repoName
stringlengths 7
77
| tree
stringlengths 0
2.85M
| readme
stringlengths 0
4.9M
|
---|---|---|
near-examples_cross-contract-hello-js | .github
workflows
tests.yml
.gitpod.yml
README.md
contract
README.md
build.sh
deploy.sh
package.json
src
contract.ts
tsconfig.json
integration-tests
package.json
src
main.ava.ts
package.json
| # 📞 Cross-Contract Hello
[](https://docs.near.org/tutorials/welcome)
[](https://gitpod.io/#/https://github.com/near-examples/xcc-js)
[](https://docs.near.org/develop/contracts/anatomy)
[](#)
[](https://docs.near.org/develop/integrate/frontend)
The smart contract implements the simplest form of cross-contract calls: it calls the [Hello NEAR example](https://docs.near.org/tutorials/examples/hello-near) to get and set a greeting.
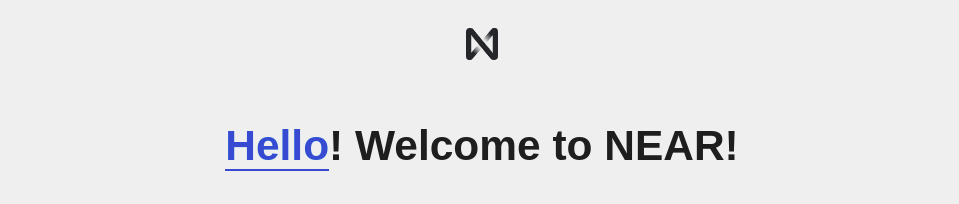
# What This Example Shows
1. How to query information from an external contract.
2. How to interact with an external contract.
<br />
# Quickstart
Clone this repository locally or [**open it in gitpod**](https://gitpod.io/#/https://github.com/near-examples/xcc-js). Then follow these steps:
### 1. Install Dependencies
```bash
npm install
```
### 2. Test the Contract
Deploy your contract in a sandbox and simulate interactions from users.
```bash
npm test
```
### 3. Deploy the Contract
Build the contract and deploy it in a testnet account
```bash
npm run deploy
```
### 4. Interact With the Contract
Ask the contract to perform a cross-contract call to query or change the greeting in Hello NEAR.
```bash
# Use near-cli to ask the contract to query te greeting
near call <dev-account> query_greeting --accountId <dev-account>
# Use near-cli to set increment the counter
near call <dev-account> change_greeting '{"new_greeting":"XCC Hi"}' --accountId <dev-account>
```
---
# Learn More
1. Learn more about the contract through its [README](./contract/README.md).
2. Check [**our documentation**](https://docs.near.org/develop/welcome).
# Cross-Contract Hello Contract
The smart contract implements the simplest form of cross-contract calls: it calls the [Hello NEAR example](https://docs.near.org/tutorials/examples/hello-near) to get and set a greeting.
```ts
@call
query_greeting() {
const call = near.promiseBatchCreate(this.hello_account);
near.promiseBatchActionFunctionCall(call, "get_greeting", bytes(JSON.stringify({})), 0, 5 * TGAS);
const then = near.promiseThen(call, near.currentAccountId(), "query_greeting_callback", bytes(JSON.stringify({})), 0, 5 * TGAS);
return near.promiseReturn(then);
}
@call
query_greeting_callback() {
assert(near.currentAccountId() === near.predecessorAccountId(), "This is a private method");
const greeting = near.promiseResult(0) as String;
return greeting.substring(1, greeting.length-1);
}
@call
change_greeting({ new_greeting }: { new_greeting: string }) {
const call = near.promiseBatchCreate(this.hello_account);
near.promiseBatchActionFunctionCall(call, "set_greeting", bytes(JSON.stringify({ greeting: new_greeting })), 0, 5 * TGAS);
const then = near.promiseThen(call, near.currentAccountId(), "change_greeting_callback", bytes(JSON.stringify({})), 0, 5 * TGAS);
return near.promiseReturn(then);
}
@call
change_greeting_callback() {
assert(near.currentAccountId() === near.predecessorAccountId(), "This is a private method");
if (near.promiseResultsCount() == BigInt(1)) {
near.log("Promise was successful!")
return true
} else {
near.log("Promise failed...")
return false
}
}
```
<br />
# Quickstart
1. Make sure you have installed [node.js](https://nodejs.org/en/download/package-manager/) >= 16.
2. Install the [`NEAR CLI`](https://github.com/near/near-cli#setup)
<br />
## 1. Build and Deploy the Contract
You can automatically compile and deploy the contract in the NEAR testnet by running:
```bash
npm run deploy
```
Once finished, check the `neardev/dev-account` file to find the address in which the contract was deployed:
```bash
cat ./neardev/dev-account # dev-1659899566943-21539992274727
```
<br />
## 2. Get the Greeting
`query_greeting` performs a cross-contract call, calling the `get_greeting()` method from `hello-nearverse.testnet`.
`Call` methods can only be invoked using a NEAR account, since the account needs to pay GAS for the transaction.
```bash
# Use near-cli to ask the contract to query the greeting
near call <dev-account> query_greeting --accountId <dev-account>
```
<br />
## 3. Set a New Greeting
`change_greeting` performs a cross-contract call, calling the `set_greeting({greeting:String})` method from `hello-nearverse.testnet`.
`Call` methods can only be invoked using a NEAR account, since the account needs to pay GAS for the transaction.
```bash
# Use near-cli to change the greeting
near call <dev-account> change_greeting '{"new_greeting":"XCC Hi"}' --accountId <dev-account>
```
**Tip:** If you would like to call `change_greeting` or `query_greeting` using your own account, first login into NEAR using:
```bash
# Use near-cli to login your NEAR account
near login
```
and then use the logged account to sign the transaction: `--accountId <your-account>`.
|
kquan99dk_challenge2-mintnft | .gitpod.yml
README.md
babel.config.js
contract
Cargo.toml
README.md
compile.js
src
approval.rs
enumeration.rs
internal.rs
lib.rs
metadata.rs
mint.rs
nft_core.rs
royalty.rs
target
.rustc_info.json
debug
.fingerprint
Inflector-9e3c62115074b9cf
lib-inflector.json
Inflector-c436f7f0ac4cac71
lib-inflector.json
ahash-1967cc04c22c18c6
lib-ahash.json
aho-corasick-dfd7d94436316217
lib-aho_corasick.json
autocfg-9256c11c5a94315c
lib-autocfg.json
autocfg-cd255646c4ed3339
lib-autocfg.json
base64-71c90ac137df990d
lib-base64.json
block-buffer-7cdf9178091a7da1
lib-block-buffer.json
block-buffer-fecb4b6366e32b09
lib-block-buffer.json
block-padding-62081e46e84fb085
lib-block-padding.json
borsh-455d723f2e7eb382
lib-borsh.json
borsh-derive-4ceaabc5dd04bd8c
lib-borsh-derive.json
borsh-derive-5ae16aa117c7f399
lib-borsh-derive.json
borsh-derive-internal-a4b50dd4391e6a8d
lib-borsh-derive-internal.json
borsh-derive-internal-d0a21fc13ecc1b98
lib-borsh-derive-internal.json
borsh-schema-derive-internal-ab395ab8b11e5be3
lib-borsh-schema-derive-internal.json
borsh-schema-derive-internal-f225755753cda604
lib-borsh-schema-derive-internal.json
bs58-69a66f084a652b72
lib-bs58.json
byte-tools-1d047ab32bad91f7
lib-byte-tools.json
byteorder-390f4de49b33fbe5
build-script-build-script-build.json
byteorder-d171a414cbddb916
lib-byteorder.json
byteorder-d77aa010d83d8b2e
run-build-script-build-script-build.json
byteorder-f262064c186a8c75
build-script-build-script-build.json
cfg-if-3cd7bda968e519ae
lib-cfg-if.json
cfg-if-d11314982639f912
lib-cfg-if.json
convert_case-6aefe2f3d987d223
lib-convert_case.json
convert_case-95e284bc56c12eb5
lib-convert_case.json
cpuid-bool-2e91630d513571a1
lib-cpuid-bool.json
derive_more-5255bfa3b62e923e
lib-derive_more.json
derive_more-69cde6e327ff0a85
lib-derive_more.json
digest-004be3906fa4cc02
lib-digest.json
digest-7c0db2823bd46ffa
lib-digest.json
generic-array-092d57466d24febe
build-script-build-script-build.json
generic-array-2ca012656fd96d03
lib-generic_array.json
generic-array-2ca68d944663b98e
build-script-build-script-build.json
generic-array-581aa29a40d86c87
lib-generic_array.json
generic-array-edaf070b8515c940
run-build-script-build-script-build.json
greeter-ca56de37fb9aeb98
test-lib-greeter.json
greeter-d872d1ed9224c2e4
lib-greeter.json
hashbrown-23b6f330e2a36dbb
run-build-script-build-script-build.json
hashbrown-376c4e72037f389f
run-build-script-build-script-build.json
hashbrown-62d2794599d0b3a5
lib-hashbrown.json
hashbrown-a3aee600bf90c851
build-script-build-script-build.json
hashbrown-a8671ea72407354d
lib-hashbrown.json
hashbrown-b14545ff43de8045
lib-hashbrown.json
hashbrown-cdcf863ec2cdd3e4
build-script-build-script-build.json
hashbrown-d487e72206b07264
lib-hashbrown.json
hex-b14a6cc6710b1be9
lib-hex.json
indexmap-1e685d288818a816
lib-indexmap.json
indexmap-8c9f9453652d0887
run-build-script-build-script-build.json
indexmap-a39c0fe53f69676f
lib-indexmap.json
indexmap-b5b2936f08563f02
run-build-script-build-script-build.json
indexmap-c3ac49ead90ef7d0
build-script-build-script-build.json
indexmap-d783966786b01c6b
build-script-build-script-build.json
indexmap-f66d4c008314b4ba
lib-indexmap.json
itoa-334a999bacc48d3a
lib-itoa.json
itoa-b06d4cff304254a3
lib-itoa.json
itoa-b43a70e1308d4059
lib-itoa.json
keccak-9486c3b3fabd92f5
lib-keccak.json
lazy_static-c4fa5978e157842d
lib-lazy_static.json
libc-776832e16d6fe779
run-build-script-build-script-build.json
libc-ab145babf30c9e9f
build-script-build-script-build.json
libc-bb09fb4e126f2d8a
lib-libc.json
memchr-035c4269a6233099
build-script-build-script-build.json
memchr-18021bf2e0504af3
build-script-build-script-build.json
memchr-88cbb3a1f07aeacc
run-build-script-build-script-build.json
memchr-c4478d63a1e0bded
lib-memchr.json
memory_units-7cdfc6b494713aeb
lib-memory_units.json
near-primitives-core-1a9927e20e4cca2f
lib-near-primitives-core.json
near-rpc-error-core-b63ef2006bff2a54
lib-near-rpc-error-core.json
near-rpc-error-core-d66a3bd0b42b0618
lib-near-rpc-error-core.json
near-rpc-error-macro-379a6a754086af95
lib-near-rpc-error-macro.json
near-rpc-error-macro-438c4d8046055ab7
lib-near-rpc-error-macro.json
near-runtime-utils-cfaecdd6308100c9
lib-near-runtime-utils.json
near-sdk-75db23ee92788818
lib-near-sdk.json
near-sdk-core-05f819364f7a560b
lib-near-sdk-core.json
near-sdk-core-f46d7222a619c60b
lib-near-sdk-core.json
near-sdk-macros-67a465e716adb526
lib-near-sdk-macros.json
near-sdk-macros-c527437a538e59b5
lib-near-sdk-macros.json
near-vm-errors-6c4e1c3aa31b46a6
lib-near-vm-errors.json
near-vm-logic-7b9cae9ded7ffed2
lib-near-vm-logic.json
num-bigint-24878def5e0a4e95
run-build-script-build-script-build.json
num-bigint-2ecc5aa16ac5fa11
build-script-build-script-build.json
num-bigint-d69df36f763339d9
