code
stringlengths 3
1.05M
| repo_name
stringlengths 4
116
| path
stringlengths 3
942
| language
stringclasses 30
values | license
stringclasses 15
values | size
int32 3
1.05M
|
---|---|---|---|---|---|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class oscilation : MonoBehaviour {
public float m_speed = 0.008f;
public int m_distance = 45;
private int compteur = 0;
private int compteur2 = 0;
// Use this for initialization
void Start () {
Vector3 yOrigin = transform.localPosition;
/*Vector3 move = new Vector3(0.0f,0.0f,0.0f);
Vector3 move2 = new Vector3(0.0f,0.0f,0.0f);
move.y = m_speed;
move2.y = -m_speed;*/
}
// Update is called once per frame
void Update () {
if (compteur < m_distance) {
Vector3 move = new Vector3(0.0f,0.0f,0.0f);
move.y = m_speed;
transform.localPosition = transform.localPosition + move;
compteur++;
} else if (compteur2 < m_distance) {
Vector3 move2 = new Vector3(0.0f,0.0f,0.0f);
move2.y = -m_speed;
compteur2++;
transform.localPosition = transform.localPosition + move2;
} else {
compteur = 0;
compteur2 = 0;
}
}
}
| Wormkil/firstProject | Assets/oscilation.cs | C# | mit | 962 |
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Threading.Tasks;
using System.Net;
using System.Web;
using System.Web.Mvc;
using Transit.Models;
namespace Transit.Controllers
{
public class RoutesController : Controller
{
private ApplicationDbContext db = new ApplicationDbContext();
// GET: Routes
public async Task<ActionResult> Index()
{
var routes = db.Routes.Include(r => r.agency).Include(r => r.type);
return View(await routes.ToListAsync());
}
// GET: Routes/Details/5
public async Task<ActionResult> Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Route route = await db.Routes.FindAsync(id);
if (route == null)
{
return HttpNotFound();
}
return View(route);
}
// GET: Routes/Create
public ActionResult Create()
{
ViewBag.agencyId = new SelectList(db.Agencies, "id", "name");
ViewBag.typeId = new SelectList(db.RouteTypes, "id", "label");
return View();
}
// POST: Routes/Create
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Create([Bind(Include = "id,agencyId,label,fullName,description,typeId,url,color,textColor")] Route route)
{
if (ModelState.IsValid)
{
db.Routes.Add(route);
await db.SaveChangesAsync();
return RedirectToAction("Index");
}
ViewBag.agencyId = new SelectList(db.Agencies, "id", "name", route.agencyId);
ViewBag.typeId = new SelectList(db.RouteTypes, "id", "label", route.typeId);
return View(route);
}
// GET: Routes/Edit/5
public async Task<ActionResult> Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Route route = await db.Routes.FindAsync(id);
if (route == null)
{
return HttpNotFound();
}
ViewBag.agencyId = new SelectList(db.Agencies, "id", "name", route.agencyId);
ViewBag.typeId = new SelectList(db.RouteTypes, "id", "label", route.typeId);
return View(route);
}
// POST: Routes/Edit/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Edit([Bind(Include = "id,agencyId,label,fullName,description,typeId,url,color,textColor")] Route route)
{
if (ModelState.IsValid)
{
db.Entry(route).State = EntityState.Modified;
await db.SaveChangesAsync();
return RedirectToAction("Index");
}
ViewBag.agencyId = new SelectList(db.Agencies, "id", "name", route.agencyId);
ViewBag.typeId = new SelectList(db.RouteTypes, "id", "label", route.typeId);
return View(route);
}
// GET: Routes/Delete/5
public async Task<ActionResult> Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Route route = await db.Routes.FindAsync(id);
if (route == null)
{
return HttpNotFound();
}
return View(route);
}
// POST: Routes/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public async Task<ActionResult> DeleteConfirmed(int id)
{
Route route = await db.Routes.FindAsync(id);
db.Routes.Remove(route);
await db.SaveChangesAsync();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
}
| cdchild/TransitApp | Transit/Controllers/RoutesController.cs | C# | mit | 4,630 |
/*
* This file is part of LuckPerms, licensed under the MIT License.
*
* Copyright (c) lucko (Luck) <[email protected]>
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package me.lucko.luckperms.nukkit.inject.server;
import me.lucko.luckperms.nukkit.LPNukkitPlugin;
import cn.nukkit.Server;
import cn.nukkit.permission.Permissible;
import cn.nukkit.plugin.PluginManager;
import java.lang.reflect.Field;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
/**
* Injects a {@link LuckPermsSubscriptionMap} into the {@link PluginManager}.
*/
public class InjectorSubscriptionMap implements Runnable {
private static final Field PERM_SUBS_FIELD;
static {
Field permSubsField = null;
try {
permSubsField = PluginManager.class.getDeclaredField("permSubs");
permSubsField.setAccessible(true);
} catch (Exception e) {
// ignore
}
PERM_SUBS_FIELD = permSubsField;
}
private final LPNukkitPlugin plugin;
public InjectorSubscriptionMap(LPNukkitPlugin plugin) {
this.plugin = plugin;
}
@Override
public void run() {
try {
LuckPermsSubscriptionMap subscriptionMap = inject();
if (subscriptionMap != null) {
this.plugin.setSubscriptionMap(subscriptionMap);
}
} catch (Exception e) {
this.plugin.getLogger().severe("Exception occurred whilst injecting LuckPerms Permission Subscription map.", e);
}
}
private LuckPermsSubscriptionMap inject() throws Exception {
Objects.requireNonNull(PERM_SUBS_FIELD, "PERM_SUBS_FIELD");
PluginManager pluginManager = this.plugin.getBootstrap().getServer().getPluginManager();
Object map = PERM_SUBS_FIELD.get(pluginManager);
if (map instanceof LuckPermsSubscriptionMap) {
if (((LuckPermsSubscriptionMap) map).plugin == this.plugin) {
return null;
}
map = ((LuckPermsSubscriptionMap) map).detach();
}
//noinspection unchecked
Map<String, Set<Permissible>> castedMap = (Map<String, Set<Permissible>>) map;
// make a new subscription map & inject it
LuckPermsSubscriptionMap newMap = new LuckPermsSubscriptionMap(this.plugin, castedMap);
PERM_SUBS_FIELD.set(pluginManager, newMap);
return newMap;
}
public static void uninject() {
try {
Objects.requireNonNull(PERM_SUBS_FIELD, "PERM_SUBS_FIELD");
PluginManager pluginManager = Server.getInstance().getPluginManager();
Object map = PERM_SUBS_FIELD.get(pluginManager);
if (map instanceof LuckPermsSubscriptionMap) {
LuckPermsSubscriptionMap lpMap = (LuckPermsSubscriptionMap) map;
PERM_SUBS_FIELD.set(pluginManager, lpMap.detach());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
| lucko/LuckPerms | nukkit/src/main/java/me/lucko/luckperms/nukkit/inject/server/InjectorSubscriptionMap.java | Java | mit | 4,057 |
jQuery(document).ready(function(){
jQuery('.carousel').carousel()
var FPS = 30;
var player = $('#player')
var pWidth = player.width();
$window = $(window)
var wWidth = $window.width();
setInterval(function() {
update();
}, 1000/FPS);
function update() {
if(keydown.space) {
player.shoot();
}
if(keydown.left) {
console.log('go left')
player.css('left', '-=10');
}
if(keydown.right) {
console.log('go right')
var x = player.position().left;
if(x + pWidth > wWidth)
{
player.css('left', '0')
}
else if(x < 0 )
{
var p = wWidth + x - pWidth;
var t = p + 'px'
player.css('left', t)
}
else {
player.css('left', '+=10');
}
}
}
$('')
})
| ni5ni6/chiptuna.com | js/main.js | JavaScript | mit | 739 |
/// <reference path="tsUnit.ts" />
/// <reference path="MockExpression.ts" />
/// <reference path="../src/EventBinding.ts" />
module jsBind {
private fireEvent(elem: any): void {
if (document.createEventObject) {
// IE 9 & 10
var event: any = document.createEventObject();
event.eventType = "onclick";
elem.fireEvent("onclick", event);
} else if (dispatchEvent) {
// For DOM
var evt = document.createEvent("HTMLEvents");
evt.initEvent("click", false, true);
elem.dispatchEvent(evt);
} else {
// IE 8-
elem.fireEvent("onclick");
}
}
export class EventBindingTests {
public testEvaluate(c: tsUnit.TestContext): void {
var elem: any = document.getElementById("Results");
var expr = new MockExpression(42);
var binding = new EventBinding(elem, "click", expr, null, null);
binding.evaluate();
fireEvent(elem);
c.isTrue(expr.wasEvaluated);
}
public testDispose(c: tsUnit.TestContext): void {
var elem: any = document.getElementById("Results");
var expr = new MockExpression(42);
var binding = new EventBinding(elem, "click", expr, null, null);
binding.evaluate();
// Check the dispose occurs
binding.dispose();
c.isTrue(expr.isDisposed);
fireEvent(elem);
c.isFalse(expr.wasEvaluated);
}
}
} | voss-tech/jsBind | Code/tests/EventBindingTests.ts | TypeScript | mit | 1,578 |
// Copyright (c) 2015 The original author or authors
//
// This software may be modified and distributed under the terms
// of the MIT license. See the LICENSE file for details.
#if UNITY_EDITOR
using SpriterDotNet;
using System;
using System.IO;
using System.Linq;
using UnityEditor;
using UnityEngine;
namespace SpriterDotNetUnity
{
public class SpriterImporter : AssetPostprocessor
{
private static readonly string[] ScmlExtensions = new string[] { ".scml" };
private static readonly float DeltaZ = -0.001f;
private static void OnPostprocessAllAssets(string[] importedAssets, string[] deletedAssets, string[] movedAssets, string[] movedFromPath)
{
foreach (string asset in importedAssets)
{
if (!IsScml(asset)) continue;
CreateSpriter(asset);
}
foreach (string asset in deletedAssets)
{
if (!IsScml(asset)) continue;
}
for (int i = 0; i < movedAssets.Length; i++)
{
string asset = movedAssets[i];
if (!IsScml(asset)) continue;
}
}
private static bool IsScml(string path)
{
return ScmlExtensions.Any(path.EndsWith);
}
private static void CreateSpriter(string path)
{
string data = File.ReadAllText(path);
Spriter spriter = SpriterParser.Parse(data);
string rootFolder = Path.GetDirectoryName(path);
foreach (SpriterEntity entity in spriter.Entities)
{
GameObject go = new GameObject();
go.name = entity.Name;
SpriterDotNetBehaviour sdnBehaviour = go.AddComponent<SpriterDotNetBehaviour>();
sdnBehaviour.Entity = entity;
sdnBehaviour.enabled = true;
LoadSprites(sdnBehaviour, spriter, rootFolder);
CreateChildren(entity, sdnBehaviour, spriter, go);
string prefabPath = rootFolder + "/" + entity.Name + ".prefab";
PrefabUtility.CreatePrefab(prefabPath, go, ReplacePrefabOptions.ConnectToPrefab);
GameObject.DestroyImmediate(go);
}
}
private static void CreateChildren(SpriterEntity entity, SpriterDotNetBehaviour sdnBehaviour, Spriter spriter, GameObject parent)
{
int maxObjects = 0;
foreach (SpriterAnimation animation in entity.Animations)
{
foreach (SpriterMainLineKey mainKey in animation.MainlineKeys)
{
maxObjects = Math.Max(maxObjects, mainKey.ObjectRefs.Length);
}
}
sdnBehaviour.Children = new GameObject[maxObjects];
sdnBehaviour.Pivots = new GameObject[maxObjects];
for (int i = 0; i < maxObjects; ++i)
{
GameObject pivot = new GameObject();
GameObject child = new GameObject();
sdnBehaviour.Pivots[i] = pivot;
sdnBehaviour.Children[i] = child;
pivot.transform.SetParent(parent.transform);
child.transform.SetParent(pivot.transform);
child.transform.localPosition = new Vector3(0, 0, DeltaZ * i);
pivot.name = "pivot " + i;
child.name = "child " + i;
child.AddComponent<SpriteRenderer>();
}
}
private static void LoadSprites(SpriterDotNetBehaviour sdnBehaviour, Spriter spriter, string rootFolder)
{
sdnBehaviour.Folders = new SdnFolder[spriter.Folders.Length];
for (int i = 0; i < spriter.Folders.Length; ++i)
{
SpriterFolder folder = spriter.Folders[i];
SdnFolder sdnFolder = new SdnFolder();
sdnFolder.Files = new Sprite[folder.Files.Length];
sdnBehaviour.Folders[i] = sdnFolder;
for (int j = 0; j < folder.Files.Length; ++j)
{
SpriterFile file = folder.Files[j];
string spritePath = rootFolder;
spritePath += "/";
spritePath += file.Name;
Sprite sprite = AssetDatabase.LoadAssetAtPath<Sprite>(spritePath);
if (sprite == null)
{
Debug.LogWarning("Unable to load sprite: " + spritePath);
continue;
}
sdnFolder.Files[j] = sprite;
}
}
}
}
}
#endif
| adamholdenyall/SpriterDotNet | SpriterDotNet.Unity/Assets/SpriterDotNet/SpriterImporter.cs | C# | mit | 4,693 |
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import base64
import json
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions
from behave import *
@step('I share first element in the history list')
def step_impl(context):
context.execute_steps(u'''
given I open History dialog
''')
history = context.browser.find_element_by_id("HistoryPopup")
entries = history.find_elements_by_xpath('.//li[not(@data-clone-template)]')
assert len(entries) > 0, "There are no entries in the history"
item = entries[0]
item.find_elements_by_xpath('.//*[@data-share-item]')[0].click()
@then('the json to share is shown with url "{url}" and contains the following headers')
def step_impl(context, url):
# Wait for modal to appear
WebDriverWait(context.browser, 10).until(
expected_conditions.visibility_of_element_located(
(By.ID, 'ShareRequestForm')))
output = context.browser.execute_script("return restman.ui.editors.get('#ShareRequestEditor').getValue();")
snippet = json.loads(output)
assert url == snippet["url"], "URL: \"{}\" not in output.\nOutput: {}".format(value, output)
for row in context.table:
assert row['key'] in snippet['headers'], "Header {} is not in output".format(row['key'])
assert row['value'] == snippet['headers'][row['key']], "Header value is not correct. Expected: {}; Actual: {}".format(value, snippet['headers'][name])
@step('I click on import request')
def step_impl(context):
context.execute_steps(u'''
given I open History dialog
''')
# Click on import
context.browser.find_element_by_id('ImportHistory').click()
WebDriverWait(context.browser, 10).until(
expected_conditions.visibility_of_element_located(
(By.ID, 'ImportRequestForm')))
@step('I write a shared request for "{url}"')
def step_impl(context, url):
req = json.dumps({
"method": "POST",
"url": url,
"headers": {
"Content-Type": "application/json",
"X-Test-Header": "shared_request"
},
"body": {
"type": "form",
"content": {
"SomeKey": "SomeValue11233",
"SomeOtherKey": "SomeOtherValue019",
}
}
})
context.browser.execute_script("return restman.ui.editors.setValue('#ImportRequestEditor', atob('{}'));".format(base64.b64encode(req)))
@step('I click on load import request')
def step_impl(context):
# Import request
context.browser.find_element_by_xpath("//*[@id='ImportRequestForm']//input[@value='Import']").click()
| jsargiot/restman | tests/steps/share.py | Python | mit | 2,709 |
---
layout: link
type: link
link: https://www.bloomberg.com/news/articles/2019-11-05/why-indonesia-failed-to-cash-in-on-the-china-u-s-trade-war
title: "Bloomberg: Why Indonesia Failed to Cash in on the China-U.S. Trade War"
category: links
tags:
- life
- "daily found"
- development
- indonesia
categories: dailyfound
published: true
comments: true
---
Dengan adanya perang dagang antara Amerika dan Tiongkok, banyak berimbas terhadap industri-industri yang ada didua negara itu, dan beberapa negara di asia tenggara bisa mengambil untung dari kondisi itu.
Sebut Vietnam, Thailand, banyak investor yang mengalihkan investasinya ke negara tersebut, alasan kenapa Indonesia tidak begitu besar terkena imbasnya.
<!--more-->
> The reasons for the poor performance are well documented: inadequate infrastructure, particularly in transport; rigid labor rules; limits on how much foreigners can invest in several industries; bureaucratic red tape and a habit of backtracking on regulations that makes it tricky to do business in the country.
Memang sangat berbelit investasi di sini, belum lagi kondisi pekerjanya yang terhitung mahal, belum juga preman-preman, ditambah isu investor asing yang berkonotasi negatif, pemerintah dianggap menjual negara ini ke asing, padahal membuka investasi.
Membangun infrastuktur yang bisa dijadikan pendukung pemerataan usaha, juga mempermudah transportasi sehingga atraktif untuk investor, tapi tetap itu dianggap sebagai suatu pemborosan.
Ada juga kalimat andalan "Negara ini kaya, masa *gak* bisa mengambil untung dari itu? tidak butuh asing untuk mengelola", dan ucapan-ucapan sejenis, yang mana tentu itu keluar dari ketidak tahuan akan kondisi, seperti penonton bola, biasanya penonton bola lebih pintar dari pemainnya, yang latihan 10 jam sehari, kalau gagal gol akan dibilang bodoh oleh penonton budiman.
Sama seperti warga yang mengomentari pemerintah, merasa lebih pintar. | dedenf/dedenf.github.io | _posts/2019-11-06-indonesia-failed-to-take-profit.md | Markdown | mit | 1,938 |
import { entryPoint } from '@rpgjs/standalone'
import globalConfigClient from './config/client'
import globalConfigServer from './config/server'
import modules from './modules'
document.addEventListener('DOMContentLoaded', function() {
entryPoint(modules, {
globalConfigClient,
globalConfigServer
}).start()
}) | RSamaium/RPG-JS | packages/sample3/src/standalone.ts | TypeScript | mit | 338 |
/* Copyright (c) MediaArea.net SARL. All Rights Reserved.
*
* Use of this source code is governed by a BSD-style license that can
* be found in the License.html file in the root of the source tree.
*/
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//
// Elements part
//
// Contributor: Lionel Duchateau, [email protected]
//
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//---------------------------------------------------------------------------
// Pre-compilation
#include "MediaInfo/PreComp.h"
#ifdef __BORLANDC__
#pragma hdrstop
#endif
//---------------------------------------------------------------------------
//---------------------------------------------------------------------------
#include "MediaInfo/Setup.h"
#include <ZenLib/Ztring.h>
#include <string>
using namespace std;
using namespace ZenLib;
//---------------------------------------------------------------------------
//***************************************************************************
// Infos
//***************************************************************************
//---------------------------------------------------------------------------
#if defined(MEDIAINFO_RIFF_YES) || defined(MEDIAINFO_MK_YES)
//---------------------------------------------------------------------------
namespace MediaInfoLib
{
//---------------------------------------------------------------------------
std::string ExtensibleWave_ChannelMask (int32u ChannelMask)
{
std::string Text;
if ((ChannelMask&0x0007)!=0x0000)
Text+="Front:";
if (ChannelMask&0x0001)
Text+=" L";
if (ChannelMask&0x0004)
Text+=" C";
if (ChannelMask&0x0002)
Text+=" R";
if ((ChannelMask&0x0600)!=0x0000)
Text+=", Side:";
if (ChannelMask&0x0200)
Text+=" L";
if (ChannelMask&0x0400)
Text+=" R";
if ((ChannelMask&0x0130)!=0x0000)
Text+=", Back:";
if (ChannelMask&0x0010)
Text+=" L";
if (ChannelMask&0x0100)
Text+=" C";
if (ChannelMask&0x0020)
Text+=" R";
if ((ChannelMask&0x0008)!=0x0000)
Text+=", LFE";
return Text;
}
//---------------------------------------------------------------------------
std::string ExtensibleWave_ChannelMask2 (int32u ChannelMask)
{
std::string Text;
int8u Count=0;
if (ChannelMask&0x0001)
Count++;
if (ChannelMask&0x0004)
Count++;
if (ChannelMask&0x0002)
Count++;
Text+=Ztring::ToZtring(Count).To_UTF8();
Count=0;
if (ChannelMask&0x0200)
Count++;
if (ChannelMask&0x0400)
Count++;
Text+="/"+Ztring::ToZtring(Count).To_UTF8();
Count=0;
if (ChannelMask&0x0010)
Count++;
if (ChannelMask&0x0100)
Count++;
if (ChannelMask&0x0020)
Count++;
Text+="/"+Ztring::ToZtring(Count).To_UTF8();
Count=0;
if (ChannelMask&0x0008)
Text+=".1";
return Text;
}
}
//---------------------------------------------------------------------------
#endif
//---------------------------------------------------------------------------
//---------------------------------------------------------------------------
#ifdef MEDIAINFO_RIFF_YES
//---------------------------------------------------------------------------
//---------------------------------------------------------------------------
#include "MediaInfo/Multiple/File_Riff.h"
#if defined(MEDIAINFO_DVDIF_YES)
#include "MediaInfo/Multiple/File_DvDif.h"
#endif
#if defined(MEDIAINFO_OGG_YES)
#include "MediaInfo/Multiple/File_Ogg.h"
#include "MediaInfo/Multiple/File_Ogg_SubElement.h"
#endif
#if defined(MEDIAINFO_FFV1_YES)
#include "MediaInfo/Video/File_Ffv1.h"
#endif
#if defined(MEDIAINFO_HUFFYUV_YES)
#include "MediaInfo/Video/File_HuffYuv.h"
#endif
#if defined(MEDIAINFO_MPEG4V_YES)
#include "MediaInfo/Video/File_Mpeg4v.h"
#endif
#if defined(MEDIAINFO_MPEGV_YES)
#include "MediaInfo/Video/File_Mpegv.h"
#endif
#if defined(MEDIAINFO_PRORES_YES)
#include "MediaInfo/Video/File_ProRes.h"
#endif
#if defined(MEDIAINFO_AVC_YES)
#include "MediaInfo/Video/File_Avc.h"
#endif
#if defined(MEDIAINFO_CANOPUS_YES)
#include "MediaInfo/Video/File_Canopus.h"
#endif
#if defined(MEDIAINFO_FRAPS_YES)
#include "MediaInfo/Video/File_Fraps.h"
#endif
#if defined(MEDIAINFO_LAGARITH_YES)
#include "MediaInfo/Video/File_Lagarith.h"
#endif
#if defined(MEDIAINFO_MPEGA_YES)
#include "MediaInfo/Audio/File_Mpega.h"
#endif
#if defined(MEDIAINFO_AAC_YES)
#include "MediaInfo/Audio/File_Aac.h"
#endif
#if defined(MEDIAINFO_AC3_YES)
#include "MediaInfo/Audio/File_Ac3.h"
#endif
#if defined(MEDIAINFO_DTS_YES)
#include "MediaInfo/Audio/File_Dts.h"
#endif
#if defined(MEDIAINFO_JPEG_YES)
#include "MediaInfo/Image/File_Jpeg.h"
#endif
#if defined(MEDIAINFO_SUBRIP_YES)
#include "MediaInfo/Text/File_SubRip.h"
#endif
#if defined(MEDIAINFO_OTHERTEXT_YES)
#include "MediaInfo/Text/File_OtherText.h"
#endif
#if defined(MEDIAINFO_ADPCM_YES)
#include "MediaInfo/Audio/File_Adpcm.h"
#endif
#if defined(MEDIAINFO_PCM_YES)
#include "MediaInfo/Audio/File_Pcm.h"
#endif
#if defined(MEDIAINFO_SMPTEST0337_YES)
#include "MediaInfo/Audio/File_SmpteSt0337.h"
#endif
#if defined(MEDIAINFO_ID3_YES)
#include "MediaInfo/Tag/File_Id3.h"
#endif
#if defined(MEDIAINFO_ID3V2_YES)
#include "MediaInfo/Tag/File_Id3v2.h"
#endif
#if defined(MEDIAINFO_GXF_YES)
#if defined(MEDIAINFO_CDP_YES)
#include "MediaInfo/Text/File_Cdp.h"
#include <cstring>
#endif
#endif //MEDIAINFO_GXF_YES
#include <vector>
#include "MediaInfo/MediaInfo_Config_MediaInfo.h"
using namespace std;
//---------------------------------------------------------------------------
namespace MediaInfoLib
{
//***************************************************************************
// Const
//***************************************************************************
namespace Elements
{
const int32u FORM=0x464F524D;
const int32u LIST=0x4C495354;
const int32u ON2_=0x4F4E3220;
const int32u RIFF=0x52494646;
const int32u RF64=0x52463634;
const int32u AIFC=0x41494643;
const int32u AIFC_COMM=0x434F4D4D;
const int32u AIFC_COMT=0x434F4D54;
const int32u AIFC_FVER=0x46564552;
const int32u AIFC_SSND=0x53534E44;
const int32u AIFF=0x41494646;
const int32u AIFF_COMM=0x434F4D4D;
const int32u AIFF_COMT=0x434F4D54;
const int32u AIFF_SSND=0x53534E44;
const int32u AIFF__c__=0x28632920;
const int32u AIFF_ANNO=0x414E4E4F;
const int32u AIFF_AUTH=0x41555448;
const int32u AIFF_NAME=0x4E414D45;
const int32u AIFF_ID3_=0x49443320;
const int32u AVI_=0x41564920;
const int32u AVI__cset=0x63736574;
const int32u AVI__Cr8r=0x43723872;
const int32u AVI__exif=0x65786966;
const int32u AVI__exif_ecor=0x65636F72;
const int32u AVI__exif_emdl=0x656D646C;
const int32u AVI__exif_emnt=0x656D6E74;
const int32u AVI__exif_erel=0x6572656C;
const int32u AVI__exif_etim=0x6574696D;
const int32u AVI__exif_eucm=0x6575636D;
const int32u AVI__exif_ever=0x65766572;
const int32u AVI__goog=0x676F6F67;
const int32u AVI__goog_GDAT=0x47444154;
const int32u AVI__GMET=0x474D4554;
const int32u AVI__hdlr=0x6864726C;
const int32u AVI__hdlr_avih=0x61766968;
const int32u AVI__hdlr_JUNK=0x4A554E4B;
const int32u AVI__hdlr_strl=0x7374726C;
const int32u AVI__hdlr_strl_indx=0x696E6478;
const int32u AVI__hdlr_strl_JUNK=0x4A554E4B;
const int32u AVI__hdlr_strl_strd=0x73747264;
const int32u AVI__hdlr_strl_strf=0x73747266;
const int32u AVI__hdlr_strl_strh=0x73747268;
const int32u AVI__hdlr_strl_strh_auds=0x61756473;
const int32u AVI__hdlr_strl_strh_iavs=0x69617673;
const int32u AVI__hdlr_strl_strh_mids=0x6D696473;
const int32u AVI__hdlr_strl_strh_vids=0x76696473;
const int32u AVI__hdlr_strl_strh_txts=0x74787473;
const int32u AVI__hdlr_strl_strn=0x7374726E;
const int32u AVI__hdlr_strl_vprp=0x76707270;
const int32u AVI__hdlr_odml=0x6F646D6C;
const int32u AVI__hdlr_odml_dmlh=0x646D6C68;
const int32u AVI__hdlr_ON2h=0x4F4E3268;
const int32u AVI__idx1=0x69647831;
const int32u AVI__INFO=0x494E464F;
const int32u AVI__INFO_IARL=0x4941524C;
const int32u AVI__INFO_IART=0x49415254;
const int32u AVI__INFO_IAS1=0x49415331;
const int32u AVI__INFO_IAS2=0x49415332;
const int32u AVI__INFO_IAS3=0x49415333;
const int32u AVI__INFO_IAS4=0x49415334;
const int32u AVI__INFO_IAS5=0x49415335;
const int32u AVI__INFO_IAS6=0x49415336;
const int32u AVI__INFO_IAS7=0x49415337;
const int32u AVI__INFO_IAS8=0x49415338;
const int32u AVI__INFO_IAS9=0x49415339;
const int32u AVI__INFO_ICDS=0x49434453;
const int32u AVI__INFO_ICMS=0x49434D53;
const int32u AVI__INFO_ICMT=0x49434D54;
const int32u AVI__INFO_ICNT=0x49434E54;
const int32u AVI__INFO_ICOP=0x49434F50;
const int32u AVI__INFO_ICNM=0x49434E4D;
const int32u AVI__INFO_ICRD=0x49435244;
const int32u AVI__INFO_ICRP=0x49435250;
const int32u AVI__INFO_IDIM=0x4944494D;
const int32u AVI__INFO_IDIT=0x49444954;
const int32u AVI__INFO_IDPI=0x49445049;
const int32u AVI__INFO_IDST=0x49445354;
const int32u AVI__INFO_IEDT=0x49454454;
const int32u AVI__INFO_IENG=0x49454E47;
const int32u AVI__INFO_IFRM=0x4946524D;
const int32u AVI__INFO_IGNR=0x49474E52;
const int32u AVI__INFO_IID3=0x49494433;
const int32u AVI__INFO_IKEY=0x494B4559;
const int32u AVI__INFO_ILGT=0x494C4754;
const int32u AVI__INFO_ILNG=0x494C4E47;
const int32u AVI__INFO_ILYC=0x494C5943;
const int32u AVI__INFO_IMED=0x494D4544;
const int32u AVI__INFO_IMP3=0x494D5033;
const int32u AVI__INFO_IMUS=0x494D5553;
const int32u AVI__INFO_INAM=0x494E414D;
const int32u AVI__INFO_IPLT=0x49504C54;
const int32u AVI__INFO_IPDS=0x49504453;
const int32u AVI__INFO_IPRD=0x49505244;
const int32u AVI__INFO_IPRT=0x49505254;
const int32u AVI__INFO_IPRO=0x4950524F;
const int32u AVI__INFO_IRTD=0x49525444;
const int32u AVI__INFO_ISBJ=0x4953424A;
const int32u AVI__INFO_ISGN=0x4953474E;
const int32u AVI__INFO_ISTD=0x49535444;
const int32u AVI__INFO_ISTR=0x49535452;
const int32u AVI__INFO_ISFT=0x49534654;
const int32u AVI__INFO_ISHP=0x49534850;
const int32u AVI__INFO_ISMP=0x49534D50;
const int32u AVI__INFO_ISRC=0x49535243;
const int32u AVI__INFO_ISRF=0x49535246;
const int32u AVI__INFO_ITCH=0x49544348;
const int32u AVI__INFO_IWEB=0x49574542;
const int32u AVI__INFO_IWRI=0x49575249;
const int32u AVI__INFO_JUNK=0x4A554E4B;
const int32u AVI__JUNK=0x4A554E4B;
const int32u AVI__MD5_=0x4D443520;
const int32u AVI__movi=0x6D6F7669;
const int32u AVI__movi_rec_=0x72656320;
const int32u AVI__movi_xxxx_____=0x00005F5F;
const int32u AVI__movi_xxxx___db=0x00006462;
const int32u AVI__movi_xxxx___dc=0x00006463;
const int32u AVI__movi_xxxx___sb=0x00007362;
const int32u AVI__movi_xxxx___tx=0x00007478;
const int32u AVI__movi_xxxx___wb=0x00007762;
const int32u AVI__PrmA=0x50726D41;
const int32u AVI__Tdat=0x54646174;
const int32u AVI__Tdat_rn_A=0x726E5F41;
const int32u AVI__Tdat_rn_O=0x726E5F4F;
const int32u AVI__Tdat_tc_A=0x74635F41;
const int32u AVI__Tdat_tc_O=0x74635F4F;
const int32u AVIX=0x41564958;
const int32u AVIX_idx1=0x69647831;
const int32u AVIX_movi=0x6D6F7669;
const int32u AVIX_movi_rec_=0x72656320;
const int32u CADP=0x43414450;
const int32u CDDA=0x43444441;
const int32u CDDA_fmt_=0x666D7420;
const int32u CMJP=0x434D4A50;
const int32u CMP4=0x434D5034;
const int32u IDVX=0x49445658;
const int32u INDX=0x494E4458;
const int32u JUNK=0x4A554E4B;
const int32u menu=0x6D656E75;
const int32u MThd=0x4D546864;
const int32u MTrk=0x4D54726B;
const int32u PAL_=0x50414C20;
const int32u QLCM=0x514C434D;
const int32u QLCM_fmt_=0x666D7420;
const int32u rcrd=0x72637264;
const int32u rcrd_desc=0x64657363;
const int32u rcrd_fld_=0x666C6420;
const int32u rcrd_fld__anc_=0x616E6320;
const int32u rcrd_fld__anc__pos_=0x706F7320;
const int32u rcrd_fld__anc__pyld=0x70796C64;
const int32u rcrd_fld__finf=0x66696E66;
const int32u RDIB=0x52444942;
const int32u RMID=0x524D4944;
const int32u RMMP=0x524D4D50;
const int32u RMP3=0x524D5033;
const int32u RMP3_data=0x64617461;
const int32u RMP3_INFO=0x494E464F;
const int32u RMP3_INFO_IID3=0x49494433;
const int32u RMP3_INFO_ILYC=0x494C5943;
const int32u RMP3_INFO_IMP3=0x494D5033;
const int32u RMP3_INFO_JUNK=0x4A554E4B;
const int32u SMV0=0x534D5630;
const int32u SMV0_xxxx=0x534D563A;
const int32u WAVE=0x57415645;
const int32u WAVE__pmx=0x20786D70;
const int32u WAVE_aXML=0x61584D4C;
const int32u WAVE_bext=0x62657874;
const int32u WAVE_cue_=0x63756520;
const int32u WAVE_data=0x64617461;
const int32u WAVE_ds64=0x64733634;
const int32u WAVE_fact=0x66616374;
const int32u WAVE_fmt_=0x666D7420;
const int32u WAVE_ID3_=0x49443320;
const int32u WAVE_id3_=0x69643320;
const int32u WAVE_INFO=0x494E464F;
const int32u WAVE_iXML=0x69584D4C;
const int32u wave=0x77617665;
const int32u wave_data=0x64617461;
const int32u wave_fmt_=0x666D7420;
const int32u W3DI=0x57334449;
#define UUID(NAME, PART1, PART2, PART3, PART4, PART5) \
const int64u NAME =0x##PART3##PART2##PART1##ULL; \
const int64u NAME##2=0x##PART4##PART5##ULL; \
UUID(QLCM_QCELP1, 5E7F6D41, B115, 11D0, BA91, 00805FB4B97E)
UUID(QLCM_QCELP2, 5E7F6D42, B115, 11D0, BA91, 00805FB4B97E)
UUID(QLCM_EVRC, E689D48D, 9076, 46B5, 91EF, 736A5100CEB4)
UUID(QLCM_SMV, 8D7C2B75, A797, ED49, 985E, D53C8CC75F84)
}
//***************************************************************************
// Format
//***************************************************************************
//---------------------------------------------------------------------------
void File_Riff::Data_Parse()
{
//Alignement specific
Element_Size-=Alignement_ExtraByte;
DATA_BEGIN
LIST(AIFC)
ATOM_BEGIN
ATOM(AIFC_COMM)
ATOM(AIFC_COMT)
ATOM(AIFC_FVER)
ATOM(AIFC_SSND)
ATOM_DEFAULT(AIFC_xxxx)
ATOM_END_DEFAULT
LIST(AIFF)
ATOM_BEGIN
ATOM(AIFF_COMM)
ATOM(AIFF_COMT)
ATOM(AIFF_ID3_)
LIST_SKIP(AIFF_SSND)
ATOM_DEFAULT(AIFF_xxxx)
ATOM_END_DEFAULT
LIST(AVI_)
ATOM_BEGIN
ATOM(AVI__Cr8r);
ATOM(AVI__cset)
LIST(AVI__exif)
ATOM_DEFAULT_ALONE(AVI__exif_xxxx)
LIST(AVI__goog)
ATOM_BEGIN
ATOM(AVI__goog_GDAT)
ATOM_END
ATOM(AVI__GMET)
LIST(AVI__hdlr)
ATOM_BEGIN
ATOM(AVI__hdlr_avih)
ATOM(AVI__hdlr_JUNK)
LIST(AVI__hdlr_strl)
ATOM_BEGIN
ATOM(AVI__hdlr_strl_indx)
ATOM(AVI__hdlr_strl_JUNK)
ATOM(AVI__hdlr_strl_strd)
ATOM(AVI__hdlr_strl_strf)
ATOM(AVI__hdlr_strl_strh)
ATOM(AVI__hdlr_strl_strn)
ATOM(AVI__hdlr_strl_vprp)
ATOM_END
LIST(AVI__hdlr_odml)
ATOM_BEGIN
ATOM(AVI__hdlr_odml_dmlh)
ATOM_END
ATOM(AVI__hdlr_ON2h)
LIST(AVI__INFO)
ATOM_BEGIN
ATOM(AVI__INFO_IID3)
ATOM(AVI__INFO_ILYC)
ATOM(AVI__INFO_IMP3)
ATOM(AVI__INFO_JUNK)
ATOM_DEFAULT(AVI__INFO_xxxx)
ATOM_END_DEFAULT
ATOM_DEFAULT(AVI__hdlr_xxxx)
ATOM_END_DEFAULT
ATOM(AVI__idx1)
LIST(AVI__INFO)
ATOM_BEGIN
ATOM(AVI__INFO_IID3)
ATOM(AVI__INFO_ILYC)
ATOM(AVI__INFO_IMP3)
ATOM(AVI__INFO_JUNK)
ATOM_DEFAULT(AVI__INFO_xxxx)
ATOM_END_DEFAULT
ATOM(AVI__JUNK)
ATOM(AVI__MD5_)
LIST(AVI__movi)
ATOM_BEGIN
LIST(AVI__movi_rec_)
ATOM_DEFAULT_ALONE(AVI__movi_xxxx)
ATOM_DEFAULT(AVI__movi_xxxx)
ATOM_END_DEFAULT
ATOM(AVI__PrmA);
LIST(AVI__Tdat)
ATOM_BEGIN
ATOM(AVI__Tdat_rn_A)
ATOM(AVI__Tdat_rn_O)
ATOM(AVI__Tdat_tc_A)
ATOM(AVI__Tdat_tc_O)
ATOM_END
ATOM_DEFAULT(AVI__xxxx)
ATOM_END_DEFAULT
LIST(AVIX) //OpenDML
ATOM_BEGIN
ATOM(AVIX_idx1)
LIST(AVIX_movi)
ATOM_BEGIN
LIST(AVIX_movi_rec_)
ATOM_DEFAULT_ALONE(AVIX_movi_xxxx)
ATOM_DEFAULT(AVIX_movi_xxxx)
ATOM_END_DEFAULT
ATOM_END
ATOM_PARTIAL(CADP)
LIST(CDDA)
ATOM_BEGIN
ATOM(CDDA_fmt_)
ATOM_END
ATOM_PARTIAL(CMJP)
ATOM(CMP4)
ATOM(IDVX)
LIST(INDX)
ATOM_DEFAULT_ALONE(INDX_xxxx)
LIST_SKIP(JUNK)
LIST_SKIP(menu)
ATOM(MThd)
LIST_SKIP(MTrk)
LIST_SKIP(PAL_)
LIST(QLCM)
ATOM_BEGIN
ATOM(QLCM_fmt_)
ATOM_END
#if defined(MEDIAINFO_GXF_YES)
LIST(rcrd)
ATOM_BEGIN
ATOM(rcrd_desc)
LIST(rcrd_fld_)
ATOM_BEGIN
LIST(rcrd_fld__anc_)
ATOM_BEGIN
ATOM(rcrd_fld__anc__pos_)
ATOM(rcrd_fld__anc__pyld)
ATOM_END
ATOM(rcrd_fld__finf)
ATOM_END
ATOM_END
#endif //defined(MEDIAINFO_GXF_YES)
LIST_SKIP(RDIB)
LIST_SKIP(RMID)
LIST_SKIP(RMMP)
LIST(RMP3)
ATOM_BEGIN
LIST(RMP3_data)
break;
LIST(RMP3_INFO)
ATOM_BEGIN
ATOM(RMP3_INFO_IID3)
ATOM(RMP3_INFO_ILYC)
ATOM(RMP3_INFO_IMP3)
ATOM(RMP3_INFO_JUNK)
ATOM_DEFAULT(RMP3_INFO_xxxx)
ATOM_END_DEFAULT
ATOM_END
ATOM(SMV0)
ATOM(SMV0_xxxx)
ATOM(W3DI)
LIST(WAVE)
ATOM_BEGIN
ATOM(WAVE__pmx)
ATOM(WAVE_aXML)
ATOM(WAVE_bext)
LIST(WAVE_data)
break;
ATOM(WAVE_cue_)
ATOM(WAVE_ds64)
ATOM(WAVE_fact)
ATOM(WAVE_fmt_)
ATOM(WAVE_ID3_)
ATOM(WAVE_id3_)
LIST(WAVE_INFO)
ATOM_DEFAULT_ALONE(WAVE_INFO_xxxx)
ATOM(WAVE_iXML)
ATOM_END
LIST(wave)
ATOM_BEGIN
LIST(wave_data)
break;
ATOM(wave_fmt_)
ATOM_END
DATA_END
if (Alignement_ExtraByte)
{
Element_Size+=Alignement_ExtraByte;
if (Element_Offset+Alignement_ExtraByte==Element_Size)
Skip_XX(Alignement_ExtraByte, "Alignement");
}
}
//***************************************************************************
// Elements
//***************************************************************************
//---------------------------------------------------------------------------
void File_Riff::AIFC()
{
Data_Accept("AIFF Compressed");
Element_Name("AIFF Compressed");
//Filling
Fill(Stream_General, 0, General_Format, "AIFF");
Stream_Prepare(Stream_Audio);
Kind=Kind_Aiff;
#if MEDIAINFO_EVENTS
StreamIDs_Width[0]=0;
#endif //MEDIAINFO_EVENTS
}
//---------------------------------------------------------------------------
void File_Riff::AIFC_COMM()
{
AIFF_COMM();
}
//---------------------------------------------------------------------------
void File_Riff::AIFC_COMT()
{
AIFF_COMT();
}
//---------------------------------------------------------------------------
void File_Riff::AIFC_FVER()
{
Element_Name("Format Version");
//Parsing
Skip_B4( "Version");
}
//---------------------------------------------------------------------------
void File_Riff::AIFC_SSND()
{
AIFF_SSND();
}
//---------------------------------------------------------------------------
void File_Riff::AIFC_xxxx()
{
AIFF_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::AIFF()
{
Data_Accept("AIFF");
Element_Name("AIFF");
//Filling
Fill(Stream_General, 0, General_Format, "AIFF");
Stream_Prepare(Stream_Audio);
Kind=Kind_Aiff;
#if MEDIAINFO_EVENTS
StreamIDs_Width[0]=0;
#endif //MEDIAINFO_EVENTS
}
//---------------------------------------------------------------------------
void File_Riff::AIFF_COMM()
{
Element_Name("Common");
int32u numSampleFrames;
int16u numChannels, sampleSize;
float80 sampleRate;
//Parsing
Get_B2 (numChannels, "numChannels");
Get_B4 (numSampleFrames, "numSampleFrames");
Get_B2 (sampleSize, "sampleSize");
Get_BF10(sampleRate, "sampleRate");
if (Data_Remain()) //AIFC
{
int32u compressionType;
Get_C4 (compressionType, "compressionType");
Skip_PA( "compressionName");
//Filling
CodecID_Fill(Ztring().From_CC4(compressionType), Stream_Audio, StreamPos_Last, InfoCodecID_Format_Mpeg4);
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, Ztring().From_CC4(compressionType));
}
else
{
//Filling
Fill(Stream_Audio, StreamPos_Last, Audio_Format, "PCM");
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, "PCM");
}
//Filling
Fill(Stream_Audio, StreamPos_Last, Audio_Channel_s_, numChannels);
Fill(Stream_Audio, StreamPos_Last, Audio_BitDepth, sampleSize);
if (sampleRate)
Fill(Stream_Audio, StreamPos_Last, Audio_Duration, numSampleFrames/sampleRate*1000);
Fill(Stream_Audio, StreamPos_Last, Audio_SamplingRate, sampleRate, 0);
//Compute the current codec ID
Element_Code=(int64u)-1;
Stream_ID=(int32u)-1;
stream_Count=1;
//Specific cases
#if defined(MEDIAINFO_SMPTEST0337_YES)
if (Retrieve(Stream_Audio, 0, Audio_CodecID).empty() && numChannels==2 && sampleSize<=32 && sampleRate==48000) //Some SMPTE ST 337 streams are hidden in PCM stream
{
File_SmpteSt0337* Parser=new File_SmpteSt0337;
Parser->Endianness='B';
Parser->Container_Bits=(int8u)sampleSize;
Parser->ShouldContinueParsing=true;
#if MEDIAINFO_DEMUX
if (Config->Demux_Unpacketize_Get())
{
Parser->Demux_Level=2; //Container
Parser->Demux_UnpacketizeContainer=true;
Demux_Level=4; //Intermediate
}
#endif //MEDIAINFO_DEMUX
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
stream& StreamItem = Stream[Stream_ID];
#if defined(MEDIAINFO_PCM_YES)
File_Pcm* Parser=new File_Pcm;
Parser->Codec=Retrieve(Stream_Audio, StreamPos_Last, Audio_CodecID);
if (Parser->Codec.empty() || Parser->Codec==__T("NONE"))
Parser->Endianness='B';
Parser->BitDepth=(int8u)sampleSize;
#if MEDIAINFO_DEMUX
if (Demux_Rate)
Parser->Frame_Count_Valid = float64_int64s(Demux_Rate);
if (Config->Demux_Unpacketize_Get())
{
Parser->Demux_Level=2; //Container
Parser->Demux_UnpacketizeContainer=true;
Demux_Level=4; //Intermediate
}
#else //MEDIAINFO_DEMUX
Parser->Frame_Count_Valid=(int64u)-1; //Disabling it, waiting for SMPTE ST 337 parser reject
#endif //MEDIAINFO_DEMUX
StreamItem.Parsers.push_back(Parser);
StreamItem.IsPcm=true;
StreamItem.StreamKind=Stream_Audio;
#endif
#if MEDIAINFO_DEMUX
BlockAlign=numChannels*sampleSize/8;
AvgBytesPerSec=(int32u)float64_int64s(BlockAlign*(float64)sampleRate);
#endif //MEDIAINFO_DEMUX
Element_Code=(int64u)-1;
Open_Buffer_Init_All();
}
//---------------------------------------------------------------------------
void File_Riff::AIFF_COMT()
{
//Parsing
int16u numComments;
Get_B2(numComments, "numComments");
for (int16u Pos=0; Pos<=numComments; Pos++)
{
Ztring text;
int16u count;
Element_Begin1("Comment");
Skip_B4( "timeStamp");
Skip_B4( "marker");
Get_B2 (count, "count");
count+=count%1; //always even
Get_Local(count, text, "text");
Element_End0();
//Filling
Fill(Stream_General, 0, General_Comment, text);
}
}
//---------------------------------------------------------------------------
void File_Riff::AIFF_SSND()
{
WAVE_data();
}
//---------------------------------------------------------------------------
void File_Riff::AIFF_SSND_Continue()
{
WAVE_data_Continue();
}
//---------------------------------------------------------------------------
void File_Riff::AIFF_xxxx()
{
#define ELEMENT_CASE(_ELEMENT, _NAME) \
case Elements::_ELEMENT : Element_Name(_NAME); Name=_NAME; break;
//Known?
