content_type
stringclasses 8
values | main_lang
stringclasses 7
values | message
stringlengths 1
50
| sha
stringlengths 40
40
| patch
stringlengths 52
962k
| file_count
int64 1
300
|
---|---|---|---|---|---|
Java | Java | introduce mockrestserviceserver builder | a56c69c9ca410b2a04d946a1199fe1dc10b89f5b | <ide><path>spring-test/src/main/java/org/springframework/test/web/client/AbstractRequestExpectationManager.java
<ide> public AbstractRequestExpectationManager(List<RequestExpectation> expectations)
<ide> }
<ide>
<ide>
<del> @Override
<ide> public List<RequestExpectation> getExpectations() {
<ide> return this.expectations;
<ide> }
<ide>
<del> @Override
<ide> public List<ClientHttpRequest> getRequests() {
<ide> return this.requests;
<ide> }
<ide><path>spring-test/src/main/java/org/springframework/test/web/client/MockRestServiceServer.java
<ide>
<ide> import java.io.IOException;
<ide> import java.net.URI;
<del>import java.util.List;
<ide>
<ide> import org.springframework.http.HttpMethod;
<ide> import org.springframework.http.client.AsyncClientHttpRequest;
<ide> */
<ide> public class MockRestServiceServer {
<ide>
<del> private RequestExpectationManager expectationManager = new OrderedRequestExpectationManager();
<add> private final RequestExpectationManager expectationManager;
<ide>
<ide>
<ide> /**
<ide> * Private constructor.
<del> * @see #createServer(RestTemplate)
<del> * @see #createServer(RestGatewaySupport)
<add> * See static builder methods and {@code createServer} shortcut methods.
<ide> */
<ide> private MockRestServiceServer() {
<add> this.expectationManager = new SimpleRequestExpectationManager();
<add> }
<add>
<add> /**
<add> * Private constructor with {@code RequestExpectationManager}.
<add> * See static builder methods and {@code createServer} shortcut methods.
<add> */
<add> private MockRestServiceServer(RequestExpectationManager expectationManager) {
<add> this.expectationManager = expectationManager;
<add> }
<add>
<add>
<add> /**
<add> * Set up a new HTTP request expectation. The returned {@link ResponseActions}
<add> * is used to set up further expectations and to define the response.
<add> * <p>This method may be invoked multiple times before starting the test, i.e. before
<add> * using the {@code RestTemplate}, to set up expectations for multiple requests.
<add> * @param matcher a request expectation, see {@link MockRestRequestMatchers}
<add> * @return used to set up further expectations or to define a response
<add> */
<add> public ResponseActions expect(RequestMatcher matcher) {
<add> return this.expectationManager.expectRequest(matcher);
<add> }
<add>
<add>
<add> /**
<add> * Verify that all expected requests set up via
<add> * {@link #expect(RequestMatcher)} were indeed performed.
<add> * @throws AssertionError when some expectations were not met
<add> */
<add> public void verify() {
<add> this.expectationManager.verify();
<ide> }
<ide>
<ide>
<ide> /**
<del> * Create a {@code MockRestServiceServer} and set up the given
<del> * {@code RestTemplate} with a mock {@link ClientHttpRequestFactory}.
<add> * Build a {@code MockRestServiceServer} with a {@code RestTemplate}.
<add> * @since 4.3
<add> */
<add> public static MockRestServiceServerBuilder restTemplate(RestTemplate restTemplate) {
<add> return new DefaultBuilder(restTemplate);
<add> }
<add>
<add> /**
<add> * Build a {@code MockRestServiceServer} with an {@code AsyncRestTemplate}.
<add> * @since 4.3
<add> */
<add> public static MockRestServiceServerBuilder asyncRestTemplate(AsyncRestTemplate asyncRestTemplate) {
<add> return new DefaultBuilder(asyncRestTemplate);
<add> }
<add>
<add> /**
<add> * Build a {@code MockRestServiceServer} with a {@code RestGateway}.
<add> * @since 4.3
<add> */
<add> public static MockRestServiceServerBuilder restGateway(RestGatewaySupport restGateway) {
<add> Assert.notNull(restGateway, "'gatewaySupport' must not be null");
<add> return new DefaultBuilder(restGateway.getRestTemplate());
<add> }
<add>
<add>
<add> /**
<add> * A shortcut for {@code restTemplate(restTemplate).build()}.
<ide> * @param restTemplate the RestTemplate to set up for mock testing
<del> * @return the created mock server
<add> * @return the mock server
<ide> */
<ide> public static MockRestServiceServer createServer(RestTemplate restTemplate) {
<del> Assert.notNull(restTemplate, "'restTemplate' must not be null");
<del> MockRestServiceServer mockServer = new MockRestServiceServer();
<del> MockClientHttpRequestFactory factory = mockServer.new MockClientHttpRequestFactory();
<del> restTemplate.setRequestFactory(factory);
<del> return mockServer;
<add> return restTemplate(restTemplate).build();
<ide> }
<ide>
<ide> /**
<del> * Create a {@code MockRestServiceServer} and set up the given
<del> * {@code AsyRestTemplate} with a mock {@link AsyncClientHttpRequestFactory}.
<add> * A shortcut for {@code asyncRestTemplate(asyncRestTemplate).build()}.
<ide> * @param asyncRestTemplate the AsyncRestTemplate to set up for mock testing
<ide> * @return the created mock server
<ide> */
<ide> public static MockRestServiceServer createServer(AsyncRestTemplate asyncRestTemplate) {
<del> Assert.notNull(asyncRestTemplate, "'asyncRestTemplate' must not be null");
<del> MockRestServiceServer mockServer = new MockRestServiceServer();
<del> MockClientHttpRequestFactory factory = mockServer.new MockClientHttpRequestFactory();
<del> asyncRestTemplate.setAsyncRequestFactory(factory);
<del> return mockServer;
<add> return asyncRestTemplate(asyncRestTemplate).build();
<ide> }
<ide>
<ide> /**
<del> * Create a {@code MockRestServiceServer} and set up the given
<del> * {@code RestGatewaySupport} with a mock {@link ClientHttpRequestFactory}.
<add> * A shortcut for {@code restGateway(restGateway).build()}.
<ide> * @param restGateway the REST gateway to set up for mock testing
<ide> * @return the created mock server
<ide> */
<ide> public static MockRestServiceServer createServer(RestGatewaySupport restGateway) {
<del> Assert.notNull(restGateway, "'gatewaySupport' must not be null");
<del> return createServer(restGateway.getRestTemplate());
<add> return restGateway(restGateway).build();
<ide> }
<ide>
<ide>
<del> /**
<del> * When this option is set, the order in which requests are executed does not
<del> * need to match the order in which expected requests are declared.
<del> */
<del> public MockRestServiceServer setIgnoreRequestOrder() {
<del> String message = "Cannot switch to unordered mode after actual requests are made.";
<del> Assert.state(this.expectationManager.getRequests().isEmpty(), message);
<del> List<RequestExpectation> expectations = this.expectationManager.getExpectations();
<del> this.expectationManager = new UnorderedRequestExpectationManager(expectations);
<del> return this;
<del> }
<ide>
<ide> /**
<del> * Set up a new HTTP request expectation. The returned {@link ResponseActions}
<del> * is used to set up further expectations and to define the response.
<del> * <p>This method may be invoked multiple times before starting the test, i.e. before
<del> * using the {@code RestTemplate}, to set up expectations for multiple requests.
<del> * @param matcher a request expectation, see {@link MockRestRequestMatchers}
<del> * @return used to set up further expectations or to define a response
<add> * Builder to create a {@code MockRestServiceServer}.
<add>
<ide> */
<del> public ResponseActions expect(RequestMatcher matcher) {
<del> return this.expectationManager.expectRequest(matcher);
<add> public interface MockRestServiceServerBuilder {
<add>
<add> /**
<add> * When this option is set, requests can be executed in any order, i.e.
<add> * not matching the order in which expected requests are declared.
<add> */
<add> MockRestServiceServerBuilder ignoreExpectOrder();
<add>
<add> /**
<add> * Build the {@code MockRestServiceServer} and setting up the underlying
<add> * {@code RestTemplate} or {@code AsyncRestTemplate} with a
<add> * {@link ClientHttpRequestFactory} that creates mock requests.
<add> */
<add> MockRestServiceServer build();
<add>
<ide> }
<ide>
<add> private static class DefaultBuilder implements MockRestServiceServerBuilder {
<ide>
<del> /**
<del> * Verify that all expected requests set up via
<del> * {@link #expect(RequestMatcher)} were indeed performed.
<del> * @throws AssertionError when some expectations were not met
<del> */
<del> public void verify() {
<del> this.expectationManager.verify();
<add> private final RestTemplate restTemplate;
<add>
<add> private final AsyncRestTemplate asyncRestTemplate;
<add>
<add> private boolean ignoreExpectOrder;
<add>
<add>
<add> public DefaultBuilder(RestTemplate restTemplate) {
<add> Assert.notNull(restTemplate, "'restTemplate' must not be null");
<add> this.restTemplate = restTemplate;
<add> this.asyncRestTemplate = null;
<add> }
<add>
<add> public DefaultBuilder(AsyncRestTemplate asyncRestTemplate) {
<add> Assert.notNull(asyncRestTemplate, "'asyncRestTemplate' must not be null");
<add> this.restTemplate = null;
<add> this.asyncRestTemplate = asyncRestTemplate;
<add> }
<add>
<add>
<add> @Override
<add> public MockRestServiceServerBuilder ignoreExpectOrder() {
<add> this.ignoreExpectOrder = true;
<add> return this;
<add> }
<add>
<add>
<add> @Override
<add> public MockRestServiceServer build() {
<add>
<add> MockRestServiceServer server = (this.ignoreExpectOrder ?
<add> new MockRestServiceServer(new UnorderedRequestExpectationManager()) :
<add> new MockRestServiceServer());
<add>
<add> MockClientHttpRequestFactory factory = server.new MockClientHttpRequestFactory();
<add> if (this.restTemplate != null) {
<add> this.restTemplate.setRequestFactory(factory);
<add> }
<add> if (this.asyncRestTemplate != null) {
<add> this.asyncRestTemplate.setAsyncRequestFactory(factory);
<add> }
<add>
<add> return server;
<add> }
<ide> }
<ide>
<ide>
<ide><path>spring-test/src/main/java/org/springframework/test/web/client/RequestExpectationManager.java
<ide> package org.springframework.test.web.client;
<ide>
<ide> import java.io.IOException;
<del>import java.util.List;
<ide>
<ide> import org.springframework.http.client.ClientHttpRequest;
<ide> import org.springframework.http.client.ClientHttpResponse;
<ide> */
<ide> public interface RequestExpectationManager {
<ide>
<del> /**
<del> * Return the list of declared request expectations.
<del> */
<del> List<RequestExpectation> getExpectations();
<del>
<del> /**
<del> * Return the list of actual requests.
<del> */
<del> List<ClientHttpRequest> getRequests();
<del>
<ide> /**
<ide> * Set up a new request expectation. The returned {@link ResponseActions} is
<ide> * used to add more expectations and define a response.
<add><path>spring-test/src/main/java/org/springframework/test/web/client/SimpleRequestExpectationManager.java
<del><path>spring-test/src/main/java/org/springframework/test/web/client/OrderedRequestExpectationManager.java
<ide> * @author Rossen Stoyanchev
<ide> * @since 4.3
<ide> */
<del>public class OrderedRequestExpectationManager extends AbstractRequestExpectationManager {
<add>public class SimpleRequestExpectationManager extends AbstractRequestExpectationManager {
<ide>
<ide> private Iterator<RequestExpectation> iterator;
<ide>
<ide><path>spring-test/src/test/java/org/springframework/test/web/client/OrderedRequestExpectationManagerTests.java
<ide> */
<ide> public class OrderedRequestExpectationManagerTests {
<ide>
<del> private OrderedRequestExpectationManager manager = new OrderedRequestExpectationManager();
<add> private SimpleRequestExpectationManager manager = new SimpleRequestExpectationManager();
<ide>
<ide>
<ide> @Test | 5 |
Javascript | Javascript | simplify a module in hotcase | d7605cae25bd0ed7d07ac932c5fec33f2bed35e2 | <ide><path>test/hotCases/runtime/require-disposed-module-warning/a.js
<ide> export default module;
<del>---
<del>---
<del>export default "a2"; | 1 |
Javascript | Javascript | set the url even if the browser transforms it | 51cfb61e46c18a4aac27340120a276dda1112637 | <ide><path>src/ng/browser.js
<ide> function Browser(window, document, $log, $sniffer) {
<ide> // Do the assignment again so that those two variables are referentially identical.
<ide> lastHistoryState = cachedState;
<ide> } else {
<del> if (!sameBase || pendingLocation) {
<add> if (!sameBase) {
<ide> pendingLocation = url;
<ide> }
<ide> if (replace) {
<ide> function Browser(window, document, $log, $sniffer) {
<ide> pendingLocation = url;
<ide> }
<ide> }
<add> if (pendingLocation) {
<add> pendingLocation = url;
<add> }
<ide> return self;
<ide> // getter
<ide> } else {
<ide><path>test/ng/browserSpecs.js
<ide> function MockWindow(options) {
<ide> },
<ide> replaceState: function(state, title, url) {
<ide> locationHref = url;
<add> if (!options.updateAsync) committedHref = locationHref;
<ide> mockWindow.history.state = copy(state);
<add> if (!options.updateAsync) this.flushHref();
<add> },
<add> flushHref: function() {
<add> committedHref = locationHref;
<ide> }
<ide> };
<ide> // IE 10-11 deserialize history.state on each read making subsequent reads
<ide> describe('browser', function() {
<ide>
<ide> });
<ide>
<add> describe('url (with ie 11 weirdnesses)', function() {
<add>
<add> it('url() should actually set the url, even if IE 11 is weird and replaces HTML entities in the URL', function() {
<add> // this test can not be expressed with the Jasmine spies in the previous describe block, because $browser.url()
<add> // needs to observe the change to location.href during its invocation to enter the failing code path, but the spies
<add> // are not callThrough
<add>
<add> sniffer.history = true;
<add> var originalReplace = fakeWindow.location.replace;
<add> fakeWindow.location.replace = function(url) {
<add> url = url.replace('¬', '¬');
<add> // I really don't know why IE 11 (sometimes) does this, but I am not the only one to notice:
<add> // https://connect.microsoft.com/IE/feedback/details/1040980/bug-in-ie-which-interprets-document-location-href-as-html
<add> originalReplace.call(this, url);
<add> };
<add>
<add> // the initial URL contains a lengthy oauth token in the hash
<add> var initialUrl = 'http://test.com/oauthcallback#state=xxx%3D¬-before-policy=0';
<add> fakeWindow.location.href = initialUrl;
<add> browser = new Browser(fakeWindow, fakeDocument, fakeLog, sniffer);
<add>
<add> // somehow, $location gets a version of this url where the = is no longer escaped, and tells the browser:
<add> var initialUrlFixedByLocation = initialUrl.replace('%3D', '=');
<add> browser.url(initialUrlFixedByLocation, true, null);
<add> expect(browser.url()).toEqual(initialUrlFixedByLocation);
<add>
<add> // a little later (but in the same digest cycle) the view asks $location to replace the url, which tells $browser
<add> var secondUrl = 'http://test.com/otherView';
<add> browser.url(secondUrl, true, null);
<add> expect(browser.url()).toEqual(secondUrl);
<add> });
<add>
<add> });
<add>
<ide> describe('url (when state passed)', function() {
<ide> var currentHref, pushState, replaceState, locationReplace;
<ide> | 2 |
Ruby | Ruby | update minimumsystemversion condition | 4433f4a985e92526bd77705039a3dcbb6d128956 | <ide><path>Library/Homebrew/livecheck/strategy/sparkle.rb
<ide> def self.item_from_content(content)
<ide>
<ide> next if os && os != "osx"
<ide>
<del> if OS.mac? && (minimum_system_version = (item > "minimumSystemVersion").first&.text&.strip)
<add> if (minimum_system_version = item.elements["minimumSystemVersion"]&.text&.gsub(/\A\D+|\D+\z/, ""))
<ide> macos_minimum_system_version = begin
<ide> MacOS::Version.new(minimum_system_version).strip_patch
<ide> rescue MacOSVersionError | 1 |
Javascript | Javascript | add more options | 1ee8c0c2c01186d1e7f85a592b558235596d51be | <ide><path>RNTester/js/examples/Layout/LayoutAnimationExample.js
<ide> const {
<ide> TouchableOpacity,
<ide> } = require('react-native');
<ide>
<del>class AddRemoveExample extends React.Component<{...}, $FlowFixMeState> {
<add>type ExampleViewSpec = {|
<add> key: number,
<add>|};
<add>
<add>type AddRemoveExampleState = {|
<add> views: Array<ExampleViewSpec>,
<add> nextKey: number,
<add>|};
<add>
<add>function shuffleArray(array: Array<ExampleViewSpec>) {
<add> var currentIndex: number = array.length,
<add> temporaryValue: ExampleViewSpec,
<add> randomIndex: number;
<add>
<add> // While there remain elements to shuffle...
<add> while (currentIndex !== 0) {
<add> // Pick a remaining element...
<add> randomIndex = Math.floor(Math.random() * currentIndex);
<add> currentIndex -= 1;
<add>
<add> // And swap it with the current element.
<add> temporaryValue = array[currentIndex];
<add> array[currentIndex] = array[randomIndex];
<add> array[randomIndex] = temporaryValue;
<add> }
<add>
<add> return array;
<add>}
<add>
<add>class AddRemoveExample extends React.Component<{...}, AddRemoveExampleState> {
<ide> state = {
<ide> views: [],
<add> nextKey: 1,
<ide> };
<ide>
<del> UNSAFE_componentWillUpdate() {
<del> LayoutAnimation.easeInEaseOut(args =>
<del> console.log('AddRemoveExample completed', args),
<add> configureNextAnimation() {
<add> LayoutAnimation.configureNext(
<add> {
<add> duration: 1000,
<add> create: {type: 'easeInEaseOut', property: 'opacity'},
<add> update: {type: 'easeInEaseOut', property: 'opacity'},
<add> delete: {type: 'easeInEaseOut', property: 'opacity'},
<add> },
<add> args => console.log('AddRemoveExample completed', args),
<ide> );
<ide> }
<ide>
<add> _onPressAddViewAnimated = () => {
<add> this.configureNextAnimation();
<add> this._onPressAddView();
<add> };
<add>
<add> _onPressRemoveViewAnimated = () => {
<add> this.configureNextAnimation();
<add> this._onPressRemoveView();
<add> };
<add>
<add> _onPressReorderViewsAnimated = () => {
<add> this.configureNextAnimation();
<add> this._onPressReorderViews();
<add> };
<add>
<ide> _onPressAddView = () => {
<del> this.setState(state => ({views: [...state.views, {}]}));
<add> this.setState(state => ({
<add> views: [...state.views, {key: state.nextKey}],
<add> nextKey: state.nextKey + 1,
<add> }));
<ide> };
<ide>
<ide> _onPressRemoveView = () => {
<ide> this.setState(state => ({views: state.views.slice(0, -1)}));
<ide> };
<ide>
<add> _onPressReorderViews = () => {
<add> this.setState(state => ({views: shuffleArray(state.views)}));
<add> };
<add>
<ide> render() {
<del> const views = this.state.views.map((view, i) => (
<del> <View key={i} style={styles.view}>
<del> <Text>{i}</Text>
<add> const views = this.state.views.map(({key}) => (
<add> <View
<add> key={key}
<add> style={styles.view}
<add> onLayout={evt => console.log('Box onLayout')}>
<add> <Text>{key}</Text>
<ide> </View>
<ide> ));
<ide> return (
<ide> <View style={styles.container}>
<del> <TouchableOpacity onPress={this._onPressAddView}>
<add> <TouchableOpacity onPress={this._onPressAddViewAnimated}>
<ide> <View style={styles.button}>
<ide> <Text>Add view</Text>
<ide> </View>
<ide> </TouchableOpacity>
<del> <TouchableOpacity onPress={this._onPressRemoveView}>
<add> <TouchableOpacity onPress={this._onPressRemoveViewAnimated}>
<ide> <View style={styles.button}>
<ide> <Text>Remove view</Text>
<ide> </View>
<ide> </TouchableOpacity>
<add> <TouchableOpacity onPress={this._onPressReorderViewsAnimated}>
<add> <View style={styles.button}>
<add> <Text>Reorder Views</Text>
<add> </View>
<add> </TouchableOpacity>
<add> <TouchableOpacity onPress={this._onPressAddView}>
<add> <View style={styles.button}>
<add> <Text>Add view (no animation)</Text>
<add> </View>
<add> </TouchableOpacity>
<add> <TouchableOpacity onPress={this._onPressRemoveView}>
<add> <View style={styles.button}>
<add> <Text>Remove view (no animation)</Text>
<add> </View>
<add> </TouchableOpacity>
<add> <TouchableOpacity onPress={this._onPressReorderViews}>
<add> <View style={styles.button}>
<add> <Text>Reorder Views (no animation)</Text>
<add> </View>
<add> </TouchableOpacity>
<ide> <View style={styles.viewContainer}>{views}</View>
<ide> </View>
<ide> );
<ide> const BlueSquare = () => (
<ide> </View>
<ide> );
<ide>
<del>class CrossFadeExample extends React.Component<{...}, $FlowFixMeState> {
<add>type CrossFadeExampleState = {|
<add> toggled: boolean,
<add>|};
<add>
<add>class CrossFadeExample extends React.Component<{...}, CrossFadeExampleState> {
<ide> state = {
<ide> toggled: false,
<ide> };
<ide> class CrossFadeExample extends React.Component<{...}, $FlowFixMeState> {
<ide> }
<ide> }
<ide>
<del>class LayoutUpdateExample extends React.Component<{...}, $FlowFixMeState> {
<add>type LayoutUpdateExampleState = {|
<add> width: number,
<add> height: number,
<add>|};
<add>
<add>class LayoutUpdateExample extends React.Component<
<add> {...},
<add> LayoutUpdateExampleState,
<add>> {
<ide> state = {
<ide> width: 200,
<ide> height: 100, | 1 |
Ruby | Ruby | remove m4 macro warning | c6a8dd247e4c31ca1e9e2dba613fae9b9e65b78a | <ide><path>Library/Homebrew/formula_installer.rb
<ide> def caveats
<ide> audit_lib
<ide> check_manpages
<ide> check_infopages
<del> check_m4
<ide> end
<ide>
<ide> keg = Keg.new(f.prefix)
<ide> def audit_lib
<ide> check_jars
<ide> check_non_libraries
<ide> end
<del>
<del> def check_m4
<del> # Newer versions of Xcode don't come with autotools
<del> return unless MacOS::Xcode.provides_autotools?
<del>
<del> # If the user has added our path to dirlist, don't complain
<del> return if File.open("/usr/share/aclocal/dirlist") do |dirlist|
<del> dirlist.grep(%r{^#{HOMEBREW_PREFIX}/share/aclocal$}).length > 0
<del> end rescue false
<del>
<del> # Check for installed m4 files
<del> if Dir[f.share+"aclocal/*.m4"].length > 0
<del> opoo 'm4 macros were installed to "share/aclocal".'
<del> puts "Homebrew does not append \"#{HOMEBREW_PREFIX}/share/aclocal\""
<del> puts "to \"/usr/share/aclocal/dirlist\". If an autoconf script you use"
<del> puts "requires these m4 macros, you'll need to add this path manually."
<del> @show_summary_heading = true
<del> end
<del> end
<ide> end
<ide>
<ide> | 1 |
Java | Java | fix broken test in contentassertiontests | 33ee0ea4a6eb4a47e4a4291e36124ec631a2f814 | <ide><path>spring-test-mvc/src/test/java/org/springframework/test/web/servlet/samples/standalone/resultmatchers/ContentAssertionTests.java
<ide> public void testContentType() throws Exception {
<ide> this.mockMvc.perform(get("/handleUtf8"))
<ide> .andExpect(content().contentType(MediaType.valueOf("text/plain;charset=UTF-8")))
<ide> .andExpect(content().contentType("text/plain;charset=UTF-8"))
<del> .andExpect(content().contentTypeCompatibleWith("text/plan"))
<add> .andExpect(content().contentTypeCompatibleWith("text/plain"))
<ide> .andExpect(content().contentTypeCompatibleWith(MediaType.TEXT_PLAIN));
<ide> }
<ide> | 1 |
Ruby | Ruby | fix arity warning for template handlers | b2b2f70f1f81b54b799118e8a4feb601ab480c41 | <ide><path>actionview/lib/action_view/template/handlers.rb
<ide> def register_template_handler(*extensions, handler)
<ide>
<ide> unless params.find_all { |type, _| type == :req || type == :opt }.length >= 2
<ide> ActiveSupport::Deprecation.warn <<~eowarn
<del> Single arity template handlers are deprecated. Template handlers must
<add> Single arity template handlers are deprecated. Template handlers must
<ide> now accept two parameters, the view object and the source for the view object.
<ide> Change:
<del> >> #{handler.class}#call(#{params.map(&:last).join(", ")})
<add> >> #{handler}.call(#{params.map(&:last).join(", ")})
<ide> To:
<del> >> #{handler.class}#call(#{params.map(&:last).join(", ")}, source)
<add> >> #{handler}.call(#{params.map(&:last).join(", ")}, source)
<ide> eowarn
<ide> handler = LegacyHandlerWrapper.new(handler)
<ide> end | 1 |
Go | Go | add docker tag tests | c496f24157afd81c9a26f5746175236485e97fa7 | <ide><path>integration-cli/docker_cli_tag_test.go
<ide> package main
<ide> import (
<ide> "fmt"
<ide> "os/exec"
<add> "strings"
<ide> "testing"
<ide> )
<ide>
<ide> func TestTagValidPrefixedRepo(t *testing.T) {
<ide> logDone(logMessage)
<ide> }
<ide> }
<add>
<add>// tag an image with an existed tag name without -f option should fail
<add>func TestTagExistedNameWithoutForce(t *testing.T) {
<add> if err := pullImageIfNotExist("busybox:latest"); err != nil {
<add> t.Fatal("couldn't find the busybox:latest image locally and failed to pull it")
<add> }
<add>
<add> tagCmd := exec.Command(dockerBinary, "tag", "busybox:latest", "busybox:test")
<add> if out, _, err := runCommandWithOutput(tagCmd); err != nil {
<add> t.Fatal(out, err)
<add> }
<add> tagCmd = exec.Command(dockerBinary, "tag", "busybox:latest", "busybox:test")
<add> out, _, err := runCommandWithOutput(tagCmd)
<add> if err == nil || !strings.Contains(out, "Conflict: Tag test is already set to image") {
<add> t.Fatal("tag busybox busybox:test should have failed,because busybox:test is existed")
<add> }
<add> deleteImages("busybox:test")
<add>
<add> logDone("tag - busybox with an existed tag name without -f option --> must fail")
<add>}
<add>
<add>// tag an image with an existed tag name with -f option should work
<add>func TestTagExistedNameWithForce(t *testing.T) {
<add> if err := pullImageIfNotExist("busybox:latest"); err != nil {
<add> t.Fatal("couldn't find the busybox:latest image locally and failed to pull it")
<add> }
<add>
<add> tagCmd := exec.Command(dockerBinary, "tag", "busybox:latest", "busybox:test")
<add> if out, _, err := runCommandWithOutput(tagCmd); err != nil {
<add> t.Fatal(out, err)
<add> }
<add> tagCmd = exec.Command(dockerBinary, "tag", "-f", "busybox:latest", "busybox:test")
<add> if out, _, err := runCommandWithOutput(tagCmd); err != nil {
<add> t.Fatal(out, err)
<add> }
<add> deleteImages("busybox:test")
<add>
<add> logDone("tag - busybox with an existed tag name with -f option work")
<add>} | 1 |
Javascript | Javascript | add test for frozencopy deprecation | 013f797d22d9ac71bbb01d71bacbcc5ab681ca02 | <ide><path>packages/ember-runtime/lib/mixins/copyable.js
<ide> export default Mixin.create({
<ide> @private
<ide> */
<ide> frozenCopy() {
<del> Ember.deprecate('`frozenCopy` is deprecated, use Object.freeze instead.');
<add> Ember.deprecate('`frozenCopy` is deprecated, use `Object.freeze` instead.');
<ide> if (Freezable && Freezable.detect(this)) {
<ide> return get(this, 'isFrozen') ? this : this.copy().freeze();
<ide> } else {
<ide><path>packages/ember-runtime/tests/mixins/copyable_test.js
<ide> import CopyableTests from 'ember-runtime/tests/suites/copyable';
<ide> import Copyable from 'ember-runtime/mixins/copyable';
<add>import {Freezable} from 'ember-runtime/mixins/freezable';
<ide> import EmberObject from 'ember-runtime/system/object';
<ide> import {generateGuid} from 'ember-metal/utils';
<ide> import {set} from 'ember-metal/property_set';
<ide> import {get} from 'ember-metal/property_get';
<ide>
<add>QUnit.module('Ember.Copyable.frozenCopy');
<add>
<add>QUnit.test('should be deprecated', function() {
<add> expectDeprecation('`frozenCopy` is deprecated, use `Object.freeze` instead.');
<add>
<add> var Obj = EmberObject.extend(Freezable, Copyable, {
<add> copy() {
<add> return Obj.create();
<add> }
<add> });
<add>
<add> Obj.create().frozenCopy();
<add>});
<add>
<ide> var CopyableObject = EmberObject.extend(Copyable, {
<ide>
<ide> id: null, | 2 |
Javascript | Javascript | remove redundant guards for the event methods | a873558436383beea7a05fd07db7070a30420100 | <ide><path>src/event.js
<ide> jQuery.Event.prototype = {
<ide>
<ide> this.isDefaultPrevented = returnTrue;
<ide>
<del> if ( e && e.preventDefault ) {
<add> if ( e ) {
<ide> e.preventDefault();
<ide> }
<ide> },
<ide> jQuery.Event.prototype = {
<ide>
<ide> this.isPropagationStopped = returnTrue;
<ide>
<del> if ( e && e.stopPropagation ) {
<add> if ( e ) {
<ide> e.stopPropagation();
<ide> }
<ide> },
<ide> jQuery.Event.prototype = {
<ide>
<ide> this.isImmediatePropagationStopped = returnTrue;
<ide>
<del> if ( e && e.stopImmediatePropagation ) {
<add> if ( e ) {
<ide> e.stopImmediatePropagation();
<ide> }
<ide> | 1 |
PHP | PHP | pull makes now use of array_pull | c0eaab9554bc2971d8eb92261d36944f7657349b | <ide><path>src/Illuminate/Support/Collection.php
<ide> public function push($value)
<ide> */
<ide> public function pull($key, $default = null)
<ide> {
<del> $value = $this->get($key, $default);
<del>
<del> $this->forget($key);
<del>
<del> return $value;
<add> return array_pull($this->items, $key, $default);
<ide> }
<ide>
<ide> /** | 1 |
Text | Text | fix some typos in n-api docs | 484c6c31b068fb64fa8b4bd46b82bc09a54a6a17 | <ide><path>doc/api/errors.md
<ide> value.
<ide>
<ide> On the main thread, values are removed from the queue associated with the
<ide> thread-safe function in an idle loop. This error indicates that an error
<del>has occurred when attemping to start the loop.
<add>has occurred when attempting to start the loop.
<ide>
<ide> <a id="ERR_NAPI_TSFN_STOP_IDLE_LOOP"></a>
<ide> ### ERR_NAPI_TSFN_STOP_IDLE_LOOP
<ide><path>doc/api/n-api.md
<ide> napi_create_threadsafe_function(napi_env env,
<ide> - `[in] func`: The JavaScript function to call from another thread.
<ide> - `[in] async_resource`: An optional object associated with the async work that
<ide> will be passed to possible `async_hooks` [`init` hooks][].
<del>- `[in] async_resource_name`: A javaScript string to provide an identifier for
<add>- `[in] async_resource_name`: A JavaScript string to provide an identifier for
<ide> the kind of resource that is being provided for diagnostic information exposed
<ide> by the `async_hooks` API.
<del>- `[in] max_queue_size`: Maximum size of the queue. 0 for no limit.
<add>- `[in] max_queue_size`: Maximum size of the queue. `0` for no limit.
<ide> - `[in] initial_thread_count`: The initial number of threads, including the main
<ide> thread, which will be making use of this function.
<ide> - `[in] thread_finalize_data`: Data to be passed to `thread_finalize_cb`.
<ide> napi_get_threadsafe_function_context(napi_threadsafe_function func,
<ide> ```
<ide>
<ide> - `[in] func`: The thread-safe function for which to retrieve the context.
<del>- `[out] context`: The location where to store the context.
<add>- `[out] result`: The location where to store the context.
<ide>
<ide> This API may be called from any thread which makes use of `func`.
<ide> | 2 |
Ruby | Ruby | pass chdir block to stage | 1cc983f00d552da17336405460e3adc001c95ba5 | <ide><path>Library/Homebrew/download_strategy.rb
<ide> def quiet?
<ide> # Unlike {Resource#stage}, this does not take a block.
<ide> #
<ide> # @api public
<del> def stage
<add> def stage(&block)
<ide> UnpackStrategy.detect(cached_location,
<ide> prioritise_extension: true,
<ide> ref_type: @ref_type, ref: @ref)
<ide> .extract_nestedly(basename: basename,
<ide> prioritise_extension: true,
<ide> verbose: verbose? && !quiet?)
<del> chdir
<add> chdir(&block)
<ide> end
<ide>
<del> def chdir
<add> def chdir(&block)
<ide> entries = Dir["*"]
<ide> raise "Empty archive" if entries.length.zero?
<del> return if entries.length != 1
<ide>
<del> begin
<del> Dir.chdir entries.first
<del> rescue
<del> nil
<add> if entries.length != 1
<add> yield if block
<add> return
<add> end
<add>
<add> if File.directory? entries.first
<add> Dir.chdir(entries.first, &block)
<add> elsif block
<add> yield
<ide> end
<ide> end
<ide> private :chdir
<ide><path>Library/Homebrew/resource.rb
<ide> def apply_patches
<ide> # A target or a block must be given, but not both.
<ide> def unpack(target = nil)
<ide> mktemp(download_name) do |staging|
<del> downloader.stage
<del> @source_modified_time = downloader.source_modified_time
<del> apply_patches
<del> if block_given?
<del> yield ResourceStageContext.new(self, staging)
<del> elsif target
<del> target = Pathname(target)
<del> target.install Pathname.pwd.children
<add> downloader.stage do
<add> @source_modified_time = downloader.source_modified_time
<add> apply_patches
<add> if block_given?
<add> yield ResourceStageContext.new(self, staging)
<add> elsif target
<add> target = Pathname(target)
<add> target.install Pathname.pwd.children
<add> end
<ide> end
<ide> end
<ide> end | 2 |
Python | Python | add cli argument for configuring labels | 99b189df6de71b2f01d6f72e6b1f4aa74455275b | <ide><path>examples/run_ner.py
<ide> def set_seed(args):
<ide> torch.cuda.manual_seed_all(args.seed)
<ide>
<ide>
<del>def train(args, train_dataset, model, tokenizer, pad_token_label_id):
<add>def train(args, train_dataset, model, tokenizer, labels, pad_token_label_id):
<ide> """ Train the model """
<ide> if args.local_rank in [-1, 0]:
<ide> tb_writer = SummaryWriter()
<ide> def train(args, train_dataset, model, tokenizer, pad_token_label_id):
<ide> if args.local_rank in [-1, 0] and args.logging_steps > 0 and global_step % args.logging_steps == 0:
<ide> # Log metrics
<ide> if args.local_rank == -1 and args.evaluate_during_training: # Only evaluate when single GPU otherwise metrics may not average well
<del> results = evaluate(args, model, tokenizer, pad_token_label_id)
<add> results = evaluate(args, model, tokenizer, labels, pad_token_label_id)
<ide> for key, value in results.items():
<ide> tb_writer.add_scalar("eval_{}".format(key), value, global_step)
<ide> tb_writer.add_scalar("lr", scheduler.get_lr()[0], global_step)
<ide> def train(args, train_dataset, model, tokenizer, pad_token_label_id):
<ide> output_dir = os.path.join(args.output_dir, "checkpoint-{}".format(global_step))
<ide> if not os.path.exists(output_dir):
<ide> os.makedirs(output_dir)
<del> model_to_save = model.module if hasattr(model,
<del> "module") else model # Take care of distributed/parallel training
<add> model_to_save = model.module if hasattr(model, "module") else model # Take care of distributed/parallel training
<ide> model_to_save.save_pretrained(output_dir)
<ide> torch.save(args, os.path.join(output_dir, "training_args.bin"))
<ide> logger.info("Saving model checkpoint to %s", output_dir)
<ide> def train(args, train_dataset, model, tokenizer, pad_token_label_id):
<ide> return global_step, tr_loss / global_step
<ide>
<ide>
<del>def evaluate(args, model, tokenizer, pad_token_label_id, prefix=""):
<del> eval_dataset = load_and_cache_examples(args, tokenizer, pad_token_label_id, evaluate=True)
<add>def evaluate(args, model, tokenizer, labels, pad_token_label_id, prefix=""):
<add> eval_dataset = load_and_cache_examples(args, tokenizer, labels, pad_token_label_id, evaluate=True)
<ide>
<ide> args.eval_batch_size = args.per_gpu_eval_batch_size * max(1, args.n_gpu)
<ide> # Note that DistributedSampler samples randomly
<ide> def evaluate(args, model, tokenizer, pad_token_label_id, prefix=""):
<ide> eval_loss = eval_loss / nb_eval_steps
<ide> preds = np.argmax(preds, axis=2)
<ide>
<del> label_map = {i: label for i, label in enumerate(get_labels())}
<add> label_map = {i: label for i, label in enumerate(labels)}
<ide>
<ide> out_label_list = [[] for _ in range(out_label_ids.shape[0])]
<ide> preds_list = [[] for _ in range(out_label_ids.shape[0])]
<ide> def evaluate(args, model, tokenizer, pad_token_label_id, prefix=""):
<ide> return results
<ide>
<ide>
<del>def load_and_cache_examples(args, tokenizer, pad_token_label_id, evaluate=False):
<add>def load_and_cache_examples(args, tokenizer, labels, pad_token_label_id, evaluate=False):
<ide> if args.local_rank not in [-1, 0] and not evaluate:
<ide> torch.distributed.barrier() # Make sure only the first process in distributed training process the dataset, and the others will use the cache
<ide>
<ide> def load_and_cache_examples(args, tokenizer, pad_token_label_id, evaluate=False)
<ide> features = torch.load(cached_features_file)
<ide> else:
<ide> logger.info("Creating features from dataset file at %s", args.data_dir)
<del> label_list = get_labels()
<ide> examples = read_examples_from_file(args.data_dir, evaluate=evaluate)
<del> features = convert_examples_to_features(examples, label_list, args.max_seq_length, tokenizer,
<add> features = convert_examples_to_features(examples, labels, args.max_seq_length, tokenizer,
<ide> cls_token_at_end=bool(args.model_type in ["xlnet"]),
<ide> # xlnet has a cls token at the end
<ide> cls_token=tokenizer.cls_token,
<ide> def main():
<ide> help="The output directory where the model predictions and checkpoints will be written.")
<ide>
<ide> ## Other parameters
<add> parser.add_argument("--labels", default="", type=str,
<add> help="Path to a file containing all labels. If not specified, CoNLL-2003 labels are used.")
<ide> parser.add_argument("--config_name", default="", type=str,
<ide> help="Pretrained config name or path if not the same as model_name")
<ide> parser.add_argument("--tokenizer_name", default="", type=str,
<ide> def main():
<ide> set_seed(args)
<ide>
<ide> # Prepare CONLL-2003 task
<del> label_list = get_labels()
<del> num_labels = len(label_list)
<add> labels = get_labels(args.labels)
<add> num_labels = len(labels)
<ide> # Use cross entropy ignore index as padding label id so that only real label ids contribute to the loss later
<ide> pad_token_label_id = CrossEntropyLoss().ignore_index
<ide>
<ide> def main():
<ide>
<ide> # Training
<ide> if args.do_train:
<del> train_dataset = load_and_cache_examples(args, tokenizer, pad_token_label_id, evaluate=False)
<del> global_step, tr_loss = train(args, train_dataset, model, tokenizer, pad_token_label_id)
<add> train_dataset = load_and_cache_examples(args, tokenizer, labels, pad_token_label_id, evaluate=False)
<add> global_step, tr_loss = train(args, train_dataset, model, tokenizer, labels, pad_token_label_id)
<ide> logger.info(" global_step = %s, average loss = %s", global_step, tr_loss)
<ide>
<ide> # Saving best-practices: if you use defaults names for the model, you can reload it using from_pretrained()
<ide> def main():
<ide> global_step = checkpoint.split("-")[-1] if len(checkpoints) > 1 else ""
<ide> model = model_class.from_pretrained(checkpoint)
<ide> model.to(args.device)
<del> result = evaluate(args, model, tokenizer, pad_token_label_id, prefix=global_step)
<add> result = evaluate(args, model, tokenizer, labels, pad_token_label_id, prefix=global_step)
<ide> if global_step:
<ide> result = {"{}_{}".format(global_step, k): v for k, v in result.items()}
<ide> results.update(result)
<ide><path>examples/utils_ner.py
<ide> def convert_examples_to_features(examples,
<ide> return features
<ide>
<ide>
<del>def get_labels():
<del> return ["O", "B-MISC", "I-MISC", "B-PER", "I-PER", "B-ORG", "I-ORG", "B-LOC", "I-LOC"]
<add>def get_labels(path):
<add> if path:
<add> with open(path, "r") as f:
<add> labels = f.read().splitlines()
<add> if "O" not in labels:
<add> labels = ["O"] + labels
<add> return labels
<add> else:
<add> return ["O", "B-MISC", "I-MISC", "B-PER", "I-PER", "B-ORG", "I-ORG", "B-LOC", "I-LOC"] | 2 |
Go | Go | simplify code logic | c6894aa49246183deddc367d3adf696311fb7950 | <ide><path>daemon/stats/collector_unix.go
<ide> const nanoSecondsPerSecond = 1e9
<ide> // provided. See `man 5 proc` for details on specific field
<ide> // information.
<ide> func (s *Collector) getSystemCPUUsage() (uint64, error) {
<del> var line string
<ide> f, err := os.Open("/proc/stat")
<ide> if err != nil {
<ide> return 0, err
<ide> func (s *Collector) getSystemCPUUsage() (uint64, error) {
<ide> f.Close()
<ide> }()
<ide> s.bufReader.Reset(f)
<del> err = nil
<del> for err == nil {
<del> line, err = s.bufReader.ReadString('\n')
<add>
<add> for {
<add> line, err := s.bufReader.ReadString('\n')
<ide> if err != nil {
<ide> break
<ide> } | 1 |
PHP | PHP | add removed getdatasource() call | 0bbf61ce3db3d7f0657083f6530426c269aa4c10 | <ide><path>lib/Cake/Model/CakeSchema.php
<ide> public function read($options = array()) {
<ide> if (!is_object($Object) || $Object->useTable === false) {
<ide> continue;
<ide> }
<add> $db = $Object->getDataSource();
<ide>
<ide> $fulltable = $table = $db->fullTableName($Object, false, false);
<ide> if ($prefix && strpos($table, $prefix) !== 0) { | 1 |
Javascript | Javascript | fix a bug in selector functions | 9d4b6a02fb7ef49444d0456e5861184e34267fba | <ide><path>d3.js
<ide> if (!Object.create) Object.create = function(o) {
<ide> f.prototype = o;
<ide> return new f;
<ide> };
<del>var d3_array = d3_arraySlice; // conversion for NodeLists
<del>
<del>function d3_arrayCopy(pseudoarray) {
<del> var i = -1, n = pseudoarray.length, array = [];
<del> while (++i < n) array.push(pseudoarray[i]);
<del> return array;
<del>}
<del>
<del>function d3_arraySlice(pseudoarray) {
<del> return Array.prototype.slice.call(pseudoarray);
<del>}
<del>
<del>try {
<del> d3_array(document.documentElement.childNodes)[0].nodeType;
<del>} catch(e) {
<del> d3_array = d3_arrayCopy;
<del>}
<del>
<ide> var d3_arraySubclass = [].__proto__?
<ide>
<ide> // Until ECMAScript supports array subclassing, prototype injection works well.
<ide> d3.select = function(selector) {
<ide> d3.selectAll = function(selector) {
<ide> return typeof selector === "string"
<ide> ? d3_selectionRoot.selectAll(selector)
<del> : d3_selection([d3_array(selector)]); // assume node[]
<add> : d3_selection([selector]); // assume node[]
<ide> };
<ide> function d3_selection(groups) {
<ide> d3_arraySubclass(groups, d3_selectionPrototype);
<ide> return groups;
<ide> }
<ide>
<ide> var d3_select = function(s, n) { return n.querySelector(s); },
<del> d3_selectAll = function(s, n) { return d3_array(n.querySelectorAll(s)); };
<add> d3_selectAll = function(s, n) { return n.querySelectorAll(s); };
<ide>
<ide> // Prefer Sizzle, if available.
<ide> if (typeof Sizzle === "function") {
<ide> d3_selectionPrototype.select = function(selector) {
<ide> subgroup.parentNode = (group = this[j]).parentNode;
<ide> for (var i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroup.push(subnode = selector(node));
<add> subgroup.push(subnode = selector.call(node, node.__data__, i));
<ide> if (subnode && "__data__" in node) subnode.__data__ = node.__data__;
<ide> } else {
<ide> subgroup.push(null);
<ide> d3_selectionPrototype.select = function(selector) {
<ide> };
<ide>
<ide> function d3_selection_selector(selector) {
<del> return function(node) {
<del> return d3_select(selector, node);
<add> return function() {
<add> return d3_select(selector, this);
<ide> };
<ide> }
<ide> d3_selectionPrototype.selectAll = function(selector) {
<ide> d3_selectionPrototype.selectAll = function(selector) {
<ide> for (var j = -1, m = this.length; ++j < m;) {
<ide> for (var group = this[j], i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroups.push(subgroup = selector(node));
<add> subgroups.push(subgroup = selector.call(node, node.__data__, i));
<ide> subgroup.parentNode = node;
<ide> }
<ide> }
<ide> d3_selectionPrototype.selectAll = function(selector) {
<ide> };
<ide>
<ide> function d3_selection_selectorAll(selector) {
<del> return function(node) {
<del> return d3_selectAll(selector, node);
<add> return function() {
<add> return d3_selectAll(selector, this);
<ide> };
<ide> }
<ide> d3_selectionPrototype.attr = function(name, value) {
<ide> d3_selectionPrototype.html = function(value) {
<ide> d3_selectionPrototype.append = function(name) {
<ide> name = d3.ns.qualify(name);
<ide>
<del> function append(node) {
<del> return node.appendChild(document.createElement(name));
<add> function append() {
<add> return this.appendChild(document.createElement(name));
<ide> }
<ide>
<del> function appendNS(node) {
<del> return node.appendChild(document.createElementNS(name.space, name.local));
<add> function appendNS() {
<add> return this.appendChild(document.createElementNS(name.space, name.local));
<ide> }
<ide>
<ide> return this.select(name.local ? appendNS : append);
<ide> d3_selectionPrototype.append = function(name) {
<ide> d3_selectionPrototype.insert = function(name, before) {
<ide> name = d3.ns.qualify(name);
<ide>
<del> function insert(node) {
<del> return node.insertBefore(
<add> function insert() {
<add> return this.insertBefore(
<ide> document.createElement(name),
<del> d3_select(before, node));
<add> d3_select(before, this));
<ide> }
<ide>
<del> function insertNS(node) {
<del> return node.insertBefore(
<add> function insertNS() {
<add> return this.insertBefore(
<ide> document.createElementNS(name.space, name.local),
<del> d3_select(before, node));
<add> d3_select(before, this));
<ide> }
<ide>
<ide> return this.select(name.local ? insertNS : insert);
<ide> d3_selection_enterPrototype.select = function(selector) {
<ide> subgroup.parentNode = group.parentNode;
<ide> for (var i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroup.push(upgroup[i] = subnode = selector(group.parentNode));
<add> subgroup.push(upgroup[i] = subnode = selector.call(group.parentNode, node.__data__, i));
<ide> subnode.__data__ = node.__data__;
<ide> } else {
<ide> subgroup.push(null);
<ide> d3_transitionPrototype.select = function(selector) {
<ide> for (var j = -1, m = this.length; ++j < m;) {
<ide> subgroups.push(subgroup = []);
<ide> for (var group = this[j], i = -1, n = group.length; ++i < n;) {
<del> if ((node = group[i]) && (subnode = selector(node.node))) {
<add> if ((node = group[i]) && (subnode = selector.call(node.node, node.node.__data__, i))) {
<ide> if ("__data__" in node.node) subnode.__data__ = node.node.__data__;
<ide> subgroup.push({node: subnode, delay: node.delay, duration: node.duration});
<ide> } else {
<ide> d3_transitionPrototype.selectAll = function(selector) {
<ide> for (var j = -1, m = this.length; ++j < m;) {
<ide> for (var group = this[j], i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroups.push(subgroup = selector(node.node));
<add> subgroups.push(subgroup = selector.call(node.node, node.node.__data__, i));
<ide> for (var k = -1, o = subgroup.length; ++k < o;) {
<ide> subgroup[k] = {node: subgroup[k], delay: node.delay, duration: node.duration};
<ide> }
<ide> function d3_svg_mousePoint(container, e) {
<ide> };
<ide> d3.svg.touches = function(container) {
<ide> var touches = d3.event.touches;
<del> return touches ? d3_array(touches).map(function(touch) {
<add> return touches ? Array.prototype.map.call(touches, function(touch) {
<ide> var point = d3_svg_mousePoint(container, touch);
<ide> point.identifier = touch.identifier;
<ide> return point;
<ide><path>d3.min.js
<del>(function(){function dm(a,b,c){function i(a,b){var c=a.__domain||(a.__domain=a.domain()),d=a.range().map(function(a){return(a-b)/h});a.domain(c).domain(d.map(a.invert))}var d=Math.pow(2,(da[2]=a)-c[2]),e=da[0]=b[0]-d*c[0],f=da[1]=b[1]-d*c[1],g=d3.event,h=Math.pow(2,a);d3.event={scale:h,translate:[e,f],transform:function(a,b){a&&i(a,e),b&&i(b,f)}};try{db.apply(dc,dd)}finally{d3.event=g}g.preventDefault()}function dl(){de&&(d3.event.stopPropagation(),d3.event.preventDefault(),de=!1)}function dk(){cY&&(d3_behavior_zoomMoved&&(de=!0),dj(),cY=null)}function dj(){cZ=null,cY&&(d3_behavior_zoomMoved=!0,dm(da[2],d3.svg.mouse(dc),cY))}function di(){var a=d3.svg.touches(dc);switch(a.length){case 1:var b=a[0];dm(da[2],b,c$[b.identifier]);break;case 2:var c=a[0],d=a[1],e=[(c[0]+d[0])/2,(c[1]+d[1])/2],f=c$[c.identifier],g=c$[d.identifier],h=[(f[0]+g[0])/2,(f[1]+g[1])/2,f[2]];dm(Math.log(d3.event.scale)/Math.LN2+f[2],e,h)}}function dh(){var a=d3.svg.touches(dc),b=-1,c=a.length,d;while(++b<c)c$[(d=a[b]).identifier]=df(d);return a}function dg(){cX||(cX=d3.select("body").append("div").style("visibility","hidden").style("top",0).style("height",0).style("width",0).style("overflow-y","scroll").append("div").style("height","2000px").node().parentNode);var a=d3.event,b;try{cX.scrollTop=1e3,cX.dispatchEvent(a),b=1e3-cX.scrollTop}catch(c){b=a.wheelDelta||-a.detail}return b*.005}function df(a){return[a[0]-da[0],a[1]-da[1],da[2]]}function cW(){d3.event.stopPropagation(),d3.event.preventDefault()}function cV(){cQ&&(cW(),cQ=!1)}function cU(){!cN||(cR("dragend"),d3_behavior_dragForce=cN=null,cP&&(cQ=!0,cW()))}function cT(){if(!!cN){var a=cN.parentNode;if(!a)return cU();cR("drag"),cW()}}function cS(a){return d3.event.touches?d3.svg.touches(a)[0]:d3.svg.mouse(a)}function cR(a){var b=d3.event,c=cN.parentNode,d=0,e=0;c&&(c=cS(c),d=c[0]-cO[0],e=c[1]-cO[1],cO=c,cP|=d|e);try{d3.event={dx:d,dy:e},cM[a].dispatch.apply(cN,d3_behavior_dragArguments)}finally{d3.event=b}b.preventDefault()}function cL(a,b,c){e=[];if(c&&b.length>1){var d=bp(a.domain()),e,f=-1,g=b.length,h=(b[1]-b[0])/++c,i,j;while(++f<g)for(i=c;--i>0;)(j=+b[f]-i*h)>=d[0]&&e.push(j);for(--f,i=0;++i<c&&(j=+b[f]+i*h)<d[1];)e.push(j)}return e}function cK(a,b){a.attr("transform",function(a){return"translate(0,"+b(a)+")"})}function cJ(a,b){a.attr("transform",function(a){return"translate("+b(a)+",0)"})}function cF(){return"circle"}function cE(){return 64}function cD(a,b){var c=(a.ownerSVGElement||a).createSVGPoint();if(cC<0&&(window.scrollX||window.scrollY)){var d=d3.select(document.body).append("svg:svg").style("position","absolute").style("top",0).style("left",0),e=d[0][0].getScreenCTM();cC=!e.f&&!e.e,d.remove()}cC?(c.x=b.pageX,c.y=b.pageY):(c.x=b.clientX,c.y=b.clientY),c=c.matrixTransform(a.getScreenCTM().inverse());return[c.x,c.y]}function cB(a){return function(){var b=a.apply(this,arguments),c=b[0],d=b[1]+bN;return[c*Math.cos(d),c*Math.sin(d)]}}function cA(a){return[a.x,a.y]}function cz(a){return a.endAngle}function cy(a){return a.startAngle}function cx(a){return a.radius}function cw(a){return a.target}function cv(a){return a.source}function cu(a){return function(b,c){return a[c][1]}}function ct(a){return function(b,c){return a[c][0]}}function cs(a){function i(f){if(f.length<1)return null;var i=bU(this,f,b,d),j=bU(this,f,b===c?ct(i):c,d===e?cu(i):e);return"M"+g(a(j),h)+"L"+g(a(i.reverse()),h)+"Z"}var b=bV,c=bV,d=0,e=bW,f="linear",g=bX[f],h=.7;i.x=function(a){if(!arguments.length)return c;b=c=a;return i},i.x0=function(a){if(!arguments.length)return b;b=a;return i},i.x1=function(a){if(!arguments.length)return c;c=a;return i},i.y=function(a){if(!arguments.length)return e;d=e=a;return i},i.y0=function(a){if(!arguments.length)return d;d=a;return i},i.y1=function(a){if(!arguments.length)return e;e=a;return i},i.interpolate=function(a){if(!arguments.length)return f;g=bX[f=a];return i},i.tension=function(a){if(!arguments.length)return h;h=a;return i};return i}function cr(a){var b,c=-1,d=a.length,e,f;while(++c<d)b=a[c],e=b[0],f=b[1]+bN,b[0]=e*Math.cos(f),b[1]=e*Math.sin(f);return a}function cq(a){return a.length<3?bY(a):a[0]+cc(a,cp(a))}function cp(a){var b=[],c,d,e,f,g=co(a),h=-1,i=a.length-1;while(++h<i)c=cn(a[h],a[h+1]),Math.abs(c)<1e-6?g[h]=g[h+1]=0:(d=g[h]/c,e=g[h+1]/c,f=d*d+e*e,f>9&&(f=c*3/Math.sqrt(f),g[h]=f*d,g[h+1]=f*e));h=-1;while(++h<=i)f=(a[Math.min(i,h+1)][0]-a[Math.max(0,h-1)][0])/(6*(1+g[h]*g[h])),b.push([f||0,g[h]*f||0]);return b}function co(a){var b=0,c=a.length-1,d=[],e=a[0],f=a[1],g=d[0]=cn(e,f);while(++b<c)d[b]=g+(g=cn(e=f,f=a[b+1]));d[b]=g;return d}function cn(a,b){return(b[1]-a[1])/(b[0]-a[0])}function cm(a,b,c){a.push("C",ci(cj,b),",",ci(cj,c),",",ci(ck,b),",",ci(ck,c),",",ci(cl,b),",",ci(cl,c))}function ci(a,b){return a[0]*b[0]+a[1]*b[1]+a[2]*b[2]+a[3]*b[3]}function ch(a,b){var c=a.length-1,d=a[0][0],e=a[0][1],f=a[c][0]-d,g=a[c][1]-e,h=-1,i,j;while(++h<=c)i=a[h],j=h/c,i[0]=b*i[0]+(1-b)*(d+j*f),i[1]=b*i[1]+(1-b)*(e+j*g);return ce(a)}function cg(a){var b,c=-1,d=a.length,e=d+4,f,g=[],h=[];while(++c<4)f=a[c%d],g.push(f[0]),h.push(f[1]);b=[ci(cl,g),",",ci(cl,h)],--c;while(++c<e)f=a[c%d],g.shift(),g.push(f[0]),h.shift(),h.push(f[1]),cm(b,g,h);return b.join("")}function cf(a){if(a.length<4)return bY(a);var b=[],c=-1,d=a.length,e,f=[0],g=[0];while(++c<3)e=a[c],f.push(e[0]),g.push(e[1]);b.push(ci(cl,f)+","+ci(cl,g)),--c;while(++c<d)e=a[c],f.shift(),f.push(e[0]),g.shift(),g.push(e[1]),cm(b,f,g);return b.join("")}function ce(a){if(a.length<3)return bY(a);var b=[],c=1,d=a.length,e=a[0],f=e[0],g=e[1],h=[f,f,f,(e=a[1])[0]],i=[g,g,g,e[1]];b.push(f,",",g),cm(b,h,i);while(++c<d)e=a[c],h.shift(),h.push(e[0]),i.shift(),i.push(e[1]),cm(b,h,i);c=-1;while(++c<2)h.shift(),h.push(e[0]),i.shift(),i.push(e[1]),cm(b,h,i);return b.join("")}function cd(a,b){var c=[],d=(1-b)/2,e,f=a[0],g=a[1],h=1,i=a.length;while(++h<i)e=f,f=g,g=a[h],c.push([d*(g[0]-e[0]),d*(g[1]-e[1])]);return c}function cc(a,b){if(b.length<1||a.length!=b.length&&a.length!=b.length+2)return bY(a);var c=a.length!=b.length,d="",e=a[0],f=a[1],g=b[0],h=g,i=1;c&&(d+="Q"+(f[0]-g[0]*2/3)+","+(f[1]-g[1]*2/3)+","+f[0]+","+f[1],e=a[1],i=2);if(b.length>1){h=b[1],f=a[i],i++,d+="C"+(e[0]+g[0])+","+(e[1]+g[1])+","+(f[0]-h[0])+","+(f[1]-h[1])+","+f[0]+","+f[1];for(var j=2;j<b.length;j++,i++)f=a[i],h=b[j],d+="S"+(f[0]-h[0])+","+(f[1]-h[1])+","+f[0]+","+f[1]}if(c){var k=a[i];d+="Q"+(f[0]+h[0]*2/3)+","+(f[1]+h[1]*2/3)+","+k[0]+","+k[1]}return d}function cb(a,b,c){return a.length<3?bY(a):a[0]+cc(a,cd(a,b))}function ca(a,b){return a.length<3?bY(a):a[0]+cc((a.push(a[0]),a),cd([a[a.length-2]].concat(a,[a[1]]),b))}function b_(a,b){return a.length<4?bY(a):a[1]+cc(a.slice(1,a.length-1),cd(a,b))}function b$(a){var b=[],c=0,d=a.length,e=a[0];b.push(e[0],",",e[1]);while(++c<d)b.push("H",(e=a[c])[0],"V",e[1]);return b.join("")}function bZ(a){var b=[],c=0,d=a.length,e=a[0];b.push(e[0],",",e[1]);while(++c<d)b.push("V",(e=a[c])[1],"H",e[0]);return b.join("")}function bY(a){var b=[],c=0,d=a.length,e=a[0];b.push(e[0],",",e[1]);while(++c<d)b.push("L",(e=a[c])[0],",",e[1]);return b.join("")}function bW(a){return a[1]}function bV(a){return a[0]}function bU(a,b,c,d){var e=[],f=-1,g=b.length,h=typeof c=="function",i=typeof d=="function",j;if(h&&i)while(++f<g)e.push([c.call(a,j=b[f],f),d.call(a,j,f)]);else if(h)while(++f<g)e.push([c.call(a,b[f],f),d]);else if(i)while(++f<g)e.push([c,d.call(a,b[f],f)]);else while(++f<g)e.push([c,d]);return e}function bT(a){function g(d){return d.length<1?null:"M"+e(a(bU(this,d,b,c)),f)}var b=bV,c=bW,d="linear",e=bX[d],f=.7;g.x=function(a){if(!arguments.length)return b;b=a;return g},g.y=function(a){if(!arguments.length)return c;c=a;return g},g.interpolate=function(a){if(!arguments.length)return d;e=bX[d=a];return g},g.tension=function(a){if(!arguments.length)return f;f=a;return g};return g}function bS(a){return a.endAngle}function bR(a){return a.startAngle}function bQ(a){return a.outerRadius}function bP(a){return a.innerRadius}function bM(a,b,c){function g(){d=c.length/(b-a),e=c.length-1;return f}function f(b){return c[Math.max(0,Math.min(e,Math.floor(d*(b-a))))]}var d,e;f.domain=function(c){if(!arguments.length)return[a,b];a=+c[0],b=+c[c.length-1];return g()},f.range=function(a){if(!arguments.length)return c;c=a;return g()},f.copy=function(){return bM(a,b,c)};return g()}function bL(a,b){function e(a){return isNaN(a=+a)?NaN:b[d3.bisect(c,a)]}function d(){var d=0,f=a.length,g=b.length;c=[];while(++d<g)c[d-1]=d3.quantile(a,d/g);return e}var c;e.domain=function(b){if(!arguments.length)return a;a=b.filter(function(a){return!isNaN(a)}).sort(d3.ascending);return d()},e.range=function(a){if(!arguments.length)return b;b=a;return d()},e.quantiles=function(){return c},e.copy=function(){return bL(a,b)};return d()}function bG(a,b,c){function f(c){return d[((a[c]||(a[c]=++b))-1)%d.length]}var d,e;f.domain=function(d){if(!arguments.length)return d3.keys(a);a={},b=0;var e=-1,g=d.length,h;while(++e<g)a[h=d[e]]||(a[h]=++b);return f[c.t](c.x,c.p)},f.range=function(a){if(!arguments.length)return d;d=a,e=0,c={t:"range",x:a};return f},f.rangePoints=function(a,g){arguments.length<2&&(g=0);var h=a[0],i=a[1],j=(i-h)/(b-1+g);d=b<2?[(h+i)/2]:d3.range(h+j*g/2,i+j/2,j),e=0,c={t:"rangePoints",x:a,p:g};return f},f.rangeBands=function(a,g){arguments.length<2&&(g=0);var h=a[0],i=a[1],j=(i-h)/(b+g);d=d3.range(h+j*g,i,j),e=j*(1-g),c={t:"rangeBands",x:a,p:g};return f},f.rangeRoundBands=function(a,g){arguments.length<2&&(g=0);var h=a[0],i=a[1],j=Math.floor((i-h)/(b+g)),k=i-h-(b-g)*j;d=d3.range(h+Math.round(k/2),i,j),e=Math.round(j*(1-g)),c={t:"rangeRoundBands",x:a,p:g};return f},f.rangeBand=function(){return e},f.copy=function(){var d={},e;for(e in a)d[e]=a[e];return bG(d,b,c)};return f[c.t](c.x,c.p)}function bF(a){return function(b){return b<0?-Math.pow(-b,a):Math.pow(b,a)}}function bE(a,b){function e(b){return a(c(b))}var c=bF(b),d=bF(1/b);e.invert=function(b){return d(a.invert(b))},e.domain=function(b){if(!arguments.length)return a.domain().map(d);a.domain(b.map(c));return e},e.ticks=function(a){return bw(e.domain(),a)},e.tickFormat=function(a){return bx(e.domain(),a)},e.nice=function(){return e.domain(bq(e.domain(),bu))},e.exponent=function(a){if(!arguments.length)return b;var f=e.domain();c=bF(b=a),d=bF(1/b);return e.domain(f)},e.copy=function(){return bE(a.copy(),b)};return bt(e,a)}function bD(a){return a.toPrecision(1)}function bC(a){return-Math.log(-a)/Math.LN10}function bB(a){return Math.log(a)/Math.LN10}function bA(a,b){function d(c){return a(b(c))}var c=b.pow;d.invert=function(b){return c(a.invert(b))},d.domain=function(e){if(!arguments.length)return a.domain().map(c);b=e[0]<0?bC:bB,c=b.pow,a.domain(e.map(b));return d},d.nice=function(){a.domain(bq(a.domain(),br));return d},d.ticks=function(){var d=bp(a.domain()),e=[];if(d.every(isFinite)){var f=Math.floor(d[0]),g=Math.ceil(d[1]),h=c(d[0]),i=c(d[1]);if(b===bC){e.push(c(f));for(;f++<g;)for(var j=9;j>0;j--)e.push(c(f)*j)}else{for(;f<g;f++)for(var j=1;j<10;j++)e.push(c(f)*j);e.push(c(f))}for(f=0;e[f]<h;f++);for(g=e.length;e[g-1]>i;g--);e=e.slice(f,g)}return e},d.tickFormat=function(){return bD},d.copy=function(){return bA(a.copy(),b)};return bt(d,a)}function bz(a,b,c,d){var e=[],f=[],g=0,h=a.length;while(++g<h)e.push(c(a[g-1],a[g])),f.push(d(b[g-1],b[g]));return function(b){var c=d3.bisect(a,b,1,a.length-1)-1;return f[c](e[c](b))}}function by(a,b,c,d){var e=c(a[0],a[1]),f=d(b[0],b[1]);return function(a){return f(e(a))}}function bx(a,b){return d3.format(",."+Math.max(0,-Math.floor(Math.log(bv(a,b)[2])/Math.LN10+.01))+"f")}function bw(a,b){return d3.range.apply(d3,bv(a,b))}function bv(a,b){var c=bp(a),d=c[1]-c[0],e=Math.pow(10,Math.floor(Math.log(d/b)/Math.LN10)),f=b/d*e;f<=.15?e*=10:f<=.35?e*=5:f<=.75&&(e*=2),c[0]=Math.ceil(c[0]/e)*e,c[1]=Math.floor(c[1]/e)*e+e*.5,c[2]=e;return c}function bu(a){a=Math.pow(10,Math.round(Math.log(a)/Math.LN10)-1);return{floor:function(b){return Math.floor(b/a)*a},ceil:function(b){return Math.ceil(b/a)*a}}}function bt(a,b){a.range=d3.rebind(a,b.range),a.rangeRound=d3.rebind(a,b.rangeRound),a.interpolate=d3.rebind(a,b.interpolate),a.clamp=d3.rebind(a,b.clamp);return a}function bs(a,b,c,d){function h(a){return e(a)}function g(){var g=a.length==2?by:bz,i=d?G:F;e=g(a,b,i,c),f=g(b,a,i,d3.interpolate);return h}var e,f;h.invert=function(a){return f(a)},h.domain=function(b){if(!arguments.length)return a;a=b.map(Number);return g()},h.range=function(a){if(!arguments.length)return b;b=a;return g()},h.rangeRound=function(a){return h.range(a).interpolate(d3.interpolateRound)},h.clamp=function(a){if(!arguments.length)return d;d=a;return g()},h.interpolate=function(a){if(!arguments.length)return c;c=a;return g()},h.ticks=function(b){return bw(a,b)},h.tickFormat=function(b){return bx(a,b)},h.nice=function(){bq(a,bu);return g()},h.copy=function(){return bs(a,b,c,d)};return g()}function br(){return Math}function bq(a,b){var c=0,d=a.length-1,e=a[c],f=a[d],g;f<e&&(g=c,c=d,d=g,g=e,e=f,f=g),b=b(f-e),a[c]=b.floor(e),a[d]=b.ceil(f);return a}function bp(a){var b=a[0],c=a[a.length-1];return b<c?[b,c]:[c,b]}function bo(){}function bm(){var a=null,b=bi,c=Infinity;while(b)b.flush?b=a?a.next=b.next:bi=b.next:(c=Math.min(c,b.then+b.delay),b=(a=b).next);return c}function bl(){var a,b=Date.now(),c=bi;while(c)a=b-c.then,a>=c.delay&&(c.flush=c.callback(a)),c=c.next;var d=bm()-b;d>24?(isFinite(d)&&(clearTimeout(bk),bk=setTimeout(bl,d)),bj=0):(bj=1,bn(bl))}function bh(a){for(var b=0,c=this.length;b<c;b++)for(var d=this[b],e=0,f=d.length;e<f;e++){var g=d[e];g&&a.call(g=g.node,g.__data__,e,b)}return this}function bc(a){return typeof a=="function"?function(b,c,d){var e=a.call(this,b,c)+"";return d!=e&&d3.interpolate(d,e)}:(a=a+"",function(b,c,d){return d!=a&&d3.interpolate(d,a)})}function bb(a,b){e(a,bd);var c={},d=d3.dispatch("start","end"),f=bg;a.id=b,a.tween=function(b,d){if(arguments.length<2)return c[b];d==null?delete c[b]:c[b]=d;return a},a.ease=function(b){if(!arguments.length)return f;f=typeof b=="function"?b:d3.ease.apply(d3,arguments);return a},a.each=function(b,c){if(arguments.length<2)return bh.call(a,b);d[b].add(c);return a},d3.timer(function(e){a.each(function(g,h,i){function p(a){if(n.active!==b)return!0;var c=Math.min(1,(a-l)/m),e=f(c),i=j.length;while(--i>=0)j[i].call(k,e);if(c===1){n.active=0,n.owner===b&&delete k.__transition__,bf=b,d.end.dispatch.call(k,g,h),bf=0;return!0}}function o(a){if(n.active<=b){n.active=b,d.start.dispatch.call(k,g,h);for(var e in c)(e=c[e].call(k,g,h))&&j.push(e);l-=a,d3.timer(p)}return!0}var j=[],k=this,l=a[i][h].delay-e,m=a[i][h].duration,n=k.__transition__;n?n.owner<b&&(n.owner=b):n=k.__transition__={active:0,owner:b},l<=0?o(0):d3.timer(o,l)});return!0});return a}function ba(a){arguments.length||(a=d3.ascending);return function(b,c){return a(b&&b.__data__,c&&c.__data__)}}function $(a){e(a,_);return a}function Z(a){return{__data__:a}}function Y(a){return function(b){return U(a,b)}}function X(a){return function(b){return T(a,b)}}function S(a){e(a,V);return a}function R(a,b,c){function g(a){return Math.round(f(a)*255)}function f(a){a>360?a-=360:a<0&&(a+=360);return a<60?d+(e-d)*a/60:a<180?e:a<240?d+(e-d)*(240-a)/60:d}var d,e;a=a%360,a<0&&(a+=360),b=b<0?0:b>1?1:b,c=c<0?0:c>1?1:c,e=c<=.5?c*(1+b):c+b-c*b,d=2*c-e;return H(g(a+120),g(a),g(a-120))}function Q(a,b,c){this.h=a,this.s=b,this.l=c}function P(a,b,c){return new Q(a,b,c)}function M(a){var b=parseFloat(a);return a.charAt(a.length-1)==="%"?Math.round(b*2.55):b}function L(a,b,c){var d=Math.min(a/=255,b/=255,c/=255),e=Math.max(a,b,c),f=e-d,g,h,i=(e+d)/2;f?(h=i<.5?f/(e+d):f/(2-e-d),a==e?g=(b-c)/f+(b<c?6:0):b==e?g=(c-a)/f+2:g=(a-b)/f+4,g*=60):h=g=0;return P(g,h,i)}function K(a,b,c){var d=0,e=0,f=0,g,h,i;g=/([a-z]+)\((.*)\)/i.exec(a);if(g){h=g[2].split(",");switch(g[1]){case"hsl":return c(parseFloat(h[0]),parseFloat(h[1])/100,parseFloat(h[2])/100);case"rgb":return b(M(h[0]),M(h[1]),M(h[2]))}}if(i=N[a])return b(i.r,i.g,i.b);a!=null&&a.charAt(0)==="#"&&(a.length===4?(d=a.charAt(1),d+=d,e=a.charAt(2),e+=e,f=a.charAt(3),f+=f):a.length===7&&(d=a.substring(1,3),e=a.substring(3,5),f=a.substring(5,7)),d=parseInt(d,16),e=parseInt(e,16),f=parseInt(f,16));return b(d,e,f)}function J(a){return a<16?"0"+a.toString(16):a.toString(16)}function I(a,b,c){this.r=a,this.g=b,this.b=c}function H(a,b,c){return new I(a,b,c)}function G(a,b){b=b-(a=+a)?1/(b-a):0;return function(c){return Math.max(0,Math.min(1,(c-a)*b))}}function F(a,b){b=b-(a=+a)?1/(b-a):0;return function(c){return(c-a)*b}}function E(a){return a in D||/\bcolor\b/.test(a)?d3.interpolateRgb:d3.interpolate}function B(a){return a<1/2.75?7.5625*a*a:a<2/2.75?7.5625*(a-=1.5/2.75)*a+.75:a<2.5/2.75?7.5625*(a-=2.25/2.75)*a+.9375:7.5625*(a-=2.625/2.75)*a+.984375}function A(a){a||(a=1.70158);return function(b){return b*b*((a+1)*b-a)}}function z(a,b){var c;arguments.length<2&&(b=.45),arguments.length<1?(a=1,c=b/4):c=b/(2*Math.PI)*Math.asin(1/a);return function(d){return 1+a*Math.pow(2,10*-d)*Math.sin((d-c)*2*Math.PI/b)}}function y(a){return 1-Math.sqrt(1-a*a)}function x(a){return Math.pow(2,10*(a-1))}function w(a){return 1-Math.cos(a*Math.PI/2)}function v(a){return function(b){return Math.pow(b,a)}}function u(a){return a}function t(a){return function(b){return.5*(b<.5?a(2*b):2-a(2-2*b))}}function s(a){return function(b){return 1-a(1-b)}}function n(a){var b=a.lastIndexOf("."),c=b>=0?a.substring(b):(b=a.length,""),d=[];while(b>0)d.push(a.substring(b-=3,b+3));return d.reverse().join(",")+c}function m(a){return a+""}function j(a){var b={},c=[];b.add=function(a){for(var d=0;d<c.length;d++)if(c[d].listener==a)return b;c.push({listener:a,on:!0});return b},b.remove=function(a){for(var d=0;d<c.length;d++){var e=c[d];if(e.listener==a){e.on=!1,c=c.slice(0,d).concat(c.slice(d+1));break}}return b},b.dispatch=function(){var a=c;for(var b=0,d=a.length;b<d;b++){var e=a[b];e.on&&e.listener.apply(this,arguments)}};return b}function h(a){return a.replace(/(^\s+)|(\s+$)/g,"").replace(/\s+/g," ")}function g(a){return a==null}function f(a){return a.length}function c(a){return Array.prototype.slice.call(a)}function b(a){var b=-1,c=a.length,d=[];while(++b<c)d.push(a[b]);return d}d3={version:"2.0.0"},Date.now||(Date.now=function(){return+(new Date)}),Object.create||(Object.create=function(a){function b(){}b.prototype=a;return new b});var a=c;try{a(document.documentElement.childNodes)[0].nodeType}catch(d){a=b}var e=[].__proto__?function(a,b){a.__proto__=b}:function(a,b){for(var c in b)a[c]=b[c]};d3.functor=function(a){return typeof a=="function"?a:function(){return a}},d3.rebind=function(a,b){return function(){var c=b.apply(a,arguments);return arguments.length?a:c}},d3.ascending=function(a,b){return a<b?-1:a>b?1:a>=b?0:NaN},d3.descending=function(a,b){return b<a?-1:b>a?1:b>=a?0:NaN},d3.min=function(a,b){var c=-1,d=a.length,e,f;if(arguments.length===1){while(++c<d&&((e=a[c])==null||e!=e))e=undefined;while(++c<d)(f=a[c])!=null&&e>f&&(e=f)}else{while(++c<d&&((e=b.call(a,a[c],c))==null||e!=e))e=undefined;while(++c<d)(f=b.call(a,a[c],c))!=null&&e>f&&(e=f)}return e},d3.max=function(a,b){var c=-1,d=a.length,e,f;if(arguments.length===1){while(++c<d&&((e=a[c])==null||e!