build-script-build-script-build.json
num-bigint-e6063b2824ca7f78
lib-num-bigint.json
num-integer-0dc92eae980549ce
build-script-build-script-build.json
num-integer-733a54d88eb397d7
build-script-build-script-build.json
num-integer-857b4d0c0fb1ebb2
lib-num-integer.json
num-integer-bfcb27fa88a06435
run-build-script-build-script-build.json
num-rational-53d8c28e51e4edeb
lib-num-rational.json
num-rational-5cee69414ba13397
build-script-build-script-build.json
num-rational-ae7f1682837cfa6a
build-script-build-script-build.json
num-rational-e41c1ae7c12fd05a
run-build-script-build-script-build.json
num-traits-148ddaa8ac8aed3d
build-script-build-script-build.json
num-traits-4b4399d154f5b10c
build-script-build-script-build.json
num-traits-8ada646017a565de
lib-num-traits.json
num-traits-e9e69ac574c21b5e
run-build-script-build-script-build.json
opaque-debug-40ef33ab5d5455d5
lib-opaque-debug.json
opaque-debug-9f33558de634ada2
lib-opaque-debug.json
proc-macro-crate-12334d71f27edc5f
lib-proc-macro-crate.json
proc-macro-crate-f8a29538f3d5d264
lib-proc-macro-crate.json
proc-macro2-199e7d79ea890f98
build-script-build-script-build.json
proc-macro2-3fad645d9b421e8e
run-build-script-build-script-build.json
proc-macro2-5fcfb2a46c5df79c
build-script-build-script-build.json
proc-macro2-666f73db07a53a8d
lib-proc-macro2.json
proc-macro2-bd2c37d8933f4808
run-build-script-build-script-build.json
proc-macro2-c9e8a8bd316f311f
lib-proc-macro2.json
quote-577c40b40ecd60bd
lib-quote.json
quote-9bda4ac1e186b791
lib-quote.json
regex-2da0ebf3876e69eb
lib-regex.json
regex-syntax-4674515918c0a7b3
lib-regex-syntax.json
ryu-05927dde86fed045
run-build-script-build-script-build.json
ryu-218129458e3751da
run-build-script-build-script-build.json
ryu-71b733cfce4d5fb9
build-script-build-script-build.json
ryu-78844ca83f6bd916
lib-ryu.json
ryu-9d8a66d1893ee378
lib-ryu.json
ryu-a617547c15767d1a
build-script-build-script-build.json
ryu-b54e014f019506d7
lib-ryu.json
serde-04ff27b5376387bd
lib-serde.json
serde-283b18786897374f
lib-serde.json
serde-4e5700cebad65fe6
run-build-script-build-script-build.json
serde-552ea552149e1a4d
build-script-build-script-build.json
serde-61b3756bc6d1e581
lib-serde.json
serde-b99429f97a9b8ecc
run-build-script-build-script-build.json
serde-ce79dbda8006d582
build-script-build-script-build.json
serde_derive-0735097b743a1fb7
build-script-build-script-build.json
serde_derive-3fa5b2f0604dda9d
lib-serde_derive.json
serde_derive-4f31ad7a0e5ec105
build-script-build-script-build.json
serde_derive-9dbff8f6028e8a2c
run-build-script-build-script-build.json
serde_derive-a081d5fd2c137a9a
run-build-script-build-script-build.json
serde_derive-ea52ca527bcbc75e
lib-serde_derive.json
serde_json-17657cb37f02500a
lib-serde_json.json
serde_json-5c67599c92665964
lib-serde_json.json
serde_json-8d57a558bc719086
run-build-script-build-script-build.json
serde_json-bf5b0064d1b45776
run-build-script-build-script-build.json
serde_json-c83f8bc67b0f9037
lib-serde_json.json
serde_json-e672e5dc6dad8890
build-script-build-script-build.json
serde_json-e9017e2e6ab453c7
build-script-build-script-build.json
sha2-ce147e528dceb8c1
lib-sha2.json
sha3-69ffdc009f5fc5f1
lib-sha3.json
syn-0359a400ed92222f
build-script-build-script-build.json
syn-5d4f461e709da010
lib-syn.json
syn-60a21e1eff858db6
lib-syn.json
syn-84879f3141fd595e
run-build-script-build-script-build.json
syn-a2e6dc1d550d999e
run-build-script-build-script-build.json
syn-c998c8ac30099f7a
build-script-build-script-build.json
toml-d192d9fbebd5cb61
lib-toml.json
toml-dc98d37f2aab96c5
lib-toml.json
typenum-70d0c98cc58168b3
lib-typenum.json
typenum-92573e41ef1e85e1
run-build-script-build-script-main.json
typenum-9fdca179d12f05c5
build-script-build-script-main.json
typenum-b487004bf7bc9a32
build-script-build-script-main.json
unicode-xid-1ccf9a8622388d0a
lib-unicode-xid.json
unicode-xid-a53958b7612b2e15
lib-unicode-xid.json
version_check-45c9caec9b6617f4
lib-version_check.json
version_check-c02be86861b3fab0
lib-version_check.json
wee_alloc-4c1d5fc69208e232
build-script-build-script-build.json
wee_alloc-8104e1550ed45c97
lib-wee_alloc.json
wee_alloc-b724ac321ae537f6
build-script-build-script-build.json
wee_alloc-e2cb34bd00ad2611
run-build-script-build-script-build.json
build
num-bigint-24878def5e0a4e95
out
radix_bases.rs
typenum-92573e41ef1e85e1
out
consts.rs
op.rs
tests.rs
wee_alloc-e2cb34bd00ad2611
out
wee_alloc_static_array_backend_size_bytes.txt
release
.fingerprint
Inflector-2da74efad54ec4e9
lib-inflector.json
autocfg-36d1143bb15fdaf7
lib-autocfg.json
borsh-derive-d3309b1c2945f4b4
lib-borsh-derive.json
borsh-derive-internal-4fd70805e36c12ee
lib-borsh-derive-internal.json
borsh-schema-derive-internal-6c3198d8384c335b
lib-borsh-schema-derive-internal.json
byteorder-34b699f45df0469a
build-script-build-script-build.json
convert_case-e7b041cf96916609
lib-convert_case.json
derive_more-95bf4ed2bff31aa5
lib-derive_more.json
generic-array-03d434b88d3350aa
build-script-build-script-build.json
hashbrown-66fbbd79aeb96263
run-build-script-build-script-build.json
hashbrown-767ac6df210d85c3
build-script-build-script-build.json
hashbrown-b314f4e750fcb629
lib-hashbrown.json
indexmap-5641e382683c2348
build-script-build-script-build.json
indexmap-621c0771e4d53689
lib-indexmap.json
indexmap-da6bbc563e54eefc
run-build-script-build-script-build.json
itoa-883ba7f8c78c7fb0
lib-itoa.json
memchr-571d6af0e3027553
build-script-build-script-build.json
near-rpc-error-core-3e0ebc8152da9b66
lib-near-rpc-error-core.json
near-rpc-error-macro-97041912661dd741
lib-near-rpc-error-macro.json
near-sdk-core-b9805aa4019f82e3
lib-near-sdk-core.json
near-sdk-macros-1e6184e96df0d59b
lib-near-sdk-macros.json
num-bigint-30c840a9e7063b4d
build-script-build-script-build.json
num-integer-ec839767373b3743
build-script-build-script-build.json
num-rational-f2a56683457495dd
build-script-build-script-build.json
num-traits-1d6dd1c6b98fdf86
build-script-build-script-build.json
proc-macro-crate-f4485160eb4f02d3
lib-proc-macro-crate.json
proc-macro2-3de109d2561ac664
run-build-script-build-script-build.json
proc-macro2-4094ae938d816092
lib-proc-macro2.json
proc-macro2-4d4508a2f12da927
build-script-build-script-build.json
quote-9a82d9e11303b9eb
lib-quote.json
ryu-6b9dc0114a855fde
lib-ryu.json
ryu-ef3de59efab1d079
build-script-build-script-build.json
ryu-f100889b314cbd00
run-build-script-build-script-build.json
serde-7f9925e932b711c9
run-build-script-build-script-build.json
serde-9d93eacc5f8ff8ae
lib-serde.json
serde-e4468589e8222045
build-script-build-script-build.json
serde_derive-7f877e1a14c4c4bf
build-script-build-script-build.json
serde_derive-8d327c44951ba679
lib-serde_derive.json
serde_derive-92ba21b400705006
run-build-script-build-script-build.json
serde_json-47f7bb457d89d314
run-build-script-build-script-build.json
serde_json-89f09557b69ff7b1
lib-serde_json.json
serde_json-a896ebe1f93935d3
build-script-build-script-build.json
syn-1d236f2ec0494c3d
build-script-build-script-build.json
syn-6746bc1fd932cafb
run-build-script-build-script-build.json
syn-d1a686908e03bd0b
lib-syn.json
toml-9c7331cb48354fa5
lib-toml.json
typenum-cede2f005662170f
build-script-build-script-main.json
unicode-xid-d6cea238fb245afb
lib-unicode-xid.json
version_check-ce742f3a694dc905
lib-version_check.json
wee_alloc-0c0fa5accfc438b3
build-script-build-script-build.json
rls
.rustc_info.json
debug
.fingerprint
Inflector-c436f7f0ac4cac71
lib-inflector.json
ahash-1967cc04c22c18c6
lib-ahash.json
aho-corasick-dfd7d94436316217
lib-aho_corasick.json
autocfg-9256c11c5a94315c
lib-autocfg.json
base64-71c90ac137df990d
lib-base64.json
block-buffer-7cdf9178091a7da1
lib-block-buffer.json
block-buffer-fecb4b6366e32b09
lib-block-buffer.json
block-padding-62081e46e84fb085
lib-block-padding.json
borsh-455d723f2e7eb382
lib-borsh.json
borsh-derive-4ceaabc5dd04bd8c
lib-borsh-derive.json
borsh-derive-internal-a4b50dd4391e6a8d
lib-borsh-derive-internal.json
borsh-schema-derive-internal-f225755753cda604
lib-borsh-schema-derive-internal.json
bs58-69a66f084a652b72
lib-bs58.json
byte-tools-1d047ab32bad91f7
lib-byte-tools.json
byteorder-390f4de49b33fbe5
build-script-build-script-build.json
byteorder-d171a414cbddb916
lib-byteorder.json
byteorder-d77aa010d83d8b2e
run-build-script-build-script-build.json
cfg-if-3cd7bda968e519ae
lib-cfg-if.json
cfg-if-d11314982639f912
lib-cfg-if.json
convert_case-6aefe2f3d987d223
lib-convert_case.json
cpuid-bool-2e91630d513571a1
lib-cpuid-bool.json
derive_more-5255bfa3b62e923e
lib-derive_more.json
digest-004be3906fa4cc02
lib-digest.json
digest-7c0db2823bd46ffa
lib-digest.json
generic-array-2ca012656fd96d03
lib-generic_array.json
generic-array-2ca68d944663b98e
build-script-build-script-build.json
generic-array-581aa29a40d86c87
lib-generic_array.json
generic-array-edaf070b8515c940
run-build-script-build-script-build.json
greeter-ca56de37fb9aeb98
test-lib-greeter.json
greeter-d872d1ed9224c2e4
lib-greeter.json
hashbrown-376c4e72037f389f
run-build-script-build-script-build.json
hashbrown-62d2794599d0b3a5
lib-hashbrown.json
hashbrown-a3aee600bf90c851
build-script-build-script-build.json
hashbrown-a8671ea72407354d
lib-hashbrown.json
hashbrown-b14545ff43de8045
lib-hashbrown.json
hex-b14a6cc6710b1be9
lib-hex.json
indexmap-a39c0fe53f69676f
lib-indexmap.json
indexmap-b5b2936f08563f02
run-build-script-build-script-build.json
indexmap-d783966786b01c6b
build-script-build-script-build.json
indexmap-f66d4c008314b4ba
lib-indexmap.json
itoa-334a999bacc48d3a
lib-itoa.json
itoa-b43a70e1308d4059
lib-itoa.json
keccak-9486c3b3fabd92f5
lib-keccak.json
lazy_static-c4fa5978e157842d
lib-lazy_static.json
libc-776832e16d6fe779
run-build-script-build-script-build.json
libc-ab145babf30c9e9f
build-script-build-script-build.json
libc-bb09fb4e126f2d8a
lib-libc.json
memchr-18021bf2e0504af3
build-script-build-script-build.json
memchr-88cbb3a1f07aeacc
run-build-script-build-script-build.json
