content_type
stringclasses 8
values | main_lang
stringclasses 7
values | message
stringlengths 1
50
| sha
stringlengths 40
40
| patch
stringlengths 52
962k
| file_count
int64 1
300
|
---|---|---|---|---|---|
PHP | PHP | add helper method to get request's webroot | b378834ba79d67bd500cfa63466aad486ee33b96 | <ide><path>src/View/Asset.php
<ide> use Cake\Core\Configure;
<ide> use Cake\Core\Exception\Exception;
<ide> use Cake\Core\Plugin;
<del>use Cake\Http\ServerRequest;
<ide> use Cake\Routing\Router;
<ide> use Cake\Utility\Inflector;
<ide>
<ide> public static function assetTimestamp(string $path, $timestamp = null): string
<ide> $timestampEnabled = $timestamp === 'force' || ($timestamp === true && Configure::read('debug'));
<ide> if ($timestampEnabled) {
<ide> $filepath = preg_replace(
<del> '/^' . preg_quote(static::request()->getAttribute('webroot'), '/') . '/',
<add> '/^' . preg_quote(static::requestWebroot(), '/') . '/',
<ide> '',
<ide> urldecode($path)
<ide> );
<ide> public static function assetTimestamp(string $path, $timestamp = null): string
<ide> public static function webroot(string $file, array $options = []): string
<ide> {
<ide> $options += ['theme' => null];
<del> $request = static::request();
<add> $requestWebroot = static::requestWebroot();
<ide>
<ide> $asset = explode('?', $file);
<ide> $asset[1] = isset($asset[1]) ? '?' . $asset[1] : '';
<del> $webPath = $request->getAttribute('webroot') . $asset[0];
<add> $webPath = $requestWebroot . $asset[0];
<ide> $file = $asset[0];
<ide>
<ide> $themeName = $options['theme'];
<ide> public static function webroot(string $file, array $options = []): string
<ide> }
<ide>
<ide> if (file_exists(Configure::read('App.wwwRoot') . $theme . $file)) {
<del> $webPath = $request->getAttribute('webroot') . $theme . $asset[0];
<add> $webPath = $requestWebroot . $theme . $asset[0];
<ide> } else {
<ide> $themePath = Plugin::path($themeName);
<ide> $path = $themePath . 'webroot/' . $file;
<ide> if (file_exists($path)) {
<del> $webPath = $request->getAttribute('webroot') . $theme . $asset[0];
<add> $webPath = $requestWebroot . $theme . $asset[0];
<ide> }
<ide> }
<ide> }
<ide> protected static function inflectThemeName(string $name): string
<ide> }
<ide>
<ide> /**
<del> * Get current request instance from Router.
<add> * Get webroot from request.
<ide> *
<del> * @return \Cake\Http\ServerRequest
<add> * @return string
<ide> */
<del> protected static function request(): ServerRequest
<add> protected static function requestWebroot(): string
<ide> {
<ide> $request = Router::getRequest(true);
<ide> if ($request === null) {
<ide> throw new Exception('No request instance present in Router.');
<ide> }
<ide>
<del> return $request;
<add> return $request->getAttribute('webroot');
<ide> }
<ide>
<ide> /** | 1 |
Java | Java | update webclient builder | 82a34f4b246537429a8b236f0d1790b1ae924091 | <ide><path>spring-webflux/src/main/java/org/springframework/web/reactive/function/client/DefaultWebClient.java
<ide> import java.time.ZonedDateTime;
<ide> import java.time.format.DateTimeFormatter;
<ide> import java.util.Arrays;
<add>import java.util.Map;
<ide> import java.util.function.Function;
<ide>
<ide> import org.jetbrains.annotations.NotNull;
<ide> public HeaderSpec uri(String uriTemplate, Object... uriVariables) {
<ide> return uri(getUriBuilderFactory().expand(uriTemplate, uriVariables));
<ide> }
<ide>
<add> @Override
<add> public HeaderSpec uri(String uriTemplate, Map<String, ?> uriVariables) {
<add> return uri(getUriBuilderFactory().expand(uriTemplate, uriVariables));
<add> }
<add>
<ide> @Override
<ide> public HeaderSpec uri(Function<UriBuilderFactory, URI> uriFunction) {
<ide> return uri(uriFunction.apply(getUriBuilderFactory()));
<ide><path>spring-webflux/src/main/java/org/springframework/web/reactive/function/client/DefaultWebClientBuilder.java
<ide> package org.springframework.web.reactive.function.client;
<ide>
<ide> import java.util.Arrays;
<add>import java.util.Map;
<ide>
<ide> import org.springframework.http.HttpHeaders;
<ide> import org.springframework.http.client.reactive.ClientHttpConnector;
<ide> */
<ide> class DefaultWebClientBuilder implements WebClient.Builder {
<ide>
<del> private UriBuilderFactory uriBuilderFactory;
<del>
<del> private ClientHttpConnector connector;
<add> private String baseUrl;
<ide>
<del> private ExchangeStrategies exchangeStrategies = ExchangeStrategies.withDefaults();
<add> private Map<String, ?> defaultUriVariables;
<ide>
<del> private ExchangeFunction exchangeFunction;
<add> private UriBuilderFactory uriBuilderFactory;
<ide>
<ide> private HttpHeaders defaultHeaders;
<ide>
<ide> private MultiValueMap<String, String> defaultCookies;
<ide>
<add> private ClientHttpConnector connector;
<ide>
<del> public DefaultWebClientBuilder() {
<del> this(new DefaultUriBuilderFactory());
<del> }
<del>
<del> public DefaultWebClientBuilder(String baseUrl) {
<del> this(new DefaultUriBuilderFactory(baseUrl));
<del> }
<add> private ExchangeStrategies exchangeStrategies = ExchangeStrategies.withDefaults();
<ide>
<del> public DefaultWebClientBuilder(UriBuilderFactory uriBuilderFactory) {
<del> Assert.notNull(uriBuilderFactory, "UriBuilderFactory is required.");
<del> this.uriBuilderFactory = uriBuilderFactory;
<del> }
<add> private ExchangeFunction exchangeFunction;
<ide>
<ide>
<ide> @Override
<del> public WebClient.Builder clientConnector(ClientHttpConnector connector) {
<del> this.connector = connector;
<add> public WebClient.Builder baseUrl(String baseUrl) {
<add> this.baseUrl = baseUrl;
<ide> return this;
<ide> }
<ide>
<ide> @Override
<del> public WebClient.Builder exchangeStrategies(ExchangeStrategies strategies) {
<del> Assert.notNull(strategies, "ExchangeStrategies is required.");
<del> this.exchangeStrategies = strategies;
<add> public WebClient.Builder defaultUriVariables(Map<String, ?> defaultUriVariables) {
<add> this.defaultUriVariables = defaultUriVariables;
<ide> return this;
<ide> }
<ide>
<ide> @Override
<del> public WebClient.Builder exchangeFunction(ExchangeFunction exchangeFunction) {
<del> this.exchangeFunction = exchangeFunction;
<add> public WebClient.Builder uriBuilderFactory(UriBuilderFactory uriBuilderFactory) {
<add> this.uriBuilderFactory = uriBuilderFactory;
<ide> return this;
<ide> }
<ide>
<ide> public WebClient.Builder defaultCookie(String cookieName, String... cookieValues
<ide> return this;
<ide> }
<ide>
<add> @Override
<add> public WebClient.Builder clientConnector(ClientHttpConnector connector) {
<add> this.connector = connector;
<add> return this;
<add> }
<add>
<add> @Override
<add> public WebClient.Builder exchangeStrategies(ExchangeStrategies strategies) {
<add> Assert.notNull(strategies, "ExchangeStrategies is required.");
<add> this.exchangeStrategies = strategies;
<add> return this;
<add> }
<add>
<add> @Override
<add> public WebClient.Builder exchangeFunction(ExchangeFunction exchangeFunction) {
<add> this.exchangeFunction = exchangeFunction;
<add> return this;
<add> }
<add>
<ide> @Override
<ide> public WebClient build() {
<del> return new DefaultWebClient(initExchangeFunction(),
<del> this.uriBuilderFactory, this.defaultHeaders, this.defaultCookies);
<add> return new DefaultWebClient(initExchangeFunction(), initUriBuilderFactory(),
<add> this.defaultHeaders, this.defaultCookies);
<add> }
<add>
<add> private UriBuilderFactory initUriBuilderFactory() {
<add> if (this.uriBuilderFactory != null) {
<add> return this.uriBuilderFactory;
<add> }
<add> DefaultUriBuilderFactory factory = this.baseUrl != null ?
<add> new DefaultUriBuilderFactory(this.baseUrl) : new DefaultUriBuilderFactory();
<add>
<add> factory.setDefaultUriVariables(this.defaultUriVariables);
<add> return factory;
<ide> }
<ide>
<ide> private ExchangeFunction initExchangeFunction() {
<ide><path>spring-webflux/src/main/java/org/springframework/web/reactive/function/client/WebClient.java
<ide> import java.net.URI;
<ide> import java.nio.charset.Charset;
<ide> import java.time.ZonedDateTime;
<add>import java.util.Map;
<ide> import java.util.function.Function;
<ide>
<ide> import org.reactivestreams.Publisher;
<ide> import org.springframework.http.client.reactive.ClientHttpRequest;
<ide> import org.springframework.util.MultiValueMap;
<ide> import org.springframework.web.reactive.function.BodyInserter;
<del>import org.springframework.web.util.DefaultUriBuilderFactory;
<ide> import org.springframework.web.util.UriBuilderFactory;
<ide>
<ide> /**
<ide> * The main class for performing Web requests.
<ide> *
<ide> * <pre class="code">
<ide> *
<del> * // Create ExchangeFunction (application-wide)
<add> * // Initialize the client
<ide> *
<del> * ClientHttpConnector connector = new ReactorClientHttpConnector();
<del> * ExchangeFunction exchangeFunction = ExchangeFunctions.create(connector);
<del> *
<del> * // Create WebClient (per base URI)
<del> *
<del> * String baseUri = "http://abc.com";
<del> * UriBuilderFactory factory = new DefaultUriBuilderFactory(baseUri);
<del> * WebClient operations = WebClient.create(exchangeFunction, factory);
<add> * WebClient client = WebClient.create("http://abc.com");
<ide> *
<ide> * // Perform requests...
<ide> *
<del> * Mono<String> result = operations.get()
<add> * Mono<String> result = client.get()
<ide> * .uri("/foo")
<ide> * .exchange()
<ide> * .then(response -> response.bodyToMono(String.class));
<ide> public interface WebClient {
<ide> // Static, factory methods
<ide>
<ide> /**
<del> * Shortcut for:
<del> * <pre class="code">
<del> * WebClient client = builder().build();
<del> * </pre>
<add> * Create a new {@code WebClient} with no default, shared preferences across
<add> * requests such as base URI, default headers, and others.
<ide> */
<ide> static WebClient create() {
<ide> return new DefaultWebClientBuilder().build();
<ide> }
<ide>
<ide> /**
<del> * Shortcut for:
<add> * Configure a base URI for requests performed through the client for
<add> * example to avoid repeating the same host, port, base path, or even
<add> * query parameters with every request.
<add> *
<add> * <p>Given the following initialization:
<add> * <pre class="code">
<add> * WebClient client = WebClient.create("http://abc.com/v1");
<add> * </pre>
<add> *
<add> * <p>A base URI is applied when using a URI template:
<add> * <pre class="code">
<add> *
<add> * // GET http://abc.com/v1/accounts/43
<add> *
<add> * Mono<Account> result = client.get()
<add> * .uri("/accounts/{id}", 43)
<add> * .exchange()
<add> * .then(response -> response.bodyToMono(String.class));
<add> * </pre>
<add> *
<add> * <p>It is also applied when using a {@link UriBuilderFactory}:
<ide> * <pre class="code">
<del> * WebClient client = builder(baseUrl).build();
<add>
<add> * // GET http://abc.com/v1/accounts?q=12
<add> *
<add> * Mono<Account> result = client.get()
<add> * .uri(factory -> factory.uriString("/accounts").queryParam("q", "12").build())
<add> * .exchange()
<add> * .then(response -> response.bodyToMono(String.class));
<ide> * </pre>
<add> *
<ide> * @param baseUrl the base URI for all requests
<ide> */
<ide> static WebClient create(String baseUrl) {
<del> return new DefaultWebClientBuilder(baseUrl).build();
<add> return new DefaultWebClientBuilder().baseUrl(baseUrl).build();
<ide> }
<ide>
<ide> /**
<ide> static WebClient.Builder builder() {
<ide> return new DefaultWebClientBuilder();
<ide> }
<ide>
<del> /**
<del> * Obtain a {@code WebClient} builder with a base URI to be used as the
<del> * base for expanding URI templates during exchanges. The given String
<del> * is used to create an instance of {@link DefaultUriBuilderFactory} whose
<del> * {@link DefaultUriBuilderFactory#DefaultUriBuilderFactory(String)
<del> * constructor} provides more details on how the base URI is applied.
<del> * @param baseUrl the base URI for all requests
<del> */
<del> static WebClient.Builder builder(String baseUrl) {
<del> return new DefaultWebClientBuilder(baseUrl);
<del> }
<del>
<del> /**
<del> * Obtain a {@code WebClient} builder with the {@link UriBuilderFactory}
<del> * to use for expanding URI templates during exchanges.
<del> * @param uriBuilderFactory the factory to use
<del> */
<del> static WebClient.Builder builder(UriBuilderFactory uriBuilderFactory) {
<del> return new DefaultWebClientBuilder(uriBuilderFactory);
<del> }
<del>
<ide>
<ide> /**
<ide> * A mutable builder for a {@link WebClient}.
<ide> */
<ide> interface Builder {
<ide>
<ide> /**
<del> * Configure the {@link ClientHttpConnector} to use.
<del> * <p>By default an instance of
<del> * {@link org.springframework.http.client.reactive.ReactorClientHttpConnector
<del> * ReactorClientHttpConnector} is created if this is not set. However a
<del> * shared instance may be passed instead, e.g. for use with multiple
<del> * {@code WebClient}'s targeting different base URIs.
<del> * @param connector the connector to use
<add> * Configure a base URI as described in {@link WebClient#create(String)
<add> * WebClient.create(String)}.
<add> * @see #defaultUriVariables(Map)
<add> * @see #uriBuilderFactory(UriBuilderFactory)
<ide> */
<del> Builder clientConnector(ClientHttpConnector connector);
<add> Builder baseUrl(String baseUrl);
<ide>
<ide> /**
<del> * Configure the {@link ExchangeStrategies} to use.
<del> * <p>By default {@link ExchangeStrategies#withDefaults()} is used.
<del> * @param strategies the strategies to use
<add> * Configure default URI variable values that will be used when expanding
<add> * URI templates using a {@link Map}.
<add> * @param defaultUriVariables the default values to use
<add> * @see #baseUrl(String)
<add> * @see #uriBuilderFactory(UriBuilderFactory)
<ide> */
<del> Builder exchangeStrategies(ExchangeStrategies strategies);
<add> Builder defaultUriVariables(Map<String, ?> defaultUriVariables);
<ide>
<ide> /**
<del> * Configure directly an {@link ExchangeFunction} instead of separately
<del> * providing a {@link ClientHttpConnector} and/or
<del> * {@link ExchangeStrategies}.
<del> * @param exchangeFunction the exchange function to use
<add> * Provide a pre-configured {@link UriBuilderFactory} instance. This is
<add> * an alternative to and effectively overrides the following:
<add> * <ul>
<add> * <li>{@link #baseUrl(String)}
<add> * <li>{@link #defaultUriVariables(Map)}.
<add> * </ul>
<add> * @param uriBuilderFactory the URI builder factory to use
<add> * @see #baseUrl(String)
<add> * @see #defaultUriVariables(Map)
<ide> */
<del> Builder exchangeFunction(ExchangeFunction exchangeFunction);
<add> Builder uriBuilderFactory(UriBuilderFactory uriBuilderFactory);
<ide>
<ide> /**
<ide> * Add the given header to all requests that haven't added it.
<ide> interface Builder {
<ide> */
<ide> Builder defaultCookie(String cookieName, String... cookieValues);
<ide>
<add> /**
<add> * Configure the {@link ClientHttpConnector} to use.
<add> * <p>By default an instance of
<add> * {@link org.springframework.http.client.reactive.ReactorClientHttpConnector
<add> * ReactorClientHttpConnector} is created if this is not set. However a
<add> * shared instance may be passed instead, e.g. for use with multiple
<add> * {@code WebClient}'s targeting different base URIs.
<add> * @param connector the connector to use
<add> * @see #exchangeStrategies(ExchangeStrategies)
<add> * @see #exchangeFunction(ExchangeFunction)
<add> */
<add> Builder clientConnector(ClientHttpConnector connector);
<add>
<add> /**
<add> * Configure the {@link ExchangeStrategies} to use.
<add> * <p>By default {@link ExchangeStrategies#withDefaults()} is used.
<add> * @param strategies the strategies to use
<add> * @see #clientConnector(ClientHttpConnector)
<add> * @see #exchangeFunction(ExchangeFunction)
<add> */
<add> Builder exchangeStrategies(ExchangeStrategies strategies);
<add>
<add> /**
<add> * Provide a pre-configured {@link ExchangeFunction} instance. This is
<add> * an alternative to and effectively overrides the following:
<add> * <ul>
<add> * <li>{@link #clientConnector(ClientHttpConnector)}
<add> * <li>{@link #exchangeStrategies(ExchangeStrategies)}.
<add> * </ul>
<add> * @param exchangeFunction the exchange function to use
<add> * @see #clientConnector(ClientHttpConnector)
<add> * @see #exchangeStrategies(ExchangeStrategies)
<add> */
<add> Builder exchangeFunction(ExchangeFunction exchangeFunction);
<add>
<ide> /**
<ide> * Builder the {@link WebClient} instance.
<ide> */
<ide> interface UriSpec {
<ide> * Specify the URI for the request using a URI template and URI variables.
<ide> * If a {@link UriBuilderFactory} was configured for the client (e.g.
<ide> * with a base URI) it will be used to expand the URI template.
<del> * @see #builder(String)
<ide> */
<ide> HeaderSpec uri(String uri, Object... uriVariables);
<ide>
<add> /**
<add> * Specify the URI for the request using a URI template and URI variables.
<add> * If a {@link UriBuilderFactory} was configured for the client (e.g.
<add> * with a base URI) it will be used to expand the URI template.
<add> */
<add> HeaderSpec uri(String uri, Map<String, ?> uriVariables);
<add>
<ide> /**
<ide> * Build the URI for the request using the {@link UriBuilderFactory}
<ide> * configured for this client.
<del> * @see #builder(String)
<ide> */
<ide> HeaderSpec uri(Function<UriBuilderFactory, URI> uriFunction);
<ide>
<ide><path>spring-webflux/src/test/java/org/springframework/web/reactive/function/client/DefaultWebClientTests.java
<ide> public void defaultHeaderAndCookieOverrides() throws Exception {
<ide>
<ide>
<ide> private WebClient.Builder builder() {
<del> return WebClient.builder("/base").exchangeFunction(this.exchangeFunction);
<add> return WebClient.builder().baseUrl("/base").exchangeFunction(this.exchangeFunction);
<ide> }
<ide>
<ide> private ClientRequest<?> verifyExchange() { | 4 |
Text | Text | add target option to npm readme | 148543ce2b81768cb52b303fad12b5d762799142 | <ide><path>npm-react-tools/README.md
<ide> By default JSX files with a `.js` extension are transformed. Use the `-x` option
<ide> --strip-types Strips out type annotations
<ide> --es6module Parses the file as a valid ES6 module
<ide> --non-strict-es6module Parses the file as an ES6 module, except disables implicit strict-mode (i.e. CommonJS modules et al are allowed)
<add> --target <version> Target version of ECMAScript. Valid values are "es3" and "es5". Use "es3" for legacy browsers like IE8.
<ide>
<ide> ## API
<ide>
<ide> option | values | default
<ide> `stripTypes` | `true`: strips out type annotations | `false`
<ide> `es6module` | `true`: parses the file as an ES6 module | `false`
<ide> `nonStrictEs6module` | `true`: parses the file as an ES6 module, except disables implicit strict-mode (i.e. CommonJS modules et al are allowed) | `false`
<add>`target` | `"es3"`: ECMAScript 3<br>`"es5"`: ECMAScript 5| `"es5"`
<ide>
<ide> ```js
<ide> var reactTools = require('react-tools'); | 1 |
Ruby | Ruby | fix final url spacing in debug output | 88843d2e437ba7d46c6e39659c4d07863f490deb | <ide><path>Library/Homebrew/livecheck/livecheck.rb
<ide> def latest_version(formula_or_cask, json: false, full_name: false, verbose: fals
<ide>
<ide> if debug
<ide> puts "URL (strategy): #{strategy_data[:url]}" if strategy_data[:url] != url
<del> puts "URL (final): #{strategy_data[:final_url]}" if strategy_data[:final_url]
<add> puts "URL (final): #{strategy_data[:final_url]}" if strategy_data[:final_url]
<ide> puts "Regex (strategy): #{strategy_data[:regex].inspect}" if strategy_data[:regex] != livecheck_regex
<ide> end
<ide> | 1 |
Python | Python | remove str.format to support python2.5 | 4b0241d5892f91dfac0438f9051c71e790be95ea | <ide><path>tools/getnodeversion.py
<del>import os,re;
<add>import os,re
<ide>
<ide> node_version_h = os.path.join(os.path.dirname(__file__), '..', 'src',
<ide> 'node_version.h')
<ide> if re.match('#define NODE_PATCH_VERSION', line):
<ide> patch = line.split()[2]
<ide>
<del>print '{0:s}.{1:s}.{2:s}'.format(major, minor, patch)
<add>print '%(major)s.%(minor)s.%(patch)s'% locals() | 1 |
Python | Python | test correct tokenizers after default switch | b90745c5901809faef3136ed09a689e7d733526c | <ide><path>tests/test_tokenization_auto.py
<ide> def test_parents_and_children_in_mappings(self):
<ide> self.assertFalse(issubclass(child_model_fast, parent_model_fast))
<ide>
<ide> def test_from_pretrained_use_fast_toggle(self):
<del> self.assertIsInstance(AutoTokenizer.from_pretrained("bert-base-cased"), BertTokenizerFast)
<del> self.assertIsInstance(AutoTokenizer.from_pretrained("bert-base-cased", use_fast=False), BertTokenizer)
<add> self.assertIsInstance(AutoTokenizer.from_pretrained("bert-base-cased"), BertTokenizer)
<add> self.assertIsInstance(AutoTokenizer.from_pretrained("bert-base-cased", use_fast=True), BertTokenizerFast) | 1 |
Python | Python | fix parsing file that contains multi byte char | a179d84afe1c399bc607ff6330d877527ea6a6b2 | <ide><path>airflow/models.py
<ide> def process_file(self, filepath, only_if_updated=True, safe_mode=True):
<ide>
<ide> if safe_mode and os.path.isfile(filepath):
<ide> # Skip file if no obvious references to airflow or DAG are found.
<del> with open(filepath, 'r') as f:
<add> with open(filepath, 'rb') as f:
<ide> content = f.read()
<del> if not all([s in content for s in ('DAG', 'airflow')]):
<add> if not all([s in content for s in (b'DAG', b'airflow')]):
<ide> return found_dags
<ide>
<ide> if (not only_if_updated or
<ide><path>tests/models.py
<add># -*- coding: utf-8 -*-
<ide> from __future__ import absolute_import
<ide> from __future__ import division
<ide> from __future__ import print_function
<ide> def test_get_non_existing_dag(self):
<ide> non_existing_dag_id = "non_existing_dag_id"
<ide> assert dagbag.get_dag(non_existing_dag_id) is None
<ide>
<add> def test_process_file_that_contains_multi_bytes_char(self):
<add> """
<add> test that we're able to parse file that contains multi-byte char
<add> """
<add> from tempfile import NamedTemporaryFile
<add> f = NamedTemporaryFile()
<add> f.write('\u3042'.encode('utf8')) # write multi-byte char (hiragana)
<add> f.flush()
<add>
<add> dagbag = models.DagBag(include_examples=True)
<add> assert dagbag.process_file(f.name) == []
<add>
<ide>
<ide> class TaskInstanceTest(unittest.TestCase):
<ide> | 2 |
Javascript | Javascript | fix bugs related to unmounting error boundaries | 5cdd744e0610c914aa34ec1eb5a566006d693cae | <ide><path>src/renderers/shared/fiber/ReactFiberScheduler.js
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> // The loop stops once the children have unmounted and error lifecycles are
<ide> // called. Then we return to the regular flow.
<ide>
<del> if (capturedErrors !== null && capturedErrors.size > 0) {
<add> if (
<add> capturedErrors !== null &&
<add> capturedErrors.size > 0 &&
<add> nextPriorityLevel === TaskPriority
<add> ) {
<ide> while (nextUnitOfWork !== null) {
<ide> if (hasCapturedError(nextUnitOfWork)) {
<ide> // Use a forked version of performUnitOfWork
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> commitAllWork(pendingCommit);
<ide> priorityContext = nextPriorityLevel;
<ide>
<del> if (capturedErrors === null || capturedErrors.size === 0) {
<add> if (
<add> capturedErrors === null ||
<add> capturedErrors.size === 0 ||
<add> nextPriorityLevel !== TaskPriority
<add> ) {
<ide> // There are no more unhandled errors. We can exit this special
<ide> // work loop. If there's still additional work, we'll perform it
<ide> // using one of the normal work loops.
<ide> break;
<ide> }
<ide> // The commit phase produced additional errors. Continue working.
<del> invariant(
<del> nextPriorityLevel === TaskPriority,
<del> 'Commit phase errors should be scheduled to recover with task ' +
<del> 'priority. This error is likely caused by a bug in React. ' +
<del> 'Please file an issue.',
<del> );
<ide> }
<ide> }
<ide> }
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> willRetry = true;
<ide> }
<ide> } else if (node.tag === HostRoot) {
<del> // Treat the root like a no-op error boundary.
<add> // Treat the root like a no-op error boundary
<ide> boundary = node;
<ide> }
<ide>
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> }
<ide>
<ide> function scheduleUpdate(fiber: Fiber, priorityLevel: PriorityLevel) {
<add> return scheduleUpdateImpl(fiber, priorityLevel, false);
<add> }
<add>
<add> function scheduleUpdateImpl(
<add> fiber: Fiber,
<add> priorityLevel: PriorityLevel,
<add> isErrorRecovery: boolean,
<add> ) {
<ide> if (__DEV__) {
<ide> recordScheduleUpdate();
<ide> }
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> }
<ide>
<ide> if (__DEV__) {
<del> if (fiber.tag === ClassComponent) {
<add> if (!isErrorRecovery && fiber.tag === ClassComponent) {
<ide> const instance = fiber.stateNode;
<ide> warnAboutInvalidUpdates(instance);
<ide> }
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> }
<ide> } else {
<ide> if (__DEV__) {
<del> if (fiber.tag === ClassComponent) {
<add> if (!isErrorRecovery && fiber.tag === ClassComponent) {
<ide> warnAboutUpdateOnUnmounted(fiber.stateNode);
<ide> }
<ide> }
<ide> module.exports = function<T, P, I, TI, PI, C, CX, PL>(
<ide> }
<ide>
<ide> function scheduleErrorRecovery(fiber: Fiber) {
<del> scheduleUpdate(fiber, TaskPriority);
<add> scheduleUpdateImpl(fiber, TaskPriority, true);
<ide> }
<ide>
<ide> function performWithPriority(priorityLevel: PriorityLevel, fn: Function) {
<ide><path>src/renderers/shared/fiber/__tests__/ReactIncrementalErrorHandling-test.js
<ide> describe('ReactIncrementalErrorHandling', () => {
<ide> }
<ide>
<ide> ReactNoop.flushSync(() => {
<del> ReactNoop.render(
<del> <ErrorBoundary>
<del> Before the storm.
<del> </ErrorBoundary>,
<del> );
<add> ReactNoop.render(<ErrorBoundary>Before the storm.</ErrorBoundary>);
<ide> ReactNoop.render(
<ide> <ErrorBoundary>
<ide> <BrokenRender />
<ide> describe('ReactIncrementalErrorHandling', () => {
<ide> expect(() => {
<ide> ReactNoop.flushSync(() => {
<ide> ReactNoop.render(
<del> <RethrowErrorBoundary>
<del> Before the storm.
<del> </RethrowErrorBoundary>,
<add> <RethrowErrorBoundary>Before the storm.</RethrowErrorBoundary>,
<ide> );
<ide> ReactNoop.render(
<ide> <RethrowErrorBoundary>
<ide> describe('ReactIncrementalErrorHandling', () => {
<ide> expect(ops).toEqual(['Foo']);
<ide> });
<ide>
<add> it('should not attempt to recover an unmounting error boundary', () => {
<add> class Parent extends React.Component {
<add> componentWillUnmount() {
<add> ReactNoop.yield('Parent componentWillUnmount');
<add> }
<add> render() {
<add> return <Boundary />;
<add> }
<add> }
<add>
<add> class Boundary extends React.Component {
<add> componentDidCatch(e) {
<add> ReactNoop.yield(`Caught error: ${e.message}`);
<add> }
<add> render() {
<add> return <ThrowsOnUnmount />;
<add> }
<add> }
<add>
<add> class ThrowsOnUnmount extends React.Component {
<add> componentWillUnmount() {
<add> ReactNoop.yield('ThrowsOnUnmount componentWillUnmount');
<add> throw new Error('unmount error');
<add> }
<add> render() {
<add> return null;
<add> }
<add> }
<add>
<add> ReactNoop.render(<Parent />);
<add> ReactNoop.flush();
<add> ReactNoop.render(null);
<add> expect(ReactNoop.flush()).toEqual([
<add> // Parent unmounts before the error is thrown.
<add> 'Parent componentWillUnmount',
<add> 'ThrowsOnUnmount componentWillUnmount',
<add> ]);
<add> ReactNoop.render(<Parent />);
<add> });
<add>
<add> it('can unmount an error boundary before it is handled', () => {
<add> let parent;
<add>
<add> class Parent extends React.Component {
<add> state = {step: 0};
<add> render() {
<add> parent = this;
<add> return this.state.step === 0 ? <Boundary /> : null;
<add> }
<add> }
<add>
<add> class Boundary extends React.Component {
<add> componentDidCatch() {}
<add> render() {
<add> return <Child />;
<add> }
<add> }
<add>
<add> class Child extends React.Component {
<add> componentDidUpdate() {
<add> parent.setState({step: 1});
<add> throw new Error('update error');
<add> }
<add> render() {
<add> return null;
<add> }
<add> }
<add>
<add> ReactNoop.render(<Parent />);
<add> ReactNoop.flush();
<add>
<add> ReactNoop.flushSync(() => {
<add> ReactNoop.render(<Parent />);
<add> });
<add> });
<add>
<ide> it('continues work on other roots despite caught errors', () => {
<ide> class ErrorBoundary extends React.Component {
<ide> state = {error: null};
<ide> describe('ReactIncrementalErrorHandling', () => {
<ide> }
<ide>
<ide> try {
<del> ReactNoop.render(<div><span><ErrorThrowingComponent /></span></div>);
<add> ReactNoop.render(
<add> <div>
<add> <span>
<add> <ErrorThrowingComponent />
<add> </span>
<add> </div>,
<add> );
<ide> ReactNoop.flushDeferredPri();
<ide> } catch (error) {}
<ide>
<ide> describe('ReactIncrementalErrorHandling', () => {
<ide> }
<ide>
<ide> try {
<del> ReactNoop.render(<div><span><ErrorThrowingComponent /></span></div>);
<add> ReactNoop.render(
<add> <div>
<add> <span>
<add> <ErrorThrowingComponent />
<add> </span>
<add> </div>,
<add> );
<ide> ReactNoop.flushDeferredPri();
<ide> } catch (error) {}
<ide>
<ide> describe('ReactIncrementalErrorHandling', () => {
<ide> );
<ide>
<ide> try {
<del> ReactNoop.render(<div><span><ErrorThrowingComponent /></span></div>);
<add> ReactNoop.render(
<add> <div>
<add> <span>
<add> <ErrorThrowingComponent />
<add> </span>
<add> </div>,
<add> );
<ide> ReactNoop.flushDeferredPri();
<ide> } catch (error) {}
<ide> | 2 |
Javascript | Javascript | fix lint warnings | de34ca0b649b609fcb8d2cab569239bed42e5af8 | <ide><path>src/filters.js
<ide> angularFilter.number = function(number, fractionSize) {
<ide> }
<ide>
<ide> function formatNumber(number, pattern, groupSep, decimalSep, fractionSize) {
<del> var isNegative = number < 0,
<add> var isNegative = number < 0;
<ide> number = Math.abs(number);
<ide> var numStr = number + '',
<ide> formatedText = '',
<ide> parts = [];
<ide>
<ide> if (numStr.indexOf('e') !== -1) {
<del> var formatedText = numStr;
<add> formatedText = numStr;
<ide> } else {
<ide> var fractionLen = (numStr.split(DECIMAL_SEP)[1] || '').length;
<ide> | 1 |
PHP | PHP | apply fixes from styleci | e4b54ba7d3105e8966bade0080c8d9afe556376e | <ide><path>src/Illuminate/Queue/QueueManager.php
<ide> public function connection($name = null)
<ide> *
<ide> * @param string $name
<ide> * @return \Illuminate\Contracts\Queue\Queue
<del> *
<add> *
<ide> * @throws \InvalidArgumentException
<ide> */
<ide> protected function resolve($name) | 1 |
Javascript | Javascript | implement setborderstyle for annotations | 9ba4f74370a3463e94897b46ed4770bb032cfef1 | <ide><path>src/core/annotation.js
<ide> */
<ide> /* globals PDFJS, Util, isDict, isName, stringToPDFString, warn, Dict, Stream,
<ide> stringToBytes, assert, Promise, isArray, ObjectLoader, OperatorList,
<del> isValidUrl, OPS, createPromiseCapability, AnnotationType,
<del> stringToUTF8String */
<add> isValidUrl, OPS, createPromiseCapability, AnnotationType,
<add> stringToUTF8String, AnnotationBorderStyleType */
<ide>
<ide> 'use strict';
<ide>
<ide> var Annotation = (function AnnotationClosure() {
<ide> }
<ide>
<ide> Annotation.prototype = {
<add> /**
<add> * Set the border style (as AnnotationBorderStyle object).
<add> *
<add> * @public
<add> * @memberof Annotation
<add> * @param {Dict} borderStyle - The border style dictionary
<add> */
<add> setBorderStyle: function Annotation_setBorderStyle(borderStyle) {
<add> if (!isDict(borderStyle)) {
<add> return;
<add> }
<add> if (borderStyle.has('BS')) {
<add> var dict = borderStyle.get('BS');
<add> var dictType;
<add>
<add> if (!dict.has('Type') || (isName(dictType = dict.get('Type')) &&
<add> dictType.name === 'Border')) {
<add> this.borderStyle.setWidth(dict.get('W'));
<add> this.borderStyle.setStyle(dict.get('S'));
<add> this.borderStyle.setDashArray(dict.get('D'));
<add> }
<add> } else if (borderStyle.has('Border')) {
<add> var array = borderStyle.get('Border');
<add> if (isArray(array) && array.length >= 3) {
<add> this.borderStyle.setHorizontalCornerRadius(array[0]);
<add> this.borderStyle.setVerticalCornerRadius(array[1]);
<add> this.borderStyle.setWidth(array[2]);
<add> this.borderStyle.setStyle('S');
<add>
<add> if (array.length === 4) { // Dash array available
<add> this.borderStyle.setDashArray(array[3]);
<add> }
<add> }
<add> }
<add> },
<ide>
<ide> getData: function Annotation_getData() {
<ide> return this.data; | 1 |
Ruby | Ruby | fix method alias | 5c17405982b3b600f6788a13b8ee2dbe5b054bd0 | <ide><path>Library/Homebrew/os/linux/hardware.rb
<ide> def type
<ide> def family
<ide> :dunno
<ide> end
<del> alias_method :intel_family, :cpu_family
<add> alias_method :intel_family, :family
<ide>
<ide> def cores
<ide> `grep -c ^processor /proc/cpuinfo`.to_i | 1 |
Javascript | Javascript | use texture loader to load textures in test | 0bfe511c9102c2cb8e6c2b334e210e017a2c8489 | <ide><path>test/unit/extras/ImageUtils.test.js
<ide> QUnit.test( "test load handler", function( assert ) {
<ide>
<ide> var done = assert.async();
<ide>
<del> THREE.ImageUtils.loadTexture( good_url, undefined, function ( tex ) {
<add> new THREE.TextureLoader().load(good_url, function ( tex ) {
<ide>
<ide> assert.success( "load handler should be called" );
<ide> assert.ok( tex, "texture is defined" );
<ide> assert.ok( tex.image, "texture.image is defined" );
<ide> done();
<ide>
<del> }, function () {
<add> }, undefined, function () {
<ide>
<ide> assert.fail( "error handler should not be called" );
<ide> done();
<ide>
<ide> });
<del>
<ide> });
<ide>
<ide>
<ide> QUnit.test( "test error handler", function( assert ) {
<ide>
<ide> var done = assert.async();
<ide>
<del> THREE.ImageUtils.loadTexture( bad_url, undefined, function () {
<add> new THREE.TextureLoader().load(bad_url, function () {
<ide>
<ide> assert.fail( "load handler should not be called" );
<ide> done();
<ide>
<del> }, function ( event ) {
<add> },
<add>
<add> undefined,
<add>
<add> function ( event ) {
<ide>
<ide> assert.success( "error handler should be called" );
<ide> assert.ok( event.type === 'error', "should have error event" );
<ide> QUnit.test( "test cached texture", function( assert ) {
<ide>
<ide> var done = assert.async();
<ide>
<del> var rtex1 = THREE.ImageUtils.loadTexture( good_url, undefined, function ( tex1 ) {
<add> var rtex1 = new THREE.TextureLoader().load(good_url, function ( tex1 ) {
<ide>
<ide> assert.ok( rtex1.image !== undefined, "texture 1 image is loaded" );
<ide> assert.equal( rtex1, tex1, "texture 1 callback is equal to return" );
<ide>
<del> var rtex2 = THREE.ImageUtils.loadTexture( good_url, undefined, function ( tex2 ) {
<add> var rtex2 = new THREE.TextureLoader().load(good_url, function ( tex2 ) {
<ide>
<ide> assert.ok( rtex2 !== undefined, "cached callback is async" );
<ide> assert.ok( rtex2.image !== undefined, "texture 2 image is loaded" );
<ide> QUnit.test( "test cached texture", function( assert ) {
<ide> assert.ok( rtex1.image === undefined, "texture 1 image is not loaded" );
<ide>
<ide> });
<del> | 1 |
Javascript | Javascript | update regexp grouping cheat-sheat | 8ace8073fd1ad2fab8291246fe262d11b8c0f211 | <ide><path>src/ng/directive/select.js
<ide> var ngOptionsMinErr = minErr('ngOptions');
<ide> var ngOptionsDirective = valueFn({ terminal: true });
<ide> // jshint maxlen: false
<ide> var selectDirective = ['$compile', '$parse', function($compile, $parse) {
<del> //0000111110000000000022220000000000000000000000333300000000000000444444444444444000000000555555555555555000000066666666666666600000000000000007777000000000000000000088888
<add> //000011111111110000000000022222222220000000000000000000003333333333000000000000004444444444444440000000005555555555555550000000666666666666666000000000000000777777777700000000000000000008888888888
<ide> var NG_OPTIONS_REGEXP = /^\s*([\s\S]+?)(?:\s+as\s+([\s\S]+?))?(?:\s+group\s+by\s+([\s\S]+?))?\s+for\s+(?:([\$\w][\$\w]*)|(?:\(\s*([\$\w][\$\w]*)\s*,\s*([\$\w][\$\w]*)\s*\)))\s+in\s+([\s\S]+?)(?:\s+track\s+by\s+([\s\S]+?))?$/,
<ide> nullModelCtrl = {$setViewValue: noop};
<ide> // jshint maxlen: 100 | 1 |
PHP | PHP | fix param docblock. | b6339ce45191be4c70b59ec44a1a915f0f662925 | <ide><path>src/Illuminate/Session/DatabaseSessionHandler.php
<ide> public function read($sessionId)
<ide> /**
<ide> * Determine if the session is expired.
<ide> *
<del> * @param StdClass $session
<add> * @param \StdClass $session
<ide> * @return bool
<ide> */
<ide> protected function expired($session) | 1 |
Python | Python | replace tab escapes with four spaces | 11818a2eabb299b13d9920bdad2518772c4edce0 | <ide><path>numpy/f2py/cfuncs.py
<ide> #ifdef DEBUGCFUNCS
<ide> #define CFUNCSMESS(mess) fprintf(stderr,\"debug-capi:\"mess);
<ide> #define CFUNCSMESSPY(mess,obj) CFUNCSMESS(mess) \\
<del>\tPyObject_Print((PyObject *)obj,stderr,Py_PRINT_RAW);\\
<del>\tfprintf(stderr,\"\\n\");
<add> PyObject_Print((PyObject *)obj,stderr,Py_PRINT_RAW);\\
<add> fprintf(stderr,\"\\n\");
<ide> #else
<ide> #define CFUNCSMESS(mess)
<ide> #define CFUNCSMESSPY(mess,obj)
<ide> """
<ide> cppmacros['SWAP'] = """\
<ide> #define SWAP(a,b,t) {\\
<del>\tt *c;\\
<del>\tc = a;\\
<del>\ta = b;\\
<del>\tb = c;}
<add> t *c;\\
<add> c = a;\\
<add> a = b;\\
<add> b = c;}
<ide> """
<ide> # cppmacros['ISCONTIGUOUS']='#define ISCONTIGUOUS(m) (PyArray_FLAGS(m) &
<ide> # NPY_ARRAY_C_CONTIGUOUS)'
<ide> cppmacros['PRINTPYOBJERR'] = """\
<ide> #define PRINTPYOBJERR(obj)\\
<del>\tfprintf(stderr,\"#modulename#.error is related to \");\\
<del>\tPyObject_Print((PyObject *)obj,stderr,Py_PRINT_RAW);\\
<del>\tfprintf(stderr,\"\\n\");
<add> fprintf(stderr,\"#modulename#.error is related to \");\\
<add> PyObject_Print((PyObject *)obj,stderr,Py_PRINT_RAW);\\
<add> fprintf(stderr,\"\\n\");
<ide> """
<ide> cppmacros['MINMAX'] = """\
<ide> #ifndef max
<ide> """
<ide> # cppmacros['NUMFROMARROBJ']="""\
<ide> # define NUMFROMARROBJ(typenum,ctype) \\
<del># \tif (PyArray_Check(obj)) arr = (PyArrayObject *)obj;\\
<del># \telse arr = (PyArrayObject *)PyArray_ContiguousFromObject(obj,typenum,0,0);\\
<del># \tif (arr) {\\
<del># \t\tif (PyArray_TYPE(arr)==NPY_OBJECT) {\\
<del># \t\t\tif (!ctype ## _from_pyobj(v,(PyArray_DESCR(arr)->getitem)(PyArray_DATA(arr)),\"\"))\\
<del># \t\t\tgoto capi_fail;\\
<del># \t\t} else {\\
<del># \t\t\t(PyArray_DESCR(arr)->cast[typenum])(PyArray_DATA(arr),1,(char*)v,1,1);\\
<del># \t\t}\\
<del># \t\tif ((PyObject *)arr != obj) { Py_DECREF(arr); }\\
<del># \t\treturn 1;\\
<del># \t}
<add># if (PyArray_Check(obj)) arr = (PyArrayObject *)obj;\\
<add># else arr = (PyArrayObject *)PyArray_ContiguousFromObject(obj,typenum,0,0);\\
<add># if (arr) {\\
<add># if (PyArray_TYPE(arr)==NPY_OBJECT) {\\
<add># if (!ctype ## _from_pyobj(v,(PyArray_DESCR(arr)->getitem)(PyArray_DATA(arr)),\"\"))\\
<add># goto capi_fail;\\
<add># } else {\\
<add># (PyArray_DESCR(arr)->cast[typenum])(PyArray_DATA(arr),1,(char*)v,1,1);\\
<add># }\\
<add># if ((PyObject *)arr != obj) { Py_DECREF(arr); }\\
<add># return 1;\\
<add># }
<ide> # """
<ide> # XXX: Note that CNUMFROMARROBJ is identical with NUMFROMARROBJ
<ide> # cppmacros['CNUMFROMARROBJ']="""\
<ide> # define CNUMFROMARROBJ(typenum,ctype) \\
<del># \tif (PyArray_Check(obj)) arr = (PyArrayObject *)obj;\\
<del># \telse arr = (PyArrayObject *)PyArray_ContiguousFromObject(obj,typenum,0,0);\\
<del># \tif (arr) {\\
<del># \t\tif (PyArray_TYPE(arr)==NPY_OBJECT) {\\
<del># \t\t\tif (!ctype ## _from_pyobj(v,(PyArray_DESCR(arr)->getitem)(PyArray_DATA(arr)),\"\"))\\
<del># \t\t\tgoto capi_fail;\\
<del># \t\t} else {\\
<del># \t\t\t(PyArray_DESCR(arr)->cast[typenum])((void *)(PyArray_DATA(arr)),1,(void *)(v),1,1);\\
<del># \t\t}\\
<del># \t\tif ((PyObject *)arr != obj) { Py_DECREF(arr); }\\
<del># \t\treturn 1;\\
<del># \t}
<add># if (PyArray_Check(obj)) arr = (PyArrayObject *)obj;\\
<add># else arr = (PyArrayObject *)PyArray_ContiguousFromObject(obj,typenum,0,0);\\
<add># if (arr) {\\
<add># if (PyArray_TYPE(arr)==NPY_OBJECT) {\\
<add># if (!ctype ## _from_pyobj(v,(PyArray_DESCR(arr)->getitem)(PyArray_DATA(arr)),\"\"))\\
<add># goto capi_fail;\\
<add># } else {\\
<add># (PyArray_DESCR(arr)->cast[typenum])((void *)(PyArray_DATA(arr)),1,(void *)(v),1,1);\\
<add># }\\
<add># if ((PyObject *)arr != obj) { Py_DECREF(arr); }\\
<add># return 1;\\
<add># }
<ide> # """
<ide>
<ide>
<ide> needs['GETSTRFROMPYTUPLE'] = ['STRINGCOPYN', 'PRINTPYOBJERR']
<ide> cppmacros['GETSTRFROMPYTUPLE'] = """\
<ide> #define GETSTRFROMPYTUPLE(tuple,index,str,len) {\\
<del>\t\tPyObject *rv_cb_str = PyTuple_GetItem((tuple),(index));\\
<del>\t\tif (rv_cb_str == NULL)\\
<del>\t\t\tgoto capi_fail;\\
<del>\t\tif (PyString_Check(rv_cb_str)) {\\
<del>\t\t\tstr[len-1]='\\0';\\
<del>\t\t\tSTRINGCOPYN((str),PyString_AS_STRING((PyStringObject*)rv_cb_str),(len));\\
<del>\t\t} else {\\
<del>\t\t\tPRINTPYOBJERR(rv_cb_str);\\
<del>\t\t\tPyErr_SetString(#modulename#_error,\"string object expected\");\\
<del>\t\t\tgoto capi_fail;\\
<del>\t\t}\\
<del>\t}
<add> PyObject *rv_cb_str = PyTuple_GetItem((tuple),(index));\\
<add> if (rv_cb_str == NULL)\\
<add> goto capi_fail;\\
<add> if (PyString_Check(rv_cb_str)) {\\
<add> str[len-1]='\\0';\\
<add> STRINGCOPYN((str),PyString_AS_STRING((PyStringObject*)rv_cb_str),(len));\\
<add> } else {\\
<add> PRINTPYOBJERR(rv_cb_str);\\
<add> PyErr_SetString(#modulename#_error,\"string object expected\");\\
<add> goto capi_fail;\\
<add> }\\
<add> }
<ide> """
<ide> cppmacros['GETSCALARFROMPYTUPLE'] = """\
<ide> #define GETSCALARFROMPYTUPLE(tuple,index,var,ctype,mess) {\\
<del>\t\tif ((capi_tmp = PyTuple_GetItem((tuple),(index)))==NULL) goto capi_fail;\\
<del>\t\tif (!(ctype ## _from_pyobj((var),capi_tmp,mess)))\\
<del>\t\t\tgoto capi_fail;\\
<del>\t}
<add> if ((capi_tmp = PyTuple_GetItem((tuple),(index)))==NULL) goto capi_fail;\\
<add> if (!(ctype ## _from_pyobj((var),capi_tmp,mess)))\\
<add> goto capi_fail;\\
<add> }
<ide> """
<ide>
<ide> cppmacros['FAILNULL'] = """\\
<ide> """
<ide> cppmacros['STRINGMALLOC'] = """\
<ide> #define STRINGMALLOC(str,len)\\
<del>\tif ((str = (string)malloc(sizeof(char)*(len+1))) == NULL) {\\
<del>\t\tPyErr_SetString(PyExc_MemoryError, \"out of memory\");\\
<del>\t\tgoto capi_fail;\\
<del>\t} else {\\
<del>\t\t(str)[len] = '\\0';\\
<del>\t}
<add> if ((str = (string)malloc(sizeof(char)*(len+1))) == NULL) {\\
<add> PyErr_SetString(PyExc_MemoryError, \"out of memory\");\\
<add> goto capi_fail;\\
<add> } else {\\
<add> (str)[len] = '\\0';\\
<add> }
<ide> """
<ide> cppmacros['STRINGFREE'] = """\
<ide> #define STRINGFREE(str) do {if (!(str == NULL)) free(str);} while (0)
<ide> """
<ide> cppmacros['CHECKGENERIC'] = """\
<ide> #define CHECKGENERIC(check,tcheck,name) \\
<del>\tif (!(check)) {\\
<del>\t\tPyErr_SetString(#modulename#_error,\"(\"tcheck\") failed for \"name);\\
<del>\t\t/*goto capi_fail;*/\\
<del>\t} else """
<add> if (!(check)) {\\
<add> PyErr_SetString(#modulename#_error,\"(\"tcheck\") failed for \"name);\\
<add> /*goto capi_fail;*/\\
<add> } else """
<ide> cppmacros['CHECKARRAY'] = """\
<ide> #define CHECKARRAY(check,tcheck,name) \\
<del>\tif (!(check)) {\\
<del>\t\tPyErr_SetString(#modulename#_error,\"(\"tcheck\") failed for \"name);\\
<del>\t\t/*goto capi_fail;*/\\
<del>\t} else """
<add> if (!(check)) {\\
<add> PyErr_SetString(#modulename#_error,\"(\"tcheck\") failed for \"name);\\
<add> /*goto capi_fail;*/\\
<add> } else """
<ide> cppmacros['CHECKSTRING'] = """\
<ide> #define CHECKSTRING(check,tcheck,name,show,var)\\
<del>\tif (!(check)) {\\
<del>\t\tchar errstring[256];\\
<del>\t\tsprintf(errstring, \"%s: \"show, \"(\"tcheck\") failed for \"name, slen(var), var);\\
<del>\t\tPyErr_SetString(#modulename#_error, errstring);\\
<del>\t\t/*goto capi_fail;*/\\
<del>\t} else """
<add> if (!(check)) {\\
<add> char errstring[256];\\
<add> sprintf(errstring, \"%s: \"show, \"(\"tcheck\") failed for \"name, slen(var), var);\\
<add> PyErr_SetString(#modulename#_error, errstring);\\
<add> /*goto capi_fail;*/\\
<add> } else """
<ide> cppmacros['CHECKSCALAR'] = """\
<ide> #define CHECKSCALAR(check,tcheck,name,show,var)\\
<del>\tif (!(check)) {\\
<del>\t\tchar errstring[256];\\
<del>\t\tsprintf(errstring, \"%s: \"show, \"(\"tcheck\") failed for \"name, var);\\
<del>\t\tPyErr_SetString(#modulename#_error,errstring);\\
<del>\t\t/*goto capi_fail;*/\\
<del>\t} else """
<add> if (!(check)) {\\
<add> char errstring[256];\\
<add> sprintf(errstring, \"%s: \"show, \"(\"tcheck\") failed for \"name, var);\\
<add> PyErr_SetString(#modulename#_error,errstring);\\
<add> /*goto capi_fail;*/\\
<add> } else """
<ide> # cppmacros['CHECKDIMS']="""\
<ide> # define CHECKDIMS(dims,rank) \\
<del># \tfor (int i=0;i<(rank);i++)\\
<del># \t\tif (dims[i]<0) {\\
<del># \t\t\tfprintf(stderr,\"Unspecified array argument requires a complete dimension specification.\\n\");\\
<del># \t\t\tgoto capi_fail;\\
<del># \t\t}
<add># for (int i=0;i<(rank);i++)\\
<add># if (dims[i]<0) {\\
<add># fprintf(stderr,\"Unspecified array argument requires a complete dimension specification.\\n\");\\
<add># goto capi_fail;\\
<add># }
<ide> # """
<ide> cppmacros[
<ide> 'ARRSIZE'] = '#define ARRSIZE(dims,rank) (_PyArray_multiply_list(dims,rank))'
<ide>
<ide> cfuncs['calcarrindex'] = """\
<ide> static int calcarrindex(int *i,PyArrayObject *arr) {
<del>\tint k,ii = i[0];
<del>\tfor (k=1; k < PyArray_NDIM(arr); k++)
<del>\t\tii += (ii*(PyArray_DIM(arr,k) - 1)+i[k]); /* assuming contiguous arr */
<del>\treturn ii;
<add> int k,ii = i[0];
<add> for (k=1; k < PyArray_NDIM(arr); k++)
<add> ii += (ii*(PyArray_DIM(arr,k) - 1)+i[k]); /* assuming contiguous arr */
<add> return ii;
<ide> }"""
<ide> cfuncs['calcarrindextr'] = """\
<ide> static int calcarrindextr(int *i,PyArrayObject *arr) {
<del>\tint k,ii = i[PyArray_NDIM(arr)-1];
<del>\tfor (k=1; k < PyArray_NDIM(arr); k++)
<del>\t\tii += (ii*(PyArray_DIM(arr,PyArray_NDIM(arr)-k-1) - 1)+i[PyArray_NDIM(arr)-k-1]); /* assuming contiguous arr */
<del>\treturn ii;
<add> int k,ii = i[PyArray_NDIM(arr)-1];
<add> for (k=1; k < PyArray_NDIM(arr); k++)
<add> ii += (ii*(PyArray_DIM(arr,PyArray_NDIM(arr)-k-1) - 1)+i[PyArray_NDIM(arr)-k-1]); /* assuming contiguous arr */
<add> return ii;
<ide> }"""
<ide> cfuncs['forcomb'] = """\
<ide> static struct { int nd;npy_intp *d;int *i,*i_tr,tr; } forcombcache;
<ide> needs['try_pyarr_from_string'] = ['STRINGCOPYN', 'PRINTPYOBJERR', 'string']
<ide> cfuncs['try_pyarr_from_string'] = """\
<ide> static int try_pyarr_from_string(PyObject *obj,const string str) {
<del>\tPyArrayObject *arr = NULL;
<del>\tif (PyArray_Check(obj) && (!((arr = (PyArrayObject *)obj) == NULL)))
<del>\t\t{ STRINGCOPYN(PyArray_DATA(arr),str,PyArray_NBYTES(arr)); }
<del>\treturn 1;
<add> PyArrayObject *arr = NULL;
<add> if (PyArray_Check(obj) && (!((arr = (PyArrayObject *)obj) == NULL)))
<add> { STRINGCOPYN(PyArray_DATA(arr),str,PyArray_NBYTES(arr)); }
<add> return 1;
<ide> capi_fail:
<del>\tPRINTPYOBJERR(obj);
<del>\tPyErr_SetString(#modulename#_error,\"try_pyarr_from_string failed\");
<del>\treturn 0;
<add> PRINTPYOBJERR(obj);
<add> PyErr_SetString(#modulename#_error,\"try_pyarr_from_string failed\");
<add> return 0;
<ide> }
<ide> """
<ide> needs['string_from_pyobj'] = ['string', 'STRINGMALLOC', 'STRINGCOPYN']
<ide> cfuncs['string_from_pyobj'] = """\
<ide> static int string_from_pyobj(string *str,int *len,const string inistr,PyObject *obj,const char *errmess) {
<del>\tPyArrayObject *arr = NULL;
<del>\tPyObject *tmp = NULL;
<add> PyArrayObject *arr = NULL;
<add> PyObject *tmp = NULL;
<ide> #ifdef DEBUGCFUNCS
<ide> fprintf(stderr,\"string_from_pyobj(str='%s',len=%d,inistr='%s',obj=%p)\\n\",(char*)str,*len,(char *)inistr,obj);
<ide> #endif
<del>\tif (obj == Py_None) {
<del>\t\tif (*len == -1)
<del>\t\t\t*len = strlen(inistr); /* Will this cause problems? */
<del>\t\tSTRINGMALLOC(*str,*len);
<del>\t\tSTRINGCOPYN(*str,inistr,*len+1);
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyArray_Check(obj)) {
<del>\t\tif ((arr = (PyArrayObject *)obj) == NULL)
<del>\t\t\tgoto capi_fail;
<del>\t\tif (!ISCONTIGUOUS(arr)) {
<del>\t\t\tPyErr_SetString(PyExc_ValueError,\"array object is non-contiguous.\");
<del>\t\t\tgoto capi_fail;
<del>\t\t}
<del>\t\tif (*len == -1)
<del>\t\t\t*len = (PyArray_ITEMSIZE(arr))*PyArray_SIZE(arr);
<del>\t\tSTRINGMALLOC(*str,*len);
<del>\t\tSTRINGCOPYN(*str,PyArray_DATA(arr),*len+1);
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyString_Check(obj)) {
<del>\t\ttmp = obj;
<del>\t\tPy_INCREF(tmp);
<del>\t}
<add> if (obj == Py_None) {
<add> if (*len == -1)
<add> *len = strlen(inistr); /* Will this cause problems? */
<add> STRINGMALLOC(*str,*len);
<add> STRINGCOPYN(*str,inistr,*len+1);
<add> return 1;
<add> }
<add> if (PyArray_Check(obj)) {
<add> if ((arr = (PyArrayObject *)obj) == NULL)
<add> goto capi_fail;
<add> if (!ISCONTIGUOUS(arr)) {
<add> PyErr_SetString(PyExc_ValueError,\"array object is non-contiguous.\");
<add> goto capi_fail;
<add> }
<add> if (*len == -1)
<add> *len = (PyArray_ITEMSIZE(arr))*PyArray_SIZE(arr);
<add> STRINGMALLOC(*str,*len);
<add> STRINGCOPYN(*str,PyArray_DATA(arr),*len+1);
<add> return 1;
<add> }
<add> if (PyString_Check(obj)) {
<add> tmp = obj;
<add> Py_INCREF(tmp);
<add> }
<ide> #if PY_VERSION_HEX >= 0x03000000
<del>\telse if (PyUnicode_Check(obj)) {
<del>\t\ttmp = PyUnicode_AsASCIIString(obj);
<del>\t}
<del>\telse {
<del>\t\tPyObject *tmp2;
<del>\t\ttmp2 = PyObject_Str(obj);
<del>\t\tif (tmp2) {
<del>\t\t\ttmp = PyUnicode_AsASCIIString(tmp2);
<del>\t\t\tPy_DECREF(tmp2);
<del>\t\t}
<del>\t\telse {
<del>\t\t\ttmp = NULL;
<del>\t\t}
<del>\t}
<add> else if (PyUnicode_Check(obj)) {
<add> tmp = PyUnicode_AsASCIIString(obj);
<add> }
<add> else {
<add> PyObject *tmp2;
<add> tmp2 = PyObject_Str(obj);
<add> if (tmp2) {
<add> tmp = PyUnicode_AsASCIIString(tmp2);
<add> Py_DECREF(tmp2);
<add> }
<add> else {
<add> tmp = NULL;
<add> }
<add> }
<ide> #else
<del>\telse {
<del>\t\ttmp = PyObject_Str(obj);
<del>\t}
<add> else {
<add> tmp = PyObject_Str(obj);
<add> }
<ide> #endif
<del>\tif (tmp == NULL) goto capi_fail;
<del>\tif (*len == -1)
<del>\t\t*len = PyString_GET_SIZE(tmp);
<del>\tSTRINGMALLOC(*str,*len);
<del>\tSTRINGCOPYN(*str,PyString_AS_STRING(tmp),*len+1);
<del>\tPy_DECREF(tmp);
<del>\treturn 1;
<add> if (tmp == NULL) goto capi_fail;
<add> if (*len == -1)
<add> *len = PyString_GET_SIZE(tmp);
<add> STRINGMALLOC(*str,*len);
<add> STRINGCOPYN(*str,PyString_AS_STRING(tmp),*len+1);
<add> Py_DECREF(tmp);
<add> return 1;
<ide> capi_fail:
<del>\tPy_XDECREF(tmp);
<del>\t{
<del>\t\tPyObject* err = PyErr_Occurred();
<del>\t\tif (err==NULL) err = #modulename#_error;
<del>\t\tPyErr_SetString(err,errmess);
<del>\t}
<del>\treturn 0;
<add> Py_XDECREF(tmp);
<add> {
<add> PyObject* err = PyErr_Occurred();
<add> if (err==NULL) err = #modulename#_error;
<add> PyErr_SetString(err,errmess);
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['char_from_pyobj'] = ['int_from_pyobj']
<ide> cfuncs['char_from_pyobj'] = """\
<ide> static int char_from_pyobj(char* v,PyObject *obj,const char *errmess) {
<del>\tint i=0;
<del>\tif (int_from_pyobj(&i,obj,errmess)) {
<del>\t\t*v = (char)i;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> int i=0;
<add> if (int_from_pyobj(&i,obj,errmess)) {
<add> *v = (char)i;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['signed_char_from_pyobj'] = ['int_from_pyobj', 'signed_char']
<ide> cfuncs['signed_char_from_pyobj'] = """\
<ide> static int signed_char_from_pyobj(signed_char* v,PyObject *obj,const char *errmess) {
<del>\tint i=0;
<del>\tif (int_from_pyobj(&i,obj,errmess)) {
<del>\t\t*v = (signed_char)i;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> int i=0;
<add> if (int_from_pyobj(&i,obj,errmess)) {
<add> *v = (signed_char)i;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['short_from_pyobj'] = ['int_from_pyobj']
<ide> cfuncs['short_from_pyobj'] = """\
<ide> static int short_from_pyobj(short* v,PyObject *obj,const char *errmess) {
<del>\tint i=0;
<del>\tif (int_from_pyobj(&i,obj,errmess)) {
<del>\t\t*v = (short)i;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> int i=0;
<add> if (int_from_pyobj(&i,obj,errmess)) {
<add> *v = (short)i;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> cfuncs['int_from_pyobj'] = """\
<ide> static int int_from_pyobj(int* v,PyObject *obj,const char *errmess) {
<del>\tPyObject* tmp = NULL;
<del>\tif (PyInt_Check(obj)) {
<del>\t\t*v = (int)PyInt_AS_LONG(obj);
<del>\t\treturn 1;
<del>\t}
<del>\ttmp = PyNumber_Int(obj);
<del>\tif (tmp) {
<del>\t\t*v = PyInt_AS_LONG(tmp);
<del>\t\tPy_DECREF(tmp);
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyComplex_Check(obj))
<del>\t\ttmp = PyObject_GetAttrString(obj,\"real\");
<del>\telse if (PyString_Check(obj) || PyUnicode_Check(obj))
<del>\t\t/*pass*/;
<del>\telse if (PySequence_Check(obj))
<del>\t\ttmp = PySequence_GetItem(obj,0);
<del>\tif (tmp) {
<del>\t\tPyErr_Clear();
<del>\t\tif (int_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<del>\t\tPy_DECREF(tmp);