std::string Name;
switch(Element_Code)
{
ELEMENT_CASE(AIFF__c__, "Copyright");
ELEMENT_CASE(AIFF_ANNO, "Comment");
ELEMENT_CASE(AIFF_AUTH, "Performer");
ELEMENT_CASE(AIFF_NAME, "Title");
default : Skip_XX(Element_Size, "Unknown");
return;
}
//Parsing
Ztring text;
Get_Local(Element_Size, text, "text");
//Filling
Fill(Stream_General, 0, Name.c_str(), text);
}
//---------------------------------------------------------------------------
void File_Riff::AVI_()
{
Element_Name("AVI");
//Test if there is only one AVI chunk
if (Status[IsAccepted])
{
Element_Info1("Problem: 2 AVI chunks, this is not normal");
Skip_XX(Element_TotalSize_Get(), "Data");
return;
}
Data_Accept("AVI");
//Filling
Fill(Stream_General, 0, General_Format, "AVI");
Kind=Kind_Avi;
//Configuration
Buffer_MaximumSize=64*1024*1024; //Some big frames are possible (e.g YUV 4:2:2 10 bits 1080p)
}
//---------------------------------------------------------------------------
void File_Riff::AVI__Cr8r()
{
Element_Name("Adobe Premiere Cr8r");
//Parsing
Skip_C4( "FourCC");
Skip_B4( "Size");
Skip_XX(Element_Size-Element_Offset, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__cset()
{
Element_Name("Regional settings");
//Parsing
Skip_L2( "CodePage"); //TODO: take a look about IBM/MS RIFF/MCI Specification 1.0
Skip_L2( "CountryCode");
Skip_L2( "LanguageCode");
Skip_L2( "Dialect");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__exif()
{
Element_Name("Exif (Exchangeable Image File Format)");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__exif_xxxx()
{
Element_Name("Value");
//Parsing
Ztring Value;
Get_Local(Element_Size, Value, "Value");
//Filling
switch (Element_Code)
{
case Elements::AVI__exif_ecor : Fill(Stream_General, 0, "Make", Value); break;
case Elements::AVI__exif_emdl : Fill(Stream_General, 0, "Model", Value); break;
case Elements::AVI__exif_emnt : Fill(Stream_General, 0, "MakerNotes", Value); break;
case Elements::AVI__exif_erel : Fill(Stream_General, 0, "RelatedImageFile", Value); break;
case Elements::AVI__exif_etim : Fill(Stream_General, 0, "Written_Date", Value); break;
case Elements::AVI__exif_eucm : Fill(Stream_General, 0, General_Comment, Value); break;
case Elements::AVI__exif_ever : break; //Exif version
default: Fill(Stream_General, 0, Ztring().From_CC4((int32u)Element_Code).To_Local().c_str(), Value);
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__goog()
{
Element_Name("Google specific");
//Filling
Fill(Stream_General, 0, General_Format, "Google Video", Unlimited, false, true);
}
//---------------------------------------------------------------------------
void File_Riff::AVI__goog_GDAT()
{
Element_Name("Google datas");
}
//---------------------------------------------------------------------------
// Google Metadata
//
void File_Riff::AVI__GMET()
{
Element_Name("Google Metadatas");
//Parsing
Ztring Value; Value.From_Local((const char*)(Buffer+Buffer_Offset+0), (size_t)Element_Size);
ZtringListList List;
List.Separator_Set(0, __T("\n"));
List.Separator_Set(1, __T(":"));
List.Max_Set(1, 2);
List.Write(Value);
//Details
#if MEDIAINFO_TRACE
if (Config_Trace_Level)
{
//for (size_t Pos=0; Pos<List.size(); Pos++)
// Details_Add_Info(Pos, List(Pos, 0).To_Local().c_str(), List(Pos, 1));
}
#endif //MEDIAINFO_TRACE
//Filling
for (size_t Pos=0; Pos<List.size(); Pos++)
{
if (List(Pos, 0)==__T("title")) Fill(Stream_General, 0, General_Title, List(Pos, 1));
if (List(Pos, 0)==__T("description")) Fill(Stream_General, 0, General_Title_More, List(Pos, 1));
if (List(Pos, 0)==__T("url")) Fill(Stream_General, 0, General_Title_Url, List(Pos, 1));
if (List(Pos, 0)==__T("docid")) Fill(Stream_General, 0, General_UniqueID, List(Pos, 1));
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr()
{
Element_Name("AVI Header");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_avih()
{
Element_Name("File header");
//Parsing
int32u MicrosecPerFrame, Flags;
Get_L4 (MicrosecPerFrame, "MicrosecPerFrame");
Skip_L4( "MaxBytesPerSec");
Skip_L4( "PaddingGranularity");
Get_L4 (Flags, "Flags");
Skip_Flags(Flags, 4, "HasIndex");
Skip_Flags(Flags, 5, "MustUseIndex");
Skip_Flags(Flags, 8, "IsInterleaved");
Skip_Flags(Flags, 9, "UseCKTypeToFindKeyFrames");
Skip_Flags(Flags, 11, "TrustCKType");
Skip_Flags(Flags, 16, "WasCaptureFile");
Skip_Flags(Flags, 17, "Copyrighted");
Get_L4 (avih_TotalFrame, "TotalFrames");
Skip_L4( "InitialFrames");
Skip_L4( "StreamsCount");
Skip_L4( "SuggestedBufferSize");
Skip_L4( "Width");
Skip_L4( "Height");
Skip_L4( "Reserved");
Skip_L4( "Reserved");
Skip_L4( "Reserved");
Skip_L4( "Reserved");
if(Element_Offset<Element_Size)
Skip_XX(Element_Size-Element_Offset, "Unknown");
//Filling
if (MicrosecPerFrame>0)
avih_FrameRate=1000000.0/MicrosecPerFrame;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_JUNK()
{
Element_Name("Garbage");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_odml()
{
Element_Name("OpenDML");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_odml_dmlh()
{
Element_Name("OpenDML Header");
//Parsing
Get_L4(dmlh_TotalFrame, "GrandFrames");
if (Element_Offset<Element_Size)
Skip_XX(Element_Size-Element_Offset, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_ON2h()
{
Element_Name("On2 header");
//Parsing
Skip_XX(Element_Size, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl()
{
Element_Name("Stream info");
Element_Info1(stream_Count);
//Clean up
StreamKind_Last=Stream_Max;
StreamPos_Last=(size_t)-1;
//Compute the current codec ID
Stream_ID=(('0'+stream_Count/10)*0x01000000
+('0'+stream_Count )*0x00010000);
stream_Count++;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_indx()
{
Element_Name("Index");
int32u Entry_Count, ChunkId;
int16u LongsPerEntry;
int8u IndexType, IndexSubType;
Get_L2 (LongsPerEntry, "LongsPerEntry"); //Size of each entry in aIndex array
Get_L1 (IndexSubType, "IndexSubType");
Get_L1 (IndexType, "IndexType");
Get_L4 (Entry_Count, "EntriesInUse"); //Index of first unused member in aIndex array
Get_C4 (ChunkId, "ChunkId"); //FCC of what is indexed
//Depends of size of structure...
switch (IndexType)
{
case 0x01 : //AVI_INDEX_OF_CHUNKS
switch (IndexSubType)
{
case 0x00 : AVI__hdlr_strl_indx_StandardIndex(Entry_Count, ChunkId); break;
case 0x01 : AVI__hdlr_strl_indx_FieldIndex(Entry_Count, ChunkId); break; //AVI_INDEX_2FIELD
default: Skip_XX(Element_Size-Element_Offset, "Unknown");
}
break;
case 0x0 : //AVI_INDEX_OF_INDEXES
switch (IndexSubType)
{
case 0x00 :
case 0x01 : AVI__hdlr_strl_indx_SuperIndex(Entry_Count, ChunkId); break; //AVI_INDEX_2FIELD
default: Skip_XX(Element_Size-Element_Offset, "Unknown");
}
break;
default: Skip_XX(Element_Size-Element_Offset, "Unknown");
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_indx_StandardIndex(int32u Entry_Count, int32u ChunkId)
{
Element_Name("Standard Index");
//Parsing
int64u BaseOffset, StreamSize=0;
Get_L8 (BaseOffset, "BaseOffset");
Skip_L4( "Reserved3");
for (int32u Pos=0; Pos<Entry_Count; Pos++)
{
//Is too slow
/*
Element_Begin1("Index");
int32u Offset, Size;
Get_L4 (Offset, "Offset"); //BaseOffset + this is absolute file offset
Get_L4 (Size, "Size"); //Bit 31 is set if this is NOT a keyframe
Element_Info1(Size&0x7FFFFFFF);
if (Size)
Element_Info1("KeyFrame");
Element_End0();
*/
//Faster method
if (Element_Offset+8>Element_Size)
break; //Malformed index
int32u Offset=LittleEndian2int32u(Buffer+Buffer_Offset+(size_t)Element_Offset );
int32u Size =LittleEndian2int32u(Buffer+Buffer_Offset+(size_t)Element_Offset+4)&0x7FFFFFFF;
Element_Offset+=8;
//Stream Position and size
if (Pos<300 || MediaInfoLib::Config.ParseSpeed_Get()==1.00)
{
Stream_Structure[BaseOffset+Offset-8].Name=ChunkId&0xFFFF0000;
Stream_Structure[BaseOffset+Offset-8].Size=Size;
}
StreamSize+=(Size&0x7FFFFFFF);
Stream[ChunkId&0xFFFF0000].PacketCount++;
//Interleaved
if (Pos== 0 && (ChunkId&0xFFFF0000)==0x30300000 && Interleaved0_1 ==0)
Interleaved0_1 =BaseOffset+Offset-8;
if (Pos==Entry_Count/10 && (ChunkId&0xFFFF0000)==0x30300000 && Interleaved0_10==0)
Interleaved0_10=BaseOffset+Offset-8;
if (Pos== 0 && (ChunkId&0xFFFF0000)==0x30310000 && Interleaved1_1 ==0)
Interleaved1_1 =BaseOffset+Offset-8;
if (Pos==Entry_Count/10 && (ChunkId&0xFFFF0000)==0x30310000 && Interleaved1_10==0)
Interleaved1_10=BaseOffset+Offset-8;
}
Stream[ChunkId&0xFFFF0000].StreamSize+=StreamSize;
if (Element_Offset<Element_Size)
Skip_XX(Element_Size-Element_Offset, "Garbage");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_indx_FieldIndex(int32u Entry_Count, int32u)
{
Element_Name("Field Index");
//Parsing
Skip_L8( "Offset");
Skip_L4( "Reserved2");
for (int32u Pos=0; Pos<Entry_Count; Pos++)
{
Element_Begin1("Index");
Skip_L4( "Offset"); //BaseOffset + this is absolute file offset
Skip_L4( "Size"); //Bit 31 is set if this is NOT a keyframe
Skip_L4( "OffsetField2"); //Offset to second field
Element_End0();
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_indx_SuperIndex(int32u Entry_Count, int32u ChunkId)
{
Element_Name("Index of Indexes");
//Parsing
int64u Offset;
Skip_L4( "Reserved0");
Skip_L4( "Reserved1");
Skip_L4( "Reserved2");
stream& StreamItem = Stream[Stream_ID];
for (int32u Pos=0; Pos<Entry_Count; Pos++)
{
int32u Duration;
Element_Begin1("Index of Indexes");
Get_L8 (Offset, "Offset");
Skip_L4( "Size"); //Size of index chunk at this offset
Get_L4 (Duration, "Duration"); //time span in stream ticks
Index_Pos[Offset]=ChunkId;
StreamItem.indx_Duration+=Duration;
Element_End0();
}
//We needn't anymore Old version
NeedOldIndex=false;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_JUNK()
{
Element_Name("Garbage");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strd()
{
Element_Name("Stream datas");
//Parsing
Skip_XX(Element_Size, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf()
{
Element_Name("Stream format");
//Parse depending of kind of stream
stream& StreamItem = Stream[Stream_ID];
switch (StreamItem.fccType)
{
case Elements::AVI__hdlr_strl_strh_auds : AVI__hdlr_strl_strf_auds(); break;
case Elements::AVI__hdlr_strl_strh_iavs : AVI__hdlr_strl_strf_iavs(); break;
case Elements::AVI__hdlr_strl_strh_mids : AVI__hdlr_strl_strf_mids(); break;
case Elements::AVI__hdlr_strl_strh_txts : AVI__hdlr_strl_strf_txts(); break;
case Elements::AVI__hdlr_strl_strh_vids : AVI__hdlr_strl_strf_vids(); break;
default : Element_Info1("Unknown");
}
//Registering stream
StreamItem.StreamKind=StreamKind_Last;
StreamItem.StreamPos=StreamPos_Last;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_auds()
{
Element_Info1("Audio");
//Parsing
#if !MEDIAINFO_DEMUX
int32u AvgBytesPerSec;
#endif //!MEDIAINFO_DEMUX
int16u FormatTag, Channels;
BitsPerSample=0;
Get_L2 (FormatTag, "FormatTag");
Get_L2 (Channels, "Channels");
Get_L4 (SamplesPerSec, "SamplesPerSec");
Get_L4 (AvgBytesPerSec, "AvgBytesPerSec");
#if MEDIAINFO_DEMUX
Get_L2 (BlockAlign, "BlockAlign");
#else //MEDIAINFO_DEMUX
Skip_L2( "BlockAlign");
#endif //MEDIAINFO_DEMUX
if (Element_Offset+2<=Element_Size)
Get_L2 (BitsPerSample, "BitsPerSample");
if (FormatTag==1) //Only for PCM
{
//Coherancy
if (BitsPerSample && SamplesPerSec*BitsPerSample*Channels/8==AvgBytesPerSec*8)
AvgBytesPerSec*=8; //Found in one file. TODO: Provide information to end user about such error
//Computing of missing value
if (!BitsPerSample && AvgBytesPerSec && SamplesPerSec && Channels)
BitsPerSample=(int16u)(AvgBytesPerSec*8/SamplesPerSec/Channels);
}
//Filling
Stream_Prepare(Stream_Audio);
stream& StreamItem = Stream[Stream_ID];
StreamItem.Compression=FormatTag;
Ztring Codec; Codec.From_Number(FormatTag, 16);
Codec.MakeUpperCase();
CodecID_Fill(Codec, Stream_Audio, StreamPos_Last, InfoCodecID_Format_Riff);
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, Codec); //May be replaced by codec parser
Fill(Stream_Audio, StreamPos_Last, Audio_Codec_CC, Codec);
if (Channels)
Fill(Stream_Audio, StreamPos_Last, Audio_Channel_s_, (Channels!=5 || FormatTag==0xFFFE)?Channels:6);
if (SamplesPerSec)
Fill(Stream_Audio, StreamPos_Last, Audio_SamplingRate, SamplesPerSec);
if (AvgBytesPerSec)
Fill(Stream_Audio, StreamPos_Last, Audio_BitRate, AvgBytesPerSec*8);
if (BitsPerSample)
Fill(Stream_Audio, StreamPos_Last, Audio_BitDepth, BitsPerSample);
StreamItem.AvgBytesPerSec=AvgBytesPerSec; //Saving bitrate for each stream
if (SamplesPerSec && TimeReference!=(int64u)-1)
{
Fill(Stream_Audio, 0, Audio_Delay, float64_int64s(((float64)TimeReference)*1000/SamplesPerSec));
Fill(Stream_Audio, 0, Audio_Delay_Source, "Container (bext)");
}
//Specific cases
#if defined(MEDIAINFO_DTS_YES) || defined(MEDIAINFO_SMPTEST0337_YES)
if (FormatTag==0x1 && Retrieve(Stream_General, 0, General_Format)==__T("Wave")) //Some DTS or SMPTE ST 337 streams are coded "1"
{
#if defined(MEDIAINFO_DTS_YES)
{
File_Dts* Parser=new File_Dts;
Parser->Frame_Count_Valid=2;
Parser->ShouldContinueParsing=true;
#if MEDIAINFO_DEMUX
if (Config->Demux_Unpacketize_Get() && Retrieve(Stream_General, 0, General_Format)==__T("Wave"))
{
Parser->Demux_Level=2; //Container
Parser->Demux_UnpacketizeContainer=true;
Demux_Level=4; //Intermediate
}
#endif //MEDIAINFO_DEMUX
StreamItem.Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_SMPTEST0337_YES)
{
File_SmpteSt0337* Parser=new File_SmpteSt0337;
Parser->Container_Bits=(int8u)BitsPerSample;
Parser->Aligned=true;
Parser->ShouldContinueParsing=true;
#if MEDIAINFO_DEMUX
if (Config->Demux_Unpacketize_Get() && Retrieve(Stream_General, 0, General_Format)==__T("Wave"))
{
Parser->Demux_Level=2; //Container
Parser->Demux_UnpacketizeContainer=true;
Demux_Level=4; //Intermediate
}
#endif //MEDIAINFO_DEMUX
StreamItem.Parsers.push_back(Parser);
}
#endif
}
#endif
//Creating the parser
if (0);
#if defined(MEDIAINFO_MPEGA_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("MPEG Audio"))
{
File_Mpega* Parser=new File_Mpega;
Parser->CalculateDelay=true;
Parser->ShouldContinueParsing=true;
StreamItem.Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_AC3_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("AC-3"))
{
File_Ac3* Parser=new File_Ac3;
Parser->Frame_Count_Valid=2;
Parser->CalculateDelay=true;
Parser->ShouldContinueParsing=true;
StreamItem.Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_DTS_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("DTS"))
{
File_Dts* Parser=new File_Dts;
Parser->Frame_Count_Valid=2;
Parser->ShouldContinueParsing=true;
StreamItem.Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_AAC_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("AAC"))
{
File_Aac* Parser=new File_Aac;
Parser->Mode=File_Aac::Mode_ADTS;
Parser->Frame_Count_Valid=1;
Parser->ShouldContinueParsing=true;
StreamItem.Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_PCM_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("PCM"))
{
File_Pcm* Parser=new File_Pcm;
Parser->Codec=Codec;
Parser->Endianness='L';
Parser->BitDepth=(int8u)BitsPerSample;
#if MEDIAINFO_DEMUX
if (Demux_Rate)
Parser->Frame_Count_Valid = float64_int64s(Demux_Rate);
if (Config->Demux_Unpacketize_Get() && Retrieve(Stream_General, 0, General_Format)==__T("Wave"))
{
Parser->Demux_Level=2; //Container
Parser->Demux_UnpacketizeContainer=true;
Demux_Level=4; //Intermediate
}
#else //MEDIAINFO_DEMUX
Parser->Frame_Count_Valid=(int64u)-1; //Disabling it, waiting for SMPTE ST 337 parser reject
#endif //MEDIAINFO_DEMUX
stream& StreamItem = Stream[Stream_ID];
StreamItem.Parsers.push_back(Parser);
StreamItem.IsPcm=true;
}
#endif
#if defined(MEDIAINFO_ADPCM_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("ADPCM"))
{
//Creating the parser
File_Adpcm MI;
MI.Codec=Codec;
//Parsing
Open_Buffer_Init(&MI);
Open_Buffer_Continue(&MI, 0);
//Filling
Finish(&MI);
Merge(MI, StreamKind_Last, 0, StreamPos_Last);
}
#endif
#if defined(MEDIAINFO_OGG_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("Vorbis")
&& FormatTag!=0x566F) //0x566F has config in this chunk
{
File_Ogg* Parser=new File_Ogg;
Parser->ShouldContinueParsing=true;
StreamItem.Parsers.push_back(Parser);
}
#endif
Open_Buffer_Init_All();
//Options
if (Element_Offset+2>Element_Size)
return; //No options
//Parsing
int16u Option_Size;
Get_L2 (Option_Size, "cbSize");
//Filling
if (Option_Size>0)
{
if (0);
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Codec)==__T("MPEG Audio"))
{
if (Option_Size==12)
AVI__hdlr_strl_strf_auds_Mpega();
else
Skip_XX(Option_Size, "MPEG Audio - Uknown");
}
else if (Codec==__T("AAC") || Codec==__T("FF") || Codec==__T("8180"))
AVI__hdlr_strl_strf_auds_Aac();
else if (FormatTag==0x566F) //Vorbis with Config in this chunk
AVI__hdlr_strl_strf_auds_Vorbis();
else if (FormatTag==0x6750) //Vorbis with Config in this chunk
AVI__hdlr_strl_strf_auds_Vorbis2();
else if (FormatTag==0xFFFE) //Extensible Wave
AVI__hdlr_strl_strf_auds_ExtensibleWave();
else if (Element_Offset+Option_Size<=Element_Size)
Skip_XX(Option_Size, "Unknown");
else if (Element_Offset!=Element_Size)
Skip_XX(Element_Size-Element_Offset, "Error");
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_auds_Mpega()
{
//Parsing
Element_Begin1("MPEG Audio options");
Skip_L2( "ID");
Skip_L4( "Flags");
Skip_L2( "BlockSize");
Skip_L2( "FramesPerBlock");
Skip_L2( "CodecDelay");
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_auds_Aac()
{
//Parsing
Element_Begin1("AAC options");
#if defined(MEDIAINFO_AAC_YES)
File_Aac* MI=new File_Aac();
MI->Mode=File_Aac::Mode_AudioSpecificConfig;
Open_Buffer_Init(MI);
Open_Buffer_Continue(MI);
Finish(MI);
Merge(*MI, StreamKind_Last, 0, StreamPos_Last);
delete MI; //MI=NULL;
#else //MEDIAINFO_MPEG4_YES
Skip_XX(Element_Size-Element_Offset, "(AudioSpecificConfig)");
#endif
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_auds_Vorbis()
{
//Parsing
Element_Begin1("Vorbis options");
#if defined(MEDIAINFO_OGG_YES)
File_Ogg_SubElement MI;
Open_Buffer_Init(&MI);
Element_Begin1("Element sizes");
//All elements parsing, except last one
std::vector<size_t> Elements_Size;
size_t Elements_TotalSize=0;
int8u Elements_Count;
Get_L1(Elements_Count, "Element count");
Elements_Size.resize(Elements_Count+1); //+1 for the last block
for (int8u Pos=0; Pos<Elements_Count; Pos++)
{
int8u Size;
Get_L1(Size, "Size");
Elements_Size[Pos]=Size;
Elements_TotalSize+=Size;
}
Element_End0();
if (Element_Offset+Elements_TotalSize>Element_Size)
return;
//Adding the last block
Elements_Size[Elements_Count]=(size_t)(Element_Size-(Element_Offset+Elements_TotalSize));
Elements_Count++;
//Parsing blocks
for (int8u Pos=0; Pos<Elements_Count; Pos++)
{
Open_Buffer_Continue(&MI, Elements_Size[Pos]);
Open_Buffer_Continue(&MI, 0);
Element_Offset+=Elements_Size[Pos];
}
//Finalizing
Finish(&MI);
Merge(MI, StreamKind_Last, 0, StreamPos_Last);
Clear(Stream_Audio, StreamPos_Last, Audio_BitDepth); //Resolution is not valid for Vorbis
Element_Show();
#else //MEDIAINFO_MPEG4_YES
Skip_XX(Element_Size-Element_Offset, "(Vorbis headers)");
#endif
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_auds_Vorbis2()
{
//Parsing
Skip_XX(8, "Vorbis Unknown");
Element_Begin1("Vorbis options");
#if defined(MEDIAINFO_OGG_YES)
stream& StreamItem = Stream[Stream_ID];
Open_Buffer_Continue(StreamItem.Parsers[0]);
Open_Buffer_Continue(StreamItem.Parsers[0], 0);
Finish(StreamItem.Parsers[0]);
Merge(*StreamItem.Parsers[0], StreamKind_Last, 0, StreamPos_Last);
Element_Show();
#else //MEDIAINFO_MPEG4_YES
Skip_XX(Element_Size-Element_Offset, "(Vorbis headers)");
#endif
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_auds_ExtensibleWave()
{
//Parsing
int128u SubFormat;
int32u ChannelMask;
Skip_L2( "ValidBitsPerSample / SamplesPerBlock");
Get_L4 (ChannelMask, "ChannelMask");
Get_GUID(SubFormat, "SubFormat");
FILLING_BEGIN();
if ((SubFormat.hi&0xFFFFFFFFFFFF0000LL)==0x0010000000000000LL && SubFormat.lo==0x800000AA00389B71LL)
{
CodecID_Fill(Ztring().From_Number((int16u)SubFormat.hi, 16), Stream_Audio, StreamPos_Last, InfoCodecID_Format_Riff);
Fill(Stream_Audio, StreamPos_Last, Audio_CodecID, Ztring().From_GUID(SubFormat), true);
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, MediaInfoLib::Config.Codec_Get(Ztring().From_Number((int16u)SubFormat.hi, 16)), true);
//Creating the parser
if (0);
#if defined(MEDIAINFO_PCM_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Audio, InfoCodecID_Format_Riff, Ztring().From_Number((int16u)SubFormat.hi, 16))==__T("PCM"))
{
//Creating the parser
File_Pcm* Parser=new File_Pcm;
Parser->Codec=Ztring().From_GUID(SubFormat);
Parser->Endianness='L';
Parser->Sign='S';
Parser->BitDepth=(int8u)BitsPerSample;
#if MEDIAINFO_DEMUX
if (Config->Demux_Unpacketize_Get() && Retrieve(Stream_General, 0, General_Format)==__T("Wave"))
{
Parser->Demux_Level=2; //Container
Parser->Demux_UnpacketizeContainer=true;
Demux_Level=4; //Intermediate
}
#endif //MEDIAINFO_DEMUX
stream& StreamItem = Stream[Stream_ID];
StreamItem.Parsers.push_back(Parser);
StreamItem.IsPcm=true;
}
#endif
Open_Buffer_Init_All();
}
else
{
CodecID_Fill(Ztring().From_GUID(SubFormat), Stream_Audio, StreamPos_Last, InfoCodecID_Format_Riff);
}
Fill(Stream_Audio, StreamPos_Last, Audio_ChannelPositions, ExtensibleWave_ChannelMask(ChannelMask));
Fill(Stream_Audio, StreamPos_Last, Audio_ChannelPositions_String2, ExtensibleWave_ChannelMask2(ChannelMask));
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_iavs()
{
//Standard video header before Iavs?
if (Element_Size==72)
{
Element_Begin0();
AVI__hdlr_strl_strf_vids();
Element_End0();
}
Element_Info1("Interleaved Audio/Video");
#ifdef MEDIAINFO_DVDIF_YES
if (Element_Size<8*4)
return;
//Parsing
DV_FromHeader=new File_DvDif();
Open_Buffer_Init(DV_FromHeader);
//DVAAuxSrc
((File_DvDif*)DV_FromHeader)->AuxToAnalyze=0x50; //Audio source
Open_Buffer_Continue(DV_FromHeader, 4);
//DVAAuxCtl
((File_DvDif*)DV_FromHeader)->AuxToAnalyze=0x51; //Audio control
Open_Buffer_Continue(DV_FromHeader, Buffer+Buffer_Offset+(size_t)Element_Offset, 4);
Element_Offset+=4;
//DVAAuxSrc1
Skip_L4( "DVAAuxSrc1");
//DVAAuxCtl1
Skip_L4( "DVAAuxCtl1");
//DVVAuxSrc
((File_DvDif*)DV_FromHeader)->AuxToAnalyze=0x60; //Video source
Open_Buffer_Continue(DV_FromHeader, 4);
//DVAAuxCtl
((File_DvDif*)DV_FromHeader)->AuxToAnalyze=0x61; //Video control
Open_Buffer_Continue(DV_FromHeader, 4);
//Reserved
if (Element_Offset<Element_Size)
{
Skip_L4( "DVReserved");
Skip_L4( "DVReserved");
}
Finish(DV_FromHeader);
Stream_Prepare(Stream_Video);
stream& StreamItem = Stream[Stream_ID];
StreamItem.Parsers.push_back(new File_DvDif);
Open_Buffer_Init(StreamItem.Parsers[0]);
#else //MEDIAINFO_DVDIF_YES
//Parsing
Skip_L4( "DVAAuxSrc");
Skip_L4( "DVAAuxCtl");
Skip_L4( "DVAAuxSrc1");
Skip_L4( "DVAAuxCtl1");
Skip_L4( "DVVAuxSrc");
Skip_L4( "DVVAuxCtl");
Skip_L4( "DVReserved");
Skip_L4( "DVReserved");
//Filling
Ztring Codec; Codec.From_CC4(Stream[Stream_ID].fccHandler);
Stream_Prepare(Stream_Video);
float32 FrameRate=Retrieve(Stream_Video, StreamPos_Last, Video_FrameRate).To_float32();
Fill(Stream_Video, StreamPos_Last, Video_Codec, Codec); //May be replaced by codec parser
Fill(Stream_Video, StreamPos_Last, Video_Codec_CC, Codec);
if (Codec==__T("dvsd")
|| Codec==__T("dvsl"))
{
Fill(Stream_Video, StreamPos_Last, Video_Width, 720);
if (FrameRate==25.000) Fill(Stream_Video, StreamPos_Last, Video_Height, 576);
else if (FrameRate==29.970) Fill(Stream_Video, StreamPos_Last, Video_Height, 480);
Fill(Stream_Video, StreamPos_Last, Video_DisplayAspectRatio, 4.0/3, 3, true);
}
else if (Codec==__T("dvhd"))
{
Fill(Stream_Video, StreamPos_Last, Video_Width, 1440);
if (FrameRate==25.000) Fill(Stream_Video, StreamPos_Last, Video_Height, 1152);
else if (FrameRate==30.000) Fill(Stream_Video, StreamPos_Last, Video_Height, 960);
Fill(Stream_Video, StreamPos_Last, Video_DisplayAspectRatio, 4.0/3, 3, true);
}
Stream_Prepare(Stream_Audio);
CodecID_Fill(Codec, Stream_Audio, StreamPos_Last, InfoCodecID_Format_Riff);
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, Codec); //May be replaced by codec parser
Fill(Stream_Audio, StreamPos_Last, Audio_Codec_CC, Codec);
#endif //MEDIAINFO_DVDIF_YES
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_mids()
{
Element_Info1("Midi");
//Filling
Stream_Prepare(Stream_Audio);
Fill(Stream_Audio, StreamPos_Last, Audio_Format, "MIDI");
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, "Midi");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_txts()
{
Element_Info1("Text");
//Parsing
Ztring Format;
if (Element_Size)
{
Get_Local(10, Format, "Format");
Skip_XX(22, "Unknown");
}
FILLING_BEGIN_PRECISE();
Stream_Prepare(Stream_Text);
if (Element_Size==0)
{
//Creating the parser
stream& StreamItem = Stream[Stream_ID];
#if defined(MEDIAINFO_SUBRIP_YES)
StreamItem.Parsers.push_back(new File_SubRip);
#endif
#if defined(MEDIAINFO_OTHERTEXT_YES)
StreamItem.Parsers.push_back(new File_OtherText); //For SSA
#endif
Open_Buffer_Init_All();
}
else
{
Fill(Stream_Text, StreamPos_Last, Text_Format, Format);
}
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_vids()
{
Element_Info1("Video");
//Parsing
int32u Compression, Width, Height;
int16u Resolution;
Skip_L4( "Size");
Get_L4 (Width, "Width");
Get_L4 (Height, "Height");
Skip_L2( "Planes");
Get_L2 (Resolution, "BitCount"); //Do not use it
Get_C4 (Compression, "Compression");
Skip_L4( "SizeImage");
Skip_L4( "XPelsPerMeter");
Skip_L4( "YPelsPerMeter");
Skip_L4( "ClrUsed");
Skip_L4( "ClrImportant");
//Filling
Stream[Stream_ID].Compression=Compression;
if (Compression==CC4("DXSB"))
{
//Divx.com hack for subtitle, this is a text stream in a DivX Format
Fill(Stream_General, 0, General_Format, "DivX", Unlimited, true, true);
Stream_Prepare(Stream_Text);
}
else
Stream_Prepare(Stream_Video);
//Filling
CodecID_Fill(Ztring().From_CC4(Compression), StreamKind_Last, StreamPos_Last, InfoCodecID_Format_Riff);
Fill(StreamKind_Last, StreamPos_Last, Fill_Parameter(StreamKind_Last, Generic_Codec), Ztring().From_CC4(Compression).To_Local().c_str()); //FormatTag, may be replaced by codec parser
Fill(StreamKind_Last, StreamPos_Last, Fill_Parameter(StreamKind_Last, Generic_Codec_CC), Ztring().From_CC4(Compression).To_Local().c_str()); //FormatTag
Fill(StreamKind_Last, StreamPos_Last, "Width", Width, 10, true);
Fill(StreamKind_Last, StreamPos_Last, "Height", Height>=0x80000000?(-((int32s)Height)):Height, 10, true); // AVI can use negative height for raw to signal that it's coded top-down, not bottom-up
if (Resolution==32 && Compression==0x74736363) //tscc
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", 8);
else if (Compression==0x44495633) //DIV3
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", 8);
else if (MediaInfoLib::Config.CodecID_Get(StreamKind_Last, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression)).find(__T("Canopus"))!=std::string::npos) //Canopus codecs
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", Resolution/3);
else if (Compression==0x44585342) //DXSB
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", Resolution);
else if (MediaInfoLib::Config.CodecID_Get(StreamKind_Last, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_ColorSpace).find(__T("RGBA"))!=std::string::npos) //RGB codecs
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", Resolution/4);
else if (Compression==0x00000000 //RGB
|| MediaInfoLib::Config.CodecID_Get(StreamKind_Last, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_ColorSpace).find(__T("RGB"))!=std::string::npos) //RGB codecs
{
if (Resolution==32)
{
Fill(StreamKind_Last, StreamPos_Last, Fill_Parameter(StreamKind_Last, Generic_Format), "RGBA", Unlimited, true, true);
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", Resolution/4); //With Alpha
}
else
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", Resolution<=16?8:(Resolution/3)); //indexed or normal
}
else if (Compression==0x56503632 //VP62
|| MediaInfoLib::Config.CodecID_Get(StreamKind_Last, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_Format)==__T("H.263") //H.263
|| MediaInfoLib::Config.CodecID_Get(StreamKind_Last, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_Format)==__T("VC-1")) //VC-1
Fill(StreamKind_Last, StreamPos_Last, "BitDepth", Resolution/3);
Stream[Stream_ID].StreamKind=StreamKind_Last;
//Creating the parser
if (0);
#if defined(MEDIAINFO_FFV1_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_Format)==__T("FFV1"))
{
File_Ffv1* Parser=new File_Ffv1;
Parser->Width=Width;
Parser->Height=Height;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_HUFFYUV_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_Format)==__T("HuffYUV"))
{
File_HuffYuv* Parser=new File_HuffYuv;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_MPEGV_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_Format)==__T("MPEG Video"))
{
File_Mpegv* Parser=new File_Mpegv;
Parser->FrameIsAlwaysComplete=true;
Parser->TimeCodeIsNotTrustable=true;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_MPEG4V_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("MPEG-4 Visual"))
{
File_Mpeg4v* Parser=new File_Mpeg4v;
Stream[Stream_ID].Specific_IsMpeg4v=true;
Parser->FrameIsAlwaysComplete=true;
if (MediaInfoLib::Config.ParseSpeed_Get()>=0.5)
Parser->ShouldContinueParsing=true;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_PRORES_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression), InfoCodecID_Format)==__T("ProRes"))
{
File_ProRes* Parser=new File_ProRes;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_AVC_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("AVC"))
{
File_Avc* Parser=new File_Avc;
Parser->FrameIsAlwaysComplete=true;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_CANOPUS_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("Canopus HQ"))
{
File_Canopus* Parser=new File_Canopus;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_JPEG_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("JPEG"))
{
File_Jpeg* Parser=new File_Jpeg;
Parser->StreamKind=Stream_Video;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_DVDIF_YES)
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("DV"))
{
File_DvDif* Parser=new File_DvDif;
Parser->IgnoreAudio=true;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
#if defined(MEDIAINFO_FRAPS_YES)
else if (Compression==0x46505331) //"FPS1"
{
File_Fraps* Parser=new File_Fraps;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
else if (Compression==0x48465955) //"HFUY"
{
switch (Resolution)
{
case 16 : Fill(Stream_Video, StreamPos_Last, Video_ColorSpace, "YUV"); Fill(Stream_Video, StreamPos_Last, Video_ChromaSubsampling, "4:2:2"); Fill(Stream_Video, StreamPos_Last, Video_BitDepth, 8); break;
case 24 : Fill(Stream_Video, StreamPos_Last, Video_ColorSpace, "RGB"); Fill(Stream_Video, StreamPos_Last, Video_BitDepth, 8); break;
case 32 : Fill(Stream_Video, StreamPos_Last, Video_ColorSpace, "RGBA"); Fill(Stream_Video, StreamPos_Last, Video_BitDepth, 8); break;
default : ;
}
}
#if defined(MEDIAINFO_LAGARITH_YES)
else if (Compression==0x4C414753) //"LAGS"
{
File_Lagarith* Parser=new File_Lagarith;
Stream[Stream_ID].Parsers.push_back(Parser);
}
#endif
Open_Buffer_Init_All();
//Options
if (Element_Offset>=Element_Size)
return; //No options
//Filling
if (0);
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("AVC"))
AVI__hdlr_strl_strf_vids_Avc();
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("FFV1"))
AVI__hdlr_strl_strf_vids_Ffv1();
else if (MediaInfoLib::Config.CodecID_Get(Stream_Video, InfoCodecID_Format_Riff, Ztring().From_CC4(Compression))==__T("HuffYUV"))
AVI__hdlr_strl_strf_vids_HuffYUV(Resolution, Height);
else Skip_XX(Element_Size-Element_Offset, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_vids_Avc()
{
//Parsing
Element_Begin1("AVC options");
#if defined(MEDIAINFO_AVC_YES)
//Can be sized block or with 000001
stream& StreamItem = Stream[Stream_ID];
File_Avc* Parser=(File_Avc*)StreamItem.Parsers[0];
Parser->MustParse_SPS_PPS=false;
Parser->SizedBlocks=false;
Parser->MustSynchronize=true;
int64u Element_Offset_Save=Element_Offset;
Open_Buffer_Continue(Parser);
if (!Parser->Status[IsAccepted])
{
Element_Offset=Element_Offset_Save;
delete StreamItem.Parsers[0]; StreamItem.Parsers[0]=new File_Avc;
Parser=(File_Avc*)StreamItem.Parsers[0];
Open_Buffer_Init(Parser);
Parser->FrameIsAlwaysComplete=true;
Parser->MustParse_SPS_PPS=true;
Parser->SizedBlocks=true;
Parser->MustSynchronize=false;
Open_Buffer_Continue(Parser);
Element_Show();
}
#else //MEDIAINFO_AVC_YES
Skip_XX(Element_Size-Element_Offset, "(AVC headers)");
#endif
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_vids_Ffv1()
{
//Parsing
Element_Begin1("FFV1 options");
#if defined(MEDIAINFO_FFV1_YES)
File_Ffv1* Parser=(File_Ffv1*)Stream[Stream_ID].Parsers[0];
Open_Buffer_OutOfBand(Parser);
#else //MEDIAINFO_FFV1_YES
Skip_XX(Element_Size-Element_Offset, "(FFV1 headers)");
#endif
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strf_vids_HuffYUV(int16u BitCount, int32u Height)
{
//Parsing
Element_Begin1("HuffYUV options");
#if defined(MEDIAINFO_HUFFYUV_YES)
File_HuffYuv* Parser=(File_HuffYuv*)Stream[Stream_ID].Parsers[0];
Parser->IsOutOfBandData=true;
Parser->BitCount=BitCount;
Parser->Height=Height;
Open_Buffer_Continue(Parser);
#else //MEDIAINFO_HUFFYUV_YES
Skip_XX(Element_Size-Element_Offset, "(HuffYUV headers)");
#endif
Element_End0();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strh()
{
Element_Name("Stream header");
//Parsing
int32u fccType, fccHandler, Scale, Rate, Start, Length;
int16u Left, Top, Right, Bottom;
Get_C4 (fccType, "fccType");
switch (fccType)
{
case Elements::AVI__hdlr_strl_strh_auds :
Get_L4 (fccHandler, "fccHandler");
break;
default:
Get_C4 (fccHandler, "fccHandler");
}
Skip_L4( "Flags");
Skip_L2( "Priority");
Skip_L2( "Language");
Skip_L4( "InitialFrames");
Get_L4 (Scale, "Scale");
Get_L4 (Rate, "Rate"); //Rate/Scale is stream tick rate in ticks/sec
Get_L4 (Start, "Start");
Get_L4 (Length, "Length");
Skip_L4( "SuggestedBufferSize");
Skip_L4( "Quality");
Skip_L4( "SampleSize");
Get_L2 (Left, "Frame_Left");
Get_L2 (Top, "Frame_Top");
Get_L2 (Right, "Frame_Right");
Get_L2 (Bottom, "Frame_Bottom");
if(Element_Offset<Element_Size)
Skip_XX(Element_Size-Element_Offset, "Unknown");
//Filling
float32 FrameRate=0;
if (Rate>0 && Scale>0)
{
//FrameRate (without known value detection)
FrameRate=((float32)Rate)/Scale;
if (FrameRate>1)
{
float32 Rest=FrameRate-(int32u)FrameRate;
if (Rest<0.01)
FrameRate-=Rest;
else if (Rest>0.99)
FrameRate+=1-Rest;
else
{
float32 Rest1001=FrameRate*1001/1000-(int32u)(FrameRate*1001/1000);
if (Rest1001<0.001)
FrameRate=(float32)((int32u)(FrameRate*1001/1000))*1000/1001;
if (Rest1001>0.999)
FrameRate=(float32)((int32u)(FrameRate*1001/1000)+1)*1000/1001;
}
}
//Duration
if (FrameRate)
{
int64u Duration=float32_int64s((1000*(float32)Length)/FrameRate);
if (avih_TotalFrame>0 //avih_TotalFrame is here because some files have a wrong Audio Duration if TotalFrame==0 (which is a bug, of course!)
