err.message = '[worker] ' + err.message
reject(err)
})
worker.on('message', (event) => {
if (event && event.port) {
workerAddress = 'http://localhost:' + event.port
activeWorker = worker
pendingWorker = null
resolve(workerAddress)
}
})
})
}
// App
const app = createApp()
// _nuxt and static
app.use(nitroContext._nuxt.publicPath, serveStatic(resolve(nitroContext._nuxt.buildDir, 'dist/client')))
app.use(nitroContext._nuxt.routerBase, serveStatic(resolve(nitroContext._nuxt.publicDir)))
// Dynamic Middlwware
const legacyMiddleware = createDynamicMiddleware()
const devMiddleware = createDynamicMiddleware()
app.use(legacyMiddleware.middleware)
app.use(devMiddleware.middleware)
// serve placeholder 404 assets instead of hitting SSR
app.use(nitroContext._nuxt.publicPath, servePlaceholder())
// SSR Proxy
const proxy = createProxy()
app.use((req, res) => {
if (workerAddress) {
proxy.web(req, res, { target: workerAddress }, (_err: unknown) => {
// console.error('[proxy]', err)
})
} else {
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.end('<!DOCTYPE html><html><head><meta http-equiv="refresh" content="1"><head><body>...')
}
})
// Listen
let listeners: Listener[] = []
const _listen = async (port: ListenOptions['port'], opts?: Partial<ListenOptions>) => {
const listener = await listen(app, { port, ...opts })
listeners.push(listener)
return listener
}
// Watch for dist and reload worker
const pattern = '**/*.{js,json}'
const events = ['add', 'change']
let watcher: FSWatcher
function watch () {
if (watcher) { return }
const dReload = debounce(() => reload().catch(console.warn), 200, true)
watcher = chokidar.watch([
resolve(nitroContext.output.serverDir, pattern),
resolve(nitroContext._nuxt.buildDir, 'dist/server', pattern)
]).on('all', event => events.includes(event) && dReload())
}
// Close handler
async function close () {
if (watcher) {
await watcher.close()
}
if (activeWorker) {
await activeWorker.terminate()
}
if (pendingWorker) {
await pendingWorker.terminate()
}
await Promise.all(listeners.map(l => l.close()))
listeners = []
}
nitroContext._internal.hooks.hook('close', close)
return {
reload,
listen: _listen,
app,
close,
watch,
setLegacyMiddleware: legacyMiddleware.set,
setDevMiddleware: devMiddleware.set
}
}
interface DynamicMiddleware {
set: (input: Middleware) => void
middleware: Middleware
}
function createDynamicMiddleware (): DynamicMiddleware {
let middleware: Middleware
return {
set: (input) => {
if (!Array.isArray(input)) {
middleware = input
return
}
const app: Server = require('connect')()
for (const m of input) {
app.use(m.path || m.route || '/', m.handler || m.handle!)
}
middleware = app
},
middleware: (req, res, next) =>
middleware ? middleware(req, res, next) : next()
}
}
| packages/nitro/src/server/dev.ts | 0 | https://github.com/nuxt/nuxt/commit/b53d8a77ffe1bdd69ae8dcc41da9d37a867329cc | [
0.00026174072991125286,
0.0001771303650457412,
0.0001681770954746753,
0.00017110527551267296,
0.000022016960429027677
] |
{
"id": 8,
"code_window": [
" $config: any\n",
" }\n",
" }\n",
"}\n",
"\n",
"// type export required to turn this into a module for TS augmentation purposes\n",
"export type A = {}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"replace"
],
"after_edit": [
"// export required to turn this into a module for TS augmentation purposes\n",
"export { }"
],
"file_path": "packages/nitro/src/runtime/types.d.ts",
"type": "replace",
"edit_start_line_idx": 9
} | import { defineNuxtPlugin } from '@nuxt/app'
export default defineNuxtPlugin(({ app }) => {
const { $nuxt } = app
$nuxt.hook('app:mounted', () => {
const el = document.createElement('div')
el.id = 'nuxt-progress'
document.body.appendChild(el)
el.style.position = 'fixed'
el.style.backgroundColor = 'black'
el.style.height = '2px'
el.style.top = '0px'
el.style.left = '0px'
el.style.transition = 'width 0.1s, opacity 0.4s'
const duration = 3000
const progress = 10000 / Math.floor(duration)
let timeout
let interval
$nuxt.hook('page:start', () => {
if (timeout) { return }
timeout = setTimeout(() => {
let width = 10
el.style.opacity = '100%'
el.style.width = '10%'
interval = setInterval(() => {
if (width >= 100) { return }
width = Math.floor(width + progress)
el.style.width = `${width}%`
}, 100)
}, 200)
})
$nuxt.hook('page:finish', () => {
timeout && clearTimeout(timeout)
timeout = null
interval && clearInterval(interval)
interval = null
el.style.width = '100%'
el.style.opacity = '0%'
setTimeout(() => {
el.style.width = '0%'
}, 500)
})
})
})
| packages/app/src/plugins/progress.client.ts | 0 | https://github.com/nuxt/nuxt/commit/b53d8a77ffe1bdd69ae8dcc41da9d37a867329cc | [
0.0001705239701550454,
0.0001694209931883961,
0.00016811008390504867,
0.000169797582202591,
8.892871505850053e-7
] |
{
"id": 9,
"code_window": [
" export function readAsset<T=any>(id: string): Promise<T>\n",
" export function statAsset(id: string): Promise<AssetMeta>\n",
" export function getAsset<T=any>(id: string): { read: () => Promise<T>, meta: AssetMeta }\n",
"}\n",
"\n",
"export {}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace"
],
"after_edit": [],
"file_path": "packages/nitro/types/shims.d.ts",
"type": "replace",
"edit_start_line_idx": 23
} | import Vue from 'vue'
import { $Fetch } from 'ohmyfetch'
import { Nuxt } from '../dist'
declare global {
// eslint-disable-next-line no-var
var $fetch: $Fetch
namespace NodeJS {
interface Global {
$fetch: $Fetch
}
interface Process {
browser: boolean
client: boolean
mode: 'spa' | 'universal'
server: boolean
static: boolean
}
}
interface Window {
__NUXT__?: Record<string, any>
}
}
declare module '*.vue' {
export default Vue
}
declare module 'vue' {
interface App {
$nuxt: Nuxt
}
}
export {}
| packages/app/types/shims.d.ts | 1 | https://github.com/nuxt/nuxt/commit/b53d8a77ffe1bdd69ae8dcc41da9d37a867329cc | [
0.0001732252276269719,
0.00017102740821428597,
0.00016828361549414694,
0.00017130040214397013,
0.000002089500640067854
] |
{
"id": 9,
"code_window": [
" export function readAsset<T=any>(id: string): Promise<T>\n",
" export function statAsset(id: string): Promise<AssetMeta>\n",
" export function getAsset<T=any>(id: string): { read: () => Promise<T>, meta: AssetMeta }\n",
"}\n",
"\n",
"export {}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace"
],
"after_edit": [],
"file_path": "packages/nitro/types/shims.d.ts",
"type": "replace",
"edit_start_line_idx": 23
} | import type { ModuleContainer } from '../module/container'
import { Nuxt } from './nuxt'
import { NuxtHooks } from './hooks'
export interface ModuleMeta {
/** The module name. */
name?: string
/**
* The configuration key used within `nuxt.config` for this module's options.
* For example, `@nuxtjs/axios` uses `axios`.
*/
configKey?: string
[key: string]: any
}
/** The options received */
export type ModuleOptions = Record<string, any>
/** A pre-kit Nuxt module */
export interface LegacyNuxtModule {
(this: ModuleContainer, inlineOptions?: ModuleOptions): void | Promise<void>
meta?: ModuleMeta
}
/** A Nuxt module definition */
export interface NuxtModule<T extends ModuleOptions = any> extends ModuleMeta {
defaults?: T
setup?: (this: null, resolvedOptions: T, nuxt: Nuxt) => void | Promise<void>
hooks?: Partial<NuxtHooks>
}
export type ModuleSrc = string | NuxtModule | LegacyNuxtModule
export interface ModuleInstallOptionsObj {
src: ModuleSrc,
meta: ModuleMeta
options: ModuleOptions
handler: LegacyNuxtModule
}
export type ModuleInstallOptions =
ModuleSrc |
[ModuleSrc, ModuleOptions?] |
Partial<ModuleInstallOptionsObj>
// -- Templates --
export interface TemplateOpts {
/** The target filename once the template is copied into the Nuxt buildDir */
filename?: string
/** The target filename once the template is copied into the Nuxt buildDir */
fileName?: string
/** An options object that will be accessible within the template via `<% options %>` */
options?: Record<string, any>
/** The resolved path to the source file to be templated */
src: string
}
export interface PluginTemplateOpts extends TemplateOpts {
/** @deprecated use mode */
ssr?: boolean
/** Whether the plugin will be loaded on only server-side, only client-side or on both. */
mode?: 'all' | 'server' | 'client'
}
| packages/kit/src/types/module.ts | 0 | https://github.com/nuxt/nuxt/commit/b53d8a77ffe1bdd69ae8dcc41da9d37a867329cc | [
0.00027256019529886544,
0.00020510610193014145,
0.0001688762567937374,
0.00017252285033464432,
0.000042445110011612996
] |
{
"id": 9,
"code_window": [
" export function readAsset<T=any>(id: string): Promise<T>\n",
" export function statAsset(id: string): Promise<AssetMeta>\n",
" export function getAsset<T=any>(id: string): { read: () => Promise<T>, meta: AssetMeta }\n",
"}\n",
"\n",
"export {}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace"
],
"after_edit": [],
"file_path": "packages/nitro/types/shims.d.ts",
"type": "replace",
"edit_start_line_idx": 23
} | import { defineNuxtConfig } from '@nuxt/kit'
export default defineNuxtConfig({})
| test/fixtures/basic/nuxt.config.ts | 0 | https://github.com/nuxt/nuxt/commit/b53d8a77ffe1bdd69ae8dcc41da9d37a867329cc | [
0.00017574122466612607,
0.00017574122466612607,
0.00017574122466612607,
0.00017574122466612607,
0
] |
{
"id": 9,
"code_window": [
" export function readAsset<T=any>(id: string): Promise<T>\n",
" export function statAsset(id: string): Promise<AssetMeta>\n",
" export function getAsset<T=any>(id: string): { read: () => Promise<T>, meta: AssetMeta }\n",
"}\n",
"\n",
"export {}"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace"
],
"after_edit": [],
"file_path": "packages/nitro/types/shims.d.ts",
"type": "replace",
"edit_start_line_idx": 23
} | # PM2
> How to deploy Nuxt to a Node.js host with Nuxt Nitro
- Support for ultra-minimal SSR build
- Zero millisecond cold start
- More configuration required
## Setup
Make sure another preset isn't set in `nuxt.config`.
```ts [nuxt.config.js]
export default {
nitro: {
// this is the default preset so you can also just omit it entirely
// preset: 'server'
}
}
```
## Deployment
After running `yarn build`, all you need is in the `.output` folder. Static assets are in the `public` subdirectory and the server and its dependencies are within the `server` subdirectory.
This `.output` folder can be deployed to your Node.js host and the server can be run using [`pm2`](https://pm2.keymetrics.io/docs/).
To start the server in production mode, run:
```bash
node .output/server
```
For example, using `pm2`:
```js [ecosystem.config.js]
module.exports = {
apps: [
{
name: 'NuxtAppName',
exec_mode: 'cluster',
instances: 'max',
script: './.output/server/index.js'
}
]
}
```
## More information
See [more information on the server preset](/presets/server).
| docs/content/6.deployment/platforms/pm2.md | 0 | https://github.com/nuxt/nuxt/commit/b53d8a77ffe1bdd69ae8dcc41da9d37a867329cc | [
0.00017398469208274037,
0.0001706529437797144,
0.00016680524277035147,
0.00017086406296584755,
0.0000024492733246006537
] |
{
"id": 0,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/invite`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/invite.ts",
"type": "replace",
"edit_start_line_idx": 23
} | import { NextApiRequest, NextApiResponse } from 'next'
import SqlString from 'sqlstring'
import apiWrapper from 'lib/api/apiWrapper'
import { constructHeaders } from 'lib/api/apiHelpers'
import { post } from 'lib/common/fetch'
import { tryParseInt } from 'lib/helpers'
export default (req: NextApiRequest, res: NextApiResponse) => apiWrapper(req, res, handler)
async function handler(req: NextApiRequest, res: NextApiResponse) {
const { method } = req
switch (method) {
case 'POST':
return handlePost(req, res)
default:
res.setHeader('Allow', ['POST'])
res.status(405).json({ data: null, error: { message: `Method ${method} Not Allowed` } })
}
}
const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {
const headers = constructHeaders({
'Content-Type': 'application/json',
Accept: 'application/json',
Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,
})
const url = `${process.env.SUPABASE_URL}/auth/v1/magiclink`
const payload = { email: req.body.email }
const response = await post(url, payload, { headers })
return res.status(200).json(response)
}
| studio/pages/api/auth/[ref]/magiclink.ts | 1 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.9981552958488464,
0.7484298944473267,
0.002356519689783454,
0.9966038465499878,
0.4307461977005005
] |
{
"id": 0,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/invite`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/invite.ts",
"type": "replace",
"edit_start_line_idx": 23
} | # Example: Supabase authentication client- and server-side (API routes), and SSR with auth cookie.
This example shows how to use Supabase auth both on the client and server in both [API routes](https://nextjs.org/docs/api-routes/introduction) and when using [server side rendering (SSR)](https://nextjs.org/docs/basic-features/pages#server-side-rendering).
This example is the same as [nextjs-with-supabase-auth](https://github.com/supabase/supabase/tree/master/examples/nextjs-with-supabase-auth) however it does not use the @supabase/ui Auth component.
| examples/nextjs-auth/README.md | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00016366808267775923,
0.00016366808267775923,
0.00016366808267775923,
0.00016366808267775923,
0
] |
{
"id": 0,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/invite`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/invite.ts",
"type": "replace",
"edit_start_line_idx": 23
} | import AuthLayout from './AuthLayout/AuthLayout'
import ProjectLayout from './ProjectLayout/ProjectLayout'
import TableEditorLayout from './TableEditorLayout/TableEditorLayout'
import SQLEditorLayout from './SQLEditorLayout/SQLEditorLayout'
import DatabaseLayout from './DatabaseLayout/DatabaseLayout'
import DocsLayout from './DocsLayout/DocsLayout'
import SettingsLayout from './SettingsLayout/SettingsLayout'
import StorageLayout from './StorageLayout/StorageLayout'
import AccountLayout from './AccountLayout/AccountLayout'
import WizardLayout from './WizardLayout'
import VercelIntegrationLayout from './VercelIntegrationLayout'
export {
AuthLayout,
DatabaseLayout,
DocsLayout,
TableEditorLayout,
SQLEditorLayout,
SettingsLayout,
StorageLayout,
AccountLayout,
WizardLayout,
VercelIntegrationLayout,
}
export default ProjectLayout
| studio/components/layouts/index.ts | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.0001732703240122646,
0.00017180187569465488,
0.000170670376974158,
0.0001714648969937116,
0.0000010878395642066607
] |
{
"id": 0,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/invite`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/invite.ts",
"type": "replace",
"edit_start_line_idx": 23
} | # See https://help.github.com/articles/ignoring-files/ for more about ignoring files.
# dependencies
/node_modules
/.pnp
.pnp.js
# testing
/coverage
# next.js
/.next/
/out/
# production
/build
# misc
.DS_Store
*.pem
# debug
npm-debug.log*
yarn-debug.log*
yarn-error.log*
# local env files
.env.local
.env.development.local
.env.test.local
.env.production.local
# vercel
.vercel
| examples/nextjs-todo-list/.gitignore | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.0001726546761346981,
0.00016748227062635124,
0.00016443002095911652,
0.00016642219270579517,
0.0000030968453756941017
] |
{
"id": 1,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/magiclink`\n",
" const payload = { email: req.body.email }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/magiclink.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { NextApiRequest, NextApiResponse } from 'next'
import SqlString from 'sqlstring'
import apiWrapper from 'lib/api/apiWrapper'
import { constructHeaders } from 'lib/api/apiHelpers'
import { post } from 'lib/common/fetch'
import { tryParseInt } from 'lib/helpers'
export default (req: NextApiRequest, res: NextApiResponse) => apiWrapper(req, res, handler)
async function handler(req: NextApiRequest, res: NextApiResponse) {
const { method } = req
switch (method) {
case 'POST':
return handlePost(req, res)
default:
res.setHeader('Allow', ['POST'])
res.status(405).json({ data: null, error: { message: `Method ${method} Not Allowed` } })
}
}
const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {
const headers = constructHeaders({
'Content-Type': 'application/json',
Accept: 'application/json',
Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,
})
const url = `${process.env.SUPABASE_URL}/auth/v1/recover`
const payload = { email: req.body.email }
const response = await post(url, payload, { headers })
return res.status(200).json(response)
}
| studio/pages/api/auth/[ref]/recover.ts | 1 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.9982525706291199,
0.7511687278747559,
0.01360372919589281,
0.9964093565940857,
0.4258359968662262
] |
{
"id": 1,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/magiclink`\n",
" const payload = { email: req.body.email }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/magiclink.ts",
"type": "replace",
"edit_start_line_idx": 26
} | module.exports = {
moduleNameMapper: {
'@core/(.*)': '<rootDir>/src/app/core/$1',
},
preset: 'jest-preset-angular',
setupFilesAfterEnv: ['<rootDir>/setup-jest.ts'],
}
| examples/angular-todo-list/jest.config.js | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00017320606275461614,
0.00017320606275461614,
0.00017320606275461614,
0.00017320606275461614,
0
] |
{
"id": 1,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/magiclink`\n",
" const payload = { email: req.body.email }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/magiclink.ts",
"type": "replace",
"edit_start_line_idx": 26
} | type params = { name: string; description: string; tabs: string }
const Example = ({ name, description = '', tabs = '' }: params) =>
`
### ${name}
${description}
${tabs}
`.trim()
export default Example
| web/spec/gen/components/Example.ts | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00017025686975102872,
0.00017000771185848862,
0.00016975855396594852,
0.00017000771185848862,
2.4915789254009724e-7
] |
{
"id": 1,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/magiclink`\n",
" const payload = { email: req.body.email }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/magiclink.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { newE2EPage } from '@stencil/core/testing';
describe('app-flash-message', () => {
it('renders', async () => {
const page = await newE2EPage();
await page.setContent('<app-flash-message></app-flash-message>');
const element = await page.find('app-flash-message');
expect(element).toHaveClass('hydrated');
});
});
| examples/with-stencil/src/components/app-flash-message/app-flash-message.e2e.ts | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.0001735355326673016,
0.00016991129086818546,
0.00016628704906906933,
0.00016991129086818546,
0.0000036242417991161346
] |
{
"id": 2,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/otp`\n",
" const payload = { phone: req.body.phone }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/otp.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { NextApiRequest, NextApiResponse } from 'next'
import SqlString from 'sqlstring'
import apiWrapper from 'lib/api/apiWrapper'
import { constructHeaders } from 'lib/api/apiHelpers'
import { post } from 'lib/common/fetch'
import { tryParseInt } from 'lib/helpers'
export default (req: NextApiRequest, res: NextApiResponse) => apiWrapper(req, res, handler)
async function handler(req: NextApiRequest, res: NextApiResponse) {
const { method } = req
switch (method) {
case 'POST':
return handlePost(req, res)
default:
res.setHeader('Allow', ['POST'])
res.status(405).json({ data: null, error: { message: `Method ${method} Not Allowed` } })
}
}
const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {
const headers = constructHeaders({
'Content-Type': 'application/json',
Accept: 'application/json',
Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,
})
const url = `${process.env.SUPABASE_URL}/auth/v1/otp`
const payload = { phone: req.body.phone }
const response = await post(url, payload, { headers })
return res.status(200).json(response)
}
| studio/pages/api/auth/[ref]/otp.ts | 1 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.9983872175216675,
0.7507117390632629,
0.012122624553740025,
0.9961684942245483,
0.4264281988143921
] |
{
"id": 2,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/otp`\n",
" const payload = { phone: req.body.phone }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/otp.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import React, { useState } from 'react'
import CodeBlock from '@theme/CodeBlock'
export default function CustomCodeBlock({
header,
code,
highlight,
js,
jsHighlight,
response,
language,
}) {
const [showResponse, toggleResponse] = useState(false)
let wrapperClass = ''
if (header) wrapperClass += ' code-with-header'
if (response) wrapperClass += ' code-with-response'
return (
<>
<div className={wrapperClass}>
{header && <div className="code-header">{header}</div>}
<>
{code && (
<CodeBlock className={language || 'bash'} metastring={highlight}>
{code}
</CodeBlock>
)}
{js && <CodeBlock metastring={jsHighlight}>{js}</CodeBlock>}
</>
</div>
{response && (
<>
<div className={'code-with-header repsonse-header'}>
<a
className="code-header has-hover-pointer"
style={showResponse ? styles.responseShown : styles.responseHidden}
onClick={() => toggleResponse(!showResponse)}
>
{showResponse ? 'Hide' : 'Show'} Response
</a>
{showResponse && <CodeBlock>{response}</CodeBlock>}
</div>
</>
)}
</>
)
}
const styles = {
responseShown: {
textAlign: 'right',
display: 'block',
borderRadius: '0',
color: 'var(--custom-primary)',
borderTop: '1px solid #444',
},
responseHidden: {
textAlign: 'right',
display: 'block',
borderBottom: 'none',
borderRadius: '0 0 4px 4px',
borderTop: '1px solid #444',
color: '#ccc',
},
}
| about/src/components/CustomCodeBlock.js | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00017638198914937675,
0.0001719138672342524,
0.00016779331781435758,
0.00017176124674733728,
0.0000024419605324510485
] |
{
"id": 2,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/otp`\n",
" const payload = { phone: req.body.phone }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/otp.ts",
"type": "replace",
"edit_start_line_idx": 26
} | {
"paul_copplestone": {
"name": "Paul Copplestone",
"job_title": "CEO and Co-Founder",
"username": "kiwicopple",
"avatar_url": "https://avatars0.githubusercontent.com/u/10214025?v=4"
},
"anthony_wilson": {
"name": "Anthony Wilson",
"job_title": "CTO and Co-Founder",
"username": "awalias",
"avatar_url": "https://avatars3.githubusercontent.com/u/458736?v=4"
},
"rory": {
"name": "Rory Wilding",
"job_title": "Head of Growth",
"username": "roryw10",
"avatar_url": "https://avatars0.githubusercontent.com/u/10214025?v=4"
}
}
| www/data/Authors.json | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00017865432891994715,
0.00017583118460606784,
0.00017430217121727765,
0.00017453702457714826,
0.000001998572543016053
] |
{
"id": 2,
"code_window": [
"const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {\n",
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/otp`\n",
" const payload = { phone: req.body.phone }\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/otp.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { FC, useState, useEffect } from 'react'
import { find, isUndefined } from 'lodash'
import { Query, Dictionary } from '@supabase/grid'
import { PostgresRelationship, PostgresTable, PostgresColumn } from '@supabase/postgres-meta'
import { useStore } from 'hooks'
import { RowEditor, ColumnEditor, TableEditor } from '.'
import { ImportContent } from './TableEditor/TableEditor.types'
import { ColumnField, CreateColumnPayload, UpdateColumnPayload } from './SidePanelEditor.types'
import ConfirmationModal from 'components/ui/ConfirmationModal'
interface Props {
selectedSchema: string
selectedTable?: PostgresTable
selectedRowToEdit?: Dictionary<any>
selectedColumnToEdit?: PostgresColumn
selectedTableToEdit?: PostgresTable
sidePanelKey?: 'row' | 'column' | 'table'
isDuplicating?: boolean
closePanel: () => void
onRowCreated?: (row: Dictionary<any>) => void
onRowUpdated?: (row: Dictionary<any>, idx: number) => void
// Because the panel is shared between grid editor and database pages
// Both require different responses upon success of these events
onTableCreated?: (table: PostgresTable) => void
onColumnSaved?: () => void
}
const SidePanelEditor: FC<Props> = ({
selectedSchema,
selectedTable,
selectedRowToEdit,
selectedColumnToEdit,
selectedTableToEdit,
sidePanelKey,
isDuplicating = false,
closePanel,
onRowCreated = () => {},
onRowUpdated = () => {},
onTableCreated = () => {},
onColumnSaved = () => {},
}) => {
const { meta, ui } = useStore()
const [enumTypes, setEnumTypes] = useState<any[]>([])
const [isEdited, setIsEdited] = useState<boolean>(false)
const [isClosingPanel, setIsClosingPanel] = useState<boolean>(false)
const tables = meta.tables.list()
useEffect(() => {
let cancel = false
const fetchEnumTypes = async () => {
const enumTypes = await meta.schemas.getEnums()
if (!cancel) setEnumTypes(enumTypes)
}
fetchEnumTypes()
return () => {
cancel = true
}
}, [])
const saveRow = async (
payload: any,
isNewRecord: boolean,
configuration: { identifiers: any; rowIdx: number },
resolve: any
) => {
let saveRowError = false
if (isNewRecord) {
const insertQuery = new Query()
.from(selectedTable!.name, selectedTable!.schema)
.insert([payload], { returning: true })
.toSql()
const res: any = await meta.query(insertQuery)
if (res.error) {
saveRowError = true
ui.setNotification({ category: 'error', message: res.error?.message })
} else {
onRowCreated(res[0])
}
} else {
if (selectedTable!.primary_keys.length > 0) {
const updateQuery = new Query()
.from(selectedTable!.name, selectedTable!.schema)
.update(payload, { returning: true })
.match(configuration.identifiers)
.toSql()
const res: any = await meta.query(updateQuery)
if (res.error) {
saveRowError = true
ui.setNotification({ category: 'error', message: res.error?.message })
} else {
onRowUpdated(res[0], configuration.rowIdx)
}
} else {
saveRowError = true
ui.setNotification({
category: 'error',
message:
"We can't make changes to this table because there is no primary key. Please create a primary key and try again.",
})
}
}
resolve()
if (!saveRowError) {
setIsEdited(false)
closePanel()
}
}
const saveColumn = async (
payload: CreateColumnPayload | UpdateColumnPayload,
foreignKey: Partial<PostgresRelationship> | undefined,
isNewRecord: boolean,
configuration: { columnId?: string },
resolve: any
) => {
const response = isNewRecord
? await meta.createColumn(payload as CreateColumnPayload, foreignKey)
: await meta.updateColumn(
configuration.columnId as string,
payload as UpdateColumnPayload,
selectedTable as PostgresTable,
foreignKey
)
if (response?.error) {
ui.setNotification({ category: 'error', message: response.error.message })
} else {
await meta.tables.loadById(selectedTable!.id)
onColumnSaved()
setIsEdited(false)
closePanel()
}
resolve()
}
const saveTable = async (
payload: any,
columns: ColumnField[],
isNewRecord: boolean,
configuration: {
tableId?: number
importContent?: ImportContent
isRLSEnabled: boolean
isDuplicateRows: boolean
},
resolve: any
) => {
let toastId
let saveTableError = false
const { tableId, importContent, isRLSEnabled, isDuplicateRows } = configuration
try {
if (isDuplicating) {
const duplicateTable = find(tables, { id: tableId }) as PostgresTable
toastId = ui.setNotification({
category: 'loading',
message: `Duplicating table: ${duplicateTable.name}...`,
})
const table: any = await meta.duplicateTable(payload, {
isRLSEnabled,
isDuplicateRows,
duplicateTable,
})
ui.setNotification({
id: toastId,
category: 'success',
message: `Table ${duplicateTable.name} has been successfully duplicated into ${table.name}!`,
})
onTableCreated(table)
} else if (isNewRecord) {
toastId = ui.setNotification({
category: 'loading',
message: `Creating new table: ${payload.name}...`,
})
const table = await meta.createTable(toastId, payload, isRLSEnabled, columns, importContent)
ui.setNotification({
id: toastId,
category: 'success',
message: `Table ${table.name} is good to go!`,
})
onTableCreated(table)
} else if (selectedTableToEdit) {
toastId = ui.setNotification({
category: 'loading',
message: `Updating table: ${selectedTableToEdit?.name}...`,
})
const table: any = await meta.updateTable(toastId, selectedTableToEdit, payload, columns)
ui.setNotification({
id: toastId,
category: 'success',
message: `Successfully updated ${table.name}!`,
})
}
} catch (error: any) {
saveTableError = true
ui.setNotification({ id: toastId, category: 'error', message: error.message })
}
if (!saveTableError) {
setIsEdited(false)
closePanel()
}
resolve()
}
const onClosePanel = () => {
if (isEdited) {
setIsClosingPanel(true)
} else {
closePanel()
}
}
return (
<>
{!isUndefined(selectedTable) && (
<RowEditor
row={selectedRowToEdit}
selectedTable={selectedTable}
visible={sidePanelKey === 'row'}
closePanel={onClosePanel}
saveChanges={saveRow}
updateEditorDirty={() => setIsEdited(true)}
/>
)}
{!isUndefined(selectedTable) && (
<ColumnEditor
enumTypes={enumTypes}
tables={tables}
column={selectedColumnToEdit}
selectedTable={selectedTable}
visible={sidePanelKey === 'column'}
closePanel={onClosePanel}
saveChanges={saveColumn}
updateEditorDirty={() => setIsEdited(true)}
/>
)}
<TableEditor
table={selectedTableToEdit}
tables={tables}
enumTypes={enumTypes}
selectedSchema={selectedSchema}
isDuplicating={isDuplicating}
visible={sidePanelKey === 'table'}
closePanel={onClosePanel}
saveChanges={saveTable}
updateEditorDirty={() => setIsEdited(true)}
/>
<ConfirmationModal
visible={isClosingPanel}
title="Confirm to close"
description="There are unsaved changes. Are you sure you want to close the panel? Your changes will be lost."
buttonLabel="Confirm"
onSelectCancel={() => setIsClosingPanel(false)}
onSelectConfirm={() => {
setIsClosingPanel(false)
setIsEdited(false)
closePanel()
}}
/>
</>
)
}
export default SidePanelEditor
| studio/components/interfaces/TableGridEditor/SidePanelEditor/SidePanelEditor.tsx | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.9739635586738586,
0.08712302148342133,
0.00016780100122559816,
0.00017491821199655533,
0.25658077001571655
] |
{
"id": 3,
"code_window": [
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/recover`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/recover.ts",
"type": "replace",
"edit_start_line_idx": 26
} | import { NextApiRequest, NextApiResponse } from 'next'
import apiWrapper from 'lib/api/apiWrapper'
import { constructHeaders } from 'lib/api/apiHelpers'
import { post } from 'lib/common/fetch'
export default (req: NextApiRequest, res: NextApiResponse) => apiWrapper(req, res, handler)
async function handler(req: NextApiRequest, res: NextApiResponse) {
const { method } = req
switch (method) {
case 'POST':
return handlePost(req, res)
default:
res.setHeader('Allow', ['POST'])
res.status(405).json({ data: null, error: { message: `Method ${method} Not Allowed` } })
}
}
const handlePost = async (req: NextApiRequest, res: NextApiResponse) => {
const headers = constructHeaders({
'Content-Type': 'application/json',
Accept: 'application/json',
Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,
})
const url = `${process.env.SUPABASE_URL}/auth/v1/invite`
const payload = { email: req.body.email }
const response = await post(url, payload, { headers })
return res.status(200).json(response)
}
| studio/pages/api/auth/[ref]/invite.ts | 1 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.9974357485771179,
0.2495890110731125,
0.0001659408153500408,
0.00037717429222539067,
0.4317695200443268
] |
{
"id": 3,
"code_window": [
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/recover`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/recover.ts",
"type": "replace",
"edit_start_line_idx": 26
} | # React Native Todo List example with Expo
## Requirements
- Install the [Expo CLI](https://docs.expo.io/get-started/installation/)
## Setup & run locally
### 1. Create new project
Sign up to Supabase - [https://app.supabase.io](https://app.supabase.io) and create a new project. Wait for your database to start.