<del>\t}
<del>\t{
<del>\t\tPyObject* err = PyErr_Occurred();
<del>\t\tif (err==NULL) err = #modulename#_error;
<del>\t\tPyErr_SetString(err,errmess);
<del>\t}
<del>\treturn 0;
<add> PyObject* tmp = NULL;
<add> if (PyInt_Check(obj)) {
<add> *v = (int)PyInt_AS_LONG(obj);
<add> return 1;
<add> }
<add> tmp = PyNumber_Int(obj);
<add> if (tmp) {
<add> *v = PyInt_AS_LONG(tmp);
<add> Py_DECREF(tmp);
<add> return 1;
<add> }
<add> if (PyComplex_Check(obj))
<add> tmp = PyObject_GetAttrString(obj,\"real\");
<add> else if (PyString_Check(obj) || PyUnicode_Check(obj))
<add> /*pass*/;
<add> else if (PySequence_Check(obj))
<add> tmp = PySequence_GetItem(obj,0);
<add> if (tmp) {
<add> PyErr_Clear();
<add> if (int_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<add> Py_DECREF(tmp);
<add> }
<add> {
<add> PyObject* err = PyErr_Occurred();
<add> if (err==NULL) err = #modulename#_error;
<add> PyErr_SetString(err,errmess);
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> cfuncs['long_from_pyobj'] = """\
<ide> static int long_from_pyobj(long* v,PyObject *obj,const char *errmess) {
<del>\tPyObject* tmp = NULL;
<del>\tif (PyInt_Check(obj)) {
<del>\t\t*v = PyInt_AS_LONG(obj);
<del>\t\treturn 1;
<del>\t}
<del>\ttmp = PyNumber_Int(obj);
<del>\tif (tmp) {
<del>\t\t*v = PyInt_AS_LONG(tmp);
<del>\t\tPy_DECREF(tmp);
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyComplex_Check(obj))
<del>\t\ttmp = PyObject_GetAttrString(obj,\"real\");
<del>\telse if (PyString_Check(obj) || PyUnicode_Check(obj))
<del>\t\t/*pass*/;
<del>\telse if (PySequence_Check(obj))
<del>\t\ttmp = PySequence_GetItem(obj,0);
<del>\tif (tmp) {
<del>\t\tPyErr_Clear();
<del>\t\tif (long_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<del>\t\tPy_DECREF(tmp);
<del>\t}
<del>\t{
<del>\t\tPyObject* err = PyErr_Occurred();
<del>\t\tif (err==NULL) err = #modulename#_error;
<del>\t\tPyErr_SetString(err,errmess);
<del>\t}
<del>\treturn 0;
<add> PyObject* tmp = NULL;
<add> if (PyInt_Check(obj)) {
<add> *v = PyInt_AS_LONG(obj);
<add> return 1;
<add> }
<add> tmp = PyNumber_Int(obj);
<add> if (tmp) {
<add> *v = PyInt_AS_LONG(tmp);
<add> Py_DECREF(tmp);
<add> return 1;
<add> }
<add> if (PyComplex_Check(obj))
<add> tmp = PyObject_GetAttrString(obj,\"real\");
<add> else if (PyString_Check(obj) || PyUnicode_Check(obj))
<add> /*pass*/;
<add> else if (PySequence_Check(obj))
<add> tmp = PySequence_GetItem(obj,0);
<add> if (tmp) {
<add> PyErr_Clear();
<add> if (long_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<add> Py_DECREF(tmp);
<add> }
<add> {
<add> PyObject* err = PyErr_Occurred();
<add> if (err==NULL) err = #modulename#_error;
<add> PyErr_SetString(err,errmess);
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['long_long_from_pyobj'] = ['long_long']
<ide> cfuncs['long_long_from_pyobj'] = """\
<ide> static int long_long_from_pyobj(long_long* v,PyObject *obj,const char *errmess) {
<del>\tPyObject* tmp = NULL;
<del>\tif (PyLong_Check(obj)) {
<del>\t\t*v = PyLong_AsLongLong(obj);
<del>\t\treturn (!PyErr_Occurred());
<del>\t}
<del>\tif (PyInt_Check(obj)) {
<del>\t\t*v = (long_long)PyInt_AS_LONG(obj);
<del>\t\treturn 1;
<del>\t}
<del>\ttmp = PyNumber_Long(obj);
<del>\tif (tmp) {
<del>\t\t*v = PyLong_AsLongLong(tmp);
<del>\t\tPy_DECREF(tmp);
<del>\t\treturn (!PyErr_Occurred());
<del>\t}
<del>\tif (PyComplex_Check(obj))
<del>\t\ttmp = PyObject_GetAttrString(obj,\"real\");
<del>\telse if (PyString_Check(obj) || PyUnicode_Check(obj))
<del>\t\t/*pass*/;
<del>\telse if (PySequence_Check(obj))
<del>\t\ttmp = PySequence_GetItem(obj,0);
<del>\tif (tmp) {
<del>\t\tPyErr_Clear();
<del>\t\tif (long_long_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<del>\t\tPy_DECREF(tmp);
<del>\t}
<del>\t{
<del>\t\tPyObject* err = PyErr_Occurred();
<del>\t\tif (err==NULL) err = #modulename#_error;
<del>\t\tPyErr_SetString(err,errmess);
<del>\t}
<del>\treturn 0;
<add> PyObject* tmp = NULL;
<add> if (PyLong_Check(obj)) {
<add> *v = PyLong_AsLongLong(obj);
<add> return (!PyErr_Occurred());
<add> }
<add> if (PyInt_Check(obj)) {
<add> *v = (long_long)PyInt_AS_LONG(obj);
<add> return 1;
<add> }
<add> tmp = PyNumber_Long(obj);
<add> if (tmp) {
<add> *v = PyLong_AsLongLong(tmp);
<add> Py_DECREF(tmp);
<add> return (!PyErr_Occurred());
<add> }
<add> if (PyComplex_Check(obj))
<add> tmp = PyObject_GetAttrString(obj,\"real\");
<add> else if (PyString_Check(obj) || PyUnicode_Check(obj))
<add> /*pass*/;
<add> else if (PySequence_Check(obj))
<add> tmp = PySequence_GetItem(obj,0);
<add> if (tmp) {
<add> PyErr_Clear();
<add> if (long_long_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<add> Py_DECREF(tmp);
<add> }
<add> {
<add> PyObject* err = PyErr_Occurred();
<add> if (err==NULL) err = #modulename#_error;
<add> PyErr_SetString(err,errmess);
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['long_double_from_pyobj'] = ['double_from_pyobj', 'long_double']
<ide> cfuncs['long_double_from_pyobj'] = """\
<ide> static int long_double_from_pyobj(long_double* v,PyObject *obj,const char *errmess) {
<del>\tdouble d=0;
<del>\tif (PyArray_CheckScalar(obj)){
<del>\t\tif PyArray_IsScalar(obj, LongDouble) {
<del>\t\t\tPyArray_ScalarAsCtype(obj, v);
<del>\t\t\treturn 1;
<del>\t\t}
<del>\t\telse if (PyArray_Check(obj) && PyArray_TYPE(obj)==NPY_LONGDOUBLE) {
<del>\t\t\t(*v) = *((npy_longdouble *)PyArray_DATA(obj));
<del>\t\t\treturn 1;
<del>\t\t}
<del>\t}
<del>\tif (double_from_pyobj(&d,obj,errmess)) {
<del>\t\t*v = (long_double)d;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> double d=0;
<add> if (PyArray_CheckScalar(obj)){
<add> if PyArray_IsScalar(obj, LongDouble) {
<add> PyArray_ScalarAsCtype(obj, v);
<add> return 1;
<add> }
<add> else if (PyArray_Check(obj) && PyArray_TYPE(obj)==NPY_LONGDOUBLE) {
<add> (*v) = *((npy_longdouble *)PyArray_DATA(obj));
<add> return 1;
<add> }
<add> }
<add> if (double_from_pyobj(&d,obj,errmess)) {
<add> *v = (long_double)d;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> cfuncs['double_from_pyobj'] = """\
<ide> static int double_from_pyobj(double* v,PyObject *obj,const char *errmess) {
<del>\tPyObject* tmp = NULL;
<del>\tif (PyFloat_Check(obj)) {
<add> PyObject* tmp = NULL;
<add> if (PyFloat_Check(obj)) {
<ide> #ifdef __sgi
<del>\t\t*v = PyFloat_AsDouble(obj);
<add> *v = PyFloat_AsDouble(obj);
<ide> #else
<del>\t\t*v = PyFloat_AS_DOUBLE(obj);
<add> *v = PyFloat_AS_DOUBLE(obj);
<ide> #endif
<del>\t\treturn 1;
<del>\t}
<del>\ttmp = PyNumber_Float(obj);
<del>\tif (tmp) {
<add> return 1;
<add> }
<add> tmp = PyNumber_Float(obj);
<add> if (tmp) {
<ide> #ifdef __sgi
<del>\t\t*v = PyFloat_AsDouble(tmp);
<add> *v = PyFloat_AsDouble(tmp);
<ide> #else
<del>\t\t*v = PyFloat_AS_DOUBLE(tmp);
<add> *v = PyFloat_AS_DOUBLE(tmp);
<ide> #endif
<del>\t\tPy_DECREF(tmp);
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyComplex_Check(obj))
<del>\t\ttmp = PyObject_GetAttrString(obj,\"real\");
<del>\telse if (PyString_Check(obj) || PyUnicode_Check(obj))
<del>\t\t/*pass*/;
<del>\telse if (PySequence_Check(obj))
<del>\t\ttmp = PySequence_GetItem(obj,0);
<del>\tif (tmp) {
<del>\t\tPyErr_Clear();
<del>\t\tif (double_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<del>\t\tPy_DECREF(tmp);
<del>\t}
<del>\t{
<del>\t\tPyObject* err = PyErr_Occurred();
<del>\t\tif (err==NULL) err = #modulename#_error;
<del>\t\tPyErr_SetString(err,errmess);
<del>\t}
<del>\treturn 0;
<add> Py_DECREF(tmp);
<add> return 1;
<add> }
<add> if (PyComplex_Check(obj))
<add> tmp = PyObject_GetAttrString(obj,\"real\");
<add> else if (PyString_Check(obj) || PyUnicode_Check(obj))
<add> /*pass*/;
<add> else if (PySequence_Check(obj))
<add> tmp = PySequence_GetItem(obj,0);
<add> if (tmp) {
<add> PyErr_Clear();
<add> if (double_from_pyobj(v,tmp,errmess)) {Py_DECREF(tmp); return 1;}
<add> Py_DECREF(tmp);
<add> }
<add> {
<add> PyObject* err = PyErr_Occurred();
<add> if (err==NULL) err = #modulename#_error;
<add> PyErr_SetString(err,errmess);
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['float_from_pyobj'] = ['double_from_pyobj']
<ide> cfuncs['float_from_pyobj'] = """\
<ide> static int float_from_pyobj(float* v,PyObject *obj,const char *errmess) {
<del>\tdouble d=0.0;
<del>\tif (double_from_pyobj(&d,obj,errmess)) {
<del>\t\t*v = (float)d;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> double d=0.0;
<add> if (double_from_pyobj(&d,obj,errmess)) {
<add> *v = (float)d;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['complex_long_double_from_pyobj'] = ['complex_long_double', 'long_double',
<ide> 'complex_double_from_pyobj']
<ide> cfuncs['complex_long_double_from_pyobj'] = """\
<ide> static int complex_long_double_from_pyobj(complex_long_double* v,PyObject *obj,const char *errmess) {
<del>\tcomplex_double cd={0.0,0.0};
<del>\tif (PyArray_CheckScalar(obj)){
<del>\t\tif PyArray_IsScalar(obj, CLongDouble) {
<del>\t\t\tPyArray_ScalarAsCtype(obj, v);
<del>\t\t\treturn 1;
<del>\t\t}
<del>\t\telse if (PyArray_Check(obj) && PyArray_TYPE(obj)==NPY_CLONGDOUBLE) {
<del>\t\t\t(*v).r = ((npy_clongdouble *)PyArray_DATA(obj))->real;
<del>\t\t\t(*v).i = ((npy_clongdouble *)PyArray_DATA(obj))->imag;
<del>\t\t\treturn 1;
<del>\t\t}
<del>\t}
<del>\tif (complex_double_from_pyobj(&cd,obj,errmess)) {
<del>\t\t(*v).r = (long_double)cd.r;
<del>\t\t(*v).i = (long_double)cd.i;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> complex_double cd={0.0,0.0};
<add> if (PyArray_CheckScalar(obj)){
<add> if PyArray_IsScalar(obj, CLongDouble) {
<add> PyArray_ScalarAsCtype(obj, v);
<add> return 1;
<add> }
<add> else if (PyArray_Check(obj) && PyArray_TYPE(obj)==NPY_CLONGDOUBLE) {
<add> (*v).r = ((npy_clongdouble *)PyArray_DATA(obj))->real;
<add> (*v).i = ((npy_clongdouble *)PyArray_DATA(obj))->imag;
<add> return 1;
<add> }
<add> }
<add> if (complex_double_from_pyobj(&cd,obj,errmess)) {
<add> (*v).r = (long_double)cd.r;
<add> (*v).i = (long_double)cd.i;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['complex_double_from_pyobj'] = ['complex_double']
<ide> cfuncs['complex_double_from_pyobj'] = """\
<ide> static int complex_double_from_pyobj(complex_double* v,PyObject *obj,const char *errmess) {
<del>\tPy_complex c;
<del>\tif (PyComplex_Check(obj)) {
<del>\t\tc=PyComplex_AsCComplex(obj);
<del>\t\t(*v).r=c.real, (*v).i=c.imag;
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyArray_IsScalar(obj, ComplexFloating)) {
<del>\t\tif (PyArray_IsScalar(obj, CFloat)) {
<del>\t\t\tnpy_cfloat new;
<del>\t\t\tPyArray_ScalarAsCtype(obj, &new);
<del>\t\t\t(*v).r = (double)new.real;
<del>\t\t\t(*v).i = (double)new.imag;
<del>\t\t}
<del>\t\telse if (PyArray_IsScalar(obj, CLongDouble)) {
<del>\t\t\tnpy_clongdouble new;
<del>\t\t\tPyArray_ScalarAsCtype(obj, &new);
<del>\t\t\t(*v).r = (double)new.real;
<del>\t\t\t(*v).i = (double)new.imag;
<del>\t\t}
<del>\t\telse { /* if (PyArray_IsScalar(obj, CDouble)) */
<del>\t\t\tPyArray_ScalarAsCtype(obj, v);
<del>\t\t}
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyArray_CheckScalar(obj)) { /* 0-dim array or still array scalar */
<del>\t\tPyObject *arr;
<del>\t\tif (PyArray_Check(obj)) {
<del>\t\t\tarr = PyArray_Cast((PyArrayObject *)obj, NPY_CDOUBLE);
<del>\t\t}
<del>\t\telse {
<del>\t\t\tarr = PyArray_FromScalar(obj, PyArray_DescrFromType(NPY_CDOUBLE));
<del>\t\t}
<del>\t\tif (arr==NULL) return 0;
<del>\t\t(*v).r = ((npy_cdouble *)PyArray_DATA(arr))->real;
<del>\t\t(*v).i = ((npy_cdouble *)PyArray_DATA(arr))->imag;
<del>\t\treturn 1;
<del>\t}
<del>\t/* Python does not provide PyNumber_Complex function :-( */
<del>\t(*v).i=0.0;
<del>\tif (PyFloat_Check(obj)) {
<add> Py_complex c;
<add> if (PyComplex_Check(obj)) {
<add> c=PyComplex_AsCComplex(obj);
<add> (*v).r=c.real, (*v).i=c.imag;
<add> return 1;
<add> }
<add> if (PyArray_IsScalar(obj, ComplexFloating)) {
<add> if (PyArray_IsScalar(obj, CFloat)) {
<add> npy_cfloat new;
<add> PyArray_ScalarAsCtype(obj, &new);
<add> (*v).r = (double)new.real;
<add> (*v).i = (double)new.imag;
<add> }
<add> else if (PyArray_IsScalar(obj, CLongDouble)) {
<add> npy_clongdouble new;
<add> PyArray_ScalarAsCtype(obj, &new);
<add> (*v).r = (double)new.real;
<add> (*v).i = (double)new.imag;
<add> }
<add> else { /* if (PyArray_IsScalar(obj, CDouble)) */
<add> PyArray_ScalarAsCtype(obj, v);
<add> }
<add> return 1;
<add> }
<add> if (PyArray_CheckScalar(obj)) { /* 0-dim array or still array scalar */
<add> PyObject *arr;
<add> if (PyArray_Check(obj)) {
<add> arr = PyArray_Cast((PyArrayObject *)obj, NPY_CDOUBLE);
<add> }
<add> else {
<add> arr = PyArray_FromScalar(obj, PyArray_DescrFromType(NPY_CDOUBLE));
<add> }
<add> if (arr==NULL) return 0;
<add> (*v).r = ((npy_cdouble *)PyArray_DATA(arr))->real;
<add> (*v).i = ((npy_cdouble *)PyArray_DATA(arr))->imag;
<add> return 1;
<add> }
<add> /* Python does not provide PyNumber_Complex function :-( */
<add> (*v).i=0.0;
<add> if (PyFloat_Check(obj)) {
<ide> #ifdef __sgi
<del>\t\t(*v).r = PyFloat_AsDouble(obj);
<add> (*v).r = PyFloat_AsDouble(obj);
<ide> #else
<del>\t\t(*v).r = PyFloat_AS_DOUBLE(obj);
<add> (*v).r = PyFloat_AS_DOUBLE(obj);
<ide> #endif
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyInt_Check(obj)) {
<del>\t\t(*v).r = (double)PyInt_AS_LONG(obj);
<del>\t\treturn 1;
<del>\t}
<del>\tif (PyLong_Check(obj)) {
<del>\t\t(*v).r = PyLong_AsDouble(obj);
<del>\t\treturn (!PyErr_Occurred());
<del>\t}
<del>\tif (PySequence_Check(obj) && !(PyString_Check(obj) || PyUnicode_Check(obj))) {
<del>\t\tPyObject *tmp = PySequence_GetItem(obj,0);
<del>\t\tif (tmp) {
<del>\t\t\tif (complex_double_from_pyobj(v,tmp,errmess)) {
<del>\t\t\t\tPy_DECREF(tmp);
<del>\t\t\t\treturn 1;
<del>\t\t\t}
<del>\t\t\tPy_DECREF(tmp);
<del>\t\t}
<del>\t}
<del>\t{
<del>\t\tPyObject* err = PyErr_Occurred();
<del>\t\tif (err==NULL)
<del>\t\t\terr = PyExc_TypeError;
<del>\t\tPyErr_SetString(err,errmess);
<del>\t}
<del>\treturn 0;
<add> return 1;
<add> }
<add> if (PyInt_Check(obj)) {
<add> (*v).r = (double)PyInt_AS_LONG(obj);
<add> return 1;
<add> }
<add> if (PyLong_Check(obj)) {
<add> (*v).r = PyLong_AsDouble(obj);
<add> return (!PyErr_Occurred());
<add> }
<add> if (PySequence_Check(obj) && !(PyString_Check(obj) || PyUnicode_Check(obj))) {
<add> PyObject *tmp = PySequence_GetItem(obj,0);
<add> if (tmp) {
<add> if (complex_double_from_pyobj(v,tmp,errmess)) {
<add> Py_DECREF(tmp);
<add> return 1;
<add> }
<add> Py_DECREF(tmp);
<add> }
<add> }
<add> {
<add> PyObject* err = PyErr_Occurred();
<add> if (err==NULL)
<add> err = PyExc_TypeError;
<add> PyErr_SetString(err,errmess);
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['complex_float_from_pyobj'] = [
<ide> 'complex_float', 'complex_double_from_pyobj']
<ide> cfuncs['complex_float_from_pyobj'] = """\
<ide> static int complex_float_from_pyobj(complex_float* v,PyObject *obj,const char *errmess) {
<del>\tcomplex_double cd={0.0,0.0};
<del>\tif (complex_double_from_pyobj(&cd,obj,errmess)) {
<del>\t\t(*v).r = (float)cd.r;
<del>\t\t(*v).i = (float)cd.i;
<del>\t\treturn 1;
<del>\t}
<del>\treturn 0;
<add> complex_double cd={0.0,0.0};
<add> if (complex_double_from_pyobj(&cd,obj,errmess)) {
<add> (*v).r = (float)cd.r;
<add> (*v).i = (float)cd.i;
<add> return 1;
<add> }
<add> return 0;
<ide> }
<ide> """
<ide> needs['try_pyarr_from_char'] = ['pyobj_from_char1', 'TRYPYARRAYTEMPLATE']
<ide> cfuncs[
<del> 'try_pyarr_from_char'] = 'static int try_pyarr_from_char(PyObject* obj,char* v) {\n\tTRYPYARRAYTEMPLATE(char,\'c\');\n}\n'
<add> 'try_pyarr_from_char'] = 'static int try_pyarr_from_char(PyObject* obj,char* v) {\n TRYPYARRAYTEMPLATE(char,\'c\');\n}\n'
<ide> needs['try_pyarr_from_signed_char'] = ['TRYPYARRAYTEMPLATE', 'unsigned_char']
<ide> cfuncs[
<del> 'try_pyarr_from_unsigned_char'] = 'static int try_pyarr_from_unsigned_char(PyObject* obj,unsigned_char* v) {\n\tTRYPYARRAYTEMPLATE(unsigned_char,\'b\');\n}\n'
<add> 'try_pyarr_from_unsigned_char'] = 'static int try_pyarr_from_unsigned_char(PyObject* obj,unsigned_char* v) {\n TRYPYARRAYTEMPLATE(unsigned_char,\'b\');\n}\n'
<ide> needs['try_pyarr_from_signed_char'] = ['TRYPYARRAYTEMPLATE', 'signed_char']
<ide> cfuncs[
<del> 'try_pyarr_from_signed_char'] = 'static int try_pyarr_from_signed_char(PyObject* obj,signed_char* v) {\n\tTRYPYARRAYTEMPLATE(signed_char,\'1\');\n}\n'
<add> 'try_pyarr_from_signed_char'] = 'static int try_pyarr_from_signed_char(PyObject* obj,signed_char* v) {\n TRYPYARRAYTEMPLATE(signed_char,\'1\');\n}\n'
<ide> needs['try_pyarr_from_short'] = ['pyobj_from_short1', 'TRYPYARRAYTEMPLATE']
<ide> cfuncs[
<del> 'try_pyarr_from_short'] = 'static int try_pyarr_from_short(PyObject* obj,short* v) {\n\tTRYPYARRAYTEMPLATE(short,\'s\');\n}\n'
<add> 'try_pyarr_from_short'] = 'static int try_pyarr_from_short(PyObject* obj,short* v) {\n TRYPYARRAYTEMPLATE(short,\'s\');\n}\n'
<ide> needs['try_pyarr_from_int'] = ['pyobj_from_int1', 'TRYPYARRAYTEMPLATE']
<ide> cfuncs[
<del> 'try_pyarr_from_int'] = 'static int try_pyarr_from_int(PyObject* obj,int* v) {\n\tTRYPYARRAYTEMPLATE(int,\'i\');\n}\n'
<add> 'try_pyarr_from_int'] = 'static int try_pyarr_from_int(PyObject* obj,int* v) {\n TRYPYARRAYTEMPLATE(int,\'i\');\n}\n'
<ide> needs['try_pyarr_from_long'] = ['pyobj_from_long1', 'TRYPYARRAYTEMPLATE']
<ide> cfuncs[
<del> 'try_pyarr_from_long'] = 'static int try_pyarr_from_long(PyObject* obj,long* v) {\n\tTRYPYARRAYTEMPLATE(long,\'l\');\n}\n'
<add> 'try_pyarr_from_long'] = 'static int try_pyarr_from_long(PyObject* obj,long* v) {\n TRYPYARRAYTEMPLATE(long,\'l\');\n}\n'
<ide> needs['try_pyarr_from_long_long'] = [
<ide> 'pyobj_from_long_long1', 'TRYPYARRAYTEMPLATE', 'long_long']
<ide> cfuncs[
<del> 'try_pyarr_from_long_long'] = 'static int try_pyarr_from_long_long(PyObject* obj,long_long* v) {\n\tTRYPYARRAYTEMPLATE(long_long,\'L\');\n}\n'
<add> 'try_pyarr_from_long_long'] = 'static int try_pyarr_from_long_long(PyObject* obj,long_long* v) {\n TRYPYARRAYTEMPLATE(long_long,\'L\');\n}\n'
<ide> needs['try_pyarr_from_float'] = ['pyobj_from_float1', 'TRYPYARRAYTEMPLATE']
<ide> cfuncs[
<del> 'try_pyarr_from_float'] = 'static int try_pyarr_from_float(PyObject* obj,float* v) {\n\tTRYPYARRAYTEMPLATE(float,\'f\');\n}\n'
<add> 'try_pyarr_from_float'] = 'static int try_pyarr_from_float(PyObject* obj,float* v) {\n TRYPYARRAYTEMPLATE(float,\'f\');\n}\n'
<ide> needs['try_pyarr_from_double'] = ['pyobj_from_double1', 'TRYPYARRAYTEMPLATE']
<ide> cfuncs[
<del> 'try_pyarr_from_double'] = 'static int try_pyarr_from_double(PyObject* obj,double* v) {\n\tTRYPYARRAYTEMPLATE(double,\'d\');\n}\n'
<add> 'try_pyarr_from_double'] = 'static int try_pyarr_from_double(PyObject* obj,double* v) {\n TRYPYARRAYTEMPLATE(double,\'d\');\n}\n'
<ide> needs['try_pyarr_from_complex_float'] = [
<ide> 'pyobj_from_complex_float1', 'TRYCOMPLEXPYARRAYTEMPLATE', 'complex_float']
<ide> cfuncs[
<del> 'try_pyarr_from_complex_float'] = 'static int try_pyarr_from_complex_float(PyObject* obj,complex_float* v) {\n\tTRYCOMPLEXPYARRAYTEMPLATE(float,\'F\');\n}\n'
<add> 'try_pyarr_from_complex_float'] = 'static int try_pyarr_from_complex_float(PyObject* obj,complex_float* v) {\n TRYCOMPLEXPYARRAYTEMPLATE(float,\'F\');\n}\n'
<ide> needs['try_pyarr_from_complex_double'] = [
<ide> 'pyobj_from_complex_double1', 'TRYCOMPLEXPYARRAYTEMPLATE', 'complex_double']
<ide> cfuncs[
<del> 'try_pyarr_from_complex_double'] = 'static int try_pyarr_from_complex_double(PyObject* obj,complex_double* v) {\n\tTRYCOMPLEXPYARRAYTEMPLATE(double,\'D\');\n}\n'
<add> 'try_pyarr_from_complex_double'] = 'static int try_pyarr_from_complex_double(PyObject* obj,complex_double* v) {\n TRYCOMPLEXPYARRAYTEMPLATE(double,\'D\');\n}\n'
<ide>
<ide> needs['create_cb_arglist'] = ['CFUNCSMESS', 'PRINTPYOBJERR', 'MINMAX']
<ide> cfuncs['create_cb_arglist'] = """\
<ide> static int create_cb_arglist(PyObject* fun,PyTupleObject* xa,const int maxnofargs,const int nofoptargs,int *nofargs,PyTupleObject **args,const char *errmess) {
<del>\tPyObject *tmp = NULL;
<del>\tPyObject *tmp_fun = NULL;
<del>\tint tot,opt,ext,siz,i,di=0;
<del>\tCFUNCSMESS(\"create_cb_arglist\\n\");
<del>\ttot=opt=ext=siz=0;
<del>\t/* Get the total number of arguments */
<del>\tif (PyFunction_Check(fun))
<del>\t\ttmp_fun = fun;
<del>\telse {
<del>\t\tdi = 1;
<del>\t\tif (PyObject_HasAttrString(fun,\"im_func\")) {
<del>\t\t\ttmp_fun = PyObject_GetAttrString(fun,\"im_func\");
<del>\t\t}
<del>\t\telse if (PyObject_HasAttrString(fun,\"__call__\")) {
<del>\t\t\ttmp = PyObject_GetAttrString(fun,\"__call__\");
<del>\t\t\tif (PyObject_HasAttrString(tmp,\"im_func\"))
<del>\t\t\t\ttmp_fun = PyObject_GetAttrString(tmp,\"im_func\");
<del>\t\t\telse {
<del>\t\t\t\ttmp_fun = fun; /* built-in function */
<del>\t\t\t\ttot = maxnofargs;
<del>\t\t\t\tif (xa != NULL)
<del>\t\t\t\t\ttot += PyTuple_Size((PyObject *)xa);
<del>\t\t\t}
<del>\t\t\tPy_XDECREF(tmp);
<del>\t\t}
<del>\t\telse if (PyFortran_Check(fun) || PyFortran_Check1(fun)) {
<del>\t\t\ttot = maxnofargs;
<del>\t\t\tif (xa != NULL)
<del>\t\t\t\ttot += PyTuple_Size((PyObject *)xa);
<del>\t\t\ttmp_fun = fun;
<del>\t\t}
<del>\t\telse if (F2PyCapsule_Check(fun)) {
<del>\t\t\ttot = maxnofargs;
<del>\t\t\tif (xa != NULL)
<del>\t\t\t\text = PyTuple_Size((PyObject *)xa);
<del>\t\t\tif(ext>0) {
<del>\t\t\t\tfprintf(stderr,\"extra arguments tuple cannot be used with CObject call-back\\n\");
<del>\t\t\t\tgoto capi_fail;
<del>\t\t\t}
<del>\t\t\ttmp_fun = fun;
<del>\t\t}
<del>\t}
<add> PyObject *tmp = NULL;
<add> PyObject *tmp_fun = NULL;
<add> int tot,opt,ext,siz,i,di=0;
<add> CFUNCSMESS(\"create_cb_arglist\\n\");
<add> tot=opt=ext=siz=0;
<add> /* Get the total number of arguments */
<add> if (PyFunction_Check(fun))
<add> tmp_fun = fun;
<add> else {
<add> di = 1;
<add> if (PyObject_HasAttrString(fun,\"im_func\")) {
<add> tmp_fun = PyObject_GetAttrString(fun,\"im_func\");
<add> }
<add> else if (PyObject_HasAttrString(fun,\"__call__\")) {
<add> tmp = PyObject_GetAttrString(fun,\"__call__\");
<add> if (PyObject_HasAttrString(tmp,\"im_func\"))
<add> tmp_fun = PyObject_GetAttrString(tmp,\"im_func\");
<add> else {
<add> tmp_fun = fun; /* built-in function */
<add> tot = maxnofargs;
<add> if (xa != NULL)
<add> tot += PyTuple_Size((PyObject *)xa);
<add> }
<add> Py_XDECREF(tmp);
<add> }
<add> else if (PyFortran_Check(fun) || PyFortran_Check1(fun)) {
<add> tot = maxnofargs;
<add> if (xa != NULL)
<add> tot += PyTuple_Size((PyObject *)xa);
<add> tmp_fun = fun;
<add> }
<add> else if (F2PyCapsule_Check(fun)) {
<add> tot = maxnofargs;
<add> if (xa != NULL)
<add> ext = PyTuple_Size((PyObject *)xa);
<add> if(ext>0) {
<add> fprintf(stderr,\"extra arguments tuple cannot be used with CObject call-back\\n\");
<add> goto capi_fail;
<add> }
<add> tmp_fun = fun;
<add> }
<add> }
<ide> if (tmp_fun==NULL) {
<ide> fprintf(stderr,\"Call-back argument must be function|instance|instance.__call__|f2py-function but got %s.\\n\",(fun==NULL?\"NULL\":Py_TYPE(fun)->tp_name));
<ide> goto capi_fail;
<ide> }
<ide> #if PY_VERSION_HEX >= 0x03000000
<del>\tif (PyObject_HasAttrString(tmp_fun,\"__code__\")) {
<del>\t\tif (PyObject_HasAttrString(tmp = PyObject_GetAttrString(tmp_fun,\"__code__\"),\"co_argcount\"))
<add> if (PyObject_HasAttrString(tmp_fun,\"__code__\")) {
<add> if (PyObject_HasAttrString(tmp = PyObject_GetAttrString(tmp_fun,\"__code__\"),\"co_argcount\"))
<ide> #else
<del>\tif (PyObject_HasAttrString(tmp_fun,\"func_code\")) {
<del>\t\tif (PyObject_HasAttrString(tmp = PyObject_GetAttrString(tmp_fun,\"func_code\"),\"co_argcount\"))
<add> if (PyObject_HasAttrString(tmp_fun,\"func_code\")) {
<add> if (PyObject_HasAttrString(tmp = PyObject_GetAttrString(tmp_fun,\"func_code\"),\"co_argcount\"))
<ide> #endif
<del>\t\t\ttot = PyInt_AsLong(PyObject_GetAttrString(tmp,\"co_argcount\")) - di;
<del>\t\tPy_XDECREF(tmp);
<del>\t}
<del>\t/* Get the number of optional arguments */
<add> tot = PyInt_AsLong(PyObject_GetAttrString(tmp,\"co_argcount\")) - di;
<add> Py_XDECREF(tmp);
<add> }
<add> /* Get the number of optional arguments */
<ide> #if PY_VERSION_HEX >= 0x03000000
<del>\tif (PyObject_HasAttrString(tmp_fun,\"__defaults__\")) {
<del>\t\tif (PyTuple_Check(tmp = PyObject_GetAttrString(tmp_fun,\"__defaults__\")))
<add> if (PyObject_HasAttrString(tmp_fun,\"__defaults__\")) {
<add> if (PyTuple_Check(tmp = PyObject_GetAttrString(tmp_fun,\"__defaults__\")))
<ide> #else
<del>\tif (PyObject_HasAttrString(tmp_fun,\"func_defaults\")) {
<del>\t\tif (PyTuple_Check(tmp = PyObject_GetAttrString(tmp_fun,\"func_defaults\")))
<add> if (PyObject_HasAttrString(tmp_fun,\"func_defaults\")) {
<add> if (PyTuple_Check(tmp = PyObject_GetAttrString(tmp_fun,\"func_defaults\")))
<ide> #endif
<del>\t\t\topt = PyTuple_Size(tmp);
<del>\t\tPy_XDECREF(tmp);
<del>\t}
<del>\t/* Get the number of extra arguments */
<del>\tif (xa != NULL)
<del>\t\text = PyTuple_Size((PyObject *)xa);
<del>\t/* Calculate the size of call-backs argument list */
<del>\tsiz = MIN(maxnofargs+ext,tot);
<del>\t*nofargs = MAX(0,siz-ext);
<add> opt = PyTuple_Size(tmp);
<add> Py_XDECREF(tmp);
<add> }
<add> /* Get the number of extra arguments */
<add> if (xa != NULL)
<add> ext = PyTuple_Size((PyObject *)xa);
<add> /* Calculate the size of call-backs argument list */
<add> siz = MIN(maxnofargs+ext,tot);
<add> *nofargs = MAX(0,siz-ext);
<ide> #ifdef DEBUGCFUNCS
<del>\tfprintf(stderr,\"debug-capi:create_cb_arglist:maxnofargs(-nofoptargs),tot,opt,ext,siz,nofargs=%d(-%d),%d,%d,%d,%d,%d\\n\",maxnofargs,nofoptargs,tot,opt,ext,siz,*nofargs);
<add> fprintf(stderr,\"debug-capi:create_cb_arglist:maxnofargs(-nofoptargs),tot,opt,ext,siz,nofargs=%d(-%d),%d,%d,%d,%d,%d\\n\",maxnofargs,nofoptargs,tot,opt,ext,siz,*nofargs);
<ide> #endif
<del>\tif (siz<tot-opt) {
<del>\t\tfprintf(stderr,\"create_cb_arglist: Failed to build argument list (siz) with enough arguments (tot-opt) required by user-supplied function (siz,tot,opt=%d,%d,%d).\\n\",siz,tot,opt);
<del>\t\tgoto capi_fail;
<del>\t}
<del>\t/* Initialize argument list */
<del>\t*args = (PyTupleObject *)PyTuple_New(siz);
<del>\tfor (i=0;i<*nofargs;i++) {
<del>\t\tPy_INCREF(Py_None);
<del>\t\tPyTuple_SET_ITEM((PyObject *)(*args),i,Py_None);
<del>\t}
<del>\tif (xa != NULL)
<del>\t\tfor (i=(*nofargs);i<siz;i++) {
<del>\t\t\ttmp = PyTuple_GetItem((PyObject *)xa,i-(*nofargs));
<del>\t\t\tPy_INCREF(tmp);
<del>\t\t\tPyTuple_SET_ITEM(*args,i,tmp);
<del>\t\t}
<del>\tCFUNCSMESS(\"create_cb_arglist-end\\n\");
<del>\treturn 1;
<add> if (siz<tot-opt) {
<add> fprintf(stderr,\"create_cb_arglist: Failed to build argument list (siz) with enough arguments (tot-opt) required by user-supplied function (siz,tot,opt=%d,%d,%d).\\n\",siz,tot,opt);
<add> goto capi_fail;
<add> }
<add> /* Initialize argument list */
<add> *args = (PyTupleObject *)PyTuple_New(siz);
<add> for (i=0;i<*nofargs;i++) {
<add> Py_INCREF(Py_None);
<add> PyTuple_SET_ITEM((PyObject *)(*args),i,Py_None);
<add> }
<add> if (xa != NULL)
<add> for (i=(*nofargs);i<siz;i++) {
<add> tmp = PyTuple_GetItem((PyObject *)xa,i-(*nofargs));
<add> Py_INCREF(tmp);
<add> PyTuple_SET_ITEM(*args,i,tmp);
<add> }
<add> CFUNCSMESS(\"create_cb_arglist-end\\n\");
<add> return 1;
<ide> capi_fail:
<del>\tif ((PyErr_Occurred())==NULL)
<del>\t\tPyErr_SetString(#modulename#_error,errmess);
<del>\treturn 0;
<add> if ((PyErr_Occurred())==NULL)
<add> PyErr_SetString(#modulename#_error,errmess);
<add> return 0;
<ide> }
<ide> """
<ide> | 1 |
Python | Python | fix percentile examples. closes | 5aa360321321c69d8052ef0718721baae3587900 | <ide><path>numpy/lib/function_base.py
<ide> def percentile(a, q, axis=None, out=None, overwrite_input=False):
<ide> >>> a
<ide> array([[10, 7, 4],
<ide> [ 3, 2, 1]])
<del> >>> np.percentile(a, 0.5)
<add> >>> np.percentile(a, 50)
<ide> 3.5
<ide> >>> np.percentile(a, 0.5, axis=0)
<ide> array([ 6.5, 4.5, 2.5])
<del> >>> np.percentile(a, 0.5, axis=1)
<add> >>> np.percentile(a, 50, axis=1)
<ide> array([ 7., 2.])
<ide>
<del> >>> m = np.percentile(a, 0.5, axis=0)
<add> >>> m = np.percentile(a, 50, axis=0)
<ide> >>> out = np.zeros_like(m)
<del> >>> np.percentile(a, 0.5, axis=0, out=m)
<add> >>> np.percentile(a, 50, axis=0, out=m)
<ide> array([ 6.5, 4.5, 2.5])
<ide> >>> m
<ide> array([ 6.5, 4.5, 2.5])
<ide>
<ide> >>> b = a.copy()
<del> >>> np.percentile(b, 0.5, axis=1, overwrite_input=True)
<add> >>> np.percentile(b, 50, axis=1, overwrite_input=True)
<ide> array([ 7., 2.])
<ide> >>> assert not np.all(a==b)
<ide> >>> b = a.copy()
<del> >>> np.percentile(b, 0.5, axis=None, overwrite_input=True)
<add> >>> np.percentile(b, 50, axis=None, overwrite_input=True)
<ide> 3.5
<del> >>> assert not np.all(a==b)
<ide>
<ide> """
<ide> a = np.asarray(a) | 1 |
PHP | PHP | update documentation for mixed parameters | 87a7b5687b4c5790c210c3617b2a81722bb810cc | <ide><path>src/Illuminate/Routing/UrlGenerator.php
<ide> public function previous()
<ide> * Generate a absolute URL to the given path.
<ide> *
<ide> * @param string $path
<del> * @param array $parameters
<add> * @param mixed $parameters
<ide> * @param bool $secure
<ide> * @return string
<ide> */
<ide> protected function getScheme($secure)
<ide> * Get the URL to a named route.
<ide> *
<ide> * @param string $name
<del> * @param array $parameters
<add> * @param mixed $parameters
<ide> * @param bool $absolute
<ide> * @return string
<ide> */
<ide> protected function buildParameterList($route, array $params)
<ide> * Get the URL to a controller action.
<ide> *
<ide> * @param string $action
<del> * @param array $parameters
<add> * @param mixed $parameters
<ide> * @param bool $absolute
<ide> * @return string
<ide> */ | 1 |
Mixed | Ruby | update ruby-macho to 1.1.0 | 024264c381a5dc02dea8680dea29e83bdce9d13b | <ide><path>Library/Homebrew/vendor/README.md
<ide> Vendored Dependencies
<ide>
<ide> * [plist](https://github.com/bleything/plist), version 3.1.0
<ide>
<del>* [ruby-macho](https://github.com/Homebrew/ruby-macho), version 0.2.6
<add>* [ruby-macho](https://github.com/Homebrew/ruby-macho), version 1.1.0
<ide>
<ide> ## Licenses:
<ide>
<ide> Vendored Dependencies
<ide> ### ruby-macho
<ide>
<ide> > The MIT License
<del>> Copyright (c) 2015, 2016 William Woodruff <william @ tuffbizz.com>
<add>> Copyright (c) 2015, 2016, 2017 William Woodruff <william @ tuffbizz.com>
<ide> >
<ide> > Permission is hereby granted, free of charge, to any person obtaining a copy
<ide> > of this software and associated documentation files (the "Software"), to deal
<ide><path>Library/Homebrew/vendor/macho/macho.rb
<ide> require "#{File.dirname(__FILE__)}/macho/sections"
<ide> require "#{File.dirname(__FILE__)}/macho/macho_file"
<ide> require "#{File.dirname(__FILE__)}/macho/fat_file"
<del>require "#{File.dirname(__FILE__)}/macho/open"
<ide> require "#{File.dirname(__FILE__)}/macho/exceptions"
<ide> require "#{File.dirname(__FILE__)}/macho/utils"
<ide> require "#{File.dirname(__FILE__)}/macho/tools"
<ide>
<ide> # The primary namespace for ruby-macho.
<ide> module MachO
<ide> # release version
<del> VERSION = "0.2.6".freeze
<add> VERSION = "1.1.0".freeze
<add>
<add> # Opens the given filename as a MachOFile or FatFile, depending on its magic.
<add> # @param filename [String] the file being opened
<add> # @return [MachOFile] if the file is a Mach-O
<add> # @return [FatFile] if the file is a Fat file
<add> # @raise [ArgumentError] if the given file does not exist
<add> # @raise [TruncatedFileError] if the file is too small to have a valid header
<add> # @raise [MagicError] if the file's magic is not valid Mach-O magic
<add> def self.open(filename)
<add> raise ArgumentError, "#{filename}: no such file" unless File.file?(filename)
<add> raise TruncatedFileError unless File.stat(filename).size >= 4
<add>
<add> magic = File.open(filename, "rb") { |f| f.read(4) }.unpack("N").first
<add>
<add> if Utils.fat_magic?(magic)
<add> file = FatFile.new(filename)
<add> elsif Utils.magic?(magic)
<add> file = MachOFile.new(filename)
<add> else
<add> raise MagicError, magic
<add> end
<add>
<add> file
<add> end
<ide> end
<ide><path>Library/Homebrew/vendor/macho/macho/exceptions.rb
<ide> class CPUSubtypeError < MachOError
<ide> # @param cputype [Fixnum] the CPU type of the unknown pair
<ide> # @param cpusubtype [Fixnum] the CPU sub-type of the unknown pair
<ide> def initialize(cputype, cpusubtype)
<del> super "Unrecognized CPU sub-type: 0x#{"%08x" % cpusubtype} (for CPU type: 0x#{"%08x" % cputype})"
<add> super "Unrecognized CPU sub-type: 0x#{"%08x" % cpusubtype}" \
<add> " (for CPU type: 0x#{"%08x" % cputype})"
<ide> end
<ide> end
<ide>
<ide> def initialize(cmd_sym)
<ide> end
<ide> end
<ide>
<del> # Raised when the number of arguments used to create a load command manually is wrong.
<add> # Raised when the number of arguments used to create a load command manually
<add> # is wrong.
<ide> class LoadCommandCreationArityError < MachOError
<ide> # @param cmd_sym [Symbol] the load command's symbol
<ide> # @param expected_arity [Fixnum] the number of arguments expected
<ide> # @param actual_arity [Fixnum] the number of arguments received
<ide> def initialize(cmd_sym, expected_arity, actual_arity)
<del> super "Expected #{expected_arity} arguments for #{cmd_sym} creation, got #{actual_arity}"
<add> super "Expected #{expected_arity} arguments for #{cmd_sym} creation," \
<add> " got #{actual_arity}"
<ide> end
<ide> end
<ide>
<ide> def initialize(cmd_sym)
<ide> class LCStrMalformedError < MachOError
<ide> # @param lc [MachO::LoadCommand] the load command containing the string
<ide> def initialize(lc)
<del> super "Load command #{lc.type} at offset #{lc.view.offset} contains a malformed string"
<add> super "Load command #{lc.type} at offset #{lc.view.offset} contains a" \
<add> " malformed string"
<ide> end
<ide> end
<ide>
<ide><path>Library/Homebrew/vendor/macho/macho/fat_file.rb
<add>require "forwardable"
<add>
<ide> module MachO
<ide> # Represents a "Fat" file, which contains a header, a listing of available
<ide> # architectures, and one or more Mach-O binaries.
<ide> # @see https://en.wikipedia.org/wiki/Mach-O#Multi-architecture_binaries
<del> # @see MachO::MachOFile
<add> # @see MachOFile
<ide> class FatFile
<add> extend Forwardable
<add>
<ide> # @return [String] the filename loaded from, or nil if loaded from a binary string
<ide> attr_accessor :filename
<ide>
<del> # @return [MachO::FatHeader] the file's header
<add> # @return [Headers::FatHeader] the file's header
<ide> attr_reader :header
<ide>
<del> # @return [Array<MachO::FatArch>] an array of fat architectures
<add> # @return [Array<Headers::FatArch>] an array of fat architectures
<ide> attr_reader :fat_archs
<ide>
<del> # @return [Array<MachO::MachOFile>] an array of Mach-O binaries
<add> # @return [Array<MachOFile>] an array of Mach-O binaries
<ide> attr_reader :machos
<ide>
<add> # Creates a new FatFile from the given (single-arch) Mach-Os
<add> # @param machos [Array<MachOFile>] the machos to combine
<add> # @return [FatFile] a new FatFile containing the give machos
<add> def self.new_from_machos(*machos)
<add> header = Headers::FatHeader.new(Headers::FAT_MAGIC, machos.size)
<add> offset = Headers::FatHeader.bytesize + (machos.size * Headers::FatArch.bytesize)
<add> fat_archs = []
<add> machos.each do |macho|
<add> fat_archs << Headers::FatArch.new(macho.header.cputype,
<add> macho.header.cpusubtype,
<add> offset, macho.serialize.bytesize,
<add> macho.alignment)
<add> offset += macho.serialize.bytesize
<add> end
<add>
<add> bin = header.serialize
<add> bin << fat_archs.map(&:serialize).join
<add> bin << machos.map(&:serialize).join
<add>
<add> new_from_bin(bin)
<add> end
<add>
<ide> # Creates a new FatFile instance from a binary string.
<ide> # @param bin [String] a binary string containing raw Mach-O data
<del> # @return [MachO::FatFile] a new FatFile
<add> # @return [FatFile] a new FatFile
<ide> def self.new_from_bin(bin)
<ide> instance = allocate
<ide> instance.initialize_from_bin(bin)
<ide> def initialize(filename)
<ide> end
<ide>
<ide> # Initializes a new FatFile instance from a binary string.
<del> # @see MachO::FatFile.new_from_bin
<add> # @see new_from_bin
<ide> # @api private
<ide> def initialize_from_bin(bin)
<ide> @filename = nil
<ide> def serialize
<ide> @raw_data
<ide> end
<ide>
<del> # @return [Boolean] true if the file is of type `MH_OBJECT`, false otherwise
<del> def object?
<del> machos.first.object?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_EXECUTE`, false otherwise
<del> def executable?
<del> machos.first.executable?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_FVMLIB`, false otherwise
<del> def fvmlib?
<del> machos.first.fvmlib?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_CORE`, false otherwise
<del> def core?
<del> machos.first.core?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_PRELOAD`, false otherwise
<del> def preload?
<del> machos.first.preload?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_DYLIB`, false otherwise
<del> def dylib?
<del> machos.first.dylib?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_DYLINKER`, false otherwise
<del> def dylinker?
<del> machos.first.dylinker?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_BUNDLE`, false otherwise
<del> def bundle?
<del> machos.first.bundle?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_DSYM`, false otherwise
<del> def dsym?
<del> machos.first.dsym?
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_KEXT_BUNDLE`, false otherwise
<del> def kext?
<del> machos.first.kext?
<del> end
<del>
<del> # @return [Fixnum] the file's magic number
<del> def magic
<del> header.magic
<del> end
<add> # @!method object?
<add> # @return (see MachO::MachOFile#object?)
<add> # @!method executable?
<add> # @return (see MachO::MachOFile#executable?)
<add> # @!method fvmlib?
<add> # @return (see MachO::MachOFile#fvmlib?)
<add> # @!method core?
<add> # @return (see MachO::MachOFile#core?)
<add> # @!method preload?
<add> # @return (see MachO::MachOFile#preload?)
<add> # @!method dylib?
<add> # @return (see MachO::MachOFile#dylib?)
<add> # @!method dylinker?
<add> # @return (see MachO::MachOFile#dylinker?)
<add> # @!method bundle?
<add> # @return (see MachO::MachOFile#bundle?)
<add> # @!method dsym?
<add> # @return (see MachO::MachOFile#dsym?)
<add> # @!method kext?
<add> # @return (see MachO::MachOFile#kext?)
<add> # @!method filetype
<add> # @return (see MachO::MachOFile#filetype)
<add> # @!method dylib_id
<add> # @return (see MachO::MachOFile#dylib_id)
<add> def_delegators :canonical_macho, :object?, :executable?, :fvmlib?,
<add> :core?, :preload?, :dylib?, :dylinker?, :bundle?,
<add> :dsym?, :kext?, :filetype, :dylib_id
<add>
<add> # @!method magic
<add> # @return (see MachO::Headers::FatHeader#magic)
<add> def_delegators :header, :magic
<ide>
<ide> # @return [String] a string representation of the file's magic number
<ide> def magic_string
<del> MH_MAGICS[magic]
<del> end
<del>
<del> # The file's type. Assumed to be the same for every Mach-O within.
<del> # @return [Symbol] the filetype
<del> def filetype
<del> machos.first.filetype
<add> Headers::MH_MAGICS[magic]
<ide> end
<ide>
<ide> # Populate the instance's fields with the raw Fat Mach-O data.
<ide> def populate_fields
<ide> end
<ide>
<ide> # All load commands responsible for loading dylibs in the file's Mach-O's.
<del> # @return [Array<MachO::DylibCommand>] an array of DylibCommands
<add> # @return [Array<LoadCommands::DylibCommand>] an array of DylibCommands
<ide> def dylib_load_commands
<ide> machos.map(&:dylib_load_commands).flatten
<ide> end
<ide>
<del> # The file's dylib ID. If the file is not a dylib, returns `nil`.
<del> # @example
<del> # file.dylib_id # => 'libBar.dylib'
<del> # @return [String, nil] the file's dylib ID
<del> # @see MachO::MachOFile#linked_dylibs
<del> def dylib_id
<del> machos.first.dylib_id
<del> end
<del>
<del> # Changes the file's dylib ID to `new_id`. If the file is not a dylib, does nothing.
<add> # Changes the file's dylib ID to `new_id`. If the file is not a dylib,
<add> # does nothing.
<ide> # @example
<ide> # file.change_dylib_id('libFoo.dylib')
<ide> # @param new_id [String] the new dylib ID
<ide> def dylib_id
<ide> # if false, fail only if all slices fail.
<ide> # @return [void]
<ide> # @raise [ArgumentError] if `new_id` is not a String
<del> # @see MachO::MachOFile#linked_dylibs
<add> # @see MachOFile#linked_dylibs
<ide> def change_dylib_id(new_id, options = {})
<ide> raise ArgumentError, "argument must be a String" unless new_id.is_a?(String)
<ide> return unless machos.all?(&:dylib?)
<ide> def change_dylib_id(new_id, options = {})
<ide>
<ide> # All shared libraries linked to the file's Mach-Os.
<ide> # @return [Array<String>] an array of all shared libraries
<del> # @see MachO::MachOFile#linked_dylibs
<add> # @see MachOFile#linked_dylibs
<ide> def linked_dylibs
<ide> # Individual architectures in a fat binary can link to different subsets
<ide> # of libraries, but at this point we want to have the full picture, i.e.
<ide> # the union of all libraries used by all architectures.
<ide> machos.map(&:linked_dylibs).flatten.uniq
<ide> end
<ide>
<del> # Changes all dependent shared library install names from `old_name` to `new_name`.
<del> # In a fat file, this changes install names in all internal Mach-Os.
<add> # Changes all dependent shared library install names from `old_name` to
<add> # `new_name`. In a fat file, this changes install names in all internal
<add> # Mach-Os.
<ide> # @example
<ide> # file.change_install_name('/usr/lib/libFoo.dylib', '/usr/lib/libBar.dylib')
<ide> # @param old_name [String] the shared library name being changed
<ide> def linked_dylibs
<ide> # @option options [Boolean] :strict (true) if true, fail if one slice fails.
<ide> # if false, fail only if all slices fail.
<ide> # @return [void]
<del> # @see MachO::MachOFile#change_install_name
<add> # @see MachOFile#change_install_name
<ide> def change_install_name(old_name, new_name, options = {})
<ide> each_macho(options) do |macho|
<ide> macho.change_install_name(old_name, new_name, options)
<ide> def change_install_name(old_name, new_name, options = {})
<ide>
<ide> # All runtime paths associated with the file's Mach-Os.
<ide> # @return [Array<String>] an array of all runtime paths
<del> # @see MachO::MachOFile#rpaths
<add> # @see MachOFile#rpaths
<ide> def rpaths
<ide> # Can individual architectures have different runtime paths?
<ide> machos.map(&:rpaths).flatten.uniq
<ide> def rpaths
<ide> # @option options [Boolean] :strict (true) if true, fail if one slice fails.
<ide> # if false, fail only if all slices fail.
<ide> # @return [void]
<del> # @see MachO::MachOFile#change_rpath
<add> # @see MachOFile#change_rpath
<ide> def change_rpath(old_path, new_path, options = {})
<ide> each_macho(options) do |macho|
<ide> macho.change_rpath(old_path, new_path, options)
<ide> def change_rpath(old_path, new_path, options = {})
<ide> # @option options [Boolean] :strict (true) if true, fail if one slice fails.
<ide> # if false, fail only if all slices fail.
<ide> # @return [void]
<del> # @see MachO::MachOFile#add_rpath
<add> # @see MachOFile#add_rpath
<ide> def add_rpath(path, options = {})
<ide> each_macho(options) do |macho|
<ide> macho.add_rpath(path, options)
<ide> def add_rpath(path, options = {})
<ide> # @option options [Boolean] :strict (true) if true, fail if one slice fails.
<ide> # if false, fail only if all slices fail.
<ide> # @return void
<del> # @see MachO::MachOFile#delete_rpath
<add> # @see MachOFile#delete_rpath
<ide> def delete_rpath(path, options = {})
<ide> each_macho(options) do |macho|
<ide> macho.delete_rpath(path, options)
<ide> def delete_rpath(path, options = {})
<ide> # @example
<ide> # file.extract(:i386) # => MachO::MachOFile
<ide> # @param cputype [Symbol] the CPU type of the Mach-O being extracted
<del> # @return [MachO::MachOFile, nil] the extracted Mach-O or nil if no Mach-O has the given CPU type
<add> # @return [MachOFile, nil] the extracted Mach-O or nil if no Mach-O has the given CPU type
<ide> def extract(cputype)
<ide> machos.select { |macho| macho.cputype == cputype }.first
<ide> end
<ide>
<ide> # Write all (fat) data to the given filename.
<ide> # @param filename [String] the file to write to
<add> # @return [void]
<ide> def write(filename)
<ide> File.open(filename, "wb") { |f| f.write(@raw_data) }
<ide> end
<ide>
<ide> # Write all (fat) data to the file used to initialize the instance.
<ide> # @return [void]
<del> # @raise [MachO::MachOError] if the instance was initialized without a file
<add> # @raise [MachOError] if the instance was initialized without a file
<ide> # @note Overwrites all data in the file!
<ide> def write!
<ide> if filename.nil?
<ide> def write!
<ide> private
<ide>
<ide> # Obtain the fat header from raw file data.
<del> # @return [MachO::FatHeader] the fat header
<del> # @raise [MachO::TruncatedFileError] if the file is too small to have a valid header
<del> # @raise [MachO::MagicError] if the magic is not valid Mach-O magic
<del> # @raise [MachO::MachOBinaryError] if the magic is for a non-fat Mach-O file
<del> # @raise [MachO::JavaClassFileError] if the file is a Java classfile
<add> # @return [Headers::FatHeader] the fat header
<add> # @raise [TruncatedFileError] if the file is too small to have a
<add> # valid header
<add> # @raise [MagicError] if the magic is not valid Mach-O magic
<add> # @raise [MachOBinaryError] if the magic is for a non-fat Mach-O file
<add> # @raise [JavaClassFileError] if the file is a Java classfile
<ide> # @api private
<ide> def populate_fat_header
<ide> # the smallest fat Mach-O header is 8 bytes
<ide> raise TruncatedFileError if @raw_data.size < 8
<ide>
<del> fh = FatHeader.new_from_bin(:big, @raw_data[0, FatHeader.bytesize])
<add> fh = Headers::FatHeader.new_from_bin(:big, @raw_data[0, Headers::FatHeader.bytesize])
<ide>
<ide> raise MagicError, fh.magic unless Utils.magic?(fh.magic)
<ide> raise MachOBinaryError unless Utils.fat_magic?(fh.magic)
<ide> def populate_fat_header
<ide> end
<ide>
<ide> # Obtain an array of fat architectures from raw file data.
<del> # @return [Array<MachO::FatArch>] an array of fat architectures
<add> # @return [Array<Headers::FatArch>] an array of fat architectures
<ide> # @api private
<ide> def populate_fat_archs
<ide> archs = []
<ide>
<del> fa_off = FatHeader.bytesize
<del> fa_len = FatArch.bytesize
<add> fa_off = Headers::FatHeader.bytesize
<add> fa_len = Headers::FatArch.bytesize
<ide> header.nfat_arch.times do |i|
<del> archs << FatArch.new_from_bin(:big, @raw_data[fa_off + (fa_len * i), fa_len])
<add> archs << Headers::FatArch.new_from_bin(:big, @raw_data[fa_off + (fa_len * i), fa_len])
<ide> end
<ide>
<ide> archs
<ide> end
<ide>
<ide> # Obtain an array of Mach-O blobs from raw file data.
<del> # @return [Array<MachO::MachOFile>] an array of Mach-Os
<add> # @return [Array<MachOFile>] an array of Mach-Os
<ide> # @api private
<ide> def populate_machos
<ide> machos = []
<ide> def repopulate_raw_machos
<ide> # @option options [Boolean] :strict (true) whether or not to fail loudly
<ide> # with an exception if at least one Mach-O raises an exception. If false,
<ide> # only raises an exception if *all* Mach-Os raise exceptions.
<del> # @raise [MachO::RecoverableModificationError] under the conditions of
<add> # @raise [RecoverableModificationError] under the conditions of
<ide> # the `:strict` option above.
<ide> # @api private
<ide> def each_macho(options = {})
<ide> def each_macho(options = {})
<ide> # Non-strict mode: Raise first error if *all* Mach-O slices failed.
<ide> raise errors.first if errors.size == machos.size
<ide> end
<add>
<add> # Return a single-arch Mach-O that represents this fat Mach-O for purposes
<add> # of delegation.
<add> # @return [MachOFile] the Mach-O file
<add> # @api private
<add> def canonical_macho
<add> machos.first
<add> end
<ide> end
<ide> end
<ide><path>Library/Homebrew/vendor/macho/macho/headers.rb
<ide> module MachO
<del> # big-endian fat magic
<del> # @api private
<del> FAT_MAGIC = 0xcafebabe
<del>
<del> # little-endian fat magic
<del> # this is defined, but should never appear in ruby-macho code because
<del> # fat headers are always big-endian and therefore always unpacked as such.
<del> # @api private
<del> FAT_CIGAM = 0xbebafeca
<del>
<del> # 32-bit big-endian magic
<del> # @api private
<del> MH_MAGIC = 0xfeedface
<del>
<del> # 32-bit little-endian magic
<del> # @api private
<del> MH_CIGAM = 0xcefaedfe
<del>
<del> # 64-bit big-endian magic
<del> # @api private
<del> MH_MAGIC_64 = 0xfeedfacf
<del>
<del> # 64-bit little-endian magic
<del> # @api private
<del> MH_CIGAM_64 = 0xcffaedfe
<del>
<del> # association of magic numbers to string representations
<del> # @api private
<del> MH_MAGICS = {
<del> FAT_MAGIC => "FAT_MAGIC",
<del> MH_MAGIC => "MH_MAGIC",
<del> MH_CIGAM => "MH_CIGAM",
<del> MH_MAGIC_64 => "MH_MAGIC_64",
<del> MH_CIGAM_64 => "MH_CIGAM_64",
<del> }.freeze
<del>
<del> # mask for CPUs with 64-bit architectures (when running a 64-bit ABI?)
<del> # @api private
<del> CPU_ARCH_ABI64 = 0x01000000
<del>
<del> # any CPU (unused?)
<del> # @api private
<del> CPU_TYPE_ANY = -1
<del>
<del> # m68k compatible CPUs
<del> # @api private
<del> CPU_TYPE_MC680X0 = 0x06
<del>
<del> # i386 and later compatible CPUs
<del> # @api private
<del> CPU_TYPE_I386 = 0x07
<del>
<del> # x86_64 (AMD64) compatible CPUs
<del> # @api private
<del> CPU_TYPE_X86_64 = (CPU_TYPE_I386 | CPU_ARCH_ABI64)
<del>
<del> # 32-bit ARM compatible CPUs
<del> # @api private
<del> CPU_TYPE_ARM = 0x0c
<del>
<del> # m88k compatible CPUs
<del> # @api private
<del> CPU_TYPE_MC88000 = 0xd
<del>
<del> # 64-bit ARM compatible CPUs
<del> # @api private
<del> CPU_TYPE_ARM64 = (CPU_TYPE_ARM | CPU_ARCH_ABI64)
<del>
<del> # PowerPC compatible CPUs
<del> # @api private
<del> CPU_TYPE_POWERPC = 0x12
<del>
<del> # PowerPC64 compatible CPUs
<del> # @api private
<del> CPU_TYPE_POWERPC64 = (CPU_TYPE_POWERPC | CPU_ARCH_ABI64)
<del>
<del> # association of cpu types to symbol representations
<del> # @api private
<del> CPU_TYPES = {
<del> CPU_TYPE_ANY => :any,
<del> CPU_TYPE_I386 => :i386,
<del> CPU_TYPE_X86_64 => :x86_64,
<del> CPU_TYPE_ARM => :arm,
<del> CPU_TYPE_ARM64 => :arm64,
<del> CPU_TYPE_POWERPC => :ppc,
<del> CPU_TYPE_POWERPC64 => :ppc64,
<del> }.freeze
<del>
<del> # mask for CPU subtype capabilities
<del> # @api private
<del> CPU_SUBTYPE_MASK = 0xff000000
<del>
<del> # 64-bit libraries (undocumented!)
<del> # @see http://llvm.org/docs/doxygen/html/Support_2MachO_8h_source.html
<del> # @api private
<del> CPU_SUBTYPE_LIB64 = 0x80000000
<del>
<del> # the lowest common sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_I386 = 3
<del>
<del> # the i486 sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_486 = 4
<del>
<del> # the i486SX sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_486SX = 132
<del>
<del> # the i586 (P5, Pentium) sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_586 = 5
<del>
<del> # @see CPU_SUBTYPE_586
<del> # @api private
<del> CPU_SUBTYPE_PENT = CPU_SUBTYPE_586
<del>
<del> # the Pentium Pro (P6) sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_PENTPRO = 22
<del>
<del> # the Pentium II (P6, M3?) sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_PENTII_M3 = 54
<del>
<del> # the Pentium II (P6, M5?) sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_PENTII_M5 = 86
<del>
<del> # the Pentium 4 (Netburst) sub-type for `CPU_TYPE_I386`
<del> # @api private
<del> CPU_SUBTYPE_PENTIUM_4 = 10
<del>
<del> # the lowest common sub-type for `CPU_TYPE_MC680X0`
<del> # @api private
<del> CPU_SUBTYPE_MC680X0_ALL = 1
<del>
<del> # @see CPU_SUBTYPE_MC680X0_ALL
<del> # @api private
<del> CPU_SUBTYPE_MC68030 = CPU_SUBTYPE_MC680X0_ALL
<del>
<del> # the 040 subtype for `CPU_TYPE_MC680X0`
<del> # @api private
<del> CPU_SUBTYPE_MC68040 = 2
<del>
<del> # the 030 subtype for `CPU_TYPE_MC680X0`
<del> # @api private
<del> CPU_SUBTYPE_MC68030_ONLY = 3
<del>
<del> # the lowest common sub-type for `CPU_TYPE_X86_64`
<del> # @api private
<del> CPU_SUBTYPE_X86_64_ALL = CPU_SUBTYPE_I386
<del>
<del> # the Haskell sub-type for `CPU_TYPE_X86_64`
<del> # @api private
<del> CPU_SUBTYPE_X86_64_H = 8
<del>
<del> # the lowest common sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_ALL = 0
<del>
<del> # the v4t sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V4T = 5
<del>
<del> # the v6 sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V6 = 6
<del>
<del> # the v5 sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V5TEJ = 7
<del>
<del> # the xscale (v5 family) sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_XSCALE = 8
<del>
<del> # the v7 sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V7 = 9
<del>
<del> # the v7f (Cortex A9) sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V7F = 10
<add> # Classes and constants for parsing the headers of Mach-O binaries.