=e))e=undefined;while(++c<d)(f=a[c])!=null&&f>e&&(e=f)}else{while(++c<d&&((e=b.call(a,a[c],c))==null||e!=e))e=undefined;while(++c<d)(f=b.call(a,a[c],c))!=null&&f>e&&(e=f)}return e},d3.sum=function(a,b){var c=0,d=a.length,e,f=-1;if(arguments.length===1)while(++f<d)isNaN(e=+a[f])||(c+=e);else while(++f<d)isNaN(e=+b.call(a,a[f],f))||(c+=e);return c},d3.quantile=function(a,b){var c=(a.length-1)*b+1,d=Math.floor(c),e=a[d-1],f=c-d;return f?e+f*(a[d]-e):e},d3.zip=function(){if(!(e=arguments.length))return[];for(var a=-1,b=d3.min(arguments,f),c=Array(b);++a<b;)for(var d=-1,e,g=c[a]=Array(e);++d<e;)g[d]=arguments[d][a];return c},d3.bisectLeft=function(a,b,c,d){arguments.length<3&&(c=0),arguments.length<4&&(d=a.length);while(c<d){var e=c+d>>1;a[e]<b?c=e+1:d=e}return c},d3.bisect=d3.bisectRight=function(a,b,c,d){arguments.length<3&&(c=0),arguments.length<4&&(d=a.length);while(c<d){var e=c+d>>1;b<a[e]?d=e:c=e+1}return c},d3.first=function(a,b){var c=0,d=a.length,e=a[0],f;arguments.length===1&&(b=d3.ascending);while(++c<d)b.call(a,e,f=a[c])>0&&(e=f);return e},d3.last=function(a,b){var c=0,d=a.length,e=a[0],f;arguments.length===1&&(b=d3.ascending);while(++c<d)b.call(a,e,f=a[c])<=0&&(e=f);return e},d3.nest=function(){function g(a,d){if(d>=b.length)return a;var e=[],f=c[d++],h;for(h in a)e.push({key:h,values:g(a[h],d)});f&&e.sort(function(a,b){return f(a.key,b.key)});return e}function f(c,g){if(g>=b.length)return e?e.call(a,c):d?c.sort(d):c;var h=-1,i=c.length,j=b[g++],k,l,m={};while(++h<i)(k=j(l=c[h]))in m?m[k].push(l):m[k]=[l];for(k in m)m[k]=f(m[k],g);return m}var a={},b=[],c=[],d,e;a.map=function(a){return f(a,0)},a.entries=function(a){return g(f(a,0),0)},a.key=function(c){b.push(c);return a},a.sortKeys=function(d){c[b.length-1]=d;return a},a.sortValues=function(b){d=b;return a},a.rollup=function(b){e=b;return a};return a},d3.keys=function(a){var b=[];for(var c in a)b.push(c);return b},d3.values=function(a){var b=[];for(var c in a)b.push(a[c]);return b},d3.entries=function(a){var b=[];for(var c in a)b.push({key:c,value:a[c]});return b},d3.permute=function(a,b){var c=[],d=-1,e=b.length;while(++d<e)c[d]=a[b[d]];return c},d3.merge=function(a){return Array.prototype.concat.apply([],a)},d3.split=function(a,b){var c=[],d=[],e,f=-1,h=a.length;arguments.length<2&&(b=g);while(++f<h)b.call(d,e=a[f],f)?d=[]:(d.length||c.push(d),d.push(e));return c},d3.range=function(a,b,c){arguments.length<3&&(c=1,arguments.length<2&&(b=a,a=0));if((b-a)/c==Infinity)throw new Error("infinite range");var d=[],e=-1,f;if(c<0)while((f=a+c*++e)>b)d.push(f);else while((f=a+c*++e)<b)d.push(f);return d},d3.requote=function(a){return a.replace(i,"\\$&")};var i=/[\\\^\$\*\+\?\|\[\]\(\)\.\{\}]/g;d3.round=function(a,b){return b?Math.round(a*Math.pow(10,b))*Math.pow(10,-b):Math.round(a)},d3.xhr=function(a,b,c){var d=new XMLHttpRequest;arguments.length<3?c=b:b&&d.overrideMimeType&&d.overrideMimeType(b),d.open("GET",a,!0),d.onreadystatechange=function(){d.readyState===4&&c(d.status<300?d:null)},d.send(null)},d3.text=function(a,b,c){function d(a){c(a&&a.responseText)}arguments.length<3&&(c=b,b=null),d3.xhr(a,b,d)},d3.json=function(a,b){d3.text(a,"application/json",function(a){b(a?JSON.parse(a):null)})},d3.html=function(a,b){d3.text(a,"text/html",function(a){if(a!=null){var c=document.createRange();c.selectNode(document.body),a=c.createContextualFragment(a)}b(a)})},d3.xml=function(a,b,c){function d(a){c(a&&a.responseXML)}arguments.length<3&&(c=b,b=null),d3.xhr(a,b,d)},d3.ns={prefix:{svg:"http://www.w3.org/2000/svg",xhtml:"http://www.w3.org/1999/xhtml",xlink:"http://www.w3.org/1999/xlink",xml:"http://www.w3.org/XML/1998/namespace",xmlns:"http://www.w3.org/2000/xmlns/"},qualify:function(a){var b=a.indexOf(":");return b<0?a:{space:d3.ns.prefix[a.substring(0,b)],local:a.substring(b+1)}}},d3.dispatch=function(a){var b={},c;for(var d=0,e=arguments.length;d<e;d++)c=arguments[d],b[c]=j(c);return b},d3.format=function(a){var b=k.exec(a),c=b[1]||" ",d=b[3]||"",e=b[5],f=+b[6],g=b[7],h=b[8],i=b[9],j=!1,o=!1;h&&(h=h.substring(1)),e&&(c="0",g&&(f-=Math.floor((f-1)/4)));switch(i){case"n":g=!0,i="g";break;case"%":j=!0,i="f";break;case"p":j=!0,i="r";break;case"d":o=!0,h="0"}i=l[i]||m;return function(a){var b=j?a*100:+a,k=b<0&&(b=-b)?"−":d;if(o&&b%1)return"";a=i(b,h);if(e){var l=a.length+k.length;l<f&&(a=Array(f-l+1).join(c)+a),g&&(a=n(a)),a=k+a}else{g&&(a=n(a)),a=k+a;var l=a.length;l<f&&(a=Array(f-l+1).join(c)+a)}j&&(a+="%");return a}};var k=/(?:([^{])?([<>=^]))?([+\- ])?(#)?(0)?([0-9]+)?(,)?(\.[0-9]+)?([a-zA-Z%])?/,l={g:function(a,b){return a.toPrecision(b)},e:function(a,b){return a.toExponential(b)},f:function(a,b){return a.toFixed(b)},r:function(a,b){var c=1+Math.floor(1e-15+Math.log(a)/Math.LN10);return d3.round(a,b-c).toFixed(Math.max(0,Math.min(20,b-c)))}},o=v(2),p=v(3),q={linear:function(){return u},poly:v,quad:function(){return o},cubic:function(){return p},sin:function(){return w},exp:function(){return x},circle:function(){return y},elastic:z,back:A,bounce:function(){return B}},r={"in":function(a){return a},out:s,"in-out":t,"out-in":function(a){return t(s(a))}};d3.ease=function(a){var b=a.indexOf("-"),c=b>=0?a.substring(0,b):a,d=b>=0?a.substring(b+1):"in";return r[d](q[c].apply(null,Array.prototype.slice.call(arguments,1)))},d3.event=null,d3.interpolate=function(a,b){var c=d3.interpolators.length,d;while(--c>=0&&!(d=d3.interpolators[c](a,b)));return d},d3.interpolateNumber=function(a,b){b-=a;return function(c){return a+b*c}},d3.interpolateRound=function(a,b){b-=a;return function(c){return Math.round(a+b*c)}},d3.interpolateString=function(a,b){var c,d,e,f=0,g=0,h=[],i=[],j,k;C.lastIndex=0;for(d=0;c=C.exec(b);++d)c.index&&h.push(b.substring(f,g=c.index)),i.push({i:h.length,x:c[0]}),h.push(null),f=C.lastIndex;f<b.length&&h.push(b.substring(f));for(d=0,j=i.length;(c=C.exec(a))&&d<j;++d){k=i[d];if(k.x==c[0]){if(k.i)if(h[k.i+1]==null){h[k.i-1]+=k.x,h.splice(k.i,1);for(e=d+1;e<j;++e)i[e].i--}else{h[k.i-1]+=k.x+h[k.i+1],h.splice(k.i,2);for(e=d+1;e<j;++e)i[e].i-=2}else if(h[k.i+1]==null)h[k.i]=k.x;else{h[k.i]=k.x+h[k.i+1],h.splice(k.i+1,1);for(e=d+1;e<j;++e)i[e].i--}i.splice(d,1),j--,d--}else k.x=d3.interpolateNumber(parseFloat(c[0]),parseFloat(k.x))}while(d<j)k=i.pop(),h[k.i+1]==null?h[k.i]=k.x:(h[k.i]=k.x+h[k.i+1],h.splice(k.i+1,1)),j--;return h.length===1?h[0]==null?i[0].x:function(){return b}:function(a){for(d=0;d<j;++d)h[(k=i[d]).i]=k.x(a);return h.join("")}},d3.interpolateRgb=function(a,b){a=d3.rgb(a),b=d3.rgb(b);var c=a.r,d=a.g,e=a.b,f=b.r-c,g=b.g-d,h=b.b-e;return function(a){return"rgb("+Math.round(c+f*a)+","+Math.round(d+g*a)+","+Math.round(e+h*a)+")"}},d3.interpolateHsl=function(a,b){a=d3.hsl(a),b=d3.hsl(b);var c=a.h,d=a.s,e=a.l,f=b.h-c,g=b.s-d,h=b.l-e;return function(a){return R(c+f*a,d+g*a,e+h*a).toString()}},d3.interpolateArray=function(a,b){var c=[],d=[],e=a.length,f=b.length,g=Math.min(a.length,b.length),h;for(h=0;h<g;++h)c.push(d3.interpolate(a[h],b[h]));for(;h<e;++h)d[h]=a[h];for(;h<f;++h)d[h]=b[h];return function(a){for(h=0;h<g;++h)d[h]=c[h](a);return d}},d3.interpolateObject=function(a,b){var c={},d={},e;for(e in a)e in b?c[e]=E(e)(a[e],b[e]):d[e]=a[e];for(e in b)e in a||(d[e]=b[e]);return function(a){for(e in c)d[e]=c[e](a);return d}};var C=/[-+]?(?:\d+\.\d+|\d+\.|\.\d+|\d+)(?:[eE][-]?\d+)?/g,D={background:1,fill:1,stroke:1};d3.interpolators=[d3.interpolateObject,function(a,b){return b instanceof Array&&d3.interpolateArray(a,b)},function(a,b){return typeof b=="string"&&d3.interpolateString(String(a),b)},function(a,b){return(typeof b=="string"?b in N||/^(#|rgb\(|hsl\()/.test(b):b instanceof I||b instanceof Q)&&d3.interpolateRgb(String(a),b)},function(a,b){return typeof b=="number"&&d3.interpolateNumber(+a,b)}],d3.rgb=function(a,b,c){return arguments.length===1?K(""+a,H,R):H(~~a,~~b,~~c)},I.prototype.brighter=function(a){a=Math.pow(.7,arguments.length?a:1);var b=this.r,c=this.g,d=this.b,e=30;if(!b&&!c&&!d)return H(e,e,e);b&&b<e&&(b=e),c&&c<e&&(c=e),d&&d<e&&(d=e);return H(Math.min(255,Math.floor(b/a)),Math.min(255,Math.floor(c/a)),Math.min(255,Math.floor(d/a)))},I.prototype.darker=function(a){a=Math.pow(.7,arguments.length?a:1);return H(Math.max(0,Math.floor(a*this.r)),Math.max(0,Math.floor(a*this.g)),Math.max(0,Math.floor(a*this.b)))},I.prototype.hsl=function(){return L(this.r,this.g,this.b)},I.prototype.toString=function(){return"#"+J(this.r)+J(this.g)+J(this.b)};var N={aliceblue:"#f0f8ff",antiquewhite:"#faebd7",aqua:"#00ffff",aquamarine:"#7fffd4",azure:"#f0ffff",beige:"#f5f5dc",bisque:"#ffe4c4",black:"#000000",blanchedalmond:"#ffebcd",blue:"#0000ff",blueviolet:"#8a2be2",brown:"#a52a2a",burlywood:"#deb887",cadetblue:"#5f9ea0",chartreuse:"#7fff00",chocolate:"#d2691e",coral:"#ff7f50",cornflowerblue:"#6495ed",cornsilk:"#fff8dc",crimson:"#dc143c",cyan:"#00ffff",darkblue:"#00008b",darkcyan:"#008b8b",darkgoldenrod:"#b8860b",darkgray:"#a9a9a9",darkgreen:"#006400",darkgrey:"#a9a9a9",darkkhaki:"#bdb76b",darkmagenta:"#8b008b",darkolivegreen:"#556b2f",darkorange:"#ff8c00",darkorchid:"#9932cc",darkred:"#8b0000",darksalmon:"#e9967a",darkseagreen:"#8fbc8f",darkslateblue:"#483d8b",darkslategray:"#2f4f4f",darkslategrey:"#2f4f4f",darkturquoise:"#00ced1",darkviolet:"#9400d3",deeppink:"#ff1493",deepskyblue:"#00bfff",dimgray:"#696969",dimgrey:"#696969",dodgerblue:"#1e90ff",firebrick:"#b22222",floralwhite:"#fffaf0",forestgreen:"#228b22",fuchsia:"#ff00ff",gainsboro:"#dcdcdc",ghostwhite:"#f8f8ff",gold:"#ffd700",goldenrod:"#daa520",gray:"#808080",green:"#008000",greenyellow:"#adff2f",grey:"#808080",honeydew:"#f0fff0",hotpink:"#ff69b4",indianred:"#cd5c5c",indigo:"#4b0082",ivory:"#fffff0",khaki:"#f0e68c",lavender:"#e6e6fa",lavenderblush:"#fff0f5",lawngreen:"#7cfc00",lemonchiffon:"#fffacd",lightblue:"#add8e6",lightcoral:"#f08080",lightcyan:"#e0ffff",lightgoldenrodyellow:"#fafad2",lightgray:"#d3d3d3",lightgreen:"#90ee90",lightgrey:"#d3d3d3",lightpink:"#ffb6c1",lightsalmon:"#ffa07a",lightseagreen:"#20b2aa",lightskyblue:"#87cefa",lightslategray:"#778899",lightslategrey:"#778899",lightsteelblue:"#b0c4de",lightyellow:"#ffffe0",lime:"#00ff00",limegreen:"#32cd32",linen:"#faf0e6",magenta:"#ff00ff",maroon:"#800000",mediumaquamarine:"#66cdaa",mediumblue:"#0000cd",mediumorchid:"#ba55d3",mediumpurple:"#9370db",mediumseagreen:"#3cb371",mediumslateblue:"#7b68ee",mediumspringgreen:"#00fa9a",mediumturquoise:"#48d1cc",mediumvioletred:"#c71585",midnightblue:"#191970",mintcream:"#f5fffa",mistyrose:"#ffe4e1",moccasin:"#ffe4b5",navajowhite:"#ffdead",navy:"#000080",oldlace:"#fdf5e6",olive:"#808000",olivedrab:"#6b8e23",orange:"#ffa500",orangered:"#ff4500",orchid:"#da70d6",palegoldenrod:"#eee8aa",palegreen:"#98fb98",paleturquoise:"#afeeee",palevioletred:"#db7093",papayawhip:"#ffefd5",peachpuff:"#ffdab9",peru:"#cd853f",pink:"#ffc0cb",plum:"#dda0dd",powderblue:"#b0e0e6",purple:"#800080",red:"#ff0000",rosybrown:"#bc8f8f",royalblue:"#4169e1",saddlebrown:"#8b4513",salmon:"#fa8072",sandybrown:"#f4a460",seagreen:"#2e8b57",seashell:"#fff5ee",sienna:"#a0522d",silver:"#c0c0c0",skyblue:"#87ceeb",slateblue:"#6a5acd",slategray:"#708090",slategrey:"#708090",snow:"#fffafa",springgreen:"#00ff7f",steelblue:"#4682b4",tan:"#d2b48c",teal:"#008080",thistle:"#d8bfd8",tomato:"#ff6347",turquoise:"#40e0d0",violet:"#ee82ee",wheat:"#f5deb3",white:"#ffffff",whitesmoke:"#f5f5f5",yellow:"#ffff00",yellowgreen:"#9acd32"};for(var O in N)N[O]=K(N[O],H,R);d3.hsl=function(a,b,c){return arguments.length===1?K(""+a,L,P):P(+a,+b,+c)},Q.prototype.brighter=function(a){a=Math.pow(.7,arguments.length?a:1);return P(this.h,this.s,this.l/a)},Q.prototype.darker=function(a){a=Math.pow(.7,arguments.length?a:1);return P(this.h,this.s,a*this.l)},Q.prototype.rgb=function(){return R(this.h,this.s,this.l)},Q.prototype.toString=function(){return"hsl("+this.h+","+this.s*100+"%,"+this.l*100+"%)"},d3.select=function(a){return typeof a=="string"?W.select(a):S([[a]])},d3.selectAll=function(b){return typeof b=="string"?W.selectAll(b):S([a(b)])};var T=function(a,b){return b.querySelector(a)},U=function(b,c){return a(c.querySelectorAll(b))};typeof Sizzle=="function"&&(T=function(a,b){return Sizzle(a,b)[0]},U=function(a,b){return Sizzle.uniqueSort(Sizzle(a,b))});var V=[],W=S([[document]]);W[0].parentNode=document.documentElement,d3.selection=function(){return W},d3.selection.prototype=V,V.select=function(a){var b=[],c,d,e,f;typeof a!="function"&&(a=X(a));for(var g=-1,h=this.length;++g<h;){b.push(c=[]),c.parentNode=(e=this[g]).parentNode;for(var i=-1,j=e.length;++i<j;)(f=e[i])?(c.push(d=a(f)),d&&"__data__"in f&&(d.__data__=f.__data__)):c.push(null)}return S(b)},V.selectAll=function(a){var b=[],c,d;typeof a!="function"&&(a=Y(a));for(var e=-1,f=this.length;++e<f;)for(var g=this[e],h=-1,i=g.length;++h<i;)if(d=g[h])b.push(c=a(d)),c.parentNode=d;return S(b)},V.attr=function(a,b){function i(){var c=b.apply(this,arguments);c==null?this.removeAttributeNS(a.space,a.local):this.setAttributeNS(a.space,a.local,c)}function h(){var c=b.apply(this,arguments);c==null?this.removeAttribute(a):this.setAttribute(a,c)}function g(){this.setAttributeNS(a.space,a.local,b)}function f(){this.setAttribute(a,b)}function e(){this.removeAttributeNS(a.space,a.local)}function d(){this.removeAttribute(a)}a=d3.ns.qualify(a);if(arguments.length<2){var c=this.node();return a.local?c.getAttributeNS(a.space,a.local):c.getAttribute(a)}return this.each(b==null?a.local?e:d:typeof b=="function"?a.local?i:h:a.local?g:f)},V.classed=function(a,b){function i(){(b.apply(this,arguments)?f:g).call(this)}function g(){if(b=this.classList)return b.remove(a);var b=this.className,d=b.baseVal!=null,e=d?b.baseVal:b;e=h(e.replace(c," ")),d?b.baseVal=e:this.className=e}function f(){if(b=this.classList)return b.add(a);var b=this.className
<del>,d=b.baseVal!=null,e=d?b.baseVal:b;c.lastIndex=0,c.test(e)||(e=h(e+" "+a),d?b.baseVal=e:this.className=e)}var c=new RegExp("(^|\\s+)"+d3.requote(a)+"(\\s+|$)","g");if(arguments.length<2){var d=this.node();if(e=d.classList)return e.contains(a);var e=d.className;c.lastIndex=0;return c.test(e.baseVal!=null?e.baseVal:e)}return this.each(typeof b=="function"?i:b?f:g)},V.style=function(a,b,c){function f(){var d=b.apply(this,arguments);d==null?this.style.removeProperty(a):this.style.setProperty(a,d,c)}function e(){this.style.setProperty(a,b,c)}function d(){this.style.removeProperty(a)}arguments.length<3&&(c="");return arguments.length<2?window.getComputedStyle(this.node(),null).getPropertyValue(a):this.each(b==null?d:typeof b=="function"?f:e)},V.property=function(a,b){function e(){var c=b.apply(this,arguments);c==null?delete this[a]:this[a]=c}function d(){this[a]=b}function c(){delete this[a]}return arguments.length<2?this.node()[a]:this.each(b==null?c:typeof b=="function"?e:d)},V.text=function(a){return arguments.length<1?this.node().textContent:this.each(typeof a=="function"?function(){this.textContent=a.apply(this,arguments)}:function(){this.textContent=a})},V.html=function(a){return arguments.length<1?this.node().innerHTML:this.each(typeof a=="function"?function(){this.innerHTML=a.apply(this,arguments)}:function(){this.innerHTML=a})},V.append=function(a){function c(b){return b.appendChild(document.createElementNS(a.space,a.local))}function b(b){return b.appendChild(document.createElement(a))}a=d3.ns.qualify(a);return this.select(a.local?c:b)},V.insert=function(a,b){function d(c){return c.insertBefore(document.createElementNS(a.space,a.local),T(b,c))}function c(c){return c.insertBefore(document.createElement(a),T(b,c))}a=d3.ns.qualify(a);return this.select(a.local?d:c)},V.remove=function(){return this.each(function(){var a=this.parentNode;a&&a.removeChild(this)})},V.data=function(a,b){function f(a,f){var g,h=a.length,i=f.length,j=Math.min(h,i),k=Math.max(h,i),l=[],m=[],n=[],o,p;if(b){var q={},r=[],s,t=f.length;for(g=-1;++g<h;)s=b.call(o=a[g],o.__data__,g),s in q?n[t++]=o:q[s]=o,r.push(s);for(g=-1;++g<i;)o=q[s=b.call(f,p=f[g],g)],o?(o.__data__=p,l[g]=o,m[g]=n[g]=null):(m[g]=Z(p),l[g]=n[g]=null),delete q[s];for(g=-1;++g<h;)r[g]in q&&(n[g]=a[g])}else{for(g=-1;++g<j;)o=a[g],p=f[g],o?(o.__data__=p,l[g]=o,m[g]=n[g]=null):(m[g]=Z(p),l[g]=n[g]=null);for(;g<i;++g)m[g]=Z(f[g]),l[g]=n[g]=null;for(;g<k;++g)n[g]=a[g],m[g]=l[g]=null}m.update=l,m.parentNode=l.parentNode=n.parentNode=a.parentNode,c.push(m),d.push(l),e.push(n)}var c=[],d=[],e=[],g=-1,h=this.length,i;if(typeof a=="function")while(++g<h)f(i=this[g],a.call(i,i.parentNode.__data__,g));else while(++g<h)f(i=this[g],a);var j=S(d);j.enter=function(){return $(c)},j.exit=function(){return S(e)};return j};var _=[];_.append=V.append,_.insert=V.insert,_.empty=V.empty,_.select=function(a){var b=[],c,d,e,f,g;for(var h=-1,i=this.length;++h<i;){e=(f=this[h]).update,b.push(c=[]),c.parentNode=f.parentNode;for(var j=-1,k=f.length;++j<k;)(g=f[j])?(c.push(e[j]=d=a(f.parentNode)),d.__data__=g.__data__):c.push(null)}return S(b)},V.filter=function(a){var b=[],c,d,e;for(var f=0,g=this.length;f<g;f++){b.push(c=[]),c.parentNode=(d=this[f]).parentNode;for(var h=0,i=d.length;h<i;h++)(e=d[h])&&a.call(e,e.__data__,h)&&c.push(e)}return S(b)},V.map=function(a){return this.each(function(){this.__data__=a.apply(this,arguments)})},V.sort=function(a){a=ba.apply(this,arguments);for(var b=0,c=this.length;b<c;b++)for(var d=this[b].sort(a),e=1,f=d.length,g=d[0];e<f;e++){var h=d[e];h&&(g&&g.parentNode.insertBefore(h,g.nextSibling),g=h)}return this},V.on=function(a,b,c){arguments.length<3&&(c=!1);var d="__on"+a,e=a.indexOf(".");e>0&&(a=a.substring(0,e));return arguments.length<2?(e=this.node()[d])&&e._:this.each(function(e,f){function h(a){var c=d3.event;d3.event=a;try{b.call(g,g.__data__,f)}finally{d3.event=c}}var g=this;g[d]&&g.removeEventListener(a,g[d],c),b&&g.addEventListener(a,g[d]=h,c),h._=b})},V.each=function(a){for(var b=-1,c=this.length;++b<c;)for(var d=this[b],e=-1,f=d.length;++e<f;){var g=d[e];g&&a.call(g,g.__data__,e,b)}return this},V.call=function(a){a.apply(this,(arguments[0]=this,arguments));return this},V.empty=function(){return!this.node()},V.node=function(a){for(var b=0,c=this.length;b<c;b++)for(var d=this[b],e=0,f=d.length;e<f;e++){var g=d[e];if(g)return g}return null},V.transition=function(){var a=[],b,c;for(var d=-1,e=this.length;++d<e;){a.push(b=[]);for(var f=this[d],g=-1,h=f.length;++g<h;)b.push((c=f[g])?{node:c,delay:0,duration:250}:null)}return bb(a,bf||++be)};var bd=[],be=0,bf=0,bg=d3.ease("cubic-in-out");bd.call=V.call,d3.transition=function(){return W.transition()},d3.transition.prototype=bd,bd.select=function(a){var b=[],c,d,e;typeof a!="function"&&(a=X(a));for(var f=-1,g=this.length;++f<g;){b.push(c=[]);for(var h=this[f],i=-1,j=h.length;++i<j;)(e=h[i])&&(d=a(e.node))?("__data__"in e.node&&(d.__data__=e.node.__data__),c.push({node:d,delay:e.delay,duration:e.duration})):c.push(null)}return bb(b,this.id).ease(this.ease())},bd.selectAll=function(a){var b=[],c,d;typeof a!="function"&&(a=Y(a));for(var e=-1,f=this.length;++e<f;)for(var g=this[e],h=-1,i=g.length;++h<i;)if(d=g[h]){b.push(c=a(d.node));for(var j=-1,k=c.length;++j<k;)c[j]={node:c[j],delay:d.delay,duration:d.duration}}return bb(b,this.id).ease(this.ease())},bd.attr=function(a,b){return this.attrTween(a,bc(b))},bd.attrTween=function(a,b){function d(c,d){var e=b.call(this,c,d,this.getAttributeNS(a.space,a.local));return e&&function(b){this.setAttributeNS(a.space,a.local,e(b))}}function c(c,d){var e=b.call(this,c,d,this.getAttribute(a));return e&&function(b){this.setAttribute(a,e(b))}}a=d3.ns.qualify(a);return this.tween("attr."+a,a.local?d:c)},bd.style=function(a,b,c){arguments.length<3&&(c=null);return this.styleTween(a,bc(b),c)},bd.styleTween=function(a,b,c){arguments.length<3&&(c=null);return this.tween("style."+a,function(d,e){var f=b.call(this,d,e,window.getComputedStyle(this,null).getPropertyValue(a));return f&&function(b){this.style.setProperty(a,f(b),c)}})},bd.text=function(a){return this.tween("text",function(b,c){this.textContent=typeof a=="function"?a.call(this,b,c):a})},bd.remove=function(){return this.each("end",function(){var a;!this.__transition__&&(a=this.parentNode)&&a.removeChild(this)})},bd.delay=function(a){var b=this;return b.each(typeof a=="function"?function(c,d,e){b[e][d].delay=+a.apply(this,arguments)}:(a=+a,function(c,d,e){b[e][d].delay=a}))},bd.duration=function(a){var b=this;return b.each(typeof a=="function"?function(c,d,e){b[e][d].duration=+a.apply(this,arguments)}:(a=+a,function(c,d,e){b[e][d].duration=a}))};var bi=null,bj,bk;d3.timer=function(a,b){var c=Date.now(),d=!1,e,f=bi;if(arguments.length<2)b=0;else if(!isFinite(b))return;while(f){if(f.callback===a){f.then=c,f.delay=b,d=!0;break}e=f,f=f.next}d||(bi={callback:a,then:c,delay:b,next:bi}),bj||(bk=clearTimeout(bk),bj=1,bn(bl))},d3.timer.flush=function(){var a,b=Date.now(),c=bi;while(c)a=b-c.then,c.delay||(c.flush=c.callback(a)),c=c.next;bm()};var bn=window.requestAnimationFrame||window.webkitRequestAnimationFrame||window.mozRequestAnimationFrame||window.oRequestAnimationFrame||window.msRequestAnimationFrame||function(a){setTimeout(a,17)};d3.scale={},d3.scale.linear=function(){return bs([0,1],[0,1],d3.interpolate,!1)},d3.scale.log=function(){return bA(d3.scale.linear(),bB)},bB.pow=function(a){return Math.pow(10,a)},bC.pow=function(a){return-Math.pow(10,-a)},d3.scale.pow=function(){return bE(d3.scale.linear(),1)},d3.scale.sqrt=function(){return d3.scale.pow().exponent(.5)},d3.scale.ordinal=function(){return bG({},0,{t:"range",x:[]})},d3.scale.category10=function(){return d3.scale.ordinal().range(bH)},d3.scale.category20=function(){return d3.scale.ordinal().range(bI)},d3.scale.category20b=function(){return d3.scale.ordinal().range(bJ)},d3.scale.category20c=function(){return d3.scale.ordinal().range(bK)};var bH=["#1f77b4","#ff7f0e","#2ca02c","#d62728","#9467bd","#8c564b","#e377c2","#7f7f7f","#bcbd22","#17becf"],bI=["#1f77b4","#aec7e8","#ff7f0e","#ffbb78","#2ca02c","#98df8a","#d62728","#ff9896","#9467bd","#c5b0d5","#8c564b","#c49c94","#e377c2","#f7b6d2","#7f7f7f","#c7c7c7","#bcbd22","#dbdb8d","#17becf","#9edae5"],bJ=["#393b79","#5254a3","#6b6ecf","#9c9ede","#637939","#8ca252","#b5cf6b","#cedb9c","#8c6d31","#bd9e39","#e7ba52","#e7cb94","#843c39","#ad494a","#d6616b","#e7969c","#7b4173","#a55194","#ce6dbd","#de9ed6"],bK=["#3182bd","#6baed6","#9ecae1","#c6dbef","#e6550d","#fd8d3c","#fdae6b","#fdd0a2","#31a354","#74c476","#a1d99b","#c7e9c0","#756bb1","#9e9ac8","#bcbddc","#dadaeb","#636363","#969696","#bdbdbd","#d9d9d9"];d3.scale.quantile=function(){return bL([],[])},d3.scale.quantize=function(){return bM(0,1,[0,1])},d3.svg={},d3.svg.arc=function(){function e(){var e=a.apply(this,arguments),f=b.apply(this,arguments),g=c.apply(this,arguments)+bN,h=d.apply(this,arguments)+bN,i=(h<g&&(i=g,g=h,h=i),h-g),j=i<Math.PI?"0":"1",k=Math.cos(g),l=Math.sin(g),m=Math.cos(h),n=Math.sin(h);return i>=bO?e?"M0,"+f+"A"+f+","+f+" 0 1,1 0,"+ -f+"A"+f+","+f+" 0 1,1 0,"+f+"M0,"+e+"A"+e+","+e+" 0 1,1 0,"+ -e+"A"+e+","+e+" 0 1,1 0,"+e+"Z":"M0,"+f+"A"+f+","+f+" 0 1,1 0,"+ -f+"A"+f+","+f+" 0 1,1 0,"+f+"Z":e?"M"+f*k+","+f*l+"A"+f+","+f+" 0 "+j+",1 "+f*m+","+f*n+"L"+e*m+","+e*n+"A"+e+","+e+" 0 "+j+",0 "+e*k+","+e*l+"Z":"M"+f*k+","+f*l+"A"+f+","+f+" 0 "+j+",1 "+f*m+","+f*n+"L0,0"+"Z"}var a=bP,b=bQ,c=bR,d=bS;e.innerRadius=function(b){if(!arguments.length)return a;a=d3.functor(b);return e},e.outerRadius=function(a){if(!arguments.length)return b;b=d3.functor(a);return e},e.startAngle=function(a){if(!arguments.length)return c;c=d3.functor(a);return e},e.endAngle=function(a){if(!arguments.length)return d;d=d3.functor(a);return e},e.centroid=function(){var e=(a.apply(this,arguments)+b.apply(this,arguments))/2,f=(c.apply(this,arguments)+d.apply(this,arguments))/2+bN;return[Math.cos(f)*e,Math.sin(f)*e]};return e};var bN=-Math.PI/2,bO=2*Math.PI-1e-6;d3.svg.line=function(){return bT(Object)};var bX={linear:bY,"step-before":bZ,"step-after":b$,basis:ce,"basis-open":cf,"basis-closed":cg,bundle:ch,cardinal:cb,"cardinal-open":b_,"cardinal-closed":ca,monotone:cq},cj=[0,2/3,1/3,0],ck=[0,1/3,2/3,0],cl=[0,1/6,2/3,1/6];d3.svg.line.radial=function(){var a=bT(cr);a.radius=a.x,delete a.x,a.angle=a.y,delete a.y;return a},d3.svg.area=function(){return cs(Object)},d3.svg.area.radial=function(){var a=cs(cr);a.radius=a.x,delete a.x,a.innerRadius=a.x0,delete a.x0,a.outerRadius=a.x1,delete a.x1,a.angle=a.y,delete a.y,a.startAngle=a.y0,delete a.y0,a.endAngle=a.y1,delete a.y1;return a},d3.svg.chord=function(){function j(a,b,c,d){return"Q 0,0 "+d}function i(a,b){return"A"+a+","+a+" 0 0,1 "+b}function h(a,b){return a.a0==b.a0&&a.a1==b.a1}function g(a,b,f,g){var h=b.call(a,f,g),i=c.call(a,h,g),j=d.call(a,h,g)+bN,k=e.call(a,h,g)+bN;return{r:i,a0:j,a1:k,p0:[i*Math.cos(j),i*Math.sin(j)],p1:[i*Math.cos(k),i*Math.sin(k)]}}function f(c,d){var e=g(this,a,c,d),f=g(this,b,c,d);return"M"+e.p0+i(e.r,e.p1)+(h(e,f)?j(e.r,e.p1,e.r,e.p0):j(e.r,e.p1,f.r,f.p0)+i(f.r,f.p1)+j(f.r,f.p1,e.r,e.p0))+"Z"}var a=cv,b=cw,c=cx,d=bR,e=bS;f.radius=function(a){if(!arguments.length)return c;c=d3.functor(a);return f},f.source=function(b){if(!arguments.length)return a;a=d3.functor(b);return f},f.target=function(a){if(!arguments.length)return b;b=d3.functor(a);return f},f.startAngle=function(a){if(!arguments.length)return d;d=d3.functor(a);return f},f.endAngle=function(a){if(!arguments.length)return e;e=d3.functor(a);return f};return f},d3.svg.diagonal=function(){function d(d,e){var f=a.call(this,d,e),g=b.call(this,d,e),h=(f.y+g.y)/2,i=[f,{x:f.x,y:h},{x:g.x,y:h},g];i=i.map(c);return"M"+i[0]+"C"+i[1]+" "+i[2]+" "+i[3]}var a=cv,b=cw,c=cA;d.source=function(b){if(!arguments.length)return a;a=d3.functor(b);return d},d.target=function(a){if(!arguments.length)return b;b=d3.functor(a);return d},d.projection=function(a){if(!arguments.length)return c;c=a;return d};return d},d3.svg.diagonal.radial=function(){var a=d3.svg.diagonal(),b=cA,c=a.projection;a.projection=function(a){return arguments.length?c(cB(b=a)):b};return a},d3.svg.mouse=function(a){return cD(a,d3.event)};var cC=/WebKit/.test(navigator.userAgent)?-1:0;d3.svg.touches=function(b){var c=d3.event.touches;return c?a(c).map(function(a){var c=cD(b,a);c.identifier=a.identifier;return c}):[]},d3.svg.symbol=function(){function c(c,d){return(cG[a.call(this,c,d)]||cG.circle)(b.call(this,c,d))}var a=cF,b=cE;c.type=function(b){if(!arguments.length)return a;a=d3.functor(b);return c},c.size=function(a){if(!arguments.length)return b;b=d3.functor(a);return c};return c};var cG={circle:function(a){var b=Math.sqrt(a/Math.PI);return"M0,"+b+"A"+b+","+b+" 0 1,1 0,"+ -b+"A"+b+","+b+" 0 1,1 0,"+b+"Z"},cross:function(a){var b=Math.sqrt(a/5)/2;return"M"+ -3*b+","+ -b+"H"+ -b+"V"+ -3*b+"H"+b+"V"+ -b+"H"+3*b+"V"+b+"H"+b+"V"+3*b+"H"+ -b+"V"+b+"H"+ -3*b+"Z"},diamond:function(a){var b=Math.sqrt(a/(2*cI)),c=b*cI;return"M0,"+ -b+"L"+c+",0"+" 0,"+b+" "+ -c+",0"+"Z"},square:function(a){var b=Math.sqrt(a)/2;return"M"+ -b+","+ -b+"L"+b+","+ -b+" "+b+","+b+" "+ -b+","+b+"Z"},"triangle-down":function(a){var b=Math.sqrt(a/cH),c=b*cH/2;return"M0,"+c+"L"+b+","+ -c+" "+ -b+","+ -c+"Z"},"triangle-up":function(a){var b=Math.sqrt(a/cH),c=b*cH/2;return"M0,"+ -c+"L"+b+","+c+" "+ -b+","+c+"Z"}};d3.svg.symbolTypes=d3.keys(cG);var cH=Math.sqrt(3),cI=Math.tan(30*Math.PI/180);d3.svg.axis=function(){function j(j){j.each(function(k,l,m){function F(a){return j.delay?a.transition().delay(j[m][l].delay).duration(j[m][l].duration).ease(j.ease()):a}var n=d3.select(this),o=a.ticks.apply(a,g),p=h||a.tickFormat.apply(a,g),q=cL(a,o,i),r=n.selectAll(".minor").data(q,String),s=r.enter().insert("svg:line","g").attr("class","tick minor").style("opacity",1e-6),t=F(r.exit()).style("opacity",1e-6).remove(),u=F(r).style("opacity",1),v=n.selectAll("g").data(o,String),w=v.enter().insert("svg:g","path").style("opacity",1e-6),x=F(v.exit()).style("opacity",1e-6).remove(),y=F(v).style("opacity",1),z,A=a.range(),B=n.selectAll(".domain").data([]),C=B.enter().append("svg:path").attr("class","domain"),D=F(B),E=this.__chart__||a;this.__chart__=a.copy(),w.append("svg:line").attr("class","tick"),w.append("svg:text"),y.select("text").text(p);switch(b){case"bottom":z=cJ,u.attr("y2",d),w.select("text").attr("dy",".71em").attr("text-anchor","middle"),y.select("line").attr("y2",c),y.select("text").attr("y",Math.max(c,0)+f),D.attr("d","M"+A[0]+","+e+"V0H"+A[1]+"V"+e);break;case"top":z=cJ,u.attr("y2",-d),w.select("text").attr("text-anchor","middle"),y.select("line").attr("y2",-c),y.select("text").attr("y",-(Math.max(c,0)+f)),D.attr("d","M"+A[0]+","+ -e+"V0H"+A[1]+"V"+ -e);break;case"left":z=cK,u.attr("x2",-d),w.select("text").attr("dy",".32em").attr("text-anchor","end"),y.select("line").attr("x2",-c),y.select("text").attr("x",-(Math.max(c,0)+f)),D.attr("d","M"+ -e+","+A[0]+"H0V"+A[1]+"H"+ -e);break;case"right":z=cK,u.attr("x2",d),w.select("text").attr("dy",".32em"),y.select("line").attr("x2",c),y.select("text").attr("x",Math.max(c,0)+f),D.attr("d","M"+e+","+A[0]+"H0V"+A[1]+"H"+e)}w.call(z,E),y.call(z,a),x.call(z,a),s.call(z,E),u.call(z,a),t.call(z,a)})}var a=d3.scale.linear(),b="bottom",c=6,d=6,e=6,f=3,g=[10],h,i=0;j.scale=function(b){if(!arguments.length)return a;a=b;return j},j.orient=function(a){if(!arguments.length)return b;b=a;return j},j.ticks=function(){if(!arguments.length)return g;g=arguments;return j},j.tickFormat=function(a){if(!arguments.length)return h;h=a;return j},j.tickSize=function(a,b,f){if(!arguments.length)return c;var g=arguments.length-1;c=+a,d=g>1?+b:c,e=g>0?+arguments[g]:c;return j},j.tickPadding=function(a){if(!arguments.length)return f;f=+a;return j},j.tickSubdivide=function(a){if(!arguments.length)return i;i=+a;return j};return j},d3.behavior={},d3.behavior.drag=function(){function d(){c.apply(this,arguments),cR("dragstart")}function c(){cM=a,cO=cS((cN=this).parentNode),cP=0,d3_behavior_dragArguments=arguments}function b(){this.on("mousedown.drag",d).on("touchstart.drag",d),d3.select(window).on("mousemove.drag",cT).on("touchmove.drag",cT).on("mouseup.drag",cU,!0).on("touchend.drag",cU,!0).on("click.drag",cV,!0)}var a=d3.dispatch("drag","dragstart","dragend");b.on=function(c,d){a[c].add(d);return b};return b};var cM,cN,cO,cP,cQ;d3.behavior.zoom=function(){function h(){d.apply(this,arguments);var b=dh(),c,e=Date.now();b.length===1&&e-c_<300&&dm(1+Math.floor(a[2]),c=b[0],c$[c.identifier]),c_=e}function g(){d.apply(this,arguments);var b=d3.svg.mouse(dc);dm(d3.event.shiftKey?Math.ceil(a[2]-1):Math.floor(a[2]+1),b,df(b))}function f(){d.apply(this,arguments),cZ||(cZ=df(d3.svg.mouse(dc))),dm(dg()+a[2],d3.svg.mouse(dc),cZ)}function e(){d.apply(this,arguments),cY=df(d3.svg.mouse(dc)),d3_behavior_zoomMoved=!1,d3.event.preventDefault(),window.focus()}function d(){da=a,db=b.zoom.dispatch,dc=this,dd=arguments}function c(){this.on("mousedown.zoom",e).on("mousewheel.zoom",f).on("DOMMouseScroll.zoom",g).on("dblclick.zoom",g).on("touchstart.zoom",h),d3.select(window).on("mousemove.zoom",dj).on("mouseup.zoom",dk).on("touchmove.zoom",di).on("touchend.zoom",dh).on("click.zoom",dl,!0)}var a=[0,0,0],b=d3.dispatch("zoom");c.on=function(a,d){b[a].add(d);return c};return c};var cX,cY,cZ,c$={},c_=0,da,db,dc,dd,de})()
<ide>\ No newline at end of file
<add>(function(){function di(a,b,c){function i(a,b){var c=a.__domain||(a.__domain=a.domain()),d=a.range().map(function(a){return(a-b)/h});a.domain(c).domain(d.map(a.invert))}var d=Math.pow(2,(cY[2]=a)-c[2]),e=cY[0]=b[0]-d*c[0],f=cY[1]=b[1]-d*c[1],g=d3.event,h=Math.pow(2,a);d3.event={scale:h,translate:[e,f],transform:function(a,b){a&&i(a,e),b&&i(b,f)}};try{cZ.apply(c$,c_)}finally{d3.event=g}g.preventDefault()}function dh(){da&&(d3.event.stopPropagation(),d3.event.preventDefault(),da=!1)}function dg(){cU&&(d3_behavior_zoomMoved&&(da=!0),df(),cU=null)}function df(){cV=null,cU&&(d3_behavior_zoomMoved=!0,di(cY[2],d3.svg.mouse(c$),cU))}function de(){var a=d3.svg.touches(c$);switch(a.length){case 1:var b=a[0];di(cY[2],b,cW[b.identifier]);break;case 2:var c=a[0],d=a[1],e=[(c[0]+d[0])/2,(c[1]+d[1])/2],f=cW[c.identifier],g=cW[d.identifier],h=[(f[0]+g[0])/2,(f[1]+g[1])/2,f[2]];di(Math.log(d3.event.scale)/Math.LN2+f[2],e,h)}}function dd(){var a=d3.svg.touches(c$),b=-1,c=a.length,d;while(++b<c)cW[(d=a[b]).identifier]=db(d);return a}function dc(){cT||(cT=d3.select("body").append("div").style("visibility","hidden").style("top",0).style("height",0).style("width",0).style("overflow-y","scroll").append("div").style("height","2000px").node().parentNode);var a=d3.event,b;try{cT.scrollTop=1e3,cT.dispatchEvent(a),b=1e3-cT.scrollTop}catch(c){b=a.wheelDelta||-a.detail}return b*.005}function db(a){return[a[0]-cY[0],a[1]-cY[1],cY[2]]}function cS(){d3.event.stopPropagation(),d3.event.preventDefault()}function cR(){cM&&(cS(),cM=!1)}function cQ(){!cJ||(cN("dragend"),d3_behavior_dragForce=cJ=null,cL&&(cM=!0,cS()))}function cP(){if(!!cJ){var a=cJ.parentNode;if(!a)return cQ();cN("drag"),cS()}}function cO(a){return d3.event.touches?d3.svg.touches(a)[0]:d3.svg.mouse(a)}function cN(a){var b=d3.event,c=cJ.parentNode,d=0,e=0;c&&(c=cO(c),d=c[0]-cK[0],e=c[1]-cK[1],cK=c,cL|=d|e);try{d3.event={dx:d,dy:e},cI[a].dispatch.apply(cJ,d3_behavior_dragArguments)}finally{d3.event=b}b.preventDefault()}function cH(a,b,c){e=[];if(c&&b.length>1){var d=bl(a.domain()),e,f=-1,g=b.length,h=(b[1]-b[0])/++c,i,j;while(++f<g)for(i=c;--i>0;)(j=+b[f]-i*h)>=d[0]&&e.push(j);for(--f,i=0;++i<c&&(j=+b[f]+i*h)<d[1];)e.push(j)}return e}function cG(a,b){a.attr("transform",function(a){return"translate(0,"+b(a)+")"})}function cF(a,b){a.attr("transform",function(a){return"translate("+b(a)+",0)"})}function cB(){return"circle"}function cA(){return 64}function cz(a,b){var c=(a.ownerSVGElement||a).createSVGPoint();if(cy<0&&(window.scrollX||window.scrollY)){var d=d3.select(document.body).append("svg:svg").style("position","absolute").style("top",0).style("left",0),e=d[0][0].getScreenCTM();cy=!e.f&&!e.e,d.remove()}cy?(c.x=b.pageX,c.y=b.pageY):(c.x=b.clientX,c.y=b.clientY),c=c.matrixTransform(a.getScreenCTM().inverse());return[c.x,c.y]}function cx(a){return function(){var b=a.apply(this,arguments),c=b[0],d=b[1]+bJ;return[c*Math.cos(d),c*Math.sin(d)]}}function cw(a){return[a.x,a.y]}function cv(a){return a.endAngle}function cu(a){return a.startAngle}function ct(a){return a.radius}function cs(a){return a.target}function cr(a){return a.source}function cq(a){return function(b,c){return a[c][1]}}function cp(a){return function(b,c){return a[c][0]}}function co(a){function i(f){if(f.length<1)return null;var i=bQ(this,f,b,d),j=bQ(this,f,b===c?cp(i):c,d===e?cq(i):e);return"M"+g(a(j),h)+"L"+g(a(i.reverse()),h)+"Z"}var b=bR,c=bR,d=0,e=bS,f="linear",g=bT[f],h=.7;i.x=function(a){if(!arguments.length)return c;b=c=a;return i},i.x0=function(a){if(!arguments.length)return b;b=a;return i},i.x1=function(a){if(!arguments.length)return c;c=a;return i},i.y=function(a){if(!arguments.length)return e;d=e=a;return i},i.y0=function(a){if(!arguments.length)return d;d=a;return i},i.y1=function(a){if(!arguments.length)return e;e=a;return i},i.interpolate=function(a){if(!arguments.length)return f;g=bT[f=a];return i},i.tension=function(a){if(!arguments.length)return h;h=a;return i};return i}function cn(a){var b,c=-1,d=a.length,e,f;while(++c<d)b=a[c],e=b[0],f=b[1]+bJ,b[0]=e*Math.cos(f),b[1]=e*Math.sin(f);return a}function cm(a){return a.length<3?bU(a):a[0]+b$(a,cl(a))}function cl(a){var b=[],c,d,e,f,g=ck(a),h=-1,i=a.length-1;while(++h<i)c=cj(a[h],a[h+1]),Math.abs(c)<1e-6?g[h]=g[h+1]=0:(d=g[h]/c,e=g[h+1]/c,f=d*d+e*e,f>9&&(f=c*3/Math.sqrt(f),g[h]=f*d,g[h+1]=f*e));h=-1;while(++h<=i)f=(a[Math.min(i,h+1)][0]-a[Math.max(0,h-1)][0])/(6*(1+g[h]*g[h])),b.push([f||0,g[h]*f||0]);return b}function ck(a){var b=0,c=a.length-1,d=[],e=a[0],f=a[1],g=d[0]=cj(e,f);while(++b<c)d[b]=g+(g=cj(e=f,f=a[b+1]));d[b]=g;return d}function cj(a,b){return(b[1]-a[1])/(b[0]-a[0])}function ci(a,b,c){a.push("C",ce(cf,b),",",ce(cf,c),",",ce(cg,b),",",ce(cg,c),",",ce(ch,b),",",ce(ch,c))}function ce(a,b){return a[0]*b[0]+a[1]*b[1]+a[2]*b[2]+a[3]*b[3]}function cd(a,b){var c=a.length-1,d=a[0][0],e=a[0][1],f=a[c][0]-d,g=a[c][1]-e,h=-1,i,j;while(++h<=c)i=a[h],j=h/c,i[0]=b*i[0]+(1-b)*(d+j*f),i[1]=b*i[1]+(1-b)*(e+j*g);return ca(a)}function cc(a){var b,c=-1,d=a.length,e=d+4,f,g=[],h=[];while(++c<4)f=a[c%d],g.push(f[0]),h.push(f[1]);b=[ce(ch,g),",",ce(ch,h)],--c;while(++c<e)f=a[c%d],g.shift(),g.push(f[0]),h.shift(),h.push(f[1]),ci(b,g,h);return b.join("")}function cb(a){if(a.length<4)return bU(a);var b=[],c=-1,d=a.length,e,f=[0],g=[0];while(++c<3)e=a[c],f.push(e[0]),g.push(e[1]);b.push(ce(ch,f)+","+ce(ch,g)),--c;while(++c<d)e=a[c],f.shift(),f.push(e[0]),g.shift(),g.push(e[1]),ci(b,f,g);return b.join("")}function ca(a){if(a.length<3)return bU(a);var b=[],c=1,d=a.length,e=a[0],f=e[0],g=e[1],h=[f,f,f,(e=a[1])[0]],i=[g,g,g,e[1]];b.push(f,",",g),ci(b,h,i);while(++c<d)e=a[c],h.shift(),h.push(e[0]),i.shift(),i.push(e[1]),ci(b,h,i);c=-1;while(++c<2)h.shift(),h.push(e[0]),i.shift(),i.push(e[1]),ci(b,h,i);return b.join("")}function b_(a,b){var c=[],d=(1-b)/2,e,f=a[0],g=a[1],h=1,i=a.length;while(++h<i)e=f,f=g,g=a[h],c.push([d*(g[0]-e[0]),d*(g[1]-e[1])]);return c}function b$(a,b){if(b.length<1||a.length!=b.length&&a.length!=b.length+2)return bU(a);var c=a.length!=b.length,d="",e=a[0],f=a[1],g=b[0],h=g,i=1;c&&(d+="Q"+(f[0]-g[0]*2/3)+","+(f[1]-g[1]*2/3)+","+f[0]+","+f[1],e=a[1],i=2);if(b.length>1){h=b[1],f=a[i],i++,d+="C"+(e[0]+g[0])+","+(e[1]+g[1])+","+(f[0]-h[0])+","+(f[1]-h[1])+","+f[0]+","+f[1];for(var j=2;j<b.length;j++,i++)f=a[i],h=b[j],d+="S"+(f[0]-h[0])+","+(f[1]-h[1])+","+f[0]+","+f[1]}if(c){var k=a[i];d+="Q"+(f[0]+h[0]*2/3)+","+(f[1]+h[1]*2/3)+","+k[0]+","+k[1]}return d}function bZ(a,b,c){return a.length<3?bU(a):a[0]+b$(a,b_(a,b))}function bY(a,b){return a.length<3?bU(a):a[0]+b$((a.push(a[0]),a),b_([a[a.length-2]].concat(a,[a[1]]),b))}function bX(a,b){return a.length<4?bU(a):a[1]+b$(a.slice(1,a.length-1),b_(a,b))}function bW(a){var b=[],c=0,d=a.length,e=a[0];b.push(e[0],",",e[1]);while(++c<d)b.push("H",(e=a[c])[0],"V",e[1]);return b.join("")}function bV(a){var b=[],c=0,d=a.length,e=a[0];b.push(e[0],",",e[1]);while(++c<d)b.push("V",(e=a[c])[1],"H",e[0]);return b.join("")}function bU(a){var b=[],c=0,d=a.length,e=a[0];b.push(e[0],",",e[1]);while(++c<d)b.push("L",(e=a[c])[0],",",e[1]);return b.join("")}function bS(a){return a[1]}function bR(a){return a[0]}function bQ(a,b,c,d){var e=[],f=-1,g=b.length,h=typeof c=="function",i=typeof d=="function",j;if(h&&i)while(++f<g)e.push([c.call(a,j=b[f],f),d.call(a,j,f)]);else if(h)while(++f<g)e.push([c.call(a,b[f],f),d]);else if(i)while(++f<g)e.push([c,d.call(a,b[f],f)]);else while(++f<g)e.push([c,d]);return e}function bP(a){function g(d){return d.length<1?null:"M"+e(a(bQ(this,d,b,c)),f)}var b=bR,c=bS,d="linear",e=bT[d],f=.7;g.x=function(a){if(!arguments.length)return b;b=a;return g},g.y=function(a){if(!arguments.length)return c;c=a;return g},g.interpolate=function(a){if(!arguments.length)return d;e=bT[d=a];return g},g.tension=function(a){if(!arguments.length)return f;f=a;return g};return g}function bO(a){return a.endAngle}function bN(a){return a.startAngle}function bM(a){return a.outerRadius}function bL(a){return a.innerRadius}function bI(a,b,c){function g(){d=c.length/(b-a),e=c.length-1;return f}function f(b){return c[Math.max(0,Math.min(e,Math.floor(d*(b-a))))]}var d,e;f.domain=function(c){if(!arguments.length)return[a,b];a=+c[0],b=+c[c.length-1];return g()},f.range=function(a){if(!arguments.length)return c;c=a;return g()},f.copy=function(){return bI(a,b,c)};return g()}function bH(a,b){function e(a){return isNaN(a=+a)?NaN:b[d3.bisect(c,a)]}function d(){var d=0,f=a.length,g=b.length;c=[];while(++d<g)c[d-1]=d3.quantile(a,d/g);return e}var c;e.domain=function(b){if(!arguments.length)return a;a=b.filter(function(a){return!isNaN(a)}).sort(d3.ascending);return d()},e.range=function(a){if(!arguments.length)return b;b=a;return d()},e.quantiles=function(){return c},e.copy=function(){return bH(a,b)};return d()}function bC(a,b,c){function f(c){return d[((a[c]||(a[c]=++b))-1)%d.length]}var d,e;f.domain=function(d){if(!arguments.length)return d3.keys(a);a={},b=0;var e=-1,g=d.length,h;while(++e<g)a[h=d[e]]||(a[h]=++b);return f[c.t](c.x,c.p)},f.range=function(a){if(!arguments.length)return d;d=a,e=0,c={t:"range",x:a};return f},f.rangePoints=function(a,g){arguments.length<2&&(g=0);var h=a[0],i=a[1],j=(i-h)/(b-1+g);d=b<2?[(h+i)/2]:d3.range(h+j*g/2,i+j/2,j),e=0,c={t:"rangePoints",x:a,p:g};return f},f.rangeBands=function(a,g){arguments.length<2&&(g=0);var h=a[0],i=a[1],j=(i-h)/(b+g);d=d3.range(h+j*g,i,j),e=j*(1-g),c={t:"rangeBands",x:a,p:g};return f},f.rangeRoundBands=function(a,g){arguments.length<2&&(g=0);var h=a[0],i=a[1],j=Math.floor((i-h)/(b+g)),k=i-h-(b-g)*j;d=d3.range(h+Math.round(k/2),i,j),e=Math.round(j*(1-g)),c={t:"rangeRoundBands",x:a,p:g};return f},f.rangeBand=function(){return e},f.copy=function(){var d={},e;for(e in a)d[e]=a[e];return bC(d,b,c)};return f[c.t](c.x,c.p)}function bB(a){return function(b){return b<0?-Math.pow(-b,a):Math.pow(b,a)}}function bA(a,b){function e(b){return a(c(b))}var c=bB(b),d=bB(1/b);e.invert=function(b){return d(a.invert(b))},e.domain=function(b){if(!arguments.length)return a.domain().map(d);a.domain(b.map(c));return e},e.ticks=function(a){return bs(e.domain(),a)},e.tickFormat=function(a){return bt(e.domain(),a)},e.nice=function(){return e.domain(bm(e.domain(),bq))},e.exponent=function(a){if(!arguments.length)return b;var f=e.domain();c=bB(b=a),d=bB(1/b);return e.domain(f)},e.copy=function(){return bA(a.copy(),b)};return bp(e,a)}function bz(a){return a.toPrecision(1)}function by(a){return-Math.log(-a)/Math.LN10}function bx(a){return Math.log(a)/Math.LN10}function bw(a,b){function d(c){return a(b(c))}var c=b.pow;d.invert=function(b){return c(a.invert(b))},d.domain=function(e){if(!arguments.length)return a.domain().map(c);b=e[0]<0?by:bx,c=b.pow,a.domain(e.map(b));return d},d.nice=function(){a.domain(bm(a.domain(),bn));return d},d.ticks=function(){var d=bl(a.domain()),e=[];if(d.every(isFinite)){var f=Math.floor(d[0]),g=Math.ceil(d[1]),h=c(d[0]),i=c(d[1]);if(b===by){e.push(c(f));for(;f++<g;)for(var j=9;j>0;j--)e.push(c(f)*j)}else{for(;f<g;f++)for(var j=1;j<10;j++)e.push(c(f)*j);e.push(c(f))}for(f=0;e[f]<h;f++);for(g=e.length;e[g-1]>i;g--);e=e.slice(f,g)}return e},d.tickFormat=function(){return bz},d.copy=function(){return bw(a.copy(),b)};return bp(d,a)}function bv(a,b,c,d){var e=[],f=[],g=0,h=a.length;while(++g<h)e.push(c(a[g-1],a[g])),f.push(d(b[g-1],b[g]));return function(b){var c=d3.bisect(a,b,1,a.length-1)-1;return f[c](e[c](b))}}function bu(a,b,c,d){var e=c(a[0],a[1]),f=d(b[0],b[1]);return function(a){return f(e(a))}}function bt(a,b){return d3.format(",."+Math.max(0,-Math.floor(Math.log(br(a,b)[2])/Math.LN10+.01))+"f")}function bs(a,b){return d3.range.apply(d3,br(a,b))}function br(a,b){var c=bl(a),d=c[1]-c[0],e=Math.pow(10,Math.floor(Math.log(d/b)/Math.LN10)),f=b/d*e;f<=.15?e*=10:f<=.35?e*=5:f<=.75&&(e*=2),c[0]=Math.ceil(c[0]/e)*e,c[1]=Math.floor(c[1]/e)*e+e*.5,c[2]=e;return c}function bq(a){a=Math.pow(10,Math.round(Math.log(a)/Math.LN10)-1);return{floor:function(b){return Math.floor(b/a)*a},ceil:function(b){return Math.ceil(b/a)*a}}}function bp(a,b){a.range=d3.rebind(a,b.range),a.rangeRound=d3.rebind(a,b.rangeRound),a.interpolate=d3.rebind(a,b.interpolate),a.clamp=d3.rebind(a,b.clamp);return a}function bo(a,b,c,d){function h(a){return e(a)}function g(){var g=a.length==2?bu:bv,i=d?C:B;e=g(a,b,i,c),f=g(b,a,i,d3.interpolate);return h}var e,f;h.invert=function(a){return f(a)},h.domain=function(b){if(!arguments.length)return a;a=b.map(Number);return g()},h.range=function(a){if(!arguments.length)return b;b=a;return g()},h.rangeRound=function(a){return h.range(a).interpolate(d3.interpolateRound)},h.clamp=function(a){if(!arguments.length)return d;d=a;return g()},h.interpolate=function(a){if(!arguments.length)return c;c=a;return g()},h.ticks=function(b){return bs(a,b)},h.tickFormat=function(b){return bt(a,b)},h.nice=function(){bm(a,bq);return g()},h.copy=function(){return bo(a,b,c,d)};return g()}function bn(){return Math}function bm(a,b){var c=0,d=a.length-1,e=a[c],f=a[d],g;f<e&&(g=c,c=d,d=g,g=e,e=f,f=g),b=b(f-e),a[c]=b.floor(e),a[d]=b.ceil(f);return a}function bl(a){var b=a[0],c=a[a.length-1];return b<c?[b,c]:[c,b]}function bk(){}function bi(){var a=null,b=be,c=Infinity;while(b)b.flush?b=a?a.next=b.next:be=b.next:(c=Math.min(c,b.then+b.delay),b=(a=b).next);return c}function bh(){var a,b=Date.now(),c=be;while(c)a=b-c.then,a>=c.delay&&(c.flush=c.callback(a)),c=c.next;var d=bi()-b;d>24?(isFinite(d)&&(clearTimeout(bg),bg=setTimeout(bh,d)),bf=0):(bf=1,bj(bh))}function bd(a){for(var b=0,c=this.length;b<c;b++)for(var d=this[b],e=0,f=d.length;e<f;e++){var g=d[e];g&&a.call(g=g.node,g.__data__,e,b)}return this}function $(a){return typeof a=="function"?function(b,c,d){var e=a.call(this,b,c)+"";return d!=e&&d3.interpolate(d,e)}:(a=a+"",function(b,c,d){return d!=a&&d3.interpolate(d,a)})}function Z(b,c){a(b,_);var d={},e=d3.dispatch("start","end"),f=bc;b.id=c,b.tween=function(a,c){if(arguments.length<2)return d[a];c==null?delete d[a]:d[a]=c;return b},b.ease=function(a){if(!arguments.length)return f;f=typeof a=="function"?a:d3.ease.apply(d3,arguments);return b},b.each=function(a,c){if(arguments.length<2)return bd.call(b,a);e[a].add(c);return b},d3.timer(function(a){b.each(function(g,h,i){function p(a){if(n.active!==c)return!0;var b=Math.min(1,(a-l)/m),d=f(b),i=j.length;while(--i>=0)j[i].call(k,d);if(b===1){n.active=0,n.owner===c&&delete k.__transition__,bb=c,e.end.dispatch.call(k,g,h),bb=0;return!0}}function o(a){if(n.active<=c){n.active=c,e.start.dispatch.call(k,g,h);for(var b in d)(b=d[b].call(k,g,h))&&j.push(b);l-=a,d3.timer(p)}return!0}var j=[],k=this,l=b[i][h].delay-a,m=b[i][h].duration,n=k.__transition__;n?n.owner<c&&(n.owner=c):n=k.__transition__={active:0,owner:c},l<=0?o(0):d3.timer(o,l)});return!0});return b}function Y(a){arguments.length||(a=d3.ascending);return function(b,c){return a(b&&b.__data__,c&&c.__data__)}}function W(b){a(b,X);return b}function V(a){return{__data__:a}}function U(a){return function(){return Q(a,this)}}function T(a){return function(){return P(a,this)}}function O(b){a(b,R);return b}function N(a,b,c){function g(a){return Math.round(f(a)*255)}function f(a){a>360?a-=360:a<0&&(a+=360);return a<60?d+(e-d)*a/60:a<180?e:a<240?d+(e-d)*(240-a)/60:d}var d,e;a=a%360,a<0&&(a+=360),b=b<0?0:b>1?1:b,c=c<0?0:c>1?1:c,e=c<=.5?c*(1+b):c+b-c*b,d=2*c-e;return D(g(a+120),g(a),g(a-120))}function M(a,b,c){this.h=a,this.s=b,this.l=c}function L(a,b,c){return new M(a,b,c)}function I(a){var b=parseFloat(a);return a.charAt(a.length-1)==="%"?Math.round(b*2.55):b}function H(a,b,c){var d=Math.min(a/=255,b/=255,c/=255),e=Math.max(a,b,c),f=e-d,g,h,i=(e+d)/2;f?(h=i<.5?f/(e+d):f/(2-e-d),a==e?g=(b-c)/f+(b<c?6:0):b==e?g=(c-a)/f+2:g=(a-b)/f+4,g*=60):h=g=0;return L(g,h,i)}function G(a,b,c){var d=0,e=0,f=0,g,h,i;g=/([a-z]+)\((.*)\)/i.exec(a);if(g){h=g[2].split(",");switch(g[1]){case"hsl":return c(parseFloat(h[0]),parseFloat(h[1])/100,parseFloat(h[2])/100);case"rgb":return b(I(h[0]),I(h[1]),I(h[2]))}}if(i=J[a])return b(i.r,i.g,i.b);a!=null&&a.charAt(0)==="#"&&(a.length===4?(d=a.charAt(1),d+=d,e=a.charAt(2),e+=e,f=a.charAt(3),f+=f):a.length===7&&(d=a.substring(1,3),e=a.substring(3,5),f=a.substring(5,7)),d=parseInt(d,16),e=parseInt(e,16),f=parseInt(f,16));return b(d,e,f)}function F(a){return a<16?"0"+a.toString(16):a.toString(16)}function E(a,b,c){this.r=a,this.g=b,this.b=c}function D(a,b,c){return new E(a,b,c)}function C(a,b){b=b-(a=+a)?1/(b-a):0;return function(c){return Math.max(0,Math.min(1,(c-a)*b))}}function B(a,b){b=b-(a=+a)?1/(b-a):0;return function(c){return(c-a)*b}}function A(a){return a in z||/\bcolor\b/.test(a)?d3.interpolateRgb:d3.interpolate}function x(a){return a<1/2.75?7.5625*a*a:a<2/2.75?7.5625*(a-=1.5/2.75)*a+.75:a<2.5/2.75?7.5625*(a-=2.25/2.75)*a+.9375:7.5625*(a-=2.625/2.75)*a+.984375}function w(a){a||(a=1.70158);return function(b){return b*b*((a+1)*b-a)}}function v(a,b){var c;arguments.length<2&&(b=.45),arguments.length<1?(a=1,c=b/4):c=b/(2*Math.PI)*Math.asin(1/a);return function(d){return 1+a*Math.pow(2,10*-d)*Math.sin((d-c)*2*Math.PI/b)}}function u(a){return 1-Math.sqrt(1-a*a)}function t(a){return Math.pow(2,10*(a-1))}function s(a){return 1-Math.cos(a*Math.PI/2)}function r(a){return function(b){return Math.pow(b,a)}}function q(a){return a}function p(a){return function(b){return.5*(b<.5?a(2*b):2-a(2-2*b))}}function o(a){return function(b){return 1-a(1-b)}}function j(a){var b=a.lastIndexOf("."),c=b>=0?a.substring(b):(b=a.length,""),d=[];while(b>0)d.push(a.substring(b-=3,b+3));return d.reverse().join(",")+c}function i(a){return a+""}function f(a){var b={},c=[];b.add=function(a){for(var d=0;d<c.length;d++)if(c[d].listener==a)return b;c.push({listener:a,on:!0});return b},b.remove=function(a){for(var d=0;d<c.length;d++){var e=c[d];if(e.listener==a){e.on=!1,c=c.slice(0,d).concat(c.slice(d+1));break}}return b},b.dispatch=function(){var a=c;for(var b=0,d=a.length;b<d;b++){var e=a[b];e.on&&e.listener.apply(this,arguments)}};return b}function d(a){return a.replace(/(^\s+)|(\s+$)/g,"").replace(/\s+/g," ")}function c(a){return a==null}function b(a){return a.length}d3={version:"2.0.0"},Date.now||(Date.now=function(){return+(new Date)}),Object.create||(Object.create=function(a){function b(){}b.prototype=a;return new b});var a=[].__proto__?function(a,b){a.__proto__=b}:function(a,b){for(var c in b)a[c]=b[c]};d3.functor=function(a){return typeof a=="function"?a:function(){return a}},d3.rebind=function(a,b){return function(){var c=b.apply(a,arguments);return arguments.length?a:c}},d3.ascending=function(a,b){return a<b?-1:a>b?1:a>=b?0:NaN},d3.descending=function(a,b){return b<a?-1:b>a?1:b>=a?0:NaN},d3.min=function(a,b){var c=-1,d=a.length,e,f;if(arguments.length===1){while(++c<d&&((e=a[c])==null||e!=e))e=undefined;while(++c<d)(f=a[c])!=null&&e>f&&(e=f)}else{while(++c<d&&((e=b.call(a,a[c],c))==null||e!=e))e=undefined;while(++c<d)(f=b.call(a,a[c],c))!=null&&e>f&&(e=f)}return e},d3.max=function(a,b){var c=-1,d=a.length,e,f;if(arguments.length===1){while(++c<d&&((e=a[c])==null||e!=e))e=undefined;while(++c<d)(f=a[c])!=null&&f>e&&(e=f)}else{while(++c<d&&((e=b.call(a,a[c],c))==null||e!=e))e=undefined;while(++c<d)(f=b.call(a,a[c],c))!=null&&f>e&&(e=f)}return e},d3.sum=function(a,b){var c=0,d=a.length,e,f=-1;if(arguments.length===1)while(++f<d)isNaN(e=+a[f])||(c+=e);else while(++f<d)isNaN(e=+b.call(a,a[f],f))||(c+=e);return c},d3.quantile=function(a,b){var c=(a.length-1)*b+1,d=Math.floor(c),e=a[d-1],f=c-d;return f?e+f*(a[d]-e):e},d3.zip=function(){if(!(f=arguments.length))return[];for(var a=-1,c=d3.min(arguments,b),d=Array(c);++a<c;)for(var e=-1,f,g=d[a]=Array(f);++e<f;)g[e]=arguments[e][a];return d},d3.bisectLeft=function(a,b,c,d){arguments.length<3&&(c=0),arguments.length<4&&(d=a.length);while(c<d){var e=c+d>>1;a[e]<b?c=e+1:d=e}return c},d3.bisect=d3.bisectRight=function(a,b,c,d){arguments.length<3&&(c=0),arguments.length<4&&(d=a.length);while(c<d){var e=c+d>>1;b<a[e]?d=e:c=e+1}return c},d3.first=function(a,b){var c=0,d=a.length,e=a[0],f;arguments.length===1&&(b=d3.ascending);while(++c<d)b.call(a,e,f=a[c])>0&&(e=f);return e},d3.last=function(a,b){var c=0,d=a.length,e=a[0],f;arguments.length===1&&(b=d3.ascending);while(++c<d)b.call(a,e,f=a[c])<=0&&(e=f);return e},d3.nest=function(){function g(a,d){if(d>=b.length)return a;var e=[],f=c[d++],h;for(h in a)e.push({key:h,values:g(a[h],d)});f&&e.sort(function(a,b){return f(a.key,b.key)});return e}function f(c,g){if(g>=b.length)return e?e.call(a,c):d?c.sort(d):c;var h=-1,i=c.length,j=b[g++],k,l,m={};while(++h<i)(k=j(l=c[h]))in m?m[k].push(l):m[k]=[l];for(k in m)m[k]=f(m[k],g);return m}var a={},b=[],c=[],d,e;a.map=function(a){return f(a,0)},a.entries=function(a){return g(f(a,0),0)},a.key=function(c){b.push(c);return a},a.sortKeys=function(d){c[b.length-1]=d;return a},a.sortValues=function(b){d=b;return a},a.rollup=function(b){e=b;return a};return a},d3.keys=function(a){var b=[];for(var c in a)b.push(c);return b},d3.values=function(a){var b=[];for(var c in a)b.push(a[c]);return b},d3.entries=function(a){var b=[];for(var c in a)b.push({key:c,value:a[c]});return b},d3.permute=function(a,b){var c=[],d=-1,e=b.length;while(++d<e)c[d]=a[b[d]];return c},d3.merge=function(a){return Array.prototype.concat.apply([],a)},d3.split=function(a,b){var d=[],e=[],f,g=-1,h=a.length;arguments.length<2&&(b=c);while(++g<h)b.call(e,f=a[g],g)?e=[]:(e.length||d.push(e),e.push(f));return d},d3.range=function(a,b,c){arguments.length<3&&(c=1,arguments.length<2&&(b=a,a=0));if((b-a)/c==Infinity)throw new Error("infinite range");var d=[],e=-1,f;if(c<0)while((f=a+c*++e)>b)d.push(f);else while((f=a+c*++e)<b)d.push(f);return d},d3.requote=function(a){return a.replace(e,"\\$&")};var e=/[\\\^\$\*\+\?\|\[\]\(\)\.\{\}]/g;d3.round=function(a,b){return b?Math.round(a*Math.pow(10,b))*Math.pow(10,-b):Math.round(a)},d3.xhr=function(a,b,c){var d=new XMLHttpRequest;arguments.length<3?c=b:b&&d.overrideMimeType&&d.overrideMimeType(b),d.open("GET",a,!0),d.onreadystatechange=function(){d.readyState===4&&c(d.status<300?d:null)},d.send(null)},d3.text=function(a,b,c){function d(a){c(a&&a.responseText)}arguments.length<3&&(c=b,b=null),d3.xhr(a,b,d)},d3.json=function(a,b){d3.text(a,"application/json",function(a){b(a?JSON.parse(a):null)})},d3.html=function(a,b){d3.text(a,"text/html",function(a){if(a!=null){var c=document.createRange();c.selectNode(document.body),a=c.createContextualFragment(a)}b(a)})},d3.xml=function(a,b,c){function d(a){c(a&&a.responseXML)}arguments.length<3&&(c=b,b=null),d3.xhr(a,b,d)},d3.ns={prefix:{svg:"http://www.w3.org/2000/svg",xhtml:"http://www.w3.org/1999/xhtml",xlink:"http://www.w3.org/1999/xlink",xml:"http://www.w3.org/XML/1998/namespace",xmlns:"http://www.w3.org/2000/xmlns/"},qualify:function(a){var b=a.indexOf(":");return b<0?a:{space:d3.ns.prefix[a.substring(0,b)],local:a.substring(b+1)}}},d3.dispatch=function(a){var b={},c;for(var d=0,e=arguments.length;d<e;d++)c=arguments[d],b[c]=f(c);return b},d3.format=function(a){var b=g.exec(a),c=b[1]||" ",d=b[3]||"",e=b[5],f=+b[6],k=b[7],l=b[8],m=b[9],n=!1,o=!1;l&&(l=l.substring(1)),e&&(c="0",k&&(f-=Math.floor((f-1)/4)));switch(m){case"n":k=!0,m="g";break;case"%":n=!0,m="f";break;case"p":n=!0,m="r";break;case"d":o=!0,l="0"}m=h[m]||i;return function(a){var b=n?a*100:+a,g=b<0&&(b=-b)?"−":d;if(o&&b%1)return"";a=m(b,l);if(e){var h=a.length+g.length;h<f&&(a=Array(f-h+1).join(c)+a),k&&(a=j(a)),a=g+a}else{k&&(a=j(a)),a=g+a;var h=a.length;h<f&&(a=Array(f-h+1).join(c)+a)}n&&(a+="%");return a}};var g=/(?:([^{])?([<>=^]))?([+\- ])?(#)?(0)?([0-9]+)?(,)?(\.[0-9]+)?([a-zA-Z%])?/,h={g:function(a,b){return a.toPrecision(b)},e:function(a,b){return a.toExponential(b)},f:function(a,b){return a.toFixed(b)},r:function(a,b){var c=1+Math.floor(1e-15+Math.log(a)/Math.LN10);return d3.round(a,b-c).toFixed(Math.max(0,Math.min(20,b-c)))}},k=r(2),l=r(3),m={linear:function(){return q},poly:r,quad:function(){return k},cubic:function(){return l},sin:function(){return s},exp:function(){return t},circle:function(){return u},elastic:v,back:w,bounce:function(){return x}},n={"in":function(a){return a},out:o,"in-out":p,"out-in":function(a){return p(o(a))}};d3.ease=function(a){var b=a.indexOf("-"),c=b>=0?a.substring(0,b):a,d=b>=0?a.substring(b+1):"in";return n[d](m[c].apply(null,Array.prototype.slice.call(arguments,1)))},d3.event=null,d3.interpolate=function(a,b){var c=d3.interpolators.length,d;while(--c>=0&&!(d=d3.interpolators[c](a,b)));return d},d3.interpolateNumber=function(a,b){b-=a;return function(c){return a+b*c}},d3.interpolateRound=function(a,b){b-=a;return function(c){return Math.round(a+b*c)}},d3.interpolateString=function(a,b){var c,d,e,f=0,g=0,h=[],i=[],j,k;y.lastIndex=0;for(d=0;c=y.exec(b);++d)c.index&&h.push(b.substring(f,g=c.index)),i.push({i:h.length,x:c[0]}),h.push(null),f=y.lastIndex;f<b.length&&h.push(b.substring(f));for(d=0,j=i.length;(c=y.exec(a))&&d<j;++d){k=i[d];if(k.x==c[0]){if(k.i)if(h[k.i+1]==null){h[k.i-1]+=k.x,h.splice(k.i,1);for(e=d+1;e<j;++e)i[e].i--}else{h[k.i-1]+=k.x+h[k.i+1],h.splice(k.i,2);for(e=d+1;e<j;++e)i[e].i-=2}else if(h[k.i+1]==null)h[k.i]=k.x;else{h[k.i]=k.x+h[k.i+1],h.splice(k.i+1,1);for(e=d+1;e<j;++e)i[e].i--}i.splice(d,1),j--,d--}else k.x=d3.interpolateNumber(parseFloat(c[0]),parseFloat(k.x))}while(d<j)k=i.pop(),h[k.i+1]==null?h[k.i]=k.x:(h[k.i]=k.x+h[k.i+1],h.splice(k.i+1,1)),j--;return h.length===1?h[0]==null?i[0].x:function(){return b}:function(a){for(d=0;d<j;++d)h[(k=i[d]).i]=k.x(a);return h.join("")}},d3.interpolateRgb=function(a,b){a=d3.rgb(a),b=d3.rgb(b);var c=a.r,d=a.g,e=a.b,f=b.r-c,g=b.g-d,h=b.b-e;return function(a){return"rgb("+Math.round(c+f*a)+","+Math.round(d+g*a)+","+Math.round(e+h*a)+")"}},d3.interpolateHsl=function(a,b){a=d3.hsl(a),b=d3.hsl(b);var c=a.h,d=a.s,e=a.l,f=b.h-c,g=b.s-d,h=b.l-e;return function(a){return N(c+f*a,d+g*a,e+h*a).toString()}},d3.interpolateArray=function(a,b){var c=[],d=[],e=a.length,f=b.length,g=Math.min(a.length,b.length),h;for(h=0;h<g;++h)c.push(d3.interpolate(a[h],b[h]));for(;h<e;++h)d[h]=a[h];for(;h<f;++h)d[h]=b[h];return function(a){for(h=0;h<g;++h)d[h]=c[h](a);return d}},d3.interpolateObject=function(a,b){var c={},d={},e;for(e in a)e in b?c[e]=A(e)(a[e],b[e]):d[e]=a[e];for(e in b)e in a||(d[e]=b[e]);return function(a){for(e in c)d[e]=c[e](a);return d}};var y=/[-+]?(?:\d+\.\d+|\d+\.|\.\d+|\d+)(?:[eE][-]?\d+)?/g,z={background:1,fill:1,stroke:1};d3.interpolators=[d3.interpolateObject,function(a,b){return b instanceof Array&&d3.interpolateArray(a,b)},function(a,b){return typeof b=="string"&&d3.interpolateString(String(a),b)},function(a,b){return(typeof b=="string"?b in J||/^(#|rgb\(|hsl\()/.test(b):b instanceof E||b instanceof M)&&d3.interpolateRgb(String(a),b)},function(a,b){return typeof b=="number"&&d3.interpolateNumber(+a,b)}],d3.rgb=function(a,b,c){return arguments.length===1?G(""+a,D,N):D(~~a,~~b,~~c)},E.prototype.brighter=function(a){a=Math.pow(.7,arguments.length?a:1);var b=this.r,c=this.g,d=this.b,e=30;if(!b&&!c&&!d)return D(e,e,e);b&&b<e&&(b=e),c&&c<e&&(c=e),d&&d<e&&(d=e);return D(Math.min(255,Math.floor(b/a)),Math.min(255,Math.floor(c/a)),Math.min(255,Math.floor(d/a)))},E.prototype.darker=function(a){a=Math.pow(.7,arguments.length?a:1);return D(Math.max(0,Math.floor(a*this.r)),Math.max(0,Math.floor(a*this.g)),Math.max(0,Math.floor(a*this.b)))},E.prototype.hsl=function(){return H(this.r,this.g,this.b)},E.prototype.toString=function(){return"#"+F(this.r)+F(this.g)+F(this.b)};var J={aliceblue:"#f0f8ff",antiquewhite:"#faebd7",aqua:"#00ffff",aquamarine:"#7fffd4",azure:"#f0ffff",beige:"#f5f5dc",bisque:"#ffe4c4",black:"#000000",blanchedalmond:"#ffebcd",blue:"#0000ff",blueviolet:"#8a2be2",brown:"#a52a2a",burlywood:"#deb887",cadetblue:"#5f9ea0",chartreuse:"#7fff00",chocolate:"#d2691e",coral:"#ff7f50",cornflowerblue:"#6495ed",cornsilk:"#fff8dc",crimson:"#dc143c",cyan:"#00ffff",darkblue:"#00008b",darkcyan:"#008b8b",darkgoldenrod:"#b8860b",darkgray:"#a9a9a9",darkgreen:"#006400",darkgrey:"#a9a9a9",darkkhaki:"#bdb76b",darkmagenta:"#8b008b",darkolivegreen:"#556b2f",darkorange:"#ff8c00",darkorchid:"#9932cc",darkred:"#8b0000",darksalmon:"#e9967a",darkseagreen:"#8fbc8f",darkslateblue:"#483d8b",darkslategray:"#2f4f4f",darkslategrey:"#2f4f4f",darkturquoise:"#00ced1",darkviolet:"#9400d3",deeppink:"#ff1493",deepskyblue:"#00bfff",dimgray:"#696969",dimgrey:"#696969",dodgerblue:"#1e90ff",firebrick:"#b22222",floralwhite:"#fffaf0",forestgreen:"#228b22",fuchsia:"#ff00ff",gainsboro:"#dcdcdc",ghostwhite:"#f8f8ff",gold:"#ffd700",goldenrod:"#daa520",gray:"#808080",green:"#008000",greenyellow:"#adff2f",grey:"#808080",honeydew:"#f0fff0",hotpink:"#ff69b4",indianred:"#cd5c5c",indigo:"#4b0082",ivory:"#fffff0",khaki:"#f0e68c",lavender:"#e6e6fa",lavenderblush:"#fff0f5",lawngreen:"#7cfc00",lemonchiffon:"#fffacd",lightblue:"#add8e6",lightcoral:"#f08080",lightcyan:"#e0ffff",lightgoldenrodyellow:"#fafad2",lightgray:"#d3d3d3",lightgreen:"#90ee90",lightgrey:"#d3d3d3",lightpink:"#ffb6c1",lightsalmon:"#ffa07a",lightseagreen:"#20b2aa",lightskyblue:"#87cefa",lightslategray:"#778899",lightslategrey:"#778899",lightsteelblue:"#b0c4de",lightyellow:"#ffffe0",lime:"#00ff00",limegreen:"#32cd32",linen:"#faf0e6",magenta:"#ff00ff",maroon:"#800000",mediumaquamarine:"#66cdaa",mediumblue:"#0000cd",mediumorchid:"#ba55d3",mediumpurple:"#9370db",mediumseagreen:"#3cb371",mediumslateblue:"#7b68ee",mediumspringgreen:"#00fa9a",mediumturquoise:"#48d1cc",mediumvioletred:"#c71585",midnightblue:"#191970",mintcream:"#f5fffa",mistyrose:"#ffe4e1",moccasin:"#ffe4b5",navajowhite:"#ffdead",navy:"#000080",oldlace:"#fdf5e6",olive:"#808000",olivedrab:"#6b8e23",orange:"#ffa500",orangered:"#ff4500",orchid:"#da70d6",palegoldenrod:"#eee8aa",palegreen:"#98fb98",paleturquoise:"#afeeee",palevioletred:"#db7093",papayawhip:"#ffefd5",peachpuff:"#ffdab9",peru:"#cd853f",pink:"#ffc0cb",plum:"#dda0dd",powderblue:"#b0e0e6",purple:"#800080",red:"#ff0000",rosybrown:"#bc8f8f",royalblue:"#4169e1",saddlebrown:"#8b4513",salmon:"#fa8072",sandybrown:"#f4a460",seagreen:"#2e8b57",seashell:"#fff5ee",sienna:"#a0522d",silver:"#c0c0c0",skyblue:"#87ceeb",slateblue:"#6a5acd",slategray:"#708090",slategrey:"#708090",snow:"#fffafa",springgreen:"#00ff7f",steelblue:"#4682b4",tan:"#d2b48c",teal:"#008080",thistle:"#d8bfd8",tomato:"#ff6347",turquoise:"#40e0d0",violet:"#ee82ee",wheat:"#f5deb3",white:"#ffffff",whitesmoke:"#f5f5f5",yellow:"#ffff00",yellowgreen:"#9acd32"};for(var K in J)J[K]=G(J[K],D,N);d3.hsl=function(a,b,c){return arguments.length===1?G(""+a,H,L):L(+a,+b,+c)},M.prototype.brighter=function(a){a=Math.pow(.7,arguments.length?a:1);return L(this.h,this.s,this.l/a)},M.prototype.darker=function(a){a=Math.pow(.7,arguments.length?a:1);return L(this.h,this.s,a*this.l)},M.prototype.rgb=function(){return N(this.h,this.s,this.l)},M.prototype.toString=function(){return"hsl("+this.h+","+this.s*100+"%,"+this.l*100+"%)"},d3.select=function(a){return typeof a=="string"?S.select(a):O([[a]])},d3.selectAll=function(a){return typeof a=="string"?S.selectAll(a):O([a])};var P=function(a,b){return b.querySelector(a)},Q=function(a,b){return b.querySelectorAll(a)};typeof Sizzle=="function"&&(P=function(a,b){return Sizzle(a,b)[0]},Q=function(a,b){return Sizzle.uniqueSort(Sizzle(a,b))});var R=[],S=O([[document]]);S[0].parentNode=document.documentElement,d3.selection=function(){return S},d3.selection.prototype=R,R.select=function(a){var b=[],c,d,e,f;typeof a!="function"&&(a=T(a));for(var g=-1,h=this.length;++g<h;){b.push(c=[]),c.parentNode=(e=this[g]).parentNode;for(var i=-1,j=e.length;++i<j;)(f=e[i])?(c.push(d=a.call(f,f.__data__,i)),d&&"__data__"in f&&(d.__data__=f.__data__)):c.push(null)}return O(b)},R.selectAll=function(a){var b=[],c,d;typeof a!="function"&&(a=U(a));for(var e=-1,f=this.length;++e<f;)for(var g=this[e],h=-1,i=g.length;++h<i;)if(d=g[h])b.push(c=a.call(d,d.__data__,h)),c.parentNode=d;return O(b)},R.attr=function(a,b){function i(){var c=b.apply(this,arguments);c==null?this.removeAttributeNS(a.space,a.local):this.setAttributeNS(a.space,a.local,c)}function h(){var c=b.apply(this,arguments);c==null?this.removeAttribute(a):this.setAttribute(a,c)}function g(){this.setAttributeNS(a.space,a.local,b)}function f(){this.setAttribute(a,b)}function e(){this.removeAttributeNS(a.space,a.local)}function d(){this.removeAttribute(a)}a=d3.ns.qualify(a);if(arguments.length<2){var c=this.node();return a.local?c.getAttributeNS(a.space,a.local):c.getAttribute(a)}return this.each(b==null?a.local?e:d:typeof b=="function"?a.local?i:h:a.local?g:f)},R.classed=function(a,b){function i(){(b.apply(this,arguments)?g:h).call(this)}function h(){if(b=this.classList)return b.remove(a);var b=this.className,e=b.baseVal!=null,f=e?b.baseVal:b;f=d(f.replace(c," ")),e?b.baseVal=f:this.className=f}function g(){if(b=this.classList)return b.add(a);var b=this.className,e=b.baseVal!=null,f=e?b.baseVal:b;c.lastIndex=0,c.test(f)||(f=d(f+" "+a),e?b.baseVal=f:this.className=f)}var c=new RegExp("(^|\\s+)"+d3.requote(a)+"(\\s+|$)","g")
