modelId
stringlengths 5
122
| author
stringlengths 2
42
| last_modified
timestamp[us, tz=UTC] | downloads
int64 0
738M
| likes
int64 0
11k
| library_name
stringclasses 245
values | tags
sequencelengths 1
4.05k
| pipeline_tag
stringclasses 48
values | createdAt
timestamp[us, tz=UTC] | card
stringlengths 1
901k
|
---|---|---|---|---|---|---|---|---|---|
timm/eva_giant_patch14_224.clip_ft_in1k | timm | 2024-02-10T23:28:53Z | 4,505 | 2 | timm | [
"timm",
"pytorch",
"safetensors",
"image-classification",
"arxiv:2303.15389",
"license:mit",
"region:us"
] | image-classification | 2022-12-23T02:37:40Z | ---
license: mit
library_name: timm
tags:
- image-classification
- timm
---
# Model card for eva_giant_patch14_224.clip_ft_in1k
An EVA-CLIP image classification model. Pretrained on LAION-400M with CLIP and fine-tuned on ImageNet-1k by paper authors. EVA-CLIP uses MIM pretrained image towers and pretrained text towers, FLIP patch dropout, and different optimizers and hparams to accelerate training.
NOTE: `timm` checkpoints are float32 for consistency with other models. Original checkpoints are float16 or bfloat16 in some cases, see originals if that's preferred.
## Model Details
- **Model Type:** Image classification / feature backbone
- **Model Stats:**
- Params (M): 1012.6
- GMACs: 267.2
- Activations (M): 192.6
- Image size: 224 x 224
- **Papers:**
- EVA-CLIP: Improved Training Techniques for CLIP at Scale: https://arxiv.org/abs/2303.15389
- **Original:**
- https://github.com/baaivision/EVA
- https://huggingface.co/QuanSun/EVA-CLIP
## Model Usage
### Image Classification
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model('eva_giant_patch14_224.clip_ft_in1k', pretrained=True)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # unsqueeze single image into batch of 1
top5_probabilities, top5_class_indices = torch.topk(output.softmax(dim=1) * 100, k=5)
```
### Image Embeddings
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model(
'eva_giant_patch14_224.clip_ft_in1k',
pretrained=True,
num_classes=0, # remove classifier nn.Linear
)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # output is (batch_size, num_features) shaped tensor
# or equivalently (without needing to set num_classes=0)
output = model.forward_features(transforms(img).unsqueeze(0))
# output is unpooled, a (1, 257, 1408) shaped tensor
output = model.forward_head(output, pre_logits=True)
# output is a (1, num_features) shaped tensor
```
## Model Comparison
Explore the dataset and runtime metrics of this model in timm [model results](https://github.com/huggingface/pytorch-image-models/tree/main/results).
|model |top1 |top5 |param_count|img_size|
|-----------------------------------------------|------|------|-----------|--------|
|eva02_large_patch14_448.mim_m38m_ft_in22k_in1k |90.054|99.042|305.08 |448 |
|eva02_large_patch14_448.mim_in22k_ft_in22k_in1k|89.946|99.01 |305.08 |448 |
|eva_giant_patch14_560.m30m_ft_in22k_in1k |89.792|98.992|1014.45 |560 |
|eva02_large_patch14_448.mim_in22k_ft_in1k |89.626|98.954|305.08 |448 |
|eva02_large_patch14_448.mim_m38m_ft_in1k |89.57 |98.918|305.08 |448 |
|eva_giant_patch14_336.m30m_ft_in22k_in1k |89.56 |98.956|1013.01 |336 |
|eva_giant_patch14_336.clip_ft_in1k |89.466|98.82 |1013.01 |336 |
|eva_large_patch14_336.in22k_ft_in22k_in1k |89.214|98.854|304.53 |336 |
|eva_giant_patch14_224.clip_ft_in1k |88.882|98.678|1012.56 |224 |
|eva02_base_patch14_448.mim_in22k_ft_in22k_in1k |88.692|98.722|87.12 |448 |
|eva_large_patch14_336.in22k_ft_in1k |88.652|98.722|304.53 |336 |
|eva_large_patch14_196.in22k_ft_in22k_in1k |88.592|98.656|304.14 |196 |
|eva02_base_patch14_448.mim_in22k_ft_in1k |88.23 |98.564|87.12 |448 |
|eva_large_patch14_196.in22k_ft_in1k |87.934|98.504|304.14 |196 |
|eva02_small_patch14_336.mim_in22k_ft_in1k |85.74 |97.614|22.13 |336 |
|eva02_tiny_patch14_336.mim_in22k_ft_in1k |80.658|95.524|5.76 |336 |
## Citation
```bibtex
@article{EVA-CLIP,
title={EVA-02: A Visual Representation for Neon Genesis},
author={Sun, Quan and Fang, Yuxin and Wu, Ledell and Wang, Xinlong and Cao, Yue},
journal={arXiv preprint arXiv:2303.15389},
year={2023}
}
```
```bibtex
@misc{rw2019timm,
author = {Ross Wightman},
title = {PyTorch Image Models},
year = {2019},
publisher = {GitHub},
journal = {GitHub repository},
doi = {10.5281/zenodo.4414861},
howpublished = {\url{https://github.com/huggingface/pytorch-image-models}}
}
```
|
Yntec/IdleFancy | Yntec | 2024-01-26T13:46:46Z | 4,505 | 3 | diffusers | [
"diffusers",
"safetensors",
"General",
"Photorealistic",
"Fantasy",
"LandScapes",
"DucHaiten",
"Lykon",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2024-01-25T10:14:06Z | ---
license: creativeml-openrail-m
library_name: diffusers
pipeline_tag: text-to-image
tags:
- General
- Photorealistic
- Fantasy
- LandScapes
- DucHaiten
- Lykon
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- diffusers
---
# Idle Fancy
A mix of Absolute Remix (which includes Absolute Reality 1.0 and 1.6) and DucHaitenFANCYxFANCY for a model that can manage photorealism and fantasy prompts!
Sample and prompts:

(Click for larger)
Top left: a close up portrait photo of pretty cute little girl in wastelander clothes, long haircut, pale skin, background is city overgrown, 8k uhd, dslr, soft lighting, high quality, film grain, Fujifilm XT3
Top right: Full body picture of a pretty cute girl making cake in school, detailed brown eyes, short smile, beautiful and aesthetic, intricate, neat hair, highly detailed, detailed face, smooth, sharp focus, chiaroscuro, magazine ad, 1949, 2D Game Art, anime on canvas, rossdraws, clay mann, CHIBI ART, light novel cover art
Bottom left: sitting Pretty Cute Girl, Detailed Eyes, holding coins, beautiful detailed slot machine, gorgeous detailed hair, shoes, Magazine ad, iconic, 1943, from the movie, sharp focus. visible brushstrokes by ROSSDRAWS
Bottom right: Portrait Painting, Pretty CUTE little Girl wearing tennis shoes riding a wave under clouds inside of a large jar on a table, fairy wings, beautiful detailed eyes and face, gorgeous detailed hair, Magazine ad, iconic, 1941, sharp focus. visible brushstrokes, high detail
# AbsoluteFANCYxFANCY
A different version of the same models to be used if you want to generate NSFW stuff.
Original pages:
https://civitai.com/models/81458?modelVersionId=132760 (Absolute Reality)
https://civitai.com/models/101354/duchaiten-fancyxfancy
# Recipes
SuperMerger Weight sum MBW 1,0,0,0,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,1,1,1
Model A: AbsoluteRemix
Model B: DucHaiten-FANCYxFANCY
Output: IdleFancy
SuperMerger Weight sum MBW 1,0,0,0,0,0,0,1,1,1,1,1,1,0,1,1,1,1,1,1,0,0,0,0,0,0
Model A: AbsoluteRemix
Model B: DucHaiten-FANCYxFANCY
Output: AbsoluteFANCYxFANCY |
nateraw/vit-base-patch16-224-cifar10 | nateraw | 2022-01-28T10:22:01Z | 4,504 | 7 | transformers | [
"transformers",
"pytorch",
"vit",
"image-classification",
"vision",
"dataset:cifar10",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | image-classification | 2022-03-02T23:29:05Z | ---
tags:
- image-classification
- vision
- pytorch
license: apache-2.0
datasets:
- cifar10
metrics:
- accuracy
thumbnail: https://avatars3.githubusercontent.com/u/32437151?s=460&u=4ec59abc8d21d5feea3dab323d23a5860e6996a4&v=4
---
# Vision Transformer Fine Tuned on CIFAR10
Vision Transformer (ViT) model pre-trained on ImageNet-21k (14 million images, 21,843 classes) and **fine-tuned on CIFAR10** at resolution 224x224.
Check out the code at my [my Github repo](https://github.com/nateraw/huggingface-vit-finetune).
## Usage
```python
from transformers import ViTFeatureExtractor, ViTForImageClassification
from PIL import Image
import requests
url = 'https://www.cs.toronto.edu/~kriz/cifar-10-sample/dog10.png'
image = Image.open(requests.get(url, stream=True).raw)
feature_extractor = ViTFeatureExtractor.from_pretrained('nateraw/vit-base-patch16-224-cifar10')
model = ViTForImageClassification.from_pretrained('nateraw/vit-base-patch16-224-cifar10')
inputs = feature_extractor(images=image, return_tensors="pt")
outputs = model(**inputs)
preds = outputs.logits.argmax(dim=1)
classes = [
'airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck'
]
classes[preds[0]]
```
## Model description
The Vision Transformer (ViT) is a transformer encoder model (BERT-like) pretrained on a large collection of images in a supervised fashion, namely ImageNet-21k, at a resolution of 224x224 pixels.
Images are presented to the model as a sequence of fixed-size patches (resolution 16x16), which are linearly embedded. One also adds a [CLS] token to the beginning of a sequence to use it for classification tasks. One also adds absolute position embeddings before feeding the sequence to the layers of the Transformer encoder.
Note that this model does not provide any fine-tuned heads, as these were zero'd by Google researchers. However, the model does include the pre-trained pooler, which can be used for downstream tasks (such as image classification).
By pre-training the model, it learns an inner representation of images that can then be used to extract features useful for downstream tasks: if you have a dataset of labeled images for instance, you can train a standard classifier by placing a linear layer on top of the pre-trained encoder. One typically places a linear layer on top of the [CLS] token, as the last hidden state of this token can be seen as a representation of an entire image.
|
yunconglong/Truthful_DPO_TomGrc_FusionNet_7Bx2_MoE_13B | yunconglong | 2024-02-28T02:15:25Z | 4,504 | 52 | transformers | [
"transformers",
"safetensors",
"mixtral",
"text-generation",
"moe",
"DPO",
"RL-TUNED",
"license:mit",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2024-01-21T09:01:56Z | ---
license: mit
tags:
- moe
- DPO
- RL-TUNED
---
* [DPO Trainer](https://huggingface.co/docs/trl/main/en/dpo_trainer) with dataset jondurbin/truthy-dpo-v0.1 to improve [TomGrc/FusionNet_7Bx2_MoE_14B]
```
DPO Trainer
TRL supports the DPO Trainer for training language models from preference data, as described in the paper Direct Preference Optimization: Your Language Model is Secretly a Reward Model by Rafailov et al., 2023.
``` |
LinWeizheDragon/ColBERT-v2 | LinWeizheDragon | 2024-02-27T05:21:41Z | 4,501 | 1 | transformers | [
"transformers",
"safetensors",
"flmr",
"ColBERT",
"Passage Retrieval",
"en",
"arxiv:2112.01488",
"arxiv:2104.08663",
"arxiv:2402.08327",
"license:mit",
"endpoints_compatible",
"region:us"
] | null | 2024-02-27T04:12:09Z | ---
license: mit
language:
- en
tags:
- ColBERT
- Passage Retrieval
---
# Model Card for ColBERT
<!-- Provide a quick summary of what the model is/does. -->
`ColBERT-v2` is a passage retrieval model that leverages late interaction to allow token-level interaction between query and document embeddings. It outperforms traditional retrievers (such as Dense Passage Retrieval) that encode queries and documents into one-dimensional embeddings.
With the approval of the original author, this model is re-implemented with the huggingface-compatible implementation of [PreFLMR](https://preflmr.github.io/). All the weights are imported from the [official ColBERTv2 checkpoint](https://github.com/stanford-futuredata/ColBERT?tab=readme-ov-file).
## Model Details
### Model Description
<!-- Provide a longer summary of what this model is. -->
- **Developed by:** Keshav Santhanam, Omar Khattab, Jon Saad-Falcon, Christopher Potts, Matei Zaharia
- **Re-implemented by:** Weizhe Lin, Howard Mei, Jinghong Chen, Bill Byrne
- **Model type:** FLMRModelForRetrieval
- **Language(s) (NLP):** English
- **License:** MIT License
### Model Sources
<!-- Provide the basic links for the model. -->
- **Repository:** https://github.com/stanford-futuredata/ColBERT
- **Repository of implementation:** https://github.com/LinWeizheDragon/FLMR
- **Paper:** https://arxiv.org/abs/2112.01488
## Uses
<!-- Address questions around how the model is intended to be used, including the foreseeable users of the model and those affected by the model. -->
### Direct Use
<!-- This section is for the model use without fine-tuning or plugging into a larger ecosystem/app. -->
This model can be used directly to retrieve documents from a large corpus using text queries. The retrieval usage can be found in the [PreFLMR implementation](https://github.com/LinWeizheDragon/FLMR).
### Downstream Use
<!-- This section is for the model use when fine-tuned for a task, or when plugged into a larger ecosystem/app -->
This model can be used combined with language models to create a retrieval-augmented language model.
## How to Get Started with the Model
For details of training, indexing, and performing retrieval, please refer to [here](https://github.com/LinWeizheDragon/FLMR).
1. Install the [FLMR package](https://github.com/LinWeizheDragon/FLMR).
2. A simple example use of this model:
```python
from flmr import FLMRConfig, FLMRModelForRetrieval, FLMRQueryEncoderTokenizer, FLMRContextEncoderTokenizer
checkpoint_path = "LinWeizheDragon/ColBERT-v2"
query_tokenizer = FLMRQueryEncoderTokenizer.from_pretrained(checkpoint_path, subfolder="query_tokenizer")
context_tokenizer = FLMRContextEncoderTokenizer.from_pretrained(checkpoint_path, subfolder="context_tokenizer")
model = FLMRModelForRetrieval.from_pretrained(checkpoint_path,
query_tokenizer=query_tokenizer,
context_tokenizer=context_tokenizer,
)
Q_encoding = query_tokenizer(["What is the capital of France?", "What is the capital of China?"])
D_encoding = context_tokenizer(["Paris is the capital of France.", "Beijing is the capital of China.",
"Paris is the capital of France.", "Beijing is the capital of China."])
inputs = dict(
query_input_ids=Q_encoding['input_ids'],
query_attention_mask=Q_encoding['attention_mask'],
context_input_ids=D_encoding['input_ids'],
context_attention_mask=D_encoding['attention_mask'],
use_in_batch_negatives=True,
)
res = model.forward(**inputs)
```
## Training Details
### Training Data
<!-- This should link to a Dataset Card, perhaps with a short stub of information on what the training data is all about as well as documentation related to data pre-processing or additional filtering. -->
The model was trained on [MS MARCO Passage Ranking](https://github.com/microsoft/MSMARCO-Passage-Ranking)
More details can be found in the [official ColBERT repository](https://github.com/stanford-futuredata/ColBERT?tab=readme-ov-file)
### Training Procedure
The training details can be found in the [official ColBERT repository](https://github.com/stanford-futuredata/ColBERT?tab=readme-ov-file) and the [ColBERTv2 paper](https://arxiv.org/abs/2112.01488)
## Evaluation
<!-- This section describes the evaluation protocols and provides the results. -->
### Testing Data, Factors & Metrics
#### Testing Data
The model is evaluated on a range of retrieval tasks, such as:
- [MS MARCO Passage Ranking](https://github.com/microsoft/MSMARCO-Passage-Ranking)
- [BEIR](https://arxiv.org/abs/2104.08663)
- [LoTTE](https://github.com/stanford-futuredata/ColBERT/blob/main/LoTTE.md)
- [OOD Wikipedia Open QA](https://arxiv.org/abs/2112.01488)
### Results
Find the evaluation results in the [ColBERTv2 paper](https://arxiv.org/abs/2112.01488)
## Citation
<!-- If there is a paper or blog post introducing the model, the APA and Bibtex information for that should go in this section. -->
**BibTeX:**
Please cite the original papers:
```
@inproceedings{10.1145/3397271.3401075,
author = {Khattab, Omar and Zaharia, Matei},
title = {ColBERT: Efficient and Effective Passage Search via Contextualized Late Interaction over BERT},
year = {2020},
isbn = {9781450380164},
publisher = {Association for Computing Machinery},
address = {New York, NY, USA},
url = {https://doi.org/10.1145/3397271.3401075},
doi = {10.1145/3397271.3401075},
abstract = {Recent progress in Natural Language Understanding (NLU) is driving fast-paced advances in Information Retrieval (IR), largely owed to fine-tuning deep language models (LMs) for document ranking. While remarkably effective, the ranking models based on these LMs increase computational cost by orders of magnitude over prior approaches, particularly as they must feed each query-document pair through a massive neural network to compute a single relevance score. To tackle this, we present ColBERT, a novel ranking model that adapts deep LMs (in particular, BERT) for efficient retrieval. ColBERT introduces a late interaction architecture that independently encodes the query and the document using BERT and then employs a cheap yet powerful interaction step that models their fine-grained similarity. By delaying and yet retaining this fine-granular interaction, ColBERT can leverage the expressiveness of deep LMs while simultaneously gaining the ability to pre-compute document representations offline, considerably speeding up query processing. Crucially, ColBERT's pruning-friendly interaction mechanism enables leveraging vector-similarity indexes for end-to-end retrieval directly from millions of documents. We extensively evaluate ColBERT using two recent passage search datasets. Results show that ColBERT's effectiveness is competitive with existing BERT-based models (and outperforms every non-BERT baseline), while executing two orders-of-magnitude faster and requiring up to four orders-of-magnitude fewer FLOPs per query.},
booktitle = {Proceedings of the 43rd International ACM SIGIR Conference on Research and Development in Information Retrieval},
pages = {39–48},
numpages = {10},
keywords = {bert, deep language models, efficiency, neural ir},
location = {Virtual Event, China},
series = {SIGIR '20}
}
@inproceedings{santhanam-etal-2022-colbertv2,
title = "{C}ol{BERT}v2: Effective and Efficient Retrieval via Lightweight Late Interaction",
author = "Santhanam, Keshav and
Khattab, Omar and
Saad-Falcon, Jon and
Potts, Christopher and
Zaharia, Matei",
editor = "Carpuat, Marine and
de Marneffe, Marie-Catherine and
Meza Ruiz, Ivan Vladimir",
booktitle = "Proceedings of the 2022 Conference of the North American Chapter of the Association for Computational Linguistics: Human Language Technologies",
month = jul,
year = "2022",
address = "Seattle, United States",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2022.naacl-main.272",
doi = "10.18653/v1/2022.naacl-main.272",
pages = "3715--3734",
abstract = "Neural information retrieval (IR) has greatly advanced search and other knowledge-intensive language tasks. While many neural IR methods encode queries and documents into single-vector representations, late interaction models produce multi-vector representations at the granularity of each token and decompose relevance modeling into scalable token-level computations. This decomposition has been shown to make late interaction more effective, but it inflates the space footprint of these models by an order of magnitude. In this work, we introduce ColBERTv2, a retriever that couples an aggressive residual compression mechanism with a denoised supervision strategy to simultaneously improve the quality and space footprint of late interaction. We evaluate ColBERTv2 across a wide range of benchmarks, establishing state-of-the-art quality within and outside the training domain while reducing the space footprint of late interaction models by 6{--}10x.",
}
@inproceedings{10.1145/3511808.3557325,
author = {Santhanam, Keshav and Khattab, Omar and Potts, Christopher and Zaharia, Matei},
title = {PLAID: An Efficient Engine for Late Interaction Retrieval},
year = {2022},
isbn = {9781450392365},
publisher = {Association for Computing Machinery},
address = {New York, NY, USA},
url = {https://doi.org/10.1145/3511808.3557325},
doi = {10.1145/3511808.3557325},
abstract = {Pre-trained language models are increasingly important components across multiple information retrieval (IR) paradigms. Late interaction, introduced with the ColBERT model and recently refined in ColBERTv2, is a popular paradigm that holds state-of-the-art status across many benchmarks. To dramatically speed up the search latency of late interaction, we introduce the Performance-optimized Late Interaction Driver (PLAID) engine. Without impacting quality, PLAID swiftly eliminates low-scoring passages using a novel centroid interaction mechanism that treats every passage as a lightweight bag of centroids. PLAID uses centroid interaction as well as centroid pruning, a mechanism for sparsifying the bag of centroids, within a highly-optimized engine to reduce late interaction search latency by up to 7x on a GPU and 45x on a CPU against vanilla ColBERTv2, while continuing to deliver state-of-the-art retrieval quality. This allows the PLAID engine with ColBERTv2 to achieve latency of tens of milliseconds on a GPU and tens or just few hundreds of milliseconds on a CPU at large scale, even at the largest scales we evaluate with 140M passages.},
booktitle = {Proceedings of the 31st ACM International Conference on Information \& Knowledge Management},
pages = {1747–1756},
numpages = {10},
keywords = {colbert, dynamic pruning, efficient search, late interaction, neural information retrieval},
location = {Atlanta, GA, USA},
series = {CIKM '22}
}
```
If the implementation helps your research, please cite:
```
@article{Lin_Mei_Chen_Byrne_2024,
title={PreFLMR: Scaling Up Fine-Grained Late-Interaction Multi-modal Retrievers},
url={http://arxiv.org/abs/2402.08327},
number={arXiv:2402.08327},
publisher={arXiv},
author={Lin, Weizhe and Mei, Jingbiao and Chen, Jinghong and Byrne, Bill},
year={2024}}
``` |
sentence-transformers/all-mpnet-base-v1 | sentence-transformers | 2024-03-27T09:45:46Z | 4,500 | 7 | sentence-transformers | [
"sentence-transformers",
"pytorch",
"safetensors",
"mpnet",
"fill-mask",
"feature-extraction",
"sentence-similarity",
"transformers",
"en",
"arxiv:1904.06472",
"arxiv:2102.07033",
"arxiv:2104.08727",
"arxiv:1704.05179",
"arxiv:1810.09305",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | sentence-similarity | 2022-03-02T23:29:05Z | ---
language: en
license: apache-2.0
library_name: sentence-transformers
tags:
- sentence-transformers
- feature-extraction
- sentence-similarity
- transformers
pipeline_tag: sentence-similarity
---
# all-mpnet-base-v1
This is a [sentence-transformers](https://www.SBERT.net) model: It maps sentences & paragraphs to a 768 dimensional dense vector space and can be used for tasks like clustering or semantic search.
## Usage (Sentence-Transformers)
Using this model becomes easy when you have [sentence-transformers](https://www.SBERT.net) installed:
```
pip install -U sentence-transformers
```
Then you can use the model like this:
```python
from sentence_transformers import SentenceTransformer
sentences = ["This is an example sentence", "Each sentence is converted"]
model = SentenceTransformer('sentence-transformers/all-mpnet-base-v1')
embeddings = model.encode(sentences)
print(embeddings)
```
## Usage (HuggingFace Transformers)
Without [sentence-transformers](https://www.SBERT.net), you can use the model like this: First, you pass your input through the transformer model, then you have to apply the right pooling-operation on-top of the contextualized word embeddings.
```python
from transformers import AutoTokenizer, AutoModel
import torch
import torch.nn.functional as F
#Mean Pooling - Take attention mask into account for correct averaging
def mean_pooling(model_output, attention_mask):
token_embeddings = model_output[0] #First element of model_output contains all token embeddings
input_mask_expanded = attention_mask.unsqueeze(-1).expand(token_embeddings.size()).float()
return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9)
# Sentences we want sentence embeddings for
sentences = ['This is an example sentence', 'Each sentence is converted']
# Load model from HuggingFace Hub
tokenizer = AutoTokenizer.from_pretrained('sentence-transformers/all-mpnet-base-v1')
model = AutoModel.from_pretrained('sentence-transformers/all-mpnet-base-v1')
# Tokenize sentences
encoded_input = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt')
# Compute token embeddings
with torch.no_grad():
model_output = model(**encoded_input)
# Perform pooling
sentence_embeddings = mean_pooling(model_output, encoded_input['attention_mask'])
# Normalize embeddings
sentence_embeddings = F.normalize(sentence_embeddings, p=2, dim=1)
print("Sentence embeddings:")
print(sentence_embeddings)
```
## Evaluation Results
For an automated evaluation of this model, see the *Sentence Embeddings Benchmark*: [https://seb.sbert.net](https://seb.sbert.net?model_name=sentence-transformers/all-mpnet-base-v1)
------
## Background
The project aims to train sentence embedding models on very large sentence level datasets using a self-supervised
contrastive learning objective. We used the pretrained [`microsoft/mpnet-base`](https://huggingface.co/microsoft/mpnet-base) model and fine-tuned in on a
1B sentence pairs dataset. We use a contrastive learning objective: given a sentence from the pair, the model should predict which out of a set of randomly sampled other sentences, was actually paired with it in our dataset.
We developped this model during the
[Community week using JAX/Flax for NLP & CV](https://discuss.huggingface.co/t/open-to-the-community-community-week-using-jax-flax-for-nlp-cv/7104),
organized by Hugging Face. We developped this model as part of the project:
[Train the Best Sentence Embedding Model Ever with 1B Training Pairs](https://discuss.huggingface.co/t/train-the-best-sentence-embedding-model-ever-with-1b-training-pairs/7354). We benefited from efficient hardware infrastructure to run the project: 7 TPUs v3-8, as well as intervention from Googles Flax, JAX, and Cloud team member about efficient deep learning frameworks.
## Intended uses
Our model is intented to be used as a sentence and short paragraph encoder. Given an input text, it ouptuts a vector which captures
the semantic information. The sentence vector may be used for information retrieval, clustering or sentence similarity tasks.
By default, input text longer than 128 word pieces is truncated.
## Training procedure
### Pre-training
We use the pretrained [`microsoft/mpnet-base`](https://huggingface.co/microsoft/mpnet-base). Please refer to the model card for more detailed information about the pre-training procedure.
### Fine-tuning
We fine-tune the model using a contrastive objective. Formally, we compute the cosine similarity from each possible sentence pairs from the batch.
We then apply the cross entropy loss by comparing with true pairs.
#### Hyper parameters
We trained ou model on a TPU v3-8. We train the model during 920k steps using a batch size of 512 (64 per TPU core).
We use a learning rate warm up of 500. The sequence length was limited to 128 tokens. We used the AdamW optimizer with
a 2e-5 learning rate. The full training script is accessible in this current repository: `train_script.py`.
#### Training data
We use the concatenation from multiple datasets to fine-tune our model. The total number of sentence pairs is above 1 billion sentences.
We sampled each dataset given a weighted probability which configuration is detailed in the `data_config.json` file.
| Dataset | Paper | Number of training tuples |
|--------------------------------------------------------|:----------------------------------------:|:--------------------------:|
| [Reddit comments (2015-2018)](https://github.com/PolyAI-LDN/conversational-datasets/tree/master/reddit) | [paper](https://arxiv.org/abs/1904.06472) | 726,484,430 |
| [S2ORC](https://github.com/allenai/s2orc) Citation pairs (Abstracts) | [paper](https://aclanthology.org/2020.acl-main.447/) | 116,288,806 |
| [WikiAnswers](https://github.com/afader/oqa#wikianswers-corpus) Duplicate question pairs | [paper](https://doi.org/10.1145/2623330.2623677) | 77,427,422 |
| [PAQ](https://github.com/facebookresearch/PAQ) (Question, Answer) pairs | [paper](https://arxiv.org/abs/2102.07033) | 64,371,441 |
| [S2ORC](https://github.com/allenai/s2orc) Citation pairs (Titles) | [paper](https://aclanthology.org/2020.acl-main.447/) | 52,603,982 |
| [S2ORC](https://github.com/allenai/s2orc) (Title, Abstract) | [paper](https://aclanthology.org/2020.acl-main.447/) | 41,769,185 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) (Title, Body) pairs | - | 25,316,456 |
| [MS MARCO](https://microsoft.github.io/msmarco/) triplets | [paper](https://doi.org/10.1145/3404835.3462804) | 9,144,553 |
| [GOOAQ: Open Question Answering with Diverse Answer Types](https://github.com/allenai/gooaq) | [paper](https://arxiv.org/pdf/2104.08727.pdf) | 3,012,496 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) (Title, Answer) | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 1,198,260 |
| [Code Search](https://huggingface.co/datasets/code_search_net) | - | 1,151,414 |
| [COCO](https://cocodataset.org/#home) Image captions | [paper](https://link.springer.com/chapter/10.1007%2F978-3-319-10602-1_48) | 828,395|
| [SPECTER](https://github.com/allenai/specter) citation triplets | [paper](https://doi.org/10.18653/v1/2020.acl-main.207) | 684,100 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) (Question, Answer) | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 681,164 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) (Title, Question) | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 659,896 |
| [SearchQA](https://huggingface.co/datasets/search_qa) | [paper](https://arxiv.org/abs/1704.05179) | 582,261 |
| [Eli5](https://huggingface.co/datasets/eli5) | [paper](https://doi.org/10.18653/v1/p19-1346) | 325,475 |
| [Flickr 30k](https://shannon.cs.illinois.edu/DenotationGraph/) | [paper](https://transacl.org/ojs/index.php/tacl/article/view/229/33) | 317,695 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) Duplicate questions (titles) | | 304,525 |
| AllNLI ([SNLI](https://nlp.stanford.edu/projects/snli/) and [MultiNLI](https://cims.nyu.edu/~sbowman/multinli/) | [paper SNLI](https://doi.org/10.18653/v1/d15-1075), [paper MultiNLI](https://doi.org/10.18653/v1/n18-1101) | 277,230 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) Duplicate questions (bodies) | | 250,519 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) Duplicate questions (titles+bodies) | | 250,460 |
| [Sentence Compression](https://github.com/google-research-datasets/sentence-compression) | [paper](https://www.aclweb.org/anthology/D13-1155/) | 180,000 |
| [Wikihow](https://github.com/pvl/wikihow_pairs_dataset) | [paper](https://arxiv.org/abs/1810.09305) | 128,542 |
| [Altlex](https://github.com/chridey/altlex/) | [paper](https://aclanthology.org/P16-1135.pdf) | 112,696 |
| [Quora Question Triplets](https://quoradata.quora.com/First-Quora-Dataset-Release-Question-Pairs) | - | 103,663 |
| [Simple Wikipedia](https://cs.pomona.edu/~dkauchak/simplification/) | [paper](https://www.aclweb.org/anthology/P11-2117/) | 102,225 |
| [Natural Questions (NQ)](https://ai.google.com/research/NaturalQuestions) | [paper](https://transacl.org/ojs/index.php/tacl/article/view/1455) | 100,231 |
| [SQuAD2.0](https://rajpurkar.github.io/SQuAD-explorer/) | [paper](https://aclanthology.org/P18-2124.pdf) | 87,599 |
| [TriviaQA](https://huggingface.co/datasets/trivia_qa) | - | 73,346 |
| **Total** | | **1,124,818,467** | |
RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf | RichardErkhov | 2024-06-05T18:20:40Z | 4,498 | 0 | null | [
"gguf",
"region:us"
] | null | 2024-06-05T14:33:24Z | Quantization made by Richard Erkhov.
[Github](https://github.com/RichardErkhov)
[Discord](https://discord.gg/pvy7H8DZMG)
[Request more models](https://github.com/RichardErkhov/quant_request)
OpenHermes-2.5-neural-chat-7b-v3-1-7B - GGUF
- Model creator: https://huggingface.co/Weyaxi/
- Original model: https://huggingface.co/Weyaxi/OpenHermes-2.5-neural-chat-7b-v3-1-7B/
| Name | Quant method | Size |
| ---- | ---- | ---- |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q2_K.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q2_K.gguf) | Q2_K | 2.53GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ3_XS.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ3_XS.gguf) | IQ3_XS | 2.81GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ3_S.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ3_S.gguf) | IQ3_S | 2.96GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K_S.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K_S.gguf) | Q3_K_S | 2.95GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ3_M.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ3_M.gguf) | IQ3_M | 3.06GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K.gguf) | Q3_K | 3.28GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K_M.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K_M.gguf) | Q3_K_M | 3.28GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K_L.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q3_K_L.gguf) | Q3_K_L | 3.56GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ4_XS.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ4_XS.gguf) | IQ4_XS | 3.67GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_0.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_0.gguf) | Q4_0 | 3.83GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ4_NL.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.IQ4_NL.gguf) | IQ4_NL | 3.87GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_K_S.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_K_S.gguf) | Q4_K_S | 3.86GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_K.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_K.gguf) | Q4_K | 4.07GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_K_M.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_K_M.gguf) | Q4_K_M | 4.07GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_1.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q4_1.gguf) | Q4_1 | 4.24GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_0.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_0.gguf) | Q5_0 | 4.65GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_K_S.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_K_S.gguf) | Q5_K_S | 4.65GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_K.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_K.gguf) | Q5_K | 4.78GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_K_M.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_K_M.gguf) | Q5_K_M | 4.78GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_1.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q5_1.gguf) | Q5_1 | 5.07GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q6_K.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q6_K.gguf) | Q6_K | 5.53GB |
| [OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q8_0.gguf](https://huggingface.co/RichardErkhov/Weyaxi_-_OpenHermes-2.5-neural-chat-7b-v3-1-7B-gguf/blob/main/OpenHermes-2.5-neural-chat-7b-v3-1-7B.Q8_0.gguf) | Q8_0 | 7.17GB |
Original model description:
---
license: apache-2.0
datasets:
- Open-Orca/SlimOrca
tags:
- mistral
---

Merge of [teknium/OpenHermes-2.5-Mistral-7B](https://huggingface.co/teknium/OpenHermes-2.5-Mistral-7B) and [Intel/neural-chat-7b-v3-1](https://huggingface.co/Intel/neural-chat-7b-v3-1) using ties merge.
### *Weights*
- [teknium/OpenHermes-2.5-Mistral-7B](https://huggingface.co/teknium/OpenHermes-2.5-Mistral-7B): 0.5
- [Intel/neural-chat-7b-v3-1](https://huggingface.co/Intel/neural-chat-7b-v3-1): 0.3
### *Density*
- [teknium/OpenHermes-2.5-Mistral-7B](https://huggingface.co/teknium/OpenHermes-2.5-Mistral-7B): 0.5
- [Intel/neural-chat-7b-v3-1](https://huggingface.co/Intel/neural-chat-7b-v3-1): 0.5
# Prompt Templates
You can use these prompt templates, but I recommend using ChatML.
### ChatML [(OpenHermes-2.5-Mistral-7B)](https://huggingface.co/teknium/OpenHermes-2.5-Mistral-7B):
```
<|im_start|>system
{system}<|im_end|>
<|im_start|>user
{user}<|im_end|>
<|im_start|>assistant
{asistant}<|im_end|>
```
### [neural-chat-7b-v3-1](https://huggingface.co/Intel/neural-chat-7b-v3-1):
```
### System:
{system}
### User:
{usr}
### Assistant:
```
# Quantizationed versions
Quantizationed versions of this model is available thanks to [TheBloke](https://hf.co/TheBloke).
##### GPTQ
- [TheBloke/OpenHermes-2.5-neural-chat-7B-v3-1-7B-GPTQ](https://huggingface.co/TheBloke/OpenHermes-2.5-neural-chat-7B-v3-1-7B-GPTQ)
##### GGUF
- [TheBloke/OpenHermes-2.5-neural-chat-7B-v3-1-7B-GGUF](https://huggingface.co/TheBloke/OpenHermes-2.5-neural-chat-7B-v3-1-7B-GGUF)
##### AWQ
- [TheBloke/OpenHermes-2.5-neural-chat-7B-v3-1-7B-AWQ](https://huggingface.co/TheBloke/OpenHermes-2.5-neural-chat-7B-v3-1-7B-AWQ)
# [Open LLM Leaderboard Evaluation Results](https://huggingface.co/spaces/HuggingFaceH4/open_llm_leaderboard)
Detailed results can be found [here](https://huggingface.co/datasets/open-llm-leaderboard/details_Weyaxi__OpenHermes-2.5-neural-chat-7b-v3-1-7B)
| Metric | Value |
|-----------------------|---------------------------|
| Avg. | 67.84 |
| ARC (25-shot) | 66.55 |
| HellaSwag (10-shot) | 84.47 |
| MMLU (5-shot) | 63.34 |
| TruthfulQA (0-shot) | 61.22 |
| Winogrande (5-shot) | 78.37 |
| GSM8K (5-shot) | 53.07 |
If you would like to support me:
[☕ Buy Me a Coffee](https://www.buymeacoffee.com/weyaxi)
|
unsloth/Qwen2-0.5B-bnb-4bit | unsloth | 2024-06-06T17:18:58Z | 4,498 | 1 | transformers | [
"transformers",
"safetensors",
"qwen2",
"text-generation",
"unsloth",
"conversational",
"en",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"4-bit",
"bitsandbytes",
"region:us"
] | text-generation | 2024-06-06T16:35:15Z | ---
language:
- en
license: apache-2.0
library_name: transformers
tags:
- unsloth
- transformers
- qwen2
---
# Finetune Mistral, Gemma, Llama 2-5x faster with 70% less memory via Unsloth!
We have a Google Colab Tesla T4 notebook for Qwen2 7b here: https://colab.research.google.com/drive/1mvwsIQWDs2EdZxZQF9pRGnnOvE86MVvR?usp=sharing
And a Colab notebook for [Qwen2 0.5b](https://colab.research.google.com/drive/1-7tjDdMAyeCueyLAwv6vYeBMHpoePocN?usp=sharing) and another for [Qwen2 1.5b](https://colab.research.google.com/drive/1W0j3rP8WpgxRdUgkb5l6E00EEVyjEZGk?usp=sharing)
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/Discord%20button.png" width="200"/>](https://discord.gg/u54VK8m8tk)
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/buy%20me%20a%20coffee%20button.png" width="200"/>](https://ko-fi.com/unsloth)
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/unsloth%20made%20with%20love.png" width="200"/>](https://github.com/unslothai/unsloth)
## ✨ Finetune for Free
All notebooks are **beginner friendly**! Add your dataset, click "Run All", and you'll get a 2x faster finetuned model which can be exported to GGUF, vLLM or uploaded to Hugging Face.
| Unsloth supports | Free Notebooks | Performance | Memory use |
|-----------------|--------------------------------------------------------------------------------------------------------------------------|-------------|----------|
| **Llama-3 8b** | [▶️ Start on Colab](https://colab.research.google.com/drive/135ced7oHytdxu3N2DNe1Z0kqjyYIkDXp?usp=sharing) | 2.4x faster | 58% less |
| **Gemma 7b** | [▶️ Start on Colab](https://colab.research.google.com/drive/10NbwlsRChbma1v55m8LAPYG15uQv6HLo?usp=sharing) | 2.4x faster | 58% less |
| **Mistral 7b** | [▶️ Start on Colab](https://colab.research.google.com/drive/1Dyauq4kTZoLewQ1cApceUQVNcnnNTzg_?usp=sharing) | 2.2x faster | 62% less |
| **Llama-2 7b** | [▶️ Start on Colab](https://colab.research.google.com/drive/1lBzz5KeZJKXjvivbYvmGarix9Ao6Wxe5?usp=sharing) | 2.2x faster | 43% less |
| **TinyLlama** | [▶️ Start on Colab](https://colab.research.google.com/drive/1AZghoNBQaMDgWJpi4RbffGM1h6raLUj9?usp=sharing) | 3.9x faster | 74% less |
| **CodeLlama 34b** A100 | [▶️ Start on Colab](https://colab.research.google.com/drive/1y7A0AxE3y8gdj4AVkl2aZX47Xu3P1wJT?usp=sharing) | 1.9x faster | 27% less |
| **Mistral 7b** 1xT4 | [▶️ Start on Kaggle](https://www.kaggle.com/code/danielhanchen/kaggle-mistral-7b-unsloth-notebook) | 5x faster\* | 62% less |
| **DPO - Zephyr** | [▶️ Start on Colab](https://colab.research.google.com/drive/15vttTpzzVXv_tJwEk-hIcQ0S9FcEWvwP?usp=sharing) | 1.9x faster | 19% less |
- This [conversational notebook](https://colab.research.google.com/drive/1Aau3lgPzeZKQ-98h69CCu1UJcvIBLmy2?usp=sharing) is useful for ShareGPT ChatML / Vicuna templates.
- This [text completion notebook](https://colab.research.google.com/drive/1ef-tab5bhkvWmBOObepl1WgJvfvSzn5Q?usp=sharing) is for raw text. This [DPO notebook](https://colab.research.google.com/drive/15vttTpzzVXv_tJwEk-hIcQ0S9FcEWvwP?usp=sharing) replicates Zephyr.
- \* Kaggle has 2x T4s, but we use 1. Due to overhead, 1x T4 is 5x faster. |
microsoft/wavlm-base-sv | microsoft | 2022-03-25T12:05:52Z | 4,493 | 5 | transformers | [
"transformers",
"pytorch",
"wavlm",
"audio-xvector",
"speech",
"en",
"arxiv:2110.13900",
"endpoints_compatible",
"region:us"
] | null | 2022-03-02T23:29:05Z | ---
language:
- en
tags:
- speech
---
# WavLM-Base for Speaker Verification
[Microsoft's WavLM](https://github.com/microsoft/unilm/tree/master/wavlm)
The model was pretrained on 16kHz sampled speech audio with utterance and speaker contrastive loss. When using the model, make sure that your speech input is also sampled at 16kHz.
The model was pre-trained on 960h of [Librispeech](https://huggingface.co/datasets/librispeech_asr).
[Paper: WavLM: Large-Scale Self-Supervised Pre-Training for Full Stack Speech Processing](https://arxiv.org/abs/2110.13900)
Authors: Sanyuan Chen, Chengyi Wang, Zhengyang Chen, Yu Wu, Shujie Liu, Zhuo Chen, Jinyu Li, Naoyuki Kanda, Takuya Yoshioka, Xiong Xiao, Jian Wu, Long Zhou, Shuo Ren, Yanmin Qian, Yao Qian, Jian Wu, Michael Zeng, Furu Wei
**Abstract**
*Self-supervised learning (SSL) achieves great success in speech recognition, while limited exploration has been attempted for other speech processing tasks. As speech signal contains multi-faceted information including speaker identity, paralinguistics, spoken content, etc., learning universal representations for all speech tasks is challenging. In this paper, we propose a new pre-trained model, WavLM, to solve full-stack downstream speech tasks. WavLM is built based on the HuBERT framework, with an emphasis on both spoken content modeling and speaker identity preservation. We first equip the Transformer structure with gated relative position bias to improve its capability on recognition tasks. For better speaker discrimination, we propose an utterance mixing training strategy, where additional overlapped utterances are created unsupervisely and incorporated during model training. Lastly, we scale up the training dataset from 60k hours to 94k hours. WavLM Large achieves state-of-the-art performance on the SUPERB benchmark, and brings significant improvements for various speech processing tasks on their representative benchmarks.*
The original model can be found under https://github.com/microsoft/unilm/tree/master/wavlm.
# Fine-tuning details
The model is fine-tuned on the [VoxCeleb1 dataset](https://www.robots.ox.ac.uk/~vgg/data/voxceleb/vox1.html) using an X-Vector head with an Additive Margin Softmax loss
[X-Vectors: Robust DNN Embeddings for Speaker Recognition](https://www.danielpovey.com/files/2018_icassp_xvectors.pdf)
# Usage
## Speaker Verification
```python
from transformers import Wav2Vec2FeatureExtractor, WavLMForXVector
from datasets import load_dataset
import torch
dataset = load_dataset("hf-internal-testing/librispeech_asr_demo", "clean", split="validation")
feature_extractor = Wav2Vec2FeatureExtractor.from_pretrained('microsoft/wavlm-base-sv')
model = WavLMForXVector.from_pretrained('microsoft/wavlm-base-sv')
# audio files are decoded on the fly
inputs = feature_extractor(dataset[:2]["audio"]["array"], return_tensors="pt")
embeddings = model(**inputs).embeddings
embeddings = torch.nn.functional.normalize(embeddings, dim=-1).cpu()
# the resulting embeddings can be used for cosine similarity-based retrieval
cosine_sim = torch.nn.CosineSimilarity(dim=-1)
similarity = cosine_sim(embeddings[0], embeddings[1])
threshold = 0.86 # the optimal threshold is dataset-dependent
if similarity < threshold:
print("Speakers are not the same!")
```
# License
The official license can be found [here](https://github.com/microsoft/UniSpeech/blob/main/LICENSE)
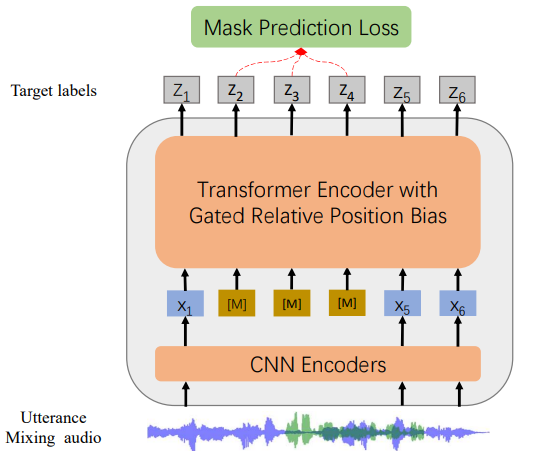 |
facebook/mask2former-swin-small-coco-instance | facebook | 2023-09-07T15:39:26Z | 4,493 | 2 | transformers | [
"transformers",
"pytorch",
"safetensors",
"mask2former",
"vision",
"image-segmentation",
"dataset:coco",
"arxiv:2112.01527",
"arxiv:2107.06278",
"license:other",
"endpoints_compatible",
"region:us"
] | image-segmentation | 2022-12-26T13:27:43Z | ---
license: other
tags:
- vision
- image-segmentation
datasets:
- coco
widget:
- src: http://images.cocodataset.org/val2017/000000039769.jpg
example_title: Cats
- src: http://images.cocodataset.org/val2017/000000039770.jpg
example_title: Castle
---
# Mask2Former
Mask2Former model trained on COCO instance segmentation (small-sized version, Swin backbone). It was introduced in the paper [Masked-attention Mask Transformer for Universal Image Segmentation
](https://arxiv.org/abs/2112.01527) and first released in [this repository](https://github.com/facebookresearch/Mask2Former/).
Disclaimer: The team releasing Mask2Former did not write a model card for this model so this model card has been written by the Hugging Face team.
## Model description
Mask2Former addresses instance, semantic and panoptic segmentation with the same paradigm: by predicting a set of masks and corresponding labels. Hence, all 3 tasks are treated as if they were instance segmentation. Mask2Former outperforms the previous SOTA,
[MaskFormer](https://arxiv.org/abs/2107.06278) both in terms of performance an efficiency by (i) replacing the pixel decoder with a more advanced multi-scale deformable attention Transformer, (ii) adopting a Transformer decoder with masked attention to boost performance without
without introducing additional computation and (iii) improving training efficiency by calculating the loss on subsampled points instead of whole masks.
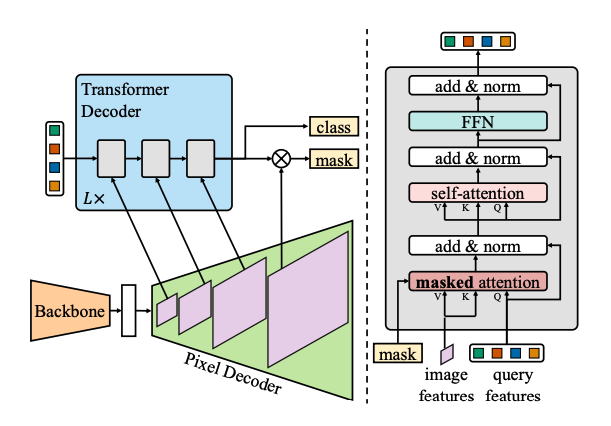
## Intended uses & limitations
You can use this particular checkpoint for instance segmentation. See the [model hub](https://huggingface.co/models?search=mask2former) to look for other
fine-tuned versions on a task that interests you.
### How to use
Here is how to use this model:
```python
import requests
import torch
from PIL import Image
from transformers import AutoImageProcessor, Mask2FormerForUniversalSegmentation
# load Mask2Former fine-tuned on COCO instance segmentation
processor = AutoImageProcessor.from_pretrained("facebook/mask2former-swin-small-coco-instance")
model = Mask2FormerForUniversalSegmentation.from_pretrained("facebook/mask2former-swin-small-coco-instance")
url = "http://images.cocodataset.org/val2017/000000039769.jpg"
image = Image.open(requests.get(url, stream=True).raw)
inputs = processor(images=image, return_tensors="pt")
with torch.no_grad():
outputs = model(**inputs)
# model predicts class_queries_logits of shape `(batch_size, num_queries)`
# and masks_queries_logits of shape `(batch_size, num_queries, height, width)`
class_queries_logits = outputs.class_queries_logits
masks_queries_logits = outputs.masks_queries_logits
# you can pass them to processor for postprocessing
result = processor.post_process_instance_segmentation(outputs, target_sizes=[image.size[::-1]])[0]
# we refer to the demo notebooks for visualization (see "Resources" section in the Mask2Former docs)
predicted_instance_map = result["segmentation"]
```
For more code examples, we refer to the [documentation](https://huggingface.co/docs/transformers/master/en/model_doc/mask2former). |
RichardErkhov/gemmathon_-_gemma-pro-3.1b-ko-v0.1-gguf | RichardErkhov | 2024-06-23T18:29:56Z | 4,492 | 0 | null | [
"gguf",
"region:us"
] | null | 2024-06-23T18:00:21Z | Entry not found |
mradermacher/Samantha-Qwen2-1.5B-GGUF | mradermacher | 2024-06-28T16:36:57Z | 4,492 | 0 | transformers | [
"transformers",
"gguf",
"en",
"dataset:macadeliccc/opus_samantha",
"dataset:teknium/OpenHermes-2.5",
"dataset:cognitivecomputations/samantha-data",
"dataset:cognitivecomputations/samantha-1.5",
"dataset:HuggingfaceH4/ultrachat_200k",
"dataset:jondurbin/airoboros-3.2",
"dataset:microsoft/orca-math-word-problems-200k",
"dataset:Sao10K/Claude-3-Opus-Instruct-15K",
"dataset:Locutusque/function-calling-chatml",
"dataset:Migtissera/Hitchhikers",
"base_model:macadeliccc/Samantha-Qwen2-1.5B",
"endpoints_compatible",
"region:us"
] | null | 2024-06-28T16:21:51Z | ---
base_model: macadeliccc/Samantha-Qwen2-1.5B
datasets:
- macadeliccc/opus_samantha
- teknium/OpenHermes-2.5
- cognitivecomputations/samantha-data
- cognitivecomputations/samantha-1.5
- HuggingfaceH4/ultrachat_200k
- jondurbin/airoboros-3.2
- microsoft/orca-math-word-problems-200k
- Sao10K/Claude-3-Opus-Instruct-15K
- Locutusque/function-calling-chatml
- Migtissera/Hitchhikers
language:
- en
library_name: transformers
quantized_by: mradermacher
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: -->
static quants of https://huggingface.co/macadeliccc/Samantha-Qwen2-1.5B
<!-- provided-files -->
weighted/imatrix quants seem not to be available (by me) at this time. If they do not show up a week or so after the static ones, I have probably not planned for them. Feel free to request them by opening a Community Discussion.
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q2_K.gguf) | Q2_K | 0.8 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.IQ3_XS.gguf) | IQ3_XS | 0.8 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q3_K_S.gguf) | Q3_K_S | 0.9 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.IQ3_S.gguf) | IQ3_S | 0.9 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.IQ3_M.gguf) | IQ3_M | 0.9 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q3_K_M.gguf) | Q3_K_M | 0.9 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q3_K_L.gguf) | Q3_K_L | 1.0 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.IQ4_XS.gguf) | IQ4_XS | 1.0 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q4_K_S.gguf) | Q4_K_S | 1.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q4_K_M.gguf) | Q4_K_M | 1.1 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q5_K_S.gguf) | Q5_K_S | 1.2 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q5_K_M.gguf) | Q5_K_M | 1.2 | |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q6_K.gguf) | Q6_K | 1.4 | very good quality |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.Q8_0.gguf) | Q8_0 | 1.7 | fast, best quality |
| [GGUF](https://huggingface.co/mradermacher/Samantha-Qwen2-1.5B-GGUF/resolve/main/Samantha-Qwen2-1.5B.f16.gguf) | f16 | 3.2 | 16 bpw, overkill |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time.
<!-- end -->
|
wkcn/TinyCLIP-ViT-40M-32-Text-19M-LAION400M | wkcn | 2024-05-08T03:12:43Z | 4,491 | 1 | transformers | [
"transformers",
"pytorch",
"safetensors",
"clip",
"zero-shot-image-classification",
"tinyclip",
"dataset:laion/laion400m",
"license:mit",
"endpoints_compatible",
"region:us"
] | zero-shot-image-classification | 2023-12-19T13:43:52Z | ---
license: mit
datasets:
- laion/laion400m
pipeline_tag: zero-shot-image-classification
tags:
- tinyclip
---
# TinyCLIP: CLIP Distillation via Affinity Mimicking and Weight Inheritance
**[ICCV 2023]** - [TinyCLIP: CLIP Distillation via Affinity Mimicking and Weight Inheritance](https://openaccess.thecvf.com/content/ICCV2023/html/Wu_TinyCLIP_CLIP_Distillation_via_Affinity_Mimicking_and_Weight_Inheritance_ICCV_2023_paper.html)
**TinyCLIP** is a novel **cross-modal distillation** method for large-scale language-image pre-trained models. The method introduces two core techniques: **affinity mimicking** and **weight inheritance**. This work unleashes the capacity of small CLIP models, fully leveraging large-scale models as well as pre-training data and striking the best trade-off between speed and accuracy.
<p align="center">
<img src="./figure/TinyCLIP.jpg" width="1000">
</p>
## Use with Transformers
```python
from PIL import Image
import requests
from transformers import CLIPProcessor, CLIPModel
model = CLIPModel.from_pretrained("wkcn/TinyCLIP-ViT-40M-32-Text-19M-LAION400M")
processor = CLIPProcessor.from_pretrained("wkcn/TinyCLIP-ViT-40M-32-Text-19M-LAION400M")
url = "http://images.cocodataset.org/val2017/000000039769.jpg"
image = Image.open(requests.get(url, stream=True).raw)
inputs = processor(text=["a photo of a cat", "a photo of a dog"], images=image, return_tensors="pt", padding=True)
outputs = model(**inputs)
logits_per_image = outputs.logits_per_image # this is the image-text similarity score
probs = logits_per_image.softmax(dim=1) # we can take the softmax to get the label probabilities
```
## Highlights
<p align="center">
<img src="./figure/fig1.jpg" width="500">
</p>
* TinyCLIP ViT-45M/32 uses only **half parameters** of ViT-B/32 to achieves **comparable zero-shot performance**.
* TinyCLIP ResNet-19M reduces the parameters by **50\%** while getting **2x** inference speedup, and obtains **56.4\%** accuracy on ImageNet.
## Model Zoo
| Model | Weight inheritance | Pretrain | IN-1K Acc@1(%) | MACs(G) | Throughput(pairs/s) | Link |
|--------------------|--------------------|---------------|----------------|---------|---------------------|------|
[TinyCLIP ViT-39M/16 Text-19M](./src/open_clip/model_configs/TinyCLIP-ViT-39M-16-Text-19M.json) | manual | YFCC-15M | 63.5 | 9.5 | 1,469 | [Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-ViT-39M-16-Text-19M-YFCC15M.pt)
[TinyCLIP ViT-8M/16 Text-3M](./src/open_clip/model_configs/TinyCLIP-ViT-8M-16-Text-3M.json) | manual | YFCC-15M | 41.1 | 2.0 | 4,150 | [Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-ViT-8M-16-Text-3M-YFCC15M.pt)
[TinyCLIP ResNet-30M Text-29M](./src/open_clip/model_configs/TinyCLIP-ResNet-30M-Text-29M.json) | manual | LAION-400M | 59.1 | 6.9 | 1,811 | [Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-ResNet-30M-Text-29M-LAION400M.pt)
[TinyCLIP ResNet-19M Text-19M](./src/open_clip/model_configs/TinyCLIP-ResNet-19M-Text-19M.json) | manual | LAION-400M | 56.4 | 4.4 | 3,024| [Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-ResNet-19M-Text-19M-LAION400M.pt)
[TinyCLIP ViT-61M/32 Text-29M](./src/open_clip/model_configs/TinyCLIP-ViT-61M-32-Text-29M.json) | manual | LAION-400M | 62.4 | 5.3 | 3,191|[Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-ViT-61M-32-Text-29M-LAION400M.pt)
[TinyCLIP ViT-40M/32 Text-19M](./src/open_clip/model_configs/TinyCLIP-ViT-40M-32-Text-19M.json) | manual | LAION-400M | 59.8 | 3.5 | 4,641|[Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-ViT-40M-32-Text-19M-LAION400M.pt)
TinyCLIP ViT-63M/32 Text-31M | auto | LAION-400M | 63.9 | 5.6 | 2,905|[Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-auto-ViT-63M-32-Text-31M-LAION400M.pt)
TinyCLIP ViT-45M/32 Text-18M | auto | LAION-400M | 61.4 | 3.7 | 3,682|[Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-auto-ViT-45M-32-Text-18M-LAION400M.pt)
TinyCLIP ViT-22M/32 Text-10M | auto | LAION-400M | 53.7 | 1.9 | 5,504|[Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-auto-ViT-22M-32-Text-10M-LAION400M.pt)
TinyCLIP ViT-63M/32 Text-31M | auto | LAION+YFCC-400M | 64.5 | 5.6| 2,909 | [Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-auto-ViT-63M-32-Text-31M-LAIONYFCC400M.pt)
TinyCLIP ViT-45M/32 Text-18M | auto | LAION+YFCC-400M | 62.7 | 1.9 | 3,685 | [Model](https://github.com/wkcn/TinyCLIP-model-zoo/releases/download/checkpoints/TinyCLIP-auto-ViT-45M-32-Text-18M-LAIONYFCC400M.pt)
Note: The configs of models with auto inheritance are generated automatically.
## Official PyTorch Implementation
https://github.com/microsoft/Cream/tree/main/TinyCLIP
## Citation
If this repo is helpful for you, please consider to cite it. :mega: Thank you! :)
```bibtex
@InProceedings{tinyclip,
title = {TinyCLIP: CLIP Distillation via Affinity Mimicking and Weight Inheritance},
author = {Wu, Kan and Peng, Houwen and Zhou, Zhenghong and Xiao, Bin and Liu, Mengchen and Yuan, Lu and Xuan, Hong and Valenzuela, Michael and Chen, Xi (Stephen) and Wang, Xinggang and Chao, Hongyang and Hu, Han},
booktitle = {Proceedings of the IEEE/CVF International Conference on Computer Vision (ICCV)},
month = {October},
year = {2023},
pages = {21970-21980}
}
```
## Acknowledge
Our code is based on [CLIP](https://github.com/openai/CLIP), [OpenCLIP](https://github.com/mlfoundations/open_clip), [CoFi](https://github.com/princeton-nlp/CoFiPruning) and [PyTorch](https://github.com/pytorch/pytorch). Thank contributors for their awesome contribution!
## License
- [License](https://github.com/microsoft/Cream/blob/main/TinyCLIP/LICENSE) |
dejanseo/LinkBERT-mini | dejanseo | 2024-06-29T12:01:04Z | 4,491 | 1 | transformers | [
"transformers",
"pytorch",
"onnx",
"albert",
"fill-mask",
"token-classification",
"en",
"license:other",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | token-classification | 2024-03-26T03:53:09Z | ---
language:
- en
license: other
license_name: link-attribution
license_link: https://dejanmarketing.com/link-attribution/
pipeline_tag: token-classification
widget:
- text: >-
LinkBERT is an advanced fine-tuned version of the albert-base-v2 model
developed by Dejan Marketing. The model is designed to predict natural link
placement within web content.
---
# LinkBERT: Fine-tuned BERT for Natural Link Prediction
LinkBERT is an advanced fine-tuned version of the [albert-base-v2](https://huggingface.co/albert/albert-base-v2) model developed by [Dejan Marketing](https://dejanmarketing.com/). The model is designed to predict natural link placement within web content. This binary classification model excels in identifying distinct token ranges that web authors are likely to choose as anchor text for links. By analyzing never-before-seen texts, LinkBERT can predict areas within the content where links might naturally occur, effectively simulating web author behavior in link creation.
# Engage Our Team
Interested in using this in an automated pipeline for bulk link prediction?
Please [book an appointment](https://dejanmarketing.com/conference/) to discuss your needs.
# Online Demo
Online demo of this model is available at https://linkbert.com/
## Applications of LinkBERT
LinkBERT's applications are vast and diverse, tailored to enhance both the efficiency and quality of web content creation and analysis:
- **Anchor Text Suggestion:** Acts as a mechanism during internal link optimization, suggesting potential anchor texts to web authors.
- **Evaluation of Existing Links:** Assesses the naturalness of link placements within existing content, aiding in the refinement of web pages.
- **Link Placement Guide:** Offers guidance to link builders by suggesting optimal placement for links within content.
- **Anchor Text Idea Generator:** Provides creative anchor text suggestions to enrich content and improve SEO strategies.
- **Spam and Inorganic SEO Detection:** Helps identify unnatural link patterns, contributing to the detection of spam and inorganic SEO tactics.
## Training and Performance
LinkBERT was fine-tuned on a dataset of organic web content and editorial links.
https://www.youtube.com/watch?v=A0ZulyVqjZo
### Source:
- [USA](https://www.owayo.com/), [Australia](https://www.owayo.com.au/), [Germany](https://www.owayo.de/), [UK](https://www.owayo.co.uk/), [Canada](https://www.owayo.ca/)
### Training Highlights:
- **Dataset:** Custom organic web content with editorial links.
- **Preprocessing:** Links annotated with `[START_LINK]` and `[END_LINK]` markup.
- **Tokenization:** Utilized input_ids, token_type_ids, attention_mask, and labels for model training, with a unique labeling system to differentiate between link/anchor text and plain text.
### Technical Specifications:
- **Batch Size:** 10, with class weights adjusted to address class imbalance between link and plain text.
- **Optimizer:** AdamW with a learning rate of 5e-5.
- **Epochs:** 5, incorporating gradient accumulation and warmup steps to optimize training outcomes.
- **Hardware:** 1 x RTX4090 24GB VRAM
- **Duration:** 32 hours
## Utilization and Integration
LinkBERT is positioned as a powerful tool for content creators, SEO specialists, and webmasters, offering unparalleled support in optimizing web content for both user engagement and search engine recognition. Its predictive capabilities not only streamline the content creation process but also offer insights into the natural integration of links, enhancing the overall quality and relevance of web content.
## Accessibility
LinkBERT leverages the robust architecture of bert-large-cased, enhancing it with capabilities specifically tailored for web content analysis. This model represents a significant advancement in the understanding and generation of web content, providing a nuanced approach to natural link prediction and anchor text suggestion.
--- |
mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF | mradermacher | 2024-06-05T08:44:20Z | 4,489 | 0 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"not-for-all-audiences",
"en",
"base_model:v000000/L3-8B-Poppy-Sunspice",
"endpoints_compatible",
"region:us"
] | null | 2024-06-04T10:22:53Z | ---
base_model: v000000/L3-8B-Poppy-Sunspice
language:
- en
library_name: transformers
quantized_by: mradermacher
tags:
- mergekit
- merge
- not-for-all-audiences
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/v000000/L3-8B-Poppy-Sunspice
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-Poppy-Sunspice-i1-GGUF/resolve/main/L3-8B-Poppy-Sunspice.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
Undi95/Xwin-MLewd-13B-V0.2-GGUF | Undi95 | 2023-10-15T02:21:05Z | 4,488 | 54 | null | [
"gguf",
"not-for-all-audiences",
"nsfw",
"license:cc-by-nc-4.0",
"region:us"
] | null | 2023-10-14T23:49:26Z | ---
license: cc-by-nc-4.0
tags:
- not-for-all-audiences
- nsfw
---