<add> module Headers
<add> # big-endian fat magic
<add> # @api private
<add> FAT_MAGIC = 0xcafebabe
<ide>
<del> # the v7s ("Swift") sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V7S = 11
<add> # little-endian fat magic
<add> # this is defined, but should never appear in ruby-macho code because
<add> # fat headers are always big-endian and therefore always unpacked as such.
<add> # @api private
<add> FAT_CIGAM = 0xbebafeca
<ide>
<del> # the v7k ("Kirkwood40") sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V7K = 12
<del>
<del> # the v6m sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V6M = 14
<del>
<del> # the v7m sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V7M = 15
<del>
<del> # the v7em sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V7EM = 16
<del>
<del> # the v8 sub-type for `CPU_TYPE_ARM`
<del> # @api private
<del> CPU_SUBTYPE_ARM_V8 = 13
<del>
<del> # the lowest common sub-type for `CPU_TYPE_ARM64`
<del> # @api private
<del> CPU_SUBTYPE_ARM64_ALL = 0
<del>
<del> # the v8 sub-type for `CPU_TYPE_ARM64`
<del> # @api private
<del> CPU_SUBTYPE_ARM64_V8 = 1
<del>
<del> # the lowest common sub-type for `CPU_TYPE_MC88000`
<del> # @api private
<del> CPU_SUBTYPE_MC88000_ALL = 0
<del>
<del> # @see CPU_SUBTYPE_MC88000_ALL
<del> # @api private
<del> CPU_SUBTYPE_MMAX_JPC = CPU_SUBTYPE_MC88000_ALL
<del>
<del> # the 100 sub-type for `CPU_TYPE_MC88000`
<del> # @api private
<del> CPU_SUBTYPE_MC88100 = 1
<del>
<del> # the 110 sub-type for `CPU_TYPE_MC88000`
<del> # @api private
<del> CPU_SUBTYPE_MC88110 = 2
<del>
<del> # the lowest common sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_ALL = 0
<del>
<del> # the 601 sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_601 = 1
<del>
<del> # the 602 sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_602 = 2
<del>
<del> # the 603 sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_603 = 3
<del>
<del> # the 603e (G2) sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_603E = 4
<del>
<del> # the 603ev sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_603EV = 5
<del>
<del> # the 604 sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_604 = 6
<del>
<del> # the 604e sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_604E = 7
<del>
<del> # the 620 sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_620 = 8
<del>
<del> # the 750 (G3) sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_750 = 9
<del>
<del> # the 7400 (G4) sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_7400 = 10
<del>
<del> # the 7450 (G4 "Voyager") sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_7450 = 11
<del>
<del> # the 970 (G5) sub-type for `CPU_TYPE_POWERPC`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC_970 = 100
<del>
<del> # any CPU sub-type for CPU type `CPU_TYPE_POWERPC64`
<del> # @api private
<del> CPU_SUBTYPE_POWERPC64_ALL = CPU_SUBTYPE_POWERPC_ALL
<del>
<del> # association of CPU types/subtype pairs to symbol representations in
<del> # (very) roughly descending order of commonness
<del> # @see https://opensource.apple.com/source/cctools/cctools-877.8/libstuff/arch.c
<del> # @api private
<del> CPU_SUBTYPES = {
<del> CPU_TYPE_I386 => {
<del> CPU_SUBTYPE_I386 => :i386,
<del> CPU_SUBTYPE_486 => :i486,
<del> CPU_SUBTYPE_486SX => :i486SX,
<del> CPU_SUBTYPE_586 => :i586, # also "pentium" in arch(3)
<del> CPU_SUBTYPE_PENTPRO => :i686, # also "pentpro" in arch(3)
<del> CPU_SUBTYPE_PENTII_M3 => :pentIIm3,
<del> CPU_SUBTYPE_PENTII_M5 => :pentIIm5,
<del> CPU_SUBTYPE_PENTIUM_4 => :pentium4,
<del> }.freeze,
<del> CPU_TYPE_X86_64 => {
<del> CPU_SUBTYPE_X86_64_ALL => :x86_64,
<del> CPU_SUBTYPE_X86_64_H => :x86_64h,
<del> }.freeze,
<del> CPU_TYPE_ARM => {
<del> CPU_SUBTYPE_ARM_ALL => :arm,
<del> CPU_SUBTYPE_ARM_V4T => :armv4t,
<del> CPU_SUBTYPE_ARM_V6 => :armv6,
<del> CPU_SUBTYPE_ARM_V5TEJ => :armv5,
<del> CPU_SUBTYPE_ARM_XSCALE => :xscale,
<del> CPU_SUBTYPE_ARM_V7 => :armv7,
<del> CPU_SUBTYPE_ARM_V7F => :armv7f,
<del> CPU_SUBTYPE_ARM_V7S => :armv7s,
<del> CPU_SUBTYPE_ARM_V7K => :armv7k,
<del> CPU_SUBTYPE_ARM_V6M => :armv6m,
<del> CPU_SUBTYPE_ARM_V7M => :armv7m,
<del> CPU_SUBTYPE_ARM_V7EM => :armv7em,
<del> CPU_SUBTYPE_ARM_V8 => :armv8,
<del> }.freeze,
<del> CPU_TYPE_ARM64 => {
<del> CPU_SUBTYPE_ARM64_ALL => :arm64,
<del> CPU_SUBTYPE_ARM64_V8 => :arm64v8,
<del> }.freeze,
<del> CPU_TYPE_POWERPC => {
<del> CPU_SUBTYPE_POWERPC_ALL => :ppc,
<del> CPU_SUBTYPE_POWERPC_601 => :ppc601,
<del> CPU_SUBTYPE_POWERPC_603 => :ppc603,
<del> CPU_SUBTYPE_POWERPC_603E => :ppc603e,
<del> CPU_SUBTYPE_POWERPC_603EV => :ppc603ev,
<del> CPU_SUBTYPE_POWERPC_604 => :ppc604,
<del> CPU_SUBTYPE_POWERPC_604E => :ppc604e,
<del> CPU_SUBTYPE_POWERPC_750 => :ppc750,
<del> CPU_SUBTYPE_POWERPC_7400 => :ppc7400,
<del> CPU_SUBTYPE_POWERPC_7450 => :ppc7450,
<del> CPU_SUBTYPE_POWERPC_970 => :ppc970,
<del> }.freeze,
<del> CPU_TYPE_POWERPC64 => {
<del> CPU_SUBTYPE_POWERPC64_ALL => :ppc64,
<del> # apparently the only exception to the naming scheme
<del> CPU_SUBTYPE_POWERPC_970 => :ppc970_64,
<del> }.freeze,
<del> CPU_TYPE_MC680X0 => {
<del> CPU_SUBTYPE_MC680X0_ALL => :m68k,
<del> CPU_SUBTYPE_MC68030 => :mc68030,
<del> CPU_SUBTYPE_MC68040 => :mc68040,
<del> },
<del> CPU_TYPE_MC88000 => {
<del> CPU_SUBTYPE_MC88000_ALL => :m88k,
<del> },
<del> }.freeze
<del>
<del> # relocatable object file
<del> # @api private
<del> MH_OBJECT = 0x1
<del>
<del> # demand paged executable file
<del> # @api private
<del> MH_EXECUTE = 0x2
<del>
<del> # fixed VM shared library file
<del> # @api private
<del> MH_FVMLIB = 0x3
<del>
<del> # core dump file
<del> # @api private
<del> MH_CORE = 0x4
<del>
<del> # preloaded executable file
<del> # @api private
<del> MH_PRELOAD = 0x5
<del>
<del> # dynamically bound shared library
<del> # @api private
<del> MH_DYLIB = 0x6
<del>
<del> # dynamic link editor
<del> # @api private
<del> MH_DYLINKER = 0x7
<del>
<del> # dynamically bound bundle file
<del> # @api private
<del> MH_BUNDLE = 0x8
<del>
<del> # shared library stub for static linking only, no section contents
<del> # @api private
<del> MH_DYLIB_STUB = 0x9
<del>
<del> # companion file with only debug sections
<del> # @api private
<del> MH_DSYM = 0xa
<del>
<del> # x86_64 kexts
<del> # @api private
<del> MH_KEXT_BUNDLE = 0xb
<del>
<del> # association of filetypes to Symbol representations
<del> # @api private
<del> MH_FILETYPES = {
<del> MH_OBJECT => :object,
<del> MH_EXECUTE => :execute,
<del> MH_FVMLIB => :fvmlib,
<del> MH_CORE => :core,
<del> MH_PRELOAD => :preload,
<del> MH_DYLIB => :dylib,
<del> MH_DYLINKER => :dylinker,
<del> MH_BUNDLE => :bundle,
<del> MH_DYLIB_STUB => :dylib_stub,
<del> MH_DSYM => :dsym,
<del> MH_KEXT_BUNDLE => :kext_bundle,
<del> }.freeze
<del>
<del> # association of mach header flag symbols to values
<del> # @api private
<del> MH_FLAGS = {
<del> :MH_NOUNDEFS => 0x1,
<del> :MH_INCRLINK => 0x2,
<del> :MH_DYLDLINK => 0x4,
<del> :MH_BINDATLOAD => 0x8,
<del> :MH_PREBOUND => 0x10,
<del> :MH_SPLIT_SEGS => 0x20,
<del> :MH_LAZY_INIT => 0x40,
<del> :MH_TWOLEVEL => 0x80,
<del> :MH_FORCE_FLAT => 0x100,
<del> :MH_NOMULTIDEFS => 0x200,
<del> :MH_NOPREFIXBINDING => 0x400,
<del> :MH_PREBINDABLE => 0x800,
<del> :MH_ALLMODSBOUND => 0x1000,
<del> :MH_SUBSECTIONS_VIA_SYMBOLS => 0x2000,
<del> :MH_CANONICAL => 0x4000,
<del> :MH_WEAK_DEFINES => 0x8000,
<del> :MH_BINDS_TO_WEAK => 0x10000,
<del> :MH_ALLOW_STACK_EXECUTION => 0x20000,
<del> :MH_ROOT_SAFE => 0x40000,
<del> :MH_SETUID_SAFE => 0x80000,
<del> :MH_NO_REEXPORTED_DYLIBS => 0x100000,
<del> :MH_PIE => 0x200000,
<del> :MH_DEAD_STRIPPABLE_DYLIB => 0x400000,
<del> :MH_HAS_TLV_DESCRIPTORS => 0x800000,
<del> :MH_NO_HEAP_EXECUTION => 0x1000000,
<del> :MH_APP_EXTENSION_SAFE => 0x02000000,
<del> }.freeze
<del>
<del> # Fat binary header structure
<del> # @see MachO::FatArch
<del> class FatHeader < MachOStructure
<del> # @return [Fixnum] the magic number of the header (and file)
<del> attr_reader :magic
<del>
<del> # @return [Fixnum] the number of fat architecture structures following the header
<del> attr_reader :nfat_arch
<del>
<del> # always big-endian
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "N2".freeze
<del>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 8
<del>
<del> # @api private
<del> def initialize(magic, nfat_arch)
<del> @magic = magic
<del> @nfat_arch = nfat_arch
<del> end
<del> end
<add> # 32-bit big-endian magic
<add> # @api private
<add> MH_MAGIC = 0xfeedface
<ide>
<del> # Fat binary header architecture structure. A Fat binary has one or more of
<del> # these, representing one or more internal Mach-O blobs.
<del> # @see MachO::FatHeader
<del> class FatArch < MachOStructure
<del> # @return [Fixnum] the CPU type of the Mach-O
<del> attr_reader :cputype
<add> # 32-bit little-endian magic
<add> # @api private
<add> MH_CIGAM = 0xcefaedfe
<ide>
<del> # @return [Fixnum] the CPU subtype of the Mach-O
<del> attr_reader :cpusubtype
<add> # 64-bit big-endian magic
<add> # @api private
<add> MH_MAGIC_64 = 0xfeedfacf
<ide>
<del> # @return [Fixnum] the file offset to the beginning of the Mach-O data
<del> attr_reader :offset
<add> # 64-bit little-endian magic
<add> # @api private
<add> MH_CIGAM_64 = 0xcffaedfe
<ide>
<del> # @return [Fixnum] the size, in bytes, of the Mach-O data
<del> attr_reader :size
<add> # association of magic numbers to string representations
<add> # @api private
<add> MH_MAGICS = {
<add> FAT_MAGIC => "FAT_MAGIC",
<add> MH_MAGIC => "MH_MAGIC",
<add> MH_CIGAM => "MH_CIGAM",
<add> MH_MAGIC_64 => "MH_MAGIC_64",
<add> MH_CIGAM_64 => "MH_CIGAM_64",
<add> }.freeze
<add>
<add> # mask for CPUs with 64-bit architectures (when running a 64-bit ABI?)
<add> # @api private
<add> CPU_ARCH_ABI64 = 0x01000000
<ide>
<del> # @return [Fixnum] the alignment, as a power of 2
<del> attr_reader :align
<add> # any CPU (unused?)
<add> # @api private
<add> CPU_TYPE_ANY = -1
<ide>
<del> # always big-endian
<del> # @see MachOStructure::FORMAT
<add> # m68k compatible CPUs
<ide> # @api private
<del> FORMAT = "N5".freeze
<add> CPU_TYPE_MC680X0 = 0x06
<ide>
<del> # @see MachOStructure::SIZEOF
<add> # i386 and later compatible CPUs
<ide> # @api private
<del> SIZEOF = 20
<add> CPU_TYPE_I386 = 0x07
<ide>
<add> # x86_64 (AMD64) compatible CPUs
<ide> # @api private
<del> def initialize(cputype, cpusubtype, offset, size, align)
<del> @cputype = cputype
<del> @cpusubtype = cpusubtype
<del> @offset = offset
<del> @size = size
<del> @align = align
<del> end
<del> end
<add> CPU_TYPE_X86_64 = (CPU_TYPE_I386 | CPU_ARCH_ABI64)
<ide>
<del> # 32-bit Mach-O file header structure
<del> class MachHeader < MachOStructure
<del> # @return [Fixnum] the magic number
<del> attr_reader :magic
<add> # 32-bit ARM compatible CPUs
<add> # @api private
<add> CPU_TYPE_ARM = 0x0c
<ide>
<del> # @return [Fixnum] the CPU type of the Mach-O
<del> attr_reader :cputype
<add> # m88k compatible CPUs
<add> # @api private
<add> CPU_TYPE_MC88000 = 0xd
<ide>
<del> # @return [Fixnum] the CPU subtype of the Mach-O
<del> attr_reader :cpusubtype
<add> # 64-bit ARM compatible CPUs
<add> # @api private
<add> CPU_TYPE_ARM64 = (CPU_TYPE_ARM | CPU_ARCH_ABI64)
<ide>
<del> # @return [Fixnum] the file type of the Mach-O
<del> attr_reader :filetype
<add> # PowerPC compatible CPUs
<add> # @api private
<add> CPU_TYPE_POWERPC = 0x12
<ide>
<del> # @return [Fixnum] the number of load commands in the Mach-O
<del> attr_reader :ncmds
<add> # PowerPC64 compatible CPUs
<add> # @api private
<add> CPU_TYPE_POWERPC64 = (CPU_TYPE_POWERPC | CPU_ARCH_ABI64)
<ide>
<del> # @return [Fixnum] the size of all load commands, in bytes, in the Mach-O
<del> attr_reader :sizeofcmds
<add> # association of cpu types to symbol representations
<add> # @api private
<add> CPU_TYPES = {
<add> CPU_TYPE_ANY => :any,
<add> CPU_TYPE_I386 => :i386,
<add> CPU_TYPE_X86_64 => :x86_64,
<add> CPU_TYPE_ARM => :arm,
<add> CPU_TYPE_ARM64 => :arm64,
<add> CPU_TYPE_POWERPC => :ppc,
<add> CPU_TYPE_POWERPC64 => :ppc64,
<add> }.freeze
<add>
<add> # mask for CPU subtype capabilities
<add> # @api private
<add> CPU_SUBTYPE_MASK = 0xff000000
<ide>
<del> # @return [Fixnum] the header flags associated with the Mach-O
<del> attr_reader :flags
<add> # 64-bit libraries (undocumented!)
<add> # @see http://llvm.org/docs/doxygen/html/Support_2MachO_8h_source.html
<add> # @api private
<add> CPU_SUBTYPE_LIB64 = 0x80000000
<ide>
<del> # @see MachOStructure::FORMAT
<add> # the lowest common sub-type for `CPU_TYPE_I386`
<ide> # @api private
<del> FORMAT = "L=7".freeze
<add> CPU_SUBTYPE_I386 = 3
<ide>
<del> # @see MachOStructure::SIZEOF
<add> # the i486 sub-type for `CPU_TYPE_I386`
<ide> # @api private
<del> SIZEOF = 28
<add> CPU_SUBTYPE_486 = 4
<ide>
<add> # the i486SX sub-type for `CPU_TYPE_I386`
<ide> # @api private
<del> def initialize(magic, cputype, cpusubtype, filetype, ncmds, sizeofcmds,
<del> flags)
<del> @magic = magic
<del> @cputype = cputype
<del> # For now we're not interested in additional capability bits also to be
<del> # found in the `cpusubtype` field. We only care about the CPU sub-type.
<del> @cpusubtype = cpusubtype & ~CPU_SUBTYPE_MASK
<del> @filetype = filetype
<del> @ncmds = ncmds
<del> @sizeofcmds = sizeofcmds
<del> @flags = flags
<del> end
<add> CPU_SUBTYPE_486SX = 132
<ide>
<del> # @example
<del> # puts "this mach-o has position-independent execution" if header.flag?(:MH_PIE)
<del> # @param flag [Symbol] a mach header flag symbol
<del> # @return [Boolean] true if `flag` is present in the header's flag section
<del> def flag?(flag)
<del> flag = MH_FLAGS[flag]
<del> return false if flag.nil?
<del> flags & flag == flag
<del> end
<del> end
<add> # the i586 (P5, Pentium) sub-type for `CPU_TYPE_I386`
<add> # @api private
<add> CPU_SUBTYPE_586 = 5
<add>
<add> # @see CPU_SUBTYPE_586
<add> # @api private
<add> CPU_SUBTYPE_PENT = CPU_SUBTYPE_586
<add>
<add> # the Pentium Pro (P6) sub-type for `CPU_TYPE_I386`
<add> # @api private
<add> CPU_SUBTYPE_PENTPRO = 22
<add>
<add> # the Pentium II (P6, M3?) sub-type for `CPU_TYPE_I386`
<add> # @api private
<add> CPU_SUBTYPE_PENTII_M3 = 54
<add>
<add> # the Pentium II (P6, M5?) sub-type for `CPU_TYPE_I386`
<add> # @api private
<add> CPU_SUBTYPE_PENTII_M5 = 86
<add>
<add> # the Pentium 4 (Netburst) sub-type for `CPU_TYPE_I386`
<add> # @api private
<add> CPU_SUBTYPE_PENTIUM_4 = 10
<add>
<add> # the lowest common sub-type for `CPU_TYPE_MC680X0`
<add> # @api private
<add> CPU_SUBTYPE_MC680X0_ALL = 1
<add>
<add> # @see CPU_SUBTYPE_MC680X0_ALL
<add> # @api private
<add> CPU_SUBTYPE_MC68030 = CPU_SUBTYPE_MC680X0_ALL
<add>
<add> # the 040 subtype for `CPU_TYPE_MC680X0`
<add> # @api private
<add> CPU_SUBTYPE_MC68040 = 2
<add>
<add> # the 030 subtype for `CPU_TYPE_MC680X0`
<add> # @api private
<add> CPU_SUBTYPE_MC68030_ONLY = 3
<add>
<add> # the lowest common sub-type for `CPU_TYPE_X86_64`
<add> # @api private
<add> CPU_SUBTYPE_X86_64_ALL = CPU_SUBTYPE_I386
<add>
<add> # the Haskell sub-type for `CPU_TYPE_X86_64`
<add> # @api private
<add> CPU_SUBTYPE_X86_64_H = 8
<add>
<add> # the lowest common sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_ALL = 0
<add>
<add> # the v4t sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V4T = 5
<add>
<add> # the v6 sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V6 = 6
<add>
<add> # the v5 sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V5TEJ = 7
<add>
<add> # the xscale (v5 family) sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_XSCALE = 8
<add>
<add> # the v7 sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V7 = 9
<add>
<add> # the v7f (Cortex A9) sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V7F = 10
<add>
<add> # the v7s ("Swift") sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V7S = 11
<add>
<add> # the v7k ("Kirkwood40") sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V7K = 12
<add>
<add> # the v6m sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V6M = 14
<add>
<add> # the v7m sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V7M = 15
<ide>
<del> # 64-bit Mach-O file header structure
<del> class MachHeader64 < MachHeader
<del> # @return [void]
<del> attr_reader :reserved
<add> # the v7em sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V7EM = 16
<add>
<add> # the v8 sub-type for `CPU_TYPE_ARM`
<add> # @api private
<add> CPU_SUBTYPE_ARM_V8 = 13
<add>
<add> # the lowest common sub-type for `CPU_TYPE_ARM64`
<add> # @api private
<add> CPU_SUBTYPE_ARM64_ALL = 0
<add>
<add> # the v8 sub-type for `CPU_TYPE_ARM64`
<add> # @api private
<add> CPU_SUBTYPE_ARM64_V8 = 1
<add>
<add> # the lowest common sub-type for `CPU_TYPE_MC88000`
<add> # @api private
<add> CPU_SUBTYPE_MC88000_ALL = 0
<add>
<add> # @see CPU_SUBTYPE_MC88000_ALL
<add> # @api private
<add> CPU_SUBTYPE_MMAX_JPC = CPU_SUBTYPE_MC88000_ALL
<add>
<add> # the 100 sub-type for `CPU_TYPE_MC88000`
<add> # @api private
<add> CPU_SUBTYPE_MC88100 = 1
<add>
<add> # the 110 sub-type for `CPU_TYPE_MC88000`
<add> # @api private
<add> CPU_SUBTYPE_MC88110 = 2
<add>
<add> # the lowest common sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_ALL = 0
<add>
<add> # the 601 sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_601 = 1
<add>
<add> # the 602 sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_602 = 2
<add>
<add> # the 603 sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_603 = 3
<add>
<add> # the 603e (G2) sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_603E = 4
<add>
<add> # the 603ev sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_603EV = 5
<add>
<add> # the 604 sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_604 = 6
<add>
<add> # the 604e sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_604E = 7
<add>
<add> # the 620 sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_620 = 8
<add>
<add> # the 750 (G3) sub-type for `CPU_TYPE_POWERPC`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC_750 = 9
<ide>
<del> # @see MachOStructure::FORMAT
<add> # the 7400 (G4) sub-type for `CPU_TYPE_POWERPC`
<ide> # @api private
<del> FORMAT = "L=8".freeze
<add> CPU_SUBTYPE_POWERPC_7400 = 10
<ide>
<del> # @see MachOStructure::SIZEOF
<add> # the 7450 (G4 "Voyager") sub-type for `CPU_TYPE_POWERPC`
<ide> # @api private
<del> SIZEOF = 32
<add> CPU_SUBTYPE_POWERPC_7450 = 11
<ide>
<add> # the 970 (G5) sub-type for `CPU_TYPE_POWERPC`
<ide> # @api private
<del> def initialize(magic, cputype, cpusubtype, filetype, ncmds, sizeofcmds,
<del> flags, reserved)
<del> super(magic, cputype, cpusubtype, filetype, ncmds, sizeofcmds, flags)
<del> @reserved = reserved
<add> CPU_SUBTYPE_POWERPC_970 = 100
<add>
<add> # any CPU sub-type for CPU type `CPU_TYPE_POWERPC64`
<add> # @api private
<add> CPU_SUBTYPE_POWERPC64_ALL = CPU_SUBTYPE_POWERPC_ALL
<add>
<add> # association of CPU types/subtype pairs to symbol representations in
<add> # (very) roughly descending order of commonness
<add> # @see https://opensource.apple.com/source/cctools/cctools-877.8/libstuff/arch.c
<add> # @api private
<add> CPU_SUBTYPES = {
<add> CPU_TYPE_I386 => {
<add> CPU_SUBTYPE_I386 => :i386,
<add> CPU_SUBTYPE_486 => :i486,
<add> CPU_SUBTYPE_486SX => :i486SX,
<add> CPU_SUBTYPE_586 => :i586, # also "pentium" in arch(3)
<add> CPU_SUBTYPE_PENTPRO => :i686, # also "pentpro" in arch(3)
<add> CPU_SUBTYPE_PENTII_M3 => :pentIIm3,
<add> CPU_SUBTYPE_PENTII_M5 => :pentIIm5,
<add> CPU_SUBTYPE_PENTIUM_4 => :pentium4,
<add> }.freeze,
<add> CPU_TYPE_X86_64 => {
<add> CPU_SUBTYPE_X86_64_ALL => :x86_64,
<add> CPU_SUBTYPE_X86_64_H => :x86_64h,
<add> }.freeze,
<add> CPU_TYPE_ARM => {
<add> CPU_SUBTYPE_ARM_ALL => :arm,
<add> CPU_SUBTYPE_ARM_V4T => :armv4t,
<add> CPU_SUBTYPE_ARM_V6 => :armv6,
<add> CPU_SUBTYPE_ARM_V5TEJ => :armv5,
<add> CPU_SUBTYPE_ARM_XSCALE => :xscale,
<add> CPU_SUBTYPE_ARM_V7 => :armv7,
<add> CPU_SUBTYPE_ARM_V7F => :armv7f,
<add> CPU_SUBTYPE_ARM_V7S => :armv7s,
<add> CPU_SUBTYPE_ARM_V7K => :armv7k,
<add> CPU_SUBTYPE_ARM_V6M => :armv6m,
<add> CPU_SUBTYPE_ARM_V7M => :armv7m,
<add> CPU_SUBTYPE_ARM_V7EM => :armv7em,
<add> CPU_SUBTYPE_ARM_V8 => :armv8,
<add> }.freeze,
<add> CPU_TYPE_ARM64 => {
<add> CPU_SUBTYPE_ARM64_ALL => :arm64,
<add> CPU_SUBTYPE_ARM64_V8 => :arm64v8,
<add> }.freeze,
<add> CPU_TYPE_POWERPC => {
<add> CPU_SUBTYPE_POWERPC_ALL => :ppc,
<add> CPU_SUBTYPE_POWERPC_601 => :ppc601,
<add> CPU_SUBTYPE_POWERPC_603 => :ppc603,
<add> CPU_SUBTYPE_POWERPC_603E => :ppc603e,
<add> CPU_SUBTYPE_POWERPC_603EV => :ppc603ev,
<add> CPU_SUBTYPE_POWERPC_604 => :ppc604,
<add> CPU_SUBTYPE_POWERPC_604E => :ppc604e,
<add> CPU_SUBTYPE_POWERPC_750 => :ppc750,
<add> CPU_SUBTYPE_POWERPC_7400 => :ppc7400,
<add> CPU_SUBTYPE_POWERPC_7450 => :ppc7450,
<add> CPU_SUBTYPE_POWERPC_970 => :ppc970,
<add> }.freeze,
<add> CPU_TYPE_POWERPC64 => {
<add> CPU_SUBTYPE_POWERPC64_ALL => :ppc64,
<add> # apparently the only exception to the naming scheme
<add> CPU_SUBTYPE_POWERPC_970 => :ppc970_64,
<add> }.freeze,
<add> CPU_TYPE_MC680X0 => {
<add> CPU_SUBTYPE_MC680X0_ALL => :m68k,
<add> CPU_SUBTYPE_MC68030 => :mc68030,
<add> CPU_SUBTYPE_MC68040 => :mc68040,
<add> },
<add> CPU_TYPE_MC88000 => {
<add> CPU_SUBTYPE_MC88000_ALL => :m88k,
<add> },
<add> }.freeze
<add>
<add> # relocatable object file
<add> # @api private
<add> MH_OBJECT = 0x1
<add>
<add> # demand paged executable file
<add> # @api private
<add> MH_EXECUTE = 0x2
<add>
<add> # fixed VM shared library file
<add> # @api private
<add> MH_FVMLIB = 0x3
<add>
<add> # core dump file
<add> # @api private
<add> MH_CORE = 0x4
<add>
<add> # preloaded executable file
<add> # @api private
<add> MH_PRELOAD = 0x5
<add>
<add> # dynamically bound shared library
<add> # @api private
<add> MH_DYLIB = 0x6
<add>
<add> # dynamic link editor
<add> # @api private
<add> MH_DYLINKER = 0x7
<add>
<add> # dynamically bound bundle file
<add> # @api private
<add> MH_BUNDLE = 0x8
<add>
<add> # shared library stub for static linking only, no section contents
<add> # @api private
<add> MH_DYLIB_STUB = 0x9
<add>
<add> # companion file with only debug sections
<add> # @api private
<add> MH_DSYM = 0xa
<add>
<add> # x86_64 kexts
<add> # @api private
<add> MH_KEXT_BUNDLE = 0xb
<add>
<add> # association of filetypes to Symbol representations
<add> # @api private
<add> MH_FILETYPES = {
<add> MH_OBJECT => :object,
<add> MH_EXECUTE => :execute,
<add> MH_FVMLIB => :fvmlib,
<add> MH_CORE => :core,
<add> MH_PRELOAD => :preload,
<add> MH_DYLIB => :dylib,
<add> MH_DYLINKER => :dylinker,
<add> MH_BUNDLE => :bundle,
<add> MH_DYLIB_STUB => :dylib_stub,
<add> MH_DSYM => :dsym,
<add> MH_KEXT_BUNDLE => :kext_bundle,
<add> }.freeze
<add>
<add> # association of mach header flag symbols to values
<add> # @api private
<add> MH_FLAGS = {
<add> :MH_NOUNDEFS => 0x1,
<add> :MH_INCRLINK => 0x2,
<add> :MH_DYLDLINK => 0x4,
<add> :MH_BINDATLOAD => 0x8,
<add> :MH_PREBOUND => 0x10,
<add> :MH_SPLIT_SEGS => 0x20,
<add> :MH_LAZY_INIT => 0x40,
<add> :MH_TWOLEVEL => 0x80,
<add> :MH_FORCE_FLAT => 0x100,
<add> :MH_NOMULTIDEFS => 0x200,
<add> :MH_NOPREFIXBINDING => 0x400,
<add> :MH_PREBINDABLE => 0x800,
<add> :MH_ALLMODSBOUND => 0x1000,
<add> :MH_SUBSECTIONS_VIA_SYMBOLS => 0x2000,
<add> :MH_CANONICAL => 0x4000,
<add> :MH_WEAK_DEFINES => 0x8000,
<add> :MH_BINDS_TO_WEAK => 0x10000,
<add> :MH_ALLOW_STACK_EXECUTION => 0x20000,
<add> :MH_ROOT_SAFE => 0x40000,
<add> :MH_SETUID_SAFE => 0x80000,
<add> :MH_NO_REEXPORTED_DYLIBS => 0x100000,
<add> :MH_PIE => 0x200000,
<add> :MH_DEAD_STRIPPABLE_DYLIB => 0x400000,
<add> :MH_HAS_TLV_DESCRIPTORS => 0x800000,
<add> :MH_NO_HEAP_EXECUTION => 0x1000000,
<add> :MH_APP_EXTENSION_SAFE => 0x02000000,
<add> }.freeze
<add>
<add> # Fat binary header structure
<add> # @see MachO::FatArch
<add> class FatHeader < MachOStructure
<add> # @return [Fixnum] the magic number of the header (and file)
<add> attr_reader :magic
<add>
<add> # @return [Fixnum] the number of fat architecture structures following the header
<add> attr_reader :nfat_arch
<add>
<add> # always big-endian
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "N2".freeze
<add>
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 8
<add>
<add> # @api private
<add> def initialize(magic, nfat_arch)
<add> @magic = magic
<add> @nfat_arch = nfat_arch
<add> end
<add>
<add> # @return [String] the serialized fields of the fat header
<add> def serialize
<add> [magic, nfat_arch].pack(FORMAT)
<add> end
<add> end
<add>
<add> # Fat binary header architecture structure. A Fat binary has one or more of
<add> # these, representing one or more internal Mach-O blobs.
<add> # @see MachO::Headers::FatHeader
<add> class FatArch < MachOStructure
<add> # @return [Fixnum] the CPU type of the Mach-O
<add> attr_reader :cputype
<add>
<add> # @return [Fixnum] the CPU subtype of the Mach-O
<add> attr_reader :cpusubtype
<add>
<add> # @return [Fixnum] the file offset to the beginning of the Mach-O data
<add> attr_reader :offset
<add>
<add> # @return [Fixnum] the size, in bytes, of the Mach-O data
<add> attr_reader :size
<add>
<add> # @return [Fixnum] the alignment, as a power of 2
<add> attr_reader :align
<add>
<add> # always big-endian
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "N5".freeze
<add>
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 20
<add>
<add> # @api private
<add> def initialize(cputype, cpusubtype, offset, size, align)
<add> @cputype = cputype
<add> @cpusubtype = cpusubtype
<add> @offset = offset
<add> @size = size
<add> @align = align
<add> end
<add>
<add> # @return [String] the serialized fields of the fat arch
<add> def serialize
<add> [cputype, cpusubtype, offset, size, align].pack(FORMAT)
<add> end
<add> end
<add>
<add> # 32-bit Mach-O file header structure
<add> class MachHeader < MachOStructure
<add> # @return [Fixnum] the magic number
<add> attr_reader :magic
<add>
<add> # @return [Fixnum] the CPU type of the Mach-O
<add> attr_reader :cputype
<add>
<add> # @return [Fixnum] the CPU subtype of the Mach-O
<add> attr_reader :cpusubtype
<add>
<add> # @return [Fixnum] the file type of the Mach-O
<add> attr_reader :filetype
<add>
<add> # @return [Fixnum] the number of load commands in the Mach-O
<add> attr_reader :ncmds
<add>
<add> # @return [Fixnum] the size of all load commands, in bytes, in the Mach-O
<add> attr_reader :sizeofcmds
<add>
<add> # @return [Fixnum] the header flags associated with the Mach-O
<add> attr_reader :flags
<add>
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=7".freeze
<add>
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 28
<add>
<add> # @api private
<add> def initialize(magic, cputype, cpusubtype, filetype, ncmds, sizeofcmds,
<add> flags)
<add> @magic = magic
<add> @cputype = cputype
<add> # For now we're not interested in additional capability bits also to be
<add> # found in the `cpusubtype` field. We only care about the CPU sub-type.
<add> @cpusubtype = cpusubtype & ~CPU_SUBTYPE_MASK
<add> @filetype = filetype
<add> @ncmds = ncmds
<add> @sizeofcmds = sizeofcmds
<add> @flags = flags
<add> end
<add>
<add> # @example
<add> # puts "this mach-o has position-independent execution" if header.flag?(:MH_PIE)
<add> # @param flag [Symbol] a mach header flag symbol
<add> # @return [Boolean] true if `flag` is present in the header's flag section
<add> def flag?(flag)
<add> flag = MH_FLAGS[flag]
<add> return false if flag.nil?
<add> flags & flag == flag
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_OBJECT`
<add> def object?
<add> filetype == Headers::MH_OBJECT
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_EXECUTE`
<add> def executable?
<add> filetype == Headers::MH_EXECUTE
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_FVMLIB`
<add> def fvmlib?
<add> filetype == Headers::MH_FVMLIB
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_CORE`
<add> def core?
<add> filetype == Headers::MH_CORE
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_PRELOAD`
<add> def preload?
<add> filetype == Headers::MH_PRELOAD
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_DYLIB`
<add> def dylib?
<add> filetype == Headers::MH_DYLIB
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_DYLINKER`
<add> def dylinker?
<add> filetype == Headers::MH_DYLINKER
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_BUNDLE`
<add> def bundle?
<add> filetype == Headers::MH_BUNDLE
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_DSYM`
<add> def dsym?
<add> filetype == Headers::MH_DSYM
<add> end
<add>
<add> # @return [Boolean] whether or not the file is of type `MH_KEXT_BUNDLE`
<add> def kext?
<add> filetype == Headers::MH_KEXT_BUNDLE
<add> end
<add>
<add> # @return [Boolean] true if the Mach-O has 32-bit magic, false otherwise
<add> def magic32?
<add> Utils.magic32?(magic)
<add> end
<add>
<add> # @return [Boolean] true if the Mach-O has 64-bit magic, false otherwise
<add> def magic64?
<add> Utils.magic64?(magic)
<add> end
<add>
<add> # @return [Fixnum] the file's internal alignment
<add> def alignment
<add> magic32? ? 4 : 8
<add> end
<add> end
<add>
<add> # 64-bit Mach-O file header structure
<add> class MachHeader64 < MachHeader
<add> # @return [void]
<add> attr_reader :reserved
<add>
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=8".freeze
<add>
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 32
<add>
<add> # @api private
<add> def initialize(magic, cputype, cpusubtype, filetype, ncmds, sizeofcmds,
<add> flags, reserved)
<add> super(magic, cputype, cpusubtype, filetype, ncmds, sizeofcmds, flags)
<add> @reserved = reserved
<add> end
<ide> end
<ide> end
<ide> end
<ide><path>Library/Homebrew/vendor/macho/macho/load_commands.rb
<ide> module MachO
<del> # load commands added after OS X 10.1 need to be bitwise ORed with
<del> # LC_REQ_DYLD to be recognized by the dynamic linder (dyld)
<del> # @api private
<del> LC_REQ_DYLD = 0x80000000
<del>
<del> # association of load commands to symbol representations
<del> # @api private
<del> LOAD_COMMANDS = {
<del> 0x1 => :LC_SEGMENT,
<del> 0x2 => :LC_SYMTAB,
<del> 0x3 => :LC_SYMSEG,
<del> 0x4 => :LC_THREAD,
<del> 0x5 => :LC_UNIXTHREAD,
<del> 0x6 => :LC_LOADFVMLIB,
<del> 0x7 => :LC_IDFVMLIB,
<del> 0x8 => :LC_IDENT,
<del> 0x9 => :LC_FVMFILE,
<del> 0xa => :LC_PREPAGE,
<del> 0xb => :LC_DYSYMTAB,
<del> 0xc => :LC_LOAD_DYLIB,
<del> 0xd => :LC_ID_DYLIB,
<del> 0xe => :LC_LOAD_DYLINKER,
<del> 0xf => :LC_ID_DYLINKER,
<del> 0x10 => :LC_PREBOUND_DYLIB,
<del> 0x11 => :LC_ROUTINES,
<del> 0x12 => :LC_SUB_FRAMEWORK,
<del> 0x13 => :LC_SUB_UMBRELLA,
<del> 0x14 => :LC_SUB_CLIENT,
<del> 0x15 => :LC_SUB_LIBRARY,
<del> 0x16 => :LC_TWOLEVEL_HINTS,
<del> 0x17 => :LC_PREBIND_CKSUM,
<del> (0x18 | LC_REQ_DYLD) => :LC_LOAD_WEAK_DYLIB,
<del> 0x19 => :LC_SEGMENT_64,
<del> 0x1a => :LC_ROUTINES_64,
<del> 0x1b => :LC_UUID,
<del> (0x1c | LC_REQ_DYLD) => :LC_RPATH,
<del> 0x1d => :LC_CODE_SIGNATURE,
<del> 0x1e => :LC_SEGMENT_SPLIT_INFO,
<del> (0x1f | LC_REQ_DYLD) => :LC_REEXPORT_DYLIB,
<del> 0x20 => :LC_LAZY_LOAD_DYLIB,
<del> 0x21 => :LC_ENCRYPTION_INFO,
<del> 0x22 => :LC_DYLD_INFO,
<del> (0x22 | LC_REQ_DYLD) => :LC_DYLD_INFO_ONLY,
<del> (0x23 | LC_REQ_DYLD) => :LC_LOAD_UPWARD_DYLIB,
<del> 0x24 => :LC_VERSION_MIN_MACOSX,
<del> 0x25 => :LC_VERSION_MIN_IPHONEOS,
<del> 0x26 => :LC_FUNCTION_STARTS,
<del> 0x27 => :LC_DYLD_ENVIRONMENT,
<del> (0x28 | LC_REQ_DYLD) => :LC_MAIN,
<del> 0x29 => :LC_DATA_IN_CODE,
<del> 0x2a => :LC_SOURCE_VERSION,
<del> 0x2b => :LC_DYLIB_CODE_SIGN_DRS,
<del> 0x2c => :LC_ENCRYPTION_INFO_64,
<del> 0x2d => :LC_LINKER_OPTION,
<del> 0x2e => :LC_LINKER_OPTIMIZATION_HINT,
<del> 0x2f => :LC_VERSION_MIN_TVOS,
<del> 0x30 => :LC_VERSION_MIN_WATCHOS,
<del> }.freeze
<del>
<del> # association of symbol representations to load command constants
<del> # @api private
<del> LOAD_COMMAND_CONSTANTS = LOAD_COMMANDS.invert.freeze
<del>
<del> # load commands responsible for loading dylibs
<del> # @api private
<del> DYLIB_LOAD_COMMANDS = [
<del> :LC_LOAD_DYLIB,
<del> :LC_LOAD_WEAK_DYLIB,
<del> :LC_REEXPORT_DYLIB,
<del> :LC_LAZY_LOAD_DYLIB,
<del> :LC_LOAD_UPWARD_DYLIB,
<del> ].freeze
<del>
<del> # load commands that can be created manually via {LoadCommand.create}
<del> # @api private
<del> CREATABLE_LOAD_COMMANDS = DYLIB_LOAD_COMMANDS + [
<del> :LC_ID_DYLIB,
<del> :LC_RPATH,
<del> :LC_LOAD_DYLINKER,
<del> ].freeze
<del>
<del> # association of load command symbols to string representations of classes
<del> # @api private
<del> LC_STRUCTURES = {
<del> :LC_SEGMENT => "SegmentCommand",
<del> :LC_SYMTAB => "SymtabCommand",
<del> :LC_SYMSEG => "SymsegCommand", # obsolete
<del> :LC_THREAD => "ThreadCommand", # seems obsolete, but not documented as such
<del> :LC_UNIXTHREAD => "ThreadCommand",
<del> :LC_LOADFVMLIB => "FvmlibCommand", # obsolete
<del> :LC_IDFVMLIB => "FvmlibCommand", # obsolete
<del> :LC_IDENT => "IdentCommand", # obsolete
<del> :LC_FVMFILE => "FvmfileCommand", # reserved for internal use only
<del> :LC_PREPAGE => "LoadCommand", # reserved for internal use only, no public struct
<del> :LC_DYSYMTAB => "DysymtabCommand",
<del> :LC_LOAD_DYLIB => "DylibCommand",
<del> :LC_ID_DYLIB => "DylibCommand",
<del> :LC_LOAD_DYLINKER => "DylinkerCommand",
<del> :LC_ID_DYLINKER => "DylinkerCommand",
<del> :LC_PREBOUND_DYLIB => "PreboundDylibCommand",
<del> :LC_ROUTINES => "RoutinesCommand",
<del> :LC_SUB_FRAMEWORK => "SubFrameworkCommand",
<del> :LC_SUB_UMBRELLA => "SubUmbrellaCommand",
<del> :LC_SUB_CLIENT => "SubClientCommand",
<del> :LC_SUB_LIBRARY => "SubLibraryCommand",
<del> :LC_TWOLEVEL_HINTS => "TwolevelHintsCommand",
<del> :LC_PREBIND_CKSUM => "PrebindCksumCommand",
<del> :LC_LOAD_WEAK_DYLIB => "DylibCommand",
<del> :LC_SEGMENT_64 => "SegmentCommand64",
<del> :LC_ROUTINES_64 => "RoutinesCommand64",
<del> :LC_UUID => "UUIDCommand",
<del> :LC_RPATH => "RpathCommand",
<del> :LC_CODE_SIGNATURE => "LinkeditDataCommand",
<del> :LC_SEGMENT_SPLIT_INFO => "LinkeditDataCommand",
<del> :LC_REEXPORT_DYLIB => "DylibCommand",
<del> :LC_LAZY_LOAD_DYLIB => "DylibCommand",
<del> :LC_ENCRYPTION_INFO => "EncryptionInfoCommand",
<del> :LC_DYLD_INFO => "DyldInfoCommand",
<del> :LC_DYLD_INFO_ONLY => "DyldInfoCommand",
<del> :LC_LOAD_UPWARD_DYLIB => "DylibCommand",
<del> :LC_VERSION_MIN_MACOSX => "VersionMinCommand",
<del> :LC_VERSION_MIN_IPHONEOS => "VersionMinCommand",
<del> :LC_FUNCTION_STARTS => "LinkeditDataCommand",
<del> :LC_DYLD_ENVIRONMENT => "DylinkerCommand",
<del> :LC_MAIN => "EntryPointCommand",
<del> :LC_DATA_IN_CODE => "LinkeditDataCommand",
<del> :LC_SOURCE_VERSION => "SourceVersionCommand",
<del> :LC_DYLIB_CODE_SIGN_DRS => "LinkeditDataCommand",
<del> :LC_ENCRYPTION_INFO_64 => "EncryptionInfoCommand64",
<del> :LC_LINKER_OPTION => "LinkerOptionCommand",
<del> :LC_LINKER_OPTIMIZATION_HINT => "LinkeditDataCommand",
<del> :LC_VERSION_MIN_TVOS => "VersionMinCommand",
<del> :LC_VERSION_MIN_WATCHOS => "VersionMinCommand",
<del> }.freeze
<del>
<del> # association of segment name symbols to names
<del> # @api private
<del> SEGMENT_NAMES = {
<del> :SEG_PAGEZERO => "__PAGEZERO",
<del> :SEG_TEXT => "__TEXT",
<del> :SEG_DATA => "__DATA",
<del> :SEG_OBJC => "__OBJC",
<del> :SEG_ICON => "__ICON",
<del> :SEG_LINKEDIT => "__LINKEDIT",
<del> :SEG_UNIXSTACK => "__UNIXSTACK",
<del> :SEG_IMPORT => "__IMPORT",
<del> }.freeze
<del>
<del> # association of segment flag symbols to values
<del> # @api private
<del> SEGMENT_FLAGS = {
<del> :SG_HIGHVM => 0x1,
<del> :SG_FVMLIB => 0x2,
<del> :SG_NORELOC => 0x4,
<del> :SG_PROTECTED_VERSION_1 => 0x8,
<del> }.freeze
<del>
<del> # Mach-O load command structure
<del> # This is the most generic load command - only cmd ID and size are
<del> # represented, and no actual data. Used when a more specific class
<del> # isn't available/implemented.
<del> class LoadCommand < MachOStructure
<del> # @return [MachO::MachOView] the raw view associated with the load command
<del> attr_reader :view
<del>
<del> # @return [Fixnum] the load command's identifying number
<del> attr_reader :cmd
<del>
<del> # @return [Fixnum] the size of the load command, in bytes
<del> attr_reader :cmdsize
<del>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2".freeze
<add> # Classes and constants for parsing load commands in Mach-O binaries.
<add> module LoadCommands
<add> # load commands added after OS X 10.1 need to be bitwise ORed with
<add> # LC_REQ_DYLD to be recognized by the dynamic linker (dyld)
<add> # @api private
<add> LC_REQ_DYLD = 0x80000000
<add>
<add> # association of load commands to symbol representations
<add> # @api private
<add> LOAD_COMMANDS = {
<add> 0x1 => :LC_SEGMENT,
<add> 0x2 => :LC_SYMTAB,
<add> 0x3 => :LC_SYMSEG,
<add> 0x4 => :LC_THREAD,
<add> 0x5 => :LC_UNIXTHREAD,
<add> 0x6 => :LC_LOADFVMLIB,
<add> 0x7 => :LC_IDFVMLIB,
<add> 0x8 => :LC_IDENT,
<add> 0x9 => :LC_FVMFILE,
<add> 0xa => :LC_PREPAGE,
<add> 0xb => :LC_DYSYMTAB,
<add> 0xc => :LC_LOAD_DYLIB,
<add> 0xd => :LC_ID_DYLIB,
<add> 0xe => :LC_LOAD_DYLINKER,
<add> 0xf => :LC_ID_DYLINKER,
<add> 0x10 => :LC_PREBOUND_DYLIB,
<add> 0x11 => :LC_ROUTINES,
<add> 0x12 => :LC_SUB_FRAMEWORK,
<add> 0x13 => :LC_SUB_UMBRELLA,
<add> 0x14 => :LC_SUB_CLIENT,
<add> 0x15 => :LC_SUB_LIBRARY,
<add> 0x16 => :LC_TWOLEVEL_HINTS,
<add> 0x17 => :LC_PREBIND_CKSUM,
<add> (0x18 | LC_REQ_DYLD) => :LC_LOAD_WEAK_DYLIB,
<add> 0x19 => :LC_SEGMENT_64,
<add> 0x1a => :LC_ROUTINES_64,
<add> 0x1b => :LC_UUID,
<add> (0x1c | LC_REQ_DYLD) => :LC_RPATH,
<add> 0x1d => :LC_CODE_SIGNATURE,
<add> 0x1e => :LC_SEGMENT_SPLIT_INFO,
<add> (0x1f | LC_REQ_DYLD) => :LC_REEXPORT_DYLIB,
<add> 0x20 => :LC_LAZY_LOAD_DYLIB,
<add> 0x21 => :LC_ENCRYPTION_INFO,
<add> 0x22 => :LC_DYLD_INFO,
<add> (0x22 | LC_REQ_DYLD) => :LC_DYLD_INFO_ONLY,
<add> (0x23 | LC_REQ_DYLD) => :LC_LOAD_UPWARD_DYLIB,
<add> 0x24 => :LC_VERSION_MIN_MACOSX,
<add> 0x25 => :LC_VERSION_MIN_IPHONEOS,
<add> 0x26 => :LC_FUNCTION_STARTS,
<add> 0x27 => :LC_DYLD_ENVIRONMENT,
<add> (0x28 | LC_REQ_DYLD) => :LC_MAIN,
<add> 0x29 => :LC_DATA_IN_CODE,
<add> 0x2a => :LC_SOURCE_VERSION,
<add> 0x2b => :LC_DYLIB_CODE_SIGN_DRS,
<add> 0x2c => :LC_ENCRYPTION_INFO_64,
<add> 0x2d => :LC_LINKER_OPTION,
<add> 0x2e => :LC_LINKER_OPTIMIZATION_HINT,
<add> 0x2f => :LC_VERSION_MIN_TVOS,
<add> 0x30 => :LC_VERSION_MIN_WATCHOS,
<add> }.freeze
<add>
<add> # association of symbol representations to load command constants
<add> # @api private
<add> LOAD_COMMAND_CONSTANTS = LOAD_COMMANDS.invert.freeze
<add>
<add> # load commands responsible for loading dylibs
<add> # @api private
<add> DYLIB_LOAD_COMMANDS = [
<add> :LC_LOAD_DYLIB,
<add> :LC_LOAD_WEAK_DYLIB,
<add> :LC_REEXPORT_DYLIB,
<add> :LC_LAZY_LOAD_DYLIB,
<add> :LC_LOAD_UPWARD_DYLIB,
<add> ].freeze
<add>
<add> # load commands that can be created manually via {LoadCommand.create}
<add> # @api private
<add> CREATABLE_LOAD_COMMANDS = DYLIB_LOAD_COMMANDS + [
<add> :LC_ID_DYLIB,
<add> :LC_RPATH,
<add> :LC_LOAD_DYLINKER,
<add> ].freeze
<add>
<add> # association of load command symbols to string representations of classes
<add> # @api private
<add> LC_STRUCTURES = {
<add> :LC_SEGMENT => "SegmentCommand",
<add> :LC_SYMTAB => "SymtabCommand",
<add> # "obsolete"
<add> :LC_SYMSEG => "SymsegCommand",
<add> # seems obsolete, but not documented as such
<add> :LC_THREAD => "ThreadCommand",
<add> :LC_UNIXTHREAD => "ThreadCommand",
<add> # "obsolete"
<add> :LC_LOADFVMLIB => "FvmlibCommand",
<add> # "obsolete"
<add> :LC_IDFVMLIB => "FvmlibCommand",
<add> # "obsolete"
<add> :LC_IDENT => "IdentCommand",
<add> # "reserved for internal use only"
<add> :LC_FVMFILE => "FvmfileCommand",
<add> # "reserved for internal use only", no public struct
<add> :LC_PREPAGE => "LoadCommand",
<add> :LC_DYSYMTAB => "DysymtabCommand",
<add> :LC_LOAD_DYLIB => "DylibCommand",
<add> :LC_ID_DYLIB => "DylibCommand",
<add> :LC_LOAD_DYLINKER => "DylinkerCommand",
<add> :LC_ID_DYLINKER => "DylinkerCommand",
<add> :LC_PREBOUND_DYLIB => "PreboundDylibCommand",
<add> :LC_ROUTINES => "RoutinesCommand",
<add> :LC_SUB_FRAMEWORK => "SubFrameworkCommand",
<add> :LC_SUB_UMBRELLA => "SubUmbrellaCommand",
<add> :LC_SUB_CLIENT => "SubClientCommand",
<add> :LC_SUB_LIBRARY => "SubLibraryCommand",
<add> :LC_TWOLEVEL_HINTS => "TwolevelHintsCommand",
<add> :LC_PREBIND_CKSUM => "PrebindCksumCommand",
<add> :LC_LOAD_WEAK_DYLIB => "DylibCommand",
<add> :LC_SEGMENT_64 => "SegmentCommand64",
<add> :LC_ROUTINES_64 => "RoutinesCommand64",
<add> :LC_UUID => "UUIDCommand",
<add> :LC_RPATH => "RpathCommand",
<add> :LC_CODE_SIGNATURE => "LinkeditDataCommand",
<add> :LC_SEGMENT_SPLIT_INFO => "LinkeditDataCommand",
<add> :LC_REEXPORT_DYLIB => "DylibCommand",
<add> :LC_LAZY_LOAD_DYLIB => "DylibCommand",
<add> :LC_ENCRYPTION_INFO => "EncryptionInfoCommand",
<add> :LC_DYLD_INFO => "DyldInfoCommand",
<add> :LC_DYLD_INFO_ONLY => "DyldInfoCommand",
<add> :LC_LOAD_UPWARD_DYLIB => "DylibCommand",
<add> :LC_VERSION_MIN_MACOSX => "VersionMinCommand",
<add> :LC_VERSION_MIN_IPHONEOS => "VersionMinCommand",
<add> :LC_FUNCTION_STARTS => "LinkeditDataCommand",
<add> :LC_DYLD_ENVIRONMENT => "DylinkerCommand",
<add> :LC_MAIN => "EntryPointCommand",
<add> :LC_DATA_IN_CODE => "LinkeditDataCommand",
<add> :LC_SOURCE_VERSION => "SourceVersionCommand",
<add> :LC_DYLIB_CODE_SIGN_DRS => "LinkeditDataCommand",
<add> :LC_ENCRYPTION_INFO_64 => "EncryptionInfoCommand64",
<add> :LC_LINKER_OPTION => "LinkerOptionCommand",
<add> :LC_LINKER_OPTIMIZATION_HINT => "LinkeditDataCommand",
<add> :LC_VERSION_MIN_TVOS => "VersionMinCommand",
<add> :LC_VERSION_MIN_WATCHOS => "VersionMinCommand",
<add> }.freeze
<add>
<add> # association of segment name symbols to names
<add> # @api private
<add> SEGMENT_NAMES = {
<add> :SEG_PAGEZERO => "__PAGEZERO",
<add> :SEG_TEXT => "__TEXT",
<add> :SEG_DATA => "__DATA",
<add> :SEG_OBJC => "__OBJC",
<add> :SEG_ICON => "__ICON",
<add> :SEG_LINKEDIT => "__LINKEDIT",
<add> :SEG_UNIXSTACK => "__UNIXSTACK",
<add> :SEG_IMPORT => "__IMPORT",
<add> }.freeze
<add>
<add> # association of segment flag symbols to values
<add> # @api private
<add> SEGMENT_FLAGS = {
<add> :SG_HIGHVM => 0x1,
<add> :SG_FVMLIB => 0x2,
<add> :SG_NORELOC => 0x4,
<add> :SG_PROTECTED_VERSION_1 => 0x8,
<add> }.freeze
<add>
<add> # Mach-O load command structure
<add> # This is the most generic load command - only cmd ID and size are
<add> # represented, and no actual data. Used when a more specific class
<add> # isn't available/implemented.
<add> class LoadCommand < MachOStructure
<add> # @return [MachO::MachOView] the raw view associated with the load command
<add> attr_reader :view
<add>
<add> # @return [Fixnum] the load command's identifying number
<add> attr_reader :cmd
<add>
<add> # @return [Fixnum] the size of the load command, in bytes
<add> attr_reader :cmdsize
<add>
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 8
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 8
<ide>
<del> # Instantiates a new LoadCommand given a view into its origin Mach-O
<del> # @param view [MachO::MachOView] the load command's raw view
<del> # @return [MachO::LoadCommand] the new load command
<del> # @api private
<del> def self.new_from_bin(view)
<del> bin = view.raw_data.slice(view.offset, bytesize)
<del> format = Utils.specialize_format(self::FORMAT, view.endianness)
<add> # Instantiates a new LoadCommand given a view into its origin Mach-O
<add> # @param view [MachO::MachOView] the load command's raw view
<add> # @return [LoadCommand] the new load command
<add> # @api private
<add> def self.new_from_bin(view)
<add> bin = view.raw_data.slice(view.offset, bytesize)
<add> format = Utils.specialize_format(self::FORMAT, view.endianness)
<ide>
<del> new(view, *bin.unpack(format))
<del> end
<add> new(view, *bin.unpack(format))
<add> end
<ide>
<del> # Creates a new (viewless) command corresponding to the symbol provided
<del> # @param cmd_sym [Symbol] the symbol of the load command being created
<del> # @param args [Array] the arguments for the load command being created
<del> def self.create(cmd_sym, *args)
<del> raise LoadCommandNotCreatableError, cmd_sym unless CREATABLE_LOAD_COMMANDS.include?(cmd_sym)
<add> # Creates a new (viewless) command corresponding to the symbol provided
<add> # @param cmd_sym [Symbol] the symbol of the load command being created
<add> # @param args [Array] the arguments for the load command being created
<add> def self.create(cmd_sym, *args)
<add> raise LoadCommandNotCreatableError, cmd_sym unless CREATABLE_LOAD_COMMANDS.include?(cmd_sym)
<ide>
<del> klass = MachO.const_get LC_STRUCTURES[cmd_sym]
<del> cmd = LOAD_COMMAND_CONSTANTS[cmd_sym]
<add> klass = LoadCommands.const_get LC_STRUCTURES[cmd_sym]
<add> cmd = LOAD_COMMAND_CONSTANTS[cmd_sym]
<ide>
<del> # cmd will be filled in, view and cmdsize will be left unpopulated
<del> klass_arity = klass.instance_method(:initialize).arity - 3
<add> # cmd will be filled in, view and cmdsize will be left unpopulated
<add> klass_arity = klass.instance_method(:initialize).arity - 3
<ide>
<del> raise LoadCommandCreationArityError.new(cmd_sym, klass_arity, args.size) if klass_arity != args.size
<add> raise LoadCommandCreationArityError.new(cmd_sym, klass_arity, args.size) if klass_arity != args.size
<ide>
<del> klass.new(nil, cmd, nil, *args)
<del> end
<add> klass.new(nil, cmd, nil, *args)
<add> end
<ide>
<del> # @param view [MachO::MachOView] the load command's raw view
<del> # @param cmd [Fixnum] the load command's identifying number
<del> # @param cmdsize [Fixnum] the size of the load command in bytes
<del> # @api private
<del> def initialize(view, cmd, cmdsize)
<del> @view = view
<del> @cmd = cmd
<del> @cmdsize = cmdsize
<del> end
<add> # @param view [MachO::MachOView] the load command's raw view
<add> # @param cmd [Fixnum] the load command's identifying number
<add> # @param cmdsize [Fixnum] the size of the load command in bytes
<add> # @api private
<add> def initialize(view, cmd, cmdsize)
<add> @view = view
<add> @cmd = cmd
<add> @cmdsize = cmdsize
<add> end
<ide>
<del> # @return [Boolean] true if the load command can be serialized, false otherwise
<del> def serializable?
<del> CREATABLE_LOAD_COMMANDS.include?(LOAD_COMMANDS[cmd])
<del> end
<add> # @return [Boolean] whether the load command can be serialized
<add> def serializable?
<add> CREATABLE_LOAD_COMMANDS.include?(LOAD_COMMANDS[cmd])
<add> end
<ide>
<del> # @param context [MachO::LoadCommand::SerializationContext] the context
<del> # to serialize into
<del> # @return [String, nil] the serialized fields of the load command, or nil
<del> # if the load command can't be serialized
<del> # @api private
<del> def serialize(context)
<del> raise LoadCommandNotSerializableError, LOAD_COMMANDS[cmd] unless serializable?
<del> format = Utils.specialize_format(FORMAT, context.endianness)
<del> [cmd, SIZEOF].pack(format)
<del> end
<add> # @param context [SerializationContext] the context
<add> # to serialize into
<add> # @return [String, nil] the serialized fields of the load command, or nil
<add> # if the load command can't be serialized
<add> # @api private
<add> def serialize(context)
<add> raise LoadCommandNotSerializableError, LOAD_COMMANDS[cmd] unless serializable?
<add> format = Utils.specialize_format(FORMAT, context.endianness)
<add> [cmd, SIZEOF].pack(format)
<add> end
<ide>
<del> # @return [Fixnum] the load command's offset in the source file
<del> # @deprecated use {#view} instead
<del> def offset
<del> view.offset
<del> end
<add> # @return [Fixnum] the load command's offset in the source file
<add> # @deprecated use {#view} instead
<add> def offset
<add> view.offset
<add> end
<ide>
<del> # @return [Symbol] a symbol representation of the load command's identifying number
<del> def type
<del> LOAD_COMMANDS[cmd]
<del> end
<add> # @return [Symbol] a symbol representation of the load command's
<add> # identifying number
<add> def type
<add> LOAD_COMMANDS[cmd]
<add> end
<ide>
<del> alias to_sym type
<add> alias to_sym type
<ide>
<del> # @return [String] a string representation of the load command's identifying number
<del> def to_s
<del> type.to_s
<del> end
<add> # @return [String] a string representation of the load command's
<add> # identifying number
<add> def to_s
<add> type.to_s
<add> end
<ide>
<del> # Represents a Load Command string. A rough analogue to the lc_str
<del> # struct used internally by OS X. This class allows ruby-macho to
<del> # pretend that strings stored in LCs are immediately available without
<del> # explicit operations on the raw Mach-O data.
<del> class LCStr
<del> # @param lc [MachO::LoadCommand] the load command
<del> # @param lc_str [Fixnum, String] the offset to the beginning of the string,
<del> # or the string itself if not being initialized with a view.
<del> # @raise [MachO::LCStrMalformedError] if the string is malformed
<del> # @todo devise a solution such that the `lc_str` parameter is not
<del> # interpreted differently depending on `lc.view`. The current behavior
<del> # is a hack to allow viewless load command creation.
<del> # @api private
<del> def initialize(lc, lc_str)
<del> view = lc.view
<del>
<del> if view
<del> lc_str_abs = view.offset + lc_str
<del> lc_end = view.offset + lc.cmdsize - 1
<del> raw_string = view.raw_data.slice(lc_str_abs..lc_end)
<del> @string, null_byte, _padding = raw_string.partition("\x00")
<del> raise LCStrMalformedError, lc if null_byte.empty?
<del> @string_offset = lc_str
<del> else
<del> @string = lc_str
<del> @string_offset = 0
<add> # Represents a Load Command string. A rough analogue to the lc_str
<add> # struct used internally by OS X. This class allows ruby-macho to
<add> # pretend that strings stored in LCs are immediately available without
<add> # explicit operations on the raw Mach-O data.
<add> class LCStr
<add> # @param lc [LoadCommand] the load command
<add> # @param lc_str [Fixnum, String] the offset to the beginning of the
<add> # string, or the string itself if not being initialized with a view.