<add>;if(arguments.length<2){var e=this.node();if(f=e.classList)return f.contains(a);var f=e.className;c.lastIndex=0;return c.test(f.baseVal!=null?f.baseVal:f)}return this.each(typeof b=="function"?i:b?g:h)},R.style=function(a,b,c){function f(){var d=b.apply(this,arguments);d==null?this.style.removeProperty(a):this.style.setProperty(a,d,c)}function e(){this.style.setProperty(a,b,c)}function d(){this.style.removeProperty(a)}arguments.length<3&&(c="");return arguments.length<2?window.getComputedStyle(this.node(),null).getPropertyValue(a):this.each(b==null?d:typeof b=="function"?f:e)},R.property=function(a,b){function e(){var c=b.apply(this,arguments);c==null?delete this[a]:this[a]=c}function d(){this[a]=b}function c(){delete this[a]}return arguments.length<2?this.node()[a]:this.each(b==null?c:typeof b=="function"?e:d)},R.text=function(a){return arguments.length<1?this.node().textContent:this.each(typeof a=="function"?function(){this.textContent=a.apply(this,arguments)}:function(){this.textContent=a})},R.html=function(a){return arguments.length<1?this.node().innerHTML:this.each(typeof a=="function"?function(){this.innerHTML=a.apply(this,arguments)}:function(){this.innerHTML=a})},R.append=function(a){function c(){return this.appendChild(document.createElementNS(a.space,a.local))}function b(){return this.appendChild(document.createElement(a))}a=d3.ns.qualify(a);return this.select(a.local?c:b)},R.insert=function(a,b){function d(){return this.insertBefore(document.createElementNS(a.space,a.local),P(b,this))}function c(){return this.insertBefore(document.createElement(a),P(b,this))}a=d3.ns.qualify(a);return this.select(a.local?d:c)},R.remove=function(){return this.each(function(){var a=this.parentNode;a&&a.removeChild(this)})},R.data=function(a,b){function f(a,f){var g,h=a.length,i=f.length,j=Math.min(h,i),k=Math.max(h,i),l=[],m=[],n=[],o,p;if(b){var q={},r=[],s,t=f.length;for(g=-1;++g<h;)s=b.call(o=a[g],o.__data__,g),s in q?n[t++]=o:q[s]=o,r.push(s);for(g=-1;++g<i;)o=q[s=b.call(f,p=f[g],g)],o?(o.__data__=p,l[g]=o,m[g]=n[g]=null):(m[g]=V(p),l[g]=n[g]=null),delete q[s];for(g=-1;++g<h;)r[g]in q&&(n[g]=a[g])}else{for(g=-1;++g<j;)o=a[g],p=f[g],o?(o.__data__=p,l[g]=o,m[g]=n[g]=null):(m[g]=V(p),l[g]=n[g]=null);for(;g<i;++g)m[g]=V(f[g]),l[g]=n[g]=null;for(;g<k;++g)n[g]=a[g],m[g]=l[g]=null}m.update=l,m.parentNode=l.parentNode=n.parentNode=a.parentNode,c.push(m),d.push(l),e.push(n)}var c=[],d=[],e=[],g=-1,h=this.length,i;if(typeof a=="function")while(++g<h)f(i=this[g],a.call(i,i.parentNode.__data__,g));else while(++g<h)f(i=this[g],a);var j=O(d);j.enter=function(){return W(c)},j.exit=function(){return O(e)};return j};var X=[];X.append=R.append,X.insert=R.insert,X.empty=R.empty,X.select=function(a){var b=[],c,d,e,f,g;for(var h=-1,i=this.length;++h<i;){e=(f=this[h]).update,b.push(c=[]),c.parentNode=f.parentNode;for(var j=-1,k=f.length;++j<k;)(g=f[j])?(c.push(e[j]=d=a.call(f.parentNode,g.__data__,j)),d.__data__=g.__data__):c.push(null)}return O(b)},R.filter=function(a){var b=[],c,d,e;for(var f=0,g=this.length;f<g;f++){b.push(c=[]),c.parentNode=(d=this[f]).parentNode;for(var h=0,i=d.length;h<i;h++)(e=d[h])&&a.call(e,e.__data__,h)&&c.push(e)}return O(b)},R.map=function(a){return this.each(function(){this.__data__=a.apply(this,arguments)})},R.sort=function(a){a=Y.apply(this,arguments);for(var b=0,c=this.length;b<c;b++)for(var d=this[b].sort(a),e=1,f=d.length,g=d[0];e<f;e++){var h=d[e];h&&(g&&g.parentNode.insertBefore(h,g.nextSibling),g=h)}return this},R.on=function(a,b,c){arguments.length<3&&(c=!1);var d="__on"+a,e=a.indexOf(".");e>0&&(a=a.substring(0,e));return arguments.length<2?(e=this.node()[d])&&e._:this.each(function(e,f){function h(a){var c=d3.event;d3.event=a;try{b.call(g,g.__data__,f)}finally{d3.event=c}}var g=this;g[d]&&g.removeEventListener(a,g[d],c),b&&g.addEventListener(a,g[d]=h,c),h._=b})},R.each=function(a){for(var b=-1,c=this.length;++b<c;)for(var d=this[b],e=-1,f=d.length;++e<f;){var g=d[e];g&&a.call(g,g.__data__,e,b)}return this},R.call=function(a){a.apply(this,(arguments[0]=this,arguments));return this},R.empty=function(){return!this.node()},R.node=function(a){for(var b=0,c=this.length;b<c;b++)for(var d=this[b],e=0,f=d.length;e<f;e++){var g=d[e];if(g)return g}return null},R.transition=function(){var a=[],b,c;for(var d=-1,e=this.length;++d<e;){a.push(b=[]);for(var f=this[d],g=-1,h=f.length;++g<h;)b.push((c=f[g])?{node:c,delay:0,duration:250}:null)}return Z(a,bb||++ba)};var _=[],ba=0,bb=0,bc=d3.ease("cubic-in-out");_.call=R.call,d3.transition=function(){return S.transition()},d3.transition.prototype=_,_.select=function(a){var b=[],c,d,e;typeof a!="function"&&(a=T(a));for(var f=-1,g=this.length;++f<g;){b.push(c=[]);for(var h=this[f],i=-1,j=h.length;++i<j;)(e=h[i])&&(d=a.call(e.node,e.node.__data__,i))?("__data__"in e.node&&(d.__data__=e.node.__data__),c.push({node:d,delay:e.delay,duration:e.duration})):c.push(null)}return Z(b,this.id).ease(this.ease())},_.selectAll=function(a){var b=[],c,d;typeof a!="function"&&(a=U(a));for(var e=-1,f=this.length;++e<f;)for(var g=this[e],h=-1,i=g.length;++h<i;)if(d=g[h]){b.push(c=a.call(d.node,d.node.__data__,h));for(var j=-1,k=c.length;++j<k;)c[j]={node:c[j],delay:d.delay,duration:d.duration}}return Z(b,this.id).ease(this.ease())},_.attr=function(a,b){return this.attrTween(a,$(b))},_.attrTween=function(a,b){function d(c,d){var e=b.call(this,c,d,this.getAttributeNS(a.space,a.local));return e&&function(b){this.setAttributeNS(a.space,a.local,e(b))}}function c(c,d){var e=b.call(this,c,d,this.getAttribute(a));return e&&function(b){this.setAttribute(a,e(b))}}a=d3.ns.qualify(a);return this.tween("attr."+a,a.local?d:c)},_.style=function(a,b,c){arguments.length<3&&(c=null);return this.styleTween(a,$(b),c)},_.styleTween=function(a,b,c){arguments.length<3&&(c=null);return this.tween("style."+a,function(d,e){var f=b.call(this,d,e,window.getComputedStyle(this,null).getPropertyValue(a));return f&&function(b){this.style.setProperty(a,f(b),c)}})},_.text=function(a){return this.tween("text",function(b,c){this.textContent=typeof a=="function"?a.call(this,b,c):a})},_.remove=function(){return this.each("end",function(){var a;!this.__transition__&&(a=this.parentNode)&&a.removeChild(this)})},_.delay=function(a){var b=this;return b.each(typeof a=="function"?function(c,d,e){b[e][d].delay=+a.apply(this,arguments)}:(a=+a,function(c,d,e){b[e][d].delay=a}))},_.duration=function(a){var b=this;return b.each(typeof a=="function"?function(c,d,e){b[e][d].duration=+a.apply(this,arguments)}:(a=+a,function(c,d,e){b[e][d].duration=a}))};var be=null,bf,bg;d3.timer=function(a,b){var c=Date.now(),d=!1,e,f=be;if(arguments.length<2)b=0;else if(!isFinite(b))return;while(f){if(f.callback===a){f.then=c,f.delay=b,d=!0;break}e=f,f=f.next}d||(be={callback:a,then:c,delay:b,next:be}),bf||(bg=clearTimeout(bg),bf=1,bj(bh))},d3.timer.flush=function(){var a,b=Date.now(),c=be;while(c)a=b-c.then,c.delay||(c.flush=c.callback(a)),c=c.next;bi()};var bj=window.requestAnimationFrame||window.webkitRequestAnimationFrame||window.mozRequestAnimationFrame||window.oRequestAnimationFrame||window.msRequestAnimationFrame||function(a){setTimeout(a,17)};d3.scale={},d3.scale.linear=function(){return bo([0,1],[0,1],d3.interpolate,!1)},d3.scale.log=function(){return bw(d3.scale.linear(),bx)},bx.pow=function(a){return Math.pow(10,a)},by.pow=function(a){return-Math.pow(10,-a)},d3.scale.pow=function(){return bA(d3.scale.linear(),1)},d3.scale.sqrt=function(){return d3.scale.pow().exponent(.5)},d3.scale.ordinal=function(){return bC({},0,{t:"range",x:[]})},d3.scale.category10=function(){return d3.scale.ordinal().range(bD)},d3.scale.category20=function(){return d3.scale.ordinal().range(bE)},d3.scale.category20b=function(){return d3.scale.ordinal().range(bF)},d3.scale.category20c=function(){return d3.scale.ordinal().range(bG)};var bD=["#1f77b4","#ff7f0e","#2ca02c","#d62728","#9467bd","#8c564b","#e377c2","#7f7f7f","#bcbd22","#17becf"],bE=["#1f77b4","#aec7e8","#ff7f0e","#ffbb78","#2ca02c","#98df8a","#d62728","#ff9896","#9467bd","#c5b0d5","#8c564b","#c49c94","#e377c2","#f7b6d2","#7f7f7f","#c7c7c7","#bcbd22","#dbdb8d","#17becf","#9edae5"],bF=["#393b79","#5254a3","#6b6ecf","#9c9ede","#637939","#8ca252","#b5cf6b","#cedb9c","#8c6d31","#bd9e39","#e7ba52","#e7cb94","#843c39","#ad494a","#d6616b","#e7969c","#7b4173","#a55194","#ce6dbd","#de9ed6"],bG=["#3182bd","#6baed6","#9ecae1","#c6dbef","#e6550d","#fd8d3c","#fdae6b","#fdd0a2","#31a354","#74c476","#a1d99b","#c7e9c0","#756bb1","#9e9ac8","#bcbddc","#dadaeb","#636363","#969696","#bdbdbd","#d9d9d9"];d3.scale.quantile=function(){return bH([],[])},d3.scale.quantize=function(){return bI(0,1,[0,1])},d3.svg={},d3.svg.arc=function(){function e(){var e=a.apply(this,arguments),f=b.apply(this,arguments),g=c.apply(this,arguments)+bJ,h=d.apply(this,arguments)+bJ,i=(h<g&&(i=g,g=h,h=i),h-g),j=i<Math.PI?"0":"1",k=Math.cos(g),l=Math.sin(g),m=Math.cos(h),n=Math.sin(h);return i>=bK?e?"M0,"+f+"A"+f+","+f+" 0 1,1 0,"+ -f+"A"+f+","+f+" 0 1,1 0,"+f+"M0,"+e+"A"+e+","+e+" 0 1,1 0,"+ -e+"A"+e+","+e+" 0 1,1 0,"+e+"Z":"M0,"+f+"A"+f+","+f+" 0 1,1 0,"+ -f+"A"+f+","+f+" 0 1,1 0,"+f+"Z":e?"M"+f*k+","+f*l+"A"+f+","+f+" 0 "+j+",1 "+f*m+","+f*n+"L"+e*m+","+e*n+"A"+e+","+e+" 0 "+j+",0 "+e*k+","+e*l+"Z":"M"+f*k+","+f*l+"A"+f+","+f+" 0 "+j+",1 "+f*m+","+f*n+"L0,0"+"Z"}var a=bL,b=bM,c=bN,d=bO;e.innerRadius=function(b){if(!arguments.length)return a;a=d3.functor(b);return e},e.outerRadius=function(a){if(!arguments.length)return b;b=d3.functor(a);return e},e.startAngle=function(a){if(!arguments.length)return c;c=d3.functor(a);return e},e.endAngle=function(a){if(!arguments.length)return d;d=d3.functor(a);return e},e.centroid=function(){var e=(a.apply(this,arguments)+b.apply(this,arguments))/2,f=(c.apply(this,arguments)+d.apply(this,arguments))/2+bJ;return[Math.cos(f)*e,Math.sin(f)*e]};return e};var bJ=-Math.PI/2,bK=2*Math.PI-1e-6;d3.svg.line=function(){return bP(Object)};var bT={linear:bU,"step-before":bV,"step-after":bW,basis:ca,"basis-open":cb,"basis-closed":cc,bundle:cd,cardinal:bZ,"cardinal-open":bX,"cardinal-closed":bY,monotone:cm},cf=[0,2/3,1/3,0],cg=[0,1/3,2/3,0],ch=[0,1/6,2/3,1/6];d3.svg.line.radial=function(){var a=bP(cn);a.radius=a.x,delete a.x,a.angle=a.y,delete a.y;return a},d3.svg.area=function(){return co(Object)},d3.svg.area.radial=function(){var a=co(cn);a.radius=a.x,delete a.x,a.innerRadius=a.x0,delete a.x0,a.outerRadius=a.x1,delete a.x1,a.angle=a.y,delete a.y,a.startAngle=a.y0,delete a.y0,a.endAngle=a.y1,delete a.y1;return a},d3.svg.chord=function(){function j(a,b,c,d){return"Q 0,0 "+d}function i(a,b){return"A"+a+","+a+" 0 0,1 "+b}function h(a,b){return a.a0==b.a0&&a.a1==b.a1}function g(a,b,f,g){var h=b.call(a,f,g),i=c.call(a,h,g),j=d.call(a,h,g)+bJ,k=e.call(a,h,g)+bJ;return{r:i,a0:j,a1:k,p0:[i*Math.cos(j),i*Math.sin(j)],p1:[i*Math.cos(k),i*Math.sin(k)]}}function f(c,d){var e=g(this,a,c,d),f=g(this,b,c,d);return"M"+e.p0+i(e.r,e.p1)+(h(e,f)?j(e.r,e.p1,e.r,e.p0):j(e.r,e.p1,f.r,f.p0)+i(f.r,f.p1)+j(f.r,f.p1,e.r,e.p0))+"Z"}var a=cr,b=cs,c=ct,d=bN,e=bO;f.radius=function(a){if(!arguments.length)return c;c=d3.functor(a);return f},f.source=function(b){if(!arguments.length)return a;a=d3.functor(b);return f},f.target=function(a){if(!arguments.length)return b;b=d3.functor(a);return f},f.startAngle=function(a){if(!arguments.length)return d;d=d3.functor(a);return f},f.endAngle=function(a){if(!arguments.length)return e;e=d3.functor(a);return f};return f},d3.svg.diagonal=function(){function d(d,e){var f=a.call(this,d,e),g=b.call(this,d,e),h=(f.y+g.y)/2,i=[f,{x:f.x,y:h},{x:g.x,y:h},g];i=i.map(c);return"M"+i[0]+"C"+i[1]+" "+i[2]+" "+i[3]}var a=cr,b=cs,c=cw;d.source=function(b){if(!arguments.length)return a;a=d3.functor(b);return d},d.target=function(a){if(!arguments.length)return b;b=d3.functor(a);return d},d.projection=function(a){if(!arguments.length)return c;c=a;return d};return d},d3.svg.diagonal.radial=function(){var a=d3.svg.diagonal(),b=cw,c=a.projection;a.projection=function(a){return arguments.length?c(cx(b=a)):b};return a},d3.svg.mouse=function(a){return cz(a,d3.event)};var cy=/WebKit/.test(navigator.userAgent)?-1:0;d3.svg.touches=function(a){var b=d3.event.touches;return b?Array.prototype.map.call(b,function(b){var c=cz(a,b);c.identifier=b.identifier;return c}):[]},d3.svg.symbol=function(){function c(c,d){return(cC[a.call(this,c,d)]||cC.circle)(b.call(this,c,d))}var a=cB,b=cA;c.type=function(b){if(!arguments.length)return a;a=d3.functor(b);return c},c.size=function(a){if(!arguments.length)return b;b=d3.functor(a);return c};return c};var cC={circle:function(a){var b=Math.sqrt(a/Math.PI);return"M0,"+b+"A"+b+","+b+" 0 1,1 0,"+ -b+"A"+b+","+b+" 0 1,1 0,"+b+"Z"},cross:function(a){var b=Math.sqrt(a/5)/2;return"M"+ -3*b+","+ -b+"H"+ -b+"V"+ -3*b+"H"+b+"V"+ -b+"H"+3*b+"V"+b+"H"+b+"V"+3*b+"H"+ -b+"V"+b+"H"+ -3*b+"Z"},diamond:function(a){var b=Math.sqrt(a/(2*cE)),c=b*cE;return"M0,"+ -b+"L"+c+",0"+" 0,"+b+" "+ -c+",0"+"Z"},square:function(a){var b=Math.sqrt(a)/2;return"M"+ -b+","+ -b+"L"+b+","+ -b+" "+b+","+b+" "+ -b+","+b+"Z"},"triangle-down":function(a){var b=Math.sqrt(a/cD),c=b*cD/2;return"M0,"+c+"L"+b+","+ -c+" "+ -b+","+ -c+"Z"},"triangle-up":function(a){var b=Math.sqrt(a/cD),c=b*cD/2;return"M0,"+ -c+"L"+b+","+c+" "+ -b+","+c+"Z"}};d3.svg.symbolTypes=d3.keys(cC);var cD=Math.sqrt(3),cE=Math.tan(30*Math.PI/180);d3.svg.axis=function(){function j(j){j.each(function(k,l,m){function F(a){return j.delay?a.transition().delay(j[m][l].delay).duration(j[m][l].duration).ease(j.ease()):a}var n=d3.select(this),o=a.ticks.apply(a,g),p=h||a.tickFormat.apply(a,g),q=cH(a,o,i),r=n.selectAll(".minor").data(q,String),s=r.enter().insert("svg:line","g").attr("class","tick minor").style("opacity",1e-6),t=F(r.exit()).style("opacity",1e-6).remove(),u=F(r).style("opacity",1),v=n.selectAll("g").data(o,String),w=v.enter().insert("svg:g","path").style("opacity",1e-6),x=F(v.exit()).style("opacity",1e-6).remove(),y=F(v).style("opacity",1),z,A=a.range(),B=n.selectAll(".domain").data([]),C=B.enter().append("svg:path").attr("class","domain"),D=F(B),E=this.__chart__||a;this.__chart__=a.copy(),w.append("svg:line").attr("class","tick"),w.append("svg:text"),y.select("text").text(p);switch(b){case"bottom":z=cF,u.attr("y2",d),w.select("text").attr("dy",".71em").attr("text-anchor","middle"),y.select("line").attr("y2",c),y.select("text").attr("y",Math.max(c,0)+f),D.attr("d","M"+A[0]+","+e+"V0H"+A[1]+"V"+e);break;case"top":z=cF,u.attr("y2",-d),w.select("text").attr("text-anchor","middle"),y.select("line").attr("y2",-c),y.select("text").attr("y",-(Math.max(c,0)+f)),D.attr("d","M"+A[0]+","+ -e+"V0H"+A[1]+"V"+ -e);break;case"left":z=cG,u.attr("x2",-d),w.select("text").attr("dy",".32em").attr("text-anchor","end"),y.select("line").attr("x2",-c),y.select("text").attr("x",-(Math.max(c,0)+f)),D.attr("d","M"+ -e+","+A[0]+"H0V"+A[1]+"H"+ -e);break;case"right":z=cG,u.attr("x2",d),w.select("text").attr("dy",".32em"),y.select("line").attr("x2",c),y.select("text").attr("x",Math.max(c,0)+f),D.attr("d","M"+e+","+A[0]+"H0V"+A[1]+"H"+e)}w.call(z,E),y.call(z,a),x.call(z,a),s.call(z,E),u.call(z,a),t.call(z,a)})}var a=d3.scale.linear(),b="bottom",c=6,d=6,e=6,f=3,g=[10],h,i=0;j.scale=function(b){if(!arguments.length)return a;a=b;return j},j.orient=function(a){if(!arguments.length)return b;b=a;return j},j.ticks=function(){if(!arguments.length)return g;g=arguments;return j},j.tickFormat=function(a){if(!arguments.length)return h;h=a;return j},j.tickSize=function(a,b,f){if(!arguments.length)return c;var g=arguments.length-1;c=+a,d=g>1?+b:c,e=g>0?+arguments[g]:c;return j},j.tickPadding=function(a){if(!arguments.length)return f;f=+a;return j},j.tickSubdivide=function(a){if(!arguments.length)return i;i=+a;return j};return j},d3.behavior={},d3.behavior.drag=function(){function d(){c.apply(this,arguments),cN("dragstart")}function c(){cI=a,cK=cO((cJ=this).parentNode),cL=0,d3_behavior_dragArguments=arguments}function b(){this.on("mousedown.drag",d).on("touchstart.drag",d),d3.select(window).on("mousemove.drag",cP).on("touchmove.drag",cP).on("mouseup.drag",cQ,!0).on("touchend.drag",cQ,!0).on("click.drag",cR,!0)}var a=d3.dispatch("drag","dragstart","dragend");b.on=function(c,d){a[c].add(d);return b};return b};var cI,cJ,cK,cL,cM;d3.behavior.zoom=function(){function h(){d.apply(this,arguments);var b=dd(),c,e=Date.now();b.length===1&&e-cX<300&&di(1+Math.floor(a[2]),c=b[0],cW[c.identifier]),cX=e}function g(){d.apply(this,arguments);var b=d3.svg.mouse(c$);di(d3.event.shiftKey?Math.ceil(a[2]-1):Math.floor(a[2]+1),b,db(b))}function f(){d.apply(this,arguments),cV||(cV=db(d3.svg.mouse(c$))),di(dc()+a[2],d3.svg.mouse(c$),cV)}function e(){d.apply(this,arguments),cU=db(d3.svg.mouse(c$)),d3_behavior_zoomMoved=!1,d3.event.preventDefault(),window.focus()}function d(){cY=a,cZ=b.zoom.dispatch,c$=this,c_=arguments}function c(){this.on("mousedown.zoom",e).on("mousewheel.zoom",f).on("DOMMouseScroll.zoom",g).on("dblclick.zoom",g).on("touchstart.zoom",h),d3.select(window).on("mousemove.zoom",df).on("mouseup.zoom",dg).on("touchmove.zoom",de).on("touchend.zoom",dd).on("click.zoom",dh,!0)}var a=[0,0,0],b=d3.dispatch("zoom");c.on=function(a,d){b[a].add(d);return c};return c};var cT,cU,cV,cW={},cX=0,cY,cZ,c$,c_,da})()
<ide>\ No newline at end of file
<ide><path>src/core/array.js
<del>var d3_array = d3_arraySlice; // conversion for NodeLists
<del>
<del>function d3_arrayCopy(pseudoarray) {
<del> var i = -1, n = pseudoarray.length, array = [];
<del> while (++i < n) array.push(pseudoarray[i]);
<del> return array;
<del>}
<del>
<del>function d3_arraySlice(pseudoarray) {
<del> return Array.prototype.slice.call(pseudoarray);
<del>}
<del>
<del>try {
<del> d3_array(document.documentElement.childNodes)[0].nodeType;
<del>} catch(e) {
<del> d3_array = d3_arrayCopy;
<del>}
<del>
<ide> var d3_arraySubclass = [].__proto__?
<ide>
<ide> // Until ECMAScript supports array subclassing, prototype injection works well.
<ide><path>src/core/selectAll.js
<ide> d3.selectAll = function(selector) {
<ide> return typeof selector === "string"
<ide> ? d3_selectionRoot.selectAll(selector)
<del> : d3_selection([d3_array(selector)]); // assume node[]
<add> : d3_selection([selector]); // assume node[]
<ide> };
<ide><path>src/core/selection-append.js
<ide> d3_selectionPrototype.append = function(name) {
<ide> name = d3.ns.qualify(name);
<ide>
<del> function append(node) {
<del> return node.appendChild(document.createElement(name));
<add> function append() {
<add> return this.appendChild(document.createElement(name));
<ide> }
<ide>
<del> function appendNS(node) {
<del> return node.appendChild(document.createElementNS(name.space, name.local));
<add> function appendNS() {
<add> return this.appendChild(document.createElementNS(name.space, name.local));
<ide> }
<ide>
<ide> return this.select(name.local ? appendNS : append);
<ide><path>src/core/selection-enter-select.js
<ide> d3_selection_enterPrototype.select = function(selector) {
<ide> subgroup.parentNode = group.parentNode;
<ide> for (var i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroup.push(upgroup[i] = subnode = selector(group.parentNode));
<add> subgroup.push(upgroup[i] = subnode = selector.call(group.parentNode, node.__data__, i));
<ide> subnode.__data__ = node.__data__;
<ide> } else {
<ide> subgroup.push(null);
<ide><path>src/core/selection-insert.js
<ide> d3_selectionPrototype.insert = function(name, before) {
<ide> name = d3.ns.qualify(name);
<ide>
<del> function insert(node) {
<del> return node.insertBefore(
<add> function insert() {
<add> return this.insertBefore(
<ide> document.createElement(name),
<del> d3_select(before, node));
<add> d3_select(before, this));
<ide> }
<ide>
<del> function insertNS(node) {
<del> return node.insertBefore(
<add> function insertNS() {
<add> return this.insertBefore(
<ide> document.createElementNS(name.space, name.local),
<del> d3_select(before, node));
<add> d3_select(before, this));
<ide> }
<ide>
<ide> return this.select(name.local ? insertNS : insert);
<ide><path>src/core/selection-select.js
<ide> d3_selectionPrototype.select = function(selector) {
<ide> subgroup.parentNode = (group = this[j]).parentNode;
<ide> for (var i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroup.push(subnode = selector(node));
<add> subgroup.push(subnode = selector.call(node, node.__data__, i));
<ide> if (subnode && "__data__" in node) subnode.__data__ = node.__data__;
<ide> } else {
<ide> subgroup.push(null);
<ide> d3_selectionPrototype.select = function(selector) {
<ide> };
<ide>
<ide> function d3_selection_selector(selector) {
<del> return function(node) {
<del> return d3_select(selector, node);
<add> return function() {
<add> return d3_select(selector, this);
<ide> };
<ide> }
<ide><path>src/core/selection-selectAll.js
<ide> d3_selectionPrototype.selectAll = function(selector) {
<ide> for (var j = -1, m = this.length; ++j < m;) {
<ide> for (var group = this[j], i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroups.push(subgroup = selector(node));
<add> subgroups.push(subgroup = selector.call(node, node.__data__, i));
<ide> subgroup.parentNode = node;
<ide> }
<ide> }
<ide> d3_selectionPrototype.selectAll = function(selector) {
<ide> };
<ide>
<ide> function d3_selection_selectorAll(selector) {
<del> return function(node) {
<del> return d3_selectAll(selector, node);
<add> return function() {
<add> return d3_selectAll(selector, this);
<ide> };
<ide> }
<ide><path>src/core/selection.js
<ide> function d3_selection(groups) {
<ide> }
<ide>
<ide> var d3_select = function(s, n) { return n.querySelector(s); },
<del> d3_selectAll = function(s, n) { return d3_array(n.querySelectorAll(s)); };
<add> d3_selectAll = function(s, n) { return n.querySelectorAll(s); };
<ide>
<ide> // Prefer Sizzle, if available.
<ide> if (typeof Sizzle === "function") {
<ide><path>src/core/transition-select.js
<ide> d3_transitionPrototype.select = function(selector) {
<ide> for (var j = -1, m = this.length; ++j < m;) {
<ide> subgroups.push(subgroup = []);
<ide> for (var group = this[j], i = -1, n = group.length; ++i < n;) {
<del> if ((node = group[i]) && (subnode = selector(node.node))) {
<add> if ((node = group[i]) && (subnode = selector.call(node.node, node.node.__data__, i))) {
<ide> if ("__data__" in node.node) subnode.__data__ = node.node.__data__;
<ide> subgroup.push({node: subnode, delay: node.delay, duration: node.duration});
<ide> } else {
<ide><path>src/core/transition-selectAll.js
<ide> d3_transitionPrototype.selectAll = function(selector) {
<ide> for (var j = -1, m = this.length; ++j < m;) {
<ide> for (var group = this[j], i = -1, n = group.length; ++i < n;) {
<ide> if (node = group[i]) {
<del> subgroups.push(subgroup = selector(node.node));
<add> subgroups.push(subgroup = selector.call(node.node, node.node.__data__, i));
<ide> for (var k = -1, o = subgroup.length; ++k < o;) {
<ide> subgroup[k] = {node: subgroup[k], delay: node.delay, duration: node.duration};
<ide> }
<ide><path>src/svg/touches.js
<ide> d3.svg.touches = function(container) {
<ide> var touches = d3.event.touches;
<del> return touches ? d3_array(touches).map(function(touch) {
<add> return touches ? Array.prototype.map.call(touches, function(touch) {
<ide> var point = d3_svg_mousePoint(container, touch);
<ide> point.identifier = touch.identifier;
<ide> return point;
<ide><path>test/core/selection-select-test.js
<ide> suite.addBatch({
<ide> assert.equal(span[0][0], null);
<ide> assert.equal(span.length, 1);
<ide> assert.equal(span[0].length, 1);
<add> },
<add> "can select via function": function(body) {
<add> body.append("foo");
<add> var d = {}, dd = [], ii = [], tt = [],
<add> s = body.data([d]).select(function(d, i) { dd.push(d); ii.push(i); tt.push(this); return this.firstChild; });
<add> assert.deepEqual(dd, [d], "expected data, got {actual}");
<add> assert.deepEqual(ii, [0], "expected index, got {actual}");
<add> assert.domEqual(tt[0], document.body, "expected this, got {actual}");
<add> assert.domEqual(s[0][0], document.body.firstChild);
<add> delete document.body.__data__;
<ide> }
<ide> }
<ide> });
<ide> suite.addBatch({
<ide> assert.equal(span[0].length, 2);
<ide> assert.isTrue(span[0][0].parentNode === div[0][0]);
<ide> assert.isNull(span[0][1]);
<add> },
<add> "can select via function": function(div) {
<add> var dd = [], ii = [], tt = [],
<add> s = div.data(["a", "b"]).select(function(d, i) { dd.push(d); ii.push(i); tt.push(this); return this.firstChild; });
<add> assert.deepEqual(dd, ["a", "b"], "expected data, got {actual}");
<add> assert.deepEqual(ii, [0, 1], "expected index, got {actual}");
<add> assert.domEqual(tt[0], div[0][0], "expected this, got {actual}");
<add> assert.domEqual(tt[1], div[0][1], "expected this, got {actual}");
<add> assert.domEqual(s[0][0], div[0][0].firstChild);
<add> assert.domEqual(s[0][1], div[0][1].firstChild);
<ide> }
<ide> }
<ide> });
<ide><path>test/core/selection-selectAll-test.js
<ide> suite.addBatch({
<ide> var span = body.selectAll("span");
<ide> assert.equal(span.length, 1);
<ide> assert.equal(span[0].length, 0);
<add> },
<add> "can select via function": function(body) {
<add> var d = {}, dd = [], ii = [], tt = [],
<add> s = body.data([d]).selectAll(function(d, i) { dd.push(d); ii.push(i); tt.push(this); return this.childNodes; });
<add> assert.deepEqual(dd, [d], "expected data, got {actual}");
<add> assert.deepEqual(ii, [0], "expected index, got {actual}");
<add> assert.domEqual(tt[0], document.body, "expected this, got {actual}");
<add> assert.domEqual(s[0][0], document.body.firstChild);
<add> assert.domEqual(s[0][1], document.body.lastChild);
<add> delete document.body.__data__;
<ide> }
<ide> }
<ide> });
<ide> suite.addBatch({
<ide> assert.equal(span[0].length, 2);
<ide> assert.isTrue(span[0][0].parentNode === div[0][0]);
<ide> assert.isTrue(span[0][1].parentNode === div[0][0]);
<add> },
<add> "can select via function": function(div) {
<add> var dd = [], ii = [], tt = [],
<add> s = div.data(["a", "b"]).selectAll(function(d, i) { dd.push(d); ii.push(i); tt.push(this); return this.childNodes; });
<add> assert.deepEqual(dd, ["a", "b"], "expected data, got {actual}");
<add> assert.deepEqual(ii, [0, 1], "expected index, got {actual}");
<add> assert.domEqual(tt[0], div[0][0], "expected this, got {actual}");
<add> assert.domEqual(tt[1], div[0][1], "expected this, got {actual}");
<add> assert.domEqual(s[0][0], div[0][0].firstChild);
<add> assert.domEqual(s[0][1], div[0][0].lastChild);
<add> assert.domEqual(s[1][0], div[0][1].firstChild);
<add> assert.domEqual(s[1][1], div[0][1].lastChild);
<ide> }
<ide> }
<ide> }); | 15 |
Javascript | Javascript | make my code prettier | dbbf30a332936b67c88631d7ccc2ba5d32114ec5 | <ide><path>src/devtools/views/WarnIfLegacyBackendDetected.js
<ide> export default function WarnIfLegacyBackendDetected(_: {||}) {
<ide> dispatch({
<ide> canBeDismissed: false,
<ide> type: 'SHOW',
<del> title:
<del> 'DevTools v4 is incompatible with this version of React',
<add> title: 'DevTools v4 is incompatible with this version of React',
<ide> content: <InvalidBackendDetected />,
<ide> });
<ide> | 1 |
PHP | PHP | fix bug in router | 87ee594cd3a164491aea13e209762b4a10c308a6 | <ide><path>src/Illuminate/Routing/Route.php
<ide> protected static function compileParameters($value, array $wheres = array())
<ide> {
<ide> $value = static::compileWhereParameters($value, $wheres);
<ide>
<del> return preg_replace('/\{([\w\-]+)\}/', static::$wildcard, $value);
<add> return preg_replace('/\{([A-Za-z\-\_]+)\}/', static::$wildcard, $value);
<ide> }
<ide>
<ide> /**
<ide> protected static function compileOptional($value, $wheres = array())
<ide> */
<ide> protected static function compileStandardOptional($value, $custom = 0)
<ide> {
<del> $value = preg_replace('/\/\{([\w\-]+)\?\}/', static::$optional, $value, -1, $count);
<add> $value = preg_replace('/\/\{([A-Za-z\-\_]+)\?\}/', static::$optional, $value, -1, $count);
<ide>
<del> $value = preg_replace('/^(\{([\w\-]+)\?\})/', static::$leadingOptional, $value, -1, $leading);
<add> $value = preg_replace('/^(\{([A-Za-z\-\_]+)\?\})/', static::$leadingOptional, $value, -1, $leading);
<ide>
<ide> $total = $leading + $count + $custom;
<ide> | 1 |
Text | Text | improve child_process `maxbuffer` text | ea5a2f4e2b477ae589a22ffc0659e244491c7d05 | <ide><path>doc/api/child_process.md
<ide> added: v0.1.90
<ide> understand the `-c` switch on UNIX or `/d /s /c` on Windows. On Windows,
<ide> command line parsing should be compatible with `cmd.exe`.)
<ide> * `timeout` {number} (Default: `0`)
<del> * [`maxBuffer`][] {number} largest amount of data (in bytes) allowed on
<del> stdout or stderr - if exceeded child process is killed (Default: `200*1024`)
<add> * `maxBuffer` {number} Largest amount of data in bytes allowed on stdout or
<add> stderr. (Default: `200*1024`) If exceeded, the child process is terminated.
<add> See caveat at [`maxBuffer` and Unicode][].
<ide> * `killSignal` {string|integer} (Default: `'SIGTERM'`)
<ide> * `uid` {number} Sets the user identity of the process. (See setuid(2).)
<ide> * `gid` {number} Sets the group identity of the process. (See setgid(2).)
<ide> added: v0.1.91
<ide> * `env` {Object} Environment key-value pairs
<ide> * `encoding` {string} (Default: `'utf8'`)
<ide> * `timeout` {number} (Default: `0`)
<del> * [`maxBuffer`][] {number} largest amount of data (in bytes) allowed on
<del> stdout or stderr - if exceeded child process is killed (Default: `200*1024`)
<add> * `maxBuffer` {number} Largest amount of data in bytes allowed on stdout or
<add> stderr. (Default: `200*1024`) If exceeded, the child process is terminated.
<add> See caveat at [`maxBuffer` and Unicode][].
<ide> * `killSignal` {string|integer} (Default: `'SIGTERM'`)
<ide> * `uid` {number} Sets the user identity of the process. (See setuid(2).)
<ide> * `gid` {number} Sets the group identity of the process. (See setgid(2).)
<ide> changes:
<ide> is allowed to run. (Default: `undefined`)
<ide> * `killSignal` {string|integer} The signal value to be used when the spawned
<ide> process will be killed. (Default: `'SIGTERM'`)
<del> * [`maxBuffer`][] {number} largest amount of data (in bytes) allowed on
<del> stdout or stderr - if exceeded child process is killed
<add> * `maxBuffer` {number} Largest amount of data in bytes allowed on stdout or
<add> stderr. (Default: `200*1024`) If exceeded, the child process is terminated.
<add> See caveat at [`maxBuffer` and Unicode][].
<ide> * `encoding` {string} The encoding used for all stdio inputs and outputs. (Default: `'buffer'`)
<ide> * Returns: {Buffer|string} The stdout from the command
<ide>
<ide> changes:
<ide> is allowed to run. (Default: `undefined`)
<ide> * `killSignal` {string|integer} The signal value to be used when the spawned
<ide> process will be killed. (Default: `'SIGTERM'`)
<del> * [`maxBuffer`][] {number} largest amount of data (in bytes) allowed on
<del> stdout or stderr - if exceeded child process is killed
<add> * `maxBuffer` {number} Largest amount of data in bytes allowed on stdout or
<add> stderr. (Default: `200*1024`) If exceeded, the child process is terminated.
<add> See caveat at [`maxBuffer` and Unicode][].
<ide> * `encoding` {string} The encoding used for all stdio inputs and outputs.
<ide> (Default: `'buffer'`)
<ide> * Returns: {Buffer|string} The stdout from the command
<ide> changes:
<ide> is allowed to run. (Default: `undefined`)
<ide> * `killSignal` {string|integer} The signal value to be used when the spawned
<ide> process will be killed. (Default: `'SIGTERM'`)
<del> * [`maxBuffer`][] {number} largest amount of data (in bytes) allowed on
<del> stdout or stderr - if exceeded child process is killed
<add> * `maxBuffer` {number} Largest amount of data in bytes allowed on stdout or
<add> stderr. (Default: `200*1024`) If exceeded, the child process is terminated.
<add> See caveat at [`maxBuffer` and Unicode][].
<ide> * `encoding` {string} The encoding used for all stdio inputs and outputs.
<ide> (Default: `'buffer'`)
<ide> * `shell` {boolean|string} If `true`, runs `command` inside of a shell. Uses
<ide> to `stdout` although there are only 4 characters.
<ide> [`Error`]: errors.html#errors_class_error
<ide> [`EventEmitter`]: events.html#events_class_eventemitter
<ide> [`JSON.stringify()`]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON/stringify
<del>[`maxBuffer`]: #child_process_maxbuffer_and_unicode
<add>[`maxBuffer` and Unicode]: #child_process_maxbuffer_and_unicode
<ide> [`net.Server`]: net.html#net_class_net_server
<ide> [`net.Socket`]: net.html#net_class_net_socket
<ide> [`options.detached`]: #child_process_options_detached | 1 |
PHP | PHP | fix tests for windows environments | 7c699802c00e798f8f9f2874d19eea6e4ea0124c | <ide><path>tests/Integration/Mail/RenderingMailWithLocaleTest.php
<ide> public function testMailableRendersInDefaultLocale()
<ide> {
<ide> $mail = new RenderedTestMail;
<ide>
<del> $this->assertEquals("name\n", $mail->render());
<add> $this->assertEquals('name'.PHP_EOL, $mail->render());
<ide> }
<ide>
<ide> public function testMailableRendersInSelectedLocale()
<ide> {
<ide> $mail = (new RenderedTestMail)->locale('es');
<ide>
<del> $this->assertEquals("nombre\n", $mail->render());
<add> $this->assertEquals('nombre'.PHP_EOL, $mail->render());
<ide> }
<ide>
<ide> public function testMailableRendersInAppSelectedLocale()
<ide> public function testMailableRendersInAppSelectedLocale()
<ide>
<ide> $mail = new RenderedTestMail;
<ide>
<del> $this->assertEquals("nombre\n", $mail->render());
<add> $this->assertEquals('nombre'.PHP_EOL, $mail->render());
<ide> }
<ide> }
<ide> | 1 |
Python | Python | improve the docstring on @action | f744da74d2878b480220ebaf9d8117ff9b79a947 | <ide><path>rest_framework/decorators.py
<ide> def action(methods=None, detail=None, url_path=None, url_name=None, **kwargs):
<ide> """
<ide> Mark a ViewSet method as a routable action.
<ide>
<del> Set the `detail` boolean to determine if this action should apply to
<del> instance/detail requests or collection/list requests.
<add> `@action`-decorated functions will be endowed with a `mapping` property,
<add> a `MethodMapper` that can be used to add additional method-based behaviors
<add> on the routed action.
<add>
<add> :param methods: A list of HTTP method names this action responds to.
<add> Defaults to GET only.
<add> :param detail: Required. Determines whether this action applies to
<add> instance/detail requests or collection/list requests.
<add> :param url_path: Define the URL segment for this action. Defaults to the
<add> name of the method decorated.
<add> :param url_name: Define the internal (`reverse`) URL name for this action.
<add> Defaults to the name of the method decorated with underscores
<add> replaced with dashes.
<add> :param kwargs: Additional properties to set on the view. This can be used
<add> to override viewset-level *_classes settings, equivalent to
<add> how the `@renderer_classes` etc. decorators work for function-
<add> based API views.
<ide> """
<ide> methods = ['get'] if (methods is None) else methods
<ide> methods = [method.lower() for method in methods]
<ide> def decorator(func):
<ide> func.detail = detail
<ide> func.url_path = url_path if url_path else func.__name__
<ide> func.url_name = url_name if url_name else func.__name__.replace('_', '-')
<add>
<add> # These kwargs will end up being passed to `ViewSet.as_view()` within
<add> # the router, which eventually delegates to Django's CBV `View`,
<add> # which assigns them as instance attributes for each request.
<ide> func.kwargs = kwargs
<ide>
<ide> # Set descriptive arguments for viewsets | 1 |
Java | Java | preserve shadowed fields in directfieldaccessor | 0e9e63e082493746657b060ead07c624b1a52e19 | <ide><path>org.springframework.beans/src/main/java/org/springframework/beans/DirectFieldAccessor.java
<ide> /*
<del> * Copyright 2002-2010 the original author or authors.
<add> * Copyright 2002-2011 the original author or authors.
<ide> *
<ide> * Licensed under the Apache License, Version 2.0 (the "License");
<ide> * you may not use this file except in compliance with the License.
<ide> public class DirectFieldAccessor extends AbstractPropertyAccessor {
<ide> * Create a new DirectFieldAccessor for the given target object.
<ide> * @param target the target object to access
<ide> */
<del> public DirectFieldAccessor(Object target) {
<add> public DirectFieldAccessor(final Object target) {
<ide> Assert.notNull(target, "Target object must not be null");
<ide> this.target = target;
<ide> ReflectionUtils.doWithFields(this.target.getClass(), new ReflectionUtils.FieldCallback() {
<ide> public void doWith(Field field) {
<del> fieldMap.put(field.getName(), field);
<add> if (fieldMap.containsKey(field.getName())) {
<add> // ignore superclass declarations of fields already found in a subclass
<add> } else {
<add> fieldMap.put(field.getName(), field);
<add> }
<ide> }
<ide> });
<ide> this.typeConverterDelegate = new TypeConverterDelegate(this, target);
<ide> public boolean isWritableProperty(String propertyName) throws BeansException {
<ide> }
<ide>
<ide> @Override
<del> public Class getPropertyType(String propertyName) throws BeansException {
<add> public Class<?> getPropertyType(String propertyName) throws BeansException {
<ide> Field field = this.fieldMap.get(propertyName);
<ide> if (field != null) {
<ide> return field.getType();
<ide><path>org.springframework.beans/src/test/java/org/springframework/beans/DirectFieldAccessorTests.java
<add>/*
<add> * Copyright 2002-2011 the original author or authors.
<add> *
<add> * Licensed under the Apache License, Version 2.0 (the "License");
<add> * you may not use this file except in compliance with the License.
<add> * You may obtain a copy of the License at
<add> *
<add> * http://www.apache.org/licenses/LICENSE-2.0
<add> *
<add> * Unless required by applicable law or agreed to in writing, software
<add> * distributed under the License is distributed on an "AS IS" BASIS,
<add> * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
<add> * See the License for the specific language governing permissions and
<add> * limitations under the License.
<add> */
<add>
<add>package org.springframework.beans;
<add>
<add>import static org.junit.Assert.assertEquals;
<add>
<add>import javax.swing.JPanel;
<add>import javax.swing.JTextField;
<add>
<add>import org.junit.Test;
<add>
<add>/**
<add> * Unit tests for {@link DirectFieldAccessor}
<add> *
<add> * @author Jose Luis Martin
<add> * @author Chris Beams
<add> */
<add>public class DirectFieldAccessorTests {
<add>
<add> @Test
<add> public void withShadowedField() throws Exception {
<add> @SuppressWarnings("serial")
<add> JPanel p = new JPanel() {
<add> @SuppressWarnings("unused")
<add> JTextField name = new JTextField();
<add> };
<add>
<add> DirectFieldAccessor dfa = new DirectFieldAccessor(p);
<add> assertEquals(JTextField.class, dfa.getPropertyType("name"));
<add> }
<add>} | 2 |
Python | Python | fix tf.name_scope support for keras nested layers | 9733982976f62c351ea3db2aeb1f28a67bb5d2fa | <ide><path>keras/engine/base_layer.py
<ide> import tensorflow.compat.v2 as tf
<ide>
<ide> import collections
<add>import contextlib
<ide> import copy
<ide> import functools
<ide> import itertools
<ide>
<ide> _is_name_scope_on_model_declaration_enabled = False
<ide>
<add>_name_scope_unnester_stack = threading.local()
<add>
<add>
<add>@contextlib.contextmanager
<add>def _name_scope_unnester(full_name_scope):
<add> """Helper to get relative name scope from fully specified nested name scopes.
<add>
<add> Args:
<add> full_name_scope: full(absolute) name scope path.
<add>
<add> Yields:
<add> Relative name scope path from the parent `_name_scope_unnester` context
<add> manager.
<add>
<add> Example:
<add> ```
<add> with _name_scope_unnester('a') as name1: # name1 == 'a'
<add> with _name_scope_unnester('a/b') as name2: # name2 == 'b'
<add> with _name_scope_unnester('a/b/c') as name3: # name3 == 'c'
<add> pass
<add> ```
<add> """
<add> if not getattr(_name_scope_unnester_stack, 'value', None):
<add> _name_scope_unnester_stack.value = ['']
<add>
<add> _name_scope_unnester_stack.value.append(full_name_scope)
<add>
<add> try:
<add> full_name_scope = _name_scope_unnester_stack.value[-1]
<add> outer_name_scope = _name_scope_unnester_stack.value[-2]
<add> relative_name_scope = full_name_scope.lstrip(outer_name_scope)
<add> relative_name_scope = relative_name_scope.lstrip('/')
<add> yield relative_name_scope
<add> finally:
<add> _name_scope_unnester_stack.value.pop()
<add>
<ide>
<ide> @keras_export('keras.layers.Layer')
<ide> class Layer(tf.Module, version_utils.LayerVersionSelector):
<ide> def __init__(self,
<ide>
<ide> # Save outer name scope at layer declaration so that it is preserved at
<ide> # the actual layer construction.
<del> self._outer_name_scope = tf.get_current_name_scope()
<add> self._name_scope_on_declaration = tf.get_current_name_scope()
<ide>
<ide> @tf.__internal__.tracking.no_automatic_dependency_tracking
<ide> @generic_utils.default
<ide> def __call__(self, *args, **kwargs):
<ide> training=training_mode):
<ide>
<ide> input_spec.assert_input_compatibility(self.input_spec, inputs, self.name)
<add>
<ide> if eager:
<ide> call_fn = self.call
<ide> name_scope = self._name
<ide> else:
<del> name_scope = self._name_scope() # Avoid autoincrementing. # pylint: disable=not-callable
<add> name_scope = self._get_unnested_name_scope()
<ide> call_fn = self._autographed_call()
<add>
<ide> call_fn = traceback_utils.inject_argument_info_in_traceback(
<ide> call_fn,
<ide> object_name=f'layer "{self.name}" (type {self.__class__.__name__})')
<add> with contextlib.ExitStack() as namescope_stack:
<add> if _is_name_scope_on_model_declaration_enabled:
<add> namescope_stack.enter_context(_name_scope_unnester(
<add> self._name_scope_on_declaration))
<add> namescope_stack.enter_context(tf.name_scope(name_scope))
<ide>
<del> with tf.name_scope(name_scope):
<ide> if not self.built:
<ide> self._maybe_build(inputs)
<ide>
<ide> def __call__(self, *args, **kwargs):
<ide>
<ide> return outputs
<ide>
<add> def _get_unnested_name_scope(self):
<add> if _is_name_scope_on_model_declaration_enabled:
<add> with _name_scope_unnester(self._name_scope_on_declaration
<add> ) as relative_name_scope_on_declaration:
<add> # To avoid `tf.name_scope` autoincrement, use absolute path.
<add> relative_name_scope = filter(
<add> None,
<add> [tf.get_current_name_scope(), relative_name_scope_on_declaration])
<add> current_name_scope = '/'.join(relative_name_scope) + '/'
<add> with tf.name_scope(current_name_scope):
<add> name_scope = self._name_scope() # Avoid autoincrementing. # pylint: disable=not-callable
<add> else:
<add> name_scope = self._name_scope()
<add>
<add> return name_scope
<add>
<ide> def _functional_construction_call(self, inputs, args, kwargs, input_list):
<ide> call_context = base_layer_utils.call_context()
<ide>
<ide> def _name_scope(self): # pylint: disable=method-hidden
<ide> if not tf.__internal__.tf2.enabled():
<ide> return self.name
<ide> name_scope = self.name
<del> if _is_name_scope_on_model_declaration_enabled and self._outer_name_scope:
<del> name_scope = self._outer_name_scope + '/' + name_scope
<ide> current_name_scope = tf.__internal__.get_name_scope()
<ide> if current_name_scope:
<ide> name_scope = current_name_scope + '/' + name_scope
<ide><path>keras/engine/base_layer_test.py
<ide> def test_apply_name_scope_on_model_declaration(self):
<ide> ])
<ide> base_layer._apply_name_scope_on_model_declaration(False)
<ide>
<add> @testing_utils.run_v2_only
<add> def test_apply_name_scope_on_nested_layer_model_declaration(self):
<add> if not tf.executing_eagerly():
<add> self.skipTest('`apply_name_scope_on_model_declaration` API is supported'
<add> ' only for V2 eager')
<add>
<add> base_layer._apply_name_scope_on_model_declaration(True)
<add>
<add> class ThreeDenses(layers.Layer):
<add>
<add> def __init__(self, name='ThreeDenses', **kwargs):
<add> super().__init__(name=name, **kwargs)
<add> self.inner_dense_1 = layers.Dense(10, name='NestedDense1')
<add> with tf.name_scope('inner1/inner2'):
<add> self.inner_dense_2 = layers.Dense(20, name='NestedDense2')
<add> self.inner_dense_3 = layers.Dense(30, name='NestedDense3')
<add>
<add> def call(self, x):
<add> x = self.inner_dense_1(x)
<add> x = self.inner_dense_2(x)
<add> x = self.inner_dense_3(x)
<add> return x
<add>
<add> inputs = input_layer.Input((3,))
<add> with tf.name_scope('outer'):
<add> x = ThreeDenses()(inputs)
<add> outputs = layers.Dense(10, name='OuterDense')(x)
<add>
<add> model = training_lib.Model(inputs, outputs)
<add> node_names = self._get_model_node_names(model, np.random.random((1, 3)),
<add> 'call_scope')
<add>
<add> self.assertListEqual(node_names, [
<add> 'call_scope/Const', 'call_scope/model/Cast',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense1/MatMul/ReadVariableOp/resource',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense1/MatMul/ReadVariableOp',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense1/MatMul',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense1/BiasAdd/ReadVariableOp/resource',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense1/BiasAdd/ReadVariableOp',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense1/BiasAdd',
<add> 'call_scope/model/outer/ThreeDenses/inner1/inner2/NestedDense2/MatMul/ReadVariableOp/resource',
<add> 'call_scope/model/outer/ThreeDenses/inner1/inner2/NestedDense2/MatMul/ReadVariableOp',
<add> 'call_scope/model/outer/ThreeDenses/inner1/inner2/NestedDense2/MatMul',
<add> 'call_scope/model/outer/ThreeDenses/inner1/inner2/NestedDense2/BiasAdd/ReadVariableOp/resource',
<add> 'call_scope/model/outer/ThreeDenses/inner1/inner2/NestedDense2/BiasAdd/ReadVariableOp',
<add> 'call_scope/model/outer/ThreeDenses/inner1/inner2/NestedDense2/BiasAdd',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense3/MatMul/ReadVariableOp/resource',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense3/MatMul/ReadVariableOp',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense3/MatMul',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense3/BiasAdd/ReadVariableOp/resource',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense3/BiasAdd/ReadVariableOp',
<add> 'call_scope/model/outer/ThreeDenses/NestedDense3/BiasAdd',
<add> 'call_scope/model/OuterDense/MatMul/ReadVariableOp/resource',
<add> 'call_scope/model/OuterDense/MatMul/ReadVariableOp',
<add> 'call_scope/model/OuterDense/MatMul',
<add> 'call_scope/model/OuterDense/BiasAdd/ReadVariableOp/resource',
<add> 'call_scope/model/OuterDense/BiasAdd/ReadVariableOp',
<add> 'call_scope/model/OuterDense/BiasAdd', 'Identity', 'NoOp'
<add> ])
<add> base_layer._apply_name_scope_on_model_declaration(False)
<add>
<ide> def _get_model_node_names(self, model, inputs, call_name_scope):
<ide> """Returns a list of model's node names."""
<ide> | 2 |
Javascript | Javascript | fix some ordering issues with transitions | 4356e4fb21538d982ff5eba750021207ffd3e53d | <ide><path>d3.chart.js
<ide> d3.chart.box = function() {
<ide> var center = g.selectAll("line.center")
<ide> .data(whiskerData ? [whiskerData] : []);
<ide>
<del> center.enter().append("svg:line")
<add> center.enter().insert("svg:line", "rect")
<ide> .attr("class", "center")
<ide> .attr("x1", width / 2)
<ide> .attr("y1", function(d) { return x0(d[0]); })
<ide> d3.chart.box = function() {
<ide> var whisker = g.selectAll("line.whisker")
<ide> .data(whiskerData || []);
<ide>
<del> whisker.enter().append("svg:line")
<add> whisker.enter().insert("svg:line", "circle, text")
<ide> .attr("class", "whisker")
<ide> .attr("x1", 0)
<ide> .attr("y1", x0)
<ide> d3.chart.box = function() {
<ide> var outlier = g.selectAll("circle.outlier")
<ide> .data(outlierIndices, Number);
<ide>
<del> outlier.enter().append("svg:circle")
<add> outlier.enter().insert("svg:circle", "text")
<ide> .attr("class", "outlier")
<ide> .attr("r", 5)
<ide> .attr("cx", width / 2)
<ide><path>d3.chart.min.js
<del>(function(){function g(a){var b=a(0);return function(c){return Math.abs(a(c)-b)}}function f(a){return function(b){return"translate("+a(b)+",0)"}}function e(a){return a.measures}function d(a){return a.markers}function c(a){return a.ranges}function b(a){var b=a.length;return[.25,.5,.75].map(function(c){c*=b;return~~c===c?(a[c]+a[c+1])/2:a[Math.round(c)]})}function a(a){return[0,a.length-1]}d3.chart={},d3.chart.box=function(){function k(a){a.each(function(a,b){a=a.map(g).sort(d3.ascending);var k=d3.select(this),l=a.length,m=a[0],n=a[l-1],o=a.quartiles=i(a),p=h&&h.call(this,a,b),q=p&&p.map(function(b){return a[b]}),r=p?d3.range(0,p[0]).concat(d3.range(p[1]+1,l)):d3.range(l),s=d3.scale.linear().domain(f&&f.call(this,a,b)||[m,n]).range([d,0]),t=this.__chart__||d3.scale.linear().domain([0,Infinity]).range(s.range());this.__chart__=s;var u=k.selectAll("line.center").data(q?[q]:[]);u.enter().append("svg:line").attr("class","center").attr("x1",c/2).attr("y1",function(a){return t(a[0])}).attr("x2",c/2).attr("y2",function(a){return t(a[1])}).style("opacity",1e-6).transition().duration(e).style("opacity",1).attr("y1",function(a){return s(a[0])}).attr("y2",function(a){return s(a[1])}),u.transition().duration(e).style("opacity",1).attr("y1",function(a){return s(a[0])}).attr("y2",function(a){return s(a[1])}),u.exit().transition().duration(e).style("opacity",1e-6).attr("y1",function(a){return s(a[0])}).attr("y2",function(a){return s(a[1])}).remove();var v=k.selectAll("rect.box").data([o]);v.enter().append("svg:rect").attr("class","box").attr("x",0).attr("y",function(a){return t(a[2])}).attr("width",c).attr("height",function(a){return t(a[0])-t(a[2])}).transition().duration(e).attr("y",function(a){return s(a[2])}).attr("height",function(a){return s(a[0])-s(a[2])}),v.transition().duration(e).attr("y",function(a){return s(a[2])}).attr("height",function(a){return s(a[0])-s(a[2])});var w=k.selectAll("line.median").data([o[1]]);w.enter().append("svg:line").attr("class","median").attr("x1",0).attr("y1",t).attr("x2",c).attr("y2",t).transition().duration(e).attr("y1",s).attr("y2",s),w.transition().duration(e).attr("y1",s).attr("y2",s);var x=k.selectAll("line.whisker").data(q||[]);x.enter().append("svg:line").attr("class","whisker").attr("x1",0).attr("y1",t).attr("x2",c).attr("y2",t).style("opacity",1e-6).transition().duration(e).attr("y1",s).attr("y2",s).style("opacity",1),x.transition().duration(e).attr("y1",s).attr("y2",s).style("opacity",1),x.exit().transition().duration(e).attr("y1",s).attr("y2",s).style("opacity",1e-6).remove();var y=k.selectAll("circle.outlier").data(r,Number);y.enter().append("svg:circle").attr("class","outlier").attr("r",5).attr("cx",c/2).attr("cy",function(b){return t(a[b])}).style("opacity",1e-6).transition().duration(e).attr("cy",function(b){return s(a[b])}).style("opacity",1),y.transition().duration(e).attr("cy",function(b){return s(a[b])}).style("opacity",1),y.exit().transition().duration(e).attr("cy",function(b){return s(a[b])}).style("opacity",1e-6).remove();var z=j||s.tickFormat(8),A=k.selectAll("text.box").data(o);A.enter().append("svg:text").attr("class","box").attr("dy",".3em").attr("dx",function(a,b){return b&1?6:-6}).attr("x",function(a,b){return b&1?c:0}).attr("y",t).attr("text-anchor",function(a,b){return b&1?"start":"end"}).text(z).transition().duration(e).attr("y",s),A.transition().duration(e).text(z).attr("y",s);var B=k.selectAll("text.whisker").data(q||[]);B.enter().append("svg:text").attr("class","whisker").attr("dy",".3em").attr("dx",6).attr("x",c).attr("y",t).text(z).style("opacity",1e-6).transition().duration(e).attr("y",s).style("opacity",1),B.transition().duration(e).text(z).attr("y",s).style("opacity",1),B.exit().transition().duration(e).attr("y",s).style("opacity",1e-6).remove()})}var c=1,d=1,e=0,f=null,g=Number,h=a,i=b,j=null;k.width=function(a){if(!arguments.length)return c;c=a;return k},k.height=function(a){if(!arguments.length)return d;d=a;return k},k.tickFormat=function(a){if(!arguments.length)return j;j=a;return k},k.duration=function(a){if(!arguments.length)return e;e=a;return k},k.domain=function(a){if(!arguments.length)return f;f=a==null?a:d3.functor(a);return k},k.value=function(a){if(!arguments.length)return g;g=a;return k},k.whiskers=function(a){if(!arguments.length)return h;h=a;return k},k.quartiles=function(a){if(!arguments.length)return i;i=a;return k};return k},d3.chart.bullet=function(){function o(a){a.each(function(a,c){var d=i.call(this,a,c).slice().sort(d3.descending),e=j.call(this,a,c).slice().sort(d3.descending),o=k.call(this,a,c).slice().sort(d3.descending),p=d3.select(this),q=d3.scale.linear().domain([0,Math.max(d[0],e[0],o[0])]).range(b?[l,0]:[0,l]),r=this.__chart__||d3.scale.linear().domain([0,Infinity]).range(q.range());this.__chart__=q;var s=g(r),t=g(q),u=p.selectAll("rect.range").data(d);u.enter().append("svg:rect").attr("class",function(a,b){return"range s"+b}).attr("width",s).attr("height",m).attr("x",b?r:0).transition().duration(h).attr("width",t).attr("x",b?q:0),u.transition().duration(h).attr("x",b?q:0).attr("width",t).attr("height",m);var v=p.selectAll("rect.measure").data(o);v.enter().append("svg:rect").attr("class",function(a,b){return"measure s"+b}).attr("width",s).attr("height",m/3).attr("x",b?r:0).attr("y",m/3).transition().duration(h).attr("width",t).attr("x",b?q:0),v.transition().duration(h).attr("width",t).attr("height",m/3).attr("x",b?q:0).attr("y",m/3);var w=p.selectAll("line.marker").data(e);w.enter().append("svg:line").attr("class","marker").attr("x1",r).attr("x2",r).attr("y1",m/6).attr("y2",m*5/6).transition().duration(h).attr("x1",q).attr("x2",q),w.transition().duration(h).attr("x1",q).attr("x2",q).attr("y1",m/6).attr("y2",m*5/6);var x=n||q.tickFormat(8),y=p.selectAll("g.tick").data(q.ticks(8),function(a){return this.textContent||x(a)}),z=y.enter().append("svg:g").attr("class","tick").attr("transform",f(r)).attr("opacity",1e-6);z.append("svg:line").attr("y1",m).attr("y2",m*7/6),z.append("svg:text").attr("text-anchor","middle").attr("dy","1em").attr("y",m*7/6).text(x),z.transition().duration(h).attr("transform",f(q)).attr("opacity",1);var A=y.transition().duration(h).attr("transform",f(q)).attr("opacity",1);A.select("line").attr("y1",m).attr("y2",m*7/6),A.select("text").attr("y",m*7/6),y.exit().transition().duration(h).attr("transform",f(q)).attr("opacity",1e-6).remove()})}var a="left",b=!1,h=0,i=c,j=d,k=e,l=380,m=30,n=null;o.orient=function(c){if(!arguments.length)return a;a=c,b=a=="right"||a=="bottom";return o},o.ranges=function(a){if(!arguments.length)return i;i=a;return o},o.markers=function(a){if(!arguments.length)return j;j=a;return o},o.measures=function(a){if(!arguments.length)return k;k=a;return o},o.width=function(a){if(!arguments.length)return l;l=a;return o},o.height=function(a){if(!arguments.length)return m;m=a;return o},o.tickFormat=function(a){if(!arguments.length)return n;n=a;return o},o.duration=function(a){if(!arguments.length)return h;h=a;return o};return o}})()
<ide>\ No newline at end of file
<add>(function(){function g(a){var b=a(0);return function(c){return Math.abs(a(c)-b)}}function f(a){return function(b){return"translate("+a(b)+",0)"}}function e(a){return a.measures}function d(a){return a.markers}function c(a){return a.ranges}function b(a){var b=a.length;return[.25,.5,.75].map(function(c){c*=b;return~~c===c?(a[c]+a[c+1])/2:a[Math.round(c)]})}function a(a){return[0,a.length-1]}d3.chart={},d3.chart.box=function(){function k(a){a.each(function(a,b){a=a.map(g).sort(d3.ascending);var k=d3.select(this),l=a.length,m=a[0],n=a[l-1],o=a.quartiles=i(a),p=h&&h.call(this,a,b),q=p&&p.map(function(b){return a[b]}),r=p?d3.range(0,p[0]).concat(d3.range(p[1]+1,l)):d3.range(l),s=d3.scale.linear().domain(f&&f.call(this,a,b)||[m,n]).range([d,0]),t=this.__chart__||d3.scale.linear().domain([0,Infinity]).range(s.range());this.__chart__=s;var u=k.selectAll("line.center").data(q?[q]:[]);u.enter().insert("svg:line","rect").attr("class","center").attr("x1",c/2).attr("y1",function(a){return t(a[0])}).attr("x2",c/2).attr("y2",function(a){return t(a[1])}).style("opacity",1e-6).transition().duration(e).style("opacity",1).attr("y1",function(a){return s(a[0])}).attr("y2",function(a){return s(a[1])}),u.transition().duration(e).style("opacity",1).attr("y1",function(a){return s(a[0])}).attr("y2",function(a){return s(a[1])}),u.exit().transition().duration(e).style("opacity",1e-6).attr("y1",function(a){return s(a[0])}).attr("y2",function(a){return s(a[1])}).remove();var v=k.selectAll("rect.box").data([o]);v.enter().append("svg:rect").attr("class","box").attr("x",0).attr("y",function(a){return t(a[2])}).attr("width",c).attr("height",function(a){return t(a[0])-t(a[2])}).transition().duration(e).attr("y",function(a){return s(a[2])}).attr("height",function(a){return s(a[0])-s(a[2])}),v.transition().duration(e).attr("y",function(a){return s(a[2])}).attr("height",function(a){return s(a[0])-s(a[2])});var w=k.selectAll("line.median").data([o[1]]);w.enter().append("svg:line").attr("class","median").attr("x1",0).attr("y1",t).attr("x2",c).attr("y2",t).transition().duration(e).attr("y1",s).attr("y2",s),w.transition().duration(e).attr("y1",s).attr("y2",s);var x=k.selectAll("line.whisker").data(q||[]);x.enter().insert("svg:line","circle, text").attr("class","whisker").attr("x1",0).attr("y1",t).attr("x2",c).attr("y2",t).style("opacity",1e-6).transition().duration(e).attr("y1",s).attr("y2",s).style("opacity",1),x.transition().duration(e).attr("y1",s).attr("y2",s).style("opacity",1),x.exit().transition().duration(e).attr("y1",s).attr("y2",s).style("opacity",1e-6).remove();var y=k.selectAll("circle.outlier").data(r,Number);y.enter().insert("svg:circle","text").attr("class","outlier").attr("r",5).attr("cx",c/2).attr("cy",function(b){return t(a[b])}).style("opacity",1e-6).transition().duration(e).attr("cy",function(b){return s(a[b])}).style("opacity",1),y.transition().duration(e).attr("cy",function(b){return s(a[b])}).style("opacity",1),y.exit().transition().duration(e).attr("cy",function(b){return s(a[b])}).style("opacity",1e-6).remove();var z=j||s.tickFormat(8),A=k.selectAll("text.box").data(o);A.enter().append("svg:text").attr("class","box").attr("dy",".3em").attr("dx",function(a,b){return b&1?6:-6}).attr("x",function(a,b){return b&1?c:0}).attr("y",t).attr("text-anchor",function(a,b){return b&1?"start":"end"}).text(z).transition().duration(e).attr("y",s),A.transition().duration(e).text(z).attr("y",s);var B=k.selectAll("text.whisker").data(q||[]);B.enter().append("svg:text").attr("class","whisker").attr("dy",".3em").attr("dx",6).attr("x",c).attr("y",t).text(z).style("opacity",1e-6).transition().duration(e).attr("y",s).style("opacity",1),B.transition().duration(e).text(z).attr("y",s).style("opacity",1),B.exit().transition().duration(e).attr("y",s).style("opacity",1e-6).remove()})}var c=1,d=1,e=0,f=null,g=Number,h=a,i=b,j=null;k.width=function(a){if(!arguments.length)return c;c=a;return k},k.height=function(a){if(!arguments.length)return d;d=a;return k},k.tickFormat=function(a){if(!arguments.length)return j;j=a;return k},k.duration=function(a){if(!arguments.length)return e;e=a;return k},k.domain=function(a){if(!arguments.length)return f;f=a==null?a:d3.functor(a);return k},k.value=function(a){if(!arguments.length)return g;g=a;return k},k.whiskers=function(a){if(!arguments.length)return h;h=a;return k},k.quartiles=function(a){if(!arguments.length)return i;i=a;return k};return k},d3.chart.bullet=function(){function o(a){a.each(function(a,c){var d=i.call(this,a,c).slice().sort(d3.descending),e=j.call(this,a,c).slice().sort(d3.descending),o=k.call(this,a,c).slice().sort(d3.descending),p=d3.select(this),q=d3.scale.linear().domain([0,Math.max(d[0],e[0],o[0])]).range(b?[l,0]:[0,l]),r=this.__chart__||d3.scale.linear().domain([0,Infinity]).range(q.range());this.__chart__=q;var s=g(r),t=g(q),u=p.selectAll("rect.range").data(d);u.enter().append("svg:rect").attr("class",function(a,b){return"range s"+b}).attr("width",s).attr("height",m).attr("x",b?r:0).transition().duration(h).attr("width",t).attr("x",b?q:0),u.transition().duration(h).attr("x",b?q:0).attr("width",t).attr("height",m);var v=p.selectAll("rect.measure").data(o);v.enter().append("svg:rect").attr("class",function(a,b){return"measure s"+b}).attr("width",s).attr("height",m/3).attr("x",b?r:0).attr("y",m/3).transition().duration(h).attr("width",t).attr("x",b?q:0),v.transition().duration(h).attr("width",t).attr("height",m/3).attr("x",b?q:0).attr("y",m/3);var w=p.selectAll("line.marker").data(e);w.enter().append("svg:line").attr("class","marker").attr("x1",r).attr("x2",r).attr("y1",m/6).attr("y2",m*5/6).transition().duration(h).attr("x1",q).attr("x2",q),w.transition().duration(h).attr("x1",q).attr("x2",q).attr("y1",m/6).attr("y2",m*5/6);var x=n||q.tickFormat(8),y=p.selectAll("g.tick").data(q.ticks(8),function(a){return this.textContent||x(a)}),z=y.enter().append("svg:g").attr("class","tick").attr("transform",f(r)).attr("opacity",1e-6);z.append("svg:line").attr("y1",m).attr("y2",m*7/6),z.append("svg:text").attr("text-anchor","middle").attr("dy","1em").attr("y",m*7/6).text(x),z.transition().duration(h).attr("transform",f(q)).attr("opacity",1);var A=y.transition().duration(h).attr("transform",f(q)).attr("opacity",1);A.select("line").attr("y1",m).attr("y2",m*7/6),A.select("text").attr("y",m*7/6),y.exit().transition().duration(h).attr("transform",f(q)).attr("opacity",1e-6).remove()})}var a="left",b=!1,h=0,i=c,j=d,k=e,l=380,m=30,n=null;o.orient=function(c){if(!arguments.length)return a;a=c,b=a=="right"||a=="bottom";return o},o.ranges=function(a){if(!arguments.length)return i;i=a;return o},o.markers=function(a){if(!arguments.length)return j;j=a;return o},o.measures=function(a){if(!arguments.length)return k;k=a;return o},o.width=function(a){if(!arguments.length)return l;l=a;return o},o.height=function(a){if(!arguments.length)return m;m=a;return o},o.tickFormat=function(a){if(!arguments.length)return n;n=a;return o},o.duration=function(a){if(!arguments.length)return h;h=a;return o};return o}})()
<ide>\ No newline at end of file
<ide><path>src/chart/box.js
<ide> d3.chart.box = function() {
<ide> var center = g.selectAll("line.center")
<ide> .data(whiskerData ? [whiskerData] : []);
<ide>
<del> center.enter().append("svg:line")
<add> center.enter().insert("svg:line", "rect")
<ide> .attr("class", "center")
<ide> .attr("x1", width / 2)
<ide> .attr("y1", function(d) { return x0(d[0]); })
<ide> d3.chart.box = function() {
<ide> var whisker = g.selectAll("line.whisker")
<ide> .data(whiskerData || []);
<ide>
<del> whisker.enter().append("svg:line")
<add> whisker.enter().insert("svg:line", "circle, text")
<ide> .attr("class", "whisker")
<ide> .attr("x1", 0)
<ide> .attr("y1", x0)
<ide> d3.chart.box = function() {
<ide> var outlier = g.selectAll("circle.outlier")
<ide> .data(outlierIndices, Number);
<ide>
<del> outlier.enter().append("svg:circle")
<add> outlier.enter().insert("svg:circle", "text")
<ide> .attr("class", "outlier")
<ide> .attr("r", 5)
<ide> .attr("cx", width / 2) | 3 |
Javascript | Javascript | fix coding style in web/pdf_find_bar.js | 2e09f14a80b26ff9f14a099f86599048ad6625ea | <ide><path>web/pdf_find_bar.js
<ide> * searching is done by PDFFindController
<ide> */
<ide> var PDFFindBar = {
<del>
<ide> opened: false,
<ide> bar: null,
<ide> toggleButton: null,
<ide> var PDFFindBar = {
<ide>
<ide> initialize: function(options) {
<ide> if(typeof PDFFindController === 'undefined' || PDFFindController === null) {
<del> throw 'PDFFindBar cannot be initialized ' +
<add> throw 'PDFFindBar cannot be initialized ' +
<ide> 'without a PDFFindController instance.';
<ide> }
<ide>
<ide> var PDFFindBar = {
<ide> },
<ide>
<ide> close: function() {
<del> if (!this.opened) return;
<del>
<add> if (!this.opened) {
<add> return;
<add> }
<ide> this.opened = false;
<ide> this.toggleButton.classList.remove('toggled');
<ide> this.bar.classList.add('hidden'); | 1 |
Python | Python | fix cut and paste error, derivative <- integral | 0402e1c6a5cc5693a1f021446f20baebe9073890 | <ide><path>numpy/polynomial/chebyshev.py
<ide> def chebint(c, m=1, k=[], lbnd=0, scl=1, axis=0):
<ide> Following each integration the result is *multiplied* by `scl`
<ide> before the integration constant is added. (Default: 1)
<ide> axis : int, optional
<del> Axis over which the derivative is taken. (Default: 0).
<add> Axis over which the integral is taken. (Default: 0).
<ide>
<ide> .. versionadded:: 1.7.0
<ide>
<ide><path>numpy/polynomial/hermite.py
<ide> def hermint(c, m=1, k=[], lbnd=0, scl=1, axis=0):
<ide> Following each integration the result is *multiplied* by `scl`
<ide> before the integration constant is added. (Default: 1)
<ide> axis : int, optional
<del> Axis over which the derivative is taken. (Default: 0).
<add> Axis over which the integral is taken. (Default: 0).
<ide>
<ide> .. versionadded:: 1.7.0
<ide>
<ide><path>numpy/polynomial/hermite_e.py
<ide> def hermeint(c, m=1, k=[], lbnd=0, scl=1, axis=0):
<ide> Following each integration the result is *multiplied* by `scl`
<ide> before the integration constant is added. (Default: 1)
<ide> axis : int, optional
<del> Axis over which the derivative is taken. (Default: 0).
<add> Axis over which the integral is taken. (Default: 0).
<ide>
<ide> .. versionadded:: 1.7.0
<ide>
<ide><path>numpy/polynomial/laguerre.py
<ide> def lagint(c, m=1, k=[], lbnd=0, scl=1, axis=0):
<ide> Following each integration the result is *multiplied* by `scl`
<ide> before the integration constant is added. (Default: 1)
<ide> axis : int, optional
<del> Axis over which the derivative is taken. (Default: 0).
<add> Axis over which the integral is taken. (Default: 0).
<ide>
<ide> .. versionadded:: 1.7.0
<ide>
<ide><path>numpy/polynomial/legendre.py
<ide> def legpow(c, pow, maxpower=16) :
<ide> Parameters
<ide> ----------
<ide> c : array_like
<del> l1-D array of Legendre series coefficients ordered from low to
<add> 1-D array of Legendre series coefficients ordered from low to
<ide> high.
<ide> pow : integer
<ide> Power to which the series will be raised
<ide> def legint(c, m=1, k=[], lbnd=0, scl=1, axis=0):
<ide> Following each integration the result is *multiplied* by `scl`
<ide> before the integration constant is added. (Default: 1)
<ide> axis : int, optional
<del> Axis over which the derivative is taken. (Default: 0).