### 2. Run "Todo List" Quickstart
Once your database has started, run the "Todo List" quickstart. Inside of your project, enter the `SQL editor` tab and scroll down until you see `TODO LIST: Build a basic todo list with Row Level Security`.
### 3. Get the URL and Key
Go to the Project Settings (the cog icon), open the API tab, and find your API URL and `anon` key, you'll need these in the next step.
The `anon` key is your client-side API key. It allows "anonymous access" to your database, until the user has logged in. Once they have logged in, the keys will switch to the user's own login token. This enables row level security for your data. Read more about this [below](#postgres-row-level-security).
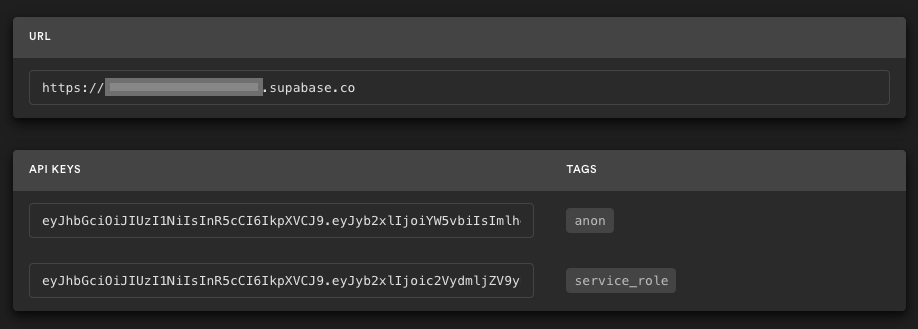
**_NOTE_**: The `service_role` key has full access to your data, bypassing any security policies. These keys have to be kept secret and are meant to be used in server environments and never on a client or browser.
Set the required environment variables:
```bash
cp .env.example .env
```
Set `REACT_NATIVE_SUPABASE_URL` and `REACT_NATIVE_SUPABASE_ANON_KEY`.
### 4. Install the dependencies & run the project:
Install the dependencies:
```bash
npm i
```
Run the project:
```bash
npm start
```
## Supabase details
### Postgres Row level security
This project uses very high-level Authorization using Postgres' Role Level Security.
When you start a Postgres database on Supabase, we populate it with an `auth` schema, and some helper functions.
When a user logs in, they are issued a JWT with the role `authenticated` and their UUID.
We can use these details to provide fine-grained control over what each user can and cannot do.
This is a trimmed-down schema, with the policies:
```sql
create table todos (
id bigint generated by default as identity primary key,
user_id uuid references auth.users not null,
task text check (char_length(task) > 3),
is_complete boolean default false,
inserted_at timestamp with time zone default timezone('utc'::text, now()) not null
);
alter table todos enable row level security;
create policy "Individuals can create todos." on todos for
insert with check (auth.uid() = user_id);
create policy "Individuals can view their own todos. " on todos for
select using (auth.uid() = user_id);
create policy "Individuals can update their own todos." on todos for
update using (auth.uid() = user_id);
create policy "Individuals can delete their own todos." on todos for
delete using (auth.uid() = user_id);
```
## Authors
- [Supabase](https://supabase.com)
Supabase is open source, we'd love for you to follow along and get involved at https://github.com/supabase/supabase
| examples/expo-todo-list/README.md | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00022381654707714915,
0.00017888833826873451,
0.00016095659520942718,
0.00016928398690652102,
0.000022021689801476896
] |
{
"id": 3,
"code_window": [
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/recover`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/recover.ts",
"type": "replace",
"edit_start_line_idx": 26
} | <?xml version="1.0" encoding="utf-8"?>
<browserconfig>
<msapplication>
<tile>
<square150x150logo src="/favicons/mstile-150x150.png"/>
<TileColor>#000000</TileColor>
</tile>
</msapplication>
</browserconfig>
| www/public/favicon/browserconfig.xml | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.00017366348765790462,
0.00017366348765790462,
0.00017366348765790462,
0.00017366348765790462,
0
] |
{
"id": 3,
"code_window": [
" const headers = constructHeaders({\n",
" 'Content-Type': 'application/json',\n",
" Accept: 'application/json',\n",
" Authorization: `Bearer ${process.env.SUPABASE_ANON_KEY}`,\n",
" })\n",
" const url = `${process.env.SUPABASE_URL}/auth/v1/recover`\n",
" const payload = { email: req.body.email }\n",
" const response = await post(url, payload, { headers })\n",
" return res.status(200).json(response)\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" Authorization: `Bearer ${process.env.SUPABASE_SERVICE_KEY}`,\n"
],
"file_path": "studio/pages/api/auth/[ref]/recover.ts",
"type": "replace",
"edit_start_line_idx": 26
} | <svg width="581" height="113" viewBox="0 0 581 113" fill="none" xmlns="http://www.w3.org/2000/svg">
<path d="M151.397 66.7608C151.996 72.3621 157.091 81.9642 171.877 81.9642C184.764 81.9642 190.959 73.7624 190.959 65.7607C190.959 58.559 186.063 52.6577 176.373 50.6571L169.379 49.1569C166.682 48.6568 164.884 47.1565 164.884 44.7559C164.884 41.9552 167.681 39.8549 171.178 39.8549C176.772 39.8549 178.87 43.5556 179.27 46.4564L190.359 43.9558C189.76 38.6546 185.064 29.7527 171.078 29.7527C160.488 29.7527 152.696 37.0543 152.696 45.8561C152.696 52.7576 156.991 58.4591 166.482 60.5594L172.976 62.0598C176.772 62.8599 178.271 64.6605 178.271 66.8609C178.271 69.4615 176.173 71.762 171.777 71.762C165.983 71.762 163.085 68.1611 162.786 64.2602L151.397 66.7608Z" fill="white"/>
<path d="M233.421 80.4639H246.109C245.909 78.7635 245.609 75.3628 245.609 71.5618V31.2529H232.321V59.8592C232.321 65.5606 228.925 69.5614 223.031 69.5614C216.837 69.5614 214.039 65.1604 214.039 59.6592V31.2529H200.752V62.3599C200.752 73.0622 207.545 81.7642 219.434 81.7642C224.628 81.7642 230.325 79.7638 233.022 75.1627C233.022 77.1631 233.221 79.4636 233.421 80.4639Z" fill="white"/>
<path d="M273.076 99.4682V75.663C275.473 78.9636 280.469 81.6644 287.263 81.6644C301.149 81.6644 310.439 70.6617 310.439 55.7584C310.439 41.1553 302.148 30.1528 287.762 30.1528C280.37 30.1528 274.875 33.4534 272.677 37.2544V31.253H259.79V99.4682H273.076ZM297.352 55.8585C297.352 64.6606 291.958 69.7616 285.164 69.7616C278.372 69.7616 272.877 64.5605 272.877 55.8585C272.877 47.1566 278.372 42.0554 285.164 42.0554C291.958 42.0554 297.352 47.1566 297.352 55.8585Z" fill="white"/>
<path d="M317.964 67.0609C317.964 74.7627 324.357 81.8643 334.848 81.8643C342.139 81.8643 346.835 78.4634 349.332 74.5625C349.332 76.463 349.532 79.1635 349.832 80.4639H362.02C361.72 78.7635 361.422 75.2627 361.422 72.6622V48.4567C361.422 38.5545 355.627 29.7527 340.043 29.7527C326.855 29.7527 319.761 38.2544 318.963 45.9562L330.751 48.4567C331.151 44.1558 334.348 40.455 340.141 40.455C345.737 40.455 348.434 43.3556 348.434 46.8564C348.434 48.5568 347.536 49.9572 344.738 50.3572L332.65 52.1576C324.458 53.3579 317.964 58.2589 317.964 67.0609ZM337.644 71.962C333.349 71.962 331.25 69.1614 331.25 66.2608C331.25 62.4599 333.947 60.5594 337.345 60.0594L348.434 58.359V60.5594C348.434 69.2615 343.239 71.962 337.644 71.962Z" fill="white"/>
<path d="M387.703 80.4641V74.4627C390.299 78.6637 395.494 81.6644 402.288 81.6644C416.276 81.6644 425.467 70.5618 425.467 55.6585C425.467 41.0552 417.174 29.9528 402.788 29.9528C395.494 29.9528 390.1 33.1535 387.902 36.6541V8.04785H374.815V80.4641H387.703ZM412.178 55.7584C412.178 64.7605 406.784 69.7616 399.99 69.7616C393.297 69.7616 387.703 64.6606 387.703 55.7584C387.703 46.7564 393.297 41.8554 399.99 41.8554C406.784 41.8554 412.178 46.7564 412.178 55.7584Z" fill="white"/>
<path d="M432.99 67.0609C432.99 74.7627 439.383 81.8643 449.873 81.8643C457.165 81.8643 461.862 78.4634 464.358 74.5625C464.358 76.463 464.559 79.1635 464.858 80.4639H477.046C476.748 78.7635 476.448 75.2627 476.448 72.6622V48.4567C476.448 38.5545 470.653 29.7527 455.068 29.7527C441.881 29.7527 434.788 38.2544 433.989 45.9562L445.776 48.4567C446.177 44.1558 449.374 40.455 455.167 40.455C460.763 40.455 463.46 43.3556 463.46 46.8564C463.46 48.5568 462.561 49.9572 459.763 50.3572L447.676 52.1576C439.484 53.3579 432.99 58.2589 432.99 67.0609ZM452.671 71.962C448.375 71.962 446.276 69.1614 446.276 66.2608C446.276 62.4599 448.973 60.5594 452.371 60.0594L463.46 58.359V60.5594C463.46 69.2615 458.265 71.962 452.671 71.962Z" fill="white"/>
<path d="M485.645 66.7608C486.243 72.3621 491.339 81.9642 506.124 81.9642C519.012 81.9642 525.205 73.7624 525.205 65.7607C525.205 58.559 520.311 52.6577 510.62 50.6571L503.626 49.1569C500.929 48.6568 499.132 47.1565 499.132 44.7559C499.132 41.9552 501.928 39.8549 505.425 39.8549C511.021 39.8549 513.118 43.5556 513.519 46.4564L524.607 43.9558C524.007 38.6546 519.312 29.7527 505.326 29.7527C494.735 29.7527 486.944 37.0543 486.944 45.8561C486.944 52.7576 491.238 58.4591 500.73 60.5594L507.224 62.0598C511.021 62.8599 512.519 64.6605 512.519 66.8609C512.519 69.4615 510.421 71.762 506.025 71.762C500.23 71.762 497.334 68.1611 497.034 64.2602L485.645 66.7608Z" fill="white"/>
<path d="M545.385 50.2571C545.685 45.7562 549.482 40.5549 556.375 40.5549C563.967 40.5549 567.165 45.3561 567.365 50.2571H545.385ZM568.664 63.0601C567.065 67.4609 563.668 70.5617 557.474 70.5617C550.88 70.5617 545.385 65.8606 545.087 59.3593H580.252C580.252 59.159 580.451 57.1587 580.451 55.2582C580.451 39.4547 571.361 29.7527 556.175 29.7527C543.588 29.7527 531.998 39.9548 531.998 55.6584C531.998 72.262 543.886 81.9642 557.374 81.9642C569.462 81.9642 577.255 74.8626 579.753 66.3607L568.664 63.0601Z" fill="white"/>
<path d="M63.7076 110.284C60.8481 113.885 55.0502 111.912 54.9813 107.314L53.9738 40.0627L99.1935 40.0627C107.384 40.0627 111.952 49.5228 106.859 55.9374L63.7076 110.284Z" fill="url(#paint0_linear)"/>
<path d="M63.7076 110.284C60.8481 113.885 55.0502 111.912 54.9813 107.314L53.9738 40.0627L99.1935 40.0627C107.384 40.0627 111.952 49.5228 106.859 55.9374L63.7076 110.284Z" fill="url(#paint1_linear)" fill-opacity="0.2"/>
<path d="M45.317 2.07103C48.1765 -1.53037 53.9745 0.442937 54.0434 5.041L54.4849 72.2922H9.83113C1.64038 72.2922 -2.92775 62.8321 2.1655 56.4175L45.317 2.07103Z" fill="#3ECF8E"/>
<defs>
<linearGradient id="paint0_linear" x1="53.9738" y1="54.974" x2="94.1635" y2="71.8295" gradientUnits="userSpaceOnUse">
<stop stop-color="#249361"/>
<stop offset="1" stop-color="#3ECF8E"/>
</linearGradient>
<linearGradient id="paint1_linear" x1="36.1558" y1="30.578" x2="54.4844" y2="65.0806" gradientUnits="userSpaceOnUse">
<stop/>
<stop offset="1" stop-opacity="0"/>
</linearGradient>
</defs>
</svg>
| www/public/images/supabase-logo-wordmark--dark.svg | 0 | https://github.com/supabase/supabase/commit/7e61af3dcb85f4013d53c409eb0e240c46113d7e | [
0.0009728562436066568,
0.00043659890070557594,
0.00016573540051467717,
0.00017120498523581773,
0.000379197794245556
] |
{
"id": 0,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return 'potato';\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: 'potato',\n",
" };\n"
],
"file_path": "integration-tests/filter/my-clowny-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | 'use strict';
module.exports = function(tests) {
return new Promise(resolve => {
setTimeout(() => {
resolve(tests.filter(t => t.indexOf('foo') !== -1));
}, 500);
});
};
| integration-tests/filter/my-filter.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.18371108174324036,
0.18371108174324036,
0.18371108174324036,
0.18371108174324036,
0
] |
{
"id": 0,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return 'potato';\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: 'potato',\n",
" };\n"
],
"file_path": "integration-tests/filter/my-clowny-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | {
"name": "not-a-haste-package",
"main": "./core.js"
}
| packages/jest-runtime/src/__tests__/test_root/node_modules/not-a-haste-package/package.json | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017591977666597813,
0.00017591977666597813,
0.00017591977666597813,
0.00017591977666597813,
0
] |
{
"id": 0,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return 'potato';\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: 'potato',\n",
" };\n"
],
"file_path": "integration-tests/filter/my-clowny-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | {
"numFailedTests": 2,
"numPassedTests": 28,
"numPendingTests": 0,
"numRuntimeErrorTestSuites": 0,
"numTotalTestSuites": 5,
"numTotalTests": 30,
"startTime": 1479669116874,
"success": false,
"testResults": [
{
"message": "",
"name": "/Users/orta/dev/projects/danger/danger-js/source/runner/_tests/Executor.test.js",
"summary": "",
"status": "passed",
"startTime": 1479669117864,
"endTime": 1479669118393,
"assertionResults": [
{
"status": "passed",
"title": "gets diff / pr info in setup",
"failureMessages": []
},
{
"status": "passed",
"title": "gets diff / pr info in setup",
"failureMessages": []
},
{
"status": "passed",
"title": "Deletes a post when there are no messages",
"failureMessages": []
},
{
"status": "passed",
"title": "Updates or Creates comments for warnings",
"failureMessages": []
}
]
},
{
"message": "",
"name": "/Users/orta/dev/projects/danger/danger-js/source/platforms/_tests/GitHub.test.js",
"summary": "",
"status": "passed",
"startTime": 1479669118460,
"endTime": 1479669118481,
"assertionResults": [
{
"status": "passed",
"title": "sets the correct paths for pull request comments",
"failureMessages": []
},
{
"status": "passed",
"title": "sets the correct paths for getPullRequestDiff",
"failureMessages": []
}
]
},
{
"message": "",
"name": "/Users/orta/dev/projects/danger/danger-js/source/ci_source/_tests/_circle.test.js",
"summary": "",
"status": "passed",
"startTime": 1479669118529,
"endTime": 1479669118558,
"assertionResults": [
{
"status": "passed",
"title": "validates when all Circle environment vars are set",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate without josh",
"failureMessages": []
},
{
"status": "passed",
"title": "validates when all circle environment vars are set",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate outside of circle",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate when CIRCLE_CI_API_TOKEN is missing",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate when CIRCLE_PROJECT_USERNAME is missing",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate when CIRCLE_PROJECT_REPONAME is missing",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate when CIRCLE_BUILD_NUM is missing",
"failureMessages": []
},
{
"status": "passed",
"title": "needs to have a PR number",
"failureMessages": []
},
{
"status": "passed",
"title": "pulls it out of the env",
"failureMessages": []
},
{
"status": "passed",
"title": "can derive it from PR Url",
"failureMessages": []
},
{
"status": "passed",
"title": "derives it from the PR Url",
"failureMessages": []
}
]
},
{
"message": "",
"name": "/Users/orta/dev/projects/danger/danger-js/source/runner/templates/_tests/github-issue-templates.test.js",
"summary": "",
"status": "passed",
"startTime": 1479669118617,
"endTime": 1479669118631,
"assertionResults": [
{
"status": "passed",
"title": "shows no tables for empty results",
"failureMessages": []
},
{
"status": "passed",
"title": "Shows the failing messages in a table",
"failureMessages": []
},
{
"status": "passed",
"title": "Shows the warning messages in a table",
"failureMessages": []
}
]
},
{
"message": "\u001b[1m\u001b[31m \u001b[1m● \u001b[1m.isCI › validates when all Travis environment vars are set and Josh K says so\u001b[39m\u001b[22m\n\n \u001b[2mexpect(\u001b[22m\u001b[31mreceived\u001b[39m\u001b[2m).toBeFalsy(\u001b[22m\u001b[2m)\u001b[22m\n \n Expected value to be falsy, instead received\n \u001b[31mtrue\u001b[39m\n\u001b[2m \n \u001b[2mat Object.<anonymous> (\u001b[2m\u001b[0m\u001b[36msource/ci_source/_tests/_travis.test.js\u001b[39m\u001b[0m\u001b[2m:12:25)\u001b[2m\n \u001b[2mat process._tickCallback (\u001b[2minternal/process/next_tick.js\u001b[2m:103:7)\u001b[2m\u001b[22m\n\n\u001b[1m\u001b[31m \u001b[1m● \u001b[1m.isPR › does not validate without josh\u001b[39m\u001b[22m\n\n \u001b[2mexpect(\u001b[22m\u001b[31mreceived\u001b[39m\u001b[2m).toEqual(\u001b[22m\u001b[32mexpected\u001b[39m\u001b[2m)\u001b[22m\n \n Expected value to equal:\n \u001b[32m\"sdasdsads\"\u001b[39m\n Received:\n \u001b[31mundefined\u001b[39m\n \n Difference:\n \n Comparing two different types of values:\n Expected: \u001b[32mstring\u001b[39m\n Received: \u001b[31mundefined\u001b[39m\n\u001b[2m \n \u001b[2mat Object.<anonymous> (\u001b[2m\u001b[0m\u001b[36msource/ci_source/_tests/_travis.test.js\u001b[39m\u001b[0m\u001b[2m:30:34)\u001b[2m\n \u001b[2mat process._tickCallback (\u001b[2minternal/process/next_tick.js\u001b[2m:103:7)\u001b[2m\u001b[22m\n",
"name": "/Users/orta/dev/projects/danger/danger-js/source/ci_source/_tests/_travis.test.js",
"summary": "",
"status": "failed",
"startTime": 1479669118690,
"endTime": 1479669118945,
"assertionResults": [
{
"status": "failed",
"title": "validates when all Travis environment vars are set and Josh K says so",
"failureMessages": [
"Error: \u001b[2mexpect(\u001b[22m\u001b[31mreceived\u001b[39m\u001b[2m).toBeFalsy(\u001b[22m\u001b[2m)\u001b[22m\n\nExpected value to be falsy, instead received\n \u001b[31mtrue\u001b[39m\n at Object.<anonymous> (/Users/orta/dev/projects/danger/danger-js/source/ci_source/_tests/_travis.test.js:12:25)\n at Object.<anonymous> (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:42:32)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:68:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at Spec.queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Spec.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:372:10)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2584:37)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2569:13)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at TreeProcessor.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2431:7)\n at Env.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:780:17)\n at jasmine2 (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/index.js:96:7)\n at runTest (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-cli/build/runTest.js:50:10)\n at promise.then (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-cli/build/TestRunner.js:304:14)\n at process._tickCallback (internal/process/next_tick.js:103:7)"
]
},
{
"status": "passed",
"title": "does not validate without josh",
"failureMessages": []
},
{
"status": "passed",
"title": "validates when all Travis environment vars are set and Josh K says so",
"failureMessages": []
},
{
"status": "failed",
"title": "does not validate without josh",
"failureMessages": [
"Error: \u001b[2mexpect(\u001b[22m\u001b[31mreceived\u001b[39m\u001b[2m).toEqual(\u001b[22m\u001b[32mexpected\u001b[39m\u001b[2m)\u001b[22m\n\nExpected value to equal:\n \u001b[32m\"sdasdsads\"\u001b[39m\nReceived:\n \u001b[31mundefined\u001b[39m\n\nDifference:\n\nComparing two different types of values:\n Expected: \u001b[32mstring\u001b[39m\n Received: \u001b[31mundefined\u001b[39m\n at Object.<anonymous> (/Users/orta/dev/projects/danger/danger-js/source/ci_source/_tests/_travis.test.js:30:34)\n at Object.<anonymous> (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:42:32)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:68:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at Spec.queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Spec.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:372:10)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2584:37)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at complete (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:384:9)\n at QueueRunner.clearStack (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:681:9)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1949:12)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.<anonymous> (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:47:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:68:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at Spec.queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Spec.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:372:10)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2584:37)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2569:13)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at onComplete (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2572:17)\n at QueueRunner.clearStack (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:681:9)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1949:12)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at complete (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:384:9)\n at QueueRunner.clearStack (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:681:9)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1949:12)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.<anonymous> (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:47:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:68:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at Spec.queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Spec.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:372:10)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2584:37)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at complete (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:384:9)\n at QueueRunner.clearStack (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:681:9)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1949:12)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1987:9)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1966:16\n at /Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1909:9\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/jasmine-async.js:68:11)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at Spec.queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Spec.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:372:10)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2584:37)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at Object.fn (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2569:13)\n at attemptAsync (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1984:24)\n at QueueRunner.run (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1939:9)\n at QueueRunner.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:1927:10)\n at queueRunnerFactory (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:718:35)\n at TreeProcessor.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:2431:7)\n at Env.execute (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/vendor/jasmine-2.5.2.js:780:17)\n at jasmine2 (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-jasmine2/build/index.js:96:7)\n at runTest (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-cli/build/runTest.js:50:10)\n at promise.then (/Users/orta/dev/projects/danger/danger-js/node_modules/jest-cli/build/TestRunner.js:304:14)\n at process._tickCallback (internal/process/next_tick.js:103:7)"
]
},
{
"status": "passed",
"title": "does not validate when TRAVIS_PULL_REQUEST is missing",
"failureMessages": []
},
{
"status": "passed",
"title": "does not validate when TRAVIS_REPO_SLUG is missing",
"failureMessages": []
},
{
"status": "passed",
"title": "needs to have a PR number",
"failureMessages": []
},
{
"status": "passed",
"title": "pulls it out of the env",
"failureMessages": []
},
{
"status": "passed",
"title": "pulls it out of the env",
"failureMessages": []
}
]
}
]
} | fixtures/failing-jsons/failing_json_multiple.json | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0003290745662525296,
0.0001757164136506617,
0.00016448725364170969,
0.0001688473130343482,
0.00003351651321281679
] |
{
"id": 0,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return 'potato';\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: 'potato',\n",
" };\n"
],
"file_path": "integration-tests/filter/my-clowny-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | // Copyright 2004-present Facebook. All Rights Reserved.
/**
* This file illustrates how to do a partial mock where a subset
* of a module's exports have been mocked and the rest
* keep their actual implementation.
*/
import defaultExport, {apple, strawberry} from '../fruit';
jest.mock('../fruit', () => {
const originalModule = require.requireActual('../fruit');
const mockedModule = jest.genMockFromModule('../fruit');
//Mock the default export and named export 'apple'.
return Object.assign({}, mockedModule, originalModule, {
apple: 'mocked apple',
default: jest.fn(() => 'mocked fruit'),
});
});
it('does a partial mock', () => {
const defaultExportResult = defaultExport();
expect(defaultExportResult).toBe('mocked fruit');
expect(defaultExport).toHaveBeenCalled();
expect(apple).toBe('mocked apple');
expect(strawberry()).toBe('strawberry');
});
| examples/module-mock/__tests__/partial_mock.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017206401389557868,
0.0001688319753156975,
0.00016599035006947815,
0.00016844157653395087,
0.0000024948826649051625
] |
{
"id": 1,
"code_window": [
"module.exports = function(tests) {\n",
" return new Promise(resolve => {\n",
" setTimeout(() => {\n",
" resolve(tests.filter(t => t.indexOf('foo') !== -1));\n",
" }, 500);\n",
" });\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [
" resolve({\n",
" filtered: tests\n",
" .filter(t => t.indexOf('foo') !== -1)\n",
" .map(test => ({message: 'some message', test})),\n",
" });\n",
" }, 100);\n"
],
"file_path": "integration-tests/filter/my-filter.js",
"type": "replace",
"edit_start_line_idx": 5
} | 'use strict';
module.exports = function(tests) {
return tests.filter(t => t.indexOf('foo') !== -1);
};
| integration-tests/filter/my-secondary-filter.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.05733494088053703,
0.05733494088053703,
0.05733494088053703,
0.05733494088053703,
0
] |
{
"id": 1,
"code_window": [
"module.exports = function(tests) {\n",
" return new Promise(resolve => {\n",
" setTimeout(() => {\n",
" resolve(tests.filter(t => t.indexOf('foo') !== -1));\n",
" }, 500);\n",
" });\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [
" resolve({\n",
" filtered: tests\n",
" .filter(t => t.indexOf('foo') !== -1)\n",
" .map(test => ({message: 'some message', test})),\n",
" });\n",
" }, 100);\n"
],
"file_path": "integration-tests/filter/my-filter.js",
"type": "replace",
"edit_start_line_idx": 5
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
const fs = require('fs');
const path = require('path');
const PACKAGES_DIR = path.resolve(__dirname, '../packages');
// Get absolute paths of all directories under packages/*
module.exports = function getPackages() {
return fs
.readdirSync(PACKAGES_DIR)
.map(file => path.resolve(PACKAGES_DIR, file))
.filter(f => fs.lstatSync(path.resolve(f)).isDirectory());
};
| scripts/getPackages.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0009581735939718783,
0.0005674104322679341,
0.00017664724146015942,
0.0005674104322679341,
0.00039076319080777466
] |
{
"id": 1,
"code_window": [
"module.exports = function(tests) {\n",
" return new Promise(resolve => {\n",
" setTimeout(() => {\n",
" resolve(tests.filter(t => t.indexOf('foo') !== -1));\n",
" }, 500);\n",
" });\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [
" resolve({\n",
" filtered: tests\n",
" .filter(t => t.indexOf('foo') !== -1)\n",
" .map(test => ({message: 'some message', test})),\n",
" });\n",
" }, 100);\n"
],
"file_path": "integration-tests/filter/my-filter.js",
"type": "replace",
"edit_start_line_idx": 5
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
module.exports = 'test';
| integration-tests/resolve-node-module/__mocks__/mock-module-alt/index.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0001685090537648648,
0.0001685090537648648,
0.0001685090537648648,
0.0001685090537648648,
0
] |
{
"id": 1,
"code_window": [
"module.exports = function(tests) {\n",
" return new Promise(resolve => {\n",
" setTimeout(() => {\n",
" resolve(tests.filter(t => t.indexOf('foo') !== -1));\n",
" }, 500);\n",
" });\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"keep",
"keep"
],
"after_edit": [
" resolve({\n",
" filtered: tests\n",
" .filter(t => t.indexOf('foo') !== -1)\n",
" .map(test => ({message: 'some message', test})),\n",
" });\n",
" }, 100);\n"
],
"file_path": "integration-tests/filter/my-filter.js",
"type": "replace",
"edit_start_line_idx": 5
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`creates a snapshot summary 1`] = `
"<bold>Snapshot Summary</>
<bold><green> › 1 snapshot written </></>from 1 test suite.
<bold><red> › 1 snapshot failed</></> from 1 test suite. <dim>Inspect your code changes or press --u to update them.</>
<bold><green> › 1 snapshot updated </></>from 1 test suite.
<bold><yellow> › 1 snapshot file obsolete </></>from 1 test suite. <dim>To remove it, press --u.</>
<bold><yellow> › 1 snapshot obsolete </></>from 1 test suite. <dim>To remove it, press --u.</>
↳ <dim>../path/to/</><bold>suite_one</>
• unchecked snapshot 1"
`;
exports[`creates a snapshot summary after an update 1`] = `
"<bold>Snapshot Summary</>
<bold><green> › 1 snapshot written </></>from 1 test suite.
<bold><red> › 1 snapshot failed</></> from 1 test suite. <dim>Inspect your code changes or press --u to update them.</>
<bold><green> › 1 snapshot updated </></>from 1 test suite.
<bold><green> › 1 snapshot file removed </></>from 1 test suite.
<bold><green> › 1 snapshot removed </></>from 1 test suite.
↳ <dim>../path/to/</><bold>suite_one</>
• unchecked snapshot 1"
`;
exports[`creates a snapshot summary with multiple snapshot being written/updated 1`] = `
"<bold>Snapshot Summary</>
<bold><green> › 2 snapshots written </></>from 2 test suites.
<bold><red> › 2 snapshots failed</></> from 2 test suites. <dim>Inspect your code changes or press --u to update them.</>
<bold><green> › 2 snapshots updated </></>from 2 test suites.