memchr-c4478d63a1e0bded
lib-memchr.json
memory_units-7cdfc6b494713aeb
lib-memory_units.json
near-primitives-core-1a9927e20e4cca2f
lib-near-primitives-core.json
near-rpc-error-core-d66a3bd0b42b0618
lib-near-rpc-error-core.json
near-rpc-error-macro-438c4d8046055ab7
lib-near-rpc-error-macro.json
near-runtime-utils-cfaecdd6308100c9
lib-near-runtime-utils.json
near-sdk-75db23ee92788818
lib-near-sdk.json
near-sdk-core-05f819364f7a560b
lib-near-sdk-core.json
near-sdk-macros-c527437a538e59b5
lib-near-sdk-macros.json
near-vm-errors-6c4e1c3aa31b46a6
lib-near-vm-errors.json
near-vm-logic-7b9cae9ded7ffed2
lib-near-vm-logic.json
num-bigint-24878def5e0a4e95
run-build-script-build-script-build.json
num-bigint-2ecc5aa16ac5fa11
build-script-build-script-build.json
num-bigint-e6063b2824ca7f78
lib-num-bigint.json
num-integer-0dc92eae980549ce
build-script-build-script-build.json
num-integer-857b4d0c0fb1ebb2
lib-num-integer.json
num-integer-bfcb27fa88a06435
run-build-script-build-script-build.json
num-rational-53d8c28e51e4edeb
lib-num-rational.json
num-rational-5cee69414ba13397
build-script-build-script-build.json
num-rational-e41c1ae7c12fd05a
run-build-script-build-script-build.json
num-traits-148ddaa8ac8aed3d
build-script-build-script-build.json
num-traits-8ada646017a565de
lib-num-traits.json
num-traits-e9e69ac574c21b5e
run-build-script-build-script-build.json
opaque-debug-40ef33ab5d5455d5
lib-opaque-debug.json
opaque-debug-9f33558de634ada2
lib-opaque-debug.json
proc-macro-crate-f8a29538f3d5d264
lib-proc-macro-crate.json
proc-macro2-199e7d79ea890f98
build-script-build-script-build.json
proc-macro2-666f73db07a53a8d
lib-proc-macro2.json
proc-macro2-bd2c37d8933f4808
run-build-script-build-script-build.json
quote-9bda4ac1e186b791
lib-quote.json
regex-2da0ebf3876e69eb
lib-regex.json
regex-syntax-4674515918c0a7b3
lib-regex-syntax.json
ryu-05927dde86fed045
run-build-script-build-script-build.json
ryu-71b733cfce4d5fb9
build-script-build-script-build.json
ryu-78844ca83f6bd916
lib-ryu.json
ryu-9d8a66d1893ee378
lib-ryu.json
serde-04ff27b5376387bd
lib-serde.json
serde-4e5700cebad65fe6
run-build-script-build-script-build.json
serde-61b3756bc6d1e581
lib-serde.json
serde-ce79dbda8006d582
build-script-build-script-build.json
serde_derive-0735097b743a1fb7
build-script-build-script-build.json
serde_derive-3fa5b2f0604dda9d
lib-serde_derive.json
serde_derive-a081d5fd2c137a9a
run-build-script-build-script-build.json
serde_json-17657cb37f02500a
lib-serde_json.json
serde_json-5c67599c92665964
lib-serde_json.json
serde_json-8d57a558bc719086
run-build-script-build-script-build.json
serde_json-e672e5dc6dad8890
build-script-build-script-build.json
sha2-ce147e528dceb8c1
lib-sha2.json
sha3-69ffdc009f5fc5f1
lib-sha3.json
syn-5d4f461e709da010
lib-syn.json
syn-a2e6dc1d550d999e
run-build-script-build-script-build.json
syn-c998c8ac30099f7a
build-script-build-script-build.json
toml-d192d9fbebd5cb61
lib-toml.json
typenum-70d0c98cc58168b3
lib-typenum.json
typenum-92573e41ef1e85e1
run-build-script-build-script-main.json
typenum-9fdca179d12f05c5
build-script-build-script-main.json
unicode-xid-a53958b7612b2e15
lib-unicode-xid.json
version_check-45c9caec9b6617f4
lib-version_check.json
wee_alloc-8104e1550ed45c97
lib-wee_alloc.json
wee_alloc-b724ac321ae537f6
build-script-build-script-build.json
wee_alloc-e2cb34bd00ad2611
run-build-script-build-script-build.json
build
byteorder-390f4de49b33fbe5
save-analysis
build_script_build-390f4de49b33fbe5.json
generic-array-2ca68d944663b98e
save-analysis
build_script_build-2ca68d944663b98e.json
hashbrown-a3aee600bf90c851
save-analysis
build_script_build-a3aee600bf90c851.json
indexmap-d783966786b01c6b
save-analysis
build_script_build-d783966786b01c6b.json
libc-ab145babf30c9e9f
save-analysis
build_script_build-ab145babf30c9e9f.json
memchr-18021bf2e0504af3
save-analysis
build_script_build-18021bf2e0504af3.json
num-bigint-24878def5e0a4e95
out
radix_bases.rs
num-bigint-2ecc5aa16ac5fa11
save-analysis
build_script_build-2ecc5aa16ac5fa11.json
num-integer-0dc92eae980549ce
save-analysis
build_script_build-0dc92eae980549ce.json
num-rational-5cee69414ba13397
save-analysis
build_script_build-5cee69414ba13397.json
num-traits-148ddaa8ac8aed3d
save-analysis
build_script_build-148ddaa8ac8aed3d.json
proc-macro2-199e7d79ea890f98
save-analysis
build_script_build-199e7d79ea890f98.json
ryu-71b733cfce4d5fb9
save-analysis
build_script_build-71b733cfce4d5fb9.json
serde-ce79dbda8006d582
save-analysis
build_script_build-ce79dbda8006d582.json
serde_derive-0735097b743a1fb7
save-analysis
build_script_build-0735097b743a1fb7.json
serde_json-e672e5dc6dad8890
save-analysis
build_script_build-e672e5dc6dad8890.json
syn-c998c8ac30099f7a
save-analysis
build_script_build-c998c8ac30099f7a.json
typenum-92573e41ef1e85e1
out
consts.rs
op.rs
tests.rs
typenum-9fdca179d12f05c5
save-analysis
build_script_main-9fdca179d12f05c5.json
wee_alloc-b724ac321ae537f6
save-analysis
build_script_build-b724ac321ae537f6.json
wee_alloc-e2cb34bd00ad2611
out
wee_alloc_static_array_backend_size_bytes.txt
deps
save-analysis
greeter-ca56de37fb9aeb98.json
libahash-1967cc04c22c18c6.json
libaho_corasick-dfd7d94436316217.json
libautocfg-9256c11c5a94315c.json
libbase64-71c90ac137df990d.json
libblock_buffer-fecb4b6366e32b09.json
libblock_padding-62081e46e84fb085.json
libborsh-455d723f2e7eb382.json
libbs58-69a66f084a652b72.json
libbyte_tools-1d047ab32bad91f7.json
libbyteorder-d171a414cbddb916.json
libderive_more-5255bfa3b62e923e.json
libdigest-004be3906fa4cc02.json
libgeneric_array-2ca012656fd96d03.json
libgreeter-d872d1ed9224c2e4.json
libhashbrown-a8671ea72407354d.json
libhashbrown-b14545ff43de8045.json
libhex-b14a6cc6710b1be9.json
libindexmap-a39c0fe53f69676f.json
libindexmap-f66d4c008314b4ba.json
libinflector-c436f7f0ac4cac71.json
libitoa-b43a70e1308d4059.json
libkeccak-9486c3b3fabd92f5.json
liblazy_static-c4fa5978e157842d.json
libmemchr-c4478d63a1e0bded.json
libmemory_units-7cdfc6b494713aeb.json
libnear_primitives_core-1a9927e20e4cca2f.json
libnear_rpc_error_core-d66a3bd0b42b0618.json
libnear_rpc_error_macro-438c4d8046055ab7.json
libnear_runtime_utils-cfaecdd6308100c9.json
libnear_sdk-75db23ee92788818.json
libnear_vm_errors-6c4e1c3aa31b46a6.json
libnear_vm_logic-7b9cae9ded7ffed2.json
libnum_bigint-e6063b2824ca7f78.json
libnum_integer-857b4d0c0fb1ebb2.json
libnum_rational-53d8c28e51e4edeb.json
libnum_traits-8ada646017a565de.json
libopaque_debug-40ef33ab5d5455d5.json
libproc_macro2-666f73db07a53a8d.json
libproc_macro_crate-f8a29538f3d5d264.json
libquote-9bda4ac1e186b791.json
libregex-2da0ebf3876e69eb.json
libryu-78844ca83f6bd916.json
libsha2-ce147e528dceb8c1.json
libsha3-69ffdc009f5fc5f1.json
libtoml-d192d9fbebd5cb61.json
libunicode_xid-a53958b7612b2e15.json
libversion_check-45c9caec9b6617f4.json
libwee_alloc-8104e1550ed45c97.json
wasm32-unknown-unknown
debug
.fingerprint
ahash-1e4f7001357845d9
lib-ahash.json
ahash-31500eed5b448aad
lib-ahash.json
aho-corasick-4e2f89079b9e00e0
lib-aho_corasick.json
aho-corasick-b89132b0381c749e
lib-aho_corasick.json
base64-24b7b79876c360ef
lib-base64.json
base64-646109cef868d84f
lib-base64.json
block-buffer-37003fcc48ccf01d
lib-block-buffer.json
block-buffer-5f9d9d8b55f1d3b1
lib-block-buffer.json
block-buffer-a4f1735d27d7a03f
lib-block-buffer.json
block-buffer-bc99728b4d693dcc
lib-block-buffer.json
block-padding-13821e343f46f753
lib-block-padding.json
block-padding-338643242f2dae2c
lib-block-padding.json
borsh-6fb510f42155d666
lib-borsh.json
borsh-dc5eef91f25dddab
lib-borsh.json
bs58-7212f4faed67cdbf
lib-bs58.json
bs58-c1405f3d470899a9
lib-bs58.json
byte-tools-135d698576218f7f
lib-byte-tools.json
byte-tools-a6cd60c7ea46575a
lib-byte-tools.json
byteorder-08f29ea8d461900c
run-build-script-build-script-build.json
byteorder-425eab9cce122222
lib-byteorder.json
byteorder-b1278529b57a037f
run-build-script-build-script-build.json
byteorder-dd42706ea898e564
lib-byteorder.json
cfg-if-53998aee5392ec20
lib-cfg-if.json
cfg-if-8cd778ce3477266f
lib-cfg-if.json
cfg-if-f1de31271fe8376a
lib-cfg-if.json
cfg-if-f786cbb548399339
lib-cfg-if.json
digest-02b66ce3a4c78c1c
lib-digest.json
digest-19541f9b7e0c7774
lib-digest.json
digest-5bb936ed42c05efb
lib-digest.json
digest-735ab6181239f6d2
lib-digest.json
generic-array-25c6d4dda83f7be7
run-build-script-build-script-build.json
generic-array-30cd0a03156a9b87
lib-generic_array.json
generic-array-6802468a8a36c271
run-build-script-build-script-build.json
generic-array-845551d223c666ba
lib-generic_array.json
generic-array-d996d3171b5da4ba
lib-generic_array.json
generic-array-fa1caae96ee63780
lib-generic_array.json
greeter-98ace302c5fafa12
lib-greeter.json
hashbrown-483ac2a52167ff2a
lib-hashbrown.json
hashbrown-51d3921aceef5ebb
run-build-script-build-script-build.json
hashbrown-9e21cf3d1d28819b
lib-hashbrown.json
hashbrown-b3cfd744f48b3b6e
lib-hashbrown.json
hashbrown-e4a4c2f7e2717426
lib-hashbrown.json
hashbrown-f3541e9aee499733
run-build-script-build-script-build.json
hex-261cfc3fcecaa4ef
lib-hex.json
hex-c604b67ad129b15c
lib-hex.json
indexmap-003f41db7a36cd3b
run-build-script-build-script-build.json
indexmap-33441b070f70d190
run-build-script-build-script-build.json
indexmap-549d81fd1b4aaa1a
lib-indexmap.json
indexmap-b8bfbab8f475b75f
lib-indexmap.json
itoa-4c06a779eef25af4
lib-itoa.json
itoa-e60cf4857d9d2913
lib-itoa.json
keccak-b0c9ccd044944503
lib-keccak.json
keccak-d4c6e65621b821f6
lib-keccak.json
lazy_static-15a36e6855247c67
lib-lazy_static.json
lazy_static-b79bf709c5f09987
lib-lazy_static.json
memchr-29e30936df02ad7c
run-build-script-build-script-build.json
memchr-8974d6d0eea4ab03
lib-memchr.json
memchr-d3aaac5f8308eb58
lib-memchr.json
memchr-f922bd3de584c24c
run-build-script-build-script-build.json
memory_units-3804df6a88cda429
lib-memory_units.json
memory_units-90906d142a260054
lib-memory_units.json
near-primitives-core-22512a59771af8d0
lib-near-primitives-core.json