<add> Axis over which the integral is taken. (Default: 0).
<ide>
<ide> .. versionadded:: 1.7.0
<ide> | 5 |
Javascript | Javascript | gatsby the academic honesty page | cc5459541436dc959e9f755d1614e4365027a495 | <ide><path>client/src/pages/academic-honesty.js
<add>import React from 'react';
<add>import { Grid } from 'react-bootstrap';
<add>
<add>import Layout from '../components/Layout';
<add>import Spacer from '../components/helpers/Spacer';
<add>import FullWidthRow from '../components/helpers/FullWidthRow';
<add>
<add>function AcademicHonesty() {
<add> return (
<add> <Layout>
<add> <Grid>
<add> <FullWidthRow>
<add> <Spacer />
<add> <h2 className='text-center'>Academic Honesty Policy</h2>
<add> <hr />
<add> <p>
<add> Before we issue our verified certification to a camper, he or she
<add> must accept our Academic Honesty Pledge, which reads:
<add> </p>
<add> <p>
<add> "I understand that plagiarism means copying someone else’s work and
<add> presenting the work as if it were my own, without clearly
<add> attributing the original author.
<add> </p>
<add> <p>
<add> "I understand that plagiarism is an act of intellectual dishonesty,
<add> and that people usually get kicked out of university or fired from
<add> their jobs if they get caught plagiarizing.
<add> </p>
<add> <p>
<add> "Aside from using open source libraries such as jQuery and
<add> Bootstrap, and short snippets of code which are clearly attributed
<add> to their original author, 100% of the code in my projects was
<add> written by me, or along with another camper with whom I was pair
<add> programming in real time.
<add> </p>
<add> <p>
<add> "I pledge that I did not plagiarize any of my freeCodeCamp.org work.
<add> I understand that freeCodeCamp.org’s team will audit my projects to
<add> confirm this."
<add> </p>
<add> <p>
<add> In the situations where we discover instances of unambiguous
<add> plagiarism, we will replace the camper in question’s certification
<add> with a message that "Upon review, this account has been flagged for
<add> academic dishonesty."
<add> </p>
<add> <p>
<add> As an academic institution that grants achievement-based
<add> certifications, we take academic honesty very seriously. If you have
<add> any questions about this policy, or suspect that someone has
<add> violated it, you can email{' '}
<add> <a href='mailto:[email protected]'>[email protected]</a>
<add>  and we will investigate.
<add> </p>
<add> </FullWidthRow>
<add> </Grid>
<add> </Layout>
<add> );
<add>}
<add>
<add>AcademicHonesty.displayName = 'AcademicHonesty';
<add>
<add>export default AcademicHonesty; | 1 |
Text | Text | fix path markdown formatting | 65a42ab8927ffc53f0188e16d33579f839b9815f | <ide><path>doc/api/path.md
<ide> path.parse('/home/user/dir/file.txt')
<ide> │ root │ │ name │ ext │
<ide> " / home/user/dir / file .txt "
<ide> └──────┴──────────────┴──────┴─────┘
<del>(all spaces in the "" line should be ignored -- they're purely for formatting)
<add>(all spaces in the "" line should be ignored -- they are purely for formatting)
<ide> ```
<ide>
<ide> On Windows:
<ide> path.parse('C:\\path\\dir\\file.txt')
<ide> │ root │ │ name │ ext │
<ide> " C:\ path\dir \ file .txt "
<ide> └──────┴──────────────┴──────┴─────┘
<del>(all spaces in the "" line should be ignored -- they're purely for formatting)
<add>(all spaces in the "" line should be ignored -- they are purely for formatting)
<ide> ```
<ide>
<ide> A [`TypeError`][] is thrown if `path` is not a string. | 1 |
PHP | PHP | fix double spaces in doc blocks | 194ee3fae7f356bdbee744c5807f6e5dc1677428 | <ide><path>src/Collection/Iterator/FilterIterator.php
<ide> public function __construct($items, callable $callback)
<ide> /**
<ide> * {@inheritDoc}
<ide> *
<del> * We perform here some strictness analysis so that the
<add> * We perform here some strictness analysis so that the
<ide> * iterator logic is bypassed entirely.
<ide> *
<ide> * @return \Iterator
<ide><path>src/Collection/Iterator/ReplaceIterator.php
<ide> public function current()
<ide> /**
<ide> * {@inheritDoc}
<ide> *
<del> * We perform here some strictness analysis so that the
<add> * We perform here some strictness analysis so that the
<ide> * iterator logic is bypassed entirely.
<ide> *
<ide> * @return \Iterator
<ide><path>src/Collection/Iterator/StoppableIterator.php
<ide> public function valid()
<ide> /**
<ide> * {@inheritDoc}
<ide> *
<del> * We perform here some strictness analysis so that the
<add> * We perform here some strictness analysis so that the
<ide> * iterator logic is bypassed entirely.
<ide> *
<ide> * @return \Iterator | 3 |
Python | Python | change serializer name for removing confusion | f5fc6937ece8c2bc70088979cc19c2c0a660c7a0 | <ide><path>rest_framework/tests/test_serializer.py
<ide> def test_writable_star_source_on_nested_serializer(self):
<ide> self.assertEqual(serializer.is_valid(), True)
<ide>
<ide> def test_writable_star_source_on_nested_serializer_with_parent_object(self):
<del> class Serializer(serializers.Serializer):
<add> class TitleSerializer(serializers.Serializer):
<ide> title = serializers.WritableField(source='title')
<ide>
<ide> class AlbumSerializer(serializers.ModelSerializer):
<del> nested = Serializer(source='*')
<add> nested = TitleSerializer(source='*')
<ide>
<ide> class Meta:
<ide> model = Album | 1 |
Go | Go | use shim v2 | 612343618dd7dad7cf023e6263d693ab37507a92 | <ide><path>daemon/daemon.go
<ide> func NewDaemon(ctx context.Context, config *config.Config, pluginStore *plugin.S
<ide> PluginStore: pluginStore,
<ide> startupDone: make(chan struct{}),
<ide> }
<add>
<ide> // Ensure the daemon is properly shutdown if there is a failure during
<ide> // initialization
<ide> defer func() {
<ide> func NewDaemon(ctx context.Context, config *config.Config, pluginStore *plugin.S
<ide> }
<ide> }
<ide>
<del> return pluginexec.New(ctx, getPluginExecRoot(config.Root), pluginCli, config.ContainerdPluginNamespace, m)
<add> return pluginexec.New(ctx, getPluginExecRoot(config.Root), pluginCli, config.ContainerdPluginNamespace, m, d.useShimV2())
<ide> }
<ide>
<ide> // Plugin system initialization should happen before restore. Do not change order.
<ide> func NewDaemon(ctx context.Context, config *config.Config, pluginStore *plugin.S
<ide>
<ide> go d.execCommandGC()
<ide>
<del> d.containerd, err = libcontainerd.NewClient(ctx, d.containerdCli, filepath.Join(config.ExecRoot, "containerd"), config.ContainerdNamespace, d)
<add> d.containerd, err = libcontainerd.NewClient(ctx, d.containerdCli, filepath.Join(config.ExecRoot, "containerd"), config.ContainerdNamespace, d, d.useShimV2())
<ide> if err != nil {
<ide> return nil, err
<ide> }
<ide><path>daemon/daemon_unix.go
<ide> func (daemon *Daemon) setupSeccompProfile() error {
<ide> }
<ide> return nil
<ide> }
<add>
<add>func (daemon *Daemon) useShimV2() bool {
<add> return cgroups.IsCgroup2UnifiedMode()
<add>}
<ide><path>daemon/daemon_windows.go
<ide> func (daemon *Daemon) initRuntimes(_ map[string]types.Runtime) error {
<ide>
<ide> func setupResolvConf(config *config.Config) {
<ide> }
<add>
<add>func (daemon *Daemon) useShimV2() bool {
<add> return true
<add>}
<ide><path>daemon/start_unix.go
<ide> import (
<ide> "path/filepath"
<ide>
<ide> "github.com/containerd/containerd/runtime/linux/runctypes"
<add> v2runcoptions "github.com/containerd/containerd/runtime/v2/runc/options"
<ide> "github.com/docker/docker/container"
<ide> "github.com/docker/docker/errdefs"
<ide> "github.com/pkg/errors"
<ide> func (daemon *Daemon) getLibcontainerdCreateOptions(container *container.Contain
<ide> if err != nil {
<ide> return nil, err
<ide> }
<add> if daemon.useShimV2() {
<add> opts := &v2runcoptions.Options{
<add> BinaryName: path,
<add> Root: filepath.Join(daemon.configStore.ExecRoot,
<add> fmt.Sprintf("runtime-%s", container.HostConfig.Runtime)),
<add> }
<add>
<add> if UsingSystemd(daemon.configStore) {
<add> opts.SystemdCgroup = true
<add> }
<add>
<add> return opts, nil
<add>
<add> }
<ide> opts := &runctypes.RuncOptions{
<ide> Runtime: path,
<ide> RuntimeRoot: filepath.Join(daemon.configStore.ExecRoot,
<ide><path>libcontainerd/libcontainerd_linux.go
<ide> import (
<ide> )
<ide>
<ide> // NewClient creates a new libcontainerd client from a containerd client
<del>func NewClient(ctx context.Context, cli *containerd.Client, stateDir, ns string, b libcontainerdtypes.Backend) (libcontainerdtypes.Client, error) {
<del> return remote.NewClient(ctx, cli, stateDir, ns, b)
<add>func NewClient(ctx context.Context, cli *containerd.Client, stateDir, ns string, b libcontainerdtypes.Backend, useShimV2 bool) (libcontainerdtypes.Client, error) {
<add> return remote.NewClient(ctx, cli, stateDir, ns, b, useShimV2)
<ide> }
<ide><path>libcontainerd/libcontainerd_windows.go
<ide> import (
<ide> )
<ide>
<ide> // NewClient creates a new libcontainerd client from a containerd client
<del>func NewClient(ctx context.Context, cli *containerd.Client, stateDir, ns string, b libcontainerdtypes.Backend) (libcontainerdtypes.Client, error) {
<add>func NewClient(ctx context.Context, cli *containerd.Client, stateDir, ns string, b libcontainerdtypes.Backend, useShimV2 bool) (libcontainerdtypes.Client, error) {
<ide> if !system.ContainerdRuntimeSupported() {
<add> // useShimV2 is ignored for windows
<ide> return local.NewClient(ctx, cli, stateDir, ns, b)
<ide> }
<del> return remote.NewClient(ctx, cli, stateDir, ns, b)
<add> return remote.NewClient(ctx, cli, stateDir, ns, b, useShimV2)
<ide> }
<ide><path>libcontainerd/remote/client.go
<ide> import (
<ide> "github.com/containerd/containerd/events"
<ide> "github.com/containerd/containerd/images"
<ide> "github.com/containerd/containerd/runtime/linux/runctypes"
<add> v2runcoptions "github.com/containerd/containerd/runtime/v2/runc/options"
<ide> "github.com/containerd/typeurl"
<ide> "github.com/docker/docker/errdefs"
<ide> "github.com/docker/docker/libcontainerd/queue"
<ide> type client struct {
<ide> logger *logrus.Entry
<ide> ns string
<ide>
<del> backend libcontainerdtypes.Backend
<del> eventQ queue.Queue
<del> oomMu sync.Mutex
<del> oom map[string]bool
<add> backend libcontainerdtypes.Backend
<add> eventQ queue.Queue
<add> oomMu sync.Mutex
<add> oom map[string]bool
<add> useShimV2 bool
<add> v2runcoptionsMu sync.Mutex
<add> // v2runcoptions is used for copying options specified on Create() to Start()
<add> v2runcoptions map[string]v2runcoptions.Options
<ide> }
<ide>
<ide> // NewClient creates a new libcontainerd client from a containerd client
<del>func NewClient(ctx context.Context, cli *containerd.Client, stateDir, ns string, b libcontainerdtypes.Backend) (libcontainerdtypes.Client, error) {
<add>func NewClient(ctx context.Context, cli *containerd.Client, stateDir, ns string, b libcontainerdtypes.Backend, useShimV2 bool) (libcontainerdtypes.Client, error) {
<ide> c := &client{
<del> client: cli,
<del> stateDir: stateDir,
<del> logger: logrus.WithField("module", "libcontainerd").WithField("namespace", ns),
<del> ns: ns,
<del> backend: b,
<del> oom: make(map[string]bool),
<add> client: cli,
<add> stateDir: stateDir,
<add> logger: logrus.WithField("module", "libcontainerd").WithField("namespace", ns),
<add> ns: ns,
<add> backend: b,
<add> oom: make(map[string]bool),
<add> useShimV2: useShimV2,
<add> v2runcoptions: make(map[string]v2runcoptions.Options),
<ide> }
<ide>
<ide> go c.processEventStream(ctx, ns)
<ide> func (c *client) Create(ctx context.Context, id string, ociSpec *specs.Spec, run
<ide> bdir := c.bundleDir(id)
<ide> c.logger.WithField("bundle", bdir).WithField("root", ociSpec.Root.Path).Debug("bundle dir created")
<ide>
<add> rt := runtimeName
<add> if c.useShimV2 {
<add> rt = shimV2RuntimeName
<add> }
<ide> newOpts := []containerd.NewContainerOpts{
<ide> containerd.WithSpec(ociSpec),
<del> containerd.WithRuntime(runtimeName, runtimeOptions),
<add> containerd.WithRuntime(rt, runtimeOptions),
<ide> WithBundle(bdir, ociSpec),
<ide> }
<ide> opts = append(opts, newOpts...)
<ide> func (c *client) Create(ctx context.Context, id string, ociSpec *specs.Spec, run
<ide> }
<ide> return wrapError(err)
<ide> }
<add> if c.useShimV2 {
<add> if x, ok := runtimeOptions.(*v2runcoptions.Options); ok {
<add> c.v2runcoptionsMu.Lock()
<add> c.v2runcoptions[id] = *x
<add> c.v2runcoptionsMu.Unlock()
<add> }
<add> }
<ide> return nil
<ide> }
<ide>
<ide> func (c *client) Start(ctx context.Context, id, checkpointDir string, withStdin
<ide>
<ide> if runtime.GOOS != "windows" {
<ide> taskOpts = append(taskOpts, func(_ context.Context, _ *containerd.Client, info *containerd.TaskInfo) error {
<del> info.Options = &runctypes.CreateOptions{
<del> IoUid: uint32(uid),
<del> IoGid: uint32(gid),
<del> NoPivotRoot: os.Getenv("DOCKER_RAMDISK") != "",
<add> if c.useShimV2 {
<add> // For v2, we need to inherit options specified on Create
<add> c.v2runcoptionsMu.Lock()
<add> opts, ok := c.v2runcoptions[id]
<add> c.v2runcoptionsMu.Unlock()
<add> if !ok {
<add> opts = v2runcoptions.Options{}
<add> }
<add> opts.IoUid = uint32(uid)
<add> opts.IoGid = uint32(gid)
<add> opts.NoPivotRoot = os.Getenv("DOCKER_RAMDISK") != ""
<add> info.Options = &opts
<add> } else {
<add> info.Options = &runctypes.CreateOptions{
<add> IoUid: uint32(uid),
<add> IoGid: uint32(gid),
<add> NoPivotRoot: os.Getenv("DOCKER_RAMDISK") != "",
<add> }
<ide> }
<add>
<ide> return nil
<ide> })
<ide> } else {
<ide> func (c *client) Delete(ctx context.Context, containerID string) error {
<ide> c.oomMu.Lock()
<ide> delete(c.oom, containerID)
<ide> c.oomMu.Unlock()
<add> c.v2runcoptionsMu.Lock()
<add> delete(c.v2runcoptions, containerID)
<add> c.v2runcoptionsMu.Unlock()
<ide> if os.Getenv("LIBCONTAINERD_NOCLEAN") != "1" {
<ide> if err := os.RemoveAll(bundle); err != nil {
<ide> c.logger.WithError(err).WithFields(logrus.Fields{
<ide><path>libcontainerd/remote/client_linux.go
<ide> import (
<ide> "github.com/sirupsen/logrus"
<ide> )
<ide>
<del>const runtimeName = "io.containerd.runtime.v1.linux"
<add>const (
<add> runtimeName = "io.containerd.runtime.v1.linux"
<add> shimV2RuntimeName = "io.containerd.runc.v2"
<add>)
<ide>
<ide> func summaryFromInterface(i interface{}) (*libcontainerdtypes.Summary, error) {
<ide> return &libcontainerdtypes.Summary{}, nil
<ide><path>libcontainerd/remote/client_windows.go
<ide> import (
<ide> "github.com/sirupsen/logrus"
<ide> )
<ide>
<del>const runtimeName = "io.containerd.runhcs.v1"
<add>const (
<add> runtimeName = "io.containerd.runhcs.v1"
<add> shimV2RuntimeName = runtimeName
<add>)
<ide>
<ide> func summaryFromInterface(i interface{}) (*libcontainerdtypes.Summary, error) {
<ide> switch pd := i.(type) {
<ide><path>plugin/executor/containerd/containerd.go
<ide> type ExitHandler interface {
<ide> }
<ide>
<ide> // New creates a new containerd plugin executor
<del>func New(ctx context.Context, rootDir string, cli *containerd.Client, ns string, exitHandler ExitHandler) (*Executor, error) {
<add>func New(ctx context.Context, rootDir string, cli *containerd.Client, ns string, exitHandler ExitHandler, useShimV2 bool) (*Executor, error) {
<ide> e := &Executor{
<ide> rootDir: rootDir,
<ide> exitHandler: exitHandler,
<ide> }
<ide>
<del> client, err := libcontainerd.NewClient(ctx, cli, rootDir, ns, e)
<add> client, err := libcontainerd.NewClient(ctx, cli, rootDir, ns, e, useShimV2)
<ide> if err != nil {
<ide> return nil, errors.Wrap(err, "error creating containerd exec client")
<ide> } | 10 |
PHP | PHP | fix some typos in phpdoc | 9eec4ca2851e81d866e32ab53d0e7584a315dd1d | <ide><path>src/Console/CommandCollection.php
<ide> protected function resolveNames(array $input): array
<ide> * - CakePHP provided commands
<ide> * - Application commands
<ide> *
<del> * Commands defined in the application will ovewrite commands with
<add> * Commands defined in the application will overwrite commands with
<ide> * the same name provided by CakePHP.
<ide> *
<ide> * @return string[] An array of command names and their classes.
<ide><path>src/Controller/Component/AuthComponent.php
<ide> class AuthComponent extends Component implements EventDispatcherInterface
<ide> use EventDispatcherTrait;
<ide>
<ide> /**
<del> * The query string key used for remembering the referrered page when getting
<add> * The query string key used for remembering the referred page when getting
<ide> * redirected to login.
<ide> */
<ide> public const QUERY_STRING_REDIRECT = 'redirect';
<ide><path>src/Database/Type/ExpressionTypeCasterTrait.php
<ide> trait ExpressionTypeCasterTrait
<ide> * if the type class implements the ExpressionTypeInterface. Otherwise,
<ide> * returns the value unmodified.
<ide> *
<del> * @param mixed $value The value to converto to ExpressionInterface
<add> * @param mixed $value The value to convert to ExpressionInterface
<ide> * @param string|null $type The type name
<ide> * @return mixed
<ide> */
<ide><path>src/Http/Middleware/EncryptedCookieMiddleware.php
<ide> use Psr\Http\Server\RequestHandlerInterface;
<ide>
<ide> /**
<del> * Middlware for encrypting & decrypting cookies.
<add> * Middleware for encrypting & decrypting cookies.
<ide> *
<ide> * This middleware layer will encrypt/decrypt the named cookies with the given key
<ide> * and cipher type. To support multiple keys/cipher types use this middleware multiple
<ide><path>src/Mailer/AbstractTransport.php
<ide> abstract class AbstractTransport
<ide> /**
<ide> * Send mail
<ide> *
<del> * @param \Cake\Mailer\Message $message Email mesage.
<add> * @param \Cake\Mailer\Message $message Email message.
<ide> * @return array
<ide> * @psalm-return array{headers: string, message: string}
<ide> */
<ide><path>src/Mailer/Email.php
<ide> public function unserialize($data): void
<ide> }
<ide>
<ide> /**
<del> * Proxy all static method calls (for methods provided by StaticConfigTrat) to Mailer.
<add> * Proxy all static method calls (for methods provided by StaticConfigTrait) to Mailer.
<ide> *
<ide> * @param string $name Method name.
<ide> * @param array $arguments Method argument.
<ide><path>src/View/Form/ContextFactory.php
<ide> public function addProvider(string $type, callable $check)
<ide> * @param \Cake\Http\ServerRequest $request Request instance.
<ide> * @param array $data The data to get a context provider for.
<ide> * @return \Cake\View\Form\ContextInterface Context provider.
<del> * @throws \RuntimeException When a context instace cannot be generated for given entity.
<add> * @throws \RuntimeException When a context instance cannot be generated for given entity.
<ide> */
<ide> public function get(ServerRequest $request, array $data = []): ContextInterface
<ide> {
<ide><path>src/View/JsonView.php
<ide> * You can also set `'serialize'` to a string or array to serialize only the
<ide> * specified view variables.
<ide> *
<del> * If you don't set the `serialize` opton, you will need a view template.
<add> * If you don't set the `serialize` option, you will need a view template.
<ide> * You can use extended views to provide layout-like functionality.
<ide> *
<ide> * You can also enable JSONP support by setting `jsonp` option to true or a | 8 |
PHP | PHP | fix bug in input class | 1c26ce8ce56f524e70c8e4b44b5d7ad7aca9e378 | <ide><path>laravel/input.php
<ide> public static function has($key)
<ide> */
<ide> public static function get($key = null, $default = null)
<ide> {
<del> $value = Request::foundation()->request->get($key);
<add> $value = array_get(Request::foundation()->request->all(), $key);
<ide>
<ide> if (is_null($value))
<ide> { | 1 |
Java | Java | remove dependency on webutils | 8ca5b830b1699608e8afefef16b1668359fdd2fe | <ide><path>spring-web-reactive/src/main/java/org/springframework/web/reactive/result/method/RequestMappingInfoHandlerMapping.java
<ide> import java.util.Map;
<ide> import java.util.Map.Entry;
<ide> import java.util.Set;
<add>import java.util.StringTokenizer;
<ide> import java.util.stream.Collectors;
<ide>
<ide> import org.springframework.http.HttpHeaders;
<ide> import org.springframework.http.MediaType;
<ide> import org.springframework.http.server.reactive.ServerHttpRequest;
<ide> import org.springframework.util.CollectionUtils;
<add>import org.springframework.util.LinkedMultiValueMap;
<ide> import org.springframework.util.MultiValueMap;
<add>import org.springframework.util.StringUtils;
<ide> import org.springframework.web.bind.annotation.RequestMethod;
<ide> import org.springframework.web.method.HandlerMethod;
<ide> import org.springframework.web.reactive.HandlerMapping;
<ide> import org.springframework.web.server.NotAcceptableStatusException;
<ide> import org.springframework.web.server.ServerWebExchange;
<ide> import org.springframework.web.server.UnsupportedMediaTypeStatusException;
<del>import org.springframework.web.util.WebUtils;
<ide>
<ide> /**
<ide> * Abstract base class for classes for which {@link RequestMappingInfo} defines
<ide> private Map<String, MultiValueMap<String, String>> extractMatrixVariables(
<ide> uriVariables.put(uriVar.getKey(), uriVarValue.substring(0, semicolonIndex));
<ide> }
<ide>
<del> MultiValueMap<String, String> vars = WebUtils.parseMatrixVariables(matrixVariables);
<add> MultiValueMap<String, String> vars = parseMatrixVariables(matrixVariables);
<ide> result.put(uriVar.getKey(), getPathHelper().decodeMatrixVariables(exchange, vars));
<ide> }
<ide> return result;
<ide> }
<ide>
<add> /**
<add> * Parse the given string with matrix variables. An example string would look
<add> * like this {@code "q1=a;q1=b;q2=a,b,c"}. The resulting map would contain
<add> * keys {@code "q1"} and {@code "q2"} with values {@code ["a","b"]} and
<add> * {@code ["a","b","c"]} respectively.
<add> * @param matrixVariables the unparsed matrix variables string
<add> * @return a map with matrix variable names and values (never {@code null})
<add> */
<add> private static MultiValueMap<String, String> parseMatrixVariables(String matrixVariables) {
<add> MultiValueMap<String, String> result = new LinkedMultiValueMap<>();
<add> if (!StringUtils.hasText(matrixVariables)) {
<add> return result;
<add> }
<add> StringTokenizer pairs = new StringTokenizer(matrixVariables, ";");
<add> while (pairs.hasMoreTokens()) {
<add> String pair = pairs.nextToken();
<add> int index = pair.indexOf('=');
<add> if (index != -1) {
<add> String name = pair.substring(0, index);
<add> String rawValue = pair.substring(index + 1);
<add> for (String value : StringUtils.commaDelimitedListToStringArray(rawValue)) {
<add> result.add(name, value);
<add> }
<add> }
<add> else {
<add> result.add(pair, "");
<add> }
<add> }
<add> return result;
<add> }
<add>
<ide> /**
<ide> * Iterate all RequestMappingInfos once again, look if any match by URL at
<ide> * least and raise exceptions accordingly.
<ide><path>spring-web-reactive/src/main/java/org/springframework/web/reactive/result/method/annotation/RequestMappingHandlerMapping.java
<ide> import org.springframework.stereotype.Controller;
<ide> import org.springframework.util.Assert;
<ide> import org.springframework.util.StringValueResolver;
<del>import org.springframework.web.accept.ContentNegotiationManager;
<ide> import org.springframework.web.bind.annotation.RequestMapping;
<del>import org.springframework.web.reactive.accept.CompositeContentTypeResolver;
<ide> import org.springframework.web.reactive.accept.CompositeContentTypeResolverBuilder;
<ide> import org.springframework.web.reactive.accept.ContentTypeResolver;
<del>import org.springframework.web.reactive.accept.HeaderContentTypeResolver;
<ide> import org.springframework.web.reactive.result.condition.RequestCondition;
<ide> import org.springframework.web.reactive.result.method.RequestMappingInfo;
<ide> import org.springframework.web.reactive.result.method.RequestMappingInfoHandlerMapping;
<ide> public void setUseTrailingSlashMatch(boolean useTrailingSlashMatch) {
<ide> }
<ide>
<ide> /**
<del> * Set the {@link ContentNegotiationManager} to use to determine requested media types.
<add> * Set the {@link ContentTypeResolver} to use to determine requested media types.
<ide> * If not set, the default constructor is used.
<ide> */
<ide> public void setContentTypeResolver(ContentTypeResolver contentTypeResolver) { | 2 |
Javascript | Javascript | fix license header to use bsd | 12808e81c18ca8e9921b606cc680f30cca0a5bab | <ide><path>src/browser/ui/dom/setTextContent.js
<ide> /**
<del> * Copyright 2013-2014 Facebook, Inc.
<add> * Copyright 2013-2014, Facebook, Inc.
<add> * All rights reserved.
<ide> *
<del> * Licensed under the Apache License, Version 2.0 (the "License");
<del> * you may not use this file except in compliance with the License.
<del> * You may obtain a copy of the License at
<del> *
<del> * http://www.apache.org/licenses/LICENSE-2.0
<del> *
<del> * Unless required by applicable law or agreed to in writing, software
<del> * distributed under the License is distributed on an "AS IS" BASIS,
<del> * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
<del> * See the License for the specific language governing permissions and
<del> * limitations under the License.
<add> * This source code is licensed under the BSD-style license found in the
<add> * LICENSE file in the root directory of this source tree. An additional grant
<add> * of patent rights can be found in the PATENTS file in the same directory.
<ide> *
<ide> * @providesModule setTextContent
<ide> */ | 1 |
Mixed | Python | add docstrings in layers | 40864d89974a2b0a2f97aaf7bfdd66659c8ceae2 | <ide><path>docs/sources/examples.md
<ide> model.fit([images, partial_captions], next_words, batch_size=16, nb_epoch=100)
<ide> ```
<ide>
<ide> In the examples folder, you will find example models for real datasets:
<add>
<ide> - CIFAR10 small images classification: Convolutional Neural Network (CNN) with realtime data augmentation
<ide> - IMDB movie review sentiment classification: LSTM over sequences of words
<ide> - Reuters newswires topic classification: Multilayer Perceptron (MLP)
<ide><path>keras/layers/advanced_activations.py
<ide> from .. import initializations
<del>from ..layers.core import Layer, MaskedLayer
<add>from ..layers.core import MaskedLayer
<ide> from .. import backend as K
<ide> import numpy as np
<ide>
<ide>
<ide> class LeakyReLU(MaskedLayer):
<add> '''Special version of a Rectified Linear Unit
<add> that allows a small gradient when the unit is not active
<add> (`f(x) = alpha*x for x < 0`).
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as the input.
<add>
<add> # Arguments
<add> alpha: float >= 0. Negative slope coefficient.
<add> '''
<ide> def __init__(self, alpha=0.3, **kwargs):
<ide> super(LeakyReLU, self).__init__(**kwargs)
<ide> self.alpha = alpha
<ide> def get_config(self):
<ide>
<ide> class PReLU(MaskedLayer):
<ide> '''
<del> Reference:
<del> Delving Deep into Rectifiers: Surpassing Human-Level
<del> Performance on ImageNet Classification
<del> http://arxiv.org/pdf/1502.01852v1.pdf
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as the input.
<add>
<add> # Arguments:
<add> init: initialization function for the weights.
<add> weights: initial weights, as a list of a single numpy array.
<add>
<add> # References:
<add> - [Delving Deep into Rectifiers: Surpassing Human-Level Performance on ImageNet Classification](http://arxiv.org/pdf/1502.01852v1.pdf)
<ide> '''
<ide> def __init__(self, init='zero', weights=None, **kwargs):
<ide> self.init = initializations.get(init)
<ide> def get_config(self):
<ide>
<ide>
<ide> class ELU(MaskedLayer):
<add> '''
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as the input.
<add>
<add> # Arguments
<add> alpha: scale for the negative factor.
<add>
<add> # References
<add> - [Fast and Accurate Deep Network Learning by Exponential Linear Units (ELUs)](http://arxiv.org/pdf/1511.07289v1.pdf)
<add> '''
<ide> def __init__(self, alpha=1.0, **kwargs):
<ide> super(ELU, self).__init__(**kwargs)
<ide> self.alpha = alpha
<ide> def get_config(self):
<ide>
<ide>
<ide> class ParametricSoftplus(MaskedLayer):
<del> '''
<del> Parametric Softplus of the form: alpha * log(1 + exp(beta * X))
<add> '''Parametric Softplus of the form: alpha * log(1 + exp(beta * X))
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as the input.
<ide>
<del> Reference:
<del> Inferring Nonlinear Neuronal Computation
<del> Based on Physiologically Plausible Inputs
<del> http://journals.plos.org/ploscompbiol/article?id=10.1371/journal.pcbi.1003143
<add> # Arguments
<add> alpha_init: float. Initial value of the alpha weights.
<add> beta_init: float. Initial values of the beta weights.
<add> weights: initial weights, as a list of 2 numpy arrays.
<add>
<add> # References:
<add> - [Inferring Nonlinear Neuronal Computation Based on Physiologically Plausible Inputs](http://journals.plos.org/ploscompbiol/article?id=10.1371/journal.pcbi.1003143)
<ide> '''
<ide> def __init__(self, alpha_init=0.2, beta_init=5.0,
<ide> weights=None, **kwargs):
<ide> def get_config(self):
<ide>
<ide>
<ide> class ThresholdedLinear(MaskedLayer):
<del> '''
<del> Thresholded Linear Activation
<add> '''Thresholded Linear Activation.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<ide>
<del> Reference:
<del> Zero-Bias Autoencoders and the Benefits of Co-Adapting Features
<del> http://arxiv.org/pdf/1402.3337.pdf
<add> # Output shape
<add> Same shape as the input.
<add>
<add> # Arguments
<add> theta: float >= 0. Threshold location of activation.
<add>
<add> # References
<add> [Zero-Bias Autoencoders and the Benefits of Co-Adapting Features](http://arxiv.org/pdf/1402.3337.pdf)
<ide> '''
<ide> def __init__(self, theta=1.0, **kwargs):
<ide> super(ThresholdedLinear, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class ThresholdedReLU(MaskedLayer):
<del> '''
<del> Thresholded Rectified Activation
<add> '''Thresholded Rectified Activation.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as the input.
<add>
<add> # Arguments
<add> theta: float >= 0. Threshold location of activation.
<ide>
<del> Reference:
<del> Zero-Bias Autoencoders and the Benefits of Co-Adapting Features
<del> http://arxiv.org/pdf/1402.3337.pdf
<add> # References
<add> [Zero-Bias Autoencoders and the Benefits of Co-Adapting Features](http://arxiv.org/pdf/1402.3337.pdf)
<ide> '''
<ide> def __init__(self, theta=1.0, **kwargs):
<ide> super(ThresholdedReLU, self).__init__(**kwargs)
<ide><path>keras/layers/containers.py
<ide>
<ide>
<ide> class Sequential(Layer):
<del> '''
<del> Simple linear stack of layers.
<add> '''The Sequential container is a linear stack of layers.
<add> Apart from the `add` methods and the `layers` constructor argument,
<add> the API is identical to that of the `Layer` class.
<ide>
<del> inherited from Layer:
<del> - get_params
<del> - get_output_mask
<del> - supports_masked_input
<del> '''
<add> This class is also the basis for the `keras.models.Sequential` model.
<ide>
<add> # Arguments
<add> layers: list of layers to be added to the container.
<add> '''
<ide> def __init__(self, layers=[]):
<ide> self.layers = []
<ide> for layer in layers:
<ide> def set_weights(self, weights):
<ide> weights = weights[nb_param:]
<ide>
<ide> def get_config(self):
<del> return {"name": self.__class__.__name__,
<del> "layers": [layer.get_config() for layer in self.layers]}
<add> return {'name': self.__class__.__name__,
<add> 'layers': [layer.get_config() for layer in self.layers]}
<ide>
<ide> def count_params(self):
<ide> return sum([layer.count_params() for layer in self.layers])
<ide>
<ide>
<ide> class Graph(Layer):
<del> '''
<del> Implement a NN graph with arbitrary layer connections,
<del> arbitrary number of inputs and arbitrary number of outputs.
<del>
<del> Note: Graph can only be used as a layer
<del> (connect, input, get_input, get_output)
<del> when it has exactly one input and one output.
<del>
<del> inherited from Layer:
<del> - get_output_mask
<del> - supports_masked_input
<del> - get_weights
<del> - set_weights
<add> '''Implement a NN graph with arbitrary layer connections,
<add> arbitrary number of inputs and arbitrary number of outputs.
<add>
<add> This class is also the basis for the `keras.models.Graph` model.
<add>
<add> Note: `Graph` can only be used as a layer
<add> (connect, input, get_input, get_output)
<add> when it has exactly one input and one output.
<ide> '''
<ide> def __init__(self):
<ide> self.namespace = set() # strings
<ide> def updates(self):
<ide>
<ide> def set_previous(self, layer, connection_map={}):
<ide> if self.nb_input != layer.nb_output:
<del> raise Exception('Cannot connect layers: input count does not match output count.')
<add> raise Exception('Cannot connect layers: '
<add> 'input count does not match output count.')
<ide> if self.nb_input == 1:
<ide> self.inputs[self.input_order[0]].set_previous(layer)
<ide> else:
<ide> if not connection_map:
<del> raise Exception('Cannot attach multi-input layer: no connection_map provided.')
<add> raise Exception('Cannot attach multi-input layer: '
<add> 'no connection_map provided.')
<ide> for k, v in connection_map.items():
<ide> if k in self.inputs and v in layer.outputs:
<ide> self.inputs[k].set_previous(layer.outputs[v])
<ide> def get_output(self, train=False):
<ide> else:
<ide> return dict([(k, v.get_output(train)) for k, v in self.outputs.items()])
<ide>
<del> def add_input(self, name, input_shape=None, batch_input_shape=None, dtype='float'):
<add> def add_input(self, name, input_shape=None,
<add> batch_input_shape=None, dtype='float'):
<add> '''Add an input to the graph.
<add>
<add> # Arguments:
<add> name: string. The name of the new input. Must be unique in the graph.
<add> input_shape: a tuple of integers, the expected shape of the input samples.
<add> Does not include the batch size.
<add> batch_input_shape: a tuple of integers, the expected shape of the
<add> whole input batch, including the batch size.
<add> dtype: 'float' or 'int'.
<add> '''
<ide> if name in self.namespace:
<ide> raise Exception('Duplicate node identifier: ' + name)
<ide> self.namespace.add(name)
<ide> def add_input(self, name, input_shape=None, batch_input_shape=None, dtype='float
<ide> def add_node(self, layer, name, input=None, inputs=[],
<ide> merge_mode='concat', concat_axis=-1, dot_axes=-1,
<ide> create_output=False):
<add> '''Add a node in the graph. It can be connected to multiple
<add> inputs, which will first be merged into one tensor
<add> according to the mode specified.
<add>
<add> # Arguments
<add> layer: the layer at the node.
<add> name: name for the node.
<add> input: when connecting the layer to a single input,
<add> this is the name of the incoming node.
<add> inputs: when connecting the layer to multiple inputs,
<add> this is a list of names of incoming nodes.
<add> merge_mode: one of {concat, sum, dot, ave, mul}
<add> concat_axis: when `merge_mode=='concat'`, this is the
<add> input concatenation axis.
<add> dot_axes: when `merge_mode='dot'`, this is the contraction axes
<add> specification; see the `Merge layer for details.
<add> create_output: boolean. Set this to `True` if you want the output
<add> of your node to be an output of the graph.
<add> '''
<ide> if name in self.namespace:
<ide> raise Exception('Duplicate node identifier: ' + name)
<ide> if input:
<ide> def add_node(self, layer, name, input=None, inputs=[],
<ide> def add_shared_node(self, layer, name, inputs=[], merge_mode=None,
<ide> concat_axis=-1, dot_axes=-1, outputs=[],
<ide> create_output=False):
<del> '''
<del> Used to shared / multi input-multi output node
<del>
<del> Arguments
<del> ------------
<del> layer - The layer to be shared across multiple inputs
<del> name - Name of the shared layer
<del> inputs - List of names of input nodes
<del> merge_mode - Similar to merge_mode argument of add_node()
<del> concat_axis - Similar to concat_axis argument of add_node()
<del> dot_axes - Similar to dot_axes argument of add_node()
<del> outputs - Names for output nodes. Used when merge_mode = None
<del> create_output - Similar to create_output argument of add_node().
<del> Output will be created only if merge_mode is given
<add> '''Used to share a same layer across multiple nodes.
<add>
<add> Supposed, for instance, that you want to apply one same `Dense`
<add> layer after to the output of two different nodes.
<add> You can then add the `Dense` layer as a shared node.
<add>
<add> # Arguments
<add> layer: The layer to be shared across multiple inputs
<add> name: Name of the shared node
<add> inputs: List of names of input nodes
<add> merge_mode: Same meaning as `merge_mode` argument of `add_node()`
<add> concat_axis: Same meaning as `concat_axis` argument of `add_node()`
<add> dot_axes: Same meaning as `dot_axes` argument of `add_node()`
<add> outputs: Used when `merge_mode=None`. Names for the output nodes.
<add> create_output: Same meaning as `create_output` argument of `add_node()`.
<add> When creating an output, `merge_mode` must be specified.
<ide> '''
<ide> if name in self.namespace:
<ide> raise Exception('Duplicate node identifier: ' + name)
<ide> def add_shared_node(self, layer, name, inputs=[], merge_mode=None,
<ide> raise Exception('Duplicate node identifier: ' + o)
<ide> if merge_mode:
<ide> if merge_mode not in {'sum', 'ave', 'mul', 'dot', 'cos', 'concat', 'join'}:
<del> raise Exception("Invalid merge mode")
<add> raise Exception('Invalid merge mode')
<ide> layers = []
<ide> for i in range(len(inputs)):
<ide> input = inputs[i]
<ide> def add_shared_node(self, layer, name, inputs=[], merge_mode=None,
<ide> layers.append(n)
<ide> else:
<ide> raise Exception('Unknown identifier: ' + input)
<del> s = Siamese(layer, layers, merge_mode, concat_axis=concat_axis, dot_axes=dot_axes)
<add> s = Siamese(layer, layers, merge_mode,
<add> concat_axis=concat_axis,
<add> dot_axes=dot_axes)
<ide> self.namespace.add(name)
<ide> self.nodes[name] = s
<ide> self.node_config.append({'name': name,
<ide> def add_shared_node(self, layer, name, inputs=[], merge_mode=None,
<ide>
<ide> if create_output and merge_mode:
<ide> if merge_mode == 'join':
<del> raise Exception("Output can not be of type OrderedDict")
<add> raise Exception('Output can not be of type OrderedDict')
<ide> self.add_output(name, input=name)
<ide>
<ide> def add_output(self, name, input=None, inputs=[],
<ide> merge_mode='concat', concat_axis=-1, dot_axes=-1):
<add> '''Add an output to the graph.
<add>
<add> This output can merge several node outputs into a single output.
<add>
<add> # Arguments
<add> name: name of the output.
<add> input: when connecting the layer to a single input,
<add> this is the name of the incoming node.
<add> inputs: when connecting the layer to multiple inputs,
<add> this is a list of names of incoming nodes.
<add> merge_mode: one of {concat, sum, dot, ave, mul}
<add> concat_axis: when `merge_mode=='concat'`, this is the
<add> input concatenation axis.
<add> dot_axes: when `merge_mode='dot'`, this is the contraction axes
<add> specification; see the `Merge layer for details.
<add> '''
<ide> if name in self.output_order:
<ide> raise Exception('Duplicate output identifier: ' + name)
<ide> if input:
<ide> def add_output(self, name, input=None, inputs=[],
<ide> 'dot_axes': dot_axes})
<ide>
<ide> def get_config(self):
<del> return {"name": self.__class__.__name__,
<del> "input_config": self.input_config,
<del> "node_config": self.node_config,
<del> "output_config": self.output_config,
<del> "input_order": self.input_order,
<del> "output_order": self.output_order,
<del> "nodes": dict([(c["name"], self.nodes[c["name"]].get_config()) for c in self.node_config])}
<add> return {'name': self.__class__.__name__,
<add> 'input_config': self.input_config,
<add> 'node_config': self.node_config,
<add> 'output_config': self.output_config,
<add> 'input_order': self.input_order,
<add> 'output_order': self.output_order,
<add> 'nodes': dict([(c['name'], self.nodes[c['name']].get_config()) for c in self.node_config])}
<ide>
<ide> def count_params(self):
<ide> return sum([layer.count_params() for layer in self.nodes.values()])
<ide><path>keras/layers/convolutional.py
<ide> def conv_output_length(input_length, filter_size, border_mode, stride):
<ide>
<ide>
<ide> class Convolution1D(Layer):
<add> '''Convolution operator for filtering neighborhoods of one-dimensional inputs.
<add> When using this layer as the first layer in a model,
<add> either provide the keyword argument `input_dim`
<add> (int, e.g. 128 for sequences of 128-dimensional vectors),
<add> or `input_shape` (tuple of integers, e.g. (10, 128) for sequences
<add> of 10 vectors of 128-dimensional vectors).
<add>
<add> # Input shape
<add> 3D tensor with shape: `(samples, steps, input_dim)`.
<add>
<add> # Output shape
<add> 3D tensor with shape: `(samples, new_steps, nb_filter)`.
<add> `steps` value might have changed due to padding.
<add>
<add> # Arguments
<add> nb_filter: Number of convolution kernels to use
<add> (dimensionality of the output).
<add> filter_length: The extension (spatial or temporal) of each filter.
<add> init: name of initialization function for the weights of the layer
<add> (see [initializations](../initializations.md)),
<add> or alternatively, Theano function to use for weights initialization.
<add> This parameter is only relevant if you don't pass a `weights` argument.
<add> activation: name of activation function to use
<add> (see [activations](../activations.md)),
<add> or alternatively, elementwise Theano function.
<add> If you don't specify anything, no activation is applied
<add> (ie. "linear" activation: a(x) = x).
<add> weights: list of numpy arrays to set as initial weights.
<add> border_mode: 'valid' or 'same'.
<add> subsample_length: factor by which to subsample output.
<add> W_regularizer: instance of [WeightRegularizer](../regularizers.md)
<add> (eg. L1 or L2 regularization), applied to the main weights matrix.
<add> b_regularizer: instance of [WeightRegularizer](../regularizers.md),
<add> applied to the bias.
<add> activity_regularizer: instance of [ActivityRegularizer](../regularizers.md),
<add> applied to the network output.
<add> W_constraint: instance of the [constraints](../constraints.md) module
<add> (eg. maxnorm, nonneg), applied to the main weights matrix.
<add> b_constraint: instance of the [constraints](../constraints.md) module,
<add> applied to the bias.
<add> input_dim: Number of channels/dimensions in the input.
<add> Either this argument or the keyword argument `input_shape`must be
<add> provided when using this layer as the first layer in a model.
<add> input_length: Length of input sequences, when it is constant.
<add> This argument is required if you are going to connect
<add> `Flatten` then `Dense` layers upstream
<add> (without it, the shape of the dense outputs cannot be computed).
<add> '''
<ide> input_ndim = 3
<ide>
<ide> def __init__(self, nb_filter, filter_length,
<ide> def get_output(self, train=False):
<ide> return output
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "nb_filter": self.nb_filter,
<del> "filter_length": self.filter_length,
<del> "init": self.init.__name__,
<del> "activation": self.activation.__name__,
<del> "border_mode": self.border_mode,
<del> "subsample_length": self.subsample_length,
<del> "W_regularizer": self.W_regularizer.get_config() if self.W_regularizer else None,
<del> "b_regularizer": self.b_regularizer.get_config() if self.b_regularizer else None,
<del> "activity_regularizer": self.activity_regularizer.get_config() if self.activity_regularizer else None,
<del> "W_constraint": self.W_constraint.get_config() if self.W_constraint else None,
<del> "b_constraint": self.b_constraint.get_config() if self.b_constraint else None,
<del> "input_dim": self.input_dim,
<del> "input_length": self.input_length}
<add> config = {'name': self.__class__.__name__,
<add> 'nb_filter': self.nb_filter,
<add> 'filter_length': self.filter_length,
<add> 'init': self.init.__name__,
<add> 'activation': self.activation.__name__,
<add> 'border_mode': self.border_mode,
<add> 'subsample_length': self.subsample_length,
<add> 'W_regularizer': self.W_regularizer.get_config() if self.W_regularizer else None,
<add> 'b_regularizer': self.b_regularizer.get_config() if self.b_regularizer else None,
<add> 'activity_regularizer': self.activity_regularizer.get_config() if self.activity_regularizer else None,
<add> 'W_constraint': self.W_constraint.get_config() if self.W_constraint else None,
<add> 'b_constraint': self.b_constraint.get_config() if self.b_constraint else None,
<add> 'input_dim': self.input_dim,
<add> 'input_length': self.input_length}
<ide> base_config = super(Convolution1D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class Convolution2D(Layer):
<add> '''Convolution operator for filtering windows of two-dimensional inputs.
<add> When using this layer as the first layer in a model,
<add> provide the keyword argument `input_shape`
<add> (tuple of integers, does not include the sample axis),
<add> e.g. `input_shape=(3, 128, 128)` for 128x128 RGB pictures.
<add>
<add> # Input shape
<add> 4D tensor with shape:
<add> `(samples, channels, rows, cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, rows, cols, channels)` if dim_ordering='tf'.
<add>
<add> # Output shape
<add> 4D tensor with shape:
<add> `(samples, nb_filter, nb_row, nb_col)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, nb_row, nb_col, nb_filter)` if dim_ordering='tf'.
<add>
<add>
<add> # Arguments
<add> nb_filter: Number of convolution filters to use.
<add> nb_row: Number of rows in the convolution kernel.
<add> nb_col: Number of columns in the convolution kernel.
<add> init: name of initialization function for the weights of the layer
<add> (see [initializations](../initializations.md)), or alternatively,
<add> Theano function to use for weights initialization.
<add> This parameter is only relevant if you don't pass
<add> a `weights` argument.
<add> activation: name of activation function to use
<add> (see [activations](../activations.md)),
<add> or alternatively, elementwise Theano function.
<add> If you don't specify anything, no activation is applied
<add> (ie. "linear" activation: a(x) = x).
<add> weights: list of numpy arrays to set as initial weights.
<add> border_mode: 'valid' or 'same'.
<add> subsample: tuple of length 2. Factor by which to subsample output.
<add> Also called strides elsewhere.
<add> W_regularizer: instance of [WeightRegularizer](../regularizers.md)
<add> (eg. L1 or L2 regularization), applied to the main weights matrix.
<add> b_regularizer: instance of [WeightRegularizer](../regularizers.md),
<add> applied to the bias.
<add> activity_regularizer: instance of [ActivityRegularizer](../regularizers.md),
<add> applied to the network output.
<add> W_constraint: instance of the [constraints](../constraints.md) module
<add> (eg. maxnorm, nonneg), applied to the main weights matrix.
<add> b_constraint: instance of the [constraints](../constraints.md) module,
<add> applied to the bias.
<add> dim_ordering: 'th' or 'tf'. In 'th' mode, the channels dimension
<add> (the depth) is at index 1, in 'tf' mode is it at index 3.
<add> '''
<ide> input_ndim = 4
<ide>
<ide> def __init__(self, nb_filter, nb_row, nb_col,
<ide> def get_output(self, train=False):
<ide> return output
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "nb_filter": self.nb_filter,
<del> "nb_row": self.nb_row,
<del> "nb_col": self.nb_col,
<del> "init": self.init.__name__,
<del> "activation": self.activation.__name__,
<del> "border_mode": self.border_mode,
<del> "subsample": self.subsample,
<del> "dim_ordering": self.dim_ordering,
<del> "W_regularizer": self.W_regularizer.get_config() if self.W_regularizer else None,
<del> "b_regularizer": self.b_regularizer.get_config() if self.b_regularizer else None,
<del> "activity_regularizer": self.activity_regularizer.get_config() if self.activity_regularizer else None,
<del> "W_constraint": self.W_constraint.get_config() if self.W_constraint else None,
<del> "b_constraint": self.b_constraint.get_config() if self.b_constraint else None}
<add> config = {'name': self.__class__.__name__,
<add> 'nb_filter': self.nb_filter,
<add> 'nb_row': self.nb_row,
<add> 'nb_col': self.nb_col,
<add> 'init': self.init.__name__,
<add> 'activation': self.activation.__name__,
<add> 'border_mode': self.border_mode,
<add> 'subsample': self.subsample,
<add> 'dim_ordering': self.dim_ordering,
<add> 'W_regularizer': self.W_regularizer.get_config() if self.W_regularizer else None,
<add> 'b_regularizer': self.b_regularizer.get_config() if self.b_regularizer else None,
<add> 'activity_regularizer': self.activity_regularizer.get_config() if self.activity_regularizer else None,
<add> 'W_constraint': self.W_constraint.get_config() if self.W_constraint else None,
<add> 'b_constraint': self.b_constraint.get_config() if self.b_constraint else None}
<ide> base_config = super(Convolution2D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<del>class Pooling1D(Layer):
<add>class _Pooling1D(Layer):
<add> '''Abstract class for different pooling 1D layers.
<add> '''
<ide> input_dim = 3
<ide>
<ide> def __init__(self, pool_length=2, stride=None,
<ide> border_mode='valid', **kwargs):
<del> super(Pooling1D, self).__init__(**kwargs)
<add> super(_Pooling1D, self).__init__(**kwargs)
<ide> if stride is None:
<ide> stride = pool_length
<ide> self.pool_length = pool_length
<ide> def output_shape(self):
<ide> self.border_mode, self.stride)
<ide> return (input_shape[0], length, input_shape[2])
<ide>
<del> def pooling_function(self, back_end, inputs, pool_size, strides,
<del> border_mode, dim_ordering):
<add> def _pooling_function(self, back_end, inputs, pool_size, strides,
<add> border_mode, dim_ordering):
<ide> raise NotImplementedError
<ide>
<ide> def get_output(self, train=False):
<ide> X = self.get_input(train)
<ide> X = K.expand_dims(X, -1) # add dummy last dimension
<ide> X = K.permute_dimensions(X, (0, 2, 1, 3))
<del> output = self.pooling_function(inputs=X, pool_size=self.pool_size,
<del> strides=self.st,
<del> border_mode=self.border_mode,
<del> dim_ordering='th')
<add> output = self._pooling_function(inputs=X, pool_size=self.pool_size,
<add> strides=self.st,
<add> border_mode=self.border_mode,
<add> dim_ordering='th')
<ide> output = K.permute_dimensions(output, (0, 2, 1, 3))
<ide> return K.squeeze(output, 3) # remove dummy last dimension
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "stride": self.stride,
<del> "pool_length": self.pool_length,
<del> "border_mode": self.border_mode}
<del> base_config = super(Pooling1D, self).get_config()
<add> config = {'name': self.__class__.__name__,
<add> 'stride': self.stride,
<add> 'pool_length': self.pool_length,
<add> 'border_mode': self.border_mode}
<add> base_config = super(_Pooling1D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<del>class MaxPooling1D(Pooling1D):
<del> def __init__(self, *args, **kwargs):
<del> super(MaxPooling1D, self).__init__(*args, **kwargs)
<add>class MaxPooling1D(_Pooling1D):
<add> '''Max pooling operation for temporal data.
<ide>
<del> def pooling_function(self, inputs, pool_size, strides,
<del> border_mode, dim_ordering):
<add> # Input shape
<add> 3D tensor with shape: `(samples, steps, features)`.
<add>
<add> # Output shape
<add> 3D tensor with shape: `(samples, downsampled_steps, features)`.
<add>
<add> # Arguments
<add> pool_length: factor by which to downscale. 2 will halve the input.
<add> stride: integer or None. Stride value.
<add> border_mode: 'valid' or 'same'.
<add> Note: 'same' will only work with TensorFlow for the time being.
<add> '''
<add> def __init__(self, pool_length=2, stride=None,
<add> border_mode='valid', **kwargs):
<add> super(MaxPooling1D, self).__init__(pool_length, stride,
<add> border_mode, **kwargs)
<add>
<add> def _pooling_function(self, inputs, pool_size, strides,
<add> border_mode, dim_ordering):
<ide> output = K.pool2d(inputs, pool_size, strides,
<ide> border_mode, dim_ordering, pool_mode='max')
<ide> return output
<ide>
<ide>
<del>class AveragePooling1D(Pooling1D):
<del> def __init__(self, *args, **kwargs):
<del> super(AveragePooling1D, self).__init__(*args, **kwargs)
<add>class AveragePooling1D(_Pooling1D):
<add> '''Average pooling for temporal data.
<add>
<add> # Input shape
<add> 3D tensor with shape: `(samples, steps, features)`.
<add>
<add> # Output shape
<add> 3D tensor with shape: `(samples, downsampled_steps, features)`.
<add>
<add> # Arguments
<add> pool_length: factor by which to downscale. 2 will halve the input.
<add> stride: integer or None. Stride value.
<add> border_mode: 'valid' or 'same'.
<add> Note: 'same' will only work with TensorFlow for the time being.
<add> '''
<add> def __init__(self, pool_length=2, stride=None,
<add> border_mode='valid', **kwargs):
<add> super(AveragePooling1D, self).__init__(pool_length, stride,
<add> border_mode, **kwargs)
<ide>
<del> def pooling_function(self, inputs, pool_size, strides,
<del> border_mode, dim_ordering):
<add> def _pooling_function(self, inputs, pool_size, strides,
<add> border_mode, dim_ordering):
<ide> output = K.pool2d(inputs, pool_size, strides,
<ide> border_mode, dim_ordering, pool_mode='avg')
<ide> return output
<ide>
<ide>
<del>class Pooling2D(Layer):
<add>class _Pooling2D(Layer):
<add> '''Abstract class for different pooling 2D layers.
<add> '''
<ide> input_ndim = 4
<ide>
<ide> def __init__(self, pool_size=(2, 2), strides=None, border_mode='valid',
<ide> dim_ordering='th', **kwargs):
<del> super(Pooling2D, self).__init__(**kwargs)
<add> super(_Pooling2D, self).__init__(**kwargs)
<ide> self.input = K.placeholder(ndim=4)
<ide> self.pool_size = tuple(pool_size)
<ide> if strides is None:
<ide> def output_shape(self):
<ide> else:
<ide> raise Exception('Invalid dim_ordering: ' + self.dim_ordering)
<ide>
<del> def pooling_function(self, inputs, pool_size, strides,
<del> border_mode, dim_ordering):
<add> def _pooling_function(self, inputs, pool_size, strides,
<add> border_mode, dim_ordering):
<ide> raise NotImplementedError
<ide>
<ide> def get_output(self, train=False):
<ide> X = self.get_input(train)
<del> output = self.pooling_function(inputs=X, pool_size=self.pool_size,
<del> strides=self.strides,
<del> border_mode=self.border_mode,
<del> dim_ordering=self.dim_ordering)
<add> output = self._pooling_function(inputs=X, pool_size=self.pool_size,
<add> strides=self.strides,
<add> border_mode=self.border_mode,
<add> dim_ordering=self.dim_ordering)
<ide> return output
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "pool_size": self.pool_size,
<del> "border_mode": self.border_mode,
<del> "strides": self.strides,
<del> "dim_ordering": self.dim_ordering}
<del> base_config = super(Pooling2D, self).get_config()
<add> config = {'name': self.__class__.__name__,
<add> 'pool_size': self.pool_size,
<add> 'border_mode': self.border_mode,
<add> 'strides': self.strides,
<add> 'dim_ordering': self.dim_ordering}
<add> base_config = super(_Pooling2D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<del>class MaxPooling2D(Pooling2D):
<del> def __init__(self, *args, **kwargs):
<del> super(MaxPooling2D, self).__init__(*args, **kwargs)
<add>class MaxPooling2D(_Pooling2D):
<add> '''Max pooling operation for spatial data.
<add>
<add> # Input shape
<add> 4D tensor with shape:
<add> `(samples, channels, rows, cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, rows, cols, channels)` if dim_ordering='tf'.
<add>
<add> # Output shape
<add> 4D tensor with shape:
<add> `(nb_samples, channels, pooled_rows, pooled_cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, pooled_rows, pooled_cols, channels)` if dim_ordering='tf'.
<add>
<add> # Arguments
<add> pool_size: tuple of 2 integers,
<add> factors by which to downscale (vertical, horizontal).
<add> (2, 2) will halve the image in each dimension.
<add> strides: tuple of 2 integers, or None. Strides values.
<add> border_mode: 'valid' or 'same'.
<add> Note: 'same' will only work with TensorFlow for the time being.
<add> dim_ordering: 'th' or 'tf'. In 'th' mode, the channels dimension
<add> (the depth) is at index 1, in 'tf' mode is it at index 3.
<add> '''
<add> def __init__(self, pool_size=(2, 2), strides=None, border_mode='valid',
<add> dim_ordering='th', **kwargs):
<add> super(MaxPooling2D, self).__init__(pool_size, strides, border_mode,
<add> dim_ordering, **kwargs)
<ide>
<del> def pooling_function(self, inputs, pool_size, strides,
<del> border_mode, dim_ordering):
<add> def _pooling_function(self, inputs, pool_size, strides,
<add> border_mode, dim_ordering):
<ide> output = K.pool2d(inputs, pool_size, strides,
<ide> border_mode, dim_ordering, pool_mode='max')
<ide> return output
<ide>
<ide>
<del>class AveragePooling2D(Pooling2D):
<del> def __init__(self, *args, **kwargs):
<del> super(AveragePooling2D, self).__init__(*args, **kwargs)
<add>class AveragePooling2D(_Pooling2D):
<add> '''Average pooling operation for spatial data.
<add>
<add> # Input shape
<add> 4D tensor with shape:
<add> `(samples, channels, rows, cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, rows, cols, channels)` if dim_ordering='tf'.
<add>
<add> # Output shape
<add> 4D tensor with shape:
<add> `(nb_samples, channels, pooled_rows, pooled_cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, pooled_rows, pooled_cols, channels)` if dim_ordering='tf'.
<add>
<add> # Arguments
<add> pool_size: tuple of 2 integers,
<add> factors by which to downscale (vertical, horizontal).
<add> (2, 2) will halve the image in each dimension.
<add> strides: tuple of 2 integers, or None. Strides values.
<add> border_mode: 'valid' or 'same'.
<add> Note: 'same' will only work with TensorFlow for the time being.
<add> dim_ordering: 'th' or 'tf'. In 'th' mode, the channels dimension
<add> (the depth) is at index 1, in 'tf' mode is it at index 3.
<add> '''
<add> def __init__(self, pool_size=(2, 2), strides=None, border_mode='valid',
<add> dim_ordering='th', **kwargs):
<add> super(AveragePooling2D, self).__init__(pool_size, strides, border_mode,
<add> dim_ordering, **kwargs)
<ide>
<del> def pooling_function(self, inputs, pool_size, strides,
<del> border_mode, dim_ordering):
<add> def _pooling_function(self, inputs, pool_size, strides,
<add> border_mode, dim_ordering):
<ide> output = K.pool2d(inputs, pool_size, strides,
<ide> border_mode, dim_ordering, pool_mode='avg')
<ide> return output
<ide>
<ide>
<ide> class UpSampling1D(Layer):
<add> '''Repeats each temporal step `length` times along the time axis.
<add>
<add> # Input shape
<add> 3D tensor with shape: `(samples, steps, features)`.
<add>
<add> # Output shape
<add> 3D tensor with shape: `(samples, upsampled_steps, features)`.
<add>
<add> # Arguments:
<add> length: integer. Upsampling factor.
<add> '''
<ide> input_ndim = 3
<ide>
<ide> def __init__(self, length=2, **kwargs):
<ide> def get_output(self, train=False):
<ide> return output
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "length": self.length}
<add> config = {'name': self.__class__.__name__,
<add> 'length': self.length}
<ide> base_config = super(UpSampling1D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class UpSampling2D(Layer):
<add> '''Repeats the rows and columns of the data
<add> by size[0] and size[1] respectively.
<add>
<add> # Input shape
<add> 4D tensor with shape:
<add> `(samples, channels, rows, cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, rows, cols, channels)` if dim_ordering='tf'.
<add>
<add> # Output shape
<add> 4D tensor with shape:
<add> `(samples, channels, upsampled_rows, upsampled_cols)` if dim_ordering='th'
<add> or 4D tensor with shape:
<add> `(samples, upsampled_rows, upsampled_cols, channels)` if dim_ordering='tf'.
<add>
<add> # Arguments
<add> size: tuple of 2 integers. The upsampling factors for rows and columns.
<add> dim_ordering: 'th' or 'tf'.
<add> In 'th' mode, the channels dimension (the depth)
<add> is at index 1, in 'tf' mode is it at index 3.
<add> '''
<ide> input_ndim = 4
<ide>
<ide> def __init__(self, size=(2, 2), dim_ordering='th', **kwargs):
<ide> def get_output(self, train=False):
<ide> return output
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "size": self.size}
<add> config = {'name': self.__class__.__name__,
<add> 'size': self.size}
<ide> base_config = super(UpSampling2D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class ZeroPadding1D(Layer):
<del> """Zero-padding layer for 1D input (e.g. temporal sequence).
<del>
<del> Input shape
<del> -----------
<del> 3D tensor with shape (samples, axis_to_pad, features)
<del>
<del> Output shape
<del> ------------
<del> 3D tensor with shape (samples, padded_axis, features)
<del>
<del> Arguments
<del> ---------
<del> padding: int
<del> How many zeros to add at the beginning and end of
<del> the padding dimension (axis 1).
<del> """
<add> '''Zero-padding layer for 1D input (e.g. temporal sequence).
<add>
<add> # Input shape
<add> 3D tensor with shape (samples, axis_to_pad, features)
<add>
<add> # Output shape
<add> 3D tensor with shape (samples, padded_axis, features)
<add>
<add> # Arguments
<add> padding: int
<add> How many zeros to add at the beginning and end of
<add> the padding dimension (axis 1).
<add> '''
<ide> input_ndim = 3
<ide>
<ide> def __init__(self, padding=1, **kwargs):
<ide> def get_output(self, train=False):
<ide> return K.temporal_padding(X, padding=self.padding)
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "padding": self.padding}
<add> config = {'name': self.__class__.__name__,
<add> 'padding': self.padding}
<ide> base_config = super(ZeroPadding1D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class ZeroPadding2D(Layer):
<del> """Zero-padding layer for 2D input (e.g. picture).
<add> '''Zero-padding layer for 2D input (e.g. picture).
<ide>
<del> Input shape
<del> -----------
<del> 4D tensor with shape:
<add> # Input shape
<add> 4D tensor with shape:
<ide> (samples, depth, first_axis_to_pad, second_axis_to_pad)
<ide>
<del> Output shape
<del> ------------
<del> 4D tensor with shape:
<add> # Output shape
<add> 4D tensor with shape:
<ide> (samples, depth, first_padded_axis, second_padded_axis)
<ide>
<del> Arguments
<del> ---------
<del> padding: tuple of int (length 2)
<del> How many zeros to add at the beginning and end of
<del> the 2 padding dimensions (axis 3 and 4).
<del> """
<add> # Arguments
<add> padding: tuple of int (length 2)
<add> How many zeros to add at the beginning and end of
<add> the 2 padding dimensions (axis 3 and 4).
<add> '''
<ide> input_ndim = 4
<ide>
<ide> def __init__(self, padding=(1, 1), dim_ordering='th', **kwargs):
<ide> def get_output(self, train=False):
<ide> dim_ordering=self.dim_ordering)
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "padding": self.padding}
<add> config = {'name': self.__class__.__name__,
<add> 'padding': self.padding}
<ide> base_config = super(ZeroPadding2D, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide><path>keras/layers/core.py
<ide>
<ide>
<ide> class Layer(object):
<add> '''Abstract base layer class.
<add>
<add> All Keras layers accept certain keyword arguments:
<add> trainable: boolean. Set to "False" before model compilation
<add> to freeze layer weights (they won't be updated further
<add> during training).
<add> input_shape: a tuple of integers specifying the expected shape
<add> of the input samples. Does not includes the batch size.
<add> (e.g. `(100,)` for 100-dimensional inputs).
<add> batch_input_shape: a tuple of integers specifying the expected
<add> shape of a batch of input samples. Includes the batch size
<add> (e.g. `(32, 100)` for a batch of 32 100-dimensional inputs).
<add> '''
<ide> def __init__(self, **kwargs):
<ide> allowed_kwargs = {'input_shape',
<ide> 'trainable',
<ide> def __call__(self, X, train=False):
<ide> return Y
<ide>
<ide> def set_previous(self, layer, connection_map={}):
<add> '''Connect a layer to its parent in the computational graph.
<add> '''
<ide> assert self.nb_input == layer.nb_output == 1, "Cannot connect layers: input count and output count should be 1."
<ide> if hasattr(self, 'input_ndim'):
<ide> assert self.input_ndim == len(layer.output_shape), "Incompatible shapes: layer expected input with ndim=" +\
<ide> def get_input(self, train=False):
<ide> 'and is not an input layer.')
<ide>
<ide> def supports_masked_input(self):
<del> ''' Whether or not this layer respects the output mask of its previous layer in its calculations. If you try
<del> to attach a layer that does *not* support masked_input to a layer that gives a non-None output_mask() that is
<del> an error'''
<add> ''' Whether or not this layer respects the output mask of its previous
<add> layer in its calculations.
<add> If you try to attach a layer that does *not* support masked_input to
<add> a layer that gives a non-None output_mask(), an error will be raised.
<add> '''
<ide> return False
<ide>
<ide> def get_output_mask(self, train=None):
<ide> '''
<del> For some models (such as RNNs) you want a way of being able to mark some output data-points as
<del> "masked", so they are not used in future calculations. In such a model, get_output_mask() should return a mask
<del> of one less dimension than get_output() (so if get_output is (nb_samples, nb_timesteps, nb_dimensions), then the mask
<del> is (nb_samples, nb_timesteps), with a one for every unmasked datapoint, and a zero for every masked one.
<del>
<del> If there is *no* masking then it shall return None. For instance if you attach an Activation layer (they support masking)
<del> to a layer with an output_mask, then that Activation shall also have an output_mask. If you attach it to a layer with no
<del> such mask, then the Activation's get_output_mask shall return None.
<del>
<del> Some layers have an output_mask even if their input is unmasked, notably Embedding which can turn the entry "0" into
<add> For some models (such as RNNs) you want a way of being able to mark
<add> some output data-points as "masked",
<add> so they are not used in future calculations.
<add> In such a model, get_output_mask() should return a mask
<add> of one less dimension than get_output()
<add> (so if get_output is (nb_samples, nb_timesteps, nb_dimensions),
<add> then the mask is (nb_samples, nb_timesteps),
<add> with a one for every unmasked datapoint,
<add> and a zero for every masked one.
<add>
<add> If there is *no* masking then it shall return None.
<add> For instance if you attach an Activation layer (they support masking)
<add> to a layer with an output_mask, then that Activation shall
<add> also have an output_mask.
<add> If you attach it to a layer with no such mask,
<add> then the Activation's get_output_mask shall return None.
<add>
<add> Some layers have an output_mask even if their input is unmasked,
<add> notably Embedding which can turn the entry "0" into
<ide> a mask.
<ide> '''
<ide> return None
<ide>
<ide> def set_weights(self, weights):
<add> '''Set the weights of the layer.
<add>
<add> # Arguments
<add> weights: a list of numpy arrays. The number
<add> of arrays and their shape must match
<add> number of the dimensions of the weights
<add> of the layer (i.e. it should match the
<add> output of `get_weights`).
<add> '''
<ide> assert len(self.params) == len(weights), 'Provided weight array does not match layer weights (' + \
<ide> str(len(self.params)) + ' layer params vs. ' + str(len(weights)) + ' provided weights)'
<ide> for p, w in zip(self.params, weights):
<ide> def set_weights(self, weights):
<ide> K.set_value(p, w)
<ide>
<ide> def get_weights(self):
<add> '''Return the weights of the layer.
<add>
<add> # Returns: list of numpy arrays.
<add> '''
<ide> weights = []
<ide> for p in self.params:
<ide> weights.append(K.get_value(p))
<ide> return weights
<ide>
<ide> def get_config(self):
<add> '''Return the parameters of the layer.
<add>
<add> # Returns:
<add> A dictionary mapping parameter names to their values.
<add> '''
<ide> config = {"name": self.__class__.__name__}
<ide> if hasattr(self, '_input_shape'):
<ide> config['input_shape'] = self._input_shape[1:]
<ide> def get_params(self):
<ide> return self.params, regularizers, consts, updates
<ide>
<ide> def count_params(self):
<add> '''Return the total number of floats (or ints)
<add> composing the weights of the layer.
<add> '''
<ide> return sum([K.count_params(p) for p in self.params])
<ide>
<ide>
<ide> class MaskedLayer(Layer):
<del> '''
<del> If your layer trivially supports masking
<add> '''If your layer trivially supports masking
<ide> (by simply copying the input mask to the output),
<ide> then subclass MaskedLayer instead of Layer,
<ide> and make sure that you incorporate the input mask
<del> into your calculation of get_output()
<add> into your calculation of get_output().
<ide> '''
<ide> def supports_masked_input(self):
<ide> return True
<ide> def get_output_mask(self, train=False):
<ide>
<ide>
<ide> class Masking(MaskedLayer):
<del> """Mask an input sequence by using a mask value to identify padding.
<add> '''Mask an input sequence by using a mask value to identify padding.
<ide>
<ide> This layer copies the input to the output layer with identified padding
<ide> replaced with 0s and creates an output mask in the process.
<ide>
<ide> At each timestep, if the values all equal `mask_value`,
<ide> then the corresponding mask value for the timestep is 0 (skipped),
<ide> otherwise it is 1.
<del>
<del> """
<add> '''
<ide> def __init__(self, mask_value=0., **kwargs):
<ide> super(Masking, self).__init__(**kwargs)
<ide> self.mask_value = mask_value
<ide> def get_config(self):
<ide>
<ide> class TimeDistributedMerge(Layer):
<ide> '''Sum/multiply/average over the outputs of a TimeDistributed layer.
<del> mode: {'sum', 'mul', 'ave'}
<del> Tensor input dimensions: (nb_sample, time, features)
<del> Tensor output dimensions: (nb_sample, features)
<add>
<add> # Input shape
<add> 3D tensor with shape: `(samples, steps, features)`.
<add>
<add> # Output shape
<add> 2D tensor with shape: `(samples, features)`.
<add>
<add> # Arguments
<add> mode: one of {'sum', 'mul', 'ave'}
<ide> '''
<ide> input_ndim = 3
<ide>
<ide> def get_config(self):
<ide>
<ide>
<ide> class Merge(Layer):
<add> '''Merge the output of a list of layers or containers into a single tensor.
<add>
<add> # Arguments
<add> mode: one of {sum, mul, concat, ave, dot}.
<add> sum: sum the outputs (shapes must match)
<add> mul: multiply the outputs element-wise (shapes must match)
<add> concat: concatenate the outputs along the axis specified by `concat_axis`
<add> ave: average the outputs (shapes must match)
<add> concat_axis: axis to use in `concat` mode.
<add> dot_axes: axis or axes to use in `dot` mode
<add> (see [the Numpy documentation](http://docs.scipy.org/doc/numpy-1.10.1/reference/generated/numpy.tensordot.html) for more details).
<add>
<add> # TensorFlow warning
<add> `dot` mode only works with Theano for the time being.
<add>
<add> # Examples
<add>
<add> ```python
<add> left = Sequential()
<add> left.add(Dense(50, input_shape=(784,)))
<add> left.add(Activation('relu'))
<add>
<add> right = Sequential()
<add> right.add(Dense(50, input_shape=(784,)))
<add> right.add(Activation('relu'))
<add>
<add> model = Sequential()
<add> model.add(Merge([left, right], mode='sum'))
<add>
<add> model.add(Dense(10))
<add> model.add(Activation('softmax'))
<add>
<add> model.compile(loss='categorical_crossentropy', optimizer='rmsprop')
<add>
<add> model.fit([X_train, X_train], Y_train, batch_size=128, nb_epoch=20,
<add> validation_data=([X_test, X_test], Y_test))
<add> ```
<add> '''
<ide> def __init__(self, layers, mode='sum', concat_axis=-1, dot_axes=-1):
<del> ''' Merge the output of a list of layers or containers into a single tensor.
<del> mode: {'sum', 'mul', 'concat', 'ave', 'join'}
<del> '''
<ide> if len(layers) < 2:
<ide> raise Exception("Please specify two or more input layers (or containers) to merge")
<ide>
<ide> def __init__(self, layers, mode='sum', concat_axis=-1, dot_axes=-1):
<ide> raise Exception("'concat' mode can only merge layers with matching " +
<ide> "output shapes except for the concat axis. " +
<ide> "Layer shapes: %s" % ([l.output_shape for l in layers]))
<del>
<ide> self.mode = mode
<ide> self.concat_axis = concat_axis
<ide> self.dot_axes = dot_axes
<ide> def get_output(self, train=False):
<ide> for i in range(len(self.layers)):
<ide> X = self.layers[i].get_output(train)
<ide> if X.name is None:
<del> raise ValueError("merge_mode='join' only works with named inputs")
<add> raise ValueError('merge_mode='join' only works with named inputs')
<ide> else:
<ide> inputs[X.name] = X
<ide> return inputs
<ide> def get_config(self):
<ide>
<ide>
<ide> class Dropout(MaskedLayer):
<del> '''
<del> Hinton's dropout.
<add> '''Apply Dropout to the input. Dropout consists in randomly setting
<add> a fraction `p` of input units to 0 at each update during training time,
<add> which helps prevent overfitting.
<add>
<add> # Arguments
<add> p: float between 0 and 1. Fraction of the input units to drop.
<add>
<add> # References
<add> - [Dropout: A Simple Way to Prevent Neural Networks from Overfitting](http://www.cs.toronto.edu/~rsalakhu/papers/srivastava14a.pdf)
<ide> '''
<ide> def __init__(self, p, **kwargs):
<ide> super(Dropout, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class Activation(MaskedLayer):
<add> '''Apply an activation function to an output.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as input.
<add>
<add> # Arguments:
<add> activation: name of activation function to use
<add> (see: [activations](../activations.md)),
<add> or alternatively, a Theano or TensorFlow operation.
<ide> '''
<del> Apply an activation function to an output.
<del> '''
<del> def __init__(self, activation, target=0, beta=0.1, **kwargs):
<add> def __init__(self, activation, **kwargs):
<ide> super(Activation, self).__init__(**kwargs)
<ide> self.activation = activations.get(activation)
<del> self.target = target
<del> self.beta = beta
<ide>
<ide> def get_output(self, train=False):
<ide> X = self.get_input(train)
<ide> return self.activation(X)
<ide>
<ide> def get_config(self):
<ide> config = {"name": self.__class__.__name__,
<del> "activation": self.activation.__name__,
<del> "target": self.target,
<del> "beta": self.beta}
<add> "activation": self.activation.__name__}
<ide> base_config = super(Activation, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class Reshape(Layer):
<del> '''
<del> Reshape an output to a certain shape.
<del> Can't be used as first layer in a model (no fixed input!)
<del> First dimension is assumed to be nb_samples.
<add> '''Reshape an output to a certain shape.
<add>
<add> # Input shape
<add> Arbitrary, although all dimensions in the input shaped must be fixed.
<add> Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> `(batch_size,) + dims`
<add>
<add> # Arguments
<add> dims: target shape. Tuple of integers,
<add> does not include the samples dimension (batch size).
<ide> '''
<ide> def __init__(self, dims, **kwargs):
<ide> super(Reshape, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class Permute(Layer):
<del> '''
<del> Permute the dimensions of the input according to the given tuple.
<add> '''Permute the dimensions of the input according to a given pattern.
<add>
<add> Useful for e.g. connecting RNNs and convnets together.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same as the input shape, but with the dimensions re-ordered according
<add> to the specified pattern.
<add>
<add> # Arguments
<add> dims: Tuple of integers. Permutation pattern, does not include the
<add> samples dimension. Indexing starts at 1.
<add> For instance, `(2, 1)` permutes the first and second dimension
<add> of the input.
<ide> '''
<ide> def __init__(self, dims, **kwargs):
<ide> super(Permute, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class Flatten(Layer):
<del> '''
<del> Reshape input to flat shape.
<del> First dimension is assumed to be nb_samples.
<add> '''Flatten the input. Does not affect the batch size.
<add>
<add> # Input shape
<add> Arbitrary, although all dimensions in the input shaped must be fixed.
<add> Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> `(batch_size,)`
<ide> '''
<ide> def __init__(self, **kwargs):
<ide> super(Flatten, self).__init__(**kwargs)
<ide> def get_output(self, train=False):
<ide>
<ide>
<ide> class RepeatVector(Layer):
<del> '''
<del> Repeat input n times.
<add> '''Repeat the input n times.
<ide>
<del> Dimensions of input are assumed to be (nb_samples, dim).
<del> Return tensor of shape (nb_samples, n, dim).
<add> # Input shape
<add> 2D tensor of shape `(nb_samples, features)`.
<add>
<add> # Output shape
<add> 3D tensor of shape `(nb_samples, n, features)`.
<add>
<add> # Arguments
<add> n: integer, repetition factor.
<ide> '''
<ide> def __init__(self, n, **kwargs):
<ide> super(RepeatVector, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class Dense(Layer):
<del> '''
<del> Just your regular fully connected NN layer.
<add> '''Just your regular fully connected NN layer.
<add>
<add> # Input shape
<add> 2D tensor with shape: `(nb_samples, input_dim)`.
<add>
<add> # Output shape
<add> 2D tensor with shape: `(nb_samples, output_dim)`.
<add>
<add> # Arguments
<add> output_dim: int > 0.
<add> init: name of initialization function for the weights of the layer
<add> (see [initializations](../initializations.md)),
<add> or alternatively, Theano function to use for weights
<add> initialization. This parameter is only relevant
<add> if you don't pass a `weights` argument.
<add> activation: name of activation function to use
<add> (see [activations](../activations.md)),
<add> or alternatively, elementwise Theano function.
<add> If you don't specify anything, no activation is applied
<add> (ie. "linear" activation: a(x) = x).
<add> weights: list of numpy arrays to set as initial weights.
<add> The list should have 1 element, of shape `(input_dim, output_dim)`.
<add> W_regularizer: instance of [WeightRegularizer](../regularizers.md)
<add> (eg. L1 or L2 regularization), applied to the main weights matrix.
<add> b_regularizer: instance of [WeightRegularizer](../regularizers.md),
<add> applied to the bias.
<add> activity_regularizer: instance of [ActivityRegularizer](../regularizers.md),
<add> applied to the network output.
<add> W_constraint: instance of the [constraints](../constraints.md) module
<add> (eg. maxnorm, nonneg), applied to the main weights matrix.
<add> b_constraint: instance of the [constraints](../constraints.md) module,
<add> applied to the bias.
<add> input_dim: dimensionality of the input (integer).
<add> This argument (or alternatively, the keyword argument `input_shape`)
<add> is required when using this layer as the first layer in a model.
<ide> '''
<ide> input_ndim = 2
<ide>
<ide> def get_config(self):
<ide>
<ide>
<ide> class ActivityRegularization(Layer):
<del> '''
<del> Layer that passes through its input unchanged, but applies an update
<del> to the cost function based on the activity.