&& (avih_FrameRate==0 || Duration<((float32)avih_TotalFrame)/avih_FrameRate*1000*1.10) //Some file have a nearly perfect header, except that the value is false, trying to detect it (false if 10% more than 1st video)
&& (avih_FrameRate==0 || Duration>((float32)avih_TotalFrame)/avih_FrameRate*1000*0.90)) //Some file have a nearly perfect header, except that the value is false, trying to detect it (false if 10% less than 1st video)
{
Fill(StreamKind_Last, StreamPos_Last, "Duration", Duration);
}
}
}
switch (fccType)
{
case Elements::AVI__hdlr_strl_strh_vids :
if (FrameRate>0) Fill(Stream_Video, StreamPos_Last, Video_FrameRate, FrameRate, 3);
if (Right-Left>0) Fill(Stream_Video, StreamPos_Last, Video_Width, Right-Left, 10, true);
if (Bottom-Top>0) Fill(Stream_Video, StreamPos_Last, Video_Height, Bottom-Top, 10, true);
break;
case Elements::AVI__hdlr_strl_strh_txts :
if (Right-Left>0) Fill(Stream_Text, StreamPos_Last, Text_Width, Right-Left, 10, true);
if (Bottom-Top>0) Fill(Stream_Text, StreamPos_Last, Text_Height, Bottom-Top, 10, true);
break;
default: ;
}
stream& StreamItem = Stream[Stream_ID];
StreamItem.fccType=fccType;
StreamItem.fccHandler=fccHandler;
StreamItem.Scale=Scale;
StreamItem.Rate=Rate;
StreamItem.Start=Start;
StreamItem.Length=Length;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_strn()
{
Element_Name("Stream name");
//Parsing
Ztring Title;
Get_Local(Element_Size, Title, "StreamName");
//Filling
Fill(StreamKind_Last, StreamPos_Last, "Title", Title);
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_strl_vprp()
{
Element_Name("Video properties");
//Parsing
int32u FieldPerFrame;
int16u FrameAspectRatio_H, FrameAspectRatio_W;
Skip_L4( "VideoFormatToken");
Skip_L4( "VideoStandard");
Skip_L4( "VerticalRefreshRate");
Skip_L4( "HTotalInT");
Skip_L4( "VTotalInLines");
Get_L2 (FrameAspectRatio_H, "FrameAspectRatio Height");
Get_L2 (FrameAspectRatio_W, "FrameAspectRatio Width");
Skip_L4( "FrameWidthInPixels");
Skip_L4( "FrameHeightInLines");
Get_L4 (FieldPerFrame, "FieldPerFrame");
vector<int32u> VideoYValidStartLines;
for (int32u Pos=0; Pos<FieldPerFrame; Pos++)
{
Element_Begin1("Field");
int32u VideoYValidStartLine;
Skip_L4( "CompressedBMHeight");
Skip_L4( "CompressedBMWidth");
Skip_L4( "ValidBMHeight");
Skip_L4( "ValidBMWidth");
Skip_L4( "ValidBMXOffset");
Skip_L4( "ValidBMYOffset");
Skip_L4( "VideoXOffsetInT");
Get_L4 (VideoYValidStartLine, "VideoYValidStartLine");
VideoYValidStartLines.push_back(VideoYValidStartLine);
Element_End0();
}
if(Element_Offset<Element_Size)
Skip_XX(Element_Size-Element_Offset, "Unknown");
FILLING_BEGIN();
if (FrameAspectRatio_H && FrameAspectRatio_W)
Fill(Stream_Video, 0, Video_DisplayAspectRatio, ((float32)FrameAspectRatio_W)/FrameAspectRatio_H, 3);
switch (FieldPerFrame)
{
case 1 :
Fill(Stream_Video, 0, Video_ScanType, "Progressive");
break;
case 2 :
Fill(Stream_Video, 0, Video_ScanType, "Interlaced");
if (VideoYValidStartLines.size()==2 && VideoYValidStartLines[0]<VideoYValidStartLines[1])
Fill(Stream_Video, 0, Video_ScanOrder, "TFF");
if (VideoYValidStartLines.size()==2 && VideoYValidStartLines[0]>VideoYValidStartLines[1])
Fill(Stream_Video, 0, Video_ScanOrder, "BFF");
default: ;
}
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__hdlr_xxxx()
{
AVI__INFO_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__idx1()
{
Element_Name("Index (old)");
//Tests
if (!NeedOldIndex || Idx1_Offset==(int64u)-1)
{
Skip_XX(Element_Size, "Data");
return;
}
//Testing malformed index (index is based on start of the file, wrong)
if (16<=Element_Size && Idx1_Offset+4==LittleEndian2int32u(Buffer+Buffer_Offset+(size_t)Element_Offset+ 8))
Idx1_Offset=0; //Fixing base of movi atom, the index think it is the start of the file
//Parsing
while (Element_Offset+16<=Element_Size)
{
//Is too slow
/*
int32u ChunkID, Offset, Size;
Element_Begin1("Index");
Get_C4 (ChunkID, "ChunkID"); //Bit 31 is set if this is NOT a keyframe
Info_L4(Flags, "Flags");
Skip_Flags(Flags, 0, "NoTime");
Skip_Flags(Flags, 1, "Lastpart");
Skip_Flags(Flags, 2, "Firstpart");
Skip_Flags(Flags, 3, "Midpart");
Skip_Flags(Flags, 4, "KeyFrame");
Get_L4 (Offset, "Offset"); //qwBaseOffset + this is absolute file offset
Get_L4 (Size, "Size"); //Bit 31 is set if this is NOT a keyframe
Element_Info1(Ztring().From_CC4(ChunkID));
Element_Info1(Size);
//Stream Pos and Size
int32u StreamID=(ChunkID&0xFFFF0000);
Stream[StreamID].StreamSize+=Size;
Stream[StreamID].PacketCount++;
Stream_Structure[Idx1_Offset+Offset].Name=StreamID;
Stream_Structure[Idx1_Offset+Offset].Size=Size;
Element_End0();
*/
//Faster method
int32u StreamID=BigEndian2int32u (Buffer+Buffer_Offset+(size_t)Element_Offset )&0xFFFF0000;
int32u Offset =LittleEndian2int32u(Buffer+Buffer_Offset+(size_t)Element_Offset+ 8);
int32u Size =LittleEndian2int32u(Buffer+Buffer_Offset+(size_t)Element_Offset+12);
stream& Stream_Item=Stream[StreamID];
Stream_Item.StreamSize+=Size;
Stream_Item.PacketCount++;
stream_structure& Stream_Structure_Item=Stream_Structure[Idx1_Offset+Offset];
Stream_Structure_Item.Name=StreamID;
Stream_Structure_Item.Size=Size;
Element_Offset+=16;
}
//Interleaved
size_t Pos0=0;
size_t Pos1=0;
for (std::map<int64u, stream_structure>::iterator Temp=Stream_Structure.begin(); Temp!=Stream_Structure.end(); ++Temp)
{
switch (Temp->second.Name)
{
case 0x30300000 :
if (Interleaved0_1==0) Interleaved0_1=Temp->first;
if (Interleaved0_10==0)
{
Pos0++;
if (Pos0>1)
Interleaved0_10=Temp->first;
}
break;
case 0x30310000 :
if (Interleaved1_1==0) Interleaved1_1=Temp->first;
if (Interleaved1_10==0)
{
Pos1++;
if (Pos1>1)
Interleaved1_10=Temp->first;
}
break;
default:;
}
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__INFO()
{
Element_Name("Tags");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__INFO_IID3()
{
Element_Name("ID3 Tag");
//Parsing
#if defined(MEDIAINFO_ID3_YES)
File_Id3 MI;
Open_Buffer_Init(&MI);
Open_Buffer_Continue(&MI);
Finish(&MI);
Merge(MI, Stream_General, 0, 0);
#endif
}
//---------------------------------------------------------------------------
void File_Riff::AVI__INFO_ILYC()
{
Element_Name("Lyrics");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__INFO_IMP3()
{
Element_Name("MP3 Information");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__INFO_JUNK()
{
Element_Name("Garbage");
}
//---------------------------------------------------------------------------
// List of information atoms
// Name X bytes, Pos=0
//
void File_Riff::AVI__INFO_xxxx()
{
//Parsing
Ztring Value;
Get_Local(Element_Size, Value, "Value");
//Filling
stream_t StreamKind=Stream_General;
size_t StreamPos=0;
size_t Parameter=(size_t)-1;
switch (Element_Code)
{
case 0x00000000 : Parameter=General_Comment; break;
case Elements::AVI__INFO_IARL : Parameter=General_Archival_Location; break;
case Elements::AVI__INFO_IART : Parameter=General_Director; break;
case Elements::AVI__INFO_IAS1 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=0; break;
case Elements::AVI__INFO_IAS2 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=1; break;
case Elements::AVI__INFO_IAS3 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=2; break;
case Elements::AVI__INFO_IAS4 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=3; break;
case Elements::AVI__INFO_IAS5 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=4; break;
case Elements::AVI__INFO_IAS6 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=5; break;
case Elements::AVI__INFO_IAS7 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=6; break;
case Elements::AVI__INFO_IAS8 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=7; break;
case Elements::AVI__INFO_IAS9 : Parameter=Audio_Language; StreamKind=Stream_Audio; StreamPos=8; break;
case Elements::AVI__INFO_ICDS : Parameter=General_CostumeDesigner; break;
case Elements::AVI__INFO_ICMS : Parameter=General_CommissionedBy; break;
case Elements::AVI__INFO_ICMT : Parameter=General_Comment; break;
case Elements::AVI__INFO_ICNM : Parameter=General_DirectorOfPhotography; break;
case Elements::AVI__INFO_ICNT : Parameter=General_Movie_Country; break;
case Elements::AVI__INFO_ICOP : Parameter=General_Copyright; break;
case Elements::AVI__INFO_ICRD : Parameter=General_Recorded_Date; Value.Date_From_String(Value.To_Local().c_str()); break;
case Elements::AVI__INFO_ICRP : Parameter=General_Cropped; break;
case Elements::AVI__INFO_IDIM : Parameter=General_Dimensions; break;
case Elements::AVI__INFO_IDIT : Parameter=General_Mastered_Date; Value.Date_From_String(Value.To_Local().c_str()); break;
case Elements::AVI__INFO_IDPI : Parameter=General_DotsPerInch; break;
case Elements::AVI__INFO_IDST : Parameter=General_DistributedBy; break;
case Elements::AVI__INFO_IEDT : Parameter=General_EditedBy; break;
case Elements::AVI__INFO_IENG : Parameter=General_EncodedBy; break;
case Elements::AVI__INFO_IGNR : Parameter=General_Genre; break;
case Elements::AVI__INFO_IFRM : Parameter=General_Part_Position_Total; break;
case Elements::AVI__INFO_IKEY : Parameter=General_Keywords; break;
case Elements::AVI__INFO_ILGT : Parameter=General_Lightness; break;
case Elements::AVI__INFO_ILNG : Parameter=Audio_Language; StreamKind=Stream_Audio; break;
case Elements::AVI__INFO_IMED : Parameter=General_OriginalSourceMedium; break;
case Elements::AVI__INFO_IMUS : Parameter=General_MusicBy; break;
case Elements::AVI__INFO_INAM : Parameter=General_Title; break;
case Elements::AVI__INFO_IPDS : Parameter=General_ProductionDesigner; break;
case Elements::AVI__INFO_IPLT : Parameter=General_OriginalSourceForm_NumColors; break;
case Elements::AVI__INFO_IPRD : Parameter=General_OriginalSourceForm_Name; break;
case Elements::AVI__INFO_IPRO : Parameter=General_Producer; break;
case Elements::AVI__INFO_IPRT : Parameter=General_Part_Position; break;
case Elements::AVI__INFO_IRTD : Parameter=General_LawRating; break;
case Elements::AVI__INFO_ISBJ : Parameter=General_Subject; break;
case Elements::AVI__INFO_ISFT : Parameter=General_Encoded_Application; break;
case Elements::AVI__INFO_ISGN : Parameter=General_Genre; break;
case Elements::AVI__INFO_ISHP : Parameter=General_OriginalSourceForm_Sharpness; break;
case Elements::AVI__INFO_ISRC : Parameter=General_OriginalSourceForm_DistributedBy; break;
case Elements::AVI__INFO_ISRF : Parameter=General_OriginalSourceForm; break;
case Elements::AVI__INFO_ISTD : Parameter=General_ProductionStudio; break;
case Elements::AVI__INFO_ISTR : Parameter=General_Performer; break;
case Elements::AVI__INFO_ITCH : Parameter=General_EncodedBy; break;
case Elements::AVI__INFO_IWEB : Parameter=General_Movie_Url; break;
case Elements::AVI__INFO_IWRI : Parameter=General_WrittenBy; break;
default : ;
}
Element_Name(MediaInfoLib::Config.Info_Get(StreamKind, Parameter, Info_Name));
Element_Info1(Value);
switch (Element_Code)
{
case Elements::AVI__INFO_ISMP : INFO_ISMP=Value;
break;
case Elements::AVI__INFO_IGNR :
{
Ztring ISGN=Retrieve(Stream_General, 0, General_Genre);
Clear(Stream_General, 0, General_Genre);
Fill(StreamKind, StreamPos, General_Genre, Value);
if (!ISGN.empty())
Fill(StreamKind, StreamPos, General_Genre, ISGN);
}
break;
default :
if (!Value.empty())
{
if (Parameter!=(size_t)-1)
Fill(StreamKind, StreamPos, Parameter, Value);
else
Fill(StreamKind, StreamPos, Ztring().From_CC4((int32u)Element_Code).To_Local().c_str(), Value, true);
}
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__JUNK()
{
Element_Name("Garbage"); //Library defined size for padding, often used to store library name
if (Element_Size<8)
{
Skip_XX(Element_Size, "Junk");
return;
}
//Detect DivX files
if (CC5(Buffer+Buffer_Offset)==CC5("DivX "))
{
Fill(Stream_General, 0, General_Format, "DivX", Unlimited, true, true);
}
//MPlayer
else if (CC8(Buffer+Buffer_Offset)==CC8("[= MPlay") && Retrieve(Stream_General, 0, General_Encoded_Library).empty())
Fill(Stream_General, 0, General_Encoded_Library, "MPlayer");
//Scenalyzer
else if (CC8(Buffer+Buffer_Offset)==CC8("scenalyz") && Retrieve(Stream_General, 0, General_Encoded_Library).empty())
Fill(Stream_General, 0, General_Encoded_Library, "Scenalyzer");
//FFMpeg broken files detection
else if (CC8(Buffer+Buffer_Offset)==CC8("odmldmlh"))
dmlh_TotalFrame=0; //this is not normal to have this string in a JUNK block!!! and in files tested, in this case TotalFrame is broken too
//VirtualDubMod
else if (CC8(Buffer+Buffer_Offset)==CC8("INFOISFT"))
{
int32u Size=LittleEndian2int32u(Buffer+Buffer_Offset+8);
if (Size>Element_Size-12)
Size=(int32u)Element_Size-12;
Fill(Stream_General, 0, General_Encoded_Library, (const char*)(Buffer+Buffer_Offset+12), Size);
}
else if (CC8(Buffer+Buffer_Offset)==CC8("INFOIENG"))
{
int32u Size=LittleEndian2int32u(Buffer+Buffer_Offset+8);
if (Size>Element_Size-12)
Size=(int32u)Element_Size-12;
Fill(Stream_General, 0, General_Encoded_Library, (const char*)(Buffer+Buffer_Offset+12), Size);
}
//Other libraries?
else if (CC1(Buffer+Buffer_Offset)>=CC1("A") && CC1(Buffer+Buffer_Offset)<=CC1("z") && Retrieve(Stream_General, 0, General_Encoded_Library).empty())
Fill(Stream_General, 0, General_Encoded_Library, (const char*)(Buffer+Buffer_Offset), (size_t)Element_Size);
Skip_XX(Element_Size, "Data");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__MD5_()
{
//Parsing
while (Element_Offset<Element_Size)
{
int128u MD5Stored;
Get_L16 (MD5Stored, "MD5");
Ztring MD5_PerItem;
MD5_PerItem.From_Number(MD5Stored, 16);
while (MD5_PerItem.size()<32)
MD5_PerItem.insert(MD5_PerItem.begin(), '0'); //Padding with 0, this must be a 32-byte string
MD5_PerItem.MakeLowerCase();
MD5s.push_back(MD5_PerItem);
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi()
{
Element_Name("Datas");
//Only the first time, no need in AVIX
if (movi_Size==0)
{
Idx1_Offset=File_Offset+Buffer_Offset-4;
BookMark_Set(); //Remenbering this place, for stream parsing in phase 2
//For each stream
std::map<int32u, stream>::iterator Temp=Stream.begin();
while (Temp!=Stream.end())
{
if ((Temp->second.Parsers.empty() || Temp->second.Parsers[0]==NULL) && Temp->second.fccType!=Elements::AVI__hdlr_strl_strh_txts)
{
Temp->second.SearchingPayload=false;
stream_Count--;
}
++Temp;
}
}
//Probing rec (with index, this is not always tested in the flow
if (Element_Size<12)
{
Element_WaitForMoreData();
return;
}
if (CC4(Buffer+Buffer_Offset+8)==0x72656320) //"rec "
rec__Present=true;
//Filling
if (!SecondPass)
movi_Size+=Element_TotalSize_Get();
//We must parse moov?
if (NeedOldIndex || (stream_Count==0 && Index_Pos.empty()))
{
//Jumping
#if MEDIAINFO_TRACE
if (Trace_Activated)
Param("Data", Ztring("(")+Ztring::ToZtring(Element_TotalSize_Get())+Ztring(" bytes)"));
#endif //MEDIAINFO_TRACE
Element_Offset=Element_TotalSize_Get(); //Not using Skip_XX() because we want to skip data we don't have, and Skip_XX() does a test on size of buffer
return;
}
//Jump to next useful data
AVI__movi_StreamJump();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_rec_()
{
Element_Name("Syncronisation");
rec__Present=true;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_rec__xxxx()
{
AVI__movi_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_xxxx()
{
if (Element_Code==Elements::AVI__JUNK)
{
Skip_XX(Element_Size, "Junk");
AVI__movi_StreamJump();
return;
}
if (Element_Code!=(int64u)-1)
Stream_ID=(int32u)(Element_Code&0xFFFF0000);
else
Stream_ID=(int32u)-1;
if (Stream_ID==0x69780000) //ix..
{
//AVI Standard Index Chunk
AVI__hdlr_strl_indx();
Stream_ID=(int32u)(Element_Code&0x0000FFFF)<<16;
AVI__movi_StreamJump();
return;
}
if ((Element_Code&0x0000FFFF)==0x00006978) //..ix (Out of specs, but found in a Adobe After Effects CS4 DV file
{
//AVI Standard Index Chunk
AVI__hdlr_strl_indx();
Stream_ID=(int32u)(Element_Code&0xFFFF0000);
AVI__movi_StreamJump();
return;
}
stream& StreamItem = Stream[Stream_ID];
#if MEDIAINFO_DEMUX
if (StreamItem.Rate) //AVI
{
int64u Element_Code_Old=Element_Code;
Element_Code=((Element_Code_Old>>24)&0xF)*10+((Element_Code_Old>>16)&0xF);
Frame_Count_NotParsedIncluded= StreamItem.PacketPos;
FrameInfo.DTS=Frame_Count_NotParsedIncluded*1000000000* StreamItem.Scale/ StreamItem.Rate;
Demux(Buffer+Buffer_Offset, (size_t)Element_Size, ContentType_MainStream);
Element_Code=Element_Code_Old;
Frame_Count_NotParsedIncluded=(int64u)-1;
}
else //WAV
{
//TODO
}
#endif //MEDIAINFO_DEMUX
StreamItem.PacketPos++;
//Finished?
if (!StreamItem.SearchingPayload)
{
Element_DoNotShow();
AVI__movi_StreamJump();
return;
}
#if MEDIAINFO_TRACE
if (Config_Trace_Level)
{
switch (Element_Code&0x0000FFFF) //2 last bytes
{
case Elements::AVI__movi_xxxx_____ : Element_Info1("DV"); break;
case Elements::AVI__movi_xxxx___db :
case Elements::AVI__movi_xxxx___dc : Element_Info1("Video"); break;
case Elements::AVI__movi_xxxx___sb :
case Elements::AVI__movi_xxxx___tx : Element_Info1("Text"); break;
case Elements::AVI__movi_xxxx___wb : Element_Info1("Audio"); break;
default : Element_Info1("Unknown"); break;
}
Element_Info1(Stream[Stream_ID].PacketPos);
}
#endif //MEDIAINFO_TRACE
//Some specific stuff
switch (Element_Code&0x0000FFFF) //2 last bytes
{
case Elements::AVI__movi_xxxx___tx : AVI__movi_xxxx___tx(); break;
default : ;
}
//Parsing
for (size_t Pos=0; Pos<StreamItem.Parsers.size(); Pos++)
if (StreamItem.Parsers[Pos])
{
if (FrameInfo.PTS!=(int64u)-1)
StreamItem.Parsers[Pos]->FrameInfo.PTS=FrameInfo.PTS;
if (FrameInfo.DTS!=(int64u)-1)
StreamItem.Parsers[Pos]->FrameInfo.DTS=FrameInfo.DTS;
Open_Buffer_Continue(StreamItem.Parsers[Pos], Buffer+Buffer_Offset+(size_t)Element_Offset, (size_t)(Element_Size-Element_Offset));
Element_Show();
if (StreamItem.Parsers.size()==1 && StreamItem.Parsers[Pos]->Buffer_Size>0)
StreamItem.ChunksAreComplete=false;
if (StreamItem.Parsers.size()>1)
{
if (!StreamItem.Parsers[Pos]->Status[IsAccepted] && StreamItem.Parsers[Pos]->Status[IsFinished])
{
delete *(StreamItem.Parsers.begin()+Pos);
StreamItem.Parsers.erase(StreamItem.Parsers.begin()+Pos);
Pos--;
}
else if (StreamItem.Parsers.size()>1 && StreamItem.Parsers[Pos]->Status[IsAccepted])
{
File__Analyze* Parser= StreamItem.Parsers[Pos];
for (size_t Pos2=0; Pos2<StreamItem.Parsers.size(); Pos2++)
{
if (Pos2!=Pos)
delete *(StreamItem.Parsers.begin()+Pos2);
}
StreamItem.Parsers.clear();
StreamItem.Parsers.push_back(Parser);
Pos=0;
}
}
#if MEDIAINFO_DEMUX
if (Config->Demux_EventWasSent)
{
Demux_Parser= StreamItem.Parsers[Pos];
return;
}
#endif //MEDIAINFO_DEMUX
}
Element_Offset=Element_Size;
//Some specific stuff
switch (Element_Code&0x0000FFFF) //2 last bytes
{
case Elements::AVI__movi_xxxx_____ :
case Elements::AVI__movi_xxxx___db :
case Elements::AVI__movi_xxxx___dc : AVI__movi_xxxx___dc(); break;
case Elements::AVI__movi_xxxx___wb : AVI__movi_xxxx___wb(); break;
default : ;
}
//We must always parse moov?
AVI__movi_StreamJump();
Element_Show();
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_xxxx___dc()
{
//Finish (if requested)
stream& StreamItem = Stream[Stream_ID];
if (StreamItem.Parsers.empty()
|| StreamItem.Parsers[0]->Status[IsFinished]
|| (StreamItem.PacketPos>=300 && MediaInfoLib::Config.ParseSpeed_Get()<1.00))
{
StreamItem.SearchingPayload=false;
stream_Count--;
return;
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_xxxx___tx()
{
//Parsing
int32u Name_Size;
Ztring Value;
int32u GAB2;
Peek_B4(GAB2);
if (GAB2==0x47414232 && Element_Size>=17)
{
Skip_C4( "GAB2");
Skip_L1( "Zero");
Skip_L2( "CodePage"); //2=Unicode
Get_L4 (Name_Size, "Name_Size");
Skip_UTF16L(Name_Size, "Name");
Skip_L2( "Four");
Skip_L4( "File_Size");
if (Element_Offset>Element_Size)
Element_Offset=Element_Size; //Problem
}
//Skip it
Stream[Stream_ID].SearchingPayload=false;
stream_Count--;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_xxxx___wb()
{
//Finish (if requested)
stream& StreamItem = Stream[Stream_ID];
if (StreamItem.PacketPos>=4 //For having the chunk alignement
&& (StreamItem.Parsers.empty()
|| StreamItem.Parsers[0]->Status[IsFinished]
|| (StreamItem.PacketPos>=300 && MediaInfoLib::Config.ParseSpeed_Get()<1.00)))
{
StreamItem.SearchingPayload=false;
stream_Count--;
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__movi_StreamJump()
{
//Jump to next useful data
if (!Index_Pos.empty())
{
if (Index_Pos.begin()->first<=File_Offset+Buffer_Offset && Element_Code!=Elements::AVI__movi)
Index_Pos.erase(Index_Pos.begin());
int64u ToJump=File_Size;
if (!Index_Pos.empty())
ToJump=Index_Pos.begin()->first;
if (ToJump>File_Size)
ToJump=File_Size;
if (ToJump>=File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2)) //We want always Element movi
{
#if MEDIAINFO_HASH
if (Config->File_Hash_Get().to_ulong() && SecondPass)
Hash_ParseUpTo=File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2);
else
#endif //MEDIAINFO_HASH
GoTo(File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2), "AVI"); //Not in this chunk
}
else if (ToJump!=File_Offset+Buffer_Offset+(Element_Code==Elements::AVI__movi?0:Element_Size))
{
#if MEDIAINFO_HASH
if (Config->File_Hash_Get().to_ulong() && SecondPass)
Hash_ParseUpTo=File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2);
else
#endif //MEDIAINFO_HASH
GoTo(ToJump, "AVI"); //Not just after
}
}
else if (stream_Count==0)
{
//Jumping
Element_Show();
if (rec__Present)
Element_End0();
Info("movi, Jumping to end of chunk");
if (SecondPass)
{
std::map<int32u, stream>::iterator Temp=Stream.begin();
while (Temp!=Stream.end())
{
for (size_t Pos=0; Pos<Temp->second.Parsers.size(); ++Pos)
{
Temp->second.Parsers[Pos]->Fill();
Temp->second.Parsers[Pos]->Open_Buffer_Unsynch();
}
++Temp;
}
Finish("AVI"); //The rest is already parsed
}
else
GoTo(File_Offset+Buffer_Offset+Element_TotalSize_Get(), "AVI");
}
else if (Stream_Structure_Temp!=Stream_Structure.end())
{
do
Stream_Structure_Temp++;
while (Stream_Structure_Temp!=Stream_Structure.end() && !(Stream[(int32u)Stream_Structure_Temp->second.Name].SearchingPayload && Config->ParseSpeed<1.0));
if (Stream_Structure_Temp!=Stream_Structure.end())
{
int64u ToJump=Stream_Structure_Temp->first;
if (ToJump>=File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2))
{
#if MEDIAINFO_HASH
if (Config->File_Hash_Get().to_ulong() && SecondPass)
Hash_ParseUpTo=File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2);
else
#endif //MEDIAINFO_HASH
GoTo(File_Offset+Buffer_Offset+Element_TotalSize_Get(Element_Level-2), "AVI"); //Not in this chunk
}
else if (ToJump!=File_Offset+Buffer_Offset+Element_Size)
{
#if MEDIAINFO_HASH
if (Config->File_Hash_Get().to_ulong() && SecondPass)
Hash_ParseUpTo=ToJump;
else
#endif //MEDIAINFO_HASH
GoTo(ToJump, "AVI"); //Not just after
}
}
else
Finish("AVI");
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__PrmA()
{
Element_Name("Adobe Premiere PrmA");
//Parsing
int32u FourCC, Size;
Get_C4 (FourCC, "FourCC");
Get_B4 (Size, "Size");
switch (FourCC)
{
case 0x50415266:
if (Size==20)
{
int32u PAR_X, PAR_Y;
Skip_B4( "Unknown");
Get_B4 (PAR_X, "PAR_X");
Get_B4 (PAR_Y, "PAR_Y");
if (PAR_Y)
PAR=((float64)PAR_X)/PAR_Y;
}
else
Skip_XX(Element_Size-Element_Offset, "Unknown");
break;
default:
for (int32u Pos=8; Pos<Size; Pos++)
Skip_B4( "Unknown");
}
}
//---------------------------------------------------------------------------
void File_Riff::AVI__Tdat()
{
Element_Name("Adobe Premiere Tdat");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__Tdat_tc_A()
{
Element_Name("tc_A");
//Parsing
Ztring Value;
Get_Local(Element_Size, Value, "Unknown");
if (Value.find_first_not_of(__T("0123456789:;"))==string::npos)
Tdat_tc_A=Value;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__Tdat_tc_O()
{
Element_Name("tc_O");
//Parsing
Ztring Value;
Get_Local(Element_Size, Value, "Unknown");
if (Value.find_first_not_of(__T("0123456789:;"))==string::npos)
Tdat_tc_O=Value;
}
//---------------------------------------------------------------------------
void File_Riff::AVI__Tdat_rn_A()
{
Element_Name("rn_A");
//Parsing
Skip_Local(Element_Size, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__Tdat_rn_O()
{
Element_Name("rn_O");
//Parsing
Skip_Local(Element_Size, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::AVI__xxxx()
{
Stream_ID=(int32u)(Element_Code&0xFFFF0000);
if (Stream_ID==0x69780000) //ix..
{
//AVI Standard Index Chunk
AVI__hdlr_strl_indx();
Stream_ID=(int32u)(Element_Code&0x0000FFFF)<<16;
AVI__movi_StreamJump();
return;
}
if ((Element_Code&0x0000FFFF)==0x00006978) //..ix (Out of specs, but found in a Adobe After Effects CS4 DV file
{
//AVI Standard Index Chunk
AVI__hdlr_strl_indx();
Stream_ID=(int32u)(Element_Code&0xFFFF0000);
AVI__movi_StreamJump();
return;
}
}
//---------------------------------------------------------------------------
void File_Riff::AVIX()
{
//Filling
Fill(Stream_General, 0, General_Format_Profile, "OpenDML", Unlimited, true, true);
}
//---------------------------------------------------------------------------
void File_Riff::AVIX_idx1()
{
AVI__idx1();
}
//---------------------------------------------------------------------------
void File_Riff::AVIX_movi()
{
AVI__movi();
}
//---------------------------------------------------------------------------
void File_Riff::AVIX_movi_rec_()
{
AVI__movi_rec_();
}
//---------------------------------------------------------------------------
void File_Riff::AVIX_movi_rec__xxxx()
{
AVIX_movi_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::AVIX_movi_xxxx()
{
AVI__movi_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::CADP()
{
Element_Name("CMP4 - ADPCM");
//Testing if we have enough data
if (Element_Size<4)
{
Element_WaitForMoreData();
return;
}
//Parsing
int32u Codec;
Get_C4 (Codec, "Codec");
#if MEDIAINFO_TRACE
if (Trace_Activated)
Param("Data", Ztring("(")+Ztring::ToZtring(Element_TotalSize_Get()-Element_Offset)+Ztring(" bytes)"));
#endif //MEDIAINFO_TRACE
Element_Offset=Element_TotalSize_Get(); //Not using Skip_XX() because we want to skip data we don't have, and Skip_XX() does a test on size of buffer
FILLING_BEGIN();
Stream_Prepare(Stream_Audio);
if (Codec==0x41647063) //Adpc
Fill(Stream_Audio, StreamPos_Last, Audio_Format, "ADPCM");
Fill(Stream_Audio, StreamPos_Last, Audio_StreamSize, Element_TotalSize_Get());
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::CDDA()
{
Element_Name("Compact Disc for Digital Audio");
//Filling
Accept("CDDA");
}
//---------------------------------------------------------------------------
void File_Riff::CDDA_fmt_()
{
//Specs: http://fr.wikipedia.org/wiki/Compact_Disc_Audio_track
//Specs: http://www.moon-soft.com/program/FORMAT/sound/cda.htm
Element_Name("Stream format");
//Parsing
int32u id;
int16u Version, tracknb=1;
int8u TPositionF=0, TPositionS=0, TPositionM=0, TDurationF=0, TDurationS=0, TDurationM=0;
Get_L2 (Version, "Version"); // Always 1
if (Version!=1)
{
//Not supported
Skip_XX(Element_Size-2, "Data");
return;
}
Get_L2 (tracknb, "Number"); // Start at 1
Get_L4 (id, "id");
Skip_L4( "offset"); // in frames //Priority of FSM format
Skip_L4( "Duration"); // in frames //Priority of FSM format
Get_L1 (TPositionF, "Track_PositionF"); // in frames
Get_L1 (TPositionS, "Track_PositionS"); // in seconds
Get_L1 (TPositionM, "Track_PositionM"); // in minutes
Skip_L1( "empty");
Get_L1 (TDurationF, "Track_DurationF"); // in frames
Get_L1 (TDurationS, "Track_DurationS"); // in seconds
Get_L1 (TDurationM, "Track_DurationM"); // in minutes
Skip_L1( "empty");
FILLING_BEGIN();
int32u TPosition=TPositionM*60*75+TPositionS*75+TPositionF;
int32u TDuration=TDurationM*60*75+TDurationS*75+TDurationF;
Fill(Stream_General, 0, General_Track_Position, tracknb);
Fill(Stream_General, 0, General_Format, "CDDA");
Fill(Stream_General, 0, General_Format_Info, "Compact Disc for Digital Audio");
Fill(Stream_General, 0, General_UniqueID, id);
Fill(Stream_General, 0, General_FileSize, File_Size+TDuration*2352, 10, true);
Stream_Prepare(Stream_Audio);
Fill(Stream_Audio, 0, Audio_Format, "PCM");
Fill(Stream_Audio, 0, Audio_Format_Settings_Endianness, "Little");
Fill(Stream_Audio, 0, Audio_BitDepth, 16);
Fill(Stream_Audio, 0, Audio_Channel_s_, 2);
Fill(Stream_Audio, 0, Audio_SamplingRate, 44100);
Fill(Stream_Audio, 0, Audio_FrameRate, (float)75);
Fill(Stream_Audio, 0, Audio_BitRate, 1411200);
Fill(Stream_Audio, 0, Audio_Compression_Mode, "Lossless");
Fill(Stream_Audio, 0, Audio_FrameCount, TDuration);
Fill(Stream_Audio, 0, Audio_Duration, float32_int32s(((float32)TDuration)*1000/75));
Fill(Stream_Audio, 0, Audio_Delay, float32_int32s(((float32)TPosition)*1000/75));
//No more need data
Finish("CDDA");
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::CMJP()
{
Element_Name("CMP4 - JPEG");
//Parsing
#ifdef MEDIAINFO_JPEG_YES
Stream_ID=0;
File_Jpeg* Parser=new File_Jpeg;
Open_Buffer_Init(Parser);
Parser->StreamKind=Stream_Video;
Open_Buffer_Continue(Parser);
Element_Offset=Element_TotalSize_Get(); //Not using Skip_XX() because we want to skip data we don't have, and Skip_XX() does a test on size of buffer
FILLING_BEGIN();
Stream_Prepare(Stream_Video);
Fill(Stream_Video, StreamPos_Last, Video_StreamSize, Element_TotalSize_Get());
Finish(Parser);
Merge(*Parser, StreamKind_Last, 0, StreamPos_Last);
FILLING_END();
Stream[Stream_ID].Parsers.push_back(Parser);
#else
Element_Offset=Element_TotalSize_Get(); //Not using Skip_XX() because we want to skip data we don't have, and Skip_XX() does a test on size of buffer
FILLING_BEGIN();
Stream_Prepare(Stream_Video);
Fill(Stream_Video, StreamPos_Last, Video_Format, "JPEG");
Fill(Stream_Video, StreamPos_Last, Video_StreamSize, Element_TotalSize_Get());
FILLING_END();
#endif
}
//---------------------------------------------------------------------------
void File_Riff::CMP4()
{
Accept("CMP4");
Element_Name("CMP4 - Header");
//Parsing
Ztring Title;
Get_Local(Element_Size, Title, "Title");
FILLING_BEGIN();
Fill(Stream_General, 0, General_Format, "CMP4");
Fill(Stream_General, 0, "Title", Title);
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::IDVX()
{
Element_Name("Tags");
}
//---------------------------------------------------------------------------
void File_Riff::INDX()
{
Element_Name("Index (from which spec?)");
}
//---------------------------------------------------------------------------
void File_Riff::INDX_xxxx()
{
Stream_ID=(int32u)(Element_Code&0xFFFF0000);
if (Stream_ID==0x69780000) //ix..
{
//Index
int32u Entry_Count, ChunkId;
int16u LongsPerEntry;
int8u IndexType, IndexSubType;
Get_L2 (LongsPerEntry, "LongsPerEntry"); //Size of each entry in aIndex array
Get_L1 (IndexSubType, "IndexSubType");
Get_L1 (IndexType, "IndexType");
Get_L4 (Entry_Count, "EntriesInUse"); //Index of first unused member in aIndex array
Get_C4 (ChunkId, "ChunkId"); //FCC of what is indexed
Skip_L4( "Unknown");
Skip_L4( "Unknown");
Skip_L4( "Unknown");
for (int32u Pos=0; Pos<Entry_Count; Pos++)
{
Skip_L8( "Offset");
Skip_L4( "Size");
Skip_L4( "Frame number?");
Skip_L4( "Frame number?");
Skip_L4( "Zero");
}
}
//Currently, we do not use the index
//TODO: use the index
Stream_Structure.clear();
}
//---------------------------------------------------------------------------
void File_Riff::JUNK()
{
Element_Name("Junk");
//Parse
#if MEDIAINFO_TRACE
if (Trace_Activated)
Param("Junk", Ztring("(")+Ztring::ToZtring(Element_TotalSize_Get())+Ztring(" bytes)"));
#endif //MEDIAINFO_TRACE
Element_Offset=Element_TotalSize_Get(); //Not using Skip_XX() because we want to skip data we don't have, and Skip_XX() does a test on size of buffer
}
//---------------------------------------------------------------------------
void File_Riff::menu()
{
Element_Name("DivX Menu");
//Filling
Stream_Prepare(Stream_Menu);
Fill(Stream_Menu, StreamPos_Last, Menu_Format, "DivX Menu");
Fill(Stream_Menu, StreamPos_Last, Menu_Codec, "DivX");
}
//---------------------------------------------------------------------------
void File_Riff::MThd()
{
Element_Name("MIDI header");
//Parsing
Skip_B2( "format");
Skip_B2( "ntrks");
Skip_B2( "division");
FILLING_BEGIN_PRECISE();
Accept("MIDI");
Fill(Stream_General, 0, General_Format, "MIDI");
FILLING_ELSE();
Reject("MIDI");
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::MTrk()
{
Element_Name("MIDI Track");
//Parsing
#if MEDIAINFO_TRACE
if (Trace_Activated)
Param("Data", Ztring("(")+Ztring::ToZtring(Element_TotalSize_Get())+Ztring(" bytes)"));
#endif //MEDIAINFO_TRACE
Element_Offset=Element_TotalSize_Get(); //Not using Skip_XX() because we want to skip data we don't have, and Skip_XX() does a test on size of buffer
FILLING_BEGIN();
Stream_Prepare(Stream_Audio);
Fill(Stream_Audio, StreamPos_Last, Audio_Format, "MIDI");
Fill(Stream_Audio, StreamPos_Last, Audio_Codec, "Midi");
Finish("MIDI");
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::PAL_()
{
Data_Accept("RIFF Palette");
Element_Name("RIFF Palette");
//Filling
Fill(Stream_General, 0, General_Format, "RIFF Palette");
}
//---------------------------------------------------------------------------
void File_Riff::QLCM()
{
Data_Accept("QLCM");
Element_Name("QLCM");
//Filling
Fill(Stream_General, 0, General_Format, "QLCM");
}
//---------------------------------------------------------------------------
void File_Riff::QLCM_fmt_()
{
//Parsing
Ztring codec_name;
int128u codec_guid;
int32u num_rates;
int16u codec_version, average_bps, packet_size, block_size, sampling_rate, sample_size;
int8u major, minor;
Get_L1 (major, "major");
Get_L1 (minor, "minor");
Get_GUID(codec_guid, "codec-guid");
Get_L2 (codec_version, "codec-version");
Get_Local(80, codec_name, "codec-name");
Get_L2 (average_bps, "average-bps");
Get_L2 (packet_size, "packet-size");
Get_L2 (block_size, "block-size");
Get_L2 (sampling_rate, "sampling-rate");
Get_L2 (sample_size, "sample-size");
Element_Begin1("rate-map-table");
Get_L4 (num_rates, "num-rates");
for (int32u rate=0; rate<num_rates; rate++)
{
Skip_L2( "rate-size");
Skip_L2( "rate-octet");
}
Element_End0();
Skip_L4( "Reserved");
Skip_L4( "Reserved");
Skip_L4( "Reserved");
Skip_L4( "Reserved");
if (Element_Offset<Element_Size)
Skip_L4( "Reserved"); //Some files don't have the 5th reserved dword
FILLING_BEGIN_PRECISE();
Stream_Prepare (Stream_Audio);
switch (codec_guid.hi)
{
case Elements::QLCM_QCELP1 :
case Elements::QLCM_QCELP2 : Fill(Stream_Audio, 0, Audio_Format, "QCELP"); Fill(Stream_Audio, 0, Audio_Codec, "QCELP"); break;
case Elements::QLCM_EVRC : Fill(Stream_Audio, 0, Audio_Format, "EVRC"); Fill(Stream_Audio, 0, Audio_Codec, "EVRC"); break;
case Elements::QLCM_SMV : Fill(Stream_Audio, 0, Audio_Format, "SMV"); Fill(Stream_Audio, 0, Audio_Codec, "SMV"); break;
default : ;
}
Fill(Stream_Audio, 0, Audio_BitRate, average_bps);
Fill(Stream_Audio, 0, Audio_SamplingRate, sampling_rate);
Fill(Stream_Audio, 0, Audio_BitDepth, sample_size);
Fill(Stream_Audio, 0, Audio_Channel_s_, 1);
FILLING_END();
}
#if defined(MEDIAINFO_GXF_YES)
//---------------------------------------------------------------------------
void File_Riff::rcrd()
{
Data_Accept("Ancillary media packets");
Element_Name("Ancillary media packets");
//Filling
if (Retrieve(Stream_General, 0, General_Format).empty())
Fill(Stream_General, 0, General_Format, "Ancillary media packets"); //GXF, RDD14-2007
//Clearing old data
if (Ancillary)
{
(*Ancillary)->FrameInfo.DTS=FrameInfo.DTS;
Open_Buffer_Continue(*Ancillary, Buffer, 0);
}
}
//---------------------------------------------------------------------------
void File_Riff::rcrd_desc()
{
Element_Name("Ancillary media packet description");
//Parsing
int32u Version;
Get_L4 (Version, "Version");
if (Version==2)
{
Skip_L4( "Number of fields");
Skip_L4( "Length of the ancillary data field descriptions");
Skip_L4( "Byte size of the complete ancillary media packet");
Skip_L4( "Format of the video");
}
else
Skip_XX(Element_Size-Element_Offset, "Unknown");
}
//---------------------------------------------------------------------------
void File_Riff::rcrd_fld_()
{
Element_Name("Ancillary data field description");
}
//---------------------------------------------------------------------------
void File_Riff::rcrd_fld__anc_()
{
Element_Name("Ancillary data sample description");
rcrd_fld__anc__pos__LineNumber=(int32u)-1;
}
//---------------------------------------------------------------------------
void File_Riff::rcrd_fld__anc__pos_()
{
Element_Name("Ancillary data sample description");
//Parsing
Get_L4 (rcrd_fld__anc__pos__LineNumber, "Video line number");
Skip_L4( "Ancillary video color difference or luma space");
Skip_L4( "Ancillary video space");
}
//---------------------------------------------------------------------------
void File_Riff::rcrd_fld__anc__pyld()
{
Element_Name("Ancillary data sample payload");
if (Ancillary)
{
(*Ancillary)->FrameInfo.DTS=FrameInfo.DTS;
(*Ancillary)->LineNumber=rcrd_fld__anc__pos__LineNumber;
Open_Buffer_Continue(*Ancillary);
}
}
//---------------------------------------------------------------------------
void File_Riff::rcrd_fld__finf()
{
Element_Name("Data field description");
//Parsing
Skip_L4( "Video field identifier");
}
#endif //MEDIAINFO_GXF_YES
//---------------------------------------------------------------------------
void File_Riff::RDIB()
{
Data_Accept("RIFF DIB");
Element_Name("RIFF DIB");
//Filling
Fill(Stream_General, 0, General_Format, "RIFF DIB");
}
//---------------------------------------------------------------------------
void File_Riff::RMID()
{
Data_Accept("RIFF MIDI");
Element_Name("RIFF MIDI");
//Filling
Fill(Stream_General, 0, General_Format, "RIFF MIDI");
}
//---------------------------------------------------------------------------
void File_Riff::RMMP()
{
Data_Accept("RIFF MMP");
Element_Name("RIFF MMP");
//Filling
Fill(Stream_General, 0, General_Format, "RIFF MMP");
}
//---------------------------------------------------------------------------
void File_Riff::RMP3()
{
Data_Accept("RMP3");
Element_Name("RMP3");
//Filling
Fill(Stream_General, 0, General_Format, "RMP3");
Kind=Kind_Rmp3;
}
//---------------------------------------------------------------------------
void File_Riff::RMP3_data()
{
Element_Name("Raw datas");
Fill(Stream_Audio, 0, Audio_StreamSize, Buffer_DataToParse_End-Buffer_DataToParse_Begin);
Stream_Prepare(Stream_Audio);
//Creating parser
#if defined(MEDIAINFO_MPEGA_YES)
File_Mpega* Parser=new File_Mpega;
Parser->CalculateDelay=true;
Parser->ShouldContinueParsing=true;
Open_Buffer_Init(Parser);
stream& StreamItem=Stream[(int32u)-1];
StreamItem.StreamKind=Stream_Audio;
StreamItem.StreamPos=0;
StreamItem.Parsers.push_back(Parser);
#else //MEDIAINFO_MPEG4_YES
Fill(Stream_Audio, 0, Audio_Format, "MPEG Audio");
Skip_XX(Buffer_DataToParse_End-Buffer_DataToParse_Begin, "Data");
#endif
}
//---------------------------------------------------------------------------
void File_Riff::RMP3_data_Continue()
{
#if MEDIAINFO_DEMUX
if (Element_Size)
{
Demux_random_access=true;
Demux(Buffer+Buffer_Offset, (size_t)Element_Size, ContentType_MainStream);
}
#endif //MEDIAINFO_DEMUX
Element_Code=(int64u)-1;
AVI__movi_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::SMV0()
{
Accept("SMV");
//Parsing
int8u Version;
Skip_C1( "Identifier (continuing)");
Get_C1 (Version, "Version");
Skip_C3( "Identifier (continuing)");
if (Version=='1')
{
int32u Width, Height, FrameRate, BlockSize, FrameCount;
Get_B3 (Width, "Width");
Get_B3 (Height, "Height");
Skip_B3( "0x000010");
Skip_B3( "0x000001");
Get_B3 (BlockSize, "Block size");
Get_B3 (FrameRate, "Frame rate");
Get_B3 (FrameCount, "Frame count");
Skip_B3( "0x000000");
Skip_B3( "0x000000");
Skip_B3( "0x000000");
Skip_B3( "0x010101");
Skip_B3( "0x010101");
Skip_B3( "0x010101");
Skip_B3( "0x010101");
//Filling
Fill(Stream_General, 0, General_Format_Profile, "SMV v1");
Stream_Prepare(Stream_Video);
Fill(Stream_Video, 0, Video_MuxingMode, "SMV v1");
Fill(Stream_Video, 0, Video_Width, Width);
Fill(Stream_Video, 0, Video_Height, Height);
Fill(Stream_Video, 0, Video_FrameRate, (float)FrameRate);
Fill(Stream_Video, 0, Video_FrameCount, FrameCount);
Finish("SMV");
}
else if (Version=='2')
{
int32u Width, Height, FrameRate;
Get_L3 (Width, "Width");
Get_L3 (Height, "Height");
Skip_L3( "0x000010");
Skip_L3( "0x000001");
Get_L3 (SMV_BlockSize, "Block size");
Get_L3 (FrameRate, "Frame rate");
Get_L3 (SMV_FrameCount, "Frame count");
Skip_L3( "0x000001");
Skip_L3( "0x000000");
Skip_L3( "Frame rate");
Skip_L3( "0x010101");
Skip_L3( "0x010101");
Skip_L3( "0x010101");
Skip_L3( "0x010101");
//Filling
SMV_BlockSize+=3;
SMV_FrameCount++;
Fill(Stream_General, 0, General_Format_Profile, "SMV v2");
Stream_Prepare(Stream_Video);
Fill(Stream_Video, 0, Video_Format, "JPEG");
Fill(Stream_Video, 0, Video_Codec, "JPEG");
Fill(Stream_Video, 0, Video_MuxingMode, "SMV v2");
Fill(Stream_Video, 0, Video_Width, Width);
Fill(Stream_Video, 0, Video_Height, Height);
Fill(Stream_Video, 0, Video_FrameRate, FrameRate);
Fill(Stream_Video, 0, Video_FrameCount, SMV_FrameCount);
Fill(Stream_Video, 0, Video_StreamSize, SMV_BlockSize*SMV_FrameCount);
}
else
Finish("SMV");
}
//---------------------------------------------------------------------------
void File_Riff::SMV0_xxxx()
{
//Parsing
int32u Size;
Get_L3 (Size, "Size");
#if defined(MEDIAINFO_JPEG_YES)
//Creating the parser
File_Jpeg MI;
Open_Buffer_Init(&MI);
//Parsing
Open_Buffer_Continue(&MI, Size);
//Filling
Finish(&MI);
Merge(MI, Stream_Video, 0, StreamPos_Last);
//Positioning
Element_Offset+=Size;
#else
//Parsing
Skip_XX(Size, "JPEG data");
#endif
Skip_XX(Element_Size-Element_Offset, "Padding");
//Filling
#if MEDIAINFO_HASH
if (Config->File_Hash_Get().to_ulong())
Element_Offset=Element_Size+(SMV_FrameCount-1)*SMV_BlockSize;
#endif //MEDIAINFO_HASH
Data_GoTo(File_Offset+Buffer_Offset+(size_t)Element_Size+(SMV_FrameCount-1)*SMV_BlockSize, "SMV");
SMV_BlockSize=0;
}
//---------------------------------------------------------------------------
void File_Riff::WAVE()
{
Data_Accept("Wave");
Element_Name("Wave");
//Filling
Fill(Stream_General, 0, General_Format, "Wave");
Kind=Kind_Wave;
#if MEDIAINFO_EVENTS
StreamIDs_Width[0]=0;
#endif //MEDIAINFO_EVENTS
}
//---------------------------------------------------------------------------
void File_Riff::WAVE__pmx()
{
Element_Name("XMP");
//Parsing
Ztring XML_Data;
Get_Local(Element_Size, XML_Data, "XML data");
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_aXML()
{
Element_Name("aXML");
//Parsing
Skip_Local(Element_Size, "XML data");
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_bext()
{
Element_Name("Broadcast extension");
//Parsing
Ztring Description, Originator, OriginatorReference, OriginationDate, OriginationTime, History;
int16u Version;
Get_Local(256, Description, "Description");
Get_Local( 32, Originator, "Originator");
Get_Local( 32, OriginatorReference, "OriginatorReference");
Get_Local( 10, OriginationDate, "OriginationDate");
Get_Local( 8, OriginationTime, "OriginationTime");
Get_L8 ( TimeReference, "TimeReference"); //To be divided by SamplesPerSec
Get_L2 ( Version, "Version");
if (Version==1)
Skip_UUID( "UMID");
Skip_XX (602-Element_Offset, "Reserved");
if (Element_Offset<Element_Size)
Get_Local(Element_Size-Element_Offset, History, "History");
FILLING_BEGIN();
Fill(Stream_General, 0, General_Description, Description);
Fill(Stream_General, 0, General_Producer, Originator);
Fill(Stream_General, 0, "Producer_Reference", OriginatorReference);
Fill(Stream_General, 0, General_Encoded_Date, OriginationDate+__T(' ')+OriginationTime);
Fill(Stream_General, 0, General_Encoded_Library_Settings, History);
if (SamplesPerSec && TimeReference!=(int64u)-1)
{
Fill(Stream_Audio, 0, Audio_Delay, float64_int64s(((float64)TimeReference)*1000/SamplesPerSec));
Fill(Stream_Audio, 0, Audio_Delay_Source, "Container (bext)");
}
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_cue_()
{
Element_Name("Cue points");
//Parsing
int32u numCuePoints;
Get_L4(numCuePoints, "numCuePoints");
for (int32u Pos=0; Pos<numCuePoints; Pos++)
{
Element_Begin1("Cue point");
Skip_L4( "ID");
Skip_L4( "Position");
Skip_C4( "DataChunkID");
Skip_L4( "ChunkStart");
Skip_L4( "BlockStart");
Skip_L4( "SampleOffset");
Element_End0();
}
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_data()
{
Element_Name("Raw datas");
if (Buffer_DataToParse_End-Buffer_DataToParse_Begin<100)
{
Skip_XX(Buffer_DataToParse_End-Buffer_Offset, "Unknown");
return; //This is maybe embeded in another container, and there is only the header (What is the junk?)