THIS MODEL IS MADE FOR LEWD
SEXUAL, CRUDE AND KINKY CONTENT IN OUTPUT CAN AND WILL HAPPEN. YOU'RE WARNED
This is MLewd merged with [Xwin-LM/Xwin-LM-13B-V0.2](https://huggingface.co/Xwin-LM/Xwin-LM-13B-V0.2)
<!-- description start -->
## Description
This repo contains quantized files of Xwin-MLewd-13B-V0.2, very hot and lewd model based on Xwin 0.2 13B.
<!-- description end -->
<!-- description start -->
## Models and loras used
- Undi95/ReMM-S-Light (base/private)
- Undi95/CreativeEngine
- Brouz/Slerpeno
- The-Face-Of-Goonery/Huginn-v3-13b
- zattio770/120-Days-of-LORA-v2-13B
- PygmalionAI/pygmalion-2-13b
- Undi95/StoryTelling
- TokenBender/sakhi_13B_roleplayer_NSFW_chat_adapter
- nRuaif/Kimiko-v2-13B
- The-Face-Of-Goonery/Huginn-13b-FP16
- lemonilia/LimaRP-Llama2-13B-v3-EXPERIMENT
- Xwin-LM/Xwin-LM-13B-V0.2
<!-- description end -->
<!-- prompt-template start -->
## Prompt template: Alpaca
```
Below is an instruction that describes a task. Write a response that appropriately completes the request.
### Instruction:
{prompt}
### Response:
```
## The secret sauce
```
slices:
- sources:
- model: Xwin-LM/Xwin-LM-13B-V0.2
layer_range: [0, 40]
- model: Undi95/MLewd-v2.4-13B
layer_range: [0, 40]
merge_method: slerp
base_model: Xwin-LM/Xwin-LM-13B-V0.2
parameters:
t:
- filter: lm_head
value: [0.55]
- filter: embed_tokens
value: [0.7]
- filter: self_attn
value: [0.65, 0.35]
- filter: mlp
value: [0.35, 0.65]
- filter: layernorm
value: [0.4, 0.6]
- filter: modelnorm
value: [0.6]
- value: 0.5 # fallback for rest of tensors
dtype: float16
```
Special thanks to Sushi and Shena ♥
If you want to support me, you can [here](https://ko-fi.com/undiai). |
arbazk/maestroqa-distilbert-negative-sentiment | arbazk | 2023-03-27T17:39:15Z | 4,486 | 2 | transformers | [
"transformers",
"pytorch",
"safetensors",
"distilbert",
"text-classification",
"generated_from_trainer",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | text-classification | 2023-03-22T21:42:45Z | ---
license: apache-2.0
tags:
- generated_from_trainer
metrics:
- accuracy
model-index:
- name: maestroqa-distilbert-negative-sentiment
results: []
---
<!-- This model card has been generated automatically according to the information the Trainer had access to. You
should probably proofread and complete it, then remove this comment. -->
# maestroqa-distilbert-negative-sentiment
This model is a fine-tuned version of [distilbert-base-uncased-finetuned-sst-2-english](https://huggingface.co/distilbert-base-uncased-finetuned-sst-2-english) on an unknown dataset.
It achieves the following results on the evaluation set:
- Loss: 0.8880
- Accuracy: 0.77
## Model description
More information needed
## Intended uses & limitations
More information needed
## Training and evaluation data
More information needed
## Training procedure
### Training hyperparameters
The following hyperparameters were used during training:
- learning_rate: 2e-05
- train_batch_size: 16
- eval_batch_size: 16
- seed: 42
- optimizer: Adam with betas=(0.9,0.999) and epsilon=1e-08
- lr_scheduler_type: linear
- num_epochs: 5
### Training results
| Training Loss | Epoch | Step | Validation Loss | Accuracy |
|:-------------:|:-----:|:----:|:---------------:|:--------:|
| No log | 1.0 | 100 | 0.5659 | 0.73 |
| No log | 2.0 | 200 | 0.5930 | 0.76 |
| No log | 3.0 | 300 | 0.7645 | 0.76 |
| No log | 4.0 | 400 | 0.8880 | 0.77 |
| 0.267 | 5.0 | 500 | 0.9724 | 0.77 |
### Framework versions
- Transformers 4.25.1
- Pytorch 2.0.0
- Datasets 2.10.1
- Tokenizers 0.13.2
|
RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf | RichardErkhov | 2024-06-06T02:37:29Z | 4,485 | 0 | null | [
"gguf",
"region:us"
] | null | 2024-06-06T00:31:56Z | Quantization made by Richard Erkhov.
[Github](https://github.com/RichardErkhov)
[Discord](https://discord.gg/pvy7H8DZMG)
[Request more models](https://github.com/RichardErkhov/quant_request)
Mistral-7B-v0.1-OKI-v20231124-1e-5 - GGUF
- Model creator: https://huggingface.co/heegyu/
- Original model: https://huggingface.co/heegyu/Mistral-7B-v0.1-OKI-v20231124-1e-5/
| Name | Quant method | Size |
| ---- | ---- | ---- |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q2_K.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q2_K.gguf) | Q2_K | 2.53GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ3_XS.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ3_XS.gguf) | IQ3_XS | 2.81GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ3_S.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ3_S.gguf) | IQ3_S | 2.96GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K_S.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K_S.gguf) | Q3_K_S | 2.95GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ3_M.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ3_M.gguf) | IQ3_M | 3.06GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K.gguf) | Q3_K | 3.28GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K_M.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K_M.gguf) | Q3_K_M | 3.28GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K_L.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q3_K_L.gguf) | Q3_K_L | 3.56GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ4_XS.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ4_XS.gguf) | IQ4_XS | 3.67GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_0.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_0.gguf) | Q4_0 | 3.83GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ4_NL.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.IQ4_NL.gguf) | IQ4_NL | 3.87GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_K_S.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_K_S.gguf) | Q4_K_S | 3.86GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_K.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_K.gguf) | Q4_K | 4.07GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_K_M.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_K_M.gguf) | Q4_K_M | 4.07GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_1.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q4_1.gguf) | Q4_1 | 4.24GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_0.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_0.gguf) | Q5_0 | 4.65GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_K_S.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_K_S.gguf) | Q5_K_S | 4.65GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_K.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_K.gguf) | Q5_K | 4.78GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_K_M.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_K_M.gguf) | Q5_K_M | 4.78GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_1.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q5_1.gguf) | Q5_1 | 5.07GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q6_K.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q6_K.gguf) | Q6_K | 5.53GB |
| [Mistral-7B-v0.1-OKI-v20231124-1e-5.Q8_0.gguf](https://huggingface.co/RichardErkhov/heegyu_-_Mistral-7B-v0.1-OKI-v20231124-1e-5-gguf/blob/main/Mistral-7B-v0.1-OKI-v20231124-1e-5.Q8_0.gguf) | Q8_0 | 7.17GB |
Original model description:
Entry not found
|
Helsinki-NLP/opus-mt-tc-big-he-en | Helsinki-NLP | 2023-10-10T10:25:39Z | 4,484 | 3 | transformers | [
"transformers",
"pytorch",
"tf",
"safetensors",
"marian",
"text2text-generation",
"translation",
"opus-mt-tc",
"en",
"he",
"license:cc-by-4.0",
"model-index",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | translation | 2022-04-13T16:27:23Z | ---
language:
- en
- he
tags:
- translation
- opus-mt-tc
license: cc-by-4.0
model-index:
- name: opus-mt-tc-big-he-en
results:
- task:
name: Translation heb-eng
type: translation
args: heb-eng
dataset:
name: flores101-devtest
type: flores_101
args: heb eng devtest
metrics:
- name: BLEU
type: bleu
value: 44.1
- task:
name: Translation heb-eng
type: translation
args: heb-eng
dataset:
name: tatoeba-test-v2021-08-07
type: tatoeba_mt
args: heb-eng
metrics:
- name: BLEU
type: bleu
value: 53.8
---
# opus-mt-tc-big-he-en
Neural machine translation model for translating from Hebrew (he) to English (en).
This model is part of the [OPUS-MT project](https://github.com/Helsinki-NLP/Opus-MT), an effort to make neural machine translation models widely available and accessible for many languages in the world. All models are originally trained using the amazing framework of [Marian NMT](https://marian-nmt.github.io/), an efficient NMT implementation written in pure C++. The models have been converted to pyTorch using the transformers library by huggingface. Training data is taken from [OPUS](https://opus.nlpl.eu/) and training pipelines use the procedures of [OPUS-MT-train](https://github.com/Helsinki-NLP/Opus-MT-train).
* Publications: [OPUS-MT – Building open translation services for the World](https://aclanthology.org/2020.eamt-1.61/) and [The Tatoeba Translation Challenge – Realistic Data Sets for Low Resource and Multilingual MT](https://aclanthology.org/2020.wmt-1.139/) (Please, cite if you use this model.)
```
@inproceedings{tiedemann-thottingal-2020-opus,
title = "{OPUS}-{MT} {--} Building open translation services for the World",
author = {Tiedemann, J{\"o}rg and Thottingal, Santhosh},
booktitle = "Proceedings of the 22nd Annual Conference of the European Association for Machine Translation",
month = nov,
year = "2020",
address = "Lisboa, Portugal",
publisher = "European Association for Machine Translation",
url = "https://aclanthology.org/2020.eamt-1.61",
pages = "479--480",
}
@inproceedings{tiedemann-2020-tatoeba,
title = "The Tatoeba Translation Challenge {--} Realistic Data Sets for Low Resource and Multilingual {MT}",
author = {Tiedemann, J{\"o}rg},
booktitle = "Proceedings of the Fifth Conference on Machine Translation",
month = nov,
year = "2020",
address = "Online",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2020.wmt-1.139",
pages = "1174--1182",
}
```
## Model info
* Release: 2022-03-13
* source language(s): heb
* target language(s): eng
* model: transformer-big
* data: opusTCv20210807+bt ([source](https://github.com/Helsinki-NLP/Tatoeba-Challenge))
* tokenization: SentencePiece (spm32k,spm32k)
* original model: [opusTCv20210807+bt_transformer-big_2022-03-13.zip](https://object.pouta.csc.fi/Tatoeba-MT-models/heb-eng/opusTCv20210807+bt_transformer-big_2022-03-13.zip)
* more information released models: [OPUS-MT heb-eng README](https://github.com/Helsinki-NLP/Tatoeba-Challenge/tree/master/models/heb-eng/README.md)
## Usage
A short example code:
```python
from transformers import MarianMTModel, MarianTokenizer
src_text = [
"היא שכחה לכתוב לו.",
"אני רוצה לדעת מיד כשמשהו יקרה."
]
model_name = "pytorch-models/opus-mt-tc-big-he-en"
tokenizer = MarianTokenizer.from_pretrained(model_name)
model = MarianMTModel.from_pretrained(model_name)
translated = model.generate(**tokenizer(src_text, return_tensors="pt", padding=True))
for t in translated:
print( tokenizer.decode(t, skip_special_tokens=True) )
# expected output:
# She forgot to write to him.
# I want to know as soon as something happens.
```
You can also use OPUS-MT models with the transformers pipelines, for example:
```python
from transformers import pipeline
pipe = pipeline("translation", model="Helsinki-NLP/opus-mt-tc-big-he-en")
print(pipe("היא שכחה לכתוב לו."))
# expected output: She forgot to write to him.
```
## Benchmarks
* test set translations: [opusTCv20210807+bt_transformer-big_2022-03-13.test.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/heb-eng/opusTCv20210807+bt_transformer-big_2022-03-13.test.txt)
* test set scores: [opusTCv20210807+bt_transformer-big_2022-03-13.eval.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/heb-eng/opusTCv20210807+bt_transformer-big_2022-03-13.eval.txt)
* benchmark results: [benchmark_results.txt](benchmark_results.txt)
* benchmark output: [benchmark_translations.zip](benchmark_translations.zip)
| langpair | testset | chr-F | BLEU | #sent | #words |
|----------|---------|-------|-------|-------|--------|
| heb-eng | tatoeba-test-v2021-08-07 | 0.68565 | 53.8 | 10519 | 77427 |
| heb-eng | flores101-devtest | 0.68116 | 44.1 | 1012 | 24721 |
## Acknowledgements
The work is supported by the [European Language Grid](https://www.european-language-grid.eu/) as [pilot project 2866](https://live.european-language-grid.eu/catalogue/#/resource/projects/2866), by the [FoTran project](https://www.helsinki.fi/en/researchgroups/natural-language-understanding-with-cross-lingual-grounding), funded by the European Research Council (ERC) under the European Union’s Horizon 2020 research and innovation programme (grant agreement No 771113), and the [MeMAD project](https://memad.eu/), funded by the European Union’s Horizon 2020 Research and Innovation Programme under grant agreement No 780069. We are also grateful for the generous computational resources and IT infrastructure provided by [CSC -- IT Center for Science](https://www.csc.fi/), Finland.
## Model conversion info
* transformers version: 4.16.2
* OPUS-MT git hash: 3405783
* port time: Wed Apr 13 19:27:12 EEST 2022
* port machine: LM0-400-22516.local
|
mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF | mradermacher | 2024-06-09T13:16:06Z | 4,480 | 1 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"en",
"base_model:bluuwhale/L3-SthenoMaid-8B-V1",
"endpoints_compatible",
"region:us"
] | null | 2024-06-09T10:33:36Z | ---
base_model: bluuwhale/L3-SthenoMaid-8B-V1
language:
- en
library_name: transformers
quantized_by: mradermacher
tags:
- mergekit
- merge
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/bluuwhale/L3-SthenoMaid-8B-V1
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-SthenoMaid-8B-V1-i1-GGUF/resolve/main/L3-SthenoMaid-8B-V1.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
Helsinki-NLP/opus-mt-ur-en | Helsinki-NLP | 2023-08-16T12:08:24Z | 4,478 | 2 | transformers | [
"transformers",
"pytorch",
"tf",
"marian",
"text2text-generation",
"translation",
"ur",
"en",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | translation | 2022-03-02T23:29:04Z | ---
language:
- ur
- en
tags:
- translation
license: apache-2.0
---
### urd-eng
* source group: Urdu
* target group: English
* OPUS readme: [urd-eng](https://github.com/Helsinki-NLP/Tatoeba-Challenge/tree/master/models/urd-eng/README.md)
* model: transformer-align
* source language(s): urd
* target language(s): eng
* model: transformer-align
* pre-processing: normalization + SentencePiece (spm32k,spm32k)
* download original weights: [opus-2020-06-17.zip](https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.zip)
* test set translations: [opus-2020-06-17.test.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.test.txt)
* test set scores: [opus-2020-06-17.eval.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.eval.txt)
## Benchmarks
| testset | BLEU | chr-F |
|-----------------------|-------|-------|
| Tatoeba-test.urd.eng | 23.2 | 0.435 |
### System Info:
- hf_name: urd-eng
- source_languages: urd
- target_languages: eng
- opus_readme_url: https://github.com/Helsinki-NLP/Tatoeba-Challenge/tree/master/models/urd-eng/README.md
- original_repo: Tatoeba-Challenge
- tags: ['translation']
- languages: ['ur', 'en']
- src_constituents: {'urd'}
- tgt_constituents: {'eng'}
- src_multilingual: False
- tgt_multilingual: False
- prepro: normalization + SentencePiece (spm32k,spm32k)
- url_model: https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.zip
- url_test_set: https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.test.txt
- src_alpha3: urd
- tgt_alpha3: eng
- short_pair: ur-en
- chrF2_score: 0.435
- bleu: 23.2
- brevity_penalty: 0.975
- ref_len: 12029.0
- src_name: Urdu
- tgt_name: English
- train_date: 2020-06-17
- src_alpha2: ur
- tgt_alpha2: en
- prefer_old: False
- long_pair: urd-eng
- helsinki_git_sha: 480fcbe0ee1bf4774bcbe6226ad9f58e63f6c535
- transformers_git_sha: 2207e5d8cb224e954a7cba69fa4ac2309e9ff30b
- port_machine: brutasse
- port_time: 2020-08-21-14:41 |
facebook/galactica-120b | facebook | 2023-01-24T17:20:48Z | 4,475 | 148 | transformers | [
"transformers",
"pytorch",
"opt",
"text-generation",
"galactica",
"arxiv:1810.03993",
"license:cc-by-nc-4.0",
"autotrain_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2022-11-16T14:49:36Z | ---
license: cc-by-nc-4.0
tags:
- galactica
widget:
- text: "The Transformer architecture [START_REF]"
- text: "The Schwarzschild radius is defined as: \\["
- text: "A force of 0.6N is applied to an object, which accelerates at 3m/s. What is its mass? <work>"
- text: "Lecture 1: The Ising Model\n\n"
- text: "[START_I_SMILES]"
- text: "[START_AMINO]GHMQSITAGQKVISKHKNGRFYQCEVVRLTTETFYEVNFDDGSFSDNLYPEDIVSQDCLQFGPPAEGEVVQVRWTDGQVYGAKFVASHPIQMYQVEFEDGSQLVVKRDDVYTLDEELP[END_AMINO] ## Keywords"
inference: false
---