<bold><yellow> › 2 snapshot files obsolete </></>from 2 test suites. <dim>To remove them all, press --u.</>
<bold><yellow> › 2 snapshots obsolete </></>from 2 test suites. <dim>To remove them all, press --u.</>
↳ <dim>../path/to/</><bold>suite_one</>
• unchecked snapshot 1
↳ <dim>../path/to/</><bold>suite_two</>
• unchecked snapshot 2"
`;
exports[`returns nothing if there are no updates 1`] = `"<bold>Snapshot Summary</>"`;
| packages/jest-cli/src/reporters/__tests__/__snapshots__/get_snapshot_summary.test.js.snap | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00021924986504018307,
0.00018584507051855326,
0.00016429665265604854,
0.0001799168821889907,
0.00002174294786527753
] |
{
"id": 2,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return tests.filter(t => t.indexOf('foo') !== -1);\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: tests.filter(t => t.indexOf('foo') !== -1).map(test => ({test})),\n",
" };\n"
],
"file_path": "integration-tests/filter/my-secondary-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
import type {Context} from 'types/Context';
import type {Glob, GlobalConfig, Path} from 'types/Config';
import type {Test} from 'types/TestRunner';
import type {ChangedFilesPromise} from 'types/ChangedFiles';
import path from 'path';
import micromatch from 'micromatch';
import DependencyResolver from 'jest-resolve-dependencies';
import testPathPatternToRegExp from './test_path_pattern_to_regexp';
import {escapePathForRegex} from 'jest-regex-util';
import {replaceRootDirInPath} from 'jest-config';
type SearchResult = {|
noSCM?: boolean,
stats?: {[key: string]: number},
collectCoverageFrom?: Array<string>,
tests: Array<Test>,
total?: number,
|};
export type TestSelectionConfig = {|
input?: string,
findRelatedTests?: boolean,
onlyChanged?: boolean,
paths?: Array<Path>,
shouldTreatInputAsPattern?: boolean,
testPathPattern?: string,
watch?: boolean,
|};
const globsToMatcher = (globs: ?Array<Glob>) => {
if (globs == null || globs.length === 0) {
return () => true;
}
const matchers = globs.map(each => micromatch.matcher(each, {dot: true}));
return path => matchers.some(each => each(path));
};
const regexToMatcher = (testRegex: string) => {
if (!testRegex) {
return () => true;
}
const regex = new RegExp(testRegex);
return path => regex.test(path);
};
const toTests = (context, tests) =>
tests.map(path => ({
context,
duration: undefined,
path,
}));
export default class SearchSource {
_context: Context;
_rootPattern: RegExp;
_testIgnorePattern: ?RegExp;
_testPathCases: {
roots: (path: Path) => boolean,
testMatch: (path: Path) => boolean,
testRegex: (path: Path) => boolean,
testPathIgnorePatterns: (path: Path) => boolean,
};
constructor(context: Context) {
const {config} = context;
this._context = context;
this._rootPattern = new RegExp(
config.roots.map(dir => escapePathForRegex(dir + path.sep)).join('|'),
);
const ignorePattern = config.testPathIgnorePatterns;
this._testIgnorePattern = ignorePattern.length
? new RegExp(ignorePattern.join('|'))
: null;
this._testPathCases = {
roots: path => this._rootPattern.test(path),
testMatch: globsToMatcher(config.testMatch),
testPathIgnorePatterns: path =>
!this._testIgnorePattern || !this._testIgnorePattern.test(path),
testRegex: regexToMatcher(config.testRegex),
};
}
_filterTestPathsWithStats(
allPaths: Array<Test>,
testPathPattern?: string,
): SearchResult {
const data = {
stats: {},
tests: [],
total: allPaths.length,
};
const testCases = Object.assign({}, this._testPathCases);
if (testPathPattern) {
const regex = testPathPatternToRegExp(testPathPattern);
testCases.testPathPattern = path => regex.test(path);
}
const testCasesKeys = Object.keys(testCases);
data.tests = allPaths.filter(test => {
return testCasesKeys.reduce((flag, key) => {
if (testCases[key](test.path)) {
data.stats[key] = ++data.stats[key] || 1;
return flag && true;
}
data.stats[key] = data.stats[key] || 0;
return false;
}, true);
});
return data;
}
_getAllTestPaths(testPathPattern: string): SearchResult {
return this._filterTestPathsWithStats(
toTests(this._context, this._context.hasteFS.getAllFiles()),
testPathPattern,
);
}
isTestFilePath(path: Path): boolean {
return Object.keys(this._testPathCases).every(key =>
this._testPathCases[key](path),
);
}
findMatchingTests(testPathPattern: string): SearchResult {
return this._getAllTestPaths(testPathPattern);
}
findRelatedTests(
allPaths: Set<Path>,
collectCoverage: boolean,
): SearchResult {
const dependencyResolver = new DependencyResolver(
this._context.resolver,
this._context.hasteFS,
);
const tests = toTests(
this._context,
dependencyResolver.resolveInverse(
allPaths,
this.isTestFilePath.bind(this),
{
skipNodeResolution: this._context.config.skipNodeResolution,
},
),
);
let collectCoverageFrom;
// If we are collecting coverage, also return collectCoverageFrom patterns
if (collectCoverage) {
collectCoverageFrom = Array.from(allPaths).map(filename => {
filename = replaceRootDirInPath(this._context.config.rootDir, filename);
return path.isAbsolute(filename)
? path.relative(this._context.config.rootDir, filename)
: filename;
});
}
return {collectCoverageFrom, tests};
}
findTestsByPaths(paths: Array<Path>): SearchResult {
return {
tests: toTests(
this._context,
paths
.map(p => path.resolve(process.cwd(), p))
.filter(this.isTestFilePath.bind(this)),
),
};
}
findRelatedTestsFromPattern(
paths: Array<Path>,
collectCoverage: boolean,
): SearchResult {
if (Array.isArray(paths) && paths.length) {
const resolvedPaths = paths.map(p => path.resolve(process.cwd(), p));
return this.findRelatedTests(new Set(resolvedPaths), collectCoverage);
}
return {tests: []};
}
async findTestRelatedToChangedFiles(
changedFilesPromise: ChangedFilesPromise,
collectCoverage: boolean,
) {
const {repos, changedFiles} = await changedFilesPromise;
// no SCM (git/hg/...) is found in any of the roots.
const noSCM = Object.keys(repos).every(scm => repos[scm].size === 0);
return noSCM
? {noSCM: true, tests: []}
: this.findRelatedTests(changedFiles, collectCoverage);
}
_getTestPaths(
globalConfig: GlobalConfig,
changedFilesPromise: ?ChangedFilesPromise,
): Promise<SearchResult> {
const paths = globalConfig.nonFlagArgs;
if (globalConfig.onlyChanged) {
if (!changedFilesPromise) {
throw new Error('This promise must be present when running with -o.');
}
return this.findTestRelatedToChangedFiles(
changedFilesPromise,
globalConfig.collectCoverage,
);
} else if (globalConfig.runTestsByPath && paths && paths.length) {
return Promise.resolve(this.findTestsByPaths(paths));
} else if (globalConfig.findRelatedTests && paths && paths.length) {
return Promise.resolve(
this.findRelatedTestsFromPattern(paths, globalConfig.collectCoverage),
);
} else if (globalConfig.testPathPattern != null) {
return Promise.resolve(
this.findMatchingTests(globalConfig.testPathPattern),
);
} else {
return Promise.resolve({tests: []});
}
}
async getTestPaths(
globalConfig: GlobalConfig,
changedFilesPromise: ?ChangedFilesPromise,
): Promise<SearchResult> {
const searchResult = await this._getTestPaths(
globalConfig,
changedFilesPromise,
);
const filterPath = globalConfig.filter;
if (filterPath && !globalConfig.skipFilter) {
const tests = searchResult.tests;
// $FlowFixMe: dynamic require.
const filter = require(filterPath);
const filtered = await filter(tests.map(test => test.path));
if (!Array.isArray(filtered)) {
throw new Error(
`Filter ${filterPath} did not return a valid test list`,
);
}
const filteredSet = new Set(filtered);
// $FlowFixMe: Object.assign with empty object causes troubles to Flow.
return Object.assign({}, searchResult, {
tests: tests.filter(test => filteredSet.has(test.path)),
});
}
return searchResult;
}
}
| packages/jest-cli/src/search_source.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0808863565325737,
0.003959120716899633,
0.00016769638750702143,
0.00017534097423776984,
0.014981906861066818
] |
{
"id": 2,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return tests.filter(t => t.indexOf('foo') !== -1);\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: tests.filter(t => t.indexOf('foo') !== -1).map(test => ({test})),\n",
" };\n"
],
"file_path": "integration-tests/filter/my-secondary-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
import cli from 'jest-cli';
module.exports = cli;
| packages/jest/src/jest.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.014643344096839428,
0.007410040125250816,
0.00017673619731795043,
0.007410040125250816,
0.007233303971588612
] |
{
"id": 2,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return tests.filter(t => t.indexOf('foo') !== -1);\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: tests.filter(t => t.indexOf('foo') !== -1).map(test => ({test})),\n",
" };\n"
],
"file_path": "integration-tests/filter/my-secondary-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
*/
// This file is a heavily modified fork of Jasmine. Original license:
/*
Copyright (c) 2008-2016 Pivotal Labs
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
"Software"), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
/* @flow */
/* eslint-disable sort-keys */
const defaultNow = (function(Date) {
return function() {
return new Date().getTime();
};
})(Date);
export default function Timer(options: Object) {
options = options || {};
const now = options.now || defaultNow;
let startTime;
this.start = function() {
startTime = now();
};
this.elapsed = function() {
return now() - startTime;
};
}
| packages/jest-jasmine2/src/jasmine/Timer.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0001789649686543271,
0.0001746907946653664,
0.00017073792696464807,
0.00017438491340726614,
0.0000028042884423484793
] |
{
"id": 2,
"code_window": [
"'use strict';\n",
"\n",
"module.exports = function(tests) {\n",
" return tests.filter(t => t.indexOf('foo') !== -1);\n",
"};"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" return {\n",
" filtered: tests.filter(t => t.indexOf('foo') !== -1).map(test => ({test})),\n",
" };\n"
],
"file_path": "integration-tests/filter/my-secondary-filter.js",
"type": "replace",
"edit_start_line_idx": 3
} | packages/jest-resolve/src/__mocks__/bar/node_modules/bar/index.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017995163216255605,
0.00017995163216255605,
0.00017995163216255605,
0.00017995163216255605,
0
] |
|
{
"id": 3,
"code_window": [
" watch?: boolean,\n",
"|};\n",
"\n",
"const globsToMatcher = (globs: ?Array<Glob>) => {\n",
" if (globs == null || globs.length === 0) {\n",
" return () => true;\n",
" }\n",
"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FilterResult = {\n",
" test: string,\n",
" message: string,\n",
"};\n",
"\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "add",
"edit_start_line_idx": 39
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
import type {Context} from 'types/Context';
import type {Glob, GlobalConfig, Path} from 'types/Config';
import type {Test} from 'types/TestRunner';
import type {ChangedFilesPromise} from 'types/ChangedFiles';
import path from 'path';
import micromatch from 'micromatch';
import DependencyResolver from 'jest-resolve-dependencies';
import testPathPatternToRegExp from './test_path_pattern_to_regexp';
import {escapePathForRegex} from 'jest-regex-util';
import {replaceRootDirInPath} from 'jest-config';
type SearchResult = {|
noSCM?: boolean,
stats?: {[key: string]: number},
collectCoverageFrom?: Array<string>,
tests: Array<Test>,
total?: number,
|};
export type TestSelectionConfig = {|
input?: string,
findRelatedTests?: boolean,
onlyChanged?: boolean,
paths?: Array<Path>,
shouldTreatInputAsPattern?: boolean,
testPathPattern?: string,
watch?: boolean,
|};
const globsToMatcher = (globs: ?Array<Glob>) => {
if (globs == null || globs.length === 0) {
return () => true;
}
const matchers = globs.map(each => micromatch.matcher(each, {dot: true}));
return path => matchers.some(each => each(path));
};
const regexToMatcher = (testRegex: string) => {
if (!testRegex) {
return () => true;
}
const regex = new RegExp(testRegex);
return path => regex.test(path);
};
const toTests = (context, tests) =>
tests.map(path => ({
context,
duration: undefined,
path,
}));
export default class SearchSource {
_context: Context;
_rootPattern: RegExp;
_testIgnorePattern: ?RegExp;
_testPathCases: {
roots: (path: Path) => boolean,
testMatch: (path: Path) => boolean,
testRegex: (path: Path) => boolean,
testPathIgnorePatterns: (path: Path) => boolean,
};
constructor(context: Context) {
const {config} = context;
this._context = context;
this._rootPattern = new RegExp(
config.roots.map(dir => escapePathForRegex(dir + path.sep)).join('|'),
);
const ignorePattern = config.testPathIgnorePatterns;
this._testIgnorePattern = ignorePattern.length
? new RegExp(ignorePattern.join('|'))
: null;
this._testPathCases = {
roots: path => this._rootPattern.test(path),
testMatch: globsToMatcher(config.testMatch),
testPathIgnorePatterns: path =>
!this._testIgnorePattern || !this._testIgnorePattern.test(path),
testRegex: regexToMatcher(config.testRegex),
};
}
_filterTestPathsWithStats(
allPaths: Array<Test>,
testPathPattern?: string,
): SearchResult {
const data = {
stats: {},
tests: [],
total: allPaths.length,
};
const testCases = Object.assign({}, this._testPathCases);
if (testPathPattern) {
const regex = testPathPatternToRegExp(testPathPattern);
testCases.testPathPattern = path => regex.test(path);
}
const testCasesKeys = Object.keys(testCases);
data.tests = allPaths.filter(test => {
return testCasesKeys.reduce((flag, key) => {
if (testCases[key](test.path)) {
data.stats[key] = ++data.stats[key] || 1;
return flag && true;
}
data.stats[key] = data.stats[key] || 0;
return false;
}, true);
});
return data;
}
_getAllTestPaths(testPathPattern: string): SearchResult {
return this._filterTestPathsWithStats(
toTests(this._context, this._context.hasteFS.getAllFiles()),
testPathPattern,
);
}
isTestFilePath(path: Path): boolean {
return Object.keys(this._testPathCases).every(key =>
this._testPathCases[key](path),
);
}
findMatchingTests(testPathPattern: string): SearchResult {
return this._getAllTestPaths(testPathPattern);
}
findRelatedTests(
allPaths: Set<Path>,
collectCoverage: boolean,
): SearchResult {
const dependencyResolver = new DependencyResolver(
this._context.resolver,
this._context.hasteFS,
);
const tests = toTests(
this._context,
dependencyResolver.resolveInverse(
allPaths,
this.isTestFilePath.bind(this),
{
skipNodeResolution: this._context.config.skipNodeResolution,
},
),
);
let collectCoverageFrom;
// If we are collecting coverage, also return collectCoverageFrom patterns
if (collectCoverage) {
collectCoverageFrom = Array.from(allPaths).map(filename => {
filename = replaceRootDirInPath(this._context.config.rootDir, filename);
return path.isAbsolute(filename)
? path.relative(this._context.config.rootDir, filename)
: filename;
});
}
return {collectCoverageFrom, tests};
}
findTestsByPaths(paths: Array<Path>): SearchResult {
return {
tests: toTests(
this._context,
paths
.map(p => path.resolve(process.cwd(), p))
.filter(this.isTestFilePath.bind(this)),
),
};
}
findRelatedTestsFromPattern(
paths: Array<Path>,
collectCoverage: boolean,
): SearchResult {
if (Array.isArray(paths) && paths.length) {
const resolvedPaths = paths.map(p => path.resolve(process.cwd(), p));
return this.findRelatedTests(new Set(resolvedPaths), collectCoverage);
}
return {tests: []};
}
async findTestRelatedToChangedFiles(
changedFilesPromise: ChangedFilesPromise,
collectCoverage: boolean,
) {
const {repos, changedFiles} = await changedFilesPromise;
// no SCM (git/hg/...) is found in any of the roots.
const noSCM = Object.keys(repos).every(scm => repos[scm].size === 0);
return noSCM
? {noSCM: true, tests: []}
: this.findRelatedTests(changedFiles, collectCoverage);
}
_getTestPaths(
globalConfig: GlobalConfig,
changedFilesPromise: ?ChangedFilesPromise,
): Promise<SearchResult> {
const paths = globalConfig.nonFlagArgs;
if (globalConfig.onlyChanged) {
if (!changedFilesPromise) {
throw new Error('This promise must be present when running with -o.');
}
return this.findTestRelatedToChangedFiles(
changedFilesPromise,
globalConfig.collectCoverage,
);
} else if (globalConfig.runTestsByPath && paths && paths.length) {
return Promise.resolve(this.findTestsByPaths(paths));
} else if (globalConfig.findRelatedTests && paths && paths.length) {
return Promise.resolve(
this.findRelatedTestsFromPattern(paths, globalConfig.collectCoverage),
);
} else if (globalConfig.testPathPattern != null) {
return Promise.resolve(
this.findMatchingTests(globalConfig.testPathPattern),
);
} else {
return Promise.resolve({tests: []});
}
}
async getTestPaths(
globalConfig: GlobalConfig,
changedFilesPromise: ?ChangedFilesPromise,
): Promise<SearchResult> {
const searchResult = await this._getTestPaths(
globalConfig,
changedFilesPromise,
);
const filterPath = globalConfig.filter;
if (filterPath && !globalConfig.skipFilter) {
const tests = searchResult.tests;
// $FlowFixMe: dynamic require.
const filter = require(filterPath);
const filtered = await filter(tests.map(test => test.path));
if (!Array.isArray(filtered)) {
throw new Error(
`Filter ${filterPath} did not return a valid test list`,
);
}
const filteredSet = new Set(filtered);
// $FlowFixMe: Object.assign with empty object causes troubles to Flow.
return Object.assign({}, searchResult, {
tests: tests.filter(test => filteredSet.has(test.path)),
});
}
return searchResult;
}
}
| packages/jest-cli/src/search_source.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.9992280006408691,
0.10705889761447906,
0.00016585666162427515,
0.00017318379832431674,
0.30819258093833923
] |
{
"id": 3,
"code_window": [
" watch?: boolean,\n",
"|};\n",
"\n",
"const globsToMatcher = (globs: ?Array<Glob>) => {\n",
" if (globs == null || globs.length === 0) {\n",
" return () => true;\n",
" }\n",
"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FilterResult = {\n",
" test: string,\n",
" message: string,\n",
"};\n",
"\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "add",
"edit_start_line_idx": 39
} | // Copyright 2004-present Facebook. All Rights Reserved.
'use strict';
jest.mock('fs');
describe('listFilesInDirectorySync', () => {
const MOCK_FILE_INFO = {
'/path/to/file1.js': 'console.log("file1 contents");',
'/path/to/file2.txt': 'file2 contents',
};
beforeEach(() => {
// Set up some mocked out file info before each test
require('fs').__setMockFiles(MOCK_FILE_INFO);
});
it('includes all files in the directory in the summary', () => {
const FileSummarizer = require('../FileSummarizer');
const fileSummary = FileSummarizer.summarizeFilesInDirectorySync(
'/path/to'
);
expect(fileSummary.length).toBe(2);
});
});
| examples/manual-mocks/__tests__/file_summarizer.test.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0001782719773473218,
0.00017394630413036793,
0.00017079069220926613,
0.00017277622828260064,
0.0000031643014608562225
] |
{
"id": 3,
"code_window": [
" watch?: boolean,\n",
"|};\n",
"\n",
"const globsToMatcher = (globs: ?Array<Glob>) => {\n",
" if (globs == null || globs.length === 0) {\n",
" return () => true;\n",
" }\n",
"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FilterResult = {\n",
" test: string,\n",
" message: string,\n",
"};\n",
"\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "add",
"edit_start_line_idx": 39
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
'use strict';
import runJest from '../runJest';
import os from 'os';
import path from 'path';
const {cleanup, writeFiles} = require('../Utils');
const SkipOnWindows = require('../../scripts/SkipOnWindows');
const DIR = path.resolve(os.tmpdir(), 'force_exit_test');
SkipOnWindows.suite();
beforeEach(() => cleanup(DIR));
afterEach(() => cleanup(DIR));
test('exits the process after test are done but before timers complete', () => {
writeFiles(DIR, {
'.watchmanconfig': '',
'__tests__/test.test.js': `
test('finishes before the timer is complete', () => {
setTimeout(() => console.log('TIMER_DONE'), 500);
});
`,
'package.json': JSON.stringify({jest: {testEnvironment: 'node'}}),
});
let stdout;
let stderr;
({stdout, stderr} = runJest(DIR));
expect(stderr).toMatch(/PASS.*test\.test\.js/);
expect(stdout).toMatch(/TIMER_DONE/);
writeFiles(DIR, {
'package.json': JSON.stringify({
jest: {forceExit: true, testEnvironment: 'node'},
}),
});
({stdout, stderr} = runJest(DIR));
expect(stderr).toMatch(/PASS.*test\.test\.js/);
expect(stdout).not.toMatch(/TIMER_DONE/);
});
| integration-tests/__tests__/force_exit.test.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0001801071484806016,
0.00017491861945018172,
0.00016827366198413074,
0.00017446381389163435,
0.000004231903403706383
] |
{
"id": 3,
"code_window": [
" watch?: boolean,\n",
"|};\n",
"\n",
"const globsToMatcher = (globs: ?Array<Glob>) => {\n",
" if (globs == null || globs.length === 0) {\n",
" return () => true;\n",
" }\n",
"\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FilterResult = {\n",
" test: string,\n",
" message: string,\n",
"};\n",
"\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "add",
"edit_start_line_idx": 39
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
import type {
MockFunctionMetadata as _MockFunctionMetadata,
ModuleMocker as _ModuleMocker,
} from 'jest-mock';
export type MockFunctionMetadata = _MockFunctionMetadata;
export type ModuleMocker = _ModuleMocker;
| types/Mock.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.000174774308106862,
0.00017312173440586776,
0.0001714691607048735,
0.00017312173440586776,
0.0000016525737009942532
] |
{
"id": 4,
"code_window": [
" if (filterPath && !globalConfig.skipFilter) {\n",
" const tests = searchResult.tests;\n",
"\n",
" // $FlowFixMe: dynamic require.\n",
" const filter = require(filterPath);\n",
" const filtered = await filter(tests.map(test => test.path));\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep"
],
"after_edit": [
" const filter: Array<FilterResult> = require(filterPath);\n",
" const filterResult = await filter(tests.map(test => test.path));\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 257
} | 'use strict';
module.exports = function(tests) {
return 'potato';
};
| integration-tests/filter/my-clowny-filter.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00041171364136971533,
0.00041171364136971533,
0.00041171364136971533,
0.00041171364136971533,
0
] |
{
"id": 4,
"code_window": [
" if (filterPath && !globalConfig.skipFilter) {\n",
" const tests = searchResult.tests;\n",
"\n",
" // $FlowFixMe: dynamic require.\n",
" const filter = require(filterPath);\n",
" const filtered = await filter(tests.map(test => test.path));\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep"
],
"after_edit": [
" const filter: Array<FilterResult> = require(filterPath);\n",
" const filterResult = await filter(tests.map(test => test.path));\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 257
} | ## 👉 [Please follow one of these issue templates](https://github.com/facebook/jest/issues/new/choose) 👈
Note: to keep the backlog clean and actionable, issues may be immediately closed
if they do not follow one of the above issue templates.
| .github/ISSUE_TEMPLATE.md | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017849172581918538,
0.00017849172581918538,
0.00017849172581918538,
0.00017849172581918538,
0
] |
{
"id": 4,
"code_window": [
" if (filterPath && !globalConfig.skipFilter) {\n",
" const tests = searchResult.tests;\n",
"\n",
" // $FlowFixMe: dynamic require.\n",
" const filter = require(filterPath);\n",
" const filtered = await filter(tests.map(test => test.path));\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep"
],
"after_edit": [
" const filter: Array<FilterResult> = require(filterPath);\n",
" const filterResult = await filter(tests.map(test => test.path));\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 257
} | // Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`for multiline test name returns test name with highlighted pattern and replaced line breaks 1`] = `"<dim>should⏎ </></>name</><dim> the ⏎function you at...</>"`;
exports[`for multiline test name returns test name with highlighted pattern and replaced line breaks 2`] = `"<dim>should⏎ </></>name</><dim> the ⏎function you at...</>"`;
exports[`for multiline test name returns test name with highlighted pattern and replaced line breaks 3`] = `"<dim>should⏎ </></>name</><dim> the ⏎function you at...</>"`;
exports[`for one line test name pattern in the middle test name with cutted tail and cutted highlighted pattern 1`] = `"<dim>should </></>nam...</>"`;
exports[`for one line test name pattern in the middle test name with cutted tail and highlighted pattern 1`] = `"<dim>should </></>name</><dim> the functi...</>"`;
exports[`for one line test name pattern in the middle test name with highlighted cutted 1`] = `"<dim>sho</></>...</>"`;
exports[`for one line test name pattern in the middle test name with highlighted pattern returns 1`] = `"<dim>should </></>name</><dim> the function you attach</>"`;
exports[`for one line test name pattern in the tail returns test name with cutted tail and cutted highlighted pattern 1`] = `"<dim>should name the function you </></>a...</>"`;
exports[`for one line test name pattern in the tail returns test name with highlighted cutted 1`] = `"<dim>sho</></>...</>"`;
exports[`for one line test name pattern in the tail returns test name with highlighted pattern 1`] = `"<dim>should name the function you </></>attach</>"`;
exports[`for one line test name with pattern in the head returns test name with cutted tail and cutted highlighted pattern 1`] = `"</>shoul...</>"`;
exports[`for one line test name with pattern in the head returns test name with cutted tail and highlighted pattern 1`] = `"</>should</><dim> name the function yo...</>"`;
exports[`for one line test name with pattern in the head returns test name with highlighted pattern 1`] = `"</>should</><dim> name the function you attach</>"`;
| packages/jest-cli/src/lib/__tests__/__snapshots__/format_test_name_by_pattern.test.js.snap | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017220992594957352,
0.0001702127483440563,
0.00016890863480512053,
0.00016951965517364442,
0.0000014340861298478558
] |
{
"id": 4,
"code_window": [
" if (filterPath && !globalConfig.skipFilter) {\n",
" const tests = searchResult.tests;\n",
"\n",
" // $FlowFixMe: dynamic require.\n",
" const filter = require(filterPath);\n",
" const filtered = await filter(tests.map(test => test.path));\n",
"\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"replace",
"keep"
],
"after_edit": [
" const filter: Array<FilterResult> = require(filterPath);\n",
" const filterResult = await filter(tests.map(test => test.path));\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 257
} | {
"jest": {
"testEnvironment": "node"
}
}
| integration-tests/custom-matcher-stack-trace/package.json | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017925498832482845,
0.00017925498832482845,
0.00017925498832482845,
0.00017925498832482845,
0
] |
{
"id": 5,
"code_window": [
"\n",
" if (!Array.isArray(filtered)) {\n",
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (!Array.isArray(filterResult.filtered)) {\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 260
} | 'use strict';
module.exports = function(tests) {
return new Promise(resolve => {
setTimeout(() => {
resolve(tests.filter(t => t.indexOf('foo') !== -1));
}, 500);
});
};
| integration-tests/filter/my-filter.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00022393801191356033,
0.00022393801191356033,
0.00022393801191356033,
0.00022393801191356033,
0
] |
{
"id": 5,
"code_window": [
"\n",
" if (!Array.isArray(filtered)) {\n",
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (!Array.isArray(filterResult.filtered)) {\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 260
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
'use strict';
module.exports = require.main;
| packages/jest-runtime/src/__tests__/test_root/modules_with_main/export_main.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017832263256423175,
0.00017789239063858986,
0.0001774621632648632,
0.00017789239063858986,
4.3023464968428016e-7
] |
{
"id": 5,
"code_window": [
"\n",
" if (!Array.isArray(filtered)) {\n",
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (!Array.isArray(filterResult.filtered)) {\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 260
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
afterAll(() => {
throw new Error('afterAll just failed!');
});
test('one', () => {});
test('two', () => {});
| integration-tests/lifecycles/__tests__/index.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017609093629289418,
0.000175426626810804,
0.00017476231732871383,
0.000175426626810804,
6.643094820901752e-7
] |
{
"id": 5,
"code_window": [
"\n",
" if (!Array.isArray(filtered)) {\n",
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n"
],
"labels": [
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" if (!Array.isArray(filterResult.filtered)) {\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 260
} | {
"name": "jest-diff",
"version": "22.4.0",
"repository": {
"type": "git",
"url": "https://github.com/facebook/jest.git"
},
"license": "MIT",
"main": "build/index.js",
"dependencies": {
"chalk": "^2.0.1",
"diff": "^3.2.0",
"jest-get-type": "^22.1.0",
"pretty-format": "^22.4.0"
}
}
| packages/jest-diff/package.json | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017418172501493245,
0.00017352262511849403,
0.00017286353977397084,
0.00017352262511849403,
6.590926204808056e-7
] |
{
"id": 6,
"code_window": [
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n",
" }\n",
"\n",
" const filteredSet = new Set(filtered);\n",
"\n",
" // $FlowFixMe: Object.assign with empty object causes troubles to Flow.\n",
" return Object.assign({}, searchResult, {\n",
" tests: tests.filter(test => filteredSet.has(test.path)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const filteredSet = new Set(\n",
" filterResult.filtered.map(result => result.test),\n",
" );\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 266
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
import type {Context} from 'types/Context';
import type {Glob, GlobalConfig, Path} from 'types/Config';
import type {Test} from 'types/TestRunner';
import type {ChangedFilesPromise} from 'types/ChangedFiles';
import path from 'path';
import micromatch from 'micromatch';
import DependencyResolver from 'jest-resolve-dependencies';
import testPathPatternToRegExp from './test_path_pattern_to_regexp';
import {escapePathForRegex} from 'jest-regex-util';
import {replaceRootDirInPath} from 'jest-config';
type SearchResult = {|
noSCM?: boolean,
stats?: {[key: string]: number},
collectCoverageFrom?: Array<string>,
tests: Array<Test>,
total?: number,
|};
export type TestSelectionConfig = {|
input?: string,
findRelatedTests?: boolean,
onlyChanged?: boolean,
paths?: Array<Path>,
shouldTreatInputAsPattern?: boolean,
testPathPattern?: string,
watch?: boolean,
|};
const globsToMatcher = (globs: ?Array<Glob>) => {
if (globs == null || globs.length === 0) {
return () => true;
}
const matchers = globs.map(each => micromatch.matcher(each, {dot: true}));
return path => matchers.some(each => each(path));
};
const regexToMatcher = (testRegex: string) => {
if (!testRegex) {
return () => true;
}
const regex = new RegExp(testRegex);
return path => regex.test(path);
};
const toTests = (context, tests) =>
tests.map(path => ({
context,
duration: undefined,
path,
}));
export default class SearchSource {
_context: Context;
_rootPattern: RegExp;
_testIgnorePattern: ?RegExp;
_testPathCases: {
roots: (path: Path) => boolean,
testMatch: (path: Path) => boolean,
testRegex: (path: Path) => boolean,
testPathIgnorePatterns: (path: Path) => boolean,
};
constructor(context: Context) {
const {config} = context;
this._context = context;
this._rootPattern = new RegExp(
config.roots.map(dir => escapePathForRegex(dir + path.sep)).join('|'),
);
const ignorePattern = config.testPathIgnorePatterns;
this._testIgnorePattern = ignorePattern.length
? new RegExp(ignorePattern.join('|'))
: null;
this._testPathCases = {
roots: path => this._rootPattern.test(path),
testMatch: globsToMatcher(config.testMatch),
testPathIgnorePatterns: path =>
!this._testIgnorePattern || !this._testIgnorePattern.test(path),
testRegex: regexToMatcher(config.testRegex),
};
}
_filterTestPathsWithStats(
allPaths: Array<Test>,
testPathPattern?: string,
): SearchResult {
const data = {
stats: {},
tests: [],
total: allPaths.length,
};
const testCases = Object.assign({}, this._testPathCases);
if (testPathPattern) {
const regex = testPathPatternToRegExp(testPathPattern);
testCases.testPathPattern = path => regex.test(path);
}
const testCasesKeys = Object.keys(testCases);
data.tests = allPaths.filter(test => {
return testCasesKeys.reduce((flag, key) => {
if (testCases[key](test.path)) {
data.stats[key] = ++data.stats[key] || 1;
return flag && true;
}
data.stats[key] = data.stats[key] || 0;
return false;
}, true);
});
return data;
}
_getAllTestPaths(testPathPattern: string): SearchResult {
return this._filterTestPathsWithStats(
toTests(this._context, this._context.hasteFS.getAllFiles()),
testPathPattern,
);
}
isTestFilePath(path: Path): boolean {
return Object.keys(this._testPathCases).every(key =>
this._testPathCases[key](path),
);
}
findMatchingTests(testPathPattern: string): SearchResult {
return this._getAllTestPaths(testPathPattern);
}
findRelatedTests(
allPaths: Set<Path>,
collectCoverage: boolean,
): SearchResult {
const dependencyResolver = new DependencyResolver(
this._context.resolver,
this._context.hasteFS,
);
const tests = toTests(
this._context,
dependencyResolver.resolveInverse(
allPaths,
this.isTestFilePath.bind(this),
{
skipNodeResolution: this._context.config.skipNodeResolution,
},
),
);
let collectCoverageFrom;
// If we are collecting coverage, also return collectCoverageFrom patterns
if (collectCoverage) {
collectCoverageFrom = Array.from(allPaths).map(filename => {
filename = replaceRootDirInPath(this._context.config.rootDir, filename);
return path.isAbsolute(filename)
? path.relative(this._context.config.rootDir, filename)
: filename;
});
}
return {collectCoverageFrom, tests};
}
findTestsByPaths(paths: Array<Path>): SearchResult {
return {
tests: toTests(
this._context,
paths
.map(p => path.resolve(process.cwd(), p))
.filter(this.isTestFilePath.bind(this)),
),
};
}
findRelatedTestsFromPattern(
paths: Array<Path>,
collectCoverage: boolean,
): SearchResult {
if (Array.isArray(paths) && paths.length) {
const resolvedPaths = paths.map(p => path.resolve(process.cwd(), p));
return this.findRelatedTests(new Set(resolvedPaths), collectCoverage);
}
return {tests: []};
}
async findTestRelatedToChangedFiles(
changedFilesPromise: ChangedFilesPromise,
collectCoverage: boolean,
) {
const {repos, changedFiles} = await changedFilesPromise;
// no SCM (git/hg/...) is found in any of the roots.