}
FILLING_BEGIN();
Fill(Stream_Audio, 0, Audio_StreamSize, Buffer_DataToParse_End-Buffer_DataToParse_Begin);
FILLING_END();
//Parsing
Element_Code=(int64u)-1;
FILLING_BEGIN();
int64u Duration=Retrieve(Stream_Audio, 0, Audio_Duration).To_int64u();
int64u BitRate=Retrieve(Stream_Audio, 0, Audio_BitRate).To_int64u();
if (Duration)
{
int64u BitRate_New=(Buffer_DataToParse_End-Buffer_DataToParse_Begin)*8*1000/Duration;
if (BitRate_New<BitRate*0.95 || BitRate_New>BitRate*1.05)
Fill(Stream_Audio, 0, Audio_BitRate, BitRate_New, 10, true); //Correcting the bitrate, it was false in the header
}
else if (BitRate)
{
if (IsSub)
//Retrieving "data" real size, in case of truncated files and/or wave header in another container
Duration=((int64u)LittleEndian2int32u(Buffer+Buffer_Offset-4))*8*1000/BitRate; //TODO: RF64 is not handled
else
Duration=(Buffer_DataToParse_End-Buffer_DataToParse_Begin)*8*1000/BitRate;
Fill(Stream_General, 0, General_Duration, Duration, 10, true);
Fill(Stream_Audio, 0, Audio_Duration, Duration, 10, true);
}
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_data_Continue()
{
#if MEDIAINFO_DEMUX
Element_Code=(int64u)-1;
if (AvgBytesPerSec && Demux_Rate)
{
FrameInfo.DTS=float64_int64s((File_Offset+Buffer_Offset-Buffer_DataToParse_Begin)*1000000000.0/AvgBytesPerSec);
FrameInfo.PTS=FrameInfo.DTS;
Frame_Count_NotParsedIncluded=float64_int64s(((float64)FrameInfo.DTS)/1000000000.0*Demux_Rate);
}
Demux_random_access=true;
Demux(Buffer+Buffer_Offset, (size_t)Element_Size, ContentType_MainStream);
Frame_Count_NotParsedIncluded=(int64u)-1;
#endif //MEDIAINFO_DEMUX
Element_Code=(int64u)-1;
AVI__movi_xxxx();
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_ds64()
{
Element_Name("DataSize64");
//Parsing
int32u tableLength;
Skip_L8( "riffSize"); //Is directly read from the header parser
Get_L8 (WAVE_data_Size, "dataSize");
Get_L8 (WAVE_fact_samplesCount, "sampleCount");
Get_L4 (tableLength, "tableLength");
for (int32u Pos=0; Pos<tableLength; Pos++)
Skip_L8( "table[]");
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_fact()
{
Element_Name("Sample count");
//Parsing
int64u SamplesCount64;
int32u SamplesCount;
Get_L4 (SamplesCount, "SamplesCount");
SamplesCount64=SamplesCount;
if (SamplesCount64==0xFFFFFFFF)
SamplesCount64=WAVE_fact_samplesCount;
FILLING_BEGIN();
int32u SamplingRate=Retrieve(Stream_Audio, 0, Audio_SamplingRate).To_int32u();
if (SamplingRate)
{
//Calculating
int64u Duration=(SamplesCount64*1000)/SamplingRate;
//Coherency test
bool IsOK=true;
if (File_Size!=(int64u)-1)
{
int64u BitRate=Retrieve(Stream_Audio, 0, Audio_BitRate).To_int64u();
if (BitRate)
{
int64u Duration_FromBitRate = File_Size * 8 * 1000 / BitRate;
if (Duration_FromBitRate > Duration*1.10 || Duration_FromBitRate < Duration*0.9)
IsOK = false;
}
}
//Filling
if (IsOK)
Fill(Stream_Audio, 0, Audio_Duration, Duration);
}
FILLING_END();
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_fmt_()
{
//Compute the current codec ID
Element_Code=(int64u)-1;
Stream_ID=(int32u)-1;
stream_Count=1;
Stream[(int32u)-1].fccType=Elements::AVI__hdlr_strl_strh_auds;
AVI__hdlr_strl_strf();
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_ID3_()
{
Element_Name("ID3v2 tags");
//Parsing
#if defined(MEDIAINFO_ID3V2_YES)
File_Id3v2 MI;
Open_Buffer_Init(&MI);
Open_Buffer_Continue(&MI);
Finish(&MI);
Merge(MI, Stream_General, 0, 0);
#endif
}
//---------------------------------------------------------------------------
void File_Riff::WAVE_iXML()
{
Element_Name("iXML");
//Parsing
Skip_Local(Element_Size, "XML data");
}
//---------------------------------------------------------------------------
void File_Riff::wave()
{
Data_Accept("Wave64");
Element_Name("Wave64");
//Filling
Fill(Stream_General, 0, General_Format, "Wave64");
}
//---------------------------------------------------------------------------
void File_Riff::W3DI()
{
Element_Name("IDVX tags (Out of specs!)");
//Parsing
int32u Size=(int32u)Element_Size;
Ztring Title, Artist, Album, Unknown, Genre, Comment;
int32u TrackPos;
Get_Local(Size, Title, "Title");
Element_Offset=(int32u)Title.size();
Size-=(int32u)Title.size();
if (Size==0) return;
Skip_L1( "Zero"); Size--; //NULL char
Get_Local(Size, Artist, "Artist");
Element_Offset=(int32u)Title.size()+1+(int32u)Artist.size();
Size-=(int32u)Artist.size();
if (Size==0) return;
Skip_L1( "Zero"); Size--; //NULL char
Get_Local(Size, Album, "Album");
Element_Offset=(int32u)Title.size()+1+(int32u)Artist.size()+1+(int32u)Album.size();
Size-=(int32u)Album.size();
if (Size==0) return;
Skip_L1( "Zero"); Size--; //NULL char
Get_Local(Size, Unknown, "Unknown");
Element_Offset=(int32u)Title.size()+1+(int32u)Artist.size()+1+(int32u)Album.size()+1+(int32u)Unknown.size();
Size-=(int32u)Unknown.size();
if (Size==0) return;
Skip_L1( "Zero"); Size--; //NULL char
Get_Local(Size, Genre, "Genre");
Element_Offset=(int32u)Title.size()+1+(int32u)Artist.size()+1+(int32u)Album.size()+1+(int32u)Unknown.size()+1+(int32u)Genre.size();
Size-=(int32u)Genre.size();
if (Size==0) return;
Skip_L1( "Zero"); Size--; //NULL char
Get_Local(Size, Comment, "Comment");
Element_Offset=(int32u)Title.size()+1+(int32u)Artist.size()+1+(int32u)Album.size()+1+(int32u)Unknown.size()+1+(int32u)Genre.size()+1+(int32u)Comment.size();
Size-=(int32u)Comment.size();
if (Size==0) return;
Skip_L1( "Zero"); Size--; //NULL char
Get_L4 (TrackPos, "Track_Position");
if(Element_Offset+8<Element_Size)
Skip_XX(Element_Size-Element_Offset, "Unknown");
Element_Begin1("Footer");
Skip_L4( "Size");
Skip_C4( "Name");
Element_End0();
//Filling
Fill(Stream_General, 0, General_Track, Title);
Fill(Stream_General, 0, General_Performer, Artist);
Fill(Stream_General, 0, General_Album, Album);
Fill(Stream_General, 0, "Unknown", Unknown);
Fill(Stream_General, 0, General_Genre, Genre);
Fill(Stream_General, 0, General_Comment, Comment);
Fill(Stream_General, 0, General_Track_Position, TrackPos);
}
void File_Riff::Open_Buffer_Init_All()
{
stream& StreamItem = Stream[Stream_ID];
for (size_t Pos = 0; Pos<StreamItem.Parsers.size(); Pos++)
Open_Buffer_Init(StreamItem.Parsers[Pos]);
}
//***************************************************************************
// C++
//***************************************************************************
} //NameSpace
#endif //MEDIAINFO_RIFF_YES
| pavel-pimenov/sandbox | mediainfo/MediaInfoLib/Source/MediaInfo/Multiple/File_Riff_Elements.cpp | C++ | mit | 147,762 |
///////////////////////////////////////////////////////////////////////////////
//
// (C) Autodesk, Inc. 2007-2011. All rights reserved.
//
// Permission to use, copy, modify, and distribute this software in
// object code form for any purpose and without fee is hereby granted,
// provided that the above copyright notice appears in all copies and
// that both that copyright notice and the limited warranty and
// restricted rights notice below appear in all supporting
// documentation.
//
// AUTODESK PROVIDES THIS PROGRAM "AS IS" AND WITH ALL FAULTS.
// AUTODESK SPECIFICALLY DISCLAIMS ANY IMPLIED WARRANTY OF
// MERCHANTABILITY OR FITNESS FOR A PARTICULAR USE. AUTODESK, INC.
// DOES NOT WARRANT THAT THE OPERATION OF THE PROGRAM WILL BE
// UNINTERRUPTED OR ERROR FREE.
//
// Use, duplication, or disclosure by the U.S. Government is subject to
// restrictions set forth in FAR 52.227-19 (Commercial Computer
// Software - Restricted Rights) and DFAR 252.227-7013(c)(1)(ii)
// (Rights in Technical Data and Computer Software), as applicable.
//
///////////////////////////////////////////////////////////////////////////////
#ifndef ATILDEFS_H
#include "AtilDefs.h"
#endif
#ifndef FORMATCODECPROPERTYINTERFACE_H
#include "FormatCodecPropertyInterface.h"
#endif
#ifndef FORMATCODECINCLUSIVEPROPERTYSETINTERFACE_H
#define FORMATCODECINCLUSIVEPROPERTYSETINTERFACE_H
#if __GNUC__ >= 4
#pragma GCC visibility push(default)
#endif
namespace Atil
{
/// <summary>
/// This class groups properties into a set of properties much
/// like a "structure" in "c".
/// </summary>
///
class FormatCodecInclusivePropertySetInterface : public FormatCodecPropertyInterface
{
public:
/// <summary>
/// The virtual destructor.
/// </summary>
///
virtual ~FormatCodecInclusivePropertySetInterface ();
/// <summary>
/// This returns the number of properties in the set.
/// </summary>
///
/// <returns>
/// The number of properties in the set.
/// </returns>
///
virtual int getNumProperties () const;
/// <summary>
/// This returns a selected property from the properties in the set.
/// </summary>
///
/// <param name="nIndex">
/// The index of the property to that is returned.
/// </param>
///
/// <returns>
/// A pointer to the property at the index. This pointer should not be freed by the caller.
/// </returns>
///
virtual FormatCodecPropertyInterface* getProperty (int nIndex) const;
protected:
/// <summary>
/// (Protected) Copy constructor.
/// </summary>
///
/// <param name="iter">
/// The instance to be copied.
/// </param>
///
FormatCodecInclusivePropertySetInterface (const FormatCodecInclusivePropertySetInterface& iter);
/// <summary>
/// (Protected) The simple constructor.
/// </summary>
///
FormatCodecInclusivePropertySetInterface ();
/// <summary>
/// (Protected) The pre-allocating constructor.
/// </summary>
///
/// <param name="nNumToAlloc">
/// The number of properties that will be in the set.
/// </param>
///
FormatCodecInclusivePropertySetInterface (int nNumToAlloc);
/// <summary>
/// (Protected) This sets the property at index in the set.
/// </summary>
///
/// <param name="nIndex">
/// The index of the property that is to be set.
/// </param>
///
/// <param name="pProperty">
/// A pointer to the property that should be placed at the index. The
/// property will be <c>clone()</c> copied. The pointer is not held by the set.
/// </param>
///
void setProperty (int nIndex, FormatCodecPropertyInterface* pProperty);
/// <summary>
/// (Protected) This adds the property to the set.
/// </summary>
///
/// <param name="pProperty">
/// A pointer to the property that should be placed at the index. The
/// property will be <c>clone()</c> copied. The pointer is not held by the set.
/// </param>
///
void appendProperty (FormatCodecPropertyInterface* pProperty);
/// <summary>
/// (Protected) The array of property pointers held by the set.
/// </summary>
///
FormatCodecPropertyInterface** mppProperties;
/// <summary>
/// (Protected) The number of properties that have been set into the set.
/// </summary>
int mnNumProperties;
/// <summary>
/// (Protected) The length of the property array.
/// </summary>
int mnArraySize;
private:
FormatCodecInclusivePropertySetInterface& operator= (const FormatCodecInclusivePropertySetInterface& from);
};
} // end of namespace Atil
#if __GNUC__ >= 4
#pragma GCC visibility pop
#endif
#endif
| kevinzhwl/ObjectARXCore | 2013/utils/Atil/Inc/codec_properties/FormatCodecInclusivePropertySetInterface.h | C | mit | 4,904 |
'use strict';
// Configuring the Articles module
angular.module('about').run(['Menus',
function(Menus) {
// Set top bar menu items
Menus.addMenuItem('mainmenu', 'About Us', 'about', 'left-margin', '/about-us', true, null, 3);
}
]);
| spiridonov-oa/people-ma | public/modules/about/config/about.client.config.js | JavaScript | mit | 257 |
package cwr;
import java.util.ArrayList;
public class RobotBite
{
//0 = time [state]
//1 = x [state]
//2 = y [state]
//3 = energy [state]
//4 = bearing radians [relative position]
//5 = distance [relative position]
//6 = heading radians [travel]
//7 = velocity [travel]
String name;
long cTime;
double cx;
double cy;
cwruBase origin;
double cEnergy;
double cBearing_radians;
double cDistance;
double cHeading_radians;
double cVelocity;
ArrayList<Projection> projec; //forward projections for x
public RobotBite(String name, long time, cwruBase self,
double energy, double bearing_radians, double distance,
double heading_radians, double velocity)
{
this.name = name;
cTime = time;
origin = self;
cEnergy = energy;
cBearing_radians = bearing_radians;
double myBearing = self.getHeadingRadians();
//System.out.println("I'm going "+self.getHeadingRadians());
double adjust_bearing = (bearing_radians+myBearing)%(2*Math.PI);
//System.out.println("input bearing "+(bearing_radians));
//System.out.println("adjust bearing "+(adjust_bearing));
//System.out.println("math bearing"+(-adjust_bearing+Math.PI/2));
cDistance = distance;
cHeading_radians = heading_radians;
//System.out.println("location heading "+heading_radians);
cVelocity = velocity;
double myX = self.getX();
double myY = self.getY();
double math_bearing = (-adjust_bearing+Math.PI/2)%(2*Math.PI);
//double math_heading = (-heading_radians+Math.PI/2)%(2*Math.PI);
/*
* 0
* 90
* -90 180 0 90
* -90
* 180
*/
double dX = distance*Math.cos(math_bearing);
//System.out.println("location dx:" + dX);
double dY = distance*Math.sin(math_bearing);
//System.out.println("location dy:" + dY);
cx = myX+dX;
cy = myY+dY;
}
public void attachProjection(ArrayList<Projection> projList)
{
projec = projList;
}
}
| buckbaskin/RoboCodeGit | old_src/cwr/RobotBite.java | Java | mit | 1,934 |
export default function closest(n, arr) {
let i
let ndx
let diff
let best = Infinity
let low = 0
let high = arr.length - 1
while (low <= high) {
// eslint-disable-next-line no-bitwise
i = low + ((high - low) >> 1)
diff = arr[i] - n
if (diff < 0) {
low = i + 1
} else if (diff > 0) {
high = i - 1
}
diff = Math.abs(diff)
if (diff < best) {
best = diff
ndx = i
}
if (arr[i] === n) break
}
return arr[ndx]
}
| octopitus/rn-sliding-up-panel | libs/closest.js | JavaScript | mit | 493 |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearScript.Installer.Demo
{
public class Class1
{
}
}
| eswann/ClearScript.Installer | src/ClearScript.Installer.Demo/Class1.cs | C# | mit | 197 |
/**
* 斐波那契数列
*/
export default function fibonacci(n){
if(n <= 2){
return 1;
}
let n1 = 1, n2 = 1, sn = 0;
for(let i = 0; i < n - 2; i ++){
sn = n1 + n2;
n1 = n2;
n2 = sn;
}
return sn;
}
| lomocc/react-boilerplate | src/utils/fibonacci.js | JavaScript | mit | 230 |
import * as UTILS from '@utils';
const app = new WHS.App([
...UTILS.appModules({
position: new THREE.Vector3(0, 40, 70)
})
]);
const halfMat = {
transparent: true,
opacity: 0.5
};
const box = new WHS.Box({
geometry: {
width: 30,
height: 2,
depth: 2
},
modules: [
new PHYSICS.BoxModule({
mass: 0
})
],
material: new THREE.MeshPhongMaterial({
color: UTILS.$colors.mesh,
...halfMat
}),
position: {
y: 40
}
});
const box2 = new WHS.Box({
geometry: {
width: 30,
height: 1,
depth: 20
},
modules: [
new PHYSICS.BoxModule({
mass: 10,
damping: 0.1
})
],
material: new THREE.MeshPhongMaterial({
color: UTILS.$colors.softbody,
...halfMat
}),
position: {
y: 38,
z: 12
}
});
const pointer = new WHS.Sphere({
modules: [
new PHYSICS.SphereModule()
],
material: new THREE.MeshPhongMaterial({
color: UTILS.$colors.mesh
})
});
console.log(pointer);
pointer.position.set(0, 60, -8);
pointer.addTo(app);
box.addTo(app);
box2.addTo(app).then(() => {
const constraint = new PHYSICS.DOFConstraint(box2, box,
new THREE.Vector3(0, 38, 1)
);
app.addConstraint(constraint);
constraint.enableAngularMotor(10, 20);
});
UTILS.addPlane(app, 250);
UTILS.addBasicLights(app);
app.start();
| WhitestormJS/whs.js | examples/physics/Constraints/DofConstraint/script.js | JavaScript | mit | 1,332 |
package unit.com.bitdubai.fermat_dmp_plugin.layer.basic_wallet.bitcoin_wallet.developer.bitdubai.version_1.structure.BitcoinWalletBasicWalletAvailableBalance;
import com.bitdubai.fermat_api.layer.dmp_basic_wallet.common.exceptions.CantRegisterDebitException;
import com.bitdubai.fermat_api.layer.dmp_basic_wallet.bitcoin_wallet.interfaces.BitcoinWalletTransactionRecord;
import com.bitdubai.fermat_api.layer.osa_android.database_system.Database;
import com.bitdubai.fermat_api.layer.osa_android.database_system.DatabaseTable;
import com.bitdubai.fermat_api.layer.osa_android.database_system.DatabaseTableRecord;
import com.bitdubai.fermat_api.layer.osa_android.database_system.DatabaseTransaction;
import com.bitdubai.fermat_api.layer.osa_android.database_system.PluginDatabaseSystem;
import com.bitdubai.fermat_api.layer.osa_android.database_system.exceptions.CantLoadTableToMemoryException;
import com.bitdubai.fermat_api.layer.osa_android.database_system.exceptions.CantOpenDatabaseException;
import com.bitdubai.fermat_api.layer.osa_android.database_system.exceptions.DatabaseNotFoundException;
import com.bitdubai.fermat_pip_api.layer.pip_platform_service.error_manager.ErrorManager;
import com.bitdubai.fermat_dmp_plugin.layer.basic_wallet.bitcoin_wallet.developer.bitdubai.version_1.structure.BitcoinWalletBasicWalletAvailableBalance;
import com.bitdubai.fermat_dmp_plugin.layer.basic_wallet.bitcoin_wallet.developer.bitdubai.version_1.structure.BitcoinWalletDatabaseConstants;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.Mock;
import org.mockito.runners.MockitoJUnitRunner;
import java.util.ArrayList;
import java.util.List;
import unit.com.bitdubai.fermat_dmp_plugin.layer.basic_wallet.bitcoin_wallet.developer.bitdubai.version_1.structure.mocks.MockBitcoinWalletTransactionRecord;
import unit.com.bitdubai.fermat_dmp_plugin.layer.basic_wallet.bitcoin_wallet.developer.bitdubai.version_1.structure.mocks.MockDatabaseTableRecord;
import static com.googlecode.catchexception.CatchException.catchException;
import static com.googlecode.catchexception.CatchException.caughtException;
import static org.fest.assertions.api.Assertions.assertThat;
import static org.fest.assertions.api.Assertions.fail;
import static org.mockito.Mockito.doThrow;
import static org.mockito.Mockito.when;
/**
* Created by jorgegonzalez on 2015.07.14..
*/
@RunWith(MockitoJUnitRunner.class)
public class DebitTest {
@Mock
private ErrorManager mockErrorManager;
@Mock
private PluginDatabaseSystem mockPluginDatabaseSystem;
@Mock
private Database mockDatabase;
@Mock
private DatabaseTable mockWalletTable;
@Mock
private DatabaseTable mockBalanceTable;
@Mock
private DatabaseTransaction mockTransaction;
private List<DatabaseTableRecord> mockRecords;
private DatabaseTableRecord mockBalanceRecord;
private DatabaseTableRecord mockWalletRecord;
private BitcoinWalletTransactionRecord mockTransactionRecord;
private BitcoinWalletBasicWalletAvailableBalance testBalance;
@Before
public void setUpMocks(){
mockTransactionRecord = new MockBitcoinWalletTransactionRecord();
mockBalanceRecord = new MockDatabaseTableRecord();
mockWalletRecord = new MockDatabaseTableRecord();
mockRecords = new ArrayList<>();
mockRecords.add(mockBalanceRecord);
setUpMockitoRules();
}
public void setUpMockitoRules(){
when(mockDatabase.getTable(BitcoinWalletDatabaseConstants.BITCOIN_WALLET_TABLE_NAME)).thenReturn(mockWalletTable);
when(mockDatabase.getTable(BitcoinWalletDatabaseConstants.BITCOIN_WALLET_BALANCE_TABLE_NAME)).thenReturn(mockBalanceTable);
when(mockBalanceTable.getRecords()).thenReturn(mockRecords);
when(mockWalletTable.getEmptyRecord()).thenReturn(mockWalletRecord);
when(mockDatabase.newTransaction()).thenReturn(mockTransaction);
}
@Before
public void setUpAvailableBalance(){
testBalance = new BitcoinWalletBasicWalletAvailableBalance(mockDatabase);
}
@Test
public void Debit_SuccesfullyInvoked_ReturnsAvailableBalance() throws Exception{
catchException(testBalance).debit(mockTransactionRecord);
assertThat(caughtException()).isNull();
}
@Test
public void Debit_OpenDatabaseCantOpenDatabase_ReturnsAvailableBalance() throws Exception{
doThrow(new CantOpenDatabaseException("MOCK", null, null, null)).when(mockDatabase).openDatabase();
catchException(testBalance).debit(mockTransactionRecord);
assertThat(caughtException())
.isNotNull()
.isInstanceOf(CantRegisterDebitException.class);
}
@Test
public void Debit_OpenDatabaseDatabaseNotFound_Throws() throws Exception{
doThrow(new DatabaseNotFoundException("MOCK", null, null, null)).when(mockDatabase).openDatabase();
catchException(testBalance).debit(mockTransactionRecord);
assertThat(caughtException())
.isNotNull()
.isInstanceOf(CantRegisterDebitException.class);
}
@Test
public void Debit_DaoCantCalculateBalanceException_ReturnsAvailableBalance() throws Exception{
doThrow(new CantLoadTableToMemoryException("MOCK", null, null, null)).when(mockWalletTable).loadToMemory();
catchException(testBalance).debit(mockTransactionRecord);
assertThat(caughtException())
.isNotNull()
.isInstanceOf(CantRegisterDebitException.class);
}
}
| fvasquezjatar/fermat-unused | DMP/plugin/basic_wallet/fermat-dmp-plugin-basic-wallet-bitcoin-wallet-bitdubai/src/test/java/unit/com/bitdubai/fermat_dmp_plugin/layer/basic_wallet/bitcoin_wallet/developer/bitdubai/version_1/structure/BitcoinWalletBasicWalletAvailableBalance/DebitTest.java | Java | mit | 5,573 |
// ******************************************************************
//
// Copyright (c) Microsoft. All rights reserved.
// This code is licensed under the MIT License (MIT).
// THE CODE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
// IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
// TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH
// THE CODE OR THE USE OR OTHER DEALINGS IN THE CODE.
//
// ******************************************************************
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Text;
using Windows.Foundation;
namespace Microsoft.Toolkit.Uwp.Services.OAuth
{
/// <summary>
/// OAuth Uri extensions.
/// </summary>
internal static class OAuthUriExtensions
{
/// <summary>
/// Get query parameters from Uri.
/// </summary>
/// <param name="uri">Uri to process.</param>
/// <returns>Dictionary of query parameters.</returns>
public static IDictionary<string, string> GetQueryParams(this Uri uri)
{
return new WwwFormUrlDecoder(uri.Query).ToDictionary(decoderEntry => decoderEntry.Name, decoderEntry => decoderEntry.Value);
}
/// <summary>
/// Get absolute Uri.
/// </summary>
/// <param name="uri">Uri to process.</param>
/// <returns>Uri without query string.</returns>
public static string AbsoluteWithoutQuery(this Uri uri)
{
if (string.IsNullOrEmpty(uri.Query))
{
return uri.AbsoluteUri;
}
return uri.AbsoluteUri.Replace(uri.Query, string.Empty);
}
/// <summary>
/// Normalize the Uri into string.
/// </summary>
/// <param name="uri">Uri to process.</param>
/// <returns>Normalized string.</returns>
public static string Normalize(this Uri uri)
{
var result = new StringBuilder(string.Format(CultureInfo.InvariantCulture, "{0}://{1}", uri.Scheme, uri.Host));
if (!((uri.Scheme == "http" && uri.Port == 80) || (uri.Scheme == "https" && uri.Port == 443)))
{
result.Append(string.Concat(":", uri.Port));
}
result.Append(uri.AbsolutePath);
return result.ToString();
}
}
}
| phmatray/UWPCommunityToolkit | Microsoft.Toolkit.Uwp.Services/OAuth/OAuthUriExtensions.cs | C# | mit | 2,612 |
<html><body>
<h4>Windows 10 x64 (18363.900)</h4><br>
<h2>_OPENCOUNT_REASON</h2>
<font face="arial"> OpenCount_SkipLogging = 0n0<br>
OpenCount_AsyncRead = 0n1<br>
OpenCount_FlushCache = 0n2<br>
OpenCount_GetDirtyPage = 0n3<br>
OpenCount_GetFlushedVDL = 0n4<br>
OpenCount_InitCachemap1 = 0n5<br>
OpenCount_InitCachemap2 = 0n6<br>
OpenCount_InitCachemap3 = 0n7<br>
OpenCount_InitCachemap4 = 0n8<br>
OpenCount_InitCachemap5 = 0n9<br>
OpenCount_MdlWrite = 0n10<br>
OpenCount_MdlWriteAbort = 0n11<br>
OpenCount_NotifyMappedWrite = 0n12<br>
OpenCount_NotifyMappedWriteCompCallback = 0n13<br>
OpenCount_PurgeCache = 0n14<br>
OpenCount_PurgeCacheActiveViews = 0n15<br>
OpenCount_ReadAhead = 0n16<br>
OpenCount_SetFileSize = 0n17<br>
OpenCount_SetFileSizeSection = 0n18<br>
OpenCount_UninitCachemapReadAhead = 0n19<br>
OpenCount_UninitCachemapReg = 0n20<br>
OpenCount_UnmapInactiveViews = 0n21<br>
OpenCount_UnmapInactiveViews1 = 0n22<br>
OpenCount_UnmapInactiveViews2 = 0n23<br>
OpenCount_UnmapInactiveViews3 = 0n24<br>
OpenCount_WriteBehind = 0n25<br>
OpenCount_WriteBehindComplete = 0n26<br>
OpenCount_WriteBehindFailAcquire = 0n27<br>
</font></body></html> | epikcraw/ggool | public/Windows 10 x64 (18363.900)/_OPENCOUNT_REASON.html | HTML | mit | 1,264 |
#ifndef BITMAP_H
#define BITMAP_H
#include <allegro5/allegro.h>
#include <memory>
#include "renderlist.h"
// This class does double duty as a renderable, and a wrapper for allegro bitmap.
class Bitmap;
using BitmapPtr = std::shared_ptr<Bitmap>;
class Bitmap : public Renderable
{
ALLEGRO_BITMAP* bitmap;
float x, y;
float scale;
public:
Bitmap() : bitmap(nullptr), x(0), y(0), scale(1.0f)
{}
Bitmap( ALLEGRO_BITMAP* _bitmap, int _x = 0 , int _y = 0 ) : bitmap(_bitmap), x(_x), y(_y), scale(1.0f)
{}
~Bitmap();
void render();
void create( int w, int h, int flags = 0 );
bool loadFromFile( const std::string& filename, int flags = 0 );
bool saveToFile( const std::string& filename );
BitmapPtr getSubBitmap( int _x, int _y, int _w, int _h );
void setBitmap( ALLEGRO_BITMAP* nb );
void setBitmap( BitmapPtr& nb );
void blit( const BitmapPtr& other, float x, float y, float scale );
float getX() { return x; }
float getY() { return y; }
float getScale() { return scale; }
void setX( int _x ) { x = _x; }
void setY( int _y ) { y = _y; }
int getWidth() { return al_get_bitmap_width( bitmap ); }
int getHeight() { return al_get_bitmap_height( bitmap ); }
void setScale( float _scale ) { scale = _scale; }
};
#endif // BITMAP_H
| merlinblack/oyunum | src/bitmap.h | C | mit | 1,343 |
# BullDawgBeds | Rayjk123/BullDawgBeds | README.md | Markdown | mit | 14 |
/*
gopm (Go Package Manager)
Copyright (c) 2012 cailei ([email protected])
The MIT License (MIT)
Permission is hereby granted, free of charge, to any person obtaining a copy of
this software and associated documentation files (the "Software"), to deal in
the Software without restriction, including without limitation the rights to
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
of the Software, and to permit persons to whom the Software is furnished to do
so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
package main
import (
"fmt"
"github.com/cailei/gopm_index/gopm/index"
"github.com/hailiang/gosocks"
"io"
"io/ioutil"
"log"
"net/http"
"net/url"
"os"
)
type Agent struct {
client *http.Client
}
func newAgent() *Agent {
client := http.DefaultClient
// check if using a proxy
proxy_addr := os.Getenv("GOPM_PROXY")
if proxy_addr != "" {
fmt.Printf("NOTE: Using socks5 proxy: %v\n", proxy_addr)
proxy := socks.DialSocksProxy(socks.SOCKS5, proxy_addr)
transport := &http.Transport{Dial: proxy}
client = &http.Client{Transport: transport}
}
return &Agent{client}
}
func (agent *Agent) getFullIndexReader() io.Reader {
request := remote_db_host + "/all"
return agent._get_body_reader(request)
}
func (agent *Agent) uploadPackage(meta index.PackageMeta) {
request := fmt.Sprintf("%v/publish", remote_db_host)
// marshal PackageMeta to json
json, err := meta.ToJson()
if err != nil {
log.Fatalln(err)
}
// create a POST request
response, err := http.PostForm(request, url.Values{"pkg": {string(json)}})
if err != nil {
log.Fatalln(err)
}
body, err := ioutil.ReadAll(response.Body)
defer response.Body.Close()
if err != nil {
log.Fatalln(err)
}
if len(body) > 0 {
fmt.Println(string(body))
}
// check response
if response.StatusCode != 200 {
log.Fatalln(response.Status)
}
}
func (agent *Agent) _get_body_reader(request string) io.ReadCloser {
// GET the index content
response, err := agent.client.Get(request)
if err != nil {
log.Fatalln(err)
}
// check response
if response.StatusCode != 200 {
body, err := ioutil.ReadAll(response.Body)
if err != nil {
log.Fatalln(err)
}
if len(body) > 0 {
fmt.Println(string(body))
}
log.Fatalln(response.Status)
}
return response.Body
}
| cailei/gopm | gopm/agent.go | GO | mit | 3,137 |
package org.usfirst.frc.team6135.robot.subsystems;
import java.awt.geom.Arc2D.Double;
import org.usfirst.frc.team6135.robot.RobotMap;
import org.usfirst.frc.team6135.robot.commands.teleopDrive;
import com.kauailabs.navx.frc.AHRS;
import edu.wpi.first.wpilibj.ADXRS450_Gyro;
import edu.wpi.first.wpilibj.RobotDrive;
import edu.wpi.first.wpilibj.SerialPort;
import edu.wpi.first.wpilibj.VictorSP;
import edu.wpi.first.wpilibj.command.Subsystem;
public class Drive extends Subsystem {
//Constants
private static final boolean rReverse = true;
private static final boolean lReverse = false;
private static final double kA=0.03;
//Objects
private VictorSP leftDrive = null;
private VictorSP rightDrive = null;
public ADXRS450_Gyro gyro=null;
public AHRS ahrs=null;
public RobotDrive robotDrive=null;
//Constructors
public Drive() {
gyro=RobotMap.gyro;
ahrs=new AHRS(SerialPort.Port.kUSB1);
robotDrive=new RobotDrive(RobotMap.leftDriveVictor,RobotMap.rightDriveVictor);
leftDrive = RobotMap.leftDriveVictor;
rightDrive = RobotMap.rightDriveVictor;
leftDrive.set(0);
rightDrive.set(0);
leftDrive.setInverted(lReverse);
rightDrive.setInverted(rReverse);
ahrs.reset();
}
//Direct object access methods
public void setMotors(double l, double r) {//sets motor speeds accounting for directions of motors
leftDrive.set(l);
rightDrive.set(r);
}
public void setLeft(double d) {
leftDrive.set(d);
}
public void setRight(double d) {
rightDrive.set(d);
}
//Teleop Driving methods
private static final double accBoundX = 0.7; //The speed after which the drive starts to accelerate over time
private static final int accLoopX = 15; //The number of loops for the bot to accelerate to max speed
private int accLoopCountX = 0;
public double accCalcX(double input) {//applies a delay for motors to reach full speed for larger joystick inputs
if(input > accBoundX && accLoopCountX < accLoopX) {//positive inputs
return accBoundX + (input - accBoundX) * (accLoopCountX++ / (double) accLoopX);
}
else if(input < -accBoundX && accLoopCountX < accLoopX) {//negative inputs
return -accBoundX + (input + accBoundX) * (accLoopCountX++ / (double) accLoopX);
}
else if(Math.abs(input) <= accBoundX) {
accLoopCountX = 0;
}
return input;
}
private static final double accBoundY = 0.7; //The speed after which the drive starts to accelerate over time
private static final int accLoopY = 15; //The number of loops for the bot to accelerate to max speed
private int accLoopCountY = 0;
public double accCalcY(double input) {//applies a delay for motors to reach full speed for larger joystick inputs
if(input > accBoundY && accLoopCountY < accLoopY) {//positive inputs
return accBoundY + (input - accBoundY) * (accLoopCountY++ / (double) accLoopY);
}
else if(input < -accBoundY && accLoopCountY < accLoopY) {//negative inputs
return -accBoundY + (input + accBoundY) * (accLoopCountY++ / (double) accLoopY);
}
else if(Math.abs(input) <= accBoundY) {
accLoopCountY = 0;
}
return input;
}
private static final double accBoundZ = 0.7; //The speed after which the drive starts to accelerate over time
private static final int accLoopZ = 15; //The number of loops for the bot to accelerate to max speed
private int accLoopCountZ = 0;
public double accCalcZ(double input) {//applies a delay for motors to reach full speed for larger joystick inputs
if(input > accBoundZ && accLoopCountZ < accLoopZ) {//positive inputs
return accBoundZ + (input - accBoundZ) * (accLoopCountZ++ / (double) accLoopZ);
}
else if(input < -accBoundZ && accLoopCountZ < accLoopZ) {//negative inputs
return -accBoundZ + (input + accBoundZ) * (accLoopCountZ++ / (double) accLoopZ);
}
else if(Math.abs(input) <= accBoundZ) {
accLoopCountZ = 0;
}
return input;
}
public double sensitivityCalc(double input) {//Squares magnitude of input to reduce magnitude of smaller joystick inputs
if (input >= 0.0) {
return (input * input);
}
else {
return -(input * input);
}
}
public void reverse() {
leftDrive.setInverted(!leftDrive.getInverted());
rightDrive.setInverted(!rightDrive.getInverted());
VictorSP temp1 = leftDrive;
VictorSP temp2 = rightDrive;
rightDrive = temp1;
leftDrive = temp2;
}
public double getGyroAngle()
{
return gyro.getAngle();
}
public double getNAVXAngle()
{
return ahrs.getAngle();
}
public void driveStraight()
{
robotDrive.drive(0.6, -gyro.getAngle()*kA);
}
@Override
protected void initDefaultCommand() {
setDefaultCommand(new teleopDrive());
}
}
| Arctos6135/frc-2017 | src/org/usfirst/frc/team6135/robot/subsystems/Drive.java | Java | mit | 4,676 |
module Shopping
class LineItem < ActiveRecord::Base
extend Shopping::AttributeAccessibleHelper
belongs_to :cart
belongs_to :source, polymorphic: true
validate :unique_source_and_cart, on: :create
validate :unpurchased_cart
validates :quantity, allow_nil: true, numericality: {only_integer: true, greater_than: -1}
validates :cart_id, presence: true
validates :source_id, presence: true
validates :source_type, presence: true
attr_accessible :cart_id, :source, :source_id, :source_type, :quantity, :list_price, :sale_price, :options
before_create :set_prices, :set_name
def unique_source_and_cart
other = Shopping::LineItem.where(source_id: self.source_id, source_type: self.source_type, cart_id: self.cart_id)
if other.count > 0
self.errors.add(:source, "A line item with identical source and cart exists, update quantity instead (id=#{other.first.id})")
end
end
def unpurchased_cart
if cart && cart.purchased?
changes.keys.each do |k|
self.errors.add(k, "Cannot change `#{k}', cart is purchased")
end
end
end
def set_name
self.source_name ||= source.try(:name)
end
def set_prices
self.list_price ||= source.try(:price)
self.sale_price ||= source.try(:price)
end
end
end
| barkbox/shopping | app/models/shopping/line_item.rb | Ruby | mit | 1,352 |
<?php
use Symfony\Component\HttpKernel\Kernel;
use Symfony\Component\Config\Loader\LoaderInterface;
class AppKernel extends Kernel
{
public function registerBundles()
{
$bundles = array(
new Symfony\Bundle\FrameworkBundle\FrameworkBundle(),
new Symfony\Bundle\SecurityBundle\SecurityBundle(),
new Symfony\Bundle\TwigBundle\TwigBundle(),
new Symfony\Bundle\MonologBundle\MonologBundle(),
new Symfony\Bundle\SwiftmailerBundle\SwiftmailerBundle(),
new Symfony\Bundle\AsseticBundle\AsseticBundle(),
new Doctrine\Bundle\DoctrineBundle\DoctrineBundle(),
new Sensio\Bundle\FrameworkExtraBundle\SensioFrameworkExtraBundle(),
new Tuhn\HelloBundle\TuhnHelloBundle(),
new Trungnd\StoreBundle\TrungndStoreBundle(),
);
if (in_array($this->getEnvironment(), array('dev', 'test'))) {
$bundles[] = new Acme\DemoBundle\AcmeDemoBundle();
$bundles[] = new Symfony\Bundle\WebProfilerBundle\WebProfilerBundle();
$bundles[] = new Sensio\Bundle\DistributionBundle\SensioDistributionBundle();
$bundles[] = new Sensio\Bundle\GeneratorBundle\SensioGeneratorBundle();
}
return $bundles;
}
public function registerContainerConfiguration(LoaderInterface $loader)
{
$loader->load(__DIR__.'/config/config_'.$this->getEnvironment().'.yml');
}
}
| longran/project1 | app/AppKernel.php | PHP | mit | 1,458 |
#!/usr/bin/env python
"""
This script is used to run tests, create a coverage report and output the
statistics at the end of the tox run.
To run this script just execute ``tox``
"""
import re
from fabric.api import local, warn
from fabric.colors import green, red
if __name__ == '__main__':
# Kept some files for backwards compatibility. If support is dropped,
# remove it here
deprecated_files = '*utils_email*,*utils_log*'
local('flake8 --ignore=E126 --ignore=W391 --statistics'
' --exclude=submodules,migrations,build .')
local('coverage run --source="django_libs" manage.py test -v 2'
' --traceback --failfast --settings=django_libs.tests.settings'
' --pattern="*_tests.py"')
local('coverage html -d coverage'
' --omit="*__init__*,*/settings/*,*/migrations/*,*/tests/*,'
'*admin*,{}"'.format(deprecated_files))
total_line = local('grep -n pc_cov coverage/index.html', capture=True)
percentage = float(re.findall(r'(\d+)%', total_line)[-1])
if percentage < 100:
warn(red('Coverage is {0}%'.format(percentage)))
else:
print(green('Coverage is {0}%'.format(percentage)))
| bitmazk/django-libs | runtests.py | Python | mit | 1,182 |
using System;
namespace KeyWatcher.Reactive
{
internal sealed class ObservingKeyWatcher
: IObserver<char>
{
private readonly string id;
internal ObservingKeyWatcher(string id) =>
this.id = id;
public void OnCompleted() =>
Console.Out.WriteLine($"{this.id} - {nameof(this.OnCompleted)}");
public void OnError(Exception error) =>
Console.Out.WriteLine($"{this.id} - {nameof(this.OnError)} - {error.Message}");
public void OnNext(char value) =>
Console.Out.WriteLine($"{this.id} - {nameof(this.OnNext)} - {value}");
}
}
| rockfordlhotka/DistributedComputingDemo | src/KeyWatcher - Core/KeyWatcher.Reactive/ObservingKeyWatcher.cs | C# | mit | 552 |
from baroque.entities.event import Event
class EventCounter:
"""A counter of events."""
def __init__(self):
self.events_count = 0
self.events_count_by_type = dict()
def increment_counting(self, event):
"""Counts an event
Args:
event (:obj:`baroque.entities.event.Event`): the event to be counted
"""
assert isinstance(event, Event)
self.events_count += 1
t = type(event.type)
if t in self.events_count_by_type:
self.events_count_by_type[t] += 1
else:
self.events_count_by_type[t] = 1
def count_all(self):
"""Tells how many events have been counted globally
Returns:
int
"""
return self.events_count
def count(self, eventtype):
"""Tells how many events have been counted of the specified type
Args:
eventtype (:obj:`baroque.entities.eventtype.EventType`): the type of events to be counted
Returns:
int
"""
return self.events_count_by_type.get(type(eventtype), 0)
| baroquehq/baroque | baroque/datastructures/counters.py | Python | mit | 1,120 |
/*
Fontname: -Adobe-Times-Medium-R-Normal--11-80-100-100-P-54-ISO10646-1
Copyright: Copyright (c) 1984, 1987 Adobe Systems Incorporated. All Rights Reserved. Copyright (c) 1988, 1991 Digital Equipment Corporation. All Rights Reserved.