<add> '''Layer that passes through its input unchanged, but applies an update
<add> to the cost function based on the activity.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as input.
<add>
<add> # Arguments
<add> l1: L1 regularization factor.
<add> l2: L2 regularization factor.
<ide> '''
<ide> def __init__(self, l1=0., l2=0., **kwargs):
<ide> super(ActivityRegularization, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class TimeDistributedDense(MaskedLayer):
<del> '''
<del> Apply a same Dense layer for each dimension[1] (time_dimension) input.
<del> Especially useful after a recurrent network with 'return_sequence=True'.
<del> Tensor input dimensions: (nb_sample, time_dimension, input_dim)
<del> Tensor output dimensions: (nb_sample, time_dimension, output_dim)
<del>
<add> '''Apply a same Dense layer for each dimension[1] (time_dimension) input.
<add> Especially useful after a recurrent network with 'return_sequence=True'.
<add>
<add> # Input shape
<add> 3D tensor with shape `(nb_sample, time_dimension, input_dim)`.
<add>
<add> # Output shape
<add> 3D tensor with shape `(nb_sample, time_dimension, output_dim)`.
<add>
<add> # Arguments
<add> output_dim: int > 0.
<add> init: name of initialization function for the weights of the layer
<add> (see [initializations](../initializations.md)),
<add> or alternatively, Theano function to use for weights
<add> initialization. This parameter is only relevant
<add> if you don't pass a `weights` argument.
<add> activation: name of activation function to use
<add> (see [activations](../activations.md)),
<add> or alternatively, elementwise Theano function.
<add> If you don't specify anything, no activation is applied
<add> (ie. "linear" activation: a(x) = x).
<add> weights: list of numpy arrays to set as initial weights.
<add> The list should have 1 element, of shape `(input_dim, output_dim)`.
<add> W_regularizer: instance of [WeightRegularizer](../regularizers.md)
<add> (eg. L1 or L2 regularization), applied to the main weights matrix.
<add> b_regularizer: instance of [WeightRegularizer](../regularizers.md),
<add> applied to the bias.
<add> activity_regularizer: instance of [ActivityRegularizer](../regularizers.md),
<add> applied to the network output.
<add> W_constraint: instance of the [constraints](../constraints.md) module
<add> (eg. maxnorm, nonneg), applied to the main weights matrix.
<add> b_constraint: instance of the [constraints](../constraints.md) module,
<add> applied to the bias.
<add> input_dim: dimensionality of the input (integer).
<add> This argument (or alternatively, the keyword argument `input_shape`)
<add> is required when using this layer as the first layer in a model.
<ide> '''
<ide> input_ndim = 3
<ide>
<ide> def get_config(self):
<ide> class AutoEncoder(Layer):
<ide> '''A customizable autoencoder model.
<ide>
<del> Tensor input dimensions: same as encoder input
<del> Tensor output dimensions:
<del> if output_reconstruction:
<del> same as encoder output
<del> else:
<del> same as decoder output
<add> # Input shape
<add> Same as encoder input.
<add>
<add> # Output shape
<add> If `output_reconstruction = True` then dim(input) = dim(output)
<add> else dim(output) = dim(hidden).
<add>
<add> # Arguments
<add> encoder: A [layer](./) or [layer container](./containers.md).
<add> decoder: A [layer](./) or [layer container](./containers.md).
<add> output_reconstruction: If this is `False`,
<add> the output of the autoencoder is the output of
<add> the deepest hidden layer.
<add> Otherwise, the output of the final decoder layer is returned.
<add> weights: list of numpy arrays to set as initial weights.
<add>
<add> # Examples
<add> ```python
<add> from keras.layers import containers
<add>
<add> # input shape: (nb_samples, 32)
<add> encoder = containers.Sequential([Dense(16, input_dim=32), Dense(8)])
<add> decoder = containers.Sequential([Dense(16, input_dim=8), Dense(32)])
<add>
<add> autoencoder = Sequential()
<add> autoencoder.add(AutoEncoder(encoder=encoder, decoder=decoder,
<add> output_reconstruction=False))
<add> ```
<ide> '''
<del> def __init__(self, encoder, decoder, output_reconstruction=True, weights=None, **kwargs):
<add> def __init__(self, encoder, decoder, output_reconstruction=True,
<add> weights=None, **kwargs):
<ide> super(AutoEncoder, self).__init__(**kwargs)
<ide>
<ide> self.output_reconstruction = output_reconstruction
<ide> def get_config(self):
<ide>
<ide>
<ide> class MaxoutDense(Layer):
<del> '''
<del> Max-out layer, nb_feature is the number of pieces in the piecewise linear approx.
<del> Refer to http://arxiv.org/pdf/1302.4389.pdf
<add> '''A dense maxout layer.
<add>
<add> A `MaxoutDense` layer takes the element-wise maximum of
<add> `nb_feature` `Dense(input_dim, output_dim)` linear layers.
<add> This allows the layer to learn a convex,
<add> piecewise linear activation function over the inputs.
<add>
<add> Note that this is a *linear* layer;
<add> if you wish to apply activation function
<add> (you shouldn't need to --they are universal function approximators),
<add> an `Activation` layer must be added after.
<add>
<add> # Input shape
<add> 2D tensor with shape: `(nb_samples, input_dim)`.
<add>
<add> # Output shape
<add> 2D tensor with shape: `(nb_samples, output_dim)`.
<add>
<add> # References
<add> - [Maxout Networks](http://arxiv.org/pdf/1302.4389.pdf)
<ide> '''
<ide> input_ndim = 2
<ide>
<del> def __init__(self, output_dim, nb_feature=4, init='glorot_uniform', weights=None,
<add> def __init__(self, output_dim, nb_feature=4,
<add> init='glorot_uniform', weights=None,
<ide> W_regularizer=None, b_regularizer=None, activity_regularizer=None,
<ide> W_constraint=None, b_constraint=None, input_dim=None, **kwargs):
<ide> self.output_dim = output_dim
<ide> def get_output(self, train=False):
<ide> return output
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "output_dim": self.output_dim,
<del> "init": self.init.__name__,
<del> "nb_feature": self.nb_feature,
<del> "W_regularizer": self.W_regularizer.get_config() if self.W_regularizer else None,
<del> "b_regularizer": self.b_regularizer.get_config() if self.b_regularizer else None,
<del> "activity_regularizer": self.activity_regularizer.get_config() if self.activity_regularizer else None,
<del> "W_constraint": self.W_constraint.get_config() if self.W_constraint else None,
<del> "b_constraint": self.b_constraint.get_config() if self.b_constraint else None,
<del> "input_dim": self.input_dim}
<add> config = {'name': self.__class__.__name__,
<add> 'output_dim': self.output_dim,
<add> 'init': self.init.__name__,
<add> 'nb_feature': self.nb_feature,
<add> 'W_regularizer': self.W_regularizer.get_config() if self.W_regularizer else None,
<add> 'b_regularizer': self.b_regularizer.get_config() if self.b_regularizer else None,
<add> 'activity_regularizer': self.activity_regularizer.get_config() if self.activity_regularizer else None,
<add> 'W_constraint': self.W_constraint.get_config() if self.W_constraint else None,
<add> 'b_constraint': self.b_constraint.get_config() if self.b_constraint else None,
<add> 'input_dim': self.input_dim}
<ide> base_config = super(MaxoutDense, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class Lambda(Layer):
<del> """Lambda layer for evaluating arbitrary function
<del>
<del> Input shape
<del> -----------
<del> output_shape of previous layer
<del>
<del> Output shape
<del> ------------
<del> Specified by output_shape argument
<del>
<del> Arguments
<del> ---------
<del> function - The function to be evaluated. Takes one argument : output of previous layer
<del> output_shape - Expected output shape from function. Could be a tuple or a function of the shape of the input
<del> """
<del>
<add> '''Used for evaluating an arbitrary Theano / TensorFlow expression
<add> on the output of the previous layer.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument input_shape
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Specified by `output_shape` argument.
<add>
<add> # Arguments
<add> function: The function to be evaluated.
<add> Takes one argument: the output of previous layer
<add> output_shape: Expected output shape from function.
<add> Could be a tuple or a function of the shape of the input
<add> '''
<ide> def __init__(self, function, output_shape=None, **kwargs):
<ide> super(Lambda, self).__init__(**kwargs)
<ide> py3 = sys.version_info[0] == 3
<ide> class MaskedLambda(MaskedLayer, Lambda):
<ide>
<ide>
<ide> class LambdaMerge(Lambda):
<del> """LambdaMerge layer for evaluating arbitrary function over multiple inputs
<del>
<del> Input shape
<del> -----------
<del> None
<del>
<del> Output shape
<del> ------------
<del> Specified by output_shape argument
<del>
<del> Arguments
<del> ---------
<del> layers - Input layers. Similar to layers argument of Merge
<del> function - The function to be evaluated. Takes one argument:
<del> list of outputs from input layers
<del> output_shape - Expected output shape from function.
<del> Could be a tuple or a function of list of input shapes
<del> """
<add> '''LambdaMerge layer for evaluating an arbitrary Theano / TensorFlow
<add> function over multiple inputs.
<add>
<add> # Output shape
<add> Specified by output_shape argument
<add>
<add> # Arguments
<add> layers - Input layers. Similar to layers argument of Merge
<add> function - The function to be evaluated. Takes one argument:
<add> list of outputs from input layers
<add> output_shape - Expected output shape from function.
<add> Could be a tuple or a function of list of input shapes
<add> '''
<ide> def __init__(self, layers, function, output_shape=None):
<ide> if len(layers) < 2:
<del> raise Exception("Please specify two or more input layers (or containers) to merge")
<add> raise Exception('Please specify two or more input layers '
<add> '(or containers) to merge')
<ide> self.layers = layers
<ide> self.params = []
<ide> self.regularizers = []
<ide> def output_shape(self):
<ide> output_shape_func = types.FunctionType(output_shape_func, globals())
<ide> shape = output_shape_func(input_shapes)
<ide> if type(shape) not in {list, tuple}:
<del> raise Exception("output_shape function must return a tuple")
<add> raise Exception('output_shape function must return a tuple')
<ide> return tuple(shape)
<ide>
<ide> def get_params(self):
<ide> def set_weights(self, weights):
<ide> weights = weights[nb_param:]
<ide>
<ide> def get_config(self):
<del> config = {"name": self.__class__.__name__,
<del> "layers": [l.get_config() for l in self.layers],
<del> "function": self.function,
<del> "output_shape": self._output_shape
<del> }
<add> config = {'name': self.__class__.__name__,
<add> 'layers': [l.get_config() for l in self.layers],
<add> 'function': self.function,
<add> 'output_shape': self._output_shape}
<ide> base_config = super(LambdaMerge, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide>
<ide> class Siamese(Layer):
<del> '''Shared layer with multiple inputs
<del>
<del> Output shape
<del> ------------
<del> Depends on merge_mode argument
<del>
<del> Arguments
<del> ---------
<del> layer - The layer to be shared across multiple inputs
<del> inputs - inputs to the shared layer
<del> merge_mode - Similar to mode argument of Merge layer
<del> concat_axis - Similar to concat_axis argument of Merge layer
<del> dot_axes - Similar to dot_axes argument of Merge layer
<add> '''Share a layer accross multiple inputs.
<add>
<add> For instance, this allows you to applied e.g.
<add> a same `Dense` layer to the output of two
<add> different layers in a graph.
<add>
<add> # Output shape
<add> Depends on merge_mode argument
<add>
<add> # Arguments
<add> layer: The layer to be shared across multiple inputs
<add> inputs: Inputs to the shared layer
<add> merge_mode: Same meaning as `mode` argument of Merge layer
<add> concat_axis: Same meaning as `concat_axis` argument of Merge layer
<add> dot_axes: Same meaning as `dot_axes` argument of Merge layer
<ide> '''
<ide> def __init__(self, layer, inputs, merge_mode='concat',
<ide> concat_axis=1, dot_axes=-1):
<ide> if merge_mode not in ['sum', 'mul', 'concat', 'ave',
<ide> 'join', 'cos', 'dot', None]:
<del> raise Exception("Invalid merge mode: " + str(merge_mode))
<add> raise Exception('Invalid merge mode: ' + str(merge_mode))
<ide>
<ide> if merge_mode in {'cos', 'dot'}:
<ide> if len(inputs) > 2:
<del> raise Exception(merge_mode + " merge takes exactly 2 layers")
<add> raise Exception(merge_mode + ' merge takes exactly 2 layers')
<ide>
<ide> self.layer = layer
<ide> self.inputs = inputs
<ide> def get_output_join(self, train=False):
<ide> for i in range(len(self.inputs)):
<ide> X = self.get_output_at(i, train)
<ide> if X.name is None:
<del> raise ValueError("merge_mode='join' only works with named inputs")
<add> raise ValueError('merge_mode="join" '
<add> 'only works with named inputs')
<ide> o[X.name] = X
<ide> return o
<ide>
<ide> def set_weights(self, weights):
<ide> weights = weights[nb_param:]
<ide>
<ide> def get_config(self):
<del>
<del> config = {"name": self.__class__.__name__,
<del> "layer": self.layer.get_config(),
<del> "inputs": [m.get_config() for m in self.inputs],
<del> "merge_mode": self.merge_mode,
<del> "concat_axis": self.concat_axis,
<del> "dot_axes": self.dot_axes
<del> }
<add> config = {'name': self.__class__.__name__,
<add> 'layer': self.layer.get_config(),
<add> 'inputs': [m.get_config() for m in self.inputs],
<add> 'merge_mode': self.merge_mode,
<add> 'concat_axis': self.concat_axis,
<add> 'dot_axes': self.dot_axes}
<ide> base_config = super(Siamese, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide>
<ide> class SiameseHead(Layer):
<ide> Outputs the output of the Siamese layer at a given index,
<ide> specified by the head argument
<ide>
<del> Arguments
<del> ---------
<del> head - The index at which the output of the Siamese layer
<del> should be obtained
<add> # Arguments
<add> head: The index at which the output of the Siamese layer
<add> should be obtained
<ide> '''
<ide> def __init__(self, head):
<ide> self.head = head
<ide> def get_input(self, train=False):
<ide>
<ide> def get_config(self):
<ide>
<del> config = {"name": self.__class__.__name__,
<del> "head": self.head}
<add> config = {'name': self.__class__.__name__,
<add> 'head': self.head}
<ide>
<ide> base_config = super(SiameseHead, self).get_config()
<ide> return dict(list(base_config.items()) + list(config.items()))
<ide> def set_previous(self, layer):
<ide>
<ide>
<ide> def add_shared_layer(layer, inputs):
<del> '''
<del> Use this function to add a shared layer across multiple Sequential models
<del> without merging the outputs
<add> '''Use this function to add a shared layer across
<add> multiple Sequential models without merging the outputs
<ide> '''
<ide> input_layers = [l.layers[-1] for l in inputs]
<ide> s = Siamese(layer, input_layers, merge_mode=None)
<ide><path>keras/layers/embeddings.py
<ide>
<ide>
<ide> class Embedding(Layer):
<del> '''
<del> Turn positive integers (indexes) into denses vectors of fixed size.
<del> eg. [[4], [20]] -> [[0.25, 0.1], [0.6, -0.2]]
<del>
<del> @input_dim: size of vocabulary (highest input integer + 1)
<del> @out_dim: size of dense representation
<add> '''Turn positive integers (indexes) into denses vectors of fixed size.
<add> eg. [[4], [20]] -> [[0.25, 0.1], [0.6, -0.2]]
<add>
<add> This layer can only be used as the first layer in a model.
<add>
<add> # Input shape
<add> 2D tensor with shape: `(nb_samples, sequence_length)`.
<add>
<add> # Output shape
<add> 3D tensor with shape: `(nb_samples, sequence_length, output_dim)`.
<add>
<add> # Arguments
<add> input_dim: int >= 0. Size of the vocabulary, ie.
<add> 1 + maximum integer index occurring in the input data.
<add> output_dim: int >= 0. Dimension of the dense embedding.
<add> init: name of initialization function for the weights
<add> of the layer (see: [initializations](../initializations.md)),
<add> or alternatively, Theano function to use for weights initialization.
<add> This parameter is only relevant if you don't pass a `weights` argument.
<add> weights: list of numpy arrays to set as initial weights.
<add> The list should have 1 element, of shape `(input_dim, output_dim)`.
<add> W_regularizer: instance of the [regularizers](../regularizers.md) module
<add> (eg. L1 or L2 regularization), applied to the embedding matrix.
<add> W_constraint: instance of the [constraints](../constraints.md) module
<add> (eg. maxnorm, nonneg), applied to the embedding matrix.
<add> mask_zero: Whether or not the input value 0 is a special "padding"
<add> value that should be masked out.
<add> This is useful for [recurrent layers](recurrent.md) which may take
<add> variable length input. If this is `True` then all subsequent layers
<add> in the model need to support masking or an exception will be raised.
<add> input_length: Length of input sequences, when it is constantself.
<add> This argument is required if you are going to connect
<add> `Flatten` then `Dense` layers upstream
<add> (without it, the shape of the dense outputs cannot be computed).
<ide> '''
<ide> input_ndim = 2
<ide>
<ide><path>keras/layers/noise.py
<ide>
<ide>
<ide> class GaussianNoise(MaskedLayer):
<del> '''
<del> Corruption process with GaussianNoise
<add> '''Apply to the input an additive zero-centred gaussian noise with
<add> standard deviation `sigma`. This is useful to mitigate overfitting
<add> (you could see it as a kind of random data augmentation).
<add> Gaussian Noise (GS) is a natural choice as corruption process
<add> for real valued inputs.
<add>
<add> As it is a regularization layer, it is only active at training time.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as input.
<add>
<add> # Arguments
<add> sigma: float, standard deviation of the noise distribution.
<ide> '''
<ide> def __init__(self, sigma, **kwargs):
<ide> super(GaussianNoise, self).__init__(**kwargs)
<ide> def get_config(self):
<ide>
<ide>
<ide> class GaussianDropout(MaskedLayer):
<del> '''
<del> Multiplicative Gaussian Noise
<del> Reference:
<del> Dropout: A Simple Way to Prevent Neural Networks from Overfitting
<del> Srivastava, Hinton, et al. 2014
<del> http://www.cs.toronto.edu/~rsalakhu/papers/srivastava14a.pdf
<add> '''Apply to the input an multiplicative one-centred gaussian noise
<add> with standard deviation `sqrt(p/(1-p))`.
<add>
<add> As it is a regularization layer, it is only active at training time.
<add>
<add> # Arguments
<add> p: float, drop probability (as with `Dropout`).
<add>
<add> # References:
<add> [Dropout: A Simple Way to Prevent Neural Networks from Overfitting Srivastava, Hinton, et al. 2014](http://www.cs.toronto.edu/~rsalakhu/papers/srivastava14a.pdf)
<ide> '''
<ide> def __init__(self, p, **kwargs):
<ide> super(GaussianDropout, self).__init__(**kwargs)
<ide><path>keras/layers/normalization.py
<ide>
<ide>
<ide> class BatchNormalization(Layer):
<del> '''
<del> Reference:
<del> Batch Normalization: Accelerating Deep Network Training
<del> by Reducing Internal Covariate Shift
<del> http://arxiv.org/pdf/1502.03167v3.pdf
<add> '''Normalize the activations of the previous layer at each batch.
<add>
<add> # Input shape
<add> Arbitrary. Use the keyword argument `input_shape`
<add> (tuple of integers, does not include the samples axis)
<add> when using this layer as the first layer in a model.
<add>
<add> # Output shape
<add> Same shape as input.
<ide>
<del> mode: 0 -> featurewise normalization
<del> 1 -> samplewise normalization
<del> (may sometimes outperform featurewise mode)
<add> # Arguments
<add> epsilon: small float > 0. Fuzz parameter.
<add> mode: integer, 0 or 1.
<add> - 0: feature-wise normalization.
<add> - 1: sample-wise normalization.
<add> momentum: momentum in the computation of the
<add> exponential average of the mean and standard deviation
<add> of the data, for feature-wise normalization.
<add> weights: Initialization weights.
<add> List of 2 numpy arrays, with shapes:
<add> `[(input_shape,), (input_shape,)]`
<ide>
<del> momentum: momentum term in the computation
<del> of a running estimate of the mean and std of the data
<add> # References
<add> - [Batch Normalization: Accelerating Deep Network Training by Reducing Internal Covariate Shift](http://arxiv.org/pdf/1502.03167v3.pdf)
<ide> '''
<ide> def __init__(self, epsilon=1e-6, mode=0, momentum=0.9,
<ide> weights=None, **kwargs):
<ide><path>keras/layers/recurrent.py
<ide>
<ide>
<ide> class Recurrent(MaskedLayer):
<add> '''Abstract base class for recurrent layers.
<add> Do not use in a model -- it's not a functional layer!
<add>
<add> All recurrent layers (GRU, LSTM, SimpleRNN) also
<add> follow the specifications of this class and accept
<add> the keyword arguments listed below.
<add>
<add> # Input shape
<add> 3D tensor with shape `(nb_samples, timesteps, input_dim)`.
<add>
<add> # Output shape
<add> - if `return_sequences`: 3D tensor with shape
<add> `(nb_samples, timesteps, output_dim)`.
<add> - else, 2D tensor with shape `(nb_samples, output_dim)`.
<add>
<add> # Arguments
<add> weights: list of numpy arrays to set as initial weights.
<add> The list should have 3 elements, of shapes:
<add> `[(input_dim, output_dim), (output_dim, output_dim), (output_dim,)]`.
<add> return_sequences: Boolean. Whether to return the last output
<add> in the output sequence, or the full sequence.
<add> go_backwards: Boolean (default False).
<add> If True, rocess the input sequence backwards.
<add> stateful: Boolean (default False). If True, the last state
<add> for each sample at index i in a batch will be used as initial
<add> state for the sample of index i in the following batch.
<add> input_dim: dimensionality of the input (integer).
<add> This argument (or alternatively, the keyword argument `input_shape`)
<add> is required when using this layer as the first layer in a model.
<add> input_length: Length of input sequences, to be specified
<add> when it is constant.
<add> This argument is required if you are going to connect
<add> `Flatten` then `Dense` layers upstream
<add> (without it, the shape of the dense outputs cannot be computed).
<add> Note that if the recurrent layer is not the first layer
<add> in your model, you would need to specify the input Length
<add> at the level of the first layer
<add> (e.g. via the `input_shape` argument)
<add>
<add> # Masking
<add> This layer supports masking for input data with a variable number
<add> of timesteps. To introduce masks to your data,
<add> use an [Embedding](embeddings.md) layer with the `mask_zero` parameter
<add> set to `True`.
<add> **Note:** for the time being, masking is only supported with Theano.
<add>
<add> # TensorFlow warning
<add> For the time being, when using the TensorFlow backend,
<add> the number of timesteps used must be specified in your model.
<add> Make sure to pass an `input_length` int argument to your
<add> recurrent layer (if it comes first in your model),
<add> or to pass a complete `input_shape` argument to the first layer
<add> in your model otherwise.
<add>
<add>
<add> # Note on using statefulness in RNNs
<add> You can set RNN layers to be 'stateful', which means that the states
<add> computed for the samples in one batch will be reused as initial states
<add> for the samples in the next batch.
<add> This assumes a one-to-one mapping between
<add> samples in different successive batches.
<add>
<add> To enable statefulness:
<add> - specify `stateful=True` in the layer constructor.
<add> - specify a fixed batch size for your model, by passing
<add> a `batch_input_size=(...)` to the first layer in your model.
<add> This is the expected shape of your inputs *including the batch size*.
<add> It should be a tuple of integers, e.g. `(32, 10, 100)`.
<add>
<add> To reset the states of your model, call `.reset_states()` on either
<add> a specific layer, or on your entire model.
<add> '''
<ide> input_ndim = 3
<ide>
<ide> def __init__(self, weights=None,
<ide> def get_config(self):
<ide>
<ide>
<ide> class SimpleRNN(Recurrent):
<del> '''
<del> Fully-connected RNN where the output is to fed back to input.
<del> Takes inputs with shape:
<del> (nb_samples, max_sample_length, input_dim)
<del> (samples shorter than `max_sample_length`
<del> are padded with zeros at the end)
<del> and returns outputs with shape:
<del> if not return_sequences:
<del> (nb_samples, output_dim)
<del> if return_sequences:
<del> (nb_samples, max_sample_length, output_dim)
<add> '''Fully-connected RNN where the output is to fed back to input.
<add>
<add> # Arguments
<add> output_dim: dimension of the internal projections and the final output.
<add> init: weight initialization function.
<add> Can be the name of an existing function (str),
<add> or a Theano function (see: [initializations](../initializations.md)).
<add> inner_init: initialization function of the inner cells.
<add> activation: activation function.
<add> Can be the name of an existing function (str),
<add> or a Theano function (see: [activations](../activations.md)).
<ide> '''
<ide> def __init__(self, output_dim,
<ide> init='glorot_uniform', inner_init='orthogonal',
<ide> def get_config(self):
<ide>
<ide>
<ide> class GRU(Recurrent):
<del> '''
<del> Gated Recurrent Unit - Cho et al. 2014
<del> Acts as a spatiotemporal projection,
<del> turning a sequence of vectors into a single vector.
<del> Takes inputs with shape:
<del> (nb_samples, max_sample_length, input_dim)
<del> (samples shorter than `max_sample_length`
<del> are padded with zeros at the end)
<del> and returns outputs with shape:
<del> if not return_sequences:
<del> (nb_samples, output_dim)
<del> if return_sequences:
<del> (nb_samples, max_sample_length, output_dim)
<del> References:
<del> On the Properties of Neural Machine Translation:
<del> Encoder–Decoder Approaches
<del> http://www.aclweb.org/anthology/W14-4012
<del> Empirical Evaluation of Gated Recurrent Neural Networks
<del> on Sequence Modeling
<del> http://arxiv.org/pdf/1412.3555v1.pdf
<add> '''Gated Recurrent Unit - Cho et al. 2014.
<add>
<add> # Arguments
<add> output_dim: dimension of the internal projections and the final output.
<add> init: weight initialization function.
<add> Can be the name of an existing function (str),
<add> or a Theano function (see: [initializations](../initializations.md)).
<add> inner_init: initialization function of the inner cells.
<add> activation: activation function.
<add> Can be the name of an existing function (str),
<add> or a Theano function (see: [activations](../activations.md)).
<add> inner_activation: activation function for the inner cells.
<add>
<add> # References
<add> - [On the Properties of Neural Machine Translation: Encoder–Decoder Approaches](http://www.aclweb.org/anthology/W14-4012)
<add> - [Empirical Evaluation of Gated Recurrent Neural Networks on Sequence Modeling](http://arxiv.org/pdf/1412.3555v1.pdf)
<ide> '''
<ide> def __init__(self, output_dim,
<ide> init='glorot_uniform', inner_init='orthogonal',
<ide> def get_config(self):
<ide>
<ide>
<ide> class LSTM(Recurrent):
<del> '''
<del> Acts as a spatiotemporal projection,
<del> turning a sequence of vectors into a single vector.
<del> Takes inputs with shape:
<del> (nb_samples, max_sample_length, input_dim)
<del> (samples shorter than `max_sample_length`
<del> are padded with zeros at the end)
<del> and returns outputs with shape:
<del> if not return_sequences:
<del> (nb_samples, output_dim)
<del> if return_sequences:
<del> (nb_samples, max_sample_length, output_dim)
<del> For a step-by-step description of the algorithm, see:
<del> http://deeplearning.net/tutorial/lstm.html
<del> References:
<del> Long short-term memory (original 97 paper)
<del> http://deeplearning.cs.cmu.edu/pdfs/Hochreiter97_lstm.pdf
<del> Learning to forget: Continual prediction with LSTM
<del> http://www.mitpressjournals.org/doi/pdf/10.1162/089976600300015015
<del> Supervised sequence labelling with recurrent neural networks
<del> http://www.cs.toronto.edu/~graves/preprint.pdf
<add> '''Long-Short Term Memory unit - Hochreiter 1997.
<add>
<add> For a step-by-step description of the algorithm, see
<add> [this tutorial](http://deeplearning.net/tutorial/lstm.html).
<add>
<add> # Arguments
<add> output_dim: dimension of the internal projections and the final output.
<add> init: weight initialization function.
<add> Can be the name of an existing function (str),
<add> or a Theano function (see: [initializations](../initializations.md)).
<add> inner_init: initialization function of the inner cells.
<add> forget_bias_init: initialization function for the bias of the forget gate.
<add> [Jozefowicz et al.](http://www.jmlr.org/proceedings/papers/v37/jozefowicz15.pdf)
<add> recommend initializing with ones.
<add> activation: activation function.
<add> Can be the name of an existing function (str),
<add> or a Theano function (see: [activations](../activations.md)).
<add> inner_activation: activation function for the inner cells.
<add>
<add> # References
<add> - [Long short-term memory](http://deeplearning.cs.cmu.edu/pdfs/Hochreiter97_lstm.pdf) (original 1997 paper)
<add> - [Learning to forget: Continual prediction with LSTM](http://www.mitpressjournals.org/doi/pdf/10.1162/089976600300015015)
<add> - [Supervised sequence labelling with recurrent neural networks](http://www.cs.toronto.edu/~graves/preprint.pdf)
<ide> '''
<ide> def __init__(self, output_dim,
<ide> init='glorot_uniform', inner_init='orthogonal', | 9 |
Javascript | Javascript | fix spelling error in tagalog test | 76e1b2cf89fdb6e291f18a8002ed9f0424e91e0a | <ide><path>test/lang/tl-ph.js
<ide> exports["lang:tl-ph"] = {
<ide>
<ide> test.done();
<ide> },
<del>
<add>
<ide> "returns the name of the language" : function (test) {
<del> if (typeof module !== 'undefied' && module.exports) {
<add> if (typeof module !== 'undefined' && module.exports) {
<ide> test.equal(require('../../lang/tl-ph'), 'tl-ph', "module should export tl-ph");
<ide> }
<del>
<add>
<ide> test.done();
<ide> }
<ide> }; | 1 |
Javascript | Javascript | use the `domcanvasfactory` in the api unit-tests | e6db75b2ef5aeabf6f7157f2dbd4b226f9649e57 | <ide><path>test/unit/api_spec.js
<ide>
<ide> var PDFJS = displayGlobal.PDFJS;
<ide> var createPromiseCapability = sharedUtil.createPromiseCapability;
<add>var DOMCanvasFactory = displayDOMUtils.DOMCanvasFactory;
<ide> var RenderingCancelledException = displayDOMUtils.RenderingCancelledException;
<ide> var PDFDocumentProxy = displayApi.PDFDocumentProxy;
<ide> var InvalidPDFException = sharedUtil.InvalidPDFException;
<ide> var FontType = sharedUtil.FontType;
<ide> describe('api', function() {
<ide> var basicApiUrl = new URL('../pdfs/basicapi.pdf', window.location).href;
<ide> var basicApiFileLength = 105779; // bytes
<add> var CanvasFactory;
<add>
<add> beforeAll(function(done) {
<add> CanvasFactory = new DOMCanvasFactory();
<add> done();
<add> });
<add>
<add> afterAll(function () {
<add> CanvasFactory = null;
<add> });
<ide>
<ide> function waitSome(callback) {
<ide> var WAIT_TIMEOUT = 10;
<ide> describe('api', function() {
<ide> });
<ide>
<ide> it('cancels rendering of page', function(done) {
<del> var canvas = document.createElement('canvas');
<ide> var viewport = page.getViewport(1);
<add> var canvasAndCtx = CanvasFactory.create(viewport.width, viewport.height);
<ide>
<ide> var renderTask = page.render({
<del> canvasContext: canvas.getContext('2d'),
<add> canvasContext: canvasAndCtx.context,
<ide> viewport: viewport,
<ide> });
<ide> renderTask.cancel();
<ide> describe('api', function() {
<ide> }).catch(function (error) {
<ide> expect(error instanceof RenderingCancelledException).toEqual(true);
<ide> expect(error.type).toEqual('canvas');
<add> CanvasFactory.destroy(canvasAndCtx);
<ide> done();
<ide> });
<ide> });
<ide> describe('api', function() {
<ide> pdfDocuments.push(pdf);
<ide> return pdf.getPage(1);
<ide> }).then(function(page) {
<del> var c = document.createElement('canvas');
<del> var v = page.getViewport(1.2);
<del> c.width = v.width;
<del> c.height = v.height;
<add> var viewport = page.getViewport(1.2);
<add> var canvasAndCtx = CanvasFactory.create(viewport.width,
<add> viewport.height);
<ide> return page.render({
<del> canvasContext: c.getContext('2d'),
<del> viewport: v,
<add> canvasContext: canvasAndCtx.context,
<add> viewport: viewport,
<ide> }).then(function() {
<del> return c.toDataURL();
<add> var data = canvasAndCtx.canvas.toDataURL();
<add> CanvasFactory.destroy(canvasAndCtx);
<add> return data;
<ide> });
<ide> });
<ide> } | 1 |
Text | Text | add changelog for batch touch records | f5b553c94d9169f24f7149640b4afd0663327ee2 | <ide><path>activerecord/CHANGELOG.md
<ide>
<ide> ## Rails 5.0.0.beta1 (December 18, 2015) ##
<ide>
<add>* Limit record touching to once per transaction.
<add>
<add> If you have a parent/grand-parent relation like:
<add>
<add> Comment belongs_to :message, touch: true
<add> Message belongs_to :project, touch: true
<add> Project belongs_to :account, touch: true
<add>
<add> When the lowest entry(`Comment`) is saved, now, it won't repeat the touch
<add> call multiple times for the parent records.
<add>
<add> Related #18606.
<add>
<add> *arthurnn*
<add>
<ide> * Order the result of `find(ids)` to match the passed array, if the relation
<ide> has no explicit order defined.
<ide> | 1 |
Javascript | Javascript | avoid unneccessary array copies in timer callbacks | bcc6f9b5e43938ca06149ce96ee6acac9856529c | <ide><path>Libraries/Core/Timers/JSTimers.js
<ide> function _callTimer(timerID: number, frameTime: number, didTimeout: ?boolean) {
<ide> }
<ide>
<ide> if (__DEV__) {
<del> Systrace.beginEvent('Systrace.callTimer: ' + type);
<add> Systrace.beginEvent(type + ' [invoke]');
<ide> }
<ide>
<ide> // Clear the metadata
<del> if (
<del> type === 'setTimeout' ||
<del> type === 'setImmediate' ||
<del> type === 'requestAnimationFrame' ||
<del> type === 'requestIdleCallback'
<del> ) {
<add> if (type !== 'setInterval') {
<ide> _clearIndex(timerIndex);
<ide> }
<ide>
<ide> function _callTimer(timerID: number, frameTime: number, didTimeout: ?boolean) {
<ide> * more immediates are queued up (can be used as a condition a while loop).
<ide> */
<ide> function _callImmediatesPass() {
<add> if (immediates.length === 0) {
<add> return false;
<add> }
<add>
<ide> if (__DEV__) {
<ide> Systrace.beginEvent('callImmediatesPass()');
<ide> }
<ide>
<ide> // The main reason to extract a single pass is so that we can track
<ide> // in the system trace
<del> if (immediates.length > 0) {
<del> const passImmediates = immediates.slice();
<del> immediates = [];
<del>
<del> // Use for loop rather than forEach as per @vjeux's advice
<del> // https://github.com/facebook/react-native/commit/c8fd9f7588ad02d2293cac7224715f4af7b0f352#commitcomment-14570051
<del> for (let i = 0; i < passImmediates.length; ++i) {
<del> _callTimer(passImmediates[i], 0);
<del> }
<add> const passImmediates = immediates;
<add> immediates = [];
<add>
<add> // Use for loop rather than forEach as per @vjeux's advice
<add> // https://github.com/facebook/react-native/commit/c8fd9f7588ad02d2293cac7224715f4af7b0f352#commitcomment-14570051
<add> for (let i = 0; i < passImmediates.length; ++i) {
<add> _callTimer(passImmediates[i], 0);
<ide> }
<ide>
<ide> if (__DEV__) {
<ide> const JSTimers = {
<ide> 'Cannot call `callTimers` with an empty list of IDs.',
<ide> );
<ide>
<del> // $FlowFixMe: optionals do not allow assignment from null
<del> errors = null;
<add> errors = (null: ?Array<Error>);
<ide> for (let i = 0; i < timersToCall.length; i++) {
<ide> _callTimer(timersToCall[i], 0);
<ide> }
<ide> const JSTimers = {
<ide> return;
<ide> }
<ide>
<del> // $FlowFixMe: optionals do not allow assignment from null
<del> errors = null;
<add> errors = (null: ?Array<Error>);
<ide> if (requestIdleCallbacks.length > 0) {
<del> const passIdleCallbacks = requestIdleCallbacks.slice();
<add> const passIdleCallbacks = requestIdleCallbacks;
<ide> requestIdleCallbacks = [];
<ide>
<ide> for (let i = 0; i < passIdleCallbacks.length; ++i) {
<ide> const JSTimers = {
<ide> * before we hand control back to native.
<ide> */
<ide> callImmediates() {
<del> errors = null;
<add> errors = (null: ?Array<Error>);
<ide> while (_callImmediatesPass()) {}
<ide> if (errors) {
<ide> errors.forEach(error =>
<ide> let ExportedJSTimers: {|
<ide> setInterval: (func: any, duration: number, ...args: any) => number,
<ide> setTimeout: (func: any, duration: number, ...args: any) => number,
<ide> |};
<add>
<ide> if (!NativeTiming) {
<ide> console.warn("Timing native module is not available, can't set timers.");
<ide> // $FlowFixMe: we can assume timers are generally available
<ide> if (!NativeTiming) {
<ide> ExportedJSTimers = JSTimers;
<ide> }
<ide>
<del>BatchedBridge.setImmediatesCallback(
<del> ExportedJSTimers.callImmediates.bind(ExportedJSTimers),
<del>);
<add>BatchedBridge.setImmediatesCallback(JSTimers.callImmediates);
<ide>
<ide> module.exports = ExportedJSTimers;
<ide><path>Libraries/Core/Timers/__tests__/JSTimers-test.js
<add>/**
<add> * Copyright (c) Facebook, Inc. and its affiliates.
<add> *
<add> * This source code is licensed under the MIT license found in the
<add> * LICENSE file in the root directory of this source tree.
<add> *
<add> * @format
<add> * @emails oncall+react_native
<add> */
<add>
<add>'use strict';
<add>
<add>const NativeTiming = {
<add> createTimer: jest.fn(),
<add> deleteTimer: jest.fn(),
<add> setSendIdleEvents: jest.fn(),
<add>};
<add>
<add>const warning = jest.fn();
<add>
<add>jest
<add> .enableAutomock()
<add> .mock('fbjs/lib/warning', () => warning, {virtual: true})
<add> .mock('../NativeTiming', () => ({
<add> __esModule: true,
<add> default: NativeTiming,
<add> }))
<add> .unmock('../JSTimers');
<add>
<add>const JSTimers = require('../JSTimers');
<add>
<add>describe('JSTimers', function() {
<add> const firstArgumentOfTheLastCallTo = function(func) {
<add> return func.mock.calls[func.mock.calls.length - 1][0];
<add> };
<add>
<add> beforeEach(function() {
<add> global.setTimeout = JSTimers.setTimeout;
<add> });
<add>
<add> it('should call function with setTimeout', function() {
<add> let didCall = false;
<add> const id = JSTimers.setTimeout(function() {
<add> didCall = true;
<add> });
<add> JSTimers.callTimers([id]);
<add> expect(didCall).toBe(true);
<add> });
<add>
<add> it('should call nested setTimeout when cleared', function() {
<add> let id1, id2, id3;
<add> let callCount = 0;
<add>
<add> id1 = JSTimers.setTimeout(function() {
<add> JSTimers.clearTimeout(id1);
<add> id2 = JSTimers.setTimeout(function() {
<add> JSTimers.clearTimeout(id2);
<add> id3 = JSTimers.setTimeout(function() {
<add> callCount += 1;
<add> });
<add> });
<add> });
<add> JSTimers.callTimers([id1]);
<add> JSTimers.callTimers([id2]);
<add> JSTimers.callTimers([id3]);
<add>
<add> expect(callCount).toBe(1);
<add> });
<add>
<add> it('should call nested setImmediate when cleared', function() {
<add> let id1, id2, id3;
<add> let callCount = 0;
<add>
<add> id1 = JSTimers.setImmediate(function() {
<add> JSTimers.clearImmediate(id1);
<add> id2 = JSTimers.setImmediate(function() {
<add> JSTimers.clearImmediate(id2);
<add> id3 = JSTimers.setImmediate(function() {
<add> callCount += 1;
<add> });
<add> });
<add> });
<add> JSTimers.callTimers([id1]);
<add> JSTimers.callTimers([id2]);
<add> JSTimers.callTimers([id3]);
<add>
<add> expect(callCount).toBe(1);
<add> });
<add>
<add> it('should call nested requestAnimationFrame when cleared', function() {
<add> let id1, id2, id3;
<add> let callCount = 0;
<add>
<add> id1 = JSTimers.requestAnimationFrame(function() {
<add> JSTimers.cancelAnimationFrame(id1);
<add> id2 = JSTimers.requestAnimationFrame(function() {
<add> JSTimers.cancelAnimationFrame(id2);
<add> id3 = JSTimers.requestAnimationFrame(function() {
<add> callCount += 1;
<add> });
<add> });
<add> });
<add> JSTimers.callTimers([id1]);
<add> JSTimers.callTimers([id2]);
<add> JSTimers.callTimers([id3]);
<add>
<add> expect(callCount).toBe(1);
<add> });
<add>
<add> it('should call nested setInterval when cleared', function() {
<add> let id1, id2, id3;
<add> let callCount = 0;
<add>
<add> id1 = JSTimers.setInterval(function() {
<add> JSTimers.clearInterval(id1);
<add> id2 = JSTimers.setInterval(function() {
<add> JSTimers.clearInterval(id2);
<add> id3 = JSTimers.setInterval(function() {
<add> callCount += 1;
<add> });
<add> });
<add> });
<add> JSTimers.callTimers([id1]);
<add> JSTimers.callTimers([id2]);
<add> JSTimers.callTimers([id3]);
<add>
<add> expect(callCount).toBe(1);
<add> });
<add>
<add> it('should call function with setInterval', function() {
<add> const callback = jest.fn();
<add> const id = JSTimers.setInterval(callback);
<add> JSTimers.callTimers([id]);
<add> expect(callback).toBeCalledTimes(1);
<add> });
<add>
<add> it('should call function with setImmediate', function() {
<add> const callback = jest.fn();
<add> JSTimers.setImmediate(callback);
<add> JSTimers.callImmediates();
<add> expect(callback).toBeCalledTimes(1);
<add> });
<add>
<add> it('should not call function with clearImmediate', function() {
<add> const callback = jest.fn();
<add> const id = JSTimers.setImmediate(callback);
<add> JSTimers.clearImmediate(id);
<add> JSTimers.callImmediates();
<add> expect(callback).not.toBeCalled();
<add> });
<add>
<add> it('should call functions in the right order with setImmediate', function() {
<add> let count = 0;
<add> let firstCalled = null;
<add> let secondCalled = null;
<add> JSTimers.setImmediate(function() {
<add> firstCalled = count++;
<add> });
<add> JSTimers.setImmediate(function() {
<add> secondCalled = count++;
<add> });
<add> JSTimers.callImmediates();
<add> expect(firstCalled).toBe(0);
<add> expect(secondCalled).toBe(1);
<add> });
<add>
<add> it('should call functions in the right order with nested setImmediate', function() {
<add> let count = 0;
<add> let firstCalled = null;
<add> let secondCalled = null;
<add> let thirdCalled = null;
<add> JSTimers.setImmediate(function() {
<add> firstCalled = count++;
<add> JSTimers.setImmediate(function() {
<add> thirdCalled = count++;
<add> });
<add> secondCalled = count++;
<add> });
<add> JSTimers.callImmediates();
<add> expect(firstCalled).toBe(0);
<add> expect(secondCalled).toBe(1);
<add> expect(thirdCalled).toBe(2);
<add> });
<add>
<add> it('should call nested setImmediate', function() {
<add> let firstCalled = false;
<add> let secondCalled = false;
<add> JSTimers.setImmediate(function() {
<add> firstCalled = true;
<add> JSTimers.setImmediate(function() {
<add> secondCalled = true;
<add> });
<add> });
<add> JSTimers.callImmediates();
<add> expect(firstCalled).toBe(true);
<add> expect(secondCalled).toBe(true);
<add> });
<add>
<add> it('should call function with requestAnimationFrame', function() {
<add> const callback = jest.fn();
<add> const id = JSTimers.requestAnimationFrame(callback);
<add> JSTimers.callTimers([id]);
<add> expect(callback).toBeCalledTimes(1);
<add> });
<add>
<add> it("should not call function if we don't callTimers", function() {
<add> const callback = jest.fn();
<add> JSTimers.setTimeout(callback, 10);
<add> expect(callback).not.toBeCalled();
<add> JSTimers.setInterval(callback, 10);
<add> expect(callback).not.toBeCalled();
<add> JSTimers.requestAnimationFrame(callback);
<add> expect(callback).not.toBeCalled();
<add> });
<add>
<add> it('should call setInterval as many times as callTimers is called', function() {
<add> const callback = jest.fn();
<add> const id = JSTimers.setInterval(callback, 10);
<add> JSTimers.callTimers([id]);
<add> JSTimers.callTimers([id]);
<add> JSTimers.callTimers([id]);
<add> JSTimers.callTimers([id]);
<add> expect(callback).toBeCalledTimes(4);
<add> });
<add>
<add> it("should only call the function who's id we pass in", function() {
<add> let firstCalled = false;
<add> let secondCalled = false;
<add> JSTimers.setTimeout(function() {
<add> firstCalled = true;
<add> });
<add> const secondID = JSTimers.setTimeout(function() {
<add> secondCalled = true;
<add> });
<add> JSTimers.callTimers([secondID]);
<add> expect(firstCalled).toBe(false);
<add> expect(secondCalled).toBe(true);
<add> });
<add>
<add> it('should work with calling multiple timers', function() {
<add> let firstCalled = false;
<add> let secondCalled = false;
<add> const firstID = JSTimers.setTimeout(function() {
<add> firstCalled = true;
<add> });
<add> const secondID = JSTimers.setTimeout(function() {
<add> secondCalled = true;
<add> });
<add> JSTimers.callTimers([firstID, secondID]);
<add> expect(firstCalled).toBe(true);
<add> expect(secondCalled).toBe(true);
<add> });
<add>
<add> it('should still execute all callbacks even if one throws', function() {
<add> const firstID = JSTimers.setTimeout(function() {
<add> throw new Error('error');
<add> }, 10);
<add> let secondCalled = false;
<add> const secondID = JSTimers.setTimeout(function() {
<add> secondCalled = true;
<add> }, 10);
<add> expect(JSTimers.callTimers.bind(null, [firstID, secondID])).toThrow();
<add> expect(secondCalled).toBe(true);
<add> });
<add>
<add> it('should clear timers even if callback throws', function() {
<add> const timerID = JSTimers.setTimeout(function() {
<add> throw new Error('error');
<add> }, 10);
<add> expect(JSTimers.callTimers.bind(null, [timerID])).toThrow('error');
<add> JSTimers.callTimers.bind(null, [timerID]);
<add> });
<add>
<add> it('should not warn if callback is called on cancelled timer', function() {
<add> const callback = jest.fn();
<add> const timerID = JSTimers.setTimeout(callback, 10);
<add> JSTimers.clearTimeout(timerID);
<add> JSTimers.callTimers([timerID]);
<add> expect(callback).not.toBeCalled();
<add> expect(firstArgumentOfTheLastCallTo(warning)).toBe(true);
<add> });
<add>
<add> it('should warn when callTimers is called with garbage timer id', function() {
<add> JSTimers.callTimers([1337]);
<add> expect(firstArgumentOfTheLastCallTo(warning)).toBe(false);
<add> });
<add>
<add> it('should only call callback once for setTimeout', function() {
<add> const callback = jest.fn();
<add> const timerID = JSTimers.setTimeout(callback, 10);
<add> // First time the timer fires, should call callback
<add> JSTimers.callTimers([timerID]);
<add> expect(callback).toBeCalledTimes(1);
<add> // Second time it should be ignored
<add> JSTimers.callTimers([timerID]);
<add> expect(callback).toBeCalledTimes(1);
<add> expect(firstArgumentOfTheLastCallTo(warning)).toBe(true);
<add> });
<add>
<add> it('should only call callback once for requestAnimationFrame', function() {
<add> const callback = jest.fn();
<add> const timerID = JSTimers.requestAnimationFrame(callback, 10);
<add> // First time the timer fires, should call callback
<add> JSTimers.callTimers([timerID]);
<add> expect(callback).toBeCalledTimes(1);
<add> // Second time it should be ignored
<add> JSTimers.callTimers([timerID]);
<add> expect(callback).toBeCalledTimes(1);
<add> expect(firstArgumentOfTheLastCallTo(warning)).toBe(true);
<add> });
<add>
<add> it('should re-throw first exception', function() {
<add> const timerID1 = JSTimers.setTimeout(function() {
<add> throw new Error('first error');
<add> });
<add> const timerID2 = JSTimers.setTimeout(function() {
<add> throw new Error('second error');
<add> });
<add> expect(JSTimers.callTimers.bind(null, [timerID1, timerID2])).toThrowError(
<add> 'first error',
<add> );
<add> });
<add>
<add> it('should pass along errors thrown from setImmediate', function() {
<add> JSTimers.setImmediate(function() {
<add> throw new Error('error within setImmediate');
<add> });
<add>
<add> NativeTiming.createTimer = jest.fn();
<add> JSTimers.callImmediates();
<add>
<add> // The remaining errors should be called within setTimeout, in case there
<add> // are a series of them
<add> expect(NativeTiming.createTimer).toBeCalled();
<add> const timerID = NativeTiming.createTimer.mock.calls[0][0];
<add> expect(JSTimers.callTimers.bind(null, [timerID])).toThrowError(
<add> 'error within setImmediate',
<add> );
<add> });
<add>
<add> it('should throw all errors from setImmediate', function() {
<add> JSTimers.setImmediate(function() {
<add> throw new Error('first error');
<add> });
<add>
<add> JSTimers.setImmediate(function() {
<add> throw new Error('second error');
<add> });
<add>
<add> NativeTiming.createTimer = jest.fn();
<add> JSTimers.callImmediates();
<add>
<add> expect(NativeTiming.createTimer.mock.calls.length).toBe(2);
<add>
<add> const firstTimerID = NativeTiming.createTimer.mock.calls[0][0];
<add> expect(JSTimers.callTimers.bind(null, [firstTimerID])).toThrowError(
<add> 'first error',
<add> );
<add>
<add> const secondTimerID = NativeTiming.createTimer.mock.calls[1][0];
<add> expect(JSTimers.callTimers.bind(null, [secondTimerID])).toThrowError(
<add> 'second error',
<add> );
<add> });
<add>
<add> it('should pass along errors thrown from setTimeout', function() {
<add> const timerID = JSTimers.setTimeout(function() {
<add> throw new Error('error within setTimeout');
<add> });
<add>
<add> expect(JSTimers.callTimers.bind(null, [timerID])).toThrowError(
<add> 'error within setTimeout',
<add> );
<add> });
<add>
<add> it('should throw all errors from setTimeout', function() {
<add> const firstTimerID = JSTimers.setTimeout(function() {
<add> throw new Error('first error');
<add> });
<add> const secondTimerID = JSTimers.setTimeout(function() {
<add> throw new Error('second error');
<add> });
<add>
<add> NativeTiming.createTimer = jest.fn();
<add> expect(
<add> JSTimers.callTimers.bind(null, [firstTimerID, secondTimerID]),
<add> ).toThrowError('first error');
<add>
<add> expect(NativeTiming.createTimer.mock.calls.length).toBe(1);
<add> const thirdTimerID = NativeTiming.createTimer.mock.calls[0][0];
<add> expect(JSTimers.callTimers.bind(null, [thirdTimerID])).toThrowError(
<add> 'second error',
<add> );
<add> });
<add>
<add> it('should pass along errors thrown from setInterval', function() {
<add> const timerID = JSTimers.setInterval(function() {
<add> throw new Error('error within setInterval');
<add> });
<add> expect(JSTimers.callTimers.bind(null, [timerID])).toThrowError(
<add> 'error within setInterval',
<add> );
<add> });
<add>
<add> it('should not call to native when clearing a null timer', function() {
<add> const timerID = JSTimers.setTimeout(() => {});
<add> JSTimers.clearTimeout(timerID);
<add> NativeTiming.deleteTimer = jest.fn();
<add>
<add> JSTimers.clearTimeout(null);
<add> expect(NativeTiming.deleteTimer.mock.calls.length).toBe(0);
<add> });
<add>}); | 2 |
Python | Python | add method to update / reset pkuseg user dict | c963e269bac9c41222d81abf82131b1937912325 | <ide><path>spacy/lang/zh/__init__.py
<ide> def __call__(self, text):
<ide> (words, spaces) = util.get_words_and_spaces(words, text)
<ide> return Doc(self.vocab, words=words, spaces=spaces)
<ide>
<add> def pkuseg_update_user_dict(self, words, reset=False):
<add> if self.pkuseg_seg:
<add> if reset:
<add> try:
<add> import pkuseg
<add> self.pkuseg_seg.preprocesser = pkuseg.Preprocesser(None)
<add> except ImportError:
<add> if self.use_pkuseg:
<add> msg = (
<add> "pkuseg not installed: unable to reset pkuseg "
<add> "user dict. Please " + _PKUSEG_INSTALL_MSG
<add> )
<add> raise ImportError(msg)
<add> for word in words:
<add> self.pkuseg_seg.preprocesser.insert(word.strip(), '')
<add>
<ide> def _get_config(self):
<ide> config = OrderedDict(
<ide> (
<ide><path>spacy/tests/lang/zh/test_tokenizer.py
<ide> from __future__ import unicode_literals
<ide>
<ide> import pytest
<add>from spacy.lang.zh import _get_pkuseg_trie_data
<ide>
<ide>
<ide> # fmt: off
<ide> def test_zh_tokenizer_pkuseg(zh_tokenizer_pkuseg, text, expected_tokens):
<ide> assert tokens == expected_tokens
<ide>
<ide>
<add>def test_zh_tokenizer_pkuseg_user_dict(zh_tokenizer_pkuseg):
<add> user_dict = _get_pkuseg_trie_data(zh_tokenizer_pkuseg.pkuseg_seg.preprocesser.trie)
<add> zh_tokenizer_pkuseg.pkuseg_update_user_dict(["nonsense_asdf"])
<add> updated_user_dict = _get_pkuseg_trie_data(zh_tokenizer_pkuseg.pkuseg_seg.preprocesser.trie)
<add> assert len(user_dict) == len(updated_user_dict) - 1
<add>
<add> # reset user dict
<add> zh_tokenizer_pkuseg.pkuseg_update_user_dict([], reset=True)
<add> reset_user_dict = _get_pkuseg_trie_data(zh_tokenizer_pkuseg.pkuseg_seg.preprocesser.trie)
<add> assert len(reset_user_dict) == 0
<add>
<add>
<ide> def test_extra_spaces(zh_tokenizer_char):
<ide> # note: three spaces after "I"
<ide> tokens = zh_tokenizer_char("I like cheese.") | 2 |
Go | Go | remove debug logging | bc8acc5b08e5aac5ba8d968e101660dded7d1870 | <ide><path>pkg/fileutils/fileutils.go
<ide> import (
<ide> "regexp"
<ide> "strings"
<ide> "text/scanner"
<del>
<del> "github.com/sirupsen/logrus"
<ide> )
<ide>
<ide> // PatternMatcher allows checking paths against a list of patterns
<ide> func (pm *PatternMatcher) Matches(file string) (bool, error) {
<ide> }
<ide> }
<ide>
<del> if matched {
<del> logrus.Debugf("Skipping excluded path: %s", file)
<del> }
<del>
<ide> return matched, nil
<ide> }
<ide> | 1 |
Javascript | Javascript | replace fixturesdir with fixtures module | 261ae7f58a9ec670f7c6c7dbb911619685105068 | <ide><path>test/sequential/test-debugger-debug-brk.js
<ide> 'use strict';
<ide> const common = require('../common');
<ide> common.skipIfInspectorDisabled();
<add>const fixtures = require('../common/fixtures');
<ide> const assert = require('assert');
<ide> const spawn = require('child_process').spawn;
<ide>
<del>const script = `${common.fixturesDir}/empty.js`;
<add>// file name here doesn't actually matter since
<add>// debugger will connect regardless of file name arg
<add>const script = fixtures.path('empty.js');
<ide>
<ide> function test(arg) {
<ide> const child = spawn(process.execPath, ['--inspect', arg, script]); | 1 |
Go | Go | update native driver to use labels from opts | 6c9a47f01c583e9c22b831eb426192148d29d792 | <ide><path>runtime/execdriver/native/create.go
<ide> package native
<ide>
<ide> import (
<ide> "fmt"
<add> "github.com/dotcloud/docker/pkg/label"
<ide> "github.com/dotcloud/docker/pkg/libcontainer"
<ide> "github.com/dotcloud/docker/runtime/execdriver"
<ide> "github.com/dotcloud/docker/runtime/execdriver/native/configuration"
<ide> func (d *driver) createContainer(c *execdriver.Command) (*libcontainer.Container
<ide> if err := d.setupMounts(container, c); err != nil {
<ide> return nil, err
<ide> }
<add> if err := d.setupLabels(container, c); err != nil {
<add> return nil, err
<add> }
<ide> if err := configuration.ParseConfiguration(container, d.activeContainers, c.Config["native"]); err != nil {
<ide> return nil, err
<ide> }
<ide> func (d *driver) setupMounts(container *libcontainer.Container, c *execdriver.Co
<ide> }
<ide> return nil
<ide> }
<add>
<add>func (d *driver) setupLabels(container *libcontainer.Container, c *execdriver.Command) error {
<add> labels := c.Config["label"]
<add> if len(labels) > 0 {
<add> process, mount, err := label.GenLabels(labels[0])
<add> if err != nil {
<add> return err
<add> }
<add> container.Context["mount_label"] = mount
<add> container.Context["process_label"] = process
<add> }
<add> return nil
<add>} | 1 |
Python | Python | allow graceful recovery for no compiler | fc398de0f13fd709079e666b8c2a03db76316c1d | <ide><path>numpy/distutils/tests/test_system_info.py
<ide> import os
<ide> import shutil
<ide> from tempfile import mkstemp, mkdtemp
<add>from subprocess import Popen, PIPE
<add>from distutils.errors import DistutilsError
<ide>
<ide> from numpy.distutils import ccompiler
<ide> from numpy.testing import TestCase, run_module_suite, assert_, assert_equal
<ide> def get_class(name, notfound_action=1):
<ide> }
<ide> """
<ide>
<add>def have_compiler():
<add> """ Return True if there appears to be an executable compiler
<add> """
<add> compiler = ccompiler.new_compiler()
<add> try:
<add> cmd = compiler.compiler # Unix compilers
<add> except AttributeError:
<add> try:
<add> compiler.initialize() # MSVC is different
<add> except DistutilsError:
<add> return False
<add> cmd = [compiler.cc]
<add> try:
<add> Popen(cmd, stdout=PIPE, stderr=PIPE)
<add> except OSError:
<add> return False
<add> return True
<add>
<add>
<add>HAVE_COMPILER = have_compiler()
<add>
<ide>
<ide> class test_system_info(system_info):
<ide>
<ide> def test_temp2(self):
<ide> extra = tsi.calc_extra_info()
<ide> assert_equal(extra['extra_link_args'], ['-Wl,-rpath=' + self._lib2])
<ide>
<add> @skipif(not HAVE_COMPILER)
<ide> def test_compile1(self):
<ide> # Compile source and link the first source
<ide> c = ccompiler.new_compiler()
<add> previousDir = os.getcwd()
<ide> try:
<ide> # Change directory to not screw up directories
<del> previousDir = os.getcwd()
<ide> os.chdir(self._dir1)
<ide> c.compile([os.path.basename(self._src1)], output_dir=self._dir1)
<ide> # Ensure that the object exists
<ide> assert_(os.path.isfile(self._src1.replace('.c', '.o')) or
<ide> os.path.isfile(self._src1.replace('.c', '.obj')))
<add> finally:
<ide> os.chdir(previousDir)
<del> except OSError:
<del> pass
<ide>
<add> @skipif(not HAVE_COMPILER)
<ide> @skipif('msvc' in repr(ccompiler.new_compiler()))
<ide> def test_compile2(self):
<ide> # Compile source and link the second source
<ide> tsi = self.c_temp2
<ide> c = ccompiler.new_compiler()
<ide> extra_link_args = tsi.calc_extra_info()['extra_link_args']
<add> previousDir = os.getcwd()
<ide> try:
<ide> # Change directory to not screw up directories
<del> previousDir = os.getcwd()
<ide> os.chdir(self._dir2)
<ide> c.compile([os.path.basename(self._src2)], output_dir=self._dir2,
<ide> extra_postargs=extra_link_args)
<ide> # Ensure that the object exists
<ide> assert_(os.path.isfile(self._src2.replace('.c', '.o')))
<add> finally:
<ide> os.chdir(previousDir)
<del> except OSError:
<del> pass
<add>
<ide>
<ide> if __name__ == '__main__':
<ide> run_module_suite() | 1 |
Javascript | Javascript | use private fields in a few more viewer classes | e7a6e7393a710cf4603aafc55a8cadbe916f1eec | <ide><path>web/download_manager.js
<ide> function download(blobUrl, filename) {
<ide> * @implements {IDownloadManager}
<ide> */
<ide> class DownloadManager {
<del> constructor() {
<del> this._openBlobUrls = new WeakMap();
<del> }
<add> #openBlobUrls = new WeakMap();
<ide>
<ide> downloadUrl(url, filename) {
<ide> if (!createValidAbsoluteUrl(url, "http://example.com")) {
<ide> class DownloadManager {
<ide> const contentType = isPdfData ? "application/pdf" : "";
<ide>
<ide> if (isPdfData) {
<del> let blobUrl = this._openBlobUrls.get(element);
<add> let blobUrl = this.#openBlobUrls.get(element);
<ide> if (!blobUrl) {
<ide> blobUrl = URL.createObjectURL(new Blob([data], { type: contentType }));
<del> this._openBlobUrls.set(element, blobUrl);
<add> this.#openBlobUrls.set(element, blobUrl);
<ide> }
<ide> let viewerUrl;
<ide> if (typeof PDFJSDev === "undefined" || PDFJSDev.test("GENERIC")) {
<ide> class DownloadManager {
<ide> // Release the `blobUrl`, since opening it failed, and fallback to
<ide> // downloading the PDF file.
<ide> URL.revokeObjectURL(blobUrl);
<del> this._openBlobUrls.delete(element);
<add> this.#openBlobUrls.delete(element);
<ide> }
<ide> }
<ide>
<ide><path>web/event_utils.js
<ide> function waitOnEventOrTimeout({ target, name, delay = 0 }) {
<ide> * and `off` methods. To raise an event, the `dispatch` method shall be used.
<ide> */
<ide> class EventBus {
<del> constructor() {
<del> this._listeners = Object.create(null);
<del> }
<add> #listeners = Object.create(null);
<ide>
<ide> /**
<ide> * @param {string} eventName
<ide> class EventBus {
<ide> * @param {Object} data
<ide> */
<ide> dispatch(eventName, data) {
<del> const eventListeners = this._listeners[eventName];
<add> const eventListeners = this.#listeners[eventName];
<ide> if (!eventListeners || eventListeners.length === 0) {
<ide> return;
<ide> }
<ide> class EventBus {
<ide> * @ignore
<ide> */
<ide> _on(eventName, listener, options = null) {
<del> const eventListeners = (this._listeners[eventName] ||= []);
<add> const eventListeners = (this.#listeners[eventName] ||= []);
<ide> eventListeners.push({
<ide> listener,
<ide> external: options?.external === true,
<ide> class EventBus {
<ide> * @ignore
<ide> */
<ide> _off(eventName, listener, options = null) {
<del> const eventListeners = this._listeners[eventName];
<add> const eventListeners = this.#listeners[eventName];
<ide> if (!eventListeners) {
<ide> return;
<ide> }
<ide><path>web/firefoxcom.js
<ide> class FirefoxCom {
<ide> }
<ide>
<ide> class DownloadManager {
<del> constructor() {
<del> this._openBlobUrls = new WeakMap();
<del> }
<add> #openBlobUrls = new WeakMap();
<ide>
<ide> downloadUrl(url, filename) {
<ide> FirefoxCom.request("download", {
<ide> class DownloadManager {
<ide> const contentType = isPdfData ? "application/pdf" : "";
<ide>
<ide> if (isPdfData) {
<del> let blobUrl = this._openBlobUrls.get(element);
<add> let blobUrl = this.#openBlobUrls.get(element);
<ide> if (!blobUrl) {
<ide> blobUrl = URL.createObjectURL(new Blob([data], { type: contentType }));
<del> this._openBlobUrls.set(element, blobUrl);
<add> this.#openBlobUrls.set(element, blobUrl);
<ide> }
<ide> // Let Firefox's content handler catch the URL and display the PDF.
<ide> const viewerUrl = blobUrl + "#filename=" + encodeURIComponent(filename);
<ide> class DownloadManager {
<ide> // Release the `blobUrl`, since opening it failed, and fallback to
<ide> // downloading the PDF file.
<ide> URL.revokeObjectURL(blobUrl);
<del> this._openBlobUrls.delete(element);
<add> this.#openBlobUrls.delete(element);
<ide> }
<ide> }
<ide> | 3 |
Ruby | Ruby | update errorsubscriber signature | dd80ab07a869fa7b6ef17ab081d71fc4ccc23523 | <ide><path>activesupport/lib/active_support/error_reporter.rb
<ide> module ActiveSupport
<ide> # +severity+ can be one of +:error+, +:warning+, or +:info+. Handled errors default to the +:warning+
<ide> # severity, and unhandled ones to +:error+.
<ide> #
<add> # A +source+ can also be specified, describing where the error originates from. Error subscribers can
<add> # use this to ignore certain errors. For instance, ActiveSupport may report internal errors
<add> # such as cache failures with a source like "redis_cache_store.active_support".
<add> # The default +source+ is "application".
<add> #
<ide> # Both +handle+ and +record+ pass through the return value from the block. In the case of +handle+
<ide> # rescuing an error, a fallback can be provided. The fallback must be a callable whose result will
<ide> # be returned when the block raises and is handled:
<ide> def handle(error_class = StandardError, severity: :warning, context: {}, fallbac
<ide> fallback.call if fallback
<ide> end
<ide>
<add> # Report any unhandled exception, but do not swallow it.
<add> #
<add> # Rails.error.record do
<add> # # Will report the TypeError to all subscribers and then raise it.
<add> # 1 + '1'
<add> # end
<add> #
<ide> def record(error_class = StandardError, severity: :error, context: {}, source: DEFAULT_SOURCE)
<ide> yield
<ide> rescue error_class => error
<ide> def record(error_class = StandardError, severity: :error, context: {}, source: D
<ide>
<ide> # Register a new error subscriber. The subscriber must respond to
<ide> #
<del> # report(Exception, handled: Boolean, context: Hash)
<add> # report(Exception, handled: Boolean, severity: (:error OR :warning OR :info), context: Hash, source: String)
<ide> #
<ide> # The +report+ method +should+ never raise an error.
<ide> def subscribe(subscriber)
<ide> def subscribe(subscriber)
<ide> end
<ide>
<ide> # Prevent a subscriber from being notified of errors for the
<del> # duration of the block.
<add> # duration of the block. You may pass in the subscriber itself, or its class.
<ide> #
<del> # It can be used by error reporting service integration when they wish
<del> # to handle the error higher in the stack.
<add> # This can be helpful for error reporting service integrations, when they wish
<add> # to handle any errors higher in the stack.
<ide> def disable(subscriber)
<ide> disabled_subscribers = (ActiveSupport::IsolatedExecutionState[self] ||= [])
<ide> disabled_subscribers << subscriber
<ide> def disable(subscriber)
<ide> #
<ide> # Rails.error.set_context(section: "checkout", user_id: @user.id)
<ide> #
<add> # Any context passed to +handle+, +record+, or +report+ will be merged with the context set here.
<ide> # See +ActiveSupport::ExecutionContext.set+
<ide> def set_context(...)
<ide> ActiveSupport::ExecutionContext.set(...) | 1 |
Go | Go | add integration tests for build with network opts | e3b48fca0db71cc24270dcf254590541279980d4 | <ide><path>integration-cli/docker_cli_build_test.go
<ide> func (s *DockerSuite) TestBuildCacheFrom(c *check.C) {
<ide> }
<ide> c.Assert(layers1[len(layers1)-1], checker.Not(checker.Equals), layers2[len(layers1)-1])
<ide> }
<add>
<add>func (s *DockerSuite) TestBuildNetNone(c *check.C) {
<add> testRequires(c, DaemonIsLinux)
<add>
<add> name := "testbuildnetnone"
<add> _, out, err := buildImageWithOut(name, `
<add> FROM busybox
<add> RUN ping -c 1 8.8.8.8
<add> `, true, "--network=none")
<add> c.Assert(err, checker.NotNil)
<add> c.Assert(out, checker.Contains, "unreachable")
<add>}
<add>
<add>func (s *DockerSuite) TestBuildNetContainer(c *check.C) {
<add> testRequires(c, DaemonIsLinux)
<add>
<add> id, _ := dockerCmd(c, "run", "--hostname", "foobar", "-d", "busybox", "nc", "-ll", "-p", "1234", "-e", "hostname")
<add>
<add> name := "testbuildnetcontainer"
<add> out, err := buildImage(name, `
<add> FROM busybox
<add> RUN nc localhost 1234 > /otherhost
<add> `, true, "--network=container:"+strings.TrimSpace(id))
<add> c.Assert(err, checker.IsNil, check.Commentf("out: %v", out))
<add>
<add> host, _ := dockerCmd(c, "run", "testbuildnetcontainer", "cat", "/otherhost")
<add> c.Assert(strings.TrimSpace(host), check.Equals, "foobar")
<add>} | 1 |
Python | Python | avoid double key lookup on callback.py | b6ca3ef051107f544b97cdb315eaf1b8e12665d9 | <ide><path>keras/callbacks.py
<ide> def on_train_begin(self, logs={}):
<ide> def on_epoch_end(self, epoch, logs={}):
<ide> self.epoch.append(epoch)
<ide> for k, v in logs.items():
<del> if k not in self.history:
<del> self.history[k] = []
<del> self.history[k].append(v)
<del>
<add> self.history.setdefault(k, []).append(v)
<ide>
<ide> class ModelCheckpoint(Callback):
<ide> '''Save the model after every epoch. | 1 |
Javascript | Javascript | convert the overlay manager to es6 syntax | e7a04fc82dc7a65007662a048ea754628e0366fa | <ide><path>web/app.js
<ide> var PDFViewerApplication = {
<ide> store: null,
<ide> /** @type {DownloadManager} */
<ide> downloadManager: null,
<add> /** @type {OverlayManager} */
<add> overlayManager: null,
<ide> /** @type {Preferences} */
<ide> preferences: null,
<ide> /** @type {Toolbar} */
<ide> var PDFViewerApplication = {
<ide> let appConfig = this.appConfig;
<ide>
<ide> return new Promise((resolve, reject) => {
<add> this.overlayManager = new OverlayManager();
<add>
<ide> let eventBus = appConfig.eventBus || getGlobalEventBus();
<ide> this.eventBus = eventBus;
<ide>
<ide> var PDFViewerApplication = {
<ide>
<ide> this.pdfViewer.setFindController(this.findController);
<ide>
<del> // FIXME better PDFFindBar constructor parameters
<add> // TODO: improve `PDFFindBar` constructor parameter passing
<ide> let findBarConfig = Object.create(appConfig.findBar);
<ide> findBarConfig.findController = this.findController;
<ide> findBarConfig.eventBus = eventBus;
<ide> this.findBar = new PDFFindBar(findBarConfig);
<ide>
<del> this.overlayManager = OverlayManager;
<del>
<ide> this.pdfDocumentProperties =
<del> new PDFDocumentProperties(appConfig.documentProperties);
<add> new PDFDocumentProperties(appConfig.documentProperties,
<add> this.overlayManager);
<ide>
<ide> this.pdfCursorTools = new PDFCursorTools({
<ide> container,
<ide> var PDFViewerApplication = {
<ide> });
<ide> }
<ide>
<del> this.passwordPrompt = new PasswordPrompt(appConfig.passwordOverlay);
<add> this.passwordPrompt = new PasswordPrompt(appConfig.passwordOverlay,
<add> this.overlayManager);
<ide>
<ide> this.pdfOutlineViewer = new PDFOutlineViewer({
<ide> container: appConfig.sidebar.outlineView,
<ide> var PDFViewerApplication = {
<ide> downloadManager,
<ide> });
<ide>
<del> // FIXME better PDFSidebar constructor parameters
<add> // TODO: improve `PDFSidebar` constructor parameter passing
<ide> let sidebarConfig = Object.create(appConfig.sidebar);
<ide> sidebarConfig.pdfViewer = this.pdfViewer;
<ide> sidebarConfig.pdfThumbnailViewer = this.pdfThumbnailViewer;
<ide> function webViewerClick(evt) {
<ide> }
<ide>
<ide> function webViewerKeyDown(evt) {
<del> if (OverlayManager.active) {
<add> if (PDFViewerApplication.overlayManager.active) {
<ide> return;
<ide> }
<ide>
<ide><path>web/chromecom.js
<ide> import { DefaultExternalServices, PDFViewerApplication } from './app';
<ide> import { BasePreferences } from './preferences';
<ide> import { DownloadManager } from './download_manager';
<del>import { OverlayManager } from './overlay_manager';
<ide> import { PDFJS } from './pdfjs';
<ide>
<ide> if (typeof PDFJSDev === 'undefined' || !PDFJSDev.test('CHROME')) {
<ide> ChromeCom.request = function ChromeCom_request(action, data, callback) {
<ide> /**
<ide> * Resolves a PDF file path and attempts to detects length.
<ide> *
<del> * @param {String} file Absolute URL of PDF file.
<del> * @param {Function} callback A callback with resolved URL and file length.
<add> * @param {String} file - Absolute URL of PDF file.
<add> * @param {OverlayManager} overlayManager - Manager for the viewer overlays.
<add> * @param {Function} callback - A callback with resolved URL and file length.
<ide> */
<del>ChromeCom.resolvePDFFile = function ChromeCom_resolvePDFFile(file, callback) {
<add>ChromeCom.resolvePDFFile = function(file, overlayManager, callback) {
<ide> // Expand drive:-URLs to filesystem URLs (Chrome OS)
<ide> file = file.replace(/^drive:/i,
<ide> 'filesystem:' + location.origin + '/external/');
<ide> ChromeCom.resolvePDFFile = function ChromeCom_resolvePDFFile(file, callback) {
<ide> if (isAllowedAccess) {
<ide> callback(file);
<ide> } else {
<del> requestAccessToLocalFile(file);
<add> requestAccessToLocalFile(file, overlayManager);
<ide> }
<ide> });
<ide> });
<ide> function reloadIfRuntimeIsUnavailable() {
<ide> }
<ide>
<ide> var chromeFileAccessOverlayPromise;
<del>function requestAccessToLocalFile(fileUrl) {
<add>function requestAccessToLocalFile(fileUrl, overlayManager) {
<ide> var onCloseOverlay = null;
<ide> if (top !== window) {
<ide> // When the extension reloads after receiving new permissions, the pages
<ide> function requestAccessToLocalFile(fileUrl) {
<ide> onCloseOverlay = function() {
<ide> window.removeEventListener('focus', reloadIfRuntimeIsUnavailable);
<ide> reloadIfRuntimeIsUnavailable();
<del> OverlayManager.close('chromeFileAccessOverlay');
<add> overlayManager.close('chromeFileAccessOverlay');
<ide> };
<ide> }
<ide> if (!chromeFileAccessOverlayPromise) {
<del> chromeFileAccessOverlayPromise = OverlayManager.register(
<add> chromeFileAccessOverlayPromise = overlayManager.register(
<ide> 'chromeFileAccessOverlay',
<ide> document.getElementById('chromeFileAccessOverlay'),
<ide> onCloseOverlay, true);
<ide> function requestAccessToLocalFile(fileUrl) {
<ide> // why this permission request is shown.
<ide> document.getElementById('chrome-url-of-local-file').textContent = fileUrl;
<ide>
<del> OverlayManager.open('chromeFileAccessOverlay');
<add> overlayManager.open('chromeFileAccessOverlay');
<ide> });
<ide> }
<ide>
<ide> class ChromePreferences extends BasePreferences {
<ide>
<ide> var ChromeExternalServices = Object.create(DefaultExternalServices);
<ide> ChromeExternalServices.initPassiveLoading = function (callbacks) {
<del> var appConfig = PDFViewerApplication.appConfig;
<del> ChromeCom.resolvePDFFile(appConfig.defaultUrl,
<add> let { appConfig, overlayManager, } = PDFViewerApplication;
<add> ChromeCom.resolvePDFFile(appConfig.defaultUrl, overlayManager,
<ide> function (url, length, originalURL) {
<ide> callbacks.onOpenWithURL(url, length, originalURL);
<ide> });
<ide><path>web/overlay_manager.js
<ide> * limitations under the License.
<ide> */
<ide>
<del>var OverlayManager = {
<del> overlays: {},
<del> active: null,
<add>class OverlayManager {
<add> constructor() {
<add> this._overlays = {};
<add> this._active = null;
<add> this._keyDownBound = this._keyDown.bind(this);
<add> }
<add>
<add> get active() {
<add> return this._active;
<add> }
<ide>
<ide> /**
<del> * @param {string} name The name of the overlay that is registered.
<del> * @param {HTMLDivElement} element The overlay's DOM element.
<del> * @param {function} callerCloseMethod (optional) The method that, if present,
<del> * will call OverlayManager.close from the Object
<add> * @param {string} name - The name of the overlay that is registered.
<add> * @param {HTMLDivElement} element - The overlay's DOM element.
<add> * @param {function} callerCloseMethod - (optional) The method that, if
<add> * present, calls `OverlayManager.close` from the object
<ide> * registering the overlay. Access to this method is
<ide> * necessary in order to run cleanup code when e.g.
<del> * the overlay is force closed. The default is null.
<del> * @param {boolean} canForceClose (optional) Indicates if opening the overlay
<del> * will close an active overlay. The default is false.
<add> * the overlay is force closed. The default is `null`.
<add> * @param {boolean} canForceClose - (optional) Indicates if opening the
<add> * overlay closes an active overlay. The default is `false`.
<ide> * @returns {Promise} A promise that is resolved when the overlay has been
<ide> * registered.
<ide> */
<del> register(name, element, callerCloseMethod, canForceClose) {
<add> register(name, element, callerCloseMethod = null, canForceClose = false) {
<ide> return new Promise((resolve) => {
<del> var container;
<add> let container;
<ide> if (!name || !element || !(container = element.parentNode)) {
<ide> throw new Error('Not enough parameters.');
<del> } else if (this.overlays[name]) {
<add> } else if (this._overlays[name]) {
<ide> throw new Error('The overlay is already registered.');
<ide> }
<del> this.overlays[name] = {
<add> this._overlays[name] = {
<ide> element,
<ide> container,
<del> callerCloseMethod: (callerCloseMethod || null),
<del> canForceClose: (canForceClose || false),
<add> callerCloseMethod,
<add> canForceClose,
<ide> };
<ide> resolve();
<ide> });
<del> },
<add> }
<ide>
<ide> /**
<del> * @param {string} name The name of the overlay that is unregistered.
<add> * @param {string} name - The name of the overlay that is unregistered.
<ide> * @returns {Promise} A promise that is resolved when the overlay has been
<ide> * unregistered.
<ide> */
<ide> unregister(name) {
<ide> return new Promise((resolve) => {
<del> if (!this.overlays[name]) {
<add> if (!this._overlays[name]) {
<ide> throw new Error('The overlay does not exist.');
<del> } else if (this.active === name) {
<add> } else if (this._active === name) {
<ide> throw new Error('The overlay cannot be removed while it is active.');
<ide> }
<del> delete this.overlays[name];
<del>
<add> delete this._overlays[name];
<ide> resolve();
<ide> });
<del> },
<add> }
<ide>
<ide> /**
<del> * @param {string} name The name of the overlay that should be opened.
<add> * @param {string} name - The name of the overlay that should be opened.
<ide> * @returns {Promise} A promise that is resolved when the overlay has been
<ide> * opened.
<ide> */
<ide> open(name) {
<ide> return new Promise((resolve) => {
<del> if (!this.overlays[name]) {
<add> if (!this._overlays[name]) {
<ide> throw new Error('The overlay does not exist.');
<del> } else if (this.active) {
<del> if (this.overlays[name].canForceClose) {
<add> } else if (this._active) {
<add> if (this._overlays[name].canForceClose) {
<ide> this._closeThroughCaller();
<del> } else if (this.active === name) {
<add> } else if (this._active === name) {
<ide> throw new Error('The overlay is already active.');
<ide> } else {
<ide> throw new Error('Another overlay is currently active.');
<ide> }
<ide> }
<del> this.active = name;
<del> this.overlays[this.active].element.classList.remove('hidden');
<del> this.overlays[this.active].container.classList.remove('hidden');
<add> this._active = name;
<add> this._overlays[this._active].element.classList.remove('hidden');
<add> this._overlays[this._active].container.classList.remove('hidden');
<ide>
<del> window.addEventListener('keydown', this._keyDown);
<add> window.addEventListener('keydown', this._keyDownBound);
<ide> resolve();
<ide> });
<del> },
<add> }
<ide>
<ide> /**
<del> * @param {string} name The name of the overlay that should be closed.