const noSCM = Object.keys(repos).every(scm => repos[scm].size === 0);
return noSCM
? {noSCM: true, tests: []}
: this.findRelatedTests(changedFiles, collectCoverage);
}
_getTestPaths(
globalConfig: GlobalConfig,
changedFilesPromise: ?ChangedFilesPromise,
): Promise<SearchResult> {
const paths = globalConfig.nonFlagArgs;
if (globalConfig.onlyChanged) {
if (!changedFilesPromise) {
throw new Error('This promise must be present when running with -o.');
}
return this.findTestRelatedToChangedFiles(
changedFilesPromise,
globalConfig.collectCoverage,
);
} else if (globalConfig.runTestsByPath && paths && paths.length) {
return Promise.resolve(this.findTestsByPaths(paths));
} else if (globalConfig.findRelatedTests && paths && paths.length) {
return Promise.resolve(
this.findRelatedTestsFromPattern(paths, globalConfig.collectCoverage),
);
} else if (globalConfig.testPathPattern != null) {
return Promise.resolve(
this.findMatchingTests(globalConfig.testPathPattern),
);
} else {
return Promise.resolve({tests: []});
}
}
async getTestPaths(
globalConfig: GlobalConfig,
changedFilesPromise: ?ChangedFilesPromise,
): Promise<SearchResult> {
const searchResult = await this._getTestPaths(
globalConfig,
changedFilesPromise,
);
const filterPath = globalConfig.filter;
if (filterPath && !globalConfig.skipFilter) {
const tests = searchResult.tests;
// $FlowFixMe: dynamic require.
const filter = require(filterPath);
const filtered = await filter(tests.map(test => test.path));
if (!Array.isArray(filtered)) {
throw new Error(
`Filter ${filterPath} did not return a valid test list`,
);
}
const filteredSet = new Set(filtered);
// $FlowFixMe: Object.assign with empty object causes troubles to Flow.
return Object.assign({}, searchResult, {
tests: tests.filter(test => filteredSet.has(test.path)),
});
}
return searchResult;
}
}
| packages/jest-cli/src/search_source.js | 1 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.9983898401260376,
0.044183190912008286,
0.0001643453724682331,
0.0002111380163114518,
0.18809345364570618
] |
{
"id": 6,
"code_window": [
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n",
" }\n",
"\n",
" const filteredSet = new Set(filtered);\n",
"\n",
" // $FlowFixMe: Object.assign with empty object causes troubles to Flow.\n",
" return Object.assign({}, searchResult, {\n",
" tests: tests.filter(test => filteredSet.has(test.path)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const filteredSet = new Set(\n",
" filterResult.filtered.map(result => result.test),\n",
" );\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 266
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow
*/
import type {
AggregatedResult,
AssertionResult,
CodeCoverageFormatter,
CodeCoverageReporter,
FormattedAssertionResult,
FormattedTestResult,
FormattedTestResults,
TestResult,
} from 'types/TestResult';
const formatResult = (
testResult: TestResult,
codeCoverageFormatter: CodeCoverageFormatter,
reporter: CodeCoverageReporter,
): FormattedTestResult => {
const now = Date.now();
const output: FormattedTestResult = {
assertionResults: [],
coverage: {},
endTime: now,
message: '',
name: testResult.testFilePath,
startTime: now,
status: 'failed',
summary: '',
};
if (testResult.testExecError) {
output.message = testResult.testExecError.message;
output.coverage = {};
} else {
const allTestsPassed = testResult.numFailingTests === 0;
output.status = allTestsPassed ? 'passed' : 'failed';
output.startTime = testResult.perfStats.start;
output.endTime = testResult.perfStats.end;
output.coverage = codeCoverageFormatter(testResult.coverage, reporter);
}
output.assertionResults = testResult.testResults.map(formatTestAssertion);
if (testResult.failureMessage) {
output.message = testResult.failureMessage;
}
return output;
};
function formatTestAssertion(
assertion: AssertionResult,
): FormattedAssertionResult {
const result: FormattedAssertionResult = {
ancestorTitles: assertion.ancestorTitles,
failureMessages: null,
fullName: assertion.fullName,
location: assertion.location,
status: assertion.status,
title: assertion.title,
};
if (assertion.failureMessages) {
result.failureMessages = assertion.failureMessages;
}
return result;
}
export default function formatTestResults(
results: AggregatedResult,
codeCoverageFormatter?: CodeCoverageFormatter,
reporter?: CodeCoverageReporter,
): FormattedTestResults {
const formatter = codeCoverageFormatter || (coverage => coverage);
const testResults = results.testResults.map(testResult =>
formatResult(testResult, formatter, reporter),
);
return Object.assign((Object.create(null): any), results, {
testResults,
});
}
| packages/jest-util/src/format_test_results.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.0002370272413827479,
0.000185783181223087,
0.00016403601330239326,
0.0001748096547089517,
0.000026324716600356624
] |
{
"id": 6,
"code_window": [
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n",
" }\n",
"\n",
" const filteredSet = new Set(filtered);\n",
"\n",
" // $FlowFixMe: Object.assign with empty object causes troubles to Flow.\n",
" return Object.assign({}, searchResult, {\n",
" tests: tests.filter(test => filteredSet.has(test.path)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const filteredSet = new Set(\n",
" filterResult.filtered.map(result => result.test),\n",
" );\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 266
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
/* eslint-disable */
var expect = require('../../packages/expect/build-es5/index.js');
var mock = require('../../packages/jest-mock/build-es5/index.js');
var prettyFormat = require('../../packages/pretty-format/build-es5/index.js');
describe('es5 builds in browser', function() {
it('runs assertions', function() {
expect(1).toBe(1);
});
it('runs mocks', function() {
var someMockFunction = mock.fn();
expect(someMockFunction).not.toHaveBeenCalled();
someMockFunction();
expect(someMockFunction).toHaveBeenCalledTimes(1);
});
it('pretty formats a string', function() {
expect(prettyFormat('obj')).toBe('"obj"');
});
});
| integration-tests/browser-support/browser-test.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017698932788334787,
0.0001759250444592908,
0.00017409039719495922,
0.00017669542285148054,
0.000001302831833527307
] |
{
"id": 6,
"code_window": [
" throw new Error(\n",
" `Filter ${filterPath} did not return a valid test list`,\n",
" );\n",
" }\n",
"\n",
" const filteredSet = new Set(filtered);\n",
"\n",
" // $FlowFixMe: Object.assign with empty object causes troubles to Flow.\n",
" return Object.assign({}, searchResult, {\n",
" tests: tests.filter(test => filteredSet.has(test.path)),\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" const filteredSet = new Set(\n",
" filterResult.filtered.map(result => result.test),\n",
" );\n"
],
"file_path": "packages/jest-cli/src/search_source.js",
"type": "replace",
"edit_start_line_idx": 266
} | /**
* Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
'use strict';
const loader = require('../loader');
test('loader should load a module', () => {
expect(loader('../example.js')).toBeTruthy();
});
| integration-tests/require-main/__tests__/loader.test.js | 0 | https://github.com/jestjs/jest/commit/bbbffcf8c7ca0786016cc7747c76107b1ff8339c | [
0.00017805452807806432,
0.00017542773275636137,
0.0001728009374346584,
0.00017542773275636137,
0.000002626795321702957
] |
{
"id": 0,
"code_window": [
"<a>\n",
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<a class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.1.html",
"type": "replace",
"edit_start_line_idx": 5
} | <a routerLink="/">
<h1>My Store</h1>
</a>
<!-- #docregion cart-route -->
<a routerLink="/cart" class="button fancy-button">
<i class="material-icons">shopping_cart</i>Checkout
</a>
<!-- #enddocregion cart-route -->
| aio/content/examples/getting-started/src/app/top-bar/top-bar.component.html | 1 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.15996001660823822,
0.15996001660823822,
0.15996001660823822,
0.15996001660823822,
0
] |
{
"id": 0,
"code_window": [
"<a>\n",
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<a class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.1.html",
"type": "replace",
"edit_start_line_idx": 5
} | template: function MyComponent_Template(rf, ctx) {
…
if (rf & 2) {
$r3$.ɵɵattributeInterpolate1("tabindex", "prefix-", 0 + 3, "");
$r3$.ɵɵattributeInterpolate2("aria-label", "hello-", 1 + 3, "-", 2 + 3, "");
$r3$.ɵɵattribute("title", 1)("id", 2);
}
}
| packages/compiler-cli/test/compliance/test_cases/r3_view_compiler_bindings/attribute_bindings/chain_bindings_with_interpolations.js | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00016874363063834608,
0.00016874363063834608,
0.00016874363063834608,
0.00016874363063834608,
0
] |
{
"id": 0,
"code_window": [
"<a>\n",
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<a class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.1.html",
"type": "replace",
"edit_start_line_idx": 5
} | /**
* @license
* Copyright Google LLC All Rights Reserved.
*
* Use of this source code is governed by an MIT-style license that can be
* found in the LICENSE file at https://angular.io/license
*/
import {initMockFileSystem} from '@angular/compiler-cli/src/ngtsc/file_system/testing';
import {assertFileNames, assertTextSpans, createModuleAndProjectWithDeclarations, humanizeDocumentSpanLike, LanguageServiceTestEnv, OpenBuffer, Project} from '../testing';
describe('definitions', () => {
it('gets definition for template reference in overridden template', () => {
initMockFileSystem('Native');
const files = {
'app.html': '',
'app.ts': `
import {Component} from '@angular/core';
@Component({templateUrl: '/app.html'})
export class AppCmp {}
`,
};
const env = LanguageServiceTestEnv.setup();
const project = createModuleAndProjectWithDeclarations(env, 'test', files);
const template = project.openFile('app.html');
template.contents = '<input #myInput /> {{myInput.value}}';
project.expectNoSourceDiagnostics();
template.moveCursorToText('{{myIn¦put.value}}');
const {definitions} = getDefinitionsAndAssertBoundSpan(env, template);
expect(definitions![0].name).toEqual('myInput');
assertFileNames(Array.from(definitions!), ['app.html']);
});
it('returns the pipe definitions when checkTypeOfPipes is false', () => {
initMockFileSystem('Native');
const files = {
'app.ts': `
import {Component, NgModule} from '@angular/core';
import {CommonModule} from '@angular/common';
@Component({templateUrl: 'app.html'})
export class AppCmp {}
`,
'app.html': '{{"1/1/2020" | date}}'
};
// checkTypeOfPipes is set to false when strict templates is false
const env = LanguageServiceTestEnv.setup();
const project =
createModuleAndProjectWithDeclarations(env, 'test', files, {strictTemplates: false});
const template = project.openFile('app.html');
project.expectNoSourceDiagnostics();
template.moveCursorToText('da¦te');
const {textSpan, definitions} = getDefinitionsAndAssertBoundSpan(env, template);
expect(template.contents.slice(textSpan.start, textSpan.start + textSpan.length))
.toEqual('date');
expect(definitions.length).toEqual(3);
assertTextSpans(definitions, ['transform']);
assertFileNames(definitions, ['index.d.ts']);
});
it('gets definitions for all inputs when attribute matches more than one', () => {
initMockFileSystem('Native');
const files = {
'app.ts': `
import {Component, NgModule} from '@angular/core';
import {CommonModule} from '@angular/common';
@Component({templateUrl: 'app.html'})
export class AppCmp {}
`,
'app.html': '<div dir inputA="abc"></div>',
'dir.ts': `
import {Directive, Input} from '@angular/core';
@Directive({selector: '[dir]'})
export class MyDir {
@Input() inputA!: any;
}`,
'dir2.ts': `
import {Directive, Input} from '@angular/core';
@Directive({selector: '[dir]'})
export class MyDir2 {
@Input() inputA!: any;
}`
};
const env = LanguageServiceTestEnv.setup();
const project = createModuleAndProjectWithDeclarations(env, 'test', files);
const template = project.openFile('app.html');
template.moveCursorToText('inpu¦tA="abc"');
const {textSpan, definitions} = getDefinitionsAndAssertBoundSpan(env, template);
expect(template.contents.slice(textSpan.start, textSpan.start + textSpan.length))
.toEqual('inputA');
expect(definitions!.length).toEqual(2);
const [def, def2] = definitions!;
expect(def.textSpan).toContain('inputA');
expect(def2.textSpan).toContain('inputA');
// TODO(atscott): investigate why the text span includes more than just 'inputA'
// assertTextSpans([def, def2], ['inputA']);
assertFileNames([def, def2], ['dir2.ts', 'dir.ts']);
});
it('gets definitions for all outputs when attribute matches more than one', () => {
initMockFileSystem('Native');
const files = {
'app.html': '<div dir (someEvent)="doSomething()"></div>',
'dir.ts': `
import {Directive, Output, EventEmitter} from '@angular/core';
@Directive({selector: '[dir]'})
export class MyDir {
@Output() someEvent = new EventEmitter<void>();
}`,
'dir2.ts': `
import {Directive, Output, EventEmitter} from '@angular/core';
@Directive({selector: '[dir]'})
export class MyDir2 {
@Output() someEvent = new EventEmitter<void>();
}`,
'app.ts': `
import {Component, NgModule} from '@angular/core';
import {CommonModule} from '@angular/common';
@Component({templateUrl: 'app.html'})
export class AppCmp {
doSomething() {}
}
`
};
const env = LanguageServiceTestEnv.setup();
const project = createModuleAndProjectWithDeclarations(env, 'test', files);
const template = project.openFile('app.html');
template.moveCursorToText('(someEv¦ent)');
const {textSpan, definitions} = getDefinitionsAndAssertBoundSpan(env, template);
expect(template.contents.slice(textSpan.start, textSpan.start + textSpan.length))
.toEqual('someEvent');
expect(definitions.length).toEqual(2);
const [def, def2] = definitions;
expect(def.textSpan).toContain('someEvent');
expect(def2.textSpan).toContain('someEvent');
// TODO(atscott): investigate why the text span includes more than just 'someEvent'
// assertTextSpans([def, def2], ['someEvent']);
assertFileNames([def, def2], ['dir2.ts', 'dir.ts']);
});
it('should go to the pre-compiled style sheet', () => {
initMockFileSystem('Native');
const files = {
'app.ts': `
import {Component} from '@angular/core';
@Component({
template: '',
styleUrls: ['./style.css'],
})
export class AppCmp {}
`,
'style.scss': '',
};
const env = LanguageServiceTestEnv.setup();
const project = createModuleAndProjectWithDeclarations(env, 'test', files);
const appFile = project.openFile('app.ts');
appFile.moveCursorToText(`['./styl¦e.css']`);
const {textSpan, definitions} = getDefinitionsAndAssertBoundSpan(env, appFile);
expect(appFile.contents.slice(textSpan.start, textSpan.start + textSpan.length))
.toEqual('./style.css');
expect(definitions.length).toEqual(1);
assertFileNames(definitions, ['style.scss']);
});
it('gets definition for property of variable declared in template', () => {
initMockFileSystem('Native');
const files = {
'app.html': `
<ng-container *ngIf="{prop: myVal} as myVar">
{{myVar.prop.name}}
</ng-container>
`,
'app.ts': `
import {Component} from '@angular/core';
@Component({templateUrl: '/app.html'})
export class AppCmp {
myVal = {name: 'Andrew'};
}
`,
};
const env = LanguageServiceTestEnv.setup();
const project = createModuleAndProjectWithDeclarations(env, 'test', files);
const template = project.openFile('app.html');
project.expectNoSourceDiagnostics();
template.moveCursorToText('{{myVar.pro¦p.name}}');
const {definitions} = getDefinitionsAndAssertBoundSpan(env, template);
expect(definitions![0].name).toEqual('"prop"');
assertFileNames(Array.from(definitions!), ['app.html']);
});
function getDefinitionsAndAssertBoundSpan(env: LanguageServiceTestEnv, file: OpenBuffer) {
env.expectNoSourceDiagnostics();
const definitionAndBoundSpan = file.getDefinitionAndBoundSpan();
const {textSpan, definitions} = definitionAndBoundSpan!;
expect(definitions).toBeTruthy();
return {textSpan, definitions: definitions!.map(d => humanizeDocumentSpanLike(d, env))};
}
});
describe('definitions', () => {
let env: LanguageServiceTestEnv;
describe('when an input has a dollar sign', () => {
const files = {
'app.ts': `
import {Component, NgModule, Input} from '@angular/core';
@Component({selector: 'dollar-cmp', template: ''})
export class DollarCmp {
@Input() obs$!: string;
}
@Component({template: '<dollar-cmp [obs$]="greeting"></dollar-cmp>'})
export class AppCmp {
greeting = 'hello';
}
@NgModule({declarations: [AppCmp, DollarCmp]})
export class AppModule {}
`,
};
beforeEach(() => {
initMockFileSystem('Native');
env = LanguageServiceTestEnv.setup();
});
it('can get definitions for input', () => {
const project = env.addProject('test', files, {strictTemplates: false});
const definitions = getDefinitionsAndAssertBoundSpan(project, 'app.ts', '[o¦bs$]="greeting"');
expect(definitions!.length).toEqual(1);
assertTextSpans(definitions, ['obs$']);
assertFileNames(definitions, ['app.ts']);
});
it('can get definitions for component', () => {
const project = env.addProject('test', files, {strictTemplates: false});
const definitions = getDefinitionsAndAssertBoundSpan(project, 'app.ts', '<dollar-cm¦p');
expect(definitions!.length).toEqual(1);
assertTextSpans(definitions, ['DollarCmp']);
assertFileNames(definitions, ['app.ts']);
});
});
describe('when a selector and input of a directive have a dollar sign', () => {
it('can get definitions', () => {
initMockFileSystem('Native');
env = LanguageServiceTestEnv.setup();
const files = {
'app.ts': `
import {Component, Directive, NgModule, Input} from '@angular/core';
@Directive({selector: '[dollar\\\\$]', template: ''})
export class DollarDir {
@Input() dollar$!: string;
}
@Component({template: '<div [dollar$]="greeting"></div>'})
export class AppCmp {
greeting = 'hello';
}
@NgModule({declarations: [AppCmp, DollarDir]})
export class AppModule {}
`,
};
const project = env.addProject('test', files, {strictTemplates: false});
const definitions =
getDefinitionsAndAssertBoundSpan(project, 'app.ts', '[dollar¦$]="greeting"');
expect(definitions!.length).toEqual(2);
assertTextSpans(definitions, ['dollar$', 'DollarDir']);
assertFileNames(definitions, ['app.ts']);
});
});
function getDefinitionsAndAssertBoundSpan(project: Project, file: string, targetText: string) {
const template = project.openFile(file);
env.expectNoSourceDiagnostics();
project.expectNoTemplateDiagnostics('app.ts', 'AppCmp');
template.moveCursorToText(targetText);
const defAndBoundSpan = template.getDefinitionAndBoundSpan();
expect(defAndBoundSpan).toBeTruthy();
expect(defAndBoundSpan!.definitions).toBeTruthy();
return defAndBoundSpan!.definitions!.map(d => humanizeDocumentSpanLike(d, env));
}
});
| packages/language-service/test/definitions_spec.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017484650015830994,
0.00017004419350996614,
0.0001661214482737705,
0.00016959273489192128,
0.0000022609949610341573
] |
{
"id": 0,
"code_window": [
"<a>\n",
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<a class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.1.html",
"type": "replace",
"edit_start_line_idx": 5
} | load("//tools:defaults.bzl", "jasmine_node_test", "ts_library")
ts_library(
name = "test_lib",
testonly = True,
srcs = glob(
["**/*.ts"],
),
deps = [
"//packages:types",
"//packages/compiler",
"//packages/compiler/test/expression_parser/utils",
"//packages/core",
],
)
jasmine_node_test(
name = "test",
bootstrap = ["//tools/testing:node"],
deps = [
":test_lib",
],
)
| packages/compiler/test/render3/BUILD.bazel | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017030932940542698,
0.0001686814212007448,
0.00016659351240377873,
0.0001691413635853678,
0.000001551450395709253
] |
{
"id": 1,
"code_window": [
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<!-- #docregion cart-route -->\n",
"<a routerLink=\"/cart\" class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>\n",
"<!-- #enddocregion cart-route -->"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.html",
"type": "replace",
"edit_start_line_idx": 6
} | {% extends 'base.template.html' -%}
{% macro listItems(items, title, overridePath) %}
{% set filteredItems = items | filterByPropertyValue('internal', undefined) %}
{% if filteredItems.length %}
<section class="export-list">
<h3>{$ title $}</h3>
<table class="is-full-width list-table">
{% for item in filteredItems %}
<tr>
<td><code class="code-anchor{% if item.deprecated %} deprecated-api-item{% endif %}"><a href="{$ overridePath or item.path $}"{%- if item.deprecated != undefined %} class="deprecated-api-item"{% endif %}>{$ item.name | escape $}</a></code></td>
<td>
{% if item.deprecated !== undefined %}{$ ('**Deprecated:** ' + item.deprecated) | marked $}{% endif %}
{% if item.shortDescription %}{$ item.shortDescription | marked $}{% endif %}
</td>
</tr>
{% endfor %}
</table>
</section>
{% endif %}
{% endmacro %}
{% block header %}
<header class="api-header">
<h1>{$ doc.name $}</h1>
{% if doc.isPrimaryPackage %}<label class="api-type-label package">package</label>{% else %}<label class="api-type-label {$ entry-point $}">entry-point</label>{% endif %}
{% if doc.packageDeprecated or (not doc.isPrimaryPackage and doc.deprecated !== undefined) %}<label class="api-status-label deprecated">deprecated</label>{% endif %}
{% if doc.security !== undefined %}<label class="api-status-label security">security</label>{% endif %}
{% if doc.pipeOptions.pure === 'false' %}<label class="api-status-label impure-pipe">impure</label>{% endif %}
</header>
{% endblock %}
{% block body -%}
{$ doc.shortDescription | marked $}
{% if doc.description %}{$ doc.description | marked $}{% endif %}
{% include "includes/see-also.html" %}
{% if doc.isPrimaryPackage %}
<h2>Entry points</h2>
{$ listItems([doc.packageInfo.primary], 'Primary', '#primary-entry-point-exports') $}
{$ listItems(doc.packageInfo.secondary, 'Secondary') $}
{% endif %}
<h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>
{% if not doc.hasPublicExports %}
<p><i>No public exports.</i></p>
{% endif %}
{% include "includes/deprecation.html" %}
{$ listItems(doc.ngmodules, 'NgModules') $}
{$ listItems(doc.classes, 'Classes') $}
{$ listItems(doc.decorators, 'Decorators') $}
{$ listItems(doc.functions, 'Functions') $}
{$ listItems(doc.structures, 'Structures') $}
{$ listItems(doc.directives, 'Directives') $}
{$ listItems(doc.elements, 'Elements') $}
{$ listItems(doc.pipes, 'Pipes') $}
{$ listItems(doc.types, 'Types') $}
{%- endblock %}
| aio/tools/transforms/templates/api/package.template.html | 1 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017317848687525839,
0.00016665237490087748,
0.00016335687541868538,
0.00016555005277041346,
0.0000034920549296657555
] |
{
"id": 1,
"code_window": [
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<!-- #docregion cart-route -->\n",
"<a routerLink=\"/cart\" class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>\n",
"<!-- #enddocregion cart-route -->"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.html",
"type": "replace",
"edit_start_line_idx": 6
} | /**
* @license
* Copyright Google LLC All Rights Reserved.
*
* Use of this source code is governed by an MIT-style license that can be
* found in the LICENSE file at https://angular.io/license
*/
import {isRootView} from '../interfaces/type_checks';
import {FLAGS, LView, LViewFlags} from '../interfaces/view';
import {getLViewParent} from '../util/view_traversal_utils';
/**
* Marks current view and all ancestors dirty.
*
* Returns the root view because it is found as a byproduct of marking the view tree
* dirty, and can be used by methods that consume markViewDirty() to easily schedule
* change detection. Otherwise, such methods would need to traverse up the view tree
* an additional time to get the root view and schedule a tick on it.
*
* @param lView The starting LView to mark dirty
* @returns the root LView
*/
export function markViewDirty(lView: LView): LView|null {
while (lView) {
lView[FLAGS] |= LViewFlags.Dirty;
const parent = getLViewParent(lView);
// Stop traversing up as soon as you find a root view that wasn't attached to any container
if (isRootView(lView) && !parent) {
return lView;
}
// continue otherwise
lView = parent!;
}
return null;
}
| packages/core/src/render3/instructions/mark_view_dirty.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.0001742908643791452,
0.00016993522876873612,
0.00016508731641806662,
0.00017018135986290872,
0.0000032936804927885532
] |
{
"id": 1,
"code_window": [
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<!-- #docregion cart-route -->\n",
"<a routerLink=\"/cart\" class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>\n",
"<!-- #enddocregion cart-route -->"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.html",
"type": "replace",
"edit_start_line_idx": 6
} | import { Component } from '@angular/core';
@Component({
selector: 'sg-app',
template: '<input type="text" tohValidate>'
})
export class AppComponent { }
| aio/content/examples/styleguide/src/02-08/app/app.component.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017014180775731802,
0.00017014180775731802,
0.00017014180775731802,
0.00017014180775731802,
0
] |
{
"id": 1,
"code_window": [
" <h1>My Store</h1>\n",
"</a>\n",
"\n",
"<!-- #docregion cart-route -->\n",
"<a routerLink=\"/cart\" class=\"button fancy-button\">\n",
" <i class=\"material-icons\">shopping_cart</i>Checkout\n",
"</a>\n",
"<!-- #enddocregion cart-route -->"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep"
],
"after_edit": [
" <em class=\"material-icons\">shopping_cart</em>Checkout\n"
],
"file_path": "aio/content/examples/getting-started/src/app/top-bar/top-bar.component.html",
"type": "replace",
"edit_start_line_idx": 6
} | var INLINE_LINK = /(\S+)(?:\s+([\s\S]+))?/;
/**
* @dgService linkInlineTagDef
* @description
* Process inline link tags (of the form {@link some/uri Some Title}), replacing them with HTML anchors
* @kind function
* @param {Object} url The url to match
* @param {Function} docs error message
* @return {String} The html link information
*
* @property {boolean} failOnBadLink Whether to throw an error (aborting the processing) if a link is invalid.