# GALACTICA 120 B (huge)
Model card from the original [repo](https://github.com/paperswithcode/galai/blob/main/docs/model_card.md)
Following [Mitchell et al. (2018)](https://arxiv.org/abs/1810.03993), this model card provides information about the GALACTICA model, how it was trained, and the intended use cases. Full details about how the model was trained and evaluated can be found in the [release paper](https://galactica.org/paper.pdf).
**This model checkpoint was integrated into the Hub by [Manuel Romero](https://huggingface.co/mrm8488)**
## Model Details
The GALACTICA models are trained on a large-scale scientific corpus. The models are designed to perform scientific tasks, including but not limited to citation prediction, scientific QA, mathematical reasoning, summarization, document generation, molecular property prediction and entity extraction. The models were developed by the Papers with Code team at Meta AI to study the use of language models for the automatic organization of science. We train models with sizes ranging from 125M to 120B parameters. Below is a summary of the released models:
| Size | Parameters |
|:-----------:|:-----------:|
| `mini` | 125 M |
| `base` | 1.3 B |
| `standard` | 6.7 B |
| `large` | 30 B |
| `huge` | 120 B |
## Release Date
November 2022
## Model Type
Transformer based architecture in a decoder-only setup with a few modifications (see paper for more details).
## Paper & Demo
[Paper](https://galactica.org/paper.pdf) / [Demo](https://galactica.org)
## Model Use
The primary intended users of the GALACTICA models are researchers studying language models applied to the scientific domain. We also anticipate the model will be useful for developers who wish to build scientific tooling. However, we caution against production use without safeguards given the potential of language models to hallucinate.
The models are made available under a non-commercial CC BY-NC 4.0 license. More information about how to use the model can be found in the README.md of this repository.
## Training Data
The GALACTICA models are trained on 106 billion tokens of open-access scientific text and data. This includes papers, textbooks, scientific websites, encyclopedias, reference material, knowledge bases, and more. We tokenize different modalities to provide a natural langauge interface for different tasks. See the README.md for more information. See the paper for full information on the training data.
## How to use
Find below some example scripts on how to use the model in `transformers`:
## Using the Pytorch model
### Running the model on a CPU
<details>
<summary> Click to expand </summary>
```python
from transformers import AutoTokenizer, OPTForCausalLM
tokenizer = AutoTokenizer.from_pretrained("facebook/galactica-120b")
model = OPTForCausalLM.from_pretrained("facebook/galactica-120b")
input_text = "The Transformer architecture [START_REF]"
input_ids = tokenizer(input_text, return_tensors="pt").input_ids
outputs = model.generate(input_ids)
print(tokenizer.decode(outputs[0]))
```
</details>
### Running the model on a GPU
<details>
<summary> Click to expand </summary>
```python
# pip install accelerate
from transformers import AutoTokenizer, OPTForCausalLM
tokenizer = AutoTokenizer.from_pretrained("facebook/galactica-120b")
model = OPTForCausalLM.from_pretrained("facebook/galactica-120b", device_map="auto")
input_text = "The Transformer architecture [START_REF]"
input_ids = tokenizer(input_text, return_tensors="pt").input_ids.to("cuda")
outputs = model.generate(input_ids)
print(tokenizer.decode(outputs[0]))
```
</details>
### Running the model on a GPU using different precisions
#### FP16
<details>
<summary> Click to expand </summary>
```python
# pip install accelerate
import torch
from transformers import AutoTokenizer, OPTForCausalLM
tokenizer = AutoTokenizer.from_pretrained("facebook/galactica-120b")
model = OPTForCausalLM.from_pretrained("facebook/galactica-120b", device_map="auto", torch_dtype=torch.float16)
input_text = "The Transformer architecture [START_REF]"
input_ids = tokenizer(input_text, return_tensors="pt").input_ids.to("cuda")
outputs = model.generate(input_ids)
print(tokenizer.decode(outputs[0]))
```
</details>
#### INT8
<details>
<summary> Click to expand </summary>
```python
# pip install bitsandbytes accelerate
from transformers import AutoTokenizer, OPTForCausalLM
tokenizer = AutoTokenizer.from_pretrained("facebook/galactica-120b")
model = OPTForCausalLM.from_pretrained("facebook/galactica-120b", device_map="auto", load_in_8bit=True)
input_text = "The Transformer architecture [START_REF]"
input_ids = tokenizer(input_text, return_tensors="pt").input_ids.to("cuda")
outputs = model.generate(input_ids)
print(tokenizer.decode(outputs[0]))
```
</details>
## Performance and Limitations
The model outperforms several existing language models on a range of knowledge probes, reasoning, and knowledge-intensive scientific tasks. This also extends to general NLP tasks, where GALACTICA outperforms other open source general language models. That being said, we note a number of limitations in this section.
As with other language models, GALACTICA is often prone to hallucination - and training on a high-quality academic corpus does not prevent this, especially for less popular and less cited scientific concepts. There are no guarantees of truthful output when generating from the model. This extends to specific modalities such as citation prediction. While GALACTICA's citation behaviour approaches the ground truth citation behaviour with scale, the model continues to exhibit a popularity bias at larger scales.
In addition, we evaluated the model on several types of benchmarks related to stereotypes and toxicity. Overall, the model exhibits substantially lower toxicity rates compared to other large language models. That being said, the model continues to exhibit bias on certain measures (see the paper for details). So we recommend care when using the model for generations.
## Broader Implications
GALACTICA can potentially be used as a new way to discover academic literature. We also expect a lot of downstream use for application to particular domains, such as mathematics, biology, and chemistry. In the paper, we demonstrated several examples of the model acting as alternative to standard search tools. We expect a new generation of scientific tools to be built upon large language models such as GALACTICA.
We encourage researchers to investigate beneficial and new use cases for these models. That being said, it is important to be aware of the current limitations of large language models. Researchers should pay attention to common issues such as hallucination and biases that could emerge from using these models.
## Citation
```bibtex
@inproceedings{GALACTICA,
title={GALACTICA: A Large Language Model for Science},
author={Ross Taylor and Marcin Kardas and Guillem Cucurull and Thomas Scialom and Anthony Hartshorn and Elvis Saravia and Andrew Poulton and Viktor Kerkez and Robert Stojnic},
year={2022}
}
``` |
manu/sentence_croissant_alpha_v0.3 | manu | 2024-04-28T18:41:08Z | 4,475 | 2 | sentence-transformers | [
"sentence-transformers",
"safetensors",
"llama",
"feature-extraction",
"sentence-similarity",
"mteb",
"model-index",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | sentence-similarity | 2024-04-26T13:09:06Z | ---
library_name: sentence-transformers
pipeline_tag: sentence-similarity
tags:
- sentence-transformers
- feature-extraction
- sentence-similarity
- mteb
model-index:
- name: sentence_croissant_alpha_v0.3
results:
- task:
type: Clustering
dataset:
type: lyon-nlp/alloprof
name: MTEB AlloProfClusteringP2P
config: default
split: test
revision: 392ba3f5bcc8c51f578786c1fc3dae648662cb9b
metrics:
- type: v_measure
value: 56.72912207023513
- type: v_measures
value: [0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886, 0.5320130285438164, 0.5262623550285312, 0.5801017400160106, 0.5959165699319396, 0.5834996150492608, 0.5569839493118243, 0.6099665491090271, 0.5780727185697752, 0.4988023041518384, 0.6112933773114886]
- task:
type: Clustering
dataset:
type: lyon-nlp/alloprof
name: MTEB AlloProfClusteringS2S
config: default
split: test
revision: 392ba3f5bcc8c51f578786c1fc3dae648662cb9b
metrics:
- type: v_measure
value: 37.62128894914382
- type: v_measures
value: [0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827, 0.35401974034534334, 0.3980248155652733, 0.4010417412014714, 0.3771452994293956, 0.3279249606358475, 0.45943544754326515, 0.40148836454988795, 0.36775719216316904, 0.3211924643714899, 0.35409886910923827]
- task:
type: Reranking
dataset:
type: lyon-nlp/mteb-fr-reranking-alloprof-s2p
name: MTEB AlloprofReranking
config: default
split: test
revision: e40c8a63ce02da43200eccb5b0846fcaa888f562
metrics:
- type: map
value: 68.30621526032894
- type: mrr
value: 69.67719384817829
- task:
type: Retrieval
dataset:
type: lyon-nlp/alloprof
name: MTEB AlloprofRetrieval
config: default
split: test
revision: fcf295ea64c750f41fadbaa37b9b861558e1bfbd
metrics:
- type: map_at_1
value: 32.254
- type: map_at_10
value: 43.834
- type: map_at_100
value: 44.728
- type: map_at_1000
value: 44.769999999999996
- type: map_at_20
value: 44.361
- type: map_at_3
value: 40.753
- type: map_at_5
value: 42.486000000000004
- type: mrr_at_1
value: 32.254
- type: mrr_at_10
value: 43.834
- type: mrr_at_100
value: 44.728
- type: mrr_at_1000
value: 44.769999999999996
- type: mrr_at_20
value: 44.361
- type: mrr_at_3
value: 40.753
- type: mrr_at_5
value: 42.486000000000004
- type: ndcg_at_1
value: 32.254
- type: ndcg_at_10
value: 49.845
- type: ndcg_at_100
value: 54.37800000000001
- type: ndcg_at_1000
value: 55.498000000000005
- type: ndcg_at_20
value: 51.772
- type: ndcg_at_3
value: 43.486000000000004
- type: ndcg_at_5
value: 46.594
- type: precision_at_1
value: 32.254
- type: precision_at_10
value: 6.891
- type: precision_at_100
value: 0.905
- type: precision_at_1000
value: 0.099
- type: precision_at_20
value: 3.8280000000000003
- type: precision_at_3
value: 17.127
- type: precision_at_5
value: 11.779
- type: recall_at_1
value: 32.254
- type: recall_at_10
value: 68.91199999999999
- type: recall_at_100
value: 90.501
- type: recall_at_1000
value: 99.309
- type: recall_at_20
value: 76.554
- type: recall_at_3
value: 51.382000000000005
- type: recall_at_5
value: 58.894999999999996
- task:
type: Classification
dataset:
type: mteb/amazon_reviews_multi
name: MTEB AmazonReviewsClassification (fr)
config: fr
split: test
revision: 1399c76144fd37290681b995c656ef9b2e06e26d
metrics:
- type: accuracy
value: 35.106
- type: f1
value: 34.825583560299656
- task:
type: Retrieval
dataset:
type: maastrichtlawtech/bsard
name: MTEB BSARDRetrieval
config: default
split: test
revision: 5effa1b9b5fa3b0f9e12523e6e43e5f86a6e6d59
metrics:
- type: map_at_1
value: 0.0
- type: map_at_10
value: 0.15
- type: map_at_100
value: 0.19499999999999998
- type: map_at_1000
value: 0.243
- type: map_at_20
value: 0.18
- type: map_at_3
value: 0.15
- type: map_at_5
value: 0.15
- type: mrr_at_1
value: 0.0
- type: mrr_at_10
value: 0.15
- type: mrr_at_100
value: 0.19499999999999998
- type: mrr_at_1000
value: 0.243
- type: mrr_at_20
value: 0.18
- type: mrr_at_3
value: 0.15
- type: mrr_at_5
value: 0.15
- type: ndcg_at_1
value: 0.0
- type: ndcg_at_10
value: 0.22499999999999998
- type: ndcg_at_100
value: 0.545
- type: ndcg_at_1000
value: 2.622
- type: ndcg_at_20
value: 0.338
- type: ndcg_at_3
value: 0.22499999999999998
- type: ndcg_at_5
value: 0.22499999999999998
- type: precision_at_1
value: 0.0
- type: precision_at_10
value: 0.045
- type: precision_at_100
value: 0.023
- type: precision_at_1000
value: 0.02
- type: precision_at_20
value: 0.045
- type: precision_at_3
value: 0.15
- type: precision_at_5
value: 0.09
- type: recall_at_1
value: 0.0
- type: recall_at_10
value: 0.44999999999999996
- type: recall_at_100
value: 2.252
- type: recall_at_1000
value: 20.27
- type: recall_at_20
value: 0.901
- type: recall_at_3
value: 0.44999999999999996
- type: recall_at_5
value: 0.44999999999999996
- task:
type: Retrieval
dataset:
type: manu/fquad2_test
name: MTEB FQuADRetrieval
config: default
split: test
revision: 5384ce827bbc2156d46e6fcba83d75f8e6e1b4a6
metrics:
- type: map_at_1
value: 53.0
- type: map_at_10
value: 65.393
- type: map_at_100
value: 65.791
- type: map_at_1000
value: 65.79899999999999
- type: map_at_20
value: 65.644
- type: map_at_3
value: 62.74999999999999
- type: map_at_5
value: 64.075
- type: mrr_at_1
value: 53.0
- type: mrr_at_10
value: 65.393
- type: mrr_at_100
value: 65.791
- type: mrr_at_1000
value: 65.79899999999999
- type: mrr_at_20
value: 65.644
- type: mrr_at_3
value: 62.74999999999999
- type: mrr_at_5
value: 64.075
- type: ndcg_at_1
value: 53.0
- type: ndcg_at_10
value: 71.38600000000001
- type: ndcg_at_100
value: 73.275
- type: ndcg_at_1000
value: 73.42
- type: ndcg_at_20
value: 72.28099999999999
- type: ndcg_at_3
value: 65.839
- type: ndcg_at_5
value: 68.217
- type: precision_at_1
value: 53.0
- type: precision_at_10
value: 9.025
- type: precision_at_100
value: 0.9900000000000001
- type: precision_at_1000
value: 0.1
- type: precision_at_20
value: 4.688
- type: precision_at_3
value: 24.917
- type: precision_at_5
value: 16.1
- type: recall_at_1
value: 53.0
- type: recall_at_10
value: 90.25
- type: recall_at_100
value: 99.0
- type: recall_at_1000
value: 100.0
- type: recall_at_20
value: 93.75
- type: recall_at_3
value: 74.75
- type: recall_at_5
value: 80.5
- task:
type: Clustering
dataset:
type: lyon-nlp/clustering-hal-s2s
name: MTEB HALClusteringS2S
config: default
split: test
revision: e06ebbbb123f8144bef1a5d18796f3dec9ae2915
metrics:
- type: v_measure
value: 25.756762769106768
- type: v_measures
value: [0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097, 0.27992488796059395, 0.2764506771771727, 0.3243693580623437, 0.2779803803468105, 0.23611069524035522, 0.20081340028652678, 0.20920466845471178, 0.24107059599883462, 0.2301770805728178, 0.2995745328105097]
- task:
type: Clustering
dataset:
type: mlsum
name: MTEB MLSUMClusteringP2P
config: fr
split: test
revision: b5d54f8f3b61ae17845046286940f03c6bc79bc7
metrics:
- type: v_measure
value: 41.82320155017088
- type: v_measures
value: [0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863, 0.4222470060684208, 0.4358887035247771, 0.4288044607351393, 0.4278211144128014, 0.3701492929109167, 0.42700292743096274, 0.4396567571802029, 0.41889525107583575, 0.4178682135997126, 0.39398642807831863]
- task:
type: Clustering
dataset:
type: mlsum
name: MTEB MLSUMClusteringS2S
config: fr
split: test
revision: b5d54f8f3b61ae17845046286940f03c6bc79bc7
metrics:
- type: v_measure
value: 41.83097630331037
- type: v_measures
value: [0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202, 0.40556802081735377, 0.43455329357006206, 0.43325754900068963, 0.417432483055725, 0.37833136631959036, 0.43947993212701897, 0.4446524983622663, 0.4197600281286437, 0.4123873856320672, 0.3976750733176202]
- task:
type: Classification
dataset:
type: mteb/mtop_domain
name: MTEB MTOPDomainClassification (fr)
config: fr
split: test
revision: d80d48c1eb48d3562165c59d59d0034df9fff0bf
metrics:
- type: accuracy
value: 88.52489821484498
- type: f1
value: 88.39995340840026
- task:
type: Classification
dataset:
type: mteb/mtop_intent
name: MTEB MTOPIntentClassification (fr)
config: fr
split: test
revision: ae001d0e6b1228650b7bd1c2c65fb50ad11a8aba
metrics:
- type: accuracy
value: 65.92546194801128
- type: f1
value: 46.53109996877417
- task:
type: Classification
dataset:
type: masakhane/masakhanews
name: MTEB MasakhaNEWSClassification (fra)
config: fra
split: test
revision: 8ccc72e69e65f40c70e117d8b3c08306bb788b60
metrics:
- type: accuracy
value: 75.1658767772512
- type: f1
value: 71.02734472473519
- task:
type: Clustering
dataset:
type: masakhane/masakhanews
name: MTEB MasakhaNEWSClusteringP2P (fra)
config: fra
split: test
revision: 8ccc72e69e65f40c70e117d8b3c08306bb788b60
metrics:
- type: v_measure
value: 42.63310602457368
- type: v_measures
value: [1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835, 1.0, 0.06369976992079955, 0.5883538895545949, 0.18727949529930651, 0.2923221464539835]
- task:
type: Clustering
dataset:
type: masakhane/masakhanews
name: MTEB MasakhaNEWSClusteringS2S (fra)
config: fra
split: test
revision: 8ccc72e69e65f40c70e117d8b3c08306bb788b60
metrics:
- type: v_measure
value: 36.39726683344252
- type: v_measures
value: [1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356, 1.0, 0.08178119837705045, 0.23052387187167908, 0.4044733130065427, 0.10308495841685356]
- task:
type: Classification
dataset:
type: mteb/amazon_massive_intent
name: MTEB MassiveIntentClassification (fr)
config: fr
split: test
revision: 31efe3c427b0bae9c22cbb560b8f15491cc6bed7
metrics:
- type: accuracy
value: 66.48285137861467
- type: f1
value: 64.74690799351637
- task:
type: Classification
dataset:
type: mteb/amazon_massive_scenario
name: MTEB MassiveScenarioClassification (fr)
config: fr
split: test
revision: 7d571f92784cd94a019292a1f45445077d0ef634
metrics:
- type: accuracy
value: 71.47276395427033
- type: f1
value: 71.9261164692627
- task:
type: Retrieval
dataset:
type: jinaai/mintakaqa
name: MTEB MintakaRetrieval (fr)
config: fr
split: test
revision: efa78cc2f74bbcd21eff2261f9e13aebe40b814e
metrics:
- type: map_at_1
value: 16.134
- type: map_at_10
value: 26.040000000000003
- type: map_at_100
value: 27.233
- type: map_at_1000
value: 27.315
- type: map_at_20
value: 26.741999999999997
- type: map_at_3
value: 23.219
- type: map_at_5
value: 24.962999999999997
- type: mrr_at_1
value: 16.134
- type: mrr_at_10
value: 26.040000000000003
- type: mrr_at_100
value: 27.233
- type: mrr_at_1000
value: 27.315
- type: mrr_at_20
value: 26.741999999999997
- type: mrr_at_3
value: 23.219
- type: mrr_at_5
value: 24.962999999999997
- type: ndcg_at_1
value: 16.134
- type: ndcg_at_10
value: 31.255
- type: ndcg_at_100
value: 37.462
- type: ndcg_at_1000
value: 39.85
- type: ndcg_at_20
value: 33.853
- type: ndcg_at_3
value: 25.513
- type: ndcg_at_5
value: 28.653000000000002
- type: precision_at_1
value: 16.134
- type: precision_at_10
value: 4.779
- type: precision_at_100
value: 0.777
- type: precision_at_1000
value: 0.097
- type: precision_at_20
value: 2.907
- type: precision_at_3
value: 10.715
- type: precision_at_5
value: 7.951999999999999
- type: recall_at_1
value: 16.134
- type: recall_at_10
value: 47.789
- type: recall_at_100
value: 77.682
- type: recall_at_1000
value: 96.929
- type: recall_at_20
value: 58.148999999999994
- type: recall_at_3
value: 32.146
- type: recall_at_5
value: 39.762
- task:
type: PairClassification
dataset:
type: GEM/opusparcus
name: MTEB OpusparcusPC (fr)
config: fr
split: test
revision: 9e9b1f8ef51616073f47f306f7f47dd91663f86a
metrics:
- type: cos_sim_accuracy
value: 84.40054495912807
- type: cos_sim_ap
value: 93.71617772707746
- type: cos_sim_f1
value: 89.00624099855978
- type: cos_sim_precision
value: 86.15241635687732
- type: cos_sim_recall
value: 92.05561072492551
- type: dot_accuracy
value: 82.35694822888283
- type: dot_ap
value: 92.22992449042768
- type: dot_f1
value: 87.84786641929499
- type: dot_precision
value: 82.4194952132289
- type: dot_recall
value: 94.04170804369414
- type: euclidean_accuracy
value: 82.90190735694823
- type: euclidean_ap
value: 93.27345126956494
- type: euclidean_f1
value: 87.82608695652175
- type: euclidean_precision
value: 85.51269990592662
- type: euclidean_recall
value: 90.26812313803376
- type: manhattan_accuracy
value: 82.9700272479564
- type: manhattan_ap
value: 93.34994137379041
- type: manhattan_f1
value: 87.776708373436
- type: manhattan_precision
value: 85.15406162464986
- type: manhattan_recall
value: 90.56603773584906
- type: max_accuracy
value: 84.40054495912807
- type: max_ap
value: 93.71617772707746
- type: max_f1
value: 89.00624099855978
- task:
type: PairClassification
dataset:
type: paws-x
name: MTEB PawsX (fr)
config: fr
split: test
revision: 8a04d940a42cd40658986fdd8e3da561533a3646
metrics:
- type: cos_sim_accuracy
value: 63.849999999999994
- type: cos_sim_ap
value: 65.42493744372587
- type: cos_sim_f1
value: 63.87434554973822
- type: cos_sim_precision
value: 52.69978401727862
- type: cos_sim_recall
value: 81.06312292358804
- type: dot_accuracy
value: 55.35
- type: dot_ap
value: 48.9364958676423
- type: dot_f1
value: 62.491349480968864
- type: dot_precision
value: 45.44539506794162
- type: dot_recall
value: 100.0
- type: euclidean_accuracy
value: 64.4
- type: euclidean_ap
value: 65.8622099022063
- type: euclidean_f1
value: 63.762044407205686
- type: euclidean_precision
value: 51.280323450134766
- type: euclidean_recall
value: 84.27464008859357
- type: manhattan_accuracy
value: 64.5
- type: manhattan_ap
value: 65.89565256625798
- type: manhattan_f1
value: 63.75364128173118
- type: manhattan_precision
value: 51.06666666666667
- type: manhattan_recall
value: 84.82834994462901
- type: max_accuracy
value: 64.5
- type: max_ap
value: 65.89565256625798
- type: max_f1
value: 63.87434554973822
- task:
type: STS
dataset:
type: Lajavaness/SICK-fr
name: MTEB SICKFr
config: default
split: test
revision: e077ab4cf4774a1e36d86d593b150422fafd8e8a
metrics:
- type: cos_sim_pearson
value: 79.55191450239424
- type: cos_sim_spearman
value: 71.89209513298714
- type: euclidean_pearson
value: 74.18063891200164
- type: euclidean_spearman
value: 69.61463203410928
- type: manhattan_pearson
value: 74.26272426503743
- type: manhattan_spearman
value: 69.64261630235363
- task:
type: STS
dataset:
type: mteb/sts22-crosslingual-sts
name: MTEB STS22 (fr)
config: fr
split: test
revision: eea2b4fe26a775864c896887d910b76a8098ad3f
metrics:
- type: cos_sim_pearson
value: 79.18370529169849
- type: cos_sim_spearman
value: 80.80074537316342
- type: euclidean_pearson
value: 72.62308682855334
- type: euclidean_spearman
value: 75.64665618431559
- type: manhattan_pearson
value: 72.75806349827452
- type: manhattan_spearman
value: 75.34151992740627
- task:
type: STS
dataset:
type: PhilipMay/stsb_multi_mt
name: MTEB STSBenchmarkMultilingualSTS (fr)
config: fr
split: test
revision: 93d57ef91790589e3ce9c365164337a8a78b7632
metrics:
- type: cos_sim_pearson
value: 81.29611168887834
- type: cos_sim_spearman
value: 80.23434765396613
- type: euclidean_pearson
value: 77.85740285296822
- type: euclidean_spearman
value: 78.42089083386267
- type: manhattan_pearson
value: 77.85850984492824
- type: manhattan_spearman
value: 78.42578976788568
- task:
type: Summarization
dataset:
type: lyon-nlp/summarization-summeval-fr-p2p
name: MTEB SummEvalFr
config: default
split: test
revision: b385812de6a9577b6f4d0f88c6a6e35395a94054
metrics:
- type: cos_sim_pearson
value: 31.143644380271375
- type: cos_sim_spearman
value: 32.45645175142292
- type: dot_pearson
value: 28.46685825407204
- type: dot_spearman
value: 29.164040487051512
- task:
type: Reranking
dataset:
type: lyon-nlp/mteb-fr-reranking-syntec-s2p
name: MTEB SyntecReranking
config: default
split: test
revision: b205c5084a0934ce8af14338bf03feb19499c84d
metrics:
- type: map
value: 82.65
- type: mrr
value: 82.65
- task:
type: Retrieval
dataset:
type: lyon-nlp/mteb-fr-retrieval-syntec-s2p
name: MTEB SyntecRetrieval
config: default
split: test
revision: 19661ccdca4dfc2d15122d776b61685f48c68ca9
metrics:
- type: map_at_1
value: 56.99999999999999
- type: map_at_10
value: 70.6
- type: map_at_100
value: 70.814
- type: map_at_1000
value: 70.814
- type: map_at_20
value: 70.733
- type: map_at_3
value: 67.833
- type: map_at_5
value: 70.18299999999999
- type: mrr_at_1
value: 56.99999999999999
- type: mrr_at_10
value: 70.6
- type: mrr_at_100
value: 70.814
- type: mrr_at_1000
value: 70.814
- type: mrr_at_20
value: 70.733
- type: mrr_at_3
value: 67.833
- type: mrr_at_5
value: 70.18299999999999
- type: ndcg_at_1
value: 56.99999999999999
- type: ndcg_at_10
value: 76.626
- type: ndcg_at_100
value: 77.69500000000001
- type: ndcg_at_1000
value: 77.69500000000001
- type: ndcg_at_20
value: 77.12400000000001
- type: ndcg_at_3
value: 71.464
- type: ndcg_at_5
value: 75.639
- type: precision_at_1
value: 56.99999999999999
- type: precision_at_10
value: 9.5
- type: precision_at_100
value: 1.0
- type: precision_at_1000
value: 0.1
- type: precision_at_20
value: 4.8500000000000005
- type: precision_at_3
value: 27.333000000000002
- type: precision_at_5
value: 18.4
- type: recall_at_1
value: 56.99999999999999
- type: recall_at_10
value: 95.0
- type: recall_at_100
value: 100.0
- type: recall_at_1000
value: 100.0
- type: recall_at_20
value: 97.0
- type: recall_at_3
value: 82.0
- type: recall_at_5
value: 92.0
- task:
type: Retrieval
dataset:
type: jinaai/xpqa
name: MTEB XPQARetrieval (fr)
config: fr
split: test
revision: c99d599f0a6ab9b85b065da6f9d94f9cf731679f
metrics:
- type: map_at_1
value: 39.217
- type: map_at_10
value: 60.171
- type: map_at_100
value: 61.736999999999995
- type: map_at_1000
value: 61.787000000000006
- type: map_at_20
value: 61.211000000000006
- type: map_at_3
value: 53.43
- type: map_at_5
value: 57.638
- type: mrr_at_1
value: 62.617
- type: mrr_at_10
value: 69.32300000000001
- type: mrr_at_100
value: 69.95400000000001
- type: mrr_at_1000
value: 69.968
- type: mrr_at_20
value: 69.77799999999999
- type: mrr_at_3
value: 67.423
- type: mrr_at_5
value: 68.445
- type: ndcg_at_1
value: 62.617
- type: ndcg_at_10
value: 66.55499999999999
- type: ndcg_at_100
value: 71.521
- type: ndcg_at_1000
value: 72.32300000000001
- type: ndcg_at_20
value: 69.131
- type: ndcg_at_3
value: 60.88099999999999
- type: ndcg_at_5
value: 62.648
- type: precision_at_1
value: 62.617
- type: precision_at_10
value: 15.540999999999999
- type: precision_at_100
value: 1.9529999999999998
- type: precision_at_1000
value: 0.20600000000000002
- type: precision_at_20
value: 8.658000000000001
- type: precision_at_3
value: 36.805
- type: precision_at_5
value: 26.622
- type: recall_at_1
value: 39.217
- type: recall_at_10
value: 75.547
- type: recall_at_100
value: 94.226
- type: recall_at_1000
value: 99.433
- type: recall_at_20
value: 83.883
- type: recall_at_3
value: 57.867999999999995
- type: recall_at_5
value: 66.08800000000001
---
# {MODEL_NAME}
This is a [sentence-transformers](https://www.SBERT.net) model: It maps sentences & paragraphs to a 2048 dimensional dense vector space and can be used for tasks like clustering or semantic search.
<!--- Describe your model here -->
## Usage (Sentence-Transformers)
Using this model becomes easy when you have [sentence-transformers](https://www.SBERT.net) installed:
```
pip install -U sentence-transformers
```
Then you can use the model like this:
```python
from sentence_transformers import SentenceTransformer
sentences = ["This is an example sentence", "Each sentence is converted"]
model = SentenceTransformer('{MODEL_NAME}')
embeddings = model.encode(sentences)
print(embeddings)
```
## Evaluation Results
<!--- Describe how your model was evaluated -->
For an automated evaluation of this model, see the *Sentence Embeddings Benchmark*: [https://seb.sbert.net](https://seb.sbert.net?model_name={MODEL_NAME})
## Training
The model was trained with the parameters:
**DataLoader**:
`torch.utils.data.dataloader.DataLoader` of length 1401 with parameters:
```
{'batch_size': 256, 'sampler': 'torch.utils.data.sampler.SequentialSampler', 'batch_sampler': 'torch.utils.data.sampler.BatchSampler'}
```
**Loss**:
`__main__.MultipleNegativesRankingLoss_with_logging`
Parameters of the fit()-Method:
```
{
"epochs": 3,
"evaluation_steps": 0,
"evaluator": "NoneType",
"max_grad_norm": 1,
"optimizer_class": "<class 'torch.optim.adamw.AdamW'>",
"optimizer_params": {
"lr": 2e-05
},
"scheduler": "WarmupLinear",
"steps_per_epoch": null,
"warmup_steps": 100,
"weight_decay": 0.01
}
```
## Full Model Architecture
```
SentenceTransformer(
(0): Transformer({'max_seq_length': 1024, 'do_lower_case': False}) with Transformer model: LlamaModel
(1): Pooling({'word_embedding_dimension': 2048, 'pooling_mode_cls_token': False, 'pooling_mode_mean_tokens': False, 'pooling_mode_max_tokens': False, 'pooling_mode_mean_sqrt_len_tokens': False, 'pooling_mode_weightedmean_tokens': True, 'pooling_mode_lasttoken': False, 'include_prompt': True})
)
```
## Citing & Authors
<!--- Describe where people can find more information --> |
CIDAS/clipseg-rd64 | CIDAS | 2023-01-04T12:00:35Z | 4,474 | 3 | transformers | [
"transformers",
"pytorch",
"clipseg",
"vision",
"image-segmentation",
"arxiv:2112.10003",
"license:apache-2.0",
"region:us"
] | image-segmentation | 2022-11-04T09:47:58Z | ---
license: apache-2.0
tags:
- vision
- image-segmentation
inference: false
---
# CLIPSeg model
CLIPSeg model with reduce dimension 64. It was introduced in the paper [Image Segmentation Using Text and Image Prompts](https://arxiv.org/abs/2112.10003) by Lüddecke et al. and first released in [this repository](https://github.com/timojl/clipseg).
# Intended use cases
This model is intended for zero-shot and one-shot image segmentation.
# Usage
Refer to the [documentation](https://huggingface.co/docs/transformers/main/en/model_doc/clipseg). |
QuantFactory/Meta-Llama-3-70B-Instruct-GGUF | QuantFactory | 2024-04-20T16:19:20Z | 4,470 | 40 | null | [
"gguf",
"facebook",
"meta",
"pytorch",
"llama",
"llama-3",
"text-generation",
"en",
"base_model:meta-llama/Meta-Llama-3-70B-Instruct",
"license:other",
"region:us"
] | text-generation | 2024-04-19T09:14:03Z | ---
language:
- en
pipeline_tag: text-generation
tags:
- facebook
- meta
- pytorch
- llama
- llama-3
license: other
license_name: llama3
license_link: LICENSE
base_model: meta-llama/Meta-Llama-3-70B-Instruct
extra_gated_prompt: >-
### META LLAMA 3 COMMUNITY LICENSE AGREEMENT
Meta Llama 3 Version Release Date: April 18, 2024
"Agreement" means the terms and conditions for use, reproduction, distribution and modification of the
Llama Materials set forth herein.
"Documentation" means the specifications, manuals and documentation accompanying Meta Llama 3
distributed by Meta at https://llama.meta.com/get-started/.
"Licensee" or "you" means you, or your employer or any other person or entity (if you are entering into
this Agreement on such person or entity’s behalf), of the age required under applicable laws, rules or
regulations to provide legal consent and that has legal authority to bind your employer or such other
person or entity if you are entering in this Agreement on their behalf.
"Meta Llama 3" means the foundational large language models and software and algorithms, including
machine-learning model code, trained model weights, inference-enabling code, training-enabling code,
fine-tuning enabling code and other elements of the foregoing distributed by Meta at
https://llama.meta.com/llama-downloads.
"Llama Materials" means, collectively, Meta’s proprietary Meta Llama 3 and Documentation (and any
portion thereof) made available under this Agreement.
"Meta" or "we" means Meta Platforms Ireland Limited (if you are located in or, if you are an entity, your
principal place of business is in the EEA or Switzerland) and Meta Platforms, Inc. (if you are located
outside of the EEA or Switzerland).
1. License Rights and Redistribution.
a. Grant of Rights. You are granted a non-exclusive, worldwide, non-transferable and royalty-free
limited license under Meta’s intellectual property or other rights owned by Meta embodied in the Llama
Materials to use, reproduce, distribute, copy, create derivative works of, and make modifications to the
Llama Materials.
b. Redistribution and Use.
i. If you distribute or make available the Llama Materials (or any derivative works
thereof), or a product or service that uses any of them, including another AI model, you shall (A) provide
a copy of this Agreement with any such Llama Materials; and (B) prominently display “Built with Meta
Llama 3” on a related website, user interface, blogpost, about page, or product documentation. If you
use the Llama Materials to create, train, fine tune, or otherwise improve an AI model, which is
distributed or made available, you shall also include “Llama 3” at the beginning of any such AI model
name.
ii. If you receive Llama Materials, or any derivative works thereof, from a Licensee as part
of an integrated end user product, then Section 2 of this Agreement will not apply to you.
iii. You must retain in all copies of the Llama Materials that you distribute the following
attribution notice within a “Notice” text file distributed as a part of such copies: “Meta Llama 3 is
licensed under the Meta Llama 3 Community License, Copyright © Meta Platforms, Inc. All Rights
Reserved.”
iv. Your use of the Llama Materials must comply with applicable laws and regulations
(including trade compliance laws and regulations) and adhere to the Acceptable Use Policy for the Llama
Materials (available at https://llama.meta.com/llama3/use-policy), which is hereby incorporated by
reference into this Agreement.
v. You will not use the Llama Materials or any output or results of the Llama Materials to
improve any other large language model (excluding Meta Llama 3 or derivative works thereof).
2. Additional Commercial Terms. If, on the Meta Llama 3 version release date, the monthly active users
of the products or services made available by or for Licensee, or Licensee’s affiliates, is greater than 700
million monthly active users in the preceding calendar month, you must request a license from Meta,
which Meta may grant to you in its sole discretion, and you are not authorized to exercise any of the
rights under this Agreement unless or until Meta otherwise expressly grants you such rights.
3. Disclaimer of Warranty. UNLESS REQUIRED BY APPLICABLE LAW, THE LLAMA MATERIALS AND ANY
OUTPUT AND RESULTS THEREFROM ARE PROVIDED ON AN “AS IS” BASIS, WITHOUT WARRANTIES OF
ANY KIND, AND META DISCLAIMS ALL WARRANTIES OF ANY KIND, BOTH EXPRESS AND IMPLIED,
INCLUDING, WITHOUT LIMITATION, ANY WARRANTIES OF TITLE, NON-INFRINGEMENT,
MERCHANTABILITY, OR FITNESS FOR A PARTICULAR PURPOSE. YOU ARE SOLELY RESPONSIBLE FOR
DETERMINING THE APPROPRIATENESS OF USING OR REDISTRIBUTING THE LLAMA MATERIALS AND
ASSUME ANY RISKS ASSOCIATED WITH YOUR USE OF THE LLAMA MATERIALS AND ANY OUTPUT AND
RESULTS.
4. Limitation of Liability. IN NO EVENT WILL META OR ITS AFFILIATES BE LIABLE UNDER ANY THEORY OF
LIABILITY, WHETHER IN CONTRACT, TORT, NEGLIGENCE, PRODUCTS LIABILITY, OR OTHERWISE, ARISING
OUT OF THIS AGREEMENT, FOR ANY LOST PROFITS OR ANY INDIRECT, SPECIAL, CONSEQUENTIAL,
INCIDENTAL, EXEMPLARY OR PUNITIVE DAMAGES, EVEN IF META OR ITS AFFILIATES HAVE BEEN ADVISED
OF THE POSSIBILITY OF ANY OF THE FOREGOING.
5. Intellectual Property.
a. No trademark licenses are granted under this Agreement, and in connection with the Llama
Materials, neither Meta nor Licensee may use any name or mark owned by or associated with the other
or any of its affiliates, except as required for reasonable and customary use in describing and
redistributing the Llama Materials or as set forth in this Section 5(a). Meta hereby grants you a license to
use “Llama 3” (the “Mark”) solely as required to comply with the last sentence of Section 1.b.i. You will
comply with Meta’s brand guidelines (currently accessible at
https://about.meta.com/brand/resources/meta/company-brand/ ). All goodwill arising out of your use
of the Mark will inure to the benefit of Meta.
b. Subject to Meta’s ownership of Llama Materials and derivatives made by or for Meta, with
respect to any derivative works and modifications of the Llama Materials that are made by you, as
between you and Meta, you are and will be the owner of such derivative works and modifications.
c. If you institute litigation or other proceedings against Meta or any entity (including a
cross-claim or counterclaim in a lawsuit) alleging that the Llama Materials or Meta Llama 3 outputs or
results, or any portion of any of the foregoing, constitutes infringement of intellectual property or other
rights owned or licensable by you, then any licenses granted to you under this Agreement shall
terminate as of the date such litigation or claim is filed or instituted. You will indemnify and hold
harmless Meta from and against any claim by any third party arising out of or related to your use or
distribution of the Llama Materials.
6. Term and Termination. The term of this Agreement will commence upon your acceptance of this
Agreement or access to the Llama Materials and will continue in full force and effect until terminated in
accordance with the terms and conditions herein. Meta may terminate this Agreement if you are in
breach of any term or condition of this Agreement. Upon termination of this Agreement, you shall delete
and cease use of the Llama Materials. Sections 3, 4 and 7 shall survive the termination of this
Agreement.
7. Governing Law and Jurisdiction. This Agreement will be governed and construed under the laws of
the State of California without regard to choice of law principles, and the UN Convention on Contracts
for the International Sale of Goods does not apply to this Agreement. The courts of California shall have
exclusive jurisdiction of any dispute arising out of this Agreement.
### Meta Llama 3 Acceptable Use Policy
Meta is committed to promoting safe and fair use of its tools and features, including Meta Llama 3. If you
access or use Meta Llama 3, you agree to this Acceptable Use Policy (“Policy”). The most recent copy of
this policy can be found at [https://llama.meta.com/llama3/use-policy](https://llama.meta.com/llama3/use-policy)
#### Prohibited Uses
We want everyone to use Meta Llama 3 safely and responsibly. You agree you will not use, or allow
others to use, Meta Llama 3 to:
1. Violate the law or others’ rights, including to:
1. Engage in, promote, generate, contribute to, encourage, plan, incite, or further illegal or unlawful activity or content, such as:
1. Violence or terrorism
2. Exploitation or harm to children, including the solicitation, creation, acquisition, or dissemination of child exploitative content or failure to report Child Sexual Abuse Material
3. Human trafficking, exploitation, and sexual violence
4. The illegal distribution of information or materials to minors, including obscene materials, or failure to employ legally required age-gating in connection with such information or materials.
5. Sexual solicitation
6. Any other criminal activity
2. Engage in, promote, incite, or facilitate the harassment, abuse, threatening, or bullying of individuals or groups of individuals
3. Engage in, promote, incite, or facilitate discrimination or other unlawful or harmful conduct in the provision of employment, employment benefits, credit, housing, other economic benefits, or other essential goods and services
4. Engage in the unauthorized or unlicensed practice of any profession including, but not limited to, financial, legal, medical/health, or related professional practices
5. Collect, process, disclose, generate, or infer health, demographic, or other sensitive personal or private information about individuals without rights and consents required by applicable laws
6. Engage in or facilitate any action or generate any content that infringes, misappropriates, or otherwise violates any third-party rights, including the outputs or results of any products or services using the Llama Materials
7. Create, generate, or facilitate the creation of malicious code, malware, computer viruses or do anything else that could disable, overburden, interfere with or impair the proper working, integrity, operation or appearance of a website or computer system
2. Engage in, promote, incite, facilitate, or assist in the planning or development of activities that present a risk of death or bodily harm to individuals, including use of Meta Llama 3 related to the following:
1. Military, warfare, nuclear industries or applications, espionage, use for materials or activities that are subject to the International Traffic Arms Regulations (ITAR) maintained by the United States Department of State
2. Guns and illegal weapons (including weapon development)
3. Illegal drugs and regulated/controlled substances
4. Operation of critical infrastructure, transportation technologies, or heavy machinery
5. Self-harm or harm to others, including suicide, cutting, and eating disorders
6. Any content intended to incite or promote violence, abuse, or any infliction of bodily harm to an individual
3. Intentionally deceive or mislead others, including use of Meta Llama 3 related to the following:
1. Generating, promoting, or furthering fraud or the creation or promotion of disinformation
2. Generating, promoting, or furthering defamatory content, including the creation of defamatory statements, images, or other content
3. Generating, promoting, or further distributing spam
4. Impersonating another individual without consent, authorization, or legal right
5. Representing that the use of Meta Llama 3 or outputs are human-generated
6. Generating or facilitating false online engagement, including fake reviews and other means of fake online engagement
4. Fail to appropriately disclose to end users any known dangers of your AI system
Please report any violation of this Policy, software “bug,” or other problems that could lead to a violation
of this Policy through one of the following means:
* Reporting issues with the model: [https://github.com/meta-llama/llama3](https://github.com/meta-llama/llama3)
* Reporting risky content generated by the model:
developers.facebook.com/llama_output_feedback
* Reporting bugs and security concerns: facebook.com/whitehat/info
* Reporting violations of the Acceptable Use Policy or unlicensed uses of Meta Llama 3: [email protected]
extra_gated_fields:
First Name: text
Last Name: text
Date of birth: date_picker
Country: country
Affiliation: text
geo: ip_location
By clicking Submit below I accept the terms of the license and acknowledge that the information I provide will be collected stored processed and shared in accordance with the Meta Privacy Policy: checkbox
extra_gated_description: The information you provide will be collected, stored, processed and shared in accordance with the [Meta Privacy Policy](https://www.facebook.com/privacy/policy/).
extra_gated_button_content: Submit
widget:
- example_title: Winter holidays
messages:
- role: system
content: You are a helpful and honest assistant. Please, respond concisely and truthfully.
- role: user
content: Can you recommend a good destination for Winter holidays?
- example_title: Programming assistant
messages:
- role: system
content: You are a helpful and honest code and programming assistant. Please, respond concisely and truthfully.
- role: user
content: Write a function that computes the nth fibonacci number.
inference:
parameters:
max_new_tokens: 300
stop:
- <|end_of_text|>
- <|eot_id|>
---
# Meta-Llama-3-70B-Instruct-GGUF
- This is GGUF quantized version of [meta-llama/Meta-Llama-3-70B-Instruct](https://huggingface.co/meta-llama/Meta-Llama-3-70B-Instruct) created using llama.cpp
- **Re-uploaded with new end token**
## Model Details
Meta developed and released the Meta Llama 3 family of large language models (LLMs), a collection of pretrained and instruction tuned generative text models in 8 and 70B sizes. The Llama 3 instruction tuned models are optimized for dialogue use cases and outperform many of the available open source chat models on common industry benchmarks. Further, in developing these models, we took great care to optimize helpfulness and safety.
**Model developers** Meta
**Variations** Llama 3 comes in two sizes — 8B and 70B parameters — in pre-trained and instruction tuned variants.
**Input** Models input text only.
**Output** Models generate text and code only.
**Model Architecture** Llama 3 is an auto-regressive language model that uses an optimized transformer architecture. The tuned versions use supervised fine-tuning (SFT) and reinforcement learning with human feedback (RLHF) to align with human preferences for helpfulness and safety.
<table>
<tr>
<td>
</td>
<td><strong>Training Data</strong>
</td>
<td><strong>Params</strong>
</td>
<td><strong>Context length</strong>
</td>
<td><strong>GQA</strong>
</td>
<td><strong>Token count</strong>
</td>
<td><strong>Knowledge cutoff</strong>
</td>
</tr>
<tr>
<td rowspan="2" >Llama 3
</td>
<td rowspan="2" >A new mix of publicly available online data.
</td>
<td>8B
</td>
<td>8k
</td>
<td>Yes
</td>
<td rowspan="2" >15T+
</td>
<td>March, 2023
</td>
</tr>
<tr>
<td>70B
</td>
<td>8k
</td>
<td>Yes
</td>
<td>December, 2023
</td>
</tr>
</table>
**Llama 3 family of models**. Token counts refer to pretraining data only. Both the 8 and 70B versions use Grouped-Query Attention (GQA) for improved inference scalability.
**Model Release Date** April 18, 2024.
**Status** This is a static model trained on an offline dataset. Future versions of the tuned models will be released as we improve model safety with community feedback.
**License** A custom commercial license is available at: [https://llama.meta.com/llama3/license](https://llama.meta.com/llama3/license)
Where to send questions or comments about the model Instructions on how to provide feedback or comments on the model can be found in the model [README](https://github.com/meta-llama/llama3). For more technical information about generation parameters and recipes for how to use Llama 3 in applications, please go [here](https://github.com/meta-llama/llama-recipes).
## Intended Use
**Intended Use Cases** Llama 3 is intended for commercial and research use in English. Instruction tuned models are intended for assistant-like chat, whereas pretrained models can be adapted for a variety of natural language generation tasks.
**Out-of-scope** Use in any manner that violates applicable laws or regulations (including trade compliance laws). Use in any other way that is prohibited by the Acceptable Use Policy and Llama 3 Community License. Use in languages other than English**.
**Note: Developers may fine-tune Llama 3 models for languages beyond English provided they comply with the Llama 3 Community License and the Acceptable Use Policy.
## How to use
This repository contains two versions of Meta-Llama-3-70B-Instruct, for use with transformers and with the original `llama3` codebase.
### Use with transformers
See the snippet below for usage with Transformers:
```python
import transformers
import torch
model_id = "meta-llama/Meta-Llama-3-70B-Instruct"
pipeline = transformers.pipeline(
"text-generation",
model=model_id,
model_kwargs={"torch_dtype": torch.bfloat16},
device="auto",
)
messages = [
{"role": "system", "content": "You are a pirate chatbot who always responds in pirate speak!"},
{"role": "user", "content": "Who are you?"},
]
prompt = pipeline.tokenizer.apply_chat_template(
messages,
tokenize=False,
add_generation_prompt=True
)
terminators = [
pipeline.tokenizer.eos_token_id,
pipeline.tokenizer.convert_tokens_to_ids("<|eot_id|>")
]
outputs = pipeline(
prompt,
max_new_tokens=256,
eos_token_id=terminators,
do_sample=True,
temperature=0.6,
top_p=0.9,
)
print(outputs[0]["generated_text"][len(prompt):])
```
### Use with `llama3`
Please, follow the instructions in the [repository](https://github.com/meta-llama/llama3).
To download Original checkpoints, see the example command below leveraging `huggingface-cli`:
```
huggingface-cli download meta-llama/Meta-Llama-3-70B-Instruct --include "original/*" --local-dir Meta-Llama-3-70B-Instruct
```
For Hugging Face support, we recommend using transformers or TGI, but a similar command works.
## Hardware and Software
**Training Factors** We used custom training libraries, Meta's Research SuperCluster, and production clusters for pretraining. Fine-tuning, annotation, and evaluation were also performed on third-party cloud compute.
**Carbon Footprint Pretraining utilized a cumulative** 7.7M GPU hours of computation on hardware of type H100-80GB (TDP of 700W). Estimated total emissions were 2290 tCO2eq, 100% of which were offset by Meta’s sustainability program.
<table>
<tr>
<td>
</td>
<td><strong>Time (GPU hours)</strong>
</td>
<td><strong>Power Consumption (W)</strong>
</td>
<td><strong>Carbon Emitted(tCO2eq)</strong>
</td>
</tr>
<tr>
<td>Llama 3 8B
</td>
<td>1.3M
</td>
<td>700
</td>
<td>390
</td>
</tr>
<tr>
<td>Llama 3 70B
</td>
<td>6.4M
</td>
<td>700
</td>
<td>1900
</td>
</tr>
<tr>
<td>Total
</td>
<td>7.7M
</td>
<td>
</td>
<td>2290
</td>
</tr>
</table>
**CO2 emissions during pre-training**. Time: total GPU time required for training each model. Power Consumption: peak power capacity per GPU device for the GPUs used adjusted for power usage efficiency. 100% of the emissions are directly offset by Meta's sustainability program, and because we are openly releasing these models, the pretraining costs do not need to be incurred by others.
## Training Data
**Overview** Llama 3 was pretrained on over 15 trillion tokens of data from publicly available sources. The fine-tuning data includes publicly available instruction datasets, as well as over 10M human-annotated examples. Neither the pretraining nor the fine-tuning datasets include Meta user data.
**Data Freshness** The pretraining data has a cutoff of March 2023 for the 7B and December 2023 for the 70B models respectively.
## Benchmarks
In this section, we report the results for Llama 3 models on standard automatic benchmarks. For all the evaluations, we use our internal evaluations library. For details on the methodology see [here](https://github.com/meta-llama/llama3/blob/main/eval_methodology.md).
### Base pretrained models
<table>
<tr>
<td><strong>Category</strong>
</td>
<td><strong>Benchmark</strong>
</td>
<td><strong>Llama 3 8B</strong>
</td>
<td><strong>Llama2 7B</strong>
</td>
<td><strong>Llama2 13B</strong>
</td>
<td><strong>Llama 3 70B</strong>
</td>
<td><strong>Llama2 70B</strong>
</td>
</tr>
<tr>
<td rowspan="6" >General
</td>
<td>MMLU (5-shot)
</td>
<td>66.6
</td>
<td>45.7
</td>
<td>53.8
</td>
<td>79.5
</td>
<td>69.7
</td>
</tr>
<tr>
<td>AGIEval English (3-5 shot)
</td>
<td>45.9
</td>
<td>28.8
</td>
<td>38.7
</td>
<td>63.0
</td>
<td>54.8
</td>
</tr>
<tr>
<td>CommonSenseQA (7-shot)
</td>
<td>72.6
</td>
<td>57.6
</td>
<td>67.6
</td>
<td>83.8
</td>
<td>78.7
</td>
</tr>
<tr>
<td>Winogrande (5-shot)
</td>
<td>76.1
</td>
<td>73.3
</td>
<td>75.4
</td>
<td>83.1
</td>
<td>81.8
</td>
</tr>
<tr>
<td>BIG-Bench Hard (3-shot, CoT)
</td>
<td>61.1
</td>
<td>38.1
</td>
<td>47.0
</td>
<td>81.3
</td>
<td>65.7
</td>
</tr>
<tr>
<td>ARC-Challenge (25-shot)
</td>
<td>78.6
</td>
<td>53.7
</td>
<td>67.6
</td>
<td>93.0
</td>
<td>85.3
</td>
</tr>
<tr>
<td>Knowledge reasoning
</td>
<td>TriviaQA-Wiki (5-shot)
</td>
<td>78.5
</td>
<td>72.1
</td>
<td>79.6
</td>
<td>89.7
</td>
<td>87.5
</td>
</tr>
<tr>
<td rowspan="4" >Reading comprehension
</td>
<td>SQuAD (1-shot)
</td>
<td>76.4
</td>
<td>72.2
</td>
<td>72.1
</td>
<td>85.6
</td>
<td>82.6
</td>
</tr>
<tr>
<td>QuAC (1-shot, F1)
</td>
<td>44.4
</td>
<td>39.6
</td>
<td>44.9
</td>
<td>51.1
</td>
<td>49.4
</td>
</tr>
<tr>
<td>BoolQ (0-shot)
</td>
<td>75.7
</td>
<td>65.5
</td>
<td>66.9
</td>
<td>79.0
</td>
<td>73.1
</td>
</tr>
<tr>
<td>DROP (3-shot, F1)
</td>
<td>58.4
</td>
<td>37.9
</td>
<td>49.8
</td>
<td>79.7
</td>
<td>70.2
</td>
</tr>
</table>
### Instruction tuned models
<table>
<tr>
<td><strong>Benchmark</strong>
</td>
<td><strong>Llama 3 8B</strong>
</td>
<td><strong>Llama 2 7B</strong>
</td>
<td><strong>Llama 2 13B</strong>
</td>
<td><strong>Llama 3 70B</strong>
</td>
<td><strong>Llama 2 70B</strong>
</td>
</tr>
<tr>
<td>MMLU (5-shot)
</td>
<td>68.4
</td>
<td>34.1
</td>
<td>47.8
</td>
<td>82.0
</td>
<td>52.9
</td>
</tr>
<tr>
<td>GPQA (0-shot)
</td>
<td>34.2
</td>
<td>21.7
</td>
<td>22.3
</td>
<td>39.5
</td>
<td>21.0
</td>
</tr>
<tr>
<td>HumanEval (0-shot)
</td>
<td>62.2
</td>
<td>7.9
</td>
<td>14.0
</td>
<td>81.7
</td>
<td>25.6
</td>
</tr>
<tr>
<td>GSM-8K (8-shot, CoT)
</td>
<td>79.6
</td>
<td>25.7
</td>
<td>77.4
</td>
<td>93.0
</td>
<td>57.5
</td>
</tr>
<tr>
<td>MATH (4-shot, CoT)
</td>
<td>30.0
</td>
<td>3.8
</td>
<td>6.7
</td>
<td>50.4
</td>
<td>11.6
</td>
</tr>
</table>
### Responsibility & Safety
We believe that an open approach to AI leads to better, safer products, faster innovation, and a bigger overall market. We are committed to Responsible AI development and took a series of steps to limit misuse and harm and support the open source community.
Foundation models are widely capable technologies that are built to be used for a diverse range of applications. They are not designed to meet every developer preference on safety levels for all use cases, out-of-the-box, as those by their nature will differ across different applications.
Rather, responsible LLM-application deployment is achieved by implementing a series of safety best practices throughout the development of such applications, from the model pre-training, fine-tuning and the deployment of systems composed of safeguards to tailor the safety needs specifically to the use case and audience.
As part of the Llama 3 release, we updated our [Responsible Use Guide](https://llama.meta.com/responsible-use-guide/) to outline the steps and best practices for developers to implement model and system level safety for their application. We also provide a set of resources including [Meta Llama Guard 2](https://llama.meta.com/purple-llama/) and [Code Shield](https://llama.meta.com/purple-llama/) safeguards. These tools have proven to drastically reduce residual risks of LLM Systems, while maintaining a high level of helpfulness. We encourage developers to tune and deploy these safeguards according to their needs and we provide a [reference implementation](https://github.com/meta-llama/llama-recipes/tree/main/recipes/responsible_ai) to get you started.
#### Llama 3-Instruct
As outlined in the Responsible Use Guide, some trade-off between model helpfulness and model alignment is likely unavoidable. Developers should exercise discretion about how to weigh the benefits of alignment and helpfulness for their specific use case and audience. Developers should be mindful of residual risks when using Llama models and leverage additional safety tools as needed to reach the right safety bar for their use case.
<span style="text-decoration:underline;">Safety</span>
For our instruction tuned model, we conducted extensive red teaming exercises, performed adversarial evaluations and implemented safety mitigations techniques to lower residual risks. As with any Large Language Model, residual risks will likely remain and we recommend that developers assess these risks in the context of their use case. In parallel, we are working with the community to make AI safety benchmark standards transparent, rigorous and interpretable.
<span style="text-decoration:underline;">Refusals</span>
In addition to residual risks, we put a great emphasis on model refusals to benign prompts. Over-refusing not only can impact the user experience but could even be harmful in certain contexts as well. We’ve heard the feedback from the developer community and improved our fine tuning to ensure that Llama 3 is significantly less likely to falsely refuse to answer prompts than Llama 2.
We built internal benchmarks and developed mitigations to limit false refusals making Llama 3 our most helpful model to date.
#### Responsible release
In addition to responsible use considerations outlined above, we followed a rigorous process that requires us to take extra measures against misuse and critical risks before we make our release decision.
Misuse
If you access or use Llama 3, you agree to the Acceptable Use Policy. The most recent copy of this policy can be found at [https://llama.meta.com/llama3/use-policy/](https://llama.meta.com/llama3/use-policy/).
#### Critical risks
<span style="text-decoration:underline;">CBRNE</span> (Chemical, Biological, Radiological, Nuclear, and high yield Explosives)
We have conducted a two fold assessment of the safety of the model in this area:
* Iterative testing during model training to assess the safety of responses related to CBRNE threats and other adversarial risks.
* Involving external CBRNE experts to conduct an uplift test assessing the ability of the model to accurately provide expert knowledge and reduce barriers to potential CBRNE misuse, by reference to what can be achieved using web search (without the model).
### <span style="text-decoration:underline;">Cyber Security </span>
We have evaluated Llama 3 with CyberSecEval, Meta’s cybersecurity safety eval suite, measuring Llama 3’s propensity to suggest insecure code when used as a coding assistant, and Llama 3’s propensity to comply with requests to help carry out cyber attacks, where attacks are defined by the industry standard MITRE ATT&CK cyber attack ontology. On our insecure coding and cyber attacker helpfulness tests, Llama 3 behaved in the same range or safer than models of [equivalent coding capability](https://huggingface.co/spaces/facebook/CyberSecEval).
### <span style="text-decoration:underline;">Child Safety</span>
Child Safety risk assessments were conducted using a team of experts, to assess the model’s capability to produce outputs that could result in Child Safety risks and inform on any necessary and appropriate risk mitigations via fine tuning. We leveraged those expert red teaming sessions to expand the coverage of our evaluation benchmarks through Llama 3 model development. For Llama 3, we conducted new in-depth sessions using objective based methodologies to assess the model risks along multiple attack vectors. We also partnered with content specialists to perform red teaming exercises assessing potentially violating content while taking account of market specific nuances or experiences.
### Community
Generative AI safety requires expertise and tooling, and we believe in the strength of the open community to accelerate its progress. We are active members of open consortiums, including the AI Alliance, Partnership in AI and MLCommons, actively contributing to safety standardization and transparency. We encourage the community to adopt taxonomies like the MLCommons Proof of Concept evaluation to facilitate collaboration and transparency on safety and content evaluations. Our Purple Llama tools are open sourced for the community to use and widely distributed across ecosystem partners including cloud service providers. We encourage community contributions to our [Github repository](https://github.com/meta-llama/PurpleLlama).
Finally, we put in place a set of resources including an [output reporting mechanism](https://developers.facebook.com/llama_output_feedback) and [bug bounty program](https://www.facebook.com/whitehat) to continuously improve the Llama technology with the help of the community.
## Ethical Considerations and Limitations
The core values of Llama 3 are openness, inclusivity and helpfulness. It is meant to serve everyone, and to work for a wide range of use cases. It is thus designed to be accessible to people across many different backgrounds, experiences and perspectives. Llama 3 addresses users and their needs as they are, without insertion unnecessary judgment or normativity, while reflecting the understanding that even content that may appear problematic in some cases can serve valuable purposes in others. It respects the dignity and autonomy of all users, especially in terms of the values of free thought and expression that power innovation and progress.
But Llama 3 is a new technology, and like any new technology, there are risks associated with its use. Testing conducted to date has been in English, and has not covered, nor could it cover, all scenarios. For these reasons, as with all LLMs, Llama 3’s potential outputs cannot be predicted in advance, and the model may in some instances produce inaccurate, biased or other objectionable responses to user prompts. Therefore, before deploying any applications of Llama 3 models, developers should perform safety testing and tuning tailored to their specific applications of the model. As outlined in the Responsible Use Guide, we recommend incorporating [Purple Llama](https://github.com/facebookresearch/PurpleLlama) solutions into your workflows and specifically [Llama Guard](https://ai.meta.com/research/publications/llama-guard-llm-based-input-output-safeguard-for-human-ai-conversations/) which provides a base model to filter input and output prompts to layer system-level safety on top of model-level safety.
Please see the Responsible Use Guide available at [http://llama.meta.com/responsible-use-guide](http://llama.meta.com/responsible-use-guide)
## Citation instructions
@article{llama3modelcard,
title={Llama 3 Model Card},
author={AI@Meta},
year={2024},
url = {https://github.com/meta-llama/llama3/blob/main/MODEL_CARD.md}
}
## Contributors
Aaditya Singh; Aaron Grattafiori; Abhimanyu Dubey; Abhinav Jauhri; Abhinav Pandey; Abhishek Kadian; Adam Kelsey; Adi Gangidi; Ahmad Al-Dahle; Ahuva Goldstand; Aiesha Letman; Ajay Menon; Akhil Mathur; Alan Schelten; Alex Vaughan; Amy Yang; Andrei Lupu; Andres Alvarado; Andrew Gallagher; Andrew Gu; Andrew Ho; Andrew Poulton; Andrew Ryan; Angela Fan; Ankit Ramchandani; Anthony Hartshorn; Archi Mitra; Archie Sravankumar; Artem Korenev; Arun Rao; Ashley Gabriel; Ashwin Bharambe; Assaf Eisenman; Aston Zhang; Aurelien Rodriguez; Austen Gregerson; Ava Spataru; Baptiste Roziere; Ben Maurer; Benjamin Leonhardi; Bernie Huang; Bhargavi Paranjape; Bing Liu; Binh Tang; Bobbie Chern; Brani Stojkovic; Brian Fuller; Catalina Mejia Arenas; Chao Zhou; Charlotte Caucheteux; Chaya Nayak; Ching-Hsiang Chu; Chloe Bi; Chris Cai; Chris Cox; Chris Marra; Chris McConnell; Christian Keller; Christoph Feichtenhofer; Christophe Touret; Chunyang Wu; Corinne Wong; Cristian Canton Ferrer; Damien Allonsius; Daniel Kreymer; Daniel Haziza; Daniel Li; Danielle Pintz; Danny Livshits; Danny Wyatt; David Adkins; David Esiobu; David Xu; Davide Testuggine; Delia David; Devi Parikh; Dhruv Choudhary; Dhruv Mahajan; Diana Liskovich; Diego Garcia-Olano; Diego Perino; Dieuwke Hupkes; Dingkang Wang; Dustin Holland; Egor Lakomkin; Elina Lobanova; Xiaoqing Ellen Tan; Emily Dinan; Eric Smith; Erik Brinkman; Esteban Arcaute; Filip Radenovic; Firat Ozgenel; Francesco Caggioni; Frank Seide; Frank Zhang; Gabriel Synnaeve; Gabriella Schwarz; Gabrielle Lee; Gada Badeer; Georgia Anderson; Graeme Nail; Gregoire Mialon; Guan Pang; Guillem Cucurell; Hailey Nguyen; Hannah Korevaar; Hannah Wang; Haroun Habeeb; Harrison Rudolph; Henry Aspegren; Hu Xu; Hugo Touvron; Iga Kozlowska; Igor Molybog; Igor Tufanov; Iliyan Zarov; Imanol Arrieta Ibarra; Irina-Elena Veliche; Isabel Kloumann; Ishan Misra; Ivan Evtimov; Jacob Xu; Jade Copet; Jake Weissman; Jan Geffert; Jana Vranes; Japhet Asher; Jason Park; Jay Mahadeokar; Jean-Baptiste Gaya; Jeet Shah; Jelmer van der Linde; Jennifer Chan; Jenny Hong; Jenya Lee; Jeremy Fu; Jeremy Teboul; Jianfeng Chi; Jianyu Huang; Jie Wang; Jiecao Yu; Joanna Bitton; Joe Spisak; Joelle Pineau; Jon Carvill; Jongsoo Park; Joseph Rocca; Joshua Johnstun; Junteng Jia; Kalyan Vasuden Alwala; Kam Hou U; Kate Plawiak; Kartikeya Upasani; Kaushik Veeraraghavan; Ke Li; Kenneth Heafield; Kevin Stone; Khalid El-Arini; Krithika Iyer; Kshitiz Malik; Kuenley Chiu; Kunal Bhalla; Kyle Huang; Lakshya Garg; Lauren Rantala-Yeary; Laurens van der Maaten; Lawrence Chen; Leandro Silva; Lee Bell; Lei Zhang; Liang Tan; Louis Martin; Lovish Madaan; Luca Wehrstedt; Lukas Blecher; Luke de Oliveira; Madeline Muzzi; Madian Khabsa; Manav Avlani; Mannat Singh; Manohar Paluri; Mark Zuckerberg; Marcin Kardas; Martynas Mankus; Mathew Oldham; Mathieu Rita; Matthew Lennie; Maya Pavlova; Meghan Keneally; Melanie Kambadur; Mihir Patel; Mikayel Samvelyan; Mike Clark; Mike Lewis; Min Si; Mitesh Kumar Singh; Mo Metanat; Mona Hassan; Naman Goyal; Narjes Torabi; Nicolas Usunier; Nikolay Bashlykov; Nikolay Bogoychev; Niladri Chatterji; Ning Dong; Oliver Aobo Yang; Olivier Duchenne; Onur Celebi; Parth Parekh; Patrick Alrassy; Paul Saab; Pavan Balaji; Pedro Rittner; Pengchuan Zhang; Pengwei Li; Petar Vasic; Peter Weng; Polina Zvyagina; Prajjwal Bhargava; Pratik Dubal; Praveen Krishnan; Punit Singh Koura; Qing He; Rachel Rodriguez; Ragavan Srinivasan; Rahul Mitra; Ramon Calderer; Raymond Li; Robert Stojnic; Roberta Raileanu; Robin Battey; Rocky Wang; Rohit Girdhar; Rohit Patel; Romain Sauvestre; Ronnie Polidoro; Roshan Sumbaly; Ross Taylor; Ruan Silva; Rui Hou; Rui Wang; Russ Howes; Ruty Rinott; Saghar Hosseini; Sai Jayesh Bondu; Samyak Datta; Sanjay Singh; Sara Chugh; Sargun Dhillon; Satadru Pan; Sean Bell; Sergey Edunov; Shaoliang Nie; Sharan Narang; Sharath Raparthy; Shaun Lindsay; Sheng Feng; Sheng Shen; Shenghao Lin; Shiva Shankar; Shruti Bhosale; Shun Zhang; Simon Vandenhende; Sinong Wang; Seohyun Sonia Kim; Soumya Batra; Sten Sootla; Steve Kehoe; Suchin Gururangan; Sumit Gupta; Sunny Virk; Sydney Borodinsky; Tamar Glaser; Tamar Herman; Tamara Best; Tara Fowler; Thomas Georgiou; Thomas Scialom; Tianhe Li; Todor Mihaylov; Tong Xiao; Ujjwal Karn; Vedanuj Goswami; Vibhor Gupta; Vignesh Ramanathan; Viktor Kerkez; Vinay Satish Kumar; Vincent Gonguet; Vish Vogeti; Vlad Poenaru; Vlad Tiberiu Mihailescu; Vladan Petrovic; Vladimir Ivanov; Wei Li; Weiwei Chu; Wenhan Xiong; Wenyin Fu; Wes Bouaziz; Whitney Meers; Will Constable; Xavier Martinet; Xiaojian Wu; Xinbo Gao; Xinfeng Xie; Xuchao Jia; Yaelle Goldschlag; Yann LeCun; Yashesh Gaur; Yasmine Babaei; Ye Qi; Yenda Li; Yi Wen; Yiwen Song; Youngjin Nam; Yuchen Hao; Yuchen Zhang; Yun Wang; Yuning Mao; Yuzi He; Zacharie Delpierre Coudert; Zachary DeVito; Zahra Hankir; Zhaoduo Wen; Zheng Yan; Zhengxing Chen; Zhenyu Yang; Zoe Papakipos |
Lykon/absolute-reality-1.6525 | Lykon | 2023-12-06T00:56:26Z | 4,463 | 1 | diffusers | [
"diffusers",
"safetensors",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"art",
"artistic",
"absolute-realism",
"en",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-08-27T16:05:47Z | ---
language:
- en
license: creativeml-openrail-m
tags:
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- art
- artistic
- diffusers
- absolute-realism
duplicated_from: lykon/absolute-reality-1.6525
---
# Absolute reality 1 6525
`lykon/absolute-reality-1-6525` is a Stable Diffusion model that has been fine-tuned on [runwayml/stable-diffusion-v1-5](https://huggingface.co/runwayml/stable-diffusion-v1-5).
Please consider supporting me:
- on [Patreon](https://www.patreon.com/Lykon275)
- or [buy me a coffee](https://snipfeed.co/lykon)
## Diffusers
For more general information on how to run text-to-image models with 🧨 Diffusers, see [the docs](https://huggingface.co/docs/diffusers/using-diffusers/conditional_image_generation).
1. Installation
```
pip install diffusers transformers accelerate
```
2. Run
```py
from diffusers import AutoPipelineForText2Image, DEISMultistepScheduler
import torch
pipe = AutoPipelineForText2Image.from_pretrained('lykon/absolute-reality-1-6525', torch_dtype=torch.float16, variant="fp16")
pipe.scheduler = DEISMultistepScheduler.from_config(pipe.scheduler.config)
pipe = pipe.to("cuda")
prompt = "portrait photo of muscular bearded guy in a worn mech suit, light bokeh, intricate, steel metal, elegant, sharp focus, soft lighting, vibrant colors"
generator = torch.manual_seed(33)
image = pipe(prompt, generator=generator, num_inference_steps=25).images[0]
image.save("./image.png")
```
 |
mradermacher/Llama-Guard-2-8B-German-i1-GGUF | mradermacher | 2024-06-13T18:16:43Z | 4,462 | 0 | transformers | [
"transformers",
"gguf",
"facebook",
"meta",
"pytorch",
"llama",
"llama-3",
"en",
"base_model:felfri/Llama-Guard-2-8B-German",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-13T15:46:43Z | ---
base_model: felfri/Llama-Guard-2-8B-German
extra_gated_button_content: Submit
extra_gated_fields:
Affiliation: text
? By clicking Submit below I accept the terms of the license and acknowledge that
the information I provide will be collected stored processed and shared in accordance
with the Meta Privacy Policy
: checkbox
Country: country
Date of birth: date_picker
First Name: text
Last Name: text
geo: ip_location
extra_gated_prompt: "### META LLAMA 3 COMMUNITY LICENSE AGREEMENT\nMeta Llama 3 Version
Release Date: April 18, 2024\n\"Agreement\" means the terms and conditions for use,
reproduction, distribution and modification of the Llama Materials set forth herein.\n\"Documentation\"
means the specifications, manuals and documentation accompanying Meta Llama 3 distributed
by Meta at https://llama.meta.com/get-started/.\n\"Licensee\" or \"you\" means you,
or your employer or any other person or entity (if you are entering into this Agreement
on such person or entity’s behalf), of the age required under applicable laws, rules
or regulations to provide legal consent and that has legal authority to bind your
employer or such other person or entity if you are entering in this Agreement on
their behalf.\n\"Meta Llama 3\" means the foundational large language models and
software and algorithms, including machine-learning model code, trained model weights,
inference-enabling code, training-enabling code, fine-tuning enabling code and other
elements of the foregoing distributed by Meta at https://llama.meta.com/llama-downloads.\n\"Llama
Materials\" means, collectively, Meta’s proprietary Meta Llama 3 and Documentation
(and any portion thereof) made available under this Agreement.\n\"Meta\" or \"we\"
means Meta Platforms Ireland Limited (if you are located in or, if you are an entity,
your principal place of business is in the EEA or Switzerland) and Meta Platforms,
Inc. (if you are located outside of the EEA or Switzerland).\n \n1. License Rights
and Redistribution.\na. Grant of Rights. You are granted a non-exclusive, worldwide,
non-transferable and royalty-free limited license under Meta’s intellectual property
or other rights owned by Meta embodied in the Llama Materials to use, reproduce,
distribute, copy, create derivative works of, and make modifications to the Llama
Materials.\nb. Redistribution and Use.\ni. If you distribute or make available the
Llama Materials (or any derivative works thereof), or a product or service that
uses any of them, including another AI model, you shall (A) provide a copy of this
Agreement with any such Llama Materials; and (B) prominently display “Built with
Meta Llama 3” on a related website, user interface, blogpost, about page, or product
documentation. If you use the Llama Materials to create, train, fine tune, or otherwise
improve an AI model, which is distributed or made available, you shall also include
“Llama 3” at the beginning of any such AI model name.\nii. If you receive Llama
Materials, or any derivative works thereof, from a Licensee as part of an integrated
end user product, then Section 2 of this Agreement will not apply to you.\niii.
You must retain in all copies of the Llama Materials that you distribute the following
attribution notice within a “Notice” text file distributed as a part of such copies:
“Meta Llama 3 is licensed under the Meta Llama 3 Community License, Copyright ©
Meta Platforms, Inc. All Rights Reserved.”\niv. Your use of the Llama Materials
must comply with applicable laws and regulations (including trade compliance laws
and regulations) and adhere to the Acceptable Use Policy for the Llama Materials
(available at https://llama.meta.com/llama3/use-policy), which is hereby incorporated
by reference into this Agreement.\nv. You will not use the Llama Materials or any
output or results of the Llama Materials to improve any other large language model
(excluding Meta Llama 3 or derivative works thereof).\n2. Additional Commercial
Terms. If, on the Meta Llama 3 version release date, the monthly active users of
the products or services made available by or for Licensee, or Licensee’s affiliates,
is greater than 700 million monthly active users in the preceding calendar month,
you must request a license from Meta, which Meta may grant to you in its sole discretion,
and you are not authorized to exercise any of the rights under this Agreement unless
or until Meta otherwise expressly grants you such rights.\n3. Disclaimer of Warranty.
UNLESS REQUIRED BY APPLICABLE LAW, THE LLAMA MATERIALS AND ANY OUTPUT AND RESULTS
THEREFROM ARE PROVIDED ON AN “AS IS” BASIS, WITHOUT WARRANTIES OF ANY KIND, AND
META DISCLAIMS ALL WARRANTIES OF ANY KIND, BOTH EXPRESS AND IMPLIED, INCLUDING,
WITHOUT LIMITATION, ANY WARRANTIES OF TITLE, NON-INFRINGEMENT, MERCHANTABILITY,
OR FITNESS FOR A PARTICULAR PURPOSE. YOU ARE SOLELY RESPONSIBLE FOR DETERMINING
THE APPROPRIATENESS OF USING OR REDISTRIBUTING THE LLAMA MATERIALS AND ASSUME ANY
RISKS ASSOCIATED WITH YOUR USE OF THE LLAMA MATERIALS AND ANY OUTPUT AND RESULTS.\n4.
Limitation of Liability. IN NO EVENT WILL META OR ITS AFFILIATES BE LIABLE UNDER
ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, TORT, NEGLIGENCE, PRODUCTS LIABILITY,
OR OTHERWISE, ARISING OUT OF THIS AGREEMENT, FOR ANY LOST PROFITS OR ANY INDIRECT,
SPECIAL, CONSEQUENTIAL, INCIDENTAL, EXEMPLARY OR PUNITIVE DAMAGES, EVEN IF META
OR ITS AFFILIATES HAVE BEEN ADVISED OF THE POSSIBILITY OF ANY OF THE FOREGOING.\n5.
Intellectual Property.\na. No trademark licenses are granted under this Agreement,
and in connection with the Llama Materials, neither Meta nor Licensee may use any
name or mark owned by or associated with the other or any of its affiliates, except
as required for reasonable and customary use in describing and redistributing the
Llama Materials or as set forth in this Section 5(a). Meta hereby grants you a license
to use “Llama 3” (the “Mark”) solely as required to comply with the last sentence
of Section 1.b.i. You will comply with Meta’s brand guidelines (currently accessible
at https://about.meta.com/brand/resources/meta/company-brand/ ). All goodwill arising
out of your use of the Mark will inure to the benefit of Meta.\nb. Subject to Meta’s
ownership of Llama Materials and derivatives made by or for Meta, with respect to
any derivative works and modifications of the Llama Materials that are made by you,
as between you and Meta, you are and will be the owner of such derivative works
and modifications.\nc. If you institute litigation or other proceedings against
Meta or any entity (including a cross-claim or counterclaim in a lawsuit) alleging
that the Llama Materials or Meta Llama 3 outputs or results, or any portion of any
of the foregoing, constitutes infringement of intellectual property or other rights
owned or licensable by you, then any licenses granted to you under this Agreement
shall terminate as of the date such litigation or claim is filed or instituted.
You will indemnify and hold harmless Meta from and against any claim by any third
party arising out of or related to your use or distribution of the Llama Materials.\n6.
Term and Termination. The term of this Agreement will commence upon your acceptance
of this Agreement or access to the Llama Materials and will continue in full force
and effect until terminated in accordance with the terms and conditions herein.
Meta may terminate this Agreement if you are in breach of any term or condition
of this Agreement. Upon termination of this Agreement, you shall delete and cease
use of the Llama Materials. Sections 3, 4 and 7 shall survive the termination of
this Agreement.\n7. Governing Law and Jurisdiction. This Agreement will be governed
and construed under the laws of the State of California without regard to choice
of law principles, and the UN Convention on Contracts for the International Sale
of Goods does not apply to this Agreement. The courts of California shall have exclusive
jurisdiction of any dispute arising out of this Agreement.\n### Meta Llama 3 Acceptable
Use Policy\nMeta is committed to promoting safe and fair use of its tools and features,
including Meta Llama 3. If you access or use Meta Llama 3, you agree to this Acceptable
Use Policy (“Policy”). The most recent copy of this policy can be found at [https://llama.meta.com/llama3/use-policy](https://llama.meta.com/llama3/use-policy)\n####
Prohibited Uses\nWe want everyone to use Meta Llama 3 safely and responsibly. You
agree you will not use, or allow others to use, Meta Llama 3 to: 1. Violate the
law or others’ rights, including to:\n 1. Engage in, promote, generate, contribute
to, encourage, plan, incite, or further illegal or unlawful activity or content,
such as:\n 1. Violence or terrorism\n 2. Exploitation or harm to children,
including the solicitation, creation, acquisition, or dissemination of child exploitative
content or failure to report Child Sexual Abuse Material\n 3. Human trafficking,
exploitation, and sexual violence\n 4. The illegal distribution of information
or materials to minors, including obscene materials, or failure to employ legally
required age-gating in connection with such information or materials.\n 5.
Sexual solicitation\n 6. Any other criminal activity\n 2. Engage in, promote,
incite, or facilitate the harassment, abuse, threatening, or bullying of individuals
or groups of individuals\n 3. Engage in, promote, incite, or facilitate discrimination
or other unlawful or harmful conduct in the provision of employment, employment
benefits, credit, housing, other economic benefits, or other essential goods and
services\n 4. Engage in the unauthorized or unlicensed practice of any profession
including, but not limited to, financial, legal, medical/health, or related professional
practices\n 5. Collect, process, disclose, generate, or infer health, demographic,
or other sensitive personal or private information about individuals without rights
and consents required by applicable laws\n 6. Engage in or facilitate any action
or generate any content that infringes, misappropriates, or otherwise violates any
third-party rights, including the outputs or results of any products or services
using the Llama Materials\n 7. Create, generate, or facilitate the creation of
malicious code, malware, computer viruses or do anything else that could disable,
overburden, interfere with or impair the proper working, integrity, operation or
appearance of a website or computer system\n2. Engage in, promote, incite, facilitate,
or assist in the planning or development of activities that present a risk of death
or bodily harm to individuals, including use of Meta Llama 3 related to the following:\n
\ 1. Military, warfare, nuclear industries or applications, espionage, use for
materials or activities that are subject to the International Traffic Arms Regulations
(ITAR) maintained by the United States Department of State\n 2. Guns and illegal
weapons (including weapon development)\n 3. Illegal drugs and regulated/controlled
substances\n 4. Operation of critical infrastructure, transportation technologies,
or heavy machinery\n 5. Self-harm or harm to others, including suicide, cutting,
and eating disorders\n 6. Any content intended to incite or promote violence,
abuse, or any infliction of bodily harm to an individual\n3. Intentionally deceive
or mislead others, including use of Meta Llama 3 related to the following:\n 1.
Generating, promoting, or furthering fraud or the creation or promotion of disinformation\n
\ 2. Generating, promoting, or furthering defamatory content, including the creation
of defamatory statements, images, or other content\n 3. Generating, promoting,
or further distributing spam\n 4. Impersonating another individual without consent,
authorization, or legal right\n 5. Representing that the use of Meta Llama 3
or outputs are human-generated\n 6. Generating or facilitating false online engagement,
including fake reviews and other means of fake online engagement\n4. Fail to appropriately
disclose to end users any known dangers of your AI system\nPlease report any violation
of this Policy, software “bug,” or other problems that could lead to a violation
of this Policy through one of the following means:\n * Reporting issues with
the model: [https://github.com/meta-llama/llama3](https://github.com/meta-llama/llama3)\n
\ * Reporting risky content generated by the model:\n developers.facebook.com/llama_output_feedback\n
\ * Reporting bugs and security concerns: facebook.com/whitehat/info\n * Reporting
violations of the Acceptable Use Policy or unlicensed uses of Meta Llama 3: [email protected]"
language:
- en
library_name: transformers
license: llama3
quantized_by: mradermacher
tags:
- facebook
- meta
- pytorch
- llama
- llama-3
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/felfri/Llama-Guard-2-8B-German
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-Guard-2-8B-German-i1-GGUF/resolve/main/Llama-Guard-2-8B-German.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
timm/vit_base_patch16_224.augreg_in1k | timm | 2023-05-06T00:00:30Z | 4,460 | 1 | timm | [
"timm",
"pytorch",
"safetensors",
"image-classification",
"dataset:imagenet-1k",
"arxiv:2106.10270",
"arxiv:2010.11929",
"license:apache-2.0",
"region:us"
] | image-classification | 2022-12-22T07:24:52Z | ---
tags:
- image-classification
- timm
library_name: timm
license: apache-2.0
datasets:
- imagenet-1k
---
# Model card for vit_base_patch16_224.augreg_in1k
A Vision Transformer (ViT) image classification model. Trained on ImageNet-1k (with additional augmentation and regularization) in JAX by paper authors, ported to PyTorch by Ross Wightman.
## Model Details
- **Model Type:** Image classification / feature backbone
- **Model Stats:**
- Params (M): 86.6
- GMACs: 16.9
- Activations (M): 16.5
- Image size: 224 x 224
- **Papers:**
- How to train your ViT? Data, Augmentation, and Regularization in Vision Transformers: https://arxiv.org/abs/2106.10270
- An Image is Worth 16x16 Words: Transformers for Image Recognition at Scale: https://arxiv.org/abs/2010.11929v2
- **Dataset:** ImageNet-1k
- **Original:** https://github.com/google-research/vision_transformer
## Model Usage
### Image Classification
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model('vit_base_patch16_224.augreg_in1k', pretrained=True)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # unsqueeze single image into batch of 1
top5_probabilities, top5_class_indices = torch.topk(output.softmax(dim=1) * 100, k=5)
```
### Image Embeddings
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model(
'vit_base_patch16_224.augreg_in1k',
pretrained=True,
num_classes=0, # remove classifier nn.Linear
)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # output is (batch_size, num_features) shaped tensor
# or equivalently (without needing to set num_classes=0)
output = model.forward_features(transforms(img).unsqueeze(0))
# output is unpooled, a (1, 197, 768) shaped tensor
output = model.forward_head(output, pre_logits=True)
# output is a (1, num_features) shaped tensor
```
## Model Comparison
Explore the dataset and runtime metrics of this model in timm [model results](https://github.com/huggingface/pytorch-image-models/tree/main/results).
## Citation
```bibtex
@article{steiner2021augreg,
title={How to train your ViT? Data, Augmentation, and Regularization in Vision Transformers},
author={Steiner, Andreas and Kolesnikov, Alexander and and Zhai, Xiaohua and Wightman, Ross and Uszkoreit, Jakob and Beyer, Lucas},
journal={arXiv preprint arXiv:2106.10270},
year={2021}
}
```
```bibtex
@article{dosovitskiy2020vit,
title={An Image is Worth 16x16 Words: Transformers for Image Recognition at Scale},
author={Dosovitskiy, Alexey and Beyer, Lucas and Kolesnikov, Alexander and Weissenborn, Dirk and Zhai, Xiaohua and Unterthiner, Thomas and Dehghani, Mostafa and Minderer, Matthias and Heigold, Georg and Gelly, Sylvain and Uszkoreit, Jakob and Houlsby, Neil},
journal={ICLR},
year={2021}
}
```
```bibtex
@misc{rw2019timm,
author = {Ross Wightman},
title = {PyTorch Image Models},
year = {2019},
publisher = {GitHub},
journal = {GitHub repository},
doi = {10.5281/zenodo.4414861},
howpublished = {\url{https://github.com/huggingface/pytorch-image-models}}
}
```
|
unicamp-dl/ptt5-base-portuguese-vocab | unicamp-dl | 2024-04-10T17:48:42Z | 4,458 | 32 | transformers | [
"transformers",
"pytorch",
"tf",
"safetensors",
"t5",
"text2text-generation",
"tensorflow",
"pt",
"pt-br",
"dataset:brWaC",
"license:mit",
"autotrain_compatible",
"text-generation-inference",
"region:us"
] | text2text-generation | 2022-03-02T23:29:05Z | ---
language: pt
license: mit
tags:
- t5
- pytorch
- tensorflow
- pt
- pt-br
datasets:
- brWaC
widget:
- text: "Texto de exemplo em português"
inference: false
---
# Portuguese T5 (aka "PTT5")
## Introduction
PTT5 is a T5 model pretrained in the BrWac corpus, a large collection of web pages in Portuguese, improving T5's performance on Portuguese sentence similarity and entailment tasks. It's available in three sizes (small, base and large) and two vocabularies (Google's T5 original and ours, trained on Portuguese Wikipedia).
For further information or requests, please go to [PTT5 repository](https://github.com/unicamp-dl/PTT5).
## Available models
| Model | Size | #Params | Vocabulary |
| :-: | :-: | :-: | :-: |
| [unicamp-dl/ptt5-small-t5-vocab](https://huggingface.co/unicamp-dl/ptt5-small-t5-vocab) | small | 60M | Google's T5 |
| [unicamp-dl/ptt5-base-t5-vocab](https://huggingface.co/unicamp-dl/ptt5-base-t5-vocab) | base | 220M | Google's T5 |
| [unicamp-dl/ptt5-large-t5-vocab](https://huggingface.co/unicamp-dl/ptt5-large-t5-vocab) | large | 740M | Google's T5 |
| [unicamp-dl/ptt5-small-portuguese-vocab](https://huggingface.co/unicamp-dl/ptt5-small-portuguese-vocab) | small | 60M | Portuguese |
| **[unicamp-dl/ptt5-base-portuguese-vocab](https://huggingface.co/unicamp-dl/ptt5-base-portuguese-vocab)** **(Recommended)** | **base** | **220M** | **Portuguese** |
| [unicamp-dl/ptt5-large-portuguese-vocab](https://huggingface.co/unicamp-dl/ptt5-large-portuguese-vocab) | large | 740M | Portuguese |
## Usage
```python
# Tokenizer
from transformers import T5Tokenizer
# PyTorch (bare model, baremodel + language modeling head)
from transformers import T5Model, T5ForConditionalGeneration
# Tensorflow (bare model, baremodel + language modeling head)
from transformers import TFT5Model, TFT5ForConditionalGeneration
model_name = 'unicamp-dl/ptt5-base-portuguese-vocab'
tokenizer = T5Tokenizer.from_pretrained(model_name)
# PyTorch
model_pt = T5ForConditionalGeneration.from_pretrained(model_name)
# TensorFlow
model_tf = TFT5ForConditionalGeneration.from_pretrained(model_name)
```
# Citation
If you use PTT5, please cite:
@article{ptt5_2020,
title={PTT5: Pretraining and validating the T5 model on Brazilian Portuguese data},
author={Carmo, Diedre and Piau, Marcos and Campiotti, Israel and Nogueira, Rodrigo and Lotufo, Roberto},
journal={arXiv preprint arXiv:2008.09144},
year={2020}
}
|
keremberke/yolov8m-building-segmentation | keremberke | 2023-02-22T12:59:32Z | 4,458 | 1 | ultralytics | [
"ultralytics",
"tensorboard",
"v8",
"ultralyticsplus",
"yolov8",
"yolo",
"vision",
"image-segmentation",
"pytorch",
"awesome-yolov8-models",
"dataset:keremberke/satellite-building-segmentation",
"model-index",
"region:us"
] | image-segmentation | 2023-01-27T01:30:25Z |
---
tags:
- ultralyticsplus
- yolov8
- ultralytics
- yolo
- vision
- image-segmentation
- pytorch
- awesome-yolov8-models
library_name: ultralytics
library_version: 8.0.20
inference: false
datasets:
- keremberke/satellite-building-segmentation
model-index:
- name: keremberke/yolov8m-building-segmentation
results:
- task:
type: image-segmentation
dataset:
type: keremberke/satellite-building-segmentation
name: satellite-building-segmentation
split: validation
metrics:
- type: precision # since [email protected] is not available on hf.co/metrics
value: 0.62261 # min: 0.0 - max: 1.0
name: [email protected](box)
- type: precision # since [email protected] is not available on hf.co/metrics
value: 0.61275 # min: 0.0 - max: 1.0
name: [email protected](mask)
---
<div align="center">
<img width="640" alt="keremberke/yolov8m-building-segmentation" src="https://huggingface.co/keremberke/yolov8m-building-segmentation/resolve/main/thumbnail.jpg">
</div>
### Supported Labels
```
['Building']
```
### How to use
- Install [ultralyticsplus](https://github.com/fcakyon/ultralyticsplus):
```bash
pip install ultralyticsplus==0.0.21
```
- Load model and perform prediction:
```python
from ultralyticsplus import YOLO, render_result
# load model
model = YOLO('keremberke/yolov8m-building-segmentation')
# set model parameters
model.overrides['conf'] = 0.25 # NMS confidence threshold
model.overrides['iou'] = 0.45 # NMS IoU threshold
model.overrides['agnostic_nms'] = False # NMS class-agnostic
model.overrides['max_det'] = 1000 # maximum number of detections per image
# set image
image = 'https://github.com/ultralytics/yolov5/raw/master/data/images/zidane.jpg'
# perform inference
results = model.predict(image)
# observe results
print(results[0].boxes)
print(results[0].masks)
render = render_result(model=model, image=image, result=results[0])
render.show()
```
**More models available at: [awesome-yolov8-models](https://yolov8.xyz)** |
digiplay/AingDiffusion7.5 | digiplay | 2023-11-02T13:54:16Z | 4,458 | 3 | diffusers | [
"diffusers",
"safetensors",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"license:other",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-11-02T13:28:15Z | ---
license: other
tags:
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- diffusers
inference: true
---
https://civitai.com/models/34553?modelVersionId=116371
|
Infinirc/Infinirc-Llama3-8B-GGUF-fp16-v1.0 | Infinirc | 2024-06-30T06:17:56Z | 4,458 | 1 | transformers | [
"transformers",
"gguf",
"zhtw",
"zh",
"en",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-30T04:02:01Z | ---
license: llama3
language:
- zh
- en
library_name: transformers
tags:
- zhtw
---
# Infinirc-Llama3-8B-GGUF-fp16-v1.0
## 模型詳情
**開發者**:陳昭儒 [Infinirc.com](https://infinirc.com)
**模型版本**:1.0
**模型類型**:Llama3
**訓練數據源**:採用與台灣文化相關的資料集,包括台灣新聞、文學作品、網路文章等。
## 目的和用途
這款 Llama3 8B 模型專為更好地理解和生成與台灣文化相關的文本而設計和微調。目標是提供一個能捕捉台灣特有文化元素和語言習慣的強大語言模型,適用於文本生成、自動回答等多種應用。
## 模型架構
**基礎模型**:Llama3 8B
**調整策略**:對模型進行微調,使用與台灣文化相關的具體資料集,以增強模型對於本地化內容的理解和生成能力。
## 性能指標
該模型在一系列 NLP 基準測試中展示了優異的性能,特別是在文本生成和語意理解方面具有較高的準確度。
## 使用和限制
**使用建議**:推薦於需要深度理解或生成與台灣文化相關內容的應用場景中使用。
**注意**:僅供學術用途,不可商用。
## 風險與倫理考量
使用本模型時應注意確保生成的內容不包含歧視性或有害信息。模型的開發和使用應遵循倫理準則和社會責任。
## 聯絡方式
如有任何問題或需要進一步的信息,請透過下方聯絡方式與我聯繫:
Email: [[email protected]](mailto:[email protected])
網站: [https://infinirc.com](https://infinirc.com)
|
dbmdz/bert-base-italian-xxl-uncased | dbmdz | 2023-09-06T22:18:38Z | 4,455 | 8 | transformers | [
"transformers",
"pytorch",
"tf",
"jax",
"safetensors",
"bert",
"fill-mask",
"it",
"dataset:wikipedia",
"license:mit",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | fill-mask | 2022-03-02T23:29:05Z | ---
language: it
license: mit
datasets:
- wikipedia
---
# 🤗 + 📚 dbmdz BERT and ELECTRA models
In this repository the MDZ Digital Library team (dbmdz) at the Bavarian State
Library open sources Italian BERT and ELECTRA models 🎉
# Italian BERT
The source data for the Italian BERT model consists of a recent Wikipedia dump and
various texts from the [OPUS corpora](http://opus.nlpl.eu/) collection. The final
training corpus has a size of 13GB and 2,050,057,573 tokens.
For sentence splitting, we use NLTK (faster compared to spacy).
Our cased and uncased models are training with an initial sequence length of 512
subwords for ~2-3M steps.
For the XXL Italian models, we use the same training data from OPUS and extend
it with data from the Italian part of the [OSCAR corpus](https://traces1.inria.fr/oscar/).
Thus, the final training corpus has a size of 81GB and 13,138,379,147 tokens.
Note: Unfortunately, a wrong vocab size was used when training the XXL models.