<add> # @raise [MachO::LCStrMalformedError] if the string is malformed
<add> # @todo devise a solution such that the `lc_str` parameter is not
<add> # interpreted differently depending on `lc.view`. The current behavior
<add> # is a hack to allow viewless load command creation.
<add> # @api private
<add> def initialize(lc, lc_str)
<add> view = lc.view
<add>
<add> if view
<add> lc_str_abs = view.offset + lc_str
<add> lc_end = view.offset + lc.cmdsize - 1
<add> raw_string = view.raw_data.slice(lc_str_abs..lc_end)
<add> @string, null_byte, _padding = raw_string.partition("\x00")
<add> raise LCStrMalformedError, lc if null_byte.empty?
<add> @string_offset = lc_str
<add> else
<add> @string = lc_str
<add> @string_offset = 0
<add> end
<ide> end
<del> end
<ide>
<del> # @return [String] a string representation of the LCStr
<del> def to_s
<del> @string
<add> # @return [String] a string representation of the LCStr
<add> def to_s
<add> @string
<add> end
<add>
<add> # @return [Fixnum] the offset to the beginning of the string in the
<add> # load command
<add> def to_i
<add> @string_offset
<add> end
<ide> end
<ide>
<del> # @return [Fixnum] the offset to the beginning of the string in the load command
<del> def to_i
<del> @string_offset
<add> # Represents the contextual information needed by a load command to
<add> # serialize itself correctly into a binary string.
<add> class SerializationContext
<add> # @return [Symbol] the endianness of the serialized load command
<add> attr_reader :endianness
<add>
<add> # @return [Fixnum] the constant alignment value used to pad the
<add> # serialized load command
<add> attr_reader :alignment
<add>
<add> # @param macho [MachO::MachOFile] the file to contextualize
<add> # @return [SerializationContext] the
<add> # resulting context
<add> def self.context_for(macho)
<add> new(macho.endianness, macho.alignment)
<add> end
<add>
<add> # @param endianness [Symbol] the endianness of the context
<add> # @param alignment [Fixnum] the alignment of the context
<add> # @api private
<add> def initialize(endianness, alignment)
<add> @endianness = endianness
<add> @alignment = alignment
<add> end
<ide> end
<ide> end
<ide>
<del> # Represents the contextual information needed by a load command to
<del> # serialize itself correctly into a binary string.
<del> class SerializationContext
<del> # @return [Symbol] the endianness of the serialized load command
<del> attr_reader :endianness
<add> # A load command containing a single 128-bit unique random number
<add> # identifying an object produced by static link editor. Corresponds to
<add> # LC_UUID.
<add> class UUIDCommand < LoadCommand
<add> # @return [Array<Fixnum>] the UUID
<add> attr_reader :uuid
<ide>
<del> # @return [Fixnum] the constant alignment value used to pad the serialized load command
<del> attr_reader :alignment
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2a16".freeze
<ide>
<del> # @param macho [MachO::MachOFile] the file to contextualize
<del> # @return [MachO::LoadCommand::SerializationContext] the resulting context
<del> def self.context_for(macho)
<del> new(macho.endianness, macho.alignment)
<del> end
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 24
<ide>
<del> # @param endianness [Symbol] the endianness of the context
<del> # @param alignment [Fixnum] the alignment of the context
<ide> # @api private
<del> def initialize(endianness, alignment)
<del> @endianness = endianness
<del> @alignment = alignment
<add> def initialize(view, cmd, cmdsize, uuid)
<add> super(view, cmd, cmdsize)
<add> @uuid = uuid.unpack("C16") # re-unpack for the actual UUID array
<ide> end
<del> end
<del> end
<del>
<del> # A load command containing a single 128-bit unique random number identifying
<del> # an object produced by static link editor. Corresponds to LC_UUID.
<del> class UUIDCommand < LoadCommand
<del> # @return [Array<Fixnum>] the UUID
<del> attr_reader :uuid
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2a16".freeze
<del>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 24
<add> # @return [String] a string representation of the UUID
<add> def uuid_string
<add> hexes = uuid.map { |e| "%02x" % e }
<add> segs = [
<add> hexes[0..3].join, hexes[4..5].join, hexes[6..7].join,
<add> hexes[8..9].join, hexes[10..15].join
<add> ]
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, uuid)
<del> super(view, cmd, cmdsize)
<del> @uuid = uuid.unpack("C16") # re-unpack for the actual UUID array
<add> segs.join("-")
<add> end
<ide> end
<ide>
<del> # @return [String] a string representation of the UUID
<del> def uuid_string
<del> hexes = uuid.map { |e| "%02x" % e }
<del> segs = [
<del> hexes[0..3].join, hexes[4..5].join, hexes[6..7].join,
<del> hexes[8..9].join, hexes[10..15].join
<del> ]
<add> # A load command indicating that part of this file is to be mapped into
<add> # the task's address space. Corresponds to LC_SEGMENT.
<add> class SegmentCommand < LoadCommand
<add> # @return [String] the name of the segment
<add> attr_reader :segname
<ide>
<del> segs.join("-")
<del> end
<del> end
<add> # @return [Fixnum] the memory address of the segment
<add> attr_reader :vmaddr
<ide>
<del> # A load command indicating that part of this file is to be mapped into
<del> # the task's address space. Corresponds to LC_SEGMENT.
<del> class SegmentCommand < LoadCommand
<del> # @return [String] the name of the segment
<del> attr_reader :segname
<add> # @return [Fixnum] the memory size of the segment
<add> attr_reader :vmsize
<ide>
<del> # @return [Fixnum] the memory address of the segment
<del> attr_reader :vmaddr
<add> # @return [Fixnum] the file offset of the segment
<add> attr_reader :fileoff
<ide>
<del> # @return [Fixnum] the memory size of the segment
<del> attr_reader :vmsize
<add> # @return [Fixnum] the amount to map from the file
<add> attr_reader :filesize
<ide>
<del> # @return [Fixnum] the file offset of the segment
<del> attr_reader :fileoff
<add> # @return [Fixnum] the maximum VM protection
<add> attr_reader :maxprot
<ide>
<del> # @return [Fixnum] the amount to map from the file
<del> attr_reader :filesize
<add> # @return [Fixnum] the initial VM protection
<add> attr_reader :initprot
<ide>
<del> # @return [Fixnum] the maximum VM protection
<del> attr_reader :maxprot
<add> # @return [Fixnum] the number of sections in the segment
<add> attr_reader :nsects
<ide>
<del> # @return [Fixnum] the initial VM protection
<del> attr_reader :initprot
<add> # @return [Fixnum] any flags associated with the segment
<add> attr_reader :flags
<ide>
<del> # @return [Fixnum] the number of sections in the segment
<del> attr_reader :nsects
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2a16L=4l=2L=2".freeze
<ide>
<del> # @return [Fixnum] any flags associated with the segment
<del> attr_reader :flags
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 56
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2a16L=4l=2L=2".freeze
<add> # @api private
<add> def initialize(view, cmd, cmdsize, segname, vmaddr, vmsize, fileoff,
<add> filesize, maxprot, initprot, nsects, flags)
<add> super(view, cmd, cmdsize)
<add> @segname = segname.delete("\x00")
<add> @vmaddr = vmaddr
<add> @vmsize = vmsize
<add> @fileoff = fileoff
<add> @filesize = filesize
<add> @maxprot = maxprot
<add> @initprot = initprot
<add> @nsects = nsects
<add> @flags = flags
<add> end
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 56
<add> # All sections referenced within this segment.
<add> # @return [Array<MachO::Sections::Section>] if the Mach-O is 32-bit
<add> # @return [Array<MachO::Sections::Section64>] if the Mach-O is 64-bit
<add> def sections
<add> klass = case self
<add> when SegmentCommand64
<add> MachO::Sections::Section64
<add> when SegmentCommand
<add> MachO::Sections::Section
<add> end
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, segname, vmaddr, vmsize, fileoff,
<del> filesize, maxprot, initprot, nsects, flags)
<del> super(view, cmd, cmdsize)
<del> @segname = segname.delete("\x00")
<del> @vmaddr = vmaddr
<del> @vmsize = vmsize
<del> @fileoff = fileoff
<del> @filesize = filesize
<del> @maxprot = maxprot
<del> @initprot = initprot
<del> @nsects = nsects
<del> @flags = flags
<del> end
<add> offset = view.offset + self.class.bytesize
<add> length = nsects * klass.bytesize
<ide>
<del> # All sections referenced within this segment.
<del> # @return [Array<MachO::Section>] if the Mach-O is 32-bit
<del> # @return [Array<MachO::Section64>] if the Mach-O is 64-bit
<del> def sections
<del> klass = case self
<del> when MachO::SegmentCommand64
<del> MachO::Section64
<del> when MachO::SegmentCommand
<del> MachO::Section
<add> bins = view.raw_data[offset, length]
<add> bins.unpack("a#{klass.bytesize}" * nsects).map do |bin|
<add> klass.new_from_bin(view.endianness, bin)
<add> end
<ide> end
<ide>
<del> bins = view.raw_data[view.offset + self.class.bytesize, nsects * klass.bytesize]
<del> bins.unpack("a#{klass.bytesize}" * nsects).map do |bin|
<del> klass.new_from_bin(view.endianness, bin)
<add> # @example
<add> # puts "this segment relocated in/to it" if sect.flag?(:SG_NORELOC)
<add> # @param flag [Symbol] a segment flag symbol
<add> # @return [Boolean] true if `flag` is present in the segment's flag field
<add> def flag?(flag)
<add> flag = SEGMENT_FLAGS[flag]
<add> return false if flag.nil?
<add> flags & flag == flag
<ide> end
<ide> end
<ide>
<del> # @example
<del> # puts "this segment relocated in/to it" if sect.flag?(:SG_NORELOC)
<del> # @param flag [Symbol] a segment flag symbol
<del> # @return [Boolean] true if `flag` is present in the segment's flag field
<del> def flag?(flag)
<del> flag = SEGMENT_FLAGS[flag]
<del> return false if flag.nil?
<del> flags & flag == flag
<del> end
<del> end
<del>
<del> # A load command indicating that part of this file is to be mapped into
<del> # the task's address space. Corresponds to LC_SEGMENT_64.
<del> class SegmentCommand64 < SegmentCommand
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2a16Q=4l=2L=2".freeze
<add> # A load command indicating that part of this file is to be mapped into
<add> # the task's address space. Corresponds to LC_SEGMENT_64.
<add> class SegmentCommand64 < SegmentCommand
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2a16Q=4l=2L=2".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 72
<del> end
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 72
<add> end
<ide>
<del> # A load command representing some aspect of shared libraries, depending
<del> # on filetype. Corresponds to LC_ID_DYLIB, LC_LOAD_DYLIB, LC_LOAD_WEAK_DYLIB,
<del> # and LC_REEXPORT_DYLIB.
<del> class DylibCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the library's path name as an LCStr
<del> attr_reader :name
<add> # A load command representing some aspect of shared libraries, depending
<add> # on filetype. Corresponds to LC_ID_DYLIB, LC_LOAD_DYLIB,
<add> # LC_LOAD_WEAK_DYLIB, and LC_REEXPORT_DYLIB.
<add> class DylibCommand < LoadCommand
<add> # @return [LCStr] the library's path
<add> # name as an LCStr
<add> attr_reader :name
<ide>
<del> # @return [Fixnum] the library's build time stamp
<del> attr_reader :timestamp
<add> # @return [Fixnum] the library's build time stamp
<add> attr_reader :timestamp
<ide>
<del> # @return [Fixnum] the library's current version number
<del> attr_reader :current_version
<add> # @return [Fixnum] the library's current version number
<add> attr_reader :current_version
<ide>
<del> # @return [Fixnum] the library's compatibility version number
<del> attr_reader :compatibility_version
<add> # @return [Fixnum] the library's compatibility version number
<add> attr_reader :compatibility_version
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=6".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=6".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 24
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 24
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, name, timestamp, current_version, compatibility_version)
<del> super(view, cmd, cmdsize)
<del> @name = LCStr.new(self, name)
<del> @timestamp = timestamp
<del> @current_version = current_version
<del> @compatibility_version = compatibility_version
<del> end
<add> # @api private
<add> def initialize(view, cmd, cmdsize, name, timestamp, current_version,
<add> compatibility_version)
<add> super(view, cmd, cmdsize)
<add> @name = LCStr.new(self, name)
<add> @timestamp = timestamp
<add> @current_version = current_version
<add> @compatibility_version = compatibility_version
<add> end
<ide>
<del> # @param context [MachO::LoadCcommand::SerializationContext] the context
<del> # @return [String] the serialized fields of the load command
<del> # @api private
<del> def serialize(context)
<del> format = Utils.specialize_format(FORMAT, context.endianness)
<del> string_payload, string_offsets = Utils.pack_strings(SIZEOF, context.alignment, :name => name.to_s)
<del> cmdsize = SIZEOF + string_payload.bytesize
<del> [cmd, cmdsize, string_offsets[:name], timestamp, current_version,
<del> compatibility_version].pack(format) + string_payload
<add> # @param context [SerializationContext]
<add> # the context
<add> # @return [String] the serialized fields of the load command
<add> # @api private
<add> def serialize(context)
<add> format = Utils.specialize_format(FORMAT, context.endianness)
<add> string_payload, string_offsets = Utils.pack_strings(SIZEOF,
<add> context.alignment,
<add> :name => name.to_s)
<add> cmdsize = SIZEOF + string_payload.bytesize
<add> [cmd, cmdsize, string_offsets[:name], timestamp, current_version,
<add> compatibility_version].pack(format) + string_payload
<add> end
<ide> end
<del> end
<ide>
<del> # A load command representing some aspect of the dynamic linker, depending
<del> # on filetype. Corresponds to LC_ID_DYLINKER, LC_LOAD_DYLINKER, and
<del> # LC_DYLD_ENVIRONMENT.
<del> class DylinkerCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the dynamic linker's path name as an LCStr
<del> attr_reader :name
<add> # A load command representing some aspect of the dynamic linker, depending
<add> # on filetype. Corresponds to LC_ID_DYLINKER, LC_LOAD_DYLINKER, and
<add> # LC_DYLD_ENVIRONMENT.
<add> class DylinkerCommand < LoadCommand
<add> # @return [LCStr] the dynamic linker's
<add> # path name as an LCStr
<add> attr_reader :name
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, name)
<del> super(view, cmd, cmdsize)
<del> @name = LCStr.new(self, name)
<del> end
<add> # @api private
<add> def initialize(view, cmd, cmdsize, name)
<add> super(view, cmd, cmdsize)
<add> @name = LCStr.new(self, name)
<add> end
<ide>
<del> # @param context [MachO::LoadCcommand::SerializationContext] the context
<del> # @return [String] the serialized fields of the load command
<del> # @api private
<del> def serialize(context)
<del> format = Utils.specialize_format(FORMAT, context.endianness)
<del> string_payload, string_offsets = Utils.pack_strings(SIZEOF, context.alignment, :name => name.to_s)
<del> cmdsize = SIZEOF + string_payload.bytesize
<del> [cmd, cmdsize, string_offsets[:name]].pack(format) + string_payload
<add> # @param context [SerializationContext]
<add> # the context
<add> # @return [String] the serialized fields of the load command
<add> # @api private
<add> def serialize(context)
<add> format = Utils.specialize_format(FORMAT, context.endianness)
<add> string_payload, string_offsets = Utils.pack_strings(SIZEOF,
<add> context.alignment,
<add> :name => name.to_s)
<add> cmdsize = SIZEOF + string_payload.bytesize
<add> [cmd, cmdsize, string_offsets[:name]].pack(format) + string_payload
<add> end
<ide> end
<del> end
<ide>
<del> # A load command used to indicate dynamic libraries used in prebinding.
<del> # Corresponds to LC_PREBOUND_DYLIB.
<del> class PreboundDylibCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the library's path name as an LCStr
<del> attr_reader :name
<add> # A load command used to indicate dynamic libraries used in prebinding.
<add> # Corresponds to LC_PREBOUND_DYLIB.
<add> class PreboundDylibCommand < LoadCommand
<add> # @return [LCStr] the library's path
<add> # name as an LCStr
<add> attr_reader :name
<ide>
<del> # @return [Fixnum] the number of modules in the library
<del> attr_reader :nmodules
<add> # @return [Fixnum] the number of modules in the library
<add> attr_reader :nmodules
<ide>
<del> # @return [Fixnum] a bit vector of linked modules
<del> attr_reader :linked_modules
<add> # @return [Fixnum] a bit vector of linked modules
<add> attr_reader :linked_modules
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=5".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=5".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 20
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 20
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, name, nmodules, linked_modules)
<del> super(view, cmd, cmdsize)
<del> @name = LCStr.new(self, name)
<del> @nmodules = nmodules
<del> @linked_modules = linked_modules
<add> # @api private
<add> def initialize(view, cmd, cmdsize, name, nmodules, linked_modules)
<add> super(view, cmd, cmdsize)
<add> @name = LCStr.new(self, name)
<add> @nmodules = nmodules
<add> @linked_modules = linked_modules
<add> end
<ide> end
<del> end
<ide>
<del> # A load command used to represent threads.
<del> # @note cctools-870 has all fields of thread_command commented out except common ones (cmd, cmdsize)
<del> class ThreadCommand < LoadCommand
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2".freeze
<add> # A load command used to represent threads.
<add> # @note cctools-870 and onwards have all fields of thread_command commented
<add> # out except the common ones (cmd, cmdsize)
<add> class ThreadCommand < LoadCommand
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 8
<del> end
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 8
<add> end
<ide>
<del> # A load command containing the address of the dynamic shared library
<del> # initialization routine and an index into the module table for the module
<del> # that defines the routine. Corresponds to LC_ROUTINES.
<del> class RoutinesCommand < LoadCommand
<del> # @return [Fixnum] the address of the initialization routine
<del> attr_reader :init_address
<add> # A load command containing the address of the dynamic shared library
<add> # initialization routine and an index into the module table for the module
<add> # that defines the routine. Corresponds to LC_ROUTINES.
<add> class RoutinesCommand < LoadCommand
<add> # @return [Fixnum] the address of the initialization routine
<add> attr_reader :init_address
<ide>
<del> # @return [Fixnum] the index into the module table that the init routine is defined in
<del> attr_reader :init_module
<add> # @return [Fixnum] the index into the module table that the init routine
<add> # is defined in
<add> attr_reader :init_module
<ide>
<del> # @return [void]
<del> attr_reader :reserved1
<add> # @return [void]
<add> attr_reader :reserved1
<ide>
<del> # @return [void]
<del> attr_reader :reserved2
<add> # @return [void]
<add> attr_reader :reserved2
<ide>
<del> # @return [void]
<del> attr_reader :reserved3
<add> # @return [void]
<add> attr_reader :reserved3
<ide>
<del> # @return [void]
<del> attr_reader :reserved4
<add> # @return [void]
<add> attr_reader :reserved4
<ide>
<del> # @return [void]
<del> attr_reader :reserved5
<add> # @return [void]
<add> attr_reader :reserved5
<ide>
<del> # @return [void]
<del> attr_reader :reserved6
<add> # @return [void]
<add> attr_reader :reserved6
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=10".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=10".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 40
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 40
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, init_address, init_module, reserved1,
<del> reserved2, reserved3, reserved4, reserved5, reserved6)
<del> super(view, cmd, cmdsize)
<del> @init_address = init_address
<del> @init_module = init_module
<del> @reserved1 = reserved1
<del> @reserved2 = reserved2
<del> @reserved3 = reserved3
<del> @reserved4 = reserved4
<del> @reserved5 = reserved5
<del> @reserved6 = reserved6
<add> # @api private
<add> def initialize(view, cmd, cmdsize, init_address, init_module, reserved1,
<add> reserved2, reserved3, reserved4, reserved5, reserved6)
<add> super(view, cmd, cmdsize)
<add> @init_address = init_address
<add> @init_module = init_module
<add> @reserved1 = reserved1
<add> @reserved2 = reserved2
<add> @reserved3 = reserved3
<add> @reserved4 = reserved4
<add> @reserved5 = reserved5
<add> @reserved6 = reserved6
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing the address of the dynamic shared library
<del> # initialization routine and an index into the module table for the module
<del> # that defines the routine. Corresponds to LC_ROUTINES_64.
<del> class RoutinesCommand64 < RoutinesCommand
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2Q=8".freeze
<add> # A load command containing the address of the dynamic shared library
<add> # initialization routine and an index into the module table for the module
<add> # that defines the routine. Corresponds to LC_ROUTINES_64.
<add> class RoutinesCommand64 < RoutinesCommand
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2Q=8".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 72
<del> end
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 72
<add> end
<ide>
<del> # A load command signifying membership of a subframework containing the name
<del> # of an umbrella framework. Corresponds to LC_SUB_FRAMEWORK.
<del> class SubFrameworkCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the umbrella framework name as an LCStr
<del> attr_reader :umbrella
<add> # A load command signifying membership of a subframework containing the name
<add> # of an umbrella framework. Corresponds to LC_SUB_FRAMEWORK.
<add> class SubFrameworkCommand < LoadCommand
<add> # @return [LCStr] the umbrella framework name as an LCStr
<add> attr_reader :umbrella
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, umbrella)
<del> super(view, cmd, cmdsize)
<del> @umbrella = LCStr.new(self, umbrella)
<add> # @api private
<add> def initialize(view, cmd, cmdsize, umbrella)
<add> super(view, cmd, cmdsize)
<add> @umbrella = LCStr.new(self, umbrella)
<add> end
<ide> end
<del> end
<ide>
<del> # A load command signifying membership of a subumbrella containing the name
<del> # of an umbrella framework. Corresponds to LC_SUB_UMBRELLA.
<del> class SubUmbrellaCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the subumbrella framework name as an LCStr
<del> attr_reader :sub_umbrella
<add> # A load command signifying membership of a subumbrella containing the name
<add> # of an umbrella framework. Corresponds to LC_SUB_UMBRELLA.
<add> class SubUmbrellaCommand < LoadCommand
<add> # @return [LCStr] the subumbrella framework name as an LCStr
<add> attr_reader :sub_umbrella
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, sub_umbrella)
<del> super(view, cmd, cmdsize)
<del> @sub_umbrella = LCStr.new(self, sub_umbrella)
<add> # @api private
<add> def initialize(view, cmd, cmdsize, sub_umbrella)
<add> super(view, cmd, cmdsize)
<add> @sub_umbrella = LCStr.new(self, sub_umbrella)
<add> end
<ide> end
<del> end
<ide>
<del> # A load command signifying a sublibrary of a shared library. Corresponds
<del> # to LC_SUB_LIBRARY.
<del> class SubLibraryCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the sublibrary name as an LCStr
<del> attr_reader :sub_library
<add> # A load command signifying a sublibrary of a shared library. Corresponds
<add> # to LC_SUB_LIBRARY.
<add> class SubLibraryCommand < LoadCommand
<add> # @return [LCStr] the sublibrary name as an LCStr
<add> attr_reader :sub_library
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, sub_library)
<del> super(view, cmd, cmdsize)
<del> @sub_library = LCStr.new(self, sub_library)
<add> # @api private
<add> def initialize(view, cmd, cmdsize, sub_library)
<add> super(view, cmd, cmdsize)
<add> @sub_library = LCStr.new(self, sub_library)
<add> end
<ide> end
<del> end
<ide>
<del> # A load command signifying a shared library that is a subframework of
<del> # an umbrella framework. Corresponds to LC_SUB_CLIENT.
<del> class SubClientCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the subclient name as an LCStr
<del> attr_reader :sub_client
<add> # A load command signifying a shared library that is a subframework of
<add> # an umbrella framework. Corresponds to LC_SUB_CLIENT.
<add> class SubClientCommand < LoadCommand
<add> # @return [LCStr] the subclient name as an LCStr
<add> attr_reader :sub_client
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, sub_client)
<del> super(view, cmd, cmdsize)
<del> @sub_client = LCStr.new(self, sub_client)
<add> # @api private
<add> def initialize(view, cmd, cmdsize, sub_client)
<add> super(view, cmd, cmdsize)
<add> @sub_client = LCStr.new(self, sub_client)
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing the offsets and sizes of the link-edit 4.3BSD
<del> # "stab" style symbol table information. Corresponds to LC_SYMTAB.
<del> class SymtabCommand < LoadCommand
<del> # @return [Fixnum] the symbol table's offset
<del> attr_reader :symoff
<add> # A load command containing the offsets and sizes of the link-edit 4.3BSD
<add> # "stab" style symbol table information. Corresponds to LC_SYMTAB.
<add> class SymtabCommand < LoadCommand
<add> # @return [Fixnum] the symbol table's offset
<add> attr_reader :symoff
<ide>
<del> # @return [Fixnum] the number of symbol table entries
<del> attr_reader :nsyms
<add> # @return [Fixnum] the number of symbol table entries
<add> attr_reader :nsyms
<ide>
<del> # @return the string table's offset
<del> attr_reader :stroff
<add> # @return the string table's offset
<add> attr_reader :stroff
<ide>
<del> # @return the string table size in bytes
<del> attr_reader :strsize
<add> # @return the string table size in bytes
<add> attr_reader :strsize
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=6".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=6".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 24
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 24
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, symoff, nsyms, stroff, strsize)
<del> super(view, cmd, cmdsize)
<del> @symoff = symoff
<del> @nsyms = nsyms
<del> @stroff = stroff
<del> @strsize = strsize
<add> # @api private
<add> def initialize(view, cmd, cmdsize, symoff, nsyms, stroff, strsize)
<add> super(view, cmd, cmdsize)
<add> @symoff = symoff
<add> @nsyms = nsyms
<add> @stroff = stroff
<add> @strsize = strsize
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing symbolic information needed to support data
<del> # structures used by the dynamic link editor. Corresponds to LC_DYSYMTAB.
<del> class DysymtabCommand < LoadCommand
<del> # @return [Fixnum] the index to local symbols
<del> attr_reader :ilocalsym
<add> # A load command containing symbolic information needed to support data
<add> # structures used by the dynamic link editor. Corresponds to LC_DYSYMTAB.
<add> class DysymtabCommand < LoadCommand
<add> # @return [Fixnum] the index to local symbols
<add> attr_reader :ilocalsym
<ide>
<del> # @return [Fixnum] the number of local symbols
<del> attr_reader :nlocalsym
<add> # @return [Fixnum] the number of local symbols
<add> attr_reader :nlocalsym
<ide>
<del> # @return [Fixnum] the index to externally defined symbols
<del> attr_reader :iextdefsym
<add> # @return [Fixnum] the index to externally defined symbols
<add> attr_reader :iextdefsym
<ide>
<del> # @return [Fixnum] the number of externally defined symbols
<del> attr_reader :nextdefsym
<add> # @return [Fixnum] the number of externally defined symbols
<add> attr_reader :nextdefsym
<ide>
<del> # @return [Fixnum] the index to undefined symbols
<del> attr_reader :iundefsym
<add> # @return [Fixnum] the index to undefined symbols
<add> attr_reader :iundefsym
<ide>
<del> # @return [Fixnum] the number of undefined symbols
<del> attr_reader :nundefsym
<add> # @return [Fixnum] the number of undefined symbols
<add> attr_reader :nundefsym
<ide>
<del> # @return [Fixnum] the file offset to the table of contents
<del> attr_reader :tocoff
<add> # @return [Fixnum] the file offset to the table of contents
<add> attr_reader :tocoff
<ide>
<del> # @return [Fixnum] the number of entries in the table of contents
<del> attr_reader :ntoc
<add> # @return [Fixnum] the number of entries in the table of contents
<add> attr_reader :ntoc
<ide>
<del> # @return [Fixnum] the file offset to the module table
<del> attr_reader :modtaboff
<add> # @return [Fixnum] the file offset to the module table
<add> attr_reader :modtaboff
<ide>
<del> # @return [Fixnum] the number of entries in the module table
<del> attr_reader :nmodtab
<add> # @return [Fixnum] the number of entries in the module table
<add> attr_reader :nmodtab
<ide>
<del> # @return [Fixnum] the file offset to the referenced symbol table
<del> attr_reader :extrefsymoff
<add> # @return [Fixnum] the file offset to the referenced symbol table
<add> attr_reader :extrefsymoff
<ide>
<del> # @return [Fixnum] the number of entries in the referenced symbol table
<del> attr_reader :nextrefsyms
<add> # @return [Fixnum] the number of entries in the referenced symbol table
<add> attr_reader :nextrefsyms
<ide>
<del> # @return [Fixnum] the file offset to the indirect symbol table
<del> attr_reader :indirectsymoff
<add> # @return [Fixnum] the file offset to the indirect symbol table
<add> attr_reader :indirectsymoff
<ide>
<del> # @return [Fixnum] the number of entries in the indirect symbol table
<del> attr_reader :nindirectsyms
<add> # @return [Fixnum] the number of entries in the indirect symbol table
<add> attr_reader :nindirectsyms
<ide>
<del> # @return [Fixnum] the file offset to the external relocation entries
<del> attr_reader :extreloff
<add> # @return [Fixnum] the file offset to the external relocation entries
<add> attr_reader :extreloff
<ide>
<del> # @return [Fixnum] the number of external relocation entries
<del> attr_reader :nextrel
<add> # @return [Fixnum] the number of external relocation entries
<add> attr_reader :nextrel
<ide>
<del> # @return [Fixnum] the file offset to the local relocation entries
<del> attr_reader :locreloff
<add> # @return [Fixnum] the file offset to the local relocation entries
<add> attr_reader :locreloff
<ide>
<del> # @return [Fixnum] the number of local relocation entries
<del> attr_reader :nlocrel
<add> # @return [Fixnum] the number of local relocation entries
<add> attr_reader :nlocrel
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=20".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=20".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 80
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 80
<ide>
<del> # ugh
<del> # @api private
<del> def initialize(view, cmd, cmdsize, ilocalsym, nlocalsym, iextdefsym,
<del> nextdefsym, iundefsym, nundefsym, tocoff, ntoc, modtaboff,
<del> nmodtab, extrefsymoff, nextrefsyms, indirectsymoff,
<del> nindirectsyms, extreloff, nextrel, locreloff, nlocrel)
<del> super(view, cmd, cmdsize)
<del> @ilocalsym = ilocalsym
<del> @nlocalsym = nlocalsym
<del> @iextdefsym = iextdefsym
<del> @nextdefsym = nextdefsym
<del> @iundefsym = iundefsym
<del> @nundefsym = nundefsym
<del> @tocoff = tocoff
<del> @ntoc = ntoc
<del> @modtaboff = modtaboff
<del> @nmodtab = nmodtab
<del> @extrefsymoff = extrefsymoff
<del> @nextrefsyms = nextrefsyms
<del> @indirectsymoff = indirectsymoff
<del> @nindirectsyms = nindirectsyms
<del> @extreloff = extreloff
<del> @nextrel = nextrel
<del> @locreloff = locreloff
<del> @nlocrel = nlocrel
<add> # ugh
<add> # @api private
<add> def initialize(view, cmd, cmdsize, ilocalsym, nlocalsym, iextdefsym,
<add> nextdefsym, iundefsym, nundefsym, tocoff, ntoc, modtaboff,
<add> nmodtab, extrefsymoff, nextrefsyms, indirectsymoff,
<add> nindirectsyms, extreloff, nextrel, locreloff, nlocrel)
<add> super(view, cmd, cmdsize)
<add> @ilocalsym = ilocalsym
<add> @nlocalsym = nlocalsym
<add> @iextdefsym = iextdefsym
<add> @nextdefsym = nextdefsym
<add> @iundefsym = iundefsym
<add> @nundefsym = nundefsym
<add> @tocoff = tocoff
<add> @ntoc = ntoc
<add> @modtaboff = modtaboff
<add> @nmodtab = nmodtab
<add> @extrefsymoff = extrefsymoff
<add> @nextrefsyms = nextrefsyms
<add> @indirectsymoff = indirectsymoff
<add> @nindirectsyms = nindirectsyms
<add> @extreloff = extreloff
<add> @nextrel = nextrel
<add> @locreloff = locreloff
<add> @nlocrel = nlocrel
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing the offset and number of hints in the two-level
<del> # namespace lookup hints table. Corresponds to LC_TWOLEVEL_HINTS.
<del> class TwolevelHintsCommand < LoadCommand
<del> # @return [Fixnum] the offset to the hint table
<del> attr_reader :htoffset
<add> # A load command containing the offset and number of hints in the two-level
<add> # namespace lookup hints table. Corresponds to LC_TWOLEVEL_HINTS.
<add> class TwolevelHintsCommand < LoadCommand
<add> # @return [Fixnum] the offset to the hint table
<add> attr_reader :htoffset
<ide>
<del> # @return [Fixnum] the number of hints in the hint table
<del> attr_reader :nhints
<add> # @return [Fixnum] the number of hints in the hint table
<add> attr_reader :nhints
<ide>
<del> # @return [MachO::TwolevelHintsCommand::TwolevelHintTable] the hint table
<del> attr_reader :table
<add> # @return [TwolevelHintsTable]
<add> # the hint table
<add> attr_reader :table
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=4".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=4".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 16
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 16
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, htoffset, nhints)
<del> super(view, cmd, cmdsize)
<del> @htoffset = htoffset
<del> @nhints = nhints
<del> @table = TwolevelHintsTable.new(view, htoffset, nhints)
<del> end
<add> # @api private
<add> def initialize(view, cmd, cmdsize, htoffset, nhints)
<add> super(view, cmd, cmdsize)
<add> @htoffset = htoffset
<add> @nhints = nhints
<add> @table = TwolevelHintsTable.new(view, htoffset, nhints)
<add> end
<ide>
<del> # A representation of the two-level namespace lookup hints table exposed
<del> # by a {TwolevelHintsCommand} (`LC_TWOLEVEL_HINTS`).
<del> class TwolevelHintsTable
<del> # @return [Array<MachO::TwoLevelHintsTable::TwoLevelHint>] all hints in the table
<del> attr_reader :hints
<add> # A representation of the two-level namespace lookup hints table exposed
<add> # by a {TwolevelHintsCommand} (`LC_TWOLEVEL_HINTS`).
<add> class TwolevelHintsTable
<add> # @return [Array<TwolevelHint>] all hints in the table
<add> attr_reader :hints
<ide>
<del> # @param view [MachO::MachOView] the view into the current Mach-O
<del> # @param htoffset [Fixnum] the offset of the hints table
<del> # @param nhints [Fixnum] the number of two-level hints in the table
<del> # @api private
<del> def initialize(view, htoffset, nhints)
<del> format = Utils.specialize_format("L=#{nhints}", view.endianness)
<del> raw_table = view.raw_data[htoffset, nhints * 4]
<del> blobs = raw_table.unpack(format)
<add> # @param view [MachO::MachOView] the view into the current Mach-O
<add> # @param htoffset [Fixnum] the offset of the hints table
<add> # @param nhints [Fixnum] the number of two-level hints in the table
<add> # @api private
<add> def initialize(view, htoffset, nhints)
<add> format = Utils.specialize_format("L=#{nhints}", view.endianness)
<add> raw_table = view.raw_data[htoffset, nhints * 4]
<add> blobs = raw_table.unpack(format)
<ide>
<del> @hints = blobs.map { |b| TwolevelHint.new(b) }
<del> end
<add> @hints = blobs.map { |b| TwolevelHint.new(b) }
<add> end
<ide>
<del> # An individual two-level namespace lookup hint.
<del> class TwolevelHint
<del> # @return [Fixnum] the index into the sub-images
<del> attr_reader :isub_image
<add> # An individual two-level namespace lookup hint.
<add> class TwolevelHint
<add> # @return [Fixnum] the index into the sub-images
<add> attr_reader :isub_image
<ide>
<del> # @return [Fixnum] the index into the table of contents
<del> attr_reader :itoc
<add> # @return [Fixnum] the index into the table of contents
<add> attr_reader :itoc
<ide>
<del> # @param blob [Fixnum] the 32-bit number containing the lookup hint
<del> # @api private
<del> def initialize(blob)
<del> @isub_image = blob >> 24
<del> @itoc = blob & 0x00FFFFFF
<add> # @param blob [Fixnum] the 32-bit number containing the lookup hint
<add> # @api private
<add> def initialize(blob)
<add> @isub_image = blob >> 24
<add> @itoc = blob & 0x00FFFFFF
<add> end
<ide> end
<ide> end
<ide> end
<del> end
<ide>
<del> # A load command containing the value of the original checksum for prebound
<del> # files, or zero. Corresponds to LC_PREBIND_CKSUM.
<del> class PrebindCksumCommand < LoadCommand
<del> # @return [Fixnum] the checksum or 0
<del> attr_reader :cksum
<add> # A load command containing the value of the original checksum for prebound
<add> # files, or zero. Corresponds to LC_PREBIND_CKSUM.
<add> class PrebindCksumCommand < LoadCommand
<add> # @return [Fixnum] the checksum or 0
<add> attr_reader :cksum
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, cksum)
<del> super(view, cmd, cmdsize)
<del> @cksum = cksum
<add> # @api private
<add> def initialize(view, cmd, cmdsize, cksum)
<add> super(view, cmd, cmdsize)
<add> @cksum = cksum
<add> end
<ide> end
<del> end
<ide>
<del> # A load command representing an rpath, which specifies a path that should
<del> # be added to the current run path used to find @rpath prefixed dylibs.
<del> # Corresponds to LC_RPATH.
<del> class RpathCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the path to add to the run path as an LCStr
<del> attr_reader :path
<add> # A load command representing an rpath, which specifies a path that should
<add> # be added to the current run path used to find @rpath prefixed dylibs.
<add> # Corresponds to LC_RPATH.
<add> class RpathCommand < LoadCommand
<add> # @return [LCStr] the path to add to the run path as an LCStr
<add> attr_reader :path
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, path)
<del> super(view, cmd, cmdsize)
<del> @path = LCStr.new(self, path)
<del> end
<add> # @api private
<add> def initialize(view, cmd, cmdsize, path)
<add> super(view, cmd, cmdsize)
<add> @path = LCStr.new(self, path)
<add> end
<ide>
<del> # @param context [MachO::LoadCcommand::SerializationContext] the context
<del> # @return [String] the serialized fields of the load command
<del> # @api private
<del> def serialize(context)
<del> format = Utils.specialize_format(FORMAT, context.endianness)
<del> string_payload, string_offsets = Utils.pack_strings(SIZEOF, context.alignment, :path => path.to_s)
<del> cmdsize = SIZEOF + string_payload.bytesize
<del> [cmd, cmdsize, string_offsets[:path]].pack(format) + string_payload
<add> # @param context [SerializationContext] the context
<add> # @return [String] the serialized fields of the load command
<add> # @api private
<add> def serialize(context)
<add> format = Utils.specialize_format(FORMAT, context.endianness)
<add> string_payload, string_offsets = Utils.pack_strings(SIZEOF,
<add> context.alignment,
<add> :path => path.to_s)
<add> cmdsize = SIZEOF + string_payload.bytesize
<add> [cmd, cmdsize, string_offsets[:path]].pack(format) + string_payload
<add> end
<ide> end
<del> end
<ide>
<del> # A load command representing the offsets and sizes of a blob of data in
<del> # the __LINKEDIT segment. Corresponds to LC_CODE_SIGNATURE, LC_SEGMENT_SPLIT_INFO,
<del> # LC_FUNCTION_STARTS, LC_DATA_IN_CODE, LC_DYLIB_CODE_SIGN_DRS, and LC_LINKER_OPTIMIZATION_HINT.
<del> class LinkeditDataCommand < LoadCommand
<del> # @return [Fixnum] offset to the data in the __LINKEDIT segment
<del> attr_reader :dataoff
<add> # A load command representing the offsets and sizes of a blob of data in
<add> # the __LINKEDIT segment. Corresponds to LC_CODE_SIGNATURE,
<add> # LC_SEGMENT_SPLIT_INFO, LC_FUNCTION_STARTS, LC_DATA_IN_CODE,
<add> # LC_DYLIB_CODE_SIGN_DRS, and LC_LINKER_OPTIMIZATION_HINT.
<add> class LinkeditDataCommand < LoadCommand
<add> # @return [Fixnum] offset to the data in the __LINKEDIT segment
<add> attr_reader :dataoff
<ide>
<del> # @return [Fixnum] size of the data in the __LINKEDIT segment
<del> attr_reader :datasize
<add> # @return [Fixnum] size of the data in the __LINKEDIT segment
<add> attr_reader :datasize
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=4".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=4".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 16
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 16
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, dataoff, datasize)
<del> super(view, cmd, cmdsize)
<del> @dataoff = dataoff
<del> @datasize = datasize
<add> # @api private
<add> def initialize(view, cmd, cmdsize, dataoff, datasize)
<add> super(view, cmd, cmdsize)
<add> @dataoff = dataoff
<add> @datasize = datasize
<add> end
<ide> end
<del> end
<ide>
<del> # A load command representing the offset to and size of an encrypted
<del> # segment. Corresponds to LC_ENCRYPTION_INFO.
<del> class EncryptionInfoCommand < LoadCommand
<del> # @return [Fixnum] the offset to the encrypted segment
<del> attr_reader :cryptoff
<add> # A load command representing the offset to and size of an encrypted
<add> # segment. Corresponds to LC_ENCRYPTION_INFO.
<add> class EncryptionInfoCommand < LoadCommand
<add> # @return [Fixnum] the offset to the encrypted segment
<add> attr_reader :cryptoff
<ide>
<del> # @return [Fixnum] the size of the encrypted segment
<del> attr_reader :cryptsize
<add> # @return [Fixnum] the size of the encrypted segment
<add> attr_reader :cryptsize
<ide>
<del> # @return [Fixnum] the encryption system, or 0 if not encrypted yet
<del> attr_reader :cryptid
<add> # @return [Fixnum] the encryption system, or 0 if not encrypted yet
<add> attr_reader :cryptid
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=5".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=5".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 20
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 20
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, cryptoff, cryptsize, cryptid)
<del> super(view, cmd, cmdsize)
<del> @cryptoff = cryptoff
<del> @cryptsize = cryptsize
<del> @cryptid = cryptid
<add> # @api private
<add> def initialize(view, cmd, cmdsize, cryptoff, cryptsize, cryptid)
<add> super(view, cmd, cmdsize)
<add> @cryptoff = cryptoff
<add> @cryptsize = cryptsize
<add> @cryptid = cryptid
<add> end
<ide> end
<del> end
<ide>
<del> # A load command representing the offset to and size of an encrypted
<del> # segment. Corresponds to LC_ENCRYPTION_INFO_64.
<del> class EncryptionInfoCommand64 < LoadCommand
<del> # @return [Fixnum] the offset to the encrypted segment
<del> attr_reader :cryptoff
<add> # A load command representing the offset to and size of an encrypted
<add> # segment. Corresponds to LC_ENCRYPTION_INFO_64.
<add> class EncryptionInfoCommand64 < LoadCommand
<add> # @return [Fixnum] the offset to the encrypted segment
<add> attr_reader :cryptoff
<ide>
<del> # @return [Fixnum] the size of the encrypted segment
<del> attr_reader :cryptsize
<add> # @return [Fixnum] the size of the encrypted segment
<add> attr_reader :cryptsize
<ide>
<del> # @return [Fixnum] the encryption system, or 0 if not encrypted yet
<del> attr_reader :cryptid
<add> # @return [Fixnum] the encryption system, or 0 if not encrypted yet
<add> attr_reader :cryptid
<ide>
<del> # @return [Fixnum] 64-bit padding value
<del> attr_reader :pad
<add> # @return [Fixnum] 64-bit padding value
<add> attr_reader :pad
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=6".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=6".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 24
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 24
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, cryptoff, cryptsize, cryptid, pad)
<del> super(view, cmd, cmdsize)
<del> @cryptoff = cryptoff
<del> @cryptsize = cryptsize
<del> @cryptid = cryptid
<del> @pad = pad
<add> # @api private
<add> def initialize(view, cmd, cmdsize, cryptoff, cryptsize, cryptid, pad)
<add> super(view, cmd, cmdsize)
<add> @cryptoff = cryptoff
<add> @cryptsize = cryptsize
<add> @cryptid = cryptid
<add> @pad = pad
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing the minimum OS version on which the binary
<del> # was built to run. Corresponds to LC_VERSION_MIN_MACOSX and LC_VERSION_MIN_IPHONEOS.
<del> class VersionMinCommand < LoadCommand
<del> # @return [Fixnum] the version X.Y.Z packed as x16.y8.z8
<del> attr_reader :version
<add> # A load command containing the minimum OS version on which the binary
<add> # was built to run. Corresponds to LC_VERSION_MIN_MACOSX and
<add> # LC_VERSION_MIN_IPHONEOS.
<add> class VersionMinCommand < LoadCommand
<add> # @return [Fixnum] the version X.Y.Z packed as x16.y8.z8
<add> attr_reader :version
<ide>
<del> # @return [Fixnum] the SDK version X.Y.Z packed as x16.y8.z8
<del> attr_reader :sdk
<add> # @return [Fixnum] the SDK version X.Y.Z packed as x16.y8.z8
<add> attr_reader :sdk
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=4".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=4".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 16
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 16
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, version, sdk)
<del> super(view, cmd, cmdsize)
<del> @version = version
<del> @sdk = sdk
<del> end
<add> # @api private
<add> def initialize(view, cmd, cmdsize, version, sdk)
<add> super(view, cmd, cmdsize)
<add> @version = version
<add> @sdk = sdk
<add> end
<ide>
<del> # A string representation of the binary's minimum OS version.
<del> # @return [String] a string representing the minimum OS version.
<del> def version_string
<del> binary = "%032b" % version
<del> segs = [
<del> binary[0..15], binary[16..23], binary[24..31]
<del> ].map { |s| s.to_i(2) }
<add> # A string representation of the binary's minimum OS version.
<add> # @return [String] a string representing the minimum OS version.
<add> def version_string
<add> binary = "%032b" % version
<add> segs = [
<add> binary[0..15], binary[16..23], binary[24..31]
<add> ].map { |s| s.to_i(2) }
<ide>
<del> segs.join(".")
<del> end
<add> segs.join(".")
<add> end
<ide>
<del> # A string representation of the binary's SDK version.
<del> # @return [String] a string representing the SDK version.
<del> def sdk_string
<del> binary = "%032b" % sdk
<del> segs = [
<del> binary[0..15], binary[16..23], binary[24..31]
<del> ].map { |s| s.to_i(2) }
<add> # A string representation of the binary's SDK version.
<add> # @return [String] a string representing the SDK version.
<add> def sdk_string
<add> binary = "%032b" % sdk
<add> segs = [
<add> binary[0..15], binary[16..23], binary[24..31]
<add> ].map { |s| s.to_i(2) }
<ide>
<del> segs.join(".")
<add> segs.join(".")
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing the file offsets and sizes of the new
<del> # compressed form of the information dyld needs to load the image.
<del> # Corresponds to LC_DYLD_INFO and LC_DYLD_INFO_ONLY.
<del> class DyldInfoCommand < LoadCommand
<del> # @return [Fixnum] the file offset to the rebase information
<del> attr_reader :rebase_off
<add> # A load command containing the file offsets and sizes of the new
<add> # compressed form of the information dyld needs to load the image.
<add> # Corresponds to LC_DYLD_INFO and LC_DYLD_INFO_ONLY.
<add> class DyldInfoCommand < LoadCommand
<add> # @return [Fixnum] the file offset to the rebase information
<add> attr_reader :rebase_off
<ide>
<del> # @return [Fixnum] the size of the rebase information
<del> attr_reader :rebase_size
<add> # @return [Fixnum] the size of the rebase information
<add> attr_reader :rebase_size
<ide>
<del> # @return [Fixnum] the file offset to the binding information
<del> attr_reader :bind_off
<add> # @return [Fixnum] the file offset to the binding information
<add> attr_reader :bind_off
<ide>
<del> # @return [Fixnum] the size of the binding information
<del> attr_reader :bind_size
<add> # @return [Fixnum] the size of the binding information
<add> attr_reader :bind_size
<ide>
<del> # @return [Fixnum] the file offset to the weak binding information
<del> attr_reader :weak_bind_off
<add> # @return [Fixnum] the file offset to the weak binding information
<add> attr_reader :weak_bind_off
<ide>
<del> # @return [Fixnum] the size of the weak binding information
<del> attr_reader :weak_bind_size
<add> # @return [Fixnum] the size of the weak binding information
<add> attr_reader :weak_bind_size
<ide>
<del> # @return [Fixnum] the file offset to the lazy binding information
<del> attr_reader :lazy_bind_off
<add> # @return [Fixnum] the file offset to the lazy binding information
<add> attr_reader :lazy_bind_off
<ide>
<del> # @return [Fixnum] the size of the lazy binding information
<del> attr_reader :lazy_bind_size
<add> # @return [Fixnum] the size of the lazy binding information
<add> attr_reader :lazy_bind_size
<ide>
<del> # @return [Fixnum] the file offset to the export information
<del> attr_reader :export_off
<add> # @return [Fixnum] the file offset to the export information
<add> attr_reader :export_off
<ide>
<del> # @return [Fixnum] the size of the export information
<del> attr_reader :export_size
<add> # @return [Fixnum] the size of the export information
<add> attr_reader :export_size
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=12".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=12".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 48
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 48
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, rebase_off, rebase_size, bind_off,
<del> bind_size, weak_bind_off, weak_bind_size, lazy_bind_off,
<del> lazy_bind_size, export_off, export_size)
<del> super(view, cmd, cmdsize)
<del> @rebase_off = rebase_off
<del> @rebase_size = rebase_size
<del> @bind_off = bind_off
<del> @bind_size = bind_size
<del> @weak_bind_off = weak_bind_off
<del> @weak_bind_size = weak_bind_size
<del> @lazy_bind_off = lazy_bind_off
<del> @lazy_bind_size = lazy_bind_size
<del> @export_off = export_off
<del> @export_size = export_size
<add> # @api private
<add> def initialize(view, cmd, cmdsize, rebase_off, rebase_size, bind_off,
<add> bind_size, weak_bind_off, weak_bind_size, lazy_bind_off,
<add> lazy_bind_size, export_off, export_size)
<add> super(view, cmd, cmdsize)
<add> @rebase_off = rebase_off
<add> @rebase_size = rebase_size
<add> @bind_off = bind_off
<add> @bind_size = bind_size
<add> @weak_bind_off = weak_bind_off
<add> @weak_bind_size = weak_bind_size
<add> @lazy_bind_off = lazy_bind_off
<add> @lazy_bind_size = lazy_bind_size
<add> @export_off = export_off
<add> @export_size = export_size
<add> end
<ide> end
<del> end
<ide>
<del> # A load command containing linker options embedded in object files.
<del> # Corresponds to LC_LINKER_OPTION.
<del> class LinkerOptionCommand < LoadCommand
<del> # @return [Fixnum] the number of strings
<del> attr_reader :count
<add> # A load command containing linker options embedded in object files.
<add> # Corresponds to LC_LINKER_OPTION.
<add> class LinkerOptionCommand < LoadCommand
<add> # @return [Fixnum] the number of strings
<add> attr_reader :count
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=3".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=3".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 12
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 12
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, count)
<del> super(view, cmd, cmdsize)
<del> @count = count
<add> # @api private
<add> def initialize(view, cmd, cmdsize, count)
<add> super(view, cmd, cmdsize)
<add> @count = count
<add> end
<ide> end
<del> end
<ide>
<del> # A load command specifying the offset of main(). Corresponds to LC_MAIN.
<del> class EntryPointCommand < LoadCommand
<del> # @return [Fixnum] the file (__TEXT) offset of main()
<del> attr_reader :entryoff
<add> # A load command specifying the offset of main(). Corresponds to LC_MAIN.
<add> class EntryPointCommand < LoadCommand
<add> # @return [Fixnum] the file (__TEXT) offset of main()
<add> attr_reader :entryoff
<ide>
<del> # @return [Fixnum] if not 0, the initial stack size.
<del> attr_reader :stacksize
<add> # @return [Fixnum] if not 0, the initial stack size.
<add> attr_reader :stacksize
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2Q=2".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2Q=2".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 24
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 24
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, entryoff, stacksize)
<del> super(view, cmd, cmdsize)
<del> @entryoff = entryoff
<del> @stacksize = stacksize
<add> # @api private
<add> def initialize(view, cmd, cmdsize, entryoff, stacksize)
<add> super(view, cmd, cmdsize)
<add> @entryoff = entryoff
<add> @stacksize = stacksize
<add> end
<ide> end
<del> end
<ide>
<del> # A load command specifying the version of the sources used to build the
<del> # binary. Corresponds to LC_SOURCE_VERSION.
<del> class SourceVersionCommand < LoadCommand
<del> # @return [Fixnum] the version packed as a24.b10.c10.d10.e10
<del> attr_reader :version
<add> # A load command specifying the version of the sources used to build the
<add> # binary. Corresponds to LC_SOURCE_VERSION.
<add> class SourceVersionCommand < LoadCommand
<add> # @return [Fixnum] the version packed as a24.b10.c10.d10.e10
<add> attr_reader :version
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2Q=1".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2Q=1".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 16
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 16
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, version)
<del> super(view, cmd, cmdsize)
<del> @version = version
<del> end
<add> # @api private
<add> def initialize(view, cmd, cmdsize, version)
<add> super(view, cmd, cmdsize)
<add> @version = version
<add> end
<ide>
<del> # A string representation of the sources used to build the binary.
<del> # @return [String] a string representation of the version
<del> def version_string
<del> binary = "%064b" % version
<del> segs = [
<del> binary[0..23], binary[24..33], binary[34..43], binary[44..53],
<del> binary[54..63]
<del> ].map { |s| s.to_i(2) }
<add> # A string representation of the sources used to build the binary.
<add> # @return [String] a string representation of the version
<add> def version_string
<add> binary = "%064b" % version
<add> segs = [
<add> binary[0..23], binary[24..33], binary[34..43], binary[44..53],
<add> binary[54..63]
<add> ].map { |s| s.to_i(2) }
<ide>
<del> segs.join(".")
<add> segs.join(".")
<add> end
<ide> end
<del> end
<ide>
<del> # An obsolete load command containing the offset and size of the (GNU style)
<del> # symbol table information. Corresponds to LC_SYMSEG.
<del> class SymsegCommand < LoadCommand
<del> # @return [Fixnum] the offset to the symbol segment
<del> attr_reader :offset
<add> # An obsolete load command containing the offset and size of the (GNU style)
<add> # symbol table information. Corresponds to LC_SYMSEG.
<add> class SymsegCommand < LoadCommand
<add> # @return [Fixnum] the offset to the symbol segment
<add> attr_reader :offset
<ide>
<del> # @return [Fixnum] the size of the symbol segment in bytes
<del> attr_reader :size
<add> # @return [Fixnum] the size of the symbol segment in bytes
<add> attr_reader :size
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=4".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=4".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 16
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 16
<ide>
<del> # @api private
<del> def initialize(view, cmd, cmdsize, offset, size)
<del> super(view, cmd, cmdsize)
<del> @offset = offset
<del> @size = size
<add> # @api private
<add> def initialize(view, cmd, cmdsize, offset, size)
<add> super(view, cmd, cmdsize)
<add> @offset = offset
<add> @size = size
<add> end
<ide> end
<del> end
<ide>
<del> # An obsolete load command containing a free format string table. Each string
<del> # is null-terminated and the command is zero-padded to a multiple of 4.
<del> # Corresponds to LC_IDENT.
<del> class IdentCommand < LoadCommand
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=2".freeze
<add> # An obsolete load command containing a free format string table. Each
<add> # string is null-terminated and the command is zero-padded to a multiple of
<add> # 4. Corresponds to LC_IDENT.
<add> class IdentCommand < LoadCommand
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=2".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 8
<del> end
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 8
<add> end
<ide>
<del> # An obsolete load command containing the path to a file to be loaded into
<del> # memory. Corresponds to LC_FVMFILE.
<del> class FvmfileCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the pathname of the file being loaded
<del> attr_reader :name
<add> # An obsolete load command containing the path to a file to be loaded into
<add> # memory. Corresponds to LC_FVMFILE.
<add> class FvmfileCommand < LoadCommand
<add> # @return [LCStr] the pathname of the file being loaded
<add> attr_reader :name
<ide>
<del> # @return [Fixnum] the virtual address being loaded at
<del> attr_reader :header_addr
<add> # @return [Fixnum] the virtual address being loaded at
<add> attr_reader :header_addr
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=4".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=4".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 16
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 16
<ide>
<del> def initialize(view, cmd, cmdsize, name, header_addr)
<del> super(view, cmd, cmdsize)
<del> @name = LCStr.new(self, name)
<del> @header_addr = header_addr
<add> def initialize(view, cmd, cmdsize, name, header_addr)
<add> super(view, cmd, cmdsize)
<add> @name = LCStr.new(self, name)
<add> @header_addr = header_addr
<add> end
<ide> end
<del> end
<ide>
<del> # An obsolete load command containing the path to a library to be loaded into
<del> # memory. Corresponds to LC_LOADFVMLIB and LC_IDFVMLIB.
<del> class FvmlibCommand < LoadCommand
<del> # @return [MachO::LoadCommand::LCStr] the library's target pathname
<del> attr_reader :name
<add> # An obsolete load command containing the path to a library to be loaded
<add> # into memory. Corresponds to LC_LOADFVMLIB and LC_IDFVMLIB.
<add> class FvmlibCommand < LoadCommand
<add> # @return [LCStr] the library's target pathname
<add> attr_reader :name
<ide>
<del> # @return [Fixnum] the library's minor version number
<del> attr_reader :minor_version
<add> # @return [Fixnum] the library's minor version number
<add> attr_reader :minor_version
<ide>
<del> # @return [Fixnum] the library's header address
<del> attr_reader :header_addr
<add> # @return [Fixnum] the library's header address
<add> attr_reader :header_addr
<ide>
<del> # @see MachOStructure::FORMAT
<del> # @api private
<del> FORMAT = "L=5".freeze
<add> # @see MachOStructure::FORMAT
<add> # @api private
<add> FORMAT = "L=5".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> # @api private
<del> SIZEOF = 20
<add> # @see MachOStructure::SIZEOF
<add> # @api private
<add> SIZEOF = 20
<ide>
<del> def initialize(view, cmd, cmdsize, name, minor_version, header_addr)
<del> super(view, cmd, cmdsize)
<del> @name = LCStr.new(self, name)
<del> @minor_version = minor_version
<del> @header_addr = header_addr
<add> def initialize(view, cmd, cmdsize, name, minor_version, header_addr)
<add> super(view, cmd, cmdsize)
<add> @name = LCStr.new(self, name)
<add> @minor_version = minor_version
<add> @header_addr = header_addr
<add> end
<ide> end
<ide> end
<ide> end
<ide><path>Library/Homebrew/vendor/macho/macho/macho_file.rb
<add>require "forwardable"
<add>
<ide> module MachO
<ide> # Represents a Mach-O file, which contains a header and load commands
<ide> # as well as binary executable instructions. Mach-O binaries are
<ide> # architecture specific.
<ide> # @see https://en.wikipedia.org/wiki/Mach-O
<del> # @see MachO::FatFile
<add> # @see FatFile
<ide> class MachOFile
<del> # @return [String] the filename loaded from, or nil if loaded from a binary string
<add> extend Forwardable
<add>
<add> # @return [String] the filename loaded from, or nil if loaded from a binary
<add> # string
<ide> attr_accessor :filename
<ide>
<ide> # @return [Symbol] the endianness of the file, :big or :little
<ide> attr_reader :endianness
<ide>
<del> # @return [MachO::MachHeader] if the Mach-O is 32-bit
<del> # @return [MachO::MachHeader64] if the Mach-O is 64-bit
<add> # @return [Headers::MachHeader] if the Mach-O is 32-bit
<add> # @return [Headers::MachHeader64] if the Mach-O is 64-bit
<ide> attr_reader :header
<ide>
<del> # @return [Array<MachO::LoadCommand>] an array of the file's load commands
<add> # @return [Array<LoadCommands::LoadCommand>] an array of the file's load
<add> # commands
<ide> # @note load commands are provided in order of ascending offset.
<ide> attr_reader :load_commands
<ide>
<ide> # Creates a new MachOFile instance from a binary string.
<ide> # @param bin [String] a binary string containing raw Mach-O data
<del> # @return [MachO::MachOFile] a new MachOFile
<add> # @return [MachOFile] a new MachOFile
<ide> def self.new_from_bin(bin)
<ide> instance = allocate
<ide> instance.initialize_from_bin(bin)
<ide> def serialize
<ide> @raw_data
<ide> end
<ide>
<del> # @return [Boolean] true if the Mach-O has 32-bit magic, false otherwise
<del> def magic32?
<del> Utils.magic32?(header.magic)
<del> end
<del>
<del> # @return [Boolean] true if the Mach-O has 64-bit magic, false otherwise
<del> def magic64?
<del> Utils.magic64?(header.magic)
<del> end
<del>
<del> # @return [Fixnum] the file's internal alignment
<del> def alignment
<del> magic32? ? 4 : 8
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_OBJECT`, false otherwise
<del> def object?
<del> header.filetype == MH_OBJECT
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_EXECUTE`, false otherwise
<del> def executable?
<del> header.filetype == MH_EXECUTE
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_FVMLIB`, false otherwise
<del> def fvmlib?
<del> header.filetype == MH_FVMLIB
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_CORE`, false otherwise
<del> def core?
<del> header.filetype == MH_CORE
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_PRELOAD`, false otherwise
<del> def preload?
<del> header.filetype == MH_PRELOAD
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_DYLIB`, false otherwise
<del> def dylib?
<del> header.filetype == MH_DYLIB
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_DYLINKER`, false otherwise
<del> def dylinker?
<del> header.filetype == MH_DYLINKER
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_BUNDLE`, false otherwise
<del> def bundle?
<del> header.filetype == MH_BUNDLE
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_DSYM`, false otherwise
<del> def dsym?
<del> header.filetype == MH_DSYM
<del> end
<del>
<del> # @return [Boolean] true if the file is of type `MH_KEXT_BUNDLE`, false otherwise
<del> def kext?
<del> header.filetype == MH_KEXT_BUNDLE
<del> end
<del>
<del> # @return [Fixnum] the file's magic number
<del> def magic
<del> header.magic
<del> end
<add> # @!method magic
<add> # @return (see MachO::Headers::MachHeader#magic)
<add> # @!method ncmds
<add> # @return (see MachO::Headers::MachHeader#ncmds)
<add> # @!method sizeofcmds
<add> # @return (see MachO::Headers::MachHeader#sizeofcmds)
<add> # @!method flags
<add> # @return (see MachO::Headers::MachHeader#flags)
<add> # @!method object?
<add> # @return (see MachO::Headers::MachHeader#object?)
<add> # @!method executable?
<add> # @return (see MachO::Headers::MachHeader#executable?)
<add> # @!method fvmlib?
<add> # @return (see MachO::Headers::MachHeader#fvmlib?)
<add> # @!method core?
<add> # @return (see MachO::Headers::MachHeader#core?)
<add> # @!method preload?
<add> # @return (see MachO::Headers::MachHeader#preload?)
<add> # @!method dylib?
<add> # @return (see MachO::Headers::MachHeader#dylib?)
<add> # @!method dylinker?
<add> # @return (see MachO::Headers::MachHeader#dylinker?)
<add> # @!method bundle?
<add> # @return (see MachO::Headers::MachHeader#bundle?)
<add> # @!method dsym?
<add> # @return (see MachO::Headers::MachHeader#dsym?)
<add> # @!method kext?
<add> # @return (see MachO::Headers::MachHeader#kext?)
<add> # @!method magic32?
<add> # @return (see MachO::Headers::MachHeader#magic32?)
<add> # @!method magic64?
<add> # @return (see MachO::Headers::MachHeader#magic64?)
<add> # @!method alignment
<add> # @return (see MachO::Headers::MachHeader#alignment)
<add> def_delegators :header, :magic, :ncmds, :sizeofcmds, :flags, :object?,
<add> :executable?, :fvmlib?, :core?, :preload?, :dylib?,
<add> :dylinker?, :bundle?, :dsym?, :kext?, :magic32?, :magic64?,
<add> :alignment
<ide>
<ide> # @return [String] a string representation of the file's magic number
<ide> def magic_string
<del> MH_MAGICS[magic]
<add> Headers::MH_MAGICS[magic]
<ide> end
<ide>
<ide> # @return [Symbol] a string representation of the Mach-O's filetype
<ide> def filetype
<del> MH_FILETYPES[header.filetype]
<add> Headers::MH_FILETYPES[header.filetype]
<ide> end
<ide>
<ide> # @return [Symbol] a symbol representation of the Mach-O's CPU type
<ide> def cputype
<del> CPU_TYPES[header.cputype]
<add> Headers::CPU_TYPES[header.cputype]
<ide> end
<ide>
<ide> # @return [Symbol] a symbol representation of the Mach-O's CPU subtype
<ide> def cpusubtype
<del> CPU_SUBTYPES[header.cputype][header.cpusubtype]
<del> end
<del>
<del> # @return [Fixnum] the number of load commands in the Mach-O's header
<del> def ncmds
<del> header.ncmds
<del> end
<del>
<del> # @return [Fixnum] the size of all load commands, in bytes
<del> def sizeofcmds
<del> header.sizeofcmds
<del> end
<del>
<del> # @return [Fixnum] execution flags set by the linker
<del> def flags
<del> header.flags
<add> Headers::CPU_SUBTYPES[header.cputype][header.cpusubtype]
<ide> end
<ide>
<ide> # All load commands of a given name.