<add> * @param {string} name - The name of the overlay that should be closed.
<ide> * @returns {Promise} A promise that is resolved when the overlay has been
<ide> * closed.
<ide> */
<ide> close(name) {
<ide> return new Promise((resolve) => {
<del> if (!this.overlays[name]) {
<add> if (!this._overlays[name]) {
<ide> throw new Error('The overlay does not exist.');
<del> } else if (!this.active) {
<add> } else if (!this._active) {
<ide> throw new Error('The overlay is currently not active.');
<del> } else if (this.active !== name) {
<add> } else if (this._active !== name) {
<ide> throw new Error('Another overlay is currently active.');
<ide> }
<del> this.overlays[this.active].container.classList.add('hidden');
<del> this.overlays[this.active].element.classList.add('hidden');
<del> this.active = null;
<add> this._overlays[this._active].container.classList.add('hidden');
<add> this._overlays[this._active].element.classList.add('hidden');
<add> this._active = null;
<ide>
<del> window.removeEventListener('keydown', this._keyDown);
<add> window.removeEventListener('keydown', this._keyDownBound);
<ide> resolve();
<ide> });
<del> },
<add> }
<ide>
<ide> /**
<ide> * @private
<ide> */
<ide> _keyDown(evt) {
<del> var self = OverlayManager;
<del> if (self.active && evt.keyCode === 27) { // Esc key.
<del> self._closeThroughCaller();
<add> if (this._active && evt.keyCode === 27) { // Esc key.
<add> this._closeThroughCaller();
<ide> evt.preventDefault();
<ide> }
<del> },
<add> }
<ide>
<ide> /**
<ide> * @private
<ide> */
<ide> _closeThroughCaller() {
<del> if (this.overlays[this.active].callerCloseMethod) {
<del> this.overlays[this.active].callerCloseMethod();
<add> if (this._overlays[this._active].callerCloseMethod) {
<add> this._overlays[this._active].callerCloseMethod();
<ide> }
<del> if (this.active) {
<del> this.close(this.active);
<add> if (this._active) {
<add> this.close(this._active);
<ide> }
<ide> }
<del>};
<add>}
<ide>
<ide> export {
<ide> OverlayManager,
<ide><path>web/password_prompt.js
<ide> */
<ide>
<ide> import { mozL10n } from './ui_utils';
<del>import { OverlayManager } from './overlay_manager';
<ide> import { PasswordResponses } from './pdfjs';
<ide>
<ide> /**
<ide> import { PasswordResponses } from './pdfjs';
<ide> class PasswordPrompt {
<ide> /**
<ide> * @param {PasswordPromptOptions} options
<add> * @param {OverlayManager} overlayManager - Manager for the viewer overlays.
<ide> */
<del> constructor(options) {
<add> constructor(options, overlayManager) {
<ide> this.overlayName = options.overlayName;
<ide> this.container = options.container;
<ide> this.label = options.label;
<ide> this.input = options.input;
<ide> this.submitButton = options.submitButton;
<ide> this.cancelButton = options.cancelButton;
<add> this.overlayManager = overlayManager;
<ide>
<ide> this.updateCallback = null;
<ide> this.reason = null;
<ide> class PasswordPrompt {
<ide> }
<ide> });
<ide>
<del> OverlayManager.register(this.overlayName, this.container,
<del> this.close.bind(this), true);
<add> this.overlayManager.register(this.overlayName, this.container,
<add> this.close.bind(this), true);
<ide> }
<ide>
<ide> open() {
<del> OverlayManager.open(this.overlayName).then(() => {
<add> this.overlayManager.open(this.overlayName).then(() => {
<ide> this.input.focus();
<ide>
<ide> var promptString = mozL10n.get('password_label', null,
<ide> class PasswordPrompt {
<ide> }
<ide>
<ide> close() {
<del> OverlayManager.close(this.overlayName).then(() => {
<add> this.overlayManager.close(this.overlayName).then(() => {
<ide> this.input.value = '';
<ide> });
<ide> }
<ide><path>web/pdf_document_properties.js
<ide>
<ide> import { cloneObj, getPDFFileNameFromURL, mozL10n } from './ui_utils';
<ide> import { createPromiseCapability } from './pdfjs';
<del>import { OverlayManager } from './overlay_manager';
<ide>
<ide> const DEFAULT_FIELD_CONTENT = '-';
<ide>
<ide> const DEFAULT_FIELD_CONTENT = '-';
<ide> class PDFDocumentProperties {
<ide> /**
<ide> * @param {PDFDocumentPropertiesOptions} options
<add> * @param {OverlayManager} overlayManager - Manager for the viewer overlays.
<ide> */
<del> constructor({ overlayName, fields, container, closeButton, }) {
<add> constructor({ overlayName, fields, container, closeButton, },
<add> overlayManager) {
<ide> this.overlayName = overlayName;
<ide> this.fields = fields;
<ide> this.container = container;
<add> this.overlayManager = overlayManager;
<ide>
<ide> this._reset();
<ide>
<ide> if (closeButton) { // Bind the event listener for the Close button.
<ide> closeButton.addEventListener('click', this.close.bind(this));
<ide> }
<del> OverlayManager.register(this.overlayName, this.container,
<del> this.close.bind(this));
<add> this.overlayManager.register(this.overlayName, this.container,
<add> this.close.bind(this));
<ide> }
<ide>
<ide> /**
<ide> class PDFDocumentProperties {
<ide> });
<ide> };
<ide>
<del> Promise.all([OverlayManager.open(this.overlayName),
<add> Promise.all([this.overlayManager.open(this.overlayName),
<ide> this._dataAvailableCapability.promise]).then(() => {
<ide> // If the document properties were previously fetched (for this PDF file),
<ide> // just update the dialog immediately to avoid redundant lookups.
<ide> class PDFDocumentProperties {
<ide> * Close the document properties overlay.
<ide> */
<ide> close() {
<del> OverlayManager.close(this.overlayName);
<add> this.overlayManager.close(this.overlayName);
<ide> }
<ide>
<ide> /**
<ide> class PDFDocumentProperties {
<ide> }
<ide> return;
<ide> }
<del> if (OverlayManager.active !== this.overlayName) {
<add> if (this.overlayManager.active !== this.overlayName) {
<ide> // Don't bother updating the dialog if has already been closed,
<ide> // since it will be updated the next time `this.open` is called.
<ide> return;
<ide><path>web/pdf_print_service.js
<ide> */
<ide>
<ide> import { CSS_UNITS, mozL10n } from './ui_utils';
<del>import { OverlayManager } from './overlay_manager';
<add>import { PDFPrintServiceFactory, PDFViewerApplication } from './app';
<ide> import { PDFJS } from './pdfjs';
<del>import { PDFPrintServiceFactory } from './app';
<ide>
<del>var activeService = null;
<add>let activeService = null;
<add>let overlayManager = null;
<ide>
<ide> // Renders the page to the canvas of the given print service, and returns
<ide> // the suggested dimensions of the output page.
<ide> PDFPrintService.prototype = {
<ide> this.scratchCanvas = null;
<ide> activeService = null;
<ide> ensureOverlay().then(function () {
<del> if (OverlayManager.active !== 'printServiceOverlay') {
<add> if (overlayManager.active !== 'printServiceOverlay') {
<ide> return; // overlay was already closed
<ide> }
<del> OverlayManager.close('printServiceOverlay');
<add> overlayManager.close('printServiceOverlay');
<ide> });
<ide> },
<ide>
<ide> window.print = function print() {
<ide> }
<ide> ensureOverlay().then(function () {
<ide> if (activeService) {
<del> OverlayManager.open('printServiceOverlay');
<add> overlayManager.open('printServiceOverlay');
<ide> }
<ide> });
<ide>
<ide> window.print = function print() {
<ide> } finally {
<ide> if (!activeService) {
<ide> console.error('Expected print service to be initialized.');
<del> if (OverlayManager.active === 'printServiceOverlay') {
<del> OverlayManager.close('printServiceOverlay');
<del> }
<add> ensureOverlay().then(function () {
<add> if (overlayManager.active === 'printServiceOverlay') {
<add> overlayManager.close('printServiceOverlay');
<add> }
<add> });
<ide> return; // eslint-disable-line no-unsafe-finally
<ide> }
<ide> var activeServiceOnEntry = activeService;
<ide> if ('onbeforeprint' in window) {
<ide> var overlayPromise;
<ide> function ensureOverlay() {
<ide> if (!overlayPromise) {
<del> overlayPromise = OverlayManager.register('printServiceOverlay',
<add> overlayManager = PDFViewerApplication.overlayManager;
<add> if (!overlayManager) {
<add> throw new Error('The overlay manager has not yet been initialized.');
<add> }
<add>
<add> overlayPromise = overlayManager.register('printServiceOverlay',
<ide> document.getElementById('printServiceOverlay'), abort, true);
<ide> document.getElementById('printCancel').onclick = abort;
<ide> } | 6 |
Javascript | Javascript | fix persistent caching timeout | 767ac32aa27bc52cbe3911c0107ab15f824f7f73 | <ide><path>test/PersistentCaching.test.js
<ide> export default ${files.map((_, i) => `f${i}`).join(" + ")};
<ide> await compile(configAdditions);
<ide> await expect(execute()).resolves.toEqual({ ok: true });
<ide> });
<del>});
<add>}, 60000); | 1 |
Javascript | Javascript | remove unnecessary flag check | ece5295e5af992d30256f26ca428abdad514f862 | <ide><path>packages/react-reconciler/src/ReactFiberBeginWork.new.js
<ide> function updateHostRoot(current, workInProgress, renderLanes) {
<ide> } else {
<ide> // The outermost shell has not hydrated yet. Start hydrating.
<ide> enterHydrationState(workInProgress);
<del> if (enableUseMutableSource && supportsHydration) {
<add> if (enableUseMutableSource) {
<ide> const mutableSourceEagerHydrationData =
<ide> root.mutableSourceEagerHydrationData;
<ide> if (mutableSourceEagerHydrationData != null) {
<ide><path>packages/react-reconciler/src/ReactFiberBeginWork.old.js
<ide> function updateHostRoot(current, workInProgress, renderLanes) {
<ide> } else {
<ide> // The outermost shell has not hydrated yet. Start hydrating.
<ide> enterHydrationState(workInProgress);
<del> if (enableUseMutableSource && supportsHydration) {
<add> if (enableUseMutableSource) {
<ide> const mutableSourceEagerHydrationData =
<ide> root.mutableSourceEagerHydrationData;
<ide> if (mutableSourceEagerHydrationData != null) { | 2 |
Javascript | Javascript | use es6 to update let & const | a1bd070f87bfb7d32ef9ddee5c121d8eaf2f0c2b | <ide><path>test/parallel/test-child-process-stdout-flush-exit.js
<ide> 'use strict';
<del>var common = require('../common');
<del>var assert = require('assert');
<add>const common = require('../common');
<add>const assert = require('assert');
<ide>
<ide> // if child process output to console and exit
<ide> if (process.argv[2] === 'child') {
<ide> console.log('hello');
<del> for (var i = 0; i < 200; i++) {
<add> for (let i = 0; i < 200; i++) {
<ide> console.log('filler');
<ide> }
<ide> console.log('goodbye');
<ide> process.exit(0);
<ide> } else {
<ide> // parent process
<del> var spawn = require('child_process').spawn;
<add> const spawn = require('child_process').spawn;
<ide>
<ide> // spawn self as child
<del> var child = spawn(process.argv[0], [process.argv[1], 'child']);
<add> const child = spawn(process.argv[0], [process.argv[1], 'child']);
<ide>
<del> var stdout = '';
<add> let stdout = '';
<ide>
<ide> child.stderr.setEncoding('utf8');
<ide> child.stderr.on('data', function(data) {
<ide> if (process.argv[2] === 'child') {
<ide> });
<ide>
<ide> child.on('close', common.mustCall(function() {
<del> assert.equal(stdout.slice(0, 6), 'hello\n');
<del> assert.equal(stdout.slice(stdout.length - 8), 'goodbye\n');
<add> assert.strictEqual(stdout.slice(0, 6), 'hello\n');
<add> assert.strictEqual(stdout.slice(stdout.length - 8), 'goodbye\n');
<ide> }));
<ide> }
<ide><path>test/parallel/test-crypto-cipher-decipher.js
<ide> 'use strict';
<del>var common = require('../common');
<del>var assert = require('assert');
<add>const common = require('../common');
<ide>
<ide> if (!common.hasCrypto) {
<ide> common.skip('missing crypto');
<ide> if (common.hasFipsCrypto) {
<ide> common.skip('not supported in FIPS mode');
<ide> return;
<ide> }
<del>var crypto = require('crypto');
<add>const crypto = require('crypto');
<add>const assert = require('assert');
<ide>
<ide> function testCipher1(key) {
<ide> // Test encryption and decryption
<del> var plaintext = 'Keep this a secret? No! Tell everyone about node.js!';
<del> var cipher = crypto.createCipher('aes192', key);
<add> const plaintext = 'Keep this a secret? No! Tell everyone about node.js!';
<add> const cipher = crypto.createCipher('aes192', key);
<ide>
<ide> // encrypt plaintext which is in utf8 format
<ide> // to a ciphertext which will be in hex
<del> var ciph = cipher.update(plaintext, 'utf8', 'hex');
<add> let ciph = cipher.update(plaintext, 'utf8', 'hex');
<ide> // Only use binary or hex, not base64.
<ide> ciph += cipher.final('hex');
<ide>
<del> var decipher = crypto.createDecipher('aes192', key);
<del> var txt = decipher.update(ciph, 'hex', 'utf8');
<add> const decipher = crypto.createDecipher('aes192', key);
<add> let txt = decipher.update(ciph, 'hex', 'utf8');
<ide> txt += decipher.final('utf8');
<ide>
<ide> assert.strictEqual(txt, plaintext, 'encryption and decryption');
<ide> function testCipher1(key) {
<ide> // NB: In real life, it's not guaranteed that you can get all of it
<ide> // in a single read() like this. But in this case, we know it's
<ide> // quite small, so there's no harm.
<del> var cStream = crypto.createCipher('aes192', key);
<add> const cStream = crypto.createCipher('aes192', key);
<ide> cStream.end(plaintext);
<ide> ciph = cStream.read();
<ide>
<del> var dStream = crypto.createDecipher('aes192', key);
<add> const dStream = crypto.createDecipher('aes192', key);
<ide> dStream.end(ciph);
<ide> txt = dStream.read().toString('utf8');
<ide>
<ide> function testCipher1(key) {
<ide> function testCipher2(key) {
<ide> // encryption and decryption with Base64
<ide> // reported in https://github.com/joyent/node/issues/738
<del> var plaintext =
<add> const plaintext =
<ide> '32|RmVZZkFUVmpRRkp0TmJaUm56ZU9qcnJkaXNNWVNpTTU*|iXmckfRWZBGWWELw' +
<ide> 'eCBsThSsfUHLeRe0KCsK8ooHgxie0zOINpXxfZi/oNG7uq9JWFVCk70gfzQH8ZUJ' +
<ide> 'jAfaFg**';
<del> var cipher = crypto.createCipher('aes256', key);
<add> const cipher = crypto.createCipher('aes256', key);
<ide>
<ide> // encrypt plaintext which is in utf8 format
<ide> // to a ciphertext which will be in Base64
<del> var ciph = cipher.update(plaintext, 'utf8', 'base64');
<add> let ciph = cipher.update(plaintext, 'utf8', 'base64');
<ide> ciph += cipher.final('base64');
<ide>
<del> var decipher = crypto.createDecipher('aes256', key);
<del> var txt = decipher.update(ciph, 'base64', 'utf8');
<add> const decipher = crypto.createDecipher('aes256', key);
<add> let txt = decipher.update(ciph, 'base64', 'utf8');
<ide> txt += decipher.final('utf8');
<ide>
<ide> assert.strictEqual(txt, plaintext, 'encryption and decryption with Base64');
<ide> testCipher2(Buffer.from('0123456789abcdef'));
<ide> const key = '0123456789abcdef';
<ide> const plaintext = 'Top secret!!!';
<ide> const c = crypto.createCipher('aes192', key);
<del> var ciph = c.update(plaintext, 'utf16le', 'base64');
<add> let ciph = c.update(plaintext, 'utf16le', 'base64');
<ide> ciph += c.final('base64');
<ide>
<del> var decipher = crypto.createDecipher('aes192', key);
<add> let decipher = crypto.createDecipher('aes192', key);
<ide>
<del> var txt;
<add> let txt;
<ide> assert.doesNotThrow(() => txt = decipher.update(ciph, 'base64', 'ucs2'));
<ide> assert.doesNotThrow(() => txt += decipher.final('ucs2'));
<ide> assert.strictEqual(txt, plaintext, 'decrypted result in ucs2');
<ide><path>test/parallel/test-https-timeout-server.js
<ide> 'use strict';
<del>var common = require('../common');
<del>var assert = require('assert');
<add>const common = require('../common');
<ide>
<ide> if (!common.hasCrypto) {
<ide> common.skip('missing crypto');
<ide> return;
<ide> }
<del>var https = require('https');
<add>const assert = require('assert');
<add>const https = require('https');
<ide>
<del>var net = require('net');
<del>var fs = require('fs');
<add>const net = require('net');
<add>const fs = require('fs');
<ide>
<del>var options = {
<add>const options = {
<ide> key: fs.readFileSync(common.fixturesDir + '/keys/agent1-key.pem'),
<ide> cert: fs.readFileSync(common.fixturesDir + '/keys/agent1-cert.pem'),
<ide> handshakeTimeout: 50
<ide> };
<ide>
<del>var server = https.createServer(options, common.fail);
<add>const server = https.createServer(options, common.fail);
<ide>
<ide> server.on('clientError', common.mustCall(function(err, conn) {
<ide> // Don't hesitate to update the asserts if the internal structure of
<ide> // the cleartext object ever changes. We're checking that the https.Server
<ide> // has closed the client connection.
<del> assert.equal(conn._secureEstablished, false);
<add> assert.strictEqual(conn._secureEstablished, false);
<ide> server.close();
<ide> conn.destroy();
<ide> }));
<ide><path>test/parallel/test-tls-on-empty-socket.js
<ide> 'use strict';
<del>var common = require('../common');
<del>var assert = require('assert');
<add>const common = require('../common');
<ide>
<ide> if (!common.hasCrypto) {
<ide> common.skip('missing crypto');
<ide> return;
<ide> }
<del>var tls = require('tls');
<add>const assert = require('assert');
<add>const tls = require('tls');
<ide>
<del>var fs = require('fs');
<del>var net = require('net');
<add>const fs = require('fs');
<add>const net = require('net');
<ide>
<del>var out = '';
<add>let out = '';
<ide>
<del>var server = tls.createServer({
<add>const server = tls.createServer({
<ide> key: fs.readFileSync(common.fixturesDir + '/keys/agent1-key.pem'),
<ide> cert: fs.readFileSync(common.fixturesDir + '/keys/agent1-cert.pem')
<ide> }, function(c) {
<ide> c.end('hello');
<ide> }).listen(0, function() {
<del> var socket = new net.Socket();
<add> const socket = new net.Socket();
<ide>
<del> var s = tls.connect({
<add> const s = tls.connect({
<ide> socket: socket,
<ide> rejectUnauthorized: false
<ide> }, function() {
<ide> var server = tls.createServer({
<ide> });
<ide>
<ide> process.on('exit', function() {
<del> assert.equal(out, 'hello');
<add> assert.strictEqual(out, 'hello');
<ide> }); | 4 |
Javascript | Javascript | fix formdata to properly handle appended arrays. | d2e8e7d58e680e0bb3b4da1f820dd4dd840639f5 | <ide><path>Libraries/Network/FormData.js
<ide> class FormData {
<ide> // an object with a `uri` attribute. Optionally, it can also
<ide> // have a `name` and `type` attribute to specify filename and
<ide> // content type (cf. web Blob interface.)
<del> if (typeof value === 'object' && value) {
<add> if (typeof value === 'object' && !Array.isArray(value) && value) {
<ide> if (typeof value.name === 'string') {
<ide> headers['content-disposition'] += '; filename="' + value.name + '"';
<ide> }
<ide><path>Libraries/Network/__tests__/FormData-test.js
<ide> describe('FormData', function () {
<ide> };
<ide> expect(formData.getParts()[0]).toMatchObject(expectedPart);
<ide> });
<add>
<add> it('should return non blob array', function () {
<add> formData.append('array', [
<add> true,
<add> false,
<add> undefined,
<add> null,
<add> {},
<add> [],
<add> 'string',
<add> 0,
<add> ]);
<add>
<add> const expectedPart = {
<add> string: 'true,false,,,[object Object],,string,0',
<add> headers: {
<add> 'content-disposition': 'form-data; name="array"',
<add> },
<add> fieldName: 'array',
<add> };
<add> expect(formData.getParts()[0]).toMatchObject(expectedPart);
<add> });
<ide> }); | 2 |
Python | Python | hide nan warnings for internal ma computations | fd19bf398ad716cb36476b4fbe3df1ae75466fe9 | <ide><path>numpy/ma/core.py
<ide> def __init__(self, a, b):
<ide>
<ide> def __call__(self, x):
<ide> "Execute the call behavior."
<del> return umath.logical_or(umath.greater(x, self.b),
<del> umath.less(x, self.a))
<add> # nans at masked positions cause RuntimeWarnings, even though
<add> # they are masked. To avoid this we suppress warnings.
<add> with np.errstate(invalid='ignore'):
<add> return umath.logical_or(umath.greater(x, self.b),
<add> umath.less(x, self.a))
<ide>
<ide>
<ide> class _DomainTan:
<ide> def __init__(self, eps):
<ide>
<ide> def __call__(self, x):
<ide> "Executes the call behavior."
<del> return umath.less(umath.absolute(umath.cos(x)), self.eps)
<add> with np.errstate(invalid='ignore'):
<add> return umath.less(umath.absolute(umath.cos(x)), self.eps)
<ide>
<ide>
<ide> class _DomainSafeDivide:
<ide> def __call__(self, a, b):
<ide> self.tolerance = np.finfo(float).tiny
<ide> # don't call ma ufuncs from __array_wrap__ which would fail for scalars
<ide> a, b = np.asarray(a), np.asarray(b)
<del> return umath.absolute(a) * self.tolerance >= umath.absolute(b)
<add> with np.errstate(invalid='ignore'):
<add> return umath.absolute(a) * self.tolerance >= umath.absolute(b)
<ide>
<ide>
<ide> class _DomainGreater:
<ide> def __init__(self, critical_value):
<ide>
<ide> def __call__(self, x):
<ide> "Executes the call behavior."
<del> return umath.less_equal(x, self.critical_value)
<add> with np.errstate(invalid='ignore'):
<add> return umath.less_equal(x, self.critical_value)
<ide>
<ide>
<ide> class _DomainGreaterEqual:
<ide> def __init__(self, critical_value):
<ide>
<ide> def __call__(self, x):
<ide> "Executes the call behavior."
<del> return umath.less(x, self.critical_value)
<add> with np.errstate(invalid='ignore'):
<add> return umath.less(x, self.critical_value)
<ide>
<ide>
<ide> class _MaskedUnaryOperation:
<ide> def __call__(self, a, *args, **kwargs):
<ide> # Deal with domain
<ide> if self.domain is not None:
<ide> # Case 1.1. : Domained function
<add> # nans at masked positions cause RuntimeWarnings, even though
<add> # they are masked. To avoid this we suppress warnings.
<ide> with np.errstate(divide='ignore', invalid='ignore'):
<ide> result = self.f(d, *args, **kwargs)
<ide> # Make a mask
<ide> def __call__(self, a, *args, **kwargs):
<ide> else:
<ide> # Case 1.2. : Function without a domain
<ide> # Get the result and the mask
<del> result = self.f(d, *args, **kwargs)
<add> with np.errstate(divide='ignore', invalid='ignore'):
<add> result = self.f(d, *args, **kwargs)
<ide> m = getmask(a)
<ide>
<ide> if not result.ndim:
<ide> def __call__(self, a, b, *args, **kwargs):
<ide> # Apply the domain
<ide> domain = ufunc_domain.get(self.f, None)
<ide> if domain is not None:
<del> m |= filled(domain(da, db), True)
<add> m |= domain(da, db)
<ide> # Take care of the scalar case first
<ide> if (not m.ndim):
<ide> if m:
<ide> def __array_wrap__(self, obj, context=None):
<ide> if domain is not None:
<ide> # Take the domain, and make sure it's a ndarray
<ide> if len(args) > 2:
<del> d = filled(reduce(domain, args), True)
<add> with np.errstate(divide='ignore', invalid='ignore'):
<add> d = filled(reduce(domain, args), True)
<ide> else:
<del> d = filled(domain(*args), True)
<add> with np.errstate(divide='ignore', invalid='ignore'):
<add> d = filled(domain(*args), True)
<ide> # Fill the result where the domain is wrong
<ide> try:
<ide> # Binary domain: take the last value
<ide> def allclose(a, b, masked_equal=True, rtol=1e-5, atol=1e-8):
<ide> return False
<ide> # No infs at all
<ide> if not np.any(xinf):
<del> d = filled(umath.less_equal(umath.absolute(x - y),
<del> atol + rtol * umath.absolute(y)),
<add> d = filled(less_equal(absolute(x - y), atol + rtol * absolute(y)),
<ide> masked_equal)
<ide> return np.all(d)
<ide>
<ide> def allclose(a, b, masked_equal=True, rtol=1e-5, atol=1e-8):
<ide> x = x[~xinf]
<ide> y = y[~xinf]
<ide>
<del> d = filled(umath.less_equal(umath.absolute(x - y),
<del> atol + rtol * umath.absolute(y)),
<add> d = filled(less_equal(absolute(x - y), atol + rtol * absolute(y)),
<ide> masked_equal)
<ide>
<ide> return np.all(d)
<ide><path>numpy/ma/tests/test_core.py
<ide> )
<ide> from numpy.ma.core import (
<ide> MAError, MaskError, MaskType, MaskedArray, abs, absolute, add, all,
<del> allclose, allequal, alltrue, angle, anom, arange, arccos, arctan2,
<add> allclose, allequal, alltrue, angle, anom, arange, arccos, arccosh, arctan2,
<ide> arcsin, arctan, argsort, array, asarray, choose, concatenate,
<ide> conjugate, cos, cosh, count, default_fill_value, diag, divide, empty,
<ide> empty_like, equal, exp, flatten_mask, filled, fix_invalid,
<ide> def __rdiv__(self, other):
<ide> assert_(a * me_too == "Me2rmul")
<ide> assert_(a / me_too == "Me2rdiv")
<ide>
<add> def test_no_masked_nan_warnings(self):
<add> # check that a nan in masked position does not
<add> # cause ufunc warnings
<add>
<add> m = np.ma.array([0.5, np.nan], mask=[0,1])
<add>
<add> with warnings.catch_warnings():
<add> warnings.filterwarnings("error")
<add>
<add> # test unary and binary ufuncs
<add> exp(m)
<add> add(m, 1)
<add> m > 0
<add>
<add> # test different unary domains
<add> sqrt(m)
<add> log(m)
<add> tan(m)
<add> arcsin(m)
<add> arccos(m)
<add> arccosh(m)
<add>
<add> # test binary domains
<add> divide(m, 2)
<add>
<add> # also check that allclose uses ma ufuncs, to avoid warning
<add> allclose(m, 0.5)
<ide>
<ide> class TestMaskedArrayInPlaceArithmetics(TestCase):
<ide> # Test MaskedArray Arithmetics | 2 |
Text | Text | add a note about confidential stuff in err | 1e28f04fec70affd8fae57f4bdd23a84853f4efc | <ide><path>docs/extend/authorization.md
<ide> Name | Type | Description
<ide> --------|--------|----------------------------------------------------------------------------------
<ide> Allow | bool | Boolean value indicating whether the request is allowed or denied
<ide> Msg | string | Authorization message (will be returned to the client in case the access is denied)
<del>Err | string | Error message (will be returned to the client in case the plugin encounter an error)
<add>Err | string | Error message (will be returned to the client in case the plugin encounter an error. The string value supplied may appear in logs, so should not include confidential information)
<ide>
<ide> ### Response authorization
<ide>
<ide> Name | Type | Description
<ide> --------|--------|----------------------------------------------------------------------------------
<ide> Allow | bool | Boolean value indicating whether the response is allowed or denied
<ide> Msg | string | Authorization message (will be returned to the client in case the access is denied)
<del>Err | string | Error message (will be returned to the client in case the plugin encounter an error)
<add>Err | string | Error message (will be returned to the client in case the plugin encounter an error. The string value supplied may appear in logs, so should not include confidential information) | 1 |
Python | Python | fix deprecation warning in `to_categorical` | 9bfbe6ae3e3d516bbb44871d1451a775795784b8 | <ide><path>keras/utils/np_utils.py
<ide> def to_categorical(y, nb_classes=None):
<ide> # Returns
<ide> A binary matrix representation of the input.
<ide> '''
<add> y = np.array(y, dtype='int')
<ide> if not nb_classes:
<ide> nb_classes = np.max(y)+1
<ide> Y = np.zeros((len(y), nb_classes)) | 1 |
Python | Python | fix a slow test. | 04683c0659aacf31a1e1df8aa2e6cf7b447a6f12 | <ide><path>tests/test_pipelines_audio_classification.py
<ide> def test_large_model_pt(self):
<ide> audio = np.array(dataset[3]["speech"], dtype=np.float32)
<ide> output = audio_classifier(audio, top_k=4)
<ide> self.assertEqual(
<del> nested_simplify(output, decimals=4),
<add> nested_simplify(output, decimals=3),
<ide> [
<del> {"score": 0.9809, "label": "go"},
<del> {"score": 0.0073, "label": "up"},
<del> {"score": 0.0064, "label": "_unknown_"},
<del> {"score": 0.0015, "label": "down"},
<add> {"score": 0.981, "label": "go"},
<add> {"score": 0.007, "label": "up"},
<add> {"score": 0.006, "label": "_unknown_"},
<add> {"score": 0.001, "label": "down"},
<ide> ],
<ide> )
<ide> | 1 |
Mixed | Javascript | use windowinsetscompat for keyboard events | 1e48274223ee647ac4fc2c21822b5240f3c47e4c | <ide><path>Libraries/Components/Keyboard/Keyboard.js
<ide> class Keyboard {
<ide> * - `keyboardWillChangeFrame`
<ide> * - `keyboardDidChangeFrame`
<ide> *
<del> * Note that if you set `android:windowSoftInputMode` to `adjustResize` or `adjustNothing`,
<del> * only `keyboardDidShow` and `keyboardDidHide` events will be available on Android.
<del> * `keyboardWillShow` as well as `keyboardWillHide` are generally not available on Android
<del> * since there is no native corresponding event.
<add> * Android versions prior to API 30 rely on observing layout changes when
<add> * `android:windowSoftInputMode` is set to `adjustResize` or `adjustPan`.
<add> *
<add> * `keyboardWillShow` as well as `keyboardWillHide` are not available on Android since there is
<add> * no native corresponding event.
<ide> *
<ide> * @param {function} callback function to be called when the event fires.
<ide> */
<ide><path>ReactAndroid/src/main/java/com/facebook/react/ReactRootView.java
<ide> import static com.facebook.react.uimanager.common.UIManagerType.FABRIC;
<ide> import static com.facebook.systrace.Systrace.TRACE_TAG_REACT_JAVA_BRIDGE;
<ide>
<add>import android.app.Activity;
<ide> import android.content.Context;
<ide> import android.graphics.Canvas;
<ide> import android.graphics.Point;
<ide> import android.view.WindowManager;
<ide> import android.widget.FrameLayout;
<ide> import androidx.annotation.Nullable;
<add>import androidx.annotation.RequiresApi;
<add>import androidx.core.graphics.Insets;
<add>import androidx.core.view.WindowInsetsCompat;
<ide> import com.facebook.common.logging.FLog;
<ide> import com.facebook.infer.annotation.Assertions;
<ide> import com.facebook.infer.annotation.ThreadConfined;
<ide> public void runApplication() {
<ide>
<ide> @VisibleForTesting
<ide> /* package */ void simulateCheckForKeyboardForTesting() {
<del> getCustomGlobalLayoutListener().checkForKeyboardEvents();
<add> if (Build.VERSION.SDK_INT >= 23) {
<add> getCustomGlobalLayoutListener().checkForKeyboardEvents();
<add> } else {
<add> getCustomGlobalLayoutListener().checkForKeyboardEventsLegacy();
<add> }
<ide> }
<ide>
<ide> private CustomGlobalLayoutListener getCustomGlobalLayoutListener() {
<ide> private class CustomGlobalLayoutListener implements ViewTreeObserver.OnGlobalLay
<ide> private final Rect mVisibleViewArea;
<ide> private final int mMinKeyboardHeightDetected;
<ide>
<del> private int mKeyboardHeight = 0;
<add> private boolean mKeyboardIsVisible = false;
<add> private int mKeyboardHeight = 0; // Only used in checkForKeyboardEventsLegacy path
<ide> private int mDeviceRotation = 0;
<ide>
<ide> /* package */ CustomGlobalLayoutListener() {
<ide> public void onGlobalLayout() {
<ide> || mReactInstanceManager.getCurrentReactContext() == null) {
<ide> return;
<ide> }
<del> checkForKeyboardEvents();
<add>
<add> // WindowInsetsCompat IME measurement is reliable for API level 23+.
<add> // https://developer.android.com/jetpack/androidx/releases/core#1.5.0-alpha02
<add> if (Build.VERSION.SDK_INT >= 23) {
<add> checkForKeyboardEvents();
<add> } else {
<add> checkForKeyboardEventsLegacy();
<add> }
<add>
<ide> checkForDeviceOrientationChanges();
<ide> checkForDeviceDimensionsChanges();
<ide> }
<ide>
<add> @RequiresApi(api = Build.VERSION_CODES.M)
<ide> private void checkForKeyboardEvents() {
<ide> getRootView().getWindowVisibleDisplayFrame(mVisibleViewArea);
<add> WindowInsets rootInsets = getRootView().getRootWindowInsets();
<add> WindowInsetsCompat compatRootInsets = WindowInsetsCompat.toWindowInsetsCompat(rootInsets);
<add>
<add> boolean keyboardIsVisible = compatRootInsets.isVisible(WindowInsetsCompat.Type.ime());
<add> if (keyboardIsVisible != mKeyboardIsVisible) {
<add> mKeyboardIsVisible = keyboardIsVisible;
<add>
<add> if (keyboardIsVisible) {
<add> Insets imeInsets = compatRootInsets.getInsets(WindowInsetsCompat.Type.ime());
<add> Insets barInsets = compatRootInsets.getInsets(WindowInsetsCompat.Type.systemBars());
<add> int height = imeInsets.bottom - barInsets.bottom;
<add>
<add> int softInputMode = ((Activity) getContext()).getWindow().getAttributes().softInputMode;
<add> int screenY =
<add> softInputMode == WindowManager.LayoutParams.SOFT_INPUT_ADJUST_NOTHING
<add> ? mVisibleViewArea.bottom - height
<add> : mVisibleViewArea.bottom;
<add>
<add> sendEvent(
<add> "keyboardDidShow",
<add> createKeyboardEventPayload(
<add> PixelUtil.toDIPFromPixel(screenY),
<add> PixelUtil.toDIPFromPixel(mVisibleViewArea.left),
<add> PixelUtil.toDIPFromPixel(mVisibleViewArea.width()),
<add> PixelUtil.toDIPFromPixel(height)));
<add> } else {
<add> sendEvent(
<add> "keyboardDidHide",
<add> createKeyboardEventPayload(
<add> PixelUtil.toDIPFromPixel(mLastHeight),
<add> 0,
<add> PixelUtil.toDIPFromPixel(mVisibleViewArea.width()),
<add> 0));
<add> }
<add> }
<add> }
<add>
<add> private void checkForKeyboardEventsLegacy() {
<add> getRootView().getWindowVisibleDisplayFrame(mVisibleViewArea);
<add>
<ide> int notchHeight = 0;
<ide> if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {
<ide> WindowInsets insets = getRootView().getRootWindowInsets();
<ide> private void checkForKeyboardEvents() {
<ide>
<ide> boolean isKeyboardShowingOrKeyboardHeightChanged =
<ide> mKeyboardHeight != heightDiff && heightDiff > mMinKeyboardHeightDetected;
<add>
<ide> if (isKeyboardShowingOrKeyboardHeightChanged) {
<ide> mKeyboardHeight = heightDiff;
<add> mKeyboardIsVisible = true;
<ide> sendEvent(
<ide> "keyboardDidShow",
<ide> createKeyboardEventPayload(
<ide> private void checkForKeyboardEvents() {
<ide> boolean isKeyboardHidden = mKeyboardHeight != 0 && heightDiff <= mMinKeyboardHeightDetected;
<ide> if (isKeyboardHidden) {
<ide> mKeyboardHeight = 0;
<add> mKeyboardIsVisible = false;
<ide> sendEvent(
<ide> "keyboardDidHide",
<ide> createKeyboardEventPayload( | 2 |
Javascript | Javascript | avoid allocations in snapshotoptimization | 96aca0e4d7ab239d0b61ffb0b3ea372d1aa11456 | <ide><path>lib/FileSystemInfo.js
<ide> class SnapshotOptimization {
<ide> * @param {function(Snapshot): boolean} has has value
<ide> * @param {function(Snapshot): Map<string, T> | Set<string>} get get value
<ide> * @param {function(Snapshot, Map<string, T> | Set<string>): void} set set value
<add> * @param {boolean=} useStartTime use the start time of snapshots
<ide> * @param {boolean=} isSet value is an Set instead of a Map
<ide> */
<del> constructor(has, get, set, isSet = false) {
<add> constructor(has, get, set, useStartTime = true, isSet = false) {
<ide> this._has = has;
<ide> this._get = get;
<ide> this._set = set;
<add> this._useStartTime = useStartTime;
<ide> this._isSet = isSet;
<ide> /** @type {Map<string, SnapshotOptimizationEntry>} */
<ide> this._map = new Map();
<ide> class SnapshotOptimization {
<ide> this._statReusedSharedSnapshots = 0;
<ide> }
<ide>
<del> storeUnsharedSnapshot(snapshot, locations) {
<del> if (locations === undefined) return;
<del> const optimizationEntry = {
<del> snapshot,
<del> shared: 0,
<del> snapshotContent: undefined,
<del> children: undefined
<del> };
<del> for (const path of locations) {
<del> this._map.set(path, optimizationEntry);
<del> }
<del> }
<del>
<del> optimize(capturedFiles, startTime, children) {
<del> /** @type {Set<string>} */
<del> const unsetOptimizationEntries = new Set();
<del> /** @type {Set<SnapshotOptimizationEntry>} */
<del> const checkedOptimizationEntries = new Set();
<add> /**
<add> * @param {Snapshot} newSnapshot snapshot
<add> * @param {Set<string>} capturedFiles files to snapshot/share
<add> * @returns {void}
<add> */
<add> optimize(newSnapshot, capturedFiles) {
<ide> /**
<ide> * @param {SnapshotOptimizationEntry} entry optimization entry
<ide> * @returns {void}
<ide> class SnapshotOptimization {
<ide> capturedFiles.delete(path);
<ide> }
<ide> };
<add>
<add> /** @type {SnapshotOptimizationEntry} */
<add> let newOptimizationEntry = undefined;
<add>
<ide> const capturedFilesSize = capturedFiles.size;
<del> capturedFiles: for (const path of capturedFiles) {
<add>
<add> /** @type {Set<SnapshotOptimizationEntry> | undefined} */
<add> const optimizationEntries = new Set();
<add>
<add> for (const path of capturedFiles) {
<ide> const optimizationEntry = this._map.get(path);
<ide> if (optimizationEntry === undefined) {
<del> unsetOptimizationEntries.add(path);
<add> if (newOptimizationEntry === undefined) {
<add> newOptimizationEntry = {
<add> snapshot: newSnapshot,
<add> shared: 0,
<add> snapshotContent: undefined,
<add> children: undefined
<add> };
<add> }
<add> this._map.set(path, newOptimizationEntry);
<ide> continue;
<add> } else {
<add> optimizationEntries.add(optimizationEntry);
<ide> }
<del> if (checkedOptimizationEntries.has(optimizationEntry)) continue;
<add> }
<add>
<add> optimizationEntries: for (const optimizationEntry of optimizationEntries) {
<ide> const snapshot = optimizationEntry.snapshot;
<ide> if (optimizationEntry.shared > 0) {
<ide> // It's a shared snapshot
<ide> // We can't change it, so we can only use it when all files match
<ide> // and startTime is compatible
<ide> if (
<del> startTime &&
<del> (!snapshot.startTime || snapshot.startTime > startTime)
<add> this._useStartTime &&
<add> newSnapshot.startTime &&
<add> (!snapshot.startTime || snapshot.startTime > newSnapshot.startTime)
<ide> ) {
<ide> continue;
<ide> }
<ide> class SnapshotOptimization {
<ide> if (!snapshotEntries.has(path)) {
<ide> // File is not shared and can't be removed from the snapshot
<ide> // because it's in a child of the snapshot
<del> checkedOptimizationEntries.add(optimizationEntry);
<del> continue capturedFiles;
<add> continue optimizationEntries;
<ide> }
<ide> nonSharedFiles.add(path);
<ide> continue;
<ide> class SnapshotOptimization {
<ide> if (nonSharedFiles.size === 0) {
<ide> // The complete snapshot is shared
<ide> // add it as child
<del> children.add(snapshot);
<add> newSnapshot.addChild(snapshot);
<ide> increaseSharedAndStoreOptimizationEntry(optimizationEntry);
<ide> this._statReusedSharedSnapshots++;
<ide> } else {
<ide> // Only a part of the snapshot is shared
<ide> const sharedCount = snapshotContent.size - nonSharedFiles.size;
<ide> if (sharedCount < MIN_COMMON_SNAPSHOT_SIZE) {
<ide> // Common part it too small
<del> checkedOptimizationEntries.add(optimizationEntry);
<del> continue capturedFiles;
<add> continue optimizationEntries;
<ide> }
<ide> // Extract common timestamps from both snapshots
<ide> let commonMap;
<ide> class SnapshotOptimization {
<ide> }
<ide> // Create and attach snapshot
<ide> const commonSnapshot = new Snapshot();
<del> commonSnapshot.setMergedStartTime(startTime, snapshot);
<add> if (this._useStartTime) {
<add> commonSnapshot.setMergedStartTime(newSnapshot.startTime, snapshot);
<add> }
<ide> this._set(commonSnapshot, commonMap);
<del> children.add(commonSnapshot);
<add> newSnapshot.addChild(commonSnapshot);
<ide> snapshot.addChild(commonSnapshot);
<ide> // Create optimization entry
<ide> const newEntry = {
<ide> class SnapshotOptimization {
<ide> // We can extract a common shared snapshot
<ide> // with all common files
<ide> const snapshotEntries = this._get(snapshot);
<add> if (snapshotEntries === undefined) {
<add> // Incomplete snapshot, that can't be used
<add> continue optimizationEntries;
<add> }
<ide> let commonMap;
<ide> if (this._isSet) {
<ide> commonMap = new Set();
<ide> class SnapshotOptimization {
<ide>
<ide> if (commonMap.size < MIN_COMMON_SNAPSHOT_SIZE) {
<ide> // Common part it too small
<del> checkedOptimizationEntries.add(optimizationEntry);
<del> continue capturedFiles;
<add> continue optimizationEntries;
<ide> }
<ide> // Create and attach snapshot
<ide> const commonSnapshot = new Snapshot();
<del> commonSnapshot.setMergedStartTime(startTime, snapshot);
<add> if (this._useStartTime) {
<add> commonSnapshot.setMergedStartTime(newSnapshot.startTime, snapshot);
<add> }
<ide> this._set(commonSnapshot, commonMap);
<del> children.add(commonSnapshot);
<add> newSnapshot.addChild(commonSnapshot);
<ide> snapshot.addChild(commonSnapshot);
<ide> // Remove files from snapshot
<ide> for (const path of commonMap.keys()) snapshotEntries.delete(path);
<ide> class SnapshotOptimization {
<ide> });
<ide> this._statSharedSnapshots++;
<ide> }
<del> checkedOptimizationEntries.add(optimizationEntry);
<ide> }
<ide> const unshared = capturedFiles.size;
<ide> this._statItemsUnshared += unshared;
<ide> this._statItemsShared += capturedFilesSize - unshared;
<del> return unsetOptimizationEntries;
<ide> }
<ide> }
<ide>
<ide> class FileSystemInfo {
<ide> this._fileHashesOptimization = new SnapshotOptimization(
<ide> s => s.hasFileHashes(),
<ide> s => s.fileHashes,
<del> (s, v) => s.setFileHashes(v)
<add> (s, v) => s.setFileHashes(v),
<add> false
<ide> );
<ide> this._fileTshsOptimization = new SnapshotOptimization(
<ide> s => s.hasFileTshs(),
<ide> class FileSystemInfo {
<ide> this._contextHashesOptimization = new SnapshotOptimization(
<ide> s => s.hasContextHashes(),
<ide> s => s.contextHashes,
<del> (s, v) => s.setContextHashes(v)
<add> (s, v) => s.setContextHashes(v),
<add> false
<ide> );
<ide> this._contextTshsOptimization = new SnapshotOptimization(
<ide> s => s.hasContextTshs(),
<ide> class FileSystemInfo {
<ide> this._missingExistenceOptimization = new SnapshotOptimization(
<ide> s => s.hasMissingExistence(),
<ide> s => s.missingExistence,
<del> (s, v) => s.setMissingExistence(v)
<add> (s, v) => s.setMissingExistence(v),
<add> false
<ide> );
<ide> this._managedItemInfoOptimization = new SnapshotOptimization(
<ide> s => s.hasManagedItemInfo(),
<ide> s => s.managedItemInfo,
<del> (s, v) => s.setManagedItemInfo(v)
<add> (s, v) => s.setManagedItemInfo(v),
<add> false
<ide> );
<ide> this._managedFilesOptimization = new SnapshotOptimization(
<ide> s => s.hasManagedFiles(),
<ide> s => s.managedFiles,
<ide> (s, v) => s.setManagedFiles(v),
<add> false,
<ide> true
<ide> );
<ide> this._managedContextsOptimization = new SnapshotOptimization(
<ide> s => s.hasManagedContexts(),
<ide> s => s.managedContexts,
<ide> (s, v) => s.setManagedContexts(v),
<add> false,
<ide> true
<ide> );
<ide> this._managedMissingOptimization = new SnapshotOptimization(
<ide> s => s.hasManagedMissing(),
<ide> s => s.managedMissing,
<ide> (s, v) => s.setManagedMissing(v),
<add> false,
<ide> true
<ide> );
<ide> /** @type {StackedCacheMap<string, FileSystemInfoEntry | "ignore" | null>} */
<ide> class FileSystemInfo {
<ide> /** @type {Set<Snapshot>} */
<ide> const children = new Set();
<ide>
<del> /** @type {Set<string>} */
<del> let unsharedFileTimestamps;
<del> /** @type {Set<string>} */
<del> let unsharedFileHashes;
<del> /** @type {Set<string>} */
<del> let unsharedFileTshs;
<del> /** @type {Set<string>} */
<del> let unsharedContextTimestamps;
<del> /** @type {Set<string>} */
<del> let unsharedContextHashes;
<del> /** @type {Set<string>} */
<del> let unsharedContextTshs;
<del> /** @type {Set<string>} */
<del> let unsharedMissingExistence;
<del> /** @type {Set<string>} */
<del> let unsharedManagedItemInfo;
<add> const snapshot = new Snapshot();
<add> if (startTime) snapshot.setStartTime(startTime);
<ide>
<ide> /** @type {Set<string>} */
<ide> const managedItems = new Set();
<ide> class FileSystemInfo {
<ide> let jobs = 1;
<ide> const jobDone = () => {
<ide> if (--jobs === 0) {
<del> const snapshot = new Snapshot();
<del> if (startTime) snapshot.setStartTime(startTime);
<ide> if (fileTimestamps.size !== 0) {
<ide> snapshot.setFileTimestamps(fileTimestamps);
<del> this._fileTimestampsOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedFileTimestamps
<del> );
<ide> }
<ide> if (fileHashes.size !== 0) {
<ide> snapshot.setFileHashes(fileHashes);
<del> this._fileHashesOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedFileHashes
<del> );
<ide> }
<ide> if (fileTshs.size !== 0) {
<ide> snapshot.setFileTshs(fileTshs);
<del> this._fileTshsOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedFileTshs
<del> );
<ide> }
<ide> if (contextTimestamps.size !== 0) {
<ide> snapshot.setContextTimestamps(contextTimestamps);
<del> this._contextTimestampsOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedContextTimestamps
<del> );
<ide> }
<ide> if (contextHashes.size !== 0) {
<ide> snapshot.setContextHashes(contextHashes);
<del> this._contextHashesOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedContextHashes
<del> );
<ide> }
<ide> if (contextTshs.size !== 0) {
<ide> snapshot.setContextTshs(contextTshs);
<del> this._contextTshsOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedContextTshs
<del> );
<ide> }
<ide> if (missingExistence.size !== 0) {
<ide> snapshot.setMissingExistence(missingExistence);
<del> this._missingExistenceOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedMissingExistence
<del> );
<ide> }
<ide> if (managedItemInfo.size !== 0) {
<ide> snapshot.setManagedItemInfo(managedItemInfo);
<del> this._managedItemInfoOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedManagedItemInfo
<del> );
<ide> }
<del> const unsharedManagedFiles = this._managedFilesOptimization.optimize(
<del> managedFiles,
<del> undefined,
<del> children
<del> );
<add> this._managedFilesOptimization.optimize(snapshot, managedFiles);
<ide> if (managedFiles.size !== 0) {
<ide> snapshot.setManagedFiles(managedFiles);
<del> this._managedFilesOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedManagedFiles
<del> );
<ide> }
<del> const unsharedManagedContexts =
<del> this._managedContextsOptimization.optimize(
<del> managedContexts,
<del> undefined,
<del> children
<del> );
<add> this._managedContextsOptimization.optimize(snapshot, managedContexts);
<ide> if (managedContexts.size !== 0) {
<ide> snapshot.setManagedContexts(managedContexts);
<del> this._managedContextsOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedManagedContexts
<del> );
<ide> }
<del> const unsharedManagedMissing =
<del> this._managedMissingOptimization.optimize(
<del> managedMissing,
<del> undefined,
<del> children
<del> );
<add> this._managedMissingOptimization.optimize(snapshot, managedMissing);
<ide> if (managedMissing.size !== 0) {
<ide> snapshot.setManagedMissing(managedMissing);
<del> this._managedMissingOptimization.storeUnsharedSnapshot(
<del> snapshot,
<del> unsharedManagedMissing
<del> );
<ide> }
<ide> if (children.size !== 0) {
<ide> snapshot.setChildren(children);
<ide> class FileSystemInfo {
<ide> const capturedFiles = captureNonManaged(files, managedFiles);
<ide> switch (mode) {
<ide> case 3:
<del> unsharedFileTshs = this._fileTshsOptimization.optimize(
<del> capturedFiles,
<del> undefined,
<del> children
<del> );
<add> this._fileTshsOptimization.optimize(snapshot, capturedFiles);
<ide> for (const path of capturedFiles) {
<ide> const cache = this._fileTshs.get(path);
<ide> if (cache !== undefined) {
<ide> class FileSystemInfo {
<ide> }
<ide> break;
<ide> case 2:
<del> unsharedFileHashes = this._fileHashesOptimization.optimize(
<del> capturedFiles,
<del> undefined,
<del> children
<del> );
<add> this._fileHashesOptimization.optimize(snapshot, capturedFiles);
<ide> for (const path of capturedFiles) {
<ide> const cache = this._fileHashes.get(path);
<ide> if (cache !== undefined) {
<ide> class FileSystemInfo {
<ide> }
<ide> break;
<ide> case 1:
<del> unsharedFileTimestamps = this._fileTimestampsOptimization.optimize(
<del> capturedFiles,
<del> startTime,
<del> children
<del> );
<add> this._fileTimestampsOptimization.optimize(snapshot, capturedFiles);
<ide> for (const path of capturedFiles) {
<ide> const cache = this._fileTimestamps.get(path);
<ide> if (cache !== undefined) {
<ide> class FileSystemInfo {
<ide> );
<ide> switch (mode) {
<ide> case 3:
<del> unsharedContextTshs = this._contextTshsOptimization.optimize(
<del> capturedDirectories,
<del> undefined,
<del> children
<del> );
<add> this._contextTshsOptimization.optimize(snapshot, capturedDirectories);
<ide> for (const path of capturedDirectories) {
<ide> const cache = this._contextTshs.get(path);
<ide> let resolved;
<ide> class FileSystemInfo {
<ide> }
<ide> break;
<ide> case 2:
<del> unsharedContextHashes = this._contextHashesOptimization.optimize(
<del> capturedDirectories,
<del> undefined,
<del> children
<add> this._contextHashesOptimization.optimize(
<add> snapshot,
<add> capturedDirectories
<ide> );
<ide> for (const path of capturedDirectories) {
<ide> const cache = this._contextHashes.get(path);
<ide> class FileSystemInfo {
<ide> }
<ide> break;
<ide> case 1:
<del> unsharedContextTimestamps =
<del> this._contextTimestampsOptimization.optimize(
<del> capturedDirectories,
<del> startTime,
<del> children
<del> );
<add> this._contextTimestampsOptimization.optimize(
<add> snapshot,
<add> capturedDirectories
<add> );
<ide> for (const path of capturedDirectories) {
<ide> const cache = this._contextTimestamps.get(path);
<ide> let resolved;
<ide> class FileSystemInfo {
<ide> }
<ide> if (missing) {
<ide> const capturedMissing = captureNonManaged(missing, managedMissing);
<del> unsharedMissingExistence = this._missingExistenceOptimization.optimize(
<del> capturedMissing,
<del> startTime,
<del> children
<del> );
<add> this._missingExistenceOptimization.optimize(snapshot, capturedMissing);
<ide> for (const path of capturedMissing) {
<ide> const cache = this._fileTimestamps.get(path);
<ide> if (cache !== undefined) {
<ide> class FileSystemInfo {
<ide> }
<ide> }
<ide> }
<del> unsharedManagedItemInfo = this._managedItemInfoOptimization.optimize(
<del> managedItems,
<del> undefined,
<del> children
<del> );
<add> this._managedItemInfoOptimization.optimize(snapshot, managedItems);
<ide> for (const path of managedItems) {
<ide> const cache = this._managedItems.get(path);
<ide> if (cache !== undefined) { | 1 |
Python | Python | add comment to usage of m2m_reverse_field_name | 3a338d00ec0d32d54faa0bf88409d02638eae60d | <ide><path>django/db/backends/schema.py
<ide> def _alter_many_to_many(self, model, old_field, new_field, strict):
<ide> # Repoint the FK to the other side
<ide> self.alter_field(
<ide> new_field.rel.through,
<add> # We need the field that points to the target model, so we can tell alter_field to change it -
<add> # this is m2m_reverse_field_name() (as opposed to m2m_field_name, which points to our model)
<ide> old_field.rel.through._meta.get_field_by_name(old_field.m2m_reverse_field_name())[0],
<ide> new_field.rel.through._meta.get_field_by_name(new_field.m2m_reverse_field_name())[0],
<ide> ) | 1 |
Javascript | Javascript | use pre-defined displays for html and body | a7724186c98734529b06bebb8f2dc7fc2b641011 | <ide><path>src/css/defaultDisplay.js
<ide> define([
<ide> ], function( jQuery ) {
<ide>
<ide> var iframe,
<del> elemdisplay = {};
<add> elemdisplay = {
<add>
<add> // Support: Firefox
<add> // We have to pre-define this values for FF (#10227)
<add> HTML: "block",
<add> BODY: "block"
<add> };
<ide>
<ide> /**
<ide> * Retrieve the actual display of a element | 1 |
Javascript | Javascript | update documentation for emberarray.find | 9f6bece75648d11222c54b828c18b47477bcdb1a | <ide><path>packages/@ember/-internals/runtime/lib/mixins/array.js
<ide> const ArrayMixin = Mixin.create(Enumerable, {
<ide> Note that in addition to a callback, you can also pass an optional target
<ide> object that will be set as `this` on the context. This is a good way
<ide> to give your iterator function access to the current object.
<add>
<add> Example Usage:
<add>
<add> ```javascript
<add> let users = [
<add> { id: 1, name: 'Yehuda' },
<add> { id: 2, name: 'Tom' },
<add> { id: 3, name: 'Melanie' },
<add> { id: 4, name: 'Leah' }
<add> ];
<add>
<add> users.find((user) => user.name == 'Tom'); // [{ id: 2, name: 'Tom' }]
<add> users.find(({ id }) => id == 3); // [{ id: 3, name: 'Melanie' }]
<add> ```
<ide>
<ide> @method find
<ide> @param {Function} callback The callback to execute | 1 |
Python | Python | handle the case when title is none | 88b3a91e6149fe62cd3d08b1954abb0f1c74dba7 | <ide><path>examples/rag/use_own_knowledge_dataset.py
<ide> def split_documents(documents: dict) -> dict:
<ide> """Split documents into passages"""
<ide> titles, texts = [], []
<ide> for title, text in zip(documents["title"], documents["text"]):
<del> for passage in split_text(text):
<del> titles.append(title)
<del> texts.append(passage)
<add> if text is not None:
<add> for passage in split_text(text):
<add> titles.append(title if title is not None else "")
<add> texts.append(passage)
<ide> return {"title": titles, "text": texts}
<ide>
<ide> | 1 |
Javascript | Javascript | fix debugger repl tests | ec378aaa690dee25b34a696bd893f646ab4616d1 | <ide><path>test/fixtures/breakpoints_utf8.js
<ide> b();
<ide>
<ide> setInterval(function() {
<ide> }, 5000);
<add>
<add>
<add>now = new Date();
<add>debugger;
<ide><path>test/simple/test-debugger-repl-utf8.js
<ide> // OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
<ide> // USE OR OTHER DEALINGS IN THE SOFTWARE.
<ide>
<del>
<del>process.env.NODE_DEBUGGER_TIMEOUT = 2000;
<ide> var common = require('../common');
<del>var assert = require('assert');
<del>var spawn = require('child_process').spawn;
<del>var debug = require('_debugger');
<del>
<del>var port = common.PORT + 1337;
<del>
<ide> var script = common.fixturesDir + '/breakpoints_utf8.js';
<add>process.env.NODE_DEBUGGER_TEST_SCRIPT = script;
<ide>
<del>var child = spawn(process.execPath, ['debug', '--port=' + port, script]);
<del>
<del>console.error('./node', 'debug', '--port=' + port, script);
<del>
<del>var buffer = '';
<del>child.stdout.setEncoding('utf-8');
<del>child.stdout.on('data', function(data) {
<del> data = (buffer + data.toString()).split(/\n/g);
<del> buffer = data.pop();
<del> data.forEach(function(line) {
<del> child.emit('line', line);
<del> });
<del>});
<del>child.stderr.pipe(process.stdout);
<del>
<del>var expected = [];
<del>
<del>child.on('line', function(line) {
<del> line = line.replace(/^(debug> )+/, 'debug> ');
<del> console.error('line> ' + line);
<del> assert.ok(expected.length > 0, 'Got unexpected line: ' + line);
<del>
<del> var expectedLine = expected[0].lines.shift();
<del> assert.ok(line.match(expectedLine) !== null, line + ' != ' + expectedLine);
<del>
<del> if (expected[0].lines.length === 0) {
<del> var callback = expected[0].callback;
<del> expected.shift();
<del> callback && callback();
<del> }
<del>});
<del>
<del>function addTest(input, output) {
<del> function next() {
<del> if (expected.length > 0) {
<del> child.stdin.write(expected[0].input + '\n');
<del>
<del> if (!expected[0].lines) {
<del> setTimeout(function() {
<del> var callback = expected[0].callback;
<del> expected.shift();
<del>
<del> callback && callback();
<del> }, 50);
<del> }
<del> } else {
<del> finish();
<del> }
<del> };
<del> expected.push({input: input, lines: output, callback: next});
<del>}
<del>
<del>// Initial lines
<del>addTest(null, [
<del> /listening on port \d+/,
<del> /connecting... ok/,
<del> /break in .*:1/,
<del> /1/, /2/, /3/
<del>]);
<del>
<del>// Next
<del>addTest('n', [
<del> /break in .*:11/,
<del> /9/, /10/, /11/, /12/, /13/
<del>]);
<del>
<del>// Watch
<del>addTest('watch("\'x\'")');
<del>
<del>// Continue
<del>addTest('c', [
<del> /break in .*:5/,
<del> /Watchers/,
<del> /0:\s+'x' = "x"/,
<del> /()/,
<del> /3/, /4/, /5/, /6/, /7/
<del>]);
<del>
<del>// Show watchers
<del>addTest('watchers', [
<del> /0:\s+'x' = "x"/
<del>]);
<del>
<del>// Unwatch
<del>addTest('unwatch("\'x\'")');
<del>
<del>// Step out
<del>addTest('o', [
<del> /break in .*:12/,
<del> /10/, /11/, /12/, /13/, /14/
<del>]);
<del>
<del>// Continue
<del>addTest('c', [
<del> /break in .*:5/,
<del> /3/, /4/, /5/, /6/, /7/
<del>]);
<del>
<del>// Set breakpoint by function name
<del>addTest('sb("setInterval()", "!(setInterval.flag++)")', [
<del> /1/, /2/, /3/, /4/, /5/, /6/, /7/, /8/, /9/, /10/
<del>]);
<del>
<del>// Continue
<del>addTest('c', [
<del> /break in node.js:\d+/,
<del> /\d/, /\d/, /\d/, /\d/, /\d/
<del>]);
<del>
<del>function finish() {
<del> process.exit(0);
<del>}
<del>
<del>function quit() {
<del> if (quit.called) return;
<del> quit.called = true;
<del> child.stdin.write('quit');
<del>}
<del>
<del>setTimeout(function() {
<del> console.error('dying badly');
<del> var err = 'Timeout';
<del> if (expected.length > 0 && expected[0].lines) {
<del> err = err + '. Expected: ' + expected[0].lines.shift();
<del> }
<del> quit();
<del> child.kill('SIGINT');
<del> child.kill('SIGTERM');
<del>
<del> // give the sigkill time to work.
<del> setTimeout(function() {
<del> throw new Error(err);
<del> }, 100);
<del>
<del>}, 5000);
<del>
<del>process.once('uncaughtException', function(e) {
<del> quit();
<del> console.error(e.toString());
<del> process.exit(1);
<del>});
<add>require('./test-debugger-repl.js');
<ide>
<del>process.on('exit', function(code) {
<del> quit();
<del> if (code === 0) {
<del> assert.equal(expected.length, 0);
<del> }
<del>});
<ide><path>test/simple/test-debugger-repl.js
<ide> // OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
<ide> // USE OR OTHER DEALINGS IN THE SOFTWARE.
<ide>
<del>
<ide> process.env.NODE_DEBUGGER_TIMEOUT = 2000;
<ide> var common = require('../common');
<ide> var assert = require('assert');
<ide> var debug = require('_debugger');
<ide>
<ide> var port = common.PORT + 1337;
<ide>
<del>var script = common.fixturesDir + '/breakpoints.js';
<add>var script = process.env.NODE_DEBUGGER_TEST_SCRIPT ||
<add> common.fixturesDir + '/breakpoints.js';
<ide>
<del>var child = spawn(process.execPath, ['debug', '--port=' + port, script], {
<del> env: { NODE_FORCE_READLINE: 1 }
<del>});
<add>var child = spawn(process.execPath, ['debug', '--port=' + port, script]);
<ide>
<ide> console.error('./node', 'debug', '--port=' + port, script);
<ide>
<ide> child.stdout.on('data', function(data) {
<ide> child.emit('line', line);
<ide> });
<ide> });
<del>child.stderr.pipe(process.stdout);
<add>child.stderr.pipe(process.stderr);
<ide>
<ide> var expected = [];
<ide>
<ide> child.on('line', function(line) {
<del> line = line.replace(/^(debug> )+/, 'debug> ');
<del> line = line.replace(/\u001b\[\d+\w/g, '');
<del> console.error('line> ' + line);
<add> line = line.replace(/^(debug> *)+/, '');
<add> console.log(line);
<ide> assert.ok(expected.length > 0, 'Got unexpected line: ' + line);
<ide>
<ide> var expectedLine = expected[0].lines.shift();
<ide> child.on('line', function(line) {
<ide> function addTest(input, output) {
<ide> function next() {
<ide> if (expected.length > 0) {
<del> var res = child.stdin.write(expected[0].input + '\n'),
<del> callback;
<add> console.log('debug> ' + expected[0].input);
<add> child.stdin.write(expected[0].input + '\n');
<ide>
<ide> if (!expected[0].lines) {
<del> callback = expected[0].callback;
<add> var callback = expected[0].callback;
<ide> expected.shift();
<del> }
<ide>
<del> if (callback) {
<del> if (res !== true) {
<del> child.stdin.on('drain', callback);
<del> } else {
<del> process.nextTick(callback);
<del> }
<add> callback && callback();
<ide> }
<ide> } else {
<del> finish();
<add> quit();
<ide> }
<ide> };
<ide> expected.push({input: input, lines: output, callback: next});
<ide> addTest(null, [
<ide>
<ide> // Next
<ide> addTest('n', [
<del> /debug> n/,
<ide> /break in .*:11/,
<ide> /9/, /10/, /11/, /12/, /13/
<ide> ]);
<ide>
<ide> // Watch
<del>addTest('watch("\'x\'"), true', [/debug>/, /true/]);
<add>addTest('watch("\'x\'")');
<ide>
<ide> // Continue
<ide> addTest('c', [
<del> /debug>/,
<ide> /break in .*:5/,
<ide> /Watchers/,
<ide> /0:\s+'x' = "x"/,
<ide> addTest('c', [
<ide>
<ide> // Show watchers
<ide> addTest('watchers', [
<del> /debug>/,
<ide> /0:\s+'x' = "x"/
<ide> ]);
<ide>
<ide> // Unwatch
<del>addTest('unwatch("\'x\'"), true', [/debug>/, /true/]);
<add>addTest('unwatch("\'x\'")');
<ide>
<ide> // Step out
<ide> addTest('o', [
<del> /debug>/,
<ide> /break in .*:12/,
<ide> /10/, /11/, /12/, /13/, /14/
<ide> ]);
<ide>
<ide> // Continue
<ide> addTest('c', [
<del> /debug>/,
<ide> /break in .*:5/,
<ide> /3/, /4/, /5/, /6/, /7/
<ide> ]);
<ide>
<ide> // Set breakpoint by function name
<ide> addTest('sb("setInterval()", "!(setInterval.flag++)")', [
<del> /debug>/,
<ide> /1/, /2/, /3/, /4/, /5/, /6/, /7/, /8/, /9/, /10/
<ide> ]);
<ide>
<ide> // Continue
<ide> addTest('c', [
<del> /debug>/,
<ide> /break in node.js:\d+/,
<ide> /\d/, /\d/, /\d/, /\d/, /\d/
<ide> ]);
<ide>
<del>// Repeat last command
<del>addTest('', [
<del> /debug>/,
<del> /break in .*breakpoints.js:\d+/,
<del> /\d/, /\d/, /\d/, /\d/, /\d/
<del>]);
<del>
<del>addTest('repl', [
<del> /debug>/,
<del> /Press Ctrl \+ C to leave debug repl/
<del>]);
<del>
<del>addTest('now', [
<del> /> now/,
<del> /\w* \w* \d* \d* \d*:\d*:\d* GMT[+-]\d* (\w*)/
<del>]);
<del>
<del>function finish() {
<del> // Exit debugger repl
<del> child.kill('SIGINT');
<del> child.kill('SIGINT');
<add>addTest('quit', []);
<ide>
<del> // Exit debugger
<del> child.kill('SIGINT');
<del> process.exit(0);
<del>}
<add>var childClosed = false;
<add>child.on('close', function(code) {
<add> assert(!code);
<add> childClosed = true;
<add>});
<ide>
<add>var quitCalled = false;
<ide> function quit() {
<del> if (quit.called) return;
<del> quit.called = true;
<add> if (quitCalled || childClosed) return;
<add> quitCalled = true;
<ide> child.stdin.write('quit');
<add> child.kill('SIGTERM');
<ide> }
<ide>
<ide> setTimeout(function() {
<add> console.error('dying badly buffer=%j', buffer);
<ide> var err = 'Timeout';
<ide> if (expected.length > 0 && expected[0].lines) {
<ide> err = err + '. Expected: ' + expected[0].lines.shift();
<ide> }
<del> quit();
<del> child.kill('SIGINT');
<del> child.kill('SIGTERM');
<ide>
<del> // give the sigkill time to work.
<del> setTimeout(function() {
<add> child.on('close', function() {
<add> console.error('child is closed');
<ide> throw new Error(err);
<del> }, 100);
<add> });
<ide>
<del>}, 5000);
<add> quit();
<add>}, 5000).unref();
<ide>
<ide> process.once('uncaughtException', function(e) {
<add> console.error('UncaughtException', e, e.stack);
<ide> quit();
<ide> console.error(e.toString());
<ide> process.exit(1);
<ide> });
<ide>
<ide> process.on('exit', function(code) {
<add> console.error('process exit', code);
<ide> quit();
<del> if (code === 0) {
<del> assert.equal(expected.length, 0);
<del> }
<add> if (code === 0)
<add> assert(childClosed);
<ide> }); | 3 |
Text | Text | unify installation scripts for example apps | c47f3d84c4065b87d7ea7a0e1bbfe0f4f14dc08e | <ide><path>examples/amp-story/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example amp-story amp-app
<add>npx create-next-app --example amp-story amp-story-app
<ide> # or
<del>yarn create next-app --example amp-story amp-app
<add>yarn create next-app --example amp-story amp-story-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/auth0/README.md
<ide> Read more: [https://auth0.com/blog/ultimate-guide-nextjs-authentication-auth0/](
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example auth0 auth0
<add>npx create-next-app --example auth0 auth0-app
<ide> # or
<del>yarn create next-app --example auth0 auth0
<add>yarn create next-app --example auth0 auth0-app
<ide> ```
<ide>
<ide> ## Configuring Auth0
<ide><path>examples/fast-refresh-demo/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example fast-refresh-demo fast-refresh-demo
<add>npx create-next-app --example fast-refresh-demo fast-refresh-demo-app
<ide> # or
<del>yarn create next-app --example fast-refresh-demo fast-refresh-demo
<add>yarn create next-app --example fast-refresh-demo fast-refresh-demo-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-draft-js/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-draft-js
<add>npx create-next-app --example with-draft-js with-draft-js-app
<ide> # or
<ide> yarn create next-app --example with-draft-js with-draft-js-app
<ide> ```
<ide><path>examples/with-env-from-next-config-js/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-env-from-next-config-js
<add>npx create-next-app --example with-env-from-next-config-js with-env-from-next-config-js-app
<ide> # or
<del>yarn create next-app --example with-env-from-next-config-js
<add>yarn create next-app --example with-env-from-next-config-js with-env-from-next-config-js-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-framer-motion/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-framer-motion with-framer-motion
<add>npx create-next-app --example with-framer-motion with-framer-motion-app
<ide> # or
<del>yarn create next-app --example with-framer-motion with-framer-motion
<add>yarn create next-app --example with-framer-motion with-framer-motion-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-graphql-faunadb/README.md
<ide> At the end, a `.env.local` [file](https://nextjs.org/docs/basic-features/environ
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```
<del>npx create-next-app --example with-graphql-faunadb with-graphql-faunadb
<add>npx create-next-app --example with-graphql-faunadb with-graphql-faunadb-app
<ide> # or
<del>yarn create next-app --example with-graphql-faunadb with-graphql-faunadb
<add>yarn create next-app --example with-graphql-faunadb with-graphql-faunadb-app
<ide> ```
<ide>
<ide> ### Run locally
<ide><path>examples/with-i18n-rosetta/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-i18n-rosetta with-i18n-rosetta
<add>npx create-next-app --example with-i18n-rosetta with-i18n-rosetta-app
<ide> # or
<del>yarn create next-app --example with-i18n-rosetta with-i18n-rosetta
<add>yarn create next-app --example with-i18n-rosetta with-i18n-rosetta-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-jest/README.md
<ide> Quickly get started using [Create Next App](https://github.com/vercel/next.js/tr
<ide> In your terminal, run the following command:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-jest
<add>npx create-next-app --example with-jest with-jest-app
<add># or
<add>yarn create next-app --example with-jest with-jest-app
<ide> ```
<ide>
<ide> ## Run Jest Tests
<ide><path>examples/with-knex/README.md
<ide> Once you have access to the environment variables you'll need, deploy the exampl
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-knex with-knex
<add>npx create-next-app --example with-knex with-knex-app
<ide> # or
<del>yarn create next-app --example with-knex with-knex
<add>yarn create next-app --example with-knex with-knex-app
<ide> ```
<ide>
<ide> ## Configuration
<ide><path>examples/with-mongodb/README.md
<ide> Once you have access to the environment variables you'll need, deploy the exampl
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-mongodb with-mongodb
<add>npx create-next-app --example with-mongodb with-mongodb-app
<ide> # or
<del>yarn create next-app --example with-mongodb with-mongodb
<add>yarn create next-app --example with-mongodb with-mongodb-app
<ide> ```
<ide>
<ide> ## Configuration
<ide><path>examples/with-next-page-transitions/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-next-page-transitions with-next-page-transitions
<add>npx create-next-app --example with-next-page-transitions with-next-page-transitions-app
<ide> # or
<del>yarn create next-app --example with-next-page-transitions with-next-page-transitions
<add>yarn create next-app --example with-next-page-transitions with-next-page-transitions-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-portals-ssr/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-portals-ssr with-portals-ssr
<add>npx create-next-app --example with-portals-ssr with-portals-ssr-app
<ide> # or
<del>yarn create next-app --example with-portals-ssr with-portals-ssr
<add>yarn create next-app --example with-portals-ssr with-portals-ssr-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-react-native-web/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-react-native-web
<add>npx create-next-app --example with-react-native-web with-react-native-web-app
<ide> # or
<ide> yarn create next-app --example with-react-native-web with-react-native-web-app
<ide> ```
<ide><path>examples/with-reason-relay/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-reason-relay with-reason-relay
<add>npx create-next-app --example with-reason-relay with-reason-relay-app
<ide> # or
<del>yarn create next-app --example with-reason-relay with-reason-relay
<add>yarn create next-app --example with-reason-relay with-reason-relay-app
<ide> ```
<ide>
<ide> Download schema introspection data from configured Relay endpoint:
<ide><path>examples/with-sentry/README.md
<ide> Once you have access to your [Sentry DSN](#step-1-enable-error-tracking), deploy
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-sentry with-sentry
<add>npx create-next-app --example with-sentry with-sentry-app
<ide> # or
<del>yarn create next-app --example with-sentry with-sentry
<add>yarn create next-app --example with-sentry with-sentry-app
<ide> ```
<ide>
<ide> ## Configuration
<ide><path>examples/with-stitches/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [Create Next App](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-stitches
<add>npx create-next-app --example with-stitches with-stitches-app
<ide> # or
<del>yarn create next-app --example with-stitches
<add>yarn create next-app --example with-stitches with-stitches-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
<ide><path>examples/with-typescript-styled-components/README.md
<ide> Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_mediu
<ide> Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
<ide>
<ide> ```bash
<del>npx create-next-app --example with-typescript-styled-components with-typescript-styled-components
<add>npx create-next-app --example with-typescript-styled-components with-typescript-styled-components-app
<ide> # or
<del>yarn create next-app --example with-typescript-styled-components with-typescript-styled-components
<add>yarn create next-app --example with-typescript-styled-components with-typescript-styled-components-app
<ide> ```
<ide>
<ide> Deploy it to the cloud with [Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)). | 18 |
Text | Text | update description of 'clienterror' event | 54cb3c5759919745c25554daffc613dbee230d37 | <ide><path>doc/api/http.md
<ide> changes:
<ide>
<ide> If a client connection emits an `'error'` event, it will be forwarded here.
<ide> Listener of this event is responsible for closing/destroying the underlying
<del>socket. For example, one may wish to more gracefully close the socket with an
<del>HTTP '400 Bad Request' response instead of abruptly severing the connection.
<add>socket. For example, one may wish to more gracefully close the socket with a
<add>custom HTTP response instead of abruptly severing the connection.
<ide>
<del>Default behavior is to destroy the socket immediately on malformed request.
<add>Default behavior is to close the socket with an HTTP '400 Bad Request' response
<add>if possible, otherwise the socket is immediately destroyed.
<ide>
<ide> `socket` is the [`net.Socket`][] object that the error originated from.
<ide> | 1 |
Javascript | Javascript | show proper differences | 0cdc87778e9c871e8bbbb081f4dc52fb17248e58 | <ide><path>lib/internal/errors.js
<ide> function createErrDiff(actual, expected, operator) {
<ide> var skipped = false;
<ide> const util = lazyUtil();
<ide> const actualLines = util
<del> .inspect(actual, { compact: false }).split('\n');
<add> .inspect(actual, { compact: false, customInspect: false }).split('\n');
<ide> const expectedLines = util
<del> .inspect(expected, { compact: false }).split('\n');
<add> .inspect(expected, { compact: false, customInspect: false }).split('\n');
<ide> const msg = `Input A expected to ${operator} input B:\n` +
<ide> `${green}+ expected${white} ${red}- actual${white}`;
<ide> const skippedMsg = ' ... Lines skipped';
<ide> class AssertionError extends Error {
<ide> } else if (errorDiff === 1) {
<ide> // In case the objects are equal but the operator requires unequal, show
<ide> // the first object and say A equals B
<del> const res = util
<del> .inspect(actual, { compact: false }).split('\n');
<add> const res = util.inspect(
<add> actual,
<add> { compact: false, customInspect: false }
<add> ).split('\n');
<ide>
<ide> if (res.length > 20) {
<ide> res[19] = '...';
<ide><path>test/parallel/test-assert.js
<ide> common.expectsError(
<ide> message: `${start}\n` +
<ide> `${actExp}\n` +
<ide> '\n' +
<del> ' {}'
<add> `${minus} {}\n` +
<add> `${plus} {\n` +
<add> `${plus} loop: 'forever',\n` +
<add> `${plus} [Symbol(util.inspect.custom)]: [Function]\n` +
<add> `${plus} }`
<ide> });
<ide>
<ide> // notDeepEqual tests | 2 |
Javascript | Javascript | remove common module from test it thwarts | 4885982ee270429e1d54602d6abb7ee1b174cb1b | <ide><path>test/parallel/test-global-console-exists.js
<ide> /* eslint-disable required-modules */
<del>// ordinarily test files must require('common') but that action causes
<del>// the global console to be compiled, defeating the purpose of this test
<ide>
<ide> 'use strict';
<ide>
<del>const common = require('../common');
<add>// Ordinarily test files must require('common') but that action causes
<add>// the global console to be compiled, defeating the purpose of this test.
<add>
<ide> const assert = require('assert');
<ide> const EventEmitter = require('events');
<ide> const leakWarning = /EventEmitter memory leak detected\. 2 hello listeners/;
<ide>
<del>common.hijackStderr(common.mustCall(function(data) {
<del> if (process.stderr.writeTimes === 0) {
<del> assert.ok(leakWarning.test(data));
<del> } else {
<del> assert.fail('stderr.write should be called only once');
<del> }
<del>}));
<del>
<del>process.on('warning', function(warning) {
<add>let writeTimes = 0;
<add>let warningTimes = 0;
<add>process.on('warning', () => {
<ide> // This will be called after the default internal
<ide> // process warning handler is called. The default
<ide> // process warning writes to the console, which will
<ide> // invoke the monkeypatched process.stderr.write
<ide> // below.
<del> assert.strictEqual(process.stderr.writeTimes, 1);
<add> assert.strictEqual(writeTimes, 1);
<ide> EventEmitter.defaultMaxListeners = oldDefault;
<del> // when we get here, we should be done
<add> warningTimes++;
<add>});
<add>
<add>process.on('exit', () => {
<add> assert.strictEqual(warningTimes, 1);
<ide> });
<ide>
<add>process.stderr.write = (data) => {
<add> if (writeTimes === 0)
<add> assert.ok(leakWarning.test(data));
<add> else
<add> assert.fail('stderr.write should be called only once');
<add>
<add> writeTimes++;
<add>};
<add>
<ide> const oldDefault = EventEmitter.defaultMaxListeners;
<ide> EventEmitter.defaultMaxListeners = 1;
<ide>
<ide> const e = new EventEmitter();
<del>e.on('hello', common.noop);
<del>e.on('hello', common.noop);
<add>e.on('hello', () => {});
<add>e.on('hello', () => {});
<ide>
<ide> // TODO: Figure out how to validate console. Currently,
<ide> // there is no obvious way of validating that console | 1 |
Go | Go | fix typos in pkg | aef02273d9cdf8144b95c9c9a8d1e119d24b2d9d | <ide><path>pkg/parsers/kernel/uname_linux.go
<ide> import (
<ide> )
<ide>
<ide> // Utsname represents the system name structure.