This explains the mismatch of the "real" vocab size of 31102, compared to the
vocab size specified in `config.json`. However, the model is working and all
evaluations were done under those circumstances.
See [this issue](https://github.com/dbmdz/berts/issues/7) for more information.
The Italian ELECTRA model was trained on the "XXL" corpus for 1M steps in total using a batch
size of 128. We pretty much following the ELECTRA training procedure as used for
[BERTurk](https://github.com/stefan-it/turkish-bert/tree/master/electra).
## Model weights
Currently only PyTorch-[Transformers](https://github.com/huggingface/transformers)
compatible weights are available. If you need access to TensorFlow checkpoints,
please raise an issue!
| Model | Downloads
| ---------------------------------------------------- | ---------------------------------------------------------------------------------------------------------------
| `dbmdz/bert-base-italian-cased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-cased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-cased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-cased/vocab.txt)
| `dbmdz/bert-base-italian-uncased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-uncased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-uncased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-uncased/vocab.txt)
| `dbmdz/bert-base-italian-xxl-cased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-cased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-cased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-cased/vocab.txt)
| `dbmdz/bert-base-italian-xxl-uncased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-uncased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-uncased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-uncased/vocab.txt)
| `dbmdz/electra-base-italian-xxl-cased-discriminator` | [`config.json`](https://s3.amazonaws.com/models.huggingface.co/bert/dbmdz/electra-base-italian-xxl-cased-discriminator/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-discriminator/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-discriminator/vocab.txt)
| `dbmdz/electra-base-italian-xxl-cased-generator` | [`config.json`](https://s3.amazonaws.com/models.huggingface.co/bert/dbmdz/electra-base-italian-xxl-cased-generator/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-generator/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-generator/vocab.txt)
## Results
For results on downstream tasks like NER or PoS tagging, please refer to
[this repository](https://github.com/stefan-it/italian-bertelectra).
## Usage
With Transformers >= 2.3 our Italian BERT models can be loaded like:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/bert-base-italian-cased"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
```
To load the (recommended) Italian XXL BERT models, just use:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/bert-base-italian-xxl-cased"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
```
To load the Italian XXL ELECTRA model (discriminator), just use:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/electra-base-italian-xxl-cased-discriminator"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelWithLMHead.from_pretrained(model_name)
```
# Huggingface model hub
All models are available on the [Huggingface model hub](https://huggingface.co/dbmdz).
# Contact (Bugs, Feedback, Contribution and more)
For questions about our BERT/ELECTRA models just open an issue
[here](https://github.com/dbmdz/berts/issues/new) 🤗
# Acknowledgments
Research supported with Cloud TPUs from Google's TensorFlow Research Cloud (TFRC).
Thanks for providing access to the TFRC ❤️
Thanks to the generous support from the [Hugging Face](https://huggingface.co/) team,
it is possible to download both cased and uncased models from their S3 storage 🤗
|
redstonehero/ReV_Animated_Inpainting | redstonehero | 2023-04-29T05:51:56Z | 4,454 | 0 | diffusers | [
"diffusers",
"text-to-image",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-04-29T04:27:15Z | ---
license: creativeml-openrail-m
library_name: diffusers
pipeline_tag: text-to-image
--- |
stablediffusionapi/strongly-styled | stablediffusionapi | 2023-11-27T07:47:51Z | 4,453 | 1 | diffusers | [
"diffusers",
"stablediffusionapi.com",
"stable-diffusion-api",
"text-to-image",
"ultra-realistic",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionXLPipeline",
"region:us"
] | text-to-image | 2023-11-27T07:42:59Z | ---
license: creativeml-openrail-m
tags:
- stablediffusionapi.com
- stable-diffusion-api
- text-to-image
- ultra-realistic
pinned: true
---
# Strongly styled API Inference
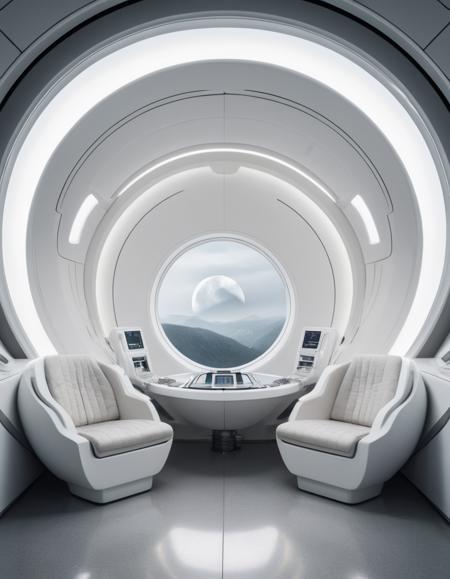
## Get API Key
Get API key from [Stable Diffusion API](http://stablediffusionapi.com/), No Payment needed.
Replace Key in below code, change **model_id** to "strongly-styled"
Coding in PHP/Node/Java etc? Have a look at docs for more code examples: [View docs](https://stablediffusionapi.com/docs)
Try model for free: [Generate Images](https://stablediffusionapi.com/models/strongly-styled)
Model link: [View model](https://stablediffusionapi.com/models/strongly-styled)
Credits: [View credits](https://civitai.com/?query=Strongly%20styled)
View all models: [View Models](https://stablediffusionapi.com/models)
import requests
import json
url = "https://stablediffusionapi.com/api/v4/dreambooth"
payload = json.dumps({
"key": "your_api_key",
"model_id": "strongly-styled",
"prompt": "ultra realistic close up portrait ((beautiful pale cyberpunk female with heavy black eyeliner)), blue eyes, shaved side haircut, hyper detail, cinematic lighting, magic neon, dark red city, Canon EOS R3, nikon, f/1.4, ISO 200, 1/160s, 8K, RAW, unedited, symmetrical balance, in-frame, 8K",
"negative_prompt": "painting, extra fingers, mutated hands, poorly drawn hands, poorly drawn face, deformed, ugly, blurry, bad anatomy, bad proportions, extra limbs, cloned face, skinny, glitchy, double torso, extra arms, extra hands, mangled fingers, missing lips, ugly face, distorted face, extra legs, anime",
"width": "512",
"height": "512",
"samples": "1",
"num_inference_steps": "30",
"safety_checker": "no",
"enhance_prompt": "yes",
"seed": None,
"guidance_scale": 7.5,
"multi_lingual": "no",
"panorama": "no",
"self_attention": "no",
"upscale": "no",
"embeddings": "embeddings_model_id",
"lora": "lora_model_id",
"webhook": None,
"track_id": None
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
> Use this coupon code to get 25% off **DMGG0RBN** |
cardiffnlp/twitter-roberta-large-hate-latest | cardiffnlp | 2024-03-07T12:17:23Z | 4,451 | 2 | transformers | [
"transformers",
"safetensors",
"roberta",
"text-classification",
"en",
"dataset:cardiffnlp/super_tweeteval",
"arxiv:2310.14757",
"license:mit",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | text-classification | 2023-11-09T10:42:56Z | ---
language:
- en
license: mit
datasets:
- cardiffnlp/super_tweeteval
pipeline_tag: text-classification
---
# cardiffnlp/twitter-roberta-large-hate-latest
This is a RoBERTa-large model trained on 154M tweets until the end of December 2022 and finetuned for hate speech detection (multiclass classification) on the _TweetHate_ dataset of [SuperTweetEval](https://huggingface.co/datasets/cardiffnlp/super_tweeteval).
The original Twitter-based RoBERTa model can be found [here](https://huggingface.co/cardiffnlp/twitter-roberta-large-2022-154m).
# Labels
<code>
"id2label": {
"0": "hate_gender",
"1": "hate_race",
"2": "hate_sexuality",
"3": "hate_religion",
"4": "hate_origin",
"5": "hate_disability",
"6": "hate_age",
"7": "not_hate"
}
</code>
## Example
```python
from transformers import pipeline
text = 'Eid Mubarak Everyone!!! ❤ May Allah unite all Muslims, show us the right path, and bless us with good health.❣'
pipe = pipeline('text-classification', model="cardiffnlp/twitter-roberta-large-hate-latest")
pipe(text)
>> [{'label': 'not_hate', 'score': 0.9997966885566711}]
```
## Citation Information
Please cite the [reference paper](https://arxiv.org/abs/2310.14757) if you use this model.
```bibtex
@inproceedings{antypas2023supertweeteval,
title={SuperTweetEval: A Challenging, Unified and Heterogeneous Benchmark for Social Media NLP Research},
author={Dimosthenis Antypas and Asahi Ushio and Francesco Barbieri and Leonardo Neves and Kiamehr Rezaee and Luis Espinosa-Anke and Jiaxin Pei and Jose Camacho-Collados},
booktitle={Findings of the Association for Computational Linguistics: EMNLP 2023},
year={2023}
}
``` |
zhyever/patchfusion_depth_anything_vitl14 | zhyever | 2024-03-16T10:48:07Z | 4,448 | 1 | transformers | [
"transformers",
"pytorch",
"license:mit",
"endpoints_compatible",
"region:us"
] | null | 2024-03-16T10:46:58Z | ---
license: mit
---
|
trituenhantaoio/bert-base-vietnamese-uncased | trituenhantaoio | 2021-05-20T08:06:49Z | 4,447 | 4 | transformers | [
"transformers",
"pytorch",
"tf",
"jax",
"bert",
"endpoints_compatible",
"region:us"
] | null | 2022-03-02T23:29:05Z | ## Usage
```python
from transformers import BertForSequenceClassification
from transformers import BertTokenizer
model = BertForSequenceClassification.from_pretrained("trituenhantaoio/bert-base-vietnamese-uncased")
tokenizer = BertTokenizer.from_pretrained("trituenhantaoio/bert-base-vietnamese-uncased")
```
### References
```
@article{ttnt2020bert,
title={Vietnamese BERT: Pretrained on News and Wiki},
author={trituenhantao.io},
year = {2020},
publisher = {GitHub},
journal = {GitHub repository},
howpublished = {\url{https://github.com/trituenhantaoio/vn-bert-base-uncased}},
}
```
[trituenhantao.io](https://trituenhantao.io) |
stablediffusionapi/zavychromaxlv3 | stablediffusionapi | 2023-12-10T00:34:54Z | 4,447 | 1 | diffusers | [
"diffusers",
"stablediffusionapi.com",
"stable-diffusion-api",
"text-to-image",
"ultra-realistic",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionXLPipeline",
"region:us"
] | text-to-image | 2023-12-10T00:33:00Z | ---
license: creativeml-openrail-m
tags:
- stablediffusionapi.com
- stable-diffusion-api
- text-to-image
- ultra-realistic
pinned: true
---
# ZavyChromaXL_v3 API Inference
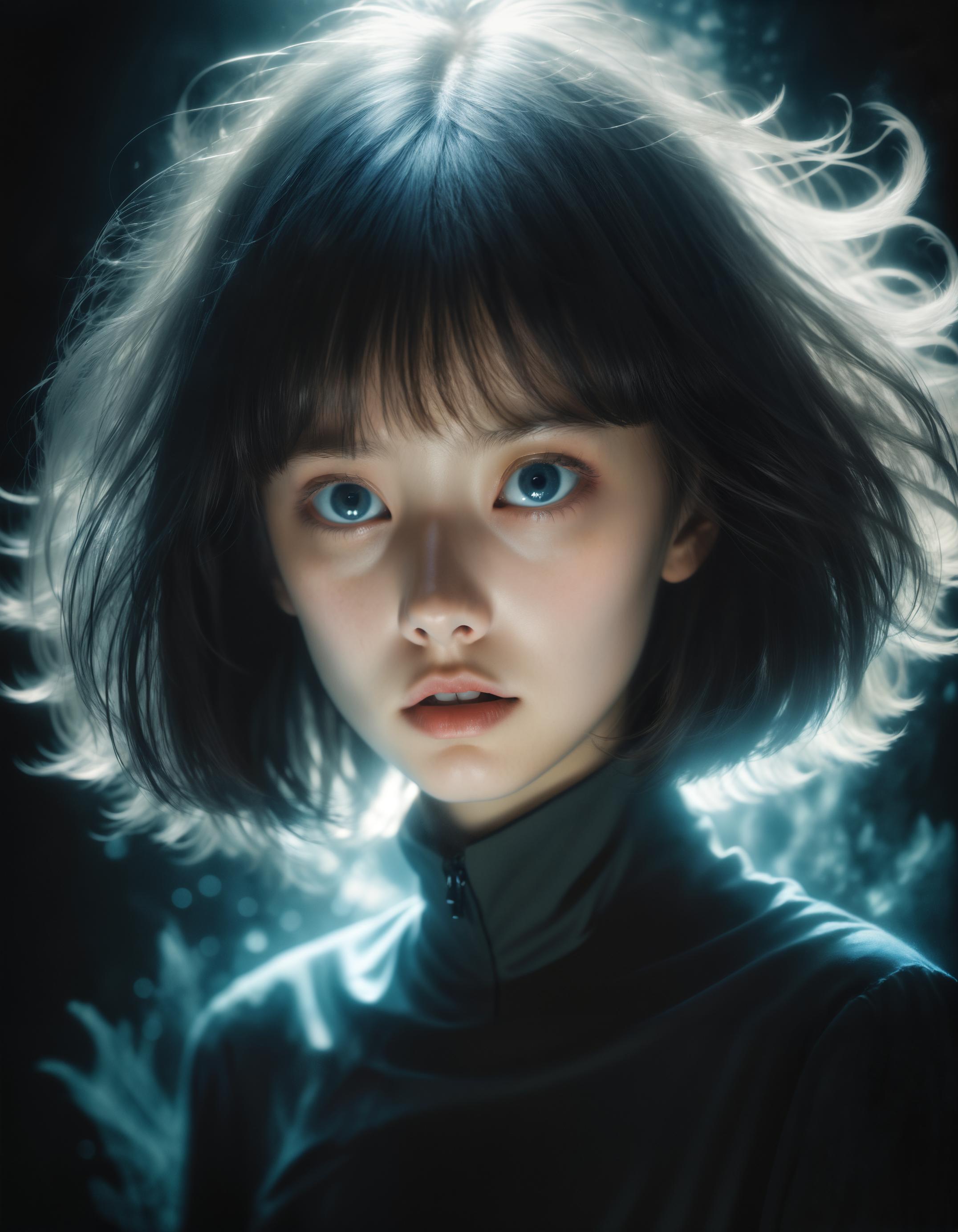
## Get API Key
Get API key from [Stable Diffusion API](http://stablediffusionapi.com/), No Payment needed.
Replace Key in below code, change **model_id** to "zavychromaxlv3"
Coding in PHP/Node/Java etc? Have a look at docs for more code examples: [View docs](https://stablediffusionapi.com/docs)
Try model for free: [Generate Images](https://stablediffusionapi.com/models/zavychromaxlv3)
Model link: [View model](https://stablediffusionapi.com/models/zavychromaxlv3)
Credits: [View credits](https://civitai.com/?query=ZavyChromaXL_v3)
View all models: [View Models](https://stablediffusionapi.com/models)
import requests
import json
url = "https://stablediffusionapi.com/api/v4/dreambooth"
payload = json.dumps({
"key": "your_api_key",
"model_id": "zavychromaxlv3",
"prompt": "ultra realistic close up portrait ((beautiful pale cyberpunk female with heavy black eyeliner)), blue eyes, shaved side haircut, hyper detail, cinematic lighting, magic neon, dark red city, Canon EOS R3, nikon, f/1.4, ISO 200, 1/160s, 8K, RAW, unedited, symmetrical balance, in-frame, 8K",
"negative_prompt": "painting, extra fingers, mutated hands, poorly drawn hands, poorly drawn face, deformed, ugly, blurry, bad anatomy, bad proportions, extra limbs, cloned face, skinny, glitchy, double torso, extra arms, extra hands, mangled fingers, missing lips, ugly face, distorted face, extra legs, anime",
"width": "512",
"height": "512",
"samples": "1",
"num_inference_steps": "30",
"safety_checker": "no",
"enhance_prompt": "yes",
"seed": None,
"guidance_scale": 7.5,
"multi_lingual": "no",
"panorama": "no",
"self_attention": "no",
"upscale": "no",
"embeddings": "embeddings_model_id",
"lora": "lora_model_id",
"webhook": None,
"track_id": None
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
> Use this coupon code to get 25% off **DMGG0RBN** |
mradermacher/T-850-8B-i1-GGUF | mradermacher | 2024-06-18T15:56:18Z | 4,446 | 1 | transformers | [
"transformers",
"gguf",
"not-for-all-audiences",
"en",
"dataset:MinervaAI/Aesir-Preview",
"base_model:ChaoticNeutrals/T-850-8B",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-18T13:39:20Z | ---
base_model: ChaoticNeutrals/T-850-8B
datasets:
- MinervaAI/Aesir-Preview
language:
- en
library_name: transformers
license: apache-2.0
quantized_by: mradermacher
tags:
- not-for-all-audiences
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/ChaoticNeutrals/T-850-8B
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/T-850-8B-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/T-850-8B-i1-GGUF/resolve/main/T-850-8B.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
sngsng/llama-3-8b-Instruct-bnb-4bit-Taigi-translator-trial | sngsng | 2024-06-25T15:21:47Z | 4,445 | 0 | transformers | [
"transformers",
"gguf",
"text-generation-inference",
"unsloth",
"llama",
"en",
"base_model:unsloth/llama-3-8b-Instruct-bnb-4bit",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-25T08:34:14Z | ---
base_model: unsloth/llama-3-8b-Instruct-bnb-4bit
language:
- en
license: apache-2.0
tags:
- text-generation-inference
- transformers
- unsloth
- llama
- gguf
---
# Uploaded model
- **Developed by:** sngsng
- **License:** apache-2.0
- **Finetuned from model :** unsloth/llama-3-8b-Instruct-bnb-4bit
This llama model was trained 2x faster with [Unsloth](https://github.com/unslothai/unsloth) and Huggingface's TRL library.
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/unsloth%20made%20with%20love.png" width="200"/>](https://github.com/unslothai/unsloth)
|
Norod78/distilgpt2-base-pretrained-he | Norod78 | 2023-03-26T12:07:13Z | 4,443 | 1 | transformers | [
"transformers",
"pytorch",
"tf",
"jax",
"coreml",
"onnx",
"safetensors",
"gpt2",
"text-generation",
"he",
"license:mit",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2022-03-02T23:29:04Z | ---
language: he
thumbnail: https://avatars1.githubusercontent.com/u/3617152?norod.jpg
widget:
- text: "האיש האחרון עלי אדמות ישב לבד בחדרו כשלפתע נשמעה נקישה"
- text: "שלום, קרואים לי"
- text: "הארי פוטר חייך חיוך נבוך"
- text: "החתול שלך מאוד חמוד ו"
license: mit
---
# distilgpt2-base-pretrained-he
A tiny GPT2 based Hebrew text generation model initially trained on a TPUv3-8 which was made avilable to me via the [TPU Research Cloud](https://sites.research.google/trc/) Program. Then was further fine-tuned on GPU.
## Dataset
### oscar (unshuffled deduplicated he) - [Homepage](https://oscar-corpus.com) | [Dataset Permalink](https://huggingface.co/datasets/viewer/?dataset=oscar&config=unshuffled_deduplicated_he)
The Open Super-large Crawled ALMAnaCH coRpus is a huge multilingual corpus obtained by language classification and filtering of the Common Crawl corpus using the goclassy architecture.
### CC-100 (he) - [HomePage](https://data.statmt.org/cc-100/)
This corpus comprises of monolingual data for 100+ languages and also includes data for romanized languages. This was constructed using the urls and paragraph indices provided by the CC-Net repository by processing January-December 2018 Commoncrawl snapshots. Each file comprises of documents separated by double-newlines and paragraphs within the same document separated by a newline. The data is generated using the open source CC-Net repository.
### Misc
* Hebrew Twitter
* Wikipedia
* Various other sources
## Training
* Done on a TPUv3-8 VM using [Huggingface's clm-flax example script](https://github.com/huggingface/transformers/blob/master/examples/flax/language-modeling/run_clm_flax.py) <BR>
* I have made a list of items which might make it easier for other to use this script. The list was posted to [This discussion forum](https://discuss.huggingface.co/t/ideas-for-beginner-friendlier-tpu-vm-clm-training/8351)
* Further training was performed on GPU
## Usage
#### Simple usage sample code
```python
from transformers import AutoTokenizer, AutoModelForCausalLM, pipeline
def main():
model_name="Norod78/distilgpt2-base-pretrained-he"
prompt_text = "שלום, קוראים לי"
generated_max_length = 192
print("Loading model...")
model = AutoModelForCausalLM.from_pretrained(model_name)
print('Loading Tokenizer...')
tokenizer = AutoTokenizer.from_pretrained(model_name)
text_generator = pipeline(task="text-generation", model=model, tokenizer=tokenizer)
print("Generating text...")
result = text_generator(prompt_text, num_return_sequences=1, batch_size=1, do_sample=True, top_k=40, top_p=0.92, temperature = 1, repetition_penalty=5.0, max_length = generated_max_length)
print("result = " + str(result))
if __name__ == '__main__':
main()
```
|
stablediffusionapi/disney-pixal-cartoon | stablediffusionapi | 2024-04-17T03:31:30Z | 4,442 | 22 | diffusers | [
"diffusers",
"safetensors",
"stablediffusionapi.com",
"stable-diffusion-api",
"text-to-image",
"ultra-realistic",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-06-01T04:35:53Z | ---
license: creativeml-openrail-m
tags:
- stablediffusionapi.com
- stable-diffusion-api
- text-to-image
- ultra-realistic
pinned: true
---
# Disney Pixal Cartoon Type API Inference
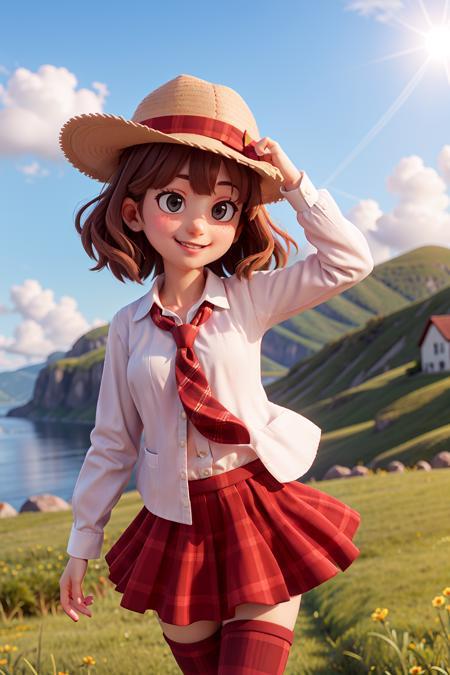
## Get API Key
Get API key from [Stable Diffusion API](http://stablediffusionapi.com/), No Payment needed.
Replace Key in below code, change **model_id** to "disney-pixal-cartoon"
Coding in PHP/Node/Java etc? Have a look at docs for more code examples: [View docs](https://stablediffusionapi.com/docs)
Try model for free: [Generate Images](https://stablediffusionapi.com/models/disney-pixal-cartoon)
Model link: [View model](https://stablediffusionapi.com/models/disney-pixal-cartoon)
Credits: [View credits](https://civitai.com/?query=Disney%20Pixal%20Cartoon%20Type)
View all models: [View Models](https://stablediffusionapi.com/models)
import requests
import json
url = "https://stablediffusionapi.com/api/v3/dreambooth"
payload = json.dumps({
"key": "your_api_key",
"model_id": "disney-pixal-cartoon",
"prompt": "ultra realistic close up portrait ((beautiful pale cyberpunk female with heavy black eyeliner)), blue eyes, shaved side haircut, hyper detail, cinematic lighting, magic neon, dark red city, Canon EOS R3, nikon, f/1.4, ISO 200, 1/160s, 8K, RAW, unedited, symmetrical balance, in-frame, 8K",
"negative_prompt": "painting, extra fingers, mutated hands, poorly drawn hands, poorly drawn face, deformed, ugly, blurry, bad anatomy, bad proportions, extra limbs, cloned face, skinny, glitchy, double torso, extra arms, extra hands, mangled fingers, missing lips, ugly face, distorted face, extra legs, anime",
"width": "512",
"height": "512",
"samples": "1",
"num_inference_steps": "30",
"safety_checker": "no",
"enhance_prompt": "yes",
"seed": None,
"guidance_scale": 7.5,
"multi_lingual": "no",
"panorama": "no",
"self_attention": "no",
"upscale": "no",
"embeddings": "embeddings_model_id",
"lora": "lora_model_id",
"webhook": None,
"track_id": None
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
> Use this coupon code to get 25% off **DMGG0RBN** |
mradermacher/Smaura_8B-i1-GGUF | mradermacher | 2024-06-14T13:28:22Z | 4,442 | 0 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"en",
"base_model:jeiku/Smaura_8B",
"endpoints_compatible",
"region:us"
] | null | 2024-06-14T10:47:03Z | ---
base_model: jeiku/Smaura_8B
language:
- en
library_name: transformers
quantized_by: mradermacher
tags:
- mergekit
- merge
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/jeiku/Smaura_8B
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/Smaura_8B-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/Smaura_8B-i1-GGUF/resolve/main/Smaura_8B.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
mohammad2928git/medical_v4_gguf | mohammad2928git | 2024-06-29T06:09:39Z | 4,442 | 0 | transformers | [
"transformers",
"gguf",
"llama",
"text-generation-inference",
"unsloth",
"en",
"base_model:ruslanmv/Medical-Llama3-8B",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-29T06:02:21Z | ---
base_model: ruslanmv/Medical-Llama3-8B
language:
- en
license: apache-2.0
tags:
- text-generation-inference
- transformers
- unsloth
- llama
- gguf
---
# Uploaded model
- **Developed by:** mohammad2928git
- **License:** apache-2.0
- **Finetuned from model :** ruslanmv/Medical-Llama3-8B
This llama model was trained 2x faster with [Unsloth](https://github.com/unslothai/unsloth) and Huggingface's TRL library.
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/unsloth%20made%20with%20love.png" width="200"/>](https://github.com/unslothai/unsloth)
|
mradermacher/L3-8B-MegaSerpentine-i1-GGUF | mradermacher | 2024-06-19T09:52:50Z | 4,441 | 2 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"llama",
"not-for-all-audiences",
"en",
"base_model:v000000/L3-8B-MegaSerpentine",
"endpoints_compatible",
"region:us"
] | null | 2024-06-18T15:16:02Z | ---
base_model: v000000/L3-8B-MegaSerpentine
language:
- en
library_name: transformers
quantized_by: mradermacher
tags:
- mergekit
- merge
- llama
- not-for-all-audiences
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/v000000/L3-8B-MegaSerpentine
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-MegaSerpentine-i1-GGUF/resolve/main/L3-8B-MegaSerpentine.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
Luyu/co-condenser-marco | Luyu | 2021-08-13T13:54:21Z | 4,439 | 3 | transformers | [
"transformers",
"pytorch",
"bert",
"fill-mask",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | fill-mask | 2022-03-02T23:29:04Z | Entry not found |
OpenAssistant/codellama-13b-oasst-sft-v10 | OpenAssistant | 2023-08-29T19:16:10Z | 4,439 | 66 | transformers | [
"transformers",
"safetensors",
"llama",
"text-generation",
"custom_code",
"en",
"dataset:OpenAssistant/oasst1",
"dataset:shahules786/orca-best",
"license:llama2",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2023-08-26T10:13:29Z | ---
license: llama2
datasets:
- OpenAssistant/oasst1
- shahules786/orca-best
language:
- en
---
# Open-Assistant CodeLlama 13B SFT v10
This model is an Open-Assistant fine-tuning of Meta's CodeLlama 13B LLM.
**Note**: Due to the new RoPE Theta value (1e6 instead of 1e4), for correct results you must load this model with `trust_remote_code=True` or use the latest main branch of Huggingface transformers (until version 4.33 is released).
## Model Details
- **Finetuned from:** [codellama/CodeLlama-7b-hf](https://huggingface.co/codellama/CodeLlama-7b-hf) via [epfLLM/Megatron-LLM](https://github.com/epfLLM/Megatron-LLM)
- **Model type:** Causal decoder-only transformer language model
- **Language:** English
- **Weights & Biases training logs:** 6123 steps, BS 64 [run56_oa_llamacode](https://wandb.ai/open-assistant/public-sft/runs/run56_oa_llamacode)
- **Demo:** [Continuations for 250 random prompts (without system message)](https://open-assistant.github.io/oasst-model-eval/?f=https%3A%2F%2Fraw.githubusercontent.com%2FOpen-Assistant%2Foasst-model-eval%2Fmain%2Fsampling_reports%2Foasst-sft%2F2023-08-26_OpenAssistant_codellama-13b-oasst-sft-v10_sampling_noprefix2.json)
- **License:** [LLAMA 2 COMMUNITY LICENSE AGREEMENT](https://huggingface.co/meta-llama/Llama-2-70b/raw/main/LICENSE.txt)
- **Contact:** [Open-Assistant Discord](https://ykilcher.com/open-assistant-discord)
## Prompting / Prompt Template
Due to public demand (see [survey](https://twitter.com/erhartford/status/1682403597525430272)) we changed the prompt-template for this model from custom prompter/assistant tokens to OpenAI's [chatml](https://github.com/openai/openai-python/blob/main/chatml.md) standard prompt format.
We hope that this leads to greater compatibility with chat inference/frontend applications.
Prompt dialogue template:
```
"""
<|im_start|>system
{system_message}<|im_end|>
<|im_start|>user
{prompt}<|im_end|>
<|im_start|>assistant
"""
```
The model input can contain multiple conversation turns between user and assistant, e.g.
```
<|im_start|>user
{prompt 1}<|im_end|>
<|im_start|>assistant
{reply 1}<|im_end|>
<|im_start|>user
{prompt 2}<|im_end|>
<|im_start|>assistant
(...)
```
The model was partly trained with orca system messages.
For inference we recommend to use the official [Llama2 system message](https://github.com/facebookresearch/llama/blob/ea9f33d6d3ea8ed7d560d270986407fd6c2e52b7/example_chat_completion.py#L57-L61):
```
<|im_start|>system
You are a helpful, respectful and honest assistant. Always answer as helpfully as possible, while being safe. Your answers should not include any harmful, unethical, racist, sexist, toxic, dangerous, or illegal content. Please ensure that your responses are socially unbiased and positive in nature.
If a question does not make any sense, or is not factually coherent, explain why instead of answering something not correct. If you don't know the answer to a question, please don't share false information.
<|im_end|>
```
### Credits & Special Thanks
- Thanks to [Meta AI](https://ai.meta.com/) for training and releasing the CodeLLlama model.
- Distributed training support was provided by EPFL's [Machine Learning and Optimization Laboratory](https://www.epfl.ch/labs/mlo/), and [Natural Language Processing Lab](https://nlp.epfl.ch/).
- The open-source [epfLLM/Megatron-LLM](https://github.com/epfLLM/Megatron-LLM) trainer was used for fine-tuning.
- [rombodawg](https://huggingface.co/rombodawg) curated the [LosslessMegaCodeTrainingV2_1m_Evol_Uncensored](https://huggingface.co/datasets/rombodawg/LosslessMegaCodeTrainingV2_1m_Evol_Uncensored) dataset.
- [ehartford](https://huggingface.co/ehartford) generated and published the [ehartford/dolphin](https://huggingface.co/datasets/ehartford/dolphin).
- [shahules786](https://github.com/shahules786) de-duped and filtered the Dolphin and Megacode dataset with a clustering/controid approach and generated orca-best & bestofmegacode.
- [andreaskoepf](https://github.com/andreaskoepf/) prepared & orchestrated the training.
## Ethical Considerations and Limitations
Testing conducted to date has been in English, and has not covered, nor could it cover all scenarios.
For these reasons, as with all LLMs, the potential outputs of codellama-13b-oasst-sft-v10 cannot be predicted
in advance, and the model may in some instances produce inaccurate, biased or other objectionable responses
to user prompts. Therefore, before deploying any applications of codellama-13b-oasst-sft-v10, developers should
perform safety testing and tuning tailored to their specific applications of the model.
Please see Meta's [Responsible Use Guide](https://ai.meta.com/llama/responsible-use-guide/).
## Configuration Details
The "pretokenizer" utility used to tokenize the datamix is part of the Open-Assistant github repository and can be found here: [model/pretokenizer](https://github.com/LAION-AI/Open-Assistant/tree/main/model/pretokenizer).
### Pretokenizer Configuration
```
orca_megacode_oasst_best:
datasets:
- orca-chat:
val_split: 0.01
max_val_set: 1000
- bestofmegacode:
val_split: 0.01
max_val_set: 1000
- oasst_export:
lang: "bg,ca,cs,da,de,en,es,fr,hr,hu,it,nl,pl,pt,ro,ru,sl,sr,sv,uk"
#hf_dataset_name: OpenAssistant/oasst1
input_file_path: 2023-08-25_oasst_ready.jsonl.gz
top_k: 1
val_split: 0.025
output_dir: "output/orca_megacode_oasst_best"
filename_prefix: "orca_megacode_oasst_best"
min_assistant_tokens: 1
```
|
michaelbenayoun/llama-2-tiny-4kv-heads-4layers-random | michaelbenayoun | 2024-04-18T10:25:46Z | 4,437 | 0 | transformers | [
"transformers",
"safetensors",
"llama",
"feature-extraction",
"arxiv:1910.09700",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | feature-extraction | 2024-03-28T10:06:36Z | ---
library_name: transformers
tags: []
---
# Model Card for Model ID
<!-- Provide a quick summary of what the model is/does. -->
## Model Details
### Model Description
<!-- Provide a longer summary of what this model is. -->
This is the model card of a 🤗 transformers model that has been pushed on the Hub. This model card has been automatically generated.
- **Developed by:** [More Information Needed]
- **Funded by [optional]:** [More Information Needed]
- **Shared by [optional]:** [More Information Needed]
- **Model type:** [More Information Needed]
- **Language(s) (NLP):** [More Information Needed]
- **License:** [More Information Needed]
- **Finetuned from model [optional]:** [More Information Needed]
### Model Sources [optional]
<!-- Provide the basic links for the model. -->
- **Repository:** [More Information Needed]
- **Paper [optional]:** [More Information Needed]
- **Demo [optional]:** [More Information Needed]
## Uses
<!-- Address questions around how the model is intended to be used, including the foreseeable users of the model and those affected by the model. -->
### Direct Use
<!-- This section is for the model use without fine-tuning or plugging into a larger ecosystem/app. -->
[More Information Needed]
### Downstream Use [optional]
<!-- This section is for the model use when fine-tuned for a task, or when plugged into a larger ecosystem/app -->
[More Information Needed]
### Out-of-Scope Use
<!-- This section addresses misuse, malicious use, and uses that the model will not work well for. -->
[More Information Needed]
## Bias, Risks, and Limitations
<!-- This section is meant to convey both technical and sociotechnical limitations. -->
[More Information Needed]
### Recommendations
<!-- This section is meant to convey recommendations with respect to the bias, risk, and technical limitations. -->
Users (both direct and downstream) should be made aware of the risks, biases and limitations of the model. More information needed for further recommendations.
## How to Get Started with the Model
Use the code below to get started with the model.
[More Information Needed]
## Training Details
### Training Data
<!-- This should link to a Dataset Card, perhaps with a short stub of information on what the training data is all about as well as documentation related to data pre-processing or additional filtering. -->
[More Information Needed]
### Training Procedure
<!-- This relates heavily to the Technical Specifications. Content here should link to that section when it is relevant to the training procedure. -->
#### Preprocessing [optional]
[More Information Needed]
#### Training Hyperparameters
- **Training regime:** [More Information Needed] <!--fp32, fp16 mixed precision, bf16 mixed precision, bf16 non-mixed precision, fp16 non-mixed precision, fp8 mixed precision -->
#### Speeds, Sizes, Times [optional]
<!-- This section provides information about throughput, start/end time, checkpoint size if relevant, etc. -->
[More Information Needed]
## Evaluation
<!-- This section describes the evaluation protocols and provides the results. -->
### Testing Data, Factors & Metrics
#### Testing Data
<!-- This should link to a Dataset Card if possible. -->
[More Information Needed]
#### Factors
<!-- These are the things the evaluation is disaggregating by, e.g., subpopulations or domains. -->
[More Information Needed]
#### Metrics
<!-- These are the evaluation metrics being used, ideally with a description of why. -->
[More Information Needed]
### Results
[More Information Needed]
#### Summary
## Model Examination [optional]
<!-- Relevant interpretability work for the model goes here -->
[More Information Needed]
## Environmental Impact
<!-- Total emissions (in grams of CO2eq) and additional considerations, such as electricity usage, go here. Edit the suggested text below accordingly -->
Carbon emissions can be estimated using the [Machine Learning Impact calculator](https://mlco2.github.io/impact#compute) presented in [Lacoste et al. (2019)](https://arxiv.org/abs/1910.09700).
- **Hardware Type:** [More Information Needed]
- **Hours used:** [More Information Needed]
- **Cloud Provider:** [More Information Needed]
- **Compute Region:** [More Information Needed]
- **Carbon Emitted:** [More Information Needed]
## Technical Specifications [optional]
### Model Architecture and Objective
[More Information Needed]
### Compute Infrastructure
[More Information Needed]
#### Hardware
[More Information Needed]
#### Software
[More Information Needed]
## Citation [optional]
<!-- If there is a paper or blog post introducing the model, the APA and Bibtex information for that should go in this section. -->
**BibTeX:**
[More Information Needed]
**APA:**
[More Information Needed]
## Glossary [optional]
<!-- If relevant, include terms and calculations in this section that can help readers understand the model or model card. -->
[More Information Needed]
## More Information [optional]
[More Information Needed]
## Model Card Authors [optional]
[More Information Needed]
## Model Card Contact
[More Information Needed] |
stablediffusionapi/uber-realistic-porn-merge | stablediffusionapi | 2023-12-08T03:41:58Z | 4,434 | 12 | diffusers | [
"diffusers",
"safetensors",
"stablediffusionapi.com",
"stable-diffusion-api",
"text-to-image",
"ultra-realistic",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-09-30T13:03:55Z | ---
license: creativeml-openrail-m
tags:
- stablediffusionapi.com
- stable-diffusion-api
- text-to-image
- ultra-realistic
pinned: true
---
# Uber Realistic Porn Merge (URPM) [LEGACY Version] API Inference

## Get API Key
Get API key from [Stable Diffusion API](http://stablediffusionapi.com/), No Payment needed.
Replace Key in below code, change **model_id** to "uber-realistic-porn-merge"
Coding in PHP/Node/Java etc? Have a look at docs for more code examples: [View docs](https://stablediffusionapi.com/docs)
Try model for free: [Generate Images](https://stablediffusionapi.com/models/uber-realistic-porn-merge)
Model link: [View model](https://stablediffusionapi.com/models/uber-realistic-porn-merge)
Credits: [View credits](https://civitai.com/?query=Uber%20Realistic%20Porn%20Merge%20%28URPM%29%20%5BLEGACY%20Version%5D)
View all models: [View Models](https://stablediffusionapi.com/models)
import requests
import json
url = "https://stablediffusionapi.com/api/v4/dreambooth"
payload = json.dumps({
"key": "your_api_key",
"model_id": "uber-realistic-porn-merge",
"prompt": "ultra realistic close up portrait ((beautiful pale cyberpunk female with heavy black eyeliner)), blue eyes, shaved side haircut, hyper detail, cinematic lighting, magic neon, dark red city, Canon EOS R3, nikon, f/1.4, ISO 200, 1/160s, 8K, RAW, unedited, symmetrical balance, in-frame, 8K",
"negative_prompt": "painting, extra fingers, mutated hands, poorly drawn hands, poorly drawn face, deformed, ugly, blurry, bad anatomy, bad proportions, extra limbs, cloned face, skinny, glitchy, double torso, extra arms, extra hands, mangled fingers, missing lips, ugly face, distorted face, extra legs, anime",
"width": "512",
"height": "512",
"samples": "1",
"num_inference_steps": "30",
"safety_checker": "no",
"enhance_prompt": "yes",
"seed": None,
"guidance_scale": 7.5,
"multi_lingual": "no",
"panorama": "no",
"self_attention": "no",
"upscale": "no",
"embeddings": "embeddings_model_id",
"lora": "lora_model_id",
"webhook": None,
"track_id": None
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
> Use this coupon code to get 25% off **DMGG0RBN** |
mradermacher/L3-8B-sunfall-v0.3-i1-GGUF | mradermacher | 2024-06-14T02:58:02Z | 4,431 | 0 | transformers | [
"transformers",
"gguf",
"not-for-all-audiences",
"en",
"base_model:crestf411/L3-8B-sunfall-v0.3",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-14T01:31:31Z | ---
base_model: crestf411/L3-8B-sunfall-v0.3
language:
- en
library_name: transformers
license: llama3
license_link: LICENSE
license_name: llama3
quantized_by: mradermacher
tags:
- not-for-all-audiences
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: -->
weighted/imatrix quants of https://huggingface.co/crestf411/L3-8B-sunfall-v0.3
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-v0.3-i1-GGUF/resolve/main/L3-8B-sunfall-v0.3.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time.
<!-- end -->
|
vaishali/multitabqa-base | vaishali | 2024-02-20T20:38:26Z | 4,429 | 4 | transformers | [
"transformers",
"pytorch",
"safetensors",
"bart",
"text2text-generation",
"multitabqa",
"multi-table-question-answering",
"table-question-answering",
"en",
"dataset:vaishali/spider-tableQA",
"arxiv:2305.12820",
"license:mit",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | table-question-answering | 2023-07-05T16:49:51Z | ---
language: en
tags:
- multitabqa
- multi-table-question-answering
license: mit
pipeline_tag: table-question-answering
datasets:
- vaishali/spider-tableQA
---
# MultiTabQA (base-sized model)
MultiTabQA was proposed in [MultiTabQA: Generating Tabular Answers for Multi-Table Question Answering](https://arxiv.org/abs/2305.12820) by Vaishali Pal, Andrew Yates, Evangelos Kanoulas, Maarten de Rijke. The original repo can be found [here](https://github.com/kolk/MultiTabQA).
## Model description
MultiTabQA is a tableQA model which generates the answer table from multiple-input tables. It can handle multi-table operators such as UNION, INTERSECT, EXCEPT, JOINS, etc.
MultiTabQA is based on the TAPEX(BART) architecture, which is a bidirectional (BERT-like) encoder and an autoregressive (GPT-like) decoder.
## Intended Uses
You can use the raw model SQL execution over multiple input tables. The model has been finetuned on Spider dataset where it answers natural language questions over multiple input tables.
### How to Use
Here is how to use this model in transformers:
```python
from transformers import AutoTokenizer, AutoModelForSeq2SeqLM
import pandas as pd
tokenizer = AutoTokenizer.from_pretrained("vaishali/multitabqa-base")
model = AutoModelForSeq2SeqLM.from_pretrained("vaishali/multitabqa-base")
question = "How many departments are led by heads who are not mentioned?"
table_names = ['department', 'management']
tables=[{"columns":["Department_ID","Name","Creation","Ranking","Budget_in_Billions","Num_Employees"],
"index":[0,1,2,3,4,5,6,7,8,9,10,11,12,13,14],
"data":[
[1,"State","1789",1,9.96,30266.0],
[2,"Treasury","1789",2,11.1,115897.0],
[3,"Defense","1947",3,439.3,3000000.0],
[4,"Justice","1870",4,23.4,112557.0],
[5,"Interior","1849",5,10.7,71436.0],
[6,"Agriculture","1889",6,77.6,109832.0],
[7,"Commerce","1903",7,6.2,36000.0],
[8,"Labor","1913",8,59.7,17347.0],
[9,"Health and Human Services","1953",9,543.2,67000.0],
[10,"Housing and Urban Development","1965",10,46.2,10600.0],
[11,"Transportation","1966",11,58.0,58622.0],
[12,"Energy","1977",12,21.5,116100.0],
[13,"Education","1979",13,62.8,4487.0],
[14,"Veterans Affairs","1989",14,73.2,235000.0],
[15,"Homeland Security","2002",15,44.6,208000.0]
]
},
{"columns":["department_ID","head_ID","temporary_acting"],
"index":[0,1,2,3,4],
"data":[
[2,5,"Yes"],
[15,4,"Yes"],
[2,6,"Yes"],
[7,3,"No"],
[11,10,"No"]
]
}]
input_tables = [pd.read_json(table, orient="split") for table in tables]
# flatten the model inputs in the format: query + " " + <table_name> : table_name1 + flattened_table1 + <table_name> : table_name2 + flattened_table2 + ...
#flattened_input = question + " " + [f"<table_name> : {table_name} linearize_table(table) for table_name, table in zip(table_names, tables)]
model_input_string = """How many departments are led by heads who are not mentioned? <table_name> : department col : Department_ID | Name | Creation | Ranking | Budget_in_Billions | Num_Employees row 1 : 1 | State | 1789 | 1 | 9.96 | 30266 row 2 : 2 | Treasury | 1789 | 2 | 11.1 | 115897 row 3 : 3 | Defense | 1947 | 3 | 439.3 | 3000000 row 4 : 4 | Justice | 1870 | 4 | 23.4 | 112557 row 5 : 5 | Interior | 1849 | 5 | 10.7 | 71436 row 6 : 6 | Agriculture | 1889 | 6 | 77.6 | 109832 row 7 : 7 | Commerce | 1903 | 7 | 6.2 | 36000 row 8 : 8 | Labor | 1913 | 8 | 59.7 | 17347 row 9 : 9 | Health and Human Services | 1953 | 9 | 543.2 | 67000 row 10 : 10 | Housing and Urban Development | 1965 | 10 | 46.2 | 10600 row 11 : 11 | Transportation | 1966 | 11 | 58.0 | 58622 row 12 : 12 | Energy | 1977 | 12 | 21.5 | 116100 row 13 : 13 | Education | 1979 | 13 | 62.8 | 4487 row 14 : 14 | Veterans Affairs | 1989 | 14 | 73.2 | 235000 row 15 : 15 | Homeland Security | 2002 | 15 | 44.6 | 208000 <table_name> : management col : department_ID | head_ID | temporary_acting row 1 : 2 | 5 | Yes row 2 : 15 | 4 | Yes row 3 : 2 | 6 | Yes row 4 : 7 | 3 | No row 5 : 11 | 10 | No"""
inputs = tokenizer(model_input_string, return_tensors="pt")
outputs = model.generate(**inputs)
print(tokenizer.batch_decode(outputs, skip_special_tokens=True))
# 'col : count(*) row 1 : 11'
```
### How to Fine-tuning
Please find the fine-tuning script [here](https://github.com/kolk/MultiTabQA).
### BibTeX entry and citation info
```bibtex
@inproceedings{pal-etal-2023-multitabqa,
title = "{M}ulti{T}ab{QA}: Generating Tabular Answers for Multi-Table Question Answering",
author = "Pal, Vaishali and
Yates, Andrew and
Kanoulas, Evangelos and
de Rijke, Maarten",
booktitle = "Proceedings of the 61st Annual Meeting of the Association for Computational Linguistics (Volume 1: Long Papers)",
month = jul,
year = "2023",
address = "Toronto, Canada",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2023.acl-long.348",
doi = "10.18653/v1/2023.acl-long.348",
pages = "6322--6334",
abstract = "Recent advances in tabular question answering (QA) with large language models are constrained in their coverage and only answer questions over a single table. However, real-world queries are complex in nature, often over multiple tables in a relational database or web page. Single table questions do not involve common table operations such as set operations, Cartesian products (joins), or nested queries. Furthermore, multi-table operations often result in a tabular output, which necessitates table generation capabilities of tabular QA models. To fill this gap, we propose a new task of answering questions over multiple tables. Our model, MultiTabQA, not only answers questions over multiple tables, but also generalizes to generate tabular answers. To enable effective training, we build a pre-training dataset comprising of 132,645 SQL queries and tabular answers. Further, we evaluate the generated tables by introducing table-specific metrics of varying strictness assessing various levels of granularity of the table structure. MultiTabQA outperforms state-of-the-art single table QA models adapted to a multi-table QA setting by finetuning on three datasets: Spider, Atis and GeoQuery.",
}
``` |
echarlaix/tiny-random-t5 | echarlaix | 2024-05-31T16:35:01Z | 4,429 | 0 | transformers | [
"transformers",
"pytorch",
"tf",
"t5",
"feature-extraction",
"license:apache-2.0",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | feature-extraction | 2024-05-31T16:33:46Z | ---
license: apache-2.0
---
|
dlb/electra-base-portuguese-uncased-brwac | dlb | 2021-12-10T12:33:58Z | 4,427 | 6 | transformers | [
"transformers",
"pytorch",
"electra",
"pretraining",
"pt",
"dataset:brwac",
"endpoints_compatible",
"region:us"
] | null | 2022-03-02T23:29:05Z | ---
language: pt
tags:
- electra
- pretraining
- pytorch
datasets:
- brwac
---
|
RichardErkhov/Gryphe_-_MythoMist-7b-gguf | RichardErkhov | 2024-06-03T10:44:10Z | 4,426 | 0 | null | [
"gguf",
"region:us"
] | null | 2024-06-03T08:08:54Z | Quantization made by Richard Erkhov.
[Github](https://github.com/RichardErkhov)
[Discord](https://discord.gg/pvy7H8DZMG)
[Request more models](https://github.com/RichardErkhov/quant_request)
MythoMist-7b - GGUF
- Model creator: https://huggingface.co/Gryphe/
- Original model: https://huggingface.co/Gryphe/MythoMist-7b/
| Name | Quant method | Size |
| ---- | ---- | ---- |
| [MythoMist-7b.Q2_K.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q2_K.gguf) | Q2_K | 2.53GB |
| [MythoMist-7b.IQ3_XS.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.IQ3_XS.gguf) | IQ3_XS | 2.81GB |
| [MythoMist-7b.IQ3_S.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.IQ3_S.gguf) | IQ3_S | 2.96GB |
| [MythoMist-7b.Q3_K_S.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q3_K_S.gguf) | Q3_K_S | 2.95GB |
| [MythoMist-7b.IQ3_M.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.IQ3_M.gguf) | IQ3_M | 3.06GB |
| [MythoMist-7b.Q3_K.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q3_K.gguf) | Q3_K | 3.28GB |
| [MythoMist-7b.Q3_K_M.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q3_K_M.gguf) | Q3_K_M | 3.28GB |
| [MythoMist-7b.Q3_K_L.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q3_K_L.gguf) | Q3_K_L | 3.56GB |
| [MythoMist-7b.IQ4_XS.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.IQ4_XS.gguf) | IQ4_XS | 3.67GB |
| [MythoMist-7b.Q4_0.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q4_0.gguf) | Q4_0 | 3.83GB |
| [MythoMist-7b.IQ4_NL.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.IQ4_NL.gguf) | IQ4_NL | 3.87GB |
| [MythoMist-7b.Q4_K_S.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q4_K_S.gguf) | Q4_K_S | 3.86GB |
| [MythoMist-7b.Q4_K.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q4_K.gguf) | Q4_K | 4.07GB |
| [MythoMist-7b.Q4_K_M.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q4_K_M.gguf) | Q4_K_M | 4.07GB |
| [MythoMist-7b.Q4_1.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q4_1.gguf) | Q4_1 | 4.24GB |
| [MythoMist-7b.Q5_0.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q5_0.gguf) | Q5_0 | 4.65GB |
| [MythoMist-7b.Q5_K_S.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q5_K_S.gguf) | Q5_K_S | 4.65GB |
| [MythoMist-7b.Q5_K.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q5_K.gguf) | Q5_K | 4.78GB |
| [MythoMist-7b.Q5_K_M.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q5_K_M.gguf) | Q5_K_M | 4.78GB |
| [MythoMist-7b.Q5_1.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q5_1.gguf) | Q5_1 | 5.07GB |
| [MythoMist-7b.Q6_K.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q6_K.gguf) | Q6_K | 5.53GB |
| [MythoMist-7b.Q8_0.gguf](https://huggingface.co/RichardErkhov/Gryphe_-_MythoMist-7b-gguf/blob/main/MythoMist-7b.Q8_0.gguf) | Q8_0 | 7.17GB |
Original model description:
---
license: other
language:
- en
---
MythoMist 7b is, as always, a highly experimental Mistral-based merge based on my latest algorithm, which actively benchmarks the model as it's being built in pursuit of a goal set by the user.
**Addendum (2023-11-23)**: A more thorough investigation revealed a flaw in my original algorithm that has since been resolved. I've considered deleting this model as it did not follow its original objective completely but since there are plenty of folks enjoying it I'll be keeping it around. Keep a close eye [on my MergeMonster repo](https://huggingface.co/Gryphe/MergeMonster) for further developments and releases of merges produced by the Merge Monster.
The primary purpose for MythoMist was to reduce usage of the word anticipation, ministrations and other variations we've come to associate negatively with ChatGPT roleplaying data. This algorithm cannot outright ban these words, but instead strives to minimize the usage.
[The script has now been made available on my Github. Warning - Plenty of VRAM is needed.](https://github.com/Gryphe/MergeMonster/)
Quantized models are available from TheBloke: [GGUF](https://huggingface.co/TheBloke/MythoMist-7B-GGUF) - [GPTQ](https://huggingface.co/TheBloke/MythoMist-7B-GPTQ) - [AWQ](https://huggingface.co/TheBloke/MythoMist-7B-AWQ) (You're the best!)
## Final merge composition
After processing 12 models my algorithm ended up with the following (approximated) final composition:
| Model | Contribution |
|--------------------------|--------------|
| Neural-chat-7b-v3-1 | 26% |
| Synatra-7B-v0.3-RP | 22% |
| Airoboros-m-7b-3.1.2 | 10% |
| Toppy-M-7B | 10% |
| Zephyr-7b-beta | 7% |
| Nous-Capybara-7B-V1.9 | 5% |
| OpenHermes-2.5-Mistral-7B| 5% |
| Dolphin-2.2.1-mistral-7b | 4% |
| Noromaid-7b-v0.1.1 | 4% |
| SynthIA-7B-v1.3 | 3% |
| Mistral-7B-v0.1 | 2% |
| Openchat_3.5 | 2% |
There is no real logic in how these models were divided throughout the merge - Small bits and pieces were taken from each and then mixed in with other models on a layer by layer basis, using a pattern similar to my MythoMax recipe in which underlying tensors are mixed in a criss-cross manner.
This new process only decides on the model's layers, not the singular lm_head and embed_tokens layers which influence much of the model's output. I ran a seperate script for that, picking the singular tensors that resulted in the longest responses, which settled on Toppy-M-7B.
## Prompt Format
Due to the wide variation in prompt formats used in this merge I (for now) recommend using Alpaca as the prompt template for compatibility reasons:
```
### Instruction:
Your instruction or question here.
### Response:
```
---
license: other
---
|
Niggendar/prefectPonyXL_v10 | Niggendar | 2024-05-22T13:11:01Z | 4,424 | 2 | diffusers | [
"diffusers",
"safetensors",
"arxiv:1910.09700",
"endpoints_compatible",
"diffusers:StableDiffusionXLPipeline",
"region:us"
] | text-to-image | 2024-05-22T13:01:29Z | ---
library_name: diffusers
---
# Model Card for Model ID
<!-- Provide a quick summary of what the model is/does. -->
## Model Details
### Model Description
<!-- Provide a longer summary of what this model is. -->
This is the model card of a 🧨 diffusers model that has been pushed on the Hub. This model card has been automatically generated.
- **Developed by:** [More Information Needed]
- **Funded by [optional]:** [More Information Needed]
- **Shared by [optional]:** [More Information Needed]
- **Model type:** [More Information Needed]
- **Language(s) (NLP):** [More Information Needed]
- **License:** [More Information Needed]
- **Finetuned from model [optional]:** [More Information Needed]
### Model Sources [optional]
<!-- Provide the basic links for the model. -->
- **Repository:** [More Information Needed]
- **Paper [optional]:** [More Information Needed]
- **Demo [optional]:** [More Information Needed]
## Uses
<!-- Address questions around how the model is intended to be used, including the foreseeable users of the model and those affected by the model. -->
### Direct Use
<!-- This section is for the model use without fine-tuning or plugging into a larger ecosystem/app. -->
[More Information Needed]
### Downstream Use [optional]
<!-- This section is for the model use when fine-tuned for a task, or when plugged into a larger ecosystem/app -->
[More Information Needed]
### Out-of-Scope Use
<!-- This section addresses misuse, malicious use, and uses that the model will not work well for. -->
[More Information Needed]
## Bias, Risks, and Limitations
<!-- This section is meant to convey both technical and sociotechnical limitations. -->
[More Information Needed]
### Recommendations
<!-- This section is meant to convey recommendations with respect to the bias, risk, and technical limitations. -->
Users (both direct and downstream) should be made aware of the risks, biases and limitations of the model. More information needed for further recommendations.
## How to Get Started with the Model
Use the code below to get started with the model.
[More Information Needed]
## Training Details
### Training Data
<!-- This should link to a Dataset Card, perhaps with a short stub of information on what the training data is all about as well as documentation related to data pre-processing or additional filtering. -->
[More Information Needed]
### Training Procedure
<!-- This relates heavily to the Technical Specifications. Content here should link to that section when it is relevant to the training procedure. -->
#### Preprocessing [optional]
[More Information Needed]
#### Training Hyperparameters
- **Training regime:** [More Information Needed] <!--fp32, fp16 mixed precision, bf16 mixed precision, bf16 non-mixed precision, fp16 non-mixed precision, fp8 mixed precision -->
#### Speeds, Sizes, Times [optional]
<!-- This section provides information about throughput, start/end time, checkpoint size if relevant, etc. -->
[More Information Needed]
## Evaluation
<!-- This section describes the evaluation protocols and provides the results. -->
### Testing Data, Factors & Metrics
#### Testing Data
<!-- This should link to a Dataset Card if possible. -->
[More Information Needed]
#### Factors
<!-- These are the things the evaluation is disaggregating by, e.g., subpopulations or domains. -->
[More Information Needed]
#### Metrics
<!-- These are the evaluation metrics being used, ideally with a description of why. -->
[More Information Needed]
### Results
[More Information Needed]
#### Summary
## Model Examination [optional]
<!-- Relevant interpretability work for the model goes here -->
[More Information Needed]
## Environmental Impact
<!-- Total emissions (in grams of CO2eq) and additional considerations, such as electricity usage, go here. Edit the suggested text below accordingly -->
Carbon emissions can be estimated using the [Machine Learning Impact calculator](https://mlco2.github.io/impact#compute) presented in [Lacoste et al. (2019)](https://arxiv.org/abs/1910.09700).
- **Hardware Type:** [More Information Needed]
- **Hours used:** [More Information Needed]
- **Cloud Provider:** [More Information Needed]
- **Compute Region:** [More Information Needed]
- **Carbon Emitted:** [More Information Needed]
## Technical Specifications [optional]
### Model Architecture and Objective
[More Information Needed]
### Compute Infrastructure
[More Information Needed]
#### Hardware
[More Information Needed]
#### Software
[More Information Needed]
## Citation [optional]
<!-- If there is a paper or blog post introducing the model, the APA and Bibtex information for that should go in this section. -->
**BibTeX:**
[More Information Needed]
**APA:**
[More Information Needed]
## Glossary [optional]
<!-- If relevant, include terms and calculations in this section that can help readers understand the model or model card. -->
[More Information Needed]
## More Information [optional]
[More Information Needed]
## Model Card Authors [optional]
[More Information Needed]
## Model Card Contact
[More Information Needed] |
mradermacher/T-800-8B-i1-GGUF | mradermacher | 2024-06-17T06:05:37Z | 4,424 | 0 | transformers | [
"transformers",
"gguf",
"not-for-all-audiences",
"en",
"dataset:Dogge/freedom-rp-alpaca",
"dataset:ResplendentAI/BluemoonAlpaca",
"dataset:ResplendentAI/Synthetic_Soul_1k",
"dataset:ResplendentAI/NSFW_Format_Test",
"dataset:ResplendentAI/Alpaca_NSFW_Shuffled",
"dataset:ResplendentAI/theory_of_mind_fixed_output",
"dataset:jeiku/JeikuL3v2",
"dataset:ResplendentAI/Sissification_Hypno_1k",
"base_model:ChaoticNeutrals/T-800-8B",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-17T01:10:08Z | ---
base_model: ChaoticNeutrals/T-800-8B
datasets:
- Dogge/freedom-rp-alpaca
- ResplendentAI/BluemoonAlpaca
- ResplendentAI/Synthetic_Soul_1k
- ResplendentAI/NSFW_Format_Test
- ResplendentAI/Alpaca_NSFW_Shuffled
- ResplendentAI/theory_of_mind_fixed_output
- jeiku/JeikuL3v2
- ResplendentAI/Sissification_Hypno_1k
language:
- en
library_name: transformers
license: apache-2.0
quantized_by: mradermacher
tags:
- not-for-all-audiences
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/ChaoticNeutrals/T-800-8B
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/T-800-8B-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/T-800-8B-i1-GGUF/resolve/main/T-800-8B.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
mradermacher/Oumuamua-7b-instruct-v2-GGUF | mradermacher | 2024-06-15T08:29:06Z | 4,423 | 2 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"ja",
"en",
"base_model:nitky/Oumuamua-7b-instruct-v2",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-14T19:19:30Z | ---
base_model: nitky/Oumuamua-7b-instruct-v2
language:
- ja
- en
library_name: transformers
license: apache-2.0
quantized_by: mradermacher
tags:
- mergekit
- merge
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: -->
static quants of https://huggingface.co/nitky/Oumuamua-7b-instruct-v2
<!-- provided-files -->
weighted/imatrix quants are available at https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-i1-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q2_K.gguf) | Q2_K | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.IQ3_XS.gguf) | IQ3_XS | 3.2 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q3_K_S.gguf) | Q3_K_S | 3.3 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.IQ3_S.gguf) | IQ3_S | 3.3 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.IQ3_M.gguf) | IQ3_M | 3.4 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q3_K_M.gguf) | Q3_K_M | 3.7 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q3_K_L.gguf) | Q3_K_L | 4.0 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.IQ4_XS.gguf) | IQ4_XS | 4.1 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q4_K_S.gguf) | Q4_K_S | 4.3 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q4_K_M.gguf) | Q4_K_M | 4.5 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q5_K_S.gguf) | Q5_K_S | 5.2 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q5_K_M.gguf) | Q5_K_M | 5.3 | |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q6_K.gguf) | Q6_K | 6.1 | very good quality |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.Q8_0.gguf) | Q8_0 | 7.9 | fast, best quality |
| [GGUF](https://huggingface.co/mradermacher/Oumuamua-7b-instruct-v2-GGUF/resolve/main/Oumuamua-7b-instruct-v2.f16.gguf) | f16 | 14.8 | 16 bpw, overkill |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time.
<!-- end -->
|
misri/realismEngineSDXL_v30VAE | misri | 2024-01-12T10:19:06Z | 4,422 | 2 | diffusers | [
"diffusers",
"safetensors",
"license:unknown",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionXLPipeline",
"region:us"
] | text-to-image | 2024-01-11T14:29:26Z | ---
license: unknown
---
|
shayantreylon2/no2_model5 | shayantreylon2 | 2024-06-26T16:44:53Z | 4,422 | 0 | transformers | [
"transformers",
"gguf",
"llama",
"text-generation-inference",
"unsloth",
"en",
"base_model:unsloth/llama-3-8b-bnb-4bit",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-26T16:36:14Z | ---
base_model: unsloth/llama-3-8b-bnb-4bit
language:
- en
license: apache-2.0
tags:
- text-generation-inference
- transformers
- unsloth
- llama
- gguf
---
# Uploaded model
- **Developed by:** shayantreylon2
- **License:** apache-2.0
- **Finetuned from model :** unsloth/llama-3-8b-bnb-4bit
This llama model was trained 2x faster with [Unsloth](https://github.com/unslothai/unsloth) and Huggingface's TRL library.
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/unsloth%20made%20with%20love.png" width="200"/>](https://github.com/unslothai/unsloth)
|
lighteternal/gpt2-finetuned-greek | lighteternal | 2021-05-23T08:33:11Z | 4,419 | 6 | transformers | [
"transformers",
"pytorch",
"jax",
"gpt2",
"text-generation",
"causal-lm",
"el",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2022-03-02T23:29:05Z |
---
language:
- el
tags:
- pytorch
- causal-lm
widget:
- text: "Το αγαπημένο μου μέρος είναι"
license: apache-2.0
---
# Greek (el) GPT2 model
<img src="https://huggingface.co/lighteternal/gpt2-finetuned-greek-small/raw/main/GPT2el.png" width="600"/>
### By the Hellenic Army Academy (SSE) and the Technical University of Crete (TUC)
* language: el
* licence: apache-2.0
* dataset: ~23.4 GB of Greek corpora
* model: GPT2 (12-layer, 768-hidden, 12-heads, 117M parameters. OpenAI GPT-2 English model, finetuned for the Greek language)
* pre-processing: tokenization + BPE segmentation
* metrics: perplexity
### Model description
A text generation (autoregressive) model, using Huggingface transformers and fastai based on the English GPT-2.
Finetuned with gradual layer unfreezing. This is a more efficient and sustainable alternative compared to training from scratch, especially for low-resource languages.
Based on the work of Thomas Dehaene (ML6) for the creation of a Dutch GPT2: https://colab.research.google.com/drive/1Y31tjMkB8TqKKFlZ5OJ9fcMp3p8suvs4?usp=sharing
### How to use
```
from transformers import pipeline
model = "lighteternal/gpt2-finetuned-greek"
generator = pipeline(
'text-generation',
device=0,
model=f'{model}',
tokenizer=f'{model}')
text = "Μια φορά κι έναν καιρό"
print("\
".join([x.get("generated_text") for x in generator(
text,
max_length=len(text.split(" "))+15,
do_sample=True,
top_k=50,
repetition_penalty = 1.2,
add_special_tokens=False,
num_return_sequences=5,
temperature=0.95,
top_p=0.95)]))
```
## Training data
We used a 23.4GB sample from a consolidated Greek corpus from CC100, Wikimatrix, Tatoeba, Books, SETIMES and GlobalVoices containing long senquences.
This is a better version of our GPT-2 small model (https://huggingface.co/lighteternal/gpt2-finetuned-greek-small)
## Metrics
| Metric | Value |
| ----------- | ----------- |
| Train Loss | 3.67 |
| Validation Loss | 3.83 |
| Perplexity | 39.12 |
### Acknowledgement
The research work was supported by the Hellenic Foundation for Research and Innovation (HFRI) under the HFRI PhD Fellowship grant (Fellowship Number:50, 2nd call)
Based on the work of Thomas Dehaene (ML6): https://blog.ml6.eu/dutch-gpt2-autoregressive-language-modelling-on-a-budget-cff3942dd020
|
mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF | mradermacher | 2024-06-13T21:05:56Z | 4,418 | 1 | transformers | [
"transformers",
"gguf",
"merge",
"mergekit",
"lazymergekit",
"not-for-all-audiences",
"nsfw",
"rp",
"roleplay",
"role-play",
"en",
"base_model:Casual-Autopsy/L3-Umbral-Mind-RP-8B",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-12T15:57:01Z | ---
base_model: Casual-Autopsy/L3-Umbral-Mind-RP-8B
language:
- en
library_name: transformers
license: llama3
quantized_by: mradermacher
tags:
- merge
- mergekit
- lazymergekit
- not-for-all-audiences
- nsfw
- rp
- roleplay
- role-play
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/Casual-Autopsy/L3-Umbral-Mind-RP-8B
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-Umbral-Mind-RP-8B-i1-GGUF/resolve/main/L3-Umbral-Mind-RP-8B.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
KETI-AIR-Downstream/long-ke-t5-base-summarization_e10 | KETI-AIR-Downstream | 2023-09-18T01:28:33Z | 4,417 | 0 | transformers | [
"transformers",
"pytorch",
"safetensors",
"longt5",
"text2text-generation",
"generated_from_trainer",
"dataset:jsonl_dataset_sum.py",
"base_model:KETI-AIR/long-ke-t5-base",
"model-index",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | text2text-generation | 2023-06-05T04:24:59Z | ---
tags:
- generated_from_trainer
datasets:
- jsonl_dataset_sum.py
metrics:
- rouge
widget:
- text: 'summarization-num_lines-1: 현대자동차는 18일(현지 시간) 이탈리아 레이크 코모에서 개최된 ''현대 리유니온''
행사에서 ''포니 쿠페 콘셉트'' 복원 모델을 세계에 첫 공개했습니다. 이 프로젝트는 현대차의 창업자인 정주영 선대 회장의 수출보국(輸出報國)
정신과 포니 쿠페를 통한 글로벌 브랜드 정립에 대한 끊임없는 열정과 도전 정신을 재조명하기 위한 것입니다. 현대차에 따르면, 이번 현대 리유니온
행사는 회사의 역사를 다시 돌아보며 변하지 않는 미래 지향적인 비전과 방향성을 공유하는 브랜드 유산 행사입니다.'
example_title: sample 1
base_model: KETI-AIR/long-ke-t5-base
model-index:
- name: summarization_all
results:
- task:
type: summarization
name: Summarization
dataset:
name: jsonl_dataset_sum.py
type: jsonl_dataset_sum.py
config: 'null'
split: None
metrics:
- type: rouge
value: 21.9857
name: Rouge1
---
<!-- This model card has been generated automatically according to the information the Trainer had access to. You
should probably proofread and complete it, then remove this comment. -->
# summarization_all
This model is a fine-tuned version of [KETI-AIR/long-ke-t5-base](https://huggingface.co/KETI-AIR/long-ke-t5-base) on the jsonl_dataset_sum.py dataset.
It achieves the following results on the evaluation set:
- Loss: 1.1442
- Rouge1: 21.9857
- Rouge2: 10.2876
- Rougel: 21.4026
- Rougelsum: 21.4278
- Gen Len: 86.2560
## Model description
More information needed
## Intended uses & limitations
More information needed
## Training and evaluation data
More information needed
## Training procedure
### Training hyperparameters
The following hyperparameters were used during training:
- learning_rate: 0.001
- train_batch_size: 1
- eval_batch_size: 1
- seed: 42
- distributed_type: multi-GPU
- num_devices: 8
- total_train_batch_size: 8
- total_eval_batch_size: 8
- optimizer: Adam with betas=(0.9,0.999) and epsilon=1e-08
- lr_scheduler_type: linear
- num_epochs: 10.0
### Training results
| Training Loss | Epoch | Step | Validation Loss | Rouge1 | Rouge2 | Rougel | Rougelsum | Gen Len |
|:-------------:|:-----:|:-------:|:---------------:|:-------:|:-------:|:-------:|:---------:|:-------:|
| 1.2503 | 1.0 | 184670 | 1.2439 | 20.2525 | 9.1467 | 19.7454 | 19.771 | 87.1766 |
| 1.1629 | 2.0 | 369340 | 1.1773 | 21.0068 | 9.6691 | 20.4565 | 20.4888 | 89.6074 |
| 1.1087 | 3.0 | 554010 | 1.1431 | 21.0216 | 9.6545 | 20.489 | 20.5108 | 85.5895 |
| 1.056 | 4.0 | 738680 | 1.1247 | 21.6776 | 10.1424 | 21.09 | 21.1168 | 89.6576 |
| 1.0199 | 5.0 | 923350 | 1.1179 | 21.6563 | 10.0965 | 21.0814 | 21.1056 | 89.2454 |
| 0.9652 | 6.0 | 1108020 | 1.1122 | 21.6209 | 10.0725 | 21.0623 | 21.0864 | 86.7079 |
| 0.92 | 7.0 | 1292690 | 1.1136 | 21.9396 | 10.2734 | 21.3465 | 21.3745 | 86.5547 |
| 0.8804 | 8.0 | 1477360 | 1.1228 | 21.8457 | 10.1858 | 21.2552 | 21.278 | 87.6413 |
| 0.8447 | 9.0 | 1662030 | 1.1327 | 21.92 | 10.2635 | 21.3415 | 21.3633 | 86.4453 |
| 0.7678 | 10.0 | 1846700 | 1.1442 | 21.9857 | 10.2876 | 21.4026 | 21.4278 | 86.2560 |
### Framework versions
- Transformers 4.25.1
- Pytorch 1.12.0
- Datasets 2.8.0
- Tokenizers 0.13.2
|
aubmindlab/bert-base-arabertv02-twitter | aubmindlab | 2023-03-23T16:26:59Z | 4,416 | 5 | transformers | [
"transformers",
"pytorch",
"tensorboard",
"safetensors",
"bert",
"fill-mask",
"ar",
"arxiv:2003.00104",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | fill-mask | 2022-03-02T23:29:05Z | ---
language: ar
datasets:
- wikipedia
- Osian
- 1.5B-Arabic-Corpus
- oscar-arabic-unshuffled
- Assafir(private)
- Twitter(private)
widget:
- text: " عاصمة لبنان هي [MASK] ."
---
<img src="https://raw.githubusercontent.com/aub-mind/arabert/master/arabert_logo.png" width="100" align="center"/>
# AraBERTv0.2-Twitter
AraBERTv0.2-Twitter-base/large are two new models for Arabic dialects and tweets, trained by continuing the pre-training using the MLM task on ~60M Arabic tweets (filtered from a collection on 100M).
The two new models have had emojies added to their vocabulary in addition to common words that weren't at first present. The pre-training was done with a max sentence length of 64 only for 1 epoch.
**AraBERT** is an Arabic pretrained language model based on [Google's BERT architechture](https://github.com/google-research/bert). AraBERT uses the same BERT-Base config. More details are available in the [AraBERT Paper](https://arxiv.org/abs/2003.00104) and in the [AraBERT Meetup](https://github.com/WissamAntoun/pydata_khobar_meetup)
## Other Models
Model | HuggingFace Model Name | Size (MB/Params)| Pre-Segmentation | DataSet (Sentences/Size/nWords) |
---|:---:|:---:|:---:|:---:
AraBERTv0.2-base | [bert-base-arabertv02](https://huggingface.co/aubmindlab/bert-base-arabertv02) | 543MB / 136M | No | 200M / 77GB / 8.6B |
AraBERTv0.2-large| [bert-large-arabertv02](https://huggingface.co/aubmindlab/bert-large-arabertv02) | 1.38G / 371M | No | 200M / 77GB / 8.6B |
AraBERTv2-base| [bert-base-arabertv2](https://huggingface.co/aubmindlab/bert-base-arabertv2) | 543MB / 136M | Yes | 200M / 77GB / 8.6B |
AraBERTv2-large| [bert-large-arabertv2](https://huggingface.co/aubmindlab/bert-large-arabertv2) | 1.38G / 371M | Yes | 200M / 77GB / 8.6B |
AraBERTv0.1-base| [bert-base-arabertv01](https://huggingface.co/aubmindlab/bert-base-arabertv01) | 543MB / 136M | No | 77M / 23GB / 2.7B |
AraBERTv1-base| [bert-base-arabert](https://huggingface.co/aubmindlab/bert-base-arabert) | 543MB / 136M | Yes | 77M / 23GB / 2.7B |
AraBERTv0.2-Twitter-base| [bert-base-arabertv02-twitter](https://huggingface.co/aubmindlab/bert-base-arabertv02-twitter) | 543MB / 136M | No | Same as v02 + 60M Multi-Dialect Tweets|
AraBERTv0.2-Twitter-large| [bert-large-arabertv02-twitter](https://huggingface.co/aubmindlab/bert-large-arabertv02-twitter) | 1.38G / 371M | No | Same as v02 + 60M Multi-Dialect Tweets|
# Preprocessing
**The model is trained on a sequence length of 64, using max length beyond 64 might result in degraded performance**
It is recommended to apply our preprocessing function before training/testing on any dataset.
The preprocessor will keep and space out emojis when used with a "twitter" model.
```python
from arabert.preprocess import ArabertPreprocessor
from transformers import AutoTokenizer, AutoModelForMaskedLM
model_name="aubmindlab/bert-base-arabertv02-twitter"
arabert_prep = ArabertPreprocessor(model_name=model_name)
text = "ولن نبالغ إذا قلنا إن هاتف أو كمبيوتر المكتب في زمننا هذا ضروري"
arabert_prep.preprocess(text)
tokenizer = AutoTokenizer.from_pretrained("aubmindlab/bert-base-arabertv02-twitter")
model = AutoModelForMaskedLM.from_pretrained("aubmindlab/bert-base-arabertv02-twitter")
```
# If you used this model please cite us as :
Google Scholar has our Bibtex wrong (missing name), use this instead
```
@inproceedings{antoun2020arabert,
title={AraBERT: Transformer-based Model for Arabic Language Understanding},
author={Antoun, Wissam and Baly, Fady and Hajj, Hazem},
booktitle={LREC 2020 Workshop Language Resources and Evaluation Conference 11--16 May 2020},
pages={9}
}
```
# Acknowledgments
Thanks to TensorFlow Research Cloud (TFRC) for the free access to Cloud TPUs, couldn't have done it without this program, and to the [AUB MIND Lab](https://sites.aub.edu.lb/mindlab/) Members for the continuous support. Also thanks to [Yakshof](https://www.yakshof.com/#/) and Assafir for data and storage access. Another thanks for Habib Rahal (https://www.behance.net/rahalhabib), for putting a face to AraBERT.
# Contacts
**Wissam Antoun**: [Linkedin](https://www.linkedin.com/in/wissam-antoun-622142b4/) | [Twitter](https://twitter.com/wissam_antoun) | [Github](https://github.com/WissamAntoun) | <[email protected]> | <[email protected]>
**Fady Baly**: [Linkedin](https://www.linkedin.com/in/fadybaly/) | [Twitter](https://twitter.com/fadybaly) | [Github](https://github.com/fadybaly) | <[email protected]> | <[email protected]>
|
OpenSourceEnjoyer/LLaMA-3-8B-Function-Calling-GGUF | OpenSourceEnjoyer | 2024-06-27T04:00:15Z | 4,415 | 0 | transformers | [
"transformers",
"gguf",
"llama",
"text-generation-inference",
"unsloth",
"en",
"base_model:unsloth/llama-3-8b-bnb-4bit",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-27T03:45:27Z | ---
base_model: unsloth/llama-3-8b-bnb-4bit
language:
- en
license: apache-2.0
tags:
- text-generation-inference
- transformers
- unsloth
- llama
- gguf
---
# Uploaded model
- **Developed by:** OpenSourceEnjoyer
- **License:** apache-2.0
- **Finetuned from model :** unsloth/llama-3-8b-bnb-4bit
This llama model was trained 2x faster with [Unsloth](https://github.com/unslothai/unsloth) and Huggingface's TRL library.
[<img src="https://raw.githubusercontent.com/unslothai/unsloth/main/images/unsloth%20made%20with%20love.png" width="200"/>](https://github.com/unslothai/unsloth)
|
Yntec/ReVAnimatedRemix | Yntec | 2024-05-25T20:44:29Z | 4,414 | 1 | diffusers | [
"diffusers",
"safetensors",
"Anime",
"Illustration",
"Cartoon",
"90s",
"Retro",
"Vintage",
"s6yx",
"andite",
"Clumsy_Trainer",
"OneRing",
"DucHaiten",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"license:creativeml-openrail-m",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2024-05-20T17:03:36Z | ---
license: creativeml-openrail-m
library_name: diffusers
pipeline_tag: text-to-image
tags:
- Anime
- Illustration
- Cartoon
- 90s
- Retro
- Vintage
- s6yx
- andite
- Clumsy_Trainer
- OneRing
- DucHaiten
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- diffusers
---
# ReVAnimated Remix
ReVAnimated 1.0 mixed with AnythingNostalgic to expand what it can draw.
Samples and prompts:

Top left: pretty cute little girls, Library Background,action,Pigtails,Crew Jeans,final fantasy, chibi, masterpiece, detailed, highres, 90s eyes
Top right: retro funk 70s style color movie still of beautiful face, young pretty Audrey Hepburn voluptuous at a neon convenience storefront
Bottom left: Pretty detailed CUTE Girl, Cartoon, sitting on a computer monitor, holding antique TV, DETAILED CHIBI EYES, gorgeous detailed hair, Magazine ad, iconic, 1940, sharp focus. Illustration By KlaysMoji and artgerm and Clay Mann and and leyendecker and kyoani
Bottom right: blonde pretty Princess Peach wearing crown in the mushroom kingdom
Original pages:
https://huggingface.co/xyn-ai/anything-v4.0
https://civitai.com/models/137781?modelVersionId=152147 (Esthetic Retro Anime)
https://huggingface.co/Yntec/KIDSILLUSTRATIONS
KIDS ILLUSTRATIONS V2 - https://civitai.com/models/60724?modelVersionId=67980
DucHaiten-GoldenLife - https://tensor.art/models/628276277415133426
https://huggingface.co/Yntec/NostalgicLife
https://huggingface.co/Yntec/GoodLife
https://huggingface.co/Yntec/Nostalgic
# Recipe:
- SuperMerger Weight Sum Use MBW 0,0,0,0,0,0,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1
Model A:
RevAnimated
Model B:
AnythingNostalgic
Output:
ReVAnimatedRemix |
togethercomputer/RedPajama-INCITE-7B-Base | togethercomputer | 2023-06-06T02:44:34Z | 4,412 | 93 | transformers | [
"transformers",
"pytorch",
"gpt_neox",
"text-generation",
"en",
"dataset:togethercomputer/RedPajama-Data-1T",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2023-05-04T05:50:06Z | ---
license: apache-2.0
language:
- en
datasets:
- togethercomputer/RedPajama-Data-1T
---
# RedPajama-INCITE-7B-Base
RedPajama-INCITE-7B-Base was developed by Together and leaders from the open-source AI community including Ontocord.ai, ETH DS3Lab, AAI CERC, Université de Montréal, MILA - Québec AI Institute, Stanford Center for Research on Foundation Models (CRFM), Stanford Hazy Research research group and LAION.
The training was done on 3,072 V100 GPUs provided as part of the INCITE 2023 project on Scalable Foundation Models for Transferrable Generalist AI, awarded to MILA, LAION, and EleutherAI in fall 2022, with support from the Oak Ridge Leadership Computing Facility (OLCF) and INCITE program.
- Base Model: [RedPajama-INCITE-7B-Base](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base)
- Instruction-tuned Version: [RedPajama-INCITE-7B-Instruct](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Instruct)
- Chat Version: [RedPajama-INCITE-7B-Chat](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Chat)
## Model Details
- **Developed by**: Together Computer.
- **Model type**: Language Model
- **Language(s)**: English
- **License**: Apache 2.0
- **Model Description**: A 6.9B parameter pretrained language model.
# Quick Start
Please note that the model requires `transformers` version >= 4.25.1.
## GPU Inference
This requires a GPU with 16GB memory.
```python
import torch
import transformers
from transformers import AutoTokenizer, AutoModelForCausalLM
MIN_TRANSFORMERS_VERSION = '4.25.1'
# check transformers version
assert transformers.__version__ >= MIN_TRANSFORMERS_VERSION, f'Please upgrade transformers to version {MIN_TRANSFORMERS_VERSION} or higher.'
# init
tokenizer = AutoTokenizer.from_pretrained("togethercomputer/RedPajama-INCITE-7B-Base")
model = AutoModelForCausalLM.from_pretrained("togethercomputer/RedPajama-INCITE-7B-Base", torch_dtype=torch.float16)
model = model.to('cuda:0')
# infer
prompt = "Alan Turing is"
inputs = tokenizer(prompt, return_tensors='pt').to(model.device)
input_length = inputs.input_ids.shape[1]
outputs = model.generate(
**inputs, max_new_tokens=128, do_sample=True, temperature=0.7, top_p=0.7, top_k=50, return_dict_in_generate=True
)
token = outputs.sequences[0, input_length:]
output_str = tokenizer.decode(token)
print(output_str)
"""
widely considered to be the father of modern computer science and artificial intelligence. He was a brilliant mathematician and cryptographer, who worked for the British government during World War II. He was instrumental in breaking the German Enigma code, and is credited with helping to shorten the war by two years...
"""
```
## GPU Inference in Int8
This requires a GPU with 12GB memory.
To run inference with int8, please ensure you have installed accelerate and bitandbytes. You can install them with the following command:
```bash
pip install accelerate
pip install bitsandbytes
```
Then you can run inference with int8 as follows:
```python
import torch
import transformers
from transformers import AutoTokenizer, AutoModelForCausalLM
MIN_TRANSFORMERS_VERSION = '4.25.1'
# check transformers version
assert transformers.__version__ >= MIN_TRANSFORMERS_VERSION, f'Please upgrade transformers to version {MIN_TRANSFORMERS_VERSION} or higher.'
# init
tokenizer = AutoTokenizer.from_pretrained("togethercomputer/RedPajama-INCITE-7B-Base")
model = AutoModelForCausalLM.from_pretrained("togethercomputer/RedPajama-INCITE-7B-Base", device_map='auto', torch_dtype=torch.float16, load_in_8bit=True)
# infer
prompt = "Alan Turing is"
inputs = tokenizer(prompt, return_tensors='pt').to(model.device)
input_length = inputs.input_ids.shape[1]
outputs = model.generate(
**inputs, max_new_tokens=128, do_sample=True, temperature=0.7, top_p=0.7, top_k=50, return_dict_in_generate=True
)
token = outputs.sequences[0, input_length:]
output_str = tokenizer.decode(token)
print(output_str)
"""
a very well-known name in the world of computer science. It is named after the mathematician Alan Turing. He is famous for his work on the Enigma machine, which was used by the Germans during World War II....
"""```
## CPU Inference
```python
import torch
import transformers
from transformers import AutoTokenizer, AutoModelForCausalLM
MIN_TRANSFORMERS_VERSION = '4.25.1'
# check transformers version
assert transformers.__version__ >= MIN_TRANSFORMERS_VERSION, f'Please upgrade transformers to version {MIN_TRANSFORMERS_VERSION} or higher.'
# init
tokenizer = AutoTokenizer.from_pretrained("togethercomputer/RedPajama-INCITE-7B-Base")
model = AutoModelForCausalLM.from_pretrained("togethercomputer/RedPajama-INCITE-7B-Base", torch_dtype=torch.bfloat16)
# infer
prompt = "Alan Turing is"
inputs = tokenizer(prompt, return_tensors='pt').to(model.device)
input_length = inputs.input_ids.shape[1]
outputs = model.generate(
**inputs, max_new_tokens=128, do_sample=True, temperature=0.7, top_p=0.7, top_k=50, return_dict_in_generate=True
)
token = outputs.sequences[0, input_length:]
output_str = tokenizer.decode(token)
print(output_str)
"""
one of the most important figures in the history of computing. He is best known for his work on the development of the modern computer and for his code-breaking work during World War II. He was also a brilliant mathematician and philosopher.
"""
```
Please note that since `LayerNormKernelImpl` is not implemented in fp16 for CPU, we use `bfloat16` for CPU inference.
# Uses
## Direct Use
Excluded uses are described below.
### Misuse, Malicious Use, and Out-of-Scope Use
It is the responsibility of the end user to ensure that the model is used in a responsible and ethical manner.
#### Out-of-Scope Use
`RedPajama-INCITE-7B-Base` is a language model and may not perform well for other use cases outside of its intended scope.
For example, it may not be suitable for use in safety-critical applications or for making decisions that have a significant impact on individuals or society.
It is important to consider the limitations of the model and to only use it for its intended purpose.
#### Misuse and Malicious Use
`RedPajama-INCITE-7B-Base` is designed for language modeling.
Misuse of the model, such as using it to engage in illegal or unethical activities, is strictly prohibited and goes against the principles of the project.
Using the model to generate content that is cruel to individuals is a misuse of this model. This includes, but is not limited to:
- Generating fake news, misinformation, or propaganda
- Promoting hate speech, discrimination, or violence against individuals or groups
- Impersonating individuals or organizations without their consent
- Engaging in cyberbullying or harassment
- Defamatory content
- Spamming or scamming
- Sharing confidential or sensitive information without proper authorization
- Violating the terms of use of the model or the data used to train it
- Creating automated bots for malicious purposes such as spreading malware, phishing scams, or spamming
## Limitations
`RedPajama-INCITE-7B-Base`, like other language models, has limitations that should be taken into consideration.
For example, the model may not always provide accurate or relevant answers, particularly for questions that are complex, ambiguous, or outside of its training data.
We therefore welcome contributions from individuals and organizations, and encourage collaboration towards creating a more robust and inclusive chatbot.
## Training
**Training Data**
Please refer to [togethercomputer/RedPajama-Data-1T](https://huggingface.co/datasets/togethercomputer/RedPajama-Data-1T)
**Training Procedure**
- **Hardware:** 512 nodes of 6xV100 (IBM Power9), on the OLCF Summit cluster
- **Optimizer:** Apex FusedAdam
- **Parallelism:** Pipeline parallel 12, tensor parallel 2
- **Gradient Accumulations**: 8 (global batch size 4M tokens)
- **Num of Tokens:** 1.001T Tokens
- **Learning rate:** 0.00012
## Benchmark
Please refer to our [blog post](https://together.xyz) for benchmark results.
## Intermediate Checkpoints
We provide 11 intermediate checkpoints that have been released for study.
The checkpoints are organized based on the number of tokens they contain, ranging from 240 billion tokens to 1 trillion tokens.
- [240b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/240b_tokens)
- [280b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/280b_tokens)
- [400b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/400b_tokens)
- [440b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/440b_tokens)
- [500b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/500b_tokens)
- [600b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/600b_tokens)
- [700b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/700b_tokens)
- [720b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/720b_tokens)
- [960b_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/960b_tokens)
- [1t_tokens](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/1t_tokens)
- [latest](https://huggingface.co/togethercomputer/RedPajama-INCITE-7B-Base/tree/main)
## Community
Join us on [Together Discord](https://discord.gg/6ZVDU8tTD4) |
Habana/llama | Habana | 2024-05-01T17:10:32Z | 4,410 | 0 | null | [
"optimum_habana",
"license:apache-2.0",
"region:us"
] | null | 2024-05-01T17:05:55Z | ---
license: apache-2.0
---
[Optimum Habana](https://github.com/huggingface/optimum-habana) is the interface between the Hugging Face Transformers and Diffusers libraries and Habana's Gaudi processor (HPU).
It provides a set of tools enabling easy and fast model loading, training and inference on single- and multi-HPU settings for different downstream tasks.
Learn more about how to take advantage of the power of Habana HPUs to train and deploy Transformers and Diffusers models at [hf.co/hardware/habana](https://huggingface.co/hardware/habana).
## Llama model HPU configuration
This model only contains the `GaudiConfig` file for running [Llama models](https://huggingface.co/meta-llama) on Habana's Gaudi processors (HPU).
**This model contains no model weights, only a GaudiConfig.**
This enables to specify:
- `use_fused_adam`: whether to use Habana's custom AdamW implementation
- `use_fused_clip_norm`: whether to use Habana's fused gradient norm clipping operator
- `use_torch_autocast`: whether to use PyTorch's autocast mixed precision
## Usage
The model is instantiated the same way as in the Transformers library.
The only difference is that there are a few new training arguments specific to HPUs.
[Here](https://github.com/huggingface/optimum-habana/blob/main/examples/language-modeling/run_clm.py) is a causal language modeling example script to pre-train/fine-tune a model. You can run it with Llama with the following command:
```bash
python3 run_lora_clm.py \
--model_name_or_path huggyllama/llama-7b \
--dataset_name tatsu-lab/alpaca \
--bf16 True \
--output_dir ./model_lora_llama \
--num_train_epochs 3 \
--per_device_train_batch_size 16 \
--evaluation_strategy "no" \
--save_strategy "no" \
--learning_rate 1e-4 \
--warmup_ratio 0.03 \
--lr_scheduler_type "constant" \
--max_grad_norm 0.3 \
--logging_steps 1 \
--do_train \
--do_eval \
--use_habana \
--use_lazy_mode \
--throughput_warmup_steps 3 \
--lora_rank=8 \
--lora_alpha=16 \
--lora_dropout=0.05 \
--lora_target_modules "q_proj" "v_proj" \
--dataset_concatenation \
--max_seq_length 512 \
--low_cpu_mem_usage True \
--validation_split_percentage 4 \
--adam_epsilon 1e-08
```
You will need to install the [PEFT](https://huggingface.co/docs/peft/index) library with `pip install peft` to run the command above.
Check the [documentation](https://huggingface.co/docs/optimum/habana/index) out for more advanced usage and examples.
|
stablediffusionapi/deliberate-v2 | stablediffusionapi | 2023-12-27T14:32:56Z | 4,409 | 10 | diffusers | [
"diffusers",
"safetensors",
"modelslab.com",
"stable-diffusion-api",
"text-to-image",
"ultra-realistic",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-03-27T14:45:13Z | ---
license: creativeml-openrail-m
tags:
- modelslab.com
- stable-diffusion-api
- text-to-image
- ultra-realistic
pinned: true
---
# model_name API Inference
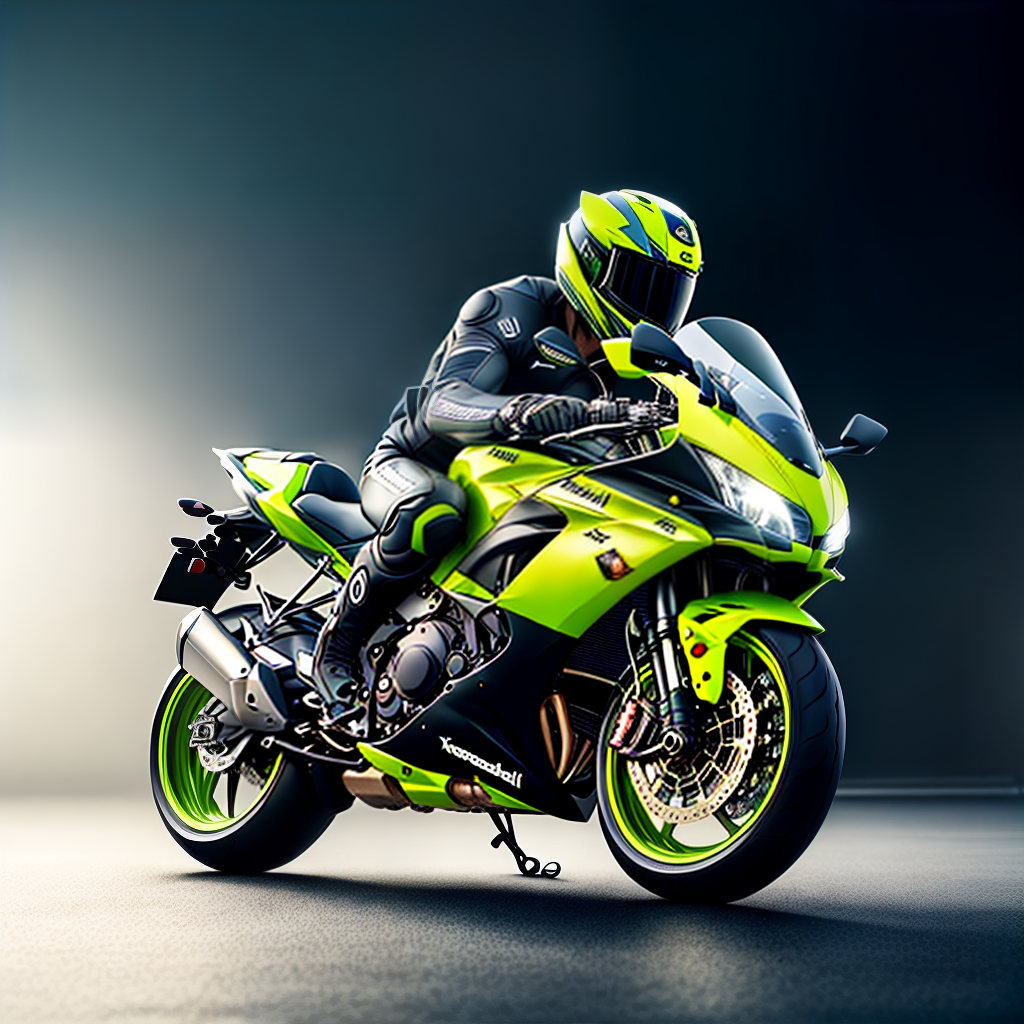
## Get API Key
Get API key from [ModelsLab API](http://modelslab.com), No Payment needed.
Replace Key in below code, change **model_id** to "deliberate-v2"
Coding in PHP/Node/Java etc? Have a look at docs for more code examples: [View docs](https://modelslab.com/docs)
Try model for free: [Generate Images](https://modelslab.com/models/deliberate-v2)
Model link: [View model](https://modelslab.com/models/deliberate-v2)
View all models: [View Models](https://modelslab.com/models)
import requests
import json
url = "https://modelslab.com/api/v6/images/text2img"
payload = json.dumps({
"key": "your_api_key",
"model_id": "deliberate-v2",
"prompt": "ultra realistic close up portrait ((beautiful pale cyberpunk female with heavy black eyeliner)), blue eyes, shaved side haircut, hyper detail, cinematic lighting, magic neon, dark red city, Canon EOS R3, nikon, f/1.4, ISO 200, 1/160s, 8K, RAW, unedited, symmetrical balance, in-frame, 8K",
"negative_prompt": "painting, extra fingers, mutated hands, poorly drawn hands, poorly drawn face, deformed, ugly, blurry, bad anatomy, bad proportions, extra limbs, cloned face, skinny, glitchy, double torso, extra arms, extra hands, mangled fingers, missing lips, ugly face, distorted face, extra legs, anime",
"width": "512",
"height": "512",
"samples": "1",
"num_inference_steps": "30",
"safety_checker": "no",
"enhance_prompt": "yes",
"seed": None,
"guidance_scale": 7.5,
"multi_lingual": "no",
"panorama": "no",
"self_attention": "no",
"upscale": "no",
"embeddings": "embeddings_model_id",
"lora": "lora_model_id",
"webhook": None,
"track_id": None
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
> Use this coupon code to get 25% off **DMGG0RBN** |
RichardErkhov/freecs_-_ThetaWave-7B-v0.1-gguf | RichardErkhov | 2024-06-16T13:02:52Z | 4,406 | 0 | null | [
"gguf",
"region:us"
] | null | 2024-06-16T09:58:49Z | Entry not found |
urchade/gliner_large-v2.1 | urchade | 2024-04-10T10:09:30Z | 4,405 | 16 | gliner | [
"gliner",
"pytorch",
"token-classification",
"multilingual",
"dataset:urchade/pile-mistral-v0.1",
"arxiv:2311.08526",
"license:apache-2.0",
"region:us"
] | token-classification | 2024-04-10T10:04:07Z | ---
license: apache-2.0
language:
- multilingual
library_name: gliner
datasets:
- urchade/pile-mistral-v0.1
pipeline_tag: token-classification
---
# About
GLiNER is a Named Entity Recognition (NER) model capable of identifying any entity type using a bidirectional transformer encoder (BERT-like). It provides a practical alternative to traditional NER models, which are limited to predefined entities, and Large Language Models (LLMs) that, despite their flexibility, are costly and large for resource-constrained scenarios.
## Links
* Paper: https://arxiv.org/abs/2311.08526
* Repository: https://github.com/urchade/GLiNER
## Available models
| Release | Model Name | # of Parameters | Language | License |
| - | - | - | - | - |
| v0 | [urchade/gliner_base](https://huggingface.co/urchade/gliner_base)<br>[urchade/gliner_multi](https://huggingface.co/urchade/gliner_multi) | 209M<br>209M | English<br>Multilingual | cc-by-nc-4.0 |
| v1 | [urchade/gliner_small-v1](https://huggingface.co/urchade/gliner_small-v1)<br>[urchade/gliner_medium-v1](https://huggingface.co/urchade/gliner_medium-v1)<br>[urchade/gliner_large-v1](https://huggingface.co/urchade/gliner_large-v1) | 166M<br>209M<br>459M | English <br> English <br> English | cc-by-nc-4.0 |
| v2 | [urchade/gliner_small-v2](https://huggingface.co/urchade/gliner_small-v2)<br>[urchade/gliner_medium-v2](https://huggingface.co/urchade/gliner_medium-v2)<br>[urchade/gliner_large-v2](https://huggingface.co/urchade/gliner_large-v2) | 166M<br>209M<br>459M | English <br> English <br> English | apache-2.0 |
| v2.1 | [urchade/gliner_small-v2.1](https://huggingface.co/urchade/gliner_small-v2.1)<br>[urchade/gliner_medium-v2.1](https://huggingface.co/urchade/gliner_medium-v2.1)<br>[urchade/gliner_large-v2.1](https://huggingface.co/urchade/gliner_large-v2.1) <br>[urchade/gliner_multi-v2.1](https://huggingface.co/urchade/gliner_multi-v2.1) | 166M<br>209M<br>459M<br>209M | English <br> English <br> English <br> Multilingual | apache-2.0 |
## Installation
To use this model, you must install the GLiNER Python library:
```
!pip install gliner
```
## Usage
Once you've downloaded the GLiNER library, you can import the GLiNER class. You can then load this model using `GLiNER.from_pretrained` and predict entities with `predict_entities`.
```python
from gliner import GLiNER
model = GLiNER.from_pretrained("urchade/gliner_large-v2.1")
text = """
Cristiano Ronaldo dos Santos Aveiro (Portuguese pronunciation: [kɾiʃˈtjɐnu ʁɔˈnaldu]; born 5 February 1985) is a Portuguese professional footballer who plays as a forward for and captains both Saudi Pro League club Al Nassr and the Portugal national team. Widely regarded as one of the greatest players of all time, Ronaldo has won five Ballon d'Or awards,[note 3] a record three UEFA Men's Player of the Year Awards, and four European Golden Shoes, the most by a European player. He has won 33 trophies in his career, including seven league titles, five UEFA Champions Leagues, the UEFA European Championship and the UEFA Nations League. Ronaldo holds the records for most appearances (183), goals (140) and assists (42) in the Champions League, goals in the European Championship (14), international goals (128) and international appearances (205). He is one of the few players to have made over 1,200 professional career appearances, the most by an outfield player, and has scored over 850 official senior career goals for club and country, making him the top goalscorer of all time.
"""
labels = ["person", "award", "date", "competitions", "teams"]
entities = model.predict_entities(text, labels)
for entity in entities:
print(entity["text"], "=>", entity["label"])
```
```
Cristiano Ronaldo dos Santos Aveiro => person
5 February 1985 => date
Al Nassr => teams
Portugal national team => teams
Ballon d'Or => award
UEFA Men's Player of the Year Awards => award
European Golden Shoes => award
UEFA Champions Leagues => competitions
UEFA European Championship => competitions
UEFA Nations League => competitions
Champions League => competitions
European Championship => competitions
```
## Named Entity Recognition benchmark result