Glyphs: 191/913
BBX Build Mode: 0
*/
const uint8_t u8g2_font_timR08_tf[2170] U8G2_FONT_SECTION("u8g2_font_timR08_tf") =
"\277\0\3\2\4\4\2\4\5\13\15\377\375\7\376\7\376\1H\2\277\10a \5\0\246\4!\7q\343"
"\304\240\4\42\7#\66E\242\4#\16ubM)I\6\245\62(\245$\1$\14\224^U\64D\242"
"i\310\22\0%\21w\42\316\60$Q\322\226$RRJ\332\22\0&\20x\42\226\232\244\312\264D\221"
"\226)\311\242\0'\6!\266\204\0(\13\223\32U\22%Q-\312\2)\14\223\32E\26eQ%J"
"\42\0*\7\63sE\322\1+\12U\242U\30\15R\30\1,\6\42\336\204\22-\6\23*\305\0."
"\6\21\343D\0/\12s\342T%\252D\21\0\60\12tb\215\22yJ\24\0\61\10scM\42u"
"\31\62\14tb\215\22eQ\26EC\0\63\14tb\215\22e\211\230\15\11\0\64\14ub]&%"
"\245d\320\302\4\65\13tb\315\22\215Y\66$\0\66\13tb\225\22-\221)Q\0\67\12tb\305"
" \325\242\254\4\70\14tb\215\22I\211\22I\211\2\71\13tb\215\22\231\222)\221\0:\6Q\343"
"D\26;\7b\336L\254\4<\7ScUR+=\10\65\246\305\240\16\2>\10SbEV)\1"
"?\13s\42\305\220DI\224&\0@\24\230Z\326\220\205I\264(Q\242D\211\222\230\332\221A\1A"
"\15w\42^:&a\222\15R\226\34B\15u\242\305\20U\242d\252D\203\2C\14v\342\315\60d"
"jUK\206\4D\20v\342\305 EJ\226dI\226D\303\220\0E\14u\242\305\240DI\70\205\321"
"\60F\14u\242\305\240DI\70\205\331\4G\16v\342\315\60dj\64\204[\62$\0H\17w\42\306"
"\262dQ\26\15R\26e\311\1I\10s\42\305\22u\31J\12t\42\315\224\265T$\0K\16v\342"
"\305\242D\225LL\262(Y\4L\11u\242\305\26v\32\6M\21y\242\306\266hR\322\224\64%K"
"\324\262$\3N\20w\42\306\262H\225RR\212\244H\231\22\0O\14v\342\315\220H\243qR\206\4"
"P\14u\242\305\20U\242d\12\263\11Q\14\226\332\315\220H\243qRV\3R\15v\342\305\220EM"
"[\222E\311\42S\13tb\315\20m\242\64$\0T\12u\242\305\245\24\266-\0U\17w\42\306\262"
"dQ\26eQ\26)\332\4V\17w\42\306\262d\221\242%a\222\306\31\0W\22{\241\306r\311J"
"R\244%K\230\264fq\226\1X\14w\42\306\262d\225\264\222U\16Y\14w\42\306\262d\225p\215"
"\323\11Z\10u\242\305\255\267a[\10\222\332\304\322\27\1\134\11s\342D\224E\265(]\10\222\332\204"
"\322\227\1^\7\63sME\11_\6\25V\305 `\6\42\372D\24a\10S\42\205\226\34\2b\14"
"ua\205\30N\225(\211\222\5c\7S\42\315T\23d\15ub\225\30-Q\22%Q\244\4e\7"
"S\42\315%\23f\12t\42\225\22MYi\1g\14tZ\315\220\64eJ\64$\0h\13va\205"
"\232nQO\212\0i\7r\342L\244tj\11\223\331T(uZ\0k\13va\205Z\252d[T"
"\22l\10s\42\205\324\313\0m\14X\42\306\42\265D\225\250\342\0n\12Ub\305T\211\222(\61o"
"\11Tb\215\22\231\22\5p\16\205U\305\20U\242$J\246\60\233\0q\14\205V\315\22%Q\22E"
"cmr\11S\42E\222(Q\62s\10S\42\315\226\15\1t\11d\42M\64eE\1u\14Ub"
"\205\62%Q\22EJ\0v\14Va\305\242DY\222\251\21\0w\15Y!\306b\211jIQQ\223"
"\14x\12U\242\205\242\324*\211\1y\14vY\205\262D\225P\14\65\21z\11Tb\305\20\65\15\2"
"{\12\223\32U\22U\262\250\26|\6\221\232\304\3}\13\223\32E\26\325\222\250\22\1~\7&\352\215"
"d\1\240\5\0\246\4\241\7q\333D\62\10\242\14t^U\64DZ\224H\21\0\243\14ub\225\224"
"D\331\226I\203\2\244\14efE\226LI\224DK\26\245\15ubE\226TL\321 e\13\0\246"
"\6q\242\304\62\247\17\224Z\315\20%QRJJI\64$\0\250\6\23wE\22\251\16wc\326V"
"\211\24%\223\224Z\66\1\252\10S*\205\246d\3\253\11DfM\242\64%\1\254\7%\347\305 \6"
"\255\6\23*\305\0\256\15wc\326VI\26\223\322-\233\0\257\6\23\66\305\0\260\11D.\215\22I"
"\211\2\261\14u\242U\30\15R\230\3\203\0\262\7C\356\314R\31\263\10C\356\304\222-\0\264\6\42"
"\366\214\2\265\16uZE\224DI\224D\311\42\206\0\266\23\226\232\315\60(\311\222,\221\222%Y\222"
"%Y\222\0\267\6\21\252D\0\270\7\63\26M\266\0\271\10C\356L\42%\3\272\7S*M\307\1"
"\273\12DfE\22%\25%\1\274\21w\42N\226HY\24\15I\226h\203\222%\0\275\17w\42N"
"\226HY\24\15\211\224\224\232\6\276\17w\42\306\324\230DJ-\321\6%K\0\277\12s\32M\32%"
"Q\62\4\300\20\247\42V\16\344p:&a\222\15R\226\34\301\17\247\42f\232\303\351\230\204I\66H"
"Yr\302\20\247\42^\232\344h:&a\222\15R\226\34\303\21\247\42^\222%\71\232\216I\230d\203"
"\224%\7\304\17\227\42V\222\243\351\230\204I\66HYr\305\20\247\42^\232\244q:&a\222\15R"
"\226\34\306\20xb\336 M\225\64\231\206\60\212\206d\10\307\17\246\326\315\60djUK\206,\316\64"
"\0\310\17\245\242M\232\3\203\22%\341\24F\303\0\311\16\245\242]\35\30\224(\11\247\60\32\6\312\17"
"\245\242U\226\304\203\22%\341\24F\303\0\313\15\225\242MyP\242$\234\302h\30\314\12\243\42E\26"
".Q\227\1\315\12\243\42U\22.Q\227\1\316\11\243\42M\333\22u\31\317\12\223\42E\222-Q\227"
"\1\320\20v\342\305 EJ\66DI\226D\303\220\0\321\23\247\42^\222%\71$-R$UJI"
")R\246\4\322\17\246\342U\234CC\42\215\306I\31\22\0\323\17\246\342]\230cC\42\215\306I\31"
"\22\0\324\17\246\342]\230\344\310\220H\243qR\206\4\325\20\246\342U\22%\71\64$\322h\234\224!"
"\1\326\17\226\342M\224#C\42\215\306I\31\22\0\327\12U\242E\226\324*\265\0\330\22\230\35>\20"
"\15\222\251\22\205Q\22E\246A\312\1\331\22\247\42V\16\344\330\262dQ\26eQ\26)\332\4\332\22"
"\247\42f\232c\313\222EY\224EY\244h\23\0\333\22\247\42^\232\344\320\262dQ\26eQ\26)"
"\332\4\334\22\227\42V\222C\313\222EY\224EY\244h\23\0\335\16\247\42f\232c\226\254\22\256q"
":\1\336\13u\242\305\26N\225)\233\0\337\13tbU\245EJ*C\2\340\11\203\42E\26j\311"
"!\341\11\203\42U\22j\311!\342\11\203\42M\233\226\34\2\343\14\204\42M\242\244b\244T\26\0\344"
"\11s\42E\222i\311!\345\11\203\42M\27-\71\4\346\13U\242\205\42%\311RR\4\347\11\203\26"
"\315TS\262\5\350\11\203\42E\226.K&\351\11\203\42U\222.K&\352\11\203\42M\343\262d\2"
"\353\11s\42E\22.K&\354\10\202\342D\24)\35\355\11\203\342T\22J]\0\356\10\203\342L\233"
"\324\5\357\11s\342D\222I]\0\360\14\204bM\66$\321\20\231\22\5\361\14\205bMw`\252D"
"I\224\30\362\13\204bM\30+\221)Q\0\363\13\204bU\35P\42S\242\0\364\14\204bM\224\304"
"JdJ\24\0\365\14\204bM\242\304JdJ\24\0\366\13tbE\22+\221)Q\0\367\12U\242"
"U\16\14:\20\1\370\12v]m\64\365iJ\1\371\17\205bM\232\3Q\22%Q\22EJ\0\372"
"\17\205bU\226#Q\22%Q\22EJ\0\373\17\205bU\226\304Q\22%Q\22EJ\0\374\15u"
"bM\71J\242$J\242H\11\375\17\246Y]\230C\312\22UB\61\324D\0\376\17\245U\205\30N"
"\225(\211\222)\314&\0\377\16\226YM\35Q\226\250\22\212\241&\2\0\0\0";
| WiseLabCMU/gridballast | Source/framework/main/u8g2/tools/font/build/single_font_files/u8g2_font_timR08_tf.c | C | mit | 6,149 |
import React from 'react'
import { PlanItineraryContainer } from './index'
const PlanMapRoute = () => {
return (
<div>
<PlanItineraryContainer />
</div>
)
}
export default PlanMapRoute
| in-depth/indepth-demo | src/shared/views/plans/planItinerary/PlanItineraryRoute.js | JavaScript | mit | 206 |
//
// Reliant.h
// Reliant
//
// Created by Michael Seghers on 18/09/14.
//
//
#ifndef Reliant_Header____FILEEXTENSION___
#define Reliant_Header____FILEEXTENSION___
#import "OCSObjectContext.h"
#import "OCSConfiguratorFromClass.h"
#import "NSObject+OCSReliantContextBinding.h"
#import "NSObject+OCSReliantInjection.h"
#endif
| appfoundry/Reliant | Reliant/Classes/Reliant.h | C | mit | 331 |
// ==========================================================================
// Squidex Headless CMS
// ==========================================================================
// Copyright (c) Squidex UG (haftungsbeschraenkt)
// All rights reserved. Licensed under the MIT license.
// ==========================================================================
using System.Collections.Immutable;
using OpenIddict.Abstractions;
using OpenIddict.Server;
using static OpenIddict.Server.OpenIddictServerEvents;
namespace Squidex.Areas.IdentityServer.Config
{
public sealed class AlwaysAddTokenHandler : IOpenIddictServerHandler<ProcessSignInContext>
{
public ValueTask HandleAsync(ProcessSignInContext context)
{
if (context == null)
{
return default;
}
if (!string.IsNullOrWhiteSpace(context.Response.AccessToken))
{
var scopes = context.AccessTokenPrincipal?.GetScopes() ?? ImmutableArray<string>.Empty;
context.Response.Scope = string.Join(" ", scopes);
}
return default;
}
}
}
| Squidex/squidex | backend/src/Squidex/Areas/IdentityServer/Config/AlwaysAddTokenHandler.cs | C# | mit | 1,160 |
using System.Diagnostics.CodeAnalysis;
namespace Npoi.Mapper
{
/// <summary>
/// Information for one row that read from file.
/// </summary>
/// <typeparam name="TTarget">The target mapping type for a row.</typeparam>
[SuppressMessage("ReSharper", "UnusedAutoPropertyAccessor.Global")]
public class RowInfo<TTarget> : IRowInfo
{
#region Properties
/// <summary>
/// Row number.
/// </summary>
public int RowNumber { get; set; }
/// <summary>
/// Constructed value object from the row.
/// </summary>
public TTarget Value { get; set; }
/// <summary>
/// Column index of the first error.
/// </summary>
public int ErrorColumnIndex { get; set; }
/// <summary>
/// Error message for the first error.
/// </summary>
public string ErrorMessage { get; set; }
#endregion
#region Constructors
/// <summary>
/// Initialize a new RowData object.
/// </summary>
/// <param name="rowNumber">The row number</param>
/// <param name="value">Constructed value object from the row</param>
/// <param name="errorColumnIndex">Column index of the first error cell</param>
/// <param name="errorMessage">The error message</param>
public RowInfo(int rowNumber, TTarget value, int errorColumnIndex, string errorMessage)
{
RowNumber = rowNumber;
Value = value;
ErrorColumnIndex = errorColumnIndex;
ErrorMessage = errorMessage;
}
#endregion
}
}
| donnytian/Npoi.Mapper | Npoi.Mapper/src/Npoi.Mapper/RowInfo.cs | C# | mit | 1,652 |
const path = require('path');
module.exports = {
lazyLoad: true,
pick: {
posts(markdownData) {
return {
meta: markdownData.meta,
description: markdownData.description,
};
},
},
plugins: [path.join(__dirname, '..', 'node_modules', 'bisheng-plugin-description')],
routes: [{
path: '/',
component: './template/Archive',
}, {
path: '/posts/:post',
dataPath: '/:post',
component: './template/Post',
}, {
path: '/tags',
component: './template/TagCloud',
}],
};
| benjycui/bisheng | packages/bisheng-theme-one/src/index.js | JavaScript | mit | 536 |
<?php
namespace WindowsAzure\DistributionBundle\Deployment;
/**
* @author Stéphane Escandell <[email protected]>
*/
interface CustomIteratorInterface
{
/**
* @param array $dirs
* @param array $subdirs
*/
public function getIterator(array $dirs, array $subdirs);
} | beberlei/AzureDistributionBundle | Deployment/CustomIteratorInterface.php | PHP | mit | 302 |
import { Injectable } from '@angular/core';
import {Http,Response,ConnectionBackend,Headers,RequestMethod} from '@angular/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
import 'rxjs/add/operator/toPromise';
import{AppHttpUtil} from '../appUtil/httpUtil';
import {AppPermService} from "./permService";
import {AppUrlConfig} from "../appConfig/urlConfig";
@Injectable()
export class AppLoginService {
constructor(private httpUtil: AppHttpUtil,private urlConfig:AppUrlConfig){
}
/**
* 用户登录
*/
login(_name:string,_pass:string){
return this.httpUtil.ajax({
url:this.urlConfig.emarketService+'system/login/',
method:RequestMethod.Post,
body:{
loginName:_name,
password:_pass
}
});
}
}
| inherents/efeNew | app/appService/loginService.ts | TypeScript | mit | 841 |
/****************************************************************************
Copyright (c) 2010-2012 cocos2d-x.org
Copyright (c) 2013-2016 zilongshanren
http://www.cocos2d-x.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
****************************************************************************/
#import <AppKit/AppKit.h>
#include "ui/UIEditBox/Mac/CCUITextInput.h"
@interface CCUIPasswordTextField : NSTextField<CCUITextInput>
{
NSMutableDictionary* _placeholderAttributes;
}
@end
| MaiyaT/cocosStudy | Eliminate/builds/jsb-default/frameworks/cocos2d-x/cocos/ui/UIEditBox/Mac/CCUIPasswordTextField.h | C | mit | 1,500 |
// Init ES2015 + .jsx environments for .require()
require('babel-register');
var express = require('express');
var fluxexapp = require('./fluxexapp');
var serverAction = require('./actions/server');
var fluxexServerExtra = require('fluxex/extra/server');
var app = express();
// Provide /static/js/main.js
fluxexServerExtra.initStatic(app);
// Mount test page at /test
app.use('/product', fluxexServerExtra.createMiddlewareByAction(fluxexapp, serverAction.samplePage));
// Start server
app.listen(process.env.TESTPORT || 3000);
console.log('Fluxex started! Go http://localhost:3001/product?id=124');
| zordius/fluxex | examples/01-history-api/server.js | JavaScript | mit | 604 |
import numpy as np
class Surface(object):
def __init__(self, image, edge_points3d, edge_points2d):
"""
Constructor for a surface defined by a texture image and
4 boundary points. Choose the first point as the origin
of the surface's coordinate system.
:param image: image array
:param edge_points3d: array of 3d coordinates of 4 corner points in clockwise direction
:param edge_points2d: array of 2d coordinates of 4 corner points in clockwise direction
"""
assert len(edge_points3d) == 4 and len(edge_points2d) == 4
self.image = image
self.edge_points3d = edge_points3d
self.edge_points2d = np.float32(edge_points2d) # This is required for using cv2's getPerspectiveTransform
self.normal = self._get_normal_vector()
def top_left_corner3d(self):
return self.edge_points3d[0]
def top_right_corner3d(self):
return self.edge_points3d[1]
def bottom_right_corner3d(self):
return self.edge_points3d[2]
def bottom_left_corner3d(self):
return self.edge_points3d[3]
def distance_to_point(self, point):
point_to_surface = point - self.top_left_corner3d()
distance_to_surface = self.normal.dot(point_to_surface)
return distance_to_surface
def _get_normal_vector(self):
"""
:return: the normal vector of the surface. It determined the front side
of the surface and it's not necessarily a unit vector
"""
p0 = self.edge_points3d[0]
p1 = self.edge_points3d[1]
p3 = self.edge_points3d[3]
v1 = p3 - p0
v2 = p1 - p0
normal = np.cross(v1, v2)
norm = np.linalg.norm(normal)
return normal / norm
class Polyhedron(object):
def __init__(self, surfaces):
self.surfaces = surfaces
class Space(object):
def __init__(self, models=None):
self.models = models or []
def add_model(self, model):
assert isinstance(model, Polyhedron)
self.models.append(model)
class Line2D(object):
def __init__(self, point1, point2):
"""
Using the line equation a*x + b*y + c = 0 with b >= 0
:param point1: starting point
:param point2: ending point
:return: a Line object
"""
assert len(point1) == 2 and len(point2) == 2
self.a = point2[1] - point1[1]
self.b = point1[0] - point2[0]
self.c = point1[1] * point2[0] - point1[0] * point2[1]
if self.b < 0:
self.a = -self.a
self.b = -self.b
self.c = -self.c
def is_point_on_left(self, point):
return self.a * point[0] + self.b * point[1] + self.c > 0
def is_point_on_right(self, point):
return self.a * point[0] + self.b * point[1] + self.c < 0
def is_point_on_line(self, point):
return self.a * point[0] + self.b * point[1] + self.c == 0
def get_y_from_x(self, x):
if self.b == 0:
return 0.0
return 1.0 * (-self.c - self.a * x) / self.b
def get_x_from_y(self, y):
if self.a == 0:
return 0.0
return 1.0 * (-self.c - self.b * y) / self.a
| yangshun/cs4243-project | app/surface.py | Python | mit | 3,220 |
# Sparse Arrays
Internally the V8 engine can represent `Array`s following one of two approaches:
- **Fast Elements**: linear storage for compact keys sets.
- **Dictionary Elements**: hash table storage (more expensive to access on runtime).
If you want V8 to represent your `Array` in the `Fast Elements` form, you need to take into account the following:
- Use contiguous keys starting at `0`.
- Don't pre-allocate large `Array` (e.g. *> 64K* elements) that you don't use.
- Don't delete elements, specially in numeric `Array`s.
- Don't load uninitialized or deleted elements.
Another consideration: any name used as property name that is not a `String` will be serialized, read more at [Properties Names](v8-tips/properties-names).
| Kikobeats/js-mythbusters | docs/v8-tips/sparse-arrays.md | Markdown | mit | 739 |
<?php
return [
/**
*--------------------------------------------------------------------------
* Logging Configuration
*--------------------------------------------------------------------------
*
* Here you may configure the log settings for your application. Out of
* the box, the application uses the Monolog PHP logging library. This gives
* you a variety of powerful log handlers / formatters to utilize.
*
* Available Settings: "single", "daily", "syslog", "errorlog"
*
*/
'log' => env('APP_LOG', 'single'),
'log_level' => env('APP_LOG_LEVEL', 'debug'),
];
| lioneil/pluma | config/logging.php | PHP | mit | 633 |
# [Demo](https://keaws.github.io/git-api-client/)
## Install
```
npm i
npm start
```
| Keaws/Keaws.github.io | git-api-client/README.md | Markdown | mit | 86 |
A PvPGNChatParser converts raw messages received from PvPGNChat to ChatMessage objects | serpi90/PvPGNChat | PvPGNChat-Model.package/PvPGNChatParser.class/README.md | Markdown | mit | 86 |
import { fullSrc } from '../element/element';
import { pixels } from '../math/unit';
import { Clone } from './clone';
import { Container } from './container';
import { Image } from './image';
import { Overlay } from './overlay';
import { Wrapper } from './wrapper';
export class ZoomDOM {
static useExisting(element: HTMLImageElement, parent: HTMLElement, grandparent: HTMLElement): ZoomDOM {
let overlay = Overlay.create();
let wrapper = new Wrapper(grandparent);
let container = new Container(parent);
let image = new Image(element);
let src = fullSrc(element);
if (src === element.src) {
return new ZoomDOM(overlay, wrapper, container, image);
} else {
return new ZoomDOM(overlay, wrapper, container, image, new Clone(container.clone()));
}
}
static create(element: HTMLImageElement): ZoomDOM {
let overlay = Overlay.create();
let wrapper = Wrapper.create();
let container = Container.create();
let image = new Image(element);
let src = fullSrc(element);
if (src === element.src) {
return new ZoomDOM(overlay, wrapper, container, image);
} else {
return new ZoomDOM(overlay, wrapper, container, image, Clone.create(src));
}
}
readonly overlay: Overlay;
readonly wrapper: Wrapper;
readonly container: Container;
readonly image: Image;
readonly clone?: Clone;
constructor(overlay: Overlay, wrapper: Wrapper, container: Container, image: Image, clone?: Clone) {
this.overlay = overlay;
this.wrapper = wrapper;
this.container = container;
this.image = image;
this.clone = clone;
}
appendContainerToWrapper(): void {
this.wrapper.element.appendChild(this.container.element);
}
replaceImageWithWrapper(): void {
let parent = this.image.element.parentElement as HTMLElement;
parent.replaceChild(this.wrapper.element, this.image.element);
}
appendImageToContainer(): void {
this.container.element.appendChild(this.image.element);
}
appendCloneToContainer(): void {
if (this.clone !== undefined) {
this.container.element.appendChild(this.clone.element);
}
}
replaceImageWithClone(): void {
if (this.clone !== undefined) {
this.clone.show();
this.image.hide();
}
}
replaceCloneWithImage(): void {
if (this.clone !== undefined) {
this.image.show();
this.clone.hide();
}
}
fixWrapperHeight(): void {
this.wrapper.element.style.height = pixels(this.image.element.height);
}
collapsed(): void {
this.overlay.removeFrom(document.body);
this.image.deactivate();
this.wrapper.finishCollapsing();
}
/**
* Called at the end of an expansion to check if the clone loaded before our expansion finished. If it did, and is
* still not visible, we can now show it to the client.
*/
showCloneIfLoaded(): void {
if (this.clone !== undefined && this.clone.isLoaded() && this.clone.isHidden()) {
this.replaceImageWithClone();
}
}
}
| MikeBull94/zoom.ts | src/dom/zoom-dom.ts | TypeScript | mit | 3,272 |
define(function(){
return {"乙":"乚乛",
"人":"亻",
"刀":"刂",
"卩":"㔾",
"尢":"尣𡯂兀",
"巛":"巜川",
"己":"已巳",
"彐":"彑",
"心":"忄",
"手":"扌",
"攴":"攵",
"无":"旡",
"歹":"歺",
"水":"氵氺",
"爪":"爫",
"火":"灬",
"":"户",
"牛":"牜",
"犬":"犭",
"玉":"王",
"疋":"",
"目":"",
"示":"礻",
"玄":"𤣥",
"糸":"糹",
"网":"冈",
"艸":"艹",
"竹":"",
"衣":"衤",
"襾":"西覀",
"老":"",
"聿":"",
"肉":"⺼",
"言":"訁",
"足":"",
"辵":"辶",
"邑":"⻏",
"阜":"阝",
"金":"釒",
"長":"镸",
"食":"飠",
"金":"釒",
"廾":"廾",
"爿":"丬"};
}); | yapcheahshen/ksanapc | node_webkit/jslib/cjk/radicalvariants.js | JavaScript | mit | 688 |
"use strict";
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
var qs = require("querystring");
var partial = require("lodash.partial");
var endpoints = require("./stats-endpoints");
var dicts = require("./dicts");
var translateKeys = require("./util/translate-keys");
var transport = require("./get-json");
var translate = partial(translateKeys, dicts.jsToNbaMap);
var stats = Object.create({
setTransport: function setTransport(_transport) {
transport = _transport;
},
getTransport: function getTransport() {
return transport;
}
});
Object.keys(endpoints).forEach(function (key) {
stats[key] = makeStatsMethod(endpoints[key]);
});
function makeStatsMethod(endpoint) {
return function statsMethod(query, callback) {
if (typeof query === "function") {
callback = query;
query = {};
}
if (typeof callback !== "function") {
throw new TypeError("Must pass a callback function.");
}
var params = _extends({}, endpoint.defaults, translate(query));
transport(endpoint.url, params, function (err, response) {
if (err) return callback(err);
if (response == null) return callback();
// response is something like "GameID is required"
if (typeof response === "string") return callback(new Error(response));
if (endpoint.transform) return callback(null, endpoint.transform(response));
callback(null, response);
});
};
}
module.exports = stats; | weixiyen/nba | lib/stats.js | JavaScript | mit | 1,662 |
<?php
/* TwigBundle:Exception:exception.json.twig */
class __TwigTemplate_8d9b12e119daada0de361618d51aaa01 extends Twig_Template
{
public function __construct(Twig_Environment $env)
{
parent::__construct($env);
$this->parent = false;
$this->blocks = array(
);
}
protected function doDisplay(array $context, array $blocks = array())
{
// line 1
if (isset($context["exception"])) { $_exception_ = $context["exception"]; } else { $_exception_ = null; }
echo twig_jsonencode_filter($this->getAttribute($_exception_, "toarray"));
echo "
";
}
public function getTemplateName()
{
return "TwigBundle:Exception:exception.json.twig";
}
public function isTraitable()
{
return false;
}
public function getDebugInfo()
{
return array ( 47 => 8, 30 => 4, 27 => 3, 22 => 4, 117 => 22, 112 => 21, 109 => 20, 104 => 19, 96 => 18, 84 => 14, 80 => 12, 68 => 9, 44 => 7, 26 => 4, 23 => 3, 20 => 2, 17 => 1, 92 => 39, 86 => 6, 79 => 40, 57 => 22, 46 => 7, 37 => 8, 33 => 7, 29 => 6, 24 => 4, 19 => 1, 144 => 54, 138 => 50, 130 => 46, 124 => 24, 121 => 41, 115 => 40, 111 => 38, 108 => 37, 99 => 32, 94 => 29, 91 => 17, 88 => 16, 85 => 26, 77 => 39, 74 => 20, 71 => 19, 65 => 16, 62 => 15, 58 => 8, 54 => 11, 51 => 10, 42 => 9, 38 => 8, 35 => 5, 31 => 4, 28 => 3,);
}
}
| waltertschwe/kids-reading-network | app/cache/prod/twig/8d/9b/12e119daada0de361618d51aaa01.php | PHP | mit | 1,455 |
<div id="menu-logo-wrapper" class="animated slideInDown">
<div class="main-menu">
<div class="pull-left">
<div class="toggle-menu-container">
<div class="toggle-menu">
<a href="javascript:void(0)">
<span class="nav-bar"></span>
<span class="nav-bar"></span>
<span class="nav-bar"></span>
</a>
</div>
</div>
<div class="main-nav-wrapper">
<ul class="main-nav">
<li><a href="<?php echo View::url('/'); ?>#about">About</a></li>
<li><a href="<?php echo View::url('/'); ?>#flavours">Flavours</a></li>
<li><a href="<?php echo View::url('/'); ?>#news">News</a></li>
<li><a href="<?php echo View::url('/'); ?>#locate">Locate</a></li>
<li><a href="<?php echo View::url('/'); ?>#wholesale">Wholesale</a></li>
</ul>
</div>
</div>
<a href="<?php echo View::url('/'); ?>" class="logo pull-right"><img src="<?= $view->getThemePath() ?>/images/logos/main-logo.png" alt=""></a>
</div>
</div> | zawzawzaw/scoop | application/themes/scooptherapy/elements/menu.php | PHP | mit | 988 |
[](https://travis-ci.org/practicefusion/ember-background-update)
# Ember-background-updates
This README outlines the details of collaborating on this Ember addon.
## Installation
* `git clone` this repository
* `npm install`
* `bower install`
## Running
* `ember server`
* Visit your app at http://localhost:4200.
## Running Tests
* `npm test` (Runs `ember try:testall` to test your addon against multiple Ember versions)
* `ember test`
* `ember test --server`
## Building
* `ember build`
For more information on using ember-cli, visit [http://www.ember-cli.com/](http://www.ember-cli.com/).
| practicefusion/ember-background-update | README.md | Markdown | mit | 684 |
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.vmwarecloudsimple.models;
import com.azure.resourcemanager.vmwarecloudsimple.fluent.models.CustomizationPolicyInner;
/** An immutable client-side representation of CustomizationPolicy. */
public interface CustomizationPolicy {
/**
* Gets the id property: Customization policy azure id.
*
* @return the id value.
*/
String id();
/**
* Gets the location property: Azure region.
*
* @return the location value.
*/
String location();
/**
* Gets the name property: Customization policy name.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type property.
*
* @return the type value.
*/
String type();
/**
* Gets the description property: Policy description.
*
* @return the description value.
*/
String description();
/**
* Gets the privateCloudId property: The Private cloud id.
*
* @return the privateCloudId value.
*/
String privateCloudId();
/**
* Gets the specification property: Detailed customization policy specification.
*
* @return the specification value.
*/
CustomizationSpecification specification();
/**
* Gets the typePropertiesType property: The type of customization (Linux or Windows).
*
* @return the typePropertiesType value.
*/
CustomizationPolicyPropertiesType typePropertiesType();
/**
* Gets the version property: Policy version.
*
* @return the version value.
*/
String version();
/**
* Gets the inner com.azure.resourcemanager.vmwarecloudsimple.fluent.models.CustomizationPolicyInner object.
*
* @return the inner object.
*/
CustomizationPolicyInner innerModel();
}
| Azure/azure-sdk-for-java | sdk/vmwarecloudsimple/azure-resourcemanager-vmwarecloudsimple/src/main/java/com/azure/resourcemanager/vmwarecloudsimple/models/CustomizationPolicy.java | Java | mit | 2,001 |
import React from 'react';
import ErrorIcon from './ErrorIcon';
export const symbols = {
'ErrorIcon -> with filled': <ErrorIcon filled={true} />,
'ErrorIcon -> without filled': <ErrorIcon filled={false} />
};
| seekinternational/seek-asia-style-guide | react/ErrorIcon/ErrorIcon.iconSketch.js | JavaScript | mit | 214 |
{% extends "base.html"%}
{% block title %}Sequence{% endblock %}
<!---- css/js dependencies ---->
{% block head %}
<link rel="stylesheet" type="text/css" href="{{ static_file('css/rhizo/app.css') }}">
<script type="text/javascript" src="/static/js/moment.min.js"></script>
<script type="text/javascript" src="/static/js/paho-mqtt.js"></script>
<script type="text/javascript" src="{{ static_file('js/rhizo/messages.js') }}"></script>
<script type="text/javascript" src="{{ static_file('js/manyplot.js') }}"></script>
<style>
div#plot {
display: none;
margin-bottom: 20px;
}
</style>
{% endblock head %}
<!---- js code ---->
{% block script %}
<script>
var g_resource = {{ resource|safe }};
var g_resourcePath = '{{ resource_path|safe }}'; // assume includes leading slash
var g_thumbnailResourcePath = '{{ thumbnail_resource_path|safe }}'; // assume includes leading slash
var g_timestamps = {{ timestamps|safe }};
var g_values = {{ values|safe }};
var g_thumbnailRevs = {{ thumbnail_revs|safe }};
var g_fullImageRevs = {{ full_image_revs|safe }};
var g_plotHandler = null;
var g_xData = null;
var g_yData = null;
var g_tableData = null;
$(function() {
// add a menus
var menuData = createMenuData();
menuData.add('Download', downloadHistory);
menuData.add('Summary', dataSummary);
menuData.add('Delete Data', deleteAllData);
createDropDownMenu({id: 'dataMenu', label: 'Data', compact: true, menuData: menuData}).appendTo($('#menuArea'));
// add summary area
var dataTypeName = '';
switch (g_resource.system_attributes.data_type) {
case 1: dataTypeName = 'numeric'; break;
case 2: dataTypeName = 'text'; break;
case 3: dataTypeName = 'image'; break;
}
var nvd = createNameValueData();
nvd.add('ID', g_resource.id);
nvd.add('Name', g_resource.name);
nvd.add('Data Type', dataTypeName);
nvd.add('Decimal Places', g_resource.system_attributes.decimal_places);
nvd.add('Units', g_resource.system_attributes.units);
nvd.add('Max History', g_resource.system_attributes.max_history);
nvd.add('Min Storage Interval', g_resource.system_attributes.min_storage_interval ? g_resource.system_attributes.min_storage_interval + ' seconds' : '0');
createNameValueView(nvd).appendTo($('#sequenceInfo'));
var decimalPlaces = g_resource.decimal_places;
var format = '.' + decimalPlaces + 'f';
// create plot (for numeric sequences)
if (g_resource.system_attributes.data_type == 1) {
var valuesRev = g_values.slice(0).reverse(); // fix(faster): do we need to make a copy if we're reversing?
var timestampsRev = g_timestamps.slice(0).reverse();
var canvas = document.getElementById('canvas');
// make sure all values are numeric (or null)
// fix(faster): combine with reverse (or do on server)
var len = valuesRev.length;
for (var i = 0; i < len; i++) {
var val = valuesRev[i];
if (val !== null) {
valuesRev[i] = +val; // convert to number
}
}
// create plot using manyplot library
g_plotHandler = createPlotHandler(canvas);
g_xData = createDataColumn('timestamp', timestampsRev);
g_xData.type = 'timestamp';
g_yData = createDataColumn('value', valuesRev);
g_yData.name = g_resource.name;
var dataPairs = [
{
'xData': g_xData,
'yData': g_yData,
}
];
g_plotHandler.plotter.setData(dataPairs);
g_plotHandler.drawPlot(null, null);
$('#plot').show();
}
// create table of historical values
if (g_values.length) {
// prep data
var tableLength = 200;
var timestamps = g_timestamps.slice(0, tableLength);
for (var i = 0; i < timestamps.length; i++) {
timestamps[i] *= 1000; // fix(later): do this automatically in table? standardize on unix or unix * 1000?
}
var values = [];
if (g_resource.system_attributes.data_type == 3) {
values = [];
for (var i = 0; i < tableLength; i++) {
var fullImageUrl = '/api/v1/resources' + g_resourcePath + '?rev=' + g_fullImageRevs[i];
if (g_thumbnailRevs[i]) {
var thumbnailUrl = '/api/v1/resources' + g_thumbnailResourcePath + '?rev=' + g_thumbnailRevs[i];
var link = $('<a>', {href: fullImageUrl});
$('<img>', {src: thumbnailUrl}).appendTo(link);
} else {
var link = createLink({'text': 'image', 'href': fullImageUrl});
}
values.push(link);
}
} else {
values = g_values.slice(0, tableLength);
}
// create table
var div = $('#history');
$('<h2>', {html: 'Recent History'}).appendTo(div);
g_tableData = createTableData();
g_tableData.addColumn('Timestamp', timestamps);
g_tableData.addColumn('Value', values);
g_tableData.setFormat('Timestamp', 'timestamp');
createTable(g_tableData).appendTo(div);
}
// subscribe to updates
var lastSlashPos = g_resourcePath.lastIndexOf('/');
var folderName = g_resourcePath.slice(0, lastSlashPos);
subscribeToFolder(folderName);
connectWebSocket();
g_wsh.addSequenceHandler(function(path, timestamp, value) {
if (path == g_resourcePath) {
var unixTimestamp = timestamp.unix();
// update plot
if (g_resource.system_attributes.data_type == 1) {
g_xData.data.push(unixTimestamp);
g_yData.data.push(value);
g_plotHandler.plotter.autoBounds();
g_plotHandler.drawPlot(null, null);
}
// update image
// note: not every sequence update will be stored in DB, so not every image update message will include revision_ids
if (g_resource.system_attributes.data_type == 3 && params.revision_id && params.thumbnail_revision_id) {
var fullImageUrl = '/api/v1/resources' + g_resourcePath + '?rev=' + params.revision_id;
var thumbnailUrl = '/api/v1/resources' + g_thumbnailResourcePath + '?rev=' + params.thumbnail_revision_id;
var link = $('<a>', {href: fullImageUrl});
$('<img>', {src: thumbnailUrl}).appendTo(link);
value = link;
}
// update table
// fix(faster): just add row?
if (value) {
g_tableData.column('Timestamp').values.unshift(unixTimestamp * 1000);
g_tableData.column('Value').values.unshift(value);
var div = $('#history');
div.html('');
$('<h2>', {html: 'Recent History'}).appendTo(div);
createTable(g_tableData).appendTo(div);
}
}
});
});
function dataSummary() {
var handler = function(data) {
var len = data.length;
var messages = [];
var counts = [];
for (var i = 0; i < len; i++) {
var item = data[i];
messages.push(item[0]);
counts.push(item[1]);
}
var div = $('#history');
div.html(''); // clear out old html
$('<h2>', {html: 'History Summary'}).appendTo(div);
var tableData = createTableData();
tableData.addColumn('Message', messages);
tableData.addColumn('Count', counts);
createTable(tableData).appendTo(div);
}
var params = {
'summary': 1,
'prefix_length': 10,
'count': 10000,
'csrf_token': g_csrfToken,
};
$.get('/api/v1/resources' + g_resourcePath, params, handler);
}
function downloadHistory() {
// create a modal
var modalDiv = createBasicModal('downloadHistory', 'Download History');
modalDiv.appendTo($('body'));
// add date range selectors
var todayStr = moment().format('YYYY-MM-DD');
var fg = createFormGroup({id: 'startDate', label: 'Start Date'}).appendTo($('#downloadHistory-body'));
createTextInput({id: 'startDate', value: todayStr}).appendTo(fg);
var fg = createFormGroup({id: 'endDate', label: 'End Date'}).appendTo($('#downloadHistory-body'));
createTextInput({id: 'endDate', value: todayStr}).appendTo(fg);
// handle user choosing to proceed
$('#downloadHistory-ok').click(function() {
var startDate = $('#startDate').val();
var endDate = $('#endDate').val();
var startMoment = moment(startDate);
var endMoment = moment(endDate);
if (!startMoment.isValid()) {
alert('Please enter a valid start date.');
$('#startDate').focus();
} else if (!endMoment.isValid()) {
alert('Please enter a valid end date.');
$('#endDate').focus();
} else {
var startTimestamp = startMoment.startOf('day').toISOString();
var endTimestamp = endMoment.endOf('day').toISOString();
$('#downloadHistory').modal('hide');
window.location.href = '/api/v1/resources' + g_resourcePath + '?download=1&start_timestamp=' + startTimestamp + '&end_timestamp=' + endTimestamp;
}
});
// display the modal
$('#downloadHistory').modal('show');
}
function deleteAllData() {
modalConfirm({
title: 'Delete Sequence Data',
'prompt': 'Are you sure you want to <b>delete all data</b> for sequence <b>' + g_resource.name + '</b>?',
yesFunc: function() {
$.ajax({
url: '/api/v1/resources' + g_resourcePath,
data: {'csrf_token': g_csrfToken, 'data_only': 1},
type: 'DELETE',
success: function() {window.location.reload();}, // fix(later): make more graceful
});
}
});
}
</script>
{% endblock %}
<!---- page content ---->
{% block content %}
<h2>Sequence</h2>
<div id="sequenceInfo"></div>
<div id="plot">
<canvas id="canvas" width="900" height="400"></canvas>
<br>
<button class="btn" onclick="g_plotHandler.zoomIn()">Zoom In</button>
<button class="btn" onclick="g_plotHandler.zoomOut()">Zoom Out</button>
</div>
<div id="history"></div>
{% endblock %}
| rhizolab/rhizo-server | main/templates/resources/sequence.html | HTML | mit | 9,049 |
<!DOCTYPE HTML>
<?php include '../inc/draft_config.php'; ?><!-- Use relative path to find config file -->
<html lang="">
<head>
<base href="<?php echo BASE_URL; ?>">
<meta http-equiv="content-type" content="text/html; charset=utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title>Project Draft — 404</title>
<meta name="robots" content="noindex, nofollow">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<?php include INC_PATH . 'pages_resources.php'; ?>
<link rel="icon" sizes="16x16" href="assets/favicon-16.png">
<link rel="icon" sizes="32x32" href="assets/favicon-32.png">
<link rel="icon" sizes="48x48" href="assets/favicon-48.png">
<link rel="icon" sizes="64x64" href="assets/favicon-64.png">
<link rel="icon" sizes="128x128" href="assets/favicon-128.png">
<link rel="icon" sizes="192x192" href="assets/favicon-192.png">
</head>
<body class="error-404">
<?php $page = 'error-404'; include INC_PATH . 'pages_overhead.php'; ?>
<!-- Example 404 content (markup based on Bootstrap 3 framework) -->
<div class="container">
<div class="row">
<div class="col-md-12">
<h1>Error 404
<br>Page not found!</h1>
<p>Oops! Seems like the page you are looking for no longer exists.
<br>You may go <a href='javascript:history.back(1)'>back</a> or <a href='<?php echo BASE_URL; ?>'>start over</a>.</p>
<hr>
<p class="small text-muted">Rename and edit <code>htaccess</code> file to activate custom error pages.</p>
</div>
</div>
</div>
<!-- END of example content -->
<?php include INC_PATH . 'pages_scripts.php'; ?>
</body>
</html>
| Kiriniy/Project_Draft | dist/assets/errors/404.php | PHP | mit | 1,764 |
#entry {
margin: 10px auto 10px auto;
font-size: 10pt;
width: 100%;
height: 70px;
display: table;
border-radius: 10px;
background-color: #301E19;
border: 10px;
text-align: center;
vertical-align: middle;
}
.coffeeType {
width: 265px;
height: 34px;
margin: 0 auto 0 auto;
border-bottom: solid;
border-width: 1px;
line-height: 30px;
display: table;
}
.coffeeTypeCell {
display: table-cell;
}
.coffeeTime {
height: 35px;
margin: 0 auto 0 auto;
line-height: 30px;
display: table;
}
.coffeeTimeCell {
display: table-cell;
width: 1000px;
} | maxxgl/maxxgl.github.io | app/entry.css | CSS | mit | 633 |
package commands
import (
"compress/gzip"
"errors"
"fmt"
"io"
"os"
"path/filepath"
"strings"
core "github.com/ipfs/go-ipfs/core"
cmdenv "github.com/ipfs/go-ipfs/core/commands/cmdenv"
e "github.com/ipfs/go-ipfs/core/commands/e"
tar "gx/ipfs/QmQine7gvHncNevKtG9QXxf3nXcwSj6aDDmMm52mHofEEp/tar-utils"
uarchive "gx/ipfs/QmSMJ4rZbCJaih3y82Ebq7BZqK6vU2FHsKcWKQiE1DPTpS/go-unixfs/archive"
"gx/ipfs/QmWGm4AbZEbnmdgVTza52MSNpEmBdFVqzmAysRbjrRyGbH/go-ipfs-cmds"
path "gx/ipfs/QmWqh9oob7ZHQRwU5CdTqpnC8ip8BEkFNrwXRxeNo5Y7vA/go-path"
"gx/ipfs/QmYWB8oH6o7qftxoyqTTZhzLrhKCVT7NYahECQTwTtqbgj/pb"
dag "gx/ipfs/Qmb2UEG2TAeVrEJSjqsZF7Y2he7wRDkrdt6c3bECxwZf4k/go-merkledag"
"gx/ipfs/Qmde5VP1qUkyQXKCfmEUA7bP64V2HAptbJ7phuPp7jXWwg/go-ipfs-cmdkit"
)
var ErrInvalidCompressionLevel = errors.New("compression level must be between 1 and 9")
const (
outputOptionName = "output"
archiveOptionName = "archive"
compressOptionName = "compress"
compressionLevelOptionName = "compression-level"
)
var GetCmd = &cmds.Command{
Helptext: cmdkit.HelpText{
Tagline: "Download IPFS objects.",
ShortDescription: `
Stores to disk the data contained an IPFS or IPNS object(s) at the given path.
By default, the output will be stored at './<ipfs-path>', but an alternate
path can be specified with '--output=<path>' or '-o=<path>'.
To output a TAR archive instead of unpacked files, use '--archive' or '-a'.
To compress the output with GZIP compression, use '--compress' or '-C'. You
may also specify the level of compression by specifying '-l=<1-9>'.