<ide> # @example
<ide> # file.command("LC_LOAD_DYLIB")
<ide> # file[:LC_LOAD_DYLIB]
<ide> # @param [String, Symbol] name the load command ID
<del> # @return [Array<MachO::LoadCommand>] an array of LoadCommands corresponding to `name`
<add> # @return [Array<LoadCommands::LoadCommand>] an array of load commands
<add> # corresponding to `name`
<ide> def command(name)
<ide> load_commands.select { |lc| lc.type == name.to_sym }
<ide> end
<ide> def command(name)
<ide>
<ide> # Inserts a load command at the given offset.
<ide> # @param offset [Fixnum] the offset to insert at
<del> # @param lc [MachO::LoadCommand] the load command to insert
<add> # @param lc [LoadCommands::LoadCommand] the load command to insert
<ide> # @param options [Hash]
<ide> # @option options [Boolean] :repopulate (true) whether or not to repopulate
<ide> # the instance fields
<del> # @raise [MachO::OffsetInsertionError] if the offset is not in the load command region
<del> # @raise [MachO::HeaderPadError] if the new command exceeds the header pad buffer
<add> # @raise [OffsetInsertionError] if the offset is not in the load command region
<add> # @raise [HeaderPadError] if the new command exceeds the header pad buffer
<ide> # @note Calling this method with an arbitrary offset in the load command
<ide> # region **will leave the object in an inconsistent state**.
<ide> def insert_command(offset, lc, options = {})
<del> context = LoadCommand::SerializationContext.context_for(self)
<add> context = LoadCommands::LoadCommand::SerializationContext.context_for(self)
<ide> cmd_raw = lc.serialize(context)
<ide>
<ide> if offset < header.class.bytesize || offset + cmd_raw.bytesize > low_fileoff
<ide> def insert_command(offset, lc, options = {})
<ide> end
<ide>
<ide> # Replace a load command with another command in the Mach-O, preserving location.
<del> # @param old_lc [MachO::LoadCommand] the load command being replaced
<del> # @param new_lc [MachO::LoadCommand] the load command being added
<add> # @param old_lc [LoadCommands::LoadCommand] the load command being replaced
<add> # @param new_lc [LoadCommands::LoadCommand] the load command being added
<ide> # @return [void]
<del> # @raise [MachO::HeaderPadError] if the new command exceeds the header pad buffer
<del> # @see {#insert_command}
<add> # @raise [HeaderPadError] if the new command exceeds the header pad buffer
<add> # @see #insert_command
<ide> # @note This is public, but methods like {#dylib_id=} should be preferred.
<ide> def replace_command(old_lc, new_lc)
<del> context = LoadCommand::SerializationContext.context_for(self)
<add> context = LoadCommands::LoadCommand::SerializationContext.context_for(self)
<ide> cmd_raw = new_lc.serialize(context)
<ide> new_sizeofcmds = sizeofcmds + cmd_raw.bytesize - old_lc.cmdsize
<ide> if header.class.bytesize + new_sizeofcmds > low_fileoff
<ide> def replace_command(old_lc, new_lc)
<ide> end
<ide>
<ide> # Appends a new load command to the Mach-O.
<del> # @param lc [MachO::LoadCommand] the load command being added
<add> # @param lc [LoadCommands::LoadCommand] the load command being added
<ide> # @param options [Hash]
<ide> # @option options [Boolean] :repopulate (true) whether or not to repopulate
<ide> # the instance fields
<ide> # @return [void]
<del> # @see {#insert_command}
<add> # @see #insert_command
<ide> # @note This is public, but methods like {#add_rpath} should be preferred.
<ide> # Setting `repopulate` to false **will leave the instance in an
<ide> # inconsistent state** unless {#populate_fields} is called **immediately**
<ide> def add_command(lc, options = {})
<ide> end
<ide>
<ide> # Delete a load command from the Mach-O.
<del> # @param lc [MachO::LoadCommand] the load command being deleted
<add> # @param lc [LoadCommands::LoadCommand] the load command being deleted
<ide> # @param options [Hash]
<ide> # @option options [Boolean] :repopulate (true) whether or not to repopulate
<ide> # the instance fields
<ide> def populate_fields
<ide> end
<ide>
<ide> # All load commands responsible for loading dylibs.
<del> # @return [Array<MachO::DylibCommand>] an array of DylibCommands
<add> # @return [Array<LoadCommands::DylibCommand>] an array of DylibCommands
<ide> def dylib_load_commands
<del> load_commands.select { |lc| DYLIB_LOAD_COMMANDS.include?(lc.type) }
<add> load_commands.select { |lc| LoadCommands::DYLIB_LOAD_COMMANDS.include?(lc.type) }
<ide> end
<ide>
<ide> # All segment load commands in the Mach-O.
<del> # @return [Array<MachO::SegmentCommand>] if the Mach-O is 32-bit
<del> # @return [Array<MachO::SegmentCommand64>] if the Mach-O is 64-bit
<add> # @return [Array<LoadCommands::SegmentCommand>] if the Mach-O is 32-bit
<add> # @return [Array<LoadCommands::SegmentCommand64>] if the Mach-O is 64-bit
<ide> def segments
<ide> if magic32?
<ide> command(:LC_SEGMENT)
<ide> def change_dylib_id(new_id, _options = {})
<ide> old_lc = command(:LC_ID_DYLIB).first
<ide> raise DylibIdMissingError unless old_lc
<ide>
<del> new_lc = LoadCommand.create(:LC_ID_DYLIB, new_id,
<del> old_lc.timestamp,
<del> old_lc.current_version,
<del> old_lc.compatibility_version)
<add> new_lc = LoadCommands::LoadCommand.create(:LC_ID_DYLIB, new_id,
<add> old_lc.timestamp,
<add> old_lc.current_version,
<add> old_lc.compatibility_version)
<ide>
<ide> replace_command(old_lc, new_lc)
<ide> end
<ide> def linked_dylibs
<ide>
<ide> # Changes the shared library `old_name` to `new_name`
<ide> # @example
<del> # file.change_install_name("/usr/lib/libWhatever.dylib", "/usr/local/lib/libWhatever2.dylib")
<add> # file.change_install_name("abc.dylib", "def.dylib")
<ide> # @param old_name [String] the shared library's old name
<ide> # @param new_name [String] the shared library's new name
<ide> # @param _options [Hash]
<ide> # @return [void]
<del> # @raise [MachO::DylibUnknownError] if no shared library has the old name
<add> # @raise [DylibUnknownError] if no shared library has the old name
<ide> # @note `_options` is currently unused and is provided for signature
<ide> # compatibility with {MachO::FatFile#change_install_name}
<ide> def change_install_name(old_name, new_name, _options = {})
<ide> old_lc = dylib_load_commands.find { |d| d.name.to_s == old_name }
<ide> raise DylibUnknownError, old_name if old_lc.nil?
<ide>
<del> new_lc = LoadCommand.create(old_lc.type, new_name,
<del> old_lc.timestamp,
<del> old_lc.current_version,
<del> old_lc.compatibility_version)
<add> new_lc = LoadCommands::LoadCommand.create(old_lc.type, new_name,
<add> old_lc.timestamp,
<add> old_lc.current_version,
<add> old_lc.compatibility_version)
<ide>
<ide> replace_command(old_lc, new_lc)
<ide> end
<ide> def rpaths
<ide> # @param new_path [String] the new runtime path
<ide> # @param _options [Hash]
<ide> # @return [void]
<del> # @raise [MachO::RpathUnknownError] if no such old runtime path exists
<del> # @raise [MachO::RpathExistsError] if the new runtime path already exists
<add> # @raise [RpathUnknownError] if no such old runtime path exists
<add> # @raise [RpathExistsError] if the new runtime path already exists
<ide> # @note `_options` is currently unused and is provided for signature
<ide> # compatibility with {MachO::FatFile#change_rpath}
<ide> def change_rpath(old_path, new_path, _options = {})
<ide> old_lc = command(:LC_RPATH).find { |r| r.path.to_s == old_path }
<ide> raise RpathUnknownError, old_path if old_lc.nil?
<ide> raise RpathExistsError, new_path if rpaths.include?(new_path)
<ide>
<del> new_lc = LoadCommand.create(:LC_RPATH, new_path)
<add> new_lc = LoadCommands::LoadCommand.create(:LC_RPATH, new_path)
<ide>
<ide> delete_rpath(old_path)
<ide> insert_command(old_lc.view.offset, new_lc)
<ide> def change_rpath(old_path, new_path, _options = {})
<ide> # @param path [String] the new runtime path
<ide> # @param _options [Hash]
<ide> # @return [void]
<del> # @raise [MachO::RpathExistsError] if the runtime path already exists
<add> # @raise [RpathExistsError] if the runtime path already exists
<ide> # @note `_options` is currently unused and is provided for signature
<ide> # compatibility with {MachO::FatFile#add_rpath}
<ide> def add_rpath(path, _options = {})
<ide> raise RpathExistsError, path if rpaths.include?(path)
<ide>
<del> rpath_cmd = LoadCommand.create(:LC_RPATH, path)
<add> rpath_cmd = LoadCommands::LoadCommand.create(:LC_RPATH, path)
<ide> add_command(rpath_cmd)
<ide> end
<ide>
<ide> def add_rpath(path, _options = {})
<ide> # @param path [String] the runtime path to delete
<ide> # @param _options [Hash]
<ide> # @return void
<del> # @raise [MachO::RpathUnknownError] if no such runtime path exists
<add> # @raise [RpathUnknownError] if no such runtime path exists
<ide> # @note `_options` is currently unused and is provided for signature
<ide> # compatibility with {MachO::FatFile#delete_rpath}
<ide> def delete_rpath(path, _options = {})
<ide> def delete_rpath(path, _options = {})
<ide> populate_fields
<ide> end
<ide>
<del> # All sections of the segment `segment`.
<del> # @param segment [MachO::SegmentCommand, MachO::SegmentCommand64] the segment being inspected
<del> # @return [Array<MachO::Section>] if the Mach-O is 32-bit
<del> # @return [Array<MachO::Section64>] if the Mach-O is 64-bit
<del> # @deprecated use {MachO::SegmentCommand#sections} instead
<del> def sections(segment)
<del> segment.sections
<del> end
<del>
<ide> # Write all Mach-O data to the given filename.
<ide> # @param filename [String] the file to write to
<ide> # @return [void]
<ide> def write(filename)
<ide>
<ide> # Write all Mach-O data to the file used to initialize the instance.
<ide> # @return [void]
<del> # @raise [MachO::MachOError] if the instance was initialized without a file
<add> # @raise [MachOError] if the instance was initialized without a file
<ide> # @note Overwrites all data in the file!
<ide> def write!
<ide> if @filename.nil?
<ide> def write!
<ide> private
<ide>
<ide> # The file's Mach-O header structure.
<del> # @return [MachO::MachHeader] if the Mach-O is 32-bit
<del> # @return [MachO::MachHeader64] if the Mach-O is 64-bit
<del> # @raise [MachO::TruncatedFileError] if the file is too small to have a valid header
<add> # @return [Headers::MachHeader] if the Mach-O is 32-bit
<add> # @return [Headers::MachHeader64] if the Mach-O is 64-bit
<add> # @raise [TruncatedFileError] if the file is too small to have a valid header
<ide> # @api private
<ide> def populate_mach_header
<ide> # the smallest Mach-O header is 28 bytes
<ide> raise TruncatedFileError if @raw_data.size < 28
<ide>
<ide> magic = populate_and_check_magic
<del> mh_klass = Utils.magic32?(magic) ? MachHeader : MachHeader64
<add> mh_klass = Utils.magic32?(magic) ? Headers::MachHeader : Headers::MachHeader64
<ide> mh = mh_klass.new_from_bin(endianness, @raw_data[0, mh_klass.bytesize])
<ide>
<ide> check_cputype(mh.cputype)
<ide> def populate_mach_header
<ide>
<ide> # Read just the file's magic number and check its validity.
<ide> # @return [Fixnum] the magic
<del> # @raise [MachO::MagicError] if the magic is not valid Mach-O magic
<del> # @raise [MachO::FatBinaryError] if the magic is for a Fat file
<add> # @raise [MagicError] if the magic is not valid Mach-O magic
<add> # @raise [FatBinaryError] if the magic is for a Fat file
<ide> # @api private
<ide> def populate_and_check_magic
<ide> magic = @raw_data[0..3].unpack("N").first
<ide> def populate_and_check_magic
<ide>
<ide> # Check the file's CPU type.
<ide> # @param cputype [Fixnum] the CPU type
<del> # @raise [MachO::CPUTypeError] if the CPU type is unknown
<add> # @raise [CPUTypeError] if the CPU type is unknown
<ide> # @api private
<ide> def check_cputype(cputype)
<del> raise CPUTypeError, cputype unless CPU_TYPES.key?(cputype)
<add> raise CPUTypeError, cputype unless Headers::CPU_TYPES.key?(cputype)
<ide> end
<ide>
<ide> # Check the file's CPU type/subtype pair.
<ide> # @param cpusubtype [Fixnum] the CPU subtype
<del> # @raise [MachO::CPUSubtypeError] if the CPU sub-type is unknown
<add> # @raise [CPUSubtypeError] if the CPU sub-type is unknown
<ide> # @api private
<ide> def check_cpusubtype(cputype, cpusubtype)
<ide> # Only check sub-type w/o capability bits (see `populate_mach_header`).
<del> raise CPUSubtypeError.new(cputype, cpusubtype) unless CPU_SUBTYPES[cputype].key?(cpusubtype)
<add> raise CPUSubtypeError.new(cputype, cpusubtype) unless Headers::CPU_SUBTYPES[cputype].key?(cpusubtype)
<ide> end
<ide>
<ide> # Check the file's type.
<ide> # @param filetype [Fixnum] the file type
<del> # @raise [MachO::FiletypeError] if the file type is unknown
<add> # @raise [FiletypeError] if the file type is unknown
<ide> # @api private
<ide> def check_filetype(filetype)
<del> raise FiletypeError, filetype unless MH_FILETYPES.key?(filetype)
<add> raise FiletypeError, filetype unless Headers::MH_FILETYPES.key?(filetype)
<ide> end
<ide>
<ide> # All load commands in the file.
<del> # @return [Array<MachO::LoadCommand>] an array of load commands
<del> # @raise [MachO::LoadCommandError] if an unknown load command is encountered
<add> # @return [Array<LoadCommands::LoadCommand>] an array of load commands
<add> # @raise [LoadCommandError] if an unknown load command is encountered
<ide> # @api private
<ide> def populate_load_commands
<ide> offset = header.class.bytesize
<ide> def populate_load_commands
<ide> header.ncmds.times do
<ide> fmt = Utils.specialize_format("L=", endianness)
<ide> cmd = @raw_data.slice(offset, 4).unpack(fmt).first
<del> cmd_sym = LOAD_COMMANDS[cmd]
<add> cmd_sym = LoadCommands::LOAD_COMMANDS[cmd]
<ide>
<ide> raise LoadCommandError, cmd if cmd_sym.nil?
<ide>
<ide> # why do I do this? i don't like declaring constants below
<ide> # classes, and i need them to resolve...
<del> klass = MachO.const_get LC_STRUCTURES[cmd_sym]
<add> klass = LoadCommands.const_get LoadCommands::LC_STRUCTURES[cmd_sym]
<ide> view = MachOView.new(@raw_data, endianness, offset)
<ide> command = klass.new_from_bin(view)
<ide>
<ide><path>Library/Homebrew/vendor/macho/macho/open.rb
<del>module MachO
<del> # Opens the given filename as a MachOFile or FatFile, depending on its magic.
<del> # @param filename [String] the file being opened
<del> # @return [MachO::MachOFile] if the file is a Mach-O
<del> # @return [MachO::FatFile] if the file is a Fat file
<del> # @raise [ArgumentError] if the given file does not exist
<del> # @raise [MachO::TruncatedFileError] if the file is too small to have a valid header
<del> # @raise [MachO::MagicError] if the file's magic is not valid Mach-O magic
<del> def self.open(filename)
<del> raise ArgumentError, "#{filename}: no such file" unless File.file?(filename)
<del> raise TruncatedFileError unless File.stat(filename).size >= 4
<del>
<del> magic = File.open(filename, "rb") { |f| f.read(4) }.unpack("N").first
<del>
<del> if Utils.fat_magic?(magic)
<del> file = FatFile.new(filename)
<del> elsif Utils.magic?(magic)
<del> file = MachOFile.new(filename)
<del> else
<del> raise MagicError, magic
<del> end
<del>
<del> file
<del> end
<del>end
<ide><path>Library/Homebrew/vendor/macho/macho/sections.rb
<ide> module MachO
<del> # type mask
<del> SECTION_TYPE = 0x000000ff
<del>
<del> # attributes mask
<del> SECTION_ATTRIBUTES = 0xffffff00
<del>
<del> # user settable attributes mask
<del> SECTION_ATTRIBUTES_USR = 0xff000000
<del>
<del> # system settable attributes mask
<del> SECTION_ATTRIBUTES_SYS = 0x00ffff00
<del>
<del> # association of section flag symbols to values
<del> # @api private
<del> SECTION_FLAGS = {
<del> :S_REGULAR => 0x0,
<del> :S_ZEROFILL => 0x1,
<del> :S_CSTRING_LITERALS => 0x2,
<del> :S_4BYTE_LITERALS => 0x3,
<del> :S_8BYTE_LITERALS => 0x4,
<del> :S_LITERAL_POINTERS => 0x5,
<del> :S_NON_LAZY_SYMBOL_POINTERS => 0x6,
<del> :S_LAZY_SYMBOL_POINTERS => 0x7,
<del> :S_SYMBOL_STUBS => 0x8,
<del> :S_MOD_INIT_FUNC_POINTERS => 0x9,
<del> :S_MOD_TERM_FUNC_POINTERS => 0xa,
<del> :S_COALESCED => 0xb,
<del> :S_GB_ZEROFILE => 0xc,
<del> :S_INTERPOSING => 0xd,
<del> :S_16BYTE_LITERALS => 0xe,
<del> :S_DTRACE_DOF => 0xf,
<del> :S_LAZY_DYLIB_SYMBOL_POINTERS => 0x10,
<del> :S_THREAD_LOCAL_REGULAR => 0x11,
<del> :S_THREAD_LOCAL_ZEROFILL => 0x12,
<del> :S_THREAD_LOCAL_VARIABLES => 0x13,
<del> :S_THREAD_LOCAL_VARIABLE_POINTERS => 0x14,
<del> :S_THREAD_LOCAL_INIT_FUNCTION_POINTERS => 0x15,
<del> :S_ATTR_PURE_INSTRUCTIONS => 0x80000000,
<del> :S_ATTR_NO_TOC => 0x40000000,
<del> :S_ATTR_STRIP_STATIC_SYMS => 0x20000000,
<del> :S_ATTR_NO_DEAD_STRIP => 0x10000000,
<del> :S_ATTR_LIVE_SUPPORT => 0x08000000,
<del> :S_ATTR_SELF_MODIFYING_CODE => 0x04000000,
<del> :S_ATTR_DEBUG => 0x02000000,
<del> :S_ATTR_SOME_INSTRUCTIONS => 0x00000400,
<del> :S_ATTR_EXT_RELOC => 0x00000200,
<del> :S_ATTR_LOC_RELOC => 0x00000100,
<del> }.freeze
<del>
<del> # association of section name symbols to names
<del> # @api private
<del> SECTION_NAMES = {
<del> :SECT_TEXT => "__text",
<del> :SECT_FVMLIB_INIT0 => "__fvmlib_init0",
<del> :SECT_FVMLIB_INIT1 => "__fvmlib_init1",
<del> :SECT_DATA => "__data",
<del> :SECT_BSS => "__bss",
<del> :SECT_COMMON => "__common",
<del> :SECT_OBJC_SYMBOLS => "__symbol_table",
<del> :SECT_OBJC_MODULES => "__module_info",
<del> :SECT_OBJC_STRINGS => "__selector_strs",
<del> :SECT_OBJC_REFS => "__selector_refs",
<del> :SECT_ICON_HEADER => "__header",
<del> :SECT_ICON_TIFF => "__tiff",
<del> }.freeze
<del>
<del> # Represents a section of a segment for 32-bit architectures.
<del> class Section < MachOStructure
<del> # @return [String] the name of the section, including null pad bytes
<del> attr_reader :sectname
<del>
<del> # @return [String] the name of the segment's section, including null pad bytes
<del> attr_reader :segname
<del>
<del> # @return [Fixnum] the memory address of the section
<del> attr_reader :addr
<del>
<del> # @return [Fixnum] the size, in bytes, of the section
<del> attr_reader :size
<del>
<del> # @return [Fixnum] the file offset of the section
<del> attr_reader :offset
<del>
<del> # @return [Fixnum] the section alignment (power of 2) of the section
<del> attr_reader :align
<del>
<del> # @return [Fixnum] the file offset of the section's relocation entries
<del> attr_reader :reloff
<del>
<del> # @return [Fixnum] the number of relocation entries
<del> attr_reader :nreloc
<del>
<del> # @return [Fixnum] flags for type and attributes of the section
<del> attr_reader :flags
<del>
<del> # @return [void] reserved (for offset or index)
<del> attr_reader :reserved1
<del>
<del> # @return [void] reserved (for count or sizeof)
<del> attr_reader :reserved2
<del>
<del> # @see MachOStructure::FORMAT
<del> FORMAT = "a16a16L=9".freeze
<del>
<del> # @see MachOStructure::SIZEOF
<del> SIZEOF = 68
<add> # Classes and constants for parsing sections in Mach-O binaries.
<add> module Sections
<add> # type mask
<add> SECTION_TYPE = 0x000000ff
<ide>
<del> # @api private
<del> def initialize(sectname, segname, addr, size, offset, align, reloff,
<del> nreloc, flags, reserved1, reserved2)
<del> @sectname = sectname
<del> @segname = segname
<del> @addr = addr
<del> @size = size
<del> @offset = offset
<del> @align = align
<del> @reloff = reloff
<del> @nreloc = nreloc
<del> @flags = flags
<del> @reserved1 = reserved1
<del> @reserved2 = reserved2
<del> end
<add> # attributes mask
<add> SECTION_ATTRIBUTES = 0xffffff00
<ide>
<del> # @return [String] the section's name, with any trailing NULL characters removed
<del> def section_name
<del> sectname.delete("\x00")
<del> end
<del>
<del> # @return [String] the parent segment's name, with any trailing NULL characters removed
<del> def segment_name
<del> segname.delete("\x00")
<del> end
<add> # user settable attributes mask
<add> SECTION_ATTRIBUTES_USR = 0xff000000
<ide>
<del> # @return [Boolean] true if the section has no contents (i.e, `size` is 0)
<del> def empty?
<del> size.zero?
<del> end
<add> # system settable attributes mask
<add> SECTION_ATTRIBUTES_SYS = 0x00ffff00
<ide>
<del> # @example
<del> # puts "this section is regular" if sect.flag?(:S_REGULAR)
<del> # @param flag [Symbol] a section flag symbol
<del> # @return [Boolean] true if `flag` is present in the section's flag field
<del> def flag?(flag)
<del> flag = SECTION_FLAGS[flag]
<del> return false if flag.nil?
<del> flags & flag == flag
<add> # association of section flag symbols to values
<add> # @api private
<add> SECTION_FLAGS = {
<add> :S_REGULAR => 0x0,
<add> :S_ZEROFILL => 0x1,
<add> :S_CSTRING_LITERALS => 0x2,
<add> :S_4BYTE_LITERALS => 0x3,
<add> :S_8BYTE_LITERALS => 0x4,
<add> :S_LITERAL_POINTERS => 0x5,
<add> :S_NON_LAZY_SYMBOL_POINTERS => 0x6,
<add> :S_LAZY_SYMBOL_POINTERS => 0x7,
<add> :S_SYMBOL_STUBS => 0x8,
<add> :S_MOD_INIT_FUNC_POINTERS => 0x9,
<add> :S_MOD_TERM_FUNC_POINTERS => 0xa,
<add> :S_COALESCED => 0xb,
<add> :S_GB_ZEROFILE => 0xc,
<add> :S_INTERPOSING => 0xd,
<add> :S_16BYTE_LITERALS => 0xe,
<add> :S_DTRACE_DOF => 0xf,
<add> :S_LAZY_DYLIB_SYMBOL_POINTERS => 0x10,
<add> :S_THREAD_LOCAL_REGULAR => 0x11,
<add> :S_THREAD_LOCAL_ZEROFILL => 0x12,
<add> :S_THREAD_LOCAL_VARIABLES => 0x13,
<add> :S_THREAD_LOCAL_VARIABLE_POINTERS => 0x14,
<add> :S_THREAD_LOCAL_INIT_FUNCTION_POINTERS => 0x15,
<add> :S_ATTR_PURE_INSTRUCTIONS => 0x80000000,
<add> :S_ATTR_NO_TOC => 0x40000000,
<add> :S_ATTR_STRIP_STATIC_SYMS => 0x20000000,
<add> :S_ATTR_NO_DEAD_STRIP => 0x10000000,
<add> :S_ATTR_LIVE_SUPPORT => 0x08000000,
<add> :S_ATTR_SELF_MODIFYING_CODE => 0x04000000,
<add> :S_ATTR_DEBUG => 0x02000000,
<add> :S_ATTR_SOME_INSTRUCTIONS => 0x00000400,
<add> :S_ATTR_EXT_RELOC => 0x00000200,
<add> :S_ATTR_LOC_RELOC => 0x00000100,
<add> }.freeze
<add>
<add> # association of section name symbols to names
<add> # @api private
<add> SECTION_NAMES = {
<add> :SECT_TEXT => "__text",
<add> :SECT_FVMLIB_INIT0 => "__fvmlib_init0",
<add> :SECT_FVMLIB_INIT1 => "__fvmlib_init1",
<add> :SECT_DATA => "__data",
<add> :SECT_BSS => "__bss",
<add> :SECT_COMMON => "__common",
<add> :SECT_OBJC_SYMBOLS => "__symbol_table",
<add> :SECT_OBJC_MODULES => "__module_info",
<add> :SECT_OBJC_STRINGS => "__selector_strs",
<add> :SECT_OBJC_REFS => "__selector_refs",
<add> :SECT_ICON_HEADER => "__header",
<add> :SECT_ICON_TIFF => "__tiff",
<add> }.freeze
<add>
<add> # Represents a section of a segment for 32-bit architectures.
<add> class Section < MachOStructure
<add> # @return [String] the name of the section, including null pad bytes
<add> attr_reader :sectname
<add>
<add> # @return [String] the name of the segment's section, including null
<add> # pad bytes
<add> attr_reader :segname
<add>
<add> # @return [Fixnum] the memory address of the section
<add> attr_reader :addr
<add>
<add> # @return [Fixnum] the size, in bytes, of the section
<add> attr_reader :size
<add>
<add> # @return [Fixnum] the file offset of the section
<add> attr_reader :offset
<add>
<add> # @return [Fixnum] the section alignment (power of 2) of the section
<add> attr_reader :align
<add>
<add> # @return [Fixnum] the file offset of the section's relocation entries
<add> attr_reader :reloff
<add>
<add> # @return [Fixnum] the number of relocation entries
<add> attr_reader :nreloc
<add>
<add> # @return [Fixnum] flags for type and attributes of the section
<add> attr_reader :flags
<add>
<add> # @return [void] reserved (for offset or index)
<add> attr_reader :reserved1
<add>
<add> # @return [void] reserved (for count or sizeof)
<add> attr_reader :reserved2
<add>
<add> # @see MachOStructure::FORMAT
<add> FORMAT = "a16a16L=9".freeze
<add>
<add> # @see MachOStructure::SIZEOF
<add> SIZEOF = 68
<add>
<add> # @api private
<add> def initialize(sectname, segname, addr, size, offset, align, reloff,
<add> nreloc, flags, reserved1, reserved2)
<add> @sectname = sectname
<add> @segname = segname
<add> @addr = addr
<add> @size = size
<add> @offset = offset
<add> @align = align
<add> @reloff = reloff
<add> @nreloc = nreloc
<add> @flags = flags
<add> @reserved1 = reserved1
<add> @reserved2 = reserved2
<add> end
<add>
<add> # @return [String] the section's name, with any trailing NULL characters
<add> # removed
<add> def section_name
<add> sectname.delete("\x00")
<add> end
<add>
<add> # @return [String] the parent segment's name, with any trailing NULL
<add> # characters removed
<add> def segment_name
<add> segname.delete("\x00")
<add> end
<add>
<add> # @return [Boolean] whether the section is empty (i.e, {size} is 0)
<add> def empty?
<add> size.zero?
<add> end
<add>
<add> # @example
<add> # puts "this section is regular" if sect.flag?(:S_REGULAR)
<add> # @param flag [Symbol] a section flag symbol
<add> # @return [Boolean] whether the flag is present in the section's {flags}
<add> def flag?(flag)
<add> flag = SECTION_FLAGS[flag]
<add> return false if flag.nil?
<add> flags & flag == flag
<add> end
<ide> end
<del> end
<ide>
<del> # Represents a section of a segment for 64-bit architectures.
<del> class Section64 < Section
<del> # @return [void] reserved
<del> attr_reader :reserved3
<add> # Represents a section of a segment for 64-bit architectures.
<add> class Section64 < Section
<add> # @return [void] reserved
<add> attr_reader :reserved3
<ide>
<del> # @see MachOStructure::FORMAT
<del> FORMAT = "a16a16Q=2L=8".freeze
<add> # @see MachOStructure::FORMAT
<add> FORMAT = "a16a16Q=2L=8".freeze
<ide>
<del> # @see MachOStructure::SIZEOF
<del> SIZEOF = 80
<add> # @see MachOStructure::SIZEOF
<add> SIZEOF = 80
<ide>
<del> # @api private
<del> def initialize(sectname, segname, addr, size, offset, align, reloff,
<del> nreloc, flags, reserved1, reserved2, reserved3)
<del> super(sectname, segname, addr, size, offset, align, reloff,
<del> nreloc, flags, reserved1, reserved2)
<del> @reserved3 = reserved3
<add> # @api private
<add> def initialize(sectname, segname, addr, size, offset, align, reloff,
<add> nreloc, flags, reserved1, reserved2, reserved3)
<add> super(sectname, segname, addr, size, offset, align, reloff,
<add> nreloc, flags, reserved1, reserved2)
<add> @reserved3 = reserved3
<add> end
<ide> end
<ide> end
<ide> end
<ide><path>Library/Homebrew/vendor/macho/macho/structure.rb
<ide> def self.bytesize
<ide>
<ide> # @param endianness [Symbol] either `:big` or `:little`
<ide> # @param bin [String] the string to be unpacked into the new structure
<del> # @return [MachO::MachOStructure] a new MachOStructure initialized with `bin`
<add> # @return [MachO::MachOStructure] the resulting structure
<ide> # @api private
<ide> def self.new_from_bin(endianness, bin)
<ide> format = Utils.specialize_format(self::FORMAT, endianness)
<ide><path>Library/Homebrew/vendor/macho/macho/tools.rb
<ide> module MachO
<del> # A collection of convenient methods for common operations on Mach-O and Fat binaries.
<add> # A collection of convenient methods for common operations on Mach-O and Fat
<add> # binaries.
<ide> module Tools
<ide> # @param filename [String] the Mach-O or Fat binary being read
<ide> # @return [Array<String>] an array of all dylibs linked to the binary
<ide> def self.dylibs(filename)
<ide> file.linked_dylibs
<ide> end
<ide>
<del> # Changes the dylib ID of a Mach-O or Fat binary, overwriting the source file.
<add> # Changes the dylib ID of a Mach-O or Fat binary, overwriting the source
<add> # file.
<ide> # @param filename [String] the Mach-O or Fat binary being modified
<ide> # @param new_id [String] the new dylib ID for the binary
<ide> # @param options [Hash]
<ide> def self.change_dylib_id(filename, new_id, options = {})
<ide> file.write!
<ide> end
<ide>
<del> # Changes a shared library install name in a Mach-O or Fat binary, overwriting the source file.
<add> # Changes a shared library install name in a Mach-O or Fat binary,
<add> # overwriting the source file.
<ide> # @param filename [String] the Mach-O or Fat binary being modified
<ide> # @param old_name [String] the old shared library name
<ide> # @param new_name [String] the new shared library name
<ide> def self.change_install_name(filename, old_name, new_name, options = {})
<ide> file.write!
<ide> end
<ide>
<del> # Changes a runtime path in a Mach-O or Fat binary, overwriting the source file.
<add> # Changes a runtime path in a Mach-O or Fat binary, overwriting the source
<add> # file.
<ide> # @param filename [String] the Mach-O or Fat binary being modified
<ide> # @param old_path [String] the old runtime path
<ide> # @param new_path [String] the new runtime path
<ide> def self.add_rpath(filename, new_path, options = {})
<ide> file.write!
<ide> end
<ide>
<del> # Delete a runtime path from a Mach-O or Fat binary, overwriting the source file.
<add> # Delete a runtime path from a Mach-O or Fat binary, overwriting the source
<add> # file.
<ide> # @param filename [String] the Mach-O or Fat binary being modified
<ide> # @param old_path [String] the old runtime path
<ide> # @param options [Hash]
<ide> def self.delete_rpath(filename, old_path, options = {})
<ide> file.delete_rpath(old_path, options)
<ide> file.write!
<ide> end
<add>
<add> # Merge multiple Mach-Os into one universal (Fat) binary.
<add> # @param filename [String] the fat binary to create
<add> # @param files [Array<MachO::MachOFile, MachO::FatFile>] the files to merge
<add> # @return [void]
<add> def self.merge_machos(filename, *files)
<add> machos = files.map do |file|
<add> macho = MachO.open(file)
<add> case macho
<add> when MachO::MachOFile
<add> macho
<add> else
<add> macho.machos
<add> end
<add> end.flatten
<add>
<add> fat_macho = MachO::FatFile.new_from_machos(*machos)
<add> fat_macho.write(filename)
<add> end
<ide> end
<ide> end
<ide><path>Library/Homebrew/vendor/macho/macho/utils.rb
<ide> module Utils
<ide> # @param value [Fixnum] the number being rounded
<ide> # @param round [Fixnum] the number being rounded with
<ide> # @return [Fixnum] the rounded value
<del> # @see https://www.opensource.apple.com/source/cctools/cctools-870/libstuff/rnd.c
<add> # @see http://www.opensource.apple.com/source/cctools/cctools-870/libstuff/rnd.c
<ide> def self.round(value, round)
<ide> round -= 1
<ide> value += round
<ide> value &= ~round
<ide> value
<ide> end
<ide>
<del> # Returns the number of bytes needed to pad the given size to the given alignment.
<add> # Returns the number of bytes needed to pad the given size to the given
<add> # alignment.
<ide> # @param size [Fixnum] the unpadded size
<ide> # @param alignment [Fixnum] the number to alignment the size with
<ide> # @return [Fixnum] the number of pad bytes required
<ide> def self.padding_for(size, alignment)
<ide> round(size, alignment) - size
<ide> end
<ide>
<del> # Converts an abstract (native-endian) String#unpack format to big or little.
<add> # Converts an abstract (native-endian) String#unpack format to big or
<add> # little.
<ide> # @param format [String] the format string being converted
<ide> # @param endianness [Symbol] either `:big` or `:little`
<ide> # @return [String] the converted string
<ide> def self.specialize_format(format, endianness)
<ide> end
<ide>
<ide> # Packs tagged strings into an aligned payload.
<del> # @param fixed_offset [Fixnum] the baseline offset for the first packed string
<add> # @param fixed_offset [Fixnum] the baseline offset for the first packed
<add> # string
<ide> # @param alignment [Fixnum] the alignment value to use for packing
<ide> # @param strings [Hash] the labeled strings to pack
<ide> # @return [Array<String, Hash>] the packed string and labeled offsets
<ide> def self.pack_strings(fixed_offset, alignment, strings = {})
<ide>
<ide> # Compares the given number to valid Mach-O magic numbers.
<ide> # @param num [Fixnum] the number being checked
<del> # @return [Boolean] true if `num` is a valid Mach-O magic number, false otherwise
<add> # @return [Boolean] whether `num` is a valid Mach-O magic number
<ide> def self.magic?(num)
<del> MH_MAGICS.key?(num)
<add> Headers::MH_MAGICS.key?(num)
<ide> end
<ide>
<ide> # Compares the given number to valid Fat magic numbers.
<ide> # @param num [Fixnum] the number being checked
<del> # @return [Boolean] true if `num` is a valid Fat magic number, false otherwise
<add> # @return [Boolean] whether `num` is a valid Fat magic number
<ide> def self.fat_magic?(num)
<del> num == FAT_MAGIC
<add> num == Headers::FAT_MAGIC
<ide> end
<ide>
<ide> # Compares the given number to valid 32-bit Mach-O magic numbers.
<ide> # @param num [Fixnum] the number being checked
<del> # @return [Boolean] true if `num` is a valid 32-bit magic number, false otherwise
<add> # @return [Boolean] whether `num` is a valid 32-bit magic number
<ide> def self.magic32?(num)
<del> num == MH_MAGIC || num == MH_CIGAM
<add> num == Headers::MH_MAGIC || num == Headers::MH_CIGAM
<ide> end
<ide>
<ide> # Compares the given number to valid 64-bit Mach-O magic numbers.
<ide> # @param num [Fixnum] the number being checked
<del> # @return [Boolean] true if `num` is a valid 64-bit magic number, false otherwise
<add> # @return [Boolean] whether `num` is a valid 64-bit magic number
<ide> def self.magic64?(num)
<del> num == MH_MAGIC_64 || num == MH_CIGAM_64
<add> num == Headers::MH_MAGIC_64 || num == Headers::MH_CIGAM_64
<ide> end
<ide>
<ide> # Compares the given number to valid little-endian magic numbers.
<ide> # @param num [Fixnum] the number being checked
<del> # @return [Boolean] true if `num` is a valid little-endian magic number, false otherwise
<add> # @return [Boolean] whether `num` is a valid little-endian magic number
<ide> def self.little_magic?(num)
<del> num == MH_CIGAM || num == MH_CIGAM_64
<add> num == Headers::MH_CIGAM || num == Headers::MH_CIGAM_64
<ide> end
<ide>
<ide> # Compares the given number to valid big-endian magic numbers.
<ide> # @param num [Fixnum] the number being checked
<del> # @return [Boolean] true if `num` is a valid big-endian magic number, false otherwise
<add> # @return [Boolean] whether `num` is a valid big-endian magic number
<ide> def self.big_magic?(num)
<del> num == MH_CIGAM || num == MH_CIGAM_64
<add> num == Headers::MH_CIGAM || num == Headers::MH_CIGAM_64
<ide> end
<ide> end
<ide> end | 12 |
Ruby | Ruby | remove empty directories during uninstall | 11953cbcb9fe33afcc8fff46622208523f3930e9 | <ide><path>Library/Homebrew/keg.rb
<ide> def unlink
<ide> next unless dst.symlink?
<ide> dst.uninstall_info if dst.to_s =~ INFOFILE_RX and ENV['HOMEBREW_KEEP_INFO']
<ide> dst.unlink
<add> dst.parent.rmdir_if_possible
<ide> n+=1
<ide> Find.prune if src.directory?
<ide> end | 1 |
Ruby | Ruby | eliminate warning with layout is unset | 1fcf32f8fef8fb5a63a66edacf556a107d12c049 | <ide><path>actionpack/lib/abstract_controller/layouts.rb
<ide> def _implied_layout_name
<ide> # name, return that string. Otherwise, use the superclass'
<ide> # layout (which might also be implied)
<ide> def _write_layout_method
<del> case @_layout
<add> case defined?(@_layout) ? @_layout : nil
<ide> when String
<ide> self.class_eval %{def _layout(details) #{@_layout.inspect} end}
<ide> when Symbol | 1 |
Java | Java | fix typo in generated yoga classes | 43dedbbd63ca4a8eb0ea0aedb5b93325e3ef122c | <ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaAlign.java
<ide> public static YogaAlign fromInt(int value) {
<ide> case 3: return FLEX_END;
<ide> case 4: return STRETCH;
<ide> case 5: return BASELINE;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaDimension.java
<ide> public static YogaDimension fromInt(int value) {
<ide> switch (value) {
<ide> case 0: return WIDTH;
<ide> case 1: return HEIGHT;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaDirection.java
<ide> public static YogaDirection fromInt(int value) {
<ide> case 0: return INHERIT;
<ide> case 1: return LTR;
<ide> case 2: return RTL;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaEdge.java
<ide> public static YogaEdge fromInt(int value) {
<ide> case 6: return HORIZONTAL;
<ide> case 7: return VERTICAL;
<ide> case 8: return ALL;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaExperimentalFeature.java
<ide> public static YogaExperimentalFeature fromInt(int value) {
<ide> switch (value) {
<ide> case 0: return ROUNDING;
<ide> case 1: return WEB_FLEX_BASIS;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaFlexDirection.java
<ide> public static YogaFlexDirection fromInt(int value) {
<ide> case 1: return COLUMN_REVERSE;
<ide> case 2: return ROW;
<ide> case 3: return ROW_REVERSE;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaJustify.java
<ide> public static YogaJustify fromInt(int value) {
<ide> case 2: return FLEX_END;
<ide> case 3: return SPACE_BETWEEN;
<ide> case 4: return SPACE_AROUND;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaLogLevel.java
<ide> public static YogaLogLevel fromInt(int value) {
<ide> case 2: return INFO;
<ide> case 3: return DEBUG;
<ide> case 4: return VERBOSE;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaMeasureMode.java
<ide> public static YogaMeasureMode fromInt(int value) {
<ide> case 0: return UNDEFINED;
<ide> case 1: return EXACTLY;
<ide> case 2: return AT_MOST;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaOverflow.java
<ide> public static YogaOverflow fromInt(int value) {
<ide> case 0: return VISIBLE;
<ide> case 1: return HIDDEN;
<ide> case 2: return SCROLL;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaPositionType.java
<ide> public static YogaPositionType fromInt(int value) {
<ide> switch (value) {
<ide> case 0: return RELATIVE;
<ide> case 1: return ABSOLUTE;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaPrintOptions.java
<ide> public static YogaPrintOptions fromInt(int value) {
<ide> case 1: return LAYOUT;
<ide> case 2: return STYLE;
<ide> case 4: return CHILDREN;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaUnit.java
<ide> public static YogaUnit fromInt(int value) {
<ide> case 0: return UNDEFINED;
<ide> case 1: return PIXEL;
<ide> case 2: return PERCENT;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> }
<ide><path>ReactAndroid/src/main/java/com/facebook/yoga/YogaWrap.java
<ide> public static YogaWrap fromInt(int value) {
<ide> switch (value) {
<ide> case 0: return NO_WRAP;
<ide> case 1: return WRAP;
<del> default: throw new IllegalArgumentException("Unkown enum value: " + value);
<add> default: throw new IllegalArgumentException("Unknown enum value: " + value);
<ide> }
<ide> }
<ide> } | 14 |
Java | Java | delete unused imports in spring-messaging | bf0703e07e2c968c6d777101c10b01f4f4650a91 | <ide><path>spring-messaging/src/main/java/org/springframework/messaging/simp/stomp/Reactor11TcpStompClient.java
<ide> import org.springframework.messaging.tcp.TcpOperations;
<ide> import org.springframework.messaging.tcp.reactor.Reactor11TcpClient;
<ide> import org.springframework.util.concurrent.ListenableFuture;
<del>import org.springframework.util.concurrent.SettableListenableFuture;
<ide>
<ide> /**
<ide> * A STOMP over TCP client that uses
<ide><path>spring-messaging/src/main/java/org/springframework/messaging/simp/stomp/StompHeaders.java
<ide> package org.springframework.messaging.simp.stomp;
<ide>
<ide> import java.io.Serializable;
<del>import java.util.Arrays;
<ide> import java.util.Collection;
<ide> import java.util.Collections;
<ide> import java.util.LinkedHashMap;
<ide><path>spring-messaging/src/test/java/org/springframework/messaging/simp/broker/DefaultSubscriptionRegistryTests.java
<ide> public void findSubscriptionsNoMatches() {
<ide> // SPR-12665
<ide>
<ide> @Test
<add> @SuppressWarnings("rawtypes")
<ide> public void findSubscriptionsReturnsMapSafeToIterate() throws Exception {
<ide> this.registry.registerSubscription(subscribeMessage("sess1", "1", "/foo"));
<ide> this.registry.registerSubscription(subscribeMessage("sess2", "1", "/foo"));
<ide><path>spring-messaging/src/test/java/org/springframework/messaging/simp/stomp/DefaultStompSessionTests.java
<ide> import static org.mockito.Matchers.any;
<ide> import static org.mockito.Mockito.eq;
<ide> import static org.mockito.Mockito.*;
<del>import static org.mockito.Mockito.same;
<ide>
<ide> import java.nio.charset.Charset;
<ide> import java.util.Date;
<ide> public void receiptReceivedBeforeTaskAdded() throws Exception {
<ide> assertTrue(received.get());
<ide> }
<ide>
<del> @SuppressWarnings("unchecked")
<ide> @Test
<add> @SuppressWarnings({ "unchecked", "rawtypes" })
<ide> public void receiptNotReceived() throws Exception {
<ide>
<ide> TaskScheduler taskScheduler = mock(TaskScheduler.class);
<ide><path>spring-messaging/src/test/java/org/springframework/messaging/simp/stomp/StompClientSupportTests.java
<ide> */
<ide> package org.springframework.messaging.simp.stomp;
<ide>
<del>import static org.junit.Assert.*;
<del>import static org.mockito.Matchers.same;
<del>
<ide> import org.junit.Before;
<del>import org.junit.Rule;
<ide> import org.junit.Test;
<del>import org.junit.rules.ExpectedException;
<del>import org.mockito.MockitoAnnotations;
<ide>
<add>import static org.junit.Assert.*;
<ide>
<ide> /**
<ide> * Unit tests for {@@link StompClientSupport}. | 5 |
Ruby | Ruby | add test for register_alias | 27f3619cd5d3700b2ff16f7c153c3549316b9410 | <ide><path>actionpack/test/dispatch/mime_type_test.rb
<ide> class MimeTypeTest < ActiveSupport::TestCase
<ide> end
<ide> end
<ide>
<add> test "register alias" do
<add> begin
<add> Mime::Type.register_alias "application/xhtml+xml", :foobar
<add> assert_equal Mime::HTML, Mime::EXTENSION_LOOKUP['foobar']
<add> ensure
<add> Mime::Type.unregister(:FOOBAR)
<add> end
<add> end
<add>
<ide> test "type should be equal to symbol" do
<ide> assert_equal Mime::HTML, 'application/xhtml+xml'
<ide> assert_equal Mime::HTML, :html | 1 |
Python | Python | add a deprecation note | 8f94cc6aecaa5aab1a42be99008a6db76d7eee86 | <ide><path>numpy/core/numerictypes.py
<ide> def sctype2char(sctype):
<ide> 'All':'?bhilqpBHILQPefdgFDGSUVOMm'}
<ide>
<ide> # backwards compatibility --- deprecated name
<add># Formal deprecation: Numpy 1.20.0, 2020-10-19
<ide> typeDict = sctypeDict
<ide>
<ide> # b -> boolean | 1 |
Python | Python | fix typo in np.insert docstring | c1a3fcc07c6b0de34668831676813b96e355fffd | <ide><path>numpy/lib/function_base.py
<ide> def insert(arr, obj, values, axis=None):
<ide> [3, 5, 3]])
<ide>
<ide> Difference between sequence and scalars:
<add>
<ide> >>> np.insert(a, [1], [[1],[2],[3]], axis=1)
<ide> array([[1, 1, 1],
<ide> [2, 2, 2], | 1 |
Text | Text | add prisma.io examples list | 5247807da0a53379997cc514cd8c93b53ae005f7 | <ide><path>examples/with-prisma/README.md
<add>Prisma.io maintains it's own Next.Js examples
<add>
<add>- [Javascript](https://github.com/prisma/prisma-examples/tree/master/javascript/rest-nextjs)
<add>- [Typescript](https://github.com/prisma/prisma-examples/tree/master/typescript/rest-nextjs)
<add>- [Typescript-GraphQL](https://github.com/prisma/prisma-examples/tree/master/typescript/graphql-nextjs) | 1 |
Ruby | Ruby | use coreformularepository abstraction | ba147818cb4144e579a35cac949ca97b1253d50e | <ide><path>Library/Homebrew/test/test_formulary.rb
<ide> def test_class_naming
<ide> class FormularyFactoryTest < Homebrew::TestCase
<ide> def setup
<ide> @name = "testball_bottle"
<del> @path = HOMEBREW_PREFIX/"Library/Formula/#{@name}.rb"
<add> @path = CoreFormulaRepository.new.formula_dir/"#{@name}.rb"
<ide> @bottle_dir = Pathname.new("#{File.expand_path("..", __FILE__)}/bottles")
<ide> @bottle = @bottle_dir/"testball_bottle-0.1.#{bottle_tag}.bottle.tar.gz"
<ide> @path.write <<-EOS.undent
<ide> def test_factory_ambiguity_tap_formulae
<ide> class FormularyTapPriorityTest < Homebrew::TestCase
<ide> def setup
<ide> @name = "foo"
<del> @core_path = HOMEBREW_PREFIX/"Library/Formula/#{@name}.rb"
<add> @core_path = CoreFormulaRepository.new.formula_dir/"#{@name}.rb"
<ide> @tap = Tap.new "homebrew", "foo"
<ide> @tap_path = @tap.path/"#{@name}.rb"
<ide> code = <<-EOS.undent | 1 |
Ruby | Ruby | implement required #encode_with | b8e8096a89b0405544c8004c9c445a7894cf7ec9 | <ide><path>activerecord/lib/active_record/relation/delegation.rb
<ide> def respond_to?(method, include_private = false)
<ide> arel.respond_to?(method, include_private)
<ide> end
<ide>
<add> def encode_with(coder)
<add> coder.represent_seq(nil, to_a)
<add> end
<add>
<ide> protected
<ide>
<ide> def array_delegable?(method)
<ide><path>activerecord/test/cases/yaml_serialization_test.rb
<ide> require 'cases/helper'
<ide> require 'models/topic'
<add>require 'models/reply'
<ide> require 'models/post'
<ide> require 'models/author'
<ide> | 2 |
Javascript | Javascript | add a test using stop propagation | b8b23d00decf77b6aabec167d36df79eda298705 | <ide><path>test/unit/plugins.js
<ide> test('Plugins should get events in registration order', function() {
<ide> (function (name) {
<ide> vjs.plugin(name, function (opts) {
<ide> this.on('test', function (event) {
<del> order.push(name)
<add> order.push(name);
<ide> });
<ide> });
<ide> player[name]({});
<ide> test('Plugins should get events in registration order', function() {
<ide>
<ide> player['testerPlugin']({});
<ide>
<del> deepEqual(order, expectedOrder, "plugins should receive events in order of initialization")
<add> deepEqual(order, expectedOrder, "plugins should receive events in order of initialization");
<ide> player.dispose();
<ide> });
<add>
<add>test('Plugins should not get events after stopImmediatePropagation is called', function () {
<add> var order = [];
<add> var expectedOrder = [];
<add> var pluginName = 'orderPlugin';
<add> var i = 0;
<add> var name;
<add> var player = PlayerTest.makePlayer({});
<add>
<add> for (; i < 3; i++ ) {
<add> name = pluginName + i;
<add> expectedOrder.push(name);
<add> (function (name) {
<add> vjs.plugin(name, function (opts) {
<add> this.on('test', function (event) {
<add> order.push(name);
<add> event.stopImmediatePropagation();
<add> });
<add> });
<add> player[name]({});
<add> })(name);
<add> }
<add>
<add> vjs.plugin("testerPlugin", function (opts) {
<add> this.trigger('test');
<add> });
<add>
<add> player['testerPlugin']({});
<add>
<add> deepEqual(order, expectedOrder.slice(0, order.length), "plugins should receive events in order of initialization, until stopImmediatePropagation");
<add> player.dispose();
<add>
<add>}) | 1 |
Javascript | Javascript | remove framestopop from yellowbox | cf4d45ec2bcd301be7793d5840de21ec7d02275b | <ide><path>Libraries/YellowBox/Data/YellowBoxRegistry.js
<ide> function handleUpdate(): void {
<ide> const YellowBoxRegistry = {
<ide> add({
<ide> args,
<del> framesToPop,
<ide> }: $ReadOnly<{|
<ide> args: $ReadOnlyArray<mixed>,
<del> framesToPop: number,
<ide> |}>): void {
<ide> if (typeof args[0] === 'string' && args[0].startsWith('(ADVICE)')) {
<ide> return;
<ide> }
<ide> const {category, message, stack} = YellowBoxWarning.parse({
<ide> args,
<del> framesToPop: framesToPop + 1,
<ide> });
<ide>
<ide> let warnings = registry.get(category);
<ide><path>Libraries/YellowBox/Data/YellowBoxSymbolication.js
<ide> const sanitize = (maybeStack: mixed): Stack => {
<ide> if (typeof maybeFrame.methodName !== 'string') {
<ide> throw new Error('Expected stack frame `methodName` to be a string.');
<ide> }
<add> let collapse = false;
<add> if ('collapse' in maybeFrame) {
<add> if (typeof maybeFrame.collapse !== 'boolean') {
<add> throw new Error('Expected stack frame `collapse` to be a boolean.');
<add> }
<add> collapse = maybeFrame.collapse;
<add> }
<ide> stack.push({
<ide> column: maybeFrame.column,
<ide> file: maybeFrame.file,
<ide> lineNumber: maybeFrame.lineNumber,
<ide> methodName: maybeFrame.methodName,
<add> collapse,
<ide> });
<ide> }
<ide> return stack;
<ide><path>Libraries/YellowBox/Data/YellowBoxWarning.js
<ide> export type SymbolicationRequest = $ReadOnly<{|
<ide> class YellowBoxWarning {
<ide> static parse({
<ide> args,
<del> framesToPop,
<ide> }: $ReadOnly<{|
<ide> args: $ReadOnlyArray<mixed>,
<del> framesToPop: number,
<ide> |}>): {|
<ide> category: Category,
<ide> message: Message,
<ide> class YellowBoxWarning {
<ide>
<ide> return {
<ide> ...YellowBoxCategory.parse(mutableArgs),
<del> stack: createStack({framesToPop: framesToPop + 1}),
<add> // TODO: Use Error.captureStackTrace on Hermes
<add> stack: parseErrorStack(new Error()),
<ide> };
<ide> }
<ide>
<ide> class YellowBoxWarning {
<ide> }
<ide> }
<ide>
<del>function createStack({framesToPop}: $ReadOnly<{|framesToPop: number|}>): Stack {
<del> const error: any = new Error();
<del> error.framesToPop = framesToPop + 1;
<del> return parseErrorStack(error);
<del>}
<del>
<ide> module.exports = YellowBoxWarning;
<ide><path>Libraries/YellowBox/Data/__tests__/YellowBoxRegistry-test.js
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('adds and deletes warnings', () => {
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide> const {category: categoryA} = YellowBoxCategory.parse(['A']);
<ide>
<ide> expect(registry().size).toBe(1);
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('clears all warnings', () => {
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['B'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['C'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<add> YellowBoxRegistry.add({args: ['B']});
<add> YellowBoxRegistry.add({args: ['C']});
<ide>
<ide> expect(registry().size).toBe(3);
<ide>
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('sorts warnings in chronological order', () => {
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['B'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['C'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<add> YellowBoxRegistry.add({args: ['B']});
<add> YellowBoxRegistry.add({args: ['C']});
<ide>
<ide> const {category: categoryA} = YellowBoxCategory.parse(['A']);
<ide> const {category: categoryB} = YellowBoxCategory.parse(['B']);
<ide> describe('YellowBoxRegistry', () => {
<ide> categoryC,
<ide> ]);
<ide>
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide>
<ide> // Expect `A` to be hoisted to the end of the registry.
<ide> expect(Array.from(registry().keys())).toEqual([
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('ignores warnings matching patterns', () => {
<del> YellowBoxRegistry.add({args: ['A!'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['B?'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['C!'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A!']});
<add> YellowBoxRegistry.add({args: ['B?']});
<add> YellowBoxRegistry.add({args: ['C!']});
<ide> expect(registry().size).toBe(3);
<ide>
<ide> YellowBoxRegistry.addIgnorePatterns(['!']);
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('ignores warnings matching regexs or pattern', () => {
<del> YellowBoxRegistry.add({args: ['There are 4 dogs'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['There are 3 cats'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['There are H cats'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['There are 4 dogs']});
<add> YellowBoxRegistry.add({args: ['There are 3 cats']});
<add> YellowBoxRegistry.add({args: ['There are H cats']});
<ide> expect(registry().size).toBe(3);
<ide>
<ide> YellowBoxRegistry.addIgnorePatterns(['dogs']);
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('ignores all warnings when disabled', () => {
<del> YellowBoxRegistry.add({args: ['A!'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['B?'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['C!'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A!']});
<add> YellowBoxRegistry.add({args: ['B?']});
<add> YellowBoxRegistry.add({args: ['C!']});
<ide> expect(registry().size).toBe(3);
<ide>
<ide> YellowBoxRegistry.setDisabled(true);
<ide> describe('YellowBoxRegistry', () => {
<ide> });
<ide>
<ide> it('groups warnings by simple categories', () => {
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide> expect(registry().size).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide> expect(registry().size).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['B']});
<ide> expect(registry().size).toBe(2);
<ide> });
<ide>
<ide> it('groups warnings by format string categories', () => {
<del> YellowBoxRegistry.add({args: ['%s', 'A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['%s', 'A']});
<ide> expect(registry().size).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['%s', 'B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['%s', 'B']});
<ide> expect(registry().size).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide> expect(registry().size).toBe(2);
<ide>
<del> YellowBoxRegistry.add({args: ['B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['B']});
<ide> expect(registry().size).toBe(3);
<ide> });
<ide>
<ide> it('groups warnings with consideration for arguments', () => {
<del> YellowBoxRegistry.add({args: ['A', 'B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A', 'B']});
<ide> expect(registry().size).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A', 'B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A', 'B']});
<ide> expect(registry().size).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A', 'C'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A', 'C']});
<ide> expect(registry().size).toBe(2);
<ide>
<del> YellowBoxRegistry.add({args: ['%s', 'A', 'A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['%s', 'A', 'A']});
<ide> expect(registry().size).toBe(3);
<ide>
<del> YellowBoxRegistry.add({args: ['%s', 'B', 'A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['%s', 'B', 'A']});
<ide> expect(registry().size).toBe(3);
<ide>
<del> YellowBoxRegistry.add({args: ['%s', 'B', 'B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['%s', 'B', 'B']});
<ide> expect(registry().size).toBe(4);
<ide> });
<ide>
<ide> it('ignores warnings starting with "(ADVICE)"', () => {
<del> YellowBoxRegistry.add({args: ['(ADVICE) ...'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['(ADVICE) ...']});
<ide> expect(registry().size).toBe(0);
<ide> });
<ide>
<ide> it('does not ignore warnings formatted to start with "(ADVICE)"', () => {
<del> YellowBoxRegistry.add({args: ['%s ...', '(ADVICE)'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['%s ...', '(ADVICE)']});
<ide> expect(registry().size).toBe(1);
<ide> });
<ide>
<ide> describe('YellowBoxRegistry', () => {
<ide> const {observer} = observe();
<ide> expect(observer.mock.calls.length).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<del> YellowBoxRegistry.add({args: ['B'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<add> YellowBoxRegistry.add({args: ['B']});
<ide> jest.runAllImmediates();
<ide> expect(observer.mock.calls.length).toBe(2);
<ide> });
<ide> describe('YellowBoxRegistry', () => {
<ide> const {observer} = observe();
<ide> expect(observer.mock.calls.length).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide> jest.runAllImmediates();
<ide> expect(observer.mock.calls.length).toBe(2);
<ide>
<ide> describe('YellowBoxRegistry', () => {
<ide> const {observer} = observe();
<ide> expect(observer.mock.calls.length).toBe(1);
<ide>
<del> YellowBoxRegistry.add({args: ['A'], framesToPop: 0});
<add> YellowBoxRegistry.add({args: ['A']});
<ide> jest.runAllImmediates();
<ide> expect(observer.mock.calls.length).toBe(2);
<ide>
<ide><path>Libraries/YellowBox/UI/YellowBoxInspector.js
<ide> class YellowBoxInspector extends React.Component<Props, State> {
<ide> />
<ide> </View>
<ide> {warning.getAvailableStack().map((frame, index) => {
<del> const {file, lineNumber} = frame;
<add> const {file, lineNumber, collapse = false} = frame;
<add> if (collapse) {
<add> return null;
<add> }
<ide> return (
<ide> <YellowBoxInspectorStackFrame
<ide> key={index}
<ide><path>Libraries/YellowBox/YellowBox.js
<ide> if (__DEV__) {
<ide> };
<ide>
<ide> const registerWarning = (...args): void => {
<del> // YellowBox should ignore the top 3-4 stack frames:
<del> // 1: registerWarning() itself
<del> // 2: YellowBox's own console override (in this file)
<del> // 3: React DevTools console.error override (to add component stack)
<del> // (The override feature may be disabled by a runtime preference.)
<del> // 4: The actual console method itself.
<del> // $FlowFixMe This prop is how the DevTools override is observable.
<del> const isDevToolsOvveride = !!console.warn
<del> .__REACT_DEVTOOLS_ORIGINAL_METHOD__;
<del> YellowBoxRegistry.add({args, framesToPop: isDevToolsOvveride ? 4 : 3});
<add> YellowBoxRegistry.add({args});
<ide> };
<ide> } else {
<ide> YellowBox = class extends React.Component<Props, State> { | 6 |
Python | Python | remove google from the bucket name | 0930d16718e8bfa8509937de8fceb8dd04c7bec8 | <ide><path>tests/system/providers/google/ads/example_ads.py
<ide>
<ide> DAG_ID = "example_google_ads"
<ide>
<del>BUCKET_NAME = f"bucket_{DAG_ID}_{ENV_ID}"
<add>BUCKET_NAME = f"bucket_ads_{ENV_ID}"
<ide> CLIENT_IDS = ["1111111111", "2222222222"]
<ide> GCS_OBJ_PATH = "folder_name/google-ads-api-results.csv"
<ide> GCS_ACCOUNTS_CSV = "folder_name/accounts.csv" | 1 |
Javascript | Javascript | add todo() for shading pattern | 20b0fb9dc084f40f14fad95edd5267db062eba51 | <ide><path>pdf.js
<ide> var CanvasGraphics = (function() {
<ide> error("Unable to find pattern resource");
<ide>
<ide> var pattern = xref.fetchIfRef(patternRes.get(patternName.name));
<del>
<del> const types = [null, this.tilingFill];
<del> var typeNum = pattern.dict.get("PatternType");
<add> var patternDict = IsStream(pattern) ? pattern.dict : pattern;
<add> const types = [null, this.tilingFill,
<add> function() { TODO("Shading Patterns"); }];
<add> var typeNum = patternDict.get("PatternType");
<ide> var patternFn = types[typeNum];
<ide> if (!patternFn)
<ide> error("Unhandled pattern type");
<del> patternFn.call(this, pattern);
<add> patternFn.call(this, pattern, patternDict);
<ide> }
<ide> } else {
<ide> // TODO real impl | 1 |
Ruby | Ruby | fix undefined attribute method with attr_readonly | 429f0a7d67ae85395e549a0d33269d1c58175a29 | <ide><path>activerecord/lib/active_record/associations/collection_association.rb
<ide> def merge_target_lists(persisted, memory)
<ide> persisted.map! do |record|
<ide> if mem_record = memory.delete(record)
<ide>
<del> ((record.attribute_names & mem_record.attribute_names) - mem_record.changed_attribute_names_to_save).each do |name|
<add> ((record.attribute_names & mem_record.attribute_names) - mem_record.changed_attribute_names_to_save - mem_record.class._attr_readonly).each do |name|
<ide> mem_record._write_attribute(name, record[name])
<ide> end
<ide>
<ide><path>activerecord/lib/active_record/readonly_attributes.rb
<ide> module ClassMethods
<ide> # post.title = "a different title" # raises ActiveRecord::ReadonlyAttributeError
<ide> # post.update(title: "a different title") # raises ActiveRecord::ReadonlyAttributeError
<ide> def attr_readonly(*attributes)
<del> new_attributes = attributes.map(&:to_s).reject { |a| _attr_readonly.include?(a) }
<add> self._attr_readonly |= attributes.map(&:to_s)
<ide>
<ide> if ActiveRecord.raise_on_assign_to_attr_readonly
<del> new_attributes.each do |attribute|
<del> define_method("#{attribute}=") do |value|
<del> raise ReadonlyAttributeError.new(attribute) unless new_record?