## Model Authors
The model authors are:
* [Urchade Zaratiana](https://huggingface.co/urchade)
* Nadi Tomeh
* Pierre Holat
* Thierry Charnois
## Citation
```bibtex
@misc{zaratiana2023gliner,
title={GLiNER: Generalist Model for Named Entity Recognition using Bidirectional Transformer},
author={Urchade Zaratiana and Nadi Tomeh and Pierre Holat and Thierry Charnois},
year={2023},
eprint={2311.08526},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
``` |
digiplay/K-main_NEO | digiplay | 2024-04-12T14:50:02Z | 4,404 | 4 | diffusers | [
"diffusers",
"safetensors",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"license:other",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2024-04-12T09:01:33Z | ---
license: other
tags:
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- diffusers
inference: true
---
Model info:
https://civitai.com/models/87906/k-main
https://civitai.com/models/87906?modelVersionId=355353
|
mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF | mradermacher | 2024-06-23T12:03:15Z | 4,403 | 0 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"en",
"base_model:grimjim/Llama-3-Luminurse-v0.2-OAS-8B",
"license:cc-by-nc-4.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-08T08:45:59Z | ---
base_model: grimjim/Llama-3-Luminurse-v0.2-OAS-8B
language:
- en
library_name: transformers
license: cc-by-nc-4.0
license_link: LICENSE
quantized_by: mradermacher
tags:
- mergekit
- merge
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/grimjim/Llama-3-Luminurse-v0.2-OAS-8B
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-Luminurse-v0.2-OAS-8B-i1-GGUF/resolve/main/Llama-3-Luminurse-v0.2-OAS-8B.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
MaziyarPanahi/Mistral-7B-v0.3 | MaziyarPanahi | 2024-05-22T17:47:19Z | 4,399 | 5 | transformers | [
"transformers",
"safetensors",
"mistral",
"text-generation",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2024-05-22T17:34:35Z | ---
license: apache-2.0
---
# Model Card for Mistral-7B-v0.3
The Mistral-7B-v0.3 Large Language Model (LLM) is a Mistral-7B-v0.2 with extended vocabulary.
Mistral-7B-v0.3 has the following changes compared to [Mistral-7B-v0.2](https://huggingface.co/mistralai/Mistral-7B-v0.2/edit/main/README.md)
- Extended vocabulary to 32768
## Installation
It is recommended to use `mistralai/Mistral-7B-v0.3` with [mistral-inference](https://github.com/mistralai/mistral-inference). For HF transformers code snippets, please keep scrolling.
```
pip install mistral_inference
```
## Download
```py
from huggingface_hub import snapshot_download
from pathlib import Path
mistral_models_path = Path.home().joinpath('mistral_models', '7B-v0.3')
mistral_models_path.mkdir(parents=True, exist_ok=True)
snapshot_download(repo_id="mistralai/Mistral-7B-v0.3", allow_patterns=["params.json", "consolidated.safetensors", "tokenizer.model.v3"], local_dir=mistral_models_path)
```
### Demo
After installing `mistral_inference`, a `mistral-demo` CLI command should be available in your environment.
```
mistral-demo $HOME/mistral_models/7B-v0.3
```
Should give something along the following lines:
```
This is a test of the emergency broadcast system. This is only a test.
If this were a real emergency, you would be told what to do.
This is a test
=====================
This is another test of the new blogging software. I’m not sure if I’m going to keep it or not. I’m not sure if I’m going to keep
=====================
This is a third test, mistral AI is very good at testing. 🙂
This is a third test, mistral AI is very good at testing. 🙂
This
=====================
```
## Generate with `transformers`
If you want to use Hugging Face `transformers` to generate text, you can do something like this.
```py
from transformers import AutoModelForCausalLM, AutoTokenizer
model_id = "mistralai/Mistral-7B-v0.3"
tokenizer = AutoTokenizer.from_pretrained(model_id)
model = AutoModelForCausalLM.from_pretrained(model_id)
inputs = tokenizer("Hello my name is", return_tensors="pt")
outputs = model.generate(**inputs, max_new_tokens=20)
print(tokenizer.decode(outputs[0], skip_special_tokens=True))
```
## Limitations
The Mistral 7B Instruct model is a quick demonstration that the base model can be easily fine-tuned to achieve compelling performance.
It does not have any moderation mechanisms. We're looking forward to engaging with the community on ways to
make the model finely respect guardrails, allowing for deployment in environments requiring moderated outputs.
## The Mistral AI Team
Albert Jiang, Alexandre Sablayrolles, Alexis Tacnet, Antoine Roux, Arthur Mensch, Audrey Herblin-Stoop, Baptiste Bout, Baudouin de Monicault, Blanche Savary, Bam4d, Caroline Feldman, Devendra Singh Chaplot, Diego de las Casas, Eleonore Arcelin, Emma Bou Hanna, Etienne Metzger, Gianna Lengyel, Guillaume Bour, Guillaume Lample, Harizo Rajaona, Jean-Malo Delignon, Jia Li, Justus Murke, Louis Martin, Louis Ternon, Lucile Saulnier, Lélio Renard Lavaud, Margaret Jennings, Marie Pellat, Marie Torelli, Marie-Anne Lachaux, Nicolas Schuhl, Patrick von Platen, Pierre Stock, Sandeep Subramanian, Sophia Yang, Szymon Antoniak, Teven Le Scao, Thibaut Lavril, Timothée Lacroix, Théophile Gervet, Thomas Wang, Valera Nemychnikova, William El Sayed, William Marshall |
mrm8488/bert-small-finetuned-squadv2 | mrm8488 | 2021-05-20T00:33:09Z | 4,398 | 1 | transformers | [
"transformers",
"pytorch",
"jax",
"bert",
"question-answering",
"en",
"arxiv:1908.08962",
"endpoints_compatible",
"region:us"
] | question-answering | 2022-03-02T23:29:05Z | ---
language: en
thumbnail:
---
# BERT-Small fine-tuned on SQuAD v2
[BERT-Small](https://github.com/google-research/bert/) created by [Google Research](https://github.com/google-research) and fine-tuned on [SQuAD 2.0](https://rajpurkar.github.io/SQuAD-explorer/) for **Q&A** downstream task.
**Mode size** (after training): **109.74 MB**
## Details of BERT-Small and its 'family' (from their documentation)
Released on March 11th, 2020
This is model is a part of 24 smaller BERT models (English only, uncased, trained with WordPiece masking) referenced in [Well-Read Students Learn Better: On the Importance of Pre-training Compact Models](https://arxiv.org/abs/1908.08962).
The smaller BERT models are intended for environments with restricted computational resources. They can be fine-tuned in the same manner as the original BERT models. However, they are most effective in the context of knowledge distillation, where the fine-tuning labels are produced by a larger and more accurate teacher.
## Details of the downstream task (Q&A) - Dataset
[SQuAD2.0](https://rajpurkar.github.io/SQuAD-explorer/) combines the 100,000 questions in SQuAD1.1 with over 50,000 unanswerable questions written adversarially by crowdworkers to look similar to answerable ones. To do well on SQuAD2.0, systems must not only answer questions when possible, but also determine when no answer is supported by the paragraph and abstain from answering.
| Dataset | Split | # samples |
| -------- | ----- | --------- |
| SQuAD2.0 | train | 130k |
| SQuAD2.0 | eval | 12.3k |
## Model training
The model was trained on a Tesla P100 GPU and 25GB of RAM.
The script for fine tuning can be found [here](https://github.com/huggingface/transformers/blob/master/examples/question-answering/run_squad.py)
## Results:
| Metric | # Value |
| ------ | --------- |
| **EM** | **60.49** |
| **F1** | **64.21** |
## Comparison:
| Model | EM | F1 score | SIZE (MB) |
| ------------------------------------------------------------------------------------------- | --------- | --------- | --------- |
| [bert-tiny-finetuned-squadv2](https://huggingface.co/mrm8488/bert-tiny-finetuned-squadv2) | 48.60 | 49.73 | **16.74** |
| [bert-mini-finetuned-squadv2](https://huggingface.co/mrm8488/bert-mini-finetuned-squadv2) | 56.31 | 59.65 | 42.63 |
| [bert-small-finetuned-squadv2](https://huggingface.co/mrm8488/bert-small-finetuned-squadv2) | **60.49** | **64.21** | 109.74 |
## Model in action
Fast usage with **pipelines**:
```python
from transformers import pipeline
qa_pipeline = pipeline(
"question-answering",
model="mrm8488/bert-small-finetuned-squadv2",
tokenizer="mrm8488/bert-small-finetuned-squadv2"
)
qa_pipeline({
'context': "Manuel Romero has been working hardly in the repository hugginface/transformers lately",
'question': "Who has been working hard for hugginface/transformers lately?"
})
# Output:
```
```json
{
"answer": "Manuel Romero",
"end": 13,
"score": 0.9939319924374637,
"start": 0
}
```
### Yes! That was easy 🎉 Let's try with another example
```python
qa_pipeline({
'context': "Manuel Romero has been working hardly in the repository hugginface/transformers lately",
'question': "For which company has worked Manuel Romero?"
})
# Output:
```
```json
{
"answer": "hugginface/transformers",
"end": 79,
"score": 0.6024888734447131,
"start": 56
}
```
### It works!! 🎉 🎉 🎉
> Created by [Manuel Romero/@mrm8488](https://twitter.com/mrm8488) | [LinkedIn](https://www.linkedin.com/in/manuel-romero-cs/)
> Made with <span style="color: #e25555;">♥</span> in Spain
|
AI-Sweden-Models/Llama-3-8B-instruct | AI-Sweden-Models | 2024-06-26T09:01:47Z | 4,397 | 8 | transformers | [
"transformers",
"safetensors",
"gguf",
"llama",
"text-generation",
"conversational",
"sv",
"da",
"no",
"base_model:AI-Sweden-Models/Llama-3-8B",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2024-06-01T12:47:33Z | ---
language:
- sv
- da
- 'no'
license: apache-2.0
base_model: AI-Sweden-Models/Llama-3-8B
---
# Checkpoint 1
## Training setup
The training was perfomed on the [LUMI supercomputer](https://lumi-supercomputer.eu/) within the [DeployAI EU project](https://www.ai.se/en/project/deployai).
Based of the base model [AI-Sweden-Models/Llama-3-8B](https://huggingface.co/AI-Sweden-Models/Llama-3-8B).
## Dataset
A data recipe by: [42 Labs](https://huggingface.co/four-two-labs)