near-primitives-core-8eab4af63289cca4
lib-near-primitives-core.json
near-runtime-utils-305f43dd617f4a70
lib-near-runtime-utils.json
near-runtime-utils-3357838d53825c1b
lib-near-runtime-utils.json
near-sdk-b965d2f0da3bed34
lib-near-sdk.json
near-sdk-f66bacd755fc9d77
lib-near-sdk.json
near-vm-errors-20e6f8caa2eb3f21
lib-near-vm-errors.json
near-vm-errors-e5fbb2c274b4a5ef
lib-near-vm-errors.json
near-vm-logic-26e22134f5e27306
lib-near-vm-logic.json
near-vm-logic-afd430776e895057
lib-near-vm-logic.json
num-bigint-5fedcba82e247578
lib-num-bigint.json
num-bigint-692978290ad6ac51
run-build-script-build-script-build.json
num-bigint-7e7d1d90c83f30cc
lib-num-bigint.json
num-bigint-a699a4a251dccc5e
run-build-script-build-script-build.json
num-integer-6bdbe1733947e393
run-build-script-build-script-build.json
num-integer-72c4894d57403887
lib-num-integer.json
num-integer-8d01ee791cd20c62
lib-num-integer.json
num-integer-e00df3a90a9271c0
run-build-script-build-script-build.json
num-rational-abf9bfbe7cbd8dd6
lib-num-rational.json
num-rational-c333a4fa265eb85d
run-build-script-build-script-build.json
num-rational-e5b980680ebaaecb
run-build-script-build-script-build.json
num-rational-f4f592a6b0c04ec3
lib-num-rational.json
num-traits-28f28195512e15b0
lib-num-traits.json
num-traits-838e9f4f81aae4f8
run-build-script-build-script-build.json
num-traits-c1660e0226ce670c
run-build-script-build-script-build.json
num-traits-e0f409119940623e
lib-num-traits.json
opaque-debug-19d0f9d6d6732dda
lib-opaque-debug.json
opaque-debug-20397d3162c70a7a
lib-opaque-debug.json
opaque-debug-67e11037907b0a62
lib-opaque-debug.json
opaque-debug-d4218c184d36d52a
lib-opaque-debug.json
regex-348a33fe5c87c03d
lib-regex.json
regex-e489d254d1e7e12d
lib-regex.json
regex-syntax-bcaa825332c5ba50
lib-regex-syntax.json
regex-syntax-cd626ce4deb5f357
lib-regex-syntax.json
ryu-1c89b4c0977fa398
lib-ryu.json
ryu-52a388757c15c1a6
run-build-script-build-script-build.json
ryu-618a27724935a027
run-build-script-build-script-build.json
ryu-a7a81e23b9bcb06b
lib-ryu.json
serde-6d59abfee35ae43b
run-build-script-build-script-build.json
serde-c480407a95773cc0
lib-serde.json
serde-ebe94b1241f5047e
lib-serde.json
serde-f83c67a06a5b12c5
run-build-script-build-script-build.json
serde_json-1da7a0c73c632567
lib-serde_json.json
serde_json-2effd68d351abc28
lib-serde_json.json
serde_json-6c28b4927146d49d
run-build-script-build-script-build.json
serde_json-c3b71e6248b2b8a1
run-build-script-build-script-build.json
sha2-0f67ed3ef159a91a
lib-sha2.json
sha2-bdebeba22d9faa70
lib-sha2.json
sha3-2517d071adfa8028
lib-sha3.json
sha3-4cf15448deb657a1
lib-sha3.json
typenum-4976863587ad7c9e
run-build-script-build-script-main.json
typenum-765fe82de63af7f0
run-build-script-build-script-main.json
typenum-b3f9023e815d815e
lib-typenum.json
typenum-eee99a2ed0f1494e
lib-typenum.json
wee_alloc-1aec1ccd611d0139
run-build-script-build-script-build.json
wee_alloc-6b415af9b59e77f3
lib-wee_alloc.json
wee_alloc-70e83ed96f4a461b
lib-wee_alloc.json
wee_alloc-96614ccff1c83e60
run-build-script-build-script-build.json
build
num-bigint-692978290ad6ac51
out
radix_bases.rs
num-bigint-a699a4a251dccc5e
out
radix_bases.rs
typenum-4976863587ad7c9e
out
consts.rs
op.rs
tests.rs
typenum-765fe82de63af7f0
out
consts.rs
op.rs
tests.rs
wee_alloc-1aec1ccd611d0139
out
wee_alloc_static_array_backend_size_bytes.txt
wee_alloc-96614ccff1c83e60
out
wee_alloc_static_array_backend_size_bytes.txt
release
.fingerprint
ahash-c39a1557b0cb64fd
lib-ahash.json
aho-corasick-cddd1d2769bbc24c
lib-aho_corasick.json
base64-0b7ec90a02269293
lib-base64.json
block-buffer-3adf35a631626521
lib-block-buffer.json
block-buffer-7667b3843146785f
lib-block-buffer.json
block-padding-add315c1a10297ab
lib-block-padding.json
borsh-20f80cee6bb6ec9c
lib-borsh.json
bs58-7c9614b1e7bf825e
lib-bs58.json
byte-tools-78a50f0bb79f4220
lib-byte-tools.json
byteorder-49b8d6e679c1e64e
run-build-script-build-script-build.json
byteorder-87dc9d029147e43c
lib-byteorder.json
cfg-if-1c5136e600ed0421
lib-cfg-if.json
cfg-if-a1f5f75da1d819bb
lib-cfg-if.json
digest-26443559f0b8ab0c
lib-digest.json
digest-47255a1a287092ff
lib-digest.json
generic-array-8a6609a86a263663
run-build-script-build-script-build.json
generic-array-aeaec4f020f3d126
lib-generic_array.json
generic-array-f75a4be0bb981076
lib-generic_array.json
greeter-98ace302c5fafa12
lib-greeter.json
hashbrown-030c6a3a4817b33d
lib-hashbrown.json
hashbrown-323acde7cb1b3303
lib-hashbrown.json
hashbrown-726d24c31ecf2221
run-build-script-build-script-build.json
hex-d8ef8d74602b7b40
lib-hex.json
indexmap-26aa6e17137aa197
lib-indexmap.json
indexmap-c9d688ec2b5e6183
run-build-script-build-script-build.json
itoa-f08dc1fc9126aefd
lib-itoa.json
keccak-71984737368ee5c0
lib-keccak.json
lazy_static-bd10a22929086909
lib-lazy_static.json
memchr-975969f7a5c8ad0e
lib-memchr.json
memchr-a9fc1d76fb65bb32
run-build-script-build-script-build.json
memory_units-b90fcde6ee21ae92
lib-memory_units.json
near-primitives-core-0d072222326a6e39
lib-near-primitives-core.json
near-runtime-utils-0dcaf8cff4281f50
lib-near-runtime-utils.json
near-sdk-729cdab4924d53a0
lib-near-sdk.json
near-vm-errors-639978f6ebdf5530
lib-near-vm-errors.json
near-vm-logic-8f4e35fe57a69f32
lib-near-vm-logic.json
num-bigint-00a18bfc5e6bcf5d
lib-num-bigint.json
num-bigint-dd2b12ef358ad150
run-build-script-build-script-build.json
num-integer-20fc3c5b6715fc29
lib-num-integer.json
num-integer-7bbca57a59f2c64d
run-build-script-build-script-build.json
num-rational-75a878223dda610e
lib-num-rational.json
num-rational-e245fb60ae5d228f
run-build-script-build-script-build.json
num-traits-fc3edba68694a94a
lib-num-traits.json
num-traits-fceda933139e35d0
run-build-script-build-script-build.json
opaque-debug-b2242681a00bd93a
lib-opaque-debug.json
opaque-debug-ddcd1b07edf68a37
lib-opaque-debug.json
regex-4f0c6a2bc6131dcf
lib-regex.json
regex-syntax-9455a93df8c78607
lib-regex-syntax.json
ryu-05a79e24ef72bed6
run-build-script-build-script-build.json
ryu-ce46002e78d0049e
lib-ryu.json
serde-5e009a63aa5e09e9
run-build-script-build-script-build.json
serde-82b8b5f0a44b45e0
lib-serde.json
serde_json-3a1344f733a9e713
run-build-script-build-script-build.json
serde_json-6e0448f6309c187f
lib-serde_json.json
sha2-9b68277cd7b2095b
lib-sha2.json
sha3-555bfa621f506fdc
lib-sha3.json
typenum-306e31ff236dbc19
lib-typenum.json
typenum-39cdaa6935864a49
run-build-script-build-script-main.json
wee_alloc-73a4a3d36eaed7a5
lib-wee_alloc.json
wee_alloc-eee7ea5cef997a34
run-build-script-build-script-build.json
build
num-bigint-dd2b12ef358ad150
out
radix_bases.rs
typenum-39cdaa6935864a49
out
consts.rs
op.rs
tests.rs
wee_alloc-eee7ea5cef997a34
out
wee_alloc_static_array_backend_size_bytes.txt
|
|","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1038,"byte_end":1133,"line_start":22,"line_end":22,"column_start":1,"column_end":96}},{"value":"
| `encode` | Returns a new `String` | Always |","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1134,"byte_end":1229,"line_start":23,"line_end":23,"column_start":1,"column_end":96}},{"value":"
| `encode_config` | Returns a new `String` | Always |","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1230,"byte_end":1325,"line_start":24,"line_end":24,"column_start":1,"column_end":96}},{"value":"
| `encode_config_buf` | Appends to provided `String` | Only if `String` needs to grow |","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1326,"byte_end":1421,"line_start":25,"line_end":25,"column_start":1,"column_end":96}},{"value":"
| `encode_config_slice` | Writes to provided `&[u8]` | Never |","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1422,"byte_end":1517,"line_start":26,"line_end":26,"column_start":1,"column_end":96}},{"value":"
","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1518,"byte_end":1521,"line_start":27,"line_end":27,"column_start":1,"column_end":4}},{"value":"
All of the encoding functions that take a `Config` will pad as per the config.","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1522,"byte_end":1604,"line_start":28,"line_end":28,"column_start":1,"column_end":83}},{"value":"
","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1605,"byte_end":1608,"line_start":29,"line_end":29,"column_start":1,"column_end":4}},{"value":"
# Decoding","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1609,"byte_end":1623,"line_start":30,"line_end":30,"column_start":1,"column_end":15}},{"value":"
","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1624,"byte_end":1627,"line_start":31,"line_end":31,"column_start":1,"column_end":4}},{"value":"
Just as for encoding, there are different decoding functions available.","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1628,"byte_end":1703,"line_start":32,"line_end":32,"column_start":1,"column_end":76}},{"value":"
","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1704,"byte_end":1707,"line_start":33,"line_end":33,"column_start":1,"column_end":4}},{"value":"
| Function | Output | Allocates |","span":{"file_name":"
Users
iliashuianov
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1708,"byte_end":1804,"line_start":34,"line_end":34,"column_start":1,"column_end":97}},{"value":"
|
package.json
src
App.js
Components
MintingTool.js
login.js
__mocks__
fileMock.js
assets
logo-black.svg
logo-white.svg
config.js
global.css
index.html
index.js
jest.init.js
main.test.js
utils.js
wallet
login
index.html
| my-nft
==================
This [React] app was initialized with [create-near-app]
Quick Start
===========
To run this project locally:
1. Prerequisites: Make sure you've installed [Node.js] ≥ 12
2. Install dependencies: `yarn install`
3. Run the local development server: `yarn dev` (see `package.json` for a
full list of `scripts` you can run with `yarn`)
Now you'll have a local development environment backed by the NEAR TestNet!