*/
module.exports = function linkInlineTagDef(getLinkInfo, createDocMessage, log) {
return {
name: 'link',
failOnBadLink: true,
description:
'Process inline link tags (of the form {@link some/uri Some Title}), replacing them with HTML anchors',
handler(doc, tagName, tagDescription) {
// Parse out the uri and title
return tagDescription.replace(INLINE_LINK, (match, uri, title) => {
var linkInfo = getLinkInfo(uri, title, doc);
if (!linkInfo.valid) {
const message = createDocMessage(`Error in {@${tagName} ${tagDescription}} - ${linkInfo.error}`, doc);
if (this.failOnBadLink) {
throw new Error(message);
} else {
log.warn(message);
}
}
return '<a href=\'' + linkInfo.url + '\'>' + linkInfo.title + '</a>';
});
}
};
}; | aio/tools/transforms/links-package/inline-tag-defs/link.js | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017355075397063047,
0.00016843753110151738,
0.00016394064004998654,
0.00016857268929015845,
0.0000032456125609314768
] |
{
"id": 2,
"code_window": [
"interface WindowWithAnalytics extends Window {\n",
" dataLayer?: any[];\n",
" gtag?(...args: any[]): void;\n",
" /** Legacy Universal Analytics `analytics.js` field. */\n",
" ga?(...args: any[]): void;\n",
"};\n",
"\n",
"@Injectable()\n",
"/**\n",
" * Google Analytics Service - captures app behaviors and sends them to Google Analytics.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"}\n"
],
"file_path": "aio/src/app/shared/analytics.service.ts",
"type": "replace",
"edit_start_line_idx": 14
} | {% extends 'base.template.html' -%}
{% macro listItems(items, title, overridePath) %}
{% set filteredItems = items | filterByPropertyValue('internal', undefined) %}
{% if filteredItems.length %}
<section class="export-list">
<h3>{$ title $}</h3>
<table class="is-full-width list-table">
{% for item in filteredItems %}
<tr>
<td><code class="code-anchor{% if item.deprecated %} deprecated-api-item{% endif %}"><a href="{$ overridePath or item.path $}"{%- if item.deprecated != undefined %} class="deprecated-api-item"{% endif %}>{$ item.name | escape $}</a></code></td>
<td>
{% if item.deprecated !== undefined %}{$ ('**Deprecated:** ' + item.deprecated) | marked $}{% endif %}
{% if item.shortDescription %}{$ item.shortDescription | marked $}{% endif %}
</td>
</tr>
{% endfor %}
</table>
</section>
{% endif %}
{% endmacro %}
{% block header %}
<header class="api-header">
<h1>{$ doc.name $}</h1>
{% if doc.isPrimaryPackage %}<label class="api-type-label package">package</label>{% else %}<label class="api-type-label {$ entry-point $}">entry-point</label>{% endif %}
{% if doc.packageDeprecated or (not doc.isPrimaryPackage and doc.deprecated !== undefined) %}<label class="api-status-label deprecated">deprecated</label>{% endif %}
{% if doc.security !== undefined %}<label class="api-status-label security">security</label>{% endif %}
{% if doc.pipeOptions.pure === 'false' %}<label class="api-status-label impure-pipe">impure</label>{% endif %}
</header>
{% endblock %}
{% block body -%}
{$ doc.shortDescription | marked $}
{% if doc.description %}{$ doc.description | marked $}{% endif %}
{% include "includes/see-also.html" %}
{% if doc.isPrimaryPackage %}
<h2>Entry points</h2>
{$ listItems([doc.packageInfo.primary], 'Primary', '#primary-entry-point-exports') $}
{$ listItems(doc.packageInfo.secondary, 'Secondary') $}
{% endif %}
<h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>
{% if not doc.hasPublicExports %}
<p><i>No public exports.</i></p>
{% endif %}
{% include "includes/deprecation.html" %}
{$ listItems(doc.ngmodules, 'NgModules') $}
{$ listItems(doc.classes, 'Classes') $}
{$ listItems(doc.decorators, 'Decorators') $}
{$ listItems(doc.functions, 'Functions') $}
{$ listItems(doc.structures, 'Structures') $}
{$ listItems(doc.directives, 'Directives') $}
{$ listItems(doc.elements, 'Elements') $}
{$ listItems(doc.pipes, 'Pipes') $}
{$ listItems(doc.types, 'Types') $}
{%- endblock %}
| aio/tools/transforms/templates/api/package.template.html | 1 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017826604016590863,
0.00017701253818813711,
0.00017535990627948195,
0.0001770424423739314,
0.0000010518145927562728
] |
{
"id": 2,
"code_window": [
"interface WindowWithAnalytics extends Window {\n",
" dataLayer?: any[];\n",
" gtag?(...args: any[]): void;\n",
" /** Legacy Universal Analytics `analytics.js` field. */\n",
" ga?(...args: any[]): void;\n",
"};\n",
"\n",
"@Injectable()\n",
"/**\n",
" * Google Analytics Service - captures app behaviors and sends them to Google Analytics.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"}\n"
],
"file_path": "aio/src/app/shared/analytics.service.ts",
"type": "replace",
"edit_start_line_idx": 14
} | LifecycleComp.ɵcmp = /*@__PURE__*/ $r3$.ɵɵdefineComponent({
type: LifecycleComp,
selectors: [["lifecycle-comp"]],
inputs: {nameMin: ["name", "nameMin"]},
features: [$r3$.ɵɵNgOnChangesFeature],
decls: 0,
vars: 0,
template: function LifecycleComp_Template(rf, ctx) {},
encapsulation: 2
});
| packages/compiler-cli/test/compliance/test_cases/r3_compiler_compliance/components_and_directives/lifecycle_hooks/lifecycle_hooks_lifecycle_comp_def.js | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017563920118846,
0.0001738865685183555,
0.00017213392129633576,
0.0001738865685183555,
0.0000017526399460621178
] |
{
"id": 2,
"code_window": [
"interface WindowWithAnalytics extends Window {\n",
" dataLayer?: any[];\n",
" gtag?(...args: any[]): void;\n",
" /** Legacy Universal Analytics `analytics.js` field. */\n",
" ga?(...args: any[]): void;\n",
"};\n",
"\n",
"@Injectable()\n",
"/**\n",
" * Google Analytics Service - captures app behaviors and sends them to Google Analytics.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"}\n"
],
"file_path": "aio/src/app/shared/analytics.service.ts",
"type": "replace",
"edit_start_line_idx": 14
} | <!DOCTYPE html>
<html>
<head>
<!-- Prevent the browser from requesting any favicon. -->
<link rel="icon" href="data:," />
</head>
<body>
<h2>Params</h2>
<form>
Depth:
<input type="number" name="depth" placeholder="depth" value="10" />
<br />
<button>Apply</button>
</form>
<h2>Ng2 Static Tree Benchmark</h2>
<p>
<button id="destroyDom">destroyDom</button>
<button id="createDom">createDom</button>
<button id="updateDomProfile">profile updateDom</button>
<button id="createDomProfile">profile createDom</button>
</p>
<div>
<tree id="root"></tree>
</div>
<!-- BEGIN-EXTERNAL -->
<script src="/npm/node_modules/reflect-metadata/Reflect.js"></script>
<script src="/angular/packages/zone.js/bundles/zone.umd.js"></script>
<!-- END-EXTERNAL -->
<!-- Needs to be named `app_bundle` for sync into Google. -->
<script src="/app_bundle.js"></script>
</body>
</html>
| modules/benchmarks/src/tree/ng2_static/index.html | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017575193487573415,
0.00017356879834551364,
0.00016962282825261354,
0.00017445022240281105,
0.000002479749127815012
] |
{
"id": 2,
"code_window": [
"interface WindowWithAnalytics extends Window {\n",
" dataLayer?: any[];\n",
" gtag?(...args: any[]): void;\n",
" /** Legacy Universal Analytics `analytics.js` field. */\n",
" ga?(...args: any[]): void;\n",
"};\n",
"\n",
"@Injectable()\n",
"/**\n",
" * Google Analytics Service - captures app behaviors and sends them to Google Analytics.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"}\n"
],
"file_path": "aio/src/app/shared/analytics.service.ts",
"type": "replace",
"edit_start_line_idx": 14
} | // #docregion
import { Injectable } from '@angular/core';
import { HeroJobAdComponent } from './hero-job-ad.component';
import { HeroProfileComponent } from './hero-profile.component';
import { AdItem } from './ad-item';
@Injectable()
export class AdService {
getAds() {
return [
new AdItem(
HeroProfileComponent,
{ name: 'Bombasto', bio: 'Brave as they come' }
),
new AdItem(
HeroProfileComponent,
{ name: 'Dr. IQ', bio: 'Smart as they come' }
),
new AdItem(
HeroJobAdComponent,
{ headline: 'Hiring for several positions', body: 'Submit your resume today!' }
),
new AdItem(
HeroJobAdComponent,
{ headline: 'Openings in all departments', body: 'Apply today' }
)
];
}
}
| aio/content/examples/dynamic-component-loader/src/app/ad.service.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017685604689177126,
0.0001725921465549618,
0.00016656809020787477,
0.00017347221728414297,
0.000003860116976284189
] |
{
"id": 3,
"code_window": [
" {% endif %}\n",
"\n",
" <h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>\n",
" {% if not doc.hasPublicExports %}\n",
" <p><i>No public exports.</i></p>\n",
" {% endif %}\n",
" {% include \"includes/deprecation.html\" %}\n",
" {$ listItems(doc.ngmodules, 'NgModules') $}\n",
" {$ listItems(doc.classes, 'Classes') $}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <p><em>No public exports.</em></p>\n"
],
"file_path": "aio/tools/transforms/templates/api/package.template.html",
"type": "replace",
"edit_start_line_idx": 46
} | import { Inject, Injectable } from '@angular/core';
import { trustedResourceUrl, unwrapResourceUrl } from 'safevalues';
import { formatErrorEventForAnalytics } from './analytics-format-error';
import { WindowToken } from '../shared/window';
import { environment } from '../../environments/environment';
/** Extension of `Window` with potential Google Analytics fields. */
interface WindowWithAnalytics extends Window {
dataLayer?: any[];
gtag?(...args: any[]): void;
/** Legacy Universal Analytics `analytics.js` field. */
ga?(...args: any[]): void;
};
@Injectable()
/**
* Google Analytics Service - captures app behaviors and sends them to Google Analytics.
*
* Note: Presupposes that the legacy `analytics.js` script has been loaded on the
* host web page.
*
* Associates data with properties determined from the environment configurations:
* - Data is uploaded to a legacy Universal Analytics property
* - Data is uploaded to our main Google Analytics 4+ property.
*/
export class AnalyticsService {
/** Whether the application runs in e2e tests using Protractor. */
private readonly isProtractor = this.window.name.includes('NG_DEFER_BOOTSTRAP');
/** Previously reported URL. Cached to allow for duplicates being filtered. */
private previousUrl: string;
constructor(@Inject(WindowToken) private window: WindowWithAnalytics) {
this._installGlobalSiteTag();
this._installWindowErrorHandler();
// TODO: Remove this when we fully switch to Google Analytics 4+.
this._legacyGa('create', environment.legacyUniversalAnalyticsId , 'auto');
this._legacyGa('set', 'anonymizeIp', true);
}
reportError(description: string, fatal = true) {
// Limit descriptions to maximum of 150 characters.
// See: https://developers.google.com/analytics/devguides/collection/protocol/v1/parameters#exd.
description = description.substring(0, 150);
this._legacyGa('send', 'exception', {exDescription: description, exFatal: fatal});
this._gtag('event', 'exception', {description, fatal});
}
locationChanged(url: string) {
this._sendPage(url);
}
sendEvent(name: string, parameters: Record<string, string|boolean|number>) {
this._gtag('event', name, parameters);
}
private _sendPage(url: string) {
// Won't re-send if the url hasn't changed.
if (url === this.previousUrl) { return; }
this.previousUrl = url;
this._legacyGa('set', 'page', '/' + url);
this._legacyGa('send', 'pageview');
}
private _gtag(...args: any[]) {
if (this.window.gtag) {
this.window.gtag(...args);
}
}
private _legacyGa(...args: any[]) {
if (this.window.ga) {
this.window.ga(...args);
}
}
private _installGlobalSiteTag() {
const window = this.window;
const url: TrustedScriptURL =
trustedResourceUrl`https://www.googletagmanager.com/gtag/js?id=${environment.googleAnalyticsId}`;
// Note: This cannot be an arrow function as `gtag.js` expects an actual `Arguments`
// instance with e.g. `callee` to be set. Do not attempt to change this and keep this
// as much as possible in sync with the tracking code snippet suggested by the Google
// Analytics 4 web UI under `Data Streams`.
window.dataLayer = this.window.dataLayer || [];
window.gtag = function() { window.dataLayer?.push(arguments); };
window.gtag('js', new Date());
// Configure properties before loading the script. This is necessary to avoid
// loading multiple instances of the gtag JS scripts.
window.gtag('config', environment.googleAnalyticsId);
// do not load the library when we are running in an e2e protractor test.
if (this.isProtractor) {
return;
}
const el = window.document.createElement('script');
el.async = true;
el.src = unwrapResourceUrl(url) as string;
window.document.head.appendChild(el);
}
private _installWindowErrorHandler() {
this.window.addEventListener('error', event =>
this.reportError(formatErrorEventForAnalytics(event), true));
}
}
| aio/src/app/shared/analytics.service.ts | 1 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017558997205924243,
0.00017212315287906677,
0.00016585744742769748,
0.00017309561371803284,
0.0000032113334782479797
] |
{
"id": 3,
"code_window": [
" {% endif %}\n",
"\n",
" <h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>\n",
" {% if not doc.hasPublicExports %}\n",
" <p><i>No public exports.</i></p>\n",
" {% endif %}\n",
" {% include \"includes/deprecation.html\" %}\n",
" {$ listItems(doc.ngmodules, 'NgModules') $}\n",
" {$ listItems(doc.classes, 'Classes') $}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <p><em>No public exports.</em></p>\n"
],
"file_path": "aio/tools/transforms/templates/api/package.template.html",
"type": "replace",
"edit_start_line_idx": 46
} | import { Component } from '@angular/core';
import { HomeComponent } from './home/home.component';
@Component({
selector: 'app-root',
standalone: true,
imports: [
HomeComponent,
],
template: `
<main>
<header class="brand-name">
<img class="brand-logo" src="/assets/logo.svg" alt="logo" aria-hidden="true">
</header>
<section class="content">
<app-home></app-home>
</section>
</main>
`,
styleUrls: ['./app.component.css'],
})
export class AppComponent {
title = 'homes';
}
| aio/content/examples/first-app-lesson-09/src/app/app.component.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.0001756278215907514,
0.00017211704107467085,
0.0001678461121628061,
0.00017287723312620074,
0.000003222023451598943
] |
{
"id": 3,
"code_window": [
" {% endif %}\n",
"\n",
" <h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>\n",
" {% if not doc.hasPublicExports %}\n",
" <p><i>No public exports.</i></p>\n",
" {% endif %}\n",
" {% include \"includes/deprecation.html\" %}\n",
" {$ listItems(doc.ngmodules, 'NgModules') $}\n",
" {$ listItems(doc.classes, 'Classes') $}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <p><em>No public exports.</em></p>\n"
],
"file_path": "aio/tools/transforms/templates/api/package.template.html",
"type": "replace",
"edit_start_line_idx": 46
} | The `<ng-content>` element specifies where to project content inside a component template.
@elementAttribute select="selector"
Only select elements from the projected content that match the given CSS `selector`.
Angular supports [selectors](https://developer.mozilla.org/docs/Web/CSS/CSS_Selectors) for any combination of tag name, attribute, CSS class, and the `:not` pseudo-class. | aio/content/special-elements/core/ng-content.md | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00016960744687821716,
0.00016960744687821716,
0.00016960744687821716,
0.00016960744687821716,
0
] |
{
"id": 3,
"code_window": [
" {% endif %}\n",
"\n",
" <h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>\n",
" {% if not doc.hasPublicExports %}\n",
" <p><i>No public exports.</i></p>\n",
" {% endif %}\n",
" {% include \"includes/deprecation.html\" %}\n",
" {$ listItems(doc.ngmodules, 'NgModules') $}\n",
" {$ listItems(doc.classes, 'Classes') $}\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" <p><em>No public exports.</em></p>\n"
],
"file_path": "aio/tools/transforms/templates/api/package.template.html",
"type": "replace",
"edit_start_line_idx": 46
} | /**
* Split the description (of selected docs) into:
* * `shortDescription`: the first paragraph
* * `description`: the rest of the paragraphs
*/
module.exports = function splitDescription() {
return {
$runAfter: ['tags-extracted'],
$runBefore: ['processing-docs'],
docTypes: [],
$process(docs) {
docs.forEach(doc => {
if (this.docTypes.indexOf(doc.docType) !== -1 && doc.description !== undefined) {
const description = doc.description.trim();
const endOfParagraph = description.search(/\n\s*\n/);
if (endOfParagraph === -1) {
doc.shortDescription = description;
doc.description = '';
} else {
doc.shortDescription = description.slice(0, endOfParagraph).trim();
doc.description = description.slice(endOfParagraph).trim();
}
}
});
}
};
};
| aio/tools/transforms/angular-base-package/processors/splitDescription.js | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.004621118772774935,
0.0016645031282678246,
0.00017352693248540163,
0.00019886373775079846,
0.002090668538585305
] |
{
"id": 4,
"code_window": [
"{% import \"lib/githubLinks.html\" as github -%}\n",
"{% import \"api/lib/memberHelpers.html\" as members -%}\n",
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><i class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</i></a>{% endmacro %}\n",
"{% macro label(test, class, text) %}{% if test %}<label class=\"{$ class $}\">{$ text $}</label>{% endif %}{% endmacro %}\n",
"{% macro renderLabels(doc) -%}\n",
" {$ label(doc.notYetDocumented, 'no-doc', 'UNDOCUMENTED') $}\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><em class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</em></a>{% endmacro %}\n"
],
"file_path": "aio/tools/transforms/templates/overview-dump.template.html",
"type": "replace",
"edit_start_line_idx": 2
} | {% extends 'base.template.html' -%}
{% macro listItems(items, title, overridePath) %}
{% set filteredItems = items | filterByPropertyValue('internal', undefined) %}
{% if filteredItems.length %}
<section class="export-list">
<h3>{$ title $}</h3>
<table class="is-full-width list-table">
{% for item in filteredItems %}
<tr>
<td><code class="code-anchor{% if item.deprecated %} deprecated-api-item{% endif %}"><a href="{$ overridePath or item.path $}"{%- if item.deprecated != undefined %} class="deprecated-api-item"{% endif %}>{$ item.name | escape $}</a></code></td>
<td>
{% if item.deprecated !== undefined %}{$ ('**Deprecated:** ' + item.deprecated) | marked $}{% endif %}
{% if item.shortDescription %}{$ item.shortDescription | marked $}{% endif %}
</td>
</tr>
{% endfor %}
</table>
</section>
{% endif %}
{% endmacro %}
{% block header %}
<header class="api-header">
<h1>{$ doc.name $}</h1>
{% if doc.isPrimaryPackage %}<label class="api-type-label package">package</label>{% else %}<label class="api-type-label {$ entry-point $}">entry-point</label>{% endif %}
{% if doc.packageDeprecated or (not doc.isPrimaryPackage and doc.deprecated !== undefined) %}<label class="api-status-label deprecated">deprecated</label>{% endif %}
{% if doc.security !== undefined %}<label class="api-status-label security">security</label>{% endif %}
{% if doc.pipeOptions.pure === 'false' %}<label class="api-status-label impure-pipe">impure</label>{% endif %}
</header>
{% endblock %}
{% block body -%}
{$ doc.shortDescription | marked $}
{% if doc.description %}{$ doc.description | marked $}{% endif %}
{% include "includes/see-also.html" %}
{% if doc.isPrimaryPackage %}
<h2>Entry points</h2>
{$ listItems([doc.packageInfo.primary], 'Primary', '#primary-entry-point-exports') $}
{$ listItems(doc.packageInfo.secondary, 'Secondary') $}
{% endif %}
<h2>{% if doc.isPrimaryPackage %}Primary entry{% else %}Entry{% endif %} point exports</h2>
{% if not doc.hasPublicExports %}
<p><i>No public exports.</i></p>
{% endif %}
{% include "includes/deprecation.html" %}
{$ listItems(doc.ngmodules, 'NgModules') $}
{$ listItems(doc.classes, 'Classes') $}
{$ listItems(doc.decorators, 'Decorators') $}
{$ listItems(doc.functions, 'Functions') $}
{$ listItems(doc.structures, 'Structures') $}
{$ listItems(doc.directives, 'Directives') $}
{$ listItems(doc.elements, 'Elements') $}
{$ listItems(doc.pipes, 'Pipes') $}
{$ listItems(doc.types, 'Types') $}
{%- endblock %}
| aio/tools/transforms/templates/api/package.template.html | 1 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.0005369095597416162,
0.00025874769198708236,
0.00016980632790364325,
0.0001847652019932866,
0.0001316193229286
] |
{
"id": 4,
"code_window": [
"{% import \"lib/githubLinks.html\" as github -%}\n",
"{% import \"api/lib/memberHelpers.html\" as members -%}\n",
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><i class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</i></a>{% endmacro %}\n",
"{% macro label(test, class, text) %}{% if test %}<label class=\"{$ class $}\">{$ text $}</label>{% endif %}{% endmacro %}\n",
"{% macro renderLabels(doc) -%}\n",
" {$ label(doc.notYetDocumented, 'no-doc', 'UNDOCUMENTED') $}\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><em class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</em></a>{% endmacro %}\n"
],
"file_path": "aio/tools/transforms/templates/overview-dump.template.html",
"type": "replace",
"edit_start_line_idx": 2
} | /*
* A collection of demo components showing different ways to provide services
* in @Component metadata
*/
import { Component, Inject, Injectable, OnInit } from '@angular/core';
import {
APP_CONFIG,
AppConfig,
HERO_DI_CONFIG } from './app.config';
import { HeroService } from './heroes/hero.service';
import { heroServiceProvider } from './heroes/hero.service.provider';
import { Logger } from './logger.service';
import { UserService } from './user.service';
const template = '{{log}}';
@Component({
selector: 'provider-1',
template,
// #docregion providers-logger
providers: [Logger]
// #enddocregion providers-logger
})
export class Provider1Component {
log: string;
constructor(logger: Logger) {
logger.log('Hello from logger provided with Logger class');
this.log = logger.logs[0];
}
}
//////////////////////////////////////////
@Component({
selector: 'provider-3',
template,
providers:
// #docregion providers-3
[{ provide: Logger, useClass: Logger }]
// #enddocregion providers-3
})
export class Provider3Component {
log: string;
constructor(logger: Logger) {
logger.log('Hello from logger provided with useClass:Logger');
this.log = logger.logs[0];
}
}
//////////////////////////////////////////
export class BetterLogger extends Logger {}
@Component({
selector: 'provider-4',
template,
providers:
// #docregion providers-4
[{ provide: Logger, useClass: BetterLogger }]
// #enddocregion providers-4
})
export class Provider4Component {
log: string;
constructor(logger: Logger) {
logger.log('Hello from logger provided with useClass:BetterLogger');
this.log = logger.logs[0];
}
}
//////////////////////////////////////////
// #docregion EvenBetterLogger
@Injectable()
export class EvenBetterLogger extends Logger {
constructor(private userService: UserService) { super(); }
override log(message: string) {
const name = this.userService.user.name;
super.log(`Message to ${name}: ${message}`);
}
}
// #enddocregion EvenBetterLogger
@Component({
selector: 'provider-5',
template,
providers:
// #docregion providers-5
[ UserService,
{ provide: Logger, useClass: EvenBetterLogger }]
// #enddocregion providers-5
})
export class Provider5Component {
log: string;
constructor(logger: Logger) {
logger.log('Hello from EvenBetterlogger');
this.log = logger.logs[0];
}
}
//////////////////////////////////////////
export class NewLogger extends Logger {}
export class OldLogger {
logs: string[] = [];
log(message: string) {
throw new Error('Should not call the old logger!');
}
}
@Component({
selector: 'provider-6a',
template,
providers:
[ NewLogger,
// Not aliased! Creates two instances of `NewLogger`
{ provide: OldLogger, useClass: NewLogger}]
})
export class Provider6aComponent {
log: string;
constructor(newLogger: NewLogger, oldLogger: OldLogger) {
if (newLogger === oldLogger) {
throw new Error('expected the two loggers to be different instances');
}
oldLogger.log('Hello OldLogger (but we want NewLogger)');
// The newLogger wasn't called so no logs[]
// display the logs of the oldLogger.
this.log = newLogger.logs[0] || oldLogger.logs[0];
}
}
@Component({
selector: 'provider-6b',
template,
providers:
// #docregion providers-6b
[ NewLogger,
// Alias OldLogger w/ reference to NewLogger
{ provide: OldLogger, useExisting: NewLogger}]
// #enddocregion providers-6b
})
export class Provider6bComponent {
log: string;
constructor(newLogger: NewLogger, oldLogger: OldLogger) {
if (newLogger !== oldLogger) {
throw new Error('expected the two loggers to be the same instance');
}
oldLogger.log('Hello from NewLogger (via aliased OldLogger)');
this.log = newLogger.logs[0];
}
}
//////////////////////////////////////////
// An object in the shape of the logger service
function silentLoggerFn() {}
export const SilentLogger = {
logs: ['Silent logger says "Shhhhh!". Provided via "useValue"'],
log: silentLoggerFn
};
@Component({
selector: 'provider-7',
template,
providers:
[{ provide: Logger, useValue: SilentLogger }]
})
export class Provider7Component {
log: string;
constructor(logger: Logger) {
logger.log('Hello from logger provided with useValue');
this.log = logger.logs[0];
}
}
/////////////////
@Component({
selector: 'provider-8',
template,
providers: [heroServiceProvider, Logger, UserService]
})
export class Provider8Component {
// must be true else this component would have blown up at runtime
log = 'Hero service injected successfully via heroServiceProvider';
constructor(heroService: HeroService) { }
}
/////////////////
@Component({
selector: 'provider-9',
template,
/*
// #docregion providers-9-interface
// Can't use interface as provider token
[{ provide: AppConfig, useValue: HERO_DI_CONFIG })]
// #enddocregion providers-9-interface
*/
// #docregion providers-9
providers: [{ provide: APP_CONFIG, useValue: HERO_DI_CONFIG }]
// #enddocregion providers-9
})
export class Provider9Component implements OnInit {
log = '';
/*
// #docregion provider-9-ctor-interface
// Can't inject using the interface as the parameter type
constructor(private config: AppConfig){ }
// #enddocregion provider-9-ctor-interface
*/
constructor(@Inject(APP_CONFIG) private config: AppConfig) { }
ngOnInit() {
this.log = 'APP_CONFIG Application title is ' + this.config.title;
}
}
//////////////////////////////////////////
// Sample providers 1 to 7 illustrate a required logger dependency.
// Optional logger, can be null
import { Optional } from '@angular/core';
const someMessage = 'Hello from the injected logger';
@Component({
selector: 'provider-10',
template,
providers: [{ provide: Logger, useValue: null }]
})
export class Provider10Component implements OnInit {
log = '';
constructor(@Optional() private logger?: Logger) {
if (this.logger) {
this.logger.log(someMessage);
}
}
ngOnInit() {
this.log = this.logger ? this.logger.logs[0] : 'Optional logger was not available';
}
}
/////////////////
@Component({
selector: 'app-providers',
template: `
<h2>Provider variations</h2>
<div id="p1"><provider-1></provider-1></div>
<div id="p3"><provider-3></provider-3></div>
<div id="p4"><provider-4></provider-4></div>
<div id="p5"><provider-5></provider-5></div>
<div id="p6a"><provider-6a></provider-6a></div>
<div id="p6b"><provider-6b></provider-6b></div>
<div id="p7"><provider-7></provider-7></div>
<div id="p8"><provider-8></provider-8></div>
<div id="p9"><provider-9></provider-9></div>
<div id="p10"><provider-10></provider-10></div>
`
})
export class ProvidersComponent { }
| aio/content/examples/dependency-injection/src/app/providers.component.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00018717744387686253,
0.0001695522660156712,
0.0001640277187107131,
0.00016873156710062176,
0.000004456949682207778
] |
{
"id": 4,
"code_window": [
"{% import \"lib/githubLinks.html\" as github -%}\n",
"{% import \"api/lib/memberHelpers.html\" as members -%}\n",
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><i class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</i></a>{% endmacro %}\n",
"{% macro label(test, class, text) %}{% if test %}<label class=\"{$ class $}\">{$ text $}</label>{% endif %}{% endmacro %}\n",
"{% macro renderLabels(doc) -%}\n",
" {$ label(doc.notYetDocumented, 'no-doc', 'UNDOCUMENTED') $}\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><em class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</em></a>{% endmacro %}\n"
],
"file_path": "aio/tools/transforms/templates/overview-dump.template.html",
"type": "replace",
"edit_start_line_idx": 2
} | /**
* @license
* Copyright Google LLC All Rights Reserved.
*
* Use of this source code is governed by an MIT-style license that can be
* found in the LICENSE file at https://angular.io/license
*/
import {PrefetchAssetGroup} from '../src/assets';
import {CacheDatabase} from '../src/db-cache';
import {IdleScheduler} from '../src/idle';
import {MockCache} from '../testing/cache';
import {MockExtendableEvent} from '../testing/events';
import {MockRequest} from '../testing/fetch';
import {MockFileSystemBuilder, MockServerStateBuilder, tmpHashTable, tmpManifestSingleAssetGroup} from '../testing/mock';
import {SwTestHarnessBuilder} from '../testing/scope';
import {envIsSupported} from '../testing/utils';
(function() {
// Skip environments that don't support the minimum APIs needed to run the SW tests.
if (!envIsSupported()) {
return;
}
const dist = new MockFileSystemBuilder()
.addFile('/foo.txt', 'this is foo', {Vary: 'Accept'})
.addFile('/bar.txt', 'this is bar')
.build();
const manifest = tmpManifestSingleAssetGroup(dist);
const server = new MockServerStateBuilder().withStaticFiles(dist).withManifest(manifest).build();
const scope = new SwTestHarnessBuilder().withServerState(server).build();
const db = new CacheDatabase(scope);
const testEvent = new MockExtendableEvent('test');
describe('prefetch assets', () => {
let group: PrefetchAssetGroup;
let idle: IdleScheduler;
beforeEach(() => {
idle = new IdleScheduler(null!, 3000, 30000, {
log: (v, ctx = '') => console.error(v, ctx),
});
group = new PrefetchAssetGroup(
scope, scope, idle, manifest.assetGroups![0], tmpHashTable(manifest), db, 'test');
});
it('initializes without crashing', async () => {
await group.initializeFully();
});
it('fully caches the two files', async () => {
await group.initializeFully();
scope.updateServerState();
const res1 = await group.handleFetch(scope.newRequest('/foo.txt'), testEvent);
const res2 = await group.handleFetch(scope.newRequest('/bar.txt'), testEvent);
expect(await res1!.text()).toEqual('this is foo');
expect(await res2!.text()).toEqual('this is bar');
});
it('persists the cache across restarts', async () => {
await group.initializeFully();
const freshScope =
new SwTestHarnessBuilder().withCacheState(scope.caches.original.dehydrate()).build();
group = new PrefetchAssetGroup(
freshScope, freshScope, idle, manifest.assetGroups![0], tmpHashTable(manifest),
new CacheDatabase(freshScope), 'test');
await group.initializeFully();
const res1 = await group.handleFetch(scope.newRequest('/foo.txt'), testEvent);
const res2 = await group.handleFetch(scope.newRequest('/bar.txt'), testEvent);
expect(await res1!.text()).toEqual('this is foo');
expect(await res2!.text()).toEqual('this is bar');
});
it('caches properly if resources are requested before initialization', async () => {
const res1 = await group.handleFetch(scope.newRequest('/foo.txt'), testEvent);
const res2 = await group.handleFetch(scope.newRequest('/bar.txt'), testEvent);
expect(await res1!.text()).toEqual('this is foo');
expect(await res2!.text()).toEqual('this is bar');
scope.updateServerState();
await group.initializeFully();
});
it('throws if the server-side content does not match the manifest hash', async () => {
const badHashFs = dist.extend().addFile('/foo.txt', 'corrupted file').build();
const badServer =
new MockServerStateBuilder().withManifest(manifest).withStaticFiles(badHashFs).build();
const badScope = new SwTestHarnessBuilder().withServerState(badServer).build();
group = new PrefetchAssetGroup(
badScope, badScope, idle, manifest.assetGroups![0], tmpHashTable(manifest),
new CacheDatabase(badScope), 'test');
const err = await errorFrom(group.initializeFully());
expect(err.message).toContain('Hash mismatch');
});
it('CacheQueryOptions are passed through', async () => {
await group.initializeFully();
const matchSpy = spyOn(MockCache.prototype, 'match').and.callThrough();
await group.handleFetch(scope.newRequest('/foo.txt'), testEvent);
expect(matchSpy).toHaveBeenCalledWith(new MockRequest('/foo.txt'), {ignoreVary: true});
});
});
})();
function errorFrom(promise: Promise<any>): Promise<any> {
return promise.catch(err => err);
}
| packages/service-worker/worker/test/prefetch_spec.ts | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.00017809341079555452,
0.00017342383216600865,
0.00016788452921900898,
0.00017460058734286577,
0.0000027707467324944446
] |
{
"id": 4,
"code_window": [
"{% import \"lib/githubLinks.html\" as github -%}\n",
"{% import \"api/lib/memberHelpers.html\" as members -%}\n",
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><i class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</i></a>{% endmacro %}\n",
"{% macro label(test, class, text) %}{% if test %}<label class=\"{$ class $}\">{$ text $}</label>{% endif %}{% endmacro %}\n",
"{% macro renderLabels(doc) -%}\n",
" {$ label(doc.notYetDocumented, 'no-doc', 'UNDOCUMENTED') $}\n"
],
"labels": [
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"{% macro goToCode(doc) %}<a href=\"{$ github.githubViewHref(doc, versionInfo) $}\" class=\"go-to-code\" title=\"Go to source code\"><em class=\"material-icons\" aria-hidden=\"true\" role=\"img\">code</em></a>{% endmacro %}\n"
],
"file_path": "aio/tools/transforms/templates/overview-dump.template.html",
"type": "replace",
"edit_start_line_idx": 2
} | .errorMessage{
color:red;
}
.form-row{
margin-top: 10px;
} | aio/content/examples/dynamic-form/src/assets/sample.css | 0 | https://github.com/angular/angular/commit/70c2e39b0b70c05744765cd9f1dc5f9c87518e8a | [
0.0001660009438637644,
0.0001660009438637644,
0.0001660009438637644,
0.0001660009438637644,
0
] |
{
"id": 0,
"code_window": [
"// Type definitions for lolex 3.1\n",
"// Project: https://github.com/sinonjs/lolex\n",
"// Definitions by: Wim Looman <https://github.com/Nemo157>\n",
"// Josh Goldberg <https://github.com/joshuakgoldberg>\n",
"// Rogier Schouten <https://github.com/rogierschouten>\n",
"// Yishai Zehavi <https://github.com/zyishai>\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// Type definitions for lolex 5.1\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 0
} | // Type definitions for lolex 3.1
// Project: https://github.com/sinonjs/lolex
// Definitions by: Wim Looman <https://github.com/Nemo157>
// Josh Goldberg <https://github.com/joshuakgoldberg>
// Rogier Schouten <https://github.com/rogierschouten>
// Yishai Zehavi <https://github.com/zyishai>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
/**
* Names of clock methods that may be faked by install.