## How to use
```python
import transformers
import torch
model_id = "AI-Sweden-Models/Llama-3-8B-instruct"
pipeline = transformers.pipeline(
"text-generation",
model=model_id,
model_kwargs={"torch_dtype": torch.bfloat16},
device_map="auto",
)
messages = [
{"role": "system", "content": "Du är en hjälpsam assistant som svarar klokt och vänligt."},
{"role": "user", "content": "Hur gör man pannkakor? Och vad behöver man handla? Undrar också vad 5+6 är.."},
]
terminators = [
pipeline.tokenizer.eos_token_id,
pipeline.tokenizer.convert_tokens_to_ids("<|eot_id|>")
]
outputs = pipeline(
messages,
max_new_tokens=256,
eos_token_id=terminators,
do_sample=True,
temperature=0.6,
top_p=0.9,
)
print(outputs[0]["generated_text"][-1])
```
```python
>>> "För att göra pannkakor behöver du följande ingredienser:
- 1 kopp vetemjöl
- 1 tesked bakpulver
- 1/4 tesked salt
- 1 kopp mjölk
- 1 stort ägg
- 2 matskedar smält smör eller olja
För att börja, blanda vetemjölet, bakpulvret och saltet i en bunke. I en annan skål, vispa ihop mjölken, ägget och smöret eller oljan.
Tillsätt de våta ingredienserna till de torra ingredienserna och blanda tills det är väl blandat.
Låt smeten vila i cirka 10 minuter.
För att göra pannkakorna, värm en non-stick-panna eller stekpanna över medelvärme.
När den är varm, häll smeten på pannan och grädda tills kanterna börjar torka ut och toppen är fast.
Vänd pannkakan med en stekspade och grädda den andra sidan tills den är gyllenbrun.
Upprepa med resten av smeten.
När det gäller 5+6 är svaret 11."
``` |
princeton-nlp/unsup-simcse-bert-base-uncased | princeton-nlp | 2022-11-11T20:04:07Z | 4,396 | 3 | transformers | [
"transformers",
"pytorch",
"jax",
"bert",
"feature-extraction",
"arxiv:2104.08821",
"arxiv:1910.09700",
"endpoints_compatible",
"text-embeddings-inference",
"region:us"
] | feature-extraction | 2022-03-02T23:29:05Z | ---
tags:
- feature-extraction
- bert
---
# Model Card for unsup-simcse-bert-base-uncased
# Model Details
## Model Description
More information needed
- **Developed by:** Princeton NLP group
- **Shared by [Optional]:** Hugging Face
- **Model type:** Feature Extraction
- **Language(s) (NLP):** More information needed
- **License:** More information needed
- **Related Models:**
- **Parent Model:** BERT
- **Resources for more information:**
- [GitHub Repo](https://github.com/princeton-nlp/SimCSE)
- [Model Space](https://huggingface.co/spaces/mteb/leaderboard)
- [Associated Paper](https://arxiv.org/abs/2104.08821)
# Uses
## Direct Use
This model can be used for the task of Feature Engineering.
## Downstream Use [Optional]
More information needed
## Out-of-Scope Use
The model should not be used to intentionally create hostile or alienating environments for people.
# Bias, Risks, and Limitations
Significant research has explored bias and fairness issues with language models (see, e.g., [Sheng et al. (2021)](https://aclanthology.org/2021.acl-long.330.pdf) and [Bender et al. (2021)](https://dl.acm.org/doi/pdf/10.1145/3442188.3445922)). Predictions generated by the model may include disturbing and harmful stereotypes across protected classes; identity characteristics; and sensitive, social, and occupational groups.
## Recommendations
Users (both direct and downstream) should be made aware of the risks, biases and limitations of the model. More information needed for further recommendations.
# Training Details
## Training Data
The model craters note in the [Github Repository](https://github.com/princeton-nlp/SimCSE/blob/main/README.md)
> We train unsupervised SimCSE on 106 randomly sampled sentences from English Wikipedia, and train supervised SimCSE on the combination of MNLI and SNLI datasets (314k).
## Training Procedure
### Preprocessing
More information needed
### Speeds, Sizes, Times
More information needed
# Evaluation
## Testing Data, Factors & Metrics
### Testing Data
The model craters note in the [associated paper](https://arxiv.org/pdf/2104.08821.pdf)
> Our evaluation code for sentence embeddings is based on a modified version of [SentEval](https://github.com/facebookresearch/SentEval). It evaluates sentence embeddings on semantic textual similarity (STS) tasks and downstream transfer tasks. For STS tasks, our evaluation takes the "all" setting, and report Spearman's correlation. See [associated paper](https://arxiv.org/pdf/2104.08821.pdf) (Appendix B) for evaluation details.
### Factors
More information needed
### Metrics
More information needed
## Results
More information needed
# Model Examination
The model craters note in the [associated paper](https://arxiv.org/pdf/2104.08821.pdf)
> **Uniformity and alignment.**
We also observe that (1) though pre-trained embeddings have good alignment, their uniformity is poor (i.e., the embeddings are highly anisotropic); (2) post-processing methods like BERT-flow and BERT-whitening greatly improve uniformity but also suffer a degeneration in alignment; (3) unsupervised SimCSE effectively improves uniformity of pre-trained embeddings whereas keeping a good alignment;(4) incorporating supervised data in SimCSE further amends alignment.
# Environmental Impact
Carbon emissions can be estimated using the [Machine Learning Impact calculator](https://mlco2.github.io/impact#compute) presented in [Lacoste et al. (2019)](https://arxiv.org/abs/1910.09700).
- **Hardware Type:** Nvidia 3090 GPUs with CUDA 11
- **Hours used:** More information needed
- **Cloud Provider:** More information needed
- **Compute Region:** More information needed
- **Carbon Emitted:** More information needed
# Technical Specifications [optional]
## Model Architecture and Objective
More information needed
## Compute Infrastructure
More information needed
### Hardware
More information needed
### Software
More information needed
# Citation
**BibTeX:**
```bibtex
@inproceedings{gao2021simcse,
title={{SimCSE}: Simple Contrastive Learning of Sentence Embeddings},
author={Gao, Tianyu and Yao, Xingcheng and Chen, Danqi},
booktitle={Empirical Methods in Natural Language Processing (EMNLP)},
year={2021}
}
```
# Glossary [optional]
More information needed
# More Information [optional]
More information needed
# Model Card Authors [optional]
Princeton NLP group in collaboration with Ezi Ozoani and the Hugging Face team
# Model Card Contact
If you have any questions related to the code or the paper, feel free to email Tianyu (`[email protected]`) and Xingcheng (`[email protected]`). If you encounter any problems when using the code, or want to report a bug, you can open an issue. Please try to specify the problem with details so we can help you better and quicker!
# How to Get Started with the Model
Use the code below to get started with the model.
<details>
<summary> Click to expand </summary>
```python
from transformers import AutoTokenizer, AutoModel
tokenizer = AutoTokenizer.from_pretrained("princeton-nlp/unsup-simcse-bert-base-uncased")
model = AutoModel.from_pretrained("princeton-nlp/unsup-simcse-bert-base-uncased")
```
</details>
|
mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF | mradermacher | 2024-06-12T16:15:53Z | 4,393 | 0 | transformers | [
"transformers",
"gguf",
"facebook",
"meta",
"pytorch",
"llama",
"llama-3",
"en",
"base_model:failspy/Llama-3-8B-Instruct-MopeyMule",
"license:other",
"endpoints_compatible",
"region:us"
] | null | 2024-06-12T11:28:34Z | ---
base_model: failspy/Llama-3-8B-Instruct-MopeyMule
language:
- en
library_name: transformers
license: other
license_name: llama3
quantized_by: mradermacher
tags:
- facebook
- meta
- pytorch
- llama
- llama-3
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/failspy/Llama-3-8B-Instruct-MopeyMule
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/Llama-3-8B-Instruct-MopeyMule-i1-GGUF/resolve/main/Llama-3-8B-Instruct-MopeyMule.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
SargeZT/t2i-adapter-sdxl-segmentation | SargeZT | 2023-08-12T16:24:16Z | 4,390 | 9 | diffusers | [
"diffusers",
"safetensors",
"stable-diffusion-xl",
"stable-diffusion-xl-diffusers",
"text-to-image",
"t2i_adapter",
"base_model:stabilityai/stable-diffusion-xl-base-1.0",
"license:creativeml-openrail-m",
"region:us"
] | text-to-image | 2023-08-02T17:24:57Z |
---
license: creativeml-openrail-m
base_model: stabilityai/stable-diffusion-xl-base-1.0
tags:
- stable-diffusion-xl
- stable-diffusion-xl-diffusers
- text-to-image
- diffusers
- t2i_adapter
inference: true
---
# T2I_Adapter-SargeZT/t2i-adapter-sdxl-segmentation
These are T2I-adapter weights trained on stabilityai/stable-diffusion-xl-base-1.0. Please note this uses custom code to initialize the T2I. [You can find the adapter, pipeline, and training code here.](https://github.com/AMorporkian/T2IAdapter-SDXL-Diffusers)
You can find some example images below.
prompt: ['a cat laying on top of a blanket on a bed']