<del>
<del> super(value)
<del> end
<del> end
<del>
<ide> include(HasReadonlyAttributes)
<ide> end
<del>
<del> self._attr_readonly = Set.new(new_attributes) + _attr_readonly
<ide> end
<ide>
<ide> # Returns an array of all the attributes that have been specified as readonly.
<ide> def readonly_attribute?(name) # :nodoc:
<ide> end
<ide>
<ide> module HasReadonlyAttributes # :nodoc:
<del> def write_attribute(attr_name, value)
<del> if !new_record? && self.class.readonly_attribute?(attr_name.to_s)
<del> raise ReadonlyAttributeError.new(attr_name)
<del> end
<add> [:write_attribute, :_write_attribute].each do |name|
<add> define_method(name) do |attr_name, value|
<add> if !new_record? && self.class.readonly_attribute?(attr_name.to_s)
<add> raise ReadonlyAttributeError.new(attr_name)
<add> end
<ide>
<del> super
<add> super(attr_name, value)
<add> end
<ide> end
<ide> end
<ide> end
<ide><path>activerecord/test/cases/base_test.rb
<ide> class ReadonlyTitlePost < Post
<ide> attr_readonly :title
<ide> end
<ide>
<add>class ReadonlyTitleAbstractPost < ActiveRecord::Base
<add> self.abstract_class = true
<add>
<add> attr_readonly :title
<add>end
<add>
<add>class ReadonlyTitlePostWithAbstractParent < ReadonlyTitleAbstractPost
<add> self.table_name = "posts"
<add>end
<add>
<ide> previous_value, ActiveRecord.raise_on_assign_to_attr_readonly = ActiveRecord.raise_on_assign_to_attr_readonly, false
<ide>
<ide> class NonRaisingPost < Post
<ide> def test_comparison_with_different_objects_in_array
<ide> end
<ide>
<ide> def test_readonly_attributes
<del> assert_equal Set.new([ "title" ]), ReadonlyTitlePost.readonly_attributes
<add> assert_equal [ "title" ], ReadonlyTitlePost.readonly_attributes
<ide>
<ide> post = ReadonlyTitlePost.create(title: "cannot change this", body: "changeable")
<ide> assert_equal "cannot change this", post.title
<ide> def test_readonly_attributes
<ide> end
<ide>
<ide> def test_readonly_attributes_on_a_new_record
<del> assert_equal Set.new([ "title" ]), ReadonlyTitlePost.readonly_attributes
<add> assert_equal [ "title" ], ReadonlyTitlePost.readonly_attributes
<ide>
<ide> post = ReadonlyTitlePost.new(title: "can change this until you save", body: "changeable")
<ide> assert_equal "can change this until you save", post.title
<ide> def test_readonly_attributes_on_a_new_record
<ide> assert_equal "changed via write_attribute", post.title
<ide> assert_equal "changed via write_attribute", post.body
<ide>
<del>
<ide> post.assign_attributes(body: "changed via assign_attributes", title: "changed via assign_attributes")
<ide> assert_equal "changed via assign_attributes", post.title
<ide> assert_equal "changed via assign_attributes", post.body
<ide> def test_readonly_attributes_on_a_new_record
<ide> assert_equal "changed via []=", post.body
<ide> end
<ide>
<add> def test_readonly_attributes_in_abstract_class_descendant
<add> assert_equal [ "title" ], ReadonlyTitlePostWithAbstractParent.readonly_attributes
<add>
<add> assert_nothing_raised do
<add> ReadonlyTitlePostWithAbstractParent.new(title: "can change this until you save")
<add> end
<add> end
<add>
<ide> def test_readonly_attributes_when_configured_to_not_raise
<del> assert_equal Set.new([ "title" ]), NonRaisingPost.readonly_attributes
<add> assert_equal [ "title" ], NonRaisingPost.readonly_attributes
<ide>
<ide> post = NonRaisingPost.create(title: "cannot change this", body: "changeable")
<ide> assert_equal "cannot change this", post.title
<ide><path>activerecord/test/cases/locking_test.rb
<ide> def test_lock_with_custom_column_without_default_queries_count
<ide> end
<ide>
<ide> def test_readonly_attributes
<del> assert_equal Set.new([ "name" ]), ReadonlyNameShip.readonly_attributes
<add> assert_equal [ "name" ], ReadonlyNameShip.readonly_attributes
<ide>
<ide> s = ReadonlyNameShip.create(name: "unchangeable name")
<ide> s.reload | 4 |
Go | Go | remove overly spewy debugf | f7e374fb3a51bd25e61ae6c4343838a0ca756fba | <ide><path>devmapper/deviceset_devmapper.go
<ide> func (devices *DeviceSetDM) SetInitialized(hash string) error {
<ide> }
<ide>
<ide> func (devices *DeviceSetDM) ensureInit() error {
<del> utils.Debugf("ensureInit(). Initialized: %v", devices.initialized)
<ide> if !devices.initialized {
<ide> devices.initialized = true
<ide> if err := devices.initDevmapper(); err != nil { | 1 |
Javascript | Javascript | fix whitespace in object3d.js | 340f82394fb41ab1df5527ce7979c779ab459363 | <ide><path>src/core/Object3D.js
<ide> THREE.Object3D.prototype = {
<ide> },
<ide>
<ide> removeChild: function ( child ) {
<del> var scene = this;
<add> var scene = this;
<ide>
<ide> var childIndex = this.children.indexOf( child );
<ide>
<ide> if ( childIndex !== - 1 ) {
<ide>
<del> child.parent = undefined;
<del> this.children.splice( childIndex, 1 );
<add> child.parent = undefined;
<add> this.children.splice( childIndex, 1 );
<ide>
<del> // remove from scene
<add> // remove from scene
<ide>
<del> while ( scene.parent !== undefined ) {
<add> while ( scene.parent !== undefined ) {
<ide>
<del> scene = scene.parent;
<add> scene = scene.parent;
<ide>
<del> }
<add> }
<ide>
<del> if ( scene !== undefined && scene instanceof THREE.Scene ) {
<add> if ( scene !== undefined && scene instanceof THREE.Scene ) {
<ide>
<del> scene.removeChildRecurse( child );
<add> scene.removeChildRecurse( child );
<ide>
<del> }
<add> }
<ide>
<del> }
<add> }
<ide>
<ide> },
<ide> | 1 |
Javascript | Javascript | fix imageloader.getsize jest mock | 7be829f2c9756f8973401644579dd2910b5d3209 | <ide><path>jest/setup.js
<ide> jest
<ide> reload: jest.fn(),
<ide> },
<ide> ImageLoader: {
<del> getSize: jest.fn(url => Promise.resolve({width: 320, height: 240})),
<add> getSize: jest.fn(url => Promise.resolve([320, 240])),
<ide> prefetchImage: jest.fn(),
<ide> },
<ide> ImageViewManager: { | 1 |
PHP | PHP | add illuminate\support\arr to compiled class | aa1eada9c212ef879c03e9f71c93ad2b14c55614 | <ide><path>src/Illuminate/Foundation/Console/Optimize/config.php
<ide> $basePath.'/vendor/laravel/framework/src/Illuminate/Events/EventServiceProvider.php',
<ide> $basePath.'/vendor/laravel/framework/src/Illuminate/Support/Facades/Facade.php',
<ide> $basePath.'/vendor/laravel/framework/src/Illuminate/Support/Traits/MacroableTrait.php',
<add> $basePath.'/vendor/laravel/framework/src/Illuminate/Support/Arr.php',
<ide> $basePath.'/vendor/laravel/framework/src/Illuminate/Support/Str.php',
<ide> $basePath.'/vendor/symfony/debug/Symfony/Component/Debug/ErrorHandler.php',
<ide> $basePath.'/vendor/symfony/http-kernel/Symfony/Component/HttpKernel/Debug/ErrorHandler.php', | 1 |
PHP | PHP | apply fixes from styleci | 915c93d093323496446003bace29ce16d2e52da7 | <ide><path>src/Illuminate/Database/Schema/Grammars/SQLiteGrammar.php
<ide> protected function getForeignKey($foreign)
<ide> // We need to columnize the columns that the foreign key is being defined for
<ide> // so that it is a properly formatted list. Once we have done this, we can
<ide> // return the foreign key SQL declaration to the calling method for use.
<del> return sprintf(", foreign key(%s) references %s(%s)",
<add> return sprintf(', foreign key(%s) references %s(%s)',
<ide> $this->columnize($foreign->columns),
<ide> $this->wrapTable($foreign->on),
<ide> $this->columnize((array) $foreign->references)
<ide> public function compileAdd(Blueprint $blueprint, Fluent $command)
<ide> */
<ide> public function compileUnique(Blueprint $blueprint, Fluent $command)
<ide> {
<del> return sprintf("create unique index %s on %s (%s)",
<add> return sprintf('create unique index %s on %s (%s)',
<ide> $this->wrap($command->index),
<ide> $this->wrapTable($blueprint),
<ide> $this->columnize($command->columns)
<ide> public function compileUnique(Blueprint $blueprint, Fluent $command)
<ide> */
<ide> public function compileIndex(Blueprint $blueprint, Fluent $command)
<ide> {
<del> return sprintf("create index %s on %s (%s)",
<add> return sprintf('create index %s on %s (%s)',
<ide> $this->wrap($command->index),
<ide> $this->wrapTable($blueprint),
<ide> $this->columnize($command->columns) | 1 |
Mixed | Python | fix typos in sorts and bit_manipulation | 50485f7c8e33b0a3bf6e603cdae3505d40b1d97a | <ide><path>bit_manipulation/README.md
<del>https://docs.python.org/3/reference/expressions.html#binary-bitwise-operations
<del>https://docs.python.org/3/reference/expressions.html#unary-arithmetic-and-bitwise-operations
<del>https://docs.python.org/3/library/stdtypes.html#bitwise-operations-on-integer-types
<del>
<del>https://wiki.python.org/moin/BitManipulation
<del>https://wiki.python.org/moin/BitwiseOperators
<del>https://www.tutorialspoint.com/python3/bitwise_operators_example.htm
<add>* https://docs.python.org/3/reference/expressions.html#binary-bitwise-operations
<add>* https://docs.python.org/3/reference/expressions.html#unary-arithmetic-and-bitwise-operations
<add>* https://docs.python.org/3/library/stdtypes.html#bitwise-operations-on-integer-types
<add>* https://wiki.python.org/moin/BitManipulation
<add>* https://wiki.python.org/moin/BitwiseOperators
<add>* https://www.tutorialspoint.com/python3/bitwise_operators_example.htm
<ide><path>bit_manipulation/binary_and_operator.py
<ide> def binary_and(a: int, b: int) -> str:
<ide> >>> binary_and(0, -1)
<ide> Traceback (most recent call last):
<ide> ...
<del> ValueError: the value of both input must be positive
<add> ValueError: the value of both inputs must be positive
<ide> >>> binary_and(0, 1.1)
<ide> Traceback (most recent call last):
<ide> ...
<ide> def binary_and(a: int, b: int) -> str:
<ide> TypeError: '<' not supported between instances of 'str' and 'int'
<ide> """
<ide> if a < 0 or b < 0:
<del> raise ValueError("the value of both input must be positive")
<add> raise ValueError("the value of both inputs must be positive")
<ide>
<ide> a_binary = str(bin(a))[2:] # remove the leading "0b"
<ide> b_binary = str(bin(b))[2:] # remove the leading "0b"
<ide><path>bit_manipulation/binary_or_operator.py
<ide> def binary_or(a: int, b: int) -> str:
<ide> >>> binary_or(0, -1)
<ide> Traceback (most recent call last):
<ide> ...
<del> ValueError: the value of both input must be positive
<add> ValueError: the value of both inputs must be positive
<ide> >>> binary_or(0, 1.1)
<ide> Traceback (most recent call last):
<ide> ...
<ide> def binary_or(a: int, b: int) -> str:
<ide> TypeError: '<' not supported between instances of 'str' and 'int'
<ide> """
<ide> if a < 0 or b < 0:
<del> raise ValueError("the value of both input must be positive")
<add> raise ValueError("the value of both inputs must be positive")
<ide> a_binary = str(bin(a))[2:] # remove the leading "0b"
<ide> b_binary = str(bin(b))[2:]
<ide> max_len = max(len(a_binary), len(b_binary))
<ide><path>bit_manipulation/binary_xor_operator.py
<ide> def binary_xor(a: int, b: int) -> str:
<ide> >>> binary_xor(0, -1)
<ide> Traceback (most recent call last):
<ide> ...
<del> ValueError: the value of both input must be positive
<add> ValueError: the value of both inputs must be positive
<ide> >>> binary_xor(0, 1.1)
<ide> Traceback (most recent call last):
<ide> ...
<ide> def binary_xor(a: int, b: int) -> str:
<ide> TypeError: '<' not supported between instances of 'str' and 'int'
<ide> """
<ide> if a < 0 or b < 0:
<del> raise ValueError("the value of both input must be positive")
<add> raise ValueError("the value of both inputs must be positive")
<ide>
<ide> a_binary = str(bin(a))[2:] # remove the leading "0b"
<ide> b_binary = str(bin(b))[2:] # remove the leading "0b"
<ide><path>sorts/bead_sort.py
<ide> def bead_sort(sequence: list) -> list:
<ide> >>> bead_sort([1, .9, 0.0, 0, -1, -.9])
<ide> Traceback (most recent call last):
<ide> ...
<del> TypeError: Sequence must be list of nonnegative integers
<add> TypeError: Sequence must be list of non-negative integers
<ide>
<ide> >>> bead_sort("Hello world")
<ide> Traceback (most recent call last):
<ide> ...
<del> TypeError: Sequence must be list of nonnegative integers
<add> TypeError: Sequence must be list of non-negative integers
<ide> """
<ide> if any(not isinstance(x, int) or x < 0 for x in sequence):
<del> raise TypeError("Sequence must be list of nonnegative integers")
<add> raise TypeError("Sequence must be list of non-negative integers")
<ide> for _ in range(len(sequence)):
<ide> for i, (rod_upper, rod_lower) in enumerate(zip(sequence, sequence[1:])):
<ide> if rod_upper > rod_lower:
<ide><path>sorts/double_sort.py
<ide> def double_sort(lst):
<del> """this sorting algorithm sorts an array using the principle of bubble sort,
<del> but does it both from left to right and right to left,
<del> hence i decided to call it "double sort"
<add> """This sorting algorithm sorts an array using the principle of bubble sort,
<add> but does it both from left to right and right to left.
<add> Hence, it's called "Double sort"
<ide> :param collection: mutable ordered sequence of elements
<ide> :return: the same collection in ascending order
<ide> Examples: | 6 |
Text | Text | add new hint to break up problem into small chunks | 1ba28c9af60e8ec32b10468a5a1fa9aaf23a841c | <ide><path>guide/english/certifications/javascript-algorithms-and-data-structures/javascript-algorithms-and-data-structures-projects/telephone-number-validator/index.md
<ide> Start by trying to get it to validate each format from the example, each one sho
<ide>
<ide> > _try to solve the problem now_
<ide>
<add>##  Hint: 4
<add> Think through what you are trying to solve in a step by step fashion. Below are the different Booleans you could set up. Once you have these set up, you can create small regex tests for each variable.
<add> This will lead to a much longer solution than those contained in the spoilers. However it will be easier to decipher and generate.
<add>```js
<add> // Set up your Booleans here
<add> let hasTenDigits = false;
<add> let hasElevenDigits = false;
<add> let startsWithOne = false;
<add> let hasPermittedCharsOnly = false;
<add> let hasCorrectParentheses = false;
<add>
<add> // Write regular expressions here so that the Booleans contain the correct values
<add> // INSERT CODE WITH REGEX HERE
<add>
<add> // Use the Booleans to return true or false, without needing to string together one complex regular expression
<add> if (!hasTenDigits && !hasElevenDigits) {
<add> return false;
<add> } else if (!hasPermittedCharsOnly || !hasCorrectParentheses) {
<add> return false;
<add> } else if (hasElevenDigits && !startsWithOne) {
<add> return false;
<add> } else {
<add> return true;
<add> }
<add>```
<add> > _try to solve the problem now_
<add>
<ide> ## Spoiler Alert!
<ide>
<ide> 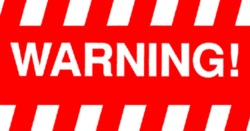 | 1 |
Mixed | Javascript | expose boxwidth and boxheight on tooltip | e1796d361fe7a942168a4193e2f7ec14b7ee1c49 | <ide><path>docs/configuration/tooltip.md
<ide> The tooltip configuration is passed into the `options.tooltips` namespace. The g
<ide> | `cornerRadius` | `number` | `6` | Radius of tooltip corner curves.
<ide> | `multiKeyBackground` | `Color` | `'#fff'` | Color to draw behind the colored boxes when multiple items are in the tooltip.
<ide> | `displayColors` | `boolean` | `true` | If true, color boxes are shown in the tooltip.
<add>| `boxWidth` | `number` | `bodyFontSize` | Width of the color box if displayColors is true.
<add>| `boxHeight` | `number` | `bodyFontSize` | Height of the color box if displayColors is true.
<ide> | `borderColor` | `Color` | `'rgba(0, 0, 0, 0)'` | Color of the border.
<ide> | `borderWidth` | `number` | `0` | Size of the border.
<ide> | `rtl` | `boolean` | | `true` for rendering the legends from right to left.
<ide><path>src/plugins/plugin.tooltip.js
<ide> function resolveOptions(options) {
<ide> options.bodyFontStyle = valueOrDefault(options.bodyFontStyle, defaults.fontStyle);
<ide> options.bodyFontSize = valueOrDefault(options.bodyFontSize, defaults.fontSize);
<ide>
<add> options.boxHeight = valueOrDefault(options.boxHeight, options.bodyFontSize);
<add> options.boxWidth = valueOrDefault(options.boxWidth, options.bodyFontSize);
<add>
<ide> options.titleFontFamily = valueOrDefault(options.titleFontFamily, defaults.fontFamily);
<ide> options.titleFontStyle = valueOrDefault(options.titleFontStyle, defaults.fontStyle);
<ide> options.titleFontSize = valueOrDefault(options.titleFontSize, defaults.fontSize);
<ide> function resolveOptions(options) {
<ide> function getTooltipSize(tooltip) {
<ide> const ctx = tooltip._chart.ctx;
<ide> const {body, footer, options, title} = tooltip;
<del> const {bodyFontSize, footerFontSize, titleFontSize} = options;
<add> const {bodyFontSize, footerFontSize, titleFontSize, boxWidth, boxHeight} = options;
<ide> const titleLineCount = title.length;
<ide> const footerLineCount = footer.length;
<add> const bodyLineItemCount = body.length;
<ide>
<ide> let height = options.yPadding * 2; // Tooltip Padding
<ide> let width = 0;
<ide> function getTooltipSize(tooltip) {
<ide> + options.titleMarginBottom;
<ide> }
<ide> if (combinedBodyLength) {
<del> height += combinedBodyLength * bodyFontSize
<add> // Body lines may include some extra height depending on boxHeight
<add> const bodyLineHeight = options.displayColors ? Math.max(boxHeight, bodyFontSize) : bodyFontSize;
<add> height += bodyLineItemCount * bodyLineHeight
<add> + (combinedBodyLength - bodyLineItemCount) * bodyFontSize
<ide> + (combinedBodyLength - 1) * options.bodySpacing;
<ide> }
<ide> if (footerLineCount) {
<ide> function getTooltipSize(tooltip) {
<ide> helpers.each(tooltip.beforeBody.concat(tooltip.afterBody), maxLineWidth);
<ide>
<ide> // Body lines may include some extra width due to the color box
<del> widthPadding = options.displayColors ? (bodyFontSize + 2) : 0;
<add> widthPadding = options.displayColors ? (boxWidth + 2) : 0;
<ide> helpers.each(body, (bodyItem) => {
<ide> helpers.each(bodyItem.before, maxLineWidth);
<ide> helpers.each(bodyItem.lines, maxLineWidth);
<ide> export class Tooltip extends Element {
<ide> const me = this;
<ide> const options = me.options;
<ide> const labelColors = me.labelColors[i];
<del> const bodyFontSize = options.bodyFontSize;
<add> const {boxHeight, boxWidth, bodyFontSize} = options;
<ide> const colorX = getAlignedX(me, 'left');
<ide> const rtlColorX = rtlHelper.x(colorX);
<add> const yOffSet = boxHeight < bodyFontSize ? (bodyFontSize - boxHeight) / 2 : 0;
<add> const colorY = pt.y + yOffSet;
<ide>
<ide> // Fill a white rect so that colours merge nicely if the opacity is < 1
<ide> ctx.fillStyle = options.multiKeyBackground;
<del> ctx.fillRect(rtlHelper.leftForLtr(rtlColorX, bodyFontSize), pt.y, bodyFontSize, bodyFontSize);
<add> ctx.fillRect(rtlHelper.leftForLtr(rtlColorX, boxWidth), colorY, boxWidth, boxHeight);
<ide>
<ide> // Border
<ide> ctx.lineWidth = 1;
<ide> ctx.strokeStyle = labelColors.borderColor;
<del> ctx.strokeRect(rtlHelper.leftForLtr(rtlColorX, bodyFontSize), pt.y, bodyFontSize, bodyFontSize);
<add> ctx.strokeRect(rtlHelper.leftForLtr(rtlColorX, boxWidth), colorY, boxWidth, boxHeight);
<ide>
<ide> // Inner square
<ide> ctx.fillStyle = labelColors.backgroundColor;
<del> ctx.fillRect(rtlHelper.leftForLtr(rtlHelper.xPlus(rtlColorX, 1), bodyFontSize - 2), pt.y + 1, bodyFontSize - 2, bodyFontSize - 2);
<add> ctx.fillRect(rtlHelper.leftForLtr(rtlHelper.xPlus(rtlColorX, 1), boxWidth - 2), colorY + 1, boxWidth - 2, boxHeight - 2);
<ide>
<ide> // restore fillStyle
<ide> ctx.fillStyle = me.labelTextColors[i];
<ide> export class Tooltip extends Element {
<ide> drawBody(pt, ctx) {
<ide> const me = this;
<ide> const {body, options} = me;
<del> const {bodyFontSize, bodySpacing, bodyAlign, displayColors} = options;
<add> const {bodyFontSize, bodySpacing, bodyAlign, displayColors, boxHeight, boxWidth} = options;
<add> let bodyLineHeight = bodyFontSize;
<ide> let xLinePadding = 0;
<ide>
<ide> const rtlHelper = getRtlHelper(options.rtl, me.x, me.width);
<ide>
<ide> const fillLineOfText = function(line) {
<del> ctx.fillText(line, rtlHelper.x(pt.x + xLinePadding), pt.y + bodyFontSize / 2);
<del> pt.y += bodyFontSize + bodySpacing;
<add> ctx.fillText(line, rtlHelper.x(pt.x + xLinePadding), pt.y + bodyLineHeight / 2);
<add> pt.y += bodyLineHeight + bodySpacing;
<ide> };
<ide>
<ide> const bodyAlignForCalculation = rtlHelper.textAlign(bodyAlign);
<ide> export class Tooltip extends Element {
<ide> helpers.each(me.beforeBody, fillLineOfText);
<ide>
<ide> xLinePadding = displayColors && bodyAlignForCalculation !== 'right'
<del> ? bodyAlign === 'center' ? (bodyFontSize / 2 + 1) : (bodyFontSize + 2)
<add> ? bodyAlign === 'center' ? (boxWidth / 2 + 1) : (boxWidth + 2)
<ide> : 0;
<ide>
<ide> // Draw body lines now
<ide> export class Tooltip extends Element {
<ide> // Draw Legend-like boxes if needed
<ide> if (displayColors && lines.length) {
<ide> me._drawColorBox(ctx, pt, i, rtlHelper);
<add> bodyLineHeight = Math.max(bodyFontSize, boxHeight);
<ide> }
<ide>
<ide> for (j = 0, jlen = lines.length; j < jlen; ++j) {
<ide> fillLineOfText(lines[j]);
<add> // Reset for any lines that don't include colorbox
<add> bodyLineHeight = bodyFontSize;
<ide> }
<ide>
<ide> helpers.each(bodyItem.after, fillLineOfText);
<ide> }
<ide>
<ide> // Reset back to 0 for after body
<ide> xLinePadding = 0;
<add> bodyLineHeight = bodyFontSize;
<ide>
<ide> // After body lines
<ide> helpers.each(me.afterBody, fillLineOfText); | 2 |
Javascript | Javascript | allow bound constructor fns as controllers | d17fbc3862e0a2e646db1222f184dbe663da4a1f | <ide><path>src/auto/injector.js
<ide> function createInjector(modulesToLoad, strictDi) {
<ide> // Check if Type is annotated and use just the given function at n-1 as parameter
<ide> // e.g. someModule.factory('greeter', ['$window', function(renamed$window) {}]);
<ide> // Object creation: http://jsperf.com/create-constructor/2
<del> var instance = Object.create((isArray(Type) ? Type[Type.length - 1] : Type).prototype);
<add> var instance = Object.create((isArray(Type) ? Type[Type.length - 1] : Type).prototype || null);
<ide> var returnedValue = invoke(Type, instance, locals, serviceName);
<ide>
<ide> return isObject(returnedValue) || isFunction(returnedValue) ? returnedValue : instance;
<ide><path>src/ng/controller.js
<ide> function $ControllerProvider() {
<ide> // Object creation: http://jsperf.com/create-constructor/2
<ide> var controllerPrototype = (isArray(expression) ?
<ide> expression[expression.length - 1] : expression).prototype;
<del> instance = Object.create(controllerPrototype);
<add> instance = Object.create(controllerPrototype || null);
<ide>
<ide> if (identifier) {
<ide> addIdentifier(locals, identifier, instance, constructor || expression.name);
<ide><path>test/ng/controllerSpec.js
<ide> describe('$controller', function() {
<ide> expect(ctrl instanceof FooCtrl).toBe(true);
<ide> });
<ide>
<add> it('should allow registration of bound controller functions', function() {
<add> var FooCtrl = function($scope) { $scope.foo = 'bar'; },
<add> scope = {},
<add> ctrl;
<add>
<add> var BoundFooCtrl = FooCtrl.bind(null);
<add>
<add> $controllerProvider.register('FooCtrl', ['$scope', BoundFooCtrl]);
<add> ctrl = $controller('FooCtrl', {$scope: scope});
<add>
<add> expect(scope.foo).toBe('bar');
<add> });
<ide>
<ide> it('should allow registration of map of controllers', function() {
<ide> var FooCtrl = function($scope) { $scope.foo = 'foo'; },
<ide><path>test/ng/directive/ngControllerSpec.js
<ide> describe('ngController', function() {
<ide> this.mark = 'works';
<ide> });
<ide>
<add> var Foo = function($scope) {
<add> $scope.mark = 'foo';
<add> };
<add> $controllerProvider.register('BoundFoo', ['$scope', Foo.bind(null)]);
<ide> }));
<ide>
<ide> afterEach(function() {
<ide> describe('ngController', function() {
<ide> expect(element.text()).toBe('Hello Misko!');
<ide> }));
<ide>
<add> it('should instantiate bound constructor functions', inject(function($compile, $rootScope) {
<add> element = $compile('<div ng-controller="BoundFoo">{{mark}}</div>')($rootScope);
<add> $rootScope.$digest();
<add> expect(element.text()).toBe('foo');
<add> }));
<ide>
<ide> it('should publish controller into scope', inject(function($compile, $rootScope) {
<ide> element = $compile('<div ng-controller="Public as p">{{p.mark}}</div>')($rootScope); | 4 |
PHP | PHP | add root url to elixir | 18dfea3a1c47116407bb7cefd6897318b9f2087a | <ide><path>src/Illuminate/Foundation/helpers.php
<ide> function elixir($file)
<ide> }
<ide>
<ide> if (isset($manifest[$file])) {
<del> return '/build/'.$manifest[$file];
<add> return asset('build/'.$manifest[$file]);
<ide> }
<ide>
<ide> throw new InvalidArgumentException("File {$file} not defined in asset manifest."); | 1 |
PHP | PHP | fix coding standard issues with fixtures | fab9547ac903e1a1320ab041a588188b13c398b3 | <ide><path>lib/Cake/Test/Fixture/AccountFixture.php
<ide> class AccountFixture extends CakeTestFixture {
<ide> * @var string 'Aco'
<ide> */
<ide> public $name = 'Account';
<add>
<ide> public $table = 'Accounts';
<ide>
<ide> /**
<ide><path>lib/Cake/Test/Fixture/AssertTagsTestCase.php
<ide> public function testNumericValuesInExpectationForAssertTags() {
<ide> $this->assertTags($input, $pattern);
<ide> }
<ide>
<del> /**
<add>/**
<ide> * testBadAssertTags
<ide> *
<ide> * @return void
<ide><path>lib/Cake/Test/Fixture/BookFixture.php
<ide> class BookFixture extends CakeTestFixture {
<ide> public $fields = array(
<ide> 'id' => array('type' => 'integer', 'key' => 'primary'),
<ide> 'isbn' => array('type' => 'string', 'length' => 13),
<del> 'title' => array('type' => 'string', 'length' => 255),
<add> 'title' => array('type' => 'string', 'length' => 255),
<ide> 'author' => array('type' => 'string', 'length' => 255),
<ide> 'year' => array('type' => 'integer', 'null' => true),
<ide> 'pages' => array('type' => 'integer', 'null' => true)
<ide><path>lib/Cake/Test/Fixture/CdFixture.php
<ide> class CdFixture extends CakeTestFixture {
<ide> */
<ide> public $fields = array(
<ide> 'id' => array('type' => 'integer', 'key' => 'primary'),
<del> 'title' => array('type' => 'string', 'length' => 255),
<add> 'title' => array('type' => 'string', 'length' => 255),
<ide> 'artist' => array('type' => 'string', 'length' => 255, 'null' => true),
<ide> 'genre' => array('type' => 'string', 'length' => 255, 'null' => true)
<ide> );
<ide><path>lib/Cake/Test/Fixture/ContentAccountFixture.php
<ide> class ContentAccountFixture extends CakeTestFixture {
<ide> * @var string 'Aco'
<ide> */
<ide> public $name = 'ContentAccount';
<add>
<ide> public $table = 'ContentAccounts';
<ide>
<ide> /**
<ide> class ContentAccountFixture extends CakeTestFixture {
<ide> */
<ide> public $fields = array(
<ide> 'iContentAccountsId' => array('type' => 'integer', 'key' => 'primary'),
<del> 'iContentId' => array('type' => 'integer'),
<del> 'iAccountId' => array('type' => 'integer')
<add> 'iContentId' => array('type' => 'integer'),
<add> 'iAccountId' => array('type' => 'integer')
<ide> );
<ide>
<ide> /**
<ide><path>lib/Cake/Test/Fixture/ContentFixture.php
<ide> class ContentFixture extends CakeTestFixture {
<ide> * @var string 'Aco'
<ide> */
<ide> public $name = 'Content';
<add>
<ide> public $table = 'Content';
<ide>
<ide> /**
<ide> class ContentFixture extends CakeTestFixture {
<ide> * @var array
<ide> */
<ide> public $fields = array(
<del> 'iContentId' => array('type' => 'integer', 'key' => 'primary'),
<del> 'cDescription' => array('type' => 'string', 'length' => 50, 'null' => true)
<add> 'iContentId' => array('type' => 'integer', 'key' => 'primary'),
<add> 'cDescription' => array('type' => 'string', 'length' => 50, 'null' => true)
<ide> );
<ide>
<ide> /**
<ide><path>lib/Cake/Test/Fixture/DataTestFixture.php
<ide> class DataTestFixture extends CakeTestFixture {
<ide> 'id' => array('type' => 'integer', 'key' => 'primary'),
<ide> 'count' => array('type' => 'integer', 'default' => 0),
<ide> 'float' => array('type' => 'float', 'default' => 0),
<del> //'timestamp' => array('type' => 'timestamp', 'default' => null, 'null' => true),
<ide> 'created' => array('type' => 'datetime', 'default' => null),
<ide> 'updated' => array('type' => 'datetime', 'default' => null)
<ide> );
<ide><path>lib/Cake/Test/Fixture/FixturizedTestCase.php
<ide> public function testSkipIfFalse() {
<ide> * test that a fixtures are unoaded even if the test throws exceptions
<ide> *
<ide> * @return void
<add> * @throws Exception
<ide> */
<ide> public function testThrowException() {
<ide> throw new Exception();
<ide><path>lib/Cake/Test/Fixture/FruitFixture.php
<ide> class FruitFixture extends CakeTestFixture {
<ide> 'id' => array('type' => 'string', 'length' => 36, 'key' => 'primary'),
<ide> 'name' => array('type' => 'string', 'length' => 255),
<ide> 'color' => array('type' => 'string', 'length' => 13),
<del> 'shape' => array('type' => 'string', 'length' => 255),
<add> 'shape' => array('type' => 'string', 'length' => 255),
<ide> 'taste' => array('type' => 'string', 'length' => 255)
<ide> );
<ide>
<ide><path>lib/Cake/Test/Fixture/GroupUpdateAllFixture.php
<ide> * @package Cake.Test.Fixture
<ide> */
<ide> class GroupUpdateAllFixture extends CakeTestFixture {
<add>
<ide> public $name = 'GroupUpdateAll';
<add>
<ide> public $table = 'group_update_all';
<ide>
<ide> public $fields = array(
<del> 'id' => array('type' => 'integer', 'null' => false, 'default' => NULL, 'key' => 'primary'),
<add> 'id' => array('type' => 'integer', 'null' => false, 'default' => null, 'key' => 'primary'),
<ide> 'name' => array('type' => 'string', 'null' => false, 'length' => 29),
<ide> 'code' => array('type' => 'integer', 'null' => false, 'length' => 4),
<ide> 'indexes' => array('PRIMARY' => array('column' => 'id', 'unique' => 1))
<ide> );
<add>
<ide> public $records = array(
<ide> array(
<ide> 'id' => 1,
<ide><path>lib/Cake/Test/Fixture/PrefixTestFixture.php
<ide> class PrefixTestFixture extends CakeTestFixture {
<ide>
<ide> public $name = 'PrefixTest';
<add>
<ide> public $table = 'prefix_prefix_tests';
<ide>
<ide> public $fields = array(
<ide> 'id' => array('type' => 'integer', 'key' => 'primary'),
<ide> );
<add>
<ide> }
<ide><path>lib/Cake/Test/Fixture/ProductUpdateAllFixture.php
<ide> * @package Cake.Test.Fixture
<ide> */
<ide> class ProductUpdateAllFixture extends CakeTestFixture {
<add>
<ide> public $name = 'ProductUpdateAll';
<add>
<ide> public $table = 'product_update_all';
<ide>
<ide> public $fields = array(
<del> 'id' => array('type' => 'integer', 'null' => false, 'default' => NULL, 'key' => 'primary'),
<add> 'id' => array('type' => 'integer', 'null' => false, 'default' => null, 'key' => 'primary'),
<ide> 'name' => array('type' => 'string', 'null' => false, 'length' => 29),
<ide> 'groupcode' => array('type' => 'integer', 'null' => false, 'length' => 4),
<ide> 'group_id' => array('type' => 'integer', 'null' => false, 'length' => 8),
<ide> 'indexes' => array('PRIMARY' => array('column' => 'id', 'unique' => 1))
<ide> );
<add>
<ide> public $records = array(
<ide> array(
<ide> 'id' => 1,
<ide><path>lib/Cake/Test/Fixture/SessionFixture.php
<ide> class SessionFixture extends CakeTestFixture {
<ide> */
<ide> public $name = 'Session';
<ide>
<del>/**
<del> * table property.
<del> *
<del> * @var string
<del> */
<del> // public $table = 'sessions';
<del>
<ide> /**
<ide> * fields property
<ide> *
<ide><path>lib/Cake/Test/Fixture/TranslateWithPrefixFixture.php
<ide> <?php
<del>/* SVN FILE: $Id$ */
<ide> /**
<ide> * Short description for file.
<ide> *
<ide> * @package Cake.Test.Fixture
<ide> */
<ide> class TranslateWithPrefixFixture extends CakeTestFixture {
<add>
<ide> /**
<ide> * name property
<ide> *
<ide><path>lib/Cake/Test/Fixture/UnderscoreFieldFixture.php
<ide> class UnderscoreFieldFixture extends CakeTestFixture {
<ide> * @var string 'UnderscoreField'
<ide> */
<ide> public $name = 'UnderscoreField';
<del> /**
<add>
<add>/**
<ide> * fields property
<ide> *
<ide> * @var array
<ide> class UnderscoreFieldFixture extends CakeTestFixture {
<ide> 'published' => array('type' => 'string', 'length' => 1, 'default' => 'N'),
<ide> 'another_field' => array('type' => 'integer', 'length' => 3),
<ide> );
<del> /**
<add>
<add>/**
<ide> * records property
<ide> *
<ide> * @var array
<ide><path>lib/Cake/Test/Fixture/UuidTagFixture.php
<ide> class UuidTagFixture extends CakeTestFixture {
<ide> 'id' => array('type' => 'string', 'length' => 36, 'key' => 'primary'),
<ide> 'name' => array('type' => 'string', 'length' => 255),
<ide> 'created' => array('type' => 'datetime')
<del>);
<add> );
<ide>
<ide> /**
<ide> * records property | 16 |
Python | Python | use morph hash in lemmatizer cache key | 8008e2f75b93505734018a072e4131650459ec3a | <ide><path>spacy/pipeline/lemmatizer.py
<ide> def rule_lemmatize(self, token: Token) -> List[str]:
<ide>
<ide> DOCS: https://spacy.io/api/lemmatizer#rule_lemmatize
<ide> """
<del> cache_key = (token.orth, token.pos, token.morph)
<add> cache_key = (token.orth, token.pos, token.morph.key)
<ide> if cache_key in self.cache:
<ide> return self.cache[cache_key]
<ide> string = token.text
<ide><path>spacy/tests/pipeline/test_lemmatizer.py
<ide> import pytest
<ide> import logging
<ide> import mock
<add>import pickle
<ide> from spacy import util, registry
<ide> from spacy.lang.en import English
<ide> from spacy.lookups import Lookups
<ide> def cope_lookups():
<ide> doc2 = nlp2.make_doc("coping")
<ide> doc2[0].pos_ = "VERB"
<ide> assert doc2[0].lemma_ == ""
<del> doc2 = lemmatizer(doc2)
<add> doc2 = lemmatizer2(doc2)
<ide> assert doc2[0].text == "coping"
<ide> assert doc2[0].lemma_ == "cope"
<add>
<add> # Make sure that lemmatizer cache can be pickled
<add> b = pickle.dumps(lemmatizer2) | 2 |
Python | Python | fix a little typo | 9e1775dd2346d68dfdb882ff1581ed1d7e0ad15d | <ide><path>src/transformers/convert_graph_to_onnx.py
<ide> def convert(
<ide> def optimize(onnx_model_path: Path) -> Path:
<ide> """
<ide> Load the model at the specified path and let onnxruntime look at transformations on the graph to enable all the
<del> optimizations possibl
<add> optimizations possible
<ide>
<ide> Args:
<ide> onnx_model_path: filepath where the model binary description is stored | 1 |
Python | Python | freeze feature encoder in flaxspeechencoderdecoder | fde901877a9c876799fa4df5ebf36a2b344ef924 | <ide><path>src/transformers/models/speech_encoder_decoder/modeling_flax_speech_encoder_decoder.py
<ide> def _get_projection_module(self):
<ide> def _get_decoder_module(self):
<ide> return self.decoder
<ide>
<del> def freeze_feature_encoder(self):
<del> """
<del> Calling this function will disable the gradient computation for the feature encoder of the speech encoder in
<del> order that its parameters are not updated during training.
<del> """
<del> self.encoder.freeze_feature_encoder()
<del>
<ide> def __call__(
<ide> self,
<ide> inputs,
<ide> def __call__(
<ide> output_hidden_states: bool = False,
<ide> return_dict: bool = True,
<ide> deterministic: bool = True,
<add> freeze_feature_encoder: bool = False,
<ide> ):
<ide> if encoder_outputs is None:
<ide> encoder_outputs = self.encoder(
<ide> def __call__(
<ide> output_hidden_states=output_hidden_states,
<ide> return_dict=return_dict,
<ide> deterministic=deterministic,
<add> freeze_feature_encoder=freeze_feature_encoder,
<ide> )
<ide>
<ide> encoder_hidden_states = encoder_outputs[0]
<ide> def encode(
<ide> output_hidden_states: Optional[bool] = None,
<ide> return_dict: Optional[bool] = None,
<ide> train: bool = False,
<add> freeze_feature_encoder: bool = False,
<ide> params: dict = None,
<ide> dropout_rng: PRNGKey = None,
<ide> ):
<ide> def _encoder_forward(module, inputs, attention_mask, **kwargs):
<ide> output_hidden_states=output_hidden_states,
<ide> return_dict=return_dict,
<ide> deterministic=not train,
<add> freeze_feature_encoder=freeze_feature_encoder,
<ide> rngs=rngs,
<ide> method=_encoder_forward,
<ide> )
<ide> def __call__(
<ide> output_hidden_states: Optional[bool] = None,
<ide> return_dict: Optional[bool] = None,
<ide> train: bool = False,
<add> freeze_feature_encoder: bool = False,
<ide> params: dict = None,
<ide> dropout_rng: PRNGKey = None,
<ide> ):
<ide> def __call__(
<ide> output_hidden_states=output_hidden_states,
<ide> return_dict=return_dict,
<ide> deterministic=not train,
<add> freeze_feature_encoder=freeze_feature_encoder,
<ide> rngs=rngs,
<ide> )
<ide>
<ide><path>tests/speech_encoder_decoder/test_modeling_flax_speech_encoder_decoder.py
<ide>
<ide>
<ide> if is_flax_available():
<add> import jax
<add> import jax.numpy as jnp
<add> from flax.training.common_utils import onehot
<add> from flax.traverse_util import flatten_dict
<ide> from transformers import (
<ide> FlaxBartForCausalLM,
<ide> FlaxGPT2LMHeadModel,
<ide> def check_encoder_decoder_model_generate(self, inputs, config, decoder_config, *
<ide> generated_sequences = generated_output.sequences
<ide> self.assertEqual(generated_sequences.shape, (inputs.shape[0],) + (decoder_config.max_length,))
<ide>
<add> def check_freeze_feature_encoder(
<add> self,
<add> config,
<add> inputs,
<add> attention_mask,
<add> encoder_hidden_states,
<add> decoder_config,
<add> decoder_input_ids,
<add> decoder_attention_mask,
<add> **kwargs
<add> ):
<add> encoder_decoder_config = SpeechEncoderDecoderConfig.from_encoder_decoder_configs(config, decoder_config)
<add> enc_dec_model = FlaxSpeechEncoderDecoderModel(encoder_decoder_config)
<add> params = enc_dec_model.params
<add>
<add> def cross_entropy(logits, labels):
<add> return -jnp.sum(labels * jax.nn.log_softmax(logits, axis=-1), axis=-1)
<add>
<add> # define a dummy loss function for computing the loss over a forward pass
<add> def compute_loss(
<add> params,
<add> inputs,
<add> attention_mask,
<add> decoder_input_ids,
<add> decoder_attention_mask,
<add> freeze_feature_encoder: bool = False,
<add> ):
<add> outputs_enc_dec = enc_dec_model(
<add> inputs=inputs,
<add> attention_mask=attention_mask,
<add> decoder_input_ids=decoder_input_ids,
<add> decoder_attention_mask=decoder_attention_mask,
<add> freeze_feature_encoder=freeze_feature_encoder,
<add> params=params,
<add> )
<add> logits = outputs_enc_dec.logits
<add> vocab_size = logits.shape[-1]
<add> loss = cross_entropy(logits, onehot(labels=decoder_input_ids, num_classes=vocab_size)).sum()
<add> return loss
<add>
<add> # transform the loss function to get the gradients
<add> grad_fn = jax.value_and_grad(compute_loss)
<add>
<add> # compute the loss and gradients for the unfrozen model
<add> loss, grads = grad_fn(
<add> params, inputs, attention_mask, decoder_input_ids, decoder_attention_mask, freeze_feature_encoder=False
<add> )
<add>
<add> # compare to the loss and gradients for the frozen model
<add> loss_frozen, grads_frozen = grad_fn(
<add> params, inputs, attention_mask, decoder_input_ids, decoder_attention_mask, freeze_feature_encoder=True
<add> )
<add>
<add> self.assert_almost_equals(loss, loss_frozen, 1e-5)
<add>
<add> grads = flatten_dict(grads)
<add> grads_frozen = flatten_dict(grads_frozen)
<add>
<add> # ensure that the dicts of gradients contain the same keys
<add> self.assertEqual(grads.keys(), grads_frozen.keys())
<add>
<add> # ensure that the gradients of the frozen layers are precisely zero and that they differ to the gradients of the unfrozen layers
<add> feature_extractor_grads = tuple(grads[k] for k in grads if "feature_extractor" in k)
<add> feature_extractor_grads_frozen = tuple(grads_frozen[k] for k in grads_frozen if "feature_extractor" in k)
<add>
<add> for feature_extractor_grad, feature_extractor_grad_frozen in zip(
<add> feature_extractor_grads, feature_extractor_grads_frozen
<add> ):
<add> self.assertTrue((feature_extractor_grad_frozen == 0.0).all())
<add> self.assert_difference(feature_extractor_grad, feature_extractor_grad_frozen, 1e-8)
<add>
<add> # ensure that the gradients of all unfrozen layers remain equal, i.e. all layers excluding the frozen 'feature_extractor'
<add> grads = tuple(grads[k] for k in grads if "feature_extractor" not in k)
<add> grads_frozen = tuple(grads_frozen[k] for k in grads_frozen if "feature_extractor" not in k)
<add>
<add> for grad, grad_frozen in zip(grads, grads_frozen):
<add> self.assert_almost_equals(grad, grad_frozen, 1e-8)
<add>
<ide> def check_pt_flax_equivalence(self, pt_model, fx_model, inputs_dict):
<ide>
<ide> pt_model.to(torch_device)
<ide> def test_encoder_decoder_model_output_attentions(self):
<ide> input_ids_dict = self.prepare_config_and_inputs()
<ide> self.check_encoder_decoder_model_output_attentions(**input_ids_dict)
<ide>
<add> def test_freeze_feature_encoder(self):
<add> input_ids_dict = self.prepare_config_and_inputs()
<add> self.check_freeze_feature_encoder(**input_ids_dict)
<add>
<ide> def test_encoder_decoder_model_generate(self):
<ide> input_ids_dict = self.prepare_config_and_inputs()
<ide> self.check_encoder_decoder_model_generate(**input_ids_dict)
<ide>
<ide> def assert_almost_equals(self, a: np.ndarray, b: np.ndarray, tol: float):
<ide> diff = np.abs((a - b)).max()
<del> self.assertLessEqual(diff, tol, f"Difference between torch and flax is {diff} (>= {tol}).")
<add> self.assertLessEqual(diff, tol, f"Difference between arrays is {diff} (>= {tol}).")
<add>
<add> def assert_difference(self, a: np.ndarray, b: np.ndarray, tol: float):
<add> diff = np.abs((a - b)).min()
<add> self.assertGreaterEqual(diff, tol, f"Difference between arrays is {diff} (<= {tol}).")
<ide>
<ide> @is_pt_flax_cross_test
<ide> def test_pt_flax_equivalence(self): | 2 |
Ruby | Ruby | show realpath for pyenv and rbenv | 6d786e7dbbeb9c1c473ec80aabbf4a61ecaca1a1 | <ide><path>Library/Homebrew/cmd/config.rb
<ide> def describe_perl
<ide> end
<ide>
<ide> def describe_python
<del> describe_path(which 'python')
<add> python = which 'python'
<add> if %r{/shims/python$} =~ python && which('pyenv')
<add> "#{python} => #{Pathname.new(`pyenv which python`.strip).realpath}" rescue describe_path(python)
<add> else
<add> describe_path(python)
<add> end
<ide> end
<ide>
<ide> def describe_ruby
<del> describe_path(which 'ruby')
<add> ruby = which 'ruby'
<add> if %r{/shims/ruby$} =~ ruby && which('rbenv')
<add> "#{ruby} => #{Pathname.new(`rbenv which ruby`.strip).realpath}" rescue describe_path(ruby)
<add> else
<add> describe_path(ruby)
<add> end
<ide> end
<ide>
<ide> def hardware | 1 |
Java | Java | fix maybetimber by using scheduler and unit | 56d5586f5ed8b08576418f6e8c8a2ea9c39ccb46 | <ide><path>src/main/java/io/reactivex/internal/operators/completable/CompletableTimer.java
<ide> package io.reactivex.internal.operators.completable;
<ide>
<ide> import java.util.concurrent.TimeUnit;
<add>import java.util.concurrent.atomic.AtomicReference;
<ide>
<ide> import io.reactivex.*;
<del>import io.reactivex.internal.disposables.SequentialDisposable;
<add>import io.reactivex.disposables.Disposable;
<add>import io.reactivex.internal.disposables.DisposableHelper;
<ide>
<add>/**
<add> * Signals an {@code onCompleted} event after the specified delay
<add> */
<ide> public final class CompletableTimer extends Completable {
<ide>
<ide> final long delay;
<ide> public CompletableTimer(long delay, TimeUnit unit, Scheduler scheduler) {
<ide> this.scheduler = scheduler;
<ide> }
<ide>
<del>
<del>
<ide> @Override
<ide> protected void subscribeActual(final CompletableObserver s) {
<del> SequentialDisposable sd = new SequentialDisposable();
<del> s.onSubscribe(sd);
<del> if (!sd.isDisposed()) {
<del> sd.replace(scheduler.scheduleDirect(new Runnable() {
<del> @Override
<del> public void run() {
<del> s.onComplete();
<del> }
<del> }, delay, unit));
<del> }
<add> TimerDisposable parent = new TimerDisposable(s);
<add> s.onSubscribe(parent);
<add> parent.setFuture(scheduler.scheduleDirect(parent, delay, unit));
<ide> }
<ide>
<add> static final class TimerDisposable extends AtomicReference<Disposable> implements Disposable, Runnable {
<add> /** */
<add> private static final long serialVersionUID = 3167244060586201109L;
<add> final CompletableObserver actual;
<add>
<add> TimerDisposable(final CompletableObserver actual) {
<add> this.actual = actual;
<add> }
<add>
<add> @Override
<add> public void run() {
<add> actual.onComplete();
<add> }
<add>
<add> @Override
<add> public void dispose() {
<add> DisposableHelper.dispose(this);
<add> }
<add>
<add> @Override
<add> public boolean isDisposed() {
<add> return DisposableHelper.isDisposed(get());
<add> }
<add>
<add> void setFuture(Disposable d) {
<add> DisposableHelper.replace(this, d);
<add> }
<add> }
<ide> }
<ide><path>src/main/java/io/reactivex/internal/operators/maybe/MaybeTimer.java
<ide> import io.reactivex.internal.disposables.DisposableHelper;
<ide>
<ide> /**
<del> * Signals a 0L after the specified delay
<add> * Signals a {@code 0L} after the specified delay
<ide> */
<ide> public final class MaybeTimer extends Maybe<Long> {
<ide>
<ide> public MaybeTimer(long delay, TimeUnit unit, Scheduler scheduler) {
<ide> }
<ide>
<ide> @Override
<del> protected void subscribeActual(MaybeObserver<? super Long> observer) {
<add> protected void subscribeActual(final MaybeObserver<? super Long> observer) {
<ide> TimerDisposable parent = new TimerDisposable(observer);
<ide> observer.onSubscribe(parent);
<del>
<del> parent.setFuture(scheduler.scheduleDirect(parent));
<add> parent.setFuture(scheduler.scheduleDirect(parent, delay, unit));
<ide> }
<ide>
<ide> static final class TimerDisposable extends AtomicReference<Disposable> implements Disposable, Runnable {
<del>
<ide> /** */
<ide> private static final long serialVersionUID = 2875964065294031672L;
<ide> final MaybeObserver<? super Long> actual;
<ide>
<del> public TimerDisposable(MaybeObserver<? super Long> actual) {
<add> TimerDisposable(final MaybeObserver<? super Long> actual) {
<ide> this.actual = actual;
<ide> }
<ide>
<ide><path>src/main/java/io/reactivex/internal/operators/single/SingleTimer.java
<ide> package io.reactivex.internal.operators.single;
<ide>
<ide> import java.util.concurrent.TimeUnit;
<add>import java.util.concurrent.atomic.AtomicReference;
<ide>
<ide> import io.reactivex.*;
<del>import io.reactivex.internal.disposables.SequentialDisposable;
<add>import io.reactivex.disposables.Disposable;
<add>import io.reactivex.internal.disposables.DisposableHelper;
<ide>
<add>/**
<add> * Signals a {@code 0L} after the specified delay
<add> */
<ide> public final class SingleTimer extends Single<Long> {
<ide>
<ide> final long delay;
<ide> public SingleTimer(long delay, TimeUnit unit, Scheduler scheduler) {
<ide>
<ide> @Override
<ide> protected void subscribeActual(final SingleObserver<? super Long> s) {
<del> SequentialDisposable sd = new SequentialDisposable();
<add> TimerDisposable parent = new TimerDisposable(s);
<add> s.onSubscribe(parent);
<add> parent.setFuture(scheduler.scheduleDirect(parent, delay, unit));
<add> }
<ide>
<del> s.onSubscribe(sd);
<add> static final class TimerDisposable extends AtomicReference<Disposable> implements Disposable, Runnable {
<add> /** */
<add> private static final long serialVersionUID = 8465401857522493082L;
<add> final SingleObserver<? super Long> actual;
<ide>
<del> sd.replace(scheduler.scheduleDirect(new Runnable() {
<del> @Override
<del> public void run() {
<del> s.onSuccess(0L);
<del> }
<del> }, delay, unit));
<del> }
<add> TimerDisposable(final SingleObserver<? super Long> actual) {
<add> this.actual = actual;
<add> }
<ide>
<add> @Override
<add> public void run() {
<add> actual.onSuccess(0L);
<add> }
<add>
<add> @Override
<add> public void dispose() {
<add> DisposableHelper.dispose(this);
<add> }
<add>
<add> @Override
<add> public boolean isDisposed() {
<add> return DisposableHelper.isDisposed(get());
<add> }
<add>
<add> void setFuture(Disposable d) {
<add> DisposableHelper.replace(this, d);
<add> }
<add> }
<ide> }
<ide><path>src/test/java/io/reactivex/completable/CompletableTimerTest.java
<add>/**
<add> * Copyright 2016 Netflix, Inc.
<add> *
<add> * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
<add> * compliance with the License. You may obtain a copy of the License at
<add> *
<add> * http://www.apache.org/licenses/LICENSE-2.0
<add> *
<add> * Unless required by applicable law or agreed to in writing, software distributed under the License is
<add> * distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
<add> * the License for the specific language governing permissions and limitations under the License.
<add> */
<add>
<add>package io.reactivex.completable;
<add>
<add>import org.junit.Test;
<add>
<add>import java.util.concurrent.TimeUnit;
<add>import java.util.concurrent.atomic.AtomicLong;
<add>
<add>import io.reactivex.Completable;
<add>import io.reactivex.functions.Action;
<add>import io.reactivex.schedulers.TestScheduler;
<add>
<add>import static org.junit.Assert.assertEquals;
<add>
<add>public class CompletableTimerTest {
<add> @Test
<add> public void timer() {
<add> final TestScheduler testScheduler = new TestScheduler();
<add>
<add> final AtomicLong atomicLong = new AtomicLong();
<add> Completable.timer(2, TimeUnit.SECONDS, testScheduler).subscribe(new Action() {
<add> @Override
<add> public void run() throws Exception {
<add> atomicLong.incrementAndGet();
<add> }
<add> });
<add>
<add> assertEquals(0, atomicLong.get());
<add>
<add> testScheduler.advanceTimeBy(1, TimeUnit.SECONDS);
<add>
<add> assertEquals(0, atomicLong.get());
<add>
<add> testScheduler.advanceTimeBy(1, TimeUnit.SECONDS);
<add>
<add> assertEquals(1, atomicLong.get());
<add> }
<add>}
<ide><path>src/test/java/io/reactivex/maybe/MaybeTimerTest.java
<add>/**
<add> * Copyright 2016 Netflix, Inc.
<add> *
<add> * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
<add> * compliance with the License. You may obtain a copy of the License at
<add> *
<add> * http://www.apache.org/licenses/LICENSE-2.0
<add> *
<add> * Unless required by applicable law or agreed to in writing, software distributed under the License is
<add> * distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
<add> * the License for the specific language governing permissions and limitations under the License.
<add> */
<add>
<add>package io.reactivex.maybe;
<add>
<add>import org.junit.Test;
<add>
<add>import java.util.concurrent.TimeUnit;
<add>import java.util.concurrent.atomic.AtomicLong;
<add>
<add>import io.reactivex.Maybe;
<add>import io.reactivex.functions.Consumer;
<add>import io.reactivex.schedulers.TestScheduler;
<add>
<add>import static org.junit.Assert.assertEquals;
<add>
<add>public class MaybeTimerTest {
<add> @Test
<add> public void timer() {
<add> final TestScheduler testScheduler = new TestScheduler();
<add>
<add> final AtomicLong atomicLong = new AtomicLong();
<add> Maybe.timer(2, TimeUnit.SECONDS, testScheduler).subscribe(new Consumer<Long>() {
<add> @Override
<add> public void accept(final Long value) throws Exception {
<add> atomicLong.incrementAndGet();
<add> }
<add> });
<add>
<add> assertEquals(0, atomicLong.get());
<add>
<add> testScheduler.advanceTimeBy(1, TimeUnit.SECONDS);
<add>
<add> assertEquals(0, atomicLong.get());
<add>
<add> testScheduler.advanceTimeBy(1, TimeUnit.SECONDS);
<add>
<add> assertEquals(1, atomicLong.get());
<add> }
<add>}
<ide><path>src/test/java/io/reactivex/single/SingleTimerTest.java
<add>/**
<add> * Copyright 2016 Netflix, Inc.
<add> *
<add> * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
<add> * compliance with the License. You may obtain a copy of the License at
<add> *
<add> * http://www.apache.org/licenses/LICENSE-2.0
<add> *
<add> * Unless required by applicable law or agreed to in writing, software distributed under the License is
<add> * distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
<add> * the License for the specific language governing permissions and limitations under the License.
<add> */
<add>
<add>package io.reactivex.single;
<add>
<add>import org.junit.Test;
<add>
<add>import java.util.concurrent.TimeUnit;
<add>import java.util.concurrent.atomic.AtomicLong;
<add>
<add>import io.reactivex.Single;
<add>import io.reactivex.functions.Consumer;
<add>import io.reactivex.schedulers.TestScheduler;
<add>
<add>import static org.junit.Assert.assertEquals;
<add>
<add>public class SingleTimerTest {
<add> @Test
<add> public void timer() {
<add> final TestScheduler testScheduler = new TestScheduler();
<add>
<add> final AtomicLong atomicLong = new AtomicLong();
<add> Single.timer(2, TimeUnit.SECONDS, testScheduler).subscribe(new Consumer<Long>() {
<add> @Override
<add> public void accept(final Long value) throws Exception {
<add> atomicLong.incrementAndGet();
<add> }
<add> });
<add>
<add> assertEquals(0, atomicLong.get());
<add>
<add> testScheduler.advanceTimeBy(1, TimeUnit.SECONDS);
<add>
<add> assertEquals(0, atomicLong.get());
<add>
<add> testScheduler.advanceTimeBy(1, TimeUnit.SECONDS);
<add>
<add> assertEquals(1, atomicLong.get());
<add> }
<add>} | 6 |
PHP | PHP | fix password check | 4436662a1ee19fc5e9eb76a0651d0de1aedb3ee2 | <ide><path>src/Illuminate/Auth/EloquentUserProvider.php
<ide> public function retrieveByCredentials(array $credentials)
<ide> {
<ide> if (empty($credentials) ||
<ide> (count($credentials) === 1 &&
<del> array_key_exists('password', $credentials))) {
<add> Str::contains($this->firstCredentialKey($credentials), 'password'))) {
<ide> return;
<ide> }
<ide>
<ide> public function retrieveByCredentials(array $credentials)
<ide> return $query->first();
<ide> }
<ide>
<add> /**
<add> * Get the first key from the credential array.
<add> *
<add> * @param array $credentials
<add> * @return string|null
<add> */
<add> protected function firstCredentialKey(array $credentials)
<add> {
<add> foreach ($credentials as $key => $value) {
<add> return $key;
<add> }
<add> }
<add>
<ide> /**
<ide> * Validate a user against the given credentials.
<ide> * | 1 |
Text | Text | fix inconsistencies in code style | 28d9485c25a0ddeb8817525e25d4c7bb1ee5013b | <ide><path>doc/api/assert.md
<ide> const obj3 = {
<ide> a : {
<ide> b : 1
<ide> }
<del>}
<add>};
<ide> const obj4 = Object.create(obj1);
<ide>
<ide> assert.deepEqual(obj1, obj1);
<ide> const assert = require('assert');
<ide>
<ide> assert.ifError(0); // OK
<ide> assert.ifError(1); // Throws 1
<del>assert.ifError('error') // Throws 'error'
<add>assert.ifError('error'); // Throws 'error'
<ide> assert.ifError(new Error()); // Throws Error
<ide> ```
<ide>
<ide><path>doc/api/buffer.md
<ide> Buffers can be iterated over using the ECMAScript 2015 (ES6) `for..of` syntax:
<ide> const buf = Buffer.from([1, 2, 3]);
<ide>
<ide> for (var b of buf)
<del> console.log(b)
<add> console.log(b);
<ide>
<ide> // Prints:
<ide> // 1
<ide><path>doc/api/crypto.md
<ide> var decrypted = '';
<ide> decipher.on('readable', () => {
<ide> var data = decipher.read();
<ide> if (data)
<del> decrypted += data.toString('utf8');
<add> decrypted += data.toString('utf8');
<ide> });
<ide> decipher.on('end', () => {
<ide> console.log(decrypted);
<ide><path>doc/api/fs.md
<ide> will be returned._
<ide> // OS X and Linux
<ide> fs.open('<directory>', 'a+', (err, fd) => {
<ide> // => [Error: EISDIR: illegal operation on a directory, open <directory>]
<del>})
<add>});
<ide>
<ide> // Windows and FreeBSD
<ide> fs.open('<directory>', 'a+', (err, fd) => {
<ide> // => null, <fd>
<del>})
<add>});
<ide> ```
<ide>
<ide> ## fs.openSync(path, flags[, mode])
<ide><path>doc/api/http.md
<ide> http.get({
<ide> agent: false // create a new agent just for this one request
<ide> }, (res) => {
<ide> // Do stuff with response
<del>})
<add>});
<ide> ```
<ide>
<ide> ### new Agent([options])
<ide> var req = http.request(options, (res) => {
<ide> console.log(`BODY: ${chunk}`);
<ide> });
<ide> res.on('end', () => {
<del> console.log('No more data in response.')
<del> })
<add> console.log('No more data in response.');
<add> });
<ide> });
<ide>
<ide> req.on('error', (e) => {
<ide><path>doc/api/https.md
<ide> options.agent = new https.Agent(options);
<ide>
<ide> var req = https.request(options, (res) => {
<ide> ...
<del>}
<add>});
<ide> ```
<ide>
<ide> Alternatively, opt out of connection pooling by not using an `Agent`.
<ide> var options = {
<ide>
<ide> var req = https.request(options, (res) => {
<ide> ...
<del>}
<add>});
<ide> ```
<ide>
<ide> [`Agent`]: #https_class_https_agent
<ide><path>doc/api/path.md
<ide> path.format({
<ide> base : "file.txt",
<ide> ext : ".txt",
<ide> name : "file"
<del>})
<add>});
<ide> // returns 'C:\\path\\dir\\file.txt'
<ide> ```
<ide>
<ide><path>doc/api/readline.md
<ide> For instance: `[[substr1, substr2, ...], originalsubstring]`.