*/
type FakeMethod = "setTimeout" | "clearTimeout" | "setImmediate" | "clearImmediate" | "setInterval" | "clearInterval" | "Date" | "nextTick" | "hrtime" | "requestAnimationFrame" | "cancelAnimationFrame" | "requestIdleCallback" | "cancelIdleCallback";
/**
* Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).
*/
export interface GlobalTimers<TTimerId extends TimerId> {
/**
* Schedules a callback to be fired once timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait to run the callback.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setTimeout: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setTimeout.
*
* @param id Timer ID or object.
*/
clearTimeout: (id: TimerId) => void;
/**
* Schedules a callback to be fired every time timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait between callbacks.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setInterval: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setInterval.
*
* @param id Timer ID or object.
*/
clearInterval: (id: TTimerId) => void;
/**
* Schedules the callback to be fired once 0 milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @remarks You'll still have to call clock.tick() for the callback to fire.
* @remarks If called during a tick the callback won't fire until 1 millisecond has ticked by.
*/
setImmediate: (callback: () => void) => TTimerId;
/**
* Clears a timer, as long as it was created using setImmediate.
*
* @param id Timer ID or object.
*/
clearImmediate: (id: TTimerId) => void;
/**
* Implements the Date object but using this clock to provide the correct time.
*/
Date: typeof Date;
}
/**
* Timer object used in node.
*/
export interface NodeTimer {
/**
* Stub method call. Does nothing.
*/
ref(): void;
/**
* Stub method call. Does nothing.
*/
unref(): void;
}
/**
* Timer identifier for clock scheduling.
*/
export type TimerId = number | NodeTimer;
/**
* Controls the flow of time.
*/
export interface LolexClock<TTimerId extends TimerId> extends GlobalTimers<TTimerId> {
/**
* Current clock time.
*/
now: number;
/**
* Don't know what this prop is for, but it was included in the clocks that `createClock` or
* `install` return (it is never used in the code, for now).
*/
timeouts: {};
/**
* Maximum number of timers that will be run when calling runAll().
*/
loopLimit: number;
/**
* Schedule callback to run in the next animation frame.
*
* @param callback Callback to be fired.
* @returns Request id.
*/
requestAnimationFrame: (callback: (time: number) => void) => TTimerId;
/**
* Cancel animation frame request.
*
* @param id The id returned from requestAnimationFrame method.
*/
cancelAnimationFrame: (id: TTimerId) => void;
/**
* Queues the callback to be fired during idle periods to perform background and low priority work on the main event loop.
*
* @param callback Callback to be fired.
* @param timeout The maximum number of ticks before the callback must be fired.
* @remarks Callbacks which have a timeout option will be fired no later than time in milliseconds.
*/
requestIdleCallback: (callback: () => void, timeout?: number) => TTimerId;
/**
* Clears a timer, as long as it was created using requestIdleCallback.
*
* @param id Timer ID or object.
*/
cancelIdleCallback: (id: TTimerId) => void;
/**
* Get the number of waiting timers.
*
* @returns number of waiting timers.
*/
countTimers: () => number;
/**
* Advances the clock to the the moment of the first scheduled timer, firing it.
*/
next: () => void;
/**
* Advance the clock, firing callbacks if necessary.
*
* @param time How many ticks to advance by.
*/
tick: (time: number | string) => void;
/**
* Removes all timers and tick without firing them and restore now to its original value.
*/
reset: () => void;
/**
* Runs all pending timers until there are none remaining.
*
* @remarks If new timers are added while it is executing they will be run as well.
*/
runAll: () => void;
/**
* Advanced the clock to the next animation frame while firing all scheduled callbacks.
*/
runToFrame: () => void;
/**
* Takes note of the last scheduled timer when it is run, and advances the clock to
* that time firing callbacks as necessary.
*/
runToLast: () => void;
/**
* Simulates a user changing the system clock.
*
* @param now New system time.
* @remarks This affects the current time but it does not in itself cause timers to fire.
*/
setSystemTime: (now?: number | Date) => void;
}
/**
* Lolex clock for a browser environment.
*/
type BrowserClock = LolexClock<number> & {
/**
* Mimics performance.now().
*/
performance: {
now: () => number;
}
};
/**
* Lolex clock for a Node environment.
*/
type NodeClock = LolexClock<NodeTimer> & {
/**
* Mimicks process.hrtime().
*
* @param prevTime Previous system time to calculate time elapsed.
* @returns High resolution real time as [seconds, nanoseconds].
*/
hrtime(prevTime?: [number, number]): [number, number];
/**
* Mimics process.nextTick() explicitly dropping additional arguments.
*/
queueMicrotask: (callback: () => void) => void;
/**
* Simulates process.nextTick().
*/
nextTick: (callback: () => void) => void;
/**
* Run all pending microtasks scheduled with nextTick.
*/
runMicrotasks: () => void;
};
/**
* Clock object created by lolex.
*/
type Clock = BrowserClock | NodeClock;
/**
* Additional methods that installed clock have.
*/
type InstalledMethods = {
/**
* Restores the original methods on the context that was passed to lolex.install,
* or the native timers if no context was given.
*/
uninstall: () => void;
methods: FakeMethod[];
};
/**
* Clock object created by calling `install();`.
*
* @type TClock type of base clock (e.g BrowserClock).
*/
type InstalledClock<TClock extends Clock = Clock> = TClock & InstalledMethods;
/**
* Creates a clock.
*
* @param now Current time for the clock.
* @param loopLimit Maximum number of timers that will be run when calling runAll()
* before assuming that we have an infinite loop and throwing an error
* (by default, 1000).
* @type TClock Type of clock to create.
* @remarks The default epoch is 0.
*/
export declare function createClock<TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number): TClock;
export interface LolexInstallOpts {
/**
* Installs lolex onto the specified target context (default: global)
*/
target?: any;
/**
* Installs lolex with the specified unix epoch (default: 0)
*/
now?: number | Date;
/**
* An array with explicit function names to hijack. When not set, lolex will automatically fake all methods except nextTick
* e.g., lolex.install({ toFake: ["setTimeout", "nextTick"]}) will fake only setTimeout and nextTick
*/
toFake?: FakeMethod[];
/**
* The maximum number of timers that will be run when calling runAll() (default: 1000)
*/
loopLimit?: number;
/**
* Tells lolex to increment mocked time automatically based on the real system time shift (e.g. the mocked time will be incremented by
* 20ms for every 20ms change in the real system time) (default: false)
*/
shouldAdvanceTime?: boolean;
/**
* Relevant only when using with shouldAdvanceTime: true. increment mocked time by advanceTimeDelta ms every advanceTimeDelta ms change
* in the real system time (default: 20)
*/
advanceTimeDelta?: number;
}
/**
* Creates a clock and installs it globally.
*
* @param now Current time for the clock, as with lolex.createClock().
* @param toFake Names of methods that should be faked.
* @type TClock Type of clock to create.
*/
export declare function install<TClock extends Clock = Clock>(opts?: LolexInstallOpts): InstalledClock<TClock>;
export interface LolexWithContext {
timers: GlobalTimers<TimerId>;
createClock: <TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number) => TClock;
install: <TClock extends Clock = Clock>(opts?: LolexInstallOpts) => InstalledClock<TClock>;
withGlobal: (global: Object) => LolexWithContext;
}
/**
* Apply new context to lolex.
*
* @param global New context to apply like `window` (in browsers) or `global` (in node).
*/
export declare function withGlobal(global: Object): LolexWithContext;
export declare const timers: GlobalTimers<TimerId>;
| types/lolex/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.9423108100891113,
0.02788730151951313,
0.00016115563630592078,
0.00016689163749106228,
0.15918070077896118
] |
{
"id": 0,
"code_window": [
"// Type definitions for lolex 3.1\n",
"// Project: https://github.com/sinonjs/lolex\n",
"// Definitions by: Wim Looman <https://github.com/Nemo157>\n",
"// Josh Goldberg <https://github.com/joshuakgoldberg>\n",
"// Rogier Schouten <https://github.com/rogierschouten>\n",
"// Yishai Zehavi <https://github.com/zyishai>\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// Type definitions for lolex 5.1\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 0
} | {
"extends": "dtslint/dt.json",
"rules": {
"unified-signatures": false,
"npm-naming": false
}
} | types/ember/tslint.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017355786985717714,
0.00017355786985717714,
0.00017355786985717714,
0.00017355786985717714,
0
] |
{
"id": 0,
"code_window": [
"// Type definitions for lolex 3.1\n",
"// Project: https://github.com/sinonjs/lolex\n",
"// Definitions by: Wim Looman <https://github.com/Nemo157>\n",
"// Josh Goldberg <https://github.com/joshuakgoldberg>\n",
"// Rogier Schouten <https://github.com/rogierschouten>\n",
"// Yishai Zehavi <https://github.com/zyishai>\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// Type definitions for lolex 5.1\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 0
} | {
"files": [
"index.d.ts",
"node-tests.ts"
],
"compilerOptions": {
"module": "commonjs",
"target": "esnext",
"lib": [
"es6",
"dom"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"baseUrl": "../../",
"typeRoots": [
"../../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
}
}
| types/node/ts3.2/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001723977184155956,
0.00017099139222409576,
0.00017009524162858725,
0.00017048123118001968,
0.000001006827233140939
] |
{
"id": 0,
"code_window": [
"// Type definitions for lolex 3.1\n",
"// Project: https://github.com/sinonjs/lolex\n",
"// Definitions by: Wim Looman <https://github.com/Nemo157>\n",
"// Josh Goldberg <https://github.com/joshuakgoldberg>\n",
"// Rogier Schouten <https://github.com/rogierschouten>\n",
"// Yishai Zehavi <https://github.com/zyishai>\n"
],
"labels": [
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
"// Type definitions for lolex 5.1\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 0
} | import uid2 = require('uid2');
uid2(20); // $ExpectType string
uid2(20, (error, result) => {
error; // $ExpectType Error | null
result; // $ExpectType string | undefined
});
| types/uid2/uid2-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017079751705750823,
0.00017079751705750823,
0.00017079751705750823,
0.00017079751705750823,
0
] |
{
"id": 1,
"code_window": [
"/**\n",
" * Names of clock methods that may be faked by install.\n",
" */\n",
"type FakeMethod = \"setTimeout\" | \"clearTimeout\" | \"setImmediate\" | \"clearImmediate\" | \"setInterval\" | \"clearInterval\" | \"Date\" | \"nextTick\" | \"hrtime\" | \"requestAnimationFrame\" | \"cancelAnimationFrame\" | \"requestIdleCallback\" | \"cancelIdleCallback\";\n",
"\n",
"/**\n",
" * Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FakeMethod =\n",
" | 'setTimeout'\n",
" | 'clearTimeout'\n",
" | 'setImmediate'\n",
" | 'clearImmediate'\n",
" | 'setInterval'\n",
" | 'clearInterval'\n",
" | 'Date'\n",
" | 'nextTick'\n",
" | 'hrtime'\n",
" | 'requestAnimationFrame'\n",
" | 'cancelAnimationFrame'\n",
" | 'requestIdleCallback'\n",
" | 'cancelIdleCallback';\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 12
} | // Type definitions for lolex 3.1
// Project: https://github.com/sinonjs/lolex
// Definitions by: Wim Looman <https://github.com/Nemo157>
// Josh Goldberg <https://github.com/joshuakgoldberg>
// Rogier Schouten <https://github.com/rogierschouten>
// Yishai Zehavi <https://github.com/zyishai>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
/**
* Names of clock methods that may be faked by install.
*/
type FakeMethod = "setTimeout" | "clearTimeout" | "setImmediate" | "clearImmediate" | "setInterval" | "clearInterval" | "Date" | "nextTick" | "hrtime" | "requestAnimationFrame" | "cancelAnimationFrame" | "requestIdleCallback" | "cancelIdleCallback";
/**
* Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).
*/
export interface GlobalTimers<TTimerId extends TimerId> {
/**
* Schedules a callback to be fired once timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait to run the callback.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setTimeout: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setTimeout.
*
* @param id Timer ID or object.
*/
clearTimeout: (id: TimerId) => void;
/**
* Schedules a callback to be fired every time timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait between callbacks.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setInterval: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setInterval.
*
* @param id Timer ID or object.
*/
clearInterval: (id: TTimerId) => void;
/**
* Schedules the callback to be fired once 0 milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @remarks You'll still have to call clock.tick() for the callback to fire.
* @remarks If called during a tick the callback won't fire until 1 millisecond has ticked by.
*/
setImmediate: (callback: () => void) => TTimerId;
/**
* Clears a timer, as long as it was created using setImmediate.
*
* @param id Timer ID or object.
*/
clearImmediate: (id: TTimerId) => void;
/**
* Implements the Date object but using this clock to provide the correct time.
*/
Date: typeof Date;
}
/**
* Timer object used in node.
*/
export interface NodeTimer {
/**
* Stub method call. Does nothing.
*/
ref(): void;
/**
* Stub method call. Does nothing.
*/
unref(): void;
}
/**
* Timer identifier for clock scheduling.
*/
export type TimerId = number | NodeTimer;
/**
* Controls the flow of time.
*/
export interface LolexClock<TTimerId extends TimerId> extends GlobalTimers<TTimerId> {
/**
* Current clock time.
*/
now: number;
/**
* Don't know what this prop is for, but it was included in the clocks that `createClock` or
* `install` return (it is never used in the code, for now).
*/
timeouts: {};
/**
* Maximum number of timers that will be run when calling runAll().
*/
loopLimit: number;
/**
* Schedule callback to run in the next animation frame.
*
* @param callback Callback to be fired.
* @returns Request id.
*/
requestAnimationFrame: (callback: (time: number) => void) => TTimerId;
/**
* Cancel animation frame request.
*
* @param id The id returned from requestAnimationFrame method.
*/
cancelAnimationFrame: (id: TTimerId) => void;
/**
* Queues the callback to be fired during idle periods to perform background and low priority work on the main event loop.
*
* @param callback Callback to be fired.
* @param timeout The maximum number of ticks before the callback must be fired.
* @remarks Callbacks which have a timeout option will be fired no later than time in milliseconds.
*/
requestIdleCallback: (callback: () => void, timeout?: number) => TTimerId;
/**
* Clears a timer, as long as it was created using requestIdleCallback.
*
* @param id Timer ID or object.
*/
cancelIdleCallback: (id: TTimerId) => void;
/**
* Get the number of waiting timers.
*
* @returns number of waiting timers.
*/
countTimers: () => number;
/**
* Advances the clock to the the moment of the first scheduled timer, firing it.
*/
next: () => void;
/**
* Advance the clock, firing callbacks if necessary.
*
* @param time How many ticks to advance by.
*/
tick: (time: number | string) => void;
/**
* Removes all timers and tick without firing them and restore now to its original value.
*/
reset: () => void;
/**
* Runs all pending timers until there are none remaining.
*
* @remarks If new timers are added while it is executing they will be run as well.
*/
runAll: () => void;
/**
* Advanced the clock to the next animation frame while firing all scheduled callbacks.
*/
runToFrame: () => void;
/**
* Takes note of the last scheduled timer when it is run, and advances the clock to
* that time firing callbacks as necessary.
*/
runToLast: () => void;
/**
* Simulates a user changing the system clock.
*
* @param now New system time.
* @remarks This affects the current time but it does not in itself cause timers to fire.
*/
setSystemTime: (now?: number | Date) => void;
}
/**
* Lolex clock for a browser environment.
*/
type BrowserClock = LolexClock<number> & {
/**
* Mimics performance.now().
*/
performance: {
now: () => number;
}
};
/**
* Lolex clock for a Node environment.
*/
type NodeClock = LolexClock<NodeTimer> & {
/**
* Mimicks process.hrtime().
*
* @param prevTime Previous system time to calculate time elapsed.
* @returns High resolution real time as [seconds, nanoseconds].
*/
hrtime(prevTime?: [number, number]): [number, number];
/**
* Mimics process.nextTick() explicitly dropping additional arguments.
*/
queueMicrotask: (callback: () => void) => void;
/**
* Simulates process.nextTick().
*/
nextTick: (callback: () => void) => void;
/**
* Run all pending microtasks scheduled with nextTick.
*/
runMicrotasks: () => void;
};
/**
* Clock object created by lolex.
*/
type Clock = BrowserClock | NodeClock;
/**
* Additional methods that installed clock have.
*/
type InstalledMethods = {
/**
* Restores the original methods on the context that was passed to lolex.install,
* or the native timers if no context was given.
*/
uninstall: () => void;
methods: FakeMethod[];
};
/**
* Clock object created by calling `install();`.
*
* @type TClock type of base clock (e.g BrowserClock).
*/
type InstalledClock<TClock extends Clock = Clock> = TClock & InstalledMethods;
/**
* Creates a clock.
*
* @param now Current time for the clock.
* @param loopLimit Maximum number of timers that will be run when calling runAll()
* before assuming that we have an infinite loop and throwing an error
* (by default, 1000).
* @type TClock Type of clock to create.
* @remarks The default epoch is 0.
*/
export declare function createClock<TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number): TClock;
export interface LolexInstallOpts {
/**
* Installs lolex onto the specified target context (default: global)
*/
target?: any;
/**
* Installs lolex with the specified unix epoch (default: 0)
*/
now?: number | Date;
/**
* An array with explicit function names to hijack. When not set, lolex will automatically fake all methods except nextTick
* e.g., lolex.install({ toFake: ["setTimeout", "nextTick"]}) will fake only setTimeout and nextTick
*/
toFake?: FakeMethod[];
/**
* The maximum number of timers that will be run when calling runAll() (default: 1000)
*/
loopLimit?: number;
/**
* Tells lolex to increment mocked time automatically based on the real system time shift (e.g. the mocked time will be incremented by
* 20ms for every 20ms change in the real system time) (default: false)
*/
shouldAdvanceTime?: boolean;
/**
* Relevant only when using with shouldAdvanceTime: true. increment mocked time by advanceTimeDelta ms every advanceTimeDelta ms change
* in the real system time (default: 20)
*/
advanceTimeDelta?: number;
}
/**
* Creates a clock and installs it globally.
*
* @param now Current time for the clock, as with lolex.createClock().
* @param toFake Names of methods that should be faked.
* @type TClock Type of clock to create.
*/
export declare function install<TClock extends Clock = Clock>(opts?: LolexInstallOpts): InstalledClock<TClock>;
export interface LolexWithContext {
timers: GlobalTimers<TimerId>;
createClock: <TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number) => TClock;
install: <TClock extends Clock = Clock>(opts?: LolexInstallOpts) => InstalledClock<TClock>;
withGlobal: (global: Object) => LolexWithContext;
}
/**
* Apply new context to lolex.
*
* @param global New context to apply like `window` (in browsers) or `global` (in node).
*/
export declare function withGlobal(global: Object): LolexWithContext;
export declare const timers: GlobalTimers<TimerId>;
| types/lolex/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.9963735938072205,
0.07474887371063232,
0.00016535766189917922,
0.0003182878717780113,
0.2438058704137802
] |
{
"id": 1,
"code_window": [
"/**\n",
" * Names of clock methods that may be faked by install.\n",
" */\n",
"type FakeMethod = \"setTimeout\" | \"clearTimeout\" | \"setImmediate\" | \"clearImmediate\" | \"setInterval\" | \"clearInterval\" | \"Date\" | \"nextTick\" | \"hrtime\" | \"requestAnimationFrame\" | \"cancelAnimationFrame\" | \"requestIdleCallback\" | \"cancelIdleCallback\";\n",
"\n",
"/**\n",
" * Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FakeMethod =\n",
" | 'setTimeout'\n",
" | 'clearTimeout'\n",
" | 'setImmediate'\n",
" | 'clearImmediate'\n",
" | 'setInterval'\n",
" | 'clearInterval'\n",
" | 'Date'\n",
" | 'nextTick'\n",
" | 'hrtime'\n",
" | 'requestAnimationFrame'\n",
" | 'cancelAnimationFrame'\n",
" | 'requestIdleCallback'\n",
" | 'cancelIdleCallback';\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 12
} |
url();
url('domain');
url(1);
url('domain', 'test.www.example.com/path/here');
url(-1, 'test.www.example.com/path/here');
| types/js-url/js-url-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001642487186472863,
0.0001642487186472863,
0.0001642487186472863,
0.0001642487186472863,
0
] |
{
"id": 1,
"code_window": [
"/**\n",
" * Names of clock methods that may be faked by install.\n",
" */\n",
"type FakeMethod = \"setTimeout\" | \"clearTimeout\" | \"setImmediate\" | \"clearImmediate\" | \"setInterval\" | \"clearInterval\" | \"Date\" | \"nextTick\" | \"hrtime\" | \"requestAnimationFrame\" | \"cancelAnimationFrame\" | \"requestIdleCallback\" | \"cancelIdleCallback\";\n",
"\n",
"/**\n",
" * Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FakeMethod =\n",
" | 'setTimeout'\n",
" | 'clearTimeout'\n",
" | 'setImmediate'\n",
" | 'clearImmediate'\n",
" | 'setInterval'\n",
" | 'clearInterval'\n",
" | 'Date'\n",
" | 'nextTick'\n",
" | 'hrtime'\n",
" | 'requestAnimationFrame'\n",
" | 'cancelAnimationFrame'\n",
" | 'requestIdleCallback'\n",
" | 'cancelIdleCallback';\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 12
} | // Type definitions for non-npm package jquery.stickem.js 1.4
// Project: https://github.com/davist11/jQuery-Stickem
// Definitions by: David Paz <https://github.com/davidmpaz>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
/// <reference types="jquery"/>
interface StickemSettings {
/**
* selector for element to make sticky
*/
item?: string;
/**
* selector for content container, sizes matched with `item`
*/
container?: string;
/**
* css class used to apply
*/
stickClass?: string;
/**
* css class used to apply when ending sticky
*/
endStickClass?: string;
/**
* offset to use for the sticky element in the parent element
*/
offset?: number | string;
/**
* where to place sticky element
*/
start?: number | string;
/**
* Callback to execute when in stick state
*/
onStick?: () => void;
/**
* Callback to execute when getting out of stick state
*/
onUnstick?: () => void;
}
interface JQuery {
stickem(settings?: StickemSettings): JQuery;
}
| types/jquery.stickem/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001747350033838302,
0.0001683274022070691,
0.00016592323663644493,
0.0001672699290793389,
0.000002935927113867365
] |
{
"id": 1,
"code_window": [
"/**\n",
" * Names of clock methods that may be faked by install.\n",
" */\n",
"type FakeMethod = \"setTimeout\" | \"clearTimeout\" | \"setImmediate\" | \"clearImmediate\" | \"setInterval\" | \"clearInterval\" | \"Date\" | \"nextTick\" | \"hrtime\" | \"requestAnimationFrame\" | \"cancelAnimationFrame\" | \"requestIdleCallback\" | \"cancelIdleCallback\";\n",
"\n",
"/**\n",
" * Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
"type FakeMethod =\n",
" | 'setTimeout'\n",
" | 'clearTimeout'\n",
" | 'setImmediate'\n",
" | 'clearImmediate'\n",
" | 'setInterval'\n",
" | 'clearInterval'\n",
" | 'Date'\n",
" | 'nextTick'\n",
" | 'hrtime'\n",
" | 'requestAnimationFrame'\n",
" | 'cancelAnimationFrame'\n",
" | 'requestIdleCallback'\n",
" | 'cancelIdleCallback';\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 12
} | /**
* Created by kubosho_ on 6/16/2014.
*/
Flipsnap('', {
maxPoint: 3,
distance: 230,
transitionDuration: 500,
disableTouch: true,
disable3d: false
});
| types/flipsnap/flipsnap-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00018651026766747236,
0.00017547036986798048,
0.00016443048662040383,
0.00017547036986798048,
0.000011039890523534268
] |
{
"id": 2,
"code_window": [
" */\n",
" cancelIdleCallback: (id: TTimerId) => void;\n",
"\n",
"\t/**\n",
"\t * Get the number of waiting timers.\n",
"\t *\n",
"\t * @returns number of waiting timers.\n",
"\t */\n",
"\tcountTimers: () => number;\n",
"\n",
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Get the number of waiting timers.\n",
" *\n",
" * @returns number of waiting timers.\n",
" */\n",
" countTimers: () => number;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 145
} | import lolex = require("lolex");
const global: lolex.LolexWithContext = lolex.withGlobal({});
const timers: lolex.GlobalTimers<lolex.TimerId> = lolex.timers;
const lolexTimeout: lolex.TimerId = timers.setTimeout(() => {}, 42);
const lolexInterval: lolex.TimerId = timers.setInterval(() => {}, 42);
const lolexImmediate: lolex.TimerId = timers.setImmediate(() => {});
const lolexDate: Date = new timers.Date();
timers.clearTimeout(lolexTimeout);
timers.clearInterval(lolexInterval);
timers.clearImmediate(lolexImmediate);
let browserClock: lolex.BrowserClock = lolex.createClock() as lolex.BrowserClock;
let nodeClock: lolex.NodeClock = lolex.createClock() as lolex.NodeClock;
browserClock = lolex.createClock<lolex.BrowserClock>();
nodeClock = lolex.createClock<lolex.NodeClock>();
lolex.createClock<lolex.BrowserClock>(7);
lolex.createClock<lolex.BrowserClock>(new Date());
lolex.createClock<lolex.BrowserClock>(7, 9001);
lolex.createClock<lolex.BrowserClock>(new Date(), 9001);
lolex.createClock<lolex.NodeClock>(7);
lolex.createClock<lolex.NodeClock>(new Date());
lolex.createClock<lolex.NodeClock>(7, 9001);
lolex.createClock<lolex.NodeClock>(new Date(), 9001);
const browserInstalledClock = lolex.install<lolex.BrowserClock>({
advanceTimeDelta: 20,
loopLimit: 10,
now: 0,
shouldAdvanceTime: true,
target: {},
toFake: ["setTimeout", "nextTick", "hrtime"]
});
const nodeInstalledClock = lolex.install<lolex.NodeClock>({
advanceTimeDelta: 20,
loopLimit: 10,
now: new Date(0),
shouldAdvanceTime: true,
target: {},
toFake: ["setTimeout", "nextTick", "hrtime"]
});
const browserNow: number = browserClock.now;
const browserTimeouts: Object = browserClock.timeouts;
const browserLoopLimit: number = browserClock.loopLimit;
const browserDate: Date = new browserClock.Date();
const browserPerformanceNow: number = browserClock.performance.now();
const nodeNow: number = nodeClock.now;
const nodeDate: Date = new nodeClock.Date();
const browserTimeout: number = browserClock.setTimeout(() => {}, 7);
const browserInterval: number = browserClock.setInterval(() => {}, 7);
const browserImmediate: number = browserClock.setImmediate(() => {});
const browserAnimationFrame: number = browserClock.requestAnimationFrame(() => {});
const browserIdleCallback: number = browserClock.requestIdleCallback(() => {});
const browserIdleCallbackWithTimeout: number = browserClock.requestIdleCallback(() => {}, 7);
const nodeTimeout: lolex.NodeTimer = nodeClock.setTimeout(() => {}, 7);
const nodeInterval: lolex.NodeTimer = nodeClock.setInterval(() => {}, 7);
const nodeImmediate: lolex.NodeTimer = nodeClock.setImmediate(() => {});
const nodeAnimationFrame: lolex.NodeTimer = nodeClock.requestAnimationFrame(() => {});
const nodeIdleCallback: lolex.NodeTimer = nodeClock.requestIdleCallback(() => {});
const nodeIdleCallbackWithTimeout: lolex.NodeTimer = nodeClock.requestIdleCallback(() => {}, 7);
nodeTimeout.ref();
nodeTimeout.unref();
browserClock.clearTimeout(browserTimeout);
browserClock.clearInterval(browserInterval);
browserClock.clearImmediate(browserImmediate);
browserClock.cancelAnimationFrame(browserAnimationFrame);
browserClock.cancelIdleCallback(browserIdleCallback);
browserClock.cancelIdleCallback(browserIdleCallbackWithTimeout);
nodeClock.clearTimeout(nodeTimeout);
nodeClock.clearInterval(nodeInterval);
nodeClock.clearImmediate(nodeImmediate);
nodeClock.cancelAnimationFrame(nodeAnimationFrame);
nodeClock.cancelIdleCallback(nodeIdleCallback);
nodeClock.cancelIdleCallback(nodeIdleCallbackWithTimeout);
browserClock.tick(7);
browserClock.tick("08");
nodeClock.tick(7);
nodeClock.tick("08:03");
browserClock.next();
nodeClock.next();
browserClock.reset();
nodeClock.reset();
browserClock.runAll();
nodeClock.runAll();
nodeClock.runMicrotasks();
browserClock.runToFrame();
nodeClock.runToFrame();
browserClock.runToLast();
nodeClock.runToLast();
browserClock.setSystemTime();
browserClock.setSystemTime(7);
browserClock.setSystemTime(new Date());
nodeClock.setSystemTime();
nodeClock.setSystemTime(7);
nodeClock.setSystemTime(new Date());
nodeClock.nextTick(() => undefined);
nodeClock.queueMicrotask(() => {});
const browserTimersCount: number = browserClock.countTimers();
const nodeTimersCount: number = nodeClock.countTimers();
let [secs, nanos] = nodeClock.hrtime([0, 0]);
[secs, nanos] = nodeClock.hrtime();
// shows that typescript successfully infer the return values as numbers.
secs.toFixed();
nanos.toExponential();
browserInstalledClock.performance.now();
nodeInstalledClock.nextTick(() => {});
browserInstalledClock.uninstall();
nodeInstalledClock.uninstall();
// Clocks should be typed to have unbound method signatures that can be passed around
const { clearTimeout } = browserClock;
clearTimeout(0);
// TClock of InstalledClock<TClock> is optional.