`,
},
Arguments: []cmdkit.Argument{
cmdkit.StringArg("ipfs-path", true, false, "The path to the IPFS object(s) to be outputted.").EnableStdin(),
},
Options: []cmdkit.Option{
cmdkit.StringOption(outputOptionName, "o", "The path where the output should be stored."),
cmdkit.BoolOption(archiveOptionName, "a", "Output a TAR archive."),
cmdkit.BoolOption(compressOptionName, "C", "Compress the output with GZIP compression."),
cmdkit.IntOption(compressionLevelOptionName, "l", "The level of compression (1-9)."),
},
PreRun: func(req *cmds.Request, env cmds.Environment) error {
_, err := getCompressOptions(req)
return err
},
Run: func(req *cmds.Request, res cmds.ResponseEmitter, env cmds.Environment) error {
cmplvl, err := getCompressOptions(req)
if err != nil {
return err
}
node, err := cmdenv.GetNode(env)
if err != nil {
return err
}
p := path.Path(req.Arguments[0])
ctx := req.Context
dn, err := core.Resolve(ctx, node.Namesys, node.Resolver, p)
if err != nil {
return err
}
switch dn := dn.(type) {
case *dag.ProtoNode:
size, err := dn.Size()
if err != nil {
return err
}
res.SetLength(size)
case *dag.RawNode:
res.SetLength(uint64(len(dn.RawData())))
default:
return err
}
archive, _ := req.Options[archiveOptionName].(bool)
reader, err := uarchive.DagArchive(ctx, dn, p.String(), node.DAG, archive, cmplvl)
if err != nil {
return err
}
return res.Emit(reader)
},
PostRun: cmds.PostRunMap{
cmds.CLI: func(res cmds.Response, re cmds.ResponseEmitter) error {
req := res.Request()
v, err := res.Next()
if err != nil {
return err
}
outReader, ok := v.(io.Reader)
if !ok {
return e.New(e.TypeErr(outReader, v))
}
outPath := getOutPath(req)
cmplvl, err := getCompressOptions(req)
if err != nil {
return err
}
archive, _ := req.Options[archiveOptionName].(bool)
gw := getWriter{
Out: os.Stdout,
Err: os.Stderr,
Archive: archive,
Compression: cmplvl,
Size: int64(res.Length()),
}
return gw.Write(outReader, outPath)
},
},
}
type clearlineReader struct {
io.Reader
out io.Writer
}
func (r *clearlineReader) Read(p []byte) (n int, err error) {
n, err = r.Reader.Read(p)
if err == io.EOF {
// callback
fmt.Fprintf(r.out, "\033[2K\r") // clear progress bar line on EOF
}
return
}
func progressBarForReader(out io.Writer, r io.Reader, l int64) (*pb.ProgressBar, io.Reader) {
bar := makeProgressBar(out, l)
barR := bar.NewProxyReader(r)
return bar, &clearlineReader{barR, out}
}
func makeProgressBar(out io.Writer, l int64) *pb.ProgressBar {
// setup bar reader
// TODO: get total length of files
bar := pb.New64(l).SetUnits(pb.U_BYTES)
bar.Output = out
// the progress bar lib doesn't give us a way to get the width of the output,
// so as a hack we just use a callback to measure the output, then git rid of it
bar.Callback = func(line string) {
terminalWidth := len(line)
bar.Callback = nil
log.Infof("terminal width: %v\n", terminalWidth)
}
return bar
}
func getOutPath(req *cmds.Request) string {
outPath, _ := req.Options[outputOptionName].(string)
if outPath == "" {
trimmed := strings.TrimRight(req.Arguments[0], "/")
_, outPath = filepath.Split(trimmed)
outPath = filepath.Clean(outPath)
}
return outPath
}
type getWriter struct {
Out io.Writer // for output to user
Err io.Writer // for progress bar output
Archive bool
Compression int
Size int64
}
func (gw *getWriter) Write(r io.Reader, fpath string) error {
if gw.Archive || gw.Compression != gzip.NoCompression {
return gw.writeArchive(r, fpath)
}
return gw.writeExtracted(r, fpath)
}
func (gw *getWriter) writeArchive(r io.Reader, fpath string) error {
// adjust file name if tar
if gw.Archive {
if !strings.HasSuffix(fpath, ".tar") && !strings.HasSuffix(fpath, ".tar.gz") {
fpath += ".tar"
}
}
// adjust file name if gz
if gw.Compression != gzip.NoCompression {
if !strings.HasSuffix(fpath, ".gz") {
fpath += ".gz"
}
}
// create file
file, err := os.Create(fpath)
if err != nil {
return err
}
defer file.Close()
fmt.Fprintf(gw.Out, "Saving archive to %s\n", fpath)
bar, barR := progressBarForReader(gw.Err, r, gw.Size)
bar.Start()
defer bar.Finish()
_, err = io.Copy(file, barR)
return err
}
func (gw *getWriter) writeExtracted(r io.Reader, fpath string) error {
fmt.Fprintf(gw.Out, "Saving file(s) to %s\n", fpath)
bar := makeProgressBar(gw.Err, gw.Size)
bar.Start()
defer bar.Finish()
defer bar.Set64(gw.Size)
extractor := &tar.Extractor{Path: fpath, Progress: bar.Add64}
return extractor.Extract(r)
}
func getCompressOptions(req *cmds.Request) (int, error) {
cmprs, _ := req.Options[compressOptionName].(bool)
cmplvl, cmplvlFound := req.Options[compressionLevelOptionName].(int)
switch {
case !cmprs:
return gzip.NoCompression, nil
case cmprs && !cmplvlFound:
return gzip.DefaultCompression, nil
case cmprs && (cmplvl < 1 || cmplvl > 9):
return gzip.NoCompression, ErrInvalidCompressionLevel
}
return cmplvl, nil
}
| chriscool/go-ipfs | core/commands/get.go | GO | mit | 6,703 |
package org.reasm.m68k.assembly.internal;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.Immutable;
import org.reasm.Value;
import org.reasm.ValueToBooleanVisitor;
/**
* The <code>WHILE</code> directive.
*
* @author Francis Gagné
*/
@Immutable
class WhileDirective extends Mnemonic {
@Nonnull
static final WhileDirective WHILE = new WhileDirective();
private WhileDirective() {
}
@Override
void assemble(M68KAssemblyContext context) {
context.sizeNotAllowed();
final Object blockState = context.getParentBlock();
if (!(blockState instanceof WhileBlockState)) {
throw new AssertionError();
}
final WhileBlockState whileBlockState = (WhileBlockState) blockState;
// The WHILE directive is assembled on every iteration.
// Parse the condition operand on the first iteration only.
if (!whileBlockState.parsedCondition) {
if (context.requireNumberOfOperands(1)) {
whileBlockState.conditionExpression = parseExpressionOperand(context, 0);
}
whileBlockState.parsedCondition = true;
}
final Value condition;
if (whileBlockState.conditionExpression != null) {
condition = whileBlockState.conditionExpression.evaluate(context.getEvaluationContext());
} else {
condition = null;
}
final Boolean result = Value.accept(condition, ValueToBooleanVisitor.INSTANCE);
if (!(result != null && result.booleanValue())) {
// Skip the block body and stop the iteration.
whileBlockState.iterator.next();
whileBlockState.hasNextIteration = false;
}
}
}
| reasm/reasm-m68k | src/main/java/org/reasm/m68k/assembly/internal/WhileDirective.java | Java | mit | 1,747 |
/*
* This file is part of FlexibleLogin
*
* The MIT License (MIT)
*
* Copyright (c) 2015-2018 contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.github.games647.flexiblelogin.listener.prevent;
import com.flowpowered.math.vector.Vector3i;
import com.github.games647.flexiblelogin.FlexibleLogin;
import com.github.games647.flexiblelogin.PomData;
import com.github.games647.flexiblelogin.config.Settings;
import com.google.inject.Inject;
import java.util.List;
import java.util.Optional;
import org.spongepowered.api.command.CommandManager;
import org.spongepowered.api.command.CommandMapping;
import org.spongepowered.api.command.CommandResult;
import org.spongepowered.api.entity.living.player.Player;
import org.spongepowered.api.event.Listener;
import org.spongepowered.api.event.Order;
import org.spongepowered.api.event.block.InteractBlockEvent;
import org.spongepowered.api.event.command.SendCommandEvent;
import org.spongepowered.api.event.entity.DamageEntityEvent;
import org.spongepowered.api.event.entity.InteractEntityEvent;
import org.spongepowered.api.event.entity.MoveEntityEvent;
import org.spongepowered.api.event.filter.Getter;
import org.spongepowered.api.event.filter.cause.First;
import org.spongepowered.api.event.filter.cause.Root;
import org.spongepowered.api.event.filter.type.Exclude;
import org.spongepowered.api.event.item.inventory.ChangeInventoryEvent;
import org.spongepowered.api.event.item.inventory.ClickInventoryEvent;
import org.spongepowered.api.event.item.inventory.ClickInventoryEvent.NumberPress;
import org.spongepowered.api.event.item.inventory.DropItemEvent;
import org.spongepowered.api.event.item.inventory.InteractInventoryEvent;
import org.spongepowered.api.event.item.inventory.InteractItemEvent;
import org.spongepowered.api.event.item.inventory.UseItemStackEvent;
import org.spongepowered.api.event.message.MessageChannelEvent;
public class PreventListener extends AbstractPreventListener {
private final CommandManager commandManager;
@Inject PreventListener(FlexibleLogin plugin, Settings settings, CommandManager commandManager) {
super(plugin, settings);
this.commandManager = commandManager;
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onPlayerMove(MoveEntityEvent playerMoveEvent, @First Player player) {
if (playerMoveEvent instanceof MoveEntityEvent.Teleport) {
return;
}
Vector3i oldLocation = playerMoveEvent.getFromTransform().getPosition().toInt();
Vector3i newLocation = playerMoveEvent.getToTransform().getPosition().toInt();
if (oldLocation.getX() != newLocation.getX() || oldLocation.getZ() != newLocation.getZ()) {
checkLoginStatus(playerMoveEvent, player);
}
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onChat(MessageChannelEvent.Chat chatEvent, @First Player player) {
checkLoginStatus(chatEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onCommand(SendCommandEvent commandEvent, @First Player player) {
String command = commandEvent.getCommand();
Optional<? extends CommandMapping> commandOpt = commandManager.get(command);
if (commandOpt.isPresent()) {
CommandMapping mapping = commandOpt.get();
command = mapping.getPrimaryAlias();
//do not blacklist our own commands
if (commandManager.getOwner(mapping)
.map(pc -> pc.getId().equals(PomData.ARTIFACT_ID))
.orElse(false)) {
return;
}
}
commandEvent.setResult(CommandResult.empty());
if (settings.getGeneral().isCommandOnlyProtection()) {
List<String> protectedCommands = settings.getGeneral().getProtectedCommands();
if (protectedCommands.contains(command) && !plugin.getDatabase().isLoggedIn(player)) {
player.sendMessage(settings.getText().getProtectedCommand());
commandEvent.setCancelled(true);
}
} else {
checkLoginStatus(commandEvent, player);
}
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onPlayerItemDrop(DropItemEvent dropItemEvent, @First Player player) {
checkLoginStatus(dropItemEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onPlayerItemPickup(ChangeInventoryEvent.Pickup pickupItemEvent, @Root Player player) {
checkLoginStatus(pickupItemEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onItemConsume(UseItemStackEvent.Start itemConsumeEvent, @First Player player) {
checkLoginStatus(itemConsumeEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onItemInteract(InteractItemEvent interactItemEvent, @First Player player) {
checkLoginStatus(interactItemEvent, player);
}
// Ignore number press events, because Sponge before this commit
// https://github.com/SpongePowered/SpongeForge/commit/f0605fb0bd62ca2f958425378776608c41f16cca
// has a duplicate bug. Using this exclude we can ignore it, but still cancel the movement of the item
// it appears to be fixed using SpongeForge 4005 (fixed) and 4004 with this change
@Exclude(NumberPress.class)
@Listener(order = Order.FIRST, beforeModifications = true)
public void onInventoryChange(ChangeInventoryEvent changeInventoryEvent, @First Player player) {
checkLoginStatus(changeInventoryEvent, player);
}
@Exclude(NumberPress.class)
@Listener(order = Order.FIRST, beforeModifications = true)
public void onInventoryInteract(InteractInventoryEvent interactInventoryEvent, @First Player player) {
checkLoginStatus(interactInventoryEvent, player);
}
@Exclude(NumberPress.class)
@Listener(order = Order.FIRST, beforeModifications = true)
public void onInventoryClick(ClickInventoryEvent clickInventoryEvent, @First Player player) {
checkLoginStatus(clickInventoryEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onBlockInteract(InteractBlockEvent interactBlockEvent, @First Player player) {
checkLoginStatus(interactBlockEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onPlayerInteractEntity(InteractEntityEvent interactEntityEvent, @First Player player) {
checkLoginStatus(interactEntityEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onPlayerDamage(DamageEntityEvent damageEntityEvent, @First Player player) {
//player is damage source
checkLoginStatus(damageEntityEvent, player);
}
@Listener(order = Order.FIRST, beforeModifications = true)
public void onDamagePlayer(DamageEntityEvent damageEntityEvent, @Getter("getTargetEntity") Player player) {
//player is damage target
checkLoginStatus(damageEntityEvent, player);
}
}
| games647/FlexibleLogin | src/main/java/com/github/games647/flexiblelogin/listener/prevent/PreventListener.java | Java | mit | 8,213 |
<div class="card">
<div class="card-header">
<span class="h3">
Chat Page!!
</span>
</div>
<div class="card-block">
<div class="panel-body">
<ngb-alert [dismissible]="false">
<strong>Warning!</strong> You need to login to see contextual data in chat!!
</ngb-alert>
<ul class="chat">
<li *ngFor="let message of messages" class="right clearfix"><span class="chat-img" [class.pull-right]="message.isSelf" >
<img src="http://placehold.it/50/FA6F57/fff&text={{message.user}}" alt="User Avatar" class="img-circle"/>
</span>
<div class="chat-body clearfix">
<div class="header">
<small class=" text-muted"><span class="glyphicon glyphicon-time"></span>{{message.timestamp}}</small>
<strong [class.pull-right]="message.isSelf" class="primary-font">{{message.user}}</strong>
</div>
<p>
{{message.content}}
<a *ngIf="message.isNavigation" [routerLink]="['/indoor-map', {fromLocationId: message.meta.fromLocationId, toLocationId: message.meta.toLocationId }]" >Here</a>
</p>
</div>
</li>
</ul>
</div>
<div class="panel-footer">
<div class="input-group">
<input [(ngModel)]="newMessage.content" id="btn-input" type="text" class="form-control input-sm" placeholder="Type your message here...">
<span class="input-group-btn">
<button class="btn btn-warning btn-sm" id="btn-chat" (click)="send(newMessage)">
Send</button>
</span>
</div>
</div>
</div>
</div>
| rajagopal28/Donna | src/app/chat-bot/chat-bot.component.html | HTML | mit | 1,744 |
# coding: utf-8
"""
ORCID Member
No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
OpenAPI spec version: Latest
Generated by: https://github.com/swagger-api/swagger-codegen.git
"""
from pprint import pformat
from six import iteritems
import re
class ContributorOrcid(object):
"""
NOTE: This class is auto generated by the swagger code generator program.
Do not edit the class manually.
"""
def __init__(self, uri=None, path=None, host=None):
"""
ContributorOrcid - a model defined in Swagger
:param dict swaggerTypes: The key is attribute name
and the value is attribute type.
:param dict attributeMap: The key is attribute name
and the value is json key in definition.
"""
self.swagger_types = {
'uri': 'str',
'path': 'str',
'host': 'str'
}
self.attribute_map = {
'uri': 'uri',
'path': 'path',
'host': 'host'
}
self._uri = uri
self._path = path
self._host = host
@property
def uri(self):
"""
Gets the uri of this ContributorOrcid.
:return: The uri of this ContributorOrcid.
:rtype: str
"""
return self._uri
@uri.setter
def uri(self, uri):
"""
Sets the uri of this ContributorOrcid.
:param uri: The uri of this ContributorOrcid.
:type: str
"""
self._uri = uri
@property
def path(self):
"""
Gets the path of this ContributorOrcid.
:return: The path of this ContributorOrcid.
:rtype: str
"""
return self._path
@path.setter
def path(self, path):
"""
Sets the path of this ContributorOrcid.
:param path: The path of this ContributorOrcid.
:type: str
"""
self._path = path
@property
def host(self):
"""
Gets the host of this ContributorOrcid.
:return: The host of this ContributorOrcid.
:rtype: str
"""
return self._host
@host.setter
def host(self, host):
"""
Sets the host of this ContributorOrcid.
:param host: The host of this ContributorOrcid.
:type: str
"""
self._host = host
def to_dict(self):
"""
Returns the model properties as a dict
"""
result = {}
for attr, _ in iteritems(self.swagger_types):
value = getattr(self, attr)
if isinstance(value, list):
result[attr] = list(map(
lambda x: x.to_dict() if hasattr(x, "to_dict") else x,
value
))
elif hasattr(value, "to_dict"):
result[attr] = value.to_dict()
elif isinstance(value, dict):
result[attr] = dict(map(
lambda item: (item[0], item[1].to_dict())
if hasattr(item[1], "to_dict") else item,
value.items()
))
else:
result[attr] = value
return result
def to_str(self):
"""
Returns the string representation of the model
"""
return pformat(self.to_dict())
def __repr__(self):
"""
For `print` and `pprint`
"""
return self.to_str()
def __eq__(self, other):
"""
Returns true if both objects are equal
"""
if not isinstance(other, ContributorOrcid):
return False
return self.__dict__ == other.__dict__
def __ne__(self, other):
"""
Returns true if both objects are not equal
"""
return not self == other
| Royal-Society-of-New-Zealand/NZ-ORCID-Hub | orcid_api/models/contributor_orcid.py | Python | mit | 3,922 |
package com.contexthub.storageapp;
import android.os.Bundle;
import android.support.v4.app.FragmentManager;
import android.support.v4.view.MenuItemCompat;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.widget.SearchView;
import android.view.Menu;
import android.view.MenuItem;
import com.chaione.contexthub.sdk.model.VaultDocument;
import com.contexthub.storageapp.fragments.AboutFragment;
import com.contexthub.storageapp.fragments.EditVaultItemFragment;
import com.contexthub.storageapp.fragments.VaultItemListFragment;
import com.contexthub.storageapp.models.Person;
public class MainActivity extends ActionBarActivity implements VaultItemListFragment.Listener, FragmentManager.OnBackStackChangedListener {
private MenuItem menuSearch;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if(savedInstanceState == null) {
getSupportFragmentManager().beginTransaction()
.replace(android.R.id.content, new VaultItemListFragment())
.commit();
getSupportFragmentManager().addOnBackStackChangedListener(this);
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
setupSearchView(menu.findItem(R.id.action_search));
return true;
}
private void setupSearchView(final MenuItem menuSearch) {
this.menuSearch = menuSearch;
SearchView searchView = (SearchView) MenuItemCompat.getActionView(menuSearch);
SearchView.SearchAutoComplete searchAutoComplete = (SearchView.SearchAutoComplete) searchView.findViewById(R.id.search_src_text);
searchAutoComplete.setHint(R.string.search_hint);
searchView.setOnQueryTextListener(new android.support.v7.widget.SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
menuSearch.collapseActionView();
getSupportFragmentManager().beginTransaction()
.addToBackStack(null)
.replace(android.R.id.content, VaultItemListFragment.newInstance(query))
.commit();
return true;
}
@Override
public boolean onQueryTextChange(String query) {
return false;
}
});
}
@Override
public boolean onPrepareOptionsMenu(Menu menu) {
boolean isMainFragment = getSupportFragmentManager().getBackStackEntryCount() <= 0;
menu.findItem(R.id.action_search).setVisible(isMainFragment);
menu.findItem(R.id.action_add).setVisible(isMainFragment);
menu.findItem(R.id.action_about).setVisible(isMainFragment);
return super.onPrepareOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
menuSearch.collapseActionView();
switch(item.getItemId()) {
case R.id.action_add:
launchEditVaultItemFragment(null);
return true;
case R.id.action_about:
getSupportFragmentManager().beginTransaction()
.addToBackStack(null)
.replace(android.R.id.content, new AboutFragment())
.commit();
return true;
default:
return super.onOptionsItemSelected(item);
}
}
private void launchEditVaultItemFragment(VaultDocument<Person> document) {
EditVaultItemFragment fragment = document == null ? new EditVaultItemFragment() :
EditVaultItemFragment.newInstance(document);
getSupportFragmentManager().beginTransaction()
.addToBackStack(null)
.replace(android.R.id.content, fragment)
.commit();
}
@Override
public void onItemClick(VaultDocument<Person> document) {
menuSearch.collapseActionView();
launchEditVaultItemFragment(document);
}
@Override
public void onBackStackChanged() {
supportInvalidateOptionsMenu();
}
}
| contexthub/storage-android | StorageApp/app/src/main/java/com/contexthub/storageapp/MainActivity.java | Java | mit | 4,259 |
// --------------------------------------------------------------------------------------------------------------------
// <copyright file="ModelAppServiceInfoProviderTest.cs" company="Kephas Software SRL">
// Copyright (c) Kephas Software SRL. All rights reserved.
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
// </copyright>
// --------------------------------------------------------------------------------------------------------------------
namespace Kephas.Model.Tests
{
using System.Collections.Generic;
using System.Reflection;
using System.Threading.Tasks;
using Kephas.Composition.Conventions;
using Kephas.Composition.Lite;
using Kephas.Model.Elements;
using Kephas.Services;
using Kephas.Testing.Model;
using NUnit.Framework;
[TestFixture]
public class ModelAppServiceInfoProviderTest : ModelTestBase
{
public override IEnumerable<Assembly> GetDefaultConventionAssemblies()
{
return new List<Assembly>(base.GetDefaultConventionAssemblies())
{
typeof(IModelSpace).Assembly,
};
}
[Test]
public async Task GetAppServiceInfos_EnsureModelSpaceRegistered()
{
var ambientServices = new AmbientServices()
.RegisterMultiple<IAppServiceInfoProvider>(b => b.WithType<ModelAppServiceInfoProvider>());
var container = this.CreateContainer(ambientServices);
var provider = container.GetExport<IModelSpaceProvider>();
await provider.InitializeAsync(new Context(container));
var modelSpace = container.GetExport<IModelSpace>();
Assert.IsInstanceOf<DefaultModelSpace>(modelSpace);
}
}
} | quartz-software/kephas | src/Tests/Kephas.Model.Tests/ModelAppServiceInfoProviderTest.cs | C# | mit | 1,803 |
<?php
/**
* @author Victor Demin <[email protected]>
* @copyright (c) 2015, Victor Demin <[email protected]>
*/
namespace GlenDemon\ZabbixApi\Repository;
use \GlenDemon\ZabbixApi\Entity\HostGroup;
/**
* HostGroup repository.
*/
class HostGroupRepository extends AbstractRepository
{
/**
* Finds an object by its primary key / identifier.
*
* @param mixed $id The identifier.
*
* @return HostGroup The object.
*/
public function find($id)
{
return parent::find($id);
}
/**
* Finds all objects in the repository.
*
* @return HostGroup[] The objects.
*/
public function findAll()
{
return parent::findAll();
}
/**
* @inherit
*/
protected function getRawData(array $params)
{
return $this->api->hostgroupGet($params);
}
/**
* Finds a single object by a set of criteria.
*
* @param array $criteria The criteria.
*
* @return HostGroup|null The object.
*/
public function findOneBy(array $criteria)
{
return parent::findOneBy($criteria);
}
}
| glendemon/zabbix-api | src/Repository/HostGroupRepository.php | PHP | mit | 1,128 |
// Copyright Aleksey Gurtovoy 2000-2004
//
// Distributed under the Boost Software License, Version 1.0.
// (See accompanying file LICENSE_1_0.txt or copy at
// http://www.lslboost.org/LICENSE_1_0.txt)
//
// Preprocessed version of "lslboost/mpl/list/list10_c.hpp" header
// -- DO NOT modify by hand!
namespace lslboost { namespace mpl {
template<
typename T
, T C0
>
struct list1_c
: l_item<
long_<1>
, integral_c< T,C0 >
, l_end
>
{
typedef list1_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1
>
struct list2_c
: l_item<
long_<2>
, integral_c< T,C0 >
, list1_c< T,C1 >
>
{
typedef list2_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2
>
struct list3_c
: l_item<
long_<3>
, integral_c< T,C0 >
, list2_c< T,C1,C2 >
>
{
typedef list3_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3
>
struct list4_c
: l_item<
long_<4>
, integral_c< T,C0 >
, list3_c< T,C1,C2,C3 >
>
{
typedef list4_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3, T C4
>
struct list5_c
: l_item<
long_<5>
, integral_c< T,C0 >
, list4_c< T,C1,C2,C3,C4 >
>
{
typedef list5_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3, T C4, T C5
>
struct list6_c
: l_item<
long_<6>
, integral_c< T,C0 >
, list5_c< T,C1,C2,C3,C4,C5 >
>
{
typedef list6_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3, T C4, T C5, T C6
>
struct list7_c
: l_item<
long_<7>
, integral_c< T,C0 >
, list6_c< T,C1,C2,C3,C4,C5,C6 >
>
{
typedef list7_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3, T C4, T C5, T C6, T C7
>
struct list8_c
: l_item<
long_<8>
, integral_c< T,C0 >
, list7_c< T,C1,C2,C3,C4,C5,C6,C7 >
>
{
typedef list8_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3, T C4, T C5, T C6, T C7, T C8
>
struct list9_c
: l_item<
long_<9>
, integral_c< T,C0 >
, list8_c< T,C1,C2,C3,C4,C5,C6,C7,C8 >
>
{
typedef list9_c type;
typedef T value_type;
};
template<
typename T
, T C0, T C1, T C2, T C3, T C4, T C5, T C6, T C7, T C8, T C9
>
struct list10_c
: l_item<
long_<10>
, integral_c< T,C0 >
, list9_c< T,C1,C2,C3,C4,C5,C6,C7,C8,C9 >
>
{
typedef list10_c type;
typedef T value_type;
};
}}
| gazzlab/LSL-gazzlab-branch | liblsl/external/lslboost/mpl/list/aux_/preprocessed/plain/list10_c.hpp | C++ | mit | 2,868 |
module CardHelper
def card_element_properties(node, options = {})
{ class: dom_class(node, :card),
itemscope: '',
itemtype: 'http://schema.org/Thing',
itemid: node.uuid,
itemtype: node.class.schema_path }.merge(options)
end
def cardtec_header(node)
content_tag(:span, node.uuid, itemprop: :uuid) <<
content_tag(:span, node.ident, itemprop: :ident) <<
content_tag(:span, node.class.name, itemprop: :class_name) <<
content_tag(:span, node.path, itemprop: :path) <<
content_tag(:span, node.created_at, itemprop: :created_at) <<
content_tag(:span, node.updated_at, itemprop: :updated_at)
end
end
| dinge/dinghub | app/helpers/card_helper.rb | Ruby | mit | 705 |
/*
* The MIT License
*
* Copyright 2014 Jon Arney, Ensor Robotics.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.ensor.robots.roboclawdriver;
/**
*
* @author jona
*/
class CommandReadMainBatteryVoltage extends CommandReadBatteryVoltage {
protected CommandReadMainBatteryVoltage(RoboClaw aRoboClaw) {
super(aRoboClaw);
}
@Override
protected byte getCommandByte() {
return (byte)24;
}
@Override
protected void onResponse(double voltage) {
mRoboClaw.setMainBatteryVoltage(voltage);
}
}
| jarney/snackbot | src/main/java/org/ensor/robots/roboclawdriver/CommandReadMainBatteryVoltage.java | Java | mit | 1,602 |
package org.kalnee.trivor.insights.web.rest;
import com.codahale.metrics.annotation.Timed;
import org.kalnee.trivor.insights.domain.insights.Insights;
import org.kalnee.trivor.insights.service.InsightService;
import org.kalnee.trivor.nlp.domain.ChunkFrequency;
import org.kalnee.trivor.nlp.domain.PhrasalVerbUsage;
import org.kalnee.trivor.nlp.domain.SentenceFrequency;
import org.kalnee.trivor.nlp.domain.WordUsage;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Map;
import java.util.Set;
@RestController
@RequestMapping(value = "/api/insights")
public class InsightsResource {
private final InsightService insightService;
public InsightsResource(InsightService insightService) {
this.insightService = insightService;
}
@GetMapping
public ResponseEntity<Page<Insights>> findByInsightAndImdb(@RequestParam("imdbId") String imdbId,
Pageable pageable) {
return ResponseEntity.ok().body(insightService.findByImdbId(imdbId, pageable));
}
@GetMapping("/summary")
@Timed
public ResponseEntity<Map<String, Object>> getInsightsSummary(@RequestParam("imdbId") String imdbId) {
return ResponseEntity.ok().body(insightService.getInsightsSummary(imdbId));
}
@GetMapping("/sentences/frequency")
@Timed
public ResponseEntity<List<SentenceFrequency>> findSentencesFrequency(@RequestParam("imdbId") String imdbId,
@RequestParam(value = "limit", required = false) Integer limit) {
return ResponseEntity.ok().body(
insightService.findSentencesFrequencyByImdbId(imdbId, limit)
);
}
@GetMapping("/chunks/frequency")
@Timed
public ResponseEntity<List<ChunkFrequency>> findChunksFrequency(@RequestParam("imdbId") String imdbId,
@RequestParam(value = "limit", required = false) Integer limit) {
return ResponseEntity.ok().body(
insightService.findChunksFrequencyByImdbId(imdbId, limit)
);
}
@GetMapping("/phrasal-verbs/usage")
@Timed
public ResponseEntity<List<PhrasalVerbUsage>> findPhrasalVerbsUsageByImdbId(@RequestParam("imdbId") String imdbId) {
return ResponseEntity.ok().body(insightService.findPhrasalVerbsUsageByImdbId(imdbId));
}
@GetMapping("/vocabulary/{vocabulary}/frequency")
@Timed
public ResponseEntity<Map<String, Integer>> findVocabularyFrequencyByImdbId(
@PathVariable("vocabulary") String vocabulary,
@RequestParam("imdbId") String imdbId,
@RequestParam(value = "limit", required = false) Integer limit) {
return ResponseEntity.ok().body(insightService.findVocabularyFrequencyByImdbId(vocabulary, imdbId, limit));
}
@GetMapping("/vocabulary/{vocabulary}/usage")
@Timed
public ResponseEntity<List<WordUsage>> findVocabularyUsageByImdbAndSeasonAndEpisode(
@PathVariable("vocabulary") String vocabulary,
@RequestParam("imdbId") String imdbId,
@RequestParam(value = "season", required = false) Integer season,
@RequestParam(value = "episode", required = false) Integer episode) {
return ResponseEntity.ok().body(
insightService.findVocabularyUsageByImdbAndSeasonAndEpisode(vocabulary, imdbId, season, episode)
);
}
@GetMapping("/{insight}/genres/{genre}")
@Timed
public ResponseEntity<List<Insights>> findInsightsByGenre(
@PathVariable("genre") String genre) {
return ResponseEntity.ok().body(insightService.findInsightsByInsightAndGenre(genre));
}
@GetMapping("/{insight}/keywords/{keyword}")
@Timed
public ResponseEntity<List<Insights>> findInsightsByKeyword(
@PathVariable("keyword") String keyword) {
return ResponseEntity.ok().body(insightService.findInsightsByInsightAndKeyword(keyword));
}
}
| kalnee/trivor | insights/src/main/java/org/kalnee/trivor/insights/web/rest/InsightsResource.java | Java | mit | 4,162 |
// --------------------------------------------------------------------------------------------------------------------
// <copyright file="GlobalSuppressions.cs">
// Copyright (c) 2017. All rights reserved. Licensed under the MIT license. See LICENSE file in
// the project root for full license information.
// </copyright>
// --------------------------------------------------------------------------------------------------------------------
// This file is used by Code Analysis to maintain SuppressMessage attributes that are applied to this
// project. Project-level suppressions either have no target or are given a specific target and
// scoped to a namespace, type, member, etc.
[assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Style", "IDE0002:Simplify Member Access", Justification = "This is an auto-generated file.", Scope = "member", Target = "~P:Spritely.Foundations.WebApi.Messages.ResourceManager")]
[assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Globalization", "CA1303:Do not pass literals as localized parameters", MessageId = "Spritely.Foundations.WebApi.WriteLog.Invoke(System.String)", Scope = "member", Target = "Spritely.Foundations.WebApi.BasicWebApiLogPolicy.#.cctor()", Justification = "Colon is used as a separator for log file output - overkill to move this into resources.")]
| spritely/Foundations.WebApi | Foundations.WebApi/GlobalSuppressions.cs | C# | mit | 1,356 |
#ifndef _VSARDUINO_H_
#define _VSARDUINO_H_
//Board = Arduino Nano w/ ATmega328
#define __AVR_ATmega328p__
#define __AVR_ATmega328P__
#define ARDUINO 105
#define ARDUINO_MAIN
#define __AVR__
#define __avr__
#define F_CPU 16000000L
#define __cplusplus
#define __inline__
#define __asm__(x)
#define __extension__
#define __ATTR_PURE__
#define __ATTR_CONST__
#define __inline__
#define __asm__
#define __volatile__
#define __builtin_va_list
#define __builtin_va_start
#define __builtin_va_end
#define __DOXYGEN__
#define __attribute__(x)
#define NOINLINE __attribute__((noinline))
#define prog_void
#define PGM_VOID_P int
typedef unsigned char byte;
extern "C" void __cxa_pure_virtual() {;}
int serial_putc(char c, FILE *);
void printf_begin(void);
static void dhcp_status_cb(int s, const uint16_t *dnsaddr);
static void resolv_found_cb(char *name, uint16_t *addr);
void ___dhcp_status(int s, const uint16_t *);
//
//
void webclient_datahandler(char *data, u16_t len);
void webclient_connected(void);
void webclient_timedout(void);
void webclient_aborted(void);
void webclient_closed(void);
#include "D:\arduino\arduino-1.0.5\hardware\arduino\cores\arduino\arduino.h"
#include "D:\arduino\arduino-1.0.5\hardware\arduino\variants\eightanaloginputs\pins_arduino.h"
#include "D:\Projects\arduino-sensor-network\nrf24l01\RF24SensorTestServerMQTT2\RF24SensorTestServerMQTT2.ino"
#endif
| radzio/arduino-sensor-network | nrf24l01/RF24SensorTestServerMQTT2/Visual Micro/.RF24SensorTestServerMQTT2.vsarduino.h | C | mit | 1,396 |
# encoding: utf-8
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is
# regenerated.
module Azure::Network::Mgmt::V2018_04_01
module Models
#
# List of Vpn-Sites
#
class GetVpnSitesConfigurationRequest
include MsRestAzure
# @return [Array<SubResource>] List of resource-ids of the vpn-sites for
# which config is to be downloaded.
attr_accessor :vpn_sites
# @return [String] The sas-url to download the configurations for
# vpn-sites
attr_accessor :output_blob_sas_url
#
# Mapper for GetVpnSitesConfigurationRequest class as Ruby Hash.
# This will be used for serialization/deserialization.
#
def self.mapper()
{
client_side_validation: true,
required: false,
serialized_name: 'GetVpnSitesConfigurationRequest',
type: {
name: 'Composite',
class_name: 'GetVpnSitesConfigurationRequest',
model_properties: {
vpn_sites: {
client_side_validation: true,
required: false,
serialized_name: 'vpnSites',
type: {
name: 'Sequence',
element: {
client_side_validation: true,
required: false,
serialized_name: 'SubResourceElementType',
type: {
name: 'Composite',
class_name: 'SubResource'
}
}
}
},
output_blob_sas_url: {
client_side_validation: true,
required: false,
serialized_name: 'outputBlobSasUrl',
type: {
name: 'String'
}
}
}
}
}
end
end
end
end
| Azure/azure-sdk-for-ruby | management/azure_mgmt_network/lib/2018-04-01/generated/azure_mgmt_network/models/get_vpn_sites_configuration_request.rb | Ruby | mit | 1,986 |
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=US-ASCII">
<title>Confidence Intervals on the Standard Deviation</title>
<link rel="stylesheet" href="../../../../math.css" type="text/css">
<meta name="generator" content="DocBook XSL Stylesheets V1.77.1">
<link rel="home" href="../../../../index.html" title="Math Toolkit 2.2.1">
<link rel="up" href="../cs_eg.html" title="Chi Squared Distribution Examples">
<link rel="prev" href="../cs_eg.html" title="Chi Squared Distribution Examples">
<link rel="next" href="chi_sq_test.html" title="Chi-Square Test for the Standard Deviation">
</head>
<body bgcolor="white" text="black" link="#0000FF" vlink="#840084" alink="#0000FF">
<table cellpadding="2" width="100%"><tr>
<td valign="top"><img alt="Boost C++ Libraries" width="277" height="86" src="../../../../../../../../boost.png"></td>
<td align="center"><a href="../../../../../../../../index.html">Home</a></td>
<td align="center"><a href="../../../../../../../../libs/libraries.htm">Libraries</a></td>
<td align="center"><a href="http://www.boost.org/users/people.html">People</a></td>
<td align="center"><a href="http://www.boost.org/users/faq.html">FAQ</a></td>
<td align="center"><a href="../../../../../../../../more/index.htm">More</a></td>
</tr></table>
<hr>
<div class="spirit-nav">
<a accesskey="p" href="../cs_eg.html"><img src="../../../../../../../../doc/src/images/prev.png" alt="Prev"></a><a accesskey="u" href="../cs_eg.html"><img src="../../../../../../../../doc/src/images/up.png" alt="Up"></a><a accesskey="h" href="../../../../index.html"><img src="../../../../../../../../doc/src/images/home.png" alt="Home"></a><a accesskey="n" href="chi_sq_test.html"><img src="../../../../../../../../doc/src/images/next.png" alt="Next"></a>
</div>
<div class="section">
<div class="titlepage"><div><div><h5 class="title">
<a name="math_toolkit.stat_tut.weg.cs_eg.chi_sq_intervals"></a><a class="link" href="chi_sq_intervals.html" title="Confidence Intervals on the Standard Deviation">Confidence
Intervals on the Standard Deviation</a>
</h5></div></div></div>
<p>
Once you have calculated the standard deviation for your data, a legitimate
question to ask is "How reliable is the calculated standard deviation?".
For this situation the Chi Squared distribution can be used to calculate
confidence intervals for the standard deviation.
</p>
<p>
The full example code & sample output is in <a href="../../../../../../example/chi_square_std_dev_test.cpp" target="_top">chi_square_std_dev_test.cpp</a>.
</p>
<p>
We'll begin by defining the procedure that will calculate and print out
the confidence intervals:
</p>
<pre class="programlisting"><span class="keyword">void</span> <span class="identifier">confidence_limits_on_std_deviation</span><span class="special">(</span>
<span class="keyword">double</span> <span class="identifier">Sd</span><span class="special">,</span> <span class="comment">// Sample Standard Deviation</span>
<span class="keyword">unsigned</span> <span class="identifier">N</span><span class="special">)</span> <span class="comment">// Sample size</span>
<span class="special">{</span>
</pre>
<p>
We'll begin by printing out some general information:
</p>
<pre class="programlisting"><span class="identifier">cout</span> <span class="special"><<</span>
<span class="string">"________________________________________________\n"</span>
<span class="string">"2-Sided Confidence Limits For Standard Deviation\n"</span>
<span class="string">"________________________________________________\n\n"</span><span class="special">;</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">setprecision</span><span class="special">(</span><span class="number">7</span><span class="special">);</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">setw</span><span class="special">(</span><span class="number">40</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">left</span> <span class="special"><<</span> <span class="string">"Number of Observations"</span> <span class="special"><<</span> <span class="string">"= "</span> <span class="special"><<</span> <span class="identifier">N</span> <span class="special"><<</span> <span class="string">"\n"</span><span class="special">;</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">setw</span><span class="special">(</span><span class="number">40</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">left</span> <span class="special"><<</span> <span class="string">"Standard Deviation"</span> <span class="special"><<</span> <span class="string">"= "</span> <span class="special"><<</span> <span class="identifier">Sd</span> <span class="special"><<</span> <span class="string">"\n"</span><span class="special">;</span>
</pre>
<p>
and then define a table of significance levels for which we'll calculate
intervals:
</p>
<pre class="programlisting"><span class="keyword">double</span> <span class="identifier">alpha</span><span class="special">[]</span> <span class="special">=</span> <span class="special">{</span> <span class="number">0.5</span><span class="special">,</span> <span class="number">0.25</span><span class="special">,</span> <span class="number">0.1</span><span class="special">,</span> <span class="number">0.05</span><span class="special">,</span> <span class="number">0.01</span><span class="special">,</span> <span class="number">0.001</span><span class="special">,</span> <span class="number">0.0001</span><span class="special">,</span> <span class="number">0.00001</span> <span class="special">};</span>
</pre>
<p>
The distribution we'll need to calculate the confidence intervals is
a Chi Squared distribution, with N-1 degrees of freedom:
</p>
<pre class="programlisting"><span class="identifier">chi_squared</span> <span class="identifier">dist</span><span class="special">(</span><span class="identifier">N</span> <span class="special">-</span> <span class="number">1</span><span class="special">);</span>
</pre>
<p>
For each value of alpha, the formula for the confidence interval is given
by:
</p>
<p>
<span class="inlinemediaobject"><img src="../../../../../equations/chi_squ_tut1.svg"></span>
</p>
<p>
Where <span class="inlinemediaobject"><img src="../../../../../equations/chi_squ_tut2.svg"></span> is the upper critical value, and <span class="inlinemediaobject"><img src="../../../../../equations/chi_squ_tut3.svg"></span> is
the lower critical value of the Chi Squared distribution.
</p>
<p>
In code we begin by printing out a table header:
</p>
<pre class="programlisting"><span class="identifier">cout</span> <span class="special"><<</span> <span class="string">"\n\n"</span>
<span class="string">"_____________________________________________\n"</span>
<span class="string">"Confidence Lower Upper\n"</span>
<span class="string">" Value (%) Limit Limit\n"</span>
<span class="string">"_____________________________________________\n"</span><span class="special">;</span>
</pre>
<p>
and then loop over the values of alpha and calculate the intervals for
each: remember that the lower critical value is the same as the quantile,
and the upper critical value is the same as the quantile from the complement
of the probability:
</p>
<pre class="programlisting"><span class="keyword">for</span><span class="special">(</span><span class="keyword">unsigned</span> <span class="identifier">i</span> <span class="special">=</span> <span class="number">0</span><span class="special">;</span> <span class="identifier">i</span> <span class="special"><</span> <span class="keyword">sizeof</span><span class="special">(</span><span class="identifier">alpha</span><span class="special">)/</span><span class="keyword">sizeof</span><span class="special">(</span><span class="identifier">alpha</span><span class="special">[</span><span class="number">0</span><span class="special">]);</span> <span class="special">++</span><span class="identifier">i</span><span class="special">)</span>
<span class="special">{</span>
<span class="comment">// Confidence value:</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">fixed</span> <span class="special"><<</span> <span class="identifier">setprecision</span><span class="special">(</span><span class="number">3</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">setw</span><span class="special">(</span><span class="number">10</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">right</span> <span class="special"><<</span> <span class="number">100</span> <span class="special">*</span> <span class="special">(</span><span class="number">1</span><span class="special">-</span><span class="identifier">alpha</span><span class="special">[</span><span class="identifier">i</span><span class="special">]);</span>
<span class="comment">// Calculate limits:</span>
<span class="keyword">double</span> <span class="identifier">lower_limit</span> <span class="special">=</span> <span class="identifier">sqrt</span><span class="special">((</span><span class="identifier">N</span> <span class="special">-</span> <span class="number">1</span><span class="special">)</span> <span class="special">*</span> <span class="identifier">Sd</span> <span class="special">*</span> <span class="identifier">Sd</span> <span class="special">/</span> <span class="identifier">quantile</span><span class="special">(</span><span class="identifier">complement</span><span class="special">(</span><span class="identifier">dist</span><span class="special">,</span> <span class="identifier">alpha</span><span class="special">[</span><span class="identifier">i</span><span class="special">]</span> <span class="special">/</span> <span class="number">2</span><span class="special">)));</span>
<span class="keyword">double</span> <span class="identifier">upper_limit</span> <span class="special">=</span> <span class="identifier">sqrt</span><span class="special">((</span><span class="identifier">N</span> <span class="special">-</span> <span class="number">1</span><span class="special">)</span> <span class="special">*</span> <span class="identifier">Sd</span> <span class="special">*</span> <span class="identifier">Sd</span> <span class="special">/</span> <span class="identifier">quantile</span><span class="special">(</span><span class="identifier">dist</span><span class="special">,</span> <span class="identifier">alpha</span><span class="special">[</span><span class="identifier">i</span><span class="special">]</span> <span class="special">/</span> <span class="number">2</span><span class="special">));</span>
<span class="comment">// Print Limits:</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">fixed</span> <span class="special"><<</span> <span class="identifier">setprecision</span><span class="special">(</span><span class="number">5</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">setw</span><span class="special">(</span><span class="number">15</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">right</span> <span class="special"><<</span> <span class="identifier">lower_limit</span><span class="special">;</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">fixed</span> <span class="special"><<</span> <span class="identifier">setprecision</span><span class="special">(</span><span class="number">5</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">setw</span><span class="special">(</span><span class="number">15</span><span class="special">)</span> <span class="special"><<</span> <span class="identifier">right</span> <span class="special"><<</span> <span class="identifier">upper_limit</span> <span class="special"><<</span> <span class="identifier">endl</span><span class="special">;</span>
<span class="special">}</span>
<span class="identifier">cout</span> <span class="special"><<</span> <span class="identifier">endl</span><span class="special">;</span>
</pre>
<p>
To see some example output we'll use the <a href="http://www.itl.nist.gov/div898/handbook/eda/section3/eda3581.htm" target="_top">gear
data</a> from the <a href="http://www.itl.nist.gov/div898/handbook/" target="_top">NIST/SEMATECH
e-Handbook of Statistical Methods.</a>. The data represents measurements
of gear diameter from a manufacturing process.