Go ahead and play with the app and the code. As you make code changes, the app will automatically reload.
Exploring The Code
==================
1. The "backend" code lives in the `/contract` folder. See the README there for
more info.
2. The frontend code lives in the `/src` folder. `/src/index.html` is a great
place to start exploring. Note that it loads in `/src/index.js`, where you
can learn how the frontend connects to the NEAR blockchain.
3. Tests: there are different kinds of tests for the frontend and the smart
contract. See `contract/README` for info about how it's tested. The frontend
code gets tested with [jest]. You can run both of these at once with `yarn
run test`.
Deploy
======
Every smart contract in NEAR has its [own associated account][NEAR accounts]. When you run `yarn dev`, your smart contract gets deployed to the live NEAR TestNet with a throwaway account. When you're ready to make it permanent, here's how.
Step 0: Install near-cli (optional)
-------------------------------------
[near-cli] is a command line interface (CLI) for interacting with the NEAR blockchain. It was installed to the local `node_modules` folder when you ran `yarn install`, but for best ergonomics you may want to install it globally:
yarn install --global near-cli
Or, if you'd rather use the locally-installed version, you can prefix all `near` commands with `npx`
Ensure that it's installed with `near --version` (or `npx near --version`)
Step 1: Create an account for the contract
------------------------------------------
Each account on NEAR can have at most one contract deployed to it. If you've already created an account such as `your-name.testnet`, you can deploy your contract to `my-nft.your-name.testnet`. Assuming you've already created an account on [NEAR Wallet], here's how to create `my-nft.your-name.testnet`:
1. Authorize NEAR CLI, following the commands it gives you:
near login
2. Create a subaccount (replace `YOUR-NAME` below with your actual account name):
near create-account my-nft.YOUR-NAME.testnet --masterAccount YOUR-NAME.testnet
Step 2: set contract name in code
---------------------------------
Modify the line in `src/config.js` that sets the account name of the contract. Set it to the account id you used above.
const CONTRACT_NAME = process.env.CONTRACT_NAME || 'my-nft.YOUR-NAME.testnet'
Step 3: deploy!
---------------
One command:
yarn deploy
As you can see in `package.json`, this does two things:
1. builds & deploys smart contract to NEAR TestNet
2. builds & deploys frontend code to GitHub using [gh-pages]. This will only work if the project already has a repository set up on GitHub. Feel free to modify the `deploy` script in `package.json` to deploy elsewhere.
Troubleshooting
===============
On Windows, if you're seeing an error containing `EPERM` it may be related to spaces in your path. Please see [this issue](https://github.com/zkat/npx/issues/209) for more details.
[React]: https://reactjs.org/
[create-near-app]: https://github.com/near/create-near-app
[Node.js]: https://nodejs.org/en/download/package-manager/
[jest]: https://jestjs.io/
[NEAR accounts]: https://docs.near.org/docs/concepts/account
[NEAR Wallet]: https://wallet.testnet.near.org/
[near-cli]: https://github.com/near/near-cli
[gh-pages]: https://github.com/tschaub/gh-pages
my-nft Smart Contract
==================
A [smart contract] written in [Rust] for an app initialized with [create-near-app]
Quick Start
===========
Before you compile this code, you will need to install Rust with [correct target]
Exploring The Code
==================
1. The main smart contract code lives in `src/lib.rs`. You can compile it with
the `./compile` script.
2. Tests: You can run smart contract tests with the `./test` script. This runs
standard Rust tests using [cargo] with a `--nocapture` flag so that you
can see any debug info you print to the console.
[smart contract]: https://docs.near.org/docs/develop/contracts/overview
[Rust]: https://www.rust-lang.org/
[create-near-app]: https://github.com/near/create-near-app
[correct target]: https://github.com/near/near-sdk-rs#pre-requisites
[cargo]: https://doc.rust-lang.org/book/ch01-03-hello-cargo.html
|
Kikimora-Labs_gonear-name | .github
workflows
main.yml
README.md
economics
rewards.py
escrow_contract
Cargo.toml
build.sh
compile_and_test.sh
expensive.sh
src
action.rs
lib.rs
proposal.rs
tests
general.rs
frontend
README.md
package.json
public
browserconfig.xml
index.html
robots.txt
safari-pinned-tab.svg
src
assets
fonts
Poppins
OFL.txt
images
light.svg
helpers
api.ts
config.ts
hooks.ts
mappers.ts
media.ts
near.ts
routes.ts
walletConnection.ts
react-app-env.d.ts
tsconfig.json
lock_unlock_account_contract
Cargo.toml
LIST_OF_HASHES.md
build.sh
src
lib.rs
marketplace_contract
Cargo.toml
build.sh
compile_and_test.sh
expensive.sh
src
action.rs
bid.rs
lib.rs
profile.rs
tests
general.rs
| # TBD
# NEAR Protocol Accounts Marketplace dApp
## Quick start
To run this locally:
1. Config envs: `cp .env.example .env`
2. Install dependencies via `yarn install`
3. Start the development server using `yarn start`
4. Open up http://localhost:8080
## Running tests
There are no any tests :–(.
## Deployment
Project deploys on Github Pages via Github Actions. Look through `.github/workflows` to learn more about our CI/CD.
## Links
TBD.
test
|
ippishio_near-cat-nft | README.md
browserconfig.xml
index.html
main.js
near.js
nft-contract
Cargo.toml
README.md
build.sh
src
approval.rs
enumeration.rs
internal.rs
lib.rs
metadata.rs
mint.rs
nft_core.rs
royalty.rs
target
.rustc_info.json
release
.fingerprint
Inflector-960a11a1bb9b0b12
lib-inflector.json
borsh-derive-9716af4d796c49fb
lib-borsh-derive.json
borsh-derive-internal-1a2124d8de9961f4
lib-borsh-derive-internal.json
borsh-schema-derive-internal-8155710b558af6ce
lib-borsh-schema-derive-internal.json
near-sdk-macros-ee696373e39c2f5e
lib-near-sdk-macros.json
proc-macro-crate-d70091033d4a0551
lib-proc-macro-crate.json
proc-macro2-1ebdcc67ef8f8deb
run-build-script-build-script-build.json
proc-macro2-4c32726517dc6db4
build-script-build-script-build.json
proc-macro2-ac14bfa0bcfd21ea
lib-proc-macro2.json
quote-c9c79feb515f4b78
lib-quote.json
ryu-bd573eab492ed36b
build-script-build-script-build.json
serde-3bc4bb0098066134
run-build-script-build-script-build.json
serde-7c3951e5294c5be9
build-script-build-script-build.json
serde-d3f3fd5e30d75da3
build-script-build-script-build.json
serde-d66fcce83c310ecb
lib-serde.json
serde_derive-4de387af8789c500
lib-serde_derive.json
serde_derive-77a3bddd2c8f6568
build-script-build-script-build.json
serde_derive-a3933a103b38c66b
run-build-script-build-script-build.json
serde_json-bab05d69821c8adc
build-script-build-script-build.json
syn-179f7a3f2c7c4f73
run-build-script-build-script-build.json
syn-3e6e20baa85c8b7b
build-script-build-script-build.json
syn-ace682f0d4ac75cf
lib-syn.json
toml-4976a4381be275b5
lib-toml.json
unicode-xid-4500716f7413df0f
lib-unicode-xid.json
wee_alloc-3059e8679fcf3f87
build-script-build-script-build.json
rls
.rustc_info.json
debug
.fingerprint
Inflector-d7846ac18fccc54a
lib-inflector.json
ahash-ea756eae10ed06fa
lib-ahash.json
aho-corasick-605c2fc4423212b9
lib-aho_corasick.json
autocfg-054c764b6e2dde70
lib-autocfg.json
base64-67ca47b261bc1218
lib-base64.json
block-buffer-8f18644fac76287b
lib-block-buffer.json
block-padding-bb70e0ce09c7c18a
lib-block-padding.json
borsh-d578fda74c1f5fe4
lib-borsh.json
borsh-derive-a56b11869ff4a914
lib-borsh-derive.json
borsh-derive-internal-0a7c8d2a61e46c67
lib-borsh-derive-internal.json
borsh-schema-derive-internal-179fd76cd65f9ba1
lib-borsh-schema-derive-internal.json
bs58-a876b3bfe7fdfbb2
lib-bs58.json
byteorder-2290de94bb3170df
lib-byteorder.json
cfg-if-7d42709f3d54d907
lib-cfg-if.json
cfg-if-946e885e71060f52
lib-cfg-if.json
cpuid-bool-7870d413d03df8e6
lib-cpuid-bool.json
derive_more-48d160c843093db6
lib-derive_more.json
digest-db8488b404d5a67e
lib-digest.json
generic-array-9d0faf177ca55a7f
lib-generic_array.json
generic-array-d45e25a518da2990
run-build-script-build-script-build.json
generic-array-e34cb9c8696f9dd7
build-script-build-script-build.json
hashbrown-b246c2681abb2a40
lib-hashbrown.json
hashbrown-bab87b5fa9ee644c
lib-hashbrown.json
hex-e3ef3d0deba940d2
lib-hex.json
indexmap-13e3ac522ca1a795
build-script-build-script-build.json
indexmap-2a5577ad4e88c4e6
lib-indexmap.json
indexmap-ebd6102278340f3f
run-build-script-build-script-build.json
itoa-8f44d7ad2d7fc6ec
lib-itoa.json
itoa-cfd94be669bf17b8
lib-itoa.json
keccak-c714784b3a2b37d2
lib-keccak.json
lazy_static-6a4f05fd7715fb09
lib-lazy_static.json
libc-5021878a9336ac06
run-build-script-build-script-build.json
libc-94349327ca7f1ff9
build-script-build-script-build.json
libc-ed0346412ab912bf
lib-libc.json
memchr-6f6b27353c2ae857
run-build-script-build-script-build.json
memchr-a0a2d74a7d35cda0
lib-memchr.json
memchr-df2fece483f5c21d
build-script-build-script-build.json
memory_units-93b980468bca74ec
lib-memory_units.json
near-primitives-core-18fe7722a1075959
lib-near-primitives-core.json
near-rpc-error-core-0514b8d6a412afe8
lib-near-rpc-error-core.json
near-rpc-error-macro-6e39ba1c97441183
lib-near-rpc-error-macro.json
near-runtime-utils-d518c65a7267d6b3
lib-near-runtime-utils.json
near-sdk-8fe05f5a4832ad5e
lib-near-sdk.json
near-sdk-macros-f4d31ded00ed54cf
lib-near-sdk-macros.json
near-sys-8890c0c8561d9125
lib-near-sys.json
near-vm-errors-ad553f3b28b1e2f5
lib-near-vm-errors.json
near-vm-logic-b6e43dc9518f7571
lib-near-vm-logic.json
nft_simple-9b113c1c51dabbc1
lib-nft_simple.json
nft_simple-9f32599b2aa4cf30
test-lib-nft_simple.json
num-bigint-50c4d06a651a7285
run-build-script-build-script-build.json
num-bigint-5633504c86cfd51e
build-script-build-script-build.json
num-bigint-9583ee0d762e97c6
lib-num-bigint.json
num-integer-4e58a38361f03b55
run-build-script-build-script-build.json
num-integer-8df6aff2d36bf732
build-script-build-script-build.json
num-integer-f72b388cd8eac043