<del>// It is passthgrouh for syscall.Utsname in order to make it portable with
<add>// It is passthrough for syscall.Utsname in order to make it portable with
<ide> // other platforms where it is not available.
<ide> type Utsname syscall.Utsname
<ide>
<ide><path>pkg/platform/architecture_freebsd.go
<ide> import (
<ide> "os/exec"
<ide> )
<ide>
<del>// runtimeArchitecture get the name of the current architecture (x86, x86_64, …)
<add>// runtimeArchitecture gets the name of the current architecture (x86, x86_64, …)
<ide> func runtimeArchitecture() (string, error) {
<ide> cmd := exec.Command("uname", "-m")
<ide> machine, err := cmd.Output()
<ide><path>pkg/platform/architecture_linux.go
<ide> import (
<ide> "syscall"
<ide> )
<ide>
<del>// runtimeArchitecture get the name of the current architecture (x86, x86_64, …)
<add>// runtimeArchitecture gets the name of the current architecture (x86, x86_64, …)
<ide> func runtimeArchitecture() (string, error) {
<ide> utsname := &syscall.Utsname{}
<ide> if err := syscall.Uname(utsname); err != nil {
<ide><path>pkg/platform/architecture_windows.go
<ide> const (
<ide>
<ide> var sysinfo systeminfo
<ide>
<del>// runtimeArchitecture get the name of the current architecture (x86, x86_64, …)
<add>// runtimeArchitecture gets the name of the current architecture (x86, x86_64, …)
<ide> func runtimeArchitecture() (string, error) {
<ide> syscall.Syscall(procGetSystemInfo.Addr(), 1, uintptr(unsafe.Pointer(&sysinfo)), 0, 0)
<ide> switch sysinfo.wProcessorArchitecture {
<ide><path>pkg/platform/platform.go
<ide> func init() {
<ide> var err error
<ide> Architecture, err = runtimeArchitecture()
<ide> if err != nil {
<del> logrus.Errorf("Could no read system architecture info: %v", err)
<add> logrus.Errorf("Could not read system architecture info: %v", err)
<ide> }
<ide> OSType = runtime.GOOS
<ide> }
<ide><path>pkg/reexec/reexec.go
<ide> var registeredInitializers = make(map[string]func())
<ide> // Register adds an initialization func under the specified name
<ide> func Register(name string, initializer func()) {
<ide> if _, exists := registeredInitializers[name]; exists {
<del> panic(fmt.Sprintf("reexec func already registred under name %q", name))
<add> panic(fmt.Sprintf("reexec func already registered under name %q", name))
<ide> }
<ide>
<ide> registeredInitializers[name] = initializer
<ide><path>pkg/registrar/registrar.go
<ide> var (
<ide> ErrNoSuchKey = errors.New("provided key does not exist")
<ide> )
<ide>
<del>// Registrar stores indexes a list of keys and their registered names as well as indexes names and the key that they are registred to
<add>// Registrar stores indexes a list of keys and their registered names as well as indexes names and the key that they are registered to
<ide> // Names must be unique.
<ide> // Registrar is safe for concurrent access.
<ide> type Registrar struct {
<ide><path>pkg/stringid/stringid.go
<ide> func IsShortID(id string) bool {
<ide> // TruncateID returns a shorthand version of a string identifier for convenience.
<ide> // A collision with other shorthands is very unlikely, but possible.
<ide> // In case of a collision a lookup with TruncIndex.Get() will fail, and the caller
<del>// will need to use a langer prefix, or the full-length Id.
<add>// will need to use a longer prefix, or the full-length Id.
<ide> func TruncateID(id string) string {
<ide> if i := strings.IndexRune(id, ':'); i >= 0 {
<ide> id = id[i+1:]
<ide> func generateID(crypto bool) string {
<ide> }
<ide> }
<ide>
<del>// GenerateRandomID returns an unique id.
<add>// GenerateRandomID returns a unique id.
<ide> func GenerateRandomID() string {
<ide> return generateID(true)
<ide>
<ide><path>pkg/stringutils/stringutils.go
<ide> func GenerateRandomAlphaOnlyString(n int) string {
<ide> return string(b)
<ide> }
<ide>
<del>// GenerateRandomASCIIString generates an ASCII random stirng with length n.
<add>// GenerateRandomASCIIString generates an ASCII random string with length n.
<ide> func GenerateRandomASCIIString(n int) string {
<ide> chars := "abcdefghijklmnopqrstuvwxyz" +
<ide> "ABCDEFGHIJKLMNOPQRSTUVWXYZ" +
<ide> func quote(word string, buf *bytes.Buffer) {
<ide> }
<ide>
<ide> // ShellQuoteArguments takes a list of strings and escapes them so they will be
<del>// handled right when passed as arguments to an program via a shell
<add>// handled right when passed as arguments to a program via a shell
<ide> func ShellQuoteArguments(args []string) string {
<ide> var buf bytes.Buffer
<ide> for i, arg := range args {
<ide><path>pkg/system/umask.go
<ide> import (
<ide> )
<ide>
<ide> // Umask sets current process's file mode creation mask to newmask
<del>// and return oldmask.
<add>// and returns oldmask.
<ide> func Umask(newmask int) (oldmask int, err error) {
<ide> return syscall.Umask(newmask), nil
<ide> } | 10 |
Javascript | Javascript | use synthetic events | 88e90d5601aa29e606ed2c2e248f34df46ecad6f | <add><path>src/core/__tests__/ReactEventEmitter-test.js
<del><path>src/core/__tests__/ReactEvent-test.js
<ide> describe('ReactEventEmitter', function() {
<ide>
<ide> it('should invoke handlers that were removed while bubbling', function() {
<ide> var handleParentClick = mocks.getMockFunction();
<del> var handleChildClick = function(abstractEvent) {
<add> var handleChildClick = function(event) {
<ide> ReactEventEmitter.deleteAllListeners(PARENT.id);
<ide> };
<ide> ReactEventEmitter.putListener(CHILD.id, ON_CLICK_KEY, handleChildClick);
<ide> describe('ReactEventEmitter', function() {
<ide>
<ide> it('should not invoke newly inserted handlers while bubbling', function() {
<ide> var handleParentClick = mocks.getMockFunction();
<del> var handleChildClick = function(abstractEvent) {
<add> var handleChildClick = function(event) {
<ide> ReactEventEmitter.putListener(PARENT.id, ON_CLICK_KEY, handleParentClick);
<ide> };
<ide> ReactEventEmitter.putListener(CHILD.id, ON_CLICK_KEY, handleChildClick);
<ide><path>src/event/AbstractEvent.js
<del>/**
<del> * Copyright 2013 Facebook, Inc.
<del> *
<del> * Licensed under the Apache License, Version 2.0 (the "License");
<del> * you may not use this file except in compliance with the License.
<del> * You may obtain a copy of the License at
<del> *
<del> * http://www.apache.org/licenses/LICENSE-2.0
<del> *
<del> * Unless required by applicable law or agreed to in writing, software
<del> * distributed under the License is distributed on an "AS IS" BASIS,
<del> * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
<del> * See the License for the specific language governing permissions and
<del> * limitations under the License.
<del> *
<del> * @providesModule AbstractEvent
<del> */
<del>
<del>"use strict";
<del>
<del>var BrowserEnv = require('BrowserEnv');
<del>var PooledClass = require('PooledClass');
<del>var TouchEventUtils = require('TouchEventUtils');
<del>
<del>var emptyFunction = require('emptyFunction');
<del>
<del>var MAX_POOL_SIZE = 20;
<del>
<del>/**
<del> * AbstractEvent copy constructor. @see `PooledClass`. Provides a single place
<del> * to define all cross browser normalization of DOM events. Does not attempt to
<del> * extend a native event, rather creates a completely new object that has a
<del> * reference to the nativeEvent through .nativeEvent member. The property .data
<del> * should hold all data that is extracted from the event in a cross browser
<del> * manner. Application code should use the data field when possible, not the
<del> * unreliable native event.
<del> */
<del>function AbstractEvent(
<del> reactEventType,
<del> reactTargetID, // Allows the abstract target to differ from native.
<del> nativeEvent,
<del> data) {
<del> this.reactEventType = reactEventType;
<del> this.reactTargetID = reactTargetID || '';
<del> this.nativeEvent = nativeEvent;
<del> this.data = data;
<del> // TODO: Deprecate storing target - doesn't always make sense for some types
<del> this.target = nativeEvent && nativeEvent.target;
<del>
<del> /**
<del> * As a performance optimization, we tag the existing event with the listeners
<del> * (or listener [singular] if only one). This avoids having to package up an
<del> * abstract event along with the set of listeners into a wrapping "dispatch"
<del> * object. No one should ever read this property except event system and
<del> * plugin/dispatcher code. We also tag the abstract event with a parallel
<del> * ID array. _dispatchListeners[i] is being dispatched to a DOM node at ID
<del> * _dispatchIDs[i]. The lengths should never, ever, ever be different.
<del> */
<del> this._dispatchListeners = null;
<del> this._dispatchIDs = null;
<del>
<del> this.isPropagationStopped = false;
<del> this.isPersistent = emptyFunction.thatReturnsFalse;
<del>}
<del>
<del>/** `PooledClass` looks for this. */
<del>AbstractEvent.poolSize = MAX_POOL_SIZE;
<del>
<del>/**
<del> * `PooledClass` looks for `destructor` on each instance it releases. We need to
<del> * ensure that we remove all references to listeners which could trap large
<del> * amounts of memory in their closures.
<del> */
<del>AbstractEvent.prototype.destructor = function() {
<del> this.target = null;
<del> this._dispatchListeners = null;
<del> this._dispatchIDs = null;
<del>};
<del>
<del>/**
<del> * Enhance the `AbstractEvent` class to have pooling abilities. We instruct
<del> * `PooledClass` that our copy constructor accepts five arguments (this is just
<del> * a performance optimization). These objects are instantiated frequently.
<del> */
<del>PooledClass.addPoolingTo(AbstractEvent, PooledClass.fiveArgumentPooler);
<del>
<del>AbstractEvent.prototype.stopPropagation = function() {
<del> this.isPropagationStopped = true;
<del> if (this.nativeEvent.stopPropagation) {
<del> this.nativeEvent.stopPropagation();
<del> }
<del> // IE8 only understands cancelBubble, not stopPropagation().
<del> this.nativeEvent.cancelBubble = true;
<del>};
<del>
<del>AbstractEvent.prototype.preventDefault = function() {
<del> AbstractEvent.preventDefaultOnNativeEvent(this.nativeEvent);
<del>};
<del>
<del>/**
<del> * We clear out all dispatched `AbstractEvent`s after each event loop, adding
<del> * them back into the pool. This allows a way to hold onto a reference that
<del> * won't be added back into the pool.
<del> */
<del>AbstractEvent.prototype.persist = function() {
<del> this.isPersistent = emptyFunction.thatReturnsTrue;
<del>};
<del>
<del>/**
<del> * Utility function for preventing default in cross browser manner.
<del> */
<del>AbstractEvent.preventDefaultOnNativeEvent = function(nativeEvent) {
<del> if (nativeEvent.preventDefault) {
<del> nativeEvent.preventDefault();
<del> } else {
<del> nativeEvent.returnValue = false;
<del> }
<del>};
<del>
<del>/**
<del> * @param {Element} target The target element.
<del> */
<del>AbstractEvent.normalizeScrollDataFromTarget = function(target) {
<del> return {
<del> scrollTop: target.scrollTop,
<del> scrollLeft: target.scrollLeft,
<del> clientWidth: target.clientWidth,
<del> clientHeight: target.clientHeight,
<del> scrollHeight: target.scrollHeight,
<del> scrollWidth: target.scrollWidth
<del> };
<del>};
<del>
<del>/*
<del> * There are some normalizations that need to happen for various browsers. In
<del> * addition to replacing the general event fixing with a framework such as
<del> * jquery, we need to normalize mouse events here. Code below is mostly borrowed
<del> * from: jScrollPane/script/jquery.mousewheel.js
<del> */
<del>AbstractEvent.normalizeMouseWheelData = function(nativeEvent) {
<del> var delta = 0;
<del> var deltaX = 0;
<del> var deltaY = 0;
<del>
<del> /* traditional scroll wheel data */
<del> if ( nativeEvent.wheelDelta ) { delta = nativeEvent.wheelDelta/120; }
<del> if ( nativeEvent.detail ) { delta = -nativeEvent.detail/3; }
<del>
<del> /* Multidimensional scroll (touchpads) with deltas */
<del> deltaY = delta;
<del>
<del> /* Gecko based browsers */
<del> if (nativeEvent.axis !== undefined &&
<del> nativeEvent.axis === nativeEvent.HORIZONTAL_AXIS ) {
<del> deltaY = 0;
<del> deltaX = -delta;
<del> }
<del>
<del> /* Webkit based browsers */
<del> if (nativeEvent.wheelDeltaY !== undefined ) {
<del> deltaY = nativeEvent.wheelDeltaY/120;
<del> }
<del> if (nativeEvent.wheelDeltaX !== undefined ) {
<del> deltaX = -nativeEvent.wheelDeltaX/120;
<del> }
<del>
<del> return { delta: delta, deltaX: deltaX, deltaY: deltaY };
<del>};
<del>
<del>/**
<del> * I <3 Quirksmode.org:
<del> * http://www.quirksmode.org/js/events_properties.html
<del> */
<del>AbstractEvent.isNativeClickEventRightClick = function(nativeEvent) {
<del> return nativeEvent.which ? nativeEvent.which === 3 :
<del> nativeEvent.button ? nativeEvent.button === 2 :
<del> false;
<del>};
<del>
<del>AbstractEvent.normalizePointerData = function(nativeEvent) {
<del> return {
<del> globalX: AbstractEvent.eventPageX(nativeEvent),
<del> globalY: AbstractEvent.eventPageY(nativeEvent),
<del> rightMouseButton:
<del> AbstractEvent.isNativeClickEventRightClick(nativeEvent)
<del> };
<del>};
<del>
<del>AbstractEvent.normalizeDragEventData =
<del> function(nativeEvent, globalX, globalY, startX, startY) {
<del> return {
<del> globalX: globalX,
<del> globalY: globalY,
<del> startX: startX,
<del> startY: startY
<del> };
<del> };
<del>
<del>/**
<del> * Warning: It is possible to move your finger on a touch surface, yet not
<del> * effect the `eventPageX/Y` because the touch had caused a scroll that
<del> * compensated for your movement. To track movements across the page, prevent
<del> * default to avoid scrolling, and control scrolling in javascript.
<del> */
<del>
<del>/**
<del> * Gets the exact position of a touch/mouse event on the page with respect to
<del> * the document body. The only reason why this method is needed instead of using
<del> * `TouchEventUtils.extractSingleTouch` is to support IE8-. Mouse events in all
<del> * browsers except IE8- contain a pageY. IE8 and below require clientY
<del> * computation:
<del> *
<del> * @param {Event} nativeEvent Native event, possibly touch or mouse.
<del> * @return {number} Coordinate with respect to document body.
<del> */
<del>AbstractEvent.eventPageY = function(nativeEvent) {
<del> var singleTouch = TouchEventUtils.extractSingleTouch(nativeEvent);
<del> if (singleTouch) {
<del> return singleTouch.pageY;
<del> } else if (typeof nativeEvent.pageY !== 'undefined') {
<del> return nativeEvent.pageY;
<del> } else {
<del> return nativeEvent.clientY + BrowserEnv.currentPageScrollTop;
<del> }
<del>};
<del>
<del>/**
<del> * @see `AbstractEvent.eventPageY`.
<del> *
<del> * @param {Event} nativeEvent Native event, possibly touch or mouse.
<del> * @return {number} Coordinate with respect to document body.
<del> */
<del>AbstractEvent.eventPageX = function(nativeEvent) {
<del> var singleTouch = TouchEventUtils.extractSingleTouch(nativeEvent);
<del> if (singleTouch) {
<del> return singleTouch.pageX;
<del> } else if (typeof nativeEvent.pageX !== 'undefined') {
<del> return nativeEvent.pageX;
<del> } else {
<del> return nativeEvent.clientX + BrowserEnv.currentPageScrollLeft;
<del> }
<del>};
<del>
<del>/**
<del> * @deprecated
<del> */
<del>AbstractEvent.persistentCloneOf = function(abstractEvent) {
<del> abstractEvent.persist();
<del> return abstractEvent;
<del>};
<del>
<del>module.exports = AbstractEvent;
<del>
<ide><path>src/event/EventPluginHub.js
<ide> var executeDispatchesAndRelease = function(event) {
<ide> * Required. When a top-level event is fired, this method is expected to
<ide> * extract synthetic events that will in turn be queued and dispatched.
<ide> *
<del> * `abstractEventTypes` {object}
<add> * `eventTypes` {object}
<ide> * Optional, plugins that fire events must publish a mapping of registration
<ide> * names that are used to register listeners. Values of this mapping must
<ide> * be objects that contain `registrationName` or `phasedRegistrationNames`.
<ide><path>src/event/EventPluginRegistry.js
<ide> function recomputePluginOrdering() {
<ide> pluginName
<ide> );
<ide> EventPluginRegistry.plugins[pluginIndex] = PluginModule;
<del> var publishedEvents = PluginModule.abstractEventTypes;
<add> var publishedEvents = PluginModule.eventTypes;
<ide> for (var eventName in publishedEvents) {
<ide> invariant(
<ide> publishEventForPlugin(publishedEvents[eventName], PluginModule),
<ide> var EventPluginRegistry = {
<ide> * @internal
<ide> */
<ide> getPluginModuleForEvent: function(event) {
<del> var dispatchConfig = event.reactEventType;
<add> var dispatchConfig = event.dispatchConfig;
<ide> if (dispatchConfig.registrationName) {
<ide> return EventPluginRegistry.registrationNames[
<ide> dispatchConfig.registrationName
<ide><path>src/event/EventPluginUtils.js
<ide> "use strict";
<ide>
<ide> var EventConstants = require('EventConstants');
<del>var AbstractEvent = require('AbstractEvent');
<ide>
<ide> var invariant = require('invariant');
<ide>
<ide> function isStartish(topLevelType) {
<ide> topLevelType === topLevelTypes.topTouchStart;
<ide> }
<ide>
<del>function storePageCoordsIn(obj, nativeEvent) {
<del> var pageX = AbstractEvent.eventPageX(nativeEvent);
<del> var pageY = AbstractEvent.eventPageY(nativeEvent);
<del> obj.pageX = pageX;
<del> obj.pageY = pageY;
<del>}
<del>
<del>function eventDistance(coords, nativeEvent) {
<del> var pageX = AbstractEvent.eventPageX(nativeEvent);
<del> var pageY = AbstractEvent.eventPageY(nativeEvent);
<del> return Math.pow(
<del> Math.pow(pageX - coords.pageX, 2) + Math.pow(pageY - coords.pageY, 2),
<del> 0.5
<del> );
<del>}
<del>
<ide> var validateEventDispatches;
<ide> if (__DEV__) {
<del> validateEventDispatches = function(abstractEvent) {
<del> var dispatchListeners = abstractEvent._dispatchListeners;
<del> var dispatchIDs = abstractEvent._dispatchIDs;
<add> validateEventDispatches = function(event) {
<add> var dispatchListeners = event._dispatchListeners;
<add> var dispatchIDs = event._dispatchIDs;
<ide>
<ide> var listenersIsArr = Array.isArray(dispatchListeners);
<ide> var idsIsArr = Array.isArray(dispatchIDs);
<ide> if (__DEV__) {
<ide>
<ide> invariant(
<ide> idsIsArr === listenersIsArr && IDsLen === listenersLen,
<del> 'EventPluginUtils: Invalid `abstractEvent`.'
<add> 'EventPluginUtils: Invalid `event`.'
<ide> );
<ide> };
<ide> }
<ide>
<ide> /**
<del> * Invokes `cb(abstractEvent, listener, id)`. Avoids using call if no scope is
<add> * Invokes `cb(event, listener, id)`. Avoids using call if no scope is
<ide> * provided. The `(listener,id)` pair effectively forms the "dispatch" but are
<ide> * kept separate to conserve memory.
<ide> */
<del>function forEachEventDispatch(abstractEvent, cb) {
<del> var dispatchListeners = abstractEvent._dispatchListeners;
<del> var dispatchIDs = abstractEvent._dispatchIDs;
<add>function forEachEventDispatch(event, cb) {
<add> var dispatchListeners = event._dispatchListeners;
<add> var dispatchIDs = event._dispatchIDs;
<ide> if (__DEV__) {
<del> validateEventDispatches(abstractEvent);
<add> validateEventDispatches(event);
<ide> }
<ide> if (Array.isArray(dispatchListeners)) {
<del> var i;
<del> for (
<del> i = 0;
<del> i < dispatchListeners.length && !abstractEvent.isPropagationStopped;
<del> i++) {
<add> for (var i = 0; i < dispatchListeners.length; i++) {
<add> if (event.isPropagationStopped()) {
<add> break;
<add> }
<ide> // Listeners and IDs are two parallel arrays that are always in sync.
<del> cb(abstractEvent, dispatchListeners[i], dispatchIDs[i]);
<add> cb(event, dispatchListeners[i], dispatchIDs[i]);
<ide> }
<ide> } else if (dispatchListeners) {
<del> cb(abstractEvent, dispatchListeners, dispatchIDs);
<add> cb(event, dispatchListeners, dispatchIDs);
<ide> }
<ide> }
<ide>
<ide> /**
<ide> * Default implementation of PluginModule.executeDispatch().
<del> * @param {AbstractEvent} AbstractEvent to handle
<add> * @param {SyntheticEvent} SyntheticEvent to handle
<ide> * @param {function} Application-level callback
<ide> * @param {string} domID DOM id to pass to the callback.
<ide> */
<del>function executeDispatch(abstractEvent, listener, domID) {
<del> listener(abstractEvent, domID);
<add>function executeDispatch(event, listener, domID) {
<add> listener(event, domID);
<ide> }
<ide>
<ide> /**
<ide> * Standard/simple iteration through an event's collected dispatches.
<ide> */
<del>function executeDispatchesInOrder(abstractEvent, executeDispatch) {
<del> forEachEventDispatch(abstractEvent, executeDispatch);
<del> abstractEvent._dispatchListeners = null;
<del> abstractEvent._dispatchIDs = null;
<add>function executeDispatchesInOrder(event, executeDispatch) {
<add> forEachEventDispatch(event, executeDispatch);
<add> event._dispatchListeners = null;
<add> event._dispatchIDs = null;
<ide> }
<ide>
<ide> /**
<ide> function executeDispatchesInOrder(abstractEvent, executeDispatch) {
<ide> * @returns id of the first dispatch execution who's listener returns true, or
<ide> * null if no listener returned true.
<ide> */
<del>function executeDispatchesInOrderStopAtTrue(abstractEvent) {
<del> var dispatchListeners = abstractEvent._dispatchListeners;
<del> var dispatchIDs = abstractEvent._dispatchIDs;
<add>function executeDispatchesInOrderStopAtTrue(event) {
<add> var dispatchListeners = event._dispatchListeners;
<add> var dispatchIDs = event._dispatchIDs;
<ide> if (__DEV__) {
<del> validateEventDispatches(abstractEvent);
<add> validateEventDispatches(event);
<ide> }
<ide> if (Array.isArray(dispatchListeners)) {
<del> var i;
<del> for (
<del> i = 0;
<del> i < dispatchListeners.length && !abstractEvent.isPropagationStopped;
<del> i++) {
<add> for (var i = 0; i < dispatchListeners.length; i++) {
<add> if (event.isPropagationStopped()) {
<add> break;
<add> }
<ide> // Listeners and IDs are two parallel arrays that are always in sync.
<del> if (dispatchListeners[i](abstractEvent, dispatchIDs[i])) {
<add> if (dispatchListeners[i](event, dispatchIDs[i])) {
<ide> return dispatchIDs[i];
<ide> }
<ide> }
<ide> } else if (dispatchListeners) {
<del> if (dispatchListeners(abstractEvent, dispatchIDs)) {
<add> if (dispatchListeners(event, dispatchIDs)) {
<ide> return dispatchIDs;
<ide> }
<ide> }
<ide> function executeDispatchesInOrderStopAtTrue(abstractEvent) {
<ide> *
<ide> * @returns The return value of executing the single dispatch.
<ide> */
<del>function executeDirectDispatch(abstractEvent) {
<add>function executeDirectDispatch(event) {
<ide> if (__DEV__) {
<del> validateEventDispatches(abstractEvent);
<add> validateEventDispatches(event);
<ide> }
<del> var dispatchListener = abstractEvent._dispatchListeners;
<del> var dispatchID = abstractEvent._dispatchIDs;
<add> var dispatchListener = event._dispatchListeners;
<add> var dispatchID = event._dispatchIDs;
<ide> invariant(
<ide> !Array.isArray(dispatchListener),
<del> 'executeDirectDispatch(...): Invalid `abstractEvent`.'
<add> 'executeDirectDispatch(...): Invalid `event`.'
<ide> );
<ide> var res = dispatchListener ?
<del> dispatchListener(abstractEvent, dispatchID) :
<add> dispatchListener(event, dispatchID) :
<ide> null;
<del> abstractEvent._dispatchListeners = null;
<del> abstractEvent._dispatchIDs = null;
<add> event._dispatchListeners = null;
<add> event._dispatchIDs = null;
<ide> return res;
<ide> }
<ide>
<ide> /**
<del> * @param {AbstractEvent} abstractEvent
<add> * @param {SyntheticEvent} event
<ide> * @returns {bool} True iff number of dispatches accumulated is greater than 0.
<ide> */
<del>function hasDispatches(abstractEvent) {
<del> return !!abstractEvent._dispatchListeners;
<add>function hasDispatches(event) {
<add> return !!event._dispatchListeners;
<ide> }
<ide>
<ide> /**
<ide> var EventPluginUtils = {
<ide> isEndish: isEndish,
<ide> isMoveish: isMoveish,
<ide> isStartish: isStartish,
<del> storePageCoordsIn: storePageCoordsIn,
<del> eventDistance: eventDistance,
<ide> executeDispatchesInOrder: executeDispatchesInOrder,
<ide> executeDispatchesInOrderStopAtTrue: executeDispatchesInOrderStopAtTrue,
<ide> executeDirectDispatch: executeDirectDispatch,
<ide><path>src/event/EventPropagators.js
<ide> var injection = {
<ide> * Some event types have a notion of different registration names for different
<ide> * "phases" of propagation. This finds listeners by a given phase.
<ide> */
<del>function listenerAtPhase(id, abstractEvent, propagationPhase) {
<add>function listenerAtPhase(id, event, propagationPhase) {
<ide> var registrationName =
<del> abstractEvent.reactEventType.phasedRegistrationNames[propagationPhase];
<add> event.dispatchConfig.phasedRegistrationNames[propagationPhase];
<ide> return getListener(id, registrationName);
<ide> }
<ide>
<ide> /**
<del> * Tags an `AbstractEvent` with dispatched listeners. Creating this function
<add> * Tags a `SyntheticEvent` with dispatched listeners. Creating this function
<ide> * here, allows us to not have to bind or create functions for each event.
<ide> * Mutating the event's members allows us to not have to create a wrapping
<ide> * "dispatch" object that pairs the event with the listener.
<ide> */
<del>function accumulateDirectionalDispatches(domID, upwards, abstractEvent) {
<add>function accumulateDirectionalDispatches(domID, upwards, event) {
<ide> if (__DEV__) {
<ide> if (!domID) {
<ide> throw new Error('Dispatching id must not be null');
<ide> }
<ide> injection.validate();
<ide> }
<ide> var phase = upwards ? PropagationPhases.bubbled : PropagationPhases.captured;
<del> var listener = listenerAtPhase(domID, abstractEvent, phase);
<add> var listener = listenerAtPhase(domID, event, phase);
<ide> if (listener) {
<del> abstractEvent._dispatchListeners =
<del> accumulate(abstractEvent._dispatchListeners, listener);
<del> abstractEvent._dispatchIDs = accumulate(abstractEvent._dispatchIDs, domID);
<add> event._dispatchListeners = accumulate(event._dispatchListeners, listener);
<add> event._dispatchIDs = accumulate(event._dispatchIDs, domID);
<ide> }
<ide> }
<ide>
<ide> function accumulateDirectionalDispatches(domID, upwards, abstractEvent) {
<ide> * single traversal for the entire collection of events because each event may
<ide> * have a different target.
<ide> */
<del>function accumulateTwoPhaseDispatchesSingle(abstractEvent) {
<del> if (abstractEvent && abstractEvent.reactEventType.phasedRegistrationNames) {
<add>function accumulateTwoPhaseDispatchesSingle(event) {
<add> if (event && event.dispatchConfig.phasedRegistrationNames) {
<ide> injection.InstanceHandle.traverseTwoPhase(
<del> abstractEvent.reactTargetID,
<add> event.dispatchMarker,
<ide> accumulateDirectionalDispatches,
<del> abstractEvent
<add> event
<ide> );
<ide> }
<ide> }
<ide> function accumulateTwoPhaseDispatchesSingle(abstractEvent) {
<ide> /**
<ide> * Accumulates without regard to direction, does not look for phased
<ide> * registration names. Same as `accumulateDirectDispatchesSingle` but without
<del> * requiring that the `reactTargetID` be the same as the dispatched ID.
<add> * requiring that the `dispatchMarker` be the same as the dispatched ID.
<ide> */
<del>function accumulateDispatches(id, ignoredDirection, abstractEvent) {
<del> if (abstractEvent && abstractEvent.reactEventType.registrationName) {
<del> var registrationName = abstractEvent.reactEventType.registrationName;
<add>function accumulateDispatches(id, ignoredDirection, event) {
<add> if (event && event.dispatchConfig.registrationName) {
<add> var registrationName = event.dispatchConfig.registrationName;
<ide> var listener = getListener(id, registrationName);
<ide> if (listener) {
<del> abstractEvent._dispatchListeners =
<del> accumulate(abstractEvent._dispatchListeners, listener);
<del> abstractEvent._dispatchIDs = accumulate(abstractEvent._dispatchIDs, id);
<add> event._dispatchListeners = accumulate(event._dispatchListeners, listener);
<add> event._dispatchIDs = accumulate(event._dispatchIDs, id);
<ide> }
<ide> }
<ide> }
<ide>
<ide> /**
<del> * Accumulates dispatches on an `AbstractEvent`, but only for the
<del> * `reactTargetID`.
<del> * @param {AbstractEvent} abstractEvent
<add> * Accumulates dispatches on an `SyntheticEvent`, but only for the
<add> * `dispatchMarker`.
<add> * @param {SyntheticEvent} event
<ide> */
<del>function accumulateDirectDispatchesSingle(abstractEvent) {
<del> if (abstractEvent && abstractEvent.reactEventType.registrationName) {
<del> accumulateDispatches(abstractEvent.reactTargetID, null, abstractEvent);
<add>function accumulateDirectDispatchesSingle(event) {
<add> if (event && event.dispatchConfig.registrationName) {
<add> accumulateDispatches(event.dispatchMarker, null, event);
<ide> }
<ide> }
<ide>
<del>function accumulateTwoPhaseDispatches(abstractEvents) {
<add>function accumulateTwoPhaseDispatches(events) {
<ide> if (__DEV__) {
<ide> injection.validate();
<ide> }
<del> forEachAccumulated(abstractEvents, accumulateTwoPhaseDispatchesSingle);
<add> forEachAccumulated(events, accumulateTwoPhaseDispatchesSingle);
<ide> }
<ide>
<ide> function accumulateEnterLeaveDispatches(leave, enter, fromID, toID) {
<ide> function accumulateEnterLeaveDispatches(leave, enter, fromID, toID) {
<ide> }
<ide>
<ide>
<del>function accumulateDirectDispatches(abstractEvents) {
<add>function accumulateDirectDispatches(events) {
<ide> if (__DEV__) {
<ide> injection.validate();
<ide> }
<del> forEachAccumulated(abstractEvents, accumulateDirectDispatchesSingle);
<add> forEachAccumulated(events, accumulateDirectDispatchesSingle);
<ide> }
<ide>
<ide>
<ide><path>src/event/__tests__/EventPluginRegistry-test.js
<ide> describe('EventPluginRegistry', function() {
<ide>
<ide> it('should publish registration names of injected plugins', function() {
<ide> var OnePlugin = createPlugin({
<del> abstractEventTypes: {
<add> eventTypes: {
<ide> click: {registrationName: 'onClick'},
<ide> focus: {registrationName: 'onFocus'}
<ide> }
<ide> });
<ide> var TwoPlugin = createPlugin({
<del> abstractEventTypes: {
<add> eventTypes: {
<ide> magic: {
<ide> phasedRegistrationNames: {
<ide> bubbled: 'onMagicBubble',
<ide> describe('EventPluginRegistry', function() {
<ide>
<ide> it('should throw if multiple registration names collide', function() {
<ide> var OnePlugin = createPlugin({
<del> abstractEventTypes: {
<add> eventTypes: {
<ide> photoCapture: {registrationName: 'onPhotoCapture'}
<ide> }
<ide> });
<ide> var TwoPlugin = createPlugin({
<del> abstractEventTypes: {
<add> eventTypes: {
<ide> photo: {
<ide> phasedRegistrationNames: {
<ide> bubbled: 'onPhotoBubble',
<ide> describe('EventPluginRegistry', function() {
<ide>
<ide> it('should throw if an invalid event is published', function() {
<ide> var OnePlugin = createPlugin({
<del> abstractEventTypes: {
<add> eventTypes: {
<ide> badEvent: {/* missing configuration */}
<ide> }
<ide> });
<ide> describe('EventPluginRegistry', function() {
<ide> };
<ide>
<ide> var OnePlugin = createPlugin({
<del> abstractEventTypes: {
<add> eventTypes: {
<ide> click: clickDispatchConfig,
<ide> magic: magicDispatchConfig
<ide> }
<ide> });
<ide>
<del> var clickEvent = {reactEventType: clickDispatchConfig};
<del> var magicEvent = {reactEventType: magicDispatchConfig};
<add> var clickEvent = {dispatchConfig: clickDispatchConfig};
<add> var magicEvent = {dispatchConfig: magicDispatchConfig};
<ide>
<ide> expect(EventPluginRegistry.getPluginModuleForEvent(clickEvent)).toBe(null);
<ide> expect(EventPluginRegistry.getPluginModuleForEvent(magicEvent)).toBe(null);
<ide><path>src/eventPlugins/AnalyticsEventPluginFactory.js
<ide> if (__DEV__) {
<ide> }
<ide>
<ide> /**
<del> * This plugin does not really extract any abstract events. Rather it just looks
<del> * at the top level event and bumps up counters as appropriate
<add> * This plugin does not really extract any synthetic events. Rather it just
<add> * looks at the top-level event and bumps up counters as appropriate
<ide> *
<ide> * @param {string} topLevelType Record from `EventConstants`.
<ide> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<ide> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<ide> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of `AbstractEvent`s.
<add> * @return {*} An accumulation of synthetic events.
<ide> * @see {EventPluginHub.extractEvents}
<ide> */
<ide> function extractEvents(
<ide><path>src/eventPlugins/EnterLeaveEventPlugin.js
<ide>
<ide> "use strict";
<ide>
<add>var EventConstants = require('EventConstants');
<ide> var EventPropagators = require('EventPropagators');
<ide> var ExecutionEnvironment = require('ExecutionEnvironment');
<del>var AbstractEvent = require('AbstractEvent');
<del>var EventConstants = require('EventConstants');
<ide> var ReactInstanceHandles = require('ReactInstanceHandles');
<add>var SyntheticMouseEvent = require('SyntheticMouseEvent');
<ide>
<ide> var getDOMNodeID = require('getDOMNodeID');
<ide> var keyOf = require('keyOf');
<ide>
<ide> var topLevelTypes = EventConstants.topLevelTypes;
<ide> var getFirstReactDOM = ReactInstanceHandles.getFirstReactDOM;
<ide>
<del>var abstractEventTypes = {
<add>var eventTypes = {
<ide> mouseEnter: {registrationName: keyOf({onMouseEnter: null})},
<ide> mouseLeave: {registrationName: keyOf({onMouseLeave: null})}
<ide> };
<ide>
<del>/**
<del> * For almost every interaction we care about, there will be a top-level
<del> * `mouseover` and `mouseout` event that occurs so only pay attention to one of
<del> * the two (to avoid duplicate events). We use the `mouseout` event.
<del> *
<del> * However, there's one interaction where there will be no `mouseout` event to
<del> * rely on - mousing from outside the browser *into* the chrome. We detect this
<del> * scenario and only in that case, we use the `mouseover` event.
<del> *
<del> * @param {string} topLevelType Record from `EventConstants`.
<del> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<del> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<del> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of `AbstractEvent`s.
<del> * @see {EventPluginHub.extractEvents}
<del> */
<del>var extractEvents = function(
<del> topLevelType,
<del> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent) {
<del> if (topLevelType === topLevelTypes.topMouseOver &&
<del> (nativeEvent.relatedTarget || nativeEvent.fromElement)) {
<del> return null;
<del> }
<del> if (topLevelType !== topLevelTypes.topMouseOut &&
<del> topLevelType !== topLevelTypes.topMouseOver) {
<del> return null; // Must not be a mouse in or mouse out - ignoring.
<del> }
<add>var EnterLeaveEventPlugin = {
<ide>
<del> var to, from;
<del> if (topLevelType === topLevelTypes.topMouseOut) {
<del> to = getFirstReactDOM(nativeEvent.relatedTarget || nativeEvent.toElement) ||
<del> ExecutionEnvironment.global;
<del> from = topLevelTarget;
<del> } else {
<del> to = topLevelTarget;
<del> from = ExecutionEnvironment.global;
<del> }
<add> eventTypes: eventTypes,
<ide>
<del> // Nothing pertains to our managed components.
<del> if (from === to) {
<del> return null;
<del> }
<add> /**
<add> * For almost every interaction we care about, there will be both a top-level
<add> * `mouseover` and `mouseout` event that occurs. Only use `mouseout` so that
<add> * we do not extract duplicate events. However, moving the mouse into the
<add> * browser from outside will not fire a `mouseout` event. In this case, we use
<add> * the `mouseover` top-level event.
<add> *
<add> * @param {string} topLevelType Record from `EventConstants`.
<add> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<add> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<add> * @param {object} nativeEvent Native browser event.
<add> * @return {*} An accumulation of synthetic events.
<add> * @see {EventPluginHub.extractEvents}
<add> */
<add> extractEvents: function(
<add> topLevelType,
<add> topLevelTarget,
<add> topLevelTargetID,
<add> nativeEvent) {
<add> if (topLevelType === topLevelTypes.topMouseOver &&
<add> (nativeEvent.relatedTarget || nativeEvent.fromElement)) {
<add> return null;
<add> }
<add> if (topLevelType !== topLevelTypes.topMouseOut &&
<add> topLevelType !== topLevelTypes.topMouseOver) {
<add> // Must not be a mouse in or mouse out - ignoring.
<add> return null;
<add> }
<ide>
<del> var fromID = from ? getDOMNodeID(from) : '';
<del> var toID = to ? getDOMNodeID(to) : '';
<add> var from, to;
<add> if (topLevelType === topLevelTypes.topMouseOut) {
<add> from = topLevelTarget;
<add> to = getFirstReactDOM(nativeEvent.relatedTarget || nativeEvent.toElement)
<add> || ExecutionEnvironment.global;
<add> } else {
<add> from = ExecutionEnvironment.global;
<add> to = topLevelTarget;
<add> }
<ide>
<del> var leave = AbstractEvent.getPooled(
<del> abstractEventTypes.mouseLeave,
<del> fromID,
<del> nativeEvent
<del> );
<del> var enter = AbstractEvent.getPooled(
<del> abstractEventTypes.mouseEnter,
<del> toID,
<del> nativeEvent
<del> );
<add> if (from === to) {
<add> // Nothing pertains to our managed components.
<add> return null;
<add> }
<ide>
<del> EventPropagators.accumulateEnterLeaveDispatches(leave, enter, fromID, toID);
<add> var fromID = from ? getDOMNodeID(from) : '';
<add> var toID = to ? getDOMNodeID(to) : '';
<ide>
<del> return [leave, enter];
<del>};
<add> var leave = SyntheticMouseEvent.getPooled(
<add> eventTypes.mouseLeave,
<add> fromID,
<add> nativeEvent
<add> );
<add> var enter = SyntheticMouseEvent.getPooled(
<add> eventTypes.mouseEnter,
<add> toID,
<add> nativeEvent
<add> );
<add>
<add> EventPropagators.accumulateEnterLeaveDispatches(leave, enter, fromID, toID);
<add> return [leave, enter];
<add> }
<ide>
<del>var EnterLeaveEventPlugin = {
<del> abstractEventTypes: abstractEventTypes,
<del> extractEvents: extractEvents
<ide> };
<ide>
<ide> module.exports = EnterLeaveEventPlugin;
<ide><path>src/eventPlugins/ResponderEventPlugin.js
<ide>
<ide> "use strict";
<ide>
<del>var AbstractEvent = require('AbstractEvent');
<ide> var EventConstants = require('EventConstants');
<ide> var EventPluginUtils = require('EventPluginUtils');
<ide> var EventPropagators = require('EventPropagators');
<add>var SyntheticEvent = require('SyntheticEvent');
<ide>
<ide> var accumulate = require('accumulate');
<ide> var keyOf = require('keyOf');
<ide> var executeDispatchesInOrderStopAtTrue =
<ide> var responderID = null;
<ide> var isPressing = false;
<ide>
<del>var getResponderID = function() {
<del> return responderID;
<del>};
<del>
<del>var abstractEventTypes = {
<add>var eventTypes = {
<ide> /**
<ide> * On a `touchStart`/`mouseDown`, is it desired that this element become the
<ide> * responder?
<ide> var abstractEventTypes = {
<ide> * `extractEvents()`.
<ide> * - These events that are returned from `extractEvents` are "deferred
<ide> * dispatched events".
<del> * - When returned from `extractEvents`, deferred dispatched events
<del> * contain an "accumulation" of deferred dispatches.
<del> * -- These deferred dispatches are accumulated/collected before they are
<del> * returned, but processed at a later time by the `EventPluginHub` (hence the
<del> * name deferred).
<add> * - When returned from `extractEvents`, deferred-dispatched events contain an
<add> * "accumulation" of deferred dispatches.
<add> * - These deferred dispatches are accumulated/collected before they are
<add> * returned, but processed at a later time by the `EventPluginHub` (hence the
<add> * name deferred).
<ide> *
<del> * In the process of returning their deferred dispatched events, event plugins
<add> * In the process of returning their deferred-dispatched events, event plugins
<ide> * themselves can dispatch events on-demand without returning them from
<del> * `extractEvents`. Plugins might want to do this, so that they can use
<del> * event dispatching as a tool that helps them decide which events should be
<del> * extracted in the first place.
<add> * `extractEvents`. Plugins might want to do this, so that they can use event
<add> * dispatching as a tool that helps them decide which events should be extracted
<add> * in the first place.
<ide> *
<ide> * "On-Demand-Dispatched Events":
<ide> *
<del> * - On-demand dispatched are not returned from `extractEvents`.
<del> * - On-demand dispatched events are dispatched during the process of returning
<add> * - On-demand-dispatched events are not returned from `extractEvents`.
<add> * - On-demand-dispatched events are dispatched during the process of returning
<ide> * the deferred-dispatched events.
<ide> * - They should not have side effects.
<ide> * - They should be avoided, and/or eventually be replaced with another
<ide> var abstractEventTypes = {
<ide> * - `touchStart` (`EventPluginHub` dispatches as usual)
<ide> * - `responderGrant/Reject` (`EventPluginHub` dispatches as usual)
<ide> *
<del> * @returns {Accumulation<AbstractEvent>}
<del> */
<del>
<del>/**
<ide> * @param {string} topLevelType Record from `EventConstants`.
<del> * @param {string} renderedTargetID ID of deepest React rendered element.
<add> * @param {string} topLevelTargetID ID of deepest React rendered element.
<ide> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of extracted `AbstractEvent`s.
<add> * @return {*} An accumulation of synthetic events.
<ide> */
<del>var setResponderAndExtractTransfer =
<del> function(topLevelType, renderedTargetID, nativeEvent) {
<del> var type;
<del> var shouldSetEventType =
<del> isStartish(topLevelType) ? abstractEventTypes.startShouldSetResponder :
<del> isMoveish(topLevelType) ? abstractEventTypes.moveShouldSetResponder :
<del> abstractEventTypes.scrollShouldSetResponder;
<add>function setResponderAndExtractTransfer(
<add> topLevelType,
<add> topLevelTargetID,
<add> nativeEvent) {
<add> var shouldSetEventType =
<add> isStartish(topLevelType) ? eventTypes.startShouldSetResponder :
<add> isMoveish(topLevelType) ? eventTypes.moveShouldSetResponder :
<add> eventTypes.scrollShouldSetResponder;
<ide>
<del> var bubbleShouldSetFrom = responderID || renderedTargetID;
<del> var shouldSetEvent = AbstractEvent.getPooled(
<del> shouldSetEventType,
<del> bubbleShouldSetFrom,
<del> topLevelType,
<del> nativeEvent,
<del> AbstractEvent.normalizePointerData(nativeEvent)
<del> );
<del> EventPropagators.accumulateTwoPhaseDispatches(shouldSetEvent);
<del> var wantsResponderID = executeDispatchesInOrderStopAtTrue(shouldSetEvent);
<del> AbstractEvent.release(shouldSetEvent);
<add> var bubbleShouldSetFrom = responderID || topLevelTargetID;
<add> var shouldSetEvent = SyntheticEvent.getPooled(
<add> shouldSetEventType,
<add> bubbleShouldSetFrom,
<add> nativeEvent
<add> );
<add> EventPropagators.accumulateTwoPhaseDispatches(shouldSetEvent);
<add> var wantsResponderID = executeDispatchesInOrderStopAtTrue(shouldSetEvent);
<add> if (!shouldSetEvent.isPersistent()) {
<add> shouldSetEvent.constructor.release(shouldSetEvent);
<add> }
<ide>
<del> if (!wantsResponderID || wantsResponderID === responderID) {
<del> return null;
<del> }
<del> var extracted;
<del> var grantEvent = AbstractEvent.getPooled(
<del> abstractEventTypes.responderGrant,
<del> wantsResponderID,
<del> topLevelType,
<add> if (!wantsResponderID || wantsResponderID === responderID) {
<add> return null;
<add> }
<add> var extracted;
<add> var grantEvent = SyntheticEvent.getPooled(
<add> eventTypes.responderGrant,
<add> wantsResponderID,
<add> nativeEvent
<add> );
<add>
<add> EventPropagators.accumulateDirectDispatches(grantEvent);
<add> if (responderID) {
<add> var terminationRequestEvent = SyntheticEvent.getPooled(
<add> eventTypes.responderTerminationRequest,
<add> responderID,
<ide> nativeEvent
<ide> );
<add> EventPropagators.accumulateDirectDispatches(terminationRequestEvent);
<add> var shouldSwitch = !hasDispatches(terminationRequestEvent) ||
<add> executeDirectDispatch(terminationRequestEvent);
<add> if (!terminationRequestEvent.isPersistent()) {
<add> terminationRequestEvent.constructor.release(terminationRequestEvent);
<add> }
<ide>
<del> EventPropagators.accumulateDirectDispatches(grantEvent);
<del> if (responderID) {
<del> type = abstractEventTypes.responderTerminationRequest;
<del> var terminationRequestEvent = AbstractEvent.getPooled(type, responderID);
<del> EventPropagators.accumulateDirectDispatches(terminationRequestEvent);
<del> var shouldSwitch = !hasDispatches(terminationRequestEvent) ||
<del> executeDirectDispatch(terminationRequestEvent);
<del> AbstractEvent.release(terminationRequestEvent);
<del> if (shouldSwitch) {
<del> var terminateType = abstractEventTypes.responderTerminate;
<del> var terminateEvent = AbstractEvent.getPooled(
<del> terminateType,
<del> responderID,
<del> topLevelType,
<del> nativeEvent
<del> );
<del> EventPropagators.accumulateDirectDispatches(terminateEvent);
<del> extracted = accumulate(extracted, [grantEvent, terminateEvent]);
<del> responderID = wantsResponderID;
<del> } else {
<del> var rejectEvent = AbstractEvent.getPooled(
<del> abstractEventTypes.responderReject,
<del> wantsResponderID,
<del> topLevelType,
<del> nativeEvent
<del> );
<del> EventPropagators.accumulateDirectDispatches(rejectEvent);
<del> extracted = accumulate(extracted, rejectEvent);
<del> }
<del> } else {
<del> extracted = accumulate(extracted, grantEvent);
<add> if (shouldSwitch) {
<add> var terminateType = eventTypes.responderTerminate;
<add> var terminateEvent = SyntheticEvent.getPooled(
<add> terminateType,
<add> responderID,
<add> nativeEvent
<add> );
<add> EventPropagators.accumulateDirectDispatches(terminateEvent);
<add> extracted = accumulate(extracted, [grantEvent, terminateEvent]);
<ide> responderID = wantsResponderID;
<add> } else {
<add> var rejectEvent = SyntheticEvent.getPooled(
<add> eventTypes.responderReject,
<add> wantsResponderID,
<add> nativeEvent
<add> );
<add> EventPropagators.accumulateDirectDispatches(rejectEvent);
<add> extracted = accumulate(extracted, rejectEvent);
<ide> }
<del> return extracted;
<del> };
<add> } else {
<add> extracted = accumulate(extracted, grantEvent);
<add> responderID = wantsResponderID;
<add> }
<add> return extracted;
<add>}
<ide>
<ide> /**
<ide> * A transfer is a negotiation between a currently set responder and the next
<ide> var setResponderAndExtractTransfer =
<ide> * currently a responder set (in other words as long as the user is pressing
<ide> * down).
<ide> *
<del> * @param {EventConstants.topLevelTypes} topLevelType
<del> * @return {boolean} Whether or not a transfer of responder could possibly
<del> * occur.
<add> * @param {string} topLevelType Record from `EventConstants`.
<add> * @return {boolean} True if a transfer of responder could possibly occur.
<ide> */
<ide> function canTriggerTransfer(topLevelType) {
<ide> return topLevelType === EventConstants.topLevelTypes.topScroll ||
<ide> function canTriggerTransfer(topLevelType) {
<ide> }
<ide>
<ide> /**
<del> * @param {string} topLevelType Record from `EventConstants`.
<del> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<del> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<del> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of `AbstractEvent`s.
<del> * @see {EventPluginHub.extractEvents}
<add> * Event plugin for formalizing the negotiation between claiming locks on
<add> * receiving touches.
<ide> */
<del>var extractEvents = function(
<del> topLevelType,
<del> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent) {
<del> var extracted;
<del> // Must have missed an end event - reset the state here.
<del> if (responderID && isStartish(topLevelType)) {
<del> responderID = null;
<del> }
<del> if (isStartish(topLevelType)) {
<del> isPressing = true;
<del> } else if (isEndish(topLevelType)) {
<del> isPressing = false;
<del> }
<del> if (canTriggerTransfer(topLevelType)) {
<del> var transfer = setResponderAndExtractTransfer(
<add>var ResponderEventPlugin = {
<add>
<add> getResponderID: function() {
<add> return responderID;
<add> },
<add>
<add> eventTypes: eventTypes,
<add>
<add> /**
<add> * @param {string} topLevelType Record from `EventConstants`.
<add> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<add> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<add> * @param {object} nativeEvent Native browser event.
<add> * @return {*} An accumulation of synthetic events.
<add> * @see {EventPluginHub.extractEvents}
<add> */
<add> extractEvents: function(
<ide> topLevelType,
<add> topLevelTarget,
<ide> topLevelTargetID,
<del> nativeEvent
<del> );
<del> if (transfer) {
<del> extracted = accumulate(extracted, transfer);
<add> nativeEvent) {
<add> var extracted;
<add> // Must have missed an end event - reset the state here.
<add> if (responderID && isStartish(topLevelType)) {
<add> responderID = null;
<ide> }
<add> if (isStartish(topLevelType)) {
<add> isPressing = true;
<add> } else if (isEndish(topLevelType)) {
<add> isPressing = false;
<add> }
<add> if (canTriggerTransfer(topLevelType)) {
<add> var transfer = setResponderAndExtractTransfer(
<add> topLevelType,
<add> topLevelTargetID,
<add> nativeEvent
<add> );
<add> if (transfer) {
<add> extracted = accumulate(extracted, transfer);
<add> }
<add> }
<add> // Now that we know the responder is set correctly, we can dispatch
<add> // responder type events (directly to the responder).
<add> var type = isMoveish(topLevelType) ? eventTypes.responderMove :
<add> isEndish(topLevelType) ? eventTypes.responderRelease :
<add> isStartish(topLevelType) ? eventTypes.responderStart : null;
<add> if (type) {
<add> var gesture = SyntheticEvent.getPooled(
<add> type,
<add> responderID || '',
<add> nativeEvent
<add> );
<add> EventPropagators.accumulateDirectDispatches(gesture);
<add> extracted = accumulate(extracted, gesture);
<add> }
<add> if (type === eventTypes.responderRelease) {
<add> responderID = null;
<add> }
<add> return extracted;
<ide> }
<del> // Now that we know the responder is set correctly, we can dispatch
<del> // responder type events (directly to the responder).
<del> var type = isMoveish(topLevelType) ? abstractEventTypes.responderMove :
<del> isEndish(topLevelType) ? abstractEventTypes.responderRelease :
<del> isStartish(topLevelType) ? abstractEventTypes.responderStart : null;
<del> if (type) {
<del> var data = AbstractEvent.normalizePointerData(nativeEvent);
<del> var gesture = AbstractEvent.getPooled(
<del> type,
<del> responderID,
<del> nativeEvent,
<del> data
<del> );
<del> EventPropagators.accumulateDirectDispatches(gesture);
<del> extracted = accumulate(extracted, gesture);
<del> }
<del> if (type === abstractEventTypes.responderRelease) {
<del> responderID = null;
<del> }
<del> return extracted;
<del>};
<ide>
<del>/**
<del> * Event plugin for formalizing the negotiation between claiming locks on
<del> * receiving touches.
<del> */
<del>var ResponderEventPlugin = {
<del> abstractEventTypes: abstractEventTypes,
<del> extractEvents: extractEvents,
<del> getResponderID: getResponderID
<ide> };
<ide>
<ide> module.exports = ResponderEventPlugin;
<ide><path>src/eventPlugins/SimpleEventPlugin.js
<ide>
<ide> "use strict";
<ide>
<del>var AbstractEvent = require('AbstractEvent');
<ide> var EventConstants = require('EventConstants');
<ide> var EventPropagators = require('EventPropagators');
<add>var SyntheticEvent = require('SyntheticEvent');
<add>var SyntheticFocusEvent = require('SyntheticFocusEvent');
<add>var SyntheticKeyboardEvent = require('SyntheticKeyboardEvent');
<add>var SyntheticMouseEvent = require('SyntheticMouseEvent');
<add>var SyntheticMutationEvent = require('SyntheticMutationEvent');
<add>var SyntheticTouchEvent = require('SyntheticTouchEvent');
<add>var SyntheticUIEvent = require('SyntheticUIEvent');
<add>var SyntheticWheelEvent = require('SyntheticWheelEvent');
<ide>
<add>var invariant = require('invariant');
<ide> var keyOf = require('keyOf');
<ide>
<ide> var topLevelTypes = EventConstants.topLevelTypes;
<ide>
<del>var SimpleEventPlugin = {
<del> abstractEventTypes: {
<del> // Note: We do not allow listening to mouseOver events. Instead, use the
<del> // onMouseEnter/onMouseLeave created by `EnterLeaveEventPlugin`.
<del> mouseDown: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onMouseDown: true}),
<del> captured: keyOf({onMouseDownCapture: true})
<del> }
<del> },
<del> mouseUp: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onMouseUp: true}),
<del> captured: keyOf({onMouseUpCapture: true})
<del> }
<del> },
<del> mouseMove: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onMouseMove: true}),
<del> captured: keyOf({onMouseMoveCapture: true})
<del> }
<del> },
<del> doubleClick: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDoubleClick: true}),
<del> captured: keyOf({onDoubleClickCapture: true})
<del> }
<del> },
<del> click: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onClick: true}),
<del> captured: keyOf({onClickCapture: true})
<del> }
<del> },
<del> wheel: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onWheel: true}),
<del> captured: keyOf({onWheelCapture: true})
<del> }
<del> },
<del> touchStart: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onTouchStart: true}),
<del> captured: keyOf({onTouchStartCapture: true})
<del> }
<del> },
<del> touchEnd: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onTouchEnd: true}),
<del> captured: keyOf({onTouchEndCapture: true})
<del> }
<del> },
<del> touchCancel: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onTouchCancel: true}),
<del> captured: keyOf({onTouchCancelCapture: true})
<del> }
<del> },
<del> touchMove: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onTouchMove: true}),
<del> captured: keyOf({onTouchMoveCapture: true})
<del> }
<del> },
<del> keyUp: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onKeyUp: true}),
<del> captured: keyOf({onKeyUpCapture: true})
<del> }
<del> },
<del> keyPress: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onKeyPress: true}),
<del> captured: keyOf({onKeyPressCapture: true})
<del> }
<del> },
<del> keyDown: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onKeyDown: true}),
<del> captured: keyOf({onKeyDownCapture: true})
<del> }
<del> },
<del> input: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onInput: true}),
<del> captured: keyOf({onInputCapture: true})
<del> }
<del> },
<del> focus: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onFocus: true}),
<del> captured: keyOf({onFocusCapture: true})
<del> }
<del> },
<del> blur: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onBlur: true}),
<del> captured: keyOf({onBlurCapture: true})
<del> }
<del> },
<del> scroll: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onScroll: true}),
<del> captured: keyOf({onScrollCapture: true})
<del> }
<del> },
<del> change: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onChange: true}),
<del> captured: keyOf({onChangeCapture: true})
<del> }
<del> },
<del> submit: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onSubmit: true}),
<del> captured: keyOf({onSubmitCapture: true})
<del> }
<del> },
<del> DOMCharacterDataModified: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDOMCharacterDataModified: true}),
<del> captured: keyOf({onDOMCharacterDataModifiedCapture: true})
<del> }
<del> },
<del> drag: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDrag: true}),
<del> captured: keyOf({onDragCapture: true})
<del> }
<del> },
<del> dragEnd: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDragEnd: true}),
<del> captured: keyOf({onDragEndCapture: true})
<del> }
<del> },
<del> dragEnter: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDragEnter: true}),
<del> captured: keyOf({onDragEnterCapture: true})
<del> }
<del> },
<del> dragExit: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDragExit: true}),
<del> captured: keyOf({onDragExitCapture: true})
<del> }
<del> },
<del> dragLeave: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDragLeave: true}),
<del> captured: keyOf({onDragLeaveCapture: true})
<del> }
<del> },
<del> dragOver: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDragOver: true}),
<del> captured: keyOf({onDragOverCapture: true})
<del> }
<del> },
<del> dragStart: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDragStart: true}),
<del> captured: keyOf({onDragStartCapture: true})
<del> }
<del> },
<del> drop: {
<del> phasedRegistrationNames: {
<del> bubbled: keyOf({onDrop: true}),
<del> captured: keyOf({onDropCapture: true})
<del> }
<add>var eventTypes = {
<add> blur: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onBlur: true}),
<add> captured: keyOf({onBlurCapture: true})
<add> }
<add> },
<add> change: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onChange: true}),
<add> captured: keyOf({onChangeCapture: true})
<add> }
<add> },
<add> click: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onClick: true}),
<add> captured: keyOf({onClickCapture: true})
<add> }
<add> },
<add> doubleClick: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDoubleClick: true}),
<add> captured: keyOf({onDoubleClickCapture: true})
<add> }
<add> },
<add> drag: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDrag: true}),
<add> captured: keyOf({onDragCapture: true})
<add> }
<add> },
<add> dragEnd: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDragEnd: true}),
<add> captured: keyOf({onDragEndCapture: true})
<add> }
<add> },
<add> dragEnter: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDragEnter: true}),
<add> captured: keyOf({onDragEnterCapture: true})
<add> }
<add> },
<add> dragExit: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDragExit: true}),
<add> captured: keyOf({onDragExitCapture: true})
<add> }
<add> },
<add> dragLeave: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDragLeave: true}),
<add> captured: keyOf({onDragLeaveCapture: true})
<add> }
<add> },
<add> dragOver: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDragOver: true}),
<add> captured: keyOf({onDragOverCapture: true})
<add> }
<add> },
<add> dragStart: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDragStart: true}),
<add> captured: keyOf({onDragStartCapture: true})
<add> }
<add> },
<add> drop: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onDrop: true}),
<add> captured: keyOf({onDropCapture: true})
<add> }
<add> },
<add> focus: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onFocus: true}),
<add> captured: keyOf({onFocusCapture: true})
<add> }
<add> },
<add> input: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onInput: true}),
<add> captured: keyOf({onInputCapture: true})
<add> }
<add> },
<add> keyDown: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onKeyDown: true}),
<add> captured: keyOf({onKeyDownCapture: true})
<add> }
<add> },
<add> keyPress: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onKeyPress: true}),
<add> captured: keyOf({onKeyPressCapture: true})
<add> }
<add> },
<add> keyUp: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onKeyUp: true}),
<add> captured: keyOf({onKeyUpCapture: true})
<add> }
<add> },
<add> // Note: We do not allow listening to mouseOver events. Instead, use the
<add> // onMouseEnter/onMouseLeave created by `EnterLeaveEventPlugin`.
<add> mouseDown: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onMouseDown: true}),
<add> captured: keyOf({onMouseDownCapture: true})
<add> }
<add> },
<add> mouseMove: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onMouseMove: true}),
<add> captured: keyOf({onMouseMoveCapture: true})
<add> }
<add> },
<add> mouseUp: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onMouseUp: true}),
<add> captured: keyOf({onMouseUpCapture: true})
<add> }
<add> },
<add> scroll: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onScroll: true}),
<add> captured: keyOf({onScrollCapture: true})
<add> }
<add> },
<add> submit: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onSubmit: true}),
<add> captured: keyOf({onSubmitCapture: true})
<ide> }
<ide> },
<add> touchCancel: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onTouchCancel: true}),
<add> captured: keyOf({onTouchCancelCapture: true})
<add> }
<add> },
<add> touchEnd: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onTouchEnd: true}),
<add> captured: keyOf({onTouchEndCapture: true})
<add> }
<add> },
<add> touchMove: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onTouchMove: true}),
<add> captured: keyOf({onTouchMoveCapture: true})
<add> }
<add> },
<add> touchStart: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onTouchStart: true}),
<add> captured: keyOf({onTouchStartCapture: true})
<add> }
<add> },
<add> wheel: {
<add> phasedRegistrationNames: {
<add> bubbled: keyOf({onWheel: true}),
<add> captured: keyOf({onWheelCapture: true})
<add> }
<add> }
<add>};
<add>
<add>var topLevelEventsToDispatchConfig = {
<add> topBlur: eventTypes.blur,
<add> topChange: eventTypes.change,
<add> topClick: eventTypes.click,
<add> topDoubleClick: eventTypes.doubleClick,
<add> topDOMCharacterDataModified: eventTypes.DOMCharacterDataModified,
<add> topDrag: eventTypes.drag,
<add> topDragEnd: eventTypes.dragEnd,
<add> topDragEnter: eventTypes.dragEnter,
<add> topDragExit: eventTypes.dragExit,
<add> topDragLeave: eventTypes.dragLeave,
<add> topDragOver: eventTypes.dragOver,
<add> topDragStart: eventTypes.dragStart,
<add> topDrop: eventTypes.drop,
<add> topFocus: eventTypes.focus,
<add> topInput: eventTypes.input,
<add> topKeyDown: eventTypes.keyDown,
<add> topKeyPress: eventTypes.keyPress,
<add> topKeyUp: eventTypes.keyUp,
<add> topMouseDown: eventTypes.mouseDown,
<add> topMouseMove: eventTypes.mouseMove,
<add> topMouseUp: eventTypes.mouseUp,
<add> topScroll: eventTypes.scroll,
<add> topSubmit: eventTypes.submit,
<add> topTouchCancel: eventTypes.touchCancel,
<add> topTouchEnd: eventTypes.touchEnd,
<add> topTouchMove: eventTypes.touchMove,
<add> topTouchStart: eventTypes.touchStart,
<add> topWheel: eventTypes.wheel
<add>};
<add>
<add>var SimpleEventPlugin = {
<add>
<add> eventTypes: eventTypes,
<ide>
<ide> /**
<ide> * Same as the default implementation, except cancels the event when return
<ide> * value is false.
<ide> *
<del> * @param {AbstractEvent} AbstractEvent to handle
<del> * @param {function} Application-level callback
<del> * @param {string} domID DOM id to pass to the callback.
<add> * @param {object} Event to be dispatched.
<add> * @param {function} Application-level callback.
<add> * @param {string} domID DOM ID to pass to the callback.
<ide> */
<del> executeDispatch: function(abstractEvent, listener, domID) {
<del> var returnValue = listener(abstractEvent, domID);
<add> executeDispatch: function(event, listener, domID) {
<add> var returnValue = listener(event, domID);
<ide> if (returnValue === false) {
<del> abstractEvent.stopPropagation();
<del> abstractEvent.preventDefault();
<add> event.stopPropagation();
<add> event.preventDefault();
<ide> }
<ide> },
<ide>
<ide> var SimpleEventPlugin = {
<ide> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<ide> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<ide> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of `AbstractEvent`s.
<add> * @return {*} An accumulation of synthetic events.
<ide> * @see {EventPluginHub.extractEvents}
<ide> */
<ide> extractEvents: function(
<ide> topLevelType,
<ide> topLevelTarget,
<ide> topLevelTargetID,
<ide> nativeEvent) {
<del> var data;
<del> var abstractEventType =
<del> SimpleEventPlugin.topLevelTypesToAbstract[topLevelType];
<del> if (!abstractEventType) {
<add> var dispatchConfig = topLevelEventsToDispatchConfig[topLevelType];
<add> if (!dispatchConfig) {
<ide> return null;
<ide> }
<add> var EventConstructor;
<ide> switch(topLevelType) {
<del> case topLevelTypes.topWheel:
<del> data = AbstractEvent.normalizeMouseWheelData(nativeEvent);
<add> case topLevelTypes.topChange:
<add> case topLevelTypes.topInput:
<add> case topLevelTypes.topSubmit:
<add> // HTML Events
<add> // @see http://www.w3.org/TR/html5/index.html#events-0
<add> EventConstructor = SyntheticEvent;
<ide> break;
<del> case topLevelTypes.topScroll:
<del> data = AbstractEvent.normalizeScrollDataFromTarget(topLevelTarget);
<add> case topLevelTypes.topKeyDown:
<add> case topLevelTypes.topKeyPress:
<add> case topLevelTypes.topKeyUp:
<add> EventConstructor = SyntheticKeyboardEvent;
<add> break;
<add> case topLevelTypes.topBlur:
<add> case topLevelTypes.topFocus:
<add> EventConstructor = SyntheticFocusEvent;
<ide> break;
<ide> case topLevelTypes.topClick:
<ide> case topLevelTypes.topDoubleClick:
<del> case topLevelTypes.topChange:
<del> case topLevelTypes.topDOMCharacterDataModified:
<del> case topLevelTypes.topMouseDown:
<del> case topLevelTypes.topMouseUp:
<del> case topLevelTypes.topMouseMove:
<del> case topLevelTypes.topTouchMove:
<del> case topLevelTypes.topTouchStart:
<del> case topLevelTypes.topTouchEnd:
<ide> case topLevelTypes.topDrag:
<ide> case topLevelTypes.topDragEnd:
<ide> case topLevelTypes.topDragEnter:
<ide> var SimpleEventPlugin = {
<ide> case topLevelTypes.topDragOver:
<ide> case topLevelTypes.topDragStart:
<ide> case topLevelTypes.topDrop:
<del> data = AbstractEvent.normalizePointerData(nativeEvent);
<del> // todo: Use AbstractEvent.normalizeDragEventData for drag/drop?
<add> case topLevelTypes.topMouseDown:
<add> case topLevelTypes.topMouseMove:
<add> case topLevelTypes.topMouseUp:
<add> EventConstructor = SyntheticMouseEvent;
<add> break;
<add> case topLevelTypes.topDOMCharacterDataModified:
<add> EventConstructor = SyntheticMutationEvent;
<add> break;
<add> case topLevelTypes.topTouchCancel:
<add> case topLevelTypes.topTouchEnd:
<add> case topLevelTypes.topTouchMove:
<add> case topLevelTypes.topTouchStart:
<add> EventConstructor = SyntheticTouchEvent;
<add> break;
<add> case topLevelTypes.topScroll:
<add> EventConstructor = SyntheticUIEvent;
<add> break;
<add> case topLevelTypes.topWheel:
<add> EventConstructor = SyntheticWheelEvent;
<ide> break;
<del> default:
<del> data = null;
<ide> }
<del> var abstractEvent = AbstractEvent.getPooled(
<del> abstractEventType,
<add> invariant(
<add> EventConstructor,
<add> 'SimpleEventPlugin: Unhandled event type, `%s`.',
<add> topLevelType
<add> );
<add> var event = EventConstructor.getPooled(
<add> dispatchConfig,
<ide> topLevelTargetID,
<del> nativeEvent,
<del> data
<add> nativeEvent
<ide> );
<del> EventPropagators.accumulateTwoPhaseDispatches(abstractEvent);
<del> return abstractEvent;
<add> EventPropagators.accumulateTwoPhaseDispatches(event);
<add> return event;
<ide> }
<del>};
<ide>
<del>SimpleEventPlugin.topLevelTypesToAbstract = {
<del> topMouseDown: SimpleEventPlugin.abstractEventTypes.mouseDown,
<del> topMouseUp: SimpleEventPlugin.abstractEventTypes.mouseUp,
<del> topMouseMove: SimpleEventPlugin.abstractEventTypes.mouseMove,
<del> topClick: SimpleEventPlugin.abstractEventTypes.click,
<del> topDoubleClick: SimpleEventPlugin.abstractEventTypes.doubleClick,
<del> topWheel: SimpleEventPlugin.abstractEventTypes.wheel,
<del> topTouchStart: SimpleEventPlugin.abstractEventTypes.touchStart,
<del> topTouchEnd: SimpleEventPlugin.abstractEventTypes.touchEnd,
<del> topTouchMove: SimpleEventPlugin.abstractEventTypes.touchMove,
<del> topTouchCancel: SimpleEventPlugin.abstractEventTypes.touchCancel,
<del> topKeyUp: SimpleEventPlugin.abstractEventTypes.keyUp,
<del> topKeyPress: SimpleEventPlugin.abstractEventTypes.keyPress,
<del> topKeyDown: SimpleEventPlugin.abstractEventTypes.keyDown,
<del> topInput: SimpleEventPlugin.abstractEventTypes.input,
<del> topFocus: SimpleEventPlugin.abstractEventTypes.focus,
<del> topBlur: SimpleEventPlugin.abstractEventTypes.blur,
<del> topScroll: SimpleEventPlugin.abstractEventTypes.scroll,
<del> topChange: SimpleEventPlugin.abstractEventTypes.change,
<del> topSubmit: SimpleEventPlugin.abstractEventTypes.submit,
<del> topDOMCharacterDataModified:
<del> SimpleEventPlugin.abstractEventTypes.DOMCharacterDataModified,
<del> topDrag: SimpleEventPlugin.abstractEventTypes.drag,
<del> topDragEnd: SimpleEventPlugin.abstractEventTypes.dragEnd,
<del> topDragEnter: SimpleEventPlugin.abstractEventTypes.dragEnter,
<del> topDragExit: SimpleEventPlugin.abstractEventTypes.dragExit,
<del> topDragLeave: SimpleEventPlugin.abstractEventTypes.dragLeave,
<del> topDragOver: SimpleEventPlugin.abstractEventTypes.dragOver,
<del> topDragStart: SimpleEventPlugin.abstractEventTypes.dragStart,
<del> topDrop: SimpleEventPlugin.abstractEventTypes.drop
<ide> };
<ide>
<ide> module.exports = SimpleEventPlugin;
<ide><path>src/eventPlugins/TapEventPlugin.js
<ide>
<ide> "use strict";
<ide>
<del>var AbstractEvent = require('AbstractEvent');
<add>var BrowserEnv = require('BrowserEnv');
<ide> var EventPluginUtils = require('EventPluginUtils');
<ide> var EventPropagators = require('EventPropagators');
<add>var SyntheticUIEvent = require('SyntheticUIEvent');
<add>var TouchEventUtils = require('TouchEventUtils');
<ide>
<ide> var keyOf = require('keyOf');
<ide>
<ide> var isStartish = EventPluginUtils.isStartish;
<ide> var isEndish = EventPluginUtils.isEndish;
<del>var storePageCoordsIn = EventPluginUtils.storePageCoordsIn;
<del>var eventDistance = EventPluginUtils.eventDistance;
<ide>
<ide> /**
<del> * The number of pixels that are tolerated in between a touchStart and
<del> * touchEnd in order to still be considered a 'tap' event.
<add> * Number of pixels that are tolerated in between a `touchStart` and `touchEnd`
<add> * in order to still be considered a 'tap' event.
<ide> */
<ide> var tapMoveThreshold = 10;
<ide> var startCoords = {x: null, y: null};
<ide>
<del>var abstractEventTypes = {
<add>var Axis = {
<add> x: {page: 'pageX', client: 'clientX', envScroll: 'currentPageScrollLeft'},
<add> y: {page: 'pageY', client: 'clientY', envScroll: 'currentPageScrollTop'}
<add>};
<add>
<add>function getAxisCoordOfEvent(axis, nativeEvent) {
<add> var singleTouch = TouchEventUtils.extractSingleTouch(nativeEvent);
<add> if (singleTouch) {
<add> return singleTouch[axis.page];
<add> }
<add> return axis.page in nativeEvent ?
<add> nativeEvent[axis.page] :
<add> nativeEvent[axis.client] + BrowserEnv[axis.envScroll];
<add>}
<add>
<add>function getDistance(coords, nativeEvent) {
<add> var pageX = getAxisCoordOfEvent(Axis.x, nativeEvent);
<add> var pageY = getAxisCoordOfEvent(Axis.y, nativeEvent);
<add> return Math.pow(
<add> Math.pow(pageX - coords.x, 2) + Math.pow(pageY - coords.y, 2),
<add> 0.5
<add> );
<add>}
<add>
<add>var eventTypes = {
<ide> touchTap: {
<ide> phasedRegistrationNames: {
<ide> bubbled: keyOf({onTouchTap: null}),
<ide> var abstractEventTypes = {
<ide> }
<ide> };
<ide>
<del>/**
<del> * @param {string} topLevelType Record from `EventConstants`.
<del> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<del> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<del> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of `AbstractEvent`s.
<del> * @see {EventPluginHub.extractEvents}
<del> */
<del>var extractEvents = function(
<del> topLevelType,
<del> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent) {
<del> if (!isStartish(topLevelType) && !isEndish(topLevelType)) {
<del> return;
<del> }
<del> var abstractEvent;
<del> var dist = eventDistance(startCoords, nativeEvent);
<del> if (isEndish(topLevelType) && dist < tapMoveThreshold) {
<del> abstractEvent = AbstractEvent.getPooled(
<del> abstractEventTypes.touchTap,
<add>var TapEventPlugin = {
<add>
<add> tapMoveThreshold: tapMoveThreshold,
<add>
<add> eventTypes: eventTypes,
<add>
<add> /**
<add> * @param {string} topLevelType Record from `EventConstants`.
<add> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<add> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<add> * @param {object} nativeEvent Native browser event.
<add> * @return {*} An accumulation of synthetic events.
<add> * @see {EventPluginHub.extractEvents}
<add> */
<add> extractEvents: function(
<add> topLevelType,
<add> topLevelTarget,
<ide> topLevelTargetID,
<del> nativeEvent
<del> );
<del> }
<del> if (isStartish(topLevelType)) {
<del> storePageCoordsIn(startCoords, nativeEvent);
<del> } else if (isEndish(topLevelType)) {
<del> startCoords.x = 0;
<del> startCoords.y = 0;
<add> nativeEvent) {
<add> if (!isStartish(topLevelType) && !isEndish(topLevelType)) {
<add> return null;
<add> }
<add> var event = null;
<add> var distance = getDistance(startCoords, nativeEvent);
<add> if (isEndish(topLevelType) && distance < tapMoveThreshold) {
<add> event = SyntheticUIEvent.getPooled(
<add> eventTypes.touchTap,
<add> topLevelTargetID,
<add> nativeEvent
<add> );
<add> }
<add> if (isStartish(topLevelType)) {
<add> startCoords.x = getAxisCoordOfEvent(Axis.x, nativeEvent);
<add> startCoords.y = getAxisCoordOfEvent(Axis.y, nativeEvent);
<add> } else if (isEndish(topLevelType)) {
<add> startCoords.x = 0;
<add> startCoords.y = 0;
<add> }
<add> EventPropagators.accumulateTwoPhaseDispatches(event);
<add> return event;
<ide> }
<del> EventPropagators.accumulateTwoPhaseDispatches(abstractEvent);
<del> return abstractEvent;
<del>};
<ide>
<del>var TapEventPlugin = {
<del> tapMoveThreshold: tapMoveThreshold,
<del> startCoords: startCoords,
<del> abstractEventTypes: abstractEventTypes,
<del> extractEvents: extractEvents
<ide> };
<ide>
<ide> module.exports = TapEventPlugin;
<ide><path>src/eventPlugins/TextChangeEventPlugin.js
<ide>
<ide> "use strict";
<ide>
<del>var AbstractEvent = require('AbstractEvent');
<ide> var EventConstants = require('EventConstants');
<ide> var EventPluginHub = require('EventPluginHub');
<ide> var EventPropagators = require('EventPropagators');
<ide> var ExecutionEnvironment = require('ExecutionEnvironment');
<add>var SyntheticEvent = require('SyntheticEvent');
<ide>
<ide> var isEventSupported = require('isEventSupported');
<ide> var keyOf = require('keyOf');
<ide>
<ide> var topLevelTypes = EventConstants.topLevelTypes;
<ide>
<del>var abstractEventTypes = {
<add>var eventTypes = {
<ide> textChange: {
<ide> phasedRegistrationNames: {
<ide> bubbled: keyOf({onTextChange: null}),
<ide> var stopWatching = function() {
<ide> * the value of the active element has changed.
<ide> */
<ide> var handlePropertyChange = function(nativeEvent) {
<del> var value;
<del> var abstractEvent;
<del>
<del> if (nativeEvent.propertyName === "value") {
<del> value = nativeEvent.srcElement.value;
<del> if (value !== activeElementValue) {
<del> activeElementValue = value;
<del>
<del> abstractEvent = AbstractEvent.getPooled(
<del> abstractEventTypes.textChange,
<del> activeElementID,
<del> nativeEvent
<del> );
<del> EventPropagators.accumulateTwoPhaseDispatches(abstractEvent);
<del>
<del> // If propertychange bubbled, we'd just bind to it like all the other
<del> // events and have it go through ReactEventTopLevelCallback. Since it
<del> // doesn't, we manually listen for the propertychange event and so we
<del> // have to enqueue and process the abstract event manually.
<del> EventPluginHub.enqueueEvents(abstractEvent);
<del> EventPluginHub.processEventQueue();
<del> }
<add> if (nativeEvent.propertyName !== "value") {
<add> return;
<add> }
<add> var value = nativeEvent.srcElement.value;
<add> if (value === activeElementValue) {
<add> return;
<ide> }
<add> activeElementValue = value;
<add>
<add> var event = SyntheticEvent.getPooled(
<add> eventTypes.textChange,
<add> activeElementID,
<add> nativeEvent
<add> );
<add> EventPropagators.accumulateTwoPhaseDispatches(event);
<add>
<add> // If propertychange bubbled, we'd just bind to it like all the other events
<add> // and have it go through ReactEventTopLevelCallback. Since it doesn't, we
<add> // manually listen for the propertychange event and so we have to enqueue and
<add> // process the abstract event manually.
<add> EventPluginHub.enqueueEvents(event);
<add> EventPluginHub.processEventQueue();
<ide> };
<ide>
<ide> /**
<ide> if (isInputSupported) {
<ide> targetIDForTextChangeEvent = function(
<ide> topLevelType,
<ide> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent) {
<add> topLevelTargetID) {
<ide> if (topLevelType === topLevelTypes.topInput) {
<ide> // In modern browsers (i.e., not IE8 or IE9), the input event is exactly
<ide> // what we want so fall through here and trigger an abstract event...
<ide> if (isInputSupported) {
<ide> targetIDForTextChangeEvent = function(
<ide> topLevelType,
<ide> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent) {
<add> topLevelTargetID) {
<ide> if (topLevelType === topLevelTypes.topFocus) {
<ide> // In IE8, we can capture almost all .value changes by adding a
<ide> // propertychange handler and looking for events with propertyName
<ide> if (isInputSupported) {
<ide> };
<ide> }
<ide>
<del>/**
<del> * @param {string} topLevelType Record from `EventConstants`.