prompt: ['two elephants are walking in a zoo enclosure']

prompt: ['a variety of items are laid out on a table']

prompt: ['a sandwich and french fries on a tray']

prompt: ['a crowd of people flying kites on a beach']

prompt: ['a man holding a rainbow colored umbrella in front of a crowd']

prompt: ['a man riding skis down a snow covered slope']

prompt: ['a yellow fire hydrant sitting in the middle of a sidewalk']

## License
[SDXL 1.0 License](https://huggingface.co/stabilityai/stable-diffusion-xl-base-1.0/blob/main/LICENSE.md)
|
digiplay/xiaomeige_outlineColor_v10 | digiplay | 2024-04-01T13:10:08Z | 4,390 | 2 | diffusers | [
"diffusers",
"safetensors",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"license:other",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2024-04-01T12:37:25Z | ---
license: other
tags:
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- diffusers
inference: true
---
Model info: Outline Color | 勾线彩画凤
https://civitai.com/models/378117/outline-color-or
outlineColor_v10.safetensors
Author Civitai.com Page:
https://civitai.com/user/xiaomeige
Author's DEMO images:

|
euclaise/falcon_1b_stage1 | euclaise | 2023-09-17T20:30:14Z | 4,388 | 0 | transformers | [
"transformers",
"pytorch",
"falcon",
"text-generation",
"generated_from_trainer",
"custom_code",
"base_model:tiiuae/falcon-rw-1b",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2023-09-15T22:07:48Z | ---
license: apache-2.0
base_model: tiiuae/falcon-rw-1b
tags:
- generated_from_trainer
model-index:
- name: falcon_1b_stage1
results: []
---
<!-- This model card has been generated automatically according to the information the Trainer had access to. You
should probably proofread and complete it, then remove this comment. -->
# falcon_1b_stage1
This model is a fine-tuned version of [tiiuae/falcon-rw-1b](https://huggingface.co/tiiuae/falcon-rw-1b) on the None dataset.
It achieves the following results on the evaluation set:
- Loss: 2.0640
## Model description
More information needed
## Intended uses & limitations
More information needed
## Training and evaluation data
More information needed
## Training procedure
### Training hyperparameters
The following hyperparameters were used during training:
- learning_rate: 1e-05
- train_batch_size: 16
- eval_batch_size: 16
- seed: 42
- gradient_accumulation_steps: 8.0
- total_train_batch_size: 128.0
- optimizer: Adam with betas=(0.9,0.999) and epsilon=1e-08
- lr_scheduler_type: linear
- lr_scheduler_warmup_ratio: 0.2
- num_epochs: 1
### Training results
| Training Loss | Epoch | Step | Validation Loss |
|:-------------:|:-----:|:----:|:---------------:|
| 2.0522 | 1.0 | 652 | 2.0640 |
### Framework versions
- Transformers 4.33.2
- Pytorch 2.0.1+cu117
- Datasets 2.14.5
- Tokenizers 0.13.3
|
Harveenchadha/vakyansh-wav2vec2-tamil-tam-250 | Harveenchadha | 2021-09-22T07:55:33Z | 4,387 | 1 | transformers | [
"transformers",
"pytorch",
"wav2vec2",
"automatic-speech-recognition",
"audio",
"speech",
"ta",
"arxiv:2107.07402",
"license:mit",
"model-index",
"endpoints_compatible",
"region:us"
] | automatic-speech-recognition | 2022-03-02T23:29:04Z | ---
language: ta
#datasets:
#- Interspeech 2021
metrics:
- wer
tags:
- audio
- automatic-speech-recognition
- speech
license: mit
model-index:
- name: Wav2Vec2 Vakyansh Tamil Model by Harveen Chadha
results:
- task:
name: Speech Recognition
type: automatic-speech-recognition
dataset:
name: Common Voice ta
type: common_voice
args: ta
metrics:
- name: Test WER
type: wer
value: 53.64
---
## Pretrained Model
Fine-tuned on Multilingual Pretrained Model [CLSRIL-23](https://arxiv.org/abs/2107.07402). The original fairseq checkpoint is present [here](https://github.com/Open-Speech-EkStep/vakyansh-models). When using this model, make sure that your speech input is sampled at 16kHz.
**Note: The result from this model is without a language model so you may witness a higher WER in some cases.**
## Dataset
This model was trained on 4200 hours of Hindi Labelled Data. The labelled data is not present in public domain as of now.
## Training Script
Models were trained using experimental platform setup by Vakyansh team at Ekstep. Here is the [training repository](https://github.com/Open-Speech-EkStep/vakyansh-wav2vec2-experimentation).
In case you want to explore training logs on wandb they are [here](https://wandb.ai/harveenchadha/tamil-finetuning-multilingual).
## [Colab Demo](https://github.com/harveenchadha/bol/blob/main/demos/hf/tamil/hf_tamil_tnm_4200_demo.ipynb)
## Usage
The model can be used directly (without a language model) as follows:
```python
import soundfile as sf
import torch
from transformers import Wav2Vec2ForCTC, Wav2Vec2Processor
import argparse
def parse_transcription(wav_file):
# load pretrained model
processor = Wav2Vec2Processor.from_pretrained("Harveenchadha/vakyansh-wav2vec2-tamil-tam-250")
model = Wav2Vec2ForCTC.from_pretrained("Harveenchadha/vakyansh-wav2vec2-tamil-tam-250")
# load audio
audio_input, sample_rate = sf.read(wav_file)
# pad input values and return pt tensor
input_values = processor(audio_input, sampling_rate=sample_rate, return_tensors="pt").input_values
# INFERENCE
# retrieve logits & take argmax
logits = model(input_values).logits
predicted_ids = torch.argmax(logits, dim=-1)
# transcribe
transcription = processor.decode(predicted_ids[0], skip_special_tokens=True)
print(transcription)
```
## Evaluation
The model can be evaluated as follows on the hindi test data of Common Voice.
```python
import torch
import torchaudio
from datasets import load_dataset, load_metric
from transformers import Wav2Vec2ForCTC, Wav2Vec2Processor
import re
test_dataset = load_dataset("common_voice", "ta", split="test")
wer = load_metric("wer")
processor = Wav2Vec2Processor.from_pretrained("Harveenchadha/vakyansh-wav2vec2-tamil-tam-250")
model = Wav2Vec2ForCTC.from_pretrained("Harveenchadha/vakyansh-wav2vec2-tamil-tam-250")
model.to("cuda")
resampler = torchaudio.transforms.Resample(48_000, 16_000)
chars_to_ignore_regex = '[\,\?\.\!\-\;\:\"\“]'
# Preprocessing the datasets.
# We need to read the aduio files as arrays
def speech_file_to_array_fn(batch):
batch["sentence"] = re.sub(chars_to_ignore_regex, '', batch["sentence"]).lower()
speech_array, sampling_rate = torchaudio.load(batch["path"])
batch["speech"] = resampler(speech_array).squeeze().numpy()
return batch
test_dataset = test_dataset.map(speech_file_to_array_fn)
# Preprocessing the datasets.
# We need to read the aduio files as arrays
def evaluate(batch):
inputs = processor(batch["speech"], sampling_rate=16_000, return_tensors="pt", padding=True)
with torch.no_grad():
logits = model(inputs.input_values.to("cuda")).logits
pred_ids = torch.argmax(logits, dim=-1)
batch["pred_strings"] = processor.batch_decode(pred_ids, skip_special_tokens=True)
return batch
result = test_dataset.map(evaluate, batched=True, batch_size=8)
print("WER: {:2f}".format(100 * wer.compute(predictions=result["pred_strings"], references=result["sentence"])))
```
**Test Result**: 53.64 %
[**Colab Evaluation**](https://github.com/harveenchadha/bol/blob/main/demos/hf/tamil/hf_vakyansh_tamil_tnm_4200_evaluation_common_voice.ipynb)
## Credits
Thanks to Ekstep Foundation for making this possible. The vakyansh team will be open sourcing speech models in all the Indic Languages. |
mradermacher/llama-3-fantasy-writer-8b-i1-GGUF | mradermacher | 2024-06-15T23:29:23Z | 4,387 | 0 | transformers | [
"transformers",
"gguf",
"en",
"base_model:maldv/llama-3-fantasy-writer-8b",
"license:cc-by-nc-4.0",
"endpoints_compatible",
"region:us"
] | null | 2024-06-15T19:13:55Z | ---
base_model: maldv/llama-3-fantasy-writer-8b
language:
- en
library_name: transformers
license: cc-by-nc-4.0
quantized_by: mradermacher
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/maldv/llama-3-fantasy-writer-8b
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/llama-3-fantasy-writer-8b-i1-GGUF/resolve/main/llama-3-fantasy-writer-8b.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF | mradermacher | 2024-06-05T08:44:13Z | 4,382 | 2 | transformers | [
"transformers",
"gguf",
"not-for-all-audiences",
"llama3",
"en",
"base_model:Vdr1/L3-8B-sunfall-Stheno-v3.1",
"endpoints_compatible",
"region:us"
] | null | 2024-06-04T10:25:06Z | ---
base_model: Vdr1/L3-8B-sunfall-Stheno-v3.1
language:
- en
library_name: transformers
quantized_by: mradermacher
tags:
- not-for-all-audiences
- llama3
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/Vdr1/L3-8B-sunfall-Stheno-v3.1
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-Stheno-v3.1-i1-GGUF/resolve/main/L3-8B-sunfall-Stheno-v3.1.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
apple/OpenELM-450M | apple | 2024-05-02T00:55:46Z | 4,381 | 22 | transformers | [
"transformers",
"safetensors",
"openelm",
"text-generation",
"custom_code",
"arxiv:2404.14619",
"license:other",
"autotrain_compatible",
"region:us"
] | text-generation | 2024-04-12T21:48:16Z | ---
license: other
license_name: apple-sample-code-license
license_link: LICENSE
---
# OpenELM
*Sachin Mehta, Mohammad Hossein Sekhavat, Qingqing Cao, Maxwell Horton, Yanzi Jin, Chenfan Sun, Iman Mirzadeh, Mahyar Najibi, Dmitry Belenko, Peter Zatloukal, Mohammad Rastegari*
We introduce **OpenELM**, a family of **Open** **E**fficient **L**anguage **M**odels. OpenELM uses a layer-wise scaling strategy to efficiently allocate parameters within each layer of the transformer model, leading to enhanced accuracy. We pretrained OpenELM models using the [CoreNet](https://github.com/apple/corenet) library. We release both pretrained and instruction tuned models with 270M, 450M, 1.1B and 3B parameters.
Our pre-training dataset contains RefinedWeb, deduplicated PILE, a subset of RedPajama, and a subset of Dolma v1.6, totaling approximately 1.8 trillion tokens. Please check license agreements and terms of these datasets before using them.
## Usage
We have provided an example function to generate output from OpenELM models loaded via [HuggingFace Hub](https://huggingface.co/docs/hub/) in `generate_openelm.py`.
You can try the model by running the following command:
```
python generate_openelm.py --model apple/OpenELM-450M --hf_access_token [HF_ACCESS_TOKEN] --prompt 'Once upon a time there was' --generate_kwargs repetition_penalty=1.2
```
Please refer to [this link](https://huggingface.co/docs/hub/security-tokens) to obtain your hugging face access token.
Additional arguments to the hugging face generate function can be passed via `generate_kwargs`. As an example, to speedup the inference, you can try [lookup token speculative generation](https://huggingface.co/docs/transformers/generation_strategies) by passing the `prompt_lookup_num_tokens` argument as follows:
```
python generate_openelm.py --model apple/OpenELM-450M --hf_access_token [HF_ACCESS_TOKEN] --prompt 'Once upon a time there was' --generate_kwargs repetition_penalty=1.2 prompt_lookup_num_tokens=10
```
Alternatively, try model-wise speculative generation with an [assistive model](https://huggingface.co/blog/assisted-generation) by passing a smaller model through the `assistant_model` argument, for example:
```
python generate_openelm.py --model apple/OpenELM-450M --hf_access_token [HF_ACCESS_TOKEN] --prompt 'Once upon a time there was' --generate_kwargs repetition_penalty=1.2 --assistant_model [SMALLER_MODEL]
```
## Main Results
### Zero-Shot
| **Model Size** | **ARC-c** | **ARC-e** | **BoolQ** | **HellaSwag** | **PIQA** | **SciQ** | **WinoGrande** | **Average** |
|-----------------------------------------------------------------------------|-----------|-----------|-----------|---------------|-----------|-----------|----------------|-------------|
| [OpenELM-270M](https://huggingface.co/apple/OpenELM-270M) | 26.45 | 45.08 | **53.98** | 46.71 | 69.75 | **84.70** | **53.91** | 54.37 |
| [OpenELM-270M-Instruct](https://huggingface.co/apple/OpenELM-270M-Instruct) | **30.55** | **46.68** | 48.56 | **52.07** | **70.78** | 84.40 | 52.72 | **55.11** |
| [OpenELM-450M](https://huggingface.co/apple/OpenELM-450M) | 27.56 | 48.06 | 55.78 | 53.97 | 72.31 | 87.20 | 58.01 | 57.56 |
| [OpenELM-450M-Instruct](https://huggingface.co/apple/OpenELM-450M-Instruct) | **30.38** | **50.00** | **60.37** | **59.34** | **72.63** | **88.00** | **58.96** | **59.95** |
| [OpenELM-1_1B](https://huggingface.co/apple/OpenELM-1_1B) | 32.34 | **55.43** | 63.58 | 64.81 | **75.57** | **90.60** | 61.72 | 63.44 |
| [OpenELM-1_1B-Instruct](https://huggingface.co/apple/OpenELM-1_1B-Instruct) | **37.97** | 52.23 | **70.00** | **71.20** | 75.03 | 89.30 | **62.75** | **65.50** |
| [OpenELM-3B](https://huggingface.co/apple/OpenELM-3B) | 35.58 | 59.89 | 67.40 | 72.44 | 78.24 | **92.70** | 65.51 | 67.39 |
| [OpenELM-3B-Instruct](https://huggingface.co/apple/OpenELM-3B-Instruct) | **39.42** | **61.74** | **68.17** | **76.36** | **79.00** | 92.50 | **66.85** | **69.15** |
### LLM360
| **Model Size** | **ARC-c** | **HellaSwag** | **MMLU** | **TruthfulQA** | **WinoGrande** | **Average** |
|-----------------------------------------------------------------------------|-----------|---------------|-----------|----------------|----------------|-------------|
| [OpenELM-270M](https://huggingface.co/apple/OpenELM-270M) | 27.65 | 47.15 | 25.72 | **39.24** | **53.83** | 38.72 |
| [OpenELM-270M-Instruct](https://huggingface.co/apple/OpenELM-270M-Instruct) | **32.51** | **51.58** | **26.70** | 38.72 | 53.20 | **40.54** |
| [OpenELM-450M](https://huggingface.co/apple/OpenELM-450M) | 30.20 | 53.86 | **26.01** | 40.18 | 57.22 | 41.50 |
| [OpenELM-450M-Instruct](https://huggingface.co/apple/OpenELM-450M-Instruct) | **33.53** | **59.31** | 25.41 | **40.48** | **58.33** | **43.41** |
| [OpenELM-1_1B](https://huggingface.co/apple/OpenELM-1_1B) | 36.69 | 65.71 | **27.05** | 36.98 | 63.22 | 45.93 |
| [OpenELM-1_1B-Instruct](https://huggingface.co/apple/OpenELM-1_1B-Instruct) | **41.55** | **71.83** | 25.65 | **45.95** | **64.72** | **49.94** |
| [OpenELM-3B](https://huggingface.co/apple/OpenELM-3B) | 42.24 | 73.28 | **26.76** | 34.98 | 67.25 | 48.90 |
| [OpenELM-3B-Instruct](https://huggingface.co/apple/OpenELM-3B-Instruct) | **47.70** | **76.87** | 24.80 | **38.76** | **67.96** | **51.22** |
### OpenLLM Leaderboard
| **Model Size** | **ARC-c** | **CrowS-Pairs** | **HellaSwag** | **MMLU** | **PIQA** | **RACE** | **TruthfulQA** | **WinoGrande** | **Average** |
|-----------------------------------------------------------------------------|-----------|-----------------|---------------|-----------|-----------|-----------|----------------|----------------|-------------|
| [OpenELM-270M](https://huggingface.co/apple/OpenELM-270M) | 27.65 | **66.79** | 47.15 | 25.72 | 69.75 | 30.91 | **39.24** | **53.83** | 45.13 |
| [OpenELM-270M-Instruct](https://huggingface.co/apple/OpenELM-270M-Instruct) | **32.51** | 66.01 | **51.58** | **26.70** | **70.78** | 33.78 | 38.72 | 53.20 | **46.66** |
| [OpenELM-450M](https://huggingface.co/apple/OpenELM-450M) | 30.20 | **68.63** | 53.86 | **26.01** | 72.31 | 33.11 | 40.18 | 57.22 | 47.69 |
| [OpenELM-450M-Instruct](https://huggingface.co/apple/OpenELM-450M-Instruct) | **33.53** | 67.44 | **59.31** | 25.41 | **72.63** | **36.84** | **40.48** | **58.33** | **49.25** |
| [OpenELM-1_1B](https://huggingface.co/apple/OpenELM-1_1B) | 36.69 | **71.74** | 65.71 | **27.05** | **75.57** | 36.46 | 36.98 | 63.22 | 51.68 |
| [OpenELM-1_1B-Instruct](https://huggingface.co/apple/OpenELM-1_1B-Instruct) | **41.55** | 71.02 | **71.83** | 25.65 | 75.03 | **39.43** | **45.95** | **64.72** | **54.40** |
| [OpenELM-3B](https://huggingface.co/apple/OpenELM-3B) | 42.24 | **73.29** | 73.28 | **26.76** | 78.24 | **38.76** | 34.98 | 67.25 | 54.35 |
| [OpenELM-3B-Instruct](https://huggingface.co/apple/OpenELM-3B-Instruct) | **47.70** | 72.33 | **76.87** | 24.80 | **79.00** | 38.47 | **38.76** | **67.96** | **55.73** |
See the technical report for more results and comparison.
## Evaluation
### Setup
Install the following dependencies:
```bash
# install public lm-eval-harness
harness_repo="public-lm-eval-harness"
git clone https://github.com/EleutherAI/lm-evaluation-harness ${harness_repo}
cd ${harness_repo}
# use main branch on 03-15-2024, SHA is dc90fec
git checkout dc90fec
pip install -e .
cd ..
# 66d6242 is the main branch on 2024-04-01
pip install datasets@git+https://github.com/huggingface/datasets.git@66d6242
pip install tokenizers>=0.15.2 transformers>=4.38.2 sentencepiece>=0.2.0
```
### Evaluate OpenELM
```bash
# OpenELM-450M
hf_model=apple/OpenELM-450M
# this flag is needed because lm-eval-harness set add_bos_token to False by default, but OpenELM uses LLaMA tokenizer which requires add_bos_token to be True
tokenizer=meta-llama/Llama-2-7b-hf
add_bos_token=True
batch_size=1
mkdir lm_eval_output
shot=0
task=arc_challenge,arc_easy,boolq,hellaswag,piqa,race,winogrande,sciq,truthfulqa_mc2
lm_eval --model hf \
--model_args pretrained=${hf_model},trust_remote_code=True,add_bos_token=${add_bos_token},tokenizer=${tokenizer} \
--tasks ${task} \
--device cuda:0 \
--num_fewshot ${shot} \
--output_path ./lm_eval_output/${hf_model//\//_}_${task//,/_}-${shot}shot \
--batch_size ${batch_size} 2>&1 | tee ./lm_eval_output/eval-${hf_model//\//_}_${task//,/_}-${shot}shot.log
shot=5
task=mmlu,winogrande
lm_eval --model hf \
--model_args pretrained=${hf_model},trust_remote_code=True,add_bos_token=${add_bos_token},tokenizer=${tokenizer} \
--tasks ${task} \
--device cuda:0 \
--num_fewshot ${shot} \
--output_path ./lm_eval_output/${hf_model//\//_}_${task//,/_}-${shot}shot \
--batch_size ${batch_size} 2>&1 | tee ./lm_eval_output/eval-${hf_model//\//_}_${task//,/_}-${shot}shot.log
shot=25
task=arc_challenge,crows_pairs_english
lm_eval --model hf \
--model_args pretrained=${hf_model},trust_remote_code=True,add_bos_token=${add_bos_token},tokenizer=${tokenizer} \
--tasks ${task} \
--device cuda:0 \
--num_fewshot ${shot} \
--output_path ./lm_eval_output/${hf_model//\//_}_${task//,/_}-${shot}shot \
--batch_size ${batch_size} 2>&1 | tee ./lm_eval_output/eval-${hf_model//\//_}_${task//,/_}-${shot}shot.log
shot=10
task=hellaswag
lm_eval --model hf \
--model_args pretrained=${hf_model},trust_remote_code=True,add_bos_token=${add_bos_token},tokenizer=${tokenizer} \
--tasks ${task} \
--device cuda:0 \
--num_fewshot ${shot} \
--output_path ./lm_eval_output/${hf_model//\//_}_${task//,/_}-${shot}shot \
--batch_size ${batch_size} 2>&1 | tee ./lm_eval_output/eval-${hf_model//\//_}_${task//,/_}-${shot}shot.log
```
## Bias, Risks, and Limitations
The release of OpenELM models aims to empower and enrich the open research community by providing access to state-of-the-art language models. Trained on publicly available datasets, these models are made available without any safety guarantees. Consequently, there exists the possibility of these models producing outputs that are inaccurate, harmful, biased, or objectionable in response to user prompts. Thus, it is imperative for users and developers to undertake thorough safety testing and implement appropriate filtering mechanisms tailored to their specific requirements.
## Citation
If you find our work useful, please cite:
```BibTex
@article{mehtaOpenELMEfficientLanguage2024,
title = {{OpenELM}: {An} {Efficient} {Language} {Model} {Family} with {Open} {Training} and {Inference} {Framework}},
shorttitle = {{OpenELM}},
url = {https://arxiv.org/abs/2404.14619v1},
language = {en},
urldate = {2024-04-24},
journal = {arXiv.org},
author = {Mehta, Sachin and Sekhavat, Mohammad Hossein and Cao, Qingqing and Horton, Maxwell and Jin, Yanzi and Sun, Chenfan and Mirzadeh, Iman and Najibi, Mahyar and Belenko, Dmitry and Zatloukal, Peter and Rastegari, Mohammad},
month = apr,
year = {2024},
}
@inproceedings{mehta2022cvnets,
author = {Mehta, Sachin and Abdolhosseini, Farzad and Rastegari, Mohammad},
title = {CVNets: High Performance Library for Computer Vision},
year = {2022},
booktitle = {Proceedings of the 30th ACM International Conference on Multimedia},
series = {MM '22}
}
```
|
facebook/esm1b_t33_650M_UR50S | facebook | 2022-12-02T14:40:11Z | 4,379 | 16 | transformers | [
"transformers",
"pytorch",
"tf",
"esm",
"fill-mask",
"arxiv:1907.11692",
"arxiv:1810.04805",
"arxiv:1603.05027",
"license:mit",
"autotrain_compatible",
"endpoints_compatible",
"region:us"
] | fill-mask | 2022-10-17T15:06:20Z | ---
license: mit
widget:
- text: "MQIFVKTLTGKTITLEVEPS<mask>TIENVKAKIQDKEGIPPDQQRLIFAGKQLEDGRTLSDYNIQKESTLHLVLRLRGG"
---
# **ESM-1b**
ESM-1b ([paper](https://www.pnas.org/content/118/15/e2016239118#:~:text=https%3A//doi.org/10.1073/pnas.2016239118), [repository](https://github.com/facebookresearch/esm)) is a transformer protein language model, trained on protein sequence data without label supervision. The model is pretrained on Uniref50 with an unsupervised masked language modeling (MLM) objective, meaning the model is trained to predict amino acids from the surrounding sequence context. This pretraining objective allows ESM-1b to learn generally useful features which can be transferred to downstream prediction tasks. ESM-1b has been evaluated on a variety of tasks related to protein structure and function, including remote homology detection, secondary structure prediction, contact prediction, and prediction of the effects of mutations on function, producing state-of-the-art results.
**Important note**: ESM-2 is now available in a range of checkpoint sizes. For most tasks, ESM-2 performance will be superior to ESM-1 and ESM-1b, and so we recommend using it instead unless your goal is explicitly to compare against ESM-1b. The ESM-2 checkpoint closest in size to ESM-1b is [esm2_t33_650M_UR50D](https://huggingface.co/facebook/esm2_t33_650M_UR50D).
## **Model description**
The ESM-1b model is based on the [RoBERTa](https://arxiv.org/abs/1907.11692) architecture and training procedure, using the Uniref50 2018_03 database of protein sequences. Note that the pretraining is on the raw protein sequences only. The training is purely unsupervised -- during training no labels are given related to structure or function.
Training is with the masked language modeling objective. The masking follows the procedure of [Devlin et al. 2019](https://arxiv.org/abs/1810.04805), randomly masking 15% of the amino acids in the input, and includes the pass-through and random token noise. One architecture difference from the RoBERTa model is that ESM-1b uses [pre-activation layer normalization](https://arxiv.org/abs/1603.05027).
The learned representations can be used as features for downstream tasks. For example if you have a dataset of measurements of protein activity you can fit a regression model on the features output by ESM-1b to predict the activity of new sequences. The model can also be fine-tuned.
ESM-1b can infer information about the structure and function of proteins without further supervision, i.e. it is capable of zero-shot transfer to structure and function prediction. [Rao et al. 2020](https://openreview.net/pdf?id=fylclEqgvgd) found that the attention heads of ESM-1b directly represent contacts in the 3d structure of the protein. [Meier et al. 2021](https://openreview.net/pdf?id=uXc42E9ZPFs) found that ESM-1b can be used to score the effect of sequence variations on protein function.
## **Intended uses & limitations**
The model can be used for feature extraction, fine-tuned on downstream tasks, or used directly to make inferences about the structure and function of protein sequences, like any other masked language model. For full examples, please see [our notebook on fine-tuning protein models](https://colab.research.google.com/github/huggingface/notebooks/blob/main/examples/protein_language_modeling.ipynb)
## **Training data**
The ESM-1b model was pretrained on [Uniref50](https://www.uniprot.org/downloads) 2018-03, a dataset consisting of approximately 30 million protein sequences.
## **Training procedure**
### **Preprocessing**
The protein sequences are uppercased and tokenized using a single space and a vocabulary size of 21. The inputs of the model are then of the form:
```
<cls> Protein Sequence A
```
During training, sequences longer than 1023 tokens (without CLS) are randomly cropped to a length of 1023.
The details of the masking procedure for each sequence follow Devlin et al. 2019:
* 15% of the amino acids are masked.
* In 80% of the cases, the masked amino acids are replaced by `<mask>`.
* In 10% of the cases, the masked amino acids are replaced by a random amino acid (different) from the one they replace.
* In the 10% remaining cases, the masked amino acids are left as is.
### **Pretraining**
The model was trained on 128 NVIDIA v100 GPUs for 500K updates, using sequence length 1024 (131,072 tokens per batch). The optimizer used is Adam (betas=[0.9, 0.999]) with a learning rate of 1e-4, a weight decay of 0, learning rate warmup for 16k steps and inverse square root decay of the learning rate after. |
mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF | mradermacher | 2024-06-04T05:50:16Z | 4,379 | 0 | transformers | [
"transformers",
"gguf",
"en",
"base_model:zeroblu3/NeuralPoppy-EVO-L3-8B",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-03T06:30:49Z | ---
base_model: zeroblu3/NeuralPoppy-EVO-L3-8B
language:
- en
library_name: transformers
license: llama3
quantized_by: mradermacher
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/zeroblu3/NeuralPoppy-EVO-L3-8B
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/NeuralPoppy-EVO-L3-8B-i1-GGUF/resolve/main/NeuralPoppy-EVO-L3-8B.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF | mradermacher | 2024-06-26T20:51:02Z | 4,377 | 0 | transformers | [
"transformers",
"gguf",
"mergekit",
"merge",
"en",
"base_model:saishf/Long-SOVL-Experiment-8B-L3-262K",
"endpoints_compatible",
"region:us"
] | null | 2024-06-03T10:36:13Z | ---
base_model: saishf/Long-SOVL-Experiment-8B-L3-262K
language:
- en
library_name: transformers
quantized_by: mradermacher
tags:
- mergekit
- merge
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/saishf/Long-SOVL-Experiment-8B-L3-262K
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/Long-SOVL-Experiment-8B-L3-262K-i1-GGUF/resolve/main/Long-SOVL-Experiment-8B-L3-262K.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF | mradermacher | 2024-06-06T21:49:53Z | 4,377 | 0 | transformers | [
"transformers",
"gguf",
"not-for-all-audiences",
"en",
"base_model:crestf411/L3-8B-sunfall-abliterated-v0.2",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-05T08:10:21Z | ---
base_model: crestf411/L3-8B-sunfall-abliterated-v0.2
language:
- en
library_name: transformers
license: llama3
license_link: LICENSE
license_name: llama3
quantized_by: mradermacher
tags:
- not-for-all-audiences
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/crestf411/L3-8B-sunfall-abliterated-v0.2
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/L3-8B-sunfall-abliterated-v0.2-i1-GGUF/resolve/main/L3-8B-sunfall-abliterated-v0.2.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
Yntec/mistoonAnime2 | Yntec | 2023-10-05T19:14:03Z | 4,375 | 9 | diffusers | [
"diffusers",
"safetensors",
"Anime",
"Cartoon",
"Colorful",
"Inzaniak",
"stable-diffusion",
"stable-diffusion-diffusers",
"text-to-image",
"license:creativeml-openrail-m",
"autotrain_compatible",
"endpoints_compatible",
"diffusers:StableDiffusionPipeline",
"region:us"
] | text-to-image | 2023-10-05T13:09:06Z | ---
license: creativeml-openrail-m
library_name: diffusers
pipeline_tag: text-to-image
tags:
- Anime
- Cartoon
- Colorful
- Inzaniak
- stable-diffusion
- stable-diffusion-diffusers
- text-to-image
- diffusers
---
Original page: https://civitai.com/models/24149?modelVersionId=108545
Comparison:

Samples and prompt:

videogames, robert jordan pepperoni pizza, josephine wall winner, hidari, roll20 illumination, radiant light, sitting Pretty CUTE girl, gorgeous hair, DETAILED EYES, Magazine ad, iconic, 1943, Cartoon, sharp focus, 4k, towel. comic art on canvas by kyoani and watched and ROSSDRAWS. elementary |
timm/xception41.tf_in1k | timm | 2023-04-21T23:43:10Z | 4,374 | 1 | timm | [
"timm",
"pytorch",
"safetensors",
"image-classification",
"dataset:imagenet-1k",
"arxiv:1802.02611",
"arxiv:1610.02357",
"license:apache-2.0",
"region:us"
] | image-classification | 2023-04-21T23:42:47Z | ---
tags:
- image-classification
- timm
library_name: timm
license: apache-2.0
datasets:
- imagenet-1k
---
# Model card for xception41.tf_in1k
An Aligned Xception image classification model. Trained on ImageNet-1k in Tensorflow and ported to PyTorch by Ross Wightman.
## Model Details
- **Model Type:** Image classification / feature backbone
- **Model Stats:**
- Params (M): 27.0
- GMACs: 9.3
- Activations (M): 39.9
- Image size: 299 x 299
- **Papers:**
- Encoder-Decoder with Atrous Separable Convolution for Semantic Image Segmentation: https://arxiv.org/abs/1802.02611
- Xception: Deep Learning with Depthwise Separable Convolutions: https://arxiv.org/abs/1610.02357
- **Dataset:** ImageNet-1k
- **Original:** https://github.com/tensorflow/models/blob/master/research/deeplab/
## Model Usage
### Image Classification
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model('xception41.tf_in1k', pretrained=True)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # unsqueeze single image into batch of 1
top5_probabilities, top5_class_indices = torch.topk(output.softmax(dim=1) * 100, k=5)
```
### Feature Map Extraction
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model(
'xception41.tf_in1k',
pretrained=True,
features_only=True,
)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # unsqueeze single image into batch of 1
for o in output:
# print shape of each feature map in output
# e.g.:
# torch.Size([1, 128, 150, 150])
# torch.Size([1, 256, 75, 75])
# torch.Size([1, 728, 38, 38])
# torch.Size([1, 1024, 19, 19])
# torch.Size([1, 2048, 10, 10])
print(o.shape)
```
### Image Embeddings
```python
from urllib.request import urlopen
from PIL import Image
import timm
img = Image.open(urlopen(
'https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/beignets-task-guide.png'
))
model = timm.create_model(
'xception41.tf_in1k',
pretrained=True,
num_classes=0, # remove classifier nn.Linear
)
model = model.eval()
# get model specific transforms (normalization, resize)
data_config = timm.data.resolve_model_data_config(model)
transforms = timm.data.create_transform(**data_config, is_training=False)
output = model(transforms(img).unsqueeze(0)) # output is (batch_size, num_features) shaped tensor
# or equivalently (without needing to set num_classes=0)
output = model.forward_features(transforms(img).unsqueeze(0))
# output is unpooled, a (1, 2048, 10, 10) shaped tensor
output = model.forward_head(output, pre_logits=True)
# output is a (1, num_features) shaped tensor
```
## Citation
```bibtex
@inproceedings{deeplabv3plus2018,
title={Encoder-Decoder with Atrous Separable Convolution for Semantic Image Segmentation},
author={Liang-Chieh Chen and Yukun Zhu and George Papandreou and Florian Schroff and Hartwig Adam},
booktitle={ECCV},
year={2018}
}
```
```bibtex
@misc{chollet2017xception,
title={Xception: Deep Learning with Depthwise Separable Convolutions},
author={François Chollet},
year={2017},
eprint={1610.02357},
archivePrefix={arXiv},
primaryClass={cs.CV}
}
```
|
Qwen/Qwen-VL | Qwen | 2024-01-25T15:16:24Z | 4,373 | 179 | transformers | [
"transformers",
"pytorch",
"qwen",
"text-generation",
"custom_code",
"zh",
"en",
"arxiv:2308.12966",
"autotrain_compatible",
"region:us"
] | text-generation | 2023-08-18T02:20:59Z | ---
language:
- zh
- en
tags:
- qwen
pipeline_tag: text-generation
inference: false
---
# Qwen-VL
<br>
<p align="center">
<img src="https://qianwen-res.oss-cn-beijing.aliyuncs.com/logo_vl.jpg" width="400"/>
<p>
<br>
<p align="center">
Qwen-VL
<a href="https://huggingface.co/Qwen/Qwen-VL">🤗</a>
<a href="https://modelscope.cn/models/qwen/Qwen-VL/summary">🤖</a>  |
Qwen-VL-Chat
<a href="https://huggingface.co/Qwen/Qwen-VL-Chat">🤗</a>
<a href="https://modelscope.cn/models/qwen/Qwen-VL-Chat/summary">🤖</a> 
(Int4:
<a href="https://huggingface.co/Qwen/Qwen-VL-Chat-Int4">🤗</a>
<a href="https://modelscope.cn/models/qwen/Qwen-VL-Chat-Int4/summary">🤖</a> ) |
Qwen-VL-Plus
<a href="https://huggingface.co/spaces/Qwen/Qwen-VL-Plus">🤗</a>
<a href="https://modelscope.cn/studios/qwen/Qwen-VL-Chat-Demo/summary">🤖</a>  |
Qwen-VL-Max
<a href="https://huggingface.co/spaces/Qwen/Qwen-VL-Max">🤗</a>
<a href="https://modelscope.cn/studios/qwen/Qwen-VL-Max/summary">🤖</a> 
<br>
<a href="https://tongyi.aliyun.com/qianwen">Web</a>   |   
<a href="https://help.aliyun.com/zh/dashscope/developer-reference/vl-plus-quick-start">API</a>   |   
<a href="assets/wechat.png">WeChat</a>   |   
<a href="https://discord.gg/z3GAxXZ9Ce">Discord</a>   |   
<a href="https://arxiv.org/abs/2308.12966">Paper</a>   |   
<a href="TUTORIAL.md">Tutorial</a>
</p>
<br>
**Qwen-VL** 是阿里云研发的大规模视觉语言模型(Large Vision Language Model, LVLM)。Qwen-VL 可以以图像、文本、检测框作为输入,并以文本和检测框作为输出。Qwen-VL 系列模型性能强大,具备多语言对话、多图交错对话等能力,并支持中文开放域定位和细粒度图像识别与理解。
**Qwen-VL** (Qwen Large Vision Language Model) is the visual multimodal version of the large model series, Qwen (abbr. Tongyi Qianwen), proposed by Alibaba Cloud. Qwen-VL accepts image, text, and bounding box as inputs, outputs text and bounding box. The features of Qwen-VL include:
目前,我们提供了Qwen-VL和Qwen-VL-Chat两个模型,分别为预训练模型和Chat模型。如果想了解更多关于模型的信息,请点击[链接](https://github.com/QwenLM/Qwen-VL/blob/master/visual_memo.md)查看我们的技术备忘录。本仓库为Qwen-VL-Chat仓库。
We release Qwen-VL and Qwen-VL-Chat, which are pretrained model and Chat model respectively. For more details about Qwen-VL, please refer to our [technical memo](https://github.com/QwenLM/Qwen-VL/blob/master/visual_memo.md). This repo is the one for Qwen-VL.
<br>
## 安装要求 (Requirements)
* python 3.8及以上版本
* pytorch 1.12及以上版本,推荐2.0及以上版本
* 建议使用CUDA 11.4及以上(GPU用户需考虑此选项)
* python 3.8 and above
* pytorch 1.12 and above, 2.0 and above are recommended
* CUDA 11.4 and above are recommended (this is for GPU users)
<br>
## 快速开始 (Quickstart)
我们提供简单的示例来说明如何利用 🤗 Transformers 快速使用 Qwen-VL。
在开始前,请确保你已经配置好环境并安装好相关的代码包。最重要的是,确保你满足上述要求,然后安装相关的依赖库。
Below, we provide simple examples to show how to use Qwen-VL with 🤗 Transformers.
Before running the code, make sure you have setup the environment and installed the required packages. Make sure you meet the above requirements, and then install the dependent libraries.
```bash
pip install -r requirements.txt
```
接下来你可以开始使用Transformers来使用我们的模型。关于视觉模块的更多用法,请参考[教程](TUTORIAL.md)。
Now you can start with Transformers. More usage aboue vision encoder, please refer to [tutorial](TUTORIAL_zh.md).
#### 🤗 Transformers
To use Qwen-VL for the inference, all you need to do is to input a few lines of codes as demonstrated below. However, **please make sure that you are using the latest code.**
```python
from transformers import AutoModelForCausalLM, AutoTokenizer
from transformers.generation import GenerationConfig
import torch
torch.manual_seed(1234)
tokenizer = AutoTokenizer.from_pretrained("Qwen/Qwen-VL", trust_remote_code=True)
# use bf16
# model = AutoModelForCausalLM.from_pretrained("Qwen/Qwen-VL", device_map="auto", trust_remote_code=True, bf16=True).eval()
# use fp16
# model = AutoModelForCausalLM.from_pretrained("Qwen/Qwen-VL", device_map="auto", trust_remote_code=True, fp16=True).eval()
# use cpu only
# model = AutoModelForCausalLM.from_pretrained("Qwen/Qwen-VL", device_map="cpu", trust_remote_code=True).eval()
# use cuda device
model = AutoModelForCausalLM.from_pretrained("Qwen/Qwen-VL", device_map="cuda", trust_remote_code=True).eval()
# Specify hyperparameters for generation (No need to do this if you are using transformers>=4.32.0)
# model.generation_config = GenerationConfig.from_pretrained("Qwen/Qwen-VL", trust_remote_code=True)
query = tokenizer.from_list_format([
{'image': 'https://qianwen-res.oss-cn-beijing.aliyuncs.com/Qwen-VL/assets/demo.jpeg'},
{'text': 'Generate the caption in English with grounding:'},
])
inputs = tokenizer(query, return_tensors='pt')
inputs = inputs.to(model.device)
pred = model.generate(**inputs)
response = tokenizer.decode(pred.cpu()[0], skip_special_tokens=False)
print(response)
# <img>https://qianwen-res.oss-cn-beijing.aliyuncs.com/Qwen-VL/assets/demo.jpeg</img>Generate the caption in English with grounding:<ref> Woman</ref><box>(451,379),(731,806)</box> and<ref> her dog</ref><box>(219,424),(576,896)</box> playing on the beach<|endoftext|>
image = tokenizer.draw_bbox_on_latest_picture(response)
if image:
image.save('2.jpg')
else:
print("no box")
```
<p align="center">
<img src="https://qianwen-res.oss-cn-beijing.aliyuncs.com/Qwen-VL/assets/demo_spotting_caption.jpg" width="500"/>
<p>
<br>
## 评测
我们从两个角度评测了两个模型的能力:
1. 在**英文标准 Benchmark** 上评测模型的基础任务能力。目前评测了四大类多模态任务:
- Zero-shot Caption: 评测模型在未见过数据集上的零样本图片描述能力;
- General VQA: 评测模型的通用问答能力,例如判断题、颜色、个数、类目等问答能力;
- Text-based VQA:评测模型对于图片中文字相关的识别/问答能力,例如文档问答、图表问答、文字问答等;
- Referring Expression Compression:评测模型给定物体描述画检测框的能力;
2. **试金石 (TouchStone)**:为了评测模型整体的图文对话能力和人类对齐水平。我们为此构建了一个基于 GPT4 打分来评测 LVLM 模型的 Benchmark:TouchStone。在 TouchStone-v0.1 中:
- 评测基准总计涵盖 300+张图片、800+道题目、27个类别。包括基础属性问答、人物地标问答、影视作品问答、视觉推理、反事实推理、诗歌创作、故事写作,商品比较、图片解题等**尽可能广泛的类别**。
- 为了弥补目前 GPT4 无法直接读取图片的缺陷,我们给所有的带评测图片提供了**人工标注的充分详细描述**,并且将图片的详细描述、问题和模型的输出结果一起交给 GPT4 打分。
- 评测同时包含英文版本和中文版本。
评测结果如下:
We evaluated the model's ability from two perspectives:
1. **Standard Benchmarks**: We evaluate the model's basic task capabilities on four major categories of multimodal tasks:
- Zero-shot Caption: Evaluate model's zero-shot image captioning ability on unseen datasets;
- General VQA: Evaluate the general question-answering ability of pictures, such as the judgment, color, number, category, etc;
- Text-based VQA: Evaluate the model's ability to recognize text in pictures, such as document QA, chart QA, etc;
- Referring Expression Comprehension: Evaluate the ability to localize a target object in an image described by a referring expression.
2. **TouchStone**: To evaluate the overall text-image dialogue capability and alignment level with humans, we have constructed a benchmark called TouchStone, which is based on scoring with GPT4 to evaluate the LVLM model.
- The TouchStone benchmark covers a total of 300+ images, 800+ questions, and 27 categories. Such as attribute-based Q&A, celebrity recognition, writing poetry, summarizing multiple images, product comparison, math problem solving, etc;
- In order to break the current limitation of GPT4 in terms of direct image input, TouchStone provides fine-grained image annotations by human labeling. These detailed annotations, along with the questions and the model's output, are then presented to GPT4 for scoring.
- The benchmark includes both English and Chinese versions.
The results of the evaluation are as follows:
Qwen-VL outperforms current SOTA generalist models on multiple VL tasks and has a more comprehensive coverage in terms of capability range.
<p align="center">
<img src="https://qianwen-res.oss-cn-beijing.aliyuncs.com/Qwen-VL/assets/radar.png" width="600"/>
<p>
### 零样本图像描述 & 通用视觉问答 (Zero-shot Captioning & General VQA)
<table>
<thead>
<tr>
<th rowspan="2">Model type</th>
<th rowspan="2">Model</th>
<th colspan="2">Zero-shot Captioning</th>
<th colspan="5">General VQA</th>
</tr>
<tr>
<th>NoCaps</th>
<th>Flickr30K</th>
<th>VQAv2<sup>dev</sup></th>
<th>OK-VQA</th>
<th>GQA</th>
<th>SciQA-Img<br>(0-shot)</th>
<th>VizWiz<br>(0-shot)</th>
</tr>
</thead>
<tbody align="center">
<tr>
<td rowspan="10">Generalist<br>Models</td>
<td>Flamingo-9B</td>
<td>-</td>
<td>61.5</td>
<td>51.8</td>
<td>44.7</td>
<td>-</td>
<td>-</td>
<td>28.8</td>
</tr>
<tr>
<td>Flamingo-80B</td>
<td>-</td>
<td>67.2</td>
<td>56.3</td>
<td>50.6</td>
<td>-</td>
<td>-</td>
<td>31.6</td>
</tr>
<tr>
<td>Unified-IO-XL</td>
<td>100.0</td>
<td>-</td>
<td>77.9</td>
<td>54.0</td>
<td>-</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td>Kosmos-1</td>
<td>-</td>
<td>67.1</td>
<td>51.0</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>29.2</td>
</tr>
<tr>
<td>Kosmos-2</td>
<td>-</td>
<td>66.7</td>
<td>45.6</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td>BLIP-2 (Vicuna-13B)</td>
<td>103.9</td>
<td>71.6</td>
<td>65.0</td>
<td>45.9</td>
<td>32.3</td>
<td>61.0</td>
<td>19.6</td>
</tr>
<tr>
<td>InstructBLIP (Vicuna-13B)</td>
<td><strong>121.9</strong></td>
<td>82.8</td>
<td>-</td>
<td>-</td>
<td>49.5</td>
<td>63.1</td>
<td>33.4</td>
</tr>
<tr>
<td>Shikra (Vicuna-13B)</td>
<td>-</td>
<td>73.9</td>
<td>77.36</td>
<td>47.16</td>
<td>-</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td><strong>Qwen-VL (Qwen-7B)</strong></td>
<td>121.4</td>
<td><b>85.8</b></td>
<td><b>78.8</b></td>
<td><b>58.6</b></td>
<td><b>59.3</b></td>
<td>67.1</td>
<td>35.2</td>
</tr>
<!-- <tr>
<td>Qwen-VL (4-shot)</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>63.6</td>
<td>-</td>
<td>-</td>
<td>39.1</td>
</tr> -->
<tr>
<td>Qwen-VL-Chat</td>
<td>120.2</td>
<td>81.0</td>
<td>78.2</td>
<td>56.6</td>
<td>57.5</td>
<td><b>68.2</b></td>
<td><b>38.9</b></td>
</tr>
<!-- <tr>
<td>Qwen-VL-Chat (4-shot)</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>60.6</td>
<td>-</td>
<td>-</td>
<td>44.45</td>
</tr> -->
<tr>
<td>Previous SOTA<br>(Per Task Fine-tuning)</td>
<td>-</td>
<td>127.0<br>(PALI-17B)</td>
<td>84.5<br>(InstructBLIP<br>-FlanT5-XL)</td>
<td>86.1<br>(PALI-X<br>-55B)</td>
<td>66.1<br>(PALI-X<br>-55B)</td>
<td>72.1<br>(CFR)</td>
<td>92.53<br>(LLaVa+<br>GPT-4)</td>
<td>70.9<br>(PALI-X<br>-55B)</td>
</tr>
</tbody>
</table>
- 在 Zero-shot Caption 中,Qwen-VL 在 Flickr30K 数据集上取得了 **SOTA** 的结果,并在 Nocaps 数据集上取得了和 InstructBlip 可竞争的结果。
- 在 General VQA 中,Qwen-VL 取得了 LVLM 模型同等量级和设定下 **SOTA** 的结果。
- For zero-shot image captioning, Qwen-VL achieves the **SOTA** on Flickr30K and competitive results on Nocaps with InstructBlip.
- For general VQA, Qwen-VL achieves the **SOTA** under the same generalist LVLM scale settings.
### 文本导向的视觉问答 (Text-oriented VQA)
<table>
<thead>
<tr>
<th>Model type</th>
<th>Model</th>
<th>TextVQA</th>
<th>DocVQA</th>
<th>ChartQA</th>
<th>AI2D</th>
<th>OCR-VQA</th>
</tr>
</thead>
<tbody align="center">
<tr>
<td rowspan="5">Generalist Models</td>
<td>BLIP-2 (Vicuna-13B)</td>
<td>42.4</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td>InstructBLIP (Vicuna-13B)</td>
<td>50.7</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td>mPLUG-DocOwl (LLaMA-7B)</td>
<td>52.6</td>
<td>62.2</td>
<td>57.4</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td>Pic2Struct-Large (1.3B)</td>
<td>-</td>
<td><b>76.6</b></td>
<td>58.6</td>
<td>42.1</td>
<td>71.3</td>
</tr>
<tr>
<td>Qwen-VL (Qwen-7B)</td>
<td><b>63.8</b></td>
<td>65.1</td>
<td><b>65.7</b></td>
<td><b>62.3</b></td>
<td><b>75.7</b></td>
</tr>
<tr>
<td>Specialist SOTAs<br>(Specialist/Finetuned)</td>
<td>PALI-X-55B (Single-task FT)<br>(Without OCR Pipeline)</td>
<td>71.44</td>
<td>80.0</td>
<td>70.0</td>
<td>81.2</td>
<td>75.0</td>
</tr>
</tbody>
</table>
- 在文字相关的识别/问答评测上,取得了当前规模下通用 LVLM 达到的最好结果。
- 分辨率对上述某几个评测非常重要,大部分 224 分辨率的开源 LVLM 模型无法完成以上评测,或只能通过切图的方式解决。Qwen-VL 将分辨率提升到 448,可以直接以端到端的方式进行以上评测。Qwen-VL 在很多任务上甚至超过了 1024 分辨率的 Pic2Struct-Large 模型。
- In text-related recognition/QA evaluation, Qwen-VL achieves the SOTA under the generalist LVLM scale settings.
- Resolution is important for several above evaluations. While most open-source LVLM models with 224 resolution are incapable of these evaluations or can only solve these by cutting images, Qwen-VL scales the resolution to 448 so that it can be evaluated end-to-end. Qwen-VL even outperforms Pic2Struct-Large models of 1024 resolution on some tasks.
### 细粒度视觉定位 (Referring Expression Comprehension)
<table>
<thead>
<tr>
<th rowspan="2">Model type</th>
<th rowspan="2">Model</th>
<th colspan="3">RefCOCO</th>
<th colspan="3">RefCOCO+</th>
<th colspan="2">RefCOCOg</th>
<th>GRIT</th>
</tr>
<tr>
<th>val</th>
<th>test-A</th>
<th>test-B</th>
<th>val</th>
<th>test-A</th>
<th>test-B</th>
<th>val-u</th>
<th>test-u</th>
<th>refexp</th>
</tr>
</thead>
<tbody align="center">
<tr>
<td rowspan="8">Generalist Models</td>
<td>GPV-2</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>51.50</td>
</tr>
<tr>
<td>OFA-L*</td>
<td>79.96</td>
<td>83.67</td>
<td>76.39</td>
<td>68.29</td>
<td>76.00</td>
<td>61.75</td>
<td>67.57</td>
<td>67.58</td>
<td>61.70</td>
</tr>
<tr>
<td>Unified-IO</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td><b>78.61</b></td>
</tr>
<tr>
<td>VisionLLM-H</td>
<td></td>
<td>86.70</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
<td>-</td>
</tr>
<tr>
<td>Shikra-7B</td>
<td>87.01</td>
<td>90.61</td>
<td>80.24 </td>
<td>81.60</td>
<td>87.36</td>
<td>72.12</td>
<td>82.27</td>
<td>82.19</td>
<td>69.34</td>
</tr>
<tr>
<td>Shikra-13B</td>
<td>87.83 </td>
<td>91.11</td>
<td>81.81</td>
<td>82.89</td>
<td>87.79</td>
<td>74.41</td>
<td>82.64</td>
<td>83.16</td>
<td>69.03</td>
</tr>
<tr>
<td>Qwen-VL-7B</td>
<td><b>89.36</b></td>
<td>92.26</td>
<td><b>85.34</b></td>
<td><b>83.12</b></td>
<td>88.25</td>
<td><b>77.21</b></td>
<td>85.58</td>
<td>85.48</td>
<td>78.22</td>
</tr>
<tr>
<td>Qwen-VL-7B-Chat</td>
<td>88.55</td>
<td><b>92.27</b></td>
<td>84.51</td>
<td>82.82</td>
<td><b>88.59</b></td>
<td>76.79</td>
<td><b>85.96</b></td>
<td><b>86.32</b></td>
<td>-</td>
<tr>
<td rowspan="3">Specialist SOTAs<br>(Specialist/Finetuned)</td>
<td>G-DINO-L</td>
<td>90.56 </td>
<td>93.19</td>
<td>88.24</td>
<td>82.75</td>
<td>88.95</td>
<td>75.92</td>
<td>86.13</td>
<td>87.02</td>
<td>-</td>
</tr>
<tr>
<td>UNINEXT-H</td>
<td>92.64 </td>
<td>94.33</td>
<td>91.46</td>
<td>85.24</td>
<td>89.63</td>
<td>79.79</td>
<td>88.73</td>
<td>89.37</td>
<td>-</td>
</tr>
<tr>
<td>ONE-PEACE</td>
<td>92.58 </td>
<td>94.18</td>
<td>89.26</td>
<td>88.77</td>
<td>92.21</td>
<td>83.23</td>
<td>89.22</td>
<td>89.27</td>
<td>-</td>
</tr>
</tbody>
</table>
- 在定位任务上,Qwen-VL 全面超过 Shikra-13B,取得了目前 Generalist LVLM 模型上在 Refcoco 上的 **SOTA**。
- Qwen-VL 并没有在任何中文定位数据上训练过,但通过中文 Caption 数据和 英文 Grounding 数据的训练,可以 Zero-shot 泛化出中文 Grounding 能力。
我们提供了以上**所有**评测脚本以供复现我们的实验结果。请阅读 [eval/EVALUATION.md](eval/EVALUATION.md) 了解更多信息。
- Qwen-VL achieves the **SOTA** in all above referring expression comprehension benchmarks.
- Qwen-VL has not been trained on any Chinese grounding data, but it can still generalize to the Chinese Grounding tasks in a zero-shot way by training Chinese Caption data and English Grounding data.
We provide all of the above evaluation scripts for reproducing our experimental results. Please read [eval/EVALUATION.md](eval/EVALUATION.md) for more information.
### 闲聊能力测评 (Chat Evaluation)
TouchStone 是一个基于 GPT4 打分来评测 LVLM 模型的图文对话能力和人类对齐水平的基准。它涵盖了 300+张图片、800+道题目、27个类别,包括基础属性、人物地标、视觉推理、诗歌创作、故事写作、商品比较、图片解题等**尽可能广泛的类别**。关于 TouchStone 的详细介绍,请参考[touchstone/README_CN.md](touchstone/README_CN.md)了解更多信息。
TouchStone is a benchmark based on scoring with GPT4 to evaluate the abilities of the LVLM model on text-image dialogue and alignment levels with humans. It covers a total of 300+ images, 800+ questions, and 27 categories, such as attribute-based Q&A, celebrity recognition, writing poetry, summarizing multiple images, product comparison, math problem solving, etc. Please read [touchstone/README_CN.md](touchstone/README.md) for more information.
#### 英语 (English)
| Model | Score |
|---------------|-------|
| PandaGPT | 488.5 |
| MiniGPT4 | 531.7 |
| InstructBLIP | 552.4 |
| LLaMA-AdapterV2 | 590.1 |
| mPLUG-Owl | 605.4 |
| LLaVA | 602.7 |
| Qwen-VL-Chat | 645.2 |
#### 中文 (Chinese)
| Model | Score |
|---------------|-------|
| VisualGLM | 247.1 |
| Qwen-VL-Chat | 401.2 |
Qwen-VL-Chat 模型在中英文的对齐评测中均取得当前 LVLM 模型下的最好结果。
Qwen-VL-Chat has achieved the best results in both Chinese and English alignment evaluation.
<br>
## 常见问题 (FAQ)
如遇到问题,敬请查阅 [FAQ](https://github.com/QwenLM/Qwen-VL/blob/master/FAQ_zh.md)以及issue区,如仍无法解决再提交issue。
If you meet problems, please refer to [FAQ](https://github.com/QwenLM/Qwen-VL/blob/master/FAQ.md) and the issues first to search a solution before you launch a new issue.
<br>
## 使用协议 (License Agreement)
研究人员与开发者可使用Qwen-VL和Qwen-VL-Chat或进行二次开发。我们同样允许商业使用,具体细节请查看[LICENSE](https://github.com/QwenLM/Qwen-VL/blob/master/LICENSE)。如需商用,请填写[问卷](https://dashscope.console.aliyun.com/openModelApply/qianwen)申请。
Researchers and developers are free to use the codes and model weights of both Qwen-VL and Qwen-VL-Chat. We also allow their commercial use. Check our license at [LICENSE](LICENSE) for more details.
<br>
## 引用 (Citation)[](https://)
如果你觉得我们的论文和代码对你的研究有帮助,请考虑:star: 和引用 :pencil: :)
If you find our paper and code useful in your research, please consider giving a star :star: and citation :pencil: :)
```BibTeX
@article{Qwen-VL,
title={Qwen-VL: A Frontier Large Vision-Language Model with Versatile Abilities},
author={Bai, Jinze and Bai, Shuai and Yang, Shusheng and Wang, Shijie and Tan, Sinan and Wang, Peng and Lin, Junyang and Zhou, Chang and Zhou, Jingren},
journal={arXiv preprint arXiv:2308.12966},
year={2023}
}
```
<br>
## 联系我们 (Contact Us)
如果你想给我们的研发团队和产品团队留言,请通过邮件([email protected])联系我们。
If you are interested to leave a message to either our research team or product team, feel free to send an email to [email protected].
|
facebook/hubert-large-ll60k | facebook | 2021-11-05T12:42:57Z | 4,370 | 19 | transformers | [
"transformers",
"pytorch",
"tf",
"hubert",
"feature-extraction",
"speech",
"en",
"dataset:libri-light",
"arxiv:2106.07447",
"license:apache-2.0",
"endpoints_compatible",
"region:us"
] | feature-extraction | 2022-03-02T23:29:05Z | ---
language: en
datasets:
- libri-light
tags:
- speech
license: apache-2.0
---
# Hubert-Large
[Facebook's Hubert](https://ai.facebook.com/blog/hubert-self-supervised-representation-learning-for-speech-recognition-generation-and-compression)
The large model pretrained on 16kHz sampled speech audio. When using the model make sure that your speech input is also sampled at 16Khz.
**Note**: This model does not have a tokenizer as it was pretrained on audio alone. In order to use this model **speech recognition**, a tokenizer should be created and the model should be fine-tuned on labeled text data. Check out [this blog](https://huggingface.co/blog/fine-tune-wav2vec2-english) for more in-detail explanation of how to fine-tune the model.
The model was pretrained on [Libri-Light](https://github.com/facebookresearch/libri-light).
[Paper](https://arxiv.org/abs/2106.07447)
Authors: Wei-Ning Hsu, Benjamin Bolte, Yao-Hung Hubert Tsai, Kushal Lakhotia, Ruslan Salakhutdinov, Abdelrahman Mohamed
**Abstract**
Self-supervised approaches for speech representation learning are challenged by three unique problems: (1) there are multiple sound units in each input utterance, (2) there is no lexicon of input sound units during the pre-training phase, and (3) sound units have variable lengths with no explicit segmentation. To deal with these three problems, we propose the Hidden-Unit BERT (HuBERT) approach for self-supervised speech representation learning, which utilizes an offline clustering step to provide aligned target labels for a BERT-like prediction loss. A key ingredient of our approach is applying the prediction loss over the masked regions only, which forces the model to learn a combined acoustic and language model over the continuous inputs. HuBERT relies primarily on the consistency of the unsupervised clustering step rather than the intrinsic quality of the assigned cluster labels. Starting with a simple k-means teacher of 100 clusters, and using two iterations of clustering, the HuBERT model either matches or improves upon the state-of-the-art wav2vec 2.0 performance on the Librispeech (960h) and Libri-light (60,000h) benchmarks with 10min, 1h, 10h, 100h, and 960h fine-tuning subsets. Using a 1B parameter model, HuBERT shows up to 19% and 13% relative WER reduction on the more challenging dev-other and test-other evaluation subsets.
The original model can be found under https://github.com/pytorch/fairseq/tree/master/examples/hubert .
# Usage
See [this blog](https://huggingface.co/blog/fine-tune-wav2vec2-english) for more information on how to fine-tune the model. Note that the class `Wav2Vec2ForCTC` has to be replaced by `HubertForCTC`. |
utrobinmv/t5_translate_en_ru_zh_large_1024 | utrobinmv | 2024-03-13T08:25:09Z | 4,370 | 69 | transformers | [
"transformers",
"safetensors",
"t5",
"text2text-generation",
"translation",
"ru",
"zh",
"en",
"dataset:ccmatrix",
"license:apache-2.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | translation | 2024-01-19T11:59:57Z | ---
language:
- ru
- zh
- en
tags:
- translation
license: apache-2.0
datasets:
- ccmatrix
metrics:
- sacrebleu
widget:
- example_title: translate zh-ru
text: >
translate to ru: 开发的目的是为用户提供个人同步翻译。
- example_title: translate ru-en
text: >
translate to en: Цель разработки — предоставить пользователям личного синхронного переводчика.
- example_title: translate en-ru
text: >
translate to ru: The purpose of the development is to provide users with a personal synchronized interpreter.
- example_title: translate en-zh
text: >
translate to zh: The purpose of the development is to provide users with a personal synchronized interpreter.
- example_title: translate zh-en
text: >
translate to en: 开发的目的是为用户提供个人同步解释器。
- example_title: translate ru-zh
text: >
translate to zh: Цель разработки — предоставить пользователям личного синхронного переводчика.
---
# T5 English, Russian and Chinese multilingual machine translation
This model represents a conventional T5 transformer in multitasking mode for translation into the required language, precisely configured for machine translation for pairs: ru-zh, zh-ru, en-zh, zh-en, en-ru, ru-en.
The model can perform direct translation between any pair of Russian, Chinese or English languages. For translation into the target language, the target language identifier is specified as a prefix 'translate to <lang>:'. In this case, the source language may not be specified, in addition, the source text may be multilingual.
Example translate Russian to Chinese
```python
from transformers import T5ForConditionalGeneration, T5Tokenizer
device = 'cuda' #or 'cpu' for translate on cpu
model_name = 'utrobinmv/t5_translate_en_ru_zh_large_1024'
model = T5ForConditionalGeneration.from_pretrained(model_name)
model.to(device)
tokenizer = T5Tokenizer.from_pretrained(model_name)
prefix = 'translate to zh: '
src_text = prefix + "Съешь ещё этих мягких французских булок."
# translate Russian to Chinese
input_ids = tokenizer(src_text, return_tensors="pt")
generated_tokens = model.generate(**input_ids.to(device))
result = tokenizer.batch_decode(generated_tokens, skip_special_tokens=True)
print(result)
# 再吃这些法国的甜蜜的面包。
```
and Example translate Chinese to Russian
```python
from transformers import T5ForConditionalGeneration, T5Tokenizer
device = 'cuda' #or 'cpu' for translate on cpu
model_name = 'utrobinmv/t5_translate_en_ru_zh_large_1024'
model = T5ForConditionalGeneration.from_pretrained(model_name)
model.to(device)
tokenizer = T5Tokenizer.from_pretrained(model_name)
prefix = 'translate to ru: '
src_text = prefix + "再吃这些法国的甜蜜的面包。"
# translate Russian to Chinese
input_ids = tokenizer(src_text, return_tensors="pt")
generated_tokens = model.generate(**input_ids.to(device))
result = tokenizer.batch_decode(generated_tokens, skip_special_tokens=True)
print(result)
# Съешьте этот сладкий хлеб из Франции.
```
##
## Languages covered
Russian (ru_RU), Chinese (zh_CN), English (en_US)
|
RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf | RichardErkhov | 2024-06-30T01:14:03Z | 4,370 | 0 | null | [
"gguf",
"region:us"
] | null | 2024-06-30T00:52:04Z | Quantization made by Richard Erkhov.
[Github](https://github.com/RichardErkhov)
[Discord](https://discord.gg/pvy7H8DZMG)
[Request more models](https://github.com/RichardErkhov/quant_request)
pip-library-etl-1.3b - GGUF
- Model creator: https://huggingface.co/PipableAI/
- Original model: https://huggingface.co/PipableAI/pip-library-etl-1.3b/
| Name | Quant method | Size |
| ---- | ---- | ---- |
| [pip-library-etl-1.3b.Q2_K.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q2_K.gguf) | Q2_K | 0.52GB |
| [pip-library-etl-1.3b.IQ3_XS.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.IQ3_XS.gguf) | IQ3_XS | 0.57GB |
| [pip-library-etl-1.3b.IQ3_S.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.IQ3_S.gguf) | IQ3_S | 0.6GB |
| [pip-library-etl-1.3b.Q3_K_S.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q3_K_S.gguf) | Q3_K_S | 0.6GB |
| [pip-library-etl-1.3b.IQ3_M.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.IQ3_M.gguf) | IQ3_M | 0.63GB |
| [pip-library-etl-1.3b.Q3_K.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q3_K.gguf) | Q3_K | 0.66GB |
| [pip-library-etl-1.3b.Q3_K_M.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q3_K_M.gguf) | Q3_K_M | 0.66GB |
| [pip-library-etl-1.3b.Q3_K_L.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q3_K_L.gguf) | Q3_K_L | 0.69GB |
| [pip-library-etl-1.3b.IQ4_XS.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.IQ4_XS.gguf) | IQ4_XS | 0.7GB |
| [pip-library-etl-1.3b.Q4_0.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q4_0.gguf) | Q4_0 | 0.72GB |
| [pip-library-etl-1.3b.IQ4_NL.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.IQ4_NL.gguf) | IQ4_NL | 0.73GB |
| [pip-library-etl-1.3b.Q4_K_S.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q4_K_S.gguf) | Q4_K_S | 0.76GB |
| [pip-library-etl-1.3b.Q4_K.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q4_K.gguf) | Q4_K | 0.81GB |
| [pip-library-etl-1.3b.Q4_K_M.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q4_K_M.gguf) | Q4_K_M | 0.81GB |
| [pip-library-etl-1.3b.Q4_1.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q4_1.gguf) | Q4_1 | 0.8GB |
| [pip-library-etl-1.3b.Q5_0.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q5_0.gguf) | Q5_0 | 0.87GB |
| [pip-library-etl-1.3b.Q5_K_S.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q5_K_S.gguf) | Q5_K_S | 0.89GB |
| [pip-library-etl-1.3b.Q5_K.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q5_K.gguf) | Q5_K | 0.93GB |
| [pip-library-etl-1.3b.Q5_K_M.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q5_K_M.gguf) | Q5_K_M | 0.93GB |
| [pip-library-etl-1.3b.Q5_1.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q5_1.gguf) | Q5_1 | 0.95GB |
| [pip-library-etl-1.3b.Q6_K.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q6_K.gguf) | Q6_K | 1.09GB |
| [pip-library-etl-1.3b.Q8_0.gguf](https://huggingface.co/RichardErkhov/PipableAI_-_pip-library-etl-1.3b-gguf/blob/main/pip-library-etl-1.3b.Q8_0.gguf) | Q8_0 | 1.33GB |
Original model description:
---
language:
- en
license: apache-2.0
library_name: transformers
tags:
- python
- java
- cpp
- sql
- function calling
- unit tests
- causalLM
- codeLLAMA modified archi
- document
- code
- code2doc
- instruction_tuned
- basemodel
- pytorch
- docstring
- documentation
- text-generation-inference
metrics:
- accuracy
pipeline_tag: text-generation
widget:
- text: '<example_response>--code:def function_divide2(x): return x / 2--question:Document
the code--doc:Description:This function takes a number and divides it by 2.Parameters:-
x (numeric): The input value to be divided by 2.Returns:- float: The result of
x divided by 2.Example:To call the function, use the following code:function_divide2(1.0)</example_response><function_code>def
_plot_bounding_polygon(polygons_coordinates, output_html_path=bounding_polygon_map.html):map_center
= [sum([coord[0]for polygon_coords in polygons_coordinatesfor coord in polygon_coords])/
sum([len(polygon_coords) for polygon_coords in polygons_coordinates]),sum([coord[1]for
polygon_coords in polygons_coordinatesfor coord in polygon_coords])/ sum([len(polygon_coords)
for polygon_coords in polygons_coordinates]),]my_map = folium.Map(location=map_center,
zoom_start=12)for polygon_coords in polygons_coordinates:folium.Polygon(locations=polygon_coords,color=blue,fill=True,fill_color=blue,fill_opacity=0.2,).add_to(my_map)marker_cluster
= MarkerCluster().add_to(my_map)for polygon_coords in polygons_coordinates:for
coord in polygon_coords:folium.Marker(location=[coord[0], coord[1]], popup=fCoordinates:
{coord}).add_to(marker_cluster)draw = Draw(export=True)draw.add_to(my_map)my_map.save(output_html_path)return
output_html_path</function_code><question>Document the python code above giving
function description ,parameters and return type and example how to call the function</question><doc>'
example_title: example
---
# pip-library-etl-1.3b
[pipableAi](https://www.pipable.ai/)
[colab_notebook](https://colab.research.google.com/drive/10av3SxFf0Psx_IkmZbcUhiVznStV5pVS?usp=sharing)
[pip etl](https://github.com/PipableAI/pip-library-etl)
## How we built it?
We used softmax cross entropy and a modified form of policy grad along with Q loss, optimized in an EM set up.
The performance for the metioned tasks are comparable to much bigger LLMs and GPT-3.5
## License
The model is open source under apache 2.0. License
## Usage
### NOTE:
If you wish to try this model without utilizing your GPU, we have hosted the model on our end. To execute the library using the hosted playground model, initialize the generator as shown below:
```python
from pip_library_etl import PipEtl
generator = PipEtl(device="cloud")
```
We have hosted the model at https://playground.pipable.ai/infer. Hence, one can also make a POST request to this endpoint with the following payload:
```json
{
"model_name": "PipableAI/pip-library-etl-1.3b",
"prompt": "prompt",
"max_new_tokens": "400"
}
```
```bash
curl -X 'POST' \
'https://playground.pipable.ai/infer' \
-H 'accept: application/json' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'model_name=PipableAI%2Fpip-library-etl-1.3b&prompt="YOUR PROMPT"&max_new_tokens=400'
```
Alternatively, you can directly access UI endpoint at https://playground.pipable.ai/docs#/default/infer_infer_post.
### Library use
For directly using the capabilities of model without putting extra efforts on schems and prompts try to use [pip library_etl](https://github.com/PipableAI/pip-library-etl.git).
Here's a brief overview of what can be achieved using the PipEtl library:
- `Function Call Generation` : The generate_function_call method facilitates the generation of Python function calls based on provided questions and either docstrings or undocumented code. This feature can be useful for generating example function calls or for prototyping code snippets.
- `Automated Documentation Generation` : With the generate_docstring method, users can automatically generate comprehensive docstrings for Python functions. This feature aids in maintaining well-documented codebases and adhering to best practices.
- `Module Documentation` : The generate_module_docstrings method allows for generating documentation for all methods and functions within a given module or package. This capability streamlines the documentation process, especially for large codebases with numerous functions.
- `SQL Query Generation` : Users can leverage the generate_sql method to automatically generate SQL queries based on provided schemas and questions. This functionality simplifies the process of creating SQL queries, particularly for data-related tasks.
For detailed usage refer to the [colab_notebook](https://colab.research.google.com/drive/17PyMU_3QN9LROy7x-jmaema0cuLRzBvc?usp=sharing)
### Installation
```bash
pip install transformers
```
### Prompt
```python
prompt = f"""<example_response>{--question , --query}</example_response><function_code>{code}</function_code>
<question>Give one line description of the python code above in natural language.</question>
<doc>"""
prompt = f"""<example_response>{example of some --question: , --query}</example_response><schema>{schema with cols described}</schema>
<question>Write a sql query to ....</question>
<sql>"""
```
### PyTorch
```python
from transformers import AutoModelForCausalLM, AutoTokenizer
device = "cuda"
model = AutoModelForCausalLM.from_pretrained("PipableAI/pip-library-etl-1.3b").to(device)
tokenizer = AutoTokenizer.from_pretrained("PipableAI/pip-library-etl-1.3b")
prompt = f"""
<example_response>
--code:def divide_by_two(x: float) -> float: return x / 2
--question:Document the python code above giving function description ,parameters and return type and example on how to call the function
--doc:
Description: This function divides a given number by 2.
Parameters:
- x (float): The input value to be divided by 2.
Returns:
- float: The result of x divided by 2.
Example:
divide_by_two(1.0)
</example_response>
<function_code>
def download_file(shared_url, destination):
try:
if not shared_url.startswith("https://drive.google.com"):
raise ValueError("Please provde a valid google drive link.")
file_id = shared_url.split("/d/")[1]
file_id = file_id.split("/")[0]
url = f"https://drive.google.com/uc?id={file_id}"
gdown.download(url, destination, quiet=False)
except Exception as e:
print(f"Error downloading file from Google Drive as {e}")
raise e
</function_code>
<instructions>
1. In the examples while calling function use the name mentioned after `def ` in the above function_code.
2. In the generated docs use valid python type hints as per PEP 484.
</instructions>
<question>Document the python code above giving function description ,parameters and return type and example how to call the function.</question>
<doc>
"""
inputs = tokenizer(prompt, return_tensors="pt").to("cuda")
outputs = model.generate(**inputs, max_new_tokens=450)
doc = (
tokenizer.decode(outputs[0], skip_special_tokens=True)
.split("<doc>")[-1]
.split("</doc>")[0]
)
doc = (
doc.replace("<p>", "")
.replace("</p>", "")
.replace("<function_description>", "")
.replace("</function_description>", "")
)
print(doc)
```
## Examples
### 1. Code Documentation
### prompt
```python
prompt ='''<example_response>
--code:def divide_by_two(x: float) -> float: return x / 2
--question:Document the python code above giving function description ,parameters and return type and example on how to call the function
--doc:
Description: This function divides a given number by 2.
Parameters:
- x (float): The input value to be divided by 2.
Returns:
- float: The result of x divided by 2.
Example:
divide_by_two(1.0)
</example_response>
<function_code>def _plot_bounding_polygon(
polygons_coordinates, output_html_path="bounding_polygon_map.html"
):
# Create a Folium map centered at the average coordinates of all bounding boxes
map_center = [
sum(
[
coord[0]
for polygon_coords in polygons_coordinates
for coord in polygon_coords
]
)
/ sum([len(polygon_coords) for polygon_coords in polygons_coordinates]),
sum(
[
coord[1]
for polygon_coords in polygons_coordinates
for coord in polygon_coords
]
)
/ sum([len(polygon_coords) for polygon_coords in polygons_coordinates]),
]
my_map = folium.Map(location=map_center, zoom_start=12)
# Add each bounding polygon to the map
for polygon_coords in polygons_coordinates:
folium.Polygon(
locations=polygon_coords,
color="blue",
fill=True,
fill_color="blue",
fill_opacity=0.2,
).add_to(my_map)
# Add bounding boxes as markers to the map
marker_cluster = MarkerCluster().add_to(my_map)
for polygon_coords in polygons_coordinates:
for coord in polygon_coords:
folium.Marker(
location=[coord[0], coord[1]], popup=f"Coordinates: {coord}"
).add_to(marker_cluster)
# Add draw control to allow users to draw additional polygons
draw = Draw(export=True)
draw.add_to(my_map)
# Save the map as an HTML file
my_map.save(output_html_path)
return output_html_path
</function_code>
<instructions>
1. In the examples while calling function use the name mentioned after `def ` in the above function_code.
2. In the generated docs use valid python type hints as per PEP 484.
</instructions>
<question>Document the python code above giving function description ,parameters and return type and example how to call the function</question><doc>'''
```
### Response
```txt
Description:This function generates a map of the bounding polygons and saves it as an HTML file.
Parameters:
- polygons_coordinates (list of lists of tuples): A list of lists of tuples representing the coordinates of the polygons. Each polygon is a list of coordinates.
- output_html_path (str, optional): The path where the HTML file should be saved. Defaults to "bounding_polygon_map.html".
Returns:
- str: The path to the saved HTML file.
Example:
To call the function, use the following code:
plot_bounding_polygon([[(0, 0), (1, 0), (1, 1), (0, 1)], [(2, 2), (3, 2), (3, 3), (2, 3)]], "my_map.html").
```
### 2. SQL Generation
### prompt
```python
prompt = """Generate a simple SQL query from the schema mentioned for the following question.
<schema>
CREATE TABLE department (
Department_ID number, -- Unique identifier for the department
Name text, -- Name of the department
Creation text, -- Date of creation or establishment
Ranking number, -- Ranking of the department
Budget_in_Billions number, -- Budget of the department in billions
Num_Employees number -- Number of employees in the department
);
CREATE TABLE head (
head_ID number, -- Unique identifier for the head
name text, -- Name of the head
born_state text, -- State where the head was born
age number -- Age of the head
);
CREATE TABLE management (
department_ID number, -- Foreign key referencing Department_ID in department table
head_ID number, -- Foreign key referencing head_ID in head table
temporary_acting text -- Indicates if the head is temporarily acting
);
</schema>
<question>What are the names of the heads who are born outside the California state?</question>
<sql>
"""
```
### response
```sql
SELECT head.name FROM head WHERE head.born_state <> 'California';
```
### 3. Performance Schema Monitoring
### prompt
```python
prompt = """Generate the SQL query for SkySQL performance schema for the following question.
<example>
--question: What are the top 10 most frequently used queries/statements?
--sql: SELECT DIGEST_TEXT, COUNT(*) as frequency FROM performance_schema.events_statements_summary_by_digest GROUP BY DIGEST_TEXT ORDER BY frequency DESC LIMIT 10;
</example>
<schema>
CREATE TABLE `accounts` (`USER` char(128) DEFAULT NULL -- 'The connection''s client user name for the connection, or NULL if an internal thread.',
`HOST` char(255) DEFAULT NULL -- 'The connection client''s host name, or NULL if an internal thread.',
`CURRENT_CONNECTIONS` bigint(20) NOT NULL -- 'Current connections for the account.',\n
`TOTAL_CONNECTIONS` bigint(20) NOT NULL -- 'Total connections for the account.'
) ;
</schema>
<question>
Tell me the number of active connections each user has.
</question>
<sql>
"""
```
### response
```sql
SELECT USER, CURRENT_CONNECTIONS FROM accounts;
```
### prompt
```python
prompt = """Generate the SQL query for SkySQL performance schema for the following question.
<example>
--question: What are the top 10 most frequently used queries/statements?
--sql: SELECT DIGEST_TEXT, COUNT(*) as frequency FROM performance_schema.events_statements_summary_by_digest GROUP BY DIGEST_TEXT ORDER BY frequency DESC LIMIT 10;
</example>
<schema>
CREATE TABLE `file_summary_by_instance` (
`FILE_NAME` varchar(512) NOT NULL -- 'File name.',
`EVENT_NAME` varchar(128) NOT NULL -- 'Event name.',
`OBJECT_INSTANCE_BEGIN` bigint(20) unsigned NOT NULL -- 'Address in memory. Together with FILE_NAME and EVENT_NAME uniquely identifies a row.',
`COUNT_STAR` bigint(20) unsigned NOT NULL -- 'Number of summarized events',
`SUM_TIMER_WAIT` bigint(20) unsigned NOT NULL -- 'Total wait time of the summarized events that are timed.',
`MIN_TIMER_WAIT` bigint(20) unsigned NOT NULL -- 'Minimum wait time of the summarized events that are timed.',
`AVG_TIMER_WAIT` bigint(20) unsigned NOT NULL -- 'Average wait time of the summarized events that are timed.',
`MAX_TIMER_WAIT` bigint(20) unsigned NOT NULL -- 'Maximum wait time of the summarized events that are timed.',
`COUNT_READ` bigint(20) unsigned NOT NULL -- 'Number of all read operations, including FGETS, FGETC, FREAD, and READ.',
`SUM_TIMER_READ` bigint(20) unsigned NOT NULL -- 'Total wait time of all read operations that are timed.',
`MIN_TIMER_READ` bigint(20) unsigned NOT NULL -- 'Minimum wait time of all read operations that are timed.',
`AVG_TIMER_READ` bigint(20) unsigned NOT NULL -- 'Average wait time of all read operations that are timed.',
`MAX_TIMER_READ` bigint(20) unsigned NOT NULL -- 'Maximum wait time of all read operations that are timed.',
`SUM_NUMBER_OF_BYTES_READ` bigint(20) NOT NULL -- 'Bytes read by read operations.',
`COUNT_WRITE` bigint(20) unsigned NOT NULL -- 'Number of all write operations, including FPUTS, FPUTC, FPRINTF, VFPRINTF, FWRITE, and PWRITE.',
`SUM_TIMER_WRITE` bigint(20) unsigned NOT NULL -- 'Total wait time of all write operations that are timed.',
`MIN_TIMER_WRITE` bigint(20) unsigned NOT NULL -- 'Minimum wait time of all write operations that are timed.',
`AVG_TIMER_WRITE` bigint(20) unsigned NOT NULL -- 'Average wait time of all write operations that are timed.',
`MAX_TIMER_WRITE` bigint(20) unsigned NOT NULL -- 'Maximum wait time of all write operations that are timed.',
`SUM_NUMBER_OF_BYTES_WRITE` bigint(20) NOT NULL -- 'Bytes written by write operations.',
`COUNT_MISC` bigint(20) unsigned NOT NULL -- 'Number of all miscellaneous operations not counted above, including CREATE, DELETE, OPEN, CLOSE, STREAM_OPEN, STREAM_CLOSE, SEEK, TELL, FLUSH, STAT, FSTAT, CHSIZE, RENAME, and SYNC.',
`SUM_TIMER_MISC` bigint(20) unsigned NOT NULL -- 'Total wait time of all miscellaneous operations that are timed.',
`MIN_TIMER_MISC` bigint(20) unsigned NOT NULL -- 'Minimum wait time of all miscellaneous operations that are timed.',
`AVG_TIMER_MISC` bigint(20) unsigned NOT NULL -- 'Average wait time of all miscellaneous operations that are timed.',
`MAX_TIMER_MISC` bigint(20) unsigned NOT NULL -- 'Maximum wait time of all miscellaneous operations that are timed.'
);
</schema>
<question>
List out 10 names of the files with the most read and writes
</question>
<sql>
"""
```
### response
```sql
SELECT FILE_NAME FROM file_summary_by_instance ORDER BY SUM_NUMBER_OF_BYTES_READ DESC, SUM_NUMBER_OF_BYTES_WRITE DESC LIMIT 10;
```
### 4. Function Calling
### prompt
```python
prompt = """
Give a function call in python langugae for the following question:
<example_response>
--doc: Description: This function logs a curl command in debug mode.
Parameters:
- method (str): The HTTP method to use for the request.
- url (str): The URL to send the request to.
- data (dict, optional): The data to send in the request. Defaults to None.
- headers (dict, optional): The headers to send with the request. Defaults to None.
- level (int, optional): The log level to use for this log message. Defaults to logging.DEBUG.
Returns:
- None
Example:
log_curl_debug('GET', 'https://example.com')
--question: log a curl PUT request for url https://web.io/
--function_call: log_curl_debug(method='PUT', url = 'https://web.io')
</example_response>
<doc>
Function Name: make_get_req()
Description: This function is used to make a GET request.
Parameters:
- path (str): The path of the URL to be requested.
- data (dict): The data to be sent in the body of the request.
- flags (dict): The flags to be sent in the request.
- params (dict): The parameters to be sent in the request.
- headers (dict): The headers to be sent in the request.
- not_json_response (bool): OPTIONAL: If set to True, the function will return the raw response content instead of trying to parse it as JSON.
- trailing (str): OPTIONAL: For wrapping slash symbol in the end of string.
- absolute (bool): OPTIONAL: If set to True, the function will not prefix the URL with the base URL.
- advanced_mode (bool): OPTIONAL: If set to True, the function will return the raw response instead of trying to parse it as JSON.
Returns:
- Union[str, dict, list, None]: The response content as a string, a dictionary, a list, or None if the response was not successful.
</doc>
<instruction>
1. Strictly use named parameters mentioned in the doc to generate function calls.
2. Only return the response as python parsable string version of function call.
3. mention the 'self' parameter if required.
</instruction>
<question>
Make a GET request for the URL parameter using variable_2. For the params parameter, use 'weight' as one of the keys with variable_3 as its value, and 'width' as another key with a value of 10. For the data parameter, use variable_1. Prefix the URL with the base URL, and ensure the response is in raw format.
</question>
<function_call>
"""
```
### response
```python
make_get_req(path='https://example.com/api/v1/users', data=variable_1, params={'weight': variable_3, 'width': 10}, headers={'Content-Type': 'application/json'}, not_json_response=True, absolute=True)
```
### prompt
```python
prompt = """
Give only function call in python langugae as response for the following question:
<example_response>
--doc:
Function:
Help on function head in module pandas.core.generic:
head(self, n: 'int' = 5) -> 'Self'
Return the first `n` rows.
This function returns the first `n` rows for the object based
on position. It is useful for quickly testing if your object
has the right type of data in it.
For negative values of `n`, this function returns all rows except
the last `|n|` rows, equivalent to ``df[:n]``.
If n is larger than the number of rows, this function returns all rows.
Parameters
----------
n : int, default 5
Number of rows to select.
Returns
-------
same type as caller
The first `n` rows of the caller object.
See Also
--------
DataFrame.tail: Returns the last `n` rows.
Examples
--------
>>> df = pd.DataFrame({'animal': ['alligator', 'bee', 'falcon', 'lion',
... 'monkey', 'parrot', 'shark', 'whale', 'zebra']})
>>> df
animal
0 alligator
--question: Get the top 5 rows with the highest Engagement_Score. Parameter Description: Use 5 as Number of rows to return ,Use variable_3 as Sorted DataFrame, Do not call any other function, Pass variable to self parameter for method calls
--function_call: head(self=variable_3, n=5)
</example_response>
<doc>
Function: sort_values
sort_values in module pandas.core.frame:
sort_values(self, by: 'IndexLabel', *, axis: 'Axis' = 0, ascending: 'bool | list[bool] | tuple[bool, ...]' = True, inplace: 'bool' = False, kind: 'SortKind' = 'quicksort', na_position: 'str' = 'last', ignore_index: 'bool' = False, key: 'ValueKeyFunc | None' = None) -> 'DataFrame | None'
Sort by the values along either axis.
Parameters
----------
by : str or list of str
Name or list of names to sort by.
- if `axis` is 0 or `'index'` then `by` may contain index
levels and/or column labels.
- if `axis` is 1 or `'columns'` then `by` may contain column
levels and/or index labels.
axis : "{0 or 'index', 1 or 'columns'}", default 0
Axis to be sorted.
ascending : bool or list of bool, default True
Sort ascending vs. descending. Specify list for multiple sort
orders. If this is a list of bools, must match the length of
the
</doc>
<instruction>
1. Strictly use named parameters mentioned in the doc to generate function calls.
2. Only return the response as python parsable string version of function call.
3. Use the 'self' parameter if required in the function call with it's value in named keyword format.
</instruction>
<question>
Using the above function, Sort the DataFrame by the Engagement_Score in descending order. Parameter Description: Use Engagement_Score as Column name to sort by ,Use False as Sort in descending order ,Use variable_1 as DataFrame to sort, Do not call any other function, Pass variable to self parameter for method calls
</question>
<function_call>
"""
```
### response
```python
sort_values(self=variable_1, by='Engagement_Score', ascending=False)
```
### Team
Avi Kothari, Gyan Ranjan, Pratham Gupta, Ritvik Aryan Kalra, Soham Acharya
|
csebuetnlp/banglabert | csebuetnlp | 2022-12-23T18:49:36Z | 4,364 | 16 | transformers | [
"transformers",
"pytorch",
"electra",
"pretraining",
"bn",
"endpoints_compatible",
"region:us"
] | null | 2022-03-02T23:29:05Z | ---
language:
- bn
licenses:
- cc-by-nc-sa-4.0
---
# BanglaBERT
This repository contains the pretrained discriminator checkpoint of the model **BanglaBERT**. This is an [ELECTRA](https://openreview.net/pdf?id=r1xMH1BtvB) discriminator model pretrained with the Replaced Token Detection (RTD) objective. Finetuned models using this checkpoint achieve state-of-the-art results on many of the NLP tasks in bengali.
For finetuning on different downstream tasks such as `Sentiment classification`, `Named Entity Recognition`, `Natural Language Inference` etc., refer to the scripts in the official GitHub [repository](https://github.com/csebuetnlp/banglabert).
**Note**: This model was pretrained using a specific normalization pipeline available [here](https://github.com/csebuetnlp/normalizer). All finetuning scripts in the official GitHub repository uses this normalization by default. If you need to adapt the pretrained model for a different task make sure the text units are normalized using this pipeline before tokenizing to get best results. A basic example is given below:
## Using this model as a discriminator in `transformers` (tested on 4.11.0.dev0)
```python
from transformers import AutoModelForPreTraining, AutoTokenizer
from normalizer import normalize # pip install git+https://github.com/csebuetnlp/normalizer
import torch
model = AutoModelForPreTraining.from_pretrained("csebuetnlp/banglabert")
tokenizer = AutoTokenizer.from_pretrained("csebuetnlp/banglabert")
original_sentence = "আমি কৃতজ্ঞ কারণ আপনি আমার জন্য অনেক কিছু করেছেন।"
fake_sentence = "আমি হতাশ কারণ আপনি আমার জন্য অনেক কিছু করেছেন।"
fake_sentence = normalize(fake_sentence) # this normalization step is required before tokenizing the text
fake_tokens = tokenizer.tokenize(fake_sentence)
fake_inputs = tokenizer.encode(fake_sentence, return_tensors="pt")
discriminator_outputs = model(fake_inputs).logits
predictions = torch.round((torch.sign(discriminator_outputs) + 1) / 2)
[print("%7s" % token, end="") for token in fake_tokens]
print("\n" + "-" * 50)
[print("%7s" % int(prediction), end="") for prediction in predictions.squeeze().tolist()[1:-1]]
print("\n" + "-" * 50)
```
## Benchmarks
* Zero-shot cross-lingual transfer-learning
| Model | Params | SC (macro-F1) | NLI (accuracy) | NER (micro-F1) | QA (EM/F1) | BangLUE score |
|----------------|-----------|-----------|-----------|-----------|-----------|-----------|
|[mBERT](https://huggingface.co/bert-base-multilingual-cased) | 180M | 27.05 | 62.22 | 39.27 | 59.01/64.18 | 50.35 |
|[XLM-R (base)](https://huggingface.co/xlm-roberta-base) | 270M | 42.03 | 72.18 | 45.37 | 55.03/61.83 | 55.29 |
|[XLM-R (large)](https://huggingface.co/xlm-roberta-large) | 550M | 49.49 | 78.13 | 56.48 | 71.13/77.70 | 66.59 |
|[BanglishBERT](https://huggingface.co/csebuetnlp/banglishbert) | 110M | 48.39 | 75.26 | 55.56 | 72.87/78.63 | 66.14 |
* Supervised fine-tuning
| Model | Params | SC (macro-F1) | NLI (accuracy) | NER (micro-F1) | QA (EM/F1) | BangLUE score |
|----------------|-----------|-----------|-----------|-----------|-----------|-----------|
|[mBERT](https://huggingface.co/bert-base-multilingual-cased) | 180M | 67.59 | 75.13 | 68.97 | 67.12/72.64 | 70.29 |
|[XLM-R (base)](https://huggingface.co/xlm-roberta-base) | 270M | 69.54 | 78.46 | 73.32 | 68.09/74.27 | 72.82 |
|[XLM-R (large)](https://huggingface.co/xlm-roberta-large) | 550M | 70.97 | 82.40 | 78.39 | 73.15/79.06 | 76.79 |
|[sahajBERT](https://huggingface.co/neuropark/sahajBERT) | 18M | 71.12 | 76.92 | 70.94 | 65.48/70.69 | 71.03 |
|[BanglishBERT](https://huggingface.co/csebuetnlp/banglishbert) | 110M | 70.61 | 80.95 | 76.28 | 72.43/78.40 | 75.73 |
|[BanglaBERT](https://huggingface.co/csebuetnlp/banglabert) | 110M | 72.89 | 82.80 | 77.78 | 72.63/79.34 | **77.09** |
The benchmarking datasets are as follows:
* **SC:** **[Sentiment Classification](https://aclanthology.org/2021.findings-emnlp.278)**
* **NER:** **[Named Entity Recognition](https://multiconer.github.io/competition)**
* **NLI:** **[Natural Language Inference](https://github.com/csebuetnlp/banglabert/#datasets)**
* **QA:** **[Question Answering](https://github.com/csebuetnlp/banglabert/#datasets)**
## Citation
If you use this model, please cite the following paper:
```
@inproceedings{bhattacharjee-etal-2022-banglabert,
title = "{B}angla{BERT}: Language Model Pretraining and Benchmarks for Low-Resource Language Understanding Evaluation in {B}angla",
author = "Bhattacharjee, Abhik and
Hasan, Tahmid and
Ahmad, Wasi and
Mubasshir, Kazi Samin and
Islam, Md Saiful and
Iqbal, Anindya and
Rahman, M. Sohel and
Shahriyar, Rifat",
booktitle = "Findings of the Association for Computational Linguistics: NAACL 2022",
month = jul,
year = "2022",
address = "Seattle, United States",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2022.findings-naacl.98",
pages = "1318--1327",
abstract = "In this work, we introduce BanglaBERT, a BERT-based Natural Language Understanding (NLU) model pretrained in Bangla, a widely spoken yet low-resource language in the NLP literature. To pretrain BanglaBERT, we collect 27.5 GB of Bangla pretraining data (dubbed {`}Bangla2B+{'}) by crawling 110 popular Bangla sites. We introduce two downstream task datasets on natural language inference and question answering and benchmark on four diverse NLU tasks covering text classification, sequence labeling, and span prediction. In the process, we bring them under the first-ever Bangla Language Understanding Benchmark (BLUB). BanglaBERT achieves state-of-the-art results outperforming multilingual and monolingual models. We are making the models, datasets, and a leaderboard publicly available at \url{https://github.com/csebuetnlp/banglabert} to advance Bangla NLP.",
}
```
If you use the normalization module, please cite the following paper:
```
@inproceedings{hasan-etal-2020-low,
title = "Not Low-Resource Anymore: Aligner Ensembling, Batch Filtering, and New Datasets for {B}engali-{E}nglish Machine Translation",
author = "Hasan, Tahmid and
Bhattacharjee, Abhik and
Samin, Kazi and
Hasan, Masum and
Basak, Madhusudan and
Rahman, M. Sohel and
Shahriyar, Rifat",
booktitle = "Proceedings of the 2020 Conference on Empirical Methods in Natural Language Processing (EMNLP)",
month = nov,
year = "2020",
address = "Online",
publisher = "Association for Computational Linguistics",
url = "https://www.aclweb.org/anthology/2020.emnlp-main.207",
doi = "10.18653/v1/2020.emnlp-main.207",
pages = "2612--2623",
abstract = "Despite being the seventh most widely spoken language in the world, Bengali has received much less attention in machine translation literature due to being low in resources. Most publicly available parallel corpora for Bengali are not large enough; and have rather poor quality, mostly because of incorrect sentence alignments resulting from erroneous sentence segmentation, and also because of a high volume of noise present in them. In this work, we build a customized sentence segmenter for Bengali and propose two novel methods for parallel corpus creation on low-resource setups: aligner ensembling and batch filtering. With the segmenter and the two methods combined, we compile a high-quality Bengali-English parallel corpus comprising of 2.75 million sentence pairs, more than 2 million of which were not available before. Training on neural models, we achieve an improvement of more than 9 BLEU score over previous approaches to Bengali-English machine translation. We also evaluate on a new test set of 1000 pairs made with extensive quality control. We release the segmenter, parallel corpus, and the evaluation set, thus elevating Bengali from its low-resource status. To the best of our knowledge, this is the first ever large scale study on Bengali-English machine translation. We believe our study will pave the way for future research on Bengali-English machine translation as well as other low-resource languages. Our data and code are available at https://github.com/csebuetnlp/banglanmt.",
}
```
|
deepseek-ai/deepseek-vl-7b-chat | deepseek-ai | 2024-03-15T07:04:05Z | 4,364 | 204 | transformers | [
"transformers",
"safetensors",
"multi_modality",
"image-text-to-text",
"arxiv:2403.05525",
"license:other",
"endpoints_compatible",
"region:us"
] | image-text-to-text | 2024-03-07T06:14:29Z | ---
license: other
license_name: deepseek
license_link: LICENSE
pipeline_tag: image-text-to-text
---
## 1. Introduction
Introducing DeepSeek-VL, an open-source Vision-Language (VL) Model designed for real-world vision and language understanding applications. DeepSeek-VL possesses general multimodal understanding capabilities, capable of processing logical diagrams, web pages, formula recognition, scientific literature, natural images, and embodied intelligence in complex scenarios.
[DeepSeek-VL: Towards Real-World Vision-Language Understanding](https://arxiv.org/abs/2403.05525)
[**Github Repository**](https://github.com/deepseek-ai/DeepSeek-VL)
Haoyu Lu*, Wen Liu*, Bo Zhang**, Bingxuan Wang, Kai Dong, Bo Liu, Jingxiang Sun, Tongzheng Ren, Zhuoshu Li, Hao Yang, Yaofeng Sun, Chengqi Deng, Hanwei Xu, Zhenda Xie, Chong Ruan (*Equal Contribution, **Project Lead)

### 2. Model Summary
DeepSeek-VL-7b-base uses the [SigLIP-L](https://huggingface.co/timm/ViT-L-16-SigLIP-384) and [SAM-B](https://huggingface.co/facebook/sam-vit-base) as the hybrid vision encoder supporting 1024 x 1024 image input
and is constructed based on the DeepSeek-LLM-7b-base which is trained on an approximate corpus of 2T text tokens. The whole DeepSeek-VL-7b-base model is finally trained around 400B vision-language tokens.
DeekSeel-VL-7b-chat is an instructed version based on [DeepSeek-VL-7b-base](https://huggingface.co/deepseek-ai/deepseek-vl-7b-base).
## 3. Quick Start
### Installation
On the basis of `Python >= 3.8` environment, install the necessary dependencies by running the following command:
```shell
git clone https://github.com/deepseek-ai/DeepSeek-VL
cd DeepSeek-VL
pip install -e .
```
### Simple Inference Example
```python
import torch
from transformers import AutoModelForCausalLM
from deepseek_vl.models import VLChatProcessor, MultiModalityCausalLM
from deepseek_vl.utils.io import load_pil_images
# specify the path to the model
model_path = "deepseek-ai/deepseek-vl-7b-chat"
vl_chat_processor: VLChatProcessor = VLChatProcessor.from_pretrained(model_path)
tokenizer = vl_chat_processor.tokenizer
vl_gpt: MultiModalityCausalLM = AutoModelForCausalLM.from_pretrained(model_path, trust_remote_code=True)
vl_gpt = vl_gpt.to(torch.bfloat16).cuda().eval()
conversation = [
{
"role": "User",
"content": "<image_placeholder>Describe each stage of this image.",
"images": ["./images/training_pipelines.png"]
},
{
"role": "Assistant",
"content": ""
}
]
# load images and prepare for inputs
pil_images = load_pil_images(conversation)
prepare_inputs = vl_chat_processor(
conversations=conversation,
images=pil_images,
force_batchify=True
).to(vl_gpt.device)
# run image encoder to get the image embeddings
inputs_embeds = vl_gpt.prepare_inputs_embeds(**prepare_inputs)
# run the model to get the response
outputs = vl_gpt.language_model.generate(
inputs_embeds=inputs_embeds,
attention_mask=prepare_inputs.attention_mask,
pad_token_id=tokenizer.eos_token_id,
bos_token_id=tokenizer.bos_token_id,
eos_token_id=tokenizer.eos_token_id,
max_new_tokens=512,
do_sample=False,
use_cache=True
)
answer = tokenizer.decode(outputs[0].cpu().tolist(), skip_special_tokens=True)
print(f"{prepare_inputs['sft_format'][0]}", answer)
```
### CLI Chat
```bash
python cli_chat.py --model_path "deepseek-ai/deepseek-vl-7b-chat"
# or local path
python cli_chat.py --model_path "local model path"
```
## 4. License
This code repository is licensed under [the MIT License](https://github.com/deepseek-ai/DeepSeek-LLM/blob/HEAD/LICENSE-CODE). The use of DeepSeek-VL Base/Chat models is subject to [DeepSeek Model License](https://github.com/deepseek-ai/DeepSeek-LLM/blob/HEAD/LICENSE-MODEL). DeepSeek-VL series (including Base and Chat) supports commercial use.
## 5. Citation
```
@misc{lu2024deepseekvl,
title={DeepSeek-VL: Towards Real-World Vision-Language Understanding},
author={Haoyu Lu and Wen Liu and Bo Zhang and Bingxuan Wang and Kai Dong and Bo Liu and Jingxiang Sun and Tongzheng Ren and Zhuoshu Li and Yaofeng Sun and Chengqi Deng and Hanwei Xu and Zhenda Xie and Chong Ruan},
year={2024},
eprint={2403.05525},
archivePrefix={arXiv},
primaryClass={cs.AI}
}
```
## 6. Contact
If you have any questions, please raise an issue or contact us at [[email protected]](mailto:[email protected]). |
luffycodes/vicuna-class-shishya-7b-ep3 | luffycodes | 2023-12-14T15:41:15Z | 4,362 | 0 | transformers | [
"transformers",
"pytorch",
"llama",
"text-generation",
"arxiv:2305.13272",
"license:llama2",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2023-12-14T15:20:40Z | ---
license: llama2
---
If you use this work, please cite:
CLASS Meet SPOCK: An Education Tutoring Chatbot based on Learning Science Principles
https://arxiv.org/abs/2305.13272
```
@misc{sonkar2023class,
title={CLASS Meet SPOCK: An Education Tutoring Chatbot based on Learning Science Principles},
author={Shashank Sonkar and Lucy Liu and Debshila Basu Mallick and Richard G. Baraniuk},
year={2023},
eprint={2305.13272},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
``` |
LDCC/LDCC-SOLAR-10.7B | LDCC | 2024-02-08T01:59:04Z | 4,360 | 14 | transformers | [
"transformers",
"safetensors",
"llama",
"text-generation",
"ko",
"arxiv:2312.15166",
"license:cc-by-nc-4.0",
"autotrain_compatible",
"endpoints_compatible",
"text-generation-inference",
"region:us"
] | text-generation | 2024-01-03T03:43:58Z | ---
license: cc-by-nc-4.0
language:
- ko
---
# Model Card for LDCC-SOLAR-10.7B
## Developed by : Wonchul Kim ([Lotte Data Communication](https://www.ldcc.co.kr) AI Technical Team)
## Hardware and Software
* **Hardware**: We utilized an A100x4 * 1 for training our model
* **Training Factors**: We fine-tuned this model using a combination of the [DeepSpeed library](https://github.com/microsoft/DeepSpeed) and the [HuggingFace TRL Trainer](https://huggingface.co/docs/trl/trainer) / [HuggingFace Accelerate](https://huggingface.co/docs/accelerate/index)
## Method
- This model was trained using the learning method introduced in the [SOLAR paper](https://arxiv.org/pdf/2312.15166.pdf).
## Base Model
- [yanolja/KoSOLAR-10.7B-v0.1](https://huggingface.co/yanolja/KoSOLAR-10.7B-v0.1) (This model is no longer supported due to a tokenizer issue.)
## Caution
- If you want to fine-tune this model, it is recommended to use the [tokenizer.json](https://huggingface.co/LDCC/LDCC-SOLAR-10.7B/blob/v1.1/tokenizer.json) and [tokenizer_config.json](https://huggingface.co/LDCC/LDCC-SOLAR-10.7B/blob/v1.1/tokenizer_config.json) files from revision v1.1. |
mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF | mradermacher | 2024-06-04T05:49:01Z | 4,360 | 0 | transformers | [
"transformers",
"gguf",
"en",
"base_model:Hastagaras/Halu-8B-Llama3-CR-v0.45",
"license:llama3",
"endpoints_compatible",
"region:us"
] | null | 2024-06-03T20:44:02Z | ---
base_model: Hastagaras/Halu-8B-Llama3-CR-v0.45
language:
- en
library_name: transformers
license: llama3
quantized_by: mradermacher
---
## About
<!-- ### quantize_version: 2 -->
<!-- ### output_tensor_quantised: 1 -->
<!-- ### convert_type: hf -->
<!-- ### vocab_type: -->
<!-- ### tags: nicoboss -->
weighted/imatrix quants of https://huggingface.co/Hastagaras/Halu-8B-Llama3-CR-v0.45
<!-- provided-files -->
static quants are available at https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-GGUF
## Usage
If you are unsure how to use GGUF files, refer to one of [TheBloke's
READMEs](https://huggingface.co/TheBloke/KafkaLM-70B-German-V0.1-GGUF) for
more details, including on how to concatenate multi-part files.
## Provided Quants
(sorted by size, not necessarily quality. IQ-quants are often preferable over similar sized non-IQ quants)
| Link | Type | Size/GB | Notes |
|:-----|:-----|--------:|:------|
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ1_S.gguf) | i1-IQ1_S | 2.1 | for the desperate |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ1_M.gguf) | i1-IQ1_M | 2.3 | mostly desperate |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ2_XXS.gguf) | i1-IQ2_XXS | 2.5 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ2_XS.gguf) | i1-IQ2_XS | 2.7 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ2_S.gguf) | i1-IQ2_S | 2.9 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ2_M.gguf) | i1-IQ2_M | 3.0 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q2_K.gguf) | i1-Q2_K | 3.3 | IQ3_XXS probably better |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ3_XXS.gguf) | i1-IQ3_XXS | 3.4 | lower quality |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ3_XS.gguf) | i1-IQ3_XS | 3.6 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q3_K_S.gguf) | i1-Q3_K_S | 3.8 | IQ3_XS probably better |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ3_S.gguf) | i1-IQ3_S | 3.8 | beats Q3_K* |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ3_M.gguf) | i1-IQ3_M | 3.9 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q3_K_M.gguf) | i1-Q3_K_M | 4.1 | IQ3_S probably better |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q3_K_L.gguf) | i1-Q3_K_L | 4.4 | IQ3_M probably better |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-IQ4_XS.gguf) | i1-IQ4_XS | 4.5 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q4_0.gguf) | i1-Q4_0 | 4.8 | fast, low quality |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q4_K_S.gguf) | i1-Q4_K_S | 4.8 | optimal size/speed/quality |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q4_K_M.gguf) | i1-Q4_K_M | 5.0 | fast, recommended |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q5_K_S.gguf) | i1-Q5_K_S | 5.7 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q5_K_M.gguf) | i1-Q5_K_M | 5.8 | |
| [GGUF](https://huggingface.co/mradermacher/Halu-8B-Llama3-CR-v0.45-i1-GGUF/resolve/main/Halu-8B-Llama3-CR-v0.45.i1-Q6_K.gguf) | i1-Q6_K | 6.7 | practically like static Q6_K |
Here is a handy graph by ikawrakow comparing some lower-quality quant
types (lower is better):

And here are Artefact2's thoughts on the matter:
https://gist.github.com/Artefact2/b5f810600771265fc1e39442288e8ec9
## FAQ / Model Request
See https://huggingface.co/mradermacher/model_requests for some answers to
questions you might have and/or if you want some other model quantized.
## Thanks
I thank my company, [nethype GmbH](https://www.nethype.de/), for letting
me use its servers and providing upgrades to my workstation to enable
this work in my free time. Additional thanks to [@nicoboss](https://huggingface.co/nicoboss) for giving me access to his hardware for calculating the imatrix for these quants.
<!-- end -->
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.