lib-num-integer.json
num-rational-118ccdc387676ecc
lib-num-rational.json
num-rational-55ada24dca21e189
build-script-build-script-build.json
num-rational-a77ec9ea1dcae64f
run-build-script-build-script-build.json
num-traits-571a5f9a58b358d1
lib-num-traits.json
num-traits-5a950220f325e130
run-build-script-build-script-build.json
num-traits-e15f1c4fdc5627ae
build-script-build-script-build.json
once_cell-8eda0f4467fe6074
lib-once_cell.json
opaque-debug-e0b9da328b624eef
lib-opaque-debug.json
proc-macro-crate-b5597a1d444622d7
lib-proc-macro-crate.json
proc-macro2-20eea9ee85e1b29b
run-build-script-build-script-build.json
proc-macro2-852747da0a4bfd54
build-script-build-script-build.json
proc-macro2-c95b77c8edde12a1
lib-proc-macro2.json
quote-fcd22b39084cd4b0
lib-quote.json
regex-7d239c115e61eebd
lib-regex.json
regex-syntax-05bd8cbfa4902ce2
lib-regex-syntax.json
ryu-10101ea116e40bdd
lib-ryu.json
ryu-25c40b8833883533
build-script-build-script-build.json
ryu-fe0a48ad916f6cd5
lib-ryu.json
ryu-fe763814b6e2cf9e
run-build-script-build-script-build.json
serde-23ca0e3c5b712315
lib-serde.json
serde-c6dd786dd855770b
lib-serde.json
serde-ca378e34f3a56144
run-build-script-build-script-build.json
serde-d45fdeca565d1a22
build-script-build-script-build.json
serde_derive-413d1b37a8706aa8
build-script-build-script-build.json
serde_derive-9f2676edab5163dc
lib-serde_derive.json
serde_derive-c15848797502099e
run-build-script-build-script-build.json
serde_json-32b53a992d738e61
run-build-script-build-script-build.json
serde_json-59856343dde6bf0a
build-script-build-script-build.json
serde_json-5e8a697724fba638
run-build-script-build-script-build.json
serde_json-86a16eb1e2401541
lib-serde_json.json
serde_json-dc7bcd09c921189c
lib-serde_json.json
serde_json-fc38e8ee01abca4c
build-script-build-script-build.json
sha2-c8413ea0721b16f1
lib-sha2.json
sha3-19bd4fcc2db00535
lib-sha3.json
syn-3f74edf56d8a81a0
lib-syn.json
syn-515545dd0ddad3b3
run-build-script-build-script-build.json
syn-64c38b1ea456d05a
build-script-build-script-build.json
thread_local-6c59f363c8c67451
lib-thread_local.json
toml-b9132b21fa720de4
lib-toml.json
typenum-1948c84d5636f8ed
run-build-script-build-script-main.json
typenum-e9e5a6fdbddcdf4e
lib-typenum.json
typenum-f99a0afb39bbe014
build-script-build-script-main.json
unicode-xid-40a58482a0cdcda6
lib-unicode-xid.json
version_check-21c0f9acc8eafaf4
lib-version_check.json
wee_alloc-79bb5db00e84c17e
run-build-script-build-script-build.json
wee_alloc-9fb56260a1c682d5
build-script-build-script-build.json
wee_alloc-e96b7d7a567a76bf
lib-wee_alloc.json
build
generic-array-e34cb9c8696f9dd7
save-analysis
build_script_build-e34cb9c8696f9dd7.json
indexmap-13e3ac522ca1a795
save-analysis
build_script_build-13e3ac522ca1a795.json
libc-94349327ca7f1ff9
save-analysis
build_script_build-94349327ca7f1ff9.json
memchr-df2fece483f5c21d
save-analysis
build_script_build-df2fece483f5c21d.json
num-bigint-50c4d06a651a7285
out
radix_bases.rs
num-bigint-5633504c86cfd51e
save-analysis
build_script_build-5633504c86cfd51e.json
num-integer-8df6aff2d36bf732
save-analysis
build_script_build-8df6aff2d36bf732.json
num-rational-55ada24dca21e189
save-analysis
build_script_build-55ada24dca21e189.json
num-traits-e15f1c4fdc5627ae
save-analysis
build_script_build-e15f1c4fdc5627ae.json
proc-macro2-852747da0a4bfd54
save-analysis
build_script_build-852747da0a4bfd54.json
ryu-25c40b8833883533
save-analysis
build_script_build-25c40b8833883533.json
serde-d45fdeca565d1a22
save-analysis
build_script_build-d45fdeca565d1a22.json
serde_derive-413d1b37a8706aa8
save-analysis
build_script_build-413d1b37a8706aa8.json
serde_json-59856343dde6bf0a
save-analysis
build_script_build-59856343dde6bf0a.json
serde_json-fc38e8ee01abca4c
save-analysis
build_script_build-fc38e8ee01abca4c.json
syn-64c38b1ea456d05a
save-analysis
build_script_build-64c38b1ea456d05a.json
typenum-1948c84d5636f8ed
out
consts.rs
op.rs
tests.rs
typenum-f99a0afb39bbe014
save-analysis
build_script_main-f99a0afb39bbe014.json
wee_alloc-79bb5db00e84c17e
out
wee_alloc_static_array_backend_size_bytes.txt
wee_alloc-9fb56260a1c682d5
save-analysis
build_script_build-9fb56260a1c682d5.json
deps
save-analysis
libahash-ea756eae10ed06fa.json
libaho_corasick-605c2fc4423212b9.json
libautocfg-054c764b6e2dde70.json
libbase64-67ca47b261bc1218.json
libblock_buffer-8f18644fac76287b.json
libblock_padding-bb70e0ce09c7c18a.json
libborsh-d578fda74c1f5fe4.json
libbs58-a876b3bfe7fdfbb2.json
libbyteorder-2290de94bb3170df.json
libderive_more-48d160c843093db6.json
libdigest-db8488b404d5a67e.json
libgeneric_array-9d0faf177ca55a7f.json
libhashbrown-b246c2681abb2a40.json
libhex-e3ef3d0deba940d2.json
libindexmap-2a5577ad4e88c4e6.json
libinflector-d7846ac18fccc54a.json
libitoa-cfd94be669bf17b8.json
libkeccak-c714784b3a2b37d2.json
liblazy_static-6a4f05fd7715fb09.json
libmemchr-a0a2d74a7d35cda0.json
libmemory_units-93b980468bca74ec.json
libnear_primitives_core-18fe7722a1075959.json
libnear_rpc_error_core-0514b8d6a412afe8.json
libnear_rpc_error_macro-6e39ba1c97441183.json
libnear_runtime_utils-d518c65a7267d6b3.json
libnear_sdk-8fe05f5a4832ad5e.json
libnear_sys-8890c0c8561d9125.json
libnear_vm_errors-ad553f3b28b1e2f5.json
libnear_vm_logic-b6e43dc9518f7571.json
libnft_simple-9b113c1c51dabbc1.json
libnum_bigint-9583ee0d762e97c6.json
libnum_integer-f72b388cd8eac043.json
libnum_rational-118ccdc387676ecc.json
libnum_traits-571a5f9a58b358d1.json
libonce_cell-8eda0f4467fe6074.json
libopaque_debug-e0b9da328b624eef.json
libproc_macro2-c95b77c8edde12a1.json
libproc_macro_crate-b5597a1d444622d7.json
libquote-fcd22b39084cd4b0.json
libregex-7d239c115e61eebd.json
libryu-10101ea116e40bdd.json
libryu-fe0a48ad916f6cd5.json
libsha2-c8413ea0721b16f1.json
libsha3-19bd4fcc2db00535.json
libthread_local-6c59f363c8c67451.json
libtoml-b9132b21fa720de4.json
libunicode_xid-40a58482a0cdcda6.json
libversion_check-21c0f9acc8eafaf4.json
libwee_alloc-e96b7d7a567a76bf.json
nft_simple-9f32599b2aa4cf30.json
wasm32-unknown-unknown
release
.fingerprint
ahash-399a40215a5a6369
lib-ahash.json
base64-54eec6bc3a2e281a
lib-base64.json
borsh-e2cbfb1aadddee61
lib-borsh.json
bs58-4f2061a8295f0e79
lib-bs58.json
cfg-if-b4aa0ed23f0cba7d
lib-cfg-if.json
hashbrown-ed40e67e8b1ddc4e
lib-hashbrown.json
itoa-6fd5b19c7fa6477b
lib-itoa.json
memory_units-2825d28615e564a4
lib-memory_units.json
near-sdk-d2ccc76f4eda272d
lib-near-sdk.json
near-sys-b1e388795f25b4fc
lib-near-sys.json
nft_simple-322841616f66c026
lib-nft_simple.json
ryu-7238508d6fbfb755
lib-ryu.json
ryu-c14cb36d8f13b8d3
run-build-script-build-script-build.json
serde-bdac925e69dd0523
lib-serde.json
serde-fbb9d892d3ad2866
run-build-script-build-script-build.json
serde_json-cdc2354079746d8e
run-build-script-build-script-build.json
serde_json-faad7932bab30118
lib-serde_json.json
wee_alloc-1e0d214c0b3fb3e8
lib-wee_alloc.json
wee_alloc-a931c3171d428f84
run-build-script-build-script-build.json
build
wee_alloc-a931c3171d428f84
out
wee_alloc_static_array_backend_size_bytes.txt
|
|","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1038,"byte_end":1133,"line_start":22,"line_end":22,"column_start":1,"column_end":96}},{"value":"
| `encode` | Returns a new `String` | Always |","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1134,"byte_end":1229,"line_start":23,"line_end":23,"column_start":1,"column_end":96}},{"value":"
| `encode_config` | Returns a new `String` | Always |","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1230,"byte_end":1325,"line_start":24,"line_end":24,"column_start":1,"column_end":96}},{"value":"
| `encode_config_buf` | Appends to provided `String` | Only if `String` needs to grow |","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1326,"byte_end":1421,"line_start":25,"line_end":25,"column_start":1,"column_end":96}},{"value":"
| `encode_config_slice` | Writes to provided `&[u8]` | Never |","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1422,"byte_end":1517,"line_start":26,"line_end":26,"column_start":1,"column_end":96}},{"value":"
","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1518,"byte_end":1521,"line_start":27,"line_end":27,"column_start":1,"column_end":4}},{"value":"
All of the encoding functions that take a `Config` will pad as per the config.","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1522,"byte_end":1604,"line_start":28,"line_end":28,"column_start":1,"column_end":83}},{"value":"
","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1605,"byte_end":1608,"line_start":29,"line_end":29,"column_start":1,"column_end":4}},{"value":"
# Decoding","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1609,"byte_end":1623,"line_start":30,"line_end":30,"column_start":1,"column_end":15}},{"value":"
","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1624,"byte_end":1627,"line_start":31,"line_end":31,"column_start":1,"column_end":4}},{"value":"
Just as for encoding, there are different decoding functions available.","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1628,"byte_end":1703,"line_start":32,"line_end":32,"column_start":1,"column_end":76}},{"value":"
","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1704,"byte_end":1707,"line_start":33,"line_end":33,"column_start":1,"column_end":4}},{"value":"
| Function | Output | Allocates |","span":{"file_name":"
home
ippishio
.cargo
registry
src
github.com-1ecc6299db9ec823
base64-0.13.0
src
lib.rs","byte_start":1708,"byte_end":1804,"line_start":34,"line_end":34,"column_start":1,"column_end":97}},{"value":"
|
nft-uploader
package-lock.json
package.json
package-lock.json
package.json
safari-pinned-tab.svg
style.css
| # near-cat-nft
Challenge #3
#### NEAR CATS NFT Collection contains 50 images of cats, personally drawn by my hand :)
#### Because this isn't real NFT collection, you can mint tokens as many as you want.
## Project structure
Repository contains two folders:
- nft-uploader
- nft-contract
#### nft-uploader
Contains script, that is used to upload all 50 images to nft.storage using API.