<del> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<del> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<del> * @param {object} nativeEvent Native browser event.
<del> * @return {*} An accumulation of `AbstractEvent`s.
<del> * @see {EventPluginHub.extractEvents}
<del> */
<del>var extractEvents = function(
<del> topLevelType,
<del> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent) {
<del> var targetID = targetIDForTextChangeEvent(
<del> topLevelType,
<del> topLevelTarget,
<del> topLevelTargetID,
<del> nativeEvent
<del> );
<add>var TextChangeEventPlugin = {
<ide>
<del> if (targetID) {
<del> var abstractEvent = AbstractEvent.getPooled(
<del> abstractEventTypes.textChange,
<del> targetID,
<del> nativeEvent
<add> eventTypes: eventTypes,
<add>
<add> /**
<add> * @param {string} topLevelType Record from `EventConstants`.
<add> * @param {DOMEventTarget} topLevelTarget The listening component root node.
<add> * @param {string} topLevelTargetID ID of `topLevelTarget`.
<add> * @param {object} nativeEvent Native browser event.
<add> * @return {*} An accumulation of synthetic events.
<add> * @see {EventPluginHub.extractEvents}
<add> */
<add> extractEvents: function(
<add> topLevelType,
<add> topLevelTarget,
<add> topLevelTargetID,
<add> nativeEvent) {
<add> var targetID = targetIDForTextChangeEvent(
<add> topLevelType,
<add> topLevelTarget,
<add> topLevelTargetID
<ide> );
<del> EventPropagators.accumulateTwoPhaseDispatches(abstractEvent);
<del> return abstractEvent;
<add>
<add> if (targetID) {
<add> var event = SyntheticEvent.getPooled(
<add> eventTypes.textChange,
<add> targetID,
<add> nativeEvent
<add> );
<add> EventPropagators.accumulateTwoPhaseDispatches(event);
<add> return event;
<add> }
<ide> }
<del>};
<ide>
<del>var TextChangeEventPlugin = {
<del> abstractEventTypes: abstractEventTypes,
<del> extractEvents: extractEvents
<ide> };
<ide>
<ide> module.exports = TextChangeEventPlugin;
<ide><path>src/eventPlugins/__tests__/ResponderEventPlugin-test.js
<ide> var EventConstants;
<ide> var EventPropagators;
<ide> var ReactInstanceHandles;
<ide> var ResponderEventPlugin;
<del>var AbstractEvent;
<add>var SyntheticEvent;
<ide>
<ide> var GRANDPARENT_ID = '.reactRoot[0]';
<ide> var PARENT_ID = '.reactRoot[0].0';
<ide> var CHILD_ID = '.reactRoot[0].0.0';
<ide>
<ide> var topLevelTypes;
<del>var responderAbstractEventTypes;
<add>var responderEventTypes;
<ide> var spies;
<ide>
<ide> var DUMMY_NATIVE_EVENT = {};
<ide> var DUMMY_RENDERED_TARGET = {};
<ide>
<ide> var onStartShouldSetResponder = function(id, cb, capture) {
<del> var registrationNames = responderAbstractEventTypes
<add> var registrationNames = responderEventTypes
<ide> .startShouldSetResponder
<ide> .phasedRegistrationNames;
<ide> CallbackRegistry.putListener(
<ide> var onStartShouldSetResponder = function(id, cb, capture) {
<ide> };
<ide>
<ide> var onScrollShouldSetResponder = function(id, cb, capture) {
<del> var registrationNames = responderAbstractEventTypes
<add> var registrationNames = responderEventTypes
<ide> .scrollShouldSetResponder
<ide> .phasedRegistrationNames;
<ide> CallbackRegistry.putListener(
<ide> var onScrollShouldSetResponder = function(id, cb, capture) {
<ide> };
<ide>
<ide> var onMoveShouldSetResponder = function(id, cb, capture) {
<del> var registrationNames = responderAbstractEventTypes
<add> var registrationNames = responderEventTypes
<ide> .moveShouldSetResponder
<ide> .phasedRegistrationNames;
<ide> CallbackRegistry.putListener(
<ide> var onMoveShouldSetResponder = function(id, cb, capture) {
<ide> var onResponderGrant = function(id, cb) {
<ide> CallbackRegistry.putListener(
<ide> id,
<del> responderAbstractEventTypes.responderGrant.registrationName,
<add> responderEventTypes.responderGrant.registrationName,
<ide> cb
<ide> );
<ide> };
<ide> var existsInExtraction = function(extracted, test) {
<ide> * Helper validators.
<ide> */
<ide> function assertGrantEvent(id, extracted) {
<del> var test = function(abstractEvent) {
<del> return abstractEvent instanceof AbstractEvent &&
<del> abstractEvent.reactEventType ===
<del> responderAbstractEventTypes.responderGrant &&
<del> abstractEvent.reactTargetID === id;
<add> var test = function(event) {
<add> return event instanceof SyntheticEvent &&
<add> event.dispatchConfig === responderEventTypes.responderGrant &&
<add> event.dispatchMarker === id;
<ide> };
<ide> expect(ResponderEventPlugin.getResponderID()).toBe(id);
<ide> expect(existsInExtraction(extracted, test)).toBe(true);
<ide> }
<ide>
<ide> function assertResponderMoveEvent(id, extracted) {
<del> var test = function(abstractEvent) {
<del> return abstractEvent instanceof AbstractEvent &&
<del> abstractEvent.reactEventType ===
<del> responderAbstractEventTypes.responderMove &&
<del> abstractEvent.reactTargetID === id;
<add> var test = function(event) {
<add> return event instanceof SyntheticEvent &&
<add> event.dispatchConfig === responderEventTypes.responderMove &&
<add> event.dispatchMarker === id;
<ide> };
<ide> expect(ResponderEventPlugin.getResponderID()).toBe(id);
<ide> expect(existsInExtraction(extracted, test)).toBe(true);
<ide> }
<ide>
<ide> function assertTerminateEvent(id, extracted) {
<del> var test = function(abstractEvent) {
<del> return abstractEvent instanceof AbstractEvent &&
<del> abstractEvent.reactEventType ===
<del> responderAbstractEventTypes.responderTerminate &&
<del> abstractEvent.reactTargetID === id;
<add> var test = function(event) {
<add> return event instanceof SyntheticEvent &&
<add> event.dispatchConfig === responderEventTypes.responderTerminate &&
<add> event.dispatchMarker === id;
<ide> };
<ide> expect(ResponderEventPlugin.getResponderID()).not.toBe(id);
<ide> expect(existsInExtraction(extracted, test)).toBe(true);
<ide> }
<ide>
<ide> function assertRelease(id, extracted) {
<del> var test = function(abstractEvent) {
<del> return abstractEvent instanceof AbstractEvent &&
<del> abstractEvent.reactEventType ===
<del> responderAbstractEventTypes.responderRelease &&
<del> abstractEvent.reactTargetID === id;
<add> var test = function(event) {
<add> return event instanceof SyntheticEvent &&
<add> event.dispatchConfig === responderEventTypes.responderRelease &&
<add> event.dispatchMarker === id;
<ide> };
<ide> expect(ResponderEventPlugin.getResponderID()).toBe(null);
<ide> expect(existsInExtraction(extracted, test)).toBe(true);
<ide> describe('ResponderEventPlugin', function() {
<ide> beforeEach(function() {
<ide> require('mock-modules').dumpCache();
<ide>
<del> AbstractEvent = require('AbstractEvent');
<ide> CallbackRegistry = require('CallbackRegistry');
<ide> EventConstants = require('EventConstants');
<ide> EventPropagators = require('EventPropagators');
<ide> ReactInstanceHandles = require('ReactInstanceHandles');
<ide> ResponderEventPlugin = require('ResponderEventPlugin');
<add> SyntheticEvent = require('SyntheticEvent');
<ide> EventPropagators.injection.injectInstanceHandle(ReactInstanceHandles);
<ide>
<ide> // dumpCache, in open-source tests, only resets existing mocks. It does not
<ide> describe('ResponderEventPlugin', function() {
<ide> CallbackRegistry.__purge();
<ide>
<ide> topLevelTypes = EventConstants.topLevelTypes;
<del> responderAbstractEventTypes = ResponderEventPlugin.abstractEventTypes;
<add> responderEventTypes = ResponderEventPlugin.eventTypes;
<ide>
<ide> spies = {
<ide> onStartShouldSetResponderChild: function() {},
<ide><path>src/test/ReactTestUtils.js
<ide> var ReactTestUtils = {
<ide> * on and `Element` node.
<ide> * @param topLevelType {Object} A type from `EventConstants.topLevelTypes`
<ide> * @param {!Element} node The dom to simulate an event occurring on.
<del> * @param {?Event} fakeNativeEvent Fake native event to pass to ReactEvent.
<add> * @param {?Event} fakeNativeEvent Fake native event to use in SyntheticEvent.
<ide> */
<ide> simulateEventOnNode: function(topLevelType, node, fakeNativeEvent) {
<ide> var virtualHandler =
<ide> var ReactTestUtils = {
<ide> * on the `ReactNativeComponent` `comp`.
<ide> * @param topLevelType {Object} A type from `EventConstants.topLevelTypes`.
<ide> * @param comp {!ReactNativeComponent}
<del> * @param {?Event} fakeNativeEvent Fake native event to pass to ReactEvent.
<add> * @param {?Event} fakeNativeEvent Fake native event to use in SyntheticEvent.
<ide> */
<ide> simulateEventOnDOMComponent: function(topLevelType, comp, fakeNativeEvent) {
<ide> var reactRootID = comp._rootNodeID || comp._rootDomId;
<ide> for (eventType in topLevelTypes) {
<ide> eventType.charAt(3).toLowerCase() + eventType.substr(4) : eventType;
<ide> /**
<ide> * @param {!Element || ReactNativeComponent} domComponentOrNode
<del> * @param {?Event} nativeEventData Fake native event to pass to ReactEvent.
<add> * @param {?Event} nativeEventData Fake native event to use in SyntheticEvent.
<ide> */
<ide> ReactTestUtils.Simulate[convenienceName] = makeSimulator(eventType);
<ide> } | 15 |
Javascript | Javascript | return meaningful error when no devices available | ad4aee75199efbab8d028403d0459b74bf9d3816 | <ide><path>local-cli/runIOS/runIOS.js
<ide> function runIOS(argv, config, args) {
<ide> );
<ide> if (args.device) {
<ide> const selectedDevice = matchingDevice(devices, args.device);
<del> if (selectedDevice){
<add> if (selectedDevice) {
<ide> return runOnDevice(selectedDevice, scheme, xcodeProject, args.configuration, args.packager);
<ide> } else {
<del> if (devices){
<add> if (devices && devices.length > 0) {
<ide> console.log('Could not find device with the name: "' + args.device + '".');
<ide> console.log('Choose one of the following:');
<ide> printFoundDevices(devices);
<ide> function runIOS(argv, config, args) {
<ide>
<ide> function runOnDeviceByUdid(args, scheme, xcodeProject, devices) {
<ide> const selectedDevice = matchingDeviceByUdid(devices, args.udid);
<del> if (selectedDevice){
<add> if (selectedDevice) {
<ide> return runOnDevice(selectedDevice, scheme, xcodeProject, args.configuration, args.packager);
<ide> } else {
<del> if (devices){
<add> if (devices && devices.length > 0) {
<ide> console.log('Could not find device with the udid: "' + args.udid + '".');
<ide> console.log('Choose one of the following:');
<ide> printFoundDevices(devices);
<ide> function runOnDeviceByUdid(args, scheme, xcodeProject, devices) {
<ide> }
<ide> }
<ide>
<del>function runOnSimulator(xcodeProject, args, scheme){
<add>function runOnSimulator(xcodeProject, args, scheme) {
<ide> return new Promise((resolve) => {
<ide> try {
<ide> var simulators = JSON.parse(
<ide> function formattedDeviceName(simulator) {
<ide> return `${simulator.name} (${simulator.version})`;
<ide> }
<ide>
<del>function printFoundDevices(devices){
<add>function printFoundDevices(devices) {
<ide> for (let i = devices.length - 1; i >= 0; i--) {
<ide> console.log(devices[i].name + ' Udid: ' + devices[i].udid);
<ide> } | 1 |
Text | Text | add هنيئا لك | 1b987f265fb9d6866f35d24843360c00273b3db7 | <ide><path>guide/arabic/react/hello-world/index.md
<ide> ---
<ide> title: Hello World
<ide> localeTitle: مرحبا بالعالم
<del>---
<ide>## مرحبا بالعالم !!
<del>
<del>يبدأ كل تعلم لغة بمثال Traditional Hello World. هنا ، يمكنك التعرف على React مع نفس برنامج HelloWorld.
<del>
<del>كل شيء في React مكون.
<del>
<del>ولكن قبل ذلك ، نحتاج إلى التأكد من تثبيت node.js و npm في جهاز الكمبيوتر. بشكل اختياري ، يمكننا استخدام CRA (إنشاء تطبيق React) وهو عبارة عن أداة تم تصميمها بواسطة المطورين على Facebook لمساعدتك على بناء تطبيقات React. إنه يوفر عليك من الإعداد والتهيئة المستهلكة للوقت. يمكنك ببساطة تشغيل أمر واحد وإنشاء تطبيق رد يعمل على إعداد الأدوات التي تحتاجها لبدء مشروع React.
<del>
<del>يمكننا تثبيته من خلال الأوامر التالية
<del>
<del> `npm install -g create-react-app
<del>
<del> create-react-app my-app
<del>
<del> cd my-app
<del> npm start
<del>`
<del>
<del>يجب أن يمنحك سطر الأوامر مخرجات حيث يمكنك العثور على التطبيق في المستعرض. يجب أن يكون الإعداد الافتراضي هو localhost: 8080. إذا كنت تستخدم IE أو Edge فقط على جهاز يعمل بنظام التشغيل Windows ، فيمكنني أن أوصيك بتثبيت Chrome أيضًا للوصول إلى بيئة مطوّري البرامج وأدوات React Developer التي تتوفر كإضافة Chrome.
<del>
<del>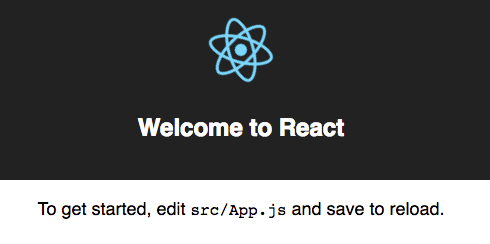
<del>
<del>#### SRC / App.js
<del>
<del>نسخ الرمز أدناه ولصقه في src / App.js
<del>
<del> ` import React from 'react';
<del>
<del> class App extends React.Component{
<del> constructor(props) {
<del> super(props);
<del> }
<del>
<del> render(){
<del> return(
<del> <div>
<del> <p>Hello World !!</p>
<del> </div>
<del> );
<del> }
<del> }
<del>
<del> export default App;
<del>`
<del>
<del>إذا تحققنا من ملف index.js في المجلد src ، نجد أن تطبيق App.js أعلاه يسمى index.js ثم تم تقديمه.
<del>
<del> `// Other code
<del> import App from './App'; // The App component is imported
<del>
<del> // Other code
<del> ReactDOM.render(<App />,
<del> document.getElementById('root')); //The <App /> is the way components are called in react after importing them
<del>
<del> // Other code
<del>`
<del>
<del>في ما سبق ، يسمى App.js بمكون. عادة ، نقوم بعمل مكونات متعددة ونضعها معًا في App.js والتي سيتم بعد ذلك تقديمها في index.js والتي يتم بعد ذلك تحويلها إلى div root الموجود في index.html.
<del>
<del>مبروك !! لقد أنشأت أول تطبيق لـ React Hello world. تعلم المزيد عن رد الفعل في المقالات القادمة.
<del>
<del>الترميز سعيدة!
<ide>\ No newline at end of file
<add>---
<add>## مرحبا بالعالم !!
<add>
<add>يبدأ كل تعلم لغة بمثال Traditional Hello World. هنا ، يمكنك التعرف على React مع نفس برنامج HelloWorld.
<add>
<add>كل شيء في React مكون.
<add>
<add>ولكن قبل ذلك ، نحتاج إلى التأكد من تثبيت node.js و npm في جهاز الكمبيوتر. بشكل اختياري ، يمكننا استخدام CRA (إنشاء تطبيق React) وهو عبارة عن أداة تم تصميمها بواسطة المطورين على Facebook لمساعدتك على بناء تطبيقات React. إنه يوفر عليك من الإعداد والتهيئة المستهلكة للوقت. يمكنك ببساطة تشغيل أمر واحد وإنشاء تطبيق رد يعمل على إعداد الأدوات التي تحتاجها لبدء مشروع React.
<add>
<add>يمكننا تثبيته من خلال الأوامر التالية
<add>
<add> ```shell
<add> npm install -g create-react-app
<add>
<add> create-react-app my-app
<add>
<add> cd my-app
<add> npm start
<add>```
<add>
<add>يجب أن يمنحك سطر الأوامر مخرجات حيث يمكنك العثور على التطبيق في المستعرض. يجب أن يكون الإعداد الافتراضي هو localhost: 8080. إذا كنت تستخدم IE أو Edge فقط على جهاز يعمل بنظام التشغيل Windows ، فيمكنني أن أوصيك بتثبيت Chrome أيضًا للوصول إلى بيئة مطوّري البرامج وأدوات React Developer التي تتوفر كإضافة Chrome.
<add>
<add>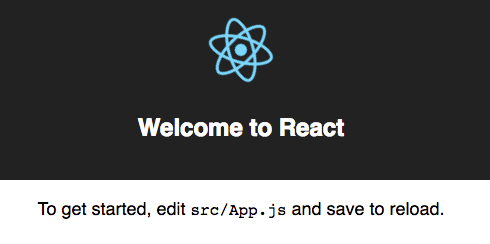
<add>
<add>#### SRC / App.js
<add>
<add>نسخ الرمز أدناه ولصقه في src / App.js
<add>
<add>```jsx
<add> import React from 'react';
<add>
<add> class App extends React.Component{
<add> constructor(props) {
<add> super(props);
<add> }
<add>
<add> render(){
<add> return(
<add> <div>
<add> <p>Hello World !!</p>
<add> </div>
<add> );
<add> }
<add> }
<add>
<add> export default App;
<add>```
<add>
<add>إذا تحققنا من ملف index.js في المجلد src ، نجد أن تطبيق App.js أعلاه يسمى index.js ثم تم تقديمه.
<add>
<add>```jsx
<add>// Other code
<add> import App from './App'; // The App component is imported
<add>
<add> // Other code
<add> ReactDOM.render(<App />,
<add> document.getElementById('root')); //The <App /> is the way components are called in react after importing them
<add>
<add> // Other code
<add>```
<add>
<add>في ما سبق ، يسمى App.js بمكون. عادة ، نقوم بعمل مكونات متعددة ونضعها معًا في App.js والتي سيتم بعد ذلك تقديمها في index.js والتي يتم بعد ذلك تحويلها إلى div root الموجود في index.html.
<add>
<add>مبروك !! لقد أنشأت أول تطبيق لـ React Hello world. تعلم المزيد عن رد الفعل في المقالات القادمة.
<add>
<add>الترميز سعيدة!
<add>*هنيئا لك | 1 |
PHP | PHP | move exceptions into internal array | 841b36cc005ee5c400f1276175db9e2692d1e167 | <ide><path>src/Illuminate/Foundation/Exceptions/Handler.php
<ide> class Handler implements ExceptionHandlerContract
<ide> */
<ide> protected $dontReport = [];
<ide>
<add> /**
<add> * A list of the internal exception types that should not be reported.
<add> *
<add> * @var array
<add> */
<add> protected $internalDontReport = [
<add> \Illuminate\Auth\AuthenticationException::class,
<add> \Illuminate\Auth\Access\AuthorizationException::class,
<add> \Symfony\Component\HttpKernel\Exception\HttpException::class,
<add> HttpResponseException::class,
<add> \Illuminate\Database\Eloquent\ModelNotFoundException::class,
<add> \Illuminate\Session\TokenMismatchException::class,
<add> \Illuminate\Validation\ValidationException::class,
<add> ];
<add>
<ide> /**
<ide> * Create a new exception handler instance.
<ide> *
<ide> public function shouldReport(Exception $e)
<ide> */
<ide> protected function shouldntReport(Exception $e)
<ide> {
<del> $dontReport = array_merge($this->dontReport, [HttpResponseException::class]);
<add> $dontReport = array_merge($this->dontReport, $this->internalDontReport);
<ide>
<ide> return ! is_null(collect($dontReport)->first(function ($type) use ($e) {
<ide> return $e instanceof $type; | 1 |
Javascript | Javascript | add comment for type3 bypass | 17ba5e3f68bd187a526c3ab11043c929ca69b0d5 | <ide><path>pdf.js
<ide> var PartialEvaluator = (function partialEvaluator() {
<ide> // This case is here for compatibility.
<ide> var descriptor = xref.fetchIfRef(dict.get('FontDescriptor'));
<ide> if (!descriptor) {
<add> // Note for Type3 fonts: it has no no base font, feeding default
<add> // font name and trying to get font metrics as the same way as for
<add> // a font without descriptor.
<ide> var baseFontName = dict.get('BaseFont') || new Name('sans-serif');
<ide>
<ide> // Using base font name as a font name. | 1 |
Javascript | Javascript | add page with next/image to stats-app | 556216b1adb39d4b0e67d7cad2f204234c46aa6b | <ide><path>test/.stats-app/image.js
<add>import Image from 'next/image'
<add>import logo from './nextjs.png'
<add>
<add>function ImagePage(props) {
<add> return (
<add> <>
<add> <h1>next/image example</h1>
<add> <Image src={logo} placeholder="blur" />
<add> </>
<add> )
<add>}
<add>
<add>// we add getServerSideProps to prevent static optimization
<add>// to allow us to compare server-side changes
<add>export const getServerSideProps = () => {
<add> return {
<add> props: {},
<add> }
<add>}
<add>
<add>export default ImagePage
<ide><path>test/.stats-app/pages/index.js
<ide> const Page = () => 'Hello world 👋'
<ide>
<del>Page.getInitialProps = () => ({})
<add>// we add getServerSideProps to prevent static optimization
<add>// to allow us to compare server-side changes
<add>export const getServerSideProps = () => {
<add> return {
<add> props: {},
<add> }
<add>}
<ide>
<ide> export default Page
<ide><path>test/.stats-app/pages/link.js
<ide> function aLink(props) {
<ide> )
<ide> }
<ide>
<del>aLink.getInitialProps = () => ({})
<add>// we add getServerSideProps to prevent static optimization
<add>// to allow us to compare server-side changes
<add>export const getServerSideProps = () => {
<add> return {
<add> props: {},
<add> }
<add>}
<ide>
<ide> export default aLink
<ide><path>test/.stats-app/pages/routerDirect.js
<ide> function routerDirect(props) {
<ide> return <div>I import the router directly</div>
<ide> }
<ide>
<del>routerDirect.getInitialProps = () => ({})
<add>// we add getServerSideProps to prevent static optimization
<add>// to allow us to compare server-side changes
<add>export const getServerSideProps = () => {
<add> return {
<add> props: {},
<add> }
<add>}
<ide>
<ide> export default routerDirect
<ide><path>test/.stats-app/pages/withRouter.js
<ide> function useWithRouter(props) {
<ide> return <div>I use withRouter</div>
<ide> }
<ide>
<del>useWithRouter.getInitialProps = () => ({})
<add>// we add getServerSideProps to prevent static optimization
<add>// to allow us to compare server-side changes
<add>export const getServerSideProps = () => {
<add> return {
<add> props: {},
<add> }
<add>}
<ide>
<ide> export default withRouter(useWithRouter)
<ide><path>test/.stats-app/stats-config.js
<add>const fs = require('fs')
<add>const path = require('path')
<add>// this page is conditionally added when not testing
<add>// in webpack 4 mode since it's not supported for webpack 4
<add>const imagePageData = fs.readFileSync(
<add> path.join(__dirname, './image.js'),
<add> 'utf8'
<add>)
<add>
<ide> const clientGlobs = [
<ide> {
<ide> name: 'Client Bundles (main, webpack, commons)',
<ide> const clientGlobs = [
<ide> },
<ide> {
<ide> name: 'Client Pages',
<del> globs: ['.next/static/*/pages/**/*', '.next/static/css/**/*'],
<add> globs: ['.next/static/BUILD_ID/pages/**/*.js', '.next/static/css/**/*'],
<ide> },
<ide> {
<ide> name: 'Client Build Manifests',
<del> globs: ['.next/static/*/_buildManifest*'],
<add> globs: ['.next/static/BUILD_ID/_buildManifest*'],
<ide> },
<ide> {
<ide> name: 'Rendered Page Sizes',
<ide> const clientGlobs = [
<ide>
<ide> const renames = [
<ide> {
<del> srcGlob: '.next/static/*/pages',
<add> srcGlob: '.next/static/chunks/pages',
<ide> dest: '.next/static/BUILD_ID/pages',
<ide> },
<ide> {
<del> srcGlob: '.next/static/*/pages/**/*',
<add> srcGlob: '.next/static/BUILD_ID/pages/**/*.js',
<ide> removeHash: true,
<ide> },
<ide> {
<del> srcGlob: '.next/static/runtime/*',
<add> srcGlob: '.next/static/runtime/*.js',
<ide> removeHash: true,
<ide> },
<ide> {
<del> srcGlob: '.next/static/chunks/*',
<add> srcGlob: '.next/static/chunks/*.js',
<ide> removeHash: true,
<ide> },
<ide> {
<ide> module.exports = {
<ide> title: 'Default Build',
<ide> diff: 'onOutputChange',
<ide> diffConfigFiles: [
<add> {
<add> path: 'pages/image.js',
<add> content: imagePageData,
<add> },
<ide> {
<ide> path: 'next.config.js',
<ide> content: `
<ide> module.exports = {
<ide> // renames to apply to make file names deterministic
<ide> renames,
<ide> configFiles: [
<add> {
<add> path: 'pages/image.js',
<add> content: imagePageData,
<add> },
<ide> {
<ide> path: 'next.config.js',
<ide> content: ` | 6 |
Text | Text | fix typos in buffer doc | 59cad32b519438bb6f296bb6f8c3391eb4060ecc | <ide><path>doc/api/buffer.md
<ide> string into a `Buffer` as decoding.
<ide> tabs, and new lines contained within the base64-encoded string are ignored.
<ide>
<ide> * `'hex'`: Encode each byte as two hexadecimal characters. Data truncation
<del> may occur when decoding string that do exclusively contain valid hexadecimal
<add> may occur when decoding strings that do exclusively contain valid hexadecimal
<ide> characters. See below for an example.
<ide>
<ide> The following legacy character encodings are also supported:
<ide>
<ide> * `'ascii'`: For 7-bit [ASCII][] data only. When encoding a string into a
<ide> `Buffer`, this is equivalent to using `'latin1'`. When decoding a `Buffer`
<del> into a string, using encoding this will additionally unset the highest bit of
<add> into a string, using this encoding will additionally unset the highest bit of
<ide> each byte before decoding as `'latin1'`.
<ide> Generally, there should be no reason to use this encoding, as `'utf8'`
<ide> (or, if the data is known to always be ASCII-only, `'latin1'`) will be a
<ide> In particular:
<ide> There are two ways to create new [`TypedArray`][] instances from a `Buffer`:
<ide>
<ide> * Passing a `Buffer` to a [`TypedArray`][] constructor will copy the `Buffer`s
<del> contents, interpreted an array array of integers, and not as a byte sequence
<add> contents, interpreted as an array of integers, and not as a byte sequence
<ide> of the target type.
<ide>
<ide> ```js | 1 |
Ruby | Ruby | fix equality comparison raising error bug | e7834214a668cde0a4f7757f7f4a3d78f73f2fd8 | <ide><path>activemodel/lib/active_model/error.rb
<ide> def strict_match?(attribute, type, **options)
<ide> end
<ide>
<ide> def ==(other)
<del> attributes_for_hash == other.attributes_for_hash
<add> other.is_a?(self.class) && attributes_for_hash == other.attributes_for_hash
<ide> end
<ide> alias eql? ==
<ide>
<ide><path>activemodel/test/cases/error_test.rb
<ide> def test_initialize
<ide> assert error != ActiveModel::Error.new(person, :title, foo: :bar)
<ide> assert error != ActiveModel::Error.new(Person.new, :name, foo: :bar)
<ide> end
<add>
<add> test "comparing against different class would not raise error" do
<add> person = Person.new
<add> error = ActiveModel::Error.new(person, :name, foo: :bar)
<add>
<add> assert error != person
<add> end
<ide> end | 2 |
Ruby | Ruby | simplify implementation of throughreflection#chain | 39a6f4f25d958783c73377ac52886c9edc19632e | <ide><path>activerecord/lib/active_record/reflection.rb
<ide> def through_reflection
<ide> # Returns an array of reflections which are involved in this association. Each item in the
<ide> # array corresponds to a table which will be part of the query for this association.
<ide> #
<del> # If the source reflection is itself a ThroughReflection, then we don't include self in
<del> # the chain, but just defer to the source reflection.
<del> #
<ide> # The chain is built by recursively calling #chain on the source reflection and the through
<ide> # reflection. The base case for the recursion is a normal association, which just returns
<ide> # [self] as its #chain.
<ide> def chain
<ide> @chain ||= begin
<del> if source_reflection.source_reflection
<del> # If the source reflection has its own source reflection, then the chain must start
<del> # by getting us to that source reflection.
<del> chain = source_reflection.chain
<del> else
<del> # If the source reflection does not go through another reflection, then we can get
<del> # to this reflection directly, and so start the chain here
<del> #
<del> # It is important to use self, rather than the source_reflection, because self
<del> # may has a :source_type option which needs to be used.
<del> #
<del> # FIXME: Not sure this is correct justification now that we have #conditions
<del> chain = [self]
<del> end
<del>
<del> # Recursively build the rest of the chain
<del> chain += through_reflection.chain
<del>
<del> # Finally return the completed chain
<add> chain = source_reflection.chain + through_reflection.chain
<add> chain[0] = self # Use self so we don't lose the information from :source_type
<ide> chain
<ide> end
<ide> end
<ide><path>activerecord/test/cases/reflection_test.rb
<ide> def test_has_many_through_reflection
<ide>
<ide> def test_chain
<ide> expected = [
<del> Author.reflect_on_association(:essay_categories),
<add> Organization.reflect_on_association(:author_essay_categories),
<ide> Author.reflect_on_association(:essays),
<ide> Organization.reflect_on_association(:authors)
<ide> ] | 2 |
Text | Text | add changelog for 2.10.2 [ci skip] | c5268257b5ee6e8a2268cb961a995c2be239cedc | <ide><path>CHANGELOG.md
<ide> - [#14441](https://github.com/emberjs/ember.js/pull/14441) [DEPRECATION] Deprecate remaining usage of the `{{render}}` helper.
<ide> - [#14482](https://github.com/emberjs/ember.js/pull/14482) [DEPRECATION] Deprecate `Component#renderToElement`.
<ide>
<add>### 2.10.2 (December 19, 2016)
<add>
<add>- [#14685](https://github.com/emberjs/ember.js/pull/14685) [BUGFIX] Fix `this.$()` returning `undefined` in `willDestroyElement`.
<add>- [#14717](https://github.com/emberjs/ember.js/pull/14717) [BUGFIX] Fix an issue with block params named `attrs`.
<add>
<ide> ### 2.10.1 (December 13, 2016)
<ide>
<ide> - [#14671](https://github.com/emberjs/ember.js/pull/14671) [BUGFIX] Fix an issue with the list attribute in <input> elements. | 1 |
Python | Python | add cost regions for ecs | c222bb12a1465b92b1f76888338a773a1276a3d0 | <ide><path>libcloud/compute/drivers/ecs.py
<ide> ECS_API_ENDPOINT = 'ecs.aliyuncs.com'
<ide> DEFAULT_SIGNATURE_VERSION = '1.0'
<ide>
<add>COST_REGIONS = ['ecs-ap-northeast-1', 'ecs-ap-south-1', 'ecs-ap-southeast-1',
<add> 'ecs-ap-southeast-2', 'ecs-ap-southeast-3', 'ecs-ap-southeast-5',
<add> 'ecs-cn-beijing', 'ecs-cn-chengdu', 'ecs-cn-hangzhou', 'ecs-cn-hongkong',
<add> 'ecs-cn-huhehaote', 'ecs-cn-qingdao', 'ecs-cn-shanghai', 'ecs-cn-shenzhen',
<add> 'ecs-cn-zhangjiakou', 'ecs-eu-central-1', 'ecs-eu-west-1', 'ecs-me-east-1',
<add> 'ecs-us-east-1', 'ecs-us-west-1']
<add>
<ide>
<ide> def _parse_bool(value):
<ide> if isinstance(value, bool): | 1 |
Javascript | Javascript | fix sporadic hang and partial reads | 671b5be6e9d74fec9e94b1ab88d2b2648c540078 | <ide><path>lib/tls.js
<ide> CryptoStream.prototype._read = function read(size) {
<ide> var bytesRead = 0,
<ide> start = this._buffer.offset;
<ide> do {
<del> var read = this._buffer.use(this.pair.ssl, out, size);
<add> var read = this._buffer.use(this.pair.ssl, out, size - bytesRead);
<ide> if (read > 0) {
<ide> bytesRead += read;
<del> size -= read;
<ide> }
<ide>
<ide> // Handle and report errors | 1 |
Javascript | Javascript | fix linting errors | debb7eb046ca167fa627fd873fd6bbd7e188b221 | <ide><path>src/materials/ShaderMaterial.js
<ide> ShaderMaterial.prototype.copy = function ( source ) {
<ide>
<ide> this.extensions = Object.assign( {}, source.extensions );
<ide>
<del> this.glslVersion = source.glslVersion
<add> this.glslVersion = source.glslVersion;
<ide>
<ide> return this;
<ide>
<ide> ShaderMaterial.prototype.toJSON = function ( meta ) {
<ide>
<ide> const data = Material.prototype.toJSON.call( this, meta );
<ide>
<del> data.glslVersion = this.glslVersion
<add> data.glslVersion = this.glslVersion;
<ide> data.uniforms = {};
<del>
<add>
<ide> for ( const name in this.uniforms ) {
<ide>
<ide> const uniform = this.uniforms[ name ];
<ide><path>src/renderers/webgl/WebGLProgram.js
<ide> function WebGLProgram( renderer, cacheKey, parameters, bindingStates ) {
<ide> const program = gl.createProgram();
<ide>
<ide> let prefixVertex, prefixFragment;
<del> let versionString = parameters.glslVersion ? '#version ' + parameters.glslVersion : ''
<add> let versionString = parameters.glslVersion ? '#version ' + parameters.glslVersion : '';
<ide>
<ide> if ( parameters.isRawShaderMaterial ) {
<ide> | 2 |
Python | Python | fix isort errors | 4a1ab3c18c7c85c9d962e7b6605e7d57423d29fe | <ide><path>rest_framework/fields.py
<ide> from django.conf import settings
<ide> from django.core.exceptions import ValidationError as DjangoValidationError
<ide> from django.core.exceptions import ObjectDoesNotExist
<del>from django.core.validators import EmailValidator, RegexValidator, ip_address_validators, URLValidator
<add>from django.core.validators import (
<add> EmailValidator, RegexValidator, URLValidator, ip_address_validators
<add>)
<ide> from django.forms import FilePathField as DjangoFilePathField
<ide> from django.forms import ImageField as DjangoImageField
<ide> from django.utils import six, timezone
<ide> from rest_framework import ISO_8601
<ide> from rest_framework.compat import (
<ide> MaxLengthValidator, MaxValueValidator, MinLengthValidator,
<del> MinValueValidator, OrderedDict, duration_string,
<del> parse_duration, unicode_repr, unicode_to_repr
<add> MinValueValidator, OrderedDict, duration_string, parse_duration,
<add> unicode_repr, unicode_to_repr
<ide> )
<ide> from rest_framework.exceptions import ValidationError
<ide> from rest_framework.settings import api_settings | 1 |
Python | Python | fix obvious mistake in testing/decorators warning | d06b690a330d05dd3997b154bbed7527333db1bb | <ide><path>numpy/testing/decorators.py
<ide> """
<ide> import warnings
<ide>
<del>warnings.warn(ImportWarning,
<del> "Import from numpy.testing, not numpy.testing.decorators")
<add>warnings.warn("Import from numpy.testing, not numpy.testing.decorators",
<add> ImportWarning)
<ide>
<ide> from ._private.decorators import * | 1 |
Javascript | Javascript | fix copy and clone methods | a99eac6138a368f88c6639b4888d33c5a6c518ce | <ide><path>src/objects/Line.js
<ide> Line.prototype = Object.assign( Object.create( Object3D.prototype ), {
<ide>
<ide> }() ),
<ide>
<add> copy: function ( source ) {
<add>
<add> Object3D.prototype.copy.call( this, source );
<add>
<add> this.geometry.copy( source.geometry );
<add> this.material.copy( source.material );
<add>
<add> return this;
<add>
<add> },
<add>
<ide> clone: function () {
<ide>
<del> return new this.constructor( this.geometry, this.material ).copy( this );
<add> return new this.constructor().copy( this );
<ide>
<ide> }
<ide> | 1 |
Javascript | Javascript | add clear connections to secure-pair | fc553fd8213f81267d5bec76a9f9d9db1cda52a9 | <ide><path>benchmark/net/net-pipe.js
<ide> const net = require('net');
<ide> const PORT = common.PORT;
<ide>
<ide> const bench = common.createBenchmark(main, {
<del> len: [64, 102400, 1024 * 1024 * 16],
<add> len: [2, 64, 102400, 1024 * 1024 * 16],
<ide> type: ['utf', 'asc', 'buf'],
<ide> dur: [5],
<ide> });
<ide><path>benchmark/tls/secure-pair.js
<ide> const common = require('../common.js');
<ide> const bench = common.createBenchmark(main, {
<ide> dur: [5],
<del> securing: ['SecurePair', 'TLSSocket'],
<del> size: [2, 1024, 1024 * 1024]
<add> securing: ['SecurePair', 'TLSSocket', 'clear'],
<add> size: [2, 100, 1024, 1024 * 1024]
<ide> });
<ide>
<ide> const fixtures = require('../../test/common/fixtures');
<ide> function main({ dur, size, securing }) {
<ide> isServer: false,
<ide> rejectUnauthorized: false,
<ide> };
<del> const conn = tls.connect(clientOptions, () => {
<add> const network = securing === 'clear' ? net : tls;
<add> const conn = network.connect(clientOptions, () => {
<ide> setTimeout(() => {
<ide> const mbits = (received * 8) / (1024 * 1024);
<ide> bench.end(mbits);
<ide> function main({ dur, size, securing }) {
<ide> case 'TLSSocket':
<ide> secureTLSSocket(conn, client);
<ide> break;
<add> case 'clear':
<add> conn.pipe(client);
<add> break;
<ide> default:
<ide> throw new Error('Invalid securing method');
<ide> } | 2 |
PHP | PHP | add the laravel start time to the benchmarker | a474850071ca4ba2c0ec7e5dca6e761fb714e8cd | <ide><path>public/index.php
<ide> * @link http://laravel.com
<ide> */
<ide>
<del>$t = microtime(true);
<add>// --------------------------------------------------------------
<add>// Get the framework start time.
<add>// --------------------------------------------------------------
<add>$start = microtime(true);
<ide>
<ide> // --------------------------------------------------------------
<ide> // The path to the application directory.
<ide> }
<ide> });
<ide>
<add>// --------------------------------------------------------------
<add>// Register the framework starting time with the Benchmarker.
<add>// --------------------------------------------------------------
<add>System\Benchmark::$marks['laravel'] = $start;
<add>
<ide> // --------------------------------------------------------------
<ide> // Set the error reporting and display levels.
<ide> // -------------------------------------------------------------- | 1 |
Text | Text | update portuguese translation on typeof | a794645084f29437467d271e077015e952898d66 | <ide><path>guide/portuguese/javascript/typeof/index.md
<ide> localeTitle: Tipo de
<ide>
<ide> `typeof` é uma palavra-chave JavaScript que retornará o tipo de uma variável quando você a chamar. Você pode usar isso para validar parâmetros de função ou verificar se variáveis estão definidas. Existem outros usos também.
<ide>
<del>O operador `typeof` é útil porque é uma maneira fácil de verificar o tipo de uma variável em seu código. Isso é importante porque o JavaScript é uma [linguagem digitada dinamicamente](https://stackoverflow.com/questions/2690544/what-is-the-difference-between-a-strongly-typed-language-and-a-statically-typed) . Isso significa que você não é obrigado a atribuir tipos a variáveis ao criá-los. Como uma variável não é restrita dessa maneira, seu tipo pode mudar durante o tempo de execução de um programa.
<add>O operador `typeof` é útil porque é uma maneira fácil de verificar o tipo de uma variável em seu código. Isso é importante porque o JavaScript é uma [linguagem dinamicamente tipada](https://stackoverflow.com/questions/2690544/what-is-the-difference-between-a-strongly-typed-language-and-a-statically-typed) . Isso significa que você não é obrigado a atribuir tipos a variáveis ao criá-los. Como uma variável não é restrita dessa maneira, seu tipo pode mudar durante o tempo de execução de um programa.
<ide>
<ide> Por exemplo:
<ide>
<del>```javascript
<add>```js
<ide> var x = 12345; // number
<del> x = 'string'; // string
<del> x = { key: 'value' }; // object
<add>x = 'string'; // string
<add>x = { key: 'value' }; // object
<ide> ```
<ide>
<del>Como você pode ver no exemplo acima, uma variável em JavaScript pode alterar os tipos durante a execução de um programa. Isso pode ser difícil de controlar como um programador, e é aí que o operador `typeof` é útil.
<add>Como você pode ver no exemplo acima, uma variável em JavaScript pode ter outro tipo durante a execução de um programa. Isso pode ser difícil de controlar como um programador, e é aí que o operador `typeof` é útil.
<ide>
<ide> O operador `typeof` retorna uma string que representa o tipo atual de uma variável. Você pode usá-lo digitando `typeof(variable)` ou `typeof variable` . Voltando ao exemplo anterior, você pode usá-lo para verificar o tipo da variável `x` em cada estágio:
<ide>
<ide> function(x){
<ide> ```
<ide>
<ide> A saída do operador `typeof` pode nem sempre ser o que você espera quando você verifica um número.
<del>Os números podem se transformar no valor [NaN (não um número)](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/NaN) por vários motivos.
<add>Os números podem se transformar no valor [NaN (não é um número)](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/NaN) por vários motivos.
<ide>
<ide> ```javascript
<ide> console.log(typeof NaN); //"number"
<ide> function isNumber(data) {
<ide> }
<ide> ```
<ide>
<del>Mesmo pensando que este é um método de validação útil, temos que ter cuidado, porque o JavaScript tem algumas partes estranhas e uma delas é o resultado do `typeof` instruções específicas. Por exemplo, em JavaScript muitas coisas são apenas `objects` assim você encontrará.
<add>Mesmo pensando que este é um método de validação útil, temos que ter cuidado, porque o JavaScript tem algumas partes estranhas e uma delas é o resultado do `typeof` em instruções específicas. Por exemplo, em JavaScript muitas coisas são apenas `objects` assim você encontrará.
<ide>
<ide> ```javascript
<ide> var x = [1,2,3,4];
<ide> var x = [1,2,3,4];
<ide>
<ide> ### Mais Informações:
<ide>
<del>[Documentação MDN para typeof](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof)
<ide>\ No newline at end of file
<add>[Documentação MDN para typeof](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof) | 1 |
Text | Text | fix minor typo in docs | 45368779f86c2f0e78a6703bf64a0b149251b8b5 | <ide><path>docs/reference/commandline/volume_create.md
<ide> parent = "smn_cli"
<ide> --name= Specify volume name
<ide> -o, --opt=map[] Set driver specific options
<ide>
<del>Creates a new volume that containers can can consume and store data in. If a name is not specified, Docker generates a random name. You create a volume and then configure the container to use it, for example:
<add>Creates a new volume that containers can consume and store data in. If a name is not specified, Docker generates a random name. You create a volume and then configure the container to use it, for example:
<ide>
<ide> $ docker volume create --name hello
<ide> hello | 1 |
Javascript | Javascript | fix _final and 'prefinish' timing | ea87809bb6696e2c1ec5d470031f137e18641183 | <ide><path>lib/_stream_transform.js
<ide> function Transform(options) {
<ide> this._flush = options.flush;
<ide> }
<ide>
<del> // TODO(ronag): Unfortunately _final is invoked asynchronously.
<del> // Use `prefinish` hack. `prefinish` is emitted synchronously when
<del> // and only when `_final` is not defined. Implementing `_final`
<del> // to a Transform should be an error.
<add> // When the writable side finishes, then flush out anything remaining.
<add> // Backwards compat. Some Transform streams incorrectly implement _final
<add> // instead of or in addition to _flush. By using 'prefinish' instead of
<add> // implementing _final we continue supporting this unfortunate use case.
<ide> this.on('prefinish', prefinish);
<ide> }
<ide>
<ide><path>lib/_stream_writable.js
<ide> function needFinish(state) {
<ide> }
<ide>
<ide> function callFinal(stream, state) {
<add> state.sync = true;
<add> state.pendingcb++;
<ide> stream._final((err) => {
<ide> state.pendingcb--;
<ide> if (err) {
<del> errorOrDestroy(stream, err);
<del> } else {
<add> errorOrDestroy(stream, err, state.sync);
<add> } else if (needFinish(state)) {
<ide> state.prefinished = true;
<ide> stream.emit('prefinish');
<del> finishMaybe(stream, state);
<add> // Backwards compat. Don't check state.sync here.
<add> // Some streams assume 'finish' will be emitted
<add> // asynchronously relative to _final callback.
<add> state.pendingcb++;
<add> process.nextTick(finish, stream, state);
<ide> }
<ide> });
<add> state.sync = false;
<ide> }
<ide>
<ide> function prefinish(stream, state) {
<ide> if (!state.prefinished && !state.finalCalled) {
<ide> if (typeof stream._final === 'function' && !state.destroyed) {
<del> state.pendingcb++;
<ide> state.finalCalled = true;
<del> process.nextTick(callFinal, stream, state);
<add> callFinal(stream, state);
<ide> } else {
<ide> state.prefinished = true;
<ide> stream.emit('prefinish');
<ide> function prefinish(stream, state) {
<ide> }
<ide>
<ide> function finishMaybe(stream, state, sync) {
<del> const need = needFinish(state);
<del> if (need) {
<add> if (needFinish(state)) {
<ide> prefinish(stream, state);
<del> if (state.pendingcb === 0) {
<add> if (state.pendingcb === 0 && needFinish(state)) {
<ide> state.pendingcb++;
<ide> if (sync) {
<ide> process.nextTick(finish, stream, state);
<ide> function finishMaybe(stream, state, sync) {
<ide> }
<ide> }
<ide> }
<del> return need;
<ide> }
<ide>
<ide> function finish(stream, state) {
<ide> state.pendingcb--;
<ide> if (state.errorEmitted)
<ide> return;
<ide>
<add> // TODO(ronag): This could occur after 'close' is emitted.
<add>
<ide> state.finished = true;
<ide> stream.emit('finish');
<ide>
<ide><path>lib/internal/http2/core.js
<ide> function streamOnPause() {
<ide> }
<ide>
<ide> function afterShutdown(status) {
<add> const stream = this.handle[kOwner];
<add> if (stream) {
<add> stream.on('finish', () => {
<add> stream[kMaybeDestroy]();
<add> });
<add> }
<ide> // Currently this status value is unused
<ide> this.callback();
<del> const stream = this.handle[kOwner];
<del> if (stream)
<del> stream[kMaybeDestroy]();
<ide> }
<ide>
<ide> function finishSendTrailers(stream, headersList) {
<ide><path>test/parallel/test-stream-transform-final-sync.js
<ide> const t = new stream.Transform({
<ide> process.nextTick(function() {
<ide> state++;
<ide> // fluchCallback part 2
<del> assert.strictEqual(state, 15);
<add> assert.strictEqual(state, 13);
<ide> done();
<ide> });
<ide> }, 1)
<ide> });
<ide> t.on('finish', common.mustCall(function() {
<ide> state++;
<ide> // finishListener
<del> assert.strictEqual(state, 13);
<add> assert.strictEqual(state, 14);
<ide> }, 1));
<ide> t.on('end', common.mustCall(function() {
<ide> state++;
<ide> t.write(4);
<ide> t.end(7, common.mustCall(function() {
<ide> state++;
<ide> // endMethodCallback
<del> assert.strictEqual(state, 14);
<add> assert.strictEqual(state, 15);
<ide> }, 1));
<ide><path>test/parallel/test-stream-transform-final.js
<ide> const t = new stream.Transform({
<ide> process.nextTick(function() {
<ide> state++;
<ide> // flushCallback part 2
<del> assert.strictEqual(state, 15);
<add> assert.strictEqual(state, 13);
<ide> done();
<ide> });
<ide> }, 1)
<ide> });
<ide> t.on('finish', common.mustCall(function() {
<ide> state++;
<ide> // finishListener
<del> assert.strictEqual(state, 13);
<add> assert.strictEqual(state, 14);
<ide> }, 1));
<ide> t.on('end', common.mustCall(function() {
<ide> state++;
<ide> t.write(4);
<ide> t.end(7, common.mustCall(function() {
<ide> state++;
<ide> // endMethodCallback
<del> assert.strictEqual(state, 14);
<add> assert.strictEqual(state, 15);
<ide> }, 1));
<ide><path>test/parallel/test-stream-writable-finished.js
<ide> const assert = require('assert');
<ide> }
<ide>
<ide> {
<del> // Emit finish asynchronously
<add> // Emit finish asynchronously.
<ide>
<ide> const w = new Writable({
<ide> write(chunk, encoding, cb) {
<ide> const assert = require('assert');
<ide> w.end();
<ide> w.on('finish', common.mustCall());
<ide> }
<add>
<add>{
<add> // Emit prefinish synchronously.
<add>
<add> const w = new Writable({
<add> write(chunk, encoding, cb) {
<add> cb();
<add> }
<add> });
<add>
<add> let sync = true;
<add> w.on('prefinish', common.mustCall(() => {
<add> assert.strictEqual(sync, true);
<add> }));
<add> w.end();
<add> sync = false;
<add>}
<add>
<add>{
<add> // Emit prefinish synchronously w/ final.
<add>
<add> const w = new Writable({
<add> write(chunk, encoding, cb) {
<add> cb();
<add> },
<add> final(cb) {
<add> cb();
<add> }
<add> });
<add>
<add> let sync = true;
<add> w.on('prefinish', common.mustCall(() => {
<add> assert.strictEqual(sync, true);
<add> }));
<add> w.end();
<add> sync = false;
<add>}
<add>
<add>
<add>{
<add> // Call _final synchronously.
<add>
<add> let sync = true;
<add> const w = new Writable({
<add> write(chunk, encoding, cb) {
<add> cb();
<add> },
<add> final: common.mustCall((cb) => {
<add> assert.strictEqual(sync, true);
<add> cb();
<add> })
<add> });
<add>
<add> w.end();
<add> sync = false;
<add>} | 6 |
Python | Python | update several examples to work with the new api | eec61d9d4921fb7a62926e217fbfe43cd7062ac4 | <ide><path>examples/imdb_cnn.py
<ide> from keras.models import Sequential
<ide> from keras.layers import Dense, Dropout, Activation
<ide> from keras.layers import Embedding
<del>from keras.layers import Convolution1D, GlobalMaxPooling1D
<add>from keras.layers import Conv1D, GlobalMaxPooling1D
<ide> from keras.datasets import imdb
<ide>
<ide>
<ide> batch_size = 32
<ide> embedding_dims = 50
<ide> filters = 250
<del>filter_length = 3
<add>kernel_size = 3
<ide> hidden_dims = 250
<ide> epochs = 2
<ide>
<ide> # our vocab indices into embedding_dims dimensions
<ide> model.add(Embedding(max_features,
<ide> embedding_dims,
<del> input_length=maxlen,
<del> dropout=0.2))
<add> input_length=maxlen))
<add>model.add(Dropout(0.2))
<ide>
<ide> # we add a Convolution1D, which will learn filters
<ide> # word group filters of size filter_length:
<del>model.add(Convolution1D(filters=filters,
<del> filter_length=filter_length,
<del> border_mode='valid',
<del> activation='relu',
<del> subsample_length=1))
<add>model.add(Conv1D(filters,
<add> kernel_size,
<add> padding='valid',
<add> activation='relu',
<add> strides=1))
<ide> # we use max pooling:
<ide> model.add(GlobalMaxPooling1D())
<ide>
<ide><path>examples/imdb_cnn_lstm.py
<ide> from keras.layers import Dense, Dropout, Activation
<ide> from keras.layers import Embedding
<ide> from keras.layers import LSTM
<del>from keras.layers import Convolution1D, MaxPooling1D
<add>from keras.layers import Conv1D, MaxPooling1D
<ide> from keras.datasets import imdb
<ide>
<ide>
<ide> embedding_size = 128
<ide>
<ide> # Convolution
<del>filter_length = 5
<add>kernel_size = 5
<ide> filters = 64
<del>pool_length = 4
<add>pool_size = 4
<ide>
<ide> # LSTM
<ide> lstm_output_size = 70
<ide> model = Sequential()
<ide> model.add(Embedding(max_features, embedding_size, input_length=maxlen))
<ide> model.add(Dropout(0.25))
<del>model.add(Convolution1D(filters=filters,
<del> filter_length=filter_length,
<del> border_mode='valid',
<del> activation='relu',
<del> subsample_length=1))
<del>model.add(MaxPooling1D(pool_length=pool_length))
<add>model.add(Conv1D(filters,
<add> kernel_size,
<add> padding='valid',
<add> activation='relu',
<add> strides=1))
<add>model.add(MaxPooling1D(pool_size=pool_size))
<ide> model.add(LSTM(lstm_output_size))
<ide> model.add(Dense(1))
<ide> model.add(Activation('sigmoid'))
<ide><path>examples/lstm_benchmark.py
<ide> '''Compare LSTM implementations on the IMDB sentiment classification task.
<ide>
<del>consume_less='cpu' preprocesses input to the LSTM which typically results in
<add>implementation=0 preprocesses input to the LSTM which typically results in
<ide> faster computations at the expense of increased peak memory usage as the
<ide> preprocessed input must be kept in memory.
<ide>
<del>consume_less='mem' does away with the preprocessing, meaning that it might take
<add>implementation=1 does away with the preprocessing, meaning that it might take
<ide> a little longer, but should require less peak memory.
<ide>
<del>consume_less='gpu' concatenates the input, output and forget gate's weights
<add>implementation=2 concatenates the input, output and forget gate's weights
<ide> into one, large matrix, resulting in faster computation time as the GPU can
<ide> utilize more cores, at the expense of reduced regularization because the same
<ide> dropout is shared across the gates.
<ide>
<ide> from keras.preprocessing import sequence
<ide> from keras.models import Sequential
<del>from keras.layers import Embedding, Dense, LSTM
<add>from keras.layers import Embedding, Dense, LSTM, Dropout
<ide> from keras.datasets import imdb
<ide>
<ide> max_features = 20000
<ide> max_length = 80
<ide> embedding_dim = 256
<ide> batch_size = 128
<ide> epochs = 10
<del>modes = ['cpu', 'mem', 'gpu']
<add>modes = [0, 1, 2]
<ide>
<ide> print('Loading data...')
<ide> (X_train, y_train), (X_test, y_test) = imdb.load_data(num_words=max_features)
<ide> # Compile and train different models while meauring performance.
<ide> results = []
<ide> for mode in modes:
<del> print('Testing mode: consume_less="{}"'.format(mode))
<add> print('Testing mode: implementation={}'.format(mode))
<ide>
<ide> model = Sequential()
<del> model.add(Embedding(max_features, embedding_dim, input_length=max_length, dropout=0.2))
<del> model.add(LSTM(embedding_dim, dropout_W=0.2, dropout_U=0.2, consume_less=mode))
<add> model.add(Embedding(max_features, embedding_dim, input_length=max_length))
<add> model.add(Dropout(0.2))
<add> model.add(LSTM(embedding_dim, dropout=0.2, recurrent_dropout=0.2, implementation=mode))
<ide> model.add(Dense(1, activation='sigmoid'))
<ide> model.compile(loss='binary_crossentropy',
<ide> optimizer='adam',
<ide><path>examples/mnist_acgan.py
<ide> def build_generator(latent_size):
<ide>
<ide> # upsample to (..., 14, 14)
<ide> cnn.add(UpSampling2D(size=(2, 2)))
<del> cnn.add(Convolution2D(256, 5, 5, border_mode='same',
<del> activation='relu', init='glorot_normal'))
<add> cnn.add(Convolution2D(256, 5, padding='same',
<add> activation='relu', kernel_initializer='glorot_normal'))
<ide>
<ide> # upsample to (..., 28, 28)
<ide> cnn.add(UpSampling2D(size=(2, 2)))
<del> cnn.add(Convolution2D(128, 5, 5, border_mode='same',
<del> activation='relu', init='glorot_normal'))
<add> cnn.add(Convolution2D(128, 5, padding='same',
<add> activation='relu', kernel_initializer='glorot_normal'))
<ide>
<ide> # take a channel axis reduction
<del> cnn.add(Convolution2D(1, 2, 2, border_mode='same',
<del> activation='tanh', init='glorot_normal'))
<add> cnn.add(Convolution2D(1, 2, padding='same',
<add> activation='tanh', kernel_initializer='glorot_normal'))
<ide>
<ide> # this is the z space commonly refered to in GAN papers
<ide> latent = Input(shape=(latent_size, ))
<ide> def build_generator(latent_size):
<ide>
<ide> # 10 classes in MNIST
<ide> cls = Flatten()(Embedding(10, latent_size,
<del> init='glorot_normal')(image_class))
<add> embeddings_initializer='glorot_normal')(image_class))
<ide>
<ide> # hadamard product between z-space and a class conditional embedding
<ide> h = merge([latent, cls], mode='mul')
<ide>
<ide> fake_image = cnn(h)
<ide>
<del> return Model(input=[latent, image_class], output=fake_image)
<add> return Model([latent, image_class], fake_image)
<ide>
<ide>
<ide> def build_discriminator():
<ide> # build a relatively standard conv net, with LeakyReLUs as suggested in
<ide> # the reference paper
<ide> cnn = Sequential()
<ide>
<del> cnn.add(Convolution2D(32, 3, 3, border_mode='same', subsample=(2, 2),
<add> cnn.add(Convolution2D(32, 3, padding='same', strides=2,
<ide> input_shape=(1, 28, 28)))
<ide> cnn.add(LeakyReLU())
<ide> cnn.add(Dropout(0.3))
<ide>
<del> cnn.add(Convolution2D(64, 3, 3, border_mode='same', subsample=(1, 1)))
<add> cnn.add(Convolution2D(64, 3, padding='same', strides=2))
<ide> cnn.add(LeakyReLU())
<ide> cnn.add(Dropout(0.3))
<ide>
<del> cnn.add(Convolution2D(128, 3, 3, border_mode='same', subsample=(2, 2)))
<add> cnn.add(Convolution2D(128, 3, padding='same', strides=2))
<ide> cnn.add(LeakyReLU())
<ide> cnn.add(Dropout(0.3))
<ide>
<del> cnn.add(Convolution2D(256, 3, 3, border_mode='same', subsample=(1, 1)))
<add> cnn.add(Convolution2D(256, 3, padding='same', strides=1))
<ide> cnn.add(LeakyReLU())
<ide> cnn.add(Dropout(0.3))
<ide>
<ide> def build_discriminator():
<ide> fake = Dense(1, activation='sigmoid', name='generation')(features)
<ide> aux = Dense(10, activation='softmax', name='auxiliary')(features)
<ide>
<del> return Model(input=image, output=[fake, aux])
<add> return Model(image, [fake, aux])
<ide>
<ide> if __name__ == '__main__':
<ide>
<ide> # batch and latent size taken from the paper
<del> epochss = 50
<add> epochs = 50
<ide> batch_size = 100
<ide> latent_size = 100
<ide>
<ide> def build_discriminator():
<ide> # we only want to be able to train generation for the combined model
<ide> discriminator.trainable = False
<ide> fake, aux = discriminator(fake)
<del> combined = Model(input=[latent, image_class], output=[fake, aux])
<add> combined = Model([latent, image_class], [fake, aux])
<ide>
<ide> combined.compile(
<ide> optimizer=Adam(lr=adam_lr, beta_1=adam_beta_1),
<ide> def build_discriminator():
<ide> train_history = defaultdict(list)
<ide> test_history = defaultdict(list)
<ide>
<del> for epoch in range(epochss):
<del> print('Epoch {} of {}'.format(epoch + 1, epochss))
<add> for epoch in range(epochs):
<add> print('Epoch {} of {}'.format(epoch + 1, epochs))
<ide>
<ide> num_batches = int(X_train.shape[0] / batch_size)
<ide> progress_bar = Progbar(target=num_batches)
<ide><path>examples/mnist_hierarchical_rnn.py
<ide> # Training parameters.
<ide> batch_size = 32
<ide> num_classes = 10
<del>epochss = 5
<add>epochs = 5
<ide>
<ide> # Embedding dimensions.
<ide> row_hidden = 128
<ide> col_hidden = 128
<ide>
<ide> # The data, shuffled and split between train and test sets.
<del>(X_train, y_train), (X_test, y_test) = mnist.load_data()
<add>(x_train, y_train), (x_test, y_test) = mnist.load_data()
<ide>
<ide> # Reshapes data to 4D for Hierarchical RNN.
<del>X_train = X_train.reshape(X_train.shape[0], 28, 28, 1)
<del>X_test = X_test.reshape(X_test.shape[0], 28, 28, 1)
<del>X_train = X_train.astype('float32')
<del>X_test = X_test.astype('float32')
<del>X_train /= 255
<del>X_test /= 255
<del>print('X_train shape:', X_train.shape)
<del>print(X_train.shape[0], 'train samples')
<del>print(X_test.shape[0], 'test samples')
<add>x_train = x_train.reshape(x_train.shape[0], 28, 28, 1)
<add>x_test = x_test.reshape(x_test.shape[0], 28, 28, 1)
<add>x_train = x_train.astype('float32')
<add>x_test = x_test.astype('float32')
<add>x_train /= 255
<add>x_test /= 255
<add>print('x_train shape:', x_train.shape)
<add>print(x_train.shape[0], 'train samples')
<add>print(x_test.shape[0], 'test samples')
<ide>
<ide> # Converts class vectors to binary class matrices.
<ide> Y_train = np_utils.to_categorical(y_train, num_classes)
<ide> Y_test = np_utils.to_categorical(y_test, num_classes)
<ide>
<del>row, col, pixel = X_train.shape[1:]
<add>row, col, pixel = x_train.shape[1:]
<ide>
<ide> # 4D input.
<ide> x = Input(shape=(row, col, pixel))
<ide>
<ide> # Encodes a row of pixels using TimeDistributed Wrapper.
<del>encoded_rows = TimeDistributed(LSTM(output_dim=row_hidden))(x)
<add>encoded_rows = TimeDistributed(LSTM(row_hidden))(x)
<ide>
<ide> # Encodes columns of encoded rows.
<ide> encoded_columns = LSTM(col_hidden)(encoded_rows)
<ide>
<ide> # Final predictions and model.
<ide> prediction = Dense(num_classes, activation='softmax')(encoded_columns)
<del>model = Model(input=x, output=prediction)
<add>model = Model(x, prediction)
<ide> model.compile(loss='categorical_crossentropy',
<ide> optimizer='rmsprop',
<ide> metrics=['accuracy'])
<ide>
<ide> # Training.
<del>model.fit(X_train, Y_train, batch_size=batch_size, epochs=epochss,
<del> verbose=1, validation_data=(X_test, Y_test))
<add>model.fit(x_train, Y_train, batch_size=batch_size, epochs=epochs,
<add> verbose=1, validation_data=(x_test, Y_test))
<ide>
<ide> # Evaluation.
<del>scores = model.evaluate(X_test, Y_test, verbose=0)
<add>scores = model.evaluate(x_test, Y_test, verbose=0)
<ide> print('Test loss:', scores[0])
<ide> print('Test accuracy:', scores[1])
<ide><path>examples/mnist_irnn.py
<ide>
<ide> batch_size = 32
<ide> num_classes = 10
<del>epochss = 200
<add>epochs = 200
<ide> hidden_units = 100
<ide>
<ide> learning_rate = 1e-6
<ide>
<ide> print('Evaluate IRNN...')
<ide> model = Sequential()
<del>model.add(SimpleRNN(output_dim=hidden_units,
<del> init=initializers.RandomNormal(stddev=0.001),
<del> inner_init=initializers.Identity(gain=1.0),
<add>model.add(SimpleRNN(hidden_units,
<add> kernel_initializer=initializers.RandomNormal(stddev=0.001),
<add> recurrent_initializer=initializers.Identity(gain=1.0),
<ide> activation='relu',
<ide> input_shape=X_train.shape[1:]))
<ide> model.add(Dense(num_classes))
<ide> optimizer=rmsprop,
<ide> metrics=['accuracy'])
<ide>
<del>model.fit(X_train, Y_train, batch_size=batch_size, epochs=epochss,
<add>model.fit(X_train, Y_train, batch_size=batch_size, epochs=epochs,
<ide> verbose=1, validation_data=(X_test, Y_test))
<ide>
<ide> scores = model.evaluate(X_test, Y_test, verbose=0)
<ide><path>examples/mnist_siamese_graph.py
<ide> def compute_accuracy(predictions, labels):
<ide>
<ide>
<ide> # the data, shuffled and split between train and test sets
<del>(X_train, y_train), (X_test, y_test) = mnist.load_data()
<del>X_train = X_train.reshape(60000, 784)
<del>X_test = X_test.reshape(10000, 784)
<del>X_train = X_train.astype('float32')
<del>X_test = X_test.astype('float32')
<del>X_train /= 255
<del>X_test /= 255
<add>(x_train, y_train), (x_test, y_test) = mnist.load_data()
<add>x_train = x_train.reshape(60000, 784)
<add>x_test = x_test.reshape(10000, 784)
<add>x_train = x_train.astype('float32')
<add>x_test = x_test.astype('float32')
<add>x_train /= 255
<add>x_test /= 255
<ide> input_dim = 784
<ide> epochs = 20
<ide>
<ide> # create training+test positive and negative pairs
<ide> digit_indices = [np.where(y_train == i)[0] for i in range(10)]
<del>tr_pairs, tr_y = create_pairs(X_train, digit_indices)
<add>tr_pairs, tr_y = create_pairs(x_train, digit_indices)
<ide>
<ide> digit_indices = [np.where(y_test == i)[0] for i in range(10)]
<del>te_pairs, te_y = create_pairs(X_test, digit_indices)
<add>te_pairs, te_y = create_pairs(x_test, digit_indices)
<ide>
<ide> # network definition
<ide> base_network = create_base_network(input_dim)
<ide> def compute_accuracy(predictions, labels):
<ide>
<ide> distance = Lambda(euclidean_distance, output_shape=eucl_dist_output_shape)([processed_a, processed_b])
<ide>
<del>model = Model(input=[input_a, input_b], output=distance)
<add>model = Model([input_a, input_b], distance)
<ide>
<ide> # train
<ide> rms = RMSprop()
<ide><path>examples/mnist_transfer_cnn.py
<ide> from keras.datasets import mnist
<ide> from keras.models import Sequential
<ide> from keras.layers import Dense, Dropout, Activation, Flatten
<del>from keras.layers import Convolution2D, MaxPooling2D
<add>from keras.layers import Conv2D, MaxPooling2D
<ide> from keras.utils import np_utils
<ide> from keras import backend as K
<ide>
<ide> # input image dimensions
<ide> img_rows, img_cols = 28, 28
<ide> # number of convolutional filters to use
<del>filterss = 32
<add>filters = 32
<ide> # size of pooling area for max pooling
<ide> pool_size = 2
<ide> # convolution kernel size
<ide>
<ide>
<ide> def train_model(model, train, test, num_classes):
<del> X_train = train[0].reshape((train[0].shape[0],) + input_shape)
<del> X_test = test[0].reshape((test[0].shape[0],) + input_shape)
<del> X_train = X_train.astype('float32')
<del> X_test = X_test.astype('float32')
<del> X_train /= 255
<del> X_test /= 255
<del> print('X_train shape:', X_train.shape)
<del> print(X_train.shape[0], 'train samples')
<del> print(X_test.shape[0], 'test samples')
<add> x_train = train[0].reshape((train[0].shape[0],) + input_shape)
<add> x_test = test[0].reshape((test[0].shape[0],) + input_shape)
<add> x_train = x_train.astype('float32')
<add> x_test = x_test.astype('float32')
<add> x_train /= 255
<add> x_test /= 255
<add> print('x_train shape:', x_train.shape)
<add> print(x_train.shape[0], 'train samples')
<add> print(x_test.shape[0], 'test samples')
<ide>
<ide> # convert class vectors to binary class matrices
<del> Y_train = np_utils.to_categorical(train[1], num_classes)
<del> Y_test = np_utils.to_categorical(test[1], num_classes)
<add> y_train = np_utils.to_categorical(train[1], num_classes)
<add> y_test = np_utils.to_categorical(test[1], num_classes)
<ide>
<ide> model.compile(loss='categorical_crossentropy',
<ide> optimizer='adadelta',
<ide> metrics=['accuracy'])
<ide>
<ide> t = now()
<del> model.fit(X_train, Y_train,
<add> model.fit(x_train, y_train,
<ide> batch_size=batch_size, epochs=epochs,
<ide> verbose=1,
<del> validation_data=(X_test, Y_test))
<add> validation_data=(x_test, y_test))
<ide> print('Training time: %s' % (now() - t))
<del> score = model.evaluate(X_test, Y_test, verbose=0)
<add> score = model.evaluate(x_test, y_test, verbose=0)
<ide> print('Test score:', score[0])
<ide> print('Test accuracy:', score[1])
<ide>
<ide>
<ide> # the data, shuffled and split between train and test sets
<del>(X_train, y_train), (X_test, y_test) = mnist.load_data()
<add>(x_train, y_train), (x_test, y_test) = mnist.load_data()
<ide>
<ide> # create two datasets one with digits below 5 and one with 5 and above
<del>X_train_lt5 = X_train[y_train < 5]
<add>x_train_lt5 = x_train[y_train < 5]
<ide> y_train_lt5 = y_train[y_train < 5]
<del>X_test_lt5 = X_test[y_test < 5]
<add>x_test_lt5 = x_test[y_test < 5]
<ide> y_test_lt5 = y_test[y_test < 5]
<ide>
<del>X_train_gte5 = X_train[y_train >= 5]
<add>x_train_gte5 = x_train[y_train >= 5]
<ide> y_train_gte5 = y_train[y_train >= 5] - 5 # make classes start at 0 for
<del>X_test_gte5 = X_test[y_test >= 5] # np_utils.to_categorical
<add>x_test_gte5 = x_test[y_test >= 5] # np_utils.to_categorical
<ide> y_test_gte5 = y_test[y_test >= 5] - 5
<ide>
<ide> # define two groups of layers: feature (convolutions) and classification (dense)
<ide> feature_layers = [
<del> Convolution2D(filterss, kernel_size, kernel_size,
<del> border_mode='valid',
<del> input_shape=input_shape),
<add> Conv2D(filters, kernel_size,
<add> padding='valid',
<add> input_shape=input_shape),
<ide> Activation('relu'),
<del> Convolution2D(filterss, kernel_size, kernel_size),
<add> Conv2D(filters, kernel_size),
<ide> Activation('relu'),
<del> MaxPooling2D(pool_size=(pool_size, pool_size)),
<add> MaxPooling2D(pool_size=pool_size),
<ide> Dropout(0.25),
<ide> Flatten(),
<ide> ]
<add>
<ide> classification_layers = [
<ide> Dense(128),
<ide> Activation('relu'),
<ide> def train_model(model, train, test, num_classes):
<ide>
<ide> # train model for 5-digit classification [0..4]
<ide> train_model(model,
<del> (X_train_lt5, y_train_lt5),
<del> (X_test_lt5, y_test_lt5), num_classes)
<add> (x_train_lt5, y_train_lt5),
<add> (x_test_lt5, y_test_lt5), num_classes)
<ide>
<ide> # freeze feature layers and rebuild model
<ide> for l in feature_layers:
<ide> l.trainable = False
<ide>
<ide> # transfer: train dense layers for new classification task [5..9]
<ide> train_model(model,
<del> (X_train_gte5, y_train_gte5),
<del> (X_test_gte5, y_test_gte5), num_classes)
<add> (x_train_gte5, y_train_gte5),
<add> (x_test_gte5, y_test_gte5), num_classes) | 8 |
Ruby | Ruby | remove ruby 1.8 checking in constantize method | 6e5ab54b608d3c681263c5574ed4b0167a26b641 | <ide><path>activesupport/lib/active_support/inflector/methods.rb
<ide> def foreign_key(class_name, separate_class_name_and_id_with_underscore = true)
<ide> underscore(demodulize(class_name)) + (separate_class_name_and_id_with_underscore ? "_id" : "id")
<ide> end
<ide>
<del> # Ruby 1.9 introduces an inherit argument for Module#const_get and
<del> # #const_defined? and changes their default behavior.
<del> if Module.method(:const_get).arity == 1
<del> # Tries to find a constant with the name specified in the argument string:
<del> #
<del> # "Module".constantize # => Module
<del> # "Test::Unit".constantize # => Test::Unit
<del> #
<del> # The name is assumed to be the one of a top-level constant, no matter whether
<del> # it starts with "::" or not. No lexical context is taken into account:
<del> #
<del> # C = 'outside'
<del> # module M
<del> # C = 'inside'
<del> # C # => 'inside'
<del> # "C".constantize # => 'outside', same as ::C
<del> # end
<del> #
<del> # NameError is raised when the name is not in CamelCase or the constant is
<del> # unknown.
<del> def constantize(camel_cased_word)
<del> names = camel_cased_word.split('::')
<del> names.shift if names.empty? || names.first.empty?
<del>
<del> constant = Object
<del> names.each do |name|
<del> constant = constant.const_defined?(name) ? constant.const_get(name) : constant.const_missing(name)
<del> end
<del> constant
<del> end
<del> else
<del> def constantize(camel_cased_word) #:nodoc:
<del> names = camel_cased_word.split('::')
<del> names.shift if names.empty? || names.first.empty?
<add> # Tries to find a constant with the name specified in the argument string:
<add> #
<add> # "Module".constantize # => Module
<add> # "Test::Unit".constantize # => Test::Unit
<add> #
<add> # The name is assumed to be the one of a top-level constant, no matter whether
<add> # it starts with "::" or not. No lexical context is taken into account:
<add> #
<add> # C = 'outside'
<add> # module M
<add> # C = 'inside'
<add> # C # => 'inside'
<add> # "C".constantize # => 'outside', same as ::C
<add> # end
<add> #
<add> # NameError is raised when the name is not in CamelCase or the constant is
<add> # unknown.
<add> def constantize(camel_cased_word) #:nodoc:
<add> names = camel_cased_word.split('::')
<add> names.shift if names.empty? || names.first.empty?
<ide>
<del> constant = Object
<del> names.each do |name|
<del> constant = constant.const_defined?(name, false) ? constant.const_get(name) : constant.const_missing(name)
<del> end
<del> constant
<add> constant = Object
<add> names.each do |name|
<add> constant = constant.const_defined?(name, false) ? constant.const_get(name) : constant.const_missing(name)
<ide> end
<add> constant
<ide> end
<ide>
<ide> # Tries to find a constant with the name specified in the argument string: | 1 |
Text | Text | add rlidwka as collaborator | 7192b6688c36553ac8b338ae160a4a02ef2d9a2d | <ide><path>README.md
<ide> information about the governance of the io.js project, see
<ide> * **Roman Reiss** ([@silverwind](https://github.com/silverwind)) <[email protected]>
<ide> * **Petka Antonov** ([@petkaantonov](https://github.com/petkaantonov)) <[email protected]>
<ide> * **Yosuke Furukawa** ([@yosuke-furukawa](https://github.com/yosuke-furukawa)) <[email protected]>
<add>* **Alex Kocharin** ([@rlidwka](https://github.com/rlidwka)) <[email protected]>
<ide>
<ide> Collaborators follow the [COLLABORATOR_GUIDE.md](./COLLABORATOR_GUIDE.md) in
<ide> maintaining the io.js project. | 1 |
Python | Python | fix output_token_type in glue | 789ea72037219332e20e66e626b6c4c32851f0dc | <ide><path>pytorch_transformers/data/processors/glue.py
<ide> def glue_convert_examples_to_features(examples, label_list, max_seq_length,
<ide> example.text_a,
<ide> example.text_b,
<ide> add_special_tokens=True,
<del> output_token_type=True,
<ide> max_length=max_seq_length,
<ide> truncate_first_sequence=True # We're truncating the first sequence as a priority
<ide> ) | 1 |
Javascript | Javascript | make require() from the repl be relative to cwd | a26f7d753dca57cfcf84867631de66da2ae58aa9 | <ide><path>lib/repl.js
<ide> var evalcx = process.binding('evals').Script.runInNewContext;
<ide> var path = require("path");
<ide> var scope;
<ide>
<add>function cwdRequire (id) {
<add> if (id.match(/^\.\.\//) || id.match(/^\.\//)) {
<add> id = path.join(process.cwd(), id);
<add> }
<add> return require(id);
<add>}
<add>
<ide> function setScope (self) {
<ide> scope = {};
<ide> for (var i in global) scope[i] = global[i];
<ide> scope.module = module;
<del> scope.require = require;
<add> scope.require = cwdRequire;
<ide> }
<ide>
<ide> | 1 |
PHP | PHP | fix coding standards for whitespaces | 8f78b1e05b2d16ed6ec71ab27fe37f1310a95a7d | <ide><path>lib/Cake/Console/Command/Task/FixtureTask.php
<ide> protected function _generateRecords($tableInfo, $recordCount = 1) {
<ide> } else {
<ide> $insert = "Lorem ipsum dolor sit amet";
<ide> if (!empty($fieldInfo['length'])) {
<del> $insert = substr($insert, 0, (int)$fieldInfo['length'] - 2);
<add> $insert = substr($insert, 0, (int)$fieldInfo['length'] - 2);
<ide> }
<ide> }
<ide> break;
<ide><path>lib/Cake/Model/Datasource/CakeSession.php
<ide> protected static function _setPath($base = null) {
<ide> return;
<ide> }
<ide> if (strpos($base, 'index.php') !== false) {
<del> $base = str_replace('index.php', '', $base);
<add> $base = str_replace('index.php', '', $base);
<ide> }
<ide> if (strpos($base, '?') !== false) {
<del> $base = str_replace('?', '', $base);
<add> $base = str_replace('?', '', $base);
<ide> }
<ide> self::$path = $base;
<ide> }
<ide><path>lib/Cake/Model/Datasource/Database/Postgres.php
<ide> public function connect() {
<ide> $this->setEncoding($config['encoding']);
<ide> }
<ide> if (!empty($config['schema'])) {
<del> $this->_execute('SET search_path TO ' . $config['schema']);
<add> $this->_execute('SET search_path TO ' . $config['schema']);
<ide> }
<ide> } catch (PDOException $e) {
<ide> throw new MissingConnectionException(array(
<ide><path>lib/Cake/Model/Datasource/Database/Sqlite.php
<ide> public function resultSet($results) {
<ide> continue;
<ide> }
<ide> if (preg_match('/\bAS\s+(.*)/i', $selects[$j], $matches)) {
<del> $columnName = trim($matches[1], '"');
<add> $columnName = trim($matches[1], '"');
<ide> } else {
<ide> $columnName = trim(str_replace('"', '', $selects[$j]));
<ide> }
<ide><path>lib/Cake/Model/Datasource/DboSource.php
<ide> public function lastNumRows($source = null) {
<ide> * @return resource Result resource identifier.
<ide> */
<ide> public function query() {
<del> $args = func_get_args();
<del> $fields = null;
<del> $order = null;
<del> $limit = null;
<del> $page = null;
<add> $args = func_get_args();
<add> $fields = null;
<add> $order = null;
<add> $limit = null;
<add> $page = null;
<ide> $recursive = null;
<ide>
<ide> if (count($args) === 1) {
<ide> public function name($data) {
<ide> }
<ide> if (preg_match('/^([\w-]+)\((.*)\)$/', $data, $matches)) { // Functions
<ide> return $this->cacheMethod(__FUNCTION__, $cacheKey,
<del> $matches[1] . '(' . $this->name($matches[2]) . ')'
<add> $matches[1] . '(' . $this->name($matches[2]) . ')'
<ide> );
<ide> }
<ide> if ( | 5 |
Python | Python | adapt import to new structure | cae394c8faac229d43df7559476cfbdd745f8c41 | <ide><path>tests/sagemaker/scripts/tensorflow/run_tf_dist.py
<ide> from tqdm import tqdm
<ide>
<ide> from transformers import AutoTokenizer, TFAutoModelForSequenceClassification
<del>from transformers.file_utils import is_sagemaker_dp_enabled
<add>from transformers.utils import is_sagemaker_dp_enabled
<ide>
<ide>
<ide> if os.environ.get("SDP_ENABLED") or is_sagemaker_dp_enabled(): | 1 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.