let installedClock: lolex.InstalledClock;
installedClock = nodeInstalledClock;
installedClock = browserInstalledClock;
| types/lolex/lolex-tests.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.9985204339027405,
0.07050812244415283,
0.00016842648619785905,
0.00018544503836892545,
0.24816244840621948
] |
{
"id": 2,
"code_window": [
" */\n",
" cancelIdleCallback: (id: TTimerId) => void;\n",
"\n",
"\t/**\n",
"\t * Get the number of waiting timers.\n",
"\t *\n",
"\t * @returns number of waiting timers.\n",
"\t */\n",
"\tcountTimers: () => number;\n",
"\n",
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Get the number of waiting timers.\n",
" *\n",
" * @returns number of waiting timers.\n",
" */\n",
" countTimers: () => number;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 145
} | import { overArgs } from "../fp";
export = overArgs;
| types/lodash/fp/overArgs.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017168265185318887,
0.00017168265185318887,
0.00017168265185318887,
0.00017168265185318887,
0
] |
{
"id": 2,
"code_window": [
" */\n",
" cancelIdleCallback: (id: TTimerId) => void;\n",
"\n",
"\t/**\n",
"\t * Get the number of waiting timers.\n",
"\t *\n",
"\t * @returns number of waiting timers.\n",
"\t */\n",
"\tcountTimers: () => number;\n",
"\n",
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Get the number of waiting timers.\n",
" *\n",
" * @returns number of waiting timers.\n",
" */\n",
" countTimers: () => number;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 145
} | {
"compilerOptions": {
"module": "commonjs",
"lib": [
"es6",
"dom"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"baseUrl": "../",
"typeRoots": [
"../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
},
"files": [
"index.d.ts",
"global.d.ts",
"semantic-ui-site-tests.ts"
]
} | types/semantic-ui-site/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001693574304226786,
0.00016890547703951597,
0.00016847081133164465,
0.00016888817481230944,
3.62167327239149e-7
] |
{
"id": 2,
"code_window": [
" */\n",
" cancelIdleCallback: (id: TTimerId) => void;\n",
"\n",
"\t/**\n",
"\t * Get the number of waiting timers.\n",
"\t *\n",
"\t * @returns number of waiting timers.\n",
"\t */\n",
"\tcountTimers: () => number;\n",
"\n",
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Get the number of waiting timers.\n",
" *\n",
" * @returns number of waiting timers.\n",
" */\n",
" countTimers: () => number;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 145
} | {
"compilerOptions": {
"module": "commonjs",
"lib": [
"es6"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"baseUrl": "../",
"typeRoots": [
"../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
},
"files": [
"index.d.ts",
"gulp-image-tests.ts"
]
}
| types/gulp-image/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00016932387370616198,
0.00016913302533794194,
0.0001690251228865236,
0.0001690501085249707,
1.353282925720123e-7
] |
{
"id": 3,
"code_window": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" */\n",
" next: () => void;\n",
"\n",
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" nextAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 157
} | import lolex = require("lolex");
const global: lolex.LolexWithContext = lolex.withGlobal({});
const timers: lolex.GlobalTimers<lolex.TimerId> = lolex.timers;
const lolexTimeout: lolex.TimerId = timers.setTimeout(() => {}, 42);
const lolexInterval: lolex.TimerId = timers.setInterval(() => {}, 42);
const lolexImmediate: lolex.TimerId = timers.setImmediate(() => {});
const lolexDate: Date = new timers.Date();
timers.clearTimeout(lolexTimeout);
timers.clearInterval(lolexInterval);
timers.clearImmediate(lolexImmediate);
let browserClock: lolex.BrowserClock = lolex.createClock() as lolex.BrowserClock;
let nodeClock: lolex.NodeClock = lolex.createClock() as lolex.NodeClock;
browserClock = lolex.createClock<lolex.BrowserClock>();
nodeClock = lolex.createClock<lolex.NodeClock>();
lolex.createClock<lolex.BrowserClock>(7);
lolex.createClock<lolex.BrowserClock>(new Date());
lolex.createClock<lolex.BrowserClock>(7, 9001);
lolex.createClock<lolex.BrowserClock>(new Date(), 9001);
lolex.createClock<lolex.NodeClock>(7);
lolex.createClock<lolex.NodeClock>(new Date());
lolex.createClock<lolex.NodeClock>(7, 9001);
lolex.createClock<lolex.NodeClock>(new Date(), 9001);
const browserInstalledClock = lolex.install<lolex.BrowserClock>({
advanceTimeDelta: 20,
loopLimit: 10,
now: 0,
shouldAdvanceTime: true,
target: {},
toFake: ["setTimeout", "nextTick", "hrtime"]
});
const nodeInstalledClock = lolex.install<lolex.NodeClock>({
advanceTimeDelta: 20,
loopLimit: 10,
now: new Date(0),
shouldAdvanceTime: true,
target: {},
toFake: ["setTimeout", "nextTick", "hrtime"]
});
const browserNow: number = browserClock.now;
const browserTimeouts: Object = browserClock.timeouts;
const browserLoopLimit: number = browserClock.loopLimit;
const browserDate: Date = new browserClock.Date();
const browserPerformanceNow: number = browserClock.performance.now();
const nodeNow: number = nodeClock.now;
const nodeDate: Date = new nodeClock.Date();
const browserTimeout: number = browserClock.setTimeout(() => {}, 7);
const browserInterval: number = browserClock.setInterval(() => {}, 7);
const browserImmediate: number = browserClock.setImmediate(() => {});
const browserAnimationFrame: number = browserClock.requestAnimationFrame(() => {});
const browserIdleCallback: number = browserClock.requestIdleCallback(() => {});
const browserIdleCallbackWithTimeout: number = browserClock.requestIdleCallback(() => {}, 7);
const nodeTimeout: lolex.NodeTimer = nodeClock.setTimeout(() => {}, 7);
const nodeInterval: lolex.NodeTimer = nodeClock.setInterval(() => {}, 7);
const nodeImmediate: lolex.NodeTimer = nodeClock.setImmediate(() => {});
const nodeAnimationFrame: lolex.NodeTimer = nodeClock.requestAnimationFrame(() => {});
const nodeIdleCallback: lolex.NodeTimer = nodeClock.requestIdleCallback(() => {});
const nodeIdleCallbackWithTimeout: lolex.NodeTimer = nodeClock.requestIdleCallback(() => {}, 7);
nodeTimeout.ref();
nodeTimeout.unref();
browserClock.clearTimeout(browserTimeout);
browserClock.clearInterval(browserInterval);
browserClock.clearImmediate(browserImmediate);
browserClock.cancelAnimationFrame(browserAnimationFrame);
browserClock.cancelIdleCallback(browserIdleCallback);
browserClock.cancelIdleCallback(browserIdleCallbackWithTimeout);
nodeClock.clearTimeout(nodeTimeout);
nodeClock.clearInterval(nodeInterval);
nodeClock.clearImmediate(nodeImmediate);
nodeClock.cancelAnimationFrame(nodeAnimationFrame);
nodeClock.cancelIdleCallback(nodeIdleCallback);
nodeClock.cancelIdleCallback(nodeIdleCallbackWithTimeout);
browserClock.tick(7);
browserClock.tick("08");
nodeClock.tick(7);
nodeClock.tick("08:03");
browserClock.next();
nodeClock.next();
browserClock.reset();
nodeClock.reset();
browserClock.runAll();
nodeClock.runAll();
nodeClock.runMicrotasks();
browserClock.runToFrame();
nodeClock.runToFrame();
browserClock.runToLast();
nodeClock.runToLast();
browserClock.setSystemTime();
browserClock.setSystemTime(7);
browserClock.setSystemTime(new Date());
nodeClock.setSystemTime();
nodeClock.setSystemTime(7);
nodeClock.setSystemTime(new Date());
nodeClock.nextTick(() => undefined);
nodeClock.queueMicrotask(() => {});
const browserTimersCount: number = browserClock.countTimers();
const nodeTimersCount: number = nodeClock.countTimers();
let [secs, nanos] = nodeClock.hrtime([0, 0]);
[secs, nanos] = nodeClock.hrtime();
// shows that typescript successfully infer the return values as numbers.
secs.toFixed();
nanos.toExponential();
browserInstalledClock.performance.now();
nodeInstalledClock.nextTick(() => {});
browserInstalledClock.uninstall();
nodeInstalledClock.uninstall();
// Clocks should be typed to have unbound method signatures that can be passed around
const { clearTimeout } = browserClock;
clearTimeout(0);
// TClock of InstalledClock<TClock> is optional.
let installedClock: lolex.InstalledClock;
installedClock = nodeInstalledClock;
installedClock = browserInstalledClock;
| types/lolex/lolex-tests.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0090065011754632,
0.0007813355186954141,
0.00016774612595327199,
0.00017680243763606995,
0.002198590897023678
] |
{
"id": 3,
"code_window": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" */\n",
" next: () => void;\n",
"\n",
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" nextAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 157
} | // Type definitions for vue2-hammer 2.1
// Project: https://github.com/bsdfzzzy/vue2-hammer
// Definitions by: Peter Tandler <https://github.com/ptandler>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 3.2
import { PluginObject } from "vue";
export interface VueHammer extends PluginObject<any> {
config: any;
}
export const VueHammer: VueHammer;
| types/vue2-hammer/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017297516751568764,
0.0001712237426545471,
0.00016947230324149132,
0.0001712237426545471,
0.0000017514321370981634
] |
{
"id": 3,
"code_window": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" */\n",
" next: () => void;\n",
"\n",
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" nextAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 157
} | { "extends": "dtslint/dt.json" }
| types/end-of-stream/tslint.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00016950299323070794,
0.00016950299323070794,
0.00016950299323070794,
0.00016950299323070794,
0
] |
{
"id": 3,
"code_window": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" */\n",
" next: () => void;\n",
"\n",
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advances the clock to the the moment of the first scheduled timer, firing it.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" nextAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 157
} | /// <reference types="angular" />
var app = angular.module('app', ['highcharts-ng']);
class AppController {
chartConfig: HighChartsNGConfig = {
options: {
chart: {
type: 'bar'
},
tooltip: {
style: {
padding: 10,
fontWeight: 'bold'
}
},
credits: {
enabled: false
},
plotOptions: {}
},
series: [
{
data: [10, 15, 12, 8, 7]
},
{
data: [[0, 10], [1, 15], [2, 12], [3, 8], [4, 7]]
},
{
data: [{ name: "A", y: 10 }, { name: "B", y: 15 }, { name: "C", y: 12 }, { name: "D", y: 8 }, { name: "E", y: 7 }]
}
],
title: {
text: 'My Awesome Chart'
},
loading: true,
noData: 'No data here'
};
constructor($timeout: ng.ITimeoutService) {
var vm = this;
$timeout(function() {
//Some async action
vm.chartConfig.loading = false;
});
}
}
app.controller("AppController", AppController);
| types/highcharts-ng/highcharts-ng-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00016936649626586586,
0.00016852094267960638,
0.0001676357933320105,
0.0001686440664343536,
6.447982627832971e-7
] |
{
"id": 4,
"code_window": [
" */\n",
" tick: (time: number | string) => void;\n",
"\n",
" /**\n",
" * Removes all timers and tick without firing them and restore now to its original value.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @param time How many ticks to advance by.\n",
" */\n",
" tickAsync: (time: number | string) => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 164
} | // Type definitions for lolex 3.1
// Project: https://github.com/sinonjs/lolex
// Definitions by: Wim Looman <https://github.com/Nemo157>
// Josh Goldberg <https://github.com/joshuakgoldberg>
// Rogier Schouten <https://github.com/rogierschouten>
// Yishai Zehavi <https://github.com/zyishai>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
/**
* Names of clock methods that may be faked by install.
*/
type FakeMethod = "setTimeout" | "clearTimeout" | "setImmediate" | "clearImmediate" | "setInterval" | "clearInterval" | "Date" | "nextTick" | "hrtime" | "requestAnimationFrame" | "cancelAnimationFrame" | "requestIdleCallback" | "cancelIdleCallback";
/**
* Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).
*/
export interface GlobalTimers<TTimerId extends TimerId> {
/**
* Schedules a callback to be fired once timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait to run the callback.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setTimeout: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setTimeout.
*
* @param id Timer ID or object.
*/
clearTimeout: (id: TimerId) => void;
/**
* Schedules a callback to be fired every time timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait between callbacks.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setInterval: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setInterval.
*
* @param id Timer ID or object.
*/
clearInterval: (id: TTimerId) => void;
/**
* Schedules the callback to be fired once 0 milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @remarks You'll still have to call clock.tick() for the callback to fire.
* @remarks If called during a tick the callback won't fire until 1 millisecond has ticked by.
*/
setImmediate: (callback: () => void) => TTimerId;
/**
* Clears a timer, as long as it was created using setImmediate.
*
* @param id Timer ID or object.
*/
clearImmediate: (id: TTimerId) => void;
/**
* Implements the Date object but using this clock to provide the correct time.
*/
Date: typeof Date;
}
/**
* Timer object used in node.
*/
export interface NodeTimer {
/**
* Stub method call. Does nothing.
*/
ref(): void;
/**
* Stub method call. Does nothing.
*/
unref(): void;
}
/**
* Timer identifier for clock scheduling.
*/
export type TimerId = number | NodeTimer;
/**
* Controls the flow of time.
*/
export interface LolexClock<TTimerId extends TimerId> extends GlobalTimers<TTimerId> {
/**
* Current clock time.
*/
now: number;
/**
* Don't know what this prop is for, but it was included in the clocks that `createClock` or
* `install` return (it is never used in the code, for now).
*/
timeouts: {};
/**
* Maximum number of timers that will be run when calling runAll().
*/
loopLimit: number;
/**
* Schedule callback to run in the next animation frame.
*
* @param callback Callback to be fired.
* @returns Request id.
*/
requestAnimationFrame: (callback: (time: number) => void) => TTimerId;
/**
* Cancel animation frame request.
*
* @param id The id returned from requestAnimationFrame method.
*/
cancelAnimationFrame: (id: TTimerId) => void;
/**
* Queues the callback to be fired during idle periods to perform background and low priority work on the main event loop.
*
* @param callback Callback to be fired.
* @param timeout The maximum number of ticks before the callback must be fired.
* @remarks Callbacks which have a timeout option will be fired no later than time in milliseconds.
*/
requestIdleCallback: (callback: () => void, timeout?: number) => TTimerId;
/**
* Clears a timer, as long as it was created using requestIdleCallback.
*
* @param id Timer ID or object.
*/
cancelIdleCallback: (id: TTimerId) => void;
/**
* Get the number of waiting timers.
*
* @returns number of waiting timers.
*/
countTimers: () => number;
/**
* Advances the clock to the the moment of the first scheduled timer, firing it.
*/
next: () => void;
/**
* Advance the clock, firing callbacks if necessary.
*
* @param time How many ticks to advance by.
*/
tick: (time: number | string) => void;
/**
* Removes all timers and tick without firing them and restore now to its original value.
*/
reset: () => void;
/**
* Runs all pending timers until there are none remaining.
*
* @remarks If new timers are added while it is executing they will be run as well.
*/
runAll: () => void;
/**
* Advanced the clock to the next animation frame while firing all scheduled callbacks.
*/
runToFrame: () => void;
/**
* Takes note of the last scheduled timer when it is run, and advances the clock to
* that time firing callbacks as necessary.
*/
runToLast: () => void;
/**
* Simulates a user changing the system clock.
*
* @param now New system time.
* @remarks This affects the current time but it does not in itself cause timers to fire.
*/
setSystemTime: (now?: number | Date) => void;
}
/**
* Lolex clock for a browser environment.
*/
type BrowserClock = LolexClock<number> & {
/**
* Mimics performance.now().
*/
performance: {
now: () => number;
}
};
/**
* Lolex clock for a Node environment.
*/
type NodeClock = LolexClock<NodeTimer> & {
/**
* Mimicks process.hrtime().
*
* @param prevTime Previous system time to calculate time elapsed.
* @returns High resolution real time as [seconds, nanoseconds].
*/
hrtime(prevTime?: [number, number]): [number, number];
/**
* Mimics process.nextTick() explicitly dropping additional arguments.
*/
queueMicrotask: (callback: () => void) => void;
/**
* Simulates process.nextTick().
*/
nextTick: (callback: () => void) => void;
/**
* Run all pending microtasks scheduled with nextTick.
*/
runMicrotasks: () => void;
};
/**
* Clock object created by lolex.
*/
type Clock = BrowserClock | NodeClock;
/**
* Additional methods that installed clock have.
*/
type InstalledMethods = {
/**
* Restores the original methods on the context that was passed to lolex.install,
* or the native timers if no context was given.
*/
uninstall: () => void;
methods: FakeMethod[];
};
/**
* Clock object created by calling `install();`.
*
* @type TClock type of base clock (e.g BrowserClock).
*/
type InstalledClock<TClock extends Clock = Clock> = TClock & InstalledMethods;
/**
* Creates a clock.
*
* @param now Current time for the clock.
* @param loopLimit Maximum number of timers that will be run when calling runAll()
* before assuming that we have an infinite loop and throwing an error
* (by default, 1000).
* @type TClock Type of clock to create.
* @remarks The default epoch is 0.
*/
export declare function createClock<TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number): TClock;
export interface LolexInstallOpts {
/**
* Installs lolex onto the specified target context (default: global)
*/
target?: any;
/**
* Installs lolex with the specified unix epoch (default: 0)
*/
now?: number | Date;
/**
* An array with explicit function names to hijack. When not set, lolex will automatically fake all methods except nextTick
* e.g., lolex.install({ toFake: ["setTimeout", "nextTick"]}) will fake only setTimeout and nextTick
*/
toFake?: FakeMethod[];
/**
* The maximum number of timers that will be run when calling runAll() (default: 1000)
*/
loopLimit?: number;
/**
* Tells lolex to increment mocked time automatically based on the real system time shift (e.g. the mocked time will be incremented by
* 20ms for every 20ms change in the real system time) (default: false)
*/
shouldAdvanceTime?: boolean;
/**
* Relevant only when using with shouldAdvanceTime: true. increment mocked time by advanceTimeDelta ms every advanceTimeDelta ms change
* in the real system time (default: 20)
*/
advanceTimeDelta?: number;
}
/**
* Creates a clock and installs it globally.
*
* @param now Current time for the clock, as with lolex.createClock().
* @param toFake Names of methods that should be faked.
* @type TClock Type of clock to create.
*/
export declare function install<TClock extends Clock = Clock>(opts?: LolexInstallOpts): InstalledClock<TClock>;
export interface LolexWithContext {
timers: GlobalTimers<TimerId>;
createClock: <TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number) => TClock;
install: <TClock extends Clock = Clock>(opts?: LolexInstallOpts) => InstalledClock<TClock>;
withGlobal: (global: Object) => LolexWithContext;
}
/**
* Apply new context to lolex.
*
* @param global New context to apply like `window` (in browsers) or `global` (in node).
*/
export declare function withGlobal(global: Object): LolexWithContext;
export declare const timers: GlobalTimers<TimerId>;
| types/lolex/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.012331429868936539,
0.0012931468663737178,
0.000167548525496386,
0.0007669716724194586,
0.002062513493001461
] |
{
"id": 4,
"code_window": [
" */\n",
" tick: (time: number | string) => void;\n",
"\n",
" /**\n",
" * Removes all timers and tick without firing them and restore now to its original value.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @param time How many ticks to advance by.\n",
" */\n",
" tickAsync: (time: number | string) => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 164
} | {
"extends": "dtslint/dt.json"
} | types/balanced-match/tslint.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001672915241215378,
0.0001672915241215378,
0.0001672915241215378,
0.0001672915241215378,
0
] |
{
"id": 4,
"code_window": [
" */\n",
" tick: (time: number | string) => void;\n",
"\n",
" /**\n",
" * Removes all timers and tick without firing them and restore now to its original value.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @param time How many ticks to advance by.\n",
" */\n",
" tickAsync: (time: number | string) => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 164
} | {
"extends": "dtslint/dt.json",
"rules": {
"adjacent-overload-signatures": false,
"array-type": false,
"arrow-return-shorthand": false,
"ban-types": false,
"callable-types": false,
"comment-format": false,
"dt-header": false,
"npm-naming": false,
"eofline": false,
"export-just-namespace": false,
"import-spacing": false,
"interface-name": false,
"interface-over-type-literal": false,
"jsdoc-format": false,
"max-line-length": false,
"member-access": false,
"new-parens": false,
"no-any-union": false,
"no-boolean-literal-compare": false,
"no-conditional-assignment": false,
"no-consecutive-blank-lines": false,
"no-construct": false,
"no-declare-current-package": false,
"no-duplicate-imports": false,
"no-duplicate-variable": false,
"no-empty-interface": false,
"no-for-in-array": false,
"no-inferrable-types": false,
"no-internal-module": false,
"no-irregular-whitespace": false,
"no-mergeable-namespace": false,
"no-misused-new": false,
"no-namespace": false,
"no-object-literal-type-assertion": false,
"no-padding": false,
"no-redundant-jsdoc": false,
"no-redundant-jsdoc-2": false,
"no-redundant-undefined": false,
"no-reference-import": false,
"no-relative-import-in-test": false,
"no-self-import": false,
"no-single-declare-module": false,
"no-string-throw": false,
"no-unnecessary-callback-wrapper": false,
"no-unnecessary-class": false,
"no-unnecessary-generics": false,
"no-unnecessary-qualifier": false,
"no-unnecessary-type-assertion": false,
"no-useless-files": false,
"no-var-keyword": false,
"no-var-requires": false,
"no-void-expression": false,
"no-trailing-whitespace": false,
"object-literal-key-quotes": false,
"object-literal-shorthand": false,
"one-line": false,
"one-variable-per-declaration": false,
"only-arrow-functions": false,
"prefer-conditional-expression": false,
"prefer-const": false,
"prefer-declare-function": false,
"prefer-for-of": false,
"prefer-method-signature": false,
"prefer-template": false,
"radix": false,
"semicolon": false,
"space-before-function-paren": false,
"space-within-parens": false,
"strict-export-declare-modifiers": false,
"trim-file": false,
"triple-equals": false,
"typedef-whitespace": false,
"unified-signatures": false,
"void-return": false,
"whitespace": false
}
}
| types/ref-union/tslint.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017197839042637497,
0.0001667066680965945,
0.00016513785521965474,
0.00016634493658784777,
0.0000019909707589249592
] |
{
"id": 4,
"code_window": [
" */\n",
" tick: (time: number | string) => void;\n",
"\n",
" /**\n",
" * Removes all timers and tick without firing them and restore now to its original value.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Advance the clock, firing callbacks if necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @param time How many ticks to advance by.\n",
" */\n",
" tickAsync: (time: number | string) => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 164
} | import * as fs from "fs";
import temp = require("promised-temp");
function testCleanup() {
temp.cleanup().then((result: boolean | temp.Stats) => {
if (typeof result === "boolean") {
const x = result;
} else {
const { files, dirs } = result;
files.toPrecision(4);
}
});
}
function testOpen() {
temp.open({ dir: "tempDir", prefix: "pref", suffix: "suff" }).then((result: temp.OpenFile) => {
const { path, fd } = result;
path.length;
fd.toPrecision(5);
});
temp.open("strPrefix").then((result: temp.OpenFile) => {
const { path, fd } = result;
path.length;
fd.toPrecision(5);
});
}
function testCreateWriteStream() {
const stream =
temp.createWriteStream("HelloStreamAffix")
.then((stream: fs.WriteStream) => stream.write("data"));
const stream2 = temp.createWriteStream();
}
function testMkdir() {
temp.mkdir("prefix").then((dirPath: string) => {
dirPath.length;
});
}
function testPath() {
const p = temp.path({ suffix: "justSuffix" }, "defaultPrefix");
p.length;
const p2: string = temp.path("prefix");
const p3: string = temp.path({ prefix: "prefix" });
}
function testTrack() {
const tempChained = temp.track().track(true).track(false);
tempChained.dir;
tempChained.cleanup();
}
| types/promised-temp/promised-temp-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00026187128969468176,
0.00019048993999604136,
0.0001673539081821218,
0.00017240522720385343,
0.00003386361640878022
] |
{
"id": 5,
"code_window": [
" */\n",
" runAll: () => void;\n",
"\n",
"\t/**\n",
"\t * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
"\t */\n",
"\trunToFrame: () => void;\n",
"\n",
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Runs all pending timers until there are none remaining.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @remarks If new timers are added while it is executing they will be run as well.\n",
" */\n",
" runAllAsync: () => Promise<void>;\n",
"\n",
" /**\n",
" * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
" */\n",
" runToFrame: () => void;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 176
} | // Type definitions for lolex 3.1
// Project: https://github.com/sinonjs/lolex
// Definitions by: Wim Looman <https://github.com/Nemo157>
// Josh Goldberg <https://github.com/joshuakgoldberg>
// Rogier Schouten <https://github.com/rogierschouten>
// Yishai Zehavi <https://github.com/zyishai>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
/**
* Names of clock methods that may be faked by install.
*/
type FakeMethod = "setTimeout" | "clearTimeout" | "setImmediate" | "clearImmediate" | "setInterval" | "clearInterval" | "Date" | "nextTick" | "hrtime" | "requestAnimationFrame" | "cancelAnimationFrame" | "requestIdleCallback" | "cancelIdleCallback";
/**
* Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).
*/
export interface GlobalTimers<TTimerId extends TimerId> {
/**
* Schedules a callback to be fired once timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait to run the callback.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setTimeout: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setTimeout.
*
* @param id Timer ID or object.
*/
clearTimeout: (id: TimerId) => void;
/**
* Schedules a callback to be fired every time timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait between callbacks.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setInterval: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setInterval.
*
* @param id Timer ID or object.
*/
clearInterval: (id: TTimerId) => void;
/**
* Schedules the callback to be fired once 0 milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @remarks You'll still have to call clock.tick() for the callback to fire.
* @remarks If called during a tick the callback won't fire until 1 millisecond has ticked by.
*/
setImmediate: (callback: () => void) => TTimerId;
/**
* Clears a timer, as long as it was created using setImmediate.
*
* @param id Timer ID or object.
*/
clearImmediate: (id: TTimerId) => void;
/**
* Implements the Date object but using this clock to provide the correct time.
*/
Date: typeof Date;
}
/**
* Timer object used in node.
*/
export interface NodeTimer {
/**
* Stub method call. Does nothing.
*/
ref(): void;
/**
* Stub method call. Does nothing.
*/
unref(): void;
}
/**
* Timer identifier for clock scheduling.
*/
export type TimerId = number | NodeTimer;
/**
* Controls the flow of time.
*/
export interface LolexClock<TTimerId extends TimerId> extends GlobalTimers<TTimerId> {
/**
* Current clock time.
*/
now: number;
/**
* Don't know what this prop is for, but it was included in the clocks that `createClock` or
* `install` return (it is never used in the code, for now).
*/
timeouts: {};
/**
* Maximum number of timers that will be run when calling runAll().
*/
loopLimit: number;
/**
* Schedule callback to run in the next animation frame.
*
* @param callback Callback to be fired.
* @returns Request id.
*/
requestAnimationFrame: (callback: (time: number) => void) => TTimerId;
/**
* Cancel animation frame request.
*
* @param id The id returned from requestAnimationFrame method.
*/
cancelAnimationFrame: (id: TTimerId) => void;
/**
* Queues the callback to be fired during idle periods to perform background and low priority work on the main event loop.
*
* @param callback Callback to be fired.
* @param timeout The maximum number of ticks before the callback must be fired.
* @remarks Callbacks which have a timeout option will be fired no later than time in milliseconds.
*/
requestIdleCallback: (callback: () => void, timeout?: number) => TTimerId;
/**
* Clears a timer, as long as it was created using requestIdleCallback.
*
* @param id Timer ID or object.
*/
cancelIdleCallback: (id: TTimerId) => void;
/**
* Get the number of waiting timers.
*
* @returns number of waiting timers.
*/
countTimers: () => number;
/**
* Advances the clock to the the moment of the first scheduled timer, firing it.
*/
next: () => void;
/**
* Advance the clock, firing callbacks if necessary.
*
* @param time How many ticks to advance by.
*/
tick: (time: number | string) => void;
/**
* Removes all timers and tick without firing them and restore now to its original value.
*/
reset: () => void;
/**
* Runs all pending timers until there are none remaining.
*
* @remarks If new timers are added while it is executing they will be run as well.
*/
runAll: () => void;
/**
* Advanced the clock to the next animation frame while firing all scheduled callbacks.
*/
runToFrame: () => void;
/**
* Takes note of the last scheduled timer when it is run, and advances the clock to
* that time firing callbacks as necessary.
*/
runToLast: () => void;
/**
* Simulates a user changing the system clock.
*
* @param now New system time.
* @remarks This affects the current time but it does not in itself cause timers to fire.
*/
setSystemTime: (now?: number | Date) => void;
}
/**
* Lolex clock for a browser environment.
*/
type BrowserClock = LolexClock<number> & {
/**
* Mimics performance.now().
*/
performance: {
now: () => number;
}
};
/**
* Lolex clock for a Node environment.
*/
type NodeClock = LolexClock<NodeTimer> & {
/**
* Mimicks process.hrtime().
*
* @param prevTime Previous system time to calculate time elapsed.
* @returns High resolution real time as [seconds, nanoseconds].
*/
hrtime(prevTime?: [number, number]): [number, number];
/**
* Mimics process.nextTick() explicitly dropping additional arguments.
*/
queueMicrotask: (callback: () => void) => void;
/**
* Simulates process.nextTick().
*/
nextTick: (callback: () => void) => void;
/**
* Run all pending microtasks scheduled with nextTick.
*/
runMicrotasks: () => void;
};
/**
* Clock object created by lolex.
*/
type Clock = BrowserClock | NodeClock;
/**
* Additional methods that installed clock have.
*/
type InstalledMethods = {
/**
* Restores the original methods on the context that was passed to lolex.install,
* or the native timers if no context was given.
*/
uninstall: () => void;
methods: FakeMethod[];
};
/**
* Clock object created by calling `install();`.
*
* @type TClock type of base clock (e.g BrowserClock).
*/
type InstalledClock<TClock extends Clock = Clock> = TClock & InstalledMethods;
/**
* Creates a clock.
*
* @param now Current time for the clock.
* @param loopLimit Maximum number of timers that will be run when calling runAll()
* before assuming that we have an infinite loop and throwing an error
* (by default, 1000).
* @type TClock Type of clock to create.
* @remarks The default epoch is 0.
*/
export declare function createClock<TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number): TClock;
export interface LolexInstallOpts {
/**
* Installs lolex onto the specified target context (default: global)
*/
target?: any;
/**
* Installs lolex with the specified unix epoch (default: 0)
*/
now?: number | Date;
/**
* An array with explicit function names to hijack. When not set, lolex will automatically fake all methods except nextTick
* e.g., lolex.install({ toFake: ["setTimeout", "nextTick"]}) will fake only setTimeout and nextTick
*/
toFake?: FakeMethod[];
/**
* The maximum number of timers that will be run when calling runAll() (default: 1000)
*/
loopLimit?: number;
/**
* Tells lolex to increment mocked time automatically based on the real system time shift (e.g. the mocked time will be incremented by
* 20ms for every 20ms change in the real system time) (default: false)
*/
shouldAdvanceTime?: boolean;
/**
* Relevant only when using with shouldAdvanceTime: true. increment mocked time by advanceTimeDelta ms every advanceTimeDelta ms change
* in the real system time (default: 20)
*/
advanceTimeDelta?: number;
}
/**
* Creates a clock and installs it globally.