#### nft-contract
Contains smart contract source code, which was builded and deployed to nft.ippishio.testnet
#### nft-frontend(located in root)
Contains all logic behind frontend page using THREE.js and near-api-js libraries. Near.js contains all blokchain integration logic, and Main.js for all other. When mint button is pressed, Near.js generates randID(used for pick random nft from collection), and passes it to the nft_mint() contract method. After approving transaction, there is transactionHash in URL args, which contains response from mint_nft() with the same randID, that was passed. After this, randID is used to render NFT image in THREE.js
# TBD
|
Peersyst_xrp-evm-safe-client-gateway | .github
ISSUE_TEMPLATE
bug_report.md
feature_request.md
action-rs
grcov.yml
dependabot.yml
landing_page
index.html
pull_request_template.md
workflows
cla.yml
rust.yml
Cargo.toml
README.md
add_rustfmt_git_hook.sh
docker-compose.yml
rust-toolchain.toml
rustfmt.toml
scripts
autodeploy.sh
deploy_docker.sh
generate_test_tx.sh
src
cache
cache_op_executors.rs
cache_operations.rs
inner_cache.rs
manager.rs
mod.rs
redis.rs
tests
cache_inner.rs
cache_op_executors.rs
cache_operations.rs
mod.rs
common
converters
data_decoded.rs
mod.rs
page_metadata.rs
tests
balances.rs
balances_v2.rs
data_decoded.rs
get_address_ex_from_any_source.rs
get_transfer_direction.rs
mod.rs
page_metadata.rs
safe_app.rs
transfer_erc20.rs
transfer_erc721.rs
transfer_ether.rs
transfers.rs
transfers.rs
mod.rs
models
addresses.rs
backend
about.rs
balances.rs
balances_v2.rs
chains.rs
hooks.rs
mod.rs
notifications.rs
safe_apps.rs
safes.rs
transactions.rs
transfers.rs
data_decoded.rs
mod.rs
page.rs
routes
authorization.rs
mod.rs
tests
common.rs
mod.rs
config
mod.rs
tests
mod.rs
macros.rs
main.rs
monitoring
mod.rs
performance.rs
tests
mod.rs
path_patterns.rs
providers
address_info.rs
ext.rs
fiat.rs
info.rs
mod.rs
tests
fiat.rs
info.rs
mod.rs
routes
about
handlers.rs
mod.rs
models.rs
routes.rs
tests
mod.rs
routes.rs
balances
converters.rs
converters_v2.rs
handlers.rs
handlers_v2.rs
mod.rs
models.rs
routes.rs
chains
converters.rs
handlers.rs
mod.rs
models.rs
routes.rs
tests
chains.rs
json
backend_chains_info_page.json
expected_chains_info_page.json
mod.rs
routes.rs
collectibles
handlers.rs
mod.rs
models.rs
routes.rs
tests
mod.rs
routes.rs
contracts
handlers.rs
mod.rs
models.rs
routes.rs
tests
mod.rs
routes.rs
delegates
handlers.rs
mod.rs
models.rs
routes.rs
tests
json
backend_create_delegate.json
backend_delete_delegate.json
backend_delete_delegate_safe.json
backend_list_delegates_of_safe.json
expected_list_delegates_of_safe.json
mod.rs
routes.rs
health
mod.rs
routes.rs
tests
mod.rs
routes.rs
hooks
handlers.rs
mod.rs
routes.rs
tests
invalidate_caches.rs
mod.rs
routes.rs
safes.rs
messages
backend_models.rs
create_message.rs
frontend_models.rs
get_message.rs
get_messages.rs
message_mapper.rs
mod.rs
update_message.rs
mod.rs
notifications
handlers.rs
mod.rs
models.rs
routes.rs
tests
mod.rs
routes.rs
safe_apps
converters.rs
handlers.rs
mod.rs
models.rs
routes.rs
tests
json
response_safe_apps.json
response_safe_apps_url_query.json
response_safe_apps_with_tags.json
mod.rs
routes.rs
safes
converters.rs
handlers
estimations.rs
mod.rs
safes.rs
mod.rs
models.rs
routes.rs
tests
json
last_collectible_transfer.json
last_history_tx.json
last_queued_tx.json
safe_state.json
mod.rs
routes.rs
transactions
converters
details.rs
mod.rs
safe_app_info.rs
summary.rs
tests
check_sender_or_receiver.rs
data_size_calculation.rs
details.rs
is_cancellation.rs
map_status.rs
missing_signers.rs
mod.rs
safe_app_info.rs
summary.rs
transaction_id.rs
transaction_types.rs
transfer_type_checks.rs
transaction_id.rs
filters
mod.rs
module.rs
multisig.rs
tests
mod.rs
transfer.rs
handlers
commons.rs
details.rs
history.rs
mod.rs
module.rs
multisig.rs
preview.rs
proposal.rs
queued.rs
tests
mod.rs
parse_id.rs
transactions_history.rs
transactions_queued.rs
transfers.rs
transfers.rs
mod.rs
models
details.rs
mod.rs
requests.rs
summary.rs
routes.rs
tests
json
chain_response.json
contract_info_BID.json
contracts_response.json
multisig_tx_details.json
post_confirmation_result.json
preview_response.json
preview_response_data_decoded_error.json
mod.rs
preview.rs
routes.rs
tests
backend_url.rs
json
balances
balance_compound_ether.json
balance_ether.json
chains
polygon.json
rinkeby.json
rinkeby_disabled_wallets.json
rinkeby_enabled_features.json
rinkeby_fixed_gas_price.json
rinkeby_multiple_gas_price.json
rinkeby_no_gas_price.json
rinkeby_rpc_auth_unknown.json
rinkeby_rpc_no_auth.json
rinkeby_unknown_gas_price.json
collectibles
collectibles_page.json
collectibles_paginated_empty_cgw.json
collectibles_paginated_empty_txs.json
collectibles_paginated_page_1_cgw.json
collectibles_paginated_page_1_txs.json
collectibles_paginated_page_2_cgw.json
collectibles_paginated_page_2_txs.json
commons
DOCTORED_data_decoded_multi_send_nested_delegate.json
DOCTORED_data_decoded_nested_multi_sends.json
data_decoded_add_owner_with_threshold.json
data_decoded_approve.json
data_decoded_change_master_copy.json
data_decoded_change_threshold.json
data_decoded_delete_guard.json
data_decoded_disable_module.json
data_decoded_enable_module.json
data_decoded_exec_transaction_from_module.json
data_decoded_multi_send.json
data_decoded_multi_send_single_inner_transaction.json
data_decoded_nested_safe_interaction.json
data_decoded_remove_owner.json
data_decoded_set_fallback_handler.json
data_decoded_set_guard.json
data_decoded_swap_array_values.json
data_decoded_swap_owner.json
empty_page.json
contracts
contract_info_BID.json
exchange
currency_rates.json
master_copies
polygon_master_copies.json
mod.rs
results
tx_details_with_origin.json
safe_apps
polygon_safe_app_url_query.json
polygon_safe_apps.json
polygon_safe_apps_with_tags.json
safes
with_guard_safe_v130_l2.json
with_module_transactions.json
with_modules.json
with_modules_and_high_nonce.json
with_threshold_two.json
tokens
bat.json
crypto_kitties.json
dai.json
pv_memorial_token.json
usdt.json
transactions
backend_history_transaction_list_page.json
backend_multisig_transfer_tx.json
backend_queued_transaction_list_page_conflicts_393.json
backend_queued_transaction_list_page_conflicts_394.json
backend_queued_transaction_list_page_no_conflicts.json
creation_transaction.json
ethereum_inconsistent_token_types.json
module_addOwnerWithThreshold_settings_change.json
module_erc20_transfer.json
module_erc721_transfer.json
module_ether_transfer.json
module_newAndDifferentAddOwnerWithThreshold_settings_change.json
module_transaction.json
module_transaction_failed.json
multisig_addOwnerWithThreshold_settings_change.json
multisig_approve_custom.json
multisig_awaiting_confirmations.json
multisig_awaiting_confirmations_empty.json
multisig_awaiting_confirmations_null.json
multisig_awaiting_confirmations_required_null.json
multisig_awaiting_execution.json
multisig_cancellation_transaction.json
multisig_confirmations_null.json
multisig_erc20_transfer.json
multisig_erc20_transfer_delegate.json
multisig_erc20_transfer_invalid_to_and_from.json
multisig_erc20_transfer_with_value.json
multisig_erc721_transfer.json
multisig_erc721_transfer_cancelled.json
multisig_erc721_transfer_invalid_to_and_from.json
multisig_ether_transfer.json
multisig_failed_transfer.json
multisig_newAndDifferentAddOwnerWithThreshold_settings_change.json
multisig_with_origin.json
transfers
erc20_transfer_with_erc721_token_info.json
erc_20_transfer_unexpected_param_names.json
erc_20_transfer_with_token_info_incoming.json
erc_20_transfer_with_token_info_outgoing.json
erc_20_transfer_without_token_info.json
erc_721_transfer_with_token_info_incoming.json
erc_721_transfer_with_token_info_outgoing.json
erc_721_transfer_without_token_info.json
ether_transfer_incoming.json
ether_transfer_outgoing.json
main.rs
mod.rs
utils
context.rs
cors.rs
errors.rs
http_client.rs
json.rs
mod.rs
tests
data_decoded_utils.rs
errors.rs
json.rs
macros.rs
method_names.rs
mod.rs
transactions.rs
transactions.rs
urls.rs
| # Safe Client Gateway
[](https://github.com/safe-global/safe-client-gateway/actions)
[](https://coveralls.io/github/safe-global/safe-client-gateway)
## Motivation
This project is a gateway between the Safe clients ([Android](https://github.com/safe-global/safe-android)/ [iOS](https://github.com/safe-global/safe-ios)/ [web](https://github.com/safe-global/safe-react)) and the Safe backend services ([transaction service](https://github.com/safe-global/safe-transaction-service) and Ethereum nodes). It is providing a more UI-oriented mapping and multi-sourced data structures for ease of integration and rendering.
## Documentation
- [Client Gateway Docs](https://safe.global/safe-client-gateway/)
- [Swagger](https://safe-client.safe.global/index.html)
- [Safe developer documentation](https://docs.gnosis-safe.io/)
## Quickstart
This project requires `rustup` and `redis`
```bash
git clone https://github.com/safe-global/safe-client-gateway.git
cd safe-client-gateway
cp .env.sample .env
redis-server
cargo run
./add_rustfmt_git_hook.sh # It installs a git precommit hook that will autoformat the code on every commit
```
After doing any change code must be formatted using [Rustfmt](https://github.com/rust-lang/rustfmt)
- `cargo +nightly fmt --all`
Auto formatting can also [be configured in the most common code editors](https://github.com/rust-lang/rustfmt#running-rustfmt-from-your-editor)
## Configuration
Rocket specific configurations (including databases) can be configured via the `Rocket.toml` for local development (see https://rocket.rs/v0.4/guide/configuration/#rockettoml).
For configurations specific to this service the `.env` file can be used. See next section.
## Environment
Place a `.env` file in the root of the project containing URL pointing to the environment in which you want the gateway to run.
The contents of the file should be the following (see `.env.sample` for an example)
## Tests
In order to run the test suite of the project:
1. Have an instance of Redis running (as some of them test the integration with Redis).
```bash
redis-server
```
2. Make sure that the required environment variables are set (the following example assumes that Redis is runnning on the default port `6379`):
```bash
export REDIS_URI=redis://localhost:6379
export REDIS_URI_MAINNET=redis://localhost:6379
```
3. Run the tests
```bash
cargo test -- --test-threads 1
```
By default, `cargo test` will execute the tests in the test suite in parallel. Because some of the tests update some shared local state (eg.: environment variables) the tests should be executed on a single thread – thus `--test-threads 1`.
|
mustafabalci_near-smart-contract-practice-1 | README.md
as-pect.config.js
asconfig.json
package.json
scripts
1.dev-deploy.sh
2.use-contract.sh
3.cleanup.sh
README.md
src
as_types.d.ts
simple
__tests__
as-pect.d.ts
index.unit.spec.ts
asconfig.json
assembly
index.ts
singleton
__tests__
as-pect.d.ts
index.unit.spec.ts
asconfig.json
assembly
index.ts
tsconfig.json
utils.ts
| # `near-sdk-as` Starter Kit
This is a good project to use as a starting point for your AssemblyScript project.
## Samples
This repository includes a complete project structure for AssemblyScript contracts targeting the NEAR platform.
The example here is very basic. It's a simple contract demonstrating the following concepts:
- a single contract
- the difference between `view` vs. `change` methods
- basic contract storage
There are 2 AssemblyScript contracts in this project, each in their own folder:
- **simple** in the `src/simple` folder
- **singleton** in the `src/singleton` folder
### Simple
We say that an AssemblyScript contract is written in the "simple style" when the `index.ts` file (the contract entry point) includes a series of exported functions.
In this case, all exported functions become public contract methods.