<ide>
<ide> ```js
<ide> function completer(line) {
<del> var completions = '.help .error .exit .quit .q'.split(' ')
<del> var hits = completions.filter((c) => { return c.indexOf(line) == 0 })
<add> var completions = '.help .error .exit .quit .q'.split(' ');
<add> var hits = completions.filter((c) => { return c.indexOf(line) == 0 });
<ide> // show all completions if none found
<del> return [hits.length ? hits : completions, line]
<add> return [hits.length ? hits : completions, line];
<ide> }
<ide> ```
<ide>
<ide><path>doc/api/repl.md
<ide> net.createServer((socket) => {
<ide> output: socket
<ide> }).on('exit', () => {
<ide> socket.end();
<del> })
<add> });
<ide> }).listen('/tmp/node-repl-sock');
<ide>
<ide> net.createServer((socket) => {
<ide><path>doc/api/stream.md
<ide> const Writable = require('stream').Writable;
<ide> const myWritable = new Writable({
<ide> write(chunk, encoding, callback) {
<ide> if (chunk.toString().indexOf('a') >= 0) {
<del> callback(new Error('chunk is invalid'))
<add> callback(new Error('chunk is invalid'));
<ide> } else {
<del> callback()
<add> callback();
<ide> }
<ide> }
<ide> });
<ide> class MyWritable extends Writable {
<ide>
<ide> _write(chunk, encoding, callback) {
<ide> if (chunk.toString().indexOf('a') >= 0) {
<del> callback(new Error('chunk is invalid'))
<add> callback(new Error('chunk is invalid'));
<ide> } else {
<del> callback()
<add> callback();
<ide> }
<ide> }
<ide> }
<ide><path>doc/api/util.md
<ide> const util = require('util');
<ide> const EventEmitter = require('events');
<ide>
<ide> function MyStream() {
<del> EventEmitter.call(this);
<add> EventEmitter.call(this);
<ide> }
<ide>
<ide> util.inherits(MyStream, EventEmitter);
<ide>
<ide> MyStream.prototype.write = function(data) {
<del> this.emit('data', data);
<del>}
<add> this.emit('data', data);
<add>};
<ide>
<ide> const stream = new MyStream();
<ide>
<ide> console.log(MyStream.super_ === EventEmitter); // true
<ide>
<ide> stream.on('data', (data) => {
<ide> console.log(`Received data: "${data}"`);
<del>})
<add>});
<ide> stream.write('It works!'); // Received data: "It works!"
<ide> ```
<ide> | 11 |
Java | Java | simplify reactornettytcpclient input | b874692452d63c61b25ccad591662a7e1e269e56 | <ide><path>spring-messaging/src/main/java/org/springframework/messaging/simp/stomp/ReactorNettyTcpStompClient.java
<ide>
<ide> package org.springframework.messaging.simp.stomp;
<ide>
<del>import java.util.List;
<del>import java.util.function.BiConsumer;
<del>import java.util.function.Function;
<del>
<del>import io.netty.buffer.ByteBuf;
<del>import reactor.core.scheduler.Schedulers;
<del>
<del>import org.springframework.messaging.Message;
<ide> import org.springframework.messaging.tcp.TcpOperations;
<ide> import org.springframework.messaging.tcp.reactor.ReactorNettyTcpClient;
<add>import org.springframework.util.Assert;
<ide> import org.springframework.util.concurrent.ListenableFuture;
<ide>
<ide> /**
<ide> public class ReactorNettyTcpStompClient extends StompClientSupport {
<ide>
<ide> private final TcpOperations<byte[]> tcpClient;
<ide>
<add>
<ide> /**
<ide> * Create an instance with host "127.0.0.1" and port 61613.
<ide> */
<ide> public ReactorNettyTcpStompClient() {
<ide> this("127.0.0.1", 61613);
<ide> }
<ide>
<del>
<ide> /**
<ide> * Create an instance with the given host and port.
<ide> * @param host the host
<ide> * @param port the port
<ide> */
<del> public ReactorNettyTcpStompClient(final String host, final int port) {
<del> this.tcpClient = create(host, port, new StompDecoder());
<add> public ReactorNettyTcpStompClient(String host, int port) {
<add> this.tcpClient = new ReactorNettyTcpClient<byte[]>(host, port, new StompReactorNettyCodec());
<ide> }
<ide>
<ide> /**
<ide> * Create an instance with a pre-configured TCP client.
<ide> * @param tcpClient the client to use
<ide> */
<ide> public ReactorNettyTcpStompClient(TcpOperations<byte[]> tcpClient) {
<add> Assert.notNull(tcpClient, "'tcpClient' is required");
<ide> this.tcpClient = tcpClient;
<ide> }
<ide>
<ide> public void shutdown() {
<ide> this.tcpClient.shutdown();
<ide> }
<ide>
<del> /**
<del> * Create a new {@link ReactorNettyTcpClient} with Stomp specific configuration for
<del> * encoding, decoding and hand-off.
<del> *
<del> * @param host target host
<del> * @param port target port
<del> * @param decoder {@link StompDecoder} to use
<del> * @return a new {@link TcpOperations}
<del> */
<del> protected static TcpOperations<byte[]> create(String host, int port, StompDecoder decoder) {
<del> return new ReactorNettyTcpClient<>(host, port,
<del> new ReactorNettyTcpClient.MessageHandlerConfiguration<>(
<del> new DecodingFunction(decoder),
<del> new EncodingConsumer(new StompEncoder()),
<del> 128,
<del> Schedulers.newParallel("StompClient")));
<del> }
<del>
<del> private static final class EncodingConsumer implements BiConsumer<ByteBuf, Message<byte[]>> {
<del>
<del> private final StompEncoder encoder;
<del>
<del> public EncodingConsumer(StompEncoder encoder) {
<del> this.encoder = encoder;
<del> }
<del>
<del> @Override
<del> public void accept(ByteBuf byteBuf, Message<byte[]> message) {
<del> byteBuf.writeBytes(this.encoder.encode(message));
<del> }
<del> }
<del>
<del> private static final class DecodingFunction implements Function<ByteBuf, List<Message<byte[]>>> {
<del>
<del> private final StompDecoder decoder;
<del>
<del> public DecodingFunction(StompDecoder decoder) {
<del> this.decoder = decoder;
<del> }
<del>
<del> @Override
<del> public List<Message<byte[]>> apply(ByteBuf buffer) {
<del> return this.decoder.decode(buffer.nioBuffer());
<del> }
<del> }
<ide> }
<ide><path>spring-messaging/src/main/java/org/springframework/messaging/simp/stomp/StompBrokerRelayMessageHandler.java
<ide> import org.springframework.messaging.tcp.TcpConnection;
<ide> import org.springframework.messaging.tcp.TcpConnectionHandler;
<ide> import org.springframework.messaging.tcp.TcpOperations;
<add>import org.springframework.messaging.tcp.reactor.ReactorNettyCodec;
<ide> import org.springframework.messaging.tcp.reactor.ReactorNettyTcpClient;
<ide> import org.springframework.util.Assert;
<ide> import org.springframework.util.concurrent.ListenableFuture;
<ide> protected void startInternal() {
<ide> if (this.tcpClient == null) {
<ide> StompDecoder decoder = new StompDecoder();
<ide> decoder.setHeaderInitializer(getHeaderInitializer());
<del>
<del> this.tcpClient = ReactorNettyTcpStompClient.create(this.relayHost, this.relayPort, decoder);
<add> ReactorNettyCodec<byte[]> codec = new StompReactorNettyCodec(decoder);
<add> this.tcpClient = new ReactorNettyTcpClient<>(this.relayHost, this.relayPort, codec);
<ide> }
<ide>
<ide> if (logger.isInfoEnabled()) {
<ide><path>spring-messaging/src/main/java/org/springframework/messaging/simp/stomp/StompReactorNettyCodec.java
<add>/*
<add> * Copyright 2002-2016 the original author or authors.
<add> *
<add> * Licensed under the Apache License, Version 2.0 (the "License");
<add> * you may not use this file except in compliance with the License.
<add> * You may obtain a copy of the License at
<add> *
<add> * http://www.apache.org/licenses/LICENSE-2.0
<add> *
<add> * Unless required by applicable law or agreed to in writing, software
<add> * distributed under the License is distributed on an "AS IS" BASIS,
<add> * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
<add> * See the License for the specific language governing permissions and
<add> * limitations under the License.
<add> */
<add>package org.springframework.messaging.simp.stomp;
<add>
<add>import org.springframework.messaging.tcp.reactor.ReactorNettyCodec;
<add>
<add>/**
<add> * {@code ReactorNettyCodec} that delegates to {@link StompDecoder} and
<add> * {@link StompEncoder}.
<add> *
<add> * @author Rossen Stoyanchev
<add> * @since 5.0
<add> */
<add>class StompReactorNettyCodec extends ReactorNettyCodec<byte[]> {
<add>
<add> public StompReactorNettyCodec() {
<add> this(new StompDecoder(), new StompEncoder());
<add> }
<add>
<add> public StompReactorNettyCodec(StompDecoder decoder) {
<add> this(decoder, new StompEncoder());
<add> }
<add>
<add> public StompReactorNettyCodec(StompDecoder decoder, StompEncoder encoder) {
<add> super(byteBuf -> decoder.decode(byteBuf.nioBuffer()),
<add> (byteBuf, message) -> byteBuf.writeBytes(encoder.encode(message)));
<add> }
<add>
<add>}
<ide><path>spring-messaging/src/main/java/org/springframework/messaging/tcp/reactor/ReactorNettyCodec.java
<add>/*
<add> * Copyright 2002-2016 the original author or authors.
<add> *
<add> * Licensed under the Apache License, Version 2.0 (the "License");
<add> * you may not use this file except in compliance with the License.
<add> * You may obtain a copy of the License at
<add> *
<add> * http://www.apache.org/licenses/LICENSE-2.0
<add> *
<add> * Unless required by applicable law or agreed to in writing, software
<add> * distributed under the License is distributed on an "AS IS" BASIS,
<add> * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
<add> * See the License for the specific language governing permissions and
<add> * limitations under the License.
<add> */
<add>package org.springframework.messaging.tcp.reactor;
<add>
<add>import java.util.Collection;
<add>import java.util.function.BiConsumer;
<add>import java.util.function.Function;
<add>
<add>import io.netty.buffer.ByteBuf;
<add>
<add>import org.springframework.messaging.Message;
<add>import org.springframework.util.Assert;
<add>
<add>/**
<add> * Simple holder for a decoding {@link Function} and an encoding
<add> * {@link BiConsumer} to use with Reactor Netty.
<add> *
<add> * @author Rossen Stoyanchev
<add> * @since 5.0
<add> */
<add>public class ReactorNettyCodec<P> {
<add>
<add> private final Function<? super ByteBuf, ? extends Collection<Message<P>>> decoder;
<add>
<add> private final BiConsumer<? super ByteBuf, ? super Message<P>> encoder;
<add>
<add>
<add> public ReactorNettyCodec(Function<? super ByteBuf, ? extends Collection<Message<P>>> decoder,
<add> BiConsumer<? super ByteBuf, ? super Message<P>> encoder) {
<add>
<add> Assert.notNull(decoder, "'decoder' is required");
<add> Assert.notNull(encoder, "'encoder' is required");
<add> this.decoder = decoder;
<add> this.encoder = encoder;
<add> }
<add>
<add> public Function<? super ByteBuf, ? extends Collection<Message<P>>> getDecoder() {
<add> return this.decoder;
<add> }
<add>
<add> public BiConsumer<? super ByteBuf, ? super Message<P>> getEncoder() {
<add> return this.encoder;
<add> }
<add>
<add>}
<ide><path>spring-messaging/src/main/java/org/springframework/messaging/tcp/reactor/ReactorNettyTcpClient.java
<ide> package org.springframework.messaging.tcp.reactor;
<ide>
<ide> import java.util.Collection;
<del>import java.util.function.BiConsumer;
<ide> import java.util.function.BiFunction;
<ide> import java.util.function.Consumer;
<ide> import java.util.function.Function;
<ide>
<del>import io.netty.buffer.ByteBuf;
<ide> import io.netty.channel.group.ChannelGroup;
<ide> import io.netty.channel.group.DefaultChannelGroup;
<ide> import io.netty.util.concurrent.ImmediateEventExecutor;
<ide> import reactor.core.publisher.Mono;
<ide> import reactor.core.publisher.MonoProcessor;
<ide> import reactor.core.scheduler.Scheduler;
<add>import reactor.core.scheduler.Schedulers;
<ide> import reactor.ipc.netty.ChannelFutureMono;
<ide> import reactor.ipc.netty.NettyContext;
<ide> import reactor.ipc.netty.NettyInbound;
<ide>
<ide> private final TcpClient tcpClient;
<ide>
<del> private final MessageHandlerConfiguration<P> configuration;
<add> private final ReactorNettyCodec<P> codec;
<add>
<add> private final Scheduler scheduler = Schedulers.newParallel("ReactorNettyTcpClient");
<ide>
<ide> private final ChannelGroup group;
<ide>
<ide> * the {@code reactor.tcp.ioThreadCount} System property. The network I/O
<ide> * threads will be shared amongst the active clients.
<ide> * <p>Also see the constructor accepting a {@link Consumer} of
<del> * {@link ClientOptions} for advanced tuning.
<add> * {@link ClientOptions} for additional options.
<ide> *
<ide> * @param host the host to connect to
<ide> * @param port the port to connect to
<del> * @param configuration the client configuration
<add> * @param codec for encoding and decoding messages
<ide> */
<del> public ReactorNettyTcpClient(String host, int port, MessageHandlerConfiguration<P> configuration) {
<del> this(opts -> opts.connect(host, port), configuration);
<add> public ReactorNettyTcpClient(String host, int port, ReactorNettyCodec<P> codec) {
<add> this(opts -> opts.connect(host, port), codec);
<ide> }
<ide>
<ide> /**
<ide> public ReactorNettyTcpClient(String host, int port, MessageHandlerConfiguration<
<ide> * configuration.
<ide> *
<ide> * @param tcpOptions callback for configuring shared {@link ClientOptions}
<del> * @param configuration the client configuration
<add> * @param codec for encoding and decoding messages
<ide> */
<ide> public ReactorNettyTcpClient(Consumer<? super ClientOptions> tcpOptions,
<del> MessageHandlerConfiguration<P> configuration) {
<add> ReactorNettyCodec<P> codec) {
<ide>
<del> Assert.notNull(configuration, "'configuration' is required");
<add> Assert.notNull(codec, "'codec' is required");
<ide> this.group = new DefaultChannelGroup(ImmediateEventExecutor.INSTANCE);
<ide> this.tcpClient = TcpClient.create(opts -> tcpOptions.accept(opts.channelGroup(group)));
<del> this.configuration = configuration;
<add> this.codec = codec;
<ide> }
<ide>
<ide>
<ide> public ListenableFuture<Void> connect(final TcpConnectionHandler<P> handler) {
<ide> }
<ide>
<ide> Mono<Void> connectMono = this.tcpClient
<del> .newHandler(new MessageHandler<>(handler, this.configuration))
<add> .newHandler(new MessageHandler<>(handler, this.codec, this.scheduler))
<ide> .doOnError(handler::afterConnectFailure)
<ide> .then();
<ide>
<ide> public ListenableFuture<Void> connect(TcpConnectionHandler<P> handler, Reconnect
<ide>
<ide> MonoProcessor<Void> connectMono = MonoProcessor.create();
<ide>
<del> this.tcpClient.newHandler(new MessageHandler<>(handler, this.configuration))
<add> this.tcpClient.newHandler(new MessageHandler<>(handler, this.codec, this.scheduler))
<ide> .doOnNext(item -> {
<ide> if (!connectMono.isTerminated()) {
<ide> connectMono.onComplete();
<ide> public ListenableFuture<Void> shutdown() {
<ide>
<ide> this.stopping = true;
<ide>
<del> Mono<Void> completion = ChannelFutureMono.from(this.group.close());
<del>
<del> if (this.configuration.scheduler != null) {
<del> completion = completion.doAfterTerminate((x, e) -> configuration.scheduler.shutdown());
<del> }
<add> Mono<Void> completion = ChannelFutureMono.from(this.group.close())
<add> .doAfterTerminate((x, e) -> this.scheduler.shutdown());
<ide>
<ide> return new MonoToListenableFutureAdapter<>(completion);
<ide> }
<ide>
<ide>
<del> /**
<del> * A configuration holder
<del> */
<del> public static final class MessageHandlerConfiguration<P> {
<del>
<del> private final Function<? super ByteBuf, ? extends Collection<Message<P>>> decoder;
<del>
<del> private final BiConsumer<? super ByteBuf, ? super Message<P>> encoder;
<del>
<del> private final int backlog;
<del>
<del> private final Scheduler scheduler;
<del>
<del>
<del> public MessageHandlerConfiguration(
<del> Function<? super ByteBuf, ? extends Collection<Message<P>>> decoder,
<del> BiConsumer<? super ByteBuf, ? super Message<P>> encoder,
<del> int backlog, Scheduler scheduler) {
<del>
<del> this.decoder = decoder;
<del> this.encoder = encoder;
<del> this.backlog = backlog > 0 ? backlog : QueueSupplier.SMALL_BUFFER_SIZE;
<del> this.scheduler = scheduler;
<del> }
<del> }
<del>
<ide> private static final class MessageHandler<P>
<ide> implements BiFunction<NettyInbound, NettyOutbound, Publisher<Void>> {
<ide>
<ide> private final TcpConnectionHandler<P> connectionHandler;
<ide>
<del> private final MessageHandlerConfiguration<P> configuration;
<add> private final ReactorNettyCodec<P> codec;
<ide>
<add> private final Scheduler scheduler;
<add>
<add>
<add> MessageHandler(TcpConnectionHandler<P> handler, ReactorNettyCodec<P> codec,
<add> Scheduler scheduler) {
<ide>
<del> MessageHandler(TcpConnectionHandler<P> handler, MessageHandlerConfiguration<P> config) {
<ide> this.connectionHandler = handler;
<del> this.configuration = config;
<add> this.codec = codec;
<add> this.scheduler = scheduler;
<ide> }
<ide>
<ide> @Override
<ide> public Publisher<Void> apply(NettyInbound in, NettyOutbound out) {
<del> Flux<Collection<Message<P>>> inbound = in.receive().map(configuration.decoder);
<add> Flux<Collection<Message<P>>> inbound = in.receive().map(this.codec.getDecoder());
<ide>
<ide> DirectProcessor<Void> closeProcessor = DirectProcessor.create();
<add>
<ide> TcpConnection<P> tcpConnection =
<del> new ReactorNettyTcpConnection<>(in, out, configuration.encoder, closeProcessor);
<add> new ReactorNettyTcpConnection<>(in, out, this.codec.getEncoder(), closeProcessor);
<ide>
<del> if (configuration.scheduler != null) {
<del> configuration.scheduler.schedule(() -> connectionHandler.afterConnected(tcpConnection));
<del> inbound = inbound.publishOn(configuration.scheduler, configuration.backlog);
<del> }
<del> else {
<del> connectionHandler.afterConnected(tcpConnection);
<del> }
<add> this.scheduler.schedule(() -> connectionHandler.afterConnected(tcpConnection));
<add> inbound = inbound.publishOn(this.scheduler, QueueSupplier.SMALL_BUFFER_SIZE);
<ide>
<ide> inbound.flatMapIterable(Function.identity())
<del> .subscribe(connectionHandler::handleMessage,
<del> connectionHandler::handleFailure,
<del> connectionHandler::afterConnectionClosed);
<add> .subscribe(
<add> connectionHandler::handleMessage,
<add> connectionHandler::handleFailure,
<add> connectionHandler::afterConnectionClosed);
<ide>
<ide> return closeProcessor;
<ide> } | 5 |
PHP | PHP | use hmac for token hashes to avoid collisions | 22b8fc4a247201865714e5ffd4cd68f7ffb3a38c | <ide><path>src/Controller/Component/SecurityComponent.php
<ide> protected function _validatePost(Controller $controller)
<ide> {
<ide> $token = $this->_validToken($controller);
<ide> $hashParts = $this->_hashParts($controller);
<del> $check = Security::hash(implode('', $hashParts), 'sha1');
<add> $check = hash_hmac('sha1', implode('', $hashParts), Security::getSalt());
<ide>
<del> if ($token === $check) {
<add> if (hash_equals($check, $token)) {
<ide> return true;
<ide> }
<ide>
<ide> protected function _hashParts(Controller $controller)
<ide> return [
<ide> $controller->request->here(),
<ide> serialize($fieldList),
<del> $unlocked,
<del> Security::getSalt()
<add> $unlocked
<ide> ];
<ide> }
<ide>
<ide><path>src/View/Helper/SecureFieldTokenTrait.php
<ide> protected function _buildFieldToken($url, $fields, $unlockedFields = [])
<ide> $hashParts = [
<ide> $url,
<ide> serialize($fields),
<del> $unlocked,
<del> Security::getSalt()
<add> $unlocked
<ide> ];
<del> $fields = Security::hash(implode('', $hashParts), 'sha1');
<add> $fields = hash_hmac('sha1', implode('', $hashParts), Security::getSalt());
<ide>
<ide> return [
<ide> 'fields' => urlencode($fields . ':' . $locked),
<ide><path>tests/TestCase/Controller/Component/SecurityComponentTest.php
<ide> public function testValidatePost()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = '68730b0747d4889ec2766f9117405f9635f5fd5e%3AModel.valid';
<add> $fields = 'b9b68375cbfc0476f175561c07b5e04d8908f3af%3AModel.valid';
<ide> $unlocked = '';
<ide> $debug = '';
<ide>
<ide> public function testValidatePostIgnoresCsrfToken()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = '8e26ef05379e5402c2c619f37ee91152333a0264%3A';
<add> $fields = 'c0e09f0b7329e2e5873a7caaa687679e7ceec58b%3A';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostArray()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = '8e26ef05379e5402c2c619f37ee91152333a0264%3A';
<add> $fields = 'c0e09f0b7329e2e5873a7caaa687679e7ceec58b%3A';
<ide> $unlocked = '';
<ide> $debug = urlencode(json_encode([
<ide> 'some-action',
<ide> public function testValidateIntFieldName()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = '4a221010dd7a23f7166cb10c38bc21d81341c387%3A';
<add> $fields = 'b1af0affe0a36eb80f212082fd58fcda1e9e9451%3A';
<ide> $unlocked = '';
<ide> $debug = urlencode(json_encode([
<ide> 'some-action',
<ide> public function testValidatePostNoModel()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = 'a1c3724b7ba85e7022413611e30ba2c6181d5aba%3A';
<add> $fields = '1463016f332f870ae1446a3e499ec39fa51647b1%3A';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostSimple()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = 'b0914d06dfb04abf1fada53e16810e87d157950b%3A';
<add> $fields = '1af7c2889ad58b14038d1552c6127a918bb5bcb7%3A';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostComplex()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = 'b65c7463e44a61d8d2eaecce2c265b406c9c4742%3AAddresses.0.id%7CAddresses.1.id';
<add> $fields = '4dda4baf4525f1593217aaa4f71fb83fbb947f52%3AAddresses.0.id%7CAddresses.1.id';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostMultipleSelect()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = '8d8da68ba03b3d6e7e145b948abfe26741422169%3A';
<add> $fields = 'c8d0d4939f3d84e0cccc2387ca39c722922eef2d%3A';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostMultipleSelect()
<ide> $result = $this->validatePost();
<ide> $this->assertTrue($result);
<ide>
<del> $fields = 'eae2adda1628b771a30cc133342d16220c6520fe%3A';
<add> $fields = '176eb445b102cf7f85e38a7fc56f7e050143b664%3A';
<ide> $this->Controller->request->data = [
<ide> 'User.password' => 'bar', 'User.name' => 'foo', 'User.is_valid' => '1',
<ide> 'Tag' => ['Tag' => [1]],
<ide> public function testValidatePostCheckbox()
<ide> {
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<del> $fields = '68730b0747d4889ec2766f9117405f9635f5fd5e%3AModel.valid';
<add> $fields = 'b9b68375cbfc0476f175561c07b5e04d8908f3af%3AModel.valid';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostCheckbox()
<ide> $result = $this->validatePost();
<ide> $this->assertTrue($result);
<ide>
<del> $fields = 'f63e4a69b2edd31f064e8e602a04dd59307cfe9c%3A';
<add> $fields = 'e3704fbe647a57f5a99876335cb81ff424f46dc3%3A';
<ide>
<ide> $this->Controller->request->data = [
<ide> 'Model' => ['username' => '', 'password' => '', 'valid' => '0'],
<ide> public function testValidatePostHidden()
<ide> {
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<del> $fields = '973a8939a68ac014cc6f7666cec9aa6268507350%3AModel.hidden%7CModel.other_hidden';
<add> $fields = 'cbe3dc7c09e1ba2412316d1a1eff70d7f3791e1b%3AModel.hidden%7CModel.other_hidden';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostWithDisabledFields()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->config('disabledFields', ['Model.username', 'Model.password']);
<ide> $this->Security->startup($event);
<del> $fields = '1c59acfbca98bd870c11fb544d545cbf23215880%3AModel.hidden';
<add> $fields = 'aab7b67fccb5a8fec8e5bc7f4585568e45cdecd1%3AModel.hidden';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidatePostDisabledFieldsInData()
<ide> $this->Security->startup($event);
<ide> $unlocked = 'Model.username';
<ide> $fields = ['Model.hidden', 'Model.password'];
<del> $fields = urlencode(Security::hash('/articles/index' . serialize($fields) . $unlocked . Security::salt()));
<add> $fields = urlencode(
<add> hash_hmac('sha1', '/articles/index' . serialize($fields) . $unlocked, Security::salt())
<add> );
<ide> $debug = 'not used';
<ide>
<ide> $this->Controller->request->data = [
<ide> public function testValidateHiddenMultipleModel()
<ide> {
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<del> $fields = '075ca6c26c38a09a78d871201df89faf52cbbeb8%3AModel.valid%7CModel2.valid%7CModel3.valid';
<add> $fields = '1292df5b24f8c646cd93751bb9fb69d7972e69d0%3AModel.valid%7CModel2.valid%7CModel3.valid';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide>
<ide> public function testValidateHasManyModel()
<ide> {
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<del> $fields = '24a753fb62ef7839389987b58e3f7108f564e529%3AModel.0.hidden%7CModel.0.valid';
<add> $fields = '203a106a01f120823baaab668112e30c4616e3a7%3AModel.0.hidden%7CModel.0.valid';
<ide> $fields .= '%7CModel.1.hidden%7CModel.1.valid';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide> public function testValidateHasManyRecordsPass()
<ide> {
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<del> $fields = '8f7d82bf7656cf068822d9bdab109ebed1be1825%3AAddress.0.id%7CAddress.0.primary%7C';
<add> $fields = '2f086ffee2237435a13a88f38b91b0868c86ae40%3AAddress.0.id%7CAddress.0.primary%7C';
<ide> $fields .= 'Address.1.id%7CAddress.1.primary';
<ide> $unlocked = '';
<ide> $debug = 'not used';
<ide> public function testValidateNestedNumericSets()
<ide> $this->Security->startup($event);
<ide> $unlocked = '';
<ide> $hashFields = ['TaxonomyData'];
<del> $fields = urlencode(Security::hash('/articles/index' . serialize($hashFields) . $unlocked . Security::salt()));
<add> $fields = urlencode(
<add> hash_hmac('sha1', '/articles/index' . serialize($hashFields) . $unlocked, Security::salt())
<add> );
<ide> $debug = 'not used';
<ide>
<ide> $this->Controller->request->data = [
<ide> public function testFormDisabledFields()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide>
<ide> $this->Security->startup($event);
<del> $fields = '9da2b3fa2b5b8ac0bfbc1bbce145e58059629125%3An%3A0%3A%7B%7D';
<add> $fields = '882b9a1226dd9fe8a2c8eed541dd3d059ba27d12%3An%3A0%3A%7B%7D';
<ide> $unlocked = '';
<ide> $debug = urlencode(json_encode([
<ide> '/articles/index',
<ide> public function testValidatePostRadio()
<ide> {
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<del> $fields = 'c2226a8879c3f4b513691295fc2519a29c44c8bb%3An%3A0%3A%7B%7D';
<add> $fields = '14dc53654a1bfe184e921f0cb09a082003da1401%3An%3A0%3A%7B%7D';
<ide> $unlocked = '';
<ide> $debug = urlencode(json_encode([
<ide> '/articles/index',
<ide> public function testValidatePostUrlAsHashInput()
<ide> $event = new Event('Controller.startup', $this->Controller);
<ide> $this->Security->startup($event);
<ide>
<del> $fields = 'b0914d06dfb04abf1fada53e16810e87d157950b%3A';
<add> $fields = '1af7c2889ad58b14038d1552c6127a918bb5bcb7%3A';
<ide> $unlocked = '';
<ide> $debug = urlencode(json_encode([
<ide> 'another-url',
<ide><path>tests/TestCase/View/Helper/FormHelperTest.php
<ide> public function testValidateHashNoModel()
<ide> $this->Form->request->params['_Token'] = 'foo';
<ide>
<ide> $result = $this->Form->secure(['anything']);
<del> $this->assertRegExp('/540ac9c60d323c22bafe997b72c0790f39a8bdef/', $result);
<add> $this->assertRegExp('/b9731869b9915e3dee6250db1a1fad464371fb94/', $result);
<ide> }
<ide>
<ide> /**
<ide> public function testFormSecurityFields()
<ide> $this->Form->request->params['_Token'] = 'testKey';
<ide> $result = $this->Form->secure($fields);
<ide>
<del> $hash = Security::hash(serialize($fields) . Security::salt());
<add> $hash = hash_hmac('sha1', serialize($fields), Security::salt());
<ide> $hash .= ':' . 'Model.valid';
<ide> $hash = urlencode($hash);
<ide> $tokenDebug = urlencode(json_encode([
<ide> public function testFormSecurityFieldsNoDebugMode()
<ide> $this->Form->request->params['_Token'] = 'testKey';
<ide> $result = $this->Form->secure($fields);
<ide>
<del> $hash = Security::hash(serialize($fields) . Security::salt());
<add> $hash = hash_hmac('sha1', serialize($fields), Security::salt());
<ide> $hash .= ':' . 'Model.valid';
<ide> $hash = urlencode($hash);
<ide> $expected = [
<ide> public function testFormSecurityMultipleFields()
<ide> $result = $this->Form->secure($fields);
<ide>
<ide> $hash = '51e3b55a6edd82020b3f29c9ae200e14bbeb7ee5%3AModel.0.hidden%7CModel.0.valid';
<add> $hash = '16e544e04f6d3007231e3e23f8f73427a53272d4%3AModel.0.hidden%7CModel.0.valid';
<ide> $hash .= '%7CModel.1.hidden%7CModel.1.valid';
<ide> $tokenDebug = urlencode(json_encode([
<ide> '',
<ide> public function testFormSecurityMultipleControlFields()
<ide> $this->Form->control('Addresses.1.primary', ['type' => 'checkbox']);
<ide>
<ide> $result = $this->Form->secure($this->Form->fields);
<del>
<del> $hash = '8bd3911b07b507408b1a969b31ee90c47b7d387e%3AAddresses.0.id%7CAddresses.1.id';
<add> $hash = '587942c6810603a6d5a07a394316dda455580227%3AAddresses.0.id%7CAddresses.1.id';
<ide> $tokenDebug = urlencode(json_encode([
<ide> '/articles/add',
<ide> [
<ide> public function testFormSecurityMultipleControlDisabledFields()
<ide> $this->Form->text('Addresses.1.phone');
<ide>
<ide> $result = $this->Form->secure($this->Form->fields);
<del> $hash = '4fb10b46873df4ddd4ef5c3a19944a2f29b38991%3AAddresses.0.id%7CAddresses.1.id';
<add> $hash = '8db4b5f1a912dfafd9c264964df7aa598ea322c0%3AAddresses.0.id%7CAddresses.1.id';
<ide> $tokenDebug = urlencode(json_encode([
<ide> '/articles/add',
<ide> [
<ide> public function testFormSecurityControlUnlockedFields()
<ide>
<ide> $result = $this->Form->secure($expected, ['data-foo' => 'bar']);
<ide>
<del> $hash = 'a303becbdd99cb42ca14a1cf7e63dfd48696a3c5%3AAddresses.id';
<add> $hash = 'cdc8fa2dd2aa2804c12cd17279c39747f1c57354%3AAddresses.id';
<ide> $tokenDebug = urlencode(json_encode([
<ide> '/articles/add',
<ide> [
<ide> public function testFormSecurityControlUnlockedFieldsDebugSecurityTrue()
<ide> $this->assertEquals($expected, $result);
<ide> $result = $this->Form->secure($expected, ['data-foo' => 'bar', 'debugSecurity' => true]);
<ide>
<del> $hash = 'a303becbdd99cb42ca14a1cf7e63dfd48696a3c5%3AAddresses.id';
<add> $hash = 'cdc8fa2dd2aa2804c12cd17279c39747f1c57354%3AAddresses.id';
<ide> $tokenDebug = urlencode(json_encode([
<ide> '/articles/add',
<ide> [
<ide> public function testFormSecurityControlUnlockedFieldsDebugSecurityDebugFalse()
<ide> Configure::write('debug', false);
<ide> $result = $this->Form->secure($expected, ['data-foo' => 'bar', 'debugSecurity' => true]);
<ide>
<del> $hash = 'a303becbdd99cb42ca14a1cf7e63dfd48696a3c5%3AAddresses.id';
<add> $hash = 'cdc8fa2dd2aa2804c12cd17279c39747f1c57354%3AAddresses.id';
<ide> $expected = [
<ide> 'div' => ['style' => 'display:none;'],
<ide> ['input' => [
<ide> public function testFormSecurityControlUnlockedFieldsDebugSecurityFalse()
<ide>
<ide> $result = $this->Form->secure($expected, ['data-foo' => 'bar', 'debugSecurity' => false]);
<ide>
<del> $hash = 'a303becbdd99cb42ca14a1cf7e63dfd48696a3c5%3AAddresses.id';
<add> $hash = 'cdc8fa2dd2aa2804c12cd17279c39747f1c57354%3AAddresses.id';
<ide>
<ide> $expected = [
<ide> 'div' => ['style' => 'display:none;'],
<ide> public function testSecuredFormUrlIgnoresHost()
<ide> {
<ide> $this->Form->request->params['_Token'] = ['key' => 'testKey'];
<ide>
<del> $expected = '0ff0c85cd70584d8fd18fa136846d22c66c21e2d%3A';
<add> $expected = '8312b8faa7e74c6f36e05c8d188eda58b39fab20%3A';
<ide> $this->Form->create($this->article, [
<ide> 'url' => ['controller' => 'articles', 'action' => 'view', 1, '?' => ['page' => 1]]
<ide> ]);
<ide> public function testSecuredFormUrlHasHtmlAndIdentifier()
<ide> {
<ide> $this->Form->request->params['_Token'] = ['key' => 'testKey'];
<ide>
<del> $expected = 'ece0693fb1b19ca116133db1832ac29baaf41ce5%3A';
<add> $expected = '93acdc2336947d62cf057a17275264c1fecc2443%3A';
<ide> $this->Form->create($this->article, [
<ide> 'url' => [
<ide> 'controller' => 'articles',
<ide> public function testSelectMultipleCheckboxSecurity()
<ide> $this->assertEquals(['Model.multi_field'], $this->Form->fields);
<ide>
<ide> $result = $this->Form->secure($this->Form->fields);
<del> $key = 'f7d573650a295b94e0938d32b323fde775e5f32b%3A';
<add> $key = '3cecbba5b65c8792d963b0498c67741466e61d47%3A';
<ide> $this->assertRegExp('/"' . $key . '"/', $result);
<ide> }
<ide>
<ide> public function testPostLinkWithData()
<ide> */
<ide> public function testPostLinkSecurityHash()
<ide> {
<del> $hash = Security::hash(
<del> '/posts/delete/1' .
<del> serialize(['id' => '1']) .
<del> '' .
<del> Security::salt()
<del> );
<add> $hash = hash_hmac('sha1', '/posts/delete/1' . serialize(['id' => '1']), Security::getSalt());
<ide> $hash .= '%3Aid';
<ide> $this->Form->request->params['_Token']['key'] = 'test';
<ide>
<ide> public function testPostLinkSecurityHash()
<ide> */
<ide> public function testPostLinkSecurityHashBlockMode()
<ide> {
<del> $hash = Security::hash(
<del> '/posts/delete/1' .
<del> serialize([]) .
<del> '' .
<del> Security::salt()
<del> );
<add> $hash = hash_hmac('sha1', '/posts/delete/1' . serialize([]), Security::getSalt());
<ide> $hash .= '%3A';
<ide> $this->Form->request->params['_Token']['key'] = 'test';
<ide>
<ide> public function testPostLinkSecurityHashBlockMode()
<ide> public function testPostLinkSecurityHashNoDebugMode()
<ide> {
<ide> Configure::write('debug', false);
<del> $hash = Security::hash(
<del> '/posts/delete/1' .
<del> serialize(['id' => '1']) .
<del> '' .
<del> Security::salt()
<del> );
<add> $hash = hash_hmac('sha1', '/posts/delete/1' . serialize(['id' => '1']), Security::getSalt());
<ide> $hash .= '%3Aid';
<ide> $this->Form->request->params['_Token']['key'] = 'test';
<ide> | 4 |
Ruby | Ruby | raise failures and handle later | 15761a283d4dca679f28ea1141f3da7a0b3247ef | <ide><path>Library/Homebrew/cleanup.rb
<ide> def cleanup_cask(cask, ds_store: true)
<ide> end
<ide>
<ide> def cleanup_keg(keg)
<del> cleanup_path(keg) { keg.uninstall }
<add> cleanup_path(keg) { keg.uninstall(raise_failures: true) }
<ide> rescue Errno::EACCES => e
<ide> opoo e.message
<ide> unremovable_kegs << keg
<ide><path>Library/Homebrew/keg.rb
<ide> def remove_opt_record
<ide> opt_record.parent.rmdir_if_possible
<ide> end
<ide>
<del> def uninstall
<add> def uninstall(raise_failures: false)
<ide> CacheStoreDatabase.use(:linkage) do |db|
<ide> break unless db.created?
<ide>
<ide> def uninstall
<ide> remove_old_aliases
<ide> remove_oldname_opt_record
<ide> rescue Errno::EACCES, Errno::ENOTEMPTY
<add> raise if raise_failures
<add>
<ide> odie <<~EOS
<ide> Could not remove #{name} keg! Do so manually:
<ide> sudo rm -rf #{path} | 2 |
Text | Text | fix indentation on http2 doc entry | 99580bd311b29ffd6a16aa01320bedca1697d7cd | <ide><path>doc/api/http2.md
<ide> the request body.
<ide> When this event is emitted and handled, the [`'request'`][] event will
<ide> not be emitted.
<ide>
<del>### Event: `'connection'`
<add>#### Event: `'connection'`
<ide> <!-- YAML
<ide> added: v8.4.0
<ide> -->
<ide> the request body.
<ide> When this event is emitted and handled, the [`'request'`][] event will
<ide> not be emitted.
<ide>
<del>### Event: `'connection'`
<add>#### Event: `'connection'`
<ide> <!-- YAML
<ide> added: v8.4.0
<ide> --> | 1 |
Go | Go | add key migration to daemon | 007ef161b45dd91afcfb7ef9cd32e6c88dbf196e | <ide><path>docker/daemon.go
<ide> package main
<ide>
<ide> import (
<add> "fmt"
<add> "io"
<add> "os"
<add> "path/filepath"
<add>
<ide> log "github.com/Sirupsen/logrus"
<ide> "github.com/docker/docker/builder"
<ide> "github.com/docker/docker/builtins"
<ide> import (
<ide> flag "github.com/docker/docker/pkg/mflag"
<ide> "github.com/docker/docker/pkg/signal"
<ide> "github.com/docker/docker/registry"
<add> "github.com/docker/docker/utils"
<ide> )
<ide>
<ide> const CanDaemon = true
<ide> func init() {
<ide> registryCfg.InstallFlags()
<ide> }
<ide>
<add>func migrateKey() error {
<add> // Migrate trust key if exists at ~/.docker/key.json and owned by current user
<add> oldPath := filepath.Join(getHomeDir(), ".docker", defaultTrustKeyFile)
<add> newPath := filepath.Join(getDaemonConfDir(), defaultTrustKeyFile)
<add> if _, err := os.Stat(newPath); os.IsNotExist(err) && utils.IsFileOwner(oldPath) {
<add> if err := os.MkdirAll(getDaemonConfDir(), os.FileMode(0644)); err != nil {
<add> return fmt.Errorf("Unable to create daemon configuraiton directory: %s", err)
<add> }
<add>
<add> newFile, err := os.OpenFile(newPath, os.O_RDWR|os.O_CREATE|os.O_TRUNC, 0600)
<add> if err != nil {
<add> return fmt.Errorf("error creating key file %q: %s", newPath, err)
<add> }
<add> defer newFile.Close()
<add>
<add> oldFile, err := os.Open(oldPath)
<add> if err != nil {
<add> return fmt.Errorf("error opening open key file %q: %s", oldPath, err)
<add> }
<add>
<add> if _, err := io.Copy(newFile, oldFile); err != nil {
<add> return fmt.Errorf("error copying key: %s", err)
<add> }
<add>
<add> oldFile.Close()
<add> log.Debugf("Migrated key from %s to %s", oldPath, newPath)
<add> return os.Remove(oldPath)
<add> }
<add>
<add> return nil
<add>}
<add>
<ide> func mainDaemon() {
<ide> if flag.NArg() != 0 {
<ide> flag.Usage()
<ide> func mainDaemon() {
<ide> eng := engine.New()
<ide> signal.Trap(eng.Shutdown)
<ide>
<add> if err := migrateKey(); err != nil {
<add> log.Fatal(err)
<add> }
<ide> daemonCfg.TrustKeyPath = *flTrustKey
<ide>
<ide> // Load builtins
<ide><path>utils/utils_daemon.go
<ide> func TreeSize(dir string) (size int64, err error) {
<ide> })
<ide> return
<ide> }
<add>
<add>// IsFileOwner checks whether the current user is the owner of the given file.
<add>func IsFileOwner(f string) bool {
<add> if fileInfo, err := os.Stat(f); err == nil && fileInfo != nil {
<add> if stat, ok := fileInfo.Sys().(*syscall.Stat_t); ok && int(stat.Uid) == os.Getuid() {
<add> return true
<add> }
<add> }
<add> return false
<add>} | 2 |
PHP | PHP | add tests for only/except multiple arguments | a0b5cd6d6af1ed5f599a81d4005168e134b150a7 | <ide><path>tests/Support/SupportCollectionTest.php
<ide> public function testExcept()
<ide> $data = new Collection(['first' => 'Taylor', 'last' => 'Otwell', 'email' => '[email protected]']);
<ide>
<ide> $this->assertEquals(['first' => 'Taylor'], $data->except(['last', 'email', 'missing'])->all());
<add> $this->assertEquals(['first' => 'Taylor'], $data->except('last', 'email', 'missing')->all());
<add>
<ide> $this->assertEquals(['first' => 'Taylor', 'email' => '[email protected]'], $data->except(['last'])->all());
<add> $this->assertEquals(['first' => 'Taylor', 'email' => '[email protected]'], $data->except('last')->all());
<ide> }
<ide>
<ide> public function testPluckWithArrayAndObjectValues()
<ide> public function testOnly()
<ide> $data = new Collection(['first' => 'Taylor', 'last' => 'Otwell', 'email' => '[email protected]']);
<ide>
<ide> $this->assertEquals(['first' => 'Taylor'], $data->only(['first', 'missing'])->all());
<add> $this->assertEquals(['first' => 'Taylor'], $data->only('first', 'missing')->all());
<add>
<ide> $this->assertEquals(['first' => 'Taylor', 'email' => '[email protected]'], $data->only(['first', 'email'])->all());
<add> $this->assertEquals(['first' => 'Taylor', 'email' => '[email protected]'], $data->only('first', 'email')->all());
<ide> }
<ide>
<ide> public function testGettingAvgItemsFromCollection() | 1 |
Java | Java | simplify string concatenation | 1c24dc1f27fda6736c5a7b123f0476a1bc124a94 | <ide><path>spring-test/src/main/java/org/springframework/mock/web/MockHttpServletResponse.java
<ide> public void setCharacterEncoding(String characterEncoding) {
<ide>
<ide> private void updateContentTypeHeader() {
<ide> if (this.contentType != null) {
<del> StringBuilder sb = new StringBuilder(this.contentType);
<del> if (!this.contentType.toLowerCase().contains(CHARSET_PREFIX) && this.charset) {
<del> sb.append(";").append(CHARSET_PREFIX).append(this.characterEncoding);
<add> String value = this.contentType;
<add> if (this.charset && !this.contentType.toLowerCase().contains(CHARSET_PREFIX)) {
<add> value = value + ';' + CHARSET_PREFIX + this.characterEncoding;
<ide> }
<del> doAddHeaderValue(HttpHeaders.CONTENT_TYPE, sb.toString(), true);
<add> doAddHeaderValue(HttpHeaders.CONTENT_TYPE, value, true);
<ide> }
<ide> }
<ide> | 1 |
Javascript | Javascript | remove console.logs and add more comments | a493c91d337340a71a1193923f78d486656434fd | <ide><path>test/math/random-test.js
<ide> suite.addBatch({
<ide> /**
<ide> * A macro that that takes a RNG and asserts that the values generated by the
<ide> * RNG could be generated by a random variable with cumulative distribution
<del> * function `cdf'.
<add> * function `cdf'. `n' is the number of sample points to use. Higher n = better
<add> * evaluation, slower test.
<ide> *
<ide> * Passes with P≈98%.
<ide> */
<del>function KSTest(cdf) {
<add>function KSTest(cdf, n) {
<ide> return function(rng) {
<del> var n = 1000, values = [];
<add> var n = 1000;
<add> var values = [];
<ide> for (var i = 0; i < n; i++) {
<ide> values.push(rng());
<ide> }
<ide> values.sort(function(a, b) { return a - b; });
<ide>
<ide> K_positive = -Infinity; // Identity of max() function
<ide> for (var i = 0; i < n; i++) {
<del> var edf_x = i/n, x = values[i];
<del> console.log(edf_x, cdf(x));
<del> K_positive = Math.max(K_positive, edf_x - cdf(x));
<add> var edf_i = i / n; // Empirical distribution function evaluated at x=values[i]
<add> K_positive = Math.max(K_positive, edf_i - cdf(values[i]));
<ide> }
<ide> K_positive *= Math.sqrt(n);
<ide> | 1 |
Ruby | Ruby | remove silly concatenation | 1d4699163760757826eba2d2ee971b20df20247b | <ide><path>Library/Homebrew/test/test_bucket.rb
<ide> def test_formula_funcs
<ide> assert_equal "FooBar", classname
<ide> assert_match Regexp.new("^#{HOMEBREW_PREFIX}/Library/Formula"), path.to_s
<ide>
<del> path=HOMEBREW_PREFIX+'Library'+'Formula'+"#{FOOBAR}.rb"
<add> path=HOMEBREW_PREFIX+"Library/Formula/#{FOOBAR}.rb"
<ide> path.dirname.mkpath
<ide> File.open(path, 'w') do |f|
<ide> f << %{
<ide> def test_ARGV
<ide> assert_raises(KegUnspecifiedError) { ARGV.kegs }
<ide> assert ARGV.named.empty?
<ide>
<del> (HOMEBREW_CELLAR+'mxcl'+'10.0').mkpath
<add> (HOMEBREW_CELLAR+'mxcl/10.0').mkpath
<ide>
<ide> ARGV.reset
<ide> ARGV.unshift 'mxcl' | 1 |
PHP | PHP | add test for cache instances and group config | be4cebef30ce141c27078d640dbc9c784fe2b081 | <ide><path>tests/TestCase/Cache/CacheTest.php
<ide> public function testGroupConfigs()
<ide> $this->assertEquals(['archive' => ['archive', 'page']], $result);
<ide> }
<ide>
<add> /**
<add> * testGroupConfigsWithCacheInstance method
<add> */
<add> public function testGroupConfigsWithCacheInstance()
<add> {
<add> Cache::drop('test');
<add> $cache = new FileEngine();
<add> $cache->init([
<add> 'duration' => 300,
<add> 'engine' => 'File',
<add> 'groups' => ['users', 'comments'],
<add> ]);
<add> Cache::config('cached', $cache);
<add>
<add> $result = Cache::groupConfigs('users');
<add> $this->assertEquals(['users' => ['cached']], $result);
<add> }
<add>
<ide> /**
<ide> * testGroupConfigsThrowsException method
<ide> * @expectedException \InvalidArgumentException | 1 |
Javascript | Javascript | fix missing semicolon | 53697d10efc25ff4fe2bfdd049b4967b7ab507e6 | <ide><path>src/css.js
<ide> jQuery(function() {
<ide> elem.style.display = display;
<ide> return ret;
<ide> }
<del> }
<add> };
<ide> }
<ide> });
<ide> | 1 |
PHP | PHP | remove useless reducewithkeys method | 9b4f011fb95c70444812f61d46c8e21fb5b66dd9 | <ide><path>src/Illuminate/Collections/Traits/EnumeratesValues.php
<ide> class_basename(static::class), gettype($result)
<ide> return $result;
<ide> }
<ide>
<del> /**
<del> * Reduce an associative collection to a single value.
<del> *
<del> * @template TReduceWithKeysInitial
<del> * @template TReduceWithKeysReturnType
<del> *
<del> * @param callable(TReduceWithKeysInitial|TReduceWithKeysReturnType, TValue): TReduceWithKeysReturnType $callback
<del> * @param TReduceWithKeysInitial $initial
<del> * @return TReduceWithKeysReturnType
<del> */
<del> public function reduceWithKeys(callable $callback, $initial = null)
<del> {
<del> return $this->reduce($callback, $initial);
<del> }
<del>
<ide> /**
<ide> * Create a collection of all elements that do not pass a given truth test.
<ide> *
<ide><path>tests/Support/SupportCollectionTest.php
<ide> public function testReduce($collection)
<ide> }));
<ide> }
<ide>
<del> /**
<del> * @dataProvider collectionClassProvider
<del> */
<del> public function testReduceWithKeys($collection)
<del> {
<del> $data = new $collection([
<del> 'foo' => 'bar',
<del> 'baz' => 'qux',
<del> ]);
<del> $this->assertSame('foobarbazqux', $data->reduceWithKeys(function ($carry, $element, $key) {
<del> return $carry .= $key.$element;
<del> }));
<del> }
<del>
<ide> /**
<ide> * @dataProvider collectionClassProvider
<ide> */
<ide><path>types/Support/Collection.php
<ide> return 1;
<ide> }, 0));
<ide>
<del>assertType('int', $collection
<del> ->reduceWithKeys(function ($null, $user) {
<del> assertType('User', $user);
<del> assertType('int|null', $null);
<del>
<del> return 1;
<del> }));
<del>assertType('int', $collection
<del> ->reduceWithKeys(function ($int, $user) {
<del> assertType('User', $user);
<del> assertType('int', $int);
<del>
<del> return 1;
<del> }, 0));
<del>
<ide> assertType('Illuminate\Support\Collection<int, int>', $collection::make([1])->replace([1]));
<ide> assertType('Illuminate\Support\Collection<int, User>', $collection->replace([new User]));
<ide>
<ide><path>types/Support/LazyCollection.php
<ide> return 1;
<ide> }, 0));
<ide>
<del>assertType('int', $collection
<del> ->reduceWithKeys(function ($null, $user) {
<del> assertType('User', $user);
<del> assertType('int|null', $null);
<del>
<del> return 1;
<del> }));
<del>assertType('int', $collection
<del> ->reduceWithKeys(function ($int, $user) {
<del> assertType('User', $user);
<del> assertType('int', $int);
<del>
<del> return 1;
<del> }, 0));
<del>
<ide> assertType('Illuminate\Support\LazyCollection<int, int>', $collection::make([1])->replace([1]));
<ide> assertType('Illuminate\Support\LazyCollection<int, User>', $collection->replace([new User]));
<ide> | 4 |
Javascript | Javascript | remove the tabindex attrhook. fixes | 3e2a77c5e882b5fc88bf5469ea00067833a2a059 | <ide><path>src/attributes.js
<ide> jQuery.extend({
<ide> }
<ide> });
<ide>
<del>// Add the tabIndex propHook to attrHooks for back-compat (different case is intentional)
<del>jQuery.attrHooks.tabindex = jQuery.propHooks.tabIndex;
<del>
<ide> // Hook for boolean attributes
<ide> boolHook = {
<ide> get: function( elem, name ) {
<ide> if ( !getSetAttribute ) {
<ide> }
<ide> };
<ide>
<del> // Apply the nodeHook to tabindex
<del> jQuery.attrHooks.tabindex.set = nodeHook.set;
<del>
<ide> // Set width and height to auto instead of 0 on empty string( Bug #8150 )
<ide> // This is for removals
<ide> jQuery.each([ "width", "height" ], function( i, name ) {
<ide><path>test/unit/attributes.js
<ide> test("attr(jquery_method)", function(){
<ide> });
<ide>
<ide> test("attr(String, Object) - Loaded via XML document", function() {
<del> expect(2);
<add> expect( 2 );
<ide> var xml = createDashboardXML();
<ide> var titles = [];
<ide> jQuery( "tab", xml ).each(function() {
<ide> test("attr(String, Object) - Loaded via XML document", function() {
<ide> });
<ide>
<ide> test("attr('tabindex')", function() {
<del> expect(8);
<add> expect( 8 );
<ide>
<ide> // elements not natively tabbable
<del> equal(jQuery("#listWithTabIndex").attr("tabindex"), 5, "not natively tabbable, with tabindex set to 0");
<del> equal(jQuery("#divWithNoTabIndex").attr("tabindex"), undefined, "not natively tabbable, no tabindex set");
<add> equal( jQuery("#listWithTabIndex").attr("tabindex"), 5, "not natively tabbable, with tabindex set to 0" );
<add> equal( jQuery("#divWithNoTabIndex").attr("tabindex"), undefined, "not natively tabbable, no tabindex set" );
<ide>
<ide> // anchor with href
<del> equal(jQuery("#linkWithNoTabIndex").attr("tabindex"), 0, "anchor with href, no tabindex set");
<del> equal(jQuery("#linkWithTabIndex").attr("tabindex"), 2, "anchor with href, tabindex set to 2");
<del> equal(jQuery("#linkWithNegativeTabIndex").attr("tabindex"), -1, "anchor with href, tabindex set to -1");
<add> equal( jQuery("#linkWithNoTabIndex").attr("tabindex"), undefined, "anchor with href, no tabindex set" );
<add> equal( jQuery("#linkWithTabIndex").attr("tabindex"), 2, "anchor with href, tabindex set to 2" );
<add> equal( jQuery("#linkWithNegativeTabIndex").attr("tabindex"), -1, "anchor with href, tabindex set to -1" );
<ide>
<ide> // anchor without href
<del> equal(jQuery("#linkWithNoHrefWithNoTabIndex").attr("tabindex"), undefined, "anchor without href, no tabindex set");
<del> equal(jQuery("#linkWithNoHrefWithTabIndex").attr("tabindex"), 1, "anchor without href, tabindex set to 2");
<del> equal(jQuery("#linkWithNoHrefWithNegativeTabIndex").attr("tabindex"), -1, "anchor without href, no tabindex set");
<add> equal( jQuery("#linkWithNoHrefWithNoTabIndex").attr("tabindex"), undefined, "anchor without href, no tabindex set" );
<add> equal( jQuery("#linkWithNoHrefWithTabIndex").attr("tabindex"), 1, "anchor without href, tabindex set to 2" );
<add> equal( jQuery("#linkWithNoHrefWithNegativeTabIndex").attr("tabindex"), -1, "anchor without href, no tabindex set" );
<ide> });
<ide>
<ide> test("attr('tabindex', value)", function() {
<del> expect(9);
<add> expect( 9 );
<ide>
<ide> var element = jQuery("#divWithNoTabIndex");
<del> equal(element.attr("tabindex"), undefined, "start with no tabindex");
<add> equal( element.attr("tabindex"), undefined, "start with no tabindex" );
<ide>
<ide> // set a positive string
<ide> element.attr("tabindex", "1");
<del> equal(element.attr("tabindex"), 1, "set tabindex to 1 (string)");
<add> equal( element.attr("tabindex"), 1, "set tabindex to 1 (string)" );
<ide>
<ide> // set a zero string
<ide> element.attr("tabindex", "0");
<del> equal(element.attr("tabindex"), 0, "set tabindex to 0 (string)");
<add> equal( element.attr("tabindex"), 0, "set tabindex to 0 (string)" );
<ide>
<ide> // set a negative string
<ide> element.attr("tabindex", "-1");
<del> equal(element.attr("tabindex"), -1, "set tabindex to -1 (string)");
<add> equal( element.attr("tabindex"), -1, "set tabindex to -1 (string)" );
<ide>
<ide> // set a positive number
<ide> element.attr("tabindex", 1);
<del> equal(element.attr("tabindex"), 1, "set tabindex to 1 (number)");
<add> equal( element.attr("tabindex"), 1, "set tabindex to 1 (number)" );
<ide>
<ide> // set a zero number
<ide> element.attr("tabindex", 0);
<ide> equal(element.attr("tabindex"), 0, "set tabindex to 0 (number)");
<ide>
<ide> // set a negative number
<ide> element.attr("tabindex", -1);
<del> equal(element.attr("tabindex"), -1, "set tabindex to -1 (number)");
<add> equal( element.attr("tabindex"), -1, "set tabindex to -1 (number)" );
<ide>
<ide> element = jQuery("#linkWithTabIndex");
<del> equal(element.attr("tabindex"), 2, "start with tabindex 2");
<add> equal( element.attr("tabindex"), 2, "start with tabindex 2" );
<ide>
<ide> element.attr("tabindex", -1);
<del> equal(element.attr("tabindex"), -1, "set negative tabindex");
<add> equal( element.attr("tabindex"), -1, "set negative tabindex" );
<ide> });
<ide>
<ide> test("removeAttr(String)", function() {
<ide><path>test/unit/selector.js
<ide> test("class - jQuery only", function() {
<ide> deepEqual( jQuery("p").find(".blog").get(), q("mark", "simon"), "Finding elements with a context." );
<ide> });
<ide>
<add>test("attributes - jQuery only", function() {
<add> expect( 1 );
<add>
<add> t( "Find elements with a tabindex attribute", "[tabindex]", ["listWithTabIndex", "foodWithNegativeTabIndex", "linkWithTabIndex", "linkWithNegativeTabIndex", "linkWithNoHrefWithTabIndex", "linkWithNoHrefWithNegativeTabIndex"] );
<add>});
<add>
<ide> test("pseudo - visibility", function() {
<del> expect(9);
<add> expect( 9 );
<ide>
<ide> t( "Is Visible", "div:visible:not(#qunit-testrunner-toolbar):lt(2)", ["nothiddendiv", "nothiddendivchild"] );
<ide> t( "Is Not Hidden", "#qunit-fixture:hidden", [] );
<ide> test("pseudo - visibility", function() {
<ide> });
<ide>
<ide> test("disconnected nodes", function() {
<del> expect(4);
<add> expect( 4 );
<ide> var $opt = jQuery('<option></option>').attr("value", "whipit").appendTo("#qunit-fixture").detach();
<ide> equal( $opt.val(), "whipit", "option value" );
<ide> equal( $opt.is(":selected"), false, "unselected option" );
<ide> test("disconnected nodes", function() {
<ide> });
<ide>
<ide> testIframe("selector/html5_selector", "attributes - jQuery.attr", function( jQuery, window, document ) {
<del> expect(35);
<add> expect( 35 );
<ide>
<ide> /**
<ide> * Returns an array of elements with the given IDs | 3 |
PHP | PHP | improve exception message | eb26fa0399668498c725ee13de51f9765b0b6ed2 | <ide><path>src/Log/LogEngineRegistry.php
<ide> protected function _create($class, string $alias, array $settings): LoggerInterf
<ide> return $instance;
<ide> }
<ide>
<del> throw new RuntimeException('Loggers must instanceof ' . LoggerInterface::class);
<add> throw new RuntimeException(sprintf(
<add> 'Loggers must implement %s. Found `%s` instance instead.',
<add> LoggerInterface::class,
<add> getTypeName($instance)
<add> ));
<ide> }
<ide>
<ide> /** | 1 |
Text | Text | clarify upstream versions requirement | df04c2f9faf4cc7b989094cdbe4e4347fee4dd10 | <ide><path>docs/Versions.md
<ide> Versioned formulae we include in [homebrew/core](https://github.com/homebrew/hom
<ide> * Versioned software should build on all Homebrew's supported versions of macOS.
<ide> * Versioned formulae should differ in major/minor (not patch) versions from the current stable release. This is because patch versions indicate bug or security updates, and we want to ensure you apply security updates.
<ide> * Unstable versions (alpha, beta, development versions) are not acceptable for versioned (or unversioned) formulae.
<del>* Upstream should have a release branch for each formula version, and release security updates for each version when necessary. For example, [PHP 7.0 was not a supported version but PHP 7.2 was](https://php.net/supported-versions.php) in January 2020. By contrast, most software projects are structured to only release security updates for their latest versions, so their earlier versions are not eligible for versioning.
<add>* Upstream should have a release branch for each formula version, and claim to release security updates for each version when necessary. For example, [PHP 7.0 was not a supported version but PHP 7.2 was](https://php.net/supported-versions.php) in January 2020. By contrast, most software projects are structured to only release security updates for their latest versions, so their earlier versions are not eligible for versioning.
<ide> * Versioned formulae should share a codebase with the main formula. If the project is split into a different repository, we recommend creating a new formula (`formula2` rather than `formula@2` or `formula@1`).
<ide> * Formulae that depend on versioned formulae must not depend on the same formulae at two different versions twice in their recursive dependencies. For example, if you depend on `[email protected]` and `foo`, and `foo` depends on `openssl` then you must instead use `openssl`.
<ide> * Versioned formulae should only be linkable at the same time as their non-versioned counterpart if the upstream project provides support for it, e.g. using suffixed binaries. If this is not possible, use `keg_only :versioned_formula` to allow users to have multiple versions installed at once. | 1 |
Ruby | Ruby | remove hardcoded reference to env | 16c073a1531057c7f8244a2694d0923986b79d74 | <ide><path>Library/Homebrew/extend/ENV/shared.rb
<ide> def compiler
<ide> # an alternate compiler, altering the value of environment variables.
<ide> # If no valid compiler is found, raises an exception.
<ide> def validate_cc!(formula)
<del> if formula.fails_with? ENV.compiler
<add> if formula.fails_with? compiler
<ide> send CompilerSelector.new(formula).compiler
<ide> end
<ide> end | 1 |
Text | Text | fix nits in http(s) server.headerstimeout | acedf1a55a0a1712a5b06babc34694ef718275ef | <ide><path>doc/api/http.md
<ide> added: v0.1.90
<ide>
<ide> Stops the server from accepting new connections. See [`net.Server.close()`][].
<ide>
<add>### server.headersTimeout
<add><!-- YAML
<add>added: v11.3.0
<add>-->
<add>
<add>* {number} **Default:** `40000`
<add>
<add>Limit the amount of time the parser will wait to receive the complete HTTP
<add>headers.
<add>
<add>In case of inactivity, the rules defined in [`server.timeout`][] apply. However,
<add>that inactivity based timeout would still allow the connection to be kept open
<add>if the headers are being sent very slowly (by default, up to a byte per 2
<add>minutes). In order to prevent this, whenever header data arrives an additional
<add>check is made that more than `server.headersTimeout` milliseconds has not
<add>passed since the connection was established. If the check fails, a `'timeout'`
<add>event is emitted on the server object, and (by default) the socket is destroyed.
<add>See [`server.timeout`][] for more information on how timeout behavior can be
<add>customized.
<add>
<ide> ### server.listen()
<ide>
<ide> Starts the HTTP server listening for connections.
<ide> added: v0.7.0
<ide>
<ide> Limits maximum incoming headers count. If set to 0, no limit will be applied.
<ide>
<del>### server.headersTimeout
<del><!-- YAML
<del>added: v11.3.0
<del>-->
<del>
<del>* {number} **Default:** `40000`
<del>
<del>Limit the amount of time the parser will wait to receive the complete HTTP
<del>headers.
<del>
<del>In case of inactivity, the rules defined in [server.timeout][] apply. However,
<del>that inactivity based timeout would still allow the connection to be kept open
<del>if the headers are being sent very slowly (by default, up to a byte per 2
<del>minutes). In order to prevent this, whenever header data arrives an additional
<del>check is made that more than `server.headersTimeout` milliseconds has not
<del>passed since the connection was established. If the check fails, a `'timeout'`
<del>event is emitted on the server object, and (by default) the socket is destroyed.
<del>See [server.timeout][] for more information on how timeout behaviour can be
<del>customised.
<del>
<ide> ### server.setTimeout([msecs][, callback])
<ide> <!-- YAML
<ide> added: v0.9.12
<ide><path>doc/api/https.md
<ide> added: v0.1.90
<ide>
<ide> See [`server.close()`][`http.close()`] from the HTTP module for details.