</p>
<pre class="programlisting">________________________________________________
2-Sided Confidence Limits For Standard Deviation
________________________________________________
Number of Observations = 100
Standard Deviation = 0.006278908
_____________________________________________
Confidence Lower Upper
Value (%) Limit Limit
_____________________________________________
50.000 0.00601 0.00662
75.000 0.00582 0.00685
90.000 0.00563 0.00712
95.000 0.00551 0.00729
99.000 0.00530 0.00766
99.900 0.00507 0.00812
99.990 0.00489 0.00855
99.999 0.00474 0.00895
</pre>
<p>
So at the 95% confidence level we conclude that the standard deviation
is between 0.00551 and 0.00729.
</p>
<h5>
<a name="math_toolkit.stat_tut.weg.cs_eg.chi_sq_intervals.h0"></a>
<span class="phrase"><a name="math_toolkit.stat_tut.weg.cs_eg.chi_sq_intervals.confidence_intervals_as_a_functi"></a></span><a class="link" href="chi_sq_intervals.html#math_toolkit.stat_tut.weg.cs_eg.chi_sq_intervals.confidence_intervals_as_a_functi">Confidence
intervals as a function of the number of observations</a>
</h5>
<p>
Similarly, we can also list the confidence intervals for the standard
deviation for the common confidence levels 95%, for increasing numbers
of observations.
</p>
<p>
The standard deviation used to compute these values is unity, so the
limits listed are <span class="bold"><strong>multipliers</strong></span> for any
particular standard deviation. For example, given a standard deviation
of 0.0062789 as in the example above; for 100 observations the multiplier
is 0.8780 giving the lower confidence limit of 0.8780 * 0.006728 = 0.00551.
</p>
<pre class="programlisting">____________________________________________________
Confidence level (two-sided) = 0.0500000
Standard Deviation = 1.0000000
________________________________________
Observations Lower Upper
Limit Limit
________________________________________
2 0.4461 31.9102
3 0.5207 6.2847
4 0.5665 3.7285
5 0.5991 2.8736
6 0.6242 2.4526
7 0.6444 2.2021
8 0.6612 2.0353
9 0.6755 1.9158
10 0.6878 1.8256
15 0.7321 1.5771
20 0.7605 1.4606
30 0.7964 1.3443
40 0.8192 1.2840
50 0.8353 1.2461
60 0.8476 1.2197
100 0.8780 1.1617
120 0.8875 1.1454
1000 0.9580 1.0459
10000 0.9863 1.0141
50000 0.9938 1.0062
100000 0.9956 1.0044
1000000 0.9986 1.0014
</pre>
<p>
With just 2 observations the limits are from <span class="bold"><strong>0.445</strong></span>
up to to <span class="bold"><strong>31.9</strong></span>, so the standard deviation
might be about <span class="bold"><strong>half</strong></span> the observed value
up to <span class="bold"><strong>30 times</strong></span> the observed value!
</p>
<p>
Estimating a standard deviation with just a handful of values leaves
a very great uncertainty, especially the upper limit. Note especially
how far the upper limit is skewed from the most likely standard deviation.
</p>
<p>
Even for 10 observations, normally considered a reasonable number, the
range is still from 0.69 to 1.8, about a range of 0.7 to 2, and is still
highly skewed with an upper limit <span class="bold"><strong>twice</strong></span>
the median.
</p>
<p>
When we have 1000 observations, the estimate of the standard deviation
is starting to look convincing, with a range from 0.95 to 1.05 - now
near symmetrical, but still about + or - 5%.
</p>
<p>
Only when we have 10000 or more repeated observations can we start to
be reasonably confident (provided we are sure that other factors like
drift are not creeping in).
</p>
<p>
For 10000 observations, the interval is 0.99 to 1.1 - finally a really
convincing + or -1% confidence.
</p>
</div>
<table xmlns:rev="http://www.cs.rpi.edu/~gregod/boost/tools/doc/revision" width="100%"><tr>
<td align="left"></td>
<td align="right"><div class="copyright-footer">Copyright © 2006-2010, 2012-2014 Nikhar Agrawal,
Anton Bikineev, Paul A. Bristow, Marco Guazzone, Christopher Kormanyos, Hubert
Holin, Bruno Lalande, John Maddock, Johan Råde, Gautam Sewani, Benjamin Sobotta,
Thijs van den Berg, Daryle Walker and Xiaogang Zhang<p>
Distributed under the Boost Software License, Version 1.0. (See accompanying
file LICENSE_1_0.txt or copy at <a href="http://www.boost.org/LICENSE_1_0.txt" target="_top">http://www.boost.org/LICENSE_1_0.txt</a>)
</p>
</div></td>
</tr></table>
<hr>
<div class="spirit-nav">
<a accesskey="p" href="../cs_eg.html"><img src="../../../../../../../../doc/src/images/prev.png" alt="Prev"></a><a accesskey="u" href="../cs_eg.html"><img src="../../../../../../../../doc/src/images/up.png" alt="Up"></a><a accesskey="h" href="../../../../index.html"><img src="../../../../../../../../doc/src/images/home.png" alt="Home"></a><a accesskey="n" href="chi_sq_test.html"><img src="../../../../../../../../doc/src/images/next.png" alt="Next"></a>
</div>
</body>
</html>
| Franky666/programmiersprachen-raytracer | external/boost_1_59_0/libs/math/doc/html/math_toolkit/stat_tut/weg/cs_eg/chi_sq_intervals.html | HTML | mit | 19,774 |
#pragma once
#include "messages.h"
#include "primitives/string_utils.h"
// Given pairs of pulse lens from 2 base stations, this class determines the phase for current cycle
// Phases are:
// 0) Base 1 (B), horizontal sweep
// 1) Base 1 (B), vertical sweep
// 2) Base 2 (C), horizontal sweep
// 3) Base 2 (C), vertical sweep
//
// TODO: We might want to introduce a more thorough check for a fix, using the average_error_ value.
class CyclePhaseClassifier {
public:
CyclePhaseClassifier();
// Process the pulse lengths for current cycle (given by incrementing cycle_idx).
void process_pulse_lengths(uint32_t cycle_idx, const TimeDelta (&pulse_lens)[num_base_stations]);
// Get current cycle phase. -1 if phase is not known (no fix achieved).
int get_phase(uint32_t cycle_idx);
// Reference to a pair of DataFrameBit-s
typedef DataFrameBit (&DataFrameBitPair)[num_base_stations];
// Get updated data bits from the pulse lens for current cycle.
// Both bits are always returned, but caller needs to make sure they were updated this cycle by
// checking DataFrameBit.cycle_idx == cycle_idx.
DataFrameBitPair get_data_bits(uint32_t cycle_idx, const TimeDelta (&pulse_lens)[num_base_stations]);
// Reset the state of this classifier - needs to be called if the cycle fix was lost.
void reset();
// Print debug information.
virtual bool debug_cmd(HashedWord *input_words);
virtual void debug_print(PrintStream &stream);
private:
float expected_pulse_len(bool skip, bool data, bool axis);
uint32_t prev_full_cycle_idx_;
uint32_t phase_history_;
int fix_level_;
uint32_t phase_shift_;
float pulse_base_len_;
DataFrameBit bits_[num_base_stations];
float average_error_;
bool debug_print_state_;
};
| ashtuchkin/vive-diy-position-sensor | include/cycle_phase_classifier.h | C | mit | 1,815 |
<?php
# MetInfo Enterprise Content Management System
# Copyright (C) MetInfo Co.,Ltd (http://www.metinfo.cn). All rights reserved.
require_once '../login/login_check.php';
if($action=='modify'){
$shortcut=array();
$query="select * from $met_language where value='$name' and lang='$lang'";
$lang_shortcut=$db->get_one($query);
$shortcut['name']=$lang_shortcut?'lang_'.$lang_shortcut['name']:urlencode($name);
$shortcut['url']=$url;
$shortcut['bigclass']=$bigclass;
$shortcut['field']=$field;
$shortcut['type']='2';
$shortcut['list_order']=$list_order;
$shortcut['protect']='0';
$shortcut['hidden']='0';
foreach($shortcut_list as $key=>$val){
$shortcut_list[$key][name]=$shortcut_list[$key][lang];
}
$shortcut_list[]=$shortcut;
change_met_cookie('metinfo_admin_shortcut',$shortcut_list);
save_met_cookie();
$query="update $met_admin_table set admin_shortcut='".json_encode($shortcut_list)."' where admin_id='$metinfo_admin_name'";
$db->query($query);
echo '<script type="text/javascript">parent.window.location.reload();</script>';
die();
//metsave('../system/shortcut.php?anyid='.$anyid.'&lang='.$lang.'&cs='.$cs);
}else{
$query="select * from $met_app where download=1";
$app=$db->get_all($query);
$css_url="../templates/".$met_skin."/css";
$img_url="../templates/".$met_skin."/images";
include template('system/shortcut_editor');
footer();
}
# This program is an open source system, commercial use, please consciously to purchase commercial license.
# Copyright (C) MetInfo Co., Ltd. (http://www.metinfo.cn). All rights reserved.
?> | maicong/OpenAPI | MetInfo5.2/admin/system/shortcut_editor.php | PHP | mit | 1,564 |
---
layout: post
title: 循环队列
date: 2017-05-13 10:54:37
categories: Java
---
# Java实现的循环队列
`队列`具有先进先出的特点,本文所说的`循环队列`(以下简称队列)的长度是一定的(可以扩容),如果队列满了,新来的元素会覆盖队列中最先来的元素。
它的使用场景是对内存占用有要求的情况下按序缓存一些数据,比如直播中的弹幕。
* 注意:此实现非线程安全
```java
import java.util.ArrayList;
import java.util.List;
/**
* 容量受限的队列,在队列已满的情况下,新入队的元素会覆盖最老的元素。<br>
* <font color="red">此类非线程安全</font>
*
* @author rongzhisheng
*
* @param <E>
*/
public class CircleQueue<E> {
private static final int DEFAULT_CAPACITY = 10;
private int capacity;
private int head;// 即将出队的位置
private int tail;// 即将入队的位置
private List<E> queue;
private boolean isFull;// 队列是否已满,队列空和满的时候head==tail
public CircleQueue() {
this(DEFAULT_CAPACITY);
}
public CircleQueue(int capacity) {
this.capacity = capacity;
queue = new ArrayList<>(this.capacity);
head = tail = 0;
}
/**
* 入队
*
* @param e
* @return 是否丢弃了最旧的元素
*/
public boolean add(E e) {
if (isFull) {
head = addIndex(head);
addOrSet(e, tail);
tail = head;
return true;
}
addOrSet(e, tail);
tail = addIndex(tail);
if (head == tail) {
isFull = true;
}
return false;
}
public E poll() {
if (!isFull && head == tail) {
return null;
}
E e = queue.get(head);
queue.set(head, null);
head = addIndex(head);
isFull = false;
return e;
}
public int size() {
if (!isFull && head == tail) {
return 0;
}
if (isFull) {
return capacity;
}
int i = 0;
int h = head;
while (h != tail) {
i++;
h = addIndex(h);
}
return i;
}
public int capacity() {
return capacity;
}
public E element(int index) {
if (index < 0 || index >= size()) {
throw new IllegalArgumentException();
}
index = addIndex(head + index - 1);
return queue.get(index);
}
public boolean isEmpty() {
return size() == 0;
}
public List<E> getList() {
List<E> list = new ArrayList<>();
final int size = size();
for (int i = 0; i < size; i++) {
list.add(element(i));
}
return list;
}
public void clean() {
head = tail = 0;
isFull = false;
queue.clear();
}
public void grow(int newCapacity) {
if (newCapacity <= capacity) {
return;
}
List<E> data = getList();
queue.clear();
queue.addAll(data);
head = 0;
tail = head + queue.size();
capacity = newCapacity;
isFull = false;
}
@Override
public String toString() {
return getList().toString();
}
private void addOrSet(E e, int index) {
if (queue.size() > index) {
queue.set(index, e);
return;
}
if (queue.size() == index) {
queue.add(e);
return;
}
throw new IllegalStateException();
}
private int addIndex(int i) {
return (i + 1) % capacity;
}
public static void main(String[] args) {
int a = 1;
CircleQueue<Integer> queue = new CircleQueue<Integer>(5);
queue.grow(8);
queue.grow(10);
for (int i = a; i < a + 6; i++) {
queue.add(i);
}
a += 6;
System.out.println(queue);
for (int i = a; i < a + 6; i++) {
queue.add(i);
}
a += 6;
System.out.println(queue);
queue.grow(10);
for (int i = a; i < a + 6; i++) {
queue.add(i);
}
a += 6;
System.out.println(queue);
for (int i = 0; i < 5; i++) {
System.out.println(queue.poll());
}
System.out.println(queue);
queue.clean();
for (int i = 0; i < 3; i++) {
queue.add(i);
}
System.out.println(queue);
}
}
``` | wittyLuzhishen/wittyLuzhishen.github.io | _posts/2017-05-13-circlequeue.md | Markdown | mit | 4,557 |
/*
* The MIT License (MIT)
*
* Copyright (c) 2015 maldicion069
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
'use strict';
exports.init = function(req, res){
//res.write("joder\n");
//res.write(req.csrfToken());
//res.end();
req.app.db.models.Experiment.find({})
.select("tableList name uploadedBy dateDevelopment id name neuronType") // Hay que sacar también nombre y tipo D=
.exec(function(err, exps) {
if(err) {
res.send("Error");
} else {
console.log(exps);
res.render('account/experiments/compare/stage1', {
code: req.csrfToken(),
experiments: exps
});
}
});
};
/**
* Esta función sirve para que, dado
*
*/
exports.second = function(req, res) {
console.log("BODY: " + req.body.fcbklist_value);
if(req.body.fcbklist_value.length < 2) {
res.redirect("/account/experiments/compare/");
} else {
//res.send(req.body);
var orArray = [];
req.body.fcbklist_value.forEach(function(idExp) {
orArray.push({_id: idExp});
});
console.log(orArray);
req.app.db.models.Experiment.find({$or: orArray})
.select("tableList name createdBy dateDevelopment id")
//.populate("tableList", "id")
.populate("createdBy", "username")
.exec(function(err, exps) {
console.log(err);
console.log(JSON.stringify(exps));
res.render("account/experiments/compare/stage2", {
experiments: exps,
code: req.csrfToken()
});
});
}
//["54514c6a4650426d2a9bf056","5451853c745acc0a412abf68"]
// Con esta lista de ids, lo que hacemos es sacar:
// 1. Cada experimento con:
// name uploadedBy dateDevelopment id
// y el identificador de cada tabla del experimento.
// 2. Con esos datos, montamos algo guay con radio button.
};
/**
* En este método recibimos los id's de los experimentos
* y la tabla seleccionada
* Por cada tabla, sacamos las columnas comunes entre ellas e
* imprimimos los gráficos de una vez
*/
exports.third = function(req, res) {
var peticiones = req.body.tab;
console.log(peticiones);
var arr = [];
/*var mapMatch = {
"a": null,
"b": null,
"c": null,
"Datos2": null
}*/
var params = req.app.utility.variables;
var tabs = [];
var headersPaths = [];
var data = {}; // To save the return to view
var Set = req.app.utility.Set;
var commons = new Set();
var async = require('async');
async.series([
// First read data from database and save
function(callback){
console.log("ejecuto 1");
async.each(peticiones, function(tabid, call) {
console.log(tabid);
req.app.db.models.TableExperiment.findById(tabid).populate("assignedTo", "name neuronType createdBy dateDevelopment description").exec(function(err, tab) {
var username = "";
async.series({
first: function(call2) {
console.log("Paso 1");
console.log("Jodeeer, " + tab.assignedTo.createdBy);
/*console.log(tab);
async.each(tab, function(tab_, call3) {
console.log("Buscamos username " + tab_);
req.app.db.models.User.findById(tab_.createdBy._id).select("username").exec(function(err, user) {
console.log("USER : " + user);
tab.createdBy = user.username;
call3();
});
});*/
req.app.db.models.User.findById(tab.assignedTo.createdBy).select("username").exec(function(err, user) {
console.log("USER : " + user);
tab["username"] = user.username;
username = user.username;
//tab.username = user.username;
call2();
});
},
second: function(call2) {
console.log("Paso 2");
// TODO: Controlar "err"
var tab_ = {};
tab_.assignedTo = {
_id: tab.assignedTo._id,
username: username,
createdBy: tab.assignedTo.createdBy,
name: tab.assignedTo.name,
neuronType: tab.assignedTo.neuronType,
dateDevelopment: tab.assignedTo.dateDevelopment,
description: tab.assignedTo.description
};
tab_.id = tab._id;
tab_.matrix = tab.matrix;
tab_.headers = tab.headers;
/*
assignedTo:
{ _id: 545bde715e4a5d4a4089ad21,
createdBy: 545bde535e4a5d4a4089ad1f,
name: 'Mi physiological',
neuronType: 'PHYSIOLOGICAL' },
_id: 545bde715e4a5d4a4089ad52,
__v: 0,
matrix: */
console.log("SECOND : " + tab_);
// Añadimos la tabla al sistema
//console.log("Añado " + tab);
tabs.push(tab_);
// Recorro las cabeceras y genero un mapa con k(header) y v(position)
var mapMatch = {};
console.log(tab.headers);
tab.headers.forEach(function(header, pos) {
if(params.contains(header)) {
mapMatch[header] = pos;
}
});
//console.log("Añado " + JSON.stringify(mapMatch));
headersPaths.push(mapMatch); // Guardamos el mapeo
call2();
}
}, function(err, results) {
call();
});
});
}, function(err) {
callback();
});
},
// Filter columns that I use to compare table's experiment
function(callback) {
console.log("Tengo : " + tabs.length);
console.log("Tengo : " + headersPaths.length);
// Guardamos todos los valores de "params" en "data"
data.headers = {};
params.get().forEach(function(value) {
data.headers[value] = [];//undefined;
});
console.log(JSON.stringify(data));
// Creamos el attr "exps" dentro de "data"
data.exps = [];
// Ahora por cada experimento, cargamos los datos correspondientes
headersPaths.forEach(function(headerPath, index) {
console.log("--------- Empezamos a recorrer con header " + headerPath + " ---------");
var posHeader = 0;
Object.keys(headerPath).forEach(function(key) {
console.log(key + " <=> " + headerPath[key]);
//tabs.forEach(function(tab, ii) {
tabs[index].matrix.forEach(function(matrix, area) {
//console.log("Header: " + key + "\tNº Tab: " + ii + "\tArea: " + area);
data.headers[key][area] = data.headers[key][area] || [0]; // Si existe se queda igual, si no, se añade array
data.headers[key][area].push(matrix.data[posHeader]);
});
//});
/*var infoData = [];
tabs[index].matrix.forEach(function(matrix, area) {
infoData.push(matrix.data[posHeader]);
});
//console.log(infoData);
console.log("Inserta del index " + posHeader);
data.headers[key].push(infoData);*/
posHeader++;
});
// Volcamos la información del experimento asociado a cada tabla
data.exps.push(tabs[index].assignedTo);
});
console.log("----------------------");
console.log("----------------------");
//console.log(data);
console.log("----------------------");
console.log("----------------------");
/*async.each(arr, function(tab, call) {
tab.headers.forEach(function(header, position) {
//if(mapMatch[header] != undefined) {
if(header in tab.mapMatch) {
tab.mapMatch[header] = position;
}
});
// Remove all columns that not contains in mapMatch
//tab.mapMatch.forEach(function(position) {
//});
call();
});*/
callback();
}
],
// finish callback
function(err, results){
console.log("FIN: " + arr.length);
console.log(params.intersect(commons));
//res.send(data);
/*var ret = {
data: data
};
res.send(ret);*/
console.log("----------------------");
console.log("----------------------");
console.log("----------------------");
console.log("----------------------");
console.log(JSON.stringify(data));
res.render("account/experiments/compare/stage3", {
data: data,
code: req.csrfToken(),
id: 0
});
});
};
// TODO: No funciona bien T.T
/*exports.downloadHTML = function(req, res) {
phantom = require('phantom')
phantom.create(function(ph){
ph.createPage(function(page) {
page.open("http://www.google.com", function(status) {
page.render('google.pdf', function(){
console.log('Page Rendered');
ph.exit();
});
});
});
});
};*/
| maldicion069/HBPWebNodeJs | views/account/experiments/compare/stages.js | JavaScript | mit | 9,900 |
#ifndef ECLAIR_DIRECTORY_H
# define ECLAIR_DIRECTORY_H
# include "eclair.h"
# include <sys/types.h>
# include <dirent.h>
namespace Eclair
{
struct DirectoryFile
{
std::string name;
bool is_directory;
bool is_regular_file;
};
class Directory
{
private:
std::string _dirname;
DIR *_dir = nullptr;
std::string _error_str;
DirectoryFile _file;
explicit Directory();
public:
explicit Directory(const std::string& dir);
virtual ~Directory() noexcept;
bool open() noexcept;
DirectoryFile *next() noexcept;
inline std::string error() const noexcept {
return (this->_error_str);
}
inline std::string path() const noexcept {
return (this->_dirname);
}
static inline std::string current() noexcept {
char str[1025];
return (getcwd(str, 1024));
}
};
}
#endif | alex8092/eclaircpp | include/directory.h | C | mit | 824 |
import QUnit from 'qunit';
import { registerDeprecationHandler } from '@ember/debug';
let isRegistered = false;
let deprecations = new Set();
let expectedDeprecations = new Set();
// Ignore deprecations that are not caused by our own code, and which we cannot fix easily.
const ignoredDeprecations = [
// @todo remove when we can land https://github.com/emberjs/ember-render-modifiers/pull/33 here
/Versions of modifier manager capabilities prior to 3\.22 have been deprecated/,
/Usage of the Ember Global is deprecated./,
/import .* directly from/,
/Use of `assign` has been deprecated/,
];
export default function setupNoDeprecations({ beforeEach, afterEach }) {
beforeEach(function () {
deprecations.clear();
expectedDeprecations.clear();
if (!isRegistered) {
registerDeprecationHandler((message, options, next) => {
if (!ignoredDeprecations.some((regex) => message.match(regex))) {
deprecations.add(message);
}
next(message, options);
});
isRegistered = true;
}
});
afterEach(function (assert) {
// guard in if instead of using assert.equal(), to not make assert.expect() fail
if (deprecations.size > expectedDeprecations.size) {
assert.ok(
false,
`Expected ${expectedDeprecations.size} deprecations, found: ${[...deprecations]
.map((msg) => `"${msg}"`)
.join(', ')}`
);
}
});
QUnit.assert.deprecations = function (count) {
if (count === undefined) {
this.ok(deprecations.size, 'Expected deprecations during test.');
} else {
this.equal(deprecations.size, count, `Expected ${count} deprecation(s) during test.`);
}
deprecations.forEach((d) => expectedDeprecations.add(d));
};
QUnit.assert.deprecationsInclude = function (expected) {
let found = [...deprecations].find((deprecation) => deprecation.includes(expected));
this.pushResult({
result: !!found,
actual: deprecations,
message: `expected to find \`${expected}\` deprecation. Found ${[...deprecations]
.map((d) => `"${d}"`)
.join(', ')}`,
});
if (found) {
expectedDeprecations.add(found);
}
};
}
| kaliber5/ember-bootstrap | tests/helpers/setup-no-deprecations.js | JavaScript | mit | 2,205 |
/*
The MIT License (MIT)
Copyright (c) 2014 Manni Wood
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
*/
package com.manniwood.cl4pg.v1.exceptions;
public class Cl4pgConfFileException extends Cl4pgException {
private static final long serialVersionUID = 1L;
public Cl4pgConfFileException() {
super();
}
public Cl4pgConfFileException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
}
public Cl4pgConfFileException(String message, Throwable cause) {
super(message, cause);
}
public Cl4pgConfFileException(String message) {
super(message);
}
public Cl4pgConfFileException(Throwable cause) {
super(cause);
}
}
| manniwood/cl4pg | src/main/java/com/manniwood/cl4pg/v1/exceptions/Cl4pgConfFileException.java | Java | mit | 1,781 |
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// General Information about an assembly is controlled through the following
// set of attributes. Change these attribute values to modify the information
// associated with an assembly.
[assembly: AssemblyTitle("10.TraverseDirectoryXDocument")]
[assembly: AssemblyDescription("")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("")]
[assembly: AssemblyProduct("10.TraverseDirectoryXDocument")]
[assembly: AssemblyCopyright("Copyright © 2015")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// Setting ComVisible to false makes the types in this assembly not visible
// to COM components. If you need to access a type in this assembly from
// COM, set the ComVisible attribute to true on that type.
[assembly: ComVisible(false)]
// The following GUID is for the ID of the typelib if this project is exposed to COM
[assembly: Guid("0db1c3e2-162a-4e14-b304-0f69cce18d90")]
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
[assembly: AssemblyVersion("1.0.0.0")]
[assembly: AssemblyFileVersion("1.0.0.0")]
| emilti/Telerik-Academy-My-Courses | DataBases/03.Processing Xml/Processing XML/10.TraverseDirectoryXDocument/Properties/AssemblyInfo.cs | C# | mit | 1,434 |
package com.cosium.spring.data.jpa.entity.graph.repository.support;
import com.google.common.base.MoreObjects;
import org.springframework.core.ResolvableType;
import org.springframework.data.jpa.repository.query.JpaEntityGraph;
import static java.util.Objects.requireNonNull;
/**
* Wrapper class allowing to hold a {@link JpaEntityGraph} with its associated domain class. Created
* on 23/11/16.
*
* @author Reda.Housni-Alaoui
*/
class EntityGraphBean {
private final JpaEntityGraph jpaEntityGraph;
private final Class<?> domainClass;
private final ResolvableType repositoryMethodReturnType;
private final boolean optional;
private final boolean primary;
private final boolean valid;
public EntityGraphBean(
JpaEntityGraph jpaEntityGraph,
Class<?> domainClass,
ResolvableType repositoryMethodReturnType,
boolean optional,
boolean primary) {
this.jpaEntityGraph = requireNonNull(jpaEntityGraph);
this.domainClass = requireNonNull(domainClass);
this.repositoryMethodReturnType = requireNonNull(repositoryMethodReturnType);
this.optional = optional;
this.primary = primary;
this.valid = computeValidity();
}
private boolean computeValidity() {
Class<?> resolvedReturnType = repositoryMethodReturnType.resolve();
if (Void.TYPE.equals(resolvedReturnType) || domainClass.isAssignableFrom(resolvedReturnType)) {
return true;
}
for (Class genericType : repositoryMethodReturnType.resolveGenerics()) {
if (domainClass.isAssignableFrom(genericType)) {
return true;
}
}
return false;
}
/** @return The jpa entity graph */
public JpaEntityGraph getJpaEntityGraph() {
return jpaEntityGraph;
}
/** @return The jpa entity class */
public Class<?> getDomainClass() {
return domainClass;
}
/** @return True if this entity graph is not mandatory */
public boolean isOptional() {
return optional;
}
/** @return True if this EntityGraph seems valid */
public boolean isValid() {
return valid;
}
/**
* @return True if this EntityGraph is a primary one. Default EntityGraph is an example of non
* primary EntityGraph.
*/
public boolean isPrimary() {
return primary;
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("jpaEntityGraph", jpaEntityGraph)
.add("domainClass", domainClass)
.add("repositoryMethodReturnType", repositoryMethodReturnType)
.add("optional", optional)
.toString();
}
}
| Cosium/spring-data-jpa-entity-graph | core/src/main/java/com/cosium/spring/data/jpa/entity/graph/repository/support/EntityGraphBean.java | Java | mit | 2,555 |
# -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
]
operations = [
migrations.CreateModel(
name='Page',
fields=[
('id', models.AutoField(verbose_name='ID', serialize=False, auto_created=True, primary_key=True)),
('title', models.CharField(unique=True, max_length=150)),
('slug', models.SlugField(unique=True, max_length=150)),
('posted', models.DateTimeField(auto_now_add=True, db_index=True)),
],
options={
},
bases=(models.Model,),
),
]
| vollov/i18n-django-api | page/migrations/0001_initial.py | Python | mit | 723 |
module.exports = function(grunt) {
// Add our custom tasks.
grunt.loadTasks('../../../tasks');
// Project configuration.
grunt.initConfig({
mochaTest: {
options: {
reporter: 'spec',
grep: 'tests that match grep',
invert: true
},
all: {
src: ['*.js']
}
}
});
// Default task.
grunt.registerTask('default', ['mochaTest']);
};
| quantumlicht/collarbone | node_modules/grunt-mocha-test/test/scenarios/invertOption/Gruntfile.js | JavaScript | mit | 425 |
<?php
namespace App\Service;
class Message {
public function get()
{
if (isset($_SESSION['message'])) {
$array = explode(',', $_SESSION['message']);
unset($_SESSION['message']);
return $array;
}
return '';
}
public function set($message, $type = null)
{
$_SESSION['message'] = implode(',', [$message, $type]);
}
} | QA-Games/QA-tools | app/Service/Message.php | PHP | mit | 421 |
package mqttpubsub
import (
"encoding/json"
"fmt"
"sync"
"time"
log "github.com/Sirupsen/logrus"
"github.com/brocaar/loraserver/api/gw"
"github.com/brocaar/lorawan"
"github.com/eclipse/paho.mqtt.golang"
)
// Backend implements a MQTT pub-sub backend.
type Backend struct {
conn mqtt.Client
txPacketChan chan gw.TXPacketBytes
gateways map[lorawan.EUI64]struct{}
mutex sync.RWMutex
}
// NewBackend creates a new Backend.
func NewBackend(server, username, password string) (*Backend, error) {
b := Backend{
txPacketChan: make(chan gw.TXPacketBytes),
gateways: make(map[lorawan.EUI64]struct{}),
}
opts := mqtt.NewClientOptions()
opts.AddBroker(server)
opts.SetUsername(username)
opts.SetPassword(password)
opts.SetOnConnectHandler(b.onConnected)
opts.SetConnectionLostHandler(b.onConnectionLost)
log.WithField("server", server).Info("backend: connecting to mqtt broker")
b.conn = mqtt.NewClient(opts)
if token := b.conn.Connect(); token.Wait() && token.Error() != nil {
return nil, token.Error()
}
return &b, nil
}
// Close closes the backend.
func (b *Backend) Close() {
b.conn.Disconnect(250) // wait 250 milisec to complete pending actions
}
// TXPacketChan returns the TXPacketBytes channel.
func (b *Backend) TXPacketChan() chan gw.TXPacketBytes {
return b.txPacketChan
}
// SubscribeGatewayTX subscribes the backend to the gateway TXPacketBytes
// topic (packets the gateway needs to transmit).
func (b *Backend) SubscribeGatewayTX(mac lorawan.EUI64) error {
defer b.mutex.Unlock()
b.mutex.Lock()
topic := fmt.Sprintf("gateway/%s/tx", mac.String())
log.WithField("topic", topic).Info("backend: subscribing to topic")
if token := b.conn.Subscribe(topic, 0, b.txPacketHandler); token.Wait() && token.Error() != nil {
return token.Error()
}
b.gateways[mac] = struct{}{}
return nil
}
// UnSubscribeGatewayTX unsubscribes the backend from the gateway TXPacketBytes
// topic.
func (b *Backend) UnSubscribeGatewayTX(mac lorawan.EUI64) error {
defer b.mutex.Unlock()
b.mutex.Lock()
topic := fmt.Sprintf("gateway/%s/tx", mac.String())
log.WithField("topic", topic).Info("backend: unsubscribing from topic")
if token := b.conn.Unsubscribe(topic); token.Wait() && token.Error() != nil {
return token.Error()
}
delete(b.gateways, mac)
return nil
}
// PublishGatewayRX publishes a RX packet to the MQTT broker.
func (b *Backend) PublishGatewayRX(mac lorawan.EUI64, rxPacket gw.RXPacketBytes) error {
topic := fmt.Sprintf("gateway/%s/rx", mac.String())
return b.publish(topic, rxPacket)
}
// PublishGatewayStats publishes a GatewayStatsPacket to the MQTT broker.
func (b *Backend) PublishGatewayStats(mac lorawan.EUI64, stats gw.GatewayStatsPacket) error {
topic := fmt.Sprintf("gateway/%s/stats", mac.String())
return b.publish(topic, stats)
}
func (b *Backend) publish(topic string, v interface{}) error {
bytes, err := json.Marshal(v)
if err != nil {
return err
}
log.WithField("topic", topic).Info("backend: publishing packet")
if token := b.conn.Publish(topic, 0, false, bytes); token.Wait() && token.Error() != nil {
return token.Error()
}
return nil
}
func (b *Backend) txPacketHandler(c mqtt.Client, msg mqtt.Message) {
log.WithField("topic", msg.Topic()).Info("backend: packet received")
var txPacket gw.TXPacketBytes
if err := json.Unmarshal(msg.Payload(), &txPacket); err != nil {
log.Errorf("backend: decode tx packet error: %s", err)
return
}
b.txPacketChan <- txPacket
}
func (b *Backend) onConnected(c mqtt.Client) {
defer b.mutex.RUnlock()
b.mutex.RLock()
log.Info("backend: connected to mqtt broker")
if len(b.gateways) > 0 {
for {
log.WithField("topic_count", len(b.gateways)).Info("backend: re-registering to gateway topics")
topics := make(map[string]byte)
for k := range b.gateways {
topics[fmt.Sprintf("gateway/%s/tx", k)] = 0
}
if token := b.conn.SubscribeMultiple(topics, b.txPacketHandler); token.Wait() && token.Error() != nil {
log.WithField("topic_count", len(topics)).Errorf("backend: subscribe multiple failed: %s", token.Error())
time.Sleep(time.Second)
continue
}
return
}
}
}
func (b *Backend) onConnectionLost(c mqtt.Client, reason error) {
log.Errorf("backend: mqtt connection error: %s", reason)
}
| kumara0093/loraserver | backend/mqttpubsub/backend.go | GO | mit | 4,289 |
package stage2;
public class DecafError {
int numErrors;
DecafError(){
}
public static String errorPos(Position p){
return "(L: " + p.startLine +
", Col: " + p.startCol +
") -- (L: " + p.endLine +
", Col: " + p.endCol +
")";
}
public void error(String s, Position p) {
System.out.println("Error found at location "+
errorPos(p) + ":\n"+s);
}
public boolean haveErrors() {
return (numErrors>0);
}
}
| bigfatnoob/Decaf | src/stage2/DecafError.java | Java | mit | 471 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>../plugins/plugins_2d/bar/bar.vlib.js</title>
<link rel="stylesheet" href="http://yui.yahooapis.com/3.9.1/build/cssgrids/cssgrids-min.css">
<link rel="stylesheet" href="../assets/vendor/prettify/prettify-min.css">
<link rel="stylesheet" href="../assets/css/main.css" id="site_styles">
<link rel="shortcut icon" type="image/png" href="../assets/favicon.png">
<script src="http://yui.yahooapis.com/combo?3.9.1/build/yui/yui-min.js"></script>
</head>
<body class="yui3-skin-sam">
<div id="doc">
<div id="hd" class="yui3-g header">
<div class="yui3-u-3-4">
<h1><img src="../assets/css/logo.png" title=""></h1>
</div>
<div class="yui3-u-1-4 version">
<em>API Docs for: </em>
</div>
</div>
<div id="bd" class="yui3-g">
<div class="yui3-u-1-4">
<div id="docs-sidebar" class="sidebar apidocs">
<div id="api-list">
<h2 class="off-left">APIs</h2>
<div id="api-tabview" class="tabview">
<ul class="tabs">
<li><a href="#api-classes">Classes</a></li>
<li><a href="#api-modules">Modules</a></li>
</ul>
<div id="api-tabview-filter">
<input type="search" id="api-filter" placeholder="Type to filter APIs">
</div>
<div id="api-tabview-panel">
<ul id="api-classes" class="apis classes">
<li><a href="../classes/AbstractPlugin.html">AbstractPlugin</a></li>
<li><a href="../classes/Config.html">Config</a></li>
<li><a href="../classes/Controls.html">Controls</a></li>
<li><a href="../classes/Plot.html">Plot</a></li>
<li><a href="../classes/Plugin 3D.html">Plugin 3D</a></li>
<li><a href="../classes/Plugin Axes.html">Plugin Axes</a></li>
<li><a href="../classes/Plugin BasicMaterial.html">Plugin BasicMaterial</a></li>
<li><a href="../classes/Plugin CameraControl.html">Plugin CameraControl</a></li>
<li><a href="../classes/Plugin Color.html">Plugin Color</a></li>
<li><a href="../classes/Plugin Dataset.html">Plugin Dataset</a></li>
<li><a href="../classes/Plugin File.html">Plugin File</a></li>
<li><a href="../classes/Plugin Function.html">Plugin Function</a></li>
<li><a href="../classes/Plugin Heatmap.html">Plugin Heatmap</a></li>
<li><a href="../classes/Plugin Light.html">Plugin Light</a></li>
<li><a href="../classes/Plugin LinePlot.html">Plugin LinePlot</a></li>
<li><a href="../classes/Plugin Plane.html">Plugin Plane</a></li>
<li><a href="../classes/Plugin ScatterPlot.html">Plugin ScatterPlot</a></li>
<li><a href="../classes/Plugin SurfacePlot.html">Plugin SurfacePlot</a></li>
<li><a href="../classes/Plugin WireframeMaterial.html">Plugin WireframeMaterial</a></li>
<li><a href="../classes/SceneGraph.html">SceneGraph</a></li>
<li><a href="../classes/Templates.html">Templates</a></li>
<li><a href="../classes/Toolbox.html">Toolbox</a></li>
<li><a href="../classes/UTILS.html">UTILS</a></li>
<li><a href="../classes/VLib.html">VLib</a></li>
<li><a href="../classes/VMediator.html">VMediator</a></li>
</ul>
<ul id="api-modules" class="apis modules">
<li><a href="../modules/Controls.html">Controls</a></li>
<li><a href="../modules/main.html">main</a></li>
<li><a href="../modules/Plot.html">Plot</a></li>
<li><a href="../modules/SceneGraph.html">SceneGraph</a></li>
<li><a href="../modules/Templates.html">Templates</a></li>
<li><a href="../modules/Toolbox.html">Toolbox</a></li>
<li><a href="../modules/VLib.html">VLib</a></li>
</ul>
</div>
</div>
</div>
</div>
</div>
<div class="yui3-u-3-4">
<div id="api-options">
Show:
<label for="api-show-inherited">
<input type="checkbox" id="api-show-inherited" checked>
Inherited
</label>
<label for="api-show-protected">
<input type="checkbox" id="api-show-protected">
Protected
</label>
<label for="api-show-private">
<input type="checkbox" id="api-show-private">
Private
</label>
<label for="api-show-deprecated">
<input type="checkbox" id="api-show-deprecated">
Deprecated
</label>
</div>
<div class="apidocs">
<div id="docs-main">
<div class="content">
<h1 class="file-heading">File: ../plugins/plugins_2d/bar/bar.vlib.js</h1>
<div class="file">
<pre class="code prettyprint linenums">
define(['require','config'],function(require,Config) {
var plugin = (function() {
/** ********************************** */
/** PUBLIC VARIABLES * */
/** ********************************** */
this.context = Config.PLUGINTYPE.CONTEXT_2D;
this.type = Config.PLUGINTYPE.LINETYPE;
this.name = 'bar';
/** path to plugin-template file * */
this.accepts = {
predecessors : [ Config.PLUGINTYPE.DATA ],
successors : [ ]
}
this.icon = Config.absPlugins + '/plugins_2d/bar/icon.png';
this.description = 'Requires: [ '+this.accepts.predecessors.join(', ')+' ] Accepts: [ '+this.accepts.successors.join(', ')+' ]';
/** ********************************** */
/** PUBLIC METHODS * */
/** ********************************** */
/**
/***/
this.exec = function(config) {
console.log("[ bar ] \t\t EXEC");
if(config == '' || config === undefined){
config = {area:false,bar:true};
}
return {
pType : this.type,
response : {
type : config
}
};
}
});
return plugin;
});
</pre>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script src="../assets/vendor/prettify/prettify-min.js"></script>
<script>prettyPrint();</script>