*
* @param now Current time for the clock, as with lolex.createClock().
* @param toFake Names of methods that should be faked.
* @type TClock Type of clock to create.
*/
export declare function install<TClock extends Clock = Clock>(opts?: LolexInstallOpts): InstalledClock<TClock>;
export interface LolexWithContext {
timers: GlobalTimers<TimerId>;
createClock: <TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number) => TClock;
install: <TClock extends Clock = Clock>(opts?: LolexInstallOpts) => InstalledClock<TClock>;
withGlobal: (global: Object) => LolexWithContext;
}
/**
* Apply new context to lolex.
*
* @param global New context to apply like `window` (in browsers) or `global` (in node).
*/
export declare function withGlobal(global: Object): LolexWithContext;
export declare const timers: GlobalTimers<TimerId>;
| types/lolex/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.6783453226089478,
0.021113639697432518,
0.00016702360881026834,
0.000400294316932559,
0.11443822085857391
] |
{
"id": 5,
"code_window": [
" */\n",
" runAll: () => void;\n",
"\n",
"\t/**\n",
"\t * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
"\t */\n",
"\trunToFrame: () => void;\n",
"\n",
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Runs all pending timers until there are none remaining.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @remarks If new timers are added while it is executing they will be run as well.\n",
" */\n",
" runAllAsync: () => Promise<void>;\n",
"\n",
" /**\n",
" * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
" */\n",
" runToFrame: () => void;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 176
} | import { lensProp } from '../index';
export default lensProp;
| types/ramda/src/lensProp.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001730137737467885,
0.0001730137737467885,
0.0001730137737467885,
0.0001730137737467885,
0
] |
{
"id": 5,
"code_window": [
" */\n",
" runAll: () => void;\n",
"\n",
"\t/**\n",
"\t * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
"\t */\n",
"\trunToFrame: () => void;\n",
"\n",
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Runs all pending timers until there are none remaining.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @remarks If new timers are added while it is executing they will be run as well.\n",
" */\n",
" runAllAsync: () => Promise<void>;\n",
"\n",
" /**\n",
" * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
" */\n",
" runToFrame: () => void;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 176
} | import { Extent } from './extent';
import Feature, { FeatureLike } from './Feature';
import { FeatureLoader } from './featureloader';
import FeatureFormat from './format/Feature';
import Layer from './layer/Layer';
import Projection from './proj/Projection';
import ReplayGroup from './render/ReplayGroup';
import Tile, { LoadFunction, Options } from './Tile';
import { TileCoord } from './tilecoord';
import TileState from './TileState';
export default class VectorTile extends Tile {
constructor(tileCoord: TileCoord, state: TileState, src: string, format: FeatureFormat, tileLoadFunction: LoadFunction, opt_options?: Options);
getExtent(): Extent;
getFeatures(): FeatureLike[];
getFormat(): FeatureFormat;
getProjection(): Projection;
getReplayGroup(layer: Layer, key: string): ReplayGroup;
onError(): void;
onLoad(features: Feature[], dataProjection: Projection, extent: Extent): void;
setExtent(extent: Extent): void;
setFeatures(features: Feature[]): void;
setLoader(loader: FeatureLoader): void;
setProjection(projection: Projection): void;
setReplayGroup(layer: Layer, key: string, replayGroup: ReplayGroup): void;
}
| types/ol/VectorTile.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0001729908399283886,
0.00017004252003971487,
0.0001685055176494643,
0.00016863120254129171,
0.0000020854083686572267
] |
{
"id": 5,
"code_window": [
" */\n",
" runAll: () => void;\n",
"\n",
"\t/**\n",
"\t * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
"\t */\n",
"\trunToFrame: () => void;\n",
"\n",
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"keep",
"replace",
"replace",
"replace",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Runs all pending timers until there are none remaining.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" *\n",
" * @remarks If new timers are added while it is executing they will be run as well.\n",
" */\n",
" runAllAsync: () => Promise<void>;\n",
"\n",
" /**\n",
" * Advanced the clock to the next animation frame while firing all scheduled callbacks.\n",
" */\n",
" runToFrame: () => void;\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 176
} | import * as util from 'util';
import assert = require('assert');
import { readFile } from 'fs';
{
// Old and new util.inspect APIs
util.inspect(["This is nice"], false, 5);
util.inspect(["This is nice"], false, null);
util.inspect(["This is nice"], {
colors: true,
depth: 5,
customInspect: false,
showProxy: true,
maxArrayLength: 10,
breakLength: 20,
compact: true,
sorted(a, b) {
return b.localeCompare(a);
},
getters: false,
});
util.inspect(["This is nice"], {
colors: true,
depth: null,
customInspect: false,
showProxy: true,
maxArrayLength: null,
breakLength: Infinity,
compact: false,
sorted: true,
getters: 'set',
});
util.inspect(["This is nice"], {
compact: 42,
});
assert(typeof util.inspect.custom === 'symbol');
util.inspect.replDefaults = {
colors: true,
};
util.formatWithOptions({ colors: true }, 'See object %O', { foo: 42 });
// util.callbackify
// tslint:disable-next-line no-unnecessary-class
class callbackifyTest {
static fn(): Promise<void> {
assert(arguments.length === 0);
return Promise.resolve();
}
static fnE(): Promise<void> {
assert(arguments.length === 0);
return Promise.reject(new Error('fail'));
}
static fnT1(arg1: string): Promise<void> {
assert(arguments.length === 1 && arg1 === 'parameter');
return Promise.resolve();
}
static fnT1E(arg1: string): Promise<void> {
assert(arguments.length === 1 && arg1 === 'parameter');
return Promise.reject(new Error('fail'));
}
static fnTResult(): Promise<string> {
assert(arguments.length === 0);
return Promise.resolve('result');
}
static fnTResultE(): Promise<string> {
assert(arguments.length === 0);
return Promise.reject(new Error('fail'));
}
static fnT1TResult(arg1: string): Promise<string> {
assert(arguments.length === 1 && arg1 === 'parameter');
return Promise.resolve('result');
}
static fnT1TResultE(arg1: string): Promise<string> {
assert(arguments.length === 1 && arg1 === 'parameter');
return Promise.reject(new Error('fail'));
}
static test(): void {
const cfn = util.callbackify(callbackifyTest.fn);
const cfnE = util.callbackify(callbackifyTest.fnE);
const cfnT1 = util.callbackify(callbackifyTest.fnT1);
const cfnT1E = util.callbackify(callbackifyTest.fnT1E);
const cfnTResult = util.callbackify(callbackifyTest.fnTResult);
const cfnTResultE = util.callbackify(callbackifyTest.fnTResultE);
const cfnT1TResult = util.callbackify(callbackifyTest.fnT1TResult);
const cfnT1TResultE = util.callbackify(callbackifyTest.fnT1TResultE);
cfn((err: NodeJS.ErrnoException | null, ...args: string[]) => assert(err === null && args.length === 1 && args[0] === undefined));
cfnE((err: NodeJS.ErrnoException, ...args: string[]) => assert(err.message === 'fail' && args.length === 0));
cfnT1('parameter', (err: NodeJS.ErrnoException | null, ...args: string[]) => assert(err === null && args.length === 1 && args[0] === undefined));
cfnT1E('parameter', (err: NodeJS.ErrnoException, ...args: string[]) => assert(err.message === 'fail' && args.length === 0));
cfnTResult((err: NodeJS.ErrnoException | null, ...args: string[]) => assert(err === null && args.length === 1 && args[0] === 'result'));
cfnTResultE((err: NodeJS.ErrnoException, ...args: string[]) => assert(err.message === 'fail' && args.length === 0));
cfnT1TResult('parameter', (err: NodeJS.ErrnoException | null, ...args: string[]) => assert(err === null && args.length === 1 && args[0] === 'result'));
cfnT1TResultE('parameter', (err: NodeJS.ErrnoException, ...args: string[]) => assert(err.message === 'fail' && args.length === 0));
}
}
callbackifyTest.test();
// util.promisify
const readPromised = util.promisify(readFile);
const sampleRead: Promise<any> = readPromised(__filename).then((data: Buffer): void => { }).catch((error: Error): void => { });
const arg0: () => Promise<number> = util.promisify((cb: (err: Error | null, result: number) => void): void => { });
const arg0NoResult: () => Promise<any> = util.promisify((cb: (err: Error | null) => void): void => { });
const arg1: (arg: string) => Promise<number> = util.promisify((arg: string, cb: (err: Error | null, result: number) => void): void => { });
const arg1UnknownError: (arg: string) => Promise<number> = util.promisify((arg: string, cb: (err: NodeJS.PoorMansUnknown, result: number) => void): void => { });
const arg1NoResult: (arg: string) => Promise<any> = util.promisify((arg: string, cb: (err: Error | null) => void): void => { });
const cbOptionalError: () => Promise<void | {}> = util.promisify((cb: (err?: Error | null) => void): void => { cb(); }); // tslint:disable-line void-return
assert(typeof util.promisify.custom === 'symbol');
// util.deprecate
const foo = () => {};
// $ExpectType () => void
util.deprecate(foo, 'foo() is deprecated, use bar() instead');
// $ExpectType <T extends Function>(fn: T, message: string, code?: string | undefined) => T
util.deprecate(util.deprecate, 'deprecate() is deprecated, use bar() instead');
// $ExpectType <T extends Function>(fn: T, message: string, code?: string | undefined) => T
util.deprecate(util.deprecate, 'deprecate() is deprecated, use bar() instead', 'DEP0001');
// util.isDeepStrictEqual
util.isDeepStrictEqual({foo: 'bar'}, {foo: 'bar'});
// util.TextDecoder()
const td = new util.TextDecoder();
new util.TextDecoder("utf-8");
new util.TextDecoder("utf-8", { fatal: true });
new util.TextDecoder("utf-8", { fatal: true, ignoreBOM: true });
const ignoreBom: boolean = td.ignoreBOM;
const fatal: boolean = td.fatal;
const encoding: string = td.encoding;
td.decode(new Int8Array(1));
td.decode(new Int16Array(1));
td.decode(new Int32Array(1));
td.decode(new Uint8Array(1));
td.decode(new Uint16Array(1));
td.decode(new Uint32Array(1));
td.decode(new Uint8ClampedArray(1));
td.decode(new Float32Array(1));
td.decode(new Float64Array(1));
td.decode(new DataView(new Int8Array(1).buffer));
td.decode(new ArrayBuffer(1));
td.decode(null);
td.decode(null, { stream: true });
td.decode(new Int8Array(1), { stream: true });
const decode: string = td.decode(new Int8Array(1));
// util.TextEncoder()
const te = new util.TextEncoder();
const teEncoding: string = te.encoding;
const teEncodeRes: Uint8Array = te.encode("TextEncoder");
const encIntoRes: util.EncodeIntoResult = te.encodeInto('asdf', new Uint8Array(16));
// util.types
let b: boolean;
b = util.types.isBigInt64Array(15);
b = util.types.isBigUint64Array(15);
b = util.types.isModuleNamespaceObject(15);
// tslint:disable-next-line:no-construct ban-types
const maybeBoxed: number | Number = new Number(1);
if (util.types.isBoxedPrimitive(maybeBoxed)) {
const boxed: Number = maybeBoxed;
}
const maybeBoxed2: number | Number = 1;
if (!util.types.isBoxedPrimitive(maybeBoxed2)) {
const boxed: number = maybeBoxed2;
}
}
| types/node/test/util.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0003121902118436992,
0.0001833197893574834,
0.0001675608364166692,
0.00017137941904366016,
0.00003455434489296749
] |
{
"id": 6,
"code_window": [
" * that time firing callbacks as necessary.\n",
" */\n",
" runToLast: () => void;\n",
"\n",
" /**\n",
" * Simulates a user changing the system clock.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" runToLastAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 187
} | // Type definitions for lolex 3.1
// Project: https://github.com/sinonjs/lolex
// Definitions by: Wim Looman <https://github.com/Nemo157>
// Josh Goldberg <https://github.com/joshuakgoldberg>
// Rogier Schouten <https://github.com/rogierschouten>
// Yishai Zehavi <https://github.com/zyishai>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
/**
* Names of clock methods that may be faked by install.
*/
type FakeMethod = "setTimeout" | "clearTimeout" | "setImmediate" | "clearImmediate" | "setInterval" | "clearInterval" | "Date" | "nextTick" | "hrtime" | "requestAnimationFrame" | "cancelAnimationFrame" | "requestIdleCallback" | "cancelIdleCallback";
/**
* Global methods avaliable to every clock and also as standalone methods (inside `timers` global object).
*/
export interface GlobalTimers<TTimerId extends TimerId> {
/**
* Schedules a callback to be fired once timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait to run the callback.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setTimeout: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setTimeout.
*
* @param id Timer ID or object.
*/
clearTimeout: (id: TimerId) => void;
/**
* Schedules a callback to be fired every time timeout milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @param timeout How many ticks to wait between callbacks.
* @param args Any extra arguments to pass to the callback.
* @returns Time identifier for cancellation.
*/
setInterval: (callback: () => void, timeout: number, ...args: any[]) => TTimerId;
/**
* Clears a timer, as long as it was created using setInterval.
*
* @param id Timer ID or object.
*/
clearInterval: (id: TTimerId) => void;
/**
* Schedules the callback to be fired once 0 milliseconds have ticked by.
*
* @param callback Callback to be fired.
* @remarks You'll still have to call clock.tick() for the callback to fire.
* @remarks If called during a tick the callback won't fire until 1 millisecond has ticked by.
*/
setImmediate: (callback: () => void) => TTimerId;
/**
* Clears a timer, as long as it was created using setImmediate.
*
* @param id Timer ID or object.
*/
clearImmediate: (id: TTimerId) => void;
/**
* Implements the Date object but using this clock to provide the correct time.
*/
Date: typeof Date;
}
/**
* Timer object used in node.
*/
export interface NodeTimer {
/**
* Stub method call. Does nothing.
*/
ref(): void;
/**
* Stub method call. Does nothing.
*/
unref(): void;
}
/**
* Timer identifier for clock scheduling.
*/
export type TimerId = number | NodeTimer;
/**
* Controls the flow of time.
*/
export interface LolexClock<TTimerId extends TimerId> extends GlobalTimers<TTimerId> {
/**
* Current clock time.
*/
now: number;
/**
* Don't know what this prop is for, but it was included in the clocks that `createClock` or
* `install` return (it is never used in the code, for now).
*/
timeouts: {};
/**
* Maximum number of timers that will be run when calling runAll().
*/
loopLimit: number;
/**
* Schedule callback to run in the next animation frame.
*
* @param callback Callback to be fired.
* @returns Request id.
*/
requestAnimationFrame: (callback: (time: number) => void) => TTimerId;
/**
* Cancel animation frame request.
*
* @param id The id returned from requestAnimationFrame method.
*/
cancelAnimationFrame: (id: TTimerId) => void;
/**
* Queues the callback to be fired during idle periods to perform background and low priority work on the main event loop.
*
* @param callback Callback to be fired.
* @param timeout The maximum number of ticks before the callback must be fired.
* @remarks Callbacks which have a timeout option will be fired no later than time in milliseconds.
*/
requestIdleCallback: (callback: () => void, timeout?: number) => TTimerId;
/**
* Clears a timer, as long as it was created using requestIdleCallback.
*
* @param id Timer ID or object.
*/
cancelIdleCallback: (id: TTimerId) => void;
/**
* Get the number of waiting timers.
*
* @returns number of waiting timers.
*/
countTimers: () => number;
/**
* Advances the clock to the the moment of the first scheduled timer, firing it.
*/
next: () => void;
/**
* Advance the clock, firing callbacks if necessary.
*
* @param time How many ticks to advance by.
*/
tick: (time: number | string) => void;
/**
* Removes all timers and tick without firing them and restore now to its original value.
*/
reset: () => void;
/**
* Runs all pending timers until there are none remaining.
*
* @remarks If new timers are added while it is executing they will be run as well.
*/
runAll: () => void;
/**
* Advanced the clock to the next animation frame while firing all scheduled callbacks.
*/
runToFrame: () => void;
/**
* Takes note of the last scheduled timer when it is run, and advances the clock to
* that time firing callbacks as necessary.
*/
runToLast: () => void;
/**
* Simulates a user changing the system clock.
*
* @param now New system time.
* @remarks This affects the current time but it does not in itself cause timers to fire.
*/
setSystemTime: (now?: number | Date) => void;
}
/**
* Lolex clock for a browser environment.
*/
type BrowserClock = LolexClock<number> & {
/**
* Mimics performance.now().
*/
performance: {
now: () => number;
}
};
/**
* Lolex clock for a Node environment.
*/
type NodeClock = LolexClock<NodeTimer> & {
/**
* Mimicks process.hrtime().
*
* @param prevTime Previous system time to calculate time elapsed.
* @returns High resolution real time as [seconds, nanoseconds].
*/
hrtime(prevTime?: [number, number]): [number, number];
/**
* Mimics process.nextTick() explicitly dropping additional arguments.
*/
queueMicrotask: (callback: () => void) => void;
/**
* Simulates process.nextTick().
*/
nextTick: (callback: () => void) => void;
/**
* Run all pending microtasks scheduled with nextTick.
*/
runMicrotasks: () => void;
};
/**
* Clock object created by lolex.
*/
type Clock = BrowserClock | NodeClock;
/**
* Additional methods that installed clock have.
*/
type InstalledMethods = {
/**
* Restores the original methods on the context that was passed to lolex.install,
* or the native timers if no context was given.
*/
uninstall: () => void;
methods: FakeMethod[];
};
/**
* Clock object created by calling `install();`.
*
* @type TClock type of base clock (e.g BrowserClock).
*/
type InstalledClock<TClock extends Clock = Clock> = TClock & InstalledMethods;
/**
* Creates a clock.
*
* @param now Current time for the clock.
* @param loopLimit Maximum number of timers that will be run when calling runAll()
* before assuming that we have an infinite loop and throwing an error
* (by default, 1000).
* @type TClock Type of clock to create.
* @remarks The default epoch is 0.
*/
export declare function createClock<TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number): TClock;
export interface LolexInstallOpts {
/**
* Installs lolex onto the specified target context (default: global)
*/
target?: any;
/**
* Installs lolex with the specified unix epoch (default: 0)
*/
now?: number | Date;
/**
* An array with explicit function names to hijack. When not set, lolex will automatically fake all methods except nextTick
* e.g., lolex.install({ toFake: ["setTimeout", "nextTick"]}) will fake only setTimeout and nextTick
*/
toFake?: FakeMethod[];
/**
* The maximum number of timers that will be run when calling runAll() (default: 1000)
*/
loopLimit?: number;
/**
* Tells lolex to increment mocked time automatically based on the real system time shift (e.g. the mocked time will be incremented by
* 20ms for every 20ms change in the real system time) (default: false)
*/
shouldAdvanceTime?: boolean;
/**
* Relevant only when using with shouldAdvanceTime: true. increment mocked time by advanceTimeDelta ms every advanceTimeDelta ms change
* in the real system time (default: 20)
*/
advanceTimeDelta?: number;
}
/**
* Creates a clock and installs it globally.
*
* @param now Current time for the clock, as with lolex.createClock().
* @param toFake Names of methods that should be faked.
* @type TClock Type of clock to create.
*/
export declare function install<TClock extends Clock = Clock>(opts?: LolexInstallOpts): InstalledClock<TClock>;
export interface LolexWithContext {
timers: GlobalTimers<TimerId>;
createClock: <TClock extends Clock = Clock>(now?: number | Date, loopLimit?: number) => TClock;
install: <TClock extends Clock = Clock>(opts?: LolexInstallOpts) => InstalledClock<TClock>;
withGlobal: (global: Object) => LolexWithContext;
}
/**
* Apply new context to lolex.
*
* @param global New context to apply like `window` (in browsers) or `global` (in node).
*/
export declare function withGlobal(global: Object): LolexWithContext;
export declare const timers: GlobalTimers<TimerId>;
| types/lolex/index.d.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.451511025428772,
0.013651663437485695,
0.00016874185530468822,
0.00021241925423964858,
0.07622256129980087
] |
{
"id": 6,
"code_window": [
" * that time firing callbacks as necessary.\n",
" */\n",
" runToLast: () => void;\n",
"\n",
" /**\n",
" * Simulates a user changing the system clock.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" runToLastAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 187
} | // Type definitions for gulp-copy v0.0.2
// Project: https://github.com/klaascuvelier/gulp-copy
// Definitions by: Arun Aravind <https://github.com/aravindarun>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
import through = require("through");
/**
* Copy files to destination and expose those files as source streams for the gulp pipeline.
*
* @param outDirectory The name of the destination directory. If this directory
* does not exist, it will be created atomatically.
*/
declare function gulpCopy(outDirectory: string): through.ThroughStream;
/**
* Copy files to destination and expose those files as source streams for the gulp pipeline.
*
* @param outDirectory The name of the destination directory. If this directory
* does not exist, it will be created atomatically.
* @param options Override values for available settings.
*/
declare function gulpCopy(outDirectory: string, options: gulpCopy.GulpCopyOptions): through.ThroughStream;
declare namespace gulpCopy {
export interface GulpCopyOptions {
/**
* Specifies the number of parts of the path to be ignored as path prefixes.
*/
prefix: number;
}
}
export = gulpCopy;
| types/gulp-copy/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00016913282161112875,
0.00016810093075037003,
0.00016748273628763855,
0.0001678940752753988,
6.211990353222063e-7
] |
{
"id": 6,
"code_window": [
" * that time firing callbacks as necessary.\n",
" */\n",
" runToLast: () => void;\n",
"\n",
" /**\n",
" * Simulates a user changing the system clock.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" runToLastAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 187
} | // Type definitions for express-paginate 1.0
// Project: https://github.com/niftylettuce/express-paginate
// Definitions by: Amir Tugendhaft <https://github.com/AmirTugi>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.7
import express = require("express");
declare global {
namespace Express {
interface Request {
skip?: number;
offset?: number;
}
}
}
export interface PageElement {
number: number;
url: typeof href;
}
export function middleware(limit?: number, maxLimit?: number): (req: express.Request, res: express.Response, next: express.NextFunction) => void;
export function hasNextPages(req: express.Request): (pageCount: number) => boolean;
export function href(req: express.Request): (prev: object | boolean, params: object) => string;
export function getArrayPages(req: express.Request): (limit: number, pageCount: number, currentPage: number) => PageElement[];
| types/express-paginate/index.d.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017057829245459288,
0.0001682453294051811,
0.00016397172294091433,
0.00017018594371620566,
0.0000030261312531365547
] |
{
"id": 6,
"code_window": [
" * that time firing callbacks as necessary.\n",
" */\n",
" runToLast: () => void;\n",
"\n",
" /**\n",
" * Simulates a user changing the system clock.\n",
" *\n"
],
"labels": [
"keep",
"keep",
"keep",
"add",
"keep",
"keep",
"keep"
],
"after_edit": [
" /**\n",
" * Takes note of the last scheduled timer when it is run, and advances the clock to\n",
" * that time firing callbacks as necessary.\n",
" *\n",
" * Also breaks the event loop, allowing any scheduled promise callbacks to execute _before_ running the timers.\n",
" */\n",
" runToLastAsync: () => Promise<void>;\n",
"\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "add",
"edit_start_line_idx": 187
} | import ToPrimitive = require('es-to-primitive');
import ToPrimitiveES5 = require('es-to-primitive/es5');
import ToPrimitiveES6 = require('es-to-primitive/es2015');
import ToPrimitiveES2015 = require('es-to-primitive/es2015');
const any: unknown = undefined;
ToPrimitive.ES5(any);
ToPrimitive.ES6(any);
ToPrimitive.ES2015(any);
ToPrimitiveES5(any);
ToPrimitiveES6(any);
ToPrimitiveES2015(any);
| types/es-to-primitive/ts3.1/es-to-primitive-tests.ts | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017219384608324617,
0.00017097676754929125,
0.00016975970356725156,
0.00017097676754929125,
0.0000012170712579973042
] |
{
"id": 7,
"code_window": [
" /**\n",
" * Mimics performance.now().\n",
" */\n",
" performance: {\n",
" now: () => number;\n",
" }\n",
"};\n",
"\n",
"/**\n",
" * Lolex clock for a Node environment.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" };\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 205
} | import lolex = require("lolex");
const global: lolex.LolexWithContext = lolex.withGlobal({});
const timers: lolex.GlobalTimers<lolex.TimerId> = lolex.timers;
const lolexTimeout: lolex.TimerId = timers.setTimeout(() => {}, 42);
const lolexInterval: lolex.TimerId = timers.setInterval(() => {}, 42);
const lolexImmediate: lolex.TimerId = timers.setImmediate(() => {});
const lolexDate: Date = new timers.Date();
timers.clearTimeout(lolexTimeout);
timers.clearInterval(lolexInterval);
timers.clearImmediate(lolexImmediate);
let browserClock: lolex.BrowserClock = lolex.createClock() as lolex.BrowserClock;
let nodeClock: lolex.NodeClock = lolex.createClock() as lolex.NodeClock;
browserClock = lolex.createClock<lolex.BrowserClock>();
nodeClock = lolex.createClock<lolex.NodeClock>();
lolex.createClock<lolex.BrowserClock>(7);
lolex.createClock<lolex.BrowserClock>(new Date());
lolex.createClock<lolex.BrowserClock>(7, 9001);
lolex.createClock<lolex.BrowserClock>(new Date(), 9001);
lolex.createClock<lolex.NodeClock>(7);
lolex.createClock<lolex.NodeClock>(new Date());
lolex.createClock<lolex.NodeClock>(7, 9001);
lolex.createClock<lolex.NodeClock>(new Date(), 9001);
const browserInstalledClock = lolex.install<lolex.BrowserClock>({
advanceTimeDelta: 20,
loopLimit: 10,
now: 0,
shouldAdvanceTime: true,
target: {},
toFake: ["setTimeout", "nextTick", "hrtime"]
});
const nodeInstalledClock = lolex.install<lolex.NodeClock>({
advanceTimeDelta: 20,
loopLimit: 10,
now: new Date(0),
shouldAdvanceTime: true,
target: {},
toFake: ["setTimeout", "nextTick", "hrtime"]
});
const browserNow: number = browserClock.now;
const browserTimeouts: Object = browserClock.timeouts;
const browserLoopLimit: number = browserClock.loopLimit;
const browserDate: Date = new browserClock.Date();
const browserPerformanceNow: number = browserClock.performance.now();
const nodeNow: number = nodeClock.now;
const nodeDate: Date = new nodeClock.Date();
const browserTimeout: number = browserClock.setTimeout(() => {}, 7);
const browserInterval: number = browserClock.setInterval(() => {}, 7);
const browserImmediate: number = browserClock.setImmediate(() => {});
const browserAnimationFrame: number = browserClock.requestAnimationFrame(() => {});
const browserIdleCallback: number = browserClock.requestIdleCallback(() => {});
const browserIdleCallbackWithTimeout: number = browserClock.requestIdleCallback(() => {}, 7);
const nodeTimeout: lolex.NodeTimer = nodeClock.setTimeout(() => {}, 7);
const nodeInterval: lolex.NodeTimer = nodeClock.setInterval(() => {}, 7);
const nodeImmediate: lolex.NodeTimer = nodeClock.setImmediate(() => {});
const nodeAnimationFrame: lolex.NodeTimer = nodeClock.requestAnimationFrame(() => {});
const nodeIdleCallback: lolex.NodeTimer = nodeClock.requestIdleCallback(() => {});
const nodeIdleCallbackWithTimeout: lolex.NodeTimer = nodeClock.requestIdleCallback(() => {}, 7);
nodeTimeout.ref();
nodeTimeout.unref();
browserClock.clearTimeout(browserTimeout);
browserClock.clearInterval(browserInterval);
browserClock.clearImmediate(browserImmediate);
browserClock.cancelAnimationFrame(browserAnimationFrame);
browserClock.cancelIdleCallback(browserIdleCallback);
browserClock.cancelIdleCallback(browserIdleCallbackWithTimeout);
nodeClock.clearTimeout(nodeTimeout);
nodeClock.clearInterval(nodeInterval);
nodeClock.clearImmediate(nodeImmediate);
nodeClock.cancelAnimationFrame(nodeAnimationFrame);
nodeClock.cancelIdleCallback(nodeIdleCallback);
nodeClock.cancelIdleCallback(nodeIdleCallbackWithTimeout);
browserClock.tick(7);
browserClock.tick("08");
nodeClock.tick(7);
nodeClock.tick("08:03");
browserClock.next();
nodeClock.next();
browserClock.reset();
nodeClock.reset();
browserClock.runAll();
nodeClock.runAll();
nodeClock.runMicrotasks();
browserClock.runToFrame();
nodeClock.runToFrame();
browserClock.runToLast();
nodeClock.runToLast();
browserClock.setSystemTime();
browserClock.setSystemTime(7);
browserClock.setSystemTime(new Date());
nodeClock.setSystemTime();
nodeClock.setSystemTime(7);
nodeClock.setSystemTime(new Date());
nodeClock.nextTick(() => undefined);
nodeClock.queueMicrotask(() => {});
const browserTimersCount: number = browserClock.countTimers();
const nodeTimersCount: number = nodeClock.countTimers();
let [secs, nanos] = nodeClock.hrtime([0, 0]);
[secs, nanos] = nodeClock.hrtime();
// shows that typescript successfully infer the return values as numbers.
secs.toFixed();
nanos.toExponential();
browserInstalledClock.performance.now();
nodeInstalledClock.nextTick(() => {});
browserInstalledClock.uninstall();
nodeInstalledClock.uninstall();
// Clocks should be typed to have unbound method signatures that can be passed around
const { clearTimeout } = browserClock;
clearTimeout(0);
// TClock of InstalledClock<TClock> is optional.
let installedClock: lolex.InstalledClock;
installedClock = nodeInstalledClock;
installedClock = browserInstalledClock;
| types/lolex/lolex-tests.ts | 1 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.0007461559143848717,
0.00022625944984611124,
0.00016475189477205276,
0.00017329461115878075,
0.00014145104796625674
] |
{
"id": 7,
"code_window": [
" /**\n",
" * Mimics performance.now().\n",
" */\n",
" performance: {\n",
" now: () => number;\n",
" }\n",
"};\n",
"\n",
"/**\n",
" * Lolex clock for a Node environment.\n",
" */\n"
],
"labels": [
"keep",
"keep",
"keep",
"keep",
"keep",
"replace",
"keep",
"keep",
"keep",
"keep",
"keep"
],
"after_edit": [
" };\n"
],
"file_path": "types/lolex/index.d.ts",
"type": "replace",
"edit_start_line_idx": 205
} | {
"compilerOptions": {
"module": "commonjs",
"lib": [
"es6"
],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"baseUrl": "../",
"typeRoots": [
"../"
],
"types": [],
"noEmit": true,
"forceConsistentCasingInFileNames": true
},
"files": [
"index.d.ts",
"cuint-tests.ts"
]
}
| types/cuint/tsconfig.json | 0 | https://github.com/DefinitelyTyped/DefinitelyTyped/commit/61ee030edd12a3a30459d0cdc1d9128fdf714c5c | [
0.00017410401778761297,
0.00017346329696010798,
0.0001722399756545201,
0.0001740459119901061,
8.653474310449383e-7
] |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.