<ide>
<add>### server.headersTimeout
<add><!-- YAML
<add>added: v11.3.0
<add>-->
<add>- {number} **Default:** `40000`
<add>
<add>See [`http.Server#headersTimeout`][].
<add>
<ide> ### server.listen()
<ide>
<ide> Starts the HTTPS server listening for encrypted connections.
<ide> This method is identical to [`server.listen()`][] from [`net.Server`][].
<ide>
<ide> See [`http.Server#maxHeadersCount`][].
<ide>
<del>### server.headersTimeout
<del>
<del>- {number} **Default:** `40000`
<del>
<del>See [`http.Server#headersTimeout`][].
<del>
<ide> ### server.setTimeout([msecs][, callback])
<ide> <!-- YAML
<ide> added: v0.11.2
<ide> headers: max-age=0; pin-sha256="WoiWRyIOVNa9ihaBciRSC7XHjliYS9VwUGOIud4PB18="; p
<ide> [`Agent`]: #https_class_https_agent
<ide> [`URL`]: url.html#url_the_whatwg_url_api
<ide> [`http.Agent`]: http.html#http_class_http_agent
<add>[`http.Server#headersTimeout`]: http.html#http_server_headerstimeout
<ide> [`http.Server#keepAliveTimeout`]: http.html#http_server_keepalivetimeout
<ide> [`http.Server#maxHeadersCount`]: http.html#http_server_maxheaderscount
<del>[`http.Server#headersTimeout`]: http.html#http_server_headerstimeout
<ide> [`http.Server#setTimeout()`]: http.html#http_server_settimeout_msecs_callback
<ide> [`http.Server#timeout`]: http.html#http_server_timeout
<ide> [`http.Server`]: http.html#http_class_http_server | 2 |
PHP | PHP | add crypt facade methods hints | 801cbdbbe187dee2021ba9c4f69e474a526354a6 | <ide><path>src/Illuminate/Support/Facades/Crypt.php
<ide> namespace Illuminate\Support\Facades;
<ide>
<ide> /**
<del> * @method static string encrypt($value, bool $serialize = true)
<add> * @method static bool supported(string $key, string $cipher)
<add> * @method static string generateKey(string $cipher)
<add> * @method static string encrypt(mixed $value, bool $serialize = true)
<ide> * @method static string encryptString(string $value)
<del> * @method static string decrypt($payload, bool $unserialize = true)
<add> * @method static mixed decrypt(mixed $payload, bool $unserialize = true)
<ide> * @method static string decryptString(string $payload)
<add> * @method static string getKey()
<ide> *
<ide> * @see \Illuminate\Encryption\Encrypter
<ide> */ | 1 |
Python | Python | fix default folder permissions | 662a8aaac302aae9e1768c7c55e15aa8c539208e | <ide><path>airflow/providers/sftp/hooks/sftp.py
<ide> def list_directory(self, path: str) -> list[str]:
<ide> files = sorted(conn.listdir(path))
<ide> return files
<ide>
<del> def mkdir(self, path: str, mode: int = 777) -> None:
<add> def mkdir(self, path: str, mode: int = 0o777) -> None:
<ide> """
<ide> Creates a directory on the remote system.
<add> The default mode is 0777, but on some systems, the current umask value is first masked out.
<ide>
<ide> :param path: full path to the remote directory to create
<del> :param mode: permissions to set the directory with
<add> :param mode: int permissions of octal mode for directory
<ide> """
<ide> conn = self.get_conn()
<del> conn.mkdir(path, mode=int(str(mode), 8))
<add> conn.mkdir(path, mode=mode)
<ide>
<ide> def isdir(self, path: str) -> bool:
<ide> """
<ide> def isfile(self, path: str) -> bool:
<ide> result = False
<ide> return result
<ide>
<del> def create_directory(self, path: str, mode: int = 777) -> None:
<add> def create_directory(self, path: str, mode: int = 0o777) -> None:
<ide> """
<ide> Creates a directory on the remote system.
<add> The default mode is 0777, but on some systems, the current umask value is first masked out.
<ide>
<ide> :param path: full path to the remote directory to create
<del> :param mode: int representation of octal mode for directory
<add> :param mode: int permissions of octal mode for directory
<ide> """
<ide> conn = self.get_conn()
<ide> if self.isdir(path):
<ide><path>tests/providers/sftp/hooks/test_sftp.py
<ide> def test_mkdir(self):
<ide> self.hook.mkdir(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS, new_dir_name))
<ide> output = self.hook.describe_directory(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS))
<ide> assert new_dir_name in output
<add> # test the directory has default permissions to 777 - umask
<add> umask = 0o022
<add> output = self.hook.get_conn().lstat(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS, new_dir_name))
<add> assert output.st_mode & 0o777 == 0o777 - umask
<ide>
<ide> def test_create_and_delete_directory(self):
<ide> new_dir_name = "new_dir"
<ide> self.hook.create_directory(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS, new_dir_name))
<ide> output = self.hook.describe_directory(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS))
<ide> assert new_dir_name in output
<add> # test the directory has default permissions to 777
<add> umask = 0o022
<add> output = self.hook.get_conn().lstat(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS, new_dir_name))
<add> assert output.st_mode & 0o777 == 0o777 - umask
<ide> # test directory already exists for code coverage, should not raise an exception
<ide> self.hook.create_directory(os.path.join(TMP_PATH, TMP_DIR_FOR_TESTS, new_dir_name))
<ide> # test path already exists and is a file, should raise an exception | 2 |
PHP | PHP | add old request class for bc reason | 38d7a41e0d57933365588cb26c405a8e76a1a832 | <ide><path>src/Network/Request.php
<add><?php
<add>// @deprecated Load new class and alias
<add>class_exists('Cake\Http\ServerRequest');
<add>deprecationWarning('Use Cake\Http\ServerRequest instead of Cake\Network\Request.'); | 1 |
PHP | PHP | add missing reserved names | c32c4fb35f3e1cd75ff2ad1630754712a7e0c6f3 | <ide><path>src/Illuminate/Console/GeneratorCommand.php
<ide> abstract class GeneratorCommand extends Command
<ide> 'eval',
<ide> 'exit',
<ide> 'extends',
<add> 'false',
<ide> 'final',
<ide> 'finally',
<ide> 'fn',
<ide> abstract class GeneratorCommand extends Command
<ide> 'switch',
<ide> 'throw',
<ide> 'trait',
<add> 'true',
<ide> 'try',
<ide> 'unset',
<ide> 'use', | 1 |
Python | Python | fix official.vision.detection.ops import error | 403014db9f12c0228529db2c8b292efcced5133a | <ide><path>official/vision/detection/ops/__init__.py
<add># Copyright 2019 The TensorFlow Authors. All Rights Reserved.
<add>#
<add># Licensed under the Apache License, Version 2.0 (the "License");
<add># you may not use this file except in compliance with the License.
<add># You may obtain a copy of the License at
<add>#
<add># http://www.apache.org/licenses/LICENSE-2.0
<add>#
<add># Unless required by applicable law or agreed to in writing, software
<add># distributed under the License is distributed on an "AS IS" BASIS,
<add># WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
<add># See the License for the specific language governing permissions and
<add># limitations under the License.
<add># ============================================================================== | 1 |
Text | Text | fix typos in rc11 changelog | 212f685e0634a60687cae0d0824dea64accb0b26 | <ide><path>CHANGELOG.md
<ide>
<ide> ## Breaking Changes
<ide>
<del>- **$beforeRouteChange and $routeChangeStart events were renamed to $afterRouteChange and
<add>- **$beforeRouteChange and $afterRouteChange events were renamed to $routeChangeStart and
<ide> $routeChangeSuccess**
<ide>
<ide> This was done to make the naming consistent with $location events and also get events to | 1 |
Python | Python | pass app to multiprocessing pool workers | c24011bc2a87428c36afc4b5abdacda08d5da08e | <ide><path>celery/worker/__init__.py
<ide> WORKER_SIGIGNORE = frozenset(["SIGINT"])
<ide>
<ide>
<del>def process_initializer():
<add>def process_initializer(app):
<ide> """Initializes the process so it can be used to process tasks.
<ide>
<ide> Used for multiprocessing environments.
<ide>
<ide> """
<add> app = app_or_default(app)
<ide> map(platform.reset_signal, WORKER_SIGRESET)
<ide> map(platform.ignore_signal, WORKER_SIGIGNORE)
<ide> platform.set_mp_process_title("celeryd")
<ide>
<ide> # This is for windows and other platforms not supporting
<ide> # fork(). Note that init_worker makes sure it's only
<ide> # run once per process.
<del> from celery.loaders import current_loader
<del> current_loader().init_worker()
<add> app.loader.init_worker()
<ide>
<ide> signals.worker_process_init.send(sender=None)
<ide>
<ide> def __init__(self, concurrency=None, logfile=None, loglevel=None,
<ide> self.pool = instantiate(self.pool_cls, self.concurrency,
<ide> logger=self.logger,
<ide> initializer=process_initializer,
<add> initargs=(self.app, ),
<ide> maxtasksperchild=self.max_tasks_per_child,
<ide> timeout=self.task_time_limit,
<ide> soft_timeout=self.task_soft_time_limit, | 1 |
PHP | PHP | fix errorhandler tests on php 8 | 513b3ade81fd618f993e76a4f0f82ed2edb72f47 | <ide><path>tests/TestCase/Error/ErrorHandlerTest.php
<ide> public function testHandleErrorDebugOn()
<ide> $result = ob_get_clean();
<ide>
<ide> $this->assertRegExp('/<pre class="cake-error">/', $result);
<del> $this->assertRegExp('/<b>Notice<\/b>/', $result);
<del> $this->assertRegExp('/variable:\s+wrong/', $result);
<add> if (version_compare(PHP_VERSION, '8.0.0-dev', '<')) {
<add> $this->assertRegExp('/<b>Notice<\/b>/', $result);
<add> $this->assertRegExp('/variable:\s+wrong/', $result);
<add> } else {
<add> $this->assertRegExp('/<b>Warning<\/b>/', $result);
<add> $this->assertRegExp('/variable \$wrong/', $result);
<add> }
<ide> $this->assertStringContainsString(
<del> 'ErrorHandlerTest.php, line ' . (__LINE__ - 7),
<add> 'ErrorHandlerTest.php, line ' . (__LINE__ - 12),
<ide> $result,
<ide> 'Should contain file and line reference'
<ide> );
<ide> public function testErrorMapping($error, $expected)
<ide> */
<ide> public function testErrorSuppressed()
<ide> {
<add> $this->skipIf(version_compare(PHP_VERSION, '8.0.0-dev', '>='));
<add>
<ide> $errorHandler = new ErrorHandler();
<ide> $errorHandler->register();
<ide> $this->_restoreError = true;
<ide> public function testHandleErrorDebugOff()
<ide> $out = $out + 1;
<ide>
<ide> $messages = $this->logger->read();
<del> $this->assertRegExp('/^(notice|debug)/', $messages[0]);
<del> $this->assertStringContainsString(
<del> 'Notice (8): Undefined variable: out in [' . __FILE__ . ', line ' . (__LINE__ - 5) . ']' . "\n\n",
<del> $messages[0]
<del> );
<add> $this->assertRegExp('/^(notice|debug|warning)/', $messages[0]);
<add>
<add> if (version_compare(PHP_VERSION, '8.0.0-dev', '<')) {
<add> $this->assertStringContainsString(
<add> 'Notice (8): Undefined variable: out in [' . __FILE__ . ', line ' . (__LINE__ - 7) . ']' . "\n\n",
<add> $messages[0]
<add> );
<add> } else {
<add> $this->assertStringContainsString(
<add> 'Warning (2): Undefined variable $out in [' . __FILE__ . ', line ' . (__LINE__ - 12) . ']' . "\n\n",
<add> $messages[0]
<add> );
<add> }
<ide> }
<ide>
<ide> /**
<ide> public function testHandleErrorLoggingTrace()
<ide> $out = $out + 1;
<ide>
<ide> $messages = $this->logger->read();
<del> $this->assertRegExp('/^(notice|debug)/', $messages[0]);
<del> $this->assertStringContainsString(
<del> 'Notice (8): Undefined variable: out in [' . __FILE__ . ', line ' . (__LINE__ - 5) . ']',
<del> $messages[0]
<del> );
<add> $this->assertRegExp('/^(notice|debug|warning)/', $messages[0]);
<add> if (version_compare(PHP_VERSION, '8.0.0-dev', '<')) {
<add> $this->assertStringContainsString(
<add> 'Notice (8): Undefined variable: out in [' . __FILE__ . ', line ' . (__LINE__ - 6) . ']',
<add> $messages[0]
<add> );
<add> } else {
<add> $this->assertStringContainsString(
<add> 'Warning (2): Undefined variable $out in [' . __FILE__ . ', line ' . (__LINE__ - 11) . ']',
<add> $messages[0]
<add> );
<add> }
<ide> $this->assertStringContainsString('Trace:', $messages[0]);
<ide> $this->assertStringContainsString(__NAMESPACE__ . '\ErrorHandlerTest::testHandleErrorLoggingTrace()', $messages[0]);
<ide> $this->assertStringContainsString('Request URL:', $messages[0]); | 1 |
Ruby | Ruby | simplify boolean logic into ternary | f87888066a7280430fd48f7b76bf63d31dbce81a | <ide><path>activesupport/lib/active_support/core_ext/module/delegation.rb
<ide> def delegate(*methods)
<ide> raise ArgumentError, "Can only automatically set the delegation prefix when delegating to a method."
<ide> end
<ide>
<del> prefix = options[:prefix] && "#{options[:prefix] == true ? to : options[:prefix]}_" || ''
<add> prefix = options[:prefix] ? "#{options[:prefix] == true ? to : options[:prefix]}_" : ''
<ide>
<ide> file, line = caller.first.split(':', 2)
<ide> line = line.to_i | 1 |
Ruby | Ruby | move dnsiff to the boneyard | 5123f0c80274ef483ba2359fe3b3264ea9a85d02 | <ide><path>Library/Homebrew/tap_migrations.rb
<ide> "denyhosts" => "homebrew/boneyard",
<ide> "dotwrp" => "homebrew/science",
<ide> "drizzle" => "homebrew/boneyard",
<add> "dsniff" => "homebrew/boneyard",
<ide> "grads" => "homebrew/binary",
<ide> "hwloc" => "homebrew/science",
<ide> "ipopt" => "homebrew/science", | 1 |
Javascript | Javascript | remove "klockan" in `moment.calendar()` | 341142010746c4714276b1868ef2e9b954d7cecc | <ide><path>lang/sv.js
<ide> require('../moment').lang('sv', {
<ide> LLLL : "dddd D MMMM YYYY LT"
<ide> },
<ide> calendar : {
<del> sameDay: '[Idag klockan] LT',
<del> nextDay: '[Imorgon klockan] LT',
<del> lastDay: '[Igår klockan] LT',
<del> nextWeek: 'dddd [klockan] LT',
<del> lastWeek: '[Förra] dddd[en klockan] LT',
<add> sameDay: '[Idag] LT',
<add> nextDay: '[Imorgon] LT',
<add> lastDay: '[Igår] LT',
<add> nextWeek: 'dddd LT',
<add> lastWeek: '[Förra] dddd[en] LT',
<ide> sameElse: 'L'
<ide> },
<ide> relativeTime : {
<ide><path>test/lang/sv.js
<ide> exports["lang:sv"] = {
<ide>
<ide> var a = moment().hours(2).minutes(0).seconds(0);
<ide>
<del> test.equal(moment(a).calendar(), "Idag klockan 02:00", "today at the same time");
<del> test.equal(moment(a).add({ m: 25 }).calendar(), "Idag klockan 02:25", "Now plus 25 min");
<del> test.equal(moment(a).add({ h: 1 }).calendar(), "Idag klockan 03:00", "Now plus 1 hour");
<del> test.equal(moment(a).add({ d: 1 }).calendar(), "Imorgon klockan 02:00", "tomorrow at the same time");
<del> test.equal(moment(a).subtract({ h: 1 }).calendar(), "Idag klockan 01:00", "Now minus 1 hour");
<del> test.equal(moment(a).subtract({ d: 1 }).calendar(), "Igår klockan 02:00", "yesterday at the same time");
<add> test.equal(moment(a).calendar(), "Idag 02:00", "today at the same time");
<add> test.equal(moment(a).add({ m: 25 }).calendar(), "Idag 02:25", "Now plus 25 min");
<add> test.equal(moment(a).add({ h: 1 }).calendar(), "Idag 03:00", "Now plus 1 hour");
<add> test.equal(moment(a).add({ d: 1 }).calendar(), "Imorgon 02:00", "tomorrow at the same time");
<add> test.equal(moment(a).subtract({ h: 1 }).calendar(), "Idag 01:00", "Now minus 1 hour");
<add> test.equal(moment(a).subtract({ d: 1 }).calendar(), "Igår 02:00", "yesterday at the same time");
<ide> test.done();
<ide> },
<ide>
<ide> exports["lang:sv"] = {
<ide>
<ide> for (i = 2; i < 7; i++) {
<ide> m = moment().add({ d: i });
<del> test.equal(m.calendar(), m.format('dddd [klockan] LT'), "Today + " + i + " days current time");
<add> test.equal(m.calendar(), m.format('dddd LT'), "Today + " + i + " days current time");
<ide> m.hours(0).minutes(0).seconds(0).milliseconds(0);
<del> test.equal(m.calendar(), m.format('dddd [klockan] LT'), "Today + " + i + " days beginning of day");
<add> test.equal(m.calendar(), m.format('dddd LT'), "Today + " + i + " days beginning of day");
<ide> m.hours(23).minutes(59).seconds(59).milliseconds(999);
<del> test.equal(m.calendar(), m.format('dddd [klockan] LT'), "Today + " + i + " days end of day");
<add> test.equal(m.calendar(), m.format('dddd LT'), "Today + " + i + " days end of day");
<ide> }
<ide> test.done();
<ide> },
<ide> exports["lang:sv"] = {
<ide>
<ide> for (i = 2; i < 7; i++) {
<ide> m = moment().subtract({ d: i });
<del> test.equal(m.calendar(), m.format('[Förra] dddd[en klockan] LT'), "Today - " + i + " days current time");
<add> test.equal(m.calendar(), m.format('[Förra] dddd[en] LT'), "Today - " + i + " days current time");
<ide> m.hours(0).minutes(0).seconds(0).milliseconds(0);
<del> test.equal(m.calendar(), m.format('[Förra] dddd[en klockan] LT'), "Today - " + i + " days beginning of day");
<add> test.equal(m.calendar(), m.format('[Förra] dddd[en] LT'), "Today - " + i + " days beginning of day");
<ide> m.hours(23).minutes(59).seconds(59).milliseconds(999);
<del> test.equal(m.calendar(), m.format('[Förra] dddd[en klockan] LT'), "Today - " + i + " days end of day");
<add> test.equal(m.calendar(), m.format('[Förra] dddd[en] LT'), "Today - " + i + " days end of day");
<ide> }
<ide> test.done();
<ide> }, | 2 |
Ruby | Ruby | remove unneeded test after force_ssl removal | bd87b37d4fdd6407ab8a8106f14e786d738b8ec6 | <ide><path>railties/test/application/configuration_test.rb
<ide> class D < C
<ide> assert_equal :default, Rails.configuration.debug_exception_response_format
<ide> end
<ide>
<del> test "controller force_ssl declaration can be used even if session_store is disabled" do
<del> make_basic_app do |application|
<del> application.config.session_store :disabled
<del> end
<del>
<del> class ::OmgController < ActionController::Base
<del> force_ssl
<del>
<del> def index
<del> render plain: "Yay! You're on Rails!"
<del> end
<del> end
<del>
<del> get "/"
<del>
<del> assert_equal 301, last_response.status
<del> assert_equal "https://example.org/", last_response.location
<del> end
<del>
<ide> test "ActiveRecord::Base.has_many_inversing is true by default for new apps" do
<ide> app "development"
<ide> | 1 |
Text | Text | use serial comma in webstreams docs | 595ce9dac63cdd32d893c3319328a752719644d2 | <ide><path>doc/api/webstreams.md
<ide> added: v16.6.0
<ide> * `ignoreBOM` {boolean} When `true`, the `TextDecoderStream` will include the
<ide> byte order mark in the decoded result. When `false`, the byte order mark
<ide> will be removed from the output. This option is only used when `encoding` is
<del> `'utf-8'`, `'utf-16be'` or `'utf-16le'`. **Default:** `false`.
<add> `'utf-8'`, `'utf-16be'`, or `'utf-16le'`. **Default:** `false`.
<ide>
<ide> Creates a new `TextDecoderStream` instance.
<ide> | 1 |
PHP | PHP | accept collection of models in belongtomany attach | d686c9147a87a9ad9da423a13df6c5a40471666a | <ide><path>src/Illuminate/Database/Eloquent/Relations/BelongsToMany.php
<ide> public function attach($id, array $attributes = [], $touch = true)
<ide> $id = $id->getKey();
<ide> }
<ide>
<add> if ($id instanceof Collection) {
<add> $id = $id->modelKeys();
<add> }
<add>
<ide> $query = $this->newPivotStatement();
<ide>
<ide> $query->insert($this->createAttachRecords((array) $id, $attributes));
<ide><path>tests/Database/DatabaseEloquentBelongsToManyTest.php
<ide> public function testAttachMultipleInsertsPivotTableRecord()
<ide> $relation->attach([2, 3 => ['baz' => 'boom']], ['foo' => 'bar']);
<ide> }
<ide>
<add> public function testAttachMethodConvertsCollectionToArrayOfKeys()
<add> {
<add> $relation = $this->getMock('Illuminate\Database\Eloquent\Relations\BelongsToMany', ['touchIfTouching'], $this->getRelationArguments());
<add> $query = m::mock('stdClass');
<add> $query->shouldReceive('from')->once()->with('user_role')->andReturn($query);
<add> $query->shouldReceive('insert')->once()->with(
<add> [
<add> ['user_id' => 1, 'role_id' => 1],
<add> ['user_id' => 1, 'role_id' => 2],
<add> ['user_id' => 1, 'role_id' => 3],
<add> ]
<add> )->andReturn(true);
<add> $relation->getQuery()->shouldReceive('getQuery')->andReturn($mockQueryBuilder = m::mock('StdClass'));
<add> $mockQueryBuilder->shouldReceive('newQuery')->once()->andReturn($query);
<add> $relation->expects($this->once())->method('touchIfTouching');
<add>
<add> $collection = new Collection([
<add> m::mock(['getKey' => 1]),
<add> m::mock(['getKey' => 2]),
<add> m::mock(['getKey' => 3]),
<add> ]);
<add>
<add> $relation->attach($collection);
<add> }
<add>
<ide> public function testAttachInsertsPivotTableRecordWithTimestampsWhenNecessary()
<ide> {
<ide> $relation = $this->getMock('Illuminate\Database\Eloquent\Relations\BelongsToMany', ['touchIfTouching'], $this->getRelationArguments()); | 2 |
Ruby | Ruby | avoid double call to to_s, avoid present? | 9fe94dd0c16facf2c9cfac9ae1166050b6f41cee | <ide><path>lib/arel/engines/sql/relations/table.rb
<ide> def initialize(name, options = {})
<ide> if options.is_a?(Hash)
<ide> @options = options
<ide> @engine = options[:engine] || Table.engine
<del> @table_alias = options[:as].to_s if options[:as].present? && options[:as].to_s != @name
<add>
<add> if options[:as]
<add> as = options[:as].to_s
<add> @table_alias = as unless as == @name
<add> end
<ide> else
<ide> @engine = options # Table.new('foo', engine)
<ide> end | 1 |
Text | Text | update instructions for cc | 15f92ee83708c2ecbcd999cd9bb3da848a2ad209 | <ide><path>doc/guides/security-release-process.md
<ide> information described.
<ide> * Described in the pre/post announcements
<ide>
<ide> * [ ] Pre-release announcement [email][]: ***LINK TO EMAIL***
<del> * CC: `[email protected]`
<ide> * Subject: `Node.js security updates for all active release lines, Month Year`
<ide> * Body:
<ide> ```text
<ide> information described.
<ide> ```
<ide> (Get access from existing manager: Ben Noordhuis, Rod Vagg, Michael Dawson)
<ide>
<add>* [ ] CC `[email protected]` on pre-release
<add>
<add>The google groups UI does not support adding a CC, until we figure
<add>out a better way, forward the email you receive to
<add>`[email protected]` as a CC.
<add>
<ide> * [ ] Pre-release announcement to nodejs.org blog: ***LINK TO BLOG***
<ide> (Re-PR the pre-approved branch from nodejs-private/nodejs.org-private to
<ide> nodejs/nodejs.org) | 1 |
Text | Text | improve documentation for util.deprecate() | ccc87ebb3393a7a4738ed20d9378857633e74c76 | <ide><path>doc/api/util.md
<ide> environment variable set, then it will not print anything.
<ide> Multiple comma-separated `section` names may be specified in the `NODE_DEBUG`
<ide> environment variable. For example: `NODE_DEBUG=fs,net,tls`.
<ide>
<del>## util.deprecate(function, string)
<add>## util.deprecate(fn, msg[, code])
<ide> <!-- YAML
<ide> added: v0.8.0
<ide> -->
<ide>
<del>The `util.deprecate()` method wraps the given `function` or class in such a way that
<del>it is marked as deprecated.
<add>* `fn` {Function} The function that is being deprecated.
<add>* `msg` {string} A warning message to display when the deprecated function is
<add> invoked.
<add>* `code` {string} A deprecation code. See the [list of deprecated APIs][] for a
<add> list of codes.
<add>* Returns: {Function} The deprecated function wrapped to emit a warning.
<add>
<add>The `util.deprecate()` method wraps `fn` (which may be a function or class) in
<add>such a way that it is marked as deprecated.
<ide>
<ide> <!-- eslint-disable prefer-rest-params -->
<ide> ```js
<ide> exports.puts = util.deprecate(function() {
<ide> ```
<ide>
<ide> When called, `util.deprecate()` will return a function that will emit a
<del>`DeprecationWarning` using the `process.on('warning')` event. By default,
<del>this warning will be emitted and printed to `stderr` exactly once, the first
<del>time it is called. After the warning is emitted, the wrapped `function`
<del>is called.
<add>`DeprecationWarning` using the `process.on('warning')` event. The warning will
<add>be emitted and printed to `stderr` exactly once, the first time it is called.
<add>After the warning is emitted, the wrapped function is called.
<ide>
<ide> If either the `--no-deprecation` or `--no-warnings` command line flags are
<ide> used, or if the `process.noDeprecation` property is set to `true` *prior* to
<ide> Deprecated predecessor of `console.log`.
<ide> [Internationalization]: intl.html
<ide> [WHATWG Encoding Standard]: https://encoding.spec.whatwg.org/
<ide> [constructor]: https://developer.mozilla.org/en/JavaScript/Reference/Global_Objects/Object/constructor
<add>[list of deprecated APIS]: deprecations.html#deprecations_list_of_deprecated_apis
<ide> [semantically incompatible]: https://github.com/nodejs/node/issues/4179 | 1 |
Go | Go | add name generator | 971cf56d89ac9cc4959800bdfaadb69ba2cdd06a | <ide><path>names-generator/names-generator.go
<add>package namesgenerator
<add>
<add>import (
<add> "fmt"
<add> "math/rand"
<add> "time"
<add>)
<add>
<add>type NameChecker interface {
<add> Exists(name string) bool
<add>}
<add>
<add>var (
<add> colors = [...]string{"white", "silver", "gray", "black", "blue", "green", "cyan", "yellow", "gold", "orange", "brown", "red", "violet", "pink", "magenta", "purple"}
<add> animals = [...]string{"ant", "bird", "cat", "chicken", "cow", "dog", "fish", "fox", "horse", "lion", "monkey", "pig", "sheep", "tiger", "whale", "wolf"}
<add>)
<add>
<add>func GenerateRandomName(checker NameChecker) (string, error) {
<add> retry := 5
<add> rand.Seed(time.Now().UnixNano())
<add> name := fmt.Sprintf("%s_%s", colors[rand.Intn(len(colors))], animals[rand.Intn(len(animals))])
<add> for checker != nil && checker.Exists(name) && retry > 0 {
<add> name = fmt.Sprintf("%s%d", name, rand.Intn(10))
<add> retry = retry - 1
<add> }
<add> if retry == 0 {
<add> return name, fmt.Errorf("Error generating random name")
<add> }
<add> return name, nil
<add>}
<ide><path>names-generator/names-generator_test.go
<add>package namesgenerator
<add>
<add>import (
<add> "testing"
<add>)
<add>
<add>type FalseChecker struct{}
<add>
<add>func (n *FalseChecker) Exists(name string) bool {
<add> return false
<add>}
<add>
<add>type TrueChecker struct{}
<add>
<add>func (n *TrueChecker) Exists(name string) bool {
<add> return true
<add>}
<add>
<add>func TestGenerateRandomName(t *testing.T) {
<add> if _, err := GenerateRandomName(&FalseChecker{}); err != nil {
<add> t.Error(err)
<add> }
<add>
<add> if _, err := GenerateRandomName(&TrueChecker{}); err == nil {
<add> t.Error("An error was expected")
<add> }
<add>
<add>} | 2 |
PHP | PHP | fix the fix | 7a7500860018398d7402d40e8081bf846b93b3e3 | <ide><path>src/ORM/Behavior/CounterCacheBehavior.php
<ide> protected function _processAssociation(Event $event, EntityInterface $entity, As
<ide> $count = $this->_getCount($config, $countConditions);
<ide> }
<ide> if ($count !== false) {
<del> $assoc->getTarget()->updateAll([$field => $count], updateConditions);
<add> $assoc->getTarget()->updateAll([$field => $count], $updateConditions);
<ide> }
<ide>
<ide> if (isset($updateOriginalConditions)) {
<ide> protected function _processAssociation(Event $event, EntityInterface $entity, As
<ide> $count = $this->_getCount($config, $countOriginalConditions);
<ide> }
<ide> if ($count !== false) {
<del> $assoc->getTarget()->updateAll([$field => $count], updateOriginalConditions);
<add> $assoc->getTarget()->updateAll([$field => $count], $updateOriginalConditions);
<ide> }
<ide> }
<ide> } | 1 |
Javascript | Javascript | fix jslint warnings | 07662cf0350dc8aa6df9abce2f1688ff30ce79ec | <ide><path>pdf.js
<ide> var PredictorStream = (function() {
<ide> var bits = this.bits = params.get('BitsPerComponent') || 8;
<ide> var columns = this.columns = params.get('Columns') || 1;
<ide>
<del> var pixBytes = this.pixBytes = (colors * bits + 7) >> 3;
<add> this.pixBytes = (colors * bits + 7) >> 3;
<ide> // add an extra pixByte to represent the pixel left of column 0
<del> var rowBytes = this.rowBytes = (columns * colors * bits + 7) >> 3;
<add> this.rowBytes = (columns * colors * bits + 7) >> 3;
<ide>
<ide> DecodeStream.call(this);
<ide> return this;
<ide> var PredictorStream = (function() {
<ide>
<ide> constructor.prototype.readBlockTiff = function() {
<ide> var rowBytes = this.rowBytes;
<del> var pixBytes = this.pixBytes;
<ide>
<ide> var bufferLength = this.bufferLength;
<ide> var buffer = this.ensureBuffer(bufferLength + rowBytes);
<ide> var PredictorStream = (function() {
<ide>
<ide> var rawBytes = this.stream.getBytes(rowBytes);
<ide>
<add> var inbuf = 0, outbuf = 0;
<add> var inbits = 0, outbits = 0;
<add>
<ide> if (bits === 1) {
<del> var inbuf = 0;
<ide> for (var i = 0; i < rowBytes; ++i) {
<ide> var c = rawBytes[i];
<del> inBuf = (inBuf << 8) | c;
<add> inbuf = (inbuf << 8) | c;
<ide> // bitwise addition is exclusive or
<del> // first shift inBuf and then add
<del> currentRow[i] = (c ^ (inBuf >> colors)) & 0xFF;
<del> // truncate inBuf (assumes colors < 16)
<del> inBuf &= 0xFFFF;
<add> // first shift inbuf and then add
<add> currentRow[i] = (c ^ (inbuf >> colors)) & 0xFF;
<add> // truncate inbuf (assumes colors < 16)
<add> inbuf &= 0xFFFF;
<ide> }
<ide> } else if (bits === 8) {
<ide> for (var i = 0; i < colors; ++i)
<ide> var PredictorStream = (function() {
<ide> } else {
<ide> var compArray = new Uint8Array(colors + 1);
<ide> var bitMask = (1 << bits) - 1;
<del> var inbuf = 0, outbut = 0;
<del> var inbits = 0, outbits = 0;
<ide> var j = 0, k = 0;
<ide> var columns = this.columns;
<ide> for (var i = 0; i < columns; ++i) {
<ide> var Ascii85Stream = (function() {
<ide> return;
<ide> }
<ide>
<del> var bufferLength = this.bufferLength;
<add> var bufferLength = this.bufferLength, buffer;
<ide>
<ide> // special code for z
<ide> if (c == zCode) {
<del> var buffer = this.ensureBuffer(bufferLength + 4);
<add> buffer = this.ensureBuffer(bufferLength + 4);
<ide> for (var i = 0; i < 4; ++i)
<ide> buffer[bufferLength + i] = 0;
<ide> this.bufferLength += 4;
<ide> var Ascii85Stream = (function() {
<ide> if (!c || c == tildaCode)
<ide> break;
<ide> }
<del> var buffer = this.ensureBuffer(bufferLength + i - 1);
<add> buffer = this.ensureBuffer(bufferLength + i - 1);
<ide> this.bufferLength += i - 1;
<ide>
<ide> // partial ending;
<ide> var CCITTFaxStream = (function() {
<ide> for (var i = 0; i < 4; ++i) {
<ide> code1 = this.lookBits(12);
<ide> if (code1 != 1)
<del> warning('bad rtc code');
<add> warn('bad rtc code: ' + code1);
<ide> this.eatBits(12);
<ide> if (this.encoding > 0) {
<ide> this.lookBits(1);
<ide> var CCITTFaxStream = (function() {
<ide> }
<ide> }
<ide> for (var n = 11; n <= 12; ++n) {
<del> code == this.lookBits(n);
<add> code = this.lookBits(n);
<ide> if (code == EOF)
<ide> return 1;
<ide> if (n < 12)
<ide> var Lexer = (function() {
<ide> 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 // fx
<ide> ];
<ide>
<del> var MIN_INT = (1 << 31) | 0;
<del> var MAX_INT = (MIN_INT - 1) | 0;
<del> var MIN_UINT = 0;
<del> var MAX_UINT = ((1 << 30) * 4) - 1;
<del>
<ide> function ToHexDigit(ch) {
<ide> if (ch >= '0' && ch <= '9')
<ide> return ch.charCodeAt(0) - 48;
<ide> var XRef = (function() {
<ide> },
<ide> readXRefStream: function readXRefStream(stream) {
<ide> var streamParameters = stream.parameters;
<del> var length = streamParameters.get('Length');
<ide> var byteWidths = streamParameters.get('W');
<ide> var range = streamParameters.get('Index');
<ide> if (!range)
<ide> var Page = (function() {
<ide> }
<ide> return shadow(this, 'rotate', rotate);
<ide> },
<del> startRendering: function(canvasCtx, continuation, onerror) {
<add> startRendering: function(canvasCtx, continuation) {
<ide> var self = this;
<ide> var stats = self.stats;
<ide> stats.compile = stats.fonts = stats.render = 0;
<ide> var PDFDoc = (function() {
<ide> return shadow(this, 'numPages', num);
<ide> },
<ide> getPage: function(n) {
<del> var linearization = this.linearization;
<del> // assert(!linearization, "linearized page access not implemented");
<ide> return this.catalog.getPage(n);
<ide> }
<ide> };
<ide> var PartialEvaluator = (function() {
<ide> };
<ide>
<ide> constructor.prototype = {
<del> eval: function(stream, xref, resources, fonts, images) {
<add> evaluate: function(stream, xref, resources, fonts, images) {
<ide> resources = xref.fetchIfRef(resources) || new Dict();
<ide> var xobjs = xref.fetchIfRef(resources.get('XObject')) || new Dict();
<ide> var patterns = xref.fetchIfRef(resources.get('Pattern')) || new Dict();
<ide> var PartialEvaluator = (function() {
<ide> var dict = IsStream(pattern) ? pattern.dict : pattern;
<ide> var typeNum = dict.get('PatternType');
<ide> if (typeNum == 1) {
<del> patternName.code = this.eval(pattern, xref,
<del> dict.get('Resources'), fonts);
<add> patternName.code = this.evaluate(pattern, xref,
<add> dict.get('Resources'),
<add> fonts);
<ide> }
<ide> }
<ide> }
<ide> var PartialEvaluator = (function() {
<ide> );
<ide>
<ide> if ('Form' == type.name) {
<del> args[0].code = this.eval(xobj, xref, xobj.dict.get('Resources'),
<del> fonts, images);
<add> args[0].code = this.evaluate(xobj, xref,
<add> xobj.dict.get('Resources'), fonts,
<add> images);
<ide> }
<ide> if (xobj instanceof JpegStream)
<ide> images.bind(xobj); // monitoring image load
<ide> var PartialEvaluator = (function() {
<ide> },
<ide>
<ide> extractEncoding: function(dict, xref, properties) {
<del> var type = properties.type;
<add> var type = properties.type, encoding;
<ide> if (properties.composite) {
<ide> if (type == 'CIDFontType2') {
<ide> var defaultWidth = xref.fetchIfRef(dict.get('DW')) || 1000;
<ide> var PartialEvaluator = (function() {
<ide> var glyphsData = glyphsStream.getBytes(0);
<ide>
<ide> // Glyph ids are big-endian 2-byte values
<del> var encoding = properties.encoding;
<add> encoding = properties.encoding;
<ide>
<ide> // Set encoding 0 to later verify the font has an encoding
<ide> encoding[0] = { unicode: 0, width: 0 };
<ide> var PartialEvaluator = (function() {
<ide> };
<ide> }
<ide> } else if (type == 'CIDFontType0') {
<del> var encoding = xref.fetchIfRef(dict.get('Encoding'));
<add> encoding = xref.fetchIfRef(dict.get('Encoding'));
<ide> if (IsName(encoding)) {
<ide> // Encoding is a predefined CMap
<ide> if (encoding.name == 'Identity-H') {
<ide> var PartialEvaluator = (function() {
<ide> var map = properties.encoding;
<ide> var baseEncoding = null;
<ide> if (dict.has('Encoding')) {
<del> var encoding = xref.fetchIfRef(dict.get('Encoding'));
<add> encoding = xref.fetchIfRef(dict.get('Encoding'));
<ide> if (IsDict(encoding)) {
<ide> var baseName = encoding.get('BaseEncoding');
<ide> if (baseName)
<ide> var CanvasGraphics = (function() {
<ide>
<ide> compile: function(stream, xref, resources, fonts, images) {
<ide> var pe = new PartialEvaluator();
<del> return pe.eval(stream, xref, resources, fonts, images);
<add> return pe.evaluate(stream, xref, resources, fonts, images);
<ide> },
<ide>
<ide> execute: function(code, xref, resources) {
<ide> var CanvasGraphics = (function() {
<ide> this.ctx.mozDashOffset = dashPhase;
<ide> },
<ide> setRenderingIntent: function(intent) {
<del> TODO('set rendering intent');
<add> TODO('set rendering intent: ' + intent);
<ide> },
<ide> setFlatness: function(flatness) {
<del> TODO('set flatness');
<add> TODO('set flatness: ' + flatness);
<ide> },
<ide> setGState: function(dictName) {
<del> TODO('set graphics state from dict');
<add> TODO('set graphics state from dict: ' + dictName);
<ide> },
<ide> save: function() {
<ide> this.ctx.save();
<ide> var CanvasGraphics = (function() {
<ide> }
<ide> },
<ide> setTextRenderingMode: function(mode) {
<del> TODO('text rendering mode');
<add> TODO('text rendering mode: ' + mode);
<ide> },
<ide> setTextRise: function(rise) {
<del> TODO('text rise');
<add> TODO('text rise: ' + rise);
<ide> },
<ide> moveText: function(x, y) {
<ide> this.current.x = this.current.lineX += x;
<ide> var CanvasGraphics = (function() {
<ide>
<ide> // Type3 fonts
<ide> setCharWidth: function(xWidth, yWidth) {
<del> TODO("type 3 fonts ('d0' operator)");
<add> TODO('type 3 fonts ("d0" operator) xWidth: ' + xWidth + ' yWidth: ' +
<add> yWidth);
<ide> },
<ide> setCharWidthAndBounds: function(xWidth, yWidth, llx, lly, urx, ury) {
<del> TODO("type 3 fonts ('d1' operator)");
<add> TODO('type 3 fonts ("d1" operator) xWidth: ' + xWidth + ' yWidth: ' +
<add> yWidth + ' llx: ' + llx + ' lly: ' + lly + ' urx: ' + urx +
<add> ' ury ' + ury);
<ide> },
<ide>
<ide> // Color
<ide> var ColorSpace = (function() {
<ide> var lookup = xref.fetchIfRef(cs[3]);
<ide> return new IndexedCS(base, hiVal, lookup);
<ide> case 'Separation':
<del> var name = cs[1];
<ide> var alt = ColorSpace.parse(cs[2], xref, res);
<ide> var tintFn = new PDFFunction(xref, xref.fetchIfRef(cs[3]));
<ide> return new SeparationCS(alt, tintFn);
<ide> var SeparationCS = (function() {
<ide> var base = this.base;
<ide> var scale = 1 / ((1 << bits) - 1);
<ide>
<del> var length = 3 * input.length;
<add> var length = input.length;
<ide> var pos = 0;
<ide>
<ide> var numComps = base.numComps;
<del> var baseBuf = new Uint8Array(numComps * input.length);
<del> for (var i = 0, ii = input.length; i < ii; ++i) {
<add> var baseBuf = new Uint8Array(numComps * length);
<add> for (var i = 0; i < length; ++i) {
<ide> var scaled = input[i] * scale;
<ide> var tinted = tintFn.func([scaled]);
<ide> for (var j = 0; j < numComps; ++j)
<ide> var DummyShading = (function() {
<ide> var RadialAxialShading = (function() {
<ide> function constructor(dict, matrix, xref, res, ctx) {
<ide> this.matrix = matrix;
<del> var bbox = dict.get('BBox');
<del> var background = dict.get('Background');
<ide> this.coordsArr = dict.get('Coords');
<ide> this.shadingType = dict.get('ShadingType');
<ide> this.type = 'Pattern';
<ide> var RadialAxialShading = (function() {
<ide> getPattern: function() {
<ide> var coordsArr = this.coordsArr;
<ide> var type = this.shadingType;
<add> var p0, p1, r0, r1;
<ide> if (type == 2) {
<del> var p0 = [coordsArr[0], coordsArr[1]];
<del> var p1 = [coordsArr[2], coordsArr[3]];
<add> p0 = [coordsArr[0], coordsArr[1]];
<add> p1 = [coordsArr[2], coordsArr[3]];
<ide> } else if (type == 3) {
<del> var p0 = [coordsArr[0], coordsArr[1]];
<del> var p1 = [coordsArr[3], coordsArr[4]];
<del> var r0 = coordsArr[2], r1 = coordsArr[5];
<add> p0 = [coordsArr[0], coordsArr[1]];
<add> p1 = [coordsArr[3], coordsArr[4]];
<add> r0 = coordsArr[2];
<add> r1 = coordsArr[5];
<ide> } else {
<del> error();
<add> error('getPattern type unknown: ' + type);
<ide> }
<ide>
<ide> var matrix = this.matrix;
<ide> var RadialAxialShading = (function() {
<ide> p1 = Util.applyTransform(p1, userMatrix);
<ide> }
<ide>
<del> var colorStops = this.colorStops;
<add> var colorStops = this.colorStops, grad;
<ide> if (type == 2)
<del> var grad = ctx.createLinearGradient(p0[0], p0[1], p1[0], p1[1]);
<add> grad = ctx.createLinearGradient(p0[0], p0[1], p1[0], p1[1]);
<ide> else if (type == 3)
<del> var grad = ctx.createRadialGradient(p0[0], p0[1], r0, p1[0], p1[1], r1);
<add> grad = ctx.createRadialGradient(p0[0], p0[1], r0, p1[0], p1[1], r1);
<ide>
<ide> for (var i = 0, ii = colorStops.length; i < ii; ++i) {
<ide> var c = colorStops[i];
<ide> var PDFImage = (function() {
<ide> var bufferPos = 0;
<ide> var output = bpc <= 8 ? new Uint8Array(length) :
<ide> bpc <= 16 ? new Uint16Array(length) : new Uint32Array(length);
<add> var rowComps = width * numComps;
<ide>
<ide> if (bpc == 1) {
<ide> var valueZero = 0, valueOne = 1;
<ide> if (decodeMap) {
<ide> valueZero = decodeMap[0] ? 1 : 0;
<ide> valueOne = decodeMap[1] ? 1 : 0;
<ide> }
<del> var rowComps = width * numComps;
<ide> var mask = 0;
<ide> var buf = 0;
<ide>
<ide> var PDFImage = (function() {
<ide> } else {
<ide> if (decodeMap != null)
<ide> TODO('interpolate component values');
<del> var rowComps = width * numComps;
<del> var bits, buf;
<add> var bits = 0, buf = 0;
<ide> for (var i = 0, ii = length; i < ii; ++i) {
<ide> if (i % rowComps == 0) {
<ide> buf = 0;
<ide> var PDFFunction = (function() {
<ide> floor *= outputSize;
<ide> ceil *= outputSize;
<ide>
<del> var output = [];
<add> var output = [], v = 0;
<ide> for (var i = 0; i < outputSize; ++i) {
<ide> if (ceil == floor) {
<del> var v = samples[ceil + i];
<add> v = samples[ceil + i];
<ide> } else {
<ide> var low = samples[floor + i];
<ide> var high = samples[ceil + i];
<del> var v = low * scale + high * (1 - scale);
<add> v = low * scale + high * (1 - scale);
<ide> }
<ide>
<ide> var i2 = i * 2; | 1 |
Python | Python | move another test within testnestediter | 526a9f9f49567b499de4335ac7851d6932853fa7 | <ide><path>numpy/core/tests/test_nditer.py
<ide> def test_dtype_buffered(self):
<ide> y[...] += 1
<ide> assert_equal(a, [[1, 2, 3], [4, 5, 6]])
<ide>
<add> def test_0d(self):
<add> a = np.arange(12).reshape(2, 3, 2)
<add> i, j = np.nested_iters(a, [[], [1, 0, 2]])
<add> vals = []
<add> for x in i:
<add> vals.append([y for y in j])
<add> assert_equal(vals, [[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]])
<add>
<add> i, j = np.nested_iters(a, [[1, 0, 2], []])
<add> vals = []
<add> for x in i:
<add> vals.append([y for y in j])
<add> assert_equal(vals, [[0], [1], [2], [3], [4], [5], [6], [7], [8], [9], [10], [11]])
<add>
<add> i, j, k = np.nested_iters(a, [[2, 0], [], [1]])
<add> vals = []
<add> for x in i:
<add> for y in j:
<add> vals.append([z for z in k])
<add> assert_equal(vals, [[0, 2, 4], [1, 3, 5], [6, 8, 10], [7, 9, 11]])
<add>
<add>
<ide> def test_iter_reduction_error():
<ide>
<ide> a = np.arange(6)
<ide> def test_0d_iter():
<ide> assert_equal(vals['d'], 0.5)
<ide>
<ide>
<del>def test_0d_nested_iter():
<del> a = np.arange(12).reshape(2, 3, 2)
<del> i, j = np.nested_iters(a, [[], [1, 0, 2]])
<del> vals = []
<del> for x in i:
<del> vals.append([y for y in j])
<del> assert_equal(vals, [[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]])
<del>
<del> i, j = np.nested_iters(a, [[1, 0, 2], []])
<del> vals = []
<del> for x in i:
<del> vals.append([y for y in j])
<del> assert_equal(vals, [[0], [1], [2], [3], [4], [5], [6], [7], [8], [9], [10], [11]])
<del>
<del> i, j, k = np.nested_iters(a, [[2, 0], [], [1]])
<del> vals = []
<del> for x in i:
<del> for y in j:
<del> vals.append([z for z in k])
<del> assert_equal(vals, [[0, 2, 4], [1, 3, 5], [6, 8, 10], [7, 9, 11]])
<del>
<del>
<ide> def test_iter_too_large():
<ide> # The total size of the iterator must not exceed the maximum intp due
<ide> # to broadcasting. Dividing by 1024 will keep it small enough to | 1 |
Text | Text | fix `eventtarget.dispatchevent` docs | ffda9a8953ccf5afe099322d038eb23f2cfe6d82 | <ide><path>doc/api/events.md
<ide> target.removeEventListener('foo', handler, { capture: true });
<ide> added: v14.5.0
<ide> -->
<ide>
<del>* `event` {Object|Event}
<add>* `event` {Event}
<add>* Returns: {boolean} `true` if either event’s `cancelable` attribute value is
<add> false or its `preventDefault()` method was not invoked, otherwise `false`.
<ide>
<del>Dispatches the `event` to the list of handlers for `event.type`. The `event`
<del>may be an `Event` object or any object with a `type` property whose value is
<del>a `string`.
<add>Dispatches the `event` to the list of handlers for `event.type`.
<ide>
<ide> The registered event listeners is synchronously invoked in the order they
<ide> were registered. | 1 |
Python | Python | fix old markings for chord tests | ea2a803d57524db1edf0ecf81164e3c61fc8935b | <ide><path>t/integration/test_canvas.py
<del>import os
<ide> from datetime import datetime, timedelta
<ide> from time import sleep
<ide>
<ide> import pytest
<ide>
<ide> from celery import chain, chord, group, signature
<ide> from celery.backends.base import BaseKeyValueStoreBackend
<del>from celery.exceptions import ChordError, TimeoutError
<add>from celery.exceptions import TimeoutError
<ide> from celery.result import AsyncResult, GroupResult, ResultSet
<ide>
<ide> from .conftest import get_active_redis_channels, get_redis_connection
<ide> def test_eager_chord_inside_task(self, manager):
<ide>
<ide> chord_add.app.conf.task_always_eager = prev
<ide>
<del> @flaky
<ide> def test_group_chain(self, manager):
<del> if not manager.app.conf.result_backend.startswith('redis'):
<del> raise pytest.skip('Requires redis result backend.')
<add> try:
<add> manager.app.backend.ensure_chords_allowed()
<add> except NotImplementedError as e:
<add> raise pytest.skip(e.args[0])
<add>
<ide> c = (
<ide> add.s(2, 2) |
<ide> group(add.s(i) for i in range(4)) |
<ide> def test_group_chain(self, manager):
<ide> res = c()
<ide> assert res.get(timeout=TIMEOUT) == [12, 13, 14, 15]
<ide>
<del> @flaky
<del> @pytest.mark.xfail(os.environ['TEST_BACKEND'] == 'cache+pylibmc://',
<del> reason="Not supported yet by the cache backend.",
<del> strict=True,
<del> raises=ChordError)
<ide> def test_nested_group_chain(self, manager):
<ide> try:
<ide> manager.app.backend.ensure_chords_allowed() | 1 |
Text | Text | add proper indentation to example code | 9621e372d0776524b2ba6d697d45749f5725e260 | <ide><path>guide/english/javascript/global-variables/index.md
<ide> title: Global Variables
<ide> Global variables are declared outside of a function for accessibility throughout the program, while local variables are stored within a function using `var` for use only within that function's [scope](https://developer.mozilla.org/en-US/docs/Glossary/Scope). If you declare a variable without using `var`, even if it's inside a function, it will still be seen as global:
<ide>
<ide> ```javascript
<del>var x = 5; //global
<add>var x = 5; //global variable
<ide> function someThing(y) {
<del>var z = x + y;
<del>console.log(z);
<add> var z = x + y;
<add> console.log(z);
<ide> }
<ide>
<ide> function someThing(y) {
<del>x = 5; //still global!
<del>var z = x + y;
<del>console.log(z);
<add> x = 5; //still a global variable!
<add> var z = x + y;
<add> console.log(z);
<ide> }
<ide>
<del>
<ide> function someThing(y) {
<del>var x = 5; //local
<del>var z = x + y;
<del>console.log(z);
<add> var x = 5; //local variable
<add> var z = x + y;
<add> console.log(z);
<ide> }
<ide> ```
<ide> A global variable is also an object of the current scope, such as the browser window: | 1 |
Python | Python | fix minor issues in debug-data | 3ac4e8eb7a6c688ddc7abd205e2ed7060cbf0798 | <ide><path>spacy/cli/debug_data.py
<ide> def debug_data(
<ide> msg.text("{} training docs".format(len(train_dataset)))
<ide> msg.text("{} evaluation docs".format(len(gold_dev_data)))
<ide>
<add> if not len(gold_dev_data):
<add> msg.fail("No evaluation docs")
<ide> overlap = len(train_texts.intersection(dev_texts))
<ide> if overlap:
<ide> msg.warn("{} training examples also in evaluation data".format(overlap))
<ide> def debug_data(
<ide> if "ner" in pipeline:
<ide> # Get all unique NER labels present in the data
<ide> labels = set(
<del> label for label in gold_train_data["ner"] if label not in ("O", "-")
<add> label for label in gold_train_data["ner"] if label not in ("O", "-", None)
<ide> )
<ide> label_counts = gold_train_data["ner"]
<ide> model_labels = _get_labels_from_model(nlp, "ner")
<ide> def _format_labels(labels, counts=False):
<ide> def _get_examples_without_label(data, label):
<ide> count = 0
<ide> for ex in data:
<del> labels = [label.split("-")[1] for label in ex.gold.ner if label not in ("O", "-")]
<add> labels = [label.split("-")[1] for label in ex.gold.ner if label not in ("O", "-", None)]
<ide> if label not in labels:
<ide> count += 1
<ide> return count | 1 |
PHP | PHP | fix php flaw around intval with custom base | 3e25282d4c3f9940a7c9939bd189b89340c9d181 | <ide><path>lib/Cake/Utility/Folder.php
<ide> public function chmod($path, $mode = false, $recursive = true, $exceptions = arr
<ide>
<ide> if ($recursive === false && is_dir($path)) {
<ide> //@codingStandardsIgnoreStart
<del> if (@chmod($path, intval($mode, 8))) {
<add> if (@chmod($path, intval((string)$mode, 8))) {
<ide> //@codingStandardsIgnoreEnd
<ide> $this->_messages[] = __d('cake_dev', '%s changed to %s', $path, $mode);
<ide> return true;
<ide> public function chmod($path, $mode = false, $recursive = true, $exceptions = arr
<ide> }
<ide>
<ide> //@codingStandardsIgnoreStart
<del> if (@chmod($fullpath, intval($mode, 8))) {
<add> if (@chmod($fullpath, intval((string)$mode, 8))) {
<ide> //@codingStandardsIgnoreEnd
<ide> $this->_messages[] = __d('cake_dev', '%s changed to %s', $fullpath, $mode);
<ide> } else {
<ide> public function copy($options) {
<ide> $from = Folder::addPathElement($fromDir, $item);
<ide> if (is_file($from) && (!is_file($to) || $options['scheme'] != Folder::SKIP)) {
<ide> if (copy($from, $to)) {
<del> chmod($to, intval($mode, 8));
<add> chmod($to, intval((string)$mode, 8));
<ide> touch($to, filemtime($from));
<ide> $this->_messages[] = __d('cake_dev', '%s copied to %s', $from, $to);
<ide> } else { | 1 |
Ruby | Ruby | consolidate stringify keys in `predicatebuilder` | 8714b359b2c6d3b402cdbaa3f12d2690417e53f4 | <ide><path>activerecord/lib/active_record/relation/predicate_builder.rb
<ide> def initialize(table)
<ide> end
<ide>
<ide> def build_from_hash(attributes, &block)
<del> attributes = attributes.stringify_keys
<ide> attributes = convert_dot_notation_to_hash(attributes)
<del>
<ide> expand_from_hash(attributes, &block)
<ide> end
<ide>
<ide> def self.references(attributes)
<del> attributes.map do |key, value|
<del> key = key.to_s
<add> attributes.each_with_object([]) do |(key, value), result|
<ide> if value.is_a?(Hash)
<del> key
<del> else
<del> key.split(".").first if key.include?(".")
<add> result << key
<add> elsif key.include?(".")
<add> result << key.split(".").first
<ide> end
<del> end.compact
<add> end
<ide> end
<ide>
<ide> # Define how a class is converted to Arel nodes when passed to +where+.
<ide><path>activerecord/lib/active_record/relation/query_methods.rb
<ide> def build_subquery(subquery_alias, select_value) # :nodoc:
<ide>
<ide> def build_where_clause(opts, rest = []) # :nodoc:
<ide> opts = sanitize_forbidden_attributes(opts)
<del> self.references_values |= PredicateBuilder.references(opts) if Hash === opts
<ide>
<ide> case opts
<ide> when String, Array
<ide> parts = [klass.sanitize_sql(rest.empty? ? opts : [opts, *rest])]
<ide> when Hash
<add> opts = opts.stringify_keys
<add> references = PredicateBuilder.references(opts)
<add> self.references_values |= references unless references.empty?
<add>
<ide> parts = predicate_builder.build_from_hash(opts) do |table_name|
<ide> lookup_reflection_from_join_dependencies(table_name)
<ide> end | 2 |
Javascript | Javascript | remove return pointer mutation | bf7b7aeb1070f5184733ece11e67902d1ace85bd | <ide><path>packages/react-reconciler/src/ReactFiberTreeReflection.js
<ide> export function findCurrentFiberUsingSlowPath(fiber: Fiber): Fiber | null {
<ide>
<ide> export function findCurrentHostFiber(parent: Fiber): Fiber | null {
<ide> const currentParent = findCurrentFiberUsingSlowPath(parent);
<del> if (!currentParent) {
<del> return null;
<del> }
<add> return currentParent !== null
<add> ? findCurrentHostFiberImpl(currentParent)
<add> : null;
<add>}
<ide>
<add>function findCurrentHostFiberImpl(node: Fiber) {
<ide> // Next we'll drill down this component to find the first HostComponent/Text.
<del> let node: Fiber = currentParent;
<del> while (true) {
<del> if (node.tag === HostComponent || node.tag === HostText) {
<del> return node;
<del> } else if (node.child) {
<del> node.child.return = node;
<del> node = node.child;
<del> continue;
<del> }
<del> if (node === currentParent) {
<del> return null;
<del> }
<del> while (!node.sibling) {
<del> if (!node.return || node.return === currentParent) {
<del> return null;
<del> }
<del> node = node.return;
<add> if (node.tag === HostComponent || node.tag === HostText) {
<add> return node;
<add> }
<add>
<add> let child = node.child;
<add> while (child !== null) {
<add> const match = findCurrentHostFiberImpl(child);
<add> if (match !== null) {
<add> return match;
<ide> }
<del> node.sibling.return = node.return;
<del> node = node.sibling;
<add> child = child.sibling;
<ide> }
<del> // Flow needs the return null here, but ESLint complains about it.
<del> // eslint-disable-next-line no-unreachable
<add>
<ide> return null;
<ide> }
<ide>
<ide> export function findCurrentHostFiberWithNoPortals(parent: Fiber): Fiber | null {
<ide> const currentParent = findCurrentFiberUsingSlowPath(parent);
<del> if (!currentParent) {
<del> return null;
<del> }
<add> return currentParent !== null
<add> ? findCurrentHostFiberWithNoPortalsImpl(currentParent)
<add> : null;
<add>}
<ide>
<add>function findCurrentHostFiberWithNoPortalsImpl(node: Fiber) {
<ide> // Next we'll drill down this component to find the first HostComponent/Text.
<del> let node: Fiber = currentParent;
<del> while (true) {
<del> if (
<del> node.tag === HostComponent ||
<del> node.tag === HostText ||
<del> (enableFundamentalAPI && node.tag === FundamentalComponent)
<del> ) {
<del> return node;
<del> } else if (node.child && node.tag !== HostPortal) {
<del> node.child.return = node;
<del> node = node.child;
<del> continue;
<del> }
<del> if (node === currentParent) {
<del> return null;
<del> }
<del> while (!node.sibling) {
<del> if (!node.return || node.return === currentParent) {
<del> return null;
<add> if (
<add> node.tag === HostComponent ||
<add> node.tag === HostText ||
<add> (enableFundamentalAPI && node.tag === FundamentalComponent)
<add> ) {
<add> return node;
<add> }
<add>
<add> let child = node.child;
<add> while (child !== null) {
<add> if (child.tag !== HostPortal) {
<add> const match = findCurrentHostFiberWithNoPortalsImpl(child);
<add> if (match !== null) {
<add> return match;
<ide> }
<del> node = node.return;
<ide> }
<del> node.sibling.return = node.return;
<del> node = node.sibling;
<add> child = child.sibling;
<ide> }
<del> // Flow needs the return null here, but ESLint complains about it.
<del> // eslint-disable-next-line no-unreachable
<add>
<ide> return null;
<ide> }
<ide> | 1 |
PHP | PHP | move env() to foundation helper | e6b368f7413d72b2095e445283df81827fcb47a4 | <ide><path>src/Illuminate/Foundation/Bootstrap/DetectEnvironment.php
<ide> public function bootstrap(Application $app)
<ide>
<ide> $app->detectEnvironment(function()
<ide> {
<del> return getenv('APP_ENV') ?: 'production';
<add> return env('APP_ENV', 'production');
<ide> });
<ide> }
<ide>
<ide><path>src/Illuminate/Foundation/helpers.php
<ide> function view($view = null, $data = array(), $mergeData = array())
<ide> }
<ide> }
<ide>
<add>if ( ! function_exists('env'))
<add>{
<add> /**
<add> * Gets the value of an environment variable. Supports boolean, empty and null.
<add> *
<add> * @param string $key
<add> * @param mixed $default
<add> * @return mixed
<add> */
<add> function env($key, $default = null)
<add> {
<add> $value = getenv($key);
<add>
<add> if ($value === false) return value($default);
<add>
<add> switch (strtolower($value))
<add> {
<add> case 'true':
<add> case '(true)':
<add> return true;
<add>
<add> case 'false':
<add> case '(false)':
<add> return false;
<add>
<add> case '(null)':
<add> return null;
<add>
<add> case '(empty)':
<add> return '';
<add> }
<add>
<add> return $value;
<add> }
<add>}
<add>
<ide> if ( ! function_exists('elixir'))
<ide> {
<ide> /**
<ide><path>src/Illuminate/Support/helpers.php
<ide> function ends_with($haystack, $needles)
<ide> }
<ide> }
<ide>
<del>if ( ! function_exists('env'))
<del>{
<del> /**
<del> * Gets the value of an environment variable. Supports boolean, empty and null.
<del> *
<del> * @param string $key
<del> * @param mixed $default
<del> * @return mixed
<del> */
<del> function env($key, $default = null)
<del> {
<del> $value = getenv($key);
<del>
<del> if ($value === false) return value($default);
<del>
<del> switch (strtolower($value))
<del> {
<del> case 'true':
<del> case '(true)':
<del> return true;
<del>
<del> case 'false':
<del> case '(false)':
<del> return false;
<del>
<del> case '(null)':
<del> return null;
<del>
<del> case '(empty)':
<del> return '';
<del> }
<del>
<del> return $value;
<del> }
<del>}
<del>
<ide> if ( ! function_exists('head'))
<ide> {
<ide> /** | 3 |
Text | Text | add joaocgreis as a collaborator | b612f085ec12a1937e07867e7d23c5d58022b8ea | <ide><path>README.md
<ide> information about the governance of the io.js project, see
<ide> * **Сковорода Никита Андреевич** <[email protected]> ([@ChALkeR](https://github.com/ChALkeR))
<ide> * **Sakthipriyan Vairamani** <[email protected]> ([@thefourtheye](https://github.com/thefourtheye))
<ide> * **Michaël Zasso** <[email protected]> ([@targos](https://github.com/targos))
<add>* **João Reis** <[email protected]> ([@joaocgreis](https://github.com/joaocgreis))
<ide>
<ide> Collaborators & TSC members follow the [COLLABORATOR_GUIDE.md](./COLLABORATOR_GUIDE.md) in
<ide> maintaining the io.js project. | 1 |
Ruby | Ruby | remove unused variable | 1ea4a892a835ada735ba2943b20bdbfd39120e8c | <ide><path>activerecord/test/cases/base_test.rb
<ide> def test_comparison_with_different_objects
<ide>
<ide> def test_comparison_with_different_objects_in_array
<ide> topic = Topic.create
<del> category = Category.create(:name => "comparison")
<ide> assert_raises(ArgumentError) do
<ide> [1, topic].sort
<ide> end | 1 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.