<script src="../assets/js/yui-prettify.js"></script>
<script src="../assets/../api.js"></script>
<script src="../assets/js/api-filter.js"></script>
<script src="../assets/js/api-list.js"></script>
<script src="../assets/js/api-search.js"></script>
<script src="../assets/js/apidocs.js"></script>
</body>
</html>
| ndyGit/vPlot | wp-vlib/js/VLib/docu/out/files/.._plugins_plugins_2d_bar_bar.vlib.js.html | HTML | mit | 7,249 |
<!DOCTYPE html>
<!DOCTYPE html>
<html lang=sl>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Kje lahko najdem datoteke katerih varnostno kopijo želim ustvariti?</title>
<script type="text/javascript" src="jquery.js"></script><script type="text/javascript" src="jquery.syntax.js"></script><script type="text/javascript" src="yelp.js"></script><link rel="shortcut icon" href="https://www.ubuntu.si/favicon.ico">
<link rel="stylesheet" type="text/css" href="../vodnik_1404.css">
</head>
<body id="home"><div id="wrapper" class="hfeed">
<div id="header">
<div id="branding">
<div id="blog-title"><span><a rel="home" title="Ubuntu Slovenija | Uradna spletna stran slovenske skupnosti Linux distribucije Ubuntu" href="//www.ubuntu.si">Ubuntu Slovenija | Uradna spletna stran slovenske skupnosti Linux distribucije Ubuntu</a></span></div>
<h1 id="blog-description"></h1>
</div>
<div id="access"><div id="loco-header-menu"><ul id="primary-header-menu"><li class="widget-container widget_nav_menu" id="nav_menu-3"><div class="menu-glavni-meni-container"><ul class="menu" id="menu-glavni-meni">
<li id="menu-item-15" class="menu-item menu-item-type-custom menu-item-object-custom menu-item-15"><a href="//www.ubuntu.si">Domov</a></li>
<li id="menu-item-2776" class="menu-item menu-item-type-custom menu-item-object-custom menu-item-2776"><a href="//www.ubuntu.si/category/novice/">Novice</a></li>
<li id="menu-item-16" class="menu-item menu-item-type-custom menu-item-object-custom menu-item-16"><a href="//www.ubuntu.si/forum/">Forum</a></li>
<li id="menu-item-19" class="menu-item menu-item-type-post_type menu-item-object-page menu-item-19"><a href="//www.ubuntu.si/kaj-je-ubuntu/">Kaj je Ubuntu?</a></li>
<li id="menu-item-20" class="menu-item menu-item-type-post_type menu-item-object-page menu-item-20"><a href="//www.ubuntu.si/pogosta_vprasanja/">Pogosta vprašanja</a></li>
<li id="menu-item-17" class="menu-item menu-item-type-post_type menu-item-object-page menu-item-17"><a href="//www.ubuntu.si/skupnost/">Skupnost</a></li>
<li id="menu-item-18" class="menu-item menu-item-type-post_type menu-item-object-page menu-item-18"><a href="//www.ubuntu.si/povezave/">Povezave</a></li>
</ul></div></li></ul></div></div>
</div>
<div id="main"><div id="cwt-content" class="clearfix content-area"><div id="page">
<div class="trails" role="navigation"><div class="trail">
<span style="color: #333">Ubuntu 16.10</span> » <a class="trail" href="index.html" title="Namizni vodnik Ubuntu"><span class="media"><span class="media media-image"><img src="figures/ubuntu-logo.png" height="16" width="16" class="media media-inline" alt="Pomoč"></span></span> Namizni vodnik Ubuntu</a> » <a class="trail" href="files.html" title="Datoteke, mape in iskanje">Datoteke</a> › <a class="trail" href="files.html#backup" title="Ustvarjanje varnostnih kopij">Ustvarjanje varnostnih kopij</a> » </div></div>
<div id="content">
<div class="hgroup"><h1 class="title"><span class="title">Kje lahko najdem datoteke katerih varnostno kopijo želim ustvariti?</span></h1></div>
<div class="region">
<div class="contents">
<p class="p">Spodaj so izpisana najbolj pogosta mesta pomembnih datotek in nastavitev, ki si jih morda želite varnostno kopirati.</p>
<div class="list"><div class="inner"><div class="region"><ul class="list">
<li class="list">
<p class="p">Osebne datoteke (dokumenti, glasba, fotografije in videoposnetki)</p>
<p class="p">Te so ponavadi shranjene v vaši domači mapi (<span class="file">/home/vaše_ime</span>). Lahko so v podmapah kot je Namizje, Dokumenti, Slike, Glasba in Videi.</p>
<p class="p">Če je na vašem mediju varnostne kopije dovolj prostora (na primer če je to zunanji trdi disk), razmislite o ustvarjanju varnostne kopije celotne Domače mape. Koliko prostora zavzema Domača mapa lahko ugotovite z orodjem <span class="app">Preučevalnik porabe diska</span>.</p>
</li>
<li class="list">
<p class="p">Skrite datoteke</p>
<p class="p">Katerokoli ime datoteke ali mape, ki se začne s piko (.), je privzeto skrito. Za ogled skritih datotek kliknite <span class="guiseq"><span class="gui">Pogled</span> ▸ <span class="gui">Pokaži skrite datoteke</span></span> ali pritisnite <span class="keyseq"><span class="key"><kbd>Ctrl</kbd></span>+<span class="key"><kbd>H</kbd></span></span>.</p>
</li>
<li class="list">
<p class="p">Osebne nastavitve (možnosti namizja, teme in nastavitve programov)</p>
<p class="p">Večina programov shrani svoje nastavitve v skrite mape v vaši Domači mapi (glejte zgoraj za podrobnosti o skritih datotekah).</p>
<p class="p">Večina vaših nastavitev programov je shranjena v skritih mapah <span class="file">.config</span>, <span class="file">.gconf</span>, <span class="file">.gnome2</span> in <span class="file">.local</span> v vaši Domači mapi.</p>
</li>
<li class="list">
<p class="p">Sistemske nastavitve</p>
<p class="p">Nastavitve pomembnih delov sistema niso shranjene v Domači mapi. Obstajajo številna mesta, kjer so lahko shranjena, vendar jih je večina shranjena v mapi <span class="file">/etc</span>. V splošnem vam varnostne kopije teh datotek na domačem računalniku ni treba ustvariti. Če imate strežnik, je pametno ustvariti varnostno kopijo datotek za storitve, ki jih strežnik poganja.</p>
</li>
</ul></div></div></div>
</div>
<div class="sect sect-links" role="navigation">
<div class="hgroup"></div>
<div class="contents"><div class="links guidelinks"><div class="inner">
<div class="title"><h2><span class="title">Več podrobnosti</span></h2></div>
<div class="region"><ul><li class="links "><a href="files.html#backup" title="Ustvarjanje varnostnih kopij">Ustvarjanje varnostnih kopij</a></li></ul></div>
</div></div></div>
</div>
</div>
<div class="clear"></div>
</div>
</div></div></div>
<div id="footer">
<img src="https://piwik-ubuntusi.rhcloud.com/piwik.php?idsite=1&rec=1" style="border:0" alt=""><div id="siteinfo"><p>Material v tem dokumentu je na voljo pod prosto licenco. To je prevod dokumentacije Ubuntu, ki jo je sestavila <a href="https://wiki.ubuntu.com/DocumentationTeam">Ubuntu dokumentacijska ekipa za Ubuntu</a>. V slovenščino jo je prevedla skupina <a href="https://wiki.lugos.si/slovenjenje:ubuntu">Ubuntu prevajalcev</a>. Za poročanje napak v prevodih v tej dokumentaciji ali Ubuntuju pošljite sporočilo na <a href="mailto:[email protected]?subject=Prijava%20napak%20v%20prevodih">dopisni seznam</a>.</p></div>
</div>
</div></body>
</html>
| ubuntu-si/ubuntu.si | vodnik/16.10/backup-thinkabout.html | HTML | mit | 6,504 |
"use strict";
define("ace/mode/asciidoc_highlight_rules", ["require", "exports", "module", "ace/lib/oop", "ace/mode/text_highlight_rules"], function (require, exports, module) {
"use strict";
var oop = require("../lib/oop");
var TextHighlightRules = require("./text_highlight_rules").TextHighlightRules;
var AsciidocHighlightRules = function AsciidocHighlightRules() {
var identifierRe = "[a-zA-Z\xA1-\uFFFF]+\\b";
this.$rules = {
"start": [{ token: "empty", regex: /$/ }, { token: "literal", regex: /^\.{4,}\s*$/, next: "listingBlock" }, { token: "literal", regex: /^-{4,}\s*$/, next: "literalBlock" }, { token: "string", regex: /^\+{4,}\s*$/, next: "passthroughBlock" }, { token: "keyword", regex: /^={4,}\s*$/ }, { token: "text", regex: /^\s*$/ }, { token: "empty", regex: "", next: "dissallowDelimitedBlock" }],
"dissallowDelimitedBlock": [{ include: "paragraphEnd" }, { token: "comment", regex: '^//.+$' }, { token: "keyword", regex: "^(?:NOTE|TIP|IMPORTANT|WARNING|CAUTION):" }, { include: "listStart" }, { token: "literal", regex: /^\s+.+$/, next: "indentedBlock" }, { token: "empty", regex: "", next: "text" }],
"paragraphEnd": [{ token: "doc.comment", regex: /^\/{4,}\s*$/, next: "commentBlock" }, { token: "tableBlock", regex: /^\s*[|!]=+\s*$/, next: "tableBlock" }, { token: "keyword", regex: /^(?:--|''')\s*$/, next: "start" }, { token: "option", regex: /^\[.*\]\s*$/, next: "start" }, { token: "pageBreak", regex: /^>{3,}$/, next: "start" }, { token: "literal", regex: /^\.{4,}\s*$/, next: "listingBlock" }, { token: "titleUnderline", regex: /^(?:={2,}|-{2,}|~{2,}|\^{2,}|\+{2,})\s*$/, next: "start" }, { token: "singleLineTitle", regex: /^={1,5}\s+\S.*$/, next: "start" }, { token: "otherBlock", regex: /^(?:\*{2,}|_{2,})\s*$/, next: "start" }, { token: "optionalTitle", regex: /^\.[^.\s].+$/, next: "start" }],
"listStart": [{ token: "keyword", regex: /^\s*(?:\d+\.|[a-zA-Z]\.|[ixvmIXVM]+\)|\*{1,5}|-|\.{1,5})\s/, next: "listText" }, { token: "meta.tag", regex: /^.+(?::{2,4}|;;)(?: |$)/, next: "listText" }, { token: "support.function.list.callout", regex: /^(?:<\d+>|\d+>|>) /, next: "text" }, { token: "keyword", regex: /^\+\s*$/, next: "start" }],
"text": [{ token: ["link", "variable.language"], regex: /((?:https?:\/\/|ftp:\/\/|file:\/\/|mailto:|callto:)[^\s\[]+)(\[.*?\])/ }, { token: "link", regex: /(?:https?:\/\/|ftp:\/\/|file:\/\/|mailto:|callto:)[^\s\[]+/ }, { token: "link", regex: /\b[\w\.\/\-]+@[\w\.\/\-]+\b/ }, { include: "macros" }, { include: "paragraphEnd" }, { token: "literal", regex: /\+{3,}/, next: "smallPassthrough" }, { token: "escape", regex: /\((?:C|TM|R)\)|\.{3}|->|<-|=>|<=|&#(?:\d+|x[a-fA-F\d]+);|(?: |^)--(?=\s+\S)/ }, { token: "escape", regex: /\\[_*'`+#]|\\{2}[_*'`+#]{2}/ }, { token: "keyword", regex: /\s\+$/ }, { token: "text", regex: identifierRe }, { token: ["keyword", "string", "keyword"],
regex: /(<<[\w\d\-$]+,)(.*?)(>>|$)/ }, { token: "keyword", regex: /<<[\w\d\-$]+,?|>>/ }, { token: "constant.character", regex: /\({2,3}.*?\){2,3}/ }, { token: "keyword", regex: /\[\[.+?\]\]/ }, { token: "support", regex: /^\[{3}[\w\d =\-]+\]{3}/ }, { include: "quotes" }, { token: "empty", regex: /^\s*$/, next: "start" }],
"listText": [{ include: "listStart" }, { include: "text" }],
"indentedBlock": [{ token: "literal", regex: /^[\s\w].+$/, next: "indentedBlock" }, { token: "literal", regex: "", next: "start" }],
"listingBlock": [{ token: "literal", regex: /^\.{4,}\s*$/, next: "dissallowDelimitedBlock" }, { token: "constant.numeric", regex: '<\\d+>' }, { token: "literal", regex: '[^<]+' }, { token: "literal", regex: '<' }],
"literalBlock": [{ token: "literal", regex: /^-{4,}\s*$/, next: "dissallowDelimitedBlock" }, { token: "constant.numeric", regex: '<\\d+>' }, { token: "literal", regex: '[^<]+' }, { token: "literal", regex: '<' }],
"passthroughBlock": [{ token: "literal", regex: /^\+{4,}\s*$/, next: "dissallowDelimitedBlock" }, { token: "literal", regex: identifierRe + "|\\d+" }, { include: "macros" }, { token: "literal", regex: "." }],
"smallPassthrough": [{ token: "literal", regex: /[+]{3,}/, next: "dissallowDelimitedBlock" }, { token: "literal", regex: /^\s*$/, next: "dissallowDelimitedBlock" }, { token: "literal", regex: identifierRe + "|\\d+" }, { include: "macros" }],
"commentBlock": [{ token: "doc.comment", regex: /^\/{4,}\s*$/, next: "dissallowDelimitedBlock" }, { token: "doc.comment", regex: '^.*$' }],
"tableBlock": [{ token: "tableBlock", regex: /^\s*\|={3,}\s*$/, next: "dissallowDelimitedBlock" }, { token: "tableBlock", regex: /^\s*!={3,}\s*$/, next: "innerTableBlock" }, { token: "tableBlock", regex: /\|/ }, { include: "text", noEscape: true }],
"innerTableBlock": [{ token: "tableBlock", regex: /^\s*!={3,}\s*$/, next: "tableBlock" }, { token: "tableBlock", regex: /^\s*|={3,}\s*$/, next: "dissallowDelimitedBlock" }, { token: "tableBlock", regex: /\!/ }],
"macros": [{ token: "macro", regex: /{[\w\-$]+}/ }, { token: ["text", "string", "text", "constant.character", "text"], regex: /({)([\w\-$]+)(:)?(.+)?(})/ }, { token: ["text", "markup.list.macro", "keyword", "string"], regex: /(\w+)(footnote(?:ref)?::?)([^\s\[]+)?(\[.*?\])?/ }, { token: ["markup.list.macro", "keyword", "string"], regex: /([a-zA-Z\-][\w\.\/\-]*::?)([^\s\[]+)(\[.*?\])?/ }, { token: ["markup.list.macro", "keyword"], regex: /([a-zA-Z\-][\w\.\/\-]+::?)(\[.*?\])/ }, { token: "keyword", regex: /^:.+?:(?= |$)/ }],
"quotes": [{ token: "string.italic", regex: /__[^_\s].*?__/ }, { token: "string.italic", regex: quoteRule("_") }, { token: "keyword.bold", regex: /\*\*[^*\s].*?\*\*/ }, { token: "keyword.bold", regex: quoteRule("\\*") }, { token: "literal", regex: quoteRule("\\+") }, { token: "literal", regex: /\+\+[^+\s].*?\+\+/ }, { token: "literal", regex: /\$\$.+?\$\$/ }, { token: "literal", regex: quoteRule("`") }, { token: "keyword", regex: quoteRule("^") }, { token: "keyword", regex: quoteRule("~") }, { token: "keyword", regex: /##?/ }, { token: "keyword", regex: /(?:\B|^)``|\b''/ }]
};
function quoteRule(ch) {
var prefix = /\w/.test(ch) ? "\\b" : "(?:\\B|^)";
return prefix + ch + "[^" + ch + "].*?" + ch + "(?![\\w*])";
}
var tokenMap = {
macro: "constant.character",
tableBlock: "doc.comment",
titleUnderline: "markup.heading",
singleLineTitle: "markup.heading",
pageBreak: "string",
option: "string.regexp",
otherBlock: "markup.list",
literal: "support.function",
optionalTitle: "constant.numeric",
escape: "constant.language.escape",
link: "markup.underline.list"
};
for (var state in this.$rules) {
var stateRules = this.$rules[state];
for (var i = stateRules.length; i--;) {
var rule = stateRules[i];
if (rule.include || typeof rule == "string") {
var args = [i, 1].concat(this.$rules[rule.include || rule]);
if (rule.noEscape) {
args = args.filter(function (x) {
return !x.next;
});
}
stateRules.splice.apply(stateRules, args);
} else if (rule.token in tokenMap) {
rule.token = tokenMap[rule.token];
}
}
}
};
oop.inherits(AsciidocHighlightRules, TextHighlightRules);
exports.AsciidocHighlightRules = AsciidocHighlightRules;
});
define("ace/mode/folding/asciidoc", ["require", "exports", "module", "ace/lib/oop", "ace/mode/folding/fold_mode", "ace/range"], function (require, exports, module) {
"use strict";
var oop = require("../../lib/oop");
var BaseFoldMode = require("./fold_mode").FoldMode;
var Range = require("../../range").Range;
var FoldMode = exports.FoldMode = function () {};
oop.inherits(FoldMode, BaseFoldMode);
(function () {
this.foldingStartMarker = /^(?:\|={10,}|[\.\/=\-~^+]{4,}\s*$|={1,5} )/;
this.singleLineHeadingRe = /^={1,5}(?=\s+\S)/;
this.getFoldWidget = function (session, foldStyle, row) {
var line = session.getLine(row);
if (!this.foldingStartMarker.test(line)) return "";
if (line[0] == "=") {
if (this.singleLineHeadingRe.test(line)) return "start";
if (session.getLine(row - 1).length != session.getLine(row).length) return "";
return "start";
}
if (session.bgTokenizer.getState(row) == "dissallowDelimitedBlock") return "end";
return "start";
};
this.getFoldWidgetRange = function (session, foldStyle, row) {
var line = session.getLine(row);
var startColumn = line.length;
var maxRow = session.getLength();
var startRow = row;
var endRow = row;
if (!line.match(this.foldingStartMarker)) return;
var token;
function getTokenType(row) {
token = session.getTokens(row)[0];
return token && token.type;
}
var levels = ["=", "-", "~", "^", "+"];
var heading = "markup.heading";
var singleLineHeadingRe = this.singleLineHeadingRe;
function getLevel() {
var match = token.value.match(singleLineHeadingRe);
if (match) return match[0].length;
var level = levels.indexOf(token.value[0]) + 1;
if (level == 1) {
if (session.getLine(row - 1).length != session.getLine(row).length) return Infinity;
}
return level;
}
if (getTokenType(row) == heading) {
var startHeadingLevel = getLevel();
while (++row < maxRow) {
if (getTokenType(row) != heading) continue;
var level = getLevel();
if (level <= startHeadingLevel) break;
}
var isSingleLineHeading = token && token.value.match(this.singleLineHeadingRe);
endRow = isSingleLineHeading ? row - 1 : row - 2;
if (endRow > startRow) {
while (endRow > startRow && (!getTokenType(endRow) || token.value[0] == "[")) {
endRow--;
}
}
if (endRow > startRow) {
var endColumn = session.getLine(endRow).length;
return new Range(startRow, startColumn, endRow, endColumn);
}
} else {
var state = session.bgTokenizer.getState(row);
if (state == "dissallowDelimitedBlock") {
while (row-- > 0) {
if (session.bgTokenizer.getState(row).lastIndexOf("Block") == -1) break;
}
endRow = row + 1;
if (endRow < startRow) {
var endColumn = session.getLine(row).length;
return new Range(endRow, 5, startRow, startColumn - 5);
}
} else {
while (++row < maxRow) {
if (session.bgTokenizer.getState(row) == "dissallowDelimitedBlock") break;
}
endRow = row;
if (endRow > startRow) {
var endColumn = session.getLine(row).length;
return new Range(startRow, 5, endRow, endColumn - 5);
}
}
}
};
}).call(FoldMode.prototype);
});
define("ace/mode/asciidoc", ["require", "exports", "module", "ace/lib/oop", "ace/mode/text", "ace/mode/asciidoc_highlight_rules", "ace/mode/folding/asciidoc"], function (require, exports, module) {
"use strict";
var oop = require("../lib/oop");
var TextMode = require("./text").Mode;
var AsciidocHighlightRules = require("./asciidoc_highlight_rules").AsciidocHighlightRules;
var AsciidocFoldMode = require("./folding/asciidoc").FoldMode;
var Mode = function Mode() {
this.HighlightRules = AsciidocHighlightRules;
this.foldingRules = new AsciidocFoldMode();
};
oop.inherits(Mode, TextMode);
(function () {
this.type = "text";
this.getNextLineIndent = function (state, line, tab) {
if (state == "listblock") {
var match = /^((?:.+)?)([-+*][ ]+)/.exec(line);
if (match) {
return new Array(match[1].length + 1).join(" ") + match[2];
} else {
return "";
}
} else {
return this.$getIndent(line);
}
};
this.$id = "ace/mode/asciidoc";
}).call(Mode.prototype);
exports.Mode = Mode;
}); | IonicaBizau/arc-assembler | clients/ace-builds/src/mode-asciidoc.js | JavaScript | mit | 13,207 |
/*
*
* Copyright (c) 2013 - 2014 INT - National Institute of Technology & COPPE - Alberto Luiz Coimbra Institute
- Graduate School and Research in Engineering.
* See the file license.txt for copyright permission.
*
*/
package cargaDoSistema;
import modelo.TipoUsuario;
import modelo.Usuario;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import service.TipoUsuarioAppService;
import service.UsuarioAppService;
import service.controleTransacao.FabricaDeAppService;
import service.exception.AplicacaoException;
import util.JPAUtil;
/**
* Classe responsável pela inclusão de Tipos de Usuário e de Usuário.
* É usada na carga do sistema e deve ser a primeira a ser executada.
* Está criando um usuário para cada tipo. (dma)
*
* @author marques
*
*/
public class CargaUsuario {
// Services
public TipoUsuarioAppService tipoUsuarioService;
public UsuarioAppService usuarioService;
@BeforeClass
public void setupClass(){
try {
tipoUsuarioService = FabricaDeAppService.getAppService(TipoUsuarioAppService.class);
usuarioService = FabricaDeAppService.getAppService(UsuarioAppService.class);
} catch (Exception e) {
e.printStackTrace();
}
}
@Test
public void incluirTiposDeUsuario() {
TipoUsuario tipoUsuarioAdmin = new TipoUsuario();
TipoUsuario tipoUsuarioAluno = new TipoUsuario();
TipoUsuario tipoUsuarioGestor = new TipoUsuario();
TipoUsuario tipoUsuarioEngenheiro = new TipoUsuario();
tipoUsuarioAdmin.setTipoUsuario(TipoUsuario.ADMINISTRADOR);
tipoUsuarioAdmin.setDescricao("O usuário ADMINISTRADOR pode realizar qualquer operação no Sistema.");
tipoUsuarioAluno.setTipoUsuario(TipoUsuario.ALUNO);
tipoUsuarioAluno.setDescricao("O usuário ALUNO pode realizar apenas consultas e impressão de relatórios nas telas " +
"relativas ao Horizonte de Planejamento (HP,Periodo PMP, Periodo PAP) e não acessa " +
"Administração e Eng. Conhecimento");
tipoUsuarioGestor.setTipoUsuario(TipoUsuario.GESTOR);
tipoUsuarioGestor.setDescricao("O usuário GESTOR pode realizar qualquer operação no Sistema, porém não possui acesso" +
"as áreas de Administração e Engenharia de Conhecimento.");
tipoUsuarioEngenheiro.setTipoUsuario(TipoUsuario.ENGENHEIRO_DE_CONHECIMENTO);
tipoUsuarioEngenheiro.setDescricao("O usuário ENGENHEIRO pode realizar a parte de Logica Fuzzy (Engenharia de Conhecimento)" +
"no Sistema. Porém, não possui acesso a área Administrativa.");
tipoUsuarioService.inclui(tipoUsuarioAdmin);
tipoUsuarioService.inclui(tipoUsuarioAluno);
tipoUsuarioService.inclui(tipoUsuarioGestor);
tipoUsuarioService.inclui(tipoUsuarioEngenheiro);
Usuario usuarioAdmin = new Usuario();
Usuario usuarioAluno = new Usuario();
Usuario usuarioGestor = new Usuario();
Usuario usuarioEngenheiro = new Usuario();
usuarioAdmin.setNome("Administrador");
usuarioAdmin.setLogin("dgep");
usuarioAdmin.setSenha("admgesplan2@@8");
usuarioAdmin.setTipoUsuario(tipoUsuarioAdmin);
usuarioAluno.setNome("Alberto da Silva");
usuarioAluno.setLogin("alberto");
usuarioAluno.setSenha("alberto");
usuarioAluno.setTipoUsuario(tipoUsuarioAluno);
usuarioEngenheiro.setNome("Bernadete da Silva");
usuarioEngenheiro.setLogin("bernadete");
usuarioEngenheiro.setSenha("bernadete");
usuarioEngenheiro.setTipoUsuario(tipoUsuarioEngenheiro);
usuarioGestor.setNome("Carlos da Silva");
usuarioGestor.setLogin("carlos");
usuarioGestor.setSenha("carlos");
usuarioGestor.setTipoUsuario(tipoUsuarioGestor);
try {
usuarioService.inclui(usuarioAdmin, usuarioAdmin.getSenha());
usuarioService.inclui(usuarioEngenheiro, usuarioEngenheiro.getSenha());
usuarioService.inclui(usuarioGestor, usuarioGestor.getSenha());
usuarioService.inclui(usuarioAluno, usuarioAluno.getSenha());
} catch (AplicacaoException e) {
//e.printStackTrace();
System.out.println("Erro na inclusao do usuario: "+ e.getMessage());
}
}
}
| dayse/gesplan | test/cargaDoSistema/CargaUsuario.java | Java | mit | 4,187 |
import * as React from 'react'
import {Component, ComponentClass, createElement} from 'react'
import * as PropTypes from 'prop-types'
import {connect} from 'react-redux'
import {Store} from '../store'
import ComputedState from '../model/ComputedState'
function connectToStore<P>(component:ComponentClass<P>):ComponentClass<P> {
type PS = P & {store:Store}
const mapStateToProps = (state:ComputedState, ownProps:PS):P => ({
...Object(ownProps),
...state
})
const WrappedComponent = (props:P) => createElement(component, props)
const ConnectedComponent = connect(mapStateToProps)(WrappedComponent)
return class ConnectToStore extends Component<P, any> {
static contextTypes = {
rrnhStore: PropTypes.object.isRequired
}
render() {
const {rrnhStore} = this.context
return <ConnectedComponent store={rrnhStore} {...this.props} />
}
}
}
export default connectToStore | kenfehling/react-router-nested-history | src/react/connectToStore.tsx | TypeScript | mit | 921 |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!--NewPage-->
<HTML>
<HEAD>
<!-- Generated by javadoc (build 1.4.2_05) on Thu Sep 30 22:16:01 GMT+01:00 2004 -->
<TITLE>
org.springframework.web.multipart.support Class Hierarchy (Spring Framework)
</TITLE>
<LINK REL ="stylesheet" TYPE="text/css" HREF="../../../../../stylesheet.css" TITLE="Style">
<SCRIPT type="text/javascript">
function windowTitle()
{
parent.document.title="org.springframework.web.multipart.support Class Hierarchy (Spring Framework)";
}
</SCRIPT>
</HEAD>
<BODY BGCOLOR="white" onload="windowTitle();">
<!-- ========= START OF TOP NAVBAR ======= -->
<A NAME="navbar_top"><!-- --></A>
<A HREF="#skip-navbar_top" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=3 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_top_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <FONT CLASS="NavBarFont1">Class</FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <FONT CLASS="NavBarFont1">Use</FONT> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Tree</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../org/springframework/web/multipart/cos/package-tree.html"><B>PREV</B></A>
<A HREF="../../../../../org/springframework/web/servlet/package-tree.html"><B>NEXT</B></A></FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../index.html" target="_top"><B>FRAMES</B></A>
<A HREF="package-tree.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_top"></A>
<!-- ========= END OF TOP NAVBAR ========= -->
<HR>
<CENTER>
<H2>
Hierarchy For Package org.springframework.web.multipart.support
</H2>
</CENTER>
<DL>
<DT><B>Package Hierarchies:</B><DD><A HREF="../../../../../overview-tree.html">All Packages</A></DL>
<HR>
<H2>
Class Hierarchy
</H2>
<UL>
<LI TYPE="circle">class java.lang.Object<UL>
<LI TYPE="circle">class org.springframework.web.filter.<A HREF="../../../../../org/springframework/web/filter/GenericFilterBean.html" title="class in org.springframework.web.filter"><B>GenericFilterBean</B></A> (implements javax.servlet.Filter)
<UL>
<LI TYPE="circle">class org.springframework.web.filter.<A HREF="../../../../../org/springframework/web/filter/OncePerRequestFilter.html" title="class in org.springframework.web.filter"><B>OncePerRequestFilter</B></A><UL>
<LI TYPE="circle">class org.springframework.web.multipart.support.<A HREF="../../../../../org/springframework/web/multipart/support/MultipartFilter.html" title="class in org.springframework.web.multipart.support"><B>MultipartFilter</B></A></UL>
</UL>
<LI TYPE="circle">class java.beans.PropertyEditorSupport (implements java.beans.PropertyEditor)
<UL>
<LI TYPE="circle">class org.springframework.web.multipart.support.<A HREF="../../../../../org/springframework/web/multipart/support/ByteArrayMultipartFileEditor.html" title="class in org.springframework.web.multipart.support"><B>ByteArrayMultipartFileEditor</B></A><LI TYPE="circle">class org.springframework.web.multipart.support.<A HREF="../../../../../org/springframework/web/multipart/support/StringMultipartFileEditor.html" title="class in org.springframework.web.multipart.support"><B>StringMultipartFileEditor</B></A></UL>
<LI TYPE="circle">class javax.servlet.ServletRequestWrapper (implements javax.servlet.ServletRequest)
<UL>
<LI TYPE="circle">class javax.servlet.http.HttpServletRequestWrapper (implements javax.servlet.http.HttpServletRequest)
<UL>
<LI TYPE="circle">class org.springframework.web.multipart.support.<A HREF="../../../../../org/springframework/web/multipart/support/AbstractMultipartHttpServletRequest.html" title="class in org.springframework.web.multipart.support"><B>AbstractMultipartHttpServletRequest</B></A> (implements org.springframework.web.multipart.<A HREF="../../../../../org/springframework/web/multipart/MultipartHttpServletRequest.html" title="interface in org.springframework.web.multipart">MultipartHttpServletRequest</A>)
<UL>
<LI TYPE="circle">class org.springframework.web.multipart.support.<A HREF="../../../../../org/springframework/web/multipart/support/DefaultMultipartHttpServletRequest.html" title="class in org.springframework.web.multipart.support"><B>DefaultMultipartHttpServletRequest</B></A></UL>
</UL>
</UL>
</UL>
</UL>
<HR>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<A NAME="navbar_bottom"><!-- --></A>
<A HREF="#skip-navbar_bottom" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=3 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_bottom_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <FONT CLASS="NavBarFont1">Class</FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <FONT CLASS="NavBarFont1">Use</FONT> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Tree</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../org/springframework/web/multipart/cos/package-tree.html"><B>PREV</B></A>
<A HREF="../../../../../org/springframework/web/servlet/package-tree.html"><B>NEXT</B></A></FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../index.html" target="_top"><B>FRAMES</B></A>
<A HREF="package-tree.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_bottom"></A>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<HR>
<i>Copyright (C) 2003-2004 The Spring Framework Project.</i>
</BODY>
</HTML>
| dachengxi/spring1.1.1_source | docs/api/org/springframework/web/multipart/support/package-tree.html | HTML | mit | 8,329 |
---
published: true
author: Robin Wen
layout: post
title: MacBook Pro 更换电池
category: 工具
summary: "自从今年大修了一次主力 MacBook 后,笔者考虑准备一台随时可以上阵的备用机。笔者除了主力的 2018 年款 MacBook Pro,还有一台 2015 年出厂的 MacBook Pro。2015 年的这台机器可用性极强,外壳没有磕过,性能也还不错,唯一美中不足的电池鼓包严重,导致笔记本盖子都不能完全合上。不得不感慨,苹果的设备做工是真得精细啊。笔者一直认为,2015 年的 Retina MacBook Pro 是最好用的苹果笔记本(性能稳定、散热稳定、转接口方便、剪刀式键盘、没有鸡肋的 Touch Bar、有灵魂的发光的 Logo),电池续航不行了,还能动手更换。2016 年之后的 MacBook 要自己更换就没那么容易了。"
tags:
- 工具
- MacBook
- 比特币
- Bitcoin
- 水龙头
- ExinEarn
---
`文/Robin`
***
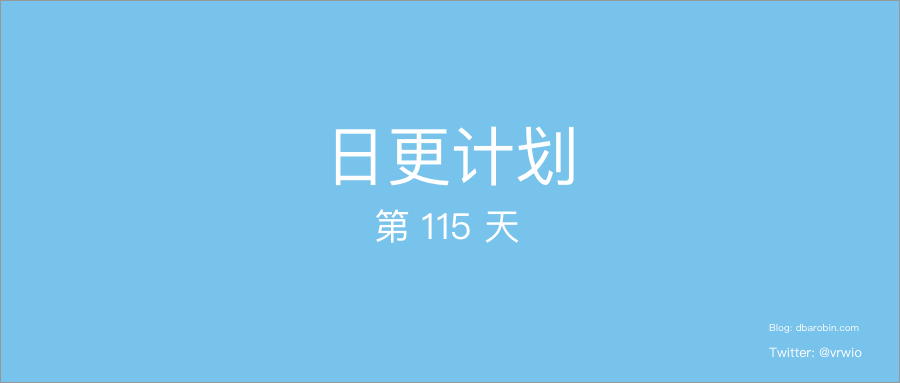
自从今年大修了一次主力 MacBook 后,笔者考虑准备一台随时可以上阵的备用机。笔者除了主力的 2018 年款 MacBook Pro,还有一台 2015 年出厂的 MacBook Pro。2015 年的这台机器可用性极强,外壳没有磕过,性能也还不错,唯一美中不足的电池鼓包严重,导致笔记本盖子都不能完全合上。
电池鼓包严重,那就要想办法解决。只是笔者的拖延症严重,导致一直没有时间去更换。最近看到一些群里聊了这个话题,于是去研究了下,发现官方渠道更换电池的费用在 3000~4000 元(2015 年的机子早已过保)。于是又找了些其他渠道,线下的一些非官方渠道在 600~1000 元不等,闪电修 588 元。
由于之前笔者在闪电修更换过几次 iPhone 电池(苹果的设备除了电池,是真耐用),所以对于他们的服务还是比较满意的。于是我在闪电修下了个上门更换电池的订单,没多久他们告知这个服务是才开通的,暂时不能上门,需要送到福田他们的门店维修。虽然是备用机,但是寄修对于我而言,是不能接受的,于是放弃了这个渠道。
线下的非官方渠道,水挺深的,师傅的技术也是因人而异,于是这个渠道也不考虑了。最后就只剩下「**自己动手,丰衣足食**」了。笔者去京东找了下适配机型的电池,发现绿巨能有一款评价不错,还附赠工具,价格 359 元,于是下了单,使用「水龙头」还返 20 元等值的比特币。第二天一到,就去寻找一些教程,准备着手更换。
iFixit 网站上有一个非常详细的教程,步骤多达 36 步。笔者看了下犯愁了,绿巨能的电池送的工具就一把塑胶铲子、两把螺丝刀,挺多工具都没有啊。于是笔者去 B 站重新找了个视频教程,看了一遍,没那么难啊,就是关键的几步注意下就行了。于是按照这个视频教程开始动手更换电池。打开背壳,发现这电池膨胀太吓人了,什么时候爆炸都难说啊。如果读者发现电池鼓包了,得尽快处理。整个过程耗时 40 分钟左右,除了拆卸电池比较费劲之外,其他的都挺容易的。除了更换电池,笔者还是用压缩空气对电路板除了下尘。
更换电池之后,开机,查看电池性能,测试电池充放,一切正常。MacBook Pro 就这样满血复活了。不过有一点需要注意,为了最低地降低风险,在更换电池之前,先打开您的 MacBook 直到完全耗尽电池电量。
不得不感慨,苹果的设备做工是真的精细啊。笔者一直认为,2015 年的 Retina MacBook Pro 是最好用的苹果笔记本(性能稳定、散热稳定、转接口方便、剪刀式键盘、没有鸡肋的 Touch Bar、有灵魂的发光的 Logo),电池续航不行了,还能动手更换。2016 年之后的 MacBook 要自己更换就没那么容易了。
这就是笔者动手更换电池的经验,希望对读者有所帮助。有任何问题,欢迎交流。
对了,关于文中提到的绿巨能笔记本电池(适配 2015 款 13 英寸 Retina MacBook Pro,型号是 A1502),如果读者有需求的话,可以扫描以下二维码购买。
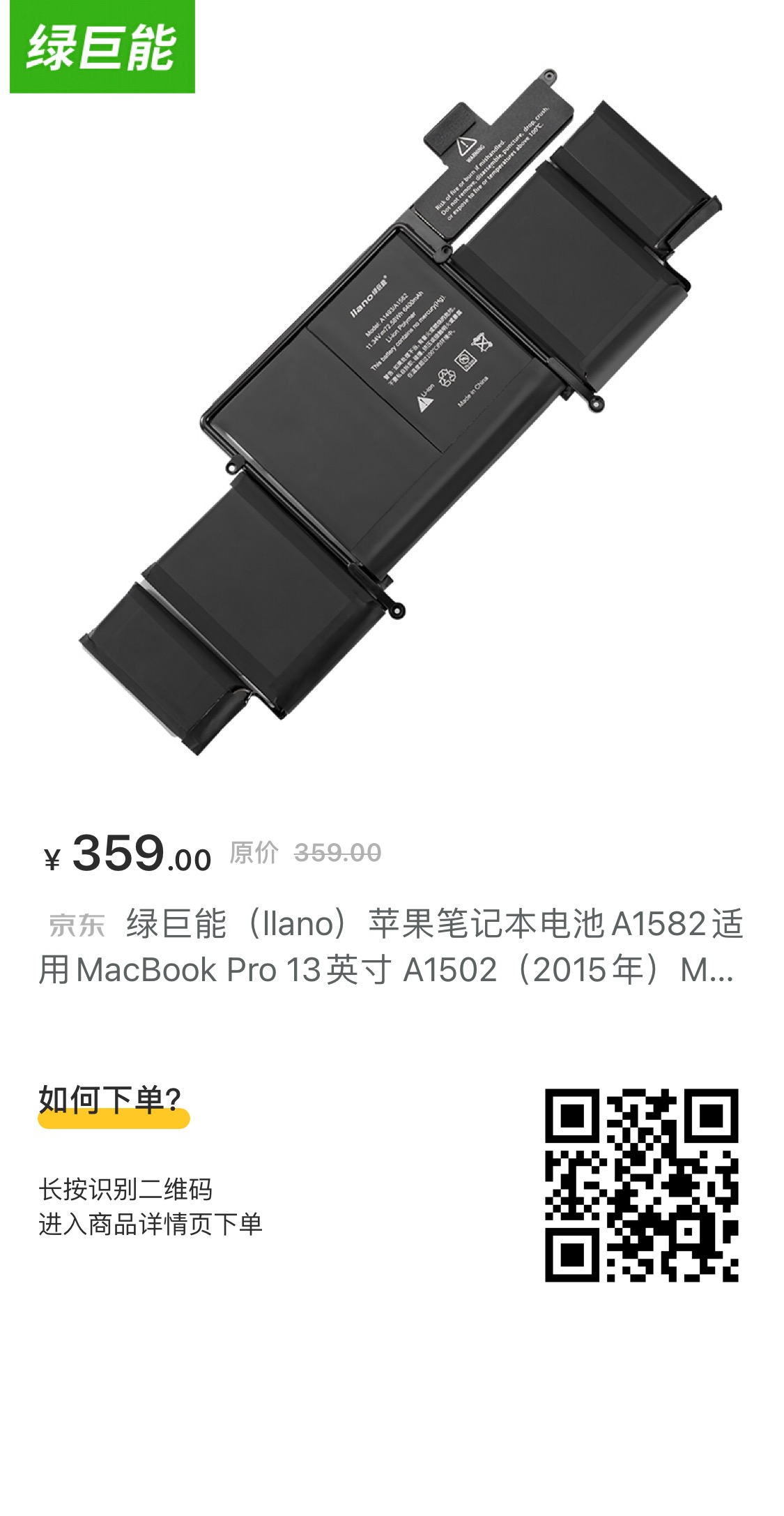
最后,本文提到的水龙头,App Store、各大安卓市场均有下载,关键词「水龙头」,也可以扫描下图的二维码注册。我的邀请码:**3XKXJB**。
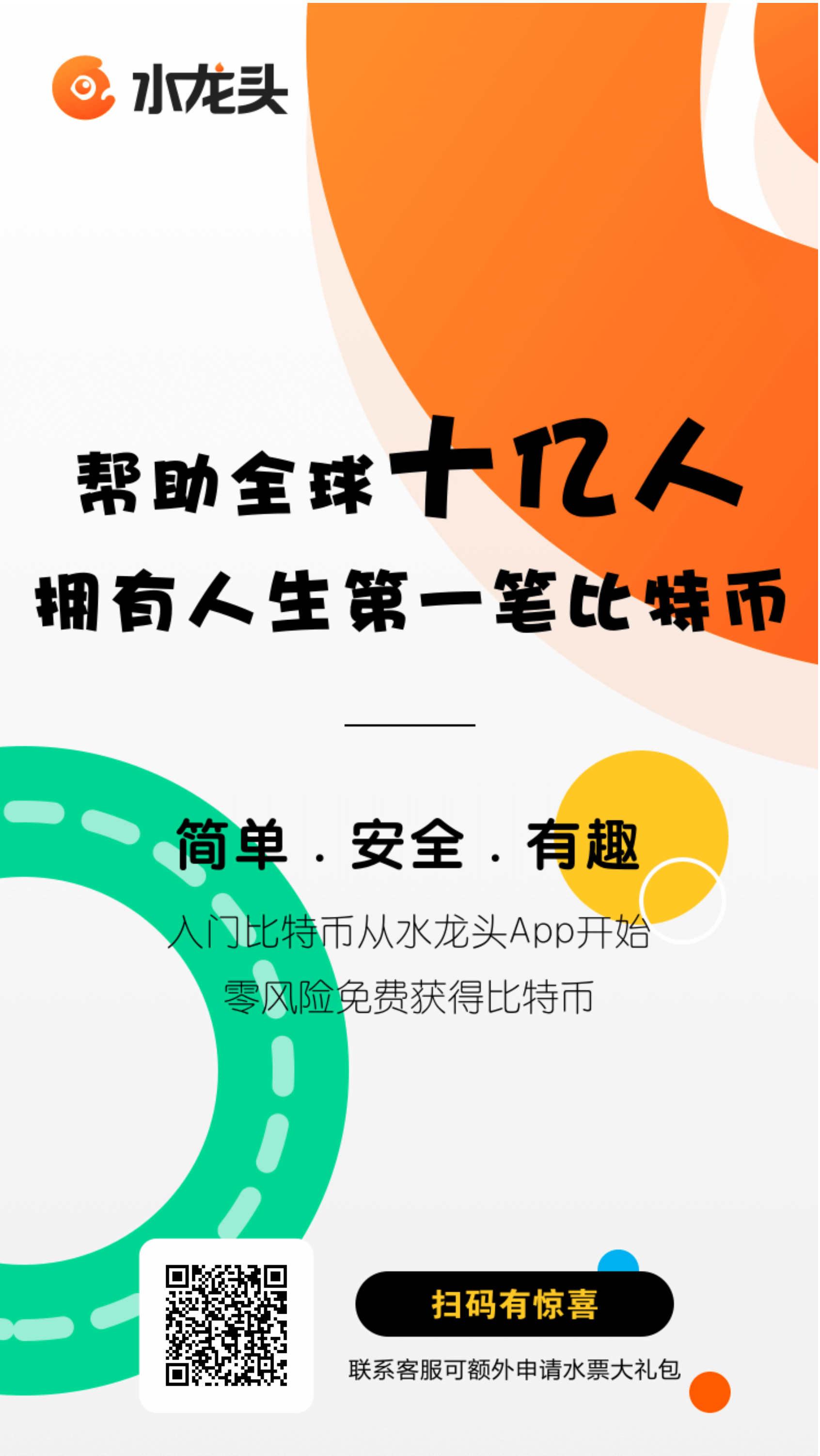
***
我是区块链罗宾,博客 [dbarobin.com](https://dbarobin.com/)
如果您想和我交流,我的 Mixin: **26930**
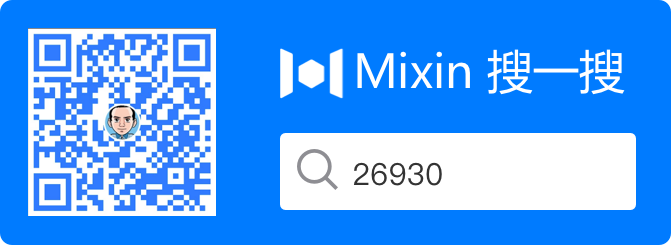
***
**本站推广**
币安是全球领先的数字货币交易平台,提供比特币、以太坊、BNB 以及 USDT 交易。
> 币安注册: [https://accounts.binancezh.io/cn/register/?ref=11190872](https://accounts.binancezh.io/cn/register/?ref=11190872)
> 邀请码: **11190872**
***
本博客开通了 Donate Cafe 打赏,支持 Mixin Messenger、Blockin Wallet、imToken、Blockchain Wallet、Ownbit、Cobo Wallet、bitpie、DropBit、BRD、Pine、Secrypto 等任意钱包扫码转账。
<center>
<div class="--donate-button"
data-button-id="f8b9df0d-af9a-460d-8258-d3f435445075"
></div>
</center>
***
–EOF–
版权声明:[自由转载-非商用-非衍生-保持署名(创意共享4.0许可证)](http://creativecommons.org/licenses/by-nc-nd/4.0/deed.zh) | dbarobin/dbarobin.github.io | _posts/工具/2020-10-28-battery.md | Markdown | mit | 5,549 |
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { enableProdMode } from '@angular/core';
import { AppModule } from './app/app.module';
enableProdMode();
platformBrowserDynamic().bootstrapModule(AppModule);
| fabricadecodigo/angular2-examples | ToDoAppWithFirebase/src/main.ts | TypeScript | mit | 242 |
from behave import given, when, then
from genosdb.models import User
from genosdb.exceptions import UserNotFound
# 'mongodb://localhost:27017/')
@given('a valid user with values {username}, {password}, {email}, {first_name}, {last_name}')
def step_impl(context, username, password, email, first_name, last_name):
context.base_user = User(username=username, email=email, password=password, first_name=first_name,
last_name=last_name)
@when('I add the user to the collection')
def step_impl(context):
context.user_service.save(context.base_user)
@then('I check {user_name} exists')
def step_impl(context, user_name):
user_exists = context.user_service.exists(user_name)
assert context.base_user.username == user_exists['username']
assert context.base_user.password == user_exists['password']
assert context.base_user.email == user_exists['email']
assert context.base_user.first_name == user_exists['first_name']
assert context.base_user.last_name == user_exists['last_name']
assert user_exists['_id'] is not None
@given('I update {username} {field} with {value}')
def step_impl(context, username, field, value):
user = context.user_service.exists(username)
if user is not None:
user[field] = value
context.user_service.update(user.to_json())
else:
raise UserNotFound(username, "User was not found")
@then('I check {username} {field} is {value}')
def step_impl(context, username, field, value):
user = context.user_service.exists(username)
if user is not None:
assert user[field] == value
else:
raise UserNotFound(username, "User was not found")
| jonrf93/genos | dbservices/tests/functional_tests/steps/user_service_steps.py | Python | mit | 1,685 |
using Mokkosu.AST;
using System.Collections.Generic;
using System.Text;
namespace Mokkosu.ClosureConversion
{
class ClosureConversionResult
{
public Dictionary<string, MExpr> FunctionTable { get; private set; }
public MExpr Main { get; private set; }
public ClosureConversionResult(Dictionary<string, MExpr> table, MExpr main)
{
FunctionTable = table;
Main = main;
}
public override string ToString()
{
var sb = new StringBuilder();
foreach (var item in FunctionTable)
{
sb.AppendFormat("=== {0} ===\n", item.Key);
sb.Append(item.Value);
sb.Append("\n");
}
sb.Append("=== Main ===\n");
sb.Append(Main);
return sb.ToString();
}
}
}
| lambdataro/Mokkosu | VS2013/MokkosuCore/ClosureConversion/ClosureConversionResult.cs | C# | mit | 870 |
package com.ov3rk1ll.kinocast.ui.util.glide;
import com.ov3rk1ll.kinocast.data.ViewModel;
public class ViewModelGlideRequest {
private ViewModel viewModel;
private int screenWidthPx;
private String type;
public ViewModelGlideRequest(ViewModel viewModel, int screenWidthPx, String type) {
this.viewModel = viewModel;
this.screenWidthPx = screenWidthPx;
this.type = type;
}
ViewModel getViewModel() {
return viewModel;
}
int getScreenWidthPx() {
return screenWidthPx;
}
public String getType() {
return type;
}
} | ov3rk1ll/KinoCast | app/src/main/java/com/ov3rk1ll/kinocast/ui/util/glide/ViewModelGlideRequest.java | Java | mit | 609 |
# core-data-ipc
Share a Core Data store between multiple processes
| ddeville/core-data-ipc | README.md | Markdown | mit | 67 |
package stat
import (
"fmt"
"time"
// "encoding/json"
)
type RevStat struct {
RevId string `json:"RevId"`
UserName string `json:"UserName"`
WordCount int `json:"WordCount"`
ModDate string `json:"ModDate"`
WordFreq []WordPair `json:"WordFreq"`
}
type DocStat struct {
FileId string `json:"FileId"`
Title string `json:"Title"`
LastMod string `json:"LastMod"`
RevList []RevStat `json:"RevList"`
}
func (rev RevStat) GetTime() string {
x, _ := time.Parse("2006-01-02T15:04:05.000Z", rev.ModDate)
return x.Format("15:04")
}
func (rev RevStat) String() string {
return fmt.Sprintf("[%s %s] %d words by %s. \n\t Words [%s]", rev.ModDate, rev.RevId, rev.WordCount, rev.UserName, rev.WordFreq)
}
func (doc DocStat) String() string {
s := fmt.Sprintf("[%s] '%s' last mod on %s with revs\n", doc.FileId, doc.Title, doc.LastMod)
for i, v := range doc.RevList {
s += fmt.Sprintf("\t %d:%s\n", i, v)
}
return s
}
| Kimau/GoDriveTracker | stat/stat.go | GO | mit | 964 |
<html>
<head>
<meta charset="utf-8">
<title>Example React.js using NPM, Babel6 and Webpack</title>
</head>
<body>
<div id="app" />
<script src="public/bundle.js" type="text/javascript"></script>
</body>
</html>
| Tabares/react-example-webpack-babel | src/client/index.html | HTML | mit | 235 |
package ru.lanbilling.webservice.wsdl;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Generated;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* <p>Java class for anonymous complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ret" type="{urn:api3}soapDocument" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"ret"
})
@XmlRootElement(name = "getClientDocumentsResponse")
@Generated(value = "com.sun.tools.xjc.Driver", date = "2015-10-25T05:29:34+06:00", comments = "JAXB RI v2.2.11")
public class GetClientDocumentsResponse {
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", date = "2015-10-25T05:29:34+06:00", comments = "JAXB RI v2.2.11")
protected List<SoapDocument> ret;
/**
* Gets the value of the ret property.
*
* <p>
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a <CODE>set</CODE> method for the ret property.
*
* <p>
* For example, to add a new item, do as follows:
* <pre>
* getRet().add(newItem);
* </pre>
*
*
* <p>
* Objects of the following type(s) are allowed in the list
* {@link SoapDocument }
*
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", date = "2015-10-25T05:29:34+06:00", comments = "JAXB RI v2.2.11")
public List<SoapDocument> getRet() {
if (ret == null) {
ret = new ArrayList<SoapDocument>();
}
return this.ret;
}
}
| kanonirov/lanb-client | src/main/java/ru/lanbilling/webservice/wsdl/GetClientDocumentsResponse.java | Java | mit | 2,281 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.