```ts
// return the string 'hello world'
export function helloWorld(): string {}
// read the given key from account (contract) storage
export function read(key: string): string {}
// write the given value at the given key to account (contract) storage
export function write(key: string, value: string): string {}
// private helper method used by read() and write() above
private storageReport(): string {}
```
### Singleton
We say that an AssemblyScript contract is written in the "singleton style" when the `index.ts` file (the contract entry point) has a single exported class (the name of the class doesn't matter) that is decorated with `@nearBindgen`.
In this case, all methods on the class become public contract methods unless marked `private`. Also, all instance variables are stored as a serialized instance of the class under a special storage key named `STATE`. AssemblyScript uses JSON for storage serialization (as opposed to Rust contracts which use a custom binary serialization format called borsh).
```ts
@nearBindgen
export class Contract {
// return the string 'hello world'
helloWorld(): string {}
// read the given key from account (contract) storage
read(key: string): string {}
// write the given value at the given key to account (contract) storage
@mutateState()
write(key: string, value: string): string {}
// private helper method used by read() and write() above
private storageReport(): string {}
}
```
## Usage
### Getting started
(see below for video recordings of each of the following steps)
INSTALL `NEAR CLI` first like this: `npm i -g near-cli`
1. clone this repo to a local folder
2. run `yarn`
3. run `./scripts/1.dev-deploy.sh`
3. run `./scripts/2.use-contract.sh`
4. run `./scripts/2.use-contract.sh` (yes, run it to see changes)
5. run `./scripts/3.cleanup.sh`
### Videos
**`1.dev-deploy.sh`**
This video shows the build and deployment of the contract.
[](https://asciinema.org/a/409575)
**`2.use-contract.sh`**
This video shows contract methods being called. You should run the script twice to see the effect it has on contract state.
[](https://asciinema.org/a/409577)
**`3.cleanup.sh`**
This video shows the cleanup script running. Make sure you add the `BENEFICIARY` environment variable. The script will remind you if you forget.
```sh
export BENEFICIARY=<your-account-here> # this account receives contract account balance
```
[](https://asciinema.org/a/409580)
### Other documentation
- See `./scripts/README.md` for documentation about the scripts
- Watch this video where Willem Wyndham walks us through refactoring a simple example of a NEAR smart contract written in AssemblyScript
https://youtu.be/QP7aveSqRPo
```
There are 2 "styles" of implementing AssemblyScript NEAR contracts:
- the contract interface can either be a collection of exported functions
- or the contract interface can be the methods of a an exported class
We call the second style "Singleton" because there is only one instance of the class which is serialized to the blockchain storage. Rust contracts written for NEAR do this by default with the contract struct.
0:00 noise (to cut)
0:10 Welcome
0:59 Create project starting with "npm init"
2:20 Customize the project for AssemblyScript development
9:25 Import the Counter example and get unit tests passing
18:30 Adapt the Counter example to a Singleton style contract
21:49 Refactoring unit tests to access the new methods
24:45 Review and summary
```
## The file system
```sh
├── README.md # this file
├── as-pect.config.js # configuration for as-pect (AssemblyScript unit testing)
├── asconfig.json # configuration for AssemblyScript compiler (supports multiple contracts)
├── package.json # NodeJS project manifest
├── scripts
│ ├── 1.dev-deploy.sh # helper: build and deploy contracts
│ ├── 2.use-contract.sh # helper: call methods on ContractPromise
│ ├── 3.cleanup.sh # helper: delete build and deploy artifacts
│ └── README.md # documentation for helper scripts
├── src
│ ├── as_types.d.ts # AssemblyScript headers for type hints
│ ├── simple # Contract 1: "Simple example"
│ │ ├── __tests__
│ │ │ ├── as-pect.d.ts # as-pect unit testing headers for type hints
│ │ │ └── index.unit.spec.ts # unit tests for contract 1
│ │ ├── asconfig.json # configuration for AssemblyScript compiler (one per contract)
│ │ └── assembly
│ │ └── index.ts # contract code for contract 1
│ ├── singleton # Contract 2: "Singleton-style example"
│ │ ├── __tests__
│ │ │ ├── as-pect.d.ts # as-pect unit testing headers for type hints
│ │ │ └── index.unit.spec.ts # unit tests for contract 2
│ │ ├── asconfig.json # configuration for AssemblyScript compiler (one per contract)
│ │ └── assembly
│ │ └── index.ts # contract code for contract 2
│ ├── tsconfig.json # Typescript configuration
│ └── utils.ts # common contract utility functions
└── yarn.lock # project manifest version lock
```
You may clone this repo to get started OR create everything from scratch.
Please note that, in order to create the AssemblyScript and tests folder structure, you may use the command `asp --init` which will create the following folders and files:
```
./assembly/
./assembly/tests/
./assembly/tests/example.spec.ts
./assembly/tests/as-pect.d.ts
```
## Setting up your terminal
The scripts in this folder are designed to help you demonstrate the behavior of the contract(s) in this project.
It uses the following setup:
```sh
# set your terminal up to have 2 windows, A and B like this:
┌─────────────────────────────────┬─────────────────────────────────┐
│ │ │
│ │ │
│ A │ B │
│ │ │
│ │ │
└─────────────────────────────────┴─────────────────────────────────┘
```
### Terminal **A**
*This window is used to compile, deploy and control the contract*
- Environment
```sh
export CONTRACT= # depends on deployment
export OWNER= # any account you control
# for example
# export CONTRACT=dev-1615190770786-2702449
# export OWNER=sherif.testnet
```
- Commands
_helper scripts_
```sh
1.dev-deploy.sh # helper: build and deploy contracts
2.use-contract.sh # helper: call methods on ContractPromise
3.cleanup.sh # helper: delete build and deploy artifacts
```
### Terminal **B**
*This window is used to render the contract account storage*
- Environment
```sh
export CONTRACT= # depends on deployment
# for example
# export CONTRACT=dev-1615190770786-2702449
```
- Commands
```sh
# monitor contract storage using near-account-utils
# https://github.com/near-examples/near-account-utils
watch -d -n 1 yarn storage $CONTRACT
```
---
## OS Support
### Linux
- The `watch` command is supported natively on Linux
- To learn more about any of these shell commands take a look at [explainshell.com](https://explainshell.com)
### MacOS
- Consider `brew info visionmedia-watch` (or `brew install watch`)
### Windows
- Consider this article: [What is the Windows analog of the Linux watch command?](https://superuser.com/questions/191063/what-is-the-windows-analog-of-the-linuo-watch-command#191068)
|
manognaaaa_blockyourvote | .gitpod.yml
README.md
contract
README.md
babel.config.json
build.sh
build
builder.c
code.h
hello_near.js
methods.h
deploy.sh
neardev
dev-account.env
package-lock.json
package.json
src
contract.ts
tsconfig.json
frontend
App.js
Components
AdminLogin.js
Comingsoon.js
Home.js
NewPoll.js
NewVoter.js
PollingStation.js
ShowResult.js
UserLogin.js
assets
global.css
logo-black.svg
logo-white.svg
dist
index.ab0485ab.css
index.html
index.html
index.js
near-wallet.js
package-lock.json
package.json
start.sh
ui-components.js
integration-tests
package-lock.json
package.json
src
main.ava.ts
package-lock.json
package.json
| # BlockYourVote
# Hello NEAR Contract
The smart contract exposes two methods to enable storing and retrieving a greeting in the NEAR network.
```ts
@NearBindgen({})
class HelloNear {
greeting: string = "Hello";
@view // This method is read-only and can be called for free
get_greeting(): string {
return this.greeting;
}
@call // This method changes the state, for which it cost gas
set_greeting({ greeting }: { greeting: string }): void {
// Record a log permanently to the blockchain!
near.log(`Saving greeting ${greeting}`);
this.greeting = greeting;
}
}
```
<br />
# Quickstart
1. Make sure you have installed [node.js](https://nodejs.org/en/download/package-manager/) >= 16.
2. Install the [`NEAR CLI`](https://github.com/near/near-cli#setup)
<br />
## 1. Build and Deploy the Contract
You can automatically compile and deploy the contract in the NEAR testnet by running:
```bash
npm run deploy
```
Once finished, check the `neardev/dev-account` file to find the address in which the contract was deployed:
```bash
cat ./neardev/dev-account
# e.g. dev-1659899566943-21539992274727
```
<br />
## 2. Retrieve the Greeting
`get_greeting` is a read-only method (aka `view` method).
`View` methods can be called for **free** by anyone, even people **without a NEAR account**!
```bash
# Use near-cli to get the greeting
near view <dev-account> get_greeting
```
<br />
## 3. Store a New Greeting
`set_greeting` changes the contract's state, for which it is a `call` method.
`Call` methods can only be invoked using a NEAR account, since the account needs to pay GAS for the transaction.
```bash
# Use near-cli to set a new greeting
near call <dev-account> set_greeting '{"greeting":"howdy"}' --accountId <dev-account>
```
**Tip:** If you would like to call `set_greeting` using your own account, first login into NEAR using:
```bash
# Use near-cli to login your NEAR account
near login
```
and then use the logged account to sign the transaction: `--accountId <your-account>`.
|
EvmosGov_tracker | .eslintrc.json
.github
ISSUE_TEMPLATE
config.yml
new_issue.yml
README.md
jest.config.js
next-env.d.ts
next.config.js
package.json
postcss.config.js
public
js
mobile.js
vercel.svg
src
config.ts
features
api-routes
api
github
__tests__
utils.test.ts
index.ts
types.ts
utils.ts
handlers
bounties
index.ts
issues
index.ts
utils.ts
types.ts
bounties
api.ts
hooks
useAddBountyMutation.ts
types.ts
common
constants.ts
hooks
useGuildQueries.ts
useWalletQueries.ts
types.ts
evmos
api.ts
issues
api.ts
constants.ts
hooks
useIssuesQueries.ts
types.ts
near
api.ts
types.ts
tokens
hooks
useTokensQueries.ts
types.ts
pages
api
bounties
index.ts
comment
addComment.ts
getVoteCount.ts
hello.ts
issues
[issueNumber].ts
index.ts
styles
fonts.css
globals.css
utils
helpers.js
tailwind.config.js
test
mocks
github.ts
tsconfig.json
| # Evmos Govnernance Issue Tracker
## Dev
Checkout the repo and set the required environmental variables by copying `./.env.example` into `./.env.local`.
Next, install the dependencies:
```bash
npm install
# or
yarn install
```
Run the development server:
```bash
npm run dev
# or
yarn dev
```
|
NEAR-Hispano_Artemis-Elearning | .history
README_20220518134301.md
README_20220518134317.md
README_20220518134353.md
README_20220518134426.md
README_20220601130356.md
README_20220601130813.md
README_20220601130825.md
README_20220601130829.md
README_20220601130832.md
README_20220601130834.md
README_20220601130841.md
README_20220601130843.md
README_20220601130845.md
README_20220601130852.md
README_20220601130854.md
README_20220601130901.md
Artemis-Contract
Cargo.toml
README.md
build.sh
src
lib.rs
Artemis-ContractNTF
Cargo.toml
src
event.rs
lib.rs
Artemis-Front
CHANGELOG.md
README.md
babel.config.js
certificado-artemis
index.html
package-lock.json
package.json
public
index.html
themes
dark
theme.css
light
theme.css
src
Routes.js
components
Layout
i18n.js
config.js
main.js
plugins
i18n.js
vuetify.js
store
index.js
vue.config.js
Artemis-Ipfs
README.md
app
controllers
admin.js
helpers
utils.js
routes
admin.js
index.js
gulpfile.js
package-lock.json
package.json
server.bundle.js
server.js
webpack.config.js
Artemis_Back_Django
mysite
backend
__init__.py
admin.py
apps.py
migrations
0001_initial.py
0002_answer_profile_question_test_delete_user_and_more.py
0003_alter_answer_question_alter_question_test.py
0004_alter_answer_question_alter_question_test.py
0005_alter_question_pregunta.py
0006_alter_answer_respuesta.py
0007_profile_firma.py
0008_alter_profile_firma.py
0009_alter_profile_firma.py
0010_alter_profile_firma.py
0011_alter_profile_firma.py
__init__.py
models.py
serializers.py
tests.py
urls.py
views.py
manage.py
mysite
__init__.py
asgi.py
settings.py
settings2.py
urls.py
wsgi.py
requirements.txt
README.md
|